title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
Python 3: Hashmap Solution - O(N) Time Complexity | swap-for-longest-repeated-character-substring | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxRepOpt1(self, text: str) -> int:\n\n # aaabaaadaaaaaaab\n # l\n # m\n # rrrr\n\n # charMap - alphabet: [starting index, count]\n charMap = defaultdict(list)\n\n l = 0\n for r in range(len(text)):\n if text[r] != text[l]:\n charMap[text[l]].append([l, r - l])\n l = r\n charMap[text[-1]].append([l, r - l + 1])\n\n # corner case: if there is only one element -> return length of the text\n if len(charMap) == 1:\n return len(text)\n\n res = 0\n for c, lst in charMap.items():\n prevStart, prevCount = lst[0]\n res = max(res, prevCount + (1 if len(lst) >= 2 else 0))\n if len(lst) >= 2:\n for curStart, curCount in lst[1:]:\n # if can connect\n one = len(lst) > 2\n if prevStart + prevCount + 1 == curStart: # 0 + 1 + 1 == 2\n res = max(res, prevCount + curCount + one)\n else:\n res = max(res, curCount + 1)\n\n prevStart, prevCount = curStart, curCount\n return res \n\n``` | 0 | You are given a string `text`. You can swap two of the characters in the `text`.
Return _the length of the longest substring with repeated characters_.
**Example 1:**
**Input:** text = "ababa "
**Output:** 3
**Explanation:** We can swap the first 'b' with the last 'a', or the last 'b' with the first 'a'. Then, the longest repeated character substring is "aaa " with length 3.
**Example 2:**
**Input:** text = "aaabaaa "
**Output:** 6
**Explanation:** Swap 'b' with the last 'a' (or the first 'a'), and we get longest repeated character substring "aaaaaa " with length 6.
**Example 3:**
**Input:** text = "aaaaa "
**Output:** 5
**Explanation:** No need to swap, longest repeated character substring is "aaaaa " with length is 5.
**Constraints:**
* `1 <= text.length <= 2 * 104`
* `text` consist of lowercase English characters only. | Split the string into words, then look at adjacent triples of words. |
Python 3: Hashmap Solution - O(N) Time Complexity | swap-for-longest-repeated-character-substring | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxRepOpt1(self, text: str) -> int:\n\n # aaabaaadaaaaaaab\n # l\n # m\n # rrrr\n\n # charMap - alphabet: [starting index, count]\n charMap = defaultdict(list)\n\n l = 0\n for r in range(len(text)):\n if text[r] != text[l]:\n charMap[text[l]].append([l, r - l])\n l = r\n charMap[text[-1]].append([l, r - l + 1])\n\n # corner case: if there is only one element -> return length of the text\n if len(charMap) == 1:\n return len(text)\n\n res = 0\n for c, lst in charMap.items():\n prevStart, prevCount = lst[0]\n res = max(res, prevCount + (1 if len(lst) >= 2 else 0))\n if len(lst) >= 2:\n for curStart, curCount in lst[1:]:\n # if can connect\n one = len(lst) > 2\n if prevStart + prevCount + 1 == curStart: # 0 + 1 + 1 == 2\n res = max(res, prevCount + curCount + one)\n else:\n res = max(res, curCount + 1)\n\n prevStart, prevCount = curStart, curCount\n return res \n\n``` | 0 | Given a binary tree with the following rules:
1. `root.val == 0`
2. If `treeNode.val == x` and `treeNode.left != null`, then `treeNode.left.val == 2 * x + 1`
3. If `treeNode.val == x` and `treeNode.right != null`, then `treeNode.right.val == 2 * x + 2`
Now the binary tree is contaminated, which means all `treeNode.val` have been changed to `-1`.
Implement the `FindElements` class:
* `FindElements(TreeNode* root)` Initializes the object with a contaminated binary tree and recovers it.
* `bool find(int target)` Returns `true` if the `target` value exists in the recovered binary tree.
**Example 1:**
**Input**
\[ "FindElements ", "find ", "find "\]
\[\[\[-1,null,-1\]\],\[1\],\[2\]\]
**Output**
\[null,false,true\]
**Explanation**
FindElements findElements = new FindElements(\[-1,null,-1\]);
findElements.find(1); // return False
findElements.find(2); // return True
**Example 2:**
**Input**
\[ "FindElements ", "find ", "find ", "find "\]
\[\[\[-1,-1,-1,-1,-1\]\],\[1\],\[3\],\[5\]\]
**Output**
\[null,true,true,false\]
**Explanation**
FindElements findElements = new FindElements(\[-1,-1,-1,-1,-1\]);
findElements.find(1); // return True
findElements.find(3); // return True
findElements.find(5); // return False
**Example 3:**
**Input**
\[ "FindElements ", "find ", "find ", "find ", "find "\]
\[\[\[-1,null,-1,-1,null,-1\]\],\[2\],\[3\],\[4\],\[5\]\]
**Output**
\[null,true,false,false,true\]
**Explanation**
FindElements findElements = new FindElements(\[-1,null,-1,-1,null,-1\]);
findElements.find(2); // return True
findElements.find(3); // return False
findElements.find(4); // return False
findElements.find(5); // return True
**Constraints:**
* `TreeNode.val == -1`
* The height of the binary tree is less than or equal to `20`
* The total number of nodes is between `[1, 104]`
* Total calls of `find()` is between `[1, 104]`
* `0 <= target <= 106` | There are two cases: a block of characters, or two blocks of characters between one different character.
By keeping a run-length encoded version of the string, we can easily check these cases. |
0(n) Run Time Solution | swap-for-longest-repeated-character-substring | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxRepOpt1(self, text: str) -> int:\n from collections import defaultdict\n hm = defaultdict(int)\n hm1 = defaultdict(int)\n max_len,i = 1,0\n \n\n for k in range(len(text)):\n hm[text[k]] += 1\n \n\n for j in range(len(text)):\n hm1[text[j]] += 1\n max_val = max(hm1.values()) \n max_val_keys = [k for k, v in hm1.items() if v == max_val]\n \n max_char = max([(k, hm[k]) for k in max_val_keys], key = itemgetter(1))[0]\n\n # print(max_val_keys)\n if (j-i+1 > max(hm1.values()) +1 ) and (hm[max_char] >= hm1[max_char]):\n if hm[max_char] == hm1[max_char] :\n max_len = max(max_len,max(hm1.values()))\n else :\n max_len = max(max_len,max(hm1.values()) +1 )\n while((j-i+1 > max(hm1.values()) +1 ) and (hm[max_char] >= hm1[max_char])):\n if hm1[text[i]] == 1 : \n hm1.pop(text[i])\n else :\n hm1[text[i]] -= 1\n i += 1\n\n max_char = max(hm1, key = hm1.get)\n\n if (j-i+1 <= max(hm1.values()) +1 ) and (hm[max_char] == hm1[max_char]) :\n max_len = max(max_len,max(hm1.values()))\n elif (j-i+1 <= max(hm1.values()) +1 ) and (hm[max_char] > hm1[max_char]) :\n max_len = max(max_len,max(hm1.values())+1)\n \n return max_len\n``` | 0 | You are given a string `text`. You can swap two of the characters in the `text`.
Return _the length of the longest substring with repeated characters_.
**Example 1:**
**Input:** text = "ababa "
**Output:** 3
**Explanation:** We can swap the first 'b' with the last 'a', or the last 'b' with the first 'a'. Then, the longest repeated character substring is "aaa " with length 3.
**Example 2:**
**Input:** text = "aaabaaa "
**Output:** 6
**Explanation:** Swap 'b' with the last 'a' (or the first 'a'), and we get longest repeated character substring "aaaaaa " with length 6.
**Example 3:**
**Input:** text = "aaaaa "
**Output:** 5
**Explanation:** No need to swap, longest repeated character substring is "aaaaa " with length is 5.
**Constraints:**
* `1 <= text.length <= 2 * 104`
* `text` consist of lowercase English characters only. | Split the string into words, then look at adjacent triples of words. |
0(n) Run Time Solution | swap-for-longest-repeated-character-substring | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxRepOpt1(self, text: str) -> int:\n from collections import defaultdict\n hm = defaultdict(int)\n hm1 = defaultdict(int)\n max_len,i = 1,0\n \n\n for k in range(len(text)):\n hm[text[k]] += 1\n \n\n for j in range(len(text)):\n hm1[text[j]] += 1\n max_val = max(hm1.values()) \n max_val_keys = [k for k, v in hm1.items() if v == max_val]\n \n max_char = max([(k, hm[k]) for k in max_val_keys], key = itemgetter(1))[0]\n\n # print(max_val_keys)\n if (j-i+1 > max(hm1.values()) +1 ) and (hm[max_char] >= hm1[max_char]):\n if hm[max_char] == hm1[max_char] :\n max_len = max(max_len,max(hm1.values()))\n else :\n max_len = max(max_len,max(hm1.values()) +1 )\n while((j-i+1 > max(hm1.values()) +1 ) and (hm[max_char] >= hm1[max_char])):\n if hm1[text[i]] == 1 : \n hm1.pop(text[i])\n else :\n hm1[text[i]] -= 1\n i += 1\n\n max_char = max(hm1, key = hm1.get)\n\n if (j-i+1 <= max(hm1.values()) +1 ) and (hm[max_char] == hm1[max_char]) :\n max_len = max(max_len,max(hm1.values()))\n elif (j-i+1 <= max(hm1.values()) +1 ) and (hm[max_char] > hm1[max_char]) :\n max_len = max(max_len,max(hm1.values())+1)\n \n return max_len\n``` | 0 | Given a binary tree with the following rules:
1. `root.val == 0`
2. If `treeNode.val == x` and `treeNode.left != null`, then `treeNode.left.val == 2 * x + 1`
3. If `treeNode.val == x` and `treeNode.right != null`, then `treeNode.right.val == 2 * x + 2`
Now the binary tree is contaminated, which means all `treeNode.val` have been changed to `-1`.
Implement the `FindElements` class:
* `FindElements(TreeNode* root)` Initializes the object with a contaminated binary tree and recovers it.
* `bool find(int target)` Returns `true` if the `target` value exists in the recovered binary tree.
**Example 1:**
**Input**
\[ "FindElements ", "find ", "find "\]
\[\[\[-1,null,-1\]\],\[1\],\[2\]\]
**Output**
\[null,false,true\]
**Explanation**
FindElements findElements = new FindElements(\[-1,null,-1\]);
findElements.find(1); // return False
findElements.find(2); // return True
**Example 2:**
**Input**
\[ "FindElements ", "find ", "find ", "find "\]
\[\[\[-1,-1,-1,-1,-1\]\],\[1\],\[3\],\[5\]\]
**Output**
\[null,true,true,false\]
**Explanation**
FindElements findElements = new FindElements(\[-1,-1,-1,-1,-1\]);
findElements.find(1); // return True
findElements.find(3); // return True
findElements.find(5); // return False
**Example 3:**
**Input**
\[ "FindElements ", "find ", "find ", "find ", "find "\]
\[\[\[-1,null,-1,-1,null,-1\]\],\[2\],\[3\],\[4\],\[5\]\]
**Output**
\[null,true,false,false,true\]
**Explanation**
FindElements findElements = new FindElements(\[-1,null,-1,-1,null,-1\]);
findElements.find(2); // return True
findElements.find(3); // return False
findElements.find(4); // return False
findElements.find(5); // return True
**Constraints:**
* `TreeNode.val == -1`
* The height of the binary tree is less than or equal to `20`
* The total number of nodes is between `[1, 104]`
* Total calls of `find()` is between `[1, 104]`
* `0 <= target <= 106` | There are two cases: a block of characters, or two blocks of characters between one different character.
By keeping a run-length encoded version of the string, we can easily check these cases. |
[Python3] Run-Length Solution | swap-for-longest-repeated-character-substring | 0 | 1 | # Code\n```\nclass Solution:\n def maxRepOpt1(self, text: str) -> int:\n chCnt = [0]*26\n for ch in text:\n chCnt[ord(ch)-ord(\'a\')]+=1\n \n runLength = []\n ans = 1\n prev = \'$\'\n cnt = 1\n for ch in text:\n if prev != ch:\n runLength.append((prev, cnt))\n ans = max(ans, cnt+1 if prev != \'$\' and chCnt[ord(prev)-ord(\'a\')] > cnt else cnt)\n prev = ch\n cnt = 1\n else:\n cnt += 1\n\n runLength.append((prev, cnt))\n ans = max(ans, cnt+1 if chCnt[ord(prev)-ord(\'a\')] > cnt else cnt)\n for i in range(0, len(runLength)-2):\n if runLength[i][0]==runLength[i+2][0] and runLength[i+1][1]==1 and runLength[i][1] + runLength[i+2][1] <= chCnt[ord(runLength[i][0])-ord(\'a\')]:\n if runLength[i][1] + runLength[i+2][1] == chCnt[ord(runLength[i][0])-ord(\'a\')]:\n ans = max(ans, runLength[i][1] + runLength[i+2][1])\n else:\n ans = max(ans, runLength[i][1] + runLength[i+2][1] + 1)\n\n return ans\n``` | 0 | You are given a string `text`. You can swap two of the characters in the `text`.
Return _the length of the longest substring with repeated characters_.
**Example 1:**
**Input:** text = "ababa "
**Output:** 3
**Explanation:** We can swap the first 'b' with the last 'a', or the last 'b' with the first 'a'. Then, the longest repeated character substring is "aaa " with length 3.
**Example 2:**
**Input:** text = "aaabaaa "
**Output:** 6
**Explanation:** Swap 'b' with the last 'a' (or the first 'a'), and we get longest repeated character substring "aaaaaa " with length 6.
**Example 3:**
**Input:** text = "aaaaa "
**Output:** 5
**Explanation:** No need to swap, longest repeated character substring is "aaaaa " with length is 5.
**Constraints:**
* `1 <= text.length <= 2 * 104`
* `text` consist of lowercase English characters only. | Split the string into words, then look at adjacent triples of words. |
[Python3] Run-Length Solution | swap-for-longest-repeated-character-substring | 0 | 1 | # Code\n```\nclass Solution:\n def maxRepOpt1(self, text: str) -> int:\n chCnt = [0]*26\n for ch in text:\n chCnt[ord(ch)-ord(\'a\')]+=1\n \n runLength = []\n ans = 1\n prev = \'$\'\n cnt = 1\n for ch in text:\n if prev != ch:\n runLength.append((prev, cnt))\n ans = max(ans, cnt+1 if prev != \'$\' and chCnt[ord(prev)-ord(\'a\')] > cnt else cnt)\n prev = ch\n cnt = 1\n else:\n cnt += 1\n\n runLength.append((prev, cnt))\n ans = max(ans, cnt+1 if chCnt[ord(prev)-ord(\'a\')] > cnt else cnt)\n for i in range(0, len(runLength)-2):\n if runLength[i][0]==runLength[i+2][0] and runLength[i+1][1]==1 and runLength[i][1] + runLength[i+2][1] <= chCnt[ord(runLength[i][0])-ord(\'a\')]:\n if runLength[i][1] + runLength[i+2][1] == chCnt[ord(runLength[i][0])-ord(\'a\')]:\n ans = max(ans, runLength[i][1] + runLength[i+2][1])\n else:\n ans = max(ans, runLength[i][1] + runLength[i+2][1] + 1)\n\n return ans\n``` | 0 | Given a binary tree with the following rules:
1. `root.val == 0`
2. If `treeNode.val == x` and `treeNode.left != null`, then `treeNode.left.val == 2 * x + 1`
3. If `treeNode.val == x` and `treeNode.right != null`, then `treeNode.right.val == 2 * x + 2`
Now the binary tree is contaminated, which means all `treeNode.val` have been changed to `-1`.
Implement the `FindElements` class:
* `FindElements(TreeNode* root)` Initializes the object with a contaminated binary tree and recovers it.
* `bool find(int target)` Returns `true` if the `target` value exists in the recovered binary tree.
**Example 1:**
**Input**
\[ "FindElements ", "find ", "find "\]
\[\[\[-1,null,-1\]\],\[1\],\[2\]\]
**Output**
\[null,false,true\]
**Explanation**
FindElements findElements = new FindElements(\[-1,null,-1\]);
findElements.find(1); // return False
findElements.find(2); // return True
**Example 2:**
**Input**
\[ "FindElements ", "find ", "find ", "find "\]
\[\[\[-1,-1,-1,-1,-1\]\],\[1\],\[3\],\[5\]\]
**Output**
\[null,true,true,false\]
**Explanation**
FindElements findElements = new FindElements(\[-1,-1,-1,-1,-1\]);
findElements.find(1); // return True
findElements.find(3); // return True
findElements.find(5); // return False
**Example 3:**
**Input**
\[ "FindElements ", "find ", "find ", "find ", "find "\]
\[\[\[-1,null,-1,-1,null,-1\]\],\[2\],\[3\],\[4\],\[5\]\]
**Output**
\[null,true,false,false,true\]
**Explanation**
FindElements findElements = new FindElements(\[-1,null,-1,-1,null,-1\]);
findElements.find(2); // return True
findElements.find(3); // return False
findElements.find(4); // return False
findElements.find(5); // return True
**Constraints:**
* `TreeNode.val == -1`
* The height of the binary tree is less than or equal to `20`
* The total number of nodes is between `[1, 104]`
* Total calls of `find()` is between `[1, 104]`
* `0 <= target <= 106` | There are two cases: a block of characters, or two blocks of characters between one different character.
By keeping a run-length encoded version of the string, we can easily check these cases. |
Python O(n) approach using spans. | swap-for-longest-repeated-character-substring | 0 | 1 | \n# Code\n```\nclass Solution:\n def maxRepOpt1(self, text: str) -> int:\n p = text[0]\n rec = dict()\n\n # Get spans \n left, right = 0, 1\n for i_, c in enumerate(text[1:]+\' \'):\n i = i_ + 1\n if c == p:\n right += 1\n else:\n spans = rec.get(p, [])\n spans = spans + [(left, right)]\n rec[p] = spans\n p = c\n left, right = i, i+1\n\n # Examine spans\n # Append potential span length after switching to lens\n lens = []\n for p, spans in rec.items():\n # Only one span - record its length\n if len(spans) == 1:\n lens.append(spans[0][1] - spans[0][0])\n else:\n # If multiple spans, examine each ajacent span pairs\n for i in range(len(spans)-1):\n l1, r1 = spans[i]\n l2, r2 = spans[i+1]\n # If only separated by one character, and there is a third span\n if l2 - r1 == 1 and len(spans) >= 3:\n lens.append(r1-l1 + r2-l2 + 1)\n # If only separated by one character, but no third span exists\n elif l2 - r1 == 1:\n lens.append(r1-l1 + r2-l2)\n # If separated by more than one char\n else:\n lens.append(max(r1-l1, r2-l2) + 1)\n # Return the maximum length\n return max(lens)\n\n``` | 0 | You are given a string `text`. You can swap two of the characters in the `text`.
Return _the length of the longest substring with repeated characters_.
**Example 1:**
**Input:** text = "ababa "
**Output:** 3
**Explanation:** We can swap the first 'b' with the last 'a', or the last 'b' with the first 'a'. Then, the longest repeated character substring is "aaa " with length 3.
**Example 2:**
**Input:** text = "aaabaaa "
**Output:** 6
**Explanation:** Swap 'b' with the last 'a' (or the first 'a'), and we get longest repeated character substring "aaaaaa " with length 6.
**Example 3:**
**Input:** text = "aaaaa "
**Output:** 5
**Explanation:** No need to swap, longest repeated character substring is "aaaaa " with length is 5.
**Constraints:**
* `1 <= text.length <= 2 * 104`
* `text` consist of lowercase English characters only. | Split the string into words, then look at adjacent triples of words. |
Python O(n) approach using spans. | swap-for-longest-repeated-character-substring | 0 | 1 | \n# Code\n```\nclass Solution:\n def maxRepOpt1(self, text: str) -> int:\n p = text[0]\n rec = dict()\n\n # Get spans \n left, right = 0, 1\n for i_, c in enumerate(text[1:]+\' \'):\n i = i_ + 1\n if c == p:\n right += 1\n else:\n spans = rec.get(p, [])\n spans = spans + [(left, right)]\n rec[p] = spans\n p = c\n left, right = i, i+1\n\n # Examine spans\n # Append potential span length after switching to lens\n lens = []\n for p, spans in rec.items():\n # Only one span - record its length\n if len(spans) == 1:\n lens.append(spans[0][1] - spans[0][0])\n else:\n # If multiple spans, examine each ajacent span pairs\n for i in range(len(spans)-1):\n l1, r1 = spans[i]\n l2, r2 = spans[i+1]\n # If only separated by one character, and there is a third span\n if l2 - r1 == 1 and len(spans) >= 3:\n lens.append(r1-l1 + r2-l2 + 1)\n # If only separated by one character, but no third span exists\n elif l2 - r1 == 1:\n lens.append(r1-l1 + r2-l2)\n # If separated by more than one char\n else:\n lens.append(max(r1-l1, r2-l2) + 1)\n # Return the maximum length\n return max(lens)\n\n``` | 0 | Given a binary tree with the following rules:
1. `root.val == 0`
2. If `treeNode.val == x` and `treeNode.left != null`, then `treeNode.left.val == 2 * x + 1`
3. If `treeNode.val == x` and `treeNode.right != null`, then `treeNode.right.val == 2 * x + 2`
Now the binary tree is contaminated, which means all `treeNode.val` have been changed to `-1`.
Implement the `FindElements` class:
* `FindElements(TreeNode* root)` Initializes the object with a contaminated binary tree and recovers it.
* `bool find(int target)` Returns `true` if the `target` value exists in the recovered binary tree.
**Example 1:**
**Input**
\[ "FindElements ", "find ", "find "\]
\[\[\[-1,null,-1\]\],\[1\],\[2\]\]
**Output**
\[null,false,true\]
**Explanation**
FindElements findElements = new FindElements(\[-1,null,-1\]);
findElements.find(1); // return False
findElements.find(2); // return True
**Example 2:**
**Input**
\[ "FindElements ", "find ", "find ", "find "\]
\[\[\[-1,-1,-1,-1,-1\]\],\[1\],\[3\],\[5\]\]
**Output**
\[null,true,true,false\]
**Explanation**
FindElements findElements = new FindElements(\[-1,-1,-1,-1,-1\]);
findElements.find(1); // return True
findElements.find(3); // return True
findElements.find(5); // return False
**Example 3:**
**Input**
\[ "FindElements ", "find ", "find ", "find ", "find "\]
\[\[\[-1,null,-1,-1,null,-1\]\],\[2\],\[3\],\[4\],\[5\]\]
**Output**
\[null,true,false,false,true\]
**Explanation**
FindElements findElements = new FindElements(\[-1,null,-1,-1,null,-1\]);
findElements.find(2); // return True
findElements.find(3); // return False
findElements.find(4); // return False
findElements.find(5); // return True
**Constraints:**
* `TreeNode.val == -1`
* The height of the binary tree is less than or equal to `20`
* The total number of nodes is between `[1, 104]`
* Total calls of `find()` is between `[1, 104]`
* `0 <= target <= 106` | There are two cases: a block of characters, or two blocks of characters between one different character.
By keeping a run-length encoded version of the string, we can easily check these cases. |
Python Groupby | swap-for-longest-repeated-character-substring | 0 | 1 | # Code\n```\nclass Solution:\n def maxRepOpt1(self, S):\n # We get the group\'s key and length first, e.g. \'aaabaaa\' -> [[a , 3], [b, 1], [a, 3]\n A = [[c, len(list(g))] for c, g in itertools.groupby(S)]\n # We also generate a count dict for easy look up e.g. \'aaabaaa\' -> {a: 6, b: 1}\n count = collections.Counter(S)\n # only extend 1 more, use min here to avoid the case that there\'s no extra char to extend\n res = max(min(k + 1, count[c]) for c, k in A)\n # merge 2 groups together\n for i in range(1, len(A) - 1):\n # if both sides have the same char and are separated by only 1 char\n if A[i - 1][0] == A[i + 1][0] and A[i][1] == 1:\n # min here serves the same purpose\n res = max(res, min(A[i - 1][1] + A[i + 1][1] + 1, count[A[i + 1][0]]))\n return res\n``` | 0 | You are given a string `text`. You can swap two of the characters in the `text`.
Return _the length of the longest substring with repeated characters_.
**Example 1:**
**Input:** text = "ababa "
**Output:** 3
**Explanation:** We can swap the first 'b' with the last 'a', or the last 'b' with the first 'a'. Then, the longest repeated character substring is "aaa " with length 3.
**Example 2:**
**Input:** text = "aaabaaa "
**Output:** 6
**Explanation:** Swap 'b' with the last 'a' (or the first 'a'), and we get longest repeated character substring "aaaaaa " with length 6.
**Example 3:**
**Input:** text = "aaaaa "
**Output:** 5
**Explanation:** No need to swap, longest repeated character substring is "aaaaa " with length is 5.
**Constraints:**
* `1 <= text.length <= 2 * 104`
* `text` consist of lowercase English characters only. | Split the string into words, then look at adjacent triples of words. |
Python Groupby | swap-for-longest-repeated-character-substring | 0 | 1 | # Code\n```\nclass Solution:\n def maxRepOpt1(self, S):\n # We get the group\'s key and length first, e.g. \'aaabaaa\' -> [[a , 3], [b, 1], [a, 3]\n A = [[c, len(list(g))] for c, g in itertools.groupby(S)]\n # We also generate a count dict for easy look up e.g. \'aaabaaa\' -> {a: 6, b: 1}\n count = collections.Counter(S)\n # only extend 1 more, use min here to avoid the case that there\'s no extra char to extend\n res = max(min(k + 1, count[c]) for c, k in A)\n # merge 2 groups together\n for i in range(1, len(A) - 1):\n # if both sides have the same char and are separated by only 1 char\n if A[i - 1][0] == A[i + 1][0] and A[i][1] == 1:\n # min here serves the same purpose\n res = max(res, min(A[i - 1][1] + A[i + 1][1] + 1, count[A[i + 1][0]]))\n return res\n``` | 0 | Given a binary tree with the following rules:
1. `root.val == 0`
2. If `treeNode.val == x` and `treeNode.left != null`, then `treeNode.left.val == 2 * x + 1`
3. If `treeNode.val == x` and `treeNode.right != null`, then `treeNode.right.val == 2 * x + 2`
Now the binary tree is contaminated, which means all `treeNode.val` have been changed to `-1`.
Implement the `FindElements` class:
* `FindElements(TreeNode* root)` Initializes the object with a contaminated binary tree and recovers it.
* `bool find(int target)` Returns `true` if the `target` value exists in the recovered binary tree.
**Example 1:**
**Input**
\[ "FindElements ", "find ", "find "\]
\[\[\[-1,null,-1\]\],\[1\],\[2\]\]
**Output**
\[null,false,true\]
**Explanation**
FindElements findElements = new FindElements(\[-1,null,-1\]);
findElements.find(1); // return False
findElements.find(2); // return True
**Example 2:**
**Input**
\[ "FindElements ", "find ", "find ", "find "\]
\[\[\[-1,-1,-1,-1,-1\]\],\[1\],\[3\],\[5\]\]
**Output**
\[null,true,true,false\]
**Explanation**
FindElements findElements = new FindElements(\[-1,-1,-1,-1,-1\]);
findElements.find(1); // return True
findElements.find(3); // return True
findElements.find(5); // return False
**Example 3:**
**Input**
\[ "FindElements ", "find ", "find ", "find ", "find "\]
\[\[\[-1,null,-1,-1,null,-1\]\],\[2\],\[3\],\[4\],\[5\]\]
**Output**
\[null,true,false,false,true\]
**Explanation**
FindElements findElements = new FindElements(\[-1,null,-1,-1,null,-1\]);
findElements.find(2); // return True
findElements.find(3); // return False
findElements.find(4); // return False
findElements.find(5); // return True
**Constraints:**
* `TreeNode.val == -1`
* The height of the binary tree is less than or equal to `20`
* The total number of nodes is between `[1, 104]`
* Total calls of `find()` is between `[1, 104]`
* `0 <= target <= 106` | There are two cases: a block of characters, or two blocks of characters between one different character.
By keeping a run-length encoded version of the string, we can easily check these cases. |
Python (Simple Maths) | online-majority-element-in-subarray | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass MajorityChecker:\n def __init__(self, arr):\n self.arr = arr\n self.dict1 = defaultdict(list)\n\n for i,x in enumerate(arr):\n self.dict1[x].append(i)\n\n def query(self, left, right, threshold):\n for _ in range(10):\n k = random.randint(left,right)\n res = self.arr[k]\n lo = bisect.bisect_left(self.dict1[res],left)\n hi = bisect.bisect_right(self.dict1[res],right)\n if hi-lo >= threshold: return res\n\n return -1\n\n\n\n\n\n \n\n``` | 1 | Design a data structure that efficiently finds the **majority element** of a given subarray.
The **majority element** of a subarray is an element that occurs `threshold` times or more in the subarray.
Implementing the `MajorityChecker` class:
* `MajorityChecker(int[] arr)` Initializes the instance of the class with the given array `arr`.
* `int query(int left, int right, int threshold)` returns the element in the subarray `arr[left...right]` that occurs at least `threshold` times, or `-1` if no such element exists.
**Example 1:**
**Input**
\[ "MajorityChecker ", "query ", "query ", "query "\]
\[\[\[1, 1, 2, 2, 1, 1\]\], \[0, 5, 4\], \[0, 3, 3\], \[2, 3, 2\]\]
**Output**
\[null, 1, -1, 2\]
**Explanation**
MajorityChecker majorityChecker = new MajorityChecker(\[1, 1, 2, 2, 1, 1\]);
majorityChecker.query(0, 5, 4); // return 1
majorityChecker.query(0, 3, 3); // return -1
majorityChecker.query(2, 3, 2); // return 2
**Constraints:**
* `1 <= arr.length <= 2 * 104`
* `1 <= arr[i] <= 2 * 104`
* `0 <= left <= right < arr.length`
* `threshold <= right - left + 1`
* `2 * threshold > right - left + 1`
* At most `104` calls will be made to `query`. | Consider a DFS traversal of the tree. You can keep track of the current path sum from root to this node, and you can also use DFS to return the minimum value of any path from this node to the leaf. This will tell you if this node is insufficient. |
Python (Simple Maths) | online-majority-element-in-subarray | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass MajorityChecker:\n def __init__(self, arr):\n self.arr = arr\n self.dict1 = defaultdict(list)\n\n for i,x in enumerate(arr):\n self.dict1[x].append(i)\n\n def query(self, left, right, threshold):\n for _ in range(10):\n k = random.randint(left,right)\n res = self.arr[k]\n lo = bisect.bisect_left(self.dict1[res],left)\n hi = bisect.bisect_right(self.dict1[res],right)\n if hi-lo >= threshold: return res\n\n return -1\n\n\n\n\n\n \n\n``` | 1 | Given an integer array `nums`, return _the **maximum possible sum** of elements of the array such that it is divisible by three_.
**Example 1:**
**Input:** nums = \[3,6,5,1,8\]
**Output:** 18
**Explanation:** Pick numbers 3, 6, 1 and 8 their sum is 18 (maximum sum divisible by 3).
**Example 2:**
**Input:** nums = \[4\]
**Output:** 0
**Explanation:** Since 4 is not divisible by 3, do not pick any number.
**Example 3:**
**Input:** nums = \[1,2,3,4,4\]
**Output:** 12
**Explanation:** Pick numbers 1, 3, 4 and 4 their sum is 12 (maximum sum divisible by 3).
**Constraints:**
* `1 <= nums.length <= 4 * 104`
* `1 <= nums[i] <= 104` | What's special about a majority element ? A majority element appears more than half the length of the array number of times. If we tried a random index of the array, what's the probability that this index has a majority element ? It's more than 50% if that array has a majority element. Try a random index for a proper number of times so that the probability of not finding the answer tends to zero. |
Python O(logn) with explanation | online-majority-element-in-subarray | 0 | 1 | ## Intuition\nLet\'s imagine that we\'re solving a simpler problem. Write a class that gets initialized with an array `num` and then calls `verify_num_threshold(left: int, right: int, num: int, threshold: int)`. \n#### PART 1: Verify Candidate [O(logn)]\nOur function should return `true` if `num` occurs `>= threshold` times in `nums[left..right]`. We could answer that query in `O(logn)` time by mapping every occurance of `num` in `nums` to an array of all it\'s `indices`.\nEx:\n```\nnums = [7, 4, 3, 3, 4, 4]\nnums_idx = { 7: [0], 4: [1, 4, 5], 3: [2, 3] }\n```\nNow, we can answer query by binary searching for `left` and `right` in `nums_idx[num]` and finding `l` and `r` such that `nums_idx[num][l] == left` and `nums_idx[num][r] == right`. We will return true if `r - l >= threshold`. This is effectively an LC medium problem.\n\nBut now we have to generate candidates in `nums` to verify. Let\'s consider our options.\n### Part 2: Generate candidates\n##### Option A: Sample numbers randomly from `nums[left..right]` [O(1)]\nIf we know that `threshold` is at minimum a strict majority of `nums[left..right]`, then each random `nums[i]` will have a `> 50%` chance of being our candidate. If we sample randomly `20` times, our chance of "missing" our candidate would be `< 0.00009536743%`. Those are pretty good odds. But let\'s consider other options.\n##### Option B: Pick most common numbers in `nums` [O(n)]\nWe could count the occurances of each element of `nums` and then iterate through those candidates in sorted order from highest to lowest. Because we eventually try every candidate in `nums`, we\'re guaranateed to eventually find the correct answer. But also because we try every candidate in `nums`, our runtime complexity is very poor at `O(n)`.\n##### Option C: Bitwise Prefix Sum [O(1)]\n**Intuition**\nThe problem specifies that `threshold` is always at minimum a *strict majority* of `right-left+1`. That means, we can only ever have one candidate that crosses `threshold`. In cases where you only need to retrieve one candidate `int` in an array of `ints`, bit hacking should be considered as an approach.\nSee: [136. Single Number](https://leetcode.com/problems/single-number/) ; [137. Single Number II](https://leetcode.com/problems/single-number-ii/) ; [260. Single Number III](https://leetcode.com/problems/single-number-iii/)\nIn `__init__`, we can precompute a bitwise prefix sum. `bit_sum[i][b]` is the sum of the b-th bit for all nums in range `[0..i)`. Our index `i` is offset by 1 so that we can query the edge case `bit_sum[0]` representing the start of the array.\nIn `query`, we reconstruct our candidate number\nAs long as `threshold` is a strict majority of `right-left+1`, we can be guaranteed that we only have 1 number that meets `threshold`. But it\'s also possible that no number meets `threshold`. In that case, we should return `-1`. Let\'s consider these 2 cases:\n##### There exists some num in nums[left..right] where count(num) >= threshold\nIn this case, each set bit (`1`) in `num` will occur *at least* `threshold` times in `nums[left..right]` and no unset bit (`0`) in `num` will occur `threshold` times because only one number can cross `threshold`.\nLikewise, each `0` bit will be `1` *less than* `threshold` times in `nums[left..right]`.\nTherefor, we can reconstruct the majority number in `nums[left..right]` simply by adding every bit in our prefix sum `bit_sum[right+1] - bit_sum[left]` where the number of `1`s crosses our threshold.\n##### There does not exist some num where count(num) >= threshold\nIf no num occurs a *majority* of times in `nums[left..right]`, then our reconstructed candidate `num` will simply be a random assortment of bits that happened to occur `>= threshold` times. So this is a problem, right? Not really. We already have our verification step constructed from Step 1. We can simply re-use that to validate whether `num` (effectively a random int) occurs `>= threshold` times and the answer will be no, so we\'ll return `-1`.\n\nIn this case, we\'ve only attempted to verify 1 candidate and that verification takes `O(logn)`. We also generated our candidate in `O(1)` time, so our total runtime complexity is `O(logn)` and our total space complexity is `O(n)` for storing both the `nums_idx` dict and the `bit_sum` prefix sum array.\n\n#### Code\nNow let\'s put part1 and part2 together in code.\n```\nMAX_N = 2*10**4\nMAX_BIT = MAX_N.bit_length()\nclass MajorityChecker:\n\n def __init__(self, nums: List[int]):\n n = len(nums)\n self.bit_sum = [[0] * MAX_BIT for _ in range(n+1)]\n for i in range(1, n+1):\n for b in range(MAX_BIT):\n self.bit_sum[i][b] = self.bit_sum[i-1][b] + ((nums[i-1] >> b) & 1)\n self.num_idx = defaultdict(list)\n for i in range(n):\n self.num_idx[nums[i]].append(i)\n\n def query(self, left: int, right: int, threshold: int) -> int:\n num = 0\n for b in range(MAX_BIT):\n if self.bit_sum[right+1][b] - self.bit_sum[left][b] >= threshold:\n num |= 1 << b\n l = bisect.bisect_left(self.num_idx[num], left)\n r = bisect.bisect_right(self.num_idx[num], right)\n if r-l >= threshold:\n return num\n return -1\n``` | 5 | Design a data structure that efficiently finds the **majority element** of a given subarray.
