title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
[Python3] Divide and Conquer: Recursion + Memoization | different-ways-to-add-parentheses | 0 | 1 | ```\nclass Solution(object):\n def diffWaysToCompute(self, s, memo=dict()):\n if s in memo:\n return memo[s]\n if s.isdigit(): # base case\n return [int(s)]\n calculate = {\'*\': lambda x, y: x * y,\n \'+\': lambda x, y: x + y,\n \'-\': lambda x, y: x - y\n }\n result = []\n for i, c in enumerate(s):\n if c in \'+-*\':\n left = self.diffWaysToCompute(s[:i], memo)\n right = self.diffWaysToCompute(s[i+1:], memo)\n for l in left:\n for r in right:\n result.append(calculate[c](l, r))\n memo[s] = result\n return result\n \n``` | 6 | Given a string `expression` of numbers and operators, return _all possible results from computing all the different possible ways to group numbers and operators_. You may return the answer in **any order**.
The test cases are generated such that the output values fit in a 32-bit integer and the number of different results does not exceed `104`.
**Example 1:**
**Input:** expression = "2-1-1 "
**Output:** \[0,2\]
**Explanation:**
((2-1)-1) = 0
(2-(1-1)) = 2
**Example 2:**
**Input:** expression = "2\*3-4\*5 "
**Output:** \[-34,-14,-10,-10,10\]
**Explanation:**
(2\*(3-(4\*5))) = -34
((2\*3)-(4\*5)) = -14
((2\*(3-4))\*5) = -10
(2\*((3-4)\*5)) = -10
(((2\*3)-4)\*5) = 10
**Constraints:**
* `1 <= expression.length <= 20`
* `expression` consists of digits and the operator `'+'`, `'-'`, and `'*'`.
* All the integer values in the input expression are in the range `[0, 99]`. | null |
Easy Solution Ever with Comment | different-ways-to-add-parentheses | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def diffWaysToCompute(self, expression: str) -> List[int]:\n # Recursive function to evaluate an expression\n def evaluate(expression):\n if expression.isdigit(): # Base case: single number\n return [int(expression)]\n \n results = [] # List to store the results\n \n for i in range(len(expression)):\n if expression[i] in [\'+\', \'-\', \'*\']:\n left_results = evaluate(expression[:i]) # Evaluate left side\n right_results = evaluate(expression[i+1:]) # Evaluate right side\n \n # Combine results using the operator\n for left in left_results:\n for right in right_results:\n if expression[i] == \'+\':\n results.append(left + right)\n elif expression[i] == \'-\':\n results.append(left - right)\n elif expression[i] == \'*\':\n results.append(left * right)\n \n return results\n \n return evaluate(expression) # Start the evaluation with the given expression\n\n``` | 4 | Given a string `expression` of numbers and operators, return _all possible results from computing all the different possible ways to group numbers and operators_. You may return the answer in **any order**.
The test cases are generated such that the output values fit in a 32-bit integer and the number of different results does not exceed `104`.
**Example 1:**
**Input:** expression = "2-1-1 "
**Output:** \[0,2\]
**Explanation:**
((2-1)-1) = 0
(2-(1-1)) = 2
**Example 2:**
**Input:** expression = "2\*3-4\*5 "
**Output:** \[-34,-14,-10,-10,10\]
**Explanation:**
(2\*(3-(4\*5))) = -34
((2\*3)-(4\*5)) = -14
((2\*(3-4))\*5) = -10
(2\*((3-4)\*5)) = -10
(((2\*3)-4)\*5) = 10
**Constraints:**
* `1 <= expression.length <= 20`
* `expression` consists of digits and the operator `'+'`, `'-'`, and `'*'`.
* All the integer values in the input expression are in the range `[0, 99]`. | null |
Detailed Python solution with comments. | different-ways-to-add-parentheses | 0 | 1 | ```\nclass Solution(object):\n def diffWaysToCompute(self, expression):\n \n res=[]\n def fn(s,memo={}):\n res=[]\n if s in memo:\n return memo[s]\n if s.isdigit():\n return [int(s)] #if string containsonly numbers return that\n syms=[\'-\',\'+\',\'*\',\'/\']\n for i in range(len(s)):\n symbol=s[i]\n if s[i] in syms: #if the character is a operator\n left=fn(s[:i],memo) #possible values from left\n right=fn(s[i+1:],memo) #possible values from right\n for l in left: #Using nested for loops, calculate all possible combinations of left and right values applying exp[i] operator\n for r in right:\n if symbol==\'+\':\n res.append(l+r)\n elif symbol==\'-\':\n res.append(l-r)\n elif symbol==\'*\':\n res.append(l*r)\n else:\n res.append(l//r)\n memo[s]=res\n return res\n return fn(expression)\n \n \n \n \n \n \n``` | 1 | Given a string `expression` of numbers and operators, return _all possible results from computing all the different possible ways to group numbers and operators_. You may return the answer in **any order**.
The test cases are generated such that the output values fit in a 32-bit integer and the number of different results does not exceed `104`.
**Example 1:**
**Input:** expression = "2-1-1 "
**Output:** \[0,2\]
**Explanation:**
((2-1)-1) = 0
(2-(1-1)) = 2
**Example 2:**
**Input:** expression = "2\*3-4\*5 "
**Output:** \[-34,-14,-10,-10,10\]
**Explanation:**
(2\*(3-(4\*5))) = -34
((2\*3)-(4\*5)) = -14
((2\*(3-4))\*5) = -10
(2\*((3-4)\*5)) = -10
(((2\*3)-4)\*5) = 10
**Constraints:**
* `1 <= expression.length <= 20`
* `expression` consists of digits and the operator `'+'`, `'-'`, and `'*'`.
* All the integer values in the input expression are in the range `[0, 99]`. | null |
67% Tc and 55% Sc easy python solution | different-ways-to-add-parentheses | 0 | 1 | ```\ndef diffWaysToCompute(self, ex: str) -> List[int]:\n\tcurr = ""\n\tarr = []\n\tfor i in ex:\n\t\tif(i in ["+","-","*"]):\n\t\t\tarr.append(curr)\n\t\t\tarr.append(i)\n\t\t\tcurr = ""\n\t\telse: curr += i\n\tarr.append(curr)\n\t@lru_cache(None)\n\tdef solve(i, j):\n\t\tif(i == j): return [int(arr[i])]\n\t\tif((i, j) in d): return d[(i, j)]\n\t\ttemp = []\n\t\tfor mid in range(i+1, j, 2):\n\t\t\tl = solve(i, mid-1)\n\t\t\tr = solve(mid+1, j)\n\t\t\tfor x in l:\n\t\t\t\tfor y in r:\n\t\t\t\t\ttemp.append(eval(str(x) + arr[mid] + str(y)))\n\t\td[(i, j)] = temp\n\t\treturn temp\n\n\td = dict()\n\treturn solve(0, len(arr)-1)\n``` | 1 | Given a string `expression` of numbers and operators, return _all possible results from computing all the different possible ways to group numbers and operators_. You may return the answer in **any order**.
The test cases are generated such that the output values fit in a 32-bit integer and the number of different results does not exceed `104`.
**Example 1:**
**Input:** expression = "2-1-1 "
**Output:** \[0,2\]
**Explanation:**
((2-1)-1) = 0
(2-(1-1)) = 2
**Example 2:**
**Input:** expression = "2\*3-4\*5 "
**Output:** \[-34,-14,-10,-10,10\]
**Explanation:**
(2\*(3-(4\*5))) = -34
((2\*3)-(4\*5)) = -14
((2\*(3-4))\*5) = -10
(2\*((3-4)\*5)) = -10
(((2\*3)-4)\*5) = 10
**Constraints:**
* `1 <= expression.length <= 20`
* `expression` consists of digits and the operator `'+'`, `'-'`, and `'*'`.
* All the integer values in the input expression are in the range `[0, 99]`. | null |
Python | Recursive | Concise Solution | different-ways-to-add-parentheses | 0 | 1 | ```\nclass Solution:\n def diffWaysToCompute(self, expression: str) -> List[int]:\n ops = {\n "+": lambda x, y : x + y,\n "-": lambda x, y : x - y,\n "*": lambda x, y : x * y\n }\n res = []\n for x, char in enumerate(expression):\n if char in ops:\n leftResults = self.diffWaysToCompute(expression[:x])\n rightResults = self.diffWaysToCompute(expression[x + 1:])\n \n for leftNum in leftResults:\n for rightNum in rightResults:\n res.append(ops[char](leftNum, rightNum))\n \n # no operations means expression is a sole number\n if not res:\n res.append(int(expression))\n return res\n``` | 4 | Given a string `expression` of numbers and operators, return _all possible results from computing all the different possible ways to group numbers and operators_. You may return the answer in **any order**.
The test cases are generated such that the output values fit in a 32-bit integer and the number of different results does not exceed `104`.
**Example 1:**
**Input:** expression = "2-1-1 "
**Output:** \[0,2\]
**Explanation:**
((2-1)-1) = 0
(2-(1-1)) = 2
**Example 2:**
**Input:** expression = "2\*3-4\*5 "
**Output:** \[-34,-14,-10,-10,10\]
**Explanation:**
(2\*(3-(4\*5))) = -34
((2\*3)-(4\*5)) = -14
((2\*(3-4))\*5) = -10
(2\*((3-4)\*5)) = -10
(((2\*3)-4)\*5) = 10
**Constraints:**
* `1 <= expression.length <= 20`
* `expression` consists of digits and the operator `'+'`, `'-'`, and `'*'`.
* All the integer values in the input expression are in the range `[0, 99]`. | null |
[Python] Memoization Solution | different-ways-to-add-parentheses | 0 | 1 | ```\nclass Solution:\n def diffWaysToCompute(self, expression: str) -> List[int]:\n \n def clac(a, b, operator):\n if operator == "+": return a + b\n if operator == "-": return a - b\n if operator == "*": return a * b\n \n memo = {} \n def solve(expr):\n if expr.isdigit(): return [int(expr)]\n if expr in memo: return memo[expr]\n res = []\n for i in range(len(expr)):\n if expr[i] in "+-*":\n left = solve(expr[:i])\n right = solve(expr[i+1:])\n for a in left:\n for b in right:\n res.append(clac(a, b, expr[i]))\n memo[expr] = res\n return memo[expr]\n \n return solve(expression)\n``` | 1 | Given a string `expression` of numbers and operators, return _all possible results from computing all the different possible ways to group numbers and operators_. You may return the answer in **any order**.
The test cases are generated such that the output values fit in a 32-bit integer and the number of different results does not exceed `104`.
**Example 1:**
**Input:** expression = "2-1-1 "
**Output:** \[0,2\]
**Explanation:**
((2-1)-1) = 0
(2-(1-1)) = 2
**Example 2:**
**Input:** expression = "2\*3-4\*5 "
**Output:** \[-34,-14,-10,-10,10\]
**Explanation:**
(2\*(3-(4\*5))) = -34
((2\*3)-(4\*5)) = -14
((2\*(3-4))\*5) = -10
(2\*((3-4)\*5)) = -10
(((2\*3)-4)\*5) = 10
**Constraints:**
* `1 <= expression.length <= 20`
* `expression` consists of digits and the operator `'+'`, `'-'`, and `'*'`.
* All the integer values in the input expression are in the range `[0, 99]`. | null |
【Video】Give me 5 minutes - 4 solutions - How we think about a solution | valid-anagram | 1 | 1 | # Intuition\nCount all characters in each string\n\n---\n\n# Solution Video\n\nhttps://youtu.be/UR9geRGBvhk\n\n\u25A0 Timeline of the video\n\n`0:03` Explain Solution 1\n`2:52` Coding of Solution 1\n`4:38` Time Complexity and Space Complexity of Solution 1\n`5:04` Step by step algorithm of my solution code of Solution 1\n`5:11` Explain Solution 2\n`8:20` Coding of Solution 2\n`10:10` Time Complexity and Space Complexity of Solution 2\n`10:21` Step by step algorithm of my solution code of Solution 2\n`10:38` Coding of Solution 3\n`12:18` Time Complexity and Space Complexity of Solution 3\n`13:15` Coding of Solution 4\n`13:37` Time Complexity and Space Complexity of Solution 4\n`13:52` Step by step algorithm of my solution code of Solution 3\n\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 3,488\nMy first goal is 10,000 (It\'s far from done \uD83D\uDE05)\nThank you for your support!\n\n---\n\n# Approach\n\n## How we think about a solution\n\nMy first solution is\n```\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool: \n return Counter(s) == Counter(t)\n```\nBut it is algorithm platform, so let\'s delve a bit deeper into the algorithm for anagrams. \n\n\n---\n\n# Solution 1\n\nFirst of all, simply the length of two strings is different, we can\'t create anagram.\n\n```\nlen(s) != len(t) \u2192 False\n```\nIf not that case, there is possiblity that we can create anagram.\n\nNext step is simply to count frequency of each character.\n\n```\nInput: s = "anagram", t = "nagaram"\n```\n\nWe use `HashMap` because we can compare frequency of character easily with `key character`.\n\n```\ns = "anagram"\n\u2193\ncounter = {"a": 3, "g": 1, "m": 1, "n": 1, "r": 1}\n```\n\nNext, we iterate through all characters in t string. If we find current character in `HashMap`, reduce `1` from the same characters.\n\n---\n\n\u2B50\uFE0F Points\n\n**In other words, if we don\'t find the same character or frequecy of character in `HashMap` is ready `0`, there is not current character in s string.**\n\nIn that case, we should return `False`, because they are not anagram.\n\n---\n\n\nLet\'s see one by one.\n\n```\nt = "nagaram"\ncounter = {"a": 3, "g": 1, "m": 1, "n": 1, "r": 1}\n\n```\n```\nt = "nagaram"\n \u2191\n\nreduce 1 from n\ncounter = {"a": 3, "g": 1, "m": 1, "n": 0, "r": 1}\n```\n```\nt = "nagaram"\n \u2191\n\nreduce 1 from a\ncounter = {"a": 2, "g": 1, "m": 1, "n": 0, "r": 1}\n```\n```\nt = "nagaram"\n \u2191\n\nreduce 1 from g\ncounter = {"a": 2, "g": 0, "m": 1, "n": 0, "r": 1}\n```\n```\nt = "nagaram"\n \u2191\n\nreduce 1 from a\ncounter = {"a": 1, "g": 0, "m": 1, "n": 0, "r": 1}\n```\n```\nt = "nagaram"\n \u2191\n\nreduce 1 from r\ncounter = {"a": 1, "g": 0, "m": 1, "n": 0, "r": 0}\n```\n```\nt = "nagaram"\n \u2191\n\nreduce 1 from a\ncounter = {"a": 0, "g": 0, "m": 1, "n": 0, "r": 0}\n```\n```\nt = "nagaram"\n \u2191\n\nreduce 1 from m\ncounter = {"a": 0, "g": 0, "m": 0, "n": 0, "r": 0}\n```\nFinish. We successfully iterate through all characters in t string. That means two input strings are anagram.\n\nEasy!\uD83D\uDE04\nLet\'s see a real algorithm!\n\n\n---\n\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$ O(26)$$ \u2192 $$O(1)$$\nIf two input strings are consisted of lowercase alpahbet characters.\n\n```python []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool: \n if len(s) != len(t):\n return False\n\n counter = {}\n\n for char in s:\n counter[char] = counter.get(char, 0) + 1\n\n for char in t:\n if char not in counter or counter[char] == 0:\n return False\n counter[char] -= 1\n\n return True\n```\n```javascript []\nvar isAnagram = function(s, t) {\n if (s.length !== t.length) {\n return false;\n }\n\n const counter = new Map();\n\n for (let char of s) {\n counter.set(char, (counter.get(char) || 0) + 1);\n }\n\n for (let char of t) {\n if (!counter.has(char) || counter.get(char) === 0) {\n return false;\n }\n counter.set(char, counter.get(char) - 1);\n }\n\n return true; \n};\n```\n```java []\nclass Solution {\n public boolean isAnagram(String s, String t) {\n if (s.length() != t.length()) {\n return false;\n }\n\n Map<Character, Integer> counter = new HashMap<>();\n\n for (int i = 0; i < s.length(); i++) {\n char ch = s.charAt(i);\n counter.put(ch, counter.getOrDefault(ch, 0) + 1);\n }\n\n for (int i = 0; i < t.length(); i++) {\n char ch = t.charAt(i);\n if (!counter.containsKey(ch) || counter.get(ch) == 0) {\n return false;\n }\n counter.put(ch, counter.get(ch) - 1);\n }\n\n return true; \n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n if (s.length() != t.length()) {\n return false;\n }\n\n unordered_map<char, int> counter;\n\n for (char ch : s) {\n counter[ch] = counter[ch] + 1;\n }\n\n for (char ch : t) {\n if (counter.find(ch) == counter.end() || counter[ch] == 0) {\n return false;\n }\n counter[ch] = counter[ch] - 1;\n }\n\n return true; \n }\n};\n```\n\n## Step by step algorithm\n\n1. **Check Lengths:**\n - If the lengths of strings `s` and `t` are not equal, return `False`.\n ```python\n if len(s) != len(t):\n return False\n ```\n\n2. **Count Characters in `s`:**\n - Create a dictionary `counter` to store the count of each character in string `s`.\n - Loop through each character `char` in string `s`.\n - Use `counter.get(char, 0)` to retrieve the current count of `char` in the dictionary. If `char` is not in the dictionary, default to 0.\n - Increment the count of `char` in the dictionary by 1.\n ```python\n counter = {}\n for char in s:\n counter[char] = counter.get(char, 0) + 1\n ```\n\n3. **Check Characters in `t`:**\n - Loop through each character `char` in string `t`.\n - Check if `char` is not in the `counter` dictionary or if its count is already 0. If either condition is met, return `False`.\n - Decrement the count of `char` in the dictionary by 1.\n ```python\n for char in t:\n if char not in counter or counter[char] == 0:\n return False\n counter[char] -= 1\n ```\n\n4. **Final Result:**\n - If the loops complete without returning `False`, it means all characters in `s` and `t` match in count, and the function returns `True`.\n ```python\n return True\n ```\n\nThis algorithm uses a HashMap (`counter`) to keep track of character counts in `s`. It then checks if the characters in `t` match the counts in the HashMap, effectively determining whether the two strings are anagrams.\n\n\n---\n\n# Solution 2\n\nMy second solution involves using ASCII values.\n\n```\n"a": 97\n"b": 98\n"c": 99\n.\n.\n.\n"y": 121 \n"z": 122 \n\n"z" - "a"\n= 122 - 97\n= 25\n```\nSo, seems like we can use the subtraction for index number from `0` to `25` (= `26` characters).\n\nApproach is very simple. First of all create array with length of `26`, then iterate through s string. Every time we caulcuate subtraction with ASCII values.\n```\nASCII value of current character - ASCII value of "a".\n```\n- Why "a"?\n\nThat\'s because "a" is the smallest ASCII value and the ASCII values of all lowercase letters are sequential. If we subtract ASCII value of "a" from ASCII value of current character, you should be able to retrieve the order of those alphabets.\n\nIn the end,\n\n```\nInput: s = "anagram", t = "nagaram"\n```\n\n```\nInput: s = "anagram"\n\n a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z\ncount = [3,0,0,0,0,0,1,0,0,0,0,0,1,1,0,0,0,1,0,0,0,0,0,0,0,0]\n\na = 97 - 97 = index 0\ng = 103 - 97 = index 6\nm = 109 - 97 = index 12\nn = 110 - 97 = index 13\nr = 114 - 97 = index 17\n\n```\n\nNext step is actually the same idea of solution 1. We iterate throught t string then\n\n---\n\n\u2B50\uFE0F Points\n\nIf target index is arealdy `0`, we should return False. If not that case, subtract 1 from the target index.\n\n---\n\nLet\'s see one by one. Let me skip calculate of ASCII value. lol\n\n```\nt = "nagaram"\n\n a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z\ncount = [3,0,0,0,0,0,1,0,0,0,0,0,1,1,0,0,0,1,0,0,0,0,0,0,0,0]\n```\n```\nt = "nagaram"\n \u2191\n\nsubtract 1 from n\n\n a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z\ncount = [3,0,0,0,0,0,1,0,0,0,0,0,1,0,0,0,0,1,0,0,0,0,0,0,0,0]\n```\n```\nt = "nagaram"\n \u2191\n\nsubtract 1 from a\n\n a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z\ncount = [2,0,0,0,0,0,1,0,0,0,0,0,1,0,0,0,0,1,0,0,0,0,0,0,0,0]\n```\n```\nt = "nagaram"\n \u2191\n\nsubtract 1 from g\n\n a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z\ncount = [2,0,0,0,0,0,0,0,0,0,0,0,1,0,0,0,0,1,0,0,0,0,0,0,0,0]\n```\n```\nt = "nagaram"\n \u2191\n\nsubtract 1 from a\n\n a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z\ncount = [1,0,0,0,0,0,0,0,0,0,0,0,1,0,0,0,0,1,0,0,0,0,0,0,0,0]\n```\n```\nt = "nagaram"\n \u2191\n\nsubtract 1 from r\n\n a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z\ncount = [1,0,0,0,0,0,0,0,0,0,0,0,1,0,0,0,0,0,0,0,0,0,0,0,0,0]\n```\n```\nt = "nagaram"\n \u2191\n\nsubtract 1 from a\n\n a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z\ncount = [0,0,0,0,0,0,0,0,0,0,0,0,1,0,0,0,0,0,0,0,0,0,0,0,0,0]\n```\n```\nt = "nagaram"\n \u2191\n\nsubtract 1 from m\n\n a,b,c,d,e,f,g,h,i,j,k,l,m,n,o,p,q,r,s,t,u,v,w,x,y,z\ncount = [0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0]\n```\n```\nOuput: True\n```\n\nEasy!\uD83D\uDE04\nLet\'s see a real algorithm!\n\n---\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$ O(26)$$ \u2192 $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n\n\n```python []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool: \n if len(s) != len(t):\n return False\n\n count = [0] * 26\n\n for char in s:\n count[ord(char) - ord(\'a\')] += 1\n\n for char in t:\n if count[ord(char) - ord(\'a\')] == 0:\n return False\n count[ord(char) - ord(\'a\')] -= 1\n\n return True\n```\n```javascript []\nvar isAnagram = function(s, t) {\n if (s.length !== t.length) {\n return false;\n }\n\n const count = Array(26).fill(0);\n\n for (let char of s) {\n count[char.charCodeAt(0) - \'a\'.charCodeAt(0)] += 1;\n }\n\n for (let char of t) {\n if (count[char.charCodeAt(0) - \'a\'.charCodeAt(0)] === 0) {\n return false;\n }\n count[char.charCodeAt(0) - \'a\'.charCodeAt(0)] -= 1;\n }\n\n return true; \n};\n```\n```java []\nclass Solution {\n public boolean isAnagram(String s, String t) {\n if (s.length() != t.length()) {\n return false;\n }\n\n int[] count = new int[26];\n\n for (int i = 0; i < s.length(); i++) {\n count[s.charAt(i) - \'a\'] += 1;\n }\n\n for (int i = 0; i < t.length(); i++) {\n if (count[t.charAt(i) - \'a\'] == 0) {\n return false;\n }\n count[t.charAt(i) - \'a\'] -= 1;\n }\n\n return true; \n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n if (s.length() != t.length()) {\n return false;\n }\n\n vector<int> count(26, 0);\n\n for (char c : s) {\n count[c - \'a\'] += 1;\n }\n\n for (char c : t) {\n if (count[c - \'a\'] == 0) {\n return false;\n }\n count[c - \'a\'] -= 1;\n }\n\n return true; \n }\n};\n```\n\n## step by step algorithm\n\n1. **Check Lengths:** Compare the lengths of strings `s` and `t`. If they are not equal, return `False` because anagrams must have the same length.\n \n ```python\n if len(s) != len(t):\n return False\n ```\n\n2. **Initialize Count Array:** Create an array `count` of size 26 (assuming the input strings are composed of lowercase English letters). Initialize all elements of `count` to 0.\n\n ```python\n count = [0] * 26\n ```\n\n3. **Count Occurrences in `s`:** Iterate through each character `char` in string `s`. Increment the count of the corresponding letter in the `count` array.\n\n ```python\n for char in s:\n count[ord(char) - ord(\'a\')] += 1\n ```\n\n - `ord(char)` returns the ASCII value of the character.\n - `ord(char) - ord(\'a\')` maps \'a\' to 0, \'b\' to 1, and so on.\n\n4. **Check Occurrences in `t`:** Iterate through each character `char` in string `t`. If the count of the corresponding letter in the `count` array is already 0, return `False` because it means `t` has more occurrences of that letter than `s`.\n\n ```python\n for char in t:\n if count[ord(char) - ord(\'a\')] == 0:\n return False\n count[ord(char) - ord(\'a\')] -= 1\n ```\n\n - Decrement the count of the corresponding letter in `count` because it has been matched with a letter in `t`.\n\n5. **Check Remaining Counts:** After both loops, check if all counts in the `count` array are zero. If so, return `True` because every letter in `s` has been matched with a letter in `t`.\n\n ```python\n return True\n ```\n\nThis algorithm efficiently determines whether two strings are anagrams by comparing the counts of each letter.\n\n\n---\n\nBe careful\n\nThere is follow up question. It says "What if the inputs contain Unicode characters? How would you adapt your solution to such a case?"\n\nIn that case, use HashMap instead of fixed size of array, because we will have more than 26 charcters. HashMap can adapt to any range of characters.\n\n---\n\n\n\n---\n\n# Solution 3\n\nI think you arealdy enough knowledge to solve this question. We can also do like this.\n\nWe know that after checking length of the two strings at first step, they have the same length, so we can count each character in both strings at the same time, I mean single loop.\n\nAt last, we just compare two HashMap.\n\nIf you are JavaScript guy, it would be wiser not to use this algorithm because \n\n```\nreturn JSON.stringify({a:1,b:2,c:3}) === JSON.stringify({b:2,c:3,a:1});\n\u2192 False\n```\nWe need extra effort. If you have good idea, please leave your comment below.\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$ O(26) + O(26)$$ \u2192 $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n```python []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool: \n if len(s) != len(t):\n return False\n \n s_count = {}\n t_count = {}\n \n for i in range(len(s)):\n s_count[s[i]] = 1 + s_count.get(s[i], 0)\n t_count[t[i]] = 1 + t_count.get(t[i], 0)\n \n return s_count == t_count\n```\n```Java []\nclass Solution {\n public boolean isAnagram(String s, String t) {\n if (s.length() != t.length()) {\n return false;\n }\n\n HashMap<Character, Integer> sCount = new HashMap<>();\n HashMap<Character, Integer> tCount = new HashMap<>();\n\n for (int i = 0; i < s.length(); i++) {\n sCount.put(s.charAt(i), 1 + sCount.getOrDefault(s.charAt(i), 0));\n tCount.put(t.charAt(i), 1 + tCount.getOrDefault(t.charAt(i), 0));\n }\n\n return sCount.equals(tCount); \n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n if (s.length() != t.length()) {\n return false;\n }\n\n unordered_map<char, int> sCount, tCount;\n\n for (int i = 0; i < s.length(); i++) {\n sCount[s[i]] = 1 + sCount[s[i]];\n tCount[t[i]] = 1 + tCount[t[i]];\n }\n\n return sCount == tCount; \n }\n};\n```\n\nI wrote JavaScript version.\n```javascript []\nvar isAnagram = function(s, t) {\n if (s.length !== t.length) {\n return false;\n }\n\n const sCount = {};\n const tCount = {};\n\n for (let i = 0; i < s.length; i++) {\n sCount[s[i]] = 1 + (sCount[s[i]] || 0);\n tCount[t[i]] = 1 + (tCount[t[i]] || 0);\n }\n\n const sortedSCount = Object.entries(sCount).sort();\n const sortedTCount = Object.entries(tCount).sort();\n\n return JSON.stringify(sortedSCount) === JSON.stringify(sortedTCount);\n};\n```\n\n## Step by step algorithm\n\n1. **Check Lengths:**\n - Compare the lengths of strings `s` and `t`. If they are not equal, return `False` because an anagram must have the same number of characters.\n\n```python\nif len(s) != len(t):\n return False\n```\n\n2. **Count Character Occurrences:**\n - Initialize empty dictionaries `s_count` and `t_count` to store the count of each character in strings `s` and `t`, respectively.\n - Loop through each character in the strings:\n - If the character is already in the dictionary, increment its count by 1.\n - If the character is not in the dictionary, set its count to 1.\n\n```python\ns_count = {}\nt_count = {}\n\nfor i in range(len(s)):\n s_count[s[i]] = 1 + s_count.get(s[i], 0)\n t_count[t[i]] = 1 + t_count.get(t[i], 0)\n```\n\n3. **Compare Counts:**\n - Check if the count dictionaries for both strings (`s_count` and `t_count`) are equal. If they are, it means both strings have the same character counts, and thus, they are anagrams.\n\n```python\nreturn s_count == t_count\n```\n\nIn summary, this algorithm compares the character counts in two strings and returns `True` if they are anagrams (have the same character counts), and `False` otherwise.\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n\u25A0 Subscribe URL\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\n\u25A0 Twitter\nhttps://twitter.com/CodingNinjaAZ\n\n### My next daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/design-a-food-rating-system/solutions/4414691/give-me-10-minutes-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/F6RAuglUOSg\n\n\u25A0 Timeline of the video\n\n`0:04` Explain constructor\n`1:21` Why -rating instead of rating?\n`4:09` Talk about changeRating\n`5:19` Time Complexity and Space Complexity\n`6:36` Step by step algorithm of my solution code\n`6:39` Python Tips - SortedList\n\n### My previous daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/destination-city/solutions/4406091/video-give-me-3-minutes-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/tjILACCjQI0\n\n\u25A0 Timeline of the video\n\n`0:04` Explain how we can solve this question\n`1:29` Coding\n`2:40` Time Complexity and Space Complexity\n`3:13` Step by step algorithm of my solution code\n | 42 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
