title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
O(k(m+n)). Python Solution with monotonically decreasing stack. Commented for clarity. | create-maximum-number | 0 | 1 | This solution is pretty efficient and intuitive.\nTime complexity is **O(k(n+m))** where n and m is length of each list. \n\n\nTo understand this solution you must also do other problems concerning monotonic stack like LC-402 which are pre-requisites for this problem.\n\nDo give me a thumbs up if u like it.\n```\nclass Solution:\n def maxNumber(self, nums1: List[int], nums2: List[int], k: int) -> List[int]:\n def maximum_num_each_list(nums: List[int], k_i: int) -> List[int]:\n # monotonically decreasing stack\n s = []\n m = len(nums) - k_i\n for n in nums:\n while s and s[-1] < n and m > 0:\n s.pop()\n m -= 1\n s.append(n)\n s = s[:len(s)-m] # very important\n return s\n def greater(a, b, i , j): # get the number which is lexiographically greater\n while i< len(a) or j < len(b): \n if i == len(a): return False\n if j == len(b): return True\n if a[i] > b[j]: return True\n if a[i] < b[j]: return False\n i += 1 # we increment until each of their elements are same\n j += 1\n \n def merge(x_num, y_num):\n n = len(x_num)\n m = len(y_num)\n i = 0\n j = 0\n s = []\n while i < n or j < m:\n a = x_num[i] if i < n else float("-inf") \n b = y_num[j] if j < m else float("-inf") \n\n if a > b or greater(x_num, y_num, i , j):\n# greater(x_num, y_num, i , j): this function is meant for check which list has element lexicographically greater means it will iterate through both arrays incrementing both at the same time until one of them is greater than other.\n chosen = a\n i += 1\n else:\n chosen = b\n j += 1\n s.append(chosen)\n return s\n\n max_num_arr = []\n for i in range(k+1): # we check for all values of k and find the maximum number we can create for that value of k and we repeat this for all values of k and then at eacch time merge the numbers to check if arrive at optimal solution\n first = maximum_num_each_list(nums1, i)\n second = maximum_num_each_list(nums2, k-i)\n merged = merge(first, second)\n # these two conditions are required because list comparison in python only compares the elements even if one of their lengths is greater, so I had to add these conditions to compare elements only if length is equal.\n\t\t\t# Alternatively you can avoid this and convert them both to int and then compare, but I wanted to this as it is somewhat more efficient.\n if len(merged) == len(max_num_arr) and merged > max_num_arr:\n max_num_arr = merged\n elif len(merged) > len(max_num_arr):\n max_num_arr = merged\n return max_num_arr\n\n```\nFeel free to ask any questions in the comments.\nDo suggest improvements if any. | 3 | You are given two integer arrays `nums1` and `nums2` of lengths `m` and `n` respectively. `nums1` and `nums2` represent the digits of two numbers. You are also given an integer `k`.
Create the maximum number of length `k <= m + n` from digits of the two numbers. The relative order of the digits from the same array must be preserved.
Return an array of the `k` digits representing the answer.
**Example 1:**
**Input:** nums1 = \[3,4,6,5\], nums2 = \[9,1,2,5,8,3\], k = 5
**Output:** \[9,8,6,5,3\]
**Example 2:**
**Input:** nums1 = \[6,7\], nums2 = \[6,0,4\], k = 5
**Output:** \[6,7,6,0,4\]
**Example 3:**
**Input:** nums1 = \[3,9\], nums2 = \[8,9\], k = 3
**Output:** \[9,8,9\]
**Constraints:**
* `m == nums1.length`
* `n == nums2.length`
* `1 <= m, n <= 500`
* `0 <= nums1[i], nums2[i] <= 9`
* `1 <= k <= m + n` | null |
python 1 hard = 2 easy + 1 medium problems | create-maximum-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIf there is only one array, how to solve it, use monotonic stack.\nIf we can find subarray in nums1 and nums2, then merge them.\nThe ans is all combinations of the merge\n# Approach\n<!-- Describe your approach to solving the problem. -->\nmonotonic stack is a medium problem\nmerge is an easy one\nfind combination should be an easy problem.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\nk* O(n^2)\n\nmonotonic stack is O(n)\nmerge is O(n)\nk loops\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(n)\n# Code\n```\nclass Solution:\n def maxNumber(self, nums1: List[int], nums2: List[int], k: int) -> List[int]:\n def getMaxOrder(nums, n, total, res): # monotonic stack\n for i, v in enumerate(nums):\n while res and len(res) + total - i > n and res[-1] < v:\n res.pop()\n if len(res) < n:\n res.append(nums[i])\n return res\n\n def merge(nums1, nums2, ans): # check lexicographically greater\n while nums1 or nums2:\n if nums1 > nums2:\n ans.append(nums1[0])\n nums1 = nums1[1:]\n else:\n ans.append(nums2[0])\n nums2 = nums2[1:]\n return ans\n\n n1, n2 = len(nums1), len(nums2)\n res = []\n for i in range(max(0, k - n2), min(k, n1) + 1): # find all combinations of nums1 and nums2\n ans = merge(getMaxOrder(nums1, i, n1, []), getMaxOrder(nums2, k - i, n2, []), [])\n res = max(ans, res)\n return res\n``` | 1 | You are given two integer arrays `nums1` and `nums2` of lengths `m` and `n` respectively. `nums1` and `nums2` represent the digits of two numbers. You are also given an integer `k`.
Create the maximum number of length `k <= m + n` from digits of the two numbers. The relative order of the digits from the same array must be preserved.
Return an array of the `k` digits representing the answer.
**Example 1:**
**Input:** nums1 = \[3,4,6,5\], nums2 = \[9,1,2,5,8,3\], k = 5
**Output:** \[9,8,6,5,3\]
**Example 2:**
**Input:** nums1 = \[6,7\], nums2 = \[6,0,4\], k = 5
**Output:** \[6,7,6,0,4\]
**Example 3:**
**Input:** nums1 = \[3,9\], nums2 = \[8,9\], k = 3
**Output:** \[9,8,9\]
**Constraints:**
* `m == nums1.length`
* `n == nums2.length`
* `1 <= m, n <= 500`
* `0 <= nums1[i], nums2[i] <= 9`
* `1 <= k <= m + n` | null |
Solution | create-maximum-number | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n #define MIN(a,b) (a<b?a:b)\n #define MAX(a,b) (a>b?a:b)\n void getMax(int* num, int& len, int* result, int& t, int& sortedLen)\n {\n \tint n, top = 0;\n \tresult[0] = num[0];\n \tconst int need2drop = len - t;\n \tfor (int i = 1; i < len; ++i){\n \t\tn = num[i];\n \t\twhile (top >= 0 && result[top] < n && (i - top) <= need2drop) --top;\n \t\tif (i - top > need2drop){\n \t\t\tsortedLen = MAX(1,top);\n \t\t\twhile (++top < t) result[top] = num[i++];\n \t\t\treturn;\n \t\t}\n \t\tif (++top < t) result[top] = n;\n \t\telse top = t - 1;\n \t}\n }\n void dp(int *num, int len, int&sortedLen, int& minL, int& maxL, int *res, int &k){\n \tint j, *head, *prevhead = res;\n \tconst int soi = sizeof(int);\n \tgetMax(num, len, res, maxL,sortedLen);\n \tfor (int l = maxL; l > MAX(minL,1); --l){\n \t\thead = prevhead + k;\n \t\tmemcpy(head, prevhead, l*soi);\n \t\tfor (j = sortedLen; j < l; ++j){\n \t\t\tif (head[j] > head[j - 1]){\n \t\t\t\tsortedLen = MAX(1, j - 1);\n \t\t\t\tmemcpy(head + j - 1, prevhead + j, soi*(l - j));\n \t\t\t\tbreak;\n \t\t\t}\n \t\t}\n \t\tif (j == l) sortedLen = l;\n \t\tprevhead = head;\n \t}\n }\n void merge(int* num1,int len1,int* num2,int len2,int* result,int& resSize){\n \tint i = 0, j = 0, k = 0;\n \twhile (i < resSize){\n \t\tif (j < len1 && k < len2){\n \t\t\tif (num1[j] > num2[k])\n \t\t\t\tresult[i++] = num1[j++];\n \t\t\telse if (num1[j] < num2[k])\n \t\t\t\tresult[i++] = num2[k++];\n \t\t\telse{\n \t\t\t\tint remaining1 = len1 - j, remaining2 = len2 - k, tmp = num1[j];\n \t\t\t\tint flag = memcmp(num1 + j, num2 + k, sizeof(int) * MIN(remaining1, remaining2));\n \t\t\t\tflag = (flag == 0 ? (remaining1>remaining2 ? 1 : -1) : flag);\n \t\t\t\tint * num = flag > 0 ? num1 : num2;\n \t\t\t\tint & cnt = flag > 0 ? j : k;\n \t\t\t\tint len = flag > 0 ? len1 : len2;\n \t\t\t\twhile (num[cnt]==tmp && cnt < len && i<resSize) result[i++] = num[cnt++];\n \t\t\t}\n \t\t}\n \t\telse if (j < len1) result[i++] = num1[j++];\n \t\telse result[i++] = num2[k++];\n \t}\n }\n vector<int> maxNumber(vector<int>& nums1, vector<int>& nums2, int k){\n \tint soi = sizeof(int), len1 = nums1.size(), len2 = nums2.size(), step = k*soi;\n \tint minL1 = MAX(0, k - len2), maxL1 = MIN(k, len1), minL2 = k - maxL1, maxL2 = k - minL1, range = maxL1 - minL1 + 1;\n \tint * res = new int[range * k * 2 + 2 * k], *dp1 = res + k, *dp2 = res + range*k+k, *tmp=res+range*2*k+k;\n \tmemset(res, 0, step);\n \tint sortedLen1 = 1, sortedLen2 = 1;\n \tif (len1 == 0 && len2 > 0) getMax(&nums2[0], len2, res, k, sortedLen2);\n \telse if (len1 > 0 && len2 == 0) getMax(&nums1[0], len1, res, k, sortedLen2);\n \telse if (len1 > 0 && len2 > 0){\n \t\tdp(&nums1[0], len1, sortedLen1, minL1, maxL1, dp1,k);\n \t\tdp(&nums2[0], len2, sortedLen2, minL2, maxL2, dp2,k);\n \t\tif (sortedLen1 + sortedLen2 > k){\n \t\t\tmerge(dp1 + k*(maxL1 - sortedLen1), sortedLen1, dp2 + k*(maxL2 - sortedLen2), sortedLen2, tmp, k);\n \t\t\tvector<int> resv(tmp, tmp + k);\n \t\t\tdelete[] res;\n \t\t\treturn resv;\n \t\t}\n \t\tfor (int i = minL1; i <= maxL1; ++i){\n \t\t\tmerge(dp1+k*(maxL1-i), i, dp2+k*(maxL2-k+i), (k-i), tmp,k);\n \t\t\tif (memcmp(res, tmp, step) < 0) memcpy(res, tmp, step);\n \t\t}\n \t}\n \tvector<int> resv(res, res + k);\n \tdelete[] res;\n \treturn resv;\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def maxNumber(self, nums1: List[int], nums2: List[int], k: int) -> List[int]:\n \n def get_max_subarray(nums, k):\n stack = []\n drop = len(nums) - k\n for num in nums:\n while drop and stack and stack[-1] < num:\n stack.pop()\n drop -= 1\n stack.append(num)\n return stack[:k]\n\n max_num = []\n\n for i in range(max(0, k - len(nums2)), min(k, len(nums1)) + 1):\n max_subarray1 = get_max_subarray(nums1, i)\n max_subarray2 = get_max_subarray(nums2, k - i)\n\n candidate = []\n while max_subarray1 or max_subarray2:\n if max_subarray1 > max_subarray2:\n candidate.append(max_subarray1.pop(0))\n else:\n candidate.append(max_subarray2.pop(0))\n\n if candidate > max_num:\n max_num = candidate\n\n return max_num\n```\n\n```Java []\nclass Solution {\n public int[] maxNumber(int[] nums1, int[] nums2, int k) {\n\n int m = nums1.length, n = nums2.length;\n int[] ans = new int[k];\n \n for (int i = Math.max(0, k-n); i <= Math.min(k, m); i++) {\n int j = k - i;\n int[] maxNum1 = getMax(nums1, i);\n int[] maxNum2 = getMax(nums2, j);\n int[] merged = merge(maxNum1, maxNum2);\n if (compare(merged, 0, ans, 0) > 0) {\n ans = merged;\n }\n }\n return ans;\n}\nprivate int[] getMax(int[] nums, int k) {\n int n = nums.length;\n int[] stack = new int[k];\n int top = -1, remaining = n - k;\n \n for (int i = 0; i < n; i++) {\n int num = nums[i];\n while (top >= 0 && stack[top] < num && remaining > 0) {\n top--;\n remaining--;\n }\n if (top < k - 1) {\n stack[++top] = num;\n } else {\n remaining--;\n }\n }\n return stack;\n}\nprivate int[] merge(int[] nums1, int[] nums2) {\n int m = nums1.length, n = nums2.length;\n int[] merged = new int[m + n];\n int i = 0, j = 0, k = 0;\n \n while (i < m && j < n) {\n if (compare(nums1, i, nums2, j) > 0) {\n merged[k++] = nums1[i++];\n } else {\n merged[k++] = nums2[j++];\n }\n }\n while (i < m) {\n merged[k++] = nums1[i++];\n }\n while (j < n) {\n merged[k++] = nums2[j++];\n }\n return merged;\n}\nprivate int compare(int[] nums1, int i, int[] nums2, int j) {\n int m = nums1.length, n = nums2.length;\n while (i < m && j < n) {\n int diff = nums1[i] - nums2[j];\n if (diff != 0) {\n return diff;\n }\n i++;\n j++;\n }\n return (m - i) - (n - j);\n}\n}\n```\n | 1 | You are given two integer arrays `nums1` and `nums2` of lengths `m` and `n` respectively. `nums1` and `nums2` represent the digits of two numbers. You are also given an integer `k`.
Create the maximum number of length `k <= m + n` from digits of the two numbers. The relative order of the digits from the same array must be preserved.
Return an array of the `k` digits representing the answer.
**Example 1:**
**Input:** nums1 = \[3,4,6,5\], nums2 = \[9,1,2,5,8,3\], k = 5
**Output:** \[9,8,6,5,3\]
**Example 2:**
**Input:** nums1 = \[6,7\], nums2 = \[6,0,4\], k = 5
**Output:** \[6,7,6,0,4\]
**Example 3:**
**Input:** nums1 = \[3,9\], nums2 = \[8,9\], k = 3
**Output:** \[9,8,9\]
**Constraints:**
* `m == nums1.length`
* `n == nums2.length`
* `1 <= m, n <= 500`
* `0 <= nums1[i], nums2[i] <= 9`
* `1 <= k <= m + n` | null |
[Python3] greedy | create-maximum-number | 0 | 1 | \n```\nclass Solution:\n def maxNumber(self, nums1: List[int], nums2: List[int], k: int) -> List[int]:\n \n def fn(arr, k):\n """Return largest sub-sequence of arr of size k."""\n ans = []\n for i, x in enumerate(arr): \n while ans and ans[-1] < x and len(ans) + len(arr) - i > k: ans.pop()\n if len(ans) < k: ans.append(x)\n return ans\n \n ans = [0] * k\n for i in range(k+1): \n if k - len(nums2) <= i <= len(nums1): \n val1 = fn(nums1, i)\n val2 = fn(nums2, k-i)\n cand = []\n i1 = i2 = 0\n while i1 < len(val1) or i2 < len(val2): \n if val1[i1:] >= val2[i2:]: \n cand.append(val1[i1])\n i1 += 1\n else: \n cand.append(val2[i2])\n i2 += 1\n ans = max(ans, cand)\n return ans \n``` | 2 | You are given two integer arrays `nums1` and `nums2` of lengths `m` and `n` respectively. `nums1` and `nums2` represent the digits of two numbers. You are also given an integer `k`.
Create the maximum number of length `k <= m + n` from digits of the two numbers. The relative order of the digits from the same array must be preserved.
Return an array of the `k` digits representing the answer.
**Example 1:**
**Input:** nums1 = \[3,4,6,5\], nums2 = \[9,1,2,5,8,3\], k = 5
**Output:** \[9,8,6,5,3\]
**Example 2:**
**Input:** nums1 = \[6,7\], nums2 = \[6,0,4\], k = 5
**Output:** \[6,7,6,0,4\]
**Example 3:**
**Input:** nums1 = \[3,9\], nums2 = \[8,9\], k = 3
**Output:** \[9,8,9\]
**Constraints:**
* `m == nums1.length`
* `n == nums2.length`
* `1 <= m, n <= 500`
* `0 <= nums1[i], nums2[i] <= 9`
* `1 <= k <= m + n` | null |
Python3 | Monotonic Stack | Greedy | create-maximum-number | 0 | 1 | ```\nclass Solution:\n def maxNumber(self, nums1: List[int], nums2: List[int], k: int) -> List[int]:\n \n def find_k_max_number_in_an_array(nums, k):\n drop_possible = len(nums) - k\n n = len(nums)\n stack = []\n for i, val in enumerate(nums):\n while stack and drop_possible and stack[-1] < val:\n drop_possible -= 1\n stack.pop()\n \n stack.append(val)\n \n return stack[:k]\n \n \n def merge_two_array(arr1, arr2):\n #print(arr1, arr2)\n return [max(arr1, arr2).pop(0) for _ in arr1 + arr2]\n\n def compare_two_array(arr1, arr2):\n """\n determine whether arr1 is greater than arr2\n """\n if not arr2:\n return True\n i = j = 0\n n = len(arr1)\n while i < n and j < n:\n if arr1[i] > arr2[j]:\n return True\n elif arr1[i] < arr2[j]:\n return False\n i += 1\n j += 1\n \n return True\n \n ans = 0\n for i in range(k + 1):\n p = k - i\n \n if i > len(nums1) or p > len(nums2):\n continue\n \n # get this two array by solving function find_k_max_number_in_an_array\n # using similar concept of 402. Remove K Digits\n first_arr = find_k_max_number_in_an_array(nums1, i)\n second_arr = find_k_max_number_in_an_array(nums2, p)\n \n # merge two array with everytime taking lexicographily larger list\n # https://leetcode.com/problems/create-maximum-number/discuss/77286/Short-Python-Ruby-C%2B%2B\n # see explanation\n curr_arr = merge_two_array(first_arr, second_arr)\n #print(curr_arr)\n \n # can be directly use python max function\n if compare_two_array(curr_arr, ans):\n ans = curr_arr\n # ans = max(ans, curr_arr) if ans else curr_arr\n \n #print(ans)\n \n return ans\n\n``` | 1 | You are given two integer arrays `nums1` and `nums2` of lengths `m` and `n` respectively. `nums1` and `nums2` represent the digits of two numbers. You are also given an integer `k`.
Create the maximum number of length `k <= m + n` from digits of the two numbers. The relative order of the digits from the same array must be preserved.
Return an array of the `k` digits representing the answer.
**Example 1:**
**Input:** nums1 = \[3,4,6,5\], nums2 = \[9,1,2,5,8,3\], k = 5
**Output:** \[9,8,6,5,3\]
**Example 2:**
**Input:** nums1 = \[6,7\], nums2 = \[6,0,4\], k = 5
**Output:** \[6,7,6,0,4\]
**Example 3:**
**Input:** nums1 = \[3,9\], nums2 = \[8,9\], k = 3
**Output:** \[9,8,9\]
**Constraints:**
* `m == nums1.length`
* `n == nums2.length`
* `1 <= m, n <= 500`
* `0 <= nums1[i], nums2[i] <= 9`
* `1 <= k <= m + n` | null |
Python | Easy to understand | Greedy + Dynamic Programminng | create-maximum-number | 0 | 1 | To solve the problem, we can define the subproblem "getMaxNumberString(i, j, length)": get the max number string with "length" from index "i" in nums1 and index "j" in nums2.\n\ngetMaxNumberString(i, j, length)\n- if nums1 has bigger digit, getMaxNumberString(i, j, length) = str(nums1[index1]) + getMaxNumberString(index1 + 1, j, length - 1)\n- if nums2 has bigger digit, getMaxNumberString(i, j, length) = str(nums2[index2]) + getMaxNumberString(i, index2 + 1, length - 1)\n- if nums1 and nums2 have the same big digit, getMaxNumberString(i, j, length) = max(str(nums1[index1]) + getMaxNumberString(index1 + 1, j, length - 1), str(nums2[index2]) + getMaxNumberString(i, index2 + 1, length - 1))\n\n```\nclass Solution:\n def maxNumber(self, nums1: List[int], nums2: List[int], k: int) -> List[int]:\n m = len(nums1)\n n = len(nums2)\n dp = {}\n \n # get the max number string with "length" from index "i" in nums1 and index "j" in nums2\n # using number string to easy to compare\n def getMaxNumberString(i, j, length):\n if length == 0:\n return ""\n \n # using memoization to optimize for the overlapping subproblems\n key = (i, j, length)\n if key in dp:\n return dp[key]\n \n # greedy to find the possible max digit from nums1 and nums2\n # 1) bigger digit in the higher position of the number will get bigger number\n # 2) at the same time, we need to ensure that we still have enough digits to form a number with "length" digits\n \n # try to find the possible max digit from index i in nums1\n index1 = None\n for ii in range(i, m):\n if (m - ii + n - j) < length:\n break\n if index1 is None or nums1[index1] < nums1[ii]:\n index1 = ii\n \n # try to find the possible max digit from index j in nums2\n index2 = None\n for jj in range(j, n):\n if (m - i + n - jj) < length:\n break\n if index2 is None or nums2[index2] < nums2[jj]:\n index2 = jj\n \n maxNumberStr = None\n if index1 is not None and index2 is not None:\n if nums1[index1] > nums2[index2]:\n maxNumberStr = str(nums1[index1]) + getMaxNumberString(index1 + 1, j, length - 1)\n elif nums1[index1] < nums2[index2]:\n maxNumberStr = str(nums2[index2]) + getMaxNumberString(i, index2 + 1, length - 1)\n else:\n # get the same digit from nums1 and nums2, so need to try two cases and get the max one \n maxNumberStr = max(str(nums1[index1]) + getMaxNumberString(index1 + 1, j, length - 1), str(nums2[index2]) + getMaxNumberString(i, index2 + 1, length - 1))\n elif index1 is not None:\n maxNumberStr = str(nums1[index1]) + getMaxNumberString(index1 + 1, j, length - 1)\n elif index2 is not None:\n maxNumberStr = str(nums2[index2]) + getMaxNumberString(i, index2 + 1, length - 1)\n \n dp[key] = maxNumberStr\n return maxNumberStr\n\n result_str = getMaxNumberString(0, 0, k)\n \n # number string to digit array\n result = []\n for c in result_str:\n result.append(int(c))\n \n return result\n``` | 1 | You are given two integer arrays `nums1` and `nums2` of lengths `m` and `n` respectively. `nums1` and `nums2` represent the digits of two numbers. You are also given an integer `k`.
Create the maximum number of length `k <= m + n` from digits of the two numbers. The relative order of the digits from the same array must be preserved.
Return an array of the `k` digits representing the answer.
**Example 1:**
**Input:** nums1 = \[3,4,6,5\], nums2 = \[9,1,2,5,8,3\], k = 5
**Output:** \[9,8,6,5,3\]
**Example 2:**
**Input:** nums1 = \[6,7\], nums2 = \[6,0,4\], k = 5
**Output:** \[6,7,6,0,4\]
**Example 3:**
**Input:** nums1 = \[3,9\], nums2 = \[8,9\], k = 3
**Output:** \[9,8,9\]
**Constraints:**
* `m == nums1.length`
* `n == nums2.length`
* `1 <= m, n <= 500`
* `0 <= nums1[i], nums2[i] <= 9`
* `1 <= k <= m + n` | null |
DP || MEMOIZATION || EASY | coin-change | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def coinChange(self, coins: List[int], amount: int) -> int:\n cache={}\n def dp(i,k):\n if (i,k) in cache:\n return cache[(i,k)]\n if i>len(coins)-1:\n cache[(i,k)]=float(\'inf\')\n return float(\'inf\')\n if k<0:\n cache[(i,k)]=float(\'inf\')\n return float(\'inf\')\n if k==0:\n cache[(i,k)]=0\n return 0\n take=1+dp(i,k-coins[i])\n ntake=dp(i+1,k)\n cache[(i,k)]=min(take,ntake)\n return min(take,ntake)\n return -1 if dp(0,amount)==float(\'inf\') else dp(0,amount)\n``` | 1 | You are given an integer array `coins` representing coins of different denominations and an integer `amount` representing a total amount of money.
Return _the fewest number of coins that you need to make up that amount_. If that amount of money cannot be made up by any combination of the coins, return `-1`.
You may assume that you have an infinite number of each kind of coin.
**Example 1:**
**Input:** coins = \[1,2,5\], amount = 11
**Output:** 3
**Explanation:** 11 = 5 + 5 + 1
**Example 2:**
**Input:** coins = \[2\], amount = 3
**Output:** -1
**Example 3:**
**Input:** coins = \[1\], amount = 0
**Output:** 0
**Constraints:**
* `1 <= coins.length <= 12`
* `1 <= coins[i] <= 231 - 1`
* `0 <= amount <= 104` | null |
Bottom-up DP with 1D array for memoization | coin-change | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def coinChange(self, coins: List[int], amount: int) -> int:\n \n def returnFewestCoinsForAmount(amount):\n if amount == 0:\n return 0\n fewestCoins = float("INF")\n for coin in coins:\n amountLeft = amount - coin\n if amountLeft >= 0:\n fewestCoinsForAmountLeft = returnFewestCoinsForAmount(amountLeft)\n if fewestCoinsForAmountLeft != -1:\n fewestCoins = min(fewestCoins, fewestCoinsForAmountLeft + 1)\n if fewestCoins == float("INF"):\n return -1\n return fewestCoins\n \n fewestCoinsForAmount = [-1 for _ in range(amount + 1)]\n fewestCoinsForAmount[0] = 0\n for coin in coins:\n if coin < amount:\n fewestCoinsForAmount[coin] = 1\n \n for amount in range(len(fewestCoinsForAmount)):\n fewestCoins = float("INF")\n for coin in coins:\n amountLeft = amount - coin\n if amountLeft >= 0:\n fewestCoinsForAmountLeft = fewestCoinsForAmount[amountLeft]\n if fewestCoinsForAmountLeft != -1:\n fewestCoins = min(fewestCoins, fewestCoinsForAmountLeft + 1)\n if fewestCoins == float("INF"):\n continue\n fewestCoinsForAmount[amount] = fewestCoins\n \n\n return fewestCoinsForAmount[amount]\n``` | 1 | You are given an integer array `coins` representing coins of different denominations and an integer `amount` representing a total amount of money.
Return _the fewest number of coins that you need to make up that amount_. If that amount of money cannot be made up by any combination of the coins, return `-1`.
You may assume that you have an infinite number of each kind of coin.
