title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
Click this if you're confused. | pacific-atlantic-water-flow | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nWe can naively perform traversal on each tile, then check if that tile can flow to both pacific and atlantic oceans. However, this would have a time complexity of \u200AMathjax\u200A since we may traverse the majority of the grid for each tile in the grid.\n\nWe know that the top and left tiles can flow to the pacific ocean and the same for the bottom and right tiles. So, we can simply traverse from these tiles and mark all tiles that we can reach\u2014tiles with greater than or equal to heights, since we\'re going in reverse direction\u2014for each ocean. Then, each tile that has been marked for both oceans would be our desired output.\n\n# Complexity\n- Time complexity: $O(n * m)$ since we will explore every tile twice, once for each ocean\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $O(n * m)$ since in worst case, we could end up traversing the whole grid, e.g. if all tiles are equal\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def pacificAtlantic(self, heights: List[List[int]]) -> List[List[int]]:\n n_rows, n_cols = len(heights), len(heights[0])\n pac, atl = set(), set()\n\n def dfs(r, c, ocean, prevH):\n # we try to reach all positions on island reachable from ocean positions\n if (r < 0 or c < 0\n or r >= n_rows or c >= n_cols\n or (r, c) in ocean # prevent infinite loop\n or heights[r][c] < prevH): # current tile couldn\'t have flowed to prev\n return\n \n ocean.add((r, c)) # current tile can flow to this ocean\n dfs(r + 1, c, ocean, heights[r][c])\n dfs(r - 1, c, ocean, heights[r][c])\n dfs(r, c + 1, ocean, heights[r][c])\n dfs(r, c - 1, ocean, heights[r][c])\n \n # we want to find all positions reachable from each of the pac and atl oceans\n for r in range(n_rows):\n dfs(r, 0, pac, heights[r][0]) # left col\n dfs(r, n_cols - 1, atl, heights[r][n_cols - 1]) # right col\n # can\'t skip first and last cols, since the loop above only accounts for pac ocean for first col and atl for last col, the corners are part of both\n for c in range(0, n_cols):\n dfs(0, c, pac, heights[0][c]) # top row\n dfs(n_rows - 1, c, atl, heights[n_rows - 1][c]) # bottom row\n \n res = []\n for r in range(n_rows):\n for c in range(n_cols):\n if (r, c) in pac and (r, c) in atl:\n res.append((r, c))\n return res\n\n\n``` | 1 | There is an `m x n` rectangular island that borders both the **Pacific Ocean** and **Atlantic Ocean**. The **Pacific Ocean** touches the island's left and top edges, and the **Atlantic Ocean** touches the island's right and bottom edges.
The island is partitioned into a grid of square cells. You are given an `m x n` integer matrix `heights` where `heights[r][c]` represents the **height above sea level** of the cell at coordinate `(r, c)`.
The island receives a lot of rain, and the rain water can flow to neighboring cells directly north, south, east, and west if the neighboring cell's height is **less than or equal to** the current cell's height. Water can flow from any cell adjacent to an ocean into the ocean.
Return _a **2D list** of grid coordinates_ `result` _where_ `result[i] = [ri, ci]` _denotes that rain water can flow from cell_ `(ri, ci)` _to **both** the Pacific and Atlantic oceans_.
**Example 1:**
**Input:** heights = \[\[1,2,2,3,5\],\[3,2,3,4,4\],\[2,4,5,3,1\],\[6,7,1,4,5\],\[5,1,1,2,4\]\]
**Output:** \[\[0,4\],\[1,3\],\[1,4\],\[2,2\],\[3,0\],\[3,1\],\[4,0\]\]
**Explanation:** The following cells can flow to the Pacific and Atlantic oceans, as shown below:
\[0,4\]: \[0,4\] -> Pacific Ocean
\[0,4\] -> Atlantic Ocean
\[1,3\]: \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,3\] -> \[1,4\] -> Atlantic Ocean
\[1,4\]: \[1,4\] -> \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,4\] -> Atlantic Ocean
\[2,2\]: \[2,2\] -> \[1,2\] -> \[0,2\] -> Pacific Ocean
\[2,2\] -> \[2,3\] -> \[2,4\] -> Atlantic Ocean
\[3,0\]: \[3,0\] -> Pacific Ocean
\[3,0\] -> \[4,0\] -> Atlantic Ocean
\[3,1\]: \[3,1\] -> \[3,0\] -> Pacific Ocean
\[3,1\] -> \[4,1\] -> Atlantic Ocean
\[4,0\]: \[4,0\] -> Pacific Ocean
\[4,0\] -> Atlantic Ocean
Note that there are other possible paths for these cells to flow to the Pacific and Atlantic oceans.
**Example 2:**
**Input:** heights = \[\[1\]\]
**Output:** \[\[0,0\]\]
**Explanation:** The water can flow from the only cell to the Pacific and Atlantic oceans.
**Constraints:**
* `m == heights.length`
* `n == heights[r].length`
* `1 <= m, n <= 200`
* `0 <= heights[r][c] <= 105` | null |
time/space -> O(m*n) | pacific-atlantic-water-flow | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def pacificAtlantic(self, heights: List[List[int]]) -> List[List[int]]:\n ROWS,COLS = len(heights),len(heights[0])\n pac,atl = set(),set()\n\n def dfs(r,c,visit,prevHeight):\n if (r < 0 or r == ROWS or c < 0 or c == COLS or \n heights[r][c] < prevHeight or (r,c)in visit):\n return\n \n visit.add((r,c))\n\n dfs(r+1,c,visit,heights[r][c])\n dfs(r-1,c,visit,heights[r][c])\n dfs(r,c+1,visit,heights[r][c])\n dfs(r,c-1,visit,heights[r][c])\n\n for c in range(COLS):\n dfs(0,c,pac,heights[0][c])\n dfs(ROWS-1,c,atl,heights[ROWS-1][c])\n\n for r in range(ROWS):\n dfs(r,0,pac,heights[r][0])\n dfs(r,COLS-1,atl,heights[r][COLS-1])\n\n\n res = []\n for r in range(ROWS):\n for c in range(COLS):\n if (r,c) in pac and (r,c) in atl:\n res.append([r,c])\n return res \n\n# time/space -> O(m*n)\n``` | 1 | There is an `m x n` rectangular island that borders both the **Pacific Ocean** and **Atlantic Ocean**. The **Pacific Ocean** touches the island's left and top edges, and the **Atlantic Ocean** touches the island's right and bottom edges.
The island is partitioned into a grid of square cells. You are given an `m x n` integer matrix `heights` where `heights[r][c]` represents the **height above sea level** of the cell at coordinate `(r, c)`.
The island receives a lot of rain, and the rain water can flow to neighboring cells directly north, south, east, and west if the neighboring cell's height is **less than or equal to** the current cell's height. Water can flow from any cell adjacent to an ocean into the ocean.
Return _a **2D list** of grid coordinates_ `result` _where_ `result[i] = [ri, ci]` _denotes that rain water can flow from cell_ `(ri, ci)` _to **both** the Pacific and Atlantic oceans_.
**Example 1:**
**Input:** heights = \[\[1,2,2,3,5\],\[3,2,3,4,4\],\[2,4,5,3,1\],\[6,7,1,4,5\],\[5,1,1,2,4\]\]
**Output:** \[\[0,4\],\[1,3\],\[1,4\],\[2,2\],\[3,0\],\[3,1\],\[4,0\]\]
**Explanation:** The following cells can flow to the Pacific and Atlantic oceans, as shown below:
\[0,4\]: \[0,4\] -> Pacific Ocean
\[0,4\] -> Atlantic Ocean
\[1,3\]: \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,3\] -> \[1,4\] -> Atlantic Ocean
\[1,4\]: \[1,4\] -> \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,4\] -> Atlantic Ocean
\[2,2\]: \[2,2\] -> \[1,2\] -> \[0,2\] -> Pacific Ocean
\[2,2\] -> \[2,3\] -> \[2,4\] -> Atlantic Ocean
\[3,0\]: \[3,0\] -> Pacific Ocean
\[3,0\] -> \[4,0\] -> Atlantic Ocean
\[3,1\]: \[3,1\] -> \[3,0\] -> Pacific Ocean
\[3,1\] -> \[4,1\] -> Atlantic Ocean
\[4,0\]: \[4,0\] -> Pacific Ocean
\[4,0\] -> Atlantic Ocean
Note that there are other possible paths for these cells to flow to the Pacific and Atlantic oceans.
**Example 2:**
**Input:** heights = \[\[1\]\]
**Output:** \[\[0,0\]\]
**Explanation:** The water can flow from the only cell to the Pacific and Atlantic oceans.
**Constraints:**
* `m == heights.length`
* `n == heights[r].length`
* `1 <= m, n <= 200`
* `0 <= heights[r][c] <= 105` | null |
Python BFS | pacific-atlantic-water-flow | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nBFS first, and then take the intersection.\n1. BFS from the nodes that are on the coast of the Pacific to find the nodes that can flow to the Pacific;\n2. BFS from the nodes that are on the coast of the Atlantic to find the nodes that can flow to the Atlantic;\n3. If both the result of the two BFS are returned as sets, take the intersection, and then convert if to list.\n\n# Code\n```\nclass Solution:\n def pacificAtlantic(self, heights: List[List[int]]) -> List[List[int]]:\n m = len(heights)\n n = len(heights[0])\n p = deque() # pacific\n a = deque() # atlantic\n directions = {(1, 0), (-1, 0), (0, 1), (0, -1)}\n\n for i in range(m):\n p.append((i, 0))\n a.append((i, n - 1))\n \n for j in range(n):\n p.append((0, j))\n a.append((m - 1, j))\n \n def bfs(q):\n can_reach = set()\n while q:\n x, y = q.popleft()\n can_reach.add((x, y))\n for dx,dy in directions:\n nx, ny = x + dx, y + dy\n if 0 <= nx < m and 0 <= ny < n and heights[nx][ny] >= heights[x][y]:\n if (nx, ny) not in can_reach:\n q.append((nx, ny))\n \n return can_reach\n \n to_pacific = bfs(p)\n to_atlantic = bfs(a)\n return list(to_pacific.intersection(to_atlantic))\n\n``` | 1 | There is an `m x n` rectangular island that borders both the **Pacific Ocean** and **Atlantic Ocean**. The **Pacific Ocean** touches the island's left and top edges, and the **Atlantic Ocean** touches the island's right and bottom edges.
The island is partitioned into a grid of square cells. You are given an `m x n` integer matrix `heights` where `heights[r][c]` represents the **height above sea level** of the cell at coordinate `(r, c)`.
The island receives a lot of rain, and the rain water can flow to neighboring cells directly north, south, east, and west if the neighboring cell's height is **less than or equal to** the current cell's height. Water can flow from any cell adjacent to an ocean into the ocean.
Return _a **2D list** of grid coordinates_ `result` _where_ `result[i] = [ri, ci]` _denotes that rain water can flow from cell_ `(ri, ci)` _to **both** the Pacific and Atlantic oceans_.
**Example 1:**
**Input:** heights = \[\[1,2,2,3,5\],\[3,2,3,4,4\],\[2,4,5,3,1\],\[6,7,1,4,5\],\[5,1,1,2,4\]\]
**Output:** \[\[0,4\],\[1,3\],\[1,4\],\[2,2\],\[3,0\],\[3,1\],\[4,0\]\]
**Explanation:** The following cells can flow to the Pacific and Atlantic oceans, as shown below:
\[0,4\]: \[0,4\] -> Pacific Ocean
\[0,4\] -> Atlantic Ocean
\[1,3\]: \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,3\] -> \[1,4\] -> Atlantic Ocean
\[1,4\]: \[1,4\] -> \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,4\] -> Atlantic Ocean
\[2,2\]: \[2,2\] -> \[1,2\] -> \[0,2\] -> Pacific Ocean
\[2,2\] -> \[2,3\] -> \[2,4\] -> Atlantic Ocean
\[3,0\]: \[3,0\] -> Pacific Ocean
\[3,0\] -> \[4,0\] -> Atlantic Ocean
\[3,1\]: \[3,1\] -> \[3,0\] -> Pacific Ocean
\[3,1\] -> \[4,1\] -> Atlantic Ocean
\[4,0\]: \[4,0\] -> Pacific Ocean
\[4,0\] -> Atlantic Ocean
Note that there are other possible paths for these cells to flow to the Pacific and Atlantic oceans.
**Example 2:**
**Input:** heights = \[\[1\]\]
**Output:** \[\[0,0\]\]
**Explanation:** The water can flow from the only cell to the Pacific and Atlantic oceans.
**Constraints:**
* `m == heights.length`
* `n == heights[r].length`
* `1 <= m, n <= 200`
* `0 <= heights[r][c] <= 105` | null |
🥇 PYTHON || EXPLAINED || ; ] | pacific-atlantic-water-flow | 0 | 1 | **UPVOTE IF HELPFuuL**\n\n**HERE I HAVE CREATED TWO SEPRATE FUNCTION , ONE FUNCTION COULD BE MADE , THIS IS JUST DONE FOR CLARITY.**\n\nWe need to count the number of cells from which waer could flow to both the oceans.\nWater can go to **left,top** for pacific and to **right,down** for atlantic.\n\n**APPROACH**\n\nWe just do what is asked for.\n\nWhen at cell ```ht[i][j]``` :\n* we check that water can flow to **left,top** and also to **right,down**.\n* It is similar to finding a path with non-increasing values.\n* To prevent repeation, we use memoization to store the result for certain ```ht[i][j]```.\n\n*How memoization helps :*\n* Let water can flow to both oceans from cell ```ht[i][j]```, so while checking for its adjacent cells, we need not find the complete path to oceans, **we just find a path that leads to a cell that reaches both oceans.**\n\n**Finding water can reach both oceans :**\n* As we going for memoization, we create two ```2-D array for atlantic and pacific``` that stores that whether water can flow to ocean for each ocean respectively.\n* For pacific, we created ```pac``` and water initially water can flow for top and left cells.\n* For atlanic, we created ```atl``` and water initially water can flow for bottom and right cells.\n* Then for each cell, we check whether water flows off :\n* * For cell ```ht[i][j]``` we recurcively call for its adjacent cells, if neightbouring height is less or equal to current cell.\n* * Water can flow off if we reach a cell for which we already have solution.\n* * * For pacific we already made top,left cells ```true``` and same for atlantic.\n\n* Whenever water reaches, make all cells in that path ```true``` so they need not to be calculated in future.\n\n\n\n**UPVOTE IF HELPFuuL**\n\n*Here i created and pdated the memoization table first and calculated number of cells afterwards, can be combined to single step.*\n\n**PYTHOON**\n```\nclass Solution:\n def pacificAtlantic(self, ht: List[List[int]]) -> List[List[int]]:\n \n def pac(i,j):\n if rp[i][j]:\n return True\n k=False\n h=ht[i][j]\n ht[i][j]=100001\n if ht[i-1][j]<=h:\n k=k or pac(i-1,j)\n \n if ht[i][j-1]<=h:\n k=k or pac(i,j-1)\n \n if i<m-1 and ht[i+1][j]<=h:\n k=k or pac(i+1,j)\n \n if j<n-1 and ht[i][j+1]<=h:\n k=k or pac(i,j+1)\n \n ht[i][j]=h\n rp[i][j]=k\n return k\n \n def ant(i,j):\n if ra[i][j]:\n return True\n k=False\n h=ht[i][j]\n ht[i][j]=100001\n if i>0 and ht[i-1][j]<=h:\n k=k or ant(i-1,j)\n \n if j>0 and ht[i][j-1]<=h:\n k=k or ant(i,j-1)\n \n if ht[i+1][j]<=h:\n k=k or ant(i+1,j)\n \n if ht[i][j+1]<=h:\n k=k or ant(i,j+1)\n \n ht[i][j]=h\n ra[i][j]=k\n return k\n \n m=len(ht)\n n=len(ht[0])\n rp=[[False for i in range(n)] for j in range(m)]\n ra=[[False for i in range(n)] for j in range(m)]\n \n for i in range(m):\n rp[i][0]=True\n ra[i][-1]=True\n for i in range(n):\n rp[0][i]=True\n ra[-1][i]=True\n \n for i in range(m):\n for j in range(n):\n pac(i,j)\n ant(i,j)\n res=[]\n for i in range(m):\n for j in range(n):\n if rp[i][j] and ra[i][j]:\n res.append([i,j])\n return res\n```\n\n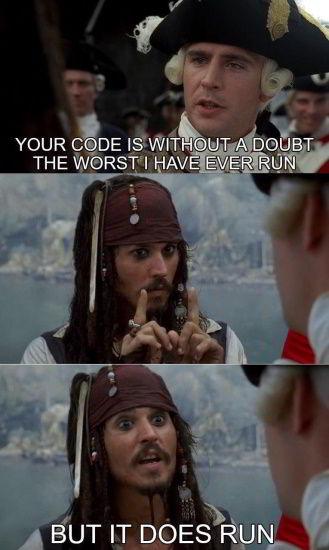\n | 23 | There is an `m x n` rectangular island that borders both the **Pacific Ocean** and **Atlantic Ocean**. The **Pacific Ocean** touches the island's left and top edges, and the **Atlantic Ocean** touches the island's right and bottom edges.
The island is partitioned into a grid of square cells. You are given an `m x n` integer matrix `heights` where `heights[r][c]` represents the **height above sea level** of the cell at coordinate `(r, c)`.
The island receives a lot of rain, and the rain water can flow to neighboring cells directly north, south, east, and west if the neighboring cell's height is **less than or equal to** the current cell's height. Water can flow from any cell adjacent to an ocean into the ocean.
Return _a **2D list** of grid coordinates_ `result` _where_ `result[i] = [ri, ci]` _denotes that rain water can flow from cell_ `(ri, ci)` _to **both** the Pacific and Atlantic oceans_.
**Example 1:**
**Input:** heights = \[\[1,2,2,3,5\],\[3,2,3,4,4\],\[2,4,5,3,1\],\[6,7,1,4,5\],\[5,1,1,2,4\]\]
**Output:** \[\[0,4\],\[1,3\],\[1,4\],\[2,2\],\[3,0\],\[3,1\],\[4,0\]\]
**Explanation:** The following cells can flow to the Pacific and Atlantic oceans, as shown below:
\[0,4\]: \[0,4\] -> Pacific Ocean
\[0,4\] -> Atlantic Ocean
\[1,3\]: \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,3\] -> \[1,4\] -> Atlantic Ocean
\[1,4\]: \[1,4\] -> \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,4\] -> Atlantic Ocean
\[2,2\]: \[2,2\] -> \[1,2\] -> \[0,2\] -> Pacific Ocean
\[2,2\] -> \[2,3\] -> \[2,4\] -> Atlantic Ocean
\[3,0\]: \[3,0\] -> Pacific Ocean
\[3,0\] -> \[4,0\] -> Atlantic Ocean
\[3,1\]: \[3,1\] -> \[3,0\] -> Pacific Ocean
\[3,1\] -> \[4,1\] -> Atlantic Ocean
\[4,0\]: \[4,0\] -> Pacific Ocean
\[4,0\] -> Atlantic Ocean
Note that there are other possible paths for these cells to flow to the Pacific and Atlantic oceans.
**Example 2:**
**Input:** heights = \[\[1\]\]
**Output:** \[\[0,0\]\]
**Explanation:** The water can flow from the only cell to the Pacific and Atlantic oceans.
**Constraints:**
* `m == heights.length`
* `n == heights[r].length`
* `1 <= m, n <= 200`
* `0 <= heights[r][c] <= 105` | null |
Python very concise solution using dfs + set (128ms). | pacific-atlantic-water-flow | 0 | 1 | ```python\ndef pacificAtlantic(self, matrix: List[List[int]]) -> List[List[int]]:\n if not matrix:\n return []\n p_land = set()\n a_land = set()\n R, C = len(matrix), len(matrix[0])\n def spread(i, j, land):\n land.add((i, j))\n for x, y in ((i+1, j), (i, j+1), (i-1, j), (i, j-1)):\n if (0<=x<R and 0<=y<C and matrix[x][y] >= matrix[i][j]\n and (x, y) not in land):\n spread(x, y, land)\n \n for i in range(R):\n spread(i, 0, p_land)\n spread(i, C-1, a_land)\n for j in range(C):\n spread(0, j, p_land)\n spread(R-1, j, a_land)\n return list(p_land & a_land)\n``` | 80 | There is an `m x n` rectangular island that borders both the **Pacific Ocean** and **Atlantic Ocean**. The **Pacific Ocean** touches the island's left and top edges, and the **Atlantic Ocean** touches the island's right and bottom edges.
The island is partitioned into a grid of square cells. You are given an `m x n` integer matrix `heights` where `heights[r][c]` represents the **height above sea level** of the cell at coordinate `(r, c)`.
The island receives a lot of rain, and the rain water can flow to neighboring cells directly north, south, east, and west if the neighboring cell's height is **less than or equal to** the current cell's height. Water can flow from any cell adjacent to an ocean into the ocean.
Return _a **2D list** of grid coordinates_ `result` _where_ `result[i] = [ri, ci]` _denotes that rain water can flow from cell_ `(ri, ci)` _to **both** the Pacific and Atlantic oceans_.
**Example 1:**
**Input:** heights = \[\[1,2,2,3,5\],\[3,2,3,4,4\],\[2,4,5,3,1\],\[6,7,1,4,5\],\[5,1,1,2,4\]\]
**Output:** \[\[0,4\],\[1,3\],\[1,4\],\[2,2\],\[3,0\],\[3,1\],\[4,0\]\]
**Explanation:** The following cells can flow to the Pacific and Atlantic oceans, as shown below:
\[0,4\]: \[0,4\] -> Pacific Ocean
\[0,4\] -> Atlantic Ocean
\[1,3\]: \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,3\] -> \[1,4\] -> Atlantic Ocean
\[1,4\]: \[1,4\] -> \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,4\] -> Atlantic Ocean
\[2,2\]: \[2,2\] -> \[1,2\] -> \[0,2\] -> Pacific Ocean
\[2,2\] -> \[2,3\] -> \[2,4\] -> Atlantic Ocean
\[3,0\]: \[3,0\] -> Pacific Ocean
\[3,0\] -> \[4,0\] -> Atlantic Ocean
\[3,1\]: \[3,1\] -> \[3,0\] -> Pacific Ocean
\[3,1\] -> \[4,1\] -> Atlantic Ocean
\[4,0\]: \[4,0\] -> Pacific Ocean
\[4,0\] -> Atlantic Ocean
Note that there are other possible paths for these cells to flow to the Pacific and Atlantic oceans.
**Example 2:**
**Input:** heights = \[\[1\]\]
**Output:** \[\[0,0\]\]
**Explanation:** The water can flow from the only cell to the Pacific and Atlantic oceans.
**Constraints:**
* `m == heights.length`
* `n == heights[r].length`
* `1 <= m, n <= 200`
* `0 <= heights[r][c] <= 105` | null |
Simple Solution | pacific-atlantic-water-flow | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def pacificAtlantic(self, h: List[List[int]]) -> List[List[int]]:\n n, m = len(h), len(h[0])\n isPacc = [[True if i == 0 or j == 0 else False for j in range(m)] for i in range(n)]\n isAtl = [[True if i == n - 1 or j == m - 1 else False for j in range(m)] for i in range(n)]\n \n def Pac(i, j, isPac):\n val = h[i][j]\n for x, y in [(i+1, j), (i-1, j), (i, j+1), (i, j-1)]:\n if 0 <= x < n and 0 <= y < m and not isPac[x][y] and h[x][y] >= val:\n isPac[x][y] = True\n Pac(x, y, isPac)\n \n for i in range(n): Pac(i, 0, isPacc)\n for j in range(m): Pac(0, j, isPacc)\n for i in range(n): Pac(i, m-1, isAtl)\n for j in range(m): Pac(n-1, j, isAtl)\n \n return [[i, j] for i in range(n) for j in range(m) if isPacc[i][j] and isAtl[i][j]]\n\n``` | 6 | There is an `m x n` rectangular island that borders both the **Pacific Ocean** and **Atlantic Ocean**. The **Pacific Ocean** touches the island's left and top edges, and the **Atlantic Ocean** touches the island's right and bottom edges.
The island is partitioned into a grid of square cells. You are given an `m x n` integer matrix `heights` where `heights[r][c]` represents the **height above sea level** of the cell at coordinate `(r, c)`.
The island receives a lot of rain, and the rain water can flow to neighboring cells directly north, south, east, and west if the neighboring cell's height is **less than or equal to** the current cell's height. Water can flow from any cell adjacent to an ocean into the ocean.
Return _a **2D list** of grid coordinates_ `result` _where_ `result[i] = [ri, ci]` _denotes that rain water can flow from cell_ `(ri, ci)` _to **both** the Pacific and Atlantic oceans_.
**Example 1:**
**Input:** heights = \[\[1,2,2,3,5\],\[3,2,3,4,4\],\[2,4,5,3,1\],\[6,7,1,4,5\],\[5,1,1,2,4\]\]
**Output:** \[\[0,4\],\[1,3\],\[1,4\],\[2,2\],\[3,0\],\[3,1\],\[4,0\]\]
**Explanation:** The following cells can flow to the Pacific and Atlantic oceans, as shown below:
\[0,4\]: \[0,4\] -> Pacific Ocean
\[0,4\] -> Atlantic Ocean
\[1,3\]: \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,3\] -> \[1,4\] -> Atlantic Ocean
\[1,4\]: \[1,4\] -> \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,4\] -> Atlantic Ocean
\[2,2\]: \[2,2\] -> \[1,2\] -> \[0,2\] -> Pacific Ocean
\[2,2\] -> \[2,3\] -> \[2,4\] -> Atlantic Ocean
\[3,0\]: \[3,0\] -> Pacific Ocean
\[3,0\] -> \[4,0\] -> Atlantic Ocean
\[3,1\]: \[3,1\] -> \[3,0\] -> Pacific Ocean
\[3,1\] -> \[4,1\] -> Atlantic Ocean
\[4,0\]: \[4,0\] -> Pacific Ocean
\[4,0\] -> Atlantic Ocean
Note that there are other possible paths for these cells to flow to the Pacific and Atlantic oceans.
**Example 2:**
**Input:** heights = \[\[1\]\]
**Output:** \[\[0,0\]\]
**Explanation:** The water can flow from the only cell to the Pacific and Atlantic oceans.
**Constraints:**
* `m == heights.length`
* `n == heights[r].length`
* `1 <= m, n <= 200`
* `0 <= heights[r][c] <= 105` | null |
417: Time 96.50% and Space 94.12%, Solution with step by step explanation | pacific-atlantic-water-flow | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. Check if the input matrix is empty. If it is, return an empty list.\n\n2. Determine the number of rows and columns in the input matrix.\n\n3. Create two visited matrices of the same size as the input matrix, one for each ocean.\n\n4. Create two empty queues, one for each ocean.\n\n5. Add all the cells in the first and last rows to the Pacific queue, and mark them as visited in the Pacific visited matrix. Add all the cells in the first and last columns to the Atlantic queue, and mark them as visited in the Atlantic visited matrix.\n\n6. Define a helper function, bfs, that takes a queue and a visited matrix as input, and runs a breadth-first search on the input matrix starting from the cells in the queue. The function visits each cell that can flow to the ocean and adds it to the queue.\n\n7. Run bfs on both oceans, starting from the boundary cells.\n\n8. Find the cells that can flow to both oceans by iterating through all cells in the input matrix, and adding the ones that are marked as visited in both the Pacific and Atlantic visited matrices to the result list.\n\n9. Return the result list.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def pacificAtlantic(self, heights: List[List[int]]) -> List[List[int]]:\n if not heights:\n return []\n \n rows, cols = len(heights), len(heights[0])\n \n # Create visited matrices for both oceans\n pacific_visited = [[False] * cols for _ in range(rows)]\n atlantic_visited = [[False] * cols for _ in range(rows)]\n \n # Create queue for both oceans\n pacific_queue = deque()\n atlantic_queue = deque()\n \n # Add cells in the first and last rows to their respective queues\n for col in range(cols):\n pacific_queue.append((0, col))\n atlantic_queue.append((rows-1, col))\n pacific_visited[0][col] = True\n atlantic_visited[rows-1][col] = True\n \n # Add cells in the first and last columns to their respective queues\n for row in range(rows):\n pacific_queue.append((row, 0))\n atlantic_queue.append((row, cols-1))\n pacific_visited[row][0] = True\n atlantic_visited[row][cols-1] = True\n \n # Define helper function to check if a cell can flow to an ocean\n def bfs(queue, visited):\n while queue:\n row, col = queue.popleft()\n # Check adjacent cells\n for dr, dc in [(0, 1), (0, -1), (1, 0), (-1, 0)]:\n r, c = row + dr, col + dc\n # Check if cell is within bounds and hasn\'t been visited yet\n if 0 <= r < rows and 0 <= c < cols and not visited[r][c]:\n # Check if cell can flow to the ocean\n if heights[r][c] >= heights[row][col]:\n visited[r][c] = True\n queue.append((r, c))\n \n # Run BFS on both oceans starting from the boundary cells\n bfs(pacific_queue, pacific_visited)\n bfs(atlantic_queue, atlantic_visited)\n \n # Find the cells that can flow to both oceans\n result = []\n for row in range(rows):\n for col in range(cols):\n if pacific_visited[row][col] and atlantic_visited[row][col]:\n result.append([row, col])\n \n return result\n\n``` | 12 | There is an `m x n` rectangular island that borders both the **Pacific Ocean** and **Atlantic Ocean**. The **Pacific Ocean** touches the island's left and top edges, and the **Atlantic Ocean** touches the island's right and bottom edges.
The island is partitioned into a grid of square cells. You are given an `m x n` integer matrix `heights` where `heights[r][c]` represents the **height above sea level** of the cell at coordinate `(r, c)`.
The island receives a lot of rain, and the rain water can flow to neighboring cells directly north, south, east, and west if the neighboring cell's height is **less than or equal to** the current cell's height. Water can flow from any cell adjacent to an ocean into the ocean.
Return _a **2D list** of grid coordinates_ `result` _where_ `result[i] = [ri, ci]` _denotes that rain water can flow from cell_ `(ri, ci)` _to **both** the Pacific and Atlantic oceans_.