The **majority element** of a subarray is an element that occurs `threshold` times or more in the subarray.
Implementing the `MajorityChecker` class:
* `MajorityChecker(int[] arr)` Initializes the instance of the class with the given array `arr`.
* `int query(int left, int right, int threshold)` returns the element in the subarray `arr[left...right]` that occurs at least `threshold` times, or `-1` if no such element exists.
**Example 1:**
**Input**
\[ "MajorityChecker ", "query ", "query ", "query "\]
\[\[\[1, 1, 2, 2, 1, 1\]\], \[0, 5, 4\], \[0, 3, 3\], \[2, 3, 2\]\]
**Output**
\[null, 1, -1, 2\]
**Explanation**
MajorityChecker majorityChecker = new MajorityChecker(\[1, 1, 2, 2, 1, 1\]);
majorityChecker.query(0, 5, 4); // return 1
majorityChecker.query(0, 3, 3); // return -1
majorityChecker.query(2, 3, 2); // return 2
**Constraints:**
* `1 <= arr.length <= 2 * 104`
* `1 <= arr[i] <= 2 * 104`
* `0 <= left <= right < arr.length`
* `threshold <= right - left + 1`
* `2 * threshold > right - left + 1`
* At most `104` calls will be made to `query`. | Consider a DFS traversal of the tree. You can keep track of the current path sum from root to this node, and you can also use DFS to return the minimum value of any path from this node to the leaf. This will tell you if this node is insufficient. |
Python O(logn) with explanation | online-majority-element-in-subarray | 0 | 1 | ## Intuition\nLet\'s imagine that we\'re solving a simpler problem. Write a class that gets initialized with an array `num` and then calls `verify_num_threshold(left: int, right: int, num: int, threshold: int)`. \n#### PART 1: Verify Candidate [O(logn)]\nOur function should return `true` if `num` occurs `>= threshold` times in `nums[left..right]`. We could answer that query in `O(logn)` time by mapping every occurance of `num` in `nums` to an array of all it\'s `indices`.\nEx:\n```\nnums = [7, 4, 3, 3, 4, 4]\nnums_idx = { 7: [0], 4: [1, 4, 5], 3: [2, 3] }\n```\nNow, we can answer query by binary searching for `left` and `right` in `nums_idx[num]` and finding `l` and `r` such that `nums_idx[num][l] == left` and `nums_idx[num][r] == right`. We will return true if `r - l >= threshold`. This is effectively an LC medium problem.\n\nBut now we have to generate candidates in `nums` to verify. Let\'s consider our options.\n### Part 2: Generate candidates\n##### Option A: Sample numbers randomly from `nums[left..right]` [O(1)]\nIf we know that `threshold` is at minimum a strict majority of `nums[left..right]`, then each random `nums[i]` will have a `> 50%` chance of being our candidate. If we sample randomly `20` times, our chance of "missing" our candidate would be `< 0.00009536743%`. Those are pretty good odds. But let\'s consider other options.\n##### Option B: Pick most common numbers in `nums` [O(n)]\nWe could count the occurances of each element of `nums` and then iterate through those candidates in sorted order from highest to lowest. Because we eventually try every candidate in `nums`, we\'re guaranateed to eventually find the correct answer. But also because we try every candidate in `nums`, our runtime complexity is very poor at `O(n)`.\n##### Option C: Bitwise Prefix Sum [O(1)]\n**Intuition**\nThe problem specifies that `threshold` is always at minimum a *strict majority* of `right-left+1`. That means, we can only ever have one candidate that crosses `threshold`. In cases where you only need to retrieve one candidate `int` in an array of `ints`, bit hacking should be considered as an approach.\nSee: [136. Single Number](https://leetcode.com/problems/single-number/) ; [137. Single Number II](https://leetcode.com/problems/single-number-ii/) ; [260. Single Number III](https://leetcode.com/problems/single-number-iii/)\nIn `__init__`, we can precompute a bitwise prefix sum. `bit_sum[i][b]` is the sum of the b-th bit for all nums in range `[0..i)`. Our index `i` is offset by 1 so that we can query the edge case `bit_sum[0]` representing the start of the array.\nIn `query`, we reconstruct our candidate number\nAs long as `threshold` is a strict majority of `right-left+1`, we can be guaranteed that we only have 1 number that meets `threshold`. But it\'s also possible that no number meets `threshold`. In that case, we should return `-1`. Let\'s consider these 2 cases:\n##### There exists some num in nums[left..right] where count(num) >= threshold\nIn this case, each set bit (`1`) in `num` will occur *at least* `threshold` times in `nums[left..right]` and no unset bit (`0`) in `num` will occur `threshold` times because only one number can cross `threshold`.\nLikewise, each `0` bit will be `1` *less than* `threshold` times in `nums[left..right]`.\nTherefor, we can reconstruct the majority number in `nums[left..right]` simply by adding every bit in our prefix sum `bit_sum[right+1] - bit_sum[left]` where the number of `1`s crosses our threshold.\n##### There does not exist some num where count(num) >= threshold\nIf no num occurs a *majority* of times in `nums[left..right]`, then our reconstructed candidate `num` will simply be a random assortment of bits that happened to occur `>= threshold` times. So this is a problem, right? Not really. We already have our verification step constructed from Step 1. We can simply re-use that to validate whether `num` (effectively a random int) occurs `>= threshold` times and the answer will be no, so we\'ll return `-1`.\n\nIn this case, we\'ve only attempted to verify 1 candidate and that verification takes `O(logn)`. We also generated our candidate in `O(1)` time, so our total runtime complexity is `O(logn)` and our total space complexity is `O(n)` for storing both the `nums_idx` dict and the `bit_sum` prefix sum array.\n\n#### Code\nNow let\'s put part1 and part2 together in code.\n```\nMAX_N = 2*10**4\nMAX_BIT = MAX_N.bit_length()\nclass MajorityChecker:\n\n def __init__(self, nums: List[int]):\n n = len(nums)\n self.bit_sum = [[0] * MAX_BIT for _ in range(n+1)]\n for i in range(1, n+1):\n for b in range(MAX_BIT):\n self.bit_sum[i][b] = self.bit_sum[i-1][b] + ((nums[i-1] >> b) & 1)\n self.num_idx = defaultdict(list)\n for i in range(n):\n self.num_idx[nums[i]].append(i)\n\n def query(self, left: int, right: int, threshold: int) -> int:\n num = 0\n for b in range(MAX_BIT):\n if self.bit_sum[right+1][b] - self.bit_sum[left][b] >= threshold:\n num |= 1 << b\n l = bisect.bisect_left(self.num_idx[num], left)\n r = bisect.bisect_right(self.num_idx[num], right)\n if r-l >= threshold:\n return num\n return -1\n``` | 5 | Given an integer array `nums`, return _the **maximum possible sum** of elements of the array such that it is divisible by three_.
**Example 1:**
**Input:** nums = \[3,6,5,1,8\]
**Output:** 18
**Explanation:** Pick numbers 3, 6, 1 and 8 their sum is 18 (maximum sum divisible by 3).
**Example 2:**
**Input:** nums = \[4\]
**Output:** 0
**Explanation:** Since 4 is not divisible by 3, do not pick any number.
**Example 3:**
**Input:** nums = \[1,2,3,4,4\]
**Output:** 12
**Explanation:** Pick numbers 1, 3, 4 and 4 their sum is 12 (maximum sum divisible by 3).
**Constraints:**
* `1 <= nums.length <= 4 * 104`
* `1 <= nums[i] <= 104` | What's special about a majority element ? A majority element appears more than half the length of the array number of times. If we tried a random index of the array, what's the probability that this index has a majority element ? It's more than 50% if that array has a majority element. Try a random index for a proper number of times so that the probability of not finding the answer tends to zero. |
Naïve working solution in Python | online-majority-element-in-subarray | 0 | 1 | # Approach\nCache the counter for subarrays.\n\n# Code\n```\nclass MajorityChecker:\n\n def __init__(self, arr: List[int]):\n self.arr = arr\n \n @functools.cache\n def sub_arr_ctr(self, left: int, right: int):\n return collections.Counter(self.arr[left:right+1])\n\n def query(self, left: int, right: int, threshold: int) -> int:\n c = self.sub_arr_ctr(left, right).most_common(1)[0]\n return c[0] if c[1] >= threshold else -1\n``` | 0 | Design a data structure that efficiently finds the **majority element** of a given subarray.
The **majority element** of a subarray is an element that occurs `threshold` times or more in the subarray.
Implementing the `MajorityChecker` class:
* `MajorityChecker(int[] arr)` Initializes the instance of the class with the given array `arr`.
* `int query(int left, int right, int threshold)` returns the element in the subarray `arr[left...right]` that occurs at least `threshold` times, or `-1` if no such element exists.
**Example 1:**
**Input**
\[ "MajorityChecker ", "query ", "query ", "query "\]
\[\[\[1, 1, 2, 2, 1, 1\]\], \[0, 5, 4\], \[0, 3, 3\], \[2, 3, 2\]\]
**Output**
\[null, 1, -1, 2\]
**Explanation**
MajorityChecker majorityChecker = new MajorityChecker(\[1, 1, 2, 2, 1, 1\]);
majorityChecker.query(0, 5, 4); // return 1
majorityChecker.query(0, 3, 3); // return -1
majorityChecker.query(2, 3, 2); // return 2
**Constraints:**
* `1 <= arr.length <= 2 * 104`
* `1 <= arr[i] <= 2 * 104`
* `0 <= left <= right < arr.length`
* `threshold <= right - left + 1`
* `2 * threshold > right - left + 1`
* At most `104` calls will be made to `query`. | Consider a DFS traversal of the tree. You can keep track of the current path sum from root to this node, and you can also use DFS to return the minimum value of any path from this node to the leaf. This will tell you if this node is insufficient. |
Naïve working solution in Python | online-majority-element-in-subarray | 0 | 1 | # Approach\nCache the counter for subarrays.\n\n# Code\n```\nclass MajorityChecker:\n\n def __init__(self, arr: List[int]):\n self.arr = arr\n \n @functools.cache\n def sub_arr_ctr(self, left: int, right: int):\n return collections.Counter(self.arr[left:right+1])\n\n def query(self, left: int, right: int, threshold: int) -> int:\n c = self.sub_arr_ctr(left, right).most_common(1)[0]\n return c[0] if c[1] >= threshold else -1\n``` | 0 | Given an integer array `nums`, return _the **maximum possible sum** of elements of the array such that it is divisible by three_.
**Example 1:**
**Input:** nums = \[3,6,5,1,8\]
**Output:** 18
**Explanation:** Pick numbers 3, 6, 1 and 8 their sum is 18 (maximum sum divisible by 3).
**Example 2:**
**Input:** nums = \[4\]
**Output:** 0
**Explanation:** Since 4 is not divisible by 3, do not pick any number.
**Example 3:**
**Input:** nums = \[1,2,3,4,4\]
**Output:** 12
**Explanation:** Pick numbers 1, 3, 4 and 4 their sum is 12 (maximum sum divisible by 3).
**Constraints:**
* `1 <= nums.length <= 4 * 104`
* `1 <= nums[i] <= 104` | What's special about a majority element ? A majority element appears more than half the length of the array number of times. If we tried a random index of the array, what's the probability that this index has a majority element ? It's more than 50% if that array has a majority element. Try a random index for a proper number of times so that the probability of not finding the answer tends to zero. |
Python3 FT 100%: Query Planning! TC O(sqrt(N*log(N))) Per Query, SC O(N) | online-majority-element-in-subarray | 0 | 1 | # Intuition\n\nWelcome to Big Chungus\'s ~4th ever FT 100% solution. Strap yourself in.\n\nThis is by far the most "fun" time complexity of any solution I\'ve submitted. $O\\left(\\sqrt{N \\log(N)}\\right)$ per query is a weird one. I\'ll prove it later.\n\n## Option 1: The Obvious\n\nWe can find the frequency of all elements in `[left, right]`, then compare to `threshold`.\n\n`threshold` is more than half the number of elements in the range, so we only need to look at the most common element. See `Counter.most_common(1)`.\n\n## Option 2: Huge Range\n\nIf the range is huge, then the obvious approach is O(N). But we have 1e4 queries.\n\nAt first we look like we\'re out of luck.\n\nBut we also know that if the interval size is O(N), then threshold is more than half, and thus also O(N).\n\n**There are at most O(1) elements with frequency O(N)**. If there were more, e.g. O(log(N)), we\'d have O(N log(N)) elements total - an obvious contradiction to having N elements.\n\nSo if the range is huge, we can do this\n* iterate over the most frequent element down to the least frequent\n* if the next frequency is less than threshold, break and return -1 immediately\n* count up how many copies of this value we have in `[left,right]`\n * we can do this by storing the indices of each value\n * then we can use binary search to find the position of the first index >= left and first index > right\n * the difference in these positions is the number of copies in `[left,right]`\n\n# Approach\n\nWhen the range is small, we use Option 1.\n\nWhen the range is huge, we use Option 2.\n\nWhat about the middle? We have to pick one. Now introducing: **query planning**\n\n## Query Planning\n\nQuick background: SQL engines do this same approach. When you provide a query, there are usually several ways a SQL engine can process the results. It can do multiple scans over a table, or do just one scan over a table and store some intermediate results. It can create a hashmap or a tree map. And so on. Cost estimates for each of these can be computed based on parameters. The SQL engines *query planner* evaluates the costs of these options and chooses the cheapest one.\n\n**Side note:** if you use SQL a lot, *pay attention to the SQL server\'s query planning parameters.* Example: if you have a ton of memory, or even host your table from a ramdisk, Postgres recommends making a variety of changes to the query planning parameters. Especially the planner\'s cost parameters for random access overhead. Similarly, be careful around HDDs vs SSDs and their random access.\n\n### Cost Estimate of Option 1\n\nWe need to do an O(range) scan, followed by finding the most frequent element among O(range) unique values. This costs about 2*range in the worst case.\n\n### Cost Estimate of Option 2\n\nThere are at most about `N // threshold` values in `arr` with at least `threshold` copies.\n\nFor each of these `O(N//threshold)` values, we need to do two binary searches to get the number of ranges.\n\nI add a `self.const` parameter to account for the prefactor of binary search versus direct iteration - complexity analysis only gives us the asymptotic cost.\n\n### Minimal Cost Path and Complexity Analysis\n\nFor a range of length `L`, option one costs about 2L.\n\nOption 2 costs about const * N//L * log(N) for the N//L binary searches.\n\n**So we pick the smaller of `2L` and `const*N//L*log(N)`**\n\nSolving for `L` we get the `L <= sqrt(const/2 * N * log(N))`.\n* if L is less than this, we\'ll do option 1: direct iteration\n* if L is larger than this, we\'ll do option 2: trying the most frequent numbers\n\n**For option 1: cost is `O(L) <= O(sqrt(N*log(N)))`**\n\nFor option 2, that will mean the cost is\n```P\ncost = const*N*log(N) / sqrt(1/2)*sqrt(const*N*log(N))\n = sqrt(2*const*N*log(N))\n = O(sqrt(N*log(N)))\n```\n\n# Complexity\n- Time complexity: $O\\left( \\sqrt{N \\log(N)} \\right)$, see the query planning stuff above\n\n- Space complexity: $O(N)$ because we store the array, indices of all elements, and the frequencies of all elements.\n\n# Code\n```\nclass MajorityChecker:\n\n def __init__(self, arr: List[int]):\n # idea:\n # for query left,right,threshold: we obviously need at least threshold copies total\n # so for large thresholds there will only be a few elements\n # for small thresholds, the range is small so we can fall back to direct iteration\n\n # threshold is O(N): there are only O(1) numbers to consider: cumulative counts\n # threshold is O(sqrt(N)): there are O(sqrt(N)) numbers to consider, and O(sqrt(N)) numbers in the range: uncanny valley\n # threshold is O(1): there are O(N) numbers to consider, but only O(1) numbers in the range: direct iteration\n \n # For uncanny valley: O(Q sqrt(N)) is 1e4 * 1e2 roughly is 1e6, doable\n\n # for cumulative counts, we want quick access to count of c in [l,r]\n # binary search? So it\'s really sqrt(N)*log(N)\n\n # I considered a variety of other approaches, but\n # divide-and-conquer is rough because most common element of two subranges can combine in many ways,\n # i.e. top 2 of two intervals combined can be neither of the top two in individual intervals\n\n counts = Counter(arr)\n counts = list((f, v) for v, f in counts.items())\n counts.sort(reverse=True) # by freq desc.\n\n locs = defaultdict(list)\n for i,a in enumerate(arr):\n locs[a].append(i)\n\n self.arr = arr\n self.locs = locs # locs[x] is all indices of x, for log(N) frequency in interval\n self.counts = counts # (f,v) pairs ordered by f desc.\n self.const = 1.0 # lower const: prefer direct iteration over most-to-least-frequent trials\n\n def query(self, left: int, right: int, threshold: int) -> int:\n L = right-left+1\n\n # Query cost: O(L) for direct iteration\n # len(arr)//threshold for numbers with at least `threshold` values\n # * log(L) to get cumulative counts in left,right for each\n if 2*L < self.const*(len(self.arr)//threshold)*log(len(self.arr)):\n # brute force: direct iteration, get counts then get max count and compare to threshold\n counts = Counter(self.arr[i] for i in range(left, right+1))\n v, f = counts.most_common(1)[0]\n return v if f >= threshold else -1\n else:\n # check the few numbers with at least `threshold` copies\n # use indices-of-left/right-index to get count of copies in [left, right]\n for f,v in self.counts:\n if f < threshold: break\n\n idxs = self.locs[v]\n kl = bisect_left(idxs, left)\n if kl == len(idxs): continue\n # idxs[kl] is left or higher\n kr = bisect_right(idxs, right)\n # idxs[kr] is 1 past end, or first value above right\n \n if kr-kl >= threshold: return v\n\n # we tried all numbers with at least threshold counts\n return -1\n \n\n\n\n# Your MajorityChecker object will be instantiated and called as such:\n# obj = MajorityChecker(arr)\n# param_1 = obj.query(left,right,threshold)\n``` | 0 | Design a data structure that efficiently finds the **majority element** of a given subarray.
The **majority element** of a subarray is an element that occurs `threshold` times or more in the subarray.
Implementing the `MajorityChecker` class:
* `MajorityChecker(int[] arr)` Initializes the instance of the class with the given array `arr`.
* `int query(int left, int right, int threshold)` returns the element in the subarray `arr[left...right]` that occurs at least `threshold` times, or `-1` if no such element exists.
**Example 1:**
**Input**
\[ "MajorityChecker ", "query ", "query ", "query "\]
\[\[\[1, 1, 2, 2, 1, 1\]\], \[0, 5, 4\], \[0, 3, 3\], \[2, 3, 2\]\]
**Output**
\[null, 1, -1, 2\]
**Explanation**
MajorityChecker majorityChecker = new MajorityChecker(\[1, 1, 2, 2, 1, 1\]);
majorityChecker.query(0, 5, 4); // return 1
majorityChecker.query(0, 3, 3); // return -1
majorityChecker.query(2, 3, 2); // return 2
**Constraints:**
* `1 <= arr.length <= 2 * 104`
* `1 <= arr[i] <= 2 * 104`
* `0 <= left <= right < arr.length`
* `threshold <= right - left + 1`
* `2 * threshold > right - left + 1`
* At most `104` calls will be made to `query`. | Consider a DFS traversal of the tree. You can keep track of the current path sum from root to this node, and you can also use DFS to return the minimum value of any path from this node to the leaf. This will tell you if this node is insufficient. |
Python3 FT 100%: Query Planning! TC O(sqrt(N*log(N))) Per Query, SC O(N) | online-majority-element-in-subarray | 0 | 1 | # Intuition\n\nWelcome to Big Chungus\'s ~4th ever FT 100% solution. Strap yourself in.\n\nThis is by far the most "fun" time complexity of any solution I\'ve submitted. $O\\left(\\sqrt{N \\log(N)}\\right)$ per query is a weird one. I\'ll prove it later.\n\n## Option 1: The Obvious\n\nWe can find the frequency of all elements in `[left, right]`, then compare to `threshold`.\n\n`threshold` is more than half the number of elements in the range, so we only need to look at the most common element. See `Counter.most_common(1)`.\n\n## Option 2: Huge Range\n\nIf the range is huge, then the obvious approach is O(N). But we have 1e4 queries.\n\nAt first we look like we\'re out of luck.\n\nBut we also know that if the interval size is O(N), then threshold is more than half, and thus also O(N).\n\n**There are at most O(1) elements with frequency O(N)**. If there were more, e.g. O(log(N)), we\'d have O(N log(N)) elements total - an obvious contradiction to having N elements.\n\nSo if the range is huge, we can do this\n* iterate over the most frequent element down to the least frequent\n* if the next frequency is less than threshold, break and return -1 immediately\n* count up how many copies of this value we have in `[left,right]`\n * we can do this by storing the indices of each value\n * then we can use binary search to find the position of the first index >= left and first index > right\n * the difference in these positions is the number of copies in `[left,right]`\n\n# Approach\n\nWhen the range is small, we use Option 1.\n\nWhen the range is huge, we use Option 2.\n\nWhat about the middle? We have to pick one. Now introducing: **query planning**\n\n## Query Planning\n\nQuick background: SQL engines do this same approach. When you provide a query, there are usually several ways a SQL engine can process the results. It can do multiple scans over a table, or do just one scan over a table and store some intermediate results. It can create a hashmap or a tree map. And so on. Cost estimates for each of these can be computed based on parameters. The SQL engines *query planner* evaluates the costs of these options and chooses the cheapest one.\n\n**Side note:** if you use SQL a lot, *pay attention to the SQL server\'s query planning parameters.* Example: if you have a ton of memory, or even host your table from a ramdisk, Postgres recommends making a variety of changes to the query planning parameters. Especially the planner\'s cost parameters for random access overhead. Similarly, be careful around HDDs vs SSDs and their random access.\n\n### Cost Estimate of Option 1\n\nWe need to do an O(range) scan, followed by finding the most frequent element among O(range) unique values. This costs about 2*range in the worst case.\n\n### Cost Estimate of Option 2\n\nThere are at most about `N // threshold` values in `arr` with at least `threshold` copies.\n\nFor each of these `O(N//threshold)` values, we need to do two binary searches to get the number of ranges.\n\nI add a `self.const` parameter to account for the prefactor of binary search versus direct iteration - complexity analysis only gives us the asymptotic cost.\n\n### Minimal Cost Path and Complexity Analysis\n\nFor a range of length `L`, option one costs about 2L.\n\nOption 2 costs about const * N//L * log(N) for the N//L binary searches.\n\n**So we pick the smaller of `2L` and `const*N//L*log(N)`**\n\nSolving for `L` we get the `L <= sqrt(const/2 * N * log(N))`.\n* if L is less than this, we\'ll do option 1: direct iteration\n* if L is larger than this, we\'ll do option 2: trying the most frequent numbers\n\n**For option 1: cost is `O(L) <= O(sqrt(N*log(N)))`**\n\nFor option 2, that will mean the cost is\n```P\ncost = const*N*log(N) / sqrt(1/2)*sqrt(const*N*log(N))\n = sqrt(2*const*N*log(N))\n = O(sqrt(N*log(N)))\n```\n\n# Complexity\n- Time complexity: $O\\left( \\sqrt{N \\log(N)} \\right)$, see the query planning stuff above\n\n- Space complexity: $O(N)$ because we store the array, indices of all elements, and the frequencies of all elements.\n\n# Code\n```\nclass MajorityChecker:\n\n def __init__(self, arr: List[int]):\n # idea:\n # for query left,right,threshold: we obviously need at least threshold copies total\n # so for large thresholds there will only be a few elements\n # for small thresholds, the range is small so we can fall back to direct iteration\n\n # threshold is O(N): there are only O(1) numbers to consider: cumulative counts\n # threshold is O(sqrt(N)): there are O(sqrt(N)) numbers to consider, and O(sqrt(N)) numbers in the range: uncanny valley\n # threshold is O(1): there are O(N) numbers to consider, but only O(1) numbers in the range: direct iteration\n \n # For uncanny valley: O(Q sqrt(N)) is 1e4 * 1e2 roughly is 1e6, doable\n\n # for cumulative counts, we want quick access to count of c in [l,r]\n # binary search? So it\'s really sqrt(N)*log(N)\n\n # I considered a variety of other approaches, but\n # divide-and-conquer is rough because most common element of two subranges can combine in many ways,\n # i.e. top 2 of two intervals combined can be neither of the top two in individual intervals\n\n counts = Counter(arr)\n counts = list((f, v) for v, f in counts.items())\n counts.sort(reverse=True) # by freq desc.\n\n locs = defaultdict(list)\n for i,a in enumerate(arr):\n locs[a].append(i)\n\n self.arr = arr\n self.locs = locs # locs[x] is all indices of x, for log(N) frequency in interval\n self.counts = counts # (f,v) pairs ordered by f desc.\n self.const = 1.0 # lower const: prefer direct iteration over most-to-least-frequent trials\n\n def query(self, left: int, right: int, threshold: int) -> int:\n L = right-left+1\n\n # Query cost: O(L) for direct iteration\n # len(arr)//threshold for numbers with at least `threshold` values\n # * log(L) to get cumulative counts in left,right for each\n if 2*L < self.const*(len(self.arr)//threshold)*log(len(self.arr)):\n # brute force: direct iteration, get counts then get max count and compare to threshold\n counts = Counter(self.arr[i] for i in range(left, right+1))\n v, f = counts.most_common(1)[0]\n return v if f >= threshold else -1\n else:\n # check the few numbers with at least `threshold` copies\n # use indices-of-left/right-index to get count of copies in [left, right]\n for f,v in self.counts:\n if f < threshold: break\n\n idxs = self.locs[v]\n kl = bisect_left(idxs, left)\n if kl == len(idxs): continue\n # idxs[kl] is left or higher\n kr = bisect_right(idxs, right)\n # idxs[kr] is 1 past end, or first value above right\n \n if kr-kl >= threshold: return v\n\n # we tried all numbers with at least threshold counts\n return -1\n \n\n\n\n# Your MajorityChecker object will be instantiated and called as such:\n# obj = MajorityChecker(arr)\n# param_1 = obj.query(left,right,threshold)\n``` | 0 | Given an integer array `nums`, return _the **maximum possible sum** of elements of the array such that it is divisible by three_.
**Example 1:**
**Input:** nums = \[3,6,5,1,8\]
**Output:** 18
**Explanation:** Pick numbers 3, 6, 1 and 8 their sum is 18 (maximum sum divisible by 3).
**Example 2:**
**Input:** nums = \[4\]
**Output:** 0
**Explanation:** Since 4 is not divisible by 3, do not pick any number.
**Example 3:**
**Input:** nums = \[1,2,3,4,4\]
**Output:** 12
**Explanation:** Pick numbers 1, 3, 4 and 4 their sum is 12 (maximum sum divisible by 3).
**Constraints:**
* `1 <= nums.length <= 4 * 104`
* `1 <= nums[i] <= 104` | What's special about a majority element ? A majority element appears more than half the length of the array number of times. If we tried a random index of the array, what's the probability that this index has a majority element ? It's more than 50% if that array has a majority element. Try a random index for a proper number of times so that the probability of not finding the answer tends to zero. |
1157. Online Majority Element In Subarray | online-majority-element-in-subarray | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Node:\n def __init__(self):\n self.l = self.r = 0\n self.x = self.cnt = 0\n\n\nclass SegmentTree:\n def __init__(self, nums):\n self.nums = nums\n n = len(nums)\n self.tr = [Node() for _ in range(n << 2)]\n self.build(1, 1, n)\n\n def build(self, u, l, r):\n self.tr[u].l, self.tr[u].r = l, r\n if l == r:\n self.tr[u].x = self.nums[l - 1]\n self.tr[u].cnt = 1\n return\n mid = (l + r) >> 1\n self.build(u << 1, l, mid)\n self.build(u << 1 | 1, mid + 1, r)\n self.pushup(u)\n\n def query(self, u, l, r):\n if self.tr[u].l >= l and self.tr[u].r <= r:\n return self.tr[u].x, self.tr[u].cnt\n mid = (self.tr[u].l + self.tr[u].r) >> 1\n if r <= mid:\n return self.query(u << 1, l, r)\n if l > mid:\n return self.query(u << 1 | 1, l, r)\n x1, cnt1 = self.query(u << 1, l, r)\n x2, cnt2 = self.query(u << 1 | 1, l, r)\n if x1 == x2:\n return x1, cnt1 + cnt2\n if cnt1 >= cnt2:\n return x1, cnt1 - cnt2\n else:\n return x2, cnt2 - cnt1\n\n def pushup(self, u):\n if self.tr[u << 1].x == self.tr[u << 1 | 1].x:\n self.tr[u].x = self.tr[u << 1].x\n self.tr[u].cnt = self.tr[u << 1].cnt + self.tr[u << 1 | 1].cnt\n elif self.tr[u << 1].cnt >= self.tr[u << 1 | 1].cnt:\n self.tr[u].x = self.tr[u << 1].x\n self.tr[u].cnt = self.tr[u << 1].cnt - self.tr[u << 1 | 1].cnt\n else:\n self.tr[u].x = self.tr[u << 1 | 1].x\n self.tr[u].cnt = self.tr[u << 1 | 1].cnt - self.tr[u << 1].cnt\n\n\nclass MajorityChecker:\n def __init__(self, arr: List[int]):\n self.tree = SegmentTree(arr)\n self.d = defaultdict(list)\n for i, x in enumerate(arr):\n self.d[x].append(i)\n\n def query(self, left: int, right: int, threshold: int) -> int:\n x, _ = self.tree.query(1, left + 1, right + 1)\n l = bisect_left(self.d[x], left)\n r = bisect_left(self.d[x], right + 1)\n return x if r - l >= threshold else -1\n\n\n# Your MajorityChecker object will be instantiated and called as such:\n# obj = MajorityChecker(arr)\n# param_1 = obj.query(left,right,threshold)\n``` | 0 | Design a data structure that efficiently finds the **majority element** of a given subarray.
The **majority element** of a subarray is an element that occurs `threshold` times or more in the subarray.
Implementing the `MajorityChecker` class:
* `MajorityChecker(int[] arr)` Initializes the instance of the class with the given array `arr`.
* `int query(int left, int right, int threshold)` returns the element in the subarray `arr[left...right]` that occurs at least `threshold` times, or `-1` if no such element exists.
**Example 1:**
**Input**
\[ "MajorityChecker ", "query ", "query ", "query "\]
\[\[\[1, 1, 2, 2, 1, 1\]\], \[0, 5, 4\], \[0, 3, 3\], \[2, 3, 2\]\]
**Output**
\[null, 1, -1, 2\]
**Explanation**
MajorityChecker majorityChecker = new MajorityChecker(\[1, 1, 2, 2, 1, 1\]);
majorityChecker.query(0, 5, 4); // return 1
majorityChecker.query(0, 3, 3); // return -1
majorityChecker.query(2, 3, 2); // return 2
**Constraints:**
* `1 <= arr.length <= 2 * 104`
* `1 <= arr[i] <= 2 * 104`
* `0 <= left <= right < arr.length`
* `threshold <= right - left + 1`
* `2 * threshold > right - left + 1`
* At most `104` calls will be made to `query`. | Consider a DFS traversal of the tree. You can keep track of the current path sum from root to this node, and you can also use DFS to return the minimum value of any path from this node to the leaf. This will tell you if this node is insufficient. |
1157. Online Majority Element In Subarray | online-majority-element-in-subarray | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Node:\n def __init__(self):\n self.l = self.r = 0\n self.x = self.cnt = 0\n\n\nclass SegmentTree:\n def __init__(self, nums):\n self.nums = nums\n n = len(nums)\n self.tr = [Node() for _ in range(n << 2)]\n self.build(1, 1, n)\n\n def build(self, u, l, r):\n self.tr[u].l, self.tr[u].r = l, r\n if l == r:\n self.tr[u].x = self.nums[l - 1]\n self.tr[u].cnt = 1\n return\n mid = (l + r) >> 1\n self.build(u << 1, l, mid)\n self.build(u << 1 | 1, mid + 1, r)\n self.pushup(u)\n\n def query(self, u, l, r):\n if self.tr[u].l >= l and self.tr[u].r <= r:\n return self.tr[u].x, self.tr[u].cnt\n mid = (self.tr[u].l + self.tr[u].r) >> 1\n if r <= mid:\n return self.query(u << 1, l, r)\n if l > mid:\n return self.query(u << 1 | 1, l, r)\n x1, cnt1 = self.query(u << 1, l, r)\n x2, cnt2 = self.query(u << 1 | 1, l, r)\n if x1 == x2:\n return x1, cnt1 + cnt2\n if cnt1 >= cnt2:\n return x1, cnt1 - cnt2\n else:\n return x2, cnt2 - cnt1\n\n def pushup(self, u):\n if self.tr[u << 1].x == self.tr[u << 1 | 1].x:\n self.tr[u].x = self.tr[u << 1].x\n self.tr[u].cnt = self.tr[u << 1].cnt + self.tr[u << 1 | 1].cnt\n elif self.tr[u << 1].cnt >= self.tr[u << 1 | 1].cnt:\n self.tr[u].x = self.tr[u << 1].x\n self.tr[u].cnt = self.tr[u << 1].cnt - self.tr[u << 1 | 1].cnt\n else:\n self.tr[u].x = self.tr[u << 1 | 1].x\n self.tr[u].cnt = self.tr[u << 1 | 1].cnt - self.tr[u << 1].cnt\n\n\nclass MajorityChecker:\n def __init__(self, arr: List[int]):\n self.tree = SegmentTree(arr)\n self.d = defaultdict(list)\n for i, x in enumerate(arr):\n self.d[x].append(i)\n\n def query(self, left: int, right: int, threshold: int) -> int:\n x, _ = self.tree.query(1, left + 1, right + 1)\n l = bisect_left(self.d[x], left)\n r = bisect_left(self.d[x], right + 1)\n return x if r - l >= threshold else -1\n\n\n# Your MajorityChecker object will be instantiated and called as such:\n# obj = MajorityChecker(arr)\n# param_1 = obj.query(left,right,threshold)\n``` | 0 | Given an integer array `nums`, return _the **maximum possible sum** of elements of the array such that it is divisible by three_.