💯Faster✅💯 Lesser✅4 Methods🔥Sorting🔥Hash Table🔥Character Array🔥Counter🔥Python🐍Java☕C++✅C📈 | valid-anagram | 1 | 1 | # \uD83D\uDE80 Hi, I\'m [Mohammed Raziullah Ansari](https://leetcode.com/Mohammed_Raziullah_Ansari/), and I\'m excited to share 4 ways to solve this question with detailed explanation of each approach:\n\n# Problem Explaination: \nThe `Valid Anagram` problem typically asks us to determine whether two given strings are anagrams of each other. An anagram is a word or phrase formed by rearranging the letters of another. In this context, an anagram of a string is another string with the same characters, but possibly in a different order.\n\n# \uD83D\uDD0D Methods To Solve This Problem:\nI\'ll be covering four different methods to solve this problem:\n1. Sorting\n2. Hash Table\n3. Character Array\n4. Counter\n\n# 1. Sorting: \n- Sort both strings.\n- Compare the sorted strings to check if they are equal.\n# Complexity\n* \u23F1\uFE0F Time Complexity: `O(n log n) `- Due to the sorting operation.\n\n* \uD83D\uDE80 Space Complexity: `O(1)` - No extra space used other than the input strings.\n\n# Code\n```Python []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n return sorted(s) == sorted(t)\n```\n```Java []\nclass Solution {\n public boolean isAnagram(String s, String t) {\n char[] charArrayS = s.toCharArray();\n char[] charArrayT = t.toCharArray();\n Arrays.sort(charArrayS);\n Arrays.sort(charArrayT);\n return Arrays.equals(charArrayS, charArrayT);\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n sort(s.begin(), s.end());\n sort(t.begin(), t.end());\n return s == t;\n }\n};\n```\n# 2. Hash Table:\n- Create a hash table (dictionary) to store the frequency of characters in the first string.\n- Iterate through the second string and decrement the corresponding frequency in the hash table.\n- If the hash table becomes empty, the strings are anagrams.\n# Complexity\n* \u23F1\uFE0F Time Complexity: `O(n)` - Iterate through both strings.\n\n* \uD83D\uDE80 Space Complexity: `O(n)` - Space required for the hash table.\n\n# Code\n```Python []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n if len(s) != len(t):\n return False\n char_count = {}\n for char in s:\n char_count[char] = char_count.get(char, 0) + 1\n for char in t:\n if char_count.get(char, 0) == 0:\n return False\n char_count[char] -= 1\n return True\n```\n```Java []\nclass Solution {\n public boolean isAnagram(String s, String t) {\n if (s.length() != t.length()) {\n return false;\n }\n Map<Character, Integer> charCount = new HashMap<>();\n for (char c : s.toCharArray()) {\n charCount.put(c, charCount.getOrDefault(c, 0) + 1);\n for (char c : t.toCharArray()) {\n if (charCount.getOrDefault(c, 0) == 0) {\n return false;\n }\n charCount.put(c, charCount.get(c) - 1);\n }\n return true;\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n if (s.length() != t.length()) {\n return false;\n }\n unordered_map<char, int> charCount;\n for (char c : s) {\n charCount[c]++;\n }\n for (char c : t) {\n if (charCount[c] == 0) {\n return false;\n }\n charCount[c]--;\n }\n return true;\n }\n};\n```\n```C []\nbool isAnagram(char* s, char* t) {\n if (strlen(s) != strlen(t)) {\n return false;\n }\n int len = strlen(s);\n int* charCountS = (int*)calloc(26, sizeof(int));\n int* charCountT = (int*)calloc(26, sizeof(int));\n for (int i = 0; i < len; i++) {\n charCountS[s[i] - \'a\']++;\n }\n for (int i = 0; i < len; i++) {\n charCountT[t[i] - \'a\']++;\n }\n bool result = true;\n for (int i = 0; i < 26; i++) {\n if (charCountS[i] != charCountT[i]) {\n result = false;\n break;\n }\n }\n free(charCountS);\n free(charCountT);\n return result;\n}\n```\n# 3. Character Array:\n- Create two arrays of size 26 to represent the count of each character in the alphabet for both strings.\n- Iterate through both strings, updating the count in the arrays.\n- Compare the arrays to check if they are equal.\n# Complexity\n* \u23F1\uFE0F Time Complexity: `O(n)` - Iterate through both strings.\n\n* \uD83D\uDE80 Space Complexity: `O(1)` - Fixed-size character arrays.\n\n# Code\n```Python []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n if len(s) != len(t):\n return False\n char_count_s = [0] * 26\n char_count_t = [0] * 26\n for char in s:\n char_count_s[ord(char) - ord(\'a\')] += 1\n for char in t:\n char_count_t[ord(char) - ord(\'a\')] += 1\n return char_count_s == char_count_t\n```\n```Java []\nclass Solution {\n public boolean isAnagram(String s, String t) {\n if (s.length() != t.length()) {\n return false;\n }\n int[] charCountS = new int[26];\n int[] charCountT = new int[26];\n for (char c : s.toCharArray()) {\n charCountS[c - \'a\']++;\n }\n for (char c : t.toCharArray()) {\n charCountT[c - \'a\']++;\n }\n return Arrays.equals(charCountS, charCountT);\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n if (s.length() != t.length()) {\n return false;\n }\n int charCountS[26] = {0};\n int charCountT[26] = {0};\n for (char c : s) {\n charCountS[c - \'a\']++;\n }\n for (char c : t) {\n charCountT[c - \'a\']++;\n }\n return memcmp(charCountS, charCountT, sizeof(charCountS)) == 0;\n }\n};\n```\n```C []\nbool isAnagram(char* s, char* t) {\n if (strlen(s) != strlen(t)) {\n return false;\n }\n int len = strlen(s);\n int charCountS[26] = {0};\n int charCountT[26] = {0};\n for (int i = 0; i < len; i++) {\n charCountS[s[i] - \'a\']++;\n }\n for (int i = 0; i < len; i++) {\n charCountT[t[i] - \'a\']++;\n }\n return memcmp(charCountS, charCountT, sizeof(charCountS)) == 0;\n}\n```\n# 4. Counter:\n- Use the Counter class from the collections module to get character counts for both strings.\n- Compare the Counters to check if they are equal.\n# Complexity\n* \u23F1\uFE0F Time Complexity: `O(n)` - The Counter class internally iterates through the strings. \n\n* \uD83D\uDE80 Space Complexity: `O(n)` - Space required for the Counters.\n\n# Code\n```Python []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n return Counter(s) == Counter(t)\n```\n```Java []\nclass Solution {\n public boolean isAnagram(String s, String t) {\n Map<Character, Integer> counterS = new HashMap<>();\n Map<Character, Integer> counterT = new HashMap<>();\n for (char c : s.toCharArray()) {\n counterS.put(c, counterS.getOrDefault(c, 0) + 1);\n }\n for (char c : t.toCharArray()) {\n counterT.put(c, counterT.getOrDefault(c, 0) + 1);\n }\n return counterS.equals(counterT);\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n unordered_map<char, int> counterS;\n unordered_map<char, int> counterT;\n for (char c : s) {\n counterS[c]++;\n }\n for (char c : t) {\n counterT[c]++;\n }\n return counterS == counterT;\n }\n};\n```\n```C []\ntypedef struct {\n char key;\n int value;\n} KeyValuePair;\n\nbool isAnagram(char* s, char* t) {\n int len_s = strlen(s);\n int len_t = strlen(t);\n if (len_s != len_t) {\n return false;\n }\n KeyValuePair* counterS = (KeyValuePair*)malloc(26 * sizeof(KeyValuePair));\n KeyValuePair* counterT = (KeyValuePair*)malloc(26 * sizeof(KeyValuePair));\n\n for (int i = 0; i < 26; i++) {\n counterS[i].key = i + \'a\';\n counterS[i].value = 0;\n counterT[i].key = i + \'a\';\n counterT[i].value = 0;\n }\n for (int i = 0; i < len_s; i++) {\n counterS[s[i] - \'a\'].value++;\n }\n for (int i = 0; i < len_t; i++) {\n counterT[t[i] - \'a\'].value++;\n }\n bool result = memcmp(counterS, counterT, 26 * sizeof(KeyValuePair)) == 0;\n free(counterS);\n free(counterT);\n return result;\n}\n```\n# \uD83C\uDFC6Conclusion: \nHash table approach is a good balance between time and space complexity. It has a time complexity of `O(n)` and a space complexity of `O(n)`, where n is the length of the input string.\n\n# \uD83D\uDCA1 I invite you to check out my profile for detailed explanations and code for each method. Happy coding and learning! \uD83D\uDCDA | 35 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
Frequency Count vs 1-line||0ms Beats 100% | valid-anagram | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFrequency Count. No hash\n# Approach\n<!-- Describe your approach to solving the problem. -->\ns is anagram of t $\\iff$ `freq(s)==freq(t)`\nIn other words, s is a permutation of t.\n \nC/C++/Python codes are implemented. The revised C code uses 1 loop by the suggestion of @Sergei which is fast, runs in 0ms & beats 100%.\n\n[Please turn on English subtitles if necessary]\n[https://youtu.be/DDSI3xzWCto?si=DmIRBD8beeKv5a98](https://youtu.be/DDSI3xzWCto?si=DmIRBD8beeKv5a98)\n\nPython 1 line code uses `sorted` with TC O(n log n)\nC++ 1 line code uses `multiset<char>` with TC O(n log n)\nThanks to @wawaroutas mentioning `std::ranges::is_permutation`, a similar C++ 1-line code is provided.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(26)$$\n# Both of C &C++ Codes run 0ms Beat 100%\n```C++ []\n#pragma GCC optimize("O3", "unroll-loops")\nclass Solution {\npublic:\n vector<int> freq(string& s){\n vector<int> ans(26, 0);\n for(char c: s){\n ans[c-\'a\']++;\n }\n return ans;\n }\n bool isAnagram(string s, string t) {\n return freq(s)==freq(t);\n }\n};\n```\n```python []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n def freq(s):\n ans=[0]*26\n for c in s:\n ans[ord(c)-ord(\'a\')]+=1\n return ans\n\n return freq(s)==freq(t)\n \n```\n```C []\n#pragma GCC optimize("O3", "unroll-loops")\nbool isAnagram(char* s, char* t) {\n int f[26]={0};\n register int i;\n for(i=0; s[i]!=\'\\0\' && t[i]!=\'\\0\'; i++){\n f[s[i]-\'a\']++; \n f[t[i]-\'a\']--;\n }\n if (s[i]!=\'\\0\' || t[i]!=\'\\0\') \n return 0;\n for(register i=0; i<26; i++)\n if (f[i]!=0) return 0;\n return 1;\n}\n```\n # Python 1 line using sorted TC O(n log n)\n```\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n return sorted(s)==sorted(t)\n \n```\n# C++ 1 line using multiset<char>\n```\nclass Solution {\npublic:\n bool isAnagram(string& s, string& t) {\n return multiset<char>(s.begin(), s.end())==multiset<char>(t.begin(), t.end());\n }\n};\n```\n# C++ 1 line using is_permutation\n```\nclass Solution {\npublic:\n bool isAnagram(string& s, string& t) {\n return is_permutation(s.begin(), s.end(), t.begin(), t.end());\n }\n};\n```\n\n | 12 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
✅3 Method's 🤯 || C++ || JAVA || PYTHON || Beginner Friendly🔥🔥🔥 | valid-anagram | 1 | 1 | \n# Intuition:\n\nThe Intuition is to determine if two strings are anagrams, compare the characters in both strings and check if they have the same characters but in a different order. By tracking the count of each character, if the counts match for all characters, the strings are anagrams; otherwise, they are not.\n\n# Approach 1: Sorting\n# Explanation:\n\n1. `sort(s.begin(), s.end());` sorts the characters in string `s` in ascending order. This rearranges the characters in `s` so that they are in alphabetical order.\n2. `sort(t.begin(), t.end());` sorts the characters in string `t` in ascending order. Similarly, this rearranges the characters in `t` to be in alphabetical order.\n3. `return s == t;` compares the sorted strings `s` and `t` using the equality operator (`==`). If the sorted strings are equal, it means that the original strings `s` and `t` have the same characters in the same order, indicating that they are anagrams. In this case, the function returns `true`. Otherwise, if the sorted strings are not equal, the function returns `false`, indicating that the strings are not anagrams.\n\nThis code takes advantage of the fact that anagrams have the same characters, but in different orders. By sorting the characters, the code transforms the problem into a comparison of the sorted strings, simplifying the anagram check.\n\nHowever, it\'s worth noting that this approach has a time complexity of O(n log n) due to the sorting operation, where n is the length of the strings.\n\n# Code\n```C++ []\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n sort(s.begin(), s.end());\n sort(t.begin(), t.end());\n return s == t;\n }\n};\n```\n```Java []\nimport java.util.Arrays;\n\nclass Solution {\n public boolean isAnagram(String s, String t) {\n char[] sChars = s.toCharArray();\n char[] tChars = t.toCharArray();\n \n Arrays.sort(sChars);\n Arrays.sort(tChars);\n \n return Arrays.equals(sChars, tChars);\n }\n}\n```\n```Python3 []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n sorted_s = sorted(s)\n sorted_t = sorted(t)\n return sorted_s == sorted_t\n```\n\n# Approach 2: Hash Table\n# Explanation:\n1. Create an unordered map `count` to store the character frequencies. The key of the map represents a character, and the value represents its frequency.\n2. Iterate over each character `x` in string `s`. For each character, increment its frequency in the `count` map by using the `count[x]++` expression.\n3. Iterate over each character `x` in string `t`. For each character, decrement its frequency in the `count` map by using the `count[x]--` expression.\n4. Iterate over each pair `x` in the `count` map. Each pair consists of a character and its corresponding frequency. Check if any frequency (`x.second`) is non-zero. If any frequency is non-zero, it means there is a character that appears more times in one string than the other, indicating that the strings are not anagrams. In that case, return `false`.\n5. If all frequencies in the `count` map are zero, it means the strings `s` and `t` have the same characters in the same frequencies, making them anagrams. In this case, the function returns `true`.\n\nThis approach counts the frequency of characters in one string and then adjusts the count by decrementing for the other string. If the strings are anagrams, the frequency of each character will cancel out, resulting in a map with all zero frequencies.\n\nThe time complexity of this solution is O(n), where n is the total number of characters in both strings. It iterates over each character once to count the frequencies and then compares the frequencies in the map, making it an efficient solution for the problem.\n\n# Code\n```C++ []\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n unordered_map<char, int> count;\n \n // Count the frequency of characters in string s\n for (auto x : s) {\n count[x]++;\n }\n \n // Decrement the frequency of characters in string t\n for (auto x : t) {\n count[x]--;\n }\n \n // Check if any character has non-zero frequency\n for (auto x : count) {\n if (x.second != 0) {\n return false;\n }\n }\n \n return true;\n }\n};\n```\n```Java []\nclass Solution {\n public boolean isAnagram(String s, String t) {\n Map<Character, Integer> count = new HashMap<>();\n \n // Count the frequency of characters in string s\n for (char x : s.toCharArray()) {\n count.put(x, count.getOrDefault(x, 0) + 1);\n }\n \n // Decrement the frequency of characters in string t\n for (char x : t.toCharArray()) {\n count.put(x, count.getOrDefault(x, 0) - 1);\n }\n \n // Check if any character has non-zero frequency\n for (int val : count.values()) {\n if (val != 0) {\n return false;\n }\n }\n \n return true;\n }\n}\n```\n```Python3 []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n count = defaultdict(int)\n \n # Count the frequency of characters in string s\n for x in s:\n count[x] += 1\n \n # Decrement the frequency of characters in string t\n for x in t:\n count[x] -= 1\n \n # Check if any character has non-zero frequency\n for val in count.values():\n if val != 0:\n return False\n \n return True\n```\n\n# Approach 3: Hash Table (Using Array)\n# Code\n```C++ []\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n int count[26] = {0};\n \n // Count the frequency of characters in string s\n for (char x : s) {\n count[x - \'a\']++;\n }\n \n // Decrement the frequency of characters in string t\n for (char x : t) {\n count[x - \'a\']--;\n }\n \n // Check if any character has non-zero frequency\n for (int val : count) {\n if (val != 0) {\n return false;\n }\n }\n \n return true;\n }\n};\n```\n```Java []\nclass Solution {\n public boolean isAnagram(String s, String t) {\n int[] count = new int[26];\n \n // Count the frequency of characters in string s\n for (char x : s.toCharArray()) {\n count[x - \'a\']++;\n }\n \n // Decrement the frequency of characters in string t\n for (char x : t.toCharArray()) {\n count[x - \'a\']--;\n }\n \n // Check if any character has non-zero frequency\n for (int val : count) {\n if (val != 0) {\n return false;\n }\n }\n \n return true;\n }\n}\n```\n```Python3 []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n count = [0] * 26\n \n # Count the frequency of characters in string s\n for x in s:\n count[ord(x) - ord(\'a\')] += 1\n \n # Decrement the frequency of characters in string t\n for x in t:\n count[ord(x) - ord(\'a\')] -= 1\n \n # Check if any character has non-zero frequency\n for val in count:\n if val != 0:\n return False\n \n return True\n```\n\n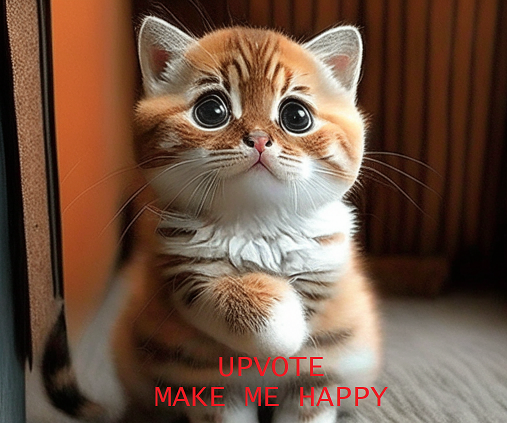\n\n**If you are a beginner solve these problems which makes concepts clear for future coding:**\n1. [Two Sum](https://leetcode.com/problems/two-sum/solutions/3619262/3-method-s-c-java-python-beginner-friendly/)\n2. [Roman to Integer](https://leetcode.com/problems/roman-to-integer/solutions/3651672/best-method-c-java-python-beginner-friendly/)\n3. [Palindrome Number](https://leetcode.com/problems/palindrome-number/solutions/3651712/2-method-s-c-java-python-beginner-friendly/)\n4. [Maximum Subarray](https://leetcode.com/problems/maximum-subarray/solutions/3666304/beats-100-c-java-python-beginner-friendly/)\n5. [Remove Element](https://leetcode.com/problems/remove-element/solutions/3670940/best-100-c-java-python-beginner-friendly/)\n6. [Contains Duplicate](https://leetcode.com/problems/contains-duplicate/solutions/3672475/4-method-s-c-java-python-beginner-friendly/)\n7. [Add Two Numbers](https://leetcode.com/problems/add-two-numbers/solutions/3675747/beats-100-c-java-python-beginner-friendly/)\n8. [Majority Element](https://leetcode.com/problems/majority-element/solutions/3676530/3-methods-beats-100-c-java-python-beginner-friendly/)\n9. [Remove Duplicates from Sorted Array](https://leetcode.com/problems/remove-duplicates-from-sorted-array/solutions/3676877/best-method-100-c-java-python-beginner-friendly/)\n10. [Valid Anagram](https://leetcode.com/problems/valid-anagram/solutions/3687854/3-methods-c-java-python-beginner-friendly/)\n11. [Group Anagrams](https://leetcode.com/problems/group-anagrams/solutions/3687735/beats-100-c-java-python-beginner-friendly/)\n12. **Practice them in a row for better understanding and please Upvote for more questions.**\n\n\n**If you found my solution helpful, I would greatly appreciate your upvote, as it would motivate me to continue sharing more solutions.** | 906 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
3 Different Approaches | 700ms to 0ms 🔥| Beats 100% in both Time and Space 😎 | valid-anagram | 1 | 1 | # Intuition\nThe given problem is to determine whether two strings, `s` and `t`, are anagrams of each other or not. An anagram is a word or phrase formed by rearranging the letters of another word or phrase. The code uses an array to keep track of the frequency of each letter in both strings and then checks if the frequency arrays are equal.\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. Initialize two arrays, `freqS` and `freqT`, of size 26 to represent the frequency of each lowercase English letter. Initialize all elements of both arrays to 0.\n2. Iterate through each character in string `s` and update the frequency array `freqS` accordingly.\n3. Iterate through each character in string `t` and update the frequency array `freqT` accordingly.\n4. Compare the frequency arrays `freqS` and `freqT` for each letter. If they are not equal, return false, indicating that the strings are not anagrams.\n5. If the loop completes without returning false, return true, indicating that the strings are anagrams.\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(N)\n- Let n be the length of the input strings `s` and `t`. The code has three loops:\n- The first two loops iterate through each character in strings `s` and `t` once, each taking O(n) time.\n- The third loop iterates through the frequency arrays of size 26, which takes constant time.\nTherefore, the overall time complexity is O(n).\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n- The code uses two arrays of size 26 to store the frequency of each letter. Therefore, the space complexity is O(1) since the size of the arrays is constant regardless of the input size.\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code - 0ms\n```\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n int freqS[26] = {0};\n int freqT[26] = {0};\n\n for(auto x : s) freqS[x - \'a\']++;\n for(auto x : t) freqT[x - \'a\']++;\n\n for(int i = 0; i < 26; i++)\n {\n if(freqS[i] != freqT[i])\n return false;\n }\n \n return true;\n }\n};\n```\n# Code - 12 ms\n```\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n sort(s.begin(),s.end());\n sort(t.begin(),t.end());\n return (s == t);\n }\n};\n```\n# Code - 726ms\n```\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n \n if(s.length() != t.length())\n return false;\n \n int n = s.length();\n for(int i = 0; i < n; i++)\n {\n char curr = s[i];\n int index = t.find(curr);\n if(index != string::npos)\n t.erase(index,1);\n }\n if(t.length() > 0)\n return false;\n \n return true;\n \n }\n};\n```\n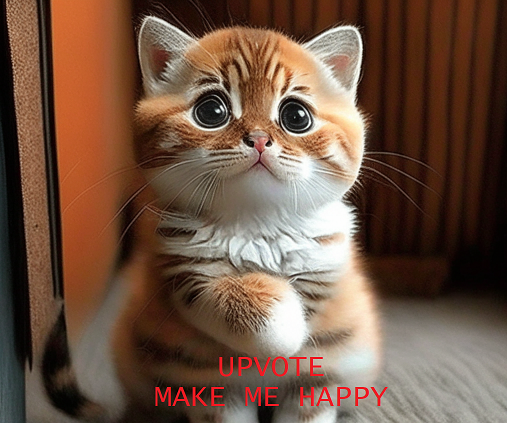\n | 5 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
🚀🚀🚀THREE LiNES OF CODE🚀🚀🚀by PRODONiK (Java, C++, Python, Go, JS, Ruby, C#) | valid-anagram | 1 | 1 | # Intuition\nThe intuition behind this solution is to determine if two strings are anagrams by comparing their sorted representations. Anagrams are words or phrases formed by rearranging the letters of another, and sorting the characters in each string allows us to easily check if they contain the same set of characters.\n\n# Approach\nThe approach involves converting the input strings `s` and `t` into character arrays `ss` and `tt` using the `toCharArray()` method. These arrays are then sorted using `Arrays.sort()` to arrange the characters in ascending order. Finally, the `Arrays.equals()` method is used to check if the sorted arrays are equal, which indicates that the original strings are anagrams.\n\n# Complexity\n- Time complexity: $$O(n \\log n)$$, where $$n$$ is the length of the input strings. The dominant factor is the sorting operation.\n- Space complexity: $$O(n)$$, as additional space is used to store the character arrays.\n\nThe space complexity is determined by the size of the character arrays, and the time complexity is influenced by the sorting operation.\n\n# Code\n```Java []\nclass Solution {\n public boolean isAnagram(String s, String t) {\n char [] ss = s.toCharArray(), tt = t.toCharArray();\n Arrays.sort(ss); Arrays.sort(tt);\n return Arrays.equals(ss, tt);\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n bool isAnagram(std::string s, std::string t) {\n // Convert strings to char arrays\n std::sort(s.begin(), s.end());\n std::sort(t.begin(), t.end());\n\n // Check if the sorted char arrays are equal\n return s == t;\n }\n};\n```\n```Python []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n # Convert strings to sorted lists of characters\n ss = sorted(s)\n tt = sorted(t)\n\n # Check if the sorted lists are equal\n return ss == tt\n```\n```C# []\npublic class Solution {\n public bool IsAnagram(string s, string t) {\n // Convert strings to char arrays\n char[] ss = s.ToCharArray();\n char[] tt = t.ToCharArray();\n \n // Sort the char arrays\n Array.Sort(ss);\n Array.Sort(tt);\n \n // Check if the sorted char arrays are equal\n return new string(ss) == new string(tt);\n }\n}\n```\n```Go []\nfunc (s *Solution) IsAnagram(str1 string, str2 string) bool {\n\t// Convert strings to sorted slices of characters\n\tss := strings.Split(str1, "")\n\ttt := strings.Split(str2, "")\n\tsort.Strings(ss)\n\tsort.Strings(tt)\n\n\t// Check if the sorted slices are equal\n\treturn strings.Join(ss, "") == strings.Join(tt, "")\n}\n```\n```Ruby []\nclass Solution\n def is_anagram(s, t)\n # Convert strings to arrays of characters and sort them\n ss = s.chars.sort\n tt = t.chars.sort\n\n # Check if the sorted arrays are equal\n ss == tt\n end\nend\n```\n```JavaScript []\nclass Solution {\n isAnagram(s, t) {\n // Convert strings to arrays of characters and sort them\n const ss = s.split(\'\').sort();\n const tt = t.split(\'\').sort();\n\n // Check if the sorted arrays are equal\n return ss.join(\'\') === tt.join(\'\');\n }\n}\n | 5 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
Rust/C++/Python linear time. Count number of each character. Python 1 line | valid-anagram | 0 | 1 | # Intuition\nAll you need is to keep track how many times each character was seen in both strings. Then the values should be equal.\n\nYou can also do short-circuit to stop if lenghts are not equal. In python you can do everything in one line with counter.\n\n# Complexity\n- Time complexity: $O(n)$\n- Space complexity: $O(1)$\n\n# Code\n```C++ []\nclass Solution {\npublic:\n bool isAnagram(string s1, string s2) {\n if (s1.size() != s2.size()) return false;\n \n vector<int> data(30);\n for (auto &c: s1) data[c - \'a\']++;\n for (auto &c: s2) data[c - \'a\']--;\n \n for (auto const &n: data) {\n if (n != 0) return false;\n }\n \n return true;\n }\n};\n```\n```python []\nfrom collections import Counter\n\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n return len(s) == len(t) and Counter(s) == Counter(t)\n```\n```Rust []\nimpl Solution {\n pub fn is_anagram(s: String, t: String) -> bool {\n let mut cnt = [0; 26];\n for v in s.as_bytes() {\n cnt[*v as usize - 97] += 1;\n }\n for v in t.as_bytes() {\n cnt[*v as usize - 97] -= 1;\n }\n\n for v in cnt {\n if v != 0 {\n return false;\n }\n }\n return true;\n }\n}\n```\n | 2 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
Leetcode daily problems | C++ | PYTHON | 100% fast 🔥 solutions ✔| 4 processes | valid-anagram | 0 | 1 | # Intuition\nWhen we approach the anagram check problem, a few initial thoughts come to mind. The answer of an anagram lies in the rearrangement of characters, meaning that two anagrams will share the same set of characters with the same frequencies. Here are some intuitive strategies for solving this problem:\n\n***Sorting:***\n\nOne straightforward approach involves sorting both strings. Anagrams, when sorted, will result in the same string. Hence, by comparing the sorted versions of the two strings, we can quickly determine if they are anagrams.\n- Sorting is generally O(n log n), making it less efficient than linear time approaches.\n\n***Frequency Counting:***\n\nAnother intuitive approach is to count the frequency of each character in both strings. Anagrams will have the same counts for each character. Utilizing data structures like dictionaries or unordered maps can help efficiently keep track of character frequencies.\n- The frequency counting approach is efficient, with linear time complexity.\n\n***Character Cancellation:***\n\nThis approach involves canceling out each character\'s occurrence in one string with its occurrence in the other. If, after canceling, the count for each character becomes zero, the strings are anagrams.\n- It is similar to the frequency counting approach but eliminates the need for additional data structures.\n\n# Approach\n# ***Solution 1: Sorting Strings***\nThis solution utilizes the fact that anagrams have the same characters with the same frequency but potentially in different orders. It sorts both strings and checks if they are equal after sorting. If the lengths are different, the strings cannot be anagrams.\n\n# Complexity\n- Time complexity:\n - Sorting both strings takes O(n log n) time, where n is the length of the strings.\n - Comparing the sorted strings takes O(n) time.\n\n- Space complexity:\n - The sorting is in-place, so the space complexity is O(1).\n\n# Code\n```CPP\n// C++ Solution\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n if (s.size() != t.size()) return false;\n sort(s.begin(), s.end());\n sort(t.begin(), t.end());\n return s == t;\n }\n};\n\n```\n```python\n# PYTHON solution\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n # Check if lengths are different\n if len(s) != len(t):\n return False\n \n # Sort both strings\n sorted_s = \'\'.join(sorted(s))\n sorted_t = \'\'.join(sorted(t))\n \n # Compare sorted strings\n return sorted_s == sorted_t\n```\n\n# Approach\n# ***Solution 2: Frequency Counter***\nThis solution employs a frequency counter approach using dictionaries. It counts the occurrences of each character in both strings and compares the counters. If the lengths are different, the strings cannot be anagrams.\n\n# Complexity\n- Time complexity:\n - Constructing the frequency counters takes O(n) time, where n is the length of the strings.\n - Comparing the counters takes O(n) time.\n\n- Space complexity:\n - The space complexity is O(n) due to the storage of frequency counters.\n\n# Code\n```PYTHON\n# PYTHON solution\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n if len(s) != len(t):\n return False\n \n s_counter = {}\n t_counter = {}\n \n for char in s:\n s_counter[char] = s_counter.get(char, 0) + 1\n \n for char in t:\n t_counter[char] = t_counter.get(char, 0) + 1\n \n return s_counter == t_counter\n\n```\n# Approach\n# ***Solution 3: Unordered Map***\nThis solution uses an unordered map to keep track of character frequencies. It increments the count for each character in the first string and decrements it for each character in the second string. It then checks if all counts are zero.\n\nTime Complexity:\n\n# Complexity\n- Time complexity:\n - Iterating through both strings and updating the map takes O(n) time, where n is the length of the strings.\n - Checking the map for non-zero counts also takes O(n) time.\n\n- Space complexity:\n - The space complexity is O(k), where k is the number of unique characters in both strings.\n\n# Code\n```CPP\n// C++ Solution\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n unordered_map<char, int> count;\n for (auto it : s) {\n count[it]++;\n }\n for (auto it : t) {\n count[it]--;\n }\n for (auto it : count) {\n if (it.second != 0) {\n return false;\n }\n }\n return true;\n }\n};\n```\n# Approach\n# ***Most Efficient Solution: Frequency Counter***\nThis solution employs a frequency counter approach using the Counter class from the collections module. It counts the occurrences of each character in both strings and compares the frequency counters. If the lengths are different, the strings cannot be anagrams.\n\nTime Complexity:\n\n# Complexity\n- Time complexity:\n - Constructing the frequency counters takes O(n) time, where n is the length of the strings.\n - Comparing the counters takes O(k) time, where k is the number of unique characters in both strings.\n\n- Space complexity:\n - The space complexity is O(k), where k is the number of unique characters in both strings. This is due to the storage of frequency counters.\n\n# Code\n```PYTHON\n# PYTHON solution\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n # Check if lengths are different\n if len(s) != len(t):\n return False\n \n # Use Counter to count character frequencies\n s_counter = Counter(s)\n t_counter = Counter(t)\n \n # Compare the frequency counters\n return s_counter == t_counter\n\n```\n\n\nwe used 2 frequency counter approach (approach 2 and 4)\n\n# Main difference between approach 2 and approach 4\n\n### ***Data Structure:***\n\n- ***Previous Approach:*** Uses a standard dictionary to maintain frequency counters.\n\n- **Most Efficient Approach:** Utilizes the Counter class from the collections module, a specialized container for counting hashable objects.\n\n### ***Implementation:***\n\n- **Previous Approach:** Manually increments counters using get method for each character.\n- ***Most Efficient Approach:*** Relies on the Counter class, providing a more concise and expressive way to count frequencies. | 2 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
