title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
Python - dict, deque, and chain star | diagonal-traverse-ii | 0 | 1 | ```\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n res = defaultdict(deque)\n for i, row in enumerate(nums):\n for j, x in enumerate(row):\n res[i + j].appendleft(x)\n return chain(*res.values())\n``` | 6 | Given a 2D integer array `nums`, return _all elements of_ `nums` _in diagonal order as shown in the below images_.
**Example 1:**
**Input:** nums = \[\[1,2,3\],\[4,5,6\],\[7,8,9\]\]
**Output:** \[1,4,2,7,5,3,8,6,9\]
**Example 2:**
**Input:** nums = \[\[1,2,3,4,5\],\[6,7\],\[8\],\[9,10,11\],\[12,13,14,15,16\]\]
**Output:** \[1,6,2,8,7,3,9,4,12,10,5,13,11,14,15,16\]
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i].length <= 105`
* `1 <= sum(nums[i].length) <= 105`
* `1 <= nums[i][j] <= 105` | Use Breadth First Search (BFS) to traverse all possible boxes you can open. Only push to the queue the boxes the you have with their keys. |
Python - dict, deque, and chain star | diagonal-traverse-ii | 0 | 1 | ```\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n res = defaultdict(deque)\n for i, row in enumerate(nums):\n for j, x in enumerate(row):\n res[i + j].appendleft(x)\n return chain(*res.values())\n``` | 6 | Given an array `arr` of positive integers sorted in a **strictly increasing order**, and an integer `k`.
Return _the_ `kth` _**positive** integer that is **missing** from this array._
**Example 1:**
**Input:** arr = \[2,3,4,7,11\], k = 5
**Output:** 9
**Explanation:** The missing positive integers are \[1,5,6,8,9,10,12,13,...\]. The 5th missing positive integer is 9.
**Example 2:**
**Input:** arr = \[1,2,3,4\], k = 2
**Output:** 6
**Explanation:** The missing positive integers are \[5,6,7,...\]. The 2nd missing positive integer is 6.
**Constraints:**
* `1 <= arr.length <= 1000`
* `1 <= arr[i] <= 1000`
* `1 <= k <= 1000`
* `arr[i] < arr[j]` for `1 <= i < j <= arr.length`
**Follow up:**
Could you solve this problem in less than O(n) complexity? | Notice that numbers with equal sums of row and column indexes belong to the same diagonal. Store them in tuples (sum, row, val), sort them, and then regroup the answer. |
(●'◡'●) Dicts are OP💕.py | diagonal-traverse-ii | 0 | 1 | # Intuition\nWe can definitely think on something like (i+j) elements are together\n# Code\n```js\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n mp,ans={},[]\n for i in range(len(nums)):\n for j in range(len(nums[i])):\n if i+j not in mp:mp[i+j]=[nums[i][j]]\n else:mp[i+j].append(nums[i][j])\n for i in mp:ans.extend(mp[i][::-1])\n return ans\n```\n\n | 11 | Given a 2D integer array `nums`, return _all elements of_ `nums` _in diagonal order as shown in the below images_.
**Example 1:**
**Input:** nums = \[\[1,2,3\],\[4,5,6\],\[7,8,9\]\]
**Output:** \[1,4,2,7,5,3,8,6,9\]
**Example 2:**
**Input:** nums = \[\[1,2,3,4,5\],\[6,7\],\[8\],\[9,10,11\],\[12,13,14,15,16\]\]
**Output:** \[1,6,2,8,7,3,9,4,12,10,5,13,11,14,15,16\]
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i].length <= 105`
* `1 <= sum(nums[i].length) <= 105`
* `1 <= nums[i][j] <= 105` | Use Breadth First Search (BFS) to traverse all possible boxes you can open. Only push to the queue the boxes the you have with their keys. |
(●'◡'●) Dicts are OP💕.py | diagonal-traverse-ii | 0 | 1 | # Intuition\nWe can definitely think on something like (i+j) elements are together\n# Code\n```js\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n mp,ans={},[]\n for i in range(len(nums)):\n for j in range(len(nums[i])):\n if i+j not in mp:mp[i+j]=[nums[i][j]]\n else:mp[i+j].append(nums[i][j])\n for i in mp:ans.extend(mp[i][::-1])\n return ans\n```\n\n | 11 | Given an array `arr` of positive integers sorted in a **strictly increasing order**, and an integer `k`.
Return _the_ `kth` _**positive** integer that is **missing** from this array._
**Example 1:**
**Input:** arr = \[2,3,4,7,11\], k = 5
**Output:** 9
**Explanation:** The missing positive integers are \[1,5,6,8,9,10,12,13,...\]. The 5th missing positive integer is 9.
**Example 2:**
**Input:** arr = \[1,2,3,4\], k = 2
**Output:** 6
**Explanation:** The missing positive integers are \[5,6,7,...\]. The 2nd missing positive integer is 6.
**Constraints:**
* `1 <= arr.length <= 1000`
* `1 <= arr[i] <= 1000`
* `1 <= k <= 1000`
* `arr[i] < arr[j]` for `1 <= i < j <= arr.length`
**Follow up:**
Could you solve this problem in less than O(n) complexity? | Notice that numbers with equal sums of row and column indexes belong to the same diagonal. Store them in tuples (sum, row, val), sort them, and then regroup the answer. |
✅ One Line Solution | diagonal-traverse-ii | 0 | 1 | # Complexity\n- Time complexity: $$O(n*log(n))$$.\n\n- Space complexity: $$O(n)$$.\n\n# Code - Oneliner\n```\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n return [num for _, _, num in sorted((i+j, -i, nums[i][j]) for i in range(len(nums)) for j in range(len(nums[i])))]\n```\n\n# Code - Unwrapped\n```\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n result = []\n for i in range(len(nums)):\n for j in range(len(nums[i])):\n result.append((i+j, -i, nums[i][j]))\n \n return [num for _, _, num in sorted(result)]\n``` | 6 | Given a 2D integer array `nums`, return _all elements of_ `nums` _in diagonal order as shown in the below images_.
**Example 1:**
**Input:** nums = \[\[1,2,3\],\[4,5,6\],\[7,8,9\]\]
**Output:** \[1,4,2,7,5,3,8,6,9\]
**Example 2:**
**Input:** nums = \[\[1,2,3,4,5\],\[6,7\],\[8\],\[9,10,11\],\[12,13,14,15,16\]\]
**Output:** \[1,6,2,8,7,3,9,4,12,10,5,13,11,14,15,16\]
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i].length <= 105`
* `1 <= sum(nums[i].length) <= 105`
* `1 <= nums[i][j] <= 105` | Use Breadth First Search (BFS) to traverse all possible boxes you can open. Only push to the queue the boxes the you have with their keys. |
✅ One Line Solution | diagonal-traverse-ii | 0 | 1 | # Complexity\n- Time complexity: $$O(n*log(n))$$.\n\n- Space complexity: $$O(n)$$.\n\n# Code - Oneliner\n```\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n return [num for _, _, num in sorted((i+j, -i, nums[i][j]) for i in range(len(nums)) for j in range(len(nums[i])))]\n```\n\n# Code - Unwrapped\n```\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n result = []\n for i in range(len(nums)):\n for j in range(len(nums[i])):\n result.append((i+j, -i, nums[i][j]))\n \n return [num for _, _, num in sorted(result)]\n``` | 6 | Given an array `arr` of positive integers sorted in a **strictly increasing order**, and an integer `k`.
Return _the_ `kth` _**positive** integer that is **missing** from this array._
**Example 1:**
**Input:** arr = \[2,3,4,7,11\], k = 5
**Output:** 9
**Explanation:** The missing positive integers are \[1,5,6,8,9,10,12,13,...\]. The 5th missing positive integer is 9.
**Example 2:**
**Input:** arr = \[1,2,3,4\], k = 2
**Output:** 6
**Explanation:** The missing positive integers are \[5,6,7,...\]. The 2nd missing positive integer is 6.
**Constraints:**
* `1 <= arr.length <= 1000`
* `1 <= arr[i] <= 1000`
* `1 <= k <= 1000`
* `arr[i] < arr[j]` for `1 <= i < j <= arr.length`
**Follow up:**
Could you solve this problem in less than O(n) complexity? | Notice that numbers with equal sums of row and column indexes belong to the same diagonal. Store them in tuples (sum, row, val), sort them, and then regroup the answer. |
Simple Solution || Beginner Friendly || Easy to Understand ✅ | diagonal-traverse-ii | 1 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\n$$Initialization:$$\n- Initialize a variable `count` to keep track of the total number of elements in the result array.\n- Create an ArrayList of ArrayLists called `lists` to store the elements diagonally.\n\n$$Traversing the Matrix Diagonally:$$\n- Use two nested loops to traverse each element in the matrix `(nums)`.\n- For each element at position `(i, j)`, calculate the diagonal index `idx` by adding `i` and `j`.\n- If the size of lists is less than `idx + 1`, it means we are encountering a new diagonal, so add a new ArrayList to lists.\n- Add the current element to the corresponding diagonal ArrayList in `lists`.\n- Increment the `count` to keep track of the total number of elements.\n\n$$Flattening Diagonal Lists to Result Array:$$\n- Create an array `res` of size `count` to store the final result.\n- Initialize an index `idx` to keep track of the position in the result array.\n\n$$Flatten Diagonals in Reverse Order:$$\n- Iterate over each diagonal ArrayList in `lists`.\n- For each diagonal, iterate in reverse order and add the elements to the result array `(res)`.\n- Increment the index `idx` for each added element.\n\n$$Return Result Array:$$\n- Return the final result array `res`.\n\n\n\n# Complexity\n- Time complexity: `O(n)`\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: `O(n)`\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```java []\nclass Solution {\n public int[] findDiagonalOrder(List<List<Integer>> nums) {\n int count = 0;\n\n List<List<Integer>> lists = new ArrayList<>();\n for (int i = 0; i < nums.size(); i++) {\n List<Integer> row = nums.get(i);\n\n for (int j = 0; j < row.size(); j++) {\n int idx = i + j;\n\n if (lists.size() < idx + 1) {\n lists.add(new ArrayList<>());\n }\n lists.get(idx).add(row.get(j));\n \n count ++;\n }\n }\n\n int[] res = new int[count];\n int idx = 0;\n for (List<Integer> list : lists) {\n for (int i = list.size() - 1; i >= 0; i--) {\n res[idx++] = list.get(i); \n }\n }\n return res;\n }\n}\n```\n```python []\nclass Solution:\n def findDiagonalOrder(self, nums):\n diagonals = [deque() for _ in range(len(nums) + max(len(row) for row in nums) - 1)]\n for row_id, row in enumerate(nums):\n for col_id in range(len(row)):\n diagonals[row_id + col_id].appendleft(row[col_id])\n return list(chain(*diagonals))\n```\n```python3 []\nclass Solution:\n def findDiagonalOrder(self, A: List[List[int]]) -> List[int]:\n d = defaultdict(list)\n for i in range(len(A)):\n for j in range(len(A[i])):\n d[i+j].append(A[i][j])\n return [v for k in d.keys() for v in reversed(d[k])]\n```\n```C++ []\nclass Solution {\npublic:\n vector<int> findDiagonalOrder(vector<vector<int>>& nums) {\n int m=nums.size(),n=0;\n \n //find max value and store in n;\n \n for(int i=0;i<m;i++){\n if(n<nums[i].size())\n n=nums[i].size();\n }\n vector<vector<int>>temp(m+n);\n vector<int>ans;\n \n // convert mat into adjacency list and keep value in temp\n \n for(int i=0;i<m;i++){\n for(int j=0;j<nums[i].size();j++){\n temp[i+j].push_back(nums[i][j]);\n }\n }\n \n // here we reverse matrix\n \n for(int i=0;i<m+n;i++){\n reverse(temp[i].begin(),temp[i].end());\n }\n \n // all value of temp in ans vector\n \n for(int i=0;i<m+n;i++){\n for(int j=0;j<temp[i].size();j++){\n ans.push_back(temp[i][j]);\n }\n }\n return ans;\n }\n};\n```\n```Javascript []\nvar findDiagonalOrder = function(nums) {\n const result = [];\n\n for (let row = 0; row < nums.length; row++) {\n for (let col = 0; col < nums[row].length; col++) {\n const num = nums[row][col];\n if (!num) continue;\n const current = result[row + col];\n\n current \n ? current.unshift(num)\n : result[row + col] = [num];\n }\n }\n return result.flat();\n};\n```\n```C []\nclass Solution {\npublic:\n vector<int> findDiagonalOrder(vector<vector<int>>& nums) {\n int m=nums.size(),n=0;\n \n //find max value and store in n;\n \n for(int i=0;i<m;i++){\n if(n<nums[i].size())\n n=nums[i].size();\n }\n vector<vector<int>>temp(m+n);\n vector<int>ans;\n \n // convert mat into adjacency list and keep value in temp\n \n for(int i=0;i<m;i++){\n for(int j=0;j<nums[i].size();j++){\n temp[i+j].push_back(nums[i][j]);\n }\n }\n \n // here we reverse matrix\n \n for(int i=0;i<m+n;i++){\n reverse(temp[i].begin(),temp[i].end());\n }\n \n // all value of temp in ans vector\n \n for(int i=0;i<m+n;i++){\n for(int j=0;j<temp[i].size();j++){\n ans.push_back(temp[i][j]);\n }\n }\n return ans;\n }\n};\n```\n# If you like the solution please UPVOTE !!\n\n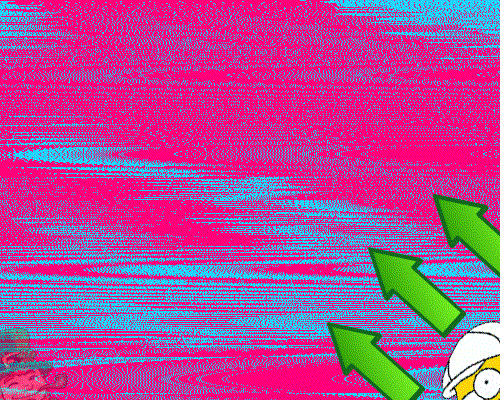\n\n\n\n\n | 87 | Given a 2D integer array `nums`, return _all elements of_ `nums` _in diagonal order as shown in the below images_.
**Example 1:**
**Input:** nums = \[\[1,2,3\],\[4,5,6\],\[7,8,9\]\]
**Output:** \[1,4,2,7,5,3,8,6,9\]
**Example 2:**
**Input:** nums = \[\[1,2,3,4,5\],\[6,7\],\[8\],\[9,10,11\],\[12,13,14,15,16\]\]
**Output:** \[1,6,2,8,7,3,9,4,12,10,5,13,11,14,15,16\]
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i].length <= 105`
* `1 <= sum(nums[i].length) <= 105`
* `1 <= nums[i][j] <= 105` | Use Breadth First Search (BFS) to traverse all possible boxes you can open. Only push to the queue the boxes the you have with their keys. |
Simple Solution || Beginner Friendly || Easy to Understand ✅ | diagonal-traverse-ii | 1 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\n$$Initialization:$$\n- Initialize a variable `count` to keep track of the total number of elements in the result array.\n- Create an ArrayList of ArrayLists called `lists` to store the elements diagonally.\n\n$$Traversing the Matrix Diagonally:$$\n- Use two nested loops to traverse each element in the matrix `(nums)`.\n- For each element at position `(i, j)`, calculate the diagonal index `idx` by adding `i` and `j`.\n- If the size of lists is less than `idx + 1`, it means we are encountering a new diagonal, so add a new ArrayList to lists.\n- Add the current element to the corresponding diagonal ArrayList in `lists`.\n- Increment the `count` to keep track of the total number of elements.\n\n$$Flattening Diagonal Lists to Result Array:$$\n- Create an array `res` of size `count` to store the final result.\n- Initialize an index `idx` to keep track of the position in the result array.\n\n$$Flatten Diagonals in Reverse Order:$$\n- Iterate over each diagonal ArrayList in `lists`.\n- For each diagonal, iterate in reverse order and add the elements to the result array `(res)`.\n- Increment the index `idx` for each added element.\n\n$$Return Result Array:$$\n- Return the final result array `res`.\n\n\n\n# Complexity\n- Time complexity: `O(n)`\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: `O(n)`\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```java []\nclass Solution {\n public int[] findDiagonalOrder(List<List<Integer>> nums) {\n int count = 0;\n\n List<List<Integer>> lists = new ArrayList<>();\n for (int i = 0; i < nums.size(); i++) {\n List<Integer> row = nums.get(i);\n\n for (int j = 0; j < row.size(); j++) {\n int idx = i + j;\n\n if (lists.size() < idx + 1) {\n lists.add(new ArrayList<>());\n }\n lists.get(idx).add(row.get(j));\n \n count ++;\n }\n }\n\n int[] res = new int[count];\n int idx = 0;\n for (List<Integer> list : lists) {\n for (int i = list.size() - 1; i >= 0; i--) {\n res[idx++] = list.get(i); \n }\n }\n return res;\n }\n}\n```\n```python []\nclass Solution:\n def findDiagonalOrder(self, nums):\n diagonals = [deque() for _ in range(len(nums) + max(len(row) for row in nums) - 1)]\n for row_id, row in enumerate(nums):\n for col_id in range(len(row)):\n diagonals[row_id + col_id].appendleft(row[col_id])\n return list(chain(*diagonals))\n```\n```python3 []\nclass Solution:\n def findDiagonalOrder(self, A: List[List[int]]) -> List[int]:\n d = defaultdict(list)\n for i in range(len(A)):\n for j in range(len(A[i])):\n d[i+j].append(A[i][j])\n return [v for k in d.keys() for v in reversed(d[k])]\n```\n```C++ []\nclass Solution {\npublic:\n vector<int> findDiagonalOrder(vector<vector<int>>& nums) {\n int m=nums.size(),n=0;\n \n //find max value and store in n;\n \n for(int i=0;i<m;i++){\n if(n<nums[i].size())\n n=nums[i].size();\n }\n vector<vector<int>>temp(m+n);\n vector<int>ans;\n \n // convert mat into adjacency list and keep value in temp\n \n for(int i=0;i<m;i++){\n for(int j=0;j<nums[i].size();j++){\n temp[i+j].push_back(nums[i][j]);\n }\n }\n \n // here we reverse matrix\n \n for(int i=0;i<m+n;i++){\n reverse(temp[i].begin(),temp[i].end());\n }\n \n // all value of temp in ans vector\n \n for(int i=0;i<m+n;i++){\n for(int j=0;j<temp[i].size();j++){\n ans.push_back(temp[i][j]);\n }\n }\n return ans;\n }\n};\n```\n```Javascript []\nvar findDiagonalOrder = function(nums) {\n const result = [];\n\n for (let row = 0; row < nums.length; row++) {\n for (let col = 0; col < nums[row].length; col++) {\n const num = nums[row][col];\n if (!num) continue;\n const current = result[row + col];\n\n current \n ? current.unshift(num)\n : result[row + col] = [num];\n }\n }\n return result.flat();\n};\n```\n```C []\nclass Solution {\npublic:\n vector<int> findDiagonalOrder(vector<vector<int>>& nums) {\n int m=nums.size(),n=0;\n \n //find max value and store in n;\n \n for(int i=0;i<m;i++){\n if(n<nums[i].size())\n n=nums[i].size();\n }\n vector<vector<int>>temp(m+n);\n vector<int>ans;\n \n // convert mat into adjacency list and keep value in temp\n \n for(int i=0;i<m;i++){\n for(int j=0;j<nums[i].size();j++){\n temp[i+j].push_back(nums[i][j]);\n }\n }\n \n // here we reverse matrix\n \n for(int i=0;i<m+n;i++){\n reverse(temp[i].begin(),temp[i].end());\n }\n \n // all value of temp in ans vector\n \n for(int i=0;i<m+n;i++){\n for(int j=0;j<temp[i].size();j++){\n ans.push_back(temp[i][j]);\n }\n }\n return ans;\n }\n};\n```\n# If you like the solution please UPVOTE !!\n\n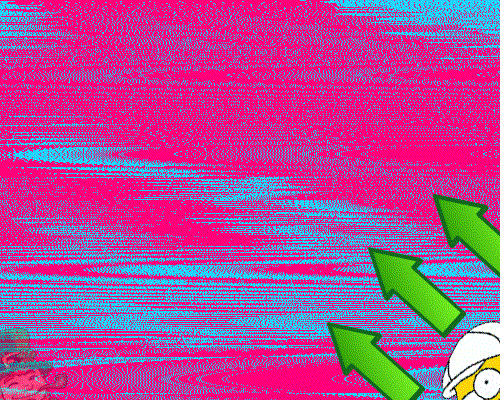\n\n\n\n\n | 87 | Given an array `arr` of positive integers sorted in a **strictly increasing order**, and an integer `k`.
Return _the_ `kth` _**positive** integer that is **missing** from this array._
**Example 1:**
**Input:** arr = \[2,3,4,7,11\], k = 5
**Output:** 9
**Explanation:** The missing positive integers are \[1,5,6,8,9,10,12,13,...\]. The 5th missing positive integer is 9.
**Example 2:**
**Input:** arr = \[1,2,3,4\], k = 2
**Output:** 6
**Explanation:** The missing positive integers are \[5,6,7,...\]. The 2nd missing positive integer is 6.
**Constraints:**
* `1 <= arr.length <= 1000`
* `1 <= arr[i] <= 1000`
* `1 <= k <= 1000`
* `arr[i] < arr[j]` for `1 <= i < j <= arr.length`
**Follow up:**
Could you solve this problem in less than O(n) complexity? | Notice that numbers with equal sums of row and column indexes belong to the same diagonal. Store them in tuples (sum, row, val), sort them, and then regroup the answer. |
EEzz simple Python solution using Hashmap/deafulatdict(list)! | diagonal-traverse-ii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n(i+j) for same\xA0diagonal elements is equal. \nThey will be added to a result(res) list after being stored in a hashmap. (To obtain the upward diagonal traversal, reverse each list in the hashmap.)\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n d , res = defaultdict(list) , []\n for i in range(len(nums)):\n for j in range(len(nums[i])):\n d[i+j].append(nums[i][j])\n for k in d.values():\n k = k[::-1]\n res.extend(k)\n return res\n``` | 3 | Given a 2D integer array `nums`, return _all elements of_ `nums` _in diagonal order as shown in the below images_.
**Example 1:**
**Input:** nums = \[\[1,2,3\],\[4,5,6\],\[7,8,9\]\]
**Output:** \[1,4,2,7,5,3,8,6,9\]
**Example 2:**
**Input:** nums = \[\[1,2,3,4,5\],\[6,7\],\[8\],\[9,10,11\],\[12,13,14,15,16\]\]
**Output:** \[1,6,2,8,7,3,9,4,12,10,5,13,11,14,15,16\]
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i].length <= 105`
* `1 <= sum(nums[i].length) <= 105`
* `1 <= nums[i][j] <= 105` | Use Breadth First Search (BFS) to traverse all possible boxes you can open. Only push to the queue the boxes the you have with their keys. |
EEzz simple Python solution using Hashmap/deafulatdict(list)! | diagonal-traverse-ii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n(i+j) for same\xA0diagonal elements is equal. \nThey will be added to a result(res) list after being stored in a hashmap. (To obtain the upward diagonal traversal, reverse each list in the hashmap.)\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n d , res = defaultdict(list) , []\n for i in range(len(nums)):\n for j in range(len(nums[i])):\n d[i+j].append(nums[i][j])\n for k in d.values():\n k = k[::-1]\n res.extend(k)\n return res\n``` | 3 | Given an array `arr` of positive integers sorted in a **strictly increasing order**, and an integer `k`.
Return _the_ `kth` _**positive** integer that is **missing** from this array._
**Example 1:**
**Input:** arr = \[2,3,4,7,11\], k = 5
**Output:** 9
**Explanation:** The missing positive integers are \[1,5,6,8,9,10,12,13,...\]. The 5th missing positive integer is 9.
**Example 2:**
**Input:** arr = \[1,2,3,4\], k = 2
**Output:** 6
**Explanation:** The missing positive integers are \[5,6,7,...\]. The 2nd missing positive integer is 6.
**Constraints:**
* `1 <= arr.length <= 1000`
* `1 <= arr[i] <= 1000`
* `1 <= k <= 1000`
* `arr[i] < arr[j]` for `1 <= i < j <= arr.length`
**Follow up:**
Could you solve this problem in less than O(n) complexity? | Notice that numbers with equal sums of row and column indexes belong to the same diagonal. Store them in tuples (sum, row, val), sort them, and then regroup the answer. |
[Python, Rust] Elegant & Short | O(n) | Ordered Map | diagonal-traverse-ii | 0 | 1 | # Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(n)$$\n\n# Code\n\n```python []\nfrom collections import defaultdict, deque\n\nclass Solution:\n def findDiagonalOrder(self, matrix: list[list[int]]) -> list[int]:\n diagonals = defaultdict(deque)\n\n for i, row in enumerate(matrix):\n for j, num in enumerate(row):\n diagonals[i + j].appendleft(num)\n\n return [num for diag in diagonals.values() for num in diag]\n```\n```rust []\nuse std::collections::{BTreeMap, VecDeque};\n\nimpl Solution {\n pub fn find_diagonal_order(matrix: Vec<Vec<i32>>) -> Vec<i32> {\n let mut diagonals: BTreeMap<usize, VecDeque<i32>> = BTreeMap::new();\n\n for (i, row) in matrix.iter().enumerate() {\n for (j, &num) in row.iter().enumerate() {\n diagonals.entry(i + j).or_insert_with(VecDeque::new).push_front(num);\n }\n }\n\n diagonals.values().flat_map(|diag| diag.iter().cloned()).collect()\n }\n}\n```\n | 2 | Given a 2D integer array `nums`, return _all elements of_ `nums` _in diagonal order as shown in the below images_.
**Example 1:**
**Input:** nums = \[\[1,2,3\],\[4,5,6\],\[7,8,9\]\]
**Output:** \[1,4,2,7,5,3,8,6,9\]
**Example 2:**
**Input:** nums = \[\[1,2,3,4,5\],\[6,7\],\[8\],\[9,10,11\],\[12,13,14,15,16\]\]
**Output:** \[1,6,2,8,7,3,9,4,12,10,5,13,11,14,15,16\]
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i].length <= 105`
* `1 <= sum(nums[i].length) <= 105`
* `1 <= nums[i][j] <= 105` | Use Breadth First Search (BFS) to traverse all possible boxes you can open. Only push to the queue the boxes the you have with their keys. |
[Python, Rust] Elegant & Short | O(n) | Ordered Map | diagonal-traverse-ii | 0 | 1 | # Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(n)$$\n\n# Code\n\n```python []\nfrom collections import defaultdict, deque\n\nclass Solution:\n def findDiagonalOrder(self, matrix: list[list[int]]) -> list[int]:\n diagonals = defaultdict(deque)\n\n for i, row in enumerate(matrix):\n for j, num in enumerate(row):\n diagonals[i + j].appendleft(num)\n\n return [num for diag in diagonals.values() for num in diag]\n```\n```rust []\nuse std::collections::{BTreeMap, VecDeque};\n\nimpl Solution {\n pub fn find_diagonal_order(matrix: Vec<Vec<i32>>) -> Vec<i32> {\n let mut diagonals: BTreeMap<usize, VecDeque<i32>> = BTreeMap::new();\n\n for (i, row) in matrix.iter().enumerate() {\n for (j, &num) in row.iter().enumerate() {\n diagonals.entry(i + j).or_insert_with(VecDeque::new).push_front(num);\n }\n }\n\n diagonals.values().flat_map(|diag| diag.iter().cloned()).collect()\n }\n}\n```\n | 2 | Given an array `arr` of positive integers sorted in a **strictly increasing order**, and an integer `k`.
Return _the_ `kth` _**positive** integer that is **missing** from this array._
**Example 1:**
**Input:** arr = \[2,3,4,7,11\], k = 5
**Output:** 9
**Explanation:** The missing positive integers are \[1,5,6,8,9,10,12,13,...\]. The 5th missing positive integer is 9.
**Example 2:**
**Input:** arr = \[1,2,3,4\], k = 2
**Output:** 6
**Explanation:** The missing positive integers are \[5,6,7,...\]. The 2nd missing positive integer is 6.
**Constraints:**
* `1 <= arr.length <= 1000`
* `1 <= arr[i] <= 1000`
* `1 <= k <= 1000`
* `arr[i] < arr[j]` for `1 <= i < j <= arr.length`
**Follow up:**
Could you solve this problem in less than O(n) complexity? | Notice that numbers with equal sums of row and column indexes belong to the same diagonal. Store them in tuples (sum, row, val), sort them, and then regroup the answer. |
【Video】Give me 9 minutes - How we think about a solution | diagonal-traverse-ii | 1 | 1 | # Intuition\nUsing BFS\n\n---\n\n# Solution Video\n\nhttps://youtu.be/4wkt4kGip64\n\n\u25A0 Timeline of the video\n`0:04` How we think about a solution\n`1:08` How can you put numbers into queue with right order?\n`2:34` Demonstrate how it works\n`6:24` Summary of main idea\n`6:47` Coding\n`8:40` Time Complexity and Space Complexity\n`8:57` Summary of the algorithm with my solution code\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 3,180\nMy first goal is 10,000 (It\'s far from done \uD83D\uDE05)\nThank you for your support!\n\n**My channel reached 3,000 subscribers these days. Thank you so much for your support!**\n\n---\n\n# Approach\n## How we think about a solution\n\nWe know order of output, so problem is that how we can take target numbers with the right order.\n\nLet\'s use Example 2 and make input array small.\n\n```\nInput: nums = [[1,2,3],[4],[5,6]]\n\n1,2,3\n4, ,\n5,6,\n```\n```\nOutput:[1,4,2,5,3,6]\n```\nWe can see that by changing our perspective on the array, we can traverse it in a manner similar to BFS.\n\n```\n 1\n 4 2\n 5 3\n 6\n```\nThat\'s why we use `Queue` to solve the problem.\n\n- How we can put numbers into queue with right order?\n\n```\n1,2,3\n4, ,\n5,6,\n```\nAcutually, we keep row and col position of each number in `queue` to calculate neighbor positions.\n\nAs you may know typical BFS, we put left child and right child into queue from parent, so when we traverse `1`, we try to put next positions in the next line staring with `4` into `queue`.\n\nBasically, we put current `right` position into `queue`, because we know that right adjacent number is a number for next line. But how can we jump to the next line?\n\nIn the example above, we want to traverse `1,4,2,5,3,6`. In other words, how can you start new lines? For example, from `2` to `5`.\n\nMy answer is when we are `col = 0`, we put a `bottom` position first, then check `right` position, so that we can start new lines with numbers in `col \n= 0`.\n\nLet\'s see one by one.\n\n```\n1,2,3\n4, ,\n5,6,\n\nq = [(0,0)] (= (0,0) is start position)\n\n(0,0): 1\n```\nTake the most left data in `queue`.\n```\n1,2,3\n4, ,\n5,6,\n\nrow = 0\ncol = 0\nq = []\nres = [1] \n\nNow col = 0, so add bottom position to queue\nq = [(1,0)]\n\nThen check right side, find 2. Add position to queue\nq = [(1,0),(0,1)]\n\n(1,0): 4\n(0,1): 2\n```\nTake the most left data in `queue`.\n```\n1,2,3\n4, ,\n5,6,\n\nrow = 1\ncol = 0\nq = [(0,1)]\nres = [1,4] \n\nNow col = 0, so add bottom position to queue\nq = [(0,1),(2,0)]\n\nThen check right side, there is no number, so skip\nq = [(0,1),(2,0)]\n\n(0,1): 2\n(2,0): 5\n```\nTake the most left data in `queue`.\n```\n1,2,3\n4, ,\n5,6,\n\nrow = 0\ncol = 1\nq = [(2,0)]\nres = [1,4,2] \n\nNow col != 0, so skip\nq = [(2,0)]\n\nThen check right side, find 3. Add position to queue\nq = [(2,0),(0,2)]\n\n(2,0): 5\n(0,2): 3\n```\nTake the most left data in `queue`.\n```\n1,2,3\n4, ,\n5,6,\n\nrow = 2\ncol = 0\nq = [(0,2)]\nres = [1,4,2,5]\n\nNow col = 0, but next bottom(3,0) is out of bounds, so skip\nq = [(0,2)]\n\nThen check right side, Find 6. Add position to queue\nq = [(0,2),(2,1)]\n\n(0,2): 3\n(2,1): 6\n```\nAt bottom from row 2, col = 0, there is no number but there is 6 on the right side. so this 6 will be start number in the next line.\n\nTake the most left data in `queue`.\n```\n1,2,3\n4, ,\n5,6,\n\nrow = 0\ncol = 2\nq = [(2,1)]\nres = [1,4,2,5,3]\n\nNow col != 0, so skip\nq = [(2,1)]\n\nThen check right side, there is no number, so skip\nq = [(2,1)]\n\n```\nTake the most left data in `queue`.\n```\n1,2,3\n4, ,\n5,6,\n\nrow = 2\ncol = 1\nq = []\nres = [1,4,2,5,3,6]\n\nNow col != 0, so skip\nq = []\n\nThen check right side, there is no number, so skip\nq = []\n\n```\n\n```\nOutput: [1,4,2,5,3,6]\n```\n\n---\n\n\u2B50\uFE0F Points\n\n- When col is equal to 0, then check bottom position, so that we can start new lines with right order\n- Check right position from every position\n\n---\n\nEasy!\uD83D\uDE04\nLet\'s see a real algorithm!\n\n\n### Algorithm Overview:\n1. Initialization\n2. BFS Traversal\n3. Return Result\n\n### Detailed Explanation:\n\n**Step 1: Initialization**\n```python\n# Create an empty list to store the result\nres = []\n\n# Initialize a queue with the starting point (0, 0)\nq = deque([(0, 0)])\n```\n\n**Explanation:** In this step, we set up the data structures needed for the algorithm. The `res` list will store the final result, and the `q` queue will be used for the breadth-first search (BFS) traversal. We start the traversal from the top-left corner, so the initial point `(0, 0)` is enqueued.\n\n**Step 2: BFS Traversal**\n```python\n# While the queue is not empty, perform the following steps\nwhile q:\n # Dequeue the front element\n row, col = q.popleft()\n\n # Append the current element to the result\n res.append(nums[row][col])\n\n # Enqueue the next element below if applicable\n if col == 0 and row + 1 < len(nums):\n q.append((row + 1, 0))\n\n # Enqueue the next element to the right if applicable\n if col + 1 < len(nums[row]):\n q.append((row, col + 1))\n```\n\n**Explanation:** This is the core of the algorithm, where the BFS traversal takes place. While the queue is not empty, we dequeue the front element, append the corresponding element from the input array `nums` to the result, and enqueue the next elements based on the conditions. If `col` is at the leftmost position (0), we enqueue the element below if there is a row below. Additionally, if there is a column to the right, we enqueue the element to the right.\n\n**Step 3: Return Result**\n```python\n# Return the final result list\nreturn res\n```\n\n**Explanation:** Once the BFS traversal is complete, the function returns the final result list containing the elements traversed diagonally in the 2D array.\n\nIn summary, the algorithm utilizes BFS to traverse the given 2D array diagonally, starting from the top-left corner and moving towards the bottom-right corner. The result is stored in the `res` list, which is then returned.\n\n\n# Complexity\n- Time complexity: $$O(N)$$\n`N` is number of position we visit.\n\n- Space complexity: $$O(N)$$\n\n\n```python []\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n res = []\n q = deque([(0, 0)])\n \n while q:\n row, col = q.popleft()\n res.append(nums[row][col])\n\n if col == 0 and row + 1 < len(nums):\n q.append((row + 1, 0))\n\n if col + 1 < len(nums[row]):\n q.append((row, col + 1))\n\n return res\n```\n```javascript []\nvar findDiagonalOrder = function(nums) {\n const res = [];\n const q = [[0, 0]];\n\n while (q.length > 0) {\n const [row, col] = q.shift();\n res.push(nums[row][col]);\n\n if (col === 0 && row + 1 < nums.length) {\n q.push([row + 1, 0]);\n }\n\n if (col + 1 < nums[row].length) {\n q.push([row, col + 1]);\n }\n }\n\n return res; \n};\n```\n```java []\nclass Solution {\n public int[] findDiagonalOrder(List<List<Integer>> nums) {\n List<Integer> resList = new ArrayList<>();\n LinkedList<int[]> q = new LinkedList<>();\n q.offer(new int[]{0, 0});\n\n while (!q.isEmpty()) {\n int[] p = q.poll();\n resList.add(nums.get(p[0]).get(p[1]));\n\n if (p[1] == 0 && p[0] + 1 < nums.size()) {\n q.offer(new int[]{p[0] + 1, 0});\n }\n\n if (p[1] + 1 < nums.get(p[0]).size()) {\n q.offer(new int[]{p[0], p[1] + 1});\n }\n }\n\n // Convert List<Integer> to int[]\n int[] res = new int[resList.size()];\n for (int i = 0; i < resList.size(); i++) {\n res[i] = resList.get(i);\n }\n\n return res; \n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n vector<int> findDiagonalOrder(vector<vector<int>>& nums) {\n vector<int> res;\n queue<pair<int, int>> q;\n q.push({0, 0});\n\n while (!q.empty()) {\n auto p = q.front();\n q.pop();\n res.push_back(nums[p.first][p.second]);\n\n if (p.second == 0 && p.first + 1 < nums.size()) {\n q.push({p.first + 1, 0});\n }\n\n if (p.second + 1 < nums[p.first].size()) {\n q.push({p.first, p.second + 1});\n }\n }\n\n return res; \n }\n};\n```\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n\u25A0 Subscribe URL\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\n\u25A0 Twitter\nhttps://twitter.com/CodingNinjaAZ\n\n### My next daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/arithmetic-subarrays/solutions/4321453/video-give-me-6-minutes-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/bE6YT9q16K8\n\n\u25A0 Timeline of the video\n`0:04` What does the formula mean?\n`1:46` Demonstrate how it works\n`3:39` Coding\n`5:40` Time Complexity and Space Complexity\n`6:06` Summary of the algorithm with my solution code\n\n### My previous daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/count-nice-pairs-in-an-array/solutions/4312501/video-give-me-6-minutes-hashmap-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/424EOdu4TeY\n\n\u25A0 Timeline of the video\n\n`0:04` Think about how we calculate the answer\n`0:58` Demonstrate how it works\n`3:58` Coding\n`5:53` Time Complexity and Space Complexity\n`6:11` Summary of the algorithm with my solution code\n | 38 | Given a 2D integer array `nums`, return _all elements of_ `nums` _in diagonal order as shown in the below images_.