**Example 1:**
**Input:** nums = \[3,6,5,1,8\]
**Output:** 18
**Explanation:** Pick numbers 3, 6, 1 and 8 their sum is 18 (maximum sum divisible by 3).
**Example 2:**
**Input:** nums = \[4\]
**Output:** 0
**Explanation:** Since 4 is not divisible by 3, do not pick any number.
**Example 3:**
**Input:** nums = \[1,2,3,4,4\]
**Output:** 12
**Explanation:** Pick numbers 1, 3, 4 and 4 their sum is 12 (maximum sum divisible by 3).
**Constraints:**
* `1 <= nums.length <= 4 * 104`
* `1 <= nums[i] <= 104` | What's special about a majority element ? A majority element appears more than half the length of the array number of times. If we tried a random index of the array, what's the probability that this index has a majority element ? It's more than 50% if that array has a majority element. Try a random index for a proper number of times so that the probability of not finding the answer tends to zero. |
Solution using Counter and lru_cache | online-majority-element-in-subarray | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nMy first thought was, this is a question involving counting, so\nwhy not use Python\'s nifty ```Counter``` class.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nFor each query, construct a Counter using the elements in \nsubarray ```arr[left,right+1]```, then take its ```most_common``` element and return it. I tried this initially and ran out of time\non a large test case. I then noticed that for this case, the same values for ```left``` and ```right``` were used in many (all?) of the queries. So, to speed up this case, I wrapped the call to construct a counter from ```arr[left,right+1]``` and take its ```most_common``` element. Then, I prefixed that function with ```lru_cache( None )```,\nwhich will cache a return the values, bypassing the construction, etc.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe time complexity for ```query``` without caching is $$O(n)$$. With caching, it is somewhat less depending on the amount of repetition in the queries. \n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity varies conversely: the more repetition of the subarrays, the less the storage, and vice versa. Worst case, it will vary as the number of queries.\n# Code\n```\nclass MajorityChecker:\n\n def __init__(self, arr: List[int]):\n self.arr = arr \n\n def query(self, left: int, right: int, threshold: int) -> int:\n element, count = self.cachedCounter( left, right )\n if count >= threshold:\n return element\n else:\n return -1\n\n @lru_cache\n def cachedCounter( self, left, right ):\n return Counter( self.arr[ left : right + 1 ] ).most_common( 1 )[ 0 ] \n\n\n# Your MajorityChecker object will be instantiated and called as such:\n# obj = MajorityChecker(arr)\n# param_1 = obj.query(left,right,threshold)\n``` | 0 | Design a data structure that efficiently finds the **majority element** of a given subarray.
The **majority element** of a subarray is an element that occurs `threshold` times or more in the subarray.
Implementing the `MajorityChecker` class:
* `MajorityChecker(int[] arr)` Initializes the instance of the class with the given array `arr`.
* `int query(int left, int right, int threshold)` returns the element in the subarray `arr[left...right]` that occurs at least `threshold` times, or `-1` if no such element exists.
**Example 1:**
**Input**
\[ "MajorityChecker ", "query ", "query ", "query "\]
\[\[\[1, 1, 2, 2, 1, 1\]\], \[0, 5, 4\], \[0, 3, 3\], \[2, 3, 2\]\]
**Output**
\[null, 1, -1, 2\]
**Explanation**
MajorityChecker majorityChecker = new MajorityChecker(\[1, 1, 2, 2, 1, 1\]);
majorityChecker.query(0, 5, 4); // return 1
majorityChecker.query(0, 3, 3); // return -1
majorityChecker.query(2, 3, 2); // return 2
**Constraints:**
* `1 <= arr.length <= 2 * 104`
* `1 <= arr[i] <= 2 * 104`
* `0 <= left <= right < arr.length`
* `threshold <= right - left + 1`
* `2 * threshold > right - left + 1`
* At most `104` calls will be made to `query`. | Consider a DFS traversal of the tree. You can keep track of the current path sum from root to this node, and you can also use DFS to return the minimum value of any path from this node to the leaf. This will tell you if this node is insufficient. |
Solution using Counter and lru_cache | online-majority-element-in-subarray | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nMy first thought was, this is a question involving counting, so\nwhy not use Python\'s nifty ```Counter``` class.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nFor each query, construct a Counter using the elements in \nsubarray ```arr[left,right+1]```, then take its ```most_common``` element and return it. I tried this initially and ran out of time\non a large test case. I then noticed that for this case, the same values for ```left``` and ```right``` were used in many (all?) of the queries. So, to speed up this case, I wrapped the call to construct a counter from ```arr[left,right+1]``` and take its ```most_common``` element. Then, I prefixed that function with ```lru_cache( None )```,\nwhich will cache a return the values, bypassing the construction, etc.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe time complexity for ```query``` without caching is $$O(n)$$. With caching, it is somewhat less depending on the amount of repetition in the queries. \n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity varies conversely: the more repetition of the subarrays, the less the storage, and vice versa. Worst case, it will vary as the number of queries.\n# Code\n```\nclass MajorityChecker:\n\n def __init__(self, arr: List[int]):\n self.arr = arr \n\n def query(self, left: int, right: int, threshold: int) -> int:\n element, count = self.cachedCounter( left, right )\n if count >= threshold:\n return element\n else:\n return -1\n\n @lru_cache\n def cachedCounter( self, left, right ):\n return Counter( self.arr[ left : right + 1 ] ).most_common( 1 )[ 0 ] \n\n\n# Your MajorityChecker object will be instantiated and called as such:\n# obj = MajorityChecker(arr)\n# param_1 = obj.query(left,right,threshold)\n``` | 0 | Given an integer array `nums`, return _the **maximum possible sum** of elements of the array such that it is divisible by three_.
**Example 1:**
**Input:** nums = \[3,6,5,1,8\]
**Output:** 18
**Explanation:** Pick numbers 3, 6, 1 and 8 their sum is 18 (maximum sum divisible by 3).
**Example 2:**
**Input:** nums = \[4\]
**Output:** 0
**Explanation:** Since 4 is not divisible by 3, do not pick any number.
**Example 3:**
**Input:** nums = \[1,2,3,4,4\]
**Output:** 12
**Explanation:** Pick numbers 1, 3, 4 and 4 their sum is 12 (maximum sum divisible by 3).
**Constraints:**
* `1 <= nums.length <= 4 * 104`
* `1 <= nums[i] <= 104` | What's special about a majority element ? A majority element appears more than half the length of the array number of times. If we tried a random index of the array, what's the probability that this index has a majority element ? It's more than 50% if that array has a majority element. Try a random index for a proper number of times so that the probability of not finding the answer tends to zero. |
Python Easy Solution | online-majority-element-in-subarray | 0 | 1 | # Code\n```\nclass MajorityChecker(object):\n\n def __init__(self, A):\n a2i = collections.defaultdict(list)\n for i, x in enumerate(A):\n a2i[x].append(i)\n self.A, self.a2i = A, a2i\n\n def query(self, left, right, threshold):\n for _ in range(20):\n a = self.A[random.randint(left, right)]\n l = bisect.bisect_left(self.a2i[a], left)\n r = bisect.bisect_right(self.a2i[a], right)\n if r - l >= threshold:\n return a\n return -1\n``` | 0 | Design a data structure that efficiently finds the **majority element** of a given subarray.
The **majority element** of a subarray is an element that occurs `threshold` times or more in the subarray.
Implementing the `MajorityChecker` class:
* `MajorityChecker(int[] arr)` Initializes the instance of the class with the given array `arr`.
* `int query(int left, int right, int threshold)` returns the element in the subarray `arr[left...right]` that occurs at least `threshold` times, or `-1` if no such element exists.
**Example 1:**
**Input**
\[ "MajorityChecker ", "query ", "query ", "query "\]
\[\[\[1, 1, 2, 2, 1, 1\]\], \[0, 5, 4\], \[0, 3, 3\], \[2, 3, 2\]\]
**Output**
\[null, 1, -1, 2\]
**Explanation**
MajorityChecker majorityChecker = new MajorityChecker(\[1, 1, 2, 2, 1, 1\]);
majorityChecker.query(0, 5, 4); // return 1
majorityChecker.query(0, 3, 3); // return -1
majorityChecker.query(2, 3, 2); // return 2
**Constraints:**
* `1 <= arr.length <= 2 * 104`
* `1 <= arr[i] <= 2 * 104`
* `0 <= left <= right < arr.length`
* `threshold <= right - left + 1`
* `2 * threshold > right - left + 1`
* At most `104` calls will be made to `query`. | Consider a DFS traversal of the tree. You can keep track of the current path sum from root to this node, and you can also use DFS to return the minimum value of any path from this node to the leaf. This will tell you if this node is insufficient. |
Python Easy Solution | online-majority-element-in-subarray | 0 | 1 | # Code\n```\nclass MajorityChecker(object):\n\n def __init__(self, A):\n a2i = collections.defaultdict(list)\n for i, x in enumerate(A):\n a2i[x].append(i)\n self.A, self.a2i = A, a2i\n\n def query(self, left, right, threshold):\n for _ in range(20):\n a = self.A[random.randint(left, right)]\n l = bisect.bisect_left(self.a2i[a], left)\n r = bisect.bisect_right(self.a2i[a], right)\n if r - l >= threshold:\n return a\n return -1\n``` | 0 | Given an integer array `nums`, return _the **maximum possible sum** of elements of the array such that it is divisible by three_.
**Example 1:**
**Input:** nums = \[3,6,5,1,8\]
**Output:** 18
**Explanation:** Pick numbers 3, 6, 1 and 8 their sum is 18 (maximum sum divisible by 3).
**Example 2:**
**Input:** nums = \[4\]
**Output:** 0
**Explanation:** Since 4 is not divisible by 3, do not pick any number.
**Example 3:**
**Input:** nums = \[1,2,3,4,4\]
**Output:** 12
**Explanation:** Pick numbers 1, 3, 4 and 4 their sum is 12 (maximum sum divisible by 3).
**Constraints:**
* `1 <= nums.length <= 4 * 104`
* `1 <= nums[i] <= 104` | What's special about a majority element ? A majority element appears more than half the length of the array number of times. If we tried a random index of the array, what's the probability that this index has a majority element ? It's more than 50% if that array has a majority element. Try a random index for a proper number of times so that the probability of not finding the answer tends to zero. |
【Video】Give me 5 minutes - How we think about a solution | find-words-that-can-be-formed-by-characters | 1 | 1 | # Intuition\nCount frequency of each character.\n\n---\n\n# Solution Video\n\nhttps://youtu.be/6LrW0uIlqX8\n\n\u25A0 Timeline of the video\n\n`0:05` How we can solve "Find Words That Can Be Formed by Characters"\n`3:48` What if we have extra character when specific character was already 0 in HashMap?\n`4:17` Coding\n`7:45` Time Complexity and Space Complexity\n`8:17` Step by step algorithm with my solution code\n\n### \u2B50\uFE0F\u2B50\uFE0F Don\'t forget to subscribe to my channel! \u2B50\uFE0F\u2B50\uFE0F\n\n**\u25A0 Subscribe URL**\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\nSubscribers: 3,351\nMy first goal is 10,000 (It\'s far from done \uD83D\uDE05)\nThank you for your support!\n\n**My channel reached 3,000 subscribers these days. Thank you so much for your support!**\n\n---\n\n# Approach\n\n## How we think about a solution\n\nFirst of all, we need to know how many each character we have, so count each character with `chars` input.\n\n```\nInput: words = ["cat","bt","hat","tree"], chars = "atach"\n```\nI use `HashMap`.\n```\nch = {\'a\': 2, \'t\': 1, \'c\': 1, \'h\': 1}\n```\nThen check characters of `words` input one by one. If we find the same character in `ch`, countdown values with `-1`. But problem is every time we start to check a new word, we want to use `ch`.\n\nIf we do,\n```\ncopy = ch\n```\nthen, we change `copy`. In that case, `ch` is also affected by the change.\n\nTo avoid the problem, use \n```\ncopy = ch.copy()\n```\nso that `ch` is not affected by the change in this case.\n\n---\n\n\u2B50\uFE0F Points\n\nUse \n```\ncopy = ch.copy()\n```\ninstead of \n```\ncopy = ch\n```\n---\n\nLet\'s check one by one.\n```\nInput: words = ["cat","bt","hat","tree"], chars = "atach"\n```\n```\nch = {\'a\': 2, \'t\': 1, \'c\': 1, \'h\': 1}\nword = cat\n\nFind `c`, `a`, `t`.\nso,\nch = {\'a\': 2, \'t\': 1, \'c\': 0, \'h\': 1} (= Find `c`)\nch = {\'a\': 1, \'t\': 1, \'c\': 0, \'h\': 1} (= Find `a`)\nch = {\'a\': 1, \'t\': 0, \'c\': 0, \'h\': 1} (= Find `t`)\n\nWe can create cat with atach.\n\nres = 3\n```\nNext\n```\nch = {\'a\': 2, \'t\': 1, \'c\': 1, \'h\': 1}\nword = bt\n\nFind `b`, `t`.\nso, but no `b` in ch\n\nWe can\'t create cat with atach.\n\nres = 3\n```\nNext\n```\nch = {\'a\': 2, \'t\': 1, \'c\': 1, \'h\': 1}\nword = hat\n\nFind `h`, `a`, \'t\'.\nch = {\'a\': 2, \'t\': 1, \'c\': 1, \'h\': 0} (= Find `h`)\nch = {\'a\': 1, \'t\': 1, \'c\': 1, \'h\': 0} (= Find `a`)\nch = {\'a\': 1, \'t\': 0, \'c\': 1, \'h\': 0} (= Find `t`)\n\nWe can create cat with atach.\n\nres = 6\n```\nNext\n```\nch = {\'a\': 2, \'t\': 1, \'c\': 1, \'h\': 1}\nword = tree\n\nFind `t`, `r`, \'e\', \'e\'.\nch = {\'a\': 2, \'t\': 0, \'c\': 1, \'h\': 1} (= Find `t`)\nbut no `r`\n\nWe can\'t create cat with atach.\n\nres = 6\n```\n```\nOutput: 6\n```\nWhat if we have extra character when specific character was already `0` in HashMap?\n\nLet\'s say next character is `a` but current HashMap is\n```\nch = {\'a\': 0, \'t\': 1, \'c\': 1, \'h\': 1}\n```\n\nIn this case, we can\'t create the word because it has at least `3` a, so we don\'t add length of the word.\n\n\n---\n\n\n\nLet\'s break down the algorithm step by step:\n\n1. **Initialize a dictionary `ch` to store the character counts in `chars`:**\n ```python\n ch = {}\n ```\n\n2. **Count the occurrences of each character in `chars` and store it in the dictionary `ch`:**\n ```python\n for c in chars:\n ch[c] = 1 + ch.get(c, 0)\n ```\n This loop iterates over each character in `chars`. If the character `c` is already in the dictionary `ch`, its count is incremented by 1. If it\'s not present, its count is set to 1.\n\n3. **Initialize a variable `res` to store the result:**\n ```python\n res = 0\n ```\n\n4. **Iterate over each word in the `words` array:**\n ```python\n for word in words:\n ```\n This loop goes through each word in the `words` array.\n\n5. **Create a copy of the `ch` dictionary for each word:**\n ```python\n copy = ch.copy()\n ```\n This creates a shallow copy of the original dictionary `ch` for each word.\n\n6. **Iterate over each character in the current word:**\n ```python\n for c in word:\n ```\n This loop goes through each character in the current word.\n\n7. **Check if the character is in the copy dictionary and has a count greater than 0:**\n ```python\n if c in copy and copy[c] != 0:\n ```\n If the character is present in the copy dictionary and its count is not zero, it means the character is available for use in the word.\n\n8. **Decrement the count of the character in the copy dictionary:**\n ```python\n copy[c] -= 1\n ```\n\n9. **If the character is not in the copy dictionary or its count is zero:**\n ```python\n else:\n res -= len(word)\n break\n ```\n If the character is not present in the copy dictionary or its count is already zero, subtract the length of the current word from the result (`res`) and break out of the loop.\n\n The reason why we subtract current length of word from `res` is that in step 10, we add current length of word to `res`, so if we subtract current length before break, we don\'t have to use if statement in step 10. Of course you can use flag or something with if statement. \n\n10. **Add the length of the word to the result:**\n ```python\n res += len(word)\n ```\n If the loop completes without breaking, add the length of the word to the result.\n\n11. **Return the final result:**\n ```python\n return res\n ```\n The function returns the accumulated result.\n\nThis algorithm essentially checks if the characters in the words can be formed using the characters in the `chars` string, considering their counts. If yes, it increments the result by the length of the word; otherwise, it decrements the result by the length of the word. The final result represents the total length of words that can be formed.\n\n\n---\n\n\n\n# Complexity\n- Time complexity: $$O(nm + mk)$$\nIterating over words: This takes $$O(m)$$ time, where m is the number of words.\nCopying the dictionary ch: This takes $$O(n)$$ time.\nIterating over word: This takes $$O(k)$$ time, where k is the length of the current word.\n\n- Space complexity: $$O(n)$$\n`ch` requires space to store the character frequencies.\n\n```python []\nclass Solution:\n def countCharacters(self, words: List[str], chars: str) -> int:\n ch = {}\n\n for c in chars:\n ch[c] = 1 + ch.get(c, 0)\n\n res = 0\n for word in words:\n copy = ch.copy()\n\n for c in word:\n if c in copy and copy[c] != 0:\n copy[c] -= 1\n else:\n res -= len(word) \n break\n \n res += len(word)\n \n return res\n```\n```javascript []\nvar countCharacters = function(words, chars) {\n const ch = {};\n\n for (const c of chars) {\n ch[c] = 1 + (ch[c] || 0);\n }\n\n let res = 0;\n for (const word of words) {\n const copy = { ...ch };\n\n for (const c of word) {\n if (c in copy && copy[c] !== 0) {\n copy[c] -= 1;\n } else {\n res -= word.length;\n break;\n }\n }\n\n res += word.length;\n }\n\n return res; \n};\n```\n```java []\nclass Solution {\n public int countCharacters(String[] words, String chars) {\n Map<Character, Integer> ch = new HashMap<>();\n\n for (char c : chars.toCharArray()) {\n ch.put(c, 1 + ch.getOrDefault(c, 0));\n }\n\n int res = 0;\n for (String word : words) {\n Map<Character, Integer> copy = new HashMap<>(ch);\n\n for (char c : word.toCharArray()) {\n if (copy.containsKey(c) && copy.get(c) != 0) {\n copy.put(c, copy.get(c) - 1);\n } else {\n res -= word.length();\n break;\n }\n }\n\n res += word.length();\n }\n\n return res; \n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int countCharacters(vector<string>& words, string chars) {\n unordered_map<char, int> ch;\n\n for (char c : chars) {\n ch[c] = 1 + ch[c];\n }\n\n int res = 0;\n for (const string& word : words) {\n unordered_map<char, int> copy = ch;\n\n for (char c : word) {\n if (copy.find(c) != copy.end() && copy[c] != 0) {\n copy[c] -= 1;\n } else {\n res -= word.length();\n break;\n }\n }\n\n res += word.length();\n }\n\n return res; \n }\n};\n```\n\n---\n\nThank you for reading my post.\n\u2B50\uFE0F Please upvote it and don\'t forget to subscribe to my channel!\n\n\u25A0 Subscribe URL\nhttp://www.youtube.com/channel/UC9RMNwYTL3SXCP6ShLWVFww?sub_confirmation=1\n\n\u25A0 Twitter\nhttps://twitter.com/CodingNinjaAZ\n\n### My previous daily coding challenge post and video.\n\npost\nhttps://leetcode.com/problems/check-if-two-string-arrays-are-equivalent/solutions/4348512/video-give-me-5-minutes-how-we-think-about-a-solution/\n\nvideo\nhttps://youtu.be/K83jwzyPyQU\n\n\u25A0 Timeline of the video\n\n`0:05` Simple way to solve this question\n`1:07` Demonstrate how it works\n`4:55` Coding\n`8:00` Time Complexity and Space Complexity\n`8:26` Step by step algorithm with my solution code\n | 22 | You are given an array of strings `words` and a string `chars`.
A string is **good** if it can be formed by characters from chars (each character can only be used once).
Return _the sum of lengths of all good strings in words_.
**Example 1:**
**Input:** words = \[ "cat ", "bt ", "hat ", "tree "\], chars = "atach "
**Output:** 6
**Explanation:** The strings that can be formed are "cat " and "hat " so the answer is 3 + 3 = 6.
**Example 2:**
**Input:** words = \[ "hello ", "world ", "leetcode "\], chars = "welldonehoneyr "
**Output:** 10
**Explanation:** The strings that can be formed are "hello " and "world " so the answer is 5 + 5 = 10.
**Constraints:**
* `1 <= words.length <= 1000`
* `1 <= words[i].length, chars.length <= 100`
* `words[i]` and `chars` consist of lowercase English letters. | Try to build the string with a backtracking DFS by considering what you can put in every position. |
✅☑[C++/Java/Python/JavaScript] || 2 Approaches || EXPLAINED🔥 | find-words-that-can-be-formed-by-characters | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n**(Also explained in the code)**\n\n#### ***Approach 1(Count With Hash Map)***\n1. **Initialization:**\n\n - The function initializes an unordered map `counts` to store the count of characters in the `chars` string.\n1. **Counting Characters:**\n\n - It iterates through each character in the `chars` string and increments the count of each character in the `counts` map.\n1. **Iterating Through Words:**\n\n - The function then initializes a variable `ans` to accumulate the count of characters from `words` that can be formed using the characters in `chars`.\n1. **Nested Loop - Word Counting:**\n\n - For each word in the `words` vector, it initializes another unordered map `wordCount` to count the frequency of characters in that word.\n1. **Check for Valid Words:**\n\n - It iterates through each character in the `word` and increments its count in `wordCount`.\n1. **Validation - Character Comparison:**\n\n - It checks if the characters in the current word can be formed using the characters available in `chars`.\n - For each character in the `word`, it compares the frequency of that character in wordCount with the frequency of that character in `counts`.\n1. **Accumulating Valid Words:**\n\n - If the current word can be formed using the available characters in `chars`, it adds the length of that word to the `ans`.\n1. **Return Result:**\n\n - Finally, the function returns the accumulated count `ans`, which represents the total length of words that can be formed using the characters in `chars`.\n\n# Complexity\n- *Time complexity:*\n $$O(n+m\u22C5k)$$\n \n\n- *Space complexity:*\n $$O(1)$$\n \n\n\n# Code\n```C++ []\n\n\nclass Solution {\npublic:\n int countCharacters(std::vector<std::string>& words, std::string chars) {\n // Create a map to store character counts in the \'chars\' string\n std::unordered_map<char, int> counts;\n \n // Count the frequency of each character in the \'chars\' string\n for (char c : chars) {\n counts[c]++;\n }\n \n int ans = 0;\n // Loop through each word in the \'words\' vector\n for (std::string word : words) {\n // Create a map to store character counts in the current \'word\'\n std::unordered_map<char, int> wordCount;\n \n // Count the frequency of each character in the current word\n for (char c : word) {\n wordCount[c]++;\n }\n \n bool good = true;\n // Check if the current word can be formed from the characters in \'chars\'\n for (auto& [c, freq] : wordCount) {\n // If the count of a character in \'chars\' is less than the count in the current word\n if (counts[c] < freq) {\n good = false; // The word cannot be formed, set \'good\' flag to false\n break; // Exit the loop as the word is not valid\n }\n }\n \n // If the word can be formed from the characters in \'chars\', add its length to \'ans\'\n if (good) {\n ans += word.size();\n }\n }\n \n return ans; // Return the total length of words that can be formed\n }\n};\n\n\n\n```\n```C []\n\n\n\nint countCharacters(char** words, int wordsSize, char* chars) {\n int counts[26] = {0}; // Array to store character counts, assuming lowercase English alphabet\n\n // Count the frequency of each character in \'chars\'\n for (int i = 0; chars[i] != \'\\0\'; i++) {\n counts[chars[i] - \'a\']++;\n }\n\n int ans = 0;\n\n // Loop through each word in the \'words\' array\n for (int j = 0; j < wordsSize; j++) {\n int wordCount[26] = {0}; // Array to store character counts in the current \'word\'\n\n // Count the frequency of each character in the current word\n for (int k = 0; words[j][k] != \'\\0\'; k++) {\n wordCount[words[j][k] - \'a\']++;\n }\n\n int good = 1;\n\n // Check if the current word can be formed from the characters in \'chars\'\n for (int l = 0; l < 26; l++) {\n if (wordCount[l] > counts[l]) {\n good = 0; // The word cannot be formed, set \'good\' flag to 0\n break; // Exit the loop as the word is not valid\n }\n }\n\n // If the word can be formed from the characters in \'chars\', add its length to \'ans\'\n if (good) {\n ans += strlen(words[j]);\n }\n }\n\n return ans; // Return the total length of words that can be formed\n}\n\n\n\n```\n```Java []\nclass Solution {\n public int countCharacters(String[] words, String chars) {\n Map<Character, Integer> counts = new HashMap();\n for (Character c : chars.toCharArray()) {\n counts.put(c, counts.getOrDefault(c, 0) + 1);\n }\n \n int ans = 0;\n for (String word : words) {\n Map<Character, Integer> wordCount = new HashMap();\n for (Character c : word.toCharArray()) {\n wordCount.put(c, wordCount.getOrDefault(c, 0) + 1);\n }\n \n boolean good = true;\n for (Character c : wordCount.keySet()) {\n if (counts.getOrDefault(c, 0) < wordCount.get(c)) {\n good = false;\n break;\n }\n }\n \n if (good) {\n ans += word.length();\n }\n }\n \n return ans;\n }\n}\n\n\n```\n```python3 []\nclass Solution:\n def countCharacters(self, words: List[str], chars: str) -> int:\n counts = defaultdict(int)\n for c in chars:\n counts[c] += 1\n \n ans = 0\n for word in words:\n word_count = defaultdict(int)\n for c in word:\n word_count[c] += 1\n \n good = True\n for c, freq in word_count.items():\n if counts[c] < freq:\n good = False\n break\n \n if good:\n ans += len(word)\n \n return ans\n\n\n```\n```javascript []\nfunction countCharacters(words, chars) {\n const counts = {};\n\n // Count the frequency of each character in \'chars\'\n for (const char of chars) {\n counts[char] = (counts[char] || 0) + 1;\n }\n\n let ans = 0;\n\n // Loop through each word in the \'words\' array\n for (const word of words) {\n const wordCount = {};\n\n // Count the frequency of each character in the current word\n for (const char of word) {\n wordCount[char] = (wordCount[char] || 0) + 1;\n }\n\n let good = true;\n\n // Check if the current word can be formed from the characters in \'chars\'\n for (const char in wordCount) {\n if (!counts[char] || wordCount[char] > counts[char]) {\n good = false; // The word cannot be formed\n break; // Exit the loop as the word is not valid\n }\n }\n\n // If the word can be formed from the characters in \'chars\', add its length to \'ans\'\n if (good) {\n ans += word.length;\n }\n }\n\n return ans; // Return the total length of words that can be formed\n}\n\n\n```\n\n---\n\n#### ***Approach 2(Count With Array)***\n1. **Character Counting:**\n\n - `counts` vector is created to store the count of characters from \'a\' to \'z\'.\n - The frequency of each character in the `chars` string is calculated and stored in the `counts` vector using ASCII values.\n1. **Checking Words:**\n\n - Each word in the `words` vector is checked if it can be formed from characters in `chars`.\n - A separate vector `wordCount` is created for each word to count the frequency of characters.\n1. **Comparison:**\n\n - Character counts in `wordCount` and `counts` are compared.\n - If any character count in `wordCount` exceeds the count in `counts`, the word cannot be formed.\n1. **Summing Length:**\n\n - If the word can be formed from the characters in `chars`, the length of the word is added to `ans`.\n1. **Returning Result:**\n\n - Finally, the total length of words that can be formed is returned as `ans`.\n\n# Complexity\n- *Time complexity:*\n $$O(n+m\u22C5k)$$\n \n\n- *Space complexity:*\n $$O(1)$$\n \n\n\n# Code\n```C++ []\n\n\nclass Solution {\npublic:\n int countCharacters(std::vector<std::string>& words, std::string chars) {\n // Create a vector to store character counts in the \'chars\' string\n std::vector<int> counts(26, 0);\n\n // Count the frequency of each character in the \'chars\' string\n for (char c : chars) {\n counts[c - \'a\']++;\n }\n \n int ans = 0;\n\n // Loop through each word in the \'words\' vector\n for (std::string word : words) {\n // Create a vector to store character counts in the current \'word\'\n std::vector<int> wordCount(26, 0);\n\n // Count the frequency of each character in the current word\n for (char c : word) {\n wordCount[c - \'a\']++;\n }\n \n bool good = true;\n\n // Compare the character counts of \'chars\' and the current \'word\'\n for (int i = 0; i < 26; i++) {\n if (counts[i] < wordCount[i]) {\n good = false; // The word cannot be formed\n break; // Exit the loop as the word is not valid\n }\n }\n\n // If the word can be formed from the characters in \'chars\', add its length to \'ans\'\n if (good) {\n ans += word.size();\n }\n }\n \n return ans; // Return the total length of words that can be formed\n }\n};\n\n\n\n```\n```C []\n\n\nint countCharacters(char** words, int wordsSize, char* chars) {\n int counts[26] = {0}; // Array to store character counts, assuming lowercase English alphabet\n\n // Count the frequency of each character in \'chars\'\n for (int i = 0; chars[i] != \'\\0\'; i++) {\n counts[chars[i] - \'a\']++;\n }\n\n int ans = 0;\n\n // Loop through each word in the \'words\' array\n for (int j = 0; j < wordsSize; j++) {\n int wordCount[26] = {0}; // Array to store character counts in the current \'word\'\n\n // Count the frequency of each character in the current word\n for (int k = 0; words[j][k] != \'\\0\'; k++) {\n wordCount[words[j][k] - \'a\']++;\n }\n\n int good = 1;\n\n // Check if the current word can be formed from the characters in \'chars\'\n for (int l = 0; l < 26; l++) {\n if (wordCount[l] > counts[l]) {\n good = 0; // The word cannot be formed, set \'good\' flag to 0\n break; // Exit the loop as the word is not valid\n }\n }\n\n // If the word can be formed from the characters in \'chars\', add its length to \'ans\'\n if (good) {\n ans += strlen(words[j]);\n }\n }\n\n return ans; // Return the total length of words that can be formed\n}\n\n\n\n\n```\n```Java []\n\nclass Solution {\n public int countCharacters(String[] words, String chars) {\n int[] counts = new int[26];\n for (Character c : chars.toCharArray()) {\n counts[c - \'a\']++;\n }\n \n int ans = 0;\n for (String word : words) {\n int[] wordCount = new int[26];\n for (Character c : word.toCharArray()) {\n wordCount[c - \'a\']++;\n }\n \n boolean good = true;\n for (int i = 0; i < 26; i++) {\n if (counts[i] < wordCount[i]) {\n good = false;\n break;\n }\n }\n \n if (good) {\n ans += word.length();\n }\n }\n \n return ans;\n }\n}\n\n```\n```python3 []\nclass Solution:\n def countCharacters(self, words: List[str], chars: str) -> int:\n counts = [0] * 26\n for c in chars:\n counts[ord(c) - ord("a")] += 1\n \n ans = 0\n for word in words:\n word_count = [0] * 26\n for c in word:\n word_count[ord(c) - ord("a")] += 1\n \n good = True\n for i in range(26):\n if counts[i] < word_count[i]:\n good = False\n break\n \n if good:\n ans += len(word)\n \n return ans\n\n\n```\n```javascript []\nfunction countCharacters(words, chars) {\n const counts = {};\n\n // Count the frequency of each character in \'chars\'\n for (const char of chars) {\n counts[char] = (counts[char] || 0) + 1;\n }\n\n let ans = 0;\n\n // Loop through each word in the \'words\' array\n for (const word of words) {\n const wordCount = {};\n\n // Count the frequency of each character in the current word\n for (const char of word) {\n wordCount[char] = (wordCount[char] || 0) + 1;\n }\n\n let good = true;\n\n // Check if the current word can be formed from the characters in \'chars\'\n for (const char in wordCount) {\n if (!counts[char] || wordCount[char] > counts[char]) {\n good = false; // The word cannot be formed\n break; // Exit the loop as the word is not valid\n }\n }\n\n // If the word can be formed from the characters in \'chars\', add its length to \'ans\'\n if (good) {\n ans += word.length;\n }\n }\n\n return ans; // Return the total length of words that can be formed\n}\n\n\n```\n\n---\n\n\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n--- | 3 | You are given an array of strings `words` and a string `chars`.
A string is **good** if it can be formed by characters from chars (each character can only be used once).
Return _the sum of lengths of all good strings in words_.
**Example 1:**
**Input:** words = \[ "cat ", "bt ", "hat ", "tree "\], chars = "atach "
**Output:** 6
**Explanation:** The strings that can be formed are "cat " and "hat " so the answer is 3 + 3 = 6.
**Example 2:**
**Input:** words = \[ "hello ", "world ", "leetcode "\], chars = "welldonehoneyr "
**Output:** 10
**Explanation:** The strings that can be formed are "hello " and "world " so the answer is 5 + 5 = 10.
**Constraints:**
* `1 <= words.length <= 1000`
* `1 <= words[i].length, chars.length <= 100`
* `words[i]` and `chars` consist of lowercase English letters. | Try to build the string with a backtracking DFS by considering what you can put in every position. |
Beats 99.51% of users with Python3 | Simple Solution | find-words-that-can-be-formed-by-characters | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countCharacters(self, words: List[str], chars: str) -> int:\n result = []\n for i in words:\n for j in i:\n if chars.count(j) < i.count(j):\n break\n else:\n result.append(len(i)) \n return sum(result)\n\n \n \n``` | 9 | You are given an array of strings `words` and a string `chars`.
A string is **good** if it can be formed by characters from chars (each character can only be used once).
Return _the sum of lengths of all good strings in words_.