✅☑[C++/C/Java/Python/JavaScript] || 3 Approaches || Beats 100% || EXPLAINED🔥 | valid-anagram | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n*(Also explained in the code)*\n\n#### ***Approach 1(Sort)***\n1. **Sorting Strings:**\n\n - The code sorts both input strings `s` and `t` using the `sort` function. Sorting rearranges the characters in each string in ascending order.\n1. **Comparison:**\n\n - After sorting, the code checks if the sorted strings `s` and `t` are equal using the `==` operator.\n - If the sorted strings are equal, it means that the original strings contain the same characters in the same frequency, thus they are anagrams. It returns `true`.\n - If the sorted strings are not equal, it indicates that the original strings differ in character frequency, meaning they are not anagrams. It returns `false`.\n\n# Complexity\n- Time complexity:\n $$O(nlogn)$$\n \n\n- Space complexity:\n $$O(nlogn)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n // Function to check if two strings s and t are anagrams of each other\n bool isAnagram(string s, string t) {\n sort(s.begin(), s.end()); // Sort the characters in string s in ascending order\n sort(t.begin(), t.end()); // Sort the characters in string t in ascending order\n \n // Check if the sorted strings s and t are equal\n if (s == t) {\n return true; // If the sorted strings are equal, s and t are anagrams\n }\n return false; // If the sorted strings are not equal, s and t are not anagrams\n }\n};\n\n\n\n```\n```C []\n\n\nint compareChars(const void *a, const void *b) {\n return (*(char *)a - *(char *)b);\n}\n\nint isAnagram(char *s, char *t) {\n int lenS = strlen(s);\n int lenT = strlen(t);\n\n if (lenS != lenT) {\n return 0; // If lengths differ, strings cannot be anagrams\n }\n\n qsort(s, lenS, sizeof(char), compareChars);\n qsort(t, lenT, sizeof(char), compareChars);\n\n return strcmp(s, t) == 0; // If sorted strings are equal, they are anagrams\n}\n\n\n```\n```Java []\nimport java.util.Arrays;\n\npublic class Main {\n public static boolean isAnagram(String s, String t) {\n char[] sChars = s.toCharArray();\n char[] tChars = t.toCharArray();\n\n Arrays.sort(sChars);\n Arrays.sort(tChars);\n\n return Arrays.equals(sChars, tChars);\n }\n\n \n}\n\n\n\n```\n```python3 []\n\ndef isAnagram(s, t):\n return sorted(s) == sorted(t)\n\n```\n```javascript []\nfunction isAnagram(s, t) {\n s = s.split(\'\').sort().join(\'\');\n t = t.split(\'\').sort().join(\'\');\n\n return s === t;\n}\n\n\n```\n---\n#### ***Approach 2(Hash table 1)***\n1. The code utilizes an array `count` of size 26 (representing lowercase English letters) to count the frequency of characters.\n1. The first loop iterates through string `s` and increments the count of each character by using its ASCII value as the index in the `count` array.\n1. The second loop iterates through string `t` and decrements the count of each character by using its ASCII value as the index in the `count` array.\n1. After counting the frequencies of characters in both strings, it checks if any character has a non-zero frequency in the `count` array.\n1. If any character has a non-zero frequency, it returns `false`, indicating that the strings are not anagrams. Otherwise, it returns `true`, indicating that the strings are anagrams.\n\n# Complexity\n- Time complexity:\n $$O(n)$$\n \n\n- Space complexity:\n $$O(26)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n // Function to check if two strings s and t are anagrams of each other\n bool isAnagram(string s, string t) {\n int count[26] = {0}; // Array to store character frequencies for lowercase English letters\n \n // Count the frequency of characters in string s\n for (char x : s) {\n count[x - \'a\']++; // Increment the count of the character (x - \'a\') in the array\n }\n \n // Decrement the frequency of characters in string t\n for (char x : t) {\n count[x - \'a\']--; // Decrement the count of the character (x - \'a\') in the array\n }\n \n // Check if any character has non-zero frequency (indicating unequal frequencies of characters)\n for (int val : count) {\n if (val != 0) {\n return false; // If any character\'s frequency is non-zero, return false (not anagrams)\n }\n }\n \n return true; // If all characters have zero frequency (equal frequencies), return true (anagrams)\n }\n};\n\n\n\n```\n```C []\n#include <stdbool.h>\n\nbool isAnagram(char * s, char * t) {\n int count[26] = {0}; // Array to store character frequencies for lowercase English letters\n \n // Count the frequency of characters in string s\n for (; *s != \'\\0\'; s++) {\n count[*s - \'a\']++; // Increment the count of the character (*s - \'a\') in the array\n }\n \n // Decrement the frequency of characters in string t\n for (; *t != \'\\0\'; t++) {\n count[*t - \'a\']--; // Decrement the count of the character (*t - \'a\') in the array\n }\n \n // Check if any character has non-zero frequency (indicating unequal frequencies of characters)\n for (int i = 0; i < 26; i++) {\n if (count[i] != 0) {\n return false; // If any character\'s frequency is non-zero, return false (not anagrams)\n }\n }\n \n return true; // If all characters have zero frequency (equal frequencies), return true (anagrams)\n}\n\n\n\n\n```\n```Java []\nclass Solution {\n public boolean isAnagram(String s, String t) {\n int[] count = new int[26]; // Array to store character frequencies for lowercase English letters\n \n // Count the frequency of characters in string s\n for (char x : s.toCharArray()) {\n count[x - \'a\']++; // Increment the count of the character (x - \'a\') in the array\n }\n \n // Decrement the frequency of characters in string t\n for (char x : t.toCharArray()) {\n count[x - \'a\']--; // Decrement the count of the character (x - \'a\') in the array\n }\n \n // Check if any character has non-zero frequency (indicating unequal frequencies of characters)\n for (int val : count) {\n if (val != 0) {\n return false; // If any character\'s frequency is non-zero, return false (not anagrams)\n }\n }\n \n return true; // If all characters have zero frequency (equal frequencies), return true (anagrams)\n }\n}\n\n\n\n```\n```python3 []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n count = [0] * 26 # List to store character frequencies for lowercase English letters\n \n # Count the frequency of characters in string s\n for x in s:\n count[ord(x) - ord(\'a\')] += 1 # Increment the count of the character (ord(x) - ord(\'a\')) in the list\n \n # Decrement the frequency of characters in string t\n for x in t:\n count[ord(x) - ord(\'a\')] -= 1 # Decrement the count of the character (ord(x) - ord(\'a\')) in the list\n \n # Check if any character has non-zero frequency (indicating unequal frequencies of characters)\n for val in count:\n if val != 0:\n return False # If any character\'s frequency is non-zero, return False (not anagrams)\n \n return True # If all characters have zero frequency (equal frequencies), return True (anagrams)\n\n\n\n```\n```javascript []\nvar isAnagram = function(s, t) {\n const count = new Array(26).fill(0); // Array to store character frequencies for lowercase English letters\n \n // Count the frequency of characters in string s\n for (let i = 0; i < s.length; i++) {\n count[s.charCodeAt(i) - 97]++; // Increment the count of the character (s.charCodeAt(i) - 97) in the array\n }\n \n // Decrement the frequency of characters in string t\n for (let i = 0; i < t.length; i++) {\n count[t.charCodeAt(i) - 97]--; // Decrement the count of the character (t.charCodeAt(i) - 97) in the array\n }\n \n // Check if any character has non-zero frequency (indicating unequal frequencies of characters)\n for (let val of count) {\n if (val !== 0) {\n return false; // If any character\'s frequency is non-zero, return false (not anagrams)\n }\n }\n \n return true; // If all characters have zero frequency (equal frequencies), return true (anagrams)\n};\n\n\n\n```\n---\n#### ***Approach 3(Hash Table 2)***\n1. The code uses an unordered map `count` to store character frequencies.\n1. It iterates through string `s` and increments the count for each character encountered.\n1. Then, it iterates through string `t` and decrements the count for each character encountered.\n1. After that, it checks the count map. If any character has a non-zero frequency, it returns `false` as they are not anagrams. Otherwise, it returns `true`, indicating that the strings are anagrams.\n\n# Complexity\n- Time complexity:\n $$O(n)$$\n \n\n- Space complexity:\n $$O(n)$$\n \n\n\n# Code\n```C++ []\n\n\nclass Solution {\npublic:\n // Function to check if two strings s and t are anagrams of each other\n bool isAnagram(string s, string t) {\n unordered_map<char, int> count; // Create an unordered_map to store character frequencies\n \n // Count the frequency of characters in string s\n for (auto x : s) {\n count[x]++; // Increment the count for each character in string s\n }\n \n // Decrement the frequency of characters in string t\n for (auto x : t) {\n count[x]--; // Decrement the count for each character in string t\n }\n \n // Check if any character has non-zero frequency\n for (auto x : count) {\n if (x.second != 0) { // If any character has a non-zero count, strings s and t are not anagrams\n return false;\n }\n }\n \n return true; // If all characters have zero frequency, strings s and t are anagrams\n }\n};\n\n\n\n```\n```C []\n\n#include <stdio.h>\n#include <string.h>\n\nint isAnagram(char *s, char *t) {\n int count[256] = {0}; // Assuming ASCII characters\n \n // Count the frequency of characters in string s\n for (int i = 0; s[i] != \'\\0\'; i++) {\n count[s[i]]++;\n }\n \n // Decrement the frequency of characters in string t\n for (int i = 0; t[i] != \'\\0\'; i++) {\n count[t[i]]--;\n }\n \n // Check if any character has non-zero frequency\n for (int i = 0; i < 256; i++) {\n if (count[i] != 0) {\n return 0; // If any character\'s frequency is non-zero, return false (not anagrams)\n }\n }\n \n return 1; // If all characters have zero frequency (equal frequencies), return true (anagrams)\n}\n\n\n\n```\n```Java []\n\nclass Solution {\n public boolean isAnagram(String s, String t) {\n int[] count = new int[256]; // Assuming ASCII characters\n \n // Count the frequency of characters in string s\n for (char c : s.toCharArray()) {\n count[c]++;\n }\n \n // Decrement the frequency of characters in string t\n for (char c : t.toCharArray()) {\n count[c]--;\n }\n \n // Check if any character has non-zero frequency\n for (int i = 0; i < 256; i++) {\n if (count[i] != 0) {\n return false; // If any character\'s frequency is non-zero, return false (not anagrams)\n }\n }\n \n return true; // If all characters have zero frequency (equal frequencies), return true (anagrams)\n }\n}\n\n\n```\n```python3 []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n count = [0] * 256 # Assuming ASCII characters\n \n # Count the frequency of characters in string s\n for char in s:\n count[ord(char)] += 1\n \n # Decrement the frequency of characters in string t\n for char in t:\n count[ord(char)] -= 1\n \n # Check if any character has non-zero frequency\n for val in count:\n if val != 0:\n return False # If any character\'s frequency is non-zero, return False (not anagrams)\n \n return True # If all characters have zero frequency (equal frequencies), return True (anagrams)\n\n\n\n```\n```javascript []\nvar isAnagram = function(s, t) {\n let count = Array(256).fill(0); // Assuming ASCII characters\n \n // Count the frequency of characters in string s\n for (let char of s) {\n count[char.charCodeAt(0)]++;\n }\n \n // Decrement the frequency of characters in string t\n for (let char of t) {\n count[char.charCodeAt(0)]--;\n }\n \n // Check if any character has non-zero frequency\n for (let val of count) {\n if (val !== 0) {\n return false; // If any character\'s frequency is non-zero, return false (not anagrams)\n }\n }\n \n return true; // If all characters have zero frequency (equal frequencies), return true (anagrams)\n};\n\n\n\n```\n---\n\n\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n--- | 2 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
solution of Valid anagram | valid-anagram | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n<!-- Describe your approach to solving the problem. -->\nBasically An anagram is a word or phrase that is formed by rearranging the letters of another word or phrase. For example, "triangle" is an anagram of "integral". \n\nfirst convert both string into list and then by using sort function sort both list and then check they are equal or not .if they are equal return True otherwise return False.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nfirst convert both string into list and then by using sort function sort both list and then check they are equal or not .if they are equal return True otherwise return False.\n\n# Complexity\n- Time complexity: O(1) \n- this code will run in constant time \n\n- Space complexity: O(1)\n\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n sa=sorted(list(s))\n ta=sorted(list(t))\n \n if(sa==ta):\n return True\n else:\n return False\n\n\n\n\n\n\n \n``` | 1 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
Easy Python. Beats 100% Time and Space. 😏🔥 | valid-anagram | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n ls1=list(s)\n ls2=list(t)\n ls1.sort()\n ls2.sort()\n \n if ls1==ls2:\n return True\n return False\n \n``` | 1 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
Easiest Solution || Python | valid-anagram | 0 | 1 | # Intuition\nA very simple way of solving this will be to check the length of the 2 strings and then checking if the frequency of letters in both the strings are equal.\n\n\n# Code\n```\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n if len(s) == len(t):\n counts={}\n countt={}\n for char in s:\n counts[char] = counts.get(char,0)+1\n for char in t:\n countt[char] = countt.get(char,0)+1\n if countt == counts:\n return True\n return False\n\n \n```\n# **PLEASE DO UPVOTE!!!\uD83E\uDD79** | 1 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
Two approaches || Simple solution with HashTable in Python3 | valid-anagram | 0 | 1 | # Intuition\nHere we have two strings, `s` and `t` respectively.\nOur goal is to check, if `t` is **anagram** of `s`.\n\n# Approach\nThere\'re **three things to notice**:\n- if strings have **different** lengths, **they\'re not equal**\n- you can sort them in any direction, and compare (**O(N log N)**)\n- you can use **HashMap** instead, to improve TC, and for each character from `s` you can **increment** frequency of this char, and for `s` - **decrement** (**O(N)**)\n\n# Complexity\n- Time complexity: **O(N log N)** for sorting, and **O(N)** for HashMap.\n\n- Space complexity: **O(N)** for both cases, **O(1)** for sorting in Python3.\n\n# Python3\n```typescript []\nfunction isAnagram(s: string, t: string): boolean {\n if (s.length !== t.length) {\n return false\n }\n\n // 1. Sorting\n const newS = s.split(\'\')\n const newT = t.split(\'\')\n newS.sort()\n newT.sort()\n \n return newS.join() === newT.join()\n\n // 2. HashMap\n\n const freqs = Array(26).fill(0)\n\n const toOrder = (char: string) => char.charCodeAt(0) - 97\n\n for (let i = 0; i < s.length; i += 1) {\n freqs[toOrder(s[i])] += 1\n freqs[toOrder(t[i])] -= 1\n }\n\n return freqs.every(freq => freq === 0)\n};\n```\n```python []\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n if len(s) != len(t):\n return False\n\n # 1. Sorting \n return sorted(s) == sorted(t)\n\n # 2. HashMap\n map = defaultdict(int)\n \n for i in range(len(s)):\n map[s[i]] += 1\n map[t[i]] -= 1\n \n for key in map:\n if map[key] != 0:\n return False\n \n return True\n``` | 1 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
Python3 Solution | valid-anagram | 0 | 1 | \n```\n"""class Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n \n if len(s) !=len(t):\n return False \n countS,countT={},{}\n \n for i in range(len(s)):\n countS[s[i]]=1+countS.get(s[i],0)\n countT[t[i]]=1+countT.get(t[i],0) \n for c in countS:\n if countS[c]!=countT.get(c,0):\n return False\n \n return True """\n\n"""class Solution:\n def isAnagram(self,s:str,t:str)->bool:\n return collections.Counter(s)==collections.Counter(t) """\n"""class Solution:\n def isAnagram(self,s:str,t:str)->bool:\n return sorted(s)==sorted(t) """\n\nclass Solution:\n def isAnagram(self,s:str,t:str)->bool:\n return sorted(s)==sorted(t) \n``` | 1 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
Valid Anagram - Python One line, easy to understand | valid-anagram | 0 | 1 | # Approach\nSort the strings using sorted(), and compare them. If they are same after the sort, t is the valid anagram of the string s.\n**Example:**\ns = anagram t = nagaram\nsorted s = aaagmnr \nsorted t = aaagmnr \n\n\n# Code\n```\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n return sorted(s)==sorted(t)\n \n``` | 1 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
1 line solutiuon | valid-anagram | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n return sorted(s) == sorted(t)\n``` | 0 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
✅✅✅ Very easy python3 solution. | valid-anagram | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n if sorted(s) == sorted(t):\n return True\n else:\n return False \n\n \n``` | 1 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
Python3 Best 100% | valid-anagram | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n return Counter(s) == Counter(t)\n \n``` | 1 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
[VIDEO] Visualization of Using Hash Tables Frequencies | valid-anagram | 0 | 1 | https://youtu.be/vYNRXZ4GXPg\n\nThe simplest approach would be to sort each string and compare them to each other. If they are the same, then they are anagrams. The drawback to this method is that since the cost of sorting can be no less than O(n log n), if the length of the longer string is n, then this strategy runs in O(n log n) time.\n\nThere\'s actually no reason why the strings need to be in sorted order though. All we need to know are the character frequencies of each string. In other words, if each string uses the same number of each character, then they are anagrams of each other.\n\nWe can use a hash table (dictionary) to keep track of the character frequencies of each string. Then, if the contents of each dictionary are equal to each other, then the two strings are anagrams. Since we only have to iterate through each string once, this runs in O(n) time.\n\nVariations:\nIf you wanted to save space, you could use one dictionary instead of two. Then, for the second string, you would decrement the character counts instead of incrementing them. If all the values end up as 0, then the strings are anagrams\n\nYou could also use an array instead to avoid the complex hash calculations required when using hash tables. "a" would map to index 0, "b" maps to index 1, etc.\n\n# Code\n```\nclass Solution:\n def isAnagram(self, s: str, t: str) -> bool:\n if len(s) != len(t):\n return False\n\n s_freq = {}\n t_freq = {}\n for char in s:\n s_freq[char] = s_freq.get(char, 0) + 1\n for char in t:\n t_freq[char] = t_freq.get(char, 0) + 1\n\n return s_freq == t_freq\n``` | 4 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
Very Easy || 100% || Fully Explained || C++, Java, Python, JavaScript, Python3 | valid-anagram | 1 | 1 | # **Java Solution:**\n```\n// If two strings are anagrams then the frequency of every char in both of the strings are same.\nclass Solution {\n public boolean isAnagram(String s, String t) {\n // Base case: if the two strings are empty...\n if(s == null || t == null) return false;\n // In case of different length of those two string...\n if(s.length() != t.length()) return false;\n // To count freq we make an array of size 26...\n int[] counter = new int[26];\n // Traverse all elements through a loop...\n for(int idx = 0; idx < s.length(); idx++){\n counter[s.charAt(idx)-\'a\']++;\n counter[t.charAt(idx)-\'a\']--;\n }\n // Above iteration provides us with count array having all values to zero then we can say we found an anagram.\n // Every element of count has to be equal to 0.\n // If it is greater than 0 it means s has a character whose occurrence is greater than its occurrence in t.\n // And if its less than 0 then, s has a character whose occurrence is smaller than its occurrence in t.\n for(int idx: counter){\n if(idx != 0)\n return false;\n }\n return true;\n }\n}\n```\n\n# **C++ Solution:**\n```\n// If two strings are anagrams then the frequency of every char in both of the strings are same.\nclass Solution {\npublic:\n bool isAnagram(string s, string t) {\n // In case of different length of thpse two strings...\n if(s.length() != t.length()) return false;\n // Count the freq of every character in s & t...\n vector<int> counter1(26,0), counter2(26,0);\n // Traverse through the loop...\n for(int idx = 0; idx < s.length(); idx++) {\n counter1[s[idx]-\'a\']++;\n counter2[t[idx]-\'a\']++;\n }\n // Compare freq1[i] and freq2[i] for every index i from 0 to 26...\n for(int idx = 0; idx < 26; idx++) {\n // If they are different, return false...\n if(counter1[idx] != counter2[idx]) return false;\n }\n return true; // Otherwise, return true...\n\n }\n};\n```\n\n# **Python/Python3 Solution:**\n```\nclass Solution(object):\n def isAnagram(self, s, t):\n # In case of different length of thpse two strings...\n if len(s) != len(t):\n return False\n for idx in set(s):\n # Compare s.count(l) and t.count(l) for every index i from 0 to 26...\n # If they are different, return false...\n if s.count(idx) != t.count(idx):\n return False\n return True # Otherwise, return true...\n```\n \n# **JavaScript Solution:**\n```\nvar isAnagram = function(s, t) {\n // To count freq we make an array of size 26...\n const counter = new Array(26).fill(0);\n // Traverse all elements through a loop...\n for(let idx = 0; idx < s.length; idx++){\n counter[s.charCodeAt(idx)-97]++;\n }\n for(let idx = 0; idx < t.length; idx++){\n counter[t.charCodeAt(idx)-97]--;\n }\n // Above iteration provides us with count array having all values to zero then we can say we found an anagram.\n // Every element of count has to be equal to 0.\n // If it is greater than 0 it means s has a character whose occurrence is greater than its occurrence in t.\n // And if its less than 0 then, s has a character whose occurrence is smaller than its occurrence in t.\n for (let idx = 0; idx < 26; idx++) {\n if(counter[idx] != 0)\n return false;\n }\n return true;\n};\n```\n**I am working hard for you guys...\nPlease upvote if you found any help with this code...** | 147 | Given two strings `s` and `t`, return `true` _if_ `t` _is an anagram of_ `s`_, and_ `false` _otherwise_.
An **Anagram** is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once.
**Example 1:**
**Input:** s = "anagram", t = "nagaram"
**Output:** true
**Example 2:**
**Input:** s = "rat", t = "car"
**Output:** false
**Constraints:**
* `1 <= s.length, t.length <= 5 * 104`
* `s` and `t` consist of lowercase English letters.
**Follow up:** What if the inputs contain Unicode characters? How would you adapt your solution to such a case? | null |
Python Easy Solution || 100% || Recursion || Beats 98% || | binary-tree-paths | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def __init__(self):\n self.res=[]\n\n def binaryTreePaths(self, root: Optional[TreeNode]) -> List[str]:\n arr=\'\'\n if not root:\n return []\n self.checkSm(root, arr)\n return self.res\n \n def checkSm(self, node, arr):\n newarr=arr+str(node.val)\n if not node.left and not node.right:\n self.res.append(newarr)\n return\n else:\n newarr=newarr+\'->\'\n if node.left:\n self.checkSm(node.left, newarr)\n if node.right:\n self.checkSm(node.right, newarr)\n return\n``` | 2 | Given the `root` of a binary tree, return _all root-to-leaf paths in **any order**_.
A **leaf** is a node with no children.
**Example 1:**
**Input:** root = \[1,2,3,null,5\]
**Output:** \[ "1->2->5 ", "1->3 "\]
**Example 2:**
**Input:** root = \[1\]
**Output:** \[ "1 "\]
**Constraints:**
* The number of nodes in the tree is in the range `[1, 100]`.
* `-100 <= Node.val <= 100` | null |
Python3 using queue (Level-Order Traversal) | binary-tree-paths | 0 | 1 | ```\nclass Node:\n def __init__(self, value):\n self.value = value\n self.next = None\n \nclass LinkedList:\n def __init__(self):\n self.head = None\n self.tail = None\n self.length = 0\n\n def __len__(self):\n return self.length\n\n def add(self, value):\n node = Node(value)\n if self.head:\n self.tail.next = node\n self.tail = node\n else:\n self.head = node\n self.tail = node\n self.length += 1\n\n def remove_first(self):\n if self.head:\n value = self.head.value\n self.head = self.head.next\n self.length -= 1\n return value\n\nclass Queue:\n def __init__(self, size = 0):\n self.list = LinkedList()\n self.size = size\n\n def enqueue(self, value):\n self.list.add(value)\n\n def dequeue(self):\n return self.list.remove_first()\n \n def is_empty(self):\n return self.list.head == None\n\nclass Solution:\n def binaryTreePaths(self, rootNode: Optional[TreeNode]) -> List[str]:\n if not rootNode:\n return\n else:\n queue = Queue()\n queue.enqueue(rootNode)\n paths = {\n f"0-{rootNode.val}": str(rootNode.val)\n }\n next_counter = 0\n current_counter = 0\n while not queue.is_empty():\n current = queue.dequeue()\n current_parent = paths[f"{current_counter}-{current.val}"]\n if current.left:\n next_counter += 1\n paths[f\'{next_counter}-{current.left.val}\'] = f\'{current_parent}->{current.left.val}\'\n queue.enqueue(current.left)\n if current.right:\n next_counter += 1\n paths[f\'{next_counter}-{current.right.val}\'] = f\'{current_parent}->{current.right.val}\'\n queue.enqueue(current.right)\n if current.right or current.left: \n del paths[f"{current_counter}-{current.val}"]\n current_counter += 1\n return list(paths.values())\n``` | 2 | Given the `root` of a binary tree, return _all root-to-leaf paths in **any order**_.
A **leaf** is a node with no children.
**Example 1:**
**Input:** root = \[1,2,3,null,5\]
**Output:** \[ "1->2->5 ", "1->3 "\]
**Example 2:**
**Input:** root = \[1\]
**Output:** \[ "1 "\]
**Constraints:**
* The number of nodes in the tree is in the range `[1, 100]`.
