title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
Using O(sqrt N) for division and N for nums | four-divisors | 0 | 1 | upvote if you like\n# Code\n```\nclass Solution:\n def sumFourDivisors(self, nums: List[int]) -> int:\n def divisors(n):\n result = set()\n for i in range(1, int(n**0.5)+1):\n if n % i == 0:\n result.add(i)\n result.add(n//i)\n if len(result) > 4: return []\n else: return list(result) if len(result) == 4 else []\n s = 0\n for n in nums:\n s+=sum(divisors(n))\n return s\n```\n | 0 | Given an integer array `nums`, return _the sum of divisors of the integers in that array that have exactly four divisors_. If there is no such integer in the array, return `0`.
**Example 1:**
**Input:** nums = \[21,4,7\]
**Output:** 32
**Explanation:**
21 has 4 divisors: 1, 3, 7, 21
4 has 3 divisors: 1, 2, 4
7 has 2 divisors: 1, 7
The answer is the sum of divisors of 21 only.
**Example 2:**
**Input:** nums = \[21,21\]
**Output:** 64
**Example 3:**
**Input:** nums = \[1,2,3,4,5\]
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 104`
* `1 <= nums[i] <= 105` | null |
Using O(sqrt N) for division and N for nums | four-divisors | 0 | 1 | upvote if you like\n# Code\n```\nclass Solution:\n def sumFourDivisors(self, nums: List[int]) -> int:\n def divisors(n):\n result = set()\n for i in range(1, int(n**0.5)+1):\n if n % i == 0:\n result.add(i)\n result.add(n//i)\n if len(result) > 4: return []\n else: return list(result) if len(result) == 4 else []\n s = 0\n for n in nums:\n s+=sum(divisors(n))\n return s\n```\n | 0 | Given a `m x n` binary matrix `mat`. In one step, you can choose one cell and flip it and all the four neighbors of it if they exist (Flip is changing `1` to `0` and `0` to `1`). A pair of cells are called neighbors if they share one edge.
Return the _minimum number of steps_ required to convert `mat` to a zero matrix or `-1` if you cannot.
A **binary matrix** is a matrix with all cells equal to `0` or `1` only.
A **zero matrix** is a matrix with all cells equal to `0`.
**Example 1:**
**Input:** mat = \[\[0,0\],\[0,1\]\]
**Output:** 3
**Explanation:** One possible solution is to flip (1, 0) then (0, 1) and finally (1, 1) as shown.
**Example 2:**
**Input:** mat = \[\[0\]\]
**Output:** 0
**Explanation:** Given matrix is a zero matrix. We do not need to change it.
**Example 3:**
**Input:** mat = \[\[1,0,0\],\[1,0,0\]\]
**Output:** -1
**Explanation:** Given matrix cannot be a zero matrix.
**Constraints:**
* `m == mat.length`
* `n == mat[i].length`
* `1 <= m, n <= 3`
* `mat[i][j]` is either `0` or `1`. | Find the divisors of each element in the array. You only need to loop to the square root of a number to find its divisors. |
Python3 prime Beats 99.70% | four-divisors | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nd = set([2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179, 181, 191, 193, 197, 199, 211, 223, 227, 229, 233, 239, 241, 251, 257, 263, 269, 271, 277, 281, 283, 293, 307, 311, 313])\nclass Solution:\n def sumFourDivisors(self, nums: List[int]) -> int:\n n = Counter(nums)\n res = 0\n for i,v in n.items():\n if i in d:\n continue\n for j in d:\n if i % j == 0:\n k = i // j\n if k == j :\n break\n if k == j * j or k in d:\n res += (1 + i + j + k) * v\n break\n if all(k % i for i in d):\n res += (1 + i + j + k) * v\n break\n return res\n\n\n\n \n\n\n\n \n``` | 0 | Given an integer array `nums`, return _the sum of divisors of the integers in that array that have exactly four divisors_. If there is no such integer in the array, return `0`.
**Example 1:**
**Input:** nums = \[21,4,7\]
**Output:** 32
**Explanation:**
21 has 4 divisors: 1, 3, 7, 21
4 has 3 divisors: 1, 2, 4
7 has 2 divisors: 1, 7
The answer is the sum of divisors of 21 only.
**Example 2:**
**Input:** nums = \[21,21\]
**Output:** 64
**Example 3:**
**Input:** nums = \[1,2,3,4,5\]
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 104`
* `1 <= nums[i] <= 105` | null |
Python3 prime Beats 99.70% | four-divisors | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nd = set([2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71, 73, 79, 83, 89, 97, 101, 103, 107, 109, 113, 127, 131, 137, 139, 149, 151, 157, 163, 167, 173, 179, 181, 191, 193, 197, 199, 211, 223, 227, 229, 233, 239, 241, 251, 257, 263, 269, 271, 277, 281, 283, 293, 307, 311, 313])\nclass Solution:\n def sumFourDivisors(self, nums: List[int]) -> int:\n n = Counter(nums)\n res = 0\n for i,v in n.items():\n if i in d:\n continue\n for j in d:\n if i % j == 0:\n k = i // j\n if k == j :\n break\n if k == j * j or k in d:\n res += (1 + i + j + k) * v\n break\n if all(k % i for i in d):\n res += (1 + i + j + k) * v\n break\n return res\n\n\n\n \n\n\n\n \n``` | 0 | Given a `m x n` binary matrix `mat`. In one step, you can choose one cell and flip it and all the four neighbors of it if they exist (Flip is changing `1` to `0` and `0` to `1`). A pair of cells are called neighbors if they share one edge.
Return the _minimum number of steps_ required to convert `mat` to a zero matrix or `-1` if you cannot.
A **binary matrix** is a matrix with all cells equal to `0` or `1` only.
A **zero matrix** is a matrix with all cells equal to `0`.
**Example 1:**
**Input:** mat = \[\[0,0\],\[0,1\]\]
**Output:** 3
**Explanation:** One possible solution is to flip (1, 0) then (0, 1) and finally (1, 1) as shown.
**Example 2:**
**Input:** mat = \[\[0\]\]
**Output:** 0
**Explanation:** Given matrix is a zero matrix. We do not need to change it.
**Example 3:**
**Input:** mat = \[\[1,0,0\],\[1,0,0\]\]
**Output:** -1
**Explanation:** Given matrix cannot be a zero matrix.
**Constraints:**
* `m == mat.length`
* `n == mat[i].length`
* `1 <= m, n <= 3`
* `mat[i][j]` is either `0` or `1`. | Find the divisors of each element in the array. You only need to loop to the square root of a number to find its divisors. |
by PRODONiK (C++, Java, C#, Python, Ruby) | four-divisors | 1 | 1 | # Intuition\n The problem requires finding the sum of four-divisor integers in an array. A four-divisor integer is an integer with exactly four divisors. Our intuition is to iterate through the array, count divisors for each number, and calculate the sum of divisors if the count is exactly 4.\n\n# Approach\n Our approach involves two functions:\n - The `sumFourDivisors` function iterates through the input array `nums` and accumulates the results of the `countDivisors` function for each number in the array. It returns the final result.\n - The `countDivisors` function takes an integer `n`, counts its divisors, and calculates their sum. It returns this sum if the count is exactly 4, otherwise, it returns 0. We use a for loop to check divisors up to the square root of `n`, as divisors occur in pairs.\n\n# Complexity\n- Time complexity:\n The time complexity of this solution is O(n * sqrt(max_value)), where `n` is the number of elements in the array and `max_value` is the maximum value in the array.\n\n- Space complexity:\n The space complexity is O(1) as we only use a constant amount of additional memory for variables. \n\n# Code\n``` Java []\nclass Solution {\n public int sumFourDivisors(int[] nums) {\n int result = 0;\n for (int number : nums) {\n result += countDivisors(number, 0, 0);\n }\n return result;\n }\n public static int countDivisors(int n, int count, int sum) {\n for (int i = 1; i * i < n + 1; i++) {\n if (n % i == 0) {\n count += 2;\n sum += ((n / i) + i);\n if (count > 4) {\n return 0;\n }\n }\n }\n if (n % Math.sqrt(n) == 0) {\n count--;\n }\n if (count == 4) {\n return sum;\n }\n return 0;\n }\n}\n```\n``` C++ []\nclass Solution {\npublic:\n int sumFourDivisors(vector<int>& nums) {\n int result = 0;\n\n int countDivisors(int n, int count, int sum) {\n for (int i = 1; i * i <= n; i++) {\n if (n % i == 0) {\n count += 2;\n sum += ((n / i) + i);\n if (count > 4) {\n return 0;\n }\n }\n }\n if (n % sqrt(n) == 0) {\n count--;\n }\n if (count == 4) {\n return sum;\n }\n return 0;\n }\n\n for (int number : nums) {\n result += countDivisors(number, 0, 0);\n }\n \n return result;\n }\n};\n\n```\n``` Python []\nclass Solution:\n def sumFourDivisors(self, nums):\n result = 0\n\n def countDivisors(n, count, _sum):\n for i in range(1, int(n**0.5) + 1):\n if n % i == 0:\n count += 2\n _sum += (n // i + i)\n if count > 4:\n return 0\n if n % (n**0.5) == 0:\n count -= 1\n if count == 4:\n return _sum\n return 0\n\n for number in nums:\n result += countDivisors(number, 0, 0)\n \n return result\n```\n``` C# []\npublic class Solution {\n public int SumFourDivisors(int[] nums) {\n int result = 0;\n\n int CountDivisors(int n, int count, int sum) {\n for (int i = 1; i * i <= n; i++) {\n if (n % i == 0) {\n count += 2;\n sum += ((n / i) + i);\n if (count > 4) {\n return 0;\n }\n }\n }\n if (n % Math.Sqrt(n) == 0) {\n count--;\n }\n if (count == 4) {\n return sum;\n }\n return 0;\n }\n\n foreach (int number in nums) {\n result += CountDivisors(number, 0, 0);\n }\n \n return result;\n }\n}\n```\n``` Ruby []\nclass Solution\n def sum_four_divisors(nums)\n result = 0\n\n def count_divisors(n, count, sum)\n (1..Math.sqrt(n).to_i).each do |i|\n if n % i == 0\n count += 2\n sum += (n / i + i)\n return 0 if count > 4\n end\n end\n count -= 1 if n % Math.sqrt(n).to_i == 0\n return sum if count == 4\n 0\n end\n\n nums.each do |number|\n result += count_divisors(number, 0, 0)\n end\n\n result\n end\nend\n\n``` | 0 | Given an integer array `nums`, return _the sum of divisors of the integers in that array that have exactly four divisors_. If there is no such integer in the array, return `0`.
**Example 1:**
**Input:** nums = \[21,4,7\]
**Output:** 32
**Explanation:**
21 has 4 divisors: 1, 3, 7, 21
4 has 3 divisors: 1, 2, 4
7 has 2 divisors: 1, 7
The answer is the sum of divisors of 21 only.
**Example 2:**
**Input:** nums = \[21,21\]
**Output:** 64
**Example 3:**
**Input:** nums = \[1,2,3,4,5\]
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 104`
* `1 <= nums[i] <= 105` | null |
by PRODONiK (C++, Java, C#, Python, Ruby) | four-divisors | 1 | 1 | # Intuition\n The problem requires finding the sum of four-divisor integers in an array. A four-divisor integer is an integer with exactly four divisors. Our intuition is to iterate through the array, count divisors for each number, and calculate the sum of divisors if the count is exactly 4.\n\n# Approach\n Our approach involves two functions:\n - The `sumFourDivisors` function iterates through the input array `nums` and accumulates the results of the `countDivisors` function for each number in the array. It returns the final result.\n - The `countDivisors` function takes an integer `n`, counts its divisors, and calculates their sum. It returns this sum if the count is exactly 4, otherwise, it returns 0. We use a for loop to check divisors up to the square root of `n`, as divisors occur in pairs.\n\n# Complexity\n- Time complexity:\n The time complexity of this solution is O(n * sqrt(max_value)), where `n` is the number of elements in the array and `max_value` is the maximum value in the array.\n\n- Space complexity:\n The space complexity is O(1) as we only use a constant amount of additional memory for variables. \n\n# Code\n``` Java []\nclass Solution {\n public int sumFourDivisors(int[] nums) {\n int result = 0;\n for (int number : nums) {\n result += countDivisors(number, 0, 0);\n }\n return result;\n }\n public static int countDivisors(int n, int count, int sum) {\n for (int i = 1; i * i < n + 1; i++) {\n if (n % i == 0) {\n count += 2;\n sum += ((n / i) + i);\n if (count > 4) {\n return 0;\n }\n }\n }\n if (n % Math.sqrt(n) == 0) {\n count--;\n }\n if (count == 4) {\n return sum;\n }\n return 0;\n }\n}\n```\n``` C++ []\nclass Solution {\npublic:\n int sumFourDivisors(vector<int>& nums) {\n int result = 0;\n\n int countDivisors(int n, int count, int sum) {\n for (int i = 1; i * i <= n; i++) {\n if (n % i == 0) {\n count += 2;\n sum += ((n / i) + i);\n if (count > 4) {\n return 0;\n }\n }\n }\n if (n % sqrt(n) == 0) {\n count--;\n }\n if (count == 4) {\n return sum;\n }\n return 0;\n }\n\n for (int number : nums) {\n result += countDivisors(number, 0, 0);\n }\n \n return result;\n }\n};\n\n```\n``` Python []\nclass Solution:\n def sumFourDivisors(self, nums):\n result = 0\n\n def countDivisors(n, count, _sum):\n for i in range(1, int(n**0.5) + 1):\n if n % i == 0:\n count += 2\n _sum += (n // i + i)\n if count > 4:\n return 0\n if n % (n**0.5) == 0:\n count -= 1\n if count == 4:\n return _sum\n return 0\n\n for number in nums:\n result += countDivisors(number, 0, 0)\n \n return result\n```\n``` C# []\npublic class Solution {\n public int SumFourDivisors(int[] nums) {\n int result = 0;\n\n int CountDivisors(int n, int count, int sum) {\n for (int i = 1; i * i <= n; i++) {\n if (n % i == 0) {\n count += 2;\n sum += ((n / i) + i);\n if (count > 4) {\n return 0;\n }\n }\n }\n if (n % Math.Sqrt(n) == 0) {\n count--;\n }\n if (count == 4) {\n return sum;\n }\n return 0;\n }\n\n foreach (int number in nums) {\n result += CountDivisors(number, 0, 0);\n }\n \n return result;\n }\n}\n```\n``` Ruby []\nclass Solution\n def sum_four_divisors(nums)\n result = 0\n\n def count_divisors(n, count, sum)\n (1..Math.sqrt(n).to_i).each do |i|\n if n % i == 0\n count += 2\n sum += (n / i + i)\n return 0 if count > 4\n end\n end\n count -= 1 if n % Math.sqrt(n).to_i == 0\n return sum if count == 4\n 0\n end\n\n nums.each do |number|\n result += count_divisors(number, 0, 0)\n end\n\n result\n end\nend\n\n``` | 0 | Given a `m x n` binary matrix `mat`. In one step, you can choose one cell and flip it and all the four neighbors of it if they exist (Flip is changing `1` to `0` and `0` to `1`). A pair of cells are called neighbors if they share one edge.
Return the _minimum number of steps_ required to convert `mat` to a zero matrix or `-1` if you cannot.
A **binary matrix** is a matrix with all cells equal to `0` or `1` only.
A **zero matrix** is a matrix with all cells equal to `0`.
**Example 1:**
**Input:** mat = \[\[0,0\],\[0,1\]\]
**Output:** 3
**Explanation:** One possible solution is to flip (1, 0) then (0, 1) and finally (1, 1) as shown.
**Example 2:**
**Input:** mat = \[\[0\]\]
**Output:** 0
**Explanation:** Given matrix is a zero matrix. We do not need to change it.
**Example 3:**
**Input:** mat = \[\[1,0,0\],\[1,0,0\]\]
**Output:** -1
**Explanation:** Given matrix cannot be a zero matrix.
**Constraints:**
* `m == mat.length`
* `n == mat[i].length`
* `1 <= m, n <= 3`
* `mat[i][j]` is either `0` or `1`. | Find the divisors of each element in the array. You only need to loop to the square root of a number to find its divisors. |
Python3 Clean Solution | four-divisors | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def sumFourDivisors(self, nums: List[int]) -> int:\n \n \n @cache\n def f(x):\n c=sm=0\n for i in range(1,isqrt(x)+1):\n if x%i!=0:\n continue\n if i*i==x:\n c+=1\n sm+=i\n else:\n c+=2\n sm+=i\n sm+=(x//i)\n if c>4:\n break\n\n return sm if c==4 else 0\n \n \n return sum(f(val) for val in nums)\n \n``` | 0 | Given an integer array `nums`, return _the sum of divisors of the integers in that array that have exactly four divisors_. If there is no such integer in the array, return `0`.
**Example 1:**
**Input:** nums = \[21,4,7\]
**Output:** 32
**Explanation:**
21 has 4 divisors: 1, 3, 7, 21
4 has 3 divisors: 1, 2, 4
7 has 2 divisors: 1, 7
The answer is the sum of divisors of 21 only.
**Example 2:**
**Input:** nums = \[21,21\]
**Output:** 64
**Example 3:**
**Input:** nums = \[1,2,3,4,5\]
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 104`
* `1 <= nums[i] <= 105` | null |
Python3 Clean Solution | four-divisors | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def sumFourDivisors(self, nums: List[int]) -> int:\n \n \n @cache\n def f(x):\n c=sm=0\n for i in range(1,isqrt(x)+1):\n if x%i!=0:\n continue\n if i*i==x:\n c+=1\n sm+=i\n else:\n c+=2\n sm+=i\n sm+=(x//i)\n if c>4:\n break\n\n return sm if c==4 else 0\n \n \n return sum(f(val) for val in nums)\n \n``` | 0 | Given a `m x n` binary matrix `mat`. In one step, you can choose one cell and flip it and all the four neighbors of it if they exist (Flip is changing `1` to `0` and `0` to `1`). A pair of cells are called neighbors if they share one edge.
Return the _minimum number of steps_ required to convert `mat` to a zero matrix or `-1` if you cannot.
A **binary matrix** is a matrix with all cells equal to `0` or `1` only.
A **zero matrix** is a matrix with all cells equal to `0`.
**Example 1:**
**Input:** mat = \[\[0,0\],\[0,1\]\]
**Output:** 3
**Explanation:** One possible solution is to flip (1, 0) then (0, 1) and finally (1, 1) as shown.
**Example 2:**
**Input:** mat = \[\[0\]\]
**Output:** 0
**Explanation:** Given matrix is a zero matrix. We do not need to change it.
**Example 3:**
**Input:** mat = \[\[1,0,0\],\[1,0,0\]\]
**Output:** -1
**Explanation:** Given matrix cannot be a zero matrix.
**Constraints:**
* `m == mat.length`
* `n == mat[i].length`
* `1 <= m, n <= 3`
* `mat[i][j]` is either `0` or `1`. | Find the divisors of each element in the array. You only need to loop to the square root of a number to find its divisors. |
[100% Faster] I don't think anyone has done this yet 😂 | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | # Intuition\nJust do as the question says.\n\n# Complexity\n- Time complexity:\n$$O(N)$$ *since each cell is visited at most once*\n\n- Space complexity:\n$$O(N)$$ *for queue*\n\n# Code\n```\nclass Solution:\n def hasValidPath(self, grid: List[List[int]]) -> bool:\n m,n = len(grid),len(grid[0])\n paths = {\n 1: ["L","R"],\n 2: ["U","D"],\n 3: ["L","D"],\n 4: ["R","D"],\n 5: ["L","U"],\n 6: ["R","U"]\n }\n directions = {\n "R": (0,1),\n "L": (0,-1),\n "D": (1,0),\n "U": (-1,0)\n }\n opposites = {\n "R": "L",\n "L": "R",\n "U": "D",\n "D": "U"\n }\n\n visited = set()\n queue = deque()\n queue.append(((0,0),None))\n\n while queue:\n cur,prev = queue.popleft()\n x,y = cur\n \n if x<0 or x>=m or y<0 or y>=n: continue\n \n street = grid[x][y]\n if prev and prev not in paths[street]: continue\n if x == m-1 and y == n-1: return True\n\n for path in paths[street]:\n if path == prev: continue\n dx,dy = directions[path]\n newx,newy = x+dx,y+dy\n if (newx,newy) not in visited:\n visited.add((newx,newy))\n queue.append(((newx,newy),opposites[path]))\n\n return False\n\n``` | 1 | You are given an `m x n` `grid`. Each cell of `grid` represents a street. The street of `grid[i][j]` can be:
* `1` which means a street connecting the left cell and the right cell.
* `2` which means a street connecting the upper cell and the lower cell.
* `3` which means a street connecting the left cell and the lower cell.
* `4` which means a street connecting the right cell and the lower cell.
* `5` which means a street connecting the left cell and the upper cell.
* `6` which means a street connecting the right cell and the upper cell.
You will initially start at the street of the upper-left cell `(0, 0)`. A valid path in the grid is a path that starts from the upper left cell `(0, 0)` and ends at the bottom-right cell `(m - 1, n - 1)`. **The path should only follow the streets**.
**Notice** that you are **not allowed** to change any street.
Return `true` _if there is a valid path in the grid or_ `false` _otherwise_.
**Example 1:**
**Input:** grid = \[\[2,4,3\],\[6,5,2\]\]
**Output:** true
**Explanation:** As shown you can start at cell (0, 0) and visit all the cells of the grid to reach (m - 1, n - 1).
**Example 2:**
**Input:** grid = \[\[1,2,1\],\[1,2,1\]\]
**Output:** false
**Explanation:** As shown you the street at cell (0, 0) is not connected with any street of any other cell and you will get stuck at cell (0, 0)
**Example 3:**
**Input:** grid = \[\[1,1,2\]\]
**Output:** false
**Explanation:** You will get stuck at cell (0, 1) and you cannot reach cell (0, 2).
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 300`
* `1 <= grid[i][j] <= 6` | Use hashset to store all elements. Loop again to count all valid elements. |
[100% Faster] I don't think anyone has done this yet 😂 | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | # Intuition\nJust do as the question says.\n\n# Complexity\n- Time complexity:\n$$O(N)$$ *since each cell is visited at most once*\n\n- Space complexity:\n$$O(N)$$ *for queue*\n\n# Code\n```\nclass Solution:\n def hasValidPath(self, grid: List[List[int]]) -> bool:\n m,n = len(grid),len(grid[0])\n paths = {\n 1: ["L","R"],\n 2: ["U","D"],\n 3: ["L","D"],\n 4: ["R","D"],\n 5: ["L","U"],\n 6: ["R","U"]\n }\n directions = {\n "R": (0,1),\n "L": (0,-1),\n "D": (1,0),\n "U": (-1,0)\n }\n opposites = {\n "R": "L",\n "L": "R",\n "U": "D",\n "D": "U"\n }\n\n visited = set()\n queue = deque()\n queue.append(((0,0),None))\n\n while queue:\n cur,prev = queue.popleft()\n x,y = cur\n \n if x<0 or x>=m or y<0 or y>=n: continue\n \n street = grid[x][y]\n if prev and prev not in paths[street]: continue\n if x == m-1 and y == n-1: return True\n\n for path in paths[street]:\n if path == prev: continue\n dx,dy = directions[path]\n newx,newy = x+dx,y+dy\n if (newx,newy) not in visited:\n visited.add((newx,newy))\n queue.append(((newx,newy),opposites[path]))\n\n return False\n\n``` | 1 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
Python Solution with dfs and Untion Find | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | \n**Title: Valid Path in a Grid - Union-Find Solution\n**\nDescription:\n\nThe problem asks us to determine whether there exists a valid path in a given grid, where each cell represents a street with specific connections. We are not allowed to change any streets, and the valid path should start from the upper-left cell and end at the bottom-right cell.\n\nThis solution utilizes the Union-Find algorithm to efficiently determine the validity of the path. The approach begins by initializing a parent dictionary using a defaultdict and assigning each cell as its own parent. Additionally, a rank matrix is created to keep track of the rank of each cell.\n\nNext, the valid connections for right and down directions are defined using dictionaries. These dictionaries map each street type to the set of valid connections it has with neighboring cells. For example, street 1 can connect with streets 1, 3, and 5 to the right.\n\nThen, a nested loop iterates through each cell in the grid. For each cell, the algorithm checks the valid connections in the right and down directions. If a valid connection is found, the algorithm performs the union operation using the Union-Find data structure. This operation merges the sets containing the cells to connect them.\n\nFinally, the algorithm checks if the upper-left cell (0, 0) and the bottom-right cell (m-1, n-1) are in the same connected component. This is done by calling the find function on both cells and comparing their roots. If they belong to the same component, it indicates the existence of a valid path from the start to the end.\n\nThe solution efficiently handles the grid\'s connectivity using the Union-Find algorithm, allowing for quick determination of a valid path. By exploring the valid connections and performing unions between cells, the algorithm accurately determines if a valid path exists in the given grid.\n\nThe overall time complexity of this solution is O(m * n * \u03B1(m * n)), where m and n are the dimensions of the grid, and \u03B1 denotes the inverse Ackermann function. This time complexity arises from the union-find operations performed on the cells in the grid.\n\nOverall, this solution provides an efficient approach to solve the problem by leveraging the Union-Find algorithm and considering the valid connections between the cells in the grid.\n\n```\nclass Solution:\n def hasValidPath(self, grid: List[List[int]]) -> bool:\n parent=defaultdict(int)\n for i in range(len(grid)):\n for j in range(len(grid[i])):\n parent[(i,j)]=(i,j)\n \n rank=[[0 for j in range(len(grid[0]))] for i in range(len(grid))]\n directions=[(1,0),(0,1)]\n def inbound(row,col):\n return 0<=row<=len(grid)-1 and 0<=col<=len(grid[0])-1\n def find(x):\n root=x\n while root!=parent[root]:\n root=parent[root]\n \n while x!=root:\n temp=parent[x]\n parent[x]=root\n x=temp\n return root\n \n def union(pair1,pair2):\n parentX=find(pair1)\n parentY=find(pair2)\n if parentX==parentY:return\n if rank[parentX[0]][parentX[1]]==rank[parentY[0]][parentY[1]]:\n rank[parentX[0]][parentX[1]]+=1\n if rank[parentX[0]][parentX[1]]>rank[parentY[0]][parentY[1]]:\n parent[parentY]=parentX\n else:\n parent[parentX]=parentY\n right = {1: {1, 3, 5}, 2: {}, 3: {}, 4: {1, 3, 5}, 5: {}, 6: {1, 3, 5}}\n down = {1: {}, 2: {2, 5, 6}, 3: {2, 5, 6}, 4: {2, 5, 6}, 5: {}, 6: {}}\n for row in range(len(grid)):\n for col in range(len(grid[i])):\n for change_row,change_col in directions:\n new_row=row+change_row\n new_col=col+change_col\n if inbound(new_row,new_col)and grid[new_row][new_col] in right[grid[row][col]] and new_col-col==1:\n \n union((row,col),(new_row,new_col))\n if inbound(new_row,new_col)and grid[new_row][new_col] in down[grid[row][col]] and new_row-row==1:\n union((row,col),(new_row,new_col))\n return find((0,0))==find((len(grid)-1,len(grid[-1])-1))\n \n \n \n``` | 2 | You are given an `m x n` `grid`. Each cell of `grid` represents a street. The street of `grid[i][j]` can be:
* `1` which means a street connecting the left cell and the right cell.
* `2` which means a street connecting the upper cell and the lower cell.
* `3` which means a street connecting the left cell and the lower cell.
* `4` which means a street connecting the right cell and the lower cell.
* `5` which means a street connecting the left cell and the upper cell.
* `6` which means a street connecting the right cell and the upper cell.
You will initially start at the street of the upper-left cell `(0, 0)`. A valid path in the grid is a path that starts from the upper left cell `(0, 0)` and ends at the bottom-right cell `(m - 1, n - 1)`. **The path should only follow the streets**.
**Notice** that you are **not allowed** to change any street.
Return `true` _if there is a valid path in the grid or_ `false` _otherwise_.
