title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
Python Recursion | maximize-number-of-nice-divisors | 0 | 1 | The idea is similar to other posts but eliminating some IF condition to make it cleaner. \n`3*3 > 2*2*2` is the most important fact to solve the problem. So we would always prefer to use `3` as the factor.\n```\nMOD = 10**9 + 7\nclass Solution:\n def maxNiceDivisors(self, n: int) -> int:\n if n <= 2: return n\n i, c = divmod(n, 3)\n if not c: return pow(3, i, MOD)\n return (self.maxNiceDivisors(n-2)*2) % MOD\n``` | 2 | You are given a positive integer `primeFactors`. You are asked to construct a positive integer `n` that satisfies the following conditions:
* The number of prime factors of `n` (not necessarily distinct) is **at most** `primeFactors`.
* The number of nice divisors of `n` is maximized. Note that a divisor of `n` is **nice** if it is divisible by every prime factor of `n`. For example, if `n = 12`, then its prime factors are `[2,2,3]`, then `6` and `12` are nice divisors, while `3` and `4` are not.
Return _the number of nice divisors of_ `n`. Since that number can be too large, return it **modulo** `109 + 7`.
Note that a prime number is a natural number greater than `1` that is not a product of two smaller natural numbers. The prime factors of a number `n` is a list of prime numbers such that their product equals `n`.
**Example 1:**
**Input:** primeFactors = 5
**Output:** 6
**Explanation:** 200 is a valid value of n.
It has 5 prime factors: \[2,2,2,5,5\], and it has 6 nice divisors: \[10,20,40,50,100,200\].
There is not other value of n that has at most 5 prime factors and more nice divisors.
**Example 2:**
**Input:** primeFactors = 8
**Output:** 18
**Constraints:**
* `1 <= primeFactors <= 109` | The constraints are small enough for an N^2 solution. Try using dynamic programming. |
Python Recursion | maximize-number-of-nice-divisors | 0 | 1 | The idea is similar to other posts but eliminating some IF condition to make it cleaner. \n`3*3 > 2*2*2` is the most important fact to solve the problem. So we would always prefer to use `3` as the factor.\n```\nMOD = 10**9 + 7\nclass Solution:\n def maxNiceDivisors(self, n: int) -> int:\n if n <= 2: return n\n i, c = divmod(n, 3)\n if not c: return pow(3, i, MOD)\n return (self.maxNiceDivisors(n-2)*2) % MOD\n``` | 2 | You are given a **strictly increasing** integer array `rungs` that represents the **height** of rungs on a ladder. You are currently on the **floor** at height `0`, and you want to reach the last rung.
You are also given an integer `dist`. You can only climb to the next highest rung if the distance between where you are currently at (the floor or on a rung) and the next rung is **at most** `dist`. You are able to insert rungs at any positive **integer** height if a rung is not already there.
Return _the **minimum** number of rungs that must be added to the ladder in order for you to climb to the last rung._
**Example 1:**
**Input:** rungs = \[1,3,5,10\], dist = 2
**Output:** 2
**Explanation:**
You currently cannot reach the last rung.
Add rungs at heights 7 and 8 to climb this ladder.
The ladder will now have rungs at \[1,3,5,7,8,10\].
**Example 2:**
**Input:** rungs = \[3,6,8,10\], dist = 3
**Output:** 0
**Explanation:**
This ladder can be climbed without adding additional rungs.
**Example 3:**
**Input:** rungs = \[3,4,6,7\], dist = 2
**Output:** 1
**Explanation:**
You currently cannot reach the first rung from the ground.
Add a rung at height 1 to climb this ladder.
The ladder will now have rungs at \[1,3,4,6,7\].
**Constraints:**
* `1 <= rungs.length <= 105`
* `1 <= rungs[i] <= 109`
* `1 <= dist <= 109`
* `rungs` is **strictly increasing**. | The number of nice divisors is equal to the product of the count of each prime factor. Then the problem is reduced to: given n, find a sequence of numbers whose sum equals n and whose product is maximized. This sequence can have no numbers that are larger than 4. Proof: if it contains a number x that is larger than 4, then you can replace x with floor(x/2) and ceil(x/2), and floor(x/2) * ceil(x/2) > x. You can also replace 4s with two 2s. Hence, there will always be optimal solutions with only 2s and 3s. If there are three 2s, you can replace them with two 3s to get a better product. Hence, you'll never have more than two 2s. Keep adding 3s as long as n ≥ 5. |
[Python3] math | maximize-number-of-nice-divisors | 0 | 1 | \n```\nclass Solution:\n def maxNiceDivisors(self, primeFactors: int) -> int:\n mod = 1_000_000_007\n if primeFactors % 3 == 0: return pow(3, primeFactors//3, mod)\n if primeFactors % 3 == 1: return 1 if primeFactors == 1 else 4*pow(3, (primeFactors-4)//3, mod) % mod\n return 2*pow(3, primeFactors//3, mod) % mod\n``` | 2 | You are given a positive integer `primeFactors`. You are asked to construct a positive integer `n` that satisfies the following conditions:
* The number of prime factors of `n` (not necessarily distinct) is **at most** `primeFactors`.
* The number of nice divisors of `n` is maximized. Note that a divisor of `n` is **nice** if it is divisible by every prime factor of `n`. For example, if `n = 12`, then its prime factors are `[2,2,3]`, then `6` and `12` are nice divisors, while `3` and `4` are not.
Return _the number of nice divisors of_ `n`. Since that number can be too large, return it **modulo** `109 + 7`.
Note that a prime number is a natural number greater than `1` that is not a product of two smaller natural numbers. The prime factors of a number `n` is a list of prime numbers such that their product equals `n`.
**Example 1:**
**Input:** primeFactors = 5
**Output:** 6
**Explanation:** 200 is a valid value of n.
It has 5 prime factors: \[2,2,2,5,5\], and it has 6 nice divisors: \[10,20,40,50,100,200\].
There is not other value of n that has at most 5 prime factors and more nice divisors.
**Example 2:**
**Input:** primeFactors = 8
**Output:** 18
**Constraints:**
* `1 <= primeFactors <= 109` | The constraints are small enough for an N^2 solution. Try using dynamic programming. |
[Python3] math | maximize-number-of-nice-divisors | 0 | 1 | \n```\nclass Solution:\n def maxNiceDivisors(self, primeFactors: int) -> int:\n mod = 1_000_000_007\n if primeFactors % 3 == 0: return pow(3, primeFactors//3, mod)\n if primeFactors % 3 == 1: return 1 if primeFactors == 1 else 4*pow(3, (primeFactors-4)//3, mod) % mod\n return 2*pow(3, primeFactors//3, mod) % mod\n``` | 2 | You are given a **strictly increasing** integer array `rungs` that represents the **height** of rungs on a ladder. You are currently on the **floor** at height `0`, and you want to reach the last rung.
You are also given an integer `dist`. You can only climb to the next highest rung if the distance between where you are currently at (the floor or on a rung) and the next rung is **at most** `dist`. You are able to insert rungs at any positive **integer** height if a rung is not already there.
Return _the **minimum** number of rungs that must be added to the ladder in order for you to climb to the last rung._
**Example 1:**
**Input:** rungs = \[1,3,5,10\], dist = 2
**Output:** 2
**Explanation:**
You currently cannot reach the last rung.
Add rungs at heights 7 and 8 to climb this ladder.
The ladder will now have rungs at \[1,3,5,7,8,10\].
**Example 2:**
**Input:** rungs = \[3,6,8,10\], dist = 3
**Output:** 0
**Explanation:**
This ladder can be climbed without adding additional rungs.
**Example 3:**
**Input:** rungs = \[3,4,6,7\], dist = 2
**Output:** 1
**Explanation:**
You currently cannot reach the first rung from the ground.
Add a rung at height 1 to climb this ladder.
The ladder will now have rungs at \[1,3,4,6,7\].
**Constraints:**
* `1 <= rungs.length <= 105`
* `1 <= rungs[i] <= 109`
* `1 <= dist <= 109`
* `rungs` is **strictly increasing**. | The number of nice divisors is equal to the product of the count of each prime factor. Then the problem is reduced to: given n, find a sequence of numbers whose sum equals n and whose product is maximized. This sequence can have no numbers that are larger than 4. Proof: if it contains a number x that is larger than 4, then you can replace x with floor(x/2) and ceil(x/2), and floor(x/2) * ceil(x/2) > x. You can also replace 4s with two 2s. Hence, there will always be optimal solutions with only 2s and 3s. If there are three 2s, you can replace them with two 3s to get a better product. Hence, you'll never have more than two 2s. Keep adding 3s as long as n ≥ 5. |
Python || 3 Lines || Eazy to understand beat 98% 🚀 | determine-color-of-a-chessboard-square | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def squareIsWhite(self, coordinates: str) -> bool:\n if (ord(coordinates[0])+int(coordinates[1]))%2 == 0:\n return False\n return True\n \n``` | 1 | You are given `coordinates`, a string that represents the coordinates of a square of the chessboard. Below is a chessboard for your reference.
Return `true` _if the square is white, and_ `false` _if the square is black_.
The coordinate will always represent a valid chessboard square. The coordinate will always have the letter first, and the number second.
**Example 1:**
**Input:** coordinates = "a1 "
**Output:** false
**Explanation:** From the chessboard above, the square with coordinates "a1 " is black, so return false.
**Example 2:**
**Input:** coordinates = "h3 "
**Output:** true
**Explanation:** From the chessboard above, the square with coordinates "h3 " is white, so return true.
**Example 3:**
**Input:** coordinates = "c7 "
**Output:** false
**Constraints:**
* `coordinates.length == 2`
* `'a' <= coordinates[0] <= 'h'`
* `'1' <= coordinates[1] <= '8'` | Discard all the spaces and dashes. Use a while loop. While the string still has digits, check its length and see which rule to apply. |
Python || 3 Lines || Eazy to understand beat 98% 🚀 | determine-color-of-a-chessboard-square | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def squareIsWhite(self, coordinates: str) -> bool:\n if (ord(coordinates[0])+int(coordinates[1]))%2 == 0:\n return False\n return True\n \n``` | 1 | Given a **zero-based permutation** `nums` (**0-indexed**), build an array `ans` of the **same length** where `ans[i] = nums[nums[i]]` for each `0 <= i < nums.length` and return it.
A **zero-based permutation** `nums` is an array of **distinct** integers from `0` to `nums.length - 1` (**inclusive**).
**Example 1:**
**Input:** nums = \[0,2,1,5,3,4\]
**Output:** \[0,1,2,4,5,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[0\], nums\[2\], nums\[1\], nums\[5\], nums\[3\], nums\[4\]\]
= \[0,1,2,4,5,3\]
**Example 2:**
**Input:** nums = \[5,0,1,2,3,4\]
**Output:** \[4,5,0,1,2,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[5\], nums\[0\], nums\[1\], nums\[2\], nums\[3\], nums\[4\]\]
= \[4,5,0,1,2,3\]
**Constraints:**
* `1 <= nums.length <= 1000`
* `0 <= nums[i] < nums.length`
* The elements in `nums` are **distinct**.
**Follow-up:** Can you solve it without using an extra space (i.e., `O(1)` memory)? | Convert the coordinates to (x, y) - that is, "a1" is (1, 1), "d7" is (4, 7). Try add the numbers together and look for a pattern. |
Easiest to Understand Python Solution, beats 99.6% Memory | determine-color-of-a-chessboard-square | 0 | 1 | \n# Code\n```\nclass Solution:\n def squareIsWhite(self, coordinates: str) -> bool:\n alfa = "abcdefghijklmnopqrstuvwxyz"\n if alfa.index(coordinates[0]) % 2 == 0:\n if int(coordinates[1]) % 2 == 0:\n return True\n else:\n return False\n else:\n if int(coordinates[1]) % 2 == 0:\n return False\n else:\n return True \n``` | 1 | You are given `coordinates`, a string that represents the coordinates of a square of the chessboard. Below is a chessboard for your reference.
Return `true` _if the square is white, and_ `false` _if the square is black_.
The coordinate will always represent a valid chessboard square. The coordinate will always have the letter first, and the number second.
**Example 1:**
**Input:** coordinates = "a1 "
**Output:** false
**Explanation:** From the chessboard above, the square with coordinates "a1 " is black, so return false.
**Example 2:**
**Input:** coordinates = "h3 "
**Output:** true
**Explanation:** From the chessboard above, the square with coordinates "h3 " is white, so return true.
**Example 3:**
**Input:** coordinates = "c7 "
**Output:** false
**Constraints:**
* `coordinates.length == 2`
* `'a' <= coordinates[0] <= 'h'`
* `'1' <= coordinates[1] <= '8'` | Discard all the spaces and dashes. Use a while loop. While the string still has digits, check its length and see which rule to apply. |
Easiest to Understand Python Solution, beats 99.6% Memory | determine-color-of-a-chessboard-square | 0 | 1 | \n# Code\n```\nclass Solution:\n def squareIsWhite(self, coordinates: str) -> bool:\n alfa = "abcdefghijklmnopqrstuvwxyz"\n if alfa.index(coordinates[0]) % 2 == 0:\n if int(coordinates[1]) % 2 == 0:\n return True\n else:\n return False\n else:\n if int(coordinates[1]) % 2 == 0:\n return False\n else:\n return True \n``` | 1 | Given a **zero-based permutation** `nums` (**0-indexed**), build an array `ans` of the **same length** where `ans[i] = nums[nums[i]]` for each `0 <= i < nums.length` and return it.
A **zero-based permutation** `nums` is an array of **distinct** integers from `0` to `nums.length - 1` (**inclusive**).
**Example 1:**
**Input:** nums = \[0,2,1,5,3,4\]
**Output:** \[0,1,2,4,5,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[0\], nums\[2\], nums\[1\], nums\[5\], nums\[3\], nums\[4\]\]
= \[0,1,2,4,5,3\]
**Example 2:**
**Input:** nums = \[5,0,1,2,3,4\]
**Output:** \[4,5,0,1,2,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[5\], nums\[0\], nums\[1\], nums\[2\], nums\[3\], nums\[4\]\]
= \[4,5,0,1,2,3\]
**Constraints:**
* `1 <= nums.length <= 1000`
* `0 <= nums[i] < nums.length`
* The elements in `nums` are **distinct**.
**Follow-up:** Can you solve it without using an extra space (i.e., `O(1)` memory)? | Convert the coordinates to (x, y) - that is, "a1" is (1, 1), "d7" is (4, 7). Try add the numbers together and look for a pattern. |
Determine color of a chessboard square | determine-color-of-a-chessboard-square | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n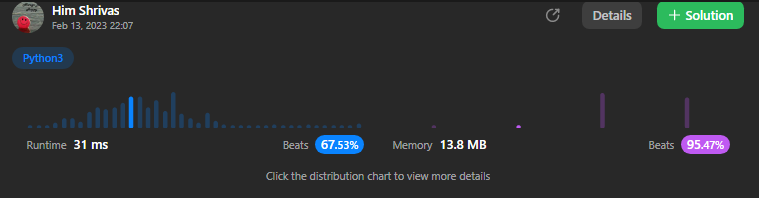\n\n# Code\n```\nclass Solution:\n def squareIsWhite(self, coordinates: str) -> bool:\n alpha=[\'a\',\'b\',\'c\',\'d\',\'e\',\'f\',\'g\',\'h\']\n for i in range(len(alpha)):\n for j in range(1,9):\n if alpha[i]+str(j)==coordinates:\n if (i+j)%2==0:\n return True\n else:\n return False\n``` | 1 | You are given `coordinates`, a string that represents the coordinates of a square of the chessboard. Below is a chessboard for your reference.
Return `true` _if the square is white, and_ `false` _if the square is black_.
The coordinate will always represent a valid chessboard square. The coordinate will always have the letter first, and the number second.
**Example 1:**
**Input:** coordinates = "a1 "
**Output:** false
**Explanation:** From the chessboard above, the square with coordinates "a1 " is black, so return false.
**Example 2:**
**Input:** coordinates = "h3 "
**Output:** true
**Explanation:** From the chessboard above, the square with coordinates "h3 " is white, so return true.
**Example 3:**
**Input:** coordinates = "c7 "
**Output:** false
**Constraints:**
* `coordinates.length == 2`
* `'a' <= coordinates[0] <= 'h'`
* `'1' <= coordinates[1] <= '8'` | Discard all the spaces and dashes. Use a while loop. While the string still has digits, check its length and see which rule to apply. |
Determine color of a chessboard square | determine-color-of-a-chessboard-square | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n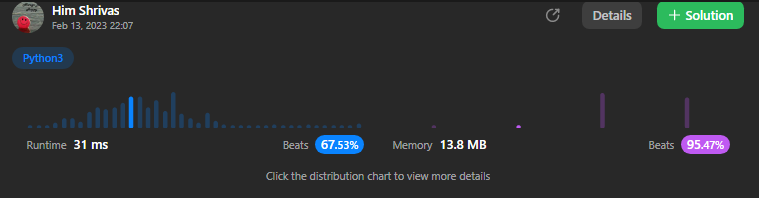\n\n# Code\n```\nclass Solution:\n def squareIsWhite(self, coordinates: str) -> bool:\n alpha=[\'a\',\'b\',\'c\',\'d\',\'e\',\'f\',\'g\',\'h\']\n for i in range(len(alpha)):\n for j in range(1,9):\n if alpha[i]+str(j)==coordinates:\n if (i+j)%2==0:\n return True\n else:\n return False\n``` | 1 | Given a **zero-based permutation** `nums` (**0-indexed**), build an array `ans` of the **same length** where `ans[i] = nums[nums[i]]` for each `0 <= i < nums.length` and return it.
A **zero-based permutation** `nums` is an array of **distinct** integers from `0` to `nums.length - 1` (**inclusive**).
**Example 1:**
**Input:** nums = \[0,2,1,5,3,4\]
**Output:** \[0,1,2,4,5,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[0\], nums\[2\], nums\[1\], nums\[5\], nums\[3\], nums\[4\]\]
= \[0,1,2,4,5,3\]
**Example 2:**
**Input:** nums = \[5,0,1,2,3,4\]
**Output:** \[4,5,0,1,2,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[5\], nums\[0\], nums\[1\], nums\[2\], nums\[3\], nums\[4\]\]
= \[4,5,0,1,2,3\]
**Constraints:**
* `1 <= nums.length <= 1000`
* `0 <= nums[i] < nums.length`
* The elements in `nums` are **distinct**.
**Follow-up:** Can you solve it without using an extra space (i.e., `O(1)` memory)? | Convert the coordinates to (x, y) - that is, "a1" is (1, 1), "d7" is (4, 7). Try add the numbers together and look for a pattern. |
A simple one liner with runtime of only 30 ms and easy to understand (I think so !). | determine-color-of-a-chessboard-square | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def squareIsWhite(self, c: str) -> bool:\n return (ord(c[0])+int(c[1]))%2!=0\n``` | 3 | You are given `coordinates`, a string that represents the coordinates of a square of the chessboard. Below is a chessboard for your reference.
Return `true` _if the square is white, and_ `false` _if the square is black_.
The coordinate will always represent a valid chessboard square. The coordinate will always have the letter first, and the number second.
**Example 1:**
**Input:** coordinates = "a1 "
**Output:** false
**Explanation:** From the chessboard above, the square with coordinates "a1 " is black, so return false.
**Example 2:**
**Input:** coordinates = "h3 "
**Output:** true
**Explanation:** From the chessboard above, the square with coordinates "h3 " is white, so return true.
**Example 3:**
**Input:** coordinates = "c7 "
**Output:** false
**Constraints:**
* `coordinates.length == 2`
* `'a' <= coordinates[0] <= 'h'`
* `'1' <= coordinates[1] <= '8'` | Discard all the spaces and dashes. Use a while loop. While the string still has digits, check its length and see which rule to apply. |
A simple one liner with runtime of only 30 ms and easy to understand (I think so !). | determine-color-of-a-chessboard-square | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def squareIsWhite(self, c: str) -> bool:\n return (ord(c[0])+int(c[1]))%2!=0\n``` | 3 | Given a **zero-based permutation** `nums` (**0-indexed**), build an array `ans` of the **same length** where `ans[i] = nums[nums[i]]` for each `0 <= i < nums.length` and return it.
A **zero-based permutation** `nums` is an array of **distinct** integers from `0` to `nums.length - 1` (**inclusive**).
**Example 1:**
**Input:** nums = \[0,2,1,5,3,4\]
**Output:** \[0,1,2,4,5,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[0\], nums\[2\], nums\[1\], nums\[5\], nums\[3\], nums\[4\]\]
= \[0,1,2,4,5,3\]
**Example 2:**
**Input:** nums = \[5,0,1,2,3,4\]
**Output:** \[4,5,0,1,2,3\]
**Explanation:** The array ans is built as follows:
ans = \[nums\[nums\[0\]\], nums\[nums\[1\]\], nums\[nums\[2\]\], nums\[nums\[3\]\], nums\[nums\[4\]\], nums\[nums\[5\]\]\]
= \[nums\[5\], nums\[0\], nums\[1\], nums\[2\], nums\[3\], nums\[4\]\]
= \[4,5,0,1,2,3\]
**Constraints:**
* `1 <= nums.length <= 1000`
* `0 <= nums[i] < nums.length`
* The elements in `nums` are **distinct**.
**Follow-up:** Can you solve it without using an extra space (i.e., `O(1)` memory)? | Convert the coordinates to (x, y) - that is, "a1" is (1, 1), "d7" is (4, 7). Try add the numbers together and look for a pattern. |
Python beats 100% | TC: O(s1 + s2) | SC: O(s1 + s2) | sentence-similarity-iii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nOnly single sentence can be inserted so only three positions possible\n\n#### Insert Left\nEx: s1 = "now", s2 = "Eating right now"\n\n__"Eating right"__ + "now == "Eating right now"\n\n\n#### Insert Right\nEx: s1 = "Eating", s2 = "Eating right now"\n\n"Eating" + __"right now"__ == "Eating right now"\n\n#### Insert Middle\nEx: s1 = "Eating now", s2 = "Eating right now"\n\n"Eating" + __"right"__ + "now" == "Eating right now"\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nLet smaller of sentence be sentence1\nTake a pointer i = 0\n\nMove the pointer till s1[i] == s2[i] (covering the left part)\n\nThen skip the pointer by n2 - n1 in s2 distance to skip different words (check insert left example) then again\n\nMove the pointer till s1[i] == s2[n2 - n1 + i] (covering the right part)\n\nIf i == len(s1) means we covered all the words and we can insert a sentence to make s1 == s2\n\n# Complexity\n- Time complexity: O(S1 + S2) \n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n\n- Space complexity: O(S1 + S2)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def areSentencesSimilar(self, sentence1: str, sentence2: str) -> bool:\n l1 = sentence1.split()\n l2 = sentence2.split()\n n1 = len(l1)\n n2 = len(l2)\n if n1 > n2:\n n1, n2 = n2, n1\n l1, l2 = l2[:], l1[:]\n\n i = 0\n while i < n1 and l1[i] == l2[i]:\n i += 1\n while i < n1 and l1[i] == l2[n2 - n1 + i]:\n i += 1\n\n return i == n1\n\n``` | 1 | A sentence is a list of words that are separated by a single space with no leading or trailing spaces. For example, `"Hello World "`, `"HELLO "`, `"hello world hello world "` are all sentences. Words consist of **only** uppercase and lowercase English letters.
Two sentences `sentence1` and `sentence2` are **similar** if it is possible to insert an arbitrary sentence **(possibly empty)** inside one of these sentences such that the two sentences become equal. For example, `sentence1 = "Hello my name is Jane "` and `sentence2 = "Hello Jane "` can be made equal by inserting `"my name is "` between `"Hello "` and `"Jane "` in `sentence2`.
Given two sentences `sentence1` and `sentence2`, return `true` _if_ `sentence1` _and_ `sentence2` _are similar._ Otherwise, return `false`.
**Example 1:**
**Input:** sentence1 = "My name is Haley ", sentence2 = "My Haley "
**Output:** true
**Explanation:** sentence2 can be turned to sentence1 by inserting "name is " between "My " and "Haley ".
**Example 2:**
**Input:** sentence1 = "of ", sentence2 = "A lot of words "
**Output:** false
**Explanation:** No single sentence can be inserted inside one of the sentences to make it equal to the other.
**Example 3:**
**Input:** sentence1 = "Eating right now ", sentence2 = "Eating "
**Output:** true
**Explanation:** sentence2 can be turned to sentence1 by inserting "right now " at the end of the sentence.
**Constraints:**
* `1 <= sentence1.length, sentence2.length <= 100`
* `sentence1` and `sentence2` consist of lowercase and uppercase English letters and spaces.
* The words in `sentence1` and `sentence2` are separated by a single space. | The main point here is for the subarray to contain unique elements for each index. Only the first subarrays starting from that index have unique elements. This can be solved using the two pointers technique |
Python beats 100% | TC: O(s1 + s2) | SC: O(s1 + s2) | sentence-similarity-iii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nOnly single sentence can be inserted so only three positions possible\n\n#### Insert Left\nEx: s1 = "now", s2 = "Eating right now"\n\n__"Eating right"__ + "now == "Eating right now"\n\n\n#### Insert Right\nEx: s1 = "Eating", s2 = "Eating right now"\n\n"Eating" + __"right now"__ == "Eating right now"\n\n#### Insert Middle\nEx: s1 = "Eating now", s2 = "Eating right now"\n\n"Eating" + __"right"__ + "now" == "Eating right now"\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nLet smaller of sentence be sentence1\nTake a pointer i = 0\n\nMove the pointer till s1[i] == s2[i] (covering the left part)\n\nThen skip the pointer by n2 - n1 in s2 distance to skip different words (check insert left example) then again\n\nMove the pointer till s1[i] == s2[n2 - n1 + i] (covering the right part)\n\nIf i == len(s1) means we covered all the words and we can insert a sentence to make s1 == s2\n\n# Complexity\n- Time complexity: O(S1 + S2) \n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n\n- Space complexity: O(S1 + S2)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def areSentencesSimilar(self, sentence1: str, sentence2: str) -> bool:\n l1 = sentence1.split()\n l2 = sentence2.split()\n n1 = len(l1)\n n2 = len(l2)\n if n1 > n2:\n n1, n2 = n2, n1\n l1, l2 = l2[:], l1[:]\n\n i = 0\n while i < n1 and l1[i] == l2[i]:\n i += 1\n while i < n1 and l1[i] == l2[n2 - n1 + i]:\n i += 1\n\n return i == n1\n\n``` | 1 | There is a country of `n` cities numbered from `0` to `n - 1`. In this country, there is a road connecting **every pair** of cities.
There are `m` friends numbered from `0` to `m - 1` who are traveling through the country. Each one of them will take a path consisting of some cities. Each path is represented by an integer array that contains the visited cities in order. The path may contain a city **more than once**, but the same city will not be listed consecutively.
Given an integer `n` and a 2D integer array `paths` where `paths[i]` is an integer array representing the path of the `ith` friend, return _the length of the **longest common subpath** that is shared by **every** friend's path, or_ `0` _if there is no common subpath at all_.
A **subpath** of a path is a contiguous sequence of cities within that path.
**Example 1:**
**Input:** n = 5, paths = \[\[0,1,2,3,4\],
\[2,3,4\],
\[4,0,1,2,3\]\]
**Output:** 2
**Explanation:** The longest common subpath is \[2,3\].
**Example 2:**
**Input:** n = 3, paths = \[\[0\],\[1\],\[2\]\]
**Output:** 0
**Explanation:** There is no common subpath shared by the three paths.
**Example 3:**
**Input:** n = 5, paths = \[\[0,1,2,3,4\],
\[4,3,2,1,0\]\]
**Output:** 1
**Explanation:** The possible longest common subpaths are \[0\], \[1\], \[2\], \[3\], and \[4\]. All have a length of 1.
**Constraints:**
* `1 <= n <= 105`
* `m == paths.length`
* `2 <= m <= 105`
* `sum(paths[i].length) <= 105`
* `0 <= paths[i][j] < n`
* The same city is not listed multiple times consecutively in `paths[i]`. | One way to look at it is to find one sentence as a concatenation of a prefix and suffix from the other sentence. Get the longest common prefix between them and the longest common suffix. |
USE QUEUE EXPLANATION || PYTHON | sentence-similarity-iii | 0 | 1 | # Intuition\nThe basic concept of the question is to fit one sentence in mid of another sentence \ni.e the bigger sentence remaining chars should be the all mid element and smaller sentence takes up the edges words\nso there are 2 conditions to check\neither the smaller sentence(s2) start word is present at s1 start\nor s2 last word is present at s1 end\nif s2 gets empty it means that s1 can fit completely in mid of s2 thus return True \nor at any point none of the condition is satisfied thus it cant fit hence return False.\n\n# Code\n```\nclass Solution:\n def areSentencesSimilar(self, sentence1: str, sentence2: str) -> bool:\n s1 = deque(sentence1.split(\' \'))\n s2 = deque(sentence2.split(\' \'))\n \n while s2 and s1:\n if s2[0] == s1[0]:\n s2.popleft()\n s1.popleft()\n continue\n elif s2[-1] == s1[-1]:\n s2.pop()\n s1.pop()\n continue\n return False\n return True\n \n\n \n \n``` | 0 | A sentence is a list of words that are separated by a single space with no leading or trailing spaces. For example, `"Hello World "`, `"HELLO "`, `"hello world hello world "` are all sentences. Words consist of **only** uppercase and lowercase English letters.
Two sentences `sentence1` and `sentence2` are **similar** if it is possible to insert an arbitrary sentence **(possibly empty)** inside one of these sentences such that the two sentences become equal. For example, `sentence1 = "Hello my name is Jane "` and `sentence2 = "Hello Jane "` can be made equal by inserting `"my name is "` between `"Hello "` and `"Jane "` in `sentence2`.
Given two sentences `sentence1` and `sentence2`, return `true` _if_ `sentence1` _and_ `sentence2` _are similar._ Otherwise, return `false`.
**Example 1:**
**Input:** sentence1 = "My name is Haley ", sentence2 = "My Haley "
**Output:** true
**Explanation:** sentence2 can be turned to sentence1 by inserting "name is " between "My " and "Haley ".
**Example 2:**
**Input:** sentence1 = "of ", sentence2 = "A lot of words "
**Output:** false
**Explanation:** No single sentence can be inserted inside one of the sentences to make it equal to the other.
**Example 3:**
**Input:** sentence1 = "Eating right now ", sentence2 = "Eating "
**Output:** true
**Explanation:** sentence2 can be turned to sentence1 by inserting "right now " at the end of the sentence.
**Constraints:**
* `1 <= sentence1.length, sentence2.length <= 100`
* `sentence1` and `sentence2` consist of lowercase and uppercase English letters and spaces.
* The words in `sentence1` and `sentence2` are separated by a single space. | The main point here is for the subarray to contain unique elements for each index. Only the first subarrays starting from that index have unique elements. This can be solved using the two pointers technique |
USE QUEUE EXPLANATION || PYTHON | sentence-similarity-iii | 0 | 1 | # Intuition\nThe basic concept of the question is to fit one sentence in mid of another sentence \ni.e the bigger sentence remaining chars should be the all mid element and smaller sentence takes up the edges words\nso there are 2 conditions to check\neither the smaller sentence(s2) start word is present at s1 start\nor s2 last word is present at s1 end\nif s2 gets empty it means that s1 can fit completely in mid of s2 thus return True \nor at any point none of the condition is satisfied thus it cant fit hence return False.\n\n# Code\n```\nclass Solution:\n def areSentencesSimilar(self, sentence1: str, sentence2: str) -> bool:\n s1 = deque(sentence1.split(\' \'))\n s2 = deque(sentence2.split(\' \'))\n \n while s2 and s1:\n if s2[0] == s1[0]:\n s2.popleft()\n s1.popleft()\n continue\n elif s2[-1] == s1[-1]:\n s2.pop()\n s1.pop()\n continue\n return False\n return True\n \n\n \n \n``` | 0 | There is a country of `n` cities numbered from `0` to `n - 1`. In this country, there is a road connecting **every pair** of cities.
There are `m` friends numbered from `0` to `m - 1` who are traveling through the country. Each one of them will take a path consisting of some cities. Each path is represented by an integer array that contains the visited cities in order. The path may contain a city **more than once**, but the same city will not be listed consecutively.
Given an integer `n` and a 2D integer array `paths` where `paths[i]` is an integer array representing the path of the `ith` friend, return _the length of the **longest common subpath** that is shared by **every** friend's path, or_ `0` _if there is no common subpath at all_.
A **subpath** of a path is a contiguous sequence of cities within that path.
**Example 1:**
**Input:** n = 5, paths = \[\[0,1,2,3,4\],
\[2,3,4\],
\[4,0,1,2,3\]\]
**Output:** 2
**Explanation:** The longest common subpath is \[2,3\].
**Example 2:**
**Input:** n = 3, paths = \[\[0\],\[1\],\[2\]\]
**Output:** 0
**Explanation:** There is no common subpath shared by the three paths.
**Example 3:**
**Input:** n = 5, paths = \[\[0,1,2,3,4\],
\[4,3,2,1,0\]\]
**Output:** 1
**Explanation:** The possible longest common subpaths are \[0\], \[1\], \[2\], \[3\], and \[4\]. All have a length of 1.
**Constraints:**
* `1 <= n <= 105`
* `m == paths.length`
* `2 <= m <= 105`
* `sum(paths[i].length) <= 105`
* `0 <= paths[i][j] < n`
* The same city is not listed multiple times consecutively in `paths[i]`. | One way to look at it is to find one sentence as a concatenation of a prefix and suffix from the other sentence. Get the longest common prefix between them and the longest common suffix. |
Python3 sol. | sentence-similarity-iii | 0 | 1 | \n# Code\n```\nclass Solution:\n def areSentencesSimilar(self, s1: str, s2: str) -> bool:\n def check(a,b):\n a=a.split()\n b=b.split()\n while len(a)>0 and len(b)>0 and a[0]==b[0]:\n a.pop(0)\n b.pop(0)\n while len(a)>0 and len(b)>0 and a[-1]==b[-1]:\n a.pop(-1)\n b.pop(-1)\n if len(a)==0:\n return True\n return check(s1,s2) or check(s2,s1)\n``` | 0 | A sentence is a list of words that are separated by a single space with no leading or trailing spaces. For example, `"Hello World "`, `"HELLO "`, `"hello world hello world "` are all sentences. Words consist of **only** uppercase and lowercase English letters.
Two sentences `sentence1` and `sentence2` are **similar** if it is possible to insert an arbitrary sentence **(possibly empty)** inside one of these sentences such that the two sentences become equal. For example, `sentence1 = "Hello my name is Jane "` and `sentence2 = "Hello Jane "` can be made equal by inserting `"my name is "` between `"Hello "` and `"Jane "` in `sentence2`.
Given two sentences `sentence1` and `sentence2`, return `true` _if_ `sentence1` _and_ `sentence2` _are similar._ Otherwise, return `false`.