**Example 1:**
**Input:** heights = \[\[1,2,2,3,5\],\[3,2,3,4,4\],\[2,4,5,3,1\],\[6,7,1,4,5\],\[5,1,1,2,4\]\]
**Output:** \[\[0,4\],\[1,3\],\[1,4\],\[2,2\],\[3,0\],\[3,1\],\[4,0\]\]
**Explanation:** The following cells can flow to the Pacific and Atlantic oceans, as shown below:
\[0,4\]: \[0,4\] -> Pacific Ocean
\[0,4\] -> Atlantic Ocean
\[1,3\]: \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,3\] -> \[1,4\] -> Atlantic Ocean
\[1,4\]: \[1,4\] -> \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,4\] -> Atlantic Ocean
\[2,2\]: \[2,2\] -> \[1,2\] -> \[0,2\] -> Pacific Ocean
\[2,2\] -> \[2,3\] -> \[2,4\] -> Atlantic Ocean
\[3,0\]: \[3,0\] -> Pacific Ocean
\[3,0\] -> \[4,0\] -> Atlantic Ocean
\[3,1\]: \[3,1\] -> \[3,0\] -> Pacific Ocean
\[3,1\] -> \[4,1\] -> Atlantic Ocean
\[4,0\]: \[4,0\] -> Pacific Ocean
\[4,0\] -> Atlantic Ocean
Note that there are other possible paths for these cells to flow to the Pacific and Atlantic oceans.
**Example 2:**
**Input:** heights = \[\[1\]\]
**Output:** \[\[0,0\]\]
**Explanation:** The water can flow from the only cell to the Pacific and Atlantic oceans.
**Constraints:**
* `m == heights.length`
* `n == heights[r].length`
* `1 <= m, n <= 200`
* `0 <= heights[r][c] <= 105` | null |
Python Elegant & Short | DFS | 99.21% faster | pacific-atlantic-water-flow | 0 | 1 | \n\n```\nclass Solution:\n\t"""\n\tTime: O(n*m)\n\tMemory: O(n*m)\n\t"""\n\n\tMOVES = [(-1, 0), (0, -1), (1, 0), (0, 1)]\n\n\tdef pacificAtlantic(self, heights: List[List[int]]) -> Set[Tuple[int, int]]:\n\t\tdef dfs(i: int, j: int, visited: set):\n\t\t\tvisited.add((i, j))\n\t\t\tfor di, dj in self.MOVES:\n\t\t\t\tx, y = i + di, j + dj\n\t\t\t\tif 0 <= x < n and 0 <= y < m and (x, y) not in visited and heights[i][j] <= heights[x][y]:\n\t\t\t\t\tdfs(x, y, visited)\n\n\t\tn, m = len(heights), len(heights[0])\n\n\t\tatl_visited = set()\n\t\tpas_visited = set()\n\n\t\tfor i in range(n):\n\t\t\tdfs(i, 0, pas_visited)\n\t\t\tdfs(i, m - 1, atl_visited)\n\n\t\tfor j in range(m):\n\t\t\tdfs( 0, j, pas_visited)\n\t\t\tdfs(n - 1, j, atl_visited)\n\n\t\treturn atl_visited & pas_visited\n```\n\nIf you like this solution remember to **upvote it** to let me know. | 14 | There is an `m x n` rectangular island that borders both the **Pacific Ocean** and **Atlantic Ocean**. The **Pacific Ocean** touches the island's left and top edges, and the **Atlantic Ocean** touches the island's right and bottom edges.
The island is partitioned into a grid of square cells. You are given an `m x n` integer matrix `heights` where `heights[r][c]` represents the **height above sea level** of the cell at coordinate `(r, c)`.
The island receives a lot of rain, and the rain water can flow to neighboring cells directly north, south, east, and west if the neighboring cell's height is **less than or equal to** the current cell's height. Water can flow from any cell adjacent to an ocean into the ocean.
Return _a **2D list** of grid coordinates_ `result` _where_ `result[i] = [ri, ci]` _denotes that rain water can flow from cell_ `(ri, ci)` _to **both** the Pacific and Atlantic oceans_.
**Example 1:**
**Input:** heights = \[\[1,2,2,3,5\],\[3,2,3,4,4\],\[2,4,5,3,1\],\[6,7,1,4,5\],\[5,1,1,2,4\]\]
**Output:** \[\[0,4\],\[1,3\],\[1,4\],\[2,2\],\[3,0\],\[3,1\],\[4,0\]\]
**Explanation:** The following cells can flow to the Pacific and Atlantic oceans, as shown below:
\[0,4\]: \[0,4\] -> Pacific Ocean
\[0,4\] -> Atlantic Ocean
\[1,3\]: \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,3\] -> \[1,4\] -> Atlantic Ocean
\[1,4\]: \[1,4\] -> \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,4\] -> Atlantic Ocean
\[2,2\]: \[2,2\] -> \[1,2\] -> \[0,2\] -> Pacific Ocean
\[2,2\] -> \[2,3\] -> \[2,4\] -> Atlantic Ocean
\[3,0\]: \[3,0\] -> Pacific Ocean
\[3,0\] -> \[4,0\] -> Atlantic Ocean
\[3,1\]: \[3,1\] -> \[3,0\] -> Pacific Ocean
\[3,1\] -> \[4,1\] -> Atlantic Ocean
\[4,0\]: \[4,0\] -> Pacific Ocean
\[4,0\] -> Atlantic Ocean
Note that there are other possible paths for these cells to flow to the Pacific and Atlantic oceans.
**Example 2:**
**Input:** heights = \[\[1\]\]
**Output:** \[\[0,0\]\]
**Explanation:** The water can flow from the only cell to the Pacific and Atlantic oceans.
**Constraints:**
* `m == heights.length`
* `n == heights[r].length`
* `1 <= m, n <= 200`
* `0 <= heights[r][c] <= 105` | null |
95% Faster, Python | pacific-atlantic-water-flow | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def pacificAtlantic(self, heights: List[List[int]]) -> List[List[int]]:\n #dfs video , watch neetcode, consider moving from pacific to inside same for atlantic\n row, cols = len(heights), len(heights[0])\n pacific_set, atlantic_set = set(), set()\n\n def dfs(i, j, visited_set, previous_height):\n if i<0 or i>row-1 or j<0 or j>cols-1 or (i, j) in visited_set or previous_height > heights[i][j]:\n return\n \n visited_set.add((i, j))\n dfs(i + 1, j, visited_set, heights[i][j])\n dfs(i - 1, j, visited_set, heights[i][j])\n dfs(i, j + 1, visited_set, heights[i][j])\n dfs(i, j - 1, visited_set, heights[i][j])\n return\n\n # moving from pacific row and atlantic row to inside the grid to find co-ordinate that can flow to pacific\n for j in range(cols):\n dfs(0, j, pacific_set, heights[0][j])\n dfs(row - 1, j, atlantic_set, heights[row - 1][j])\n \n # moving from pacific col and atlantic col to inside the grid to find co-ordinate that can flow to pacific\n for i in range(row):\n dfs(i, 0, pacific_set, heights[i][0])\n dfs(i, cols - 1, atlantic_set, heights[i][cols-1])\n \n return list(pacific_set & atlantic_set) # returning the intersection grid which flos both in pacific and atlantic ocean.\n\n\n``` | 1 | There is an `m x n` rectangular island that borders both the **Pacific Ocean** and **Atlantic Ocean**. The **Pacific Ocean** touches the island's left and top edges, and the **Atlantic Ocean** touches the island's right and bottom edges.
The island is partitioned into a grid of square cells. You are given an `m x n` integer matrix `heights` where `heights[r][c]` represents the **height above sea level** of the cell at coordinate `(r, c)`.
The island receives a lot of rain, and the rain water can flow to neighboring cells directly north, south, east, and west if the neighboring cell's height is **less than or equal to** the current cell's height. Water can flow from any cell adjacent to an ocean into the ocean.
Return _a **2D list** of grid coordinates_ `result` _where_ `result[i] = [ri, ci]` _denotes that rain water can flow from cell_ `(ri, ci)` _to **both** the Pacific and Atlantic oceans_.
**Example 1:**
**Input:** heights = \[\[1,2,2,3,5\],\[3,2,3,4,4\],\[2,4,5,3,1\],\[6,7,1,4,5\],\[5,1,1,2,4\]\]
**Output:** \[\[0,4\],\[1,3\],\[1,4\],\[2,2\],\[3,0\],\[3,1\],\[4,0\]\]
**Explanation:** The following cells can flow to the Pacific and Atlantic oceans, as shown below:
\[0,4\]: \[0,4\] -> Pacific Ocean
\[0,4\] -> Atlantic Ocean
\[1,3\]: \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,3\] -> \[1,4\] -> Atlantic Ocean
\[1,4\]: \[1,4\] -> \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,4\] -> Atlantic Ocean
\[2,2\]: \[2,2\] -> \[1,2\] -> \[0,2\] -> Pacific Ocean
\[2,2\] -> \[2,3\] -> \[2,4\] -> Atlantic Ocean
\[3,0\]: \[3,0\] -> Pacific Ocean
\[3,0\] -> \[4,0\] -> Atlantic Ocean
\[3,1\]: \[3,1\] -> \[3,0\] -> Pacific Ocean
\[3,1\] -> \[4,1\] -> Atlantic Ocean
\[4,0\]: \[4,0\] -> Pacific Ocean
\[4,0\] -> Atlantic Ocean
Note that there are other possible paths for these cells to flow to the Pacific and Atlantic oceans.
**Example 2:**
**Input:** heights = \[\[1\]\]
**Output:** \[\[0,0\]\]
**Explanation:** The water can flow from the only cell to the Pacific and Atlantic oceans.
**Constraints:**
* `m == heights.length`
* `n == heights[r].length`
* `1 <= m, n <= 200`
* `0 <= heights[r][c] <= 105` | null |
Easy understand DFS solution beat 97% | pacific-atlantic-water-flow | 0 | 1 | 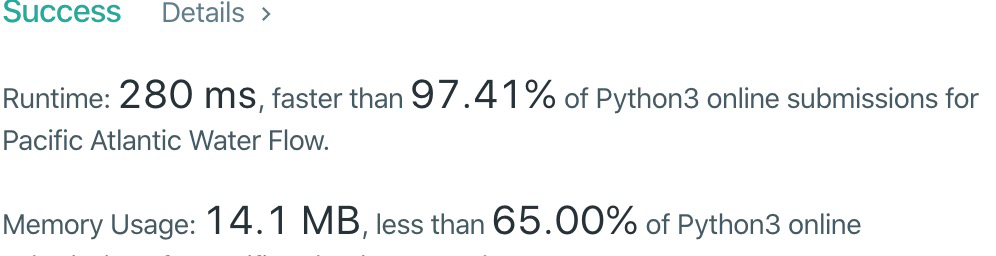\n\n```Python\ndef pacificAtlantic(self, matrix: List[List[int]]) -> List[List[int]]:\n if not matrix or not matrix[0]:return []\n m, n = len(matrix),len(matrix[0])\n p_visited = set()\n a_visited = set()\n directions = [(-1, 0), (1, 0), (0, 1), (0, -1)]\n def dfs(visited, x,y):\n visited.add((x,y))\n for dx, dy in directions:\n new_x, new_y = x+dx, y+dy\n if 0<=new_x<m and 0<=new_y<n and (new_x,new_y) not in visited and matrix[new_x][new_y]>=matrix[x][y]:\n dfs(visited, new_x,new_y)\n #iterate from left border and right border\n for i in range(m):\n dfs(p_visited,i,0)\n dfs(a_visited,i,n-1)\n #iterate from up border and bottom border\n for j in range(n):\n dfs(p_visited,0,j)\n dfs(a_visited,m-1,j)\n #The intersections of two sets are coordinates where water can flow to both P and A\n return list(p_visited.intersection(a_visited))\n``` | 31 | There is an `m x n` rectangular island that borders both the **Pacific Ocean** and **Atlantic Ocean**. The **Pacific Ocean** touches the island's left and top edges, and the **Atlantic Ocean** touches the island's right and bottom edges.
The island is partitioned into a grid of square cells. You are given an `m x n` integer matrix `heights` where `heights[r][c]` represents the **height above sea level** of the cell at coordinate `(r, c)`.
The island receives a lot of rain, and the rain water can flow to neighboring cells directly north, south, east, and west if the neighboring cell's height is **less than or equal to** the current cell's height. Water can flow from any cell adjacent to an ocean into the ocean.
Return _a **2D list** of grid coordinates_ `result` _where_ `result[i] = [ri, ci]` _denotes that rain water can flow from cell_ `(ri, ci)` _to **both** the Pacific and Atlantic oceans_.
**Example 1:**
**Input:** heights = \[\[1,2,2,3,5\],\[3,2,3,4,4\],\[2,4,5,3,1\],\[6,7,1,4,5\],\[5,1,1,2,4\]\]
**Output:** \[\[0,4\],\[1,3\],\[1,4\],\[2,2\],\[3,0\],\[3,1\],\[4,0\]\]
**Explanation:** The following cells can flow to the Pacific and Atlantic oceans, as shown below:
\[0,4\]: \[0,4\] -> Pacific Ocean
\[0,4\] -> Atlantic Ocean
\[1,3\]: \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,3\] -> \[1,4\] -> Atlantic Ocean
\[1,4\]: \[1,4\] -> \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,4\] -> Atlantic Ocean
\[2,2\]: \[2,2\] -> \[1,2\] -> \[0,2\] -> Pacific Ocean
\[2,2\] -> \[2,3\] -> \[2,4\] -> Atlantic Ocean
\[3,0\]: \[3,0\] -> Pacific Ocean
\[3,0\] -> \[4,0\] -> Atlantic Ocean
\[3,1\]: \[3,1\] -> \[3,0\] -> Pacific Ocean
\[3,1\] -> \[4,1\] -> Atlantic Ocean
\[4,0\]: \[4,0\] -> Pacific Ocean
\[4,0\] -> Atlantic Ocean
Note that there are other possible paths for these cells to flow to the Pacific and Atlantic oceans.
**Example 2:**
**Input:** heights = \[\[1\]\]
**Output:** \[\[0,0\]\]
**Explanation:** The water can flow from the only cell to the Pacific and Atlantic oceans.
**Constraints:**
* `m == heights.length`
* `n == heights[r].length`
* `1 <= m, n <= 200`
* `0 <= heights[r][c] <= 105` | null |
Python DFS and BFS Solution | pacific-atlantic-water-flow | 0 | 1 | ```\n\nDFS\n-------------------------------\nclass Solution:\n def pacificAtlantic(self, heights: List[List[int]]) -> List[List[int]]:\n rowlen = len(heights)\n collen = len(heights[0])\n pacific = set({})\n atlantic = set({})\n \n def dfs(row, col, s):\n x = heights[row][col]\n heights[row][col] = \'#\'\n # up\n if row-1 >= 0 and row-1 < rowlen and col >= 0 and col < collen and heights[row-1][col] != \'#\':\n if heights[row-1][col] >= x:\n if (row-1, col) not in s:\n s.add((row-1,col))\n dfs(row-1, col, s)\n # right\n if row >= 0 and row < rowlen and col+1 >= 0 and col+1 < collen and heights[row][col+1] != \'#\':\n if heights[row][col+1] >= x:\n if (row, col+1) not in s:\n s.add((row,col+1))\n dfs(row, col+1, s)\n # down\n if row+1 >= 0 and row+1 < rowlen and col >= 0 and col < collen and heights[row+1][col] != \'#\':\n if heights[row+1][col] >= x:\n if (row+1, col) not in s:\n s.add((row+1,col))\n dfs(row+1, col, s)\n # left\n if row >= 0 and row < rowlen and col-1 >= 0 and col-1 < collen and heights[row][col-1] != \'#\':\n if heights[row][col-1] >= x:\n if (row, col-1) not in s:\n s.add((row,col-1))\n dfs(row, col-1, s)\n \n heights[row][col] = x\n \n # pacific\n for i in range(0,collen):\n if (0,i) not in pacific:\n \n pacific.add((0,i))\n dfs(0,i,pacific)\n for i in range(0,rowlen):\n if (i,0) not in pacific:\n \n pacific.add((i,0))\n dfs(i,0,pacific)\n \n \n # atlantic\n for i in range(0,collen):\n if (rowlen-1, i) not in atlantic:\n \n atlantic.add((rowlen-1, i))\n dfs(rowlen-1, i, atlantic)\n for i in range(0,rowlen):\n if (i,collen-1) not in atlantic:\n \n atlantic.add((i,collen-1))\n dfs(i,collen-1, atlantic)\n \n return list(pacific.intersection(atlantic))\n\nBFS\n--------------------------------------------\n\nfrom collections import deque\nclass Solution:\n \n def pacificAtlantic(self, heights: List[List[int]]) -> List[List[int]]:\n \'\'\'\n we are suppose to find all the cells from which water can flow to both pacific and \n atlantic ocean, instead of doing exhaustive dfs search from all the cells and \n checking if water flows in both oceans, we can start reverse and perform bfs \n from cells that touch atlantic and pecific oceans and maintain set for both oceans \n cells, intersecting cells are the ones from whoch water\n will reach both the oceans\n \n \'\'\'\n rowlen = len(heights)\n collen = len(heights[0])\n pacific = set({})\n atlantic = set({})\n \n def bfs(queue, s):\n while queue:\n ce = queue.popleft()\n if ce == \'N\':\n if len(queue) == 0:\n pass\n else:\n queue.append(\'N\')\n else:\n # check if all the elements fall in the category\n x = [(-1,0),(0,1),(1,0),(0,-1)]\n for i in x:\n nr = ce[0] + i[0]\n nc = ce[1] + i[1]\n if nr >= 0 and nr < rowlen and nc >= 0 and nc < collen and (nr,nc) not in s and heights[nr][nc] >= heights[ce[0]][ce[1]]:\n s.add((nr,nc))\n queue.append((nr,nc)) \n return s\n \n \n q1 = deque() \n \n # pacific\n for i in range(0,collen):\n if (0,i) not in pacific:\n \n pacific.add((0,i))\n q1.append((0,i))\n \n for i in range(0,rowlen):\n if (i,0) not in pacific:\n pacific.add((i,0))\n q1.append((i,0))\n x = bfs(q1, pacific)\n print(x)\n \n \n \n # atlantic\n q2 = deque()\n for i in range(0,collen):\n if (rowlen-1, i) not in atlantic:\n \n atlantic.add((rowlen-1, i))\n q2.append((rowlen-1, i))\n \n for i in range(0,rowlen):\n if (i,collen-1) not in atlantic:\n \n atlantic.add((i,collen-1))\n q2.append((i,collen-1))\n y = bfs(q2, atlantic)\n print(y)\n return list(x.intersection(y))\n \n \n \n | 1 | There is an `m x n` rectangular island that borders both the **Pacific Ocean** and **Atlantic Ocean**. The **Pacific Ocean** touches the island's left and top edges, and the **Atlantic Ocean** touches the island's right and bottom edges.
The island is partitioned into a grid of square cells. You are given an `m x n` integer matrix `heights` where `heights[r][c]` represents the **height above sea level** of the cell at coordinate `(r, c)`.
The island receives a lot of rain, and the rain water can flow to neighboring cells directly north, south, east, and west if the neighboring cell's height is **less than or equal to** the current cell's height. Water can flow from any cell adjacent to an ocean into the ocean.
Return _a **2D list** of grid coordinates_ `result` _where_ `result[i] = [ri, ci]` _denotes that rain water can flow from cell_ `(ri, ci)` _to **both** the Pacific and Atlantic oceans_.
**Example 1:**
**Input:** heights = \[\[1,2,2,3,5\],\[3,2,3,4,4\],\[2,4,5,3,1\],\[6,7,1,4,5\],\[5,1,1,2,4\]\]
**Output:** \[\[0,4\],\[1,3\],\[1,4\],\[2,2\],\[3,0\],\[3,1\],\[4,0\]\]
**Explanation:** The following cells can flow to the Pacific and Atlantic oceans, as shown below:
\[0,4\]: \[0,4\] -> Pacific Ocean
\[0,4\] -> Atlantic Ocean
\[1,3\]: \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,3\] -> \[1,4\] -> Atlantic Ocean
\[1,4\]: \[1,4\] -> \[1,3\] -> \[0,3\] -> Pacific Ocean
\[1,4\] -> Atlantic Ocean
\[2,2\]: \[2,2\] -> \[1,2\] -> \[0,2\] -> Pacific Ocean
\[2,2\] -> \[2,3\] -> \[2,4\] -> Atlantic Ocean
\[3,0\]: \[3,0\] -> Pacific Ocean
\[3,0\] -> \[4,0\] -> Atlantic Ocean
\[3,1\]: \[3,1\] -> \[3,0\] -> Pacific Ocean
\[3,1\] -> \[4,1\] -> Atlantic Ocean
\[4,0\]: \[4,0\] -> Pacific Ocean
\[4,0\] -> Atlantic Ocean
Note that there are other possible paths for these cells to flow to the Pacific and Atlantic oceans.
**Example 2:**
**Input:** heights = \[\[1\]\]
**Output:** \[\[0,0\]\]
**Explanation:** The water can flow from the only cell to the Pacific and Atlantic oceans.
**Constraints:**
* `m == heights.length`
* `n == heights[r].length`
* `1 <= m, n <= 200`
* `0 <= heights[r][c] <= 105` | null |
Python | O(NM) one-liner Solution without modifying board | battleships-in-a-board | 0 | 1 | - Full solution:\n```python\nclass Solution:\n def countBattleships(self, board: List[List[str]]) -> int:\n count = 0\n \n for i, row in enumerate(board):\n for j, cell in enumerate(row):\n if cell == "X":\n if (i == 0 or board[i - 1][j] == ".") and\\\n (j == 0 or board[i][j - 1] == "."):\n count += 1\n \n return count\n```\n\n- One-liner version:\n```python\nclass Solution:\n def countBattleships(self, board: List[List[str]]) -> int:\n return sum(1 for i, row in enumerate(board) for j, cell in enumerate(row) if cell == "X" and (i == 0 or board[i - 1][j] == ".") and (j == 0 or board[i][j - 1] == "."))\n```\n\n**Time compexity:** `O(n * m)`\n**Space complexity:**`O(1)` | 17 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
Simple python solution Beats 99% | battleships-in-a-board | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem is asking us to count the number of battleships on the board. A battleship is represented by a \'X\' on the board, and it can be either a single \'X\' or a group of \'X\' that are horizontally or vertically adjacent to each other.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe can use a nested for loop to iterate through the entire board and check for \'X\' characters. If we find an \'X\', we will check its surrounding cells to see if it is a new battleship or a part of a previously counted battleship. We can do this by checking if the cell to the left or above the current cell is a \'.\' character. If it is, then it means that the current \'X\' is a new battleship and we increment our count.\n# Complexity\n- Time complexity: O(m*n) \n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countBattleships(self, board: List[List[str]]) -> int:\n count = 0\n for i in range(len(board)):\n for j in range(len(board[0])):\n if board[i][j] == \'X\':\n if i == 0 and j == 0:\n count += 1\n elif i == 0 and j > 0:\n if board[i][j-1] == \'.\':\n count += 1\n elif i > 0 and j == 0:\n if board[i-1][j] == \'.\':\n count += 1\n else:\n if board[i][j-1] == \'.\' and board[i-1][j] == \'.\':\n count += 1\n return count\n``` | 2 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
419: Solution with step by step explanation | battleships-in-a-board | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. Define a function called countBattleships that takes a 2D board as input and returns an integer.\n\n2. Initialize a variable called count to 0, which will hold the number of battleships found.\n\n3. Loop over the rows and columns of the board using two nested for loops. For each cell (i, j), check if the following conditions are true:\na. board[i][j] is an "X" (i.e., part of a battleship).\nb. The cell to the left or above (i-1, j) or (i, j-1) does not contain an "X" (i.e., it\'s the beginning of a new battleship).\nc. The cell to the right or below (i+1, j) or (i, j+1) does not contain an "X" (i.e., the battleship has ended).\n\n4. If the above conditions are true, increment count by 1.\n\n5. Return count.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countBattleships(self, board: List[List[str]]) -> int:\n count = 0\n # Loop over rows and columns\n for i in range(len(board)):\n for j in range(len(board[0])):\n # Check if current cell is part of a battleship\n if board[i][j] == "X":\n # Check if it\'s the beginning of a new battleship\n if (i == 0 or board[i-1][j] == ".") and (j == 0 or board[i][j-1] == "."):\n count += 1\n \n return count\n\n``` | 8 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
Simple Easy to Understand Python O(1) extra memory | battleships-in-a-board | 0 | 1 | Loop through each element in the 2D array and if the element is \'X\', check up and to the left for \'X\'. If this exists then you do not add one because it is a part of another battleship you already counted.\n```\nclass Solution:\n def countBattleships(self, board: List[List[str]]) -> int:\n count = 0\n for r in range(len(board)):\n for c in range(len(board[0])):\n if board[r][c] == \'X\':\n var = 1\n if (r > 0 and board[r-1][c] == \'X\') or (c > 0 and board[r][c-1] == \'X\'):\n var = 0\n count += var\n return count | 9 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
Easy Python Follow Up Solution with O(1) Extra Memory | battleships-in-a-board | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nSince we only need the total number of ships, we can only focus on counting the heads of each ship. Now how do we determine if a position X is head of the ship or part of the ship. Lets say the board is like this\n . . . . X . \n . . . . X .\n . . . . X .\n . X X . . . \n\nNow if start from the top left and scan from left to right row by row, if we encounter a X we check if there is a X to the left of it or to the top of it, if there isn\'t it means its the start of the ship and we can add it to the count. \nWhen we reach the X on second row it will see a X on top and thus will not be added in the answer. Same of the boat on the last row, the head will get added since there is not X to the left or right indicating it\'s the first part of the boat and the second part won\'t be added since it has a X to the left.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nAt each index in the grid we need to make two checks we can do this without extra variables, the code gets more readable therefore I added the left and right variable\n# Complexity\n- Time complexity: O(m*n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countBattleships(self, grid: List[List[str]]) -> int:\n ans = 0\n rows = len(grid)\n cols = len(grid[0])\n\n for row in range(rows):\n for col in range(cols):\n left = grid[row][col-1] if col - 1 >= 0 else \'.\' \n top = grid[row-1][col] if row - 1 >= 0 else \'.\' \n if(grid[row][col] == "X" and left != \'X\' and top != \'X\'):\n ans +=1\n \n return ans\n\n \n\n\n\n``` | 4 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
Python3 O(N ^ 2) solution with DFS (94.55% Runtime) | battleships-in-a-board | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n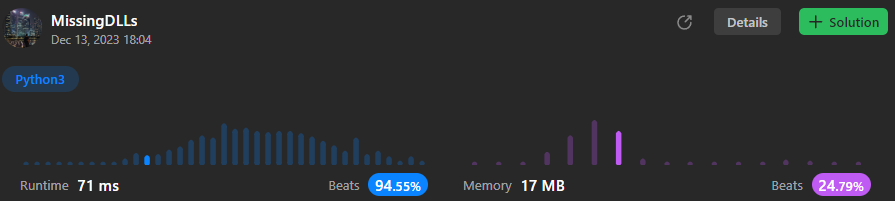\nUtilize depth first search to sort out cells belong to the same boat\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n- Define a depth first search traversal logic:\n - If given cell is out of bounds, return\n - If given cell\'s value is not "X" (not belong to a ship), return\n - Otherwise, change current value from "X" to "." (any) and continue traversal\n- Iterate through the given matrix while:\n - If you encounter a mark "X", increment your ship count:\n - Perfrom DFS to deal with cells belong to the same boat to avoid duplicated count\n- Return your final ship counts\n\n# Complexity\n- Time complexity: O(N ^ 2)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(N ^ 2)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\nIf this solution is similar to yours or helpful, upvote me if you don\'t mind\n```\nclass Solution:\n def countBattleships(self, board: List[List[str]]) -> int:\n self.m = len(board)\n self.n = len(board[0])\n\n def dfs(y: int, x: int):\n if y < 0 or x < 0 or y >= self.m or x >= self.n or board[y][x] != "X":\n return\n\n board[y][x] = "."\n dfs(y+1, x)\n dfs(y-1, x)\n dfs(y, x+1)\n dfs(y, x-1)\n\n c = 0\n for i in range(self.m):\n for j in range(self.n):\n if board[i][j] == "X":\n c += 1\n dfs(i, j)\n\n return c\n``` | 0 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
Simple straight solution, beats 43%, 45% | battleships-in-a-board | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countBattleships(self, board: List[List[str]]) -> int:\n visited = set()\n m, n = len(board), len(board[0])\n output = 0\n for i in range(m):\n for j in range(n):\n if board[i][j] == \'X\' and (i, j) not in visited:\n output += 1\n r, c = i, j\n while r < m-1 and board[r+1][c] == \'X\':\n visited.add((r+1, c))\n r += 1\n r, c = i, j\n while c < n-1 and board[r][c+1] == \'X\':\n visited.add((r, c+1))\n c += 1\n\n return output\n``` | 0 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
One-liner python solution. O(m*n) time and O(1) extra space. | battleships-in-a-board | 0 | 1 | # Intuition\nCount the top left hand corner of every battleship\n\n# Approach\nLoop through each cell on the board and only count an \'X\' cell if it does not have another \'X\' to it\'s direct top or left\n\n# Complexity\n- Time complexity:\nO(m*n)\n\n- Space complexity:\nO(1) extra space\n\n# Code\n```\nclass Solution:\n def countBattleships(self, board: List[List[str]]) -> int:\n return sum(board[i][j] == "X" and (i == 0 or board[i-1][j] == ".") and (j == 0 or board[i][j-1] == ".") for i in range(len(board)) for j in range(len(board[0])))\n``` | 0 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
DFS approach with O(MN) space complexity | battleships-in-a-board | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nImplemented a one-direction DFS helper to traverse either right or cells below and mark them as visited. We don\'t need to care about cells on the left and above since they\'ll be visited by DFS or for loops already.\n\n# Complexity\n- Time complexity: O(MN(M+N)) -> faster than 86%\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(MN)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countBattleships(self, board: list[list[str]]) -> int:\n # One-direction DFS approach: O(mn(m+n)) -> O(m^2n)time and O(mn) space complexity\n count = 0\n m = len(board)\n n = len(board[0])\n\n visited = [[0 for i in range(n)] for j in range(m)]\n\n def dfs(i: int, j: int, direction: str) -> None:\n # check if index out of bound\n if j >= n or i >= m: return\n \n # if not mark as visited continue DFS\n visited[i][j] = 1\n if board[i][j] == "X":\n if direction == "right": dfs(i, j + 1, direction="right")\n if direction == "below": dfs(i + 1, j, direction="below")\n else:\n return\n\n\n for i, row in enumerate(board):\n for j, cell in enumerate(row):\n # if it\'s been visited, or it\'s empty then continue\n if visited[i][j] or cell == ".":\n continue\n else:\n # if not then DFS the cells on the RHS and below\n visited[i][j] = 1\n count += 1\n dfs(i, j + 1, direction="right")\n dfs(i + 1, j, direction="below")\n\n return count\n``` | 0 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
Time: O(N), Space: O(1) | battleships-in-a-board | 0 | 1 | # Intuition\nIf any cell previous to current cell by 1 row or 1 column is X then we already have counted the ship on current cell.\n\n# Complexity\n- Time complexity: O(N)\n\n- Space complexity: O(1)\n\n# Code\n```\nclass Solution:\n def countBattleships(self, board: List[List[str]]) -> int:\n\n M, N = len(board), len(board[0])\n \n res = 0\n for i in range(M):\n for j in range(N):\n if board[i][j] == \'X\':\n if (i > 0 and board[i-1][j]) == \'X\' or (j > 0 and board[i][j-1] == \'X\'):\n continue\n else:\n res += 1\n\n return res\n``` | 0 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
Simple Intuative DFS | battleships-in-a-board | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def countBattleships(self, board: List[List[str]]) -> int:\n ans=0\n visited=set()\n def recursive_dfs(row,col,board,visited):\n pos=(row,col)\n if pos in visited:\n return\n if row<0 or row>=len(board) or col<0 or col>=len(board[row]):\n return\n if board[row][col]==".":\n return\n visited.add(pos)\n recursive_dfs(row+1,col,board,visited)\n recursive_dfs(row-1,col,board,visited)\n recursive_dfs(row,col+1,board,visited)\n recursive_dfs(row,col-1,board,visited)\n\n for i in range(0,len(board)):\n for j in range(0,len(board[i])):\n if board[i][j]=="X" and (i,j) not in visited:\n recursive_dfs(i,j,board,visited)\n ans+=1 \n return ans \n\n \n``` | 0 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
Follow up solution: Python3 | battleships-in-a-board | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n The problem is similar to number of islands. As we see "X", we \n increase the count of battleships and mark all the adjacents \n horizontal or vertical "X" as "."\n# Approach\n<!-- Describe your approach to solving the problem. -->\n For the follow up: We traverse left to right and then move a row\n down. The problem constarints means that for any cell with "X",\n either the cell just above or just before will have "X". Using\n this constraint and our traversing route, we process each\n cell.\n If we see "X", we increment if its a new battelship. To check if\n its a new battleship, we check the top cell and before cell. If\n any one of them has "X", that means we have already counted this\n battleship.\n \n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n O(N), traversing the matrix just once\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n O(1)\n# Code\n```\nclass Solution:\n def countBattleships(self, board: List[List[str]]) -> int:\n\n\n ct = 0\n for i in range(len(board)):\n for j in range(len(board[0])):\n if board[i][j] == "X":\n if j - 1 < 0 and i - 1 < 0:\n ct += 1\n elif j - 1 >= 0 and i - 1 < 0:\n if board[i][j-1] != "X":\n ct+=1\n elif j - 1 < 0:\n if board[i-1][j] != "X":\n ct+=1\n else:\n if board[i][j-1] != "X" and board[i-1][j]!= "X":\n ct+=1\n\n return ct\n``` | 0 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
Simple Python: Count top-left corners | O(m.n) TC + O(1) SC | battleships-in-a-board | 0 | 1 | # Intuition / Approach\nCount only the top-left corners of the battleships. A top-left corner of battleship is the cell which doesn\'t have any immediate adjacent \'X\' to its top or left.\n\n# Complexity\n- Time complexity:\n$$O(m.n)$$\n\n- Space complexity:\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def countBattleships(self, board: List[List[str]]) -> int:\n m, n = len(board), len(board[0])\n ans = 0\n for i in range(m):\n for j in range(n):\n if board[i][j] == \'.\':\n continue\n if i > 0 and board[i-1][j] == \'X\':\n continue\n if j > 0 and board[i][j-1] == \'X\':\n continue\n ans += 1\n return ans\n``` | 0 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
Easy python solution using BFS | battleships-in-a-board | 0 | 1 | # Intuition\nUse BFS to find the connected components from each ship\n\n# Approach\ninitialize `component`, `ships`, `visited` to store the number of ships, all possible ships and visited nodes respectively. for each ship apply `BFS` to find the connected component and increment the `component`. finally return the `component`.\n\nto find the connections i have defined the `neighbors`.\n\n# Complexity\n- Time complexity:\nO(mn)\n\n- Space complexity:\nO(mn)\n\n# Code\n```py\nclass Solution:\n def neighbors(self, board, node) : \n directions = [(1, 0), (-1, 0), (0, 1), (0, -1)]\n output = []\n for dx, dy in directions : \n new_x, new_y = node[0] + dx, node[1] + dy\n if 0 <= new_x < len(board) and 0 <= new_y < len(board[0]) and board[new_x][new_y] == \'X\' : \n output.append((new_x, new_y))\n\n return output \n\n def countBattleships(self, board: List[List[str]]) -> int:\n component, ships, visited = 0, [], {}\n for i in range(len(board)) :\n for j in range(len(board[0])) :\n if board[i][j] == \'X\' : \n ships.append((i, j))\n visited[(i, j)] = False \n\n for ship in ships : \n q = deque()\n if not visited[ship] :\n q.append(ship)\n component, visited[ship] = component + 1, True \n\n while q :\n node = q.popleft()\n\n neighbors = self.neighbors(board, node)\n for nbr in neighbors : \n if not visited[nbr] :\n q.append(nbr)\n visited[nbr] = True \n\n return component \n``` | 0 | Given an `m x n` matrix `board` where each cell is a battleship `'X'` or empty `'.'`, return _the number of the **battleships** on_ `board`.