**Example 1:**
**Input:** coins = \[1,2,5\], amount = 11
**Output:** 3
**Explanation:** 11 = 5 + 5 + 1
**Example 2:**
**Input:** coins = \[2\], amount = 3
**Output:** -1
**Example 3:**
**Input:** coins = \[1\], amount = 0
**Output:** 0
**Constraints:**
* `1 <= coins.length <= 12`
* `1 <= coins[i] <= 231 - 1`
* `0 <= amount <= 104` | null |
[VIDEO] Visualization of Bottom-Up Dynamic Programming Solution | coin-change | 0 | 1 | https://youtu.be/SIHLJdF4F8A?si=1zjA4TRmWfCUQp1Y\n\nFor an overview of the greedy approach and the brute force recursive solution, please see the video. This explanation will focus on the dynamic programming solution.\n\nWe start by initializing an array called `dp`. Each element of this array will contain the fewest number of coins needed to make an amount of that index. We start by putting a 0 at index 0, because you need 0 coins to make an amount of 0.\n\nThe outer `i` loop will interate over the array, and for each one of those elements, the inner `coin` loop will try subtracting each coin amount from that index. This represents "trying" each coin by subtracting it from the index amount, and so the difference of `coin - i` represents the remaining amount. We\'ll then use the `dp` array to figure out the fewest number of coins needed to make up the remaining amount, and we build the `dp` array all the way up to the last index. When both loops are done, the element at the last index will be the final answer to the problem.\n\n# Code\n```\nclass Solution:\n def coinChange(self, coins: List[int], amount: int) -> int:\n dp = [0] + ([float(\'inf\')] * amount)\n for i in range(1, amount + 1):\n for coin in coins:\n if coin <= i:\n dp[i] = min(dp[i], dp[i - coin] + 1)\n\n if dp[-1] == float(\'inf\'):\n return -1\n return dp[-1]\n\n``` | 5 | You are given an integer array `coins` representing coins of different denominations and an integer `amount` representing a total amount of money.
Return _the fewest number of coins that you need to make up that amount_. If that amount of money cannot be made up by any combination of the coins, return `-1`.
You may assume that you have an infinite number of each kind of coin.
**Example 1:**
**Input:** coins = \[1,2,5\], amount = 11
**Output:** 3
**Explanation:** 11 = 5 + 5 + 1
**Example 2:**
**Input:** coins = \[2\], amount = 3
**Output:** -1
**Example 3:**
**Input:** coins = \[1\], amount = 0
**Output:** 0
**Constraints:**
* `1 <= coins.length <= 12`
* `1 <= coins[i] <= 231 - 1`
* `0 <= amount <= 104` | null |
Python Easy 2 DP approaches | coin-change | 0 | 1 | 1. ##### **Bottom Up DP(Tabulation)**\n\n```\nclass Solution:\n def coinChange(self, coins: List[int], amount: int) -> int: \n dp=[math.inf] * (amount+1)\n dp[0]=0\n \n for coin in coins:\n for i in range(coin, amount+1):\n if i-coin>=0:\n dp[i]=min(dp[i], dp[i-coin]+1)\n \n return -1 if dp[-1]==math.inf else dp[-1]\n \n```\n\n2. ##### **Top Down DP(Memoization)**\n```\nclass Solution:\n def coinChange(self, coins: List[int], amount: int) -> int: \n def coinChangeInner(rem, cache):\n if rem < 0:\n return math.inf\n if rem == 0: \n return 0 \n if rem in cache:\n return cache[rem]\n \n cache[rem] = min(coinChangeInner(rem-x, cache) + 1 for x in coins) \n return cache[rem] \n \n ans = coinChangeInner(amount, {})\n return -1 if ans == math.inf else ans \n```\n\nIf `S` is the `amount` and `n` is the length of input `coins`, then below is the complexity of my solution:\n**Time - O(S*n)**\n**Space - O(S)**\n\n\n---\n\n***Please upvote if you find it useful*** | 57 | You are given an integer array `coins` representing coins of different denominations and an integer `amount` representing a total amount of money.
Return _the fewest number of coins that you need to make up that amount_. If that amount of money cannot be made up by any combination of the coins, return `-1`.
You may assume that you have an infinite number of each kind of coin.
**Example 1:**
**Input:** coins = \[1,2,5\], amount = 11
**Output:** 3
**Explanation:** 11 = 5 + 5 + 1
**Example 2:**
**Input:** coins = \[2\], amount = 3
**Output:** -1
**Example 3:**
**Input:** coins = \[1\], amount = 0
**Output:** 0
**Constraints:**
* `1 <= coins.length <= 12`
* `1 <= coins[i] <= 231 - 1`
* `0 <= amount <= 104` | null |
DP Solution | coin-change | 0 | 1 | \n# Code\n```\nclass Solution:\n def coinChange(self, coins: List[int], amount: int) -> int:\n def change(n):\n if F[n] != -1:\n return F[n]\n value = float(\'inf\')\n for coin in coins:\n if coin <= n:\n value = min(change(n-coin),value)\n F[n] = value + 1 if value != -1 else -1\n return F[n]\n if amount == 0:\n return 0\n F = [-1 for _ in range(amount+1)]\n F[0] = 0\n change(amount)\n return F[-1] if F[-1] != float(\'inf\') else -1\n\n``` | 1 | You are given an integer array `coins` representing coins of different denominations and an integer `amount` representing a total amount of money.
Return _the fewest number of coins that you need to make up that amount_. If that amount of money cannot be made up by any combination of the coins, return `-1`.
You may assume that you have an infinite number of each kind of coin.
**Example 1:**
**Input:** coins = \[1,2,5\], amount = 11
**Output:** 3
**Explanation:** 11 = 5 + 5 + 1
**Example 2:**
**Input:** coins = \[2\], amount = 3
**Output:** -1
**Example 3:**
**Input:** coins = \[1\], amount = 0
**Output:** 0
**Constraints:**
* `1 <= coins.length <= 12`
* `1 <= coins[i] <= 231 - 1`
* `0 <= amount <= 104` | null |
324: Time 99.2% and Space 99.16%, Solution with step by step explanation | wiggle-sort-ii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. We first sort the array nums in ascending order.\n2. We then find the median of the array (i.e., the middle element if the array has odd length, or one of the two middle elements if the array has even length). We can use (n - 1) // 2 to find the index of the median element.\n3. We then create two subarrays: one containing the larger half of the sorted nums array, and one containing the smaller half. We can use slicing to achieve this: nums[mid::-1] gives us the elements from the median index backwards to the start of the array, and nums[:mid:-1] gives us the elements from the median index forwards to the end of the array, in reverse order.\n4. Finally, we use slicing again to interleave the elements of the two subarrays. We use nums[::2] to get every second element starting from the first element, and nums[1::2] to get every second element starting from the second element. We assign the elements from the larger subarray to the even indices, and the elements from the smaller subarray to the odd indices.\n\nHere\'s a step-by-step explanation of how the code works:\n\n1. n = len(nums): Gets the length of the input array nums.\n\n2. nums.sort(): Sorts the array in ascending order. This is necessary because the algorithm needs to rearrange the array in a specific pattern.\n\n3. mid = (n - 1) // 2: Calculates the index of the middle element in the sorted array.\n\n4. nums[::2], nums[1::2] = nums[mid::-1], nums[:mid:-1]: This is where the main algorithm takes place.\n\n- nums[::2]: Slices the array starting from the first element and selects every second element (i.e. elements with even indices).\n- nums[1::2]: Slices the array starting from the second element and selects every second element (i.e. elements with odd indices).\n- nums[mid::-1]: Slices the array starting from the middle element and selects every element backwards (i.e. in descending order).\n- nums[:mid:-1]: Slices the array starting from the element after the middle element and selects every element backwards (i.e. in descending order).\nBy doing this slicing and reassignment, we\'re essentially rearranging the array in a "wiggle" pattern. Specifically, the even-indexed elements are the larger half of the sorted array, and the odd-indexed elements are the smaller half of the sorted array. This ensures that adjacent elements alternate between being larger and smaller.\n\n5. The original array nums is now sorted in a wiggle pattern.\n\nOverall, the time complexity of this solution is O(n log n) due to the sorting operation. The space complexity is O(n) due to the slicing and reassignment of nums.\n\n# Complexity\n- Time complexity:\n99.2%\n\n- Space complexity:\n99.16%\n\n# Code\n```\nclass Solution:\n def wiggleSort(self, nums: List[int]) -> None:\n n = len(nums)\n nums.sort()\n mid = (n - 1) // 2\n nums[::2], nums[1::2] = nums[mid::-1], nums[:mid:-1]\n\n``` | 15 | Given an integer array `nums`, reorder it such that `nums[0] < nums[1] > nums[2] < nums[3]...`.
You may assume the input array always has a valid answer.
**Example 1:**
**Input:** nums = \[1,5,1,1,6,4\]
**Output:** \[1,6,1,5,1,4\]
**Explanation:** \[1,4,1,5,1,6\] is also accepted.
**Example 2:**
**Input:** nums = \[1,3,2,2,3,1\]
**Output:** \[2,3,1,3,1,2\]
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `0 <= nums[i] <= 5000`
* It is guaranteed that there will be an answer for the given input `nums`.
**Follow Up:** Can you do it in `O(n)` time and/or **in-place** with `O(1)` extra space? | null |
Very easy solution in 5 lines using python | wiggle-sort-ii | 0 | 1 | **1. We have to copy array into temporary space\n2. Sort temporary array in ascending order\n3. Then run loop of size 2\n4. During loop we have to swap elements of orignal array with temporary array\n5. Swap temporary array values form end (j index point to last till i ! = end of array) and keep assigning value in orignal array start from i = 1 with jump of 2.\n6. Then reduce i - 1 -> i = 0 and start filling value where we left the j and keep assigning values to original array from i = 0 index till end with jump of 2.**\n\n```\nclass Solution:\n def wiggleSort(self, nums: List[int]) -> None:\n """\n Do not return anything, modify nums in-place instead.\n """\n tmp = nums.copy()\n tmp.sort() \n n = len(nums)\n i, j = 1, n - 1\n for _ in range(2): \n for k in range(i, n, 2): # when i == 1 then gt elements and when i == 0 then sm elements with jump of 2\n nums[k] = tmp[j] # sorted array tmp reverse order values assignment\n j -= 1\n i -= 1\n\n``` | 5 | Given an integer array `nums`, reorder it such that `nums[0] < nums[1] > nums[2] < nums[3]...`.
You may assume the input array always has a valid answer.
**Example 1:**
**Input:** nums = \[1,5,1,1,6,4\]
**Output:** \[1,6,1,5,1,4\]
**Explanation:** \[1,4,1,5,1,6\] is also accepted.
**Example 2:**
**Input:** nums = \[1,3,2,2,3,1\]
**Output:** \[2,3,1,3,1,2\]
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `0 <= nums[i] <= 5000`
* It is guaranteed that there will be an answer for the given input `nums`.
**Follow Up:** Can you do it in `O(n)` time and/or **in-place** with `O(1)` extra space? | null |
O(n) time, O(1) space. Python code + explanation. | wiggle-sort-ii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nAs the quesiton description, we have:\n```\nnums[0] < nums[1] > nums[2]\nnums[2] < nums[3] > nums[4]\nnums[4] < nums[5] > nums[6]\n...\n...\n...\n```\n\nWe can simply split the array into an odd-idx array and an even-idx array where each elements in the odd-idx array is >= every element in the even array.\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Since we need to split it into 2 half, we first find the median.\nThere\'s an avg O(n) time complexity solution which is QuickSelect.\n(Question 215: Kth Largest Element in an Array)\n2. Run a loop on even indexes, swap it to odd index if it\'s > median.\n3. Repeat step 2 for odd indexes.\n4. Now we have the smaller half on even indexes and the greater half on odd indexes, however, there might be more than 1 element which has the median value. For example, ```[1, 1, 0, 2]``` is an invalid result because ```nums[0] == nums[1]``` To fix this issue, simply move all medians on the even indexes to the left and all medians on the odd even indexes to the right.\n\nNote: For step4, we can\'t do the other way round because even[0] is always less then only odd[0], however, even[-1] may be less then both odd[-1] and odd[-2].\nExample: ```[1,2,1,2,2,3]``` is invalid and ```[2,3,1,2,1,2]``` is valid.\n\n\n\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findKthSmallest(self, nums: List[int], k: int) -> int:\n # code from: 215. Kth Largest Element in an Array\n # modified from kth largest -> kth smallest\n start, end = 0, len(nums) - 1\n while True:\n i, j = start, end\n pivot = nums[end]\n while i < j:\n if nums[i] > pivot:\n while j > i and nums[j] > pivot:\n j -= 1\n nums[i], nums[j] = nums[j], nums[i]\n j -= 1\n else:\n i += 1\n if nums[j] > pivot:\n j -= 1\n if j == k:\n return pivot\n if j < k:\n start = j\n while start < k and nums[start] <= pivot:\n start += 1\n else:\n end = j\n while end > k and nums[end] == pivot:\n end -= 1\n\n def wiggleSort(self, nums: List[int]) -> None:\n # get the median. avg O(n) time and constant space\n median = self.findKthSmallest(nums, len(nums) // 2)\n\n idx_less, idx_greater = 0, 1\n # swap elements to the correct list (even or odd index)\n # this whole process is O(n) because each element moves at most once\n for idx in range(0, len(nums), 2): # even idx. swap until the element <= median\n while nums[idx] > median:\n nums[idx], nums[idx_greater] = nums[idx_greater], nums[idx]\n idx_greater += 2\n for idx in range(idx_greater, len(nums), 2): # odd idx\n while nums[idx] < median:\n nums[idx], nums[idx_less] = nums[idx_less], nums[idx]\n idx_less += 2\n\n # move all medians on the even list to the left\n i, j = 0, len(nums) - 1\n if j & 1:\n j -= 1 # make sure j is even\n while j > 0 and nums[j] != median:\n j -= 2 # find the last non-median elelment\n\n while i < j:\n if nums[i] == median:\n i += 2\n else:\n nums[i], nums[j] = nums[j], nums[i]\n j -= 2\n\n # move all medians on the odd list to the right\n i, j = 1, len(nums) - 1\n if (j & 1) == 0:\n j -= 1 # make sure j is odd\n while i < j and nums[i] != median:\n i += 2 # find the first non-median element\n\n while i < j:\n if nums[j] == median:\n j -= 2\n else:\n nums[i], nums[j] = nums[j], nums[i]\n i += 2\n\n``` | 2 | Given an integer array `nums`, reorder it such that `nums[0] < nums[1] > nums[2] < nums[3]...`.
You may assume the input array always has a valid answer.
**Example 1:**
**Input:** nums = \[1,5,1,1,6,4\]
**Output:** \[1,6,1,5,1,4\]
**Explanation:** \[1,4,1,5,1,6\] is also accepted.
**Example 2:**
**Input:** nums = \[1,3,2,2,3,1\]
**Output:** \[2,3,1,3,1,2\]
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `0 <= nums[i] <= 5000`
* It is guaranteed that there will be an answer for the given input `nums`.
**Follow Up:** Can you do it in `O(n)` time and/or **in-place** with `O(1)` extra space? | null |
Python Solution || Easy Solution using priority queue | wiggle-sort-ii | 0 | 1 | \n# Code\n```\nclass Solution:\n def wiggleSort(self, nums: List[int]) -> None:\n """\n Do not return anything, modify nums in-place instead.\n """\n\n nums.sort()\n n = len(nums)\n\n # divide the nums into 2 sorted array\n # then each time take the maximum from both the heap using max heap\n # for even no of terms there will be no prbm\n \'\'\'\n first max ele in the first half \n then max ele in the second half\n \'\'\'\n # for odd no of terms intially add the mid ele \n \'\'\'\n first max ele in the second half \n then max ele in the first half\n \'\'\'\n\n if n&1 :\n ans = []\n mid = n//2 \n ans += [nums[mid]]\n a = [-x for x in nums[:mid]]\n b = [-x for x in nums[mid+1:]]\n heapq.heapify(a)\n heapq.heapify(b)\n cnt = mid \n while(cnt) :\n ans += [-heapq.heappop(b)]\n ans += [ -heapq.heappop(a)]\n cnt -= 1 \n nums[::] = ans[::]\n else:\n ans = []\n mid = n//2 \n a = [-x for x in nums[:mid]]\n b = [-x for x in nums[mid:]]\n heapq.heapify(a)\n heapq.heapify(b)\n cnt = mid \n while(cnt) :\n ans += [ -heapq.heappop(a)]\n ans += [-heapq.heappop(b)]\n cnt -= 1 \n nums[::] = ans[::]\n \n\n\n``` | 1 | Given an integer array `nums`, reorder it such that `nums[0] < nums[1] > nums[2] < nums[3]...`.
You may assume the input array always has a valid answer.
**Example 1:**
**Input:** nums = \[1,5,1,1,6,4\]
**Output:** \[1,6,1,5,1,4\]
**Explanation:** \[1,4,1,5,1,6\] is also accepted.
**Example 2:**
**Input:** nums = \[1,3,2,2,3,1\]
**Output:** \[2,3,1,3,1,2\]
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `0 <= nums[i] <= 5000`
* It is guaranteed that there will be an answer for the given input `nums`.
**Follow Up:** Can you do it in `O(n)` time and/or **in-place** with `O(1)` extra space? | null |
Python3 O(N) time O(1) space - Wiggle Sort II | wiggle-sort-ii | 0 | 1 | Note that the performance actually sucks. Because it\'s CPython. If a builtin is written in C and it\'s O(NlgN), its performance may be better than an O(N) implementation in pure Python.\n\n```\n# Inspired by https://leetcode.com/problems/wiggle-sort-ii/discuss/77677/O(n)%2BO(1)-after-median-Virtual-Indexing\nclass Solution:\n def wiggleSort(self, nums: List[int]) -> None:\n """\n Do not return anything, modify nums in-place instead.\n """\n mid = len(nums) // 2\n def quickselect(arr, lo, hi, k):\n pivot = random.randint(lo, hi)\n arr[pivot], arr[hi] = arr[hi], arr[pivot]\n pivot = lo\n for i in range(lo, hi):\n if arr[i] < arr[hi]:\n arr[i], arr[pivot] = arr[pivot], arr[i]\n pivot += 1\n arr[pivot], arr[hi] = arr[hi], arr[pivot]\n if k == pivot:\n return arr[pivot]\n elif k < pivot:\n return quickselect(arr, lo, pivot-1, k)\n else:\n return quickselect(arr, pivot+1, hi, k)\n median = quickselect(nums, 0, len(nums)-1, mid)\n \n # virtual index\n vi = lambda x: x * 2 + 1 if x < mid else (x - mid) * 2\n i, j, k = 0, 0, len(nums) - 1\n while j <= k:\n if nums[vi(j)] < median:\n nums[vi(j)], nums[vi(k)] = nums[vi(k)], nums[vi(j)]\n k -= 1\n elif nums[vi(j)] > median:\n nums[vi(j)], nums[vi(i)] = nums[vi(i)], nums[vi(j)]\n i += 1\n j += 1\n else:\n j += 1\n``` | 11 | Given an integer array `nums`, reorder it such that `nums[0] < nums[1] > nums[2] < nums[3]...`.
You may assume the input array always has a valid answer.
**Example 1:**
**Input:** nums = \[1,5,1,1,6,4\]
**Output:** \[1,6,1,5,1,4\]
**Explanation:** \[1,4,1,5,1,6\] is also accepted.
**Example 2:**
**Input:** nums = \[1,3,2,2,3,1\]
**Output:** \[2,3,1,3,1,2\]
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `0 <= nums[i] <= 5000`
* It is guaranteed that there will be an answer for the given input `nums`.
**Follow Up:** Can you do it in `O(n)` time and/or **in-place** with `O(1)` extra space? | null |
just code : ) | wiggle-sort-ii | 0 | 1 | \n# Code\n```\nclass Solution:\n def wiggleSort(self, nums: List[int]) -> None:\n # sorted copy of original array\n array = sorted(nums)\n # take the biggest elements and insert them into odd positions\n for i in range(1, len(nums), 2):\n nums[i] = array.pop()\n # insert lowest elements into even positions\n for i in range(0, len(nums), 2):\n nums[i] = array.pop()\n``` | 0 | Given an integer array `nums`, reorder it such that `nums[0] < nums[1] > nums[2] < nums[3]...`.
You may assume the input array always has a valid answer.
**Example 1:**
**Input:** nums = \[1,5,1,1,6,4\]
**Output:** \[1,6,1,5,1,4\]
**Explanation:** \[1,4,1,5,1,6\] is also accepted.
**Example 2:**
**Input:** nums = \[1,3,2,2,3,1\]
**Output:** \[2,3,1,3,1,2\]
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `0 <= nums[i] <= 5000`
* It is guaranteed that there will be an answer for the given input `nums`.
**Follow Up:** Can you do it in `O(n)` time and/or **in-place** with `O(1)` extra space? | null |
sorting based approach | wiggle-sort-ii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n$$O(nlog(n))$$\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nclass Solution:\n def wiggleSort(self, nums: List[int]) -> None:\n # sorted copy of original array\n array = sorted(nums)\n # take the biggest elements and insert them into odd positions\n for i in range(1, len(nums), 2):\n nums[i] = array.pop()\n # insert lowest elements into even positions\n for i in range(0, len(nums), 2):\n nums[i] = array.pop()\n``` | 0 | Given an integer array `nums`, reorder it such that `nums[0] < nums[1] > nums[2] < nums[3]...`.
You may assume the input array always has a valid answer.
**Example 1:**
**Input:** nums = \[1,5,1,1,6,4\]
**Output:** \[1,6,1,5,1,4\]
**Explanation:** \[1,4,1,5,1,6\] is also accepted.
**Example 2:**
**Input:** nums = \[1,3,2,2,3,1\]
**Output:** \[2,3,1,3,1,2\]
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `0 <= nums[i] <= 5000`
* It is guaranteed that there will be an answer for the given input `nums`.
**Follow Up:** Can you do it in `O(n)` time and/or **in-place** with `O(1)` extra space? | null |
Python with O(n) time and O(1) space | wiggle-sort-ii | 0 | 1 | # Intuition\nUse a fixed size array and assign values to even and odd indexes separately\n\n# Approach\nAssign a fixed size array of size 5000 (as 0 <= nums[i] <= 5000) and put count of numbers present in nums in the array. Then execute two for loops to put values in odd and even indexes.\n\n# Complexity\n- Time complexity:\nO(n) \n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def wiggleSort(self, nums: List[int]) -> None:\n """\n Do not return anything, modify nums in-place instead.\n """\n store = [0] * 5000\n maxNum = 0\n # O(n)\n for num in nums:\n store[num] += 1\n if(num > maxNum):\n maxNum = num\n\n # For odd indexes\n idx = 1\n l = len(nums)\n # O(n) is only one value per index.\n # if all values are same then for will not execute due to break condition\n for i in range(maxNum,-1,-1):\n while(store[i] != 0 and idx<l):\n nums[idx] = i\n store[i] -= 1\n idx += 2\n if(idx >= l):\n break\n\n # For even indexes\n idx = 0\n l = len(nums)\n for i in range(maxNum,-1,-1):\n while(store[i] != 0 and idx<l):\n nums[idx] = i\n store[i] -= 1\n idx += 2\n if(idx >= l):\n break\n\n\n``` | 0 | Given an integer array `nums`, reorder it such that `nums[0] < nums[1] > nums[2] < nums[3]...`.
You may assume the input array always has a valid answer.
**Example 1:**
**Input:** nums = \[1,5,1,1,6,4\]
**Output:** \[1,6,1,5,1,4\]
**Explanation:** \[1,4,1,5,1,6\] is also accepted.
**Example 2:**
**Input:** nums = \[1,3,2,2,3,1\]
**Output:** \[2,3,1,3,1,2\]
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `0 <= nums[i] <= 5000`
* It is guaranteed that there will be an answer for the given input `nums`.
**Follow Up:** Can you do it in `O(n)` time and/or **in-place** with `O(1)` extra space? | null |
[Python3] 2-liner | wiggle-sort-ii | 0 | 1 | # Code\n```\nclass Solution:\n def wiggleSort(self, nums: List[int]) -> None:\n nums.sort(reverse=True)\n nums[::2], nums[1::2] = nums[len(nums)//2:], nums[:len(nums)//2]\n\n \n``` | 0 | Given an integer array `nums`, reorder it such that `nums[0] < nums[1] > nums[2] < nums[3]...`.
You may assume the input array always has a valid answer.
**Example 1:**
**Input:** nums = \[1,5,1,1,6,4\]
**Output:** \[1,6,1,5,1,4\]
**Explanation:** \[1,4,1,5,1,6\] is also accepted.
**Example 2:**
**Input:** nums = \[1,3,2,2,3,1\]
**Output:** \[2,3,1,3,1,2\]
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `0 <= nums[i] <= 5000`
* It is guaranteed that there will be an answer for the given input `nums`.
**Follow Up:** Can you do it in `O(n)` time and/or **in-place** with `O(1)` extra space? | null |
( | wiggle-sort-ii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def wiggleSort(self, nums: List[int]) -> None:\n """\n Do not return anything, modify nums in-place instead.\n """\n nums.sort()\n a = [0] * len(nums)\n l = len(nums)\n j = l-1\n for i in range(1,l,2):\n a[i] = nums[j]\n j -= 1\n for i in range(0,l,2):\n a[i] = nums[j]\n j -= 1\n for i in range(l):\n nums[i] = a[i]\n \n``` | 0 | Given an integer array `nums`, reorder it such that `nums[0] < nums[1] > nums[2] < nums[3]...`.
You may assume the input array always has a valid answer.
**Example 1:**
**Input:** nums = \[1,5,1,1,6,4\]
**Output:** \[1,6,1,5,1,4\]
**Explanation:** \[1,4,1,5,1,6\] is also accepted.
**Example 2:**
**Input:** nums = \[1,3,2,2,3,1\]
**Output:** \[2,3,1,3,1,2\]
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `0 <= nums[i] <= 5000`
* It is guaranteed that there will be an answer for the given input `nums`.
**Follow Up:** Can you do it in `O(n)` time and/or **in-place** with `O(1)` extra space? | null |
Binary Search PYTHON ! | power-of-three | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isPowerOfThree(self, n: int) -> bool:\n start = 0\n end = min(151,n//3)\n while start<=end:\n mid = start+(end -start)//2\n temp = 3**mid\n if temp == n:\n return True\n elif temp>n:\n end = mid-1\n else:\n start = mid+1\n return False\n``` | 1 | Given an integer `n`, return _`true` if it is a power of three. Otherwise, return `false`_.
An integer `n` is a power of three, if there exists an integer `x` such that `n == 3x`.
**Example 1:**
**Input:** n = 27
**Output:** true
**Explanation:** 27 = 33
**Example 2:**
**Input:** n = 0
**Output:** false
**Explanation:** There is no x where 3x = 0.
**Example 3:**
**Input:** n = -1
**Output:** false
**Explanation:** There is no x where 3x = (-1).
**Constraints:**
* `-231 <= n <= 231 - 1`
**Follow up:** Could you solve it without loops/recursion? | null |
python3 code | power-of-three | 0 | 1 | # Intuition\nIt first checks if the input integer n is greater than 0. If so, it calculates the logarithm of n to the base 3 using the log() function from the math module. It then rounds the result to one decimal place using the round() function and stores it in the variable x.\n\nFinally, it checks if 3 raised to the power of x is equal to n. If it is, then it returns True, indicating that n is a power of 3. Otherwise, it returns False\n\n# Approach\nThe approach taken in the code is as follows:\n\n1. Check if the input integer `n` is greater than 0.\n2. If `n` is greater than 0, calculate the logarithm of `n` to the base 3 using the `log()` function from the `math` module. Store the result in the variable `e`.\n3. Round the value of `e` to one decimal place using the `round()` function and store it in the variable `x`.\n4. Check if 3 raised to the power of `x` is equal to `n`.\n5. If the condition in step 4 is true, return `True`, indicating that `n` is a power of 3.\n6. If the condition in step 4 is false, return `False`, indicating that `n` is not a power of 3.\n\nOverall, the code checks if a given integer is a power of 3 by calculating its logarithm to the base 3 and comparing it with the original value.\n\n# Complexity\n- Time complexity:\n O(1)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def isPowerOfThree(self, n: int) -> bool:\n import math\n if n>0:\n e = log(n,3)\n x = round(e,1)\n if(pow(3,x)==n):\n return True \n else:\n return False\n \n``` | 1 | Given an integer `n`, return _`true` if it is a power of three. Otherwise, return `false`_.