**Example 1:**
**Input:** grid = \[\[2,4,3\],\[6,5,2\]\]
**Output:** true
**Explanation:** As shown you can start at cell (0, 0) and visit all the cells of the grid to reach (m - 1, n - 1).
**Example 2:**
**Input:** grid = \[\[1,2,1\],\[1,2,1\]\]
**Output:** false
**Explanation:** As shown you the street at cell (0, 0) is not connected with any street of any other cell and you will get stuck at cell (0, 0)
**Example 3:**
**Input:** grid = \[\[1,1,2\]\]
**Output:** false
**Explanation:** You will get stuck at cell (0, 1) and you cannot reach cell (0, 2).
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 300`
* `1 <= grid[i][j] <= 6` | Use hashset to store all elements. Loop again to count all valid elements. |
Python Solution with dfs and Untion Find | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | \n**Title: Valid Path in a Grid - Union-Find Solution\n**\nDescription:\n\nThe problem asks us to determine whether there exists a valid path in a given grid, where each cell represents a street with specific connections. We are not allowed to change any streets, and the valid path should start from the upper-left cell and end at the bottom-right cell.\n\nThis solution utilizes the Union-Find algorithm to efficiently determine the validity of the path. The approach begins by initializing a parent dictionary using a defaultdict and assigning each cell as its own parent. Additionally, a rank matrix is created to keep track of the rank of each cell.\n\nNext, the valid connections for right and down directions are defined using dictionaries. These dictionaries map each street type to the set of valid connections it has with neighboring cells. For example, street 1 can connect with streets 1, 3, and 5 to the right.\n\nThen, a nested loop iterates through each cell in the grid. For each cell, the algorithm checks the valid connections in the right and down directions. If a valid connection is found, the algorithm performs the union operation using the Union-Find data structure. This operation merges the sets containing the cells to connect them.\n\nFinally, the algorithm checks if the upper-left cell (0, 0) and the bottom-right cell (m-1, n-1) are in the same connected component. This is done by calling the find function on both cells and comparing their roots. If they belong to the same component, it indicates the existence of a valid path from the start to the end.\n\nThe solution efficiently handles the grid\'s connectivity using the Union-Find algorithm, allowing for quick determination of a valid path. By exploring the valid connections and performing unions between cells, the algorithm accurately determines if a valid path exists in the given grid.\n\nThe overall time complexity of this solution is O(m * n * \u03B1(m * n)), where m and n are the dimensions of the grid, and \u03B1 denotes the inverse Ackermann function. This time complexity arises from the union-find operations performed on the cells in the grid.\n\nOverall, this solution provides an efficient approach to solve the problem by leveraging the Union-Find algorithm and considering the valid connections between the cells in the grid.\n\n```\nclass Solution:\n def hasValidPath(self, grid: List[List[int]]) -> bool:\n parent=defaultdict(int)\n for i in range(len(grid)):\n for j in range(len(grid[i])):\n parent[(i,j)]=(i,j)\n \n rank=[[0 for j in range(len(grid[0]))] for i in range(len(grid))]\n directions=[(1,0),(0,1)]\n def inbound(row,col):\n return 0<=row<=len(grid)-1 and 0<=col<=len(grid[0])-1\n def find(x):\n root=x\n while root!=parent[root]:\n root=parent[root]\n \n while x!=root:\n temp=parent[x]\n parent[x]=root\n x=temp\n return root\n \n def union(pair1,pair2):\n parentX=find(pair1)\n parentY=find(pair2)\n if parentX==parentY:return\n if rank[parentX[0]][parentX[1]]==rank[parentY[0]][parentY[1]]:\n rank[parentX[0]][parentX[1]]+=1\n if rank[parentX[0]][parentX[1]]>rank[parentY[0]][parentY[1]]:\n parent[parentY]=parentX\n else:\n parent[parentX]=parentY\n right = {1: {1, 3, 5}, 2: {}, 3: {}, 4: {1, 3, 5}, 5: {}, 6: {1, 3, 5}}\n down = {1: {}, 2: {2, 5, 6}, 3: {2, 5, 6}, 4: {2, 5, 6}, 5: {}, 6: {}}\n for row in range(len(grid)):\n for col in range(len(grid[i])):\n for change_row,change_col in directions:\n new_row=row+change_row\n new_col=col+change_col\n if inbound(new_row,new_col)and grid[new_row][new_col] in right[grid[row][col]] and new_col-col==1:\n \n union((row,col),(new_row,new_col))\n if inbound(new_row,new_col)and grid[new_row][new_col] in down[grid[row][col]] and new_row-row==1:\n union((row,col),(new_row,new_col))\n return find((0,0))==find((len(grid)-1,len(grid[-1])-1))\n \n \n \n``` | 2 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
[Python] SUPER EASY Idea: just walk the maze based on the rule | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | 0 means going right, 1 means upwards, 2 means downwards, 3 means going left. \n\nAt cell with type 1, you can either enter it with right direction and leave it keeping the same direction, or enter it with left and leave for the left cell. So the rule for cell \'1\' is `{0: 0, 3: 3}`, and we can do the same transition rule for the other types.\n\nThen we can start walking in the maze. The initial position is (0, 0), and the initial direction can have two cases. If we walk outside of the maze, walk to a visited position or reach a pixel which does not permit the current direction, we exit the loop and return `False`.\n\nRuntime: 1460 ms, faster than 87.50% of Python3 online submissions for Check if There is a Valid Path in a Grid.\nMemory Usage: 27.6 MB, less than 100.00% of Python3 online submissions for Check if There is a Valid Path in a Grid.\n\n```\n def hasValidPath(self, grid: List[List[int]]) -> bool:\n m, n = len(grid), len(grid[0])\n \n # dict for transition rule\n rules = {\n 1: {0: 0, 3: 3},\n 2: {1: 1, 2: 2},\n 3: {0: 2, 1: 3},\n 4: {1: 0, 3: 2},\n 5: {0: 1, 2: 3},\n 6: {2: 0, 3: 1},\n }\n \n # dict for update rule\n moves = {\n 0: (0, 1),\n 1: (-1, 0),\n 2: (1, 0),\n 3: (0, -1),\n }\n \n x, y = (0, 0)\n k = grid[0][0]\n d = list(rules[k].keys())\n d1, d2 = d[0], d[1]\n \n def walkMaze(x, y, d):\n visited = set([(x, y)])\n while True:\n if x < 0 or x >= m or y < 0 or y >= n:\n return False\n \n k = grid[x][y]\n if d not in rules[k]:\n return False\n \n if (x, y) == (m - 1, n - 1):\n return True\n \n d = rules[k][d]\n x, y = (x + moves[d][0], y + moves[d][1])\n if (x, y) in visited: # there is a loop\n return False\n visited.add((x, y))\n \n return walkMaze(x, y, d1) or walkMaze(x, y, d2)\n\n``` | 9 | You are given an `m x n` `grid`. Each cell of `grid` represents a street. The street of `grid[i][j]` can be:
* `1` which means a street connecting the left cell and the right cell.
* `2` which means a street connecting the upper cell and the lower cell.
* `3` which means a street connecting the left cell and the lower cell.
* `4` which means a street connecting the right cell and the lower cell.
* `5` which means a street connecting the left cell and the upper cell.
* `6` which means a street connecting the right cell and the upper cell.
You will initially start at the street of the upper-left cell `(0, 0)`. A valid path in the grid is a path that starts from the upper left cell `(0, 0)` and ends at the bottom-right cell `(m - 1, n - 1)`. **The path should only follow the streets**.
**Notice** that you are **not allowed** to change any street.
Return `true` _if there is a valid path in the grid or_ `false` _otherwise_.
**Example 1:**
**Input:** grid = \[\[2,4,3\],\[6,5,2\]\]
**Output:** true
**Explanation:** As shown you can start at cell (0, 0) and visit all the cells of the grid to reach (m - 1, n - 1).
**Example 2:**
**Input:** grid = \[\[1,2,1\],\[1,2,1\]\]
**Output:** false
**Explanation:** As shown you the street at cell (0, 0) is not connected with any street of any other cell and you will get stuck at cell (0, 0)
**Example 3:**
**Input:** grid = \[\[1,1,2\]\]
**Output:** false
**Explanation:** You will get stuck at cell (0, 1) and you cannot reach cell (0, 2).
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 300`
* `1 <= grid[i][j] <= 6` | Use hashset to store all elements. Loop again to count all valid elements. |
[Python] SUPER EASY Idea: just walk the maze based on the rule | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | 0 means going right, 1 means upwards, 2 means downwards, 3 means going left. \n\nAt cell with type 1, you can either enter it with right direction and leave it keeping the same direction, or enter it with left and leave for the left cell. So the rule for cell \'1\' is `{0: 0, 3: 3}`, and we can do the same transition rule for the other types.\n\nThen we can start walking in the maze. The initial position is (0, 0), and the initial direction can have two cases. If we walk outside of the maze, walk to a visited position or reach a pixel which does not permit the current direction, we exit the loop and return `False`.\n\nRuntime: 1460 ms, faster than 87.50% of Python3 online submissions for Check if There is a Valid Path in a Grid.\nMemory Usage: 27.6 MB, less than 100.00% of Python3 online submissions for Check if There is a Valid Path in a Grid.\n\n```\n def hasValidPath(self, grid: List[List[int]]) -> bool:\n m, n = len(grid), len(grid[0])\n \n # dict for transition rule\n rules = {\n 1: {0: 0, 3: 3},\n 2: {1: 1, 2: 2},\n 3: {0: 2, 1: 3},\n 4: {1: 0, 3: 2},\n 5: {0: 1, 2: 3},\n 6: {2: 0, 3: 1},\n }\n \n # dict for update rule\n moves = {\n 0: (0, 1),\n 1: (-1, 0),\n 2: (1, 0),\n 3: (0, -1),\n }\n \n x, y = (0, 0)\n k = grid[0][0]\n d = list(rules[k].keys())\n d1, d2 = d[0], d[1]\n \n def walkMaze(x, y, d):\n visited = set([(x, y)])\n while True:\n if x < 0 or x >= m or y < 0 or y >= n:\n return False\n \n k = grid[x][y]\n if d not in rules[k]:\n return False\n \n if (x, y) == (m - 1, n - 1):\n return True\n \n d = rules[k][d]\n x, y = (x + moves[d][0], y + moves[d][1])\n if (x, y) in visited: # there is a loop\n return False\n visited.add((x, y))\n \n return walkMaze(x, y, d1) or walkMaze(x, y, d2)\n\n``` | 9 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
Ugly DFS Solution | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | # Intuition\nUgly DFS Solution\n\n# Approach\nUse previous coordinates and current coordinate to determine if the current road is reachable from the previous road.\n\nSome example scenarios to consider: \n- Coordinate (0, 0) cannot be of street type 5\n- If the current cell is street type 3, the previous cell must have been left of it (in which case we continue by traversing to the cell beneath) or vice-versa\n\n# Complexity\n- Time complexity:\n$$O(mn)$$ because we just need to visit each cell once\n\n- Space complexity:\n$$O(mn)$$ from the visited set\n\n# Code\n```\nclass Solution:\n def hasValidPath(self, grid: List[List[int]]) -> bool:\n\n visited = set()\n\n def inBounds(i, j):\n return i >= 0 and i < len(grid) and j >= 0 and j < len(grid[0])\n\n def dfs(i, j, pI, pJ):\n nonlocal visited\n if (i, j) in visited or not inBounds(i, j):\n return\n \n if grid[i][j] == 1:\n if pI == None and pJ == None:\n visited.add((i, j))\n dfs(i, j + 1, i, j)\n elif i == pI:\n visited.add((i, j))\n dfs(i, j + 1, i, j)\n dfs(i, j - 1, i, j)\n elif grid[i][j] == 2:\n if pI == None and pJ == None:\n visited.add((i, j))\n dfs(i + 1, j, i, j)\n elif j == pJ:\n visited.add((i, j))\n dfs(i + 1, j, i, j)\n dfs(i - 1, j, i, j)\n elif grid[i][j] == 3:\n if pI == None and pJ == None:\n visited.add((i, j))\n dfs(i + 1, j, i, j)\n elif pJ + 1 == j and i == pI:\n visited.add((i, j))\n dfs(i + 1, j, i, j)\n elif pJ == j and i + 1 == pI:\n visited.add((i, j))\n dfs(i, j - 1, i, j)\n elif grid[i][j] == 4:\n if pI == None and pJ == None:\n visited.add((i, j))\n dfs(i + 1, j, i, j)\n dfs(i, j + 1, i, j)\n elif i + 1 == pI and pJ == j:\n visited.add((i, j))\n dfs(i, j + 1, i, j)\n elif j + 1 == pJ and pI == i:\n visited.add((i, j))\n dfs(i + 1, j, i, j)\n elif grid[i][j] == 5:\n if pI == None and pJ == None:\n return\n elif pI + 1 == i and pJ == j:\n visited.add((i, j))\n dfs(i, j - 1, i, j)\n elif pI == i and pJ + 1 == j:\n visited.add((i, j))\n dfs(i - 1, j, i, j)\n elif grid[i][j] == 6:\n if pI == None and pJ == None:\n visited.add((i, j))\n dfs(i, j + 1, i, j)\n elif pI + 1 == i and pJ == j:\n visited.add((i, j))\n dfs(i, j + 1, i, j)\n elif pI == i and j + 1 == pJ:\n visited.add((i, j))\n dfs(i - 1, j, i, j)\n \n visited = set()\n dfs(0, 0, None, None)\n return (len(grid) - 1, len(grid[0]) - 1) in visited\n\n``` | 0 | You are given an `m x n` `grid`. Each cell of `grid` represents a street. The street of `grid[i][j]` can be:
* `1` which means a street connecting the left cell and the right cell.
* `2` which means a street connecting the upper cell and the lower cell.
* `3` which means a street connecting the left cell and the lower cell.
* `4` which means a street connecting the right cell and the lower cell.
* `5` which means a street connecting the left cell and the upper cell.
* `6` which means a street connecting the right cell and the upper cell.
You will initially start at the street of the upper-left cell `(0, 0)`. A valid path in the grid is a path that starts from the upper left cell `(0, 0)` and ends at the bottom-right cell `(m - 1, n - 1)`. **The path should only follow the streets**.
**Notice** that you are **not allowed** to change any street.
Return `true` _if there is a valid path in the grid or_ `false` _otherwise_.
**Example 1:**
**Input:** grid = \[\[2,4,3\],\[6,5,2\]\]
**Output:** true
**Explanation:** As shown you can start at cell (0, 0) and visit all the cells of the grid to reach (m - 1, n - 1).
**Example 2:**
**Input:** grid = \[\[1,2,1\],\[1,2,1\]\]
**Output:** false
**Explanation:** As shown you the street at cell (0, 0) is not connected with any street of any other cell and you will get stuck at cell (0, 0)
**Example 3:**
**Input:** grid = \[\[1,1,2\]\]
**Output:** false
**Explanation:** You will get stuck at cell (0, 1) and you cannot reach cell (0, 2).
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 300`
* `1 <= grid[i][j] <= 6` | Use hashset to store all elements. Loop again to count all valid elements. |
Ugly DFS Solution | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | # Intuition\nUgly DFS Solution\n\n# Approach\nUse previous coordinates and current coordinate to determine if the current road is reachable from the previous road.\n\nSome example scenarios to consider: \n- Coordinate (0, 0) cannot be of street type 5\n- If the current cell is street type 3, the previous cell must have been left of it (in which case we continue by traversing to the cell beneath) or vice-versa\n\n# Complexity\n- Time complexity:\n$$O(mn)$$ because we just need to visit each cell once\n\n- Space complexity:\n$$O(mn)$$ from the visited set\n\n# Code\n```\nclass Solution:\n def hasValidPath(self, grid: List[List[int]]) -> bool:\n\n visited = set()\n\n def inBounds(i, j):\n return i >= 0 and i < len(grid) and j >= 0 and j < len(grid[0])\n\n def dfs(i, j, pI, pJ):\n nonlocal visited\n if (i, j) in visited or not inBounds(i, j):\n return\n \n if grid[i][j] == 1:\n if pI == None and pJ == None:\n visited.add((i, j))\n dfs(i, j + 1, i, j)\n elif i == pI:\n visited.add((i, j))\n dfs(i, j + 1, i, j)\n dfs(i, j - 1, i, j)\n elif grid[i][j] == 2:\n if pI == None and pJ == None:\n visited.add((i, j))\n dfs(i + 1, j, i, j)\n elif j == pJ:\n visited.add((i, j))\n dfs(i + 1, j, i, j)\n dfs(i - 1, j, i, j)\n elif grid[i][j] == 3:\n if pI == None and pJ == None:\n visited.add((i, j))\n dfs(i + 1, j, i, j)\n elif pJ + 1 == j and i == pI:\n visited.add((i, j))\n dfs(i + 1, j, i, j)\n elif pJ == j and i + 1 == pI:\n visited.add((i, j))\n dfs(i, j - 1, i, j)\n elif grid[i][j] == 4:\n if pI == None and pJ == None:\n visited.add((i, j))\n dfs(i + 1, j, i, j)\n dfs(i, j + 1, i, j)\n elif i + 1 == pI and pJ == j:\n visited.add((i, j))\n dfs(i, j + 1, i, j)\n elif j + 1 == pJ and pI == i:\n visited.add((i, j))\n dfs(i + 1, j, i, j)\n elif grid[i][j] == 5:\n if pI == None and pJ == None:\n return\n elif pI + 1 == i and pJ == j:\n visited.add((i, j))\n dfs(i, j - 1, i, j)\n elif pI == i and pJ + 1 == j:\n visited.add((i, j))\n dfs(i - 1, j, i, j)\n elif grid[i][j] == 6:\n if pI == None and pJ == None:\n visited.add((i, j))\n dfs(i, j + 1, i, j)\n elif pI + 1 == i and pJ == j:\n visited.add((i, j))\n dfs(i, j + 1, i, j)\n elif pI == i and j + 1 == pJ:\n visited.add((i, j))\n dfs(i - 1, j, i, j)\n \n visited = set()\n dfs(0, 0, None, None)\n return (len(grid) - 1, len(grid[0]) - 1) in visited\n\n``` | 0 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
Simple DFS solution | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | ```\nclass Solution:\n def hasValidPath(self, grid: List[List[int]]) -> bool:\n step = {(0, 1):{1, 3, 5}, (0, -1):{1, 4, 6}, (1, 0):{2, 5, 6}, (-1, 0):{2, 3, 4}}\n direct = {1:[(0,-1), (0,1)], 2:[(-1,0), (1,0)], 3:[(0,-1), (1,0)], 4:[(0,1), (1,0)], 5:[(0,-1), (-1,0)], 6:[(0,1), (-1,0)]}\n n, m = len(grid), len(grid[0])\n stack = [(0, 0)]\n while stack:\n x, y = stack.pop()\n for i, j in direct.get(grid[x][y], []):\n ii, jj = x+i, y+j\n if 0 <= ii < n and 0 <= jj < m and grid[ii][jj] in step[(i, j)]:\n stack.append((ii, jj))\n grid[x][y] = 0 \n return not grid[n-1][m-1] \n``` | 0 | You are given an `m x n` `grid`. Each cell of `grid` represents a street. The street of `grid[i][j]` can be:
* `1` which means a street connecting the left cell and the right cell.
* `2` which means a street connecting the upper cell and the lower cell.
* `3` which means a street connecting the left cell and the lower cell.
* `4` which means a street connecting the right cell and the lower cell.
* `5` which means a street connecting the left cell and the upper cell.
* `6` which means a street connecting the right cell and the upper cell.
You will initially start at the street of the upper-left cell `(0, 0)`. A valid path in the grid is a path that starts from the upper left cell `(0, 0)` and ends at the bottom-right cell `(m - 1, n - 1)`. **The path should only follow the streets**.
**Notice** that you are **not allowed** to change any street.
Return `true` _if there is a valid path in the grid or_ `false` _otherwise_.
**Example 1:**
**Input:** grid = \[\[2,4,3\],\[6,5,2\]\]
**Output:** true
**Explanation:** As shown you can start at cell (0, 0) and visit all the cells of the grid to reach (m - 1, n - 1).
**Example 2:**
**Input:** grid = \[\[1,2,1\],\[1,2,1\]\]
**Output:** false
**Explanation:** As shown you the street at cell (0, 0) is not connected with any street of any other cell and you will get stuck at cell (0, 0)
**Example 3:**
**Input:** grid = \[\[1,1,2\]\]
**Output:** false
**Explanation:** You will get stuck at cell (0, 1) and you cannot reach cell (0, 2).
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 300`
* `1 <= grid[i][j] <= 6` | Use hashset to store all elements. Loop again to count all valid elements. |
Simple DFS solution | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | ```\nclass Solution:\n def hasValidPath(self, grid: List[List[int]]) -> bool:\n step = {(0, 1):{1, 3, 5}, (0, -1):{1, 4, 6}, (1, 0):{2, 5, 6}, (-1, 0):{2, 3, 4}}\n direct = {1:[(0,-1), (0,1)], 2:[(-1,0), (1,0)], 3:[(0,-1), (1,0)], 4:[(0,1), (1,0)], 5:[(0,-1), (-1,0)], 6:[(0,1), (-1,0)]}\n n, m = len(grid), len(grid[0])\n stack = [(0, 0)]\n while stack:\n x, y = stack.pop()\n for i, j in direct.get(grid[x][y], []):\n ii, jj = x+i, y+j\n if 0 <= ii < n and 0 <= jj < m and grid[ii][jj] in step[(i, j)]:\n stack.append((ii, jj))\n grid[x][y] = 0 \n return not grid[n-1][m-1] \n``` | 0 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
Easy Python DFS | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | \n# Code\n```\nclass Solution:\n def dfs(self,grid,i,j):\n z=grid[i][j]\n grid[i][j]=-1\n \n \n \n if z==1:\n q=i\n w=j+1\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [1,3,5]:\n self.dfs(grid,q,w)\n q=i\n w=j-1\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [1,4,6]:\n self.dfs(grid,q,w)\n if z==2:\n \n q=i-1\n w=j\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [2,3,4]:\n self.dfs(grid,q,w)\n q=i+1\n w=j\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [2,5,6]:\n self.dfs(grid,q,w)\n if z==3:\n \n q=i\n w=j-1\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [1,4,6]:\n self.dfs(grid,q,w)\n q=i+1\n w=j\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [2,5,6]:\n self.dfs(grid,q,w)\n if z==4:\n \n q=i\n w=j+1\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [1,3,5]:\n self.dfs(grid,q,w)\n q=i+1\n w=j\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [2,5,6]:\n self.dfs(grid,q,w)\n if z==5:\n \n q=i-1\n w=j\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [2,3,4]:\n self.dfs(grid,q,w)\n q=i\n w=j-1\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [1,4,6]:\n self.dfs(grid,q,w)\n if z==6:\n \n q=i-1\n w=j\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [2,4,3]:\n self.dfs(grid,q,w)\n q=i\n w=j+1\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [1,5,3]:\n self.dfs(grid,q,w)\n\n\n \n def hasValidPath(self, grid: List[List[int]]) -> bool:\n self.dfs(grid,0,0)\n if grid[-1][-1]==-1:\n return True\n return False\n\n \n``` | 0 | You are given an `m x n` `grid`. Each cell of `grid` represents a street. The street of `grid[i][j]` can be:
* `1` which means a street connecting the left cell and the right cell.
* `2` which means a street connecting the upper cell and the lower cell.
* `3` which means a street connecting the left cell and the lower cell.
* `4` which means a street connecting the right cell and the lower cell.
* `5` which means a street connecting the left cell and the upper cell.
* `6` which means a street connecting the right cell and the upper cell.
You will initially start at the street of the upper-left cell `(0, 0)`. A valid path in the grid is a path that starts from the upper left cell `(0, 0)` and ends at the bottom-right cell `(m - 1, n - 1)`. **The path should only follow the streets**.
**Notice** that you are **not allowed** to change any street.
Return `true` _if there is a valid path in the grid or_ `false` _otherwise_.
**Example 1:**
**Input:** grid = \[\[2,4,3\],\[6,5,2\]\]
**Output:** true
**Explanation:** As shown you can start at cell (0, 0) and visit all the cells of the grid to reach (m - 1, n - 1).
**Example 2:**
**Input:** grid = \[\[1,2,1\],\[1,2,1\]\]
**Output:** false
**Explanation:** As shown you the street at cell (0, 0) is not connected with any street of any other cell and you will get stuck at cell (0, 0)
**Example 3:**
**Input:** grid = \[\[1,1,2\]\]
**Output:** false
**Explanation:** You will get stuck at cell (0, 1) and you cannot reach cell (0, 2).
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 300`
* `1 <= grid[i][j] <= 6` | Use hashset to store all elements. Loop again to count all valid elements. |
Easy Python DFS | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | \n# Code\n```\nclass Solution:\n def dfs(self,grid,i,j):\n z=grid[i][j]\n grid[i][j]=-1\n \n \n \n if z==1:\n q=i\n w=j+1\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [1,3,5]:\n self.dfs(grid,q,w)\n q=i\n w=j-1\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [1,4,6]:\n self.dfs(grid,q,w)\n if z==2:\n \n q=i-1\n w=j\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [2,3,4]:\n self.dfs(grid,q,w)\n q=i+1\n w=j\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [2,5,6]:\n self.dfs(grid,q,w)\n if z==3:\n \n q=i\n w=j-1\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [1,4,6]:\n self.dfs(grid,q,w)\n q=i+1\n w=j\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [2,5,6]:\n self.dfs(grid,q,w)\n if z==4:\n \n q=i\n w=j+1\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [1,3,5]:\n self.dfs(grid,q,w)\n q=i+1\n w=j\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [2,5,6]:\n self.dfs(grid,q,w)\n if z==5:\n \n q=i-1\n w=j\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [2,3,4]:\n self.dfs(grid,q,w)\n q=i\n w=j-1\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [1,4,6]:\n self.dfs(grid,q,w)\n if z==6:\n \n q=i-1\n w=j\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [2,4,3]:\n self.dfs(grid,q,w)\n q=i\n w=j+1\n if q>=0 and w>=0 and q<len(grid) and w<len(grid[0]) and grid[q][w] in [1,5,3]:\n self.dfs(grid,q,w)\n\n\n \n def hasValidPath(self, grid: List[List[int]]) -> bool:\n self.dfs(grid,0,0)\n if grid[-1][-1]==-1:\n return True\n return False\n\n \n``` | 0 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
Python solution beated 99% | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n- O(N*M)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n- O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def hasValidPath(self, grid: List[List[int]]) -> bool:\n L, R, U, D = 0, 1, 2, 3\n exits = {\n (L, 1): L,\n (R, 1): R,\n (U, 2): U,\n (D, 2): D,\n (L, 3): U,\n (D, 3): R,\n (R, 4): U,\n (D, 4): L,\n (L, 5): D,\n (U, 5): R,\n (U, 6): L,\n (R, 6): D,\n }\n n, m = len(grid), len(grid[0])\n def solve(dir, curr):\n x, y = 0, 0\n while x != n - 1 or y != m - 1:\n next_dir = exits[(dir, curr)]\n if next_dir == L:\n y += 1\n elif next_dir == R:\n y -= 1\n elif next_dir == U:\n x += 1\n elif next_dir == D:\n x -= 1\n if x < 0 or x >= n:\n return False\n if y < 0 or y >= m:\n return False\n if x == 0 and y == 0:\n return False\n if (next_dir, grid[x][y]) not in exits:\n return False\n dir, curr = next_dir, grid[x][y]\n return True \n if grid[0][0] == 1:\n return solve(L, 1)\n if grid[0][0] == 2:\n return solve(U, 2)\n if grid[0][0] == 3:\n return solve(L, 3)\n if grid[0][0] == 4:\n return solve(R, 4) or solve(D, 4)\n if grid[0][0] == 6:\n return solve(U, 6)\n return False\n``` | 0 | You are given an `m x n` `grid`. Each cell of `grid` represents a street. The street of `grid[i][j]` can be:
* `1` which means a street connecting the left cell and the right cell.
* `2` which means a street connecting the upper cell and the lower cell.
* `3` which means a street connecting the left cell and the lower cell.
* `4` which means a street connecting the right cell and the lower cell.
* `5` which means a street connecting the left cell and the upper cell.
* `6` which means a street connecting the right cell and the upper cell.
You will initially start at the street of the upper-left cell `(0, 0)`. A valid path in the grid is a path that starts from the upper left cell `(0, 0)` and ends at the bottom-right cell `(m - 1, n - 1)`. **The path should only follow the streets**.
**Notice** that you are **not allowed** to change any street.
Return `true` _if there is a valid path in the grid or_ `false` _otherwise_.
**Example 1:**
**Input:** grid = \[\[2,4,3\],\[6,5,2\]\]
**Output:** true
**Explanation:** As shown you can start at cell (0, 0) and visit all the cells of the grid to reach (m - 1, n - 1).
**Example 2:**
**Input:** grid = \[\[1,2,1\],\[1,2,1\]\]
**Output:** false
**Explanation:** As shown you the street at cell (0, 0) is not connected with any street of any other cell and you will get stuck at cell (0, 0)
**Example 3:**
**Input:** grid = \[\[1,1,2\]\]
**Output:** false
**Explanation:** You will get stuck at cell (0, 1) and you cannot reach cell (0, 2).
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 300`
* `1 <= grid[i][j] <= 6` | Use hashset to store all elements. Loop again to count all valid elements. |
Python solution beated 99% | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n- O(N*M)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n- O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def hasValidPath(self, grid: List[List[int]]) -> bool:\n L, R, U, D = 0, 1, 2, 3\n exits = {\n (L, 1): L,\n (R, 1): R,\n (U, 2): U,\n (D, 2): D,\n (L, 3): U,\n (D, 3): R,\n (R, 4): U,\n (D, 4): L,\n (L, 5): D,\n (U, 5): R,\n (U, 6): L,\n (R, 6): D,\n }\n n, m = len(grid), len(grid[0])\n def solve(dir, curr):\n x, y = 0, 0\n while x != n - 1 or y != m - 1:\n next_dir = exits[(dir, curr)]\n if next_dir == L:\n y += 1\n elif next_dir == R:\n y -= 1\n elif next_dir == U:\n x += 1\n elif next_dir == D:\n x -= 1\n if x < 0 or x >= n:\n return False\n if y < 0 or y >= m:\n return False\n if x == 0 and y == 0:\n return False\n if (next_dir, grid[x][y]) not in exits:\n return False\n dir, curr = next_dir, grid[x][y]\n return True \n if grid[0][0] == 1:\n return solve(L, 1)\n if grid[0][0] == 2:\n return solve(U, 2)\n if grid[0][0] == 3:\n return solve(L, 3)\n if grid[0][0] == 4:\n return solve(R, 4) or solve(D, 4)\n if grid[0][0] == 6:\n return solve(U, 6)\n return False\n``` | 0 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
Python | DFS Solution | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def hasValidPath(self, grid: List[List[int]]) -> bool: \n n=len(grid)\n m=len(grid[0])\n a=[0]\n v=set() \n \n d={\n \'down\':[\n [2,3,4],[2,5,6]\n ],\n \'right\':[\n [1,4,6],[1,3,5]\n ],\n \'up\':[\n [2,5,6],[2,3,4]\n ],\n \'left\':[\n [1,3,5],[1,4,6]\n ]\n } \n\n def dfs(i,j):\n if (i,j) in v:\n return\n if i==n-1 and j==m-1:\n a[0]=1\n return\n v.add((i,j))\n \n if i-1>=0:\n if grid[i][j] in d[\'up\'][0] and grid[i-1][j] in d[\'up\'][1]:\n dfs(i-1,j)\n\n if i+1<n:\n if grid[i][j] in d[\'down\'][0] and grid[i+1][j] in d[\'down\'][1]:\n dfs(i+1,j)\n\n if j-1>=0:\n if grid[i][j] in d[\'left\'][0] and grid[i][j-1] in d[\'left\'][1]:\n dfs(i,j-1)\n\n if j+1<m:\n if grid[i][j] in d[\'right\'][0] and grid[i][j+1] in d[\'right\'][1]:\n dfs(i,j+1)\n\n dfs(0,0)\n return a[0]\n``` | 0 | You are given an `m x n` `grid`. Each cell of `grid` represents a street. The street of `grid[i][j]` can be:
* `1` which means a street connecting the left cell and the right cell.
* `2` which means a street connecting the upper cell and the lower cell.
* `3` which means a street connecting the left cell and the lower cell.
* `4` which means a street connecting the right cell and the lower cell.
* `5` which means a street connecting the left cell and the upper cell.
* `6` which means a street connecting the right cell and the upper cell.
You will initially start at the street of the upper-left cell `(0, 0)`. A valid path in the grid is a path that starts from the upper left cell `(0, 0)` and ends at the bottom-right cell `(m - 1, n - 1)`. **The path should only follow the streets**.
**Notice** that you are **not allowed** to change any street.
Return `true` _if there is a valid path in the grid or_ `false` _otherwise_.