**Battleships** can only be placed horizontally or vertically on `board`. In other words, they can only be made of the shape `1 x k` (`1` row, `k` columns) or `k x 1` (`k` rows, `1` column), where `k` can be of any size. At least one horizontal or vertical cell separates between two battleships (i.e., there are no adjacent battleships).
**Example 1:**
**Input:** board = \[\[ "X ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\],\[ ". ", ". ", ". ", "X "\]\]
**Output:** 2
**Example 2:**
**Input:** board = \[\[ ". "\]\]
**Output:** 0
**Constraints:**
* `m == board.length`
* `n == board[i].length`
* `1 <= m, n <= 200`
* `board[i][j]` is either `'.'` or `'X'`.
**Follow up:** Could you do it in one-pass, using only `O(1)` extra memory and without modifying the values `board`? | null |
✅ [video walkthrough] 🔥 beats 95.82% || short and intuitive solution | strong-password-checker | 0 | 1 | Please upvote if you find the walkthrough and/or code helpful :)\n\nhttps://youtu.be/9CxpKVR14ps\n\n```\nimport string\n\nclass Solution: \n def strongPasswordChecker(self, s):\n lowercase = set(string.ascii_lowercase)\n uppercase = set(string.ascii_uppercase)\n digits = set([str(elem) for elem in range(10)])\n\n num_deletions = max(0, len(s) - 20)\n\n has_lowercase = any([character in lowercase for character in s])\n has_uppercase = any([character in uppercase for character in s])\n has_digits = any([character in digits for character in s])\n num_missing_types = (not has_lowercase) + (not has_uppercase) + (not has_digits)\n\n substring_lengths = self.count_substring_lengths(s)\n self.break_substrings_with_deletions(substring_lengths, num_deletions)\n num_substring_breaks = sum([length // 3 for length in substring_lengths if length >= 3])\n\n num_insertions = max(0, 6 - len(s))\n \n return num_deletions + max(num_missing_types, num_substring_breaks, num_insertions) \n\n \n def count_substring_lengths(self, s):\n # "aaabbc" => [3, 2, 1]\n result = [1]\n last_seen_character = s[0]\n for idx in range(1, len(s)):\n if s[idx] == last_seen_character:\n result[-1] += 1\n else:\n result.append(1)\n last_seen_character = s[idx]\n return result\n \n def break_substrings_with_deletions(self, substring_lengths, num_deletions):\n while num_deletions > 0:\n best_tuple_to_delete = min(enumerate(substring_lengths), key = lambda pair: pair[1] % 3 if pair[1] >= 3 else float("inf"))\n best_idx_to_delete = best_tuple_to_delete[0]\n substring_lengths[best_idx_to_delete] -= 1\n num_deletions -= 1\n``` | 214 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
Dynamic Programming | strong-password-checker | 0 | 1 | # Intuition\n\nThe state is a tuple (position in old password, new password length, mask of present characters, repeating suffix). Key insight is that optimal insertions and replacements require at most two distinct characters from each category (lowercase, uppercase, digits): `MAGIC = "abAB01"`.\n\n# Approach\n\nWhen inserting or replacing we primarily care about the third condition -- there should be no three repeating characters in a row. To guarantee this it is sufficient to take a character that differs from both of its (new) neighbors. This already justifies the usage of `MAGIC = "abcABC012"`, since among three characters at most two are banned, meaning that at least one isn\'t. This value of `MAGIC` passes the current test suite but is rather on the edge.\n\nTo see why `MAGIC = "abAB01"` also works, notice that the only reason to insert/replace a character at a specific position is to break an existing repeating group. In such a group both of our neighbors are equal, meaning that at least one of the two charaters isn\'t banned.\n\nYou can further speed up the solution by selecting one character that is different from both neighbors via caseworking but it\'s rather troublesome and the whole point of dp is to avoid excessive casework.\n\n# Complexity\n\n- Time complexity: $$O(n \\cdot m \\cdot k)$$ where $$n \\le 50$$ is old password length, $$m = 20$$ is maximum length of a new password and $$k = 26 + 26 + 10$$ is alphabet size. There are $$O(1)$$ transitions per state due to the key observation.\n\n- Space complexity: $$O(n \\cdot m \\cdot k)$$ dp states.\n\n# Code\n\n```py\nMAGIC = "abAB01"\n\n\ndef new_mask(mask: int, c: str) -> int:\n if c in string.ascii_lowercase:\n return mask | 1\n if c in string.ascii_uppercase:\n return mask | 2\n return mask | 4\n\n\ndef new_repeat(repeat: str, c: str) -> str:\n if not repeat or repeat[-1] != c:\n return c\n return repeat + c\n\n\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n mx = 70\n\n @functools.cache\n def dp(position: int, length: int, mask: int, repeat: str) -> int:\n if length > 20 or len(repeat) >= 3:\n return mx\n\n if position == len(password):\n if 6 <= length and mask == 0b111:\n return 0\n return mx\n\n # no change\n ans = dp(position + 1, length + 1, new_mask(mask, password[position]), new_repeat(repeat, password[position]))\n\n # insert\n for c in MAGIC:\n ans = min(ans, 1 + dp(position, length + 1, new_mask(mask, c), new_repeat(repeat, c)))\n\n # delete\n ans = min(ans, 1 + dp(position + 1, length, mask, repeat))\n\n # replace\n for c in MAGIC:\n ans = min(ans, 1 + dp(position + 1, length + 1, new_mask(mask, c), new_repeat(repeat, c)))\n\n return ans\n\n return dp(0, 0, 0, \'\')\n``` | 6 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
Python 3 || 14 lines, w/ brief explanation || T/M: T/M: 91% / 91% | strong-password-checker | 0 | 1 | Here\'s the plan:\nWe divide the problem into three cases based on `n = len(password)`:\n- Case 1: `n < 6` : We add enough chars to ensure 1a) the length of the password is greater than 6 and 1b) each category of chars (uppercase, lowercase, digit) are present. \n- Case 2: `n > 20` : We eliminate `n - 20` chars starting with any triples (ex: \'aaa\'), which reduces this case to Case 3, which is...\n- Case 3: `6 <= n <= 20` : We fix any triples and any missing categories of chars \n\n```\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n\n pSet, n = set(password), len(password)\n\n catCt = 3- (bool(pSet & set(ascii_lowercase))+ \n bool(pSet & set(ascii_uppercase))+\n bool(pSet & set(\'0123456789\' )))\n\n if n < 6: return max(6 - n, catCt) # Case 1\n\n repCt = [len(list(g)) for _, g in groupby(password)]\n repCt = [r for r in repCt if r > 2]\n\n if n > 20: # Case 2: reduce to 6<= n <= 20\n # by eliminating triples\n repCt = [(r%3, r) for r in repCt]\n heapify(repCt)\n\n for i in range(n-20): \n\n if not repCt: break\n\n _, r = heappop(repCt)\n if r > 3: heappush(repCt, ((r-1)%3, r-1))\n\n repCt = [r for _,r in repCt]\n \n return max(catCt, sum(r//3 for r in repCt))+max(0,n-20) # Case3\n```\n[https://leetcode.com/problems/strong-password-checker/submissions/862809225/](http://)\n\nI could be wrong, but I think that time is *O*(*N*) and space is *O*(*N*). | 6 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
Python shortest solution | strong-password-checker | 0 | 1 | # Intuition and Approach\n<!-- Describe your first thoughts on how to solve this problem. -->\n\nThis solution provides a method to determine the minimum number of steps required to make a given password strong, according to the set of requirements described in the problem.\n\nThe solution first checks if the password contains at least one digit, one lowercase letter, and one uppercase letter. If any of these conditions are not met, it adds 1 to a counter variable "ans" for each missing condition.\n\nThen, the solution checks the length of the password. If it is less than 6 characters, the solution returns the maximum value between 0 and the difference between 6 and the length of the password plus the value of "ans".\n\nNext, the solution identifies any consecutive groups of three or more repeating characters in the password and stores the lengths of these groups in a list "g".\n\nIf the length of the password is greater than 20 characters, the solution reduces the length of the password by removing characters from the groups in "g" with the largest lengths, until the length of the password is 20 or less. It does this by sorting the groups by their lengths modulo 3 and iteratively removing one character from the group with the largest length until the length of the password is 20 or less.\n\nFinally, the solution returns the maximum value between "ans" and the sum of the integer division of each element in "g" by 3, which represents the number of steps required to remove consecutive groups of repeating characters. It also adds the maximum value between 0 and the difference between the length of the password and 20, which represents the number of steps required to make the password length no more than 20 characters.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\nO(n)\n\n- Space complexity:\nO(1)\n\nThe time complexity of this solution depends on the length of the password and the number of consecutive groups of repeating characters in the password.\n\nThe solution iterates over the length of the password three times to check if it contains at least one digit, one lowercase letter, and one uppercase letter, which takes O(n) time, where n is the length of the password.\n\nThe solution then identifies consecutive groups of repeating characters in the password and stores their lengths in a list "g". The time complexity of this step is also O(n), as it involves iterating over the length of the password.\n\nIf the length of the password is greater than 20, the solution reduces the length of the password by removing characters from the groups in "g" with the largest lengths until the length of the password is 20 or less. This step involves sorting "g" and iterating over its elements, which takes O(n log n) time in the worst case scenario.\n\nFinally, the solution returns the maximum value between "ans" and the sum of the integer division of each element in "g" by 3, which represents the number of steps required to remove consecutive groups of repeating characters. This step also takes O(n) time, as it involves iterating over the length of "g".\n\nTherefore, the overall time complexity of this solution is O(n log n) in the worst case scenario when the password is longer than 20 characters and contains multiple consecutive groups of repeating characters, and O(n) in other cases.\n\nThe space complexity of this solution is O(n), as it stores the lengths of the consecutive groups of repeating characters in the password in a list "g". The other variables used in the solution, such as "ans" and "i", require constant space.\n\n# Code\n```\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n ans = 0 if any([password[i].isdigit() for i in range(len(password))]) else 1\n ans += 0 if any([password[i].islower() for i in range(len(password))]) else 1\n ans += 0 if any([password[i].isupper() for i in range(len(password))]) else 1\n\n if len(password) < 6: return max(6 - len(password), ans) \n\n g = [len(list(g)) for _, g in groupby(password)]\n g = [r for r in g if r > 2]\n\n if len(password) > 20: \n g = [(r%3, r) for r in g]\n heapify(g)\n for i in range(len(password)-20): \n if not g: break\n _, r = heappop(g)\n if r > 3: heappush(g, ((r-1)%3, r-1))\n g = [r for _,r in g]\n \n return max(ans, sum(r//3 for r in g))+max(0,len(password)-20) \n``` | 2 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
A readable solution in Python | strong-password-checker | 0 | 1 | In principle, this code just checks one rule after the other ithout taking major shortcuts besides one: The `len(s) < 6` case is not treated rigorously as it has the special case that as long as rules (1) and (2) are satisfied by inserting and replacing things, rule (3) is guaranteed to be satisfied.\nThis solution emphasizes readability over microoptimizations, especially for the deletion-case which is just implemented in a straightforward iterative manner. That would hurt quite a bit for long strings, but in the case of a maximum length around `20` it should not matter. Feel free to use this code as a starting-point to speed things up :).\n\n```python\nimport itertools\n\nclass Solution:\n lowercase = set(\'abcdefghijklmnopqrstuvwxyz\')\n uppercase = set(\'ABCDEFGHIJKLMNOPQRSTUFVWXYZ\')\n digit = set(\'0123456789\')\n \n def strongPasswordChecker(self, s: str) -> int:\n characters = set(s)\n \n # Check rule (2)\n needs_lowercase = not (characters & self.lowercase)\n needs_uppercase = not (characters & self.uppercase)\n needs_digit = not (characters & self.digit)\n num_required_type_replaces = int(needs_lowercase + needs_uppercase + needs_digit)\n \n # Check rule (1)\n num_required_inserts = max(0, 6 - len(s))\n num_required_deletes = max(0, len(s) - 20)\n \n # Check rule (3)\n # Convert s to a list of repetitions for us to manipulate\n # For s = \'11aaabB\' we have groups = [2, 3, 1, 1]\n groups = [len(list(grp)) for _, grp in itertools.groupby(s)]\n \n # We apply deletions iteratively and always choose the best one.\n # This should be fine for short passwords :)\n # A delete is better the closer it gets us to removing a group of three.\n # Thus, a group needs to be (a) larger than 3 and (b) minimal wrt modulo 3.\n def apply_best_delete():\n argmin, _ = min(\n enumerate(groups),\n # Ignore groups of length < 3 as long as others are available.\n key=lambda it: it[1] % 3 if it[1] >= 3 else 10 - it[1],\n )\n groups[argmin] -= 1\n \n for _ in range(num_required_deletes):\n apply_best_delete()\n \n # On the finished groups, we need one repace per 3 consecutive letters.\n num_required_group_replaces = sum(\n group // 3\n for group in groups\n )\n \n return (\n # Deletes need to be done anyway\n num_required_deletes\n # Type replaces can be eaten up by inserts or group replaces.\n # Note that because of the interplay of rules (1) and (2), the required number of group replaces\n # can never be greater than the number of type replaces and inserts for candidates of length < 6.\n + max(\n num_required_type_replaces,\n num_required_group_replaces,\n num_required_inserts,\n )\n )\n``` | 29 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
[Python3] greedy | strong-password-checker | 0 | 1 | Based on the excellent solution of @zhichenggu. \n\n```\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n digit = lower = upper = 1\n for ch in password: \n if ch.isdigit(): digit = 0 \n elif ch.islower(): lower = 0\n elif ch.isupper(): upper = 0 \n missing = digit + lower + upper \n reps = one = two = 0\n i = 2\n while i < len(password):\n if password[i-2] == password[i-1] == password[i]:\n sz = 3\n while i+1 < len(password) and password[i] == password[i+1]:\n sz += 1\n i += 1\n reps += sz // 3\n if sz % 3 == 0: one += 1\n elif sz % 3 == 1: two += 1\n i += 1\n if len(password) < 6: return max(missing, 6 - len(password))\n elif len(password) <= 20: return max(missing, reps)\n else: \n dels = len(password) - 20\n reps -= min(dels, one)\n reps -= min(max(dels - one, 0), two * 2) // 2\n reps -= max(dels - one - 2 * two, 0) // 3\n return dels + max(missing, reps)\n``` | 1 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
python solution | strong-password-checker | 0 | 1 | # Intuition\nThe intuition behind this code is to check the strength of a password. A strong password is one that is not easily guessable and includes a mix of lowercase letters, uppercase letters, digits, and does not contain three repeating characters in a row.\n\n# Approach\nThe approach is to first check the presence of lowercase letters, uppercase letters, and digits in the password. If any of these are missing, we increment a missing_type counter.\n\nNext, we iterate over the password and count the number of repeating characters. If a sequence of three repeating characters is found, we increment a change counter. We also keep track of sequences that can be changed with one or two deletions.\n\nIf the password length is less than 6, we return the maximum of missing_type and the difference between 6 and the password length. If the password length is less than or equal to 20, we return the maximum of missing_type and change.\n\nIf the password length is greater than 20, we calculate the number of delete operations needed to bring the length down to 20. We then update change based on the number of delete operations. Finally, we return the sum of delete_operations and change.\n\n# Complexity\n- Time complexity:\nThe time complexity of this code is O(n), where n is the length of the password. This is because we are iterating over the password once to check for the presence of lowercase letters, uppercase letters, and digits. We then iterate over the password again to count the number of repeating characters.\n\n- Space complexity:\nThe space complexity of this code is O(1), because we are not using any data structures that scale with the input size. We are only using a few integer variables to keep track of the operations and counts.\n\n# Code\n```\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n # Initialize total operations and missing type count\n total_operations = 0\n missing_type = 3\n\n # Check for presence of lowercase, uppercase, and digit\n if any(\'a\' <= c <= \'z\' for c in password):\n missing_type -= 1\n if any(\'A\' <= c <= \'Z\' for c in password):\n missing_type -= 1\n if any(c.isdigit() for c in password):\n missing_type -= 1\n\n # Initialize change, one_change, and two_change\n change = 0\n one_change = 0\n two_change = 0\n\n # Iterate over the password\n i = 2\n while i < len(password):\n # If the current character, previous character, and character before previous are the same\n if password[i] == password[i-1] == password[i-2]:\n length = 2\n # Count the length of the repeating sequence\n while i < len(password) and password[i] == password[i-1]:\n length += 1\n i += 1\n\n # Update change and one_change, two_change\n change += length // 3\n if length % 3 == 0:\n one_change += 1\n elif length % 3 == 1:\n two_change += 1\n\n else:\n i += 1\n\n # If the length of the password is less than 6\n if len(password) < 6:\n return max(missing_type, 6 - len(password))\n\n # If the length of the password is less than or equal to 20\n elif len(password) <= 20:\n return max(missing_type, change)\n\n else:\n # Calculate the number of delete operations\n delete_operations = len(password) - 20\n\n # Update change considering delete operations\n change -= min(delete_operations, one_change * 1) // 1\n change -= min(max(delete_operations - one_change, 0), two_change * 2) // 2\n change -= max(delete_operations - one_change - 2 * two_change, 0) // 3\n\n # Return the total operations\n return delete_operations + max(missing_type, change)\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
Tiny, easy, and relatively efficient solution in Python3 (4 LoC, actual "shortest" solution) | strong-password-checker | 0 | 1 | *If you found this helpful, please upvote it. If you see a mistake please let me know via a comment and I will do my best to fix it* \uD83D\uDE0A.\n\n# Intuition\nTo solve this problem, we need to focus on 3 types of operations: \n- Deletions or insertions needed to fit the password length within the 6-20 character bounds.\n- Insertions needed to disrupt repetitive character sequences.\n- Insertions required to ensure the password contains all mandated character types (uppercase, lowercase, and digits).\n\nUpon dissecting the problem, a few insights emerge:\n- The number of deletions required to trim the password to 20 characters or fewer remains constant; it\'s a non-negotiable step if the password exceeds this length.\n- Insertions can serve multiple purposes. A single insertion can simultaneously introduce a new character type, eliminate a repetitive sequence, and push the password length towards the minimum of 6 characters.\n\nTherefore, the solution only needs to consider 2 primary operations: **deletions** for length regulation and targeted **insertions** for the remaining conditions.\n\n# Approach\n*Here, we\'ll be using `p` to represent our password string.*\n\nTo minimize the steps required for transforming a given password to a "strong" password, we\'ll first initiate sets for **lowercase, uppercase, and digits** which lets us identify character types within the password. We\'ll use boolean operations to find out which types are missing since they result in 0 or 1:\n```python\nl, u, d = set("abcdefghijklmnopqrstuvwxyz"), set("ABCDEFGHIJKLMNOPQRSTUVWXYZ"), set("0123456789")\nmissing = (not any(c in l for c in p)) + (not any(c in u for c in p)) + (not any(c in d for c in p))\n```\n\nNext, we\'ll calculate **length-reducing deletions** for passwords exceeding 20 characters with a `max` operation:\n```python\ndels = max(0, len(p) - 20)\n```\n\nNext, we can iterate over the password to create an array, `sub_lens`, that holds **the lengths of each contiguous substring of identical characters.** This will help determine the number of operations to break long sequences ($$\\geq 3$$):\n```python\nsub_lens, last = [1], p[0]\nfor c in p[1:]:\n if c == last: sub_lens[-1] += 1\n else: sub_lens.append(1)\n last = c\n```\n\nNext, using the populated `sub_lens` array, we can iteratively **identify which substrings to shorten** based on the provided constraints in the problem:\n```python\nfor _ in range(dels):\n i, _ = min(enumerate(sub_lens), key = lambda s: s[1] % 3 if s[1] >= 3 else float("inf"))\n sub_lens[i] -= 1\n```\n\nThe sorting key `lambda s: s[1] % 3 if s[1] >= 3` aims to **find substrings whose lengths are just over multiples of 3 (modulo closer to 0).** Non-candidates are excluded via `float("inf")`.\n\nFinally, we\'ll calculate **the number of breaks needed for each substring** with length $$\\geq 3$$ as well as any required insertions to reach the minimum length of 6 characters:\n```python\nbreaks = sum(lens // 3 for lens in sub_lens if lens >= 3)\nins = max(0, 6 - len(p))\n```\n\nAfter getting all the required values, we can determine that the total operations needed are the **deletions plus the maximum of the missing types, breaks, and insertions:**\n```python\nreturn dels + max(missing, breaks, ins)\n```\n\n# Complexity\n- Time complexity: $$O(n\\times m)\\text{ where }n=|\\text{p}|\\text{ and }m=|\\text{sub\\_lens}|$$\n - Creation of `l`, `u`, and `d` is $$O(n)$$\n - Calculating `dels` and `missing` is $$O(n)$$\n - Counting `sub_lens` is $$O(n)$$\n - Counting needed breaks is $$O(n\\times m)$$\n - Calculating `breaks` and `ins` is $$O(n)$$\n- Space complexity: $$O(m)\\text{ where }m=|\\text{sub\\_lens}|$$\n - Charsets `l`, `u`, and `d` are $$O(1)$$\n - Variables `dels`, `missing`, `breaks`, and `ins` are $$O(1)$$\n - Array `sub_lens` is $$O(m)\\text{ where }m\\leq n\\text{ and }n=|\\text{p}|$$\n\n# Code\nFrom the above workflow, we\'re left with the following as our solution. This is sufficient for solving the problem, but as a challenge we can work to shorten it even further.\n- Lines (excluding comments and keywords like `def` and `class`): 13\n```python\nclass Solution:\n def strongPasswordChecker(self, p: str) -> int:\n l, u, d = set("abcdefghijklmnopqrstuvwxyz"), set("ABCDEFGHIJKLMNOPQRSTUVWXYZ"), set("0123456789")\n dels = max(0, len(p) - 20)\n missing = (not any(c in l for c in p)) + (not any(c in u for c in p)) + (not any(c in d for c in p))\n sub_lens, last = [1], p[0]\n for c in p[1:]:\n if c == last: sub_lens[-1] += 1\n else: sub_lens.append(1)\n last = c\n for _ in range(dels):\n i, _ = min(enumerate(sub_lens), key = lambda s: s[1] % 3 if s[1] >= 3 else float("inf"))\n sub_lens[i] -= 1\n breaks, ins = sum(lens // 3 for lens in sub_lens if lens >= 3), max(0, 6 - len(p))\n return dels + max(missing, breaks, ins)\n```\n\nFrom this, we can actually derive an even smaller version which performs the same functionality at slight cost to readability.\n- Lines (excluding comments and keywords like `def` and `class`): 7\n```python\nclass Solution:\n def strongPasswordChecker(self, p: str) -> int:\n l, u, d = set("abcdefghijklmnopqrstuvwxyz"), set("ABCDEFGHIJKLMNOPQRSTUVWXYZ"), set("0123456789")\n dels, missing = max(0, len(p) - 20), sum(not any(c in s for c in p) for s in [l, u, d])\n sub_lens = [sum(1 for _ in g) for _, g in groupby(p)]\n for _ in range(dels):\n i, _ = min(enumerate(sub_lens), key = lambda s: s[1] % 3 if s[1] >= 3 else float("inf"))\n sub_lens[i] = sub_lens[i] - 1 if sub_lens[i] > 1 else 0\n return dels + max(missing, sum(x // 3 for x in sub_lens if x > 2), max(0, 6 - len(p)))\n```\n\nIf we care not about the readability of our code, we can perform the same operations in just 4 lines (excluding keywords), although this is pushing it.\n- Lines (excluding comments and keywords like `def` and `class`): 4\n```python\nclass Solution:\n def strongPasswordChecker(self, p: str) -> int:\n s = [set("abcdefghijklmnopqrstuvwxyz"), set("ABCDEFGHIJKLMNOPQRSTUVWXYZ"), set("0123456789")]\n l, d, m = [sum(1 for _ in g) for _, g in groupby(p)], max(0, len(p) - 20), sum(not any(c in x for c in p) for x in s)\n for _ in range(d): l[min(range(len(l)), key = lambda i: (l[i] % 3 if l[i] > 2 else float("inf"), l[i]), default = 0)] -= 1\n return d + max(m, sum(x // 3 for x in l if x > 2), max(0, 6 - len(p)))\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
Python | strong-password-checker | 0 | 1 | Not the cleanest solution but it seems to work\n\n# Complexity\n- Time complexity:\n$$O(n)$$\n\n- Space complexity:\n$$O(n)$$\n\n# Code\n```\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n hasdigits = any(char.isdigit() for char in password)\n hasupper = any(char.isupper() for char in password)\n haslower = any(char.islower() for char in password)\n # positive if we need to add characters, negative if we need to delete, 0 for replacements\n additions = max(0, 6-len(password)) - max(0, len(password)-20) \n \n # list all repeating sequence lengths\n cp = None\n seq = []\n n = 1\n for c in password:\n if c == cp:\n n += 1\n if n == 3:\n seq.append(n)\n elif n > 3:\n seq[-1] = n\n else:\n n = 1\n cp = c\n seq.sort()\n edits = 0\n # keep making edits until all repeating sequences are gone\n while(len(seq) > 0):\n print(seq)\n # replace characters (additions==0), or add (>0)\n if additions >= 0:\n # if needed, we replace/add a required character\n if not hasdigits:\n hasdigits = True\n elif not haslower: \n haslower = True\n elif not hasupper:\n hasupper = True\n # take the longest sequence and start splitting it into pieces of len 2\n # this means we actually just remove 3 characters from the sequence\n seq[-1] -= 3\n if(seq[-1] < 3): del seq[-1]\n additions = max(0, additions-1) # remove one addition\n # delete or replace characters (additions < 0)\n else:\n # if we can remove all repeating sequences by only deleting characters, we start deleting\n if(sum(seq) - 2*len(seq) <= -additions):\n edits += len(seq)\n additions = min(0, additions+len(seq))\n break\n #seq[0] -= 1\n #if(seq[0] < 3): del seq[0]\n #additions = min(0, additions+1)\n # if not, it\'s more efficient to first start breaking them up with replacements\n else:\n # if needed, we replace with a required character\n if not hasdigits:\n hasdigits = True\n elif not haslower: \n haslower = True\n elif not hasupper:\n hasupper = True\n seq[-1] -= 3\n if(seq[-1] < 3): del seq[-1]\n seq.sort() # sort so the longest sequence is at the top of the stack again\n edits += 1 # add to the edit counter\n\n # if we have any additions left, we make sure we first add required characters\n for i in range(additions):\n if not hasdigits:\n hasdigits = True\n elif not haslower: \n haslower = True\n else:\n hasupper = True\n \n # add all leftover additions and required character changes to the edits \n edits += abs(additions) + (not hasdigits) + (not hasupper) + (not haslower)\n\n return edits\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
How strong is THIS password checker? | strong-password-checker | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n n = len(password)\n \n # Check for lowercase, uppercase, and digit\n has_lower = any(c.islower() for c in password)\n has_upper = any(c.isupper() for c in password)\n has_digit = any(c.isdigit() for c in password)\n \n # Total types of characters we need to add to satisfy the requirements\n types_needed = 3 - (has_lower + has_upper + has_digit)\n \n # Check for repeating characters\n repeat_replace = 0\n one_mod = two_mod = 0 # Counters for characters that repeat modulo 3\n \n i = 2\n while i < n:\n if password[i] == password[i-1] == password[i-2]:\n length = 2\n while i < n and password[i] == password[i-1]:\n length += 1\n i += 1\n \n repeat_replace += length // 3\n if length % 3 == 0:\n one_mod += 1\n elif length % 3 == 1:\n two_mod += 1\n else:\n i += 1\n \n # If the length is less than 6\n if n < 6:\n return max(types_needed, 6 - n)\n \n # If the length is between 6 and 20\n elif n <= 20:\n return max(types_needed, repeat_replace)\n \n # If the length is more than 20\n else:\n delete_needed = n - 20\n repeat_replace -= min(delete_needed, one_mod * 1) // 1\n repeat_replace -= min(max(delete_needed - one_mod, 0), two_mod * 2) // 2\n repeat_replace -= max(delete_needed - one_mod - 2 * two_mod, 0) // 3\n \n return delete_needed + max(types_needed, repeat_replace)\n\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