* `-100 <= Node.val <= 100` | null |
😎Brute Force to Efficient Method🤩 | Java | C++ | Python | JavaScript | C# | binary-tree-paths | 1 | 1 | \n# 1st Method :- Brute Force\n<!-- Describe your first thoughts on how to solve this problem. -->\n> This brute force solution would be to perform a depth-first traversal of the binary tree and, at each node, generate all possible paths from the root to that node. We can use a recursive approach to traverse the tree and construct paths as we go.\n\n### Steps\n1. Start at the root of the tree.\n2. Initialize an empty list (ArrayList) to store the paths.\n3. Define a recursive function (`dfs`) that takes the current node, the current path, and the list of paths as parameters.\n4. In the `dfs` function:\n - If the current node is null, return.\n - Append the current node\'s value to the current path as a string.\n - If the current node is a leaf node (both left and right children are null):\n - Convert the current path to a string and add it to the list of paths.\n - Recursively call `dfs` on the left child with the updated path.\n - Recursively call `dfs` on the right child with the updated path.\n5. Call the `dfs` function starting from the root node with an empty path and the list of paths.\n6. Return the list of paths.\n\n# Code\n``` Java []\nclass Solution {\n public List<String> binaryTreePaths(TreeNode root) {\n List<String> result = new ArrayList<>();\n dfs(root, "", result);\n return result;\n }\n\n private void dfs(TreeNode node, String path, List<String> result) {\n if (node == null) return;\n //Append the current node\'s value to the path.\n path += node.val;\n\n //If it\'s a leaf node, add the path to the result list.\n if (node.left == null && node.right == null) {\n result.add(path);\n } else {\n path += "->";// Separate nodes in the path.\n dfs(node.left, path, result);\n dfs(node.right, path, result);\n }\n }\n}\n```\n``` C++ []\nclass Solution {\n public:\n vector<string> binaryTreePaths(TreeNode* root) {\n vector<string> result;\n dfs(root, "", result);\n return result;\n }\n\n void dfs(TreeNode* node, string path, vector<string>& result) {\n if (!node) return;\n path += to_string(node->val);\n if (!node->left && !node->right) {\n result.push_back(path);\n } else {\n dfs(node->left, path + "->", result);\n dfs(node->right, path + "->", result);\n }\n }\n};\n```\n``` Python []\nclass Solution:\n def binaryTreePaths(self, root: Optional[TreeNode]) -> List[str]:\n def dfs(node, path, result):\n if not node:\n return\n path += str(node.val)\n if not node.left and not node.right:\n result.append(path)\n else:\n dfs(node.left, path + \'->\', result)\n dfs(node.right, path + \'->\', result)\n\n result = []\n dfs(root, \'\', result)\n return result \n```\n``` Javascript []\nvar binaryTreePaths = function(root) {\n const paths = [];\n\n function dfs(node, path) {\n if (!node) return;\n path.push(node.val.toString());\n if (!node.left && !node.right) {\n paths.push(path.join(\'->\'));\n } else {\n dfs(node.left, path.slice()); // Copy the path array\n dfs(node.right, path.slice()); // Copy the path array\n }\n }\n \n dfs(root, []);\n return paths;\n};\n```\n```C# []\npublic class Solution {\n public IList<string> BinaryTreePaths(TreeNode root) {\n List<string> result = new List<string>();\n DFS(root, "", result);\n return result;\n }\n\n private void DFS(TreeNode node, string path, List<string> result) {\n if (node == null) return;\n path += node.val.ToString();\n if (node.left == null && node.right == null) {\n result.Add(path);\n } else {\n DFS(node.left, path + "->", result);\n DFS(node.right, path + "->", result);\n }\n }\n}\n```\n# if(UpVote)? Good Bless You :(\n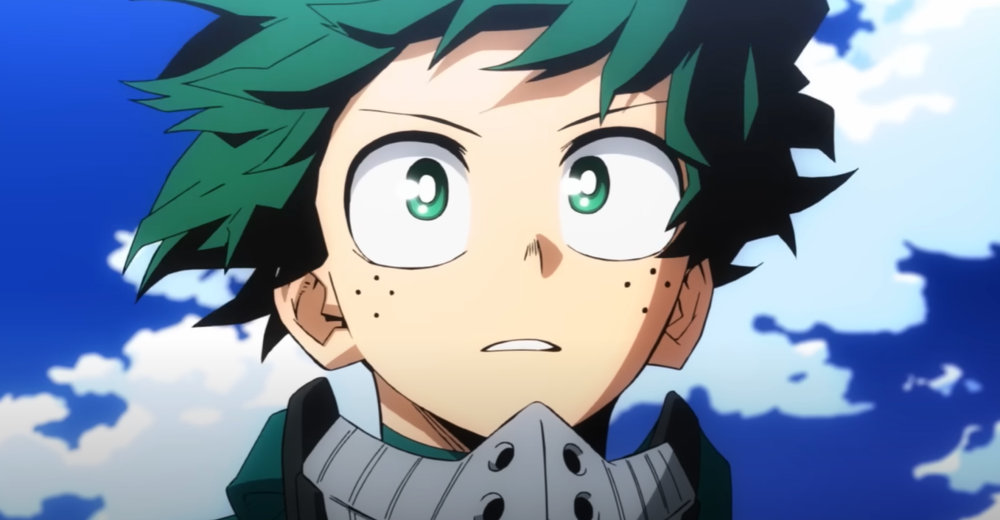\n\n\n# 2nd Method :- Efficient Method\nIn the efficient approach, we aim to minimize unnecessary string concatenations and improve overall performance. Here are the steps:\n### Steps\n1. Start at the root of the tree.\n2. Initialize an empty list (ArrayList) to store the paths and an empty StringBuilder to represent the current path.\n3. Define a recursive function (dfs) that takes the current node, the current path, and the list of paths as parameters.\n4. In the dfs function:\n - If the current node is null, return.\n - Append the current node\'s value to the current path using StringBuilder.\n - If the current node is a leaf node (both left and right children are null):\n - Convert the current StringBuilder to a string and add it to the list of paths.\n - Recursively call dfs on the left child with the updated path StringBuilder.\n - Recursively call dfs on the right child with the updated path StringBuilder.\n5. Call the dfs function starting from the root node with an empty path StringBuilder and the list of paths.\n6. Return the list of paths.\n\n# Code \n``` java []\nclass Solution {\n public List<String> binaryTreePaths(TreeNode root) {\n List<String> result = new ArrayList<>();\n dfs(root, new StringBuilder(), result);\n return result;\n }\n\n private void dfs(TreeNode node, StringBuilder path, List<String> result) {\n if (node == null) return;\n int len = path.length();\n if (len > 0) {\n path.append("->");\n }\n path.append(node.val);\n if (node.left == null && node.right == null) {\n result.add(path.toString());\n } else {\n dfs(node.left, path, result);\n dfs(node.right, path, result);\n }\n path.setLength(len); // Backtrack by resetting the StringBuilder\n }\n}\n```\n``` C++ []\nclass Solution {\n public:\n vector<string> binaryTreePaths(TreeNode* root) {\n vector<string> result;\n vector<int> path;\n dfs(root, path, result);\n return result;\n }\n void dfs(TreeNode* node, vector<int>& path, vector<string>& result) {\n if (!node) return;\n path.push_back(node->val);\n if (!node->left && !node->right) {\n string pathStr;\n for (int i = 0; i < path.size(); ++i) {\n pathStr += to_string(path[i]);\n if (i < path.size() - 1) {\n pathStr += "->";\n }\n }\n result.push_back(pathStr);\n } else {\n dfs(node->left, path, result);\n dfs(node->right, path, result);\n }\n path.pop_back();\n }\n\n};\n```\n``` Python []\nclass Solution:\n def binaryTreePaths(self, root: Optional[TreeNode]) -> List[str]:\n def dfs(node, path, result):\n if not node:\n return\n path.append(str(node.val))\n if not node.left and not node.right:\n result.append(\'->\'.join(path))\n else:\n dfs(node.left, path, result)\n dfs(node.right, path, result)\n path.pop() # Backtrack by removing the last element\n\n result = []\n dfs(root, [], result)\n return result\n```\n``` Javascript []\nvar binaryTreePaths = function(root) {\n const paths = [];\n\n function dfs(node, path) {\n if (!node) return;\n path.push(node.val.toString());\n if (!node.left && !node.right) {\n paths.push(path.join(\'->\'));\n } else {\n if (node.left) {\n dfs(node.left, path);\n path.pop();\n }\n if (node.right) {\n dfs(node.right, path);\n path.pop();\n }\n }\n }\n\n dfs(root, []);\n return paths;\n};\n```\n```C# []\npublic class Solution {\n public IList<string> BinaryTreePaths(TreeNode root) {\n List<string> result = new List<string>();\n List<int> path = new List<int>();\n DFS(root, path, result);\n return result;\n }\n\n private void DFS(TreeNode node, List<int> path, List<string> result) {\n if (node == null) return;\n path.Add(node.val);\n if (node.left == null && node.right == null) {\n result.Add(string.Join("->", path));\n } else {\n DFS(node.left, path, result);\n DFS(node.right, path, result);\n }\n path.RemoveAt(path.Count - 1);\n }\n}\n```\n\n\n\n\n\n# Java, detail explanation for brute force \n\n### Steps\n1. ***Method Signature***: Define a method called binaryTreePaths that takes the root of the binary tree as its input and returns a list of strings representing the paths.\n\n2. ***Initialize Result List***: Create an empty ArrayList called paths to store the paths that we find in the binary tree.\n\n3. ***Recursive Helper Function***: Define a recursive helper function called constructPaths. This function will be responsible for constructing paths as we traverse the binary tree.\n\n4. ***Base Case***: Inside the constructPaths function, check if the current node is null. If it is, return, as there\'s nothing to do for a null node.\n\n5. ***Path Construction***: \n - Append the value of the current node to the currentPath string. This represents adding the current node to the path.\n - If the current node is a leaf node (both left and right children are null), add the currentPath to the paths list. We\'ve found a complete path from the root to a leaf node.\n - If the current node is not a leaf node, add the arrow symbol "->" to the currentPath to separate nodes in the path.\n\n6. ***Recursive Calls***: Recursively call the constructPaths function for the left and right children of the current node. This step explores all possible paths in the binary tree.\n\n7. ***Backtracking***: After processing the left and right subtrees of the current node, backtrack by removing the current node\'s value (and the "->" separator, if added) from the currentPath. This is important to avoid mixing paths when backtracking to different nodes.\n\n8. ***Return Result***: Finally, after traversing the entire tree and adding paths to the paths list, return the paths list as the output.\n\n# Java, detail explanation for Efficient Method\n\n1. ***Method Signature***: Define a method called binaryTreePaths that takes the root of the binary tree as its input and returns a list of strings representing the paths.\n\n2. ***Initialize Result List***: Create an empty ArrayList called paths to store the paths that we find in the binary tree.\n\n3. ***Initialize StringBuilder***: Create a StringBuilder called currentPath to keep track of the current path as we traverse the tree. StringBuilder is used because it allows efficient string manipulation (append and remove).\n\n4. ***Recursive Helper Function***: Define a recursive helper function called constructPaths. This function will be responsible for constructing paths as we traverse the binary tree.\n\n5. ***Base Case***: Inside the constructPaths function, check if the current node is null. If it is, return, as there\'s nothing to do for a null node.\n\n6. ***Path Construction***:\n - Check the length of the currentPath. If it\'s not empty, add the arrow symbol "->" to separate nodes in the path.\n - Append the value of the current node to the currentPath using StringBuilder. This represents adding the current node to the path.\n - If the current node is a leaf node (both left and right children are null), add the currentPath.toString() to the paths list. We\'ve found a complete path from the root to a leaf node.\n\n7. ***Recursive Calls***: Recursively call the constructPaths function for the left and right children of the current node. This step explores all possible paths in the binary tree.\n\n8. ***Backtracking***: After processing the left and right subtrees of the current node, backtrack by setting the length of the currentPath back to its previous length. This removes the current node\'s value (and the "->" separator, if added) from the currentPath. This is important to avoid mixing paths when backtracking to different nodes.\n\n9. ***Return Result***: Finally, after traversing the entire tree and adding paths to the paths list, return the paths list as the output. | 12 | Given the `root` of a binary tree, return _all root-to-leaf paths in **any order**_.
A **leaf** is a node with no children.
**Example 1:**
**Input:** root = \[1,2,3,null,5\]
**Output:** \[ "1->2->5 ", "1->3 "\]
**Example 2:**
**Input:** root = \[1\]
**Output:** \[ "1 "\]
**Constraints:**
* The number of nodes in the tree is in the range `[1, 100]`.
* `-100 <= Node.val <= 100` | null |
Simple DFS in Python | binary-tree-paths | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nTo find all the root-to-leaf paths, we can use a depth-first search (DFS) algorithm. We\'ll implement a recursive helper function `solve()` that takes two parameters: the current node and the current path string. The `solve()` function will traverse the tree in a depth-first manner and append the root-to-leaf paths to the `ans` list.\n\nIn the `solve()` function:\n1. Append the string representation of the current node\'s value to the path string (`s += str(root.val)`).\n2. Check if the current node is a leaf node (i.e., it has no left or right child). If so, it means we have reached the end of a root-to-leaf path. In this case, append the current path string to the `ans` list and return.\n3. If the current node has a right child, recursively call `solve()` with the right child and the updated path string (`solve(root.right, s + "->")`).\n4. If the current node has a left child, recursively call `solve()` with the left child and the updated path string (`solve(root.left, s + "->")`).\n\nAfter defining the `solve()` function, we initialize an empty `ans` list. Then, we call `solve()` with the `root` node and an empty path string (`""`). Finally, we return the `ans` list, which contains all the root-to-leaf paths.\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def binaryTreePaths(self, root: Optional[TreeNode]) -> List[str]:\n ans = []\n def solve(root, s):\n s += str(root.val)\n if not root.right and not root.left:\n ans.append(s)\n return\n if root.right:\n solve(root.right, s+"->")\n if root.left:\n solve(root.left, s+"->")\n solve(root, "")\n return ans\n``` | 8 | Given the `root` of a binary tree, return _all root-to-leaf paths in **any order**_.
A **leaf** is a node with no children.
**Example 1:**
**Input:** root = \[1,2,3,null,5\]
**Output:** \[ "1->2->5 ", "1->3 "\]
**Example 2:**
**Input:** root = \[1\]
**Output:** \[ "1 "\]
**Constraints:**
* The number of nodes in the tree is in the range `[1, 100]`.
* `-100 <= Node.val <= 100` | null |
[Python 3] DFS + path backtracking | binary-tree-paths | 0 | 1 | # Code\n```python3 []\nclass Solution:\n def binaryTreePaths(self, root: Optional[TreeNode]) -> List[str]:\n paths = []\n def dfs(node, path):\n if not node: return\n path.append(node.val)\n if not (node.left or node.right): # leaf\n paths.append(path.copy())\n else:\n dfs(node.left, path)\n dfs(node.right, path)\n path.pop()\n dfs(root, [])\n\n return [\'->\'.join(map(str, path)) for path in paths]\n```\n```python3 []\nclass Solution:\n def binaryTreePaths(self, root: Optional[TreeNode]) -> List[str]:\n paths = []\n def dfs(node, path):\n if not node: return\n path = path + [node.val]\n if not (node.left or node.right):\n return paths.append(path)\n dfs(node.left, path)\n dfs(node.right, path)\n dfs(root, [])\n\n return [\'->\'.join(map(str, path)) for path in paths]\n```\n\n | 7 | Given the `root` of a binary tree, return _all root-to-leaf paths in **any order**_.
A **leaf** is a node with no children.
**Example 1:**
**Input:** root = \[1,2,3,null,5\]
**Output:** \[ "1->2->5 ", "1->3 "\]
**Example 2:**
**Input:** root = \[1\]
**Output:** \[ "1 "\]
**Constraints:**
* The number of nodes in the tree is in the range `[1, 100]`.
* `-100 <= Node.val <= 100` | null |
257: Time 96.78%, Solution with step by step explanation | binary-tree-paths | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n Here\'s a step-by-step explanation:\n```\nclass Solution:\n def binaryTreePaths(self, root: Optional[TreeNode]) -> List[str]:\n ans = []\n```\nThe solution defines a class Solution with a method binaryTreePaths. It takes in the root node of a binary tree, represented as a TreeNode object, and returns a list of strings representing all possible paths from root to leaf nodes.\n\nThe variable ans is initialized as an empty list, which will store the answer.\n\n```\n def dfs(root: Optional[TreeNode], path: List[str]) -> None:\n if not root:\n return\n```\nA depth-first search (DFS) helper function dfs is defined, which takes in a node root and a path represented as a list of strings. If the node root is None, the function returns and does nothing.\n```\n if not root.left and not root.right:\n ans.append(\'\'.join(path) + str(root.val))\n return\n```\nIf root is a leaf node (i.e. has no left or right child), the function appends the current path joined by -> and the value of the leaf node to ans. Then, the function returns and does nothing.\n\n``` path.append(str(root.val) + \'->\')\n dfs(root.left, path)\n dfs(root.right, path)\n path.pop()\n```\nIf root is not a leaf node, the function appends the current node value followed by -> to the path. It then recursively calls dfs on the left and right children of the node. After these recursive calls are complete, the current node value is popped from the path.\n\n```\n dfs(root, [])\n return ans\n```\n\nFinally, the dfs function is called on the root node with an empty path list, and ans is returned as the answer to the problem.\n\n# Complexity\n- Time complexity:\n96.78%\n\n- Space complexity:\n63.68%\n\n# Code\n```\nclass Solution:\n def binaryTreePaths(self, root: Optional[TreeNode]) -> List[str]:\n ans = []\n\n def dfs(root: Optional[TreeNode], path: List[str]) -> None:\n if not root:\n return\n if not root.left and not root.right:\n ans.append(\'\'.join(path) + str(root.val))\n return\n\n path.append(str(root.val) + \'->\')\n dfs(root.left, path)\n dfs(root.right, path)\n path.pop()\n\n dfs(root, [])\n return ans\n\n``` | 8 | Given the `root` of a binary tree, return _all root-to-leaf paths in **any order**_.
A **leaf** is a node with no children.
**Example 1:**
**Input:** root = \[1,2,3,null,5\]
**Output:** \[ "1->2->5 ", "1->3 "\]
**Example 2:**
**Input:** root = \[1\]
**Output:** \[ "1 "\]
**Constraints:**
* The number of nodes in the tree is in the range `[1, 100]`.
* `-100 <= Node.val <= 100` | null |
Binbin's another brilliant idea | binary-tree-paths | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def binaryTreePaths(self, root: Optional[TreeNode]) -> List[str]:\n if root.left is None and root.right is None:\n return [str(root.val)]\n def findpath(node,l,L):\n if node.left is None and node.right is None:\n l += str(node.val)\n L.append(l)\n if node.left is not None:\n findpath(node.left,l+str(node.val)+"->",L)\n if node.right is not None:\n findpath(node.right,l+str(node.val)+"->",L)\n return L \n \n return findpath(root,"",[])\n\n\n \n``` | 3 | Given the `root` of a binary tree, return _all root-to-leaf paths in **any order**_.
A **leaf** is a node with no children.
**Example 1:**
**Input:** root = \[1,2,3,null,5\]
**Output:** \[ "1->2->5 ", "1->3 "\]
**Example 2:**
**Input:** root = \[1\]
**Output:** \[ "1 "\]
**Constraints:**
* The number of nodes in the tree is in the range `[1, 100]`.
* `-100 <= Node.val <= 100` | null |
Recursive solution by Leo | binary-tree-paths | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def binaryTreePaths(self, root):\n if not root:\n return []\n\n if not root.left and not root.right:\n return [str(root.val)]\n\n paths = []\n if root.left:\n paths += [str(root.val) + \'->\' + path for path in self.binaryTreePaths(root.left)]\n if root.right:\n paths += [str(root.val) + \'->\' + path for path in self.binaryTreePaths(root.right)]\n\n return paths\n``` | 3 | Given the `root` of a binary tree, return _all root-to-leaf paths in **any order**_.
A **leaf** is a node with no children.
**Example 1:**
**Input:** root = \[1,2,3,null,5\]
**Output:** \[ "1->2->5 ", "1->3 "\]
**Example 2:**
**Input:** root = \[1\]
**Output:** \[ "1 "\]
**Constraints:**
* The number of nodes in the tree is in the range `[1, 100]`.
* `-100 <= Node.val <= 100` | null |
✔️ [Python3] =͟͟͞͞( ✌°∀° )☛ ITERATIVE, Explained | add-digits | 0 | 1 | **UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.**\n\nThe simplest way to solve this problem is using bruteforce solution. We convert `num` into digits, sum them together and then repeate the process until the `num` becomes less than 10.\n\nTime: **O(ceil(log10(n))^2)** - for iteration over digits recursively\nSoace: **O(1)** - nothing is stored\n\nRuntime: 28 ms, faster than **94.22%** of Python3 online submissions for Add Digits.\nMemory Usage: 13.9 MB, less than **81.53%** of Python3 online submissions for Add Digits.\n\n```\ndef addDigits(self, num: int) -> int:\n\twhile num > 9:\n\t\tsum = 0\n\t\twhile num:\n\t\t\tsum += num%10\n\t\t\tnum = num//10\n\t\t\t\n\t\tnum = sum\n\n\treturn num\n```\n\n**UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.** | 54 | Given an integer `num`, repeatedly add all its digits until the result has only one digit, and return it.
**Example 1:**
**Input:** num = 38
**Output:** 2
**Explanation:** The process is
38 --> 3 + 8 --> 11
11 --> 1 + 1 --> 2
Since 2 has only one digit, return it.
**Example 2:**
**Input:** num = 0
**Output:** 0
**Constraints:**
* `0 <= num <= 231 - 1`
**Follow up:** Could you do it without any loop/recursion in `O(1)` runtime? | A naive implementation of the above process is trivial. Could you come up with other methods? What are all the possible results? How do they occur, periodically or randomly? You may find this Wikipedia article useful. |
"🔢✨ Mastering the Digital Root: Quick Trick to Summarize Digits Using 3 lines of code! 💡🧮"| O(1) | add-digits | 0 | 1 | # Intuition\nWhen solving this problem, my first intuition was to iterate through the digits of the number and sum them up until i get a single-digit result. However, upon further analysis and observation, I noticed patterns in the digital roots of numbers and come up with the congruence formula as an elegant way to calculate the digital root directly without the need for iteration. This formula simplifies the process and improves the time complexity to O(1).\n\n# Approach\nI used a simple formula to get the desired result. The formula is derived from the concept of congruence in modular arithmetic. It\'s a mathematical property that can be used to simplify the calculation of the digital root.\n\n# Complexity\n- Time complexity:\nO(1)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def addDigits(self, num: int) -> int:\n if num == 0:\n return 0\n return 1 + (num - 1) % 9\n\n``` | 2 | Given an integer `num`, repeatedly add all its digits until the result has only one digit, and return it.
**Example 1:**
**Input:** num = 38
**Output:** 2
**Explanation:** The process is
38 --> 3 + 8 --> 11
11 --> 1 + 1 --> 2
Since 2 has only one digit, return it.
**Example 2:**
**Input:** num = 0
**Output:** 0
**Constraints:**
* `0 <= num <= 231 - 1`
**Follow up:** Could you do it without any loop/recursion in `O(1)` runtime? | A naive implementation of the above process is trivial. Could you come up with other methods? What are all the possible results? How do they occur, periodically or randomly? You may find this Wikipedia article useful. |
[Java/C++/Python] Efficient Digital Root Calculation with Math Insights Explained | add-digits | 1 | 1 | # EXPLANATION\n1. **Divisibility by 9 Rule**:\n - Any number whose digits add up to 9 is divisible by 9.\n - Examples include 18, 27, 36, 45, 54, 63, 72, 81, 90, etc.\n2. **Digital Root for Divisible by 9 Numbers:**\n - The digital root (sum of digits until a single digit is obtained) for any number divisible by 9 is always 9.\n - Example: For 99, 9 + 9 = 18, and 1 + 8 = 9, confirming the digital root is always 9.\n3. **Digital Root for 0:**\n - The digital root of 0 is always 0.\n4. **Digital Root for Other Numbers:**\n - For numbers that aren\'t 0 and aren\'t divisible by 9, the digital root is obtained by taking the number modulo 9.\n - Formula: Digital Root = n mod 9\n5. **Examples of Modulo 9 Calculation:**\n - 100 mod 9 = 1 (one greater than 99, which is divisible by 9).\n - 101 mod 9 = 2\n - 102 mod 9 = 3 and so on\n6. **Optimizing the Digital Root Calculation:**\n - This method avoids the traditional approach of repeatedly adding digits until only one digit remains.\n - It provides a more efficient way to find the digital root, especially for larger numbers.\n7. **LeetCode Perspective:**\n - Acknowledges that understanding and applying such mathematical tricks is a valuable skill in solving problems on platforms like LeetCode.\n - Emphasizes that the given explanation may seem unrelated to the traditional method but showcases the importance of different problem-solving approaches and mathematical insights.\n\n###### Remember, these points collectively highlight the mathematical principles behind the digital root calculation, providing a concise and clear understanding of the process.\n\n\n# Java\n```\nclass Solution {\n public int addDigits(int num) {\n if(num == 0) {\n return 0;\n }\n else if(num % 9 == 0) {\n return 9;\n }\n else {\n return num % 9;\n }\n }\n}\n```\n\n# C++\n```\nclass Solution {\npublic:\n int addDigits(int num) {\n if(num == 0){\n return 0;\n }\n else if(num % 9 == 0) {\n return 9;\n }\n else return {\n num % 9;\n }\n }\n};\n```\n\n# Python\n```\nclass Solution:\n def addDigits(self, num: int) -> int:\n if num == 0 : return 0\n if num % 9 == 0 : return 9\n else : return (num % 9)\n```\n | 1 | Given an integer `num`, repeatedly add all its digits until the result has only one digit, and return it.
**Example 1:**
**Input:** num = 38
**Output:** 2
**Explanation:** The process is
38 --> 3 + 8 --> 11
11 --> 1 + 1 --> 2
Since 2 has only one digit, return it.
**Example 2:**
**Input:** num = 0
**Output:** 0
**Constraints:**
* `0 <= num <= 231 - 1`
**Follow up:** Could you do it without any loop/recursion in `O(1)` runtime? | A naive implementation of the above process is trivial. Could you come up with other methods? What are all the possible results? How do they occur, periodically or randomly? You may find this Wikipedia article useful. |
My easy to understand method, Python3 Array Usage | add-digits | 0 | 1 | \n# Code\n```\nclass Solution:\n def addDigits(self, num: int) -> int:\n if len(str(num)) == 1:\n return num\n q = [num]\n while len(str(q[-1])) != 1:\n c = 0\n for i in str(q[-1]):\n c += int(i)\n q.append(c)\n return q[-1]\n``` | 1 | Given an integer `num`, repeatedly add all its digits until the result has only one digit, and return it.
**Example 1:**
**Input:** num = 38
**Output:** 2
**Explanation:** The process is
38 --> 3 + 8 --> 11
11 --> 1 + 1 --> 2
Since 2 has only one digit, return it.
**Example 2:**
**Input:** num = 0
**Output:** 0
**Constraints:**
* `0 <= num <= 231 - 1`
**Follow up:** Could you do it without any loop/recursion in `O(1)` runtime? | A naive implementation of the above process is trivial. Could you come up with other methods? What are all the possible results? How do they occur, periodically or randomly? You may find this Wikipedia article useful. |
Recursive Python Solution | Beats 93% Runtime | add-digits | 0 | 1 | # Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def addDigits(self, num: int) -> int:\n if num >= 10:\n output = num // 10 + num % 10\n if output < 10:\n return output\n else:\n return self.addDigits(output)\n else:\n return num\n``` | 1 | Given an integer `num`, repeatedly add all its digits until the result has only one digit, and return it.
**Example 1:**
**Input:** num = 38
**Output:** 2
**Explanation:** The process is
38 --> 3 + 8 --> 11
11 --> 1 + 1 --> 2
Since 2 has only one digit, return it.
**Example 2:**
**Input:** num = 0
**Output:** 0
**Constraints:**
* `0 <= num <= 231 - 1`
**Follow up:** Could you do it without any loop/recursion in `O(1)` runtime? | A naive implementation of the above process is trivial. Could you come up with other methods? What are all the possible results? How do they occur, periodically or randomly? You may find this Wikipedia article useful. |
Python shortest 1-liner. | add-digits | 0 | 1 | # Approach\nTL;DR, Similar to the [Editorial solution](https://leetcode.com/problems/add-digits/editorial/) but condensed into short 1-liner.\n\n# Complexity\n- Time complexity: $$O(1)$$\n\n- Space complexity: $$O(1)$$\n\n# Code\n```python\nclass Solution:\n def addDigits(self, num: int) -> int:\n return mod(num, 9) or min(num, 9)\n\n\n``` | 2 | Given an integer `num`, repeatedly add all its digits until the result has only one digit, and return it.
**Example 1:**
**Input:** num = 38
**Output:** 2
**Explanation:** The process is
38 --> 3 + 8 --> 11
11 --> 1 + 1 --> 2
Since 2 has only one digit, return it.
**Example 2:**
**Input:** num = 0
**Output:** 0
**Constraints:**
* `0 <= num <= 231 - 1`
**Follow up:** Could you do it without any loop/recursion in `O(1)` runtime? | A naive implementation of the above process is trivial. Could you come up with other methods? What are all the possible results? How do they occur, periodically or randomly? You may find this Wikipedia article useful. |
[c++ & Python] brute force | add-digits | 0 | 1 | \n# Complexity\n- Time complexity: $$O(log10(n))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# C++\n```\nclass Solution {\npublic:\n int addDigits(int n) {\n int sum = 0;\n while(n) {\n sum += (n % 10);\n n /= 10;\n if(n == 0) {\n if(sum < 10) return sum;\n else {\n n = sum;\n sum = 0;\n }\n }\n }\n return 0;\n }\n};\n```\n\n# Python / Python3\n```\nclass Solution:\n def addDigits(self, n: int) -> int:\n sum = 0\n while n:\n sum += int(n % 10)\n n = int(n / 10)\n if n == 0 and sum < 10:\n return sum\n elif n == 0 and sum >= 10:\n n = sum\n sum = 0\n return 0\n``` | 1 | Given an integer `num`, repeatedly add all its digits until the result has only one digit, and return it.
**Example 1:**
**Input:** num = 38
**Output:** 2
**Explanation:** The process is
38 --> 3 + 8 --> 11
11 --> 1 + 1 --> 2
Since 2 has only one digit, return it.
**Example 2:**
**Input:** num = 0
**Output:** 0
**Constraints:**
* `0 <= num <= 231 - 1`
**Follow up:** Could you do it without any loop/recursion in `O(1)` runtime? | A naive implementation of the above process is trivial. Could you come up with other methods? What are all the possible results? How do they occur, periodically or randomly? You may find this Wikipedia article useful. |
Speed is higher than 95% in Python3 | add-digits | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def addDigits(self, num: int) -> int:\n while num // 10 > 0:\n sum = 0\n for i in str(num):\n sum += int(i)\n num = sum\n\n return num\n\n \n``` | 1 | Given an integer `num`, repeatedly add all its digits until the result has only one digit, and return it.
**Example 1:**
**Input:** num = 38
**Output:** 2
**Explanation:** The process is
38 --> 3 + 8 --> 11
11 --> 1 + 1 --> 2
Since 2 has only one digit, return it.
**Example 2:**
**Input:** num = 0
**Output:** 0
**Constraints:**
* `0 <= num <= 231 - 1`
**Follow up:** Could you do it without any loop/recursion in `O(1)` runtime? | A naive implementation of the above process is trivial. Could you come up with other methods? What are all the possible results? How do they occur, periodically or randomly? You may find this Wikipedia article useful. |
Three Language Python3,Go,Java | add-digits | 1 | 1 | # Python Solution\n```\nclass Solution:\n def addDigits(self, num: int) -> int:\n if num<10:\n return num\n if num%9==0:\n return 9\n return num%9\n```\n# Go Solution\n```\nfunc addDigits(num int) int {\n if num<10{\n return num\n }\n if num%9==0{\n return 9\n }\n return num%9\n \n}\n```\n# Java Solution\n```\nclass Solution {\n public int addDigits(int num) {\n if (num<10){\n return num;\n }\n if (num%9==0){\n return 9;\n }\n return num%9;\n \n }\n}\n```\n# please upvote me it would encourage me alot\n | 4 | Given an integer `num`, repeatedly add all its digits until the result has only one digit, and return it.
**Example 1:**
**Input:** num = 38
**Output:** 2
**Explanation:** The process is
38 --> 3 + 8 --> 11
11 --> 1 + 1 --> 2
Since 2 has only one digit, return it.
**Example 2:**
**Input:** num = 0
**Output:** 0
**Constraints:**
* `0 <= num <= 231 - 1`
**Follow up:** Could you do it without any loop/recursion in `O(1)` runtime? | A naive implementation of the above process is trivial. Could you come up with other methods? What are all the possible results? How do they occur, periodically or randomly? You may find this Wikipedia article useful. |
Python3 Alterative Counter solution One-Liner (Not for interview) | single-number-iii | 0 | 1 | \n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def singleNumber(self, nums: List[int]) -> List[int]:\n a = []\n for i, j in Counter(nums).items():\n if j == 1:\n a.append(i)\n return a\n```\n\n- One-Liner\n\n```\nreturn [i for i, j in Counter(nums).items() if j == 1]\n\n``` | 1 | Given an integer array `nums`, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. You can return the answer in **any order**.
