title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
PYTHON SIMPLE DP ᓚᘏᗢ | form-largest-integer-with-digits-that-add-up-to-target | 0 | 1 | # Approach\ndp which is easy to see and read :) <3\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n\n\n# Code\n```\nclass Solution:\n def largestNumber(self, cost: List[int], target: int) -> str:\n dp=[""]*(target+1)\n for i in range(0,target+1):\n for j in range(1,10):\n if i+cost[j-1]<=target :\n if dp[i]=="" and i!=0:\n continue\n res="".join(sorted(dp[i]+str(j),reverse=True))\n if len(res)>len(dp[i+cost[j-1]]):\n dp[i+cost[j-1]]=res\n elif len(res)==len(dp[i+cost[j-1]]) and res>dp[i+cost[j-1]]:\n dp[i+cost[j-1]]=res\n return [dp[target],"0"][dp[target]==""]\n``` | 0 | Given two positive integers `n` and `k`, the binary string `Sn` is formed as follows:
* `S1 = "0 "`
* `Si = Si - 1 + "1 " + reverse(invert(Si - 1))` for `i > 1`
Where `+` denotes the concatenation operation, `reverse(x)` returns the reversed string `x`, and `invert(x)` inverts all the bits in `x` (`0` changes to `1` and `1` changes to `0`).
For example, the first four strings in the above sequence are:
* `S1 = "0 "`
* `S2 = "0**1**1 "`
* `S3 = "011**1**001 "`
* `S4 = "0111001**1**0110001 "`
Return _the_ `kth` _bit_ _in_ `Sn`. It is guaranteed that `k` is valid for the given `n`.
**Example 1:**
**Input:** n = 3, k = 1
**Output:** "0 "
**Explanation:** S3 is "**0**111001 ".
The 1st bit is "0 ".
**Example 2:**
**Input:** n = 4, k = 11
**Output:** "1 "
**Explanation:** S4 is "0111001101**1**0001 ".
The 11th bit is "1 ".
**Constraints:**
* `1 <= n <= 20`
* `1 <= k <= 2n - 1` | Use dynamic programming to find the maximum digits to paint given a total cost. Build the largest number possible using this DP table. |
Python DP Solution | Easy to Understand | Faster than 75% | form-largest-integer-with-digits-that-add-up-to-target | 0 | 1 | # Approach\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe follow a simple take/leave type Memoization DFS where we choose either to take or leave a certain digit. If it\'s not possibe, we return a generic string ```"N"```. Then we compare strings formed in both the cases and return the largest one\n\n\n\n\n# Code\n```\nclass Solution:\n def largestNumber(self, cost: List[int], target: int) -> str:\n sz = len(cost)\n costToDigit = defaultdict(list)\n for digit in range(sz - 1, -1, -1):\n costToDigit[cost[digit]].append(digit)\n \n @cache\n def dp(digit: int, target: int) -> int:\n if target == 0: return ""\n if digit == sz or target < 0: return "N"\n leave = dp(digit + 1, target)\n take = dp(digit, target - cost[digit]) + str(costToDigit[cost[digit]][0] + 1)\n res = []\n if "N" not in leave: \n res.append(leave)\n if "N" not in take: \n res.append(take)\n if not res: return "N"\n if len(res) == 1: return res[0]\n if len(leave) > len(take):\n return leave\n elif len(take) > len(leave):\n return take\n else:\n return leave if leave > take else take\n \n ans = dp(0, target)\n return "".join(sorted(ans, reverse = True)) if ans != "N" else "0"\n``` | 0 | Given an array of integers `cost` and an integer `target`, return _the **maximum** integer you can paint under the following rules_:
* The cost of painting a digit `(i + 1)` is given by `cost[i]` (**0-indexed**).
* The total cost used must be equal to `target`.
* The integer does not have `0` digits.
Since the answer may be very large, return it as a string. If there is no way to paint any integer given the condition, return `"0 "`.
**Example 1:**
**Input:** cost = \[4,3,2,5,6,7,2,5,5\], target = 9
**Output:** "7772 "
**Explanation:** The cost to paint the digit '7' is 2, and the digit '2' is 3. Then cost( "7772 ") = 2\*3+ 3\*1 = 9. You could also paint "977 ", but "7772 " is the largest number.
**Digit cost**
1 -> 4
2 -> 3
3 -> 2
4 -> 5
5 -> 6
6 -> 7
7 -> 2
8 -> 5
9 -> 5
**Example 2:**
**Input:** cost = \[7,6,5,5,5,6,8,7,8\], target = 12
**Output:** "85 "
**Explanation:** The cost to paint the digit '8' is 7, and the digit '5' is 5. Then cost( "85 ") = 7 + 5 = 12.
**Example 3:**
**Input:** cost = \[2,4,6,2,4,6,4,4,4\], target = 5
**Output:** "0 "
**Explanation:** It is impossible to paint any integer with total cost equal to target.
**Constraints:**
* `cost.length == 9`
* `1 <= cost[i], target <= 5000` | Use the maximum length of words to determine the length of the returned answer. However, don't forget to remove trailing spaces. |
Python DP Solution | Easy to Understand | Faster than 75% | form-largest-integer-with-digits-that-add-up-to-target | 0 | 1 | # Approach\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe follow a simple take/leave type Memoization DFS where we choose either to take or leave a certain digit. If it\'s not possibe, we return a generic string ```"N"```. Then we compare strings formed in both the cases and return the largest one\n\n\n\n\n# Code\n```\nclass Solution:\n def largestNumber(self, cost: List[int], target: int) -> str:\n sz = len(cost)\n costToDigit = defaultdict(list)\n for digit in range(sz - 1, -1, -1):\n costToDigit[cost[digit]].append(digit)\n \n @cache\n def dp(digit: int, target: int) -> int:\n if target == 0: return ""\n if digit == sz or target < 0: return "N"\n leave = dp(digit + 1, target)\n take = dp(digit, target - cost[digit]) + str(costToDigit[cost[digit]][0] + 1)\n res = []\n if "N" not in leave: \n res.append(leave)\n if "N" not in take: \n res.append(take)\n if not res: return "N"\n if len(res) == 1: return res[0]\n if len(leave) > len(take):\n return leave\n elif len(take) > len(leave):\n return take\n else:\n return leave if leave > take else take\n \n ans = dp(0, target)\n return "".join(sorted(ans, reverse = True)) if ans != "N" else "0"\n``` | 0 | Given two positive integers `n` and `k`, the binary string `Sn` is formed as follows:
* `S1 = "0 "`
* `Si = Si - 1 + "1 " + reverse(invert(Si - 1))` for `i > 1`
Where `+` denotes the concatenation operation, `reverse(x)` returns the reversed string `x`, and `invert(x)` inverts all the bits in `x` (`0` changes to `1` and `1` changes to `0`).
For example, the first four strings in the above sequence are:
* `S1 = "0 "`
* `S2 = "0**1**1 "`
* `S3 = "011**1**001 "`
* `S4 = "0111001**1**0110001 "`
Return _the_ `kth` _bit_ _in_ `Sn`. It is guaranteed that `k` is valid for the given `n`.
**Example 1:**
**Input:** n = 3, k = 1
**Output:** "0 "
**Explanation:** S3 is "**0**111001 ".
The 1st bit is "0 ".
**Example 2:**
**Input:** n = 4, k = 11
**Output:** "1 "
**Explanation:** S4 is "0111001101**1**0001 ".
The 11th bit is "1 ".
**Constraints:**
* `1 <= n <= 20`
* `1 <= k <= 2n - 1` | Use dynamic programming to find the maximum digits to paint given a total cost. Build the largest number possible using this DP table. |
Easy python solution 🎉 | number-of-students-doing-homework-at-a-given-time | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->First we run a loop till the length of any one the given array.Then as we know the length of both the arrays are equal so we check the elements of both array at once and check if we find our queryTime in between the range of these elements.Once we find our queryTime we increase our count value and return it.\n\n# Complexity\n- Time complexity:44ms\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->Beats 56.12%of users with Python3\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->Beats 68.62%of users with Python3\n\n# Code\n```\nclass Solution:\n def busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n c=0\n for i in range(0,len(startTime)):\n if(queryTime in range(startTime[i],endTime[i]+1)):\n c+=1\n return c\n``` | 9 | Given two integer arrays `startTime` and `endTime` and given an integer `queryTime`.
The `ith` student started doing their homework at the time `startTime[i]` and finished it at time `endTime[i]`.
Return _the number of students_ doing their homework at time `queryTime`. More formally, return the number of students where `queryTime` lays in the interval `[startTime[i], endTime[i]]` inclusive.
**Example 1:**
**Input:** startTime = \[1,2,3\], endTime = \[3,2,7\], queryTime = 4
**Output:** 1
**Explanation:** We have 3 students where:
The first student started doing homework at time 1 and finished at time 3 and wasn't doing anything at time 4.
The second student started doing homework at time 2 and finished at time 2 and also wasn't doing anything at time 4.
The third student started doing homework at time 3 and finished at time 7 and was the only student doing homework at time 4.
**Example 2:**
**Input:** startTime = \[4\], endTime = \[4\], queryTime = 4
**Output:** 1
**Explanation:** The only student was doing their homework at the queryTime.
**Constraints:**
* `startTime.length == endTime.length`
* `1 <= startTime.length <= 100`
* `1 <= startTime[i] <= endTime[i] <= 1000`
* `1 <= queryTime <= 1000` | Use the DFS to reconstruct the tree such that no leaf node is equal to the target. If the leaf node is equal to the target, return an empty object instead. |
Easy python solution 🎉 | number-of-students-doing-homework-at-a-given-time | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->First we run a loop till the length of any one the given array.Then as we know the length of both the arrays are equal so we check the elements of both array at once and check if we find our queryTime in between the range of these elements.Once we find our queryTime we increase our count value and return it.\n\n# Complexity\n- Time complexity:44ms\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->Beats 56.12%of users with Python3\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->Beats 68.62%of users with Python3\n\n# Code\n```\nclass Solution:\n def busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n c=0\n for i in range(0,len(startTime)):\n if(queryTime in range(startTime[i],endTime[i]+1)):\n c+=1\n return c\n``` | 9 | Given an integer `n` and an integer array `rounds`. We have a circular track which consists of `n` sectors labeled from `1` to `n`. A marathon will be held on this track, the marathon consists of `m` rounds. The `ith` round starts at sector `rounds[i - 1]` and ends at sector `rounds[i]`. For example, round 1 starts at sector `rounds[0]` and ends at sector `rounds[1]`
Return _an array of the most visited sectors_ sorted in **ascending** order.
Notice that you circulate the track in ascending order of sector numbers in the counter-clockwise direction (See the first example).
**Example 1:**
**Input:** n = 4, rounds = \[1,3,1,2\]
**Output:** \[1,2\]
**Explanation:** The marathon starts at sector 1. The order of the visited sectors is as follows:
1 --> 2 --> 3 (end of round 1) --> 4 --> 1 (end of round 2) --> 2 (end of round 3 and the marathon)
We can see that both sectors 1 and 2 are visited twice and they are the most visited sectors. Sectors 3 and 4 are visited only once.
**Example 2:**
**Input:** n = 2, rounds = \[2,1,2,1,2,1,2,1,2\]
**Output:** \[2\]
**Example 3:**
**Input:** n = 7, rounds = \[1,3,5,7\]
**Output:** \[1,2,3,4,5,6,7\]
**Constraints:**
* `2 <= n <= 100`
* `1 <= m <= 100`
* `rounds.length == m + 1`
* `1 <= rounds[i] <= n`
* `rounds[i] != rounds[i + 1]` for `0 <= i < m` | Imagine that startTime[i] and endTime[i] form an interval (i.e. [startTime[i], endTime[i]]). The answer is how many times the queryTime laid in those mentioned intervals. |
Very Simple Python ||O(N) | number-of-students-doing-homework-at-a-given-time | 0 | 1 | Time Complexcity O(N)\nSpace Complexcity O(1)\n```\nclass Solution:\n def busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n count=0\n n=len(endTime)\n for i in range(n):\n if endTime[i]>=queryTime and startTime[i]<=queryTime:\n count+=1\n return count\n \n \n``` | 2 | Given two integer arrays `startTime` and `endTime` and given an integer `queryTime`.
The `ith` student started doing their homework at the time `startTime[i]` and finished it at time `endTime[i]`.
Return _the number of students_ doing their homework at time `queryTime`. More formally, return the number of students where `queryTime` lays in the interval `[startTime[i], endTime[i]]` inclusive.
**Example 1:**
**Input:** startTime = \[1,2,3\], endTime = \[3,2,7\], queryTime = 4
**Output:** 1
**Explanation:** We have 3 students where:
The first student started doing homework at time 1 and finished at time 3 and wasn't doing anything at time 4.
The second student started doing homework at time 2 and finished at time 2 and also wasn't doing anything at time 4.
The third student started doing homework at time 3 and finished at time 7 and was the only student doing homework at time 4.
**Example 2:**
**Input:** startTime = \[4\], endTime = \[4\], queryTime = 4
**Output:** 1
**Explanation:** The only student was doing their homework at the queryTime.
**Constraints:**
* `startTime.length == endTime.length`
* `1 <= startTime.length <= 100`
* `1 <= startTime[i] <= endTime[i] <= 1000`
* `1 <= queryTime <= 1000` | Use the DFS to reconstruct the tree such that no leaf node is equal to the target. If the leaf node is equal to the target, return an empty object instead. |
Very Simple Python ||O(N) | number-of-students-doing-homework-at-a-given-time | 0 | 1 | Time Complexcity O(N)\nSpace Complexcity O(1)\n```\nclass Solution:\n def busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n count=0\n n=len(endTime)\n for i in range(n):\n if endTime[i]>=queryTime and startTime[i]<=queryTime:\n count+=1\n return count\n \n \n``` | 2 | Given an integer `n` and an integer array `rounds`. We have a circular track which consists of `n` sectors labeled from `1` to `n`. A marathon will be held on this track, the marathon consists of `m` rounds. The `ith` round starts at sector `rounds[i - 1]` and ends at sector `rounds[i]`. For example, round 1 starts at sector `rounds[0]` and ends at sector `rounds[1]`
Return _an array of the most visited sectors_ sorted in **ascending** order.
Notice that you circulate the track in ascending order of sector numbers in the counter-clockwise direction (See the first example).
**Example 1:**
**Input:** n = 4, rounds = \[1,3,1,2\]
**Output:** \[1,2\]
**Explanation:** The marathon starts at sector 1. The order of the visited sectors is as follows:
1 --> 2 --> 3 (end of round 1) --> 4 --> 1 (end of round 2) --> 2 (end of round 3 and the marathon)
We can see that both sectors 1 and 2 are visited twice and they are the most visited sectors. Sectors 3 and 4 are visited only once.
**Example 2:**
**Input:** n = 2, rounds = \[2,1,2,1,2,1,2,1,2\]
**Output:** \[2\]
**Example 3:**
**Input:** n = 7, rounds = \[1,3,5,7\]
**Output:** \[1,2,3,4,5,6,7\]
**Constraints:**
* `2 <= n <= 100`
* `1 <= m <= 100`
* `rounds.length == m + 1`
* `1 <= rounds[i] <= n`
* `rounds[i] != rounds[i + 1]` for `0 <= i < m` | Imagine that startTime[i] and endTime[i] form an interval (i.e. [startTime[i], endTime[i]]). The answer is how many times the queryTime laid in those mentioned intervals. |
Python Simple Solution | Zip & Iterate - 86% 37ms | number-of-students-doing-homework-at-a-given-time | 0 | 1 | There are a lot of different ways to solve this problem, including a multitude of one liners, but I didn\'t see this method posted. Generally a fan of one liners as it helps condense code a bit, but there comes a point where they\'re *too* long.\n```\nclass Solution:\n def busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n count = 0 # If a value meets the criteria, one will be added here.\n\n for x, y in zip(startTime, endTime): # Zipping the two lists to allow us to iterate over them using x,y as our variables.\n if x <= queryTime <= y: # Checking if the queryTime number is between startTime and endTime, adding one to count if it is.\n count += 1\n return count # Returning the value in count\n``` | 5 | Given two integer arrays `startTime` and `endTime` and given an integer `queryTime`.
The `ith` student started doing their homework at the time `startTime[i]` and finished it at time `endTime[i]`.
Return _the number of students_ doing their homework at time `queryTime`. More formally, return the number of students where `queryTime` lays in the interval `[startTime[i], endTime[i]]` inclusive.
**Example 1:**
**Input:** startTime = \[1,2,3\], endTime = \[3,2,7\], queryTime = 4
**Output:** 1
**Explanation:** We have 3 students where:
The first student started doing homework at time 1 and finished at time 3 and wasn't doing anything at time 4.
The second student started doing homework at time 2 and finished at time 2 and also wasn't doing anything at time 4.
The third student started doing homework at time 3 and finished at time 7 and was the only student doing homework at time 4.
**Example 2:**
**Input:** startTime = \[4\], endTime = \[4\], queryTime = 4
**Output:** 1
**Explanation:** The only student was doing their homework at the queryTime.
**Constraints:**
* `startTime.length == endTime.length`
* `1 <= startTime.length <= 100`
* `1 <= startTime[i] <= endTime[i] <= 1000`
* `1 <= queryTime <= 1000` | Use the DFS to reconstruct the tree such that no leaf node is equal to the target. If the leaf node is equal to the target, return an empty object instead. |
Python Simple Solution | Zip & Iterate - 86% 37ms | number-of-students-doing-homework-at-a-given-time | 0 | 1 | There are a lot of different ways to solve this problem, including a multitude of one liners, but I didn\'t see this method posted. Generally a fan of one liners as it helps condense code a bit, but there comes a point where they\'re *too* long.\n```\nclass Solution:\n def busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n count = 0 # If a value meets the criteria, one will be added here.\n\n for x, y in zip(startTime, endTime): # Zipping the two lists to allow us to iterate over them using x,y as our variables.\n if x <= queryTime <= y: # Checking if the queryTime number is between startTime and endTime, adding one to count if it is.\n count += 1\n return count # Returning the value in count\n``` | 5 | Given an integer `n` and an integer array `rounds`. We have a circular track which consists of `n` sectors labeled from `1` to `n`. A marathon will be held on this track, the marathon consists of `m` rounds. The `ith` round starts at sector `rounds[i - 1]` and ends at sector `rounds[i]`. For example, round 1 starts at sector `rounds[0]` and ends at sector `rounds[1]`
Return _an array of the most visited sectors_ sorted in **ascending** order.
Notice that you circulate the track in ascending order of sector numbers in the counter-clockwise direction (See the first example).
**Example 1:**
**Input:** n = 4, rounds = \[1,3,1,2\]
**Output:** \[1,2\]
**Explanation:** The marathon starts at sector 1. The order of the visited sectors is as follows:
1 --> 2 --> 3 (end of round 1) --> 4 --> 1 (end of round 2) --> 2 (end of round 3 and the marathon)
We can see that both sectors 1 and 2 are visited twice and they are the most visited sectors. Sectors 3 and 4 are visited only once.
**Example 2:**
**Input:** n = 2, rounds = \[2,1,2,1,2,1,2,1,2\]
**Output:** \[2\]
**Example 3:**
**Input:** n = 7, rounds = \[1,3,5,7\]
**Output:** \[1,2,3,4,5,6,7\]
**Constraints:**
* `2 <= n <= 100`
* `1 <= m <= 100`
* `rounds.length == m + 1`
* `1 <= rounds[i] <= n`
* `rounds[i] != rounds[i + 1]` for `0 <= i < m` | Imagine that startTime[i] and endTime[i] form an interval (i.e. [startTime[i], endTime[i]]). The answer is how many times the queryTime laid in those mentioned intervals. |
Python One-Liner | number-of-students-doing-homework-at-a-given-time | 0 | 1 | ```\nclass Solution:\n def busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n return sum([queryTime>=i and queryTime<=j for i, j in zip(startTime, endTime)])\n \n``` | 11 | Given two integer arrays `startTime` and `endTime` and given an integer `queryTime`.
The `ith` student started doing their homework at the time `startTime[i]` and finished it at time `endTime[i]`.
Return _the number of students_ doing their homework at time `queryTime`. More formally, return the number of students where `queryTime` lays in the interval `[startTime[i], endTime[i]]` inclusive.
**Example 1:**
**Input:** startTime = \[1,2,3\], endTime = \[3,2,7\], queryTime = 4
**Output:** 1
**Explanation:** We have 3 students where:
The first student started doing homework at time 1 and finished at time 3 and wasn't doing anything at time 4.
The second student started doing homework at time 2 and finished at time 2 and also wasn't doing anything at time 4.
The third student started doing homework at time 3 and finished at time 7 and was the only student doing homework at time 4.
**Example 2:**
**Input:** startTime = \[4\], endTime = \[4\], queryTime = 4
**Output:** 1
**Explanation:** The only student was doing their homework at the queryTime.
**Constraints:**
* `startTime.length == endTime.length`
* `1 <= startTime.length <= 100`
* `1 <= startTime[i] <= endTime[i] <= 1000`
* `1 <= queryTime <= 1000` | Use the DFS to reconstruct the tree such that no leaf node is equal to the target. If the leaf node is equal to the target, return an empty object instead. |
Python One-Liner | number-of-students-doing-homework-at-a-given-time | 0 | 1 | ```\nclass Solution:\n def busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n return sum([queryTime>=i and queryTime<=j for i, j in zip(startTime, endTime)])\n \n``` | 11 | Given an integer `n` and an integer array `rounds`. We have a circular track which consists of `n` sectors labeled from `1` to `n`. A marathon will be held on this track, the marathon consists of `m` rounds. The `ith` round starts at sector `rounds[i - 1]` and ends at sector `rounds[i]`. For example, round 1 starts at sector `rounds[0]` and ends at sector `rounds[1]`
Return _an array of the most visited sectors_ sorted in **ascending** order.
Notice that you circulate the track in ascending order of sector numbers in the counter-clockwise direction (See the first example).
**Example 1:**
**Input:** n = 4, rounds = \[1,3,1,2\]
**Output:** \[1,2\]
**Explanation:** The marathon starts at sector 1. The order of the visited sectors is as follows:
1 --> 2 --> 3 (end of round 1) --> 4 --> 1 (end of round 2) --> 2 (end of round 3 and the marathon)
We can see that both sectors 1 and 2 are visited twice and they are the most visited sectors. Sectors 3 and 4 are visited only once.
**Example 2:**
**Input:** n = 2, rounds = \[2,1,2,1,2,1,2,1,2\]
**Output:** \[2\]
**Example 3:**
**Input:** n = 7, rounds = \[1,3,5,7\]
**Output:** \[1,2,3,4,5,6,7\]
**Constraints:**
* `2 <= n <= 100`
* `1 <= m <= 100`
* `rounds.length == m + 1`
* `1 <= rounds[i] <= n`
* `rounds[i] != rounds[i + 1]` for `0 <= i < m` | Imagine that startTime[i] and endTime[i] form an interval (i.e. [startTime[i], endTime[i]]). The answer is how many times the queryTime laid in those mentioned intervals. |
Python one line solution | number-of-students-doing-homework-at-a-given-time | 0 | 1 | **Python :**\n\n```\ndef busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n\treturn sum([i <= queryTime and queryTime <= j for i, j in zip(startTime, endTime)])\n```\n\n**Like it ? please upvote !** | 4 | Given two integer arrays `startTime` and `endTime` and given an integer `queryTime`.
The `ith` student started doing their homework at the time `startTime[i]` and finished it at time `endTime[i]`.
Return _the number of students_ doing their homework at time `queryTime`. More formally, return the number of students where `queryTime` lays in the interval `[startTime[i], endTime[i]]` inclusive.
**Example 1:**
**Input:** startTime = \[1,2,3\], endTime = \[3,2,7\], queryTime = 4
**Output:** 1
**Explanation:** We have 3 students where:
The first student started doing homework at time 1 and finished at time 3 and wasn't doing anything at time 4.
The second student started doing homework at time 2 and finished at time 2 and also wasn't doing anything at time 4.
The third student started doing homework at time 3 and finished at time 7 and was the only student doing homework at time 4.
**Example 2:**
**Input:** startTime = \[4\], endTime = \[4\], queryTime = 4
**Output:** 1
**Explanation:** The only student was doing their homework at the queryTime.
**Constraints:**
* `startTime.length == endTime.length`
* `1 <= startTime.length <= 100`
* `1 <= startTime[i] <= endTime[i] <= 1000`
* `1 <= queryTime <= 1000` | Use the DFS to reconstruct the tree such that no leaf node is equal to the target. If the leaf node is equal to the target, return an empty object instead. |
Python one line solution | number-of-students-doing-homework-at-a-given-time | 0 | 1 | **Python :**\n\n```\ndef busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n\treturn sum([i <= queryTime and queryTime <= j for i, j in zip(startTime, endTime)])\n```\n\n**Like it ? please upvote !** | 4 | Given an integer `n` and an integer array `rounds`. We have a circular track which consists of `n` sectors labeled from `1` to `n`. A marathon will be held on this track, the marathon consists of `m` rounds. The `ith` round starts at sector `rounds[i - 1]` and ends at sector `rounds[i]`. For example, round 1 starts at sector `rounds[0]` and ends at sector `rounds[1]`
Return _an array of the most visited sectors_ sorted in **ascending** order.
Notice that you circulate the track in ascending order of sector numbers in the counter-clockwise direction (See the first example).
**Example 1:**
**Input:** n = 4, rounds = \[1,3,1,2\]
**Output:** \[1,2\]
**Explanation:** The marathon starts at sector 1. The order of the visited sectors is as follows:
1 --> 2 --> 3 (end of round 1) --> 4 --> 1 (end of round 2) --> 2 (end of round 3 and the marathon)
We can see that both sectors 1 and 2 are visited twice and they are the most visited sectors. Sectors 3 and 4 are visited only once.
**Example 2:**
**Input:** n = 2, rounds = \[2,1,2,1,2,1,2,1,2\]
**Output:** \[2\]
**Example 3:**
**Input:** n = 7, rounds = \[1,3,5,7\]
**Output:** \[1,2,3,4,5,6,7\]
**Constraints:**
* `2 <= n <= 100`
* `1 <= m <= 100`
* `rounds.length == m + 1`
* `1 <= rounds[i] <= n`
* `rounds[i] != rounds[i + 1]` for `0 <= i < m` | Imagine that startTime[i] and endTime[i] form an interval (i.e. [startTime[i], endTime[i]]). The answer is how many times the queryTime laid in those mentioned intervals. |
Python simple solution | number-of-students-doing-homework-at-a-given-time | 0 | 1 | ```\nclass Solution:\n def busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n ans = 0\n for i in range(len(startTime)):\n if startTime[i] <= queryTime <= endTime[i]:\n ans += 1\n return ans\n``` | 1 | Given two integer arrays `startTime` and `endTime` and given an integer `queryTime`.
The `ith` student started doing their homework at the time `startTime[i]` and finished it at time `endTime[i]`.
Return _the number of students_ doing their homework at time `queryTime`. More formally, return the number of students where `queryTime` lays in the interval `[startTime[i], endTime[i]]` inclusive.
**Example 1:**
**Input:** startTime = \[1,2,3\], endTime = \[3,2,7\], queryTime = 4
**Output:** 1
**Explanation:** We have 3 students where:
The first student started doing homework at time 1 and finished at time 3 and wasn't doing anything at time 4.
The second student started doing homework at time 2 and finished at time 2 and also wasn't doing anything at time 4.
The third student started doing homework at time 3 and finished at time 7 and was the only student doing homework at time 4.
**Example 2:**
**Input:** startTime = \[4\], endTime = \[4\], queryTime = 4
**Output:** 1
**Explanation:** The only student was doing their homework at the queryTime.
**Constraints:**
* `startTime.length == endTime.length`
* `1 <= startTime.length <= 100`
* `1 <= startTime[i] <= endTime[i] <= 1000`
* `1 <= queryTime <= 1000` | Use the DFS to reconstruct the tree such that no leaf node is equal to the target. If the leaf node is equal to the target, return an empty object instead. |
Python simple solution | number-of-students-doing-homework-at-a-given-time | 0 | 1 | ```\nclass Solution:\n def busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n ans = 0\n for i in range(len(startTime)):\n if startTime[i] <= queryTime <= endTime[i]:\n ans += 1\n return ans\n``` | 1 | Given an integer `n` and an integer array `rounds`. We have a circular track which consists of `n` sectors labeled from `1` to `n`. A marathon will be held on this track, the marathon consists of `m` rounds. The `ith` round starts at sector `rounds[i - 1]` and ends at sector `rounds[i]`. For example, round 1 starts at sector `rounds[0]` and ends at sector `rounds[1]`
Return _an array of the most visited sectors_ sorted in **ascending** order.
Notice that you circulate the track in ascending order of sector numbers in the counter-clockwise direction (See the first example).
**Example 1:**
**Input:** n = 4, rounds = \[1,3,1,2\]
**Output:** \[1,2\]
**Explanation:** The marathon starts at sector 1. The order of the visited sectors is as follows:
1 --> 2 --> 3 (end of round 1) --> 4 --> 1 (end of round 2) --> 2 (end of round 3 and the marathon)
We can see that both sectors 1 and 2 are visited twice and they are the most visited sectors. Sectors 3 and 4 are visited only once.
**Example 2:**
**Input:** n = 2, rounds = \[2,1,2,1,2,1,2,1,2\]
**Output:** \[2\]
**Example 3:**
**Input:** n = 7, rounds = \[1,3,5,7\]
**Output:** \[1,2,3,4,5,6,7\]
**Constraints:**
* `2 <= n <= 100`
* `1 <= m <= 100`
* `rounds.length == m + 1`
* `1 <= rounds[i] <= n`
* `rounds[i] != rounds[i + 1]` for `0 <= i < m` | Imagine that startTime[i] and endTime[i] form an interval (i.e. [startTime[i], endTime[i]]). The answer is how many times the queryTime laid in those mentioned intervals. |
Simple python solution | number-of-students-doing-homework-at-a-given-time | 0 | 1 | ```\nclass Solution:\n def busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n count = 0\n for i in range(len(startTime)):\n if queryTime <= endTime[i] and queryTime >= startTime[i]:\n count += 1\n return count\n``` | 2 | Given two integer arrays `startTime` and `endTime` and given an integer `queryTime`.
The `ith` student started doing their homework at the time `startTime[i]` and finished it at time `endTime[i]`.
Return _the number of students_ doing their homework at time `queryTime`. More formally, return the number of students where `queryTime` lays in the interval `[startTime[i], endTime[i]]` inclusive.
**Example 1:**
**Input:** startTime = \[1,2,3\], endTime = \[3,2,7\], queryTime = 4
**Output:** 1
**Explanation:** We have 3 students where:
The first student started doing homework at time 1 and finished at time 3 and wasn't doing anything at time 4.
The second student started doing homework at time 2 and finished at time 2 and also wasn't doing anything at time 4.
The third student started doing homework at time 3 and finished at time 7 and was the only student doing homework at time 4.
**Example 2:**
**Input:** startTime = \[4\], endTime = \[4\], queryTime = 4
**Output:** 1
**Explanation:** The only student was doing their homework at the queryTime.
**Constraints:**
* `startTime.length == endTime.length`
* `1 <= startTime.length <= 100`
* `1 <= startTime[i] <= endTime[i] <= 1000`
* `1 <= queryTime <= 1000` | Use the DFS to reconstruct the tree such that no leaf node is equal to the target. If the leaf node is equal to the target, return an empty object instead. |
Simple python solution | number-of-students-doing-homework-at-a-given-time | 0 | 1 | ```\nclass Solution:\n def busyStudent(self, startTime: List[int], endTime: List[int], queryTime: int) -> int:\n count = 0\n for i in range(len(startTime)):\n if queryTime <= endTime[i] and queryTime >= startTime[i]:\n count += 1\n return count\n``` | 2 | Given an integer `n` and an integer array `rounds`. We have a circular track which consists of `n` sectors labeled from `1` to `n`. A marathon will be held on this track, the marathon consists of `m` rounds. The `ith` round starts at sector `rounds[i - 1]` and ends at sector `rounds[i]`. For example, round 1 starts at sector `rounds[0]` and ends at sector `rounds[1]`
Return _an array of the most visited sectors_ sorted in **ascending** order.
Notice that you circulate the track in ascending order of sector numbers in the counter-clockwise direction (See the first example).
**Example 1:**
**Input:** n = 4, rounds = \[1,3,1,2\]
**Output:** \[1,2\]
**Explanation:** The marathon starts at sector 1. The order of the visited sectors is as follows:
1 --> 2 --> 3 (end of round 1) --> 4 --> 1 (end of round 2) --> 2 (end of round 3 and the marathon)
We can see that both sectors 1 and 2 are visited twice and they are the most visited sectors. Sectors 3 and 4 are visited only once.
**Example 2:**
**Input:** n = 2, rounds = \[2,1,2,1,2,1,2,1,2\]
**Output:** \[2\]
**Example 3:**
**Input:** n = 7, rounds = \[1,3,5,7\]
**Output:** \[1,2,3,4,5,6,7\]
**Constraints:**
* `2 <= n <= 100`
* `1 <= m <= 100`
* `rounds.length == m + 1`
* `1 <= rounds[i] <= n`
* `rounds[i] != rounds[i + 1]` for `0 <= i < m` | Imagine that startTime[i] and endTime[i] form an interval (i.e. [startTime[i], endTime[i]]). The answer is how many times the queryTime laid in those mentioned intervals. |
[Python 3] Simple | Easy To Understand | Fast | rearrange-words-in-a-sentence | 0 | 1 | # Code\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n a = []\n for x in text.split(" "):\n a.append(x.lower())\n return " ".join(sorted(a, key=len)).capitalize()\n```\n\n# One Liner\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n return " ".join(sorted(text.split(" "), key=len)).capitalize()\n``` | 2 | Given a sentence `text` (A _sentence_ is a string of space-separated words) in the following format:
* First letter is in upper case.
* Each word in `text` are separated by a single space.
Your task is to rearrange the words in text such that all words are rearranged in an increasing order of their lengths. If two words have the same length, arrange them in their original order.
Return the new text following the format shown above.
**Example 1:**
**Input:** text = "Leetcode is cool "
**Output:** "Is cool leetcode "
**Explanation:** There are 3 words, "Leetcode " of length 8, "is " of length 2 and "cool " of length 4.
Output is ordered by length and the new first word starts with capital letter.
**Example 2:**
**Input:** text = "Keep calm and code on "
**Output:** "On and keep calm code "
**Explanation:** Output is ordered as follows:
"On " 2 letters.
"and " 3 letters.
"keep " 4 letters in case of tie order by position in original text.
"calm " 4 letters.
"code " 4 letters.
**Example 3:**
**Input:** text = "To be or not to be "
**Output:** "To be or to be not "
**Constraints:**
* `text` begins with a capital letter and then contains lowercase letters and single space between words.
* `1 <= text.length <= 10^5` | Create intervals of the area covered by each tap, sort intervals by the left end. We need to cover the interval [0, n]. we can start with the first interval and out of all intervals that intersect with it we choose the one that covers the farthest point to the right. What if there is a gap between intervals that is not covered ? we should stop and return -1 as there is some interval that cannot be covered. |
[Python 3] Simple | Easy To Understand | Fast | rearrange-words-in-a-sentence | 0 | 1 | # Code\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n a = []\n for x in text.split(" "):\n a.append(x.lower())\n return " ".join(sorted(a, key=len)).capitalize()\n```\n\n# One Liner\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n return " ".join(sorted(text.split(" "), key=len)).capitalize()\n``` | 2 | There are `3n` piles of coins of varying size, you and your friends will take piles of coins as follows:
* In each step, you will choose **any** `3` piles of coins (not necessarily consecutive).
* Of your choice, Alice will pick the pile with the maximum number of coins.
* You will pick the next pile with the maximum number of coins.
* Your friend Bob will pick the last pile.
* Repeat until there are no more piles of coins.
Given an array of integers `piles` where `piles[i]` is the number of coins in the `ith` pile.
Return the maximum number of coins that you can have.
**Example 1:**
**Input:** piles = \[2,4,1,2,7,8\]
**Output:** 9
**Explanation:** Choose the triplet (2, 7, 8), Alice Pick the pile with 8 coins, you the pile with **7** coins and Bob the last one.
Choose the triplet (1, 2, 4), Alice Pick the pile with 4 coins, you the pile with **2** coins and Bob the last one.
The maximum number of coins which you can have are: 7 + 2 = 9.
On the other hand if we choose this arrangement (1, **2**, 8), (2, **4**, 7) you only get 2 + 4 = 6 coins which is not optimal.
**Example 2:**
**Input:** piles = \[2,4,5\]
**Output:** 4
**Example 3:**
**Input:** piles = \[9,8,7,6,5,1,2,3,4\]
**Output:** 18
**Constraints:**
* `3 <= piles.length <= 105`
* `piles.length % 3 == 0`
* `1 <= piles[i] <= 104` | Store each word and their relative position. Then, sort them by length of words in case of tie by their original order. |
[Python3] one line | rearrange-words-in-a-sentence | 0 | 1 | A few string operations chained together to get the job done. \n\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n return " ".join(sorted(text.split(), key=len)).capitalize()\n``` | 45 | Given a sentence `text` (A _sentence_ is a string of space-separated words) in the following format:
* First letter is in upper case.
* Each word in `text` are separated by a single space.
Your task is to rearrange the words in text such that all words are rearranged in an increasing order of their lengths. If two words have the same length, arrange them in their original order.
Return the new text following the format shown above.
**Example 1:**
**Input:** text = "Leetcode is cool "
**Output:** "Is cool leetcode "
**Explanation:** There are 3 words, "Leetcode " of length 8, "is " of length 2 and "cool " of length 4.
Output is ordered by length and the new first word starts with capital letter.
**Example 2:**
**Input:** text = "Keep calm and code on "
**Output:** "On and keep calm code "
**Explanation:** Output is ordered as follows:
"On " 2 letters.
"and " 3 letters.
"keep " 4 letters in case of tie order by position in original text.
"calm " 4 letters.
"code " 4 letters.
**Example 3:**
**Input:** text = "To be or not to be "
**Output:** "To be or to be not "
**Constraints:**
* `text` begins with a capital letter and then contains lowercase letters and single space between words.
* `1 <= text.length <= 10^5` | Create intervals of the area covered by each tap, sort intervals by the left end. We need to cover the interval [0, n]. we can start with the first interval and out of all intervals that intersect with it we choose the one that covers the farthest point to the right. What if there is a gap between intervals that is not covered ? we should stop and return -1 as there is some interval that cannot be covered. |
[Python3] one line | rearrange-words-in-a-sentence | 0 | 1 | A few string operations chained together to get the job done. \n\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n return " ".join(sorted(text.split(), key=len)).capitalize()\n``` | 45 | There are `3n` piles of coins of varying size, you and your friends will take piles of coins as follows:
* In each step, you will choose **any** `3` piles of coins (not necessarily consecutive).
* Of your choice, Alice will pick the pile with the maximum number of coins.
* You will pick the next pile with the maximum number of coins.
* Your friend Bob will pick the last pile.
* Repeat until there are no more piles of coins.
Given an array of integers `piles` where `piles[i]` is the number of coins in the `ith` pile.
Return the maximum number of coins that you can have.
**Example 1:**
**Input:** piles = \[2,4,1,2,7,8\]
**Output:** 9
**Explanation:** Choose the triplet (2, 7, 8), Alice Pick the pile with 8 coins, you the pile with **7** coins and Bob the last one.
Choose the triplet (1, 2, 4), Alice Pick the pile with 4 coins, you the pile with **2** coins and Bob the last one.
The maximum number of coins which you can have are: 7 + 2 = 9.
On the other hand if we choose this arrangement (1, **2**, 8), (2, **4**, 7) you only get 2 + 4 = 6 coins which is not optimal.
**Example 2:**
**Input:** piles = \[2,4,5\]
**Output:** 4
**Example 3:**
**Input:** piles = \[9,8,7,6,5,1,2,3,4\]
**Output:** 18
**Constraints:**
* `3 <= piles.length <= 105`
* `piles.length % 3 == 0`
* `1 <= piles[i] <= 104` | Store each word and their relative position. Then, sort them by length of words in case of tie by their original order. |
Python | Easy Solution✅ | rearrange-words-in-a-sentence | 0 | 1 | ```\ndef arrangeWords(self, text: str) -> str:\n seen = {}\n output = ""\n text_list = text.split()\n for word in text_list:\n n = len(word)\n if n in seen:\n value = seen[n]\n value.append(word.lower())\n seen[n] = value\n else:\n seen[n] = [word]\n # seen = {4: [\'Keep\', \'calm\', \'code\'], 3: [\'and\'], 2: [\'on\']}\n for key in sorted(seen):\n output += " ".join(seen[key]) + " "\n return output[:len(output)-1].capitalize()\n``` | 7 | Given a sentence `text` (A _sentence_ is a string of space-separated words) in the following format:
* First letter is in upper case.
* Each word in `text` are separated by a single space.
Your task is to rearrange the words in text such that all words are rearranged in an increasing order of their lengths. If two words have the same length, arrange them in their original order.
Return the new text following the format shown above.
**Example 1:**
**Input:** text = "Leetcode is cool "
**Output:** "Is cool leetcode "
**Explanation:** There are 3 words, "Leetcode " of length 8, "is " of length 2 and "cool " of length 4.
Output is ordered by length and the new first word starts with capital letter.
**Example 2:**
**Input:** text = "Keep calm and code on "
**Output:** "On and keep calm code "
**Explanation:** Output is ordered as follows:
"On " 2 letters.
"and " 3 letters.
"keep " 4 letters in case of tie order by position in original text.
"calm " 4 letters.
"code " 4 letters.
**Example 3:**
**Input:** text = "To be or not to be "
**Output:** "To be or to be not "
**Constraints:**
* `text` begins with a capital letter and then contains lowercase letters and single space between words.
* `1 <= text.length <= 10^5` | Create intervals of the area covered by each tap, sort intervals by the left end. We need to cover the interval [0, n]. we can start with the first interval and out of all intervals that intersect with it we choose the one that covers the farthest point to the right. What if there is a gap between intervals that is not covered ? we should stop and return -1 as there is some interval that cannot be covered. |
Python | Easy Solution✅ | rearrange-words-in-a-sentence | 0 | 1 | ```\ndef arrangeWords(self, text: str) -> str:\n seen = {}\n output = ""\n text_list = text.split()\n for word in text_list:\n n = len(word)\n if n in seen:\n value = seen[n]\n value.append(word.lower())\n seen[n] = value\n else:\n seen[n] = [word]\n # seen = {4: [\'Keep\', \'calm\', \'code\'], 3: [\'and\'], 2: [\'on\']}\n for key in sorted(seen):\n output += " ".join(seen[key]) + " "\n return output[:len(output)-1].capitalize()\n``` | 7 | There are `3n` piles of coins of varying size, you and your friends will take piles of coins as follows:
* In each step, you will choose **any** `3` piles of coins (not necessarily consecutive).
* Of your choice, Alice will pick the pile with the maximum number of coins.
* You will pick the next pile with the maximum number of coins.
* Your friend Bob will pick the last pile.
* Repeat until there are no more piles of coins.
Given an array of integers `piles` where `piles[i]` is the number of coins in the `ith` pile.
Return the maximum number of coins that you can have.
**Example 1:**
**Input:** piles = \[2,4,1,2,7,8\]
**Output:** 9
**Explanation:** Choose the triplet (2, 7, 8), Alice Pick the pile with 8 coins, you the pile with **7** coins and Bob the last one.
Choose the triplet (1, 2, 4), Alice Pick the pile with 4 coins, you the pile with **2** coins and Bob the last one.
The maximum number of coins which you can have are: 7 + 2 = 9.
On the other hand if we choose this arrangement (1, **2**, 8), (2, **4**, 7) you only get 2 + 4 = 6 coins which is not optimal.
**Example 2:**
**Input:** piles = \[2,4,5\]
**Output:** 4
**Example 3:**
**Input:** piles = \[9,8,7,6,5,1,2,3,4\]
**Output:** 18
**Constraints:**
* `3 <= piles.length <= 105`
* `piles.length % 3 == 0`
* `1 <= piles[i] <= 104` | Store each word and their relative position. Then, sort them by length of words in case of tie by their original order. |
Python Easy Solution Using Dictionary and Sorting | rearrange-words-in-a-sentence | 0 | 1 | ```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n a=text.split()\n d,t={},\'\'\n for i in a:\n l=len(i)\n if l in d:\n d[l]+=" "+i\n else:\n d[l]=i\n for i in sorted(d):\n t+=" "+d[i]\n t=t.lstrip().capitalize()\n return t\n```\n\n**Upvote if you like the solution or ask if there is any query**\n\t | 2 | Given a sentence `text` (A _sentence_ is a string of space-separated words) in the following format:
* First letter is in upper case.
* Each word in `text` are separated by a single space.
Your task is to rearrange the words in text such that all words are rearranged in an increasing order of their lengths. If two words have the same length, arrange them in their original order.
Return the new text following the format shown above.
**Example 1:**
**Input:** text = "Leetcode is cool "
**Output:** "Is cool leetcode "
**Explanation:** There are 3 words, "Leetcode " of length 8, "is " of length 2 and "cool " of length 4.
Output is ordered by length and the new first word starts with capital letter.
**Example 2:**
**Input:** text = "Keep calm and code on "
**Output:** "On and keep calm code "
**Explanation:** Output is ordered as follows:
"On " 2 letters.
"and " 3 letters.
"keep " 4 letters in case of tie order by position in original text.
"calm " 4 letters.
"code " 4 letters.
**Example 3:**
**Input:** text = "To be or not to be "
**Output:** "To be or to be not "
**Constraints:**
* `text` begins with a capital letter and then contains lowercase letters and single space between words.