**Example 1:**
**Input:** grid = \[\[2,4,3\],\[6,5,2\]\]
**Output:** true
**Explanation:** As shown you can start at cell (0, 0) and visit all the cells of the grid to reach (m - 1, n - 1).
**Example 2:**
**Input:** grid = \[\[1,2,1\],\[1,2,1\]\]
**Output:** false
**Explanation:** As shown you the street at cell (0, 0) is not connected with any street of any other cell and you will get stuck at cell (0, 0)
**Example 3:**
**Input:** grid = \[\[1,1,2\]\]
**Output:** false
**Explanation:** You will get stuck at cell (0, 1) and you cannot reach cell (0, 2).
**Constraints:**
* `m == grid.length`
* `n == grid[i].length`
* `1 <= m, n <= 300`
* `1 <= grid[i][j] <= 6` | Use hashset to store all elements. Loop again to count all valid elements. |
Python | DFS Solution | check-if-there-is-a-valid-path-in-a-grid | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def hasValidPath(self, grid: List[List[int]]) -> bool: \n n=len(grid)\n m=len(grid[0])\n a=[0]\n v=set() \n \n d={\n \'down\':[\n [2,3,4],[2,5,6]\n ],\n \'right\':[\n [1,4,6],[1,3,5]\n ],\n \'up\':[\n [2,5,6],[2,3,4]\n ],\n \'left\':[\n [1,3,5],[1,4,6]\n ]\n } \n\n def dfs(i,j):\n if (i,j) in v:\n return\n if i==n-1 and j==m-1:\n a[0]=1\n return\n v.add((i,j))\n \n if i-1>=0:\n if grid[i][j] in d[\'up\'][0] and grid[i-1][j] in d[\'up\'][1]:\n dfs(i-1,j)\n\n if i+1<n:\n if grid[i][j] in d[\'down\'][0] and grid[i+1][j] in d[\'down\'][1]:\n dfs(i+1,j)\n\n if j-1>=0:\n if grid[i][j] in d[\'left\'][0] and grid[i][j-1] in d[\'left\'][1]:\n dfs(i,j-1)\n\n if j+1<m:\n if grid[i][j] in d[\'right\'][0] and grid[i][j+1] in d[\'right\'][1]:\n dfs(i,j+1)\n\n dfs(0,0)\n return a[0]\n``` | 0 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
Dynamic programming solution | longest-happy-prefix | 0 | 1 | # Intuition\nThe idea is to compute prefix function for each substring in a given \nstring, storing the result in a dp array of size = s.length()\n\n# Approach\n1. The base case is a substring of length 1, s[0], and its prefix function\n= 0, i. e. an empty string. dp[0] = 0;\n2. Starting from i = 1 to (s.length() - 1), iterate over all possible substrings to get their prefix functions;\n3. To compute the value for dp[i] we need to compare s[i] and s[k], putting\ninto k previously calculated value, dp[i - 1];\n4. k stands for the length of prefix/suffix of a substring, s[0,k) == s[i\u2212k,i). So, on each step we need to compare the only 2 symbols, which extend out overlapping from both ends;\n5. If s[i] == s[k], then dp[i] = k + 1, otherwise k = dp[k - 1];\n6. Then while k > 0 and the current k doesn\u2019t work, keep setting k to dp[k\u22121];\n\nThe result will be in the last element of the dp array, as for the prefix function value of the whole string s.\n \n# Complexity\n- Time complexity:\nEven if we still have at most O(n) string comparisons, each one only compares two characters, so it\u2019s now O(1) per comparison. That means we can compute the whole prefix function in O(n).\n\n- Space complexity:\nWe need a dp array of size O(n) to store the results of the prefix function computation.\n\n# Code\n```\nclass Solution:\n def longestPrefix(self, s: str) -> str:\n n = [0] + [None] * (len(s) - 1)\n\n for i in range(1, len(s)):\n k = n[i - 1] # trying length k + 1\n while (k > 0) and (s[i] != s[k]):\n k = n[k - 1]\n if s[i] == s[k]:\n k += 1\n n[i] = k\n happy_border = n[-1]\n return s[:happy_border]\n``` | 1 | You are given the array `nums` consisting of `n` positive integers. You computed the sum of all non-empty continuous subarrays from the array and then sorted them in non-decreasing order, creating a new array of `n * (n + 1) / 2` numbers.
_Return the sum of the numbers from index_ `left` _to index_ `right` (**indexed from 1**)_, inclusive, in the new array._ Since the answer can be a huge number return it modulo `109 + 7`.
**Example 1:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 5
**Output:** 13
**Explanation:** All subarray sums are 1, 3, 6, 10, 2, 5, 9, 3, 7, 4. After sorting them in non-decreasing order we have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 1 to ri = 5 is 1 + 2 + 3 + 3 + 4 = 13.
**Example 2:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 3, right = 4
**Output:** 6
**Explanation:** The given array is the same as example 1. We have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 3 to ri = 4 is 3 + 3 = 6.
**Example 3:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 10
**Output:** 50
**Constraints:**
* `n == nums.length`
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 100`
* `1 <= left <= right <= n * (n + 1) / 2` | Use Longest Prefix Suffix (KMP-table) or String Hashing. |
Dynamic programming solution | longest-happy-prefix | 0 | 1 | # Intuition\nThe idea is to compute prefix function for each substring in a given \nstring, storing the result in a dp array of size = s.length()\n\n# Approach\n1. The base case is a substring of length 1, s[0], and its prefix function\n= 0, i. e. an empty string. dp[0] = 0;\n2. Starting from i = 1 to (s.length() - 1), iterate over all possible substrings to get their prefix functions;\n3. To compute the value for dp[i] we need to compare s[i] and s[k], putting\ninto k previously calculated value, dp[i - 1];\n4. k stands for the length of prefix/suffix of a substring, s[0,k) == s[i\u2212k,i). So, on each step we need to compare the only 2 symbols, which extend out overlapping from both ends;\n5. If s[i] == s[k], then dp[i] = k + 1, otherwise k = dp[k - 1];\n6. Then while k > 0 and the current k doesn\u2019t work, keep setting k to dp[k\u22121];\n\nThe result will be in the last element of the dp array, as for the prefix function value of the whole string s.\n \n# Complexity\n- Time complexity:\nEven if we still have at most O(n) string comparisons, each one only compares two characters, so it\u2019s now O(1) per comparison. That means we can compute the whole prefix function in O(n).\n\n- Space complexity:\nWe need a dp array of size O(n) to store the results of the prefix function computation.\n\n# Code\n```\nclass Solution:\n def longestPrefix(self, s: str) -> str:\n n = [0] + [None] * (len(s) - 1)\n\n for i in range(1, len(s)):\n k = n[i - 1] # trying length k + 1\n while (k > 0) and (s[i] != s[k]):\n k = n[k - 1]\n if s[i] == s[k]:\n k += 1\n n[i] = k\n happy_border = n[-1]\n return s[:happy_border]\n``` | 1 | Given two 0-indexed integer arrays nums1 and nums2, return a list answer of size 2 where: Note that the integers in the lists may be returned in any order. | For each integer in nums1, check if it exists in nums2. Do the same for each integer in nums2. |
easy-solution👁️ | well-explained✅ | O(M+N) | Microsoft🔥 | longest-happy-prefix | 0 | 1 | # Please upvote if it is helpful ^_^\n***6Companies30days #ReviseWithArsh Challenge 2023\nDay2\nQ12. Longest Happy Prefix***\n\n**Approach:** *KMP Approach, DP*\n\n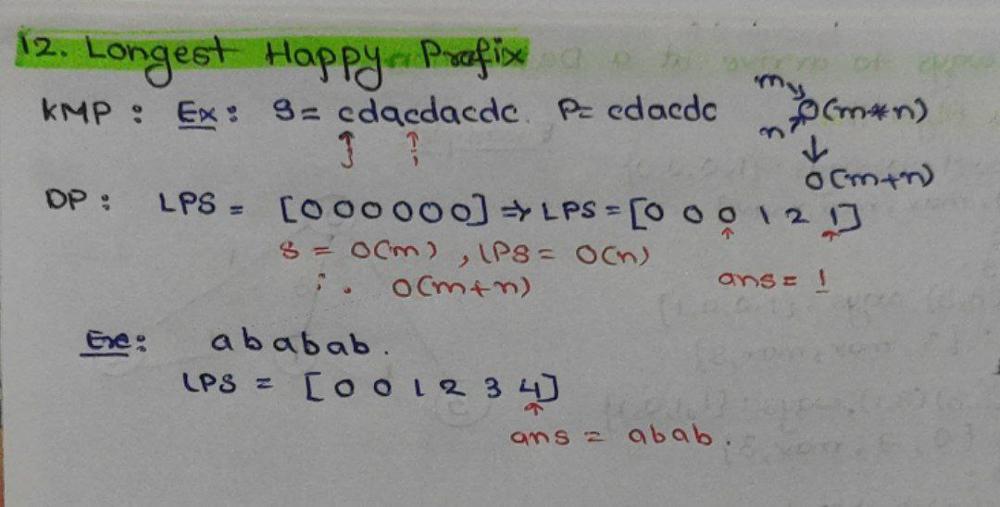\n\n**Complexity:** *O(M+N)*\n\n# Code\n**Python3:**\n```\nclass Solution:\n def longestPrefix(self, s: str) -> str:\n n=len(s)\n lps=[0]*n\n j=0\n for i in range(1,n):\n while s[i]!=s[j] and j>0:\n j=lps[j-1]\n\n if s[i]==s[j]:\n lps[i]=j+1\n j+=1\n\n return s[:lps[-1]]\n``` | 4 | You are given the array `nums` consisting of `n` positive integers. You computed the sum of all non-empty continuous subarrays from the array and then sorted them in non-decreasing order, creating a new array of `n * (n + 1) / 2` numbers.
_Return the sum of the numbers from index_ `left` _to index_ `right` (**indexed from 1**)_, inclusive, in the new array._ Since the answer can be a huge number return it modulo `109 + 7`.
**Example 1:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 5
**Output:** 13
**Explanation:** All subarray sums are 1, 3, 6, 10, 2, 5, 9, 3, 7, 4. After sorting them in non-decreasing order we have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 1 to ri = 5 is 1 + 2 + 3 + 3 + 4 = 13.
**Example 2:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 3, right = 4
**Output:** 6
**Explanation:** The given array is the same as example 1. We have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 3 to ri = 4 is 3 + 3 = 6.
**Example 3:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 10
**Output:** 50
**Constraints:**
* `n == nums.length`
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 100`
* `1 <= left <= right <= n * (n + 1) / 2` | Use Longest Prefix Suffix (KMP-table) or String Hashing. |
easy-solution👁️ | well-explained✅ | O(M+N) | Microsoft🔥 | longest-happy-prefix | 0 | 1 | # Please upvote if it is helpful ^_^\n***6Companies30days #ReviseWithArsh Challenge 2023\nDay2\nQ12. Longest Happy Prefix***\n\n**Approach:** *KMP Approach, DP*\n\n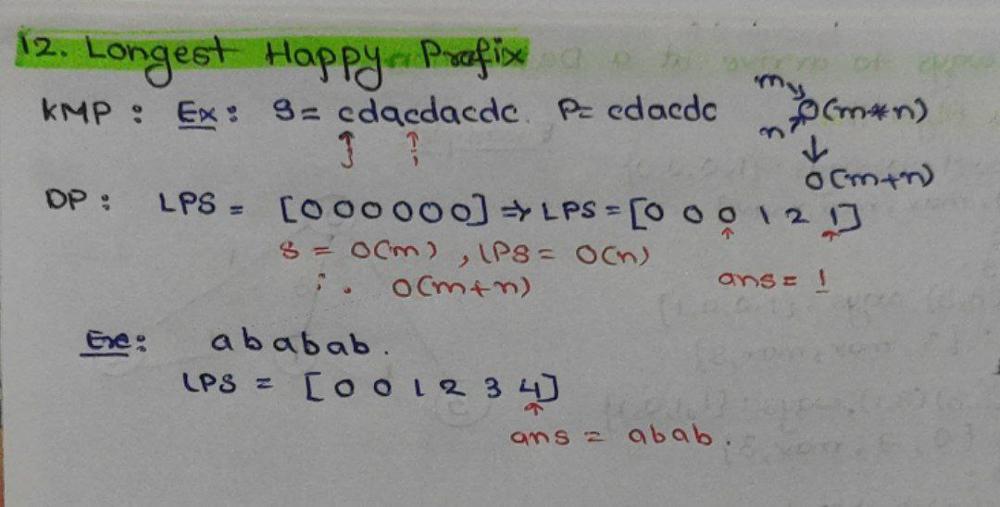\n\n**Complexity:** *O(M+N)*\n\n# Code\n**Python3:**\n```\nclass Solution:\n def longestPrefix(self, s: str) -> str:\n n=len(s)\n lps=[0]*n\n j=0\n for i in range(1,n):\n while s[i]!=s[j] and j>0:\n j=lps[j-1]\n\n if s[i]==s[j]:\n lps[i]=j+1\n j+=1\n\n return s[:lps[-1]]\n``` | 4 | Given two 0-indexed integer arrays nums1 and nums2, return a list answer of size 2 where: Note that the integers in the lists may be returned in any order. | For each integer in nums1, check if it exists in nums2. Do the same for each integer in nums2. |
longest-happy-prefix | longest-happy-prefix | 0 | 1 | # Code\n```\nclass Solution:\n def longestPrefix(self, s: str) -> str:\n b = len(s)\n t = 0\n p = ""\n for i in range(len(s)-1):\n if s[:i+1]==s[b-1-i:]:\n if i+1>t:\n p = s[:i+1]\n t= i+1 \n return p\n\n\n \n``` | 1 | You are given the array `nums` consisting of `n` positive integers. You computed the sum of all non-empty continuous subarrays from the array and then sorted them in non-decreasing order, creating a new array of `n * (n + 1) / 2` numbers.
_Return the sum of the numbers from index_ `left` _to index_ `right` (**indexed from 1**)_, inclusive, in the new array._ Since the answer can be a huge number return it modulo `109 + 7`.
**Example 1:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 5
**Output:** 13
**Explanation:** All subarray sums are 1, 3, 6, 10, 2, 5, 9, 3, 7, 4. After sorting them in non-decreasing order we have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 1 to ri = 5 is 1 + 2 + 3 + 3 + 4 = 13.
**Example 2:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 3, right = 4
**Output:** 6
**Explanation:** The given array is the same as example 1. We have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 3 to ri = 4 is 3 + 3 = 6.
**Example 3:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 10
**Output:** 50
**Constraints:**
* `n == nums.length`
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 100`
* `1 <= left <= right <= n * (n + 1) / 2` | Use Longest Prefix Suffix (KMP-table) or String Hashing. |
longest-happy-prefix | longest-happy-prefix | 0 | 1 | # Code\n```\nclass Solution:\n def longestPrefix(self, s: str) -> str:\n b = len(s)\n t = 0\n p = ""\n for i in range(len(s)-1):\n if s[:i+1]==s[b-1-i:]:\n if i+1>t:\n p = s[:i+1]\n t= i+1 \n return p\n\n\n \n``` | 1 | Given two 0-indexed integer arrays nums1 and nums2, return a list answer of size 2 where: Note that the integers in the lists may be returned in any order. | For each integer in nums1, check if it exists in nums2. Do the same for each integer in nums2. |
KMP approach | longest-happy-prefix | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nKMP approach\n\n# Approach\nCompute LPS for the string and take the last index to find the starting point of the prefix and print till end since it is always a suffix.\n\n# Complexity\n- Time complexity: O(M)\n\n- Space complexity: O(M)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def longestPrefix(self, s: str) -> str:\n lps = [0] * len(s)\n prevlps = 0\n i = 1\n while i < len(s):\n if s[i] == s[prevlps]:\n lps[i] = prevlps + 1\n prevlps+=1\n i+=1\n\n elif prevlps == 0:\n lps[i] = 0\n i+=1\n else:\n prevlps = lps[prevlps-1]\n\n upperIdx = lps[-1]\n stIdx = len(s) - upperIdx\n return s[stIdx:]\n\n\n\n\n\n \n``` | 0 | You are given the array `nums` consisting of `n` positive integers. You computed the sum of all non-empty continuous subarrays from the array and then sorted them in non-decreasing order, creating a new array of `n * (n + 1) / 2` numbers.
_Return the sum of the numbers from index_ `left` _to index_ `right` (**indexed from 1**)_, inclusive, in the new array._ Since the answer can be a huge number return it modulo `109 + 7`.
**Example 1:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 5
**Output:** 13
**Explanation:** All subarray sums are 1, 3, 6, 10, 2, 5, 9, 3, 7, 4. After sorting them in non-decreasing order we have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 1 to ri = 5 is 1 + 2 + 3 + 3 + 4 = 13.
**Example 2:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 3, right = 4
**Output:** 6
**Explanation:** The given array is the same as example 1. We have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 3 to ri = 4 is 3 + 3 = 6.
**Example 3:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 10
**Output:** 50
**Constraints:**
* `n == nums.length`
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 100`
* `1 <= left <= right <= n * (n + 1) / 2` | Use Longest Prefix Suffix (KMP-table) or String Hashing. |
KMP approach | longest-happy-prefix | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nKMP approach\n\n# Approach\nCompute LPS for the string and take the last index to find the starting point of the prefix and print till end since it is always a suffix.\n\n# Complexity\n- Time complexity: O(M)\n\n- Space complexity: O(M)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def longestPrefix(self, s: str) -> str:\n lps = [0] * len(s)\n prevlps = 0\n i = 1\n while i < len(s):\n if s[i] == s[prevlps]:\n lps[i] = prevlps + 1\n prevlps+=1\n i+=1\n\n elif prevlps == 0:\n lps[i] = 0\n i+=1\n else:\n prevlps = lps[prevlps-1]\n\n upperIdx = lps[-1]\n stIdx = len(s) - upperIdx\n return s[stIdx:]\n\n\n\n\n\n \n``` | 0 | Given two 0-indexed integer arrays nums1 and nums2, return a list answer of size 2 where: Note that the integers in the lists may be returned in any order. | For each integer in nums1, check if it exists in nums2. Do the same for each integer in nums2. |
Python Easy to understand code Beats 100% Memory , and Runtime . | find-lucky-integer-in-an-array | 0 | 1 | # Intuition\nThe code appears to be trying to find a "lucky" integer in a given list, where a "lucky" integer is defined as an integer that appears exactly as many times as its value in the list.\n\n# Approach\nThe code uses a brute-force approach to find the lucky integer. It iterates through the input list `arr`, counts the number of times each integer appears in the list, and checks if the count is equal to the value of the integer itself. If it is, the integer is considered lucky, and it is appended to the `f` list. Finally, if there are lucky integers in the list, it returns the largest lucky integer; otherwise, it returns -1.\n\n# Complexity\n- Time complexity: The code uses a nested loop with `arr.count(arr[i])`, which has a time complexity of O(n^2), where n is the length of the input list `arr`. Sorting the `f` list adds an additional O(n*log(n)) time complexity. So, the overall time complexity is O(n^2 + n*log(n)), which simplifies to O(n^2) because the quadratic term dominates.\n \n- Space complexity: The code uses additional space to store the `f` list, which can have a maximum of n elements, where n is the length of the input list `arr`. Therefore, the space complexity is O(n).\n\nOverall, the code can be optimized to achieve better time complexity by using a more efficient approach.[]()\n# Code\n```\nclass Solution:\n def findLucky(self, arr: List[int]) -> int:\n x=0\n f=[]\n for i in range(len(arr)):\n if arr.count(arr[i])==arr[i]:\n x+=1\n f.append(arr[i])\n if x==0:\n return -1\n else:\n f=sorted(f)\n return f[-1]\n``` | 3 | Given an array of integers `arr`, a **lucky integer** is an integer that has a frequency in the array equal to its value.
Return _the largest **lucky integer** in the array_. If there is no **lucky integer** return `-1`.
**Example 1:**
**Input:** arr = \[2,2,3,4\]
**Output:** 2
**Explanation:** The only lucky number in the array is 2 because frequency\[2\] == 2.
**Example 2:**
**Input:** arr = \[1,2,2,3,3,3\]
**Output:** 3
**Explanation:** 1, 2 and 3 are all lucky numbers, return the largest of them.
**Example 3:**
**Input:** arr = \[2,2,2,3,3\]
**Output:** -1
**Explanation:** There are no lucky numbers in the array.
**Constraints:**
* `1 <= arr.length <= 500`
* `1 <= arr[i] <= 500` | null |
Python Easy to understand code Beats 100% Memory , and Runtime . | find-lucky-integer-in-an-array | 0 | 1 | # Intuition\nThe code appears to be trying to find a "lucky" integer in a given list, where a "lucky" integer is defined as an integer that appears exactly as many times as its value in the list.\n\n# Approach\nThe code uses a brute-force approach to find the lucky integer. It iterates through the input list `arr`, counts the number of times each integer appears in the list, and checks if the count is equal to the value of the integer itself. If it is, the integer is considered lucky, and it is appended to the `f` list. Finally, if there are lucky integers in the list, it returns the largest lucky integer; otherwise, it returns -1.\n\n# Complexity\n- Time complexity: The code uses a nested loop with `arr.count(arr[i])`, which has a time complexity of O(n^2), where n is the length of the input list `arr`. Sorting the `f` list adds an additional O(n*log(n)) time complexity. So, the overall time complexity is O(n^2 + n*log(n)), which simplifies to O(n^2) because the quadratic term dominates.\n \n- Space complexity: The code uses additional space to store the `f` list, which can have a maximum of n elements, where n is the length of the input list `arr`. Therefore, the space complexity is O(n).\n\nOverall, the code can be optimized to achieve better time complexity by using a more efficient approach.[]()\n# Code\n```\nclass Solution:\n def findLucky(self, arr: List[int]) -> int:\n x=0\n f=[]\n for i in range(len(arr)):\n if arr.count(arr[i])==arr[i]:\n x+=1\n f.append(arr[i])\n if x==0:\n return -1\n else:\n f=sorted(f)\n return f[-1]\n``` | 3 | Alice and Bob take turns playing a game, with Alice starting first.
Initially, there are `n` stones in a pile. On each player's turn, that player makes a _move_ consisting of removing **any** non-zero **square number** of stones in the pile.
Also, if a player cannot make a move, he/she loses the game.
Given a positive integer `n`, return `true` if and only if Alice wins the game otherwise return `false`, assuming both players play optimally.
**Example 1:**
**Input:** n = 1
**Output:** true
**Explanation:** Alice can remove 1 stone winning the game because Bob doesn't have any moves.
**Example 2:**
**Input:** n = 2
**Output:** false
**Explanation:** Alice can only remove 1 stone, after that Bob removes the last one winning the game (2 -> 1 -> 0).
**Example 3:**
**Input:** n = 4
**Output:** true
**Explanation:** n is already a perfect square, Alice can win with one move, removing 4 stones (4 -> 0).
**Constraints:**
* `1 <= n <= 105` | Count the frequency of each integer in the array. Get all lucky numbers and return the largest of them. |
python3 code | find-lucky-integer-in-an-array | 0 | 1 | \n\n# Complexity\n- Time complexity:\nO(n^2)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution:\n def findLucky(self, arr: List[int]) -> int:\n l=[]\n for i in arr:\n x = arr.count(i)\n if(x==i):\n l.append(i)\n else:\n l.append(-1)\n return max(l)\n \n``` | 1 | Given an array of integers `arr`, a **lucky integer** is an integer that has a frequency in the array equal to its value.
Return _the largest **lucky integer** in the array_. If there is no **lucky integer** return `-1`.
**Example 1:**
**Input:** arr = \[2,2,3,4\]
**Output:** 2
**Explanation:** The only lucky number in the array is 2 because frequency\[2\] == 2.
**Example 2:**
**Input:** arr = \[1,2,2,3,3,3\]
**Output:** 3
**Explanation:** 1, 2 and 3 are all lucky numbers, return the largest of them.
**Example 3:**
**Input:** arr = \[2,2,2,3,3\]
**Output:** -1
**Explanation:** There are no lucky numbers in the array.
**Constraints:**
* `1 <= arr.length <= 500`
* `1 <= arr[i] <= 500` | null |
python3 code | find-lucky-integer-in-an-array | 0 | 1 | \n\n# Complexity\n- Time complexity:\nO(n^2)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution:\n def findLucky(self, arr: List[int]) -> int:\n l=[]\n for i in arr:\n x = arr.count(i)\n if(x==i):\n l.append(i)\n else:\n l.append(-1)\n return max(l)\n \n``` | 1 | Alice and Bob take turns playing a game, with Alice starting first.
Initially, there are `n` stones in a pile. On each player's turn, that player makes a _move_ consisting of removing **any** non-zero **square number** of stones in the pile.
Also, if a player cannot make a move, he/she loses the game.
Given a positive integer `n`, return `true` if and only if Alice wins the game otherwise return `false`, assuming both players play optimally.
**Example 1:**
**Input:** n = 1
**Output:** true
**Explanation:** Alice can remove 1 stone winning the game because Bob doesn't have any moves.
**Example 2:**
**Input:** n = 2
**Output:** false
**Explanation:** Alice can only remove 1 stone, after that Bob removes the last one winning the game (2 -> 1 -> 0).
**Example 3:**
**Input:** n = 4
**Output:** true
**Explanation:** n is already a perfect square, Alice can win with one move, removing 4 stones (4 -> 0).
**Constraints:**
* `1 <= n <= 105` | Count the frequency of each integer in the array. Get all lucky numbers and return the largest of them. |
Python code to return the largest lucky integer in the array. (TC & SC: O(n)) | find-lucky-integer-in-an-array | 0 | 1 | \n\n# Approach\nExplanation of the approach used in the code:\n\nInitialize two variables, x and d. x will be used to store the largest lucky number found, and d is a dictionary that will be used to count the occurrences of each element in the input array arr.\n\nIterate through the elements of the arr list using a for loop.\n\nFor each element i in arr, check if it is already a key in the dictionary d.\n\nIf i is not in d, add it as a key with a value of 1.\nIf i is already in d, increment its corresponding value by 1 to keep track of the count.\nAfter processing all elements in the arr, the dictionary d will contain counts of each unique element in the list.\n\nIterate through the keys and values in the dictionary using a for loop with key and value variables.\n\nFor each key-value pair, check if the key (element) is equal to the value (count). If they are equal, update the x variable with the key if it\'s greater than the current x.\n\nFinally, return x.\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution(object):\n def findLucky(self, arr):\n x = -1\n d = dict()\n for i in arr:\n if i not in d:\n d[i] = 1\n else:\n d[i] = d[i] + 1\n for key,value in d.items():\n if key == value:\n x = key\n return x\n \n``` | 1 | Given an array of integers `arr`, a **lucky integer** is an integer that has a frequency in the array equal to its value.
Return _the largest **lucky integer** in the array_. If there is no **lucky integer** return `-1`.
**Example 1:**
**Input:** arr = \[2,2,3,4\]
**Output:** 2
**Explanation:** The only lucky number in the array is 2 because frequency\[2\] == 2.
**Example 2:**
**Input:** arr = \[1,2,2,3,3,3\]
**Output:** 3
**Explanation:** 1, 2 and 3 are all lucky numbers, return the largest of them.
**Example 3:**
**Input:** arr = \[2,2,2,3,3\]
**Output:** -1
**Explanation:** There are no lucky numbers in the array.
**Constraints:**
* `1 <= arr.length <= 500`
* `1 <= arr[i] <= 500` | null |
Python code to return the largest lucky integer in the array. (TC & SC: O(n)) | find-lucky-integer-in-an-array | 0 | 1 | \n\n# Approach\nExplanation of the approach used in the code:\n\nInitialize two variables, x and d. x will be used to store the largest lucky number found, and d is a dictionary that will be used to count the occurrences of each element in the input array arr.\n\nIterate through the elements of the arr list using a for loop.\n\nFor each element i in arr, check if it is already a key in the dictionary d.\n\nIf i is not in d, add it as a key with a value of 1.\nIf i is already in d, increment its corresponding value by 1 to keep track of the count.\nAfter processing all elements in the arr, the dictionary d will contain counts of each unique element in the list.\n\nIterate through the keys and values in the dictionary using a for loop with key and value variables.\n\nFor each key-value pair, check if the key (element) is equal to the value (count). If they are equal, update the x variable with the key if it\'s greater than the current x.\n\nFinally, return x.\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(n)\n\n# Code\n```\nclass Solution(object):\n def findLucky(self, arr):\n x = -1\n d = dict()\n for i in arr:\n if i not in d:\n d[i] = 1\n else:\n d[i] = d[i] + 1\n for key,value in d.items():\n if key == value:\n x = key\n return x\n \n``` | 1 | Alice and Bob take turns playing a game, with Alice starting first.
Initially, there are `n` stones in a pile. On each player's turn, that player makes a _move_ consisting of removing **any** non-zero **square number** of stones in the pile.
Also, if a player cannot make a move, he/she loses the game.
Given a positive integer `n`, return `true` if and only if Alice wins the game otherwise return `false`, assuming both players play optimally.
**Example 1:**
**Input:** n = 1
**Output:** true
**Explanation:** Alice can remove 1 stone winning the game because Bob doesn't have any moves.
**Example 2:**
**Input:** n = 2
**Output:** false
**Explanation:** Alice can only remove 1 stone, after that Bob removes the last one winning the game (2 -> 1 -> 0).
**Example 3:**
**Input:** n = 4
**Output:** true
**Explanation:** n is already a perfect square, Alice can win with one move, removing 4 stones (4 -> 0).
**Constraints:**
* `1 <= n <= 105` | Count the frequency of each integer in the array. Get all lucky numbers and return the largest of them. |
Find lucky integer in an array | find-lucky-integer-in-an-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findLucky(self, arr: List[int]) -> int:\n max_num=-1\n for i in arr:\n if arr.count(i)==i:\n max_freq=i\n if max_num<max_freq:\n max_num=max_freq\n return max_num\n``` | 1 | Given an array of integers `arr`, a **lucky integer** is an integer that has a frequency in the array equal to its value.
Return _the largest **lucky integer** in the array_. If there is no **lucky integer** return `-1`.
**Example 1:**
**Input:** arr = \[2,2,3,4\]
**Output:** 2
**Explanation:** The only lucky number in the array is 2 because frequency\[2\] == 2.
**Example 2:**
**Input:** arr = \[1,2,2,3,3,3\]
**Output:** 3
**Explanation:** 1, 2 and 3 are all lucky numbers, return the largest of them.
**Example 3:**
**Input:** arr = \[2,2,2,3,3\]
**Output:** -1
**Explanation:** There are no lucky numbers in the array.
**Constraints:**
* `1 <= arr.length <= 500`
* `1 <= arr[i] <= 500` | null |
Find lucky integer in an array | find-lucky-integer-in-an-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findLucky(self, arr: List[int]) -> int:\n max_num=-1\n for i in arr:\n if arr.count(i)==i:\n max_freq=i\n if max_num<max_freq:\n max_num=max_freq\n return max_num\n``` | 1 | Alice and Bob take turns playing a game, with Alice starting first.
Initially, there are `n` stones in a pile. On each player's turn, that player makes a _move_ consisting of removing **any** non-zero **square number** of stones in the pile.
Also, if a player cannot make a move, he/she loses the game.
Given a positive integer `n`, return `true` if and only if Alice wins the game otherwise return `false`, assuming both players play optimally.
**Example 1:**
**Input:** n = 1
**Output:** true
**Explanation:** Alice can remove 1 stone winning the game because Bob doesn't have any moves.
**Example 2:**
**Input:** n = 2
**Output:** false
**Explanation:** Alice can only remove 1 stone, after that Bob removes the last one winning the game (2 -> 1 -> 0).
**Example 3:**
**Input:** n = 4
**Output:** true
**Explanation:** n is already a perfect square, Alice can win with one move, removing 4 stones (4 -> 0).
**Constraints:**
* `1 <= n <= 105` | Count the frequency of each integer in the array. Get all lucky numbers and return the largest of them. |
Python | Easy Solution✅ | find-lucky-integer-in-an-array | 0 | 1 | ```\ndef findLucky(self, arr: List[int]) -> int:\n large = -1\n unique = set(arr)\n for item in unique:\n count = arr.count(item)\n if item == count:\n large = max(large, item) \n return large\n``` | 4 | Given an array of integers `arr`, a **lucky integer** is an integer that has a frequency in the array equal to its value.
Return _the largest **lucky integer** in the array_. If there is no **lucky integer** return `-1`.