You must write an algorithm that runs in linear runtime complexity and uses only constant extra space.
**Example 1:**
**Input:** nums = \[1,2,1,3,2,5\]
**Output:** \[3,5\]
**Explanation: ** \[5, 3\] is also a valid answer.
**Example 2:**
**Input:** nums = \[-1,0\]
**Output:** \[-1,0\]
**Example 3:**
**Input:** nums = \[0,1\]
**Output:** \[1,0\]
**Constraints:**
* `2 <= nums.length <= 3 * 104`
* `-231 <= nums[i] <= 231 - 1`
* Each integer in `nums` will appear twice, only two integers will appear once. | null |
😎Brute Force to Efficient Method🤩 | Java | C++ | Python | JavaScript | single-number-iii | 1 | 1 | Java\nC++\nPython\nJavaScript\n\n# Java\n# Java 1st Method :- Brute force\n1. Initialize an empty set or list to keep track of elements with counts.\n2. Iterate through the given array.\n3. For each element in the array:\n - If it\'s not in the set or list, add it.\n - If it\'s already in the set or list, remove it (because it appears twice).\n4. At the end of the iteration, you will be left with two unique elements in the set or list.\n5. Convert the set or list to an array and return the result.\n\n# Code\n``` Java []\nclass Solution {\n public int[] singleNumber(int[] nums) {\n Set<Integer> set = new HashSet<>();\n\n for (int num : nums) {\n if (set.contains(num)) {\n set.remove(num);\n } else {\n set.add(num);\n }\n }\n\n int[] result = new int[2];\n int index = 0;\n for (int num : set) {\n result[index++] = num;\n }\n \n return result;\n }\n}\n```\n# Complexity\n- Time complexity: O(n), where n is the number of elements in the array.\n\n- Space complexity: O(n)\n\n# Java 2nd Method :- Efficient Method\n> An efficient approach to solving this problem without using extra space is to use bitwise XOR. Here are the steps:\n\n1. Initialize two variables, `xor` and `mask`, to 0. These will be used to store the XOR result of the two unique numbers and a bitmask to separate them.\n2. Iterate through the given array.\n3. For each element in the array, calculate the XOR of `xor` and the element and update `xor`.\n4. Calculate the mask as the rightmost set bit in `xor`. This can be done by finding the rightmost 1-bit in `xor` and then creating a bitmask with only that bit set to 1.\n5. Initialize two variables, `result1` and `result2`, to 0. These will be used to store the two unique numbers.\n6. Iterate through the array again.\n7. For each element in the array, check if the `mask` bit is set. If it is, XOR it with `result1`; otherwise, XOR it with `result2`.\n8. After the iteration, `result1` and `result2` will contain the two unique numbers.\n9. Return an array containing `result1` and `result2`.\n\n# Code\n```Java []\nclass Solution {\n public int[] singleNumber(int[] nums) {\n int xor = 0;\n for (int num : nums) {\n xor ^= num;\n }\n \n int mask = xor & -xor;\n \n int result1 = 0, result2 = 0;\n for (int num : nums) {\n if ((num & mask) == 0) {\n result1 ^= num;\n } else {\n result2 ^= num;\n }\n }\n \n return new int[] { result1, result2 };\n }\n}\n```\n# Complexity\n- Time complexity: O(n), where n is the number of elements in the array.\n\n- Space complexity: O(1)\n---\n# C++ #\n# C++ 1st Method :- Brute force\n>The brute force approach involves using extra space to keep track of the counts of each number in the array and then iterating through the counts to find the two numbers that appear only once. Here are the steps:\n\n1. Create a hash map (unordered_map in C++) to store the count of each number in the array.\n2. Iterate through the input array, and for each number, increment its count in the hash map.\n3. Iterate through the hash map, and for each key-value pair, if the value is 1 (indicating the number appears only once), add the key to a result vector.\n4. Return the result vector containing the two distinct numbers.\n\n# Code\n``` C++ []\nclass Solution {\n public:\n vector<int> singleNumber(vector<int>& nums) {\n unordered_map<int, int> numCount;\n vector<int> result;\n\n // Count the occurrences of each number\n for (int num : nums) {\n numCount[num]++;\n }\n\n // Find the numbers that appear only once\n for (auto& pair : numCount) {\n if (pair.second == 1) {\n result.push_back(pair.first);\n }\n }\n\n return result;\n }\n\n};\n```\n# Complexity\n- Time complexity: O(n)\n- Space complexity: O(n)\n\n# C++ 2nd Method :- Efficient Method\n>The efficient approach takes advantage of bitwise XOR (^) to solve the problem in linear time complexity without using extra space. The key idea is to XOR all the numbers in the array, which will result in a XOR value that represents the XOR of the two distinct numbers. Here are the steps:\n\n1. Initialize a variable xorResult to 0.\n2. Iterate through the input array, and for each number, XOR it with xorResult.\n3. Find the rightmost set bit (1) in the xorResult. You can do this by finding the first bit position where xorResult & -xorResult is non-zero. This bit position will help separate the two distinct numbers.\n4. Initialize two variables, num1 and num2, to 0.\n5. Iterate through the input array again, and for each number:\n - Check if the rightmost set bit of the number is set (i.e., (num & mask) != 0, where mask represents the rightmost set bit).\n - If the rightmost set bit is set, XOR the number with num1.\n - If the rightmost set bit is not set, XOR the number with num2.\n6. num1 and num2 will now contain the two distinct numbers.\n\n# Code\n``` C++ []\nclass Solution {\n public:\n vector<int> singleNumber(vector<int>& nums) {\n int xorResult = 0;\n\n // XOR all numbers to find the XOR of the two distinct numbers\n for (int num : nums) {\n xorResult ^= num;\n }\n\n // Find the rightmost set bit\n int mask = xorResult & -xorResult;\n\n int num1 = 0, num2 = 0;\n\n // Separate the numbers based on the rightmost set bit\n for (int num : nums) {\n if ((num & mask) != 0) {\n num1 ^= num;\n } else {\n num2 ^= num;\n }\n }\n\n return {num1, num2};\n }\n};\n```\n# Complexity\n- Time complexity: O(n)\n- Space complexity: O(1)\n\n---\n# Python\n# Python 1st Method :- Brute force\n>The brute force approach involves using extra space to keep track of the counts of each number in the array and then iterating through the counts to find the two numbers that appear only once. Here are the steps:\n\n1. Create a dictionary (or defaultdict) to store the count of each number in the array.\n2. Iterate through the input array, and for each number, increment its count in the dictionary.\n3. Iterate through the dictionary, and for each key-value pair, if the value is 1 (indicating the number appears only once), add the key to a result list.\n4. Return the result list containing the two distinct numbers.\n\n# Code\n```Python []\nclass Solution:\n def singleNumber(self, nums: List[int]) -> List[int]:\n num_count = defaultdict(int)\n result = []\n\n # Count the occurrences of each number\n for num in nums:\n num_count[num] += 1\n\n # Find the numbers that appear only once\n for num, count in num_count.items():\n if count == 1:\n result.append(num)\n\n return result\n```\n\nWhile this brute force approach works, it uses extra space (the dictionary) and is not optimal in terms of space complexity.\n# Complexity\n- Time complexity: O(n)\n- Space complexity: O(n)\n\n# Python 2nd Method :- Efficient Method\n>The efficient approach takes advantage of bitwise XOR (^) to solve the problem in linear time complexity without using extra space. The key idea is to XOR all the numbers in the array, which will result in a XOR value that represents the XOR of the two distinct numbers. Here are the steps:\n\n1. Initialize a variable xor_result to 0.\n2. Iterate through the input array, and for each number, XOR it with xor_result.\n3. Find the rightmost set bit (1) in xor_result. You can do this by finding the first bit position where xor_result & -xor_result is non-zero. This bit position will help separate the two distinct numbers.\n4. Initialize two variables, num1 and num2, to 0.\n5. Iterate through the input array again, and for each number:\n - Check if the rightmost set bit of the number is set (i.e., (num & mask) != 0, where mask represents the rightmost set bit).\n - If the rightmost set bit is set, XOR the number with num1.\n - If the rightmost set bit is not set, XOR the number with num2.\n6. num1 and num2 will now contain the two distinct numbers.\n\n# Code\n```Python []\nclass Solution:\n def singleNumber(self, nums: List[int]) -> List[int]:\n xor_result = 0\n\n # XOR all numbers to find the XOR of the two distinct numbers\n for num in nums:\n xor_result ^= num\n\n # Find the rightmost set bit\n mask = xor_result & -xor_result\n\n num1, num2 = 0, 0\n\n # Separate the numbers based on the rightmost set bit\n for num in nums:\n if (num & mask) != 0:\n num1 ^= num\n else:\n num2 ^= num\n\n return [num1, num2]\n```\n# Complexity\n- Time complexity: O(n)\n- Space complexity: O(1)\n\n---\n# JavaScript\n# JS 1st Method :- Brute force\n>The brute force approach involves using extra space to keep track of the counts of each number in the array and then iterating through the counts to find the two numbers that appear only once. Here are the steps:\n\n1. Create an object or a Map to store the count of each number in the array.\n\n2. Iterate through the input array, and for each number, increment its count in the object or Map.\n\n3. Iterate through the object or Map, and for each key-value pair, if the value is 1 (indicating the number appears only once), add the key to a result array.\n\n4. Return the result array containing the two distinct numbers.\n\n# Code\n```JavaScript []\nvar singleNumber = function(nums) {\n const numCount = new Map();\n const result = [];\n\n // Count the occurrences of each number\n for (const num of nums) {\n if (numCount.has(num)) {\n numCount.set(num, numCount.get(num) + 1);\n } else {\n numCount.set(num, 1);\n }\n }\n\n // Find the numbers that appear only once\n for (const [key, value] of numCount.entries()) {\n if (value === 1) {\n result.push(key);\n }\n }\n\n return result;\n};\n```\n\n# Complexity\n- Time complexity: O(n)\n- Space complexity: O(n)\n- \n# JS 2nd Method :- Efficient Method\n>The efficient approach takes advantage of bitwise XOR (^) to solve the problem in linear time complexity without using extra space. The key idea is to XOR all the numbers in the array, which will result in a XOR value that represents the XOR of the two distinct numbers. Here are the steps:\n\n1. Initialize a variable `xorResult` to 0.\n2. Iterate through the input array, and for each number, XOR it with `xorResult`.\n3. Find the rightmost set bit (1) in the xorResult. You can do this by finding the first bit position where `xorResult & -xorResult` is non-zero. This bit position will help separate the two distinct numbers.\n4. Initialize two variables, `num1` and `num2`, to 0.\n5. Iterate through the input array again, and for each number:\n - Check if the rightmost set bit of the number is set (i.e., `(num & mask) !== 0`, where `mask` represents the rightmost set bit).\n - If the rightmost set bit is set, XOR the number with `num1`.\n - If the rightmost set bit is not set, XOR the number with `num2`.\n6. `num1` and `num2` will now contain the two distinct numbers.\n\n# Code\n``` JavaScript []\nvar singleNumber = function(nums) {\n let xorResult = 0;\n\n // XOR all numbers to find the XOR of the two distinct numbers\n for (const num of nums) {\n xorResult ^= num;\n }\n\n // Find the rightmost set bit\n const mask = xorResult & -xorResult;\n\n let num1 = 0, num2 = 0;\n\n // Separate the numbers based on the rightmost set bit\n for (const num of nums) {\n if ((num & mask) !== 0) {\n num1 ^= num;\n } else {\n num2 ^= num;\n }\n }\n\n return [num1, num2];\n};\n```\n# Complexity\n- Time complexity: O(n)\n- Space complexity: O(1) | 13 | Given an integer array `nums`, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. You can return the answer in **any order**.
You must write an algorithm that runs in linear runtime complexity and uses only constant extra space.
**Example 1:**
**Input:** nums = \[1,2,1,3,2,5\]
**Output:** \[3,5\]
**Explanation: ** \[5, 3\] is also a valid answer.
**Example 2:**
**Input:** nums = \[-1,0\]
**Output:** \[-1,0\]
**Example 3:**
**Input:** nums = \[0,1\]
**Output:** \[1,0\]
**Constraints:**
* `2 <= nums.length <= 3 * 104`
* `-231 <= nums[i] <= 231 - 1`
* Each integer in `nums` will appear twice, only two integers will appear once. | null |
Fastest And Smallest python code | single-number-iii | 0 | 1 | # Intuition\nBit Manipulation\n\n# Approach\nExplanation is too long I don\'t want to write it.\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def singleNumber(self, nums: List[int]) -> List[int]:\n p=0\n q=0\n for i in nums :\n p^=i;\n for i in nums:\n if ((p&(-p))&i)==0 :\n q^=i\n return [p^q,q];\n``` | 3 | Given an integer array `nums`, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. You can return the answer in **any order**.
You must write an algorithm that runs in linear runtime complexity and uses only constant extra space.
**Example 1:**
**Input:** nums = \[1,2,1,3,2,5\]
**Output:** \[3,5\]
**Explanation: ** \[5, 3\] is also a valid answer.
**Example 2:**
**Input:** nums = \[-1,0\]
**Output:** \[-1,0\]
**Example 3:**
**Input:** nums = \[0,1\]
**Output:** \[1,0\]
**Constraints:**
* `2 <= nums.length <= 3 * 104`
* `-231 <= nums[i] <= 231 - 1`
* Each integer in `nums` will appear twice, only two integers will appear once. | null |
Easy python solution for Beginners | single-number-iii | 0 | 1 | # Code\n```\nclass Solution:\n def singleNumber(self, nums: List[int]) -> List[int]:\n alt = []\n for num in nums:\n if num not in alt:\n alt.append(num)\n else:\n alt.remove(num)\n return alt\n``` | 1 | Given an integer array `nums`, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. You can return the answer in **any order**.
You must write an algorithm that runs in linear runtime complexity and uses only constant extra space.
**Example 1:**
**Input:** nums = \[1,2,1,3,2,5\]
**Output:** \[3,5\]
**Explanation: ** \[5, 3\] is also a valid answer.
**Example 2:**
**Input:** nums = \[-1,0\]
**Output:** \[-1,0\]
**Example 3:**
**Input:** nums = \[0,1\]
**Output:** \[1,0\]
**Constraints:**
* `2 <= nums.length <= 3 * 104`
* `-231 <= nums[i] <= 231 - 1`
* Each integer in `nums` will appear twice, only two integers will appear once. | null |
Python O(1) Space | Easy | Clean Code with explaination | SR | single-number-iii | 0 | 1 | **PLEASE DO UPVOTE IF YOU GET THIS**\n```\nimport math\nclass Solution:\n \'\'\'\n say nums = [1,2,1,3,2,5]\n x = XOR(nums) -> 6\n Binary form of 6 is 110\n\n find the first set bit position and divide the array into 2 based on that bit position\n why?\n Becoz 6 is the result obtained after 3^5 -> if we can throw these numbers into different parts \n then our smaller arrays looks like -> [find the number that will appear exactly once and \n remaining elements appear twice]\n\n Based on 2nd bit position if we partition the array it looks like\n\n Array1 -> [1,1,5]\n Array2 -> [2,3,2]\n\n Apply XOR for each part -> TADA!!!! We got what we want\n\n \'\'\'\n \n def checkBit(self, n, i):\n return (n & (1<<i))!=0\n \n def getSetBitPos(self, n):\n for i in range(32):\n if self.checkBit(n, i):\n return i\n \n def singleNumber(self, nums: List[int]) -> List[int]:\n x,n = 0,len(nums)\n if n==2:\n return nums\n \n for i in range(n):\n x^=nums[i]\n \n pos = self.getSetBitPos(x)\n x1,x2 = 0,0\n for i in range(n):\n if self.checkBit(nums[i],pos):\n x1^=nums[i]\n else:\n x2^=nums[i]\n \n return [x1, x2]\n```\n**PLEASE DO UPVOTE IF YOU GET THIS** | 24 | Given an integer array `nums`, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. You can return the answer in **any order**.
You must write an algorithm that runs in linear runtime complexity and uses only constant extra space.
**Example 1:**
**Input:** nums = \[1,2,1,3,2,5\]
**Output:** \[3,5\]
**Explanation: ** \[5, 3\] is also a valid answer.
**Example 2:**
**Input:** nums = \[-1,0\]
**Output:** \[-1,0\]
**Example 3:**
**Input:** nums = \[0,1\]
**Output:** \[1,0\]
**Constraints:**
* `2 <= nums.length <= 3 * 104`
* `-231 <= nums[i] <= 231 - 1`
* Each integer in `nums` will appear twice, only two integers will appear once. | null |
260: Time 90.93%, Solution with step by step explanation | single-number-iii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nWe can solve this problem in O(n) time complexity and O(1) space complexity by using bitwise operations.\n\n1. First, we XOR all the elements in the array to find the XOR of the two elements that appear only once. Let\'s call this XOR result as "x".\n\n2. Next, we find the rightmost set bit in the XOR result "x". We can do this by taking the two\'s complement of "x" and then doing a bitwise AND with "x". Let\'s call the result of this operation as "mask".\n\n3. We then iterate through the array and separate the elements into two groups: one group with elements having the bit at the "mask" position as 1, and the other group with elements having the bit at the "mask" position as 0.\n\n4. Finally, we XOR all the elements in each group separately to get the two elements that appear only once.\n\n# Complexity\n- Time complexity:\n90.93%\n\n- Space complexity:\n56.33%\n\n# Code\n```\nclass Solution:\n def singleNumber(self, nums: List[int]) -> List[int]:\n # Step 1: Find XOR of the two elements that appear only once\n x = 0\n for num in nums:\n x ^= num\n\n # Step 2: Find the rightmost set bit in the XOR result\n mask = x & -x\n \n # Step 3: Separate the elements into two groups\n group1, group2 = 0, 0\n for num in nums:\n if num & mask:\n group1 ^= num\n else:\n group2 ^= num\n \n # Step 4: XOR all the elements in each group separately\n return [group1, group2]\n\n``` | 8 | Given an integer array `nums`, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. You can return the answer in **any order**.
You must write an algorithm that runs in linear runtime complexity and uses only constant extra space.
**Example 1:**
**Input:** nums = \[1,2,1,3,2,5\]
**Output:** \[3,5\]
**Explanation: ** \[5, 3\] is also a valid answer.
**Example 2:**
**Input:** nums = \[-1,0\]
**Output:** \[-1,0\]
**Example 3:**
**Input:** nums = \[0,1\]
**Output:** \[1,0\]
**Constraints:**
* `2 <= nums.length <= 3 * 104`
* `-231 <= nums[i] <= 231 - 1`
* Each integer in `nums` will appear twice, only two integers will appear once. | null |
use bit operators | single-number-iii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n)\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def singleNumber(self, nums: List[int]) -> List[int]:\n if len(nums) == 2:\n return nums\n\n both = 0 \n for x in nums:\n # stores both unique num into variable `both` by xor operatons.\n # duplicate number will just cancel out each other, so only 2 unique numbers remain\n both ^= x \n\n lsb = both & -both # get the least significant bit\n n1 = 0 # try to find one number\n for x in nums:\n if lsb & x == 0: # found one uniq number\n n1 ^= x\n\n return [n1, both ^ n1]\n\n\n``` | 1 | Given an integer array `nums`, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. You can return the answer in **any order**.
You must write an algorithm that runs in linear runtime complexity and uses only constant extra space.
**Example 1:**
**Input:** nums = \[1,2,1,3,2,5\]
**Output:** \[3,5\]
**Explanation: ** \[5, 3\] is also a valid answer.
**Example 2:**
**Input:** nums = \[-1,0\]
**Output:** \[-1,0\]
**Example 3:**
**Input:** nums = \[0,1\]
**Output:** \[1,0\]
**Constraints:**
* `2 <= nums.length <= 3 * 104`
* `-231 <= nums[i] <= 231 - 1`
* Each integer in `nums` will appear twice, only two integers will appear once. | null |
Python Fast using counters | single-number-iii | 0 | 1 | ```\nclass Solution:\n def singleNumber(self, nums: List[int]) -> List[int]:\n x = Counter(nums)\n return([y for y in x if x[y] == 1])\n``` | 2 | Given an integer array `nums`, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. You can return the answer in **any order**.
You must write an algorithm that runs in linear runtime complexity and uses only constant extra space.
**Example 1:**
**Input:** nums = \[1,2,1,3,2,5\]
**Output:** \[3,5\]
**Explanation: ** \[5, 3\] is also a valid answer.
**Example 2:**
**Input:** nums = \[-1,0\]
**Output:** \[-1,0\]
**Example 3:**
**Input:** nums = \[0,1\]
**Output:** \[1,0\]
**Constraints:**
* `2 <= nums.length <= 3 * 104`
* `-231 <= nums[i] <= 231 - 1`
* Each integer in `nums` will appear twice, only two integers will appear once. | null |
PYTHON EASY SOLUTION || 100% | single-number-iii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: \n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def singleNumber(self, nums: List[int]) -> List[int]:\n res = []\n for i in set(nums):\n if len(res)<=2:\n if nums.count(i)==1:\n res.append(i)\n else:\n break\n return res\n``` | 1 | Given an integer array `nums`, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. You can return the answer in **any order**.
You must write an algorithm that runs in linear runtime complexity and uses only constant extra space.
**Example 1:**
**Input:** nums = \[1,2,1,3,2,5\]
**Output:** \[3,5\]
**Explanation: ** \[5, 3\] is also a valid answer.
**Example 2:**
**Input:** nums = \[-1,0\]
**Output:** \[-1,0\]
**Example 3:**
**Input:** nums = \[0,1\]
**Output:** \[1,0\]
**Constraints:**
* `2 <= nums.length <= 3 * 104`
* `-231 <= nums[i] <= 231 - 1`
* Each integer in `nums` will appear twice, only two integers will appear once. | null |
brute force with dictnory | single-number-iii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:n\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:n\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n\n def singleNumber(self, nums: List[int]) -> List[int]:\n dc=defaultdict(lambda:0)\n for a in(nums):\n dc[a]+=1\n ans=[]\n for a in dc:\n if(dc[a]==1):\n ans.append(a)\n return ans\n``` | 5 | Given an integer array `nums`, in which exactly two elements appear only once and all the other elements appear exactly twice. Find the two elements that appear only once. You can return the answer in **any order**.
You must write an algorithm that runs in linear runtime complexity and uses only constant extra space.
**Example 1:**
**Input:** nums = \[1,2,1,3,2,5\]
**Output:** \[3,5\]
**Explanation: ** \[5, 3\] is also a valid answer.
**Example 2:**
**Input:** nums = \[-1,0\]
**Output:** \[-1,0\]
**Example 3:**
**Input:** nums = \[0,1\]
**Output:** \[1,0\]
**Constraints:**
* `2 <= nums.length <= 3 * 104`
* `-231 <= nums[i] <= 231 - 1`
* Each integer in `nums` will appear twice, only two integers will appear once. | null |
Easy solution #Python3 | ugly-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isUgly(self, n: int) -> bool:\n if n <= 0:\n return False\n while n % 2 == 0:\n n //= 2\n while n % 3 == 0:\n n //= 3\n while n % 5 == 0:\n n //= 5\n return n == 1\n\n \n``` | 1 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
I think that it is only harinadh's version but it is actual version of all 🤣🤣 | ugly-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isUgly(self, n: int) -> bool:\n while n >= 1:\n if n%2 == 0:\n n=n//2\n elif n%3 == 0:\n n=n//3\n elif n%5 == 0:\n n=n//5\n elif n == 1:\n return True\n else:\n return False\n \n``` | 0 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
Beats 100% Easy To Understand 0ms | ugly-number | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nJust do dry run on any number, you will get the working of this code.\n\n# Complexity\n- Time complexity:\nO(N)\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution {\npublic:\n bool isUgly(int n) {\n if(n <= 0) return false;\n if(n <= 6) return true;\n\n ios_base::sync_with_stdio(false);\n cin.tie(0);\n cout.tie(0);\n\n while(n % 2 == 0) n /= 2;\n while(n % 3 == 0) n /= 3;\n while(n % 5 == 0) n /= 5;\n\n return (n == 1);\n }\n};\n``` | 3 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
Simple way, top 25% Python Solutions | ugly-number | 0 | 1 | \n# Code\n```\nclass Solution:\n def isUgly(self, n: int) -> bool:\n if n == 0:\n return False\n while n != 1:\n if n % 2 == 0:\n n /= 2\n elif n % 3 == 0:\n n /= 3\n elif n % 5 == 0:\n n /= 5\n else:\n return False\n return True\n``` | 2 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
263: Solution with step by step explanation | ugly-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nThe solution can be done by dividing the given number by 2, 3, or 5 as long as it is divisible, and then checking if the resulting number is 1 (which means it is an ugly number) or not.\n\nThe time complexity of this algorithm is O(log n), since we divide the input number by 2, 3, or 5 repeatedly until we get a result, which takes logarithmic time. The space complexity is O(1), since we are not using any additional data structures.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isUgly(self, n: int) -> bool:\n if n <= 0: # Non-positive numbers are not ugly\n return False\n while n % 2 == 0: # Check if n is divisible by 2, keep dividing until it\'s not\n n /= 2\n while n % 3 == 0: # Check if n is divisible by 3, keep dividing until it\'s not\n n /= 3\n while n % 5 == 0: # Check if n is divisible by 5, keep dividing until it\'s not\n n /= 5\n return n == 1 # If n is not 1, then it\'s not an ugly number\n\n``` | 5 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
Python ||Beats 99% ||Simple Math | ugly-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isUgly(self, n: int) -> bool:\n if n<=0:\n return False\n \n for i in [2,3,5]:\n while n%i == 0:\n n=n//i\n \n return n == 1\n``` | 2 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
Ugly Number | ugly-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isUgly(self, n: int) -> bool:\n if n == 0:\n return False\n res=[2, 3, 5]\n while n!= 1:\n for i in res:\n if n%i==0:\n n=n//i\n break\n else:\n return False\n return True\n``` | 1 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
Logarithmic Solution (Best approach) in PYTHON/PYTHON3 | ugly-number | 0 | 1 | \n\n# Approach\nThe solution can be done by dividing the given number by 2, 3, or 5 as long as it is divisible, and then checking if the resulting number is 1 (which means it is an ugly number) or not.\n\nThe time complexity of this algorithm is O(log n), since we divide the input number by 2, 3, or 5 repeatedly until we get a result, which takes logarithmic time. The space complexity is O(1), since we are not using any additional data structures.\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(nlogn)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isUgly(self, n: int) -> bool:\n if n==0:\n return 0\n for i in 2,3,5:\n while n%i==0:\n n//=i\n return n==1\n```\n\n# Upvote if you like the solution or ask if there is any query | 10 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
in log(n) time | ugly-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:log(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:1\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isUgly(self, n: int) -> bool:\n while(n%2==0 and n!=0):\n n=n//2\n while(n%3==0 and n!=0):\n n=n//3\n while(n%5==0 and n!=0):\n n=n//5\n return(n==1)\n``` | 9 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
2 Lines of Code--->Awesome Approach | ugly-number | 0 | 1 | # Math formula Approach\n```\nclass Solution:\n def isUgly(self, n: int) -> bool:\n if n<1:\n return False\n multiple=2*3*5\n return (multiple**20)%n==0\n\n //please upvote me it would encourage me alot\n\n\n```\n# Chack with each ugly factor\n```\nclass Solution:\n def isUgly(self, n: int) -> bool:\n if n<1: return False\n for num in [2,3,5]:\n while n%num==0:\n n=n//num\n return n==1\n\n //please upvote me it would encourage me alot\n\n```\n# please upvote me it would encourage me alot\n | 6 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
Python || Easy || 96.74% Faster || O(log n) Solution | ugly-number | 0 | 1 | ```\nfrom math import sqrt\nclass Solution:\n def isUgly(self, n: int) -> bool:\n if n<=0:\n return False\n while n%2==0 or n%3==0 or n%5==0:\n if n%2==0:\n n//=2\n elif n%3==0:\n n//=3\n elif n%5==0:\n n//=5\n if n==1:\n return True\n return False\n```\n\n**Upvote if you like the solution or ask if there is any query** | 2 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
Python 93.76% faster | Simplest solution with explanation | Beg to Adv | Math | ugly-number | 0 | 1 | ```python\nclass Solution:\n def isUgly(self, n: int) -> bool:\n \n prime = [2, 3, 5] # prime factors list provided in question againt which we have to check the provided number. \n \n if n == 0: # as we dont have factors for 0\n return False \n \n for p in prime: # traversing prime numbers from given prime number list.\n while n % p == 0: # here we`ll check if the number is having the factor or not. For instance 6%2==0 is true implies 2 is a factor of 6.\n n //= p # num = num//p # in this we`ll be having 3(6/2), 1(3/3). Doing this division to update our number\n return n == 1 # at last we`ll always have 1, if the number would have factors from the provided list \n```\n\nTo understand it in better way, following is the expansion way it works too:- \n```python\nclass Solution:\n def isUgly(self, n: int) -> bool:\n if n == 0: return False;\n while n%2 == 0:\n n /= 2\n while n%3 == 0:\n n /= 3\n while n%5 == 0:\n n /= 5\n return n == 1\n```\n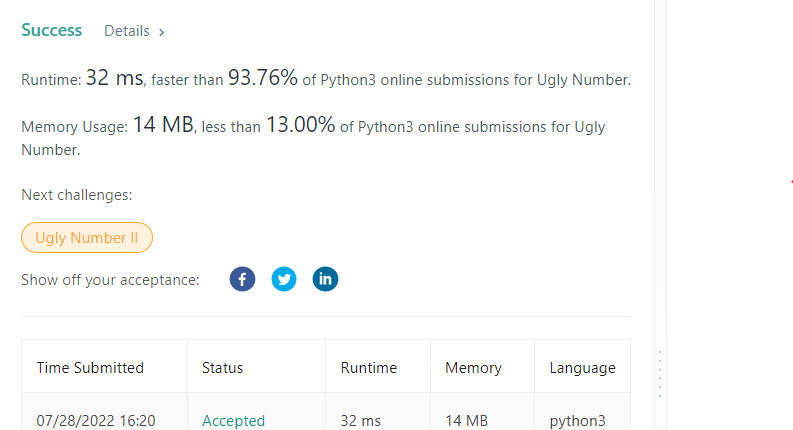\n | 3 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
Binary Search Solution beats 86.__% time and 96.__% memory | ugly-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport math\nclass Solution:\n def isUgly(self, n: int) -> bool:\n def binary(n, d, left = 0, right = 40):\n while left != right-1:\n mid = left + (right-left)//2\n if n%pow(d,mid) == 0:\n left = mid\n else:\n right = mid\n return left\n div = [2,3,5]\n for d in div:\n n = n/(pow(d,binary(n, d)))\n return n==1\n``` | 1 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
✔ Python3 | Faster solution , Basic logic with maths | ugly-number | 0 | 1 | ```\nclass Solution:\n def isUgly(self, n: int) -> bool:\n if n<=0: \n return False\n for i in [2,3,5]:\n while n%i==0:\n n=n//i\n return n==1\n``` | 17 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return `true` _if_ `n` _is an **ugly number**_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** 6 = 2 \* 3
**Example 2:**
**Input:** n = 1
**Output:** true
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Example 3:**
**Input:** n = 14
**Output:** false
**Explanation:** 14 is not ugly since it includes the prime factor 7.
**Constraints:**
* `-231 <= n <= 231 - 1` | null |
Dynamic Programming Logic Python3 | ugly-number-ii | 0 | 1 | \n\n# 1. Dynamic Programming\n```\nclass Solution:\n def nthUglyNumber(self, n: int) -> int:\n list1=[0]*n\n list1[0]=1\n a=b=c=0\n for i in range(1,n):\n list1[i]=min(list1[a]*2,list1[b]*3,list1[c]*5)\n if list1[a]*2==list1[i]:a+=1\n if list1[b]*3==list1[i]:b+=1\n if list1[c]*5==list1[i]:c+=1\n return list1[n-1]\n \n #please upvote me it would encourage me alot\n\n```\n\n# please upvote me it would encourage me alot\n | 27 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return _the_ `nth` _**ugly number**_.