**Example 1:**
**Input:** words = \[ "cat ", "bt ", "hat ", "tree "\], chars = "atach "
**Output:** 6
**Explanation:** The strings that can be formed are "cat " and "hat " so the answer is 3 + 3 = 6.
**Example 2:**
**Input:** words = \[ "hello ", "world ", "leetcode "\], chars = "welldonehoneyr "
**Output:** 10
**Explanation:** The strings that can be formed are "hello " and "world " so the answer is 5 + 5 = 10.
**Constraints:**
* `1 <= words.length <= 1000`
* `1 <= words[i].length, chars.length <= 100`
* `words[i]` and `chars` consist of lowercase English letters. | Try to build the string with a backtracking DFS by considering what you can put in every position. |
💯Faster✅💯 Lesser✅4 Methods🔥 Brute Force🔥Hash Map 🔥Counting Characters🔥Optimized Hash Map | find-words-that-can-be-formed-by-characters | 1 | 1 | # \uD83D\uDE80 Hi, I\'m [Mohammed Raziullah Ansari](https://leetcode.com/Mohammed_Raziullah_Ansari/), and I\'m excited to share 4 ways to solve this question with detailed explanation of each approach:\n\n# Problem Explaination: \nWe are given an array of strings `words` and a string `chars`. Our task is to find the sum of lengths of all words in `words` that can be formed using the characters in `chars`. We can use each character in chars only once.\n\n# \uD83D\uDD0D Methods To Solve This Problem:\nI\'ll be covering four different methods to solve this problem:\n1. Brute Force\n2. Hash Map\n3. Counting Characters\n4. Optimized Hash Map\n\n# 1. Brute Force: \n- Iterate through each word in `words`.\n- For each word, check if it can be formed using the characters in `chars`.\n- If yes, add the length of the word to the result.\n# Complexity\n- \u23F1\uFE0F Time Complexity: `O(N * M * K)`, where N is the number of words, M is the average length of a word, and K is the length of chars.\n- \uD83D\uDE80 Space Complexity: `O(1)`\n\n# Code\n```Python []\nclass Solution:\n def countCharacters(self, words: List[str], chars: str) -> int:\n def can_form(word, chars):\n char_count = Counter(chars)\n for char in word:\n if char_count[char] <= 0:\n return False\n char_count[char] -= 1\n return True\n result = 0\n for word in words:\n if can_form(word, chars):\n result += len(word)\n return result\n```\n```Java []\nclass Solution {\n public int countCharacters(String[] words, String chars) {\n int result = 0;\n for (String word : words) {\n if (canFormWord(word, chars)) {\n result += word.length();\n }\n }\n return result;\n }\n\n private boolean canFormWord(String word, String chars) {\n int[] charCount = new int[26];\n\n for (char ch : chars.toCharArray()) {\n charCount[ch - \'a\']++;\n }\n for (char ch : word.toCharArray()) {\n if (charCount[ch - \'a\'] == 0) {\n return false;\n }\n charCount[ch - \'a\']--;\n }\n return true;\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int countCharacters(vector<string>& words, string chars) {\n int result = 0;\n for (const string& word : words) {\n string tempChars = chars;\n bool valid = true;\n for (char c : word) {\n size_t pos = tempChars.find(c);\n if (pos != string::npos) {\n tempChars.erase(pos, 1);\n } else {\n valid = false;\n break;\n }\n }\n if (valid) {\n result += word.size();\n }\n }\n return result;\n }\n};\n```\n```C []\nint countCharacters(char** words, int wordsSize, char* chars) {\n int result = 0;\n for (int i = 0; i < wordsSize; ++i) {\n char* word = words[i];\n int len = strlen(word);\n int charsCopy[26] = {0};\n strcpy(charsCopy, chars);\n int j;\n for (j = 0; j < len; ++j) {\n if (--charsCopy[word[j] - \'a\'] < 0) {\n break;\n }\n }\n if (j == len) {\n result += len;\n }\n }\n return result;\n}\n```\n# 2. Hash Map:\n- Create a frequency map for the characters in `chars`.\n- Iterate through each word in `words`.\n- For each word, create a frequency map.\n- Check if the word\'s frequency map is a subset of the chars frequency map.\n- If yes, add the length of the word to the result.\n# Complexity\n- \u23F1\uFE0F Time Complexity: `O(N * (M + K))`, where N is the number of words, M is the average length of a word, and K is the length of chars.\n- \uD83D\uDE80 Space Complexity: `O(K)`\n\n# Code\n```Python []\nclass Solution:\n def countCharacters(self, words: List[str], chars: str) -> int:\n result = 0\n char_count = Counter(chars)\n for word in words:\n word_count = Counter(word)\n if all(word_count[char] <= char_count[char] for char in word):\n result += len(word)\n return result\n```\n```Java []\nimport java.util.HashMap;\nimport java.util.Map;\n\nclass Solution {\n public int countCharacters(String[] words, String chars) {\n int result = 0;\n Map<Character, Integer> charFreqMap = new HashMap<>();\n for (char ch : chars.toCharArray()) {\n charFreqMap.put(ch, charFreqMap.getOrDefault(ch, 0) + 1);\n }\n for (String word : words) {\n if (canFormWord(word, charFreqMap)) {\n result += word.length();\n }\n }\n return result;\n }\n\n private boolean canFormWord(String word, Map<Character, Integer> charFreqMap) {\n Map<Character, Integer> tempMap = new HashMap<>();\n for (char ch : word.toCharArray()) {\n tempMap.put(ch, tempMap.getOrDefault(ch, 0) + 1);\n }\n for (Map.Entry<Character, Integer> entry : tempMap.entrySet()) {\n char ch = entry.getKey();\n int count = entry.getValue();\n\n if (!charFreqMap.containsKey(ch) || charFreqMap.get(ch) < count) {\n return false;\n }\n }\n return true;\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int countCharacters(vector<string>& words, string chars) {\n int result = 0;\n unordered_map<char, int> charFreq;\n for (char c : chars) {\n charFreq[c]++;\n }\n for (const string& word : words) {\n unordered_map<char, int> wordFreq;\n bool valid = true;\n \n for (char c : word) {\n wordFreq[c]++;\n if (wordFreq[c] > charFreq[c]) {\n valid = false;\n break;\n }\n }\n \n if (valid) {\n result += word.size();\n }\n }\n return result;\n }\n};\n```\n```C []\nint countCharacters(char** words, int wordsSize, char* chars) {\n int result = 0;\n int charsCount[26] = {0};\n for (int i = 0; i < strlen(chars); ++i) {\n charsCount[chars[i] - \'a\']++;\n }\n for (int i = 0; i < wordsSize; ++i) {\n char* word = words[i];\n int len = strlen(word);\n int wordCount[26] = {0};\n for (int j = 0; j < len; ++j) {\n wordCount[word[j] - \'a\']++;\n }\n int j;\n for (j = 0; j < 26; ++j) {\n if (wordCount[j] > charsCount[j]) {\n break;\n }\n }\n if (j == 26) {\n result += len;\n }\n }\n return result;\n}\n```\n# 3. Counting Characters:\n - Create an array to count the occurrences of each character in `chars`.\n- Iterate through each word in `words`.\n- For each word, create a temporary array to count the occurrences of each character.\n- Check if the temporary array is less than or equal to the array created in step 1.\n- If yes, add the length of the word to the result.\n# Complexity\n- \u23F1\uFE0F Time Complexity: `O(N * (M + K))`, where N is the number of words, M is the average length of a word, and K is the length of chars.\n- \uD83D\uDE80 Space Complexity: `O(1)`\n\n# Code\n```Python []\nclass Solution:\n def countCharacters(self, words: List[str], chars: str) -> int:\n def count_chars(s):\n char_count = [0] * 26\n for char in s:\n char_count[ord(char) - ord(\'a\')] += 1\n return char_count\n result = 0\n chars_count = count_chars(chars)\n for word in words:\n word_count = count_chars(word)\n if all(word_count[i] <= chars_count[i] for i in range(26)):\n result += len(word) \n return result\n```\n```Java []\nclass Solution {\n public int countCharacters(String[] words, String chars) {\n int result = 0;\n int[] charCount = new int[26];\n for (char ch : chars.toCharArray()) {\n charCount[ch - \'a\']++;\n }\n for (String word : words) {\n if (canFormWord(word, charCount.clone())) {\n result += word.length();\n }\n }\n return result;\n }\n\n private boolean canFormWord(String word, int[] charCount) {\n for (char ch : word.toCharArray()) {\n if (charCount[ch - \'a\'] == 0) {\n return false;\n }\n charCount[ch - \'a\']--;\n }\n return true;\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int countCharacters(vector<string>& words, string chars) {\n int result = 0;\n vector<int> charCount(26, 0);\n for (char c : chars) {\n charCount[c - \'a\']++;\n }\n for (const string& word : words) {\n vector<int> tempCount(charCount);\n bool valid = true;\n for (char c : word) {\n if (tempCount[c - \'a\'] > 0) {\n tempCount[c - \'a\']--;\n } else {\n valid = false;\n break;\n }\n }\n if (valid) {\n result += word.size();\n }\n }\n return result;\n }\n};\n```\n```C []\nint countCharacters(char** words, int wordsSize, char* chars) {\n int result = 0;\n int charsCount[26] = {0}; \n for (int i = 0; i < strlen(chars); ++i) {\n charsCount[chars[i] - \'a\']++;\n }\n for (int i = 0; i < wordsSize; ++i) {\n char* word = words[i];\n int len = strlen(word);\n int wordCount[26] = {0};\n\n for (int j = 0; j < len; ++j) {\n wordCount[word[j] - \'a\']++;\n }\n int j;\n for (j = 0; j < 26; ++j) {\n if (wordCount[j] > charsCount[j]) {\n break;\n }\n }\n if (j == 26) {\n result += len;\n }\n }\n return result;\n}\n```\n# 4. Optimized Hash Map:\n - Create a frequency map for the characters in `chars`.\n- Iterate through each word in `words`.\n- For each word, decrement the frequency of characters in the map.\n- Check if any character\'s frequency becomes negative during this process.\n- If no negative frequencies are encountered, add the length of the word to the result.\n# Complexity\n- \u23F1\uFE0F Time Complexity: `O(N * M)`, where N is the number of words and M is the average length of a word. \n- \uD83D\uDE80 Space Complexity: `O(K)`\n\n# Code\n```Python []\nclass Solution:\n def countCharacters(self, words: List[str], chars: str) -> int:\n result = 0\n char_count = Counter(chars)\n \n for word in words:\n temp_count = char_count.copy()\n can_form_word = True\n for char in word:\n if temp_count[char] <= 0:\n can_form_word = False\n break\n temp_count[char] -= 1\n \n if can_form_word:\n result += len(word)\n \n return result\n```\n```Java []\nimport java.util.HashMap;\nimport java.util.Map;\n\nclass Solution {\n public int countCharacters(String[] words, String chars) {\n int result = 0;\n Map<Character, Integer> charFreqMap = new HashMap<>();\n for (char ch : chars.toCharArray()) {\n charFreqMap.put(ch, charFreqMap.getOrDefault(ch, 0) + 1);\n }\n for (String word : words) {\n if (canFormWord(word, new HashMap<>(charFreqMap))) {\n result += word.length();\n }\n }\n return result;\n }\n\n private boolean canFormWord(String word, Map<Character, Integer> charFreqMap) {\n for (char ch : word.toCharArray()) {\n if (!charFreqMap.containsKey(ch) || charFreqMap.get(ch) == 0) {\n return false;\n }\n charFreqMap.put(ch, charFreqMap.get(ch) - 1);\n }\n return true;\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int countCharacters(vector<string>& words, string chars) {\n int result = 0;\n unordered_map<char, int> charFreq;\n for (char c : chars) {\n charFreq[c]++;\n }\n for (const string& word : words) {\n unordered_map<char, int> tempFreq(charFreq);\n bool valid = true;\n \n for (char c : word) {\n if (tempFreq[c] > 0) {\n tempFreq[c]--;\n } else {\n valid = false;\n break;\n }\n }\n \n if (valid) {\n result += word.size();\n }\n }\n return result;\n }\n};\n```\n```C []\nint countCharacters(char** words, int wordsSize, char* chars) {\n int result = 0;\n int charsCount[26] = {0}; \n for (int i = 0; i < strlen(chars); ++i) {\n charsCount[chars[i] - \'a\']++;\n }\n for (int i = 0; i < wordsSize; ++i) {\n char* word = words[i];\n int len = strlen(word);\n int wordCount[26] = {0};\n for (int j = 0; j < len; ++j) {\n wordCount[word[j] - \'a\']++;\n }\n int j;\n for (j = 0; j < 26; ++j) {\n if (wordCount[j] > charsCount[j]) {\n break;\n }\n }\n if (j == 26) {\n result += len;\n }\n }\n return result;\n}\n```\n# \uD83D\uDCA1 I invite you to check out my profile for detailed explanations and code for each method. Happy coding and learning! \uD83D\uDCDA | 33 | You are given an array of strings `words` and a string `chars`.
A string is **good** if it can be formed by characters from chars (each character can only be used once).
Return _the sum of lengths of all good strings in words_.
**Example 1:**
**Input:** words = \[ "cat ", "bt ", "hat ", "tree "\], chars = "atach "
**Output:** 6
**Explanation:** The strings that can be formed are "cat " and "hat " so the answer is 3 + 3 = 6.
**Example 2:**
**Input:** words = \[ "hello ", "world ", "leetcode "\], chars = "welldonehoneyr "
**Output:** 10
**Explanation:** The strings that can be formed are "hello " and "world " so the answer is 5 + 5 = 10.
**Constraints:**
* `1 <= words.length <= 1000`
* `1 <= words[i].length, chars.length <= 100`
* `words[i]` and `chars` consist of lowercase English letters. | Try to build the string with a backtracking DFS by considering what you can put in every position. |
Easy Python Solution| Mapping | Beats 99% | find-words-that-can-be-formed-by-characters | 0 | 1 | # Intuition\n1) Count the frequency of characters in `chars` \n2) Compare with each individual word in `words` if at any point frequency(`chars`)< frequency(`word`) making the word is not possible\n\nNote: making a mapping of `chars` is problematic because it is possible a character was used in `word` but not in `chars` causing an error on comparison\nFor example:\n```\nchars="apple"\nchar_map={\'a\':1,\'p\':2,\'l\':1,:\'e\':1}\nword="pot"\nword_map={\'p\':1,\'o\':1,\'t\':1}\nchar_map[\'o\']<word_map[\'o\'] #It will cause problem\n```\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1)make a dictionary of all lowercase characters and update value of characters present in `chars`\n2)use a nested for loop to check character used in the individual word in `words`\n3)compare frequency of characters in `word` to characters in `chars`\n4)result += len(word) if we can make the word\n\n# Code\n```\nclass Solution:\n def countCharacters(self, words: List[str], chars: str) -> int:\n result = 0\n d={chr(i+96):0 for i in range(1,27)}\n for i in chars:\n d[i]+=1\n for i in words:\n for j in i:\n if d[j]< i.count(j):\n break\n else:\n result+=len(i)\n return result\n \n \n\n``` | 1 | You are given an array of strings `words` and a string `chars`.
A string is **good** if it can be formed by characters from chars (each character can only be used once).
Return _the sum of lengths of all good strings in words_.
**Example 1:**
**Input:** words = \[ "cat ", "bt ", "hat ", "tree "\], chars = "atach "
**Output:** 6
**Explanation:** The strings that can be formed are "cat " and "hat " so the answer is 3 + 3 = 6.
**Example 2:**
**Input:** words = \[ "hello ", "world ", "leetcode "\], chars = "welldonehoneyr "
**Output:** 10
**Explanation:** The strings that can be formed are "hello " and "world " so the answer is 5 + 5 = 10.
**Constraints:**
* `1 <= words.length <= 1000`
* `1 <= words[i].length, chars.length <= 100`
* `words[i]` and `chars` consist of lowercase English letters. | Try to build the string with a backtracking DFS by considering what you can put in every position. |
Solution of find words that can be formed by characters problem | find-words-that-can-be-formed-by-characters | 0 | 1 | # Approach\n- Step one: get out word from words list\n- Step two: ckeck out if every charachers in chars list\n- Step three: summarise length of word and add it to the counter\n\n# Complexity\n- Time complexity:\n$$O(n^2)$$ - as we use double loop\n\n- Space complexity:\n$$O(1)$$ - as no extra space is required\n\n# Code\n```\nclass Solution:\n def countCharacters(self, words: List[str], chars: str) -> int:\n count = 0\n for word in words:\n iftrue = 1\n for char in word:\n if word.count(char) > chars.count(char):\n iftrue = 0\n count += len(word) * iftrue\n return count \n \n``` | 1 | You are given an array of strings `words` and a string `chars`.
A string is **good** if it can be formed by characters from chars (each character can only be used once).
Return _the sum of lengths of all good strings in words_.
**Example 1:**
**Input:** words = \[ "cat ", "bt ", "hat ", "tree "\], chars = "atach "
**Output:** 6
**Explanation:** The strings that can be formed are "cat " and "hat " so the answer is 3 + 3 = 6.
**Example 2:**
**Input:** words = \[ "hello ", "world ", "leetcode "\], chars = "welldonehoneyr "
**Output:** 10
**Explanation:** The strings that can be formed are "hello " and "world " so the answer is 5 + 5 = 10.
**Constraints:**
* `1 <= words.length <= 1000`
* `1 <= words[i].length, chars.length <= 100`
* `words[i]` and `chars` consist of lowercase English letters. | Try to build the string with a backtracking DFS by considering what you can put in every position. |
⭐🔥One-Liner🔥CheatCode in 🐍 || Beats 66%⭐ | find-words-that-can-be-formed-by-characters | 0 | 1 | 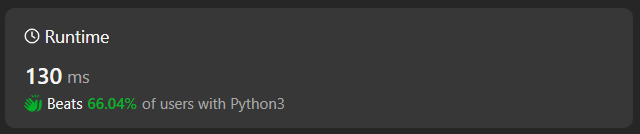\n\n# Code\n```\nfrom typing import List\nclass Solution:\n def countCharacters(self, words: List[str], chars: str) -> int:\n return sum(len(word) for word in words if all(word.count(char) <= chars.count(char) for char in set(word)))\n``` | 1 | You are given an array of strings `words` and a string `chars`.
A string is **good** if it can be formed by characters from chars (each character can only be used once).
Return _the sum of lengths of all good strings in words_.
**Example 1:**
**Input:** words = \[ "cat ", "bt ", "hat ", "tree "\], chars = "atach "
**Output:** 6
**Explanation:** The strings that can be formed are "cat " and "hat " so the answer is 3 + 3 = 6.
**Example 2:**
**Input:** words = \[ "hello ", "world ", "leetcode "\], chars = "welldonehoneyr "
**Output:** 10
**Explanation:** The strings that can be formed are "hello " and "world " so the answer is 5 + 5 = 10.
**Constraints:**
* `1 <= words.length <= 1000`
* `1 <= words[i].length, chars.length <= 100`
* `words[i]` and `chars` consist of lowercase English letters. | Try to build the string with a backtracking DFS by considering what you can put in every position. |
Python3 Solution | find-words-that-can-be-formed-by-characters | 0 | 1 | \n```\nclass Solution:\n def countCharacters(self, words: List[str], chars: str) -> int:\n return sum(len(word) for word in words if Counter(word)<=Counter(chars))\n \n``` | 1 | You are given an array of strings `words` and a string `chars`.
A string is **good** if it can be formed by characters from chars (each character can only be used once).
Return _the sum of lengths of all good strings in words_.
**Example 1:**
**Input:** words = \[ "cat ", "bt ", "hat ", "tree "\], chars = "atach "
**Output:** 6
**Explanation:** The strings that can be formed are "cat " and "hat " so the answer is 3 + 3 = 6.
**Example 2:**
**Input:** words = \[ "hello ", "world ", "leetcode "\], chars = "welldonehoneyr "
**Output:** 10
**Explanation:** The strings that can be formed are "hello " and "world " so the answer is 5 + 5 = 10.
**Constraints:**
* `1 <= words.length <= 1000`
* `1 <= words[i].length, chars.length <= 100`
* `words[i]` and `chars` consist of lowercase English letters. | Try to build the string with a backtracking DFS by considering what you can put in every position. |
Python Logic Based Approach Using Dictionary | find-words-that-can-be-formed-by-characters | 0 | 1 | \n\n# Complexity\n- Time complexity:\nO(n^2)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution:\n def countCharacters(self, words: List[str], chars: str) -> int:\n l=list(chars)\n count=0\n m={}\n for i in chars :\n if i in m :\n m[i]+=1\n else:\n m[i]=1\n for i in words : \n flag=0\n x=m.copy()\n print(x)\n for j in i : \n if j in x :\n if x[j]==0 :\n flag=1\n break\n else: \n x[j]-=1\n else:\n flag=1\n break\n if flag==0 :\n print(i)\n count+=len(i)\n return count\n``` | 1 | You are given an array of strings `words` and a string `chars`.
A string is **good** if it can be formed by characters from chars (each character can only be used once).
Return _the sum of lengths of all good strings in words_.
**Example 1:**
**Input:** words = \[ "cat ", "bt ", "hat ", "tree "\], chars = "atach "
**Output:** 6
**Explanation:** The strings that can be formed are "cat " and "hat " so the answer is 3 + 3 = 6.
**Example 2:**
**Input:** words = \[ "hello ", "world ", "leetcode "\], chars = "welldonehoneyr "
**Output:** 10
**Explanation:** The strings that can be formed are "hello " and "world " so the answer is 5 + 5 = 10.
**Constraints:**
* `1 <= words.length <= 1000`
* `1 <= words[i].length, chars.length <= 100`
* `words[i]` and `chars` consist of lowercase English letters. | Try to build the string with a backtracking DFS by considering what you can put in every position. |
✅ Explained - Simple and Clear Python3 Code✅ | maximum-level-sum-of-a-binary-tree | 0 | 1 | \n# Approach\nThe logic of the code involves recursively traversing the binary tree and maintaining a list of sums at each level. Starting from the root, the code calculates the sum of node values at each level and stores them in the list. After the traversal, it determines the maximum sum from the list and returns the corresponding level. The logic ensures that each node\'s value is added to the sum of its level and continues recursively for the left and right children, incrementing the level. Ultimately, it finds the level with the maximum sum of node values in the binary tree.\n\n\n# Complexity\n- Time complexity:\nO(n), as it has to process each node in the tree.\n- Space complexity:\nThe memory complexity of the code is O(n), as the list \'sm\' is used to store the sums at each level. The size of this list can grow up to the number of levels in the tree, which can be at most n.\n# Code\n```\nclass Solution:\n def maxLevelSum(self, root: Optional[TreeNode]) -> int:\n sm = [0]\n def cal(r,lvl):\n if len(sm)<lvl:\n sm.append(0)\n sm[lvl-1]+=r.val\n if r.right:\n cal(r.right,lvl+1)\n if r.left:\n cal(r.left,lvl+1)\n cal(root,1)\n mx=max(sm)\n for idx, x in enumerate(sm):\n if x == mx:\n return idx+1\n\n``` | 5 | Given the `root` of a binary tree, the level of its root is `1`, the level of its children is `2`, and so on.
Return the **smallest** level `x` such that the sum of all the values of nodes at level `x` is **maximal**.
**Example 1:**
**Input:** root = \[1,7,0,7,-8,null,null\]
**Output:** 2
**Explanation:**
Level 1 sum = 1.
Level 2 sum = 7 + 0 = 7.
Level 3 sum = 7 + -8 = -1.
So we return the level with the maximum sum which is level 2.
**Example 2:**
**Input:** root = \[989,null,10250,98693,-89388,null,null,null,-32127\]
**Output:** 2
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `-105 <= Node.val <= 105` | null |
Python Elegant & Short | DFS & Generators | maximum-level-sum-of-a-binary-tree | 0 | 1 | # Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(n)$$\n\n# Code\n```\nclass Solution:\n def maxLevelSum(self, root: Optional[TreeNode]) -> List[float]:\n level_sum = defaultdict(int)\n\n for lvl, val in self.inorder(root):\n level_sum[lvl + 1] += val\n\n return max(level_sum, key=lambda x: (level_sum[x], -x))\n\n @classmethod\n def inorder(cls, tree: TreeNode | None, level: int = 0):\n if tree is not None:\n yield from cls.inorder(tree.left, level + 1)\n yield level, tree.val\n yield from cls.inorder(tree.right, level + 1)\n\n``` | 2 | Given the `root` of a binary tree, the level of its root is `1`, the level of its children is `2`, and so on.
Return the **smallest** level `x` such that the sum of all the values of nodes at level `x` is **maximal**.
**Example 1:**
**Input:** root = \[1,7,0,7,-8,null,null\]
**Output:** 2
**Explanation:**
Level 1 sum = 1.
Level 2 sum = 7 + 0 = 7.
Level 3 sum = 7 + -8 = -1.
So we return the level with the maximum sum which is level 2.
**Example 2:**
**Input:** root = \[989,null,10250,98693,-89388,null,null,null,-32127\]
**Output:** 2
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `-105 <= Node.val <= 105` | null |
Level Order. Readable code - Python / C++ | maximum-level-sum-of-a-binary-tree | 0 | 1 | \n# Code\n\n```python []\nclass Solution:\n def maxLevelSum(self, root: Optional[TreeNode]) -> int:\n a = []\n\n def dfs(node, h):\n if not node:\n return\n if len(a) == h:\n a.append([])\n\n dfs(node.left, h+1)\n a[h].append(node.val)\n dfs(node.right, h+1)\n\n dfs(root, 0)\n\n level_sum = [sum(level) for level in a]\n maximal = max(level_sum)\n \n return 1 + level_sum.index(maximal)\n```\n```C++ []\nclass Solution {\nprivate:\n void dfs(TreeNode* node, int h, vector<vector<int> > &a) {\n if(!node) return;\n\n if(a.size() == h) {\n a.push_back({});\n }\n\n dfs(node->left, h+1, a);\n a[h].push_back(node->val);\n dfs(node->right, h+1, a);\n }\npublic:\n int maxLevelSum(TreeNode* root) {\n vector<vector<int>>a;\n int sm = 0;\n dfs(root, 0, a);\n\n vector<int> levelSum;\n\n for(auto &level:a) {\n for(auto ele:level) {\n sm += ele;\n }\n levelSum.push_back(sm);\n sm = 0;\n }\n\n int maxSum = *max_element(levelSum.begin(), levelSum.end());\n \n for(int i = 0; i < levelSum.size(); ++i) {\n if(levelSum[i] == maxSum) {\n return i+1;\n }\n }\n return -1;\n }\n};\n```\n | 2 | Given the `root` of a binary tree, the level of its root is `1`, the level of its children is `2`, and so on.
Return the **smallest** level `x` such that the sum of all the values of nodes at level `x` is **maximal**.
**Example 1:**
**Input:** root = \[1,7,0,7,-8,null,null\]
**Output:** 2
**Explanation:**
Level 1 sum = 1.
Level 2 sum = 7 + 0 = 7.
Level 3 sum = 7 + -8 = -1.
So we return the level with the maximum sum which is level 2.
**Example 2:**
**Input:** root = \[989,null,10250,98693,-89388,null,null,null,-32127\]
**Output:** 2
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `-105 <= Node.val <= 105` | null |
Python3 Solution | maximum-level-sum-of-a-binary-tree | 0 | 1 | \n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def helper(self,root,level):\n if root:\n self.level[level]+=root.val\n self.helper(root.left,level+1)\n self.helper(root.right,level+1)\n\n\n def maxLevelSum(self, root: Optional[TreeNode]) -> int:\n self.level=defaultdict(int)\n self.helper(root,1)\n return sorted(self.level.items(),key=lambda x:x[1],reverse=True)[0][0]\n``` | 2 | Given the `root` of a binary tree, the level of its root is `1`, the level of its children is `2`, and so on.
Return the **smallest** level `x` such that the sum of all the values of nodes at level `x` is **maximal**.
**Example 1:**
**Input:** root = \[1,7,0,7,-8,null,null\]
**Output:** 2
**Explanation:**
Level 1 sum = 1.
Level 2 sum = 7 + 0 = 7.
Level 3 sum = 7 + -8 = -1.
So we return the level with the maximum sum which is level 2.
**Example 2:**
**Input:** root = \[989,null,10250,98693,-89388,null,null,null,-32127\]
**Output:** 2
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `-105 <= Node.val <= 105` | null |
BFS Traversal | maximum-level-sum-of-a-binary-tree | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nAs to return the maximum sum of each level, its better to use BFS. Can use DFS traversal too but the solution become a little bit complex.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe will traverse each level and store the sum of all the nodes in that level to result(array to store the resultant sum of each level). Ultimately we will find the smallest index having the largest sum.\n\nNote that we are not calculating the height of the binary tree to declare a array of size equal to that if the height.\nIndeed we traverse the tree only once and store the result, each element in the queue contains the node value along with the level the node is present.\n\nModifications:\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n), where n is the number of nodes of Binary tree. We are going to traverse the tree only once.\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(n), we are going to store the nodes in the process queue.\n\n# Code\n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\n\nclass Solution:\n def maxLevelSum(self, root: Optional[TreeNode]) -> int:\n # apply BFS\n result = []\n if root == None:\n return result\n \n process = [[root, 1]] # Store the node & level\n while len(process) > 0:\n front = process[0]\n node, level = front[0], front[1]\n process.pop(0)\n\n """\n based on the length -> \n 1. insert node value if level is not added before\n 2. add the value to the corresponding level\n """\n if len(result) < level: # new level arrived\n result.append(node.val)\n else: # already the nodes in corresponding level is processed so just add to result[corresponding level]\n result[level - 1] += (node.val)\n\n\n # insert the nodes in the process\n if node.left != None:\n process.append([node.left, level + 1])\n if node.right != None:\n process.append([node.right, level + 1])\n \n return result.index(max(result)) + 1 # as 1 base indexing\n\n \n``` | 1 | Given the `root` of a binary tree, the level of its root is `1`, the level of its children is `2`, and so on.
Return the **smallest** level `x` such that the sum of all the values of nodes at level `x` is **maximal**.
**Example 1:**
**Input:** root = \[1,7,0,7,-8,null,null\]
**Output:** 2
**Explanation:**
Level 1 sum = 1.
Level 2 sum = 7 + 0 = 7.
Level 3 sum = 7 + -8 = -1.
So we return the level with the maximum sum which is level 2.
**Example 2:**
**Input:** root = \[989,null,10250,98693,-89388,null,null,null,-32127\]
**Output:** 2
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `-105 <= Node.val <= 105` | null |
Maximum level sum of a binary tree | maximum-level-sum-of-a-binary-tree | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n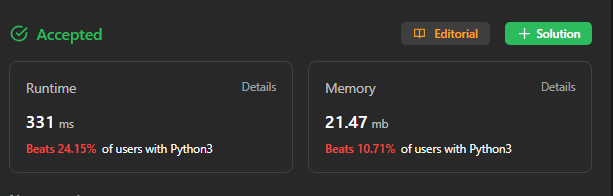\n\n# Code\n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def maxLevelSum(self, root: Optional[TreeNode]) -> int:\n l=defaultdict(list)\n def dfs(root,h):\n if root is None:\n return\n l[h].append(root.val)\n dfs(root.left,h+1)\n dfs(root.right,h+1)\n dfs(root,1)\n res=[]\n for i in l.values():\n res.append(sum(i))\n return res.index(max(res))+1\n\n``` | 1 | Given the `root` of a binary tree, the level of its root is `1`, the level of its children is `2`, and so on.
Return the **smallest** level `x` such that the sum of all the values of nodes at level `x` is **maximal**.
**Example 1:**
**Input:** root = \[1,7,0,7,-8,null,null\]
**Output:** 2
**Explanation:**
Level 1 sum = 1.
Level 2 sum = 7 + 0 = 7.
Level 3 sum = 7 + -8 = -1.
So we return the level with the maximum sum which is level 2.
**Example 2:**
**Input:** root = \[989,null,10250,98693,-89388,null,null,null,-32127\]
**Output:** 2
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `-105 <= Node.val <= 105` | null |
1161. Maximum Level Sum of a Binary Tree | maximum-level-sum-of-a-binary-tree | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe intuition behind the solution is to perform a level-order traversal of the binary tree and calculate the sum of node values at each level. By comparing the sums of different levels, we can identify the smallest level with the maximal sum. This can be achieved by using a queue to process the nodes level by level. We start with the root node and enqueue its children, then continue processing nodes in the queue until all levels are traversed. During the traversal, we keep track of the level sums and update the smallest level with the maximal sum whenever a higher sum is encountered. Finally, we return the smallest level with the maximal sum as the result. This approach ensures that we find the level with the maximal sum while minimizing the number of traversals and maintaining the order of levels using the queue data structure.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nHere\'s a step-by-step guide to solving the problem of finding the smallest level with the maximal sum in a binary tree:\n\n1. Start with the root of the binary tree.\n2. Initialize variables to track the smallest level with the maximal sum (`minLevel`) and the maximal sum found so far (`maxSum`). Set both to 1 and 0, respectively.\n3. Create an empty queue to perform level-order traversal of the binary tree.\n4. Enqueue the root node into the queue.\n5. Start a loop to process the nodes in the queue until it becomes empty.\n6. Inside the loop, get the size of the current level by checking the queue\'s size.\n7. Initialize a variable (`levelSum`) to keep track of the sum of node values at the current level. Set it to 0.\n8. Iterate through the nodes in the current level:\n - Dequeue a node from the front of the queue.\n - Add the value of the node to `levelSum`.\n - Enqueue the left and right child nodes of the current node if they exist.\n9. Check if `levelSum` is greater than `maxSum`. If so, update `maxSum` with the new value and set `minLevel` to the current level.\n10. Repeat steps 6 to 9 until all nodes are processed.\n11. Return the value of `minLevel`, which represents the smallest level with the maximal sum.\n\nBy following these steps, you can find the smallest level with the maximal sum in the given binary tree.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n`O(n)`, where n is the no. of nodes.\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n`O(n)`, where n is the no. of nodes.\n\n---\n\n# distance() in C++\n\nThe `distance()` function in C++ is a standard library function that calculates the number of elements between two iterators. It is defined in the `<iterator>` header.\n\nHere\'s an example usage of the `distance()` function:\n\n```cpp []\n#include <iostream>\n#include <vector>\n#include <iterator>\nusing namespace std;\n\nint main() {\n vector<int> nums = {1, 2, 3, 4, 5};\n\n // Get the distance between two iterators\n auto it1 = nums.begin();\n auto it2 = nums.begin() + 3;\n int dist = distance(it1, it2);\n\n cout << "Distance: " << dist << endl;\n\n return 0;\n}\n```\n\nIn this code, we have a vector `nums` with five elements. We use two iterators `it1` and `it2` to specify a range within the vector. The `distance()` function is then used to calculate the number of elements between `it1` and `it2`.\n\nThe output will be:\n```\nDistance: 3\n```\n\nThis indicates that there are 3 elements between the iterators `it1` and `it2` in the vector.\n\n---\n# Code\n``` C++ []\nclass Solution {\npublic:\n int maxLevelSum(TreeNode* root) {\n int lvl = 1;\n vector<int> sumAtLevel;\n\n queue<TreeNode*> q;\n q.push(root);\n\n int sm = INT_MIN;\n int res = 1;\n\n while(!q.empty()){\n int level_sum = 0;\n int sz = q.size();\n for(int i=0;i<sz;i++){\n TreeNode* t = q.front();\n q.pop();\n\n level_sum += t->val;\n\n if(t->left){\n q.push(t->left);\n }\n if(t->right){\n q.push(t->right);\n }\n }\n sumAtLevel.push_back(level_sum);\n }\n auto maxSum = max_element(sumAtLevel.begin(),sumAtLevel.end());\n lvl = distance(sumAtLevel.begin(),maxSum)+1;\n\n return lvl;\n }\n};\n```\n``` Python3 []\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def maxLevelSum(self, root: Optional[TreeNode]) -> int:\n minLevel = 1\n maxSum = float(\'-inf\')\n\n queue = deque()\n queue.append(root)\n\n level = 1\n\n while queue:\n levelSum = 0\n size = len(queue)\n\n for _ in range(size):\n node = queue.popleft()\n levelSum += node.val\n\n if node.left:\n queue.append(node.left)\n if node.right:\n queue.append(node.right)\n\n if levelSum > maxSum:\n maxSum = levelSum\n minLevel = level\n level += 1\n return minLevel\n``` | 1 | Given the `root` of a binary tree, the level of its root is `1`, the level of its children is `2`, and so on.
Return the **smallest** level `x` such that the sum of all the values of nodes at level `x` is **maximal**.
**Example 1:**
**Input:** root = \[1,7,0,7,-8,null,null\]
**Output:** 2
**Explanation:**
Level 1 sum = 1.
Level 2 sum = 7 + 0 = 7.
Level 3 sum = 7 + -8 = -1.
So we return the level with the maximum sum which is level 2.
**Example 2:**
**Input:** root = \[989,null,10250,98693,-89388,null,null,null,-32127\]
**Output:** 2
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `-105 <= Node.val <= 105` | null |
Python - Iterative DFS with stack | maximum-level-sum-of-a-binary-tree | 0 | 1 | # Intuition\nSimple iterative DFS approach.\n\n# Approach\n1. Init stack with root node and a default dict used to keep track of the sum for each level\n2. Traverse the tree by using a stack keeping track of the current node level\n3. Each time I pop from the stack I update the current level sum\n4. Once the traversal is completed, I need to find the max sum from the dict and its associated level (0-indexed therefore the `+ 1`)\n\n# Complexity\n- Time complexity: \n * $$O(n)$$ for DFS\n * $$O(n)$$ for finding max and its associated level\n \nTherefore: $$O(n) + O(n) = O(n)$$\n\n- Space complexity: \n * $$O(n)$$ for stack\n * $$O(n)$$ for sums\n\nTherefore: $$O(2n) = O(n)$$\n\n# Code\n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def maxLevelSum(self, root: Optional[TreeNode]) -> int:\n stack, sums = [(root, 0)], defaultdict(int)\n\n while stack:\n curr, lvl = stack.pop()\n\n sums[lvl] += curr.val\n\n if curr.left:\n stack.append([curr.left, lvl+1])\n if curr.right:\n stack.append([curr.right, lvl+1])\n \n return next(filter(lambda kv: max(sums.values()) == kv[1], sums.items()))[0] + 1\n\n # Or more readable\n # m = max(sums.values())\n # for i, v in sums.items():\n # if v == m:\n # return i+1\n``` | 1 | Given the `root` of a binary tree, the level of its root is `1`, the level of its children is `2`, and so on.