**Example 1:**
**Input:** sentence1 = "My name is Haley ", sentence2 = "My Haley "
**Output:** true
**Explanation:** sentence2 can be turned to sentence1 by inserting "name is " between "My " and "Haley ".
**Example 2:**
**Input:** sentence1 = "of ", sentence2 = "A lot of words "
**Output:** false
**Explanation:** No single sentence can be inserted inside one of the sentences to make it equal to the other.
**Example 3:**
**Input:** sentence1 = "Eating right now ", sentence2 = "Eating "
**Output:** true
**Explanation:** sentence2 can be turned to sentence1 by inserting "right now " at the end of the sentence.
**Constraints:**
* `1 <= sentence1.length, sentence2.length <= 100`
* `sentence1` and `sentence2` consist of lowercase and uppercase English letters and spaces.
* The words in `sentence1` and `sentence2` are separated by a single space. | The main point here is for the subarray to contain unique elements for each index. Only the first subarrays starting from that index have unique elements. This can be solved using the two pointers technique |
Python3 sol. | sentence-similarity-iii | 0 | 1 | \n# Code\n```\nclass Solution:\n def areSentencesSimilar(self, s1: str, s2: str) -> bool:\n def check(a,b):\n a=a.split()\n b=b.split()\n while len(a)>0 and len(b)>0 and a[0]==b[0]:\n a.pop(0)\n b.pop(0)\n while len(a)>0 and len(b)>0 and a[-1]==b[-1]:\n a.pop(-1)\n b.pop(-1)\n if len(a)==0:\n return True\n return check(s1,s2) or check(s2,s1)\n``` | 0 | There is a country of `n` cities numbered from `0` to `n - 1`. In this country, there is a road connecting **every pair** of cities.
There are `m` friends numbered from `0` to `m - 1` who are traveling through the country. Each one of them will take a path consisting of some cities. Each path is represented by an integer array that contains the visited cities in order. The path may contain a city **more than once**, but the same city will not be listed consecutively.
Given an integer `n` and a 2D integer array `paths` where `paths[i]` is an integer array representing the path of the `ith` friend, return _the length of the **longest common subpath** that is shared by **every** friend's path, or_ `0` _if there is no common subpath at all_.
A **subpath** of a path is a contiguous sequence of cities within that path.
**Example 1:**
**Input:** n = 5, paths = \[\[0,1,2,3,4\],
\[2,3,4\],
\[4,0,1,2,3\]\]
**Output:** 2
**Explanation:** The longest common subpath is \[2,3\].
**Example 2:**
**Input:** n = 3, paths = \[\[0\],\[1\],\[2\]\]
**Output:** 0
**Explanation:** There is no common subpath shared by the three paths.
**Example 3:**
**Input:** n = 5, paths = \[\[0,1,2,3,4\],
\[4,3,2,1,0\]\]
**Output:** 1
**Explanation:** The possible longest common subpaths are \[0\], \[1\], \[2\], \[3\], and \[4\]. All have a length of 1.
**Constraints:**
* `1 <= n <= 105`
* `m == paths.length`
* `2 <= m <= 105`
* `sum(paths[i].length) <= 105`
* `0 <= paths[i][j] < n`
* The same city is not listed multiple times consecutively in `paths[i]`. | One way to look at it is to find one sentence as a concatenation of a prefix and suffix from the other sentence. Get the longest common prefix between them and the longest common suffix. |
Using Dynamic Programming || Standard Algo for most of the Dynamic Programming Questions | sentence-similarity-iii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: n * m\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nIn this code we have splited the given string to array. \nso it will take less time than n * m\nWhere \nn -> len(str1)\nm -> len(str2)\n\n- Space complexity: n * m\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nWe have used memoization which is 2d. so it consumes n * m.\nWhere \nn -> len(str1)\nm -> len(str2)\n\n# Code\n```\nclass Solution:\n def areSentencesSimilar(self, sentence1: str, sentence2: str) -> bool:\n str1 = sentence1.split()\n str2 = sentence2.split()\n dp = {}\n def solve(i, j, c, flag):\n if j == len(str2):\n return c <= 1\n if i >= len(str1):\n return False\n if (i, j, c) in dp:\n return dp[(i, j, c)]\n ans = False\n if str2[j] == str1[i]:\n #ans |= solve(i+1, j, c, flag)\n ans |= solve(i+1, j+1, c, False)\n if flag:\n ans |= solve(i+1, j, c, flag)\n ans |= solve(i+1, j, c+1, True)\n dp[(i, j, c)] = ans\n return ans\n st = (str1[0] == str2[0]) or (str1[-1] == str2[-1])\n opt = solve(0, 0, 0, False) and st\n dp = {}\n str1, str2 = str2, str1\n return (solve(0, 0, 0, False) or opt) and st\n``` | 0 | A sentence is a list of words that are separated by a single space with no leading or trailing spaces. For example, `"Hello World "`, `"HELLO "`, `"hello world hello world "` are all sentences. Words consist of **only** uppercase and lowercase English letters.
Two sentences `sentence1` and `sentence2` are **similar** if it is possible to insert an arbitrary sentence **(possibly empty)** inside one of these sentences such that the two sentences become equal. For example, `sentence1 = "Hello my name is Jane "` and `sentence2 = "Hello Jane "` can be made equal by inserting `"my name is "` between `"Hello "` and `"Jane "` in `sentence2`.
Given two sentences `sentence1` and `sentence2`, return `true` _if_ `sentence1` _and_ `sentence2` _are similar._ Otherwise, return `false`.
**Example 1:**
**Input:** sentence1 = "My name is Haley ", sentence2 = "My Haley "
**Output:** true
**Explanation:** sentence2 can be turned to sentence1 by inserting "name is " between "My " and "Haley ".
**Example 2:**
**Input:** sentence1 = "of ", sentence2 = "A lot of words "
**Output:** false
**Explanation:** No single sentence can be inserted inside one of the sentences to make it equal to the other.
**Example 3:**
**Input:** sentence1 = "Eating right now ", sentence2 = "Eating "
**Output:** true
**Explanation:** sentence2 can be turned to sentence1 by inserting "right now " at the end of the sentence.
**Constraints:**
* `1 <= sentence1.length, sentence2.length <= 100`
* `sentence1` and `sentence2` consist of lowercase and uppercase English letters and spaces.
* The words in `sentence1` and `sentence2` are separated by a single space. | The main point here is for the subarray to contain unique elements for each index. Only the first subarrays starting from that index have unique elements. This can be solved using the two pointers technique |
Using Dynamic Programming || Standard Algo for most of the Dynamic Programming Questions | sentence-similarity-iii | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: n * m\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nIn this code we have splited the given string to array. \nso it will take less time than n * m\nWhere \nn -> len(str1)\nm -> len(str2)\n\n- Space complexity: n * m\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nWe have used memoization which is 2d. so it consumes n * m.\nWhere \nn -> len(str1)\nm -> len(str2)\n\n# Code\n```\nclass Solution:\n def areSentencesSimilar(self, sentence1: str, sentence2: str) -> bool:\n str1 = sentence1.split()\n str2 = sentence2.split()\n dp = {}\n def solve(i, j, c, flag):\n if j == len(str2):\n return c <= 1\n if i >= len(str1):\n return False\n if (i, j, c) in dp:\n return dp[(i, j, c)]\n ans = False\n if str2[j] == str1[i]:\n #ans |= solve(i+1, j, c, flag)\n ans |= solve(i+1, j+1, c, False)\n if flag:\n ans |= solve(i+1, j, c, flag)\n ans |= solve(i+1, j, c+1, True)\n dp[(i, j, c)] = ans\n return ans\n st = (str1[0] == str2[0]) or (str1[-1] == str2[-1])\n opt = solve(0, 0, 0, False) and st\n dp = {}\n str1, str2 = str2, str1\n return (solve(0, 0, 0, False) or opt) and st\n``` | 0 | There is a country of `n` cities numbered from `0` to `n - 1`. In this country, there is a road connecting **every pair** of cities.
There are `m` friends numbered from `0` to `m - 1` who are traveling through the country. Each one of them will take a path consisting of some cities. Each path is represented by an integer array that contains the visited cities in order. The path may contain a city **more than once**, but the same city will not be listed consecutively.
Given an integer `n` and a 2D integer array `paths` where `paths[i]` is an integer array representing the path of the `ith` friend, return _the length of the **longest common subpath** that is shared by **every** friend's path, or_ `0` _if there is no common subpath at all_.
A **subpath** of a path is a contiguous sequence of cities within that path.
**Example 1:**
**Input:** n = 5, paths = \[\[0,1,2,3,4\],
\[2,3,4\],
\[4,0,1,2,3\]\]
**Output:** 2
**Explanation:** The longest common subpath is \[2,3\].
**Example 2:**
**Input:** n = 3, paths = \[\[0\],\[1\],\[2\]\]
**Output:** 0
**Explanation:** There is no common subpath shared by the three paths.
**Example 3:**
**Input:** n = 5, paths = \[\[0,1,2,3,4\],
\[4,3,2,1,0\]\]
**Output:** 1
**Explanation:** The possible longest common subpaths are \[0\], \[1\], \[2\], \[3\], and \[4\]. All have a length of 1.
**Constraints:**
* `1 <= n <= 105`
* `m == paths.length`
* `2 <= m <= 105`
* `sum(paths[i].length) <= 105`
* `0 <= paths[i][j] < n`
* The same city is not listed multiple times consecutively in `paths[i]`. | One way to look at it is to find one sentence as a concatenation of a prefix and suffix from the other sentence. Get the longest common prefix between them and the longest common suffix. |
🔥HEART BREAKiNG SOLUTiON EVER 🔥 ✅✅✅ by PRODONIK (Java, C#, C++, Python, Ruby) 🔥 | count-nice-pairs-in-an-array | 1 | 1 | 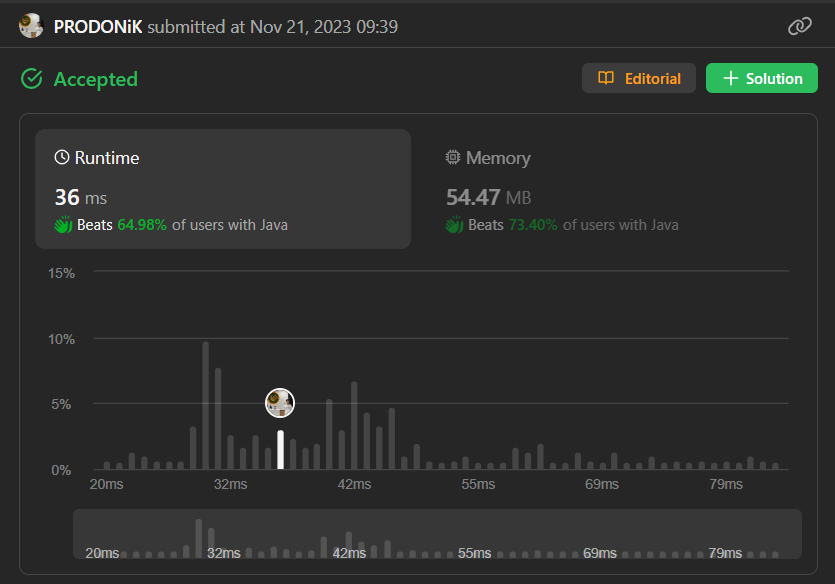\n\n# Intuition\nThe problem involves counting the number of "nice pairs" in an array, where a "nice pair" is defined as a pair of elements whose difference is equal to the difference between the reverse of the two elements. The intuition is to iterate through the array, calculate the temporary number (the difference between the element and its reverse), and maintain a count of occurrences for each temporary number.\n\n# Approach\n| Index | Element | Reversed | Modified Element |\n|-------|---------|----------|-------------------|\n| 0 | 42 | 24 | 18 |\n| 1 | 11 | 11 | 0 |\n| 2 | 1 | 1 | 0 |\n| 3 | 97 | 79 | 18 |\n- Create a HashMap to store the occurrences of temporary numbers.\n- Iterate through the array, calculate the temporary number for each element, and update the count in the HashMap.\n- Iterate through the counts in the HashMap and calculate the total number of nice pairs using the formula \\(\\frac{n \\cdot (n - 1)}{2}\\), where \\(n\\) is the count of occurrences for each temporary number.\n- Use modular arithmetic to handle large numbers.\n\n# Complexity\n- Time complexity: \\(O(n)\\), where \\(n\\) is the length of the input array. The algorithm iterates through the array once.\n- Space complexity: \\(O(n)\\), where \\(n\\) is the number of unique temporary numbers. The HashMap stores the occurrences of temporary numbers.\n\n# Code\n```java []\nimport java.util.HashMap;\nimport java.util.Collection;\nimport java.util.Map;\n\nclass Solution {\n public int countNicePairs(int[] nums) {\n /// Create a HashMap to store occurrences of temporary numbers\n Map<Integer, Integer> numbers = new HashMap<>();\n\n /// Iterate through the array\n for (int num : nums) {\n /// Calculate the temporary number\n int temporary_number = num - reverse(num);\n\n /// Update the count in the HashMap\n if (numbers.containsKey(temporary_number)) {\n numbers.put(temporary_number, numbers.get(temporary_number) + 1);\n } else {\n numbers.put(temporary_number, 1);\n }\n }\n\n /// Calculate the total number of nice pairs\n long result = 0;\n Collection<Integer> values = numbers.values();\n int mod = 1000000007;\n for (int value : values) {\n result = (result % mod + ((long) value * ((long) value - 1) / 2)) % mod;\n }\n\n // Return the result as an integer\n return (int) result;\n }\n\n /// Helper function to reverse a number\n private int reverse(int number) {\n int reversed_number = 0;\n while (number > 0) {\n reversed_number = reversed_number * 10 + number % 10;\n number /= 10;\n }\n return reversed_number;\n }\n}\n```\n```C# []\nusing System;\nusing System.Collections.Generic;\n\npublic class Solution {\n public int CountNicePairs(int[] nums) {\n Dictionary<int, int> numbers = new Dictionary<int, int>();\n\n foreach (int num in nums) {\n int temporaryNumber = num - Reverse(num);\n\n if (numbers.ContainsKey(temporaryNumber)) {\n numbers[temporaryNumber]++;\n } else {\n numbers[temporaryNumber] = 1;\n }\n }\n\n long result = 0;\n int mod = 1000000007;\n foreach (int value in numbers.Values) {\n result = (result % mod + ((long)value * ((long)value - 1) / 2)) % mod;\n }\n\n return (int)result;\n }\n\n private int Reverse(int number) {\n int reversedNumber = 0;\n while (number > 0) {\n reversedNumber = reversedNumber * 10 + number % 10;\n number /= 10;\n }\n return reversedNumber;\n }\n}\n```\n``` C++ []\n#include <unordered_map>\n#include <vector>\n\nclass Solution {\npublic:\n int countNicePairs(std::vector<int>& nums) {\n std::unordered_map<int, int> numbers;\n\n for (int num : nums) {\n int temporaryNumber = num - reverse(num);\n\n if (numbers.find(temporaryNumber) != numbers.end()) {\n numbers[temporaryNumber]++;\n } else {\n numbers[temporaryNumber] = 1;\n }\n }\n\n long result = 0;\n int mod = 1000000007;\n for (const auto& entry : numbers) {\n result = (result % mod + ((long)entry.second * ((long)entry.second - 1) / 2)) % mod;\n }\n\n return static_cast<int>(result);\n }\n\nprivate:\n int reverse(int number) {\n int reversedNumber = 0;\n while (number > 0) {\n reversedNumber = reversedNumber * 10 + number % 10;\n number /= 10;\n }\n return reversedNumber;\n }\n};\n```\n``` Python []\nclass Solution:\n def countNicePairs(self, nums):\n numbers = {}\n\n for num in nums:\n temporary_number = num - self.reverse(num)\n\n if temporary_number in numbers:\n numbers[temporary_number] += 1\n else:\n numbers[temporary_number] = 1\n\n result = 0\n mod = 1000000007\n for value in numbers.values():\n result = (result % mod + (value * (value - 1) // 2)) % mod\n\n return int(result)\n\n def reverse(self, number):\n reversed_number = 0\n while number > 0:\n reversed_number = reversed_number * 10 + number % 10\n number //= 10\n return reversed_number\n```\n``` Ruby []\nclass Solution\n def count_nice_pairs(nums)\n numbers = {}\n\n nums.each do |num|\n temporary_number = num - reverse(num)\n\n if numbers.key?(temporary_number)\n numbers[temporary_number] += 1\n else\n numbers[temporary_number] = 1\n end\n end\n\n result = 0\n numbers.values.each do |value|\n result = (result % 1_000_000_007 + (value * (value - 1) / 2) % 1_000_000_007) % 1_000_000_007\n end\n\n result.to_i\n end\n\n private\n\n def reverse(number)\n reversed_number = 0\n while number > 0\n reversed_number = reversed_number * 10 + number % 10\n number /= 10\n end\n reversed_number\n end\nend\n``` | 14 | You are given an array `nums` that consists of non-negative integers. Let us define `rev(x)` as the reverse of the non-negative integer `x`. For example, `rev(123) = 321`, and `rev(120) = 21`. A pair of indices `(i, j)` is **nice** if it satisfies all of the following conditions:
* `0 <= i < j < nums.length`
* `nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])`
Return _the number of nice pairs of indices_. Since that number can be too large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[42,11,1,97\]
**Output:** 2
**Explanation:** The two pairs are:
- (0,3) : 42 + rev(97) = 42 + 79 = 121, 97 + rev(42) = 97 + 24 = 121.
- (1,2) : 11 + rev(1) = 11 + 1 = 12, 1 + rev(11) = 1 + 11 = 12.
**Example 2:**
**Input:** nums = \[13,10,35,24,76\]
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | Let dp[i] be "the maximum score to reach the end starting at index i". The answer for dp[i] is nums[i] + max{dp[i+j]} for 1 <= j <= k. That gives an O(n*k) solution. Instead of checking every j for every i, keep track of the largest dp[i] values in a heap and calculate dp[i] from right to left. When the largest value in the heap is out of bounds of the current index, remove it and keep checking. |
🔥HEART BREAKiNG SOLUTiON EVER 🔥 ✅✅✅ by PRODONIK (Java, C#, C++, Python, Ruby) 🔥 | count-nice-pairs-in-an-array | 1 | 1 | 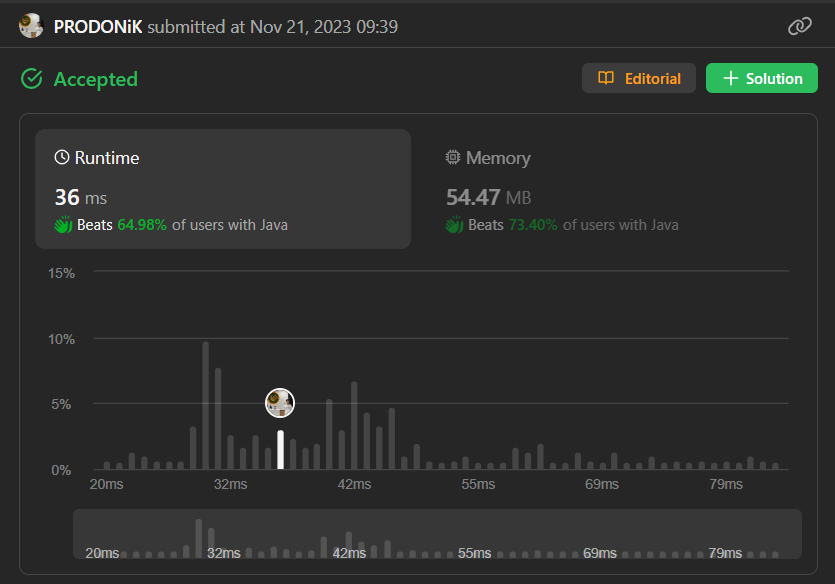\n\n# Intuition\nThe problem involves counting the number of "nice pairs" in an array, where a "nice pair" is defined as a pair of elements whose difference is equal to the difference between the reverse of the two elements. The intuition is to iterate through the array, calculate the temporary number (the difference between the element and its reverse), and maintain a count of occurrences for each temporary number.\n\n# Approach\n| Index | Element | Reversed | Modified Element |\n|-------|---------|----------|-------------------|\n| 0 | 42 | 24 | 18 |\n| 1 | 11 | 11 | 0 |\n| 2 | 1 | 1 | 0 |\n| 3 | 97 | 79 | 18 |\n- Create a HashMap to store the occurrences of temporary numbers.\n- Iterate through the array, calculate the temporary number for each element, and update the count in the HashMap.\n- Iterate through the counts in the HashMap and calculate the total number of nice pairs using the formula \\(\\frac{n \\cdot (n - 1)}{2}\\), where \\(n\\) is the count of occurrences for each temporary number.\n- Use modular arithmetic to handle large numbers.\n\n# Complexity\n- Time complexity: \\(O(n)\\), where \\(n\\) is the length of the input array. The algorithm iterates through the array once.\n- Space complexity: \\(O(n)\\), where \\(n\\) is the number of unique temporary numbers. The HashMap stores the occurrences of temporary numbers.\n\n# Code\n```java []\nimport java.util.HashMap;\nimport java.util.Collection;\nimport java.util.Map;\n\nclass Solution {\n public int countNicePairs(int[] nums) {\n /// Create a HashMap to store occurrences of temporary numbers\n Map<Integer, Integer> numbers = new HashMap<>();\n\n /// Iterate through the array\n for (int num : nums) {\n /// Calculate the temporary number\n int temporary_number = num - reverse(num);\n\n /// Update the count in the HashMap\n if (numbers.containsKey(temporary_number)) {\n numbers.put(temporary_number, numbers.get(temporary_number) + 1);\n } else {\n numbers.put(temporary_number, 1);\n }\n }\n\n /// Calculate the total number of nice pairs\n long result = 0;\n Collection<Integer> values = numbers.values();\n int mod = 1000000007;\n for (int value : values) {\n result = (result % mod + ((long) value * ((long) value - 1) / 2)) % mod;\n }\n\n // Return the result as an integer\n return (int) result;\n }\n\n /// Helper function to reverse a number\n private int reverse(int number) {\n int reversed_number = 0;\n while (number > 0) {\n reversed_number = reversed_number * 10 + number % 10;\n number /= 10;\n }\n return reversed_number;\n }\n}\n```\n```C# []\nusing System;\nusing System.Collections.Generic;\n\npublic class Solution {\n public int CountNicePairs(int[] nums) {\n Dictionary<int, int> numbers = new Dictionary<int, int>();\n\n foreach (int num in nums) {\n int temporaryNumber = num - Reverse(num);\n\n if (numbers.ContainsKey(temporaryNumber)) {\n numbers[temporaryNumber]++;\n } else {\n numbers[temporaryNumber] = 1;\n }\n }\n\n long result = 0;\n int mod = 1000000007;\n foreach (int value in numbers.Values) {\n result = (result % mod + ((long)value * ((long)value - 1) / 2)) % mod;\n }\n\n return (int)result;\n }\n\n private int Reverse(int number) {\n int reversedNumber = 0;\n while (number > 0) {\n reversedNumber = reversedNumber * 10 + number % 10;\n number /= 10;\n }\n return reversedNumber;\n }\n}\n```\n``` C++ []\n#include <unordered_map>\n#include <vector>\n\nclass Solution {\npublic:\n int countNicePairs(std::vector<int>& nums) {\n std::unordered_map<int, int> numbers;\n\n for (int num : nums) {\n int temporaryNumber = num - reverse(num);\n\n if (numbers.find(temporaryNumber) != numbers.end()) {\n numbers[temporaryNumber]++;\n } else {\n numbers[temporaryNumber] = 1;\n }\n }\n\n long result = 0;\n int mod = 1000000007;\n for (const auto& entry : numbers) {\n result = (result % mod + ((long)entry.second * ((long)entry.second - 1) / 2)) % mod;\n }\n\n return static_cast<int>(result);\n }\n\nprivate:\n int reverse(int number) {\n int reversedNumber = 0;\n while (number > 0) {\n reversedNumber = reversedNumber * 10 + number % 10;\n number /= 10;\n }\n return reversedNumber;\n }\n};\n```\n``` Python []\nclass Solution:\n def countNicePairs(self, nums):\n numbers = {}\n\n for num in nums:\n temporary_number = num - self.reverse(num)\n\n if temporary_number in numbers:\n numbers[temporary_number] += 1\n else:\n numbers[temporary_number] = 1\n\n result = 0\n mod = 1000000007\n for value in numbers.values():\n result = (result % mod + (value * (value - 1) // 2)) % mod\n\n return int(result)\n\n def reverse(self, number):\n reversed_number = 0\n while number > 0:\n reversed_number = reversed_number * 10 + number % 10\n number //= 10\n return reversed_number\n```\n``` Ruby []\nclass Solution\n def count_nice_pairs(nums)\n numbers = {}\n\n nums.each do |num|\n temporary_number = num - reverse(num)\n\n if numbers.key?(temporary_number)\n numbers[temporary_number] += 1\n else\n numbers[temporary_number] = 1\n end\n end\n\n result = 0\n numbers.values.each do |value|\n result = (result % 1_000_000_007 + (value * (value - 1) / 2) % 1_000_000_007) % 1_000_000_007\n end\n\n result.to_i\n end\n\n private\n\n def reverse(number)\n reversed_number = 0\n while number > 0\n reversed_number = reversed_number * 10 + number % 10\n number /= 10\n end\n reversed_number\n end\nend\n``` | 14 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
💯Faster✅💯 Lesser✅Simple Math🔥Hash Table🔥Simple Explanation🔥Intuition Explained🔥 | count-nice-pairs-in-an-array | 1 | 1 | # Intuition\n- We have an array of non-negative integers.\n- We\'re looking for pairs of indices (i, j) where a specific condition is satisfied.\n- The condition involves the sum of a number and its reverse (digits reversed).\n- More precisely, for indices i and j (where i < j):\n- Original condition: $$nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])$$\n- Modified condition: We simplify this by creating a new array, subtracting the reverse from each number, and storing the differences, $$nums[i] - rev(nums[i]) == nums[j] - rev(nums[j])$$.\n- For each number in the original array, we create a new array by subtracting its reverse from itself.\n- To efficiently count pairs, we sort the new array. This helps group numbers with the same differences together.\n- We count how many times each unique difference appears consecutively in the sorted array.\n- This count represents the number of pairs that satisfy the modified condition.\n- We use these counts to calculate the total number of pairs satisfying the modified condition.\n- This involves counting combinations, essentially determining how many ways we can choose pairs from the counted occurrences.\n- Since the result might be a large number, we take the answer modulo $$10^9 + 7$$ to keep it within a reasonable range.\n\n# Approach\n- Define a function reverse to reverse the digits of a non-negative integer.\n- Iterate through the array and subtract the reversed form of each number from the original number, updating the array with the differences.\n- Sort the modified array to group identical differences together.\n- Iterate through the sorted array to count the occurrences of each unique difference.\n- Use the count of occurrences to calculate the number of pairs that meet the condition.\n- Return the result modulo 10^9 + 7.\n\n# Complexity\n- Time complexity: $$O(n log n)$$\n- Space complexity: $$O(n)$$ for the modified array.\n\n\n# Code\n```Python3 []\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n mod = 1000000007\n length = len(nums)\n for i in range(length):\n nums[i] = nums[i] - self.reverse(nums[i])\n nums.sort()\n res = 0\n i = 0\n while i < length - 1:\n count = 1\n while i < length - 1 and nums[i] == nums[i + 1]:\n count += 1\n i += 1\n res = (res % mod + (count * (count - 1)) // 2) % mod\n i += 1\n\n return int(res % mod)\n def reverse(self, num: int) -> int:\n rev = 0\n while num > 0:\n rev = rev * 10 + num % 10\n num //= 10\n return rev\n```\n```Java []\nclass Solution {\n public int countNicePairs(int[] nums) {\n final int mod = 1000000007;\n int len = nums.length;\n for (int i = 0; i < len; i++) {\n nums[i] = nums[i] - reverse(nums[i]);\n }\n Arrays.sort(nums);\n long res = 0;\n for (int i = 0; i < len - 1; i++) {\n long count = 1;\n while (i < len - 1 && nums[i] == nums[i + 1]) {\n count++;\n i++;\n }\n res = (res % mod + (count * (count - 1)) / 2) % mod;\n }\n\n return (int) (res % mod);\n }\n private int reverse(int num) {\n int rev = 0;\n while (num > 0) {\n rev = rev * 10 + num % 10;\n num /= 10;\n }\n return rev;\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int countNicePairs(std::vector<int>& nums) {\n const int mod = 1000000007;\n int len = nums.size();\n for (int i = 0; i < len; i++) {\n nums[i] = nums[i] - reverse(nums[i]);\n }\n std::sort(nums.begin(), nums.end());\n long res = 0;\n for (int i = 0; i < len - 1; i++) {\n long count = 1;\n while (i < len - 1 && nums[i] == nums[i + 1]) {\n count++;\n i++;\n }\n res = (res % mod + (count * (count - 1)) / 2) % mod;\n }\n\n return static_cast<int>(res); // Add this line to return the result.\n }\n\nprivate:\n int reverse(int num) {\n int rev = 0;\n while (num > 0) {\n rev = rev * 10 + num % 10;\n num /= 10;\n }\n return rev;\n }\n};\n```\n | 57 | You are given an array `nums` that consists of non-negative integers. Let us define `rev(x)` as the reverse of the non-negative integer `x`. For example, `rev(123) = 321`, and `rev(120) = 21`. A pair of indices `(i, j)` is **nice** if it satisfies all of the following conditions:
* `0 <= i < j < nums.length`
* `nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])`
Return _the number of nice pairs of indices_. Since that number can be too large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[42,11,1,97\]
**Output:** 2
**Explanation:** The two pairs are:
- (0,3) : 42 + rev(97) = 42 + 79 = 121, 97 + rev(42) = 97 + 24 = 121.
- (1,2) : 11 + rev(1) = 11 + 1 = 12, 1 + rev(11) = 1 + 11 = 12.
**Example 2:**
**Input:** nums = \[13,10,35,24,76\]
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | Let dp[i] be "the maximum score to reach the end starting at index i". The answer for dp[i] is nums[i] + max{dp[i+j]} for 1 <= j <= k. That gives an O(n*k) solution. Instead of checking every j for every i, keep track of the largest dp[i] values in a heap and calculate dp[i] from right to left. When the largest value in the heap is out of bounds of the current index, remove it and keep checking. |
💯Faster✅💯 Lesser✅Simple Math🔥Hash Table🔥Simple Explanation🔥Intuition Explained🔥 | count-nice-pairs-in-an-array | 1 | 1 | # Intuition\n- We have an array of non-negative integers.\n- We\'re looking for pairs of indices (i, j) where a specific condition is satisfied.\n- The condition involves the sum of a number and its reverse (digits reversed).\n- More precisely, for indices i and j (where i < j):\n- Original condition: $$nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])$$\n- Modified condition: We simplify this by creating a new array, subtracting the reverse from each number, and storing the differences, $$nums[i] - rev(nums[i]) == nums[j] - rev(nums[j])$$.\n- For each number in the original array, we create a new array by subtracting its reverse from itself.\n- To efficiently count pairs, we sort the new array. This helps group numbers with the same differences together.\n- We count how many times each unique difference appears consecutively in the sorted array.\n- This count represents the number of pairs that satisfy the modified condition.\n- We use these counts to calculate the total number of pairs satisfying the modified condition.\n- This involves counting combinations, essentially determining how many ways we can choose pairs from the counted occurrences.\n- Since the result might be a large number, we take the answer modulo $$10^9 + 7$$ to keep it within a reasonable range.\n\n# Approach\n- Define a function reverse to reverse the digits of a non-negative integer.\n- Iterate through the array and subtract the reversed form of each number from the original number, updating the array with the differences.\n- Sort the modified array to group identical differences together.\n- Iterate through the sorted array to count the occurrences of each unique difference.\n- Use the count of occurrences to calculate the number of pairs that meet the condition.\n- Return the result modulo 10^9 + 7.\n\n# Complexity\n- Time complexity: $$O(n log n)$$\n- Space complexity: $$O(n)$$ for the modified array.\n\n\n# Code\n```Python3 []\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n mod = 1000000007\n length = len(nums)\n for i in range(length):\n nums[i] = nums[i] - self.reverse(nums[i])\n nums.sort()\n res = 0\n i = 0\n while i < length - 1:\n count = 1\n while i < length - 1 and nums[i] == nums[i + 1]:\n count += 1\n i += 1\n res = (res % mod + (count * (count - 1)) // 2) % mod\n i += 1\n\n return int(res % mod)\n def reverse(self, num: int) -> int:\n rev = 0\n while num > 0:\n rev = rev * 10 + num % 10\n num //= 10\n return rev\n```\n```Java []\nclass Solution {\n public int countNicePairs(int[] nums) {\n final int mod = 1000000007;\n int len = nums.length;\n for (int i = 0; i < len; i++) {\n nums[i] = nums[i] - reverse(nums[i]);\n }\n Arrays.sort(nums);\n long res = 0;\n for (int i = 0; i < len - 1; i++) {\n long count = 1;\n while (i < len - 1 && nums[i] == nums[i + 1]) {\n count++;\n i++;\n }\n res = (res % mod + (count * (count - 1)) / 2) % mod;\n }\n\n return (int) (res % mod);\n }\n private int reverse(int num) {\n int rev = 0;\n while (num > 0) {\n rev = rev * 10 + num % 10;\n num /= 10;\n }\n return rev;\n }\n}\n```\n```C++ []\nclass Solution {\npublic:\n int countNicePairs(std::vector<int>& nums) {\n const int mod = 1000000007;\n int len = nums.size();\n for (int i = 0; i < len; i++) {\n nums[i] = nums[i] - reverse(nums[i]);\n }\n std::sort(nums.begin(), nums.end());\n long res = 0;\n for (int i = 0; i < len - 1; i++) {\n long count = 1;\n while (i < len - 1 && nums[i] == nums[i + 1]) {\n count++;\n i++;\n }\n res = (res % mod + (count * (count - 1)) / 2) % mod;\n }\n\n return static_cast<int>(res); // Add this line to return the result.\n }\n\nprivate:\n int reverse(int num) {\n int rev = 0;\n while (num > 0) {\n rev = rev * 10 + num % 10;\n num /= 10;\n }\n return rev;\n }\n};\n```\n | 57 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
[Python3] Two sum approach + number of pairs n(n-1)//2 || beats 99% | count-nice-pairs-in-an-array | 0 | 1 | ### Number of pairs:\nThe formula `\uD835\uDC5B(\uD835\uDC5B\u22121)/2`for the number of pairs you can form from an `\uD835\uDC5B` element set has many derivations.\n\nOne is to imagine a room with `\uD835\uDC5B` people, each of whom shakes hands with everyone else. If you focus on just one person you see that he participates in `\uD835\uDC5B\u22121` handshakes. Since there are `\uD835\uDC5B` people, that would lead to `\uD835\uDC5B(\uD835\uDC5B\u22121)` handshakes.\n\nTo finish the problem, we should realize that the total of `\uD835\uDC5B(\uD835\uDC5B\u22121)` counts every handshake `twice`, once for each of the two people involved. So the number of handshakes is `\uD835\uDC5B(\uD835\uDC5B\u22121)/2`.\n\n###### Another way to understand the number of pairs (will be used in the second solution): \n Imagine the people entering the room one at a time and introducing themselves. The first person has nothing to do. The second person shakes one hand. The third person shakes with the two already there, and so on. The last person has `\uD835\uDC5B\u22121` hands to shake. So the total number of handshakes is `0 + 1 + 2 + ... +(\uD835\uDC5B\u22121)`.\n\n### Reverse a number:\nTo reverse a number use this: `int(str(i)[::-1]` => convert int to string, reverse and convert again to int.\n\n### Math:\nA simple math (`just move a number from left to right or right to left side with opposite sign`) and now this problem is equivalent to [1. Two Sum](https://leetcode.com/problems/two-sum/) problem.\n \n # a + b == c + d ==> a - d == c - b ==> a + b - c - d == 0\n # nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])\n # nums[i] - rev(nums[i]) == nums[j] - rev(nums[j])\n\n\n##### Bonus 2-lines and 1-lines approaches on the other tabs.\n```python3 []\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n nums = [n - int(str(n)[::-1]) for n in nums]\n res = 0\n for n in Counter(nums).values():\n res += n*(n-1)//2 \n return res % (10**9 + 7)\n```\n```python3 []\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n nums = [n - int(str(n)[::-1]) for n in nums]\n return sum(n*(n-1)//2 for v in Counter(nums).values()) % (10**9 + 7)\n```\n```python3 []\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n return sum(v*(v-1)//2 for v in Counter(n - int(str(n)[::-1]) for n in nums).values()) % (10**9 + 7)\n```\nLike a classic 2 Sum problem, but slower than code above.\nNew value `k` is making pairs with all the same values k before `d[k]`. Step by step.\n```python3 []\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n res, d = 0, defaultdict(int)\n for i, n in enumerate(nums):\n k = n - int(str(n)[::-1])\n res += d[k]\n d[k] += 1\n return res % (10**9 + 7)\n```\n\n\n### Similar problems:\n[2006. Count Number of Pairs With Absolute Difference K](https://leetcode.com/problems/count-number-of-pairs-with-absolute-difference-k/description/)\n[2176. Count Equal and Divisible Pairs in an Array](https://leetcode.com/problems/count-equal-and-divisible-pairs-in-an-array/description/)\n[1010. Pairs of Songs With Total Durations Divisible by 60](https://leetcode.com/problems/pairs-of-songs-with-total-durations-divisible-by-60/description/)\n[1711. Count Good Meals](https://leetcode.com/problems/count-good-meals/description/)\n[1814. Count Nice Pairs in an Array](https://leetcode.com/problems/count-nice-pairs-in-an-array/description/)\n[2364. Count Number of Bad Pairs](https://leetcode.com/problems/count-number-of-bad-pairs/description/)\n[2342. Max Sum of a Pair With Equal Sum of Digits](https://leetcode.com/problems/max-sum-of-a-pair-with-equal-sum-of-digits/description/)\n[2023. Number of Pairs of Strings With Concatenation Equal to Target](https://leetcode.com/problems/number-of-pairs-of-strings-with-concatenation-equal-to-target/description/)\n | 21 | You are given an array `nums` that consists of non-negative integers. Let us define `rev(x)` as the reverse of the non-negative integer `x`. For example, `rev(123) = 321`, and `rev(120) = 21`. A pair of indices `(i, j)` is **nice** if it satisfies all of the following conditions:
* `0 <= i < j < nums.length`
* `nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])`
Return _the number of nice pairs of indices_. Since that number can be too large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[42,11,1,97\]
**Output:** 2
**Explanation:** The two pairs are:
- (0,3) : 42 + rev(97) = 42 + 79 = 121, 97 + rev(42) = 97 + 24 = 121.