**Example 1:**
**Input:** n = 10
**Output:** 12
**Explanation:** \[1, 2, 3, 4, 5, 6, 8, 9, 10, 12\] is the sequence of the first 10 ugly numbers.
**Example 2:**
**Input:** n = 1
**Output:** 1
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Constraints:**
* `1 <= n <= 1690` | The naive approach is to call isUgly for every number until you reach the nth one. Most numbers are not ugly. Try to focus your effort on generating only the ugly ones. An ugly number must be multiplied by either 2, 3, or 5 from a smaller ugly number. The key is how to maintain the order of the ugly numbers. Try a similar approach of merging from three sorted lists: L1, L2, and L3. Assume you have Uk, the kth ugly number. Then Uk+1 must be Min(L1 * 2, L2 * 3, L3 * 5). |
264: Solution with step by step explanation | ugly-number-ii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nWe will use a dynamic programming approach to solve this problem. We will start with the first ugly number, which is 1, and then generate all the other ugly numbers. We will use three pointers to keep track of the next multiple of 2, 3, and 5 respectively. We will generate the next ugly number by selecting the minimum of the three next multiples, adding it to the list of ugly numbers, and updating the corresponding pointer. We will repeat this process until we have generated the nth ugly number.\n\nThe time complexity of this algorithm is O(n), and the space complexity is O(n) as we need to store the list of n ugly numbers.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def nthUglyNumber(self, n: int) -> int:\n ugly = [1] # initialize list of ugly numbers with 1\n i2, i3, i5 = 0, 0, 0 # initialize pointers for next multiples of 2, 3, and 5 respectively\n while len(ugly) < n:\n # generate the next ugly number as the minimum of the next multiples of 2, 3, and 5\n next_ugly = min(ugly[i2]*2, ugly[i3]*3, ugly[i5]*5)\n # add the next ugly number to the list\n ugly.append(next_ugly)\n # update the corresponding pointer for the next multiple\n if next_ugly == ugly[i2]*2:\n i2 += 1\n if next_ugly == ugly[i3]*3:\n i3 += 1\n if next_ugly == ugly[i5]*5:\n i5 += 1\n return ugly[-1] # return the nth ugly number\n\n``` | 17 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return _the_ `nth` _**ugly number**_.
**Example 1:**
**Input:** n = 10
**Output:** 12
**Explanation:** \[1, 2, 3, 4, 5, 6, 8, 9, 10, 12\] is the sequence of the first 10 ugly numbers.
**Example 2:**
**Input:** n = 1
**Output:** 1
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Constraints:**
* `1 <= n <= 1690` | The naive approach is to call isUgly for every number until you reach the nth one. Most numbers are not ugly. Try to focus your effort on generating only the ugly ones. An ugly number must be multiplied by either 2, 3, or 5 from a smaller ugly number. The key is how to maintain the order of the ugly numbers. Try a similar approach of merging from three sorted lists: L1, L2, and L3. Assume you have Uk, the kth ugly number. Then Uk+1 must be Min(L1 * 2, L2 * 3, L3 * 5). |
Python || 93.80% Faster || Iterative Approach || O(n) Solution | ugly-number-ii | 0 | 1 | ```\nclass Solution:\n def nthUglyNumber(self, n: int) -> int:\n ans=[1]\n prod_2=prod_3=prod_5=0\n for i in range(1,n):\n a=ans[prod_2]*2\n b=ans[prod_3]*3\n c=ans[prod_5]*5\n m=min(a,b,c)\n ans.append(m)\n if m==a:\n prod_2+=1\n if m==b:\n prod_3+=1\n if m==c:\n prod_5+=1\n return ans[-1]\n```\n\n**Upvote if you like the solution or ask if there is any query** | 12 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return _the_ `nth` _**ugly number**_.
**Example 1:**
**Input:** n = 10
**Output:** 12
**Explanation:** \[1, 2, 3, 4, 5, 6, 8, 9, 10, 12\] is the sequence of the first 10 ugly numbers.
**Example 2:**
**Input:** n = 1
**Output:** 1
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Constraints:**
* `1 <= n <= 1690` | The naive approach is to call isUgly for every number until you reach the nth one. Most numbers are not ugly. Try to focus your effort on generating only the ugly ones. An ugly number must be multiplied by either 2, 3, or 5 from a smaller ugly number. The key is how to maintain the order of the ugly numbers. Try a similar approach of merging from three sorted lists: L1, L2, and L3. Assume you have Uk, the kth ugly number. Then Uk+1 must be Min(L1 * 2, L2 * 3, L3 * 5). |
[Python] Simple DP 9 Lines | ugly-number-ii | 0 | 1 | ```\nclass Solution:\n def nthUglyNumber(self, n: int) -> int:\n k = [0] * n\n t1 = t2 = t3 = 0\n k[0] = 1\n for i in range(1,n):\n k[i] = min(k[t1]*2,k[t2]*3,k[t3]*5)\n if(k[i] == k[t1]*2): t1 += 1\n if(k[i] == k[t2]*3): t2 += 1\n if(k[i] == k[t3]*5): t3 += 1\n return k[n-1]\n```\nRuntime: 72% | 34 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return _the_ `nth` _**ugly number**_.
**Example 1:**
**Input:** n = 10
**Output:** 12
**Explanation:** \[1, 2, 3, 4, 5, 6, 8, 9, 10, 12\] is the sequence of the first 10 ugly numbers.
**Example 2:**
**Input:** n = 1
**Output:** 1
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Constraints:**
* `1 <= n <= 1690` | The naive approach is to call isUgly for every number until you reach the nth one. Most numbers are not ugly. Try to focus your effort on generating only the ugly ones. An ugly number must be multiplied by either 2, 3, or 5 from a smaller ugly number. The key is how to maintain the order of the ugly numbers. Try a similar approach of merging from three sorted lists: L1, L2, and L3. Assume you have Uk, the kth ugly number. Then Uk+1 must be Min(L1 * 2, L2 * 3, L3 * 5). |
Min Heap || with Dry Run | ugly-number-ii | 0 | 1 | \n# Code\n```\nclass Solution:\n def nthUglyNumber(self, n: int) -> int:\n # take a min heap and insert 1 into it as \n #there is no prime factor for 1\n min_heap = [1] \n #take one variable which records no of variable which have been\n # recorded already\n seen = set([1]) \n prime_no = (2,3,5)\n #iterating n-1 times\n for i in range(n-1): \n ugly_no = heapq.heappop(min_heap)\n for ele in prime_no:\n no = ugly_no * ele\n if no not in seen:\n seen.add(no)\n heapq.heappush(min_heap, no)\n return min_heap[0]\n\n```\n\n# DRY RUN\n------iteration----no------- 0\nugly 1\nproduct 2\nseen [1, 2]\nminHeap [2]\nproduct 3\nseen [1, 2, 3]\nminHeap [2, 3]\nproduct 5\nseen [1, 2, 3, 5]\nminHeap [2, 3, 5]\n------iteration----no------- 1\nugly 2\nproduct 4\nseen [1, 2, 3, 5, 4]\nminHeap [3, 5, 4]\nproduct 6\nseen [1, 2, 3, 5, 4, 6]\nminHeap [3, 5, 4, 6]\nproduct 10\nseen [1, 2, 3, 5, 4, 6, 10]\nminHeap [3, 5, 4, 6, 10]\n------iteration----no------- 2\nugly 3\nproduct 6\nproduct 9\nseen [1, 2, 3, 5, 4, 6, 10, 9]\nminHeap [4, 5, 10, 6, 9]\nproduct 15\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15]\nminHeap [4, 5, 10, 6, 9, 15]\n------iteration----no------- 3\nugly 4\nproduct 8\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15, 8]\nminHeap [5, 6, 8, 15, 9, 10]\nproduct 12\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15, 8, 12]\nminHeap [5, 6, 8, 15, 9, 10, 12]\nproduct 20\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15, 8, 12, 20]\nminHeap [5, 6, 8, 15, 9, 10, 12, 20]\n------iteration----no------- 4\nugly 5\nproduct 10\nproduct 15\nproduct 25\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15, 8, 12, 20, 25]\nminHeap [6, 9, 8, 15, 20, 10, 12, 25]\n------iteration----no------- 5\nugly 6\nproduct 12\nproduct 18\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15, 8, 12, 20, 25, 18]\nminHeap [8, 9, 10, 15, 20, 25, 12, 18]\nproduct 30\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15, 8, 12, 20, 25, 18, 30]\nminHeap [8, 9, 10, 15, 20, 25, 12, 18, 30]\n------iteration----no------- 6\nugly 8\nproduct 16\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15, 8, 12, 20, 25, 18, 30, 16]\nminHeap [9, 15, 10, 16, 20, 25, 12, 30, 18]\nproduct 24\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15, 8, 12, 20, 25, 18, 30, 16, 24]\nminHeap [9, 15, 10, 16, 20, 25, 12, 30, 18, 24]\nproduct 40\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15, 8, 12, 20, 25, 18, 30, 16, 24, 40]\nminHeap [9, 15, 10, 16, 20, 25, 12, 30, 18, 24, 40]\n------iteration----no------- 7\nugly 9\nproduct 18\nproduct 27\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15, 8, 12, 20, 25, 18, 30, 16, 24, 40, 27]\nminHeap [10, 15, 12, 16, 20, 25, 40, 30, 18, 24, 27]\nproduct 45\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15, 8, 12, 20, 25, 18, 30, 16, 24, 40, 27, 45]\nminHeap [10, 15, 12, 16, 20, 25, 40, 30, 18, 24, 27, 45]\n------iteration----no------- 8\nugly 10\nproduct 20\nproduct 30\nproduct 50\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15, 8, 12, 20, 25, 18, 30, 16, 24, 40, 27, 45, 50]\nminHeap [12, 15, 25, 16, 20, 45, 40, 30, 18, 24, 27, 50]\n\n---------------------HERE IS THE FINAL OUTPUT-------------------------\noutput 12\nseen [1, 2, 3, 5, 4, 6, 10, 9, 15, 8, 12, 20, 25, 18, 30, 16, 24, 40, 27, 45, 50]\nminheap [12, 15, 25, 16, 20, 45, 40, 30, 18, 24, 27, 50] | 3 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return _the_ `nth` _**ugly number**_.
**Example 1:**
**Input:** n = 10
**Output:** 12
**Explanation:** \[1, 2, 3, 4, 5, 6, 8, 9, 10, 12\] is the sequence of the first 10 ugly numbers.
**Example 2:**
**Input:** n = 1
**Output:** 1
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Constraints:**
* `1 <= n <= 1690` | The naive approach is to call isUgly for every number until you reach the nth one. Most numbers are not ugly. Try to focus your effort on generating only the ugly ones. An ugly number must be multiplied by either 2, 3, or 5 from a smaller ugly number. The key is how to maintain the order of the ugly numbers. Try a similar approach of merging from three sorted lists: L1, L2, and L3. Assume you have Uk, the kth ugly number. Then Uk+1 must be Min(L1 * 2, L2 * 3, L3 * 5). |
simple O(n) solution | ugly-number-ii | 0 | 1 | # Complexity\n- Time complexity:$$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def nthUglyNumber(self, n: int) -> int:\n ans = [1]\n i2, i3, i5 = 0, 0, 0\n while n > 1:\n u2, u3, u5 = 2 * ans[i2], 3 * ans[i3], 5 * ans[i5]\n mini = min((u2, u3, u5))\n if mini == u2:\n i2 += 1\n if mini == u3:\n i3 += 1\n if mini == u5:\n i5 += 1\n ans.append(mini)\n n -= 1\n \n return ans[-1]\n``` | 2 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return _the_ `nth` _**ugly number**_.
**Example 1:**
**Input:** n = 10
**Output:** 12
**Explanation:** \[1, 2, 3, 4, 5, 6, 8, 9, 10, 12\] is the sequence of the first 10 ugly numbers.
**Example 2:**
**Input:** n = 1
**Output:** 1
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Constraints:**
* `1 <= n <= 1690` | The naive approach is to call isUgly for every number until you reach the nth one. Most numbers are not ugly. Try to focus your effort on generating only the ugly ones. An ugly number must be multiplied by either 2, 3, or 5 from a smaller ugly number. The key is how to maintain the order of the ugly numbers. Try a similar approach of merging from three sorted lists: L1, L2, and L3. Assume you have Uk, the kth ugly number. Then Uk+1 must be Min(L1 * 2, L2 * 3, L3 * 5). |
Python solution using dynamic programming | ugly-number-ii | 0 | 1 | ```\nclass Solution:\n def nthUglyNumber(self, n: int) -> int:\n dp=[1]\n \n p2,p3,p5=0,0,0\n \n for i in range(n+1):\n t2=dp[p2]*2\n t3=dp[p3]*3\n t5=dp[p5]*5\n \n temp=min(t2,t3,t5)\n \n dp.append(temp)\n \n if temp==dp[p2]*2:\n p2+=1\n if temp==dp[p3]*3:\n p3+=1\n if temp==dp[p5]*5:\n p5+=1\n # print(dp) \n return dp[n-1]\n \n``` | 4 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return _the_ `nth` _**ugly number**_.
**Example 1:**
**Input:** n = 10
**Output:** 12
**Explanation:** \[1, 2, 3, 4, 5, 6, 8, 9, 10, 12\] is the sequence of the first 10 ugly numbers.
**Example 2:**
**Input:** n = 1
**Output:** 1
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Constraints:**
* `1 <= n <= 1690` | The naive approach is to call isUgly for every number until you reach the nth one. Most numbers are not ugly. Try to focus your effort on generating only the ugly ones. An ugly number must be multiplied by either 2, 3, or 5 from a smaller ugly number. The key is how to maintain the order of the ugly numbers. Try a similar approach of merging from three sorted lists: L1, L2, and L3. Assume you have Uk, the kth ugly number. Then Uk+1 must be Min(L1 * 2, L2 * 3, L3 * 5). |
Python Heap Simple Solution | ugly-number-ii | 0 | 1 | # Intuition\nUse heap to store generated ugly numbers\n\n# Approach\ngenerate more ugly numbers with the min element from the heap, insert them into heap and append the min element to the n-ugly numbers list.(see code for better intuition)\n\n# Complexity\n- Time complexity:\nO(nlog(n))\n\n- Space complexity:\no(n)\n\n# Code\n```\nclass Solution:\n def nthUglyNumber(self, n: int) -> int:\n import heapq\n el=[]\n heapq.heapify(el)\n arr=[1]\n heapq.heappush(el,2)\n heapq.heappush(el,3)\n heapq.heappush(el,5)\n d={}\n while len(arr)<n:\n \n mini=heapq.heappop(el)\n if mini*2 not in d:\n heapq.heappush(el,mini*2)\n d[mini*2]=1\n if mini*3 not in d:\n heapq.heappush(el,mini*3)\n d[mini*3]=1 \n if mini*5 not in d:\n heapq.heappush(el,mini*5)\n d[mini*5]=1\n arr.append(mini)\n return arr[-1]\n \n \n\n\n``` | 2 | An **ugly number** is a positive integer whose prime factors are limited to `2`, `3`, and `5`.
Given an integer `n`, return _the_ `nth` _**ugly number**_.
**Example 1:**
**Input:** n = 10
**Output:** 12
**Explanation:** \[1, 2, 3, 4, 5, 6, 8, 9, 10, 12\] is the sequence of the first 10 ugly numbers.
**Example 2:**
**Input:** n = 1
**Output:** 1
**Explanation:** 1 has no prime factors, therefore all of its prime factors are limited to 2, 3, and 5.
**Constraints:**
* `1 <= n <= 1690` | The naive approach is to call isUgly for every number until you reach the nth one. Most numbers are not ugly. Try to focus your effort on generating only the ugly ones. An ugly number must be multiplied by either 2, 3, or 5 from a smaller ugly number. The key is how to maintain the order of the ugly numbers. Try a similar approach of merging from three sorted lists: L1, L2, and L3. Assume you have Uk, the kth ugly number. Then Uk+1 must be Min(L1 * 2, L2 * 3, L3 * 5). |
One liners | multiple solutions | | missing-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def missingNumber(self, nums: List[int]) -> int:\n # solution1\n nums = nums + list(range(0,len(nums)+1))\n ans = 0\n for i in nums:\n ans ^= i\n return ans\n\n # solution2\n # return (list(set(range(0,len(nums)+1)) - set(nums)) or [0])[0]\n\n # solution3\n # return sum(range(0,len(nums)+1)) -sum(nums)\n\n\n\n \n\n\n\n \n\n\n\n \n``` | 1 | Given an array `nums` containing `n` distinct numbers in the range `[0, n]`, return _the only number in the range that is missing from the array._
**Example 1:**
**Input:** nums = \[3,0,1\]
**Output:** 2
**Explanation:** n = 3 since there are 3 numbers, so all numbers are in the range \[0,3\]. 2 is the missing number in the range since it does not appear in nums.
**Example 2:**
**Input:** nums = \[0,1\]
**Output:** 2
**Explanation:** n = 2 since there are 2 numbers, so all numbers are in the range \[0,2\]. 2 is the missing number in the range since it does not appear in nums.
**Example 3:**
**Input:** nums = \[9,6,4,2,3,5,7,0,1\]
**Output:** 8
**Explanation:** n = 9 since there are 9 numbers, so all numbers are in the range \[0,9\]. 8 is the missing number in the range since it does not appear in nums.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 104`
* `0 <= nums[i] <= n`
* All the numbers of `nums` are **unique**.
**Follow up:** Could you implement a solution using only `O(1)` extra space complexity and `O(n)` runtime complexity? | null |
math | missing-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def missingNumber(self, nums: List[int]) -> int:\n # nums.sort()\n # for i,x in enumerate(nums):\n # if i != x:\n # return i\n # return len(nums)\n n=sum(nums)\n m=sum(range(0,len(nums)+1))\n return m-n\n``` | 6 | Given an array `nums` containing `n` distinct numbers in the range `[0, n]`, return _the only number in the range that is missing from the array._
**Example 1:**
**Input:** nums = \[3,0,1\]
**Output:** 2
**Explanation:** n = 3 since there are 3 numbers, so all numbers are in the range \[0,3\]. 2 is the missing number in the range since it does not appear in nums.
**Example 2:**
**Input:** nums = \[0,1\]
**Output:** 2
**Explanation:** n = 2 since there are 2 numbers, so all numbers are in the range \[0,2\]. 2 is the missing number in the range since it does not appear in nums.
**Example 3:**
**Input:** nums = \[9,6,4,2,3,5,7,0,1\]
**Output:** 8
**Explanation:** n = 9 since there are 9 numbers, so all numbers are in the range \[0,9\]. 8 is the missing number in the range since it does not appear in nums.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 104`
* `0 <= nums[i] <= n`
* All the numbers of `nums` are **unique**.
**Follow up:** Could you implement a solution using only `O(1)` extra space complexity and `O(n)` runtime complexity? | null |
Easy solution sum in Python3 | missing-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def missingNumber(self, nums: List[int]) -> int:\n return sum(range(0, len(nums) + 1)) - sum(nums)\n\n \n``` | 1 | Given an array `nums` containing `n` distinct numbers in the range `[0, n]`, return _the only number in the range that is missing from the array._
**Example 1:**
**Input:** nums = \[3,0,1\]
**Output:** 2
**Explanation:** n = 3 since there are 3 numbers, so all numbers are in the range \[0,3\]. 2 is the missing number in the range since it does not appear in nums.
**Example 2:**
**Input:** nums = \[0,1\]
**Output:** 2
**Explanation:** n = 2 since there are 2 numbers, so all numbers are in the range \[0,2\]. 2 is the missing number in the range since it does not appear in nums.
**Example 3:**
**Input:** nums = \[9,6,4,2,3,5,7,0,1\]
**Output:** 8
**Explanation:** n = 9 since there are 9 numbers, so all numbers are in the range \[0,9\]. 8 is the missing number in the range since it does not appear in nums.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 104`
* `0 <= nums[i] <= n`
* All the numbers of `nums` are **unique**.
**Follow up:** Could you implement a solution using only `O(1)` extra space complexity and `O(n)` runtime complexity? | null |
90% using Math, simple 1 line | missing-number | 0 | 1 | \n# Code\n```py\nclass Solution:\n def missingNumber(self, nums: List[int]) -> int:\n return sum(range(len(nums) + 1)) - sum(nums)\n\n```\n# Line by line\n```py\nclass Solution:\n def missingNumber(self, nums: List[int]) -> int:\n # Calculate the expected sum of numbers from 0 to len(nums)\n # using the sum function on a range that starts from 0 and goes up to len(nums).\n expected_sum = sum(range(len(nums) + 1))\n\n # Calculate the actual sum of numbers in the input list (nums) using the sum function.\n actual_sum = sum(nums)\n\n # Calculate the missing number by subtracting the actual sum from the expected sum.\n return expected_sum - actual_sum\n\n```\nupvote please, thanks\uD83D\uDC40 | 4 | Given an array `nums` containing `n` distinct numbers in the range `[0, n]`, return _the only number in the range that is missing from the array._
**Example 1:**
**Input:** nums = \[3,0,1\]
**Output:** 2
**Explanation:** n = 3 since there are 3 numbers, so all numbers are in the range \[0,3\]. 2 is the missing number in the range since it does not appear in nums.
**Example 2:**
**Input:** nums = \[0,1\]
**Output:** 2
**Explanation:** n = 2 since there are 2 numbers, so all numbers are in the range \[0,2\]. 2 is the missing number in the range since it does not appear in nums.
**Example 3:**
**Input:** nums = \[9,6,4,2,3,5,7,0,1\]
**Output:** 8
**Explanation:** n = 9 since there are 9 numbers, so all numbers are in the range \[0,9\]. 8 is the missing number in the range since it does not appear in nums.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 104`
* `0 <= nums[i] <= n`
* All the numbers of `nums` are **unique**.
**Follow up:** Could you implement a solution using only `O(1)` extra space complexity and `O(n)` runtime complexity? | null |
Easy One Line. Math solution. | missing-number | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n**Use math.**\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. We know that 1 number is missing, so let\'s assume that the length of the nums must be 1 more. \n2. Using a math formula we can find the sum of all elements with missing number. `n*(n+1)/2`\n3. Find the sum of all elements from the given array.\n4. Return the difference.\n# Complexity\n- Time complexity:$$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python []\nclass Solution:\n def missingNumber(self, nums: List[int]) -> int:\n N = len(nums)\n \n sum_original = N * (N + 1) / 2\n sum_given = sum(nums)\n\n return int(sum_original - sum_given)\n```\n```javascript []\nvar missingNumber = function(nums) {\n const n = nums.length;\n\n const sumOriginal = n * (n + 1) / 2;\n const sumGiven = nums.reduce((acc, current) => acc + current, 0);\n\n return sumOriginal - sumGiven;\n};\n```\n# One Line\n```\nreturn int((len(nums)*(len(nums)+1))/2 - sum(nums))\n```\n# Please UPVOTE | 6 | Given an array `nums` containing `n` distinct numbers in the range `[0, n]`, return _the only number in the range that is missing from the array._
**Example 1:**
**Input:** nums = \[3,0,1\]
**Output:** 2
**Explanation:** n = 3 since there are 3 numbers, so all numbers are in the range \[0,3\]. 2 is the missing number in the range since it does not appear in nums.
**Example 2:**
**Input:** nums = \[0,1\]
**Output:** 2
**Explanation:** n = 2 since there are 2 numbers, so all numbers are in the range \[0,2\]. 2 is the missing number in the range since it does not appear in nums.
**Example 3:**
**Input:** nums = \[9,6,4,2,3,5,7,0,1\]
**Output:** 8
**Explanation:** n = 9 since there are 9 numbers, so all numbers are in the range \[0,9\]. 8 is the missing number in the range since it does not appear in nums.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 104`
* `0 <= nums[i] <= n`
* All the numbers of `nums` are **unique**.
**Follow up:** Could you implement a solution using only `O(1)` extra space complexity and `O(n)` runtime complexity? | null |
Easy approach with O(n) and O(1) complexities | missing-number | 0 | 1 | # Intuition\n<!-- -->The numbers in array are unique and the range of numbers is between 0 to size of array\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nSo, a number is missing in range [0,n]\n\nMissing number is : (sum of all numbers [0 to n] ) - (sum of all numbers in array)\n\n\n# Complexity\n- Time complexity:\n O(n)\n\n- Space complexity:\n O(1) \n\n# Code\n```\nclass Solution:\n def missingNumber(self, nums: List[int]) -> int:\n n=len(nums)\n return ((n*(n+1))//2)-(sum(nums))\n```\n\nplease Upvote if you like | 10 | Given an array `nums` containing `n` distinct numbers in the range `[0, n]`, return _the only number in the range that is missing from the array._
**Example 1:**
**Input:** nums = \[3,0,1\]
**Output:** 2
**Explanation:** n = 3 since there are 3 numbers, so all numbers are in the range \[0,3\]. 2 is the missing number in the range since it does not appear in nums.
**Example 2:**
**Input:** nums = \[0,1\]
**Output:** 2
**Explanation:** n = 2 since there are 2 numbers, so all numbers are in the range \[0,2\]. 2 is the missing number in the range since it does not appear in nums.
**Example 3:**
**Input:** nums = \[9,6,4,2,3,5,7,0,1\]
**Output:** 8
**Explanation:** n = 9 since there are 9 numbers, so all numbers are in the range \[0,9\]. 8 is the missing number in the range since it does not appear in nums.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 104`
* `0 <= nums[i] <= n`
* All the numbers of `nums` are **unique**.
**Follow up:** Could you implement a solution using only `O(1)` extra space complexity and `O(n)` runtime complexity? | null |
Python Easy to understand 99.5% memory | missing-number | 0 | 1 | **Plz Upvote ..if you got help from this.**\n\n# Code\n```\nclass Solution:\n def missingNumber(self, nums: List[int]) -> int:\n nums.sort()\n\n if nums[0] != 0:\n return 0\n\n for i in range(len(nums)-1):\n if nums[i] + 1 == nums[i+1]:\n continue\n else:\n return nums[i] + 1\n\n # return nums[-1] -1 if nums[-1] == 1 and len(nums) == 1 else nums[-1] +1 \n return nums[-1] + 1\n``` | 3 | Given an array `nums` containing `n` distinct numbers in the range `[0, n]`, return _the only number in the range that is missing from the array._
**Example 1:**
**Input:** nums = \[3,0,1\]
**Output:** 2
**Explanation:** n = 3 since there are 3 numbers, so all numbers are in the range \[0,3\]. 2 is the missing number in the range since it does not appear in nums.
**Example 2:**
**Input:** nums = \[0,1\]
**Output:** 2
**Explanation:** n = 2 since there are 2 numbers, so all numbers are in the range \[0,2\]. 2 is the missing number in the range since it does not appear in nums.
**Example 3:**
**Input:** nums = \[9,6,4,2,3,5,7,0,1\]
**Output:** 8
**Explanation:** n = 9 since there are 9 numbers, so all numbers are in the range \[0,9\]. 8 is the missing number in the range since it does not appear in nums.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 104`
* `0 <= nums[i] <= n`
* All the numbers of `nums` are **unique**.
**Follow up:** Could you implement a solution using only `O(1)` extra space complexity and `O(n)` runtime complexity? | null |
very easy python solution, check it ! | missing-number | 0 | 1 | # Intuition\n\n# Approach\n1. calculate sum for given range(0 to n) using formula n * (n + 1)//2\n2. and subtract sum of elements of nums\n3. resulting difference is your number\n# Complexity\n- Time complexity:\nO(n) time,because needs to iterate through the entire List.\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def missingNumber(self, nums: List[int]) -> int:\n n = len(nums)\n total = n * (n + 1)//2\n nums_Sum = sum(nums)\n return total - nums_Sum\n\n \n``` | 0 | Given an array `nums` containing `n` distinct numbers in the range `[0, n]`, return _the only number in the range that is missing from the array._
**Example 1:**
**Input:** nums = \[3,0,1\]
**Output:** 2
**Explanation:** n = 3 since there are 3 numbers, so all numbers are in the range \[0,3\]. 2 is the missing number in the range since it does not appear in nums.