* `1 <= text.length <= 10^5` | Create intervals of the area covered by each tap, sort intervals by the left end. We need to cover the interval [0, n]. we can start with the first interval and out of all intervals that intersect with it we choose the one that covers the farthest point to the right. What if there is a gap between intervals that is not covered ? we should stop and return -1 as there is some interval that cannot be covered. |
Python Easy Solution Using Dictionary and Sorting | rearrange-words-in-a-sentence | 0 | 1 | ```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n a=text.split()\n d,t={},\'\'\n for i in a:\n l=len(i)\n if l in d:\n d[l]+=" "+i\n else:\n d[l]=i\n for i in sorted(d):\n t+=" "+d[i]\n t=t.lstrip().capitalize()\n return t\n```\n\n**Upvote if you like the solution or ask if there is any query**\n\t | 2 | There are `3n` piles of coins of varying size, you and your friends will take piles of coins as follows:
* In each step, you will choose **any** `3` piles of coins (not necessarily consecutive).
* Of your choice, Alice will pick the pile with the maximum number of coins.
* You will pick the next pile with the maximum number of coins.
* Your friend Bob will pick the last pile.
* Repeat until there are no more piles of coins.
Given an array of integers `piles` where `piles[i]` is the number of coins in the `ith` pile.
Return the maximum number of coins that you can have.
**Example 1:**
**Input:** piles = \[2,4,1,2,7,8\]
**Output:** 9
**Explanation:** Choose the triplet (2, 7, 8), Alice Pick the pile with 8 coins, you the pile with **7** coins and Bob the last one.
Choose the triplet (1, 2, 4), Alice Pick the pile with 4 coins, you the pile with **2** coins and Bob the last one.
The maximum number of coins which you can have are: 7 + 2 = 9.
On the other hand if we choose this arrangement (1, **2**, 8), (2, **4**, 7) you only get 2 + 4 = 6 coins which is not optimal.
**Example 2:**
**Input:** piles = \[2,4,5\]
**Output:** 4
**Example 3:**
**Input:** piles = \[9,8,7,6,5,1,2,3,4\]
**Output:** 18
**Constraints:**
* `3 <= piles.length <= 105`
* `piles.length % 3 == 0`
* `1 <= piles[i] <= 104` | Store each word and their relative position. Then, sort them by length of words in case of tie by their original order. |
Python 3 -- Simple -- Readable -- Fast | rearrange-words-in-a-sentence | 0 | 1 | ```python\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n \n # convert string to an array\n arr = text.split()\n \n # convert the first letter of the array to a lower case letter\n arr[0] = arr[0].lower()\n \n # sort array by length\n arr.sort(key=len)\n \n # convert the first letter of the array to an upper case letter\n arr[0] = arr[0].capitalize()\n \n # convert array to string\n return " ".join(arr)\n``` | 8 | Given a sentence `text` (A _sentence_ is a string of space-separated words) in the following format:
* First letter is in upper case.
* Each word in `text` are separated by a single space.
Your task is to rearrange the words in text such that all words are rearranged in an increasing order of their lengths. If two words have the same length, arrange them in their original order.
Return the new text following the format shown above.
**Example 1:**
**Input:** text = "Leetcode is cool "
**Output:** "Is cool leetcode "
**Explanation:** There are 3 words, "Leetcode " of length 8, "is " of length 2 and "cool " of length 4.
Output is ordered by length and the new first word starts with capital letter.
**Example 2:**
**Input:** text = "Keep calm and code on "
**Output:** "On and keep calm code "
**Explanation:** Output is ordered as follows:
"On " 2 letters.
"and " 3 letters.
"keep " 4 letters in case of tie order by position in original text.
"calm " 4 letters.
"code " 4 letters.
**Example 3:**
**Input:** text = "To be or not to be "
**Output:** "To be or to be not "
**Constraints:**
* `text` begins with a capital letter and then contains lowercase letters and single space between words.
* `1 <= text.length <= 10^5` | Create intervals of the area covered by each tap, sort intervals by the left end. We need to cover the interval [0, n]. we can start with the first interval and out of all intervals that intersect with it we choose the one that covers the farthest point to the right. What if there is a gap between intervals that is not covered ? we should stop and return -1 as there is some interval that cannot be covered. |
Python 3 -- Simple -- Readable -- Fast | rearrange-words-in-a-sentence | 0 | 1 | ```python\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n \n # convert string to an array\n arr = text.split()\n \n # convert the first letter of the array to a lower case letter\n arr[0] = arr[0].lower()\n \n # sort array by length\n arr.sort(key=len)\n \n # convert the first letter of the array to an upper case letter\n arr[0] = arr[0].capitalize()\n \n # convert array to string\n return " ".join(arr)\n``` | 8 | There are `3n` piles of coins of varying size, you and your friends will take piles of coins as follows:
* In each step, you will choose **any** `3` piles of coins (not necessarily consecutive).
* Of your choice, Alice will pick the pile with the maximum number of coins.
* You will pick the next pile with the maximum number of coins.
* Your friend Bob will pick the last pile.
* Repeat until there are no more piles of coins.
Given an array of integers `piles` where `piles[i]` is the number of coins in the `ith` pile.
Return the maximum number of coins that you can have.
**Example 1:**
**Input:** piles = \[2,4,1,2,7,8\]
**Output:** 9
**Explanation:** Choose the triplet (2, 7, 8), Alice Pick the pile with 8 coins, you the pile with **7** coins and Bob the last one.
Choose the triplet (1, 2, 4), Alice Pick the pile with 4 coins, you the pile with **2** coins and Bob the last one.
The maximum number of coins which you can have are: 7 + 2 = 9.
On the other hand if we choose this arrangement (1, **2**, 8), (2, **4**, 7) you only get 2 + 4 = 6 coins which is not optimal.
**Example 2:**
**Input:** piles = \[2,4,5\]
**Output:** 4
**Example 3:**
**Input:** piles = \[9,8,7,6,5,1,2,3,4\]
**Output:** 18
**Constraints:**
* `3 <= piles.length <= 105`
* `piles.length % 3 == 0`
* `1 <= piles[i] <= 104` | Store each word and their relative position. Then, sort them by length of words in case of tie by their original order. |
Simple and Understandable solution in Python | rearrange-words-in-a-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n d = []\n for t in text.split():\n d.append((t, len(t)))\n\n sorted_words = [k.lower() for k, v in sorted(d, key=lambda item: item[1])]\n res = ""\n res += sorted_words[0].title() + " " + " ".join(sorted_words[1:])\n return res\n \n \n``` | 0 | Given a sentence `text` (A _sentence_ is a string of space-separated words) in the following format:
* First letter is in upper case.
* Each word in `text` are separated by a single space.
Your task is to rearrange the words in text such that all words are rearranged in an increasing order of their lengths. If two words have the same length, arrange them in their original order.
Return the new text following the format shown above.
**Example 1:**
**Input:** text = "Leetcode is cool "
**Output:** "Is cool leetcode "
**Explanation:** There are 3 words, "Leetcode " of length 8, "is " of length 2 and "cool " of length 4.
Output is ordered by length and the new first word starts with capital letter.
**Example 2:**
**Input:** text = "Keep calm and code on "
**Output:** "On and keep calm code "
**Explanation:** Output is ordered as follows:
"On " 2 letters.
"and " 3 letters.
"keep " 4 letters in case of tie order by position in original text.
"calm " 4 letters.
"code " 4 letters.
**Example 3:**
**Input:** text = "To be or not to be "
**Output:** "To be or to be not "
**Constraints:**
* `text` begins with a capital letter and then contains lowercase letters and single space between words.
* `1 <= text.length <= 10^5` | Create intervals of the area covered by each tap, sort intervals by the left end. We need to cover the interval [0, n]. we can start with the first interval and out of all intervals that intersect with it we choose the one that covers the farthest point to the right. What if there is a gap between intervals that is not covered ? we should stop and return -1 as there is some interval that cannot be covered. |
Simple and Understandable solution in Python | rearrange-words-in-a-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n d = []\n for t in text.split():\n d.append((t, len(t)))\n\n sorted_words = [k.lower() for k, v in sorted(d, key=lambda item: item[1])]\n res = ""\n res += sorted_words[0].title() + " " + " ".join(sorted_words[1:])\n return res\n \n \n``` | 0 | There are `3n` piles of coins of varying size, you and your friends will take piles of coins as follows:
* In each step, you will choose **any** `3` piles of coins (not necessarily consecutive).
* Of your choice, Alice will pick the pile with the maximum number of coins.
* You will pick the next pile with the maximum number of coins.
* Your friend Bob will pick the last pile.
* Repeat until there are no more piles of coins.
Given an array of integers `piles` where `piles[i]` is the number of coins in the `ith` pile.
Return the maximum number of coins that you can have.
**Example 1:**
**Input:** piles = \[2,4,1,2,7,8\]
**Output:** 9
**Explanation:** Choose the triplet (2, 7, 8), Alice Pick the pile with 8 coins, you the pile with **7** coins and Bob the last one.
Choose the triplet (1, 2, 4), Alice Pick the pile with 4 coins, you the pile with **2** coins and Bob the last one.
The maximum number of coins which you can have are: 7 + 2 = 9.
On the other hand if we choose this arrangement (1, **2**, 8), (2, **4**, 7) you only get 2 + 4 = 6 coins which is not optimal.
**Example 2:**
**Input:** piles = \[2,4,5\]
**Output:** 4
**Example 3:**
**Input:** piles = \[9,8,7,6,5,1,2,3,4\]
**Output:** 18
**Constraints:**
* `3 <= piles.length <= 105`
* `piles.length % 3 == 0`
* `1 <= piles[i] <= 104` | Store each word and their relative position. Then, sort them by length of words in case of tie by their original order. |
Python3 97 % | rearrange-words-in-a-sentence | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n text = text[0].lower()+text[1:]\n t = text.split()\n t.sort(key = len)\n t[0] = t[0].capitalize()\n return " ".join(t)\n\n\n \n``` | 0 | Given a sentence `text` (A _sentence_ is a string of space-separated words) in the following format:
* First letter is in upper case.
* Each word in `text` are separated by a single space.
Your task is to rearrange the words in text such that all words are rearranged in an increasing order of their lengths. If two words have the same length, arrange them in their original order.
Return the new text following the format shown above.
**Example 1:**
**Input:** text = "Leetcode is cool "
**Output:** "Is cool leetcode "
**Explanation:** There are 3 words, "Leetcode " of length 8, "is " of length 2 and "cool " of length 4.
Output is ordered by length and the new first word starts with capital letter.
**Example 2:**
**Input:** text = "Keep calm and code on "
**Output:** "On and keep calm code "
**Explanation:** Output is ordered as follows:
"On " 2 letters.
"and " 3 letters.
"keep " 4 letters in case of tie order by position in original text.
"calm " 4 letters.
"code " 4 letters.
**Example 3:**
**Input:** text = "To be or not to be "
**Output:** "To be or to be not "
**Constraints:**
* `text` begins with a capital letter and then contains lowercase letters and single space between words.
* `1 <= text.length <= 10^5` | Create intervals of the area covered by each tap, sort intervals by the left end. We need to cover the interval [0, n]. we can start with the first interval and out of all intervals that intersect with it we choose the one that covers the farthest point to the right. What if there is a gap between intervals that is not covered ? we should stop and return -1 as there is some interval that cannot be covered. |
Python3 97 % | rearrange-words-in-a-sentence | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n text = text[0].lower()+text[1:]\n t = text.split()\n t.sort(key = len)\n t[0] = t[0].capitalize()\n return " ".join(t)\n\n\n \n``` | 0 | There are `3n` piles of coins of varying size, you and your friends will take piles of coins as follows:
* In each step, you will choose **any** `3` piles of coins (not necessarily consecutive).
* Of your choice, Alice will pick the pile with the maximum number of coins.
* You will pick the next pile with the maximum number of coins.
* Your friend Bob will pick the last pile.
* Repeat until there are no more piles of coins.
Given an array of integers `piles` where `piles[i]` is the number of coins in the `ith` pile.
Return the maximum number of coins that you can have.
**Example 1:**
**Input:** piles = \[2,4,1,2,7,8\]
**Output:** 9
**Explanation:** Choose the triplet (2, 7, 8), Alice Pick the pile with 8 coins, you the pile with **7** coins and Bob the last one.
Choose the triplet (1, 2, 4), Alice Pick the pile with 4 coins, you the pile with **2** coins and Bob the last one.
The maximum number of coins which you can have are: 7 + 2 = 9.
On the other hand if we choose this arrangement (1, **2**, 8), (2, **4**, 7) you only get 2 + 4 = 6 coins which is not optimal.
**Example 2:**
**Input:** piles = \[2,4,5\]
**Output:** 4
**Example 3:**
**Input:** piles = \[9,8,7,6,5,1,2,3,4\]
**Output:** 18
**Constraints:**
* `3 <= piles.length <= 105`
* `piles.length % 3 == 0`
* `1 <= piles[i] <= 104` | Store each word and their relative position. Then, sort them by length of words in case of tie by their original order. |
Python3 Solution (Simple | Fast | Efficient) -> ✅ | rearrange-words-in-a-sentence | 0 | 1 | # Approach (Step by step)\n1. Split text using **split()** method and store it inside the list:\n```python\nwords = text.split()\n```\n2. Make the first word lowercase using **lower()** method:\n```python\nwords[0] = words[0].lower()\n```\n3. Sort the list by length using **sorted()** function:\n```python\nwords = sorted(words, key=len)\n```\n4. Make the first letter of the first word uppercase using **capitalize()** method:\n```python\nwords[0] = words[0].capitalize()\n```\n5. Make a string by seperating each element in a list with empty space (" "):\n```python\noutput = " ".join(words)\n```\n6. Return the string\n```python\nreturn output\n```\n\n# Resulting Code\n```python\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n words = text.split()\n words[0] = words[0].lower()\n words = sorted(words, key=len)\n words[0] = words[0].capitalize()\n output = " ".join(words)\n return output\n\n``` | 0 | Given a sentence `text` (A _sentence_ is a string of space-separated words) in the following format:
* First letter is in upper case.
* Each word in `text` are separated by a single space.
Your task is to rearrange the words in text such that all words are rearranged in an increasing order of their lengths. If two words have the same length, arrange them in their original order.
Return the new text following the format shown above.
**Example 1:**
**Input:** text = "Leetcode is cool "
**Output:** "Is cool leetcode "
**Explanation:** There are 3 words, "Leetcode " of length 8, "is " of length 2 and "cool " of length 4.
Output is ordered by length and the new first word starts with capital letter.
**Example 2:**
**Input:** text = "Keep calm and code on "
**Output:** "On and keep calm code "
**Explanation:** Output is ordered as follows:
"On " 2 letters.
"and " 3 letters.
"keep " 4 letters in case of tie order by position in original text.
"calm " 4 letters.
"code " 4 letters.
**Example 3:**
**Input:** text = "To be or not to be "
**Output:** "To be or to be not "
**Constraints:**
* `text` begins with a capital letter and then contains lowercase letters and single space between words.
* `1 <= text.length <= 10^5` | Create intervals of the area covered by each tap, sort intervals by the left end. We need to cover the interval [0, n]. we can start with the first interval and out of all intervals that intersect with it we choose the one that covers the farthest point to the right. What if there is a gap between intervals that is not covered ? we should stop and return -1 as there is some interval that cannot be covered. |
Python3 Solution (Simple | Fast | Efficient) -> ✅ | rearrange-words-in-a-sentence | 0 | 1 | # Approach (Step by step)\n1. Split text using **split()** method and store it inside the list:\n```python\nwords = text.split()\n```\n2. Make the first word lowercase using **lower()** method:\n```python\nwords[0] = words[0].lower()\n```\n3. Sort the list by length using **sorted()** function:\n```python\nwords = sorted(words, key=len)\n```\n4. Make the first letter of the first word uppercase using **capitalize()** method:\n```python\nwords[0] = words[0].capitalize()\n```\n5. Make a string by seperating each element in a list with empty space (" "):\n```python\noutput = " ".join(words)\n```\n6. Return the string\n```python\nreturn output\n```\n\n# Resulting Code\n```python\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n words = text.split()\n words[0] = words[0].lower()\n words = sorted(words, key=len)\n words[0] = words[0].capitalize()\n output = " ".join(words)\n return output\n\n``` | 0 | There are `3n` piles of coins of varying size, you and your friends will take piles of coins as follows:
* In each step, you will choose **any** `3` piles of coins (not necessarily consecutive).
* Of your choice, Alice will pick the pile with the maximum number of coins.
* You will pick the next pile with the maximum number of coins.
* Your friend Bob will pick the last pile.
* Repeat until there are no more piles of coins.
Given an array of integers `piles` where `piles[i]` is the number of coins in the `ith` pile.
Return the maximum number of coins that you can have.
**Example 1:**
**Input:** piles = \[2,4,1,2,7,8\]
**Output:** 9
**Explanation:** Choose the triplet (2, 7, 8), Alice Pick the pile with 8 coins, you the pile with **7** coins and Bob the last one.
Choose the triplet (1, 2, 4), Alice Pick the pile with 4 coins, you the pile with **2** coins and Bob the last one.
The maximum number of coins which you can have are: 7 + 2 = 9.
On the other hand if we choose this arrangement (1, **2**, 8), (2, **4**, 7) you only get 2 + 4 = 6 coins which is not optimal.
**Example 2:**
**Input:** piles = \[2,4,5\]
**Output:** 4
**Example 3:**
**Input:** piles = \[9,8,7,6,5,1,2,3,4\]
**Output:** 18
**Constraints:**
* `3 <= piles.length <= 105`
* `piles.length % 3 == 0`
* `1 <= piles[i] <= 104` | Store each word and their relative position. Then, sort them by length of words in case of tie by their original order. |
Beats 98.65% with SIMPLE Python solution. | rearrange-words-in-a-sentence | 0 | 1 | # Approach\nSplit the string into tokens. Only need to process the first token to be lower as the rest of the tokens will be the same. Sort by length. Then, make sure that the first string is capitalize correctly.\n\n# Complexity\n- Time complexity:\nO(n), where n is the length of the string\n\n- Space complexity:\nO(n), where n is the length of the string\n\n# Code\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n tokens = text.split(" ")\n tokens[0] = tokens[0].lower()\n tokens.sort(key=len)\n tokens[0] = tokens[0].capitalize()\n return " ".join(tokens)\n``` | 0 | Given a sentence `text` (A _sentence_ is a string of space-separated words) in the following format:
* First letter is in upper case.
* Each word in `text` are separated by a single space.
Your task is to rearrange the words in text such that all words are rearranged in an increasing order of their lengths. If two words have the same length, arrange them in their original order.
Return the new text following the format shown above.
**Example 1:**
**Input:** text = "Leetcode is cool "
**Output:** "Is cool leetcode "
**Explanation:** There are 3 words, "Leetcode " of length 8, "is " of length 2 and "cool " of length 4.
Output is ordered by length and the new first word starts with capital letter.
**Example 2:**
**Input:** text = "Keep calm and code on "
**Output:** "On and keep calm code "
**Explanation:** Output is ordered as follows:
"On " 2 letters.
"and " 3 letters.
"keep " 4 letters in case of tie order by position in original text.
"calm " 4 letters.
"code " 4 letters.
**Example 3:**
**Input:** text = "To be or not to be "
**Output:** "To be or to be not "
**Constraints:**
* `text` begins with a capital letter and then contains lowercase letters and single space between words.
* `1 <= text.length <= 10^5` | Create intervals of the area covered by each tap, sort intervals by the left end. We need to cover the interval [0, n]. we can start with the first interval and out of all intervals that intersect with it we choose the one that covers the farthest point to the right. What if there is a gap between intervals that is not covered ? we should stop and return -1 as there is some interval that cannot be covered. |
Beats 98.65% with SIMPLE Python solution. | rearrange-words-in-a-sentence | 0 | 1 | # Approach\nSplit the string into tokens. Only need to process the first token to be lower as the rest of the tokens will be the same. Sort by length. Then, make sure that the first string is capitalize correctly.\n\n# Complexity\n- Time complexity:\nO(n), where n is the length of the string\n\n- Space complexity:\nO(n), where n is the length of the string\n\n# Code\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n tokens = text.split(" ")\n tokens[0] = tokens[0].lower()\n tokens.sort(key=len)\n tokens[0] = tokens[0].capitalize()\n return " ".join(tokens)\n``` | 0 | There are `3n` piles of coins of varying size, you and your friends will take piles of coins as follows:
* In each step, you will choose **any** `3` piles of coins (not necessarily consecutive).
* Of your choice, Alice will pick the pile with the maximum number of coins.
* You will pick the next pile with the maximum number of coins.
* Your friend Bob will pick the last pile.
* Repeat until there are no more piles of coins.
Given an array of integers `piles` where `piles[i]` is the number of coins in the `ith` pile.
Return the maximum number of coins that you can have.
**Example 1:**
**Input:** piles = \[2,4,1,2,7,8\]
**Output:** 9
**Explanation:** Choose the triplet (2, 7, 8), Alice Pick the pile with 8 coins, you the pile with **7** coins and Bob the last one.
Choose the triplet (1, 2, 4), Alice Pick the pile with 4 coins, you the pile with **2** coins and Bob the last one.
The maximum number of coins which you can have are: 7 + 2 = 9.
On the other hand if we choose this arrangement (1, **2**, 8), (2, **4**, 7) you only get 2 + 4 = 6 coins which is not optimal.
**Example 2:**
**Input:** piles = \[2,4,5\]
**Output:** 4
**Example 3:**
**Input:** piles = \[9,8,7,6,5,1,2,3,4\]
**Output:** 18
**Constraints:**
* `3 <= piles.length <= 105`
* `piles.length % 3 == 0`
* `1 <= piles[i] <= 104` | Store each word and their relative position. Then, sort them by length of words in case of tie by their original order. |
Python O(n) solution without sorting, readable and easy to understand. | rearrange-words-in-a-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIf we can group together all the words that have the same length, then we will be able to solve this problem. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Group the words that have the same length. \n2. Iterate over the possible word stoppage indices, actually every index in the original string can be a word stoppage\n3. And finally concatenate all the words during iteration.\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport collections\n\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n result = \'\'\n strLength = len(text)\n textLenMap = collections.defaultdict(list)\n\n text = text.split(\' \')\n text[0] = text[0].lower()\n\n for word in text:\n textLenMap[len(word)].append(word)\n\n for i in range(strLength):\n for w in textLenMap[i]:\n if len(result) == 0:\n # first word. so, capitalize the first character\n # don\'t need the word separator(\' \')\n result += w.title()\n else:\n result += \' \' + w\n\n return result\n\n``` | 0 | Given a sentence `text` (A _sentence_ is a string of space-separated words) in the following format:
* First letter is in upper case.
* Each word in `text` are separated by a single space.
Your task is to rearrange the words in text such that all words are rearranged in an increasing order of their lengths. If two words have the same length, arrange them in their original order.
Return the new text following the format shown above.
**Example 1:**
**Input:** text = "Leetcode is cool "
**Output:** "Is cool leetcode "
**Explanation:** There are 3 words, "Leetcode " of length 8, "is " of length 2 and "cool " of length 4.
Output is ordered by length and the new first word starts with capital letter.
**Example 2:**
**Input:** text = "Keep calm and code on "
**Output:** "On and keep calm code "
**Explanation:** Output is ordered as follows:
"On " 2 letters.
"and " 3 letters.
"keep " 4 letters in case of tie order by position in original text.
"calm " 4 letters.
"code " 4 letters.
**Example 3:**
**Input:** text = "To be or not to be "
**Output:** "To be or to be not "
**Constraints:**
* `text` begins with a capital letter and then contains lowercase letters and single space between words.
* `1 <= text.length <= 10^5` | Create intervals of the area covered by each tap, sort intervals by the left end. We need to cover the interval [0, n]. we can start with the first interval and out of all intervals that intersect with it we choose the one that covers the farthest point to the right. What if there is a gap between intervals that is not covered ? we should stop and return -1 as there is some interval that cannot be covered. |
Python O(n) solution without sorting, readable and easy to understand. | rearrange-words-in-a-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIf we can group together all the words that have the same length, then we will be able to solve this problem. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Group the words that have the same length. \n2. Iterate over the possible word stoppage indices, actually every index in the original string can be a word stoppage\n3. And finally concatenate all the words during iteration.\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport collections\n\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n result = \'\'\n strLength = len(text)\n textLenMap = collections.defaultdict(list)\n\n text = text.split(\' \')\n text[0] = text[0].lower()\n\n for word in text:\n textLenMap[len(word)].append(word)\n\n for i in range(strLength):\n for w in textLenMap[i]:\n if len(result) == 0:\n # first word. so, capitalize the first character\n # don\'t need the word separator(\' \')\n result += w.title()\n else:\n result += \' \' + w\n\n return result\n\n``` | 0 | There are `3n` piles of coins of varying size, you and your friends will take piles of coins as follows:
* In each step, you will choose **any** `3` piles of coins (not necessarily consecutive).
* Of your choice, Alice will pick the pile with the maximum number of coins.
* You will pick the next pile with the maximum number of coins.
* Your friend Bob will pick the last pile.
* Repeat until there are no more piles of coins.
Given an array of integers `piles` where `piles[i]` is the number of coins in the `ith` pile.
Return the maximum number of coins that you can have.
**Example 1:**
**Input:** piles = \[2,4,1,2,7,8\]
**Output:** 9
**Explanation:** Choose the triplet (2, 7, 8), Alice Pick the pile with 8 coins, you the pile with **7** coins and Bob the last one.
Choose the triplet (1, 2, 4), Alice Pick the pile with 4 coins, you the pile with **2** coins and Bob the last one.
The maximum number of coins which you can have are: 7 + 2 = 9.
On the other hand if we choose this arrangement (1, **2**, 8), (2, **4**, 7) you only get 2 + 4 = 6 coins which is not optimal.
**Example 2:**
**Input:** piles = \[2,4,5\]
**Output:** 4
**Example 3:**
**Input:** piles = \[9,8,7,6,5,1,2,3,4\]
**Output:** 18
**Constraints:**
* `3 <= piles.length <= 105`
* `piles.length % 3 == 0`
* `1 <= piles[i] <= 104` | Store each word and their relative position. Then, sort them by length of words in case of tie by their original order. |
Simple python3 solution | Split + Sorting | rearrange-words-in-a-sentence | 0 | 1 | # Complexity\n- Time complexity: $$O(n \\cdot \\log(n))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n``` python3 []\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n data = [\n [len(word), i, word]\n for i, word in enumerate(text.split())\n ]\n data[0][2] = data[0][2].lower()\n data.sort()\n data[0][2] = data[0][2].title()\n\n return \' \'.join(\n word\n for _, _, word in data\n )\n``` | 0 | Given a sentence `text` (A _sentence_ is a string of space-separated words) in the following format:
* First letter is in upper case.
* Each word in `text` are separated by a single space.
Your task is to rearrange the words in text such that all words are rearranged in an increasing order of their lengths. If two words have the same length, arrange them in their original order.
Return the new text following the format shown above.
**Example 1:**
**Input:** text = "Leetcode is cool "
**Output:** "Is cool leetcode "
**Explanation:** There are 3 words, "Leetcode " of length 8, "is " of length 2 and "cool " of length 4.
Output is ordered by length and the new first word starts with capital letter.
**Example 2:**
**Input:** text = "Keep calm and code on "
**Output:** "On and keep calm code "
**Explanation:** Output is ordered as follows:
"On " 2 letters.
"and " 3 letters.
"keep " 4 letters in case of tie order by position in original text.
"calm " 4 letters.
"code " 4 letters.
**Example 3:**
**Input:** text = "To be or not to be "
**Output:** "To be or to be not "
**Constraints:**
* `text` begins with a capital letter and then contains lowercase letters and single space between words.
* `1 <= text.length <= 10^5` | Create intervals of the area covered by each tap, sort intervals by the left end. We need to cover the interval [0, n]. we can start with the first interval and out of all intervals that intersect with it we choose the one that covers the farthest point to the right. What if there is a gap between intervals that is not covered ? we should stop and return -1 as there is some interval that cannot be covered. |
Simple python3 solution | Split + Sorting | rearrange-words-in-a-sentence | 0 | 1 | # Complexity\n- Time complexity: $$O(n \\cdot \\log(n))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n``` python3 []\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n data = [\n [len(word), i, word]\n for i, word in enumerate(text.split())\n ]\n data[0][2] = data[0][2].lower()\n data.sort()\n data[0][2] = data[0][2].title()\n\n return \' \'.join(\n word\n for _, _, word in data\n )\n``` | 0 | There are `3n` piles of coins of varying size, you and your friends will take piles of coins as follows:
* In each step, you will choose **any** `3` piles of coins (not necessarily consecutive).
* Of your choice, Alice will pick the pile with the maximum number of coins.
* You will pick the next pile with the maximum number of coins.
* Your friend Bob will pick the last pile.
* Repeat until there are no more piles of coins.
Given an array of integers `piles` where `piles[i]` is the number of coins in the `ith` pile.
Return the maximum number of coins that you can have.
**Example 1:**
**Input:** piles = \[2,4,1,2,7,8\]
**Output:** 9
**Explanation:** Choose the triplet (2, 7, 8), Alice Pick the pile with 8 coins, you the pile with **7** coins and Bob the last one.
Choose the triplet (1, 2, 4), Alice Pick the pile with 4 coins, you the pile with **2** coins and Bob the last one.
The maximum number of coins which you can have are: 7 + 2 = 9.
On the other hand if we choose this arrangement (1, **2**, 8), (2, **4**, 7) you only get 2 + 4 = 6 coins which is not optimal.
**Example 2:**
**Input:** piles = \[2,4,5\]
**Output:** 4
**Example 3:**
**Input:** piles = \[9,8,7,6,5,1,2,3,4\]
**Output:** 18
**Constraints:**
* `3 <= piles.length <= 105`
* `piles.length % 3 == 0`
* `1 <= piles[i] <= 104` | Store each word and their relative position. Then, sort them by length of words in case of tie by their original order. |
Most Easiest and understandable python code | rearrange-words-in-a-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n l=[]\n for i in text.split():\n l.append(i.lower())\n l=sorted(l,key=len)\n s=l[0].capitalize()+" "\n for i in range(1,len(l)):\n s+=l[i]+" "\n return s[:-1]\n \n \n``` | 0 | Given a sentence `text` (A _sentence_ is a string of space-separated words) in the following format:
* First letter is in upper case.
* Each word in `text` are separated by a single space.
Your task is to rearrange the words in text such that all words are rearranged in an increasing order of their lengths. If two words have the same length, arrange them in their original order.
Return the new text following the format shown above.
**Example 1:**
**Input:** text = "Leetcode is cool "
**Output:** "Is cool leetcode "
**Explanation:** There are 3 words, "Leetcode " of length 8, "is " of length 2 and "cool " of length 4.
Output is ordered by length and the new first word starts with capital letter.
**Example 2:**
**Input:** text = "Keep calm and code on "
**Output:** "On and keep calm code "
**Explanation:** Output is ordered as follows:
"On " 2 letters.
"and " 3 letters.
"keep " 4 letters in case of tie order by position in original text.
"calm " 4 letters.
"code " 4 letters.
**Example 3:**
**Input:** text = "To be or not to be "
**Output:** "To be or to be not "
**Constraints:**
* `text` begins with a capital letter and then contains lowercase letters and single space between words.
* `1 <= text.length <= 10^5` | Create intervals of the area covered by each tap, sort intervals by the left end. We need to cover the interval [0, n]. we can start with the first interval and out of all intervals that intersect with it we choose the one that covers the farthest point to the right. What if there is a gap between intervals that is not covered ? we should stop and return -1 as there is some interval that cannot be covered. |
Most Easiest and understandable python code | rearrange-words-in-a-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def arrangeWords(self, text: str) -> str:\n l=[]\n for i in text.split():\n l.append(i.lower())\n l=sorted(l,key=len)\n s=l[0].capitalize()+" "\n for i in range(1,len(l)):\n s+=l[i]+" "\n return s[:-1]\n \n \n``` | 0 | There are `3n` piles of coins of varying size, you and your friends will take piles of coins as follows:
* In each step, you will choose **any** `3` piles of coins (not necessarily consecutive).
* Of your choice, Alice will pick the pile with the maximum number of coins.
* You will pick the next pile with the maximum number of coins.
* Your friend Bob will pick the last pile.
* Repeat until there are no more piles of coins.
Given an array of integers `piles` where `piles[i]` is the number of coins in the `ith` pile.
Return the maximum number of coins that you can have.
**Example 1:**
**Input:** piles = \[2,4,1,2,7,8\]
**Output:** 9
**Explanation:** Choose the triplet (2, 7, 8), Alice Pick the pile with 8 coins, you the pile with **7** coins and Bob the last one.
Choose the triplet (1, 2, 4), Alice Pick the pile with 4 coins, you the pile with **2** coins and Bob the last one.
The maximum number of coins which you can have are: 7 + 2 = 9.
On the other hand if we choose this arrangement (1, **2**, 8), (2, **4**, 7) you only get 2 + 4 = 6 coins which is not optimal.
**Example 2:**
**Input:** piles = \[2,4,5\]
**Output:** 4
**Example 3:**
**Input:** piles = \[9,8,7,6,5,1,2,3,4\]
**Output:** 18
**Constraints:**
* `3 <= piles.length <= 105`
* `piles.length % 3 == 0`
* `1 <= piles[i] <= 104` | Store each word and their relative position. Then, sort them by length of words in case of tie by their original order. |
Python Simple Solution - Using Sets!! | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Complexity\n- Time complexity: O(N*N)\n- Space complexity: O(M)\n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n \n answer: list = []\n favoriteCompanies = [set(companies) for companies in favoriteCompanies]\n\n for index, companies in enumerate(favoriteCompanies):\n controller: bool = False\n for others in favoriteCompanies:\n if companies != others and others >= companies:\n controller = True\n break\n\n if not controller: answer.append(index)\n \n answer.sort()\n return answer\n``` | 1 | Given the array `favoriteCompanies` where `favoriteCompanies[i]` is the list of favorites companies for the `ith` person (**indexed from 0**).
_Return the indices of people whose list of favorite companies is not a **subset** of any other list of favorites companies_. You must return the indices in increasing order.
**Example 1:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "google ", "microsoft "\],\[ "google ", "facebook "\],\[ "google "\],\[ "amazon "\]\]
**Output:** \[0,1,4\]
**Explanation:**
Person with index=2 has favoriteCompanies\[2\]=\[ "google ", "facebook "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] corresponding to the person with index 0.
Person with index=3 has favoriteCompanies\[3\]=\[ "google "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] and favoriteCompanies\[1\]=\[ "google ", "microsoft "\].
Other lists of favorite companies are not a subset of another list, therefore, the answer is \[0,1,4\].
**Example 2:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "leetcode ", "amazon "\],\[ "facebook ", "google "\]\]
**Output:** \[0,1\]
**Explanation:** In this case favoriteCompanies\[2\]=\[ "facebook ", "google "\] is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\], therefore, the answer is \[0,1\].
**Example 3:**
**Input:** favoriteCompanies = \[\[ "leetcode "\],\[ "google "\],\[ "facebook "\],\[ "amazon "\]\]
**Output:** \[0,1,2,3\]
**Constraints:**
* `1 <= favoriteCompanies.length <= 100`
* `1 <= favoriteCompanies[i].length <= 500`
* `1 <= favoriteCompanies[i][j].length <= 20`
* All strings in `favoriteCompanies[i]` are **distinct**.
* All lists of favorite companies are **distinct**, that is, If we sort alphabetically each list then `favoriteCompanies[i] != favoriteCompanies[j].`
* All strings consist of lowercase English letters only. | null |
Python Simple Solution - Using Sets!! | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Complexity\n- Time complexity: O(N*N)\n- Space complexity: O(M)\n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n \n answer: list = []\n favoriteCompanies = [set(companies) for companies in favoriteCompanies]\n\n for index, companies in enumerate(favoriteCompanies):\n controller: bool = False\n for others in favoriteCompanies:\n if companies != others and others >= companies:\n controller = True\n break\n\n if not controller: answer.append(index)\n \n answer.sort()\n return answer\n``` | 1 | Given an array `arr` that represents a permutation of numbers from `1` to `n`.
You have a binary string of size `n` that initially has all its bits set to zero. At each step `i` (assuming both the binary string and `arr` are 1-indexed) from `1` to `n`, the bit at position `arr[i]` is set to `1`.
You are also given an integer `m`. Find the latest step at which there exists a group of ones of length `m`. A group of ones is a contiguous substring of `1`'s such that it cannot be extended in either direction.
Return _the latest step at which there exists a group of ones of length **exactly**_ `m`. _If no such group exists, return_ `-1`.
**Example 1:**
**Input:** arr = \[3,5,1,2,4\], m = 1
**Output:** 4
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "00101 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "11101 ", groups: \[ "111 ", "1 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
The latest step at which there exists a group of size 1 is step 4.
**Example 2:**
**Input:** arr = \[3,1,5,4,2\], m = 2
**Output:** -1
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "10100 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "10111 ", groups: \[ "1 ", "111 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
No group of size 2 exists during any step.
**Constraints:**
* `n == arr.length`
* `1 <= m <= n <= 105`
* `1 <= arr[i] <= n`
* All integers in `arr` are **distinct**. | Use hashing to convert company names in numbers and then for each list check if this is a subset of any other list. In order to check if a list is a subset of another list, use two pointers technique to get a linear solution for this task. The total complexity will be O(n^2 * m) where n is the number of lists and m is the maximum number of elements in a list. |
Python | Dictionary + Bitwise operation | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nAt first sight, the naive way to do it is to use two for loops + issubset. However, the name of company could be long, which makes issubset time consuming. \n\nSo how about replace the issubset by bitwise operation? \n# Approach\n<!-- Describe your approach to solving the problem. -->\nUsing bitwise operation, we need to convert each person\'s favorite list to be a binary representation. Then still use two loops + bitwise operation to get the answer.\n\nStep 1. we find the set of all company names;\nStep 2. use dictionary to encode each company to a poistion (index);\nStep 3. map each person\'s favorite list to a binary representation;\nStep 4. use 2 for loops to check;\n \n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$O(n^2 + nm)$ where $n$ is the length of favoriteCompanies, $m$ is the length of company name.\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$O(n + k)$ where $k$ is the total number of companies (for the dictionary)\n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n\n n = len(favoriteCompanies)\n comp = []\n for f in favoriteCompanies:\n comp += f\n comp = list(set(comp))\n \n dictBit = {comp[i] : 1 << i for i in range(len(comp))}\n\n def getBit(cList):\n output = 0\n for c in cList:\n output |= dictBit[c]\n return output\n bitFav = [getBit(favoriteCompanies[i]) for i in range(n)]\n\n output = []\n for i in range(n):\n isGood = True\n for j in range(n):\n if(i != j and bitFav[i] & bitFav[j] == bitFav[i]):\n isGood = False\n break\n if(isGood):\n output.append(i)\n \n return output\n\n\n\n``` | 1 | Given the array `favoriteCompanies` where `favoriteCompanies[i]` is the list of favorites companies for the `ith` person (**indexed from 0**).
_Return the indices of people whose list of favorite companies is not a **subset** of any other list of favorites companies_. You must return the indices in increasing order.
**Example 1:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "google ", "microsoft "\],\[ "google ", "facebook "\],\[ "google "\],\[ "amazon "\]\]
**Output:** \[0,1,4\]
**Explanation:**
Person with index=2 has favoriteCompanies\[2\]=\[ "google ", "facebook "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] corresponding to the person with index 0.
Person with index=3 has favoriteCompanies\[3\]=\[ "google "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] and favoriteCompanies\[1\]=\[ "google ", "microsoft "\].
Other lists of favorite companies are not a subset of another list, therefore, the answer is \[0,1,4\].
**Example 2:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "leetcode ", "amazon "\],\[ "facebook ", "google "\]\]
**Output:** \[0,1\]
**Explanation:** In this case favoriteCompanies\[2\]=\[ "facebook ", "google "\] is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\], therefore, the answer is \[0,1\].
**Example 3:**
**Input:** favoriteCompanies = \[\[ "leetcode "\],\[ "google "\],\[ "facebook "\],\[ "amazon "\]\]
**Output:** \[0,1,2,3\]
**Constraints:**
* `1 <= favoriteCompanies.length <= 100`
* `1 <= favoriteCompanies[i].length <= 500`
* `1 <= favoriteCompanies[i][j].length <= 20`
* All strings in `favoriteCompanies[i]` are **distinct**.
* All lists of favorite companies are **distinct**, that is, If we sort alphabetically each list then `favoriteCompanies[i] != favoriteCompanies[j].`
* All strings consist of lowercase English letters only. | null |
Python | Dictionary + Bitwise operation | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nAt first sight, the naive way to do it is to use two for loops + issubset. However, the name of company could be long, which makes issubset time consuming. \n\nSo how about replace the issubset by bitwise operation? \n# Approach\n<!-- Describe your approach to solving the problem. -->\nUsing bitwise operation, we need to convert each person\'s favorite list to be a binary representation. Then still use two loops + bitwise operation to get the answer.\n\nStep 1. we find the set of all company names;\nStep 2. use dictionary to encode each company to a poistion (index);\nStep 3. map each person\'s favorite list to a binary representation;\nStep 4. use 2 for loops to check;\n \n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$O(n^2 + nm)$ where $n$ is the length of favoriteCompanies, $m$ is the length of company name.\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$O(n + k)$ where $k$ is the total number of companies (for the dictionary)\n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n\n n = len(favoriteCompanies)\n comp = []\n for f in favoriteCompanies:\n comp += f\n comp = list(set(comp))\n \n dictBit = {comp[i] : 1 << i for i in range(len(comp))}\n\n def getBit(cList):\n output = 0\n for c in cList:\n output |= dictBit[c]\n return output\n bitFav = [getBit(favoriteCompanies[i]) for i in range(n)]\n\n output = []\n for i in range(n):\n isGood = True\n for j in range(n):\n if(i != j and bitFav[i] & bitFav[j] == bitFav[i]):\n isGood = False\n break\n if(isGood):\n output.append(i)\n \n return output\n\n\n\n``` | 1 | Given an array `arr` that represents a permutation of numbers from `1` to `n`.
You have a binary string of size `n` that initially has all its bits set to zero. At each step `i` (assuming both the binary string and `arr` are 1-indexed) from `1` to `n`, the bit at position `arr[i]` is set to `1`.
You are also given an integer `m`. Find the latest step at which there exists a group of ones of length `m`. A group of ones is a contiguous substring of `1`'s such that it cannot be extended in either direction.
Return _the latest step at which there exists a group of ones of length **exactly**_ `m`. _If no such group exists, return_ `-1`.
**Example 1:**
**Input:** arr = \[3,5,1,2,4\], m = 1
**Output:** 4
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "00101 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "11101 ", groups: \[ "111 ", "1 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
The latest step at which there exists a group of size 1 is step 4.
**Example 2:**
**Input:** arr = \[3,1,5,4,2\], m = 2
**Output:** -1
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "10100 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "10111 ", groups: \[ "1 ", "111 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
No group of size 2 exists during any step.
**Constraints:**
* `n == arr.length`
* `1 <= m <= n <= 105`
* `1 <= arr[i] <= n`
* All integers in `arr` are **distinct**. | Use hashing to convert company names in numbers and then for each list check if this is a subset of any other list. In order to check if a list is a subset of another list, use two pointers technique to get a linear solution for this task. The total complexity will be O(n^2 * m) where n is the number of lists and m is the maximum number of elements in a list. |
Python3 Clean Solution , Set , For Else | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, fc: List[List[str]]) -> List[int]:\n \n \n n=len(fc)\n ans=[]\n \n for i in range(n):\n s=set(fc[i])\n \n for j in range(n):\n if i==j:\n continue\n if not s-set(fc[j]):\n break\n else:\n ans.append(i)\n \n return ans\n \n \n``` | 0 | Given the array `favoriteCompanies` where `favoriteCompanies[i]` is the list of favorites companies for the `ith` person (**indexed from 0**).
_Return the indices of people whose list of favorite companies is not a **subset** of any other list of favorites companies_. You must return the indices in increasing order.
**Example 1:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "google ", "microsoft "\],\[ "google ", "facebook "\],\[ "google "\],\[ "amazon "\]\]
**Output:** \[0,1,4\]
**Explanation:**
Person with index=2 has favoriteCompanies\[2\]=\[ "google ", "facebook "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] corresponding to the person with index 0.
Person with index=3 has favoriteCompanies\[3\]=\[ "google "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] and favoriteCompanies\[1\]=\[ "google ", "microsoft "\].
Other lists of favorite companies are not a subset of another list, therefore, the answer is \[0,1,4\].
**Example 2:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "leetcode ", "amazon "\],\[ "facebook ", "google "\]\]
**Output:** \[0,1\]
**Explanation:** In this case favoriteCompanies\[2\]=\[ "facebook ", "google "\] is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\], therefore, the answer is \[0,1\].
**Example 3:**
**Input:** favoriteCompanies = \[\[ "leetcode "\],\[ "google "\],\[ "facebook "\],\[ "amazon "\]\]
**Output:** \[0,1,2,3\]
**Constraints:**
* `1 <= favoriteCompanies.length <= 100`
* `1 <= favoriteCompanies[i].length <= 500`
* `1 <= favoriteCompanies[i][j].length <= 20`
* All strings in `favoriteCompanies[i]` are **distinct**.
* All lists of favorite companies are **distinct**, that is, If we sort alphabetically each list then `favoriteCompanies[i] != favoriteCompanies[j].`
* All strings consist of lowercase English letters only. | null |
Python3 Clean Solution , Set , For Else | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, fc: List[List[str]]) -> List[int]:\n \n \n n=len(fc)\n ans=[]\n \n for i in range(n):\n s=set(fc[i])\n \n for j in range(n):\n if i==j:\n continue\n if not s-set(fc[j]):\n break\n else:\n ans.append(i)\n \n return ans\n \n \n``` | 0 | Given an array `arr` that represents a permutation of numbers from `1` to `n`.
You have a binary string of size `n` that initially has all its bits set to zero. At each step `i` (assuming both the binary string and `arr` are 1-indexed) from `1` to `n`, the bit at position `arr[i]` is set to `1`.
You are also given an integer `m`. Find the latest step at which there exists a group of ones of length `m`. A group of ones is a contiguous substring of `1`'s such that it cannot be extended in either direction.
Return _the latest step at which there exists a group of ones of length **exactly**_ `m`. _If no such group exists, return_ `-1`.
**Example 1:**
**Input:** arr = \[3,5,1,2,4\], m = 1
**Output:** 4
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "00101 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "11101 ", groups: \[ "111 ", "1 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
The latest step at which there exists a group of size 1 is step 4.