**Example 1:**
**Input:** nums = \[\[1,2,3\],\[4,5,6\],\[7,8,9\]\]
**Output:** \[1,4,2,7,5,3,8,6,9\]
**Example 2:**
**Input:** nums = \[\[1,2,3,4,5\],\[6,7\],\[8\],\[9,10,11\],\[12,13,14,15,16\]\]
**Output:** \[1,6,2,8,7,3,9,4,12,10,5,13,11,14,15,16\]
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i].length <= 105`
* `1 <= sum(nums[i].length) <= 105`
* `1 <= nums[i][j] <= 105` | Use Breadth First Search (BFS) to traverse all possible boxes you can open. Only push to the queue the boxes the you have with their keys. |
【Video】Give me 9 minutes - How we think about a solution | diagonal-traverse-ii | 1 | 1 | # Intuition\nUsing BFS\n\n---\n\n# Solution Video\n\nhttps://youtu.be/4wkt4kGip64\n\n\u25A0 Timeline of the video\n`0:04` How we think about a solution\n`1:08` How can you put numbers into queue with right order?\n`2:34` Demonstrate how it works\n`6:24` Summary of main idea\n`6:47` Coding\n`8:40` Time Complexity and Space Complexity\n`8:57` Summary of the algorithm with my solution code\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 3,180\nMy first goal is 10,000 (It\'s far from done \uD83D\uDE05)\nThank you for your support!\n\n**My channel reached 3,000 subscribers these days. Thank you so much for your support!**\n\n---\n\n# Approach\n## How we think about a solution\n\nWe know order of output, so problem is that how we can take target numbers with the right order.\n\nLet\'s use Example 2 and make input array small.\n\n```\nInput: nums = [[1,2,3],[4],[5,6]]\n\n1,2,3\n4, ,\n5,6,\n```\n```\nOutput:[1,4,2,5,3,6]\n```\nWe can see that by changing our perspective on the array, we can traverse it in a manner similar to BFS.\n\n```\n 1\n 4 2\n 5 3\n 6\n```\nThat\'s why we use `Queue` to solve the problem.\n\n- How we can put numbers into queue with right order?\n\n```\n1,2,3\n4, ,\n5,6,\n```\nAcutually, we keep row and col position of each number in `queue` to calculate neighbor positions.\n\nAs you may know typical BFS, we put left child and right child into queue from parent, so when we traverse `1`, we try to put next positions in the next line staring with `4` into `queue`.\n\nBasically, we put current `right` position into `queue`, because we know that right adjacent number is a number for next line. But how can we jump to the next line?\n\nIn the example above, we want to traverse `1,4,2,5,3,6`. In other words, how can you start new lines? For example, from `2` to `5`.\n\nMy answer is when we are `col = 0`, we put a `bottom` position first, then check `right` position, so that we can start new lines with numbers in `col \n= 0`.\n\nLet\'s see one by one.\n\n```\n1,2,3\n4, ,\n5,6,\n\nq = [(0,0)] (= (0,0) is start position)\n\n(0,0): 1\n```\nTake the most left data in `queue`.\n```\n1,2,3\n4, ,\n5,6,\n\nrow = 0\ncol = 0\nq = []\nres = [1] \n\nNow col = 0, so add bottom position to queue\nq = [(1,0)]\n\nThen check right side, find 2. Add position to queue\nq = [(1,0),(0,1)]\n\n(1,0): 4\n(0,1): 2\n```\nTake the most left data in `queue`.\n```\n1,2,3\n4, ,\n5,6,\n\nrow = 1\ncol = 0\nq = [(0,1)]\nres = [1,4] \n\nNow col = 0, so add bottom position to queue\nq = [(0,1),(2,0)]\n\nThen check right side, there is no number, so skip\nq = [(0,1),(2,0)]\n\n(0,1): 2\n(2,0): 5\n```\nTake the most left data in `queue`.\n```\n1,2,3\n4, ,\n5,6,\n\nrow = 0\ncol = 1\nq = [(2,0)]\nres = [1,4,2] \n\nNow col != 0, so skip\nq = [(2,0)]\n\nThen check right side, find 3. Add position to queue\nq = [(2,0),(0,2)]\n\n(2,0): 5\n(0,2): 3\n```\nTake the most left data in `queue`.\n```\n1,2,3\n4, ,\n5,6,\n\nrow = 2\ncol = 0\nq = [(0,2)]\nres = [1,4,2,5]\n\nNow col = 0, but next bottom(3,0) is out of bounds, so skip\nq = [(0,2)]\n\nThen check right side, Find 6. Add position to queue\nq = [(0,2),(2,1)]\n\n(0,2): 3\n(2,1): 6\n```\nAt bottom from row 2, col = 0, there is no number but there is 6 on the right side. so this 6 will be start number in the next line.\n\nTake the most left data in `queue`.\n```\n1,2,3\n4, ,\n5,6,\n\nrow = 0\ncol = 2\nq = [(2,1)]\nres = [1,4,2,5,3]\n\nNow col != 0, so skip\nq = [(2,1)]\n\nThen check right side, there is no number, so skip\nq = [(2,1)]\n\n```\nTake the most left data in `queue`.\n```\n1,2,3\n4, ,\n5,6,\n\nrow = 2\ncol = 1\nq = []\nres = [1,4,2,5,3,6]\n\nNow col != 0, so skip\nq = []\n\nThen check right side, there is no number, so skip\nq = []\n\n```\n\n```\nOutput: [1,4,2,5,3,6]\n```\n\n---\n\n\u2B50\uFE0F Points\n\n- When col is equal to 0, then check bottom position, so that we can start new lines with right order\n- Check right position from every position\n\n---\n\nEasy!\uD83D\uDE04\nLet\'s see a real algorithm!\n\n\n### Algorithm Overview:\n1. Initialization\n2. BFS Traversal\n3. Return Result\n\n### Detailed Explanation:\n\n**Step 1: Initialization**\n```python\n# Create an empty list to store the result\nres = []\n\n# Initialize a queue with the starting point (0, 0)\nq = deque([(0, 0)])\n```\n\n**Explanation:** In this step, we set up the data structures needed for the algorithm. The `res` list will store the final result, and the `q` queue will be used for the breadth-first search (BFS) traversal. We start the traversal from the top-left corner, so the initial point `(0, 0)` is enqueued.\n\n**Step 2: BFS Traversal**\n```python\n# While the queue is not empty, perform the following steps\nwhile q:\n # Dequeue the front element\n row, col = q.popleft()\n\n # Append the current element to the result\n res.append(nums[row][col])\n\n # Enqueue the next element below if applicable\n if col == 0 and row + 1 < len(nums):\n q.append((row + 1, 0))\n\n # Enqueue the next element to the right if applicable\n if col + 1 < len(nums[row]):\n q.append((row, col + 1))\n```\n\n**Explanation:** This is the core of the algorithm, where the BFS traversal takes place. While the queue is not empty, we dequeue the front element, append the corresponding element from the input array `nums` to the result, and enqueue the next elements based on the conditions. If `col` is at the leftmost position (0), we enqueue the element below if there is a row below. Additionally, if there is a column to the right, we enqueue the element to the right.\n\n**Step 3: Return Result**\n```python\n# Return the final result list\nreturn res\n```\n\n**Explanation:** Once the BFS traversal is complete, the function returns the final result list containing the elements traversed diagonally in the 2D array.\n\nIn summary, the algorithm utilizes BFS to traverse the given 2D array diagonally, starting from the top-left corner and moving towards the bottom-right corner. The result is stored in the `res` list, which is then returned.\n\n\n# Complexity\n- Time complexity: $$O(N)$$\n`N` is number of position we visit.\n\n- Space complexity: $$O(N)$$\n\n\n```python []\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n res = []\n q = deque([(0, 0)])\n \n while q:\n row, col = q.popleft()\n res.append(nums[row][col])\n\n if col == 0 and row + 1 < len(nums):\n q.append((row + 1, 0))\n\n if col + 1 < len(nums[row]):\n q.append((row, col + 1))\n\n return res\n```\n```javascript []\nvar findDiagonalOrder = function(nums) {\n const res = [];\n const q = [[0, 0]];\n\n while (q.length > 0) {\n const [row, col] = q.shift();\n res.push(nums[row][col]);\n\n if (col === 0 && row + 1 < nums.length) {\n q.push([row + 1, 0]);\n }\n\n if (col + 1 < nums[row].length) {\n q.push([row, col + 1]);\n }\n }\n\n return res; \n};\n```\n```java []\nclass Solution {\n public int[] findDiagonalOrder(List<List<Integer>> nums) {\n List<Integer> resList = new ArrayList<>();\n LinkedList<int[]> q = new LinkedList<>();\n q.offer(new int[]{0, 0});\n\n while (!q.isEmpty()) {\n int[] p = q.poll();\n resList.add(nums.get(p[0]).get(p[1]));\n\n if (p[1] == 0 && p[0] + 1 < nums.size()) {\n q.offer(new int[]{p[0] + 1, 0});\n }\n\n if (p[1] + 1 < nums.get(p[0]).size()) {\n q.offer(new int[]{p[0], p[1] + 1});\n }\n }\n\n // Convert List<Integer> to int[]\n int[] res = new int[resList.size()];\n for (int i = 0; i < resList.size(); i++) {\n res[i] = resList.get(i);\n }\n\n return res; \n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n vector<int> findDiagonalOrder(vector<vector<int>>& nums) {\n vector<int> res;\n queue<pair<int, int>> q;\n q.push({0, 0});\n\n while (!q.empty()) {\n auto p = q.front();\n q.pop();\n res.push_back(nums[p.first][p.second]);\n\n if (p.second == 0 && p.first + 1 < nums.size()) {\n q.push({p.first + 1, 0});\n }\n\n if (p.second + 1 < nums[p.first].size()) {\n q.push({p.first, p.second + 1});\n }\n }\n\n return res; \n }\n};\n```\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n\u25A0 Subscribe URL\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\n\u25A0 Twitter\nhttps://twitter.com/CodingNinjaAZ\n\n### My next daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/arithmetic-subarrays/solutions/4321453/video-give-me-6-minutes-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/bE6YT9q16K8\n\n\u25A0 Timeline of the video\n`0:04` What does the formula mean?\n`1:46` Demonstrate how it works\n`3:39` Coding\n`5:40` Time Complexity and Space Complexity\n`6:06` Summary of the algorithm with my solution code\n\n### My previous daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/count-nice-pairs-in-an-array/solutions/4312501/video-give-me-6-minutes-hashmap-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/424EOdu4TeY\n\n\u25A0 Timeline of the video\n\n`0:04` Think about how we calculate the answer\n`0:58` Demonstrate how it works\n`3:58` Coding\n`5:53` Time Complexity and Space Complexity\n`6:11` Summary of the algorithm with my solution code\n | 38 | Given an array `arr` of positive integers sorted in a **strictly increasing order**, and an integer `k`.
Return _the_ `kth` _**positive** integer that is **missing** from this array._
**Example 1:**
**Input:** arr = \[2,3,4,7,11\], k = 5
**Output:** 9
**Explanation:** The missing positive integers are \[1,5,6,8,9,10,12,13,...\]. The 5th missing positive integer is 9.
**Example 2:**
**Input:** arr = \[1,2,3,4\], k = 2
**Output:** 6
**Explanation:** The missing positive integers are \[5,6,7,...\]. The 2nd missing positive integer is 6.
**Constraints:**
* `1 <= arr.length <= 1000`
* `1 <= arr[i] <= 1000`
* `1 <= k <= 1000`
* `arr[i] < arr[j]` for `1 <= i < j <= arr.length`
**Follow up:**
Could you solve this problem in less than O(n) complexity? | Notice that numbers with equal sums of row and column indexes belong to the same diagonal. Store them in tuples (sum, row, val), sort them, and then regroup the answer. |
All pairs of (i+j) that sums up to same value are diagonal | diagonal-traverse-ii | 0 | 1 | # Code\n```\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n n,m=len(nums),0\n for i in nums:\n m=max(m,len(i))\n ans=[[] for i in range(n+m-1)]\n for i in range(n):\n for j in range(len(nums[i])):\n ans[i+j].append(nums[i][j])\n sol=[]\n for i in ans:\n sol+=i[::-1]\n return sol\n``` | 1 | Given a 2D integer array `nums`, return _all elements of_ `nums` _in diagonal order as shown in the below images_.
**Example 1:**
**Input:** nums = \[\[1,2,3\],\[4,5,6\],\[7,8,9\]\]
**Output:** \[1,4,2,7,5,3,8,6,9\]
**Example 2:**
**Input:** nums = \[\[1,2,3,4,5\],\[6,7\],\[8\],\[9,10,11\],\[12,13,14,15,16\]\]
**Output:** \[1,6,2,8,7,3,9,4,12,10,5,13,11,14,15,16\]
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i].length <= 105`
* `1 <= sum(nums[i].length) <= 105`
* `1 <= nums[i][j] <= 105` | Use Breadth First Search (BFS) to traverse all possible boxes you can open. Only push to the queue the boxes the you have with their keys. |
All pairs of (i+j) that sums up to same value are diagonal | diagonal-traverse-ii | 0 | 1 | # Code\n```\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n n,m=len(nums),0\n for i in nums:\n m=max(m,len(i))\n ans=[[] for i in range(n+m-1)]\n for i in range(n):\n for j in range(len(nums[i])):\n ans[i+j].append(nums[i][j])\n sol=[]\n for i in ans:\n sol+=i[::-1]\n return sol\n``` | 1 | Given an array `arr` of positive integers sorted in a **strictly increasing order**, and an integer `k`.
Return _the_ `kth` _**positive** integer that is **missing** from this array._
**Example 1:**
**Input:** arr = \[2,3,4,7,11\], k = 5
**Output:** 9
**Explanation:** The missing positive integers are \[1,5,6,8,9,10,12,13,...\]. The 5th missing positive integer is 9.
**Example 2:**
**Input:** arr = \[1,2,3,4\], k = 2
**Output:** 6
**Explanation:** The missing positive integers are \[5,6,7,...\]. The 2nd missing positive integer is 6.
**Constraints:**
* `1 <= arr.length <= 1000`
* `1 <= arr[i] <= 1000`
* `1 <= k <= 1000`
* `arr[i] < arr[j]` for `1 <= i < j <= arr.length`
**Follow up:**
Could you solve this problem in less than O(n) complexity? | Notice that numbers with equal sums of row and column indexes belong to the same diagonal. Store them in tuples (sum, row, val), sort them, and then regroup the answer. |
Simple Solution for noobs | diagonal-traverse-ii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe code is about processing a 2D list (nums) diagonally and returning the elements in a specific order. It does this by first mapping elements of the 2D list to diagonals, and then sorting these diagonals based on their indices. Finally, it gathers the elements from each diagonal in reverse order.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nGrid Mapping:\n\nThe code starts by creating a dictionary (grid) to store elements based on the sum of their row and column indices. This effectively maps elements to diagonals in the 2D list.\nIterating Over the 2D List:\n\nIt then loops through each element in the 2D list (nums) and assigns it to the corresponding diagonal in the grid dictionary.\nSorting Diagonals:\n\nAfter mapping all elements to diagonals, it sorts the diagonals based on their keys (sum of row and column indices).\nBuilding Result List:\n\nFinally, it constructs the result list (res) by extending it with the elements from each diagonal, but in reverse order.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe first loop, where elements are mapped to diagonals, takes O(N) time, where N is the total number of elements in the 2D list.\nThe second loop, where diagonals are sorted, takes O(D log D) time, where D is the number of diagonals.\nThe overall time complexity is O(N + D log D).\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe additional space used is primarily for the grid dictionary, which stores elements based on their diagonals.\nThe space complexity is O(N), where N is the total number of elements in the 2D list.\n\n# Code\n```\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n grid=defaultdict(list)\n res=[]\n\n for row in range(len(nums)):\n for col in range(len(nums[row])):\n grid[row+col].append(nums[row][col])\n \n for d in sorted(grid.keys()):\n res.extend(grid[d][::-1])\n return res\n``` | 1 | Given a 2D integer array `nums`, return _all elements of_ `nums` _in diagonal order as shown in the below images_.
**Example 1:**
**Input:** nums = \[\[1,2,3\],\[4,5,6\],\[7,8,9\]\]
**Output:** \[1,4,2,7,5,3,8,6,9\]
**Example 2:**
**Input:** nums = \[\[1,2,3,4,5\],\[6,7\],\[8\],\[9,10,11\],\[12,13,14,15,16\]\]
**Output:** \[1,6,2,8,7,3,9,4,12,10,5,13,11,14,15,16\]
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i].length <= 105`
* `1 <= sum(nums[i].length) <= 105`
* `1 <= nums[i][j] <= 105` | Use Breadth First Search (BFS) to traverse all possible boxes you can open. Only push to the queue the boxes the you have with their keys. |
Simple Solution for noobs | diagonal-traverse-ii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe code is about processing a 2D list (nums) diagonally and returning the elements in a specific order. It does this by first mapping elements of the 2D list to diagonals, and then sorting these diagonals based on their indices. Finally, it gathers the elements from each diagonal in reverse order.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nGrid Mapping:\n\nThe code starts by creating a dictionary (grid) to store elements based on the sum of their row and column indices. This effectively maps elements to diagonals in the 2D list.\nIterating Over the 2D List:\n\nIt then loops through each element in the 2D list (nums) and assigns it to the corresponding diagonal in the grid dictionary.\nSorting Diagonals:\n\nAfter mapping all elements to diagonals, it sorts the diagonals based on their keys (sum of row and column indices).\nBuilding Result List:\n\nFinally, it constructs the result list (res) by extending it with the elements from each diagonal, but in reverse order.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe first loop, where elements are mapped to diagonals, takes O(N) time, where N is the total number of elements in the 2D list.\nThe second loop, where diagonals are sorted, takes O(D log D) time, where D is the number of diagonals.\nThe overall time complexity is O(N + D log D).\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe additional space used is primarily for the grid dictionary, which stores elements based on their diagonals.\nThe space complexity is O(N), where N is the total number of elements in the 2D list.\n\n# Code\n```\nclass Solution:\n def findDiagonalOrder(self, nums: List[List[int]]) -> List[int]:\n grid=defaultdict(list)\n res=[]\n\n for row in range(len(nums)):\n for col in range(len(nums[row])):\n grid[row+col].append(nums[row][col])\n \n for d in sorted(grid.keys()):\n res.extend(grid[d][::-1])\n return res\n``` | 1 | Given an array `arr` of positive integers sorted in a **strictly increasing order**, and an integer `k`.
Return _the_ `kth` _**positive** integer that is **missing** from this array._
**Example 1:**
**Input:** arr = \[2,3,4,7,11\], k = 5
**Output:** 9
**Explanation:** The missing positive integers are \[1,5,6,8,9,10,12,13,...\]. The 5th missing positive integer is 9.
**Example 2:**
**Input:** arr = \[1,2,3,4\], k = 2
**Output:** 6
**Explanation:** The missing positive integers are \[5,6,7,...\]. The 2nd missing positive integer is 6.
**Constraints:**
* `1 <= arr.length <= 1000`
* `1 <= arr[i] <= 1000`
* `1 <= k <= 1000`
* `arr[i] < arr[j]` for `1 <= i < j <= arr.length`
**Follow up:**
Could you solve this problem in less than O(n) complexity? | Notice that numbers with equal sums of row and column indexes belong to the same diagonal. Store them in tuples (sum, row, val), sort them, and then regroup the answer. |
Ordinary Heap-Based Approach | constrained-subsequence-sum | 0 | 1 | # Complexity\n- Time complexity: $$O(n*log(n))$$.\n\n- Space complexity: $$O(n)$$.\n\n# Code\n```\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n SUM, IND = 0, 1\n\n if all(num < 0 for num in nums):\n return max(nums)\n\n heap = [(0, math.inf)]\n maxSum = -math.inf\n for i, num in enumerate(nums):\n while i - heap[0][IND] > k:\n heappop(heap)\n \n summ = -heap[0][SUM] + num\n maxSum = max(maxSum, summ)\n heappush(heap, (-summ, i))\n \n return maxSum\n``` | 3 | Given an integer array `nums` and an integer `k`, return the maximum sum of a **non-empty** subsequence of that array such that for every two **consecutive** integers in the subsequence, `nums[i]` and `nums[j]`, where `i < j`, the condition `j - i <= k` is satisfied.
A _subsequence_ of an array is obtained by deleting some number of elements (can be zero) from the array, leaving the remaining elements in their original order.
**Example 1:**
**Input:** nums = \[10,2,-10,5,20\], k = 2
**Output:** 37
**Explanation:** The subsequence is \[10, 2, 5, 20\].
**Example 2:**
**Input:** nums = \[-1,-2,-3\], k = 1
**Output:** -1
**Explanation:** The subsequence must be non-empty, so we choose the largest number.
**Example 3:**
**Input:** nums = \[10,-2,-10,-5,20\], k = 2
**Output:** 23
**Explanation:** The subsequence is \[10, -2, -5, 20\].
**Constraints:**
* `1 <= k <= nums.length <= 105`
* `-104 <= nums[i] <= 104` | null |
Ordinary Heap-Based Approach | constrained-subsequence-sum | 0 | 1 | # Complexity\n- Time complexity: $$O(n*log(n))$$.\n\n- Space complexity: $$O(n)$$.\n\n# Code\n```\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n SUM, IND = 0, 1\n\n if all(num < 0 for num in nums):\n return max(nums)\n\n heap = [(0, math.inf)]\n maxSum = -math.inf\n for i, num in enumerate(nums):\n while i - heap[0][IND] > k:\n heappop(heap)\n \n summ = -heap[0][SUM] + num\n maxSum = max(maxSum, summ)\n heappush(heap, (-summ, i))\n \n return maxSum\n``` | 3 | Design the `CombinationIterator` class:
* `CombinationIterator(string characters, int combinationLength)` Initializes the object with a string `characters` of **sorted distinct** lowercase English letters and a number `combinationLength` as arguments.
* `next()` Returns the next combination of length `combinationLength` in **lexicographical order**.
* `hasNext()` Returns `true` if and only if there exists a next combination.
**Example 1:**
**Input**
\[ "CombinationIterator ", "next ", "hasNext ", "next ", "hasNext ", "next ", "hasNext "\]
\[\[ "abc ", 2\], \[\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, "ab ", true, "ac ", true, "bc ", false\]
**Explanation**
CombinationIterator itr = new CombinationIterator( "abc ", 2);
itr.next(); // return "ab "
itr.hasNext(); // return True
itr.next(); // return "ac "
itr.hasNext(); // return True
itr.next(); // return "bc "
itr.hasNext(); // return False
**Constraints:**
* `1 <= combinationLength <= characters.length <= 15`
* All the characters of `characters` are **unique**.
* At most `104` calls will be made to `next` and `hasNext`.
* It is guaranteed that all calls of the function `next` are valid. | Use dynamic programming. Let dp[i] be the solution for the prefix of the array that ends at index i, if the element at index i is in the subsequence. dp[i] = nums[i] + max(0, dp[i-k], dp[i-k+1], ..., dp[i-1]) Use a heap with the sliding window technique to optimize the dp. |
Python3 Solution | constrained-subsequence-sum | 0 | 1 | \n```\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n deque=[]\n for i,num in enumerate(nums):\n while deque and deque[0]<i-k:\n deque.pop(0)\n if deque:\n nums[i]=nums[deque[0]]+num\n while deque and nums[deque[-1]]<nums[i]:\n deque.pop()\n if nums[i]>0:\n deque.append(i)\n return max(nums) \n``` | 2 | Given an integer array `nums` and an integer `k`, return the maximum sum of a **non-empty** subsequence of that array such that for every two **consecutive** integers in the subsequence, `nums[i]` and `nums[j]`, where `i < j`, the condition `j - i <= k` is satisfied.
A _subsequence_ of an array is obtained by deleting some number of elements (can be zero) from the array, leaving the remaining elements in their original order.
**Example 1:**
**Input:** nums = \[10,2,-10,5,20\], k = 2
**Output:** 37
**Explanation:** The subsequence is \[10, 2, 5, 20\].
**Example 2:**
**Input:** nums = \[-1,-2,-3\], k = 1
**Output:** -1
**Explanation:** The subsequence must be non-empty, so we choose the largest number.
**Example 3:**
**Input:** nums = \[10,-2,-10,-5,20\], k = 2
**Output:** 23
**Explanation:** The subsequence is \[10, -2, -5, 20\].
**Constraints:**
* `1 <= k <= nums.length <= 105`
* `-104 <= nums[i] <= 104` | null |
Python3 Solution | constrained-subsequence-sum | 0 | 1 | \n```\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n deque=[]\n for i,num in enumerate(nums):\n while deque and deque[0]<i-k:\n deque.pop(0)\n if deque:\n nums[i]=nums[deque[0]]+num\n while deque and nums[deque[-1]]<nums[i]:\n deque.pop()\n if nums[i]>0:\n deque.append(i)\n return max(nums) \n``` | 2 | Design the `CombinationIterator` class:
* `CombinationIterator(string characters, int combinationLength)` Initializes the object with a string `characters` of **sorted distinct** lowercase English letters and a number `combinationLength` as arguments.
* `next()` Returns the next combination of length `combinationLength` in **lexicographical order**.
* `hasNext()` Returns `true` if and only if there exists a next combination.
**Example 1:**
**Input**
\[ "CombinationIterator ", "next ", "hasNext ", "next ", "hasNext ", "next ", "hasNext "\]
\[\[ "abc ", 2\], \[\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, "ab ", true, "ac ", true, "bc ", false\]
**Explanation**
CombinationIterator itr = new CombinationIterator( "abc ", 2);
itr.next(); // return "ab "
itr.hasNext(); // return True
itr.next(); // return "ac "
itr.hasNext(); // return True
itr.next(); // return "bc "
itr.hasNext(); // return False
**Constraints:**
* `1 <= combinationLength <= characters.length <= 15`
* All the characters of `characters` are **unique**.
* At most `104` calls will be made to `next` and `hasNext`.
* It is guaranteed that all calls of the function `next` are valid. | Use dynamic programming. Let dp[i] be the solution for the prefix of the array that ends at index i, if the element at index i is in the subsequence. dp[i] = nums[i] + max(0, dp[i-k], dp[i-k+1], ..., dp[i-1]) Use a heap with the sliding window technique to optimize the dp. |
Video Solution | Explanation With Drawings | In Depth | C++ | Java | Python 3 | constrained-subsequence-sum | 1 | 1 | # Intuition, approach and complexity discussed in detail in video solution\nhttps://youtu.be/KIB_v0d9r_E\n\n# Code\nC++\n```\n//TC : O(n), |nums | = n\n//SC : O(n)\nclass Solution {\npublic:\n int constrainedSubsetSum(vector<int>& nums, int k) {\n //maxTillThis will represent the max sum of sub seq ending at a particular index \n vector<int> maxTillThis = nums;//account for sub seq of size one i.e. the element itself\n //used to form a slding window which will in decreasing order\n deque<int> maxSbSqUptSzK;\n int res = maxTillThis[0];\n for (int indx = 0; indx < maxTillThis.size(); ++indx) {\n\n //from all subseq ending at this index what is maximum sum\n maxTillThis[indx] += maxSbSqUptSzK.size() ? maxTillThis[maxSbSqUptSzK.front()] : 0;\n \n //along that will we updating our final result\n res = max(res, maxTillThis[indx]);\n \n //remove all the sub seq sums that are lesser than current one\n while (maxSbSqUptSzK.size() && maxTillThis[indx] > maxTillThis[maxSbSqUptSzK.back()])\n maxSbSqUptSzK.pop_back();\n \n //current sub seq sum ending at \'indx\' is non zero\n if (maxTillThis[indx] > 0)\n maxSbSqUptSzK.push_back(indx);\n //remove all the sub seq sums to which current element cannot make contributions to \n if (indx >= k && maxSbSqUptSzK.size() && maxSbSqUptSzK.front() == indx - k)\n maxSbSqUptSzK.pop_front();\n \n }\n //returning maximum sum of valid sub seq\n return res;\n }\n};\n```\nJava\n```\nclass Solution {\n public int constrainedSubsetSum(int[] nums, int k) {\n int[] maxTillThis = nums.clone();\n Deque<Integer> maxSbSqUptSzK = new ArrayDeque<>();\n int res = maxTillThis[0];\n for (int indx = 0; indx < maxTillThis.length; ++indx) {\n maxTillThis[indx] += maxSbSqUptSzK.size() > 0 ? maxTillThis[maxSbSqUptSzK.peekFirst()] : 0;\n res = Math.max(res, maxTillThis[indx]);\n while (!maxSbSqUptSzK.isEmpty() && maxTillThis[indx] > maxTillThis[maxSbSqUptSzK.peekLast()]) {\n maxSbSqUptSzK.removeLast();\n }\n if (maxTillThis[indx] > 0) {\n maxSbSqUptSzK.addLast(indx);\n }\n if (indx >= k && !maxSbSqUptSzK.isEmpty() && maxSbSqUptSzK.peekFirst() == indx - k) {\n maxSbSqUptSzK.removeFirst();\n }\n }\n return res;\n }\n}\n```\nPython 3\n```\nclass Solution:\n def constrainedSubsetSum(self, nums, k):\n maxTillThis = nums.copy()\n maxSbSqUptSzK = deque()\n res = maxTillThis[0]\n for indx in range(len(maxTillThis)):\n maxTillThis[indx] += maxTillThis[maxSbSqUptSzK[0]] if len(maxSbSqUptSzK) > 0 else 0\n res = max(res, maxTillThis[indx])\n while len(maxSbSqUptSzK) > 0 and maxTillThis[indx] > maxTillThis[maxSbSqUptSzK[-1]]:\n maxSbSqUptSzK.pop()\n if maxTillThis[indx] > 0:\n maxSbSqUptSzK.append(indx)\n if indx >= k and len(maxSbSqUptSzK) > 0 and maxSbSqUptSzK[0] == indx - k:\n maxSbSqUptSzK.popleft()\n return res\n``` | 1 | Given an integer array `nums` and an integer `k`, return the maximum sum of a **non-empty** subsequence of that array such that for every two **consecutive** integers in the subsequence, `nums[i]` and `nums[j]`, where `i < j`, the condition `j - i <= k` is satisfied.
A _subsequence_ of an array is obtained by deleting some number of elements (can be zero) from the array, leaving the remaining elements in their original order.
**Example 1:**
**Input:** nums = \[10,2,-10,5,20\], k = 2
**Output:** 37
**Explanation:** The subsequence is \[10, 2, 5, 20\].
**Example 2:**
**Input:** nums = \[-1,-2,-3\], k = 1
**Output:** -1
**Explanation:** The subsequence must be non-empty, so we choose the largest number.
**Example 3:**
**Input:** nums = \[10,-2,-10,-5,20\], k = 2
**Output:** 23
**Explanation:** The subsequence is \[10, -2, -5, 20\].
**Constraints:**
* `1 <= k <= nums.length <= 105`
* `-104 <= nums[i] <= 104` | null |
Video Solution | Explanation With Drawings | In Depth | C++ | Java | Python 3 | constrained-subsequence-sum | 1 | 1 | # Intuition, approach and complexity discussed in detail in video solution\nhttps://youtu.be/KIB_v0d9r_E\n\n# Code\nC++\n```\n//TC : O(n), |nums | = n\n//SC : O(n)\nclass Solution {\npublic:\n int constrainedSubsetSum(vector<int>& nums, int k) {\n //maxTillThis will represent the max sum of sub seq ending at a particular index \n vector<int> maxTillThis = nums;//account for sub seq of size one i.e. the element itself\n //used to form a slding window which will in decreasing order\n deque<int> maxSbSqUptSzK;\n int res = maxTillThis[0];\n for (int indx = 0; indx < maxTillThis.size(); ++indx) {\n\n //from all subseq ending at this index what is maximum sum\n maxTillThis[indx] += maxSbSqUptSzK.size() ? maxTillThis[maxSbSqUptSzK.front()] : 0;\n \n //along that will we updating our final result\n res = max(res, maxTillThis[indx]);\n \n //remove all the sub seq sums that are lesser than current one\n while (maxSbSqUptSzK.size() && maxTillThis[indx] > maxTillThis[maxSbSqUptSzK.back()])\n maxSbSqUptSzK.pop_back();\n \n //current sub seq sum ending at \'indx\' is non zero\n if (maxTillThis[indx] > 0)\n maxSbSqUptSzK.push_back(indx);\n //remove all the sub seq sums to which current element cannot make contributions to \n if (indx >= k && maxSbSqUptSzK.size() && maxSbSqUptSzK.front() == indx - k)\n maxSbSqUptSzK.pop_front();\n \n }\n //returning maximum sum of valid sub seq\n return res;\n }\n};\n```\nJava\n```\nclass Solution {\n public int constrainedSubsetSum(int[] nums, int k) {\n int[] maxTillThis = nums.clone();\n Deque<Integer> maxSbSqUptSzK = new ArrayDeque<>();\n int res = maxTillThis[0];\n for (int indx = 0; indx < maxTillThis.length; ++indx) {\n maxTillThis[indx] += maxSbSqUptSzK.size() > 0 ? maxTillThis[maxSbSqUptSzK.peekFirst()] : 0;\n res = Math.max(res, maxTillThis[indx]);\n while (!maxSbSqUptSzK.isEmpty() && maxTillThis[indx] > maxTillThis[maxSbSqUptSzK.peekLast()]) {\n maxSbSqUptSzK.removeLast();\n }\n if (maxTillThis[indx] > 0) {\n maxSbSqUptSzK.addLast(indx);\n }\n if (indx >= k && !maxSbSqUptSzK.isEmpty() && maxSbSqUptSzK.peekFirst() == indx - k) {\n maxSbSqUptSzK.removeFirst();\n }\n }\n return res;\n }\n}\n```\nPython 3\n```\nclass Solution:\n def constrainedSubsetSum(self, nums, k):\n maxTillThis = nums.copy()\n maxSbSqUptSzK = deque()\n res = maxTillThis[0]\n for indx in range(len(maxTillThis)):\n maxTillThis[indx] += maxTillThis[maxSbSqUptSzK[0]] if len(maxSbSqUptSzK) > 0 else 0\n res = max(res, maxTillThis[indx])\n while len(maxSbSqUptSzK) > 0 and maxTillThis[indx] > maxTillThis[maxSbSqUptSzK[-1]]:\n maxSbSqUptSzK.pop()\n if maxTillThis[indx] > 0:\n maxSbSqUptSzK.append(indx)\n if indx >= k and len(maxSbSqUptSzK) > 0 and maxSbSqUptSzK[0] == indx - k:\n maxSbSqUptSzK.popleft()\n return res\n``` | 1 | Design the `CombinationIterator` class:
* `CombinationIterator(string characters, int combinationLength)` Initializes the object with a string `characters` of **sorted distinct** lowercase English letters and a number `combinationLength` as arguments.
* `next()` Returns the next combination of length `combinationLength` in **lexicographical order**.
* `hasNext()` Returns `true` if and only if there exists a next combination.
**Example 1:**
**Input**
\[ "CombinationIterator ", "next ", "hasNext ", "next ", "hasNext ", "next ", "hasNext "\]
\[\[ "abc ", 2\], \[\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, "ab ", true, "ac ", true, "bc ", false\]
**Explanation**
CombinationIterator itr = new CombinationIterator( "abc ", 2);
itr.next(); // return "ab "
itr.hasNext(); // return True
itr.next(); // return "ac "
itr.hasNext(); // return True
itr.next(); // return "bc "
itr.hasNext(); // return False
**Constraints:**
* `1 <= combinationLength <= characters.length <= 15`
* All the characters of `characters` are **unique**.
* At most `104` calls will be made to `next` and `hasNext`.
* It is guaranteed that all calls of the function `next` are valid. | Use dynamic programming. Let dp[i] be the solution for the prefix of the array that ends at index i, if the element at index i is in the subsequence. dp[i] = nums[i] + max(0, dp[i-k], dp[i-k+1], ..., dp[i-1]) Use a heap with the sliding window technique to optimize the dp. |
【Video】Give me 10 minutes - How we think about a solution - Python, JavaScript, Java, C++ | constrained-subsequence-sum | 1 | 1 | # Intuition\nhaving pairs (index and max value) in deque with decreasing order\n\n---\n\n# Solution Video\n\nhttps://youtu.be/KuMkwvvesgo\n\n\u25A0 Timeline of the video\n`0:04` Explain a few basic idea\n`3:21` What data we should put in deque?\n`5:10` Demonstrate how it works\n`13:27` Coding\n`16:03` Time Complexity and Space Complexity\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 2,759\nMy initial goal is 10,000\nThank you for your support!\n\n---\n\n\n# Approach\n\n## How we think about a solution\n\nIn this question, we need to find maximum sum of two numbers with the condition of `j - i <= k`.\n\nSince we need to care about range and maximum value, seems like it\'s good idea to have both data as a pair.\n\n---\n\n\u2B50\uFE0F Points\n\nWe should have pairs of `index` and `maximum sum value`, because we have to care about them for this quesiton.\n\n**Actually we can solve this question with holding only index numbers but it\'s the same as LeetCode tutorial. So I\'ll show you another way.**\n\n---\n\nYou may face a difficulty now. The question is\n\n---\n\n\u25A0 Question\n\n- How do we make sure this is maximum sum value in the `k` range?\n---\n\nSince we have range `k`, previous number might be part of the maximum sum value. So it\'s going to be tough if we move back and forth.\n\nIn this case, what data structure do you use?\n\n---\n\n\u2B50\uFE0F Points\n\nMy answer is to use `deque`.\n\nOne more important thing is that all data in `deque` are sorted in `decreasing order` of maximum sum value.\n\nIn other words, the first data(`deque[0]`) is always the maximum sum value in range `k` because all data are sorted in `decreasing order`.\n\n---\n\n- How can you keep range `k` in `deque`?\n\nI believe your next question is how you can keep range `k` in `deque`? Acutually, this is a very simple.\n\nSince we have pairs of index and maximum sum value, in every iteration, we check the index of the maximum sum values are valid or not with current index `i` - `k`.\n\nIf the index is equal to `i` - `k`, `pop` the maximum pair(= `deque[0]`) by `popleft()` because the index will be out of bounds in the next iteration, so no longer available. The reason why we use `deque` is that we can do this operation with `O(1)` instead of a list with.\n\nOf course, you can use list to keep index and maximum sum value but when you pop data from `list[0]`, it\'s going to be time comsuming because all data will shift to the next places one by one. `deque` never happens like that.\n\n- What data we should put in `deque`?\n\nBefore we discuss the question, Let me ask you one question.\n\nwe need to create the maximum sum sum, so question here is\n\n---\n\n\u25A0 Question\n\n- How can we calculate the first number(`index 0`) in input array? Because so far only one number shows up.\n\n---\n\nThis answer is also simple. This is addition, use `0` instead of `deque[0]`, because `0` doesn\'t affect any results of addition. In real solution code, use only number from `nums`.\n\nLet\'s go back to the main question. Since we calcualte the first number with `nums[0] + 0`, so we don\'t need to have negative maximum sum value in `deque`, because we can say\n\n```\nLet\'s say we are now at index 3.\n\nnums[3] + 0 > nums[3] + -1\n\n0 and -1 are maximum sum value from `deque`.\n```\n\n---\n\n\u2B50\uFE0F Points\n\nWe should have only positive maximum sum value in `deque`.\n\n---\n\nLet\'s see an example.\n\n```\nnums = [10,-2,-10,-5,20], k = 2\n```\niterate through one by one.\n\n```\nindex = 0 (= 10)\ntotal = 10 (current number + max sum value of deque(= deque[0]))\nres = 10 (this is return value)\n\nif positive maximum sum? Yes\ndeque([(0, 10)]) \u2192 (index, maximum sum value)\n```\n```\nindex = 1 (= -2)\ntotal = 8\nres = 10 (because 10 > 8)\n\nif positive maximum sum? Yes\ndeque([(0, 10), (1, 8)])\n```\n```\nindex = 2 (= -10)\ntotal = 0\nres = 10 (because 10 > 0)\n\nif positive maximum sum? No\ndeque([(0, 10), (1, 8)])\n\nif 0 == 2 - 2 (index of deque[0] == i - k)? Yes\n(0, 10) will be out of bounds in the next iteration.\n\ndeque([(1, 8)])\n```\n```\nindex = 3 (= -5)\ntotal = 3\nres = 10 (because 10 > 3)\n\nif positive maximum sum? Yes\ndeque([(1, 8), (3, 3)])\n\nif 1 == 3 - 2 (index of deque[0] == i - k)? Yes\ndeque([(3, 3)])\n```\n```\nindex = 4 (= 20)\ntotal = 23\nres = 23 (because 10 < 23)\n\nif positive maximum sum? Yes\ndeque([(3, 3), (4, 23)])\n\u2193\nkeep decreasing order of maximum sum value, pop (3, 3)\nbecause 23 is greater than 3\ndeque([(4, 23)])\n\nif 4 == 4 - 2 (index of deque[0] == i - k)? ? No\ndeque([(4, 23)])\n```\n\n```\nOutput: 23\n```\n\nThank you for reading! It took almost 2 hours to finish writing the post. lol\nLet\'s see a real algorithm.\n\n**Algorithm Overview:**\n\nThe algorithm aims to find the maximum sum of a non-empty subsequence of `nums` with a constraint that the difference between the indices of any \n consecutive elements in the subsequence must not exceed `k`.\n\n**Detailed Explanation:**\n\n1. Initialize an empty deque `q` to store indices and their corresponding total sums, and a variable `res` to negative infinity. The deque `q` will be used to efficiently maintain the maximum total sum with the constraint.\n\n2. Iterate through the elements of the `nums` list using the `enumerate` function, which provides both the index `i` and the value `num` of the current element.\n\n3. Calculate the `total` for the current element by adding the value `num` to the total sum associated with the first index in the deque, `q[0][1]`, if the deque `q` is not empty. If the deque is empty, set `total` to the value of the current element, `num`.\n\n4. Update the `res` variable with the maximum of its current value and the calculated `total`. This step keeps track of the maximum total sum seen so far.\n\n5. Check if the deque `q` is not empty and the total `total` is greater than or equal to the total sum associated with the last index in the deque, `q[-1][1]`. If this condition is met, pop the last index from the deque because it will not be needed anymore.\n\n6. If the `total` is greater than 0, append a tuple containing the current index `i` and the calculated `total` to the deque `q`. This index and its total sum will be used in the future calculations.\n\n7. Check if the deque `q` is not empty and the index associated with the first element, `q[0][0]`, is equal to `i - k`. If this condition is met, it means the index at the front of the deque is too far from the current index `i`, so pop it from the deque. This step ensures that the maximum subsequence length is limited to `k`.\n\n8. Repeat the above steps for all elements in the `nums` list.\n\n9. Finally, return the `res` variable, which holds the maximum total sum found throughout the iteration.\n\nThis algorithm efficiently maintains a deque of indices with decreasing total sums and ensures that the maximum subsequence sum with a length constraint of `k` is calculated correctly.\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(k)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n```python []\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n \n q = deque()\n res = float(\'-inf\')\n\n for i, num in enumerate(nums):\n total = num + q[0][1] if q else num\n res = max(res, total)\n\n while q and total >= q[-1][1]:\n q.pop()\n \n if total > 0:\n q.append((i, total))\n \n if q and q[0][0] == i - k:\n q.popleft()\n \n return res \n```\n```javascript []\n/**\n * @param {number[]} nums\n * @param {number} k\n * @return {number}\n */\nvar constrainedSubsetSum = function(nums, k) {\n const q = [];\n let res = -Infinity;\n\n for (let i = 0; i < nums.length; i++) {\n const num = nums[i];\n const total = num + (q.length > 0 ? q[0][1] : 0);\n res = Math.max(res, total);\n\n while (q.length > 0 && total >= q[q.length - 1][1]) {\n q.pop();\n }\n\n if (total > 0) {\n q.push([i, total]);\n }\n\n if (q.length > 0 && q[0][0] === i - k) {\n q.shift();\n }\n }\n\n return res; \n};\n```\n```java []\nclass Solution {\n public int constrainedSubsetSum(int[] nums, int k) {\n Deque<int[]> q = new LinkedList<>();\n int res = Integer.MIN_VALUE;\n\n for (int i = 0; i < nums.length; i++) {\n int num = nums[i];\n int total = num + (!q.isEmpty() ? q.getFirst()[1] : 0);\n res = Math.max(res, total);\n\n while (!q.isEmpty() && total >= q.getLast()[1]) {\n q.removeLast();\n }\n\n if (total > 0) {\n q.addLast(new int[]{i, total});\n }\n\n if (!q.isEmpty() && q.getFirst()[0] == i - k) {\n q.removeFirst();\n }\n }\n\n return res; \n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int constrainedSubsetSum(vector<int>& nums, int k) {\n deque<pair<int, int>> q;\n int res = INT_MIN;\n\n for (int i = 0; i < nums.size(); i++) {\n int num = nums[i];\n int total = num + (!q.empty() ? q.front().second : 0);\n res = max(res, total);\n\n while (!q.empty() && total >= q.back().second) {\n q.pop_back();\n }\n\n if (total > 0) {\n q.push_back(make_pair(i, total));\n }\n\n if (!q.empty() && q.front().first == i - k) {\n q.pop_front();\n }\n }\n\n return res; \n }\n};\n```\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n\u25A0 Subscribe URL\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\n### My next daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/maximum-score-of-a-good-subarray/solutions/4196248/video-give-me-10-minutes-how-we-think-about-a-solution-python-javascript-java-c/\n\nvideo\nhttps://youtu.be/ZiO47ctvu6w\n\n\u25A0 Timeline of the video\n`0:05` Easy way to solve a constraint\n`2:04` How we can move i and j pointers\n`3:59` candidates for minimum number and max score\n`4:29` Demonstrate how it works\n`8:25` Coding\n`11:04` Time Complexity and Space Complexity\n\n\n### My previous daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/flatten-nested-list-iterator/solutions/4189136/give-me-10-minutes-how-we-think-about-a-solution-python-javascript-java-c/\n\nvideo\nhttps://youtu.be/N8QehVXYSc0\n\n\u25A0 Timeline of the video\n`0:04` 2 Keys to solve this question\n`0:18` Explain the first key point\n`0:45` Explain the first key point\n`1:27` Explain the second key point\n`5:44` Coding\n`9:39` Time Complexity and Space Complexity\n | 51 | Given an integer array `nums` and an integer `k`, return the maximum sum of a **non-empty** subsequence of that array such that for every two **consecutive** integers in the subsequence, `nums[i]` and `nums[j]`, where `i < j`, the condition `j - i <= k` is satisfied.
A _subsequence_ of an array is obtained by deleting some number of elements (can be zero) from the array, leaving the remaining elements in their original order.