**Example 2:**
**Input:** nums = \[0,1\]
**Output:** 2
**Explanation:** n = 2 since there are 2 numbers, so all numbers are in the range \[0,2\]. 2 is the missing number in the range since it does not appear in nums.
**Example 3:**
**Input:** nums = \[9,6,4,2,3,5,7,0,1\]
**Output:** 8
**Explanation:** n = 9 since there are 9 numbers, so all numbers are in the range \[0,9\]. 8 is the missing number in the range since it does not appear in nums.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 104`
* `0 <= nums[i] <= n`
* All the numbers of `nums` are **unique**.
**Follow up:** Could you implement a solution using only `O(1)` extra space complexity and `O(n)` runtime complexity? | null |
✅ Python Easy One liners with Explanation | missing-number | 0 | 1 | 1. #### Gaus Formula\n\nWe can find the sum of first `n` numbers using [Gaus formula](https://nrich.maths.org/2478).\n```\n(n * (n+1))/2 where `n` is the length of input `nums`.\n```\nThen just subtract this with the sum of input `nums` to find the missing number.\n\n```\nclass Solution:\n def missingNumber(self, nums: List[int]) -> int:\n return (len(nums) * (len(nums) + 1))//2 - sum(nums)\n```\n\n---\n\n2. #### Bit Manipulation\n\nIn this approach, we will concat the input `nums` with the sequence of numbers from `[0, n]`. Then we would perform the reduce operation using an `xor` operator. The `xor` operation cancels the same elements in the list. So after the reduce operation, we would be left only with the missing number \n\n```\nclass Solution:\n def missingNumber(self, nums: List[int]) -> int:\n return reduce(lambda x,y: x ^ y, list(range(len(nums)+1)) + nums)\n```\n\n**Time - O(n)\nSpace - O(1)**\n\n---\n\n\n***Please upvote if you find it useful*** | 59 | Given an array `nums` containing `n` distinct numbers in the range `[0, n]`, return _the only number in the range that is missing from the array._
**Example 1:**
**Input:** nums = \[3,0,1\]
**Output:** 2
**Explanation:** n = 3 since there are 3 numbers, so all numbers are in the range \[0,3\]. 2 is the missing number in the range since it does not appear in nums.
**Example 2:**
**Input:** nums = \[0,1\]
**Output:** 2
**Explanation:** n = 2 since there are 2 numbers, so all numbers are in the range \[0,2\]. 2 is the missing number in the range since it does not appear in nums.
**Example 3:**
**Input:** nums = \[9,6,4,2,3,5,7,0,1\]
**Output:** 8
**Explanation:** n = 9 since there are 9 numbers, so all numbers are in the range \[0,9\]. 8 is the missing number in the range since it does not appear in nums.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 104`
* `0 <= nums[i] <= n`
* All the numbers of `nums` are **unique**.
**Follow up:** Could you implement a solution using only `O(1)` extra space complexity and `O(n)` runtime complexity? | null |
Solution | integer-to-english-words | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n string one(int num) {\n switch(num) {\n case 1: return "One";\n case 2: return "Two";\n case 3: return "Three";\n case 4: return "Four";\n case 5: return "Five";\n case 6: return "Six";\n case 7: return "Seven";\n case 8: return "Eight";\n case 9: return "Nine";\n }\n return "";\n }\n\n string twoLessThan20(int num) {\n switch(num) {\n case 10: return "Ten";\n case 11: return "Eleven";\n case 12: return "Twelve";\n case 13: return "Thirteen";\n case 14: return "Fourteen";\n case 15: return "Fifteen";\n case 16: return "Sixteen";\n case 17: return "Seventeen";\n case 18: return "Eighteen";\n case 19: return "Nineteen";\n }\n return "";\n }\n\n string ten(int num) {\n switch(num) {\n case 2: return "Twenty";\n case 3: return "Thirty";\n case 4: return "Forty";\n case 5: return "Fifty";\n case 6: return "Sixty";\n case 7: return "Seventy";\n case 8: return "Eighty";\n case 9: return "Ninety";\n }\n return "";\n }\n\n string two(int num) {\n if (num == 0)\n return "";\n else if (num < 10)\n return one(num);\n else if (num < 20)\n return twoLessThan20(num);\n else {\n int tenner = num / 10;\n int rest = num - tenner * 10;\n if (rest != 0)\n return ten(tenner) + " " + one(rest);\n else\n return ten(tenner);\n }\n }\n\n string three(int num) {\n int hundred = num / 100;\n int rest = num - hundred * 100;\n string res = "";\n if (hundred*rest != 0)\n res = one(hundred) + " Hundred " + two(rest);\n else if ((hundred == 0) && (rest != 0))\n res = two(rest);\n else if ((hundred != 0) && (rest == 0))\n res = one(hundred) + " Hundred";\n return res;\n }\n\n string numberToWords(int num) {\n if (num == 0)\n return "Zero";\n\n int billion = num / 1000000000;\n int million = (num - billion * 1000000000) / 1000000;\n int thousand = (num - billion * 1000000000 - million * 1000000) / 1000;\n int rest = num - billion * 1000000000 - million * 1000000 - thousand * 1000;\n\n string result = "";\n if (billion != 0)\n result = three(billion) + " Billion";\n if (million != 0) {\n if (result.size()!=0)\n result += " ";\n result += three(million) + " Million";\n }\n if (thousand != 0) {\n if (result.size()!=0)\n result += " ";\n result += three(thousand) + " Thousand";\n }\n if (rest != 0) {\n if (result.size()!=0)\n result += " ";\n result += three(rest);\n }\n return result;\n }\n\n};\n```\n\n```Python3 []\nclass Solution:\n def numberToWords(self, num: int) -> str:\n if num == 0:\n return "Zero"\n ones = ["", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine"]\n tens = ["", "Ten", "Twenty", "Thirty", "Forty", "Fifty", "Sixty", "Seventy", "Eighty", "Ninety"]\n teens = ["Ten", "Eleven", "Twelve", "Thirteen", "Fourteen", "Fifteen", "Sixteen", "Seventeen", "Eighteen", "Nineteen"]\n suffixes = ["", "Thousand", "Million", "Billion", "Trillion", "Quadrillion", "Quintillion", "Sextillion", "Septillion", "Octillion", "Nonillion", "Decillion"]\n words = []\n i = 0\n while num > 0:\n triplet = num % 1000\n num = num // 1000\n if triplet == 0:\n i += 1\n continue\n temp = []\n if triplet // 100 > 0:\n temp.append(ones[triplet // 100])\n temp.append("Hundred")\n if triplet % 100 >= 10 and triplet % 100 <= 19:\n temp.append(teens[triplet % 10])\n else:\n if triplet % 100 >= 20:\n temp.append(tens[triplet % 100 // 10])\n if triplet % 10 > 0:\n temp.append(ones[triplet % 10])\n if i > 0:\n temp.append(suffixes[i])\n words = temp + words\n i += 1\n return " ".join(words)\n```\n\n```Java []\nclass Solution {\n\n private final String[] belowTwenty = {"", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine",\n "Ten", "Eleven", "Twelve", "Thirteen", "Fourteen", "Fifteen", "Sixteen", "Seventeen", "Eighteen", "Nineteen"};\n private final String[] tens = {"", "", "Twenty", "Thirty", "Forty", "Fifty", "Sixty", "Seventy", "Eighty", "Ninety"};\n\n public String numberToWords(int num) {\n if (num == 0) {\n return "Zero";\n }\n return helper(num);\n }\n\n private String helper(int num) {\n StringBuilder result = new StringBuilder();\n if (num < 20) {\n result.append(belowTwenty[num]);\n } else if (num < 100) {\n result.append(tens[num / 10]).append(" ").append(belowTwenty[num % 10]);\n } else if (num < 1000) {\n result.append(helper(num / 100)).append(" Hundred ").append(helper(num % 100));\n } else if (num < 1000000) {\n result.append(helper(num / 1000)).append(" Thousand ").append(helper(num % 1000));\n } else if (num < 1000000000) {\n result.append(helper(num / 1000000)).append(" Million ").append(helper(num % 1000000));\n } else {\n result.append(helper(num / 1000000000)).append(" Billion ").append(helper(num % 1000000000));\n }\n return result.toString().trim();\n }\n}\n```\n | 436 | Convert a non-negative integer `num` to its English words representation.
**Example 1:**
**Input:** num = 123
**Output:** "One Hundred Twenty Three "
**Example 2:**
**Input:** num = 12345
**Output:** "Twelve Thousand Three Hundred Forty Five "
**Example 3:**
**Input:** num = 1234567
**Output:** "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven "
**Constraints:**
* `0 <= num <= 231 - 1` | Did you see a pattern in dividing the number into chunk of words? For example, 123 and 123000. Group the number by thousands (3 digits). You can write a helper function that takes a number less than 1000 and convert just that chunk to words. There are many edge cases. What are some good test cases? Does your code work with input such as 0? Or 1000010? (middle chunk is zero and should not be printed out) |
C++ || Recursion || Easiest Beginner Friendly Sol | integer-to-english-words | 1 | 1 | # Intuition of this Problem:\n<!-- Describe your first thoughts on how to solve this problem. -->\n**NOTE - PLEASE READ APPROACH FIRST THEN SEE THE CODE. YOU WILL DEFINITELY UNDERSTAND THE CODE LINE BY LINE AFTER SEEING THE APPROACH.**\n\n# Approach for this Problem:\n1. Declare three string arrays to hold words for numbers (0-19), ten-digit numbers (10-90), and thousands (1K, 1M, 1B).\n2. Define a helper function that takes an integer as input and returns its English representation.\n3. In the helper function, use a recursive approach to convert the input number into English words.\n4. Base cases:\n - a. If the number is less than 20, return its corresponding word from the ones array.\n - b. If the number is less than 100, return its corresponding word from the tens array plus a recursive call to the helper function with the remainder of the division by 10.\n - c. If the number is less than 1000, return its corresponding word from the ones array divided by 100, the word "Hundred", and a recursive call to the helper function with the remainder of the division by 100.\n - d. For larger numbers, loop through the thousands array from largest to smallest, and recursively call the helper function with the quotient of the input number divided by 1000 raised to the current index of the loop. Append the corresponding word from the thousands array to the result, and then recursively call the helper function with the remainder of the division by 1000 raised to the current index of the loop.\n1. In the numberToWords function, handle the edge case where the input number is zero. If it is, return the string "Zero". Otherwise, call the helper function with the input number, and remove the leading space using substr(1).\n2. Return the resulting string.\n<!-- Describe your approach to solving the problem. -->\n\n# Humble Request:\n- If my solution is helpful to you then please **UPVOTE** my solution, your **UPVOTE** motivates me to post such kind of solution.\n- Please let me know in comments if there is need to do any improvement in my approach, code....anything.\n- **Let\'s connect on** https://www.linkedin.com/in/abhinash-singh-1b851b188\n\n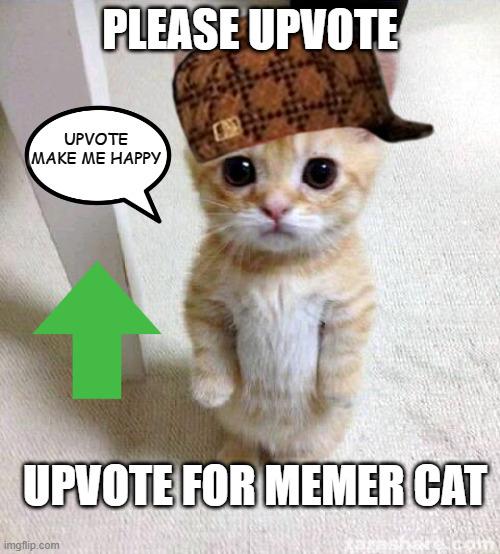\n\n# Code:\n```C++ []\nclass Solution {\npublic:\n //globally declare arrays\n string ones[20] = {"", " One", " Two", " Three", " Four", " Five", " Six", " Seven", " Eight", " Nine", " Ten", " Eleven", " Twelve", " Thirteen", " Fourteen", " Fifteen", " Sixteen", " Seventeen", " Eighteen", " Nineteen"};\n string tens[10] = {"", " Ten", " Twenty", " Thirty", " Forty", " Fifty", " Sixty", " Seventy", " Eighty", " Ninety"};\n string thousands[4] = {"", " Thousand", " Million", " Billion"};\n //helper function\n string helper(int n) {\n if (n < 20) \n return ones[n];\n if (n < 100) \n return tens[n / 10] + helper(n % 10);\n if (n < 1000) \n return helper(n / 100) + " Hundred" + helper(n % 100);\n for (int i = 3; i >= 0; i--) {\n if (n >= pow(1000, i)) {\n return helper(n / pow(1000, i)) + thousands[i] + helper(n % (int)pow(1000, i));\n }\n }\n return "";\n }\n\n string numberToWords(int num) {\n // edge case\n if (num == 0) \n return "Zero";\n return helper(num).substr(1);\n }\n};\n```\n```Java []\nclass Solution {\n //globally declare arrays\n String[] ones = {"", " One", " Two", " Three", " Four", " Five", " Six", " Seven", " Eight", " Nine", " Ten", " Eleven", " Twelve", " Thirteen", " Fourteen", " Fifteen", " Sixteen", " Seventeen", " Eighteen", " Nineteen"};\n String[] tens = {"", " Ten", " Twenty", " Thirty", " Forty", " Fifty", " Sixty", " Seventy", " Eighty", " Ninety"};\n String[] thousands = {"", " Thousand", " Million", " Billion"};\n //helper function\n public String helper(int n) {\n if (n < 20) \n return ones[n];\n if (n < 100) \n return tens[n / 10] + helper(n % 10);\n if (n < 1000) \n return helper(n / 100) + " Hundred" + helper(n % 100);\n for (int i = 3; i >= 0; i--) {\n if (n >= Math.pow(1000, i)) {\n return helper((int)(n / Math.pow(1000, i))) + thousands[i] + helper((int)(n % Math.pow(1000, i)));\n }\n }\n return "";\n }\n\n public String numberToWords(int num) {\n // edge case\n if (num == 0) \n return "Zero";\n return helper(num).substring(1);\n }\n}\n\n```\n```Python []\nclass Solution:\n def __init__(self):\n #initialize arrays\n self.ones = [\'\', \' One\', \' Two\', \' Three\', \' Four\', \' Five\', \' Six\', \' Seven\', \' Eight\', \' Nine\', \' Ten\', \' Eleven\', \' Twelve\', \' Thirteen\', \' Fourteen\', \' Fifteen\', \' Sixteen\', \' Seventeen\', \' Eighteen\', \' Nineteen\']\n self.tens = [\'\', \' Ten\', \' Twenty\', \' Thirty\', \' Forty\', \' Fifty\', \' Sixty\', \' Seventy\', \' Eighty\', \' Ninety\']\n self.thousands = [\'\', \' Thousand\', \' Million\', \' Billion\']\n \n def helper(self, n: int) -> str:\n if n < 20:\n return self.ones[n]\n elif n < 100:\n return self.tens[n // 10] + self.helper(n % 10)\n elif n < 1000:\n return self.helper(n // 100) + \' Hundred\' + self.helper(n % 100)\n else:\n for i in range(3, 0, -1):\n if n >= 1000 ** i:\n return self.helper(n // (1000 ** i)) + self.thousands[i] + self.helper(n % (1000 ** i))\n return \'\'\n \n def numberToWords(self, num: int) -> str:\n #edge case\n if num == 0:\n return \'Zero\'\n return self.helper(num).lstrip()\n\n```\n\n# Time Complexity and Space Complexity:\n- Time complexity: **O(log10(n))**, where n is the input number. This is because the number of recursive calls is proportional to the number of digits in the input number.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: **O(log10(n))**, where n is the input number. This is because the maximum amount of space used on the call stack is proportional to the number of digits in the input number.\n<!-- Add your space complexity here, e.g. $$O(n)$$ --> | 66 | Convert a non-negative integer `num` to its English words representation.
**Example 1:**
**Input:** num = 123
**Output:** "One Hundred Twenty Three "
**Example 2:**
**Input:** num = 12345
**Output:** "Twelve Thousand Three Hundred Forty Five "
**Example 3:**
**Input:** num = 1234567
**Output:** "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven "
**Constraints:**
* `0 <= num <= 231 - 1` | Did you see a pattern in dividing the number into chunk of words? For example, 123 and 123000. Group the number by thousands (3 digits). You can write a helper function that takes a number less than 1000 and convert just that chunk to words. There are many edge cases. What are some good test cases? Does your code work with input such as 0? Or 1000010? (middle chunk is zero and should not be printed out) |
Python 100% faster, simple elegant solution. | integer-to-english-words | 0 | 1 | # Intuition\nAt first glance, this problem seems complex due to how many edge cases we might find trying to represent numbers. For example, numbers from 1 to 99, like "Thirteen," have unique representations. For larger numbers, we use the same rules but add a quantity descriptor like \'Billion\' or \'Hundred\' (e.g., \'Twelve Billion Twenty Two Hundred\'). By understanding how to break down the integer \'num\' into three-digit segments and attach appropriate labels like \'Billion\', \'Million\', or \'Hundred\', we can approach this problem efficiently using modulus and division operations.\n\n# Approach\n## Representing Numbers\nThe first step is to decompose the problem into smaller, manageable subproblems. Our base case is numbers from 0 to 99, which form the foundation for larger numbers. A function is created to convert any integer value less than 99 into words. Additionally, a \'Hundreds\' function enhances code readability, though it\'s not mandatory for this approach.\n\n## Edge Cases\nSpecial attention is needed for numbers between 10 and 20, as they often present unique challenges. Arrays for units (0 - 9), teens (11 - 19), and tenths (10, 20 - 99) are utilized, applying logical indexing to select and append the correct strings.\n\n## Using Modulus and Division for Separation\nWe avoid converting the number into a string for splitting, which introduces unnecessary complexity. Instead, we utilize modulus for separation. For example, to extract \'3\' from \'143\', we use: `143 % 10 = 3`. Similarly, to extract \'123000\' from \'999123999\', we use modulus 1000000 and divide by 1000: `hundreds = (num % 1000000) // 1000`.\n\n## Main Function\nWe break the number into segments, then process these segments using our functions and append the appropriate scale (e.g., \'Millions\', \'Thousands\'). This method avoids redundancy and simplifies input handling.\n\n# Complexity\n- Time complexity: The code consists of straightforward conditional statements and operations, running in constant time **O(1)**.\n- Space complexity: The space used is proportional to the size of the output, so the complexity is **O(N)**.\n\n# Code\n```\nunits = ["", "One", "Two", "Three", "Four", "Five", "Six", \n"Seven", "Eight", "Nine"]\ntenths = [\n "", "Ten", "Twenty", "Thirty", "Forty", "Fifty", "Sixty",\n "Seventy", "Eighty", "Ninety"\n]\n\n# 1 -> 99\ndef sayTenths(x):\n unit = x % 10\n dec = x // 10\n\n if x < 10: return units[unit]\n if dec == 1:\n if x == 10: return "Ten"\n if x == 11: return "Eleven"\n if x == 12: return "Twelve"\n if x == 13: return "Thirteen"\n if x == 14: return "Fourteen"\n if x == 15: return "Fifteen"\n if x == 16: return "Sixteen"\n if x == 17: return "Seventeen"\n if x == 18: return "Eighteen"\n if x == 19: return "Nineteen"\n \n if unit > 0:\n return tenths[dec] + " " + units[unit]\n else:\n return tenths[dec]\n \n\n# 100 to 999\ndef sayHundreds(x):\n if x < 100: return sayTenths(x)\n if x == 0: return ""\n decs = x % 100\n if decs > 0:\n return sayTenths(x // 100) + " Hundred " + sayTenths(decs)\n else:\n return sayTenths(x // 100) + " Hundred"\n\nclass Solution:\n # 2,147,483,647\n def numberToWords(self, num: int) -> str:\n if num == 0: return "Zero"\n n = len(str(num))\n res = []\n if n > 9:\n bi = (num % 1000000000000) // 1000000000\n if bi != 0: \n res.append(sayTenths( bi ) + " Billion")\n if n > 6:\n thou = (num % 1000000000) // 1000000\n if thou != 0:\n res.append(sayHundreds( thou ) + " Million")\n if n > 3:\n hun = (num % 1000000) // 1000\n if hun != 0: \n res.append(sayHundreds( hun ) + " Thousand")\n if num % 1000 > 0:\n res.append(sayHundreds(num % 1000))\n\n return \' \'.join(filter(None, res))\n\n``` | 0 | Convert a non-negative integer `num` to its English words representation.
**Example 1:**
**Input:** num = 123
**Output:** "One Hundred Twenty Three "
**Example 2:**
**Input:** num = 12345
**Output:** "Twelve Thousand Three Hundred Forty Five "
**Example 3:**
**Input:** num = 1234567
**Output:** "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven "
**Constraints:**
* `0 <= num <= 231 - 1` | Did you see a pattern in dividing the number into chunk of words? For example, 123 and 123000. Group the number by thousands (3 digits). You can write a helper function that takes a number less than 1000 and convert just that chunk to words. There are many edge cases. What are some good test cases? Does your code work with input such as 0? Or 1000010? (middle chunk is zero and should not be printed out) |
Python || easy to understand | integer-to-english-words | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\n\nThe code you provided seems to be a solution for converting a given number into words based on the provided mapping. It follows a recursive approach to break down the number into its components and build the corresponding word representation.\n# Complexity\n- Time complexity: $$O(log(n))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(log(n))$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numberToWords(self, num: int) -> str:\n mapping = {\n 1000000000: \'Billion\',\n 1000000: \'Million\',\n 1000: \'Thousand\',\n 100: \'Hundred\',\n 90: \'Ninety\',\n 80: \'Eighty\',\n 70: \'Seventy\',\n 60: \'Sixty\',\n 50: \'Fifty\',\n 40: \'Forty\',\n 30: \'Thirty\',\n 20: \'Twenty\',\n 19: \'Nineteen\',\n 18: \'Eighteen\',\n 17: \'Seventeen\',\n 16: \'Sixteen\',\n 15: \'Fifteen\',\n 14: \'Fourteen\',\n 13: \'Thirteen\',\n 12: \'Twelve\',\n 11: \'Eleven\',\n 10: \'Ten\',\n 9: \'Nine\',\n 8: \'Eight\',\n 7: \'Seven\',\n 6: \'Six\',\n 5: \'Five\',\n 4: \'Four\',\n 3: \'Three\',\n 2: \'Two\',\n 1: \'One\'\n }\n if num==0:\n return "Zero"\n result=""\n \n for value, name in mapping.items():\n count=0\n if num>=value:\n count=num//value\n num%=value\n if count>1 or value>=100:\n result= result+" "+self.numberToWords(count)+" "+name\n else:\n result+=" "+name\n result=result[1:]\n return result\n\n\n``` | 7 | Convert a non-negative integer `num` to its English words representation.
**Example 1:**
**Input:** num = 123
**Output:** "One Hundred Twenty Three "
**Example 2:**
**Input:** num = 12345
**Output:** "Twelve Thousand Three Hundred Forty Five "
**Example 3:**
**Input:** num = 1234567
**Output:** "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven "
**Constraints:**
* `0 <= num <= 231 - 1` | Did you see a pattern in dividing the number into chunk of words? For example, 123 and 123000. Group the number by thousands (3 digits). You can write a helper function that takes a number less than 1000 and convert just that chunk to words. There are many edge cases. What are some good test cases? Does your code work with input such as 0? Or 1000010? (middle chunk is zero and should not be printed out) |
[Python] Solution with Interview Tip | integer-to-english-words | 0 | 1 | ```\nclass Solution:\n def numberToWords(self, num: int) -> str:\n ## RC ##\n ## APPROACH : BRUTE FORCE ##\n ## Main intent of the interviewer when asked this question is , he is testing how you are handling the test cases and how elagantly you are using sub problems to get to the final solution ## (so focus on edge cases)\n ## 1. For a two digit number if there is no ones digit 20 -> twenty + " " (number generally), dont leave the space behind, use if else case with "" (empty). Similarly for 20,000 etc.\n \n one_digit = {\n 1: \'One\',\n 2: \'Two\',\n 3: \'Three\',\n 4: \'Four\',\n 5: \'Five\',\n 6: \'Six\',\n 7: \'Seven\',\n 8: \'Eight\',\n 9: \'Nine\'\n }\n\n two_digit = {\n 10: \'Ten\',\n 11: \'Eleven\',\n 12: \'Twelve\',\n 13: \'Thirteen\',\n 14: \'Fourteen\',\n 15: \'Fifteen\',\n 16: \'Sixteen\',\n 17: \'Seventeen\',\n 18: \'Eighteen\',\n 19: \'Nineteen\'\n }\n \n tens = {\n 2: \'Twenty\',\n 3: \'Thirty\',\n 4: \'Forty\',\n 5: \'Fifty\',\n 6: \'Sixty\',\n 7: \'Seventy\',\n 8: \'Eighty\',\n 9: \'Ninety\'\n }\n \n def get_three_digit_num(num):\n if( not num ) : return ""\n if( not num// 100 ): return get_two_digit_num(num)\n return one_digit[ num // 100 ] + " Hundred" + ((" " + get_two_digit_num( num % 100 )) if( num % 100 ) else "")\n \n def get_two_digit_num( num ):\n if not num:\n return \'\'\n elif num < 10:\n return one_digit[ num ]\n elif num < 20:\n return two_digit[ num ]\n # edge case 1\n return tens[ num//10 ] + ((" " + one_digit[ num % 10 ]) if( num % 10 ) else "")\n \n # edge case\n if(num == 0): return "Zero"\n \n billion = num // 1000000000\n million = (num - billion * 1000000000) // 1000000\n thousand = (num - billion * 1000000000 - million * 1000000) // 1000\n last_three = num - billion * 1000000000 - million * 1000000 - thousand * 1000\n \n result = \'\'\n if billion: \n result = get_three_digit_num(billion) + \' Billion\'\n if million:\n # space only when prev result is not None\n result += \' \' if result else \'\' \n result += get_three_digit_num(million) + \' Million\'\n if thousand:\n result += \' \' if result else \'\'\n result += get_three_digit_num(thousand) + \' Thousand\'\n if last_three:\n result += \' \' if result else \'\'\n result += get_three_digit_num(last_three)\n return result\n``` | 36 | Convert a non-negative integer `num` to its English words representation.
**Example 1:**
**Input:** num = 123
**Output:** "One Hundred Twenty Three "
**Example 2:**
**Input:** num = 12345
**Output:** "Twelve Thousand Three Hundred Forty Five "
**Example 3:**
**Input:** num = 1234567
**Output:** "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven "
**Constraints:**
* `0 <= num <= 231 - 1` | Did you see a pattern in dividing the number into chunk of words? For example, 123 and 123000. Group the number by thousands (3 digits). You can write a helper function that takes a number less than 1000 and convert just that chunk to words. There are many edge cases. What are some good test cases? Does your code work with input such as 0? Or 1000010? (middle chunk is zero and should not be printed out) |
273: Time 86.78% and Space 94.53% , Solution with step by step explanation | integer-to-english-words | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nThis solution is similar to the previous one, but it defines the word lists and scales as instance variables of the class instead of local variables inside the function. It also defines a separate convert function to handle converting each group of three digits, and uses recursion to break down numbers greater than 100 into smaller parts. Finally, it removes any trailing spaces from the resulting string using the strip() method.\n\n# Complexity\n- Time complexity:\n86.78%\n\n- Space complexity:\n94.53%\n\n# Code\n```\nclass Solution:\n def numberToWords(self, num: int) -> str:\n if num == 0:\n return \'Zero\'\n \n # Define word lists for numbers and scales\n self.words1to19 = [\'\', \'One\', \'Two\', \'Three\', \'Four\', \'Five\', \'Six\', \'Seven\', \'Eight\', \'Nine\', \'Ten\', \'Eleven\', \'Twelve\', \'Thirteen\', \'Fourteen\', \'Fifteen\', \'Sixteen\', \'Seventeen\', \'Eighteen\', \'Nineteen\']\n self.words10to90 = [\'\', \'\', \'Twenty\', \'Thirty\', \'Forty\', \'Fifty\', \'Sixty\', \'Seventy\', \'Eighty\', \'Ninety\']\n self.scales = [\'\', \'Thousand\', \'Million\', \'Billion\']\n \n words = \'\'\n scaleIndex = 0\n while num > 0:\n if num % 1000 != 0:\n words = self.convert(num % 1000) + self.scales[scaleIndex] + \' \' + words\n num //= 1000\n scaleIndex += 1\n \n return words.strip()\n \n def convert(self, num):\n if num == 0:\n return \'\'\n elif num < 20:\n return self.words1to19[num] + \' \'\n elif num < 100:\n return self.words10to90[num // 10] + \' \' + self.convert(num % 10)\n else:\n return self.words1to19[num // 100] + \' Hundred \' + self.convert(num % 100)\n\n``` | 6 | Convert a non-negative integer `num` to its English words representation.