- (1,2) : 11 + rev(1) = 11 + 1 = 12, 1 + rev(11) = 1 + 11 = 12.
**Example 2:**
**Input:** nums = \[13,10,35,24,76\]
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | Let dp[i] be "the maximum score to reach the end starting at index i". The answer for dp[i] is nums[i] + max{dp[i+j]} for 1 <= j <= k. That gives an O(n*k) solution. Instead of checking every j for every i, keep track of the largest dp[i] values in a heap and calculate dp[i] from right to left. When the largest value in the heap is out of bounds of the current index, remove it and keep checking. |
[Python3] Two sum approach + number of pairs n(n-1)//2 || beats 99% | count-nice-pairs-in-an-array | 0 | 1 | ### Number of pairs:\nThe formula `\uD835\uDC5B(\uD835\uDC5B\u22121)/2`for the number of pairs you can form from an `\uD835\uDC5B` element set has many derivations.\n\nOne is to imagine a room with `\uD835\uDC5B` people, each of whom shakes hands with everyone else. If you focus on just one person you see that he participates in `\uD835\uDC5B\u22121` handshakes. Since there are `\uD835\uDC5B` people, that would lead to `\uD835\uDC5B(\uD835\uDC5B\u22121)` handshakes.\n\nTo finish the problem, we should realize that the total of `\uD835\uDC5B(\uD835\uDC5B\u22121)` counts every handshake `twice`, once for each of the two people involved. So the number of handshakes is `\uD835\uDC5B(\uD835\uDC5B\u22121)/2`.\n\n###### Another way to understand the number of pairs (will be used in the second solution): \n Imagine the people entering the room one at a time and introducing themselves. The first person has nothing to do. The second person shakes one hand. The third person shakes with the two already there, and so on. The last person has `\uD835\uDC5B\u22121` hands to shake. So the total number of handshakes is `0 + 1 + 2 + ... +(\uD835\uDC5B\u22121)`.\n\n### Reverse a number:\nTo reverse a number use this: `int(str(i)[::-1]` => convert int to string, reverse and convert again to int.\n\n### Math:\nA simple math (`just move a number from left to right or right to left side with opposite sign`) and now this problem is equivalent to [1. Two Sum](https://leetcode.com/problems/two-sum/) problem.\n \n # a + b == c + d ==> a - d == c - b ==> a + b - c - d == 0\n # nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])\n # nums[i] - rev(nums[i]) == nums[j] - rev(nums[j])\n\n\n##### Bonus 2-lines and 1-lines approaches on the other tabs.\n```python3 []\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n nums = [n - int(str(n)[::-1]) for n in nums]\n res = 0\n for n in Counter(nums).values():\n res += n*(n-1)//2 \n return res % (10**9 + 7)\n```\n```python3 []\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n nums = [n - int(str(n)[::-1]) for n in nums]\n return sum(n*(n-1)//2 for v in Counter(nums).values()) % (10**9 + 7)\n```\n```python3 []\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n return sum(v*(v-1)//2 for v in Counter(n - int(str(n)[::-1]) for n in nums).values()) % (10**9 + 7)\n```\nLike a classic 2 Sum problem, but slower than code above.\nNew value `k` is making pairs with all the same values k before `d[k]`. Step by step.\n```python3 []\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n res, d = 0, defaultdict(int)\n for i, n in enumerate(nums):\n k = n - int(str(n)[::-1])\n res += d[k]\n d[k] += 1\n return res % (10**9 + 7)\n```\n\n\n### Similar problems:\n[2006. Count Number of Pairs With Absolute Difference K](https://leetcode.com/problems/count-number-of-pairs-with-absolute-difference-k/description/)\n[2176. Count Equal and Divisible Pairs in an Array](https://leetcode.com/problems/count-equal-and-divisible-pairs-in-an-array/description/)\n[1010. Pairs of Songs With Total Durations Divisible by 60](https://leetcode.com/problems/pairs-of-songs-with-total-durations-divisible-by-60/description/)\n[1711. Count Good Meals](https://leetcode.com/problems/count-good-meals/description/)\n[1814. Count Nice Pairs in an Array](https://leetcode.com/problems/count-nice-pairs-in-an-array/description/)\n[2364. Count Number of Bad Pairs](https://leetcode.com/problems/count-number-of-bad-pairs/description/)\n[2342. Max Sum of a Pair With Equal Sum of Digits](https://leetcode.com/problems/max-sum-of-a-pair-with-equal-sum-of-digits/description/)\n[2023. Number of Pairs of Strings With Concatenation Equal to Target](https://leetcode.com/problems/number-of-pairs-of-strings-with-concatenation-equal-to-target/description/)\n | 21 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
✌️Maths+Maps.py🧐 | count-nice-pairs-in-an-array | 0 | 1 | # Code\n```py\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n ans,mp=0,{}\n for i in nums:\n rev=i-int((str(i)[::-1]))\n if rev not in mp:mp[rev]=1\n else:mp[rev]+=1\n print(mp)\n for i in mp:ans+=((mp[i]*(mp[i]-1)//2))%(10**9+7)\n return ans%(10**9+7)\n``` | 6 | You are given an array `nums` that consists of non-negative integers. Let us define `rev(x)` as the reverse of the non-negative integer `x`. For example, `rev(123) = 321`, and `rev(120) = 21`. A pair of indices `(i, j)` is **nice** if it satisfies all of the following conditions:
* `0 <= i < j < nums.length`
* `nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])`
Return _the number of nice pairs of indices_. Since that number can be too large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[42,11,1,97\]
**Output:** 2
**Explanation:** The two pairs are:
- (0,3) : 42 + rev(97) = 42 + 79 = 121, 97 + rev(42) = 97 + 24 = 121.
- (1,2) : 11 + rev(1) = 11 + 1 = 12, 1 + rev(11) = 1 + 11 = 12.
**Example 2:**
**Input:** nums = \[13,10,35,24,76\]
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | Let dp[i] be "the maximum score to reach the end starting at index i". The answer for dp[i] is nums[i] + max{dp[i+j]} for 1 <= j <= k. That gives an O(n*k) solution. Instead of checking every j for every i, keep track of the largest dp[i] values in a heap and calculate dp[i] from right to left. When the largest value in the heap is out of bounds of the current index, remove it and keep checking. |
✌️Maths+Maps.py🧐 | count-nice-pairs-in-an-array | 0 | 1 | # Code\n```py\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n ans,mp=0,{}\n for i in nums:\n rev=i-int((str(i)[::-1]))\n if rev not in mp:mp[rev]=1\n else:mp[rev]+=1\n print(mp)\n for i in mp:ans+=((mp[i]*(mp[i]-1)//2))%(10**9+7)\n return ans%(10**9+7)\n``` | 6 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
Easy To Understand || O(N)|| c++|| java|| python | count-nice-pairs-in-an-array | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nThe given code seems to be solving a problem related to counting "nice pairs" in an array. A "nice pair" is a pair of indices (i, j) where nums[i] - rev(nums[j]) is the same as nums[j] - rev(nums[i]). The approach involves reversing the digits of each number in the array, subtracting the reversed number from the original, and then counting the occurrences of the differences.\n\nReversing Digits: The rev function is used to reverse the digits of a number.\n\nFact Function: The fact function computes the total number of nice pairs based on occurences.\n\nCounting Nice Pairs: The main function countNicePairs subtracts the reversed number from each element in the array, counts the occurrences using a map (mp).\n\n# Complexity\n- Time complexity:\nThe time complexity is O(N) where N is the length of the input array, as each element is processed once.\n\n- Space complexity:\nThe space complexity is O(N) due to the map used to count occurrences.\n\n# Code\n```c++ []\nclass Solution {\npublic:\n int rev(int n)\n {\n int re=0;\n while(n>0)\n {\n re= re*10 + n%10;\n n/=10;\n }\n return re;\n } \n long long fact(int n)\n {\n if(n==1)\n return 1;\n return n + fact(n-1);\n }\n int countNicePairs(vector<int>& nums) {\n long count=0;\n const int mod = 1000000007;\n for(int i=0;i<nums.size();i++)\n {\n nums[i]= nums[i] - rev(nums[i]);\n }\n map<int,int> mp;\n for(auto i: nums)\n {\n mp[i]++;\n }\n for(auto i: mp)\n {\n if(i.second > 1)\n count = (count % mod + fact(i.second-1) ) % mod ;\n }\n return count ;\n }\n};\n```\n```python []\nclass Solution:\n def rev(self, n):\n re = 0\n while n > 0:\n re = re * 10 + n % 10\n n //= 10\n return re\n\n def fact(self, n):\n if n == 1:\n return 1\n return n + self.fact(n - 1)\n\n def countNicePairs(self, nums):\n count = 0\n mod = 1000000007\n\n for i in range(len(nums)):\n nums[i] = nums[i] - self.rev(nums[i])\n\n mp = Counter(nums)\n\n for key, value in mp.items():\n if value > 1:\n count = (count % mod + self.fact(value - 1)) % mod\n\n return int(count)\n```\n```java []\npublic class Solution {\n public int rev(int n) {\n int re = 0;\n while (n > 0) {\n re = re * 10 + n % 10;\n n /= 10;\n }\n return re;\n }\n\n public long fact(int n) {\n if (n == 1)\n return 1;\n return n + fact(n - 1);\n }\n\n public int countNicePairs(int[] nums) {\n long count = 0;\n final int mod = 1000000007;\n\n for (int i = 0; i < nums.length; i++) {\n nums[i] = nums[i] - rev(nums[i]);\n }\n\n Map<Integer, Integer> mp = new HashMap<>();\n for (int i : nums) {\n mp.put(i, mp.getOrDefault(i, 0) + 1);\n }\n\n for (Map.Entry<Integer, Integer> entry : mp.entrySet()) {\n if (entry.getValue() > 1) {\n count = (count % mod + fact(entry.getValue() - 1)) % mod;\n }\n }\n\n return (int) count;\n }\n}\n\n```\n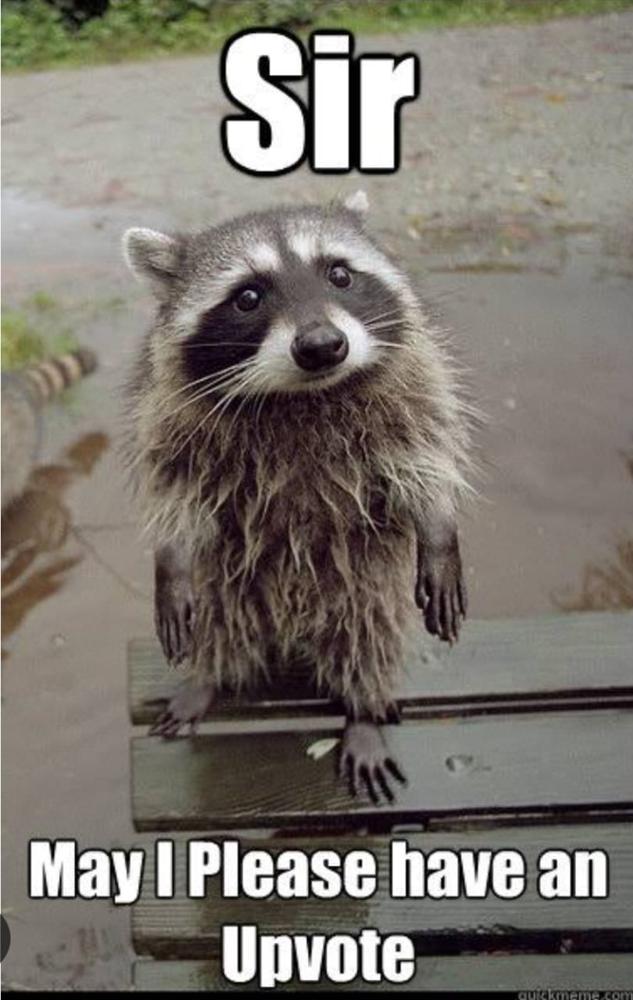\n\n | 15 | You are given an array `nums` that consists of non-negative integers. Let us define `rev(x)` as the reverse of the non-negative integer `x`. For example, `rev(123) = 321`, and `rev(120) = 21`. A pair of indices `(i, j)` is **nice** if it satisfies all of the following conditions:
* `0 <= i < j < nums.length`
* `nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])`
Return _the number of nice pairs of indices_. Since that number can be too large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[42,11,1,97\]
**Output:** 2
**Explanation:** The two pairs are:
- (0,3) : 42 + rev(97) = 42 + 79 = 121, 97 + rev(42) = 97 + 24 = 121.
- (1,2) : 11 + rev(1) = 11 + 1 = 12, 1 + rev(11) = 1 + 11 = 12.
**Example 2:**
**Input:** nums = \[13,10,35,24,76\]
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | Let dp[i] be "the maximum score to reach the end starting at index i". The answer for dp[i] is nums[i] + max{dp[i+j]} for 1 <= j <= k. That gives an O(n*k) solution. Instead of checking every j for every i, keep track of the largest dp[i] values in a heap and calculate dp[i] from right to left. When the largest value in the heap is out of bounds of the current index, remove it and keep checking. |
Easy To Understand || O(N)|| c++|| java|| python | count-nice-pairs-in-an-array | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nThe given code seems to be solving a problem related to counting "nice pairs" in an array. A "nice pair" is a pair of indices (i, j) where nums[i] - rev(nums[j]) is the same as nums[j] - rev(nums[i]). The approach involves reversing the digits of each number in the array, subtracting the reversed number from the original, and then counting the occurrences of the differences.\n\nReversing Digits: The rev function is used to reverse the digits of a number.\n\nFact Function: The fact function computes the total number of nice pairs based on occurences.\n\nCounting Nice Pairs: The main function countNicePairs subtracts the reversed number from each element in the array, counts the occurrences using a map (mp).\n\n# Complexity\n- Time complexity:\nThe time complexity is O(N) where N is the length of the input array, as each element is processed once.\n\n- Space complexity:\nThe space complexity is O(N) due to the map used to count occurrences.\n\n# Code\n```c++ []\nclass Solution {\npublic:\n int rev(int n)\n {\n int re=0;\n while(n>0)\n {\n re= re*10 + n%10;\n n/=10;\n }\n return re;\n } \n long long fact(int n)\n {\n if(n==1)\n return 1;\n return n + fact(n-1);\n }\n int countNicePairs(vector<int>& nums) {\n long count=0;\n const int mod = 1000000007;\n for(int i=0;i<nums.size();i++)\n {\n nums[i]= nums[i] - rev(nums[i]);\n }\n map<int,int> mp;\n for(auto i: nums)\n {\n mp[i]++;\n }\n for(auto i: mp)\n {\n if(i.second > 1)\n count = (count % mod + fact(i.second-1) ) % mod ;\n }\n return count ;\n }\n};\n```\n```python []\nclass Solution:\n def rev(self, n):\n re = 0\n while n > 0:\n re = re * 10 + n % 10\n n //= 10\n return re\n\n def fact(self, n):\n if n == 1:\n return 1\n return n + self.fact(n - 1)\n\n def countNicePairs(self, nums):\n count = 0\n mod = 1000000007\n\n for i in range(len(nums)):\n nums[i] = nums[i] - self.rev(nums[i])\n\n mp = Counter(nums)\n\n for key, value in mp.items():\n if value > 1:\n count = (count % mod + self.fact(value - 1)) % mod\n\n return int(count)\n```\n```java []\npublic class Solution {\n public int rev(int n) {\n int re = 0;\n while (n > 0) {\n re = re * 10 + n % 10;\n n /= 10;\n }\n return re;\n }\n\n public long fact(int n) {\n if (n == 1)\n return 1;\n return n + fact(n - 1);\n }\n\n public int countNicePairs(int[] nums) {\n long count = 0;\n final int mod = 1000000007;\n\n for (int i = 0; i < nums.length; i++) {\n nums[i] = nums[i] - rev(nums[i]);\n }\n\n Map<Integer, Integer> mp = new HashMap<>();\n for (int i : nums) {\n mp.put(i, mp.getOrDefault(i, 0) + 1);\n }\n\n for (Map.Entry<Integer, Integer> entry : mp.entrySet()) {\n if (entry.getValue() > 1) {\n count = (count % mod + fact(entry.getValue() - 1)) % mod;\n }\n }\n\n return (int) count;\n }\n}\n\n```\n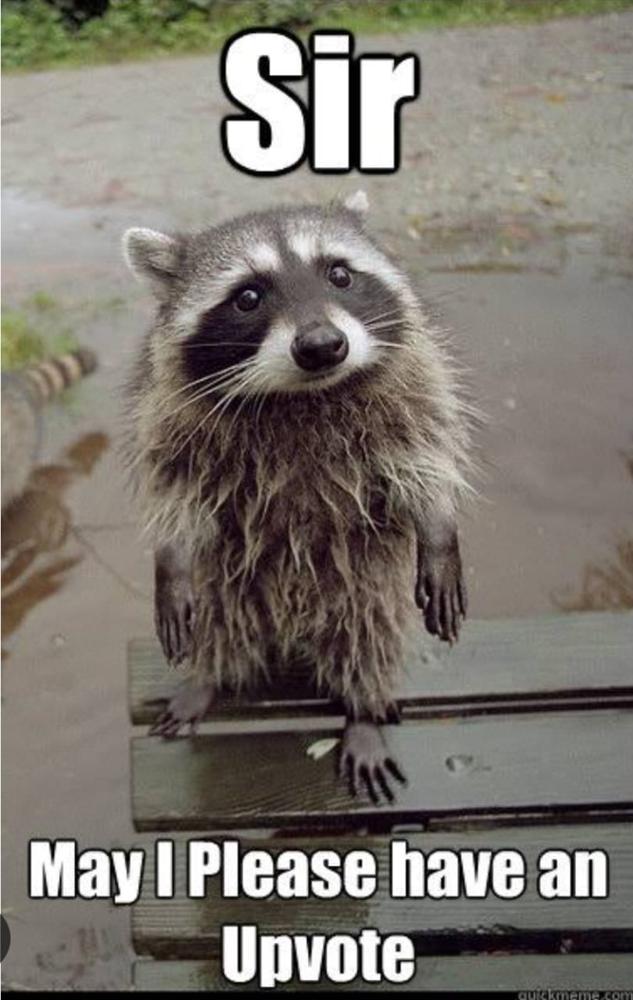\n\n | 15 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
✅ One Line Solution | count-nice-pairs-in-an-array | 0 | 1 | # Complexity\n- Time complexity: $$O(n)$$.\n\n- Space complexity: $$O(n)$$.\n\n# Code - Oneliner\n```\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n return sum(comb(cnt, 2) for cnt in Counter(num - int(str(num)[::-1]) for num in nums).values())%(10**9 + 7)\n```\n\n# Code - Unwrapped\n```\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n MOD = 10**9 + 7\n cntr = Counter(num - int(str(num)[::-1]) for num in nums)\n\n return sum(comb(cnt, 2) for cnt in cntr.values())%MOD\n``` | 3 | You are given an array `nums` that consists of non-negative integers. Let us define `rev(x)` as the reverse of the non-negative integer `x`. For example, `rev(123) = 321`, and `rev(120) = 21`. A pair of indices `(i, j)` is **nice** if it satisfies all of the following conditions:
* `0 <= i < j < nums.length`
* `nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])`
Return _the number of nice pairs of indices_. Since that number can be too large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[42,11,1,97\]
**Output:** 2
**Explanation:** The two pairs are:
- (0,3) : 42 + rev(97) = 42 + 79 = 121, 97 + rev(42) = 97 + 24 = 121.
- (1,2) : 11 + rev(1) = 11 + 1 = 12, 1 + rev(11) = 1 + 11 = 12.
**Example 2:**
**Input:** nums = \[13,10,35,24,76\]
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | Let dp[i] be "the maximum score to reach the end starting at index i". The answer for dp[i] is nums[i] + max{dp[i+j]} for 1 <= j <= k. That gives an O(n*k) solution. Instead of checking every j for every i, keep track of the largest dp[i] values in a heap and calculate dp[i] from right to left. When the largest value in the heap is out of bounds of the current index, remove it and keep checking. |
✅ One Line Solution | count-nice-pairs-in-an-array | 0 | 1 | # Complexity\n- Time complexity: $$O(n)$$.\n\n- Space complexity: $$O(n)$$.\n\n# Code - Oneliner\n```\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n return sum(comb(cnt, 2) for cnt in Counter(num - int(str(num)[::-1]) for num in nums).values())%(10**9 + 7)\n```\n\n# Code - Unwrapped\n```\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n MOD = 10**9 + 7\n cntr = Counter(num - int(str(num)[::-1]) for num in nums)\n\n return sum(comb(cnt, 2) for cnt in cntr.values())%MOD\n``` | 3 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
✅☑[C++/Java/Python/JavaScript] || 2 Approaches || EXPLAINED🔥 | count-nice-pairs-in-an-array | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n**(Also explained in the code)**\n\n### ***Approach 1(Combination)***\n1. The `rev` function reverses a given number.\n1. In `countNicePairs`, it calculates the differences between each number and its reverse, storing the frequencies of these differences in the freq map.\n1. Then, it iterates through the `freq` map, calculating the count of nice pairs using combinations and accumulates the count in `nicePairs`.\n1. Finally, it returns the total nice pairs count modulo `10^9 + 7`.\n\n# Complexity\n- *Time complexity:*\n $$O(N * K + M)$$\n \n\n- *Space complexity:*\n $$O(M)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n // Function to reverse a number\n int rev(int n){\n int ans = 0;\n while(n != 0){\n int temp = n % 10;\n ans = ans * 10 + temp;\n n = n / 10;\n }\n return ans;\n }\n\n int countNicePairs(vector<int>& nums) {\n unordered_map<int, int> freq; // To store the frequency of differences\n long long int nicePairs = 0; // To count the number of nice pairs\n\n // Calculate the differences between each number and its reverse and store their frequencies\n for(int num : nums){\n int revNum = rev(num);\n int diff = num - revNum;\n freq[diff]++;\n }\n\n // Iterate through the frequency map to calculate the nice pairs count\n for(auto &it : freq){\n int count = it.second;\n nicePairs += (long long)count * (count - 1) / 2; // Calculating combinations of count C(2)\n }\n\n return nicePairs % 1000000007; // Return the total nice pairs count modulo 10^9 + 7\n }\n};\n\n\n\n```\n```C []\n\n// Function to reverse a number\nint rev(int n){\n int ans = 0;\n while(n != 0){\n int temp = n % 10;\n ans = ans * 10 + temp;\n n = n / 10;\n }\n return ans;\n}\n\nint countNicePairs(int* nums, int numsSize) {\n int freq[100000] = {0}; // To store the frequency of differences\n long long int nicePairs = 0; // To count the number of nice pairs\n\n // Calculate the differences between each number and its reverse and store their frequencies\n for(int i = 0; i < numsSize; i++){\n int revNum = rev(nums[i]);\n int diff = nums[i] - revNum;\n freq[diff]++;\n }\n\n // Iterate through the frequency map to calculate the nice pairs count\n for(int i = 0; i < 100000; i++){\n int count = freq[i];\n nicePairs += (long long)count * (count - 1) / 2; // Calculating combinations of count C(2)\n }\n\n return nicePairs % 1000000007; // Return the total nice pairs count modulo 10^9 + 7\n}\n\n\n```\n```Java []\n\n\nclass Solution {\n // Function to reverse a number\n int rev(int n) {\n int ans = 0;\n while (n != 0) {\n int temp = n % 10;\n ans = ans * 10 + temp;\n n = n / 10;\n }\n return ans;\n }\n\n public int countNicePairs(int[] nums) {\n Map<Integer, Integer> freq = new HashMap<>(); // To store the frequency of differences\n long nicePairs = 0; // To count the number of nice pairs\n\n // Calculate the differences between each number and its reverse and store their frequencies\n for (int num : nums) {\n int revNum = rev(num);\n int diff = num - revNum;\n freq.put(diff, freq.getOrDefault(diff, 0) + 1);\n }\n\n // Iterate through the frequency map to calculate the nice pairs count\n for (Map.Entry<Integer, Integer> entry : freq.entrySet()) {\n int count = entry.getValue();\n nicePairs += (long) count * (count - 1) / 2; // Calculating combinations of count C(2)\n }\n\n return (int) (nicePairs % 1000000007); // Return the total nice pairs count modulo 10^9 + 7\n }\n}\n\n\n```\n\n```python3 []\n\nclass Solution:\n # Function to reverse a number\n def rev(self, n):\n ans = 0\n while n != 0:\n temp = n % 10\n ans = ans * 10 + temp\n n = n // 10\n return ans\n\n def countNicePairs(self, nums):\n freq = {} # To store the frequency of differences\n nicePairs = 0 # To count the number of nice pairs\n\n # Calculate the differences between each number and its reverse and store their frequencies\n for num in nums:\n revNum = self.rev(num)\n diff = num - revNum\n freq[diff] = freq.get(diff, 0) + 1\n\n # Iterate through the frequency map to calculate the nice pairs count\n for count in freq.values():\n nicePairs += count * (count - 1) // 2 # Calculating combinations of count C(2)\n\n return nicePairs % 1000000007 # Return the total nice pairs count modulo 10^9 + 7\n\n\n```\n```javascript []\nclass Solution {\n // Function to reverse a number\n rev(n) {\n let ans = 0;\n while (n !== 0) {\n let temp = n % 10;\n ans = ans * 10 + temp;\n n = Math.floor(n / 10);\n }\n return ans;\n }\n\n countNicePairs(nums) {\n let freq = new Map(); // To store the frequency of differences\n let nicePairs = 0; // To count the number of nice pairs\n\n // Calculate the differences between each number and its reverse and store their frequencies\n for (let num of nums) {\n let revNum = this.rev(num);\n let diff = num - revNum;\n freq.set(diff, (freq.get(diff) || 0) + 1);\n }\n\n // Iterate through the frequency map to calculate the nice pairs count\n for (let count of freq.values()) {\n nicePairs += count * (count - 1) / 2; // Calculating combinations of count C(2)\n }\n\n return nicePairs % 1000000007; // Return the total nice pairs count modulo 10^9 + 7\n }\n}\n\n\n```\n\n---\n\n### ***Approach 2(Counting With Hash Maps)***\n\n\n# Complexity\n- *Time complexity:*\n $$O(n)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\n\nclass Solution {\npublic:\n int countNicePairs(vector<int>& nums) {\n vector<int> arr;\n for (int i = 0; i < nums.size(); i++) {\n arr.push_back(nums[i] - rev(nums[i]));\n }\n \n unordered_map<int, int> dic;\n int ans = 0;\n int MOD = 1e9 + 7;\n for (int num : arr) {\n ans = (ans + dic[num]) % MOD;\n dic[num]++;\n }\n \n return ans;\n }\n \n int rev(int num) {\n int result = 0;\n while (num > 0) {\n result = result * 10 + num % 10;\n num /= 10;\n }\n \n return result;\n }\n};\n\n```\n```C []\n\n\nint rev(int num) {\n int result = 0;\n while (num > 0) {\n result = result * 10 + num % 10;\n num /= 10;\n }\n return result;\n}\n\nint countNicePairs(int* nums, int numsSize) {\n int* arr = (int*)malloc(numsSize * sizeof(int));\n for (int i = 0; i < numsSize; i++) {\n arr[i] = nums[i] - rev(nums[i]);\n }\n\n int* dic = (int*)calloc(numsSize, sizeof(int));\n int ans = 0;\n int MOD = 1000000007;\n for (int i = 0; i < numsSize; i++) {\n ans = (ans + dic[arr[i]]) % MOD;\n dic[arr[i]]++;\n }\n\n free(arr);\n free(dic);\n return ans;\n}\n\n\n\n```\n```Java []\nclass Solution {\n public int countNicePairs(int[] nums) {\n int[] arr = new int[nums.length];\n for (int i = 0; i < nums.length; i++) {\n arr[i] = nums[i] - rev(nums[i]);\n }\n \n Map<Integer, Integer> dic = new HashMap();\n int ans = 0;\n int MOD = (int) 1e9 + 7;\n for (int num : arr) {\n ans = (ans + dic.getOrDefault(num, 0)) % MOD;\n dic.put(num, dic.getOrDefault(num, 0) + 1);\n }\n \n return ans;\n }\n \n public int rev(int num) {\n int result = 0;\n while (num > 0) {\n result = result * 10 + num % 10;\n num /= 10;\n }\n \n return result;\n }\n}\n\n```\n\n```python3 []\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n def rev(num):\n result = 0\n while num:\n result = result * 10 + num % 10\n num //= 10\n \n return result\n\n arr = []\n for i in range(len(nums)):\n arr.append(nums[i] - rev(nums[i]))\n \n dic = defaultdict(int)\n ans = 0\n MOD = 10 ** 9 + 7\n for num in arr:\n ans = (ans + dic[num]) % MOD\n dic[num] += 1\n \n return ans\n\n\n```\n```javascript []\nfunction rev(num) {\n let result = 0;\n while (num > 0) {\n result = result * 10 + num % 10;\n num = Math.floor(num / 10);\n }\n return result;\n}\n\nfunction countNicePairs(nums) {\n let arr = [];\n for (let i = 0; i < nums.length; i++) {\n arr.push(nums[i] - rev(nums[i]));\n }\n\n let dic = new Map();\n let ans = 0;\n const MOD = 1000000007;\n for (let num of arr) {\n ans = (ans + (dic.get(num) || 0)) % MOD;\n dic.set(num, (dic.get(num) || 0) + 1);\n }\n\n return ans;\n}\n\n\n```\n\n---\n\n\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n--- | 2 | You are given an array `nums` that consists of non-negative integers. Let us define `rev(x)` as the reverse of the non-negative integer `x`. For example, `rev(123) = 321`, and `rev(120) = 21`. A pair of indices `(i, j)` is **nice** if it satisfies all of the following conditions:
* `0 <= i < j < nums.length`
* `nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])`
Return _the number of nice pairs of indices_. Since that number can be too large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[42,11,1,97\]
**Output:** 2
**Explanation:** The two pairs are:
- (0,3) : 42 + rev(97) = 42 + 79 = 121, 97 + rev(42) = 97 + 24 = 121.
- (1,2) : 11 + rev(1) = 11 + 1 = 12, 1 + rev(11) = 1 + 11 = 12.