Relatively readable ;) | strong-password-checker | 0 | 1 | # Intuition\n\nGather data first then do the necessary looping\n\n# Approach\nget to [6..20] and then do replaces as needed to avoid 3 in row and/or missing type of character\n\n# Complexity\n- Time complexity:\nmeh\n\n- Space complexity:\nlight\n\n# Code\n```\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n result = 0\n \n has_upper = False\n has_lower = False\n has_digit = False\n l = len(password)\n less_than_06 = l < 6\n more_than_20 = l > 20\n diff_len = 0\n if less_than_06: diff_len = 6 - l\n if more_than_20: diff_len = l - 20\n repeats = []\n prev_letter = \'\'\n current_counter = 0\n modifies_needed = 0\n for letter in password:\n if letter.isupper(): has_upper = True\n if letter.islower(): has_lower = True\n if letter.isdigit(): has_digit = True\n if letter == prev_letter:\n current_counter += 1\n else:\n if current_counter >= 3:\n repeats.append(current_counter)\n prev_letter = letter\n current_counter = 1\n if current_counter >= 3:\n repeats.append(current_counter)\n \n if not has_upper: modifies_needed += 1\n if not has_lower: modifies_needed += 1\n if not has_digit: modifies_needed += 1\n \n if more_than_20:\n # deletes\n while True:\n work = False\n repeats.sort(key=lambda x: x%3)\n for i in range(len(repeats)):\n if repeats[i] < 3: continue\n work = True\n repeats[i] -= 1\n diff_len -= 1\n result += 1\n break\n if not diff_len or not work: break\n result += diff_len\n if less_than_06:\n # inserts\n result += diff_len\n modifies_needed -= diff_len\n diff_len = 0\n if repeats: repeats[0] -= 2\n\n # replaces\n for i in range(len(repeats)):\n if repeats[i] < 3: continue\n replaces = repeats[i] // 3\n result += replaces\n modifies_needed -= replaces\n\n result += max(modifies_needed, 0)\n\n return result\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
36ms python3 solution | strong-password-checker | 0 | 1 | # Intuition\nTo create a strong password, the constraints require having at least one lowercase letter, one uppercase letter, one digit, a length between 6 and 20 characters, and no three repeating characters in a row. \n\n1. **Identifying Missing Types**: Start by identifying the missing character types (lowercase, uppercase, digit) that are required to make the password strong. \n\n2. **Handling Repeating Characters**: Iterate through the password to find sequences of three or more repeating characters. Divide the length of each sequence by three to find the number of replacements needed, and keep track of remainders to understand how insertions or deletions may affect these sequences.\n\n3. **Length Constraints**: If the password is shorter than 6 characters, either a missing type or an extra character should be added. If the password is longer than 20 characters, characters must be deleted.\n\n4. **Combining Steps**: Combine the missing types and the required changes to find the minimum steps needed to make the password strong. This includes using deletions to reduce the number of replacements when the password is too long.\n\nBy analyzing all constraints and calculating how to meet each one with the minimum number of steps, we can efficiently find the minimum steps required to make the password strong.\n# Approach\n1. **Figure Out What\'s Missing**: First, I checked to see what\'s missing in the password. Does it have lowercase, uppercase, and digits? If anything\'s missing, that\'s going to be something we need to fix.\n\n2. **Deal with Repeating Characters**: Next, I tackled those annoying repeating characters. If any character repeated three or more times in a row, I calculated how many changes we\'d need. I also kept tabs on the remainder of dividing by three to help figure out the best way to handle things later.\n\n3. **Too Short or Too Long?**: Then, I looked at the length of the password. If it\'s less than 6 characters, we\'ll need to add something. If it\'s more than 20, we\'ll need to delete. I used the remainder from step 2 to minimize the number of replacements needed when deleting characters.\n\n4. **Put It All Together**: Finally, I added up all the missing types, changes, deletions, or additions to get the minimum steps needed to make that password strong. It\'s like putting together a puzzle, making sure each piece fits just right.\n\nBy breaking down the problem and tackling each part methodically, I was able to create a step-by-step approach to make any password strong. It\'s a neat little solution that handles a lot of different scenarios efficiently!\n\n# Complexity\n- Time complexity:\nThe time complexity of the code is $$O(n)$$, where $$n$$ is the length of the password. The code iterates through the password string, performing constant-time operations, resulting in a linear time complexity. The reported runtime of 36ms is in line with this analysis.\n- Space complexity:\nThe space complexity of the code is $$O(1)$$, as we are only using a constant amount of extra space, irrespective of the input size.\n# Code\n```\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n missing_types = 3\n if any(\'a\' <= c <= \'z\' for c in password):\n missing_types -= 1\n if any(\'A\' <= c <= \'Z\' for c in password):\n missing_types -= 1\n if any(c.isdigit() for c in password):\n missing_types -= 1\n\n change = 0\n one, two, three = 0, 0, 0\n i = 2\n while i < len(password):\n if password[i] == password[i-1] == password[i-2]:\n length = 2\n while i < len(password) and password[i] == password[i-1]:\n length += 1\n i += 1\n \n change += length // 3\n one += length % 3 == 0\n two += length % 3 == 1\n three += length % 3 == 2\n i += 1\n\n n = len(password)\n if n < 6:\n return max(missing_types, 6 - n)\n elif n <= 20:\n return max(missing_types, change)\n else:\n deletes_needed = n - 20\n change -= min(deletes_needed, one * 1) // 1\n change -= min(max(deletes_needed - one, 0), two * 2) // 2\n change -= max(deletes_needed - one - 2 * two, 0) // 3\n\n return deletes_needed + max(missing_types, change)\n\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
Python3 with explanation | strong-password-checker | 0 | 1 | # Intuition\nChecking the strength of a password typically involves ensuring it meets certain criteria such as containing numbers, uppercase letters, lowercase letters, and not having repeated characters. The given problem seems to be an advanced form of this check, where we not only have to identify if the password is strong but also determine the minimum number of changes required to make it strong.\n\n# Approach\nType Checking:\n\n- A strong password should have three types of characters: digit, uppercase, and lowercase. We start by checking the presence of each type and keep track of the missing types.\n\nRepetitions:\n\n- Repeated characters are a sign of a weak password. We need to identify sequences of characters that are repeated.\n- For every sequence of three identical characters (e.g., \'aaa\'), one replacement is needed. For sequences of six (e.g., \'aaaaaa\'), two replacements are needed, and so on. We also keep track of sequences where the length % 3 is 0, 1, or 2, as they help in optimizing replacements later.\n\nLength Adjustments:\n\n- If the password\'s length is less than 6, we can simply add the required characters. The number of additions would be 6 - len(password).\n- If the password\'s length is between 6 and 20, we don\'t need to add or delete, just replace characters.\n- If the password\'s length is more than 20, we need to delete characters. The number of deletions is len(password) - 20. Deletions can also help in reducing the number of replacements. For instance, deleting one character from a sequence of \'aaa\' eliminates the need for a replacement.\n\nFinal Calculation:\n\n- Depending on the length of the password, we return the required operations (additions, deletions, or replacements) combined with the missing types.\n\n# Complexity\n- Time complexity:\nThe time complexity is O(n), where n is the length of the password. This is because we iterate through the password multiple times (for checking types and repeated sequences) but each operation is linear.\n\n- Space complexity:\nThe space complexity is O(1) as we are using only a constant amount of extra space regardless of the input size.\n\n# Code\n```\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n types = 3\n if any(c.isdigit() for c in password): types -= 1\n if any(c.isupper() for c in password): types -= 1\n if any(c.islower() for c in password): types -= 1\n\n change = 0\n one = two = 0\n p = 2\n while p < len(password):\n if password[p] == password[p-1] == password[p-2]:\n length = 2\n while p < len(password) and password[p] == password[p-1]:\n length += 1\n p += 1\n \n change += length // 3\n if length % 3 == 0: one += 1\n elif length % 3 == 1: two += 1\n else:\n p += 1\n\n if len(password) < 6:\n return max(types, 6 - len(password))\n elif len(password) <= 20:\n return max(types, change)\n else:\n delete = len(password) - 20\n change -= min(delete, one * 1)//1\n change -= min(max(delete - one, 0), two * 2)//2\n change -= max(delete - one - 2 * two, 0)//3\n\n return delete + max(types, change)\n\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
JS || java || Python || python3 || C++ || C || C# || PHP || Kotlin || 100% Accuracy, Runtime, Memory | strong-password-checker | 1 | 1 | # Please Upvote\n```javascript []\nconst strongPasswordChecker = (passwd) => {\n let steps = 0;\n let mustAdd = 0;\n\n if (!passwd.match(/[A-Z]/)) {\n mustAdd++;\n }\n if (!passwd.match(/[a-z]/)) {\n mustAdd++;\n }\n if (!passwd.match(/\\d/)) {\n mustAdd++;\n }\n\n let groups = passwd.match(/(.)\\1*/g).filter(x => x.length > 2);\n\n if (passwd.length <= 20) {\n groups.forEach(group => {\n steps += Math.trunc(group.length / 3);\n mustAdd -= Math.trunc(group.length / 3);\n })\n }\n\n if (passwd.length <= 20) {\n mustAdd = mustAdd > 0 ? mustAdd : 0;\n if (passwd.length + steps >= 6) {\n steps += mustAdd;\n } else {\n if (mustAdd > 6 - (passwd.length + steps)) {\n steps += mustAdd;\n } else {\n steps += 6 - (passwd.length + steps);\n }\n }\n }\n\n if (passwd.length > 20) {\n let mustRemove = passwd.length - 20;\n let lengths = [];\n let plus = [];\n let chL = 0;\n for (let i = 1; i <= 3; i++) {\n for (let k = 0; k < groups.length; k++) {\n if (plus[k] === undefined) { plus[k] = 0; }\n chL = groups[k].length - plus[k];\n if (lengths[k] === undefined) { lengths[k] = chL; }\n const rec = () => {\n if (Math.trunc((chL - i) / 3) < Math.trunc(chL / 3) && passwd.length - steps - i >= 6 && mustRemove >= i && chL > 2 && lengths[k] - i > 0) {\n steps += i;\n plus[k] += i;\n mustRemove -= i;\n chL -= i;\n lengths[k] -= i;\n rec();\n }\n }\n rec();\n }\n }\n lengths.forEach(length => {\n if (length > 2) {\n steps += Math.trunc(length / 3);\n mustAdd -= Math.trunc(length / 3);\n }\n }\n )\n\n mustRemove = mustRemove > 0 ? mustRemove : 0;\n mustAdd = mustAdd > 0 ? mustAdd : 0;\n steps += mustAdd + mustRemove;\n }\n\n return steps;\n};\n```\n```python []\nclass Solution(object):\n def strongPasswordChecker(self, s):\n """\n :type s: str\n :rtype: int\n """\n missing_type = 3\n if any(\'a\' <= c <= \'z\' for c in s): missing_type -= 1\n if any(\'A\' <= c <= \'Z\' for c in s): missing_type -= 1\n if any(c.isdigit() for c in s): missing_type -= 1\n\n change = 0\n one = two = 0\n p = 2\n while p < len(s):\n if s[p] == s[p-1] == s[p-2]:\n length = 2\n while p < len(s) and s[p] == s[p-1]:\n length += 1\n p += 1\n \n change += length / 3\n if length % 3 == 0: one += 1\n elif length % 3 == 1: two += 1\n else:\n p += 1\n \n if len(s) < 6:\n return max(missing_type, 6 - len(s))\n elif len(s) <= 20:\n return max(missing_type, change)\n else:\n delete = len(s) - 20\n \n change -= min(delete, one)\n change -= min(max(delete - one, 0), two * 2) / 2\n change -= max(delete - one - 2 * two, 0) / 3\n \n return delete + max(missing_type, change)\n```\n```Python3 []\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n steps = 0\n must_add = 0\n\n if not any(char.isupper() for char in password):\n must_add += 1\n if not any(char.islower() for char in password):\n must_add += 1\n if not any(char.isdigit() for char in password):\n must_add += 1\n\n import re\n groups = [match.group(0) for match in re.finditer(r\'(.)\\1*\', password) if len(match.group(0)) > 2]\n\n if len(password) <= 20:\n for group in groups:\n steps += len(group) // 3\n must_add -= len(group) // 3\n\n if len(password) <= 20:\n must_add = max(must_add, 0)\n if len(password) + steps >= 6:\n steps += must_add\n else:\n steps += max(must_add, 6 - (len(password) + steps))\n\n if len(password) > 20:\n must_remove = len(password) - 20\n lengths = [None] * len(groups)\n plus = [None] * len(groups)\n for i in range(1, 4):\n for k in range(len(groups)):\n if plus[k] is None:\n plus[k] = 0\n chL = len(groups[k]) - plus[k]\n if lengths[k] is None:\n lengths[k] = chL\n\n def rec():\n nonlocal steps, must_remove\n nonlocal chL, lengths\n if (chL - i) // 3 < chL // 3 and len(password) - steps - i >= 6 and must_remove >= i and chL > 2 and lengths[k] - i > 0:\n steps += i\n plus[k] += i\n must_remove -= i\n chL -= i\n lengths[k] -= i\n rec()\n\n rec()\n\n for length in lengths:\n if length > 2:\n steps += length // 3\n must_add -= length // 3\n\n must_remove = max(must_remove, 0)\n must_add = max(must_add, 0)\n steps += must_add + must_remove\n\n return steps\n```\n```JAVA []\nclass Solution {\n public int strongPasswordChecker(String s) {\n int res = 0, a = 1, A = 1, d = 1;\n char[] carr = s.toCharArray();\n int[] arr = new int[carr.length];\n \n for (int i = 0; i < arr.length;) {\n if (Character.isLowerCase(carr[i])) a = 0;\n if (Character.isUpperCase(carr[i])) A = 0;\n if (Character.isDigit(carr[i])) d = 0;\n \n int j = i;\n while (i < carr.length && carr[i] == carr[j]) i++;\n arr[j] = i - j;\n }\n \n int total_missing = (a + A + d);\n\n if (arr.length < 6) {\n res += total_missing + Math.max(0, 6 - (arr.length + total_missing));\n \n } else {\n int over_len = Math.max(arr.length - 20, 0), left_over = 0;\n res += over_len;\n \n for (int k = 1; k < 3; k++) {\n for (int i = 0; i < arr.length && over_len > 0; i++) {\n if (arr[i] < 3 || arr[i] % 3 != (k - 1)) continue;\n arr[i] -= Math.min(over_len, k);\n over_len -= k;\n }\n }\n \n for (int i = 0; i < arr.length; i++) {\n if (arr[i] >= 3 && over_len > 0) {\n int need = arr[i] - 2;\n arr[i] -= over_len;\n over_len -= need;\n }\n \n if (arr[i] >= 3) left_over += arr[i] / 3;\n }\n \n res += Math.max(total_missing, left_over);\n }\n \n return res;\n}\n}\n```\n```C++ []\nclass Solution {\npublic:\n int strongPasswordChecker(string s) {\n int deleteTarget = max(0, (int)s.length() - 20), addTarget = max(0, 6 - (int)s.length());\n int toDelete = 0, toAdd = 0, toReplace = 0, needUpper = 1, needLower = 1, needDigit = 1;\n \n ///////////////////////////////////\n // For cases of s.length() <= 20 //\n ///////////////////////////////////\n for (int l = 0, r = 0; r < s.length(); r++) {\n if (isupper(s[r])) { needUpper = 0; } \n if (islower(s[r])) { needLower = 0; }\n if (isdigit(s[r])) { needDigit = 0; }\n \n if (r - l == 2) { // if it\'s a three-letter window\n if (s[l] == s[l + 1] && s[l + 1] == s[r]) { // found a three-repeating substr\n if (toAdd < addTarget) { toAdd++, l = r; } // insert letter to break repetition if possible\n else { toReplace++, l = r + 1; } // replace current word to avoid three repeating chars\n } else { l++; } // keep the window with no more than 3 letters\n }\n }\n if (s.length() <= 20) { return max(addTarget + toReplace, needUpper + needLower + needDigit); }\n \n //////////////////////////////////\n // For cases of s.length() > 20 //\n //////////////////////////////////\n toReplace = 0; // reset toReplace\n vector<unordered_map<int, int>> lenCnts(3); // to record repetitions with (length % 3) == 0, 1 or 2\n for (int l = 0, r = 0, len; r <= s.length(); r++) { // record all repetion frequencies\n if (r == s.length() || s[l] != s[r]) {\n if ((len = r - l) > 2) { lenCnts[len % 3][len]++; } // we only care about repetions with length >= 3\n l = r;\n }\n }\n \n /*\n Use deletions to minimize replacements, following below orders:\n (1) Try to delete one letter from repetitions with (length % 3) == 0. Each deletion decreases replacement by 1\n (2) Try to delete two letters from repetitions with (length % 3) == 1. Each deletion decreases repalcement by 1\n (3) Try to delete multiple of three letters from repetions with (length % 3) == 2. Each deletion (of three \n letters) decreases repalcements by 1\n */\n for (int i = 0, numLetters, dec; i < 3; i++) { \n for (auto it = lenCnts[i].begin(); it != lenCnts[i].end(); it++) {\n if (i < 2) {\n numLetters = i + 1, dec = min(it->second, (deleteTarget - toDelete) / numLetters);\n toDelete += dec * numLetters; // dec is the number of repetitions we\'ll delete from\n it->second -= dec; // update number of repetitions left\n \n // after letters deleted, it fits in the group where (length % 3) == 2\n if (it->first - numLetters > 2) { lenCnts[2][it->first - numLetters] += dec; } \n }\n \n // record number of replacements needed\n // note if len is the length of repetition, we need (len / 3) number of replacements\n toReplace += (it->second) * ((it->first) / 3); \n } \n }\n\n int dec = (deleteTarget - toDelete) / 3; // try to delete multiple of three letters as many as possible\n toReplace -= dec, toDelete -= dec * 3;\n return deleteTarget + max(toReplace, needUpper + needLower + needDigit);\n }\n};\n\n```\n```C []\nchar letters[\'A\' + 62] = {\n [\'A\'] = 1,\n [\'B\'] = 1,\n [\'C\'] = 1,\n [\'D\'] = 1,\n [\'E\'] = 1,\n [\'F\'] = 1,\n [\'G\'] = 1,\n [\'H\'] = 1,\n [\'I\'] = 1,\n [\'J\'] = 1,\n [\'K\'] = 1,\n [\'L\'] = 1,\n [\'M\'] = 1,\n [\'N\'] = 1,\n [\'O\'] = 1,\n [\'P\'] = 1,\n [\'Q\'] = 1,\n [\'R\'] = 1,\n [\'S\'] = 1,\n [\'T\'] = 1,\n [\'U\'] = 1,\n [\'V\'] = 1,\n [\'W\'] = 1,\n [\'X\'] = 1,\n [\'Y\'] = 1,\n [\'Z\'] = 1,\n [\'a\'] = 2,\n [\'b\'] = 2,\n [\'c\'] = 2,\n [\'d\'] = 2,\n [\'e\'] = 2,\n [\'f\'] = 2,\n [\'g\'] = 2,\n [\'h\'] = 2,\n [\'i\'] = 2,\n [\'j\'] = 2,\n [\'k\'] = 2,\n [\'l\'] = 2,\n [\'m\'] = 2,\n [\'n\'] = 2,\n [\'o\'] = 2,\n [\'p\'] = 2,\n [\'q\'] = 2,\n [\'r\'] = 2,\n [\'s\'] = 2,\n [\'t\'] = 2,\n [\'u\'] = 2,\n [\'v\'] = 2,\n [\'w\'] = 2,\n [\'x\'] = 2,\n [\'y\'] = 2,\n [\'z\'] = 2,\n [\'0\'] = 3,\n [\'1\'] = 3,\n [\'2\'] = 3,\n [\'3\'] = 3,\n [\'4\'] = 3,\n [\'5\'] = 3,\n [\'6\'] = 3,\n [\'7\'] = 3,\n [\'8\'] = 3,\n [\'9\'] = 3,\n};\n\nint strongPasswordChecker(char * password){\n int length = strlen(password);\n int replace = 0;\n int one = 0;\n int two = 0;\n int cap = 1;\n int low = 1;\n int dig = 1;\n \n for(int i = 0; password[i]; i++){\n if(letters[password[i]] == 1){\n cap = 0;\n } else if(letters[password[i]] == 2) {\n low = 0;\n } else if(letters[password[i]] == 3) {\n dig = 0;\n }\n \n int repeat = 1;\n while(password[i] == password[i + 1]){\n i++;\n repeat += 1;\n }\n \n if(repeat > 2){\n replace += (repeat / 3);\n\n if(repeat % 3 == 0){\n one += 1;\n } else if(repeat % 3 == 1){\n two += 2;\n }\n }\n }\n \n if(length < 6){\n if(cap + low + dig > 6 - length){\n return cap + low + dig;\n } else {\n return 6 - length;\n }\n } else if(length <= 20){\n if(cap + low + dig > replace){\n return cap + low + dig;\n } else {\n return replace;\n }\n } else {\n int over = length - 20;\n \n if(one < over){\n replace -= one;\n } else {\n replace -= over;\n }\n \n if(over - one > 0){\n if(two < (over - one)){\n replace -= two / 2;\n } else {\n replace -= (over - one) / 2;\n }\n }\n \n if((over - one - two) > 0){\n replace -= (over - one - two) / 3;\n }\n \n if(cap + low + dig > replace){\n return over + cap + low + dig;\n } else {\n return over + replace;\n }\n }\n}\n```\n```C# []\nclass Solution {\n public int StrongPasswordChecker(string s) {\n int charSum = GetRequiredChar(s);\n if (s.Length < 6) return Math.Max(charSum, 6 - s.Length);\n int replace = 0, ones = 0, twos = 0; \n for (int i = 0; i < s.Length;) {\n int len = 1;\n while (i + len < s.Length && s[i + len] == s[i + len - 1]) len++;\n if (len >= 3) {\n replace += len / 3;\n if (len % 3 == 0) ones += 1;\n if (len % 3 == 1) twos += 2;\n }\n i += len;\n }\n if (s.Length <= 20) return Math.Max(charSum, replace);\n int deleteCount = s.Length - 20;\n replace -= Math.Min(deleteCount, ones);\n replace -= Math.Min(Math.Max(deleteCount - ones, 0), twos) / 2;\n replace -= Math.Max(deleteCount - ones - twos, 0) / 3;\n return deleteCount + Math.Max(charSum, replace);\n }\n int GetRequiredChar(string s) {\n int lowerCase = 1, upperCase = 1, digit = 1;\n foreach (var c in s) {\n if (char.IsLower(c)) lowerCase = 0;\n else if (char.IsUpper(c)) upperCase = 0;\n else if (char.IsDigit(c)) digit = 0;\n }\n return lowerCase + upperCase + digit;\n } \n}\n```\n```PHP []\nclass Solution {\n\n /**\n * @param String $password\n * @return Integer\n */\n function strongPasswordChecker($password) {\n $passwordCharList = str_split($password);\n \n $missingType = 3;\n $numberList = range(48, 57);\n $charListCapital = range(65, 90); \n $charListLowercase = range(97, 122);\n\n $numberFlag = false;\n $capitalFlag = false;\n $lowercaseFlag = false;\n\n foreach ($passwordCharList as $key => $value){\n if (!$numberFlag && in_array(ord($value), $numberList)) { \n $numberFlag = true;\n } else if (!$capitalFlag && in_array(ord($value), $charListCapital)) {\n $capitalFlag = true;\n } else if (!$lowercaseFlag && in_array(ord($value), $charListLowercase)) {\n $lowercaseFlag = true;\n }\n }\n \n $numberFlag && $missingType--;\n $capitalFlag && $missingType--;\n $lowercaseFlag && $missingType--;\n \n $change = 0;\n $first = 0;\n $second = 0;\n $charKey = 2;\n\n while ($charKey < strlen($password)){\n if ($passwordCharList[$charKey] === $passwordCharList[$charKey-1] \n && $passwordCharList[$charKey] === $passwordCharList[$charKey-2]) {\n $countOfRepeat = 2;\n\n while ($charKey<strlen($password) \n && $passwordCharList[$charKey] === $passwordCharList[$charKey-1]) {\n $countOfRepeat++;\n $charKey++;\n }\n \n $change += floor($countOfRepeat/3);\n \n $countOfRepeat % 3 === 0 && $first += 1;\n $countOfRepeat % 3 === 1 && $second += 2; \n\n continue;\n }\n \n $charKey++;\n }\n \n \n if (strlen($password) < 6) {\n return max($missingType, 6-strlen($password));\n } else if (strlen($password) >= 6 && strlen($password) <= 20) {\n return max($missingType, $change);\n }\n\n $removeKey = strlen($password) - 20;\n \n $change -= min($removeKey, $first);\n $change -= floor(min(max($removeKey - $first, 0), $second) / 2);\n $change -= floor(max($removeKey - $first - $second, 0) / 3);\n \n return $removeKey + max($missingType, $change);\n }\n}\n```\n```Kotlin []\nclass Solution {\n\n fun strongPasswordChecker(password: String): Int {\n var operations = 0\n var lower = 0\n var upper = 0\n var digits = 0\n\n val repeating = IntArray(password.length)\n\n var currentCheckIndex = 0\n while (currentCheckIndex < password.length) {\n val targetCheckIndex = currentCheckIndex // For later repeating check use\n val targetChar = password[currentCheckIndex]\n\n while (currentCheckIndex < password.length && password[currentCheckIndex] == targetChar) {\n repeating[targetCheckIndex]++\n currentCheckIndex++\n }\n }\n println("Repeating: ${repeating.joinToString(", ")}")\n for (targetChar in password) {\n if (targetChar.isUpperCase()) upper+= 1\n if (targetChar.isLowerCase()) lower+= 1\n if (targetChar.isDigit()) digits += 1\n }\n val charTypeRequirementMetCount = lower.coerceAtMost(1) + upper.coerceAtMost(1) + digits.coerceAtMost(1)\n val missingRequirements = 3 - charTypeRequirementMetCount\n\n if (password.length <= 3) { // If length is less than 3, it will be able to fix the missing requirements no matter what\n return 6 - password.length\n } else if (password.length < 6) { // If the length is 4, 5, or 6, it will be maximum value of insertion count and missing requirements count\n // We don\'t need to worry about repeating chars since we can easily fix it by inserting char in a different location\n val insertionCount = 6 - password.length\n return maxOf(insertionCount, missingRequirements)\n } else {\n println("Lowercase: $lower")\n println("Uppercase: $upper")\n println("Digits: $digits")\n\n var extraLength = password.length - 20\n\n if (extraLength > 0) {\n findMinimizedSumOfDivision(extraLength, repeating)\n operations += extraLength\n }\n\n println("After operation: " + repeating.joinToString(", "))\n\n // After reducing the length, we want to now replace all the repeating stuff.\n // Replaced "random char amount" will be stored inside a variable (For later use)\n var modifiedCharAmount = 0\n for ((index, i) in repeating.withIndex()) {\n if (i >= 3) {\n operations += i / 3\n modifiedCharAmount += i / 3\n println("Modified ${i / 3} char(s) to eliminate repeating chars")\n }\n }\n\n // At this point, "repeating" is trashed as it\'s in-accurate, and we don\'t need it in our last bit of code\n\n // Now we use the "random char amount" to decide if we have to do extra patch for char type requirements\n // If we don\'t need those (AKA we added enough random chars that will just fit those requirements), we ignore them\n // Otherwise, we add (requirements - random char amount) to operations count\n\n println("We are missing $missingRequirements requirements ($operations)")\n if (modifiedCharAmount < missingRequirements) {\n operations += (missingRequirements - modifiedCharAmount)\n }\n\n }\n\n return operations\n }\n\n\n // 63927897\n // - 11100010\n // 52827887\n // - 00002002\n // 52825885\n // - 30303333\n // - 22522552\n // - 00300330\n\n fun findMinimizedSumOfDivision(operationsLimitation: Int, nums: IntArray) {\n var operationsLeft = operationsLimitation\n var currentSubValue = 1\n var doneOperation: Boolean\n while (operationsLeft > 0) {\n doneOperation = false\n for ((i, num) in nums.withIndex()) {\n if (num >= 3) {\n if (num % 3 == currentSubValue - 1) {\n doneOperation = true\n var sub = currentSubValue\n while (operationsLeft > 0 && sub > 0) {\n operationsLeft--\n nums[i] -= 1\n sub--\n }\n }\n }\n if (operationsLeft == 0) return\n }\n if (currentSubValue == 3 && !doneOperation) break\n if (currentSubValue != 3) currentSubValue++\n }\n\n }\n}\n```\n | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
Strengthening Passwords: String Manipulation and Condition Checking | strong-password-checker | 0 | 1 | # Intuition\nThis problem involves string manipulation and checks for specific conditions. The first approach that comes to mind is to traverse the string while keeping track of the conditions that are already satisfied and the ones that aren\'t. The conditions to check are:\n\nLength of the password between 6 and 20 characters.\nAt least one lowercase letter, one uppercase letter, and one digit.\nNo three repeating characters in a row.\nTo keep track of these conditions, I\'d use counters or flags. For example, I\'d count the number of each type of character (lowercase, uppercase, digit) and the length of repeating sequences.\n\nIf the length of the password is less than 6, I\'d add the missing number of characters considering the types of characters that are still missing.\n\nIf the length of the password is more than 20, I\'d delete the excess characters. Here, I\'d prioritize deleting characters from the middle of repeating sequences of three or more characters.\n\nIf the length of the password is between 6 and 20, I\'d replace characters in the middle of repeating sequences of three or more characters considering the types of characters that are still missing.\n\n# Approach\n1. Initialize a list to store the length of each repeating sequence in the password.\n1. Traverse the password to count the repeating sequences and the types of characters.\n1. If the length of the password is less than 6, return the maximum of the number of missing types of characters and 6 minus the length.\n1. If the length of the password is between 6 and 20, return the maximum of the number of missing types of characters and the total number of characters that should be changed in the repeating sequences.\n1. If the length of the password is more than 20, perform deletions from the repeating sequences first to reduce the length to 20. Here we prioritize reducing the sequences whose lengths mod 3 equals 0, then 1, then 2. For each sequence, reduce it until its length is less than 3 or its length mod 3 is not equal to the current priority. Then, return 20 minus the original length plus the maximum of the number of missing types of characters and the total number of characters that should be changed in the repeating sequences. \n\n# Complexity\n- Time complexity:\nThe time complexity of this approach is `O(n)`, where n is the length of the password. This is because we\'re traversing the password once.\n\n- Space complexity:\nThe space complexity is `O(1)`, i.e., constant. We\'re using a fixed amount of space to store the counters and flags, which doesn\'t change with the size of the input string.\n\n# Code\n```\nclass Solution:\n def strongPasswordChecker(self, s: str) -> int:\n missing_types = 3\n if any(\'a\' <= c <= \'z\' for c in s): missing_types -= 1\n if any(\'A\' <= c <= \'Z\' for c in s): missing_types -= 1\n if any(c.isdigit() for c in s): missing_types -= 1\n\n replace = 0\n one = two = 0\n p = 2\n while p < len(s):\n if s[p] == s[p-1] == s[p-2]:\n length = 2\n while p < len(s) and s[p] == s[p-1]:\n length += 1\n p += 1\n\n replace += length // 3\n if length % 3 == 0: one += 1\n elif length % 3 == 1: two += 1\n else:\n p += 1\n\n if len(s) < 6:\n return max(missing_types, 6 - len(s))\n elif len(s) <= 20:\n return max(missing_types, replace)\n else:\n delete = len(s) - 20\n\n replace -= min(delete, one * 1)//1\n replace -= min(max(delete - one * 1, 0), two * 2) // 2\n replace -= max(delete - one * 1 - two * 2, 0) // 3\n\n return delete + max(missing_types, replace)\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