**Example 2:**
**Input:** arr = \[3,1,5,4,2\], m = 2
**Output:** -1
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "10100 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "10111 ", groups: \[ "1 ", "111 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
No group of size 2 exists during any step.
**Constraints:**
* `n == arr.length`
* `1 <= m <= n <= 105`
* `1 <= arr[i] <= n`
* All integers in `arr` are **distinct**. | Use hashing to convert company names in numbers and then for each list check if this is a subset of any other list. In order to check if a list is a subset of another list, use two pointers technique to get a linear solution for this task. The total complexity will be O(n^2 * m) where n is the number of lists and m is the maximum number of elements in a list. |
Set Theory | Commented and Explained | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nA subset is a set that contains all components of a superset (and a superset has things a subset does not, as well as everything a subset to it has). \n\nDue to the nature of sub and super sets you\'ll likely run into the issue of memory limits, and as such might need a way to work around that. Many lossless encoding schemes exist to help with this, and in this approach here we do not use them but point out WHERE you could use them and how this could help. \n\nWith the idea of subsets and supersets, we now need to figure out how we can uniquely sort the companies favorited by users in a way that allows us to \n- Minimize time complexity \n- If we so cared, minimize space complexity and utilize encoding \n- Do so in a way that is explainable easily in an interview \n\nTo start with, the larger and longer an individuals list of companies is, the more of a superset it is likely to be to smaller sets. Based on this, we can determine our first solution, which is that we need to settle on sorting our list in some order. For now, let\'s go with least to greatest number of companies, regardless of content. \n\nNow, we can turn to our final consideration, which is that the list of companies largest (longest) entry MUST be a superset. This is because of the fact that it necessarily will have as much as any others, and will have at least one more or the same. As such, it is a superset. Otherwise, it would not be there! \n\nWith this, we now turn to the first space use for time saving method, which is that we can go backwards in our list until we find our first subset! This may not be very far, but it may infact cover quite some distance. What this lets us do is skip to the very end of the story though and look there for supersets first and foremost. This lets us use our best options and to go backwards from there. \n\nAs such, we can imagine stepping back through the list to find the first subset in our list near the end. Along the way, any supersets found are added to their list and the result as needed. It must be that they are a superset of the list as a whole based on set theory at this point, and so we can be resolved of their usefulness. \n\nNext we mark the location of our first subset when the loop does terminate. If we never had to worry we have all unique sets and can simply sort the result and return. \n\nOtherwise, we will need to step forward through the list, utilizing our superset as we go to find supersets along the way. Iff an item is not a subset of the supersets, and it is not a subset of any list on the way to the supersets, then is it a superset. As such we will not be adding nearly as often, so we need to have methods in place to avoid such a prognosis. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe intution explains the approach in words. To simplify greatly \n- Keep a list of indices of supersets \n- Sort the list of favorite companies by length increasing \n- Find the supersets by going backwards over the list until your first subset \n- Find the supersets by going forwards over the list until you reach the index of your first subset from the above \n- Sort the result when done and return \n\n\n# Complexity\n- Time complexity:\n - First sort is O(C log C) -> C is size of companies list \n - Next loop is bound by C \n - Next loop is still bound by C \n - Loops again over c -> means can be treated as # * C so C \n - Final sort is O(N log N) where N is the number of supersets\n - N is at worst C, so we can interchange them \n - Total cost is O( C log C ) at worst \n\n- Space complexity:\n - Space complexity surely seems bad, however \n - Company strings could be losslessly encoded to a cipher \n - This could be done to build supersets offline and then cipher supersets and subsets by that process. \n - This would allow building to be done with storage for the ciphers, thus greatly reducing overhead \n - As such, we should consider the costs herein, but note saving momements \n - Company size list is O(C) \n - Supersets is O(N * avg length) \n - Result is O(N) \n - Total then is O(C + N * avg length + N) -> O(C) as N * avg length is sub to C \n - All of these could be done with offline storage, so you have at worst O(C) but at best O(1) \n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n # build listing \n result = [] \n status = True \n\n # sort the company size listings by size and keep as a set of comapnies with indexing \n # this allows us to use is subset alternation on future components \n # sort by length increasing so that we find all subsets quickly at start \n company_size_list = sorted([(set(companies), f_i) for f_i, companies in enumerate(favoriteCompanies)], \n key = lambda entry : len(entry[0]))\n\n # build a supersets backward \n supersets = []\n supersets.append(company_size_list[-1][0])\n result.append(company_size_list[-1][1])\n\n # by considering the set at the end to definitely be a superset of all before it \n s_j = len(company_size_list) - 2 \n s_k = 0 \n # we can then determine exactly the supersets that are uniquely supersets \n while s_j >= 0 : \n entry = company_size_list[s_j] \n for s_s in supersets : \n if entry[0].issubset(s_s) : \n # once we encounter a subset though, we need to stop here \n # this is for s_k. s_j is now set to -1 to store it \n s_k = s_j \n s_j = -1 \n break \n if s_j == -1 : \n break \n else : \n # if we do not need to break, append the entry set and indices\n supersets.append(entry[0])\n result.append(entry[1])\n if s_j != 0 : \n s_j -= 1\n else : \n break \n\n # if we need to now consider s_k \n if s_k != 0 : \n # get largest supersets first -> more likely to cause a break \n supersets.sort(key = lambda entry : len(entry), reverse=True)\n # loop on index of sorted company size list (s_i) with entry current in sorted listing \n for s_i, entry in enumerate(company_size_list) : \n # first check supersets \n for s_s in supersets : \n if entry[0].issubset(s_s) : \n status = False \n break \n # then if status is still true \n if status == True : \n # and then loop from there forward in the sorted company list \n s_j = s_i + 1 \n while s_j < s_k : \n alternate = company_size_list[s_j] \n if entry[0].issubset(alternate[0]) : \n status = False \n break \n else : \n s_j += 1 \n\n # One more check \n if status == True : \n result.append(entry[1])\n else : \n status = True\n\n if s_i + 1 == s_k : \n break \n \n # sort the result at the end of the set \n result.sort()\n return result \n \n``` | 0 | Given the array `favoriteCompanies` where `favoriteCompanies[i]` is the list of favorites companies for the `ith` person (**indexed from 0**).
_Return the indices of people whose list of favorite companies is not a **subset** of any other list of favorites companies_. You must return the indices in increasing order.
**Example 1:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "google ", "microsoft "\],\[ "google ", "facebook "\],\[ "google "\],\[ "amazon "\]\]
**Output:** \[0,1,4\]
**Explanation:**
Person with index=2 has favoriteCompanies\[2\]=\[ "google ", "facebook "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] corresponding to the person with index 0.
Person with index=3 has favoriteCompanies\[3\]=\[ "google "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] and favoriteCompanies\[1\]=\[ "google ", "microsoft "\].
Other lists of favorite companies are not a subset of another list, therefore, the answer is \[0,1,4\].
**Example 2:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "leetcode ", "amazon "\],\[ "facebook ", "google "\]\]
**Output:** \[0,1\]
**Explanation:** In this case favoriteCompanies\[2\]=\[ "facebook ", "google "\] is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\], therefore, the answer is \[0,1\].
**Example 3:**
**Input:** favoriteCompanies = \[\[ "leetcode "\],\[ "google "\],\[ "facebook "\],\[ "amazon "\]\]
**Output:** \[0,1,2,3\]
**Constraints:**
* `1 <= favoriteCompanies.length <= 100`
* `1 <= favoriteCompanies[i].length <= 500`
* `1 <= favoriteCompanies[i][j].length <= 20`
* All strings in `favoriteCompanies[i]` are **distinct**.
* All lists of favorite companies are **distinct**, that is, If we sort alphabetically each list then `favoriteCompanies[i] != favoriteCompanies[j].`
* All strings consist of lowercase English letters only. | null |
Set Theory | Commented and Explained | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nA subset is a set that contains all components of a superset (and a superset has things a subset does not, as well as everything a subset to it has). \n\nDue to the nature of sub and super sets you\'ll likely run into the issue of memory limits, and as such might need a way to work around that. Many lossless encoding schemes exist to help with this, and in this approach here we do not use them but point out WHERE you could use them and how this could help. \n\nWith the idea of subsets and supersets, we now need to figure out how we can uniquely sort the companies favorited by users in a way that allows us to \n- Minimize time complexity \n- If we so cared, minimize space complexity and utilize encoding \n- Do so in a way that is explainable easily in an interview \n\nTo start with, the larger and longer an individuals list of companies is, the more of a superset it is likely to be to smaller sets. Based on this, we can determine our first solution, which is that we need to settle on sorting our list in some order. For now, let\'s go with least to greatest number of companies, regardless of content. \n\nNow, we can turn to our final consideration, which is that the list of companies largest (longest) entry MUST be a superset. This is because of the fact that it necessarily will have as much as any others, and will have at least one more or the same. As such, it is a superset. Otherwise, it would not be there! \n\nWith this, we now turn to the first space use for time saving method, which is that we can go backwards in our list until we find our first subset! This may not be very far, but it may infact cover quite some distance. What this lets us do is skip to the very end of the story though and look there for supersets first and foremost. This lets us use our best options and to go backwards from there. \n\nAs such, we can imagine stepping back through the list to find the first subset in our list near the end. Along the way, any supersets found are added to their list and the result as needed. It must be that they are a superset of the list as a whole based on set theory at this point, and so we can be resolved of their usefulness. \n\nNext we mark the location of our first subset when the loop does terminate. If we never had to worry we have all unique sets and can simply sort the result and return. \n\nOtherwise, we will need to step forward through the list, utilizing our superset as we go to find supersets along the way. Iff an item is not a subset of the supersets, and it is not a subset of any list on the way to the supersets, then is it a superset. As such we will not be adding nearly as often, so we need to have methods in place to avoid such a prognosis. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe intution explains the approach in words. To simplify greatly \n- Keep a list of indices of supersets \n- Sort the list of favorite companies by length increasing \n- Find the supersets by going backwards over the list until your first subset \n- Find the supersets by going forwards over the list until you reach the index of your first subset from the above \n- Sort the result when done and return \n\n\n# Complexity\n- Time complexity:\n - First sort is O(C log C) -> C is size of companies list \n - Next loop is bound by C \n - Next loop is still bound by C \n - Loops again over c -> means can be treated as # * C so C \n - Final sort is O(N log N) where N is the number of supersets\n - N is at worst C, so we can interchange them \n - Total cost is O( C log C ) at worst \n\n- Space complexity:\n - Space complexity surely seems bad, however \n - Company strings could be losslessly encoded to a cipher \n - This could be done to build supersets offline and then cipher supersets and subsets by that process. \n - This would allow building to be done with storage for the ciphers, thus greatly reducing overhead \n - As such, we should consider the costs herein, but note saving momements \n - Company size list is O(C) \n - Supersets is O(N * avg length) \n - Result is O(N) \n - Total then is O(C + N * avg length + N) -> O(C) as N * avg length is sub to C \n - All of these could be done with offline storage, so you have at worst O(C) but at best O(1) \n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n # build listing \n result = [] \n status = True \n\n # sort the company size listings by size and keep as a set of comapnies with indexing \n # this allows us to use is subset alternation on future components \n # sort by length increasing so that we find all subsets quickly at start \n company_size_list = sorted([(set(companies), f_i) for f_i, companies in enumerate(favoriteCompanies)], \n key = lambda entry : len(entry[0]))\n\n # build a supersets backward \n supersets = []\n supersets.append(company_size_list[-1][0])\n result.append(company_size_list[-1][1])\n\n # by considering the set at the end to definitely be a superset of all before it \n s_j = len(company_size_list) - 2 \n s_k = 0 \n # we can then determine exactly the supersets that are uniquely supersets \n while s_j >= 0 : \n entry = company_size_list[s_j] \n for s_s in supersets : \n if entry[0].issubset(s_s) : \n # once we encounter a subset though, we need to stop here \n # this is for s_k. s_j is now set to -1 to store it \n s_k = s_j \n s_j = -1 \n break \n if s_j == -1 : \n break \n else : \n # if we do not need to break, append the entry set and indices\n supersets.append(entry[0])\n result.append(entry[1])\n if s_j != 0 : \n s_j -= 1\n else : \n break \n\n # if we need to now consider s_k \n if s_k != 0 : \n # get largest supersets first -> more likely to cause a break \n supersets.sort(key = lambda entry : len(entry), reverse=True)\n # loop on index of sorted company size list (s_i) with entry current in sorted listing \n for s_i, entry in enumerate(company_size_list) : \n # first check supersets \n for s_s in supersets : \n if entry[0].issubset(s_s) : \n status = False \n break \n # then if status is still true \n if status == True : \n # and then loop from there forward in the sorted company list \n s_j = s_i + 1 \n while s_j < s_k : \n alternate = company_size_list[s_j] \n if entry[0].issubset(alternate[0]) : \n status = False \n break \n else : \n s_j += 1 \n\n # One more check \n if status == True : \n result.append(entry[1])\n else : \n status = True\n\n if s_i + 1 == s_k : \n break \n \n # sort the result at the end of the set \n result.sort()\n return result \n \n``` | 0 | Given an array `arr` that represents a permutation of numbers from `1` to `n`.
You have a binary string of size `n` that initially has all its bits set to zero. At each step `i` (assuming both the binary string and `arr` are 1-indexed) from `1` to `n`, the bit at position `arr[i]` is set to `1`.
You are also given an integer `m`. Find the latest step at which there exists a group of ones of length `m`. A group of ones is a contiguous substring of `1`'s such that it cannot be extended in either direction.
Return _the latest step at which there exists a group of ones of length **exactly**_ `m`. _If no such group exists, return_ `-1`.
**Example 1:**
**Input:** arr = \[3,5,1,2,4\], m = 1
**Output:** 4
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "00101 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "11101 ", groups: \[ "111 ", "1 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
The latest step at which there exists a group of size 1 is step 4.
**Example 2:**
**Input:** arr = \[3,1,5,4,2\], m = 2
**Output:** -1
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "10100 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "10111 ", groups: \[ "1 ", "111 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
No group of size 2 exists during any step.
**Constraints:**
* `n == arr.length`
* `1 <= m <= n <= 105`
* `1 <= arr[i] <= n`
* All integers in `arr` are **distinct**. | Use hashing to convert company names in numbers and then for each list check if this is a subset of any other list. In order to check if a list is a subset of another list, use two pointers technique to get a linear solution for this task. The total complexity will be O(n^2 * m) where n is the number of lists and m is the maximum number of elements in a list. |
✅ The Fastest Approach Beats 100% | Explanation ✅ | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | \n\n\n# Approach\nThe main idea is to reduce nested loop time. In this case we iterates over all elements in the list that come **AFTER** the current element, instead of full iteration. It helps reduce time to become the fastest. \n\nWe don\'t need to check if **i** is subset of **j** when **len(i) > len(j)**, because bigger set cannot be subset of smaller one. In this case we can sort our list by lenght and keep original indexes.\n\n\n# Step by step explanation:\n```\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n # Final answer list and checker to use in loop\n ans, checker = [], True\n \n # We need to sort our list by lenght keeping indexes, it\'s good and easy way to do this (we also need to make set from each element):\n s = sorted([(set(i), index) for index, i in enumerate(favoriteCompanies)], key=lambda x: len(x[0]))\n # We got a list of tuples each contains set and original index. \n\n # Nested loop\n for i, check in enumerate(s):\n for look in s[i+1:]:\n\n # When we have first subset we already know that condition isn\'t fullfil\n # We need to break this loop to to save time\n if check[0].issubset(look[0]):\n checker = False\n break\n\n # If we didn\'t find any subset we can add index stores in tuple to answer list\n if checker: ans.append(check[1])\n checker = True\n\n # Our answer should be also sorted to fullfil conditions\n return sorted(ans)\n \n```\n# \u2764\uFE0F Please upvote if you found this helpfull. \u2764\uFE0F\n | 0 | Given the array `favoriteCompanies` where `favoriteCompanies[i]` is the list of favorites companies for the `ith` person (**indexed from 0**).
_Return the indices of people whose list of favorite companies is not a **subset** of any other list of favorites companies_. You must return the indices in increasing order.
**Example 1:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "google ", "microsoft "\],\[ "google ", "facebook "\],\[ "google "\],\[ "amazon "\]\]
**Output:** \[0,1,4\]
**Explanation:**
Person with index=2 has favoriteCompanies\[2\]=\[ "google ", "facebook "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] corresponding to the person with index 0.
Person with index=3 has favoriteCompanies\[3\]=\[ "google "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] and favoriteCompanies\[1\]=\[ "google ", "microsoft "\].
Other lists of favorite companies are not a subset of another list, therefore, the answer is \[0,1,4\].
**Example 2:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "leetcode ", "amazon "\],\[ "facebook ", "google "\]\]
**Output:** \[0,1\]
**Explanation:** In this case favoriteCompanies\[2\]=\[ "facebook ", "google "\] is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\], therefore, the answer is \[0,1\].
**Example 3:**
**Input:** favoriteCompanies = \[\[ "leetcode "\],\[ "google "\],\[ "facebook "\],\[ "amazon "\]\]
**Output:** \[0,1,2,3\]
**Constraints:**
* `1 <= favoriteCompanies.length <= 100`
* `1 <= favoriteCompanies[i].length <= 500`
* `1 <= favoriteCompanies[i][j].length <= 20`
* All strings in `favoriteCompanies[i]` are **distinct**.
* All lists of favorite companies are **distinct**, that is, If we sort alphabetically each list then `favoriteCompanies[i] != favoriteCompanies[j].`
* All strings consist of lowercase English letters only. | null |
✅ The Fastest Approach Beats 100% | Explanation ✅ | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | \n\n\n# Approach\nThe main idea is to reduce nested loop time. In this case we iterates over all elements in the list that come **AFTER** the current element, instead of full iteration. It helps reduce time to become the fastest. \n\nWe don\'t need to check if **i** is subset of **j** when **len(i) > len(j)**, because bigger set cannot be subset of smaller one. In this case we can sort our list by lenght and keep original indexes.\n\n\n# Step by step explanation:\n```\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n # Final answer list and checker to use in loop\n ans, checker = [], True\n \n # We need to sort our list by lenght keeping indexes, it\'s good and easy way to do this (we also need to make set from each element):\n s = sorted([(set(i), index) for index, i in enumerate(favoriteCompanies)], key=lambda x: len(x[0]))\n # We got a list of tuples each contains set and original index. \n\n # Nested loop\n for i, check in enumerate(s):\n for look in s[i+1:]:\n\n # When we have first subset we already know that condition isn\'t fullfil\n # We need to break this loop to to save time\n if check[0].issubset(look[0]):\n checker = False\n break\n\n # If we didn\'t find any subset we can add index stores in tuple to answer list\n if checker: ans.append(check[1])\n checker = True\n\n # Our answer should be also sorted to fullfil conditions\n return sorted(ans)\n \n```\n# \u2764\uFE0F Please upvote if you found this helpfull. \u2764\uFE0F\n | 0 | Given an array `arr` that represents a permutation of numbers from `1` to `n`.
You have a binary string of size `n` that initially has all its bits set to zero. At each step `i` (assuming both the binary string and `arr` are 1-indexed) from `1` to `n`, the bit at position `arr[i]` is set to `1`.
You are also given an integer `m`. Find the latest step at which there exists a group of ones of length `m`. A group of ones is a contiguous substring of `1`'s such that it cannot be extended in either direction.
Return _the latest step at which there exists a group of ones of length **exactly**_ `m`. _If no such group exists, return_ `-1`.
**Example 1:**
**Input:** arr = \[3,5,1,2,4\], m = 1
**Output:** 4
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "00101 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "11101 ", groups: \[ "111 ", "1 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
The latest step at which there exists a group of size 1 is step 4.
**Example 2:**
**Input:** arr = \[3,1,5,4,2\], m = 2
**Output:** -1
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "10100 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "10111 ", groups: \[ "1 ", "111 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
No group of size 2 exists during any step.
**Constraints:**
* `n == arr.length`
* `1 <= m <= n <= 105`
* `1 <= arr[i] <= n`
* All integers in `arr` are **distinct**. | Use hashing to convert company names in numbers and then for each list check if this is a subset of any other list. In order to check if a list is a subset of another list, use two pointers technique to get a linear solution for this task. The total complexity will be O(n^2 * m) where n is the number of lists and m is the maximum number of elements in a list. |
Python solution, readable and beats 100% memory | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nI just wanna solve stuff without the i,j and out of real world no library usages\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom itertools import combinations\n\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n\n result = []\n companies_to_companies_subsets = OrderedDict()\n\n for companies, companies_subsets in combinations(favoriteCompanies, 2):\n key1, key2 = tuple(companies), tuple(companies_subsets)\n companies_to_companies_subsets.setdefault(key1, []).append(companies_subsets)\n companies_to_companies_subsets.setdefault(key2, []).append(companies)\n\n for index, (companies, companies_subsets) in enumerate(companies_to_companies_subsets.items()):\n if not self._is_subset(companies, companies_subsets):\n result.append(index)\n\n return result\n\n def _is_subset(self, companies, companies_subsets):\n companies_set = set(companies)\n\n for companies_subset in companies_subsets:\n companies_subset_set = set(companies_subset)\n if companies_set.issubset(companies_subset_set):\n return True\n return False\n``` | 0 | Given the array `favoriteCompanies` where `favoriteCompanies[i]` is the list of favorites companies for the `ith` person (**indexed from 0**).
_Return the indices of people whose list of favorite companies is not a **subset** of any other list of favorites companies_. You must return the indices in increasing order.
**Example 1:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "google ", "microsoft "\],\[ "google ", "facebook "\],\[ "google "\],\[ "amazon "\]\]
**Output:** \[0,1,4\]
**Explanation:**
Person with index=2 has favoriteCompanies\[2\]=\[ "google ", "facebook "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] corresponding to the person with index 0.
Person with index=3 has favoriteCompanies\[3\]=\[ "google "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] and favoriteCompanies\[1\]=\[ "google ", "microsoft "\].
Other lists of favorite companies are not a subset of another list, therefore, the answer is \[0,1,4\].
**Example 2:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "leetcode ", "amazon "\],\[ "facebook ", "google "\]\]
**Output:** \[0,1\]
**Explanation:** In this case favoriteCompanies\[2\]=\[ "facebook ", "google "\] is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\], therefore, the answer is \[0,1\].
**Example 3:**
**Input:** favoriteCompanies = \[\[ "leetcode "\],\[ "google "\],\[ "facebook "\],\[ "amazon "\]\]
**Output:** \[0,1,2,3\]
**Constraints:**
* `1 <= favoriteCompanies.length <= 100`
* `1 <= favoriteCompanies[i].length <= 500`
* `1 <= favoriteCompanies[i][j].length <= 20`
* All strings in `favoriteCompanies[i]` are **distinct**.
* All lists of favorite companies are **distinct**, that is, If we sort alphabetically each list then `favoriteCompanies[i] != favoriteCompanies[j].`
* All strings consist of lowercase English letters only. | null |
Python solution, readable and beats 100% memory | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nI just wanna solve stuff without the i,j and out of real world no library usages\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom itertools import combinations\n\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n\n result = []\n companies_to_companies_subsets = OrderedDict()\n\n for companies, companies_subsets in combinations(favoriteCompanies, 2):\n key1, key2 = tuple(companies), tuple(companies_subsets)\n companies_to_companies_subsets.setdefault(key1, []).append(companies_subsets)\n companies_to_companies_subsets.setdefault(key2, []).append(companies)\n\n for index, (companies, companies_subsets) in enumerate(companies_to_companies_subsets.items()):\n if not self._is_subset(companies, companies_subsets):\n result.append(index)\n\n return result\n\n def _is_subset(self, companies, companies_subsets):\n companies_set = set(companies)\n\n for companies_subset in companies_subsets:\n companies_subset_set = set(companies_subset)\n if companies_set.issubset(companies_subset_set):\n return True\n return False\n``` | 0 | Given an array `arr` that represents a permutation of numbers from `1` to `n`.
You have a binary string of size `n` that initially has all its bits set to zero. At each step `i` (assuming both the binary string and `arr` are 1-indexed) from `1` to `n`, the bit at position `arr[i]` is set to `1`.
You are also given an integer `m`. Find the latest step at which there exists a group of ones of length `m`. A group of ones is a contiguous substring of `1`'s such that it cannot be extended in either direction.
Return _the latest step at which there exists a group of ones of length **exactly**_ `m`. _If no such group exists, return_ `-1`.
**Example 1:**
**Input:** arr = \[3,5,1,2,4\], m = 1
**Output:** 4
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "00101 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "11101 ", groups: \[ "111 ", "1 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
The latest step at which there exists a group of size 1 is step 4.
**Example 2:**
**Input:** arr = \[3,1,5,4,2\], m = 2
**Output:** -1
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "10100 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "10111 ", groups: \[ "1 ", "111 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
No group of size 2 exists during any step.
**Constraints:**
* `n == arr.length`
* `1 <= m <= n <= 105`
* `1 <= arr[i] <= n`
* All integers in `arr` are **distinct**. | Use hashing to convert company names in numbers and then for each list check if this is a subset of any other list. In order to check if a list is a subset of another list, use two pointers technique to get a linear solution for this task. The total complexity will be O(n^2 * m) where n is the number of lists and m is the maximum number of elements in a list. |
Python - 3 lines, simple with set difference | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Intuition\nConvert every list of strings to set of hash values of strings.\nDo index to index comaprison using set difference.\n\n# Complexity\n- Time complexity:\n$$O(n^2 F)$$ - F is length of list of favorites\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n N = len(favoriteCompanies)\n hs = [{ hash(f) for f in favoriteCompanies[i] } for i in range(N)]\n return [i for i in range(N) if all(i == j or hs[i] - hs[j] for j in range(N))]\n``` | 0 | Given the array `favoriteCompanies` where `favoriteCompanies[i]` is the list of favorites companies for the `ith` person (**indexed from 0**).
_Return the indices of people whose list of favorite companies is not a **subset** of any other list of favorites companies_. You must return the indices in increasing order.
**Example 1:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "google ", "microsoft "\],\[ "google ", "facebook "\],\[ "google "\],\[ "amazon "\]\]
**Output:** \[0,1,4\]
**Explanation:**
Person with index=2 has favoriteCompanies\[2\]=\[ "google ", "facebook "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] corresponding to the person with index 0.
Person with index=3 has favoriteCompanies\[3\]=\[ "google "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] and favoriteCompanies\[1\]=\[ "google ", "microsoft "\].
Other lists of favorite companies are not a subset of another list, therefore, the answer is \[0,1,4\].
**Example 2:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "leetcode ", "amazon "\],\[ "facebook ", "google "\]\]
**Output:** \[0,1\]
**Explanation:** In this case favoriteCompanies\[2\]=\[ "facebook ", "google "\] is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\], therefore, the answer is \[0,1\].
**Example 3:**
**Input:** favoriteCompanies = \[\[ "leetcode "\],\[ "google "\],\[ "facebook "\],\[ "amazon "\]\]
**Output:** \[0,1,2,3\]
**Constraints:**
* `1 <= favoriteCompanies.length <= 100`
* `1 <= favoriteCompanies[i].length <= 500`
* `1 <= favoriteCompanies[i][j].length <= 20`
* All strings in `favoriteCompanies[i]` are **distinct**.
* All lists of favorite companies are **distinct**, that is, If we sort alphabetically each list then `favoriteCompanies[i] != favoriteCompanies[j].`
* All strings consist of lowercase English letters only. | null |
Python - 3 lines, simple with set difference | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Intuition\nConvert every list of strings to set of hash values of strings.\nDo index to index comaprison using set difference.\n\n# Complexity\n- Time complexity:\n$$O(n^2 F)$$ - F is length of list of favorites\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n N = len(favoriteCompanies)\n hs = [{ hash(f) for f in favoriteCompanies[i] } for i in range(N)]\n return [i for i in range(N) if all(i == j or hs[i] - hs[j] for j in range(N))]\n``` | 0 | Given an array `arr` that represents a permutation of numbers from `1` to `n`.
You have a binary string of size `n` that initially has all its bits set to zero. At each step `i` (assuming both the binary string and `arr` are 1-indexed) from `1` to `n`, the bit at position `arr[i]` is set to `1`.
You are also given an integer `m`. Find the latest step at which there exists a group of ones of length `m`. A group of ones is a contiguous substring of `1`'s such that it cannot be extended in either direction.
Return _the latest step at which there exists a group of ones of length **exactly**_ `m`. _If no such group exists, return_ `-1`.
**Example 1:**
**Input:** arr = \[3,5,1,2,4\], m = 1
**Output:** 4
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "00101 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "11101 ", groups: \[ "111 ", "1 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
The latest step at which there exists a group of size 1 is step 4.
**Example 2:**
**Input:** arr = \[3,1,5,4,2\], m = 2
**Output:** -1
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "10100 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "10111 ", groups: \[ "1 ", "111 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
No group of size 2 exists during any step.
**Constraints:**
* `n == arr.length`
* `1 <= m <= n <= 105`
* `1 <= arr[i] <= n`
* All integers in `arr` are **distinct**. | Use hashing to convert company names in numbers and then for each list check if this is a subset of any other list. In order to check if a list is a subset of another list, use two pointers technique to get a linear solution for this task. The total complexity will be O(n^2 * m) where n is the number of lists and m is the maximum number of elements in a list. |
bad ass fuck | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, f: List[List[str]]) -> List[int]:\n dic={i:"" for i in range(len(f))}\n f=[{i[j]:"" for j in range(len(i))} for i in f]\n for i in range(len(f)) :\n arr=list(f[i].keys())\n for j in range(len(f)):\n for k in range(len(arr)):\n if i!=j:\n if f[j].get(arr[k])==None:break\n if k==len(arr)-1 and dic.get(i)!=None:dic.pop(i)\n return dic.keys()\n``` | 0 | Given the array `favoriteCompanies` where `favoriteCompanies[i]` is the list of favorites companies for the `ith` person (**indexed from 0**).
_Return the indices of people whose list of favorite companies is not a **subset** of any other list of favorites companies_. You must return the indices in increasing order.
**Example 1:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "google ", "microsoft "\],\[ "google ", "facebook "\],\[ "google "\],\[ "amazon "\]\]
**Output:** \[0,1,4\]
**Explanation:**
Person with index=2 has favoriteCompanies\[2\]=\[ "google ", "facebook "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] corresponding to the person with index 0.
Person with index=3 has favoriteCompanies\[3\]=\[ "google "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] and favoriteCompanies\[1\]=\[ "google ", "microsoft "\].
Other lists of favorite companies are not a subset of another list, therefore, the answer is \[0,1,4\].
**Example 2:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "leetcode ", "amazon "\],\[ "facebook ", "google "\]\]
**Output:** \[0,1\]
**Explanation:** In this case favoriteCompanies\[2\]=\[ "facebook ", "google "\] is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\], therefore, the answer is \[0,1\].
**Example 3:**
**Input:** favoriteCompanies = \[\[ "leetcode "\],\[ "google "\],\[ "facebook "\],\[ "amazon "\]\]
**Output:** \[0,1,2,3\]
**Constraints:**
* `1 <= favoriteCompanies.length <= 100`
* `1 <= favoriteCompanies[i].length <= 500`
* `1 <= favoriteCompanies[i][j].length <= 20`
* All strings in `favoriteCompanies[i]` are **distinct**.
* All lists of favorite companies are **distinct**, that is, If we sort alphabetically each list then `favoriteCompanies[i] != favoriteCompanies[j].`
* All strings consist of lowercase English letters only. | null |
bad ass fuck | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, f: List[List[str]]) -> List[int]:\n dic={i:"" for i in range(len(f))}\n f=[{i[j]:"" for j in range(len(i))} for i in f]\n for i in range(len(f)) :\n arr=list(f[i].keys())\n for j in range(len(f)):\n for k in range(len(arr)):\n if i!=j:\n if f[j].get(arr[k])==None:break\n if k==len(arr)-1 and dic.get(i)!=None:dic.pop(i)\n return dic.keys()\n``` | 0 | Given an array `arr` that represents a permutation of numbers from `1` to `n`.
You have a binary string of size `n` that initially has all its bits set to zero. At each step `i` (assuming both the binary string and `arr` are 1-indexed) from `1` to `n`, the bit at position `arr[i]` is set to `1`.
You are also given an integer `m`. Find the latest step at which there exists a group of ones of length `m`. A group of ones is a contiguous substring of `1`'s such that it cannot be extended in either direction.
Return _the latest step at which there exists a group of ones of length **exactly**_ `m`. _If no such group exists, return_ `-1`.
**Example 1:**
**Input:** arr = \[3,5,1,2,4\], m = 1
**Output:** 4
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "00101 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "11101 ", groups: \[ "111 ", "1 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
The latest step at which there exists a group of size 1 is step 4.
**Example 2:**
**Input:** arr = \[3,1,5,4,2\], m = 2
**Output:** -1
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "10100 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "10111 ", groups: \[ "1 ", "111 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
No group of size 2 exists during any step.
**Constraints:**
* `n == arr.length`
* `1 <= m <= n <= 105`
* `1 <= arr[i] <= n`
* All integers in `arr` are **distinct**. | Use hashing to convert company names in numbers and then for each list check if this is a subset of any other list. In order to check if a list is a subset of another list, use two pointers technique to get a linear solution for this task. The total complexity will be O(n^2 * m) where n is the number of lists and m is the maximum number of elements in a list. |
python super easy using set and map | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n\n c = collections.defaultdict(set)\n f = favoriteCompanies\n for i in range(len(f)):\n c[i] = set(f[i])\n \n\n ans = []\n for i in range(len(f)):\n for key in c:\n if key == i :\n continue\n if c[i] & c[key] == c[i]:\n break \n else:\n ans.append(i)\n return ans\n\n \n \n``` | 0 | Given the array `favoriteCompanies` where `favoriteCompanies[i]` is the list of favorites companies for the `ith` person (**indexed from 0**).
_Return the indices of people whose list of favorite companies is not a **subset** of any other list of favorites companies_. You must return the indices in increasing order.
**Example 1:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "google ", "microsoft "\],\[ "google ", "facebook "\],\[ "google "\],\[ "amazon "\]\]
**Output:** \[0,1,4\]
**Explanation:**
Person with index=2 has favoriteCompanies\[2\]=\[ "google ", "facebook "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] corresponding to the person with index 0.
Person with index=3 has favoriteCompanies\[3\]=\[ "google "\] which is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\] and favoriteCompanies\[1\]=\[ "google ", "microsoft "\].
Other lists of favorite companies are not a subset of another list, therefore, the answer is \[0,1,4\].
**Example 2:**
**Input:** favoriteCompanies = \[\[ "leetcode ", "google ", "facebook "\],\[ "leetcode ", "amazon "\],\[ "facebook ", "google "\]\]
**Output:** \[0,1\]
**Explanation:** In this case favoriteCompanies\[2\]=\[ "facebook ", "google "\] is a subset of favoriteCompanies\[0\]=\[ "leetcode ", "google ", "facebook "\], therefore, the answer is \[0,1\].
**Example 3:**
**Input:** favoriteCompanies = \[\[ "leetcode "\],\[ "google "\],\[ "facebook "\],\[ "amazon "\]\]
**Output:** \[0,1,2,3\]
**Constraints:**
* `1 <= favoriteCompanies.length <= 100`
* `1 <= favoriteCompanies[i].length <= 500`
* `1 <= favoriteCompanies[i][j].length <= 20`
* All strings in `favoriteCompanies[i]` are **distinct**.
* All lists of favorite companies are **distinct**, that is, If we sort alphabetically each list then `favoriteCompanies[i] != favoriteCompanies[j].`
* All strings consist of lowercase English letters only. | null |
python super easy using set and map | people-whose-list-of-favorite-companies-is-not-a-subset-of-another-list | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def peopleIndexes(self, favoriteCompanies: List[List[str]]) -> List[int]:\n\n c = collections.defaultdict(set)\n f = favoriteCompanies\n for i in range(len(f)):\n c[i] = set(f[i])\n \n\n ans = []\n for i in range(len(f)):\n for key in c:\n if key == i :\n continue\n if c[i] & c[key] == c[i]:\n break \n else:\n ans.append(i)\n return ans\n\n \n \n``` | 0 | Given an array `arr` that represents a permutation of numbers from `1` to `n`.
You have a binary string of size `n` that initially has all its bits set to zero. At each step `i` (assuming both the binary string and `arr` are 1-indexed) from `1` to `n`, the bit at position `arr[i]` is set to `1`.
You are also given an integer `m`. Find the latest step at which there exists a group of ones of length `m`. A group of ones is a contiguous substring of `1`'s such that it cannot be extended in either direction.
Return _the latest step at which there exists a group of ones of length **exactly**_ `m`. _If no such group exists, return_ `-1`.
**Example 1:**
**Input:** arr = \[3,5,1,2,4\], m = 1
**Output:** 4
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "00101 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "11101 ", groups: \[ "111 ", "1 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
The latest step at which there exists a group of size 1 is step 4.
**Example 2:**
**Input:** arr = \[3,1,5,4,2\], m = 2
**Output:** -1
**Explanation:**
Step 1: "00100 ", groups: \[ "1 "\]
Step 2: "10100 ", groups: \[ "1 ", "1 "\]
Step 3: "10101 ", groups: \[ "1 ", "1 ", "1 "\]
Step 4: "10111 ", groups: \[ "1 ", "111 "\]
Step 5: "11111 ", groups: \[ "11111 "\]
No group of size 2 exists during any step.
**Constraints:**
* `n == arr.length`
* `1 <= m <= n <= 105`
* `1 <= arr[i] <= n`
* All integers in `arr` are **distinct**. | Use hashing to convert company names in numbers and then for each list check if this is a subset of any other list. In order to check if a list is a subset of another list, use two pointers technique to get a linear solution for this task. The total complexity will be O(n^2 * m) where n is the number of lists and m is the maximum number of elements in a list. |
[Python3] angular sweep O(N^2 logN) | maximum-number-of-darts-inside-of-a-circular-dartboard | 0 | 1 | Algo\nPick a point, say P, from the set, and rotate a circle with fixed-radius `r`. During the rotation P lies on the circumference of the circle (note P is not the center) and maintain a count of the number of points in the circle at an angle \u0398 (between PC and x-axis, where C is the center of the circle). \n\nFor every other point Q within `2*r` distance to P, compute the angle \u0398 when Q enters the circle and the angle \u0398 when Q exits the circle with math. Sort the angles and scan through it to check the maximum points in the circle. \n\nPerform the above operation for all points to find the maximum. \n\nImplementation (time complexity `O(N^2 logN)` | space complexity `O(N)`) \n```\nclass Solution:\n def numPoints(self, points: List[List[int]], r: int) -> int:\n ans = 1\n for x, y in points: \n angles = []\n for x1, y1 in points: \n if (x1 != x or y1 != y) and (d:=sqrt((x1-x)**2 + (y1-y)**2)) <= 2*r: \n angle = atan2(y1-y, x1-x)\n delta = acos(d/(2*r))\n angles.append((angle-delta, +1)) #entry\n angles.append((angle+delta, -1)) #exit\n angles.sort(key=lambda x: (x[0], -x[1]))\n val = 1\n for _, entry in angles: \n ans = max(ans, val := val+entry)\n return ans \n``` | 42 | Alice is throwing `n` darts on a very large wall. You are given an array `darts` where `darts[i] = [xi, yi]` is the position of the `ith` dart that Alice threw on the wall.
Bob knows the positions of the `n` darts on the wall. He wants to place a dartboard of radius `r` on the wall so that the maximum number of darts that Alice throws lies on the dartboard.
Given the integer `r`, return _the maximum number of darts that can lie on the dartboard_.
**Example 1:**
**Input:** darts = \[\[-2,0\],\[2,0\],\[0,2\],\[0,-2\]\], r = 2
**Output:** 4
**Explanation:** Circle dartboard with center in (0,0) and radius = 2 contain all points.
**Example 2:**
**Input:** darts = \[\[-3,0\],\[3,0\],\[2,6\],\[5,4\],\[0,9\],\[7,8\]\], r = 5
**Output:** 5
**Explanation:** Circle dartboard with center in (0,4) and radius = 5 contain all points except the point (7,8).
**Constraints:**
* `1 <= darts.length <= 100`
* `darts[i].length == 2`
* `-104 <= xi, yi <= 104`
* All the `darts` are unique
* `1 <= r <= 5000` | null |
[Python3] angular sweep O(N^2 logN) | maximum-number-of-darts-inside-of-a-circular-dartboard | 0 | 1 | Algo\nPick a point, say P, from the set, and rotate a circle with fixed-radius `r`. During the rotation P lies on the circumference of the circle (note P is not the center) and maintain a count of the number of points in the circle at an angle \u0398 (between PC and x-axis, where C is the center of the circle). \n\nFor every other point Q within `2*r` distance to P, compute the angle \u0398 when Q enters the circle and the angle \u0398 when Q exits the circle with math. Sort the angles and scan through it to check the maximum points in the circle. \n\nPerform the above operation for all points to find the maximum. \n\nImplementation (time complexity `O(N^2 logN)` | space complexity `O(N)`) \n```\nclass Solution:\n def numPoints(self, points: List[List[int]], r: int) -> int:\n ans = 1\n for x, y in points: \n angles = []\n for x1, y1 in points: \n if (x1 != x or y1 != y) and (d:=sqrt((x1-x)**2 + (y1-y)**2)) <= 2*r: \n angle = atan2(y1-y, x1-x)\n delta = acos(d/(2*r))\n angles.append((angle-delta, +1)) #entry\n angles.append((angle+delta, -1)) #exit\n angles.sort(key=lambda x: (x[0], -x[1]))\n val = 1\n for _, entry in angles: \n ans = max(ans, val := val+entry)\n return ans \n``` | 42 | There are several stones **arranged in a row**, and each stone has an associated value which is an integer given in the array `stoneValue`.
In each round of the game, Alice divides the row into **two non-empty rows** (i.e. left row and right row), then Bob calculates the value of each row which is the sum of the values of all the stones in this row. Bob throws away the row which has the maximum value, and Alice's score increases by the value of the remaining row. If the value of the two rows are equal, Bob lets Alice decide which row will be thrown away. The next round starts with the remaining row.
The game ends when there is only **one stone remaining**. Alice's is initially **zero**.
Return _the maximum score that Alice can obtain_.
**Example 1:**
**Input:** stoneValue = \[6,2,3,4,5,5\]
**Output:** 18
**Explanation:** In the first round, Alice divides the row to \[6,2,3\], \[4,5,5\]. The left row has the value 11 and the right row has value 14. Bob throws away the right row and Alice's score is now 11.
In the second round Alice divides the row to \[6\], \[2,3\]. This time Bob throws away the left row and Alice's score becomes 16 (11 + 5).
The last round Alice has only one choice to divide the row which is \[2\], \[3\]. Bob throws away the right row and Alice's score is now 18 (16 + 2). The game ends because only one stone is remaining in the row.
**Example 2:**
**Input:** stoneValue = \[7,7,7,7,7,7,7\]
**Output:** 28
**Example 3:**
**Input:** stoneValue = \[4\]
**Output:** 0
**Constraints:**
* `1 <= stoneValue.length <= 500`
* `1 <= stoneValue[i] <= 106` | If there is an optimal solution, you can always move the circle so that two points lie on the boundary of the circle. When the radius is fixed, you can find either 0 or 1 or 2 circles that pass two given points at the same time. Loop for each pair of points and find the center of the circle, after that count the number of points inside the circle. |
Track my solution | maximum-number-of-darts-inside-of-a-circular-dartboard | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numPoints(self, darts: List[List[int]], r: int) -> int:\n ans = 0\n for i in range(len(darts)):\n angles = [(float("-inf"), 1)]\n for j in range(len(darts)):\n if i == j:\n continue\n x0, y0, x1, y1 = *darts[i], *darts[j]\n d = ((x0 - x1) ** 2 + (y0 - y1) ** 2) ** .5\n if d > 2*r:\n continue\n angle = math.atan2(y0 - y1, x0 - x1)\n delta = math.acos(d/(2*r))\n angles.append((angle - delta, 1))\n angles.append((angle + delta, -1))\n angles.sort(key=lambda x:(x[0], -x[1]))\n curr_max = max(itertools.accumulate(map(lambda x: x[1], angles)))\n if curr_max > ans:\n ans = curr_max\n return ans \n``` | 0 | Alice is throwing `n` darts on a very large wall. You are given an array `darts` where `darts[i] = [xi, yi]` is the position of the `ith` dart that Alice threw on the wall.
Bob knows the positions of the `n` darts on the wall. He wants to place a dartboard of radius `r` on the wall so that the maximum number of darts that Alice throws lies on the dartboard.
Given the integer `r`, return _the maximum number of darts that can lie on the dartboard_.
**Example 1:**
**Input:** darts = \[\[-2,0\],\[2,0\],\[0,2\],\[0,-2\]\], r = 2
**Output:** 4
**Explanation:** Circle dartboard with center in (0,0) and radius = 2 contain all points.
**Example 2:**
**Input:** darts = \[\[-3,0\],\[3,0\],\[2,6\],\[5,4\],\[0,9\],\[7,8\]\], r = 5
**Output:** 5
**Explanation:** Circle dartboard with center in (0,4) and radius = 5 contain all points except the point (7,8).
**Constraints:**
* `1 <= darts.length <= 100`
* `darts[i].length == 2`
* `-104 <= xi, yi <= 104`
* All the `darts` are unique
* `1 <= r <= 5000` | null |
Track my solution | maximum-number-of-darts-inside-of-a-circular-dartboard | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numPoints(self, darts: List[List[int]], r: int) -> int:\n ans = 0\n for i in range(len(darts)):\n angles = [(float("-inf"), 1)]\n for j in range(len(darts)):\n if i == j:\n continue\n x0, y0, x1, y1 = *darts[i], *darts[j]\n d = ((x0 - x1) ** 2 + (y0 - y1) ** 2) ** .5\n if d > 2*r:\n continue\n angle = math.atan2(y0 - y1, x0 - x1)\n delta = math.acos(d/(2*r))\n angles.append((angle - delta, 1))\n angles.append((angle + delta, -1))\n angles.sort(key=lambda x:(x[0], -x[1]))\n curr_max = max(itertools.accumulate(map(lambda x: x[1], angles)))\n if curr_max > ans:\n ans = curr_max\n return ans \n``` | 0 | There are several stones **arranged in a row**, and each stone has an associated value which is an integer given in the array `stoneValue`.