**Example 1:**
**Input:** nums = \[10,2,-10,5,20\], k = 2
**Output:** 37
**Explanation:** The subsequence is \[10, 2, 5, 20\].
**Example 2:**
**Input:** nums = \[-1,-2,-3\], k = 1
**Output:** -1
**Explanation:** The subsequence must be non-empty, so we choose the largest number.
**Example 3:**
**Input:** nums = \[10,-2,-10,-5,20\], k = 2
**Output:** 23
**Explanation:** The subsequence is \[10, -2, -5, 20\].
**Constraints:**
* `1 <= k <= nums.length <= 105`
* `-104 <= nums[i] <= 104` | null |
【Video】Give me 10 minutes - How we think about a solution - Python, JavaScript, Java, C++ | constrained-subsequence-sum | 1 | 1 | # Intuition\nhaving pairs (index and max value) in deque with decreasing order\n\n---\n\n# Solution Video\n\nhttps://youtu.be/KuMkwvvesgo\n\n\u25A0 Timeline of the video\n`0:04` Explain a few basic idea\n`3:21` What data we should put in deque?\n`5:10` Demonstrate how it works\n`13:27` Coding\n`16:03` Time Complexity and Space Complexity\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 2,759\nMy initial goal is 10,000\nThank you for your support!\n\n---\n\n\n# Approach\n\n## How we think about a solution\n\nIn this question, we need to find maximum sum of two numbers with the condition of `j - i <= k`.\n\nSince we need to care about range and maximum value, seems like it\'s good idea to have both data as a pair.\n\n---\n\n\u2B50\uFE0F Points\n\nWe should have pairs of `index` and `maximum sum value`, because we have to care about them for this quesiton.\n\n**Actually we can solve this question with holding only index numbers but it\'s the same as LeetCode tutorial. So I\'ll show you another way.**\n\n---\n\nYou may face a difficulty now. The question is\n\n---\n\n\u25A0 Question\n\n- How do we make sure this is maximum sum value in the `k` range?\n---\n\nSince we have range `k`, previous number might be part of the maximum sum value. So it\'s going to be tough if we move back and forth.\n\nIn this case, what data structure do you use?\n\n---\n\n\u2B50\uFE0F Points\n\nMy answer is to use `deque`.\n\nOne more important thing is that all data in `deque` are sorted in `decreasing order` of maximum sum value.\n\nIn other words, the first data(`deque[0]`) is always the maximum sum value in range `k` because all data are sorted in `decreasing order`.\n\n---\n\n- How can you keep range `k` in `deque`?\n\nI believe your next question is how you can keep range `k` in `deque`? Acutually, this is a very simple.\n\nSince we have pairs of index and maximum sum value, in every iteration, we check the index of the maximum sum values are valid or not with current index `i` - `k`.\n\nIf the index is equal to `i` - `k`, `pop` the maximum pair(= `deque[0]`) by `popleft()` because the index will be out of bounds in the next iteration, so no longer available. The reason why we use `deque` is that we can do this operation with `O(1)` instead of a list with.\n\nOf course, you can use list to keep index and maximum sum value but when you pop data from `list[0]`, it\'s going to be time comsuming because all data will shift to the next places one by one. `deque` never happens like that.\n\n- What data we should put in `deque`?\n\nBefore we discuss the question, Let me ask you one question.\n\nwe need to create the maximum sum sum, so question here is\n\n---\n\n\u25A0 Question\n\n- How can we calculate the first number(`index 0`) in input array? Because so far only one number shows up.\n\n---\n\nThis answer is also simple. This is addition, use `0` instead of `deque[0]`, because `0` doesn\'t affect any results of addition. In real solution code, use only number from `nums`.\n\nLet\'s go back to the main question. Since we calcualte the first number with `nums[0] + 0`, so we don\'t need to have negative maximum sum value in `deque`, because we can say\n\n```\nLet\'s say we are now at index 3.\n\nnums[3] + 0 > nums[3] + -1\n\n0 and -1 are maximum sum value from `deque`.\n```\n\n---\n\n\u2B50\uFE0F Points\n\nWe should have only positive maximum sum value in `deque`.\n\n---\n\nLet\'s see an example.\n\n```\nnums = [10,-2,-10,-5,20], k = 2\n```\niterate through one by one.\n\n```\nindex = 0 (= 10)\ntotal = 10 (current number + max sum value of deque(= deque[0]))\nres = 10 (this is return value)\n\nif positive maximum sum? Yes\ndeque([(0, 10)]) \u2192 (index, maximum sum value)\n```\n```\nindex = 1 (= -2)\ntotal = 8\nres = 10 (because 10 > 8)\n\nif positive maximum sum? Yes\ndeque([(0, 10), (1, 8)])\n```\n```\nindex = 2 (= -10)\ntotal = 0\nres = 10 (because 10 > 0)\n\nif positive maximum sum? No\ndeque([(0, 10), (1, 8)])\n\nif 0 == 2 - 2 (index of deque[0] == i - k)? Yes\n(0, 10) will be out of bounds in the next iteration.\n\ndeque([(1, 8)])\n```\n```\nindex = 3 (= -5)\ntotal = 3\nres = 10 (because 10 > 3)\n\nif positive maximum sum? Yes\ndeque([(1, 8), (3, 3)])\n\nif 1 == 3 - 2 (index of deque[0] == i - k)? Yes\ndeque([(3, 3)])\n```\n```\nindex = 4 (= 20)\ntotal = 23\nres = 23 (because 10 < 23)\n\nif positive maximum sum? Yes\ndeque([(3, 3), (4, 23)])\n\u2193\nkeep decreasing order of maximum sum value, pop (3, 3)\nbecause 23 is greater than 3\ndeque([(4, 23)])\n\nif 4 == 4 - 2 (index of deque[0] == i - k)? ? No\ndeque([(4, 23)])\n```\n\n```\nOutput: 23\n```\n\nThank you for reading! It took almost 2 hours to finish writing the post. lol\nLet\'s see a real algorithm.\n\n**Algorithm Overview:**\n\nThe algorithm aims to find the maximum sum of a non-empty subsequence of `nums` with a constraint that the difference between the indices of any \n consecutive elements in the subsequence must not exceed `k`.\n\n**Detailed Explanation:**\n\n1. Initialize an empty deque `q` to store indices and their corresponding total sums, and a variable `res` to negative infinity. The deque `q` will be used to efficiently maintain the maximum total sum with the constraint.\n\n2. Iterate through the elements of the `nums` list using the `enumerate` function, which provides both the index `i` and the value `num` of the current element.\n\n3. Calculate the `total` for the current element by adding the value `num` to the total sum associated with the first index in the deque, `q[0][1]`, if the deque `q` is not empty. If the deque is empty, set `total` to the value of the current element, `num`.\n\n4. Update the `res` variable with the maximum of its current value and the calculated `total`. This step keeps track of the maximum total sum seen so far.\n\n5. Check if the deque `q` is not empty and the total `total` is greater than or equal to the total sum associated with the last index in the deque, `q[-1][1]`. If this condition is met, pop the last index from the deque because it will not be needed anymore.\n\n6. If the `total` is greater than 0, append a tuple containing the current index `i` and the calculated `total` to the deque `q`. This index and its total sum will be used in the future calculations.\n\n7. Check if the deque `q` is not empty and the index associated with the first element, `q[0][0]`, is equal to `i - k`. If this condition is met, it means the index at the front of the deque is too far from the current index `i`, so pop it from the deque. This step ensures that the maximum subsequence length is limited to `k`.\n\n8. Repeat the above steps for all elements in the `nums` list.\n\n9. Finally, return the `res` variable, which holds the maximum total sum found throughout the iteration.\n\nThis algorithm efficiently maintains a deque of indices with decreasing total sums and ensures that the maximum subsequence sum with a length constraint of `k` is calculated correctly.\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(k)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n```python []\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n \n q = deque()\n res = float(\'-inf\')\n\n for i, num in enumerate(nums):\n total = num + q[0][1] if q else num\n res = max(res, total)\n\n while q and total >= q[-1][1]:\n q.pop()\n \n if total > 0:\n q.append((i, total))\n \n if q and q[0][0] == i - k:\n q.popleft()\n \n return res \n```\n```javascript []\n/**\n * @param {number[]} nums\n * @param {number} k\n * @return {number}\n */\nvar constrainedSubsetSum = function(nums, k) {\n const q = [];\n let res = -Infinity;\n\n for (let i = 0; i < nums.length; i++) {\n const num = nums[i];\n const total = num + (q.length > 0 ? q[0][1] : 0);\n res = Math.max(res, total);\n\n while (q.length > 0 && total >= q[q.length - 1][1]) {\n q.pop();\n }\n\n if (total > 0) {\n q.push([i, total]);\n }\n\n if (q.length > 0 && q[0][0] === i - k) {\n q.shift();\n }\n }\n\n return res; \n};\n```\n```java []\nclass Solution {\n public int constrainedSubsetSum(int[] nums, int k) {\n Deque<int[]> q = new LinkedList<>();\n int res = Integer.MIN_VALUE;\n\n for (int i = 0; i < nums.length; i++) {\n int num = nums[i];\n int total = num + (!q.isEmpty() ? q.getFirst()[1] : 0);\n res = Math.max(res, total);\n\n while (!q.isEmpty() && total >= q.getLast()[1]) {\n q.removeLast();\n }\n\n if (total > 0) {\n q.addLast(new int[]{i, total});\n }\n\n if (!q.isEmpty() && q.getFirst()[0] == i - k) {\n q.removeFirst();\n }\n }\n\n return res; \n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int constrainedSubsetSum(vector<int>& nums, int k) {\n deque<pair<int, int>> q;\n int res = INT_MIN;\n\n for (int i = 0; i < nums.size(); i++) {\n int num = nums[i];\n int total = num + (!q.empty() ? q.front().second : 0);\n res = max(res, total);\n\n while (!q.empty() && total >= q.back().second) {\n q.pop_back();\n }\n\n if (total > 0) {\n q.push_back(make_pair(i, total));\n }\n\n if (!q.empty() && q.front().first == i - k) {\n q.pop_front();\n }\n }\n\n return res; \n }\n};\n```\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n\u25A0 Subscribe URL\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\n### My next daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/maximum-score-of-a-good-subarray/solutions/4196248/video-give-me-10-minutes-how-we-think-about-a-solution-python-javascript-java-c/\n\nvideo\nhttps://youtu.be/ZiO47ctvu6w\n\n\u25A0 Timeline of the video\n`0:05` Easy way to solve a constraint\n`2:04` How we can move i and j pointers\n`3:59` candidates for minimum number and max score\n`4:29` Demonstrate how it works\n`8:25` Coding\n`11:04` Time Complexity and Space Complexity\n\n\n### My previous daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/flatten-nested-list-iterator/solutions/4189136/give-me-10-minutes-how-we-think-about-a-solution-python-javascript-java-c/\n\nvideo\nhttps://youtu.be/N8QehVXYSc0\n\n\u25A0 Timeline of the video\n`0:04` 2 Keys to solve this question\n`0:18` Explain the first key point\n`0:45` Explain the first key point\n`1:27` Explain the second key point\n`5:44` Coding\n`9:39` Time Complexity and Space Complexity\n | 51 | Design the `CombinationIterator` class:
* `CombinationIterator(string characters, int combinationLength)` Initializes the object with a string `characters` of **sorted distinct** lowercase English letters and a number `combinationLength` as arguments.
* `next()` Returns the next combination of length `combinationLength` in **lexicographical order**.
* `hasNext()` Returns `true` if and only if there exists a next combination.
**Example 1:**
**Input**
\[ "CombinationIterator ", "next ", "hasNext ", "next ", "hasNext ", "next ", "hasNext "\]
\[\[ "abc ", 2\], \[\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, "ab ", true, "ac ", true, "bc ", false\]
**Explanation**
CombinationIterator itr = new CombinationIterator( "abc ", 2);
itr.next(); // return "ab "
itr.hasNext(); // return True
itr.next(); // return "ac "
itr.hasNext(); // return True
itr.next(); // return "bc "
itr.hasNext(); // return False
**Constraints:**
* `1 <= combinationLength <= characters.length <= 15`
* All the characters of `characters` are **unique**.
* At most `104` calls will be made to `next` and `hasNext`.
* It is guaranteed that all calls of the function `next` are valid. | Use dynamic programming. Let dp[i] be the solution for the prefix of the array that ends at index i, if the element at index i is in the subsequence. dp[i] = nums[i] + max(0, dp[i-k], dp[i-k+1], ..., dp[i-1]) Use a heap with the sliding window technique to optimize the dp. |
✅☑[C++/Java/Python/JavaScript] || 3 Approaches || EXPLAINED🔥 | constrained-subsequence-sum | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n**(Also explained in the code)**\n\n#### ***Approach 1 (Heap)***\n1. We use a max heap (priority_queue) to efficiently keep track of the maximum sum of a constrained subset and the index at which it ends.\n\n1. We initialize the answer (`ans`) with the value of the first element in the input `nums` array.\n\n1. We iterate through the elements of `nums` from the second element (index 1) to the end.\n\n1. In the loop, we check whether elements in the max heap are within the constraint (at most `k` elements away from the current element).\n\n1. We remove elements from the heap that are out of range because they are no longer useful for calculating the maximum sum.\n\n1. We calculate the current sum (`curr`) by adding the value of the current element to the maximum of zero and the top element in the max heap. This ensures that we don\'t include negative values from the heap if they are not beneficial.\n\n1. We update the answer (`ans`) with the maximum of the current sum and the previous answer.\n\n1. We push the current sum and its index into the max heap for future calculations.\n\n1. After the loop, we have the maximum sum of the constrained subset in `ans`, and we return it as the result.\n\n\n# Complexity\n- *Time complexity:*\n $$O(nlogn)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int constrainedSubsetSum(vector<int>& nums, int k) {\n priority_queue<pair<int, int>> heap; // Create a max heap to keep track of the maximum sum and its index.\n heap.push({nums[0], 0}); // Push the first element into the heap with its value and index.\n int ans = nums[0]; // Initialize the answer with the first element\'s value.\n\n for (int i = 1; i < nums.size(); i++) { // Iterate through the remaining elements in nums.\n while (i - heap.top().second > k) { // While the index difference is greater than k (out of range):\n heap.pop(); // Remove elements from the heap that are out of range.\n }\n\n int curr = max(0, heap.top().first) + nums[i]; // Calculate the current sum considering the maximum possible value.\n ans = max(ans, curr); // Update the answer with the maximum of the current sum and the previous answer.\n heap.push({curr, i}); // Push the current sum and its index into the heap.\n }\n\n return ans; // Return the maximum sum of the constrained subset.\n }\n};\n\n```\n\n\n```C []\nint constrainedSubsetSum(int* nums, int numsSize, int k) {\n struct Node {\n int value;\n int index;\n };\n \n struct Node* heap = (struct Node*)malloc(numsSize * sizeof(struct Node));\n int heapSize = 0;\n \n int ans = nums[0];\n \n for (int i = 0; i < numsSize; i++) {\n while (i - heap[0].index > k) {\n // Remove elements from the heap that are out of range.\n if (heapSize > 0) {\n heap[0] = heap[heapSize - 1];\n heapSize--;\n int currIndex = 0;\n while (true) {\n int leftChild = currIndex * 2 + 1;\n int rightChild = currIndex * 2 + 2;\n int nextIndex = currIndex;\n if (leftChild < heapSize && heap[leftChild].value > heap[nextIndex].value) {\n nextIndex = leftChild;\n }\n if (rightChild < heapSize && heap[rightChild].value > heap[nextIndex].value) {\n nextIndex = rightChild;\n }\n if (nextIndex == currIndex) {\n break;\n }\n struct Node temp = heap[currIndex];\n heap[currIndex] = heap[nextIndex];\n heap[nextIndex] = temp;\n currIndex = nextIndex;\n }\n } else {\n break;\n }\n }\n \n int curr = (heapSize > 0) ? (heap[0].value + nums[i]) : nums[i];\n \n ans = (curr > ans) ? curr : ans;\n \n struct Node newNode;\n newNode.value = (curr > 0) ? curr : 0;\n newNode.index = i;\n heap[heapSize] = newNode;\n \n int currIndex = heapSize;\n while (currIndex > 0) {\n int parentIndex = (currIndex - 1) / 2;\n if (heap[currIndex].value > heap[parentIndex].value) {\n struct Node temp = heap[currIndex];\n heap[currIndex] = heap[parentIndex];\n heap[parentIndex] = temp;\n currIndex = parentIndex;\n } else {\n break;\n }\n }\n \n heapSize++;\n }\n \n free(heap);\n \n return ans;\n}\n\n\n```\n\n\n```Java []\nclass Solution {\n public int constrainedSubsetSum(int[] nums, int k) {\n PriorityQueue<int[]> heap = new PriorityQueue<>((a, b) -> {\n return b[0] - a[0];\n });\n \n heap.add(new int[] {nums[0], 0});\n int ans = nums[0];\n \n for (int i = 1; i < nums.length; i++) {\n while (i - heap.peek()[1] > k) {\n heap.remove();\n }\n \n int curr = Math.max(0, heap.peek()[0]) + nums[i];\n ans = Math.max(ans, curr);\n heap.add(new int[] {curr, i});\n }\n \n return ans;\n }\n}\n\n```\n\n```python3 []\nimport heapq\n\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n heap = [(-nums[0], 0)]\n ans = nums[0]\n \n for i in range(1, len(nums)):\n while i - heap[0][1] > k:\n heapq.heappop(heap)\n\n curr = max(0, -heap[0][0]) + nums[i]\n ans = max(ans, curr)\n heapq.heappush(heap, (-curr, i))\n\n return ans\n\n```\n\n```javascript []\nvar constrainedSubsetSum = function(nums, k) {\n let maxSum = nums[0];\n const dp = [nums[0]];\n const maxHeap = [nums[0]];\n\n for (let i = 1; i < nums.length; i++) {\n while (maxHeap.length > 0 && i - maxHeap[0][1] > k) {\n maxHeap.shift();\n }\n const current = Math.max(nums[i], nums[i] + (maxHeap.length > 0 ? maxHeap[0][0] : 0));\n maxSum = Math.max(maxSum, current);\n dp.push(current);\n while (maxHeap.length > 0 && dp[i] >= maxHeap[maxHeap.length - 1][0]) {\n maxHeap.pop();\n }\n maxHeap.push([dp[i], i]);\n }\n\n return maxSum;\n};\n\n```\n\n\n---\n\n#### ***Approach 2 (TreeMap)***\n1. Create a `map` named `window` to maintain a sliding window of values. The keys represent the sums of elements in the window, and the values represent the number of times each sum appears.\n1. Initialize `window[0]` to 0 to handle edge cases.\n1. Create a vector `dp` to store the dynamic programming values, with the same size as the input `nums`.\n1. Start iterating through the `nums` array using a `for` loop.\n1. For each element at index `i`, calculate `dp[i]` by adding the current element `nums[i]` to the maximum sum in the sliding window (`window.rbegin()->first`).\n1. Increase the count for this `dp[i]` in the `window` map to keep track of its frequency.\n1. Check if the sliding window size has exceeded `k`. If it has, remove elements from the window map that are no longer in the `window`:\n - Decrement the count of the sum corresponding to `dp[i - k]`.\n - If the count becomes 0, remove that sum from the `window` map using `window.erase()`.\n1. Continue the loop until all elements in the `nums` array are processed.\n1. After processing all elements, return the maximum value from the `dp` vector using the `*max_element(dp.begin(), dp.end())` function.\n - This maximum value represents the maximum sum of a constrained subset of at most `k` elements.\n\n\n# Complexity\n- *Time complexity:*\n $$O(nlogk)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int constrainedSubsetSum(vector<int>& nums, int k) {\n map<int, int> window;\n window[0] = 0;\n vector<int> dp(nums.size());\n \n for (int i = 0; i < nums.size(); i++) {\n dp[i] = nums[i] + window.rbegin()->first;\n window[dp[i]]++;\n \n if (i >= k) {\n window[dp[i - k]]--;\n if (window[dp[i - k]] == 0) {\n window.erase(dp[i - k]);\n }\n }\n }\n \n return *max_element(dp.begin(), dp.end());\n }\n};\n```\n\n\n```C []\n\n\nint max(int a, int b) {\n return (a > b) ? a : b;\n}\n\nint constrainedSubsetSum(int* nums, int numsSize, int k) {\n int* dp = (int*)malloc(numsSize * sizeof(int));\n int* window = (int*)malloc((numsSize + 1) * sizeof(int));\n memset(window, 0, (numsSize + 1) * sizeof(int));\n\n int maxSum = nums[0];\n dp[0] = nums[0];\n window[dp[0]] = 1;\n\n for (int i = 1; i < numsSize; i++) {\n dp[i] = nums[i] + window[maxSum];\n window[dp[i]]++;\n\n if (i >= k) {\n window[dp[i - k]]--;\n if (window[dp[i - k]] == 0) {\n window[dp[i - k]] = 0;\n }\n }\n\n maxSum = max(maxSum, dp[i]);\n }\n\n free(dp);\n free(window);\n return maxSum;\n}\n\nint main() {\n int nums[] = {10, 2, -10, 5, 20};\n int k = 2;\n int numsSize = sizeof(nums) / sizeof(nums[0]);\n int result = constrainedSubsetSum(nums, numsSize, k);\n printf("Maximum Sum: %d\\n", result);\n return 0;\n}\n\n\n```\n\n\n```Java []\nclass Solution {\n public int constrainedSubsetSum(int[] nums, int k) {\n TreeMap<Integer, Integer> window = new TreeMap();\n window.put(0, 0);\n \n int dp[] = new int[nums.length];\n \n for (int i = 0; i < nums.length; i++) {\n dp[i] = nums[i] + window.lastKey();\n window.put(dp[i], window.getOrDefault(dp[i], 0) + 1);\n \n if (i >= k) {\n window.put(dp[i - k], window.get(dp[i - k]) - 1);\n if (window.get(dp[i - k]) == 0) {\n window.remove(dp[i - k]);\n }\n }\n }\n \n int ans = Integer.MIN_VALUE;\n for (int num : dp) {\n ans = Math.max(ans, num);\n }\n \n return ans;\n }\n}\n\n```\n\n```python3 []\nfrom sortedcontainers import SortedList\n\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n window = SortedList([0])\n dp = [0] * len(nums)\n \n for i in range(len(nums)):\n dp[i] = nums[i] + window[-1]\n window.add(dp[i])\n if i >= k:\n window.remove(dp[i - k])\n \n return max(dp)\n\n```\n\n```javascript []\n`var constrainedSubsetSum = function(nums, k) {\n const dp = new Array(nums.length);\n const window = new Array(nums.length + 1).fill(0);\n\n let maxSum = nums[0];\n dp[0] = nums[0];\n window[dp[0]] = 1;\n\n for (let i = 1; i < nums.length; i++) {\n dp[i] = nums[i] + window[maxSum];\n window[dp[i]]++;\n\n if (i >= k) {\n window[dp[i - k]]--;\n if (window[dp[i - k]] === 0) {\n window[dp[i - k]] = 0;\n }\n }\n\n maxSum = Math.max(maxSum, dp[i]);\n }\n\n return maxSum;\n};\n```\n\n\n---\n\n#### ***Approach 3 (Deque)***\n1. Create a double-ended queue (`deque`) called `queue` to maintain indices of elements.\n1. Initialize a vector `dp` to store dynamic programming values, with the same size as the input `nums`.\n1. Iterate through the `nums` array using a `for` loop.\n1. Ensure that the `queue` contains only indices within the sliding window of size `k`. Remove elements that are outside the window.\n1. Calculate the value of `dp[i]`, which represents the maximum sum of a constrained subset:\n - If the `queue` is not empty, add the maximum from the previous window (front of the `queue`) to the current element at index `i`.\n - If the `queue` is empty, just consider the current element itself.\n1. Remove elements from the back of the `queue` if their corresponding `dp` values are smaller than `dp[i]`.\n - This step ensures that the `queue` maintains elements in non-increasing order based on their `dp` values.\n1. If the calculated `dp[i]` is positive, add the current index `i` to the back of the `queue`.\n - This is done because negative `dp` values will not contribute to future sums.\n1. Continue the loop until all elements in the `nums` array are processed.\n1. After processing all elements, return the maximum value from the dp vector using the `*max_element(dp.begin(), dp.end()) function`.\n - This maximum value represents the maximum sum of a constrained subset of at most k elements.\n\n\n# Complexity\n- *Time complexity:*\n $$O(n)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int constrainedSubsetSum(vector<int>& nums, int k) {\n deque<int> queue; // Create a double-ended queue (deque) to store indices of elements.\n vector<int> dp(nums.size()); // Create a vector `dp` to store dynamic programming values.\n\n for (int i = 0; i < nums.size(); i++) {\n // Ensure that the queue only contains indices within the sliding window size `k`.\n if (!queue.empty() && i - queue.front() > k) {\n queue.pop_front(); // Remove elements that are outside the window.\n }\n\n // Calculate `dp[i]` as the maximum of the current element and the maximum from the previous window.\n dp[i] = (!queue.empty() ? dp[queue.front()] : 0) + nums[i];\n\n // Remove elements from the back of the queue if their corresponding `dp` values are smaller than `dp[i]`.\n while (!queue.empty() && dp[queue.back()] < dp[i]) {\n queue.pop_back();\n }\n\n // If the calculated `dp[i]` is positive, add the current index `i` to the queue.\n if (dp[i] > 0) {\n queue.push_back(i);\n }\n }\n\n // Return the maximum value in the `dp` vector, which represents the maximum sum of a constrained subset.\n return *max_element(dp.begin(), dp.end());\n }\n};\n\n```\n\n\n```C []\n\nint max(int a, int b) {\n return (a > b) ? a : b;\n}\n\nint constrainedSubsetSum(int* nums, int numsSize, int k) {\n int* dp = (int*)malloc(numsSize * sizeof(int));\n int* queue = (int*)malloc(numsSize * sizeof(int));\n int front = 0, rear = -1;\n int ans = nums[0];\n\n for (int i = 0; i < numsSize; i++) {\n while (front <= rear && i - queue[front] > k) {\n front++;\n }\n\n dp[i] = ((front <= rear) ? dp[queue[front]] : 0) + nums[i];\n\n while (front <= rear && dp[queue[rear]] < dp[i]) {\n rear--;\n }\n\n if (dp[i] > 0) {\n queue[++rear] = i;\n }\n\n ans = max(ans, dp[i);\n }\n\n free(dp);\n free(queue);\n return ans;\n}\n\n\n```\n\n\n```Java []\nclass Solution {\n public int constrainedSubsetSum(int[] nums, int k) {\n Deque<Integer> queue = new ArrayDeque<>();\n int dp[] = new int[nums.length];\n \n for (int i = 0; i < nums.length; i++) {\n if (!queue.isEmpty() && i - queue.peek() > k) {\n queue.poll();\n }\n \n dp[i] = (!queue.isEmpty() ? dp[queue.peek()] : 0) + nums[i];\n while (!queue.isEmpty() && dp[queue.peekLast()] < dp[i]) {\n queue.pollLast();\n }\n \n if (dp[i] > 0) {\n queue.offer(i);\n }\n }\n \n int ans = Integer.MIN_VALUE;\n for (int num : dp) {\n ans = Math.max(ans, num);\n }\n \n return ans;\n }\n}\n\n```\n\n```python3 []\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n queue = deque()\n dp = [0] * len(nums)\n \n for i in range(len(nums)):\n if queue and i - queue[0] > k:\n queue.popleft()\n \n dp[i] = (dp[queue[0]] if queue else 0) + nums[i]\n while queue and dp[queue[-1]] < dp[i]:\n queue.pop()\n \n if dp[i] > 0:\n queue.append(i)\n \n return max(dp)\n\n```\n\n```javascript []\nfunction constrainedSubsetSum(nums, k) {\n const dp = new Array(nums.length);\n const queue = [];\n let ans = nums[0];\n \n for (let i = 0; i < nums.length; i++) {\n while (queue.length && i - queue[0] > k) {\n queue.shift();\n }\n \n dp[i] = (queue.length ? dp[queue[0]] : 0) + nums[i];\n \n while (queue.length && dp[queue[queue.length - 1]] < dp[i]) {\n queue.pop();\n }\n \n if (dp[i] > 0) {\n queue.push(i);\n }\n \n ans = Math.max(ans, dp[i]);\n }\n \n return ans;\n}\n\n// Example usage:\nconst nums = [10, 2, 3];\nconst k = 1;\nconsole.log(constrainedSubsetSum(nums, k)); // Output: 15\n\n```\n\n\n---\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n--- | 20 | Given an integer array `nums` and an integer `k`, return the maximum sum of a **non-empty** subsequence of that array such that for every two **consecutive** integers in the subsequence, `nums[i]` and `nums[j]`, where `i < j`, the condition `j - i <= k` is satisfied.
A _subsequence_ of an array is obtained by deleting some number of elements (can be zero) from the array, leaving the remaining elements in their original order.
**Example 1:**
**Input:** nums = \[10,2,-10,5,20\], k = 2
**Output:** 37
**Explanation:** The subsequence is \[10, 2, 5, 20\].
**Example 2:**
**Input:** nums = \[-1,-2,-3\], k = 1
**Output:** -1
**Explanation:** The subsequence must be non-empty, so we choose the largest number.
**Example 3:**
**Input:** nums = \[10,-2,-10,-5,20\], k = 2
**Output:** 23
**Explanation:** The subsequence is \[10, -2, -5, 20\].
**Constraints:**
* `1 <= k <= nums.length <= 105`
* `-104 <= nums[i] <= 104` | null |
✅☑[C++/Java/Python/JavaScript] || 3 Approaches || EXPLAINED🔥 | constrained-subsequence-sum | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n**(Also explained in the code)**\n\n#### ***Approach 1 (Heap)***\n1. We use a max heap (priority_queue) to efficiently keep track of the maximum sum of a constrained subset and the index at which it ends.\n\n1. We initialize the answer (`ans`) with the value of the first element in the input `nums` array.\n\n1. We iterate through the elements of `nums` from the second element (index 1) to the end.\n\n1. In the loop, we check whether elements in the max heap are within the constraint (at most `k` elements away from the current element).\n\n1. We remove elements from the heap that are out of range because they are no longer useful for calculating the maximum sum.\n\n1. We calculate the current sum (`curr`) by adding the value of the current element to the maximum of zero and the top element in the max heap. This ensures that we don\'t include negative values from the heap if they are not beneficial.\n\n1. We update the answer (`ans`) with the maximum of the current sum and the previous answer.\n\n1. We push the current sum and its index into the max heap for future calculations.\n\n1. After the loop, we have the maximum sum of the constrained subset in `ans`, and we return it as the result.\n\n\n# Complexity\n- *Time complexity:*\n $$O(nlogn)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int constrainedSubsetSum(vector<int>& nums, int k) {\n priority_queue<pair<int, int>> heap; // Create a max heap to keep track of the maximum sum and its index.\n heap.push({nums[0], 0}); // Push the first element into the heap with its value and index.\n int ans = nums[0]; // Initialize the answer with the first element\'s value.\n\n for (int i = 1; i < nums.size(); i++) { // Iterate through the remaining elements in nums.\n while (i - heap.top().second > k) { // While the index difference is greater than k (out of range):\n heap.pop(); // Remove elements from the heap that are out of range.\n }\n\n int curr = max(0, heap.top().first) + nums[i]; // Calculate the current sum considering the maximum possible value.\n ans = max(ans, curr); // Update the answer with the maximum of the current sum and the previous answer.\n heap.push({curr, i}); // Push the current sum and its index into the heap.\n }\n\n return ans; // Return the maximum sum of the constrained subset.\n }\n};\n\n```\n\n\n```C []\nint constrainedSubsetSum(int* nums, int numsSize, int k) {\n struct Node {\n int value;\n int index;\n };\n \n struct Node* heap = (struct Node*)malloc(numsSize * sizeof(struct Node));\n int heapSize = 0;\n \n int ans = nums[0];\n \n for (int i = 0; i < numsSize; i++) {\n while (i - heap[0].index > k) {\n // Remove elements from the heap that are out of range.\n if (heapSize > 0) {\n heap[0] = heap[heapSize - 1];\n heapSize--;\n int currIndex = 0;\n while (true) {\n int leftChild = currIndex * 2 + 1;\n int rightChild = currIndex * 2 + 2;\n int nextIndex = currIndex;\n if (leftChild < heapSize && heap[leftChild].value > heap[nextIndex].value) {\n nextIndex = leftChild;\n }\n if (rightChild < heapSize && heap[rightChild].value > heap[nextIndex].value) {\n nextIndex = rightChild;\n }\n if (nextIndex == currIndex) {\n break;\n }\n struct Node temp = heap[currIndex];\n heap[currIndex] = heap[nextIndex];\n heap[nextIndex] = temp;\n currIndex = nextIndex;\n }\n } else {\n break;\n }\n }\n \n int curr = (heapSize > 0) ? (heap[0].value + nums[i]) : nums[i];\n \n ans = (curr > ans) ? curr : ans;\n \n struct Node newNode;\n newNode.value = (curr > 0) ? curr : 0;\n newNode.index = i;\n heap[heapSize] = newNode;\n \n int currIndex = heapSize;\n while (currIndex > 0) {\n int parentIndex = (currIndex - 1) / 2;\n if (heap[currIndex].value > heap[parentIndex].value) {\n struct Node temp = heap[currIndex];\n heap[currIndex] = heap[parentIndex];\n heap[parentIndex] = temp;\n currIndex = parentIndex;\n } else {\n break;\n }\n }\n \n heapSize++;\n }\n \n free(heap);\n \n return ans;\n}\n\n\n```\n\n\n```Java []\nclass Solution {\n public int constrainedSubsetSum(int[] nums, int k) {\n PriorityQueue<int[]> heap = new PriorityQueue<>((a, b) -> {\n return b[0] - a[0];\n });\n \n heap.add(new int[] {nums[0], 0});\n int ans = nums[0];\n \n for (int i = 1; i < nums.length; i++) {\n while (i - heap.peek()[1] > k) {\n heap.remove();\n }\n \n int curr = Math.max(0, heap.peek()[0]) + nums[i];\n ans = Math.max(ans, curr);\n heap.add(new int[] {curr, i});\n }\n \n return ans;\n }\n}\n\n```\n\n```python3 []\nimport heapq\n\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n heap = [(-nums[0], 0)]\n ans = nums[0]\n \n for i in range(1, len(nums)):\n while i - heap[0][1] > k:\n heapq.heappop(heap)\n\n curr = max(0, -heap[0][0]) + nums[i]\n ans = max(ans, curr)\n heapq.heappush(heap, (-curr, i))\n\n return ans\n\n```\n\n```javascript []\nvar constrainedSubsetSum = function(nums, k) {\n let maxSum = nums[0];\n const dp = [nums[0]];\n const maxHeap = [nums[0]];\n\n for (let i = 1; i < nums.length; i++) {\n while (maxHeap.length > 0 && i - maxHeap[0][1] > k) {\n maxHeap.shift();\n }\n const current = Math.max(nums[i], nums[i] + (maxHeap.length > 0 ? maxHeap[0][0] : 0));\n maxSum = Math.max(maxSum, current);\n dp.push(current);\n while (maxHeap.length > 0 && dp[i] >= maxHeap[maxHeap.length - 1][0]) {\n maxHeap.pop();\n }\n maxHeap.push([dp[i], i]);\n }\n\n return maxSum;\n};\n\n```\n\n\n---\n\n#### ***Approach 2 (TreeMap)***\n1. Create a `map` named `window` to maintain a sliding window of values. The keys represent the sums of elements in the window, and the values represent the number of times each sum appears.\n1. Initialize `window[0]` to 0 to handle edge cases.\n1. Create a vector `dp` to store the dynamic programming values, with the same size as the input `nums`.\n1. Start iterating through the `nums` array using a `for` loop.\n1. For each element at index `i`, calculate `dp[i]` by adding the current element `nums[i]` to the maximum sum in the sliding window (`window.rbegin()->first`).\n1. Increase the count for this `dp[i]` in the `window` map to keep track of its frequency.\n1. Check if the sliding window size has exceeded `k`. If it has, remove elements from the window map that are no longer in the `window`:\n - Decrement the count of the sum corresponding to `dp[i - k]`.\n - If the count becomes 0, remove that sum from the `window` map using `window.erase()`.\n1. Continue the loop until all elements in the `nums` array are processed.\n1. After processing all elements, return the maximum value from the `dp` vector using the `*max_element(dp.begin(), dp.end())` function.\n - This maximum value represents the maximum sum of a constrained subset of at most `k` elements.\n\n\n# Complexity\n- *Time complexity:*\n $$O(nlogk)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int constrainedSubsetSum(vector<int>& nums, int k) {\n map<int, int> window;\n window[0] = 0;\n vector<int> dp(nums.size());\n \n for (int i = 0; i < nums.size(); i++) {\n dp[i] = nums[i] + window.rbegin()->first;\n window[dp[i]]++;\n \n if (i >= k) {\n window[dp[i - k]]--;\n if (window[dp[i - k]] == 0) {\n window.erase(dp[i - k]);\n }\n }\n }\n \n return *max_element(dp.begin(), dp.end());\n }\n};\n```\n\n\n```C []\n\n\nint max(int a, int b) {\n return (a > b) ? a : b;\n}\n\nint constrainedSubsetSum(int* nums, int numsSize, int k) {\n int* dp = (int*)malloc(numsSize * sizeof(int));\n int* window = (int*)malloc((numsSize + 1) * sizeof(int));\n memset(window, 0, (numsSize + 1) * sizeof(int));\n\n int maxSum = nums[0];\n dp[0] = nums[0];\n window[dp[0]] = 1;\n\n for (int i = 1; i < numsSize; i++) {\n dp[i] = nums[i] + window[maxSum];\n window[dp[i]]++;\n\n if (i >= k) {\n window[dp[i - k]]--;\n if (window[dp[i - k]] == 0) {\n window[dp[i - k]] = 0;\n }\n }\n\n maxSum = max(maxSum, dp[i]);\n }\n\n free(dp);\n free(window);\n return maxSum;\n}\n\nint main() {\n int nums[] = {10, 2, -10, 5, 20};\n int k = 2;\n int numsSize = sizeof(nums) / sizeof(nums[0]);\n int result = constrainedSubsetSum(nums, numsSize, k);\n printf("Maximum Sum: %d\\n", result);\n return 0;\n}\n\n\n```\n\n\n```Java []\nclass Solution {\n public int constrainedSubsetSum(int[] nums, int k) {\n TreeMap<Integer, Integer> window = new TreeMap();\n window.put(0, 0);\n \n int dp[] = new int[nums.length];\n \n for (int i = 0; i < nums.length; i++) {\n dp[i] = nums[i] + window.lastKey();\n window.put(dp[i], window.getOrDefault(dp[i], 0) + 1);\n \n if (i >= k) {\n window.put(dp[i - k], window.get(dp[i - k]) - 1);\n if (window.get(dp[i - k]) == 0) {\n window.remove(dp[i - k]);\n }\n }\n }\n \n int ans = Integer.MIN_VALUE;\n for (int num : dp) {\n ans = Math.max(ans, num);\n }\n \n return ans;\n }\n}\n\n```\n\n```python3 []\nfrom sortedcontainers import SortedList\n\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n window = SortedList([0])\n dp = [0] * len(nums)\n \n for i in range(len(nums)):\n dp[i] = nums[i] + window[-1]\n window.add(dp[i])\n if i >= k:\n window.remove(dp[i - k])\n \n return max(dp)\n\n```\n\n```javascript []\n`var constrainedSubsetSum = function(nums, k) {\n const dp = new Array(nums.length);\n const window = new Array(nums.length + 1).fill(0);\n\n let maxSum = nums[0];\n dp[0] = nums[0];\n window[dp[0]] = 1;\n\n for (let i = 1; i < nums.length; i++) {\n dp[i] = nums[i] + window[maxSum];\n window[dp[i]]++;\n\n if (i >= k) {\n window[dp[i - k]]--;\n if (window[dp[i - k]] === 0) {\n window[dp[i - k]] = 0;\n }\n }\n\n maxSum = Math.max(maxSum, dp[i]);\n }\n\n return maxSum;\n};\n```\n\n\n---\n\n#### ***Approach 3 (Deque)***\n1. Create a double-ended queue (`deque`) called `queue` to maintain indices of elements.\n1. Initialize a vector `dp` to store dynamic programming values, with the same size as the input `nums`.\n1. Iterate through the `nums` array using a `for` loop.\n1. Ensure that the `queue` contains only indices within the sliding window of size `k`. Remove elements that are outside the window.\n1. Calculate the value of `dp[i]`, which represents the maximum sum of a constrained subset:\n - If the `queue` is not empty, add the maximum from the previous window (front of the `queue`) to the current element at index `i`.\n - If the `queue` is empty, just consider the current element itself.\n1. Remove elements from the back of the `queue` if their corresponding `dp` values are smaller than `dp[i]`.\n - This step ensures that the `queue` maintains elements in non-increasing order based on their `dp` values.\n1. If the calculated `dp[i]` is positive, add the current index `i` to the back of the `queue`.\n - This is done because negative `dp` values will not contribute to future sums.\n1. Continue the loop until all elements in the `nums` array are processed.\n1. After processing all elements, return the maximum value from the dp vector using the `*max_element(dp.begin(), dp.end()) function`.\n - This maximum value represents the maximum sum of a constrained subset of at most k elements.\n\n\n# Complexity\n- *Time complexity:*\n $$O(n)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int constrainedSubsetSum(vector<int>& nums, int k) {\n deque<int> queue; // Create a double-ended queue (deque) to store indices of elements.\n vector<int> dp(nums.size()); // Create a vector `dp` to store dynamic programming values.\n\n for (int i = 0; i < nums.size(); i++) {\n // Ensure that the queue only contains indices within the sliding window size `k`.\n if (!queue.empty() && i - queue.front() > k) {\n queue.pop_front(); // Remove elements that are outside the window.\n }\n\n // Calculate `dp[i]` as the maximum of the current element and the maximum from the previous window.\n dp[i] = (!queue.empty() ? dp[queue.front()] : 0) + nums[i];\n\n // Remove elements from the back of the queue if their corresponding `dp` values are smaller than `dp[i]`.\n while (!queue.empty() && dp[queue.back()] < dp[i]) {\n queue.pop_back();\n }\n\n // If the calculated `dp[i]` is positive, add the current index `i` to the queue.\n if (dp[i] > 0) {\n queue.push_back(i);\n }\n }\n\n // Return the maximum value in the `dp` vector, which represents the maximum sum of a constrained subset.\n return *max_element(dp.begin(), dp.end());\n }\n};\n\n```\n\n\n```C []\n\nint max(int a, int b) {\n return (a > b) ? a : b;\n}\n\nint constrainedSubsetSum(int* nums, int numsSize, int k) {\n int* dp = (int*)malloc(numsSize * sizeof(int));\n int* queue = (int*)malloc(numsSize * sizeof(int));\n int front = 0, rear = -1;\n int ans = nums[0];\n\n for (int i = 0; i < numsSize; i++) {\n while (front <= rear && i - queue[front] > k) {\n front++;\n }\n\n dp[i] = ((front <= rear) ? dp[queue[front]] : 0) + nums[i];\n\n while (front <= rear && dp[queue[rear]] < dp[i]) {\n rear--;\n }\n\n if (dp[i] > 0) {\n queue[++rear] = i;\n }\n\n ans = max(ans, dp[i);\n }\n\n free(dp);\n free(queue);\n return ans;\n}\n\n\n```\n\n\n```Java []\nclass Solution {\n public int constrainedSubsetSum(int[] nums, int k) {\n Deque<Integer> queue = new ArrayDeque<>();\n int dp[] = new int[nums.length];\n \n for (int i = 0; i < nums.length; i++) {\n if (!queue.isEmpty() && i - queue.peek() > k) {\n queue.poll();\n }\n \n dp[i] = (!queue.isEmpty() ? dp[queue.peek()] : 0) + nums[i];\n while (!queue.isEmpty() && dp[queue.peekLast()] < dp[i]) {\n queue.pollLast();\n }\n \n if (dp[i] > 0) {\n queue.offer(i);\n }\n }\n \n int ans = Integer.MIN_VALUE;\n for (int num : dp) {\n ans = Math.max(ans, num);\n }\n \n return ans;\n }\n}\n\n```\n\n```python3 []\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n queue = deque()\n dp = [0] * len(nums)\n \n for i in range(len(nums)):\n if queue and i - queue[0] > k:\n queue.popleft()\n \n dp[i] = (dp[queue[0]] if queue else 0) + nums[i]\n while queue and dp[queue[-1]] < dp[i]:\n queue.pop()\n \n if dp[i] > 0:\n queue.append(i)\n \n return max(dp)\n\n```\n\n```javascript []\nfunction constrainedSubsetSum(nums, k) {\n const dp = new Array(nums.length);\n const queue = [];\n let ans = nums[0];\n \n for (let i = 0; i < nums.length; i++) {\n while (queue.length && i - queue[0] > k) {\n queue.shift();\n }\n \n dp[i] = (queue.length ? dp[queue[0]] : 0) + nums[i];\n \n while (queue.length && dp[queue[queue.length - 1]] < dp[i]) {\n queue.pop();\n }\n \n if (dp[i] > 0) {\n queue.push(i);\n }\n \n ans = Math.max(ans, dp[i]);\n }\n \n return ans;\n}\n\n// Example usage:\nconst nums = [10, 2, 3];\nconst k = 1;\nconsole.log(constrainedSubsetSum(nums, k)); // Output: 15\n\n```\n\n\n---\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n--- | 20 | Design the `CombinationIterator` class:
* `CombinationIterator(string characters, int combinationLength)` Initializes the object with a string `characters` of **sorted distinct** lowercase English letters and a number `combinationLength` as arguments.