420. Strong Password Checker, RunTime: 40ms Beats 88.06%, Memory: 16.21mb Beats 89.05% | strong-password-checker | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem requires finding the minimum number of steps required to make a given password strong based on specific conditions. We need to check the length of the password, whether it contains the required character types (lowercase letter, uppercase letter, and digit), and if it has repeating sequences of characters.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nTo solve the problem, we can follow these steps:\n\n1. Initialize missing_types to 3, representing the missing required character types (lowercase, uppercase, and digit).\n2. Check each character in the password and update the missing_types accordingly. If a character of a required type is found, decrease missing_types by 1 for that type.\n3. Iterate through the password to find repeating sequences of characters. While doing so, count the number of steps needed to break each repeating sequence.\n4. Based on the password length and conditions found in steps 1 and 3, calculate the minimum number of steps required to make the password strong.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe time complexity of this approach is O(n), where n is the length of the password. We go through the password once to find missing character types and repeating sequences. The other operations, such as checking for character types and breaking repeating sequences, are constant time operations.\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity is O(1) as we are using a constant amount of extra space for variables like missing_types, change_count, one_repeat, two_repeat, etc. The function does not use any additional data structures that depend on the size of the input.\n\nOverall, the provided function is an efficient and optimal solution to finding the minimum steps required to make the password strong based on the given conditions. It meets the required time and space complexity constraints for the input size.\n# Code\n```\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n missing_types = 3\n\n if any(\'a\' <= char <= \'z\' for char in password):\n missing_types -= 1\n if any(\'A\' <= char <= \'Z\' for char in password):\n missing_types -= 1\n if any(char.isdigit() for char in password):\n missing_types -= 1\n\n change_count = 0\n one_repeat, two_repeat = 0, 0\n i = 2\n while i < len(password):\n if password[i] == password[i - 1] == password[i - 2]:\n length = 2\n while i < len(password) and password[i] == password[i - 1]:\n length += 1\n i += 1\n\n change_count += length // 3\n if length % 3 == 0:\n one_repeat += 1\n elif length % 3 == 1:\n two_repeat += 1\n\n else:\n i += 1\n\n if len(password) < 6:\n return max(missing_types, 6 - len(password))\n elif len(password) <= 20:\n return max(missing_types, change_count)\n else:\n delete_count = len(password) - 20\n\n change_count -= min(delete_count, one_repeat * 1) // 1\n change_count -= min(max(delete_count - one_repeat, 0), two_repeat * 2) // 2\n change_count -= min(max(delete_count - one_repeat - 2 * two_repeat, 0), change_count * 3) // 3\n\n return delete_count + max(missing_types, change_count)\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
Easy | strong-password-checker | 0 | 1 | \n# Code\n```\nclass Solution:\n def strongPasswordChecker(self, s: str) -> int:\n missing_type = 3\n if any(\'a\' <= c <= \'z\' for c in s):\n missing_type -= 1\n if any(\'A\' <= c <= \'Z\' for c in s):\n missing_type -= 1\n if any(c.isdigit() for c in s):\n missing_type -= 1\n\n change = 0\n one = two = 0\n p = 2\n while p < len(s):\n if s[p] == s[p - 1] == s[p - 2]:\n length = 2\n while p < len(s) and s[p] == s[p - 1]:\n length += 1\n p += 1\n change += length // 3\n if length % 3 == 0:\n one += 1\n elif length % 3 == 1:\n two += 1\n else:\n p += 1\n\n if len(s) < 6:\n return max(missing_type, 6 - len(s))\n elif len(s) <= 20:\n return max(missing_type, change)\n else:\n delete = len(s) - 20\n change -= min(delete, one * 1) // 1\n change -= min(max(delete - one, 0), two * 2) // 2\n change -= max(delete - one - 2 * two, 0) // 3\n return delete + max(missing_type, change)\n\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
Strengthening Passwords: Unlocking Security with Intuition | strong-password-checker | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe task is to determine the minimum number of steps required to make a given password strong. A password is considered strong if it satisfies the following requirements:\n- It has at least 6 characters.\n- It has at least one lowercase letter, one uppercase letter, and one digit.\n- It does not contain more than three consecutive repeating characters.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe code first creates sets of lowercase, uppercase, and digit characters using the `ascii_lowercase`, `ascii_uppercase`, and `digits` constants from the `string` module.\n\nIt then determines the missing types of characters in the password by checking if the sets of characters intersect with the characters present in the password. The missing type count is calculated by subtracting the boolean results of the intersection checks from 3.\n\nNext, it calculates the number of required inserts and deletes to make the password a minimum of 6 characters long and a maximum of 20 characters long.\n\nThe code then creates a list of group lengths for consecutive repeating characters in the password using `itertools.groupby` function. \n\nTo minimize the number of actions to satisfy the repeating character constraint, the code greedily performs the best deletion by choosing the group with the minimal impact on the overall repetition pattern.\n\nFinally, it calculates the number of required group replacements by dividing each group length by 3 and taking the sum.\n\nThe return statement adds up the required deletions, the maximum number of required type replacements, group replacements, and inserts to determine the minimum steps needed to make the password strong.\n\n# Complexity\n- Time complexity: The code iterates through the password once to perform various checks and modifications. Also, `itertools.groupby` function also takes linear time. Therefore, the overall time complexity is O(n), where n is the length of the password.\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: The code uses sets and lists to store characters and group lengths, respectively. The space complexity is O(1) since the size of these sets and lists is fixed based on the nature of characters and the password, not the input size.\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nimport string\n\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n lowercase = set(string.ascii_lowercase)\n uppercase = set(string.ascii_uppercase)\n digit = set(string.digits)\n \n characters = set(password)\n \n missing_type = 3 - (bool(characters & lowercase) + bool(characters & uppercase) + bool(characters & digit))\n num_required_type_replaces = int(missing_type)\n \n num_required_inserts = max(0, 6 - len(password))\n num_required_deletes = max(0, len(password) - 20)\n \n groups = [len(list(grp)) for _, grp in itertools.groupby(password)]\n \n def apply_best_delete():\n argmin, _ = min(\n enumerate(groups),\n key=lambda it: it[1] % 3 if it[1] >= 3 else 10 - it[1],\n )\n groups[argmin] -= 1\n \n for _ in range(num_required_deletes):\n apply_best_delete()\n \n num_required_group_replaces = sum(group // 3 for group in groups)\n \n return (\n num_required_deletes +\n max(num_required_type_replaces, num_required_group_replaces, num_required_inserts)\n )\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
Effective Character Type and Length Management for Strong Password Validation | strong-password-checker | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem requires us to make a given password strong by following certain conditions. The conditions involve the length of the password, the types of characters it contains, and the absence of three repeating characters in a row. The intuition here is to handle these conditions separately and then combine the results. We need to count the missing types of characters, calculate the changes needed to eliminate sequences of three or more repeating characters, and finally adjust the length of the password if it\'s not within the range of 6 to 20 characters.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nIdentify missing character types: Check if the password contains at least one lowercase letter, one uppercase letter, and one digit. For each type that is missing, increment a counter.\nEliminate repeating sequences: Iterate through the password and look for sequences of three or more repeating characters. For each sequence found, calculate how many changes are needed to eliminate the sequence and increment a change counter accordingly.\nAdjust password length: If the length of the password is less than 6, return the maximum of the missing type counter and the difference between 6 and the length of the password. If the length is within the range of 6 to 20, return the maximum of the missing type counter and the change counter. If the length is greater than 20, calculate how many deletions are needed to reduce the length to 20, adjust the change counter based on the number of deletions, and return the sum of the deletions and the maximum of the missing type counter and the adjusted change counter.\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nThe time complexity is O(n), where n is the length of the password. This is because we only need to iterate through the password once to check for missing character types and repeating sequences.\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nThe space complexity is O(1), as we only use a fixed amount of space to store the counts of missing types, changes, and deletions, regardless of the size of the input.\n# Code\n```\nclass Solution:\n def strongPasswordChecker(self, s: str) -> int:\n missing_type = 3\n if any(\'a\' <= c <= \'z\' for c in s):\n missing_type -= 1\n if any(\'A\' <= c <= \'Z\' for c in s):\n missing_type -= 1\n if any(c.isdigit() for c in s):\n missing_type -= 1\n \n change = 0\n one = two = 0\n p = 2\n while p < len(s):\n if s[p] == s[p-1] == s[p-2]:\n length = 2\n while p < len(s) and s[p] == s[p-1]:\n length += 1\n p += 1\n \n change += length // 3\n if length % 3 == 0:\n one += 1\n elif length % 3 == 1:\n two += 1\n else:\n p += 1\n \n if len(s) < 6:\n return max(missing_type, 6 - len(s))\n elif len(s) <= 20:\n return max(missing_type, change)\n else:\n delete = len(s) - 20\n change -= min(delete, one * 1) // 1\n change -= min(max(delete - one, 0), two * 2) // 2\n change -= max(delete - one - 2 * two, 0) // 3\n \n return delete + max(missing_type, change)\n\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
Concise solution with the only external dependency being the 're' library | strong-password-checker | 0 | 1 | # Code\n```\nimport re\n\nUPPER = re.compile("[A-Z]")\nLOWER = re.compile("[a-z]")\nDIGIT = re.compile("[0-9]") \n\n\ndef diversity_penalty(password):\n score = 0\n for r in [UPPER, LOWER, DIGIT]:\n if r.search(password):\n score += 1\n return 3 - score\n\n\ndef try_deleting(password, mod3):\n curr = 1\n to_check = "$" + password + "$"\n for i in range(1, len(to_check)):\n if to_check[i] == to_check[i - 1]:\n curr += 1\n else:\n if curr >= 3 and curr % 3 == mod3:\n return password[:i - 2] + password[i - 1:]\n curr = 1\n return None\n\n\n\ndef count_replacements(password):\n curr = 1\n to_check = "$" + password + "$"\n score = 0\n for i in range(1, len(to_check)):\n if to_check[i] == to_check[i - 1]:\n curr += 1\n else:\n score += curr // 3\n curr = 1\n return score\n \n\nclass Solution:\n \n def strongPasswordChecker(self, password: str) -> int:\n\n div_penalty = diversity_penalty(password)\n\n if len(password) < 6:\n return max(6 - len(password), div_penalty)\n\n original_password = password\n\n while len(password) > 20:\n new_password = try_deleting(password, 0) or try_deleting(password, 1) or try_deleting(password, 2)\n if not new_password:\n return len(original_password) - 20 + div_penalty\n password = new_password\n \n n_replacements = count_replacements(password)\n return max(len(original_password) - 20, 0) + max(n_replacements, div_penalty) \n \n\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
re | strong-password-checker | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def strongPasswordChecker(self, password: str) -> int:\n import re\n \n \n def check_password(password: str):\n \n add, cut = 0, 0\n if len(password) < 6: add = 6 - len(password)\n elif len(password) > 20: cut = len(password) - 20\n \n up, low, dig = 1, 1, 1\n for char in password:\n if char.islower(): low = 0\n elif char.isupper(): up = 0\n elif char.isdigit(): dig = 0\n \n replace = 0\n match_list = re.findall(r\'((.)\\2{2,})+?\', password)\n can_cut = cut\n if match_list:\n match_list = [len(pair[0]) for pair in match_list]\n while can_cut:\n match_list.sort(key=lambda x: x % 3, reverse=True)\n streak = match_list.pop() \n if can_cut <= streak % 3:\n match_list.append(streak)\n break\n if streak < 3:\n continue\n dif = streak % 3 + 1\n streak -= dif\n can_cut -= dif\n match_list.append(streak)\n replace = sum([streak // 3 for streak in match_list])\n if add:\n return max(add, sum((up, low, dig)))\n if cut:\n return cut + max(replace, sum((low, up, dig)))\n return max(replace, sum((low, up, dig)))\n\n\n\n\n return check_password(password)\n\n``` | 0 | A password is considered strong if the below conditions are all met:
* It has at least `6` characters and at most `20` characters.
* It contains at least **one lowercase** letter, at least **one uppercase** letter, and at least **one digit**.
* It does not contain three repeating characters in a row (i.e., `"B**aaa**bb0 "` is weak, but `"B**aa**b**a**0 "` is strong).
Given a string `password`, return _the minimum number of steps required to make `password` strong. if `password` is already strong, return `0`._
In one step, you can:
* Insert one character to `password`,
* Delete one character from `password`, or
* Replace one character of `password` with another character.
**Example 1:**
**Input:** password = "a"
**Output:** 5
**Example 2:**
**Input:** password = "aA1"
**Output:** 3
**Example 3:**
**Input:** password = "1337C0d3"
**Output:** 0
**Constraints:**
* `1 <= password.length <= 50`
* `password` consists of letters, digits, dot `'.'` or exclamation mark `'!'`. | null |
Trie Solution Not Working - TLE (Python) | O(32n) | maximum-xor-of-two-numbers-in-an-array | 0 | 1 | As the comments before the major loops and function in the below given code tells, the allover time complexity (TC) of this code is O(n), but why is it only able to pass 41 out of 45 testcases?!\n\n```python\nclass Trie:\n def __init__(self, val: int):\n self.val = val\n self.children = [None, None]\n \n\t # O(32) = O(1) TC\n def addNum(self, val: int, bitlen: int):\n curr = self\n for i in range(bitlen, -1, -1):\n bit = (val >> i) & 1\n if not curr.children[bit]:\n curr.children[bit] = Trie(bit)\n curr = curr.children[bit]\n \n\t# O(32) = O(1) TC\n def search(self, val: int, bitlen: int):\n curr = self\n maxNum = 0\n for j in range(bitlen - 1, -1, -1):\n bit = (i >> j) & 1\n if curr.children[bit ^ 1]:\n bit ^= 1\n maxNum |= bit << j\n curr = curr.children[bit]\n return val ^ maxNum\n \nclass Solution:\n def findMaximumXOR(self, nums: List[int]) -> int:\n maxi = max(nums)\n if not maxi:\n return 0\n bitlen = int(log2(maxi) + 1)\n \n\t\t# O(32n) = O(n) TC\n tr = Trie(0)\n for i in nums:\n tr.addNum(i, bitlen - 1)\n\n\t\t# O(32n) = O(n) TC\n res = 0\n for i in nums:\n res = max(res, tr.search(i, bitlen - 1))\n return res\n``` | 0 | Given an integer array `nums`, return _the maximum result of_ `nums[i] XOR nums[j]`, where `0 <= i <= j < n`.
**Example 1:**
**Input:** nums = \[3,10,5,25,2,8\]
**Output:** 28
**Explanation:** The maximum result is 5 XOR 25 = 28.
**Example 2:**
**Input:** nums = \[14,70,53,83,49,91,36,80,92,51,66,70\]
**Output:** 127
**Constraints:**
* `1 <= nums.length <= 2 * 105`
* `0 <= nums[i] <= 231 - 1` | null |
Python || Trie Solution|| Easy to Understand | maximum-xor-of-two-numbers-in-an-array | 0 | 1 | ```\nclass Trie:\n def __init__(self):\n self.root={}\n self.m=0\n \n def insert(self,word):\n node=self.root\n for ch in word:\n if ch not in node:\n node[ch]={}\n node=node[ch]\n \n def compare(self,word,i):\n node=self.root\n t=""\n a,b=\'0\',\'1\'\n for ch in word:\n if ch==a and b in node:\n t+=b\n node=node[b]\n elif ch==b and a in node:\n t+=a\n node=node[a]\n else:\n t+=ch\n node=node[ch]\n self.m=max(self.m,int(t,2)^i)\n\nclass Solution:\n def findMaximumXOR(self, nums: List[int]) -> int:\n trie=Trie()\n for i in nums:\n word="{:032b}".format(i)\n trie.insert(word)\n for i in nums:\n word="{:032b}".format(i)\n trie.compare(word,i)\n return trie.m\n```\n**An upvote will be encouraging** | 2 | Given an integer array `nums`, return _the maximum result of_ `nums[i] XOR nums[j]`, where `0 <= i <= j < n`.
**Example 1:**
**Input:** nums = \[3,10,5,25,2,8\]
**Output:** 28
**Explanation:** The maximum result is 5 XOR 25 = 28.
**Example 2:**
**Input:** nums = \[14,70,53,83,49,91,36,80,92,51,66,70\]
**Output:** 127
**Constraints:**
* `1 <= nums.length <= 2 * 105`
* `0 <= nums[i] <= 231 - 1` | null |
421: Solution with step by step explanation | maximum-xor-of-two-numbers-in-an-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. We first find the maximum number in the input list nums using the built-in max function.\n\n2. If the maximum number is 0, it means that all the numbers in the list are also 0, and so the maximum XOR value will also be 0. So we return 0.\n\n3. Otherwise, we calculate the maximum bit position of the maximum number using the math.log2 function.\n\n4. We initialize the ans variable to 0, which will eventually hold the maximum XOR value we find.\n\n5. We also initialize a mask variable to 0, which we will use to generate a set of prefixes for each bit position.\n\n6. We loop through the bit positions in descending order from the maximum bit position to 0.\n\n7. In each iteration, we set the mask variable to the current bit position by left-shifting 1 by the bit position and subtracting 1. For example, if the bit position is 2, mask will be set to 0b00000100.\n\n8. We generate a set of prefixes for the current bit position by applying the mask to each number in the input list using the bitwise AND operator (&). This results in a set of numbers where the rightmost bits up to the current bit position are all 0.\n\n9. We initialize a candidate variable to the current ans value with the bit at the current bit position set to 1 using the bitwise OR operator (|).\n\n10. We loop through each prefix in the set of prefixes we generated in step 8.\n\n11. For each prefix, we check if there exists another prefix in the set that, when XORed with the candidate variable, results in another prefix in the set. If such a prefix exists, it means that we can use the candidate variable as the new ans value, since it indicates that we can form a larger XOR value by setting the bit at the current bit position to 1.\n\n12. If we find such a prefix, we set the ans variable to the candidate value and break out of the loop.\n\n13. We return the ans variable at the end of the loop, which should contain the maximum XOR value we found.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findMaximumXOR(self, nums: List[int]) -> int:\n maxNum = max(nums)\n if maxNum == 0:\n return 0\n maxBit = int(math.log2(maxNum))\n ans = 0\n mask = 0\n\n # If ans is 11100 when i = 2, it means that before we reach the last two\n # bits, 11100 is the maximum XOR we have, and we\'re going to explore if we\n # can get another two \'1\'s and put them into ans.\n for i in range(maxBit, -1, -1):\n # Mask grows like: 100...000, 110...000, 111...000, ..., 111...111.\n mask |= 1 << i\n # We only care about the left parts,\n # If i = 2, nums = [1110, 1011, 0111]\n # -> prefixes = [1100, 1000, 0100]\n prefixes = set([num & mask for num in nums])\n # If i = 1 and before this iteration, the ans is 10100, it means that we\n # want to grow the ans to 10100 | 1 << 1 = 10110 and we\'re looking for\n # XOR of two prefixes = candidate.\n candidate = ans | 1 << i\n for prefix in prefixes:\n if prefix ^ candidate in prefixes:\n ans = candidate\n break\n\n return ans\n\n``` | 6 | Given an integer array `nums`, return _the maximum result of_ `nums[i] XOR nums[j]`, where `0 <= i <= j < n`.
**Example 1:**
**Input:** nums = \[3,10,5,25,2,8\]
**Output:** 28
**Explanation:** The maximum result is 5 XOR 25 = 28.
**Example 2:**
**Input:** nums = \[14,70,53,83,49,91,36,80,92,51,66,70\]
**Output:** 127
**Constraints:**
* `1 <= nums.length <= 2 * 105`
* `0 <= nums[i] <= 231 - 1` | null |
Fast Python solution. Beats 99% | maximum-xor-of-two-numbers-in-an-array | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe idea behind this solution is to use a bitwise operation to find the maximum XOR value of any two numbers in the list.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nThe solution starts by initializing the variable ans to 0. Then, it loops through the bits of the numbers (from the most significant bit to the least significant bit) and for each bit, it shifts the value of ans to the left by 1, and then checks if the current bit can be set to 1 by checking if there are any two numbers in the list with different values for that bit. If there are such numbers, the bit is set to 1 and added to the ans variable.\n\nComplexity\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nwhere n is the number of elements in the list. The solution needs to loop through all the elements in the list and check their bits.\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nwhere n is the number of elements in the list. The solution uses a set to store the prefixes of the numbers in the list.\n# Code\n```\nclass Solution:\n def findMaximumXOR(self, nums: List[int]) -> int:\n ans = 0\n for i in range(32)[::-1]:\n ans <<= 1\n prefixes = {num >> i for num in nums}\n ans += any(ans^1 ^ p in prefixes for p in prefixes)\n return ans\n``` | 7 | Given an integer array `nums`, return _the maximum result of_ `nums[i] XOR nums[j]`, where `0 <= i <= j < n`.
**Example 1:**
**Input:** nums = \[3,10,5,25,2,8\]
**Output:** 28
**Explanation:** The maximum result is 5 XOR 25 = 28.
**Example 2:**
**Input:** nums = \[14,70,53,83,49,91,36,80,92,51,66,70\]
**Output:** 127
**Constraints:**
* `1 <= nums.length <= 2 * 105`
* `0 <= nums[i] <= 231 - 1` | null |
Python 3 | Standard Trie | Explanations | maximum-xor-of-two-numbers-in-an-array | 0 | 1 | ### Explanation\n- Build a trie first\n- For each number `num` in `nums`, try to find number which can generate maximum value with `num` using `XOR`\n\t- From left to right, take the reverse bit if possble, otherwise take the same bit, e.g.\n\t- say if the k th bit from left for `num` is `1`, then to make it maximum, we want to take a number whose k th bit is `0` (if possible); if not possible, we take same bit.\n- Check comments for more detail\n### Implementation\n```\nclass TrieNode:\n def __init__(self):\n self.children = dict() # children nodes\n self.val = 0 # end value \n\nclass Trie:\n def __init__(self, n):\n self.root = TrieNode() # root node\n self.n = n # max length of all numbers\n \n def add_num(self, num):\n node = self.root \n for shift in range(self.n, -1, -1): # only shift self.n bits \n val = 1 if num & (1 << shift) else 0 # verify bit from left to right \n if val not in node.children:\n node.children[val] = TrieNode()\n node = node.children[val]\n node.val = num\n \nclass Solution:\n def findMaximumXOR(self, nums: List[int]) -> int:\n max_len = len(bin(max(nums))) - 2 # get max length of all numbers\' binary\n trie = Trie(max_len)\n for num in nums: trie.add_num(num) # build trie\n \n ans = 0\n for num in nums: # for each num, find the number which can create max value with num using XOR\n node = trie.root \n for shift in range(max_len, -1, -1):\n val = 1 if num & (1 << shift) else 0 # verify bit from left to right\n node = node.children[1-val] if 1-val in node.children else node.children[val] # try opposite bit first, otherwise use same bit\n ans = max(ans, num ^ node.val) # maintain maximum\n return ans \n``` | 21 | Given an integer array `nums`, return _the maximum result of_ `nums[i] XOR nums[j]`, where `0 <= i <= j < n`.
**Example 1:**
**Input:** nums = \[3,10,5,25,2,8\]
**Output:** 28
**Explanation:** The maximum result is 5 XOR 25 = 28.
**Example 2:**
**Input:** nums = \[14,70,53,83,49,91,36,80,92,51,66,70\]
**Output:** 127
**Constraints:**
* `1 <= nums.length <= 2 * 105`
* `0 <= nums[i] <= 231 - 1` | null |
Python Bitwise Solution | maximum-xor-of-two-numbers-in-an-array | 0 | 1 | ```\nclass Solution:\n def findMaximumXOR(self, nums: List[int]) -> int:\n try: l = int(log2(max(nums)))\n except: l = 1\n\n mask, res = 0, 0\n\n for i in range(l, -1, -1):\n mask |= 1 << i\n S = set(mask & num for num in nums)\n temp = res | 1 << i\n\n for num in S:\n if num ^ temp in S:\n res = temp\n break\n\n return res\n\n``` | 1 | Given an integer array `nums`, return _the maximum result of_ `nums[i] XOR nums[j]`, where `0 <= i <= j < n`.
**Example 1:**
**Input:** nums = \[3,10,5,25,2,8\]
**Output:** 28
**Explanation:** The maximum result is 5 XOR 25 = 28.
**Example 2:**
**Input:** nums = \[14,70,53,83,49,91,36,80,92,51,66,70\]
**Output:** 127
**Constraints:**
* `1 <= nums.length <= 2 * 105`
* `0 <= nums[i] <= 231 - 1` | null |
Clean Python Solution using TRIE with Comments Easy to Understand | maximum-xor-of-two-numbers-in-an-array | 0 | 1 | Insert all the elements of nums in a trie with bit values in respective position. where every node can have 2 children either with 0 key or 1 key. \n\nAs XOR is a **inequality detector** so we try to maximize the inequality between num and node. So that the XOR of num and value of node will give the max value.\n\nSo we do the following steps While traversing the num from 31\'th bit position to 0\'th bit position:\n1. If the current bit of num is 1 then we try to move the cur pointer towards the child with 0 key.\n2. And if the current bit of num is 0 then we try to move the cur pointer towards the child with 1 key.\n\n```\n\nclass TrieNode:\n def __init__(self):\n self.children = {}\n self.val = -1 # used to store the value of entire number at the end of trie node\n\nclass Trie:\n def __init__(self):\n self.root = TrieNode() # object of trienode class\n \n def addNum(self, num):\n cur = self.root # every time start from root\n for i in range(31, -1, -1):\n bit = 1 if num & (1 << i) else 0 # bit value i\'th position of num \n if bit not in cur.children:\n cur.children[bit] = TrieNode()\n cur = cur.children[bit]\n cur.val = num # storing the value of entire num at the end of trie node\n \n\nclass Solution:\n def findMaximumXOR(self, nums: List[int]) -> int:\n trie = Trie() # creating object of Trie class\n for num in nums: # adding all num to the trie structure\n trie.addNum(num)\n \n res = 0\n for num in nums: \n cur = trie.root # every time start cur pointer from root\n for i in range(31, -1, -1):\n bit = 1 if num & (1 << i) else 0 # bit value of i\'th position of num \n if bit == 1: # try to move towards opposite key ie. 0\n if 0 in cur.children: # opposit key 0 exist then defenetly go towards the child 0\n cur = cur.children[0]\n else: # opposit key 0 not exist so we have only option to go towards what we have ie. 1\n cur = cur.children[1]\n else: # bit == 0 # try to move towards opposite key ie. 1\n if 1 in cur.children: # opposit key 1 exist then defenetly go towards the child 1\n cur = cur.children[1]\n else: # opposit key 1 not exist so we have only option to go towards what we have ie. 0\n cur = cur.children[0]\n # as we tried to maximize the inequality between cur.val and num so XOR of them will give max value\n res = max(res, cur.val ^ num)\n \n return res\n``` | 6 | Given an integer array `nums`, return _the maximum result of_ `nums[i] XOR nums[j]`, where `0 <= i <= j < n`.