In each round of the game, Alice divides the row into **two non-empty rows** (i.e. left row and right row), then Bob calculates the value of each row which is the sum of the values of all the stones in this row. Bob throws away the row which has the maximum value, and Alice's score increases by the value of the remaining row. If the value of the two rows are equal, Bob lets Alice decide which row will be thrown away. The next round starts with the remaining row.
The game ends when there is only **one stone remaining**. Alice's is initially **zero**.
Return _the maximum score that Alice can obtain_.
**Example 1:**
**Input:** stoneValue = \[6,2,3,4,5,5\]
**Output:** 18
**Explanation:** In the first round, Alice divides the row to \[6,2,3\], \[4,5,5\]. The left row has the value 11 and the right row has value 14. Bob throws away the right row and Alice's score is now 11.
In the second round Alice divides the row to \[6\], \[2,3\]. This time Bob throws away the left row and Alice's score becomes 16 (11 + 5).
The last round Alice has only one choice to divide the row which is \[2\], \[3\]. Bob throws away the right row and Alice's score is now 18 (16 + 2). The game ends because only one stone is remaining in the row.
**Example 2:**
**Input:** stoneValue = \[7,7,7,7,7,7,7\]
**Output:** 28
**Example 3:**
**Input:** stoneValue = \[4\]
**Output:** 0
**Constraints:**
* `1 <= stoneValue.length <= 500`
* `1 <= stoneValue[i] <= 106` | If there is an optimal solution, you can always move the circle so that two points lie on the boundary of the circle. When the radius is fixed, you can find either 0 or 1 or 2 circles that pass two given points at the same time. Loop for each pair of points and find the center of the circle, after that count the number of points inside the circle. |
Physical Simulation | Commented and Explained | O(P^2) Time and Space | maximum-number-of-darts-inside-of-a-circular-dartboard | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nRather than given the integer r for the radius of the circle, if we consider the points that Bob knows, we know that Bob can find the grouping (or clustering) of points that are within the best distance of the other points. This is a problem of clustering then, so we can approach it with a basis on the relationship of points to each other and the radius. \n\nFor a given circle, the radius is 2*r.\nFor a given two points in euclidean space, 2D their distance is the square root of squares of del x and del y summed. \nFor any given point i, considering all other points j of the set of points P, if point i is not point j and the distance between them is within 2 * r, the point will lie within the circle at such a time that its first angle of three sides (diameter, angle adject to point distance, angle opposite point distance) alpha defined as atan2(p1y - p2y, p1x - p2x) is comparatively smaller than its second angle beta defined as acos(distance / 2*r) where we consider the following : \n- if angle beta is positive \n - if angle alpha is negative or positive \n - the point is inside until angle of circle rotates to include alpha \n- otherwise \n - if angle alpha is negative \n - the point is never inside until angle of circle rotates to include alpha \n - otherwise \n - the point is inside until angle of circle rotates to exclude alpha \n\nThe point at which the angle alpha is included or excluded can be found by sorting the angles of the points and their relationships to a given point first by their adjusted value (alpha is alpha - beta, beta is alpha + beta), then by their type (alpha before beta on clash of adjusted values) \n\nWe then can iterate over our angles, incrementing on alpha and decrementing on finding the matching beta (as that means we have now excluded it). As we do, we maximize our points included at each step and then return maximal points found \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nTook an OOP approach for this one both for practice and to help others see the progression of points and their implementations. \n\nDuring initialization\n- Set up fields pL, max_points, distances, and angles as follows for stated reasons \n - pL is the length of our points list starts at 1 by problem definition\n - max_points starts at 0 (might be radius is too small to place circle)\n - distances is a list of lists that contains only an empty list (type match set up)\n - angles is a list of angle vectors of type alpha / beta with typing\n\nEuclidean distance function get distance \n- given points p1 and p2 find the distance \n - calculate del x as p1[0] - p2[0] \n - calculate del y as p1[1] - p2[1] \n - return math.sqrt(del x ** 2 + del y ** 2)\n\nSet up function \n- Passing in darts, set up pL, distances fields \n- set pL to length of darts \n- set distances as 0 for _ in range pL for __ in range pL (adjacency matrix) \n- for p_i in range (pL - 1) \n - for p_j in range(p_i + 1, pL) \n - get p1 as darts at p_i \n - get p2 as darts at p_j\n - distance is get distance (p1, p2) \n - set self.distances[p_i][p_j] = distance \n - set self.distances[p_j][p_i] = distance \n\nset angle vectors function \n- passing in p_i, radius, and points \n- calculate and set angle vectors as needed in relation to p_i\n- To do so, reset current angles field to an empty list \n- Then, for p_j in range(pL) \n - if p_i != p_j and distance p_i to p_j lte 2*radius \n - beta is acos(distance pi to pj / 2*radius)\n - pix, piy = points p_i \n - pjx, pjy = points p_j \n - alpha is atan2(piy - pjy, pix - pjx)\n - alpha flag is alpha - beta (include flag) \n - beta flag is alpha + beta (exclude flag)\n - append to angles (alpha_flag, False) and (beta_flag, True) \n - the boolean is so that we include before excluding any points\n\nget num points inside function \n- sort your current angles vector (see note above about booleans)\n- set num points inside to 1 and maximum num points inside to 1 \n- for angle in self.angles \n - if angle[1] is False, it\'s alpha_flag, increment num points inside \n - otherwise, it\'s a beta flag, decrement num points inside \n - set maximum num points to max of maximum num points and num points inside \n- return maximum num points \n\nnumPoints function \n- set up your solution by passing in darts \n- for p_i in range pL \n - set angle vectors by passing p_i, r, and darts \n - get current num points inside using get num points inside \n - set max points to max of max points and current num points inside \n- return max points \n\n\n# Complexity\n- Time complexity : O(P^2)\n - Takes O(P * P) to get distances set up \n - Takes O(P) to process all points in numPoints \n - within which we do O(p) to process all non-matched p_i and p_j and distances lte 2*r \n - Takes O(a * 2) to process all angles (alpha and beta angles)\n - Total then is O(P * P + P * p + 2 * a) where P is total number of points, p is number of points in range given current and not match, a is number of angles. As p is underneath P and a is underneath p, total is O(P^2) \n\n- Space complexity : O(P^2)\n - We store O(P^2) distances \n - We store O(2 * a) angles at a time \n - As a is underneath P, total is O(P^2)\n\n# Code\n```\nclass Solution:\n # initialize with fields as needed \n def __init__(self) : \n self.pL = 1 \n self.max_points = 0\n self.distances = [[]]\n self.angles = [] \n\n def get_distance(self, p1, p2) : \n # euclidean distance between two points \n del_x = p1[0] - p2[0]\n del_y = p1[1] - p2[1]\n return math.sqrt((del_x**2) + (del_y **2))\n\n def set_up(self, darts) : \n # number of points is number of darts \n self.pL = len(darts)\n # for which the distances are the same in sizing \n self.distances = [[0 for _ in range(self.pL)] for __ in range(self.pL)]\n # for point i in range up to pL - 1 \n for p_i in range(self.pL - 1) : \n # for point j in range point i + 1 up to pL \n for p_j in range(p_i + 1, self.pL) : \n # get each point \n p1 = darts[p_i]\n p2 = darts[p_j]\n # find distance \n distance = self.get_distance(p1, p2)\n # set appropriately \n self.distances[p_i][p_j] = distance \n self.distances[p_j][p_i] = distance\n\n # to get angle vectors for a given point with radius and list of points \n def set_angle_vectors(self, p_i, radius, points) : \n # reset to build \n self.angles = [] \n # for point j in range points L \n for p_j in range(self.pL) : \n # on non-match and distance in radius of 2*r \n if p_i != p_j and self.distances[p_i][p_j] <= 2 * radius : \n # get beta angle via cosine inverse \n # beta angle is angle between the distance line measure and the diameter \n beta = math.acos(self.distances[p_i][p_j] / (2*radius))\n # map in points x and y components \n pix, piy = points[p_i]\n pjx, pjy = points[p_j]\n # get alpha angle via squared tan inverse \n # this is the angle between the points that is not the right angle \n alpha = math.atan2(piy-pjy, pix - pjx)\n # set alpha flag value to result of alpha - beta and beta flag to alpha + beta \n alpha_flag = alpha - beta \n beta_flag = alpha + beta\n # append alpha with False and beta with True \n self.angles.append((alpha_flag, False))\n self.angles.append((beta_flag, True))\n \n def get_num_points_inside(self) : \n # sort the angles by their first property then their second \n # Here the alpha and beta flags are denoted by their differences \n # So long as all the alphas are less then the beta flags, which is true for positive alpha beta \n # where alpha is lte alpha, positive alpha and negative beta, negative alpha and positive beta, \n # and never for both negative we will count the alpha\'s before the betas \n # these are points that lie in the best circle placement we could consider for a given point \n # where we settle ties by having alpha appear before beta using false which evaluates to 0 \n # and true which evaluates to 1\n # We also consider the idea here that as we move the placement there is an order to the maximal placement where some points will be lost as we move it to such a position that the circle is now past the radius (the 2*r being part of the beta angle calculation).\n # our process in simplified terms is to find the number of points such that the point in question with that point has an angle alpha < 90 and beta < 90 with a length of at most 2 * r from our given point \n self.angles.sort()\n # num points inside is 1 and maximum_num_points_inside is 1 to start \n # this is because we can always at least capture the current point in question \n num_points_inside = 1 \n maximum_num_points_inside = 1 \n # for angle in self.angles \n # these are the points where the distance of points is lte 2 r \n for angle in self.angles : \n # update num points and then maximum_num_points_inside\n num_points_inside += 1 if angle[1] == False else -1\n maximum_num_points_inside = max(maximum_num_points_inside, num_points_inside)\n # return maximum found \n return maximum_num_points_inside\n\n def numPoints(self, darts: List[List[int]], r: int) -> int :\n # set up \n self.set_up(darts)\n # for each point index \n for p_i in range(self.pL) : \n # set angle vectors accordingly \n self.set_angle_vectors(p_i, r, darts)\n # get current num points inside based on angle vectors \n current_num_points_inside = self.get_num_points_inside()\n # update max points appropriately\n self.max_points = max(self.max_points, current_num_points_inside)\n # return valuation \n return self.max_points\n\n``` | 0 | Alice is throwing `n` darts on a very large wall. You are given an array `darts` where `darts[i] = [xi, yi]` is the position of the `ith` dart that Alice threw on the wall.
Bob knows the positions of the `n` darts on the wall. He wants to place a dartboard of radius `r` on the wall so that the maximum number of darts that Alice throws lies on the dartboard.
Given the integer `r`, return _the maximum number of darts that can lie on the dartboard_.
**Example 1:**
**Input:** darts = \[\[-2,0\],\[2,0\],\[0,2\],\[0,-2\]\], r = 2
**Output:** 4
**Explanation:** Circle dartboard with center in (0,0) and radius = 2 contain all points.
**Example 2:**
**Input:** darts = \[\[-3,0\],\[3,0\],\[2,6\],\[5,4\],\[0,9\],\[7,8\]\], r = 5
**Output:** 5
**Explanation:** Circle dartboard with center in (0,4) and radius = 5 contain all points except the point (7,8).
**Constraints:**
* `1 <= darts.length <= 100`
* `darts[i].length == 2`
* `-104 <= xi, yi <= 104`
* All the `darts` are unique
* `1 <= r <= 5000` | null |
Physical Simulation | Commented and Explained | O(P^2) Time and Space | maximum-number-of-darts-inside-of-a-circular-dartboard | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nRather than given the integer r for the radius of the circle, if we consider the points that Bob knows, we know that Bob can find the grouping (or clustering) of points that are within the best distance of the other points. This is a problem of clustering then, so we can approach it with a basis on the relationship of points to each other and the radius. \n\nFor a given circle, the radius is 2*r.\nFor a given two points in euclidean space, 2D their distance is the square root of squares of del x and del y summed. \nFor any given point i, considering all other points j of the set of points P, if point i is not point j and the distance between them is within 2 * r, the point will lie within the circle at such a time that its first angle of three sides (diameter, angle adject to point distance, angle opposite point distance) alpha defined as atan2(p1y - p2y, p1x - p2x) is comparatively smaller than its second angle beta defined as acos(distance / 2*r) where we consider the following : \n- if angle beta is positive \n - if angle alpha is negative or positive \n - the point is inside until angle of circle rotates to include alpha \n- otherwise \n - if angle alpha is negative \n - the point is never inside until angle of circle rotates to include alpha \n - otherwise \n - the point is inside until angle of circle rotates to exclude alpha \n\nThe point at which the angle alpha is included or excluded can be found by sorting the angles of the points and their relationships to a given point first by their adjusted value (alpha is alpha - beta, beta is alpha + beta), then by their type (alpha before beta on clash of adjusted values) \n\nWe then can iterate over our angles, incrementing on alpha and decrementing on finding the matching beta (as that means we have now excluded it). As we do, we maximize our points included at each step and then return maximal points found \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nTook an OOP approach for this one both for practice and to help others see the progression of points and their implementations. \n\nDuring initialization\n- Set up fields pL, max_points, distances, and angles as follows for stated reasons \n - pL is the length of our points list starts at 1 by problem definition\n - max_points starts at 0 (might be radius is too small to place circle)\n - distances is a list of lists that contains only an empty list (type match set up)\n - angles is a list of angle vectors of type alpha / beta with typing\n\nEuclidean distance function get distance \n- given points p1 and p2 find the distance \n - calculate del x as p1[0] - p2[0] \n - calculate del y as p1[1] - p2[1] \n - return math.sqrt(del x ** 2 + del y ** 2)\n\nSet up function \n- Passing in darts, set up pL, distances fields \n- set pL to length of darts \n- set distances as 0 for _ in range pL for __ in range pL (adjacency matrix) \n- for p_i in range (pL - 1) \n - for p_j in range(p_i + 1, pL) \n - get p1 as darts at p_i \n - get p2 as darts at p_j\n - distance is get distance (p1, p2) \n - set self.distances[p_i][p_j] = distance \n - set self.distances[p_j][p_i] = distance \n\nset angle vectors function \n- passing in p_i, radius, and points \n- calculate and set angle vectors as needed in relation to p_i\n- To do so, reset current angles field to an empty list \n- Then, for p_j in range(pL) \n - if p_i != p_j and distance p_i to p_j lte 2*radius \n - beta is acos(distance pi to pj / 2*radius)\n - pix, piy = points p_i \n - pjx, pjy = points p_j \n - alpha is atan2(piy - pjy, pix - pjx)\n - alpha flag is alpha - beta (include flag) \n - beta flag is alpha + beta (exclude flag)\n - append to angles (alpha_flag, False) and (beta_flag, True) \n - the boolean is so that we include before excluding any points\n\nget num points inside function \n- sort your current angles vector (see note above about booleans)\n- set num points inside to 1 and maximum num points inside to 1 \n- for angle in self.angles \n - if angle[1] is False, it\'s alpha_flag, increment num points inside \n - otherwise, it\'s a beta flag, decrement num points inside \n - set maximum num points to max of maximum num points and num points inside \n- return maximum num points \n\nnumPoints function \n- set up your solution by passing in darts \n- for p_i in range pL \n - set angle vectors by passing p_i, r, and darts \n - get current num points inside using get num points inside \n - set max points to max of max points and current num points inside \n- return max points \n\n\n# Complexity\n- Time complexity : O(P^2)\n - Takes O(P * P) to get distances set up \n - Takes O(P) to process all points in numPoints \n - within which we do O(p) to process all non-matched p_i and p_j and distances lte 2*r \n - Takes O(a * 2) to process all angles (alpha and beta angles)\n - Total then is O(P * P + P * p + 2 * a) where P is total number of points, p is number of points in range given current and not match, a is number of angles. As p is underneath P and a is underneath p, total is O(P^2) \n\n- Space complexity : O(P^2)\n - We store O(P^2) distances \n - We store O(2 * a) angles at a time \n - As a is underneath P, total is O(P^2)\n\n# Code\n```\nclass Solution:\n # initialize with fields as needed \n def __init__(self) : \n self.pL = 1 \n self.max_points = 0\n self.distances = [[]]\n self.angles = [] \n\n def get_distance(self, p1, p2) : \n # euclidean distance between two points \n del_x = p1[0] - p2[0]\n del_y = p1[1] - p2[1]\n return math.sqrt((del_x**2) + (del_y **2))\n\n def set_up(self, darts) : \n # number of points is number of darts \n self.pL = len(darts)\n # for which the distances are the same in sizing \n self.distances = [[0 for _ in range(self.pL)] for __ in range(self.pL)]\n # for point i in range up to pL - 1 \n for p_i in range(self.pL - 1) : \n # for point j in range point i + 1 up to pL \n for p_j in range(p_i + 1, self.pL) : \n # get each point \n p1 = darts[p_i]\n p2 = darts[p_j]\n # find distance \n distance = self.get_distance(p1, p2)\n # set appropriately \n self.distances[p_i][p_j] = distance \n self.distances[p_j][p_i] = distance\n\n # to get angle vectors for a given point with radius and list of points \n def set_angle_vectors(self, p_i, radius, points) : \n # reset to build \n self.angles = [] \n # for point j in range points L \n for p_j in range(self.pL) : \n # on non-match and distance in radius of 2*r \n if p_i != p_j and self.distances[p_i][p_j] <= 2 * radius : \n # get beta angle via cosine inverse \n # beta angle is angle between the distance line measure and the diameter \n beta = math.acos(self.distances[p_i][p_j] / (2*radius))\n # map in points x and y components \n pix, piy = points[p_i]\n pjx, pjy = points[p_j]\n # get alpha angle via squared tan inverse \n # this is the angle between the points that is not the right angle \n alpha = math.atan2(piy-pjy, pix - pjx)\n # set alpha flag value to result of alpha - beta and beta flag to alpha + beta \n alpha_flag = alpha - beta \n beta_flag = alpha + beta\n # append alpha with False and beta with True \n self.angles.append((alpha_flag, False))\n self.angles.append((beta_flag, True))\n \n def get_num_points_inside(self) : \n # sort the angles by their first property then their second \n # Here the alpha and beta flags are denoted by their differences \n # So long as all the alphas are less then the beta flags, which is true for positive alpha beta \n # where alpha is lte alpha, positive alpha and negative beta, negative alpha and positive beta, \n # and never for both negative we will count the alpha\'s before the betas \n # these are points that lie in the best circle placement we could consider for a given point \n # where we settle ties by having alpha appear before beta using false which evaluates to 0 \n # and true which evaluates to 1\n # We also consider the idea here that as we move the placement there is an order to the maximal placement where some points will be lost as we move it to such a position that the circle is now past the radius (the 2*r being part of the beta angle calculation).\n # our process in simplified terms is to find the number of points such that the point in question with that point has an angle alpha < 90 and beta < 90 with a length of at most 2 * r from our given point \n self.angles.sort()\n # num points inside is 1 and maximum_num_points_inside is 1 to start \n # this is because we can always at least capture the current point in question \n num_points_inside = 1 \n maximum_num_points_inside = 1 \n # for angle in self.angles \n # these are the points where the distance of points is lte 2 r \n for angle in self.angles : \n # update num points and then maximum_num_points_inside\n num_points_inside += 1 if angle[1] == False else -1\n maximum_num_points_inside = max(maximum_num_points_inside, num_points_inside)\n # return maximum found \n return maximum_num_points_inside\n\n def numPoints(self, darts: List[List[int]], r: int) -> int :\n # set up \n self.set_up(darts)\n # for each point index \n for p_i in range(self.pL) : \n # set angle vectors accordingly \n self.set_angle_vectors(p_i, r, darts)\n # get current num points inside based on angle vectors \n current_num_points_inside = self.get_num_points_inside()\n # update max points appropriately\n self.max_points = max(self.max_points, current_num_points_inside)\n # return valuation \n return self.max_points\n\n``` | 0 | There are several stones **arranged in a row**, and each stone has an associated value which is an integer given in the array `stoneValue`.
In each round of the game, Alice divides the row into **two non-empty rows** (i.e. left row and right row), then Bob calculates the value of each row which is the sum of the values of all the stones in this row. Bob throws away the row which has the maximum value, and Alice's score increases by the value of the remaining row. If the value of the two rows are equal, Bob lets Alice decide which row will be thrown away. The next round starts with the remaining row.
The game ends when there is only **one stone remaining**. Alice's is initially **zero**.
Return _the maximum score that Alice can obtain_.
**Example 1:**
**Input:** stoneValue = \[6,2,3,4,5,5\]
**Output:** 18
**Explanation:** In the first round, Alice divides the row to \[6,2,3\], \[4,5,5\]. The left row has the value 11 and the right row has value 14. Bob throws away the right row and Alice's score is now 11.
In the second round Alice divides the row to \[6\], \[2,3\]. This time Bob throws away the left row and Alice's score becomes 16 (11 + 5).
The last round Alice has only one choice to divide the row which is \[2\], \[3\]. Bob throws away the right row and Alice's score is now 18 (16 + 2). The game ends because only one stone is remaining in the row.
**Example 2:**
**Input:** stoneValue = \[7,7,7,7,7,7,7\]
**Output:** 28
**Example 3:**
**Input:** stoneValue = \[4\]
**Output:** 0
**Constraints:**
* `1 <= stoneValue.length <= 500`
* `1 <= stoneValue[i] <= 106` | If there is an optimal solution, you can always move the circle so that two points lie on the boundary of the circle. When the radius is fixed, you can find either 0 or 1 or 2 circles that pass two given points at the same time. Loop for each pair of points and find the center of the circle, after that count the number of points inside the circle. |
Clean Python solution with explaination | maximum-number-of-darts-inside-of-a-circular-dartboard | 0 | 1 | Step by step:\n1. The desired circle should be passed through at least 2 points out of those n points.\n\n2. Hence, let consider all pairs of those 2 points with the construction of the circle with radius r passes through those 2 points.\n\n3. Compute all possible centers (up to two). The trick in compute more faster is recognize that if there are 2 possible values -> they are in formed (x_mid + eps, y_mid + fun); (x_mid - eps, y_mid - fun) with (x_mid, y_mid) are the middle points of the choosen 2 points. \nThen we have those equations:\n\n**+) ep ^ 2 + fun ^ 2 == r ^ 2 - dist(mid, p1) ^2. // from Pythagoras equations**\n**+) ep * (p1[0] - p2[0]) + fun * (p1[1] - p2[1]) == 0. // from normal equations** \n\nwith p1, p2 are 2 choosen points, mid is the middle point of p1, p2;\n\n4. With each pairs of choosen points, iterating through the set points again to find number of points insides the circle with centers and radius r and update the result with the higher value. \n```\nimport math as m\nclass Solution(object):\n def dist(self, p1, p2):\n dx = p1[0] - p2[0]\n dy = p1[1] - p2[1]\n return m.sqrt(dx * dx + dy * dy)\n \n def intersect(self, p1, p2, r):\n res = []\n d = self.dist(p1, p2) - 2 * r\n if (d > 0):\n res = []\n elif (d == 0):\n res = [[(p1[0] + p2[0]) / 2, (p1[1] + p2[1]) / 2]] \n else:\n mid_x = (0.0 + p1[0] + p2[0]) / 2\n mid_y = (0.0 + p1[1] + p2[1]) / 2\n # ep ^ 2 + fun ^ 2 == r ^ 2 - dist(mid, p1) ^ 2;\n # ep * (p1[0] - p2[0]) + fun * (p1[1] - p2[1]) == 0 -> fun = -(p1[0] - p2[0]) / (p1[1] - p2[1]) * eps;\n if (p1[1] != p2[1]):\n ratio = - (0.0 + p1[0] - p2[0]) / (0.0 + p1[1] - p2[1])\n eps = m.sqrt((r ** 2 - self.dist([mid_x, mid_y], p1) ** 2) / (ratio ** 2 + 1))\n fun = eps * ratio\n else:\n eps = 0\n fun = m.sqrt(r ** 2 - self.dist([mid_x, mid_y], p1) ** 2)\n # res update \n res =[[mid_x + eps, mid_y + fun], [mid_x - eps, mid_y - fun]]\n return res \n \n def numPoints(self, points, r):\n l = len(points)\n result = 1\n for i in range(l):\n for j in range(i + 1, l):\n c = self.intersect(points[i], points[j], r)\n for p in c:\n au = 0\n for k in range(l):\n if (self.dist(p, points[k]) <= r): au += 1\n result = max(result, au)\n return result\n``` | 4 | Alice is throwing `n` darts on a very large wall. You are given an array `darts` where `darts[i] = [xi, yi]` is the position of the `ith` dart that Alice threw on the wall.
Bob knows the positions of the `n` darts on the wall. He wants to place a dartboard of radius `r` on the wall so that the maximum number of darts that Alice throws lies on the dartboard.
Given the integer `r`, return _the maximum number of darts that can lie on the dartboard_.
**Example 1:**
**Input:** darts = \[\[-2,0\],\[2,0\],\[0,2\],\[0,-2\]\], r = 2
**Output:** 4
**Explanation:** Circle dartboard with center in (0,0) and radius = 2 contain all points.
**Example 2:**
**Input:** darts = \[\[-3,0\],\[3,0\],\[2,6\],\[5,4\],\[0,9\],\[7,8\]\], r = 5
**Output:** 5
**Explanation:** Circle dartboard with center in (0,4) and radius = 5 contain all points except the point (7,8).
**Constraints:**
* `1 <= darts.length <= 100`
* `darts[i].length == 2`
* `-104 <= xi, yi <= 104`
* All the `darts` are unique
* `1 <= r <= 5000` | null |
Clean Python solution with explaination | maximum-number-of-darts-inside-of-a-circular-dartboard | 0 | 1 | Step by step:\n1. The desired circle should be passed through at least 2 points out of those n points.\n\n2. Hence, let consider all pairs of those 2 points with the construction of the circle with radius r passes through those 2 points.\n\n3. Compute all possible centers (up to two). The trick in compute more faster is recognize that if there are 2 possible values -> they are in formed (x_mid + eps, y_mid + fun); (x_mid - eps, y_mid - fun) with (x_mid, y_mid) are the middle points of the choosen 2 points. \nThen we have those equations:\n\n**+) ep ^ 2 + fun ^ 2 == r ^ 2 - dist(mid, p1) ^2. // from Pythagoras equations**\n**+) ep * (p1[0] - p2[0]) + fun * (p1[1] - p2[1]) == 0. // from normal equations** \n\nwith p1, p2 are 2 choosen points, mid is the middle point of p1, p2;\n\n4. With each pairs of choosen points, iterating through the set points again to find number of points insides the circle with centers and radius r and update the result with the higher value. \n```\nimport math as m\nclass Solution(object):\n def dist(self, p1, p2):\n dx = p1[0] - p2[0]\n dy = p1[1] - p2[1]\n return m.sqrt(dx * dx + dy * dy)\n \n def intersect(self, p1, p2, r):\n res = []\n d = self.dist(p1, p2) - 2 * r\n if (d > 0):\n res = []\n elif (d == 0):\n res = [[(p1[0] + p2[0]) / 2, (p1[1] + p2[1]) / 2]] \n else:\n mid_x = (0.0 + p1[0] + p2[0]) / 2\n mid_y = (0.0 + p1[1] + p2[1]) / 2\n # ep ^ 2 + fun ^ 2 == r ^ 2 - dist(mid, p1) ^ 2;\n # ep * (p1[0] - p2[0]) + fun * (p1[1] - p2[1]) == 0 -> fun = -(p1[0] - p2[0]) / (p1[1] - p2[1]) * eps;\n if (p1[1] != p2[1]):\n ratio = - (0.0 + p1[0] - p2[0]) / (0.0 + p1[1] - p2[1])\n eps = m.sqrt((r ** 2 - self.dist([mid_x, mid_y], p1) ** 2) / (ratio ** 2 + 1))\n fun = eps * ratio\n else:\n eps = 0\n fun = m.sqrt(r ** 2 - self.dist([mid_x, mid_y], p1) ** 2)\n # res update \n res =[[mid_x + eps, mid_y + fun], [mid_x - eps, mid_y - fun]]\n return res \n \n def numPoints(self, points, r):\n l = len(points)\n result = 1\n for i in range(l):\n for j in range(i + 1, l):\n c = self.intersect(points[i], points[j], r)\n for p in c:\n au = 0\n for k in range(l):\n if (self.dist(p, points[k]) <= r): au += 1\n result = max(result, au)\n return result\n``` | 4 | There are several stones **arranged in a row**, and each stone has an associated value which is an integer given in the array `stoneValue`.
In each round of the game, Alice divides the row into **two non-empty rows** (i.e. left row and right row), then Bob calculates the value of each row which is the sum of the values of all the stones in this row. Bob throws away the row which has the maximum value, and Alice's score increases by the value of the remaining row. If the value of the two rows are equal, Bob lets Alice decide which row will be thrown away. The next round starts with the remaining row.
The game ends when there is only **one stone remaining**. Alice's is initially **zero**.
Return _the maximum score that Alice can obtain_.
**Example 1:**
**Input:** stoneValue = \[6,2,3,4,5,5\]
**Output:** 18
**Explanation:** In the first round, Alice divides the row to \[6,2,3\], \[4,5,5\]. The left row has the value 11 and the right row has value 14. Bob throws away the right row and Alice's score is now 11.
In the second round Alice divides the row to \[6\], \[2,3\]. This time Bob throws away the left row and Alice's score becomes 16 (11 + 5).
The last round Alice has only one choice to divide the row which is \[2\], \[3\]. Bob throws away the right row and Alice's score is now 18 (16 + 2). The game ends because only one stone is remaining in the row.
**Example 2:**
**Input:** stoneValue = \[7,7,7,7,7,7,7\]
**Output:** 28
**Example 3:**
**Input:** stoneValue = \[4\]
**Output:** 0
**Constraints:**
* `1 <= stoneValue.length <= 500`
* `1 <= stoneValue[i] <= 106` | If there is an optimal solution, you can always move the circle so that two points lie on the boundary of the circle. When the radius is fixed, you can find either 0 or 1 or 2 circles that pass two given points at the same time. Loop for each pair of points and find the center of the circle, after that count the number of points inside the circle. |
Simple Python solution using startswith | check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def isPrefixOfWord(self, s: str, searchWord: str) -> int:\n a = s.split()\n for i in range(len(a)):\n if a[i].startswith(searchWord):\n return i+1\n return -1\n``` | 2 | Given a `sentence` that consists of some words separated by a **single space**, and a `searchWord`, check if `searchWord` is a prefix of any word in `sentence`.
Return _the index of the word in_ `sentence` _(**1-indexed**) where_ `searchWord` _is a prefix of this word_. If `searchWord` is a prefix of more than one word, return the index of the first word **(minimum index)**. If there is no such word return `-1`.
A **prefix** of a string `s` is any leading contiguous substring of `s`.
**Example 1:**
**Input:** sentence = "i love eating burger ", searchWord = "burg "
**Output:** 4
**Explanation:** "burg " is prefix of "burger " which is the 4th word in the sentence.
**Example 2:**
**Input:** sentence = "this problem is an easy problem ", searchWord = "pro "
**Output:** 2
**Explanation:** "pro " is prefix of "problem " which is the 2nd and the 6th word in the sentence, but we return 2 as it's the minimal index.
**Example 3:**
**Input:** sentence = "i am tired ", searchWord = "you "
**Output:** -1
**Explanation:** "you " is not a prefix of any word in the sentence.
**Constraints:**
* `1 <= sentence.length <= 100`
* `1 <= searchWord.length <= 10`
* `sentence` consists of lowercase English letters and spaces.
* `searchWord` consists of lowercase English letters. | Do the filtering and sort as said. Note that the id may not be the index in the array. |
Simple Python solution using startswith | check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def isPrefixOfWord(self, s: str, searchWord: str) -> int:\n a = s.split()\n for i in range(len(a)):\n if a[i].startswith(searchWord):\n return i+1\n return -1\n``` | 2 | Given an array of positive integers `arr`, find a pattern of length `m` that is repeated `k` or more times.
A **pattern** is a subarray (consecutive sub-sequence) that consists of one or more values, repeated multiple times **consecutively** without overlapping. A pattern is defined by its length and the number of repetitions.
Return `true` _if there exists a pattern of length_ `m` _that is repeated_ `k` _or more times, otherwise return_ `false`.
**Example 1:**
**Input:** arr = \[1,2,4,4,4,4\], m = 1, k = 3
**Output:** true
**Explanation:** The pattern **(4)** of length 1 is repeated 4 consecutive times. Notice that pattern can be repeated k or more times but not less.
**Example 2:**
**Input:** arr = \[1,2,1,2,1,1,1,3\], m = 2, k = 2
**Output:** true
**Explanation:** The pattern **(1,2)** of length 2 is repeated 2 consecutive times. Another valid pattern **(2,1) is** also repeated 2 times.
**Example 3:**
**Input:** arr = \[1,2,1,2,1,3\], m = 2, k = 3
**Output:** false
**Explanation:** The pattern (1,2) is of length 2 but is repeated only 2 times. There is no pattern of length 2 that is repeated 3 or more times.
**Constraints:**
* `2 <= arr.length <= 100`
* `1 <= arr[i] <= 100`
* `1 <= m <= 100`
* `2 <= k <= 100` | First extract the words of the sentence. Check for each word if searchWord occurs at index 0, if so return the index of this word (1-indexed) If searchWord doesn't exist as a prefix of any word return the default value (-1). |
python3 code | check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence | 0 | 1 | # Code\n```\nclass Solution:\n def isPrefixOfWord(self, sentence: str, searchWord: str) -> int:\n words = sentence.split()\n indicies = []\n for i, word in enumerate(words):\n if word.startswith(searchWord):\n return i + 1\n return -1\n```\n\n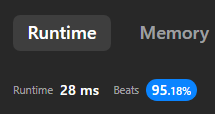 | 1 | Given a `sentence` that consists of some words separated by a **single space**, and a `searchWord`, check if `searchWord` is a prefix of any word in `sentence`.
Return _the index of the word in_ `sentence` _(**1-indexed**) where_ `searchWord` _is a prefix of this word_. If `searchWord` is a prefix of more than one word, return the index of the first word **(minimum index)**. If there is no such word return `-1`.
A **prefix** of a string `s` is any leading contiguous substring of `s`.
**Example 1:**
**Input:** sentence = "i love eating burger ", searchWord = "burg "
**Output:** 4
**Explanation:** "burg " is prefix of "burger " which is the 4th word in the sentence.
**Example 2:**
**Input:** sentence = "this problem is an easy problem ", searchWord = "pro "
**Output:** 2
**Explanation:** "pro " is prefix of "problem " which is the 2nd and the 6th word in the sentence, but we return 2 as it's the minimal index.
**Example 3:**
**Input:** sentence = "i am tired ", searchWord = "you "
**Output:** -1
**Explanation:** "you " is not a prefix of any word in the sentence.
**Constraints:**
* `1 <= sentence.length <= 100`
* `1 <= searchWord.length <= 10`
* `sentence` consists of lowercase English letters and spaces.
* `searchWord` consists of lowercase English letters. | Do the filtering and sort as said. Note that the id may not be the index in the array. |
python3 code | check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence | 0 | 1 | # Code\n```\nclass Solution:\n def isPrefixOfWord(self, sentence: str, searchWord: str) -> int:\n words = sentence.split()\n indicies = []\n for i, word in enumerate(words):\n if word.startswith(searchWord):\n return i + 1\n return -1\n```\n\n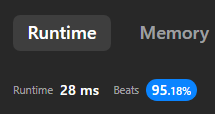 | 1 | Given an array of positive integers `arr`, find a pattern of length `m` that is repeated `k` or more times.
A **pattern** is a subarray (consecutive sub-sequence) that consists of one or more values, repeated multiple times **consecutively** without overlapping. A pattern is defined by its length and the number of repetitions.
Return `true` _if there exists a pattern of length_ `m` _that is repeated_ `k` _or more times, otherwise return_ `false`.
**Example 1:**
**Input:** arr = \[1,2,4,4,4,4\], m = 1, k = 3
**Output:** true
**Explanation:** The pattern **(4)** of length 1 is repeated 4 consecutive times. Notice that pattern can be repeated k or more times but not less.
**Example 2:**
**Input:** arr = \[1,2,1,2,1,1,1,3\], m = 2, k = 2
**Output:** true
**Explanation:** The pattern **(1,2)** of length 2 is repeated 2 consecutive times. Another valid pattern **(2,1) is** also repeated 2 times.
**Example 3:**
**Input:** arr = \[1,2,1,2,1,3\], m = 2, k = 3
**Output:** false
**Explanation:** The pattern (1,2) is of length 2 but is repeated only 2 times. There is no pattern of length 2 that is repeated 3 or more times.
**Constraints:**
* `2 <= arr.length <= 100`
* `1 <= arr[i] <= 100`
* `1 <= m <= 100`
* `2 <= k <= 100` | First extract the words of the sentence. Check for each word if searchWord occurs at index 0, if so return the index of this word (1-indexed) If searchWord doesn't exist as a prefix of any word return the default value (-1). |
python3 code | check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence | 0 | 1 | # Code\n```\nclass Solution:\n def isPrefixOfWord(self, sentence: str, searchWord: str) -> int:\n words = sentence.split()\n indicies = []\n for word in words:\n if word.startswith(searchWord):\n indicies.append(words.index(word))\n if len(indicies) > 0:\n return min(indicies) + 1\n return -1\n```\n\n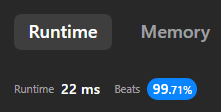 | 1 | Given a `sentence` that consists of some words separated by a **single space**, and a `searchWord`, check if `searchWord` is a prefix of any word in `sentence`.
Return _the index of the word in_ `sentence` _(**1-indexed**) where_ `searchWord` _is a prefix of this word_. If `searchWord` is a prefix of more than one word, return the index of the first word **(minimum index)**. If there is no such word return `-1`.
A **prefix** of a string `s` is any leading contiguous substring of `s`.
**Example 1:**
**Input:** sentence = "i love eating burger ", searchWord = "burg "
**Output:** 4
**Explanation:** "burg " is prefix of "burger " which is the 4th word in the sentence.
**Example 2:**
**Input:** sentence = "this problem is an easy problem ", searchWord = "pro "
**Output:** 2
**Explanation:** "pro " is prefix of "problem " which is the 2nd and the 6th word in the sentence, but we return 2 as it's the minimal index.
**Example 3:**
**Input:** sentence = "i am tired ", searchWord = "you "
**Output:** -1
**Explanation:** "you " is not a prefix of any word in the sentence.
**Constraints:**
* `1 <= sentence.length <= 100`
* `1 <= searchWord.length <= 10`
* `sentence` consists of lowercase English letters and spaces.
* `searchWord` consists of lowercase English letters. | Do the filtering and sort as said. Note that the id may not be the index in the array. |
python3 code | check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence | 0 | 1 | # Code\n```\nclass Solution:\n def isPrefixOfWord(self, sentence: str, searchWord: str) -> int:\n words = sentence.split()\n indicies = []\n for word in words:\n if word.startswith(searchWord):\n indicies.append(words.index(word))\n if len(indicies) > 0:\n return min(indicies) + 1\n return -1\n```\n\n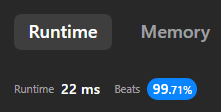 | 1 | Given an array of positive integers `arr`, find a pattern of length `m` that is repeated `k` or more times.
A **pattern** is a subarray (consecutive sub-sequence) that consists of one or more values, repeated multiple times **consecutively** without overlapping. A pattern is defined by its length and the number of repetitions.
Return `true` _if there exists a pattern of length_ `m` _that is repeated_ `k` _or more times, otherwise return_ `false`.
**Example 1:**
**Input:** arr = \[1,2,4,4,4,4\], m = 1, k = 3
**Output:** true
**Explanation:** The pattern **(4)** of length 1 is repeated 4 consecutive times. Notice that pattern can be repeated k or more times but not less.
**Example 2:**
**Input:** arr = \[1,2,1,2,1,1,1,3\], m = 2, k = 2
**Output:** true
**Explanation:** The pattern **(1,2)** of length 2 is repeated 2 consecutive times. Another valid pattern **(2,1) is** also repeated 2 times.
**Example 3:**
**Input:** arr = \[1,2,1,2,1,3\], m = 2, k = 3
**Output:** false
**Explanation:** The pattern (1,2) is of length 2 but is repeated only 2 times. There is no pattern of length 2 that is repeated 3 or more times.
**Constraints:**
* `2 <= arr.length <= 100`
* `1 <= arr[i] <= 100`
* `1 <= m <= 100`
* `2 <= k <= 100` | First extract the words of the sentence. Check for each word if searchWord occurs at index 0, if so return the index of this word (1-indexed) If searchWord doesn't exist as a prefix of any word return the default value (-1). |
Python with str.split(), 12ms | check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence | 0 | 1 | \n\t\n\tdef isPrefixOfWord(self, sentence, searchWord):\n sentence = sentence.split(\' \')\n for index,word in enumerate(sentence):\n if searchWord == word[:len(searchWord)]:\n return index+1\n return -1 | 13 | Given a `sentence` that consists of some words separated by a **single space**, and a `searchWord`, check if `searchWord` is a prefix of any word in `sentence`.
Return _the index of the word in_ `sentence` _(**1-indexed**) where_ `searchWord` _is a prefix of this word_. If `searchWord` is a prefix of more than one word, return the index of the first word **(minimum index)**. If there is no such word return `-1`.
A **prefix** of a string `s` is any leading contiguous substring of `s`.
**Example 1:**
**Input:** sentence = "i love eating burger ", searchWord = "burg "
**Output:** 4
**Explanation:** "burg " is prefix of "burger " which is the 4th word in the sentence.
**Example 2:**
**Input:** sentence = "this problem is an easy problem ", searchWord = "pro "
**Output:** 2
**Explanation:** "pro " is prefix of "problem " which is the 2nd and the 6th word in the sentence, but we return 2 as it's the minimal index.
**Example 3:**
**Input:** sentence = "i am tired ", searchWord = "you "
**Output:** -1
**Explanation:** "you " is not a prefix of any word in the sentence.
**Constraints:**
* `1 <= sentence.length <= 100`
* `1 <= searchWord.length <= 10`
* `sentence` consists of lowercase English letters and spaces.
* `searchWord` consists of lowercase English letters. | Do the filtering and sort as said. Note that the id may not be the index in the array. |
Python with str.split(), 12ms | check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence | 0 | 1 | \n\t\n\tdef isPrefixOfWord(self, sentence, searchWord):\n sentence = sentence.split(\' \')\n for index,word in enumerate(sentence):\n if searchWord == word[:len(searchWord)]:\n return index+1\n return -1 | 13 | Given an array of positive integers `arr`, find a pattern of length `m` that is repeated `k` or more times.
A **pattern** is a subarray (consecutive sub-sequence) that consists of one or more values, repeated multiple times **consecutively** without overlapping. A pattern is defined by its length and the number of repetitions.
Return `true` _if there exists a pattern of length_ `m` _that is repeated_ `k` _or more times, otherwise return_ `false`.
**Example 1:**
**Input:** arr = \[1,2,4,4,4,4\], m = 1, k = 3
**Output:** true
**Explanation:** The pattern **(4)** of length 1 is repeated 4 consecutive times. Notice that pattern can be repeated k or more times but not less.
**Example 2:**
**Input:** arr = \[1,2,1,2,1,1,1,3\], m = 2, k = 2
**Output:** true
**Explanation:** The pattern **(1,2)** of length 2 is repeated 2 consecutive times. Another valid pattern **(2,1) is** also repeated 2 times.
**Example 3:**
**Input:** arr = \[1,2,1,2,1,3\], m = 2, k = 3
**Output:** false
**Explanation:** The pattern (1,2) is of length 2 but is repeated only 2 times. There is no pattern of length 2 that is repeated 3 or more times.
**Constraints:**
* `2 <= arr.length <= 100`
* `1 <= arr[i] <= 100`
* `1 <= m <= 100`
* `2 <= k <= 100` | First extract the words of the sentence. Check for each word if searchWord occurs at index 0, if so return the index of this word (1-indexed) If searchWord doesn't exist as a prefix of any word return the default value (-1). |
Fastest 99.85% solution & Beginner friendly... Easy and simple python solution | check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence | 0 | 1 | # Approach\nJust search for the given searchWord in each of the word of the sentence by checking beginning of the word till the length of the searchWord.. As for loop starts from 0, return the index incremented by 1 in order to get the position of the word in the sentence.\nSimple............!\n<!-- Describe your approach to solving the problem. -->\n\n# Beats 99.85% of users in runtime: 19ms\n# Beats 95.54% of users in memory: 13.8mb\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isPrefixOfWord(self, sentence: str, searchWord: str) -> int:\n l = list(sentence.split(" "))\n # print(l)\n for i in range(len(l)):\n if l[i][:len(searchWord)]==searchWord:\n return i+1\n return -1\n``` | 1 | Given a `sentence` that consists of some words separated by a **single space**, and a `searchWord`, check if `searchWord` is a prefix of any word in `sentence`.
Return _the index of the word in_ `sentence` _(**1-indexed**) where_ `searchWord` _is a prefix of this word_. If `searchWord` is a prefix of more than one word, return the index of the first word **(minimum index)**. If there is no such word return `-1`.
A **prefix** of a string `s` is any leading contiguous substring of `s`.
**Example 1:**
**Input:** sentence = "i love eating burger ", searchWord = "burg "
**Output:** 4
**Explanation:** "burg " is prefix of "burger " which is the 4th word in the sentence.
**Example 2:**
**Input:** sentence = "this problem is an easy problem ", searchWord = "pro "
**Output:** 2
**Explanation:** "pro " is prefix of "problem " which is the 2nd and the 6th word in the sentence, but we return 2 as it's the minimal index.
**Example 3:**
**Input:** sentence = "i am tired ", searchWord = "you "
**Output:** -1
**Explanation:** "you " is not a prefix of any word in the sentence.
**Constraints:**
* `1 <= sentence.length <= 100`
* `1 <= searchWord.length <= 10`
* `sentence` consists of lowercase English letters and spaces.