* `next()` Returns the next combination of length `combinationLength` in **lexicographical order**.
* `hasNext()` Returns `true` if and only if there exists a next combination.
**Example 1:**
**Input**
\[ "CombinationIterator ", "next ", "hasNext ", "next ", "hasNext ", "next ", "hasNext "\]
\[\[ "abc ", 2\], \[\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, "ab ", true, "ac ", true, "bc ", false\]
**Explanation**
CombinationIterator itr = new CombinationIterator( "abc ", 2);
itr.next(); // return "ab "
itr.hasNext(); // return True
itr.next(); // return "ac "
itr.hasNext(); // return True
itr.next(); // return "bc "
itr.hasNext(); // return False
**Constraints:**
* `1 <= combinationLength <= characters.length <= 15`
* All the characters of `characters` are **unique**.
* At most `104` calls will be made to `next` and `hasNext`.
* It is guaranteed that all calls of the function `next` are valid. | Use dynamic programming. Let dp[i] be the solution for the prefix of the array that ends at index i, if the element at index i is in the subsequence. dp[i] = nums[i] + max(0, dp[i-k], dp[i-k+1], ..., dp[i-1]) Use a heap with the sliding window technique to optimize the dp. |
✅ Beats 100% 🔥 in Both || Easiest Approach || Detailed Explanation || | constrained-subsequence-sum | 1 | 1 | \n# PHP\n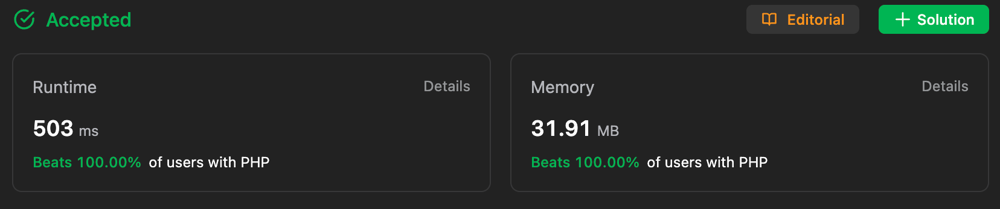\n\n# Python\n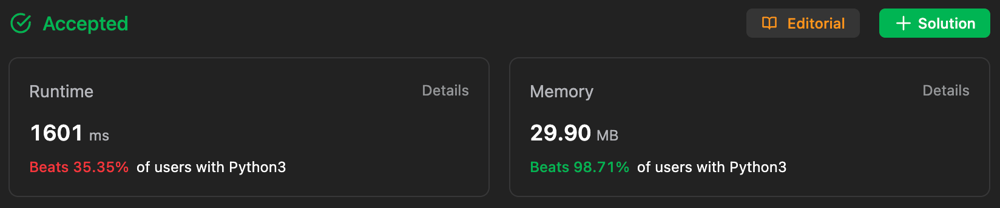\n\n# Intuition\nIf we want to get the maximum sum of a non-empty subsequence, we can use DP. dp[i] means the maximum sum of a non-empty subsequence of nums[:i+1]. So the result is max(dp). We can easily get the dp[i] with dp[i] = nums[i] + max(dp[i-k], dp[i-k+1], ..., dp[i-1]). But it will cause TLE. So we need to optimize it. \n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach : Dynamic Programming\n1. Here we use a deque to store the index of the max value in the window.\n2. The max value in the window is always the first element of the deque.\n3. If the max value is out of the window, we pop it from the deque.\n4. If the max value is smaller than the new value, we pop it from the deque.\n5. Then we append the new value to the deque.\n6. We update the dp[i] with nums[i] + dp[deque[0]].\n7. We update the result with max(result, dp[i]).\n\n# Algorithm :\n1. Initialize a deque q.\n2. Initialize a dp array with the same length of nums, and fill it with nums.\n3. Iterate the array, for each element nums[i]:\n 1. If the first element of q is out of the window, we pop it.\n 2. If the first element of q is smaller than dp[i], we pop it.\n 3. We append dp[i] to q.\n 4. We update dp[i] with nums[i] + dp[q[0]].\n 5. We update the result with max(result, dp[i]).\n4. Return the result.\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity : O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity : O(K)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n## Please Upvote \u2B06\uFE0F if you found this helpful :)\n\n# Code\n``` Python []\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n dp = copy.deepcopy(nums)\n q = deque()\n q.append(0)\n for i in range(1,len(nums)):\n while(q[-1] < i-k ):\n q.pop()\n dp[i] = max(dp[i],dp[q[-1]]+nums[i])\n while( len(q)>0 and dp[q[0]] <= dp[i] ):\n q.popleft()\n q.appendleft(i)\n return max(dp)\n```\n``` C++ []\nclass Solution {\npublic:\n int constrainedSubsetSum(vector<int>& nums, int k) {\n vector<int> dp(nums);\n deque<int> q;\n q.push_back(0);\n for (int i = 1; i < nums.size(); i++) {\n while (!q.empty() && q.back() < i - k) {\n q.pop_back();\n }\n dp[i] = max(dp[i], dp[q.back()] + nums[i]);\n while (!q.empty() && dp[q.front()] <= dp[i]) {\n q.pop_front();\n }\n q.push_front(i);\n }\n return *max_element(dp.begin(), dp.end());\n }\n};\n```\n``` Java []\nclass Solution {\n public int constrainedSubsetSum(int[] nums, int k) {\n int[] dp = Arrays.copyOf(nums, nums.length);\n Deque<Integer> q = new ArrayDeque<>();\n q.offerLast(0);\n for (int i = 1; i < nums.length; i++) {\n while (!q.isEmpty() && q.peekLast() < i - k) {\n q.pollLast();\n }\n dp[i] = Math.max(dp[i], dp[q.peekLast()] + nums[i]);\n while (!q.isEmpty() && dp[q.peekFirst()] <= dp[i]) {\n q.pollFirst();\n }\n q.offerFirst(i);\n }\n int max = Integer.MIN_VALUE;\n for (int num : dp) {\n max = Math.max(max, num);\n }\n return max;\n }\n }\n```\n``` JavaScript []\n/**\n * @param {number[]} nums\n * @param {number} k\n * @return {number}\n */\nvar constrainedSubsetSum = function(nums, k) {\n let dp = [...nums];\n let q = [];\n q.push(0);\n for (let i = 1; i < nums.length; i++) {\n while (q[q.length - 1] < i - k) {\n q.pop();\n }\n dp[i] = Math.max(dp[i], dp[q[q.length - 1]] + nums[i]);\n while (q.length > 0 && dp[q[0]] <= dp[i]) {\n q.shift();\n }\n q.unshift(i);\n }\n return Math.max(...dp);\n};\n```\n``` C# []\npublic class Solution {\n public int ConstrainedSubsetSum(int[] nums, int k) {\n int[] dp = (int[])nums.Clone();\n LinkedList<int> q = new LinkedList<int>();\n q.AddLast(0);\n for (int i = 1; i < nums.Length; i++) {\n while (q.Count > 0 && q.Last.Value < i - k) {\n q.RemoveLast();\n }\n dp[i] = Math.Max(dp[i], dp[q.Last.Value] + nums[i]);\n while (q.Count > 0 && dp[q.First.Value] <= dp[i]) {\n q.RemoveFirst();\n }\n q.AddFirst(i);\n }\n int max = int.MinValue;\n foreach (int num in dp) {\n max = Math.Max(max, num);\n }\n return max;\n }\n}\n```\n``` TypeScript []\nfunction constrainedSubsetSum(nums: number[], k: number): number {\nlet dp: number[] = [...nums];\n let q: number[] = [];\n q.push(0);\n for (let i = 1; i < nums.length; i++) {\n while (q[q.length - 1] < i - k) {\n q.pop();\n }\n dp[i] = Math.max(dp[i], dp[q[q.length - 1]] + nums[i]);\n while (q.length > 0 && dp[q[0]] <= dp[i]) {\n q.shift();\n }\n q.unshift(i);\n }\n return Math.max(...dp);\n};\n```\n``` PHP []\nclass Solution {\n\n /**\n * @param Integer[] $nums\n * @param Integer $k\n * @return Integer\n */\n function constrainedSubsetSum($nums, $k) {\n $dp = $nums;\n $q = [];\n array_push($q, 0);\n for ($i = 1; $i < count($nums); $i++) {\n while (end($q) < $i - $k) {\n array_pop($q);\n }\n $dp[$i] = max($dp[$i], $dp[end($q)] + $nums[$i]);\n while (count($q) > 0 && $dp[$q[0]] <= $dp[$i]) {\n array_shift($q);\n }\n array_unshift($q, $i);\n }\n return max($dp);\n }\n}\n```\n``` Ruby []\n# @param {Integer[]} nums\n# @param {Integer} k\n# @return {Integer}\ndef constrained_subset_sum(nums, k)\n dp = nums.dup\n q = []\n q.push(0)\n \n (1...nums.length).each do |i|\n while !q.empty? && q.last < i - k\n q.pop\n end\n \n dp[i] = [dp[i], dp[q.last] + nums[i]].max\n \n while !q.empty? && dp[q.first] <= dp[i]\n q.shift\n end\n \n q.unshift(i)\n end\n \n dp.max\nend\n```\n\n | 4 | Given an integer array `nums` and an integer `k`, return the maximum sum of a **non-empty** subsequence of that array such that for every two **consecutive** integers in the subsequence, `nums[i]` and `nums[j]`, where `i < j`, the condition `j - i <= k` is satisfied.
A _subsequence_ of an array is obtained by deleting some number of elements (can be zero) from the array, leaving the remaining elements in their original order.
**Example 1:**
**Input:** nums = \[10,2,-10,5,20\], k = 2
**Output:** 37
**Explanation:** The subsequence is \[10, 2, 5, 20\].
**Example 2:**
**Input:** nums = \[-1,-2,-3\], k = 1
**Output:** -1
**Explanation:** The subsequence must be non-empty, so we choose the largest number.
**Example 3:**
**Input:** nums = \[10,-2,-10,-5,20\], k = 2
**Output:** 23
**Explanation:** The subsequence is \[10, -2, -5, 20\].
**Constraints:**
* `1 <= k <= nums.length <= 105`
* `-104 <= nums[i] <= 104` | null |
✅ Beats 100% 🔥 in Both || Easiest Approach || Detailed Explanation || | constrained-subsequence-sum | 1 | 1 | \n# PHP\n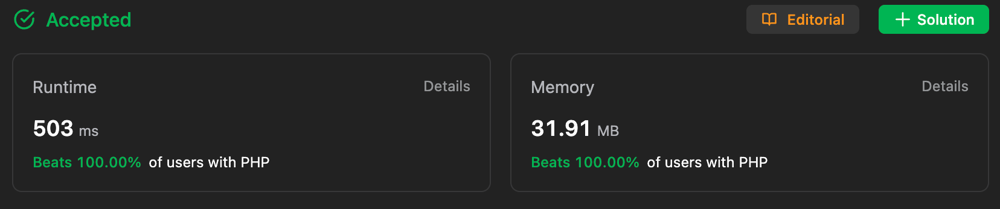\n\n# Python\n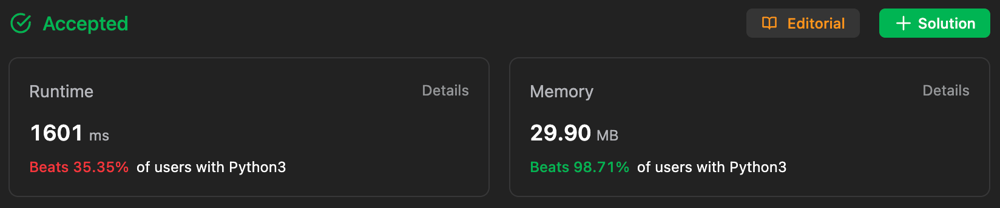\n\n# Intuition\nIf we want to get the maximum sum of a non-empty subsequence, we can use DP. dp[i] means the maximum sum of a non-empty subsequence of nums[:i+1]. So the result is max(dp). We can easily get the dp[i] with dp[i] = nums[i] + max(dp[i-k], dp[i-k+1], ..., dp[i-1]). But it will cause TLE. So we need to optimize it. \n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach : Dynamic Programming\n1. Here we use a deque to store the index of the max value in the window.\n2. The max value in the window is always the first element of the deque.\n3. If the max value is out of the window, we pop it from the deque.\n4. If the max value is smaller than the new value, we pop it from the deque.\n5. Then we append the new value to the deque.\n6. We update the dp[i] with nums[i] + dp[deque[0]].\n7. We update the result with max(result, dp[i]).\n\n# Algorithm :\n1. Initialize a deque q.\n2. Initialize a dp array with the same length of nums, and fill it with nums.\n3. Iterate the array, for each element nums[i]:\n 1. If the first element of q is out of the window, we pop it.\n 2. If the first element of q is smaller than dp[i], we pop it.\n 3. We append dp[i] to q.\n 4. We update dp[i] with nums[i] + dp[q[0]].\n 5. We update the result with max(result, dp[i]).\n4. Return the result.\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity : O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity : O(K)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n## Please Upvote \u2B06\uFE0F if you found this helpful :)\n\n# Code\n``` Python []\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n dp = copy.deepcopy(nums)\n q = deque()\n q.append(0)\n for i in range(1,len(nums)):\n while(q[-1] < i-k ):\n q.pop()\n dp[i] = max(dp[i],dp[q[-1]]+nums[i])\n while( len(q)>0 and dp[q[0]] <= dp[i] ):\n q.popleft()\n q.appendleft(i)\n return max(dp)\n```\n``` C++ []\nclass Solution {\npublic:\n int constrainedSubsetSum(vector<int>& nums, int k) {\n vector<int> dp(nums);\n deque<int> q;\n q.push_back(0);\n for (int i = 1; i < nums.size(); i++) {\n while (!q.empty() && q.back() < i - k) {\n q.pop_back();\n }\n dp[i] = max(dp[i], dp[q.back()] + nums[i]);\n while (!q.empty() && dp[q.front()] <= dp[i]) {\n q.pop_front();\n }\n q.push_front(i);\n }\n return *max_element(dp.begin(), dp.end());\n }\n};\n```\n``` Java []\nclass Solution {\n public int constrainedSubsetSum(int[] nums, int k) {\n int[] dp = Arrays.copyOf(nums, nums.length);\n Deque<Integer> q = new ArrayDeque<>();\n q.offerLast(0);\n for (int i = 1; i < nums.length; i++) {\n while (!q.isEmpty() && q.peekLast() < i - k) {\n q.pollLast();\n }\n dp[i] = Math.max(dp[i], dp[q.peekLast()] + nums[i]);\n while (!q.isEmpty() && dp[q.peekFirst()] <= dp[i]) {\n q.pollFirst();\n }\n q.offerFirst(i);\n }\n int max = Integer.MIN_VALUE;\n for (int num : dp) {\n max = Math.max(max, num);\n }\n return max;\n }\n }\n```\n``` JavaScript []\n/**\n * @param {number[]} nums\n * @param {number} k\n * @return {number}\n */\nvar constrainedSubsetSum = function(nums, k) {\n let dp = [...nums];\n let q = [];\n q.push(0);\n for (let i = 1; i < nums.length; i++) {\n while (q[q.length - 1] < i - k) {\n q.pop();\n }\n dp[i] = Math.max(dp[i], dp[q[q.length - 1]] + nums[i]);\n while (q.length > 0 && dp[q[0]] <= dp[i]) {\n q.shift();\n }\n q.unshift(i);\n }\n return Math.max(...dp);\n};\n```\n``` C# []\npublic class Solution {\n public int ConstrainedSubsetSum(int[] nums, int k) {\n int[] dp = (int[])nums.Clone();\n LinkedList<int> q = new LinkedList<int>();\n q.AddLast(0);\n for (int i = 1; i < nums.Length; i++) {\n while (q.Count > 0 && q.Last.Value < i - k) {\n q.RemoveLast();\n }\n dp[i] = Math.Max(dp[i], dp[q.Last.Value] + nums[i]);\n while (q.Count > 0 && dp[q.First.Value] <= dp[i]) {\n q.RemoveFirst();\n }\n q.AddFirst(i);\n }\n int max = int.MinValue;\n foreach (int num in dp) {\n max = Math.Max(max, num);\n }\n return max;\n }\n}\n```\n``` TypeScript []\nfunction constrainedSubsetSum(nums: number[], k: number): number {\nlet dp: number[] = [...nums];\n let q: number[] = [];\n q.push(0);\n for (let i = 1; i < nums.length; i++) {\n while (q[q.length - 1] < i - k) {\n q.pop();\n }\n dp[i] = Math.max(dp[i], dp[q[q.length - 1]] + nums[i]);\n while (q.length > 0 && dp[q[0]] <= dp[i]) {\n q.shift();\n }\n q.unshift(i);\n }\n return Math.max(...dp);\n};\n```\n``` PHP []\nclass Solution {\n\n /**\n * @param Integer[] $nums\n * @param Integer $k\n * @return Integer\n */\n function constrainedSubsetSum($nums, $k) {\n $dp = $nums;\n $q = [];\n array_push($q, 0);\n for ($i = 1; $i < count($nums); $i++) {\n while (end($q) < $i - $k) {\n array_pop($q);\n }\n $dp[$i] = max($dp[$i], $dp[end($q)] + $nums[$i]);\n while (count($q) > 0 && $dp[$q[0]] <= $dp[$i]) {\n array_shift($q);\n }\n array_unshift($q, $i);\n }\n return max($dp);\n }\n}\n```\n``` Ruby []\n# @param {Integer[]} nums\n# @param {Integer} k\n# @return {Integer}\ndef constrained_subset_sum(nums, k)\n dp = nums.dup\n q = []\n q.push(0)\n \n (1...nums.length).each do |i|\n while !q.empty? && q.last < i - k\n q.pop\n end\n \n dp[i] = [dp[i], dp[q.last] + nums[i]].max\n \n while !q.empty? && dp[q.first] <= dp[i]\n q.shift\n end\n \n q.unshift(i)\n end\n \n dp.max\nend\n```\n\n | 4 | Design the `CombinationIterator` class:
* `CombinationIterator(string characters, int combinationLength)` Initializes the object with a string `characters` of **sorted distinct** lowercase English letters and a number `combinationLength` as arguments.
* `next()` Returns the next combination of length `combinationLength` in **lexicographical order**.
* `hasNext()` Returns `true` if and only if there exists a next combination.
**Example 1:**
**Input**
\[ "CombinationIterator ", "next ", "hasNext ", "next ", "hasNext ", "next ", "hasNext "\]
\[\[ "abc ", 2\], \[\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, "ab ", true, "ac ", true, "bc ", false\]
**Explanation**
CombinationIterator itr = new CombinationIterator( "abc ", 2);
itr.next(); // return "ab "
itr.hasNext(); // return True
itr.next(); // return "ac "
itr.hasNext(); // return True
itr.next(); // return "bc "
itr.hasNext(); // return False
**Constraints:**
* `1 <= combinationLength <= characters.length <= 15`
* All the characters of `characters` are **unique**.
* At most `104` calls will be made to `next` and `hasNext`.
* It is guaranteed that all calls of the function `next` are valid. | Use dynamic programming. Let dp[i] be the solution for the prefix of the array that ends at index i, if the element at index i is in the subsequence. dp[i] = nums[i] + max(0, dp[i-k], dp[i-k+1], ..., dp[i-1]) Use a heap with the sliding window technique to optimize the dp. |
Python DP + Max Window Element ( Monotonic Queue) | constrained-subsequence-sum | 0 | 1 | \n```\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n dp = copy.deepcopy(nums)\n q = deque()\n q.append(0)\n for i in range(1,len(nums)):\n while(q[-1] < i-k ):\n q.pop()\n dp[i] = max(dp[i],dp[q[-1]]+nums[i])\n while( len(q)>0 and dp[q[0]] <= dp[i] ):\n q.popleft()\n q.appendleft(i)\n return max(dp)\n``` | 1 | Given an integer array `nums` and an integer `k`, return the maximum sum of a **non-empty** subsequence of that array such that for every two **consecutive** integers in the subsequence, `nums[i]` and `nums[j]`, where `i < j`, the condition `j - i <= k` is satisfied.
A _subsequence_ of an array is obtained by deleting some number of elements (can be zero) from the array, leaving the remaining elements in their original order.
**Example 1:**
**Input:** nums = \[10,2,-10,5,20\], k = 2
**Output:** 37
**Explanation:** The subsequence is \[10, 2, 5, 20\].
**Example 2:**
**Input:** nums = \[-1,-2,-3\], k = 1
**Output:** -1
**Explanation:** The subsequence must be non-empty, so we choose the largest number.
**Example 3:**
**Input:** nums = \[10,-2,-10,-5,20\], k = 2
**Output:** 23
**Explanation:** The subsequence is \[10, -2, -5, 20\].
**Constraints:**
* `1 <= k <= nums.length <= 105`
* `-104 <= nums[i] <= 104` | null |
Python DP + Max Window Element ( Monotonic Queue) | constrained-subsequence-sum | 0 | 1 | \n```\nclass Solution:\n def constrainedSubsetSum(self, nums: List[int], k: int) -> int:\n dp = copy.deepcopy(nums)\n q = deque()\n q.append(0)\n for i in range(1,len(nums)):\n while(q[-1] < i-k ):\n q.pop()\n dp[i] = max(dp[i],dp[q[-1]]+nums[i])\n while( len(q)>0 and dp[q[0]] <= dp[i] ):\n q.popleft()\n q.appendleft(i)\n return max(dp)\n``` | 1 | Design the `CombinationIterator` class:
* `CombinationIterator(string characters, int combinationLength)` Initializes the object with a string `characters` of **sorted distinct** lowercase English letters and a number `combinationLength` as arguments.
* `next()` Returns the next combination of length `combinationLength` in **lexicographical order**.
* `hasNext()` Returns `true` if and only if there exists a next combination.
**Example 1:**
**Input**
\[ "CombinationIterator ", "next ", "hasNext ", "next ", "hasNext ", "next ", "hasNext "\]
\[\[ "abc ", 2\], \[\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, "ab ", true, "ac ", true, "bc ", false\]
**Explanation**
CombinationIterator itr = new CombinationIterator( "abc ", 2);
itr.next(); // return "ab "
itr.hasNext(); // return True
itr.next(); // return "ac "
itr.hasNext(); // return True
itr.next(); // return "bc "
itr.hasNext(); // return False
**Constraints:**
* `1 <= combinationLength <= characters.length <= 15`
* All the characters of `characters` are **unique**.
* At most `104` calls will be made to `next` and `hasNext`.
* It is guaranteed that all calls of the function `next` are valid. | Use dynamic programming. Let dp[i] be the solution for the prefix of the array that ends at index i, if the element at index i is in the subsequence. dp[i] = nums[i] + max(0, dp[i-k], dp[i-k+1], ..., dp[i-1]) Use a heap with the sliding window technique to optimize the dp. |
Simple Python and C++ solutions. Vectors in C++ | kids-with-the-greatest-number-of-candies | 0 | 1 | # Solution in C++\n```\nclass Solution {\npublic:\n vector<bool> kidsWithCandies(vector<int>& candies, int extraCandies) {\n vector<bool>answer;\n int max_value = 0;\n for (int i = 0; i < candies.size(); i++){\n if (max_value <= candies.at(i)){max_value = candies.at(i);}\n }\n\n for (int i = 0; i < candies.size(); i++){\n if (candies.at(i) + extraCandies >= max_value){answer.push_back(true);}\n else {answer.push_back(false);}\n }\n return answer;\n }\n};\n```\n# Solution in Python\n```\nclass Solution:\n def kidsWithCandies(self, candies: List[int], extraCandies: int) -> List[bool]:\n answer = []\n max_value = 0\n for candy in candies:\n if max_value <= candy:\n max_value = candy\n\n for candy in candies:\n if candy + extraCandies >= max_value:\n answer.append(True)\n else:\n answer.append(False)\n return answer\n``` | 1 | There are `n` kids with candies. You are given an integer array `candies`, where each `candies[i]` represents the number of candies the `ith` kid has, and an integer `extraCandies`, denoting the number of extra candies that you have.
Return _a boolean array_ `result` _of length_ `n`_, where_ `result[i]` _is_ `true` _if, after giving the_ `ith` _kid all the_ `extraCandies`_, they will have the **greatest** number of candies among all the kids__, or_ `false` _otherwise_.
Note that **multiple** kids can have the **greatest** number of candies.
**Example 1:**
**Input:** candies = \[2,3,5,1,3\], extraCandies = 3
**Output:** \[true,true,true,false,true\]
**Explanation:** If you give all extraCandies to:
- Kid 1, they will have 2 + 3 = 5 candies, which is the greatest among the kids.
- Kid 2, they will have 3 + 3 = 6 candies, which is the greatest among the kids.
- Kid 3, they will have 5 + 3 = 8 candies, which is the greatest among the kids.
- Kid 4, they will have 1 + 3 = 4 candies, which is not the greatest among the kids.
- Kid 5, they will have 3 + 3 = 6 candies, which is the greatest among the kids.
**Example 2:**
**Input:** candies = \[4,2,1,1,2\], extraCandies = 1
**Output:** \[true,false,false,false,false\]
**Explanation:** There is only 1 extra candy.
Kid 1 will always have the greatest number of candies, even if a different kid is given the extra candy.
**Example 3:**
**Input:** candies = \[12,1,12\], extraCandies = 10
**Output:** \[true,false,true\]
**Constraints:**
* `n == candies.length`
* `2 <= n <= 100`
* `1 <= candies[i] <= 100`
* `1 <= extraCandies <= 50` | Consider how reversing each edge of the graph can help us. How can performing BFS/DFS on the reversed graph help us find the ancestors of every node? |
Simple Python and C++ solutions. Vectors in C++ | kids-with-the-greatest-number-of-candies | 0 | 1 | # Solution in C++\n```\nclass Solution {\npublic:\n vector<bool> kidsWithCandies(vector<int>& candies, int extraCandies) {\n vector<bool>answer;\n int max_value = 0;\n for (int i = 0; i < candies.size(); i++){\n if (max_value <= candies.at(i)){max_value = candies.at(i);}\n }\n\n for (int i = 0; i < candies.size(); i++){\n if (candies.at(i) + extraCandies >= max_value){answer.push_back(true);}\n else {answer.push_back(false);}\n }\n return answer;\n }\n};\n```\n# Solution in Python\n```\nclass Solution:\n def kidsWithCandies(self, candies: List[int], extraCandies: int) -> List[bool]:\n answer = []\n max_value = 0\n for candy in candies:\n if max_value <= candy:\n max_value = candy\n\n for candy in candies:\n if candy + extraCandies >= max_value:\n answer.append(True)\n else:\n answer.append(False)\n return answer\n``` | 1 | You are given a string `s` and an integer array `indices` of the **same length**. The string `s` will be shuffled such that the character at the `ith` position moves to `indices[i]` in the shuffled string.
Return _the shuffled string_.
**Example 1:**
**Input:** s = "codeleet ", `indices` = \[4,5,6,7,0,2,1,3\]
**Output:** "leetcode "
**Explanation:** As shown, "codeleet " becomes "leetcode " after shuffling.
**Example 2:**
**Input:** s = "abc ", `indices` = \[0,1,2\]
**Output:** "abc "
**Explanation:** After shuffling, each character remains in its position.
**Constraints:**
* `s.length == indices.length == n`
* `1 <= n <= 100`
* `s` consists of only lowercase English letters.
* `0 <= indices[i] < n`
* All values of `indices` are **unique**. | Use greedy approach. For each kid check if candies[i] + extraCandies ≥ maximum in Candies[i]. |
Shortest 1 line solution | kids-with-the-greatest-number-of-candies | 0 | 1 | # Code\n```\nclass Solution:\n def kidsWithCandies(self, c: List[int], eC: int) -> List[bool]:\n return [x+eC >= max(c)for x in c]\n```\nplease upvote :D | 9 | There are `n` kids with candies. You are given an integer array `candies`, where each `candies[i]` represents the number of candies the `ith` kid has, and an integer `extraCandies`, denoting the number of extra candies that you have.
Return _a boolean array_ `result` _of length_ `n`_, where_ `result[i]` _is_ `true` _if, after giving the_ `ith` _kid all the_ `extraCandies`_, they will have the **greatest** number of candies among all the kids__, or_ `false` _otherwise_.
Note that **multiple** kids can have the **greatest** number of candies.
**Example 1:**
**Input:** candies = \[2,3,5,1,3\], extraCandies = 3
**Output:** \[true,true,true,false,true\]
**Explanation:** If you give all extraCandies to:
- Kid 1, they will have 2 + 3 = 5 candies, which is the greatest among the kids.
- Kid 2, they will have 3 + 3 = 6 candies, which is the greatest among the kids.
- Kid 3, they will have 5 + 3 = 8 candies, which is the greatest among the kids.
- Kid 4, they will have 1 + 3 = 4 candies, which is not the greatest among the kids.
- Kid 5, they will have 3 + 3 = 6 candies, which is the greatest among the kids.
**Example 2:**
**Input:** candies = \[4,2,1,1,2\], extraCandies = 1
**Output:** \[true,false,false,false,false\]
**Explanation:** There is only 1 extra candy.
Kid 1 will always have the greatest number of candies, even if a different kid is given the extra candy.
**Example 3:**
**Input:** candies = \[12,1,12\], extraCandies = 10
**Output:** \[true,false,true\]
**Constraints:**
* `n == candies.length`
* `2 <= n <= 100`
* `1 <= candies[i] <= 100`
* `1 <= extraCandies <= 50` | Consider how reversing each edge of the graph can help us. How can performing BFS/DFS on the reversed graph help us find the ancestors of every node? |
Shortest 1 line solution | kids-with-the-greatest-number-of-candies | 0 | 1 | # Code\n```\nclass Solution:\n def kidsWithCandies(self, c: List[int], eC: int) -> List[bool]:\n return [x+eC >= max(c)for x in c]\n```\nplease upvote :D | 9 | You are given a string `s` and an integer array `indices` of the **same length**. The string `s` will be shuffled such that the character at the `ith` position moves to `indices[i]` in the shuffled string.
Return _the shuffled string_.
**Example 1:**
**Input:** s = "codeleet ", `indices` = \[4,5,6,7,0,2,1,3\]
**Output:** "leetcode "
**Explanation:** As shown, "codeleet " becomes "leetcode " after shuffling.
**Example 2:**
**Input:** s = "abc ", `indices` = \[0,1,2\]
**Output:** "abc "
**Explanation:** After shuffling, each character remains in its position.
**Constraints:**
* `s.length == indices.length == n`
* `1 <= n <= 100`
* `s` consists of only lowercase English letters.
* `0 <= indices[i] < n`
* All values of `indices` are **unique**. | Use greedy approach. For each kid check if candies[i] + extraCandies ≥ maximum in Candies[i]. |
[Java/Python 3] O(digits) w/ brief explanation and analysis. | max-difference-you-can-get-from-changing-an-integer | 1 | 1 | Convert to char array/list then process.\n\nThe following explains the algorithm of the code: -- credit to **@leetcodeCR97 and @martin20**.\n\nIf your first character `x` is \n1. neither `1` nor `9` then you will be replacing `a` with `9 `and `b` with `1 `if it matched the first character of the given number. \n2. `1` then you already have `b` starting with `1` which is least and you will have one more chance to decrease `b` to `0` which should be in MSB(Left most side). \n3. `9` then you will have one more chance to change `a` to `9` which should be in MSB(Left most side). \n\n```java\n public int maxDiff(int num) {\n char[] a = Integer.toString(num).toCharArray(), b = a.clone();\n char x = a[0], y = 0;\n for (int i = 0; i < a.length; ++i) {\n if (a[i] == x) {\n a[i] = \'9\';\n b[i] = \'1\';\n }else if (x == \'1\' && a[i] > \'0\' || x == \'9\' && a[i] < \'9\') {\n if (y == 0) {\n y = a[i];\n } \n if (y == a[i]) {\n if (x == \'1\')\n b[i] = \'0\';\n else\n a[i] = \'9\';\n }\n }\n }\n return Integer.parseInt(String.valueOf(a)) - Integer.parseInt(String.valueOf(b));\n }\n```\n```python\n def maxDiff(self, num: int) -> int:\n a, b = ([d for d in str(num)] for _ in range(2))\n x, y = a[0], \' \' \n for i, ch in enumerate(a):\n if ch == x:\n a[i] = \'9\'\n b[i] = \'1\'\n else:\n if x == \'1\' and ch != \'0\' or x == \'9\' != ch:\n if y == \' \':\n y = ch\n if y == ch:\n if x == \'1\':\n b[i] = \'0\'\n else:\n a[i] = \'9\'\n return int(\'\'.join(a)) - int(\'\'.join(b))\n```\n\n**Analysis:**\n\nTime & space: `O(n)`, where `n` is the number of the digits in `num` | 25 | You are given an integer `num`. You will apply the following steps exactly **two** times:
* Pick a digit `x (0 <= x <= 9)`.
* Pick another digit `y (0 <= y <= 9)`. The digit `y` can be equal to `x`.
* Replace all the occurrences of `x` in the decimal representation of `num` by `y`.
* The new integer **cannot** have any leading zeros, also the new integer **cannot** be 0.
Let `a` and `b` be the results of applying the operations to `num` the first and second times, respectively.
Return _the max difference_ between `a` and `b`.
**Example 1:**
**Input:** num = 555
**Output:** 888
**Explanation:** The first time pick x = 5 and y = 9 and store the new integer in a.
The second time pick x = 5 and y = 1 and store the new integer in b.
We have now a = 999 and b = 111 and max difference = 888
**Example 2:**
**Input:** num = 9
**Output:** 8
**Explanation:** The first time pick x = 9 and y = 9 and store the new integer in a.
The second time pick x = 9 and y = 1 and store the new integer in b.
We have now a = 9 and b = 1 and max difference = 8
**Constraints:**
* `1 <= num <= 10`8 | Depth-first search (DFS) with the parameters: current node in the binary tree and current position in the array of integers. When reaching at final position check if it is a leaf node. |
[Java/Python 3] O(digits) w/ brief explanation and analysis. | max-difference-you-can-get-from-changing-an-integer | 1 | 1 | Convert to char array/list then process.\n\nThe following explains the algorithm of the code: -- credit to **@leetcodeCR97 and @martin20**.\n\nIf your first character `x` is \n1. neither `1` nor `9` then you will be replacing `a` with `9 `and `b` with `1 `if it matched the first character of the given number. \n2. `1` then you already have `b` starting with `1` which is least and you will have one more chance to decrease `b` to `0` which should be in MSB(Left most side). \n3. `9` then you will have one more chance to change `a` to `9` which should be in MSB(Left most side). \n\n```java\n public int maxDiff(int num) {\n char[] a = Integer.toString(num).toCharArray(), b = a.clone();\n char x = a[0], y = 0;\n for (int i = 0; i < a.length; ++i) {\n if (a[i] == x) {\n a[i] = \'9\';\n b[i] = \'1\';\n }else if (x == \'1\' && a[i] > \'0\' || x == \'9\' && a[i] < \'9\') {\n if (y == 0) {\n y = a[i];\n } \n if (y == a[i]) {\n if (x == \'1\')\n b[i] = \'0\';\n else\n a[i] = \'9\';\n }\n }\n }\n return Integer.parseInt(String.valueOf(a)) - Integer.parseInt(String.valueOf(b));\n }\n```\n```python\n def maxDiff(self, num: int) -> int:\n a, b = ([d for d in str(num)] for _ in range(2))\n x, y = a[0], \' \' \n for i, ch in enumerate(a):\n if ch == x:\n a[i] = \'9\'\n b[i] = \'1\'\n else:\n if x == \'1\' and ch != \'0\' or x == \'9\' != ch:\n if y == \' \':\n y = ch\n if y == ch:\n if x == \'1\':\n b[i] = \'0\'\n else:\n a[i] = \'9\'\n return int(\'\'.join(a)) - int(\'\'.join(b))\n```\n\n**Analysis:**\n\nTime & space: `O(n)`, where `n` is the number of the digits in `num` | 25 | You are given a **0-indexed** binary string `target` of length `n`. You have another binary string `s` of length `n` that is initially set to all zeros. You want to make `s` equal to `target`.
In one operation, you can pick an index `i` where `0 <= i < n` and flip all bits in the **inclusive** range `[i, n - 1]`. Flip means changing `'0'` to `'1'` and `'1'` to `'0'`.
Return _the minimum number of operations needed to make_ `s` _equal to_ `target`.
**Example 1:**
**Input:** target = "10111 "
**Output:** 3
**Explanation:** Initially, s = "00000 ".
Choose index i = 2: "00000 " -> "00111 "
Choose index i = 0: "00111 " -> "11000 "
Choose index i = 1: "11000 " -> "10111 "
We need at least 3 flip operations to form target.
**Example 2:**
**Input:** target = "101 "
**Output:** 3
**Explanation:** Initially, s = "000 ".
Choose index i = 0: "000 " -> "111 "
Choose index i = 1: "111 " -> "100 "
Choose index i = 2: "100 " -> "101 "
We need at least 3 flip operations to form target.
**Example 3:**
**Input:** target = "00000 "
**Output:** 0
**Explanation:** We do not need any operations since the initial s already equals target.
**Constraints:**
* `n == target.length`
* `1 <= n <= 105`
* `target[i]` is either `'0'` or `'1'`. | We need to get the max and min value after changing num and the answer is max - min. Use brute force, try all possible changes and keep the minimum and maximum values. |
Pure Heuristics Python O(n) | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Approach\nBrute Force\n\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n l = [i for i in str(num) if i!=\'9\' ]\n if len(l)!=0: \n f = l[0]\n max_ = int(\'\'.join([n if n!=f else \'9\' for n in str(num)]))\n else: max_ = num\n\n if str(num)[0] == \'1\': \n m = \'0\'\n l = [i for i in str(num) if (i!=\'1\')&(i!=\'0\')]\n else: \n m = \'1\'\n l = [i for i in str(num) if i!=\'1\']\n if len(l)!=0:\n f = l[0]\n min_ = int(\'\'.join([n if n!=f else m for n in str(num)]))\n else: min_ = num\n return max_-min_\n``` | 0 | You are given an integer `num`. You will apply the following steps exactly **two** times:
* Pick a digit `x (0 <= x <= 9)`.
* Pick another digit `y (0 <= y <= 9)`. The digit `y` can be equal to `x`.
* Replace all the occurrences of `x` in the decimal representation of `num` by `y`.
* The new integer **cannot** have any leading zeros, also the new integer **cannot** be 0.
Let `a` and `b` be the results of applying the operations to `num` the first and second times, respectively.
Return _the max difference_ between `a` and `b`.
**Example 1:**
**Input:** num = 555
**Output:** 888
**Explanation:** The first time pick x = 5 and y = 9 and store the new integer in a.
The second time pick x = 5 and y = 1 and store the new integer in b.
We have now a = 999 and b = 111 and max difference = 888
**Example 2:**
**Input:** num = 9
**Output:** 8
**Explanation:** The first time pick x = 9 and y = 9 and store the new integer in a.
The second time pick x = 9 and y = 1 and store the new integer in b.
We have now a = 9 and b = 1 and max difference = 8
**Constraints:**
* `1 <= num <= 10`8 | Depth-first search (DFS) with the parameters: current node in the binary tree and current position in the array of integers. When reaching at final position check if it is a leaf node. |
Pure Heuristics Python O(n) | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Approach\nBrute Force\n\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n l = [i for i in str(num) if i!=\'9\' ]\n if len(l)!=0: \n f = l[0]\n max_ = int(\'\'.join([n if n!=f else \'9\' for n in str(num)]))\n else: max_ = num\n\n if str(num)[0] == \'1\': \n m = \'0\'\n l = [i for i in str(num) if (i!=\'1\')&(i!=\'0\')]\n else: \n m = \'1\'\n l = [i for i in str(num) if i!=\'1\']\n if len(l)!=0:\n f = l[0]\n min_ = int(\'\'.join([n if n!=f else m for n in str(num)]))\n else: min_ = num\n return max_-min_\n``` | 0 | You are given a **0-indexed** binary string `target` of length `n`. You have another binary string `s` of length `n` that is initially set to all zeros. You want to make `s` equal to `target`.