Return the **smallest** level `x` such that the sum of all the values of nodes at level `x` is **maximal**.
**Example 1:**
**Input:** root = \[1,7,0,7,-8,null,null\]
**Output:** 2
**Explanation:**
Level 1 sum = 1.
Level 2 sum = 7 + 0 = 7.
Level 3 sum = 7 + -8 = -1.
So we return the level with the maximum sum which is level 2.
**Example 2:**
**Input:** root = \[989,null,10250,98693,-89388,null,null,null,-32127\]
**Output:** 2
**Constraints:**
* The number of nodes in the tree is in the range `[1, 104]`.
* `-105 <= Node.val <= 105` | null |
|| 🐍 Python3 O(2n²) Easiest ✔ Solution 🔥 || Beats 80% (Runtime) & 41% (Memory) || | as-far-from-land-as-possible | 0 | 1 | # Intuition\n- Get all the index of land, check if there is only land if no, then one-by-one convert all adjacent indices(Breadth-First-Search approach) to land while incrementing the distance. At last return the last distance of land.\n\n# Approach\n1. Initializing,\n - `n` for matrix of `n x n`\n - `land` to check count of land\n - `stack` for getting index of land and distance\n2. Get all the indices of land in the matrix while appending it to stack with distance and incrementing `land` count.\n3. Check if count of land is not equal to all of matrix or an empty stack, which show the absence of water, thus returning `-1`.\n4. Iterating through the stack and one-by-one we convert the adjacent indices(Breadth-First-Search approach) to `land` and incrementing the distance. The converted indices are also added to the stack.\n5. Finally the last element\'s `distance` in stack is returned.\n\n# Complexity\n- Time complexity : $$O(2n^2)$$\n- Space complexity : $$O(n)$$\n\n# Code\n```\nclass Solution:\n def maxDistance(self, grid: List[List[int]]) -> int:\n n, land, stack= len(grid), 0, []\n\n for i in range(n):\n for j in range(n):\n if grid[i][j]:\n land+= 1\n stack.append((i, j, 0))\n\n if land== n* n or not stack: return -1\n\n for i, j, distance in stack:\n for x, y in ((i, j+ 1), (i, j- 1), (i+ 1, j), (i- 1, j)):\n if 0<= x< n and 0<= y< n and grid[x][y]== 0:\n grid[x][y]= 1\n stack.append((x, y, distance+ 1))\n \n return stack[-1][-1]\n```\n---\n\n### Happy Coding \uD83D\uDC68\u200D\uD83D\uDCBB\n\n---\n\n### If this solution helped you then do consider Upvoting \u2B06.\n#### Lets connect on LinkedIn : [Om Anand](https://www.linkedin.com/in/om-anand-38341a1ba/) \uD83D\uDD90\uD83D\uDE00\n---\n\n#### Comment your views/corrections \uD83D\uDE0A\n | 2 | Given an `n x n` `grid` containing only values `0` and `1`, where `0` represents water and `1` represents land, find a water cell such that its distance to the nearest land cell is maximized, and return the distance. If no land or water exists in the grid, return `-1`.
The distance used in this problem is the Manhattan distance: the distance between two cells `(x0, y0)` and `(x1, y1)` is `|x0 - x1| + |y0 - y1|`.
**Example 1:**
**Input:** grid = \[\[1,0,1\],\[0,0,0\],\[1,0,1\]\]
**Output:** 2
**Explanation:** The cell (1, 1) is as far as possible from all the land with distance 2.
**Example 2:**
**Input:** grid = \[\[1,0,0\],\[0,0,0\],\[0,0,0\]\]
**Output:** 4
**Explanation:** The cell (2, 2) is as far as possible from all the land with distance 4.
**Constraints:**
* `n == grid.length`
* `n == grid[i].length`
* `1 <= n <= 100`
* `grid[i][j]` is `0` or `1` | null |
Python3 using BFS with deque easy to understand. | as-far-from-land-as-possible | 0 | 1 | # Complexity\n- Time complexity:\nO(n^2)\n\n- Space complexity:\nO(n^2)\n\n# Code\n```\nclass Solution:\n def maxDistance(self, grid: List[List[int]]) -> int:\n q = deque()\n n = len(grid)\n ans = -1\n visited = set()\n\n for r in range(n):\n for c in range(n):\n if grid[r][c] == 1:\n visited.add((r, c))\n q.append((r, c, 0))\n \n while q:\n r, c, d = q.popleft()\n if grid[r][c] == 0:\n ans = max(ans, d)\n\n for nr, nc in [(r + 1, c), (r - 1, c), (r, c + 1), (r, c - 1)]:\n if nr >= 0 and nr < n and nc >= 0 and nc < n and (nr, nc) not in visited:\n visited.add((nr, nc))\n q.append((nr, nc, d + 1))\n\n return ans\n``` | 1 | Given an `n x n` `grid` containing only values `0` and `1`, where `0` represents water and `1` represents land, find a water cell such that its distance to the nearest land cell is maximized, and return the distance. If no land or water exists in the grid, return `-1`.
The distance used in this problem is the Manhattan distance: the distance between two cells `(x0, y0)` and `(x1, y1)` is `|x0 - x1| + |y0 - y1|`.
**Example 1:**
**Input:** grid = \[\[1,0,1\],\[0,0,0\],\[1,0,1\]\]
**Output:** 2
**Explanation:** The cell (1, 1) is as far as possible from all the land with distance 2.
**Example 2:**
**Input:** grid = \[\[1,0,0\],\[0,0,0\],\[0,0,0\]\]
**Output:** 4
**Explanation:** The cell (2, 2) is as far as possible from all the land with distance 4.
**Constraints:**
* `n == grid.length`
* `n == grid[i].length`
* `1 <= n <= 100`
* `grid[i][j]` is `0` or `1` | null |
Python solution beatas 99%. With explanantion | last-substring-in-lexicographical-order | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem is to find the lexicographically largest substring of a given string. My first thought is to start by comparing characters at different positions in the string and keep track of the substring with the highest lexicographic value.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe approach used in the code above is to use two pointers, i and j, to compare substrings starting at different positions in the string. A third pointer, k, is used to keep track of the current substring being compared. The code then uses a while loop to iterate through the string and compare the characters at positions i + k and j + k. If they are equal, k is incremented. If the character at i + k is greater than the character at j + k, j is moved to j + k + 1 and k is reset to 0. If the character at i + k is less than the character at j + k, i is moved to the maximum of i + k + 1 and j, and j is moved to i + 1 and k is reset to 0. The final return statement returns the substring starting at position i.\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def lastSubstring(self, s: str) -> str:\n i = 0\n j = 1\n k = 0\n n = len(s)\n while j + k < n:\n if s[i + k] == s[j + k]:\n k += 1\n elif s[i + k] > s[j + k]:\n j += k + 1\n k = 0\n elif s[i + k] < s[j + k]:\n i = max(i + k + 1, j)\n j = i + 1\n k = 0\n return s[i:]\n``` | 4 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
PYTHON | EXPLANATION WITH PHOTO | LINEAR TIME | EASY | INTUITIVE | | last-substring-in-lexicographical-order | 0 | 1 | \n# EXPLANATION\n```\nLet us start with Brute Force :\n1.Find all substring and store them in a list : list\n2.Sort the string list : list \n3.return list[-1]\n\nWe can optimize the above problem :\n\nAlways remember that "aaaba" > "aaab" so ans of this problem is always a suffix of string\n\n1. think carefully the last substring lexicographically will start from largest character in string\n2. we can have multiple occurences of such characher : say maxx \n3. find every substring starting with maxx and store them in a list and sort to find ans\n\n\nWe can give even better optimization:\n1. zaac , zaab , zaba , zaay , z , za\n2. here the largest item is "zaba" \n3. we find this by comparing each index and choosing the largest substring\n4. Note :zaaba > zaab\n5. we can use the list of indexes starting with largest character and check for each index\n6. list = [zaac,zaab,zaba,zaay,z,za]\n7. z is same for all of them\n8. gap = 1 : item "z" has no index 1 so remove it . \n9. the item having largest character at index = i + 1 are : new_list = [zaac,zaab,zaba,zaay,za]\n9. gap = 2 : item "za" has no index 2 remove it. list \n10. The item having largest character at index = i + 2 are : new_list = [zaba]\n10. Here only one substring zaba is largest at index i + 2 : hence we get our answer\n\n```\n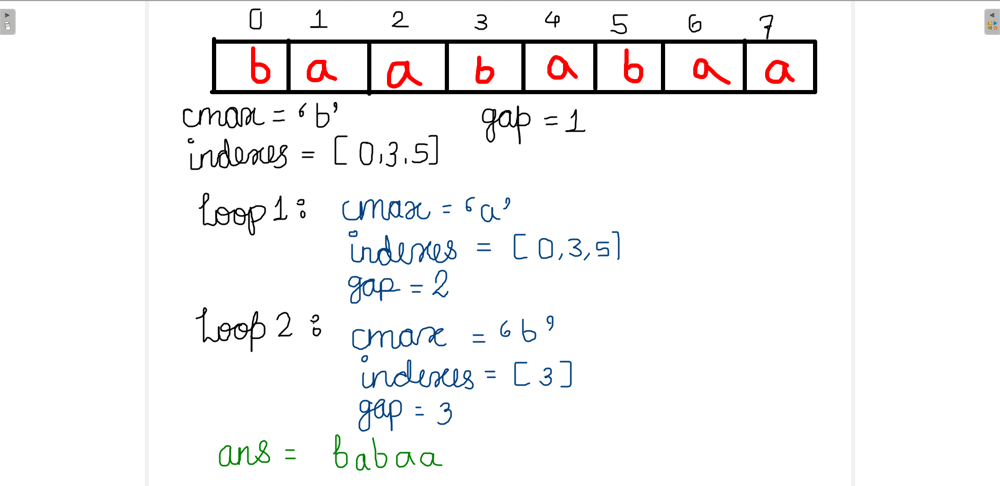\n\n```\nInstead of storing the whole substring it is preferable to store the starting index in list\n\nLast optimization\n1. When list = [a,a,a,a] \n2. we will get list =[0,1,2,3]\n3. gap = 1 : \n\t\tnew_list = [] , largest_character at index = i + 1 is a \n\t\titem at list[0] : will be added to\n\t\titem at list[1]: The character matches largest_character at gap = 1\n\t\tsince list[0] + 1 == 1( which is list[1] ) means the part is repeated i.e\n\t\tsay s = "baabaab"\n\t\tso here "baa_ _ _ _" and "_ _ _ baa _ " are same so in every case\n\t\t"baabaab" will be larger than "baab" \n\t\tsame will happen for item at list[2],list[3]\n\t\t\n\t\tHence instead of going o(n*n) we do o(n) \n\t\t\n\t\n\t\t\n```\n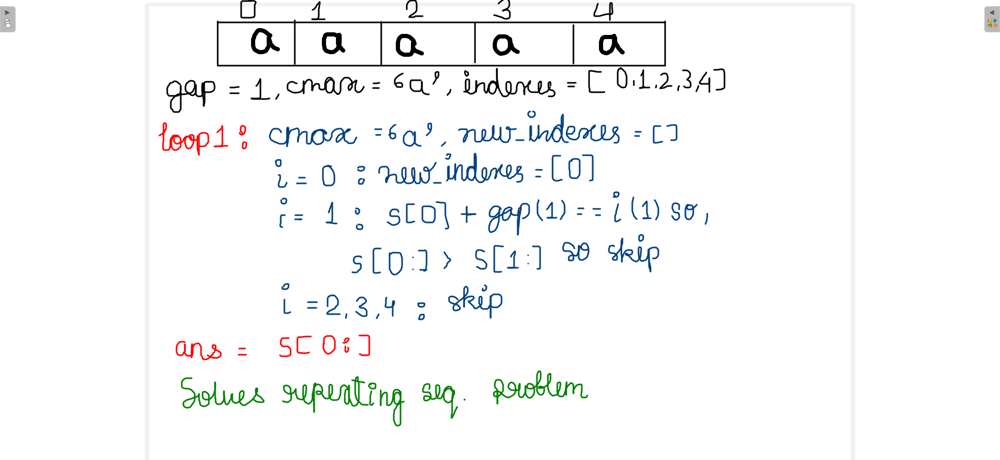\n\n\n# CODE\n```\nclass Solution:\n def lastSubstring(self, s: str) -> str:\n n = len(s)\n cmax = max(s)\n indexes = [ i for i,c in enumerate(s) if c == cmax ]\n gap = 1\n while len(indexes) > 1:\n new_indexes = []\n cmax = max(s[i+gap] for i in indexes if i+gap < n)\n for i,st in enumerate(indexes):\n if i > 0 and indexes[i-1] + gap == st: continue\n if st + gap < n and s[st + gap] == cmax:new_indexes.append(st)\n indexes = new_indexes\n gap += 1\n return s[indexes[0]:]\n``` | 1 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
One-line solution in Python | last-substring-in-lexicographical-order | 0 | 1 | # Intuition\nThe lexicographically maximum substring in `s` will start with the letter `max(s)` and continue to the end of `s`.\n\n# Code\n```\nclass Solution:\n def lastSubstring(self, s: str) -> str:\n return max(s[m.start() :] for m in re.finditer(max(s), s))\n``` | 0 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
Easy brute force in python3 that barely passes | last-substring-in-lexicographical-order | 0 | 1 | # Code\n```\nclass Solution:\n def lastSubstring(self, s: str) -> str:\n res = s\n N = len(s)\n for i in range(N):\n res = max(res,s[i:])\n return res\n\n``` | 0 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
slow | last-substring-in-lexicographical-order | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def lastSubstring(self, s: str) -> str:\n n = len(s)\n max_substring = ""\n max_char = ""\n\n for i in range(n - 1, -1, -1):\n if s[i] > max_char:\n max_char = s[i]\n max_substring = s[i:]\n elif s[i] == max_char:\n if s[i:] > max_substring:\n max_substring = s[i:]\n\n return max_substring\n\n``` | 0 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
[Python] Two pointers - 2023/06/06 | last-substring-in-lexicographical-order | 0 | 1 | # Intuition\n\nfirst, find indices of lexicographically largest character by hashmap {ch: [...indices]}\n\nsince answer must be within every possible suffix string `s[i:] where i in index[biggestCh] `\n\nlet\'s choose first 2 smallest indices since the longer the larger and maintain `i` **always smaller than** `j`\n\n```\n[XXXXXXXXX]XXXXXXX\n i k\n______[XXXXXXXXX]XXXXXX\n j k\n```\n\nand we move these two pointers by a distance `k` and keep comparing s[i+k] with s[j+k]\n\n- if s[i+k] == s[j+k]:\n - compare next character by incrementing k\n- if s[i+k] > s[j+k]:\n - it means all the s[jj:] where j < jj <= j+k are smaller than s[i:]\n - `j` start from `j+k+1` and move to next biggest character and keep comaparing s[i+k] and s[j+k]\n - reset k to 0\n- if s[i+k] > s[j+k], same as bove:\n - it means all the s[ii:] where i < ii <= i+k are smaller than s[j:]\n - `i` start from `i+k+1` and move to next biggest character and keep comaparing s[i+k] and s[j+k]\n - reset k to 0\n - special case:\n - if i == j, move j to next biggest character position since we want to compare two different suffix string\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n\n# Code\n```\nclass Solution:\n def lastSubstring(self, s):\n index = defaultdict(list)\n biggestCh = ""\n for i, c in enumerate(s):\n index[c].append(i)\n biggestCh = max(biggestCh, c)\n\n if len(index[biggestCh]) == 1:\n return s[index[biggestCh][0]:]\n\n n = len(s)\n i, j = index[biggestCh][0], index[biggestCh][1]\n k = 1\n while i+k < n and j+k < n:\n if s[i+k] == s[j+k]: # if equal, keep moving k and compare next character\n k += 1\n continue\n elif s[i+k] > s[j+k]:\n j = j+k+1\n while j < n and s[j] != biggestCh:\n j += 1\n k = 0\n else: # s[i+k] < s[j+k]\n i = i+k+1\n while i < n and s[i] != biggestCh:\n i += 1\n k = 0\n\n if i == j:\n j += 1\n while j < n and s[j] != biggestCh:\n j += 1\n return s[i:]\n``` | 0 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
Solution | last-substring-in-lexicographical-order | 1 | 1 | ```C++ []\nclass Solution {\n public:\n string lastSubstring(string s) {\n int i = 0, j = 1, k = 0, n = int(s.length());\n while ((j + k) < n) {\n if (s[i + k] == s[j + k]) k++;\n else if (s[i + k] > s[j + k]) {\n j += k + 1;\n k = 0;\n } else {\n i = std::max(i + k + 1, j);\n j = i + 1;\n k = 0;\n }\n }\n return s.substr(i);\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def lastSubstring(self, s: str) -> str:\n n = len(s)\n cmax = max(s)\n indexes = [ i for i,c in enumerate(s) if c == cmax ]\n gap = 1\n while len(indexes) > 1:\n new_indexes = []\n cmax = max(s[i+gap] for i in indexes if i+gap < n)\n for i,st in enumerate(indexes):\n if i > 0 and indexes[i-1] + gap == st: continue\n if st + gap < n and s[st + gap] == cmax:new_indexes.append(st)\n indexes = new_indexes\n gap += 1\n return s[indexes[0]:]\n```\n\n```Java []\nclass Solution {\n public String lastSubstring(String s) {\n int i = 0;\n int j = 1;\n int k = 0;\n int n = s.length();\n char[] ca = s.toCharArray();\n\n while (j + k < n) {\n if (ca[i + k] == ca[j + k]) {\n k++;\n } else if (ca[i + k] > ca[j + k]) {\n j = j + k + 1;\n k = 0;\n } else {\n i = Math.max(i + k + 1, j);\n j = i + 1;\n k = 0;\n }\n }\n return s.substring(i);\n }\n}\n``` | 0 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
Python3 easy solution | last-substring-in-lexicographical-order | 0 | 1 | # Code\n```\nclass Solution:\n def lastSubstring(self, s: str) -> str:\n i = 0\n j = 1\n k = 0\n n = len(s)\n while j + k < n:\n if s[i + k] == s[j + k]:\n k += 1\n elif s[i + k] > s[j + k]:\n j += k + 1\n k = 0\n elif s[i + k] < s[j + k]:\n i = max(i + k + 1, j)\n j = i + 1\n k = 0\n return s[i:]\n``` | 0 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
2-line solution in Python with explanation | last-substring-in-lexicographical-order | 0 | 1 | # Intuition\nThe answer will have the largest initial letter `z`. In addition, the longer the answer is the better. In other words, only the substrings `s[i:]` with `s[i] == z` ending at the end of `s` should be considered as candidates. \n\nA candidate substring `s[i:]` is always better than `s[i+1:]` where `s[i+1] == z`. Based on this property, a lot of candidates can be skipped. \n\nAs a result, only the substrings `s[i:]` with `s[i] == z` and `s[i-1] != z` should be considered as condidates. The answer is the one with the largest order. \n\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nBased on above properties, the code can be implemented in a very concise way in Python. The first line is to find the largest letter `z` from `s`, which is the initial letter of the answer. The second line picks up the largest one from the candidates `s[i:]` meet all the properties. \n\n# Code\n```\nclass Solution:\n def lastSubstring(self, s: str) -> str:\n z = max(list(s))\n return max(s[i:] for i, c in enumerate(s) if c == z and (i == 0 or s[i-1] != z))\n``` | 0 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
python3 + fast + very easy | last-substring-in-lexicographical-order | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def lastSubstring(self, s: str) -> str:\n max = 97\n for e in s:\n if ord(e) > max:\n max = ord(e)\n \n maxstr = \'\'\n for i in range(len(s)):\n if ord(s[i]) == max:\n if s[i:] > maxstr:\n maxstr = s[i:]\n \n return maxstr\n``` | 0 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
Python Brute Force Solution | last-substring-in-lexicographical-order | 0 | 1 | # Intuition\n- The answer is always suffix of the given string\n- what if store the indices of largest character and compare the substring starting from the indeces to the end of the string\n\n# Approach\n- store the indices of largest character\n- for each index in indices take the largest substring by comparing substrings starting from the index to the end of the string\n\n# Complexity\nN = len(s)\n- Time complexity:\nO(N)\n\n- Space complexity:\nO(N)\n\n# Code\n```\nclass Solution:\n def lastSubstring(self, s: str) -> str:\n left = 0\n right = 0\n index = []\n while right < len(s):\n if s[right] > s[left]:\n left = right\n if s[right] == s[left]:\n if index and s[left] != s[index[0]]:\n index = []\n index.append(right)\n right += 1\n \n largest = s[index[-1]:]\n start = index[-1]\n for i in range(len(index) - 2, -1, -1):\n if s[index[i]:] >= largest:\n largest = s[index[i]:]\n start = index[i]\n \n return s[start:]\n``` | 0 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
Python very easy solution, Please upvote and add to your favourites. | last-substring-in-lexicographical-order | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def lastSubstring(self, s: str) -> str:\n res=""\n for i in range(len(s)):\n res=max(res,s[i:])\n return res\n``` | 0 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
Python Efficient Solution | Easy to Understand | Beats 70% | last-substring-in-lexicographical-order | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe need to find optimal index of maximum element. ```s[maxIndex:]``` is the answer\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nSo the Basis of this kindof solution is the fact that the input string may not only contain unique characters, it can contain duplicate high lexicographical chars , so at that point , we need to compare the substrings from those two chars that which one is more efficient. CRUX of the problem is:\nWe iterate throught the string from right to left . (max idx = n-1 initially )\n2 Cases :\n1. If we get a higher char, we assign it to max idx.\n2. If we get a char which is same as the prev encountered high char , ie. at max idx , then we need to compaer the strings\n\n2 cases :\n1. if the prev max char is at n-1 (max idx=n-1) , we assign max idx to i ,why? ( because obv a string of greater length will be lexicographical. Higher than the same char alone)\n2. if its not at n-1 , we compare strings (till both the strings are same ), when there is a mismatch , we check if current string has a higher element , or the prev max string is outof bound .\n3. If so , we assign max idx to i.\n\nHence we return the maximum lexicographical string.\n\n\n# Code\n```\nclass Solution:\n def lastSubstring(self, s: str) -> str:\n sz = len(s)\n maxIndex = sz - 1\n for idx in range(sz - 2, -1, -1):\n if s[idx] > s[maxIndex]:\n maxIndex = idx\n elif s[idx] == s[maxIndex]:\n if maxIndex == sz-1:\n maxIndex = idx\n else:\n j = 1\n while maxIndex + j < sz and s[idx + j] == s[maxIndex + j] and (idx + j) != maxIndex:\n j += 1\n if s[idx + j] >= s[min(maxIndex + j, sz - 1)] or (maxIndex + j) >= sz:\n maxIndex = idx\n return s[maxIndex:]\n``` | 0 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
Intutive Approach with detailed explanation | last-substring-in-lexicographical-order | 0 | 1 | # Intuition\n1. Answer will must start with the biggest character in string\n\n\n# Approach\n1. Traverse string in reverse order\n2. Keep track of biggest element till that specific index also keep track of the index of that biggest element.\n3. if new character is greater than the previous big character then will update biggest characted and index\n4. if new character is less than the previous big character then don\'t need to do anything\n5. if new character is equal to the previous biggest character then compare both substring whichever is biggest will store that.\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\n# Code\n```\n##Keep track of bigest character min index O(N^2)\n##Need to slove in ()\nclass Solution:\n def lastSubstring(self, s: str) -> str:\n mx=""\n biggest=s[-1]\n index=len(s)-1\n for i in range(len(s)-2,-1,-1):\n if s[i]>biggest:\n biggest=s[i]\n index=i\n elif s[i]==biggest:\n if s[i:len(s)]>s[index:len(s)]:\n biggest=s[i]\n index=i\n return s[index:len(s)] \n``` | 0 | Given a string `s`, return _the last substring of_ `s` _in lexicographical order_.
**Example 1:**
**Input:** s = "abab "
**Output:** "bab "
**Explanation:** The substrings are \[ "a ", "ab ", "aba ", "abab ", "b ", "ba ", "bab "\]. The lexicographically maximum substring is "bab ".
**Example 2:**
**Input:** s = "leetcode "
**Output:** "tcode "
**Constraints:**
* `1 <= s.length <= 4 * 105`
* `s` contains only lowercase English letters. | null |
Simple Python Solution with Explanation | Beats 88% of Submissions | invalid-transactions | 0 | 1 | **Source**: https://leetcode.com/problems/invalid-transactions/description/\n**Author**: Hassan Abioye\n**Notes**: [[Leetcode Algorithm Notes]]\n**Date**: 2023-11-29\n**Time**: 01:39\n**Tags**: #Array #HashTable #String #Sorting \n\n---\n\n### Solution:\n```python\nclass Solution:\n def invalidTransactions(self, transactions: List[str]) -> List[str]:\n ret = set()\n dic = collections.defaultdict(list)\n for i,item in enumerate(transactions):\n name, time, amount, city = item.split(",")\n dic[name].append((int(time),city,i))\n if int(amount) > 1000:\n ret.add(i)\n for k,v in dic.items():\n v.sort()\n vleng = len(v)\n for i in range(vleng):\n old = v[i]\n for j in range(i+1,vleng):\n new = v[j]\n diff = new[0]-old[0]\n if diff <= 60:\n if new[1] != old[1]:\n ret.add(old[2])\n ret.add(new[2])\n else:\n break\n return [transactions[x] for x in ret]\n```\n\n### Explanation:\nThe solution wants a list of potentially invalid transactions. Since some transactions may be duplicates of each other, you should not use a set of strings to store the transactions. Instead, use a set of integers to store the transactions. For each transaction, you should put it in a dictionary with the name as the key and the value stored in the list as a tuple in the format `(int(time), city, transaction_index)`. If a transaction has an amount that exceeds `1000`, then you add that transaction ID to the return set. When searching through the dictionary comparing transactions, you would compare transactions with the same key to compare transactions with the same name. The list associated with each key is sorted to make comparisons faster. Using a double for loop, we compare if the difference between the time transactions is 60 or less and if the cities are different. If that is the case then the indexes for the compared transactions are added to the set. A quick optimization to make is to break out of the inner for loop if the difference is greater than 60 assuming that the items in the list are sorted by timestamps from lowest to highest. This is because the difference between a later timestamp with a current starting timestamp will always be greater. The return value will be a list of all the items in `transactions` that has an index in the return set. | 1 | A transaction is possibly invalid if:
* the amount exceeds `$1000`, or;
* if it occurs within (and including) `60` minutes of another transaction with the **same name** in a **different city**.
You are given an array of strings `transaction` where `transactions[i]` consists of comma-separated values representing the name, time (in minutes), amount, and city of the transaction.
Return a list of `transactions` that are possibly invalid. You may return the answer in **any order**.
**Example 1:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Output:** \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Explanation:** The first transaction is invalid because the second transaction occurs within a difference of 60 minutes, have the same name and is in a different city. Similarly the second one is invalid too.
**Example 2:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,1200,mtv "\]
**Output:** \[ "alice,50,1200,mtv "\]
**Example 3:**
**Input:** transactions = \[ "alice,20,800,mtv ", "bob,50,1200,mtv "\]
**Output:** \[ "bob,50,1200,mtv "\]
**Constraints:**
* `transactions.length <= 1000`
* Each `transactions[i]` takes the form `"{name},{time},{amount},{city} "`
* Each `{name}` and `{city}` consist of lowercase English letters, and have lengths between `1` and `10`.
* Each `{time}` consist of digits, and represent an integer between `0` and `1000`.
* Each `{amount}` consist of digits, and represent an integer between `0` and `2000`. | Consider the items in order from largest to smallest value, and greedily take the items if they fall under the use_limit. We can keep track of how many items of each label are used by using a hash table. |
Python Solution | Hashmap | Set | invalid-transactions | 0 | 1 | ```\nclass Solution:\n def invalidTransactions(self, transactions: List[str]) -> List[str]:\n hashmap = {}\n\t\t#Hashset is used to skip redudant transactions being added to the result\n\t\t#We will only store index of the transaction because the same transaction can repeat.\n result = set()\n for i, t in enumerate(transactions):\n name, time, amount, city = t.split(",")\n\t\t\t#If there is no transaction record for the user\n if name not in hashmap:\n hashmap[name] = []\n if int(amount) > 1000:\n result.add(i)\n\t\t\t#If there are pass transaction records\n else:\n\t\t\t\t#Fetching past transaction from hashmap\n prevTrans = hashmap[name]\n\t\t\t\t#Iterating over the past transaction of the user and finding anomalies\n for j in range(len(prevTrans)):\n prevName, prevTime, prevAmount, prevCity = transactions[prevTrans[j]].split(",")\n #Checking whether the amount exceeds $1000\n\t\t\t\t\tif int(amount) > 1000:\n result.add(i)\n\t\t\t\t\t#Checking whether it occurs within (and including) 60 minutes of another transaction with the same name in a different city.\n if abs(int(time) - int(prevTime)) <= 60 and city != prevCity:\n result.add(i)\n result.add(prevTrans[j])\n\t\t\t#Recording transaction in the hashmap for the user\n hashmap[name].append(i)\n\t\t\n\t\t#Fetching transaction using indexes stored in the result set and returning\n return [transactions[t] for t in result]\n``` | 6 | A transaction is possibly invalid if:
* the amount exceeds `$1000`, or;
* if it occurs within (and including) `60` minutes of another transaction with the **same name** in a **different city**.
You are given an array of strings `transaction` where `transactions[i]` consists of comma-separated values representing the name, time (in minutes), amount, and city of the transaction.
Return a list of `transactions` that are possibly invalid. You may return the answer in **any order**.
**Example 1:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Output:** \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Explanation:** The first transaction is invalid because the second transaction occurs within a difference of 60 minutes, have the same name and is in a different city. Similarly the second one is invalid too.
**Example 2:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,1200,mtv "\]
**Output:** \[ "alice,50,1200,mtv "\]
**Example 3:**
**Input:** transactions = \[ "alice,20,800,mtv ", "bob,50,1200,mtv "\]
**Output:** \[ "bob,50,1200,mtv "\]
**Constraints:**
* `transactions.length <= 1000`
* Each `transactions[i]` takes the form `"{name},{time},{amount},{city} "`
* Each `{name}` and `{city}` consist of lowercase English letters, and have lengths between `1` and `10`.
* Each `{time}` consist of digits, and represent an integer between `0` and `1000`.
* Each `{amount}` consist of digits, and represent an integer between `0` and `2000`. | Consider the items in order from largest to smallest value, and greedily take the items if they fall under the use_limit. We can keep track of how many items of each label are used by using a hash table. |
Python3 | Beats 98% | O(N) O(N) | invalid-transactions | 0 | 1 | # Complexity\n- Time complexity: $$O(N)$$\n\n- Space complexity: $$O(N)$$\n\n# Code\n```\nclass Solution:\n def invalidTransactions(self, transactions: List[str]) -> List[str]:\n transactions.sort(key=lambda t: int(t.split(\',\')[1]))\n ans = []\n invalid = [False]*len(transactions)\n name2q = defaultdict(lambda: deque())\n for i in range(len(transactions)):\n name, time, amount, city = transactions[i].split(\',\')\n time = int(time)\n amount = int(amount)\n q = name2q[name]\n while q and time - q[0][0] > 60:\n q.popleft()\n cur_invalid = amount > 1000\n for _, other_city, j in q:\n if city != other_city:\n cur_invalid = True\n if not invalid[j]:\n invalid[j] = True\n ans.append(transactions[j])\n if cur_invalid:\n invalid[i] = True\n ans.append(transactions[i])\n name2q[name].append((time, city, i))\n return ans\n``` | 1 | A transaction is possibly invalid if:
* the amount exceeds `$1000`, or;
* if it occurs within (and including) `60` minutes of another transaction with the **same name** in a **different city**.
You are given an array of strings `transaction` where `transactions[i]` consists of comma-separated values representing the name, time (in minutes), amount, and city of the transaction.
Return a list of `transactions` that are possibly invalid. You may return the answer in **any order**.
**Example 1:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Output:** \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Explanation:** The first transaction is invalid because the second transaction occurs within a difference of 60 minutes, have the same name and is in a different city. Similarly the second one is invalid too.
**Example 2:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,1200,mtv "\]
**Output:** \[ "alice,50,1200,mtv "\]
**Example 3:**
**Input:** transactions = \[ "alice,20,800,mtv ", "bob,50,1200,mtv "\]
**Output:** \[ "bob,50,1200,mtv "\]
**Constraints:**
* `transactions.length <= 1000`
* Each `transactions[i]` takes the form `"{name},{time},{amount},{city} "`
* Each `{name}` and `{city}` consist of lowercase English letters, and have lengths between `1` and `10`.
* Each `{time}` consist of digits, and represent an integer between `0` and `1000`.
* Each `{amount}` consist of digits, and represent an integer between `0` and `2000`. | Consider the items in order from largest to smallest value, and greedily take the items if they fall under the use_limit. We can keep track of how many items of each label are used by using a hash table. |
O(N) ?? using HashTable/HashMap | Comments added | invalid-transactions | 0 | 1 | https://github.com/paulonteri/data-structures-and-algorithms\n\n- Record all transactions done at a particular time. Recording the person and the location. \n\t```\n\t{time: {person: {location}, person2: {location1, location2}}, time: {person: {location}}}\n\t```\n\tExample:\n\t```\n\t[\'alice,20,800,mtv\', \'bob,50,1200,mtv\', \'bob,20,100,beijing\']\n \n\t{ \n\t20: {\'alice\': {\'mtv\'}, \'bob\': {\'beijing\'}}, \n\t50: {\'bob\': {\'mtv\'}}\n\t} \n\t```\n- For each transaction, check if the amount is invalid - and add it to the invalid transactions if so.\n- For each transaction, go through invalid times (+-60), check if a transaction by the same person happened in a different city - and add it to the invalid transactions if so.\n\n---\nCode\n\n```\nfrom collections import defaultdict\n\n""" \nhttps://www.notion.so/paulonteri/Hashtables-Hashsets-220d9f0e409044c58ec6c2b0e7fe0ab5#cf22995975274881a28b544b0fce4716\n"""\n\nclass Solution(object):\n def invalidTransactions(self, transactions):\n """ \n - Record all transactions done at a particular time. Recording the person and the location. Example:\n `[\'alice,20,800,mtv\',\'bob,50,1200,mtv\',\'bob,20,100,beijing\']` :\\n\n ` \n { \n 20: {\'alice\': {\'mtv\'}, \'bob\': {\'beijing\'}}, \n 50: {\'bob\': {\'mtv\'}}\n } \n ` \\n\n `{time: {person: {location}, person2: {location1, location2}}, time: {person: {location}}}`\n - For each transaction, check if the amount is invalid - and add it to the invalid transactions if so.\n - For each transaction, go through invalid times (+-60), check if a transaction by the same person happened\n in a different city - and add it to the invalid transactions if so.\n """\n invalid = []\n\n # Record all transactions done at a particular time\n # including the person and the location.\n transaction_time = defaultdict(dict)\n for transaction in transactions:\n name, str_time, amount, city = transaction.split(",")\n time = int(str_time)\n\n if name not in transaction_time[time]:\n transaction_time[time][name] = {city, }\n else:\n transaction_time[time][name].add(city)\n\n for transaction in transactions:\n name, str_time, amount, city = transaction.split(",")\n time = int(str_time)\n\n # # check amount\n if int(amount) > 1000:\n invalid.append(transaction)\n continue\n\n # # check if person did transaction within 60 minutes in a different city\n for inv_time in range(time-60, time+61):\n if inv_time not in transaction_time:\n continue\n if name not in transaction_time[inv_time]:\n continue\n\n trans_by_name_at_time = transaction_time[inv_time][name]\n\n # check if transactions were done in a different city\n if city not in trans_by_name_at_time or len(trans_by_name_at_time) > 1:\n invalid.append(transaction)\n break\n\n return invalid\n\n``` | 19 | A transaction is possibly invalid if:
* the amount exceeds `$1000`, or;
* if it occurs within (and including) `60` minutes of another transaction with the **same name** in a **different city**.