* `searchWord` consists of lowercase English letters. | Do the filtering and sort as said. Note that the id may not be the index in the array. |
Fastest 99.85% solution & Beginner friendly... Easy and simple python solution | check-if-a-word-occurs-as-a-prefix-of-any-word-in-a-sentence | 0 | 1 | # Approach\nJust search for the given searchWord in each of the word of the sentence by checking beginning of the word till the length of the searchWord.. As for loop starts from 0, return the index incremented by 1 in order to get the position of the word in the sentence.\nSimple............!\n<!-- Describe your approach to solving the problem. -->\n\n# Beats 99.85% of users in runtime: 19ms\n# Beats 95.54% of users in memory: 13.8mb\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def isPrefixOfWord(self, sentence: str, searchWord: str) -> int:\n l = list(sentence.split(" "))\n # print(l)\n for i in range(len(l)):\n if l[i][:len(searchWord)]==searchWord:\n return i+1\n return -1\n``` | 1 | Given an array of positive integers `arr`, find a pattern of length `m` that is repeated `k` or more times.
A **pattern** is a subarray (consecutive sub-sequence) that consists of one or more values, repeated multiple times **consecutively** without overlapping. A pattern is defined by its length and the number of repetitions.
Return `true` _if there exists a pattern of length_ `m` _that is repeated_ `k` _or more times, otherwise return_ `false`.
**Example 1:**
**Input:** arr = \[1,2,4,4,4,4\], m = 1, k = 3
**Output:** true
**Explanation:** The pattern **(4)** of length 1 is repeated 4 consecutive times. Notice that pattern can be repeated k or more times but not less.
**Example 2:**
**Input:** arr = \[1,2,1,2,1,1,1,3\], m = 2, k = 2
**Output:** true
**Explanation:** The pattern **(1,2)** of length 2 is repeated 2 consecutive times. Another valid pattern **(2,1) is** also repeated 2 times.
**Example 3:**
**Input:** arr = \[1,2,1,2,1,3\], m = 2, k = 3
**Output:** false
**Explanation:** The pattern (1,2) is of length 2 but is repeated only 2 times. There is no pattern of length 2 that is repeated 3 or more times.
**Constraints:**
* `2 <= arr.length <= 100`
* `1 <= arr[i] <= 100`
* `1 <= m <= 100`
* `2 <= k <= 100` | First extract the words of the sentence. Check for each word if searchWord occurs at index 0, if so return the index of this word (1-indexed) If searchWord doesn't exist as a prefix of any word return the default value (-1). |
🥇 EASY || JAVA || C++ || PYTHON || EXPLAINED WITH VIDEO || BEGINNER FRIENDLY 🔥🔥 | pseudo-palindromic-paths-in-a-binary-tree | 1 | 1 | **PLEASE LIKE AND COMMENT IF THIS SOLUTION HAS HELPED YOU**\n\n**VIDEO EXPLANATION OF SOLUTION:**\nhttps://www.youtube.com/watch?v=spC68MStRRg&ab_channel=AlgoLock\n\n**SOLUTION: JAVA**\n```java\nclass Solution {\n public int pseudoPalindromicPaths (TreeNode root) {\n return parsePalinTree(root, new int[10]);\n }\n \n public int parsePalinTree(TreeNode root, int[] arr){\n \n int count = 0; \n arr[root.val]++;\n //Base Case: Reach a leaf node\n if(root.left == null && root.right == null){\n boolean isValidPath = isPalinDromePath(arr);\n if(isValidPath) count = 1;\n }\n // Left and right subtree: \n if(root.left != null) count += parsePalinTree(root.left, arr);\n if(root.right != null) count += parsePalinTree(root.right, arr);\n \n arr[root.val]--; \n return count; \n }\n \n public boolean isPalinDromePath(int[] arr){\n boolean firstOdd = true;\n int len = arr.length;\n for(int i = 0; i < len; i++){\n if(arr[i] % 2 == 1){\n if(!firstOdd) return false;\n firstOdd = false;\n }\n }\n return true;\n }\n}\n```\n\n**SOLUTION: C++**\n```c++\nclass Solution {\npublic:\n int pseudoPalindromicPaths (TreeNode* root) {\n return calcPalindromicPaths(root, new int[10]{0}, 10);\n }\n \n int calcPalindromicPaths(TreeNode* root, int arr[], int len){\n \n if(root == NULL) return 0; \n \n int count = 0; \n arr[root->val]++;\n // Reached a leaf:\n if(root->left == NULL && root->right == NULL){\n if(isPalindromicPath(arr, 10)) count = 1;\n }\n // Left and Right paths\n if(root->left != NULL) count += calcPalindromicPaths(root->left, arr, len);\n if(root->right != NULL) count += calcPalindromicPaths(root->right, arr, len);\n \n arr[root->val]--; \n return count;\n }\n \n bool isPalindromicPath(int arr[], int len){\n \n bool sawFirstOdd = false;\n for(int i = 0; i < len; i++){\n if(arr[i] % 2 == 1){\n if(sawFirstOdd) return false;\n sawFirstOdd = true;\n }\n }\n cout << endl;\n return true;\n }\n};\n```\n\n**SOLUTION: PYTHON**\n```python\nclass Solution:\n def pseudoPalindromicPaths (self, root: Optional[TreeNode]) -> int:\n \n ## Function to check if current path has a permutation that\'s a palindrome\n def isPalindromicPath(palinArr: [int]) -> bool:\n hasSeenFirstOdd: bool = False\n for i in range(0, len(palinArr)):\n if(palinArr[i] % 2 == 1):\n if hasSeenFirstOdd: return False\n hasSeenFirstOdd = True\n return True\n \n ## Wrapper for function that calculates the number of pseudo palindromic paths\n def calcPalindromicPaths(root: Optional[TreeNode], arr: [int]) -> int:\n \n count: int = 0\n if root == None: return 0\n arr[root.val] += 1\n \n ## Leaf Node: No children\n if(root.left == None and root.right == None):\n if(isPalindromicPath(arr)): count = 1\n \n if(root.left != None): count += calcPalindromicPaths(root.left, arr)\n if(root.right != None): count += calcPalindromicPaths(root.right, arr)\n \n arr[root.val] -= 1\n return count; \n \n \n dparr: [int] = [0] * 10\n return calcPalindromicPaths(root, dparr)\n``` | 8 | Given a binary tree where node values are digits from 1 to 9. A path in the binary tree is said to be **pseudo-palindromic** if at least one permutation of the node values in the path is a palindrome.
_Return the number of **pseudo-palindromic** paths going from the root node to leaf nodes._
**Example 1:**
**Input:** root = \[2,3,1,3,1,null,1\]
**Output:** 2
**Explanation:** The figure above represents the given binary tree. There are three paths going from the root node to leaf nodes: the red path \[2,3,3\], the green path \[2,1,1\], and the path \[2,3,1\]. Among these paths only red path and green path are pseudo-palindromic paths since the red path \[2,3,3\] can be rearranged in \[3,2,3\] (palindrome) and the green path \[2,1,1\] can be rearranged in \[1,2,1\] (palindrome).
**Example 2:**
**Input:** root = \[2,1,1,1,3,null,null,null,null,null,1\]
**Output:** 1
**Explanation:** The figure above represents the given binary tree. There are three paths going from the root node to leaf nodes: the green path \[2,1,1\], the path \[2,1,3,1\], and the path \[2,1\]. Among these paths only the green path is pseudo-palindromic since \[2,1,1\] can be rearranged in \[1,2,1\] (palindrome).
**Example 3:**
**Input:** root = \[9\]
**Output:** 1
**Constraints:**
* The number of nodes in the tree is in the range `[1, 105]`.
* `1 <= Node.val <= 9` | Use DP. Try to cut the array into d non-empty sub-arrays. Try all possible cuts for the array. Use dp[i][j] where DP states are i the index of the last cut and j the number of remaining cuts. Complexity is O(n * n * d). |
🥇 EASY || JAVA || C++ || PYTHON || EXPLAINED WITH VIDEO || BEGINNER FRIENDLY 🔥🔥 | pseudo-palindromic-paths-in-a-binary-tree | 1 | 1 | **PLEASE LIKE AND COMMENT IF THIS SOLUTION HAS HELPED YOU**\n\n**VIDEO EXPLANATION OF SOLUTION:**\nhttps://www.youtube.com/watch?v=spC68MStRRg&ab_channel=AlgoLock\n\n**SOLUTION: JAVA**\n```java\nclass Solution {\n public int pseudoPalindromicPaths (TreeNode root) {\n return parsePalinTree(root, new int[10]);\n }\n \n public int parsePalinTree(TreeNode root, int[] arr){\n \n int count = 0; \n arr[root.val]++;\n //Base Case: Reach a leaf node\n if(root.left == null && root.right == null){\n boolean isValidPath = isPalinDromePath(arr);\n if(isValidPath) count = 1;\n }\n // Left and right subtree: \n if(root.left != null) count += parsePalinTree(root.left, arr);\n if(root.right != null) count += parsePalinTree(root.right, arr);\n \n arr[root.val]--; \n return count; \n }\n \n public boolean isPalinDromePath(int[] arr){\n boolean firstOdd = true;\n int len = arr.length;\n for(int i = 0; i < len; i++){\n if(arr[i] % 2 == 1){\n if(!firstOdd) return false;\n firstOdd = false;\n }\n }\n return true;\n }\n}\n```\n\n**SOLUTION: C++**\n```c++\nclass Solution {\npublic:\n int pseudoPalindromicPaths (TreeNode* root) {\n return calcPalindromicPaths(root, new int[10]{0}, 10);\n }\n \n int calcPalindromicPaths(TreeNode* root, int arr[], int len){\n \n if(root == NULL) return 0; \n \n int count = 0; \n arr[root->val]++;\n // Reached a leaf:\n if(root->left == NULL && root->right == NULL){\n if(isPalindromicPath(arr, 10)) count = 1;\n }\n // Left and Right paths\n if(root->left != NULL) count += calcPalindromicPaths(root->left, arr, len);\n if(root->right != NULL) count += calcPalindromicPaths(root->right, arr, len);\n \n arr[root->val]--; \n return count;\n }\n \n bool isPalindromicPath(int arr[], int len){\n \n bool sawFirstOdd = false;\n for(int i = 0; i < len; i++){\n if(arr[i] % 2 == 1){\n if(sawFirstOdd) return false;\n sawFirstOdd = true;\n }\n }\n cout << endl;\n return true;\n }\n};\n```\n\n**SOLUTION: PYTHON**\n```python\nclass Solution:\n def pseudoPalindromicPaths (self, root: Optional[TreeNode]) -> int:\n \n ## Function to check if current path has a permutation that\'s a palindrome\n def isPalindromicPath(palinArr: [int]) -> bool:\n hasSeenFirstOdd: bool = False\n for i in range(0, len(palinArr)):\n if(palinArr[i] % 2 == 1):\n if hasSeenFirstOdd: return False\n hasSeenFirstOdd = True\n return True\n \n ## Wrapper for function that calculates the number of pseudo palindromic paths\n def calcPalindromicPaths(root: Optional[TreeNode], arr: [int]) -> int:\n \n count: int = 0\n if root == None: return 0\n arr[root.val] += 1\n \n ## Leaf Node: No children\n if(root.left == None and root.right == None):\n if(isPalindromicPath(arr)): count = 1\n \n if(root.left != None): count += calcPalindromicPaths(root.left, arr)\n if(root.right != None): count += calcPalindromicPaths(root.right, arr)\n \n arr[root.val] -= 1\n return count; \n \n \n dparr: [int] = [0] * 10\n return calcPalindromicPaths(root, dparr)\n``` | 8 | You are given an `m x n` binary grid `grid` where `1` represents land and `0` represents water. An **island** is a maximal **4-directionally** (horizontal or vertical) connected group of `1`'s.
The grid is said to be **connected** if we have **exactly one island**, otherwise is said **disconnected**.
In one day, we are allowed to change **any** single land cell `(1)` into a water cell `(0)`.
Return _the minimum number of days to disconnect the grid_.
**Example 1:**
**Input:** grid = \[\[0,1,1,0\],\[0,1,1,0\],\[0,0,0,0\]\]
**Output:** 2
**Explanation:** We need at least 2 days to get a disconnected grid.
Change land grid\[1\]\[1\] and grid\[0\]\[2\] to water and get 2 disconnected island.
**Example 2:**
**Input:** grid = \[\[1,1\]\]
**Output:** 2
**Explanation:** Grid of full water is also disconnected (\[\[1,1\]\] -> \[\[0,0\]\]), 0 islands.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 30`
* `grid[i][j]` is either `0` or `1`. | Note that the node values of a path form a palindrome if at most one digit has an odd frequency (parity). Use a Depth First Search (DFS) keeping the frequency (parity) of the digits. Once you are in a leaf node check if at most one digit has an odd frequency (parity). |
🔥 [LeetCode The Hard Way] 🔥 Explained Line By Line | pseudo-palindromic-paths-in-a-binary-tree | 0 | 1 | Please check out [LeetCode The Hard Way](https://wingkwong.github.io/leetcode-the-hard-way/) for more solution explanations and tutorials. \nI\'ll explain my solution line by line daily and you can find the full list in my [Discord](https://discord.gg/Nqm4jJcyBf).\nIf you like it, please give a star, watch my [Github Repository](https://github.com/wingkwong/leetcode-the-hard-way) and upvote this post.\n\n---\n\n**C++**\n\n```cpp\n// Time Complexity: O(N) where N is the number of nodes\n// Space Complexity: O(H) where H is the tree height\nclass Solution {\npublic:\n int ans = 0;\n \n // the idea is that there is at most one digit with odd frequency in pseudo-palindromic path\n // e.g. [2, 3, 3] - digit 2 has odd frequency\n // e.g. [9] - digit 9 has odd frequency\n // so that the digit with odd frequency can be put in the middle, e.g. 323, 9, etc\n\t// e.g. [2,2] - or no digit with odd frequency\n\t\n int pseudoPalindromicPaths (TreeNode* root) {\n preorder(root, 0);\n return ans;\n }\n \n // if you don\'t know preorder traversal, try 144. Binary Tree Preorder Traversal first\n // Problem Link: https://leetcode.com/problems/binary-tree-preorder-traversal/\n // Explanation Link: https://leetcode.com/problems/binary-tree-preorder-traversal/discuss/2549333/LeetCode-The-Hard-Way-DFS-or-Pre-Order-or-Explained-Line-By-Line\n void preorder(TreeNode* node, int cnt) {\n // preorder traversal step 1: if node is null, then return\n if (node == NULL) return;\n // preorder traversal step 2: do something with node value here\n \n // first let\'s understand what (x << y) means \n // (x << y): shifting `x` `y` bits to the left\n // e.g. 1 << 0 = 1 (shift 0 bit - stay as it is)\n // e.g. 1 << 1 = 0b10 (shift 1 bit - becomes 2)\n // e.g. 1 << 2 = 0b100 (shift 2 bits to the left - becomes 4)\n // you may find that (1 << n) is actually just power of 2. i.e. 2 ^ n\n \n // second let\'s understand three properties of XOR\n // 1. XOR is self-inverse which means x ^ x = 0 (number XOR number evaluates to 0)\n // 2. 0 is identity element which means x ^ 0 = x (number XOR 0 remains unchanged)\n // 3. XOR is commutative, which means x ^ y = y ^ x (order doesn\'t matter)\n \n // we can use (1 << i) to set the appearance of digit i\n // but how to count the odd frequency? \n // we can use above XOR properties to achieve the following \n // if the i-bit is set, then digit i has an odd frequency\n // how? remember XOR property #1, #2, and #3?\n // if a digit appears even number of times, the bit at the end will be 0. (x ^ x = 0)\n // if a digit appears odd number of times, the bit at the will be 1. (x ^ x ^ x = (x ^ x) ^ x = 0 ^ x = x)\n cnt ^= (1 << node->val);\n // do something at the leaf\n if (!node->left && !node->right) {\n // if i-bit is set in `cnt`, that means digit `i` has an odd frequency\n // therefore, the number of 1 in `cnt` = the number of digits with an odd frequency\n // however, we only want at most one digit that has an odd frequency\n // we can use a bit trick (n & (n - 1)) to remove the rightmost set bit.\n // e.g. \n // n n n - 1 n & (n - 1)\n // -- ---- ---- -------\n // 0 0000 0111 0000\n // 1 0001 0000 0000\n // 2 0010 0001 0000\n // 3 0011 0010 0010\n // 4 0100 0011 0000\n // 5 0101 0100 0100\n // 6 0110 0101 0100\n // 7 0111 0110 0110\n // 8 1000 0111 0000 \n // 9 1001 1000 1000\n // 10 1010 1001 1000\n // 11 1011 1010 1010\n // 12 1100 1011 1000\n // 13 1101 1100 1100\n // 14 1110 1101 1100\n // 15 1111 1110 1110\n \n // if the result is 0, that means we have at most one digit that has an odd frequncy \n // hence, add one to ans\n\t\t\t// alternatively, you can use __builtin_popcount(cnt) <= 1 to check\n ans += (cnt & (cnt - 1)) == 0;\n }\n // preorder traversal step 3: traverse the left node\n preorder(node->left, cnt);\n // preorder traversal step 4: traverse the right node\n preorder(node->right, cnt);\n }\n};\n```\n\n**Python**\n\n```py\nclass Solution:\n def pseudoPalindromicPaths (self, root: Optional[TreeNode], cnt = 0) -> int:\n if not root: return 0\n cnt ^= 1 << (root.val - 1)\n if root.left is None and root.right is None:\n return 1 if cnt & (cnt - 1) == 0 else 0\n return self.pseudoPalindromicPaths(root.left, cnt) + self.pseudoPalindromicPaths(root.right, cnt)\n```\n\n**Go**\n\n```go\nfunc numberOfOnes(n int) int{\n res := 0\n for n > 0 {\n res += n & 1\n n >>= 1\n }\n return res\n}\n\nfunc preorder(root *TreeNode, cnt int) int {\n if root == nil { return 0 }\n cnt ^= (1 << root.Val)\n if root.Left == nil && root.Right == nil && numberOfOnes(cnt) <= 1 { return 1 }\n return preorder(root.Left, cnt) + preorder(root.Right, cnt)\n}\n\nfunc pseudoPalindromicPaths (root *TreeNode) int {\n return preorder(root, 0)\n}\n``` | 78 | Given a binary tree where node values are digits from 1 to 9. A path in the binary tree is said to be **pseudo-palindromic** if at least one permutation of the node values in the path is a palindrome.
_Return the number of **pseudo-palindromic** paths going from the root node to leaf nodes._
**Example 1:**
**Input:** root = \[2,3,1,3,1,null,1\]
**Output:** 2
**Explanation:** The figure above represents the given binary tree. There are three paths going from the root node to leaf nodes: the red path \[2,3,3\], the green path \[2,1,1\], and the path \[2,3,1\]. Among these paths only red path and green path are pseudo-palindromic paths since the red path \[2,3,3\] can be rearranged in \[3,2,3\] (palindrome) and the green path \[2,1,1\] can be rearranged in \[1,2,1\] (palindrome).
**Example 2:**
**Input:** root = \[2,1,1,1,3,null,null,null,null,null,1\]
**Output:** 1
**Explanation:** The figure above represents the given binary tree. There are three paths going from the root node to leaf nodes: the green path \[2,1,1\], the path \[2,1,3,1\], and the path \[2,1\]. Among these paths only the green path is pseudo-palindromic since \[2,1,1\] can be rearranged in \[1,2,1\] (palindrome).
**Example 3:**
**Input:** root = \[9\]
**Output:** 1
**Constraints:**
* The number of nodes in the tree is in the range `[1, 105]`.
* `1 <= Node.val <= 9` | Use DP. Try to cut the array into d non-empty sub-arrays. Try all possible cuts for the array. Use dp[i][j] where DP states are i the index of the last cut and j the number of remaining cuts. Complexity is O(n * n * d). |
🔥 [LeetCode The Hard Way] 🔥 Explained Line By Line | pseudo-palindromic-paths-in-a-binary-tree | 0 | 1 | Please check out [LeetCode The Hard Way](https://wingkwong.github.io/leetcode-the-hard-way/) for more solution explanations and tutorials. \nI\'ll explain my solution line by line daily and you can find the full list in my [Discord](https://discord.gg/Nqm4jJcyBf).\nIf you like it, please give a star, watch my [Github Repository](https://github.com/wingkwong/leetcode-the-hard-way) and upvote this post.\n\n---\n\n**C++**\n\n```cpp\n// Time Complexity: O(N) where N is the number of nodes\n// Space Complexity: O(H) where H is the tree height\nclass Solution {\npublic:\n int ans = 0;\n \n // the idea is that there is at most one digit with odd frequency in pseudo-palindromic path\n // e.g. [2, 3, 3] - digit 2 has odd frequency\n // e.g. [9] - digit 9 has odd frequency\n // so that the digit with odd frequency can be put in the middle, e.g. 323, 9, etc\n\t// e.g. [2,2] - or no digit with odd frequency\n\t\n int pseudoPalindromicPaths (TreeNode* root) {\n preorder(root, 0);\n return ans;\n }\n \n // if you don\'t know preorder traversal, try 144. Binary Tree Preorder Traversal first\n // Problem Link: https://leetcode.com/problems/binary-tree-preorder-traversal/\n // Explanation Link: https://leetcode.com/problems/binary-tree-preorder-traversal/discuss/2549333/LeetCode-The-Hard-Way-DFS-or-Pre-Order-or-Explained-Line-By-Line\n void preorder(TreeNode* node, int cnt) {\n // preorder traversal step 1: if node is null, then return\n if (node == NULL) return;\n // preorder traversal step 2: do something with node value here\n \n // first let\'s understand what (x << y) means \n // (x << y): shifting `x` `y` bits to the left\n // e.g. 1 << 0 = 1 (shift 0 bit - stay as it is)\n // e.g. 1 << 1 = 0b10 (shift 1 bit - becomes 2)\n // e.g. 1 << 2 = 0b100 (shift 2 bits to the left - becomes 4)\n // you may find that (1 << n) is actually just power of 2. i.e. 2 ^ n\n \n // second let\'s understand three properties of XOR\n // 1. XOR is self-inverse which means x ^ x = 0 (number XOR number evaluates to 0)\n // 2. 0 is identity element which means x ^ 0 = x (number XOR 0 remains unchanged)\n // 3. XOR is commutative, which means x ^ y = y ^ x (order doesn\'t matter)\n \n // we can use (1 << i) to set the appearance of digit i\n // but how to count the odd frequency? \n // we can use above XOR properties to achieve the following \n // if the i-bit is set, then digit i has an odd frequency\n // how? remember XOR property #1, #2, and #3?\n // if a digit appears even number of times, the bit at the end will be 0. (x ^ x = 0)\n // if a digit appears odd number of times, the bit at the will be 1. (x ^ x ^ x = (x ^ x) ^ x = 0 ^ x = x)\n cnt ^= (1 << node->val);\n // do something at the leaf\n if (!node->left && !node->right) {\n // if i-bit is set in `cnt`, that means digit `i` has an odd frequency\n // therefore, the number of 1 in `cnt` = the number of digits with an odd frequency\n // however, we only want at most one digit that has an odd frequency\n // we can use a bit trick (n & (n - 1)) to remove the rightmost set bit.\n // e.g. \n // n n n - 1 n & (n - 1)\n // -- ---- ---- -------\n // 0 0000 0111 0000\n // 1 0001 0000 0000\n // 2 0010 0001 0000\n // 3 0011 0010 0010\n // 4 0100 0011 0000\n // 5 0101 0100 0100\n // 6 0110 0101 0100\n // 7 0111 0110 0110\n // 8 1000 0111 0000 \n // 9 1001 1000 1000\n // 10 1010 1001 1000\n // 11 1011 1010 1010\n // 12 1100 1011 1000\n // 13 1101 1100 1100\n // 14 1110 1101 1100\n // 15 1111 1110 1110\n \n // if the result is 0, that means we have at most one digit that has an odd frequncy \n // hence, add one to ans\n\t\t\t// alternatively, you can use __builtin_popcount(cnt) <= 1 to check\n ans += (cnt & (cnt - 1)) == 0;\n }\n // preorder traversal step 3: traverse the left node\n preorder(node->left, cnt);\n // preorder traversal step 4: traverse the right node\n preorder(node->right, cnt);\n }\n};\n```\n\n**Python**\n\n```py\nclass Solution:\n def pseudoPalindromicPaths (self, root: Optional[TreeNode], cnt = 0) -> int:\n if not root: return 0\n cnt ^= 1 << (root.val - 1)\n if root.left is None and root.right is None:\n return 1 if cnt & (cnt - 1) == 0 else 0\n return self.pseudoPalindromicPaths(root.left, cnt) + self.pseudoPalindromicPaths(root.right, cnt)\n```\n\n**Go**\n\n```go\nfunc numberOfOnes(n int) int{\n res := 0\n for n > 0 {\n res += n & 1\n n >>= 1\n }\n return res\n}\n\nfunc preorder(root *TreeNode, cnt int) int {\n if root == nil { return 0 }\n cnt ^= (1 << root.Val)\n if root.Left == nil && root.Right == nil && numberOfOnes(cnt) <= 1 { return 1 }\n return preorder(root.Left, cnt) + preorder(root.Right, cnt)\n}\n\nfunc pseudoPalindromicPaths (root *TreeNode) int {\n return preorder(root, 0)\n}\n``` | 78 | You are given an `m x n` binary grid `grid` where `1` represents land and `0` represents water. An **island** is a maximal **4-directionally** (horizontal or vertical) connected group of `1`'s.
The grid is said to be **connected** if we have **exactly one island**, otherwise is said **disconnected**.
In one day, we are allowed to change **any** single land cell `(1)` into a water cell `(0)`.
Return _the minimum number of days to disconnect the grid_.
**Example 1:**
**Input:** grid = \[\[0,1,1,0\],\[0,1,1,0\],\[0,0,0,0\]\]
**Output:** 2
**Explanation:** We need at least 2 days to get a disconnected grid.
Change land grid\[1\]\[1\] and grid\[0\]\[2\] to water and get 2 disconnected island.
**Example 2:**
**Input:** grid = \[\[1,1\]\]
**Output:** 2
**Explanation:** Grid of full water is also disconnected (\[\[1,1\]\] -> \[\[0,0\]\]), 0 islands.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 30`
* `grid[i][j]` is either `0` or `1`. | Note that the node values of a path form a palindrome if at most one digit has an odd frequency (parity). Use a Depth First Search (DFS) keeping the frequency (parity) of the digits. Once you are in a leaf node check if at most one digit has an odd frequency (parity). |
Python | DFS & Set | With Explanation | Easy to Understand | pseudo-palindromic-paths-in-a-binary-tree | 0 | 1 | ```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def pseudoPalindromicPaths (self, root: Optional[TreeNode]) -> int:\n # traverse the tree, the set pairs maintains the number of each element\n # If you already have the same element in pairs, then remove it\n # Else, add it to pairs\n\n # In the leaf, if the set is empty, then its an even palindrome.\n # In the leaf, if the set has 1 element , its an odd palindrome.\n # In th leaf, if the set has > 1 elements, its not a palindrome.\n \n def traverse(node, pairs):\n if not node:\n return 0\n \n if node.val in pairs:\n pairs.remove(node.val)\n else:\n pairs.add(node.val)\n \n if not node.left and not node.right:\n return 1 if len(pairs) <= 1 else 0\n \n # correct!!\n left = traverse(node.left, set(pairs))\n right = traverse(node.right, set(pairs))\n \n # wrong, becasue pairs will change after we traversed node.left or node.right!\n # left = traverse(node.left, pairs)\n # right = traverse(node.right, pairs)\n \n return left + right\n \n return traverse(root, set()) | 56 | Given a binary tree where node values are digits from 1 to 9. A path in the binary tree is said to be **pseudo-palindromic** if at least one permutation of the node values in the path is a palindrome.
_Return the number of **pseudo-palindromic** paths going from the root node to leaf nodes._
**Example 1:**
**Input:** root = \[2,3,1,3,1,null,1\]
**Output:** 2
**Explanation:** The figure above represents the given binary tree. There are three paths going from the root node to leaf nodes: the red path \[2,3,3\], the green path \[2,1,1\], and the path \[2,3,1\]. Among these paths only red path and green path are pseudo-palindromic paths since the red path \[2,3,3\] can be rearranged in \[3,2,3\] (palindrome) and the green path \[2,1,1\] can be rearranged in \[1,2,1\] (palindrome).
**Example 2:**
**Input:** root = \[2,1,1,1,3,null,null,null,null,null,1\]
**Output:** 1
**Explanation:** The figure above represents the given binary tree. There are three paths going from the root node to leaf nodes: the green path \[2,1,1\], the path \[2,1,3,1\], and the path \[2,1\]. Among these paths only the green path is pseudo-palindromic since \[2,1,1\] can be rearranged in \[1,2,1\] (palindrome).
**Example 3:**
**Input:** root = \[9\]
**Output:** 1
**Constraints:**
* The number of nodes in the tree is in the range `[1, 105]`.
* `1 <= Node.val <= 9` | Use DP. Try to cut the array into d non-empty sub-arrays. Try all possible cuts for the array. Use dp[i][j] where DP states are i the index of the last cut and j the number of remaining cuts. Complexity is O(n * n * d). |
Python | DFS & Set | With Explanation | Easy to Understand | pseudo-palindromic-paths-in-a-binary-tree | 0 | 1 | ```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def pseudoPalindromicPaths (self, root: Optional[TreeNode]) -> int:\n # traverse the tree, the set pairs maintains the number of each element\n # If you already have the same element in pairs, then remove it\n # Else, add it to pairs\n\n # In the leaf, if the set is empty, then its an even palindrome.\n # In the leaf, if the set has 1 element , its an odd palindrome.\n # In th leaf, if the set has > 1 elements, its not a palindrome.\n \n def traverse(node, pairs):\n if not node:\n return 0\n \n if node.val in pairs:\n pairs.remove(node.val)\n else:\n pairs.add(node.val)\n \n if not node.left and not node.right:\n return 1 if len(pairs) <= 1 else 0\n \n # correct!!\n left = traverse(node.left, set(pairs))\n right = traverse(node.right, set(pairs))\n \n # wrong, becasue pairs will change after we traversed node.left or node.right!\n # left = traverse(node.left, pairs)\n # right = traverse(node.right, pairs)\n \n return left + right\n \n return traverse(root, set()) | 56 | You are given an `m x n` binary grid `grid` where `1` represents land and `0` represents water. An **island** is a maximal **4-directionally** (horizontal or vertical) connected group of `1`'s.
The grid is said to be **connected** if we have **exactly one island**, otherwise is said **disconnected**.
In one day, we are allowed to change **any** single land cell `(1)` into a water cell `(0)`.
Return _the minimum number of days to disconnect the grid_.
**Example 1:**
**Input:** grid = \[\[0,1,1,0\],\[0,1,1,0\],\[0,0,0,0\]\]
**Output:** 2
**Explanation:** We need at least 2 days to get a disconnected grid.
Change land grid\[1\]\[1\] and grid\[0\]\[2\] to water and get 2 disconnected island.
**Example 2:**
**Input:** grid = \[\[1,1\]\]
**Output:** 2
**Explanation:** Grid of full water is also disconnected (\[\[1,1\]\] -> \[\[0,0\]\]), 0 islands.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 30`
* `grid[i][j]` is either `0` or `1`. | Note that the node values of a path form a palindrome if at most one digit has an odd frequency (parity). Use a Depth First Search (DFS) keeping the frequency (parity) of the digits. Once you are in a leaf node check if at most one digit has an odd frequency (parity). |
Preorder Traversal python solution using mapping | pseudo-palindromic-paths-in-a-binary-tree | 0 | 1 | ```\nclass Solution:\n def pseudoPalindromicPaths (self, root: Optional[TreeNode]) -> int:\n def pre(root,dic):\n if root is None:\n return 0\n if root.left is None and root.right is None:\n if root.val not in dic:\n dic[root.val]=1\n else:\n dic[root.val]+=1\n fg=0\n for i in dic:\n if dic[i]%2==1 and fg==1:\n return 0\n if dic[i]%2==1:\n fg=1\n return 1\n if root.val not in dic:\n dic[root.val]=1\n else:\n dic[root.val]+=1\n x=pre(root.left,dic)\n if root.left:\n dic[root.left.val]-=1\n y=pre(root.right,dic)\n if root.right:\n dic[root.right.val]-=1\n return x+y\n dic={}\n return pre(root,dic)\n``` | 3 | Given a binary tree where node values are digits from 1 to 9. A path in the binary tree is said to be **pseudo-palindromic** if at least one permutation of the node values in the path is a palindrome.
_Return the number of **pseudo-palindromic** paths going from the root node to leaf nodes._
**Example 1:**
**Input:** root = \[2,3,1,3,1,null,1\]
**Output:** 2
**Explanation:** The figure above represents the given binary tree. There are three paths going from the root node to leaf nodes: the red path \[2,3,3\], the green path \[2,1,1\], and the path \[2,3,1\]. Among these paths only red path and green path are pseudo-palindromic paths since the red path \[2,3,3\] can be rearranged in \[3,2,3\] (palindrome) and the green path \[2,1,1\] can be rearranged in \[1,2,1\] (palindrome).
**Example 2:**
**Input:** root = \[2,1,1,1,3,null,null,null,null,null,1\]
**Output:** 1
**Explanation:** The figure above represents the given binary tree. There are three paths going from the root node to leaf nodes: the green path \[2,1,1\], the path \[2,1,3,1\], and the path \[2,1\]. Among these paths only the green path is pseudo-palindromic since \[2,1,1\] can be rearranged in \[1,2,1\] (palindrome).
**Example 3:**
**Input:** root = \[9\]
**Output:** 1
**Constraints:**
* The number of nodes in the tree is in the range `[1, 105]`.
* `1 <= Node.val <= 9` | Use DP. Try to cut the array into d non-empty sub-arrays. Try all possible cuts for the array. Use dp[i][j] where DP states are i the index of the last cut and j the number of remaining cuts. Complexity is O(n * n * d). |
Preorder Traversal python solution using mapping | pseudo-palindromic-paths-in-a-binary-tree | 0 | 1 | ```\nclass Solution:\n def pseudoPalindromicPaths (self, root: Optional[TreeNode]) -> int:\n def pre(root,dic):\n if root is None:\n return 0\n if root.left is None and root.right is None:\n if root.val not in dic:\n dic[root.val]=1\n else:\n dic[root.val]+=1\n fg=0\n for i in dic:\n if dic[i]%2==1 and fg==1:\n return 0\n if dic[i]%2==1:\n fg=1\n return 1\n if root.val not in dic:\n dic[root.val]=1\n else:\n dic[root.val]+=1\n x=pre(root.left,dic)\n if root.left:\n dic[root.left.val]-=1\n y=pre(root.right,dic)\n if root.right:\n dic[root.right.val]-=1\n return x+y\n dic={}\n return pre(root,dic)\n``` | 3 | You are given an `m x n` binary grid `grid` where `1` represents land and `0` represents water. An **island** is a maximal **4-directionally** (horizontal or vertical) connected group of `1`'s.
The grid is said to be **connected** if we have **exactly one island**, otherwise is said **disconnected**.
In one day, we are allowed to change **any** single land cell `(1)` into a water cell `(0)`.
Return _the minimum number of days to disconnect the grid_.
**Example 1:**
**Input:** grid = \[\[0,1,1,0\],\[0,1,1,0\],\[0,0,0,0\]\]
**Output:** 2
**Explanation:** We need at least 2 days to get a disconnected grid.
Change land grid\[1\]\[1\] and grid\[0\]\[2\] to water and get 2 disconnected island.
**Example 2:**
**Input:** grid = \[\[1,1\]\]
**Output:** 2
**Explanation:** Grid of full water is also disconnected (\[\[1,1\]\] -> \[\[0,0\]\]), 0 islands.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 30`
* `grid[i][j]` is either `0` or `1`. | Note that the node values of a path form a palindrome if at most one digit has an odd frequency (parity). Use a Depth First Search (DFS) keeping the frequency (parity) of the digits. Once you are in a leaf node check if at most one digit has an odd frequency (parity). |
✅ 90.14% Dynamic Programming Optimized | max-dot-product-of-two-subsequences | 1 | 1 | # Intuition\nWhen faced with two sequences and asked to find the maximum dot product, it becomes evident that we might have to consider various combinations of subsequences to determine the maximum value. This naturally hints towards the possibility of using dynamic programming, as there are overlapping subproblems.\n\n## Live Coding & Explain\nhttps://youtu.be/h1RAw1gEx5A?si=6zJ77Y2f9X5JLaZL\n\n# Approach\n\n1. **Dynamic Programming Approach**: \n - We can use a 2D array `dp` where `dp[i][j]` represents the maximum dot product between the first `i` elements of `nums1` and the first `j` elements of `nums2`.\n - If we decide to pick the elements `nums1[i]` and `nums2[j]`, the dot product added to the result is `nums1[i] * nums2[j]`.\n - We can also decide not to pick any element from `nums1` or `nums2`, so we will consider `dp[i-1][j]` and `dp[i][j-1]`.\n - The recurrence relation is: \n $$ dp[i][j] = \\max(dp[i-1][j], dp[i][j-1], nums1[i-1] \\times nums2[j-1] + \\max(0, dp[i-1][j-1]) $$\n\n2. **Space Optimized Approach**:\n - Instead of using a 2D array, we observe that we only need values from the current and previous row to compute the next row. Thus, two 1D arrays (current and previous) suffice.\n - At the end of processing each row, we swap the two arrays, thus making the current array act as the previous array for the next iteration.\n\n# Complexity\n- **Time complexity**: $$O(m \\times n)$$ - We have to fill up the `dp` matrix which is of size $$m \\times n$$.\n- **Space complexity**:\n - For the **DP approach**: $$O(m \\times n)$$ - The size of the `dp` matrix.\n - For the **Optimized approach**: $$O(n)$$ - We are using two 1D arrays.\n\n\n# Code Dynamic Programming\n``` Python []\nclass Solution:\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n m, n = len(nums1), len(nums2)\n \n dp = [[float(\'-inf\')] * (n + 1) for _ in range(m + 1)]\n \n for i in range(1, m + 1):\n for j in range(1, n + 1):\n curr_product = nums1[i-1] * nums2[j-1]\n \n dp[i][j] = max(dp[i][j], curr_product, dp[i-1][j], dp[i][j-1], curr_product + dp[i-1][j-1])\n \n return dp[m][n]\n\n```\n\n# Code Optimized\n``` Python []\nclass Solution:\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n m, n = len(nums1), len(nums2)\n \n current = [float(\'-inf\')] * (n + 1)\n previous = [float(\'-inf\')] * (n + 1)\n \n for i in range(1, m + 1):\n for j in range(1, n + 1):\n curr_product = nums1[i-1] * nums2[j-1]\n \n current[j] = max(curr_product, previous[j], current[j-1], curr_product + max(0, previous[j-1]))\n \n current, previous = previous, current\n \n return previous[n]\n\n```\n``` Go []\nfunc maxDotProduct(nums1 []int, nums2 []int) int {\n m, n := len(nums1), len(nums2)\n current := make([]int, n+1)\n previous := make([]int, n+1)\n for i := range current {\n current[i] = math.MinInt32\n previous[i] = math.MinInt32\n }\n\n for i := 1; i <= m; i++ {\n for j := 1; j <= n; j++ {\n currProduct := nums1[i-1] * nums2[j-1]\n current[j] = max(max(max(currProduct, previous[j]), current[j-1]), currProduct + max(0, previous[j-1]))\n }\n current, previous = previous, current\n }\n return previous[n]\n}\n\nfunc max(a, b int) int {\n if a > b {\n return a\n }\n return b\n}\n```\n``` Rust []\nimpl Solution {\n pub fn max_dot_product(nums1: Vec<i32>, nums2: Vec<i32>) -> i32 {\n let m = nums1.len();\n let n = nums2.len();\n let mut current = vec![i32::MIN; n + 1];\n let mut previous = vec![i32::MIN; n + 1];\n\n for i in 1..=m {\n for j in 1..=n {\n let curr_product = nums1[i - 1] * nums2[j - 1];\n current[j] = std::cmp::max(curr_product, std::cmp::max(previous[j], std::cmp::max(current[j - 1], curr_product + std::cmp::max(0, previous[j - 1]))));\n }\n std::mem::swap(&mut current, &mut previous);\n }\n previous[n]\n }\n}\n```\n``` C++ []\nclass Solution {\npublic:\n int maxDotProduct(std::vector<int>& nums1, std::vector<int>& nums2) {\n int m = nums1.size(), n = nums2.size();\n std::vector<int> current(n + 1, INT_MIN), previous(n + 1, INT_MIN);\n\n for (int i = 1; i <= m; ++i) {\n for (int j = 1; j <= n; ++j) {\n int curr_product = nums1[i-1] * nums2[j-1];\n current[j] = std::max({curr_product, previous[j], current[j-1], curr_product + std::max(0, previous[j-1])});\n }\n std::swap(current, previous);\n }\n return previous[n];\n }\n};\n```\n``` Java []\npublic class Solution {\n public int maxDotProduct(int[] nums1, int[] nums2) {\n int m = nums1.length, n = nums2.length;\n int[] current = new int[n + 1], previous = new int[n + 1];\n Arrays.fill(current, Integer.MIN_VALUE);\n Arrays.fill(previous, Integer.MIN_VALUE);\n\n for (int i = 1; i <= m; i++) {\n for (int j = 1; j <= n; j++) {\n int curr_product = nums1[i - 1] * nums2[j - 1];\n current[j] = Math.max(Math.max(Math.max(curr_product, previous[j]), current[j - 1]), curr_product + Math.max(0, previous[j - 1]));\n }\n int[] temp = current;\n current = previous;\n previous = temp;\n }\n return previous[n];\n }\n}\n```\n``` JavaScript []\nvar maxDotProduct = function(nums1, nums2) {\n let m = nums1.length, n = nums2.length;\n let current = new Array(n + 1).fill(Number.MIN_SAFE_INTEGER);\n let previous = new Array(n + 1).fill(Number.MIN_SAFE_INTEGER);\n\n for (let i = 1; i <= m; i++) {\n for (let j = 1; j <= n; j++) {\n let curr_product = nums1[i - 1] * nums2[j - 1];\n current[j] = Math.max(curr_product, previous[j], current[j - 1], curr_product + Math.max(0, previous[j - 1]));\n }\n [current, previous] = [previous, current];\n }\n return previous[n];\n};\n```\n``` PHP []\nclass Solution {\n function maxDotProduct($nums1, $nums2) {\n $m = count($nums1);\n $n = count($nums2);\n $current = array_fill(0, $n + 1, PHP_INT_MIN);\n $previous = array_fill(0, $n + 1, PHP_INT_MIN);\n\n for ($i = 1; $i <= $m; $i++) {\n for ($j = 1; $j <= $n; $j++) {\n $curr_product = $nums1[$i - 1] * $nums2[$j - 1];\n $current[$j] = max($curr_product, $previous[$j], $current[$j - 1], $curr_product + max(0, $previous[$j - 1]));\n }\n list($current, $previous) = array($previous, $current);\n }\n return $previous[$n];\n }\n}\n```\n``` C# []\npublic class Solution {\n public int MaxDotProduct(int[] nums1, int[] nums2) {\n int m = nums1.Length, n = nums2.Length;\n int[] current = new int[n + 1], previous = new int[n + 1];\n Array.Fill(current, int.MinValue);\n Array.Fill(previous, int.MinValue);\n\n for (int i = 1; i <= m; i++) {\n for (int j = 1; j <= n; j++) {\n int curr_product = nums1[i - 1] * nums2[j - 1];\n current[j] = Math.Max(Math.Max(Math.Max(curr_product, previous[j]), current[j - 1]), curr_product + Math.Max(0, previous[j - 1]));\n }\n (current, previous) = (previous, current);\n }\n return previous[n];\n }\n}\n```\n\n# Performance\n| Language | Execution Time (ms) | Memory Usage (MB) |\n|------------|---------------------|-------------------|\n| Rust | 0 | 2.1 |\n| Go | 3 | 2.4 |\n| Java | 8 | 40.1 |\n| C++ | 20 | 9.8 |\n| JavaScript | 61 | 43.5 |\n| PHP | 62 | 19 |\n| C# | 87 | 38.9 |\n| Python3 | 221 | 16.3 |\n\n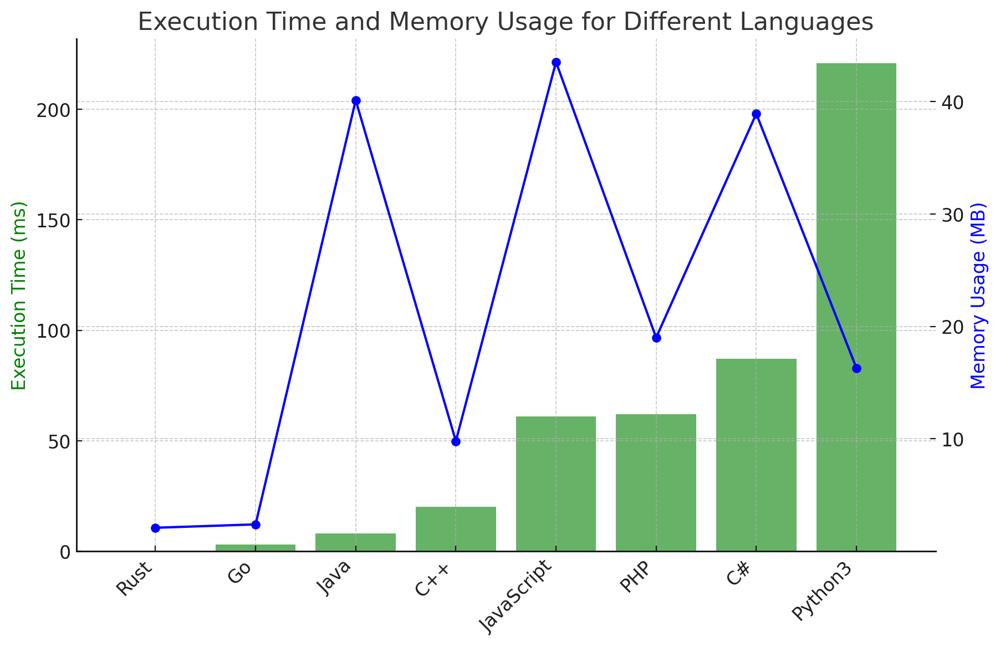\n\n\n# Why It Works?\nThe reason this solution works is that dynamic programming allows us to break the problem down into smaller subproblems. By considering all possibilities for each pair of indices `(i, j)`, we ensure that we find the maximum dot product. At each step, we make decisions based on the best possible outcome seen so far, which ensures the global maximum is achieved at the end.\n\n# What We Have Learned?\nThe problem teaches us to break a seemingly complicated problem into smaller manageable subproblems. It also emphasizes the importance of space optimization in dynamic programming problems, showcasing that often we don\'t need to store all the states.\n\n# Logic Behind the Solution\nAt every step, we consider two elements, one from each sequence. We have the choice to either consider them in the dot product or not. This decision is based on which choice yields the maximum dot product. By building our solution in this step-by-step manner, we ensure that at the end, our `dp` table\'s last cell contains the maximum dot product of any subsequence.\n\n# Why Optimized Version Works?\nThe optimized version leverages the observation that to compute the value at `dp[i][j]`, we only need values from the `i-1`th row and the `i`th row. Therefore, instead of maintaining a 2D array, we can keep track of just two rows. This significantly reduces our space requirements without affecting the logic or the outcome. | 45 | Given two arrays `nums1` and `nums2`.