An integer `n` is a power of three, if there exists an integer `x` such that `n == 3x`.
**Example 1:**
**Input:** n = 27
**Output:** true
**Explanation:** 27 = 33
**Example 2:**
**Input:** n = 0
**Output:** false
**Explanation:** There is no x where 3x = 0.
**Example 3:**
**Input:** n = -1
**Output:** false
**Explanation:** There is no x where 3x = (-1).
**Constraints:**
* `-231 <= n <= 231 - 1`
**Follow up:** Could you solve it without loops/recursion? | null |
speed was 86% in Python3 | power-of-three | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isPowerOfThree(self, n: int) -> bool:\n if n == 1:\n return True\n else:\n while n > 2:\n n = n / 3\n return n == 1\n\n``` | 2 | Given an integer `n`, return _`true` if it is a power of three. Otherwise, return `false`_.
An integer `n` is a power of three, if there exists an integer `x` such that `n == 3x`.
**Example 1:**
**Input:** n = 27
**Output:** true
**Explanation:** 27 = 33
**Example 2:**
**Input:** n = 0
**Output:** false
**Explanation:** There is no x where 3x = 0.
**Example 3:**
**Input:** n = -1
**Output:** false
**Explanation:** There is no x where 3x = (-1).
**Constraints:**
* `-231 <= n <= 231 - 1`
**Follow up:** Could you solve it without loops/recursion? | null |
Python Recursive and Iterative solution | power-of-three | 0 | 1 | # Iterative 1\n```\ndef isPowerOfThree(self, n: int) -> bool:\n if( n <=0): return False;\n x = 1;\n while(x<n): x *=3\n return x==n\n```\n# Iterative 2\n```\ndef isPowerOfThree(self, n: int) -> bool:\n if(n>1): \n while(n%3==0):n//=3\n return n==1\n```\n\n# Recursive one-liner \n```\n def isPowerOfThree(self, n: int) -> bool:\n return False if n<=0 else self.isPowerOfThree(n//3) if(n%3==0) else n==1 \n```\n | 2 | Given an integer `n`, return _`true` if it is a power of three. Otherwise, return `false`_.
An integer `n` is a power of three, if there exists an integer `x` such that `n == 3x`.
**Example 1:**
**Input:** n = 27
**Output:** true
**Explanation:** 27 = 33
**Example 2:**
**Input:** n = 0
**Output:** false
**Explanation:** There is no x where 3x = 0.
**Example 3:**
**Input:** n = -1
**Output:** false
**Explanation:** There is no x where 3x = (-1).
**Constraints:**
* `-231 <= n <= 231 - 1`
**Follow up:** Could you solve it without loops/recursion? | null |
Using Log Function | power-of-three | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n\n# Complexity\n- Time complexity: O(1)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport math\nclass Solution:\n def isPowerOfThree(self, n: int) -> bool:\n if n <= 0 :\n return False\n res = round(math.log(n,3))\n return 3**res == n\n \n \n``` | 1 | Given an integer `n`, return _`true` if it is a power of three. Otherwise, return `false`_.
An integer `n` is a power of three, if there exists an integer `x` such that `n == 3x`.
**Example 1:**
**Input:** n = 27
**Output:** true
**Explanation:** 27 = 33
**Example 2:**
**Input:** n = 0
**Output:** false
**Explanation:** There is no x where 3x = 0.
**Example 3:**
**Input:** n = -1
**Output:** false
**Explanation:** There is no x where 3x = (-1).
**Constraints:**
* `-231 <= n <= 231 - 1`
**Follow up:** Could you solve it without loops/recursion? | null |
List comprehension Python solution | power-of-three | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isPowerOfThree(self, n: int) -> bool:\n return n in [3**x for x in range(20)]\n``` | 2 | Given an integer `n`, return _`true` if it is a power of three. Otherwise, return `false`_.
An integer `n` is a power of three, if there exists an integer `x` such that `n == 3x`.
**Example 1:**
**Input:** n = 27
**Output:** true
**Explanation:** 27 = 33
**Example 2:**
**Input:** n = 0
**Output:** false
**Explanation:** There is no x where 3x = 0.
**Example 3:**
**Input:** n = -1
**Output:** false
**Explanation:** There is no x where 3x = (-1).
**Constraints:**
* `-231 <= n <= 231 - 1`
**Follow up:** Could you solve it without loops/recursion? | null |
326: Solution with step by step explanation | power-of-three | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n- The condition n > 0 is used to make sure that we only check for positive integers.\n- We check if 3 raised to the power of 19 is divisible by n. Why 19? Because 3**19 is the largest power of 3 that can fit into the integer range (2^31 - 1).\n- If n is a power of 3, then it must be a divisor of 3**19 and thus the remainder of 3**19 / n will be 0. If it\'s not a power of 3, then it won\'t be a divisor and the remainder will be non-zero.\n- Therefore, we return True if n is greater than 0 and 3**19 % n is equal to 0, and False otherwise.\n# Complexity\n- Time complexity:\n82.63%\n\n- Space complexity:\n51.99%\n\n# Code\n```\nclass Solution:\n def isPowerOfThree(self, n: int) -> bool:\n return n > 0 and 3**19 % n == 0\n``` | 21 | Given an integer `n`, return _`true` if it is a power of three. Otherwise, return `false`_.
An integer `n` is a power of three, if there exists an integer `x` such that `n == 3x`.
**Example 1:**
**Input:** n = 27
**Output:** true
**Explanation:** 27 = 33
**Example 2:**
**Input:** n = 0
**Output:** false
**Explanation:** There is no x where 3x = 0.
**Example 3:**
**Input:** n = -1
**Output:** false
**Explanation:** There is no x where 3x = (-1).
**Constraints:**
* `-231 <= n <= 231 - 1`
**Follow up:** Could you solve it without loops/recursion? | null |
Simplest Python Solution | power-of-three | 0 | 1 | \n\n# Code\n```\nclass Solution(object):\n def isPowerOfThree(self, n):\n """\n :type n: int\n :rtype: bool\n """\n if n<=0:\n return False\n while n!=1:\n if n%3!=0:\n return False\n n//=3\n return True\n``` | 2 | Given an integer `n`, return _`true` if it is a power of three. Otherwise, return `false`_.
An integer `n` is a power of three, if there exists an integer `x` such that `n == 3x`.
**Example 1:**
**Input:** n = 27
**Output:** true
**Explanation:** 27 = 33
**Example 2:**
**Input:** n = 0
**Output:** false
**Explanation:** There is no x where 3x = 0.
**Example 3:**
**Input:** n = -1
**Output:** false
**Explanation:** There is no x where 3x = (-1).
**Constraints:**
* `-231 <= n <= 231 - 1`
**Follow up:** Could you solve it without loops/recursion? | null |
Checking if an Integer is a Power of Three Using Logarithm Function. | power-of-three | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nTo check if an integer n is a power of three, we can use the logarithm function to solve it. Specifically, we can take the logarithm of n with base 3 and check if the result is an integer. If the result is an integer, then n is a power of three. Otherwise, n is not a power of three.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe approach is to use the built-in logarithm function in Python to implement this solution. We first check if n is non-positive, in which case it cannot be a power of three. Then, we take the logarithm of n with base 3 using the `math.log10()` function. We divide by the logarithm of 3 to convert the result to base 3. Finally, we check if the result is an integer using the `int()` function and compare it to the original result. If they are equal, then n is a power of three.\n# Complexity\n- Time complexity: $$O(1)$$\n\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\nThe time complexity is $$O(1)$$ since we are just taking logarithms and performing basic arithmetic operations. The space complexity is also $$O(1)$$ since we are not using any additional space apart from the input variable and a few variables to store intermediate results.\n\n# Code\n```\nimport math\nclass Solution:\n def isPowerOfThree(self, n: int) -> bool:\n if n <= 0:\n return False\n log = math.log10(n) / math.log10(3)\n return int(log) == log\n``` | 8 | Given an integer `n`, return _`true` if it is a power of three. Otherwise, return `false`_.
An integer `n` is a power of three, if there exists an integer `x` such that `n == 3x`.
**Example 1:**
**Input:** n = 27
**Output:** true
**Explanation:** 27 = 33
**Example 2:**
**Input:** n = 0
**Output:** false
**Explanation:** There is no x where 3x = 0.
**Example 3:**
**Input:** n = -1
**Output:** false
**Explanation:** There is no x where 3x = (-1).
**Constraints:**
* `-231 <= n <= 231 - 1`
**Follow up:** Could you solve it without loops/recursion? | null |
PYTHON WHILE LOOP SOLUTION | power-of-three | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isPowerOfThree(self, n: int) -> bool:\n if n <= 0 :\n return False\n if n == 1 :\n return True\n while n != 1:\n if n % 3 != 0 :\n return False\n n = n // 3\n return True\n \n``` | 2 | Given an integer `n`, return _`true` if it is a power of three. Otherwise, return `false`_.
An integer `n` is a power of three, if there exists an integer `x` such that `n == 3x`.
**Example 1:**
**Input:** n = 27
**Output:** true
**Explanation:** 27 = 33
**Example 2:**
**Input:** n = 0
**Output:** false
**Explanation:** There is no x where 3x = 0.
**Example 3:**
**Input:** n = -1
**Output:** false
**Explanation:** There is no x where 3x = (-1).
**Constraints:**
* `-231 <= n <= 231 - 1`
**Follow up:** Could you solve it without loops/recursion? | null |
Beats : 99.34% [33/145 Top Interview Question] | power-of-three | 0 | 1 | # Intuition\n*One approach to solve this is to repeatedly divide the given number by three until we get a number less than or equal to one. If the number is exactly equal to one, then it is a power of three, otherwise it is not.*\n\n*Another approach is to find the maximum power of three which is less than or equal to the given number, and check if the given number is divisible by that power of three. If yes, then the given number is a power of three, otherwise it is not.*\n\n# Approach\nThis code checks if the given integer `n` is a power of three or not. \n\nThe maximum power of 3 that can be stored in an integer is 3 to the power of 19. So the first step is to check if the given integer `n` is greater than 0 and if it is divisible by the maximum power of 3 that can be stored in an integer, i.e. `3 ** 19`. \n\nIf the above condition is true, then it means that `n` is a power of 3, otherwise, it is not. The function returns a boolean value, `True` if `n` is a power of 3, and `False` otherwise.\n\n# Complexity\n- Time complexity:\nO(1)\n\n- Space complexity:\nO(1)\n\n\nThe `time complexity` of the `isPowerOfThree` function is `O(1)` because it performs a constant number of operations, regardless of the input size. \nThe `space complexity` is also `O(1)` because it only uses a constant amount of additional memory to store the `mx` variable.\n# Code\n```\nclass Solution:\n def isPowerOfThree(self, n: int) -> bool:\n mx = 3 ** 19\n return n> 0 and mx%n == 0\n\n # class Solution:\n # def isPowerOfThree(self, n: int) -> bool:\n # res,x = 1, 0\n # while res <= n:\n # if res == n: return True\n # x += 1\n # res = 3**x\n # return False\n\n```\n\n\n | 6 | Given an integer `n`, return _`true` if it is a power of three. Otherwise, return `false`_.
An integer `n` is a power of three, if there exists an integer `x` such that `n == 3x`.
**Example 1:**
**Input:** n = 27
**Output:** true
**Explanation:** 27 = 33
**Example 2:**
**Input:** n = 0
**Output:** false
**Explanation:** There is no x where 3x = 0.
**Example 3:**
**Input:** n = -1
**Output:** false
**Explanation:** There is no x where 3x = (-1).
**Constraints:**
* `-231 <= n <= 231 - 1`
**Follow up:** Could you solve it without loops/recursion? | null |
Python different concise solutions | count-of-range-sum | 0 | 1 | The problem is similiar to [315. Count of Smaller Numbers After Self](https://leetcode.com/problems/count-of-smaller-numbers-after-self/), yoc can click **[here](https://leetcode.com/problems/count-of-smaller-numbers-after-self/discuss/408322/Python-Different-Concise-Solutions)** to check how to slolve it.\nThe difference between two probles is that you need compute the **prefix sums**, cumsum[i] means nums[:i]\'s prefix sums : \n >nums = [1,3,4]\n cumsum = [0,1,4,8]\n cumcum[1] means nums[:1]\'s prefix sums\n \nWe just need to count those where cumsum[j] - cumsum[i] is in [lower,upper].\n**Approach 1 : Prefix-sum + Hashmap**\nNaturally, utilize hashmap to reduce time time complexity\n```python\n # prefix-sum + hashmap | time complexity: O(n+(upper-lower+1)*n)\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n cumsum = [0]\n for n in nums:\n cumsum.append(cumsum[-1]+n)\n \n import collections\n record = collections.defaultdict(int)\n \n res = 0\n for csum in cumsum:\n for target in range(lower,upper+1):\n if csum - target in record:\n res += record[csum - target]\n record[csum] +=1\n return res\n```\n\n------------------------\n**Approach 2 : Prefix-sum + SegmentTree**\nInspired by [Java SegmentTree Solution, 36ms](https://leetcode.com/problems/count-of-range-sum/discuss/77987/Java-SegmentTree-Solution-36ms)\nYou can see, it was vsery similay to approach 1, SegmentTree is very useful for processing range queries, utilize SegmentTree to make query time from O(upper-lower+1) to O(logn)\n```python\nclass SegmentTreeNode:\n\tdef __init__(self,low,high):\n\t\tself.low = low\n\t\tself.high = high\n\t\tself.left = None\n\t\tself.right = None\n\t\tself.cnt = 0 \n \nclass Solution: \n def _bulid(self, left, right): \n root = SegmentTreeNode(self.cumsum[left],self.cumsum[right])\n if left == right:\n return root\n \n mid = (left+right)//2\n root.left = self._bulid(left, mid)\n root.right = self._bulid(mid+1, right)\n return root\n \n def _update(self, root, val):\n if not root:\n return \n if root.low<=val<=root.high:\n root.cnt += 1\n self._update(root.left, val)\n self._update(root.right, val)\n \n def _query(self, root, lower, upper):\n if lower <= root.low and root.high <= upper:\n return root.cnt\n if upper < root.low or root.high < lower:\n return 0\n return self._query(root.left, lower, upper) + self._query(root.right, lower, upper)\n \n # prefix-sum + SegmentTree | O(nlogn) \n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n cumsum = [0]\n for n in nums:\n cumsum.append(cumsum[-1]+n)\n \n self.cumsum = sorted(list(set(cumsum))) # need sorted\n root = self._bulid(0,len(self.cumsum)-1)\n \n res = 0\n for csum in cumsum:\n res += self._query(root, csum-upper, csum-lower)\n self._update(root, csum) \n return res\n```\n\n------------------\n**Approach 3 : Prefix-sum + Merge-sort:**\nCompute the prefix sums\nUse mergesort for counting\n\n\n```python\n # prefix-sum + merge-sort | time complexity: O(nlogn)\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n cumsum = [0]\n for n in nums:\n cumsum.append(cumsum[-1]+n)\n \n\t\t# inclusive\n def mergesort(l,r):\n if l == r:\n return 0\n mid = (l+r)//2\n cnt = mergesort(l,mid) + mergesort(mid+1,r)\n\t\t\t\n i = j = mid+1\n # O(n)\n for left in cumsum[l:mid+1]:\n while i <= r and cumsum[i] - left < lower:\n i+=1\n while j <= r and cumsum[j] - left <= upper:\n j+=1\n cnt += j-i\n \n cumsum[l:r+1] = sorted(cumsum[l:r+1])\n return cnt\n\t\t\t\n return mergesort(0,len(cumsum)-1)\n```\n\nNote\uFF1A it\'s O(n), because O(2n) = O(n).\n```python\nint j = 0;\nfor (int i = 0; i < n; i++)\n while (j < n)\n j++;\n``` | 64 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
Python bisect module | count-of-range-sum | 0 | 1 | # Python\n```\ndef countRangeSum(nums, lower, upper):\n \n prefix,cur,res = [0],0,0\n\n for n in nums:\n cur += n\n A = bisect.bisect_right(prefix, cur-lower)\n B = bisect.bisect_left(prefix, cur-upper)\n res += A - B\n bisect.insort(prefix, cur)\n \n return res\n```\n\n# Python\n```\nfrom sortedcontainers import SortedList\nclass Solution:\n def countRangeSum(self, nums, lower, upper) -> int:\n total = 0\n cumsum = 0\n cumsums = SortedList()\n for num in nums:\n cumsums.add(cumsum)\n cumsum += num\n # cumsum - lower >= x\n # cumsum - upper <= x \n total += cumsums.bisect_right(cumsum - lower) - cumsums.bisect_left(cumsum - upper)\n \n return total\n``` | 1 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
[Python] Simple solution with PrefixSum and Bisect || Documented | count-of-range-sum | 0 | 1 | ```\nimport bisect\n\nclass Solution:\n """\n Calculate Prefix Sum: \n Given an array arr[] of size n, \n its prefix sum is another array prefixSum[] of the same size, \n such that the value of prefixSum[i] is arr[0] + arr[1] + arr[2] \u2026 arr[i].\n \n Input : arr[] = {1, 2, 1}, lower = 2; upper = 3\n Output : prefixSum[] = {1, 3, 4}\n\n Count pairs where its sums is in range\n (i = 0, j = 0) = 1 - 1 = 0 -> not in range (2,3)\n \n (i = 1, j = 0) = 3 - 1 = 2 -> in range (2,3)\n (i = 1, j = 1) = 3 - 3 = 0 -> not in range (2,3)\n\n (i = 2, j = 0) = 4 - 1 = 3 -> in range (2,3)\n (i = 2, j = 1) = 4 - 2 = 2 -> in range (2,3)\n (i = 2, j = 2) = 4 - 4 = 0 -> not in range (2,3)\n """\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n # Initialize the countPairs, curSum\n countPairs, curSum = 0, 0\n\n # Initialize the prefixSum, with middle value of 0\n # there can be negateive and positive values too\n prefixSum = [0]\n for num in nums:\n \n # prefix sum\n curSum += num\n \n # bisect_left(list value)\n # Returns an index (left of same value) to insert given value to make the list sorted.\n left_index = bisect.bisect_left(prefixSum, curSum - upper)\n\n # bisect_right(list value)\n # Returns an index (right of same values) to insert given value to make the list sorted.\n right_index = bisect.bisect_right(prefixSum, curSum - lower)\n\n # count such pairs\n countPairs += right_index - left_index\n\n # add curSum into prefixSum so that prefixSum is still sorted\n # it is needed to handle negative values\n bisect.insort(prefixSum, curSum)\n\n return countPairs\n```\nUPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask. | 5 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
O(n logn) in Python faster than more than 80%, easy to understand | count-of-range-sum | 0 | 1 | ``` python\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n # First get the cumulative list\n # takes O(n)\n accumulated = nums.copy()\n for i in range(0, len(nums)):\n if i != 0:\n accumulated[i] = nums[i] + accumulated[i-1]\n\n # sort the cumulative list\n # we do this because it\'s easy for later operations\n # takes O(n logn)\n new_acc = sorted(accumulated)\n\n result = 0\n num = 0\n\n # takes O(n) \n for i in range(0, len(nums)):\n\n # get how many subarrays are within the bound\n # inside the loop, takes O(logn)\n l = bisect_left(new_acc, lower)\n r = bisect_right(new_acc, upper)\n diff = r - l\n\n result += diff\n lower += nums[num]\n upper += nums[num]\n poped = bisect_left(new_acc, accumulated[num])\n new_acc.pop(poped)\n num += 1\n\n # overall, takes O(n logn)\n return result\n``` | 1 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
327: Solution with step by step explanation | count-of-range-sum | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. The countRangeSum function takes in three parameters: nums, a list of integers; lower, an integer representing the lower bound of the sum range; and upper, an integer representing the upper bound of the sum range.\n\n2. The function initializes the length of the nums list and sets self.ans to zero.\n\n3. The function creates a prefix list by concatenating the integer list nums with a list of the cumulative sums of nums, starting with zero.\n\n4. The function calls the _mergeSort function with the prefix list, starting at index 0 and ending at the length of nums.\n\n5. The _mergeSort function recursively splits the prefix list in half until it reaches a length of 1.\n\n6. The _merge function is called for each pair of halves, sorting them and merging them back into the original prefix list.\n\n7. The _merge function initializes two variables, lo and hi, both set to the value of the middle index plus one.\n\n8. The function then loops through each index i in the range from l to m (inclusive) where l and m are the left and middle indices of the current prefix list being sorted.\n\n9. For each i, the function increments lo and hi until the difference between prefix[lo] - prefix[i] is greater than or equal to lower and prefix[hi] - prefix[i] is greater than upper, respectively.\n\n10. The function then adds the difference between hi and lo to self.ans.\n\n11. After the loop, the function initializes an empty sorted list with a length equal to the length of the current prefix list being sorted.\n\n12. The function then loops through the left and right halves of the current prefix list, adding the smallest value to the sorted list.\n\n13. If there are any remaining values in the left or right halves, the function adds them to the sorted list.\n\n14. Finally, the function replaces the values in the prefix list from l to l + len(sorted) with the values in the sorted list.\n\n15. Once all recursive calls to _mergeSort have completed, the function returns self.ans, which represents the number of subarrays of nums with a sum in the range [lower, upper].\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n n = len(nums)\n self.ans = 0\n prefix = [0] + list(itertools.accumulate(nums))\n\n self._mergeSort(prefix, 0, n, lower, upper)\n return self.ans\n\n def _mergeSort(self, prefix: List[int], l: int, r: int, lower: int, upper: int) -> None:\n if l >= r:\n return\n\n m = (l + r) // 2\n self._mergeSort(prefix, l, m, lower, upper)\n self._mergeSort(prefix, m + 1, r, lower, upper)\n self._merge(prefix, l, m, r, lower, upper)\n\n def _merge(self, prefix: List[int], l: int, m: int, r: int, lower: int, upper: int) -> None:\n lo = m + 1 # 1st index s.t. prefix[lo] - prefix[i] >= lower\n hi = m + 1 # 1st index s.t. prefix[hi] - prefix[i] > upper\n\n # For each index i in range [l, m], add hi - lo to ans\n for i in range(l, m + 1):\n while lo <= r and prefix[lo] - prefix[i] < lower:\n lo += 1\n while hi <= r and prefix[hi] - prefix[i] <= upper:\n hi += 1\n self.ans += hi - lo\n\n sorted = [0] * (r - l + 1)\n k = 0 # sorted\'s index\n i = l # left\'s index\n j = m + 1 # right\'s index\n\n while i <= m and j <= r:\n if prefix[i] < prefix[j]:\n sorted[k] = prefix[i]\n k += 1\n i += 1\n else:\n sorted[k] = prefix[j]\n k += 1\n j += 1\n\n # Put possible remaining left part to the sorted array\n while i <= m:\n sorted[k] = prefix[i]\n k += 1\n i += 1\n\n # Put possible remaining right part to the sorted array\n while j <= r:\n sorted[k] = prefix[j]\n k += 1\n j += 1\n\n prefix[l:l + len(sorted)] = sorted\n\n``` | 3 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
[Python3] 4 solutions | count-of-range-sum | 0 | 1 | divide & conquer \n```\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n prefix = [0]\n for x in nums: prefix.append(prefix[-1] + x)\n \n def fn(lo, hi): \n """Return count of range sum between prefix[lo:hi]."""\n if lo+1 >= hi: return 0 \n mid = lo + hi >> 1\n ans = fn(lo, mid) + fn(mid, hi)\n k = kk = mid \n for i in range(lo, mid): \n while k < hi and prefix[k] - prefix[i] < lower: k += 1\n while kk < hi and prefix[kk] - prefix[i] <= upper: kk += 1\n ans += kk - k \n prefix[lo:hi] = sorted(prefix[lo:hi])\n return ans \n \n return fn(0, len(prefix))\n```\n\n```\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n ans = prefix = 0 \n seen = [0]\n for x in nums: \n prefix += x \n lo = bisect_left(seen, prefix - upper)\n hi = bisect_left(seen, prefix - lower + 1) \n ans += hi - lo\n insort(seen, prefix)\n return ans \n```\n\n```\nfrom sortedcontainers import SortedList \n\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n ans = prefix = 0 \n sl = SortedList([0])\n for x in nums: \n prefix += x \n lo = sl.bisect_left(prefix - upper)\n hi = sl.bisect_left(prefix - lower + 1) - 1\n ans += hi - lo + 1\n sl.add(prefix)\n return ans \n```\n\nFenwick tree \n```\nclass Fenwick: \n def __init__(self, n): \n self.data = [0]*(n+1)\n \n def add(self, k, x): \n k += 1\n while k < len(self.data): \n self.data[k] += x\n k += k & -k \n \n def sum(self, k): \n k += 1 \n ans = 0\n while k: \n ans += self.data[k]\n k -= k & -k \n return ans \n \n\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n prefix = [0]\n for x in nums: prefix.append(prefix[-1] + x)\n prefix.sort()\n \n fen = Fenwick(len(prefix))\n fen.add(bisect_left(prefix, 0), 1)\n \n ans = val = 0\n for x in nums: \n val += x\n hi = fen.sum(bisect_left(prefix, val - lower + 1) - 1)\n lo = fen.sum(bisect_left(prefix, val - upper) - 1)\n ans += hi - lo \n fen.add(bisect_left(prefix, val), 1)\n return ans \n``` | 11 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
Just 3 Lines Python Code, SortedList, O(NlogN) | count-of-range-sum | 0 | 1 | # Intuition\nuse sortedList to keep track of pre-sums, then bisect for lower and upper bounds for each of pre-sums, any number between the bounds is a valid presum for the current presum\n\n# Code\n```\nfrom sortedcontainers import SortedList\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n sl = SortedList()\n pre = res = 0\n for n in nums:\n sl.add(pre)\n pre+=n\n res+= sl.bisect(pre-lower) - sl.bisect(pre-upper-1)\n return res\n``` | 1 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
Extremely clean solution simply using sortedlist | count-of-range-sum | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport numpy as np \nfrom sortedcontainers import SortedList\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n cs, sl = np.cumsum(nums), SortedList([0]) \n res = 0 \n for c in cs: \n res += sl.bisect_right(c - lower) - sl.bisect_left(c - upper)\n sl.add(c)\n return res \n\n\n\n``` | 0 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
Merge Sort | count-of-range-sum | 0 | 1 | \n# Code\n```\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n def mergeSort(lo, hi):\n if lo == hi:\n return 0\n\n mid = (lo + hi) // 2\n count = mergeSort(lo, mid) + mergeSort(mid + 1, hi)\n\n i = j = mid + 1\n for left in prefix_sum[lo:mid + 1]:\n while i <= hi and prefix_sum[i] - left < lower:\n i += 1\n while j <= hi and prefix_sum[j] - left <= upper:\n j += 1\n count += j - i\n\n prefix_sum[lo:hi + 1] = sorted(prefix_sum[lo:hi + 1])\n\n return count\n\n prefix_sum = [0] # Store the prefix sums\n for num in nums:\n prefix_sum.append(prefix_sum[-1] + num)\n\n return mergeSort(0, len(prefix_sum) - 1)\n``` | 0 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