In one operation, you can pick an index `i` where `0 <= i < n` and flip all bits in the **inclusive** range `[i, n - 1]`. Flip means changing `'0'` to `'1'` and `'1'` to `'0'`.
Return _the minimum number of operations needed to make_ `s` _equal to_ `target`.
**Example 1:**
**Input:** target = "10111 "
**Output:** 3
**Explanation:** Initially, s = "00000 ".
Choose index i = 2: "00000 " -> "00111 "
Choose index i = 0: "00111 " -> "11000 "
Choose index i = 1: "11000 " -> "10111 "
We need at least 3 flip operations to form target.
**Example 2:**
**Input:** target = "101 "
**Output:** 3
**Explanation:** Initially, s = "000 ".
Choose index i = 0: "000 " -> "111 "
Choose index i = 1: "111 " -> "100 "
Choose index i = 2: "100 " -> "101 "
We need at least 3 flip operations to form target.
**Example 3:**
**Input:** target = "00000 "
**Output:** 0
**Explanation:** We do not need any operations since the initial s already equals target.
**Constraints:**
* `n == target.length`
* `1 <= n <= 105`
* `target[i]` is either `'0'` or `'1'`. | We need to get the max and min value after changing num and the answer is max - min. Use brute force, try all possible changes and keep the minimum and maximum values. |
Easy To Understand Solution !! Beats 98% and solved in O(n) with extra space complexity of O(n). | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n num = str(num)\n for i in range(len(num)):\n if num[i]!="9":\n break\n for j in range(len(num)):\n if num[j]>"1":\n break\n a = num.replace(str(num[i]),str("9"))\n if num[j]!="1":\n if j==0:\n b = num.replace(str(num[j]),str("1"))\n elif j==-1:\n b = num\n else:\n b = num.replace(str(num[j]),str("0"))\n else:\n b = num\n c = int(a) - int(b)\n 9909057\n return c\n``` | 0 | You are given an integer `num`. You will apply the following steps exactly **two** times:
* Pick a digit `x (0 <= x <= 9)`.
* Pick another digit `y (0 <= y <= 9)`. The digit `y` can be equal to `x`.
* Replace all the occurrences of `x` in the decimal representation of `num` by `y`.
* The new integer **cannot** have any leading zeros, also the new integer **cannot** be 0.
Let `a` and `b` be the results of applying the operations to `num` the first and second times, respectively.
Return _the max difference_ between `a` and `b`.
**Example 1:**
**Input:** num = 555
**Output:** 888
**Explanation:** The first time pick x = 5 and y = 9 and store the new integer in a.
The second time pick x = 5 and y = 1 and store the new integer in b.
We have now a = 999 and b = 111 and max difference = 888
**Example 2:**
**Input:** num = 9
**Output:** 8
**Explanation:** The first time pick x = 9 and y = 9 and store the new integer in a.
The second time pick x = 9 and y = 1 and store the new integer in b.
We have now a = 9 and b = 1 and max difference = 8
**Constraints:**
* `1 <= num <= 10`8 | Depth-first search (DFS) with the parameters: current node in the binary tree and current position in the array of integers. When reaching at final position check if it is a leaf node. |
Easy To Understand Solution !! Beats 98% and solved in O(n) with extra space complexity of O(n). | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n num = str(num)\n for i in range(len(num)):\n if num[i]!="9":\n break\n for j in range(len(num)):\n if num[j]>"1":\n break\n a = num.replace(str(num[i]),str("9"))\n if num[j]!="1":\n if j==0:\n b = num.replace(str(num[j]),str("1"))\n elif j==-1:\n b = num\n else:\n b = num.replace(str(num[j]),str("0"))\n else:\n b = num\n c = int(a) - int(b)\n 9909057\n return c\n``` | 0 | You are given a **0-indexed** binary string `target` of length `n`. You have another binary string `s` of length `n` that is initially set to all zeros. You want to make `s` equal to `target`.
In one operation, you can pick an index `i` where `0 <= i < n` and flip all bits in the **inclusive** range `[i, n - 1]`. Flip means changing `'0'` to `'1'` and `'1'` to `'0'`.
Return _the minimum number of operations needed to make_ `s` _equal to_ `target`.
**Example 1:**
**Input:** target = "10111 "
**Output:** 3
**Explanation:** Initially, s = "00000 ".
Choose index i = 2: "00000 " -> "00111 "
Choose index i = 0: "00111 " -> "11000 "
Choose index i = 1: "11000 " -> "10111 "
We need at least 3 flip operations to form target.
**Example 2:**
**Input:** target = "101 "
**Output:** 3
**Explanation:** Initially, s = "000 ".
Choose index i = 0: "000 " -> "111 "
Choose index i = 1: "111 " -> "100 "
Choose index i = 2: "100 " -> "101 "
We need at least 3 flip operations to form target.
**Example 3:**
**Input:** target = "00000 "
**Output:** 0
**Explanation:** We do not need any operations since the initial s already equals target.
**Constraints:**
* `n == target.length`
* `1 <= n <= 105`
* `target[i]` is either `'0'` or `'1'`. | We need to get the max and min value after changing num and the answer is max - min. Use brute force, try all possible changes and keep the minimum and maximum values. |
simplest sol in python | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Intuition\nMax length of string representation of num can be 9, so can go with for loop\n\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n a,b = str(num),str(num)\n for i in range(len(a)):\n if a[i] != a[0] and a[i] != "0": \n a=a.replace(a[i],"0",-1);break\n if a[i] != "0" and a[i] != "1": \n a=a.replace(a[i],"1",-1);break\n for i in range(len(b)):\n if b[i]!="9" : \n b=b.replace(b[i],"9",-1);break\n print(a,b)\n return int(b)-int(a)\n``` | 0 | You are given an integer `num`. You will apply the following steps exactly **two** times:
* Pick a digit `x (0 <= x <= 9)`.
* Pick another digit `y (0 <= y <= 9)`. The digit `y` can be equal to `x`.
* Replace all the occurrences of `x` in the decimal representation of `num` by `y`.
* The new integer **cannot** have any leading zeros, also the new integer **cannot** be 0.
Let `a` and `b` be the results of applying the operations to `num` the first and second times, respectively.
Return _the max difference_ between `a` and `b`.
**Example 1:**
**Input:** num = 555
**Output:** 888
**Explanation:** The first time pick x = 5 and y = 9 and store the new integer in a.
The second time pick x = 5 and y = 1 and store the new integer in b.
We have now a = 999 and b = 111 and max difference = 888
**Example 2:**
**Input:** num = 9
**Output:** 8
**Explanation:** The first time pick x = 9 and y = 9 and store the new integer in a.
The second time pick x = 9 and y = 1 and store the new integer in b.
We have now a = 9 and b = 1 and max difference = 8
**Constraints:**
* `1 <= num <= 10`8 | Depth-first search (DFS) with the parameters: current node in the binary tree and current position in the array of integers. When reaching at final position check if it is a leaf node. |
simplest sol in python | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Intuition\nMax length of string representation of num can be 9, so can go with for loop\n\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n a,b = str(num),str(num)\n for i in range(len(a)):\n if a[i] != a[0] and a[i] != "0": \n a=a.replace(a[i],"0",-1);break\n if a[i] != "0" and a[i] != "1": \n a=a.replace(a[i],"1",-1);break\n for i in range(len(b)):\n if b[i]!="9" : \n b=b.replace(b[i],"9",-1);break\n print(a,b)\n return int(b)-int(a)\n``` | 0 | You are given a **0-indexed** binary string `target` of length `n`. You have another binary string `s` of length `n` that is initially set to all zeros. You want to make `s` equal to `target`.
In one operation, you can pick an index `i` where `0 <= i < n` and flip all bits in the **inclusive** range `[i, n - 1]`. Flip means changing `'0'` to `'1'` and `'1'` to `'0'`.
Return _the minimum number of operations needed to make_ `s` _equal to_ `target`.
**Example 1:**
**Input:** target = "10111 "
**Output:** 3
**Explanation:** Initially, s = "00000 ".
Choose index i = 2: "00000 " -> "00111 "
Choose index i = 0: "00111 " -> "11000 "
Choose index i = 1: "11000 " -> "10111 "
We need at least 3 flip operations to form target.
**Example 2:**
**Input:** target = "101 "
**Output:** 3
**Explanation:** Initially, s = "000 ".
Choose index i = 0: "000 " -> "111 "
Choose index i = 1: "111 " -> "100 "
Choose index i = 2: "100 " -> "101 "
We need at least 3 flip operations to form target.
**Example 3:**
**Input:** target = "00000 "
**Output:** 0
**Explanation:** We do not need any operations since the initial s already equals target.
**Constraints:**
* `n == target.length`
* `1 <= n <= 105`
* `target[i]` is either `'0'` or `'1'`. | We need to get the max and min value after changing num and the answer is max - min. Use brute force, try all possible changes and keep the minimum and maximum values. |
Python3 Naive Solution | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n \n s1=str(num)\n s2=str(num)\n \n if s1[0]!="1":\n s1=s1.replace(s1[0],"1")\n else:\n i=0\n while i<len(s1):\n if s1[i]!="1"and s2[i]!="0":\n s1=s1.replace(s1[i],"0")\n break\n i+=1\n \n if s2[0]!="9":\n s2=s2.replace(s2[0],"9")\n else:\n i=0\n while i<len(s2):\n if s2[i]!="9":\n s2=s2.replace(s2[i],"9")\n break\n i+=1\n \n return int(s2)-int(s1)\n \n \n \n``` | 0 | You are given an integer `num`. You will apply the following steps exactly **two** times:
* Pick a digit `x (0 <= x <= 9)`.
* Pick another digit `y (0 <= y <= 9)`. The digit `y` can be equal to `x`.
* Replace all the occurrences of `x` in the decimal representation of `num` by `y`.
* The new integer **cannot** have any leading zeros, also the new integer **cannot** be 0.
Let `a` and `b` be the results of applying the operations to `num` the first and second times, respectively.
Return _the max difference_ between `a` and `b`.
**Example 1:**
**Input:** num = 555
**Output:** 888
**Explanation:** The first time pick x = 5 and y = 9 and store the new integer in a.
The second time pick x = 5 and y = 1 and store the new integer in b.
We have now a = 999 and b = 111 and max difference = 888
**Example 2:**
**Input:** num = 9
**Output:** 8
**Explanation:** The first time pick x = 9 and y = 9 and store the new integer in a.
The second time pick x = 9 and y = 1 and store the new integer in b.
We have now a = 9 and b = 1 and max difference = 8
**Constraints:**
* `1 <= num <= 10`8 | Depth-first search (DFS) with the parameters: current node in the binary tree and current position in the array of integers. When reaching at final position check if it is a leaf node. |
Python3 Naive Solution | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n \n s1=str(num)\n s2=str(num)\n \n if s1[0]!="1":\n s1=s1.replace(s1[0],"1")\n else:\n i=0\n while i<len(s1):\n if s1[i]!="1"and s2[i]!="0":\n s1=s1.replace(s1[i],"0")\n break\n i+=1\n \n if s2[0]!="9":\n s2=s2.replace(s2[0],"9")\n else:\n i=0\n while i<len(s2):\n if s2[i]!="9":\n s2=s2.replace(s2[i],"9")\n break\n i+=1\n \n return int(s2)-int(s1)\n \n \n \n``` | 0 | You are given a **0-indexed** binary string `target` of length `n`. You have another binary string `s` of length `n` that is initially set to all zeros. You want to make `s` equal to `target`.
In one operation, you can pick an index `i` where `0 <= i < n` and flip all bits in the **inclusive** range `[i, n - 1]`. Flip means changing `'0'` to `'1'` and `'1'` to `'0'`.
Return _the minimum number of operations needed to make_ `s` _equal to_ `target`.
**Example 1:**
**Input:** target = "10111 "
**Output:** 3
**Explanation:** Initially, s = "00000 ".
Choose index i = 2: "00000 " -> "00111 "
Choose index i = 0: "00111 " -> "11000 "
Choose index i = 1: "11000 " -> "10111 "
We need at least 3 flip operations to form target.
**Example 2:**
**Input:** target = "101 "
**Output:** 3
**Explanation:** Initially, s = "000 ".
Choose index i = 0: "000 " -> "111 "
Choose index i = 1: "111 " -> "100 "
Choose index i = 2: "100 " -> "101 "
We need at least 3 flip operations to form target.
**Example 3:**
**Input:** target = "00000 "
**Output:** 0
**Explanation:** We do not need any operations since the initial s already equals target.
**Constraints:**
* `n == target.length`
* `1 <= n <= 105`
* `target[i]` is either `'0'` or `'1'`. | We need to get the max and min value after changing num and the answer is max - min. Use brute force, try all possible changes and keep the minimum and maximum values. |
Easy Python solution | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n str_num = str(num)\n max_num = inf\n min_num = -inf\n for digit in set(str_num):\n for new_digit in [\'0\',\'1\',\'2\',\'3\',\'4\',\'5\',\'6\',\'7\',\'8\',\'9\']:\n new_str = str_num.replace(digit, new_digit)\n if new_str[0]!="0":\n new_num = int(new_str)\n if new_num<max_num:\n max_num = new_num\n if new_num>min_num:\n min_num = new_num\n return min_num-max_num\n \n \n\n\n``` | 0 | You are given an integer `num`. You will apply the following steps exactly **two** times:
* Pick a digit `x (0 <= x <= 9)`.
* Pick another digit `y (0 <= y <= 9)`. The digit `y` can be equal to `x`.
* Replace all the occurrences of `x` in the decimal representation of `num` by `y`.
* The new integer **cannot** have any leading zeros, also the new integer **cannot** be 0.
Let `a` and `b` be the results of applying the operations to `num` the first and second times, respectively.
Return _the max difference_ between `a` and `b`.
**Example 1:**
**Input:** num = 555
**Output:** 888
**Explanation:** The first time pick x = 5 and y = 9 and store the new integer in a.
The second time pick x = 5 and y = 1 and store the new integer in b.
We have now a = 999 and b = 111 and max difference = 888
**Example 2:**
**Input:** num = 9
**Output:** 8
**Explanation:** The first time pick x = 9 and y = 9 and store the new integer in a.
The second time pick x = 9 and y = 1 and store the new integer in b.
We have now a = 9 and b = 1 and max difference = 8
**Constraints:**
* `1 <= num <= 10`8 | Depth-first search (DFS) with the parameters: current node in the binary tree and current position in the array of integers. When reaching at final position check if it is a leaf node. |
Easy Python solution | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n str_num = str(num)\n max_num = inf\n min_num = -inf\n for digit in set(str_num):\n for new_digit in [\'0\',\'1\',\'2\',\'3\',\'4\',\'5\',\'6\',\'7\',\'8\',\'9\']:\n new_str = str_num.replace(digit, new_digit)\n if new_str[0]!="0":\n new_num = int(new_str)\n if new_num<max_num:\n max_num = new_num\n if new_num>min_num:\n min_num = new_num\n return min_num-max_num\n \n \n\n\n``` | 0 | You are given a **0-indexed** binary string `target` of length `n`. You have another binary string `s` of length `n` that is initially set to all zeros. You want to make `s` equal to `target`.
In one operation, you can pick an index `i` where `0 <= i < n` and flip all bits in the **inclusive** range `[i, n - 1]`. Flip means changing `'0'` to `'1'` and `'1'` to `'0'`.
Return _the minimum number of operations needed to make_ `s` _equal to_ `target`.
**Example 1:**
**Input:** target = "10111 "
**Output:** 3
**Explanation:** Initially, s = "00000 ".
Choose index i = 2: "00000 " -> "00111 "
Choose index i = 0: "00111 " -> "11000 "
Choose index i = 1: "11000 " -> "10111 "
We need at least 3 flip operations to form target.
**Example 2:**
**Input:** target = "101 "
**Output:** 3
**Explanation:** Initially, s = "000 ".
Choose index i = 0: "000 " -> "111 "
Choose index i = 1: "111 " -> "100 "
Choose index i = 2: "100 " -> "101 "
We need at least 3 flip operations to form target.
**Example 3:**
**Input:** target = "00000 "
**Output:** 0
**Explanation:** We do not need any operations since the initial s already equals target.
**Constraints:**
* `n == target.length`
* `1 <= n <= 105`
* `target[i]` is either `'0'` or `'1'`. | We need to get the max and min value after changing num and the answer is max - min. Use brute force, try all possible changes and keep the minimum and maximum values. |
Solution in Python | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n x = int(num)\n mostSignificantDigit = -1\n while x>=1:\n if x%10 != 9 or mostSignificantDigit==-1 or x%10==mostSignificantDigit: \n mostSignificantDigit = int(x%10)\n x=int(x/10)\n x=int(num)\n maxNum = int(0)\n minNum = int(0)\n count = 0\n print(mostSignificantDigit)\n while x>=1:\n if x%10 == mostSignificantDigit:\n maxNum+=int(pow(10,count)*9)\n x=int(x/10)\n else:\n maxNum+=int(pow(10,count)*(x%10))\n x=int(x/10)\n count += 1\n print(maxNum)\n mostSignificantDigit = -1\n minNumber = 0\n x=int(num)\n while x>=1:\n if (x%10 != 1 and x%10 != 0) or mostSignificantDigit==-1 or x%10==mostSignificantDigit: \n mostSignificantDigit = int(x%10)\n if int(x/10)==0:\n minNumber = 1\n else:\n minNumber = 0\n x=int(x/10)\n x=int(num)\n count=0\n while x>=1:\n if x%10 == mostSignificantDigit:\n minNum+=int(pow(10,count)*minNumber)\n x=int(x/10)\n else:\n minNum+=int(pow(10,count)*(x%10))\n x=int(x/10)\n count += 1\n print(minNum)\n return (maxNum-minNum)\n\n \n``` | 0 | You are given an integer `num`. You will apply the following steps exactly **two** times:
* Pick a digit `x (0 <= x <= 9)`.
* Pick another digit `y (0 <= y <= 9)`. The digit `y` can be equal to `x`.
* Replace all the occurrences of `x` in the decimal representation of `num` by `y`.
* The new integer **cannot** have any leading zeros, also the new integer **cannot** be 0.
Let `a` and `b` be the results of applying the operations to `num` the first and second times, respectively.
Return _the max difference_ between `a` and `b`.
**Example 1:**
**Input:** num = 555
**Output:** 888
**Explanation:** The first time pick x = 5 and y = 9 and store the new integer in a.
The second time pick x = 5 and y = 1 and store the new integer in b.
We have now a = 999 and b = 111 and max difference = 888
**Example 2:**
**Input:** num = 9
**Output:** 8
**Explanation:** The first time pick x = 9 and y = 9 and store the new integer in a.
The second time pick x = 9 and y = 1 and store the new integer in b.
We have now a = 9 and b = 1 and max difference = 8
**Constraints:**
* `1 <= num <= 10`8 | Depth-first search (DFS) with the parameters: current node in the binary tree and current position in the array of integers. When reaching at final position check if it is a leaf node. |
Solution in Python | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n x = int(num)\n mostSignificantDigit = -1\n while x>=1:\n if x%10 != 9 or mostSignificantDigit==-1 or x%10==mostSignificantDigit: \n mostSignificantDigit = int(x%10)\n x=int(x/10)\n x=int(num)\n maxNum = int(0)\n minNum = int(0)\n count = 0\n print(mostSignificantDigit)\n while x>=1:\n if x%10 == mostSignificantDigit:\n maxNum+=int(pow(10,count)*9)\n x=int(x/10)\n else:\n maxNum+=int(pow(10,count)*(x%10))\n x=int(x/10)\n count += 1\n print(maxNum)\n mostSignificantDigit = -1\n minNumber = 0\n x=int(num)\n while x>=1:\n if (x%10 != 1 and x%10 != 0) or mostSignificantDigit==-1 or x%10==mostSignificantDigit: \n mostSignificantDigit = int(x%10)\n if int(x/10)==0:\n minNumber = 1\n else:\n minNumber = 0\n x=int(x/10)\n x=int(num)\n count=0\n while x>=1:\n if x%10 == mostSignificantDigit:\n minNum+=int(pow(10,count)*minNumber)\n x=int(x/10)\n else:\n minNum+=int(pow(10,count)*(x%10))\n x=int(x/10)\n count += 1\n print(minNum)\n return (maxNum-minNum)\n\n \n``` | 0 | You are given a **0-indexed** binary string `target` of length `n`. You have another binary string `s` of length `n` that is initially set to all zeros. You want to make `s` equal to `target`.
In one operation, you can pick an index `i` where `0 <= i < n` and flip all bits in the **inclusive** range `[i, n - 1]`. Flip means changing `'0'` to `'1'` and `'1'` to `'0'`.
Return _the minimum number of operations needed to make_ `s` _equal to_ `target`.
**Example 1:**
**Input:** target = "10111 "
**Output:** 3
**Explanation:** Initially, s = "00000 ".
Choose index i = 2: "00000 " -> "00111 "
Choose index i = 0: "00111 " -> "11000 "
Choose index i = 1: "11000 " -> "10111 "
We need at least 3 flip operations to form target.
**Example 2:**
**Input:** target = "101 "
**Output:** 3
**Explanation:** Initially, s = "000 ".
Choose index i = 0: "000 " -> "111 "
Choose index i = 1: "111 " -> "100 "
Choose index i = 2: "100 " -> "101 "
We need at least 3 flip operations to form target.
**Example 3:**
**Input:** target = "00000 "
**Output:** 0
**Explanation:** We do not need any operations since the initial s already equals target.
**Constraints:**
* `n == target.length`
* `1 <= n <= 105`
* `target[i]` is either `'0'` or `'1'`. | We need to get the max and min value after changing num and the answer is max - min. Use brute force, try all possible changes and keep the minimum and maximum values. |
(num as string)||Simplest Python solution beats 92% | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n**Max value of N is 10^8**\n**if We think here of N as Strig then the Max len is just 9 chars**\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n a,b = str(num),str(num)\n for i in range(len(a)):\n if a[i] != a[0] and a[i] != "0": \n a=a.replace(a[i],"0",-1);break\n if a[i] != "0" and a[i] != "1": \n a=a.replace(a[i],"1",-1);break\n for i in range(len(b)):\n if b[i]!="9" : \n b=b.replace(b[i],"9",-1);break\n return int(b)-int(a)\n``` | 0 | You are given an integer `num`. You will apply the following steps exactly **two** times:
* Pick a digit `x (0 <= x <= 9)`.
* Pick another digit `y (0 <= y <= 9)`. The digit `y` can be equal to `x`.
* Replace all the occurrences of `x` in the decimal representation of `num` by `y`.
* The new integer **cannot** have any leading zeros, also the new integer **cannot** be 0.
Let `a` and `b` be the results of applying the operations to `num` the first and second times, respectively.
Return _the max difference_ between `a` and `b`.
**Example 1:**
**Input:** num = 555
**Output:** 888
**Explanation:** The first time pick x = 5 and y = 9 and store the new integer in a.
The second time pick x = 5 and y = 1 and store the new integer in b.
We have now a = 999 and b = 111 and max difference = 888
**Example 2:**
**Input:** num = 9
**Output:** 8
**Explanation:** The first time pick x = 9 and y = 9 and store the new integer in a.
The second time pick x = 9 and y = 1 and store the new integer in b.
We have now a = 9 and b = 1 and max difference = 8
**Constraints:**
* `1 <= num <= 10`8 | Depth-first search (DFS) with the parameters: current node in the binary tree and current position in the array of integers. When reaching at final position check if it is a leaf node. |
(num as string)||Simplest Python solution beats 92% | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n**Max value of N is 10^8**\n**if We think here of N as Strig then the Max len is just 9 chars**\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n a,b = str(num),str(num)\n for i in range(len(a)):\n if a[i] != a[0] and a[i] != "0": \n a=a.replace(a[i],"0",-1);break\n if a[i] != "0" and a[i] != "1": \n a=a.replace(a[i],"1",-1);break\n for i in range(len(b)):\n if b[i]!="9" : \n b=b.replace(b[i],"9",-1);break\n return int(b)-int(a)\n``` | 0 | You are given a **0-indexed** binary string `target` of length `n`. You have another binary string `s` of length `n` that is initially set to all zeros. You want to make `s` equal to `target`.
In one operation, you can pick an index `i` where `0 <= i < n` and flip all bits in the **inclusive** range `[i, n - 1]`. Flip means changing `'0'` to `'1'` and `'1'` to `'0'`.
Return _the minimum number of operations needed to make_ `s` _equal to_ `target`.
**Example 1:**
**Input:** target = "10111 "
**Output:** 3
**Explanation:** Initially, s = "00000 ".
Choose index i = 2: "00000 " -> "00111 "
Choose index i = 0: "00111 " -> "11000 "
Choose index i = 1: "11000 " -> "10111 "
We need at least 3 flip operations to form target.
**Example 2:**
**Input:** target = "101 "
**Output:** 3
**Explanation:** Initially, s = "000 ".
Choose index i = 0: "000 " -> "111 "
Choose index i = 1: "111 " -> "100 "
Choose index i = 2: "100 " -> "101 "
We need at least 3 flip operations to form target.
**Example 3:**
**Input:** target = "00000 "
**Output:** 0
**Explanation:** We do not need any operations since the initial s already equals target.
**Constraints:**
* `n == target.length`
* `1 <= n <= 105`
* `target[i]` is either `'0'` or `'1'`. | We need to get the max and min value after changing num and the answer is max - min. Use brute force, try all possible changes and keep the minimum and maximum values. |
Python Easy Solution , O(n) | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n num = str(num)\n mx = float(\'-inf\')\n mn = float(\'inf\')\n for i in \'0123456789\':\n for j in \'0123456789\':\n val = num.replace(i,j)\n if val[0] == \'0\' or int(val) == 0:\n continue\n mx = max(mx,int(val))\n mn = min(mn,int(val))\n return mx - mn\n \n``` | 0 | You are given an integer `num`. You will apply the following steps exactly **two** times:
* Pick a digit `x (0 <= x <= 9)`.
* Pick another digit `y (0 <= y <= 9)`. The digit `y` can be equal to `x`.
* Replace all the occurrences of `x` in the decimal representation of `num` by `y`.
* The new integer **cannot** have any leading zeros, also the new integer **cannot** be 0.
Let `a` and `b` be the results of applying the operations to `num` the first and second times, respectively.
Return _the max difference_ between `a` and `b`.
**Example 1:**
**Input:** num = 555
**Output:** 888
**Explanation:** The first time pick x = 5 and y = 9 and store the new integer in a.
The second time pick x = 5 and y = 1 and store the new integer in b.
We have now a = 999 and b = 111 and max difference = 888
**Example 2:**
**Input:** num = 9
**Output:** 8
**Explanation:** The first time pick x = 9 and y = 9 and store the new integer in a.
The second time pick x = 9 and y = 1 and store the new integer in b.
We have now a = 9 and b = 1 and max difference = 8
**Constraints:**
* `1 <= num <= 10`8 | Depth-first search (DFS) with the parameters: current node in the binary tree and current position in the array of integers. When reaching at final position check if it is a leaf node. |
Python Easy Solution , O(n) | max-difference-you-can-get-from-changing-an-integer | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxDiff(self, num: int) -> int:\n num = str(num)\n mx = float(\'-inf\')\n mn = float(\'inf\')\n for i in \'0123456789\':\n for j in \'0123456789\':\n val = num.replace(i,j)\n if val[0] == \'0\' or int(val) == 0:\n continue\n mx = max(mx,int(val))\n mn = min(mn,int(val))\n return mx - mn\n \n``` | 0 | You are given a **0-indexed** binary string `target` of length `n`. You have another binary string `s` of length `n` that is initially set to all zeros. You want to make `s` equal to `target`.
In one operation, you can pick an index `i` where `0 <= i < n` and flip all bits in the **inclusive** range `[i, n - 1]`. Flip means changing `'0'` to `'1'` and `'1'` to `'0'`.
Return _the minimum number of operations needed to make_ `s` _equal to_ `target`.
**Example 1:**
**Input:** target = "10111 "
**Output:** 3
**Explanation:** Initially, s = "00000 ".
Choose index i = 2: "00000 " -> "00111 "
Choose index i = 0: "00111 " -> "11000 "
Choose index i = 1: "11000 " -> "10111 "
We need at least 3 flip operations to form target.
**Example 2:**
**Input:** target = "101 "
**Output:** 3
**Explanation:** Initially, s = "000 ".
Choose index i = 0: "000 " -> "111 "
Choose index i = 1: "111 " -> "100 "
Choose index i = 2: "100 " -> "101 "
We need at least 3 flip operations to form target.
**Example 3:**
**Input:** target = "00000 "
**Output:** 0
**Explanation:** We do not need any operations since the initial s already equals target.
**Constraints:**
* `n == target.length`
* `1 <= n <= 105`
* `target[i]` is either `'0'` or `'1'`. | We need to get the max and min value after changing num and the answer is max - min. Use brute force, try all possible changes and keep the minimum and maximum values. |
Python solution | check-if-a-string-can-break-another-string | 0 | 1 | \n\n# Approach\nSort the strings in descending order and check the characters\n\n# Complexity\n- Time complexity:\nO(nLogn)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:\n l1 = [i for i in s1]\n l2 = [i for i in s2]\n l1.sort(reverse=True)\n l2.sort(reverse=True)\n greater = l1\n smaller = l2\n j = 0\n while j < len(l1) and greater[j] == smaller[j]:\n j += 1\n if j == len(l1):\n return True\n if greater[j] < smaller[j]:\n greater, smaller = smaller, greater\n for i in range(j+1, len(l2)):\n if greater[i] < smaller[i]:\n return False\n return True\n \n\n``` | 0 | Given two strings: `s1` and `s2` with the same size, check if some permutation of string `s1` can break some permutation of string `s2` or vice-versa. In other words `s2` can break `s1` or vice-versa.
A string `x` can break string `y` (both of size `n`) if `x[i] >= y[i]` (in alphabetical order) for all `i` between `0` and `n-1`.
**Example 1:**
**Input:** s1 = "abc ", s2 = "xya "
**Output:** true
**Explanation:** "ayx " is a permutation of s2= "xya " which can break to string "abc " which is a permutation of s1= "abc ".
**Example 2:**
**Input:** s1 = "abe ", s2 = "acd "
**Output:** false
**Explanation:** All permutations for s1= "abe " are: "abe ", "aeb ", "bae ", "bea ", "eab " and "eba " and all permutation for s2= "acd " are: "acd ", "adc ", "cad ", "cda ", "dac " and "dca ". However, there is not any permutation from s1 which can break some permutation from s2 and vice-versa.
**Example 3:**
**Input:** s1 = "leetcodee ", s2 = "interview "
**Output:** true
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `1 <= n <= 10^5`
* All strings consist of lowercase English letters. | For encryption, use hashmap to map each char of word1 to its value. For decryption, use trie to prune when necessary. |
Python solution | check-if-a-string-can-break-another-string | 0 | 1 | \n\n# Approach\nSort the strings in descending order and check the characters\n\n# Complexity\n- Time complexity:\nO(nLogn)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:\n l1 = [i for i in s1]\n l2 = [i for i in s2]\n l1.sort(reverse=True)\n l2.sort(reverse=True)\n greater = l1\n smaller = l2\n j = 0\n while j < len(l1) and greater[j] == smaller[j]:\n j += 1\n if j == len(l1):\n return True\n if greater[j] < smaller[j]:\n greater, smaller = smaller, greater\n for i in range(j+1, len(l2)):\n if greater[i] < smaller[i]:\n return False\n return True\n \n\n``` | 0 | You are given the `root` of a binary tree and an integer `distance`. A pair of two different **leaf** nodes of a binary tree is said to be good if the length of **the shortest path** between them is less than or equal to `distance`.
Return _the number of good leaf node pairs_ in the tree.
**Example 1:**
**Input:** root = \[1,2,3,null,4\], distance = 3
**Output:** 1
**Explanation:** The leaf nodes of the tree are 3 and 4 and the length of the shortest path between them is 3. This is the only good pair.
**Example 2:**
**Input:** root = \[1,2,3,4,5,6,7\], distance = 3
**Output:** 2
**Explanation:** The good pairs are \[4,5\] and \[6,7\] with shortest path = 2. The pair \[4,6\] is not good because the length of ther shortest path between them is 4.
**Example 3:**
**Input:** root = \[7,1,4,6,null,5,3,null,null,null,null,null,2\], distance = 3
**Output:** 1
**Explanation:** The only good pair is \[2,5\].
**Constraints:**
* The number of nodes in the `tree` is in the range `[1, 210].`
* `1 <= Node.val <= 100`
* `1 <= distance <= 10` | Sort both strings and then check if one of them can break the other. |
One Liner Solution | check-if-a-string-can-break-another-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:return all(a<=b for a,b in zip(sorted(s1),sorted(s2))) or all(a>=b for a,b in zip(sorted(s1),sorted(s2)))\n\n \n \n \n``` | 0 | Given two strings: `s1` and `s2` with the same size, check if some permutation of string `s1` can break some permutation of string `s2` or vice-versa. In other words `s2` can break `s1` or vice-versa.
A string `x` can break string `y` (both of size `n`) if `x[i] >= y[i]` (in alphabetical order) for all `i` between `0` and `n-1`.
**Example 1:**
**Input:** s1 = "abc ", s2 = "xya "
**Output:** true
**Explanation:** "ayx " is a permutation of s2= "xya " which can break to string "abc " which is a permutation of s1= "abc ".
**Example 2:**
**Input:** s1 = "abe ", s2 = "acd "
**Output:** false
**Explanation:** All permutations for s1= "abe " are: "abe ", "aeb ", "bae ", "bea ", "eab " and "eba " and all permutation for s2= "acd " are: "acd ", "adc ", "cad ", "cda ", "dac " and "dca ". However, there is not any permutation from s1 which can break some permutation from s2 and vice-versa.
**Example 3:**
**Input:** s1 = "leetcodee ", s2 = "interview "
**Output:** true
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `1 <= n <= 10^5`
* All strings consist of lowercase English letters. | For encryption, use hashmap to map each char of word1 to its value. For decryption, use trie to prune when necessary. |
One Liner Solution | check-if-a-string-can-break-another-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:return all(a<=b for a,b in zip(sorted(s1),sorted(s2))) or all(a>=b for a,b in zip(sorted(s1),sorted(s2)))\n\n \n \n \n``` | 0 | You are given the `root` of a binary tree and an integer `distance`. A pair of two different **leaf** nodes of a binary tree is said to be good if the length of **the shortest path** between them is less than or equal to `distance`.
Return _the number of good leaf node pairs_ in the tree.
**Example 1:**
**Input:** root = \[1,2,3,null,4\], distance = 3
**Output:** 1
**Explanation:** The leaf nodes of the tree are 3 and 4 and the length of the shortest path between them is 3. This is the only good pair.
**Example 2:**
**Input:** root = \[1,2,3,4,5,6,7\], distance = 3
**Output:** 2
**Explanation:** The good pairs are \[4,5\] and \[6,7\] with shortest path = 2. The pair \[4,6\] is not good because the length of ther shortest path between them is 4.
**Example 3:**
**Input:** root = \[7,1,4,6,null,5,3,null,null,null,null,null,2\], distance = 3
**Output:** 1
**Explanation:** The only good pair is \[2,5\].
**Constraints:**
* The number of nodes in the `tree` is in the range `[1, 210].`
* `1 <= Node.val <= 100`
* `1 <= distance <= 10` | Sort both strings and then check if one of them can break the other. |
Python3 Solution | check-if-a-string-can-break-another-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nsort the two strings and check if one breaks the other linearly\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(nlogn + mlogm) for sorting the input string\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(n + m) for storing\n\n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:\n s1 = sorted([ord(s) for s in s1])\n s2 = sorted([ord(s) for s in s2])\n\n s1_breaks_s2 = True\n s2_breaks_s1 = True\n for i in range(len(s1)):\n if s1[i] < s2[i]:\n s1_breaks_s2 = False\n if s2[i] < s1[i]:\n s2_breaks_s1 = False\n return s2_breaks_s1 or s1_breaks_s2\n\n \n``` | 0 | Given two strings: `s1` and `s2` with the same size, check if some permutation of string `s1` can break some permutation of string `s2` or vice-versa. In other words `s2` can break `s1` or vice-versa.
A string `x` can break string `y` (both of size `n`) if `x[i] >= y[i]` (in alphabetical order) for all `i` between `0` and `n-1`.
**Example 1:**
**Input:** s1 = "abc ", s2 = "xya "
**Output:** true
**Explanation:** "ayx " is a permutation of s2= "xya " which can break to string "abc " which is a permutation of s1= "abc ".
**Example 2:**
**Input:** s1 = "abe ", s2 = "acd "
**Output:** false
**Explanation:** All permutations for s1= "abe " are: "abe ", "aeb ", "bae ", "bea ", "eab " and "eba " and all permutation for s2= "acd " are: "acd ", "adc ", "cad ", "cda ", "dac " and "dca ". However, there is not any permutation from s1 which can break some permutation from s2 and vice-versa.
**Example 3:**
**Input:** s1 = "leetcodee ", s2 = "interview "
**Output:** true
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `1 <= n <= 10^5`
* All strings consist of lowercase English letters. | For encryption, use hashmap to map each char of word1 to its value. For decryption, use trie to prune when necessary. |
Python3 Solution | check-if-a-string-can-break-another-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nsort the two strings and check if one breaks the other linearly\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(nlogn + mlogm) for sorting the input string\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(n + m) for storing\n\n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:\n s1 = sorted([ord(s) for s in s1])\n s2 = sorted([ord(s) for s in s2])\n\n s1_breaks_s2 = True\n s2_breaks_s1 = True\n for i in range(len(s1)):\n if s1[i] < s2[i]:\n s1_breaks_s2 = False\n if s2[i] < s1[i]:\n s2_breaks_s1 = False\n return s2_breaks_s1 or s1_breaks_s2\n\n \n``` | 0 | You are given the `root` of a binary tree and an integer `distance`. A pair of two different **leaf** nodes of a binary tree is said to be good if the length of **the shortest path** between them is less than or equal to `distance`.
Return _the number of good leaf node pairs_ in the tree.
**Example 1:**
**Input:** root = \[1,2,3,null,4\], distance = 3
**Output:** 1
**Explanation:** The leaf nodes of the tree are 3 and 4 and the length of the shortest path between them is 3. This is the only good pair.
**Example 2:**
**Input:** root = \[1,2,3,4,5,6,7\], distance = 3
**Output:** 2
**Explanation:** The good pairs are \[4,5\] and \[6,7\] with shortest path = 2. The pair \[4,6\] is not good because the length of ther shortest path between them is 4.
**Example 3:**
**Input:** root = \[7,1,4,6,null,5,3,null,null,null,null,null,2\], distance = 3
**Output:** 1
**Explanation:** The only good pair is \[2,5\].
**Constraints:**
* The number of nodes in the `tree` is in the range `[1, 210].`
* `1 <= Node.val <= 100`
* `1 <= distance <= 10` | Sort both strings and then check if one of them can break the other. |
Python3 Clean and Short Solution | check-if-a-string-can-break-another-string | 0 | 1 | \n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:\n \n \n sort1=sorted(s1)\n sort2=sorted(s2)\n \n return all(a<=b for a,b in zip(sort1,sort2)) or all(a>=b for a,b in zip(sort1,sort2))\n \n \n``` | 0 | Given two strings: `s1` and `s2` with the same size, check if some permutation of string `s1` can break some permutation of string `s2` or vice-versa. In other words `s2` can break `s1` or vice-versa.
A string `x` can break string `y` (both of size `n`) if `x[i] >= y[i]` (in alphabetical order) for all `i` between `0` and `n-1`.
**Example 1:**
**Input:** s1 = "abc ", s2 = "xya "
**Output:** true
**Explanation:** "ayx " is a permutation of s2= "xya " which can break to string "abc " which is a permutation of s1= "abc ".
**Example 2:**
**Input:** s1 = "abe ", s2 = "acd "
**Output:** false
**Explanation:** All permutations for s1= "abe " are: "abe ", "aeb ", "bae ", "bea ", "eab " and "eba " and all permutation for s2= "acd " are: "acd ", "adc ", "cad ", "cda ", "dac " and "dca ". However, there is not any permutation from s1 which can break some permutation from s2 and vice-versa.
**Example 3:**
**Input:** s1 = "leetcodee ", s2 = "interview "
**Output:** true
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `1 <= n <= 10^5`
* All strings consist of lowercase English letters. | For encryption, use hashmap to map each char of word1 to its value. For decryption, use trie to prune when necessary. |
Python3 Clean and Short Solution | check-if-a-string-can-break-another-string | 0 | 1 | \n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:\n \n \n sort1=sorted(s1)\n sort2=sorted(s2)\n \n return all(a<=b for a,b in zip(sort1,sort2)) or all(a>=b for a,b in zip(sort1,sort2))\n \n \n``` | 0 | You are given the `root` of a binary tree and an integer `distance`. A pair of two different **leaf** nodes of a binary tree is said to be good if the length of **the shortest path** between them is less than or equal to `distance`.
Return _the number of good leaf node pairs_ in the tree.
**Example 1:**
**Input:** root = \[1,2,3,null,4\], distance = 3
**Output:** 1
**Explanation:** The leaf nodes of the tree are 3 and 4 and the length of the shortest path between them is 3. This is the only good pair.
**Example 2:**
**Input:** root = \[1,2,3,4,5,6,7\], distance = 3
**Output:** 2
**Explanation:** The good pairs are \[4,5\] and \[6,7\] with shortest path = 2. The pair \[4,6\] is not good because the length of ther shortest path between them is 4.
**Example 3:**
**Input:** root = \[7,1,4,6,null,5,3,null,null,null,null,null,2\], distance = 3
**Output:** 1
**Explanation:** The only good pair is \[2,5\].
**Constraints:**
* The number of nodes in the `tree` is in the range `[1, 210].`
* `1 <= Node.val <= 100`
* `1 <= distance <= 10` | Sort both strings and then check if one of them can break the other. |
Easy Python Solution Using Loops | check-if-a-string-can-break-another-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:\n s1 = sorted(s1)\n s2 = sorted(s2)\n s1_can_break_s2 = True\n s2_can_break_s1 = True\n for i in range(len(s1)):\n if s1[i] < s2[i]:\n s1_can_break_s2 = False\n if s2[i] < s1[i]:\n s2_can_break_s1 = False\n return s1_can_break_s2 or s2_can_break_s1\n\n \n``` | 0 | Given two strings: `s1` and `s2` with the same size, check if some permutation of string `s1` can break some permutation of string `s2` or vice-versa. In other words `s2` can break `s1` or vice-versa.
A string `x` can break string `y` (both of size `n`) if `x[i] >= y[i]` (in alphabetical order) for all `i` between `0` and `n-1`.