**Example 1:**
**Input:** num = 123
**Output:** "One Hundred Twenty Three "
**Example 2:**
**Input:** num = 12345
**Output:** "Twelve Thousand Three Hundred Forty Five "
**Example 3:**
**Input:** num = 1234567
**Output:** "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven "
**Constraints:**
* `0 <= num <= 231 - 1` | Did you see a pattern in dividing the number into chunk of words? For example, 123 and 123000. Group the number by thousands (3 digits). You can write a helper function that takes a number less than 1000 and convert just that chunk to words. There are many edge cases. What are some good test cases? Does your code work with input such as 0? Or 1000010? (middle chunk is zero and should not be printed out) |
Easy To Follow Recursive Python | integer-to-english-words | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1-2 digits: Tens\n3 digits: Hundreds\n4-6 digits: Thousands\n7-9 digits: Millions\n10 digits: Billions\n\nUse recursion. Base case is when digits == 2. \n=\n# Code\n```\nclass Solution:\n def numberToWords(self, num: int) -> str:\n to_19 = {0:\'Zero\', 1:\'One\',2:\'Two\',3:\'Three\',4:\'Four\', 5:\'Five\', 6:\'Six\', 7: \'Seven\', 8: \'Eight\', 9: \'Nine\', 10:\'Ten\', 11: \'Eleven\', 12: \'Twelve\', 13:\'Thirteen\', 14:"Fourteen",15:"Fifteen",16:"Sixteen",17:"Seventeen",18:"Eighteen",19:"Nineteen"}\n ty = {20: \'Twenty\', 30: \'Thirty\', 40: \'Forty\', 50: \'Fifty\', 60: \'Sixty\', 70: \'Seventy\', 80:\'Eighty\', 90:\'Ninety\'}\n digs = len(str(num))\n s = ""\n if digs<=2: #tens\n if num<=19:\n s+=to_19[num]\n elif 20<=num:\n if num%10==0:\n s+=ty[num]\n else:\n s+=ty[(num//10)*10]+ \' \' + to_19[num%10]\n elif digs == 3: #hundreds\n if num%100 == 0:\n return to_19[num//100]+\' \'+\'Hundred\'\n s += to_19[num//100] + \' \' + \'Hundred\' + \' \' + self.numberToWords(num-(num//100)*100)\n elif 4<=digs<=6: #thousands\n if num%1000 == 0:\n return self.numberToWords(num//1000) + \' \' + \'Thousand\'\n s += self.numberToWords(num//1000)+ \' \' + \'Thousand\' + \' \' + self.numberToWords(num-(num//1000)*1000)\n elif 7<=digs<=9: #millions\n if num%1000000 == 0:\n return self.numberToWords(num//1000000)+\' \'+\'Million\'\n s += self.numberToWords(num//1000000)+ \' \' + \'Million\' + \' \' + self.numberToWords(num-(num//1000000)*1000000)\n elif digs == 10: #billions\n if num%1000000000 == 0:\n return to_19[num//1000000000]+\' \'+\'Billion\'\n s += to_19[num//1000000000]+\' \'+ \'Billion\' + \' \'+ self.numberToWords(num-(num//1000000000)*1000000000)\n return s\n``` | 1 | Convert a non-negative integer `num` to its English words representation.
**Example 1:**
**Input:** num = 123
**Output:** "One Hundred Twenty Three "
**Example 2:**
**Input:** num = 12345
**Output:** "Twelve Thousand Three Hundred Forty Five "
**Example 3:**
**Input:** num = 1234567
**Output:** "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven "
**Constraints:**
* `0 <= num <= 231 - 1` | Did you see a pattern in dividing the number into chunk of words? For example, 123 and 123000. Group the number by thousands (3 digits). You can write a helper function that takes a number less than 1000 and convert just that chunk to words. There are many edge cases. What are some good test cases? Does your code work with input such as 0? Or 1000010? (middle chunk is zero and should not be printed out) |
[Java/C++/Python/JavaScript/Kotlin/Swift]O(n)time/BEATS 99.97% MEMORY/SPEED 0ms APRIL 2022 | integer-to-english-words | 1 | 1 | ***Hello it would be my pleasure to introduce myself Darian.***\n\n***Java***\n```\nclass Solution {\n String[] tens = {"", "Ten", "Twenty", "Thirty", "Forty", "Fifty", "Sixty", "Seventy", "Eighty", "Ninety"};\n String[] ones = {"", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Eleven",\n "Twelve", "Thirteen", "Fourteen", "Fifteen", "Sixteen", "Seventeen", "Eighteen", "Nineteen"};\n\n public String numberToWords(int num) {\n if (num == 0)\n return "Zero";\n \n return helper(num).trim();\n }\n \n private String helper(int num) {\n StringBuilder sb=new StringBuilder();\n if (num >= 1000000000) {\n sb.append(helper(num / 1000000000)).append(" Billion ").append( helper(num % 1000000000));\n } else if (num >= 1000000) {\n sb.append(helper(num / 1000000)).append(" Million ").append( helper(num % 1000000));\n }else if (num >= 1000) {\n sb.append(helper(num / 1000)).append(" Thousand ").append( helper(num % 1000));\n }else if (num >= 100) {\n sb.append(helper(num / 100)).append(" Hundred ").append( helper(num % 100));\n }else if (num >= 20) {\n sb.append(tens[num / 10]).append(" ").append( helper(num % 10));\n } else {\n sb.append(ones[num]);\n }\n return sb.toString().trim();\n \n }\n}\n```\n\n***C++***\n```\nclass Solution {\npublic:\n \n string ones[21] = {"Zero", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Eleven", "Twelve", "Thirteen", "Fourteen", "Fifteen", "Sixteen", "Seventeen", "Eighteen", "Nineteen", "Twenty"};\n \n string tens[10] = {"##", "Ten", "Twenty", "Thirty", "Forty", "Fifty", "Sixty", "Seventy", "Eighty", "Ninety"};\n \n string pref[4] = {"##", "Thousand", "Million", "Billion"};\n \n const int billion = 1000000000;\n const int million = 1000000;\n const int thousand = 1000;\n \n string oneDigit(int num)\n {\n return (num ? ones[num] + " " : "");\n }\n \n string twoDigit(int num)\n {\n if(num == 0)\n return "";\n if(num <= 20)\n return ones[num] + " ";\n else\n return tens[num/10] + " " + (num % 10 ? ones[num%10] + " " : "");\n }\n \n string threeDigit(int num)\n {\n if(num == 0)\n return "";\n if(num / 10 == 0)\n return oneDigit(num);\n if(num / 100 == 0)\n return twoDigit(num);\n \n return ones[num/100] + " Hundred " + twoDigit(num%100);\n }\n \n string convert(int num)\n { \n if(num == 0)\n return "";\n if(num/billion)\n return threeDigit(num/billion) + "Billion " + convert(num%billion);\n if(num/million)\n return threeDigit(num/million) + "Million " + convert(num%million);\n if(num/thousand)\n return threeDigit(num/thousand) + "Thousand " + convert(num%thousand);\n return threeDigit(num);\n }\n string numberToWords(int num)\n {\n if(num == 0)\n return "Zero";\n string ans = convert(num);\n ans.pop_back();\n return ans;\n }\n};\n```\n\n***Python***\n```\nclass Solution:\n def numberToWords(self, num: int) -> str:\n mp = {1: "One", 11: "Eleven", 10: "Ten", \n 2: "Two", 12: "Twelve", 20: "Twenty", \n 3: "Three", 13: "Thirteen", 30: "Thirty", \n 4: "Four", 14: "Fourteen", 40: "Forty",\n 5: "Five", 15: "Fifteen", 50: "Fifty", \n 6: "Six", 16: "Sixteen", 60: "Sixty", \n 7: "Seven", 17: "Seventeen", 70: "Seventy", \n 8: "Eight", 18: "Eighteen", 80: "Eighty",\n 9: "Nine", 19: "Nineteen", 90: "Ninety"}\n \n def fn(n):\n """Return English words of n (0-999) in array."""\n if not n: return []\n elif n < 20: return [mp[n]]\n elif n < 100: return [mp[n//10*10]] + fn(n%10)\n else: return [mp[n//100], "Hundred"] + fn(n%100)\n \n ans = []\n for i, unit in zip((9, 6, 3, 0), ("Billion", "Million", "Thousand", "")): \n n, num = divmod(num, 10**i)\n ans.extend(fn(n))\n if n and unit: ans.append(unit)\n return " ".join(ans) or "Zero"\n```\n\n***JavaScript***\n```\nvar numberToWords = function(num) {\n if (num === 0) {\n return \'Zero\';\n }\n \n if (num <= 20) {\n return translations.get(num);\n }\n \n let result = [];\n \n for (let [value, translation] of translations) {\n const times = Math.floor(num / value);\n \n if (times === 0) {\n continue;\n }\n \n num -= times * value;\n \n if (times === 1 && value >= 100) {\n result.push(\'One\', translation);\n continue;\n }\n \n if (times === 1) {\n result.push(translation);\n continue;\n }\n \n result.push(numberToWords(times), translation);\n }\n \n return result.join(\' \');\n};\n\nconst translations = new Map([\n [1000000000, \'Billion\'],\n [1000000, \'Million\'],\n [1000, \'Thousand\'],\n [100, \'Hundred\'],\n [90, \'Ninety\'],\n [80, \'Eighty\'],\n [70, \'Seventy\'],\n [60, \'Sixty\'],\n [50, \'Fifty\'],\n [40, \'Forty\'],\n [30, \'Thirty\'],\n [20, \'Twenty\'],\n [19, \'Nineteen\'],\n [18, \'Eighteen\'],\n [17, \'Seventeen\'],\n [16, \'Sixteen\'],\n [15, \'Fifteen\'],\n [14, \'Fourteen\'],\n [13, \'Thirteen\'],\n [12, \'Twelve\'],\n [11, \'Eleven\'],\n [10, \'Ten\'],\n [9, \'Nine\'],\n [8, \'Eight\'],\n [7, \'Seven\'],\n [6, \'Six\'],\n [5, \'Five\'],\n [4, \'Four\'],\n [3, \'Three\'],\n [2, \'Two\'],\n [1, \'One\'],\n]);\n```\n\n***Kotlin***\n```\nclass Solution {\n fun numberToWords(num: Int): String {\n if (num == 0) {\n return "Zero"\n }\n \n return helper(num)\n }\n \n private fun lessTwenty(num: Int): String {\n return when(num) {\n 1 -> "One"\n 2 -> "Two"\n 3 -> "Three"\n 4 -> "Four"\n 5 -> "Five"\n 6 -> "Six"\n 7 -> "Seven"\n 8 -> "Eight"\n 9 -> "Nine"\n 10 -> "Ten"\n 11 -> "Eleven"\n 12 -> "Twelve"\n 13 -> "Thirteen"\n 14 -> "Fourteen"\n 15 -> "Fifteen"\n 16 -> "Sixteen"\n 17 -> "Seventeen"\n 18 -> "Eighteen"\n 19 -> "Nineteen"\n else -> ""\n }\n }\n \n private fun tens(num: Int): String {\n return when(num) {\n 20 -> "Twenty"\n 30 -> "Thirty"\n 40 -> "Forty"\n 50 -> "Fifty"\n 60 -> "Sixty"\n 70 -> "Seventy"\n 80 -> "Eighty"\n 90 -> "Ninety"\n else -> ""\n }\n }\n \n private fun helper(num: Int): String {\n var ans = "" // Kotlin uses StringBuilder under the hood\n \n if (num >= 1_000_000_000) {\n ans += helper(num / 1_000_000_000) + " Billion " + helper(num % 1_000_000_000)\n } else if (num >= 1_000_000) {\n ans += helper(num / 1_000_000) + " Million " + helper(num % 1_000_000)\n } else if (num >= 1_000) {\n ans += helper(num / 1_000) + " Thousand " + helper(num % 1_000)\n } else if (num >= 100) {\n ans += helper(num / 100) + " Hundred " + helper(num % 100)\n } else if (num >= 20) {\n ans += tens(num - (num % 10)) + " " + lessTwenty(num % 10)\n } else if (num < 20) {\n ans += lessTwenty(num)\n }\n \n return ans.trim()\n }\n}\n```\n\n***Swift***\n```\nclass Solution {\n \n private let kAtomicWords = [1:"One",2:"Two",3:"Three",4:"Four",5:"Five",6:"Six", \n 7:"Seven",8:"Eight",9:"Nine",10:"Ten",11:"Eleven",\n 12:"Twelve",13:"Thirteen",14:"Fourteen",15:"Fifteen",\n 16:"Sixteen",17:"Seventeen",18:"Eighteen",19:"Nineteen",\n 20:"Twenty",30:"Thirty",40:"Forty",50:"Fifty",60:"Sixty",\n 70:"Seventy",80:"Eighty",90:"Ninety"]\n\n\tprivate let kAtomicPowers = [100: "Hundred",\n 1_000: "Thousand",\n 1_000_000: "Million",\n 1_000_000_000: "Billion",\n 1_000_000_000_000: "Trillion"]\n\n func numberToWords(_ num: Int) -> String {\n guard num != 0 else { return "Zero" }\n // Remove nils and join by " "\n\t return wordFor(num).flatMap{$0}.joined(separator: " ")\n }\n\n /// Recursively build\n private func wordFor(_ num: Int) -> [String?] {\n var result = [String?]()\n \n\t // 0-19\n\t if num < 20 {\n\t\t result.append(kAtomicWords[num])\n }\n // 20-99\n else if num < 100 {\n\t let leastSig = num % 10\n\t let mostSig = (num/10) * 10\n result.append(kAtomicWords[mostSig])\n if leastSig > 0 {\n result.append(kAtomicWords[leastSig])\n }\n }\n // 1Y xx - 1YY xxx xxx xxx xxx\n else {\n\t // get largest power of number\n\t var power = 100\n\t while (num / (power*10)) > 0 {\n\t\t power *= 10\n }\n // divide by 10 until we find closest atomic word power\n\t\t\twhile kAtomicPowers[power] == nil {\n\t\t\t\tpower /= 10\n }\n \n\t var mostSig = num / power\n\t let remainder = num - (mostSig * power)\n \n result.append(contentsOf: wordFor(mostSig))\n result.append(kAtomicPowers[power])\n result.append(contentsOf: wordFor(remainder))\n }\n \n return result\n }\n}\n```\n\n***Consider upvote if useful! Hopefully it can be used in your advantage!***\n***Take care brother, peace, love!***\n***"Open your eyes."*** | 12 | Convert a non-negative integer `num` to its English words representation.
**Example 1:**
**Input:** num = 123
**Output:** "One Hundred Twenty Three "
**Example 2:**
**Input:** num = 12345
**Output:** "Twelve Thousand Three Hundred Forty Five "
**Example 3:**
**Input:** num = 1234567
**Output:** "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven "
**Constraints:**
* `0 <= num <= 231 - 1` | Did you see a pattern in dividing the number into chunk of words? For example, 123 and 123000. Group the number by thousands (3 digits). You can write a helper function that takes a number less than 1000 and convert just that chunk to words. There are many edge cases. What are some good test cases? Does your code work with input such as 0? Or 1000010? (middle chunk is zero and should not be printed out) |
PYTHON SOLUTION || EASY_TO_UNDERSTAND | integer-to-english-words | 0 | 1 | ```\nclass Solution:\n def numberToWords(self, num: int) -> str:\n if num==0:\n return "Zero"\n belowTwenty = ["", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight",\n "Nine", "Ten", "Eleven", "Twelve", "Thirteen", "Fourteen", "Fifteen",\n "Sixteen", "Seventeen", "Eighteen", "Nineteen"]\n tens = ["", "Ten", "Twenty", "Thirty", "Forty", "Fifty", "Sixty", "Seventy", \n "Eighty", "Ninety"]\n def helper(num: int) -> str:\n if num<20:\n s = belowTwenty[num]\n elif num<100:\n s = tens[num//10] + " " + belowTwenty[num%10]\n elif num<1000:\n s = helper(num//100) + " Hundred " + helper(num%100)\n elif num<1000000:\n s = helper(num//1000) + " Thousand " + helper(num%1000)\n elif num<1000000000:\n s = helper(num//1000000) + " Million " + helper(num%1000000)\n else:\n s = helper(num//1000000000) + " Billion " + helper(num%1000000000)\n return s.strip()\n return helper(num)\n```\n | 3 | Convert a non-negative integer `num` to its English words representation.
**Example 1:**
**Input:** num = 123
**Output:** "One Hundred Twenty Three "
**Example 2:**
**Input:** num = 12345
**Output:** "Twelve Thousand Three Hundred Forty Five "
**Example 3:**
**Input:** num = 1234567
**Output:** "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven "
**Constraints:**
* `0 <= num <= 231 - 1` | Did you see a pattern in dividing the number into chunk of words? For example, 123 and 123000. Group the number by thousands (3 digits). You can write a helper function that takes a number less than 1000 and convert just that chunk to words. There are many edge cases. What are some good test cases? Does your code work with input such as 0? Or 1000010? (middle chunk is zero and should not be printed out) |
short & easy to understand Python code using dictionary | integer-to-english-words | 0 | 1 | ```Python\nclass Solution: \n def numberToWords(self, num: int) -> str:\n if num == 0 : return \'Zero\' #if condition to handle zero\n d = {1000000000 : \'Billion\',1000000 : \'Million\',1000 : \'Thousand\',100 : \'Hundred\', \n\t\t90:\'Ninety\',80:\'Eighty\',70:\'Seventy\',60:\'Sixty\',50: \'Fifty\', 40 : \'Forty\', 30 : \'Thirty\', 20 : \'Twenty\',\n\t\t19 :\'Nineteen\',18 :\'Eighteen\',17:\'Seventeen\',16:\'Sixteen\',15:\'Fifteen\',14:\'Fourteen\',13:\'Thirteen\',12:\'Twelve\',11:\'Eleven\',\n\t\t10:\'Ten\',9:\'Nine\',8:\'Eight\',7:\'Seven\',6:\'Six\',5:\'Five\',4:\'Four\',3:\'Three\',2:\'Two\',1:\'One\'} #initiating a dictionary to handle number to word mapping\n ans = "" #initialising the returnable answer variable\n for key, value in d.items(): #for loop to iterate through each key-value pair in dictionary \n if num//key>0 : #checking if number is in the range of current key\n x = num//key #finding the multiple of key in num\n if key >= 100 : #logic to add the multiple number x above as word to our answer, We say "One Hundred", "One thoushand" but we don\'t say "One Fifty", we simply say "Fifty"\n ans += self.numberToWords(x) + \' \'\n ans += value + " "\n num = num%key #preparing the number for next loop i.e removing the value from num which we have already appended the words to answer.\n return ans.strip() #returning answer removing the last blank space\n``` | 9 | Convert a non-negative integer `num` to its English words representation.
**Example 1:**
**Input:** num = 123
**Output:** "One Hundred Twenty Three "
**Example 2:**
**Input:** num = 12345
**Output:** "Twelve Thousand Three Hundred Forty Five "
**Example 3:**
**Input:** num = 1234567
**Output:** "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven "
**Constraints:**
* `0 <= num <= 231 - 1` | Did you see a pattern in dividing the number into chunk of words? For example, 123 and 123000. Group the number by thousands (3 digits). You can write a helper function that takes a number less than 1000 and convert just that chunk to words. There are many edge cases. What are some good test cases? Does your code work with input such as 0? Or 1000010? (middle chunk is zero and should not be printed out) |
Python 3, Runtime 28ms, Recursive approach | integer-to-english-words | 0 | 1 | ```\nclass Solution:\n def numberToWords(self, num: int) -> str:\n self.lessThan20 = ["", "One", "Two", "Three", "Four", "Five", "Six", "Seven", "Eight", "Nine", "Ten", "Eleven", "Twelve", "Thirteen", "Fourteen", "Fifteen", "Sixteen", "Seventeen", "Eighteen", "Nineteen"]\n self.tens = ["", "", "Twenty", "Thirty", "Forty", "Fifty", "Sixty", "Seventy", "Eighty", "Ninety"]\n self.thousands = ["", "Thousand", "Million", "Billion"];\n if num == 0 :\n return "Zero"\n res = \'\'\n i = 0\n while num>0:\n if num%1000 != 0:\n res = self.helper(num%1000) + self.thousands[i] + " " + res\n num //= 1000\n i += 1\n return res.strip()\n \n def helper(self, num):\n if num == 0:\n return ""\n if num < 20:\n return self.lessThan20[num] + " "\n if num < 100:\n return self.tens[num//10] + " " + self.helper(num%10)\n if num < 1000:\n return self.lessThan20[num//100] + " Hundred " + self.helper(num%100)\n``` | 9 | Convert a non-negative integer `num` to its English words representation.
**Example 1:**
**Input:** num = 123
**Output:** "One Hundred Twenty Three "
**Example 2:**
**Input:** num = 12345
**Output:** "Twelve Thousand Three Hundred Forty Five "
**Example 3:**
**Input:** num = 1234567
**Output:** "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven "
**Constraints:**
* `0 <= num <= 231 - 1` | Did you see a pattern in dividing the number into chunk of words? For example, 123 and 123000. Group the number by thousands (3 digits). You can write a helper function that takes a number less than 1000 and convert just that chunk to words. There are many edge cases. What are some good test cases? Does your code work with input such as 0? Or 1000010? (middle chunk is zero and should not be printed out) |
Elegant Recursive Solution in Python | integer-to-english-words | 0 | 1 | ```Python\nclass Solution:\n def numberToWords(self, num: int) -> str: \n if num == 0:\n return \'Zero\'\n\n billion, million, thousand, hundred = 10 ** 9, 10 ** 6, 10 ** 3, 10 ** 2\n\n mapping = {\n 0: \'\',\n 1: \'One\',\n 2: \'Two\',\n 3: \'Three\',\n 4: \'Four\',\n 5: \'Five\',\n 6: \'Six\',\n 7: \'Seven\',\n 8: \'Eight\',\n 9: \'Nine\',\n 10: \'Ten\',\n 11: \'Eleven\',\n 12: \'Twelve\',\n 13: \'Thirteen\',\n 14: \'Fourteen\',\n 15: \'Fifteen\',\n 16: \'Sixteen\',\n 17: \'Seventeen\',\n 18: \'Eighteen\',\n 19: \'Nineteen\',\n 20: \'Twenty\',\n 30: \'Thirty\',\n 40: \'Forty\',\n 50: \'Fifty\',\n 60: \'Sixty\',\n 70: \'Seventy\',\n 80: \'Eighty\',\n 90: \'Ninety\',\n billion: \'Billion\', \n million: \'Million\', \n thousand: \'Thousand\', \n hundred: \'Hundred\',\n }\n \n for unit in [billion, million, thousand, hundred]:\n if num >= unit:\n if num % unit:\n return f"{self.numberToWords(num // unit)} {mapping[unit]} {self.numberToWords(num % unit)}"\n return f"{self.numberToWords(num // unit)} {mapping[unit]}"\n\n res = \'\'\n for i in range(99, 0, -1):\n if num // i > 0 and i in mapping:\n res = f"{mapping[i]} {mapping[num % i]}".strip()\n break\n return res \n```\n | 1 | Convert a non-negative integer `num` to its English words representation.
**Example 1:**
**Input:** num = 123
**Output:** "One Hundred Twenty Three "
**Example 2:**
**Input:** num = 12345
**Output:** "Twelve Thousand Three Hundred Forty Five "
**Example 3:**
**Input:** num = 1234567
**Output:** "One Million Two Hundred Thirty Four Thousand Five Hundred Sixty Seven "
**Constraints:**
* `0 <= num <= 231 - 1` | Did you see a pattern in dividing the number into chunk of words? For example, 123 and 123000. Group the number by thousands (3 digits). You can write a helper function that takes a number less than 1000 and convert just that chunk to words. There are many edge cases. What are some good test cases? Does your code work with input such as 0? Or 1000010? (middle chunk is zero and should not be printed out) |
Python3 One line Code 🔥🔥🔥 | h-index | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def hIndex(self, citations: List[int]) -> int:\n citations.sort(reverse=True)\n h = 0\n \n while h < len(citations) and citations[h] > h:\n h += 1\n \n return h\n``` | 6 | Given an array of integers `citations` where `citations[i]` is the number of citations a researcher received for their `ith` paper, return _the researcher's h-index_.
According to the [definition of h-index on Wikipedia](https://en.wikipedia.org/wiki/H-index): The h-index is defined as the maximum value of `h` such that the given researcher has published at least `h` papers that have each been cited at least `h` times.
**Example 1:**
**Input:** citations = \[3,0,6,1,5\]
**Output:** 3
**Explanation:** \[3,0,6,1,5\] means the researcher has 5 papers in total and each of them had received 3, 0, 6, 1, 5 citations respectively.
Since the researcher has 3 papers with at least 3 citations each and the remaining two with no more than 3 citations each, their h-index is 3.
**Example 2:**
**Input:** citations = \[1,3,1\]
**Output:** 1
**Constraints:**
* `n == citations.length`
* `1 <= n <= 5000`
* `0 <= citations[i] <= 1000` | An easy approach is to sort the array first. What are the possible values of h-index? A faster approach is to use extra space. |
✅ Explained - Simple and Clear Python3 Code✅ | h-index | 0 | 1 | # Intuition\nThe problem asks for the h-index of a researcher based on the number of citations received for their papers. The h-index is the maximum value of h such that the researcher has published at least h papers that have each been cited at least h times. To find the h-index, we need to sort the array of citations in descending order and identify the largest h-value that satisfies the given condition.\n\n\n# Approach\nThe solution begins by sorting the array of citations in reverse order, ensuring that the papers with the highest citation counts are at the beginning. Next, several conditions are checked to determine the h-index. First, if the array contains only one element and it is greater than zero, the h-index is 1. If the smallest value in the sorted array is greater than or equal to the length of the array, the h-index is equal to the array length. Otherwise, the array is traversed, and for each index i, the value at that index is compared to i+1. If the value is less than i+1, i is returned as the h-index. If none of the conditions are met, the h-index is 0.\n\n\n# Complexity\n- Time complexity:\nThe time complexity of the solution primarily depends on the sorting operation, which has a time complexity of O(n log n), where n is the length of the input array. The subsequent iterations through the array and the condition checks have a linear time complexity of O(n). Therefore, the overall time complexity is O(n log n).\n\n\n- Space complexity:\nThe solution has a space complexity of O(1) since it only uses a constant amount of extra space to store variables and does not depend on the input size. No additional data structures are employed, and the sorting operation is performed in-place.\n\n# Code\n```\nclass Solution:\n def hIndex(self, c: List[int]) -> int:\n c.sort(reverse=True)\n if len(c)==1 and c[0]>0:\n return 1\n if c[-1]>=len(c):\n return len(c)\n for i in range(len(c)):\n if c[i]<i+1:\n return i\n return 0\n``` | 22 | Given an array of integers `citations` where `citations[i]` is the number of citations a researcher received for their `ith` paper, return _the researcher's h-index_.
According to the [definition of h-index on Wikipedia](https://en.wikipedia.org/wiki/H-index): The h-index is defined as the maximum value of `h` such that the given researcher has published at least `h` papers that have each been cited at least `h` times.
**Example 1:**
**Input:** citations = \[3,0,6,1,5\]
**Output:** 3
**Explanation:** \[3,0,6,1,5\] means the researcher has 5 papers in total and each of them had received 3, 0, 6, 1, 5 citations respectively.
Since the researcher has 3 papers with at least 3 citations each and the remaining two with no more than 3 citations each, their h-index is 3.
**Example 2:**
**Input:** citations = \[1,3,1\]
**Output:** 1
**Constraints:**
* `n == citations.length`
* `1 <= n <= 5000`
* `0 <= citations[i] <= 1000` | An easy approach is to sort the array first. What are the possible values of h-index? A faster approach is to use extra space. |
Simple and easy to understand python solution with 98% runtime and 57% memory beat | h-index | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n\n# Code\n```\nclass Solution:\n def hIndex(self, citations: List[int]) -> int:\n citations.sort()\n n = len(citations)\n h = 0\n\n if n == 1 and citations[0] == 0:\n return 0\n else:\n for i in range(n):\n if citations[i] < n - i:\n h = citations[i]\n else:\n h = max(h, n-i)\n return h\n``` | 4 | Given an array of integers `citations` where `citations[i]` is the number of citations a researcher received for their `ith` paper, return _the researcher's h-index_.
According to the [definition of h-index on Wikipedia](https://en.wikipedia.org/wiki/H-index): The h-index is defined as the maximum value of `h` such that the given researcher has published at least `h` papers that have each been cited at least `h` times.
**Example 1:**
**Input:** citations = \[3,0,6,1,5\]
**Output:** 3
**Explanation:** \[3,0,6,1,5\] means the researcher has 5 papers in total and each of them had received 3, 0, 6, 1, 5 citations respectively.
Since the researcher has 3 papers with at least 3 citations each and the remaining two with no more than 3 citations each, their h-index is 3.
**Example 2:**
**Input:** citations = \[1,3,1\]
**Output:** 1
**Constraints:**
* `n == citations.length`
* `1 <= n <= 5000`
* `0 <= citations[i] <= 1000` | An easy approach is to sort the array first. What are the possible values of h-index? A faster approach is to use extra space. |
274: Time 90.70%, Solution with step by step explanation | h-index | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nTo compute the researcher\'s h-index, we can sort the citations array in non-increasing order and then iterate through the sorted array. For each citation count, we compare it to the number of papers that have at least that many citations, which can be calculated as the remaining elements in the array. If the citation count is greater than or equal to the number of papers with at least that many citations, we have found the h-index.\n\nFor example, given the citations array [3,0,6,1,5], we can sort it to obtain [6,5,3,1,0]. We then iterate through the sorted array and for each citation count, we compare it to the number of remaining elements in the array. For the first element, 6, there are 5 remaining elements in the array, all of which have at least 6 citations, so the h-index is 6. For the second element, 5, there are 4 remaining elements in the array, all of which have at least 5 citations, so the h-index is 5. For the third element, 3, there are 3 remaining elements in the array that have at least 3 citations, so the h-index is 3.\n\nTime Complexity: O(nlogn), where n is the length of the citations array. This is because we need to sort the array in non-increasing order, which takes O(nlogn) time.\n\nSpace Complexity: O(1), as we are sorting the array in-place and using only constant extra space.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def hIndex(self, citations: List[int]) -> int:\n citations.sort(reverse=True) # Sort the array in non-increasing order\n n = len(citations)\n h = 0\n \n # Iterate through the sorted array and compare each citation count to the number of papers that have at least that many citations\n for i in range(n):\n if citations[i] >= i+1: # If the citation count is greater than or equal to the number of papers with at least that many citations, we have found the h-index\n h = i+1\n \n return h\n\n``` | 11 | Given an array of integers `citations` where `citations[i]` is the number of citations a researcher received for their `ith` paper, return _the researcher's h-index_.
According to the [definition of h-index on Wikipedia](https://en.wikipedia.org/wiki/H-index): The h-index is defined as the maximum value of `h` such that the given researcher has published at least `h` papers that have each been cited at least `h` times.
**Example 1:**
**Input:** citations = \[3,0,6,1,5\]
**Output:** 3
**Explanation:** \[3,0,6,1,5\] means the researcher has 5 papers in total and each of them had received 3, 0, 6, 1, 5 citations respectively.
Since the researcher has 3 papers with at least 3 citations each and the remaining two with no more than 3 citations each, their h-index is 3.
**Example 2:**
**Input:** citations = \[1,3,1\]
**Output:** 1
**Constraints:**
* `n == citations.length`
* `1 <= n <= 5000`
* `0 <= citations[i] <= 1000` | An easy approach is to sort the array first. What are the possible values of h-index? A faster approach is to use extra space. |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.