[Python] Just use sorted list... | O(NlogN) | count-of-range-sum | 0 | 1 | # Code\n```\nfrom sortedcontainers import SortedList\nclass Solution:\n def countRangeSum(self, nums: List[int], l: int, u: int) -> int:\n sc = SortedList()\n sc.add(0);\n sm = 0\n res = 0\n for x in nums:\n sm +=x;\n lb = sm-u;\n up = sm-l;\n lf = sc.bisect_left(lb)\n rf = sc.bisect_right(up)\n res += rf-lf\n sc.add(sm)\n return res\n \n \n \n``` | 0 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
(Python) Count Range Sums | count-of-range-sum | 0 | 1 | # Intuition\nTo find the number of range sums that lie in [lower, upper] inclusive, we need to calculate the range sum S(i, j) for all possible pairs (i,j) where i <= j. Then, we count the number of sums that are between [lower, upper].\n# Approach\n1. Create an array prefix_sum of size n+1 to store the prefix sums of the array nums.\n2. Traverse the array nums, and calculate the prefix sum at each index i using the formula prefix_sum[i+1] = prefix_sum[i] + nums[i].\n3. Create an empty dictionary counter to store the count of each range sum.\n4. Traverse the prefix_sum array from left to right, and for each prefix_sum[j], calculate the number of prefix_sum[i] where i < j such that lower <= prefix_sum[j] - prefix_sum[i] <= upper.\n5. For each prefix_sum[j], add the count of such prefix_sum[i] to the counter dictionary.\n6. Finally, sum up the values in the counter dictionary and return the total count of range sums that lie in [lower, upper].\n# Complexity\n- Time complexity:\n O(nlogn) - We are traversing the prefix_sum array n times, and for each prefix_sum[j], we are searching for the count of prefix_sum[i] in the counter dictionary using binary search, which takes log(n) time. Therefore, the total time complexity is O(nlogn).\n- Space complexity:\nO(n) - We are using an array of size n+1 to store the prefix sums and a dictionary to store the count of each range sum, which can have a maximum of n entries. Therefore, the space complexity is O(n).\n# Code\n```\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n def mergeSort(lo, hi):\n if lo == hi:\n return 0\n mid = (lo + hi) // 2\n cnt = mergeSort(lo, mid) + mergeSort(mid + 1, hi)\n i = j = mid + 1\n for left in prefSum[lo:mid + 1]:\n while i <= hi and prefSum[i] - left < lower: i += 1\n while j <= hi and prefSum[j] - left <= upper: j += 1\n cnt += j - i\n prefSum[lo:hi + 1] = sorted(prefSum[lo:hi + 1])\n return cnt\n \n prefSum = [0] * (len(nums) + 1)\n for i in range(len(nums)):\n prefSum[i + 1] = prefSum[i] + nums[i]\n return mergeSort(0, len(prefSum) - 1)\n\n``` | 0 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
Solution in python | count-of-range-sum | 0 | 1 | # Approach\nUsing Merge-sort\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(nlogn)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def __init__(self):\n self.lower = 0\n self.upper = 0\n self.count = 0\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n self.lower = lower\n self.upper = upper\n # prefixSum\n sums = [0]\n temp = [0] * (len(nums)+1)\n for i in range(len(nums)):\n sums.append(sums[i]+nums[i])\n self.mergesort(sums, 0, len(sums)-1, temp)\n return self.count\n \n def mergesort(self, sums, start, end, temp):\n if start < end:\n mid = start + (end - start)//2\n self.mergesort(sums, start, mid, temp)\n self.mergesort(sums, mid + 1, end, temp)\n self.merge(sums, start, mid, end, temp)\n \n def merge(self, sums, start, mid, end, temp):\n right, low, high = mid+1, mid+1, mid+1\n index = start\n for left in range(start, mid+1):\n while low<=end and sums[low] - sums[left] < self.lower:\n low += 1\n while high<=end and sums[high] - sums[left] <= self.upper:\n high += 1\n while right<=end and sums[right] < sums[left]:\n temp[index] = sums[right]\n index+=1\n right+=1\n temp[index] = sums[left]\n self.count += high-low\n index+=1\n while right <= end:\n temp[index] = sums[right]\n index += 1\n right += 1\n for i in range(start, end+1):\n sums[i] = temp[i]\n``` | 0 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
Solution | count-of-range-sum | 1 | 1 | ```C++ []\nnamespace std {\n\ntemplate <class Fun> \nclass y_combinator_result {\n Fun fun_;\n public:\n template <class T> \n explicit y_combinator_result(T &&fun) : fun_(std::forward<T>(fun)) {}\n \n template<class ...Args> \n decltype(auto) operator()(Args &&...args) { \n return fun_(std::ref(*this), std::forward<Args>(args)...); \n }\n};\ntemplate <class Fun> \ndecltype(auto) y_combinator(Fun &&fun) { \n return y_combinator_result<std::decay_t<Fun>>(std::forward<Fun>(fun)); \n}\n}\ntemplate <typename Iter, class Comp = std::less<typename Iter::value_type>>\nvoid Merge(Iter first1, Iter last1, Iter first2, Iter last2, Comp comp = Comp()) {\n const auto m = std::distance(first1, last1);\n const auto n = std::distance(first2, last2);\n static std::vector<typename Iter::value_type> merged;\n merged.resize(m + n);\n auto it1 = first1, it2 = first2;\n auto result = merged.begin();\n while (it1 != last1 && it2 != last2) {\n if (comp(*it1, *it2)) {\n *result = *it1;\n ++it1;\n } else {\n *result = *it2;\n ++it2;\n }\n ++result;\n }\n std::copy(it1, last1, result);\n std::copy(it2, last2, result);\n result = merged.begin();\n std::copy_n(result, m, first1);\n std::copy_n(result + m, n, first2);\n}\nclass Solution {\npublic:\n int countRangeSum(vector<int>& nums, int lower, int upper) {\n int n = (int) nums.size();\n vector<long long> pref(n + 1);\n for (int i = 0; i < n; ++i) {\n pref[i + 1] = pref[i] + nums[i];\n }\n return y_combinator([&](auto&& self, auto begin, auto end) -> int {\n if (begin + 1 == end) return 0;\n auto mid = begin + (end - begin) / 2;\n int c = self(begin, mid) + self(mid, end);\n auto lb = mid, ub = mid;\n for (auto it = begin; it != mid; ++it) {\n while (lb != end && *lb - *it < lower) ++lb;\n while (ub != end && *ub - *it <= upper) ++ub;\n c += ub - lb;\n }\n Merge(begin, mid, mid, end);\n return c;\n }) (pref.begin(), pref.end());\n }\n};\n```\n\n```Python3 []\nfrom bisect import bisect_left,bisect_right\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n dp = [0]\n for i in nums:dp.append(dp[-1]+i)\n ans = 0\n go = sorted(dp)\n for i in dp[:-1]:\n del go[bisect_left(go,i)]\n ans += bisect_right(go,i+upper)-bisect_left(go,i+lower)\n return ans\n```\n\n```Java []\nclass Solution {\n int low,high;\n private void merge(long []prefix,int start1,int start2,int end2){\n int end1 = start2-1;\n int len1 = end1-start1+1,len2 = end2-start2+1;\n long []buf = new long[len1];\n for(int i = 0;i<len1;i++) buf[i] = prefix[i+start1];\n int ind1 = 0,ind2 = start2,ind = start1;\n while(ind1<len1 && ind2<=end2){\n if(buf[ind1]<=prefix[ind2]) prefix[ind++] = buf[ind1++];\n else prefix[ind++] = prefix[ind2++];\n }\n while(ind1<len1){\n prefix[ind++] = buf[ind1++];\n }\n }\n private int mergeSort(long []prefix,int start,int end){\n if(start<end){\n int mid = start+end>>1,count = 0;\n count+=mergeSort(prefix,start,mid);\n count+=mergeSort(prefix,mid+1,end);\n int lInd = mid+1,hInd = mid+1;\n for(int i = start;i<=mid;i++){\n while(lInd<=end && prefix[lInd]-prefix[i]<low) lInd++;\n while(hInd<=end && prefix[hInd]-prefix[i]<=high) hInd++;\n count+=hInd-lInd;\n }\n merge(prefix,start,mid+1,end);\n return count;\n }\n return 0;\n }\n public int countRangeSum(int[] nums, int lower, int upper) {\n low = lower;high = upper;\n int ans = 0;\n long []a = new long[nums.length];\n a[0] = nums[0];\n for(int i = 1;i<nums.length;i++) {\n a[i]=a[i-1]+nums[i];\n }\n for(long n:a) if(n>=lower && n<=upper) ans+=1;\n ans+=mergeSort(a,0,nums.length-1);\n return ans;\n }\n}\n```\n | 0 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
The most important thing to use merge sort for these similar hard problems | count-of-range-sum | 0 | 1 | # Approach\nThere are so many merge sort solution posts, and I have read all those with most votes. They all put a fake initial ZERO element in the prefix-sum array but failed to explain why.\n\nHowever, this is the most important thing for merge sort to work!\n\nThe conditions for the original problem, nums, are:\n- i <= j\n- lower <= sum of [i element, j element] <= upper\n\nFor native merge sort, we are only allowed to use it to solve these similar problems when i needs to be strictly less than j!!! This is because merge sort uses binary partition, and whenever we merge, we always merge two binary partitions. This requires i has to be strictly less than j. Otherwise, merge sort cannot work natively unless we make some special modifications so that it can handle i == j.\n\nBy adding a fake initial prefix-sum 0, we obtain presum array [0, n] from a given nums[0, n-1]. The conditions becomes:\n- i < j \n- lower <= presum[j] - presum[i] <= upper\n\nNow we can fit in merge sort method natively because the requirement becomes i < j.\n\nRemark: what if we do not add a fake initial ZERO element in the prefix sum array? We need to do a special modification to handle when i == j. This can be done during merge_sort recursion when lo == hi. See this example for reference:\n\nhttps://leetcode.com/problems/count-of-range-sum/solutions/1267104/c-simple-explanation-for-beginners-to-hard-problems/ | 0 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
Python3 || Prefix Sum + Merge Sort | count-of-range-sum | 0 | 1 | # Code\n```\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n n = len(nums)\n prefixsum = [0]*(n+1)\n for i in range(n):\n prefixsum[i+1] = prefixsum[i] + nums[i]\n \n temp = [0]*(n+1)\n count = 0\n\n def mergesort(nums, low, high):\n if low == high:\n return\n mid = (low+high) // 2\n mergesort(nums, low, mid)\n mergesort(nums, mid+1, high)\n\n return merge(nums, low, mid, high)\n\n def merge(nums, low, mid, high):\n nonlocal count\n for i in range(low, high+1):\n temp[i] = nums[i]\n\n start = end = mid + 1\n for i in range(low, mid+1):\n while start <= high and nums[start] - nums[i] < lower:\n start += 1\n while end <= high and nums[end] - nums[i] <= upper:\n end += 1\n count += end - start\n\n i, j = low, mid + 1\n for p in range(low, high+1):\n if i == mid+1:\n nums[p] = temp[j]\n j += 1\n elif j == high +1:\n nums[p] = temp[i]\n i += 1\n elif temp[i] > temp[j]:\n nums[p] = temp[j]\n j += 1\n else:\n nums[p] = temp[i]\n i += 1\n\n mergesort(prefixsum, 0, n)\n return count\n``` | 0 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
Python Segment Tree Solution. | count-of-range-sum | 0 | 1 | ```\nclass SegmentTreeNode:\n def __init__(self, min, max, left=None, right=None):\n self.min, self.max = min, max\n self.count = 0 # it marks how many sub ranges under this node\n self.left = left\n self.right = right\n\n# Conceptual Reference: \n# - https://leetcode.com/problems/count-of-range-sum/solutions/1674377/java-segment-tree-with-explanation/\n# - https://leetcode.com/problems/count-of-range-sum/solutions/77987/java-segmenttree-solution-36ms/\n\n# This is a binary tree, each node contains a range formed by "min" and "max".\n# the "min" of a parent node is determined by the minimum lower boundary of all its children\n# the "max" is determined by the maximum upper boundary of all its children.\n# And remember, the boundary value must be a sum of a certain range(i, j). And values between min and max may not corresponding to a valid sum\nclass SegmentTree:\n def _build_tree(self, low, high, nums):\n if low == high:\n return SegmentTreeNode(nums[low], nums[high])\n\n mid = (low + high) // 2\n left = self._build_tree(low, mid, nums)\n right = self._build_tree(mid + 1, high, nums)\n return SegmentTreeNode(nums[low], nums[high], left, right)\n\n def _update_node_count(self, node, val):\n if not node:\n return\n if node.max >= val >= node.min:\n node.count += 1\n self._update_node_count(node.left, val)\n self._update_node_count(node.right, val)\n\n def __init__(self, nums):\n self.root = self._build_tree(0, len(nums) - 1, nums)\n\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n def get_count(node, min, max):\n if not node:\n return 0\n if node.max < min or node.min > max:\n return 0\n if node.min >= min and node.max <= max:\n return node.count\n\n return get_count(node.left, min, max) + get_count(node.right, min, max)\n\n prefix_sums = nums[:]\n for i in range(1, len(prefix_sums)):\n prefix_sums[i] += prefix_sums[i - 1]\n\n # need sorted on unique values\n sorted_prefix_sums_set = sorted(set(prefix_sums))\n\n segment_tree = SegmentTree(sorted_prefix_sums_set)\n root = segment_tree.root\n\n res = 0\n for i in range(len(nums) - 1, -1, -1):\n segment_tree._update_node_count(root, prefix_sums[i])\n prev_prefix = prefix_sums[i - 1] if i - 1 >= 0 else 0\n\n res += get_count(root, lower + prev_prefix, upper + prev_prefix)\n\n return res\n``` | 0 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
PYTHON || BIT || EASY SOLUTION | count-of-range-sum | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nbinary indexed Tree can be use instead of segment Tree\n\n# Approach\nuse Binary Indexed Tree (BIT) for updation and setion sum\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n- o(nlog(n))\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n- o(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\ndef update(bit,i,inc):\n i+=1\n n=len(bit)\n while(i<n):\n bit[i]+=inc\n i+=i &(-i)\ndef gsum(bit,i):\n s=0\n i+=1\n while(i>0):\n s+=bit[i]\n i-=i&(-i)\n return s\ndef bs(sum,pre):\n l=0\n h=len(pre)\n while(l<h):\n m=(l+h)//2\n if(pre[m]>sum):\n h=m\n else:\n l=m+1\n return l\n\n\n\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n n=len(nums)\n pre=[0]*(n+1)\n for i in range(1,n):\n pre[i+1]=nums[i-1]+pre[i]\n pre=sorted(pre)\n bit=[0]*(len(pre)+2)\n ans=0\n sum=0\n for i in range(n):\n update(bit,bs(sum,pre),1)\n sum+=nums[i]\n ans+=(gsum(bit,bs(sum-lower,pre))-gsum(bit,bs(sum-upper-1,pre)))\n print(bit)\n print(ans)\n return ans\n\n``` | 0 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
Python with binary search better than 99% (memory) | count-of-range-sum | 0 | 1 | \n# Code\n```\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n from bisect import bisect, bisect_left\n # Counter for sum ranges\n counter = 0\n nums_sum = [0]\n # First i-elements sum elements for i in nums range\n for num in nums:\n nums_sum.append(nums_sum[-1] + num)\n # remove zero\n nums_sum.pop(0)\n # timsort for binary search\n nums_sum.sort()\n pop_target = 0\n while nums_sum:\n # count of elements between lower and upper\n low = bisect_left(nums_sum, lower)\n counter += bisect(nums_sum, upper, lo=low) - low\n nums0 = nums[0]\n # for removing nums[0] from nums_sum\n pop_target += nums0\n # Move lower and upper on nums[0]\n lower += nums0\n upper += nums0\n # Removing nums[0] from nums\n nums.pop(0)\n # Removing nums[0] from nums_sum\n nums_sum.pop(bisect_left(nums_sum, pop_target))\n return counter\n``` | 0 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
Would an interviewer accept this? | count-of-range-sum | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countRangeSum(self, nums: List[int], lower: int, upper: int) -> int:\n from sortedcontainers import SortedList\n l = SortedList()\n l.add(0)\n\n total = 0\n count = 0\n for v in nums:\n total += v\n count += (l.bisect_right(total - lower) - l.bisect_left(total - upper))\n l.add(total)\n \n return count\n\n``` | 0 | Given an integer array `nums` and two integers `lower` and `upper`, return _the number of range sums that lie in_ `[lower, upper]` _inclusive_.
Range sum `S(i, j)` is defined as the sum of the elements in `nums` between indices `i` and `j` inclusive, where `i <= j`.
**Example 1:**
**Input:** nums = \[-2,5,-1\], lower = -2, upper = 2
**Output:** 3
**Explanation:** The three ranges are: \[0,0\], \[2,2\], and \[0,2\] and their respective sums are: -2, -1, 2.
**Example 2:**
**Input:** nums = \[0\], lower = 0, upper = 0
**Output:** 1
**Constraints:**
* `1 <= nums.length <= 105`
* `-231 <= nums[i] <= 231 - 1`
* `-105 <= lower <= upper <= 105`
* The answer is **guaranteed** to fit in a **32-bit** integer. | null |
🔥Python3🔥 Two pointers | odd-even-linked-list | 0 | 1 | The problem needs to be solved with O(1) space, which leaves us no other choice but to try to use pointers to reorganize the linked list.\n(1) We use a ```odd``` pointer to link all the odd-positioned nodes, and a ```even``` pointer to link all the even-positioned nodes.\n(2) We connect the two linked lists at the end, i.e., connect the last node in the odd list to the first node in the even list.\n\nA few things we need to take care of:\n - There is a pointer that always points to the first node of the original linked list so we can return it (This is easy, just don\'t change ```head``` at all).\n - There is a pointer that always points to the second node of the original linked list so that, in the end, we can connect the last odd node to it. (This is also easy, just create ```evenHead``` points to the second node at the beginning)\n - After the iteration of connecting odd nodes to odd nodes and even nodes to even nodes, the ```odd``` pointer has to point to the last odd node (so we can connect it to ```evenHead```).\n\n ```python\nclass Solution:\n def oddEvenList(self, head: Optional[ListNode]) -> Optional[ListNode]:\n if not head: return None\n \n # odd points to the first node \n odd = head\n # even points to the second node\n # evenHead will be used at the end to connect odd and even nodes\n evenHead = even = head.next\n \n # This condition makes sure odd can never be None, since the odd node will always be the one before the even node.\n # If even is not None, then odd is not None. (odd before even)\n # If even.next is not None, then after we update odd to the next odd node, it cannot be None. (The next odd node is even.next)\n while even and even.next:\n \n # Connect the current odd node to the next odd node\n odd.next = odd.next.next\n # Move the current odd node to the next odd node\n odd = odd.next\n \n #Same thing for even node\n even.next = even.next.next\n even = even.next\n \n # Connect the last odd node to the start of the even node\n odd.next = evenHead\n\n # head never changed, so return it\n return head\n```\n\n**Upvote** if you like this post.\n\n**Connect with me on [LinkedIn](https://www.linkedin.com/in/meida-chen-938a265b/)** if you\'d like to discuss other related topics\n\nJust in case if you are working on **ML/DL 3D data-related projects** or are interested in the topic, please check out our project **[HERE](https://github.com/meidachen/STPLS3D)**\n | 35 | Given the `head` of a singly linked list, group all the nodes with odd indices together followed by the nodes with even indices, and return _the reordered list_.
The **first** node is considered **odd**, and the **second** node is **even**, and so on.
Note that the relative order inside both the even and odd groups should remain as it was in the input.
You must solve the problem in `O(1)` extra space complexity and `O(n)` time complexity.
**Example 1:**
**Input:** head = \[1,2,3,4,5\]
**Output:** \[1,3,5,2,4\]
**Example 2:**
**Input:** head = \[2,1,3,5,6,4,7\]
**Output:** \[2,3,6,7,1,5,4\]
**Constraints:**
* The number of nodes in the linked list is in the range `[0, 104]`.
* `-106 <= Node.val <= 106` | null |
Use two pointers - odd pointer and even pointer | odd-even-linked-list | 0 | 1 | # Intuition\nHave one pointer at head - odd pointer\nHave another pointer at head.next - even pointer\nFor both of the pointer we move 2 steps forward to access their type of index.\nWe also have to have access to the even head - head.next pointer.\nOnce we have connected all the odd and even head to each other.\nWe are left with connecting the end of the odd node to the start of the evne node. We do so by setting odd.next -> head of even node\n\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n# Code\n```\n# Definition for singly-linked list.\n# class ListNode:\n# def __init__(self, val=0, next=None):\n# self.val = val\n# self.next = next\nclass Solution:\n def oddEvenList(self, head: Optional[ListNode]) -> Optional[ListNode]:\n\n #for empty case\n if not head:\n return head\n \n #o-> odd pointer / e -> even pointer / heade even head pointer\n o,e,heade = head, head.next,head.next \n \n while e and e.next:\n o.next = o.next.next\n e.next = e.next.next\n o = o.next\n e = e.next \n \n #link up the last odd node to the first even node\n o.next = heade\n\n return head \n \n``` | 2 | Given the `head` of a singly linked list, group all the nodes with odd indices together followed by the nodes with even indices, and return _the reordered list_.
The **first** node is considered **odd**, and the **second** node is **even**, and so on.
Note that the relative order inside both the even and odd groups should remain as it was in the input.
You must solve the problem in `O(1)` extra space complexity and `O(n)` time complexity.
**Example 1:**
**Input:** head = \[1,2,3,4,5\]
**Output:** \[1,3,5,2,4\]
**Example 2:**
**Input:** head = \[2,1,3,5,6,4,7\]
**Output:** \[2,3,6,7,1,5,4\]
**Constraints:**
* The number of nodes in the linked list is in the range `[0, 104]`.
* `-106 <= Node.val <= 106` | null |
Python Easy Solution || Linked List | odd-even-linked-list | 0 | 1 | # Complexity\n- Time complexity:\n- O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n- O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n# Definition for singly-linked list.\n# class ListNode:\n# def __init__(self, val=0, next=None):\n# self.val = val\n# self.next = next\nclass Solution:\n def oddEvenList(self, head: Optional[ListNode]) -> Optional[ListNode]:\n if not head:\n return None\n odd = head\n even = head.next\n evenHead = even\n while even and even.next:\n odd.next = even.next\n odd = odd.next\n even.next = odd.next\n even = even.next\n odd.next = evenHead\n return head\n``` | 1 | Given the `head` of a singly linked list, group all the nodes with odd indices together followed by the nodes with even indices, and return _the reordered list_.
The **first** node is considered **odd**, and the **second** node is **even**, and so on.
Note that the relative order inside both the even and odd groups should remain as it was in the input.
You must solve the problem in `O(1)` extra space complexity and `O(n)` time complexity.
**Example 1:**
**Input:** head = \[1,2,3,4,5\]
**Output:** \[1,3,5,2,4\]
**Example 2:**
**Input:** head = \[2,1,3,5,6,4,7\]
**Output:** \[2,3,6,7,1,5,4\]
**Constraints:**
* The number of nodes in the linked list is in the range `[0, 104]`.
* `-106 <= Node.val <= 106` | null |
✅ [Python/C++/Java] next = next.next (explained) | odd-even-linked-list | 0 | 1 | **\u2705 IF YOU LIKE THIS SOLUTION, PLEASE UPVOTE.**\n****\nThis solution employs even and odd pointers to iterate through nodes in the list. Time complexity is linear: **O(N)**. Space complexity is constant: **O(1)**.\n****\n\n**Python #1.**\n```\nclass Solution:\n def oddEvenList(self, head):\n \n if not head : return head # [1] trivial case\n \n dummy_odd = odd = ListNode(0, head) # [2] initialize dummy nodes\n dummy_evn = evn = ListNode(0, head.next) # to facilitate iteration\n \n while odd.next and (odd := odd.next) and (evn := evn.next):\n \n if odd.next: odd.next = odd.next.next # [3] skip one node for \n if evn.next: evn.next = evn.next.next # both evens and odds\n \n odd.next = dummy_evn.next # [4] append evens to odds\n \n return dummy_odd.next\n```\n\n**Python #2.** A slightly modified version.\n```\nclass Solution:\n def oddEvenList(self, head):\n \n if head is None : return None\n \n odd = head\n dum = evn = head.next\n \n while evn and evn.next:\n odd.next = odd.next.next\n evn.next = evn.next.next\n odd = odd.next\n evn = evn.next\n \n odd.next = dum\n\n return head\n```\n\n**Python #3.** Compactified to the extreme.\n```\nclass Solution:\n def oddEvenList(self, head):\n \n if not head : return head\n \n odd, evn, dum = head, head.next, head.next\n \n while evn and evn.next:\n odd.next, evn.next = odd, evn = odd.next.next, evn.next.next\n \n odd.next = dum\n \n return head\n```\n\n<iframe src="https://leetcode.com/playground/G4K9Eyfq/shared" frameBorder="0" width="800" height="430"></iframe> | 22 | Given the `head` of a singly linked list, group all the nodes with odd indices together followed by the nodes with even indices, and return _the reordered list_.
The **first** node is considered **odd**, and the **second** node is **even**, and so on.
Note that the relative order inside both the even and odd groups should remain as it was in the input.
You must solve the problem in `O(1)` extra space complexity and `O(n)` time complexity.
**Example 1:**
**Input:** head = \[1,2,3,4,5\]
**Output:** \[1,3,5,2,4\]
**Example 2:**
**Input:** head = \[2,1,3,5,6,4,7\]
**Output:** \[2,3,6,7,1,5,4\]
**Constraints:**
* The number of nodes in the linked list is in the range `[0, 104]`.
* `-106 <= Node.val <= 106` | null |
IK U wont care for explanation Traitor :) | odd-even-linked-list | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nsee the code lil bit**\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nhahahahah\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n\nclass Solution(object):\n def oddEvenList(self, head):\n head1=odd=ListNode()\n head2=even=ListNode()\n t=head\n i=0\n while t:\n if i%2==0:\n even.next=t\n even=even.next\n else:\n odd.next=t\n odd=odd.next\n t=t.next\n i+=1\n odd.next=None\n even.next=head1.next\n return head2.next\n \n \n``` | 2 | Given the `head` of a singly linked list, group all the nodes with odd indices together followed by the nodes with even indices, and return _the reordered list_.
The **first** node is considered **odd**, and the **second** node is **even**, and so on.
Note that the relative order inside both the even and odd groups should remain as it was in the input.
You must solve the problem in `O(1)` extra space complexity and `O(n)` time complexity.
**Example 1:**
**Input:** head = \[1,2,3,4,5\]
**Output:** \[1,3,5,2,4\]
**Example 2:**
**Input:** head = \[2,1,3,5,6,4,7\]
**Output:** \[2,3,6,7,1,5,4\]
**Constraints:**
* The number of nodes in the linked list is in the range `[0, 104]`.