**Example 1:**
**Input:** s1 = "abc ", s2 = "xya "
**Output:** true
**Explanation:** "ayx " is a permutation of s2= "xya " which can break to string "abc " which is a permutation of s1= "abc ".
**Example 2:**
**Input:** s1 = "abe ", s2 = "acd "
**Output:** false
**Explanation:** All permutations for s1= "abe " are: "abe ", "aeb ", "bae ", "bea ", "eab " and "eba " and all permutation for s2= "acd " are: "acd ", "adc ", "cad ", "cda ", "dac " and "dca ". However, there is not any permutation from s1 which can break some permutation from s2 and vice-versa.
**Example 3:**
**Input:** s1 = "leetcodee ", s2 = "interview "
**Output:** true
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `1 <= n <= 10^5`
* All strings consist of lowercase English letters. | For encryption, use hashmap to map each char of word1 to its value. For decryption, use trie to prune when necessary. |
Easy Python Solution Using Loops | check-if-a-string-can-break-another-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:\n s1 = sorted(s1)\n s2 = sorted(s2)\n s1_can_break_s2 = True\n s2_can_break_s1 = True\n for i in range(len(s1)):\n if s1[i] < s2[i]:\n s1_can_break_s2 = False\n if s2[i] < s1[i]:\n s2_can_break_s1 = False\n return s1_can_break_s2 or s2_can_break_s1\n\n \n``` | 0 | You are given the `root` of a binary tree and an integer `distance`. A pair of two different **leaf** nodes of a binary tree is said to be good if the length of **the shortest path** between them is less than or equal to `distance`.
Return _the number of good leaf node pairs_ in the tree.
**Example 1:**
**Input:** root = \[1,2,3,null,4\], distance = 3
**Output:** 1
**Explanation:** The leaf nodes of the tree are 3 and 4 and the length of the shortest path between them is 3. This is the only good pair.
**Example 2:**
**Input:** root = \[1,2,3,4,5,6,7\], distance = 3
**Output:** 2
**Explanation:** The good pairs are \[4,5\] and \[6,7\] with shortest path = 2. The pair \[4,6\] is not good because the length of ther shortest path between them is 4.
**Example 3:**
**Input:** root = \[7,1,4,6,null,5,3,null,null,null,null,null,2\], distance = 3
**Output:** 1
**Explanation:** The only good pair is \[2,5\].
**Constraints:**
* The number of nodes in the `tree` is in the range `[1, 210].`
* `1 <= Node.val <= 100`
* `1 <= distance <= 10` | Sort both strings and then check if one of them can break the other. |
O(n) two pointer :D | check-if-a-string-can-break-another-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:\n\n\n \n \n\n\n\n def can_break(a,b):\n\n\n i = 0\n j = 0\n\n N = 26\n while True:\n\n while i < N and a[i] == 0:\n i += 1\n \n while j < N and b[j] == 0:\n j += 1\n\n if i == N and j == N:\n return True\n\n if i > j:\n return False\n else:\n a[i] -=1\n b[j] -=1\n return True\n\n \n\n ano1 = [0]*26\n ano2 = [0]*26\n for i in range(len(s1)):\n ano1[ord(s1[i])-97] += 1\n ano2[ord(s2[i])-97] += 1\n\n if can_break(ano1.copy(),ano2.copy()):\n return True\n return can_break(ano2,ano1)\n\n``` | 0 | Given two strings: `s1` and `s2` with the same size, check if some permutation of string `s1` can break some permutation of string `s2` or vice-versa. In other words `s2` can break `s1` or vice-versa.
A string `x` can break string `y` (both of size `n`) if `x[i] >= y[i]` (in alphabetical order) for all `i` between `0` and `n-1`.
**Example 1:**
**Input:** s1 = "abc ", s2 = "xya "
**Output:** true
**Explanation:** "ayx " is a permutation of s2= "xya " which can break to string "abc " which is a permutation of s1= "abc ".
**Example 2:**
**Input:** s1 = "abe ", s2 = "acd "
**Output:** false
**Explanation:** All permutations for s1= "abe " are: "abe ", "aeb ", "bae ", "bea ", "eab " and "eba " and all permutation for s2= "acd " are: "acd ", "adc ", "cad ", "cda ", "dac " and "dca ". However, there is not any permutation from s1 which can break some permutation from s2 and vice-versa.
**Example 3:**
**Input:** s1 = "leetcodee ", s2 = "interview "
**Output:** true
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `1 <= n <= 10^5`
* All strings consist of lowercase English letters. | For encryption, use hashmap to map each char of word1 to its value. For decryption, use trie to prune when necessary. |
O(n) two pointer :D | check-if-a-string-can-break-another-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:\n\n\n \n \n\n\n\n def can_break(a,b):\n\n\n i = 0\n j = 0\n\n N = 26\n while True:\n\n while i < N and a[i] == 0:\n i += 1\n \n while j < N and b[j] == 0:\n j += 1\n\n if i == N and j == N:\n return True\n\n if i > j:\n return False\n else:\n a[i] -=1\n b[j] -=1\n return True\n\n \n\n ano1 = [0]*26\n ano2 = [0]*26\n for i in range(len(s1)):\n ano1[ord(s1[i])-97] += 1\n ano2[ord(s2[i])-97] += 1\n\n if can_break(ano1.copy(),ano2.copy()):\n return True\n return can_break(ano2,ano1)\n\n``` | 0 | You are given the `root` of a binary tree and an integer `distance`. A pair of two different **leaf** nodes of a binary tree is said to be good if the length of **the shortest path** between them is less than or equal to `distance`.
Return _the number of good leaf node pairs_ in the tree.
**Example 1:**
**Input:** root = \[1,2,3,null,4\], distance = 3
**Output:** 1
**Explanation:** The leaf nodes of the tree are 3 and 4 and the length of the shortest path between them is 3. This is the only good pair.
**Example 2:**
**Input:** root = \[1,2,3,4,5,6,7\], distance = 3
**Output:** 2
**Explanation:** The good pairs are \[4,5\] and \[6,7\] with shortest path = 2. The pair \[4,6\] is not good because the length of ther shortest path between them is 4.
**Example 3:**
**Input:** root = \[7,1,4,6,null,5,3,null,null,null,null,null,2\], distance = 3
**Output:** 1
**Explanation:** The only good pair is \[2,5\].
**Constraints:**
* The number of nodes in the `tree` is in the range `[1, 210].`
* `1 <= Node.val <= 100`
* `1 <= distance <= 10` | Sort both strings and then check if one of them can break the other. |
Python beginner friendly solution O(2*N) with sort nlogn | check-if-a-string-can-break-another-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:\n #given two strings s1 and s2 with the same size, check if some permutation of string s1\n #can break some permutation of s2 or vice versa, in other words s2 can break s1 vice-versa\n #a string x can break string y both of size n if x[i] >= y[i] for all i between 0 and n-1\n \n\n s1 = list(s1)\n s2 = list(s2)\n\n s1.sort()\n s2.sort()\n\n hold1 = True\n hold2 = True\n\n for i in range(len(s1)):\n if not s1[i] >= s2[i]:\n hold1 = False\n \n for i in range(len(s1)):\n if not s2[i] >= s1[i]:\n hold2 = False\n \n return hold1 or hold2\n \n``` | 0 | Given two strings: `s1` and `s2` with the same size, check if some permutation of string `s1` can break some permutation of string `s2` or vice-versa. In other words `s2` can break `s1` or vice-versa.
A string `x` can break string `y` (both of size `n`) if `x[i] >= y[i]` (in alphabetical order) for all `i` between `0` and `n-1`.
**Example 1:**
**Input:** s1 = "abc ", s2 = "xya "
**Output:** true
**Explanation:** "ayx " is a permutation of s2= "xya " which can break to string "abc " which is a permutation of s1= "abc ".
**Example 2:**
**Input:** s1 = "abe ", s2 = "acd "
**Output:** false
**Explanation:** All permutations for s1= "abe " are: "abe ", "aeb ", "bae ", "bea ", "eab " and "eba " and all permutation for s2= "acd " are: "acd ", "adc ", "cad ", "cda ", "dac " and "dca ". However, there is not any permutation from s1 which can break some permutation from s2 and vice-versa.
**Example 3:**
**Input:** s1 = "leetcodee ", s2 = "interview "
**Output:** true
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `1 <= n <= 10^5`
* All strings consist of lowercase English letters. | For encryption, use hashmap to map each char of word1 to its value. For decryption, use trie to prune when necessary. |
Python beginner friendly solution O(2*N) with sort nlogn | check-if-a-string-can-break-another-string | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def checkIfCanBreak(self, s1: str, s2: str) -> bool:\n #given two strings s1 and s2 with the same size, check if some permutation of string s1\n #can break some permutation of s2 or vice versa, in other words s2 can break s1 vice-versa\n #a string x can break string y both of size n if x[i] >= y[i] for all i between 0 and n-1\n \n\n s1 = list(s1)\n s2 = list(s2)\n\n s1.sort()\n s2.sort()\n\n hold1 = True\n hold2 = True\n\n for i in range(len(s1)):\n if not s1[i] >= s2[i]:\n hold1 = False\n \n for i in range(len(s1)):\n if not s2[i] >= s1[i]:\n hold2 = False\n \n return hold1 or hold2\n \n``` | 0 | You are given the `root` of a binary tree and an integer `distance`. A pair of two different **leaf** nodes of a binary tree is said to be good if the length of **the shortest path** between them is less than or equal to `distance`.
Return _the number of good leaf node pairs_ in the tree.
**Example 1:**
**Input:** root = \[1,2,3,null,4\], distance = 3
**Output:** 1
**Explanation:** The leaf nodes of the tree are 3 and 4 and the length of the shortest path between them is 3. This is the only good pair.
**Example 2:**
**Input:** root = \[1,2,3,4,5,6,7\], distance = 3
**Output:** 2
**Explanation:** The good pairs are \[4,5\] and \[6,7\] with shortest path = 2. The pair \[4,6\] is not good because the length of ther shortest path between them is 4.
**Example 3:**
**Input:** root = \[7,1,4,6,null,5,3,null,null,null,null,null,2\], distance = 3
**Output:** 1
**Explanation:** The only good pair is \[2,5\].
**Constraints:**
* The number of nodes in the `tree` is in the range `[1, 210].`
* `1 <= Node.val <= 100`
* `1 <= distance <= 10` | Sort both strings and then check if one of them can break the other. |
[Python3] dp & bitmasking | number-of-ways-to-wear-different-hats-to-each-other | 0 | 1 | Algorithm:\nDefine function `fn(h, mask)` to indicate the number of ways to wear `h` (starting from 0) to last hat among people whose availability is indicated by set bit in mask. \n\nImplementation (time complexity `O(40 * 2^10)` | space complexity `O(40 * 2^10)` \n```\nfrom functools import lru_cache\n\nclass Solution:\n def numberWays(self, hats: List[List[int]]) -> int:\n ppl = dict() # mapping : hat -> people \n for i, hat in enumerate(hats):\n for x in hat: ppl.setdefault(x, []).append(i)\n \n @lru_cache(None)\n def fn(h, mask):\n """Return the number of ways to wear h to last hats among people whose \n availability is indicated by mask"""\n if mask == (1 << len(hats)) - 1: return 1 # # set bits = # people \n if h == 40: return 0 # if used all hat, \n ans = fn(h+1, mask) \n for p in ppl.get(h+1, []): # loop through all people preferring the hat\n if mask & (1 << p): continue # if taken, continue\n mask |= 1 << p # set bit\n ans += fn(h+1, mask)\n mask ^= 1 << p # reset bit\n return ans % 1_000_000_007\n \n return fn(0, 0)\n``` | 11 | There are `n` people and `40` types of hats labeled from `1` to `40`.
Given a 2D integer array `hats`, where `hats[i]` is a list of all hats preferred by the `ith` person.
Return _the number of ways that the `n` people wear different hats to each other_.
Since the answer may be too large, return it modulo `109 + 7`.
**Example 1:**
**Input:** hats = \[\[3,4\],\[4,5\],\[5\]\]
**Output:** 1
**Explanation:** There is only one way to choose hats given the conditions.
First person choose hat 3, Second person choose hat 4 and last one hat 5.
**Example 2:**
**Input:** hats = \[\[3,5,1\],\[3,5\]\]
**Output:** 4
**Explanation:** There are 4 ways to choose hats:
(3,5), (5,3), (1,3) and (1,5)
**Example 3:**
**Input:** hats = \[\[1,2,3,4\],\[1,2,3,4\],\[1,2,3,4\],\[1,2,3,4\]\]
**Output:** 24
**Explanation:** Each person can choose hats labeled from 1 to 4.
Number of Permutations of (1,2,3,4) = 24.
**Constraints:**
* `n == hats.length`
* `1 <= n <= 10`
* `1 <= hats[i].length <= 40`
* `1 <= hats[i][j] <= 40`
* `hats[i]` contains a list of **unique** integers. | Scan from right to left, in each step of the scanning check whether there is a trailing "#" 2 indexes away. |
[Python3] dp & bitmasking | number-of-ways-to-wear-different-hats-to-each-other | 0 | 1 | Algorithm:\nDefine function `fn(h, mask)` to indicate the number of ways to wear `h` (starting from 0) to last hat among people whose availability is indicated by set bit in mask. \n\nImplementation (time complexity `O(40 * 2^10)` | space complexity `O(40 * 2^10)` \n```\nfrom functools import lru_cache\n\nclass Solution:\n def numberWays(self, hats: List[List[int]]) -> int:\n ppl = dict() # mapping : hat -> people \n for i, hat in enumerate(hats):\n for x in hat: ppl.setdefault(x, []).append(i)\n \n @lru_cache(None)\n def fn(h, mask):\n """Return the number of ways to wear h to last hats among people whose \n availability is indicated by mask"""\n if mask == (1 << len(hats)) - 1: return 1 # # set bits = # people \n if h == 40: return 0 # if used all hat, \n ans = fn(h+1, mask) \n for p in ppl.get(h+1, []): # loop through all people preferring the hat\n if mask & (1 << p): continue # if taken, continue\n mask |= 1 << p # set bit\n ans += fn(h+1, mask)\n mask ^= 1 << p # reset bit\n return ans % 1_000_000_007\n \n return fn(0, 0)\n``` | 11 | [Run-length encoding](http://en.wikipedia.org/wiki/Run-length_encoding) is a string compression method that works by replacing consecutive identical characters (repeated 2 or more times) with the concatenation of the character and the number marking the count of the characters (length of the run). For example, to compress the string `"aabccc "` we replace `"aa "` by `"a2 "` and replace `"ccc "` by `"c3 "`. Thus the compressed string becomes `"a2bc3 "`.
Notice that in this problem, we are not adding `'1'` after single characters.
Given a string `s` and an integer `k`. You need to delete **at most** `k` characters from `s` such that the run-length encoded version of `s` has minimum length.
Find the _minimum length of the run-length encoded version of_ `s` _after deleting at most_ `k` _characters_.
**Example 1:**
**Input:** s = "aaabcccd ", k = 2
**Output:** 4
**Explanation:** Compressing s without deleting anything will give us "a3bc3d " of length 6. Deleting any of the characters 'a' or 'c' would at most decrease the length of the compressed string to 5, for instance delete 2 'a' then we will have s = "abcccd " which compressed is abc3d. Therefore, the optimal way is to delete 'b' and 'd', then the compressed version of s will be "a3c3 " of length 4.
**Example 2:**
**Input:** s = "aabbaa ", k = 2
**Output:** 2
**Explanation:** If we delete both 'b' characters, the resulting compressed string would be "a4 " of length 2.
**Example 3:**
**Input:** s = "aaaaaaaaaaa ", k = 0
**Output:** 3
**Explanation:** Since k is zero, we cannot delete anything. The compressed string is "a11 " of length 3.
**Constraints:**
* `1 <= s.length <= 100`
* `0 <= k <= s.length`
* `s` contains only lowercase English letters. | Dynamic programming + bitmask. dp(peopleMask, idHat) number of ways to wear different hats given a bitmask (people visited) and used hats from 1 to idHat-1. |
Updated Process | O(p_i) Time and Space | Commented / Explained | number-of-ways-to-wear-different-hats-to-each-other | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nPriorly I had worked this with a limit based on needing to consider all hats even if not present. New update allows for consideration only on number of hats as well as early stopping conditional. This gives great boost in average speed and allows for random processing for additional algorithmic boost in run time. \n\nIdea is best explained by looking at changes to variable names and progressions in the code. \n\nFirst we change \'hats\' to \'peoples_hat_preferences\' allowing readers to more easily not that the \'hats\' are really a list of people who have hat preferences. \n\nSecondly, we want to find out if we have any lonely hats (ie, any hats that NO ONE likes wearings). If we do, we cannot make any useful permutations, so we return 0. \n\nThird comes from the hint of that prior part. We have a situation where orders matter and repeats are allowed. This tells us we have 2 ** number of people in this case for our number of permutations, of which we want to consider all of them for each hat. By doing this for each hat we will cover at worst 40 * 2 ** 10 possible evaluations, which gives us a total of 40960 evaluations. This is far better if we did it the other way, which would have 10 * 2 ** 40 or approximately 10^12 evaluations! One is obviously better than the other. \n\nFourth thing to note is we only care about the prior evaluation, so we can in fact use a calculation based on only the prior, shrinking or work space to a 1D dp of size 2**10 or about 1024 spaces. \n\nFinally, since we only have at most 10 people, we can bitmask each person as the value of 1 << person for person in range number of people. This gives us unique codes for each person in an easier to store methodology.\n\nNow we turn to the approach to detail implementation. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nStart by setting up your modulo at the beginning. \nThen set up the number of people as the length of peoples hat preferences \nThen, set up a dictionary of lists called hats to person which will map which hat different people like \n\nNow, we loop over each person in our number of people \n- And for each hat in peoples hat preferences for that person \n - hats to people at hat can add that person \n\nIf at the end of this the length of our dictionary is less than our number of people, there is at least one hat that no one will wear, and we can return 0 now. This satisfies our consideration in this space. \n\nIf we proceed, proceed to set up a person mask as described in intution \n\nOur current dynamic programming array is a set of 0\'s of size 2 ** number of people, at worst 1024 spaces. We know we have at least one succesful combination based on reaching this point, so set our current dpa\'s first value to 1. \n\nNow we can loop over hat options, which we can use the existence of our dictionary to facilitate. Rather than process each in order, we can popitem from a dictionary to get an item at random, thus allowing for randomization to fuel potential speedups in algorithmic complexities. To do so, \n\nWhile we have a hats to people dictionary \n- get a random hat id and the people who like this hat by popping an item from the hats to people dictionary \n- Set up a temporary dpa of similar size and value to current\n- For each ith permutation and its value in the current dpa enumerated \n - if the permutation value is 0, continue \n - otherwise, for person in people who like this hat \n - if person mask for person & p_i is 0 \n - update temp at person_mask[person] + p_i by p_v \n - We do this to consider an option of others in a next permutation for this hat \n- set dpa current to dpa temp \nWhen done, \nreturn dpa_current[-1]%mod \n\n# Complexity\n- Time complexity : O(p_i)\n - We take O(H) where H is the number of hats at most in our outter most while loop \n - Inside which we take O(2**10) at worst for our enumeration of prior dpa, which we can consider as a O(p_i), or the number of permutations\n - Inside which we do O(avg(P)) work, where this is the average number of people who like a hat \n - Of these, H is at most 40, and avg P is at most 40, so these are far smaller than p_i, so p_i dominates and grows in relation to the people instead of the hats \n - To set up our dictionary, we take at worst O(P * avg(H)), where avg(H) is the average number of hats someone likes. Again, these are far less than p_i, so are dominated in the procession. \n\n- Space complexity : O(p_i)\n - We only store P * avg(H) in dictionary\n - We store p_i in our dpa\'s, but only one at a time \n - So O(p_i) as space based on above dominations \n\n# Code\n```\nclass Solution(object):\n def numberWays(self, peoples_hat_preferences):\n # get mod set up \n self.mod = 10**9+7\n # number of people \n number_of_people = len(peoples_hat_preferences)\n # which hats go to which persons \n hats_to_person = collections.defaultdict(list)\n # loop over person in range of hats, and hats for that person \n for person in range(number_of_people) : \n for hat in peoples_hat_preferences[person] : \n # building the list of who likes which hat \n hats_to_person[hat].append(person)\n # if there is a lonely hat, no good \n if len(hats_to_person) < number_of_people : return 0 \n # set up masking appropriately to cover each person \n person_mask = [1 << person for person in range(number_of_people)]\n # set up dynamic programming array covering 2^n permutations\n dpa_current = [0] * (2 ** number_of_people)\n # premark as 1 for the starting permutation. We know there is at least 1 by passing line 15\n dpa_current[0] = 1 \n # loop in range of hats \n while hats_to_person : \n # get a hat id and people who like this hat by popping item from dictionary \n hat_id, people_who_like_this_hat = hats_to_person.popitem()\n # set up temp dpa of same size as current, but all zeros to reflect next round\n dpa_temp = [val for val in dpa_current]\n # ith_permutation with ith_permutation_value -> p_i, p_v\n for p_i, p_v in enumerate(dpa_current) : \n # if value of permutation is 0, continue \n if not p_v : \n continue \n # otherwise then for person in people who like this hat \n for person in people_who_like_this_hat : \n # if our person mask at person and p_i is currently even \n if not (person_mask[person] & p_i) : \n # it means we had not priorly considered this person with this hat \n # and we now need to do so, and in doing so account for the number of other \n # possible succesful permutations, p_v \n dpa_temp[person_mask[person] + p_i] += p_v\n # go to the next round \n dpa_current = dpa_temp\n # return modulo valuation after considering all hats and people \n return dpa_current[-1]%self.mod\n``` | 0 | There are `n` people and `40` types of hats labeled from `1` to `40`.
Given a 2D integer array `hats`, where `hats[i]` is a list of all hats preferred by the `ith` person.
Return _the number of ways that the `n` people wear different hats to each other_.
Since the answer may be too large, return it modulo `109 + 7`.
**Example 1:**
**Input:** hats = \[\[3,4\],\[4,5\],\[5\]\]
**Output:** 1
**Explanation:** There is only one way to choose hats given the conditions.
First person choose hat 3, Second person choose hat 4 and last one hat 5.
**Example 2:**
**Input:** hats = \[\[3,5,1\],\[3,5\]\]
**Output:** 4
**Explanation:** There are 4 ways to choose hats:
(3,5), (5,3), (1,3) and (1,5)
**Example 3:**
**Input:** hats = \[\[1,2,3,4\],\[1,2,3,4\],\[1,2,3,4\],\[1,2,3,4\]\]
**Output:** 24
**Explanation:** Each person can choose hats labeled from 1 to 4.
Number of Permutations of (1,2,3,4) = 24.
**Constraints:**
* `n == hats.length`
* `1 <= n <= 10`
* `1 <= hats[i].length <= 40`
* `1 <= hats[i][j] <= 40`
* `hats[i]` contains a list of **unique** integers. | Scan from right to left, in each step of the scanning check whether there is a trailing "#" 2 indexes away. |
Updated Process | O(p_i) Time and Space | Commented / Explained | number-of-ways-to-wear-different-hats-to-each-other | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nPriorly I had worked this with a limit based on needing to consider all hats even if not present. New update allows for consideration only on number of hats as well as early stopping conditional. This gives great boost in average speed and allows for random processing for additional algorithmic boost in run time. \n\nIdea is best explained by looking at changes to variable names and progressions in the code. \n\nFirst we change \'hats\' to \'peoples_hat_preferences\' allowing readers to more easily not that the \'hats\' are really a list of people who have hat preferences. \n\nSecondly, we want to find out if we have any lonely hats (ie, any hats that NO ONE likes wearings). If we do, we cannot make any useful permutations, so we return 0. \n\nThird comes from the hint of that prior part. We have a situation where orders matter and repeats are allowed. This tells us we have 2 ** number of people in this case for our number of permutations, of which we want to consider all of them for each hat. By doing this for each hat we will cover at worst 40 * 2 ** 10 possible evaluations, which gives us a total of 40960 evaluations. This is far better if we did it the other way, which would have 10 * 2 ** 40 or approximately 10^12 evaluations! One is obviously better than the other. \n\nFourth thing to note is we only care about the prior evaluation, so we can in fact use a calculation based on only the prior, shrinking or work space to a 1D dp of size 2**10 or about 1024 spaces. \n\nFinally, since we only have at most 10 people, we can bitmask each person as the value of 1 << person for person in range number of people. This gives us unique codes for each person in an easier to store methodology.\n\nNow we turn to the approach to detail implementation. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nStart by setting up your modulo at the beginning. \nThen set up the number of people as the length of peoples hat preferences \nThen, set up a dictionary of lists called hats to person which will map which hat different people like \n\nNow, we loop over each person in our number of people \n- And for each hat in peoples hat preferences for that person \n - hats to people at hat can add that person \n\nIf at the end of this the length of our dictionary is less than our number of people, there is at least one hat that no one will wear, and we can return 0 now. This satisfies our consideration in this space. \n\nIf we proceed, proceed to set up a person mask as described in intution \n\nOur current dynamic programming array is a set of 0\'s of size 2 ** number of people, at worst 1024 spaces. We know we have at least one succesful combination based on reaching this point, so set our current dpa\'s first value to 1. \n\nNow we can loop over hat options, which we can use the existence of our dictionary to facilitate. Rather than process each in order, we can popitem from a dictionary to get an item at random, thus allowing for randomization to fuel potential speedups in algorithmic complexities. To do so, \n\nWhile we have a hats to people dictionary \n- get a random hat id and the people who like this hat by popping an item from the hats to people dictionary \n- Set up a temporary dpa of similar size and value to current\n- For each ith permutation and its value in the current dpa enumerated \n - if the permutation value is 0, continue \n - otherwise, for person in people who like this hat \n - if person mask for person & p_i is 0 \n - update temp at person_mask[person] + p_i by p_v \n - We do this to consider an option of others in a next permutation for this hat \n- set dpa current to dpa temp \nWhen done, \nreturn dpa_current[-1]%mod \n\n# Complexity\n- Time complexity : O(p_i)\n - We take O(H) where H is the number of hats at most in our outter most while loop \n - Inside which we take O(2**10) at worst for our enumeration of prior dpa, which we can consider as a O(p_i), or the number of permutations\n - Inside which we do O(avg(P)) work, where this is the average number of people who like a hat \n - Of these, H is at most 40, and avg P is at most 40, so these are far smaller than p_i, so p_i dominates and grows in relation to the people instead of the hats \n - To set up our dictionary, we take at worst O(P * avg(H)), where avg(H) is the average number of hats someone likes. Again, these are far less than p_i, so are dominated in the procession. \n\n- Space complexity : O(p_i)\n - We only store P * avg(H) in dictionary\n - We store p_i in our dpa\'s, but only one at a time \n - So O(p_i) as space based on above dominations \n\n# Code\n```\nclass Solution(object):\n def numberWays(self, peoples_hat_preferences):\n # get mod set up \n self.mod = 10**9+7\n # number of people \n number_of_people = len(peoples_hat_preferences)\n # which hats go to which persons \n hats_to_person = collections.defaultdict(list)\n # loop over person in range of hats, and hats for that person \n for person in range(number_of_people) : \n for hat in peoples_hat_preferences[person] : \n # building the list of who likes which hat \n hats_to_person[hat].append(person)\n # if there is a lonely hat, no good \n if len(hats_to_person) < number_of_people : return 0 \n # set up masking appropriately to cover each person \n person_mask = [1 << person for person in range(number_of_people)]\n # set up dynamic programming array covering 2^n permutations\n dpa_current = [0] * (2 ** number_of_people)\n # premark as 1 for the starting permutation. We know there is at least 1 by passing line 15\n dpa_current[0] = 1 \n # loop in range of hats \n while hats_to_person : \n # get a hat id and people who like this hat by popping item from dictionary \n hat_id, people_who_like_this_hat = hats_to_person.popitem()\n # set up temp dpa of same size as current, but all zeros to reflect next round\n dpa_temp = [val for val in dpa_current]\n # ith_permutation with ith_permutation_value -> p_i, p_v\n for p_i, p_v in enumerate(dpa_current) : \n # if value of permutation is 0, continue \n if not p_v : \n continue \n # otherwise then for person in people who like this hat \n for person in people_who_like_this_hat : \n # if our person mask at person and p_i is currently even \n if not (person_mask[person] & p_i) : \n # it means we had not priorly considered this person with this hat \n # and we now need to do so, and in doing so account for the number of other \n # possible succesful permutations, p_v \n dpa_temp[person_mask[person] + p_i] += p_v\n # go to the next round \n dpa_current = dpa_temp\n # return modulo valuation after considering all hats and people \n return dpa_current[-1]%self.mod\n``` | 0 | [Run-length encoding](http://en.wikipedia.org/wiki/Run-length_encoding) is a string compression method that works by replacing consecutive identical characters (repeated 2 or more times) with the concatenation of the character and the number marking the count of the characters (length of the run). For example, to compress the string `"aabccc "` we replace `"aa "` by `"a2 "` and replace `"ccc "` by `"c3 "`. Thus the compressed string becomes `"a2bc3 "`.
Notice that in this problem, we are not adding `'1'` after single characters.
Given a string `s` and an integer `k`. You need to delete **at most** `k` characters from `s` such that the run-length encoded version of `s` has minimum length.
Find the _minimum length of the run-length encoded version of_ `s` _after deleting at most_ `k` _characters_.
**Example 1:**
**Input:** s = "aaabcccd ", k = 2
**Output:** 4
**Explanation:** Compressing s without deleting anything will give us "a3bc3d " of length 6. Deleting any of the characters 'a' or 'c' would at most decrease the length of the compressed string to 5, for instance delete 2 'a' then we will have s = "abcccd " which compressed is abc3d. Therefore, the optimal way is to delete 'b' and 'd', then the compressed version of s will be "a3c3 " of length 4.
**Example 2:**
**Input:** s = "aabbaa ", k = 2
**Output:** 2
**Explanation:** If we delete both 'b' characters, the resulting compressed string would be "a4 " of length 2.
**Example 3:**
**Input:** s = "aaaaaaaaaaa ", k = 0
**Output:** 3
**Explanation:** Since k is zero, we cannot delete anything. The compressed string is "a11 " of length 3.
**Constraints:**
* `1 <= s.length <= 100`
* `0 <= k <= s.length`
* `s` contains only lowercase English letters. | Dynamic programming + bitmask. dp(peopleMask, idHat) number of ways to wear different hats given a bitmask (people visited) and used hats from 1 to idHat-1. |
Python (Simple DP + Bitmask) | number-of-ways-to-wear-different-hats-to-each-other | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numberWays(self, hats):\n ans = [[] for _ in range(41)]\n\n for i,j in enumerate(hats):\n for k in j:\n ans[k].append(i)\n\n @lru_cache(None)\n def dfs(i,mask):\n if bin(mask)[2:].count("1") == len(hats):\n return 1\n\n if i == 41:\n return 0\n\n total = dfs(i+1,mask)\n\n for j in ans[i]:\n if mask&(1<<j) == 0:\n total += dfs(i+1,mask|(1<<j))\n\n return total\n\n return dfs(0,0)%(10**9+7)\n\n\n\n \n\n \n\n \n \n``` | 0 | There are `n` people and `40` types of hats labeled from `1` to `40`.
Given a 2D integer array `hats`, where `hats[i]` is a list of all hats preferred by the `ith` person.
Return _the number of ways that the `n` people wear different hats to each other_.
Since the answer may be too large, return it modulo `109 + 7`.
**Example 1:**
**Input:** hats = \[\[3,4\],\[4,5\],\[5\]\]
**Output:** 1
**Explanation:** There is only one way to choose hats given the conditions.
First person choose hat 3, Second person choose hat 4 and last one hat 5.
**Example 2:**
**Input:** hats = \[\[3,5,1\],\[3,5\]\]
**Output:** 4
**Explanation:** There are 4 ways to choose hats:
(3,5), (5,3), (1,3) and (1,5)
**Example 3:**
**Input:** hats = \[\[1,2,3,4\],\[1,2,3,4\],\[1,2,3,4\],\[1,2,3,4\]\]
**Output:** 24
**Explanation:** Each person can choose hats labeled from 1 to 4.
Number of Permutations of (1,2,3,4) = 24.
**Constraints:**
* `n == hats.length`
* `1 <= n <= 10`
* `1 <= hats[i].length <= 40`
* `1 <= hats[i][j] <= 40`
* `hats[i]` contains a list of **unique** integers. | Scan from right to left, in each step of the scanning check whether there is a trailing "#" 2 indexes away. |
Python (Simple DP + Bitmask) | number-of-ways-to-wear-different-hats-to-each-other | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numberWays(self, hats):\n ans = [[] for _ in range(41)]\n\n for i,j in enumerate(hats):\n for k in j:\n ans[k].append(i)\n\n @lru_cache(None)\n def dfs(i,mask):\n if bin(mask)[2:].count("1") == len(hats):\n return 1\n\n if i == 41:\n return 0\n\n total = dfs(i+1,mask)\n\n for j in ans[i]:\n if mask&(1<<j) == 0:\n total += dfs(i+1,mask|(1<<j))\n\n return total\n\n return dfs(0,0)%(10**9+7)\n\n\n\n \n\n \n\n \n \n``` | 0 | [Run-length encoding](http://en.wikipedia.org/wiki/Run-length_encoding) is a string compression method that works by replacing consecutive identical characters (repeated 2 or more times) with the concatenation of the character and the number marking the count of the characters (length of the run). For example, to compress the string `"aabccc "` we replace `"aa "` by `"a2 "` and replace `"ccc "` by `"c3 "`. Thus the compressed string becomes `"a2bc3 "`.
Notice that in this problem, we are not adding `'1'` after single characters.
Given a string `s` and an integer `k`. You need to delete **at most** `k` characters from `s` such that the run-length encoded version of `s` has minimum length.
Find the _minimum length of the run-length encoded version of_ `s` _after deleting at most_ `k` _characters_.
**Example 1:**
**Input:** s = "aaabcccd ", k = 2
**Output:** 4
**Explanation:** Compressing s without deleting anything will give us "a3bc3d " of length 6. Deleting any of the characters 'a' or 'c' would at most decrease the length of the compressed string to 5, for instance delete 2 'a' then we will have s = "abcccd " which compressed is abc3d. Therefore, the optimal way is to delete 'b' and 'd', then the compressed version of s will be "a3c3 " of length 4.
**Example 2:**
**Input:** s = "aabbaa ", k = 2
**Output:** 2
**Explanation:** If we delete both 'b' characters, the resulting compressed string would be "a4 " of length 2.
**Example 3:**
**Input:** s = "aaaaaaaaaaa ", k = 0
**Output:** 3
**Explanation:** Since k is zero, we cannot delete anything. The compressed string is "a11 " of length 3.
**Constraints:**
* `1 <= s.length <= 100`
* `0 <= k <= s.length`
* `s` contains only lowercase English letters. | Dynamic programming + bitmask. dp(peopleMask, idHat) number of ways to wear different hats given a bitmask (people visited) and used hats from 1 to idHat-1. |
💯Faster✅💯 Lesser✅3 Methods🔥Using Set Operations🔥Using HashSet🔥Using Lists🔥Python🐍Java☕C++✅C📈 | destination-city | 1 | 1 | # \uD83D\uDE80 Hi, I\'m [Mohammed Raziullah Ansari](https://leetcode.com/Mohammed_Raziullah_Ansari/), and I\'m excited to share 3 ways to solve this question with detailed explanation of each approach:\n\n# Problem Explaination: \nThe `Destination City` problem typically involves a scenario where you are given a list of pairs of cities, and each pair represents a direct connection from one city to another. The task is to find the destination city, which is the city that is not the source in any pair.\n\n# \uD83D\uDD0D Methods To Solve This Problem:\nI\'ll be covering three different methods to solve this problem:\n1. Using HashSet\n2. Using Lists\n3. Using Set Operations\n\n# 1. Using HashSet: \n- Create a HashSet to store the set of source cities.\n- Iterate through the list of pairs and add each source city to the HashSet.\n- Iterate through the list of pairs again and check for the destination city that is not in the HashSet.\n# Complexity\n- \u23F1\uFE0F Time Complexity: `O(n)` where n is the number of pairs in the list.\n\n- \uD83D\uDE80 Space Complexity: `O(n)` for the HashSet.\n\n# Code\n```Python []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n source_cities = set()\n for path in paths:\n source_cities.add(path[0])\n for path in paths:\n if path[1] not in source_cities:\n return path[1]\n```\n```Java []\nclass Solution {\n public String destCity(List<List<String>> paths) {\n Set<String> sourceCities = new HashSet<>();\n for (List<String> path : paths) {\n sourceCities.add(path.get(0));\n }\n for (List<String> path : paths) {\n if (!sourceCities.contains(path.get(1))) {\n return path.get(1);\n }\n }\n \n return "";\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n string destCity(vector<vector<string>>& paths) {\n unordered_set<string> sourceCities;\n for (const auto& path : paths) {\n sourceCities.insert(path[0]);\n }\n for (const auto& path : paths) {\n if (sourceCities.find(path[1]) == sourceCities.end()) {\n return path[1];\n }\n }\n return "";\n }\n};\n\n```\n```C []\nchar* destCity(char*** paths, int pathsSize, int* pathsColSize) {\n char** sourceCities = (char**)malloc(pathsSize * sizeof(char*));\n for (int i = 0; i < pathsSize; ++i) {\n sourceCities[i] = paths[i][0];\n }\n for (int i = 0; i < pathsSize; ++i) {\n int j;\n for (j = 0; j < pathsSize; ++j) {\n if (strcmp(paths[i][1], sourceCities[j]) == 0) {\n break;\n }\n }\n if (j == pathsSize) {\n return paths[i][1];\n }\n }\n \n return "";\n}\n\n```\n# 2. Using Lists:\n- Create two lists - one for source cities and one for destination cities.\n- Iterate through the list of pairs and add each source and destination city to their respective lists.\n- Find the destination city that does not appear in the source cities list.\n# Complexity\n- \u23F1\uFE0F Time Complexity: `O(n)` where n is the number of pairs in the list.\n\n- \uD83D\uDE80 Space Complexity: `O(n)` for the two lists.\n\n# Code\n```Python []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n source_cities = set()\n destination_cities = set()\n for path in paths:\n source_cities.add(path[0])\n destination_cities.add(path[1])\n for city in destination_cities:\n if city not in source_cities:\n return city\n```\n```Java []\nclass Solution {\n public String destCity(List<List<String>> paths) {\n Set<String> sourceCities = new HashSet<>();\n Set<String> destinationCities = new HashSet<>();\n for (List<String> path : paths) {\n sourceCities.add(path.get(0));\n destinationCities.add(path.get(1));\n }\n for (String city : destinationCities) {\n if (!sourceCities.contains(city)) {\n return city;\n }\n }\n return null;\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n std::string destCity(std::vector<std::vector<std::string>>& paths) {\n unordered_set<string> sourceCities;\n unordered_set<string> destinationCities;\n for (const auto& path : paths) {\n sourceCities.insert(path[0]);\n destinationCities.insert(path[1]);\n }\n for (const auto& city : destinationCities) {\n if (sourceCities.find(city) == sourceCities.end()) {\n return city;\n }\n }\n return "";\n }\n};\n```\n```C []\nchar* destCity(char*** paths, int pathsSize, int* pathsColSize) {\n char** sourceCities = (char**)malloc(pathsSize * sizeof(char*));\n char** destinationCities = (char**)malloc(pathsSize * sizeof(char*));\n for (int i = 0; i < pathsSize; ++i) {\n sourceCities[i] = paths[i][0];\n destinationCities[i] = paths[i][1];\n }\n for (int i = 0; i < pathsSize; ++i) {\n int j;\n for (j = 0; j < pathsSize; ++j) {\n if (i != j && strcmp(destinationCities[i], sourceCities[j]) == 0) {\n break;\n }\n }\n if (j == pathsSize) {\n return destinationCities[i];\n }\n }\n return NULL;\n}\n```\n# 3. Using Set Operations:\n - Create a set of source cities.\n- Create a set of destination cities.\n- Find the difference between the two sets to get the destination city.\n# Complexity\n- \u23F1\uFE0F Time Complexity: `O(n)` where n is the number of pairs in the list.\n\n- \uD83D\uDE80 Space Complexity: `O(n)` for the two sets.\n\n# Code\n```Python []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n source_cities = set()\n destination_cities = set()\n \n for path in paths:\n source_cities.add(path[0])\n destination_cities.add(path[1])\n result = destination_cities - source_cities\n return result.pop()\n```\n```Java []\nclass Solution {\n public String destCity(List<List<String>> paths) {\n Set<String> sourceCities = new HashSet<>();\n Set<String> destinationCities = new HashSet<>();\n for (List<String> path : paths) {\n sourceCities.add(path.get(0));\n destinationCities.add(path.get(1));\n }\n destinationCities.removeAll(sourceCities);\n return destinationCities.iterator().next();\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n string destCity(vector<vector<string>>& paths) {\n unordered_set<string> sourceCities;\n unordered_set<string> destinationCities;\n for (const auto& path : paths) {\n sourceCities.insert(path[0]);\n destinationCities.insert(path[1]);\n }\n for (const auto& city : destinationCities) {\n if (sourceCities.find(city) == sourceCities.end()) {\n return city;\n }\n }\n return "";\n }\n};\n\n```\n```C []\nchar* destCity(char*** paths, int pathsSize, int* pathsColSize) {\n char* sourceCities[pathsSize];\n char* destinationCities[pathsSize];\n for (int i = 0; i < pathsSize; ++i) {\n sourceCities[i] = paths[i][0];\n destinationCities[i] = paths[i][1];\n }\n for (int i = 0; i < pathsSize; ++i) {\n int found = 0;\n for (int j = 0; j < pathsSize; ++j) {\n if (strcmp(destinationCities[i], sourceCities[j]) == 0) {\n found = 1;\n break;\n }\n }\n if (!found) {\n return destinationCities[i];\n }\n }\n return ""; \n}\n\n```\n# \uD83C\uDFC6Conclusion: \nwe explored three effective methods to solve the "Destination City" problem, which involves finding the destination city in a list of pairs representing direct connections between cities. Each method provides a different approach to tackle the problem.\n\n# \uD83D\uDCA1 I invite you to check out my profile for detailed explanations and code for each method. Happy coding and learning! \uD83D\uDCDA | 25 | You are given the array `paths`, where `paths[i] = [cityAi, cityBi]` means there exists a direct path going from `cityAi` to `cityBi`. _Return the destination city, that is, the city without any path outgoing to another city._
It is guaranteed that the graph of paths forms a line without any loop, therefore, there will be exactly one destination city.