Return the maximum dot product between **non-empty** subsequences of nums1 and nums2 with the same length.
A subsequence of a array is a new array which is formed from the original array by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (ie, `[2,3,5]` is a subsequence of `[1,2,3,4,5]` while `[1,5,3]` is not).
**Example 1:**
**Input:** nums1 = \[2,1,-2,5\], nums2 = \[3,0,-6\]
**Output:** 18
**Explanation:** Take subsequence \[2,-2\] from nums1 and subsequence \[3,-6\] from nums2.
Their dot product is (2\*3 + (-2)\*(-6)) = 18.
**Example 2:**
**Input:** nums1 = \[3,-2\], nums2 = \[2,-6,7\]
**Output:** 21
**Explanation:** Take subsequence \[3\] from nums1 and subsequence \[7\] from nums2.
Their dot product is (3\*7) = 21.
**Example 3:**
**Input:** nums1 = \[-1,-1\], nums2 = \[1,1\]
**Output:** -1
**Explanation:** Take subsequence \[-1\] from nums1 and subsequence \[1\] from nums2.
Their dot product is -1.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 500`
* `-1000 <= nums1[i], nums2[i] <= 1000` | Simulate the problem. Count the number of 1's in the binary representation of each integer. Sort by the number of 1's ascending and by the value in case of tie. |
✅ 90.14% Dynamic Programming Optimized | max-dot-product-of-two-subsequences | 1 | 1 | # Intuition\nWhen faced with two sequences and asked to find the maximum dot product, it becomes evident that we might have to consider various combinations of subsequences to determine the maximum value. This naturally hints towards the possibility of using dynamic programming, as there are overlapping subproblems.\n\n## Live Coding & Explain\nhttps://youtu.be/h1RAw1gEx5A?si=6zJ77Y2f9X5JLaZL\n\n# Approach\n\n1. **Dynamic Programming Approach**: \n - We can use a 2D array `dp` where `dp[i][j]` represents the maximum dot product between the first `i` elements of `nums1` and the first `j` elements of `nums2`.\n - If we decide to pick the elements `nums1[i]` and `nums2[j]`, the dot product added to the result is `nums1[i] * nums2[j]`.\n - We can also decide not to pick any element from `nums1` or `nums2`, so we will consider `dp[i-1][j]` and `dp[i][j-1]`.\n - The recurrence relation is: \n $$ dp[i][j] = \\max(dp[i-1][j], dp[i][j-1], nums1[i-1] \\times nums2[j-1] + \\max(0, dp[i-1][j-1]) $$\n\n2. **Space Optimized Approach**:\n - Instead of using a 2D array, we observe that we only need values from the current and previous row to compute the next row. Thus, two 1D arrays (current and previous) suffice.\n - At the end of processing each row, we swap the two arrays, thus making the current array act as the previous array for the next iteration.\n\n# Complexity\n- **Time complexity**: $$O(m \\times n)$$ - We have to fill up the `dp` matrix which is of size $$m \\times n$$.\n- **Space complexity**:\n - For the **DP approach**: $$O(m \\times n)$$ - The size of the `dp` matrix.\n - For the **Optimized approach**: $$O(n)$$ - We are using two 1D arrays.\n\n\n# Code Dynamic Programming\n``` Python []\nclass Solution:\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n m, n = len(nums1), len(nums2)\n \n dp = [[float(\'-inf\')] * (n + 1) for _ in range(m + 1)]\n \n for i in range(1, m + 1):\n for j in range(1, n + 1):\n curr_product = nums1[i-1] * nums2[j-1]\n \n dp[i][j] = max(dp[i][j], curr_product, dp[i-1][j], dp[i][j-1], curr_product + dp[i-1][j-1])\n \n return dp[m][n]\n\n```\n\n# Code Optimized\n``` Python []\nclass Solution:\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n m, n = len(nums1), len(nums2)\n \n current = [float(\'-inf\')] * (n + 1)\n previous = [float(\'-inf\')] * (n + 1)\n \n for i in range(1, m + 1):\n for j in range(1, n + 1):\n curr_product = nums1[i-1] * nums2[j-1]\n \n current[j] = max(curr_product, previous[j], current[j-1], curr_product + max(0, previous[j-1]))\n \n current, previous = previous, current\n \n return previous[n]\n\n```\n``` Go []\nfunc maxDotProduct(nums1 []int, nums2 []int) int {\n m, n := len(nums1), len(nums2)\n current := make([]int, n+1)\n previous := make([]int, n+1)\n for i := range current {\n current[i] = math.MinInt32\n previous[i] = math.MinInt32\n }\n\n for i := 1; i <= m; i++ {\n for j := 1; j <= n; j++ {\n currProduct := nums1[i-1] * nums2[j-1]\n current[j] = max(max(max(currProduct, previous[j]), current[j-1]), currProduct + max(0, previous[j-1]))\n }\n current, previous = previous, current\n }\n return previous[n]\n}\n\nfunc max(a, b int) int {\n if a > b {\n return a\n }\n return b\n}\n```\n``` Rust []\nimpl Solution {\n pub fn max_dot_product(nums1: Vec<i32>, nums2: Vec<i32>) -> i32 {\n let m = nums1.len();\n let n = nums2.len();\n let mut current = vec![i32::MIN; n + 1];\n let mut previous = vec![i32::MIN; n + 1];\n\n for i in 1..=m {\n for j in 1..=n {\n let curr_product = nums1[i - 1] * nums2[j - 1];\n current[j] = std::cmp::max(curr_product, std::cmp::max(previous[j], std::cmp::max(current[j - 1], curr_product + std::cmp::max(0, previous[j - 1]))));\n }\n std::mem::swap(&mut current, &mut previous);\n }\n previous[n]\n }\n}\n```\n``` C++ []\nclass Solution {\npublic:\n int maxDotProduct(std::vector<int>& nums1, std::vector<int>& nums2) {\n int m = nums1.size(), n = nums2.size();\n std::vector<int> current(n + 1, INT_MIN), previous(n + 1, INT_MIN);\n\n for (int i = 1; i <= m; ++i) {\n for (int j = 1; j <= n; ++j) {\n int curr_product = nums1[i-1] * nums2[j-1];\n current[j] = std::max({curr_product, previous[j], current[j-1], curr_product + std::max(0, previous[j-1])});\n }\n std::swap(current, previous);\n }\n return previous[n];\n }\n};\n```\n``` Java []\npublic class Solution {\n public int maxDotProduct(int[] nums1, int[] nums2) {\n int m = nums1.length, n = nums2.length;\n int[] current = new int[n + 1], previous = new int[n + 1];\n Arrays.fill(current, Integer.MIN_VALUE);\n Arrays.fill(previous, Integer.MIN_VALUE);\n\n for (int i = 1; i <= m; i++) {\n for (int j = 1; j <= n; j++) {\n int curr_product = nums1[i - 1] * nums2[j - 1];\n current[j] = Math.max(Math.max(Math.max(curr_product, previous[j]), current[j - 1]), curr_product + Math.max(0, previous[j - 1]));\n }\n int[] temp = current;\n current = previous;\n previous = temp;\n }\n return previous[n];\n }\n}\n```\n``` JavaScript []\nvar maxDotProduct = function(nums1, nums2) {\n let m = nums1.length, n = nums2.length;\n let current = new Array(n + 1).fill(Number.MIN_SAFE_INTEGER);\n let previous = new Array(n + 1).fill(Number.MIN_SAFE_INTEGER);\n\n for (let i = 1; i <= m; i++) {\n for (let j = 1; j <= n; j++) {\n let curr_product = nums1[i - 1] * nums2[j - 1];\n current[j] = Math.max(curr_product, previous[j], current[j - 1], curr_product + Math.max(0, previous[j - 1]));\n }\n [current, previous] = [previous, current];\n }\n return previous[n];\n};\n```\n``` PHP []\nclass Solution {\n function maxDotProduct($nums1, $nums2) {\n $m = count($nums1);\n $n = count($nums2);\n $current = array_fill(0, $n + 1, PHP_INT_MIN);\n $previous = array_fill(0, $n + 1, PHP_INT_MIN);\n\n for ($i = 1; $i <= $m; $i++) {\n for ($j = 1; $j <= $n; $j++) {\n $curr_product = $nums1[$i - 1] * $nums2[$j - 1];\n $current[$j] = max($curr_product, $previous[$j], $current[$j - 1], $curr_product + max(0, $previous[$j - 1]));\n }\n list($current, $previous) = array($previous, $current);\n }\n return $previous[$n];\n }\n}\n```\n``` C# []\npublic class Solution {\n public int MaxDotProduct(int[] nums1, int[] nums2) {\n int m = nums1.Length, n = nums2.Length;\n int[] current = new int[n + 1], previous = new int[n + 1];\n Array.Fill(current, int.MinValue);\n Array.Fill(previous, int.MinValue);\n\n for (int i = 1; i <= m; i++) {\n for (int j = 1; j <= n; j++) {\n int curr_product = nums1[i - 1] * nums2[j - 1];\n current[j] = Math.Max(Math.Max(Math.Max(curr_product, previous[j]), current[j - 1]), curr_product + Math.Max(0, previous[j - 1]));\n }\n (current, previous) = (previous, current);\n }\n return previous[n];\n }\n}\n```\n\n# Performance\n| Language | Execution Time (ms) | Memory Usage (MB) |\n|------------|---------------------|-------------------|\n| Rust | 0 | 2.1 |\n| Go | 3 | 2.4 |\n| Java | 8 | 40.1 |\n| C++ | 20 | 9.8 |\n| JavaScript | 61 | 43.5 |\n| PHP | 62 | 19 |\n| C# | 87 | 38.9 |\n| Python3 | 221 | 16.3 |\n\n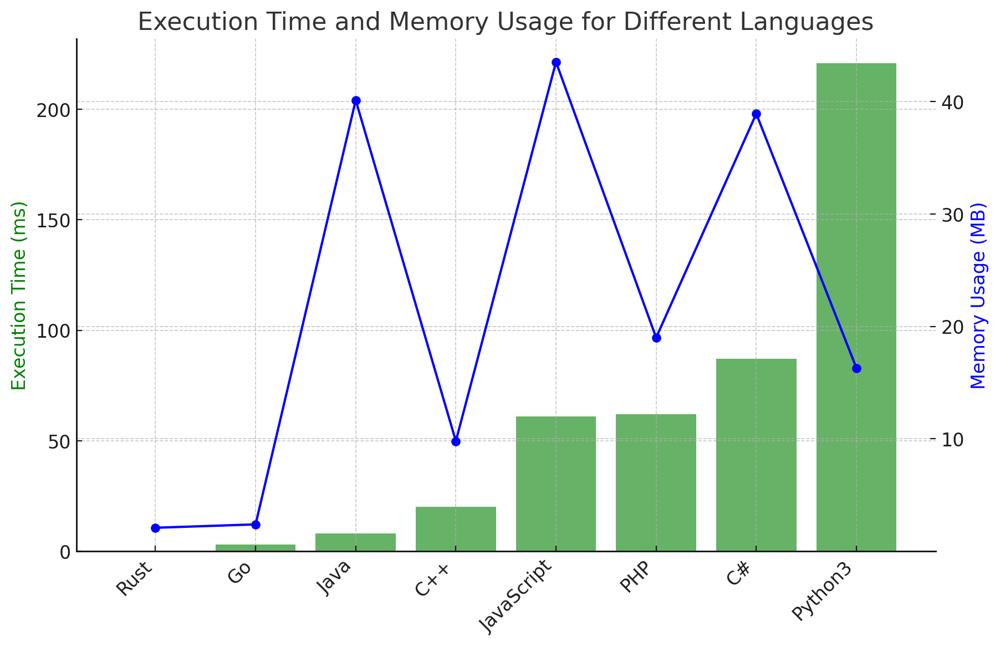\n\n\n# Why It Works?\nThe reason this solution works is that dynamic programming allows us to break the problem down into smaller subproblems. By considering all possibilities for each pair of indices `(i, j)`, we ensure that we find the maximum dot product. At each step, we make decisions based on the best possible outcome seen so far, which ensures the global maximum is achieved at the end.\n\n# What We Have Learned?\nThe problem teaches us to break a seemingly complicated problem into smaller manageable subproblems. It also emphasizes the importance of space optimization in dynamic programming problems, showcasing that often we don\'t need to store all the states.\n\n# Logic Behind the Solution\nAt every step, we consider two elements, one from each sequence. We have the choice to either consider them in the dot product or not. This decision is based on which choice yields the maximum dot product. By building our solution in this step-by-step manner, we ensure that at the end, our `dp` table\'s last cell contains the maximum dot product of any subsequence.\n\n# Why Optimized Version Works?\nThe optimized version leverages the observation that to compute the value at `dp[i][j]`, we only need values from the `i-1`th row and the `i`th row. Therefore, instead of maintaining a 2D array, we can keep track of just two rows. This significantly reduces our space requirements without affecting the logic or the outcome. | 45 | Given an array `nums` that represents a permutation of integers from `1` to `n`. We are going to construct a binary search tree (BST) by inserting the elements of `nums` in order into an initially empty BST. Find the number of different ways to reorder `nums` so that the constructed BST is identical to that formed from the original array `nums`.
* For example, given `nums = [2,1,3]`, we will have 2 as the root, 1 as a left child, and 3 as a right child. The array `[2,3,1]` also yields the same BST but `[3,2,1]` yields a different BST.
Return _the number of ways to reorder_ `nums` _such that the BST formed is identical to the original BST formed from_ `nums`.
Since the answer may be very large, **return it modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[2,1,3\]
**Output:** 1
**Explanation:** We can reorder nums to be \[2,3,1\] which will yield the same BST. There are no other ways to reorder nums which will yield the same BST.
**Example 2:**
**Input:** nums = \[3,4,5,1,2\]
**Output:** 5
**Explanation:** The following 5 arrays will yield the same BST:
\[3,1,2,4,5\]
\[3,1,4,2,5\]
\[3,1,4,5,2\]
\[3,4,1,2,5\]
\[3,4,1,5,2\]
**Example 3:**
**Input:** nums = \[1,2,3\]
**Output:** 0
**Explanation:** There are no other orderings of nums that will yield the same BST.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= nums.length`
* All integers in `nums` are **distinct**. | Use dynamic programming, define DP[i][j] as the maximum dot product of two subsequences starting in the position i of nums1 and position j of nums2. |
【Video】Give me 10 minutes - How we think about a solution - Python, JavaScript, Java, C++ | max-dot-product-of-two-subsequences | 1 | 1 | Welcome to my post! This post starts with "How we think about a solution". In other words, that is my thought process to solve the question. This post explains how I get to my solution instead of just posting solution codes or out of blue algorithms. I hope it is helpful for someone.\n\n# Intuition\nUsing Dynamic Programming\n\n---\n\n# Solution Video\n\nhttps://youtu.be/i8QNjFYSHkE\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 2,636\nMy initial goal is 10,000\nThank you for your support!\n\n---\n\n\n# Approach\n\n## How we think about a solution\n\n- **What is dot product?**\n\nFirst of all, we need to understand what dot product is.\n\n\n---\n\n\u2B50\uFE0F Points\n\nIn simple terms, the dot product is a mathematical operation between two vectors where you multiply corresponding elements of the vectors and sum up the products. When given two vectors, $$v1$$ and $$v2$$, the dot product is calculated as follows:\n\nCalculation of the dot product:\nGiven two vectors $$v1 = [a1,a2...an]$$ and $$v2 = [b1,b2...bn]$$, the dot product is calculated as:\n\n$$v1\u30FBv2 = a1 * b1 + a2 * b2 + ... + an * bn$$ \n\nIn other words, you multiply each pair of corresponding elements and sum up the results to calculate the dot product. The dot product is widely used to evaluate vector similarity and the magnitude of vectors.\n\nTo illustrate this with a more understandable example:\n\n$$Vector 1: v1 = [2,3,\u22121]$$\n$$Vector 2: v2 = [4,\u22122,5]$$\n\nTo calculate the dot product of these two vectors, you would perform the following calculation:\n\n$$v1\u30FBv2 = (2 * 4) + (3 * -2) + (-1 * 5)$$\n$$= 8 - 6 - 5$$\n$$= -3$$\n\nTherefore, the dot product of Vector 1 and Vector 2 is `-3`.\n\nYou can confirm it with `NumPy`.\n\n```\nimport numpy as np\n\nv1 = np.array([2, 3, -1])\nv2 = np.array([4, -2, 5])\nres = v1.dot(v2)\nprint(res) # -3\n```\n\n\u203B Be careful! `-3` is not a right answer of this question because we have a subsequence constraint.\n\n---\n\n- **How do we calculate number of subsequences?**\n\nOne more important thing is how we calculate number of subsequences?\n\nLet\'s think about non-empty subsequences of `[2, 1, -2, 5]` \n```\n1. [2]\n2. [1]\n3. [-2]\n4. [5]\n5. [2, 1]\n6. [2, -2]\n7. [2, 5]\n8. [1, -2]\n9. [1, 5]\n10. [-2, 5]\n11. [2, 1, -2]\n12. [2, 1, 5]\n13. [2, -2, 5]\n14. [1, -2, 5]\n15. [2, 1, -2, 5]\n```\nThe calculation of number of subsequences is `2^n` where `n` is length of the array, that\'s because we have two choices for each number, `include` or `not include`. `2` of `2^n` is coming from the two choices and you have `n` numbers.\n\nIn this case, you have 4 numbers, so\n```\n2 * 2 * 2 * 2 = 16\n```\nBut `16` includes an empty subsequence. We need to subtract `-1`, that\'s why `15`.\n\n---\n\n\u2B50\uFE0F Points\n\nWe have two choices for each number when we count subsequences. `include` or `not include`.\n\n---\n\n- **Think about subsequence cases with simple example**\n\nSeems like this question has many combinations of subsequences, so when I feel that, I always start with simple examples.\n\nLet\'s think about this case\n```\nInput: nums1 = [1], nums2 = [3,4]\n```\nIn this case, since length of `nums1` is `1`, so we have only two choice to create subsequences.\n\n`[1] & [3]` or `[1] & [4]`\n= `1 * 3` or `1 * 4`\n\n```\nOutput: 4\n```\nLet\'s call `[1] & [3]` **case1** and `[1] & [4]` ****case2****.\n\nNext, what if we have this input? \n```\nInput: nums1 = [1,2], nums2 = [3,4]\n```\nNow Let\'s check when current number is `2` in `nums1`.\n\nIn this case, we need to compare `4 cases`. Do you guess? Let\'s see one by one.\n\n`2` is at `index 1` which means length of subsequence is `2`, since dot product needs the same length of other subsequence, we also need `2 numbers` from `nums2`.\n\nIn this case, dot product is\n```\n3 + (2 * 4) = 11\n\n3 is comming from (1 * 3)\n``` \nLet\'s call this case `case3`.\n\nFor the last case(`case4`), as I told you, when we create subsequences, we have two choices...`include` or `not include`, so what if we don\'t include `1` when current number is `2` in `nums1`?\n\nIn that case,\n```\n2 * 3 or 2 * 4\n```\nThey are `case4`.\n\nNow input arrays are\n```\nInput: nums1 = [1,2], nums2 = [3,4]\n```\nThis is vice versa. we can skip `2` in `nums1` which are the same as `case1` and `case2`.\n\nThat\'s why we need compare `4 cases` every time.\n\n```\ncase1: 1 * 3 = 3\ncase2: 1 * 4 = 4\ncase3: 3 + (2 * 4) = 11\ncase4: 2 * 3 or 2 * 4 = 6 or 8\n```\n`case4` will be `case1` in next comparison if `case4` is bigger than `case1` in real program.\n```\nOutput: 11\n```\n\nTo prove why we need to consider `case 1` and `case 2` when current number is `2` in `nums1`. Let\'s think about this input.\n\n```\nInput: nums1 = [1,-2], nums2 = [3,4]\n```\n\nIn this case,\n```\ncase1: 1 * 3 = 3\ncase2: 1 * 4 = 4\ncase3: 3 + (-2 * 4) = -5\ncase4: -2 * 3 or -2 * 4 = -6 or -8\n```\n```\nOutput: 4\n```\nThis is product calculation, so we need to consider negative cases. **The number of elements alone does not guarantee a higher maximum value.**\n\nSince we need to keep previous results to compare 4 cases, seems like we can use Dynamic Programming solution.\n\nLet\'s see a real algorithm.\n\n\n---\n\nIf you are not familiar with Dynamic Programming, I created a video for Dynamic Programming.\n\nhttps://youtu.be/MUU6tgywdPY\n\n\n---\n\n\n\n\n### Algorithm Overviews:\n\n1. **Input Processing and Setup**:\n - Obtain the lengths of the input arrays, `m` and `n`.\n - Swap arrays if `nums1` is shorter than `nums2`.\n - Initialize a dynamic programming array `dp` of size `n + 1` to store maximum dot products.\n\n2. **Dynamic Programming for Maximum Dot Product**:\n - Iterate over elements in `nums1`.\n - For each element in `nums1`, iterate over elements in `nums2`.\n - Calculate the maximum dot product for the current elements from `nums1` and `nums2`, considering various cases.\n\n3. **Output**:\n - Return the maximum dot product calculated from the dynamic programming array.\n\n### Detailed Explanation:\n\n1. **Input Processing and Setup**:\n - Obtain the lengths of the input arrays, `m` and `n`.\n - Swap arrays if `nums1` is shorter than `nums2`. This ensures `nums1` is at least as long as `nums2`.\n - Initialize a dynamic programming array `dp` of size `n + 1` to store maximum dot products. Each element in `dp` corresponds to the maximum dot product for subsequences up to that point.\n\n2. **Dynamic Programming for Maximum Dot Product**:\n - Iterate over elements in `nums1`.\n - For each element in `nums1`, iterate over elements in `nums2`.\n - Calculate the maximum dot product for the current elements from `nums1` and `nums2`. The maximum dot product is calculated by considering four cases:\n - Taking the dot product of the current elements from `nums1` and `nums2`.\n - Taking the maximum dot product from the previous element in `nums1` and the current element in `nums2`.\n - Taking the maximum dot product from the current element in `nums1` and the previous element in `nums2`.\n - Taking the maximum dot product from the previous elements in both `nums1` and `nums2`.\n\n3. **Output**:\n - Return the maximum dot product calculated from the dynamic programming array, which corresponds to the maximum dot product of non-empty subsequences of `nums1` and `nums2` with the same length.\n# Complexity\n- Time complexity: $$O(mn)$$\nThe time complexity of this algorithm is O(mn), where m and n are the lengths of the input arrays nums1 and nums2, respectively. This is because there is a nested loop that iterates through both arrays, resulting in O(mn) operations.\n\n- Space complexity: $$O(n)$$\nThe space complexity is O(n), where n is the length of the shorter input array (after swapping if necessary). This is due to the dynamic programming array dp, which is of size n+1, and a few additional variables used in the function. The space used is proportional to the length of the shorter array.\n\n\n```python []\nclass Solution:\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n m, n = len(nums1), len(nums2)\n \n if m < n:\n return self.maxDotProduct(nums2, nums1)\n \n dp = [float("-inf")] * (n + 1)\n \n for i in range(m):\n prev = 0\n for j in range(n):\n tmp = dp[j + 1]\n # max(case3, case4, case1, case2)\n dp[j + 1] = max(prev + nums1[i] * nums2[j], nums1[i] * nums2[j], dp[j], dp[j + 1])\n prev = tmp\n \n return dp[-1]\n \n```\n```javascript []\n/**\n * @param {number[]} nums1\n * @param {number[]} nums2\n * @return {number}\n */\nvar maxDotProduct = function(nums1, nums2) {\n const m = nums1.length;\n const n = nums2.length;\n\n if (m < n) {\n return maxDotProduct(nums2, nums1);\n }\n\n const dp = new Array(n + 1).fill(Number.NEGATIVE_INFINITY);\n\n for (let i = 0; i < m; i++) {\n let prev = 0;\n for (let j = 0; j < n; j++) {\n const tmp = dp[j + 1];\n dp[j + 1] = Math.max(prev + nums1[i] * nums2[j], nums1[i] * nums2[j], dp[j], dp[j + 1]);\n prev = tmp;\n }\n }\n\n return dp[n]; \n};\n```\n```java []\nclass Solution {\n public int maxDotProduct(int[] nums1, int[] nums2) {\n int m = nums1.length;\n int n = nums2.length;\n\n if (m < n) {\n return maxDotProduct(nums2, nums1);\n }\n\n long[] dp = new long[n + 1];\n Arrays.fill(dp, Integer.MIN_VALUE);\n\n for (int i = 0; i < m; i++) {\n long prev = 0;\n for (int j = 0; j < n; j++) {\n long tmp = dp[j + 1];\n dp[j + 1] = Math.max(prev + nums1[i] * nums2[j], Math.max(nums1[i] * nums2[j], Math.max(dp[j], dp[j + 1])));\n prev = tmp;\n }\n }\n\n return (int)dp[n];\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int maxDotProduct(vector<int>& nums1, vector<int>& nums2) {\n int m = nums1.size();\n int n = nums2.size();\n\n if (m < n) {\n return maxDotProduct(nums2, nums1);\n }\n\n vector<long> dp(n + 1, INT_MIN);\n\n for (int i = 0; i < m; i++) {\n long prev = 0;\n for (int j = 0; j < n; j++) {\n long tmp = dp[j + 1];\n dp[j + 1] = max(prev + (long)nums1[i] * nums2[j], max((long)nums1[i] * nums2[j], max(dp[j], dp[j + 1])));\n prev = tmp;\n }\n }\n\n return (int)dp[n];\n }\n};\n```\n\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n\u2B50\uFE0F My next post and video for daily coding challenge\n\npost\nhttps://leetcode.com/problems/find-first-and-last-position-of-element-in-sorted-array/solutions/4147878/video-give-me-10-minutes-binary-search-solution-python-javascript-java-c/\n\nhttps://youtu.be/441pamgku74\n\n\u2B50\uFE0F My previous post and video\n\npost\nhttps://leetcode.com/problems/integer-break/solutions/4137225/video-give-me-10-minutes-how-we-think-about-a-solution-no-difficult-mathematics/\n\nhttps://youtu.be/q3EZpCSn6lY | 37 | Given two arrays `nums1` and `nums2`.
Return the maximum dot product between **non-empty** subsequences of nums1 and nums2 with the same length.
A subsequence of a array is a new array which is formed from the original array by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (ie, `[2,3,5]` is a subsequence of `[1,2,3,4,5]` while `[1,5,3]` is not).
**Example 1:**
**Input:** nums1 = \[2,1,-2,5\], nums2 = \[3,0,-6\]
**Output:** 18
**Explanation:** Take subsequence \[2,-2\] from nums1 and subsequence \[3,-6\] from nums2.
Their dot product is (2\*3 + (-2)\*(-6)) = 18.
**Example 2:**
**Input:** nums1 = \[3,-2\], nums2 = \[2,-6,7\]
**Output:** 21
**Explanation:** Take subsequence \[3\] from nums1 and subsequence \[7\] from nums2.
Their dot product is (3\*7) = 21.
**Example 3:**
**Input:** nums1 = \[-1,-1\], nums2 = \[1,1\]
**Output:** -1
**Explanation:** Take subsequence \[-1\] from nums1 and subsequence \[1\] from nums2.
Their dot product is -1.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 500`
* `-1000 <= nums1[i], nums2[i] <= 1000` | Simulate the problem. Count the number of 1's in the binary representation of each integer. Sort by the number of 1's ascending and by the value in case of tie. |
【Video】Give me 10 minutes - How we think about a solution - Python, JavaScript, Java, C++ | max-dot-product-of-two-subsequences | 1 | 1 | Welcome to my post! This post starts with "How we think about a solution". In other words, that is my thought process to solve the question. This post explains how I get to my solution instead of just posting solution codes or out of blue algorithms. I hope it is helpful for someone.\n\n# Intuition\nUsing Dynamic Programming\n\n---\n\n# Solution Video\n\nhttps://youtu.be/i8QNjFYSHkE\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 2,636\nMy initial goal is 10,000\nThank you for your support!\n\n---\n\n\n# Approach\n\n## How we think about a solution\n\n- **What is dot product?**\n\nFirst of all, we need to understand what dot product is.\n\n\n---\n\n\u2B50\uFE0F Points\n\nIn simple terms, the dot product is a mathematical operation between two vectors where you multiply corresponding elements of the vectors and sum up the products. When given two vectors, $$v1$$ and $$v2$$, the dot product is calculated as follows:\n\nCalculation of the dot product:\nGiven two vectors $$v1 = [a1,a2...an]$$ and $$v2 = [b1,b2...bn]$$, the dot product is calculated as:\n\n$$v1\u30FBv2 = a1 * b1 + a2 * b2 + ... + an * bn$$ \n\nIn other words, you multiply each pair of corresponding elements and sum up the results to calculate the dot product. The dot product is widely used to evaluate vector similarity and the magnitude of vectors.\n\nTo illustrate this with a more understandable example:\n\n$$Vector 1: v1 = [2,3,\u22121]$$\n$$Vector 2: v2 = [4,\u22122,5]$$\n\nTo calculate the dot product of these two vectors, you would perform the following calculation:\n\n$$v1\u30FBv2 = (2 * 4) + (3 * -2) + (-1 * 5)$$\n$$= 8 - 6 - 5$$\n$$= -3$$\n\nTherefore, the dot product of Vector 1 and Vector 2 is `-3`.\n\nYou can confirm it with `NumPy`.\n\n```\nimport numpy as np\n\nv1 = np.array([2, 3, -1])\nv2 = np.array([4, -2, 5])\nres = v1.dot(v2)\nprint(res) # -3\n```\n\n\u203B Be careful! `-3` is not a right answer of this question because we have a subsequence constraint.\n\n---\n\n- **How do we calculate number of subsequences?**\n\nOne more important thing is how we calculate number of subsequences?\n\nLet\'s think about non-empty subsequences of `[2, 1, -2, 5]` \n```\n1. [2]\n2. [1]\n3. [-2]\n4. [5]\n5. [2, 1]\n6. [2, -2]\n7. [2, 5]\n8. [1, -2]\n9. [1, 5]\n10. [-2, 5]\n11. [2, 1, -2]\n12. [2, 1, 5]\n13. [2, -2, 5]\n14. [1, -2, 5]\n15. [2, 1, -2, 5]\n```\nThe calculation of number of subsequences is `2^n` where `n` is length of the array, that\'s because we have two choices for each number, `include` or `not include`. `2` of `2^n` is coming from the two choices and you have `n` numbers.\n\nIn this case, you have 4 numbers, so\n```\n2 * 2 * 2 * 2 = 16\n```\nBut `16` includes an empty subsequence. We need to subtract `-1`, that\'s why `15`.\n\n---\n\n\u2B50\uFE0F Points\n\nWe have two choices for each number when we count subsequences. `include` or `not include`.\n\n---\n\n- **Think about subsequence cases with simple example**\n\nSeems like this question has many combinations of subsequences, so when I feel that, I always start with simple examples.\n\nLet\'s think about this case\n```\nInput: nums1 = [1], nums2 = [3,4]\n```\nIn this case, since length of `nums1` is `1`, so we have only two choice to create subsequences.\n\n`[1] & [3]` or `[1] & [4]`\n= `1 * 3` or `1 * 4`\n\n```\nOutput: 4\n```\nLet\'s call `[1] & [3]` **case1** and `[1] & [4]` ****case2****.\n\nNext, what if we have this input? \n```\nInput: nums1 = [1,2], nums2 = [3,4]\n```\nNow Let\'s check when current number is `2` in `nums1`.\n\nIn this case, we need to compare `4 cases`. Do you guess? Let\'s see one by one.\n\n`2` is at `index 1` which means length of subsequence is `2`, since dot product needs the same length of other subsequence, we also need `2 numbers` from `nums2`.\n\nIn this case, dot product is\n```\n3 + (2 * 4) = 11\n\n3 is comming from (1 * 3)\n``` \nLet\'s call this case `case3`.\n\nFor the last case(`case4`), as I told you, when we create subsequences, we have two choices...`include` or `not include`, so what if we don\'t include `1` when current number is `2` in `nums1`?\n\nIn that case,\n```\n2 * 3 or 2 * 4\n```\nThey are `case4`.\n\nNow input arrays are\n```\nInput: nums1 = [1,2], nums2 = [3,4]\n```\nThis is vice versa. we can skip `2` in `nums1` which are the same as `case1` and `case2`.\n\nThat\'s why we need compare `4 cases` every time.\n\n```\ncase1: 1 * 3 = 3\ncase2: 1 * 4 = 4\ncase3: 3 + (2 * 4) = 11\ncase4: 2 * 3 or 2 * 4 = 6 or 8\n```\n`case4` will be `case1` in next comparison if `case4` is bigger than `case1` in real program.\n```\nOutput: 11\n```\n\nTo prove why we need to consider `case 1` and `case 2` when current number is `2` in `nums1`. Let\'s think about this input.\n\n```\nInput: nums1 = [1,-2], nums2 = [3,4]\n```\n\nIn this case,\n```\ncase1: 1 * 3 = 3\ncase2: 1 * 4 = 4\ncase3: 3 + (-2 * 4) = -5\ncase4: -2 * 3 or -2 * 4 = -6 or -8\n```\n```\nOutput: 4\n```\nThis is product calculation, so we need to consider negative cases. **The number of elements alone does not guarantee a higher maximum value.**\n\nSince we need to keep previous results to compare 4 cases, seems like we can use Dynamic Programming solution.\n\nLet\'s see a real algorithm.\n\n\n---\n\nIf you are not familiar with Dynamic Programming, I created a video for Dynamic Programming.\n\nhttps://youtu.be/MUU6tgywdPY\n\n\n---\n\n\n\n\n### Algorithm Overviews:\n\n1. **Input Processing and Setup**:\n - Obtain the lengths of the input arrays, `m` and `n`.\n - Swap arrays if `nums1` is shorter than `nums2`.\n - Initialize a dynamic programming array `dp` of size `n + 1` to store maximum dot products.\n\n2. **Dynamic Programming for Maximum Dot Product**:\n - Iterate over elements in `nums1`.\n - For each element in `nums1`, iterate over elements in `nums2`.\n - Calculate the maximum dot product for the current elements from `nums1` and `nums2`, considering various cases.\n\n3. **Output**:\n - Return the maximum dot product calculated from the dynamic programming array.\n\n### Detailed Explanation:\n\n1. **Input Processing and Setup**:\n - Obtain the lengths of the input arrays, `m` and `n`.\n - Swap arrays if `nums1` is shorter than `nums2`. This ensures `nums1` is at least as long as `nums2`.\n - Initialize a dynamic programming array `dp` of size `n + 1` to store maximum dot products. Each element in `dp` corresponds to the maximum dot product for subsequences up to that point.\n\n2. **Dynamic Programming for Maximum Dot Product**:\n - Iterate over elements in `nums1`.\n - For each element in `nums1`, iterate over elements in `nums2`.\n - Calculate the maximum dot product for the current elements from `nums1` and `nums2`. The maximum dot product is calculated by considering four cases:\n - Taking the dot product of the current elements from `nums1` and `nums2`.\n - Taking the maximum dot product from the previous element in `nums1` and the current element in `nums2`.\n - Taking the maximum dot product from the current element in `nums1` and the previous element in `nums2`.\n - Taking the maximum dot product from the previous elements in both `nums1` and `nums2`.\n\n3. **Output**:\n - Return the maximum dot product calculated from the dynamic programming array, which corresponds to the maximum dot product of non-empty subsequences of `nums1` and `nums2` with the same length.\n# Complexity\n- Time complexity: $$O(mn)$$\nThe time complexity of this algorithm is O(mn), where m and n are the lengths of the input arrays nums1 and nums2, respectively. This is because there is a nested loop that iterates through both arrays, resulting in O(mn) operations.\n\n- Space complexity: $$O(n)$$\nThe space complexity is O(n), where n is the length of the shorter input array (after swapping if necessary). This is due to the dynamic programming array dp, which is of size n+1, and a few additional variables used in the function. The space used is proportional to the length of the shorter array.\n\n\n```python []\nclass Solution:\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n m, n = len(nums1), len(nums2)\n \n if m < n:\n return self.maxDotProduct(nums2, nums1)\n \n dp = [float("-inf")] * (n + 1)\n \n for i in range(m):\n prev = 0\n for j in range(n):\n tmp = dp[j + 1]\n # max(case3, case4, case1, case2)\n dp[j + 1] = max(prev + nums1[i] * nums2[j], nums1[i] * nums2[j], dp[j], dp[j + 1])\n prev = tmp\n \n return dp[-1]\n \n```\n```javascript []\n/**\n * @param {number[]} nums1\n * @param {number[]} nums2\n * @return {number}\n */\nvar maxDotProduct = function(nums1, nums2) {\n const m = nums1.length;\n const n = nums2.length;\n\n if (m < n) {\n return maxDotProduct(nums2, nums1);\n }\n\n const dp = new Array(n + 1).fill(Number.NEGATIVE_INFINITY);\n\n for (let i = 0; i < m; i++) {\n let prev = 0;\n for (let j = 0; j < n; j++) {\n const tmp = dp[j + 1];\n dp[j + 1] = Math.max(prev + nums1[i] * nums2[j], nums1[i] * nums2[j], dp[j], dp[j + 1]);\n prev = tmp;\n }\n }\n\n return dp[n]; \n};\n```\n```java []\nclass Solution {\n public int maxDotProduct(int[] nums1, int[] nums2) {\n int m = nums1.length;\n int n = nums2.length;\n\n if (m < n) {\n return maxDotProduct(nums2, nums1);\n }\n\n long[] dp = new long[n + 1];\n Arrays.fill(dp, Integer.MIN_VALUE);\n\n for (int i = 0; i < m; i++) {\n long prev = 0;\n for (int j = 0; j < n; j++) {\n long tmp = dp[j + 1];\n dp[j + 1] = Math.max(prev + nums1[i] * nums2[j], Math.max(nums1[i] * nums2[j], Math.max(dp[j], dp[j + 1])));\n prev = tmp;\n }\n }\n\n return (int)dp[n];\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int maxDotProduct(vector<int>& nums1, vector<int>& nums2) {\n int m = nums1.size();\n int n = nums2.size();\n\n if (m < n) {\n return maxDotProduct(nums2, nums1);\n }\n\n vector<long> dp(n + 1, INT_MIN);\n\n for (int i = 0; i < m; i++) {\n long prev = 0;\n for (int j = 0; j < n; j++) {\n long tmp = dp[j + 1];\n dp[j + 1] = max(prev + (long)nums1[i] * nums2[j], max((long)nums1[i] * nums2[j], max(dp[j], dp[j + 1])));\n prev = tmp;\n }\n }\n\n return (int)dp[n];\n }\n};\n```\n\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n\u2B50\uFE0F My next post and video for daily coding challenge\n\npost\nhttps://leetcode.com/problems/find-first-and-last-position-of-element-in-sorted-array/solutions/4147878/video-give-me-10-minutes-binary-search-solution-python-javascript-java-c/\n\nhttps://youtu.be/441pamgku74\n\n\u2B50\uFE0F My previous post and video\n\npost\nhttps://leetcode.com/problems/integer-break/solutions/4137225/video-give-me-10-minutes-how-we-think-about-a-solution-no-difficult-mathematics/\n\nhttps://youtu.be/q3EZpCSn6lY | 37 | Given an array `nums` that represents a permutation of integers from `1` to `n`. We are going to construct a binary search tree (BST) by inserting the elements of `nums` in order into an initially empty BST. Find the number of different ways to reorder `nums` so that the constructed BST is identical to that formed from the original array `nums`.
* For example, given `nums = [2,1,3]`, we will have 2 as the root, 1 as a left child, and 3 as a right child. The array `[2,3,1]` also yields the same BST but `[3,2,1]` yields a different BST.
Return _the number of ways to reorder_ `nums` _such that the BST formed is identical to the original BST formed from_ `nums`.
Since the answer may be very large, **return it modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[2,1,3\]
**Output:** 1
**Explanation:** We can reorder nums to be \[2,3,1\] which will yield the same BST. There are no other ways to reorder nums which will yield the same BST.