**Example 2:**
**Input:** nums = \[13,10,35,24,76\]
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | Let dp[i] be "the maximum score to reach the end starting at index i". The answer for dp[i] is nums[i] + max{dp[i+j]} for 1 <= j <= k. That gives an O(n*k) solution. Instead of checking every j for every i, keep track of the largest dp[i] values in a heap and calculate dp[i] from right to left. When the largest value in the heap is out of bounds of the current index, remove it and keep checking. |
✅☑[C++/Java/Python/JavaScript] || 2 Approaches || EXPLAINED🔥 | count-nice-pairs-in-an-array | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n**(Also explained in the code)**\n\n### ***Approach 1(Combination)***\n1. The `rev` function reverses a given number.\n1. In `countNicePairs`, it calculates the differences between each number and its reverse, storing the frequencies of these differences in the freq map.\n1. Then, it iterates through the `freq` map, calculating the count of nice pairs using combinations and accumulates the count in `nicePairs`.\n1. Finally, it returns the total nice pairs count modulo `10^9 + 7`.\n\n# Complexity\n- *Time complexity:*\n $$O(N * K + M)$$\n \n\n- *Space complexity:*\n $$O(M)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n // Function to reverse a number\n int rev(int n){\n int ans = 0;\n while(n != 0){\n int temp = n % 10;\n ans = ans * 10 + temp;\n n = n / 10;\n }\n return ans;\n }\n\n int countNicePairs(vector<int>& nums) {\n unordered_map<int, int> freq; // To store the frequency of differences\n long long int nicePairs = 0; // To count the number of nice pairs\n\n // Calculate the differences between each number and its reverse and store their frequencies\n for(int num : nums){\n int revNum = rev(num);\n int diff = num - revNum;\n freq[diff]++;\n }\n\n // Iterate through the frequency map to calculate the nice pairs count\n for(auto &it : freq){\n int count = it.second;\n nicePairs += (long long)count * (count - 1) / 2; // Calculating combinations of count C(2)\n }\n\n return nicePairs % 1000000007; // Return the total nice pairs count modulo 10^9 + 7\n }\n};\n\n\n\n```\n```C []\n\n// Function to reverse a number\nint rev(int n){\n int ans = 0;\n while(n != 0){\n int temp = n % 10;\n ans = ans * 10 + temp;\n n = n / 10;\n }\n return ans;\n}\n\nint countNicePairs(int* nums, int numsSize) {\n int freq[100000] = {0}; // To store the frequency of differences\n long long int nicePairs = 0; // To count the number of nice pairs\n\n // Calculate the differences between each number and its reverse and store their frequencies\n for(int i = 0; i < numsSize; i++){\n int revNum = rev(nums[i]);\n int diff = nums[i] - revNum;\n freq[diff]++;\n }\n\n // Iterate through the frequency map to calculate the nice pairs count\n for(int i = 0; i < 100000; i++){\n int count = freq[i];\n nicePairs += (long long)count * (count - 1) / 2; // Calculating combinations of count C(2)\n }\n\n return nicePairs % 1000000007; // Return the total nice pairs count modulo 10^9 + 7\n}\n\n\n```\n```Java []\n\n\nclass Solution {\n // Function to reverse a number\n int rev(int n) {\n int ans = 0;\n while (n != 0) {\n int temp = n % 10;\n ans = ans * 10 + temp;\n n = n / 10;\n }\n return ans;\n }\n\n public int countNicePairs(int[] nums) {\n Map<Integer, Integer> freq = new HashMap<>(); // To store the frequency of differences\n long nicePairs = 0; // To count the number of nice pairs\n\n // Calculate the differences between each number and its reverse and store their frequencies\n for (int num : nums) {\n int revNum = rev(num);\n int diff = num - revNum;\n freq.put(diff, freq.getOrDefault(diff, 0) + 1);\n }\n\n // Iterate through the frequency map to calculate the nice pairs count\n for (Map.Entry<Integer, Integer> entry : freq.entrySet()) {\n int count = entry.getValue();\n nicePairs += (long) count * (count - 1) / 2; // Calculating combinations of count C(2)\n }\n\n return (int) (nicePairs % 1000000007); // Return the total nice pairs count modulo 10^9 + 7\n }\n}\n\n\n```\n\n```python3 []\n\nclass Solution:\n # Function to reverse a number\n def rev(self, n):\n ans = 0\n while n != 0:\n temp = n % 10\n ans = ans * 10 + temp\n n = n // 10\n return ans\n\n def countNicePairs(self, nums):\n freq = {} # To store the frequency of differences\n nicePairs = 0 # To count the number of nice pairs\n\n # Calculate the differences between each number and its reverse and store their frequencies\n for num in nums:\n revNum = self.rev(num)\n diff = num - revNum\n freq[diff] = freq.get(diff, 0) + 1\n\n # Iterate through the frequency map to calculate the nice pairs count\n for count in freq.values():\n nicePairs += count * (count - 1) // 2 # Calculating combinations of count C(2)\n\n return nicePairs % 1000000007 # Return the total nice pairs count modulo 10^9 + 7\n\n\n```\n```javascript []\nclass Solution {\n // Function to reverse a number\n rev(n) {\n let ans = 0;\n while (n !== 0) {\n let temp = n % 10;\n ans = ans * 10 + temp;\n n = Math.floor(n / 10);\n }\n return ans;\n }\n\n countNicePairs(nums) {\n let freq = new Map(); // To store the frequency of differences\n let nicePairs = 0; // To count the number of nice pairs\n\n // Calculate the differences between each number and its reverse and store their frequencies\n for (let num of nums) {\n let revNum = this.rev(num);\n let diff = num - revNum;\n freq.set(diff, (freq.get(diff) || 0) + 1);\n }\n\n // Iterate through the frequency map to calculate the nice pairs count\n for (let count of freq.values()) {\n nicePairs += count * (count - 1) / 2; // Calculating combinations of count C(2)\n }\n\n return nicePairs % 1000000007; // Return the total nice pairs count modulo 10^9 + 7\n }\n}\n\n\n```\n\n---\n\n### ***Approach 2(Counting With Hash Maps)***\n\n\n# Complexity\n- *Time complexity:*\n $$O(n)$$\n \n\n- *Space complexity:*\n $$O(n)$$\n \n\n\n# Code\n```C++ []\n\nclass Solution {\npublic:\n int countNicePairs(vector<int>& nums) {\n vector<int> arr;\n for (int i = 0; i < nums.size(); i++) {\n arr.push_back(nums[i] - rev(nums[i]));\n }\n \n unordered_map<int, int> dic;\n int ans = 0;\n int MOD = 1e9 + 7;\n for (int num : arr) {\n ans = (ans + dic[num]) % MOD;\n dic[num]++;\n }\n \n return ans;\n }\n \n int rev(int num) {\n int result = 0;\n while (num > 0) {\n result = result * 10 + num % 10;\n num /= 10;\n }\n \n return result;\n }\n};\n\n```\n```C []\n\n\nint rev(int num) {\n int result = 0;\n while (num > 0) {\n result = result * 10 + num % 10;\n num /= 10;\n }\n return result;\n}\n\nint countNicePairs(int* nums, int numsSize) {\n int* arr = (int*)malloc(numsSize * sizeof(int));\n for (int i = 0; i < numsSize; i++) {\n arr[i] = nums[i] - rev(nums[i]);\n }\n\n int* dic = (int*)calloc(numsSize, sizeof(int));\n int ans = 0;\n int MOD = 1000000007;\n for (int i = 0; i < numsSize; i++) {\n ans = (ans + dic[arr[i]]) % MOD;\n dic[arr[i]]++;\n }\n\n free(arr);\n free(dic);\n return ans;\n}\n\n\n\n```\n```Java []\nclass Solution {\n public int countNicePairs(int[] nums) {\n int[] arr = new int[nums.length];\n for (int i = 0; i < nums.length; i++) {\n arr[i] = nums[i] - rev(nums[i]);\n }\n \n Map<Integer, Integer> dic = new HashMap();\n int ans = 0;\n int MOD = (int) 1e9 + 7;\n for (int num : arr) {\n ans = (ans + dic.getOrDefault(num, 0)) % MOD;\n dic.put(num, dic.getOrDefault(num, 0) + 1);\n }\n \n return ans;\n }\n \n public int rev(int num) {\n int result = 0;\n while (num > 0) {\n result = result * 10 + num % 10;\n num /= 10;\n }\n \n return result;\n }\n}\n\n```\n\n```python3 []\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n def rev(num):\n result = 0\n while num:\n result = result * 10 + num % 10\n num //= 10\n \n return result\n\n arr = []\n for i in range(len(nums)):\n arr.append(nums[i] - rev(nums[i]))\n \n dic = defaultdict(int)\n ans = 0\n MOD = 10 ** 9 + 7\n for num in arr:\n ans = (ans + dic[num]) % MOD\n dic[num] += 1\n \n return ans\n\n\n```\n```javascript []\nfunction rev(num) {\n let result = 0;\n while (num > 0) {\n result = result * 10 + num % 10;\n num = Math.floor(num / 10);\n }\n return result;\n}\n\nfunction countNicePairs(nums) {\n let arr = [];\n for (let i = 0; i < nums.length; i++) {\n arr.push(nums[i] - rev(nums[i]));\n }\n\n let dic = new Map();\n let ans = 0;\n const MOD = 1000000007;\n for (let num of arr) {\n ans = (ans + (dic.get(num) || 0)) % MOD;\n dic.set(num, (dic.get(num) || 0) + 1);\n }\n\n return ans;\n}\n\n\n```\n\n---\n\n\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n--- | 2 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
Python3 Solution | count-nice-pairs-in-an-array | 0 | 1 | \n```\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n mod=10**9+7\n ans=0\n count=collections.Counter()\n for num in nums:\n num-=int(str(num)[::-1])\n ans+=count[num]\n count[num]+=1\n return ans%mod \n``` | 2 | You are given an array `nums` that consists of non-negative integers. Let us define `rev(x)` as the reverse of the non-negative integer `x`. For example, `rev(123) = 321`, and `rev(120) = 21`. A pair of indices `(i, j)` is **nice** if it satisfies all of the following conditions:
* `0 <= i < j < nums.length`
* `nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])`
Return _the number of nice pairs of indices_. Since that number can be too large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[42,11,1,97\]
**Output:** 2
**Explanation:** The two pairs are:
- (0,3) : 42 + rev(97) = 42 + 79 = 121, 97 + rev(42) = 97 + 24 = 121.
- (1,2) : 11 + rev(1) = 11 + 1 = 12, 1 + rev(11) = 1 + 11 = 12.
**Example 2:**
**Input:** nums = \[13,10,35,24,76\]
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | Let dp[i] be "the maximum score to reach the end starting at index i". The answer for dp[i] is nums[i] + max{dp[i+j]} for 1 <= j <= k. That gives an O(n*k) solution. Instead of checking every j for every i, keep track of the largest dp[i] values in a heap and calculate dp[i] from right to left. When the largest value in the heap is out of bounds of the current index, remove it and keep checking. |
Python3 Solution | count-nice-pairs-in-an-array | 0 | 1 | \n```\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n mod=10**9+7\n ans=0\n count=collections.Counter()\n for num in nums:\n num-=int(str(num)[::-1])\n ans+=count[num]\n count[num]+=1\n return ans%mod \n``` | 2 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
Easy Hashmap + Combination approach | count-nice-pairs-in-an-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->rearrange the condition to nums[i] - rev(nums[i]) == nums[j] - rev(nums[j])\ndry run with an example \n* eg - nums = [13,10,35,24,76]\n* create a dictionary and store the value nums[i] - rev(nums[i]) as key in the dictionary and the value as it\'s frequency \n* the dictionary for the above case will be \n* d = {-18: 3, 9: 2}\n* we have 3 numbers giving us a result -18. so number of pairs giving us that combination would be (3C2 = 3) + (2C2 = 1) = 4 \n* so if there are k numbers giving a certain result then the number of nice pairs(2) for that result will be kC2.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n d , ans = {} , 0\n for i in range(len(nums)):\n rev_str = str(nums[i])[::-1]\n rev_num = int(rev_str)\n d[nums[i] - rev_num] = d.get(nums[i] - rev_num,0) + 1 \n for k in d.values():\n ans += math.comb(k,2)\n return (ans % (10**9 + 7))\n```\n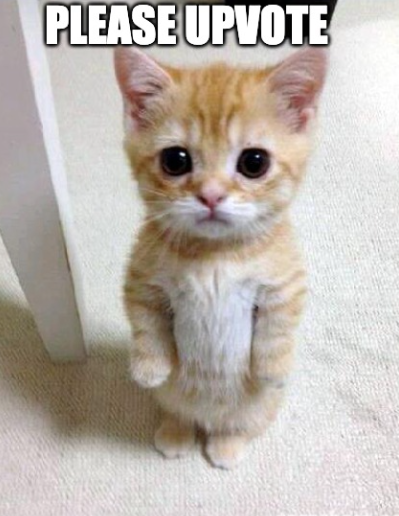\n | 2 | You are given an array `nums` that consists of non-negative integers. Let us define `rev(x)` as the reverse of the non-negative integer `x`. For example, `rev(123) = 321`, and `rev(120) = 21`. A pair of indices `(i, j)` is **nice** if it satisfies all of the following conditions:
* `0 <= i < j < nums.length`
* `nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])`
Return _the number of nice pairs of indices_. Since that number can be too large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[42,11,1,97\]
**Output:** 2
**Explanation:** The two pairs are:
- (0,3) : 42 + rev(97) = 42 + 79 = 121, 97 + rev(42) = 97 + 24 = 121.
- (1,2) : 11 + rev(1) = 11 + 1 = 12, 1 + rev(11) = 1 + 11 = 12.
**Example 2:**
**Input:** nums = \[13,10,35,24,76\]
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | Let dp[i] be "the maximum score to reach the end starting at index i". The answer for dp[i] is nums[i] + max{dp[i+j]} for 1 <= j <= k. That gives an O(n*k) solution. Instead of checking every j for every i, keep track of the largest dp[i] values in a heap and calculate dp[i] from right to left. When the largest value in the heap is out of bounds of the current index, remove it and keep checking. |
Easy Hashmap + Combination approach | count-nice-pairs-in-an-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->rearrange the condition to nums[i] - rev(nums[i]) == nums[j] - rev(nums[j])\ndry run with an example \n* eg - nums = [13,10,35,24,76]\n* create a dictionary and store the value nums[i] - rev(nums[i]) as key in the dictionary and the value as it\'s frequency \n* the dictionary for the above case will be \n* d = {-18: 3, 9: 2}\n* we have 3 numbers giving us a result -18. so number of pairs giving us that combination would be (3C2 = 3) + (2C2 = 1) = 4 \n* so if there are k numbers giving a certain result then the number of nice pairs(2) for that result will be kC2.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n d , ans = {} , 0\n for i in range(len(nums)):\n rev_str = str(nums[i])[::-1]\n rev_num = int(rev_str)\n d[nums[i] - rev_num] = d.get(nums[i] - rev_num,0) + 1 \n for k in d.values():\n ans += math.comb(k,2)\n return (ans % (10**9 + 7))\n```\n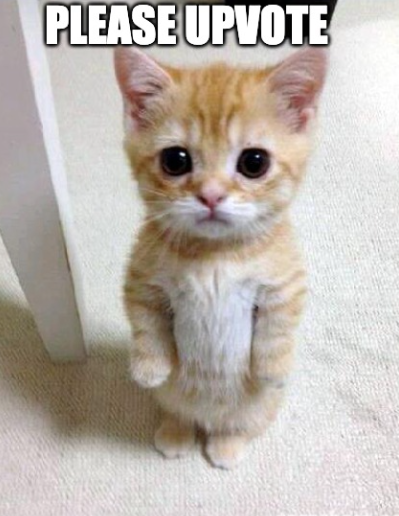\n | 2 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
1-liner | count-nice-pairs-in-an-array | 0 | 1 | # Code\n```python\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n return sum(i*(i-1)//2 for i in Counter([i-int(str(i)[::-1])for i in nums]).values()) % 1_000_000_007\n``` | 1 | You are given an array `nums` that consists of non-negative integers. Let us define `rev(x)` as the reverse of the non-negative integer `x`. For example, `rev(123) = 321`, and `rev(120) = 21`. A pair of indices `(i, j)` is **nice** if it satisfies all of the following conditions:
* `0 <= i < j < nums.length`
* `nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])`
Return _the number of nice pairs of indices_. Since that number can be too large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[42,11,1,97\]
**Output:** 2
**Explanation:** The two pairs are:
- (0,3) : 42 + rev(97) = 42 + 79 = 121, 97 + rev(42) = 97 + 24 = 121.
- (1,2) : 11 + rev(1) = 11 + 1 = 12, 1 + rev(11) = 1 + 11 = 12.
**Example 2:**
**Input:** nums = \[13,10,35,24,76\]
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | Let dp[i] be "the maximum score to reach the end starting at index i". The answer for dp[i] is nums[i] + max{dp[i+j]} for 1 <= j <= k. That gives an O(n*k) solution. Instead of checking every j for every i, keep track of the largest dp[i] values in a heap and calculate dp[i] from right to left. When the largest value in the heap is out of bounds of the current index, remove it and keep checking. |
1-liner | count-nice-pairs-in-an-array | 0 | 1 | # Code\n```python\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int:\n return sum(i*(i-1)//2 for i in Counter([i-int(str(i)[::-1])for i in nums]).values()) % 1_000_000_007\n``` | 1 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
LeetCode 1814 | Shift Terms & Combinatorics Approach | Python3 Solution | ✅99.19% | count-nice-pairs-in-an-array | 0 | 1 | # Intuition\n\n### Shift Terms\nLet\'s shift terms in this equation.\n$$n_i + rev(n_j) = n_j + rev(n_i) \\Longleftrightarrow n_i - rev(n_i) = n_j - rev(n_j)$$\n\nAssume difference be $$\\theta_i = n_i - rev(n_i)$$. As a result $$(i, j)$$ will be a nice pair if the difference of $$i$$ and $$j$$ are equal.\n$$(i, j)$$ is a nice pair $$\\Longleftrightarrow \\theta_i = \\theta_j \\Longleftrightarrow n_i - rev(n_i) = n_j - rev(n_j)$$\n\n### Combinatorics\nIf there are $$n$$ numbers that have the same difference, any of two of them will be a nice pair. Therefore, there will be $$\\binom{n}{2}$$ nice pair.\n$$\\binom{n}{2} = \\frac{n(n-1)}{2}$$\n\nWe can use a default list dictionary to collect the numbers that has the same difference.\n\n# Approach\n1. Initialize the default list dictionary.\n2. Add up the pair number of each difference.\n\n# Complexity\n- Time complexity: $$O(n)$$\n\n- Space complexity: $$O(n)$$\n\n# Code\n```python\nclass Solution:\n MODULO = 1_000_000_007\n\n def countNicePairs(self, nums: List[int]) -> int:\n # Initialize the default list dictionary.\n pairs = collections.defaultdict(list)\n for n in nums:\n difference = n - int(str(n)[::-1])\n pairs[difference].append(n)\n\n # Add up the pair number of each difference.\n answer = 0\n for numbers in pairs.values():\n answer += ((len(numbers) - 1) * len(numbers) // 2) % self.MODULO\n return answer % self.MODULO\n```\n\n# Result\n\n | 1 | You are given an array `nums` that consists of non-negative integers. Let us define `rev(x)` as the reverse of the non-negative integer `x`. For example, `rev(123) = 321`, and `rev(120) = 21`. A pair of indices `(i, j)` is **nice** if it satisfies all of the following conditions:
* `0 <= i < j < nums.length`
* `nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])`
Return _the number of nice pairs of indices_. Since that number can be too large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[42,11,1,97\]
**Output:** 2
**Explanation:** The two pairs are:
- (0,3) : 42 + rev(97) = 42 + 79 = 121, 97 + rev(42) = 97 + 24 = 121.
- (1,2) : 11 + rev(1) = 11 + 1 = 12, 1 + rev(11) = 1 + 11 = 12.
**Example 2:**
**Input:** nums = \[13,10,35,24,76\]
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | Let dp[i] be "the maximum score to reach the end starting at index i". The answer for dp[i] is nums[i] + max{dp[i+j]} for 1 <= j <= k. That gives an O(n*k) solution. Instead of checking every j for every i, keep track of the largest dp[i] values in a heap and calculate dp[i] from right to left. When the largest value in the heap is out of bounds of the current index, remove it and keep checking. |
LeetCode 1814 | Shift Terms & Combinatorics Approach | Python3 Solution | ✅99.19% | count-nice-pairs-in-an-array | 0 | 1 | # Intuition\n\n### Shift Terms\nLet\'s shift terms in this equation.\n$$n_i + rev(n_j) = n_j + rev(n_i) \\Longleftrightarrow n_i - rev(n_i) = n_j - rev(n_j)$$\n\nAssume difference be $$\\theta_i = n_i - rev(n_i)$$. As a result $$(i, j)$$ will be a nice pair if the difference of $$i$$ and $$j$$ are equal.\n$$(i, j)$$ is a nice pair $$\\Longleftrightarrow \\theta_i = \\theta_j \\Longleftrightarrow n_i - rev(n_i) = n_j - rev(n_j)$$\n\n### Combinatorics\nIf there are $$n$$ numbers that have the same difference, any of two of them will be a nice pair. Therefore, there will be $$\\binom{n}{2}$$ nice pair.\n$$\\binom{n}{2} = \\frac{n(n-1)}{2}$$\n\nWe can use a default list dictionary to collect the numbers that has the same difference.\n\n# Approach\n1. Initialize the default list dictionary.\n2. Add up the pair number of each difference.\n\n# Complexity\n- Time complexity: $$O(n)$$\n\n- Space complexity: $$O(n)$$\n\n# Code\n```python\nclass Solution:\n MODULO = 1_000_000_007\n\n def countNicePairs(self, nums: List[int]) -> int:\n # Initialize the default list dictionary.\n pairs = collections.defaultdict(list)\n for n in nums:\n difference = n - int(str(n)[::-1])\n pairs[difference].append(n)\n\n # Add up the pair number of each difference.\n answer = 0\n for numbers in pairs.values():\n answer += ((len(numbers) - 1) * len(numbers) // 2) % self.MODULO\n return answer % self.MODULO\n```\n\n# Result\n\n | 1 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
Python solution based on hint 1 || O(n) | count-nice-pairs-in-an-array | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nThe condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])).\n\n# Complexity\n- Time complexity:O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def rev(self, number):\n reversed_str = str(number)[::-1]\n reversed_number = int(reversed_str)\n return reversed_number\n\n def countNicePairs(self, nums: List[int]) -> int:\n dic = {}\n ans = 0\n for i in nums:\n rev_diff = i - self.rev(i)\n dic[rev_diff] = dic.get(rev_diff, 0) + 1\n\n for y in dic.values():\n if y > 1:\n ans += (y * (y - 1)) // 2\n ans = ans % int(1e9 + 7)\n\n return ans\n\n\n``` | 1 | You are given an array `nums` that consists of non-negative integers. Let us define `rev(x)` as the reverse of the non-negative integer `x`. For example, `rev(123) = 321`, and `rev(120) = 21`. A pair of indices `(i, j)` is **nice** if it satisfies all of the following conditions:
* `0 <= i < j < nums.length`
* `nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])`
Return _the number of nice pairs of indices_. Since that number can be too large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[42,11,1,97\]
**Output:** 2
**Explanation:** The two pairs are:
- (0,3) : 42 + rev(97) = 42 + 79 = 121, 97 + rev(42) = 97 + 24 = 121.
- (1,2) : 11 + rev(1) = 11 + 1 = 12, 1 + rev(11) = 1 + 11 = 12.
**Example 2:**
**Input:** nums = \[13,10,35,24,76\]
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | Let dp[i] be "the maximum score to reach the end starting at index i". The answer for dp[i] is nums[i] + max{dp[i+j]} for 1 <= j <= k. That gives an O(n*k) solution. Instead of checking every j for every i, keep track of the largest dp[i] values in a heap and calculate dp[i] from right to left. When the largest value in the heap is out of bounds of the current index, remove it and keep checking. |
Python solution based on hint 1 || O(n) | count-nice-pairs-in-an-array | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nThe condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])).\n\n# Complexity\n- Time complexity:O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def rev(self, number):\n reversed_str = str(number)[::-1]\n reversed_number = int(reversed_str)\n return reversed_number\n\n def countNicePairs(self, nums: List[int]) -> int:\n dic = {}\n ans = 0\n for i in nums:\n rev_diff = i - self.rev(i)\n dic[rev_diff] = dic.get(rev_diff, 0) + 1\n\n for y in dic.values():\n if y > 1:\n ans += (y * (y - 1)) // 2\n ans = ans % int(1e9 + 7)\n\n return ans\n\n\n``` | 1 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
0 Line Solution | count-nice-pairs-in-an-array | 0 | 1 | # Description\nThe entire code is ran inline for the Solution\'s Function leading to a 2 line solution (0 added lines).\n\n# Code\n```\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int: return sum([(val * (val-1)) // 2 for val in collections.Counter([num - int(str(num)[::-1]) for num in nums]).values()]) % 1000000007\n``` | 1 | You are given an array `nums` that consists of non-negative integers. Let us define `rev(x)` as the reverse of the non-negative integer `x`. For example, `rev(123) = 321`, and `rev(120) = 21`. A pair of indices `(i, j)` is **nice** if it satisfies all of the following conditions:
* `0 <= i < j < nums.length`
* `nums[i] + rev(nums[j]) == nums[j] + rev(nums[i])`
Return _the number of nice pairs of indices_. Since that number can be too large, return it **modulo** `109 + 7`.
**Example 1:**
**Input:** nums = \[42,11,1,97\]
**Output:** 2
**Explanation:** The two pairs are:
- (0,3) : 42 + rev(97) = 42 + 79 = 121, 97 + rev(42) = 97 + 24 = 121.
- (1,2) : 11 + rev(1) = 11 + 1 = 12, 1 + rev(11) = 1 + 11 = 12.
**Example 2:**
**Input:** nums = \[13,10,35,24,76\]
**Output:** 4
**Constraints:**
* `1 <= nums.length <= 105`
* `0 <= nums[i] <= 109` | Let dp[i] be "the maximum score to reach the end starting at index i". The answer for dp[i] is nums[i] + max{dp[i+j]} for 1 <= j <= k. That gives an O(n*k) solution. Instead of checking every j for every i, keep track of the largest dp[i] values in a heap and calculate dp[i] from right to left. When the largest value in the heap is out of bounds of the current index, remove it and keep checking. |
0 Line Solution | count-nice-pairs-in-an-array | 0 | 1 | # Description\nThe entire code is ran inline for the Solution\'s Function leading to a 2 line solution (0 added lines).\n\n# Code\n```\nclass Solution:\n def countNicePairs(self, nums: List[int]) -> int: return sum([(val * (val-1)) // 2 for val in collections.Counter([num - int(str(num)[::-1]) for num in nums]).values()]) % 1000000007\n``` | 1 | A **square triple** `(a,b,c)` is a triple where `a`, `b`, and `c` are **integers** and `a2 + b2 = c2`.
Given an integer `n`, return _the number of **square triples** such that_ `1 <= a, b, c <= n`.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation**: The square triples are (3,4,5) and (4,3,5).
**Example 2:**
**Input:** n = 10
**Output:** 4
**Explanation**: The square triples are (3,4,5), (4,3,5), (6,8,10), and (8,6,10).
**Constraints:**
* `1 <= n <= 250` | The condition can be rearranged to (nums[i] - rev(nums[i])) == (nums[j] - rev(nums[j])). Transform each nums[i] into (nums[i] - rev(nums[i])). Then, count the number of (i, j) pairs that have equal values. Keep a map storing the frequencies of values that you have seen so far. For each i, check if nums[i] is in the map. If it is, then add that count to the overall count. Then, increment the frequency of nums[i]. |
[Python3] No greedy, just DP | maximum-number-of-groups-getting-fresh-donuts | 0 | 1 | For each group size, we mod it with batchSize.\nThe greedy way is easy to think of, for those mod==0, make them immediately to single group.\nFor those pairs whose sum mod ==0, we also pair them to one single group.\nFor the rest, we do DP.\nBut the above 2 greedy steps do not change time complexity. Total time complexity is still decided by DP. Doing dp without greedy will still pass, and the code is much simpler.\n**dp(counter_tup,mod):**\n\t**counter_tup** stores the count of every mod, we make it touple because python "@cache" decorator supports tuple.\n\t**mod** is that left over by the previous group. If previous group has 0 left over, the a new batch can be started, the frist group in the new batch will be happy.\ndp(tup,mod) returns the maximum number of happy groups, with previous group left over \'mod\' people to fill.\n```\n def maxHappyGroups(self, batchSize: int, groups: List[int]) -> int:\n @cache\n def dp(counter_tup,mod):\n if sum(counter_tup)==0:\n return 0\n counter=list(counter_tup)\n startNewBatch = (mod==0)\n res = 0\n for i in range(len(counter)):\n if counter[i]!=0:\n counter[i]-=1\n mod2 = (mod-i)%batchSize\n nxt_res = dp(tuple(counter),mod2) + startNewBatch\n res = max(res, nxt_res)\n counter[i]+=1\n return res\n counter = [0]*batchSize\n for g in groups:\n counter[g%batchSize]+=1\n return dp(tuple(counter),0)\n``` | 2 | There is a donuts shop that bakes donuts in batches of `batchSize`. They have a rule where they must serve **all** of the donuts of a batch before serving any donuts of the next batch. You are given an integer `batchSize` and an integer array `groups`, where `groups[i]` denotes that there is a group of `groups[i]` customers that will visit the shop. Each customer will get exactly one donut.
When a group visits the shop, all customers of the group must be served before serving any of the following groups. A group will be happy if they all get fresh donuts. That is, the first customer of the group does not receive a donut that was left over from the previous group.
You can freely rearrange the ordering of the groups. Return _the **maximum** possible number of happy groups after rearranging the groups._
**Example 1:**
**Input:** batchSize = 3, groups = \[1,2,3,4,5,6\]
**Output:** 4
**Explanation:** You can arrange the groups as \[6,2,4,5,1,3\]. Then the 1st, 2nd, 4th, and 6th groups will be happy.
**Example 2:**
**Input:** batchSize = 4, groups = \[1,3,2,5,2,2,1,6\]
**Output:** 4
**Constraints:**
* `1 <= batchSize <= 9`
* `1 <= groups.length <= 30`
* `1 <= groups[i] <= 109` | All the queries are given in advance. Is there a way you can reorder the queries to avoid repeated computations? |
Modular Math and DFS of Ordering Paths | maximum-number-of-groups-getting-fresh-donuts | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFirst hint is that we have a modular math problem based on batch size. \nNext hint is that we can always satisfy those of batchsize if we put them in the right order.\n\nFirst job then is to remove these from contention and have those in our optimal count. \n\nNext job is to reduce all of the group sizes to batchSize modularity, limiting our range of values. \n\nFinally, we can get the frequencies of these modularly limited groups, and use that in our processing to find our answer. \n\nNotice from this we take the groups from 30 and lower them to 8 at most (batchSize is an option, but it would have frequency of 0). We then can have an average maximal frequency of each no more than 4 (8 * 4 is 32, more than our 30 groups originally). This limits our space dimension greatly. \n\nNow we can set up a depth first search progression to find the optimal ordering of sub groups to achieve maximal value where we consider the options recursively by removing a group at a certain time. This explores the tree of paths that reach our final maximal state. \n\nNow we turn to the implementation to describe algorithmic steps more fully. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nStart by getting the number of happy groups you should always have as thsoe that are evenly divisible by batchSize. This lets us sum up those that will always be satisfiable. \n\nNext we recast groups as the modular remainder of their group size modulo batch size if they are not an evenly divisible form of batchSize. This removes those groups in question we just counted. \n\nWe now have to set up a frequency of group sizes array of size batchSize \n\nLoop over your groups \n- if you have a frequency of the compliment of this group \n - reduce the frequency there by 1 and increment number of happy groups\n- otherwise, frequency of group sizes at g_i is incremented by 1 \n\nDoing so has now paired up all immediately complimentary groups for our problem in size O(groups) and turned our complexity to O(batchSize) for the dfs \n\nWe will cache our dfs results using an lru cache \nWe will conduct dfs by passing current group frequencies, remaining customers, and remaining number of groups to consider. While doing so \n- If the number of groups remaining is now 0, return 0 (nothing more here)\n- Otherwise, we need to calculate number that can be satisfied here starting at 0 \n- Enumerate our current group frequencies by index and frequency \n - if we have a frequency here \n - consider if we moved this group to be satisfied \n - This causes our frequency here to be one less in the next group frequencies, \n - our number of remaining customers to now be current plus groupSize modulo batch size \n - and our number of remaining groups to be one less \n - Conduct dfs with these fields, storing result as next option \n - Set number satisfied here to max of self and next option \n- At end of enumeration, return number satisfied plus int cast of if current remaining customers is strictly zero for the off by one group hinted at in the problem hint \n\n# Complexity\n- Time complexity : O(G^2)\n - O(G) to get initial value of number of happy groups and recast groups\n - O(g) to get frequency of group sizes correctly \n - O(sum(frequencies)^2) for dfs as we need to consider each frequency sum to zero for each frequency sum sub range \n - O(G) is at worst 30 \n - O(sum(frequencies)^2) is at worst 30^2 \n - Second one dominates, but is in terms of O(G) technically \n - So we have O(G^2) as Time Complexity\n\n- Space complexity : O(G) \n - Due to recursive implementation, our stack size is at worst O(G) \n - This is because though we do O(G^2) work, we never build a stack greater than O(G) for completion. This causes an accordian style grow and shrink of stack space as computations resolve. \n\n\n# Code\n```\nclass Solution:\n def maxHappyGroups(self, batchSize: int, groups: List[int]) -> int :\n # count your number of happy groups that you can perfectly satisfy \n number_of_happy_groups = sum([1 for g_i in groups if g_i%batchSize == 0])\n # remove them from groups and leave behind your non-perfect groups as modular format \n groups = [g_i%batchSize for g_i in groups if g_i%batchSize != 0]\n # set up modulor frequency of group sizes array \n frequency_of_group_sizes = [0] * batchSize\n\n # loop over unsatisfied currently and update \n for g_i in groups : \n # if we have a compliment in our frequency \n if frequency_of_group_sizes[batchSize - g_i] : \n # reduce the compliment and increment the number of happy groups \n frequency_of_group_sizes[batchSize - g_i] -= 1 \n number_of_happy_groups += 1 \n else : \n # otherwise, increment this frequency \n frequency_of_group_sizes[g_i] += 1 \n\n # the above basically accomplishes our sorting procedure for the best order, as this figures out the first best ordering\n # now we can progress over those remaining after that to determine our best ordering overall. \n # set up dfs progression using current group frequencies and current leftover customers \n @lru_cache(None)\n def dfs(current_group_frequencies, remaining_customers, num_groups_remaining) : \n if num_groups_remaining == 0 : \n return 0 \n # for our current group frequencies and remaining customers from prior operation \n number_satisfied = 0 \n # loop over those we could consider \n for g_s, g_f in enumerate(current_group_frequencies) : \n if g_f : \n # determine next group frequencies as current up to index + one less here + indices after \n next_group_frequencies = current_group_frequencies[:g_s] + (g_f-1,) + current_group_frequencies[g_s+1:]\n # consider next remaining customers as current plus this groupsize modulo batchsize \n # this is ordering shifting \n next_remaining_customers = (remaining_customers + g_s) % batchSize\n # number of groups remaining is now one less by line 34 \n # calculate next option \n next_option = dfs(next_group_frequencies, next_remaining_customers, num_groups_remaining - 1)\n # set maximally \n number_satisfied = max(number_satisfied, next_option)\n # how many did you satisfy? \n return number_satisfied + int(remaining_customers == 0)\n\n # number of happy groups is initial progression, dfs is additional ordering \n return number_of_happy_groups + dfs(tuple(frequency_of_group_sizes), 0, sum(frequency_of_group_sizes))\n\n``` | 0 | There is a donuts shop that bakes donuts in batches of `batchSize`. They have a rule where they must serve **all** of the donuts of a batch before serving any donuts of the next batch. You are given an integer `batchSize` and an integer array `groups`, where `groups[i]` denotes that there is a group of `groups[i]` customers that will visit the shop. Each customer will get exactly one donut.
When a group visits the shop, all customers of the group must be served before serving any of the following groups. A group will be happy if they all get fresh donuts. That is, the first customer of the group does not receive a donut that was left over from the previous group.