**Example 1:**
**Input:** arr = \[2,2,3,4\]
**Output:** 2
**Explanation:** The only lucky number in the array is 2 because frequency\[2\] == 2.
**Example 2:**
**Input:** arr = \[1,2,2,3,3,3\]
**Output:** 3
**Explanation:** 1, 2 and 3 are all lucky numbers, return the largest of them.
**Example 3:**
**Input:** arr = \[2,2,2,3,3\]
**Output:** -1
**Explanation:** There are no lucky numbers in the array.
**Constraints:**
* `1 <= arr.length <= 500`
* `1 <= arr[i] <= 500` | null |
Python | Easy Solution✅ | find-lucky-integer-in-an-array | 0 | 1 | ```\ndef findLucky(self, arr: List[int]) -> int:\n large = -1\n unique = set(arr)\n for item in unique:\n count = arr.count(item)\n if item == count:\n large = max(large, item) \n return large\n``` | 4 | Alice and Bob take turns playing a game, with Alice starting first.
Initially, there are `n` stones in a pile. On each player's turn, that player makes a _move_ consisting of removing **any** non-zero **square number** of stones in the pile.
Also, if a player cannot make a move, he/she loses the game.
Given a positive integer `n`, return `true` if and only if Alice wins the game otherwise return `false`, assuming both players play optimally.
**Example 1:**
**Input:** n = 1
**Output:** true
**Explanation:** Alice can remove 1 stone winning the game because Bob doesn't have any moves.
**Example 2:**
**Input:** n = 2
**Output:** false
**Explanation:** Alice can only remove 1 stone, after that Bob removes the last one winning the game (2 -> 1 -> 0).
**Example 3:**
**Input:** n = 4
**Output:** true
**Explanation:** n is already a perfect square, Alice can win with one move, removing 4 stones (4 -> 0).
**Constraints:**
* `1 <= n <= 105` | Count the frequency of each integer in the array. Get all lucky numbers and return the largest of them. |
simple solution using dictionary in python | find-lucky-integer-in-an-array | 0 | 1 | \n```\nclass Solution:\n def findLucky(self, arr: List[int]) -> int:\n dic={}\n l=[]\n for i in arr:\n if i not in dic:\n dic[i]=1\n else:\n dic[i]+=1\n for i in dic:\n if i==dic[i]:\n l.append(i)\n if(len(l)<1):\n return -1\n return max(l)\n``` | 2 | Given an array of integers `arr`, a **lucky integer** is an integer that has a frequency in the array equal to its value.
Return _the largest **lucky integer** in the array_. If there is no **lucky integer** return `-1`.
**Example 1:**
**Input:** arr = \[2,2,3,4\]
**Output:** 2
**Explanation:** The only lucky number in the array is 2 because frequency\[2\] == 2.
**Example 2:**
**Input:** arr = \[1,2,2,3,3,3\]
**Output:** 3
**Explanation:** 1, 2 and 3 are all lucky numbers, return the largest of them.
**Example 3:**
**Input:** arr = \[2,2,2,3,3\]
**Output:** -1
**Explanation:** There are no lucky numbers in the array.
**Constraints:**
* `1 <= arr.length <= 500`
* `1 <= arr[i] <= 500` | null |
simple solution using dictionary in python | find-lucky-integer-in-an-array | 0 | 1 | \n```\nclass Solution:\n def findLucky(self, arr: List[int]) -> int:\n dic={}\n l=[]\n for i in arr:\n if i not in dic:\n dic[i]=1\n else:\n dic[i]+=1\n for i in dic:\n if i==dic[i]:\n l.append(i)\n if(len(l)<1):\n return -1\n return max(l)\n``` | 2 | Alice and Bob take turns playing a game, with Alice starting first.
Initially, there are `n` stones in a pile. On each player's turn, that player makes a _move_ consisting of removing **any** non-zero **square number** of stones in the pile.
Also, if a player cannot make a move, he/she loses the game.
Given a positive integer `n`, return `true` if and only if Alice wins the game otherwise return `false`, assuming both players play optimally.
**Example 1:**
**Input:** n = 1
**Output:** true
**Explanation:** Alice can remove 1 stone winning the game because Bob doesn't have any moves.
**Example 2:**
**Input:** n = 2
**Output:** false
**Explanation:** Alice can only remove 1 stone, after that Bob removes the last one winning the game (2 -> 1 -> 0).
**Example 3:**
**Input:** n = 4
**Output:** true
**Explanation:** n is already a perfect square, Alice can win with one move, removing 4 stones (4 -> 0).
**Constraints:**
* `1 <= n <= 105` | Count the frequency of each integer in the array. Get all lucky numbers and return the largest of them. |
Easy Python Solution | find-lucky-integer-in-an-array | 1 | 1 | ```\nclass Solution:\n def findLucky(self, arr: List[int]) -> int:\n a=Counter(arr)\n l=[]\n for i in arr:\n if i==a[i]:\n l.append(i)\n if l:\n return max(l)\n return -1\n``` | 1 | Given an array of integers `arr`, a **lucky integer** is an integer that has a frequency in the array equal to its value.
Return _the largest **lucky integer** in the array_. If there is no **lucky integer** return `-1`.
**Example 1:**
**Input:** arr = \[2,2,3,4\]
**Output:** 2
**Explanation:** The only lucky number in the array is 2 because frequency\[2\] == 2.
**Example 2:**
**Input:** arr = \[1,2,2,3,3,3\]
**Output:** 3
**Explanation:** 1, 2 and 3 are all lucky numbers, return the largest of them.
**Example 3:**
**Input:** arr = \[2,2,2,3,3\]
**Output:** -1
**Explanation:** There are no lucky numbers in the array.
**Constraints:**
* `1 <= arr.length <= 500`
* `1 <= arr[i] <= 500` | null |
Easy Python Solution | find-lucky-integer-in-an-array | 1 | 1 | ```\nclass Solution:\n def findLucky(self, arr: List[int]) -> int:\n a=Counter(arr)\n l=[]\n for i in arr:\n if i==a[i]:\n l.append(i)\n if l:\n return max(l)\n return -1\n``` | 1 | Alice and Bob take turns playing a game, with Alice starting first.
Initially, there are `n` stones in a pile. On each player's turn, that player makes a _move_ consisting of removing **any** non-zero **square number** of stones in the pile.
Also, if a player cannot make a move, he/she loses the game.
Given a positive integer `n`, return `true` if and only if Alice wins the game otherwise return `false`, assuming both players play optimally.
**Example 1:**
**Input:** n = 1
**Output:** true
**Explanation:** Alice can remove 1 stone winning the game because Bob doesn't have any moves.
**Example 2:**
**Input:** n = 2
**Output:** false
**Explanation:** Alice can only remove 1 stone, after that Bob removes the last one winning the game (2 -> 1 -> 0).
**Example 3:**
**Input:** n = 4
**Output:** true
**Explanation:** n is already a perfect square, Alice can win with one move, removing 4 stones (4 -> 0).
**Constraints:**
* `1 <= n <= 105` | Count the frequency of each integer in the array. Get all lucky numbers and return the largest of them. |
JAVA|| BEATS 98.94% || 3 LINE CODE || HASHMAP | find-lucky-integer-in-an-array | 1 | 1 | **PLEASE UPVOTE THIS IF YOU LIKE IT**\n# Approach\nFinding out the frequency of each element using HashMap,then if the frequency matches the value then print that. but, since we have to return the largest so we will traverse from the last end;\n\n\n# Code\n```\nclass Solution {\n public int findLucky(int[] arr) {\n HashMap<Integer, Integer> map = new HashMap<>();\n Arrays.sort(arr);\n for (int i : arr) map.put(i, map.getOrDefault(i, 0) + 1);\n for (int j = arr.length - 1; j >= 0; j--) {\n if (map.containsKey(arr[j]) && map.get(arr[j]) == arr[j]) return arr[j];\n }\n return -1;\n }\n}\n``` | 5 | Given an array of integers `arr`, a **lucky integer** is an integer that has a frequency in the array equal to its value.
Return _the largest **lucky integer** in the array_. If there is no **lucky integer** return `-1`.
**Example 1:**
**Input:** arr = \[2,2,3,4\]
**Output:** 2
**Explanation:** The only lucky number in the array is 2 because frequency\[2\] == 2.
**Example 2:**
**Input:** arr = \[1,2,2,3,3,3\]
**Output:** 3
**Explanation:** 1, 2 and 3 are all lucky numbers, return the largest of them.
**Example 3:**
**Input:** arr = \[2,2,2,3,3\]
**Output:** -1
**Explanation:** There are no lucky numbers in the array.
**Constraints:**
* `1 <= arr.length <= 500`
* `1 <= arr[i] <= 500` | null |
JAVA|| BEATS 98.94% || 3 LINE CODE || HASHMAP | find-lucky-integer-in-an-array | 1 | 1 | **PLEASE UPVOTE THIS IF YOU LIKE IT**\n# Approach\nFinding out the frequency of each element using HashMap,then if the frequency matches the value then print that. but, since we have to return the largest so we will traverse from the last end;\n\n\n# Code\n```\nclass Solution {\n public int findLucky(int[] arr) {\n HashMap<Integer, Integer> map = new HashMap<>();\n Arrays.sort(arr);\n for (int i : arr) map.put(i, map.getOrDefault(i, 0) + 1);\n for (int j = arr.length - 1; j >= 0; j--) {\n if (map.containsKey(arr[j]) && map.get(arr[j]) == arr[j]) return arr[j];\n }\n return -1;\n }\n}\n``` | 5 | Alice and Bob take turns playing a game, with Alice starting first.
Initially, there are `n` stones in a pile. On each player's turn, that player makes a _move_ consisting of removing **any** non-zero **square number** of stones in the pile.
Also, if a player cannot make a move, he/she loses the game.
Given a positive integer `n`, return `true` if and only if Alice wins the game otherwise return `false`, assuming both players play optimally.
**Example 1:**
**Input:** n = 1
**Output:** true
**Explanation:** Alice can remove 1 stone winning the game because Bob doesn't have any moves.
**Example 2:**
**Input:** n = 2
**Output:** false
**Explanation:** Alice can only remove 1 stone, after that Bob removes the last one winning the game (2 -> 1 -> 0).
**Example 3:**
**Input:** n = 4
**Output:** true
**Explanation:** n is already a perfect square, Alice can win with one move, removing 4 stones (4 -> 0).
**Constraints:**
* `1 <= n <= 105` | Count the frequency of each integer in the array. Get all lucky numbers and return the largest of them. |
Easiest Code Ever - Python - Top 97% Speed - O( n log n ) Combinatorial | count-number-of-teams | 0 | 1 | **Easiest Code Ever - Python - Top 97% Speed - O( n log n ) Combinatorial**\n\nThe code below simply loops through the array taking an element "x" as the pivot. Using this pivot, we count separately the number of values:\n1. **Lower** than "x" to the **left** (lo_L)\n2. **Higher** than "x" to the **left** (hi_L)\n1. **Lower** than "x" to the **right** (lo_R)\n2. **Higher** than "x" to the **right** (hi_R)\n\nThese values are then combined to update our result variable:\n* result += lo_L * hi_R + hi_L * lo_R\n\nThe idea makes a lot of sense after a moment (a detailed explanation of the formula can be found below).\n\nNow, the 4 tracked variables can be obtained in two ways:\n1. The best way is to use SortedLists containing all the values to the Left and Right of our pivot. Using binary search, we can find the index of our pivot in these lists, and quickly deduce the number of elements higher or lower. This allows us to have a O( n log n ) solution.\n2. Otherwise, we can use two nested loops to build our counting variables primitively. This gives us a O(n^2) solution.\n\nBoth code versions are presented below. I hope the explanation was helpful. Cheers,\n\n```\n# Version A: [Top Speed] O(n log n) solution using SortedLists to calculate our 4 counting variables in Log(n) time.\nfrom sortedcontainers import SortedList\nclass Solution:\n def count_low_high(self,sl,x):\n lo = sl.bisect_left(x)\n hi = len(sl) - lo\n return lo, hi\n \n def numTeams(self, A):\n result = 0\n left = SortedList()\n right = SortedList(A)\n for x in A:\n right.remove(x)\n lo_L, hi_L = self.count_low_high(left ,x)\n lo_R, hi_R = self.count_low_high(right,x)\n result += lo_L*hi_R + hi_L*lo_R\n left .add(x)\n return result\n```\n\n```\n# Version B: O(n^2) solution with (primitive) nested loops for building our 4 counting variables.\nclass Solution:\n def numTeams(self, A):\n L = len(A)\n result = 0\n for j in range(1,L-1):\n x, lo_L, lo_R, hi_L, hi_R = A[j], 0, 0, 0, 0\n for i in range(j):\n if A[i]<x:\n lo_L += 1\n else:\n hi_L += 1\n for k in range(j+1,L):\n if A[k]<x:\n lo_R += 1\n else:\n hi_R += 1\n result += lo_L*hi_R + hi_L*lo_R\n return result\n```\n\n\n**PS.** Detailed explanation of the formula ``` lower_left * higher_right + higher_left * lower_right``` :\n\nThe formula comes from the fact that we need to create triplets with the form ```a<b<c``` or```a>b>c```. Since ```b``` can be regarded as the pivotal point dividing the sequence, we notice that we can form triplets by choosing:\n1) For ```a<b<c```, choose any number ```a``` (to the left) lower than ```b```, and any number ```c``` to the right which is higher. The total number of combinations for this case is ```lower_left*higher_right```.\n2) For ```a>b>c```, reverse the previous process. Choose any number ```a``` to the left higher than ```b```, and any subsequent number ```c``` which is lower. This creates a total number of combinations ```higher_left*lower_right```.\n\nAs you can see, summing the combinations for both alternatives gives us the formula ```total = lower_left*higher_right + higher_left*lower_right```. The rest of the code is just book-keeping, and choosing good Data Structures to handle the problem easily. | 69 | There are `n` soldiers standing in a line. Each soldier is assigned a **unique** `rating` value.
You have to form a team of 3 soldiers amongst them under the following rules:
* Choose 3 soldiers with index (`i`, `j`, `k`) with rating (`rating[i]`, `rating[j]`, `rating[k]`).
* A team is valid if: (`rating[i] < rating[j] < rating[k]`) or (`rating[i] > rating[j] > rating[k]`) where (`0 <= i < j < k < n`).
Return the number of teams you can form given the conditions. (soldiers can be part of multiple teams).
**Example 1:**
**Input:** rating = \[2,5,3,4,1\]
**Output:** 3
**Explanation:** We can form three teams given the conditions. (2,3,4), (5,4,1), (5,3,1).
**Example 2:**
**Input:** rating = \[2,1,3\]
**Output:** 0
**Explanation:** We can't form any team given the conditions.
**Example 3:**
**Input:** rating = \[1,2,3,4\]
**Output:** 4
**Constraints:**
* `n == rating.length`
* `3 <= n <= 1000`
* `1 <= rating[i] <= 105`
* All the integers in `rating` are **unique**.
In one second, you can either: - Move vertically by one unit, - Move horizontally by one unit, or - Move diagonally sqrt(2) units (in other words, move one unit vertically then one unit horizontally in one second). You have to visit the points in the same order as they appear in the array. You are allowed to pass through points, but they do not count as visited unless you stop on them. | To walk from point A to point B there will be an optimal strategy to walk ? Advance in diagonal as possible then after that go in straight line. Repeat the process until visiting all the points. |
Python | clean DP solution O(n^2) 92 % fast | count-number-of-teams | 0 | 1 | ```\nclass Solution:\n def numTeams(self, rating: List[int]) -> int:\n n = len(rating)\n \n up = [0] * n\n down = [0] * n\n \n teams = 0\n \n for i in range(n-1, -1, -1):\n for j in range(i+1, n):\n if rating[i] < rating[j]:\n up[i] += 1\n teams += up[j]\n else:\n down[i] += 1\n teams += down[j]\n \n return teams\n``` | 40 | There are `n` soldiers standing in a line. Each soldier is assigned a **unique** `rating` value.
You have to form a team of 3 soldiers amongst them under the following rules:
* Choose 3 soldiers with index (`i`, `j`, `k`) with rating (`rating[i]`, `rating[j]`, `rating[k]`).
* A team is valid if: (`rating[i] < rating[j] < rating[k]`) or (`rating[i] > rating[j] > rating[k]`) where (`0 <= i < j < k < n`).
Return the number of teams you can form given the conditions. (soldiers can be part of multiple teams).
**Example 1:**
**Input:** rating = \[2,5,3,4,1\]
**Output:** 3
**Explanation:** We can form three teams given the conditions. (2,3,4), (5,4,1), (5,3,1).
**Example 2:**
**Input:** rating = \[2,1,3\]
**Output:** 0
**Explanation:** We can't form any team given the conditions.
**Example 3:**
**Input:** rating = \[1,2,3,4\]
**Output:** 4
**Constraints:**
* `n == rating.length`
* `3 <= n <= 1000`
* `1 <= rating[i] <= 105`
* All the integers in `rating` are **unique**.
In one second, you can either: - Move vertically by one unit, - Move horizontally by one unit, or - Move diagonally sqrt(2) units (in other words, move one unit vertically then one unit horizontally in one second). You have to visit the points in the same order as they appear in the array. You are allowed to pass through points, but they do not count as visited unless you stop on them. | To walk from point A to point B there will be an optimal strategy to walk ? Advance in diagonal as possible then after that go in straight line. Repeat the process until visiting all the points. |
Python, O(N^2)) Time, O(N) Space | count-number-of-teams | 0 | 1 | # Intuition\nIf we know how many elements are less/greater than `rating[j]` in $$[0;j)$$ and greater/less than `rating[j]` in $$(j;n)$$ we can multiply the two numbers to get the number of triples.\n\n# Approach\nWe will keep two sorted lists: one for elements $$[0;j)$$ and one for $$(j;n)$$.\nWhile scanning from left to right we will keep updating the two via insort and slicing, then bisect (left and right) to find the number of ratings satisfying the criteria.\n\n# Complexity\n- Time complexity:\n$$O(n^2)$$, but I think we can make it $$O(n*log(n))$$ with BIT\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nclass Solution:\n def numTeams(self, rating: List[int]) -> int:\n left, right = [rating[0]], sorted(rating[1:])\n n, res = len(rating), 0\n for i in range(1, n - 1):\n r = rating[i]\n\n # remove r from right\n hi = bisect_left(right, r)\n # this is what gives it another factor of N\n # we can probably use BIT to improve this\n right = right[:hi] + right[hi + 1:]\n\n # count i < j < k\n lo = bisect_right(left, r)\n hi = bisect_left(right, r)\n res += lo * (n - i - 1 - hi)\n\n # count i > j > k\n lo = bisect_left(left, r)\n hi = bisect_right(right, r)\n res += (i - lo) * hi\n\n # add r to left\n insort(left, r)\n return res\n``` | 1 | There are `n` soldiers standing in a line. Each soldier is assigned a **unique** `rating` value.
You have to form a team of 3 soldiers amongst them under the following rules:
* Choose 3 soldiers with index (`i`, `j`, `k`) with rating (`rating[i]`, `rating[j]`, `rating[k]`).
* A team is valid if: (`rating[i] < rating[j] < rating[k]`) or (`rating[i] > rating[j] > rating[k]`) where (`0 <= i < j < k < n`).
Return the number of teams you can form given the conditions. (soldiers can be part of multiple teams).
**Example 1:**
**Input:** rating = \[2,5,3,4,1\]
**Output:** 3
**Explanation:** We can form three teams given the conditions. (2,3,4), (5,4,1), (5,3,1).
**Example 2:**
**Input:** rating = \[2,1,3\]
**Output:** 0
**Explanation:** We can't form any team given the conditions.
**Example 3:**
**Input:** rating = \[1,2,3,4\]
**Output:** 4
**Constraints:**
* `n == rating.length`
* `3 <= n <= 1000`
* `1 <= rating[i] <= 105`
* All the integers in `rating` are **unique**.
In one second, you can either: - Move vertically by one unit, - Move horizontally by one unit, or - Move diagonally sqrt(2) units (in other words, move one unit vertically then one unit horizontally in one second). You have to visit the points in the same order as they appear in the array. You are allowed to pass through points, but they do not count as visited unless you stop on them. | To walk from point A to point B there will be an optimal strategy to walk ? Advance in diagonal as possible then after that go in straight line. Repeat the process until visiting all the points. |
Python3 Solution | design-underground-system | 0 | 1 | \n```\nclass UndergroundSystem:\n\n def __init__(self):\n self.i=defaultdict(tuple)\n self.o=defaultdict(list)\n\n def checkIn(self, id: int, stationName: str, t: int) -> None:\n self.i[id]=(t,stationName)\n\n def checkOut(self, id: int, stationName: str, t: int) -> None:\n starttime,startstation=self.i[id]\n total=t-starttime\n self.o[(startstation,stationName)].append(total)\n\n def getAverageTime(self, startStation: str, endStation: str) -> float:\n return sum(self.o[(startStation,endStation)])/len(self.o[(startStation,endStation)])\n \n\n\n# Your UndergroundSystem object will be instantiated and called as such:\n# obj = UndergroundSystem()\n# obj.checkIn(id,stationName,t)\n# obj.checkOut(id,stationName,t)\n# param_3 = obj.getAverageTime(startStation,endStation)\n``` | 2 | An underground railway system is keeping track of customer travel times between different stations. They are using this data to calculate the average time it takes to travel from one station to another.
Implement the `UndergroundSystem` class:
* `void checkIn(int id, string stationName, int t)`
* A customer with a card ID equal to `id`, checks in at the station `stationName` at time `t`.
* A customer can only be checked into one place at a time.
* `void checkOut(int id, string stationName, int t)`
* A customer with a card ID equal to `id`, checks out from the station `stationName` at time `t`.
* `double getAverageTime(string startStation, string endStation)`
* Returns the average time it takes to travel from `startStation` to `endStation`.
* The average time is computed from all the previous traveling times from `startStation` to `endStation` that happened **directly**, meaning a check in at `startStation` followed by a check out from `endStation`.
* The time it takes to travel from `startStation` to `endStation` **may be different** from the time it takes to travel from `endStation` to `startStation`.
* There will be at least one customer that has traveled from `startStation` to `endStation` before `getAverageTime` is called.
You may assume all calls to the `checkIn` and `checkOut` methods are consistent. If a customer checks in at time `t1` then checks out at time `t2`, then `t1 < t2`. All events happen in chronological order.
**Example 1:**
**Input**
\[ "UndergroundSystem ", "checkIn ", "checkIn ", "checkIn ", "checkOut ", "checkOut ", "checkOut ", "getAverageTime ", "getAverageTime ", "checkIn ", "getAverageTime ", "checkOut ", "getAverageTime "\]
\[\[\],\[45, "Leyton ",3\],\[32, "Paradise ",8\],\[27, "Leyton ",10\],\[45, "Waterloo ",15\],\[27, "Waterloo ",20\],\[32, "Cambridge ",22\],\[ "Paradise ", "Cambridge "\],\[ "Leyton ", "Waterloo "\],\[10, "Leyton ",24\],\[ "Leyton ", "Waterloo "\],\[10, "Waterloo ",38\],\[ "Leyton ", "Waterloo "\]\]
**Output**
\[null,null,null,null,null,null,null,14.00000,11.00000,null,11.00000,null,12.00000\]
**Explanation**
UndergroundSystem undergroundSystem = new UndergroundSystem();
undergroundSystem.checkIn(45, "Leyton ", 3);
undergroundSystem.checkIn(32, "Paradise ", 8);
undergroundSystem.checkIn(27, "Leyton ", 10);
undergroundSystem.checkOut(45, "Waterloo ", 15); // Customer 45 "Leyton " -> "Waterloo " in 15-3 = 12
undergroundSystem.checkOut(27, "Waterloo ", 20); // Customer 27 "Leyton " -> "Waterloo " in 20-10 = 10
undergroundSystem.checkOut(32, "Cambridge ", 22); // Customer 32 "Paradise " -> "Cambridge " in 22-8 = 14
undergroundSystem.getAverageTime( "Paradise ", "Cambridge "); // return 14.00000. One trip "Paradise " -> "Cambridge ", (14) / 1 = 14
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 11.00000. Two trips "Leyton " -> "Waterloo ", (10 + 12) / 2 = 11
undergroundSystem.checkIn(10, "Leyton ", 24);
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 11.00000
undergroundSystem.checkOut(10, "Waterloo ", 38); // Customer 10 "Leyton " -> "Waterloo " in 38-24 = 14
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 12.00000. Three trips "Leyton " -> "Waterloo ", (10 + 12 + 14) / 3 = 12
**Example 2:**
**Input**
\[ "UndergroundSystem ", "checkIn ", "checkOut ", "getAverageTime ", "checkIn ", "checkOut ", "getAverageTime ", "checkIn ", "checkOut ", "getAverageTime "\]
\[\[\],\[10, "Leyton ",3\],\[10, "Paradise ",8\],\[ "Leyton ", "Paradise "\],\[5, "Leyton ",10\],\[5, "Paradise ",16\],\[ "Leyton ", "Paradise "\],\[2, "Leyton ",21\],\[2, "Paradise ",30\],\[ "Leyton ", "Paradise "\]\]
**Output**
\[null,null,null,5.00000,null,null,5.50000,null,null,6.66667\]
**Explanation**
UndergroundSystem undergroundSystem = new UndergroundSystem();
undergroundSystem.checkIn(10, "Leyton ", 3);
undergroundSystem.checkOut(10, "Paradise ", 8); // Customer 10 "Leyton " -> "Paradise " in 8-3 = 5
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 5.00000, (5) / 1 = 5
undergroundSystem.checkIn(5, "Leyton ", 10);
undergroundSystem.checkOut(5, "Paradise ", 16); // Customer 5 "Leyton " -> "Paradise " in 16-10 = 6
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 5.50000, (5 + 6) / 2 = 5.5
undergroundSystem.checkIn(2, "Leyton ", 21);
undergroundSystem.checkOut(2, "Paradise ", 30); // Customer 2 "Leyton " -> "Paradise " in 30-21 = 9
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 6.66667, (5 + 6 + 9) / 3 = 6.66667
**Constraints:**
* `1 <= id, t <= 106`
* `1 <= stationName.length, startStation.length, endStation.length <= 10`
* All strings consist of uppercase and lowercase English letters and digits.
* There will be at most `2 * 104` calls **in total** to `checkIn`, `checkOut`, and `getAverageTime`.
* Answers within `10-5` of the actual value will be accepted. | Store number of computer in each row and column. Count all servers that are not isolated. |
Python3 Solution | design-underground-system | 0 | 1 | \n```\nclass UndergroundSystem:\n\n def __init__(self):\n self.i=defaultdict(tuple)\n self.o=defaultdict(list)\n\n def checkIn(self, id: int, stationName: str, t: int) -> None:\n self.i[id]=(t,stationName)\n\n def checkOut(self, id: int, stationName: str, t: int) -> None:\n starttime,startstation=self.i[id]\n total=t-starttime\n self.o[(startstation,stationName)].append(total)\n\n def getAverageTime(self, startStation: str, endStation: str) -> float:\n return sum(self.o[(startStation,endStation)])/len(self.o[(startStation,endStation)])\n \n\n\n# Your UndergroundSystem object will be instantiated and called as such:\n# obj = UndergroundSystem()\n# obj.checkIn(id,stationName,t)\n# obj.checkOut(id,stationName,t)\n# param_3 = obj.getAverageTime(startStation,endStation)\n``` | 2 | Given an array of integers `nums`, return _the number of **good pairs**_.
A pair `(i, j)` is called _good_ if `nums[i] == nums[j]` and `i` < `j`.
**Example 1:**
**Input:** nums = \[1,2,3,1,1,3\]
**Output:** 4
**Explanation:** There are 4 good pairs (0,3), (0,4), (3,4), (2,5) 0-indexed.
**Example 2:**
**Input:** nums = \[1,1,1,1\]
**Output:** 6
**Explanation:** Each pair in the array are _good_.
**Example 3:**
**Input:** nums = \[1,2,3\]
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 100`
* `1 <= nums[i] <= 100` | Use two hash tables. The first to save the check-in time for a customer and the second to update the total time between two stations. |
Beating 86.12% Python Easiest Solution | design-underground-system | 0 | 1 | 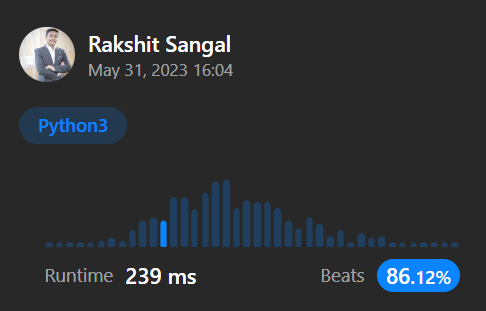\n\n\n\n# Code\n```\nclass UndergroundSystem:\n\n def __init__(self):\n self.i=defaultdict(tuple)\n self.o=defaultdict(list)\n\n def checkIn(self, id: int, stationName: str, t: int) -> None:\n self.i[id]=(t,stationName)\n\n def checkOut(self, id: int, stationName: str, t: int) -> None:\n starttime,startstation=self.i[id]\n total=t-starttime\n self.o[(startstation,stationName)].append(total)\n\n def getAverageTime(self, startStation: str, endStation: str) -> float:\n return sum(self.o[(startStation,endStation)])/len(self.o[(startStation,endStation)])\n \n\n\n# Your UndergroundSystem object will be instantiated and called as such:\n# obj = UndergroundSystem()\n# obj.checkIn(id,stationName,t)\n# obj.checkOut(id,stationName,t)\n# param_3 = obj.getAverageTime(startStation,endStation)\n``` | 1 | An underground railway system is keeping track of customer travel times between different stations. They are using this data to calculate the average time it takes to travel from one station to another.
Implement the `UndergroundSystem` class:
* `void checkIn(int id, string stationName, int t)`
* A customer with a card ID equal to `id`, checks in at the station `stationName` at time `t`.
* A customer can only be checked into one place at a time.
* `void checkOut(int id, string stationName, int t)`
* A customer with a card ID equal to `id`, checks out from the station `stationName` at time `t`.
* `double getAverageTime(string startStation, string endStation)`
* Returns the average time it takes to travel from `startStation` to `endStation`.
* The average time is computed from all the previous traveling times from `startStation` to `endStation` that happened **directly**, meaning a check in at `startStation` followed by a check out from `endStation`.
* The time it takes to travel from `startStation` to `endStation` **may be different** from the time it takes to travel from `endStation` to `startStation`.
* There will be at least one customer that has traveled from `startStation` to `endStation` before `getAverageTime` is called.
You may assume all calls to the `checkIn` and `checkOut` methods are consistent. If a customer checks in at time `t1` then checks out at time `t2`, then `t1 < t2`. All events happen in chronological order.