* `-106 <= Node.val <= 106` | null |
328: Space 98.56%, Solution with step by step explanation | odd-even-linked-list | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nWe need to group all the nodes with odd indices together followed by the nodes with even indices. The relative order inside both the even and odd groups should remain as it was in the input.\n\nTo solve the problem, we can use two pointers: odd and even. odd points to the first odd node and even points to the first even node. We also need a pointer even_head to keep track of the head of even nodes.\n\nWe traverse the linked list in pairs of odd and even nodes. In each iteration, we connect the next odd node with the current odd node and the next even node with the current even node. We then move odd and even pointers to the next odd and even nodes respectively.\n\nAfter the traversal, we connect the last odd node with the head of even nodes using odd.next = even_head. Finally, we return the head of the modified linked list.\n\n# Complexity\n- Time complexity:\n69.15%\n\n- Space complexity:\n98.56%\n\n# Code\n```\nclass Solution:\n def oddEvenList(self, head: ListNode) -> ListNode:\n # Check if linked list is empty or contains only one node\n if not head or not head.next:\n return head\n \n odd = head # Pointer to the first odd node\n even = head.next # Pointer to the first even node\n even_head = even # Pointer to the head of even nodes\n \n # Traverse the linked list in pairs of odd and even nodes\n while even and even.next:\n # Connect the next odd node with the current odd node\n odd.next = even.next\n odd = odd.next\n \n # Connect the next even node with the current even node\n even.next = odd.next\n even = even.next\n \n # Connect the last odd node with the head of even nodes\n odd.next = even_head\n \n return head\n\n``` | 16 | Given the `head` of a singly linked list, group all the nodes with odd indices together followed by the nodes with even indices, and return _the reordered list_.
The **first** node is considered **odd**, and the **second** node is **even**, and so on.
Note that the relative order inside both the even and odd groups should remain as it was in the input.
You must solve the problem in `O(1)` extra space complexity and `O(n)` time complexity.
**Example 1:**
**Input:** head = \[1,2,3,4,5\]
**Output:** \[1,3,5,2,4\]
**Example 2:**
**Input:** head = \[2,1,3,5,6,4,7\]
**Output:** \[2,3,6,7,1,5,4\]
**Constraints:**
* The number of nodes in the linked list is in the range `[0, 104]`.
* `-106 <= Node.val <= 106` | null |
Soln | odd-even-linked-list | 0 | 1 | # Code\n```\nclass Solution:\n def oddEvenList(self, head: Optional[ListNode]) -> Optional[ListNode]:\n if not head:\n return None\n odd=head\n dum=evn=head.next\n while evn and evn.next:\n odd.next=odd.next.next\n evn.next=evn.next.next\n odd=odd.next\n evn=evn.next\n odd.next=dum\n return head\n``` | 1 | Given the `head` of a singly linked list, group all the nodes with odd indices together followed by the nodes with even indices, and return _the reordered list_.
The **first** node is considered **odd**, and the **second** node is **even**, and so on.
Note that the relative order inside both the even and odd groups should remain as it was in the input.
You must solve the problem in `O(1)` extra space complexity and `O(n)` time complexity.
**Example 1:**
**Input:** head = \[1,2,3,4,5\]
**Output:** \[1,3,5,2,4\]
**Example 2:**
**Input:** head = \[2,1,3,5,6,4,7\]
**Output:** \[2,3,6,7,1,5,4\]
**Constraints:**
* The number of nodes in the linked list is in the range `[0, 104]`.
* `-106 <= Node.val <= 106` | null |
[ Python ] | Simple & Clean Code | odd-even-linked-list | 0 | 1 | \n\n# Code\n```\n# Definition for singly-linked list.\n# class ListNode:\n# def __init__(self, val=0, next=None):\n# self.val = val\n# self.next = next\nclass Solution:\n def oddEvenList(self, head: Optional[ListNode]) -> Optional[ListNode]:\n if not head or not head.next:\n return head\n\n odd_head = head\n dummy_even = head.next\n even_head = head.next\n\n while odd_head and even_head and odd_head.next and even_head.next:\n odd_head.next, even_head.next = odd_head.next.next, even_head.next.next\n odd_head = odd_head.next\n even_head = even_head.next\n\n # set even linked list at the end of odd linked list\n odd_head.next = dummy_even\n return head\n``` | 3 | Given the `head` of a singly linked list, group all the nodes with odd indices together followed by the nodes with even indices, and return _the reordered list_.
The **first** node is considered **odd**, and the **second** node is **even**, and so on.
Note that the relative order inside both the even and odd groups should remain as it was in the input.
You must solve the problem in `O(1)` extra space complexity and `O(n)` time complexity.
**Example 1:**
**Input:** head = \[1,2,3,4,5\]
**Output:** \[1,3,5,2,4\]
**Example 2:**
**Input:** head = \[2,1,3,5,6,4,7\]
**Output:** \[2,3,6,7,1,5,4\]
**Constraints:**
* The number of nodes in the linked list is in the range `[0, 104]`.
* `-106 <= Node.val <= 106` | null |
Python3 Solution | Runtime Beats 92% | DP and Memoization | longest-increasing-path-in-a-matrix | 0 | 1 | # Approach\nTo solve this problem, we can use a **dynamic programming approach** and **caching**. \nThe *"length_paths"* matrix is used for caching. This matrix stores the longest path length from each cell of the matrix *"matrix"*. \nThe dynamic programming approach is implemented by the recursive *"check_cell"* function, which stores the path length for the cell with indexes i and j in the *"length_paths"* matrix, and then returns this length+1 (this "+1" means that the current cell needs to be added to the resulting path length, but it does not need to be added to the longest path from this cell in *"length_paths"* matrix).\n\nUpvote if you like this solution. Have a nice day :D\n\n# Code\n```\nclass Solution:\n def longestIncreasingPath(self, matrix: List[List[int]]) -> int:\n m, n = len(matrix), len(matrix[0])\n length_paths = [[-1] * n for _ in range(m)]\n\n def check_cell(i: int, j: int, prev: int) -> int:\n if i < 0 or j < 0 or i >= m or j >= n or prev >= matrix[i][j]:\n return 0\n if length_paths[i][j] == -1:\n length_paths[i][j] = max(check_cell(i, j - 1, matrix[i][j]),\n check_cell(i, j + 1, matrix[i][j]),\n check_cell(i - 1, j, matrix[i][j]),\n check_cell(i + 1, j, matrix[i][j]))\n return length_paths[i][j] + 1\n\n gen = (check_cell(i, j, -1) for i in range(m) for j in range(n))\n return max(gen)\n\n``` | 5 | Given an `m x n` integers `matrix`, return _the length of the longest increasing path in_ `matrix`.
From each cell, you can either move in four directions: left, right, up, or down. You **may not** move **diagonally** or move **outside the boundary** (i.e., wrap-around is not allowed).
**Example 1:**
**Input:** matrix = \[\[9,9,4\],\[6,6,8\],\[2,1,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[1, 2, 6, 9]`.
**Example 2:**
**Input:** matrix = \[\[3,4,5\],\[3,2,6\],\[2,2,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[3, 4, 5, 6]`. Moving diagonally is not allowed.
**Example 3:**
**Input:** matrix = \[\[1\]\]
**Output:** 1
**Constraints:**
* `m == matrix.length`
* `n == matrix[i].length`
* `1 <= m, n <= 200`
* `0 <= matrix[i][j] <= 231 - 1` | null |
Solution | longest-increasing-path-in-a-matrix | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n int m, n;\n short path[200][200];\n \n int longestIncreasingPath(vector<vector<int>>& matrix) {\n m = matrix.size();\n n = matrix[0].size();\n\n memset(path, 0, sizeof(path));\n \n int max_path = 1;\n for(int i = 0; i < m; i++)\n for(int j = 0; j < n; j++)\n max_path = max(max_path, dfs(i, j, matrix));\n\n return max_path;\n }\n\n int dfs(int i, int j, vector<vector<int>>& mat) {\n if(path[i][j] > 0) return path[i][j];\n if(path[i][j] == -1) return 0;\n int max_next = 0;\n path[i][j] = -1;\n if(i > 0 && mat[i][j] < mat[i-1][j]) max_next = max(max_next, dfs(i-1, j, mat));\n if(j > 0 && mat[i][j] < mat[i][j-1]) max_next = max(max_next, dfs(i, j-1, mat));\n if(i < m-1 && mat[i][j] < mat[i+1][j]) max_next = max(max_next, dfs(i+1, j, mat));\n if(j < n-1 && mat[i][j] < mat[i][j+1]) max_next = max(max_next, dfs(i, j+1, mat));\n return path[i][j] = 1 + max_next;\n }\n\npublic:\n Solution() {\n ios_base::sync_with_stdio(false);\n cin.tie(nullptr);\n wcin.tie(nullptr);\n cerr.tie(nullptr);\n wcerr.tie(nullptr);\n clog.tie(nullptr);\n wclog.tie(nullptr);\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def longestIncreasingPath(self, matrix: List[List[int]]) -> int:\n if not matrix or not matrix[0]:\n return 0\n rows, cols = len(matrix), len(matrix[0])\n dp =[[0] * cols for i in range(rows)]\n def dfs(i, j):\n if not dp[i][j]:\n val = matrix[i][j]\n dp[i][j] = 1 + max(\n dfs(i - 1, j) if i and val > matrix[i - 1][j] else 0,\n dfs(i + 1, j) if i < rows - 1 and val > matrix[i + 1][j] else 0,\n dfs(i, j - 1) if j and val > matrix[i][j - 1] else 0,\n dfs(i, j + 1) if j < cols - 1 and val > matrix[i][j + 1] else 0)\n return dp[i][j]\n \n for r in range(rows):\n for c in range(cols):\n dfs(r,c)\n return max(max(x) for x in dp)\n```\n\n```Java []\nclass Solution {\n public int longestIncreasingPath(int[][] matrix) {\n int m = matrix.length;\n int n = matrix[0].length;\n int[][] dp = new int[m][n];\n for (int d[] : dp) Arrays.fill(d, -1);\n for (int i = 0; i < m; i++) {\n for (int j = 0; j < n; j++) {\n if (dp[i][j] == -1) dfs(matrix, dp, m, n, i, j, -1);\n }\n }\n int max = Integer.MIN_VALUE;\n for (int[] d : dp) {\n for (int i : d) max = Math.max(i, max);\n }\n return max;\n }\n\n public int dfs(\n int[][] matrix,\n int[][] dp,\n int m,\n int n,\n int i,\n int j,\n int parent\n ) {\n if (\n i >= m || j >= n || i < 0 || j < 0 || matrix[i][j] <= parent\n ) return 0;\n parent = matrix[i][j];\n if (dp[i][j] != -1) return dp[i][j];\n int left = dfs(matrix, dp, m, n, i, j - 1, parent);\n int right = dfs(matrix, dp, m, n, i, j + 1, parent);\n int bottom = dfs(matrix, dp, m, n, i + 1, j, parent);\n int top = dfs(matrix, dp, m, n, i - 1, j, parent);\n dp[i][j] = 1 + Math.max(Math.max(left, right), Math.max(top, bottom));\n return dp[i][j];\n }\n}\n```\n | 33 | Given an `m x n` integers `matrix`, return _the length of the longest increasing path in_ `matrix`.
From each cell, you can either move in four directions: left, right, up, or down. You **may not** move **diagonally** or move **outside the boundary** (i.e., wrap-around is not allowed).
**Example 1:**
**Input:** matrix = \[\[9,9,4\],\[6,6,8\],\[2,1,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[1, 2, 6, 9]`.
**Example 2:**
**Input:** matrix = \[\[3,4,5\],\[3,2,6\],\[2,2,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[3, 4, 5, 6]`. Moving diagonally is not allowed.
**Example 3:**
**Input:** matrix = \[\[1\]\]
**Output:** 1
**Constraints:**
* `m == matrix.length`
* `n == matrix[i].length`
* `1 <= m, n <= 200`
* `0 <= matrix[i][j] <= 231 - 1` | null |
dfs + dp | longest-increasing-path-in-a-matrix | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n*m)$$ \n87% beats\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(n*m)$$ \n62% beats\n\n# Code\n```\nclass Solution:\n def longestIncreasingPath(self, matrix: List[List[int]]) -> int:\n if not matrix:\n return 0\n m, n = len(matrix), len(matrix[0])\n dp=[[0]*n for i in range(m)]\n ans=0\n def dfs(i,j):\n if dp[i][j]:\n return dp[i][j]\n path=1\n for x, y in [(0, 1), (0, -1), (1, 0), (-1, 0)]: \n if 0 <= i+x < m and 0 <= j+y < n and matrix[i+x][j+y] > matrix[i][j]: \n path = max(path, 1 + dfs(i+x, j+y))\n dp[i][j]=path\n return path\n for i in range(m):\n for j in range(n):\n ans=max(ans,dfs(i,j))\n return ans\n``` | 1 | Given an `m x n` integers `matrix`, return _the length of the longest increasing path in_ `matrix`.
From each cell, you can either move in four directions: left, right, up, or down. You **may not** move **diagonally** or move **outside the boundary** (i.e., wrap-around is not allowed).
**Example 1:**
**Input:** matrix = \[\[9,9,4\],\[6,6,8\],\[2,1,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[1, 2, 6, 9]`.
**Example 2:**
**Input:** matrix = \[\[3,4,5\],\[3,2,6\],\[2,2,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[3, 4, 5, 6]`. Moving diagonally is not allowed.
**Example 3:**
**Input:** matrix = \[\[1\]\]
**Output:** 1
**Constraints:**
* `m == matrix.length`
* `n == matrix[i].length`
* `1 <= m, n <= 200`
* `0 <= matrix[i][j] <= 231 - 1` | null |
DFS with Memoization || Python | longest-increasing-path-in-a-matrix | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIt is necessary to try all possible paths and optimize the algorithm\'s complexity by using a map to store already calculated cases.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. A dictionary `memo` is declared, which will be used to store intermediate results of calculations and speed up the process.\n\n2. The function `Path` is defined, which takes row index `i` and column index `j` as arguments. This function returns the length of the longest path that can be constructed starting from position `(i, j)`.\n\n3. Inside the `Path` function, it checks if the result for position `(i, j)` is already present in the `memo` dictionary. If it is present, the stored result is directly returned.\n\n4. The variable `res` is initialized to 0 to keep track of the current path length from position `(i, j)`.\n\n5. Four checks are performed within the `Path` function to determine if it\'s possible to move in an adjacent direction (up, down, left, right) and if the number in the adjacent position is strictly greater than the current number at `(i, j)`. If these conditions are met, the length of the path from that position is calculated using recursion.\n\n6. During the calculation of the path in each direction, the `max` function is used to keep track of the maximum length among all possible directions to move. This is done to ensure considering the longest path among valid directions.\n\n7. The result `res` is stored in the `memo` dictionary to avoid recomputation in the future, and it is returned.\n\n8. Outside the `Path` function, a nested `for` loop is executed to explore each position in the `grid` matrix. The `ans` value is updated using the `max` function to obtain the maximum length of the path among all positions.\n\n9. Finally, the value `ans + 1` is returned because the length of the path is counted including the starting position.\n# Complexity\n- Time complexity: $$O(row \\cdot col)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n- Space complexity: $$O(row \\cdot col)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def longestIncreasingPath(self, grid: List[List[int]]) -> int:\n row, col, ans = len(grid), len(grid[0]), 0\n memo = {}\n \n def Path(i, j):\n if (i, j) in memo:\n return memo[(i, j)]\n\n res = 0 \n if i + 1 < row and grid[i][j] < grid[i + 1][j]:\n res = max(res,1 + Path(i + 1, j))\n \n if i - 1 >= 0 and grid[i][j] < grid[i - 1][j]:\n res = max(res, 1 + Path(i - 1, j))\n \n if j + 1 < col and grid[i][j] < grid[i][j + 1]:\n res = max(res, 1 + Path(i, j + 1))\n \n if j - 1 >= 0 and grid[i][j] < grid[i][j - 1]:\n res = max(res, 1 + Path(i, j - 1))\n \n memo[(i, j)] = res\n return memo[(i, j)]\n\n for i in range(row):\n for j in range(col):\n ans = max(ans,Path(i, j))\n \n return ans +1\n\n``` | 2 | Given an `m x n` integers `matrix`, return _the length of the longest increasing path in_ `matrix`.
From each cell, you can either move in four directions: left, right, up, or down. You **may not** move **diagonally** or move **outside the boundary** (i.e., wrap-around is not allowed).
**Example 1:**
**Input:** matrix = \[\[9,9,4\],\[6,6,8\],\[2,1,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[1, 2, 6, 9]`.
**Example 2:**
**Input:** matrix = \[\[3,4,5\],\[3,2,6\],\[2,2,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[3, 4, 5, 6]`. Moving diagonally is not allowed.
**Example 3:**
**Input:** matrix = \[\[1\]\]
**Output:** 1
**Constraints:**
* `m == matrix.length`
* `n == matrix[i].length`
* `1 <= m, n <= 200`
* `0 <= matrix[i][j] <= 231 - 1` | null |
DFS & TOPOLOGICAL SORTING CLEAR EXPLANATION | longest-increasing-path-in-a-matrix | 0 | 1 | ### DFS\n\n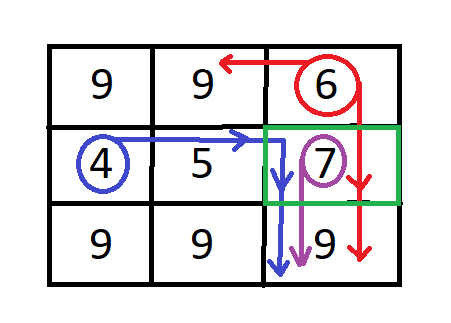\n\n```\nFrom red circle the longest sequence is 6 -> 7 -> 9. So\n matrix[0][2] (6) has 3 unit LONGEST sequence\n matrix[1][2] (7) has 2 unit LONGEST sequence\n matrix[2][2] (9) has 1 unit LONGEST sequence\n\nDuring the traversal of 6 -> 7 -> 9, not only we can find the LONGEST sequence starts from 6 but\nalso we can find about 7 and 9 as well!\n\nThe blue traversal : After 4 -> 5, 7 also valid cell to traverse but we already stored the\nlongest sequence of matrix[1][2] (7) which is 2, so 2(4 -> 5) + 2(7..) = 4.\n\nThe graph could be :\n 9 9 8 (<-- changed here)\n 4 5 7\n 9 9 9\n\nNow here 7 has the longest sequence as 7 -> 8 -> 9 -> 9. 7 has 4 branch, left + up + right +\ndown. We\'ve to take the maximum branch to find the longest sequence.\n\nSo in the DFS, from the cell we start our traversal, we\'ll compute which branch has the highest\nsequence. Then we take the maximum and add 1(to compute the total len)\nand return the longest sequence for the cell we started our DFS.\n\nin 6-> 9 and 6 -> 7 -> 9, do other cell\'s longest sequence matters? NO as whatever happens, 6\nis the mother here, it has the longest sequence! So later when we vist the other cells, we wont\nneed to do DFS from those cells.\n\nWe don\'t need \'visited array\' as we are traversing from small to strictly larger value,\na value can be traversed by multiple sequence where the largest sequence matters only. \n```\n```CPP []\nclass Solution {\npublic:\n vector<vector<int>> diff = {{0, -1}, {-1, 0}, {0, 1}, {1, 0}};\n\n int dfs(vector<vector<int>>& matrix, vector<vector<int>>& maxDist, int r, int c, int prevValue = INT_MIN)\n {\n if(r < 0 || r >= matrix.size() || c < 0 || c >= matrix[0].size() || matrix[r][c] <= prevValue)\n return 0;\n if(maxDist[r][c] > 0)\n return maxDist[r][c];\n int maxBranch = 0;\n for(const auto &d: diff)\n maxBranch = max(maxBranch, dfs(matrix, maxDist, r+d[0], c+d[1], matrix[r][c]));\n maxDist[r][c] = maxBranch + 1;\n return maxBranch + 1;\n }\n\n int longestIncreasingPath(vector<vector<int>>& matrix) \n {\n int rows = matrix.size(), cols = matrix[0].size(), maxLength = INT_MIN;\n vector<vector<int>> maxDist(rows, vector<int>(cols, 0));\n for (int r = 0; r < rows; r++)\n for (int c = 0; c < cols; c++)\n if (maxDist[r][c] == 0)\n maxLength = max(maxLength, dfs(matrix, maxDist, r, c));\n return maxLength;\n }\n};\n```\n```Python []\nclass Solution:\n def longestIncreasingPath(self, matrix: List[List[int]]) -> int:\n rows, cols = len(matrix), len(matrix[0])\n maxDist = [[0]* cols for _ in range(rows)]\n\n def dfs(r: int, c:int, prevValue: int = float(\'-inf\')) -> int :\n if r<0 or r>=rows or c<0 or c>=cols or matrix[r][c] <= prevValue:\n return 0\n if maxDist[r][c] > 0:\n return maxDist[r][c]\n MaxBranch = max(dfs(r+x, c+y, matrix[r][c]) for (x,y) in ((0, -1), (-1, 0), (0, 1), (1, 0)))\n maxDist[r][c] = MaxBranch + 1\n return MaxBranch + 1\n\n return max(dfs(r, c) for r in range(rows) for c in range(cols) if maxDist[r][c] == 0)\n```\n\n## TOPOLOGICAL SORTING\n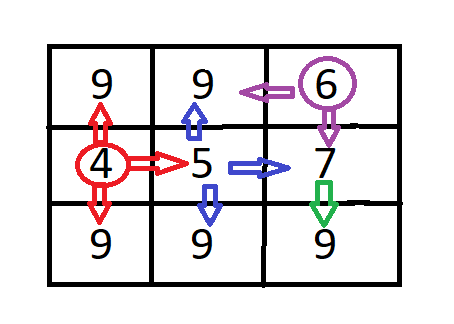\n\n```\nYou can clearly see the in-degree of red and purple circle is 0, so the longest sequence either\nstarts from 6 or 4, why? 4 -> 5 -> 7 -> 9 is the longest sequence, here the starting value 4 \nhas 0 in-degree!\n```\n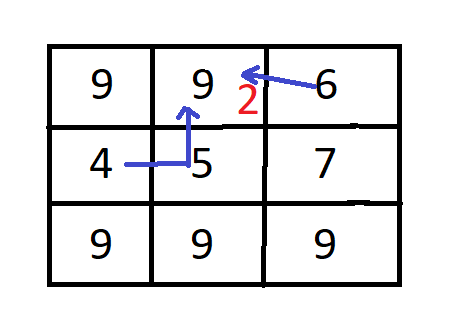\n\n```\nSo our queue has [4, 6] initially. From 4 we first reach 5, then from 6 we first reach 9. 5 has\nnot reached 9 yet but 6 did. So will we start our traversal from 9 after 6 reached 9 first\nand do again traversal from 9 after 5 will reach it? NO, it\'ll give us infinity looping.\n\nAfter reaching to 9 from 6, the in-degree of 9 is 1. As it\'s not 0, that means there is a\nsequence which still hasn\'t reached to 9 and that sequence is definitely LARGER than\n6 -> 9 which is 4 -> 5 -> 9. So after 5 reaches to 9, now the in-degree of 9 is 0 and\nwe continue our traversal from 9 as 4 -> 5 -> 9, not as 6 -> 9.\n```\n\n```PYTHON []\nclass Solution:\n def longestIncreasingPath(self, matrix: List[List[int]]) -> int:\n rows, cols, level = len(matrix), len(matrix[0]), 0\n inDegree = [ [0]* cols for _ in range(rows)]\n\n for r in range(rows):\n for c in range(cols):\n for (x,y) in ((0, -1), (-1, 0), (0, 1), (1, 0)): # For every cell visiting it\'s 4 neighbours\n tr, tc = r+x, c+y\n if tr<0 or tr>=rows or tc<0 or tc>=cols or matrix[tr][tc] <= matrix[r][c]:\n continue\n inDegree[tr][tc] += 1 # in-degree of neighbour cell increases as we CAN GO TO NEIGHBOUR\n \n q = deque([(r,c) for r in range(rows) for c in range(cols) if inDegree[r][c] == 0]) # cells with 0\n # in-degree means the longest sequence STARTS FROM EITHER ONE OF THEM \n while q:\n for _ in range(len(q)):\n pr, pc = q.popleft()\n for (x,y) in ((0, -1), (-1, 0), (0, 1), (1, 0)):\n tr, tc = pr+x, pc+y\n if tr<0 or tr>=rows or tc<0 or tc>=cols or matrix[tr][tc] <= matrix[pr][pc]:\n continue\n inDegree[tr][tc] -= 1\n if inDegree[tr][tc] == 0: # if in-degree of a cell is not 0, that means A LONGEST SEQUENCE\n q.append((tr, tc)) # HASN\'T VISITED THAT CELL YET\n level += 1\n \n return level\n```\n```\nTime complexity : O(mn)\nSpace complexity : O(mn)\n```\n\n## If there is any q, plz feel free to comment. If the post was helpful, an upvote will be appreciated. Thank youu | 1 | Given an `m x n` integers `matrix`, return _the length of the longest increasing path in_ `matrix`.
From each cell, you can either move in four directions: left, right, up, or down. You **may not** move **diagonally** or move **outside the boundary** (i.e., wrap-around is not allowed).
**Example 1:**
**Input:** matrix = \[\[9,9,4\],\[6,6,8\],\[2,1,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[1, 2, 6, 9]`.
**Example 2:**
**Input:** matrix = \[\[3,4,5\],\[3,2,6\],\[2,2,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[3, 4, 5, 6]`. Moving diagonally is not allowed.
**Example 3:**
**Input:** matrix = \[\[1\]\]
**Output:** 1
**Constraints:**
* `m == matrix.length`
* `n == matrix[i].length`
* `1 <= m, n <= 200`
* `0 <= matrix[i][j] <= 231 - 1` | null |
[Python] Solution using Memoization | longest-increasing-path-in-a-matrix | 0 | 1 | ```\nclass Solution:\n def longestIncreasingPath(self, matrix: List[List[int]]) -> int:\n row = len(matrix); col = len(matrix[0])\n res = 0\n memo = {}\n directions = [[-1, 0], [1, 0], [0, -1], [0, 1]]\n \n def helper(i, j):\n if (i,j) in memo: return memo[(i,j)]\n path = 1\n for move in directions:\n r = i + move[0]\n c = j + move[1]\n if (0<=r<row and 0<=c<col and\n matrix[r][c] != \'#\' and matrix[r][c] > matrix[i][j]):\n \n tmp = matrix[i][j]\n matrix[i][j] = \'#\'\n path = max(path, helper(r, c) + 1)\n matrix[i][j] = tmp\n \n memo[(i,j)] = path \n return memo[(i,j)]\n \n for i in range(row):\n for j in range(col):\n res = max(res, helper(i, j))\n \n return res\n\n# Time: O(N^3)\n# Space: O(N^2)\n``` | 3 | Given an `m x n` integers `matrix`, return _the length of the longest increasing path in_ `matrix`.
From each cell, you can either move in four directions: left, right, up, or down. You **may not** move **diagonally** or move **outside the boundary** (i.e., wrap-around is not allowed).
**Example 1:**
**Input:** matrix = \[\[9,9,4\],\[6,6,8\],\[2,1,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[1, 2, 6, 9]`.
**Example 2:**
**Input:** matrix = \[\[3,4,5\],\[3,2,6\],\[2,2,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[3, 4, 5, 6]`. Moving diagonally is not allowed.