**Example 1:**
**Input:** paths = \[\[ "London ", "New York "\],\[ "New York ", "Lima "\],\[ "Lima ", "Sao Paulo "\]\]
**Output:** "Sao Paulo "
**Explanation:** Starting at "London " city you will reach "Sao Paulo " city which is the destination city. Your trip consist of: "London " -> "New York " -> "Lima " -> "Sao Paulo ".
**Example 2:**
**Input:** paths = \[\[ "B ", "C "\],\[ "D ", "B "\],\[ "C ", "A "\]\]
**Output:** "A "
**Explanation:** All possible trips are:
"D " -> "B " -> "C " -> "A ".
"B " -> "C " -> "A ".
"C " -> "A ".
"A ".
Clearly the destination city is "A ".
**Example 3:**
**Input:** paths = \[\[ "A ", "Z "\]\]
**Output:** "Z "
**Constraints:**
* `1 <= paths.length <= 100`
* `paths[i].length == 2`
* `1 <= cityAi.length, cityBi.length <= 10`
* `cityAi != cityBi`
* All strings consist of lowercase and uppercase English letters and the space character. | Do BFS to find the kth level friends. Then collect movies saw by kth level friends and sort them accordingly. |
💯Faster✅💯 Lesser✅3 Methods🔥Using Set Operations🔥Using HashSet🔥Using Lists🔥Python🐍Java☕C++✅C📈 | destination-city | 1 | 1 | # \uD83D\uDE80 Hi, I\'m [Mohammed Raziullah Ansari](https://leetcode.com/Mohammed_Raziullah_Ansari/), and I\'m excited to share 3 ways to solve this question with detailed explanation of each approach:\n\n# Problem Explaination: \nThe `Destination City` problem typically involves a scenario where you are given a list of pairs of cities, and each pair represents a direct connection from one city to another. The task is to find the destination city, which is the city that is not the source in any pair.\n\n# \uD83D\uDD0D Methods To Solve This Problem:\nI\'ll be covering three different methods to solve this problem:\n1. Using HashSet\n2. Using Lists\n3. Using Set Operations\n\n# 1. Using HashSet: \n- Create a HashSet to store the set of source cities.\n- Iterate through the list of pairs and add each source city to the HashSet.\n- Iterate through the list of pairs again and check for the destination city that is not in the HashSet.\n# Complexity\n- \u23F1\uFE0F Time Complexity: `O(n)` where n is the number of pairs in the list.\n\n- \uD83D\uDE80 Space Complexity: `O(n)` for the HashSet.\n\n# Code\n```Python []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n source_cities = set()\n for path in paths:\n source_cities.add(path[0])\n for path in paths:\n if path[1] not in source_cities:\n return path[1]\n```\n```Java []\nclass Solution {\n public String destCity(List<List<String>> paths) {\n Set<String> sourceCities = new HashSet<>();\n for (List<String> path : paths) {\n sourceCities.add(path.get(0));\n }\n for (List<String> path : paths) {\n if (!sourceCities.contains(path.get(1))) {\n return path.get(1);\n }\n }\n \n return "";\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n string destCity(vector<vector<string>>& paths) {\n unordered_set<string> sourceCities;\n for (const auto& path : paths) {\n sourceCities.insert(path[0]);\n }\n for (const auto& path : paths) {\n if (sourceCities.find(path[1]) == sourceCities.end()) {\n return path[1];\n }\n }\n return "";\n }\n};\n\n```\n```C []\nchar* destCity(char*** paths, int pathsSize, int* pathsColSize) {\n char** sourceCities = (char**)malloc(pathsSize * sizeof(char*));\n for (int i = 0; i < pathsSize; ++i) {\n sourceCities[i] = paths[i][0];\n }\n for (int i = 0; i < pathsSize; ++i) {\n int j;\n for (j = 0; j < pathsSize; ++j) {\n if (strcmp(paths[i][1], sourceCities[j]) == 0) {\n break;\n }\n }\n if (j == pathsSize) {\n return paths[i][1];\n }\n }\n \n return "";\n}\n\n```\n# 2. Using Lists:\n- Create two lists - one for source cities and one for destination cities.\n- Iterate through the list of pairs and add each source and destination city to their respective lists.\n- Find the destination city that does not appear in the source cities list.\n# Complexity\n- \u23F1\uFE0F Time Complexity: `O(n)` where n is the number of pairs in the list.\n\n- \uD83D\uDE80 Space Complexity: `O(n)` for the two lists.\n\n# Code\n```Python []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n source_cities = set()\n destination_cities = set()\n for path in paths:\n source_cities.add(path[0])\n destination_cities.add(path[1])\n for city in destination_cities:\n if city not in source_cities:\n return city\n```\n```Java []\nclass Solution {\n public String destCity(List<List<String>> paths) {\n Set<String> sourceCities = new HashSet<>();\n Set<String> destinationCities = new HashSet<>();\n for (List<String> path : paths) {\n sourceCities.add(path.get(0));\n destinationCities.add(path.get(1));\n }\n for (String city : destinationCities) {\n if (!sourceCities.contains(city)) {\n return city;\n }\n }\n return null;\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n std::string destCity(std::vector<std::vector<std::string>>& paths) {\n unordered_set<string> sourceCities;\n unordered_set<string> destinationCities;\n for (const auto& path : paths) {\n sourceCities.insert(path[0]);\n destinationCities.insert(path[1]);\n }\n for (const auto& city : destinationCities) {\n if (sourceCities.find(city) == sourceCities.end()) {\n return city;\n }\n }\n return "";\n }\n};\n```\n```C []\nchar* destCity(char*** paths, int pathsSize, int* pathsColSize) {\n char** sourceCities = (char**)malloc(pathsSize * sizeof(char*));\n char** destinationCities = (char**)malloc(pathsSize * sizeof(char*));\n for (int i = 0; i < pathsSize; ++i) {\n sourceCities[i] = paths[i][0];\n destinationCities[i] = paths[i][1];\n }\n for (int i = 0; i < pathsSize; ++i) {\n int j;\n for (j = 0; j < pathsSize; ++j) {\n if (i != j && strcmp(destinationCities[i], sourceCities[j]) == 0) {\n break;\n }\n }\n if (j == pathsSize) {\n return destinationCities[i];\n }\n }\n return NULL;\n}\n```\n# 3. Using Set Operations:\n - Create a set of source cities.\n- Create a set of destination cities.\n- Find the difference between the two sets to get the destination city.\n# Complexity\n- \u23F1\uFE0F Time Complexity: `O(n)` where n is the number of pairs in the list.\n\n- \uD83D\uDE80 Space Complexity: `O(n)` for the two sets.\n\n# Code\n```Python []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n source_cities = set()\n destination_cities = set()\n \n for path in paths:\n source_cities.add(path[0])\n destination_cities.add(path[1])\n result = destination_cities - source_cities\n return result.pop()\n```\n```Java []\nclass Solution {\n public String destCity(List<List<String>> paths) {\n Set<String> sourceCities = new HashSet<>();\n Set<String> destinationCities = new HashSet<>();\n for (List<String> path : paths) {\n sourceCities.add(path.get(0));\n destinationCities.add(path.get(1));\n }\n destinationCities.removeAll(sourceCities);\n return destinationCities.iterator().next();\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n string destCity(vector<vector<string>>& paths) {\n unordered_set<string> sourceCities;\n unordered_set<string> destinationCities;\n for (const auto& path : paths) {\n sourceCities.insert(path[0]);\n destinationCities.insert(path[1]);\n }\n for (const auto& city : destinationCities) {\n if (sourceCities.find(city) == sourceCities.end()) {\n return city;\n }\n }\n return "";\n }\n};\n\n```\n```C []\nchar* destCity(char*** paths, int pathsSize, int* pathsColSize) {\n char* sourceCities[pathsSize];\n char* destinationCities[pathsSize];\n for (int i = 0; i < pathsSize; ++i) {\n sourceCities[i] = paths[i][0];\n destinationCities[i] = paths[i][1];\n }\n for (int i = 0; i < pathsSize; ++i) {\n int found = 0;\n for (int j = 0; j < pathsSize; ++j) {\n if (strcmp(destinationCities[i], sourceCities[j]) == 0) {\n found = 1;\n break;\n }\n }\n if (!found) {\n return destinationCities[i];\n }\n }\n return ""; \n}\n\n```\n# \uD83C\uDFC6Conclusion: \nwe explored three effective methods to solve the "Destination City" problem, which involves finding the destination city in a list of pairs representing direct connections between cities. Each method provides a different approach to tackle the problem.\n\n# \uD83D\uDCA1 I invite you to check out my profile for detailed explanations and code for each method. Happy coding and learning! \uD83D\uDCDA | 25 | Given a wooden stick of length `n` units. The stick is labelled from `0` to `n`. For example, a stick of length **6** is labelled as follows:
Given an integer array `cuts` where `cuts[i]` denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return _the minimum total cost_ of the cuts.
**Example 1:**
**Input:** n = 7, cuts = \[1,3,4,5\]
**Output:** 16
**Explanation:** Using cuts order = \[1, 3, 4, 5\] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be \[3, 5, 1, 4\] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
**Example 2:**
**Input:** n = 9, cuts = \[5,6,1,4,2\]
**Output:** 22
**Explanation:** If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order \[4, 6, 5, 2, 1\] has total cost = 22 which is the minimum possible.
**Constraints:**
* `2 <= n <= 106`
* `1 <= cuts.length <= min(n - 1, 100)`
* `1 <= cuts[i] <= n - 1`
* All the integers in `cuts` array are **distinct**. | Start in any city and use the path to move to the next city. Eventually, you will reach a city with no path outgoing, this is the destination city. |
✅ Navigating Urban Labyrinths: Elegant Solution to Find the Destination City 🔥 | destination-city | 1 | 1 | # Description\n\n## Intuition\nThe problem requires finding the destination city, which is the city without any outgoing path to another city. The graph is guaranteed to form a line without any loops.\n\n## Approach\nThe solution uses a dictionary (`check`) to keep track of whether a city is an "out" city (has an outgoing path) or an "in" city (has an incoming path). It iterates through the given paths, updating the status of each city accordingly. Finally, it looks for the city with an incoming path, which is the destination city.\n\n## Complexity\n- **Time complexity:** O(n), where n is the number of paths. The algorithm iterates through the given paths once.\n- **Space complexity:** O(n), where n is the number of paths. The space is used to store the status of each city in the dictionary.\n\n## Code\n```csharp []\npublic class Solution {\n public string DestCity(IList<IList<string>> paths)\n {\n Dictionary<string, string> check = new Dictionary<string, string>();\n\n foreach(var path in paths)\n {\n check[path[0]] = "out";\n if (!check.ContainsKey(path[1]))\n {\n check[path[1]] = "in";\n }\n }\n\n foreach (var item in check.Keys)\n {\n if (check[item] == "in") return item;\n }\n\n return "";\n }\n}\n```\n```java []\npublic class Solution {\n public String destCity(List<List<String>> paths) {\n HashMap<String, String> check = new HashMap<>();\n\n for (List<String> path : paths) {\n check.put(path.get(0), "out");\n if (!check.containsKey(path.get(1))) {\n check.put(path.get(1), "in");\n }\n }\n\n for (String item : check.keySet()) {\n if ("in".equals(check.get(item))) {\n return item;\n }\n }\n\n return "";\n }\n}\n```\n```python []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n check = {}\n\n for path in paths:\n check[path[0]] = "out"\n if path[1] not in check:\n check[path[1]] = "in"\n\n for item in check:\n if check[item] == "in":\n return item\n\n return ""\n```\n\n\n\n- Please upvote me !!! | 9 | You are given the array `paths`, where `paths[i] = [cityAi, cityBi]` means there exists a direct path going from `cityAi` to `cityBi`. _Return the destination city, that is, the city without any path outgoing to another city._
It is guaranteed that the graph of paths forms a line without any loop, therefore, there will be exactly one destination city.
**Example 1:**
**Input:** paths = \[\[ "London ", "New York "\],\[ "New York ", "Lima "\],\[ "Lima ", "Sao Paulo "\]\]
**Output:** "Sao Paulo "
**Explanation:** Starting at "London " city you will reach "Sao Paulo " city which is the destination city. Your trip consist of: "London " -> "New York " -> "Lima " -> "Sao Paulo ".
**Example 2:**
**Input:** paths = \[\[ "B ", "C "\],\[ "D ", "B "\],\[ "C ", "A "\]\]
**Output:** "A "
**Explanation:** All possible trips are:
"D " -> "B " -> "C " -> "A ".
"B " -> "C " -> "A ".
"C " -> "A ".
"A ".
Clearly the destination city is "A ".
**Example 3:**
**Input:** paths = \[\[ "A ", "Z "\]\]
**Output:** "Z "
**Constraints:**
* `1 <= paths.length <= 100`
* `paths[i].length == 2`
* `1 <= cityAi.length, cityBi.length <= 10`
* `cityAi != cityBi`
* All strings consist of lowercase and uppercase English letters and the space character. | Do BFS to find the kth level friends. Then collect movies saw by kth level friends and sort them accordingly. |
✅ Navigating Urban Labyrinths: Elegant Solution to Find the Destination City 🔥 | destination-city | 1 | 1 | # Description\n\n## Intuition\nThe problem requires finding the destination city, which is the city without any outgoing path to another city. The graph is guaranteed to form a line without any loops.\n\n## Approach\nThe solution uses a dictionary (`check`) to keep track of whether a city is an "out" city (has an outgoing path) or an "in" city (has an incoming path). It iterates through the given paths, updating the status of each city accordingly. Finally, it looks for the city with an incoming path, which is the destination city.\n\n## Complexity\n- **Time complexity:** O(n), where n is the number of paths. The algorithm iterates through the given paths once.\n- **Space complexity:** O(n), where n is the number of paths. The space is used to store the status of each city in the dictionary.\n\n## Code\n```csharp []\npublic class Solution {\n public string DestCity(IList<IList<string>> paths)\n {\n Dictionary<string, string> check = new Dictionary<string, string>();\n\n foreach(var path in paths)\n {\n check[path[0]] = "out";\n if (!check.ContainsKey(path[1]))\n {\n check[path[1]] = "in";\n }\n }\n\n foreach (var item in check.Keys)\n {\n if (check[item] == "in") return item;\n }\n\n return "";\n }\n}\n```\n```java []\npublic class Solution {\n public String destCity(List<List<String>> paths) {\n HashMap<String, String> check = new HashMap<>();\n\n for (List<String> path : paths) {\n check.put(path.get(0), "out");\n if (!check.containsKey(path.get(1))) {\n check.put(path.get(1), "in");\n }\n }\n\n for (String item : check.keySet()) {\n if ("in".equals(check.get(item))) {\n return item;\n }\n }\n\n return "";\n }\n}\n```\n```python []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n check = {}\n\n for path in paths:\n check[path[0]] = "out"\n if path[1] not in check:\n check[path[1]] = "in"\n\n for item in check:\n if check[item] == "in":\n return item\n\n return ""\n```\n\n\n\n- Please upvote me !!! | 9 | Given a wooden stick of length `n` units. The stick is labelled from `0` to `n`. For example, a stick of length **6** is labelled as follows:
Given an integer array `cuts` where `cuts[i]` denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return _the minimum total cost_ of the cuts.
**Example 1:**
**Input:** n = 7, cuts = \[1,3,4,5\]
**Output:** 16
**Explanation:** Using cuts order = \[1, 3, 4, 5\] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be \[3, 5, 1, 4\] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
**Example 2:**
**Input:** n = 9, cuts = \[5,6,1,4,2\]
**Output:** 22
**Explanation:** If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order \[4, 6, 5, 2, 1\] has total cost = 22 which is the minimum possible.
**Constraints:**
* `2 <= n <= 106`
* `1 <= cuts.length <= min(n - 1, 100)`
* `1 <= cuts[i] <= n - 1`
* All the integers in `cuts` array are **distinct**. | Start in any city and use the path to move to the next city. Eventually, you will reach a city with no path outgoing, this is the destination city. |
✅☑[C++/C/Java/Python/JavaScript] || 2 Approaches || EXPLAINED🔥 | destination-city | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n**(Also explained in the code)**\n\n#### ***Approach 1(Brute Force)***\n1. **Iterate Through Paths:**\n\n - The code iterates through each path in the `paths` vector.\n - It selects a candidate destination city (`candidate = paths[i][1]`) from the current path.\n1. **Check if Candidate is a Destination City:**\n\n - It checks if the candidate city is not a starting city in any other path by comparing it with all the starting cities in each path.\n - If the candidate is not found as a starting city in any other path (`good == true`), it\'s considered the destination city, and it\'s returned.\n1. **Return Default (If No Destination Found):**\n\n - If no destination city is found (which shouldn\'t happen if every path is valid), the function returns an empty string `""`.\n\n# Complexity\n- *Time complexity:*\n $$O(n^2)$$\n \n\n- *Space complexity:*\n $$O(1)$$\n \n\n\n# Code\n```C++ []\n#include <vector>\n#include <string>\n\nclass Solution {\npublic:\n // Function to find the destination city from paths\n std::string destCity(std::vector<std::vector<std::string>>& paths) {\n // Loop through each path\n for (int i = 0; i < paths.size(); i++) {\n std::string candidate = paths[i][1]; // Get the candidate destination city from the current path\n bool good = true; // Flag to determine if the candidate is the destination city\n \n // Check if the candidate city is also a starting city in other paths\n for (int j = 0; j < paths.size(); j++) {\n if (paths[j][0] == candidate) { // If the candidate is found as a starting city in another path\n good = false; // Update flag to indicate it\'s not the destination city\n break; // Exit the loop, as we\'ve found the candidate is not the destination\n }\n }\n\n if (good) {\n return candidate; // If the candidate is not a starting city in any other path, it\'s the destination city\n }\n }\n \n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n }\n};\n\n\n\n```\n```C []\n#include <stdio.h>\n#include <string.h>\n\nchar* destCity(char*** paths, int pathsSize, int* pathsColSize) {\n for (int i = 0; i < pathsSize; i++) {\n char* candidate = paths[i][1]; // Get the candidate destination city from the current path\n int good = 1; // Flag to determine if the candidate is the destination city\n \n // Check if the candidate city is also a starting city in other paths\n for (int j = 0; j < pathsSize; j++) {\n if (strcmp(paths[j][0], candidate) == 0) { // If the candidate is found as a starting city in another path\n good = 0; // Update flag to indicate it\'s not the destination city\n break; // Exit the loop, as we\'ve found the candidate is not the destination\n }\n }\n\n if (good) {\n return candidate; // If the candidate is not a starting city in any other path, it\'s the destination city\n }\n }\n \n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n}\n\n\n\n\n```\n```Java []\nimport java.util.List;\n\nclass Solution {\n public String destCity(List<List<String>> paths) {\n // Loop through each path\n for (List<String> path : paths) {\n String candidate = path.get(1); // Get the candidate destination city from the current path\n boolean good = true; // Flag to determine if the candidate is the destination city\n \n // Check if the candidate city is also a starting city in other paths\n for (List<String> otherPath : paths) {\n if (otherPath.get(0).equals(candidate)) { // If the candidate is found as a starting city in another path\n good = false; // Update flag to indicate it\'s not the destination city\n break; // Exit the loop, as we\'ve found the candidate is not the destination city\n }\n }\n\n if (good) {\n return candidate; // If the candidate is not a starting city in any other path, it\'s the destination city\n }\n }\n \n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n }\n}\n\n\n\n```\n```python3 []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n # Loop through each path\n for path in paths:\n candidate = path[1] # Get the candidate destination city from the current path\n good = True # Flag to determine if the candidate is the destination city\n \n # Check if the candidate city is also a starting city in other paths\n for other_path in paths:\n if other_path[0] == candidate: # If the candidate is found as a starting city in another path\n good = False # Update flag to indicate it\'s not the destination city\n break # Exit the loop, as we\'ve found the candidate is not the destination\n \n if good:\n return candidate # If the candidate is not a starting city in any other path, it\'s the destination city\n \n return "" # If no destination city found (shouldn\'t reach here if every path is valid)\n\n\n\n```\n```javascript []\nfunction destCity(paths) {\n for (let i = 0; i < paths.length; i++) {\n let candidate = paths[i][1]; // Get the candidate destination city from the current path\n let good = true; // Flag to determine if the candidate is the destination city\n \n // Check if the candidate city is also a starting city in other paths\n for (let j = 0; j < paths.length; j++) {\n if (paths[j][0] === candidate) { // If the candidate is found as a starting city in another path\n good = false; // Update flag to indicate it\'s not the destination city\n break; // Exit the loop, as we\'ve found the candidate is not the destination\n }\n }\n\n if (good) {\n return candidate; // If the candidate is not a starting city in any other path, it\'s the destination city\n }\n }\n \n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n}\n\n\n```\n\n---\n\n#### ***Approach 2(Sets)***\n1. **Initialization:**\n\n - A `set<string>` called `startCities` is initialized. This set will store all the starting cities from the paths.\n1. **Storing Starting Cities:**\n\n - The code iterates through each path in the input `paths`.\n - For each path, it inserts the first city (index `0` of the inner vector `path`) into the `startCities` set using `startCities.insert(path[0])`.\n1. **Finding the Destination City:**\n\n - The code then iterates through the paths again.\n - For each path, it checks if the destination city (index `1` of the inner vector `path`) is not found in the `startCities` set using `startCities.find(path[1]) == startCities.end()`.\n - If the destination city is not found among the starting cities, it means it is the actual destination, so the code returns this city using `return path[1]`.\n1. **Return Default (If No Destination Found):**\n\n - If no destination city is found (which should not happen if every path is valid), the function returns an empty string `""`.\n\n# Complexity\n- *Time complexity:*\n $$O(n)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\n\n\nclass Solution {\npublic:\n std::string destCity(std::vector<std::vector<std::string>>& paths) {\n set<string> startCities;\n\n // Store all starting cities in a set\n for (const auto& path : paths) {\n startCities.insert(path[0]);\n }\n\n // Find the destination city\n for (const auto& path : paths) {\n if (startCities.find(path[1]) == startCities.end()) {\n return path[1]; // Return the destination city\n }\n }\n\n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n }\n};\n\n\n\n```\n```C []\nchar* destCity(char paths[][2], int pathsSize) {\n char startCities[pathsSize][21]; // Assuming the maximum city name length is 20 characters\n int startCitiesCount = 0;\n\n // Store all starting cities in a set\n for (int i = 0; i < pathsSize; i++) {\n strcpy(startCities[startCitiesCount++], paths[i][0]);\n }\n\n // Find the destination city\n for (int i = 0; i < pathsSize; i++) {\n int found = 0;\n for (int j = 0; j < startCitiesCount; j++) {\n if (strcmp(paths[i][1], startCities[j]) == 0) {\n found = 1;\n break;\n }\n }\n if (!found) {\n return paths[i][1]; // Return the destination city\n }\n }\n\n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n}\n\n\n\n```\n```Java []\nimport java.util.HashSet;\nimport java.util.List;\nimport java.util.Set;\n\nclass Solution {\n public String destCity(List<List<String>> paths) {\n Set<String> startCities = new HashSet<>();\n\n // Store all starting cities in a set\n for (List<String> path : paths) {\n startCities.add(path.get(0));\n }\n\n // Find the destination city\n for (List<String> path : paths) {\n if (!startCities.contains(path.get(1))) {\n return path.get(1); // Return the destination city\n }\n }\n\n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n }\n}\n\n\n\n```\n```python3 []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n start_cities = set()\n\n # Store all starting cities in a set\n for path in paths:\n start_cities.add(path[0])\n\n # Find the destination city\n for path in paths:\n if path[1] not in start_cities:\n return path[1] # Return the destination city\n\n return "" # If no destination city found (shouldn\'t reach here if every path is valid)\n\n\n\n```\n```javascript []\nfunction destCity(paths) {\n const startCities = new Set();\n\n // Store all starting cities in a set\n for (const path of paths) {\n startCities.add(path[0]);\n }\n\n // Find the destination city\n for (const path of paths) {\n if (!startCities.has(path[1])) {\n return path[1]; // Return the destination city\n }\n }\n\n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n}\n\n\n```\n\n---\n\n\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n--- | 15 | You are given the array `paths`, where `paths[i] = [cityAi, cityBi]` means there exists a direct path going from `cityAi` to `cityBi`. _Return the destination city, that is, the city without any path outgoing to another city._
It is guaranteed that the graph of paths forms a line without any loop, therefore, there will be exactly one destination city.
**Example 1:**
**Input:** paths = \[\[ "London ", "New York "\],\[ "New York ", "Lima "\],\[ "Lima ", "Sao Paulo "\]\]
**Output:** "Sao Paulo "
**Explanation:** Starting at "London " city you will reach "Sao Paulo " city which is the destination city. Your trip consist of: "London " -> "New York " -> "Lima " -> "Sao Paulo ".
**Example 2:**
**Input:** paths = \[\[ "B ", "C "\],\[ "D ", "B "\],\[ "C ", "A "\]\]
**Output:** "A "
**Explanation:** All possible trips are:
"D " -> "B " -> "C " -> "A ".
"B " -> "C " -> "A ".
"C " -> "A ".
"A ".
Clearly the destination city is "A ".
**Example 3:**
**Input:** paths = \[\[ "A ", "Z "\]\]
**Output:** "Z "
**Constraints:**
* `1 <= paths.length <= 100`
* `paths[i].length == 2`
* `1 <= cityAi.length, cityBi.length <= 10`
* `cityAi != cityBi`
* All strings consist of lowercase and uppercase English letters and the space character. | Do BFS to find the kth level friends. Then collect movies saw by kth level friends and sort them accordingly. |
✅☑[C++/C/Java/Python/JavaScript] || 2 Approaches || EXPLAINED🔥 | destination-city | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n**(Also explained in the code)**\n\n#### ***Approach 1(Brute Force)***\n1. **Iterate Through Paths:**\n\n - The code iterates through each path in the `paths` vector.\n - It selects a candidate destination city (`candidate = paths[i][1]`) from the current path.\n1. **Check if Candidate is a Destination City:**\n\n - It checks if the candidate city is not a starting city in any other path by comparing it with all the starting cities in each path.\n - If the candidate is not found as a starting city in any other path (`good == true`), it\'s considered the destination city, and it\'s returned.\n1. **Return Default (If No Destination Found):**\n\n - If no destination city is found (which shouldn\'t happen if every path is valid), the function returns an empty string `""`.\n\n# Complexity\n- *Time complexity:*\n $$O(n^2)$$\n \n\n- *Space complexity:*\n $$O(1)$$\n \n\n\n# Code\n```C++ []\n#include <vector>\n#include <string>\n\nclass Solution {\npublic:\n // Function to find the destination city from paths\n std::string destCity(std::vector<std::vector<std::string>>& paths) {\n // Loop through each path\n for (int i = 0; i < paths.size(); i++) {\n std::string candidate = paths[i][1]; // Get the candidate destination city from the current path\n bool good = true; // Flag to determine if the candidate is the destination city\n \n // Check if the candidate city is also a starting city in other paths\n for (int j = 0; j < paths.size(); j++) {\n if (paths[j][0] == candidate) { // If the candidate is found as a starting city in another path\n good = false; // Update flag to indicate it\'s not the destination city\n break; // Exit the loop, as we\'ve found the candidate is not the destination\n }\n }\n\n if (good) {\n return candidate; // If the candidate is not a starting city in any other path, it\'s the destination city\n }\n }\n \n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n }\n};\n\n\n\n```\n```C []\n#include <stdio.h>\n#include <string.h>\n\nchar* destCity(char*** paths, int pathsSize, int* pathsColSize) {\n for (int i = 0; i < pathsSize; i++) {\n char* candidate = paths[i][1]; // Get the candidate destination city from the current path\n int good = 1; // Flag to determine if the candidate is the destination city\n \n // Check if the candidate city is also a starting city in other paths\n for (int j = 0; j < pathsSize; j++) {\n if (strcmp(paths[j][0], candidate) == 0) { // If the candidate is found as a starting city in another path\n good = 0; // Update flag to indicate it\'s not the destination city\n break; // Exit the loop, as we\'ve found the candidate is not the destination\n }\n }\n\n if (good) {\n return candidate; // If the candidate is not a starting city in any other path, it\'s the destination city\n }\n }\n \n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n}\n\n\n\n\n```\n```Java []\nimport java.util.List;\n\nclass Solution {\n public String destCity(List<List<String>> paths) {\n // Loop through each path\n for (List<String> path : paths) {\n String candidate = path.get(1); // Get the candidate destination city from the current path\n boolean good = true; // Flag to determine if the candidate is the destination city\n \n // Check if the candidate city is also a starting city in other paths\n for (List<String> otherPath : paths) {\n if (otherPath.get(0).equals(candidate)) { // If the candidate is found as a starting city in another path\n good = false; // Update flag to indicate it\'s not the destination city\n break; // Exit the loop, as we\'ve found the candidate is not the destination city\n }\n }\n\n if (good) {\n return candidate; // If the candidate is not a starting city in any other path, it\'s the destination city\n }\n }\n \n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n }\n}\n\n\n\n```\n```python3 []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n # Loop through each path\n for path in paths:\n candidate = path[1] # Get the candidate destination city from the current path\n good = True # Flag to determine if the candidate is the destination city\n \n # Check if the candidate city is also a starting city in other paths\n for other_path in paths:\n if other_path[0] == candidate: # If the candidate is found as a starting city in another path\n good = False # Update flag to indicate it\'s not the destination city\n break # Exit the loop, as we\'ve found the candidate is not the destination\n \n if good:\n return candidate # If the candidate is not a starting city in any other path, it\'s the destination city\n \n return "" # If no destination city found (shouldn\'t reach here if every path is valid)\n\n\n\n```\n```javascript []\nfunction destCity(paths) {\n for (let i = 0; i < paths.length; i++) {\n let candidate = paths[i][1]; // Get the candidate destination city from the current path\n let good = true; // Flag to determine if the candidate is the destination city\n \n // Check if the candidate city is also a starting city in other paths\n for (let j = 0; j < paths.length; j++) {\n if (paths[j][0] === candidate) { // If the candidate is found as a starting city in another path\n good = false; // Update flag to indicate it\'s not the destination city\n break; // Exit the loop, as we\'ve found the candidate is not the destination\n }\n }\n\n if (good) {\n return candidate; // If the candidate is not a starting city in any other path, it\'s the destination city\n }\n }\n \n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n}\n\n\n```\n\n---\n\n#### ***Approach 2(Sets)***\n1. **Initialization:**\n\n - A `set<string>` called `startCities` is initialized. This set will store all the starting cities from the paths.\n1. **Storing Starting Cities:**\n\n - The code iterates through each path in the input `paths`.\n - For each path, it inserts the first city (index `0` of the inner vector `path`) into the `startCities` set using `startCities.insert(path[0])`.\n1. **Finding the Destination City:**\n\n - The code then iterates through the paths again.\n - For each path, it checks if the destination city (index `1` of the inner vector `path`) is not found in the `startCities` set using `startCities.find(path[1]) == startCities.end()`.\n - If the destination city is not found among the starting cities, it means it is the actual destination, so the code returns this city using `return path[1]`.\n1. **Return Default (If No Destination Found):**\n\n - If no destination city is found (which should not happen if every path is valid), the function returns an empty string `""`.\n\n# Complexity\n- *Time complexity:*\n $$O(n)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\n\n\nclass Solution {\npublic:\n std::string destCity(std::vector<std::vector<std::string>>& paths) {\n set<string> startCities;\n\n // Store all starting cities in a set\n for (const auto& path : paths) {\n startCities.insert(path[0]);\n }\n\n // Find the destination city\n for (const auto& path : paths) {\n if (startCities.find(path[1]) == startCities.end()) {\n return path[1]; // Return the destination city\n }\n }\n\n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n }\n};\n\n\n\n```\n```C []\nchar* destCity(char paths[][2], int pathsSize) {\n char startCities[pathsSize][21]; // Assuming the maximum city name length is 20 characters\n int startCitiesCount = 0;\n\n // Store all starting cities in a set\n for (int i = 0; i < pathsSize; i++) {\n strcpy(startCities[startCitiesCount++], paths[i][0]);\n }\n\n // Find the destination city\n for (int i = 0; i < pathsSize; i++) {\n int found = 0;\n for (int j = 0; j < startCitiesCount; j++) {\n if (strcmp(paths[i][1], startCities[j]) == 0) {\n found = 1;\n break;\n }\n }\n if (!found) {\n return paths[i][1]; // Return the destination city\n }\n }\n\n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n}\n\n\n\n```\n```Java []\nimport java.util.HashSet;\nimport java.util.List;\nimport java.util.Set;\n\nclass Solution {\n public String destCity(List<List<String>> paths) {\n Set<String> startCities = new HashSet<>();\n\n // Store all starting cities in a set\n for (List<String> path : paths) {\n startCities.add(path.get(0));\n }\n\n // Find the destination city\n for (List<String> path : paths) {\n if (!startCities.contains(path.get(1))) {\n return path.get(1); // Return the destination city\n }\n }\n\n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n }\n}\n\n\n\n```\n```python3 []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n start_cities = set()\n\n # Store all starting cities in a set\n for path in paths:\n start_cities.add(path[0])\n\n # Find the destination city\n for path in paths:\n if path[1] not in start_cities:\n return path[1] # Return the destination city\n\n return "" # If no destination city found (shouldn\'t reach here if every path is valid)\n\n\n\n```\n```javascript []\nfunction destCity(paths) {\n const startCities = new Set();\n\n // Store all starting cities in a set\n for (const path of paths) {\n startCities.add(path[0]);\n }\n\n // Find the destination city\n for (const path of paths) {\n if (!startCities.has(path[1])) {\n return path[1]; // Return the destination city\n }\n }\n\n return ""; // If no destination city found (shouldn\'t reach here if every path is valid)\n}\n\n\n```\n\n---\n\n\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n--- | 15 | Given a wooden stick of length `n` units. The stick is labelled from `0` to `n`. For example, a stick of length **6** is labelled as follows:
Given an integer array `cuts` where `cuts[i]` denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return _the minimum total cost_ of the cuts.
**Example 1:**
**Input:** n = 7, cuts = \[1,3,4,5\]
**Output:** 16
**Explanation:** Using cuts order = \[1, 3, 4, 5\] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be \[3, 5, 1, 4\] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
**Example 2:**
**Input:** n = 9, cuts = \[5,6,1,4,2\]
**Output:** 22
**Explanation:** If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order \[4, 6, 5, 2, 1\] has total cost = 22 which is the minimum possible.
**Constraints:**
* `2 <= n <= 106`
* `1 <= cuts.length <= min(n - 1, 100)`
* `1 <= cuts[i] <= n - 1`
* All the integers in `cuts` array are **distinct**. | Start in any city and use the path to move to the next city. Eventually, you will reach a city with no path outgoing, this is the destination city. |
【Video】Give me 3 minutes - How we think about a solution | destination-city | 0 | 1 | # Intuition\nWe use subtraction.\n\n---\n\n# Solution Video\n\nhttps://youtu.be/tjILACCjQI0\n\n\u25A0 Timeline of the video\n\n`0:04` Explain how we can solve this question\n`1:29` Coding\n`2:40` Time Complexity and Space Complexity\n`3:13` Step by step algorithm of my solution code\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 3,469\nMy first goal is 10,000 (It\'s far from done \uD83D\uDE05)\nThank you for your support!\n\n---\n\n# Approach\n\n## How we think about a solution\n\nWe know that we have the same number of start cities and end cities. I thought if I create group of start cities and end cities, we can use subtraction.\n\n```\nInput: paths = [["B","C"],["D","B"],["C","A"]]\n```\n\nWe use `set` to create groups because we can use subtraction with set easily. Start group and end group should be\n```\nstart_cities = {"B", "D", "C"}\n```\n```\nend_cities = {"C", "B", "A"}\n```\nThen subtract `end` - `start`, which means remove `common cities` in the end group.\n\n```\nend_cities - start_cities\n= {"C", "B", "A"} - {"B", "D", "C"}\n```\nWe have "C" and "B" in both groups, so in the end, end group should be\n```\ndestination = {"A"}\n```\nThen just pop from `set`\n```\nOutput: "A"\n```\nThere is not "A" city in start group, so we are sure that "A" is definitely destination city.\n\nEasy! \uD83D\uDE04\nLet\'s see a real algorithm!\n\nI usually write 4 languages Python, JavaScript, Java and C++. But today, I don\'t have enough time. I want to create a video before I go out. \n\nIf possible, could someone convert this Python code to JavaScript, Java, and C++?\n\n\n---\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n```python []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n start_cities, end_cities = map(set, zip(*paths))\n destination = (end_cities - start_cities).pop()\n return destination\n```\n\n## Step by step algorithm\n\n1. **Create Sets for Start and End Cities:**\n - Use `zip(*paths)` to transpose the matrix of paths, separating the start cities and end cities.\n - Apply `set` to convert each set of cities to unique sets.\n\n ```python\n start_cities, end_cities = map(set, zip(*paths))\n ```\n\n2. **Find the Destination City:**\n - Use set subtraction (`end_cities - start_cities`) to find the set of cities that are destinations but not starting points.\n - Use `pop()` to retrieve any element from the set (since we expect only one element).\n\n ```python\n destination = (end_cities - start_cities).pop()\n ```\n\n3. **Return the Result:**\n - Return the found destination city.\n\n ```python\n return destination\n ```\n\nThis code essentially finds the city that is a destination but not a starting point by utilizing sets and set operations. The order of the paths and the uniqueness of cities in sets make it efficient for this specific task.\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n\u25A0 Subscribe URL\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\n\u25A0 Twitter\nhttps://twitter.com/CodingNinjaAZ\n\n### My next daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/valid-anagram/solutions/4410317/video-give-me-5-minutes-4-solutions-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/UR9geRGBvhk\n\n\u25A0 Timeline of the video\n\n`0:03` Explain Solution 1\n`2:52` Coding of Solution 1\n`4:38` Time Complexity and Space Complexity of Solution 1\n`5:04` Step by step algorithm of my solution code of Solution 1\n`5:11` Explain Solution 2\n`8:20` Coding of Solution 2\n`10:10` Time Complexity and Space Complexity of Solution 2\n`10:21` Step by step algorithm of my solution code of Solution 2\n`10:38` Coding of Solution 3\n`12:18` Time Complexity and Space Complexity of Solution 3\n`13:15` Coding of Solution 4\n`13:37` Time Complexity and Space Complexity of Solution 4\n`13:52` Step by step algorithm of my solution code of Solution 3\n\n### My previous daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/difference-between-ones-and-zeros-in-row-and-column/solutions/4401811/video-give-me-5-minutes-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/swafoNFu99o\n\n\u25A0 Timeline of the video\n\n`0:06` Create number of 1 in each row and column\n`1:16` How to calculate numbers at each position \n`4:03` Coding\n`6:32` Time Complexity and Space Complexity\n`7:02` Explain zip(*grid)\n`8:15` Step by step algorithm of my solution code\n\n | 48 | You are given the array `paths`, where `paths[i] = [cityAi, cityBi]` means there exists a direct path going from `cityAi` to `cityBi`. _Return the destination city, that is, the city without any path outgoing to another city._
It is guaranteed that the graph of paths forms a line without any loop, therefore, there will be exactly one destination city.
**Example 1:**
**Input:** paths = \[\[ "London ", "New York "\],\[ "New York ", "Lima "\],\[ "Lima ", "Sao Paulo "\]\]
**Output:** "Sao Paulo "
**Explanation:** Starting at "London " city you will reach "Sao Paulo " city which is the destination city. Your trip consist of: "London " -> "New York " -> "Lima " -> "Sao Paulo ".
**Example 2:**
**Input:** paths = \[\[ "B ", "C "\],\[ "D ", "B "\],\[ "C ", "A "\]\]
**Output:** "A "
**Explanation:** All possible trips are:
"D " -> "B " -> "C " -> "A ".
"B " -> "C " -> "A ".
"C " -> "A ".
"A ".
Clearly the destination city is "A ".
**Example 3:**
**Input:** paths = \[\[ "A ", "Z "\]\]
**Output:** "Z "
**Constraints:**
* `1 <= paths.length <= 100`
* `paths[i].length == 2`
* `1 <= cityAi.length, cityBi.length <= 10`
* `cityAi != cityBi`
* All strings consist of lowercase and uppercase English letters and the space character. | Do BFS to find the kth level friends. Then collect movies saw by kth level friends and sort them accordingly. |
【Video】Give me 3 minutes - How we think about a solution | destination-city | 0 | 1 | # Intuition\nWe use subtraction.\n\n---\n\n# Solution Video\n\nhttps://youtu.be/tjILACCjQI0\n\n\u25A0 Timeline of the video\n\n`0:04` Explain how we can solve this question\n`1:29` Coding\n`2:40` Time Complexity and Space Complexity\n`3:13` Step by step algorithm of my solution code\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 3,469\nMy first goal is 10,000 (It\'s far from done \uD83D\uDE05)\nThank you for your support!\n\n---\n\n# Approach\n\n## How we think about a solution\n\nWe know that we have the same number of start cities and end cities. I thought if I create group of start cities and end cities, we can use subtraction.\n\n```\nInput: paths = [["B","C"],["D","B"],["C","A"]]\n```\n\nWe use `set` to create groups because we can use subtraction with set easily. Start group and end group should be\n```\nstart_cities = {"B", "D", "C"}\n```\n```\nend_cities = {"C", "B", "A"}\n```\nThen subtract `end` - `start`, which means remove `common cities` in the end group.\n\n```\nend_cities - start_cities\n= {"C", "B", "A"} - {"B", "D", "C"}\n```\nWe have "C" and "B" in both groups, so in the end, end group should be\n```\ndestination = {"A"}\n```\nThen just pop from `set`\n```\nOutput: "A"\n```\nThere is not "A" city in start group, so we are sure that "A" is definitely destination city.\n\nEasy! \uD83D\uDE04\nLet\'s see a real algorithm!\n\nI usually write 4 languages Python, JavaScript, Java and C++. But today, I don\'t have enough time. I want to create a video before I go out. \n\nIf possible, could someone convert this Python code to JavaScript, Java, and C++?\n\n\n---\n\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n```python []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n start_cities, end_cities = map(set, zip(*paths))\n destination = (end_cities - start_cities).pop()\n return destination\n```\n\n## Step by step algorithm\n\n1. **Create Sets for Start and End Cities:**\n - Use `zip(*paths)` to transpose the matrix of paths, separating the start cities and end cities.\n - Apply `set` to convert each set of cities to unique sets.\n\n ```python\n start_cities, end_cities = map(set, zip(*paths))\n ```\n\n2. **Find the Destination City:**\n - Use set subtraction (`end_cities - start_cities`) to find the set of cities that are destinations but not starting points.\n - Use `pop()` to retrieve any element from the set (since we expect only one element).\n\n ```python\n destination = (end_cities - start_cities).pop()\n ```\n\n3. **Return the Result:**\n - Return the found destination city.\n\n ```python\n return destination\n ```\n\nThis code essentially finds the city that is a destination but not a starting point by utilizing sets and set operations. The order of the paths and the uniqueness of cities in sets make it efficient for this specific task.\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n\u25A0 Subscribe URL\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\n\u25A0 Twitter\nhttps://twitter.com/CodingNinjaAZ\n\n### My next daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/valid-anagram/solutions/4410317/video-give-me-5-minutes-4-solutions-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/UR9geRGBvhk\n\n\u25A0 Timeline of the video\n\n`0:03` Explain Solution 1\n`2:52` Coding of Solution 1\n`4:38` Time Complexity and Space Complexity of Solution 1\n`5:04` Step by step algorithm of my solution code of Solution 1\n`5:11` Explain Solution 2\n`8:20` Coding of Solution 2\n`10:10` Time Complexity and Space Complexity of Solution 2\n`10:21` Step by step algorithm of my solution code of Solution 2\n`10:38` Coding of Solution 3\n`12:18` Time Complexity and Space Complexity of Solution 3\n`13:15` Coding of Solution 4\n`13:37` Time Complexity and Space Complexity of Solution 4\n`13:52` Step by step algorithm of my solution code of Solution 3\n\n### My previous daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/difference-between-ones-and-zeros-in-row-and-column/solutions/4401811/video-give-me-5-minutes-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/swafoNFu99o\n\n\u25A0 Timeline of the video\n\n`0:06` Create number of 1 in each row and column\n`1:16` How to calculate numbers at each position \n`4:03` Coding\n`6:32` Time Complexity and Space Complexity\n`7:02` Explain zip(*grid)\n`8:15` Step by step algorithm of my solution code\n\n | 48 | Given a wooden stick of length `n` units. The stick is labelled from `0` to `n`. For example, a stick of length **6** is labelled as follows:
Given an integer array `cuts` where `cuts[i]` denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return _the minimum total cost_ of the cuts.