You can freely rearrange the ordering of the groups. Return _the **maximum** possible number of happy groups after rearranging the groups._
**Example 1:**
**Input:** batchSize = 3, groups = \[1,2,3,4,5,6\]
**Output:** 4
**Explanation:** You can arrange the groups as \[6,2,4,5,1,3\]. Then the 1st, 2nd, 4th, and 6th groups will be happy.
**Example 2:**
**Input:** batchSize = 4, groups = \[1,3,2,5,2,2,1,6\]
**Output:** 4
**Constraints:**
* `1 <= batchSize <= 9`
* `1 <= groups.length <= 30`
* `1 <= groups[i] <= 109` | All the queries are given in advance. Is there a way you can reorder the queries to avoid repeated computations? |
Python (Simple DP + Bitmasking) | maximum-number-of-groups-getting-fresh-donuts | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def maxHappyGroups(self, batchSize, groups):\n ans = sum([1 for i in groups if i%batchSize == 0])\n groups = sorted([i%batchSize for i in groups if i%batchSize != 0])\n\n @lru_cache(None)\n def dfs(total,groups):\n if not groups: return 0\n\n max_val = 0\n\n for i in range(len(groups)):\n max_val = max(max_val,dfs(total + groups[i],groups[:i] + groups[i+1:]))\n\n return max_val + (total%batchSize == 0)\n\n return ans + dfs(0,tuple(groups))\n\n \n\n\n\n\n\n``` | 0 | There is a donuts shop that bakes donuts in batches of `batchSize`. They have a rule where they must serve **all** of the donuts of a batch before serving any donuts of the next batch. You are given an integer `batchSize` and an integer array `groups`, where `groups[i]` denotes that there is a group of `groups[i]` customers that will visit the shop. Each customer will get exactly one donut.
When a group visits the shop, all customers of the group must be served before serving any of the following groups. A group will be happy if they all get fresh donuts. That is, the first customer of the group does not receive a donut that was left over from the previous group.
You can freely rearrange the ordering of the groups. Return _the **maximum** possible number of happy groups after rearranging the groups._
**Example 1:**
**Input:** batchSize = 3, groups = \[1,2,3,4,5,6\]
**Output:** 4
**Explanation:** You can arrange the groups as \[6,2,4,5,1,3\]. Then the 1st, 2nd, 4th, and 6th groups will be happy.
**Example 2:**
**Input:** batchSize = 4, groups = \[1,3,2,5,2,2,1,6\]
**Output:** 4
**Constraints:**
* `1 <= batchSize <= 9`
* `1 <= groups.length <= 30`
* `1 <= groups[i] <= 109` | All the queries are given in advance. Is there a way you can reorder the queries to avoid repeated computations? |
Python One Line Solution Using Split And Join Method . | truncate-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIntution is to find trunkate the Sentence given and return it.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe used the split method inside the join method for the single line easy solution .\n\n# Code\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n return \' \'.join(s.split(\' \')[:k])\n``` | 1 | A **sentence** is a list of words that are separated by a single space with no leading or trailing spaces. Each of the words consists of **only** uppercase and lowercase English letters (no punctuation).
* For example, `"Hello World "`, `"HELLO "`, and `"hello world hello world "` are all sentences.
You are given a sentence `s` and an integer `k`. You want to **truncate** `s` such that it contains only the **first** `k` words. Return `s`_ after **truncating** it._
**Example 1:**
**Input:** s = "Hello how are you Contestant ", k = 4
**Output:** "Hello how are you "
**Explanation:**
The words in s are \[ "Hello ", "how " "are ", "you ", "Contestant "\].
The first 4 words are \[ "Hello ", "how ", "are ", "you "\].
Hence, you should return "Hello how are you ".
**Example 2:**
**Input:** s = "What is the solution to this problem ", k = 4
**Output:** "What is the solution "
**Explanation:**
The words in s are \[ "What ", "is " "the ", "solution ", "to ", "this ", "problem "\].
The first 4 words are \[ "What ", "is ", "the ", "solution "\].
Hence, you should return "What is the solution ".
**Example 3:**
**Input:** s = "chopper is not a tanuki ", k = 5
**Output:** "chopper is not a tanuki "
**Constraints:**
* `1 <= s.length <= 500`
* `k` is in the range `[1, the number of words in s]`.
* `s` consist of only lowercase and uppercase English letters and spaces.
* The words in `s` are separated by a single space.
* There are no leading or trailing spaces. | Starting from the root, traverse the left and the right subtrees, checking if one of the nodes exist there. If one of the subtrees doesn't contain any given node, the LCA can be the node returned from the other subtree If both subtrees contain nodes, the LCA node is the current node. |
Python One Line Solution Using Split And Join Method . | truncate-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIntution is to find trunkate the Sentence given and return it.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe used the split method inside the join method for the single line easy solution .\n\n# Code\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n return \' \'.join(s.split(\' \')[:k])\n``` | 1 | There are `n` people standing in a queue, and they numbered from `0` to `n - 1` in **left to right** order. You are given an array `heights` of **distinct** integers where `heights[i]` represents the height of the `ith` person.
A person can **see** another person to their right in the queue if everybody in between is **shorter** than both of them. More formally, the `ith` person can see the `jth` person if `i < j` and `min(heights[i], heights[j]) > max(heights[i+1], heights[i+2], ..., heights[j-1])`.
Return _an array_ `answer` _of length_ `n` _where_ `answer[i]` _is the **number of people** the_ `ith` _person can **see** to their right in the queue_.
**Example 1:**
**Input:** heights = \[10,6,8,5,11,9\]
**Output:** \[3,1,2,1,1,0\]
**Explanation:**
Person 0 can see person 1, 2, and 4.
Person 1 can see person 2.
Person 2 can see person 3 and 4.
Person 3 can see person 4.
Person 4 can see person 5.
Person 5 can see no one since nobody is to the right of them.
**Example 2:**
**Input:** heights = \[5,1,2,3,10\]
**Output:** \[4,1,1,1,0\]
**Constraints:**
* `n == heights.length`
* `1 <= n <= 105`
* `1 <= heights[i] <= 105`
* All the values of `heights` are **unique**. | It's easier to solve this problem on an array of strings so parse the string to an array of words After return the first k words as a sentence |
Simple 2 line python code 🔥 | truncate-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n s = s.split(" ")\n S = s[0:k]\n return " ".join([str(i) for i in S]) \n\n``` \neasy code in python:\n | 1 | A **sentence** is a list of words that are separated by a single space with no leading or trailing spaces. Each of the words consists of **only** uppercase and lowercase English letters (no punctuation).
* For example, `"Hello World "`, `"HELLO "`, and `"hello world hello world "` are all sentences.
You are given a sentence `s` and an integer `k`. You want to **truncate** `s` such that it contains only the **first** `k` words. Return `s`_ after **truncating** it._
**Example 1:**
**Input:** s = "Hello how are you Contestant ", k = 4
**Output:** "Hello how are you "
**Explanation:**
The words in s are \[ "Hello ", "how " "are ", "you ", "Contestant "\].
The first 4 words are \[ "Hello ", "how ", "are ", "you "\].
Hence, you should return "Hello how are you ".
**Example 2:**
**Input:** s = "What is the solution to this problem ", k = 4
**Output:** "What is the solution "
**Explanation:**
The words in s are \[ "What ", "is " "the ", "solution ", "to ", "this ", "problem "\].
The first 4 words are \[ "What ", "is ", "the ", "solution "\].
Hence, you should return "What is the solution ".
**Example 3:**
**Input:** s = "chopper is not a tanuki ", k = 5
**Output:** "chopper is not a tanuki "
**Constraints:**
* `1 <= s.length <= 500`
* `k` is in the range `[1, the number of words in s]`.
* `s` consist of only lowercase and uppercase English letters and spaces.
* The words in `s` are separated by a single space.
* There are no leading or trailing spaces. | Starting from the root, traverse the left and the right subtrees, checking if one of the nodes exist there. If one of the subtrees doesn't contain any given node, the LCA can be the node returned from the other subtree If both subtrees contain nodes, the LCA node is the current node. |
Simple 2 line python code 🔥 | truncate-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n s = s.split(" ")\n S = s[0:k]\n return " ".join([str(i) for i in S]) \n\n``` \neasy code in python:\n | 1 | There are `n` people standing in a queue, and they numbered from `0` to `n - 1` in **left to right** order. You are given an array `heights` of **distinct** integers where `heights[i]` represents the height of the `ith` person.
A person can **see** another person to their right in the queue if everybody in between is **shorter** than both of them. More formally, the `ith` person can see the `jth` person if `i < j` and `min(heights[i], heights[j]) > max(heights[i+1], heights[i+2], ..., heights[j-1])`.
Return _an array_ `answer` _of length_ `n` _where_ `answer[i]` _is the **number of people** the_ `ith` _person can **see** to their right in the queue_.
**Example 1:**
**Input:** heights = \[10,6,8,5,11,9\]
**Output:** \[3,1,2,1,1,0\]
**Explanation:**
Person 0 can see person 1, 2, and 4.
Person 1 can see person 2.
Person 2 can see person 3 and 4.
Person 3 can see person 4.
Person 4 can see person 5.
Person 5 can see no one since nobody is to the right of them.
**Example 2:**
**Input:** heights = \[5,1,2,3,10\]
**Output:** \[4,1,1,1,0\]
**Constraints:**
* `n == heights.length`
* `1 <= n <= 105`
* `1 <= heights[i] <= 105`
* All the values of `heights` are **unique**. | It's easier to solve this problem on an array of strings so parse the string to an array of words After return the first k words as a sentence |
Python Sentence Truncation | 29ms Runtime | truncate-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe can parse the string to a list of words and then take the first `k` words as a sentence.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Parse the string to a list of words using space as a separator.\n > `str.split(sep)` \u2192 returns a list of the words in the string, using `sep` as the delimiter string\n\n2. Take the first `k` words from the list of words.\n > `list[start:stop]` \u2192 extracts a slice of elements from a list, starting from the index "**start**" and up to, but not including, the index "**stop**"\n\n3. Join these selected words back into a sentence using space as a separator.\n > `sep.join(list)` \u2192 combines the elements of a list into a single string, with each element separated by the string `sep`\n\n4. Return the truncated sentence.\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n`n` is the length of the input sentence `s`. This is because splitting the sentence and joining the words both have linear time complexity with respect to the length of the string.\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n`n` is the length of the input sentence `s`. This is because creating a list `words` to store the words after splitting the input string, and the size of this list is proportional to the length of the input string.\n\n# Code\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n words = s.split(" ")\n return " ".join(words[:k])\n``` | 3 | A **sentence** is a list of words that are separated by a single space with no leading or trailing spaces. Each of the words consists of **only** uppercase and lowercase English letters (no punctuation).
* For example, `"Hello World "`, `"HELLO "`, and `"hello world hello world "` are all sentences.
You are given a sentence `s` and an integer `k`. You want to **truncate** `s` such that it contains only the **first** `k` words. Return `s`_ after **truncating** it._
**Example 1:**
**Input:** s = "Hello how are you Contestant ", k = 4
**Output:** "Hello how are you "
**Explanation:**
The words in s are \[ "Hello ", "how " "are ", "you ", "Contestant "\].
The first 4 words are \[ "Hello ", "how ", "are ", "you "\].
Hence, you should return "Hello how are you ".
**Example 2:**
**Input:** s = "What is the solution to this problem ", k = 4
**Output:** "What is the solution "
**Explanation:**
The words in s are \[ "What ", "is " "the ", "solution ", "to ", "this ", "problem "\].
The first 4 words are \[ "What ", "is ", "the ", "solution "\].
Hence, you should return "What is the solution ".
**Example 3:**
**Input:** s = "chopper is not a tanuki ", k = 5
**Output:** "chopper is not a tanuki "
**Constraints:**
* `1 <= s.length <= 500`
* `k` is in the range `[1, the number of words in s]`.
* `s` consist of only lowercase and uppercase English letters and spaces.
* The words in `s` are separated by a single space.
* There are no leading or trailing spaces. | Starting from the root, traverse the left and the right subtrees, checking if one of the nodes exist there. If one of the subtrees doesn't contain any given node, the LCA can be the node returned from the other subtree If both subtrees contain nodes, the LCA node is the current node. |
Python Sentence Truncation | 29ms Runtime | truncate-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe can parse the string to a list of words and then take the first `k` words as a sentence.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n1. Parse the string to a list of words using space as a separator.\n > `str.split(sep)` \u2192 returns a list of the words in the string, using `sep` as the delimiter string\n\n2. Take the first `k` words from the list of words.\n > `list[start:stop]` \u2192 extracts a slice of elements from a list, starting from the index "**start**" and up to, but not including, the index "**stop**"\n\n3. Join these selected words back into a sentence using space as a separator.\n > `sep.join(list)` \u2192 combines the elements of a list into a single string, with each element separated by the string `sep`\n\n4. Return the truncated sentence.\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n`n` is the length of the input sentence `s`. This is because splitting the sentence and joining the words both have linear time complexity with respect to the length of the string.\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n`n` is the length of the input sentence `s`. This is because creating a list `words` to store the words after splitting the input string, and the size of this list is proportional to the length of the input string.\n\n# Code\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n words = s.split(" ")\n return " ".join(words[:k])\n``` | 3 | There are `n` people standing in a queue, and they numbered from `0` to `n - 1` in **left to right** order. You are given an array `heights` of **distinct** integers where `heights[i]` represents the height of the `ith` person.
A person can **see** another person to their right in the queue if everybody in between is **shorter** than both of them. More formally, the `ith` person can see the `jth` person if `i < j` and `min(heights[i], heights[j]) > max(heights[i+1], heights[i+2], ..., heights[j-1])`.
Return _an array_ `answer` _of length_ `n` _where_ `answer[i]` _is the **number of people** the_ `ith` _person can **see** to their right in the queue_.
**Example 1:**
**Input:** heights = \[10,6,8,5,11,9\]
**Output:** \[3,1,2,1,1,0\]
**Explanation:**
Person 0 can see person 1, 2, and 4.
Person 1 can see person 2.
Person 2 can see person 3 and 4.
Person 3 can see person 4.
Person 4 can see person 5.
Person 5 can see no one since nobody is to the right of them.
**Example 2:**
**Input:** heights = \[5,1,2,3,10\]
**Output:** \[4,1,1,1,0\]
**Constraints:**
* `n == heights.length`
* `1 <= n <= 105`
* `1 <= heights[i] <= 105`
* All the values of `heights` are **unique**. | It's easier to solve this problem on an array of strings so parse the string to an array of words After return the first k words as a sentence |
Python using split() and join() | truncate-sentence | 0 | 1 | # Intuition\nI figured it would be easy to convert the string into a list to get the length of the list. Once you have the length you can convert it back to a string and return it.\n\n# Approach\nFirst thing I did was take the string and convert it to a list using .split(" ") with the white space being the separator. I also made a empty list that I would use in a for loop with k so I can get the appropriate length. Once that was complete I just convert the list into a string using .join() and .map() , then return the final product.\n\n# Complexity\n- Time complexity:\nO(n)\n\n\n# Code\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n my_list = s.split(" ")\n return_list =[]\n\n for index in range(0, k):\n return_list.append(my_list[index])\n\n final = \' \'.join(map(str, return_list))\n \n return final\n``` | 0 | A **sentence** is a list of words that are separated by a single space with no leading or trailing spaces. Each of the words consists of **only** uppercase and lowercase English letters (no punctuation).
* For example, `"Hello World "`, `"HELLO "`, and `"hello world hello world "` are all sentences.
You are given a sentence `s` and an integer `k`. You want to **truncate** `s` such that it contains only the **first** `k` words. Return `s`_ after **truncating** it._
**Example 1:**
**Input:** s = "Hello how are you Contestant ", k = 4
**Output:** "Hello how are you "
**Explanation:**
The words in s are \[ "Hello ", "how " "are ", "you ", "Contestant "\].
The first 4 words are \[ "Hello ", "how ", "are ", "you "\].
Hence, you should return "Hello how are you ".
**Example 2:**
**Input:** s = "What is the solution to this problem ", k = 4
**Output:** "What is the solution "
**Explanation:**
The words in s are \[ "What ", "is " "the ", "solution ", "to ", "this ", "problem "\].
The first 4 words are \[ "What ", "is ", "the ", "solution "\].
Hence, you should return "What is the solution ".
**Example 3:**
**Input:** s = "chopper is not a tanuki ", k = 5
**Output:** "chopper is not a tanuki "
**Constraints:**
* `1 <= s.length <= 500`
* `k` is in the range `[1, the number of words in s]`.
* `s` consist of only lowercase and uppercase English letters and spaces.
* The words in `s` are separated by a single space.
* There are no leading or trailing spaces. | Starting from the root, traverse the left and the right subtrees, checking if one of the nodes exist there. If one of the subtrees doesn't contain any given node, the LCA can be the node returned from the other subtree If both subtrees contain nodes, the LCA node is the current node. |
Python using split() and join() | truncate-sentence | 0 | 1 | # Intuition\nI figured it would be easy to convert the string into a list to get the length of the list. Once you have the length you can convert it back to a string and return it.\n\n# Approach\nFirst thing I did was take the string and convert it to a list using .split(" ") with the white space being the separator. I also made a empty list that I would use in a for loop with k so I can get the appropriate length. Once that was complete I just convert the list into a string using .join() and .map() , then return the final product.\n\n# Complexity\n- Time complexity:\nO(n)\n\n\n# Code\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n my_list = s.split(" ")\n return_list =[]\n\n for index in range(0, k):\n return_list.append(my_list[index])\n\n final = \' \'.join(map(str, return_list))\n \n return final\n``` | 0 | There are `n` people standing in a queue, and they numbered from `0` to `n - 1` in **left to right** order. You are given an array `heights` of **distinct** integers where `heights[i]` represents the height of the `ith` person.
A person can **see** another person to their right in the queue if everybody in between is **shorter** than both of them. More formally, the `ith` person can see the `jth` person if `i < j` and `min(heights[i], heights[j]) > max(heights[i+1], heights[i+2], ..., heights[j-1])`.
Return _an array_ `answer` _of length_ `n` _where_ `answer[i]` _is the **number of people** the_ `ith` _person can **see** to their right in the queue_.
**Example 1:**
**Input:** heights = \[10,6,8,5,11,9\]
**Output:** \[3,1,2,1,1,0\]
**Explanation:**
Person 0 can see person 1, 2, and 4.
Person 1 can see person 2.
Person 2 can see person 3 and 4.
Person 3 can see person 4.
Person 4 can see person 5.
Person 5 can see no one since nobody is to the right of them.
**Example 2:**
**Input:** heights = \[5,1,2,3,10\]
**Output:** \[4,1,1,1,0\]
**Constraints:**
* `n == heights.length`
* `1 <= n <= 105`
* `1 <= heights[i] <= 105`
* All the values of `heights` are **unique**. | It's easier to solve this problem on an array of strings so parse the string to an array of words After return the first k words as a sentence |
Shortest 1 line solution | truncate-sentence | 0 | 1 | \n# Code\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n return " ".join(s.split(" ")[:k])\n```\n# Line by Line\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n words_list = s.split(" ")\n truncated_list = words_list[:k]\n result = " ".join(truncated_list)\n return result\n```\nplease upvote :D | 3 | A **sentence** is a list of words that are separated by a single space with no leading or trailing spaces. Each of the words consists of **only** uppercase and lowercase English letters (no punctuation).
* For example, `"Hello World "`, `"HELLO "`, and `"hello world hello world "` are all sentences.
You are given a sentence `s` and an integer `k`. You want to **truncate** `s` such that it contains only the **first** `k` words. Return `s`_ after **truncating** it._
**Example 1:**
**Input:** s = "Hello how are you Contestant ", k = 4
**Output:** "Hello how are you "
**Explanation:**
The words in s are \[ "Hello ", "how " "are ", "you ", "Contestant "\].
The first 4 words are \[ "Hello ", "how ", "are ", "you "\].
Hence, you should return "Hello how are you ".
**Example 2:**
**Input:** s = "What is the solution to this problem ", k = 4
**Output:** "What is the solution "
**Explanation:**
The words in s are \[ "What ", "is " "the ", "solution ", "to ", "this ", "problem "\].
The first 4 words are \[ "What ", "is ", "the ", "solution "\].
Hence, you should return "What is the solution ".
**Example 3:**
**Input:** s = "chopper is not a tanuki ", k = 5
**Output:** "chopper is not a tanuki "
**Constraints:**
* `1 <= s.length <= 500`
* `k` is in the range `[1, the number of words in s]`.
* `s` consist of only lowercase and uppercase English letters and spaces.
* The words in `s` are separated by a single space.
* There are no leading or trailing spaces. | Starting from the root, traverse the left and the right subtrees, checking if one of the nodes exist there. If one of the subtrees doesn't contain any given node, the LCA can be the node returned from the other subtree If both subtrees contain nodes, the LCA node is the current node. |
Shortest 1 line solution | truncate-sentence | 0 | 1 | \n# Code\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n return " ".join(s.split(" ")[:k])\n```\n# Line by Line\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n words_list = s.split(" ")\n truncated_list = words_list[:k]\n result = " ".join(truncated_list)\n return result\n```\nplease upvote :D | 3 | There are `n` people standing in a queue, and they numbered from `0` to `n - 1` in **left to right** order. You are given an array `heights` of **distinct** integers where `heights[i]` represents the height of the `ith` person.
A person can **see** another person to their right in the queue if everybody in between is **shorter** than both of them. More formally, the `ith` person can see the `jth` person if `i < j` and `min(heights[i], heights[j]) > max(heights[i+1], heights[i+2], ..., heights[j-1])`.
Return _an array_ `answer` _of length_ `n` _where_ `answer[i]` _is the **number of people** the_ `ith` _person can **see** to their right in the queue_.
**Example 1:**
**Input:** heights = \[10,6,8,5,11,9\]
**Output:** \[3,1,2,1,1,0\]
**Explanation:**
Person 0 can see person 1, 2, and 4.
Person 1 can see person 2.
Person 2 can see person 3 and 4.
Person 3 can see person 4.
Person 4 can see person 5.
Person 5 can see no one since nobody is to the right of them.
**Example 2:**
**Input:** heights = \[5,1,2,3,10\]
**Output:** \[4,1,1,1,0\]
**Constraints:**
* `n == heights.length`
* `1 <= n <= 105`
* `1 <= heights[i] <= 105`
* All the values of `heights` are **unique**. | It's easier to solve this problem on an array of strings so parse the string to an array of words After return the first k words as a sentence |
simple python 100% effective solution | truncate-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n res = []\n arr = s.split( )\n for i in range(k):\n res.append(arr[i])\n return " ".join(res)\n \n``` | 2 | A **sentence** is a list of words that are separated by a single space with no leading or trailing spaces. Each of the words consists of **only** uppercase and lowercase English letters (no punctuation).
* For example, `"Hello World "`, `"HELLO "`, and `"hello world hello world "` are all sentences.
You are given a sentence `s` and an integer `k`. You want to **truncate** `s` such that it contains only the **first** `k` words. Return `s`_ after **truncating** it._
**Example 1:**
**Input:** s = "Hello how are you Contestant ", k = 4
**Output:** "Hello how are you "
**Explanation:**
The words in s are \[ "Hello ", "how " "are ", "you ", "Contestant "\].
The first 4 words are \[ "Hello ", "how ", "are ", "you "\].
Hence, you should return "Hello how are you ".
**Example 2:**
**Input:** s = "What is the solution to this problem ", k = 4
**Output:** "What is the solution "
**Explanation:**
The words in s are \[ "What ", "is " "the ", "solution ", "to ", "this ", "problem "\].
The first 4 words are \[ "What ", "is ", "the ", "solution "\].
Hence, you should return "What is the solution ".
**Example 3:**
**Input:** s = "chopper is not a tanuki ", k = 5
**Output:** "chopper is not a tanuki "
**Constraints:**
* `1 <= s.length <= 500`
* `k` is in the range `[1, the number of words in s]`.
* `s` consist of only lowercase and uppercase English letters and spaces.
* The words in `s` are separated by a single space.
* There are no leading or trailing spaces. | Starting from the root, traverse the left and the right subtrees, checking if one of the nodes exist there. If one of the subtrees doesn't contain any given node, the LCA can be the node returned from the other subtree If both subtrees contain nodes, the LCA node is the current node. |
simple python 100% effective solution | truncate-sentence | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n res = []\n arr = s.split( )\n for i in range(k):\n res.append(arr[i])\n return " ".join(res)\n \n``` | 2 | There are `n` people standing in a queue, and they numbered from `0` to `n - 1` in **left to right** order. You are given an array `heights` of **distinct** integers where `heights[i]` represents the height of the `ith` person.
A person can **see** another person to their right in the queue if everybody in between is **shorter** than both of them. More formally, the `ith` person can see the `jth` person if `i < j` and `min(heights[i], heights[j]) > max(heights[i+1], heights[i+2], ..., heights[j-1])`.
Return _an array_ `answer` _of length_ `n` _where_ `answer[i]` _is the **number of people** the_ `ith` _person can **see** to their right in the queue_.
**Example 1:**
**Input:** heights = \[10,6,8,5,11,9\]
**Output:** \[3,1,2,1,1,0\]
**Explanation:**
Person 0 can see person 1, 2, and 4.
Person 1 can see person 2.
Person 2 can see person 3 and 4.
Person 3 can see person 4.
Person 4 can see person 5.
Person 5 can see no one since nobody is to the right of them.
**Example 2:**
**Input:** heights = \[5,1,2,3,10\]
**Output:** \[4,1,1,1,0\]
**Constraints:**
* `n == heights.length`
* `1 <= n <= 105`
* `1 <= heights[i] <= 105`
* All the values of `heights` are **unique**. | It's easier to solve this problem on an array of strings so parse the string to an array of words After return the first k words as a sentence |
3 different approach in Python | truncate-sentence | 0 | 1 | \n# 1 liner\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n return \' \'.join(s.split()[:k])\n```\n\n# Count space and do slicing\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n count=0\n for i,c in enumerate(s):\n if c==\' \':\n count+=1\n if count==k:\n return s[:i]\n return s\n```\n\n# Split the words\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n ans=""\n words=s.split()\n count=0\n for word in words:\n if count==k:\n break\n count+=1\n if count<k:\n ans+=word+" "\n else:\n ans+=word\n return ans\n``` | 9 | A **sentence** is a list of words that are separated by a single space with no leading or trailing spaces. Each of the words consists of **only** uppercase and lowercase English letters (no punctuation).
* For example, `"Hello World "`, `"HELLO "`, and `"hello world hello world "` are all sentences.
You are given a sentence `s` and an integer `k`. You want to **truncate** `s` such that it contains only the **first** `k` words. Return `s`_ after **truncating** it._
**Example 1:**
**Input:** s = "Hello how are you Contestant ", k = 4
**Output:** "Hello how are you "
**Explanation:**
The words in s are \[ "Hello ", "how " "are ", "you ", "Contestant "\].
The first 4 words are \[ "Hello ", "how ", "are ", "you "\].
Hence, you should return "Hello how are you ".
**Example 2:**
**Input:** s = "What is the solution to this problem ", k = 4
**Output:** "What is the solution "
**Explanation:**
The words in s are \[ "What ", "is " "the ", "solution ", "to ", "this ", "problem "\].
The first 4 words are \[ "What ", "is ", "the ", "solution "\].
Hence, you should return "What is the solution ".
**Example 3:**
**Input:** s = "chopper is not a tanuki ", k = 5
**Output:** "chopper is not a tanuki "
**Constraints:**
* `1 <= s.length <= 500`
* `k` is in the range `[1, the number of words in s]`.
* `s` consist of only lowercase and uppercase English letters and spaces.
* The words in `s` are separated by a single space.
* There are no leading or trailing spaces. | Starting from the root, traverse the left and the right subtrees, checking if one of the nodes exist there. If one of the subtrees doesn't contain any given node, the LCA can be the node returned from the other subtree If both subtrees contain nodes, the LCA node is the current node. |
3 different approach in Python | truncate-sentence | 0 | 1 | \n# 1 liner\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n return \' \'.join(s.split()[:k])\n```\n\n# Count space and do slicing\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n count=0\n for i,c in enumerate(s):\n if c==\' \':\n count+=1\n if count==k:\n return s[:i]\n return s\n```\n\n# Split the words\n```\nclass Solution:\n def truncateSentence(self, s: str, k: int) -> str:\n ans=""\n words=s.split()\n count=0\n for word in words:\n if count==k:\n break\n count+=1\n if count<k:\n ans+=word+" "\n else:\n ans+=word\n return ans\n``` | 9 | There are `n` people standing in a queue, and they numbered from `0` to `n - 1` in **left to right** order. You are given an array `heights` of **distinct** integers where `heights[i]` represents the height of the `ith` person.
A person can **see** another person to their right in the queue if everybody in between is **shorter** than both of them. More formally, the `ith` person can see the `jth` person if `i < j` and `min(heights[i], heights[j]) > max(heights[i+1], heights[i+2], ..., heights[j-1])`.
Return _an array_ `answer` _of length_ `n` _where_ `answer[i]` _is the **number of people** the_ `ith` _person can **see** to their right in the queue_.
**Example 1:**
**Input:** heights = \[10,6,8,5,11,9\]
**Output:** \[3,1,2,1,1,0\]
**Explanation:**
Person 0 can see person 1, 2, and 4.
Person 1 can see person 2.
Person 2 can see person 3 and 4.
Person 3 can see person 4.
Person 4 can see person 5.
Person 5 can see no one since nobody is to the right of them.
**Example 2:**
**Input:** heights = \[5,1,2,3,10\]
**Output:** \[4,1,1,1,0\]
**Constraints:**
* `n == heights.length`
* `1 <= n <= 105`
* `1 <= heights[i] <= 105`
* All the values of `heights` are **unique**. | It's easier to solve this problem on an array of strings so parse the string to an array of words After return the first k words as a sentence |
[Python 3] Using set and dictionary || beats 98% || 826ms 🥷🏼 | finding-the-users-active-minutes | 0 | 1 | ```python3 []\nclass Solution:\n def findingUsersActiveMinutes(self, logs: List[List[int]], k: int) -> List[int]:\n res, seen, d = [0] * k, set(), defaultdict(int)\n\n for user, time in logs:\n if (user, time) in seen: continue\n d[user] += 1\n seen.add((user, time))\n \n for v in d.values():\n if v <= k:\n res[v-1] += 1\n \n return res\n```\n\n | 2 | You are given the logs for users' actions on LeetCode, and an integer `k`. The logs are represented by a 2D integer array `logs` where each `logs[i] = [IDi, timei]` indicates that the user with `IDi` performed an action at the minute `timei`.
**Multiple users** can perform actions simultaneously, and a single user can perform **multiple actions** in the same minute.
The **user active minutes (UAM)** for a given user is defined as the **number of unique minutes** in which the user performed an action on LeetCode. A minute can only be counted once, even if multiple actions occur during it.
You are to calculate a **1-indexed** array `answer` of size `k` such that, for each `j` (`1 <= j <= k`), `answer[j]` is the **number of users** whose **UAM** equals `j`.
Return _the array_ `answer` _as described above_.
**Example 1:**
**Input:** logs = \[\[0,5\],\[1,2\],\[0,2\],\[0,5\],\[1,3\]\], k = 5
**Output:** \[0,2,0,0,0\]
**Explanation:**
The user with ID=0 performed actions at minutes 5, 2, and 5 again. Hence, they have a UAM of 2 (minute 5 is only counted once).
The user with ID=1 performed actions at minutes 2 and 3. Hence, they have a UAM of 2.
Since both users have a UAM of 2, answer\[2\] is 2, and the remaining answer\[j\] values are 0.
**Example 2:**
**Input:** logs = \[\[1,1\],\[2,2\],\[2,3\]\], k = 4
**Output:** \[1,1,0,0\]
**Explanation:**
The user with ID=1 performed a single action at minute 1. Hence, they have a UAM of 1.
The user with ID=2 performed actions at minutes 2 and 3. Hence, they have a UAM of 2.
There is one user with a UAM of 1 and one with a UAM of 2.
Hence, answer\[1\] = 1, answer\[2\] = 1, and the remaining values are 0.
**Constraints:**
* `1 <= logs.length <= 104`
* `0 <= IDi <= 109`
* `1 <= timei <= 105`
* `k` is in the range `[The maximum **UAM** for a user, 105]`. | Simulate the process by keeping track of how much money John is putting in and which day of the week it is, and use this information to deduce how much money John will put in the next day. |
[Python 3] Using set and dictionary || beats 98% || 826ms 🥷🏼 | finding-the-users-active-minutes | 0 | 1 | ```python3 []\nclass Solution:\n def findingUsersActiveMinutes(self, logs: List[List[int]], k: int) -> List[int]:\n res, seen, d = [0] * k, set(), defaultdict(int)\n\n for user, time in logs:\n if (user, time) in seen: continue\n d[user] += 1\n seen.add((user, time))\n \n for v in d.values():\n if v <= k:\n res[v-1] += 1\n \n return res\n```\n\n | 2 | You are given a string `s` consisting of lowercase English letters, and an integer `k`.
First, **convert** `s` into an integer by replacing each letter with its position in the alphabet (i.e., replace `'a'` with `1`, `'b'` with `2`, ..., `'z'` with `26`). Then, **transform** the integer by replacing it with the **sum of its digits**. Repeat the **transform** operation `k` **times** in total.
For example, if `s = "zbax "` and `k = 2`, then the resulting integer would be `8` by the following operations:
* **Convert**: `"zbax " ➝ "(26)(2)(1)(24) " ➝ "262124 " ➝ 262124`
* **Transform #1**: `262124 ➝ 2 + 6 + 2 + 1 + 2 + 4 ➝ 17`
* **Transform #2**: `17 ➝ 1 + 7 ➝ 8`
Return _the resulting integer after performing the operations described above_.
**Example 1:**
**Input:** s = "iiii ", k = 1
**Output:** 36
**Explanation:** The operations are as follows:
- Convert: "iiii " ➝ "(9)(9)(9)(9) " ➝ "9999 " ➝ 9999
- Transform #1: 9999 ➝ 9 + 9 + 9 + 9 ➝ 36
Thus the resulting integer is 36.
**Example 2:**
**Input:** s = "leetcode ", k = 2
**Output:** 6
**Explanation:** The operations are as follows:
- Convert: "leetcode " ➝ "(12)(5)(5)(20)(3)(15)(4)(5) " ➝ "12552031545 " ➝ 12552031545
- Transform #1: 12552031545 ➝ 1 + 2 + 5 + 5 + 2 + 0 + 3 + 1 + 5 + 4 + 5 ➝ 33
- Transform #2: 33 ➝ 3 + 3 ➝ 6
Thus the resulting integer is 6.
**Example 3:**
**Input:** s = "zbax ", k = 2
**Output:** 8
**Constraints:**
* `1 <= s.length <= 100`
* `1 <= k <= 10`
* `s` consists of lowercase English letters. | Try to find the number of different minutes when action happened for each user. For each user increase the value of the answer array index which matches the UAM for this user. |
BITMASK | EXPLOIT PYTHON | finding-the-users-active-minutes | 0 | 1 | # Note\nWe can\'t use this method in JAVA or CPP. We did use here cuz number in python have very large limit.\n# Code\n```\nclass Solution:\n def findingUsersActiveMinutes(self, logs: List[List[int]], k: int) -> List[int]:\n mp,freq,ans = {},{},[0] * k\n\n for log in logs: mp[log[0]] = mp.get(log[0],0) | (1<<log[1])\n\n for k, e in mp.items():\n cnt = bin(e).count(\'1\')\n freq[cnt] = freq.get(cnt, 0) + 1\n\n for i in range(len(ans)):\n ans[i] = freq.get(i+1, 0)\n\n return ans\n``` | 1 | You are given the logs for users' actions on LeetCode, and an integer `k`. The logs are represented by a 2D integer array `logs` where each `logs[i] = [IDi, timei]` indicates that the user with `IDi` performed an action at the minute `timei`.