**Example 3:**
**Input:** matrix = \[\[1\]\]
**Output:** 1
**Constraints:**
* `m == matrix.length`
* `n == matrix[i].length`
* `1 <= m, n <= 200`
* `0 <= matrix[i][j] <= 231 - 1` | null |
329: Solution with step by step explanation | longest-increasing-path-in-a-matrix | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nThe problem can be solved by performing a depth-first search (DFS) on each cell of the matrix and keeping track of the longest increasing path found so far. We start the search from each cell of the matrix and for each cell, we move in all four directions (left, right, up, and down) to find the increasing path. We use memoization to store the length of the longest increasing path starting from each cell to avoid recomputing it.\n\nStep-by-step algorithm:\n\n1. Create a memoization matrix of the same size as the input matrix to store the length of the longest increasing path starting from each cell.\n2. For each cell in the matrix, perform a DFS to find the length of the longest increasing path starting from that cell.\n3. During the DFS, for each unvisited neighbor of the current cell that has a greater value, perform a DFS on that neighbor and update the memoization matrix with the length of the longest increasing path starting from that neighbor.\n4. If we have already computed the length of the longest increasing path starting from a neighbor, we can use that value from the memoization matrix instead of recomputing it.\n5. During the DFS, keep track of the maximum length of the increasing path found so far and return it as the result.\n\nTime Complexity: O(mn), where m and n are the dimensions of the matrix, as we perform a DFS starting from each cell of the matrix, and each cell is visited only once.\n\nSpace Complexity: O(mn), for the memoization matrix used to store the length of the longest increasing path starting from each cell.\n\n# Complexity\n- Time complexity:\n89.87%\n\n- Space complexity:\n53.39%\n\n# Code\n```\nclass Solution:\n def longestIncreasingPath(self, matrix: List[List[int]]) -> int:\n if not matrix:\n return 0\n m, n = len(matrix), len(matrix[0])\n memo = [[0]*n for _ in range(m)] # memoization matrix to store the length of the longest increasing path starting from each cell\n res = 0 # maximum length of the increasing path found so far\n \n # DFS function to find the length of the longest increasing path starting from the current cell\n def dfs(i, j):\n if memo[i][j]:\n return memo[i][j]\n path = 1 # length of the increasing path starting from the current cell\n for x, y in [(0, 1), (0, -1), (1, 0), (-1, 0)]: # explore all four directions (right, left, down, up)\n if 0 <= i+x < m and 0 <= j+y < n and matrix[i+x][j+y] > matrix[i][j]: # if the neighbor has a greater value, move to it\n path = max(path, 1 + dfs(i+x, j+y)) # update the length of the increasing path\n memo[i][j] = path # memoize the length of the increasing path starting from the current cell\n return path\n \n for i in range(m):\n for j in range(n):\n res = max(res, dfs(i, j)) # update the maximum length of the increasing path found so far\n return res\n\n``` | 7 | Given an `m x n` integers `matrix`, return _the length of the longest increasing path in_ `matrix`.
From each cell, you can either move in four directions: left, right, up, or down. You **may not** move **diagonally** or move **outside the boundary** (i.e., wrap-around is not allowed).
**Example 1:**
**Input:** matrix = \[\[9,9,4\],\[6,6,8\],\[2,1,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[1, 2, 6, 9]`.
**Example 2:**
**Input:** matrix = \[\[3,4,5\],\[3,2,6\],\[2,2,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[3, 4, 5, 6]`. Moving diagonally is not allowed.
**Example 3:**
**Input:** matrix = \[\[1\]\]
**Output:** 1
**Constraints:**
* `m == matrix.length`
* `n == matrix[i].length`
* `1 <= m, n <= 200`
* `0 <= matrix[i][j] <= 231 - 1` | null |
Python DFS with Memoization Beats ~90% | longest-increasing-path-in-a-matrix | 0 | 1 | The solution is quite straightforward. The code just performs a dfs traversal from each cell in the grid and finds the length of valid path.\n\nBelow is the code with the comments\n```\nclass Solution:\n def longestIncreasingPath(self, grid: List[List[int]]) -> int:\n m,n=len(grid),len(grid[0])\n directions = [0, 1, 0, -1, 0] # four directions \n \n @lru_cache(maxsize=None) # using python cache lib for memoization\n def dfs(r,c):\n ans=1 \n\t\t\t# iterating through all 4 directions\n for i in range(4): \n new_row,new_col=r+directions[i], c+directions[i+1] # calculating the new cell\n\t\t\t\t# check if new cell is within the grid bounds and is an increasing sequence\n if 0<=new_row<m and 0<=new_col<n and grid[new_row][new_col]>grid[r][c]: \n ans = max(ans, dfs(new_row, new_col) + 1 ) # finding the max length of valid path from the current cell \n return ans\n \n return max(dfs(r,c) for r in range(m) for c in range(n)) \n```\n**Time - O(m.n)** - memoization helps to reduce the time as it doesn\'t visit the same cell twice.\n**Space - O(m.n)** - space required for recursive call stack and cache.\n\n---\n\n***Please upvote if you find it useful*** | 5 | Given an `m x n` integers `matrix`, return _the length of the longest increasing path in_ `matrix`.
From each cell, you can either move in four directions: left, right, up, or down. You **may not** move **diagonally** or move **outside the boundary** (i.e., wrap-around is not allowed).
**Example 1:**
**Input:** matrix = \[\[9,9,4\],\[6,6,8\],\[2,1,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[1, 2, 6, 9]`.
**Example 2:**
**Input:** matrix = \[\[3,4,5\],\[3,2,6\],\[2,2,1\]\]
**Output:** 4
**Explanation:** The longest increasing path is `[3, 4, 5, 6]`. Moving diagonally is not allowed.
**Example 3:**
**Input:** matrix = \[\[1\]\]
**Output:** 1
**Constraints:**
* `m == matrix.length`
* `n == matrix[i].length`
* `1 <= m, n <= 200`
* `0 <= matrix[i][j] <= 231 - 1` | null |
330: Solution with step by step explanation | patching-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n\nTo solve this problem, we can use a greedy approach. We start with a variable miss that represents the smallest number that cannot be formed by summing up any combination of the numbers in the array. Initially, miss is set to 1, since we can always form 1 by taking an empty combination. We also initialize a variable i to 0, which represents the index of the next number that we need to consider adding to the array.\n\nWe then loop while miss is less than or equal to n, which means that we still need to add more numbers to the array. If i is less than the length of the array and the next number in the array is less than or equal to miss, we add that number to the sum and increment i. Otherwise, we add miss to the array and update miss to be the new smallest number that cannot be formed by summing up any combination of the numbers in the array.\n\nThe reason why this greedy approach works is that whenever we add a number to the array, we can form all the numbers up to miss + number - 1. If we then update miss to be miss + number, we can now form all the numbers up to 2 * miss - 1. Therefore, we keep increasing miss until we can form all the numbers up to n.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n miss, i, patches = 1, 0, 0\n while miss <= n:\n if i < len(nums) and nums[i] <= miss:\n miss += nums[i]\n i += 1\n else:\n miss *= 2\n patches += 1\n return patches\n\n\n``` | 3 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
Python || Math | patching-array | 0 | 1 | # Intuition\nIf sum of all the numbers considered till now is x, we can form all the numbers from 1 to x. This condition is True every time.\n\n# Approach\nKeep a variable limit which tracks till what max value we can form which is nothing but sum of all values considered till now.\nIf we encounter any number i.e. num which is greater than limit+1, it means we can\'t get any number between limit+1 to num-1.So we add limit+1 to our limit to extend our current limit and increase our answer count by 1 (Internally we are adding limit+1 to our array).Continue this process untill num is greater than limit+1.Meanwhile if at any moment limit >=ssum break off the loop.\nAfter considering all numbers from array , check if limit is still less than ssum and keep adding limit+1 and incrementing answer count to our limit till limit<ssum.\nNote : We need to necessarily add limit+1 every time and not any number greater than this as we can\'t form limit+1 using any of our previously considered numbers.\n\n# Complexity\n- Time complexity:\nO(n) \n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution:\n def minPatches(self, nums: List[int], ssum: int) -> int:\n n=len(nums)\n limit,cnt=0,0\n for i in range(n):\n if nums[i]>limit+1:\n while nums[i]>limit+1:\n limit+=limit+1\n cnt+=1\n if limit>=ssum: break\n limit+=nums[i]\n if limit>=ssum: break\n while limit<ssum:\n limit+=limit+1\n cnt+=1\n return cnt\n\n``` | 2 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
Python Greedy : )) | patching-array | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def minPatches(self, nums, n):\n patches = reachable = 0\n for num in nums:\n while reachable < min(num - 1, n):\n reachable += reachable + 1\n patches += 1\n reachable += num\n \n while reachable < n:\n reachable = 2 * reachable + 1\n patches += 1 \n \n #print(reachable)\n return patches\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
Python Greedy: variant of 1798 and 2952 | patching-array | 0 | 1 | # Code\n```\nclass Solution:\n def minPatches(self, nums, n):\n patches = reachable = 0\n for num in nums:\n while reachable < min(num - 1, n):\n reachable += reachable + 1\n patches += 1\n reachable += num\n \n while reachable < n:\n reachable = 2 * reachable + 1\n patches += 1 \n \n #print(reachable)\n return patches\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
Python 3 | Solution | patching-array | 0 | 1 | # Code\n```\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n\n res = 0\n temp = 0\n indx = 0\n\n while temp < n:\n\n if indx < len(nums) and nums[indx] <= temp + 1:\n \n temp += nums[indx]\n indx += 1\n\n else:\n\n res += 1\n temp += (temp + 1)\n \n return res\n\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
✅[Python] ⏱99% Fast || Easy || Greedy ||Minimum Number of Patches | patching-array | 0 | 1 | # Intuition\nThe problem involves finding the minimum number of "patches" that need to be added to an array of positive integers (represented by the `nums` list) in order to make it contain all positive integers from 1 to `n`. The patches must be added in such a way that the array remains sorted in ascending order.\n\n# Approach\nThe provided code offers a Python implementation for solving this problem. Here\'s the approach:\n\n1. Initialize `k` to 1, which represents the current positive integer that can be formed using the given patches. Initialize `ans` to 0, which represents the count of patches added. Initialize `idx` to 1, which represents the index of the first element in the `nums` list.\n\n2. Check if the first element of `nums` is not equal to 1. If it\'s not, this means that 1 cannot be formed with the current patches, so we need to add a patch for 1. Increment `ans` by 1 and set `idx` to 0 to indicate that we have considered the first element.\n\n3. Iterate through the elements in `nums` starting from the index `idx` to the end of the list.\n\n4. For each element `x` in `nums`, check if `k` has reached `n`. If it has, we have already formed all integers from 1 to `n`, and we can exit the loop.\n\n5. If `x` is less than or equal to `k + 1`, it means that we can form the integer `k + 1` using the existing patches and the current element `x`. So, we update `k` to `k + x`.\n\n6. If `x` is greater than `k + 1`, we need to add a patch for the integer `k + 1` and increment `ans` by 1. We then iteratively increase `k` by `k + 1` until we can form the integer `x`. This ensures that we maximize the use of existing patches before adding a new one.\n\n7. After the loop, there might still be integers from `k + 1` to `n` that cannot be formed with the given patches. We add patches for these integers iteratively until `k` reaches `n`.\n\n8. Finally, return the value of `ans`, which represents the minimum number of patches required to form all integers from 1 to `n`.\n\n# Complexity\n- Time complexity: The code iterates through the `nums` list once, and the worst-case number of iterations for patching is limited by the value `n`. Therefore, the time complexity is O(n).\n- Space complexity: The code uses a constant amount of additional space for variables, so the space complexity is O(1).\n\n# Code\n```python\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n k = 1\n ans = 0\n idx = 1\n if nums[0] != 1:\n ans += 1\n idx = 0\n \n for i in range(idx, len(nums)):\n x = nums[i]\n if k >= n:\n break\n if x <= k + 1:\n k = k + x\n else:\n while k + 1 < x and k < n:\n k = k + k + 1\n ans += 1\n k = k + x\n \n while k < n:\n k = k + k + 1\n ans += 1\n \n return ans\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
python easy solution | patching-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n miss, i, patches = 1, 0, 0\n while miss <= n:\n if i < len(nums) and nums[i] <= miss:\n miss += nums[i]\n i += 1\n else:\n miss *= 2\n patches += 1\n return patches\n\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
how do i show this to the interviewer LMFAO | patching-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n covered, res, i = 0, 0, 0\n while covered < n:\n if i < len(nums) and nums[i] <= covered + 1:\n covered += nums[i]\n i += 1\n else:\n covered = 2 * covered + 1\n res += 1\n return res\n\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
i dont know how this works | patching-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n covered, res, i = 1, 0, 0\n while covered <= n:\n if i < len(nums) and nums[i] <= covered:\n covered += nums[i]\n i += 1\n else:\n covered += covered\n res += 1\n return res\n\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
Explanation for beginners like me | patching-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\ninitially, we assume that 1 is missing from the array, so we set miss to 1. let ```miss``` be the smallest sum in the```[0,n)``` that we might be missing. meaning we already can build all sums in ```[0,miss)```.\n\nif we have a number ```nums[i]<=miss``` in the given array, we can add it to miss to build all ```[0,miss+nums[i])```.\n\nhowever, if ```nums[i]>miss```, that means ```miss``` is too small to reach nums[i], so we must add a number to the array, the best choive is to add ```miss``` itself,to maximize the reach.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nexample: ```nums=[1,2,4,13,43],n=100```\nuse 1, 2, 4 we can reach ```[0,8)``` \n\nnow```miss=1(initial)+1 (nums[0])+ 2(nums[1])+3(nums[2]) = 8```\n i=3, nums[i]=13 ```nums[3]>miss```, we can not build 8 use the number in the array , so we add 8 to the array and double the miss to 16. now we can build ```[0,16)```.\n\nthan we the miss become 16+13=29 , the reach is ```[0,29)```.\n29<43, so we add 29 to the array thurn the miss to 58, the reach is ```[0,58)```.\n\nand the 43 becomes useful , the reach is ```[0,101)```.\n\nand the patches is 2.\n\n- the doubling of miss serves two purposes. \n1. **cover the current missing number**.\n2. **extend the range of the reach between** ```[miss,2*miss-1]``` **or the whole ```[0,2*miss)```.**\n\n\n\n# Code\n```\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n #track the smallest missing number in the array, first we assume 1 is missing because we start with a empty array\n miss=1\n patches=0\n i=0\n\n while miss <=n:\n if i<len(nums) and nums[i]<=miss:\n miss+=nums[i]\n i+=1\n else:\n \n miss*=2\n patches+=1\n return patches\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
Greedy Approach | patching-array | 0 | 1 | # Code\n```\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n patches = 0\n max_reachable = 0\n i = 0\n \n while max_reachable < n:\n if i < len(nums) and nums[i] <= max_reachable + 1:\n max_reachable += nums[i]\n i += 1\n else:\n patches += 1\n max_reachable += max_reachable + 1\n \n return patches\n\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
simple mathematics solution || O(N) || python | patching-array | 0 | 1 | # Intuition and Approach\n* use previous formed no. to form new numbers.\n\n# Complexity\n- Time complexity: O(N)\n\n- Space complexity:O(1)\n\n# Code\n```\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n ans=0\n pre=0\n i=0\n nextn=pre+1\n while(nextn<=n):\n if(i<len(nums) and nums[i]>nextn):\n ans+=1\n pre+=nextn\n elif(i<len(nums)):\n pre+=nums[i]\n i+=1\n else:\n ans+=1\n pre+=nextn\n nextn=pre+1\n return ans\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
(Python) Longest Increasing Path in a Matrix | patching-array | 0 | 1 | # Intuition\nOne way to solve this problem is to use a greedy approach. We can keep track of the smallest number we cannot make using the numbers we have encountered so far. We start with missing = 1, since we can always make 1 with an empty array. Then, we iterate through the given array and check if the current number is less than or equal to missing. If it is, then we can update missing to missing + nums[i], since we can now make all the numbers from missing to missing + nums[i] - 1. If the current number is greater than missing, then we need to add missing to the array to make missing + 1 up to nums[i] - 1, and then update missing to missing + nums[i].\n\n# Approach\n1. Initialize missing = 1, patches = 0, and i = 0.\n2. While missing <= n, repeat steps 3-5.\n3. If i < len(nums) and nums[i] <= missing, then update missing to missing + nums[i].\n4. Otherwise, add missing to the array and update missing to missing * 2. Also, increment patches.\n5. Increment i.\n6. Return patches.\n# Complexity\n- Time complexity:\nO(n), where n is the size of the array nums.\n- Space complexity:\nO(1). We are using constant space to keep track of variables like missing, patches, and i.\n\n# Code\n```\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n patches = 0\n missed = 1\n i = 0\n \n while missed <= n:\n if i < len(nums) and nums[i] <= missed:\n missed += nums[i]\n i += 1\n else:\n missed += missed\n patches += 1\n \n return patches\n\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
Solution | patching-array | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n int minPatches(vector<int>& nums, int n) {\n int m=nums.size();\n\n sort(nums.begin(),nums.end());\n\n long long cur=0;\n int cnt=0;\n for(int i=0;i<m;i++){\n\n while(cur<n && nums[i]>cur+1){\n cur+=cur+1;\n cnt++;\n }\n if(cur>=n)\n break;\n cur+=nums[i];\n }\n while(cur<n){\n cur+=cur+1;\n cnt++;\n }\n return cnt;\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n upper=0\n res=0\n i=0\n ln=len(nums)\n\n while upper<n:\n if i<ln:\n while nums[i]>upper+1:\n res+=1\n upper+=upper+1\n if upper>=n: return res\n upper+=nums[i]\n i+=1\n else:\n res+=1\n upper+=upper+1\n return res\n```\n\n```Java []\nclass Solution {\n public int minPatches(int[] nums, int n) {\n \n int m=nums.length;\n \n int count=0;\n \n int i=0;\n long val=1;\n long sum=0;\n \n while(i<m || sum<n){\n \n if(sum>n)break;\n \n if(i<m && nums[i]<=val){\n sum+=nums[i];\n i++; \n }\n else{\n sum+=val;\n }\n val=sum+1;\n \n count++;\n }\n return count-i;\n \n }\n}\n```\n | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
Python solution simple and fast | patching-array | 0 | 1 | \n# Code\n```\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n sum, patch, i = 0, 0, 0\n\n while sum < n:\n if i < len(nums) and nums[i] <= sum+1:\n sum += nums[i]\n i += 1\n else:\n patch += 1\n sum += sum+1\n\n return patch\n \n```\n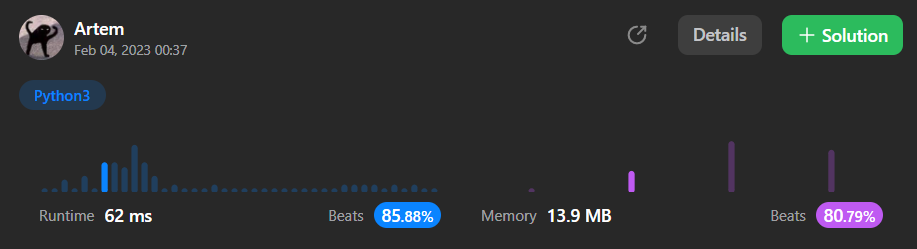\n | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
Go/Pyton O(m+log(n)) time | O(1) space | patching-array | 0 | 1 | # Complexity\n- Time complexity: $$O(m+log(n))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```golang []\nfunc minPatches(nums []int, n int) int {\n covered := 0\n i := 0\n answer := 0\n for i<len(nums) && covered < n{\n if nums[i] <= covered+1{\n covered+=nums[i]\n i+=1\n }else{\n covered+=covered+1\n answer+=1\n }\n }\n \n for covered < n{\n covered+=covered+1\n answer+=1\n }\n return answer\n}\n```\n```python []\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n covered = 0\n i = 0\n answer = 0\n while i<len(nums) and covered < n:\n if nums[i] <= covered+1:\n covered+=nums[i]\n i+=1\n else:\n covered+=covered+1\n answer+=1\n while covered < n:\n covered+=covered+1\n answer+=1\n return answer\n\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
greedy to find the next number in array or not | patching-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nthe array is sorted, and need patch to make [1, n]\n# Approach\n<!-- Describe your approach to solving the problem. -->\nif nothing: [0, 0]\nif add 1: [0, 1]\nif we need to get [1, n]\ndo we have 2 in nums, then it will become [0, 1] + [2 + 0, 2 + 1] = [0, 3]\nthe next number is added or in the nums is always depend on previous state.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def minPatches(self, nums: List[int], n: int) -> int:\n res, N, reach, i = 0, len(nums), 0, 0\n while reach < n: # if didn\'t reach n\n if i < N and nums[i] <= reach + 1: # no need to add\n reach += nums[i]\n i += 1\n else: # need to add, the nums are sorted, means others numbers after this one is not possible\n res += 1\n reach += reach + 1\n return res\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
python solution | patching-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom collections import Counter\nclass Solution:\n def minPatches(self, nums, n: int) -> int:\n ald = Counter(nums)\n cur_range = 0\n cnt = 0\n keys = sorted(ald.keys())\n idx = 0\n while cur_range < n:\n \n while idx < len(keys) and keys[idx] <= cur_range:\n added = keys[idx]\n cur_range += ald[added] * added\n idx += 1\n if cur_range >= n:\n break\n next_to_pick = cur_range + 1\n if next_to_pick in ald:\n added = keys[idx]\n cur_range += ald[added] * added\n idx += 1\n else:\n cur_range += next_to_pick\n cnt += 1\n\n \n return cnt\n``` | 0 | Given a sorted integer array `nums` and an integer `n`, add/patch elements to the array such that any number in the range `[1, n]` inclusive can be formed by the sum of some elements in the array.
Return _the minimum number of patches required_.
**Example 1:**
**Input:** nums = \[1,3\], n = 6
**Output:** 1
Explanation:
Combinations of nums are \[1\], \[3\], \[1,3\], which form possible sums of: 1, 3, 4.
Now if we add/patch 2 to nums, the combinations are: \[1\], \[2\], \[3\], \[1,3\], \[2,3\], \[1,2,3\].
Possible sums are 1, 2, 3, 4, 5, 6, which now covers the range \[1, 6\].
So we only need 1 patch.
**Example 2:**
**Input:** nums = \[1,5,10\], n = 20
**Output:** 2
Explanation: The two patches can be \[2, 4\].
**Example 3:**
**Input:** nums = \[1,2,2\], n = 5
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 104`
* `nums` is sorted in **ascending order**.
* `1 <= n <= 231 - 1` | null |
331: Time 98%, Solution with step by step explanation | verify-preorder-serialization-of-a-binary-tree | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nan explicit stack, and instead just uses a single variable degree to keep track of the outDegree (children) - inDegree (parent). It also removes the need for the stack and nodes lists used in the previous solution, which results in less memory usage and faster execution time.\n\nThe time complexity of this solution is O(n), where n is the number of nodes in the binary tree. The space complexity is O(1), as we are only using a single variable to keep track of the degree.\n\n# Complexity\n- Time complexity:\n98%\n\n- Space complexity:\n75.32%\n\n# Code\n```\nclass Solution:\n def isValidSerialization(self, preorder: str) -> bool:\n # Initialize the outDegree (children) - inDegree (parent) to 1\n degree = 1\n \n # Iterate through the nodes in the preorder traversal\n for node in preorder.split(\',\'):\n degree -= 1 # Decrement the degree by 1 for each node\n \n if degree < 0: # If the degree is negative, return False\n return False\n \n if node != \'#\': # If the node is not a leaf node\n degree += 2 # Increment the degree by 2 for each non-leaf node\n \n # If the final degree is 0, the tree is valid, else invalid\n return degree == 0\n\n``` | 8 | One way to serialize a binary tree is to use **preorder traversal**. When we encounter a non-null node, we record the node's value. If it is a null node, we record using a sentinel value such as `'#'`.
For example, the above binary tree can be serialized to the string `"9,3,4,#,#,1,#,#,2,#,6,#,# "`, where `'#'` represents a null node.
Given a string of comma-separated values `preorder`, return `true` if it is a correct preorder traversal serialization of a binary tree.
It is **guaranteed** that each comma-separated value in the string must be either an integer or a character `'#'` representing null pointer.
You may assume that the input format is always valid.
* For example, it could never contain two consecutive commas, such as `"1,,3 "`.
**Note:** You are not allowed to reconstruct the tree.
**Example 1:**
**Input:** preorder = "9,3,4,#,#,1,#,#,2,#,6,#,#"
**Output:** true
**Example 2:**
**Input:** preorder = "1,#"
**Output:** false
**Example 3:**
**Input:** preorder = "9,#,#,1"
**Output:** false
**Constraints:**
* `1 <= preorder.length <= 104`
* `preorder` consist of integers in the range `[0, 100]` and `'#'` separated by commas `','`. | null |
Python3 | O(n) Time and O(n) space | verify-preorder-serialization-of-a-binary-tree | 0 | 1 | The idea is to pop the element from the stack if you get the count of the element as 0.\n\nStack is storing the node and number of remaining branch that need to discover.\nExample [2,2] will refer a node having value 2 and we did not traverse any of the branch (left or right) but at the moment we traverse the left branch that value will change to [2,1] and after traversing through right branch it will convert into [2,0].\n\n```\nclass Solution:\n def isValidSerialization(self, preorder: str) -> bool:\n stack = []\n items = preorder.split(",")\n for i, val in enumerate(items):\n if i>0 and not stack:\n return False\n if stack:\n stack[-1][1] -= 1\n if stack[-1][1] == 0:\n stack.pop()\n if val != "#":\n stack.append([val, 2])\n return not stack\n ```\n \n If you find this good please upvote or like, and if you feel any trouble to understand then please comment here.\n Thanks | 3 | One way to serialize a binary tree is to use **preorder traversal**. When we encounter a non-null node, we record the node's value. If it is a null node, we record using a sentinel value such as `'#'`.
For example, the above binary tree can be serialized to the string `"9,3,4,#,#,1,#,#,2,#,6,#,# "`, where `'#'` represents a null node.
Given a string of comma-separated values `preorder`, return `true` if it is a correct preorder traversal serialization of a binary tree.
It is **guaranteed** that each comma-separated value in the string must be either an integer or a character `'#'` representing null pointer.
You may assume that the input format is always valid.
* For example, it could never contain two consecutive commas, such as `"1,,3 "`.
**Note:** You are not allowed to reconstruct the tree.
**Example 1:**
**Input:** preorder = "9,3,4,#,#,1,#,#,2,#,6,#,#"
**Output:** true
**Example 2:**
**Input:** preorder = "1,#"
**Output:** false
**Example 3:**
**Input:** preorder = "9,#,#,1"
**Output:** false
**Constraints:**
* `1 <= preorder.length <= 104`
* `preorder` consist of integers in the range `[0, 100]` and `'#'` separated by commas `','`. | null |
Just the code : )) | verify-preorder-serialization-of-a-binary-tree | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def isValidSerialization(self, preorder: str) -> bool:\n preorder = preorder.split(\',\')\n stack = []\n node, left, right, cur = preorder[0], None, None, \'L\'\n n = len(preorder)\n if n == 1:\n return node == \'#\'\n elif node == \'#\':\n return False\n\n for i in range(1, n):\n if preorder[i] == \'#\':\n if cur == \'L\':\n cur = \'R\'\n left = \'#\'\n else:\n if stack:\n node = stack.pop()\n left = "#"\n cur = \'R\'\n else:\n return i == n - 1\n else:\n stack.append(node)\n node, left, right = preorder[i], None, None\n\n return stack == [] and left == \'#\' and right == \'#\'\n``` | 0 | One way to serialize a binary tree is to use **preorder traversal**. When we encounter a non-null node, we record the node's value. If it is a null node, we record using a sentinel value such as `'#'`.