**Example 2:**
**Input:** nums = \[3,4,5,1,2\]
**Output:** 5
**Explanation:** The following 5 arrays will yield the same BST:
\[3,1,2,4,5\]
\[3,1,4,2,5\]
\[3,1,4,5,2\]
\[3,4,1,2,5\]
\[3,4,1,5,2\]
**Example 3:**
**Input:** nums = \[1,2,3\]
**Output:** 0
**Explanation:** There are no other orderings of nums that will yield the same BST.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= nums.length`
* All integers in `nums` are **distinct**. | Use dynamic programming, define DP[i][j] as the maximum dot product of two subsequences starting in the position i of nums1 and position j of nums2. |
🚀92.14% || DP Recursive & Iterative || Explained Intuition🚀 | max-dot-product-of-two-subsequences | 1 | 1 | # Porblem Description\n\nGiven two arrays, `nums1` and `nums2`. The task is to find the **maximum** dot product that can be obtained by taking **non-empty** **subsequences** of equal length from `nums1` and `nums2`.\n\nA **subsequence** of an array is a new array obtained by deleting some (or none) of the elements from the original array **without changing the order** of the remaining elements. \nThe **goal** is to **maximize** the dot product of the selected subsequences by choosing the elements wisely.\n\n- **Constraints:**\n - `1 <= nums1.length, nums2.length <= 500`\n - `-1000 <= nums1[i], nums2[i] <= 1000`\n\n\n---\n\n# Dynamic Programming\n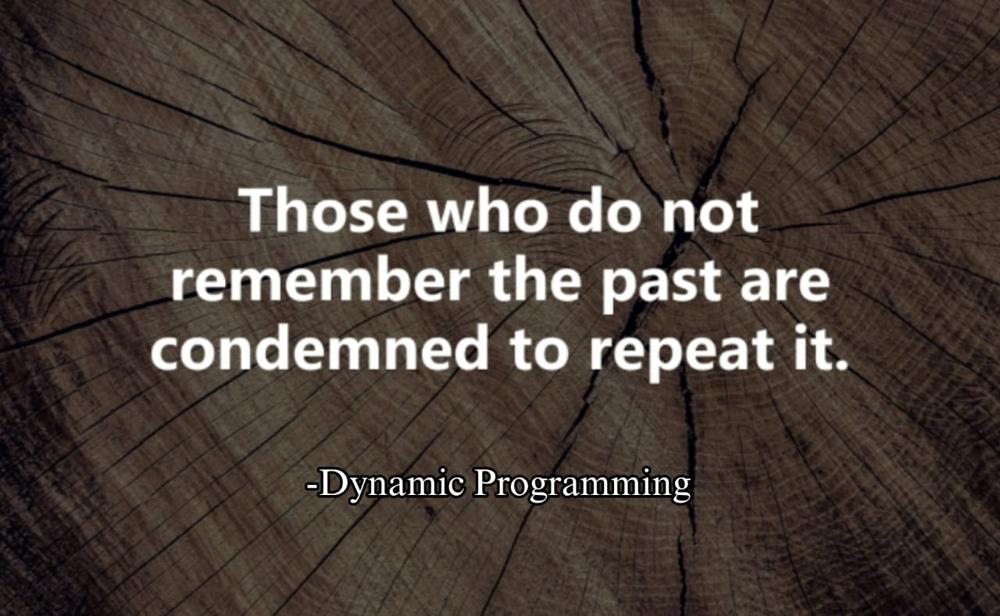\n\nI love this image. \uD83D\uDE02\n\n\n**Dynamic Programming** is a powerful technique that involves **caching** pre-computed results, thereby **eliminating** the need for redundant computations and significantly **optimizing** time complexity.\nThere are **two primary approaches** to Dynamic Programming:\n\n## Recursive Approach (Top-Down)\nWe can think of Recursive Approach as **breaking** hard bricks into **small** rocks. but, how this apply to our problem ?\nWe can **break** our **complex problem** into **easier** ones. For our problem, we can start at the **beginning** of the arrays and try to find the optimal answer for the subarrays **starting** from our current indices. until we reach the **end** of the arryas. and collect the answers together to form the optimal answer.\n\n## Iterative Approach (Bottom-Up)\nWe can think of Iterative Approach as **building** high building from small bricks. but, how this also apply to our problem ?\nWe can **solve** our **complex problem** by solving **easier** ones first and build until we reach our complex problem. For our problem, we can start from the **end** of our arrays and try to find the optimal answer for the subarrays **starting** from our current indices until the **beginning** of array. \n\n---\n\n\n\n# Intuition\n\nHi there my friends, \uD83D\uDE03\n\nLet\'s take a look on our today\'s interesting problem.\uD83D\uDC40\nThe problem to find two subsequence that their dot product is maximum.\n\nFor this problem, Their is nothing to try than trying all possible **combinations** of subsequences from the two input arrays.\nI believe that will give as the **maximum** dot product but we can see that it is **inefficient**.\uD83D\uDE14\nHow can we **optimize** it? I will pretend that I didn\'t say the approach in the title or in the previous section\uD83D\uDE02\n\nLet\'s see next example together.\n```\nEX: nums1 = [2,1,-2,5], nums2 = [3,0,-6]\nLet\'s try different subsequences: \n[2, -2] & [3, -6] -> 18\n[2, -2] & [0, -6] -> 12\n[1, -2] & [3, -6] -> 15\n[1, -2] & [0, -6] -> 13\nAnswer = 18\n```\n\nIn all **previous** subsequences, we notice something **shared** between them.\uD83E\uDD2F\nYes, The second half of all subsequences is **repeated** which mean there are repeated subproblems we can make use of.\uD83E\uDD14\n\nWe are here todya with our hero **Dynamic Programming**.\uD83E\uDDB8\u200D\u2642\uFE0F\nI think you are surprised like me \uD83D\uDE02\n\nFor the **DP** solution, we will try all **combinations** of subsequences but we won\'t **calculate** all the combinations\' results.\nWhat does that mean?\uD83E\uDD14\nLike we say in the previous example we have part of the subsequences is **repeated** so we will **cache** the optimal result for that part and whenever we encounter the same subproblem of that part again we will only return the optimal result.\uD83D\uDE03\n\n```\nEX: nums1 = [2,1,-2,5,-1,4], nums2 = [3,0,-6,5,2]\nLet\'s try different subsequences: \n[2, -2, 4] & [3, -6, 5] -> 38 = 18 + 20\n[2, -2, 4] & [0, -6, 5] -> 32 = 12 + 20\n[1, -2, 4] & [3, -6, 5] -> 35 = 15 + 20 \n[1, -2, 4] & [0, -6, 5] -> 33 = 13 + 20\nAnswer = 38\n```\nWe can see here that we added new two numbers (new subarray) in each array from the previous example.\n**BUT** we can notice that those subarrays have always the **best** answer which is `20` and won\'t change so we will **cache** it and whenever we want the best solution from them we will return simply `20`.\n\n- For the **DP** solution, we will start with **two pointers** one for each array and for the pointers we have **three** options:\n - **multiply** the numbers that the pointers point at then move the both pointers to next element indicating that we are **taking** them in the final subsequences\n - **move first** pointer to the next element indicating that we are **deleting** it from the final subsequences\n - **move second** pointer to the next element indicating that we are **deleting** it from the final subsequences\n\nThen, there is only **one edge case** that we must handle its when one of the arrays is all **negative** numbers and the other is all **positive** numbers.\nour fuction will return `0` as an answer since it won\'t take any number of the **negative** array which means **empty** subsequence which is **invalid**.\uD83D\uDE14\n```\nEX: nums1 = [-2, -3, -1, -5], nums2 = [4, 2, 1, 5]\nAnswer -> -1 * 1 = -1\n```\nThe answer is to get **maximum** element in **positive** array and **minimum** element in **negative** array then multiply them.\n\nBut what about both of the arrays are both negative numbers?\uD83E\uDD14\nsimply the dot product of any subsequence will be **positive** and we will have answer eventually unlike the previous case. \n\n\nAnd this is the solution for our today\'S problem I hope that you understood it\uD83D\uDE80\uD83D\uDE80\n\n\n---\n\n\n\n\n# Approach\n## DP Recursive (Top-Down)\n1. Initialize a dynamic programming array `dp` of size `505x505` and some variables for the **size** and **maximums** and **minimums**.\n2. Find the **maximum** and **minimum** values in both arrays to handle **special** case where all elements are negative in any of the arryas.\n3. Call the recursive function `calculateDotProduct` with initial indices `0` and `0` to compute the **maximum** dot product:\n - **Base Cases:** returns `0`, as the end of an array has been reached.\n - **Memoization:** Checks if the result for the current indices is **already** calculated and stored in `dp`.\n - **Calculates** the dot product with three options:\n - Multiply the elements at the current indices in `nums1` and `nums2`, and recursively calculate the dot product for the next indices (`idx1 + 1`, `idx2 + 1`).\n - Calculate the dot product by moving to the next index in `nums2` while keeping the index in `nums1` unchanged.\n - Calculate the dot product by moving to the next index in `nums1` while keeping the index in `nums2` unchanged.\n - **Stores** the **maximum** dot product among the three options in `dp` for the current indices (`idx1`, `idx2`) then return.\n4. Return the computed **maximum dot product** taht you got from the function.\n\n\n## Complexity\n- **Time complexity:** $$O(N * M)$$\nSince we are trying all possible options in Dynamic Programming, The complexity is the product of the sizes of the arrays which we are trying all possible combinations of then which is `O(N * M)`.\n- **Space complexity:** $$O(N * M)$$\nSince we are storing the DP matrix and its size is `N * M` then complexity is `O(M * N)`.\nwhere `N` is maximum size of first array and `M` is maximum size of second array.\n\n---\n\n## DP Iterative (Bottom-Up)\n1. Initialize a dynamic programming array `dp` of size `505x505` and some variables for the **size** and **maximums** and **minimums**.\n2. Find the **maximum** and **minimum** values in both arrays to handle **special** case where all elements are negative in any of the arryas.\n3. Iterate through `nums1` and `nums2` in **reverse** order.\n4. Calculate the dot product for each combination of elements and store the **maximum** among three options in `dotProductMatrix`.\n5. Return the maximum dot product stored in `dotProductMatrix` for the initial indices `(0, 0)`.\n\n\n## Complexity\n- **Time complexity:** $$O(N * M)$$\nSince we are trying all possible options in Dynamic Programming, The complexity is the product of the sizes of the arrays which we are trying all possible combinations of then which is `O(N * M)`.\n- **Space complexity:** $$O(N * M)$$\nSince we are storing the DP matrix and its size is `N * M` then complexity is `O(M * N)`.\nwhere `N` is maximum size of first array and `M` is maximum size of second array.\n\n\n---\n\n\n\n\n# Code\n## DP Recursive (Top-Down)\n```C++ []\nclass Solution {\npublic:\n int dp[505][505]; // Dynamic programming array\n int nums1_size, nums2_size; // Sizes of nums1 and nums2 arrays\n\n // Recursive function to calculate the maximum dot product\n int calculateDotProduct(vector<int>& nums1, vector<int>& nums2, int idx1, int idx2) {\n if (idx1 == nums1_size || idx2 == nums2_size)\n return 0;\n\n if (~dp[idx1][idx2])\n return dp[idx1][idx2];\n\n // Calculate dot product for three options\n int option1 = nums1[idx1] * nums2[idx2] + calculateDotProduct(nums1, nums2, idx1 + 1, idx2 + 1);\n int option2 = calculateDotProduct(nums1, nums2, idx1, idx2 + 1);\n int option3 = calculateDotProduct(nums1, nums2, idx1 + 1, idx2);\n\n return dp[idx1][idx2] = max({option1, option2, option3}); // Store the maximum dot product\n }\n\n // Main function to calculate the maximum dot product\n int maxDotProduct(vector<int>& nums1, vector<int>& nums2) {\n nums1_size = nums1.size();\n nums2_size = nums2.size();\n\n int firstMax = INT_MIN;\n int secondMax = INT_MIN;\n int firstMin = INT_MAX;\n int secondMin = INT_MAX;\n\n // Calculate maximum and minimum values for nums1\n for (int num : nums1) {\n firstMax = max(firstMax, num);\n firstMin = min(firstMin, num);\n }\n // Calculate maximum and minimum values for nums2\n for (int num : nums2) {\n secondMax = max(secondMax, num);\n secondMin = min(secondMin, num);\n }\n\n // Check special cases where all elements are negative\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return max(firstMax * secondMin, firstMin * secondMax);\n }\n\n memset(dp, -1, sizeof(dp)); // Initialize dp array with -1\n \n // Calculate the maximum dot product recursively\n return calculateDotProduct(nums1, nums2, 0, 0);\n }\n};\n```\n```Java []\nclass Solution {\n private int[][] dp = new int[505][505]; // Dynamic programming array\n private int nums1Size, nums2Size; // Sizes of nums1 and nums2 arrays\n\n // Recursive function to calculate the maximum dot product\n private int calculateDotProduct(int[] nums1, int[] nums2, int idx1, int idx2) {\n if (idx1 == nums1Size || idx2 == nums2Size)\n return 0;\n\n if (dp[idx1][idx2] != -1)\n return dp[idx1][idx2];\n\n // Calculate dot product for three options\n int option1 = nums1[idx1] * nums2[idx2] + calculateDotProduct(nums1, nums2, idx1 + 1, idx2 + 1);\n int option2 = calculateDotProduct(nums1, nums2, idx1, idx2 + 1);\n int option3 = calculateDotProduct(nums1, nums2, idx1 + 1, idx2);\n\n dp[idx1][idx2] = Math.max(Math.max(option1, option2), option3); // Store the maximum dot product\n return dp[idx1][idx2];\n }\n\n // Main function to calculate the maximum dot product\n public int maxDotProduct(int[] nums1, int[] nums2) {\n nums1Size = nums1.length;\n nums2Size = nums2.length;\n\n int firstMax = Integer.MIN_VALUE;\n int secondMax = Integer.MIN_VALUE;\n int firstMin = Integer.MAX_VALUE;\n int secondMin = Integer.MAX_VALUE;\n\n // Calculate maximum and minimum values for nums1\n for (int num : nums1) {\n firstMax = Math.max(firstMax, num);\n firstMin = Math.min(firstMin, num);\n }\n // Calculate maximum and minimum values for nums2\n for (int num : nums2) {\n secondMax = Math.max(secondMax, num);\n secondMin = Math.min(secondMin, num);\n }\n\n // Check special cases where all elements are negative\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return Math.max(firstMax * secondMin, firstMin * secondMax);\n }\n\n // Initialize dp array with -1\n for (int i = 0; i < 505; i++) {\n Arrays.fill(dp[i], -1);\n }\n\n // Calculate the maximum dot product recursively\n return calculateDotProduct(nums1, nums2, 0, 0);\n }\n}\n```\n```Python []\nclass Solution:\n def __init__(self):\n self.dp = [[-1] * 505 for _ in range(505)] # Dynamic programming array\n self.nums1_size = 0\n self.nums2_size = 0\n\n # Recursive function to calculate the maximum dot product\n def calculate_dot_product(self, nums1: list[int], nums2: list[int], idx1: int, idx2: int) -> int:\n if idx1 == self.nums1_size or idx2 == self.nums2_size:\n return 0\n\n if self.dp[idx1][idx2] != -1:\n return self.dp[idx1][idx2]\n\n # Calculate dot product for three options\n option1 = nums1[idx1] * nums2[idx2] + self.calculate_dot_product(nums1, nums2, idx1 + 1, idx2 + 1)\n option2 = self.calculate_dot_product(nums1, nums2, idx1, idx2 + 1)\n option3 = self.calculate_dot_product(nums1, nums2, idx1 + 1, idx2)\n\n self.dp[idx1][idx2] = max(option1, option2, option3) # Store the maximum dot product\n return self.dp[idx1][idx2]\n\n\n def maxDotProduct(self, nums1: list[int], nums2: list[int]) -> int:\n self.nums1_size = len(nums1)\n self.nums2_size = len(nums2)\n\n first_max = float(\'-inf\')\n second_max = float(\'-inf\')\n first_min = float(\'inf\')\n second_min = float(\'inf\')\n\n # Calculate maximum and minimum values for nums1\n for num in nums1:\n first_max = max(first_max, num)\n first_min = min(first_min, num)\n # Calculate maximum and minimum values for nums2\n for num in nums2:\n second_max = max(second_max, num)\n second_min = min(second_min, num)\n\n # Check special cases where all elements are negative\n if (first_max < 0 and second_min > 0) or (first_min > 0 and second_max < 0):\n return max(first_max * second_min, first_min * second_max)\n\n # Initialize dp array with -1\n for i in range(505):\n for j in range(505):\n self.dp[i][j] = -1\n\n # Calculate the maximum dot product recursively\n return self.calculate_dot_product(nums1, nums2, 0, 0)\n```\n\n\n---\n\n\n## DP Iterative (Bottom-Up)\n\n```C++ []\nclass Solution {\npublic:\n int dotProductMatrix[505][505]; // Dynamic programming array\n int nums1_size, nums2_size; // Sizes of nums1 and nums2 arrays\n\n int maxDotProduct(vector<int>& nums1, vector<int>& nums2) {\n nums1_size = nums1.size();\n nums2_size = nums2.size();\n\n int firstMax = INT_MIN;\n int secondMax = INT_MIN;\n int firstMin = INT_MAX;\n int secondMin = INT_MAX;\n\n // Calculate maximum and minimum values for nums1\n for (int num : nums1) {\n firstMax = max(firstMax, num);\n firstMin = min(firstMin, num);\n }\n // Calculate maximum and minimum values for nums2\n for (int num : nums2) {\n secondMax = max(secondMax, num);\n secondMin = min(secondMin, num);\n }\n\n // Check special cases where all elements are negative\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return max(firstMax * secondMin, firstMin * secondMax);\n }\n\n memset(dotProductMatrix, 0, sizeof dotProductMatrix); // Initialize the dot product matrix\n\n // Calculate dot products and populate the dot product matrix\n for (int i = nums1_size - 1; i >= 0; i--) {\n for (int j = nums2_size - 1; j >= 0; j--) {\n int currentDotProduct = nums1[i] * nums2[j] + dotProductMatrix[i + 1][j + 1];\n dotProductMatrix[i][j] = max({currentDotProduct, dotProductMatrix[i + 1][j], dotProductMatrix[i][j + 1]});\n }\n }\n return dotProductMatrix[0][0]; // Return the maximum dot product\n }\n};\n```\n```Java []\nclass Solution {\n int[][] dotProductMatrix = new int[505][505]; // Dynamic programming array\n int nums1Size, nums2Size; // Sizes of nums1 and nums2 arrays\n\n public int maxDotProduct(int[] nums1, int[] nums2) {\n nums1Size = nums1.length;\n nums2Size = nums2.length;\n\n int firstMax = Integer.MIN_VALUE;\n int secondMax = Integer.MIN_VALUE;\n int firstMin = Integer.MAX_VALUE;\n int secondMin = Integer.MAX_VALUE;\n\n // Calculate maximum and minimum values for nums1\n for (int num : nums1) {\n firstMax = Math.max(firstMax, num);\n firstMin = Math.min(firstMin, num);\n }\n \n // Calculate maximum and minimum values for nums2\n for (int num : nums2) {\n secondMax = Math.max(secondMax, num);\n secondMin = Math.min(secondMin, num);\n }\n\n // Check special cases where all elements are negative\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return Math.max(firstMax * secondMin, firstMin * secondMax);\n }\n\n // Initialize the dot product matrix with 0\n for (int i = 0; i < 505; i++) {\n Arrays.fill(dotProductMatrix[i], 0);\n }\n\n // Calculate dot products and populate the dot product matrix\n for (int i = nums1Size - 1; i >= 0; i--) {\n for (int j = nums2Size - 1; j >= 0; j--) {\n int currentDotProduct = nums1[i] * nums2[j] + dotProductMatrix[i + 1][j + 1];\n dotProductMatrix[i][j] = Math.max(Math.max(currentDotProduct, dotProductMatrix[i + 1][j]), dotProductMatrix[i][j + 1]);\n }\n }\n return dotProductMatrix[0][0]; // Return the maximum dot product\n }\n}\n```\n```Python []\nclass Solution:\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n dot_product_matrix = [[0] * 505 for _ in range(505)] # Dynamic programming array\n nums1_size = len(nums1)\n nums2_size = len(nums2)\n\n first_max = float(\'-inf\')\n second_max = float(\'-inf\')\n first_min = float(\'inf\')\n second_min = float(\'inf\')\n\n # Calculate maximum and minimum values for nums1\n for num in nums1:\n first_max = max(first_max, num)\n first_min = min(first_min, num)\n\n # Calculate maximum and minimum values for nums2\n for num in nums2:\n second_max = max(second_max, num)\n second_min = min(second_min, num)\n\n # Check special cases where all elements are negative\n if (first_max < 0 and second_min > 0) or (first_min > 0 and second_max < 0):\n return max(first_max * second_min, first_min * second_max)\n\n # Initialize the dot product matrix with 0\n for i in range(505):\n dot_product_matrix[i] = [0] * 505\n\n # Calculate dot products and populate the dot product matrix\n for i in range(nums1_size - 1, -1, -1):\n for j in range(nums2_size - 1, -1, -1):\n current_dot_product = nums1[i] * nums2[j] + dot_product_matrix[i + 1][j + 1]\n dot_product_matrix[i][j] = max(current_dot_product, dot_product_matrix[i + 1][j], dot_product_matrix[i][j + 1])\n\n return dot_product_matrix[0][0] # Return the maximum dot product\n```\n\n\n\n\n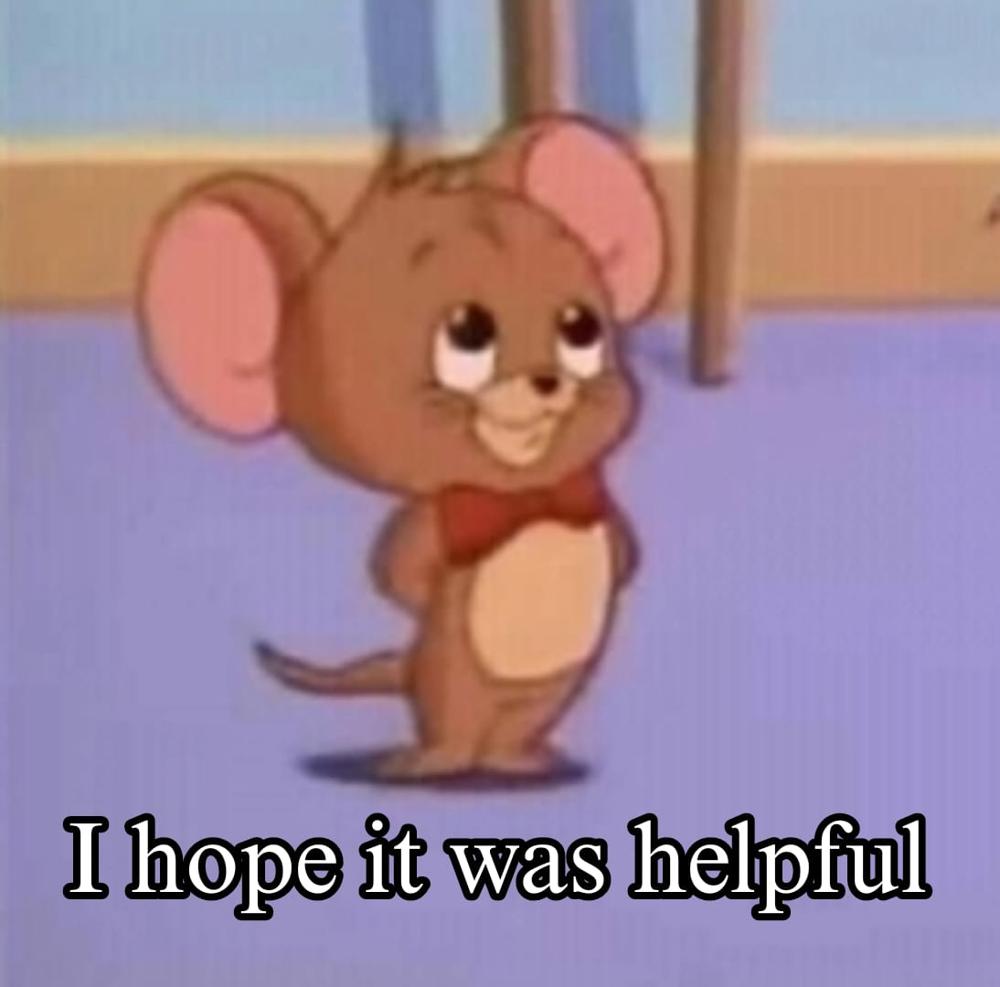\n | 42 | Given two arrays `nums1` and `nums2`.
Return the maximum dot product between **non-empty** subsequences of nums1 and nums2 with the same length.
A subsequence of a array is a new array which is formed from the original array by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (ie, `[2,3,5]` is a subsequence of `[1,2,3,4,5]` while `[1,5,3]` is not).
**Example 1:**
**Input:** nums1 = \[2,1,-2,5\], nums2 = \[3,0,-6\]
**Output:** 18
**Explanation:** Take subsequence \[2,-2\] from nums1 and subsequence \[3,-6\] from nums2.
Their dot product is (2\*3 + (-2)\*(-6)) = 18.
**Example 2:**
**Input:** nums1 = \[3,-2\], nums2 = \[2,-6,7\]
**Output:** 21
**Explanation:** Take subsequence \[3\] from nums1 and subsequence \[7\] from nums2.
Their dot product is (3\*7) = 21.
**Example 3:**
**Input:** nums1 = \[-1,-1\], nums2 = \[1,1\]
**Output:** -1
**Explanation:** Take subsequence \[-1\] from nums1 and subsequence \[1\] from nums2.
Their dot product is -1.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 500`
* `-1000 <= nums1[i], nums2[i] <= 1000` | Simulate the problem. Count the number of 1's in the binary representation of each integer. Sort by the number of 1's ascending and by the value in case of tie. |
🚀92.14% || DP Recursive & Iterative || Explained Intuition🚀 | max-dot-product-of-two-subsequences | 1 | 1 | # Porblem Description\n\nGiven two arrays, `nums1` and `nums2`. The task is to find the **maximum** dot product that can be obtained by taking **non-empty** **subsequences** of equal length from `nums1` and `nums2`.\n\nA **subsequence** of an array is a new array obtained by deleting some (or none) of the elements from the original array **without changing the order** of the remaining elements. \nThe **goal** is to **maximize** the dot product of the selected subsequences by choosing the elements wisely.\n\n- **Constraints:**\n - `1 <= nums1.length, nums2.length <= 500`\n - `-1000 <= nums1[i], nums2[i] <= 1000`\n\n\n---\n\n# Dynamic Programming\n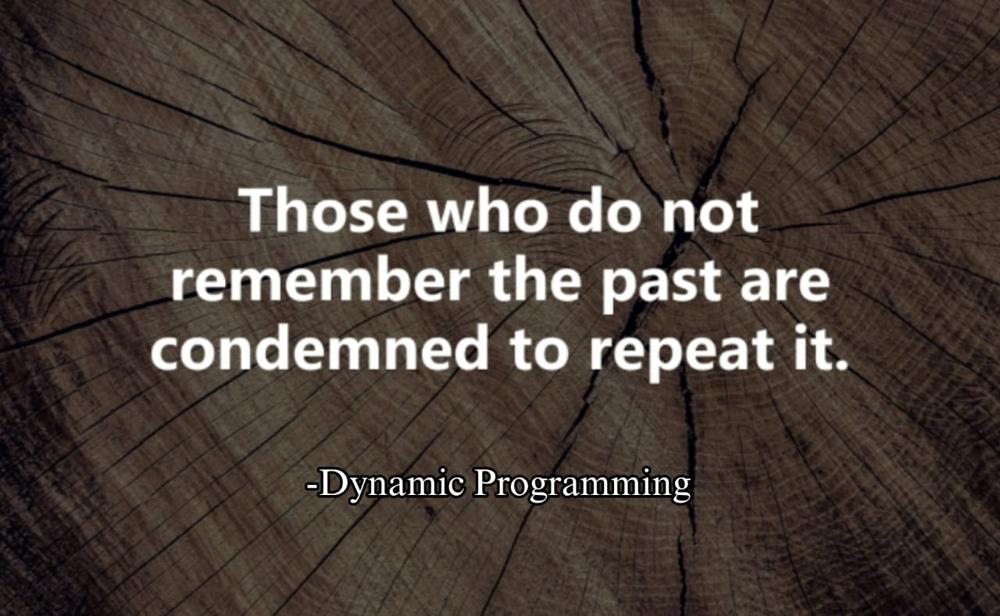\n\nI love this image. \uD83D\uDE02\n\n\n**Dynamic Programming** is a powerful technique that involves **caching** pre-computed results, thereby **eliminating** the need for redundant computations and significantly **optimizing** time complexity.\nThere are **two primary approaches** to Dynamic Programming:\n\n## Recursive Approach (Top-Down)\nWe can think of Recursive Approach as **breaking** hard bricks into **small** rocks. but, how this apply to our problem ?\nWe can **break** our **complex problem** into **easier** ones. For our problem, we can start at the **beginning** of the arrays and try to find the optimal answer for the subarrays **starting** from our current indices. until we reach the **end** of the arryas. and collect the answers together to form the optimal answer.\n\n## Iterative Approach (Bottom-Up)\nWe can think of Iterative Approach as **building** high building from small bricks. but, how this also apply to our problem ?\nWe can **solve** our **complex problem** by solving **easier** ones first and build until we reach our complex problem. For our problem, we can start from the **end** of our arrays and try to find the optimal answer for the subarrays **starting** from our current indices until the **beginning** of array. \n\n---\n\n\n\n# Intuition\n\nHi there my friends, \uD83D\uDE03\n\nLet\'s take a look on our today\'s interesting problem.\uD83D\uDC40\nThe problem to find two subsequence that their dot product is maximum.\n\nFor this problem, Their is nothing to try than trying all possible **combinations** of subsequences from the two input arrays.\nI believe that will give as the **maximum** dot product but we can see that it is **inefficient**.\uD83D\uDE14\nHow can we **optimize** it? I will pretend that I didn\'t say the approach in the title or in the previous section\uD83D\uDE02\n\nLet\'s see next example together.\n```\nEX: nums1 = [2,1,-2,5], nums2 = [3,0,-6]\nLet\'s try different subsequences: \n[2, -2] & [3, -6] -> 18\n[2, -2] & [0, -6] -> 12\n[1, -2] & [3, -6] -> 15\n[1, -2] & [0, -6] -> 13\nAnswer = 18\n```\n\nIn all **previous** subsequences, we notice something **shared** between them.\uD83E\uDD2F\nYes, The second half of all subsequences is **repeated** which mean there are repeated subproblems we can make use of.\uD83E\uDD14\n\nWe are here todya with our hero **Dynamic Programming**.\uD83E\uDDB8\u200D\u2642\uFE0F\nI think you are surprised like me \uD83D\uDE02\n\nFor the **DP** solution, we will try all **combinations** of subsequences but we won\'t **calculate** all the combinations\' results.\nWhat does that mean?\uD83E\uDD14\nLike we say in the previous example we have part of the subsequences is **repeated** so we will **cache** the optimal result for that part and whenever we encounter the same subproblem of that part again we will only return the optimal result.\uD83D\uDE03\n\n```\nEX: nums1 = [2,1,-2,5,-1,4], nums2 = [3,0,-6,5,2]\nLet\'s try different subsequences: \n[2, -2, 4] & [3, -6, 5] -> 38 = 18 + 20\n[2, -2, 4] & [0, -6, 5] -> 32 = 12 + 20\n[1, -2, 4] & [3, -6, 5] -> 35 = 15 + 20 \n[1, -2, 4] & [0, -6, 5] -> 33 = 13 + 20\nAnswer = 38\n```\nWe can see here that we added new two numbers (new subarray) in each array from the previous example.\n**BUT** we can notice that those subarrays have always the **best** answer which is `20` and won\'t change so we will **cache** it and whenever we want the best solution from them we will return simply `20`.\n\n- For the **DP** solution, we will start with **two pointers** one for each array and for the pointers we have **three** options:\n - **multiply** the numbers that the pointers point at then move the both pointers to next element indicating that we are **taking** them in the final subsequences\n - **move first** pointer to the next element indicating that we are **deleting** it from the final subsequences\n - **move second** pointer to the next element indicating that we are **deleting** it from the final subsequences\n\nThen, there is only **one edge case** that we must handle its when one of the arrays is all **negative** numbers and the other is all **positive** numbers.\nour fuction will return `0` as an answer since it won\'t take any number of the **negative** array which means **empty** subsequence which is **invalid**.\uD83D\uDE14\n```\nEX: nums1 = [-2, -3, -1, -5], nums2 = [4, 2, 1, 5]\nAnswer -> -1 * 1 = -1\n```\nThe answer is to get **maximum** element in **positive** array and **minimum** element in **negative** array then multiply them.\n\nBut what about both of the arrays are both negative numbers?\uD83E\uDD14\nsimply the dot product of any subsequence will be **positive** and we will have answer eventually unlike the previous case. \n\n\nAnd this is the solution for our today\'S problem I hope that you understood it\uD83D\uDE80\uD83D\uDE80\n\n\n---\n\n\n\n\n# Approach\n## DP Recursive (Top-Down)\n1. Initialize a dynamic programming array `dp` of size `505x505` and some variables for the **size** and **maximums** and **minimums**.\n2. Find the **maximum** and **minimum** values in both arrays to handle **special** case where all elements are negative in any of the arryas.\n3. Call the recursive function `calculateDotProduct` with initial indices `0` and `0` to compute the **maximum** dot product:\n - **Base Cases:** returns `0`, as the end of an array has been reached.\n - **Memoization:** Checks if the result for the current indices is **already** calculated and stored in `dp`.\n - **Calculates** the dot product with three options:\n - Multiply the elements at the current indices in `nums1` and `nums2`, and recursively calculate the dot product for the next indices (`idx1 + 1`, `idx2 + 1`).\n - Calculate the dot product by moving to the next index in `nums2` while keeping the index in `nums1` unchanged.\n - Calculate the dot product by moving to the next index in `nums1` while keeping the index in `nums2` unchanged.\n - **Stores** the **maximum** dot product among the three options in `dp` for the current indices (`idx1`, `idx2`) then return.\n4. Return the computed **maximum dot product** taht you got from the function.\n\n\n## Complexity\n- **Time complexity:** $$O(N * M)$$\nSince we are trying all possible options in Dynamic Programming, The complexity is the product of the sizes of the arrays which we are trying all possible combinations of then which is `O(N * M)`.\n- **Space complexity:** $$O(N * M)$$\nSince we are storing the DP matrix and its size is `N * M` then complexity is `O(M * N)`.\nwhere `N` is maximum size of first array and `M` is maximum size of second array.\n\n---\n\n## DP Iterative (Bottom-Up)\n1. Initialize a dynamic programming array `dp` of size `505x505` and some variables for the **size** and **maximums** and **minimums**.\n2. Find the **maximum** and **minimum** values in both arrays to handle **special** case where all elements are negative in any of the arryas.\n3. Iterate through `nums1` and `nums2` in **reverse** order.\n4. Calculate the dot product for each combination of elements and store the **maximum** among three options in `dotProductMatrix`.\n5. Return the maximum dot product stored in `dotProductMatrix` for the initial indices `(0, 0)`.\n\n\n## Complexity\n- **Time complexity:** $$O(N * M)$$\nSince we are trying all possible options in Dynamic Programming, The complexity is the product of the sizes of the arrays which we are trying all possible combinations of then which is `O(N * M)`.\n- **Space complexity:** $$O(N * M)$$\nSince we are storing the DP matrix and its size is `N * M` then complexity is `O(M * N)`.\nwhere `N` is maximum size of first array and `M` is maximum size of second array.\n\n\n---\n\n\n\n\n# Code\n## DP Recursive (Top-Down)\n```C++ []\nclass Solution {\npublic:\n int dp[505][505]; // Dynamic programming array\n int nums1_size, nums2_size; // Sizes of nums1 and nums2 arrays\n\n // Recursive function to calculate the maximum dot product\n int calculateDotProduct(vector<int>& nums1, vector<int>& nums2, int idx1, int idx2) {\n if (idx1 == nums1_size || idx2 == nums2_size)\n return 0;\n\n if (~dp[idx1][idx2])\n return dp[idx1][idx2];\n\n // Calculate dot product for three options\n int option1 = nums1[idx1] * nums2[idx2] + calculateDotProduct(nums1, nums2, idx1 + 1, idx2 + 1);\n int option2 = calculateDotProduct(nums1, nums2, idx1, idx2 + 1);\n int option3 = calculateDotProduct(nums1, nums2, idx1 + 1, idx2);\n\n return dp[idx1][idx2] = max({option1, option2, option3}); // Store the maximum dot product\n }\n\n // Main function to calculate the maximum dot product\n int maxDotProduct(vector<int>& nums1, vector<int>& nums2) {\n nums1_size = nums1.size();\n nums2_size = nums2.size();\n\n int firstMax = INT_MIN;\n int secondMax = INT_MIN;\n int firstMin = INT_MAX;\n int secondMin = INT_MAX;\n\n // Calculate maximum and minimum values for nums1\n for (int num : nums1) {\n firstMax = max(firstMax, num);\n firstMin = min(firstMin, num);\n }\n // Calculate maximum and minimum values for nums2\n for (int num : nums2) {\n secondMax = max(secondMax, num);\n secondMin = min(secondMin, num);\n }\n\n // Check special cases where all elements are negative\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return max(firstMax * secondMin, firstMin * secondMax);\n }\n\n memset(dp, -1, sizeof(dp)); // Initialize dp array with -1\n \n // Calculate the maximum dot product recursively\n return calculateDotProduct(nums1, nums2, 0, 0);\n }\n};\n```\n```Java []\nclass Solution {\n private int[][] dp = new int[505][505]; // Dynamic programming array\n private int nums1Size, nums2Size; // Sizes of nums1 and nums2 arrays\n\n // Recursive function to calculate the maximum dot product\n private int calculateDotProduct(int[] nums1, int[] nums2, int idx1, int idx2) {\n if (idx1 == nums1Size || idx2 == nums2Size)\n return 0;\n\n if (dp[idx1][idx2] != -1)\n return dp[idx1][idx2];\n\n // Calculate dot product for three options\n int option1 = nums1[idx1] * nums2[idx2] + calculateDotProduct(nums1, nums2, idx1 + 1, idx2 + 1);\n int option2 = calculateDotProduct(nums1, nums2, idx1, idx2 + 1);\n int option3 = calculateDotProduct(nums1, nums2, idx1 + 1, idx2);\n\n dp[idx1][idx2] = Math.max(Math.max(option1, option2), option3); // Store the maximum dot product\n return dp[idx1][idx2];\n }\n\n // Main function to calculate the maximum dot product\n public int maxDotProduct(int[] nums1, int[] nums2) {\n nums1Size = nums1.length;\n nums2Size = nums2.length;\n\n int firstMax = Integer.MIN_VALUE;\n int secondMax = Integer.MIN_VALUE;\n int firstMin = Integer.MAX_VALUE;\n int secondMin = Integer.MAX_VALUE;\n\n // Calculate maximum and minimum values for nums1\n for (int num : nums1) {\n firstMax = Math.max(firstMax, num);\n firstMin = Math.min(firstMin, num);\n }\n // Calculate maximum and minimum values for nums2\n for (int num : nums2) {\n secondMax = Math.max(secondMax, num);\n secondMin = Math.min(secondMin, num);\n }\n\n // Check special cases where all elements are negative\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return Math.max(firstMax * secondMin, firstMin * secondMax);\n }\n\n // Initialize dp array with -1\n for (int i = 0; i < 505; i++) {\n Arrays.fill(dp[i], -1);\n }\n\n // Calculate the maximum dot product recursively\n return calculateDotProduct(nums1, nums2, 0, 0);\n }\n}\n```\n```Python []\nclass Solution:\n def __init__(self):\n self.dp = [[-1] * 505 for _ in range(505)] # Dynamic programming array\n self.nums1_size = 0\n self.nums2_size = 0\n\n # Recursive function to calculate the maximum dot product\n def calculate_dot_product(self, nums1: list[int], nums2: list[int], idx1: int, idx2: int) -> int:\n if idx1 == self.nums1_size or idx2 == self.nums2_size:\n return 0\n\n if self.dp[idx1][idx2] != -1:\n return self.dp[idx1][idx2]\n\n # Calculate dot product for three options\n option1 = nums1[idx1] * nums2[idx2] + self.calculate_dot_product(nums1, nums2, idx1 + 1, idx2 + 1)\n option2 = self.calculate_dot_product(nums1, nums2, idx1, idx2 + 1)\n option3 = self.calculate_dot_product(nums1, nums2, idx1 + 1, idx2)\n\n self.dp[idx1][idx2] = max(option1, option2, option3) # Store the maximum dot product\n return self.dp[idx1][idx2]\n\n\n def maxDotProduct(self, nums1: list[int], nums2: list[int]) -> int:\n self.nums1_size = len(nums1)\n self.nums2_size = len(nums2)\n\n first_max = float(\'-inf\')\n second_max = float(\'-inf\')\n first_min = float(\'inf\')\n second_min = float(\'inf\')\n\n # Calculate maximum and minimum values for nums1\n for num in nums1:\n first_max = max(first_max, num)\n first_min = min(first_min, num)\n # Calculate maximum and minimum values for nums2\n for num in nums2:\n second_max = max(second_max, num)\n second_min = min(second_min, num)\n\n # Check special cases where all elements are negative\n if (first_max < 0 and second_min > 0) or (first_min > 0 and second_max < 0):\n return max(first_max * second_min, first_min * second_max)\n\n # Initialize dp array with -1\n for i in range(505):\n for j in range(505):\n self.dp[i][j] = -1\n\n # Calculate the maximum dot product recursively\n return self.calculate_dot_product(nums1, nums2, 0, 0)\n```\n\n\n---\n\n\n## DP Iterative (Bottom-Up)\n\n```C++ []\nclass Solution {\npublic:\n int dotProductMatrix[505][505]; // Dynamic programming array\n int nums1_size, nums2_size; // Sizes of nums1 and nums2 arrays\n\n int maxDotProduct(vector<int>& nums1, vector<int>& nums2) {\n nums1_size = nums1.size();\n nums2_size = nums2.size();\n\n int firstMax = INT_MIN;\n int secondMax = INT_MIN;\n int firstMin = INT_MAX;\n int secondMin = INT_MAX;\n\n // Calculate maximum and minimum values for nums1\n for (int num : nums1) {\n firstMax = max(firstMax, num);\n firstMin = min(firstMin, num);\n }\n // Calculate maximum and minimum values for nums2\n for (int num : nums2) {\n secondMax = max(secondMax, num);\n secondMin = min(secondMin, num);\n }\n\n // Check special cases where all elements are negative\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return max(firstMax * secondMin, firstMin * secondMax);\n }\n\n memset(dotProductMatrix, 0, sizeof dotProductMatrix); // Initialize the dot product matrix\n\n // Calculate dot products and populate the dot product matrix\n for (int i = nums1_size - 1; i >= 0; i--) {\n for (int j = nums2_size - 1; j >= 0; j--) {\n int currentDotProduct = nums1[i] * nums2[j] + dotProductMatrix[i + 1][j + 1];\n dotProductMatrix[i][j] = max({currentDotProduct, dotProductMatrix[i + 1][j], dotProductMatrix[i][j + 1]});\n }\n }\n return dotProductMatrix[0][0]; // Return the maximum dot product\n }\n};\n```\n```Java []\nclass Solution {\n int[][] dotProductMatrix = new int[505][505]; // Dynamic programming array\n int nums1Size, nums2Size; // Sizes of nums1 and nums2 arrays\n\n public int maxDotProduct(int[] nums1, int[] nums2) {\n nums1Size = nums1.length;\n nums2Size = nums2.length;\n\n int firstMax = Integer.MIN_VALUE;\n int secondMax = Integer.MIN_VALUE;\n int firstMin = Integer.MAX_VALUE;\n int secondMin = Integer.MAX_VALUE;\n\n // Calculate maximum and minimum values for nums1\n for (int num : nums1) {\n firstMax = Math.max(firstMax, num);\n firstMin = Math.min(firstMin, num);\n }\n \n // Calculate maximum and minimum values for nums2\n for (int num : nums2) {\n secondMax = Math.max(secondMax, num);\n secondMin = Math.min(secondMin, num);\n }\n\n // Check special cases where all elements are negative\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return Math.max(firstMax * secondMin, firstMin * secondMax);\n }\n\n // Initialize the dot product matrix with 0\n for (int i = 0; i < 505; i++) {\n Arrays.fill(dotProductMatrix[i], 0);\n }\n\n // Calculate dot products and populate the dot product matrix\n for (int i = nums1Size - 1; i >= 0; i--) {\n for (int j = nums2Size - 1; j >= 0; j--) {\n int currentDotProduct = nums1[i] * nums2[j] + dotProductMatrix[i + 1][j + 1];\n dotProductMatrix[i][j] = Math.max(Math.max(currentDotProduct, dotProductMatrix[i + 1][j]), dotProductMatrix[i][j + 1]);\n }\n }\n return dotProductMatrix[0][0]; // Return the maximum dot product\n }\n}\n```\n```Python []\nclass Solution:\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n dot_product_matrix = [[0] * 505 for _ in range(505)] # Dynamic programming array\n nums1_size = len(nums1)\n nums2_size = len(nums2)\n\n first_max = float(\'-inf\')\n second_max = float(\'-inf\')\n first_min = float(\'inf\')\n second_min = float(\'inf\')\n\n # Calculate maximum and minimum values for nums1\n for num in nums1:\n first_max = max(first_max, num)\n first_min = min(first_min, num)\n\n # Calculate maximum and minimum values for nums2\n for num in nums2:\n second_max = max(second_max, num)\n second_min = min(second_min, num)\n\n # Check special cases where all elements are negative\n if (first_max < 0 and second_min > 0) or (first_min > 0 and second_max < 0):\n return max(first_max * second_min, first_min * second_max)\n\n # Initialize the dot product matrix with 0\n for i in range(505):\n dot_product_matrix[i] = [0] * 505\n\n # Calculate dot products and populate the dot product matrix\n for i in range(nums1_size - 1, -1, -1):\n for j in range(nums2_size - 1, -1, -1):\n current_dot_product = nums1[i] * nums2[j] + dot_product_matrix[i + 1][j + 1]\n dot_product_matrix[i][j] = max(current_dot_product, dot_product_matrix[i + 1][j], dot_product_matrix[i][j + 1])\n\n return dot_product_matrix[0][0] # Return the maximum dot product\n```\n\n\n\n\n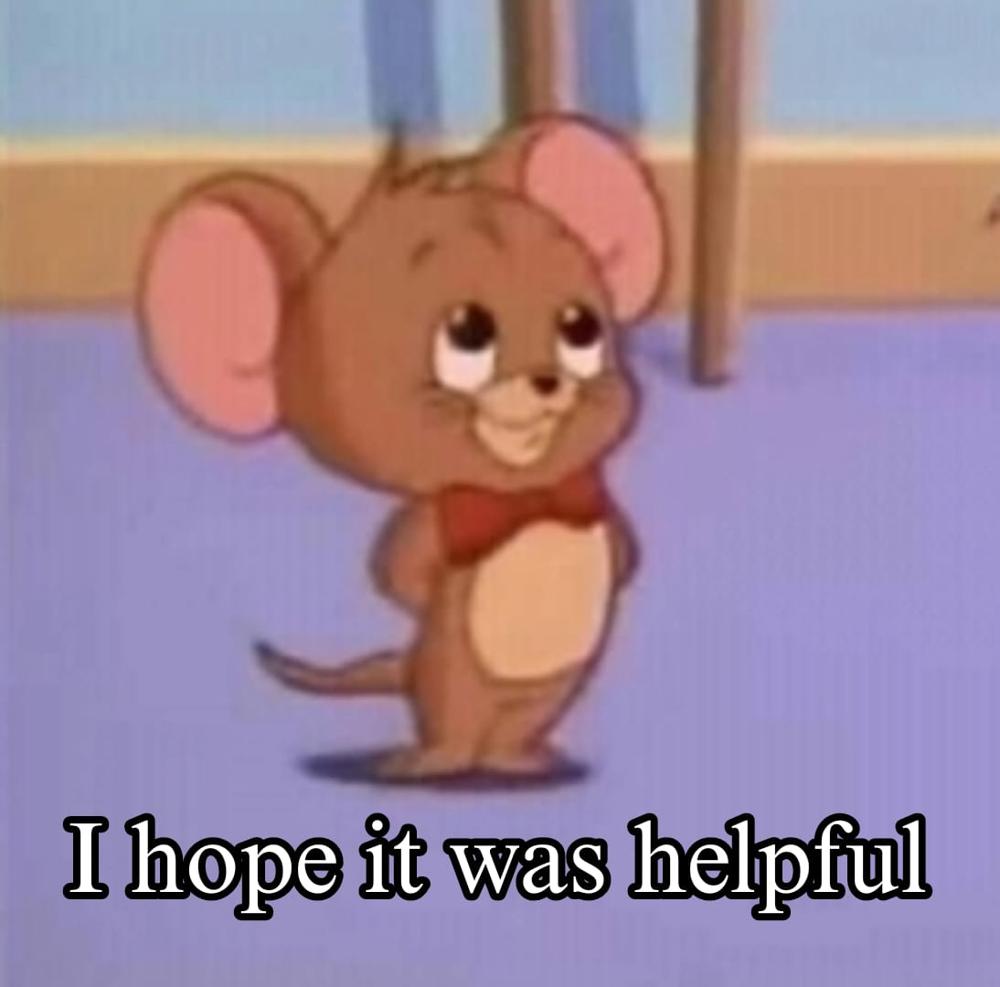\n | 42 | Given an array `nums` that represents a permutation of integers from `1` to `n`. We are going to construct a binary search tree (BST) by inserting the elements of `nums` in order into an initially empty BST. Find the number of different ways to reorder `nums` so that the constructed BST is identical to that formed from the original array `nums`.
* For example, given `nums = [2,1,3]`, we will have 2 as the root, 1 as a left child, and 3 as a right child. The array `[2,3,1]` also yields the same BST but `[3,2,1]` yields a different BST.
Return _the number of ways to reorder_ `nums` _such that the BST formed is identical to the original BST formed from_ `nums`.
Since the answer may be very large, **return it modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[2,1,3\]
**Output:** 1
**Explanation:** We can reorder nums to be \[2,3,1\] which will yield the same BST. There are no other ways to reorder nums which will yield the same BST.