**Multiple users** can perform actions simultaneously, and a single user can perform **multiple actions** in the same minute.
The **user active minutes (UAM)** for a given user is defined as the **number of unique minutes** in which the user performed an action on LeetCode. A minute can only be counted once, even if multiple actions occur during it.
You are to calculate a **1-indexed** array `answer` of size `k` such that, for each `j` (`1 <= j <= k`), `answer[j]` is the **number of users** whose **UAM** equals `j`.
Return _the array_ `answer` _as described above_.
**Example 1:**
**Input:** logs = \[\[0,5\],\[1,2\],\[0,2\],\[0,5\],\[1,3\]\], k = 5
**Output:** \[0,2,0,0,0\]
**Explanation:**
The user with ID=0 performed actions at minutes 5, 2, and 5 again. Hence, they have a UAM of 2 (minute 5 is only counted once).
The user with ID=1 performed actions at minutes 2 and 3. Hence, they have a UAM of 2.
Since both users have a UAM of 2, answer\[2\] is 2, and the remaining answer\[j\] values are 0.
**Example 2:**
**Input:** logs = \[\[1,1\],\[2,2\],\[2,3\]\], k = 4
**Output:** \[1,1,0,0\]
**Explanation:**
The user with ID=1 performed a single action at minute 1. Hence, they have a UAM of 1.
The user with ID=2 performed actions at minutes 2 and 3. Hence, they have a UAM of 2.
There is one user with a UAM of 1 and one with a UAM of 2.
Hence, answer\[1\] = 1, answer\[2\] = 1, and the remaining values are 0.
**Constraints:**
* `1 <= logs.length <= 104`
* `0 <= IDi <= 109`
* `1 <= timei <= 105`
* `k` is in the range `[The maximum **UAM** for a user, 105]`. | Simulate the process by keeping track of how much money John is putting in and which day of the week it is, and use this information to deduce how much money John will put in the next day. |
BITMASK | EXPLOIT PYTHON | finding-the-users-active-minutes | 0 | 1 | # Note\nWe can\'t use this method in JAVA or CPP. We did use here cuz number in python have very large limit.\n# Code\n```\nclass Solution:\n def findingUsersActiveMinutes(self, logs: List[List[int]], k: int) -> List[int]:\n mp,freq,ans = {},{},[0] * k\n\n for log in logs: mp[log[0]] = mp.get(log[0],0) | (1<<log[1])\n\n for k, e in mp.items():\n cnt = bin(e).count(\'1\')\n freq[cnt] = freq.get(cnt, 0) + 1\n\n for i in range(len(ans)):\n ans[i] = freq.get(i+1, 0)\n\n return ans\n``` | 1 | You are given a string `s` consisting of lowercase English letters, and an integer `k`.
First, **convert** `s` into an integer by replacing each letter with its position in the alphabet (i.e., replace `'a'` with `1`, `'b'` with `2`, ..., `'z'` with `26`). Then, **transform** the integer by replacing it with the **sum of its digits**. Repeat the **transform** operation `k` **times** in total.
For example, if `s = "zbax "` and `k = 2`, then the resulting integer would be `8` by the following operations:
* **Convert**: `"zbax " ➝ "(26)(2)(1)(24) " ➝ "262124 " ➝ 262124`
* **Transform #1**: `262124 ➝ 2 + 6 + 2 + 1 + 2 + 4 ➝ 17`
* **Transform #2**: `17 ➝ 1 + 7 ➝ 8`
Return _the resulting integer after performing the operations described above_.
**Example 1:**
**Input:** s = "iiii ", k = 1
**Output:** 36
**Explanation:** The operations are as follows:
- Convert: "iiii " ➝ "(9)(9)(9)(9) " ➝ "9999 " ➝ 9999
- Transform #1: 9999 ➝ 9 + 9 + 9 + 9 ➝ 36
Thus the resulting integer is 36.
**Example 2:**
**Input:** s = "leetcode ", k = 2
**Output:** 6
**Explanation:** The operations are as follows:
- Convert: "leetcode " ➝ "(12)(5)(5)(20)(3)(15)(4)(5) " ➝ "12552031545 " ➝ 12552031545
- Transform #1: 12552031545 ➝ 1 + 2 + 5 + 5 + 2 + 0 + 3 + 1 + 5 + 4 + 5 ➝ 33
- Transform #2: 33 ➝ 3 + 3 ➝ 6
Thus the resulting integer is 6.
**Example 3:**
**Input:** s = "zbax ", k = 2
**Output:** 8
**Constraints:**
* `1 <= s.length <= 100`
* `1 <= k <= 10`
* `s` consists of lowercase English letters. | Try to find the number of different minutes when action happened for each user. For each user increase the value of the answer array index which matches the UAM for this user. |
Python Easy Solution | finding-the-users-active-minutes | 0 | 1 | # Code\n```\nclass Solution:\n def findingUsersActiveMinutes(self, logs: List[List[int]], k: int) -> List[int]:\n dic={}\n temp=[]\n res=[0]*k\n for i in range(len(logs)):\n if logs[i][0] in dic:\n if logs[i][1] not in dic[logs[i][0]]:\n dic[logs[i][0]].append(logs[i][1])\n else:\n dic[logs[i][0]]=[logs[i][1]]\n for i in dic:\n temp.append(len(dic[i]))\n for i in temp:\n res[i-1]+=1\n return res\n``` | 1 | You are given the logs for users' actions on LeetCode, and an integer `k`. The logs are represented by a 2D integer array `logs` where each `logs[i] = [IDi, timei]` indicates that the user with `IDi` performed an action at the minute `timei`.
**Multiple users** can perform actions simultaneously, and a single user can perform **multiple actions** in the same minute.
The **user active minutes (UAM)** for a given user is defined as the **number of unique minutes** in which the user performed an action on LeetCode. A minute can only be counted once, even if multiple actions occur during it.
You are to calculate a **1-indexed** array `answer` of size `k` such that, for each `j` (`1 <= j <= k`), `answer[j]` is the **number of users** whose **UAM** equals `j`.
Return _the array_ `answer` _as described above_.
**Example 1:**
**Input:** logs = \[\[0,5\],\[1,2\],\[0,2\],\[0,5\],\[1,3\]\], k = 5
**Output:** \[0,2,0,0,0\]
**Explanation:**
The user with ID=0 performed actions at minutes 5, 2, and 5 again. Hence, they have a UAM of 2 (minute 5 is only counted once).
The user with ID=1 performed actions at minutes 2 and 3. Hence, they have a UAM of 2.
Since both users have a UAM of 2, answer\[2\] is 2, and the remaining answer\[j\] values are 0.
**Example 2:**
**Input:** logs = \[\[1,1\],\[2,2\],\[2,3\]\], k = 4
**Output:** \[1,1,0,0\]
**Explanation:**
The user with ID=1 performed a single action at minute 1. Hence, they have a UAM of 1.
The user with ID=2 performed actions at minutes 2 and 3. Hence, they have a UAM of 2.
There is one user with a UAM of 1 and one with a UAM of 2.
Hence, answer\[1\] = 1, answer\[2\] = 1, and the remaining values are 0.
**Constraints:**
* `1 <= logs.length <= 104`
* `0 <= IDi <= 109`
* `1 <= timei <= 105`
* `k` is in the range `[The maximum **UAM** for a user, 105]`. | Simulate the process by keeping track of how much money John is putting in and which day of the week it is, and use this information to deduce how much money John will put in the next day. |
Python Easy Solution | finding-the-users-active-minutes | 0 | 1 | # Code\n```\nclass Solution:\n def findingUsersActiveMinutes(self, logs: List[List[int]], k: int) -> List[int]:\n dic={}\n temp=[]\n res=[0]*k\n for i in range(len(logs)):\n if logs[i][0] in dic:\n if logs[i][1] not in dic[logs[i][0]]:\n dic[logs[i][0]].append(logs[i][1])\n else:\n dic[logs[i][0]]=[logs[i][1]]\n for i in dic:\n temp.append(len(dic[i]))\n for i in temp:\n res[i-1]+=1\n return res\n``` | 1 | You are given a string `s` consisting of lowercase English letters, and an integer `k`.
First, **convert** `s` into an integer by replacing each letter with its position in the alphabet (i.e., replace `'a'` with `1`, `'b'` with `2`, ..., `'z'` with `26`). Then, **transform** the integer by replacing it with the **sum of its digits**. Repeat the **transform** operation `k` **times** in total.
For example, if `s = "zbax "` and `k = 2`, then the resulting integer would be `8` by the following operations:
* **Convert**: `"zbax " ➝ "(26)(2)(1)(24) " ➝ "262124 " ➝ 262124`
* **Transform #1**: `262124 ➝ 2 + 6 + 2 + 1 + 2 + 4 ➝ 17`
* **Transform #2**: `17 ➝ 1 + 7 ➝ 8`
Return _the resulting integer after performing the operations described above_.
**Example 1:**
**Input:** s = "iiii ", k = 1
**Output:** 36
**Explanation:** The operations are as follows:
- Convert: "iiii " ➝ "(9)(9)(9)(9) " ➝ "9999 " ➝ 9999
- Transform #1: 9999 ➝ 9 + 9 + 9 + 9 ➝ 36
Thus the resulting integer is 36.
**Example 2:**
**Input:** s = "leetcode ", k = 2
**Output:** 6
**Explanation:** The operations are as follows:
- Convert: "leetcode " ➝ "(12)(5)(5)(20)(3)(15)(4)(5) " ➝ "12552031545 " ➝ 12552031545
- Transform #1: 12552031545 ➝ 1 + 2 + 5 + 5 + 2 + 0 + 3 + 1 + 5 + 4 + 5 ➝ 33
- Transform #2: 33 ➝ 3 + 3 ➝ 6
Thus the resulting integer is 6.
**Example 3:**
**Input:** s = "zbax ", k = 2
**Output:** 8
**Constraints:**
* `1 <= s.length <= 100`
* `1 <= k <= 10`
* `s` consists of lowercase English letters. | Try to find the number of different minutes when action happened for each user. For each user increase the value of the answer array index which matches the UAM for this user. |
Python3 O(N) solution with dictionary (91.46% Runtime) | finding-the-users-active-minutes | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\nUtilize dictionary and set to handle value updates with duplicates\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n- Define a dictionary to store unique minutes for each user\n- To store unique minutes for each user, utilize set\n- Iteratively update each user\'s active minutes\n- Construct and iteratively update an array of length k based on each users\'s unique active minutes size (length)\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(N)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\nIf this solution is similar to yours or helpful, upvote me if you don\'t mind\n```\nclass Solution:\n def findingUsersActiveMinutes(self, logs: List[List[int]], k: int) -> List[int]:\n ans = [0] * k\n d = {}\n for log in logs:\n id, min = log\n if id not in d:\n d[id] = set()\n d[id].add(min)\n\n for value in d.values():\n l = len(value)\n if l > 0:\n ans[l-1] += 1\n \n return ans\n\n``` | 1 | You are given the logs for users' actions on LeetCode, and an integer `k`. The logs are represented by a 2D integer array `logs` where each `logs[i] = [IDi, timei]` indicates that the user with `IDi` performed an action at the minute `timei`.
**Multiple users** can perform actions simultaneously, and a single user can perform **multiple actions** in the same minute.
The **user active minutes (UAM)** for a given user is defined as the **number of unique minutes** in which the user performed an action on LeetCode. A minute can only be counted once, even if multiple actions occur during it.
You are to calculate a **1-indexed** array `answer` of size `k` such that, for each `j` (`1 <= j <= k`), `answer[j]` is the **number of users** whose **UAM** equals `j`.
Return _the array_ `answer` _as described above_.
**Example 1:**
**Input:** logs = \[\[0,5\],\[1,2\],\[0,2\],\[0,5\],\[1,3\]\], k = 5
**Output:** \[0,2,0,0,0\]
**Explanation:**
The user with ID=0 performed actions at minutes 5, 2, and 5 again. Hence, they have a UAM of 2 (minute 5 is only counted once).
The user with ID=1 performed actions at minutes 2 and 3. Hence, they have a UAM of 2.
Since both users have a UAM of 2, answer\[2\] is 2, and the remaining answer\[j\] values are 0.
**Example 2:**
**Input:** logs = \[\[1,1\],\[2,2\],\[2,3\]\], k = 4
**Output:** \[1,1,0,0\]
**Explanation:**
The user with ID=1 performed a single action at minute 1. Hence, they have a UAM of 1.
The user with ID=2 performed actions at minutes 2 and 3. Hence, they have a UAM of 2.
There is one user with a UAM of 1 and one with a UAM of 2.
Hence, answer\[1\] = 1, answer\[2\] = 1, and the remaining values are 0.
**Constraints:**
* `1 <= logs.length <= 104`
* `0 <= IDi <= 109`
* `1 <= timei <= 105`
* `k` is in the range `[The maximum **UAM** for a user, 105]`. | Simulate the process by keeping track of how much money John is putting in and which day of the week it is, and use this information to deduce how much money John will put in the next day. |
Python3 O(N) solution with dictionary (91.46% Runtime) | finding-the-users-active-minutes | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\nUtilize dictionary and set to handle value updates with duplicates\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n- Define a dictionary to store unique minutes for each user\n- To store unique minutes for each user, utilize set\n- Iteratively update each user\'s active minutes\n- Construct and iteratively update an array of length k based on each users\'s unique active minutes size (length)\n\n# Complexity\n- Time complexity: O(N)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(N)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\nIf this solution is similar to yours or helpful, upvote me if you don\'t mind\n```\nclass Solution:\n def findingUsersActiveMinutes(self, logs: List[List[int]], k: int) -> List[int]:\n ans = [0] * k\n d = {}\n for log in logs:\n id, min = log\n if id not in d:\n d[id] = set()\n d[id].add(min)\n\n for value in d.values():\n l = len(value)\n if l > 0:\n ans[l-1] += 1\n \n return ans\n\n``` | 1 | You are given a string `s` consisting of lowercase English letters, and an integer `k`.
First, **convert** `s` into an integer by replacing each letter with its position in the alphabet (i.e., replace `'a'` with `1`, `'b'` with `2`, ..., `'z'` with `26`). Then, **transform** the integer by replacing it with the **sum of its digits**. Repeat the **transform** operation `k` **times** in total.
For example, if `s = "zbax "` and `k = 2`, then the resulting integer would be `8` by the following operations:
* **Convert**: `"zbax " ➝ "(26)(2)(1)(24) " ➝ "262124 " ➝ 262124`
* **Transform #1**: `262124 ➝ 2 + 6 + 2 + 1 + 2 + 4 ➝ 17`
* **Transform #2**: `17 ➝ 1 + 7 ➝ 8`
Return _the resulting integer after performing the operations described above_.
**Example 1:**
**Input:** s = "iiii ", k = 1
**Output:** 36
**Explanation:** The operations are as follows:
- Convert: "iiii " ➝ "(9)(9)(9)(9) " ➝ "9999 " ➝ 9999
- Transform #1: 9999 ➝ 9 + 9 + 9 + 9 ➝ 36
Thus the resulting integer is 36.
**Example 2:**
**Input:** s = "leetcode ", k = 2
**Output:** 6
**Explanation:** The operations are as follows:
- Convert: "leetcode " ➝ "(12)(5)(5)(20)(3)(15)(4)(5) " ➝ "12552031545 " ➝ 12552031545
- Transform #1: 12552031545 ➝ 1 + 2 + 5 + 5 + 2 + 0 + 3 + 1 + 5 + 4 + 5 ➝ 33
- Transform #2: 33 ➝ 3 + 3 ➝ 6
Thus the resulting integer is 6.
**Example 3:**
**Input:** s = "zbax ", k = 2
**Output:** 8
**Constraints:**
* `1 <= s.length <= 100`
* `1 <= k <= 10`
* `s` consists of lowercase English letters. | Try to find the number of different minutes when action happened for each user. For each user increase the value of the answer array index which matches the UAM for this user. |
Python with dictionary | Time O(n) | Space O(n + k) | finding-the-users-active-minutes | 0 | 1 | # Code\n```\nclass Solution:\n def findingUsersActiveMinutes(self, logs: List[List[int]], k: int) -> List[int]:\n # hash map of id -> set(time)\n user = {}\n # answer array with the k length\n ans = [0]*k\n\n for id, time in logs:\n # if the user id don\'t exist in user\n # create one set in the user for that id\n if id not in user:\n user[id] = set()\n # add time in that id, if it is duplicate\n # it won\'t be added\n user[id].add(time)\n \n # iterate through the user -> set(time)\n for k,v in user.items():\n # length of the user id time set - 1 index of answer\n # will get incremented\n ans[len(v)-1] += 1\n \n # return answer\n return ans\n\n \n``` | 1 | You are given the logs for users' actions on LeetCode, and an integer `k`. The logs are represented by a 2D integer array `logs` where each `logs[i] = [IDi, timei]` indicates that the user with `IDi` performed an action at the minute `timei`.
**Multiple users** can perform actions simultaneously, and a single user can perform **multiple actions** in the same minute.
The **user active minutes (UAM)** for a given user is defined as the **number of unique minutes** in which the user performed an action on LeetCode. A minute can only be counted once, even if multiple actions occur during it.
You are to calculate a **1-indexed** array `answer` of size `k` such that, for each `j` (`1 <= j <= k`), `answer[j]` is the **number of users** whose **UAM** equals `j`.
Return _the array_ `answer` _as described above_.
**Example 1:**
**Input:** logs = \[\[0,5\],\[1,2\],\[0,2\],\[0,5\],\[1,3\]\], k = 5
**Output:** \[0,2,0,0,0\]
**Explanation:**
The user with ID=0 performed actions at minutes 5, 2, and 5 again. Hence, they have a UAM of 2 (minute 5 is only counted once).
The user with ID=1 performed actions at minutes 2 and 3. Hence, they have a UAM of 2.
Since both users have a UAM of 2, answer\[2\] is 2, and the remaining answer\[j\] values are 0.
**Example 2:**
**Input:** logs = \[\[1,1\],\[2,2\],\[2,3\]\], k = 4
**Output:** \[1,1,0,0\]
**Explanation:**
The user with ID=1 performed a single action at minute 1. Hence, they have a UAM of 1.
The user with ID=2 performed actions at minutes 2 and 3. Hence, they have a UAM of 2.
There is one user with a UAM of 1 and one with a UAM of 2.
Hence, answer\[1\] = 1, answer\[2\] = 1, and the remaining values are 0.
**Constraints:**
* `1 <= logs.length <= 104`
* `0 <= IDi <= 109`
* `1 <= timei <= 105`
* `k` is in the range `[The maximum **UAM** for a user, 105]`. | Simulate the process by keeping track of how much money John is putting in and which day of the week it is, and use this information to deduce how much money John will put in the next day. |
Python with dictionary | Time O(n) | Space O(n + k) | finding-the-users-active-minutes | 0 | 1 | # Code\n```\nclass Solution:\n def findingUsersActiveMinutes(self, logs: List[List[int]], k: int) -> List[int]:\n # hash map of id -> set(time)\n user = {}\n # answer array with the k length\n ans = [0]*k\n\n for id, time in logs:\n # if the user id don\'t exist in user\n # create one set in the user for that id\n if id not in user:\n user[id] = set()\n # add time in that id, if it is duplicate\n # it won\'t be added\n user[id].add(time)\n \n # iterate through the user -> set(time)\n for k,v in user.items():\n # length of the user id time set - 1 index of answer\n # will get incremented\n ans[len(v)-1] += 1\n \n # return answer\n return ans\n\n \n``` | 1 | You are given a string `s` consisting of lowercase English letters, and an integer `k`.
First, **convert** `s` into an integer by replacing each letter with its position in the alphabet (i.e., replace `'a'` with `1`, `'b'` with `2`, ..., `'z'` with `26`). Then, **transform** the integer by replacing it with the **sum of its digits**. Repeat the **transform** operation `k` **times** in total.
For example, if `s = "zbax "` and `k = 2`, then the resulting integer would be `8` by the following operations:
* **Convert**: `"zbax " ➝ "(26)(2)(1)(24) " ➝ "262124 " ➝ 262124`
* **Transform #1**: `262124 ➝ 2 + 6 + 2 + 1 + 2 + 4 ➝ 17`
* **Transform #2**: `17 ➝ 1 + 7 ➝ 8`
Return _the resulting integer after performing the operations described above_.
**Example 1:**
**Input:** s = "iiii ", k = 1
**Output:** 36
**Explanation:** The operations are as follows:
- Convert: "iiii " ➝ "(9)(9)(9)(9) " ➝ "9999 " ➝ 9999
- Transform #1: 9999 ➝ 9 + 9 + 9 + 9 ➝ 36
Thus the resulting integer is 36.
**Example 2:**
**Input:** s = "leetcode ", k = 2
**Output:** 6
**Explanation:** The operations are as follows:
- Convert: "leetcode " ➝ "(12)(5)(5)(20)(3)(15)(4)(5) " ➝ "12552031545 " ➝ 12552031545
- Transform #1: 12552031545 ➝ 1 + 2 + 5 + 5 + 2 + 0 + 3 + 1 + 5 + 4 + 5 ➝ 33
- Transform #2: 33 ➝ 3 + 3 ➝ 6
Thus the resulting integer is 6.
**Example 3:**
**Input:** s = "zbax ", k = 2
**Output:** 8
**Constraints:**
* `1 <= s.length <= 100`
* `1 <= k <= 10`
* `s` consists of lowercase English letters. | Try to find the number of different minutes when action happened for each user. For each user increase the value of the answer array index which matches the UAM for this user. |
[Python3] hash map | finding-the-users-active-minutes | 0 | 1 | \n```\nclass Solution:\n def findingUsersActiveMinutes(self, logs: List[List[int]], k: int) -> List[int]:\n mp = {}\n for i, t in logs: \n mp.setdefault(i, set()).add(t)\n \n ans = [0]*k\n for v in mp.values(): \n if len(v) <= k: \n ans[len(v)-1] += 1\n return ans \n``` | 9 | You are given the logs for users' actions on LeetCode, and an integer `k`. The logs are represented by a 2D integer array `logs` where each `logs[i] = [IDi, timei]` indicates that the user with `IDi` performed an action at the minute `timei`.
**Multiple users** can perform actions simultaneously, and a single user can perform **multiple actions** in the same minute.
The **user active minutes (UAM)** for a given user is defined as the **number of unique minutes** in which the user performed an action on LeetCode. A minute can only be counted once, even if multiple actions occur during it.
You are to calculate a **1-indexed** array `answer` of size `k` such that, for each `j` (`1 <= j <= k`), `answer[j]` is the **number of users** whose **UAM** equals `j`.
Return _the array_ `answer` _as described above_.
**Example 1:**
**Input:** logs = \[\[0,5\],\[1,2\],\[0,2\],\[0,5\],\[1,3\]\], k = 5
**Output:** \[0,2,0,0,0\]
**Explanation:**
The user with ID=0 performed actions at minutes 5, 2, and 5 again. Hence, they have a UAM of 2 (minute 5 is only counted once).
The user with ID=1 performed actions at minutes 2 and 3. Hence, they have a UAM of 2.
Since both users have a UAM of 2, answer\[2\] is 2, and the remaining answer\[j\] values are 0.
**Example 2:**
**Input:** logs = \[\[1,1\],\[2,2\],\[2,3\]\], k = 4
**Output:** \[1,1,0,0\]
**Explanation:**
The user with ID=1 performed a single action at minute 1. Hence, they have a UAM of 1.
The user with ID=2 performed actions at minutes 2 and 3. Hence, they have a UAM of 2.
There is one user with a UAM of 1 and one with a UAM of 2.
Hence, answer\[1\] = 1, answer\[2\] = 1, and the remaining values are 0.
**Constraints:**
* `1 <= logs.length <= 104`
* `0 <= IDi <= 109`
* `1 <= timei <= 105`
* `k` is in the range `[The maximum **UAM** for a user, 105]`. | Simulate the process by keeping track of how much money John is putting in and which day of the week it is, and use this information to deduce how much money John will put in the next day. |
[Python3] hash map | finding-the-users-active-minutes | 0 | 1 | \n```\nclass Solution:\n def findingUsersActiveMinutes(self, logs: List[List[int]], k: int) -> List[int]:\n mp = {}\n for i, t in logs: \n mp.setdefault(i, set()).add(t)\n \n ans = [0]*k\n for v in mp.values(): \n if len(v) <= k: \n ans[len(v)-1] += 1\n return ans \n``` | 9 | You are given a string `s` consisting of lowercase English letters, and an integer `k`.
First, **convert** `s` into an integer by replacing each letter with its position in the alphabet (i.e., replace `'a'` with `1`, `'b'` with `2`, ..., `'z'` with `26`). Then, **transform** the integer by replacing it with the **sum of its digits**. Repeat the **transform** operation `k` **times** in total.
For example, if `s = "zbax "` and `k = 2`, then the resulting integer would be `8` by the following operations:
* **Convert**: `"zbax " ➝ "(26)(2)(1)(24) " ➝ "262124 " ➝ 262124`
* **Transform #1**: `262124 ➝ 2 + 6 + 2 + 1 + 2 + 4 ➝ 17`
* **Transform #2**: `17 ➝ 1 + 7 ➝ 8`
Return _the resulting integer after performing the operations described above_.
**Example 1:**
**Input:** s = "iiii ", k = 1
**Output:** 36
**Explanation:** The operations are as follows:
- Convert: "iiii " ➝ "(9)(9)(9)(9) " ➝ "9999 " ➝ 9999
- Transform #1: 9999 ➝ 9 + 9 + 9 + 9 ➝ 36
Thus the resulting integer is 36.
**Example 2:**
**Input:** s = "leetcode ", k = 2
**Output:** 6
**Explanation:** The operations are as follows:
- Convert: "leetcode " ➝ "(12)(5)(5)(20)(3)(15)(4)(5) " ➝ "12552031545 " ➝ 12552031545
- Transform #1: 12552031545 ➝ 1 + 2 + 5 + 5 + 2 + 0 + 3 + 1 + 5 + 4 + 5 ➝ 33
- Transform #2: 33 ➝ 3 + 3 ➝ 6
Thus the resulting integer is 6.
**Example 3:**
**Input:** s = "zbax ", k = 2
**Output:** 8
**Constraints:**
* `1 <= s.length <= 100`
* `1 <= k <= 10`
* `s` consists of lowercase English letters. | Try to find the number of different minutes when action happened for each user. For each user increase the value of the answer array index which matches the UAM for this user. |
[Python] + Fully Explained + Best Solution ✔ | minimum-absolute-sum-difference | 0 | 1 | 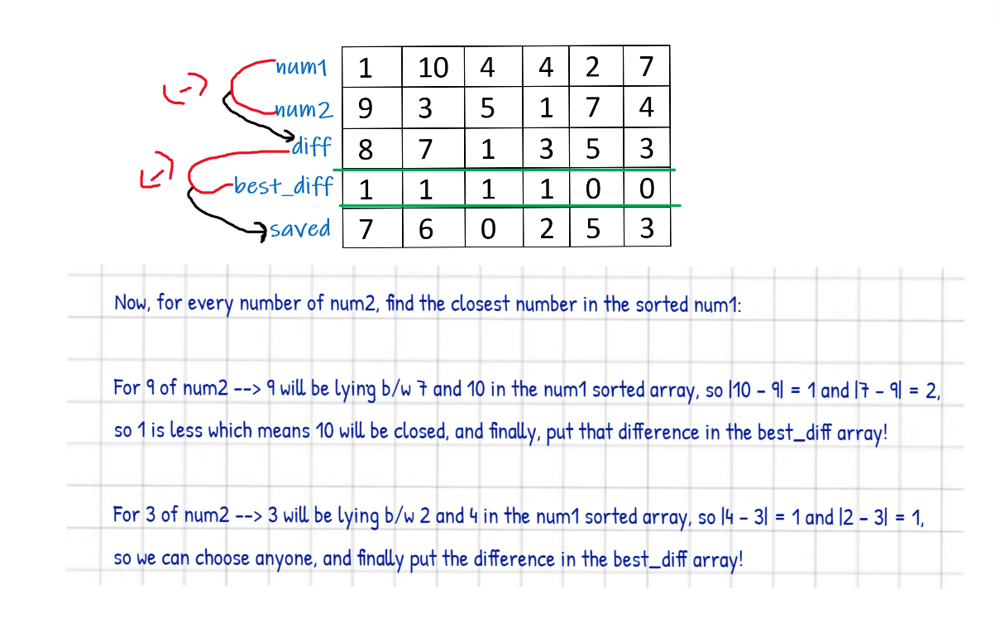\n\n\tclass Solution:\n\t\tdef minAbsoluteSumDiff(self, nums1: List[int], nums2: List[int]) -> int:\n\t\t\tn = len(nums1)\n\t\t\tdiff = []\n\t\t\tsum = 0\n\t\t\tfor i in range(n):\n\t\t\t\ttemp = abs(nums1[i]-nums2[i])\n\t\t\t\tdiff.append(temp)\n\t\t\t\tsum += temp\n\t\t\tnums1.sort()\n\t\t\tbest_diff = []\n\t\t\tfor i in range(n):\n\t\t\t\tidx = bisect.bisect_left(nums1, nums2[i])\n\t\t\t\tif idx != 0 and idx != n:\n\t\t\t\t\tbest_diff.append(\n\t\t\t\t\t\tmin(abs(nums2[i]-nums1[idx]), abs(nums2[i]-nums1[idx-1])))\n\t\t\t\telif idx == 0:\n\t\t\t\t\tbest_diff.append(abs(nums2[i]-nums1[idx]))\n\t\t\t\telse:\n\t\t\t\t\tbest_diff.append(abs(nums2[i]-nums1[idx-1]))\n\t\t\tsaved = 0\n\t\t\tfor i in range(n):\n\t\t\t\tsaved = max(saved, diff[i]-best_diff[i])\n\t\t\treturn (sum-saved) % ((10**9)+(7))\nIf you have any questions, please ask me, and if you like this approach, please **vote it up**! | 12 | You are given two positive integer arrays `nums1` and `nums2`, both of length `n`.
The **absolute sum difference** of arrays `nums1` and `nums2` is defined as the **sum** of `|nums1[i] - nums2[i]|` for each `0 <= i < n` (**0-indexed**).
You can replace **at most one** element of `nums1` with **any** other element in `nums1` to **minimize** the absolute sum difference.
Return the _minimum absolute sum difference **after** replacing at most one element in the array `nums1`._ Since the answer may be large, return it **modulo** `109 + 7`.
`|x|` is defined as:
* `x` if `x >= 0`, or
* `-x` if `x < 0`.
**Example 1:**
**Input:** nums1 = \[1,7,5\], nums2 = \[2,3,5\]
**Output:** 3
**Explanation:** There are two possible optimal solutions:
- Replace the second element with the first: \[1,**7**,5\] => \[1,**1**,5\], or
- Replace the second element with the third: \[1,**7**,5\] => \[1,**5**,5\].
Both will yield an absolute sum difference of `|1-2| + (|1-3| or |5-3|) + |5-5| =` 3.
**Example 2:**
**Input:** nums1 = \[2,4,6,8,10\], nums2 = \[2,4,6,8,10\]
**Output:** 0
**Explanation:** nums1 is equal to nums2 so no replacement is needed. This will result in an
absolute sum difference of 0.
**Example 3:**
**Input:** nums1 = \[1,10,4,4,2,7\], nums2 = \[9,3,5,1,7,4\]
**Output:** 20
**Explanation:** Replace the first element with the second: \[**1**,10,4,4,2,7\] => \[**10**,10,4,4,2,7\].
This yields an absolute sum difference of `|10-9| + |10-3| + |4-5| + |4-1| + |2-7| + |7-4| = 20`
**Constraints:**
* `n == nums1.length`
* `n == nums2.length`
* `1 <= n <= 105`
* `1 <= nums1[i], nums2[i] <= 105` | Note that it is always more optimal to take one type of substring before another You can use a stack to handle erasures |
[Python] + Fully Explained + Best Solution ✔ | minimum-absolute-sum-difference | 0 | 1 | 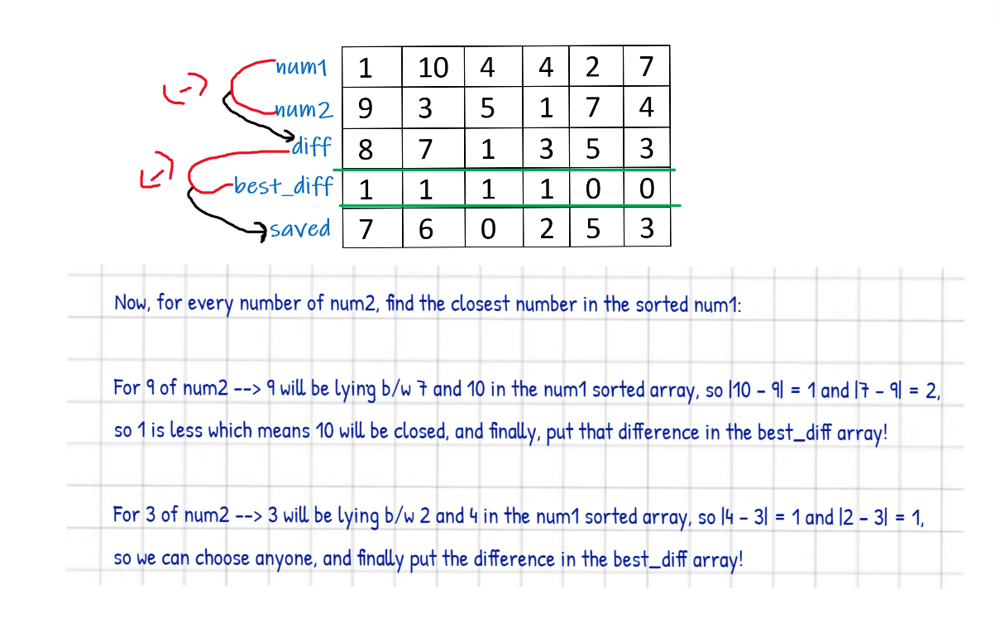\n\n\tclass Solution:\n\t\tdef minAbsoluteSumDiff(self, nums1: List[int], nums2: List[int]) -> int:\n\t\t\tn = len(nums1)\n\t\t\tdiff = []\n\t\t\tsum = 0\n\t\t\tfor i in range(n):\n\t\t\t\ttemp = abs(nums1[i]-nums2[i])\n\t\t\t\tdiff.append(temp)\n\t\t\t\tsum += temp\n\t\t\tnums1.sort()\n\t\t\tbest_diff = []\n\t\t\tfor i in range(n):\n\t\t\t\tidx = bisect.bisect_left(nums1, nums2[i])\n\t\t\t\tif idx != 0 and idx != n:\n\t\t\t\t\tbest_diff.append(\n\t\t\t\t\t\tmin(abs(nums2[i]-nums1[idx]), abs(nums2[i]-nums1[idx-1])))\n\t\t\t\telif idx == 0:\n\t\t\t\t\tbest_diff.append(abs(nums2[i]-nums1[idx]))\n\t\t\t\telse:\n\t\t\t\t\tbest_diff.append(abs(nums2[i]-nums1[idx-1]))\n\t\t\tsaved = 0\n\t\t\tfor i in range(n):\n\t\t\t\tsaved = max(saved, diff[i]-best_diff[i])\n\t\t\treturn (sum-saved) % ((10**9)+(7))\nIf you have any questions, please ask me, and if you like this approach, please **vote it up**! | 12 | You are given a string `num`, which represents a large integer. You are also given a **0-indexed** integer array `change` of length `10` that maps each digit `0-9` to another digit. More formally, digit `d` maps to digit `change[d]`.