For example, the above binary tree can be serialized to the string `"9,3,4,#,#,1,#,#,2,#,6,#,# "`, where `'#'` represents a null node.
Given a string of comma-separated values `preorder`, return `true` if it is a correct preorder traversal serialization of a binary tree.
It is **guaranteed** that each comma-separated value in the string must be either an integer or a character `'#'` representing null pointer.
You may assume that the input format is always valid.
* For example, it could never contain two consecutive commas, such as `"1,,3 "`.
**Note:** You are not allowed to reconstruct the tree.
**Example 1:**
**Input:** preorder = "9,3,4,#,#,1,#,#,2,#,6,#,#"
**Output:** true
**Example 2:**
**Input:** preorder = "1,#"
**Output:** false
**Example 3:**
**Input:** preorder = "9,#,#,1"
**Output:** false
**Constraints:**
* `1 <= preorder.length <= 104`
* `preorder` consist of integers in the range `[0, 100]` and `'#'` separated by commas `','`. | null |
Use stack, beats 95% | verify-preorder-serialization-of-a-binary-tree | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(n)\n\n# Code\n```\nclass Solution:\n def isValidSerialization(self, preorder: str) -> bool:\n preorder = preorder.split(\',\')\n stack = []\n node, left, right, cur = preorder[0], None, None, \'L\'\n n = len(preorder)\n if n == 1:\n return node == \'#\'\n elif node == \'#\':\n return False\n\n for i in range(1, n):\n if preorder[i] == \'#\':\n if cur == \'L\':\n cur = \'R\'\n left = \'#\'\n else:\n if stack:\n node = stack.pop()\n left = "#"\n cur = \'R\'\n else:\n return i == n - 1\n else:\n stack.append(node)\n node, left, right = preorder[i], None, None\n\n return stack == [] and left == \'#\' and right == \'#\'\n``` | 0 | One way to serialize a binary tree is to use **preorder traversal**. When we encounter a non-null node, we record the node's value. If it is a null node, we record using a sentinel value such as `'#'`.
For example, the above binary tree can be serialized to the string `"9,3,4,#,#,1,#,#,2,#,6,#,# "`, where `'#'` represents a null node.
Given a string of comma-separated values `preorder`, return `true` if it is a correct preorder traversal serialization of a binary tree.
It is **guaranteed** that each comma-separated value in the string must be either an integer or a character `'#'` representing null pointer.
You may assume that the input format is always valid.
* For example, it could never contain two consecutive commas, such as `"1,,3 "`.
**Note:** You are not allowed to reconstruct the tree.
**Example 1:**
**Input:** preorder = "9,3,4,#,#,1,#,#,2,#,6,#,#"
**Output:** true
**Example 2:**
**Input:** preorder = "1,#"
**Output:** false
**Example 3:**
**Input:** preorder = "9,#,#,1"
**Output:** false
**Constraints:**
* `1 <= preorder.length <= 104`
* `preorder` consist of integers in the range `[0, 100]` and `'#'` separated by commas `','`. | null |
Python easy solution | verify-preorder-serialization-of-a-binary-tree | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isValidSerialization(self, preorder: str) -> bool:\n stack = []\n for node in preorder.split(\',\'):\n stack.append(node)\n while len(stack) >= 3 and stack[-1] == \'#\' and stack[-2] == \'#\' and stack[-3] != \'#\':\n stack.pop()\n stack.pop()\n stack.pop()\n stack.append(\'#\')\n return len(stack) == 1 and stack[0] == \'#\'\n``` | 0 | One way to serialize a binary tree is to use **preorder traversal**. When we encounter a non-null node, we record the node's value. If it is a null node, we record using a sentinel value such as `'#'`.
For example, the above binary tree can be serialized to the string `"9,3,4,#,#,1,#,#,2,#,6,#,# "`, where `'#'` represents a null node.
Given a string of comma-separated values `preorder`, return `true` if it is a correct preorder traversal serialization of a binary tree.
It is **guaranteed** that each comma-separated value in the string must be either an integer or a character `'#'` representing null pointer.
You may assume that the input format is always valid.
* For example, it could never contain two consecutive commas, such as `"1,,3 "`.
**Note:** You are not allowed to reconstruct the tree.
**Example 1:**
**Input:** preorder = "9,3,4,#,#,1,#,#,2,#,6,#,#"
**Output:** true
**Example 2:**
**Input:** preorder = "1,#"
**Output:** false
**Example 3:**
**Input:** preorder = "9,#,#,1"
**Output:** false
**Constraints:**
* `1 <= preorder.length <= 104`
* `preorder` consist of integers in the range `[0, 100]` and `'#'` separated by commas `','`. | null |
✅ Fast + Easy + Accurate Solution ✅ | verify-preorder-serialization-of-a-binary-tree | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isValidSerialization(self, preorder: str) -> bool:\n stack = []\n for node in preorder.split(\',\'):\n stack.append(node)\n while len(stack) >= 3 and stack[-1] == \'#\' and stack[-2] == \'#\' and stack[-3] != \'#\':\n stack.pop()\n stack.pop()\n stack.pop()\n stack.append(\'#\')\n return len(stack) == 1 and stack[0] == \'#\'\n\n``` | 0 | One way to serialize a binary tree is to use **preorder traversal**. When we encounter a non-null node, we record the node's value. If it is a null node, we record using a sentinel value such as `'#'`.
For example, the above binary tree can be serialized to the string `"9,3,4,#,#,1,#,#,2,#,6,#,# "`, where `'#'` represents a null node.
Given a string of comma-separated values `preorder`, return `true` if it is a correct preorder traversal serialization of a binary tree.
It is **guaranteed** that each comma-separated value in the string must be either an integer or a character `'#'` representing null pointer.
You may assume that the input format is always valid.
* For example, it could never contain two consecutive commas, such as `"1,,3 "`.
**Note:** You are not allowed to reconstruct the tree.
**Example 1:**
**Input:** preorder = "9,3,4,#,#,1,#,#,2,#,6,#,#"
**Output:** true
**Example 2:**
**Input:** preorder = "1,#"
**Output:** false
**Example 3:**
**Input:** preorder = "9,#,#,1"
**Output:** false
**Constraints:**
* `1 <= preorder.length <= 104`
* `preorder` consist of integers in the range `[0, 100]` and `'#'` separated by commas `','`. | null |
Verify preorder structure using recursive simulation | verify-preorder-serialization-of-a-binary-tree | 0 | 1 | - Basic idea is to simulate the preorder construction of a complete binary tree using recursion but just leave out creating the actual nodes. \n- Edge cases:\n - There is only one item and it is an empty node \n - There are more than one item but the first item is an empty node\n- Two failure conditions:\n - Run out of nodes to complete the complete binary tree\n - Completed binary tree but there are nodes left over in the queue\n\n\n# Code\n```\nfrom collections import deque\nclass Solution:\n def isValidSerialization(self, preorder: str) -> bool:\n if len(preorder) == 1:\n return True if preorder[0] == "#" else False\n if preorder[0] == "#":\n return False\n preorder = deque(preorder.split(","))\n preorder.popleft()\n def sim():\n for _ in range(2):\n if not preorder:\n return False\n top = preorder.popleft()\n if top != "#":\n sim()\n return True\n return True if (sim() and (not preorder)) else False\n``` | 0 | One way to serialize a binary tree is to use **preorder traversal**. When we encounter a non-null node, we record the node's value. If it is a null node, we record using a sentinel value such as `'#'`.
For example, the above binary tree can be serialized to the string `"9,3,4,#,#,1,#,#,2,#,6,#,# "`, where `'#'` represents a null node.
Given a string of comma-separated values `preorder`, return `true` if it is a correct preorder traversal serialization of a binary tree.
It is **guaranteed** that each comma-separated value in the string must be either an integer or a character `'#'` representing null pointer.
You may assume that the input format is always valid.
* For example, it could never contain two consecutive commas, such as `"1,,3 "`.
**Note:** You are not allowed to reconstruct the tree.
**Example 1:**
**Input:** preorder = "9,3,4,#,#,1,#,#,2,#,6,#,#"
**Output:** true
**Example 2:**
**Input:** preorder = "1,#"
**Output:** false
**Example 3:**
**Input:** preorder = "9,#,#,1"
**Output:** false
**Constraints:**
* `1 <= preorder.length <= 104`
* `preorder` consist of integers in the range `[0, 100]` and `'#'` separated by commas `','`. | null |
using stack | verify-preorder-serialization-of-a-binary-tree | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isValidSerialization(self, preorder):\n stack = []\n for elem in preorder.split(","):\n stack.append(elem)\n while len(stack) > 2 and stack[-2:] == ["#"]*2 and stack[-3] != "#":\n stack.pop(-3)\n stack.pop(-2)\n \n return stack == ["#"]\n \n\n``` | 0 | One way to serialize a binary tree is to use **preorder traversal**. When we encounter a non-null node, we record the node's value. If it is a null node, we record using a sentinel value such as `'#'`.
For example, the above binary tree can be serialized to the string `"9,3,4,#,#,1,#,#,2,#,6,#,# "`, where `'#'` represents a null node.
Given a string of comma-separated values `preorder`, return `true` if it is a correct preorder traversal serialization of a binary tree.
It is **guaranteed** that each comma-separated value in the string must be either an integer or a character `'#'` representing null pointer.
You may assume that the input format is always valid.
* For example, it could never contain two consecutive commas, such as `"1,,3 "`.
**Note:** You are not allowed to reconstruct the tree.
**Example 1:**
**Input:** preorder = "9,3,4,#,#,1,#,#,2,#,6,#,#"
**Output:** true
**Example 2:**
**Input:** preorder = "1,#"
**Output:** false
**Example 3:**
**Input:** preorder = "9,#,#,1"
**Output:** false
**Constraints:**
* `1 <= preorder.length <= 104`
* `preorder` consist of integers in the range `[0, 100]` and `'#'` separated by commas `','`. | null |
stack with explanation | verify-preorder-serialization-of-a-binary-tree | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe idea is to delete the tree node until there is nothing to delete\n\ne.g. \n` 9\nN N\n`\nit will put into stack\nstack: [9, N, N]\nthis is a root node, but it is a leaf node as well\nif it is leaf node: delete [9, N, N] and add N, it will become [#]\nif the final is stack is [#], this means it is preorder.\nRemember, only perorder has this property, because # will be connected, if it is inorder: 9#9, if it is postorder: ##9\n\n- this is why we can use stack like this way\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isValidSerialization(self, preorder: str) -> bool:\n stack = []\n for s in preorder.split(\',\'):\n stack.append(s)\n while len(stack) > 2 and stack[-2] == \'#\' and stack[-1] == \'#\' and stack[-3] != \'#\':\n for i in range(3):\n stack.pop()\n stack.append(\'#\')\n return stack == [\'#\']\n``` | 0 | One way to serialize a binary tree is to use **preorder traversal**. When we encounter a non-null node, we record the node's value. If it is a null node, we record using a sentinel value such as `'#'`.
For example, the above binary tree can be serialized to the string `"9,3,4,#,#,1,#,#,2,#,6,#,# "`, where `'#'` represents a null node.
Given a string of comma-separated values `preorder`, return `true` if it is a correct preorder traversal serialization of a binary tree.
It is **guaranteed** that each comma-separated value in the string must be either an integer or a character `'#'` representing null pointer.
You may assume that the input format is always valid.
* For example, it could never contain two consecutive commas, such as `"1,,3 "`.
**Note:** You are not allowed to reconstruct the tree.
**Example 1:**
**Input:** preorder = "9,3,4,#,#,1,#,#,2,#,6,#,#"
**Output:** true
**Example 2:**
**Input:** preorder = "1,#"
**Output:** false
**Example 3:**
**Input:** preorder = "9,#,#,1"
**Output:** false
**Constraints:**
* `1 <= preorder.length <= 104`
* `preorder` consist of integers in the range `[0, 100]` and `'#'` separated by commas `','`. | null |
Simple Stack Python | verify-preorder-serialization-of-a-binary-tree | 0 | 1 | # Intuition\nCancel out node after finding both children\n\n# Code\n```\nclass Solution:\n def isValidSerialization(self, preorder: str) -> bool:\n # Time O(N), space O(N)\n val = preorder.split(\',\')\n stack = []\n for v in val:\n if v != \'#\':\n stack.append(val)\n else: # \'#\'\n while stack and stack[-1] == \'#\':\n stack.pop()\n if len(stack) == 0:\n return False\n stack.pop()\n stack.append(\'#\')\n\n return len(stack) == 1 and stack[0] == \'#\'\n\n``` | 0 | One way to serialize a binary tree is to use **preorder traversal**. When we encounter a non-null node, we record the node's value. If it is a null node, we record using a sentinel value such as `'#'`.
For example, the above binary tree can be serialized to the string `"9,3,4,#,#,1,#,#,2,#,6,#,# "`, where `'#'` represents a null node.
Given a string of comma-separated values `preorder`, return `true` if it is a correct preorder traversal serialization of a binary tree.
It is **guaranteed** that each comma-separated value in the string must be either an integer or a character `'#'` representing null pointer.
You may assume that the input format is always valid.
* For example, it could never contain two consecutive commas, such as `"1,,3 "`.
**Note:** You are not allowed to reconstruct the tree.
**Example 1:**
**Input:** preorder = "9,3,4,#,#,1,#,#,2,#,6,#,#"
**Output:** true
**Example 2:**
**Input:** preorder = "1,#"
**Output:** false
**Example 3:**
**Input:** preorder = "9,#,#,1"
**Output:** false
**Constraints:**
* `1 <= preorder.length <= 104`
* `preorder` consist of integers in the range `[0, 100]` and `'#'` separated by commas `','`. | null |
Python3 Solution | reconstruct-itinerary | 0 | 1 | \n```\nclass Solution:\n def findItinerary(self, tickets: List[List[str]]) -> List[str]:\n adj={}\n for ticket in tickets:\n adj[ticket[0]]=[]\n adj[ticket[1]]=[]\n\n for ticket in tickets:\n adj[ticket[0]].append(ticket[1])\n\n for l in adj.values():\n l.sort()\n\n ans=[]\n def helper(node):\n while adj[node]:\n helper(adj[node].pop(0))\n\n ans.append(node)\n helper("JFK")\n return reversed(ans) \n \n``` | 2 | You are given a list of airline `tickets` where `tickets[i] = [fromi, toi]` represent the departure and the arrival airports of one flight. Reconstruct the itinerary in order and return it.
All of the tickets belong to a man who departs from `"JFK "`, thus, the itinerary must begin with `"JFK "`. If there are multiple valid itineraries, you should return the itinerary that has the smallest lexical order when read as a single string.
* For example, the itinerary `[ "JFK ", "LGA "]` has a smaller lexical order than `[ "JFK ", "LGB "]`.
You may assume all tickets form at least one valid itinerary. You must use all the tickets once and only once.
**Example 1:**
**Input:** tickets = \[\[ "MUC ", "LHR "\],\[ "JFK ", "MUC "\],\[ "SFO ", "SJC "\],\[ "LHR ", "SFO "\]\]
**Output:** \[ "JFK ", "MUC ", "LHR ", "SFO ", "SJC "\]
**Example 2:**
**Input:** tickets = \[\[ "JFK ", "SFO "\],\[ "JFK ", "ATL "\],\[ "SFO ", "ATL "\],\[ "ATL ", "JFK "\],\[ "ATL ", "SFO "\]\]
**Output:** \[ "JFK ", "ATL ", "JFK ", "SFO ", "ATL ", "SFO "\]
**Explanation:** Another possible reconstruction is \[ "JFK ", "SFO ", "ATL ", "JFK ", "ATL ", "SFO "\] but it is larger in lexical order.
**Constraints:**
* `1 <= tickets.length <= 300`
* `tickets[i].length == 2`
* `fromi.length == 3`
* `toi.length == 3`
* `fromi` and `toi` consist of uppercase English letters.
* `fromi != toi` | null |
Python3 | reconstruct-itinerary | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findItinerary(self, tickets: List[List[str]]) -> List[str]:\n hashm=defaultdict(list)\n for i in tickets:\n if i[0] in hashm:\n hashm[i[0]].append(i[1])\n else:\n hashm[i[0]]=[i[1]]\n for i in hashm:\n hashm[i]=sorted(hashm[i], reverse=True)\n res=[]\n \n def dfs(i):\n while hashm[i]:\n dfs(hashm[i].pop())\n res.append(i)\n \n dfs(\'JFK\')\n return res[::-1]\n \n``` | 2 | You are given a list of airline `tickets` where `tickets[i] = [fromi, toi]` represent the departure and the arrival airports of one flight. Reconstruct the itinerary in order and return it.
All of the tickets belong to a man who departs from `"JFK "`, thus, the itinerary must begin with `"JFK "`. If there are multiple valid itineraries, you should return the itinerary that has the smallest lexical order when read as a single string.
* For example, the itinerary `[ "JFK ", "LGA "]` has a smaller lexical order than `[ "JFK ", "LGB "]`.
You may assume all tickets form at least one valid itinerary. You must use all the tickets once and only once.
**Example 1:**
**Input:** tickets = \[\[ "MUC ", "LHR "\],\[ "JFK ", "MUC "\],\[ "SFO ", "SJC "\],\[ "LHR ", "SFO "\]\]
**Output:** \[ "JFK ", "MUC ", "LHR ", "SFO ", "SJC "\]
**Example 2:**
**Input:** tickets = \[\[ "JFK ", "SFO "\],\[ "JFK ", "ATL "\],\[ "SFO ", "ATL "\],\[ "ATL ", "JFK "\],\[ "ATL ", "SFO "\]\]
**Output:** \[ "JFK ", "ATL ", "JFK ", "SFO ", "ATL ", "SFO "\]
**Explanation:** Another possible reconstruction is \[ "JFK ", "SFO ", "ATL ", "JFK ", "ATL ", "SFO "\] but it is larger in lexical order.
**Constraints:**
* `1 <= tickets.length <= 300`
* `tickets[i].length == 2`
* `fromi.length == 3`
* `toi.length == 3`
* `fromi` and `toi` consist of uppercase English letters.
* `fromi != toi` | null |
simple approach | reconstruct-itinerary | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom collections import defaultdict\n\nclass Solution:\n def findItinerary(self, tickets: List[List[str]]) -> List[str]:\n # Create a graph for each airport and keep list of airport reachable from it\n graph = defaultdict(list)\n for src, dst in sorted(tickets)[::-1]:\n graph[src].append(dst)\n\n route, stack = [], [\'JFK\']\n while stack:\n while graph[stack[-1]]:\n stack.append(graph[stack[-1]].pop())\n route.append(stack.pop())\n\n return route[::-1]\n\n``` | 2 | You are given a list of airline `tickets` where `tickets[i] = [fromi, toi]` represent the departure and the arrival airports of one flight. Reconstruct the itinerary in order and return it.
All of the tickets belong to a man who departs from `"JFK "`, thus, the itinerary must begin with `"JFK "`. If there are multiple valid itineraries, you should return the itinerary that has the smallest lexical order when read as a single string.
* For example, the itinerary `[ "JFK ", "LGA "]` has a smaller lexical order than `[ "JFK ", "LGB "]`.
You may assume all tickets form at least one valid itinerary. You must use all the tickets once and only once.
**Example 1:**
**Input:** tickets = \[\[ "MUC ", "LHR "\],\[ "JFK ", "MUC "\],\[ "SFO ", "SJC "\],\[ "LHR ", "SFO "\]\]
**Output:** \[ "JFK ", "MUC ", "LHR ", "SFO ", "SJC "\]
**Example 2:**
**Input:** tickets = \[\[ "JFK ", "SFO "\],\[ "JFK ", "ATL "\],\[ "SFO ", "ATL "\],\[ "ATL ", "JFK "\],\[ "ATL ", "SFO "\]\]
**Output:** \[ "JFK ", "ATL ", "JFK ", "SFO ", "ATL ", "SFO "\]
**Explanation:** Another possible reconstruction is \[ "JFK ", "SFO ", "ATL ", "JFK ", "ATL ", "SFO "\] but it is larger in lexical order.
**Constraints:**
* `1 <= tickets.length <= 300`
* `tickets[i].length == 2`
* `fromi.length == 3`
* `toi.length == 3`
* `fromi` and `toi` consist of uppercase English letters.