You are given an array of strings `transaction` where `transactions[i]` consists of comma-separated values representing the name, time (in minutes), amount, and city of the transaction.
Return a list of `transactions` that are possibly invalid. You may return the answer in **any order**.
**Example 1:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Output:** \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Explanation:** The first transaction is invalid because the second transaction occurs within a difference of 60 minutes, have the same name and is in a different city. Similarly the second one is invalid too.
**Example 2:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,1200,mtv "\]
**Output:** \[ "alice,50,1200,mtv "\]
**Example 3:**
**Input:** transactions = \[ "alice,20,800,mtv ", "bob,50,1200,mtv "\]
**Output:** \[ "bob,50,1200,mtv "\]
**Constraints:**
* `transactions.length <= 1000`
* Each `transactions[i]` takes the form `"{name},{time},{amount},{city} "`
* Each `{name}` and `{city}` consist of lowercase English letters, and have lengths between `1` and `10`.
* Each `{time}` consist of digits, and represent an integer between `0` and `1000`.
* Each `{amount}` consist of digits, and represent an integer between `0` and `2000`. | Consider the items in order from largest to smallest value, and greedily take the items if they fall under the use_limit. We can keep track of how many items of each label are used by using a hash table. |
[Python3] - Linear time Solution O(N) | invalid-transactions | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nAt first we save all the transactions in a structure where we can quickly find them again.\n\nAs we are interested in checking for transactions with the same name and for a given point in time we use a nested dictionary with exactly these keys for quick lookup.\n\nNon Python-Programmers might be confused by the defaultdict, but this is just a quick way of getting around a lot of checks, whether the nested structures in the dictionary have already been instantiated.\n\nAlso we use a set to save the city name because we need to quickly check leter whether our transaction was in a different city.\n\nDon\'t worry: I took quite a while to come up with this solution and also incorporated ideas from other solutions. **I did not find this solution on my own immediately**. I just wanted to publish a clean solution for you.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nOnce we have the structure for quick lookups we go through the transactions once again and check whether the amount is too high, which invalidates them immediately.\n\nIf that is not the case, we check if there have been any transactions with the same name 60 minutes before us or after us. We do this by iterating through all different amounts in time (time-60 until time+60).\n\nOne could use a SortedDict for the time in order to do a quicker lookup of the time scope, but this would also cost more time while inserting the cities in the first loop.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(N + 2* time_window* N) = O(N + N*120) = O(N), the 120 comes from the second loop where we go through all the points in time. One could argue that we have a high time dependency on the time window for invalidation which is correct.\n\nTo solve this we could use a SortedDict for the time points. This offers O(N* logN) time complexity in the worst case, since we need to sort all the transactions if they have they all have the same name.\n\nUsing this one could make a quicker lookup with bisect for points in time and get rid of the time loop in the second loop of transactions.\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(N) as we need to save all of the transactions in our new data structure.\n# Code\n```\nclass Solution(object):\n def invalidTransactions(self, transactions: List[str]) -> List[str]:\n \n # make a defaultdict to save the transactions first\n mapped_transactions = collections.defaultdict(lambda: collections.defaultdict(set))\n\n # go through the transactions and save all of them in the structure \n for transaction in transactions:\n name, time, amount, city = Solution.parse_transaction(transaction)\n mapped_transactions[name][time].add(city)\n \n # go through the transactions again and check for invalid ones\n result = []\n for transaction in transactions:\n\n # parse the transcation\n name, time, amount, city = Solution.parse_transaction(transaction)\n \n # check for the amount\n if amount > 1000:\n result.append(transaction)\n continue\n \n # check whether we have a transaction with the same name\n if name not in mapped_transactions:\n continue\n \n # check if other transaction exist within the invalid timeframe\n for time_point in range(time-60, time+61):\n\n # time point does not exist\n if time_point not in mapped_transactions[name]:\n continue\n\n # check if there are more cities in within the time frame and the name\n # and if it is only one check that it is not the same city!\n if len(mapped_transactions[name][time_point]) > 1 or (city not in mapped_transactions[name][time_point]):\n result.append(transaction)\n break\n \n return result\n \n @staticmethod\n def parse_transaction(transaction: str) -> Tuple[str, int, int, str]:\n name, time, amount, city = transaction.split(\',\')\n time = int(time)\n amount = int(amount)\n return name, time, amount, city\n``` | 6 | A transaction is possibly invalid if:
* the amount exceeds `$1000`, or;
* if it occurs within (and including) `60` minutes of another transaction with the **same name** in a **different city**.
You are given an array of strings `transaction` where `transactions[i]` consists of comma-separated values representing the name, time (in minutes), amount, and city of the transaction.
Return a list of `transactions` that are possibly invalid. You may return the answer in **any order**.
**Example 1:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Output:** \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Explanation:** The first transaction is invalid because the second transaction occurs within a difference of 60 minutes, have the same name and is in a different city. Similarly the second one is invalid too.
**Example 2:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,1200,mtv "\]
**Output:** \[ "alice,50,1200,mtv "\]
**Example 3:**
**Input:** transactions = \[ "alice,20,800,mtv ", "bob,50,1200,mtv "\]
**Output:** \[ "bob,50,1200,mtv "\]
**Constraints:**
* `transactions.length <= 1000`
* Each `transactions[i]` takes the form `"{name},{time},{amount},{city} "`
* Each `{name}` and `{city}` consist of lowercase English letters, and have lengths between `1` and `10`.
* Each `{time}` consist of digits, and represent an integer between `0` and `1000`.
* Each `{amount}` consist of digits, and represent an integer between `0` and `2000`. | Consider the items in order from largest to smallest value, and greedily take the items if they fall under the use_limit. We can keep track of how many items of each label are used by using a hash table. |
Python using hashmap, tricky question | invalid-transactions | 0 | 1 | \tdef invalidTransactions(self, transactions: List[str]) -> List[str]:\n out = [] #list of all the invalid transactions to return \n names = defaultdict(lambda: defaultdict(set)) #{name: {time: city}}\n \n \n for t in transactions:\n name,time,amount,city = t.split(",")\n names[name][time].add(city)\n \n #-if a certain person is at more than one city at a time then we know it\'s an invalid transaction\n\t\t#-if the city we\'re at is not in the set it means the transaction has been made at two places within the 60 min limit\n\t\t\n\t\t#I want to preface the rest of this solution by saying this has worked so far but I\'m unsure if there\'s ever a testcase\n\t\t#in which this would not function correctly. This was a very tricky question and it definitely took me a while \n\t\t#to even come up with a working solution\n \n for t in transactions:\n name, time, amount, city = t.split(",")\n if int(amount) > 1000:\n out.append(t) \n else:\n for num in names[name].keys():\n if -60 <= int(time) - int(num) <= 60 and (len(names[name][num]) > 1 or city not in names[name][num]):\n out.append(t)\n break\n \n return out | 6 | A transaction is possibly invalid if:
* the amount exceeds `$1000`, or;
* if it occurs within (and including) `60` minutes of another transaction with the **same name** in a **different city**.
You are given an array of strings `transaction` where `transactions[i]` consists of comma-separated values representing the name, time (in minutes), amount, and city of the transaction.
Return a list of `transactions` that are possibly invalid. You may return the answer in **any order**.
**Example 1:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Output:** \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Explanation:** The first transaction is invalid because the second transaction occurs within a difference of 60 minutes, have the same name and is in a different city. Similarly the second one is invalid too.
**Example 2:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,1200,mtv "\]
**Output:** \[ "alice,50,1200,mtv "\]
**Example 3:**
**Input:** transactions = \[ "alice,20,800,mtv ", "bob,50,1200,mtv "\]
**Output:** \[ "bob,50,1200,mtv "\]
**Constraints:**
* `transactions.length <= 1000`
* Each `transactions[i]` takes the form `"{name},{time},{amount},{city} "`
* Each `{name}` and `{city}` consist of lowercase English letters, and have lengths between `1` and `10`.
* Each `{time}` consist of digits, and represent an integer between `0` and `1000`.
* Each `{amount}` consist of digits, and represent an integer between `0` and `2000`. | Consider the items in order from largest to smallest value, and greedily take the items if they fall under the use_limit. We can keep track of how many items of each label are used by using a hash table. |
Python: very simple and straightforward solution | invalid-transactions | 0 | 1 | ```\nclass Solution:\n def invalidTransactions(self, transactions: List[str]) -> List[str]:\n cities = defaultdict(lambda: defaultdict(list))\n output = []\n \n\t\t#build city map. \n for t in transactions:\n name, time, amount, city = t.split(\',\')\n cities[city][name].append(time)\n\n\t\t#Check each transaction against all transactions in a given city name from a given person\n for t in transactions:\n name, time, amount, city = t.split(\',\')\n \n\t\t\t#Case 1\n if int(amount) > 1000:\n output.append(t)\n continue\n \n\t\t\t#Case 2\n for k,v in cities.items():\n if k == city:\n continue\n \n if any([abs(int(x) - int(time)) <= 60 for x in v[name]]):\n output.append(t)\n break;\n \n return output\n``` | 4 | A transaction is possibly invalid if:
* the amount exceeds `$1000`, or;
* if it occurs within (and including) `60` minutes of another transaction with the **same name** in a **different city**.
You are given an array of strings `transaction` where `transactions[i]` consists of comma-separated values representing the name, time (in minutes), amount, and city of the transaction.
Return a list of `transactions` that are possibly invalid. You may return the answer in **any order**.
**Example 1:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Output:** \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Explanation:** The first transaction is invalid because the second transaction occurs within a difference of 60 minutes, have the same name and is in a different city. Similarly the second one is invalid too.
**Example 2:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,1200,mtv "\]
**Output:** \[ "alice,50,1200,mtv "\]
**Example 3:**
**Input:** transactions = \[ "alice,20,800,mtv ", "bob,50,1200,mtv "\]
**Output:** \[ "bob,50,1200,mtv "\]
**Constraints:**
* `transactions.length <= 1000`
* Each `transactions[i]` takes the form `"{name},{time},{amount},{city} "`
* Each `{name}` and `{city}` consist of lowercase English letters, and have lengths between `1` and `10`.
* Each `{time}` consist of digits, and represent an integer between `0` and `1000`.
* Each `{amount}` consist of digits, and represent an integer between `0` and `2000`. | Consider the items in order from largest to smallest value, and greedily take the items if they fall under the use_limit. We can keep track of how many items of each label are used by using a hash table. |
Python easy to understand solution with explanation using Dictionary | invalid-transactions | 0 | 1 | ```\nclass Solution(object):\n def invalidTransactions(self, transactions):\n """\n :type transactions: List[str]\n :rtype: List[str]\n \n memo = dictionary --> create a dictionary to keep track of previous transaction \n invalid_tran = set --> to store invalid transaction / I use set to avoid duplication \n \n Iterate over transactions:\n -> if the amount is greater than 1000 add it to the invalid_tran\n -> if there is any other previous transaction done by similar person , check it from the memo\n -> bring all previous transaction done by similar person (iterate over the memo)\n -> check if the absolute time difference is less than 60 and the city is the same \n -> if that is true add it to the invalid transaction \n -> add the transaction to the dictionary (memo) - always keep track of previous transaction \n """\n import collections\n if not transactions:\n return []\n \n memo = collections.defaultdict(list) #create a dictionary to keep track of previous transaction \n invalid_tran = set() #to store invalid transaction / I use set to avoid duplication\n for i,transaction in enumerate (transactions):\n \n name, time, amount, city = (int(i) if i.isnumeric() else i for i in transaction.split(\',\'))\n \n if amount > 1000: #if the amount is greater than 1000 add it to the invalid_tran\n invalid_tran.add(transaction) \n \n if name in memo: # if there is any other previous transaction done by similar person , check it from the memo\n for tran in memo[name]: # bring all previous transaction done by similar person (iterate over the memo)\n _, prev_time, _, prev_city =(int(i) if i.isnumeric() else i for i in tran.split(\',\'))\n if abs(time-prev_time) <= 60 and prev_city != city: #check if the absolute time difference is less than 60 and the city is the same\n invalid_tran.add(tran) \n invalid_tran.add(transaction) \n \n memo[name].append(transaction) # add the transaction to the dictionary (memo) - always keep track of previous transaction \n return invalid_tran\n``` | 10 | A transaction is possibly invalid if:
* the amount exceeds `$1000`, or;
* if it occurs within (and including) `60` minutes of another transaction with the **same name** in a **different city**.
You are given an array of strings `transaction` where `transactions[i]` consists of comma-separated values representing the name, time (in minutes), amount, and city of the transaction.
Return a list of `transactions` that are possibly invalid. You may return the answer in **any order**.
**Example 1:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Output:** \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Explanation:** The first transaction is invalid because the second transaction occurs within a difference of 60 minutes, have the same name and is in a different city. Similarly the second one is invalid too.
**Example 2:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,1200,mtv "\]
**Output:** \[ "alice,50,1200,mtv "\]
**Example 3:**
**Input:** transactions = \[ "alice,20,800,mtv ", "bob,50,1200,mtv "\]
**Output:** \[ "bob,50,1200,mtv "\]
**Constraints:**
* `transactions.length <= 1000`
* Each `transactions[i]` takes the form `"{name},{time},{amount},{city} "`
* Each `{name}` and `{city}` consist of lowercase English letters, and have lengths between `1` and `10`.
* Each `{time}` consist of digits, and represent an integer between `0` and `1000`.
* Each `{amount}` consist of digits, and represent an integer between `0` and `2000`. | Consider the items in order from largest to smallest value, and greedily take the items if they fall under the use_limit. We can keep track of how many items of each label are used by using a hash table. |
Simple clean python - only 10 lines | invalid-transactions | 0 | 1 | ```python\nclass Solution:\n def invalidTransactions(self, transactions: List[str]) -> List[str]:\n invalid = []\n \n for i, t1 in enumerate(transactions):\n name1, time1, amount1, city1 = t1.split(\',\')\n if int(amount1) > 1000:\n invalid.append(t1)\n continue\n for j, t2 in enumerate(transactions):\n if i != j: \n name2, time2, amount2, city2 = t2.split(\',\')\n if name1 == name2 and city1 != city2 and abs(int(time1) - int(time2)) <= 60:\n invalid.append(t1)\n break\n \n return invalid\n \n``` | 9 | A transaction is possibly invalid if:
* the amount exceeds `$1000`, or;
* if it occurs within (and including) `60` minutes of another transaction with the **same name** in a **different city**.
You are given an array of strings `transaction` where `transactions[i]` consists of comma-separated values representing the name, time (in minutes), amount, and city of the transaction.
Return a list of `transactions` that are possibly invalid. You may return the answer in **any order**.
**Example 1:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Output:** \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Explanation:** The first transaction is invalid because the second transaction occurs within a difference of 60 minutes, have the same name and is in a different city. Similarly the second one is invalid too.
**Example 2:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,1200,mtv "\]
**Output:** \[ "alice,50,1200,mtv "\]
**Example 3:**
**Input:** transactions = \[ "alice,20,800,mtv ", "bob,50,1200,mtv "\]
**Output:** \[ "bob,50,1200,mtv "\]
**Constraints:**
* `transactions.length <= 1000`
* Each `transactions[i]` takes the form `"{name},{time},{amount},{city} "`
* Each `{name}` and `{city}` consist of lowercase English letters, and have lengths between `1` and `10`.
* Each `{time}` consist of digits, and represent an integer between `0` and `1000`.
* Each `{amount}` consist of digits, and represent an integer between `0` and `2000`. | Consider the items in order from largest to smallest value, and greedily take the items if they fall under the use_limit. We can keep track of how many items of each label are used by using a hash table. |
Python Solution Using Class, O(N^2) Complexity | invalid-transactions | 0 | 1 | # Intuition\nStore the invalid transaction using set but later figured this won\'t work as there are duplicate transactions.\n\n# Approach\nUse class to store information and add a property called valid so that I can mark a transaction to be valid or invalid.\n\n# Complexity\n- Time complexity:\nO(n^2)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Transaction:\n def __init__(self, transaction):\n self.name, self.time, self.amount, self.city = transaction.split(",")\n self.time = int(self.time)\n self.amount = int(self.amount)\n self.string = transaction\n self.valid = True\n\n def validate_amount(self):\n if self.amount > 1000:\n self.valid = False\n \n def valid_collision(self, compare_transaction):\n if compare_transaction.valid and abs(compare_transaction.time - self.time) <= 60 and compare_transaction.city != self.city:\n compare_transaction.valid = False\n self.valid = False\n\nclass Solution:\n def invalidTransactions(self, transactions: List[str]) -> List[str]:\n \n record = defaultdict(list)\n\n for transaction in transactions:\n node = Transaction(transaction)\n record[node.name].append(node)\n \n for name in record:\n for transaction in record[name]:\n transaction.validate_amount()\n for compare_transaction in record[name]:\n transaction.valid_collision(compare_transaction)\n\n result = []\n for name in record:\n for transaction in record[name]:\n if transaction.valid == False:\n result.append(transaction.string)\n\n return result\n``` | 0 | A transaction is possibly invalid if:
* the amount exceeds `$1000`, or;
* if it occurs within (and including) `60` minutes of another transaction with the **same name** in a **different city**.
You are given an array of strings `transaction` where `transactions[i]` consists of comma-separated values representing the name, time (in minutes), amount, and city of the transaction.
Return a list of `transactions` that are possibly invalid. You may return the answer in **any order**.
**Example 1:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Output:** \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Explanation:** The first transaction is invalid because the second transaction occurs within a difference of 60 minutes, have the same name and is in a different city. Similarly the second one is invalid too.
**Example 2:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,1200,mtv "\]
**Output:** \[ "alice,50,1200,mtv "\]
**Example 3:**
**Input:** transactions = \[ "alice,20,800,mtv ", "bob,50,1200,mtv "\]
**Output:** \[ "bob,50,1200,mtv "\]
**Constraints:**
* `transactions.length <= 1000`
* Each `transactions[i]` takes the form `"{name},{time},{amount},{city} "`
* Each `{name}` and `{city}` consist of lowercase English letters, and have lengths between `1` and `10`.
* Each `{time}` consist of digits, and represent an integer between `0` and `1000`.
* Each `{amount}` consist of digits, and represent an integer between `0` and `2000`. | Consider the items in order from largest to smallest value, and greedily take the items if they fall under the use_limit. We can keep track of how many items of each label are used by using a hash table. |
Python3, FT 97%: Group by Name, Two-Pointer 60 Minute Window | invalid-transactions | 0 | 1 | # Intuition\n\nThe criteria involve\n* individual transactions\n* pairs of transactions with the same name\n\nSo we first realize that we can partition/group by name to simplify the problem.\n\nThen within the transactions for each name:\n* if transaction `i` exceeds 1000, we flag it\n* if transaction `i` is within 60 minutes of another transaction `j` and `city_i != city_j`, then we flag it too.\n * the order doesn\'t matter, so we can decide that `j < i`\n * if we pick the other order, it won\'t matter: either we\'ll flag both when we process index `i`, or when we process index `i`\n \nTo quickly find all transactions within 60 minutes, we can sort transactions for each person by time ascending. Then we can use a two pointer method. For each transaction `i`:\n * iterate over `j = i-1, i-2, .., 0`\n * if `i` and `j` are in different cities, flag them both\n\nThis algorithm is technically O(T^2) for T transactions per person. In practice it\'s really O(T) if we assume that transactions have some maximum rate, e.g. no more than a transaction every minute. In this way there will be at most 60 `j` indices for each `i`, for O(60*T).\n\n# Helpful Tools\n\n## Group By\n\nIn many cases we need to process data separately by groups, where we assign each element to at most one group\n* this is a SQL `group by` clause\n* or a Pandas `.group_by` operation\n* or a Spark `partition_by` operation\n* or a Flink `keyBy` operation\n* or a JavaScript/Scala `groupBy`\n* or the keys in MapReduce\n* (are you bored yet??)\n\nThe most obvious way to group by without a built-in function is to make a `defaultdict(list)` in Python, then do `mydict[key].append(value)` for each `key, value` pair\n\n## Time Window Operations\n\nAnother common thing in time series analysis (of which this is an example) is to process events using information from other events nearby in time.\n\nThe most common way to do this is\n1. sort by time\n2. use a two-pointer approach\n\nIn the two-pointer approach we fix one index `i`, and move `j` over all other indices within some time range. It\'s pretty easy when the data is sorted.\n\nThere are several common time operations, probably not worth studying in detail unless you\'re interviewing for a data science or quant job that requires time series expertise:\n* **fixed windows**\n * **arbitrary disjoint windows**\n * **tumbling windows**\n * **sliding windows**\n* **dynamic windows**\n * **count/aggregate windows** (e.g. windows denominated by count, element sum, etc. instead of time)\n * **session windows** (windows that grow as long as the next element arrives within some maximum waiting time)\n * **temporal joins** (combining data with other data that arrived in a time interval around it)\n\n**This problem is a temporal (self-)join problem.**\n\n# Complexity\n- Time complexity: O(N^2) worst case where all N transactions are for one person within 60 minutes. Typical case O(N) if there\'s a maximum transaction rate\n - each event has at most N elements that occured in the past 60 minutes\n - so we\'ll do O(N) work per element\n - and thus the complexity is O(N^2)\n - in practice, transactions probably have a max typical rate of something like one every 5 minutes. This limits the max number of recent transactions to about 60/5 = 12, so we\'ll do O(12*N) work = O(N) work total. Although sorting is O(N log(N)) so the overall complexity would be O(N log(N))\n - although sorting can also be accelerated with a bucket sort when there is a max transaction rate\n\n- Space complexity: O(N), the big `byName` dictionary of lists\n\n# Code\n```\nclass Solution:\n def invalidTransactions(self, transactions: List[str]) -> List[str]:\n # above 1000\n # within <= 60 minutes of another tx with the same name but another city\n\n # so key by name\n # probably order by time\n # we can look at the number of distinct cities in the last 60 minutes (t-60 to t)\n # if there\'s only one then the txs are good\n # if there\'s more than one city then flag all txs\n # maybe better: two pointer\n # for each tx, scan back to t-60, if there\'s another city then flag both txs and break\n # O(T^2) for T transactions in 60 minutes, but that should be fairly low\n\n byName = defaultdict(list) # name -> (time, amt, city)\n for tx in transactions:\n name, time, amt, city = tx.split(\',\')\n time = int(time)\n amt = int(amt)\n byName[name].append((time, amt, city))\n\n ans = []\n for name, txs in byName.items():\n txs.sort(key = lambda triple: triple[0]) # by time asc.\n flagged = set()\n for i in range(len(txs)):\n if txs[i][1] > 1000: \n flagged.add(i)\n\n for j in range(i-1, -1, -1):\n if txs[j][0] < txs[i][0] - 60: break\n\n if txs[j][2] != txs[i][2]:\n # different city within 60 minutes\n flagged.add(i)\n flagged.add(j)\n # don\'t break: we may need to flag earlier j\'s\n\n for i in flagged:\n amt, time, city = txs[i]\n ans.append(f"{name},{amt},{time},{city}")\n\n return ans\n``` | 0 | A transaction is possibly invalid if:
* the amount exceeds `$1000`, or;
* if it occurs within (and including) `60` minutes of another transaction with the **same name** in a **different city**.
You are given an array of strings `transaction` where `transactions[i]` consists of comma-separated values representing the name, time (in minutes), amount, and city of the transaction.
Return a list of `transactions` that are possibly invalid. You may return the answer in **any order**.
**Example 1:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Output:** \[ "alice,20,800,mtv ", "alice,50,100,beijing "\]
**Explanation:** The first transaction is invalid because the second transaction occurs within a difference of 60 minutes, have the same name and is in a different city. Similarly the second one is invalid too.
**Example 2:**
**Input:** transactions = \[ "alice,20,800,mtv ", "alice,50,1200,mtv "\]
**Output:** \[ "alice,50,1200,mtv "\]
**Example 3:**
**Input:** transactions = \[ "alice,20,800,mtv ", "bob,50,1200,mtv "\]
**Output:** \[ "bob,50,1200,mtv "\]
**Constraints:**
* `transactions.length <= 1000`
* Each `transactions[i]` takes the form `"{name},{time},{amount},{city} "`
* Each `{name}` and `{city}` consist of lowercase English letters, and have lengths between `1` and `10`.
* Each `{time}` consist of digits, and represent an integer between `0` and `1000`.
* Each `{amount}` consist of digits, and represent an integer between `0` and `2000`. | Consider the items in order from largest to smallest value, and greedily take the items if they fall under the use_limit. We can keep track of how many items of each label are used by using a hash table. |
Solution Space (Binary Search) in Python3 / TypeScript | compare-strings-by-frequency-of-the-smallest-character | 0 | 1 | # Intuition\nFor this problem we\'re going to use **Binary Search** (Solution Space).\n\nLet\'s briefly explain why:\n```\n# 1. there are \'words\' and \'queries\'\n\n# 2. both of them contains only English lowercase letters\n\n# 3. there\'s a definition F(s), that represents\n# frequency of the smallest lexicographical character\n# of a particular string\n\n# 4. our goal is to define HOW many words satisfy \n# the next condition for queries\n# f(queries[i]) < f(w) for each w in words\n\n```\n\nIf we represent each word from `words` with the **frequency** of its characters, we can see a **frequency map**. \n\n```\n# Example\nnums = \'abacaba\'\nmap = [4,2,0,0,0....,0]\n```\n\nNext step is to **compare** frequency of a particular query with each word to find a count of words, that satisfies the condition above.\n\nBut this\'s not an effective approach.\n\nhat if the input will be **sorted?** \nWe could use the **Binary Search** to optimally find a point of insertion etc.\n\n# Approach\n1. define `extractFrequency` function, that accepts `w` as word and calculate **frequency of the lexicographically smallest character**\n2. sort mapped `words`\n3. declare an empty `ans` for storing results of **Binary Search**\n4. define `search` function to find `f(queries[i]) < f(w) for each w in words`\n5. for each query in `queries` store the result into `ans`\n6. return ans\n\n# Complexity\n- Time complexity: **O(n log n + m log n)**, this calculates Binary Search for each query and sorting of `words` after mapping with frequency\n\n- Space complexity: **O(n + m)**, where `n` is count of `words`, `m` - count of `queries`\n\n# Code in TypeScript\n```\nclass Solution:\n def numSmallerByFrequency(self, queries: List[str], words: List[str]) -> List[int]:\n def extractFrequency(w):\n min = w[0]\n\n for char in w:\n if char < min: min = char\n \n return w.count(min)\n \n words = sorted([extractFrequency(w) for w in words])\n\n def search(k):\n left, right = 0, len(words)\n\n while left < right:\n mid = (left + right) // 2\n\n if words[mid] > k: right = mid\n else: left = mid + 1\n\n return len(words) - left\n\n return [search(extractFrequency(x)) for x in queries]\n```\n# Code in TypeScript\n```\nfunction numSmallerByFrequency(queries: string[], words: string[]): number[] {\n const extractFrequency = (w) => {\n let min = w[0]\n\n for (let i = 0; i < w.length; i++) {\n if (min > w[i]) min = w[i]\n }\n\n return w.split(\'\').reduce((a, c) => a + Number(c === min), 0)\n };\n\n const mappedWords = words.map(extractFrequency);\n mappedWords.sort((a, b) => a - b);\n\n const ans: number[] = [];\n\n const search = (k) => {\n let [left, right] = [0, mappedWords.length];\n\n while (left < right) {\n const mid = (left + right) >> 1;\n\n if (mappedWords[mid] > k) {\n right = mid;\n } else {\n left = mid + 1;\n }\n }\n\n return mappedWords.length - left;\n };\n\n for (let i = 0; i < queries.length; i++) {\n ans.push(search(extractFrequency(queries[i])));\n }\n\n return ans;\n}\n``` | 1 | Let the function `f(s)` be the **frequency of the lexicographically smallest character** in a non-empty string `s`. For example, if `s = "dcce "` then `f(s) = 2` because the lexicographically smallest character is `'c'`, which has a frequency of 2.
You are given an array of strings `words` and another array of query strings `queries`. For each query `queries[i]`, count the **number of words** in `words` such that `f(queries[i])` < `f(W)` for each `W` in `words`.
Return _an integer array_ `answer`_, where each_ `answer[i]` _is the answer to the_ `ith` _query_.
**Example 1:**
**Input:** queries = \[ "cbd "\], words = \[ "zaaaz "\]
**Output:** \[1\]
**Explanation:** On the first query we have f( "cbd ") = 1, f( "zaaaz ") = 3 so f( "cbd ") < f( "zaaaz ").
**Example 2:**
**Input:** queries = \[ "bbb ", "cc "\], words = \[ "a ", "aa ", "aaa ", "aaaa "\]
**Output:** \[1,2\]
**Explanation:** On the first query only f( "bbb ") < f( "aaaa "). On the second query both f( "aaa ") and f( "aaaa ") are both > f( "cc ").
**Constraints:**
* `1 <= queries.length <= 2000`
* `1 <= words.length <= 2000`
* `1 <= queries[i].length, words[i].length <= 10`
* `queries[i][j]`, `words[i][j]` consist of lowercase English letters. | We can find the length of the longest common subsequence between str1[i:] and str2[j:] (for all (i, j)) by using dynamic programming. We can use this information to recover the longest common supersequence. |
✔ Python3 Solution | 3 Line Solution | Binary Search | compare-strings-by-frequency-of-the-smallest-character | 0 | 1 | # Complexity\n- Time complexity: $$O((n + m) * logm)$$\n- Space complexity: $$O(n)$$\n\n# Code\n```\nclass Solution:\n def numSmallerByFrequency(self, Q, W):\n f = lambda x: x.count(min(x))\n W = sorted(list(map(f, W)))\n return [len(W) - bisect_right(W, i) for i in map(f, Q)]\n``` | 2 | Let the function `f(s)` be the **frequency of the lexicographically smallest character** in a non-empty string `s`. For example, if `s = "dcce "` then `f(s) = 2` because the lexicographically smallest character is `'c'`, which has a frequency of 2.
You are given an array of strings `words` and another array of query strings `queries`. For each query `queries[i]`, count the **number of words** in `words` such that `f(queries[i])` < `f(W)` for each `W` in `words`.
Return _an integer array_ `answer`_, where each_ `answer[i]` _is the answer to the_ `ith` _query_.
**Example 1:**
**Input:** queries = \[ "cbd "\], words = \[ "zaaaz "\]
**Output:** \[1\]
**Explanation:** On the first query we have f( "cbd ") = 1, f( "zaaaz ") = 3 so f( "cbd ") < f( "zaaaz ").
**Example 2:**
**Input:** queries = \[ "bbb ", "cc "\], words = \[ "a ", "aa ", "aaa ", "aaaa "\]
**Output:** \[1,2\]
**Explanation:** On the first query only f( "bbb ") < f( "aaaa "). On the second query both f( "aaa ") and f( "aaaa ") are both > f( "cc ").
**Constraints:**
* `1 <= queries.length <= 2000`
* `1 <= words.length <= 2000`
* `1 <= queries[i].length, words[i].length <= 10`
* `queries[i][j]`, `words[i][j]` consist of lowercase English letters. | We can find the length of the longest common subsequence between str1[i:] and str2[j:] (for all (i, j)) by using dynamic programming. We can use this information to recover the longest common supersequence. |
Python3 | Solved Using Binary Search By Translating Each and Every word into Function Value | compare-strings-by-frequency-of-the-smallest-character | 0 | 1 | ```\nclass Solution:\n #Let n = len(queries) and m = len(words)\n #Time-Complexity: O(m + mlog(m) + n*log(m)) -> O(mlog(m) + nlog(m))\n #Space-Complexity: O(10*m + n*10 + m) -> O(n + m)\n def numSmallerByFrequency(self, queries: List[str], words: List[str]) -> List[int]:\n \n #Approach: Traverse linearly through each and every word in words array\n #and get its function value and append it to seperate array!\n #Then, sort the separate array in increasing order.\n #Then, for each ith query, get the function value from applying\n #function on ith query string -> look for leftmost index in\n #new array s.t. from that particular index onwards, all values\n #will be larger than the ith query function value!\n \n #This will help us get number of words in words input array that\n #have higher function value than current query!\n \n #We need to also define helper function that computes\n #function value for any non-empty string!\n def helper(s):\n hashmap = {}\n for c in s:\n if c not in hashmap:\n hashmap[c] = 1\n continue\n else:\n hashmap[c] += 1\n #iterate through each key value and put it in array of tuples,\n #where first element is letter and second element is its frequency!\n \n array = []\n for k,v in hashmap.items():\n array.append((k, v))\n #then sort the array by lexicographical order: first element of tuple!\n array.sort(key = lambda x: x[0])\n #return the first element of array: lexicographically smallest\n #char frequency!\n return array[0][1]\n ans = []\n arr = []\n for word in words:\n arr.append(helper(word))\n #sort the new array arr!\n arr.sort()\n \n #iterate through each and every query!\n for query in queries:\n requirement = helper(query)\n #initiate binary search to find leftmost index pos in arr!\n l, r = 0, (len(arr) - 1)\n leftmost_index = None\n while l <= r:\n mid = (l + r) // 2\n middle = arr[mid]\n if(middle > requirement):\n leftmost_index = mid\n r = mid - 1\n continue\n else:\n l = mid + 1 \n continue\n #once binary search is over, we should have leftmost index!\n #Append the difference val since it tells number of words\n #with function value greater than requirement!\n \n #we have to check whether leftmost_index still equals None ->\n #if none of words in words array have function val greater than\n #the requirement!\n if(leftmost_index == None):\n ans.append(0)\n continue\n ans.append(len(arr) - leftmost_index)\n return ans | 1 | Let the function `f(s)` be the **frequency of the lexicographically smallest character** in a non-empty string `s`. For example, if `s = "dcce "` then `f(s) = 2` because the lexicographically smallest character is `'c'`, which has a frequency of 2.
You are given an array of strings `words` and another array of query strings `queries`. For each query `queries[i]`, count the **number of words** in `words` such that `f(queries[i])` < `f(W)` for each `W` in `words`.
Return _an integer array_ `answer`_, where each_ `answer[i]` _is the answer to the_ `ith` _query_.
**Example 1:**
**Input:** queries = \[ "cbd "\], words = \[ "zaaaz "\]
**Output:** \[1\]
**Explanation:** On the first query we have f( "cbd ") = 1, f( "zaaaz ") = 3 so f( "cbd ") < f( "zaaaz ").
**Example 2:**
**Input:** queries = \[ "bbb ", "cc "\], words = \[ "a ", "aa ", "aaa ", "aaaa "\]
**Output:** \[1,2\]
**Explanation:** On the first query only f( "bbb ") < f( "aaaa "). On the second query both f( "aaa ") and f( "aaaa ") are both > f( "cc ").
**Constraints:**
* `1 <= queries.length <= 2000`
* `1 <= words.length <= 2000`
* `1 <= queries[i].length, words[i].length <= 10`
* `queries[i][j]`, `words[i][j]` consist of lowercase English letters. | We can find the length of the longest common subsequence between str1[i:] and str2[j:] (for all (i, j)) by using dynamic programming. We can use this information to recover the longest common supersequence. |
Python Simple Code Memory efficient | compare-strings-by-frequency-of-the-smallest-character | 0 | 1 | \tclass Solution:\n\t\tdef numSmallerByFrequency(self, queries: List[str], words: List[str]) -> List[int]:\n\t\t\tdef f(s):\n\t\t\t\tt = sorted(list(s))[0]\n\t\t\t\treturn s.count(t)\n\t\t\tquery = [f(x) for x in queries]\n\t\t\tword = [f(x) for x in words]\n\t\t\tm = []\n\t\t\tfor x in query:\n\t\t\t\tcount = 0\n\t\t\t\tfor y in word:\n\t\t\t\t\tif y>x:\n\t\t\t\t\t\tcount+=1\n\t\t\t\tm.append(count)\n\t\t\treturn m | 17 | Let the function `f(s)` be the **frequency of the lexicographically smallest character** in a non-empty string `s`. For example, if `s = "dcce "` then `f(s) = 2` because the lexicographically smallest character is `'c'`, which has a frequency of 2.