**Example 1:**
**Input:** nums = \[3,10,5,25,2,8\]
**Output:** 28
**Explanation:** The maximum result is 5 XOR 25 = 28.
**Example 2:**
**Input:** nums = \[14,70,53,83,49,91,36,80,92,51,66,70\]
**Output:** 127
**Constraints:**
* `1 <= nums.length <= 2 * 105`
* `0 <= nums[i] <= 231 - 1` | null |
70% TC and 50% SC easy python solution, with clear approach | maximum-xor-of-two-numbers-in-an-array | 0 | 1 | 1. Insert every num in the tree, and make sure that they have equal number of bits, with leading zeros.\n2. Then try every num, and find what will be the max xor of it with any other num in the array. And come up with the max xor of all.\n3. And thats done, like, if the current digit is 1, chech if 0 is avlbl at that place in the trie, and vice versa. If it is, the xor will have 1 at that bit, else 0.\n```\ndef findMaximumXOR(self, nums: List[int]) -> int:\n\tclass Trie:\n\t\tdef __init__(self):\n\t\t\tself.root = dict()\n\t\tdef insert(self, s):\n\t\t\tc = self.root\n\t\t\tfor i in s:\n\t\t\t\tif(i not in c):\n\t\t\t\t\tc[i] = dict() \n\t\t\t\tc = c[i]\n\t\t\n\tt = Trie()\n\textra = \'\'.join([\'0\'] * 31)\n\tfor num in nums:\n\t\tb = (extra + bin(num)[2:])[-31:]\n\t\tt.insert(b)\n\tans = 0\n\tfor num in nums:\n\t\tb = (extra + bin(num)[2:])[-31:]\n\t\tc = t.root\n\t\ttemp = 0\n\t\tfor d in b:\n\t\t\tif(d == "1" and "0" in c):\n\t\t\t\ttemp = 2*temp + 1\n\t\t\t\tc = c["0"]\n\t\t\telif(d == "0" and "1" in c):\n\t\t\t\ttemp = 2*temp + 1\n\t\t\t\tc = c["1"]\n\t\t\telse:\n\t\t\t\tc = c[d]\n\t\t\t\ttemp *= 2\n\t\tans = max(temp, ans)\n\treturn ans\n``` | 1 | Given an integer array `nums`, return _the maximum result of_ `nums[i] XOR nums[j]`, where `0 <= i <= j < n`.
**Example 1:**
**Input:** nums = \[3,10,5,25,2,8\]
**Output:** 28
**Explanation:** The maximum result is 5 XOR 25 = 28.
**Example 2:**
**Input:** nums = \[14,70,53,83,49,91,36,80,92,51,66,70\]
**Output:** 127
**Constraints:**
* `1 <= nums.length <= 2 * 105`
* `0 <= nums[i] <= 231 - 1` | null |
Python Simple Solution (beginners) (video + code) (Trie) | maximum-xor-of-two-numbers-in-an-array | 0 | 1 | [](https://www.youtube.com/watch?v=wSgrc98d2lI)\nhttps://www.youtube.com/watch?v=wSgrc98d2lI\n```\nclass TrieNode:\n def __init__(self):\n self.children = {}\n \nclass Solution:\n def __init__(self):\n self.root = TrieNode()\n \n def insert_bits(self, num):\n bit_num = bin(num)[2:].zfill(32)\n node = self.root\n for bit in bit_num:\n if bit not in node.children:\n node.children[bit] = TrieNode()\n node = node.children[bit]\n \n \n def find_max_xor(self, num):\n bit_num = bin(num)[2:].zfill(32)\n node = self.root\n max_xor = \'\'\n for bit in bit_num:\n if bit == \'0\':\n oppo_bit = \'1\'\n elif bit == \'1\':\n oppo_bit = \'0\'\n \n if oppo_bit in node.children:\n max_xor += oppo_bit\n node = node.children[oppo_bit]\n else:\n max_xor += bit\n node = node.children[bit]\n \n return int(max_xor, 2) ^ num\n \n \n def findMaximumXOR(self, nums: List[int]) -> int:\n for num in nums:\n self.insert_bits(num)\n \n max_ = 0\n for num in nums:\n max_ = max(max_, self.find_max_xor(num))\n \n return max_\n``` | 10 | Given an integer array `nums`, return _the maximum result of_ `nums[i] XOR nums[j]`, where `0 <= i <= j < n`.
**Example 1:**
**Input:** nums = \[3,10,5,25,2,8\]
**Output:** 28
**Explanation:** The maximum result is 5 XOR 25 = 28.
**Example 2:**
**Input:** nums = \[14,70,53,83,49,91,36,80,92,51,66,70\]
**Output:** 127
**Constraints:**
* `1 <= nums.length <= 2 * 105`
* `0 <= nums[i] <= 231 - 1` | null |
423: Solution with step by step explanation | reconstruct-original-digits-from-english | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. Create an empty dictionary called char_counts to keep track of the count of each character in the input string s.\n2. Iterate over each character c in the input string s.\n3. For each character c, update its count in char_counts by either incrementing its count if it already exists in the dictionary or adding it to the dictionary with a count of 1.\n4. Create an empty list called digit_counts of length 10 to keep track of the count of each digit in the final output.\n5. Use the get method of char_counts with a default value of 0 to update the counts of digits 0, 2, 4, 6, and 8 in digit_counts based on the counts of their corresponding characters \'z\', \'w\', \'u\', \'x\', and \'g\' in char_counts.\n6. Calculate the count of digit 1 in digit_counts by subtracting the counts of digits 0, 2, and 4 from the count of character \'o\' in char_counts.\n7. Calculate the count of digit 3 in digit_counts by subtracting the count of digit 8 from the count of character \'h\' in char_counts.\n8. Calculate the count of digit 5 in digit_counts by subtracting the count of digit 4 from the count of character \'f\' in char_counts.\n9. Calculate the count of digit 7 in digit_counts by subtracting the count of digit 6 from the count of character \'s\' in char_counts.\n10. Calculate the count of digit 9 in digit_counts by subtracting the counts of digits 5, 6, and 8 from the count of character \'i\' in char_counts.\n11. Use a list comprehension to create a string that concatenates the digits in digit_counts based on their counts. Return this string as the final output.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n char_counts = {}\n for c in s:\n if c in char_counts:\n char_counts[c] += 1\n else:\n char_counts[c] = 1\n \n digit_counts = [0] * 10\n \n digit_counts[0] = char_counts.get(\'z\', 0)\n digit_counts[2] = char_counts.get(\'w\', 0)\n digit_counts[4] = char_counts.get(\'u\', 0)\n digit_counts[6] = char_counts.get(\'x\', 0)\n digit_counts[8] = char_counts.get(\'g\', 0)\n \n digit_counts[1] = char_counts.get(\'o\', 0) - digit_counts[0] - digit_counts[2] - digit_counts[4]\n digit_counts[3] = char_counts.get(\'h\', 0) - digit_counts[8]\n digit_counts[5] = char_counts.get(\'f\', 0) - digit_counts[4]\n digit_counts[7] = char_counts.get(\'s\', 0) - digit_counts[6]\n digit_counts[9] = char_counts.get(\'i\', 0) - digit_counts[5] - digit_counts[6] - digit_counts[8]\n \n return \'\'.join(str(i) * digit_counts[i] for i in range(10))\n\n``` | 8 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Fast Python solution O(n) | reconstruct-original-digits-from-english | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe problem is asking to find the unique digits in the given string by counting the number of occurrences of each letter in the string and using that information to deduce the number of each digit.\n\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe can use a counter to count the number of occurrences of each letter in the string. We can then use this information to deduce the number of each digit by subtracting the number of occurrences of other letters that are used in the formation of that digit. For example, the number of occurrences of the letter \'o\' can be used to deduce the number of occurrences of the digit \'1\' and \'0\' since \'1\' and \'0\' both use the letter \'o\'. We can then construct the final string by concatenating the digit with the number of occurrences of that digit.\n\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nwhere n is the length of the input string. We are iterating through the string once to count the occurrences of each letter and then again to construct the final string.\n- Space complexity: O(1) \n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nwe only need a fixed number of variables to store the occurrences of each digit and the counter.\n# Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n from collections import Counter\n c = Counter(s)\n nums = [0] * 10\n nums[0] = c[\'z\']\n nums[2] = c[\'w\']\n nums[4] = c[\'u\']\n nums[6] = c[\'x\']\n nums[8] = c[\'g\']\n nums[1] = c[\'o\'] - nums[0] - nums[2] - nums[4]\n nums[3] = c[\'h\'] - nums[8]\n nums[5] = c[\'f\'] - nums[4]\n nums[7] = c[\'s\'] - nums[6]\n nums[9] = c[\'i\'] - nums[5] - nums[6] - nums[8]\n return \'\'.join(str(i) * n for i, n in enumerate(nums))\n\n``` | 4 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Python/Go O(n) by dictionary [w/ Diagram] | reconstruct-original-digits-from-english | 0 | 1 | **Hint**:\n\n1. Rebuild **even digits** first, becuase each even digit has corresponding **unique character** naturally\n\n1. Then rebuild **odd digits** from observed character-occurrence mapping.\n\n---\n\n**Diagram** for even digits (i.e., 0, 2, 4, 6, 8 ) rebuilding based on character-occurrence mapping\n\n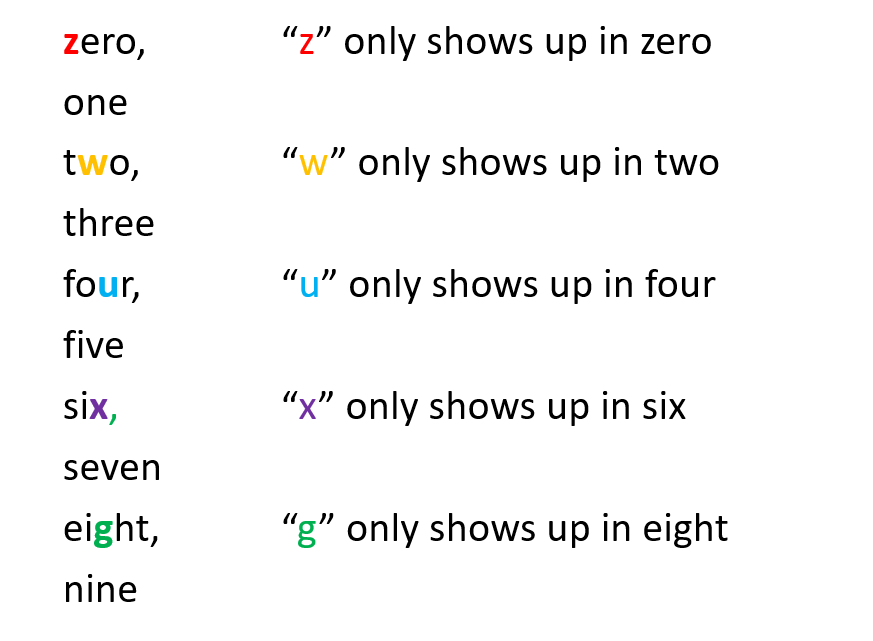\n\n---\n\n**Diagram** for odd digits (i.e., 1, 3, 5, 7, 9 ) rebuilding based on character-occurrence mapping\n\n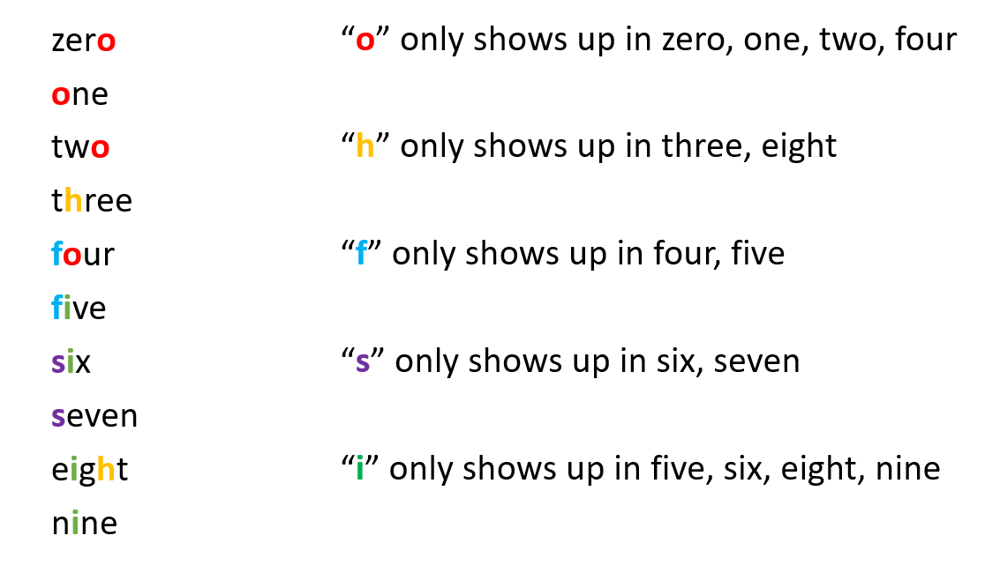\n\n\n---\n\n**Implementation** by dictioanry in Python:\n\n```\n\n\nclass Solution:\n def originalDigits(self, s: str) -> str:\n\n \n # ----------------------------------------------------------\n \n def mapping_rebuild( digit_occ_dict , char_occ_dict ):\n \n \n ## Rebuild the number and its occurrence from character frequency analysis\n \n \n # "z" only shows up in "zero"\n digit_occ_dict [0] = char_occ_dict[\'z\']\n\n # "w" only shows up in "two"\n digit_occ_dict [2] = char_occ_dict[\'w\']\n\n # "u" only shows up in "four"\n digit_occ_dict [4] = char_occ_dict[\'u\']\n\n # "x" only shows up in "six"\n digit_occ_dict [6] = char_occ_dict[\'x\']\n\n # "g" only shows up in "eight"\n digit_occ_dict [8] = char_occ_dict[\'g\']\n\n # "o" only shows up in "zero", "one", "two", "four"\n digit_occ_dict [1] = char_occ_dict[\'o\'] - digit_occ_dict [0] - digit_occ_dict [2] - digit_occ_dict [4]\n\n # "h" only shows up in "three", "eight"\n digit_occ_dict [3] = char_occ_dict[\'h\'] - digit_occ_dict [8]\n\n # "f" only shows up in "four", "five"\n digit_occ_dict [5] = char_occ_dict[\'f\'] - digit_occ_dict [4]\n\n # "s" only shows up in "six", "seven"\n digit_occ_dict [7] = char_occ_dict[\'s\'] - digit_occ_dict [6]\n\n # "i" only shows up in "five", "six", "eight", "nine"\n digit_occ_dict [9] = char_occ_dict[\'i\'] - digit_occ_dict [5] - digit_occ_dict [6] - digit_occ_dict [8]\n\n return\n # ----------------------------------------------------------\n \n ## dictionary of input s\n # key: ascii character\n # value: occurrence of ascii character\n char_occ_dict = Counter(s)\n \n ## dictionary\n # key: digit\n # value: occurrence of digit\n digit_occ_dict = defaultdict( int )\n \n # rebuild digit-occurrence mapping from input s and its char-occurrence mapping\n mapping_rebuild( digit_occ_dict , char_occ_dict)\n \n # rebuild digit string in ascending order\n digit_string = "".join( (str(digit) * digit_occ_dict [digit]) for digit in range(0, 10) )\n \n return digit_string\n \n \n```\n\n---\n\n\n\n**Implementation** by dictiaonry in Golang:\n\n```\n\nimport (\n\t"strings"\n\t"strconv"\n)\n\nfunc originalDigits(s string) string {\n \n //// dictionary of input s\n // key: ascii character\n // value: occurrence of ascii character\n charOccDict := make( map[rune]int )\n \n for _, char := range s{\n charOccDict[char] += 1\n }\n \n //// dictionary\n // key: digit\n // value: occurrence of digit \n digitOccDict := make( map[int]int )\n \n // rebuild digit-occurrence mapping from input s and its char-occurrence mapping\n mapping_rebuild( &digitOccDict, &charOccDict )\n \n \n // rebuild digit string in ascending order\n outputString := ""\n \n for digit := 0 ; digit <= 9 ; digit++{\n \n occurrence := digitOccDict[digit]\n outputString += strings.Repeat( strconv.Itoa(digit), occurrence)\n }\n \n return outputString\n}\n\n\nfunc mapping_rebuild( digitMap *map[int]int, letterMap *map[rune]int){\n // Rebuild the number and its occurrence from character frequency analysis\n \n // "z" only shows up in "zero"\n (*digitMap)[0] = (*letterMap)[\'z\']\n \n // "w" only shows up in "two"\n (*digitMap)[2] = (*letterMap)[\'w\']\n \n // "u" only shows up in "four"\n (*digitMap)[4] = (*letterMap)[\'u\']\n \n // "x" only shows up in "six"\n (*digitMap)[6] = (*letterMap)[\'x\']\n \n // "g" only shows up in "eight"\n (*digitMap)[8] = (*letterMap)[\'g\']\n \n // "o" only shows up in "zero", "one", "two", "four"\n (*digitMap)[1] = (*letterMap)[\'o\'] - (*digitMap)[0] - (*digitMap)[2] - (*digitMap)[4] \n \n // "h" only shows up in "three", "eight"\n (*digitMap)[3] = (*letterMap)[\'h\'] - (*digitMap)[8]\n \n // "f" only shows up in "four", "five"\n (*digitMap)[5] = (*letterMap)[\'f\'] - (*digitMap)[4] \n \n // "s" only shows up in "six", "seven"\n (*digitMap)[7] = (*letterMap)[\'s\'] - (*digitMap)[6]\n \n // "i" only shows up in "five", "six", "eight", "nine"\n (*digitMap)[9] = (*letterMap)[\'i\'] - (*digitMap)[5] - (*digitMap)[6] - (*digitMap)[8] \n\n}\n\n```\n\n---\n\n\nReference:\n\n[1] [Python official docs about specialized dictionary Counter( ... )](https://docs.python.org/3/library/collections.html#collections.Counter)\n\n[2] [Golang official docs about dictionary map[]](https://blog.golang.org/maps) | 17 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
[Java/C++/Python/JavaScript/Swift]O(n)time/BEATS 99.97% MEMORY/SPEED 0ms APRIL 2022 | reconstruct-original-digits-from-english | 1 | 1 | ```\n```\n\n(Note: This is part of a series of Leetcode solution explanations. If you like this solution or find it useful, ***please upvote*** this post.)\n***Take care brother, peace, love!***\n\n```\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***0ms / 38.2MB*** (beats 92.04% / 24.00%).\n* ***Java***\n```\nclass Solution {\n static final int[] DIGS = {0,2,4,6,8,5,7,3,9,1}, CHARS = {25,22,20,23,6,5,18,7,8,14};\n static final int[][] REMS = {{14},{14},{5,14},{18,8},{8,7},{8},{},{},{},{}};\n public String originalDigits(String S) {\n int[] fmap = new int[26], ans = new int[10];\n char[] SCA = S.toCharArray();\n for (char c : SCA) fmap[c - 97]++;\n for (int i = 0; i < 10; i++) {\n int count = fmap[CHARS[i]];\n for (int rem : REMS[i]) fmap[rem] -= count;\n ans[DIGS[i]] = count;\n }\n StringBuilder sb = new StringBuilder();\n for (int i = 0; i < 10; i++) {\n char c = (char)(i + 48);\n for (int j = 0; j < ans[i]; j++)\n sb.append(c);\n }\n return sb.toString();\n }\n}\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***0ms / 7.0MB*** (beats 100.00% / 100.00%).\n* ***C++***\n```\nclass Solution {\nconst int DIGS[10] = {0,2,4,6,8,5,7,3,9,1}, CHARS[10] = {25,22,20,23,6,5,18,7,8,14};\nconst vector<vector<int>> REMS = {{14},{14},{5,14},{18,8},{8,7},{8},{},{},{},{}};\npublic:\n string originalDigits(string S) {\n int fmap[26] = {0}, ans[10] = {0};\n for (char c : S) fmap[c - 97]++;\n for (int i = 0; i < 10; i++) {\n int count = fmap[CHARS[i]];\n for (int rem : REMS[i]) fmap[rem] -= count;\n ans[DIGS[i]] = count;\n }\n string ansstr;\n for (int i = 0; i < 10; i++) {\n char c = (char)(i + 48);\n for (int j = ans[i]; j; j--)\n ansstr += c;\n }\n return ansstr;\n }\n};\n```\n\n```\n```\n\n```\n```\n\n\nThe best result for the code below is ***26ms / 12.2MB*** (beats 95.42% / 82.32%).\n* ***Python***\n```\nDIGITS = [\n [0,\'z\',[]],\n [2,\'w\',[]],\n [4,\'u\',[]],\n [6,\'x\',[]],\n [8,\'g\',[]],\n [5,\'f\',[4]],\n [7,\'s\',[6]],\n [3,\'h\',[8]],\n [9,\'i\',[6,8,5]],\n [1,\'o\',[0,2,4]]\n]\nclass Solution:\n def originalDigits(self, S: str) -> str:\n fmap, ans, n = [0] * 26, [0] * 10, len(S)\n for i in range(10):\n dig, char, rems = DIGITS[i]\n count = S.count(char)\n for rem in rems: count -= ans[rem]\n ans[dig] += count\n return "".join([str(i) * ans[i] for i in range(10)])\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***51ms / 34.2MB*** (beats 100.00% / 84.12%).\n* ***JavaScript***\n```\nconst DIGITS = [\n ["0",25,[14]],\n ["2",22,[14]],\n ["4",20,[5,14]],\n ["6",23,[18,8]],\n ["8",6,[8,7]],\n ["5",5,[8]],\n ["7",18,[]],\n ["3",7,[]],\n ["9",8,[]],\n ["1",14,[]]\n]\nvar originalDigits = function(S) {\n let fmap = new Uint16Array(26),\n ans = new Array(10), len = S.length\n for (let i = 0; i < len; i++)\n fmap[S.charCodeAt(i) - 97]++\n for (let i = 0; i < 10; i++) {\n let [dig, char, rems] = DIGITS[i],\n count = fmap[char]\n for (let j = 0; j < rems.length; j++)\n fmap[rems[j]] -= count\n ans[dig] = dig.repeat(count)\n }\n return ans.join("")\n};\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***12ms / 32.2MB*** (beats 95% / 84%).\n* ***Swift***\n```\nclass Solution {\n func originalDigits(_ s: String) -> String {\n let s = Array(s).map({ Int($0.asciiValue ?? 97) - 97 }), alphabet = 26, digits = 10\n var a = [Int](repeating: 0, count: alphabet), d = [Int](repeating: 0, count: digits), result = [Int]()\n for char in s { a[char] += 1 } // count all characters in source string\n\t\t// z is unique char, count all zero\n while a[25] > 0 { d[0] += 1; a[25] -= 1; a[4] -= 1; a[17] -= 1; a[14] -= 1 }\n\t\t// w is unique char, count all two\n while a[22] > 0 { d[2] += 1; a[19] -= 1; a[22] -= 1; a[14] -= 1 }\n\t\t// u is unique char, count all four\n while a[20] > 0 { d[4] += 1; a[5] -= 1; a[14] -= 1; a[20] -= 1; a[17] -= 1 }\n\t\t// x is unique char, count all six\n while a[23] > 0 { d[6] += 1; a[18] -= 1; a[8] -= 1; a[23] -= 1 }\n\t\t// g is unique char, count all eight\n while a[6] > 0 { d[8] += 1; a[4] -= 1; a[8] -= 1; a[6] -= 1; a[7] -= 1; a[19] -= 1 }\n\t\t// now r is unique char, count all three\n while a[17] > 0 { d[3] += 1; a[19] -= 1; a[7] -= 1; a[17] -= 1; a[4] -= 2 }\n\t\t// now f is unique char, count all five\n while a[5] > 0 { d[5] += 1; a[5] -= 1; a[8] -= 1; a[21] -= 1; a[4] -= 1 }\n\t\t// now s is unique char, count all seven\n while a[18] > 0 { d[7] += 1; a[18] -= 1; a[4] -= 2; a[21] -= 1; a[13] -= 1 }\n\t\t// now o is unique char, count all one\n while a[14] > 0 { d[1] += 1; a[14] -= 1; a[13] -= 1; a[4] -= 1 }\n\t\t// now i is unique char, count all nine\n while a[8] > 0 { d[9] += 1; a[13] -= 2; a[8] -= 1; a[4] -= 1 }\n\t\t// make result\n for i in 0..<digits { for j in 0..<d[i] { result.append(i) } }\n return result.map({ "\\($0)" }).joined(separator: "")\n }\n}\n```\n\n```\n```\n\n```\n```\n\n***"Open your eyes. Expect us." - \uD835\uDCD0\uD835\uDCF7\uD835\uDCF8\uD835\uDCF7\uD835\uDD02\uD835\uDCF6\uD835\uDCF8\uD835\uDCFE\uD835\uDCFC*** | 3 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Python, Simple | reconstruct-original-digits-from-english | 0 | 1 | # Idea\nCertain chars are unique to some strings, like \'w\' in \'two\' or \'z\' in \'zero\'. As we process the digits other chars become unique, for example \'r\' is unique to \'three\' after we\'ve removed all \'four\'s and \'zero\'s. In python3 the dictionary is ordered by key insertion time by default, so the solution below works:\n```\ndef originalDigits(self, s: str) -> str:\n\tcnt = Counter(s)\n\tidchars = \\\n\t{\n\t\t\'w\': (\'two\', \'2\'),\n\t\t\'u\': (\'four\', \'4\'),\n\t\t\'x\': (\'six\', \'6\'),\n\t\t\'f\': (\'five\', \'5\'),\n\t\t\'z\': (\'zero\', \'0\'),\n\t\t\'r\': (\'three\', \'3\'), \n\t\t\'t\': (\'eight\', \'8\'),\n\t\t\'s\': (\'seven\', \'7\'),\n\t\t\'i\': (\'nine\', \'9\'),\n\t\t\'n\': (\'one\', \'1\')\n\t}\n\tdigits = []\n\tfor ch, (word, digit) in idchars.items():\n\t\tdigit_count = cnt[ch]\n\t\tdigits.append(digit * digit_count)\n\t\tfor c in word: cnt[c] -= digit_count\n\treturn \'\'.join(sorted(digits))\n``` | 7 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Python | Intuitive Solution With Explanation | reconstruct-original-digits-from-english | 0 | 1 | \n# Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n # 0 -> 2 -> 4 -> 6 -> 8 -> 1 -> 3 -> 5 ->7 -> 9\n digitEnglishMap = {\n 0: {"z": 1, "e": 1, "r": 1, "o": 1},\n 1: {"o":1, "n":1, "e":1},\n 2: {"t":1, "w":1, "o":1},\n 3: {"t":1, "h":1, "r":1, "e":2},\n 4: {"f":1, "o":1, "u":1, "r":1},\n 5: {"f":1, "i":1, "v":1, "e":1},\n 6: {"s":1, "i":1, "x":1},\n 7: {"s":1, "e":2, "v":1, "n":1},\n 8: {"e":1, "i":1, "g":1, "h":1, "t":1},\n 9: {"n":2, "i":1, "e":1}\n }\n lookup = {}\n for ch in s:\n if ch not in lookup:\n lookup[ch] = 0\n lookup[ch] += 1\n \n ans = []\n """ \n Stream is taken in this order because digits which has some unique chars should be processed first so that it doesn\'t get overriden.\n \n Example:\n s: "zeroonetwothreefourfivesixseveneightnine"\n incase of strem(0-9) 01113356 which is wrong.\n correct output: "0123456789"\n """\n for digit in (0, 2, 4, 6, 8, 1, 3, 5, 7, 9):\n digitExists = True\n while digitExists:\n # Check whether digit exists\n for ch in digitEnglishMap[digit]:\n count = digitEnglishMap[digit][ch]\n if ch not in lookup or lookup[ch] < count:\n digitExists = False\n break\n # If digit exists, add it in ans and remove from lookup\n if digitExists:\n ans.append(str(digit))\n for ch in digitEnglishMap[digit]:\n count = digitEnglishMap[digit][ch]\n lookup[ch] -= count\n return "".join(sorted(ans))\n```\n\n**If you liked the above solution then please upvote!** | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
OOP solution | reconstruct-original-digits-from-english | 0 | 1 | \n# Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n self.s = s\n self.result = \'\'\n self.digits = {\'zero\': 0, \'one\': 1, \'two\': 2, \'three\': 3, \'four\': 4, \'five\': 5, \'six\': 6, \'seven\': 7,\n \'eight\': 8, \'nine\': 9}\n\n return self.iterate()\n\n def remove_substring(self, word_to_remove):\n for letter in word_to_remove:\n self.s = self.s.replace(letter, \'\', 1)\n self.result += str(self.digits[word_to_remove])\n return self\n\n def check(self):\n if \'w\' in self.s:\n self.remove_substring(\'two\')\n elif \'u\' in self.s:\n self.remove_substring(\'four\')\n elif \'x\' in self.s:\n self.remove_substring(\'six\')\n elif \'g\' in self.s:\n self.remove_substring(\'eight\')\n elif \'z\' in self.s:\n self.remove_substring(\'zero\')\n elif \'o\' in self.s and \'n\' in self.s and \'e\' in self.s:\n self.remove_substring(\'one\')\n elif \'t\' in self.s and \'h\' in self.s and \'r\' in self.s and \'e\' in self.s and \'e\' in self.s:\n self.remove_substring(\'three\')\n elif \'f\' in self.s and \'i\' in self.s and \'v\' in self.s and \'e\' in self.s:\n self.remove_substring(\'five\')\n elif \'s\' in self.s and \'e\' in self.s and \'v\' in self.s and \'e\' in self.s and \'n\' in self.s:\n self.remove_substring(\'seven\')\n elif \'n\' in self.s and \'i\' in self.s and \'n\' in self.s and \'e\' in self.s:\n self.remove_substring(\'nine\')\n\n return self\n\n def iterate(self) -> str:\n while self.s:\n self.check()\n return \'\'.join(sorted(self.result))\n\n\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Easy but long solution | reconstruct-original-digits-from-english | 0 | 1 | # Intuition\nEliminate letters greedily in order of unique letters in the corresponding numbers. For example, the only number containing "w" between "zero" and "nine" is "two. Next, the only number containing "x" between "zero" and "nine" excluding "two" is "six". It works out nicely... Just need to sort at the end (or not, if we use another hash table to store frequencies, making runtime $O(n)$)\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: $$O(n\\log n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nfrom collections import defaultdict\n\nclass Solution:\n def originalDigits(self, s: str) -> str:\n freqs = defaultdict(int)\n\n for c in s:\n freqs[c] += 1\n\n res = ""\n\n # check 2\n while "w" in freqs:\n res += "2"\n for c in "two":\n freqs[c] -= 1\n if freqs[c] == 0:\n del freqs[c]\n \n # check 6\n while "x" in freqs:\n res += "6"\n for c in "six":\n freqs[c] -= 1\n if freqs[c] == 0:\n del freqs[c]\n \n # check 8\n while "g" in freqs:\n res += "8"\n for c in "eight":\n freqs[c] -= 1\n if freqs[c] == 0:\n del freqs[c]\n\n # check 4\n while "u" in freqs:\n res += "4"\n for c in "four":\n freqs[c] -= 1\n if freqs[c] == 0:\n del freqs[c]\n \n # check 7\n while "s" in freqs:\n res += "7"\n for c in "seven":\n freqs[c] -= 1\n if freqs[c] == 0:\n del freqs[c]\n \n # check 3\n while "h" in freqs:\n res += "3"\n for c in "three":\n freqs[c] -= 1\n if freqs[c] == 0:\n del freqs[c]\n \n # check 5\n while "v" in freqs:\n res += "5"\n for c in "five":\n freqs[c] -= 1\n if freqs[c] == 0:\n del freqs[c]\n \n # check 9\n while "i" in freqs:\n res += "9"\n for c in "nine":\n freqs[c] -= 1\n if freqs[c] == 0:\n del freqs[c]\n \n # check one\n while "n" in freqs:\n res += "1"\n for c in "one":\n freqs[c] -= 1\n if freqs[c] == 0:\n del freqs[c]\n\n # check zero\n while "z" in freqs:\n res += "0"\n for c in "zero":\n freqs[c] -= 1\n if freqs[c] == 0:\n del freqs[c]\n \n res = sorted(list(res))\n\n return "".join(res)\n\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