* `fromi != toi` | null |
✅ 95.76% DFS Recursive & Iterative | reconstruct-itinerary | 1 | 1 | # Comprehensive Guide to Solving "Reconstruct Itinerary": Navigating Airports Like a Pro\n\n## Introduction & Problem Statement\n\nHello, coding enthusiasts! Today, we\'ll tackle a problem that combines graph theory and real-world scenarios: "Reconstruct Itinerary." The problem asks you to reconstruct a trip\'s itinerary given a list of airline tickets. The catch? You start from JFK airport, and if there are multiple valid itineraries, you should return the one that has the smallest lexical order.\n\n## Key Concepts and Constraints\n\n### What Makes This Problem Unique?\n\n1. **Graph Theory**: \n The problem can be modeled as a directed graph, where each airport is a node and each ticket represents a directed edge between airports.\n \n2. **Lexical Order**: \n Among multiple valid itineraries, the one with the smallest lexical order is preferred.\n\n3. **Constraints**: \n - 1 <= tickets.length <= 300\n - Tickets are given as pairs [fromi, toi] of strings.\n - Each string consists of 3 uppercase English letters.\n\n### Strategies to Tackle the Problem\n\n1. **Recursive DFS**: \n This approach leverages the stack memory implicitly during recursion to backtrack and form the itinerary.\n \n2. **Iterative DFS**: \n This approach explicitly uses a stack to perform DFS iteratively.\n\n---\n\n## Live Coding & More in Python\nhttps://www.youtube.com/watch?v=migy5oXV1Uc\n\n## Recursive DFS Explained\n\n### Extended Explanation: Logic Behind the "Find Itinerary" Solution using Recursive DFS\n\n### What is Recursive DFS?\n\nDFS, short for Depth-First Search, is a graph-traversal algorithm. In this specific problem, we use the recursive version of DFS, which exploits the call stack to keep track of the airports (vertices) that still need exploration.\n\n### The Intricacies of Recursive DFS in "Find Itinerary"\n\n1. **Initialize the Graph**: \n - We start by initializing an adjacency list `graph` that will serve as a representation of the directed graph of flights. The graph is implemented as a defaultdict containing lists.\n \n - The adjacency list is constructed by iterating over the given list of ticket pairs, where each pair consists of a source airport and a destination airport.\n \n - These lists of destinations are sorted in reverse lexical order. This allows us to pop the last element to ensure that we are choosing the smallest lexical order when there are multiple options.\n\n2. **DFS Traversal and Exploration**: \n - The traversal starts from the JFK airport, which is our initial point. We initiate a recursive DFS function that takes the current airport as an argument.\n \n - Inside this function, we enter a loop that continues until we find an airport that has no more destinations left to visit. This is done by checking the adjacency list for each airport and popping the last element (which is the smallest in lexical order).\n \n - During this process, we recursively call the DFS function for each new destination, essentially diving deeper into the graph.\n\n3. **Backtrack and Construct Itinerary**: \n - Once we reach an airport with no outgoing edges, or in other words, no more destinations to visit, we start backtracking.\n \n - During the backtracking phase, we add the current airport to our `itinerary` list. This list was initially empty and serves as the container for our final solution.\n\n - Importantly, we are essentially building the itinerary in reverse during this phase because of the way DFS works. As we backtrack, we are popping from the call stack, revisiting the airports in the reverse order of how they will eventually appear in the itinerary.\n\n4. **Reverse to Get the Final Itinerary**: \n - Since we constructed the itinerary in reverse, the final step is to reverse this list. This gives us the correct order of airports to visit, starting from JFK, and is our final solution.\n\n### Time and Space Complexity\n\n- **Time Complexity**: $$O(N \\log N)$$ due to sorting the tickets.\n- **Space Complexity**: $$O(N)$$, for storing the graph and the itinerary.\n\n# Code Recursive \n``` Python []\nclass Solution:\n def findItinerary(self, tickets: List[List[str]]) -> List[str]:\n graph = defaultdict(list)\n \n for src, dst in sorted(tickets, reverse=True):\n graph[src].append(dst)\n \n itinerary = []\n def dfs(airport):\n while graph[airport]:\n dfs(graph[airport].pop())\n itinerary.append(airport)\n \n dfs("JFK")\n \n return itinerary[::-1]\n```\n``` Go []\nfunc findItinerary(tickets [][]string) []string {\n\tgraph := make(map[string][]string)\n\t\n\tfor _, ticket := range tickets {\n\t\tgraph[ticket[0]] = append(graph[ticket[0]], ticket[1])\n\t}\n\t\n\tfor key := range graph {\n\t\tsort.Sort(sort.Reverse(sort.StringSlice(graph[key])))\n\t}\n\t\n\tvar itinerary []string\n\t\n\tvar dfs func(airport string)\n\tdfs = func(airport string) {\n\t\tfor len(graph[airport]) > 0 {\n\t\t\tnext := graph[airport][len(graph[airport])-1]\n\t\t\tgraph[airport] = graph[airport][:len(graph[airport])-1]\n\t\t\tdfs(next)\n\t\t}\n\t\titinerary = append(itinerary, airport)\n\t}\n\t\n\tdfs("JFK")\n\t\n\tfor i := 0; i < len(itinerary)/2; i++ {\n\t\titinerary[i], itinerary[len(itinerary)-1-i] = itinerary[len(itinerary)-1-i], itinerary[i]\n\t}\n\t\n\treturn itinerary\n}\n```\n``` Rust []\nuse std::collections::HashMap;\n\nimpl Solution {\n pub fn find_itinerary(tickets: Vec<Vec<String>>) -> Vec<String> {\n let mut graph: HashMap<String, Vec<String>> = HashMap::new();\n \n for ticket in &tickets {\n let (src, dst) = (ticket[0].clone(), ticket[1].clone());\n graph.entry(src.clone()).or_insert(vec![]).push(dst);\n }\n\n for destinations in graph.values_mut() {\n destinations.sort_by(|a, b| b.cmp(a));\n }\n \n let mut itinerary = vec![];\n \n fn dfs(graph: &mut HashMap<String, Vec<String>>, airport: &str, itinerary: &mut Vec<String>) {\n while let Some(next) = graph.get_mut(airport).and_then(|dests| dests.pop()) {\n dfs(graph, &next, itinerary);\n }\n itinerary.push(airport.to_string());\n }\n \n dfs(&mut graph, "JFK", &mut itinerary);\n \n itinerary.reverse();\n \n itinerary\n }\n}\n```\n``` C++ []\nclass Solution {\npublic:\n vector<string> findItinerary(vector<vector<string>>& tickets) {\n unordered_map<string, vector<string>> graph;\n \n for (auto& ticket : tickets) {\n graph[ticket[0]].push_back(ticket[1]);\n }\n \n for (auto& [_, destinations] : graph) {\n sort(destinations.rbegin(), destinations.rend());\n }\n \n vector<string> itinerary;\n \n function<void(const string&)> dfs = [&](const string& airport) {\n while (!graph[airport].empty()) {\n string next = graph[airport].back();\n graph[airport].pop_back();\n dfs(next);\n }\n itinerary.push_back(airport);\n };\n \n dfs("JFK");\n reverse(itinerary.begin(), itinerary.end());\n \n return itinerary;\n }\n};\n```\n``` Java []\nclass Solution {\n public List<String> findItinerary(List<List<String>> tickets) {\n Map<String, PriorityQueue<String>> graph = new HashMap<>();\n \n for (List<String> ticket : tickets) {\n graph.putIfAbsent(ticket.get(0), new PriorityQueue<>());\n graph.get(ticket.get(0)).add(ticket.get(1));\n }\n \n LinkedList<String> itinerary = new LinkedList<>();\n \n dfs("JFK", graph, itinerary);\n \n return itinerary;\n }\n \n private void dfs(String airport, Map<String, PriorityQueue<String>> graph, LinkedList<String> itinerary) {\n PriorityQueue<String> nextAirports = graph.get(airport);\n while (nextAirports != null && !nextAirports.isEmpty()) {\n dfs(nextAirports.poll(), graph, itinerary);\n }\n itinerary.addFirst(airport);\n }\n}\n```\n``` C# []\npublic class Solution {\n public IList<string> FindItinerary(IList<IList<string>> tickets) {\n var graph = new Dictionary<string, List<string>>();\n \n foreach (var ticket in tickets) {\n if (!graph.ContainsKey(ticket[0])) {\n graph[ticket[0]] = new List<string>();\n }\n graph[ticket[0]].Add(ticket[1]);\n }\n \n foreach (var destinations in graph.Values) {\n destinations.Sort((a, b) => b.CompareTo(a));\n }\n \n var itinerary = new List<string>();\n \n void DFS(string airport) {\n while (graph.ContainsKey(airport) && graph[airport].Count > 0) {\n var next = graph[airport][^1];\n graph[airport].RemoveAt(graph[airport].Count - 1);\n DFS(next);\n }\n itinerary.Add(airport);\n }\n \n DFS("JFK");\n itinerary.Reverse();\n \n return itinerary;\n }\n}\n```\n``` JavaScript []\n/**\n * @param {string[][]} tickets\n * @return {string[]}\n */\nvar findItinerary = function(tickets) {\n const graph = {};\n \n for (const [src, dst] of tickets) {\n if (!graph[src]) graph[src] = [];\n graph[src].push(dst);\n }\n \n for (const key in graph) {\n graph[key].sort().reverse();\n }\n \n const itinerary = [];\n \n function dfs(airport) {\n while (graph[airport] && graph[airport].length > 0) {\n dfs(graph[airport].pop());\n }\n itinerary.push(airport);\n }\n \n dfs("JFK");\n \n return itinerary.reverse();\n};\n```\n``` PHP []\nclass Solution {\n function findItinerary($tickets) {\n $graph = [];\n \n foreach ($tickets as [$src, $dst]) {\n $graph[$src][] = $dst;\n }\n \n foreach ($graph as &$destinations) {\n rsort($destinations);\n }\n \n $itinerary = [];\n \n $dfs = function($airport) use (&$graph, &$itinerary, &$dfs) {\n while (!empty($graph[$airport])) {\n $dfs(array_pop($graph[$airport]));\n }\n $itinerary[] = $airport;\n };\n \n $dfs("JFK");\n \n return array_reverse($itinerary);\n }\n}\n```\n\n## Iterative DFS Explained\n\n### How Does Iterative DFS Work?\n\nIterative DFS also starts by initializing the graph in the same manner as the recursive approach. The difference lies in the traversal and the building of the itinerary.\n\n1. **Initialize Stack**: \n - We explicitly initialize a stack with "JFK" as the starting point.\n \n2. **Iterative DFS Traversal**: \n - We use the stack to manage the DFS traversal. For each airport on the stack, we keep visiting its next available destination until it has no outgoing edges.\n \n3. **Build Itinerary**: \n - During this process, we keep adding the current airport to the `itinerary` list.\n \n4. **Reverse the Itinerary**: \n - Finally, we reverse the `itinerary` list to get the final itinerary in correct order.\n\n### Time and Space Complexity\n\n- **Time Complexity**: $$O(N \\log N)$$, due to sorting.\n- **Space Complexity**: $$O(N)$$, for storing the graph and the itinerary.\n\n\n# Code Iterative\n``` Python []\nclass Solution:\n def findItinerary(self, tickets: List[List[str]]) -> List[str]:\n graph = defaultdict(list)\n \n for src, dst in sorted(tickets, reverse=True):\n graph[src].append(dst)\n \n stack = ["JFK"]\n itinerary = []\n \n while stack:\n while graph[stack[-1]]:\n stack.append(graph[stack[-1]].pop())\n itinerary.append(stack.pop())\n \n return itinerary[::-1]\n```\n``` Go []\nfunc findItinerary(tickets [][]string) []string {\n\tgraph := make(map[string][]string)\n\t\n\tfor _, ticket := range tickets {\n\t\tgraph[ticket[0]] = append(graph[ticket[0]], ticket[1])\n\t}\n\t\n\tfor key := range graph {\n\t\tsort.Sort(sort.Reverse(sort.StringSlice(graph[key])))\n\t}\n\t\n\tstack := []string{"JFK"}\n\tvar itinerary []string\n\t\n\tfor len(stack) > 0 {\n\t\tfor len(graph[stack[len(stack)-1]]) > 0 {\n\t\t\tlast := len(graph[stack[len(stack)-1]]) - 1\n\t\t\tstack = append(stack, graph[stack[len(stack)-1]][last])\n\t\t\tgraph[stack[len(stack)-2]] = graph[stack[len(stack)-2]][:last]\n\t\t}\n\t\titinerary = append(itinerary, stack[len(stack)-1])\n\t\tstack = stack[:len(stack)-1]\n\t}\n\t\n\tfor i := 0; i < len(itinerary)/2; i++ {\n\t\titinerary[i], itinerary[len(itinerary)-1-i] = itinerary[len(itinerary)-1-i], itinerary[i]\n\t}\n\t\n\treturn itinerary\n}\n```\n``` C++ []\nclass Solution {\npublic:\n std::vector<std::string> findItinerary(std::vector<std::vector<std::string>>& tickets) {\n std::unordered_map<std::string, std::vector<std::string>> graph;\n \n for (auto& ticket : tickets) {\n graph[ticket[0]].push_back(ticket[1]);\n }\n \n for (auto& [_, dests] : graph) {\n std::sort(dests.rbegin(), dests.rend());\n }\n \n std::vector<std::string> stack = {"JFK"};\n std::vector<std::string> itinerary;\n \n while (!stack.empty()) {\n std::string curr = stack.back();\n if (graph.find(curr) != graph.end() && !graph[curr].empty()) {\n stack.push_back(graph[curr].back());\n graph[curr].pop_back();\n } else {\n itinerary.push_back(stack.back());\n stack.pop_back();\n }\n }\n \n std::reverse(itinerary.begin(), itinerary.end());\n return itinerary;\n }\n};\n```\n``` Java []\nclass Solution {\n public List<String> findItinerary(List<List<String>> tickets) {\n Map<String, PriorityQueue<String>> graph = new HashMap<>();\n \n for (List<String> ticket : tickets) {\n graph.computeIfAbsent(ticket.get(0), k -> new PriorityQueue<>()).add(ticket.get(1));\n }\n \n LinkedList<String> stack = new LinkedList<>();\n stack.add("JFK");\n LinkedList<String> itinerary = new LinkedList<>();\n \n while (!stack.isEmpty()) {\n while (graph.getOrDefault(stack.peekLast(), new PriorityQueue<>()).size() > 0) {\n stack.add(graph.get(stack.peekLast()).poll());\n }\n itinerary.addFirst(stack.pollLast());\n }\n \n return itinerary;\n }\n}\n```\n``` JavaScript []\n/**\n * @param {string[][]} tickets\n * @return {string[]}\n */\nvar findItinerary = function(tickets) {\n const graph = {};\n \n for (const [src, dst] of tickets) {\n if (!graph[src]) graph[src] = [];\n graph[src].push(dst);\n }\n \n for (const key in graph) {\n graph[key].sort((a, b) => b.localeCompare(a));\n }\n \n const stack = [\'JFK\'];\n const itinerary = [];\n \n while (stack.length > 0) {\n while (graph[stack[stack.length - 1]] && graph[stack[stack.length - 1]].length > 0) {\n stack.push(graph[stack[stack.length - 1]].pop());\n }\n itinerary.push(stack.pop());\n }\n \n return itinerary.reverse();\n};\n```\n``` C# []\nusing System;\nusing System.Collections.Generic;\nusing System.Linq;\n\npublic class Solution {\n public IList<string> FindItinerary(IList<IList<string>> tickets) {\n var graph = new Dictionary<string, List<string>>();\n \n foreach (var ticket in tickets) {\n if (!graph.ContainsKey(ticket[0])) {\n graph[ticket[0]] = new List<string>();\n }\n graph[ticket[0]].Add(ticket[1]);\n }\n\n foreach (var key in graph.Keys) {\n graph[key].Sort((a, b) => b.CompareTo(a));\n }\n \n var stack = new Stack<string>();\n stack.Push("JFK");\n var itinerary = new List<string>();\n \n while (stack.Count > 0) {\n string curr = stack.Peek();\n if (graph.ContainsKey(curr) && graph[curr].Count > 0) {\n var next = graph[curr].Last();\n graph[curr].RemoveAt(graph[curr].Count - 1);\n stack.Push(next);\n } else {\n itinerary.Add(stack.Pop());\n }\n }\n \n itinerary.Reverse();\n return itinerary;\n }\n}\n```\n``` PHP []\nclass Solution {\n function findItinerary($tickets) {\n $graph = [];\n foreach ($tickets as $ticket) {\n $graph[$ticket[0]][] = $ticket[1];\n }\n \n foreach ($graph as &$dests) {\n rsort($dests);\n }\n \n $stack = ["JFK"];\n $itinerary = [];\n \n while (!empty($stack)) {\n while (!empty($graph[end($stack)])) {\n array_push($stack, array_pop($graph[end($stack)]));\n }\n array_push($itinerary, array_pop($stack));\n }\n \n return array_reverse($itinerary);\n }\n}\n```\n\n## Performance\n\n| Language | Time (ms) | Memory (MB) | Approach |\n|------------|-----------|-------------|-----------|\n| Rust | 5 | 2.2 | Recursive |\n| Java | 5 | 44.1 | Recursive |\n| Go | 8 | 6.8 | Recursive |\n| Go | 10 | 6.7 | Iterative |\n| C++ | 12 | 14.6 | Recursive |\n| PHP | 18 | 21 | Recursive |\n| JavaScript | 77 | 47.1 | Recursive |\n| Python3 | 82 | 16.6 | Recursive |\n| Python3 | 85 | 16.8 | Iterative |\n| C# | 178 | 57.7 | Recursive |\n\n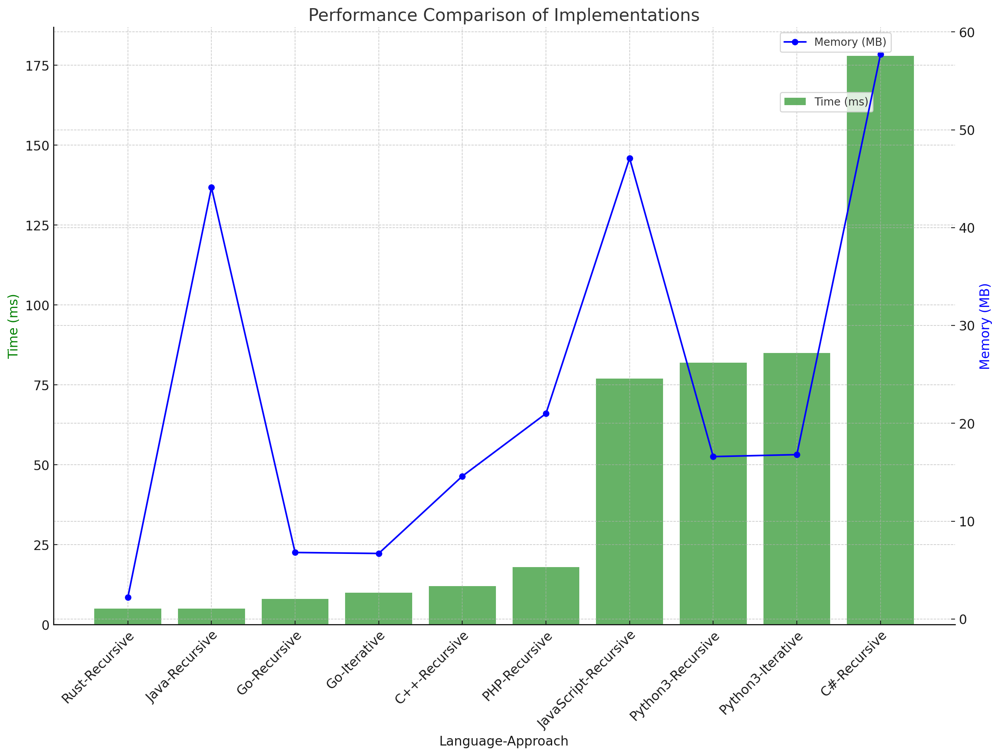\n\n\n## Live Coding+ & Go\nhttps://youtu.be/_r9OZwy5x4c?si=P-9OousNlDl-cZnJ\n\n## Code Highlights and Best Practices\n\n- The Recursive DFS is elegant but uses the system stack, making it susceptible to stack overflow for very large graphs.\n- The Iterative DFS is more flexible as it explicitly uses a stack, allowing for better control over the traversal process.\n \nMastering these approaches not only helps you solve this problem but also enhances your understanding of graph traversal algorithms, which are widely applicable in various domains. So, are you ready to construct some itineraries? Let\'s get coding! | 124 | You are given a list of airline `tickets` where `tickets[i] = [fromi, toi]` represent the departure and the arrival airports of one flight. Reconstruct the itinerary in order and return it.
All of the tickets belong to a man who departs from `"JFK "`, thus, the itinerary must begin with `"JFK "`. If there are multiple valid itineraries, you should return the itinerary that has the smallest lexical order when read as a single string.
* For example, the itinerary `[ "JFK ", "LGA "]` has a smaller lexical order than `[ "JFK ", "LGB "]`.
You may assume all tickets form at least one valid itinerary. You must use all the tickets once and only once.
**Example 1:**
**Input:** tickets = \[\[ "MUC ", "LHR "\],\[ "JFK ", "MUC "\],\[ "SFO ", "SJC "\],\[ "LHR ", "SFO "\]\]
**Output:** \[ "JFK ", "MUC ", "LHR ", "SFO ", "SJC "\]
**Example 2:**
**Input:** tickets = \[\[ "JFK ", "SFO "\],\[ "JFK ", "ATL "\],\[ "SFO ", "ATL "\],\[ "ATL ", "JFK "\],\[ "ATL ", "SFO "\]\]
**Output:** \[ "JFK ", "ATL ", "JFK ", "SFO ", "ATL ", "SFO "\]
**Explanation:** Another possible reconstruction is \[ "JFK ", "SFO ", "ATL ", "JFK ", "ATL ", "SFO "\] but it is larger in lexical order.
**Constraints:**
* `1 <= tickets.length <= 300`
* `tickets[i].length == 2`
* `fromi.length == 3`
* `toi.length == 3`
* `fromi` and `toi` consist of uppercase English letters.
* `fromi != toi` | null |
【Video】Visualized Solution - Python, JavaScript, Java, C++ | reconstruct-itinerary | 1 | 1 | # Intuition\nThis code uses airline ticket information to construct a valid itinerary starting from the departure airport "JFK" and organizing destinations into a dictionary-style graph. It manages the routes from departure to arrival using a stack, adding them to a new itinerary when there are no more destinations. The final itinerary is reversed to obtain the correct order.\n\n---\n\n# Solution Video\n\nhttps://youtu.be/7kZKIod8L94\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 2310\nThank you for your support!\n\n---\n\n# Approach\n1. Creating the Graph and Organizing Ticket Information:\n - Create a dictionary-style graph to store airports and their destinations.\n - Sort the ticket information in reverse order based on arrival airports.\n\n2. Initializing the Stack and New Itinerary:\n - Create a stack initialized with `JFK` as the departure airport.\n - Initialize a list to store the new itinerary.\n\n3. Constructing the Itinerary Using the Stack:\n - Repeat the following process as long as the stack is not empty:\n - Add potential destinations departing from the current airport to the stack.\n - When there are no more destinations, add the current airport to the new itinerary.\n\n4. Reversing the Itinerary:\n - Reverse the new itinerary to obtain the correct order of the itinerary.\n\n5. Returning the Correct Order Itinerary:\n - Return the reversed itinerary as the final result.\n\n# How it works\n\n```\nInput: tickets = [["JFK","SFO"],["JFK","ATL"],["SFO","ATL"],\n["ATL","JFK"],["ATL","SFO"]]\n```\n\n## **Step 1:** Graph Initialization\n\nIn this step, the graph is initialized. The graph is represented as a dictionary with departure points (airports) as keys and lists of arrival points (other airports) as values. In this initialization, no information has been added yet.\n\nIn this step, the provided airline ticket information is sorted in reverse order.\n\nAirline tickets sorted in reverse order for lexical order: `[[\'SFO\', \'ATL\'], [\'JFK\', \'SFO\'], [\'JFK\', \'ATL\'], [\'ATL\', \'SFO\'], [\'ATL\', \'JFK\']]`\n\nThe reason for performing reverse sorting (reverse order) in this code is to use a stack and find the "lexicographically smallest route" according to the constraints of the problem. There are two key points in the constraints:\n\n1. The departure point must be `JFK.`\n2. The lexicographically smallest route needs to be found.\n\nBy performing reverse sorting, when the departure point is `JFK` the route will start with the ticket that has the smallest destination in lexicographical order. In other words, the route that starts from `JFK` is chosen as the smallest route initially.\n\nThe other important reason is that we can use `pop()` and easily get the smallest destination in lexicographical order with `O(1)`. Therefore, reverse sorting is necessary to meet the constraints of the problem.\n\n```\ngraph = {\n "SFO": ["ATL"],\n "JFK": ["SFO", "ATL"],\n "ATL": ["SFO", "JFK"]\n}\n```\n\nThe graph has been correctly updated as a result of this step.\n\n## **Step 2:** Stack and Itinerary Initialization\n\nIn this step, the stack `st` and an empty itinerary list `new_itinerary` are initialized.\n\n```\nst: ["JFK"]\nnew_itinerary: []\n```\n\n## **Step 3:** Building the Itinerary Using the Stack\n\nIn this step, the itinerary is constructed using a stack. The following operations are repeated in each step of stack operation.\n\n1. Check the current airport at the top of the stack. The initial airport is `JFK`.\n\n - `st`: `["JFK"]`\n - `new_itinerary`: `[]`\n\n2. Find the places reachable from the current airport `JFK`. From the current airport `JFK`, you can go to `SFO` and `ATL`. Add `ATL` to the stack.\n\n - `st`: `["JFK", "ATL"]`\n - `new_itinerary`: `[]`\n\nCurrent graph:\n```\ngraph = {\n "SFO": ["ATL"],\n "JFK": ["SFO"],\n "ATL": ["SFO", "JFK"]\n}\n```\n\n4. Add the places reachable from `ATL` to the stack. From the current airport `ATL`, you can go to `SFO` and `JFK`. Add `JFK` to the stack.\n\n - `st`: `["JFK", "ATL", "JFK"]`\n - `new_itinerary`: `[]`\n\nCurrent graph:\n```\ngraph = {\n "SFO": ["ATL"],\n "JFK": ["SFO"],\n "ATL": ["SFO"]\n}\n```\n\n5. Add the places reachable from `JFK` to the stack. From the current airport `JFK`, you can go to `SFO`. Add `JFK` to the stack.\n\n - `st`: `["JFK", "ATL", "JFK", "SFO"]`\n - `new_itinerary`: `[""]`\n\nCurrent graph:\n```\ngraph = {\n "SFO": ["ATL"],\n "JFK": [""],\n "ATL": ["SFO"]\n}\n```\n\n6. Add the places reachable from `SFO` to the stack. From the current airport `SFO`, you can go to `ATL`. Add ATL` to the stack.\n\n - `st`: `["JFK", "ATL", "JFK", "SFO", "ATL"]`\n - `new_itinerary`: `[""]`\n\nCurrent graph:\n```\ngraph = {\n "SFO": [""],\n "JFK": [""],\n "ATL": ["SFO"]\n}\n```\n\n7. Add the places reachable from `ATL` to the stack. From the current airport `ATL`, you can go to `SFO`. Add `SFO` to the stack.\n\n - `st`: `["JFK", "ATL", "JFK", "SFO", "ATL", "SFO"]`\n - `new_itinerary`: `[""]`\n\nCurrent graph:\n```\ngraph = {\n "SFO": [""],\n "JFK": [""],\n "ATL": [""]\n}\n```\n\nFinally, pop data from `st` and assign it to `new_itinerary`. This operation is necessary to avoid an infinite loop in the outermost `while` loop.\n\n```\n`st`: `["JFK", "ATL", "JFK", "SFO", "ATL", "SFO"]`\n\nnew_itinerary [\'SFO\']\nnew_itinerary [\'SFO\', \'ATL\']\nnew_itinerary [\'SFO\', \'ATL\', \'SFO\']\nnew_itinerary [\'SFO\', \'ATL\', \'SFO\', \'JFK\']\nnew_itinerary [\'SFO\', \'ATL\', \'SFO\', \'JFK\', \'ATL\']\nnew_itinerary [\'SFO\', \'ATL\', \'SFO\', \'JFK\', \'ATL\', \'JFK\']\n```\n\nReverse `new_itinerary` and return it.\n\n```\nOutput: ["JFK", "ATL", "JFK", "SFO", "ATL", "SFO"]\n```\n\n# Complexity\n- Time complexity: O(N log N)\n - Let N be the number of tickets. Sorting the tickets in reverse order takes O(N log N) time.\n - Processing each ticket using the stack takes O(N) time.\n\n\n\n- Space complexity: O(N)\n - To represent the graph, O(N) space is required.\n - O(N) space is needed for the stack and the new itinerary.\n\n \n```python []\nclass Solution:\n def findItinerary(self, tickets: List[List[str]]) -> List[str]:\n # Create a graph represented as a dictionary where each airport is a key, and its destinations are values.\n graph = defaultdict(list)\n\n for departure, arrival in sorted(tickets, reverse=True):\n graph[departure].append(arrival)\n\n # Initialize the stack with the starting airport "JFK" and an empty itinerary.\n st = ["JFK"]\n new_itinerary = []\n\n while st:\n # If there are destinations from the current airport, add the next destination to the stack.\n if graph[st[-1]]:\n st.append(graph[st[-1]].pop())\n else:\n # When there are no more destinations, add the current airport to the new itinerary.\n new_itinerary.append(st.pop())\n\n # Reverse the new itinerary to get the correct order.\n return new_itinerary[::-1]\n```\n```javascript []\n/**\n * @param {string[][]} tickets\n * @return {string[]}\n */\nvar findItinerary = function(tickets) {\n const graph = new Map();\n\n for (const [departure, arrival] of tickets.sort().reverse()) {\n if (!graph.has(departure)) {\n graph.set(departure, []);\n }\n graph.get(departure).push(arrival);\n }\n\n const stack = ["JFK"];\n const newItinerary = [];\n\n while (stack.length > 0) {\n const currentAirport = stack[stack.length - 1];\n\n if (graph.has(currentAirport) && graph.get(currentAirport).length > 0) {\n stack.push(graph.get(currentAirport).pop());\n } else {\n newItinerary.push(stack.pop());\n }\n }\n\n return newItinerary.reverse(); \n};\n```\n```java []\nclass Solution {\n public List<String> findItinerary(List<List<String>> tickets) {\n Map<String, List<String>> graph = new HashMap<>();\n\n for (List<String> ticket : tickets) {\n String departure = ticket.get(0);\n String arrival = ticket.get(1);\n\n graph.computeIfAbsent(departure, k -> new ArrayList<>()).add(arrival);\n }\n\n for (List<String> destinations : graph.values()) {\n destinations.sort(Collections.reverseOrder());\n }\n\n List<String> newItinerary = new ArrayList<>();\n Deque<String> stack = new ArrayDeque<>();\n stack.push("JFK");\n\n while (!stack.isEmpty()) {\n String currentAirport = stack.peek();\n\n if (graph.containsKey(currentAirport) && !graph.get(currentAirport).isEmpty()) {\n stack.push(graph.get(currentAirport).remove(graph.get(currentAirport).size() - 1));\n } else {\n newItinerary.add(stack.pop());\n }\n }\n\n Collections.reverse(newItinerary);\n return newItinerary; \n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n vector<string> findItinerary(vector<vector<string>>& tickets) {\n unordered_map<string, priority_queue<string, vector<string>, greater<string>>> graph;\n\n for (const auto& ticket : tickets) {\n graph[ticket[0]].push(ticket[1]);\n }\n\n vector<string> newItinerary;\n stack<string> st;\n st.push("JFK");\n\n while (!st.empty()) {\n string currentAirport = st.top();\n\n if (graph.find(currentAirport) != graph.end() && !graph[currentAirport].empty()) {\n st.push(graph[currentAirport].top());\n graph[currentAirport].pop();\n } else {\n newItinerary.push_back(st.top());\n st.pop();\n }\n }\n\n reverse(newItinerary.begin(), newItinerary.end());\n return newItinerary; \n }\n};\n```\n\n\n---\n\n\n\n# Can I make a small request?\nThank you for watching my video and reading my article!\n\n\u2B50\uFE0F If you like my video and my article, please subscribe to my youtube channel and upvote the article. My initial goal for youtube is to reach 10,000 subscribers. It\'s far from done.\n\nHave a nice day! | 30 | You are given a list of airline `tickets` where `tickets[i] = [fromi, toi]` represent the departure and the arrival airports of one flight. Reconstruct the itinerary in order and return it.
All of the tickets belong to a man who departs from `"JFK "`, thus, the itinerary must begin with `"JFK "`. If there are multiple valid itineraries, you should return the itinerary that has the smallest lexical order when read as a single string.
* For example, the itinerary `[ "JFK ", "LGA "]` has a smaller lexical order than `[ "JFK ", "LGB "]`.
You may assume all tickets form at least one valid itinerary. You must use all the tickets once and only once.
**Example 1:**
**Input:** tickets = \[\[ "MUC ", "LHR "\],\[ "JFK ", "MUC "\],\[ "SFO ", "SJC "\],\[ "LHR ", "SFO "\]\]
**Output:** \[ "JFK ", "MUC ", "LHR ", "SFO ", "SJC "\]
**Example 2:**
**Input:** tickets = \[\[ "JFK ", "SFO "\],\[ "JFK ", "ATL "\],\[ "SFO ", "ATL "\],\[ "ATL ", "JFK "\],\[ "ATL ", "SFO "\]\]
**Output:** \[ "JFK ", "ATL ", "JFK ", "SFO ", "ATL ", "SFO "\]
**Explanation:** Another possible reconstruction is \[ "JFK ", "SFO ", "ATL ", "JFK ", "ATL ", "SFO "\] but it is larger in lexical order.
**Constraints:**
* `1 <= tickets.length <= 300`
* `tickets[i].length == 2`
* `fromi.length == 3`
* `toi.length == 3`
* `fromi` and `toi` consist of uppercase English letters.
* `fromi != toi` | null |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.