You are given an array of strings `words` and another array of query strings `queries`. For each query `queries[i]`, count the **number of words** in `words` such that `f(queries[i])` < `f(W)` for each `W` in `words`.
Return _an integer array_ `answer`_, where each_ `answer[i]` _is the answer to the_ `ith` _query_.
**Example 1:**
**Input:** queries = \[ "cbd "\], words = \[ "zaaaz "\]
**Output:** \[1\]
**Explanation:** On the first query we have f( "cbd ") = 1, f( "zaaaz ") = 3 so f( "cbd ") < f( "zaaaz ").
**Example 2:**
**Input:** queries = \[ "bbb ", "cc "\], words = \[ "a ", "aa ", "aaa ", "aaaa "\]
**Output:** \[1,2\]
**Explanation:** On the first query only f( "bbb ") < f( "aaaa "). On the second query both f( "aaa ") and f( "aaaa ") are both > f( "cc ").
**Constraints:**
* `1 <= queries.length <= 2000`
* `1 <= words.length <= 2000`
* `1 <= queries[i].length, words[i].length <= 10`
* `queries[i][j]`, `words[i][j]` consist of lowercase English letters. | We can find the length of the longest common subsequence between str1[i:] and str2[j:] (for all (i, j)) by using dynamic programming. We can use this information to recover the longest common supersequence. |
Easy to understand Python3 solution | compare-strings-by-frequency-of-the-smallest-character | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numSmallerByFrequency(self, queries: List[str], words: List[str]) -> List[int]:\n q_list = []\n for q in queries:\n q = list(q)\n q.sort()\n q_list.append(q.count(q[0]))\n\n w_list = []\n for w in words:\n w = list(w)\n w.sort()\n w_list.append(w.count(w[0]))\n\n res = []\n for q in q_list:\n count = 0\n for w in w_list:\n if q < w:\n count += 1\n res.append(count)\n\n return res\n \n``` | 0 | Let the function `f(s)` be the **frequency of the lexicographically smallest character** in a non-empty string `s`. For example, if `s = "dcce "` then `f(s) = 2` because the lexicographically smallest character is `'c'`, which has a frequency of 2.
You are given an array of strings `words` and another array of query strings `queries`. For each query `queries[i]`, count the **number of words** in `words` such that `f(queries[i])` < `f(W)` for each `W` in `words`.
Return _an integer array_ `answer`_, where each_ `answer[i]` _is the answer to the_ `ith` _query_.
**Example 1:**
**Input:** queries = \[ "cbd "\], words = \[ "zaaaz "\]
**Output:** \[1\]
**Explanation:** On the first query we have f( "cbd ") = 1, f( "zaaaz ") = 3 so f( "cbd ") < f( "zaaaz ").
**Example 2:**
**Input:** queries = \[ "bbb ", "cc "\], words = \[ "a ", "aa ", "aaa ", "aaaa "\]
**Output:** \[1,2\]
**Explanation:** On the first query only f( "bbb ") < f( "aaaa "). On the second query both f( "aaa ") and f( "aaaa ") are both > f( "cc ").
**Constraints:**
* `1 <= queries.length <= 2000`
* `1 <= words.length <= 2000`
* `1 <= queries[i].length, words[i].length <= 10`
* `queries[i][j]`, `words[i][j]` consist of lowercase English letters. | We can find the length of the longest common subsequence between str1[i:] and str2[j:] (for all (i, j)) by using dynamic programming. We can use this information to recover the longest common supersequence. |
Binary Search | Sorting | compare-strings-by-frequency-of-the-smallest-character | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numSmallerByFrequency(self, queries: List[str], words: List[str]) -> List[int]:\n answer = []\n for word_id, word in enumerate(words):\n word = sorted(word)\n freq = word.count(word[0])\n words[word_id] = freq\n for query_id, query in enumerate(queries):\n query = sorted(query)\n freq = query.count(query[0])\n queries[query_id] = freq\n words = sorted(words)\n for query_freq in queries:\n left, right = 0, len(words) - 1\n count = 0\n while left <= right:\n mid = (left + right) // 2\n if(words[mid] > query_freq):\n count += (right + 1) - mid \n right = mid - 1\n else:\n left = mid + 1\n answer.append(count)\n return answer\n\n\n\n\n\n\n``` | 0 | Let the function `f(s)` be the **frequency of the lexicographically smallest character** in a non-empty string `s`. For example, if `s = "dcce "` then `f(s) = 2` because the lexicographically smallest character is `'c'`, which has a frequency of 2.
You are given an array of strings `words` and another array of query strings `queries`. For each query `queries[i]`, count the **number of words** in `words` such that `f(queries[i])` < `f(W)` for each `W` in `words`.
Return _an integer array_ `answer`_, where each_ `answer[i]` _is the answer to the_ `ith` _query_.
**Example 1:**
**Input:** queries = \[ "cbd "\], words = \[ "zaaaz "\]
**Output:** \[1\]
**Explanation:** On the first query we have f( "cbd ") = 1, f( "zaaaz ") = 3 so f( "cbd ") < f( "zaaaz ").
**Example 2:**
**Input:** queries = \[ "bbb ", "cc "\], words = \[ "a ", "aa ", "aaa ", "aaaa "\]
**Output:** \[1,2\]
**Explanation:** On the first query only f( "bbb ") < f( "aaaa "). On the second query both f( "aaa ") and f( "aaaa ") are both > f( "cc ").
**Constraints:**
* `1 <= queries.length <= 2000`
* `1 <= words.length <= 2000`
* `1 <= queries[i].length, words[i].length <= 10`
* `queries[i][j]`, `words[i][j]` consist of lowercase English letters. | We can find the length of the longest common subsequence between str1[i:] and str2[j:] (for all (i, j)) by using dynamic programming. We can use this information to recover the longest common supersequence. |
Python Simple Solution | compare-strings-by-frequency-of-the-smallest-character | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n$$O(Q * W * (n + avg_w))$$\n\n Q is the number of queries.\n W is the number of words.\n n is the average length of the queries.\n avg_w is the average length of the words.\n\n\n- Space complexity:\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def numSmallerByFrequency(self, queries: List[str], words: List[str]) -> List[int]:\n def f(s):\n return s.count(min(s))\n ans = []\n for q in queries:\n count = 0\n f_query = f(q)\n for w in words:\n if f_query < f(w):\n count += 1\n ans.append(count)\n return ans\n``` | 0 | Let the function `f(s)` be the **frequency of the lexicographically smallest character** in a non-empty string `s`. For example, if `s = "dcce "` then `f(s) = 2` because the lexicographically smallest character is `'c'`, which has a frequency of 2.
You are given an array of strings `words` and another array of query strings `queries`. For each query `queries[i]`, count the **number of words** in `words` such that `f(queries[i])` < `f(W)` for each `W` in `words`.
Return _an integer array_ `answer`_, where each_ `answer[i]` _is the answer to the_ `ith` _query_.
**Example 1:**
**Input:** queries = \[ "cbd "\], words = \[ "zaaaz "\]
**Output:** \[1\]
**Explanation:** On the first query we have f( "cbd ") = 1, f( "zaaaz ") = 3 so f( "cbd ") < f( "zaaaz ").
**Example 2:**
**Input:** queries = \[ "bbb ", "cc "\], words = \[ "a ", "aa ", "aaa ", "aaaa "\]
**Output:** \[1,2\]
**Explanation:** On the first query only f( "bbb ") < f( "aaaa "). On the second query both f( "aaa ") and f( "aaaa ") are both > f( "cc ").
**Constraints:**
* `1 <= queries.length <= 2000`
* `1 <= words.length <= 2000`
* `1 <= queries[i].length, words[i].length <= 10`
* `queries[i][j]`, `words[i][j]` consist of lowercase English letters. | We can find the length of the longest common subsequence between str1[i:] and str2[j:] (for all (i, j)) by using dynamic programming. We can use this information to recover the longest common supersequence. |
python3 naive simple sol | compare-strings-by-frequency-of-the-smallest-character | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def numSmallerByFrequency(self, queries: List[str], words: List[str]) -> List[int]:\n freq = []\n for e in words:\n min = \'z\'\n for k in e:\n if k < min:\n min = k\n c = 0\n for k in e:\n if k == min:\n c+=1\n freq.append(c)\n\n res = []\n for q in queries:\n c = 0\n temp = 0\n min = \'z\'\n for k in q:\n if k < min:\n min = k\n for k in q:\n if k == min:\n temp+=1\n \n for f in freq:\n if f > temp:\n c+=1\n res.append(c)\n\n return res\n``` | 0 | Let the function `f(s)` be the **frequency of the lexicographically smallest character** in a non-empty string `s`. For example, if `s = "dcce "` then `f(s) = 2` because the lexicographically smallest character is `'c'`, which has a frequency of 2.
You are given an array of strings `words` and another array of query strings `queries`. For each query `queries[i]`, count the **number of words** in `words` such that `f(queries[i])` < `f(W)` for each `W` in `words`.
Return _an integer array_ `answer`_, where each_ `answer[i]` _is the answer to the_ `ith` _query_.
**Example 1:**
**Input:** queries = \[ "cbd "\], words = \[ "zaaaz "\]
**Output:** \[1\]
**Explanation:** On the first query we have f( "cbd ") = 1, f( "zaaaz ") = 3 so f( "cbd ") < f( "zaaaz ").
**Example 2:**
**Input:** queries = \[ "bbb ", "cc "\], words = \[ "a ", "aa ", "aaa ", "aaaa "\]
**Output:** \[1,2\]
**Explanation:** On the first query only f( "bbb ") < f( "aaaa "). On the second query both f( "aaa ") and f( "aaaa ") are both > f( "cc ").
**Constraints:**
* `1 <= queries.length <= 2000`
* `1 <= words.length <= 2000`
* `1 <= queries[i].length, words[i].length <= 10`
* `queries[i][j]`, `words[i][j]` consist of lowercase English letters. | We can find the length of the longest common subsequence between str1[i:] and str2[j:] (for all (i, j)) by using dynamic programming. We can use this information to recover the longest common supersequence. |
📌📌 Easiest Approach || Clean & Concise || Well-Explained 🐍 | remove-zero-sum-consecutive-nodes-from-linked-list | 0 | 1 | ## IDEA :\n*If we see with little focus we will come to know that we are removing the nodes with sum value 0.*\nBut here the question is how we will come to know that sum of subset nodes is 0. For this we can use **prefix sum**. If we are having sum which has already appeared once before it means that we are having sum of nodes between them = 0.\n\n**Algorithm :**\n* Firstly make a dummy Node of value 0 to connect in front of the LinkedList.\n* Using `pre` we will store node with sum value upto that node.\n\n* After storing we will again go through full linked list and if we get sum which already has stored in `dic` then we will move next pointer to that `dic` node\'s next node.\n\n\n**Implementation :**\n\'\'\'\n\n\tclass Solution:\n\t\tdef removeZeroSumSublists(self, head: Optional[ListNode]) -> Optional[ListNode]:\n\n\t\t\tdummy = ListNode(0,head)\n\t\t\tpre = 0\n\t\t\tdic = {0: dummy}\n\n\t\t\twhile head:\n\t\t\t\tpre+=head.val\n\t\t\t\tdic[pre] = head\n\t\t\t\thead = head.next\n\n\t\t\thead = dummy\n\t\t\tpre = 0\n\t\t\twhile head:\n\t\t\t\tpre+=head.val\n\t\t\t\thead.next = dic[pre].next\n\t\t\t\thead = head.next\n\n\t\t\treturn dummy.next\n\t\n**Feel free to ask if you have any doubt or want example as Explaination.** \uD83E\uDD17\n### Thanks and Upvote If you got any help or like the Idea !!\uD83E\uDD1E | 15 | Given the `head` of a linked list, we repeatedly delete consecutive sequences of nodes that sum to `0` until there are no such sequences.
After doing so, return the head of the final linked list. You may return any such answer.
(Note that in the examples below, all sequences are serializations of `ListNode` objects.)
**Example 1:**
**Input:** head = \[1,2,-3,3,1\]
**Output:** \[3,1\]
**Note:** The answer \[1,2,1\] would also be accepted.
**Example 2:**
**Input:** head = \[1,2,3,-3,4\]
**Output:** \[1,2,4\]
**Example 3:**
**Input:** head = \[1,2,3,-3,-2\]
**Output:** \[1\]
**Constraints:**
* The given linked list will contain between `1` and `1000` nodes.
* Each node in the linked list has `-1000 <= node.val <= 1000`. | Do a breadth first search to find the shortest path. |
📌📌 Easiest Approach || Clean & Concise || Well-Explained 🐍 | remove-zero-sum-consecutive-nodes-from-linked-list | 0 | 1 | ## IDEA :\n*If we see with little focus we will come to know that we are removing the nodes with sum value 0.*\nBut here the question is how we will come to know that sum of subset nodes is 0. For this we can use **prefix sum**. If we are having sum which has already appeared once before it means that we are having sum of nodes between them = 0.\n\n**Algorithm :**\n* Firstly make a dummy Node of value 0 to connect in front of the LinkedList.\n* Using `pre` we will store node with sum value upto that node.\n\n* After storing we will again go through full linked list and if we get sum which already has stored in `dic` then we will move next pointer to that `dic` node\'s next node.\n\n\n**Implementation :**\n\'\'\'\n\n\tclass Solution:\n\t\tdef removeZeroSumSublists(self, head: Optional[ListNode]) -> Optional[ListNode]:\n\n\t\t\tdummy = ListNode(0,head)\n\t\t\tpre = 0\n\t\t\tdic = {0: dummy}\n\n\t\t\twhile head:\n\t\t\t\tpre+=head.val\n\t\t\t\tdic[pre] = head\n\t\t\t\thead = head.next\n\n\t\t\thead = dummy\n\t\t\tpre = 0\n\t\t\twhile head:\n\t\t\t\tpre+=head.val\n\t\t\t\thead.next = dic[pre].next\n\t\t\t\thead = head.next\n\n\t\t\treturn dummy.next\n\t\n**Feel free to ask if you have any doubt or want example as Explaination.** \uD83E\uDD17\n### Thanks and Upvote If you got any help or like the Idea !!\uD83E\uDD1E | 15 | You are given a map of a server center, represented as a `m * n` integer matrix `grid`, where 1 means that on that cell there is a server and 0 means that it is no server. Two servers are said to communicate if they are on the same row or on the same column.
Return the number of servers that communicate with any other server.
**Example 1:**
**Input:** grid = \[\[1,0\],\[0,1\]\]
**Output:** 0
**Explanation:** No servers can communicate with others.
**Example 2:**
**Input:** grid = \[\[1,0\],\[1,1\]\]
**Output:** 3
**Explanation:** All three servers can communicate with at least one other server.
**Example 3:**
**Input:** grid = \[\[1,1,0,0\],\[0,0,1,0\],\[0,0,1,0\],\[0,0,0,1\]\]
**Output:** 4
**Explanation:** The two servers in the first row can communicate with each other. The two servers in the third column can communicate with each other. The server at right bottom corner can't communicate with any other server.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m <= 250`
* `1 <= n <= 250`
* `grid[i][j] == 0 or 1` | Convert the linked list into an array. While you can find a non-empty subarray with sum = 0, erase it. Convert the array into a linked list. |
[Python3] O(n) time solution with hashmap | remove-zero-sum-consecutive-nodes-from-linked-list | 0 | 1 | ```\nclass Solution:\n def removeZeroSumSublists(self, head: Optional[ListNode]) -> Optional[ListNode]:\n fake = ListNode(0, head)\n \n d = {0: fake}\n \n prefix_sum = 0\n while head:\n prefix_sum += head.val\n d[prefix_sum] = head\n head = head.next\n \n head = fake\n prefix_sum = 0\n while head:\n prefix_sum += head.val\n head.next = d[prefix_sum].next\n head = head.next\n \n return fake.next\n``` | 5 | Given the `head` of a linked list, we repeatedly delete consecutive sequences of nodes that sum to `0` until there are no such sequences.
After doing so, return the head of the final linked list. You may return any such answer.
(Note that in the examples below, all sequences are serializations of `ListNode` objects.)
**Example 1:**
**Input:** head = \[1,2,-3,3,1\]
**Output:** \[3,1\]
**Note:** The answer \[1,2,1\] would also be accepted.
**Example 2:**
**Input:** head = \[1,2,3,-3,4\]
**Output:** \[1,2,4\]
**Example 3:**
**Input:** head = \[1,2,3,-3,-2\]
**Output:** \[1\]
**Constraints:**
* The given linked list will contain between `1` and `1000` nodes.
* Each node in the linked list has `-1000 <= node.val <= 1000`. | Do a breadth first search to find the shortest path. |
[Python3] O(n) time solution with hashmap | remove-zero-sum-consecutive-nodes-from-linked-list | 0 | 1 | ```\nclass Solution:\n def removeZeroSumSublists(self, head: Optional[ListNode]) -> Optional[ListNode]:\n fake = ListNode(0, head)\n \n d = {0: fake}\n \n prefix_sum = 0\n while head:\n prefix_sum += head.val\n d[prefix_sum] = head\n head = head.next\n \n head = fake\n prefix_sum = 0\n while head:\n prefix_sum += head.val\n head.next = d[prefix_sum].next\n head = head.next\n \n return fake.next\n``` | 5 | You are given a map of a server center, represented as a `m * n` integer matrix `grid`, where 1 means that on that cell there is a server and 0 means that it is no server. Two servers are said to communicate if they are on the same row or on the same column.
Return the number of servers that communicate with any other server.
**Example 1:**
**Input:** grid = \[\[1,0\],\[0,1\]\]
**Output:** 0
**Explanation:** No servers can communicate with others.
**Example 2:**
**Input:** grid = \[\[1,0\],\[1,1\]\]
**Output:** 3
**Explanation:** All three servers can communicate with at least one other server.
**Example 3:**
**Input:** grid = \[\[1,1,0,0\],\[0,0,1,0\],\[0,0,1,0\],\[0,0,0,1\]\]
**Output:** 4
**Explanation:** The two servers in the first row can communicate with each other. The two servers in the third column can communicate with each other. The server at right bottom corner can't communicate with any other server.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m <= 250`
* `1 <= n <= 250`
* `grid[i][j] == 0 or 1` | Convert the linked list into an array. While you can find a non-empty subarray with sum = 0, erase it. Convert the array into a linked list. |
python3 (easy and clean) | remove-zero-sum-consecutive-nodes-from-linked-list | 0 | 1 | ```\n def removeZeroSumSublists(self, head: Optional[ListNode]) -> Optional[ListNode]:\n fake = ListNode(0, head)\n \n seen = {0: fake} \n prev = 0\n \n while head:\n prev += head.val \n seen[prev] = head\n \n head = head.next\n \n head = fake\n prev = 0\n \n while head:\n prev += head.val\n head.next = seen[prev].next\n \n head = head.next\n \n return fake.next\n``` | 5 | Given the `head` of a linked list, we repeatedly delete consecutive sequences of nodes that sum to `0` until there are no such sequences.
After doing so, return the head of the final linked list. You may return any such answer.
(Note that in the examples below, all sequences are serializations of `ListNode` objects.)
**Example 1:**
**Input:** head = \[1,2,-3,3,1\]
**Output:** \[3,1\]
**Note:** The answer \[1,2,1\] would also be accepted.
**Example 2:**
**Input:** head = \[1,2,3,-3,4\]
**Output:** \[1,2,4\]
**Example 3:**
**Input:** head = \[1,2,3,-3,-2\]
**Output:** \[1\]
**Constraints:**
* The given linked list will contain between `1` and `1000` nodes.
* Each node in the linked list has `-1000 <= node.val <= 1000`. | Do a breadth first search to find the shortest path. |
python3 (easy and clean) | remove-zero-sum-consecutive-nodes-from-linked-list | 0 | 1 | ```\n def removeZeroSumSublists(self, head: Optional[ListNode]) -> Optional[ListNode]:\n fake = ListNode(0, head)\n \n seen = {0: fake} \n prev = 0\n \n while head:\n prev += head.val \n seen[prev] = head\n \n head = head.next\n \n head = fake\n prev = 0\n \n while head:\n prev += head.val\n head.next = seen[prev].next\n \n head = head.next\n \n return fake.next\n``` | 5 | You are given a map of a server center, represented as a `m * n` integer matrix `grid`, where 1 means that on that cell there is a server and 0 means that it is no server. Two servers are said to communicate if they are on the same row or on the same column.
Return the number of servers that communicate with any other server.
**Example 1:**
**Input:** grid = \[\[1,0\],\[0,1\]\]
**Output:** 0
**Explanation:** No servers can communicate with others.
**Example 2:**
**Input:** grid = \[\[1,0\],\[1,1\]\]
**Output:** 3
**Explanation:** All three servers can communicate with at least one other server.
**Example 3:**
**Input:** grid = \[\[1,1,0,0\],\[0,0,1,0\],\[0,0,1,0\],\[0,0,0,1\]\]
**Output:** 4
**Explanation:** The two servers in the first row can communicate with each other. The two servers in the third column can communicate with each other. The server at right bottom corner can't communicate with any other server.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m <= 250`
* `1 <= n <= 250`
* `grid[i][j] == 0 or 1` | Convert the linked list into an array. While you can find a non-empty subarray with sum = 0, erase it. Convert the array into a linked list. |
| PYTHON SOL | WELL EXPLAINED | HASHMAP + PREFIX SUM| EASY | FAST | remove-zero-sum-consecutive-nodes-from-linked-list | 0 | 1 | # EXPLANATION\n```\n1. How do we know that any substring will have sum = 0\n2. The idea is if any prefix sum that had occured before occurs again we know that sum of substring\nbetween them is = 0\nexample [ 1,4,2,5,-7]\nsum will be [ 1,5,7,12,5] \nSO 5 occurs twice i.e. sum of [2,5,-7] is 0\nso we simply make 4.next = 7.next in linklist to remove [2,5,-7]\nalso we remove [2,5,-7] as node if is in hashmap\n```\n\n\n\n\n# CODE\n```\n# Definition for singly-linked list.\n# class ListNode:\n# def __init__(self, val=0, next=None):\n# self.val = val\n# self.next = next\nclass Solution:\n def removeZeroSumSublists(self, head: Optional[ListNode]) -> Optional[ListNode]:\n root = ListNode(0,head)\n summ , d , node = 0 , {} , root\n while node:\n summ += node.val\n if summ in d:\n prev = d[summ]\n tmp = prev.next\n tmp_sum = summ\n while tmp != node:\n tmp_sum += tmp.val\n if tmp_sum in d and d[tmp_sum] == tmp :d.pop(tmp_sum)\n tmp = tmp.next\n prev.next = node.next\n node = prev\n else:\n d[summ] = node\n node = node.next\n \n return root.next\n``` | 1 | Given the `head` of a linked list, we repeatedly delete consecutive sequences of nodes that sum to `0` until there are no such sequences.
After doing so, return the head of the final linked list. You may return any such answer.
(Note that in the examples below, all sequences are serializations of `ListNode` objects.)
**Example 1:**
**Input:** head = \[1,2,-3,3,1\]
**Output:** \[3,1\]
**Note:** The answer \[1,2,1\] would also be accepted.
**Example 2:**
**Input:** head = \[1,2,3,-3,4\]
**Output:** \[1,2,4\]
**Example 3:**
**Input:** head = \[1,2,3,-3,-2\]
**Output:** \[1\]
**Constraints:**
* The given linked list will contain between `1` and `1000` nodes.
* Each node in the linked list has `-1000 <= node.val <= 1000`. | Do a breadth first search to find the shortest path. |
| PYTHON SOL | WELL EXPLAINED | HASHMAP + PREFIX SUM| EASY | FAST | remove-zero-sum-consecutive-nodes-from-linked-list | 0 | 1 | # EXPLANATION\n```\n1. How do we know that any substring will have sum = 0\n2. The idea is if any prefix sum that had occured before occurs again we know that sum of substring\nbetween them is = 0\nexample [ 1,4,2,5,-7]\nsum will be [ 1,5,7,12,5] \nSO 5 occurs twice i.e. sum of [2,5,-7] is 0\nso we simply make 4.next = 7.next in linklist to remove [2,5,-7]\nalso we remove [2,5,-7] as node if is in hashmap\n```\n\n\n\n\n# CODE\n```\n# Definition for singly-linked list.\n# class ListNode:\n# def __init__(self, val=0, next=None):\n# self.val = val\n# self.next = next\nclass Solution:\n def removeZeroSumSublists(self, head: Optional[ListNode]) -> Optional[ListNode]:\n root = ListNode(0,head)\n summ , d , node = 0 , {} , root\n while node:\n summ += node.val\n if summ in d:\n prev = d[summ]\n tmp = prev.next\n tmp_sum = summ\n while tmp != node:\n tmp_sum += tmp.val\n if tmp_sum in d and d[tmp_sum] == tmp :d.pop(tmp_sum)\n tmp = tmp.next\n prev.next = node.next\n node = prev\n else:\n d[summ] = node\n node = node.next\n \n return root.next\n``` | 1 | You are given a map of a server center, represented as a `m * n` integer matrix `grid`, where 1 means that on that cell there is a server and 0 means that it is no server. Two servers are said to communicate if they are on the same row or on the same column.
Return the number of servers that communicate with any other server.
**Example 1:**
**Input:** grid = \[\[1,0\],\[0,1\]\]
**Output:** 0
**Explanation:** No servers can communicate with others.
**Example 2:**
**Input:** grid = \[\[1,0\],\[1,1\]\]
**Output:** 3
**Explanation:** All three servers can communicate with at least one other server.
**Example 3:**
**Input:** grid = \[\[1,1,0,0\],\[0,0,1,0\],\[0,0,1,0\],\[0,0,0,1\]\]
**Output:** 4
**Explanation:** The two servers in the first row can communicate with each other. The two servers in the third column can communicate with each other. The server at right bottom corner can't communicate with any other server.
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m <= 250`
* `1 <= n <= 250`
* `grid[i][j] == 0 or 1` | Convert the linked list into an array. While you can find a non-empty subarray with sum = 0, erase it. Convert the array into a linked list. |
Python Easy Solution | dinner-plate-stacks | 0 | 1 | # Code\n```\nclass DinnerPlates:\n def __init__(self, capacity):\n self.c = capacity\n self.q = [] # record the available stack, will use heap to quickly find the smallest available stack\n # if you are Java or C++ users, tree map is another good option.\n self.stacks = [] # record values of all stack of plates, its last nonempty stack are the rightmost position\n\n def push(self, val):\n # To push, we need to find the leftmost available position\n # first, let\'s remove any stacks on the left that are full\n # 1) self.q: if there is still available stack to insert plate\n # 2) self.q[0] < len(self.stacks): the leftmost available index self.q[0] is smaller than the current size of the stacks\n # 3) len(self.stacks[self.q[0]]) == self.c: the stack has reached full capacity\n while self.q and self.q[0] < len(self.stacks) and len(self.stacks[self.q[0]]) == self.c:\n # we remove the filled stack from the queue of available stacks\n heapq.heappop(self.q)\n\n # now we reach the leftmost available stack to insert\n\n # if the q is empty, meaning there are no more available stacks\n if not self.q:\n # open up a new stack to insert\n heapq.heappush(self.q, len(self.stacks))\n\n # for the newly added stack, add a new stack to self.stacks accordingly\n if self.q[0] == len(self.stacks):\n self.stacks.append([])\n\n # append the value to the leftmost available stack\n self.stacks[self.q[0]].append(val)\n\n def pop(self):\n # To pop, we need to find the rightmost nonempty stack\n # 1) stacks is not empty (self.stacks) and\n # 2) the last stack is empty\n while self.stacks and not self.stacks[-1]:\n # we throw away the last empty stack, because we can\'t pop from it\n self.stacks.pop()\n\n # now we reach the rightmost nonempty stack\n\n # we pop the plate from the last nonempty stack of self.stacks by using popAtStack function\n return self.popAtStack(len(self.stacks) - 1)\n\n def popAtStack(self, index):\n # To pop from an stack of given index, we need to make sure that it is not empty\n # 1) the index for inserting is valid and\uFF0C\n # 2) the stack of interest is not empty\n if 0 <= index < len(self.stacks) and self.stacks[index]:\n # we add the index into the available stack\n heapq.heappush(self.q, index)\n # take the top plate, pop it and return its value\n return self.stacks[index].pop()\n\n # otherwise, return -1 because we can\'t pop any plate\n return -1\n``` | 2 | You have an infinite number of stacks arranged in a row and numbered (left to right) from `0`, each of the stacks has the same maximum capacity.
Implement the `DinnerPlates` class:
* `DinnerPlates(int capacity)` Initializes the object with the maximum capacity of the stacks `capacity`.
* `void push(int val)` Pushes the given integer `val` into the leftmost stack with a size less than `capacity`.
* `int pop()` Returns the value at the top of the rightmost non-empty stack and removes it from that stack, and returns `-1` if all the stacks are empty.
* `int popAtStack(int index)` Returns the value at the top of the stack with the given index `index` and removes it from that stack or returns `-1` if the stack with that given index is empty.
**Example 1:**
**Input**
\[ "DinnerPlates ", "push ", "push ", "push ", "push ", "push ", "popAtStack ", "push ", "push ", "popAtStack ", "popAtStack ", "pop ", "pop ", "pop ", "pop ", "pop "\]
\[\[2\], \[1\], \[2\], \[3\], \[4\], \[5\], \[0\], \[20\], \[21\], \[0\], \[2\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, null, null, null, null, null, 2, null, null, 20, 21, 5, 4, 3, 1, -1\]
**Explanation:**
DinnerPlates D = DinnerPlates(2); // Initialize with capacity = 2
D.push(1);
D.push(2);
D.push(3);
D.push(4);
D.push(5); // The stacks are now: 2 4
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 2. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.push(20); // The stacks are now: 20 4
1 3 5
﹈ ﹈ ﹈
D.push(21); // The stacks are now: 20 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 20. The stacks are now: 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(2); // Returns 21. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.pop() // Returns 5. The stacks are now: 4
1 3
﹈ ﹈
D.pop() // Returns 4. The stacks are now: 1 3
﹈ ﹈
D.pop() // Returns 3. The stacks are now: 1
﹈
D.pop() // Returns 1. There are no stacks.
D.pop() // Returns -1. There are still no stacks.
**Constraints:**
* `1 <= capacity <= 2 * 104`
* `1 <= val <= 2 * 104`
* `0 <= index <= 105`
* At most `2 * 105` calls will be made to `push`, `pop`, and `popAtStack`. | null |
Heap + Hash Set Python3 Solution with Commented explanation | dinner-plate-stacks | 0 | 1 | ```\nclass DinnerPlates:\n \n \'\'\'\n \n design\n free slots: heap\n avoid repeated slots: hash set\n \n \n actions:\n push:\n - check leftmost free slot, add\n - if no free slot => create one and add to free slots\n - if curr slot reaches capacity, remove from the free slots and set\n \n pop:\n - remove element from right most\n - add that stack if not in free slots\n \n - if all stack is \n \n \n popstack:\n - check if the index exists\n - remove if there\'s a val on top of the stack\n \n \n \n \'\'\'\n\n def __init__(self, capacity: int):\n self.k = capacity\n self.row = []\n self.free = []\n self.registered = set() # registered free slots\n \n \n\n def push(self, val: int) -> None:\n # add if no stack is available\n if not self.row:\n self.row.append([])\n heapq.heappush(self.free, 0)\n self.registered.add(0)\n \n # if no free slots, add extra stack\n if not self.free:\n self.row.append([])\n heapq.heappush(self.free, len(self.row)-1)\n self.registered.add(len(self.row)-1)\n \n # get left most stack with space and push\n # if the left most free index is larger than the row size, then reset the free slots and registered and add new stack\n index = self.free[0]\n if index >= len(self.row):\n self.free = [len(self.row)]\n self.registered = set([len(self.row)])\n self.row.append([])\n index = self.free[0]\n self.row[index].append(val)\n \n # check if no space at index\n if len(self.row[index]) == self.k:\n self.registered.remove(index)\n heapq.heappop(self.free)\n \n \n\n def pop(self) -> int:\n # get the last non empty stack\n index = len(self.row)-1\n while index >= 0 and not self.row[index]:\n self.row.pop()\n index -= 1\n \n \n if index == -1:\n return -1\n \n val = self.row[index].pop()\n if index not in self.registered:\n self.registered.add(index)\n heapq.heappush(self.free, index)\n \n return val\n \n\n def popAtStack(self, index: int) -> int:\n if len(self.row) < index or not self.row[index]:\n return -1\n \n val = self.row[index].pop()\n if index not in self.registered:\n self.registered.add(index)\n heapq.heappush(self.free, index)\n \n return val\n \n\n\n# Your DinnerPlates object will be instantiated and called as such:\n# obj = DinnerPlates(capacity)\n# obj.push(val)\n# param_2 = obj.pop()\n# param_3 = obj.popAtStack(index)\n``` | 0 | You have an infinite number of stacks arranged in a row and numbered (left to right) from `0`, each of the stacks has the same maximum capacity.
Implement the `DinnerPlates` class:
* `DinnerPlates(int capacity)` Initializes the object with the maximum capacity of the stacks `capacity`.
* `void push(int val)` Pushes the given integer `val` into the leftmost stack with a size less than `capacity`.
* `int pop()` Returns the value at the top of the rightmost non-empty stack and removes it from that stack, and returns `-1` if all the stacks are empty.
* `int popAtStack(int index)` Returns the value at the top of the stack with the given index `index` and removes it from that stack or returns `-1` if the stack with that given index is empty.
**Example 1:**
**Input**
\[ "DinnerPlates ", "push ", "push ", "push ", "push ", "push ", "popAtStack ", "push ", "push ", "popAtStack ", "popAtStack ", "pop ", "pop ", "pop ", "pop ", "pop "\]
\[\[2\], \[1\], \[2\], \[3\], \[4\], \[5\], \[0\], \[20\], \[21\], \[0\], \[2\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, null, null, null, null, null, 2, null, null, 20, 21, 5, 4, 3, 1, -1\]
**Explanation:**
DinnerPlates D = DinnerPlates(2); // Initialize with capacity = 2
D.push(1);
D.push(2);
D.push(3);
D.push(4);
D.push(5); // The stacks are now: 2 4
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 2. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.push(20); // The stacks are now: 20 4
1 3 5
﹈ ﹈ ﹈
D.push(21); // The stacks are now: 20 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 20. The stacks are now: 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(2); // Returns 21. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.pop() // Returns 5. The stacks are now: 4
1 3
﹈ ﹈
D.pop() // Returns 4. The stacks are now: 1 3
﹈ ﹈
D.pop() // Returns 3. The stacks are now: 1
﹈
D.pop() // Returns 1. There are no stacks.
D.pop() // Returns -1. There are still no stacks.