You may **choose** to **mutate a single substring** of `num`. To mutate a substring, replace each digit `num[i]` with the digit it maps to in `change` (i.e. replace `num[i]` with `change[num[i]]`).
Return _a string representing the **largest** possible integer after **mutating** (or choosing not to) a **single substring** of_ `num`.
A **substring** is a contiguous sequence of characters within the string.
**Example 1:**
**Input:** num = "132 ", change = \[9,8,5,0,3,6,4,2,6,8\]
**Output:** "832 "
**Explanation:** Replace the substring "1 ":
- 1 maps to change\[1\] = 8.
Thus, "132 " becomes "832 ".
"832 " is the largest number that can be created, so return it.
**Example 2:**
**Input:** num = "021 ", change = \[9,4,3,5,7,2,1,9,0,6\]
**Output:** "934 "
**Explanation:** Replace the substring "021 ":
- 0 maps to change\[0\] = 9.
- 2 maps to change\[2\] = 3.
- 1 maps to change\[1\] = 4.
Thus, "021 " becomes "934 ".
"934 " is the largest number that can be created, so return it.
**Example 3:**
**Input:** num = "5 ", change = \[1,4,7,5,3,2,5,6,9,4\]
**Output:** "5 "
**Explanation:** "5 " is already the largest number that can be created, so return it.
**Constraints:**
* `1 <= num.length <= 105`
* `num` consists of only digits `0-9`.
* `change.length == 10`
* `0 <= change[d] <= 9` | Go through each element and test the optimal replacements. There are only 2 possible replacements for each element (higher and lower) that are optimal. |
🤯 [Python] Easy Logic | Explained | Sort + Binary Search | Codeplug | minimum-absolute-sum-difference | 0 | 1 | # Intuition\nWe first calculate the absolute differences for all the indices and the current sum of those differences.\n\nWe need to reduce this sum in the end.\n\n# Approach\nfor each value in nums2, we try to find the closest value in nums1 so that the difference becomes 0. In order to find it efficiently we can sort nums1 and use binary search. We find the maximum reduction possible.\n\nReduction at any given index = current absolute difference between the numbers - new absolute difference if we replace the num in nums1 with the closest available number to nums2.\n\nWe find the max reduction and reduce it from the current difference in the end.\n\n**Upvote if you understood the logic :)**\n\n# Complexity\n- Time complexity:\n$$O(nlogn)$$\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nclass Solution:\n def minAbsoluteSumDiff(self, nums1: List[int], nums2: List[int]) -> int:\n arr = sorted(nums1)\n n = len(arr)\n res = maxReduction = 0\n\n for i, val in enumerate(nums2):\n currDiff = abs(nums1[i] - val)\n res += currDiff\n j = bisect_right(arr, val) - 1\n op1 = abs(val - arr[j])\n op2 = None\n\n if j + 1 < n:\n op2 = abs(val - arr[j + 1])\n \n maxReduction = max(currDiff - op1, currDiff - op2 if op2 else 0, maxReduction)\n \n return (res - maxReduction) % (10 ** 9 + 7) \n``` | 3 | You are given two positive integer arrays `nums1` and `nums2`, both of length `n`.
The **absolute sum difference** of arrays `nums1` and `nums2` is defined as the **sum** of `|nums1[i] - nums2[i]|` for each `0 <= i < n` (**0-indexed**).
You can replace **at most one** element of `nums1` with **any** other element in `nums1` to **minimize** the absolute sum difference.
Return the _minimum absolute sum difference **after** replacing at most one element in the array `nums1`._ Since the answer may be large, return it **modulo** `109 + 7`.
`|x|` is defined as:
* `x` if `x >= 0`, or
* `-x` if `x < 0`.
**Example 1:**
**Input:** nums1 = \[1,7,5\], nums2 = \[2,3,5\]
**Output:** 3
**Explanation:** There are two possible optimal solutions:
- Replace the second element with the first: \[1,**7**,5\] => \[1,**1**,5\], or
- Replace the second element with the third: \[1,**7**,5\] => \[1,**5**,5\].
Both will yield an absolute sum difference of `|1-2| + (|1-3| or |5-3|) + |5-5| =` 3.
**Example 2:**
**Input:** nums1 = \[2,4,6,8,10\], nums2 = \[2,4,6,8,10\]
**Output:** 0
**Explanation:** nums1 is equal to nums2 so no replacement is needed. This will result in an
absolute sum difference of 0.
**Example 3:**
**Input:** nums1 = \[1,10,4,4,2,7\], nums2 = \[9,3,5,1,7,4\]
**Output:** 20
**Explanation:** Replace the first element with the second: \[**1**,10,4,4,2,7\] => \[**10**,10,4,4,2,7\].
This yields an absolute sum difference of `|10-9| + |10-3| + |4-5| + |4-1| + |2-7| + |7-4| = 20`
**Constraints:**
* `n == nums1.length`
* `n == nums2.length`
* `1 <= n <= 105`
* `1 <= nums1[i], nums2[i] <= 105` | Note that it is always more optimal to take one type of substring before another You can use a stack to handle erasures |
🤯 [Python] Easy Logic | Explained | Sort + Binary Search | Codeplug | minimum-absolute-sum-difference | 0 | 1 | # Intuition\nWe first calculate the absolute differences for all the indices and the current sum of those differences.\n\nWe need to reduce this sum in the end.\n\n# Approach\nfor each value in nums2, we try to find the closest value in nums1 so that the difference becomes 0. In order to find it efficiently we can sort nums1 and use binary search. We find the maximum reduction possible.\n\nReduction at any given index = current absolute difference between the numbers - new absolute difference if we replace the num in nums1 with the closest available number to nums2.\n\nWe find the max reduction and reduce it from the current difference in the end.\n\n**Upvote if you understood the logic :)**\n\n# Complexity\n- Time complexity:\n$$O(nlogn)$$\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nclass Solution:\n def minAbsoluteSumDiff(self, nums1: List[int], nums2: List[int]) -> int:\n arr = sorted(nums1)\n n = len(arr)\n res = maxReduction = 0\n\n for i, val in enumerate(nums2):\n currDiff = abs(nums1[i] - val)\n res += currDiff\n j = bisect_right(arr, val) - 1\n op1 = abs(val - arr[j])\n op2 = None\n\n if j + 1 < n:\n op2 = abs(val - arr[j + 1])\n \n maxReduction = max(currDiff - op1, currDiff - op2 if op2 else 0, maxReduction)\n \n return (res - maxReduction) % (10 ** 9 + 7) \n``` | 3 | You are given a string `num`, which represents a large integer. You are also given a **0-indexed** integer array `change` of length `10` that maps each digit `0-9` to another digit. More formally, digit `d` maps to digit `change[d]`.
You may **choose** to **mutate a single substring** of `num`. To mutate a substring, replace each digit `num[i]` with the digit it maps to in `change` (i.e. replace `num[i]` with `change[num[i]]`).
Return _a string representing the **largest** possible integer after **mutating** (or choosing not to) a **single substring** of_ `num`.
A **substring** is a contiguous sequence of characters within the string.
**Example 1:**
**Input:** num = "132 ", change = \[9,8,5,0,3,6,4,2,6,8\]
**Output:** "832 "
**Explanation:** Replace the substring "1 ":
- 1 maps to change\[1\] = 8.
Thus, "132 " becomes "832 ".
"832 " is the largest number that can be created, so return it.
**Example 2:**
**Input:** num = "021 ", change = \[9,4,3,5,7,2,1,9,0,6\]
**Output:** "934 "
**Explanation:** Replace the substring "021 ":
- 0 maps to change\[0\] = 9.
- 2 maps to change\[2\] = 3.
- 1 maps to change\[1\] = 4.
Thus, "021 " becomes "934 ".
"934 " is the largest number that can be created, so return it.
**Example 3:**
**Input:** num = "5 ", change = \[1,4,7,5,3,2,5,6,9,4\]
**Output:** "5 "
**Explanation:** "5 " is already the largest number that can be created, so return it.
**Constraints:**
* `1 <= num.length <= 105`
* `num` consists of only digits `0-9`.
* `change.length == 10`
* `0 <= change[d] <= 9` | Go through each element and test the optimal replacements. There are only 2 possible replacements for each element (higher and lower) that are optimal. |
simple - replace number with closest | minimum-absolute-sum-difference | 0 | 1 | \n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n for each pair (a,b) find the number in nums1 that is cloest to b via binary search\n after replacing a with that number keep track of the smallest absolute_sum_difference for each replacment\n\n# Code\n```\nclass Solution:\n def minAbsoluteSumDiff(self, nums1: List[int], nums2: List[int]) -> int:\n modulo = 10**9 + 7\n\n\n from bisect import bisect_left\n\n def take_closest(myList, myNumber):\n """\n Assumes myList is sorted. Returns closest value to myNumber.\n\n If two numbers are equally close, return the smallest number.\n """\n pos = bisect_left(myList, myNumber)\n if pos == 0:\n return myList[0]\n if pos == len(myList):\n return myList[-1]\n before = myList[pos - 1]\n after = myList[pos]\n if after - myNumber < myNumber - before:\n return after\n else:\n return before\n\n\n\n pairs = list(zip(nums1,nums2))\n \n absolute_sum_difference = 0\n for a,b in pairs:\n absolute_sum_difference += abs(a-b)\n\n if absolute_sum_difference == 0:\n return 0\n\n\n nums1 = sorted(nums1)\n\n\n best = absolute_sum_difference\n for a ,b in pairs:\n new_closest = take_closest(nums1,b)\n best = min(best,absolute_sum_difference - abs(abs(a-b) - abs(new_closest - b)))\n\n return best % modulo\n\n``` | 0 | You are given two positive integer arrays `nums1` and `nums2`, both of length `n`.
The **absolute sum difference** of arrays `nums1` and `nums2` is defined as the **sum** of `|nums1[i] - nums2[i]|` for each `0 <= i < n` (**0-indexed**).
You can replace **at most one** element of `nums1` with **any** other element in `nums1` to **minimize** the absolute sum difference.
Return the _minimum absolute sum difference **after** replacing at most one element in the array `nums1`._ Since the answer may be large, return it **modulo** `109 + 7`.
`|x|` is defined as:
* `x` if `x >= 0`, or
* `-x` if `x < 0`.
**Example 1:**
**Input:** nums1 = \[1,7,5\], nums2 = \[2,3,5\]
**Output:** 3
**Explanation:** There are two possible optimal solutions:
- Replace the second element with the first: \[1,**7**,5\] => \[1,**1**,5\], or
- Replace the second element with the third: \[1,**7**,5\] => \[1,**5**,5\].
Both will yield an absolute sum difference of `|1-2| + (|1-3| or |5-3|) + |5-5| =` 3.
**Example 2:**
**Input:** nums1 = \[2,4,6,8,10\], nums2 = \[2,4,6,8,10\]
**Output:** 0
**Explanation:** nums1 is equal to nums2 so no replacement is needed. This will result in an
absolute sum difference of 0.
**Example 3:**
**Input:** nums1 = \[1,10,4,4,2,7\], nums2 = \[9,3,5,1,7,4\]
**Output:** 20
**Explanation:** Replace the first element with the second: \[**1**,10,4,4,2,7\] => \[**10**,10,4,4,2,7\].
This yields an absolute sum difference of `|10-9| + |10-3| + |4-5| + |4-1| + |2-7| + |7-4| = 20`
**Constraints:**
* `n == nums1.length`
* `n == nums2.length`
* `1 <= n <= 105`
* `1 <= nums1[i], nums2[i] <= 105` | Note that it is always more optimal to take one type of substring before another You can use a stack to handle erasures |
simple - replace number with closest | minimum-absolute-sum-difference | 0 | 1 | \n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n for each pair (a,b) find the number in nums1 that is cloest to b via binary search\n after replacing a with that number keep track of the smallest absolute_sum_difference for each replacment\n\n# Code\n```\nclass Solution:\n def minAbsoluteSumDiff(self, nums1: List[int], nums2: List[int]) -> int:\n modulo = 10**9 + 7\n\n\n from bisect import bisect_left\n\n def take_closest(myList, myNumber):\n """\n Assumes myList is sorted. Returns closest value to myNumber.\n\n If two numbers are equally close, return the smallest number.\n """\n pos = bisect_left(myList, myNumber)\n if pos == 0:\n return myList[0]\n if pos == len(myList):\n return myList[-1]\n before = myList[pos - 1]\n after = myList[pos]\n if after - myNumber < myNumber - before:\n return after\n else:\n return before\n\n\n\n pairs = list(zip(nums1,nums2))\n \n absolute_sum_difference = 0\n for a,b in pairs:\n absolute_sum_difference += abs(a-b)\n\n if absolute_sum_difference == 0:\n return 0\n\n\n nums1 = sorted(nums1)\n\n\n best = absolute_sum_difference\n for a ,b in pairs:\n new_closest = take_closest(nums1,b)\n best = min(best,absolute_sum_difference - abs(abs(a-b) - abs(new_closest - b)))\n\n return best % modulo\n\n``` | 0 | You are given a string `num`, which represents a large integer. You are also given a **0-indexed** integer array `change` of length `10` that maps each digit `0-9` to another digit. More formally, digit `d` maps to digit `change[d]`.
You may **choose** to **mutate a single substring** of `num`. To mutate a substring, replace each digit `num[i]` with the digit it maps to in `change` (i.e. replace `num[i]` with `change[num[i]]`).
Return _a string representing the **largest** possible integer after **mutating** (or choosing not to) a **single substring** of_ `num`.
A **substring** is a contiguous sequence of characters within the string.
**Example 1:**
**Input:** num = "132 ", change = \[9,8,5,0,3,6,4,2,6,8\]
**Output:** "832 "
**Explanation:** Replace the substring "1 ":
- 1 maps to change\[1\] = 8.
Thus, "132 " becomes "832 ".
"832 " is the largest number that can be created, so return it.
**Example 2:**
**Input:** num = "021 ", change = \[9,4,3,5,7,2,1,9,0,6\]
**Output:** "934 "
**Explanation:** Replace the substring "021 ":
- 0 maps to change\[0\] = 9.
- 2 maps to change\[2\] = 3.
- 1 maps to change\[1\] = 4.
Thus, "021 " becomes "934 ".
"934 " is the largest number that can be created, so return it.
**Example 3:**
**Input:** num = "5 ", change = \[1,4,7,5,3,2,5,6,9,4\]
**Output:** "5 "
**Explanation:** "5 " is already the largest number that can be created, so return it.
**Constraints:**
* `1 <= num.length <= 105`
* `num` consists of only digits `0-9`.
* `change.length == 10`
* `0 <= change[d] <= 9` | Go through each element and test the optimal replacements. There are only 2 possible replacements for each element (higher and lower) that are optimal. |
[Python3] enumerate all possibilities | number-of-different-subsequences-gcds | 0 | 1 | \n```\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums: List[int]) -> int:\n nums = set(nums)\n \n ans = 0\n m = max(nums)\n for x in range(1, m+1): \n g = 0\n for xx in range(x, m+1, x): \n if xx in nums: \n g = gcd(g, xx)\n if g == x: ans += 1\n return ans \n``` | 4 | You are given an array `nums` that consists of positive integers.
The **GCD** of a sequence of numbers is defined as the greatest integer that divides **all** the numbers in the sequence evenly.
* For example, the GCD of the sequence `[4,6,16]` is `2`.
A **subsequence** of an array is a sequence that can be formed by removing some elements (possibly none) of the array.
* For example, `[2,5,10]` is a subsequence of `[1,2,1,**2**,4,1,**5**,**10**]`.
Return _the **number** of **different** GCDs among all **non-empty** subsequences of_ `nums`.
**Example 1:**
**Input:** nums = \[6,10,3\]
**Output:** 5
**Explanation:** The figure shows all the non-empty subsequences and their GCDs.
The different GCDs are 6, 10, 3, 2, and 1.
**Example 2:**
**Input:** nums = \[5,15,40,5,6\]
**Output:** 7
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 2 * 105` | Heuristic algorithm may work. |
[Python3] enumerate all possibilities | number-of-different-subsequences-gcds | 0 | 1 | \n```\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums: List[int]) -> int:\n nums = set(nums)\n \n ans = 0\n m = max(nums)\n for x in range(1, m+1): \n g = 0\n for xx in range(x, m+1, x): \n if xx in nums: \n g = gcd(g, xx)\n if g == x: ans += 1\n return ans \n``` | 4 | There is a survey that consists of `n` questions where each question's answer is either `0` (no) or `1` (yes).
The survey was given to `m` students numbered from `0` to `m - 1` and `m` mentors numbered from `0` to `m - 1`. The answers of the students are represented by a 2D integer array `students` where `students[i]` is an integer array that contains the answers of the `ith` student (**0-indexed**). The answers of the mentors are represented by a 2D integer array `mentors` where `mentors[j]` is an integer array that contains the answers of the `jth` mentor (**0-indexed**).
Each student will be assigned to **one** mentor, and each mentor will have **one** student assigned to them. The **compatibility score** of a student-mentor pair is the number of answers that are the same for both the student and the mentor.
* For example, if the student's answers were `[1, 0, 1]` and the mentor's answers were `[0, 0, 1]`, then their compatibility score is 2 because only the second and the third answers are the same.
You are tasked with finding the optimal student-mentor pairings to **maximize** the **sum of the compatibility scores**.
Given `students` and `mentors`, return _the **maximum compatibility score sum** that can be achieved._
**Example 1:**
**Input:** students = \[\[1,1,0\],\[1,0,1\],\[0,0,1\]\], mentors = \[\[1,0,0\],\[0,0,1\],\[1,1,0\]\]
**Output:** 8
**Explanation:** We assign students to mentors in the following way:
- student 0 to mentor 2 with a compatibility score of 3.
- student 1 to mentor 0 with a compatibility score of 2.
- student 2 to mentor 1 with a compatibility score of 3.
The compatibility score sum is 3 + 2 + 3 = 8.
**Example 2:**
**Input:** students = \[\[0,0\],\[0,0\],\[0,0\]\], mentors = \[\[1,1\],\[1,1\],\[1,1\]\]
**Output:** 0
**Explanation:** The compatibility score of any student-mentor pair is 0.
**Constraints:**
* `m == students.length == mentors.length`
* `n == students[i].length == mentors[j].length`
* `1 <= m, n <= 8`
* `students[i][k]` is either `0` or `1`.
* `mentors[j][k]` is either `0` or `1`. | Think of how to check if a number x is a gcd of a subsequence. If there is such subsequence, then all of it will be divisible by x. Moreover, if you divide each number in the subsequence by x , then the gcd of the resulting numbers will be 1. Adding a number to a subsequence cannot increase its gcd. So, if there is a valid subsequence for x , then the subsequence that contains all multiples of x is a valid one too. Iterate on all possiblex from 1 to 10^5, and check if there is a valid subsequence for x. |
Factor Presence Mathematics | Commented and Explained | O(max(N, maxnum log maxnum)) T O(N) S | number-of-different-subsequences-gcds | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFirst, our problem space is not ideal, so we need to restructure for that. \nThe first way to reconstruct this more usefully is to consider only unique values of nums. This is because the unique values are what will form distinct subsequences. To do this we can set cast the list and get a speed up from O(N) to O(n) where n is the unique values of N. We also get a speed up in look up time from O(N) to O(1), which is arguably more important. \n\nNext, we know we will need a variable to store the number, so we set one. \n\nThen, we know we want the max number as this is obviously the greatest factor we can reach.\n\nFrom there we loop in range 1 to max num on an outer loop to represent the range of factors possible for gcd. An inner loop goes in steps of the current factor, and if the inner loop value is present, we can then set our current gcd and check it against our current factor. On a hit, we update number of different subsequences and break out of the inner loop. \n\nThis covers our number line on the outer loop in singular steps and the inner loop in factor steps, covering the subsequence ranges. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nDetailed mainly in intuition as I needed to write all of it out first \n\n# Complexity\n- Time complexity : O( max (N, max_num log max_num )) \n - Takes O(N) where N is number of all nums in nums to make a set \n - Takes O(n) to find the max of unique nums, where n is the number of unique nums in N, bounded by N if all unique \n - Takes O(max_num) for outer loop \n - Takes O(log(max_num)) for inner loop (due to step size) \n - In total takes O(N + n + max_num log max_num)\n - Since n is bounded by N, at worst it is O(N + max_num log max_num)\n - Due to this, we can say it is O( max (N, max_num log max_num )) \n\n- Space complexity : O( N ) \n - Store n unique values in unique nums -> O(N) \n\n# Code\n```\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums: List[int]) -> int:\n # set cast nums to get unique valuation \n unique_nums = set(nums)\n # build up number of different subsequence gcd as you go along \n number_of_different_subsequence_gcd = 0 \n # get the max num from the set in O(n) \n max_num = max(unique_nums) + 1 \n \n # for n_i in range \n for n_i in range(1, max_num) : \n # set gcd to start at 0 \n n_gcd = 0 \n # for n_j in steps of n_i up to max num \n for n_j in range(n_i, max_num, n_i) : \n # if n_j is in unique nums \n if n_j in unique_nums : \n # set n_gcd to gcd of self and n_j \n n_gcd = math.gcd(n_gcd, n_j)\n # if n_gcd is now n_i \n if n_gcd == n_i : \n # increment the number of difference sequences and quit this loop \n number_of_different_subsequence_gcd += 1 \n break \n else : \n continue \n else : \n continue \n \n # when done, the subsequence is handled by the frequency of the gcd factor presence \n return number_of_different_subsequence_gcd\n\n``` | 0 | You are given an array `nums` that consists of positive integers.
The **GCD** of a sequence of numbers is defined as the greatest integer that divides **all** the numbers in the sequence evenly.
* For example, the GCD of the sequence `[4,6,16]` is `2`.
A **subsequence** of an array is a sequence that can be formed by removing some elements (possibly none) of the array.
* For example, `[2,5,10]` is a subsequence of `[1,2,1,**2**,4,1,**5**,**10**]`.
Return _the **number** of **different** GCDs among all **non-empty** subsequences of_ `nums`.
**Example 1:**
**Input:** nums = \[6,10,3\]
**Output:** 5
**Explanation:** The figure shows all the non-empty subsequences and their GCDs.
The different GCDs are 6, 10, 3, 2, and 1.
**Example 2:**
**Input:** nums = \[5,15,40,5,6\]
**Output:** 7
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 2 * 105` | Heuristic algorithm may work. |
Factor Presence Mathematics | Commented and Explained | O(max(N, maxnum log maxnum)) T O(N) S | number-of-different-subsequences-gcds | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFirst, our problem space is not ideal, so we need to restructure for that. \nThe first way to reconstruct this more usefully is to consider only unique values of nums. This is because the unique values are what will form distinct subsequences. To do this we can set cast the list and get a speed up from O(N) to O(n) where n is the unique values of N. We also get a speed up in look up time from O(N) to O(1), which is arguably more important. \n\nNext, we know we will need a variable to store the number, so we set one. \n\nThen, we know we want the max number as this is obviously the greatest factor we can reach.\n\nFrom there we loop in range 1 to max num on an outer loop to represent the range of factors possible for gcd. An inner loop goes in steps of the current factor, and if the inner loop value is present, we can then set our current gcd and check it against our current factor. On a hit, we update number of different subsequences and break out of the inner loop. \n\nThis covers our number line on the outer loop in singular steps and the inner loop in factor steps, covering the subsequence ranges. \n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nDetailed mainly in intuition as I needed to write all of it out first \n\n# Complexity\n- Time complexity : O( max (N, max_num log max_num )) \n - Takes O(N) where N is number of all nums in nums to make a set \n - Takes O(n) to find the max of unique nums, where n is the number of unique nums in N, bounded by N if all unique \n - Takes O(max_num) for outer loop \n - Takes O(log(max_num)) for inner loop (due to step size) \n - In total takes O(N + n + max_num log max_num)\n - Since n is bounded by N, at worst it is O(N + max_num log max_num)\n - Due to this, we can say it is O( max (N, max_num log max_num )) \n\n- Space complexity : O( N ) \n - Store n unique values in unique nums -> O(N) \n\n# Code\n```\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums: List[int]) -> int:\n # set cast nums to get unique valuation \n unique_nums = set(nums)\n # build up number of different subsequence gcd as you go along \n number_of_different_subsequence_gcd = 0 \n # get the max num from the set in O(n) \n max_num = max(unique_nums) + 1 \n \n # for n_i in range \n for n_i in range(1, max_num) : \n # set gcd to start at 0 \n n_gcd = 0 \n # for n_j in steps of n_i up to max num \n for n_j in range(n_i, max_num, n_i) : \n # if n_j is in unique nums \n if n_j in unique_nums : \n # set n_gcd to gcd of self and n_j \n n_gcd = math.gcd(n_gcd, n_j)\n # if n_gcd is now n_i \n if n_gcd == n_i : \n # increment the number of difference sequences and quit this loop \n number_of_different_subsequence_gcd += 1 \n break \n else : \n continue \n else : \n continue \n \n # when done, the subsequence is handled by the frequency of the gcd factor presence \n return number_of_different_subsequence_gcd\n\n``` | 0 | There is a survey that consists of `n` questions where each question's answer is either `0` (no) or `1` (yes).
The survey was given to `m` students numbered from `0` to `m - 1` and `m` mentors numbered from `0` to `m - 1`. The answers of the students are represented by a 2D integer array `students` where `students[i]` is an integer array that contains the answers of the `ith` student (**0-indexed**). The answers of the mentors are represented by a 2D integer array `mentors` where `mentors[j]` is an integer array that contains the answers of the `jth` mentor (**0-indexed**).
Each student will be assigned to **one** mentor, and each mentor will have **one** student assigned to them. The **compatibility score** of a student-mentor pair is the number of answers that are the same for both the student and the mentor.
* For example, if the student's answers were `[1, 0, 1]` and the mentor's answers were `[0, 0, 1]`, then their compatibility score is 2 because only the second and the third answers are the same.
You are tasked with finding the optimal student-mentor pairings to **maximize** the **sum of the compatibility scores**.
Given `students` and `mentors`, return _the **maximum compatibility score sum** that can be achieved._
**Example 1:**
**Input:** students = \[\[1,1,0\],\[1,0,1\],\[0,0,1\]\], mentors = \[\[1,0,0\],\[0,0,1\],\[1,1,0\]\]
**Output:** 8
**Explanation:** We assign students to mentors in the following way:
- student 0 to mentor 2 with a compatibility score of 3.
- student 1 to mentor 0 with a compatibility score of 2.
- student 2 to mentor 1 with a compatibility score of 3.
The compatibility score sum is 3 + 2 + 3 = 8.
**Example 2:**
**Input:** students = \[\[0,0\],\[0,0\],\[0,0\]\], mentors = \[\[1,1\],\[1,1\],\[1,1\]\]
**Output:** 0
**Explanation:** The compatibility score of any student-mentor pair is 0.
**Constraints:**
* `m == students.length == mentors.length`
* `n == students[i].length == mentors[j].length`
* `1 <= m, n <= 8`
* `students[i][k]` is either `0` or `1`.
* `mentors[j][k]` is either `0` or `1`. | Think of how to check if a number x is a gcd of a subsequence. If there is such subsequence, then all of it will be divisible by x. Moreover, if you divide each number in the subsequence by x , then the gcd of the resulting numbers will be 1. Adding a number to a subsequence cannot increase its gcd. So, if there is a valid subsequence for x , then the subsequence that contains all multiples of x is a valid one too. Iterate on all possiblex from 1 to 10^5, and check if there is a valid subsequence for x. |
Number of Different Subsequences GCDs | number-of-different-subsequences-gcds | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums: List[int]) -> int:\n temp = max(nums) + 1\n nums = set(nums)\n ans = 0\n \n for x in range(1, temp):\n g = 0\n for y in range(x, temp, x):\n if y in nums:\n g = gcd(g, y)\n if g == x:\n break\n\n if g == x:\n ans += 1\n\n return ans\n``` | 0 | You are given an array `nums` that consists of positive integers.
The **GCD** of a sequence of numbers is defined as the greatest integer that divides **all** the numbers in the sequence evenly.
* For example, the GCD of the sequence `[4,6,16]` is `2`.
A **subsequence** of an array is a sequence that can be formed by removing some elements (possibly none) of the array.
* For example, `[2,5,10]` is a subsequence of `[1,2,1,**2**,4,1,**5**,**10**]`.
Return _the **number** of **different** GCDs among all **non-empty** subsequences of_ `nums`.
**Example 1:**
**Input:** nums = \[6,10,3\]
**Output:** 5
**Explanation:** The figure shows all the non-empty subsequences and their GCDs.
The different GCDs are 6, 10, 3, 2, and 1.
**Example 2:**
**Input:** nums = \[5,15,40,5,6\]
**Output:** 7
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 2 * 105` | Heuristic algorithm may work. |
Number of Different Subsequences GCDs | number-of-different-subsequences-gcds | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums: List[int]) -> int:\n temp = max(nums) + 1\n nums = set(nums)\n ans = 0\n \n for x in range(1, temp):\n g = 0\n for y in range(x, temp, x):\n if y in nums:\n g = gcd(g, y)\n if g == x:\n break\n\n if g == x:\n ans += 1\n\n return ans\n``` | 0 | There is a survey that consists of `n` questions where each question's answer is either `0` (no) or `1` (yes).
The survey was given to `m` students numbered from `0` to `m - 1` and `m` mentors numbered from `0` to `m - 1`. The answers of the students are represented by a 2D integer array `students` where `students[i]` is an integer array that contains the answers of the `ith` student (**0-indexed**). The answers of the mentors are represented by a 2D integer array `mentors` where `mentors[j]` is an integer array that contains the answers of the `jth` mentor (**0-indexed**).
Each student will be assigned to **one** mentor, and each mentor will have **one** student assigned to them. The **compatibility score** of a student-mentor pair is the number of answers that are the same for both the student and the mentor.
* For example, if the student's answers were `[1, 0, 1]` and the mentor's answers were `[0, 0, 1]`, then their compatibility score is 2 because only the second and the third answers are the same.
You are tasked with finding the optimal student-mentor pairings to **maximize** the **sum of the compatibility scores**.
Given `students` and `mentors`, return _the **maximum compatibility score sum** that can be achieved._
**Example 1:**
**Input:** students = \[\[1,1,0\],\[1,0,1\],\[0,0,1\]\], mentors = \[\[1,0,0\],\[0,0,1\],\[1,1,0\]\]
**Output:** 8
**Explanation:** We assign students to mentors in the following way:
- student 0 to mentor 2 with a compatibility score of 3.
- student 1 to mentor 0 with a compatibility score of 2.
- student 2 to mentor 1 with a compatibility score of 3.
The compatibility score sum is 3 + 2 + 3 = 8.
**Example 2:**
**Input:** students = \[\[0,0\],\[0,0\],\[0,0\]\], mentors = \[\[1,1\],\[1,1\],\[1,1\]\]
**Output:** 0
**Explanation:** The compatibility score of any student-mentor pair is 0.
**Constraints:**
* `m == students.length == mentors.length`
* `n == students[i].length == mentors[j].length`
* `1 <= m, n <= 8`
* `students[i][k]` is either `0` or `1`.
* `mentors[j][k]` is either `0` or `1`. | Think of how to check if a number x is a gcd of a subsequence. If there is such subsequence, then all of it will be divisible by x. Moreover, if you divide each number in the subsequence by x , then the gcd of the resulting numbers will be 1. Adding a number to a subsequence cannot increase its gcd. So, if there is a valid subsequence for x , then the subsequence that contains all multiples of x is a valid one too. Iterate on all possiblex from 1 to 10^5, and check if there is a valid subsequence for x. |
Python (Simple Maths) | number-of-different-subsequences-gcds | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums):\n nums, total, mx = set(nums), 0, max(nums)\n\n for i in range(1,mx+1):\n gcd_ = 0\n for j in range(i,mx+1,i):\n if j in nums:\n gcd_ = math.gcd(gcd_,j)\n\n if gcd_ == i:\n total += 1\n\n return total\n\n\n\n\n\n\n\n \n\n\n\n\n\n\n``` | 0 | You are given an array `nums` that consists of positive integers.
The **GCD** of a sequence of numbers is defined as the greatest integer that divides **all** the numbers in the sequence evenly.
* For example, the GCD of the sequence `[4,6,16]` is `2`.
A **subsequence** of an array is a sequence that can be formed by removing some elements (possibly none) of the array.
* For example, `[2,5,10]` is a subsequence of `[1,2,1,**2**,4,1,**5**,**10**]`.
Return _the **number** of **different** GCDs among all **non-empty** subsequences of_ `nums`.
**Example 1:**
**Input:** nums = \[6,10,3\]
**Output:** 5
**Explanation:** The figure shows all the non-empty subsequences and their GCDs.
The different GCDs are 6, 10, 3, 2, and 1.
**Example 2:**
**Input:** nums = \[5,15,40,5,6\]
**Output:** 7
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 2 * 105` | Heuristic algorithm may work. |
Python (Simple Maths) | number-of-different-subsequences-gcds | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums):\n nums, total, mx = set(nums), 0, max(nums)\n\n for i in range(1,mx+1):\n gcd_ = 0\n for j in range(i,mx+1,i):\n if j in nums:\n gcd_ = math.gcd(gcd_,j)\n\n if gcd_ == i:\n total += 1\n\n return total\n\n\n\n\n\n\n\n \n\n\n\n\n\n\n``` | 0 | There is a survey that consists of `n` questions where each question's answer is either `0` (no) or `1` (yes).
The survey was given to `m` students numbered from `0` to `m - 1` and `m` mentors numbered from `0` to `m - 1`. The answers of the students are represented by a 2D integer array `students` where `students[i]` is an integer array that contains the answers of the `ith` student (**0-indexed**). The answers of the mentors are represented by a 2D integer array `mentors` where `mentors[j]` is an integer array that contains the answers of the `jth` mentor (**0-indexed**).
Each student will be assigned to **one** mentor, and each mentor will have **one** student assigned to them. The **compatibility score** of a student-mentor pair is the number of answers that are the same for both the student and the mentor.
* For example, if the student's answers were `[1, 0, 1]` and the mentor's answers were `[0, 0, 1]`, then their compatibility score is 2 because only the second and the third answers are the same.
You are tasked with finding the optimal student-mentor pairings to **maximize** the **sum of the compatibility scores**.