**Example 1:**
**Input**
\[ "UndergroundSystem ", "checkIn ", "checkIn ", "checkIn ", "checkOut ", "checkOut ", "checkOut ", "getAverageTime ", "getAverageTime ", "checkIn ", "getAverageTime ", "checkOut ", "getAverageTime "\]
\[\[\],\[45, "Leyton ",3\],\[32, "Paradise ",8\],\[27, "Leyton ",10\],\[45, "Waterloo ",15\],\[27, "Waterloo ",20\],\[32, "Cambridge ",22\],\[ "Paradise ", "Cambridge "\],\[ "Leyton ", "Waterloo "\],\[10, "Leyton ",24\],\[ "Leyton ", "Waterloo "\],\[10, "Waterloo ",38\],\[ "Leyton ", "Waterloo "\]\]
**Output**
\[null,null,null,null,null,null,null,14.00000,11.00000,null,11.00000,null,12.00000\]
**Explanation**
UndergroundSystem undergroundSystem = new UndergroundSystem();
undergroundSystem.checkIn(45, "Leyton ", 3);
undergroundSystem.checkIn(32, "Paradise ", 8);
undergroundSystem.checkIn(27, "Leyton ", 10);
undergroundSystem.checkOut(45, "Waterloo ", 15); // Customer 45 "Leyton " -> "Waterloo " in 15-3 = 12
undergroundSystem.checkOut(27, "Waterloo ", 20); // Customer 27 "Leyton " -> "Waterloo " in 20-10 = 10
undergroundSystem.checkOut(32, "Cambridge ", 22); // Customer 32 "Paradise " -> "Cambridge " in 22-8 = 14
undergroundSystem.getAverageTime( "Paradise ", "Cambridge "); // return 14.00000. One trip "Paradise " -> "Cambridge ", (14) / 1 = 14
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 11.00000. Two trips "Leyton " -> "Waterloo ", (10 + 12) / 2 = 11
undergroundSystem.checkIn(10, "Leyton ", 24);
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 11.00000
undergroundSystem.checkOut(10, "Waterloo ", 38); // Customer 10 "Leyton " -> "Waterloo " in 38-24 = 14
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 12.00000. Three trips "Leyton " -> "Waterloo ", (10 + 12 + 14) / 3 = 12
**Example 2:**
**Input**
\[ "UndergroundSystem ", "checkIn ", "checkOut ", "getAverageTime ", "checkIn ", "checkOut ", "getAverageTime ", "checkIn ", "checkOut ", "getAverageTime "\]
\[\[\],\[10, "Leyton ",3\],\[10, "Paradise ",8\],\[ "Leyton ", "Paradise "\],\[5, "Leyton ",10\],\[5, "Paradise ",16\],\[ "Leyton ", "Paradise "\],\[2, "Leyton ",21\],\[2, "Paradise ",30\],\[ "Leyton ", "Paradise "\]\]
**Output**
\[null,null,null,5.00000,null,null,5.50000,null,null,6.66667\]
**Explanation**
UndergroundSystem undergroundSystem = new UndergroundSystem();
undergroundSystem.checkIn(10, "Leyton ", 3);
undergroundSystem.checkOut(10, "Paradise ", 8); // Customer 10 "Leyton " -> "Paradise " in 8-3 = 5
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 5.00000, (5) / 1 = 5
undergroundSystem.checkIn(5, "Leyton ", 10);
undergroundSystem.checkOut(5, "Paradise ", 16); // Customer 5 "Leyton " -> "Paradise " in 16-10 = 6
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 5.50000, (5 + 6) / 2 = 5.5
undergroundSystem.checkIn(2, "Leyton ", 21);
undergroundSystem.checkOut(2, "Paradise ", 30); // Customer 2 "Leyton " -> "Paradise " in 30-21 = 9
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 6.66667, (5 + 6 + 9) / 3 = 6.66667
**Constraints:**
* `1 <= id, t <= 106`
* `1 <= stationName.length, startStation.length, endStation.length <= 10`
* All strings consist of uppercase and lowercase English letters and digits.
* There will be at most `2 * 104` calls **in total** to `checkIn`, `checkOut`, and `getAverageTime`.
* Answers within `10-5` of the actual value will be accepted. | Store number of computer in each row and column. Count all servers that are not isolated. |
Beating 86.12% Python Easiest Solution | design-underground-system | 0 | 1 | 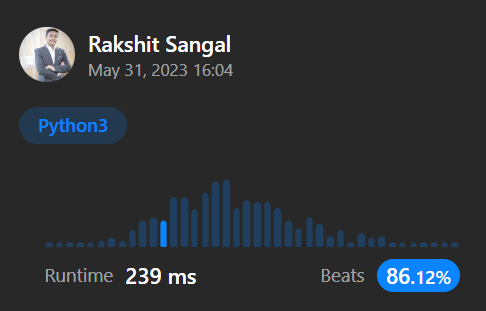\n\n\n\n# Code\n```\nclass UndergroundSystem:\n\n def __init__(self):\n self.i=defaultdict(tuple)\n self.o=defaultdict(list)\n\n def checkIn(self, id: int, stationName: str, t: int) -> None:\n self.i[id]=(t,stationName)\n\n def checkOut(self, id: int, stationName: str, t: int) -> None:\n starttime,startstation=self.i[id]\n total=t-starttime\n self.o[(startstation,stationName)].append(total)\n\n def getAverageTime(self, startStation: str, endStation: str) -> float:\n return sum(self.o[(startStation,endStation)])/len(self.o[(startStation,endStation)])\n \n\n\n# Your UndergroundSystem object will be instantiated and called as such:\n# obj = UndergroundSystem()\n# obj.checkIn(id,stationName,t)\n# obj.checkOut(id,stationName,t)\n# param_3 = obj.getAverageTime(startStation,endStation)\n``` | 1 | Given an array of integers `nums`, return _the number of **good pairs**_.
A pair `(i, j)` is called _good_ if `nums[i] == nums[j]` and `i` < `j`.
**Example 1:**
**Input:** nums = \[1,2,3,1,1,3\]
**Output:** 4
**Explanation:** There are 4 good pairs (0,3), (0,4), (3,4), (2,5) 0-indexed.
**Example 2:**
**Input:** nums = \[1,1,1,1\]
**Output:** 6
**Explanation:** Each pair in the array are _good_.
**Example 3:**
**Input:** nums = \[1,2,3\]
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 100`
* `1 <= nums[i] <= 100` | Use two hash tables. The first to save the check-in time for a customer and the second to update the total time between two stations. |
Python short and clean. | design-underground-system | 0 | 1 | # Complexity\n- Time complexity: $$O(1)$$, for each method.\n\n- Space complexity: $$O(1)$$, for each method.\n\n# Code\n```python\nclass UndergroundSystem:\n\n def __init__(self):\n self.check_ins = {} # station_in => t_in\n self.trips = defaultdict(lambda: (0, 0)) # (station_in, station_out) => (total_t, count) across all trips\n\n def checkIn(self, id: int, station_name: str, t: int) -> None:\n self.check_ins[id] = (station_name, t)\n\n def checkOut(self, id: int, station_name: str, t: int) -> None:\n s_out, t_out = station_name, t\n s_in , t_in = self.check_ins[id]\n\n self.trips[(s_in, s_out)] = tuple(map(add, self.trips[(s_in, s_out)], (t_out - t_in, 1)))\n\n def getAverageTime(self, station_in: str, station_out: str) -> float:\n return truediv(*self.trips[(station_in, station_out)])\n\n\n``` | 2 | An underground railway system is keeping track of customer travel times between different stations. They are using this data to calculate the average time it takes to travel from one station to another.
Implement the `UndergroundSystem` class:
* `void checkIn(int id, string stationName, int t)`
* A customer with a card ID equal to `id`, checks in at the station `stationName` at time `t`.
* A customer can only be checked into one place at a time.
* `void checkOut(int id, string stationName, int t)`
* A customer with a card ID equal to `id`, checks out from the station `stationName` at time `t`.
* `double getAverageTime(string startStation, string endStation)`
* Returns the average time it takes to travel from `startStation` to `endStation`.
* The average time is computed from all the previous traveling times from `startStation` to `endStation` that happened **directly**, meaning a check in at `startStation` followed by a check out from `endStation`.
* The time it takes to travel from `startStation` to `endStation` **may be different** from the time it takes to travel from `endStation` to `startStation`.
* There will be at least one customer that has traveled from `startStation` to `endStation` before `getAverageTime` is called.
You may assume all calls to the `checkIn` and `checkOut` methods are consistent. If a customer checks in at time `t1` then checks out at time `t2`, then `t1 < t2`. All events happen in chronological order.
**Example 1:**
**Input**
\[ "UndergroundSystem ", "checkIn ", "checkIn ", "checkIn ", "checkOut ", "checkOut ", "checkOut ", "getAverageTime ", "getAverageTime ", "checkIn ", "getAverageTime ", "checkOut ", "getAverageTime "\]
\[\[\],\[45, "Leyton ",3\],\[32, "Paradise ",8\],\[27, "Leyton ",10\],\[45, "Waterloo ",15\],\[27, "Waterloo ",20\],\[32, "Cambridge ",22\],\[ "Paradise ", "Cambridge "\],\[ "Leyton ", "Waterloo "\],\[10, "Leyton ",24\],\[ "Leyton ", "Waterloo "\],\[10, "Waterloo ",38\],\[ "Leyton ", "Waterloo "\]\]
**Output**
\[null,null,null,null,null,null,null,14.00000,11.00000,null,11.00000,null,12.00000\]
**Explanation**
UndergroundSystem undergroundSystem = new UndergroundSystem();
undergroundSystem.checkIn(45, "Leyton ", 3);
undergroundSystem.checkIn(32, "Paradise ", 8);
undergroundSystem.checkIn(27, "Leyton ", 10);
undergroundSystem.checkOut(45, "Waterloo ", 15); // Customer 45 "Leyton " -> "Waterloo " in 15-3 = 12
undergroundSystem.checkOut(27, "Waterloo ", 20); // Customer 27 "Leyton " -> "Waterloo " in 20-10 = 10
undergroundSystem.checkOut(32, "Cambridge ", 22); // Customer 32 "Paradise " -> "Cambridge " in 22-8 = 14
undergroundSystem.getAverageTime( "Paradise ", "Cambridge "); // return 14.00000. One trip "Paradise " -> "Cambridge ", (14) / 1 = 14
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 11.00000. Two trips "Leyton " -> "Waterloo ", (10 + 12) / 2 = 11
undergroundSystem.checkIn(10, "Leyton ", 24);
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 11.00000
undergroundSystem.checkOut(10, "Waterloo ", 38); // Customer 10 "Leyton " -> "Waterloo " in 38-24 = 14
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 12.00000. Three trips "Leyton " -> "Waterloo ", (10 + 12 + 14) / 3 = 12
**Example 2:**
**Input**
\[ "UndergroundSystem ", "checkIn ", "checkOut ", "getAverageTime ", "checkIn ", "checkOut ", "getAverageTime ", "checkIn ", "checkOut ", "getAverageTime "\]
\[\[\],\[10, "Leyton ",3\],\[10, "Paradise ",8\],\[ "Leyton ", "Paradise "\],\[5, "Leyton ",10\],\[5, "Paradise ",16\],\[ "Leyton ", "Paradise "\],\[2, "Leyton ",21\],\[2, "Paradise ",30\],\[ "Leyton ", "Paradise "\]\]
**Output**
\[null,null,null,5.00000,null,null,5.50000,null,null,6.66667\]
**Explanation**
UndergroundSystem undergroundSystem = new UndergroundSystem();
undergroundSystem.checkIn(10, "Leyton ", 3);
undergroundSystem.checkOut(10, "Paradise ", 8); // Customer 10 "Leyton " -> "Paradise " in 8-3 = 5
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 5.00000, (5) / 1 = 5
undergroundSystem.checkIn(5, "Leyton ", 10);
undergroundSystem.checkOut(5, "Paradise ", 16); // Customer 5 "Leyton " -> "Paradise " in 16-10 = 6
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 5.50000, (5 + 6) / 2 = 5.5
undergroundSystem.checkIn(2, "Leyton ", 21);
undergroundSystem.checkOut(2, "Paradise ", 30); // Customer 2 "Leyton " -> "Paradise " in 30-21 = 9
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 6.66667, (5 + 6 + 9) / 3 = 6.66667
**Constraints:**
* `1 <= id, t <= 106`
* `1 <= stationName.length, startStation.length, endStation.length <= 10`
* All strings consist of uppercase and lowercase English letters and digits.
* There will be at most `2 * 104` calls **in total** to `checkIn`, `checkOut`, and `getAverageTime`.
* Answers within `10-5` of the actual value will be accepted. | Store number of computer in each row and column. Count all servers that are not isolated. |
Python short and clean. | design-underground-system | 0 | 1 | # Complexity\n- Time complexity: $$O(1)$$, for each method.\n\n- Space complexity: $$O(1)$$, for each method.\n\n# Code\n```python\nclass UndergroundSystem:\n\n def __init__(self):\n self.check_ins = {} # station_in => t_in\n self.trips = defaultdict(lambda: (0, 0)) # (station_in, station_out) => (total_t, count) across all trips\n\n def checkIn(self, id: int, station_name: str, t: int) -> None:\n self.check_ins[id] = (station_name, t)\n\n def checkOut(self, id: int, station_name: str, t: int) -> None:\n s_out, t_out = station_name, t\n s_in , t_in = self.check_ins[id]\n\n self.trips[(s_in, s_out)] = tuple(map(add, self.trips[(s_in, s_out)], (t_out - t_in, 1)))\n\n def getAverageTime(self, station_in: str, station_out: str) -> float:\n return truediv(*self.trips[(station_in, station_out)])\n\n\n``` | 2 | Given an array of integers `nums`, return _the number of **good pairs**_.
A pair `(i, j)` is called _good_ if `nums[i] == nums[j]` and `i` < `j`.
**Example 1:**
**Input:** nums = \[1,2,3,1,1,3\]
**Output:** 4
**Explanation:** There are 4 good pairs (0,3), (0,4), (3,4), (2,5) 0-indexed.
**Example 2:**
**Input:** nums = \[1,1,1,1\]
**Output:** 6
**Explanation:** Each pair in the array are _good_.
**Example 3:**
**Input:** nums = \[1,2,3\]
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 100`
* `1 <= nums[i] <= 100` | Use two hash tables. The first to save the check-in time for a customer and the second to update the total time between two stations. |
EASY | BEGINNER FRIENDLY EXPLANATION | SPACE OPTIMISED |C++ |PYTHON3 | design-underground-system | 0 | 1 | # ADVICE\nREAD APPROACH AND TRY DOING YOURSELF .THEN SEE CODE AND UPVOTE \uD83D\uDE42.\n# Approach\nWHAT WE WANT?\nAverage Travelling time b/w two stations .\nOne person{id} is contributing some Travelling time for a particular \n{start station , end station}.\nWe will store its contribution in map .\n```\n //id StaringStationName , Checkin Time\nmap<int, pair<string , int >>chkin;\n\nmap<pair<string,string>,pair<int,int>>avg;\n```\navg Map will contain \nmap<{StartStation,EndStation},{\u2211TravellingTime,NumberOfTimes{Start-End}Station Travel has occured}>avg;\n // Here Travelling Time = Checkout Time -Checkin Time \n\nHOW WE CAN GET AVERAGE TRAVELLING TIME B/W TWO STATIONS ?\nAns. SUM OF ALL TRAVELS FROM STARTING STATION TO FINAL STATIONS DIVIDED BY TOTAL NUMBER OF {Si , Ei} TRAVELS.\n\nSince we know the ID and starting station and checkin time of persons with id I{From **map chkin**} .So at the time of checkout we will add Travelling time in our **map avg** .and Also increment the freq of {Si,Ei} Travel.\n\nSince Given in question checkOut Happens only after checkin we don\'t have to worry for other cases.\n\nFinally.\n\n```\ndouble getAverageTime(string s, string e) {\n return avg[{s,e}].first/(double)avg[{s,e}].second;\n}\n```\n\n# Code\n\n```C++ []\nclass UndergroundSystem {\n private :\n //id station name , time t1\n map<int,pair<string,int>>chkin;\n //initial station1 final station2 sum_till_now count\n map<pair<string,string>,pair<int,int>>avg;\npublic:\n UndergroundSystem(){}\n void checkIn(int id, string s, int t) {\n pair<string,int>p={s,t};\n chkin[id]=p;\n }\n void checkOut(int id, string s, int t) {\n int timetravel=t-chkin[id].second;\n avg[{chkin[id].first,s}].first+=timetravel;\n avg[{chkin[id].first,s}].second++;\n }\n double getAverageTime(string s, string e){\n return avg[{s,e}].first/(double)avg[{s,e}].second;\n }\n};\n\n```\n```python []\n\nclass UndergroundSystem:\n def __init__(self):\n self.checkIns = {}\n self.averageTimes = defaultdict(lambda: [0, 0])\n\n def checkIn(self, id: int, stationName: str, t: int) -> None:\n self.checkIns[id] = (stationName, t)\n\n def checkOut(self, id: int, stationName: str, t: int) -> None:\n startStation, startTime = self.checkIns[id]\n route = (startStation, stationName)\n timeTravel = t - startTime\n self.averageTimes[route][0] += timeTravel\n self.averageTimes[route][1] += 1\n\n def getAverageTime(self, startStation: str, endStation: str) -> float:\n totalTime, totalTrips = self.averageTimes[(startStation, endStation)]\n return totalTime / totalTrips if totalTrips > 0 else 0.0\n\n\n```\n\nTHANK YOU \nCODE BY:) AMAN MAURYA | 5 | An underground railway system is keeping track of customer travel times between different stations. They are using this data to calculate the average time it takes to travel from one station to another.
Implement the `UndergroundSystem` class:
* `void checkIn(int id, string stationName, int t)`
* A customer with a card ID equal to `id`, checks in at the station `stationName` at time `t`.
* A customer can only be checked into one place at a time.
* `void checkOut(int id, string stationName, int t)`
* A customer with a card ID equal to `id`, checks out from the station `stationName` at time `t`.
* `double getAverageTime(string startStation, string endStation)`
* Returns the average time it takes to travel from `startStation` to `endStation`.
* The average time is computed from all the previous traveling times from `startStation` to `endStation` that happened **directly**, meaning a check in at `startStation` followed by a check out from `endStation`.
* The time it takes to travel from `startStation` to `endStation` **may be different** from the time it takes to travel from `endStation` to `startStation`.
* There will be at least one customer that has traveled from `startStation` to `endStation` before `getAverageTime` is called.
You may assume all calls to the `checkIn` and `checkOut` methods are consistent. If a customer checks in at time `t1` then checks out at time `t2`, then `t1 < t2`. All events happen in chronological order.
**Example 1:**
**Input**
\[ "UndergroundSystem ", "checkIn ", "checkIn ", "checkIn ", "checkOut ", "checkOut ", "checkOut ", "getAverageTime ", "getAverageTime ", "checkIn ", "getAverageTime ", "checkOut ", "getAverageTime "\]
\[\[\],\[45, "Leyton ",3\],\[32, "Paradise ",8\],\[27, "Leyton ",10\],\[45, "Waterloo ",15\],\[27, "Waterloo ",20\],\[32, "Cambridge ",22\],\[ "Paradise ", "Cambridge "\],\[ "Leyton ", "Waterloo "\],\[10, "Leyton ",24\],\[ "Leyton ", "Waterloo "\],\[10, "Waterloo ",38\],\[ "Leyton ", "Waterloo "\]\]
**Output**
\[null,null,null,null,null,null,null,14.00000,11.00000,null,11.00000,null,12.00000\]
**Explanation**
UndergroundSystem undergroundSystem = new UndergroundSystem();
undergroundSystem.checkIn(45, "Leyton ", 3);
undergroundSystem.checkIn(32, "Paradise ", 8);
undergroundSystem.checkIn(27, "Leyton ", 10);
undergroundSystem.checkOut(45, "Waterloo ", 15); // Customer 45 "Leyton " -> "Waterloo " in 15-3 = 12
undergroundSystem.checkOut(27, "Waterloo ", 20); // Customer 27 "Leyton " -> "Waterloo " in 20-10 = 10
undergroundSystem.checkOut(32, "Cambridge ", 22); // Customer 32 "Paradise " -> "Cambridge " in 22-8 = 14
undergroundSystem.getAverageTime( "Paradise ", "Cambridge "); // return 14.00000. One trip "Paradise " -> "Cambridge ", (14) / 1 = 14
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 11.00000. Two trips "Leyton " -> "Waterloo ", (10 + 12) / 2 = 11
undergroundSystem.checkIn(10, "Leyton ", 24);
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 11.00000
undergroundSystem.checkOut(10, "Waterloo ", 38); // Customer 10 "Leyton " -> "Waterloo " in 38-24 = 14
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 12.00000. Three trips "Leyton " -> "Waterloo ", (10 + 12 + 14) / 3 = 12
**Example 2:**
**Input**
\[ "UndergroundSystem ", "checkIn ", "checkOut ", "getAverageTime ", "checkIn ", "checkOut ", "getAverageTime ", "checkIn ", "checkOut ", "getAverageTime "\]
\[\[\],\[10, "Leyton ",3\],\[10, "Paradise ",8\],\[ "Leyton ", "Paradise "\],\[5, "Leyton ",10\],\[5, "Paradise ",16\],\[ "Leyton ", "Paradise "\],\[2, "Leyton ",21\],\[2, "Paradise ",30\],\[ "Leyton ", "Paradise "\]\]
**Output**
\[null,null,null,5.00000,null,null,5.50000,null,null,6.66667\]
**Explanation**
UndergroundSystem undergroundSystem = new UndergroundSystem();
undergroundSystem.checkIn(10, "Leyton ", 3);
undergroundSystem.checkOut(10, "Paradise ", 8); // Customer 10 "Leyton " -> "Paradise " in 8-3 = 5
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 5.00000, (5) / 1 = 5
undergroundSystem.checkIn(5, "Leyton ", 10);
undergroundSystem.checkOut(5, "Paradise ", 16); // Customer 5 "Leyton " -> "Paradise " in 16-10 = 6
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 5.50000, (5 + 6) / 2 = 5.5
undergroundSystem.checkIn(2, "Leyton ", 21);
undergroundSystem.checkOut(2, "Paradise ", 30); // Customer 2 "Leyton " -> "Paradise " in 30-21 = 9
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 6.66667, (5 + 6 + 9) / 3 = 6.66667
**Constraints:**
* `1 <= id, t <= 106`
* `1 <= stationName.length, startStation.length, endStation.length <= 10`
* All strings consist of uppercase and lowercase English letters and digits.
* There will be at most `2 * 104` calls **in total** to `checkIn`, `checkOut`, and `getAverageTime`.
* Answers within `10-5` of the actual value will be accepted. | Store number of computer in each row and column. Count all servers that are not isolated. |
EASY | BEGINNER FRIENDLY EXPLANATION | SPACE OPTIMISED |C++ |PYTHON3 | design-underground-system | 0 | 1 | # ADVICE\nREAD APPROACH AND TRY DOING YOURSELF .THEN SEE CODE AND UPVOTE \uD83D\uDE42.\n# Approach\nWHAT WE WANT?\nAverage Travelling time b/w two stations .\nOne person{id} is contributing some Travelling time for a particular \n{start station , end station}.\nWe will store its contribution in map .\n```\n //id StaringStationName , Checkin Time\nmap<int, pair<string , int >>chkin;\n\nmap<pair<string,string>,pair<int,int>>avg;\n```\navg Map will contain \nmap<{StartStation,EndStation},{\u2211TravellingTime,NumberOfTimes{Start-End}Station Travel has occured}>avg;\n // Here Travelling Time = Checkout Time -Checkin Time \n\nHOW WE CAN GET AVERAGE TRAVELLING TIME B/W TWO STATIONS ?\nAns. SUM OF ALL TRAVELS FROM STARTING STATION TO FINAL STATIONS DIVIDED BY TOTAL NUMBER OF {Si , Ei} TRAVELS.\n\nSince we know the ID and starting station and checkin time of persons with id I{From **map chkin**} .So at the time of checkout we will add Travelling time in our **map avg** .and Also increment the freq of {Si,Ei} Travel.\n\nSince Given in question checkOut Happens only after checkin we don\'t have to worry for other cases.\n\nFinally.\n\n```\ndouble getAverageTime(string s, string e) {\n return avg[{s,e}].first/(double)avg[{s,e}].second;\n}\n```\n\n# Code\n\n```C++ []\nclass UndergroundSystem {\n private :\n //id station name , time t1\n map<int,pair<string,int>>chkin;\n //initial station1 final station2 sum_till_now count\n map<pair<string,string>,pair<int,int>>avg;\npublic:\n UndergroundSystem(){}\n void checkIn(int id, string s, int t) {\n pair<string,int>p={s,t};\n chkin[id]=p;\n }\n void checkOut(int id, string s, int t) {\n int timetravel=t-chkin[id].second;\n avg[{chkin[id].first,s}].first+=timetravel;\n avg[{chkin[id].first,s}].second++;\n }\n double getAverageTime(string s, string e){\n return avg[{s,e}].first/(double)avg[{s,e}].second;\n }\n};\n\n```\n```python []\n\nclass UndergroundSystem:\n def __init__(self):\n self.checkIns = {}\n self.averageTimes = defaultdict(lambda: [0, 0])\n\n def checkIn(self, id: int, stationName: str, t: int) -> None:\n self.checkIns[id] = (stationName, t)\n\n def checkOut(self, id: int, stationName: str, t: int) -> None:\n startStation, startTime = self.checkIns[id]\n route = (startStation, stationName)\n timeTravel = t - startTime\n self.averageTimes[route][0] += timeTravel\n self.averageTimes[route][1] += 1\n\n def getAverageTime(self, startStation: str, endStation: str) -> float:\n totalTime, totalTrips = self.averageTimes[(startStation, endStation)]\n return totalTime / totalTrips if totalTrips > 0 else 0.0\n\n\n```\n\nTHANK YOU \nCODE BY:) AMAN MAURYA | 5 | Given an array of integers `nums`, return _the number of **good pairs**_.
A pair `(i, j)` is called _good_ if `nums[i] == nums[j]` and `i` < `j`.
**Example 1:**
**Input:** nums = \[1,2,3,1,1,3\]
**Output:** 4
**Explanation:** There are 4 good pairs (0,3), (0,4), (3,4), (2,5) 0-indexed.
**Example 2:**
**Input:** nums = \[1,1,1,1\]
**Output:** 6
**Explanation:** Each pair in the array are _good_.
**Example 3:**
**Input:** nums = \[1,2,3\]
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 100`
* `1 <= nums[i] <= 100` | Use two hash tables. The first to save the check-in time for a customer and the second to update the total time between two stations. |
Python🔥Java🔥C++🔥Simple Solution🔥Easy to Understand | design-underground-system | 1 | 1 | **!! BIG ANNOUNCEMENT !!**\nI am currently Giving away my premium content well-structured assignments and study materials to clear interviews at top companies related to computer science and data science to my current Subscribers. This is only for first 10,000 Subscribers. **DON\'T FORGET** to Subscribe\n\n# Search \uD83D\uDC49 `Tech Wired Leetcode` to Subscribe\n\n# Video Solution\n\n# Search \uD83D\uDC49 `Design Underground System By Tech Wired` \n\n# or\n\n# Click the Link in my Profile\n\n\n# Approach:\n\nTo design the "Underground System," we can use two data structures: one to store the check-in information and another to store the travel times. Here is the overall approach:\n\n- For check-in:\nWhen a customer checks in, store their ID along with the station name and check-in time in a dictionary or map. This will allow us to retrieve the check-in information later when the customer checks out.\n- For check-out:\nWhen a customer checks out, retrieve their check-in information from the dictionary using their ID.\nCalculate the travel time by subtracting the check-in time from the current check-out time.\n- Update the travel times dictionary:\nIf the (start_station, end_station) pair already exists in the dictionary, update the total time and increment the count.\nOtherwise, create a new entry in the dictionary with the initial travel time and count.\n- For calculating average travel time:\nRetrieve the total time and count from the travel times dictionary for the given (startStation, endStation) pair.\nCalculate and return the average travel time by dividing the total time by the count.\n# Intuition:\n\nThe approach leverages the concept of storing relevant information at check-in and using it to calculate the travel times at check-out. By storing the check-in information in a dictionary or map, we can easily retrieve it when needed. Similarly, the travel times are stored in another dictionary, where the (start_station, end_station) pair serves as the key, and the total time and count are stored as values.\n\nThe use of these data structures allows for efficient retrieval and update operations. The solution optimizes both time and space complexity by removing unnecessary check-in information once the customer has checked out and by utilizing appropriate data structures to track travel times efficiently.\n\n```Python []\nclass UndergroundSystem:\n def __init__(self):\n # Dictionary to store total travel time and count for each (start_station, end_station) pair\n self.travel_times = {}\n\n # Dictionary to store check-in information with customer_id as key and (start_station, check_in_time) as value\n self.check_ins = {}\n\n def checkIn(self, id: int, stationName: str, t: int) -> None:\n self.check_ins[id] = (stationName, t)\n\n def checkOut(self, id: int, stationName: str, t: int) -> None:\n start_station, check_in_time = self.check_ins.pop(id)\n travel = (start_station, stationName)\n travel_time = t - check_in_time\n\n if travel in self.travel_times:\n total_time, count = self.travel_times[travel]\n self.travel_times[travel] = (total_time + travel_time, count + 1)\n else:\n self.travel_times[travel] = (travel_time, 1)\n\n def getAverageTime(self, startStation: str, endStation: str) -> float:\n travel = (startStation, endStation)\n total_time, count = self.travel_times[travel]\n return total_time / count\n\n```\n```Java []\n\nclass UndergroundSystem {\n private Map<Integer, CheckInInfo> checkIns;\n private Map<String, TravelInfo> travelTimes;\n\n public UndergroundSystem() {\n checkIns = new HashMap<>();\n travelTimes = new HashMap<>();\n }\n\n public void checkIn(int id, String stationName, int t) {\n checkIns.put(id, new CheckInInfo(stationName, t));\n }\n\n public void checkOut(int id, String stationName, int t) {\n CheckInInfo checkInInfo = checkIns.remove(id);\n String travel = checkInInfo.stationName + "," + stationName;\n int travelTime = t - checkInInfo.checkInTime;\n\n if (travelTimes.containsKey(travel)) {\n TravelInfo travelInfo = travelTimes.get(travel);\n travelInfo.totalTime += travelTime;\n travelInfo.count++;\n } else {\n travelTimes.put(travel, new TravelInfo(travelTime, 1));\n }\n }\n\n public double getAverageTime(String startStation, String endStation) {\n String travel = startStation + "," + endStation;\n TravelInfo travelInfo = travelTimes.get(travel);\n return (double) travelInfo.totalTime / travelInfo.count;\n }\n\n private class CheckInInfo {\n String stationName;\n int checkInTime;\n\n public CheckInInfo(String stationName, int checkInTime) {\n this.stationName = stationName;\n this.checkInTime = checkInTime;\n }\n }\n\n private class TravelInfo {\n int totalTime;\n int count;\n\n public TravelInfo(int totalTime, int count) {\n this.totalTime = totalTime;\n this.count = count;\n }\n }\n}\n\n```\n```C++ []\n\n\nclass UndergroundSystem {\npublic:\n UndergroundSystem() {}\n\n void checkIn(int id, string stationName, int t) {\n checkIns[id] = make_pair(stationName, t);\n }\n\n void checkOut(int id, string stationName, int t) {\n auto checkInInfo = checkIns[id];\n checkIns.erase(id);\n string travel = checkInInfo.first + "," + stationName;\n int travelTime = t - checkInInfo.second;\n\n if (travelTimes.find(travel) != travelTimes.end()) {\n travelTimes[travel].first += travelTime;\n travelTimes[travel].second++;\n } else {\n travelTimes[travel] = make_pair(travelTime, 1);\n }\n }\n\n double getAverageTime(string startStation, string endStation) {\n string travel = startStation + "," + endStation;\n auto travelInfo = travelTimes[travel];\n return (double) travelInfo.first / travelInfo.second;\n }\n\nprivate:\n unordered_map<int, pair<string, int>> checkIns;\n unordered_map<string, pair<int, int>> travelTimes;\n};\n\n```\n# An Upvote will be encouraging \uD83D\uDC4D\n | 32 | An underground railway system is keeping track of customer travel times between different stations. They are using this data to calculate the average time it takes to travel from one station to another.