**Example 1:**
**Input:** n = 7, cuts = \[1,3,4,5\]
**Output:** 16
**Explanation:** Using cuts order = \[1, 3, 4, 5\] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be \[3, 5, 1, 4\] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
**Example 2:**
**Input:** n = 9, cuts = \[5,6,1,4,2\]
**Output:** 22
**Explanation:** If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order \[4, 6, 5, 2, 1\] has total cost = 22 which is the minimum possible.
**Constraints:**
* `2 <= n <= 106`
* `1 <= cuts.length <= min(n - 1, 100)`
* `1 <= cuts[i] <= n - 1`
* All the integers in `cuts` array are **distinct**. | Start in any city and use the path to move to the next city. Eventually, you will reach a city with no path outgoing, this is the destination city. |
1436. Destination City => Stepwise Explained 😎| Easy To Understand 🚀 | destination-city | 1 | 1 | # Intuition\nThe code aims to find the destination city given a list of paths. It uses an unordered map to represent the paths between cities and a set to store all cities. The destination city is the one that does not have an outgoing path.\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. **Initialize Data Structures**: Create an unordered map (`m`) to store the paths and a set (`s`) to store all cities.\n2. **Populate Data Structures**: Iterate through the given paths, insert cities into the set, and update the map with the paths.\n3. **Find Destination City**: Iterate through the set to find the city that does not have an outgoing path.\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(N + M), where N is the number of paths and M is the number of unique cities.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(M), where M is the number of unique cities\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n string destCity(vector<vector<string>>& paths) {\n ios_base::sync_with_stdio(false);\n cin.tie(0);\n cout.tie(0);\n \n // Step 1: Create an unordered map to store the paths, and a set to store all cities\n unordered_map<string, string> m;\n unordered_set<string> s;\n \n // Step 2: Iterate through the paths to populate the map and set\n for(auto x : paths)\n {\n string s1 = x[0];\n string s2 = x[1];\n s.insert(s1);\n s.insert(s2);\n m[s1] = s2;\n }\n \n // Step 3: Iterate through the set to find the destination city\n for(auto x : s)\n {\n if(m.find(x) == m.end()) return x;\n }\n \n // Step 4: Return an empty string if no destination city is found (this should not happen)\n return "";\n}\n\n};\n``` | 4 | You are given the array `paths`, where `paths[i] = [cityAi, cityBi]` means there exists a direct path going from `cityAi` to `cityBi`. _Return the destination city, that is, the city without any path outgoing to another city._
It is guaranteed that the graph of paths forms a line without any loop, therefore, there will be exactly one destination city.
**Example 1:**
**Input:** paths = \[\[ "London ", "New York "\],\[ "New York ", "Lima "\],\[ "Lima ", "Sao Paulo "\]\]
**Output:** "Sao Paulo "
**Explanation:** Starting at "London " city you will reach "Sao Paulo " city which is the destination city. Your trip consist of: "London " -> "New York " -> "Lima " -> "Sao Paulo ".
**Example 2:**
**Input:** paths = \[\[ "B ", "C "\],\[ "D ", "B "\],\[ "C ", "A "\]\]
**Output:** "A "
**Explanation:** All possible trips are:
"D " -> "B " -> "C " -> "A ".
"B " -> "C " -> "A ".
"C " -> "A ".
"A ".
Clearly the destination city is "A ".
**Example 3:**
**Input:** paths = \[\[ "A ", "Z "\]\]
**Output:** "Z "
**Constraints:**
* `1 <= paths.length <= 100`
* `paths[i].length == 2`
* `1 <= cityAi.length, cityBi.length <= 10`
* `cityAi != cityBi`
* All strings consist of lowercase and uppercase English letters and the space character. | Do BFS to find the kth level friends. Then collect movies saw by kth level friends and sort them accordingly. |
1436. Destination City => Stepwise Explained 😎| Easy To Understand 🚀 | destination-city | 1 | 1 | # Intuition\nThe code aims to find the destination city given a list of paths. It uses an unordered map to represent the paths between cities and a set to store all cities. The destination city is the one that does not have an outgoing path.\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. **Initialize Data Structures**: Create an unordered map (`m`) to store the paths and a set (`s`) to store all cities.\n2. **Populate Data Structures**: Iterate through the given paths, insert cities into the set, and update the map with the paths.\n3. **Find Destination City**: Iterate through the set to find the city that does not have an outgoing path.\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(N + M), where N is the number of paths and M is the number of unique cities.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(M), where M is the number of unique cities\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution {\npublic:\n string destCity(vector<vector<string>>& paths) {\n ios_base::sync_with_stdio(false);\n cin.tie(0);\n cout.tie(0);\n \n // Step 1: Create an unordered map to store the paths, and a set to store all cities\n unordered_map<string, string> m;\n unordered_set<string> s;\n \n // Step 2: Iterate through the paths to populate the map and set\n for(auto x : paths)\n {\n string s1 = x[0];\n string s2 = x[1];\n s.insert(s1);\n s.insert(s2);\n m[s1] = s2;\n }\n \n // Step 3: Iterate through the set to find the destination city\n for(auto x : s)\n {\n if(m.find(x) == m.end()) return x;\n }\n \n // Step 4: Return an empty string if no destination city is found (this should not happen)\n return "";\n}\n\n};\n``` | 4 | Given a wooden stick of length `n` units. The stick is labelled from `0` to `n`. For example, a stick of length **6** is labelled as follows:
Given an integer array `cuts` where `cuts[i]` denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return _the minimum total cost_ of the cuts.
**Example 1:**
**Input:** n = 7, cuts = \[1,3,4,5\]
**Output:** 16
**Explanation:** Using cuts order = \[1, 3, 4, 5\] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be \[3, 5, 1, 4\] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
**Example 2:**
**Input:** n = 9, cuts = \[5,6,1,4,2\]
**Output:** 22
**Explanation:** If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order \[4, 6, 5, 2, 1\] has total cost = 22 which is the minimum possible.
**Constraints:**
* `2 <= n <= 106`
* `1 <= cuts.length <= min(n - 1, 100)`
* `1 <= cuts[i] <= n - 1`
* All the integers in `cuts` array are **distinct**. | Start in any city and use the path to move to the next city. Eventually, you will reach a city with no path outgoing, this is the destination city. |
"🧠 Intuitive Solution in C++ | ⏳ 0ms Runtime (Top 5%) | ✅ Beginner-Friendly Explanation" | destination-city | 0 | 1 | # Intuition\n<h2>\nI thought this as topological sort problem but since there is only one node with no outgoing edge therefore the solution becomes much much easier to think.<br><br>\nBut first Step is to think this as topological sort problem.\n<br><br>\n\nEdit : Topological Sort got nothing to do with the provided solution so don\'t be scared of such fancy term.\n\n<br>\n<br>\n\nThe code is designed to find the destination city in a list of paths, where each path is represented by a pair of cities [u, v], indicating a directed edge from city u to city v. The goal is to identify the city that is the ultimate destination and does not have any outgoing paths.\n\n\n\n# Approach\n**Approach to Find the Destination City:**\n\n1. **Initialize Outgoing and Incoming Sets:**\n - Create two empty sets, `outgoing` and `incoming`, to store the source and destination cities.\n\n2. **Populate Outgoing and Incoming Sets:**\n - Iterate through the given `paths` list, and for each pair [u, v]:\n - Add `u` to the `outgoing` set.\n - Add `v` to the `incoming` set.\n\n3. **Find the Destination City:**\n - Iterate through the `incoming` set.\n - For each destination city `u`:\n - Check if `u` is not present in the `outgoing` set.\n - If found, return `u` as the destination city.\n\n4. **Handle Edge Cases:**\n - If the input is well-formed, there should be exactly one destination city without any outgoing paths.\n - If no such city is found, it implies an issue with the input, and an appropriate response (e.g., an error message or default value) may be provided.(Though it is given in question that unique answer always exists but for interview pov keep this point in mind:) )\n\n**Note:**\n- The use of sets ensures constant-time membership checks, making the solution efficient.Otherwise looking-up in python list or c++ vector take O(n) time complexity. btw you can use them.\n\n**Complexity Analysis:**\n- Time Complexity: O(N), where N is the number of paths.\n- Space Complexity: O(N), as we use two sets to store the outgoing and incoming cities.\n\n\n# Code\n\n```Python []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n outgoing = set()\n incoming = set()\n for u,v in paths:\n outgoing.add(u)\n incoming.add(v)\n\n for u in incoming:\n if u not in outgoing:\n return u\n \n```\n\n```C++ []\nclass Solution {\npublic:\n string destCity(vector<vector<string>>& paths) {\n unordered_set<string> outgoing;\n unordered_set<string> incoming;\n\n for (const auto& path : paths) {\n outgoing.insert(path[0]);\n incoming.insert(path[1]);\n }\n\n for (const auto& city : incoming) {\n if (outgoing.find(city) == outgoing.end()) {\n return city;\n }\n }\n }\n};\n``` | 3 | You are given the array `paths`, where `paths[i] = [cityAi, cityBi]` means there exists a direct path going from `cityAi` to `cityBi`. _Return the destination city, that is, the city without any path outgoing to another city._
It is guaranteed that the graph of paths forms a line without any loop, therefore, there will be exactly one destination city.
**Example 1:**
**Input:** paths = \[\[ "London ", "New York "\],\[ "New York ", "Lima "\],\[ "Lima ", "Sao Paulo "\]\]
**Output:** "Sao Paulo "
**Explanation:** Starting at "London " city you will reach "Sao Paulo " city which is the destination city. Your trip consist of: "London " -> "New York " -> "Lima " -> "Sao Paulo ".
**Example 2:**
**Input:** paths = \[\[ "B ", "C "\],\[ "D ", "B "\],\[ "C ", "A "\]\]
**Output:** "A "
**Explanation:** All possible trips are:
"D " -> "B " -> "C " -> "A ".
"B " -> "C " -> "A ".
"C " -> "A ".
"A ".
Clearly the destination city is "A ".
**Example 3:**
**Input:** paths = \[\[ "A ", "Z "\]\]
**Output:** "Z "
**Constraints:**
* `1 <= paths.length <= 100`
* `paths[i].length == 2`
* `1 <= cityAi.length, cityBi.length <= 10`
* `cityAi != cityBi`
* All strings consist of lowercase and uppercase English letters and the space character. | Do BFS to find the kth level friends. Then collect movies saw by kth level friends and sort them accordingly. |
"🧠 Intuitive Solution in C++ | ⏳ 0ms Runtime (Top 5%) | ✅ Beginner-Friendly Explanation" | destination-city | 0 | 1 | # Intuition\n<h2>\nI thought this as topological sort problem but since there is only one node with no outgoing edge therefore the solution becomes much much easier to think.<br><br>\nBut first Step is to think this as topological sort problem.\n<br><br>\n\nEdit : Topological Sort got nothing to do with the provided solution so don\'t be scared of such fancy term.\n\n<br>\n<br>\n\nThe code is designed to find the destination city in a list of paths, where each path is represented by a pair of cities [u, v], indicating a directed edge from city u to city v. The goal is to identify the city that is the ultimate destination and does not have any outgoing paths.\n\n\n\n# Approach\n**Approach to Find the Destination City:**\n\n1. **Initialize Outgoing and Incoming Sets:**\n - Create two empty sets, `outgoing` and `incoming`, to store the source and destination cities.\n\n2. **Populate Outgoing and Incoming Sets:**\n - Iterate through the given `paths` list, and for each pair [u, v]:\n - Add `u` to the `outgoing` set.\n - Add `v` to the `incoming` set.\n\n3. **Find the Destination City:**\n - Iterate through the `incoming` set.\n - For each destination city `u`:\n - Check if `u` is not present in the `outgoing` set.\n - If found, return `u` as the destination city.\n\n4. **Handle Edge Cases:**\n - If the input is well-formed, there should be exactly one destination city without any outgoing paths.\n - If no such city is found, it implies an issue with the input, and an appropriate response (e.g., an error message or default value) may be provided.(Though it is given in question that unique answer always exists but for interview pov keep this point in mind:) )\n\n**Note:**\n- The use of sets ensures constant-time membership checks, making the solution efficient.Otherwise looking-up in python list or c++ vector take O(n) time complexity. btw you can use them.\n\n**Complexity Analysis:**\n- Time Complexity: O(N), where N is the number of paths.\n- Space Complexity: O(N), as we use two sets to store the outgoing and incoming cities.\n\n\n# Code\n\n```Python []\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n outgoing = set()\n incoming = set()\n for u,v in paths:\n outgoing.add(u)\n incoming.add(v)\n\n for u in incoming:\n if u not in outgoing:\n return u\n \n```\n\n```C++ []\nclass Solution {\npublic:\n string destCity(vector<vector<string>>& paths) {\n unordered_set<string> outgoing;\n unordered_set<string> incoming;\n\n for (const auto& path : paths) {\n outgoing.insert(path[0]);\n incoming.insert(path[1]);\n }\n\n for (const auto& city : incoming) {\n if (outgoing.find(city) == outgoing.end()) {\n return city;\n }\n }\n }\n};\n``` | 3 | Given a wooden stick of length `n` units. The stick is labelled from `0` to `n`. For example, a stick of length **6** is labelled as follows:
Given an integer array `cuts` where `cuts[i]` denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return _the minimum total cost_ of the cuts.
**Example 1:**
**Input:** n = 7, cuts = \[1,3,4,5\]
**Output:** 16
**Explanation:** Using cuts order = \[1, 3, 4, 5\] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be \[3, 5, 1, 4\] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
**Example 2:**
**Input:** n = 9, cuts = \[5,6,1,4,2\]
**Output:** 22
**Explanation:** If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order \[4, 6, 5, 2, 1\] has total cost = 22 which is the minimum possible.
**Constraints:**
* `2 <= n <= 106`
* `1 <= cuts.length <= min(n - 1, 100)`
* `1 <= cuts[i] <= n - 1`
* All the integers in `cuts` array are **distinct**. | Start in any city and use the path to move to the next city. Eventually, you will reach a city with no path outgoing, this is the destination city. |
pYthon3 Solution | destination-city | 0 | 1 | \n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n frm,to=zip(*paths)\n return (set(to)-set(frm)).pop()\n``` | 3 | You are given the array `paths`, where `paths[i] = [cityAi, cityBi]` means there exists a direct path going from `cityAi` to `cityBi`. _Return the destination city, that is, the city without any path outgoing to another city._
It is guaranteed that the graph of paths forms a line without any loop, therefore, there will be exactly one destination city.
**Example 1:**
**Input:** paths = \[\[ "London ", "New York "\],\[ "New York ", "Lima "\],\[ "Lima ", "Sao Paulo "\]\]
**Output:** "Sao Paulo "
**Explanation:** Starting at "London " city you will reach "Sao Paulo " city which is the destination city. Your trip consist of: "London " -> "New York " -> "Lima " -> "Sao Paulo ".
**Example 2:**
**Input:** paths = \[\[ "B ", "C "\],\[ "D ", "B "\],\[ "C ", "A "\]\]
**Output:** "A "
**Explanation:** All possible trips are:
"D " -> "B " -> "C " -> "A ".
"B " -> "C " -> "A ".
"C " -> "A ".
"A ".
Clearly the destination city is "A ".
**Example 3:**
**Input:** paths = \[\[ "A ", "Z "\]\]
**Output:** "Z "
**Constraints:**
* `1 <= paths.length <= 100`
* `paths[i].length == 2`
* `1 <= cityAi.length, cityBi.length <= 10`
* `cityAi != cityBi`
* All strings consist of lowercase and uppercase English letters and the space character. | Do BFS to find the kth level friends. Then collect movies saw by kth level friends and sort them accordingly. |
pYthon3 Solution | destination-city | 0 | 1 | \n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n frm,to=zip(*paths)\n return (set(to)-set(frm)).pop()\n``` | 3 | Given a wooden stick of length `n` units. The stick is labelled from `0` to `n`. For example, a stick of length **6** is labelled as follows:
Given an integer array `cuts` where `cuts[i]` denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return _the minimum total cost_ of the cuts.
**Example 1:**
**Input:** n = 7, cuts = \[1,3,4,5\]
**Output:** 16
**Explanation:** Using cuts order = \[1, 3, 4, 5\] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be \[3, 5, 1, 4\] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
**Example 2:**
**Input:** n = 9, cuts = \[5,6,1,4,2\]
**Output:** 22
**Explanation:** If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order \[4, 6, 5, 2, 1\] has total cost = 22 which is the minimum possible.
**Constraints:**
* `2 <= n <= 106`
* `1 <= cuts.length <= min(n - 1, 100)`
* `1 <= cuts[i] <= n - 1`
* All the integers in `cuts` array are **distinct**. | Start in any city and use the path to move to the next city. Eventually, you will reach a city with no path outgoing, this is the destination city. |
🤯 CRAZY ONE-LINE SOLUTION WITH EXPLANATION | destination-city | 0 | 1 | # Approach\n\n1. `paths`\n\n ```\n [\n ["London", "New York"],\n ["New York", "Lima"],\n ["Lima", "Sao Paulo"]\n ] \n ```\n\n2. `zip(*paths)`\n\n ```\n [\n ["London", "New York", "Lima"], \n ["New York", "Lima", "Sao Paulo"]\n ]\n ```\n\n3. `map(set, zip(*paths))`\n\n ```\n [\n {"London", "New York", "Lima"}, \n {"New York", "Lima", "Sao Paulo"}\n ]\n ```\n\n4. `reduce(lambda x, y: y - x, map(set, zip(*paths)))`\n\n ```\n {"New York", "Lima", "Sao Paulo"} \n - {"London", "New York", "Lima"} \n = {"Sao Paulo"}\n ```\n\n5. `reduce(lambda x, y: y - x, map(set, zip(*paths))).pop()`\n\n ```\n "Sao Paulo"\n ```\n\n# Complexity\n- Time complexity: $$O(N)$$\n\n- Space complexity: $$O(N)$$\n\n# Code\n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n return reduce(lambda x, y: y - x, map(set, zip(*paths))).pop()\n``` | 2 | You are given the array `paths`, where `paths[i] = [cityAi, cityBi]` means there exists a direct path going from `cityAi` to `cityBi`. _Return the destination city, that is, the city without any path outgoing to another city._
It is guaranteed that the graph of paths forms a line without any loop, therefore, there will be exactly one destination city.
**Example 1:**
**Input:** paths = \[\[ "London ", "New York "\],\[ "New York ", "Lima "\],\[ "Lima ", "Sao Paulo "\]\]
**Output:** "Sao Paulo "
**Explanation:** Starting at "London " city you will reach "Sao Paulo " city which is the destination city. Your trip consist of: "London " -> "New York " -> "Lima " -> "Sao Paulo ".
**Example 2:**
**Input:** paths = \[\[ "B ", "C "\],\[ "D ", "B "\],\[ "C ", "A "\]\]
**Output:** "A "
**Explanation:** All possible trips are:
"D " -> "B " -> "C " -> "A ".
"B " -> "C " -> "A ".
"C " -> "A ".
"A ".
Clearly the destination city is "A ".
**Example 3:**
**Input:** paths = \[\[ "A ", "Z "\]\]
**Output:** "Z "
**Constraints:**
* `1 <= paths.length <= 100`
* `paths[i].length == 2`
* `1 <= cityAi.length, cityBi.length <= 10`
* `cityAi != cityBi`
* All strings consist of lowercase and uppercase English letters and the space character. | Do BFS to find the kth level friends. Then collect movies saw by kth level friends and sort them accordingly. |
🤯 CRAZY ONE-LINE SOLUTION WITH EXPLANATION | destination-city | 0 | 1 | # Approach\n\n1. `paths`\n\n ```\n [\n ["London", "New York"],\n ["New York", "Lima"],\n ["Lima", "Sao Paulo"]\n ] \n ```\n\n2. `zip(*paths)`\n\n ```\n [\n ["London", "New York", "Lima"], \n ["New York", "Lima", "Sao Paulo"]\n ]\n ```\n\n3. `map(set, zip(*paths))`\n\n ```\n [\n {"London", "New York", "Lima"}, \n {"New York", "Lima", "Sao Paulo"}\n ]\n ```\n\n4. `reduce(lambda x, y: y - x, map(set, zip(*paths)))`\n\n ```\n {"New York", "Lima", "Sao Paulo"} \n - {"London", "New York", "Lima"} \n = {"Sao Paulo"}\n ```\n\n5. `reduce(lambda x, y: y - x, map(set, zip(*paths))).pop()`\n\n ```\n "Sao Paulo"\n ```\n\n# Complexity\n- Time complexity: $$O(N)$$\n\n- Space complexity: $$O(N)$$\n\n# Code\n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n return reduce(lambda x, y: y - x, map(set, zip(*paths))).pop()\n``` | 2 | Given a wooden stick of length `n` units. The stick is labelled from `0` to `n`. For example, a stick of length **6** is labelled as follows:
Given an integer array `cuts` where `cuts[i]` denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return _the minimum total cost_ of the cuts.
**Example 1:**
**Input:** n = 7, cuts = \[1,3,4,5\]
**Output:** 16
**Explanation:** Using cuts order = \[1, 3, 4, 5\] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be \[3, 5, 1, 4\] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
**Example 2:**
**Input:** n = 9, cuts = \[5,6,1,4,2\]
**Output:** 22
**Explanation:** If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order \[4, 6, 5, 2, 1\] has total cost = 22 which is the minimum possible.
**Constraints:**
* `2 <= n <= 106`
* `1 <= cuts.length <= min(n - 1, 100)`
* `1 <= cuts[i] <= n - 1`
* All the integers in `cuts` array are **distinct**. | Start in any city and use the path to move to the next city. Eventually, you will reach a city with no path outgoing, this is the destination city. |
Beats 99.44% runtime | Python3 | Sets | destination-city | 0 | 1 | # Approach\nWe need to find the "end destination", ie the place from where you can go any further. In the test cases, you can notice that the "end destination" is never a start (by "start" I mean the first elem of a sublist. By "destination", I mean the second elem of a sublist)\n\nSo I just find the place which is never a start. I do that by first storing all the starts in a set called "starts" (since sets have a O(1) lookup, as opposed to the O(n) lookup in lists), and then loop through every destination and check which destinaion never appears as a start\n\n# Complexity\n- Time complexity: O(2n)\n\n\n# Code\n```python3\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n starts = {i[0] for i in paths}\n\n for i in paths:\n if i[1] not in starts:\n starts.clear()\n paths.clear()\n return i[1]\n``` | 2 | You are given the array `paths`, where `paths[i] = [cityAi, cityBi]` means there exists a direct path going from `cityAi` to `cityBi`. _Return the destination city, that is, the city without any path outgoing to another city._
It is guaranteed that the graph of paths forms a line without any loop, therefore, there will be exactly one destination city.
**Example 1:**
**Input:** paths = \[\[ "London ", "New York "\],\[ "New York ", "Lima "\],\[ "Lima ", "Sao Paulo "\]\]
**Output:** "Sao Paulo "
**Explanation:** Starting at "London " city you will reach "Sao Paulo " city which is the destination city. Your trip consist of: "London " -> "New York " -> "Lima " -> "Sao Paulo ".
**Example 2:**
**Input:** paths = \[\[ "B ", "C "\],\[ "D ", "B "\],\[ "C ", "A "\]\]
**Output:** "A "
**Explanation:** All possible trips are:
"D " -> "B " -> "C " -> "A ".
"B " -> "C " -> "A ".
"C " -> "A ".
"A ".
Clearly the destination city is "A ".
**Example 3:**
**Input:** paths = \[\[ "A ", "Z "\]\]
**Output:** "Z "
**Constraints:**
* `1 <= paths.length <= 100`
* `paths[i].length == 2`
* `1 <= cityAi.length, cityBi.length <= 10`
* `cityAi != cityBi`
* All strings consist of lowercase and uppercase English letters and the space character. | Do BFS to find the kth level friends. Then collect movies saw by kth level friends and sort them accordingly. |
Beats 99.44% runtime | Python3 | Sets | destination-city | 0 | 1 | # Approach\nWe need to find the "end destination", ie the place from where you can go any further. In the test cases, you can notice that the "end destination" is never a start (by "start" I mean the first elem of a sublist. By "destination", I mean the second elem of a sublist)\n\nSo I just find the place which is never a start. I do that by first storing all the starts in a set called "starts" (since sets have a O(1) lookup, as opposed to the O(n) lookup in lists), and then loop through every destination and check which destinaion never appears as a start\n\n# Complexity\n- Time complexity: O(2n)\n\n\n# Code\n```python3\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n starts = {i[0] for i in paths}\n\n for i in paths:\n if i[1] not in starts:\n starts.clear()\n paths.clear()\n return i[1]\n``` | 2 | Given a wooden stick of length `n` units. The stick is labelled from `0` to `n`. For example, a stick of length **6** is labelled as follows:
Given an integer array `cuts` where `cuts[i]` denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return _the minimum total cost_ of the cuts.
**Example 1:**
**Input:** n = 7, cuts = \[1,3,4,5\]
**Output:** 16
**Explanation:** Using cuts order = \[1, 3, 4, 5\] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be \[3, 5, 1, 4\] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
**Example 2:**
**Input:** n = 9, cuts = \[5,6,1,4,2\]
**Output:** 22
**Explanation:** If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order \[4, 6, 5, 2, 1\] has total cost = 22 which is the minimum possible.
**Constraints:**
* `2 <= n <= 106`
* `1 <= cuts.length <= min(n - 1, 100)`
* `1 <= cuts[i] <= n - 1`
* All the integers in `cuts` array are **distinct**. | Start in any city and use the path to move to the next city. Eventually, you will reach a city with no path outgoing, this is the destination city. |
✅ One Line Solution | destination-city | 0 | 1 | # Code #1\nTime complexity: $$O(n)$$. Space complexity: $$O(n)$$.\n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n return max({B for _,B in paths} - {A for A,_ in paths})\n```\n\n# Code #2\nTime complexity: $$O(n)$$. Space complexity: $$O(n)$$.\n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n return next(B for _,B in paths if B not in {A for A,_ in paths})\n```\n\n# Code #3\nTime complexity: $$O(n)$$. Space complexity: $$O(n)$$.\n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n return max((q:=list(map(set,zip(*paths))))[1] - q[0])\n``` | 2 | You are given the array `paths`, where `paths[i] = [cityAi, cityBi]` means there exists a direct path going from `cityAi` to `cityBi`. _Return the destination city, that is, the city without any path outgoing to another city._
It is guaranteed that the graph of paths forms a line without any loop, therefore, there will be exactly one destination city.
**Example 1:**
**Input:** paths = \[\[ "London ", "New York "\],\[ "New York ", "Lima "\],\[ "Lima ", "Sao Paulo "\]\]
**Output:** "Sao Paulo "
**Explanation:** Starting at "London " city you will reach "Sao Paulo " city which is the destination city. Your trip consist of: "London " -> "New York " -> "Lima " -> "Sao Paulo ".
**Example 2:**
**Input:** paths = \[\[ "B ", "C "\],\[ "D ", "B "\],\[ "C ", "A "\]\]
**Output:** "A "
**Explanation:** All possible trips are:
"D " -> "B " -> "C " -> "A ".
"B " -> "C " -> "A ".
"C " -> "A ".
"A ".
Clearly the destination city is "A ".
**Example 3:**
**Input:** paths = \[\[ "A ", "Z "\]\]
**Output:** "Z "
**Constraints:**
* `1 <= paths.length <= 100`
* `paths[i].length == 2`
* `1 <= cityAi.length, cityBi.length <= 10`
* `cityAi != cityBi`
* All strings consist of lowercase and uppercase English letters and the space character. | Do BFS to find the kth level friends. Then collect movies saw by kth level friends and sort them accordingly. |
✅ One Line Solution | destination-city | 0 | 1 | # Code #1\nTime complexity: $$O(n)$$. Space complexity: $$O(n)$$.\n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n return max({B for _,B in paths} - {A for A,_ in paths})\n```\n\n# Code #2\nTime complexity: $$O(n)$$. Space complexity: $$O(n)$$.\n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n return next(B for _,B in paths if B not in {A for A,_ in paths})\n```\n\n# Code #3\nTime complexity: $$O(n)$$. Space complexity: $$O(n)$$.\n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n return max((q:=list(map(set,zip(*paths))))[1] - q[0])\n``` | 2 | Given a wooden stick of length `n` units. The stick is labelled from `0` to `n`. For example, a stick of length **6** is labelled as follows:
Given an integer array `cuts` where `cuts[i]` denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return _the minimum total cost_ of the cuts.
**Example 1:**
**Input:** n = 7, cuts = \[1,3,4,5\]
**Output:** 16
**Explanation:** Using cuts order = \[1, 3, 4, 5\] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be \[3, 5, 1, 4\] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
**Example 2:**
**Input:** n = 9, cuts = \[5,6,1,4,2\]
**Output:** 22
**Explanation:** If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order \[4, 6, 5, 2, 1\] has total cost = 22 which is the minimum possible.
**Constraints:**
* `2 <= n <= 106`
* `1 <= cuts.length <= min(n - 1, 100)`
* `1 <= cuts[i] <= n - 1`
* All the integers in `cuts` array are **distinct**. | Start in any city and use the path to move to the next city. Eventually, you will reach a city with no path outgoing, this is the destination city. |
Eezz Solulu without zip function | destination-city | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n* Initialize two sets dc and ac to store destination cities and arrival cities, respectively.\n* Iterate through the paths list and add each city to the corresponding set (ac for arrival cities and dc for destination cities).\n* Find the destination city by calculating the set difference (dc - ac) and extracting the only element using pop().\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n dc , ac = set() , set()\n for u,v in paths:\n ac.add(u)\n dc.add(v)\n return (dc - ac).pop()\n``` | 2 | You are given the array `paths`, where `paths[i] = [cityAi, cityBi]` means there exists a direct path going from `cityAi` to `cityBi`. _Return the destination city, that is, the city without any path outgoing to another city._
It is guaranteed that the graph of paths forms a line without any loop, therefore, there will be exactly one destination city.
**Example 1:**
**Input:** paths = \[\[ "London ", "New York "\],\[ "New York ", "Lima "\],\[ "Lima ", "Sao Paulo "\]\]
**Output:** "Sao Paulo "
**Explanation:** Starting at "London " city you will reach "Sao Paulo " city which is the destination city. Your trip consist of: "London " -> "New York " -> "Lima " -> "Sao Paulo ".
**Example 2:**
**Input:** paths = \[\[ "B ", "C "\],\[ "D ", "B "\],\[ "C ", "A "\]\]
**Output:** "A "
**Explanation:** All possible trips are:
"D " -> "B " -> "C " -> "A ".
"B " -> "C " -> "A ".
"C " -> "A ".
"A ".
Clearly the destination city is "A ".
**Example 3:**
**Input:** paths = \[\[ "A ", "Z "\]\]
**Output:** "Z "
**Constraints:**
* `1 <= paths.length <= 100`
* `paths[i].length == 2`
* `1 <= cityAi.length, cityBi.length <= 10`
* `cityAi != cityBi`
* All strings consist of lowercase and uppercase English letters and the space character. | Do BFS to find the kth level friends. Then collect movies saw by kth level friends and sort them accordingly. |
Eezz Solulu without zip function | destination-city | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n* Initialize two sets dc and ac to store destination cities and arrival cities, respectively.\n* Iterate through the paths list and add each city to the corresponding set (ac for arrival cities and dc for destination cities).\n* Find the destination city by calculating the set difference (dc - ac) and extracting the only element using pop().\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n dc , ac = set() , set()\n for u,v in paths:\n ac.add(u)\n dc.add(v)\n return (dc - ac).pop()\n``` | 2 | Given a wooden stick of length `n` units. The stick is labelled from `0` to `n`. For example, a stick of length **6** is labelled as follows:
Given an integer array `cuts` where `cuts[i]` denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return _the minimum total cost_ of the cuts.
**Example 1:**
**Input:** n = 7, cuts = \[1,3,4,5\]
**Output:** 16
**Explanation:** Using cuts order = \[1, 3, 4, 5\] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be \[3, 5, 1, 4\] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
**Example 2:**
**Input:** n = 9, cuts = \[5,6,1,4,2\]
**Output:** 22
**Explanation:** If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order \[4, 6, 5, 2, 1\] has total cost = 22 which is the minimum possible.
**Constraints:**
* `2 <= n <= 106`
* `1 <= cuts.length <= min(n - 1, 100)`
* `1 <= cuts[i] <= n - 1`
* All the integers in `cuts` array are **distinct**. | Start in any city and use the path to move to the next city. Eventually, you will reach a city with no path outgoing, this is the destination city. |
⭐One-Liner Hack in 🐍|| Two Approaches⭐ | destination-city | 0 | 1 | # Code - 1\n```py\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n return (set(city[1] for city in paths) - set(city[0] for city in paths)).pop()\n```\n---\n# Code - 2\n```py\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n return next(city[1] for city in paths if city[1] not in {path[0] for path in paths})\n```\n---\n\n# Which of these is more optimized?\n---\n_If you like it, promote it._\n\n--- | 2 | You are given the array `paths`, where `paths[i] = [cityAi, cityBi]` means there exists a direct path going from `cityAi` to `cityBi`. _Return the destination city, that is, the city without any path outgoing to another city._
It is guaranteed that the graph of paths forms a line without any loop, therefore, there will be exactly one destination city.
**Example 1:**
**Input:** paths = \[\[ "London ", "New York "\],\[ "New York ", "Lima "\],\[ "Lima ", "Sao Paulo "\]\]
**Output:** "Sao Paulo "
**Explanation:** Starting at "London " city you will reach "Sao Paulo " city which is the destination city. Your trip consist of: "London " -> "New York " -> "Lima " -> "Sao Paulo ".
**Example 2:**
**Input:** paths = \[\[ "B ", "C "\],\[ "D ", "B "\],\[ "C ", "A "\]\]
**Output:** "A "
**Explanation:** All possible trips are:
"D " -> "B " -> "C " -> "A ".
"B " -> "C " -> "A ".
"C " -> "A ".
"A ".
Clearly the destination city is "A ".
**Example 3:**
**Input:** paths = \[\[ "A ", "Z "\]\]
**Output:** "Z "
**Constraints:**
* `1 <= paths.length <= 100`
* `paths[i].length == 2`
* `1 <= cityAi.length, cityBi.length <= 10`
* `cityAi != cityBi`
* All strings consist of lowercase and uppercase English letters and the space character. | Do BFS to find the kth level friends. Then collect movies saw by kth level friends and sort them accordingly. |
⭐One-Liner Hack in 🐍|| Two Approaches⭐ | destination-city | 0 | 1 | # Code - 1\n```py\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n return (set(city[1] for city in paths) - set(city[0] for city in paths)).pop()\n```\n---\n# Code - 2\n```py\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n return next(city[1] for city in paths if city[1] not in {path[0] for path in paths})\n```\n---\n\n# Which of these is more optimized?\n---\n_If you like it, promote it._\n\n--- | 2 | Given a wooden stick of length `n` units. The stick is labelled from `0` to `n`. For example, a stick of length **6** is labelled as follows:
Given an integer array `cuts` where `cuts[i]` denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return _the minimum total cost_ of the cuts.
**Example 1:**
**Input:** n = 7, cuts = \[1,3,4,5\]
**Output:** 16
**Explanation:** Using cuts order = \[1, 3, 4, 5\] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be \[3, 5, 1, 4\] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
**Example 2:**
**Input:** n = 9, cuts = \[5,6,1,4,2\]
**Output:** 22
**Explanation:** If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order \[4, 6, 5, 2, 1\] has total cost = 22 which is the minimum possible.
**Constraints:**
* `2 <= n <= 106`
* `1 <= cuts.length <= min(n - 1, 100)`
* `1 <= cuts[i] <= n - 1`
* All the integers in `cuts` array are **distinct**. | Start in any city and use the path to move to the next city. Eventually, you will reach a city with no path outgoing, this is the destination city. |
✅ code in 2mins || simple || Python | destination-city | 0 | 1 | # Intuition\nThe problem asks to find the destination city, which is the city that is not the starting city in any route. One intuitive way to solve this is to create sets for the starting cities and destination cities, and then find the city that is in the destination set but not in the starting set.\n\n\n# Approach\n- Create two sets: **"fir"** for the starting cities and **"sec"** for the destination cities.\n- Find the set difference (**"ans"**) between the **"sec"** and **"fir"**.\n- Return the element in **"ans"**.\n\n# Complexity\n- Time complexity:\nO(n), where n is the number of routes (length of the paths list)\n- Space complexity:\nO(n), as we are creating sets for both starting and destination cities.\n# Code\n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n\n sec = set(path[1] for path in paths)\n fir = set(path[0] for path in paths)\n ans = sec - fir\n return ans.pop()\n \n``` | 6 | You are given the array `paths`, where `paths[i] = [cityAi, cityBi]` means there exists a direct path going from `cityAi` to `cityBi`. _Return the destination city, that is, the city without any path outgoing to another city._
It is guaranteed that the graph of paths forms a line without any loop, therefore, there will be exactly one destination city.
**Example 1:**
**Input:** paths = \[\[ "London ", "New York "\],\[ "New York ", "Lima "\],\[ "Lima ", "Sao Paulo "\]\]
**Output:** "Sao Paulo "
**Explanation:** Starting at "London " city you will reach "Sao Paulo " city which is the destination city. Your trip consist of: "London " -> "New York " -> "Lima " -> "Sao Paulo ".
**Example 2:**
**Input:** paths = \[\[ "B ", "C "\],\[ "D ", "B "\],\[ "C ", "A "\]\]
**Output:** "A "
**Explanation:** All possible trips are:
"D " -> "B " -> "C " -> "A ".
"B " -> "C " -> "A ".
"C " -> "A ".
"A ".
Clearly the destination city is "A ".
**Example 3:**
**Input:** paths = \[\[ "A ", "Z "\]\]
**Output:** "Z "
**Constraints:**
* `1 <= paths.length <= 100`
* `paths[i].length == 2`
* `1 <= cityAi.length, cityBi.length <= 10`
* `cityAi != cityBi`
* All strings consist of lowercase and uppercase English letters and the space character. | Do BFS to find the kth level friends. Then collect movies saw by kth level friends and sort them accordingly. |
✅ code in 2mins || simple || Python | destination-city | 0 | 1 | # Intuition\nThe problem asks to find the destination city, which is the city that is not the starting city in any route. One intuitive way to solve this is to create sets for the starting cities and destination cities, and then find the city that is in the destination set but not in the starting set.\n\n\n# Approach\n- Create two sets: **"fir"** for the starting cities and **"sec"** for the destination cities.\n- Find the set difference (**"ans"**) between the **"sec"** and **"fir"**.\n- Return the element in **"ans"**.\n\n# Complexity\n- Time complexity:\nO(n), where n is the number of routes (length of the paths list)\n- Space complexity:\nO(n), as we are creating sets for both starting and destination cities.\n# Code\n```\nclass Solution:\n def destCity(self, paths: List[List[str]]) -> str:\n\n sec = set(path[1] for path in paths)\n fir = set(path[0] for path in paths)\n ans = sec - fir\n return ans.pop()\n \n``` | 6 | Given a wooden stick of length `n` units. The stick is labelled from `0` to `n`. For example, a stick of length **6** is labelled as follows:
Given an integer array `cuts` where `cuts[i]` denotes a position you should perform a cut at.
You should perform the cuts in order, you can change the order of the cuts as you wish.
The cost of one cut is the length of the stick to be cut, the total cost is the sum of costs of all cuts. When you cut a stick, it will be split into two smaller sticks (i.e. the sum of their lengths is the length of the stick before the cut). Please refer to the first example for a better explanation.
Return _the minimum total cost_ of the cuts.
**Example 1:**
**Input:** n = 7, cuts = \[1,3,4,5\]
**Output:** 16
**Explanation:** Using cuts order = \[1, 3, 4, 5\] as in the input leads to the following scenario:
The first cut is done to a rod of length 7 so the cost is 7. The second cut is done to a rod of length 6 (i.e. the second part of the first cut), the third is done to a rod of length 4 and the last cut is to a rod of length 3. The total cost is 7 + 6 + 4 + 3 = 20.
Rearranging the cuts to be \[3, 5, 1, 4\] for example will lead to a scenario with total cost = 16 (as shown in the example photo 7 + 4 + 3 + 2 = 16).
**Example 2:**
**Input:** n = 9, cuts = \[5,6,1,4,2\]
**Output:** 22
**Explanation:** If you try the given cuts ordering the cost will be 25.
There are much ordering with total cost <= 25, for example, the order \[4, 6, 5, 2, 1\] has total cost = 22 which is the minimum possible.
**Constraints:**
* `2 <= n <= 106`
* `1 <= cuts.length <= min(n - 1, 100)`
* `1 <= cuts[i] <= n - 1`
* All the integers in `cuts` array are **distinct**. | Start in any city and use the path to move to the next city. Eventually, you will reach a city with no path outgoing, this is the destination city. |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.