Given `students` and `mentors`, return _the **maximum compatibility score sum** that can be achieved._
**Example 1:**
**Input:** students = \[\[1,1,0\],\[1,0,1\],\[0,0,1\]\], mentors = \[\[1,0,0\],\[0,0,1\],\[1,1,0\]\]
**Output:** 8
**Explanation:** We assign students to mentors in the following way:
- student 0 to mentor 2 with a compatibility score of 3.
- student 1 to mentor 0 with a compatibility score of 2.
- student 2 to mentor 1 with a compatibility score of 3.
The compatibility score sum is 3 + 2 + 3 = 8.
**Example 2:**
**Input:** students = \[\[0,0\],\[0,0\],\[0,0\]\], mentors = \[\[1,1\],\[1,1\],\[1,1\]\]
**Output:** 0
**Explanation:** The compatibility score of any student-mentor pair is 0.
**Constraints:**
* `m == students.length == mentors.length`
* `n == students[i].length == mentors[j].length`
* `1 <= m, n <= 8`
* `students[i][k]` is either `0` or `1`.
* `mentors[j][k]` is either `0` or `1`. | Think of how to check if a number x is a gcd of a subsequence. If there is such subsequence, then all of it will be divisible by x. Moreover, if you divide each number in the subsequence by x , then the gcd of the resulting numbers will be 1. Adding a number to a subsequence cannot increase its gcd. So, if there is a valid subsequence for x , then the subsequence that contains all multiples of x is a valid one too. Iterate on all possiblex from 1 to 10^5, and check if there is a valid subsequence for x. |
[Python3] - Testing all possible GCDs - Thought Process in the Comments | number-of-different-subsequences-gcds | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nAs it will be very tedious to build a ll possible subarrays and check for their gcd, we can inverse the problem and check for all possbile gcds as the number of those is limited by the maximum number.\n\nThere are severy things about the gcd listed in my comments that helped me coming up with the solution I posted here.\n\nTook me ages...\n# Approach\n<!-- Describe your approach to solving the problem. -->\nGCD rules...and gcd probing.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nI have no clue xD\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(N) for the unique numbers.\n# Code\n```\nimport math\n\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums: List[int]) -> int:\n # spontaneously two optimizations come to mind:\n #\n # 1) The highest possible gcd is the highest possible number\n # 2) The gcd will only every decrease if we add more numbers to a subarray\n # 3) It does not help to use one number multiple times (only unique numbers)\n # 4) We can exclude prime numbers (just add those to the to sequence itself)\n # 5) After one the gcd can not decrease any further by adding numbers\n # 6) If we sort the numbers we can stop after check the number equal to the current gcd\n #\n # Logical reasoning tells me that we can\'t build all subsequences\n #\n # Can we check which GCDs are possible as our numbers are of limited size?\n\n # make a set from the numbers for easy lookup\n nums = set(nums)\n\n # get the maximum number (as it represents our maximum gcd)\n maxi = max(nums)\n\n # go through every possible gcd and check whether we can build it\n result = 0\n for gcd in range(1, maxi+1):\n\n # make the current gcd\n cgcd = -1\n\n # check all multiples of gcd in our current numbers if it exists and keep updating the gcd\n for num in range(gcd, maxi+1, gcd):\n if num in nums:\n\n # set the cgcd if it is uninitialized\n if cgcd < 0:\n cgcd = num\n else:\n cgcd = math.gcd(cgcd, num)\n \n # check our current gcd whether it is correct of destroyed\n if cgcd == gcd: # we met it!\n result += 1\n break\n elif cgcd < gcd: # destroyed as gcd will never grow\n break\n return result\n``` | 0 | You are given an array `nums` that consists of positive integers.
The **GCD** of a sequence of numbers is defined as the greatest integer that divides **all** the numbers in the sequence evenly.
* For example, the GCD of the sequence `[4,6,16]` is `2`.
A **subsequence** of an array is a sequence that can be formed by removing some elements (possibly none) of the array.
* For example, `[2,5,10]` is a subsequence of `[1,2,1,**2**,4,1,**5**,**10**]`.
Return _the **number** of **different** GCDs among all **non-empty** subsequences of_ `nums`.
**Example 1:**
**Input:** nums = \[6,10,3\]
**Output:** 5
**Explanation:** The figure shows all the non-empty subsequences and their GCDs.
The different GCDs are 6, 10, 3, 2, and 1.
**Example 2:**
**Input:** nums = \[5,15,40,5,6\]
**Output:** 7
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 2 * 105` | Heuristic algorithm may work. |
[Python3] - Testing all possible GCDs - Thought Process in the Comments | number-of-different-subsequences-gcds | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nAs it will be very tedious to build a ll possible subarrays and check for their gcd, we can inverse the problem and check for all possbile gcds as the number of those is limited by the maximum number.\n\nThere are severy things about the gcd listed in my comments that helped me coming up with the solution I posted here.\n\nTook me ages...\n# Approach\n<!-- Describe your approach to solving the problem. -->\nGCD rules...and gcd probing.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nI have no clue xD\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(N) for the unique numbers.\n# Code\n```\nimport math\n\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums: List[int]) -> int:\n # spontaneously two optimizations come to mind:\n #\n # 1) The highest possible gcd is the highest possible number\n # 2) The gcd will only every decrease if we add more numbers to a subarray\n # 3) It does not help to use one number multiple times (only unique numbers)\n # 4) We can exclude prime numbers (just add those to the to sequence itself)\n # 5) After one the gcd can not decrease any further by adding numbers\n # 6) If we sort the numbers we can stop after check the number equal to the current gcd\n #\n # Logical reasoning tells me that we can\'t build all subsequences\n #\n # Can we check which GCDs are possible as our numbers are of limited size?\n\n # make a set from the numbers for easy lookup\n nums = set(nums)\n\n # get the maximum number (as it represents our maximum gcd)\n maxi = max(nums)\n\n # go through every possible gcd and check whether we can build it\n result = 0\n for gcd in range(1, maxi+1):\n\n # make the current gcd\n cgcd = -1\n\n # check all multiples of gcd in our current numbers if it exists and keep updating the gcd\n for num in range(gcd, maxi+1, gcd):\n if num in nums:\n\n # set the cgcd if it is uninitialized\n if cgcd < 0:\n cgcd = num\n else:\n cgcd = math.gcd(cgcd, num)\n \n # check our current gcd whether it is correct of destroyed\n if cgcd == gcd: # we met it!\n result += 1\n break\n elif cgcd < gcd: # destroyed as gcd will never grow\n break\n return result\n``` | 0 | There is a survey that consists of `n` questions where each question's answer is either `0` (no) or `1` (yes).
The survey was given to `m` students numbered from `0` to `m - 1` and `m` mentors numbered from `0` to `m - 1`. The answers of the students are represented by a 2D integer array `students` where `students[i]` is an integer array that contains the answers of the `ith` student (**0-indexed**). The answers of the mentors are represented by a 2D integer array `mentors` where `mentors[j]` is an integer array that contains the answers of the `jth` mentor (**0-indexed**).
Each student will be assigned to **one** mentor, and each mentor will have **one** student assigned to them. The **compatibility score** of a student-mentor pair is the number of answers that are the same for both the student and the mentor.
* For example, if the student's answers were `[1, 0, 1]` and the mentor's answers were `[0, 0, 1]`, then their compatibility score is 2 because only the second and the third answers are the same.
You are tasked with finding the optimal student-mentor pairings to **maximize** the **sum of the compatibility scores**.
Given `students` and `mentors`, return _the **maximum compatibility score sum** that can be achieved._
**Example 1:**
**Input:** students = \[\[1,1,0\],\[1,0,1\],\[0,0,1\]\], mentors = \[\[1,0,0\],\[0,0,1\],\[1,1,0\]\]
**Output:** 8
**Explanation:** We assign students to mentors in the following way:
- student 0 to mentor 2 with a compatibility score of 3.
- student 1 to mentor 0 with a compatibility score of 2.
- student 2 to mentor 1 with a compatibility score of 3.
The compatibility score sum is 3 + 2 + 3 = 8.
**Example 2:**
**Input:** students = \[\[0,0\],\[0,0\],\[0,0\]\], mentors = \[\[1,1\],\[1,1\],\[1,1\]\]
**Output:** 0
**Explanation:** The compatibility score of any student-mentor pair is 0.
**Constraints:**
* `m == students.length == mentors.length`
* `n == students[i].length == mentors[j].length`
* `1 <= m, n <= 8`
* `students[i][k]` is either `0` or `1`.
* `mentors[j][k]` is either `0` or `1`. | Think of how to check if a number x is a gcd of a subsequence. If there is such subsequence, then all of it will be divisible by x. Moreover, if you divide each number in the subsequence by x , then the gcd of the resulting numbers will be 1. Adding a number to a subsequence cannot increase its gcd. So, if there is a valid subsequence for x , then the subsequence that contains all multiples of x is a valid one too. Iterate on all possiblex from 1 to 10^5, and check if there is a valid subsequence for x. |
python iterative solution beats 100% | number-of-different-subsequences-gcds | 0 | 1 | # Intuition\nOne of the things I noticed, was that the max size of `nums` is almost as big (half) as the maximum number in nums.\nThis means, iterating over all values is approx. as expensive as iterating the array.\nWe can also construct a set from `nums` making membership testing (almost) $$O(1)$$.\n\n# Approach\nWe iterate over all possible values $i$ and check if they could be generated from the list.\nIf $i$ is in the set, we increment the counter and continue.\notherwise we check for every multiple of $i$ if it is in the set.\nIf so, we update the `gcd`.\nThe `gcd` contains information what multiple of $i$ the lowest found multiple so far is.\nwhen this reaches `1` we know, there exist some subset whose gcd is $i$ so we can increment the counter and continue with next $i$.\n\nWhile at first this seems like $O(m^2)$, it is actually $O(m*log(m))$.\nThe counter of the inner loop does only $m / i$ iterations.\nthe total complexity is \n$$m/1 + m/2 + m/3 + m/4+ ... + m/m$$ \n--> $$m*log(m)$$\n\n# Complexity\n- Time complexity:\n$$O(m*log(m))$$\n$m$ is the maximum value in `nums`\n\n- Space complexity:\n$$O(n)$$ \n(creating the set)\n\n# Code\n```\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums: List[int]) -> int:\n s = set(nums)\n count = 0\n m = max(nums)\n\n for i in range(1, m+1):\n if i in s:\n count += 1\n continue\n\n gcd = 0\n for j in range(2, m//i + 1):\n if j*i not in s:\n continue\n\n gcd = math.gcd(gcd, j)\n if gcd == 1:\n count += 1\n break\n\n return count\n``` | 0 | You are given an array `nums` that consists of positive integers.
The **GCD** of a sequence of numbers is defined as the greatest integer that divides **all** the numbers in the sequence evenly.
* For example, the GCD of the sequence `[4,6,16]` is `2`.
A **subsequence** of an array is a sequence that can be formed by removing some elements (possibly none) of the array.
* For example, `[2,5,10]` is a subsequence of `[1,2,1,**2**,4,1,**5**,**10**]`.
Return _the **number** of **different** GCDs among all **non-empty** subsequences of_ `nums`.
**Example 1:**
**Input:** nums = \[6,10,3\]
**Output:** 5
**Explanation:** The figure shows all the non-empty subsequences and their GCDs.
The different GCDs are 6, 10, 3, 2, and 1.
**Example 2:**
**Input:** nums = \[5,15,40,5,6\]
**Output:** 7
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 2 * 105` | Heuristic algorithm may work. |
python iterative solution beats 100% | number-of-different-subsequences-gcds | 0 | 1 | # Intuition\nOne of the things I noticed, was that the max size of `nums` is almost as big (half) as the maximum number in nums.\nThis means, iterating over all values is approx. as expensive as iterating the array.\nWe can also construct a set from `nums` making membership testing (almost) $$O(1)$$.\n\n# Approach\nWe iterate over all possible values $i$ and check if they could be generated from the list.\nIf $i$ is in the set, we increment the counter and continue.\notherwise we check for every multiple of $i$ if it is in the set.\nIf so, we update the `gcd`.\nThe `gcd` contains information what multiple of $i$ the lowest found multiple so far is.\nwhen this reaches `1` we know, there exist some subset whose gcd is $i$ so we can increment the counter and continue with next $i$.\n\nWhile at first this seems like $O(m^2)$, it is actually $O(m*log(m))$.\nThe counter of the inner loop does only $m / i$ iterations.\nthe total complexity is \n$$m/1 + m/2 + m/3 + m/4+ ... + m/m$$ \n--> $$m*log(m)$$\n\n# Complexity\n- Time complexity:\n$$O(m*log(m))$$\n$m$ is the maximum value in `nums`\n\n- Space complexity:\n$$O(n)$$ \n(creating the set)\n\n# Code\n```\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums: List[int]) -> int:\n s = set(nums)\n count = 0\n m = max(nums)\n\n for i in range(1, m+1):\n if i in s:\n count += 1\n continue\n\n gcd = 0\n for j in range(2, m//i + 1):\n if j*i not in s:\n continue\n\n gcd = math.gcd(gcd, j)\n if gcd == 1:\n count += 1\n break\n\n return count\n``` | 0 | There is a survey that consists of `n` questions where each question's answer is either `0` (no) or `1` (yes).
The survey was given to `m` students numbered from `0` to `m - 1` and `m` mentors numbered from `0` to `m - 1`. The answers of the students are represented by a 2D integer array `students` where `students[i]` is an integer array that contains the answers of the `ith` student (**0-indexed**). The answers of the mentors are represented by a 2D integer array `mentors` where `mentors[j]` is an integer array that contains the answers of the `jth` mentor (**0-indexed**).
Each student will be assigned to **one** mentor, and each mentor will have **one** student assigned to them. The **compatibility score** of a student-mentor pair is the number of answers that are the same for both the student and the mentor.
* For example, if the student's answers were `[1, 0, 1]` and the mentor's answers were `[0, 0, 1]`, then their compatibility score is 2 because only the second and the third answers are the same.
You are tasked with finding the optimal student-mentor pairings to **maximize** the **sum of the compatibility scores**.
Given `students` and `mentors`, return _the **maximum compatibility score sum** that can be achieved._
**Example 1:**
**Input:** students = \[\[1,1,0\],\[1,0,1\],\[0,0,1\]\], mentors = \[\[1,0,0\],\[0,0,1\],\[1,1,0\]\]
**Output:** 8
**Explanation:** We assign students to mentors in the following way:
- student 0 to mentor 2 with a compatibility score of 3.
- student 1 to mentor 0 with a compatibility score of 2.
- student 2 to mentor 1 with a compatibility score of 3.
The compatibility score sum is 3 + 2 + 3 = 8.
**Example 2:**
**Input:** students = \[\[0,0\],\[0,0\],\[0,0\]\], mentors = \[\[1,1\],\[1,1\],\[1,1\]\]
**Output:** 0
**Explanation:** The compatibility score of any student-mentor pair is 0.
**Constraints:**
* `m == students.length == mentors.length`
* `n == students[i].length == mentors[j].length`
* `1 <= m, n <= 8`
* `students[i][k]` is either `0` or `1`.
* `mentors[j][k]` is either `0` or `1`. | Think of how to check if a number x is a gcd of a subsequence. If there is such subsequence, then all of it will be divisible by x. Moreover, if you divide each number in the subsequence by x , then the gcd of the resulting numbers will be 1. Adding a number to a subsequence cannot increase its gcd. So, if there is a valid subsequence for x , then the subsequence that contains all multiples of x is a valid one too. Iterate on all possiblex from 1 to 10^5, and check if there is a valid subsequence for x. |
🌟Fully Explained Extremly Fast Beat 100% Elegant Clean Solution🌟 | number-of-different-subsequences-gcds | 0 | 1 | *Runtime: 5036 ms, faster than 100.00% of Python3 online submissions for Number of Different Subsequences GCDs.\nMemory Usage: 34.4 MB, less than 100.00% of Python3 online submissions for Number of Different Subsequences GCDs.*\n\n**Algorithm Steps:**\n1. Iterate over the possible GCD values (from 1 to maximal value in `nums`)\n2. If current gcd_candidate is indeed a GCD of values from `nums` - add it to the GCD options counter\n\nHopefully it makes sense :)\nBut how would we **know** if our gcd_candidate is indeed a GCD?\nLet\'s iterate over all values from `nums` *that are multiples of candidate* - and calculate GCD for them iteratevly - it we reach a state that the GCD is our candidate, then it\'s a valid GCD value!\n\n***Please upvote if you find the explanation helful! :)***\n\nCode:\n```\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums: List[int]) -> int:\n gcd_options = 0\n nums_set = set(nums)\n max_possible_gcd = max(nums_set)\n def get_candidate_multiples_from_nums(gcd_candidate, max_possible_gcd):\n for candidate_multiple in range(gcd_candidate, max_possible_gcd+1, gcd_candidate):\n if candidate_multiple in nums_set: \n yield candidate_multiple\n def is_candidate_a_gcd_of_numbers_from_num(gcd_candidate, max_possible_gcd):\n current_gcd = 0\n for candidate_multiple in get_candidate_multiples_from_nums(gcd_candidate, max_possible_gcd):\n if not current_gcd:\n current_gcd = candidate_multiple\n else:\n current_gcd = gcd(candidate_multiple, current_gcd)\n if current_gcd == gcd_candidate:\n return 1\n return 0\n for gcd_candidate in range(1, max_possible_gcd+1):\n if is_candidate_a_gcd_of_numbers_from_num(gcd_candidate, max_possible_gcd):\n gcd_options += 1 \n return gcd_options\n``` | 2 | You are given an array `nums` that consists of positive integers.
The **GCD** of a sequence of numbers is defined as the greatest integer that divides **all** the numbers in the sequence evenly.
* For example, the GCD of the sequence `[4,6,16]` is `2`.
A **subsequence** of an array is a sequence that can be formed by removing some elements (possibly none) of the array.
* For example, `[2,5,10]` is a subsequence of `[1,2,1,**2**,4,1,**5**,**10**]`.
Return _the **number** of **different** GCDs among all **non-empty** subsequences of_ `nums`.
**Example 1:**
**Input:** nums = \[6,10,3\]
**Output:** 5
**Explanation:** The figure shows all the non-empty subsequences and their GCDs.
The different GCDs are 6, 10, 3, 2, and 1.
**Example 2:**
**Input:** nums = \[5,15,40,5,6\]
**Output:** 7
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 2 * 105` | Heuristic algorithm may work. |
🌟Fully Explained Extremly Fast Beat 100% Elegant Clean Solution🌟 | number-of-different-subsequences-gcds | 0 | 1 | *Runtime: 5036 ms, faster than 100.00% of Python3 online submissions for Number of Different Subsequences GCDs.\nMemory Usage: 34.4 MB, less than 100.00% of Python3 online submissions for Number of Different Subsequences GCDs.*\n\n**Algorithm Steps:**\n1. Iterate over the possible GCD values (from 1 to maximal value in `nums`)\n2. If current gcd_candidate is indeed a GCD of values from `nums` - add it to the GCD options counter\n\nHopefully it makes sense :)\nBut how would we **know** if our gcd_candidate is indeed a GCD?\nLet\'s iterate over all values from `nums` *that are multiples of candidate* - and calculate GCD for them iteratevly - it we reach a state that the GCD is our candidate, then it\'s a valid GCD value!\n\n***Please upvote if you find the explanation helful! :)***\n\nCode:\n```\nclass Solution:\n def countDifferentSubsequenceGCDs(self, nums: List[int]) -> int:\n gcd_options = 0\n nums_set = set(nums)\n max_possible_gcd = max(nums_set)\n def get_candidate_multiples_from_nums(gcd_candidate, max_possible_gcd):\n for candidate_multiple in range(gcd_candidate, max_possible_gcd+1, gcd_candidate):\n if candidate_multiple in nums_set: \n yield candidate_multiple\n def is_candidate_a_gcd_of_numbers_from_num(gcd_candidate, max_possible_gcd):\n current_gcd = 0\n for candidate_multiple in get_candidate_multiples_from_nums(gcd_candidate, max_possible_gcd):\n if not current_gcd:\n current_gcd = candidate_multiple\n else:\n current_gcd = gcd(candidate_multiple, current_gcd)\n if current_gcd == gcd_candidate:\n return 1\n return 0\n for gcd_candidate in range(1, max_possible_gcd+1):\n if is_candidate_a_gcd_of_numbers_from_num(gcd_candidate, max_possible_gcd):\n gcd_options += 1 \n return gcd_options\n``` | 2 | There is a survey that consists of `n` questions where each question's answer is either `0` (no) or `1` (yes).
The survey was given to `m` students numbered from `0` to `m - 1` and `m` mentors numbered from `0` to `m - 1`. The answers of the students are represented by a 2D integer array `students` where `students[i]` is an integer array that contains the answers of the `ith` student (**0-indexed**). The answers of the mentors are represented by a 2D integer array `mentors` where `mentors[j]` is an integer array that contains the answers of the `jth` mentor (**0-indexed**).
Each student will be assigned to **one** mentor, and each mentor will have **one** student assigned to them. The **compatibility score** of a student-mentor pair is the number of answers that are the same for both the student and the mentor.
* For example, if the student's answers were `[1, 0, 1]` and the mentor's answers were `[0, 0, 1]`, then their compatibility score is 2 because only the second and the third answers are the same.
You are tasked with finding the optimal student-mentor pairings to **maximize** the **sum of the compatibility scores**.
Given `students` and `mentors`, return _the **maximum compatibility score sum** that can be achieved._
**Example 1:**
**Input:** students = \[\[1,1,0\],\[1,0,1\],\[0,0,1\]\], mentors = \[\[1,0,0\],\[0,0,1\],\[1,1,0\]\]
**Output:** 8
**Explanation:** We assign students to mentors in the following way:
- student 0 to mentor 2 with a compatibility score of 3.
- student 1 to mentor 0 with a compatibility score of 2.
- student 2 to mentor 1 with a compatibility score of 3.
The compatibility score sum is 3 + 2 + 3 = 8.
**Example 2:**
**Input:** students = \[\[0,0\],\[0,0\],\[0,0\]\], mentors = \[\[1,1\],\[1,1\],\[1,1\]\]
**Output:** 0
**Explanation:** The compatibility score of any student-mentor pair is 0.
**Constraints:**
* `m == students.length == mentors.length`
* `n == students[i].length == mentors[j].length`
* `1 <= m, n <= 8`
* `students[i][k]` is either `0` or `1`.
* `mentors[j][k]` is either `0` or `1`. | Think of how to check if a number x is a gcd of a subsequence. If there is such subsequence, then all of it will be divisible by x. Moreover, if you divide each number in the subsequence by x , then the gcd of the resulting numbers will be 1. Adding a number to a subsequence cannot increase its gcd. So, if there is a valid subsequence for x , then the subsequence that contains all multiples of x is a valid one too. Iterate on all possiblex from 1 to 10^5, and check if there is a valid subsequence for x. |
Python || easy to understand || conceptual | number-of-different-subsequences-gcds | 0 | 1 | ```\n# written by : dhruv vavliya\n\n\n\ndef gcd(a,b): # log(n)\n while b:\n a,b = b,a%b\n return a\n\t\nimport math\n\n# right but not accepted in python\ndef go(nums):\n b = max(nums)+1\n factor = [0]*b\n\n for i in range(len(nums)):\n for j in range(1,int(math.sqrt(nums[i]))+1):\n if nums[i]%j == 0:\n f1 = j\n f2 = nums[i]//j\n\n if factor[f1] == 0:\n factor[f1] = nums[i]\n else:\n factor[f1] = gcd(factor[f1] ,nums[i])\n\n if factor[f2] == 0:\n factor[f2] = nums[i]\n else:\n factor[f2] = gcd(factor[f2] ,nums[i])\n\n ans = 0\n for i in range(1,b):\n if factor[i] == i:\n ans+=1\n\n return ans\n\n\n\n# accepted in python\ndef solve(nums):\n biggest = max(nums)+1\n dp = [0]*biggest\n ans = 0\n for x in nums:\n dp[x] = True\n\n for j in range(1,biggest):\n g =0\n for x in range(j,biggest,j):\n if dp[x]:\n g = math.gcd(g,x)\n if g == j:\n break\n ans += (g==j)\n\n return ans\n\n``` | 1 | You are given an array `nums` that consists of positive integers.
The **GCD** of a sequence of numbers is defined as the greatest integer that divides **all** the numbers in the sequence evenly.
* For example, the GCD of the sequence `[4,6,16]` is `2`.
A **subsequence** of an array is a sequence that can be formed by removing some elements (possibly none) of the array.
* For example, `[2,5,10]` is a subsequence of `[1,2,1,**2**,4,1,**5**,**10**]`.
Return _the **number** of **different** GCDs among all **non-empty** subsequences of_ `nums`.
**Example 1:**
**Input:** nums = \[6,10,3\]
**Output:** 5
**Explanation:** The figure shows all the non-empty subsequences and their GCDs.
The different GCDs are 6, 10, 3, 2, and 1.
**Example 2:**
**Input:** nums = \[5,15,40,5,6\]
**Output:** 7
**Constraints:**
* `1 <= nums.length <= 105`
* `1 <= nums[i] <= 2 * 105` | Heuristic algorithm may work. |
Python || easy to understand || conceptual | number-of-different-subsequences-gcds | 0 | 1 | ```\n# written by : dhruv vavliya\n\n\n\ndef gcd(a,b): # log(n)\n while b:\n a,b = b,a%b\n return a\n\t\nimport math\n\n# right but not accepted in python\ndef go(nums):\n b = max(nums)+1\n factor = [0]*b\n\n for i in range(len(nums)):\n for j in range(1,int(math.sqrt(nums[i]))+1):\n if nums[i]%j == 0:\n f1 = j\n f2 = nums[i]//j\n\n if factor[f1] == 0:\n factor[f1] = nums[i]\n else:\n factor[f1] = gcd(factor[f1] ,nums[i])\n\n if factor[f2] == 0:\n factor[f2] = nums[i]\n else:\n factor[f2] = gcd(factor[f2] ,nums[i])\n\n ans = 0\n for i in range(1,b):\n if factor[i] == i:\n ans+=1\n\n return ans\n\n\n\n# accepted in python\ndef solve(nums):\n biggest = max(nums)+1\n dp = [0]*biggest\n ans = 0\n for x in nums:\n dp[x] = True\n\n for j in range(1,biggest):\n g =0\n for x in range(j,biggest,j):\n if dp[x]:\n g = math.gcd(g,x)\n if g == j:\n break\n ans += (g==j)\n\n return ans\n\n``` | 1 | There is a survey that consists of `n` questions where each question's answer is either `0` (no) or `1` (yes).
The survey was given to `m` students numbered from `0` to `m - 1` and `m` mentors numbered from `0` to `m - 1`. The answers of the students are represented by a 2D integer array `students` where `students[i]` is an integer array that contains the answers of the `ith` student (**0-indexed**). The answers of the mentors are represented by a 2D integer array `mentors` where `mentors[j]` is an integer array that contains the answers of the `jth` mentor (**0-indexed**).
Each student will be assigned to **one** mentor, and each mentor will have **one** student assigned to them. The **compatibility score** of a student-mentor pair is the number of answers that are the same for both the student and the mentor.
* For example, if the student's answers were `[1, 0, 1]` and the mentor's answers were `[0, 0, 1]`, then their compatibility score is 2 because only the second and the third answers are the same.
You are tasked with finding the optimal student-mentor pairings to **maximize** the **sum of the compatibility scores**.
Given `students` and `mentors`, return _the **maximum compatibility score sum** that can be achieved._
**Example 1:**
**Input:** students = \[\[1,1,0\],\[1,0,1\],\[0,0,1\]\], mentors = \[\[1,0,0\],\[0,0,1\],\[1,1,0\]\]
**Output:** 8
**Explanation:** We assign students to mentors in the following way:
- student 0 to mentor 2 with a compatibility score of 3.
- student 1 to mentor 0 with a compatibility score of 2.
- student 2 to mentor 1 with a compatibility score of 3.
The compatibility score sum is 3 + 2 + 3 = 8.
**Example 2:**
**Input:** students = \[\[0,0\],\[0,0\],\[0,0\]\], mentors = \[\[1,1\],\[1,1\],\[1,1\]\]
**Output:** 0
**Explanation:** The compatibility score of any student-mentor pair is 0.
**Constraints:**
* `m == students.length == mentors.length`
* `n == students[i].length == mentors[j].length`
* `1 <= m, n <= 8`
* `students[i][k]` is either `0` or `1`.
* `mentors[j][k]` is either `0` or `1`. | Think of how to check if a number x is a gcd of a subsequence. If there is such subsequence, then all of it will be divisible by x. Moreover, if you divide each number in the subsequence by x , then the gcd of the resulting numbers will be 1. Adding a number to a subsequence cannot increase its gcd. So, if there is a valid subsequence for x , then the subsequence that contains all multiples of x is a valid one too. Iterate on all possiblex from 1 to 10^5, and check if there is a valid subsequence for x. |
[c++ & Python] short and concise | sign-of-the-product-of-an-array | 0 | 1 | Count total number of negetive numbers in array \n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# C++\n```\nclass Solution {\npublic:\n int arraySign(vector<int>& nums) {\n int count = 0;\n for(auto i : nums){\n if(i == 0) return 0;\n else if(i < 0) count++;\n }\n if(count & 1) return -1;\n return 1;\n }\n};\n```\n\n# Python / Python3\n```\nclass Solution:\n def arraySign(self, nums: List[int]) -> int:\n count = 0\n for i in nums:\n if i == 0:\n return 0\n elif i < 1:\n count += 1\n if count & 1:\n return -1\n return 1\n``` | 1 | There is a function `signFunc(x)` that returns:
* `1` if `x` is positive.
* `-1` if `x` is negative.
* `0` if `x` is equal to `0`.
You are given an integer array `nums`. Let `product` be the product of all values in the array `nums`.
Return `signFunc(product)`.
**Example 1:**
**Input:** nums = \[-1,-2,-3,-4,3,2,1\]
**Output:** 1
**Explanation:** The product of all values in the array is 144, and signFunc(144) = 1
**Example 2:**
**Input:** nums = \[1,5,0,2,-3\]
**Output:** 0
**Explanation:** The product of all values in the array is 0, and signFunc(0) = 0
**Example 3:**
**Input:** nums = \[-1,1,-1,1,-1\]
**Output:** -1
**Explanation:** The product of all values in the array is -1, and signFunc(-1) = -1
**Constraints:**
* `1 <= nums.length <= 1000`
* `-100 <= nums[i] <= 100` | As with any good dp problem that uses palindromes, try building the palindrome from the edges The prime point is to check that no two adjacent characters are equal, so save the past character while building the palindrome. |
[c++ & Python] short and concise | sign-of-the-product-of-an-array | 0 | 1 | Count total number of negetive numbers in array \n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# C++\n```\nclass Solution {\npublic:\n int arraySign(vector<int>& nums) {\n int count = 0;\n for(auto i : nums){\n if(i == 0) return 0;\n else if(i < 0) count++;\n }\n if(count & 1) return -1;\n return 1;\n }\n};\n```\n\n# Python / Python3\n```\nclass Solution:\n def arraySign(self, nums: List[int]) -> int:\n count = 0\n for i in nums:\n if i == 0:\n return 0\n elif i < 1:\n count += 1\n if count & 1:\n return -1\n return 1\n``` | 1 | You are given an integer array `nums` of size `n`. You are asked to solve `n` queries for each integer `i` in the range `0 <= i < n`.
To solve the `ith` query:
1. Find the **minimum value** in each possible subarray of size `i + 1` of the array `nums`.
2. Find the **maximum** of those minimum values. This maximum is the **answer** to the query.
Return _a **0-indexed** integer array_ `ans` _of size_ `n` _such that_ `ans[i]` _is the answer to the_ `ith` _query_.
A **subarray** is a contiguous sequence of elements in an array.
**Example 1:**
**Input:** nums = \[0,1,2,4\]
**Output:** \[4,2,1,0\]
**Explanation:**
i=0:
- The subarrays of size 1 are \[0\], \[1\], \[2\], \[4\]. The minimum values are 0, 1, 2, 4.
- The maximum of the minimum values is 4.
i=1:
- The subarrays of size 2 are \[0,1\], \[1,2\], \[2,4\]. The minimum values are 0, 1, 2.
- The maximum of the minimum values is 2.
i=2:
- The subarrays of size 3 are \[0,1,2\], \[1,2,4\]. The minimum values are 0, 1.
- The maximum of the minimum values is 1.
i=3:
- There is one subarray of size 4, which is \[0,1,2,4\]. The minimum value is 0.
- There is only one value, so the maximum is 0.
**Example 2:**
**Input:** nums = \[10,20,50,10\]
**Output:** \[50,20,10,10\]
**Explanation:**
i=0:
- The subarrays of size 1 are \[10\], \[20\], \[50\], \[10\]. The minimum values are 10, 20, 50, 10.
- The maximum of the minimum values is 50.
i=1:
- The subarrays of size 2 are \[10,20\], \[20,50\], \[50,10\]. The minimum values are 10, 20, 10.
- The maximum of the minimum values is 20.
i=2:
- The subarrays of size 3 are \[10,20,50\], \[20,50,10\]. The minimum values are 10, 10.
- The maximum of the minimum values is 10.
i=3:
- There is one subarray of size 4, which is \[10,20,50,10\]. The minimum value is 10.
- There is only one value, so the maximum is 10.
**Constraints:**
* `n == nums.length`
* `1 <= n <= 105`
* `0 <= nums[i] <= 109` | If there is a 0 in the array the answer is 0 To avoid overflow make all the negative numbers -1 and all positive numbers 1 and calculate the prod |
[Python, Java] Elegant & Short | One Pass | sign-of-the-product-of-an-array | 0 | 1 | # Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(1)$$\n\n# Code\n\n```python []\nclass Solution:\n def arraySign(self, nums: List[int]) -> int:\n sign = 1\n\n for num in nums:\n if num < 0:\n sign *= -1\n if num == 0:\n return 0\n\n return sign\n```\n\n```java []\nclass Solution {\n public int arraySign(int[] nums) {\n int sign = 1;\n\n for (int num : nums) {\n if (num < 0)\n sign *= -1;\n else if (num == 0)\n return 0;\n }\n\n return sign;\n }\n}\n```\n | 1 | There is a function `signFunc(x)` that returns:
* `1` if `x` is positive.
* `-1` if `x` is negative.
* `0` if `x` is equal to `0`.
You are given an integer array `nums`. Let `product` be the product of all values in the array `nums`.
Return `signFunc(product)`.
**Example 1:**
**Input:** nums = \[-1,-2,-3,-4,3,2,1\]
**Output:** 1
**Explanation:** The product of all values in the array is 144, and signFunc(144) = 1
**Example 2:**
**Input:** nums = \[1,5,0,2,-3\]
**Output:** 0
**Explanation:** The product of all values in the array is 0, and signFunc(0) = 0
**Example 3:**
**Input:** nums = \[-1,1,-1,1,-1\]
**Output:** -1
**Explanation:** The product of all values in the array is -1, and signFunc(-1) = -1
**Constraints:**
* `1 <= nums.length <= 1000`
* `-100 <= nums[i] <= 100` | As with any good dp problem that uses palindromes, try building the palindrome from the edges The prime point is to check that no two adjacent characters are equal, so save the past character while building the palindrome. |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.