Implement the `UndergroundSystem` class:
* `void checkIn(int id, string stationName, int t)`
* A customer with a card ID equal to `id`, checks in at the station `stationName` at time `t`.
* A customer can only be checked into one place at a time.
* `void checkOut(int id, string stationName, int t)`
* A customer with a card ID equal to `id`, checks out from the station `stationName` at time `t`.
* `double getAverageTime(string startStation, string endStation)`
* Returns the average time it takes to travel from `startStation` to `endStation`.
* The average time is computed from all the previous traveling times from `startStation` to `endStation` that happened **directly**, meaning a check in at `startStation` followed by a check out from `endStation`.
* The time it takes to travel from `startStation` to `endStation` **may be different** from the time it takes to travel from `endStation` to `startStation`.
* There will be at least one customer that has traveled from `startStation` to `endStation` before `getAverageTime` is called.
You may assume all calls to the `checkIn` and `checkOut` methods are consistent. If a customer checks in at time `t1` then checks out at time `t2`, then `t1 < t2`. All events happen in chronological order.
**Example 1:**
**Input**
\[ "UndergroundSystem ", "checkIn ", "checkIn ", "checkIn ", "checkOut ", "checkOut ", "checkOut ", "getAverageTime ", "getAverageTime ", "checkIn ", "getAverageTime ", "checkOut ", "getAverageTime "\]
\[\[\],\[45, "Leyton ",3\],\[32, "Paradise ",8\],\[27, "Leyton ",10\],\[45, "Waterloo ",15\],\[27, "Waterloo ",20\],\[32, "Cambridge ",22\],\[ "Paradise ", "Cambridge "\],\[ "Leyton ", "Waterloo "\],\[10, "Leyton ",24\],\[ "Leyton ", "Waterloo "\],\[10, "Waterloo ",38\],\[ "Leyton ", "Waterloo "\]\]
**Output**
\[null,null,null,null,null,null,null,14.00000,11.00000,null,11.00000,null,12.00000\]
**Explanation**
UndergroundSystem undergroundSystem = new UndergroundSystem();
undergroundSystem.checkIn(45, "Leyton ", 3);
undergroundSystem.checkIn(32, "Paradise ", 8);
undergroundSystem.checkIn(27, "Leyton ", 10);
undergroundSystem.checkOut(45, "Waterloo ", 15); // Customer 45 "Leyton " -> "Waterloo " in 15-3 = 12
undergroundSystem.checkOut(27, "Waterloo ", 20); // Customer 27 "Leyton " -> "Waterloo " in 20-10 = 10
undergroundSystem.checkOut(32, "Cambridge ", 22); // Customer 32 "Paradise " -> "Cambridge " in 22-8 = 14
undergroundSystem.getAverageTime( "Paradise ", "Cambridge "); // return 14.00000. One trip "Paradise " -> "Cambridge ", (14) / 1 = 14
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 11.00000. Two trips "Leyton " -> "Waterloo ", (10 + 12) / 2 = 11
undergroundSystem.checkIn(10, "Leyton ", 24);
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 11.00000
undergroundSystem.checkOut(10, "Waterloo ", 38); // Customer 10 "Leyton " -> "Waterloo " in 38-24 = 14
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 12.00000. Three trips "Leyton " -> "Waterloo ", (10 + 12 + 14) / 3 = 12
**Example 2:**
**Input**
\[ "UndergroundSystem ", "checkIn ", "checkOut ", "getAverageTime ", "checkIn ", "checkOut ", "getAverageTime ", "checkIn ", "checkOut ", "getAverageTime "\]
\[\[\],\[10, "Leyton ",3\],\[10, "Paradise ",8\],\[ "Leyton ", "Paradise "\],\[5, "Leyton ",10\],\[5, "Paradise ",16\],\[ "Leyton ", "Paradise "\],\[2, "Leyton ",21\],\[2, "Paradise ",30\],\[ "Leyton ", "Paradise "\]\]
**Output**
\[null,null,null,5.00000,null,null,5.50000,null,null,6.66667\]
**Explanation**
UndergroundSystem undergroundSystem = new UndergroundSystem();
undergroundSystem.checkIn(10, "Leyton ", 3);
undergroundSystem.checkOut(10, "Paradise ", 8); // Customer 10 "Leyton " -> "Paradise " in 8-3 = 5
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 5.00000, (5) / 1 = 5
undergroundSystem.checkIn(5, "Leyton ", 10);
undergroundSystem.checkOut(5, "Paradise ", 16); // Customer 5 "Leyton " -> "Paradise " in 16-10 = 6
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 5.50000, (5 + 6) / 2 = 5.5
undergroundSystem.checkIn(2, "Leyton ", 21);
undergroundSystem.checkOut(2, "Paradise ", 30); // Customer 2 "Leyton " -> "Paradise " in 30-21 = 9
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 6.66667, (5 + 6 + 9) / 3 = 6.66667
**Constraints:**
* `1 <= id, t <= 106`
* `1 <= stationName.length, startStation.length, endStation.length <= 10`
* All strings consist of uppercase and lowercase English letters and digits.
* There will be at most `2 * 104` calls **in total** to `checkIn`, `checkOut`, and `getAverageTime`.
* Answers within `10-5` of the actual value will be accepted. | Store number of computer in each row and column. Count all servers that are not isolated. |
Python🔥Java🔥C++🔥Simple Solution🔥Easy to Understand | design-underground-system | 1 | 1 | **!! BIG ANNOUNCEMENT !!**\nI am currently Giving away my premium content well-structured assignments and study materials to clear interviews at top companies related to computer science and data science to my current Subscribers. This is only for first 10,000 Subscribers. **DON\'T FORGET** to Subscribe\n\n# Search \uD83D\uDC49 `Tech Wired Leetcode` to Subscribe\n\n# Video Solution\n\n# Search \uD83D\uDC49 `Design Underground System By Tech Wired` \n\n# or\n\n# Click the Link in my Profile\n\n\n# Approach:\n\nTo design the "Underground System," we can use two data structures: one to store the check-in information and another to store the travel times. Here is the overall approach:\n\n- For check-in:\nWhen a customer checks in, store their ID along with the station name and check-in time in a dictionary or map. This will allow us to retrieve the check-in information later when the customer checks out.\n- For check-out:\nWhen a customer checks out, retrieve their check-in information from the dictionary using their ID.\nCalculate the travel time by subtracting the check-in time from the current check-out time.\n- Update the travel times dictionary:\nIf the (start_station, end_station) pair already exists in the dictionary, update the total time and increment the count.\nOtherwise, create a new entry in the dictionary with the initial travel time and count.\n- For calculating average travel time:\nRetrieve the total time and count from the travel times dictionary for the given (startStation, endStation) pair.\nCalculate and return the average travel time by dividing the total time by the count.\n# Intuition:\n\nThe approach leverages the concept of storing relevant information at check-in and using it to calculate the travel times at check-out. By storing the check-in information in a dictionary or map, we can easily retrieve it when needed. Similarly, the travel times are stored in another dictionary, where the (start_station, end_station) pair serves as the key, and the total time and count are stored as values.\n\nThe use of these data structures allows for efficient retrieval and update operations. The solution optimizes both time and space complexity by removing unnecessary check-in information once the customer has checked out and by utilizing appropriate data structures to track travel times efficiently.\n\n```Python []\nclass UndergroundSystem:\n def __init__(self):\n # Dictionary to store total travel time and count for each (start_station, end_station) pair\n self.travel_times = {}\n\n # Dictionary to store check-in information with customer_id as key and (start_station, check_in_time) as value\n self.check_ins = {}\n\n def checkIn(self, id: int, stationName: str, t: int) -> None:\n self.check_ins[id] = (stationName, t)\n\n def checkOut(self, id: int, stationName: str, t: int) -> None:\n start_station, check_in_time = self.check_ins.pop(id)\n travel = (start_station, stationName)\n travel_time = t - check_in_time\n\n if travel in self.travel_times:\n total_time, count = self.travel_times[travel]\n self.travel_times[travel] = (total_time + travel_time, count + 1)\n else:\n self.travel_times[travel] = (travel_time, 1)\n\n def getAverageTime(self, startStation: str, endStation: str) -> float:\n travel = (startStation, endStation)\n total_time, count = self.travel_times[travel]\n return total_time / count\n\n```\n```Java []\n\nclass UndergroundSystem {\n private Map<Integer, CheckInInfo> checkIns;\n private Map<String, TravelInfo> travelTimes;\n\n public UndergroundSystem() {\n checkIns = new HashMap<>();\n travelTimes = new HashMap<>();\n }\n\n public void checkIn(int id, String stationName, int t) {\n checkIns.put(id, new CheckInInfo(stationName, t));\n }\n\n public void checkOut(int id, String stationName, int t) {\n CheckInInfo checkInInfo = checkIns.remove(id);\n String travel = checkInInfo.stationName + "," + stationName;\n int travelTime = t - checkInInfo.checkInTime;\n\n if (travelTimes.containsKey(travel)) {\n TravelInfo travelInfo = travelTimes.get(travel);\n travelInfo.totalTime += travelTime;\n travelInfo.count++;\n } else {\n travelTimes.put(travel, new TravelInfo(travelTime, 1));\n }\n }\n\n public double getAverageTime(String startStation, String endStation) {\n String travel = startStation + "," + endStation;\n TravelInfo travelInfo = travelTimes.get(travel);\n return (double) travelInfo.totalTime / travelInfo.count;\n }\n\n private class CheckInInfo {\n String stationName;\n int checkInTime;\n\n public CheckInInfo(String stationName, int checkInTime) {\n this.stationName = stationName;\n this.checkInTime = checkInTime;\n }\n }\n\n private class TravelInfo {\n int totalTime;\n int count;\n\n public TravelInfo(int totalTime, int count) {\n this.totalTime = totalTime;\n this.count = count;\n }\n }\n}\n\n```\n```C++ []\n\n\nclass UndergroundSystem {\npublic:\n UndergroundSystem() {}\n\n void checkIn(int id, string stationName, int t) {\n checkIns[id] = make_pair(stationName, t);\n }\n\n void checkOut(int id, string stationName, int t) {\n auto checkInInfo = checkIns[id];\n checkIns.erase(id);\n string travel = checkInInfo.first + "," + stationName;\n int travelTime = t - checkInInfo.second;\n\n if (travelTimes.find(travel) != travelTimes.end()) {\n travelTimes[travel].first += travelTime;\n travelTimes[travel].second++;\n } else {\n travelTimes[travel] = make_pair(travelTime, 1);\n }\n }\n\n double getAverageTime(string startStation, string endStation) {\n string travel = startStation + "," + endStation;\n auto travelInfo = travelTimes[travel];\n return (double) travelInfo.first / travelInfo.second;\n }\n\nprivate:\n unordered_map<int, pair<string, int>> checkIns;\n unordered_map<string, pair<int, int>> travelTimes;\n};\n\n```\n# An Upvote will be encouraging \uD83D\uDC4D\n | 32 | Given an array of integers `nums`, return _the number of **good pairs**_.
A pair `(i, j)` is called _good_ if `nums[i] == nums[j]` and `i` < `j`.
**Example 1:**
**Input:** nums = \[1,2,3,1,1,3\]
**Output:** 4
**Explanation:** There are 4 good pairs (0,3), (0,4), (3,4), (2,5) 0-indexed.
**Example 2:**
**Input:** nums = \[1,1,1,1\]
**Output:** 6
**Explanation:** Each pair in the array are _good_.
**Example 3:**
**Input:** nums = \[1,2,3\]
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 100`
* `1 <= nums[i] <= 100` | Use two hash tables. The first to save the check-in time for a customer and the second to update the total time between two stations. |
Easiest way || By using Map | design-underground-system | 1 | 1 | ```\n//Please do upvote, if you like my solution :)\n//and free to ask if have any query :)\nclass dataa{\npublic:\n string from;\n string to;\n int in;\n int out;\n};\nclass UndergroundSystem {\npublic:\n map<pair<string,string>,vector<int>> loc;\n map<int,dataa> store;\n\t\n UndergroundSystem() {\n }\n \n void checkIn(int id, string stationName, int t) {\n dataa d;\n d.from = stationName;\n d.in = t;\n store[id] = d;\n }\n \n void checkOut(int id, string stationName, int t) {\n dataa d = store[id];\n d.to = stationName;\n d.out = t;\n loc[{d.from,d.to}].push_back(d.out-d.in);\n }\n \n double getAverageTime(string startStation, string endStation) {\n auto &time = loc[{startStation,endStation}];\n double size = time.size();\n return accumulate(time.begin(),time.end(),0)/size;;\n }\n};\n``` | 26 | An underground railway system is keeping track of customer travel times between different stations. They are using this data to calculate the average time it takes to travel from one station to another.
Implement the `UndergroundSystem` class:
* `void checkIn(int id, string stationName, int t)`
* A customer with a card ID equal to `id`, checks in at the station `stationName` at time `t`.
* A customer can only be checked into one place at a time.
* `void checkOut(int id, string stationName, int t)`
* A customer with a card ID equal to `id`, checks out from the station `stationName` at time `t`.
* `double getAverageTime(string startStation, string endStation)`
* Returns the average time it takes to travel from `startStation` to `endStation`.
* The average time is computed from all the previous traveling times from `startStation` to `endStation` that happened **directly**, meaning a check in at `startStation` followed by a check out from `endStation`.
* The time it takes to travel from `startStation` to `endStation` **may be different** from the time it takes to travel from `endStation` to `startStation`.
* There will be at least one customer that has traveled from `startStation` to `endStation` before `getAverageTime` is called.
You may assume all calls to the `checkIn` and `checkOut` methods are consistent. If a customer checks in at time `t1` then checks out at time `t2`, then `t1 < t2`. All events happen in chronological order.
**Example 1:**
**Input**
\[ "UndergroundSystem ", "checkIn ", "checkIn ", "checkIn ", "checkOut ", "checkOut ", "checkOut ", "getAverageTime ", "getAverageTime ", "checkIn ", "getAverageTime ", "checkOut ", "getAverageTime "\]
\[\[\],\[45, "Leyton ",3\],\[32, "Paradise ",8\],\[27, "Leyton ",10\],\[45, "Waterloo ",15\],\[27, "Waterloo ",20\],\[32, "Cambridge ",22\],\[ "Paradise ", "Cambridge "\],\[ "Leyton ", "Waterloo "\],\[10, "Leyton ",24\],\[ "Leyton ", "Waterloo "\],\[10, "Waterloo ",38\],\[ "Leyton ", "Waterloo "\]\]
**Output**
\[null,null,null,null,null,null,null,14.00000,11.00000,null,11.00000,null,12.00000\]
**Explanation**
UndergroundSystem undergroundSystem = new UndergroundSystem();
undergroundSystem.checkIn(45, "Leyton ", 3);
undergroundSystem.checkIn(32, "Paradise ", 8);
undergroundSystem.checkIn(27, "Leyton ", 10);
undergroundSystem.checkOut(45, "Waterloo ", 15); // Customer 45 "Leyton " -> "Waterloo " in 15-3 = 12
undergroundSystem.checkOut(27, "Waterloo ", 20); // Customer 27 "Leyton " -> "Waterloo " in 20-10 = 10
undergroundSystem.checkOut(32, "Cambridge ", 22); // Customer 32 "Paradise " -> "Cambridge " in 22-8 = 14
undergroundSystem.getAverageTime( "Paradise ", "Cambridge "); // return 14.00000. One trip "Paradise " -> "Cambridge ", (14) / 1 = 14
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 11.00000. Two trips "Leyton " -> "Waterloo ", (10 + 12) / 2 = 11
undergroundSystem.checkIn(10, "Leyton ", 24);
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 11.00000
undergroundSystem.checkOut(10, "Waterloo ", 38); // Customer 10 "Leyton " -> "Waterloo " in 38-24 = 14
undergroundSystem.getAverageTime( "Leyton ", "Waterloo "); // return 12.00000. Three trips "Leyton " -> "Waterloo ", (10 + 12 + 14) / 3 = 12
**Example 2:**
**Input**
\[ "UndergroundSystem ", "checkIn ", "checkOut ", "getAverageTime ", "checkIn ", "checkOut ", "getAverageTime ", "checkIn ", "checkOut ", "getAverageTime "\]
\[\[\],\[10, "Leyton ",3\],\[10, "Paradise ",8\],\[ "Leyton ", "Paradise "\],\[5, "Leyton ",10\],\[5, "Paradise ",16\],\[ "Leyton ", "Paradise "\],\[2, "Leyton ",21\],\[2, "Paradise ",30\],\[ "Leyton ", "Paradise "\]\]
**Output**
\[null,null,null,5.00000,null,null,5.50000,null,null,6.66667\]
**Explanation**
UndergroundSystem undergroundSystem = new UndergroundSystem();
undergroundSystem.checkIn(10, "Leyton ", 3);
undergroundSystem.checkOut(10, "Paradise ", 8); // Customer 10 "Leyton " -> "Paradise " in 8-3 = 5
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 5.00000, (5) / 1 = 5
undergroundSystem.checkIn(5, "Leyton ", 10);
undergroundSystem.checkOut(5, "Paradise ", 16); // Customer 5 "Leyton " -> "Paradise " in 16-10 = 6
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 5.50000, (5 + 6) / 2 = 5.5
undergroundSystem.checkIn(2, "Leyton ", 21);
undergroundSystem.checkOut(2, "Paradise ", 30); // Customer 2 "Leyton " -> "Paradise " in 30-21 = 9
undergroundSystem.getAverageTime( "Leyton ", "Paradise "); // return 6.66667, (5 + 6 + 9) / 3 = 6.66667
**Constraints:**
* `1 <= id, t <= 106`
* `1 <= stationName.length, startStation.length, endStation.length <= 10`
* All strings consist of uppercase and lowercase English letters and digits.
* There will be at most `2 * 104` calls **in total** to `checkIn`, `checkOut`, and `getAverageTime`.
* Answers within `10-5` of the actual value will be accepted. | Store number of computer in each row and column. Count all servers that are not isolated. |
Easiest way || By using Map | design-underground-system | 1 | 1 | ```\n//Please do upvote, if you like my solution :)\n//and free to ask if have any query :)\nclass dataa{\npublic:\n string from;\n string to;\n int in;\n int out;\n};\nclass UndergroundSystem {\npublic:\n map<pair<string,string>,vector<int>> loc;\n map<int,dataa> store;\n\t\n UndergroundSystem() {\n }\n \n void checkIn(int id, string stationName, int t) {\n dataa d;\n d.from = stationName;\n d.in = t;\n store[id] = d;\n }\n \n void checkOut(int id, string stationName, int t) {\n dataa d = store[id];\n d.to = stationName;\n d.out = t;\n loc[{d.from,d.to}].push_back(d.out-d.in);\n }\n \n double getAverageTime(string startStation, string endStation) {\n auto &time = loc[{startStation,endStation}];\n double size = time.size();\n return accumulate(time.begin(),time.end(),0)/size;;\n }\n};\n``` | 26 | Given an array of integers `nums`, return _the number of **good pairs**_.
A pair `(i, j)` is called _good_ if `nums[i] == nums[j]` and `i` < `j`.
**Example 1:**
**Input:** nums = \[1,2,3,1,1,3\]
**Output:** 4
**Explanation:** There are 4 good pairs (0,3), (0,4), (3,4), (2,5) 0-indexed.
**Example 2:**
**Input:** nums = \[1,1,1,1\]
**Output:** 6
**Explanation:** Each pair in the array are _good_.
**Example 3:**
**Input:** nums = \[1,2,3\]
**Output:** 0
**Constraints:**
* `1 <= nums.length <= 100`
* `1 <= nums[i] <= 100` | Use two hash tables. The first to save the check-in time for a customer and the second to update the total time between two stations. |
Faster and less memory than all other solutions | find-all-good-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThis problem requires very, very careful counting.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe problem can be decomposed into two: counting all valid strings less than s1, then all valid strings less than s2 (and then considering s2 itself separately).\n\nCounting all valid strings less than some string s is straightforward, by recursion on n, if there is no excluded string. It is still easy when the excluded "evil" string has no overlapping prefixes and suffixes.\n\nWhen there is overlap, one also has to keep track of the number of valid strings that end in certain suffixes of the excluded string.\n\nFor example: if the excluded string is "bbb", one has to keep track of valid strings ending in "b" and "bb". If the excluded string is "zaaz", one has to keep track of valid strings ending in "zaa".\n\n# Complexity\n- Time complexity: The solution takes at most $O(nl^2)$ time, where $l$ is the length of the excluded "evil" string.\n\n- Space complexity: The solution uses no more than $O(n)$ memory.\n\n\n# Code\n```\nclass Solution:\n def findGoodStrings(self, n: int, s1: str, s2: str, evil: str) -> int:\n p=10**9+7\n ord_a=ord(\'a\')\n l=len(evil)\n dup=[]\n for i in range(1, l):\n if evil[i:]==evil[:l-i]:\n dup.append(i)\n lend=len(dup)\n def count(s):\n tmp_ct=0\n bd=1\n ind=n\n without_s=[]\n for i in range(n):\n tmp_ct*=26\n if bd:\n tmp_ct+=ord(s[i])-ord_a\n if i>=l-1 and ind>i-l and evil<s[i-l+1:i+1]:\n tmp_ct-=1\n if i>=l:\n tmp_ct-=without_s[i-l]\n if i>=l-1:\n if s[i-l+1:i+1]==evil:\n bd=0\n ind=i\n tmp_ct%=p\n tmp_with_s=0\n for j in range(lend):\n d=dup[j]\n if i>=d:\n tmp_with_s+=without_s[i-d]\n if i>=d-1 and ind>i-d and evil[:d]<s[i-d+1:i+1]:\n tmp_with_s+=1\n without_s.append(tmp_ct-tmp_with_s)\n return tmp_ct, bd\n str_ct1, bd1=count(s1)\n str_ct2, bd2=count(s2)\n return (str_ct2-str_ct1+bd2)%p\n \n``` | 1 | Given the strings `s1` and `s2` of size `n` and the string `evil`, return _the number of **good** strings_.
A **good** string has size `n`, it is alphabetically greater than or equal to `s1`, it is alphabetically smaller than or equal to `s2`, and it does not contain the string `evil` as a substring. Since the answer can be a huge number, return this **modulo** `109 + 7`.
**Example 1:**
**Input:** n = 2, s1 = "aa ", s2 = "da ", evil = "b "
**Output:** 51
**Explanation:** There are 25 good strings starting with 'a': "aa ", "ac ", "ad ",..., "az ". Then there are 25 good strings starting with 'c': "ca ", "cc ", "cd ",..., "cz " and finally there is one good string starting with 'd': "da ".
**Example 2:**
**Input:** n = 8, s1 = "leetcode ", s2 = "leetgoes ", evil = "leet "
**Output:** 0
**Explanation:** All strings greater than or equal to s1 and smaller than or equal to s2 start with the prefix "leet ", therefore, there is not any good string.
**Example 3:**
**Input:** n = 2, s1 = "gx ", s2 = "gz ", evil = "x "
**Output:** 2
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `s1 <= s2`
* `1 <= n <= 500`
* `1 <= evil.length <= 50`
* All strings consist of lowercase English letters. | Brute force is a good choice because length of the string is ≤ 1000. Binary search the answer. Use Trie data structure to store the best three matching. Traverse the Trie. |
Faster and less memory than all other solutions | find-all-good-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThis problem requires very, very careful counting.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe problem can be decomposed into two: counting all valid strings less than s1, then all valid strings less than s2 (and then considering s2 itself separately).\n\nCounting all valid strings less than some string s is straightforward, by recursion on n, if there is no excluded string. It is still easy when the excluded "evil" string has no overlapping prefixes and suffixes.\n\nWhen there is overlap, one also has to keep track of the number of valid strings that end in certain suffixes of the excluded string.\n\nFor example: if the excluded string is "bbb", one has to keep track of valid strings ending in "b" and "bb". If the excluded string is "zaaz", one has to keep track of valid strings ending in "zaa".\n\n# Complexity\n- Time complexity: The solution takes at most $O(nl^2)$ time, where $l$ is the length of the excluded "evil" string.\n\n- Space complexity: The solution uses no more than $O(n)$ memory.\n\n\n# Code\n```\nclass Solution:\n def findGoodStrings(self, n: int, s1: str, s2: str, evil: str) -> int:\n p=10**9+7\n ord_a=ord(\'a\')\n l=len(evil)\n dup=[]\n for i in range(1, l):\n if evil[i:]==evil[:l-i]:\n dup.append(i)\n lend=len(dup)\n def count(s):\n tmp_ct=0\n bd=1\n ind=n\n without_s=[]\n for i in range(n):\n tmp_ct*=26\n if bd:\n tmp_ct+=ord(s[i])-ord_a\n if i>=l-1 and ind>i-l and evil<s[i-l+1:i+1]:\n tmp_ct-=1\n if i>=l:\n tmp_ct-=without_s[i-l]\n if i>=l-1:\n if s[i-l+1:i+1]==evil:\n bd=0\n ind=i\n tmp_ct%=p\n tmp_with_s=0\n for j in range(lend):\n d=dup[j]\n if i>=d:\n tmp_with_s+=without_s[i-d]\n if i>=d-1 and ind>i-d and evil[:d]<s[i-d+1:i+1]:\n tmp_with_s+=1\n without_s.append(tmp_ct-tmp_with_s)\n return tmp_ct, bd\n str_ct1, bd1=count(s1)\n str_ct2, bd2=count(s2)\n return (str_ct2-str_ct1+bd2)%p\n \n``` | 1 | Given a binary string `s`, return _the number of substrings with all characters_ `1`_'s_. Since the answer may be too large, return it modulo `109 + 7`.
**Example 1:**
**Input:** s = "0110111 "
**Output:** 9
**Explanation:** There are 9 substring in total with only 1's characters.
"1 " -> 5 times.
"11 " -> 3 times.
"111 " -> 1 time.
**Example 2:**
**Input:** s = "101 "
**Output:** 2
**Explanation:** Substring "1 " is shown 2 times in s.
**Example 3:**
**Input:** s = "111111 "
**Output:** 21
**Explanation:** Each substring contains only 1's characters.
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is either `'0'` or `'1'`. | Use DP with 4 states (pos: Int, posEvil: Int, equalToS1: Bool, equalToS2: Bool) which compute the number of valid strings of size "pos" where the maximum common suffix with string "evil" has size "posEvil". When "equalToS1" is "true", the current valid string is equal to "S1" otherwise it is greater. In a similar way when equalToS2 is "true" the current valid string is equal to "S2" otherwise it is smaller. To update the maximum common suffix with string "evil" use KMP preprocessing. |
beats 100 and 91 % in runtime and memory , Python3 code | find-all-good-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findGoodStrings(self, n: int, s1: str, s2: str, evil: str) -> int:\n p=10**9+7\n ord_a=ord(\'a\')\n l=len(evil)\n dup=[]\n for i in range(1, l):\n if evil[i:]==evil[:l-i]:\n dup.append(i)\n lend=len(dup)\n def count(s):\n tmp_ct=0\n bd=1\n ind=n\n without_s=[]\n for i in range(n):\n tmp_ct*=26\n if bd:\n tmp_ct+=ord(s[i])-ord_a\n if i>=l-1 and ind>i-l and evil<s[i-l+1:i+1]:\n tmp_ct-=1\n if i>=l:\n tmp_ct-=without_s[i-l]\n if i>=l-1:\n if s[i-l+1:i+1]==evil:\n bd=0\n ind=i\n tmp_ct%=p\n tmp_with_s=0\n for j in range(lend):\n d=dup[j]\n if i>=d:\n tmp_with_s+=without_s[i-d]\n if i>=d-1 and ind>i-d and evil[:d]<s[i-d+1:i+1]:\n tmp_with_s+=1\n without_s.append(tmp_ct-tmp_with_s)\n return tmp_ct, bd\n str_ct1, bd1=count(s1)\n str_ct2, bd2=count(s2)\n return (str_ct2-str_ct1+bd2)%p\n``` | 0 | Given the strings `s1` and `s2` of size `n` and the string `evil`, return _the number of **good** strings_.
A **good** string has size `n`, it is alphabetically greater than or equal to `s1`, it is alphabetically smaller than or equal to `s2`, and it does not contain the string `evil` as a substring. Since the answer can be a huge number, return this **modulo** `109 + 7`.
**Example 1:**
**Input:** n = 2, s1 = "aa ", s2 = "da ", evil = "b "
**Output:** 51
**Explanation:** There are 25 good strings starting with 'a': "aa ", "ac ", "ad ",..., "az ". Then there are 25 good strings starting with 'c': "ca ", "cc ", "cd ",..., "cz " and finally there is one good string starting with 'd': "da ".
**Example 2:**
**Input:** n = 8, s1 = "leetcode ", s2 = "leetgoes ", evil = "leet "
**Output:** 0
**Explanation:** All strings greater than or equal to s1 and smaller than or equal to s2 start with the prefix "leet ", therefore, there is not any good string.
**Example 3:**
**Input:** n = 2, s1 = "gx ", s2 = "gz ", evil = "x "
**Output:** 2
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `s1 <= s2`
* `1 <= n <= 500`
* `1 <= evil.length <= 50`
* All strings consist of lowercase English letters. | Brute force is a good choice because length of the string is ≤ 1000. Binary search the answer. Use Trie data structure to store the best three matching. Traverse the Trie. |
beats 100 and 91 % in runtime and memory , Python3 code | find-all-good-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findGoodStrings(self, n: int, s1: str, s2: str, evil: str) -> int:\n p=10**9+7\n ord_a=ord(\'a\')\n l=len(evil)\n dup=[]\n for i in range(1, l):\n if evil[i:]==evil[:l-i]:\n dup.append(i)\n lend=len(dup)\n def count(s):\n tmp_ct=0\n bd=1\n ind=n\n without_s=[]\n for i in range(n):\n tmp_ct*=26\n if bd:\n tmp_ct+=ord(s[i])-ord_a\n if i>=l-1 and ind>i-l and evil<s[i-l+1:i+1]:\n tmp_ct-=1\n if i>=l:\n tmp_ct-=without_s[i-l]\n if i>=l-1:\n if s[i-l+1:i+1]==evil:\n bd=0\n ind=i\n tmp_ct%=p\n tmp_with_s=0\n for j in range(lend):\n d=dup[j]\n if i>=d:\n tmp_with_s+=without_s[i-d]\n if i>=d-1 and ind>i-d and evil[:d]<s[i-d+1:i+1]:\n tmp_with_s+=1\n without_s.append(tmp_ct-tmp_with_s)\n return tmp_ct, bd\n str_ct1, bd1=count(s1)\n str_ct2, bd2=count(s2)\n return (str_ct2-str_ct1+bd2)%p\n``` | 0 | Given a binary string `s`, return _the number of substrings with all characters_ `1`_'s_. Since the answer may be too large, return it modulo `109 + 7`.
**Example 1:**
**Input:** s = "0110111 "
**Output:** 9
**Explanation:** There are 9 substring in total with only 1's characters.
"1 " -> 5 times.
"11 " -> 3 times.
"111 " -> 1 time.