**Constraints:**
* `1 <= capacity <= 2 * 104`
* `1 <= val <= 2 * 104`
* `0 <= index <= 105`
* At most `2 * 105` calls will be made to `push`, `pop`, and `popAtStack`. | null |
Brain fck mode | dinner-plate-stacks | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass DinnerPlates:\n\n def __init__(self, capacity: int):\n self.add=dict()\n self.kick=dict()\n self.stack=[[]]\n self.cap=capacity\n def push(self, val: int) -> None:\n \n if len(self.add)==0:\n if len(self.stack)==0:\n self.stack.append([val])\n self.kick[0]=""\n if len(self.stack[-1])==self.cap:self.stack.append([])\n self.stack[-1].append(val)\n self.kick[len(self.stack)-1]=""\n else:\n min_add=min(self.add.keys()) \n self.stack[min_add].append(val)\n self.kick[min_add]=""\n if len(self.stack[min_add])==self.cap:self.add.pop(min_add)\n \n def pop(self) -> int:\n if self.stack==[]:return -1\n if self.stack[-1]==[]:\n self.stack.pop()\n item=self.stack[-1][-1]\n self.stack[-1].pop()\n if self.stack[-1]==[]:\n if self.add.get(len(self.stack)-1)!=None:self.add.pop(len(self.stack)-1)\n self.stack.pop()\n \n return item\n def popAtStack(self, index: int) -> int:\n if index>=len(self.stack) or (self.stack[index]==[] and len(self.stack)-1!=index):return -1\n elif len(self.stack)-1==index and len(self.stack[index])==0:\n self.add.pop(index)\n self.stack.pop()\n return -1\n item=self.stack[index][-1]\n self.add[index]=""\n self.stack[index].pop()\n if len(self.stack)-1==index: \n if self.stack[index]==[]:\n self.stack.pop()\n self.add.pop(index)\n return item\n\n# Your DinnerPlates object will be instantiated and called as such:\n# obj = DinnerPlates(capacity)\n# obj.push(val)\n# param_2 = obj.pop()\n# param_3 = obj.popAtStack(index)\n``` | 0 | You have an infinite number of stacks arranged in a row and numbered (left to right) from `0`, each of the stacks has the same maximum capacity.
Implement the `DinnerPlates` class:
* `DinnerPlates(int capacity)` Initializes the object with the maximum capacity of the stacks `capacity`.
* `void push(int val)` Pushes the given integer `val` into the leftmost stack with a size less than `capacity`.
* `int pop()` Returns the value at the top of the rightmost non-empty stack and removes it from that stack, and returns `-1` if all the stacks are empty.
* `int popAtStack(int index)` Returns the value at the top of the stack with the given index `index` and removes it from that stack or returns `-1` if the stack with that given index is empty.
**Example 1:**
**Input**
\[ "DinnerPlates ", "push ", "push ", "push ", "push ", "push ", "popAtStack ", "push ", "push ", "popAtStack ", "popAtStack ", "pop ", "pop ", "pop ", "pop ", "pop "\]
\[\[2\], \[1\], \[2\], \[3\], \[4\], \[5\], \[0\], \[20\], \[21\], \[0\], \[2\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, null, null, null, null, null, 2, null, null, 20, 21, 5, 4, 3, 1, -1\]
**Explanation:**
DinnerPlates D = DinnerPlates(2); // Initialize with capacity = 2
D.push(1);
D.push(2);
D.push(3);
D.push(4);
D.push(5); // The stacks are now: 2 4
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 2. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.push(20); // The stacks are now: 20 4
1 3 5
﹈ ﹈ ﹈
D.push(21); // The stacks are now: 20 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 20. The stacks are now: 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(2); // Returns 21. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.pop() // Returns 5. The stacks are now: 4
1 3
﹈ ﹈
D.pop() // Returns 4. The stacks are now: 1 3
﹈ ﹈
D.pop() // Returns 3. The stacks are now: 1
﹈
D.pop() // Returns 1. There are no stacks.
D.pop() // Returns -1. There are still no stacks.
**Constraints:**
* `1 <= capacity <= 2 * 104`
* `1 <= val <= 2 * 104`
* `0 <= index <= 105`
* At most `2 * 105` calls will be made to `push`, `pop`, and `popAtStack`. | null |
TreeSet Abstraction | Commented and Explained | dinner-plate-stacks | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFor this set up, we have a mapped tree set as the desired set up, where we can modify a set of tree sets as needed. Then, if a lazy update is used, we can get an O(1) for push with a seeming O(N) for pop at worst and O(N) for popAtStack. However, the actual implementation uses a modified version of hashing that allows us to achieve a similar result in logN time due to the use of shifted locations as we go along. This lets us have a nice method of shifting the work easily and quickly in a straightforward, though not apparent fashion. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nTo initialize \n- set up a capacity marker \n- set up a carrying marker (how many stacks are currently popped from, the additional spots we are \'carrying\' at the moment in memory) \n- set up a deque called queue to track the memory locations we are carrying in fifo order \n- mark current place within stacks to consider (we have an infinite number)\n- make a mapping of stack indices to value stored \n\nTo push \n- if we are not currently carrying any specific stacks we need to place something back on to at the moment \n - place a plate worth value at place + 1 and set place to place + 1 \n- otherwise, place a plate at the least recently used location at which we removed a plate, then decrement carrying \n\nTo pop \n- set default value of -1 \n- while place is gte 0 and the stack at place is 0 for value \n - move place back by 1, pop from queue at back and reduce carrying\n - this techinically moves the plates around and is our update phase to maintain order \n- if place is gte 0 \n - get value from mapping at place \n - set mapping at place to 0 \n - reduce place by 1 \n- at end return value \n\nTo popAtStack \n- shift index provided by capacity * index + 1 - 1 -> moves to last index to start \n- loop over capacity \n - if mapping at index shifted is not 0 \n - get value \n - set mapping to 0 \n - insert into the queue the stack position for the shifted index into the queue (takes O(N) + O(logN), uses capacity to shift spacing to provide greater likelihood of lack of clashes on updates (hashing process))\n - update carrying capacity\n - return value stored \n - otherwise, keep moving back \n- never found, return -1 \n\n# Complexity\n- Time complexity:\n - O(1) for push \n - O(logN) for pop \n - O(logN) for popAtStack \n\n- Space complexity :\n - O(Q) where Q represents the overall unique size of items utilized in the system, which is separate from each tree set\'s valuation but accounts for them in total \n\n# Code\n```\nclass DinnerPlates:\n\n def __init__(self, capacity: int) :\n # set up data structure with values for max capacity and current amount carrying in deque\n self.capacity = capacity\n self.carrying = 0\n self.queue = collections.deque()\n # current place in queue\n self.place = -1 \n # current mapping of indices to values\n self.mapping = collections.defaultdict(int)\n \n def push(self, val: int) -> None : \n # if not carrying, move place and map\n if self.carrying == 0 : \n self.place += 1 \n self.mapping[self.place] = val\n else : \n # otherwise, pop lead oldest queue indice and update to value\n self.mapping[self.queue.popleft()] = val\n # then, decrement carrying as you placed one down \n self.carrying -= 1 \n\n def pop(self) -> int : \n # default value to start at \n value = -1 \n # while place is in range and self.mapping at self.place is 0 \n while self.place >= 0 and not self.mapping[self.place] : \n # move back, pop from back, and decrement carrying \n self.place -= 1 \n _ = self.queue.pop()\n self.carrying -= 1 \n \n # if place is in range \n if self.place >= 0 : \n # get value, set to 0, and update place \n value = self.mapping[self.place]\n self.mapping[self.place] = 0 \n self.place -= 1 \n # return value after processing \n return value\n \n def popAtStack(self, index: int) -> int :\n # get index shifted by capacity \n index_shifted = self.capacity * (index + 1) - 1 \n # loop in range of capacity \n for _ in range(self.capacity) : \n # if mapping at index shifted \n if self.mapping[index_shifted] : \n # get stored value \n value = self.mapping[index_shifted]\n # update map\n self.mapping[index_shifted] = 0 \n # insert into queue at bisected position for index shifted of deque \n self.queue.insert(bisect.bisect(self.queue, index_shifted), index_shifted)\n # update carrying appropriately\n self.carrying += 1 \n # return stored value \n return value\n # move back on index shifted \n index_shifted -= 1 \n # return default \n return -1 \n \n \n\n\n# Your DinnerPlates object will be instantiated and called as such:\n# obj = DinnerPlates(capacity)\n# obj.push(val)\n# param_2 = obj.pop()\n# param_3 = obj.popAtStack(index)\n``` | 0 | You have an infinite number of stacks arranged in a row and numbered (left to right) from `0`, each of the stacks has the same maximum capacity.
Implement the `DinnerPlates` class:
* `DinnerPlates(int capacity)` Initializes the object with the maximum capacity of the stacks `capacity`.
* `void push(int val)` Pushes the given integer `val` into the leftmost stack with a size less than `capacity`.
* `int pop()` Returns the value at the top of the rightmost non-empty stack and removes it from that stack, and returns `-1` if all the stacks are empty.
* `int popAtStack(int index)` Returns the value at the top of the stack with the given index `index` and removes it from that stack or returns `-1` if the stack with that given index is empty.
**Example 1:**
**Input**
\[ "DinnerPlates ", "push ", "push ", "push ", "push ", "push ", "popAtStack ", "push ", "push ", "popAtStack ", "popAtStack ", "pop ", "pop ", "pop ", "pop ", "pop "\]
\[\[2\], \[1\], \[2\], \[3\], \[4\], \[5\], \[0\], \[20\], \[21\], \[0\], \[2\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, null, null, null, null, null, 2, null, null, 20, 21, 5, 4, 3, 1, -1\]
**Explanation:**
DinnerPlates D = DinnerPlates(2); // Initialize with capacity = 2
D.push(1);
D.push(2);
D.push(3);
D.push(4);
D.push(5); // The stacks are now: 2 4
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 2. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.push(20); // The stacks are now: 20 4
1 3 5
﹈ ﹈ ﹈
D.push(21); // The stacks are now: 20 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 20. The stacks are now: 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(2); // Returns 21. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.pop() // Returns 5. The stacks are now: 4
1 3
﹈ ﹈
D.pop() // Returns 4. The stacks are now: 1 3
﹈ ﹈
D.pop() // Returns 3. The stacks are now: 1
﹈
D.pop() // Returns 1. There are no stacks.
D.pop() // Returns -1. There are still no stacks.
**Constraints:**
* `1 <= capacity <= 2 * 104`
* `1 <= val <= 2 * 104`
* `0 <= index <= 105`
* At most `2 * 105` calls will be made to `push`, `pop`, and `popAtStack`. | null |
Beats 98.89% No Heapq! | dinner-plate-stacks | 0 | 1 | # Code\n```\nfrom bisect import bisect\nclass DinnerPlates:\n\n def __init__(self, capacity: int):\n self.capacity = capacity\n self.can_put = []\n self.max = -1\n self.my_dict = defaultdict(int)\n\n def push(self, val: int) -> None:\n if not self.can_put:\n self.max += 1\n self.my_dict[self.max] = val\n else:\n self.my_dict[self.can_put.pop(0)] = val\n\n def pop(self) -> int:\n v = -1\n while self.max >= 0 and not self.my_dict[self.max]:\n self.max -= 1\n self.can_put.pop()\n \n if self.max >= 0:\n v = self.my_dict[self.max]\n self.my_dict[self.max] = 0\n self.max -= 1\n return v\n\n def popAtStack(self, index: int) -> int:\n idx = self.capacity*(index + 1) - 1\n for _ in range(self.capacity):\n if self.my_dict[idx]:\n v = self.my_dict[idx]\n self.my_dict[idx] = 0\n self.can_put.insert(bisect(self.can_put, idx), idx)\n return v\n idx -= 1\n return -1\n``` | 0 | You have an infinite number of stacks arranged in a row and numbered (left to right) from `0`, each of the stacks has the same maximum capacity.
Implement the `DinnerPlates` class:
* `DinnerPlates(int capacity)` Initializes the object with the maximum capacity of the stacks `capacity`.
* `void push(int val)` Pushes the given integer `val` into the leftmost stack with a size less than `capacity`.
* `int pop()` Returns the value at the top of the rightmost non-empty stack and removes it from that stack, and returns `-1` if all the stacks are empty.
* `int popAtStack(int index)` Returns the value at the top of the stack with the given index `index` and removes it from that stack or returns `-1` if the stack with that given index is empty.
**Example 1:**
**Input**
\[ "DinnerPlates ", "push ", "push ", "push ", "push ", "push ", "popAtStack ", "push ", "push ", "popAtStack ", "popAtStack ", "pop ", "pop ", "pop ", "pop ", "pop "\]
\[\[2\], \[1\], \[2\], \[3\], \[4\], \[5\], \[0\], \[20\], \[21\], \[0\], \[2\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, null, null, null, null, null, 2, null, null, 20, 21, 5, 4, 3, 1, -1\]
**Explanation:**
DinnerPlates D = DinnerPlates(2); // Initialize with capacity = 2
D.push(1);
D.push(2);
D.push(3);
D.push(4);
D.push(5); // The stacks are now: 2 4
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 2. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.push(20); // The stacks are now: 20 4
1 3 5
﹈ ﹈ ﹈
D.push(21); // The stacks are now: 20 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 20. The stacks are now: 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(2); // Returns 21. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.pop() // Returns 5. The stacks are now: 4
1 3
﹈ ﹈
D.pop() // Returns 4. The stacks are now: 1 3
﹈ ﹈
D.pop() // Returns 3. The stacks are now: 1
﹈
D.pop() // Returns 1. There are no stacks.
D.pop() // Returns -1. There are still no stacks.
**Constraints:**
* `1 <= capacity <= 2 * 104`
* `1 <= val <= 2 * 104`
* `0 <= index <= 105`
* At most `2 * 105` calls will be made to `push`, `pop`, and `popAtStack`. | null |
Python (Simple Heap) | dinner-plate-stacks | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass DinnerPlates:\n\n def __init__(self, capacity):\n self.capacity = capacity\n self.stacks = [[]]\n self.avail_idx = []\n\n def push(self, val):\n if len(self.stacks[-1]) == self.capacity:\n self.stacks.append([])\n\n if not self.avail_idx:\n self.stacks[-1].append(val)\n else:\n idx = heappop(self.avail_idx)\n \n if idx < len(self.stacks):\n self.stacks[idx].append(val)\n\n def pop(self):\n while self.stacks and not self.stacks[-1]:\n self.stacks.pop()\n\n return self.popAtStack(len(self.stacks)-1)\n\n def popAtStack(self, index):\n if 0 <= index < len(self.stacks) and self.stacks[index]:\n heapq.heappush(self.avail_idx,index)\n return self.stacks[index].pop()\n\n return -1\n\n\n\n \n``` | 0 | You have an infinite number of stacks arranged in a row and numbered (left to right) from `0`, each of the stacks has the same maximum capacity.
Implement the `DinnerPlates` class:
* `DinnerPlates(int capacity)` Initializes the object with the maximum capacity of the stacks `capacity`.
* `void push(int val)` Pushes the given integer `val` into the leftmost stack with a size less than `capacity`.
* `int pop()` Returns the value at the top of the rightmost non-empty stack and removes it from that stack, and returns `-1` if all the stacks are empty.
* `int popAtStack(int index)` Returns the value at the top of the stack with the given index `index` and removes it from that stack or returns `-1` if the stack with that given index is empty.
**Example 1:**
**Input**
\[ "DinnerPlates ", "push ", "push ", "push ", "push ", "push ", "popAtStack ", "push ", "push ", "popAtStack ", "popAtStack ", "pop ", "pop ", "pop ", "pop ", "pop "\]
\[\[2\], \[1\], \[2\], \[3\], \[4\], \[5\], \[0\], \[20\], \[21\], \[0\], \[2\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, null, null, null, null, null, 2, null, null, 20, 21, 5, 4, 3, 1, -1\]
**Explanation:**
DinnerPlates D = DinnerPlates(2); // Initialize with capacity = 2
D.push(1);
D.push(2);
D.push(3);
D.push(4);
D.push(5); // The stacks are now: 2 4
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 2. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.push(20); // The stacks are now: 20 4
1 3 5
﹈ ﹈ ﹈
D.push(21); // The stacks are now: 20 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 20. The stacks are now: 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(2); // Returns 21. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.pop() // Returns 5. The stacks are now: 4
1 3
﹈ ﹈
D.pop() // Returns 4. The stacks are now: 1 3
﹈ ﹈
D.pop() // Returns 3. The stacks are now: 1
﹈
D.pop() // Returns 1. There are no stacks.
D.pop() // Returns -1. There are still no stacks.
**Constraints:**
* `1 <= capacity <= 2 * 104`
* `1 <= val <= 2 * 104`
* `0 <= index <= 105`
* At most `2 * 105` calls will be made to `push`, `pop`, and `popAtStack`. | null |
One heap, 98.7 speed 84.7 mem 839 ms | dinner-plate-stacks | 0 | 1 | # Approach\nCreate a heap of non full stacks, \npush a new value at first to the non full stack and only if there no such stacks, create a new stack.\n\n# Complexity\n- Time complexity:\nO(logn)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass DinnerPlates:\n\n def __init__(self, capacity: int):\n self.arr = []\n self.capacity = capacity\n self.nonFullSt = [] # min heap of non-full stacks\n\n def push(self, val: int) -> None:\n while self.nonFullSt:\n stID = heappop(self.nonFullSt)\n if stID < len(self.arr) and len(self.arr[stID]) < self.capacity:\n # This check is needed because the nonFullSt Id can be greater than self.arr size in case we poped many empy stacks from self.arr in a pop(self) function\n self.arr[stID].append(val)\n if len(self.arr[stID]) == self.capacity:\n heappush(self.nonFullSt, stID)\n return\n if self.arr:\n if len(self.arr[-1]) == self.capacity:\n self.arr.append([val])\n else:\n self.arr[-1].append(val)\n else:\n self.arr.append([val])\n\n\n def pop(self) -> int:\n while self.arr:\n if len(self.arr[-1]) > 0:\n return self.arr[-1].pop()\n else:\n self.arr.pop()\n return -1\n\n def popAtStack(self, index: int) -> int:\n if len(self.arr) > index and len(self.arr[index]) > 0:\n # this stack was full, add it to the nonFullSt heap\n if len(self.arr[index]) == self.capacity:\n heappush(self.nonFullSt, index)\n return self.arr[index].pop()\n return -1\n\n\n# Your DinnerPlates object will be instantiated and called as such:\n# obj = DinnerPlates(capacity)\n# obj.push(val)\n# param_2 = obj.pop()\n# param_3 = obj.popAtStack(index)\n``` | 1 | You have an infinite number of stacks arranged in a row and numbered (left to right) from `0`, each of the stacks has the same maximum capacity.
Implement the `DinnerPlates` class:
* `DinnerPlates(int capacity)` Initializes the object with the maximum capacity of the stacks `capacity`.
* `void push(int val)` Pushes the given integer `val` into the leftmost stack with a size less than `capacity`.
* `int pop()` Returns the value at the top of the rightmost non-empty stack and removes it from that stack, and returns `-1` if all the stacks are empty.
* `int popAtStack(int index)` Returns the value at the top of the stack with the given index `index` and removes it from that stack or returns `-1` if the stack with that given index is empty.
**Example 1:**
**Input**
\[ "DinnerPlates ", "push ", "push ", "push ", "push ", "push ", "popAtStack ", "push ", "push ", "popAtStack ", "popAtStack ", "pop ", "pop ", "pop ", "pop ", "pop "\]
\[\[2\], \[1\], \[2\], \[3\], \[4\], \[5\], \[0\], \[20\], \[21\], \[0\], \[2\], \[\], \[\], \[\], \[\], \[\]\]
**Output**
\[null, null, null, null, null, null, 2, null, null, 20, 21, 5, 4, 3, 1, -1\]
**Explanation:**
DinnerPlates D = DinnerPlates(2); // Initialize with capacity = 2
D.push(1);
D.push(2);
D.push(3);
D.push(4);
D.push(5); // The stacks are now: 2 4
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 2. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.push(20); // The stacks are now: 20 4
1 3 5
﹈ ﹈ ﹈
D.push(21); // The stacks are now: 20 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(0); // Returns 20. The stacks are now: 4 21
1 3 5
﹈ ﹈ ﹈
D.popAtStack(2); // Returns 21. The stacks are now: 4
1 3 5
﹈ ﹈ ﹈
D.pop() // Returns 5. The stacks are now: 4
1 3
﹈ ﹈
D.pop() // Returns 4. The stacks are now: 1 3
﹈ ﹈
D.pop() // Returns 3. The stacks are now: 1
﹈
D.pop() // Returns 1. There are no stacks.
D.pop() // Returns -1. There are still no stacks.
**Constraints:**
* `1 <= capacity <= 2 * 104`
* `1 <= val <= 2 * 104`
* `0 <= index <= 105`
* At most `2 * 105` calls will be made to `push`, `pop`, and `popAtStack`. | null |
Python Simple Intuitive Solution | prime-arrangements | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFactorial comes into mind and then concept of number of prime numbers from [2, n] inclusive is to be dealt with. Remember 1 is not prime.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nDefine an isPrime() function that tells whether an input (y) is prime or not.\nRun a for loop from 1 to n+1 and count how many primes are there.\nDefine a Factorial function to calculate x!\nNow we know that prime indexes can be arranged in c! ways so we use fac(c) and also rest of the indices i.e. n-c can be arranged in (n-c)!\nways so we use fac() to calculate these factorials and our final answer will be given after modulo operation with (10**9+7)\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->O(n**2) due to isPrime() function\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->O(1) due to the variable c.\n\n# Code\n```\nclass Solution:\n def numPrimeArrangements(self, n: int) -> int:\n def isPrime(y):\n if y == 1:\n return False\n for i in range(2, y//2+1):\n if y%i==0:\n return False\n return True\n c = 0\n for i in range(1, n+1):\n if isPrime(i) == True:\n c+=1\n def fac(x):\n f = 1\n while x > 0:\n f = (f*x)%(10**9+7)\n x-=1\n return f\n return fac(n-c)*fac(c)%(10**9+7)\n``` | 2 | Return the number of permutations of 1 to `n` so that prime numbers are at prime indices (1-indexed.)
_(Recall that an integer is prime if and only if it is greater than 1, and cannot be written as a product of two positive integers both smaller than it.)_
Since the answer may be large, return the answer **modulo `10^9 + 7`**.
**Example 1:**
**Input:** n = 5
**Output:** 12
**Explanation:** For example \[1,2,5,4,3\] is a valid permutation, but \[5,2,3,4,1\] is not because the prime number 5 is at index 1.
**Example 2:**
**Input:** n = 100
**Output:** 682289015
**Constraints:**
* `1 <= n <= 100` | Try to simulate the process. For every iteration, find the new array using the old one and the given rules. |
optimal | prime-arrangements | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numPrimeArrangements(self, n: int) -> int:\n def isPrime(n) :\n if n <= 1:\n return False\n for i in range(2, (n//2)+1):\n if n % i == 0:\n return False\n return True\n c = 1\n pc = 0\n for i in range(1, n+1):\n if isPrime(i):\n pc+=1\n npc = n-pc\n for i in range(1, n+1):\n if isPrime(i):\n c*=pc\n pc-=1\n else:\n c*=npc\n npc-=1\n return c%(10**9+7)\n``` | 0 | Return the number of permutations of 1 to `n` so that prime numbers are at prime indices (1-indexed.)
_(Recall that an integer is prime if and only if it is greater than 1, and cannot be written as a product of two positive integers both smaller than it.)_
Since the answer may be large, return the answer **modulo `10^9 + 7`**.
**Example 1:**
**Input:** n = 5
**Output:** 12
**Explanation:** For example \[1,2,5,4,3\] is a valid permutation, but \[5,2,3,4,1\] is not because the prime number 5 is at index 1.
**Example 2:**
**Input:** n = 100
**Output:** 682289015
**Constraints:**
* `1 <= n <= 100` | Try to simulate the process. For every iteration, find the new array using the old one and the given rules. |
python code simple strategy...! | prime-arrangements | 0 | 1 | \n\n# Code\n```\nimport math\nclass Solution:\n def numPrimeArrangements(self, n: int) -> int:\n primelist=[2,3]\n ans=1\n if (n<3):\n return 1\n elif (n==3):\n return 2\n else:\n for i in range(4,n+1):\n for j in range(2,i//2+1):\n if(i%j==0):\n break\n else:\n primelist.append(i)\n ans = math.factorial(len(primelist)) * math.factorial(n-len(primelist))\n return ans%(10**9+7) \n \n \n\n\n \n\n``` | 0 | Return the number of permutations of 1 to `n` so that prime numbers are at prime indices (1-indexed.)
_(Recall that an integer is prime if and only if it is greater than 1, and cannot be written as a product of two positive integers both smaller than it.)_
Since the answer may be large, return the answer **modulo `10^9 + 7`**.
**Example 1:**
**Input:** n = 5
**Output:** 12
**Explanation:** For example \[1,2,5,4,3\] is a valid permutation, but \[5,2,3,4,1\] is not because the prime number 5 is at index 1.
**Example 2:**
**Input:** n = 100
**Output:** 682289015
**Constraints:**
* `1 <= n <= 100` | Try to simulate the process. For every iteration, find the new array using the old one and the given rules. |
prime-arrangements | prime-arrangements | 0 | 1 | # Code\n```\nclass Solution:\n def numPrimeArrangements(self, n: int) -> int:\n l = []\n for i in range(2,n+1):\n count = 0\n for j in range(len(l)):\n if i%l[j]==0:\n count+=1\n break\n if count==0:\n l.append(i)\n a = len(l)\n b = n - len(l)\n count = 1\n count1 = 1\n for i in range(1,a+1):\n count*=i\n for j in range(1,b+1):\n count1*=j\n return (count*count1)%((10**9) + 7)\n\n\n \n``` | 0 | Return the number of permutations of 1 to `n` so that prime numbers are at prime indices (1-indexed.)
_(Recall that an integer is prime if and only if it is greater than 1, and cannot be written as a product of two positive integers both smaller than it.)_
Since the answer may be large, return the answer **modulo `10^9 + 7`**.
**Example 1:**
**Input:** n = 5
**Output:** 12
**Explanation:** For example \[1,2,5,4,3\] is a valid permutation, but \[5,2,3,4,1\] is not because the prime number 5 is at index 1.
**Example 2:**
**Input:** n = 100
**Output:** 682289015
**Constraints:**
* `1 <= n <= 100` | Try to simulate the process. For every iteration, find the new array using the old one and the given rules. |
Python 3: Combinatorics, TC O(n**(3/2)), SC O(1) | prime-arrangements | 0 | 1 | # Intuition\n\nUsually, order questions can be solved using combinatorics. If you\'re unfamiliar, do some studying on\n* factorial\n * you have `n` items, and you want all ways to arrange them on a line\n* the choose operation, `n choose k == n!//((n-k)!*k!)\n * you have n slots, and you want the ways to choose k of them to be filled with indistinguishable objects\n* the permutation operation `n perm k == n!//(n-k)!`\n * you have n distinguishable items, and you want the number of ways to pick k of them where order matters\n\nIn this case\n* we have `n` slots\n* the `P` primes in `1..n` must be in prime slots\n * there are `P!` different arrangements\n* the remaining `n-P` composite numbers must be in the composite indices\n * there are `(n-P)!` combinations\n\nSo the answer is `(n-P)! P!`\n\n# Approach\n\n## Checking if a Number is Prime\n\nThe basic way, presented here, is *trial division* up to `sqrt(m)`.\n\nIt\'s `sqrt(m)` because all divisors of `m` come in pairs `a*b == m`, where `a <= b` without loss of generality. \n\n`a` is at most `sqrt(m)`.\n\nTherefore you only need to check up to `sqrt(m)`; if there are no such divisors, then there can\'t be any larger ones. Because a larger divisor would be some big `b`, but you should have found a smaller `a` earlier where `a*b == m`, i.e. `a == m/b`.\n\n## Finding All Primes Up to N\n\nA faster way to find multiple primes is the *Sieve of Eratosthenes*. It\'s about the fastest multi-prime-finding algorithm you\'ll be expected to write in an interview, and afaik, the fastest one that\'s tractable.\n\nThere are faster methods, but they require more sophisticated number theory (so they aren\'t required) and involve more operations like multiplicative inverses that would take you too long to code in 20 minutes.\n\nAnyway, the idea is\n* you make an array `isPrime` with entries for `i = 0..n`\n* make the entries `True` to start\n* for k = 2, and then for `k = 3, 5, 7, ...`\n * if `isPrime[k]`, then `k` is prime. Starting with `k**2`, and every later multiple `m` of `k`, set `isPrime[m] = False`\n * if `!isPrime[k]`, continue\n\nThe complexity is TC O(n log(log(n))). The iterated logarithm is complicated, it comes from number theory. Briefly:\n* for each prime, the algorithm marks off p^2, p^2+p, .. as prime\n * this takes less than `n/p` operations per prime\n* the sum of `1/p` over all primes less than or equal to `n` is `log(log(n))`\n * you won\'t be asked to prove this, too detailed\n * [see here for a sketch proof](https://en.wikipedia.org/wiki/Divergence_of_the_sum_of_the_reciprocals_of_the_primes)\n\nSo total cost is `O(n log(log(n)))`.\n\n# Complexity\n- Time complexity: `O(n^(3/2))`, from finding primes via trial division. Again, Sieve of Eratosthenes would be faster, but for n = 100 it\'s overkill IMO. Other work done in the algorithm is at worst `O(n)`\n\n- Space complexity: `O(1)`\n\n# Code\n```\nclass Solution:\n def numPrimeArrangements(self, n: int) -> int:\n # 1. get P, the number of primes in 1..n\n\n # the n-P non-primes, and P primes, can appear in any order\n # so (n-P)! * P!\n\n def isPrime(m: int) -> bool:\n # TODO: sieve of eratosthenes??\n return m % 2 == 0 or any(m % d == 0 for d in range(3, int(sqrt(m))+1, 2))\n \n\n P = sum(isPrime(m) for m in range(1, n+1))\n\n BIG = 10**9+7\n ans = 1\n for i in range(1, n-P+1):\n ans *= i\n ans %= BIG\n\n for i in range(1, P+1):\n ans *= i\n ans %= BIG\n\n return ans\n``` | 0 | Return the number of permutations of 1 to `n` so that prime numbers are at prime indices (1-indexed.)
_(Recall that an integer is prime if and only if it is greater than 1, and cannot be written as a product of two positive integers both smaller than it.)_
Since the answer may be large, return the answer **modulo `10^9 + 7`**.
**Example 1:**
**Input:** n = 5
**Output:** 12
**Explanation:** For example \[1,2,5,4,3\] is a valid permutation, but \[5,2,3,4,1\] is not because the prime number 5 is at index 1.
**Example 2:**
**Input:** n = 100
**Output:** 682289015
**Constraints:**
* `1 <= n <= 100` | Try to simulate the process. For every iteration, find the new array using the old one and the given rules. |
Runtime Beats 98.88% and Memory Beats 96.25% | prime-arrangements | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numPrimeArrangements(self, n: int) -> int:\n def is_prime(x):\n if (x == 2) or (x == 3):\n return True\n if (x % 6 != 1) and (x % 6 != 5):\n return False\n for i in range(5, int(x ** 0.5) + 1, 6):\n if (x % i == 0) or (x % (i + 2) == 0):\n return False\n return True\n \n prime = sum(1 for i in range(2, n + 1) if is_prime(i))\n not_prime = n - prime\n\n factorial = 1\n for i in range(1, prime + 1):\n factorial = (factorial * i) % (10**9 + 7)\n \n for i in range(1, not_prime + 1):\n factorial = (factorial * i) % (10**9 + 7)\n \n return factorial\n``` | 0 | Return the number of permutations of 1 to `n` so that prime numbers are at prime indices (1-indexed.)
_(Recall that an integer is prime if and only if it is greater than 1, and cannot be written as a product of two positive integers both smaller than it.)_
Since the answer may be large, return the answer **modulo `10^9 + 7`**.
**Example 1:**
**Input:** n = 5
**Output:** 12
**Explanation:** For example \[1,2,5,4,3\] is a valid permutation, but \[5,2,3,4,1\] is not because the prime number 5 is at index 1.
**Example 2:**
**Input:** n = 100
**Output:** 682289015
**Constraints:**
* `1 <= n <= 100` | Try to simulate the process. For every iteration, find the new array using the old one and the given rules. |
[Python 3 | beats 98.92% in time | easy solution] | prime-arrangements | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThere are only 25 primes for $n \\le 100$, so we can make a list of primes. We can easily check how many primes less than equal to $n$ there are. If there are i primes in the first $n$ numbers, combinatorics tells us that there are $i! \\times (n-i)!$ number of permutations. \n# Approach\n<!-- Describe your approach to solving the problem. -->\n1) the prime numbers less than or equal to 100 are $2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97$\n2) set i = 0, and add 1 to i until prime[i] $>n$ (Then, i is the number of primes less than or equal to n)\n3) return $i! \\times (n-i)!$ divided by $10^9 + 7$\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$O(\\log n)$\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def numPrimeArrangements(self, n: int) -> int:\n prime = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, \n 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97]\n i = 0\n while i < 25 and prime[i] <= n:\n i += 1\n # i = number of primes\n\n return (factorial(i) * factorial(n-i)) % (10**9 + 7)\n \n``` | 0 | Return the number of permutations of 1 to `n` so that prime numbers are at prime indices (1-indexed.)
_(Recall that an integer is prime if and only if it is greater than 1, and cannot be written as a product of two positive integers both smaller than it.)_
Since the answer may be large, return the answer **modulo `10^9 + 7`**.
**Example 1:**
**Input:** n = 5
**Output:** 12
**Explanation:** For example \[1,2,5,4,3\] is a valid permutation, but \[5,2,3,4,1\] is not because the prime number 5 is at index 1.
**Example 2:**
**Input:** n = 100
**Output:** 682289015
**Constraints:**
* `1 <= n <= 100` | Try to simulate the process. For every iteration, find the new array using the old one and the given rules. |
PYTHON | Simple Solution | Math & Counting | prime-arrangements | 0 | 1 | # Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python\ndef factorial(n: int, to_divide: int) -> int:\n fact = 1\n while n > 0:\n fact = (fact * n) % to_divide\n n -= 1\n return fact\n\ndef is_prime(n: int) -> bool:\n if n == 1:\n return False\n for i in range(2, n // 2 + 1):\n if n % i == 0:\n return False\n return True\n\nclass Solution:\n def numPrimeArrangements(self, n: int) -> int:\n primes = 0\n for i in range(2, n + 1):\n if is_prime(i):\n primes += 1\n to_divide = 10 ** 9 + 7\n return (factorial(n - primes, to_divide) * factorial(primes, to_divide)) % to_divide\n```\n | 0 | Return the number of permutations of 1 to `n` so that prime numbers are at prime indices (1-indexed.)
_(Recall that an integer is prime if and only if it is greater than 1, and cannot be written as a product of two positive integers both smaller than it.)_
Since the answer may be large, return the answer **modulo `10^9 + 7`**.
**Example 1:**
**Input:** n = 5
**Output:** 12
**Explanation:** For example \[1,2,5,4,3\] is a valid permutation, but \[5,2,3,4,1\] is not because the prime number 5 is at index 1.
**Example 2:**
**Input:** n = 100
**Output:** 682289015
**Constraints:**
* `1 <= n <= 100` | Try to simulate the process. For every iteration, find the new array using the old one and the given rules. |
Ezreal | prime-arrangements | 0 | 1 | # Code\n```\nclass Solution:\n def numPrimeArrangements(self, n: int) -> int:\n primes = [2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97]\n p = bisect.bisect(primes,n)\n Output = factorial(p) * factorial(n - p)\n\n return Output % ( 10 ** 9 + 7 )\n \n # 20230913\n``` | 0 | Return the number of permutations of 1 to `n` so that prime numbers are at prime indices (1-indexed.)
_(Recall that an integer is prime if and only if it is greater than 1, and cannot be written as a product of two positive integers both smaller than it.)_
Since the answer may be large, return the answer **modulo `10^9 + 7`**.
**Example 1:**
**Input:** n = 5
**Output:** 12
**Explanation:** For example \[1,2,5,4,3\] is a valid permutation, but \[5,2,3,4,1\] is not because the prime number 5 is at index 1.
**Example 2:**
**Input:** n = 100
**Output:** 682289015
**Constraints:**
* `1 <= n <= 100` | Try to simulate the process. For every iteration, find the new array using the old one and the given rules. |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.