python3 solution | reconstruct-original-digits-from-english | 0 | 1 | <!-- # Intuition -->\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach : using hashmap \n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n char = Counter(s)\n count = [0] * 10\n count[0] = char[\'z\']\n count[2] = char[\'w\']\n count[4] = char[\'u\']\n count[6] = char[\'x\']\n count[7] = char[\'s\'] - count[6]\n count[8] = char[\'g\']\n count[1] = char[\'o\'] - count[0] - count[2] - count[4]\n count[3] = char[\'h\'] - count[8]\n count[5] = char[\'v\'] - count[7]\n count[9] = char[\'i\'] - count[5] - count[6] - count[8]\n result = ""\n for i in range(10):\n result += str(i) * count[i]\n return result\n\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Simple intuition, easy to understand. | reconstruct-original-digits-from-english | 0 | 1 | # Intuition\nWe need to identify the order in which we can uniquely create a number.\nFor example, only zero has z, two has w, four has u, and six has x.\n\nonce we have built six then only seven has s in it.\nonce we build all the sevens then only five has v in it\nAlso we can observe only three has r in it and once we build all 3s then eight has h and t\nand after we build all 8s then only nine has i in it and after that last number remaining is one.\n\nAnd since the string is randomly ordered we need a dictionary to store the char count and keep reducing it as we are building the numbers.\n\nThere would be further optimisations that can be done but this is easy to understand.\n\nEDIT - I have added the fastest method as well.\n\n\n# Complexity\n- Time complexity: O(n)\n\n- Space complexity: O(n) \n\n# Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n d = {}\n for i in s:\n d[i] = d.setdefault(i, 0) + 1\n ls = ["zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine"]\n\n\n ans = \'\'\n\n\n for i in [0, 2, 4, 6, 7, 5, 3, 8, 9, 1]:\n if all(i == 0 for i in d.values()):\n return ans\n\n while all(j in d and d[j] > 0 for j in ls[i]):\n ans += str(i)\n for j in ls[i]:\n d[j] -= 1\n\n return \'\'.join(sorted(ans))\n```\n\nOptimisation 1\n\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n d = {}\n for i in s:\n d[i] = d.setdefault(i, 0) + 1\n ls = ["zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine"]\n\n\n ans = \'\'\n\n\n for i in [0, 2, 4, 6, 7, 5, 3, 8, 9, 1]:\n if all(i == 0 for i in d.values()):\n return ans\n if all(j in d and d[j] > 0 for j in ls[i]):\n nums_possible = min(d[j] for j in ls[i])\n ans += str(i)*nums_possible\n for j in ls[i]:\n d[j] -= nums_possible\n # while all(j in d and d[j] > 0 for j in ls[i]):\n # ans += str(i)\n # for j in ls[i]:\n # d[j] -= 1\n\n return \'\'.join(sorted(ans))\n```\n\nOptimisation 2\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n d = {}\n for i in s:\n d[i] = d.setdefault(i, 0) + 1\n ls = ["zero", "one", "two", "three", "four", "five", "six", "seven", "eight", "nine"]\n\n\n ans = \'\'\n counts = [0 for i in range(0, 10)]\n \n for i in [0, 2, 4, 6, 7, 5, 3, 8, 9, 1]:\n if all(j in d and d[j] > 0 for j in ls[i]):\n nums_possible = min(d[j] for j in ls[i])\n for j in ls[i]:\n d[j] -= nums_possible\n counts[i] = nums_possible\n for i in range(10):\n ans += str(i)*counts[i]\n return ans\n # return \'\'.join(sorted(ans))\n```\n\nFastest\n\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n d = {}\n for i in s:\n d[i] = d.setdefault(i, 0) + 1\n\n\n ans = \'\'\n counts = [0 for i in range(0, 10)]\n counts[0] = d.setdefault(\'z\', 0)\n counts[2] = d.setdefault(\'w\', 0)\n counts[4] = d.setdefault(\'u\', 0)\n counts[6] = d.setdefault(\'x\', 0)\n counts[8] = d.setdefault(\'g\', 0)\n counts[7] = d.setdefault(\'s\', 0) - counts[6]\n counts[5] = d.setdefault(\'v\', 0) - counts[7]\n counts[3] = d.setdefault(\'r\', 0) - counts[4] - counts[0]\n \n counts[9] = d.setdefault(\'i\', 0) - counts[5] - counts[6] - counts[8]\n counts[1] = d.setdefault(\'o\', 0) - counts[0] - counts[2] - counts[4]\n \n for i in range(10):\n ans += str(i)*counts[i]\n return ans\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Easy python3 | reconstruct-original-digits-from-english | 0 | 1 | # Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n from collections import Counter\n maps_l = [{\'z\': 0, "w": 2, \'u\': 4, "x": 6, "g": 8}, {\'o\': 1, \'t\':3, \'f\': 5, \'s\': 7}]\n map_d = {0:\'zero\', 1: \'one\', 2 :\'two\', 3: \'three\', 4: \'four\', 5: \'five\', 6: \'six\', 7:\'seven\', 8:\'eight\', 9: \'nine\'}\n res = []\n s = Counter(s)\n\n # appear once\n for maps in maps_l:\n for l in maps:\n while l in s:\n res.append(maps[l])\n word = map_d[maps[l]]\n for w in word:\n s[w] -= 1\n if not s[w]:\n del s[w]\n if s:\n res += [9 for _ in range(s[\'e\'])]\n\n return \'\'.join([str(_s) for _s in sorted(res)])\n \n\n\n\n\n\n\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Python3 || Counter and Dictionary || 85% and 75% || Less than 20 lines of code | reconstruct-original-digits-from-english | 0 | 1 | # Intuition\nOn finding the count of each digits, we can find the required string.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nIntially, we find the count of each letter in the string `s` using Counter() which `letters`. Then, we calculate the no. of digits present using the counter in `digits` dictionary.\n\nNote: \nThe even digits has unique characters which are not present in other digits:\n```\n0 - \'z\'\n2 - \'w\'\n4 - \'u\'\n6 - \'x\'\n8 - \'g\'\n```\nSo, the count of these digits is simply the count of the corresponding characters. Store their count in `digits`.\n\nWe are left with digits 1, 3, 5, 7, 9\n\nThe characters unique to these alone:\n```\n1 - \'o\'\n3 - \'r\'\n5 - \'f\'\n7 - \'s\'\n```\nSo, the count of these digits is the difference between count of the corresponding characters and the sum of count of each of the even digits that contain those characters.\n(i.e.)\n0, 2 and 4 has letter \'o\' in them and thus\n`count of 1 = letters[\'o\'] - digits[\'0\'] - digits[\'2\'] - digits[\'4\']`\n\n0, 4 has letter \'r\' in them and thus\n`count of 3 = letters[\'r\'] - digits[\'0\'] - digits[\'4\']`\n\n4 has letter \'f\' in it and thus\n`count of 5 = letters[\'f\'] - digits[\'4\']`\n\n6 has letter \'s\' in it and thus\n`count of 7 = letters[\'s\'] - digits[\'6\']`\n\nFinally,\n`count of 9 = letters[\'n\'] - digits[\'7\'] - digits[\'1]`\n\nOn storing these values in `digits`, we can form the required string using for loop.\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\nFor creating Counter object O(n) and O(1) for others\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n letters = Counter(s)\n digits = defaultdict(int)\n answer = ""\n\n digits[\'0\'] = letters[\'z\']\n digits[\'2\'] = letters[\'w\']\n digits[\'4\'] = letters[\'u\']\n digits[\'6\'] = letters[\'x\']\n digits[\'8\'] = letters[\'g\']\n \n digits[\'1\'] = letters[\'o\'] - (digits[\'0\'] + digits[\'2\'] + digits[\'4\'])\n digits[\'3\'] = letters[\'r\'] - (digits[\'0\'] + digits[\'4\'])\n digits[\'5\'] = letters[\'f\'] - digits[\'4\']\n digits[\'7\'] = letters[\'s\'] - digits[\'6\']\n digits[\'9\'] = (letters[\'n\'] - digits[\'7\'] - digits[\'1\']) // 2\n\n for ch in "0123456789":\n answer += ch * digits[ch]\n\n return answer\n```\n\n# Upvote if it was helpful | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Simple Solution With Explanation | reconstruct-original-digits-from-english | 0 | 1 | # Intuition\nWe use unique characters in string representation of digits.\n\n# Approach\nWe count all characters in the string. Then we arrange digits according to unique characters in their string representation.\nFirstly we have ```(\'one\', \'two\', \'three\', \'four\', \'five\', \'six\', \'seven\', \'eight\', \'nine\', \'ten\')```. We notice that ```(\'Zero\', \'tWo\', \'foUr\', \'siX\', \'eiGht\')``` have unique characters (```ZWUXG``` respectively), so we process them first. Then we have ```(\'One\', \'tHree\', \'Five\', \'Seven\')``` with unique characters (```OHFS``` respectively). Eventually one digit ```\'nine\'``` is left.\nSo, all we need - to process digits according to the aforementioned order.\n\n# Complexity\n- Time complexity: $$O(n)$$.\n\n- Space complexity: $$O(1)$$.\n\n# Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n digChs = ((0, \'zero\'), (2, \'two\'), (4, \'four\'), (6, \'six\'), (8, \'eight\'), \n (1, \'one\'), (3, \'three\'), (5, \'five\'), (7, \'seven\'), (9, \'nine\'))\n cntrCh = Counter(s)\n cntrDig = [0]*10\n for dig, digCh in digChs:\n found = True\n while found:\n for ch in digCh:\n if cntrCh[ch] == 0:\n found = False\n break\n \n if found:\n cntrDig[dig] += 1\n for ch in digCh:\n cntrCh[ch] -= 1\n \n return \'\'.join(f\'{dig}\'*cntr for dig, cntr in enumerate(cntrDig))\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Linear Algebra Method, O(n * m) but highly generic | reconstruct-original-digits-from-english | 0 | 1 | # Why post an O(n m) solution?\n\nI dislike the solutions that have been published because they require you to spot some property of the english words `one two three four five six seven eight nine`. \n\nFor example, there\'s a couple of solutions which rely on letters being unique to words (eg. \'g\' only occurs in eight, \'u\' only occurs in four, \'x\' only occurs in six). I think of this as a slightly dirty trick that lets you weasel out of the tricky underlying problem.\n\n\n\n- When are there no solutions?\n- When are multiple solutions possible?\n\nThese are questions you cannot answer without linear algebra.\n\n\n\n# Approach\n\nConsider a mapping from numbers to words, eg. `F(12) = one two`. This can be modelled by a the matrix `Solution.arr`\n\nWe use least squares to invert this mapping.\n\n\n\n\n\n# Code\n```\nimport numpy as np\nfrom collections import Counter\nimport string\n\n\nclass Solution:\n arr = np.array(\n [\n # a b c d e f g h i j k l m n o p q r s t u v w x y z\n [ 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 1, ], # zero\n [ 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, ], # one\n [ 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, ], # two\n [ 0, 0, 0, 0, 2, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 1, 0, 0, 0, 0, 0, 0, ], # three\n [ 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, 0, ], # four\n [ 0, 0, 0, 0, 1, 1, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, ], # five\n [ 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, ], # six\n [ 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 1, 0, 0, 0, 0, ], # seven\n [ 0, 0, 0, 0, 1, 0, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, ], # eight\n [ 0, 0, 0, 0, 1, 0, 0, 0, 1, 0, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, ], # nine\n ]\n ).T\n def originalDigits(self, s: str) -> str:\n counter = Counter(s)\n x, res, _, _ = np.linalg.lstsq(self.arr, [counter[letter] for letter in string.ascii_lowercase])\n x = np.rint(x)\n return "".join( str(digit) * int(x[digit]) for digit in range(10))\n\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Reconstruct Original Digits | reconstruct-original-digits-from-english | 0 | 1 | # Intuition\nThe problem requires us to identify and count the occurrences of digits from a given string of English letters. We can use the unique patterns of English letters in each digit\'s spelling to our advantage to solve this problem.\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\nWe start by creating a count array of size 10 to keep track of the occurrences of each digit. Then, we iterate over each character of the input string and increment the corresponding count of the respective digit based on the English letter pattern of that digit.\n\nNext, we subtract the counts of the digits that can be uniquely identified using the count array of other digits. For example, we can identify the count of \'1\' by subtracting the count of \'0\', \'2\', and \'4\' from the count of \'1\'.\n\nFinally, we reconstruct the original digits by iterating over the count array and appending the digits to the result string based on their respective counts.\n\nOverall, the approach involves identifying the unique patterns of English letters in each digit\'s spelling and using this information to count and reconstruct the original digits from the input string.\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(n)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n count = [0] * 10\n\n for c in s:\n if c == \'z\':\n count[0] += 1\n if c == \'o\':\n count[1] += 1\n if c == \'w\':\n count[2] += 1\n if c == \'h\':\n count[3] += 1\n if c == \'u\':\n count[4] += 1\n if c == \'f\':\n count[5] += 1\n if c == \'x\':\n count[6] += 1\n if c == \'s\':\n count[7] += 1\n if c == \'g\':\n count[8] += 1\n if c == \'i\':\n count[9] += 1\n\n count[1] -= count[0] + count[2] + count[4]\n count[3] -= count[8]\n count[5] -= count[4]\n count[7] -= count[6]\n count[9] -= count[5] + count[6] + count[8]\n\n return \'\'.join(chr(i + ord(\'0\')) for i, c in enumerate(count) for j in range(c))\n\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
common & ordinary solution | reconstruct-original-digits-from-english | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n dic = defaultdict()\n for i in s:\n dic[i] = dic.get(i, 0) + 1\n \n zero = dic.get("z", 0)\n two = dic.get("w", 0)\n four = dic.get("u", 0)\n six = dic.get("x", 0)\n seven = dic.get("s", 0) - six\n five = dic.get("v", 0) - seven\n eight = dic.get("g", 0)\n three = dic.get("t", 0) - eight - two\n one = dic.get("o", 0) - zero - four - two\n nine = dic.get("i", 0) - eight - five - six\n\n return "0"*zero+"1"*one+"2"*two+"3"*three+"4"*four+"5"*five+"6"*six+"7"*seven+"8"*eight+"9"*nine\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Python meta-solution | reconstruct-original-digits-from-english | 0 | 1 | # Meta\n```\nN = {\'zero\', \'one\', \'two\', \'three\', \'four\', \'five\', \'six\', \'seven\', \'eight\', \'nine\'}\nwhile N:\n U = set()\n for n in N:\n this = set(n)\n other = set(\'\'.join(N - {n}))\n unique = this - other\n if unique:\n print(\'\'.join(unique), n, sep=\'\\t\')\n U.add(n)\n N -= U\n print()\n```\n```\nu four\nz zero\nx six\ng eight\nw two\n\nhtr three\no one\ns seven\nf five\n\nein nine\n```\n\n# Solution\n```\nU = (\n (\'z\', \'zero\', 0),\n (\'w\', \'two\', 2),\n (\'u\', \'four\', 4),\n (\'x\', \'six\', 6),\n (\'g\', \'eight\', 8),\n\n (\'o\', \'one\', 1),\n (\'t\', \'three\', 3),\n (\'f\', \'five\', 5),\n (\'s\', \'seven\', 7),\n\n (\'i\', \'nine\', 9),\n)\n\nclass Solution:\n def originalDigits(self, s: str) -> str:\n C = Counter(s)\n D = [0] * 10\n for u, w, i in U:\n if n := C[u]:\n D[i] = n\n for c, k in Counter(w).items():\n C[c] -= k * n\n return \'\'.join(str(i) * n for i, n in enumerate(D))\n\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Solution | reconstruct-original-digits-from-english | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n string originalDigits(string s) {\n vector<int> freq(26);\n for (char c : s) {\n freq[c - \'a\']++;\n }\n vector<int> digits(10);\n digits[0] = freq[\'z\' - \'a\'];\n digits[2] = freq[\'w\' - \'a\'];\n digits[4] = freq[\'u\' - \'a\'];\n digits[6] = freq[\'x\' - \'a\'];\n digits[8] = freq[\'g\' - \'a\'];\n digits[1] = freq[\'o\' - \'a\'] - digits[0] - digits[2] - digits[4];\n digits[3] = freq[\'h\' - \'a\'] - digits[8];\n digits[5] = freq[\'f\' - \'a\'] - digits[4];\n digits[7] = freq[\'s\' - \'a\'] - digits[6];\n digits[9] = freq[\'i\' - \'a\'] - digits[5] - digits[6] - digits[8];\n\n string result;\n for (int i = 0; i < 10; i++) {\n if (digits[i] > 0) {\n result += string(digits[i], i + \'0\');\n }\n }\n return result;\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def originalDigits(self, s: str) -> str:\n nums = [0 for x in range(10)]\n nums[0] = s.count(\'z\')\n nums[2] = s.count(\'w\')\n nums[4] = s.count(\'u\')\n nums[6] = s.count(\'x\')\n nums[8] = s.count(\'g\')\n nums[3] = s.count(\'h\') - nums[8]\n nums[7] = s.count(\'s\') - nums[6]\n nums[5] = s.count(\'v\') - nums[7]\n nums[1] = s.count(\'o\') - nums[0] - nums[2] - nums[4]\n nums[9] = (s.count(\'n\') - nums[1] - nums[7]) // 2\n result = ""\n for x in range(10):\n result += str(x) * nums[x]\n return result\n```\n\n```Java []\nclass Solution {\n static final int[] DIGS = {0,2,4,6,8,5,7,3,9,1}, CHARS = {25,22,20,23,6,5,18,7,8,14};\n static final int[][] REMS = {{14},{14},{5,14},{18,8},{8,7},{8},{},{},{},{}};\n public String originalDigits(String S) {\n int[] fmap = new int[26], ans = new int[10];\n char[] SCA = S.toCharArray();\n for (char c : SCA) fmap[c - 97]++;\n for (int i = 0; i < 10; i++) {\n int count = fmap[CHARS[i]];\n for (int rem : REMS[i]) fmap[rem] -= count;\n ans[DIGS[i]] = count;\n }\n StringBuilder sb = new StringBuilder();\n for (int i = 0; i < 10; i++) {\n char c = (char)(i + 48);\n for (int j = 0; j < ans[i]; j++)\n sb.append(c);\n }\n return sb.toString();\n }\n}\n```\n | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Another ugly python solution | reconstruct-original-digits-from-english | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n dict_freq = {}\n for letter in s:\n if letter in dict_freq:\n dict_freq[letter]+=1\n else:\n dict_freq[letter]=1\n \n \n num_ = [0]*10\n \n dict_num = {\'g\':[\'eight\',8],\'u\':[\'four\',4],\'w\':[\'two\',2],\'x\':[\'six\',6],\'z\':[\'zero\',0]}\n \n for key in dict_num:\n if key in dict_freq: \n curr_count = dict_freq[key]\n word = dict_num[key][0]\n\n number = dict_num[key][1]\n num_[number] = curr_count\n \n for letter in word:\n dict_freq[letter]-=curr_count\n if dict_freq[letter]==0:\n dict_freq.pop(letter)\n\n dict_num_2 = {\'f\':[\'five\',5],\'h\':[\'three\',3],\'o\':[\'one\',1],\'s\':[\'seven\',7]}\n \n for key in dict_num_2:\n if key in dict_freq: \n curr_count = dict_freq[key]\n word = dict_num_2[key][0]\n\n number = dict_num_2[key][1]\n num_[number] = curr_count\n \n for letter in word:\n dict_freq[letter]-=curr_count\n if dict_freq[letter]==0:\n dict_freq.pop(letter)\n if \'i\' in dict_freq:\n num_[9]=dict_freq[\'i\']\n\n List_num=[]\n for idx,count in enumerate(num_):\n for iter in range(count):\n List_num.append(str(idx))\n \n return \'\'.join(List_num)\n \n \n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
[LC-423-M | Python3] The Representative Character | reconstruct-original-digits-from-english | 0 | 1 | The occurring times of a digit word can be calculated by using its representative character.\n\n```python3 []\nclass Solution:\n def originalDigits(self, s: str) -> str:\n \'\'\'\n Owning unique character ones:\n n(\'zero\') = n(\'z\')\n n(\'two\') = n(\'w\')\n n(\'four\') = n(\'u\')\n n(\'six\') = n(\'x\')\n n(\'eight\') = n(\'g\')\n\n The rest are:\n n(\'one\') = n(\'o\') - n(\'zero\') - n(\'two\') - n(\'four\')\n n(\'three\') = n(\'r\') - n(\'zero\') - n(\'four\')\n n(\'five\') = n(\'f\') - n(\'four\')\n n(\'seven\') = n(\'s\') - n(\'six\')\n n(\'nine\') = n(\'i\') - n(\'five\') - n(\'six\') - n(\'eight\')\n \'\'\'\n \n cnter = Counter(s)\n times = [0] * 10\n times[0], times[2], times[4], times[6], times[8] = \\\n cnter[\'z\'], cnter[\'w\'], cnter[\'u\'], cnter[\'x\'], cnter[\'g\']\n times[1] = cnter[\'o\'] - times[0] - times[2] - times[4]\n times[3] = cnter[\'r\'] - times[0] - times[4]\n times[5] = cnter[\'f\'] - times[4]\n times[7] = cnter[\'s\'] - times[6]\n times[9] = cnter[\'i\'] - times[5] - times[6] - times[8]\n\n res = \'\'\n for i in range(10):\n res += str(i) * times[i]\n \n return res\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Python easy Solution | reconstruct-original-digits-from-english | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n from collections import Counter\n c = Counter(s)\n nums = [0] * 10\n nums[0] = c[\'z\']\n nums[2] = c[\'w\']\n nums[4] = c[\'u\']\n nums[6] = c[\'x\']\n nums[8] = c[\'g\']\n nums[1] = c[\'o\'] - nums[0] - nums[2] - nums[4]\n nums[3] = c[\'h\'] - nums[8]\n nums[5] = c[\'f\'] - nums[4]\n nums[7] = c[\'s\'] - nums[6]\n nums[9] = c[\'i\'] - nums[5] - nums[6] - nums[8]\n return \'\'.join(str(i) * n for i, n in enumerate(nums))\n\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Python solution | reconstruct-original-digits-from-english | 0 | 1 | # Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n charCount = {}\n targetCharCount = [0]*26\n res = [0]*10\n numString = ["zero","two","six","seven","eight","four","five","three","nine","one"]\n nums = [0,2,6,7,8,4,5,3,9,1]\n for i in range(10):\n temp = [0]*26\n for j in numString[i]:\n temp[ord(j)-ord("a")] += 1\n charCount[nums[i]] = temp\n for i in s:\n targetCharCount[ord(i)-ord("a")] += 1\n\n target_sum = sum(targetCharCount)\n i = 0\n while target_sum != 0 and i < 10:\n c = 0\n l = len(numString[i])\n t = targetCharCount.copy()\n for j in range(26):\n if charCount[nums[i]][j] <= t[j]:\n c += charCount[nums[i]][j]\n t[j] -= charCount[nums[i]][j]\n \n if c == l:\n res[nums[i]] = res[nums[i]]+1\n targetCharCount = t\n target_sum -= c\n else:\n i += 1\n resStr = ""\n for i in range(10):\n if res[i]>0:\n resStr = resStr+str(i)*res[i]\n return resStr\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
REALLY BAD BEGINNER BRUTE FORCE SOLUTION XD (IDK WHAT IM DOING) | reconstruct-original-digits-from-english | 0 | 1 | \n# Code\n```\nclass Solution:\n def originalDigits(self, s: str) -> str:\n lst = []\n h = \'\'\n n = {\'e\':0, \'g\':0, \'f\':0, \'i\':0, \'h\':0, \'o\':0, \'n\':0,\'s\':0, \'r\':0, \'u\':0, \'t\':0, \'w\':0, \'v\':0, \'x\':0, \'z\':0}\n for x in s:\n if x in n:\n n[x]+= 1\n q = []\n for z in s:\n q.append(z)\n print(n)\n # Remove all zeroes, twos, fours, six, eight if they are in the string.\n if n[\'z\'] != 0:\n while n[\'z\'] > 0:\n lst.append(\'0\')\n n[\'e\'] -= 1\n n[\'r\'] -= 1\n n[\'o\'] -= 1\n n[\'z\'] -= 1\n if n[\'w\'] != 0:\n while n[\'w\'] > 0:\n lst.append(\'2\')\n n[\'t\'] -= 1\n n[\'o\'] -= 1\n n[\'w\'] -= 1\n if n[\'u\'] != 0:\n while n[\'u\'] > 0:\n lst.append(\'4\')\n n[\'f\'] -= 1\n n[\'u\'] -= 1\n n[\'o\'] -= 1\n n[\'r\'] -= 1\n if n[\'x\'] != 0:\n while n[\'x\'] > 0:\n lst.append(\'6\')\n n[\'s\'] -= 1\n n[\'i\'] -= 1\n n[\'x\'] -= 1\n if n[\'g\'] != 0:\n while n[\'g\'] > 0:\n lst.append(\'8\')\n n[\'e\'] -= 1\n n[\'i\'] -= 1\n n[\'h\'] -= 1\n n[\'t\'] -= 1\n n[\'g\'] -= 1\n\n # AFTER REMOVAL FIND ALL ODD NUMBERS!!! ALMOST THERE!!!\n # One, Three, Five, Seven, Nine\n \n \n if n[\'o\'] != 0 and n[\'z\'] <= 0 and n[\'w\'] <= 0 and n[\'u\'] <= 0:\n while n[\'o\'] > 0:\n lst.append(\'1\')\n n[\'e\'] -= 1\n n[\'n\'] -= 1\n n[\'o\'] -= 1\n if n[\'h\'] != 0 and n[\'g\'] <= 0:\n while n[\'h\'] > 0:\n lst.append(\'3\')\n n[\'t\'] -= 1\n n[\'r\'] -= 1\n n[\'e\'] -= 1\n n[\'e\'] -= 1\n n[\'h\'] -= 1\n if n[\'f\'] != 0:\n while n[\'f\'] > 0:\n lst.append(\'5\')\n n[\'i\'] -= 1\n n[\'v\'] -= 1\n n[\'e\'] -= 1\n n[\'f\'] -= 1\n if n[\'i\'] != 0 and n[\'f\'] <= 0:\n while n[\'i\'] > 0:\n lst.append(\'9\')\n n[\'n\'] -= 1\n n[\'n\'] -= 1\n n[\'e\'] -= 1\n n[\'i\'] -= 1\n if n[\'n\'] != 0 and n[\'f\'] <= 0 and n[\'i\'] <= 0:\n while n[\'n\'] > 0:\n lst.append(\'7\')\n n[\'s\'] -= 1\n n[\'e\'] -= 1\n n[\'v\'] -= 1\n n[\'e\'] -= 1\n n[\'n\'] -= 1\n \n lst.sort()\n print(n)\n h = \'\'.join(lst)\n return h\n``` | 0 | Given a string `s` containing an out-of-order English representation of digits `0-9`, return _the digits in **ascending** order_.
**Example 1:**
**Input:** s = "owoztneoer"
**Output:** "012"
**Example 2:**
**Input:** s = "fviefuro"
**Output:** "45"
**Constraints:**
* `1 <= s.length <= 105`
* `s[i]` is one of the characters `[ "e ", "g ", "f ", "i ", "h ", "o ", "n ", "s ", "r ", "u ", "t ", "w ", "v ", "x ", "z "]`.