**Example 2:**
**Input:** nums = \[3,4,5,1,2\]
**Output:** 5
**Explanation:** The following 5 arrays will yield the same BST:
\[3,1,2,4,5\]
\[3,1,4,2,5\]
\[3,1,4,5,2\]
\[3,4,1,2,5\]
\[3,4,1,5,2\]
**Example 3:**
**Input:** nums = \[1,2,3\]
**Output:** 0
**Explanation:** There are no other orderings of nums that will yield the same BST.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= nums.length`
* All integers in `nums` are **distinct**. | Use dynamic programming, define DP[i][j] as the maximum dot product of two subsequences starting in the position i of nums1 and position j of nums2. |
✅☑[C++/C/Java/Python/JavaScript] || DP || Recursion || EXPLAINED🔥 | max-dot-product-of-two-subsequences | 1 | 1 | # *PLEASE UPVOTE IF IT HELPED*\n\n---\n\n\n# Approach\n***(Also explained in the code)***\n\n**Explanation:**\n\nThe problem can be solved using dynamic programming. The goal is to find the maximum dot product between two subsequences of the given arrays `nums1` and `nums2`. We can create a 2D DP array `dp`, where `dp[i][j]` represents the maximum dot product for `nums1` up to index `i` and `nums2` up to index `j`.\n\nHere are the steps and comments with examples:\n\n1. Initialize the DP array `dp` with a size of `(nums1_size + 1) x (nums2_size + 1)` and fill it with `-1`. We use `-1`to represent uninitialized values.\n\n1. Calculate the maximum and minimum values for `nums1` and `nums2` to handle special cases where all elements are negative.\n\n1. Check if there\'s a special case where we can return the result directly:\n\n - If `(firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0`), it means one array has all negative values, and the other has all positive values. In this case, the maximum dot product will be the product of the maximum and minimum values from each array.\n1. Define a recursive function `calculateDotProduct` that calculates the maximum dot product starting from given indices `idx1` and `idx2`. This function has three options:\n\n - **option1:** Calculate the dot product of the current elements and add it to the result of the next recursive call with both indices incremented.\n - **option2:** Skip the current element in `nums2` and call the function with `idx2` incremented.\n - **option3:** Skip the current element in `nums1` and call the function with `idx1` incremented.\n1. In the `calculateDotProduct` function, use memoization to store and reuse the calculated results.\n\n1. Call the `calculateDotProduct` function from the main function, starting with indices `0` and `0`, and return the result.\n\n**Example:**\n\nLet\'s take an example with `nums1 = [2, 1, -2, 5]` and `nums2 = [3, 0, -6]`.\n\n1. Initialize `dp` with dimensions `(5 x 4)` filled with `-1`.\n\n1. Calculate the maximum and minimum values for nums1 and nums2:\n\n - `firstMax = 5`, `firstMin = -2`\n - `secondMax = 3`, `secondMin = -6`\n1. Since we have negative and positive values, it\'s not a special case.\n\n1. Start the `calculateDotProduct` function with indices `(0, 0)`:\n\n - For `(0, 0)`, we have `option1`, which calculates `2 * 3` and calls `(1, 1)`.\n - `(1, 1)` has `option1` as well, calculating `1 * 0` and calling `(2, 2)`.\n - Continue the recursion, and at `(4, 3)`, return `40` as the maximum dot product.\n1. Return the result `40`.\n\nThe final result is `40`, which is the maximum dot product of two subsequences.\n\n\n---\n\n\n# Complexity\n- **Time complexity:**\n $$O(n*m)$$\n\n- **Space complexity:**\n $$O(n*m)$$\n\n---\n\n\n# Code\n\n```C++ []\n\nclass Solution {\npublic:\n int dp[505][505]; // Dynamic programming array\n int nums1_size, nums2_size; // Sizes of nums1 and nums2 arrays\n\n // Recursive function to calculate the maximum dot product\n int calculateDotProduct(vector<int>& nums1, vector<int>& nums2, int idx1, int idx2) {\n // Base case: If either of the indices reaches the array size, return 0.\n if (idx1 == nums1_size || idx2 == nums2_size)\n return 0;\n\n // Check if the result for this state is already calculated.\n if (~dp[idx1][idx2])\n return dp[idx1][idx2];\n\n // Calculate dot product for three options:\n // 1. Multiply current elements and add to the result of the next recursive call.\n int option1 = nums1[idx1] * nums2[idx2] + calculateDotProduct(nums1, nums2, idx1 + 1, idx2 + 1);\n // 2. Skip the current element in nums2 and call the function with idx2 incremented.\n int option2 = calculateDotProduct(nums1, nums2, idx1, idx2 + 1);\n // 3. Skip the current element in nums1 and call the function with idx1 incremented.\n int option3 = calculateDotProduct(nums1, nums2, idx1 + 1, idx2);\n\n // Store the maximum dot product among the three options in dp[idx1][idx2].\n return dp[idx1][idx2] = max({option1, option2, option3});\n }\n\n // Main function to calculate the maximum dot product\n int maxDotProduct(vector<int>& nums1, vector<int>& nums2) {\n nums1_size = nums1.size();\n nums2_size = nums2.size();\n\n int firstMax = INT_MIN;\n int secondMax = INT_MIN;\n int firstMin = INT_MAX;\n int secondMin = INT_MAX;\n\n // Calculate maximum and minimum values for nums1\n for (int num : nums1) {\n firstMax = max(firstMax, num);\n firstMin = min(firstMin, num);\n }\n // Calculate maximum and minimum values for nums2\n for (int num : nums2) {\n secondMax = max(secondMax, num);\n secondMin = min(secondMin, num);\n }\n\n // Check special cases where all elements are negative or all are positive\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return max(firstMax * secondMin, firstMin * secondMax);\n }\n\n memset(dp, -1, sizeof(dp)); // Initialize dp array with -1\n \n // Calculate the maximum dot product recursively, starting from index (0, 0).\n return calculateDotProduct(nums1, nums2, 0, 0);\n }\n};\n\n\n```\n```C []\n\n#include <limits.h>\n\nint dp[505][505];\nint nums1Size, nums2Size;\n\nint calculateDotProduct(int* nums1, int* nums2, int idx1, int idx2) {\n if (idx1 == nums1Size || idx2 == nums2Size)\n return 0;\n\n if (dp[idx1][idx2] != -1)\n return dp[idx1][idx2];\n\n int option1 = nums1[idx1] * nums2[idx2] + calculateDotProduct(nums1, nums2, idx1 + 1, idx2 + 1);\n int option2 = calculateDotProduct(nums1, nums2, idx1, idx2 + 1);\n int option3 = calculateDotProduct(nums1, nums2, idx1 + 1, idx2);\n\n dp[idx1][idx2] = (option1 > option2 ? (option1 > option3 ? option1 : option3) : (option2 > option3 ? option2 : option3));\n return dp[idx1][idx2];\n}\n\nint maxDotProduct(int* nums1, int nums1Size, int* nums2, int nums2Size) {\n nums1Size = nums1Size;\n nums2Size = nums2Size;\n memset(dp, -1, sizeof(dp));\n\n int firstMax = INT_MIN;\n int secondMax = INT_MIN;\n int firstMin = INT_MAX;\n int secondMin = INT_MAX;\n\n for (int i = 0; i < nums1Size; i++) {\n firstMax = firstMax > nums1[i] ? firstMax : nums1[i];\n firstMin = firstMin < nums1[i] ? firstMin : nums1[i];\n }\n for (int i = 0; i < nums2Size; i++) {\n secondMax = secondMax > nums2[i] ? secondMax : nums2[i];\n secondMin = secondMin < nums2[i] ? secondMin : nums2[i];\n }\n\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return (firstMax * secondMin) > (firstMin * secondMax) ? (firstMax * secondMin) : (firstMin * secondMax);\n }\n\n return calculateDotProduct(nums1, nums2, 0, 0);\n}\n\n\n```\n```Java []\n\nimport java.util.Arrays;\n\nclass Solution {\n int[][] dp;\n int nums1Size, nums2Size;\n\n public int calculateDotProduct(int[] nums1, int[] nums2, int idx1, int idx2) {\n if (idx1 == nums1Size || idx2 == nums2Size)\n return 0;\n\n if (dp[idx1][idx2] != -1)\n return dp[idx1][idx2];\n\n int option1 = nums1[idx1] * nums2[idx2] + calculateDotProduct(nums1, nums2, idx1 + 1, idx2 + 1);\n int option2 = calculateDotProduct(nums1, nums2, idx1, idx2 + 1);\n int option3 = calculateDotProduct(nums1, nums2, idx1 + 1, idx2);\n\n dp[idx1][idx2] = Math.max(Math.max(option1, option2), option3);\n return dp[idx1][idx2];\n }\n\n public int maxDotProduct(int[] nums1, int[] nums2) {\n nums1Size = nums1.length;\n nums2Size = nums2.length;\n dp = new int[nums1Size][nums2Size];\n for (int[] row : dp) {\n Arrays.fill(row, -1);\n }\n\n int firstMax = Integer.MIN_VALUE;\n int secondMax = Integer.MIN_VALUE;\n int firstMin = Integer.MAX_VALUE;\n int secondMin = Integer.MAX_VALUE;\n\n for (int num : nums1) {\n firstMax = Math.max(firstMax, num);\n firstMin = Math.min(firstMin, num);\n }\n for (int num : nums2) {\n secondMax = Math.max(secondMax, num);\n secondMin = Math.min(secondMin, num);\n }\n\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return Math.max(firstMax * secondMin, firstMin * secondMax);\n }\n\n return calculateDotProduct(nums1, nums2, 0, 0);\n }\n}\n\n\n\n```\n```python []\nclass Solution:\n def maxDotProduct(self, nums1, nums2):\n dp = [[-1] * len(nums2) for _ in range(len(nums1))]\n nums1Size = len(nums1)\n nums2Size = len(nums2)\n\n def calculateDotProduct(idx1, idx2):\n if idx1 == nums1Size or idx2 == nums2Size:\n return 0\n\n if dp[idx1][idx2] != -1:\n return dp[idx1][idx2]\n\n option1 = nums1[idx1] * nums2[idx2] + calculateDotProduct(idx1 + 1, idx2 + 1)\n option2 = calculateDotProduct(idx1, idx2 + 1)\n option3 = calculateDotProduct(idx1 + 1, idx2)\n\n dp[idx1][idx2] = max(option1, option2, option3)\n return dp[idx1][idx2]\n\n firstMax = float(\'-inf\')\n secondMax = float(\'-inf\')\n firstMin = float(\'inf\')\n secondMin = float(\'inf\')\n\n for num in nums1:\n firstMax = max(firstMax, num)\n firstMin = min(firstMin, num)\n\n for num in nums2:\n secondMax = max(secondMax, num)\n secondMin = min(secondMin, num)\n\n if (firstMax < 0 and secondMin > 0) or (firstMin > 0 and secondMax < 0):\n return max(firstMax * secondMin, firstMin * secondMax)\n\n return calculateDotProduct(0, 0)\n\n\n```\n```javascript []\nclass Solution {\n constructor() {\n this.dp = new Array(505).fill(null).map(() => new Array(505).fill(-1));\n this.nums1Size = 0;\n this.nums2Size = 0;\n }\n\n calculateDotProduct(nums1, nums2, idx1, idx2) {\n if (idx1 === this.nums1Size || idx2 === this.nums2Size)\n return 0;\n\n if (this.dp[idx1][idx2] !== -1)\n return this.dp[idx1][idx2];\n\n const option1 = nums1[idx1] * nums2[idx2] + this.calculateDotProduct(nums1, nums2, idx1 + 1, idx2 + 1);\n const option2 = this.calculateDotProduct(nums1, nums2, idx1, idx2 + 1);\n const option3 = this.calculateDotProduct(nums1, nums2, idx1 + 1, idx2);\n\n this.dp[idx1][idx2] = Math.max(option1, option2, option3);\n return this.dp[idx1][idx2];\n }\n\n maxDotProduct(nums1, nums2) {\n this.nums1Size = nums1.length;\n this.nums2Size = nums2.length;\n\n let firstMax = Number.NEGATIVE_INFINITY;\n let secondMax = Number.NEGATIVE_INFINITY;\n let firstMin = Number.POSITIVE_INFINITY;\n let secondMin = Number.POSITIVE_INFINITY;\n\n for (const num of nums1) {\n firstMax = Math.max(firstMax, num);\n firstMin = Math.min(firstMin, num);\n }\n\n for (const num of nums2) {\n secondMax = Math.max(secondMax, num);\n secondMin = Math.min(secondMin, num);\n }\n\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return Math.max(firstMax * secondMin, firstMin * secondMax);\n }\n\n for (let i = 0; i < 505; i++) {\n for (let j = 0; j < 505; j++) {\n this.dp[i][j] = -1;\n }\n }\n\n return this.calculateDotProduct(nums1, nums2, 0, 0);\n }\n}\n\n\n\n```\n\n\n# *PLEASE UPVOTE IF IT HELPED*\n\n---\n---\n\n\n---\n\n | 2 | Given two arrays `nums1` and `nums2`.
Return the maximum dot product between **non-empty** subsequences of nums1 and nums2 with the same length.
A subsequence of a array is a new array which is formed from the original array by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (ie, `[2,3,5]` is a subsequence of `[1,2,3,4,5]` while `[1,5,3]` is not).
**Example 1:**
**Input:** nums1 = \[2,1,-2,5\], nums2 = \[3,0,-6\]
**Output:** 18
**Explanation:** Take subsequence \[2,-2\] from nums1 and subsequence \[3,-6\] from nums2.
Their dot product is (2\*3 + (-2)\*(-6)) = 18.
**Example 2:**
**Input:** nums1 = \[3,-2\], nums2 = \[2,-6,7\]
**Output:** 21
**Explanation:** Take subsequence \[3\] from nums1 and subsequence \[7\] from nums2.
Their dot product is (3\*7) = 21.
**Example 3:**
**Input:** nums1 = \[-1,-1\], nums2 = \[1,1\]
**Output:** -1
**Explanation:** Take subsequence \[-1\] from nums1 and subsequence \[1\] from nums2.
Their dot product is -1.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 500`
* `-1000 <= nums1[i], nums2[i] <= 1000` | Simulate the problem. Count the number of 1's in the binary representation of each integer. Sort by the number of 1's ascending and by the value in case of tie. |
✅☑[C++/C/Java/Python/JavaScript] || DP || Recursion || EXPLAINED🔥 | max-dot-product-of-two-subsequences | 1 | 1 | # *PLEASE UPVOTE IF IT HELPED*\n\n---\n\n\n# Approach\n***(Also explained in the code)***\n\n**Explanation:**\n\nThe problem can be solved using dynamic programming. The goal is to find the maximum dot product between two subsequences of the given arrays `nums1` and `nums2`. We can create a 2D DP array `dp`, where `dp[i][j]` represents the maximum dot product for `nums1` up to index `i` and `nums2` up to index `j`.\n\nHere are the steps and comments with examples:\n\n1. Initialize the DP array `dp` with a size of `(nums1_size + 1) x (nums2_size + 1)` and fill it with `-1`. We use `-1`to represent uninitialized values.\n\n1. Calculate the maximum and minimum values for `nums1` and `nums2` to handle special cases where all elements are negative.\n\n1. Check if there\'s a special case where we can return the result directly:\n\n - If `(firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0`), it means one array has all negative values, and the other has all positive values. In this case, the maximum dot product will be the product of the maximum and minimum values from each array.\n1. Define a recursive function `calculateDotProduct` that calculates the maximum dot product starting from given indices `idx1` and `idx2`. This function has three options:\n\n - **option1:** Calculate the dot product of the current elements and add it to the result of the next recursive call with both indices incremented.\n - **option2:** Skip the current element in `nums2` and call the function with `idx2` incremented.\n - **option3:** Skip the current element in `nums1` and call the function with `idx1` incremented.\n1. In the `calculateDotProduct` function, use memoization to store and reuse the calculated results.\n\n1. Call the `calculateDotProduct` function from the main function, starting with indices `0` and `0`, and return the result.\n\n**Example:**\n\nLet\'s take an example with `nums1 = [2, 1, -2, 5]` and `nums2 = [3, 0, -6]`.\n\n1. Initialize `dp` with dimensions `(5 x 4)` filled with `-1`.\n\n1. Calculate the maximum and minimum values for nums1 and nums2:\n\n - `firstMax = 5`, `firstMin = -2`\n - `secondMax = 3`, `secondMin = -6`\n1. Since we have negative and positive values, it\'s not a special case.\n\n1. Start the `calculateDotProduct` function with indices `(0, 0)`:\n\n - For `(0, 0)`, we have `option1`, which calculates `2 * 3` and calls `(1, 1)`.\n - `(1, 1)` has `option1` as well, calculating `1 * 0` and calling `(2, 2)`.\n - Continue the recursion, and at `(4, 3)`, return `40` as the maximum dot product.\n1. Return the result `40`.\n\nThe final result is `40`, which is the maximum dot product of two subsequences.\n\n\n---\n\n\n# Complexity\n- **Time complexity:**\n $$O(n*m)$$\n\n- **Space complexity:**\n $$O(n*m)$$\n\n---\n\n\n# Code\n\n```C++ []\n\nclass Solution {\npublic:\n int dp[505][505]; // Dynamic programming array\n int nums1_size, nums2_size; // Sizes of nums1 and nums2 arrays\n\n // Recursive function to calculate the maximum dot product\n int calculateDotProduct(vector<int>& nums1, vector<int>& nums2, int idx1, int idx2) {\n // Base case: If either of the indices reaches the array size, return 0.\n if (idx1 == nums1_size || idx2 == nums2_size)\n return 0;\n\n // Check if the result for this state is already calculated.\n if (~dp[idx1][idx2])\n return dp[idx1][idx2];\n\n // Calculate dot product for three options:\n // 1. Multiply current elements and add to the result of the next recursive call.\n int option1 = nums1[idx1] * nums2[idx2] + calculateDotProduct(nums1, nums2, idx1 + 1, idx2 + 1);\n // 2. Skip the current element in nums2 and call the function with idx2 incremented.\n int option2 = calculateDotProduct(nums1, nums2, idx1, idx2 + 1);\n // 3. Skip the current element in nums1 and call the function with idx1 incremented.\n int option3 = calculateDotProduct(nums1, nums2, idx1 + 1, idx2);\n\n // Store the maximum dot product among the three options in dp[idx1][idx2].\n return dp[idx1][idx2] = max({option1, option2, option3});\n }\n\n // Main function to calculate the maximum dot product\n int maxDotProduct(vector<int>& nums1, vector<int>& nums2) {\n nums1_size = nums1.size();\n nums2_size = nums2.size();\n\n int firstMax = INT_MIN;\n int secondMax = INT_MIN;\n int firstMin = INT_MAX;\n int secondMin = INT_MAX;\n\n // Calculate maximum and minimum values for nums1\n for (int num : nums1) {\n firstMax = max(firstMax, num);\n firstMin = min(firstMin, num);\n }\n // Calculate maximum and minimum values for nums2\n for (int num : nums2) {\n secondMax = max(secondMax, num);\n secondMin = min(secondMin, num);\n }\n\n // Check special cases where all elements are negative or all are positive\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return max(firstMax * secondMin, firstMin * secondMax);\n }\n\n memset(dp, -1, sizeof(dp)); // Initialize dp array with -1\n \n // Calculate the maximum dot product recursively, starting from index (0, 0).\n return calculateDotProduct(nums1, nums2, 0, 0);\n }\n};\n\n\n```\n```C []\n\n#include <limits.h>\n\nint dp[505][505];\nint nums1Size, nums2Size;\n\nint calculateDotProduct(int* nums1, int* nums2, int idx1, int idx2) {\n if (idx1 == nums1Size || idx2 == nums2Size)\n return 0;\n\n if (dp[idx1][idx2] != -1)\n return dp[idx1][idx2];\n\n int option1 = nums1[idx1] * nums2[idx2] + calculateDotProduct(nums1, nums2, idx1 + 1, idx2 + 1);\n int option2 = calculateDotProduct(nums1, nums2, idx1, idx2 + 1);\n int option3 = calculateDotProduct(nums1, nums2, idx1 + 1, idx2);\n\n dp[idx1][idx2] = (option1 > option2 ? (option1 > option3 ? option1 : option3) : (option2 > option3 ? option2 : option3));\n return dp[idx1][idx2];\n}\n\nint maxDotProduct(int* nums1, int nums1Size, int* nums2, int nums2Size) {\n nums1Size = nums1Size;\n nums2Size = nums2Size;\n memset(dp, -1, sizeof(dp));\n\n int firstMax = INT_MIN;\n int secondMax = INT_MIN;\n int firstMin = INT_MAX;\n int secondMin = INT_MAX;\n\n for (int i = 0; i < nums1Size; i++) {\n firstMax = firstMax > nums1[i] ? firstMax : nums1[i];\n firstMin = firstMin < nums1[i] ? firstMin : nums1[i];\n }\n for (int i = 0; i < nums2Size; i++) {\n secondMax = secondMax > nums2[i] ? secondMax : nums2[i];\n secondMin = secondMin < nums2[i] ? secondMin : nums2[i];\n }\n\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return (firstMax * secondMin) > (firstMin * secondMax) ? (firstMax * secondMin) : (firstMin * secondMax);\n }\n\n return calculateDotProduct(nums1, nums2, 0, 0);\n}\n\n\n```\n```Java []\n\nimport java.util.Arrays;\n\nclass Solution {\n int[][] dp;\n int nums1Size, nums2Size;\n\n public int calculateDotProduct(int[] nums1, int[] nums2, int idx1, int idx2) {\n if (idx1 == nums1Size || idx2 == nums2Size)\n return 0;\n\n if (dp[idx1][idx2] != -1)\n return dp[idx1][idx2];\n\n int option1 = nums1[idx1] * nums2[idx2] + calculateDotProduct(nums1, nums2, idx1 + 1, idx2 + 1);\n int option2 = calculateDotProduct(nums1, nums2, idx1, idx2 + 1);\n int option3 = calculateDotProduct(nums1, nums2, idx1 + 1, idx2);\n\n dp[idx1][idx2] = Math.max(Math.max(option1, option2), option3);\n return dp[idx1][idx2];\n }\n\n public int maxDotProduct(int[] nums1, int[] nums2) {\n nums1Size = nums1.length;\n nums2Size = nums2.length;\n dp = new int[nums1Size][nums2Size];\n for (int[] row : dp) {\n Arrays.fill(row, -1);\n }\n\n int firstMax = Integer.MIN_VALUE;\n int secondMax = Integer.MIN_VALUE;\n int firstMin = Integer.MAX_VALUE;\n int secondMin = Integer.MAX_VALUE;\n\n for (int num : nums1) {\n firstMax = Math.max(firstMax, num);\n firstMin = Math.min(firstMin, num);\n }\n for (int num : nums2) {\n secondMax = Math.max(secondMax, num);\n secondMin = Math.min(secondMin, num);\n }\n\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return Math.max(firstMax * secondMin, firstMin * secondMax);\n }\n\n return calculateDotProduct(nums1, nums2, 0, 0);\n }\n}\n\n\n\n```\n```python []\nclass Solution:\n def maxDotProduct(self, nums1, nums2):\n dp = [[-1] * len(nums2) for _ in range(len(nums1))]\n nums1Size = len(nums1)\n nums2Size = len(nums2)\n\n def calculateDotProduct(idx1, idx2):\n if idx1 == nums1Size or idx2 == nums2Size:\n return 0\n\n if dp[idx1][idx2] != -1:\n return dp[idx1][idx2]\n\n option1 = nums1[idx1] * nums2[idx2] + calculateDotProduct(idx1 + 1, idx2 + 1)\n option2 = calculateDotProduct(idx1, idx2 + 1)\n option3 = calculateDotProduct(idx1 + 1, idx2)\n\n dp[idx1][idx2] = max(option1, option2, option3)\n return dp[idx1][idx2]\n\n firstMax = float(\'-inf\')\n secondMax = float(\'-inf\')\n firstMin = float(\'inf\')\n secondMin = float(\'inf\')\n\n for num in nums1:\n firstMax = max(firstMax, num)\n firstMin = min(firstMin, num)\n\n for num in nums2:\n secondMax = max(secondMax, num)\n secondMin = min(secondMin, num)\n\n if (firstMax < 0 and secondMin > 0) or (firstMin > 0 and secondMax < 0):\n return max(firstMax * secondMin, firstMin * secondMax)\n\n return calculateDotProduct(0, 0)\n\n\n```\n```javascript []\nclass Solution {\n constructor() {\n this.dp = new Array(505).fill(null).map(() => new Array(505).fill(-1));\n this.nums1Size = 0;\n this.nums2Size = 0;\n }\n\n calculateDotProduct(nums1, nums2, idx1, idx2) {\n if (idx1 === this.nums1Size || idx2 === this.nums2Size)\n return 0;\n\n if (this.dp[idx1][idx2] !== -1)\n return this.dp[idx1][idx2];\n\n const option1 = nums1[idx1] * nums2[idx2] + this.calculateDotProduct(nums1, nums2, idx1 + 1, idx2 + 1);\n const option2 = this.calculateDotProduct(nums1, nums2, idx1, idx2 + 1);\n const option3 = this.calculateDotProduct(nums1, nums2, idx1 + 1, idx2);\n\n this.dp[idx1][idx2] = Math.max(option1, option2, option3);\n return this.dp[idx1][idx2];\n }\n\n maxDotProduct(nums1, nums2) {\n this.nums1Size = nums1.length;\n this.nums2Size = nums2.length;\n\n let firstMax = Number.NEGATIVE_INFINITY;\n let secondMax = Number.NEGATIVE_INFINITY;\n let firstMin = Number.POSITIVE_INFINITY;\n let secondMin = Number.POSITIVE_INFINITY;\n\n for (const num of nums1) {\n firstMax = Math.max(firstMax, num);\n firstMin = Math.min(firstMin, num);\n }\n\n for (const num of nums2) {\n secondMax = Math.max(secondMax, num);\n secondMin = Math.min(secondMin, num);\n }\n\n if ((firstMax < 0 && secondMin > 0) || (firstMin > 0 && secondMax < 0)) {\n return Math.max(firstMax * secondMin, firstMin * secondMax);\n }\n\n for (let i = 0; i < 505; i++) {\n for (let j = 0; j < 505; j++) {\n this.dp[i][j] = -1;\n }\n }\n\n return this.calculateDotProduct(nums1, nums2, 0, 0);\n }\n}\n\n\n\n```\n\n\n# *PLEASE UPVOTE IF IT HELPED*\n\n---\n---\n\n\n---\n\n | 2 | Given an array `nums` that represents a permutation of integers from `1` to `n`. We are going to construct a binary search tree (BST) by inserting the elements of `nums` in order into an initially empty BST. Find the number of different ways to reorder `nums` so that the constructed BST is identical to that formed from the original array `nums`.
* For example, given `nums = [2,1,3]`, we will have 2 as the root, 1 as a left child, and 3 as a right child. The array `[2,3,1]` also yields the same BST but `[3,2,1]` yields a different BST.
Return _the number of ways to reorder_ `nums` _such that the BST formed is identical to the original BST formed from_ `nums`.
Since the answer may be very large, **return it modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[2,1,3\]
**Output:** 1
**Explanation:** We can reorder nums to be \[2,3,1\] which will yield the same BST. There are no other ways to reorder nums which will yield the same BST.
**Example 2:**
**Input:** nums = \[3,4,5,1,2\]
**Output:** 5
**Explanation:** The following 5 arrays will yield the same BST:
\[3,1,2,4,5\]
\[3,1,4,2,5\]
\[3,1,4,5,2\]
\[3,4,1,2,5\]
\[3,4,1,5,2\]
**Example 3:**
**Input:** nums = \[1,2,3\]
**Output:** 0
**Explanation:** There are no other orderings of nums that will yield the same BST.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= nums.length`
* All integers in `nums` are **distinct**. | Use dynamic programming, define DP[i][j] as the maximum dot product of two subsequences starting in the position i of nums1 and position j of nums2. |
EASY PYTHON SOLUTION USING DP | max-dot-product-of-two-subsequences | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def dp(self,i,j,nums1,nums2,ne,dct):\n if i==0 and j==0:\n if ne:\n return max(0,nums1[0]*nums2[0])\n return nums1[0]*nums2[0]\n if i<0 or j<0:\n if ne:\n return 0\n return float("-infinity")\n if (i,j,ne) in dct:\n return dct[(i,j,ne)]\n x=self.dp(i-1,j,nums1,nums2,ne,dct)\n y=self.dp(i,j-1,nums1,nums2,ne,dct)\n z=self.dp(i-1,j-1,nums1,nums2,True,dct)+(nums1[i]*nums2[j])\n dct[(i,j,ne)]=max(x,y,z)\n return max(x,y,z)\n\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n n1=len(nums1)\n n2=len(nums2)\n return self.dp(n1-1,n2-1,nums1,nums2,False,{})\n``` | 2 | Given two arrays `nums1` and `nums2`.
Return the maximum dot product between **non-empty** subsequences of nums1 and nums2 with the same length.
A subsequence of a array is a new array which is formed from the original array by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (ie, `[2,3,5]` is a subsequence of `[1,2,3,4,5]` while `[1,5,3]` is not).
**Example 1:**
**Input:** nums1 = \[2,1,-2,5\], nums2 = \[3,0,-6\]
**Output:** 18
**Explanation:** Take subsequence \[2,-2\] from nums1 and subsequence \[3,-6\] from nums2.
Their dot product is (2\*3 + (-2)\*(-6)) = 18.
**Example 2:**
**Input:** nums1 = \[3,-2\], nums2 = \[2,-6,7\]
**Output:** 21
**Explanation:** Take subsequence \[3\] from nums1 and subsequence \[7\] from nums2.
Their dot product is (3\*7) = 21.
**Example 3:**
**Input:** nums1 = \[-1,-1\], nums2 = \[1,1\]
**Output:** -1
**Explanation:** Take subsequence \[-1\] from nums1 and subsequence \[1\] from nums2.
Their dot product is -1.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 500`
* `-1000 <= nums1[i], nums2[i] <= 1000` | Simulate the problem. Count the number of 1's in the binary representation of each integer. Sort by the number of 1's ascending and by the value in case of tie. |
EASY PYTHON SOLUTION USING DP | max-dot-product-of-two-subsequences | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def dp(self,i,j,nums1,nums2,ne,dct):\n if i==0 and j==0:\n if ne:\n return max(0,nums1[0]*nums2[0])\n return nums1[0]*nums2[0]\n if i<0 or j<0:\n if ne:\n return 0\n return float("-infinity")\n if (i,j,ne) in dct:\n return dct[(i,j,ne)]\n x=self.dp(i-1,j,nums1,nums2,ne,dct)\n y=self.dp(i,j-1,nums1,nums2,ne,dct)\n z=self.dp(i-1,j-1,nums1,nums2,True,dct)+(nums1[i]*nums2[j])\n dct[(i,j,ne)]=max(x,y,z)\n return max(x,y,z)\n\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n n1=len(nums1)\n n2=len(nums2)\n return self.dp(n1-1,n2-1,nums1,nums2,False,{})\n``` | 2 | Given an array `nums` that represents a permutation of integers from `1` to `n`. We are going to construct a binary search tree (BST) by inserting the elements of `nums` in order into an initially empty BST. Find the number of different ways to reorder `nums` so that the constructed BST is identical to that formed from the original array `nums`.
* For example, given `nums = [2,1,3]`, we will have 2 as the root, 1 as a left child, and 3 as a right child. The array `[2,3,1]` also yields the same BST but `[3,2,1]` yields a different BST.
Return _the number of ways to reorder_ `nums` _such that the BST formed is identical to the original BST formed from_ `nums`.
Since the answer may be very large, **return it modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[2,1,3\]
**Output:** 1
**Explanation:** We can reorder nums to be \[2,3,1\] which will yield the same BST. There are no other ways to reorder nums which will yield the same BST.
**Example 2:**
**Input:** nums = \[3,4,5,1,2\]
**Output:** 5
**Explanation:** The following 5 arrays will yield the same BST:
\[3,1,2,4,5\]
\[3,1,4,2,5\]
\[3,1,4,5,2\]
\[3,4,1,2,5\]
\[3,4,1,5,2\]
**Example 3:**
**Input:** nums = \[1,2,3\]
**Output:** 0
**Explanation:** There are no other orderings of nums that will yield the same BST.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= nums.length`
* All integers in `nums` are **distinct**. | Use dynamic programming, define DP[i][j] as the maximum dot product of two subsequences starting in the position i of nums1 and position j of nums2. |
Bottom Up Python Solution | max-dot-product-of-two-subsequences | 0 | 1 | # Code\n```\nclass Solution:\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n \'\'\'\n Recurrence can be seen as dp[i][j] = max(x, x + dp[i-1][j-1], dp[i][j-1], dp[i-1][j])\n \n Where:\n 1. x: This is the product of the current elements nums1[j-1] and nums2[i-1] \n 2. x + dp[i-1][j-1]: the current product plus the best dot product we could get from the previous elements \n of both sequences\n 3. dp[i][j-1]: skip the current element from nums1 and find the best dot product using previous elements\n 4. dp[i-1][j]: skip the current element from nums2 and find the best dot product using previous elements\n \'\'\'\n\n m, n = len(nums1) + 1, len(nums2) + 1\n inf = float(\'inf\')\n dp = [[-inf] * m for _ in range(n)]\n \n for i in range(1, n):\n for j in range(1, m):\n x = nums1[j-1] * nums2[i-1]\n dp[i][j] = max(x, x + dp[i-1][j-1], dp[i][j-1], dp[i-1][j])\n\n return dp[-1][-1]\n``` | 1 | Given two arrays `nums1` and `nums2`.
Return the maximum dot product between **non-empty** subsequences of nums1 and nums2 with the same length.
A subsequence of a array is a new array which is formed from the original array by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (ie, `[2,3,5]` is a subsequence of `[1,2,3,4,5]` while `[1,5,3]` is not).
**Example 1:**
**Input:** nums1 = \[2,1,-2,5\], nums2 = \[3,0,-6\]
**Output:** 18
**Explanation:** Take subsequence \[2,-2\] from nums1 and subsequence \[3,-6\] from nums2.
Their dot product is (2\*3 + (-2)\*(-6)) = 18.
**Example 2:**
**Input:** nums1 = \[3,-2\], nums2 = \[2,-6,7\]
**Output:** 21
**Explanation:** Take subsequence \[3\] from nums1 and subsequence \[7\] from nums2.
Their dot product is (3\*7) = 21.
**Example 3:**
**Input:** nums1 = \[-1,-1\], nums2 = \[1,1\]
**Output:** -1
**Explanation:** Take subsequence \[-1\] from nums1 and subsequence \[1\] from nums2.
Their dot product is -1.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 500`
* `-1000 <= nums1[i], nums2[i] <= 1000` | Simulate the problem. Count the number of 1's in the binary representation of each integer. Sort by the number of 1's ascending and by the value in case of tie. |
Bottom Up Python Solution | max-dot-product-of-two-subsequences | 0 | 1 | # Code\n```\nclass Solution:\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n \'\'\'\n Recurrence can be seen as dp[i][j] = max(x, x + dp[i-1][j-1], dp[i][j-1], dp[i-1][j])\n \n Where:\n 1. x: This is the product of the current elements nums1[j-1] and nums2[i-1] \n 2. x + dp[i-1][j-1]: the current product plus the best dot product we could get from the previous elements \n of both sequences\n 3. dp[i][j-1]: skip the current element from nums1 and find the best dot product using previous elements\n 4. dp[i-1][j]: skip the current element from nums2 and find the best dot product using previous elements\n \'\'\'\n\n m, n = len(nums1) + 1, len(nums2) + 1\n inf = float(\'inf\')\n dp = [[-inf] * m for _ in range(n)]\n \n for i in range(1, n):\n for j in range(1, m):\n x = nums1[j-1] * nums2[i-1]\n dp[i][j] = max(x, x + dp[i-1][j-1], dp[i][j-1], dp[i-1][j])\n\n return dp[-1][-1]\n``` | 1 | Given an array `nums` that represents a permutation of integers from `1` to `n`. We are going to construct a binary search tree (BST) by inserting the elements of `nums` in order into an initially empty BST. Find the number of different ways to reorder `nums` so that the constructed BST is identical to that formed from the original array `nums`.
* For example, given `nums = [2,1,3]`, we will have 2 as the root, 1 as a left child, and 3 as a right child. The array `[2,3,1]` also yields the same BST but `[3,2,1]` yields a different BST.
Return _the number of ways to reorder_ `nums` _such that the BST formed is identical to the original BST formed from_ `nums`.
Since the answer may be very large, **return it modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[2,1,3\]
**Output:** 1
**Explanation:** We can reorder nums to be \[2,3,1\] which will yield the same BST. There are no other ways to reorder nums which will yield the same BST.
**Example 2:**
**Input:** nums = \[3,4,5,1,2\]
**Output:** 5
**Explanation:** The following 5 arrays will yield the same BST:
\[3,1,2,4,5\]
\[3,1,4,2,5\]
\[3,1,4,5,2\]
\[3,4,1,2,5\]
\[3,4,1,5,2\]
**Example 3:**
**Input:** nums = \[1,2,3\]
**Output:** 0
**Explanation:** There are no other orderings of nums that will yield the same BST.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= nums.length`
* All integers in `nums` are **distinct**. | Use dynamic programming, define DP[i][j] as the maximum dot product of two subsequences starting in the position i of nums1 and position j of nums2. |
[Python] Simplest Memoization DP Code with Approach | max-dot-product-of-two-subsequences | 0 | 1 | ### Approach:\n\n1. Base Condition - When either of the array elements are exhausted \n2. The Memoization `currentKey` should be made up of currentIndexes of both arrays\n3. Take into account all the three recursive calls:\n\ti. When both the indexes are incremented\n\tii. Two cases where one of the both indexes are incremented\n4. Taking the `currentProduct` for the current values of indexes for taking into account that it could be the last / only subsequence elements in the solution.\n5. Comparison and caching of the max value out of all our recursive calls, product & product + both increment recursive calls.\n\n\n```\nfrom sys import maxsize\nclass Solution:\n def __init__(self):\n self.cache = {}\n\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n return self.maxProduct(nums1, nums2, currentIndex1=0, currentIndex2=0)\n \n def maxProduct(self, nums1, nums2, currentIndex1, currentIndex2):\n\t # Base Condition\n if currentIndex1 >= len(nums1) or currentIndex2 >= len(nums2):\n return -maxsize\n \n\t\t# Caching / Memoization\n currentKey = (currentIndex1, currentIndex2)\n if currentKey in self.cache:\n return self.cache[currentKey]\n \n\t\t# Recursive Calls\n normal = self.maxProduct(nums1, nums2, currentIndex1 + 1, currentIndex2 + 1)\n one = self.maxProduct(nums1, nums2, currentIndex1 + 1, currentIndex2)\n two = self.maxProduct(nums1, nums2, currentIndex1, currentIndex2 + 1)\n \n\t\t# take the currentProduct\n currentProduct = nums1[currentIndex1] * nums2[currentIndex2]\n \n self.cache[currentKey] = max(\n\t\t currentProduct, \n\t\t currentProduct + normal,\n\t\t normal, one, two\n )\n \n return self.cache[currentKey]\n```\n\nLet me know if you liked the explaination of the solution / have any questions. Thanks. | 1 | Given two arrays `nums1` and `nums2`.
Return the maximum dot product between **non-empty** subsequences of nums1 and nums2 with the same length.
A subsequence of a array is a new array which is formed from the original array by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (ie, `[2,3,5]` is a subsequence of `[1,2,3,4,5]` while `[1,5,3]` is not).
**Example 1:**
**Input:** nums1 = \[2,1,-2,5\], nums2 = \[3,0,-6\]
**Output:** 18
**Explanation:** Take subsequence \[2,-2\] from nums1 and subsequence \[3,-6\] from nums2.
Their dot product is (2\*3 + (-2)\*(-6)) = 18.
**Example 2:**
**Input:** nums1 = \[3,-2\], nums2 = \[2,-6,7\]
**Output:** 21
**Explanation:** Take subsequence \[3\] from nums1 and subsequence \[7\] from nums2.
Their dot product is (3\*7) = 21.
**Example 3:**
**Input:** nums1 = \[-1,-1\], nums2 = \[1,1\]
**Output:** -1
**Explanation:** Take subsequence \[-1\] from nums1 and subsequence \[1\] from nums2.
Their dot product is -1.
**Constraints:**
* `1 <= nums1.length, nums2.length <= 500`
* `-1000 <= nums1[i], nums2[i] <= 1000` | Simulate the problem. Count the number of 1's in the binary representation of each integer. Sort by the number of 1's ascending and by the value in case of tie. |
[Python] Simplest Memoization DP Code with Approach | max-dot-product-of-two-subsequences | 0 | 1 | ### Approach:\n\n1. Base Condition - When either of the array elements are exhausted \n2. The Memoization `currentKey` should be made up of currentIndexes of both arrays\n3. Take into account all the three recursive calls:\n\ti. When both the indexes are incremented\n\tii. Two cases where one of the both indexes are incremented\n4. Taking the `currentProduct` for the current values of indexes for taking into account that it could be the last / only subsequence elements in the solution.\n5. Comparison and caching of the max value out of all our recursive calls, product & product + both increment recursive calls.\n\n\n```\nfrom sys import maxsize\nclass Solution:\n def __init__(self):\n self.cache = {}\n\n def maxDotProduct(self, nums1: List[int], nums2: List[int]) -> int:\n return self.maxProduct(nums1, nums2, currentIndex1=0, currentIndex2=0)\n \n def maxProduct(self, nums1, nums2, currentIndex1, currentIndex2):\n\t # Base Condition\n if currentIndex1 >= len(nums1) or currentIndex2 >= len(nums2):\n return -maxsize\n \n\t\t# Caching / Memoization\n currentKey = (currentIndex1, currentIndex2)\n if currentKey in self.cache:\n return self.cache[currentKey]\n \n\t\t# Recursive Calls\n normal = self.maxProduct(nums1, nums2, currentIndex1 + 1, currentIndex2 + 1)\n one = self.maxProduct(nums1, nums2, currentIndex1 + 1, currentIndex2)\n two = self.maxProduct(nums1, nums2, currentIndex1, currentIndex2 + 1)\n \n\t\t# take the currentProduct\n currentProduct = nums1[currentIndex1] * nums2[currentIndex2]\n \n self.cache[currentKey] = max(\n\t\t currentProduct, \n\t\t currentProduct + normal,\n\t\t normal, one, two\n )\n \n return self.cache[currentKey]\n```\n\nLet me know if you liked the explaination of the solution / have any questions. Thanks. | 1 | Given an array `nums` that represents a permutation of integers from `1` to `n`. We are going to construct a binary search tree (BST) by inserting the elements of `nums` in order into an initially empty BST. Find the number of different ways to reorder `nums` so that the constructed BST is identical to that formed from the original array `nums`.
* For example, given `nums = [2,1,3]`, we will have 2 as the root, 1 as a left child, and 3 as a right child. The array `[2,3,1]` also yields the same BST but `[3,2,1]` yields a different BST.
Return _the number of ways to reorder_ `nums` _such that the BST formed is identical to the original BST formed from_ `nums`.
Since the answer may be very large, **return it modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[2,1,3\]
**Output:** 1
**Explanation:** We can reorder nums to be \[2,3,1\] which will yield the same BST. There are no other ways to reorder nums which will yield the same BST.
**Example 2:**
**Input:** nums = \[3,4,5,1,2\]
**Output:** 5
**Explanation:** The following 5 arrays will yield the same BST:
\[3,1,2,4,5\]
\[3,1,4,2,5\]
\[3,1,4,5,2\]
\[3,4,1,2,5\]
\[3,4,1,5,2\]
**Example 3:**
**Input:** nums = \[1,2,3\]
**Output:** 0
**Explanation:** There are no other orderings of nums that will yield the same BST.
**Constraints:**
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= nums.length`
* All integers in `nums` are **distinct**. | Use dynamic programming, define DP[i][j] as the maximum dot product of two subsequences starting in the position i of nums1 and position j of nums2. |
Simple solution with HashMap in Python3 | make-two-arrays-equal-by-reversing-subarrays | 0 | 1 | # Intuition\nHere we have:\n- `target` and `arr` lists of integers\n- our goal is to check if we could reverse `arr` to make it as `target`\n\nAn approach is **extremely** simple:\n- create a **HashMap**, that\'ll represent a **balance** of frequencies of integers (**FOI**)\n- if FOI of each lists are **equal, then we can reverse** `arr` to make it as `target`, otherwise **it isn\'t possible**.\n\nTo avoid unnecessary space allocating, we consider each num from `target` as `+1` to **balance**, and `-1` for `arr`\n\n# Approach\n1. create **HashMap** `cache`\n2. iterate over both lists, and calculate a balance\n3. check, **if balance is established** as `particularValue == 0` from `cache`\n\n# Complexity\n- Time complexity: **O(N)**, twice iterate over `target` and `cache`\n\n- Space complexity: **O(N)**, for storing `cache`\n\n# Code\n```\nclass Solution:\n def canBeEqual(self, target: List[int], arr: List[int]) -> bool:\n cache = defaultdict(int)\n\n for i in range(len(target)):\n cache[target[i]] += 1\n cache[arr[i]] -= 1\n\n for v in cache.values():\n if v: \n return False\n\n return True\n\n``` | 1 | You are given two integer arrays of equal length `target` and `arr`. In one step, you can select any **non-empty subarray** of `arr` and reverse it. You are allowed to make any number of steps.
Return `true` _if you can make_ `arr` _equal to_ `target` _or_ `false` _otherwise_.
**Example 1:**
**Input:** target = \[1,2,3,4\], arr = \[2,4,1,3\]
**Output:** true
**Explanation:** You can follow the next steps to convert arr to target:
1- Reverse subarray \[2,4,1\], arr becomes \[1,4,2,3\]
2- Reverse subarray \[4,2\], arr becomes \[1,2,4,3\]
3- Reverse subarray \[4,3\], arr becomes \[1,2,3,4\]
There are multiple ways to convert arr to target, this is not the only way to do so.
**Example 2:**
**Input:** target = \[7\], arr = \[7\]
**Output:** true
**Explanation:** arr is equal to target without any reverses.
**Example 3:**
**Input:** target = \[3,7,9\], arr = \[3,7,11\]
**Output:** false
**Explanation:** arr does not have value 9 and it can never be converted to target.
**Constraints:**
* `target.length == arr.length`
* `1 <= target.length <= 1000`
* `1 <= target[i] <= 1000`
* `1 <= arr[i] <= 1000` | For each position we simply need to find the first occurrence of a/b/c on or after this position. So we can pre-compute three link-list of indices of each a, b, and c. |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.