**Example 2:**
**Input:** s = "101 "
**Output:** 2
**Explanation:** Substring "1 " is shown 2 times in s.
**Example 3:**
**Input:** s = "111111 "
**Output:** 21
**Explanation:** Each substring contains only 1's characters.
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is either `'0'` or `'1'`. | Use DP with 4 states (pos: Int, posEvil: Int, equalToS1: Bool, equalToS2: Bool) which compute the number of valid strings of size "pos" where the maximum common suffix with string "evil" has size "posEvil". When "equalToS1" is "true", the current valid string is equal to "S1" otherwise it is greater. In a similar way when equalToS2 is "true" the current valid string is equal to "S2" otherwise it is smaller. To update the maximum common suffix with string "evil" use KMP preprocessing. |
Python DP Solution | Using KMP Algorithm | Faster than 77% | find-all-good-strings | 0 | 1 | \n\n# Code\n```python []\nclass Solution:\n def findGoodStrings(self, n: int, s1: str, s2: str, evil: str) -> int:\n chars, lenEvil = string.ascii_lowercase, len(evil)\n lps, left = [0] * lenEvil, 0\n for right in range(1, lenEvil):\n while left and evil[left] != evil[right]:\n left = lps[left - 1]\n if evil[left] == evil[right]:\n left += 1\n lps[right] = left\n \n @cache\n def dp(charIdx: int, largerThanS1: bool, smallerThanS2: bool, evilIdx: int) -> int:\n if charIdx == n:\n return 1\n ways = 0\n for char in chars:\n if not largerThanS1 and char < s1[charIdx]: continue\n if not smallerThanS2 and char > s2[charIdx]: continue\n if evilIdx == lenEvil - 1 and char == evil[evilIdx]: continue\n if char == evil[evilIdx]:\n newEvilIdx = evilIdx + 1\n else:\n newEvilIdx = evilIdx\n while newEvilIdx and evil[newEvilIdx] != char:\n newEvilIdx = lps[newEvilIdx - 1]\n if evil[newEvilIdx] == char:\n newEvilIdx += 1\n ways += dp(charIdx + 1, largerThanS1 | (char > s1[charIdx]), smallerThanS2 | (char < s2[charIdx]), newEvilIdx)\n return ways % 1000000007\n\n return dp(0, False, False, 0)\n``` | 0 | Given the strings `s1` and `s2` of size `n` and the string `evil`, return _the number of **good** strings_.
A **good** string has size `n`, it is alphabetically greater than or equal to `s1`, it is alphabetically smaller than or equal to `s2`, and it does not contain the string `evil` as a substring. Since the answer can be a huge number, return this **modulo** `109 + 7`.
**Example 1:**
**Input:** n = 2, s1 = "aa ", s2 = "da ", evil = "b "
**Output:** 51
**Explanation:** There are 25 good strings starting with 'a': "aa ", "ac ", "ad ",..., "az ". Then there are 25 good strings starting with 'c': "ca ", "cc ", "cd ",..., "cz " and finally there is one good string starting with 'd': "da ".
**Example 2:**
**Input:** n = 8, s1 = "leetcode ", s2 = "leetgoes ", evil = "leet "
**Output:** 0
**Explanation:** All strings greater than or equal to s1 and smaller than or equal to s2 start with the prefix "leet ", therefore, there is not any good string.
**Example 3:**
**Input:** n = 2, s1 = "gx ", s2 = "gz ", evil = "x "
**Output:** 2
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `s1 <= s2`
* `1 <= n <= 500`
* `1 <= evil.length <= 50`
* All strings consist of lowercase English letters. | Brute force is a good choice because length of the string is ≤ 1000. Binary search the answer. Use Trie data structure to store the best three matching. Traverse the Trie. |
Python DP Solution | Using KMP Algorithm | Faster than 77% | find-all-good-strings | 0 | 1 | \n\n# Code\n```python []\nclass Solution:\n def findGoodStrings(self, n: int, s1: str, s2: str, evil: str) -> int:\n chars, lenEvil = string.ascii_lowercase, len(evil)\n lps, left = [0] * lenEvil, 0\n for right in range(1, lenEvil):\n while left and evil[left] != evil[right]:\n left = lps[left - 1]\n if evil[left] == evil[right]:\n left += 1\n lps[right] = left\n \n @cache\n def dp(charIdx: int, largerThanS1: bool, smallerThanS2: bool, evilIdx: int) -> int:\n if charIdx == n:\n return 1\n ways = 0\n for char in chars:\n if not largerThanS1 and char < s1[charIdx]: continue\n if not smallerThanS2 and char > s2[charIdx]: continue\n if evilIdx == lenEvil - 1 and char == evil[evilIdx]: continue\n if char == evil[evilIdx]:\n newEvilIdx = evilIdx + 1\n else:\n newEvilIdx = evilIdx\n while newEvilIdx and evil[newEvilIdx] != char:\n newEvilIdx = lps[newEvilIdx - 1]\n if evil[newEvilIdx] == char:\n newEvilIdx += 1\n ways += dp(charIdx + 1, largerThanS1 | (char > s1[charIdx]), smallerThanS2 | (char < s2[charIdx]), newEvilIdx)\n return ways % 1000000007\n\n return dp(0, False, False, 0)\n``` | 0 | Given a binary string `s`, return _the number of substrings with all characters_ `1`_'s_. Since the answer may be too large, return it modulo `109 + 7`.
**Example 1:**
**Input:** s = "0110111 "
**Output:** 9
**Explanation:** There are 9 substring in total with only 1's characters.
"1 " -> 5 times.
"11 " -> 3 times.
"111 " -> 1 time.
**Example 2:**
**Input:** s = "101 "
**Output:** 2
**Explanation:** Substring "1 " is shown 2 times in s.
**Example 3:**
**Input:** s = "111111 "
**Output:** 21
**Explanation:** Each substring contains only 1's characters.
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is either `'0'` or `'1'`. | Use DP with 4 states (pos: Int, posEvil: Int, equalToS1: Bool, equalToS2: Bool) which compute the number of valid strings of size "pos" where the maximum common suffix with string "evil" has size "posEvil". When "equalToS1" is "true", the current valid string is equal to "S1" otherwise it is greater. In a similar way when equalToS2 is "true" the current valid string is equal to "S2" otherwise it is smaller. To update the maximum common suffix with string "evil" use KMP preprocessing. |
[Python3] dp & kmp ... finally | find-all-good-strings | 0 | 1 | \n```\nclass Solution:\n def findGoodStrings(self, n: int, s1: str, s2: str, evil: str) -> int:\n lps = [0]\n k = 0 \n for i in range(1, len(evil)): \n while k and evil[k] != evil[i]: k = lps[k-1]\n if evil[k] == evil[i]: k += 1\n lps.append(k)\n \n @cache\n def fn(i, k, lower, upper): \n """Return number of good strings at position i and k prefix match."""\n if k == len(evil): return 0 # boundary condition \n if i == n: return 1 \n lo = ascii_lowercase.index(s1[i]) if lower else 0\n hi = ascii_lowercase.index(s2[i]) if upper else 25\n \n ans = 0\n for x in range(lo, hi+1): \n kk = k \n while kk and evil[kk] != ascii_lowercase[x]: \n kk = lps[kk-1]\n if evil[kk] == ascii_lowercase[x]: kk += 1\n ans += fn(i+1, kk, lower and x == lo, upper and x == hi)\n return ans \n \n return fn(0, 0, True, True) % 1_000_000_007\n``` | 4 | Given the strings `s1` and `s2` of size `n` and the string `evil`, return _the number of **good** strings_.
A **good** string has size `n`, it is alphabetically greater than or equal to `s1`, it is alphabetically smaller than or equal to `s2`, and it does not contain the string `evil` as a substring. Since the answer can be a huge number, return this **modulo** `109 + 7`.
**Example 1:**
**Input:** n = 2, s1 = "aa ", s2 = "da ", evil = "b "
**Output:** 51
**Explanation:** There are 25 good strings starting with 'a': "aa ", "ac ", "ad ",..., "az ". Then there are 25 good strings starting with 'c': "ca ", "cc ", "cd ",..., "cz " and finally there is one good string starting with 'd': "da ".
**Example 2:**
**Input:** n = 8, s1 = "leetcode ", s2 = "leetgoes ", evil = "leet "
**Output:** 0
**Explanation:** All strings greater than or equal to s1 and smaller than or equal to s2 start with the prefix "leet ", therefore, there is not any good string.
**Example 3:**
**Input:** n = 2, s1 = "gx ", s2 = "gz ", evil = "x "
**Output:** 2
**Constraints:**
* `s1.length == n`
* `s2.length == n`
* `s1 <= s2`
* `1 <= n <= 500`
* `1 <= evil.length <= 50`
* All strings consist of lowercase English letters. | Brute force is a good choice because length of the string is ≤ 1000. Binary search the answer. Use Trie data structure to store the best three matching. Traverse the Trie. |
[Python3] dp & kmp ... finally | find-all-good-strings | 0 | 1 | \n```\nclass Solution:\n def findGoodStrings(self, n: int, s1: str, s2: str, evil: str) -> int:\n lps = [0]\n k = 0 \n for i in range(1, len(evil)): \n while k and evil[k] != evil[i]: k = lps[k-1]\n if evil[k] == evil[i]: k += 1\n lps.append(k)\n \n @cache\n def fn(i, k, lower, upper): \n """Return number of good strings at position i and k prefix match."""\n if k == len(evil): return 0 # boundary condition \n if i == n: return 1 \n lo = ascii_lowercase.index(s1[i]) if lower else 0\n hi = ascii_lowercase.index(s2[i]) if upper else 25\n \n ans = 0\n for x in range(lo, hi+1): \n kk = k \n while kk and evil[kk] != ascii_lowercase[x]: \n kk = lps[kk-1]\n if evil[kk] == ascii_lowercase[x]: kk += 1\n ans += fn(i+1, kk, lower and x == lo, upper and x == hi)\n return ans \n \n return fn(0, 0, True, True) % 1_000_000_007\n``` | 4 | Given a binary string `s`, return _the number of substrings with all characters_ `1`_'s_. Since the answer may be too large, return it modulo `109 + 7`.
**Example 1:**
**Input:** s = "0110111 "
**Output:** 9
**Explanation:** There are 9 substring in total with only 1's characters.
"1 " -> 5 times.
"11 " -> 3 times.
"111 " -> 1 time.
**Example 2:**
**Input:** s = "101 "
**Output:** 2
**Explanation:** Substring "1 " is shown 2 times in s.
**Example 3:**
**Input:** s = "111111 "
**Output:** 21
**Explanation:** Each substring contains only 1's characters.
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is either `'0'` or `'1'`. | Use DP with 4 states (pos: Int, posEvil: Int, equalToS1: Bool, equalToS2: Bool) which compute the number of valid strings of size "pos" where the maximum common suffix with string "evil" has size "posEvil". When "equalToS1" is "true", the current valid string is equal to "S1" otherwise it is greater. In a similar way when equalToS2 is "true" the current valid string is equal to "S2" otherwise it is smaller. To update the maximum common suffix with string "evil" use KMP preprocessing. |
Python3 - Easy Solution Explained | count-largest-group | 0 | 1 | ```\nclass Solution:\n def countLargestGroup(self, n: int) -> int:\n\n # This method provides the sum of the digits of a number\n def sumDigits(number: int) -> int:\n\n return sum([int(d) for d in str(number)])\n \n # We initializate a dictionary to group the number by sumDigits\n freq = {}\n\n for j in range(1, n+1):\n\n sumD = sumDigits(j)\n\n if sumD in freq:\n freq[sumD] += [sumD]\n else: \n freq[sumD] = [sumD]\n \n # We look at the number of elements with a given digitSum\n groupLenght = [len(val) for val in freq.values()]\n\n # We return the number of the largest groups\n return groupLenght.count(max(groupLenght))\n``` | 1 | You are given an integer `n`.
Each number from `1` to `n` is grouped according to the sum of its digits.
Return _the number of groups that have the largest size_.
**Example 1:**
**Input:** n = 13
**Output:** 4
**Explanation:** There are 9 groups in total, they are grouped according sum of its digits of numbers from 1 to 13:
\[1,10\], \[2,11\], \[3,12\], \[4,13\], \[5\], \[6\], \[7\], \[8\], \[9\].
There are 4 groups with largest size.
**Example 2:**
**Input:** n = 2
**Output:** 2
**Explanation:** There are 2 groups \[1\], \[2\] of size 1.
**Constraints:**
* `1 <= n <= 104` | null |
Python DP O(N) 99%/100% | count-largest-group | 0 | 1 | The idea here is that we can save on calculating sums of digits for bigger numbers if we start from `1` and keep the results in `dp[]`. \n\n```\nclass Solution:\n\n def countLargestGroup(self, n: int) -> int:\n dp = {0: 0}\n counts = [0] * (4 * 9)\n for i in range(1, n + 1):\n quotient, reminder = divmod(i, 10)\n dp[i] = reminder + dp[quotient]\n counts[dp[i] - 1] += 1\n\n return counts.count(max(counts))\n``` | 52 | You are given an integer `n`.
Each number from `1` to `n` is grouped according to the sum of its digits.
Return _the number of groups that have the largest size_.
**Example 1:**
**Input:** n = 13
**Output:** 4
**Explanation:** There are 9 groups in total, they are grouped according sum of its digits of numbers from 1 to 13:
\[1,10\], \[2,11\], \[3,12\], \[4,13\], \[5\], \[6\], \[7\], \[8\], \[9\].
There are 4 groups with largest size.
**Example 2:**
**Input:** n = 2
**Output:** 2
**Explanation:** There are 2 groups \[1\], \[2\] of size 1.
**Constraints:**
* `1 <= n <= 104` | null |
Python 3 two solutions - beautiful and fast :) | count-largest-group | 0 | 1 | **Solution 1**\nThis one is short and beatiful :)\n\n```python\ndef countLargestGroup(self, n: int) -> int:\n d = {}\n for i in range(1, n + 1):\n t = sum(map(int, list(str(i))))\n d[t] = d.get(t, 0) + 1\n return sum(1 for i in d.values() if i >= max(d.values()))\n```\n\n**Solution 2**\nThis one is fastest I could get\n\n```python\ndef countLargestGroup(self, n: int) -> int:\n \n d = {}\n \n for i in range(1, n + 1):\n t = sum(map(int, list(str(i))))\n \n if t not in d:\n d[t] = 1 \n else:\n d[t] += 1\n \n m = max(d.values())\n \n return sum(1 for i in d.values() if i >= m)\n```\n\nRuntime: 108 ms, faster than 72.69% of Python3 online submissions for Count Largest Group.\nMemory Usage: 13.6 MB, less than 95.70% of Python3 online submissions for Count Largest Group.\n\n\n\nIf you like this post, please, upvote it so that others can see it too. Thanks!\n | 14 | You are given an integer `n`.
Each number from `1` to `n` is grouped according to the sum of its digits.
Return _the number of groups that have the largest size_.
**Example 1:**
**Input:** n = 13
**Output:** 4
**Explanation:** There are 9 groups in total, they are grouped according sum of its digits of numbers from 1 to 13:
\[1,10\], \[2,11\], \[3,12\], \[4,13\], \[5\], \[6\], \[7\], \[8\], \[9\].
There are 4 groups with largest size.
**Example 2:**
**Input:** n = 2
**Output:** 2
**Explanation:** There are 2 groups \[1\], \[2\] of size 1.
**Constraints:**
* `1 <= n <= 104` | null |
100% beat easy | count-largest-group | 0 | 1 | \n# Approach\nMy system for calculating sum of digits works at $$O(n)$$ time complexity versus $$O(log(n))$$ time complexity in the case where you calculating sum of digits at the every iteration.\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(log(n))$$ \n\n# Code\n```\nclass Solution:\n def countLargestGroup(self, n: int) -> int:\n d = defaultdict(int)\n num = 0\n for i in range(1, n+1):\n if i % 10 == 0:\n while i % 10 == 0:\n i //= 10\n num -= 9\n num += 1\n d[num] += 1\n mc = 0\n for k in d:\n mc = max(mc, d[k])\n count = 0\n for k in d:\n if d[k] == mc:\n count += 1\n return count\n``` | 0 | You are given an integer `n`.
Each number from `1` to `n` is grouped according to the sum of its digits.
Return _the number of groups that have the largest size_.
**Example 1:**
**Input:** n = 13
**Output:** 4
**Explanation:** There are 9 groups in total, they are grouped according sum of its digits of numbers from 1 to 13:
\[1,10\], \[2,11\], \[3,12\], \[4,13\], \[5\], \[6\], \[7\], \[8\], \[9\].
There are 4 groups with largest size.
**Example 2:**
**Input:** n = 2
**Output:** 2
**Explanation:** There are 2 groups \[1\], \[2\] of size 1.
**Constraints:**
* `1 <= n <= 104` | null |
Solution for beginners | count-largest-group | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countLargestGroup(self, n: int) -> int:\n if n<=9 :\n return n\n else :\n a=[]\n for i in range(1,10):\n a.append(1)\n for i in range(10,n+1):\n b=0\n c=str(i)\n for j in c:\n b+=int(j)\n if b>=len(a) :\n for q in range(0,b-len(a)):\n a.append(0)\n d=a[b-1]\n a[b-1]=d+1\n return a.count(max(a))\n return a.count(max(a))\n``` | 0 | You are given an integer `n`.
Each number from `1` to `n` is grouped according to the sum of its digits.
Return _the number of groups that have the largest size_.
**Example 1:**
**Input:** n = 13
**Output:** 4
**Explanation:** There are 9 groups in total, they are grouped according sum of its digits of numbers from 1 to 13:
\[1,10\], \[2,11\], \[3,12\], \[4,13\], \[5\], \[6\], \[7\], \[8\], \[9\].
There are 4 groups with largest size.
**Example 2:**
**Input:** n = 2
**Output:** 2
**Explanation:** There are 2 groups \[1\], \[2\] of size 1.
**Constraints:**
* `1 <= n <= 104` | null |
easy dictionary/ array approach | count-largest-group | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nfirst we create a dictionary with keys having all possible sum, now it\'s time to assign values to these keys. since we need to return a group maintain an array in place of values.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n> create an empty dictionary\n> find all possible sums (keys) & maintain an array for grouping (values)\n> now we again iterate but this time we store values\n> find the larget group-size, return total number having same group-size\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countLargestGroup(self, n: int) -> int:\n\n d1 = dict() # an empty dictionary\n\n # find all possible keys\n for i in range(1, n+1):\n a1 = list(str(i))\n a2 = [int(i) for i in a1] \n d1[sum(a2)] = [] # maintain an array for grouping\n \n # now we again iterate but this time we store values\n for i in range(1, n+1):\n a1 = list(str(i))\n a2 = [int(i) for i in a1]\n d1[sum(a2)].append(i) # append all possiblities to the group \n \n \'\'\'\n > store all sizes in an array\n > find the larget group-size, we can achive this by array sorting \n > return total number having same group-size\n \'\'\'\n op = [len(i) for i in list(d1.values())]\n op.sort()\n\n return op.count(op[-1])\n``` | 0 | You are given an integer `n`.
Each number from `1` to `n` is grouped according to the sum of its digits.
Return _the number of groups that have the largest size_.
**Example 1:**
**Input:** n = 13
**Output:** 4
**Explanation:** There are 9 groups in total, they are grouped according sum of its digits of numbers from 1 to 13:
\[1,10\], \[2,11\], \[3,12\], \[4,13\], \[5\], \[6\], \[7\], \[8\], \[9\].
There are 4 groups with largest size.
**Example 2:**
**Input:** n = 2
**Output:** 2
**Explanation:** There are 2 groups \[1\], \[2\] of size 1.
**Constraints:**
* `1 <= n <= 104` | null |
Python solution | count-largest-group | 0 | 1 | ```\nclass Solution:\n def countLargestGroup(self, n: int) -> int:\n hash_map, count = {}, 0\n\n for number in range(1, n + 1):\n num = sum([int(nu) for nu in str(number)])\n hash_map[num] = hash_map.get(num, 0) + 1\n\n _max = max(hash_map.values())\n\n return sum(1 for num in hash_map.values() if num == _max)\n``` | 0 | You are given an integer `n`.
Each number from `1` to `n` is grouped according to the sum of its digits.
Return _the number of groups that have the largest size_.
**Example 1:**
**Input:** n = 13
**Output:** 4
**Explanation:** There are 9 groups in total, they are grouped according sum of its digits of numbers from 1 to 13:
\[1,10\], \[2,11\], \[3,12\], \[4,13\], \[5\], \[6\], \[7\], \[8\], \[9\].
There are 4 groups with largest size.
**Example 2:**
**Input:** n = 2
**Output:** 2
**Explanation:** There are 2 groups \[1\], \[2\] of size 1.
**Constraints:**
* `1 <= n <= 104` | null |
Python Very Simple Solution!! | count-largest-group | 0 | 1 | \n# Code\n```\nclass Solution:\n def countLargestGroup(self, n: int) -> int:\n\n hashmap: dict = {}\n numbers: range = range(1, n + 1)\n for number in numbers:\n _sum: int = sum(map(int, f"{number}"))\n hashmap[_sum] = hashmap.get(_sum, 0) + 1\n\n maximum: int = max(hashmap.values())\n return sum(1 for value in hashmap.values() if value == maximum)\n \n``` | 0 | You are given an integer `n`.
Each number from `1` to `n` is grouped according to the sum of its digits.
Return _the number of groups that have the largest size_.
**Example 1:**
**Input:** n = 13
**Output:** 4
**Explanation:** There are 9 groups in total, they are grouped according sum of its digits of numbers from 1 to 13:
\[1,10\], \[2,11\], \[3,12\], \[4,13\], \[5\], \[6\], \[7\], \[8\], \[9\].
There are 4 groups with largest size.
**Example 2:**
**Input:** n = 2
**Output:** 2
**Explanation:** There are 2 groups \[1\], \[2\] of size 1.
**Constraints:**
* `1 <= n <= 104` | null |
PYTHON SIMPLE WITH EXPLANATION | count-largest-group | 0 | 1 | # Code\n```\nclass Solution:\n def countLargestGroup(self, n: int) -> int:\n # FUNCTION TO CALCULATE SUM OF DIGITS\n def getSum(n): \n return sum(int(digit) for digit in str(n))\n # CREATE A HASHMAP\n count = {}\n for i in range(1, n + 1):\n k = getSum(i)\n if k not in count:\n count[k] = 0\n count[k] += 1\n # RETURN THE MAXIMUM VALUE\n max_size = max(count.values())\n return list(count.values()).count(max_size)\n\n``` | 0 | You are given an integer `n`.
Each number from `1` to `n` is grouped according to the sum of its digits.
Return _the number of groups that have the largest size_.
**Example 1:**
**Input:** n = 13
**Output:** 4
**Explanation:** There are 9 groups in total, they are grouped according sum of its digits of numbers from 1 to 13:
\[1,10\], \[2,11\], \[3,12\], \[4,13\], \[5\], \[6\], \[7\], \[8\], \[9\].
There are 4 groups with largest size.
**Example 2:**
**Input:** n = 2
**Output:** 2
**Explanation:** There are 2 groups \[1\], \[2\] of size 1.
**Constraints:**
* `1 <= n <= 104` | null |
Rust & Python Solution | count-largest-group | 0 | 1 | # Code\n```python []\nclass Solution:\n def countLargestGroup(self, n: int) -> int:\n if n == 1:\n return 1\n\n def calculate_total(n: int) -> int:\n total = 0\n for num in str(n):\n total += int(num)\n return total\n\n map = {}\n\n for num in range(1, n + 1):\n total = calculate_total(num)\n if map.get(total):\n map[total] += 1\n else:\n map[total] = 1\n\n max_total = max(*map.values())\n return len(list(filter(lambda x: x == max_total, map.values())))\n\n```\n```rust []\nuse std::collections::HashMap;\n\nimpl Solution {\n pub fn count_largest_group(n: i32) -> i32 {\n if n == 1 {\n return 1;\n }\n\n fn calculate_total(n: i32) -> i32 {\n let mut total = 0;\n for num in format!("{}", n).chars() {\n total += num.to_digit(10).unwrap() as i32;\n }\n total\n }\n\n let mut map = HashMap::new();\n for num in 1..=n {\n let total = calculate_total(num);\n map.entry(total).and_modify(|x| *x += 1).or_insert(1);\n }\n\n let max_total = *map.values().max().unwrap();\n map.values().filter(|&&x| x == max_total).count() as i32\n }\n}\n``` | 0 | You are given an integer `n`.
Each number from `1` to `n` is grouped according to the sum of its digits.
Return _the number of groups that have the largest size_.
**Example 1:**
**Input:** n = 13
**Output:** 4
**Explanation:** There are 9 groups in total, they are grouped according sum of its digits of numbers from 1 to 13:
\[1,10\], \[2,11\], \[3,12\], \[4,13\], \[5\], \[6\], \[7\], \[8\], \[9\].
There are 4 groups with largest size.
**Example 2:**
**Input:** n = 2
**Output:** 2
**Explanation:** There are 2 groups \[1\], \[2\] of size 1.
**Constraints:**
* `1 <= n <= 104` | null |
Python3 Counter | count-largest-group | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def countLargestGroup(self, n: int) -> int:\n \n \n c1=Counter()\n \n for i in range(1,n+1):\n sm=sum(int(ch) for ch in str(i))\n c1[sm]+=1\n \n c2=Counter(c1.values())\n \n return c2[max(c2)]\n \n``` | 0 | You are given an integer `n`.
Each number from `1` to `n` is grouped according to the sum of its digits.
Return _the number of groups that have the largest size_.
**Example 1:**
**Input:** n = 13
**Output:** 4
**Explanation:** There are 9 groups in total, they are grouped according sum of its digits of numbers from 1 to 13:
\[1,10\], \[2,11\], \[3,12\], \[4,13\], \[5\], \[6\], \[7\], \[8\], \[9\].
There are 4 groups with largest size.
**Example 2:**
**Input:** n = 2
**Output:** 2
**Explanation:** There are 2 groups \[1\], \[2\] of size 1.
**Constraints:**
* `1 <= n <= 104` | null |
Clear Python 3 solution faster than 91% with explanation | construct-k-palindrome-strings | 0 | 1 | ```\nfrom collections import Counter\n\nclass Solution:\n def canConstruct(self, s: str, k: int) -> bool:\n if k > len(s):\n return False\n h = Counter(s)\n countOdd = 0\n for value in h.values():\n if value % 2:\n countOdd += 1\n if countOdd > k:\n return False\n return True\n```\nThe solution is based on the understanding that a string can be a palindrome only if it has **at most 1 character** whose frequency is odd. So if the number of characters having an odd frequency is greater than the number of palindromes we need to form, then naturally it\'s impossible to do so. | 34 | Given a string `s` and an integer `k`, return `true` _if you can use all the characters in_ `s` _to construct_ `k` _palindrome strings or_ `false` _otherwise_.
**Example 1:**
**Input:** s = "annabelle ", k = 2
**Output:** true
**Explanation:** You can construct two palindromes using all characters in s.
Some possible constructions "anna " + "elble ", "anbna " + "elle ", "anellena " + "b "
**Example 2:**
**Input:** s = "leetcode ", k = 3
**Output:** false
**Explanation:** It is impossible to construct 3 palindromes using all the characters of s.
**Example 3:**
**Input:** s = "true ", k = 4
**Output:** true
**Explanation:** The only possible solution is to put each character in a separate string.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of lowercase English letters.
* `1 <= k <= 105` | It's straightforward to check if A or B won or not, check for each row/column/diag if all the three are the same. Then if no one wins, the game is a draw iff the board is full, i.e. moves.length = 9 otherwise is pending. |
Clear Python 3 solution faster than 91% with explanation | construct-k-palindrome-strings | 0 | 1 | ```\nfrom collections import Counter\n\nclass Solution:\n def canConstruct(self, s: str, k: int) -> bool:\n if k > len(s):\n return False\n h = Counter(s)\n countOdd = 0\n for value in h.values():\n if value % 2:\n countOdd += 1\n if countOdd > k:\n return False\n return True\n```\nThe solution is based on the understanding that a string can be a palindrome only if it has **at most 1 character** whose frequency is odd. So if the number of characters having an odd frequency is greater than the number of palindromes we need to form, then naturally it\'s impossible to do so. | 34 | A sequence of numbers is called an **arithmetic progression** if the difference between any two consecutive elements is the same.
Given an array of numbers `arr`, return `true` _if the array can be rearranged to form an **arithmetic progression**. Otherwise, return_ `false`.
**Example 1:**
**Input:** arr = \[3,5,1\]
**Output:** true
**Explanation:** We can reorder the elements as \[1,3,5\] or \[5,3,1\] with differences 2 and -2 respectively, between each consecutive elements.
**Example 2:**
**Input:** arr = \[1,2,4\]
**Output:** false
**Explanation:** There is no way to reorder the elements to obtain an arithmetic progression.
**Constraints:**
* `2 <= arr.length <= 1000`
* `-106 <= arr[i] <= 106` | If the s.length < k we cannot construct k strings from s and answer is false. If the number of characters that have odd counts is > k then the minimum number of palindrome strings we can construct is > k and answer is false. Otherwise you can construct exactly k palindrome strings and answer is true (why ?). |
✔ [C++ / Python3] Solution | XOR | construct-k-palindrome-strings | 0 | 1 | # Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(1)$$\n\n# Python3\n```\nclass Solution:\n def canConstruct(self, S, K):\n return bin(reduce(operator.xor, map(lambda x: 1 << (ord(x) - 97), S))).count(\'1\') <= K <= len(S)\n```\n\n# C++\n```\nclass Solution {\npublic:\n bool canConstruct(string s, int k) {\n int val = 0;\n for(char c: s) val ^= (1 << (c - \'a\'));\n return k <= s.length() && __builtin_popcount(val) <= k;\n }\n};\n``` | 2 | Given a string `s` and an integer `k`, return `true` _if you can use all the characters in_ `s` _to construct_ `k` _palindrome strings or_ `false` _otherwise_.
**Example 1:**
**Input:** s = "annabelle ", k = 2
**Output:** true
**Explanation:** You can construct two palindromes using all characters in s.
Some possible constructions "anna " + "elble ", "anbna " + "elle ", "anellena " + "b "
**Example 2:**
**Input:** s = "leetcode ", k = 3
**Output:** false
**Explanation:** It is impossible to construct 3 palindromes using all the characters of s.
**Example 3:**
**Input:** s = "true ", k = 4
**Output:** true
**Explanation:** The only possible solution is to put each character in a separate string.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of lowercase English letters.
* `1 <= k <= 105` | It's straightforward to check if A or B won or not, check for each row/column/diag if all the three are the same. Then if no one wins, the game is a draw iff the board is full, i.e. moves.length = 9 otherwise is pending. |
✔ [C++ / Python3] Solution | XOR | construct-k-palindrome-strings | 0 | 1 | # Complexity\n- Time complexity: $$O(n)$$\n- Space complexity: $$O(1)$$\n\n# Python3\n```\nclass Solution:\n def canConstruct(self, S, K):\n return bin(reduce(operator.xor, map(lambda x: 1 << (ord(x) - 97), S))).count(\'1\') <= K <= len(S)\n```\n\n# C++\n```\nclass Solution {\npublic:\n bool canConstruct(string s, int k) {\n int val = 0;\n for(char c: s) val ^= (1 << (c - \'a\'));\n return k <= s.length() && __builtin_popcount(val) <= k;\n }\n};\n``` | 2 | A sequence of numbers is called an **arithmetic progression** if the difference between any two consecutive elements is the same.
Given an array of numbers `arr`, return `true` _if the array can be rearranged to form an **arithmetic progression**. Otherwise, return_ `false`.
**Example 1:**
**Input:** arr = \[3,5,1\]
**Output:** true
**Explanation:** We can reorder the elements as \[1,3,5\] or \[5,3,1\] with differences 2 and -2 respectively, between each consecutive elements.
**Example 2:**
**Input:** arr = \[1,2,4\]
**Output:** false
**Explanation:** There is no way to reorder the elements to obtain an arithmetic progression.
**Constraints:**
* `2 <= arr.length <= 1000`
* `-106 <= arr[i] <= 106` | If the s.length < k we cannot construct k strings from s and answer is false. If the number of characters that have odd counts is > k then the minimum number of palindrome strings we can construct is > k and answer is false. Otherwise you can construct exactly k palindrome strings and answer is true (why ?). |
Python: Using Hashmap | construct-k-palindrome-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def canConstruct(self, s: str, k: int) -> bool:\n if k>len(s):\n return False\n hash = {}\n for i in s:\n if i in hash:\n hash[i]+=1\n else:\n hash[i]=1\n count = 0\n for key,val in hash.items():\n if val%2:\n count+=1\n if count>k:\n return False\n return True\n \n\n\n \n``` | 0 | Given a string `s` and an integer `k`, return `true` _if you can use all the characters in_ `s` _to construct_ `k` _palindrome strings or_ `false` _otherwise_.
**Example 1:**
**Input:** s = "annabelle ", k = 2
**Output:** true
**Explanation:** You can construct two palindromes using all characters in s.
Some possible constructions "anna " + "elble ", "anbna " + "elle ", "anellena " + "b "
**Example 2:**
**Input:** s = "leetcode ", k = 3
**Output:** false
**Explanation:** It is impossible to construct 3 palindromes using all the characters of s.
**Example 3:**
**Input:** s = "true ", k = 4
**Output:** true
**Explanation:** The only possible solution is to put each character in a separate string.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of lowercase English letters.
* `1 <= k <= 105` | It's straightforward to check if A or B won or not, check for each row/column/diag if all the three are the same. Then if no one wins, the game is a draw iff the board is full, i.e. moves.length = 9 otherwise is pending. |
Python: Using Hashmap | construct-k-palindrome-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def canConstruct(self, s: str, k: int) -> bool:\n if k>len(s):\n return False\n hash = {}\n for i in s:\n if i in hash:\n hash[i]+=1\n else:\n hash[i]=1\n count = 0\n for key,val in hash.items():\n if val%2:\n count+=1\n if count>k:\n return False\n return True\n \n\n\n \n``` | 0 | A sequence of numbers is called an **arithmetic progression** if the difference between any two consecutive elements is the same.
Given an array of numbers `arr`, return `true` _if the array can be rearranged to form an **arithmetic progression**. Otherwise, return_ `false`.
**Example 1:**
**Input:** arr = \[3,5,1\]
**Output:** true
**Explanation:** We can reorder the elements as \[1,3,5\] or \[5,3,1\] with differences 2 and -2 respectively, between each consecutive elements.
**Example 2:**
**Input:** arr = \[1,2,4\]
**Output:** false
**Explanation:** There is no way to reorder the elements to obtain an arithmetic progression.
**Constraints:**
* `2 <= arr.length <= 1000`
* `-106 <= arr[i] <= 106` | If the s.length < k we cannot construct k strings from s and answer is false. If the number of characters that have odd counts is > k then the minimum number of palindrome strings we can construct is > k and answer is false. Otherwise you can construct exactly k palindrome strings and answer is true (why ?). |
Python3 Simple Solution | construct-k-palindrome-strings | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def canConstruct(self, s: str, x: int) -> bool:\n \n \n freq=Counter(s)\n odd=0\n \n for k,v in freq.items():\n if v%2:\n odd+=1\n \n if odd>x:\n return False\n \n return len(s)>=x\n``` | 0 | Given a string `s` and an integer `k`, return `true` _if you can use all the characters in_ `s` _to construct_ `k` _palindrome strings or_ `false` _otherwise_.
**Example 1:**
**Input:** s = "annabelle ", k = 2
**Output:** true
**Explanation:** You can construct two palindromes using all characters in s.
Some possible constructions "anna " + "elble ", "anbna " + "elle ", "anellena " + "b "
**Example 2:**
**Input:** s = "leetcode ", k = 3
**Output:** false
**Explanation:** It is impossible to construct 3 palindromes using all the characters of s.
**Example 3:**
**Input:** s = "true ", k = 4
**Output:** true
**Explanation:** The only possible solution is to put each character in a separate string.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of lowercase English letters.
* `1 <= k <= 105` | It's straightforward to check if A or B won or not, check for each row/column/diag if all the three are the same. Then if no one wins, the game is a draw iff the board is full, i.e. moves.length = 9 otherwise is pending. |
Python3 Simple Solution | construct-k-palindrome-strings | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def canConstruct(self, s: str, x: int) -> bool:\n \n \n freq=Counter(s)\n odd=0\n \n for k,v in freq.items():\n if v%2:\n odd+=1\n \n if odd>x:\n return False\n \n return len(s)>=x\n``` | 0 | A sequence of numbers is called an **arithmetic progression** if the difference between any two consecutive elements is the same.
Given an array of numbers `arr`, return `true` _if the array can be rearranged to form an **arithmetic progression**. Otherwise, return_ `false`.
**Example 1:**
**Input:** arr = \[3,5,1\]
**Output:** true
**Explanation:** We can reorder the elements as \[1,3,5\] or \[5,3,1\] with differences 2 and -2 respectively, between each consecutive elements.
**Example 2:**
**Input:** arr = \[1,2,4\]
**Output:** false
**Explanation:** There is no way to reorder the elements to obtain an arithmetic progression.
**Constraints:**
* `2 <= arr.length <= 1000`
* `-106 <= arr[i] <= 106` | If the s.length < k we cannot construct k strings from s and answer is false. If the number of characters that have odd counts is > k then the minimum number of palindrome strings we can construct is > k and answer is false. Otherwise you can construct exactly k palindrome strings and answer is true (why ?). |
Easy Python Solution | construct-k-palindrome-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nif a string has more than one letter whose frequency is odd then that string cannot form palindrome, hence keeping this in mind, we keep track of each letters frequency, if it is odd we increment num by 1 for every odd frequency. If num is Less than k value then we return true.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def canConstruct(self, s: str, k: int) -> bool:\n if k >len(s):\n return False\n num = 0\n p = Counter(s).values()\n for i in p:\n if i % 2 == 1:\n num = num+1\n if num <= k:\n return True\n return False\n``` | 0 | Given a string `s` and an integer `k`, return `true` _if you can use all the characters in_ `s` _to construct_ `k` _palindrome strings or_ `false` _otherwise_.
**Example 1:**
**Input:** s = "annabelle ", k = 2
**Output:** true
**Explanation:** You can construct two palindromes using all characters in s.
Some possible constructions "anna " + "elble ", "anbna " + "elle ", "anellena " + "b "
**Example 2:**
**Input:** s = "leetcode ", k = 3
**Output:** false
**Explanation:** It is impossible to construct 3 palindromes using all the characters of s.
**Example 3:**
**Input:** s = "true ", k = 4
**Output:** true
**Explanation:** The only possible solution is to put each character in a separate string.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of lowercase English letters.
* `1 <= k <= 105` | It's straightforward to check if A or B won or not, check for each row/column/diag if all the three are the same. Then if no one wins, the game is a draw iff the board is full, i.e. moves.length = 9 otherwise is pending. |
Easy Python Solution | construct-k-palindrome-strings | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nif a string has more than one letter whose frequency is odd then that string cannot form palindrome, hence keeping this in mind, we keep track of each letters frequency, if it is odd we increment num by 1 for every odd frequency. If num is Less than k value then we return true.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def canConstruct(self, s: str, k: int) -> bool:\n if k >len(s):\n return False\n num = 0\n p = Counter(s).values()\n for i in p:\n if i % 2 == 1:\n num = num+1\n if num <= k:\n return True\n return False\n``` | 0 | A sequence of numbers is called an **arithmetic progression** if the difference between any two consecutive elements is the same.
Given an array of numbers `arr`, return `true` _if the array can be rearranged to form an **arithmetic progression**. Otherwise, return_ `false`.
**Example 1:**
**Input:** arr = \[3,5,1\]
**Output:** true
**Explanation:** We can reorder the elements as \[1,3,5\] or \[5,3,1\] with differences 2 and -2 respectively, between each consecutive elements.
**Example 2:**
**Input:** arr = \[1,2,4\]
**Output:** false
**Explanation:** There is no way to reorder the elements to obtain an arithmetic progression.
**Constraints:**
* `2 <= arr.length <= 1000`
* `-106 <= arr[i] <= 106` | If the s.length < k we cannot construct k strings from s and answer is false. If the number of characters that have odd counts is > k then the minimum number of palindrome strings we can construct is > k and answer is false. Otherwise you can construct exactly k palindrome strings and answer is true (why ?). |
Python - Easy geometry | circle-and-rectangle-overlapping | 0 | 1 | First we have to find the nearest point on the rectangle to the center of the circle. Calculate the distance between the center of the circle with the nearest x and y coordinates. We can check the intersection by using pythagorean theorem. A point lies inside the circle if distance < radius. A point lies on the circle if d = radius. \nSo we return check if distance <= radius. \n\n# Code\n```\nclass Solution:\n def checkOverlap(self, radius: int, xCenter: int, yCenter: int, x1: int, y1: int, x2: int, y2: int) -> bool:\n nearest_x = max(x1, min(x2, xCenter))\n nearest_y = max(y1, min(y2, yCenter))\n dist_x = nearest_x - xCenter\n dist_y = nearest_y - yCenter\n\n return dist_x ** 2 + dist_y ** 2 <= radius ** 2\n``` | 2 | You are given a circle represented as `(radius, xCenter, yCenter)` and an axis-aligned rectangle represented as `(x1, y1, x2, y2)`, where `(x1, y1)` are the coordinates of the bottom-left corner, and `(x2, y2)` are the coordinates of the top-right corner of the rectangle.
Return `true` _if the circle and rectangle are overlapped otherwise return_ `false`. In other words, check if there is **any** point `(xi, yi)` that belongs to the circle and the rectangle at the same time.
**Example 1:**
**Input:** radius = 1, xCenter = 0, yCenter = 0, x1 = 1, y1 = -1, x2 = 3, y2 = 1
**Output:** true
**Explanation:** Circle and rectangle share the point (1,0).
**Example 2:**
**Input:** radius = 1, xCenter = 1, yCenter = 1, x1 = 1, y1 = -3, x2 = 2, y2 = -1
**Output:** false
**Example 3:**
**Input:** radius = 1, xCenter = 0, yCenter = 0, x1 = -1, y1 = 0, x2 = 0, y2 = 1
**Output:** true
**Constraints:**
* `1 <= radius <= 2000`
* `-104 <= xCenter, yCenter <= 104`
* `-104 <= x1 < x2 <= 104`
* `-104 <= y1 < y2 <= 104` | Can we have an answer if the number of tomatoes is odd ? If we have answer will be there multiple answers or just one answer ? Let us define number of jumbo burgers as X and number of small burgers as Y
We have to find an x and y in this equation 1. 4X + 2Y = tomato 2. X + Y = cheese |
Python, translate square by center of circle, find closest point to origin, pythagoras theorem | circle-and-rectangle-overlapping | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def checkOverlap(self, radius: int, xCenter: int, yCenter: int, x1: int, y1: int, x2: int, y2: int) -> bool:\n # (x-a)^2 + (y-b)^2 = r^2 for circle\n xdist = 0 if x1 <= xCenter <= x2 else min(abs(x1-xCenter), abs(x2-xCenter))\n ydist = 0 if y1 <= yCenter <= y2 else min(abs(y1-yCenter), abs(y2-yCenter))\n return xdist**2 + ydist**2 <= radius**2\n \n\n``` | 0 | You are given a circle represented as `(radius, xCenter, yCenter)` and an axis-aligned rectangle represented as `(x1, y1, x2, y2)`, where `(x1, y1)` are the coordinates of the bottom-left corner, and `(x2, y2)` are the coordinates of the top-right corner of the rectangle.
Return `true` _if the circle and rectangle are overlapped otherwise return_ `false`. In other words, check if there is **any** point `(xi, yi)` that belongs to the circle and the rectangle at the same time.
**Example 1:**
**Input:** radius = 1, xCenter = 0, yCenter = 0, x1 = 1, y1 = -1, x2 = 3, y2 = 1
**Output:** true
**Explanation:** Circle and rectangle share the point (1,0).
**Example 2:**
**Input:** radius = 1, xCenter = 1, yCenter = 1, x1 = 1, y1 = -3, x2 = 2, y2 = -1
**Output:** false
**Example 3:**
**Input:** radius = 1, xCenter = 0, yCenter = 0, x1 = -1, y1 = 0, x2 = 0, y2 = 1
**Output:** true
**Constraints:**
* `1 <= radius <= 2000`
* `-104 <= xCenter, yCenter <= 104`
* `-104 <= x1 < x2 <= 104`
* `-104 <= y1 < y2 <= 104` | Can we have an answer if the number of tomatoes is odd ? If we have answer will be there multiple answers or just one answer ? Let us define number of jumbo burgers as X and number of small burgers as Y
We have to find an x and y in this equation 1. 4X + 2Y = tomato 2. X + Y = cheese |
check all cases | circle-and-rectangle-overlapping | 0 | 1 | # Code\n```\nclass Solution:\n def checkOverlap(self, radius: int, xCenter: int, yCenter: int, x1: int, y1: int, x2: int, y2: int) -> bool:\n R2 = radius ** 2\n # some point on the 4 sides of the rec is inside the circle\n if R2 >= (x1 - xCenter) ** 2:\n y = yCenter + (R2 - (x1 - xCenter) ** 2) ** 0.5\n if y1 <= y <= y2:\n return True\n y = yCenter - (R2 - (x1 - xCenter) ** 2) ** 0.5\n if y1 <= y <= y2:\n return True\n if R2 >= (x2 - xCenter) ** 2:\n y = yCenter + (R2 - (x2 - xCenter) ** 2) ** 0.5\n if y1 <= y <= y2:\n return True\n y = yCenter - (R2 - (x2 - xCenter) ** 2) ** 0.5\n if y1 <= y <= y2:\n return True\n if R2 >= (y1 - yCenter) ** 2:\n x = xCenter + (R2 - (y1 - yCenter) ** 2) ** 0.5\n if x1 <= x <= x2:\n return True\n x = xCenter - (R2 - (y1 - yCenter) ** 2) ** 0.5\n if x1 <= x <= x2:\n return True\n if R2 >= (y2 - yCenter) ** 2:\n x = xCenter + (R2 - (y2 - yCenter) ** 2) ** 0.5\n if x1 <= x <= x2:\n return True\n x = xCenter - (R2 - (y2 - yCenter) ** 2) ** 0.5\n if x1 <= x <= x2:\n return True\n \n # circle inside rectangle\n if x1 <= xCenter - radius <= xCenter + radius <= x2 and y1 <= yCenter - radius <= yCenter + radius <= y2:\n return True\n \n # rec inside circle\n c1 = (x1 - xCenter) ** 2 + (y1 - yCenter) ** 2 <= R2\n c2 = (x2 - xCenter) ** 2 + (y2 - yCenter) ** 2 <= R2\n c3 = (x1 - xCenter) ** 2 + (y2 - yCenter) ** 2 <= R2\n c4 = (x2 - xCenter) ** 2 + (y1 - yCenter) ** 2 <= R2\n if c1 and c2 and c3 and c4:\n return True\n\n return False\n \n \n``` | 0 | You are given a circle represented as `(radius, xCenter, yCenter)` and an axis-aligned rectangle represented as `(x1, y1, x2, y2)`, where `(x1, y1)` are the coordinates of the bottom-left corner, and `(x2, y2)` are the coordinates of the top-right corner of the rectangle.
Return `true` _if the circle and rectangle are overlapped otherwise return_ `false`. In other words, check if there is **any** point `(xi, yi)` that belongs to the circle and the rectangle at the same time.
**Example 1:**
**Input:** radius = 1, xCenter = 0, yCenter = 0, x1 = 1, y1 = -1, x2 = 3, y2 = 1
**Output:** true
**Explanation:** Circle and rectangle share the point (1,0).
**Example 2:**
**Input:** radius = 1, xCenter = 1, yCenter = 1, x1 = 1, y1 = -3, x2 = 2, y2 = -1
**Output:** false
**Example 3:**
**Input:** radius = 1, xCenter = 0, yCenter = 0, x1 = -1, y1 = 0, x2 = 0, y2 = 1
**Output:** true
**Constraints:**
* `1 <= radius <= 2000`
* `-104 <= xCenter, yCenter <= 104`
* `-104 <= x1 < x2 <= 104`
* `-104 <= y1 < y2 <= 104` | Can we have an answer if the number of tomatoes is odd ? If we have answer will be there multiple answers or just one answer ? Let us define number of jumbo burgers as X and number of small burgers as Y
We have to find an x and y in this equation 1. 4X + 2Y = tomato 2. X + Y = cheese |
Python Beats 80% Circle and Square Intersect | circle-and-rectangle-overlapping | 0 | 1 | ```\nimport itertools\nclass Solution:\n def in_rect(corners: tuple[int, int, int, int], point: tuple[int, int]) -> bool:\n x1, y1, x2, y2 = corners\n x, y = point\n # Has to be included in both dimensions\n return x1 <= x and x <= x2 and y1 <= y and y <= y2\n def in_semi(semi: tuple[tuple[int, int], tuple[int, int]], r2: int, point: tuple[int, int]) -> bool:\n (right, cx), (up, cy) = semi\n x, y = point\n if right and x < cx or not right and x > cx:\n # Has to be to the right side of the quarter circle/s quarter-cutting radius vectors\n return False\n if up and y < cy or not up and y > cy:\n # Has to be to the right side of the quarter circle/s quarter-cutting radius vectors\n return False\n # Has to be close enough\n return (cx - x)**2 + (cy - y)**2 <= r2\n def checkOverlap(self, r: int, xCenter: int, yCenter: int, x1: int, y1: int, x2: int, y2: int) -> bool:\n # They overlap if the circle\'s center is in the rounded rectangle around the rectangle or if the\n # rectangle\'s center is in some sort of other relation with the circle... let\'s do the firs one\n horiz_cross = (x1 - r, y1, x2 + r, y2)\n vert_cross = (x1, y1 - r, x2, y2 + r)\n rects = [horiz_cross, vert_cross]\n semis = list(itertools.product(enumerate([x1, x2]), enumerate([y1, y2])))\n center = (xCenter, yCenter)\n r2 = r**2\n return (\n any([Solution.in_rect(rect, center) for rect in rects]) or\n any([Solution.in_semi(semi, r2, center) for semi in semis])\n )\n``` | 0 | You are given a circle represented as `(radius, xCenter, yCenter)` and an axis-aligned rectangle represented as `(x1, y1, x2, y2)`, where `(x1, y1)` are the coordinates of the bottom-left corner, and `(x2, y2)` are the coordinates of the top-right corner of the rectangle.
Return `true` _if the circle and rectangle are overlapped otherwise return_ `false`. In other words, check if there is **any** point `(xi, yi)` that belongs to the circle and the rectangle at the same time.
**Example 1:**
**Input:** radius = 1, xCenter = 0, yCenter = 0, x1 = 1, y1 = -1, x2 = 3, y2 = 1
**Output:** true
**Explanation:** Circle and rectangle share the point (1,0).
**Example 2:**
**Input:** radius = 1, xCenter = 1, yCenter = 1, x1 = 1, y1 = -3, x2 = 2, y2 = -1
**Output:** false
**Example 3:**
**Input:** radius = 1, xCenter = 0, yCenter = 0, x1 = -1, y1 = 0, x2 = 0, y2 = 1
**Output:** true
**Constraints:**
* `1 <= radius <= 2000`
* `-104 <= xCenter, yCenter <= 104`
* `-104 <= x1 < x2 <= 104`
* `-104 <= y1 < y2 <= 104` | Can we have an answer if the number of tomatoes is odd ? If we have answer will be there multiple answers or just one answer ? Let us define number of jumbo burgers as X and number of small burgers as Y
We have to find an x and y in this equation 1. 4X + 2Y = tomato 2. X + Y = cheese |
Simple maths solution | circle-and-rectangle-overlapping | 0 | 1 | # Intuition\nSample point from rectangle and check if in circle.\n\n# Approach\nFind a point $(x, y)$ such that $x_1 <= x <= y_2$ and $y_1 <= y <= y_2$ (i.e. in rectangle) which is closest to the circle origin.\nObviously, if $x_c$ lies within the range $[x_1, x_2]$ then $x = x_c$. If $x_c < x_1$, take $x=x_1$, conversely if $x_c > x_2$ take $x=x_2$. Do same for $y$.\nFinally test if the point lies within the circle with $(x-x_c)^2+(y-y_c)^2<=r^2$.\n\n# Complexity\n- Time complexity: $$O(1)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def checkOverlap(self, r: int, xc: int, yc: int, x1: int, y1: int, x2: int, y2: int) -> bool:\n x = xc if x1 <= xc <= x2 else x1 if xc < x1 else x2\n y = yc if y1 <= yc <= y2 else y1 if yc < y1 else y2\n return (x - xc) ** 2 + (y - yc) ** 2 <= r ** 2\n``` | 0 | You are given a circle represented as `(radius, xCenter, yCenter)` and an axis-aligned rectangle represented as `(x1, y1, x2, y2)`, where `(x1, y1)` are the coordinates of the bottom-left corner, and `(x2, y2)` are the coordinates of the top-right corner of the rectangle.
Return `true` _if the circle and rectangle are overlapped otherwise return_ `false`. In other words, check if there is **any** point `(xi, yi)` that belongs to the circle and the rectangle at the same time.
**Example 1:**
**Input:** radius = 1, xCenter = 0, yCenter = 0, x1 = 1, y1 = -1, x2 = 3, y2 = 1
**Output:** true
**Explanation:** Circle and rectangle share the point (1,0).
**Example 2:**
**Input:** radius = 1, xCenter = 1, yCenter = 1, x1 = 1, y1 = -3, x2 = 2, y2 = -1
**Output:** false
**Example 3:**
**Input:** radius = 1, xCenter = 0, yCenter = 0, x1 = -1, y1 = 0, x2 = 0, y2 = 1
**Output:** true
**Constraints:**
* `1 <= radius <= 2000`
* `-104 <= xCenter, yCenter <= 104`
* `-104 <= x1 < x2 <= 104`
* `-104 <= y1 < y2 <= 104` | Can we have an answer if the number of tomatoes is odd ? If we have answer will be there multiple answers or just one answer ? Let us define number of jumbo burgers as X and number of small burgers as Y
We have to find an x and y in this equation 1. 4X + 2Y = tomato 2. X + Y = cheese |
Python O(nlogn) solution for reference | reducing-dishes | 0 | 1 | ```\nclass Solution:\n def maxSatisfaction(self, sat: List[int]) -> int:\n sat = sorted(sat)\n \n sm, res , idx , nend = 0,0,1,-1\n for i in range(0,len(sat)):\n if(sat[i] >= 0 ):\n sm += sat[i]\n res += sat[i]*idx \n idx += 1\n if(i>0 and sat[i] >= 0 and sat[i-1] < 0 ):\n nend = i-1 ; \n \n while nend >=0 :\n if( res+sm+sat[nend] > res ):\n res = res+sm+sat[nend]\n sm = sm + sat[nend]\n nend -= 1\n else:\n break;\n return res\n \n\n``` | 2 | A chef has collected data on the `satisfaction` level of his `n` dishes. Chef can cook any dish in 1 unit of time.
**Like-time coefficient** of a dish is defined as the time taken to cook that dish including previous dishes multiplied by its satisfaction level i.e. `time[i] * satisfaction[i]`.
Return _the maximum sum of **like-time coefficient** that the chef can obtain after dishes preparation_.
Dishes can be prepared in **any** order and the chef can discard some dishes to get this maximum value.
**Example 1:**
**Input:** satisfaction = \[-1,-8,0,5,-9\]
**Output:** 14
**Explanation:** After Removing the second and last dish, the maximum total **like-time coefficient** will be equal to (-1\*1 + 0\*2 + 5\*3 = 14).
Each dish is prepared in one unit of time.
**Example 2:**
**Input:** satisfaction = \[4,3,2\]
**Output:** 20
**Explanation:** Dishes can be prepared in any order, (2\*1 + 3\*2 + 4\*3 = 20)
**Example 3:**
**Input:** satisfaction = \[-1,-4,-5\]
**Output:** 0
**Explanation:** People do not like the dishes. No dish is prepared.
**Constraints:**
* `n == satisfaction.length`
* `1 <= n <= 500`
* `-1000 <= satisfaction[i] <= 1000` | Create an additive table that counts the sum of elements of submatrix with the superior corner at (0,0). Loop over all subsquares in O(n^3) and check if the sum make the whole array to be ones, if it checks then add 1 to the answer. |
Python O(nlogn) solution for reference | reducing-dishes | 0 | 1 | ```\nclass Solution:\n def maxSatisfaction(self, sat: List[int]) -> int:\n sat = sorted(sat)\n \n sm, res , idx , nend = 0,0,1,-1\n for i in range(0,len(sat)):\n if(sat[i] >= 0 ):\n sm += sat[i]\n res += sat[i]*idx \n idx += 1\n if(i>0 and sat[i] >= 0 and sat[i-1] < 0 ):\n nend = i-1 ; \n \n while nend >=0 :\n if( res+sm+sat[nend] > res ):\n res = res+sm+sat[nend]\n sm = sm + sat[nend]\n nend -= 1\n else:\n break;\n return res\n \n\n``` | 2 | We have a wooden plank of the length `n` **units**. Some ants are walking on the plank, each ant moves with a speed of **1 unit per second**. Some of the ants move to the **left**, the other move to the **right**.
When two ants moving in two **different** directions meet at some point, they change their directions and continue moving again. Assume changing directions does not take any additional time.
When an ant reaches **one end** of the plank at a time `t`, it falls out of the plank immediately.
Given an integer `n` and two integer arrays `left` and `right`, the positions of the ants moving to the left and the right, return _the moment when the last ant(s) fall out of the plank_.
**Example 1:**
**Input:** n = 4, left = \[4,3\], right = \[0,1\]
**Output:** 4
**Explanation:** In the image above:
-The ant at index 0 is named A and going to the right.
-The ant at index 1 is named B and going to the right.
-The ant at index 3 is named C and going to the left.
-The ant at index 4 is named D and going to the left.
The last moment when an ant was on the plank is t = 4 seconds. After that, it falls immediately out of the plank. (i.e., We can say that at t = 4.0000000001, there are no ants on the plank).
**Example 2:**
**Input:** n = 7, left = \[\], right = \[0,1,2,3,4,5,6,7\]
**Output:** 7
**Explanation:** All ants are going to the right, the ant at index 0 needs 7 seconds to fall.
**Example 3:**
**Input:** n = 7, left = \[0,1,2,3,4,5,6,7\], right = \[\]
**Output:** 7
**Explanation:** All ants are going to the left, the ant at index 7 needs 7 seconds to fall.
**Constraints:**
* `1 <= n <= 104`
* `0 <= left.length <= n + 1`
* `0 <= left[i] <= n`
* `0 <= right.length <= n + 1`
* `0 <= right[i] <= n`
* `1 <= left.length + right.length <= n + 1`
* All values of `left` and `right` are unique, and each value can appear **only in one** of the two arrays. | Use dynamic programming to find the optimal solution by saving the previous best like-time coefficient and its corresponding element sum. If adding the current element to the previous best like-time coefficient and its corresponding element sum would increase the best like-time coefficient, then go ahead and add it. Otherwise, keep the previous best like-time coefficient. |
[Python3] DP + Greedy approach + explanation | reducing-dishes | 0 | 1 | # Intuition\nWe need to maximaze the satisfaction usign formulae:\n```\nsum((i+1) * dish[i])\n```\nwith some of the dishes reduced. \nWe can have negative satisfaction dishes, which reduce the overall satisfaction, apart from they add time so that further positive-satisfaction dishes contribute more satisfaction. \n\nAlso we see that we will maximize overall satisfaction if we cook best dishes last, so that their time multiplied by satisfaction is maximized.\n\nWe can use a greedy approach to maximize satisfaction by reducing some negative-satisfaction dishes.\n\nNote that if we start adding dishes, we can have overall satisfaction calculated by formulae:\n\n```\nD(i) = s(0) * (i-1) + s(1) * (i-2) + ... + s(i) * 1 = s(0) * (i-2) + s(1) * (i-3) + ... + s(i-1) + (s(0) + s(1) + ... + s(i-1)) + s(i) = D(i-1) + ps(i-1) + s(i)\n```\n\nWhere ``ps(i)`` is partial sum of first ``i`` dishes (The best ones).\n\nHere we can apply dynamic programming to solve this.\n\n# Approach\n1. Sort ``satisfaction`` in descending order\n2. Calculate ``D(i)`` and maintaining partial sum ``ps`` and updating maximum satisfaction ``result``\n3. Return the ``result``\n\n# Complexity\n- Time complexity:\n$$O(n*log(n))$$\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nclass Solution:\n def maxSatisfaction(self, satisfaction: List[int]) -> int:\n satisfaction.sort(reverse=True)\n result, current, ps = 0, 0, 0\n n = len(satisfaction)\n for i in range(n):\n current += ps + satisfaction[i]\n ps += satisfaction[i]\n result = max(result, current)\n return result\n```\n\nShall you have any questions please don\'t hesitate to ask! \n\nAnd upvote if you like this approach! | 1 | A chef has collected data on the `satisfaction` level of his `n` dishes. Chef can cook any dish in 1 unit of time.
**Like-time coefficient** of a dish is defined as the time taken to cook that dish including previous dishes multiplied by its satisfaction level i.e. `time[i] * satisfaction[i]`.
Return _the maximum sum of **like-time coefficient** that the chef can obtain after dishes preparation_.
Dishes can be prepared in **any** order and the chef can discard some dishes to get this maximum value.
**Example 1:**
**Input:** satisfaction = \[-1,-8,0,5,-9\]
**Output:** 14
**Explanation:** After Removing the second and last dish, the maximum total **like-time coefficient** will be equal to (-1\*1 + 0\*2 + 5\*3 = 14).
Each dish is prepared in one unit of time.
**Example 2:**
**Input:** satisfaction = \[4,3,2\]
**Output:** 20
**Explanation:** Dishes can be prepared in any order, (2\*1 + 3\*2 + 4\*3 = 20)
**Example 3:**
**Input:** satisfaction = \[-1,-4,-5\]
**Output:** 0
**Explanation:** People do not like the dishes. No dish is prepared.
**Constraints:**
* `n == satisfaction.length`
* `1 <= n <= 500`
* `-1000 <= satisfaction[i] <= 1000` | Create an additive table that counts the sum of elements of submatrix with the superior corner at (0,0). Loop over all subsquares in O(n^3) and check if the sum make the whole array to be ones, if it checks then add 1 to the answer. |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.