* `s` is **guaranteed** to be valid. | null |
Easy || 100% || Fully Explained || C++, Java, Python, JavaScript, Python3 || Sliding Window | longest-repeating-character-replacement | 1 | 1 | # **PROBLEM STATEMENT:**\nGiven a string s and an integer k. Choose any character of the string and change it to any other uppercase English character. Perform this operation at most k times.\nReturn the length of the longest substring containing the same letter you can get after performing the above operations.\n# **Example 1:**\n# Input: \ns = "ABAB", k = 2\n# Output: \n4\nExplanation: Replace the two \'A\'s with two \'B\'s or vice versa.\n\n# **Example 2:**\n# Input: \ns = "AABABBA", k = 1\n# Output: \n4\n# Explanation:\nReplace the one \'A\' in the middle with \'B\' and form "AABBBBA". \nThe substring "BBBB" has the longest repeating letters, which is 4.\n\n\n# **C++ Solution:**\n```\n// Time Complexity : O(n)\n// Space Complexity : O(1)\nclass Solution {\npublic:\n int characterReplacement(string s, int k) {\n // Base case...\n if (s.size() == 0) return 0;\n // Make an array...\n vector <int> arr(128);\n // Initialize largestCount & beg pointer...\n int beg = 0, largestCount = 0;\n // Traverse all characters through the loop...\n for (int end = 0; end < s.size(); end++) {\n // Get the largest count of a single, unique character in the current window...\n largestCount = max(largestCount, ++arr[s[end]]);\n // We are allowed to have at most k replacements in the window...\n // So, if max character frequency + distance between beg and end is greater than k...\n // That means we have met a largest possible sequence, we can move the window to right...\n if (end - beg + 1 - largestCount > k) // The main equation is: end - beg + 1 - largestCount...\n arr[s[beg++]]--;\n }\n // Return the sequence we have passes, which is s.length() - beg...\n return s.length() - beg;\n }\n};\n```\n\n# **Java Solution:**\n```\n// Time Complexity : O(n)\n// Space Complexity : O(1)\nclass Solution {\n public int characterReplacement(String s, int k) {\n // Make an array of size 26...\n int[] arr = new int[26];\n // Initialize largestCount, maxlen & beg pointer...\n int largestCount = 0, beg = 0, maxlen = 0;\n // Traverse all characters through the loop...\n for(int end = 0; end < s.length(); end ++){\n arr[s.charAt(end) - \'A\']++;\n // Get the largest count of a single, unique character in the current window...\n largestCount = Math.max(largestCount, arr[s.charAt(end) - \'A\']);\n // We are allowed to have at most k replacements in the window...\n // So, if max character frequency + distance between beg and end is greater than k...\n // this means we have considered changing more than k charactres. So time to shrink window...\n // Then there are more characters in the window than we can replace, and we need to shrink the window...\n if(end - beg + 1 - largestCount > k){ // The main equation is: end - beg + 1 - largestCount...\n arr[s.charAt(beg) - \'A\']--;\n beg ++;\n }\n // Get the maximum length of repeating character...\n maxlen = Math.max(maxlen, end - beg + 1); // end - beg + 1 = size of the current window...\n }\n return maxlen; // Return the maximum length of repeating character...\n }\n}\n```\n\n# **Python/Python3 Solution:**\n```\n# Time Complexity : O(n)\n# Space Complexity : O(1)\nclass Solution(object):\n def characterReplacement(self, s, k):\n maxlen, largestCount = 0, 0\n arr = collections.Counter()\n for idx in xrange(len(s)):\n arr[s[idx]] += 1\n largestCount = max(largestCount, arr[s[idx]])\n if maxlen - largestCount >= k:\n arr[s[idx - maxlen]] -= 1\n else:\n maxlen += 1\n return maxlen\n```\n \n# **JavaScript Solution:**\n```\n// Time Complexity : O(n)\n// Space Complexity : O(1)\nvar characterReplacement = function(s, k) {\n // Make a map of size 26...\n var map = [26]\n // Initialize largestCount, maxlen & beg pointer...\n let largestCount = 0, beg = 0, maxlen = 0;\n // Traverse all characters through the loop...\n for(let end = 0; end < s.length; end++){\n const c = s[end]\n map[c] = (map[c] || 0) + 1\n // Get the largest count of a single, unique character in the current window...\n largestCount = Math.max(largestCount, map[c])\n // We are allowed to have at most k replacements in the window...\n // So, if max character frequency + distance between beg and end is greater than k...\n // this means we have considered changing more than k charactres. So time to shrink window...\n // Then there are more characters in the window than we can replace, and we need to shrink the window...\n if(end - beg + 1 - largestCount > k){ // The main equation is: end - beg + 1 - largestCount...\n map[s[beg]] -= 1\n beg += 1\n }\n // Get the maximum length of repeating character...\n maxlen = Math.max(maxlen, end - beg + 1); // end - beg + 1 = size of the current window...\n }\n return maxlen; // Return the maximum length of repeating character...\n};\n```\n**I am working hard for you guys...\nPlease upvote if you found any help with this code...** | 152 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
Python: Two-Pointers + Process for coding interviews | longest-repeating-character-replacement | 0 | 1 | Hello,\n\nHere is my solution with my process for coding interview. All feedbacks are welcome.\n\n1. Problem Summary / Clarifications / TDD:\n `Ouput(ABAB, 2): 4`\n `Ouput(AABABBA, 1): 4`\n `Ouput(BAAAABBA, 1): 5`\n `Ouput(BAAAABBA, 3): 8`\n `Ouput(BAAAABBBBBA, 1): 6`\n `Ouput(CBAAAABBBBBA, 2): 6`\n `Ouput(CBAAAABBBBBA, 1): 6`\n\t\t `Ouput(CABAAAABBBBBA, 2): 7`\t\t \n \n2. Intuition:\n - Scan the array with 2 pointers: `left` and `right`\n - Store the frequency of each character\n - Compute the replacement cost: `cells count between left and right pointers - the highest frequency`\n - if the `replacement cost <= k`: update longest string size\n - if the `replacement cost > k`: decrease frequency of character at `left pointer`; increase `left pointer` and repeat\n - - - Since we are looking for the longest sub-string, we don\'t need to shrink the sliding window by more than 1 character:\n - - - When we reach a window of size `W`, we know that our target window size is higher or equal to the current one ( `>= W`).\n\t\t- - - Therefore, we could continue sliding with a window of `W` cells until we find a larger window `> W`.\n \n 0 1 2 3 4 5 6 7 8 9 10 11 12\n C A B A A A A B B B B B A, k, Replacement Cost, max len\n\t l^r 2 1 - 1 = 0 <=k 1 => increase r\n\t l^ ^r 2 2 - 1 = 1 <=k 2 => increase r\n\t l^ ^r 2 3 - 1 = 2 <=k 3 => increase r\n\t l^ ^r 2 4 - 2 = 2 <=k 4 => increase r\n\t l^ ^r 2 5 - 3 = 2 <=k 5 => increase r\n\t l^ ^r 2 6 - 4 = 2 <=k 6 => increase r\n l^ r^ 2 7 - 5 = 2 <=k 7 => increase r\n\t l^ r^ 2 8 - 5 = 3 > k 7 => increase l,r\n l^ r^ 2 8 - 5 = 3 > k 7 => increase l,r\n l^ r^ 2 8 - 4 = 4 > k 7 => increase l,r\n l^ r^ 2 8 - 4 = 4 > k 7 => increase l,r\n l^ r^ 2 8 - 5 = 3 > k 7 => increase l,r\n l^ r^ 2 8 - 4 = 4 > k 7 => STOP\n \n3. Tests: you may want to:\n\t\t- to use all test cases above\n\t\t- to add test cases based on your code (coverage): test your code as soon as you can\n\t\t- to test edge & special cases:\n\t- An empty string, \n\t- A string with 1 character, `A`\n\t- A string with same character: `AAAAA`\n\t- A string that containing distinct characters: `ABCDEFGHI`\n\n4. Analysis:\n - Time Complexity: O(26 |s|) = O(|s|)\n - Space Complexity: O(26) = O(1)\n \n```\n def characterReplacement(self, s: str, k: int) -> int:\n \n l = 0\n c_frequency = {}\n longest_str_len = 0\n for r in range(len(s)):\n \n if not s[r] in c_frequency:\n c_frequency[s[r]] = 0\n c_frequency[s[r]] += 1\n \n # Replacements cost = cells count between left and right - highest frequency\n cells_count = r - l + 1\n if cells_count - max(c_frequency.values()) <= k:\n longest_str_len = max(longest_str_len, cells_count)\n \n else:\n c_frequency[s[l]] -= 1\n if not c_frequency[s[l]]:\n c_frequency.pop(s[l])\n l += 1\n \n return longest_str_len\n\t\t\n``` | 334 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
O( n )✅ | Python (Step by step explanation)✅ | longest-repeating-character-replacement | 0 | 1 | # Intuition\nThe problem involves finding the longest substring in a string where you can replace at most \'k\' characters to make all characters in the substring the same. \n\n# Approach\n1. We maintain a sliding window approach to find the longest valid substring.\n2. We use a dictionary \'alphabets\' to keep track of the count of characters within the current window.\n3. Initialize variables \'ans\' to store the maximum length found, \'left\' and \'right\' to represent the window\'s boundaries, and \'max_len\' to track the maximum character count in the window.\n4. As we expand the window to the right, we update the counts in \'alphabets\' for each character in the window and maintain \'max_len\'.\n5. If the length of the window minus \'max_len\' (the maximum character count) exceeds \'k\', we need to shrink the window from the left side. We reduce the count of the character at the \'left\' boundary and move \'left\' to the right.\n6. We update \'ans\' with the maximum length found at each step.\n7. Finally, \'ans\' will hold the length of the longest valid substring.\n\n# Complexity\n- Time complexity: O(n), where \'n\' is the length of the input string \'s\'. We process each character once in a single pass.\n- Space complexity: O(26) = O(1), as \'alphabets\' stores counts for each alphabet character, which is a constant size.\n\n\n# Code\n```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n alphabets = {}\n\n ans = 0\n left = 0\n right = 0\n max_len = 0\n\n for right in range(len(s)) :\n alphabets[s[right]] = 1 + alphabets.get(s[right] , 0)\n max_len = max(max_len , alphabets[s[right]])\n\n if (right - left + 1) - max_len > k :\n alphabets[s[left]] -= 1\n left += 1\n else :\n ans = max(ans , (right - left + 1) ) \n\n return ans \n\n\n \n```\n\n# Please upvote the solution if you understood it.\n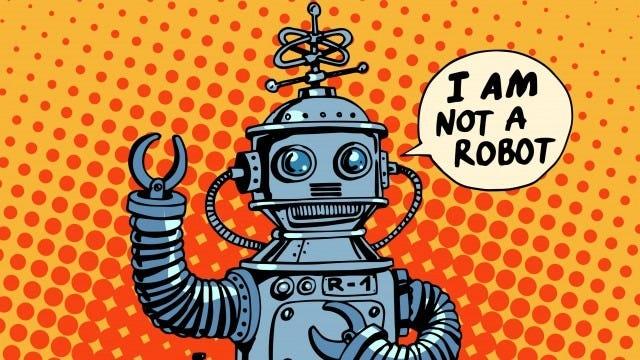\n | 2 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
O( 26*n )✅ | Python (Step by step explanation)✅ | longest-repeating-character-replacement | 0 | 1 | # Intuition\nThe problem requires finding the longest substring in a string where you can replace at most \'k\' characters to make all characters in the substring the same. \n\n# Approach\n1. We maintain a sliding window approach to find the longest valid substring.\n2. We use a dictionary \'alphabets\' to keep track of the count of characters within the current window.\n3. Initialize variables \'ans\' to store the maximum length found and \'left\' and \'right\' to represent the window\'s boundaries.\n4. As we expand the window to the right, we update the counts in \'alphabets\' for each character in the window.\n5. If the length of the window minus the maximum count in \'alphabets\' exceeds \'k\', we need to shrink the window from the left side. We reduce the count of the character at the \'left\' boundary and move \'left\' to the right.\n6. We update \'ans\' with the maximum length found at each step.\n7. Finally, \'ans\' will hold the length of the longest valid substring.\n\n# Complexity\n- Time complexity: O(n), where \'n\' is the length of the input string \'s\'. We process each character once in a single pass.\n- Space complexity: O(26) = O(1), as \'alphabets\' stores counts for each alphabet character, which is a constant size.\n\n\n# Code\n```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n alphabets = {}\n\n ans = 0\n left = 0\n right = 0\n\n for right in range(len(s)) :\n alphabets[s[right]] = 1 + alphabets.get(s[right] , 0)\n\n if (right - left + 1) - max(alphabets.values()) > k :\n alphabets[s[left]] -= 1\n left += 1\n else :\n ans = max(ans , (right - left + 1) ) \n\n return ans \n\n\n \n```\n\n# Please upvote the solution if you understood it.\n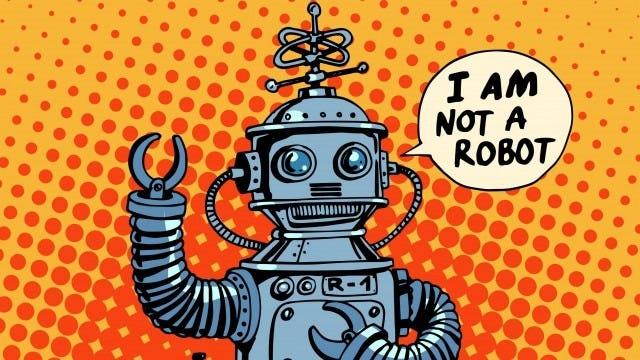\n | 4 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
Sliding Window---->Blind 75 Solution | longest-repeating-character-replacement | 0 | 1 | \n# Sliding Window ----->O(26*N)\n```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n output,left,dic=0,0,{}\n for right in range(len(s)):\n dic[s[right]]=1+dic.get(s[right],0)\n while (right-left+1)-max(dic.values())>k:\n dic[s[left]]-=1\n left+=1\n output=max(output,right-left+1)\n return output\n```\n```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n left,maxf=0,0\n dic=defaultdict(int)\n for right in range(len(s)):\n dic[s[right]]+=1\n maxf=max(maxf,dic[s[right]])\n if (right-left+1)>maxf+k:\n dic[s[left]]-=1\n left+=1\n return len(s)-left\n```\n# please upvote me it would encourage me alot\n | 16 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
✔️ 2 Ways to Solving Longest Repeating Character Replacement 🪔 | longest-repeating-character-replacement | 0 | 1 | # Solution 1:\n\n# Intuition\nThe goal is to find the length of the longest substring in which we can replace at most k characters to make all characters in the substring the same.\n\n\n# Approach\nWe maintain a sliding window to keep track of the current substring.\nFor each character encountered, we update the count of that character within the window.\nWe calculate the window length and keep track of the maximum count of any character within the window.\nIf the difference between the window length and the maximum count exceeds k, we shrink the window by moving the starting pointer to the right.\nWe continuously update the maximum length of the substring that satisfies the condition.\n\n# Complexity\n- Time complexity:O(n), where n is the length of the input string s.\n- Space complexity: O(26) or O(1), as we have a count dictionary that stores the count of characters (assuming an alphabet of size 26).\n\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n count={}\n mx=0\n\n start=0\n for end in range(len(s)):\n count[s[end]] = 1 + count.get(s[end], 0)\n windowlen = end-start+1\n maxcount = max(count.values())\n if (windowlen - maxcount) > k:\n count[s[start]]-=1\n start+=1\n mx=max(mx, end-start+1)\n return mx\n```\n# Solution 2:\n\n# Intuition\nThe objective is the same as in Code 1: finding the length of the longest substring in which we can replace at most k characters to make all characters in the substring the same.\n\n\n\n# Approach\nThis solution uses a similar sliding window approach but updates the maxcount for each character at each step.\nThe rest of the logic for maintaining the sliding window and calculating the maximum length is the same as in Code 1.\n\n# Complexity\n- Time complexity:O(n), where n is the length of the input string s.\n- Space complexity: O(26) or O(1), as we have a count dictionary that stores the count of characters (assuming an alphabet of size 26).\nBoth Code 1 and Code 2 have the same time and space complexity but use slightly different approaches to achieve the same goal.\n\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n count={}\n maxcount=0\n mx=0\n\n start=0\n for end in range(len(s)):\n count[s[end]] = 1 + count.get(s[end], 0)\n windowlen = end-start+1\n maxcount = max(maxcount, count[s[end]])\n if (windowlen - maxcount) > k:\n count[s[start]]-=1\n start+=1\n mx=max(mx, end-start+1)\n return mx return mx\n```\n | 2 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
424: Solution with step by step explanation | longest-repeating-character-replacement | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n1. Initialize variables window_start, max_length, max_count, and char_count. window_start is the start index of the sliding window. max_length is the length of the longest substring with repeating characters seen so far. max_count is the maximum count of any character seen so far. char_count is a dictionary that stores the count of each character in the current window.\n2. Traverse the string s from left to right using a for loop.\n3. Increment the count of the current character in the char_count dictionary using the get() method.\n4. Update the maximum count seen so far by taking the maximum of the current count and the previous maximum count.\n5. If the length of the current window (window_end - window_start + 1) is greater than the sum of the maximum count seen so far and k, then we need to shrink the window. To do this, decrement the count of the leftmost character (s[window_start]) in the char_count dictionary, and move the window_start index one step to the right.\n6. Update the maximum length of the substring with repeating characters seen so far by taking the maximum of the current length and the previous maximum length (window_end - window_start + 1).\n7. After the loop completes, return the maximum length of the substring with repeating characters seen so far (max_length).\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n # Initialize variables\n window_start = 0\n max_length = 0\n max_count = 0\n char_count = {}\n\n # Traverse the string s\n for window_end in range(len(s)):\n # Increment the count of the current character\n char_count[s[window_end]] = char_count.get(s[window_end], 0) + 1\n # Update the maximum count seen so far\n max_count = max(max_count, char_count[s[window_end]])\n \n # Shrink the window if required\n if window_end - window_start + 1 > max_count + k:\n char_count[s[window_start]] -= 1\n window_start += 1\n \n # Update the maximum length of the substring with repeating characters seen so far\n max_length = max(max_length, window_end - window_start + 1)\n \n return max_length\n\n``` | 7 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
Python Solution using Sliding Window | longest-repeating-character-replacement | 0 | 1 | ```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n ws=0\n freqmap={}\n maxlen=0\n maxfreq=0\n for we in range(len(s)):\n currchar=s[we]\n freqmap[currchar]=freqmap.get(currchar,0)+1\n maxfreq=max(maxfreq,freqmap[currchar])\n if((we-ws+1)-maxfreq > k):\n leftchar=s[ws]\n freqmap[leftchar]-=1\n if freqmap[leftchar]==0:\n del freqmap[leftchar]\n ws+=1\n maxlen=max(maxlen,we-ws+1)\n return maxlen\n``` | 1 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
Sliding Window Python Solution | longest-repeating-character-replacement | 0 | 1 | ```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n ws=0\n d={}\n freq=0\n maxlen=0\n for we in range(len(s)):\n c=s[we]\n d[c]=d.get(c,0)+1\n freq=max(freq,d[c])\n if we-ws+1-freq>k:\n leftchar=s[ws]\n d[leftchar]-=1\n if d[leftchar]==0:\n del d[leftchar]\n ws+=1\n maxlen=max(maxlen,we-ws+1)\n return maxlen\n \n``` | 1 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
Python3 solution with explanation || quibler7 | longest-repeating-character-replacement | 0 | 1 | # Algorithm \n\n- Initialise `count` dict to keep count of occuring char in given string. initialise `res` to keep track of length of maximum repeating window as we have to return this as ouput. set left and right pointer to 0 and `maxf` to keep count of freq of char that is occuring most in our sliding window.\n\n- Using `r` pointer we will iterate through the given string and we will update the count of chars in our dict `count`. \n\n- We will also update the `maxf` as we go along. \n\n- While number of characters need to be replaced in our window are greater than number of characters we are allowed to replace i.e. `k` , we will shrink the size of the window and and we will dec the value of that char in our hashmap. \n\n- Number of characters allowed to replace is given i.e `k` and number of characters need to be replaced from out window is found using this formula. \nSize of the current window `(r-l+1)` - max occuring char `(maxf)`\nThis gives us number of char to be replaced. \n\n- we will update the max size `res` of our window as we iterate through the string.\n\n- Lastly we will return the max length `res` of sliding window. \n\n# Code\n```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n # initialize dict to count freq of char in given string\n count = {}\n # length of maximum window in order to return later as output\n res = 0\n # left pointer\n l = 0 \n # count of most frequently occuring character \n maxf = 0\n for r in range(len(s)):\n # add each char of string to dict\n count[s[r]] = 1 + count.get(s[r], 0)\n # update the max count as we go along \n maxf = max(maxf, count[s[r]])\n # while number of characters to be replaced from window are greater than \n # number of characters we are allowed to replace\n # shrink our sliding window\n while (r-l+1)-maxf > k:\n count[s[l]] -= 1\n l += 1\n # update the length of maximum repeating char window\n res = max(res, (r-l+1))\n # return res \n return res\n\n``` | 3 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
Python code explained with approach and time complexity | longest-repeating-character-replacement | 0 | 1 | Intuition:\n\n- Initialize two pointers l and r to 0, and a dictionary count to keep track of the frequency of characters in the current window.\n- Initialize maxf and res to 0. maxf stores the frequency of the most frequent character in the current window, and res stores the length of the longest substring containing only one character seen so far.\n- Iterate over the string using the right end r of the window. For each character s[r], update its frequency in the count dictionary. Also, update maxf to be the maximum frequency of any character in the current window.\n- If the length of the window r-l+1 minus the frequency of the most frequent character maxf is greater than k, shrink the window from the left end l. Decrement the frequency of the character at s[l] in the count dictionary and increment l.\n- Continue updating res to be the maximum length of a substring containing only one character seen so far.\n- Once the entire string has been iterated over, return res.\n\nApproach:\n1. Initialize `count` to an empty dictionary, `l` to 0, `maxf` and `res` to 0.\n2. Iterate over the string using the right end `r` of the window:\n a. Update the frequency of the character `s[r]` in the `count` dictionary.\n b. Update `maxf` to be the maximum frequency of any character in the current window.\n c. If the length of the window `r-l+1` minus the frequency of the most frequent character `maxf` is greater than `k`, we need to shrink the window from the left end `l`. Decrement the frequency of the character at `s[l]` in the `count` dictionary, and increment `l`.\n d. Update `res` to be the maximum length of a substring containing only one character seen so far.\n3. Return `res`.\n\nComplexity:\n\nTime complexity: The time complexity of the algorithm is O(n), where n is the length of the string `s`. This is because we iterate over the string once using the right end `r` of the window, and each character is processed at most twice (once during expansion, and once during contraction).\n\nSpace complexity: The space complexity of the algorithm is O(1), since the size of the `count` dictionary is bounded by the number of distinct characters in the string.\n\n\n\n# Code\n```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n\n count = {}\n l=0\n maxf=0\n res=0\n\n #count.get(s[i], 0) gets the current count of the character s[i] from the count dictionary. If the character s[i] is not already a key in the dictionary, count.get(s[i], 0) returns the default value of 0. \n for r in range(len(s)):\n count[s[r]]=1+count.get(s[r],0)\n maxf = max(maxf, count[s[r]])\n \n\n if (r-l+1) - maxf >k:\n \n count[s[l]]-=1\n l+=1\n \n res = max(res,r-l+1)\n \n\n return res\n\n \n\n\n\n \n\n\n``` | 8 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
Python Sliding Window | longest-repeating-character-replacement | 0 | 1 | ```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n d = {}\n slow = 0\n ans = 0\n for fast in range(len(s)):\n if s[fast] in d:\n d[s[fast]] += 1\n else:\n d[s[fast]] = 1\n \n # get max of the window and check if \n # length of the window - max valued key <= k\n \n if ((fast-slow+1) - max(d.values())) <= k:\n pass\n else:\n # shrink the window\n while ((fast-slow+1) - max(d.values())) > k:\n d[s[slow]] -= 1\n if d[s[slow]] == 0:\n del d[s[slow]]\n slow += 1\n # calculate the length of the window\n ans = max(ans, fast - slow + 1)\n return ans\n | 3 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
Python Easiest solution With Explanation | 96.68% Faster | Beg to Adv | Sliding Window | longest-repeating-character-replacement | 0 | 1 | \n```python\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n maxf = l = 0\n count = collections.Counter() # counting the occurance of the character in the string. \n\t\t# instead of using "count = collections.Counter()", we can do the following:-\n\t\t"""\n\t\tfor r, n in enumerate(s):\n if n in hashmap:\n hashmap[n] += 1\n else:\n hashmap[n] = 1\n\t\t"""\n for r in range(len(s)):\n count[s[r]] += 1 # increasing the count of the character as per its occurance. \n maxf = max(maxf, count[s[r]]) # having a max freq of the character that is yet occurred. \n if r - l + 1 > maxf + k: # if length of sliding window is greater than max freq of the character and the allowed number of replacement.\n count[s[l]] -= 1 # then we have to decrease the occurrance of the character by 1 are we will be sliding the window. \n l += 1 # and we have to slide our window\n return len(s) - l # this will provide the length of the longest substring containing the same letter with the replacement allowed. \n```\n\n***Found helpful, Do upvote!!*** | 15 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
Sliding window - Python code with proper explanation | longest-repeating-character-replacement | 0 | 1 | ```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n """\n \n s = ABAB\n k = 2\n \n If we have no limit on k then we can say that \n (no of replacements to be done = \n length of string - count of character with maximum occurence)\n \n AAAAAABB - Here 2 replacements to be done\n ABABABABAA - Here 4 replacements to be done\n \n Let\'s say there is a restriction on the number of updates done\n no. of replacements = len of str - min (count chars with max occur , k )\n \n If we change the problem statement from String to substring then we have to\n find the sustring of max length where atmost K repelacements can be done\n \n \n s = "AABABBA"\n k = 1\n \n i\n AABABBA count of max occur = 1 length of the substr = 1 repl = 0\n j\n \n i\n AABABBA count of max occur = 2 length of substr = 2 repl = 0\n j\n \n i\n AABABBA count max occur = 2 length of substr = 3 repl = 1\n j\n \n i\n AABABBA count max occur = 3 length of substr = 4 repl = 1\n j\n \n i\n AABABBA count max occur = 3 length of substr = 5 repl = 2\n j Here 2 > k => 2 > 1\n decrease window till repl becomes 1 again\n i\n AABABBA count max occur = 2 length of substr = 4 repl = 2\n j further decrease the window\n \n i \n AABABBA count max occur = 2 length of substr = 3 repl = 1\n j\n \n i \n AABABBA count max occur = 3 length of substr = 4 repl = 1\n j\n \n i\n AABABBA count max occur = 3 length of susbstr = 5 repl = 2 increase i\n j\n i \n AABABBA length of substr = 3\n j\n \n \n \n maximum length of substring with replacements that can be done = 1 is 4\n \n At any particular moment we need (max occur,length of substr,repl)\n \n """\n # In the below line we are keeping the count of each character in the string\n count = collections.Counter()\n # i : start of the window\n # j : end of the window\n i = j = 0\n # maxLen : maximum length of the substring\n maxLen = 0\n # maxOccur will store the count of max occurring character in the window\n maxOccur = None\n while j < len(s):\n # Increase the count of characters as we are increasing the window\n count[s[j]] += 1\n # maxOccur : count maximum occurring character in the window\n maxOccur = count[max(count , key = lambda x: count[x])]\n j += 1\n # length of substring - count of character with maximum occurence <= k\n while j - i - maxOccur > k :\n # We are decreasing the count here \n count[s[i]] -= 1\n # Here we are again updating maxOccur\n maxOccur = count[max(count , key = lambda x: count[x])]\n # and then we are decreasing the window\n i += 1\n maxLen = max(maxLen , j - i)\n return maxLen\n \n # Time complexity is nearly O(n) as max operation can run for atmost 26 characters\n \n \n \n \n``` | 3 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
[Python] Simple O(N) solution with explanation | longest-repeating-character-replacement | 0 | 1 | ```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n ## RC ##\n\t\t## APPROACH : SLIDING WINDOW ##\n # Logic #\n # 1. Increase the window if the substring is valid else,\n # 2. slide the window with the same length. No need to shrink the window\n \n\t\t## TIME COMPLEXITY : O(N) ##\n\t\t## SPACE COMPLEXITY : O(N) ##\n\n freqDict = defaultdict(int)\n maxFreq = 0\n maxLength = 0\n start = end = 0\n while end < len(s):\n freqDict[s[end]] += 1\n \n # maxFreq may be invalid at some points, but this doesn\'t matter\n # maxFreq will only store the maxFreq reached till now\n maxFreq = max(maxFreq, freqDict[s[end]])\n \n # maintain the substring length and slide the window if the substring is invalid\n if ((end-start+1) - maxFreq) > k:\n freqDict[s[start]] -= 1\n start += 1\n else:\n maxLength = max(maxLength, end-start+1)\n end += 1\n return maxLength\n``` | 41 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
[Java/C++/Python/JavaScript/Kotlin/Swift]O(n)time/BEATS 99.97% MEMORY/SPEED 0ms APRIL 2022 | longest-repeating-character-replacement | 1 | 1 | ```\n```\n\n(Note: This is part of a series of Leetcode solution explanations. If you like this solution or find it useful, ***please upvote*** this post.)\n***Take care brother, peace, love!***\n\n```\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***0ms / 38.2MB*** (beats 92.04% / 24.00%).\n* ***Java***\n```\n public int characterReplacement(String s, int k) {\n\t\tMap<Character, Integer> map = new HashMap<>(); \n\n\t int left = 0, maxRepeat = 0, maxWindow = 0;\n\n\t\tfor(int right = 0; right < s.length(); right++) {\n\t\t\tchar ch = s.charAt(right);\n\t\t\tif(!map.containsKey(ch)) {\n\t\t\t\tmap.put(ch, 0);\n\t\t\t}\n\t\t\tmap.put(ch, map.get(ch) + 1);\n\t\t\t\n\t\t\t// IMPORTANT: maxRepeat is not the accurate number of dominant character, It is the historical maximum count \n\t\t\t// We do not care about it because unless it gets greater, it won\'t affect our final max window size.\n\t\t\tmaxRepeat = Math.max(maxRepeat, map.get(ch));\n\n\t\t\tif(right - left + 1 - maxRepeat > k) {\n\t\t\t\tchar remove = s.charAt(left);\n\t\t\t\tmap.put(remove, map.get(remove) - 1);\n\t\t\t\tleft++;\n\t\t\t}\n \n maxWindow = Math.max(maxWindow, right - left + 1);\n }\n \n return maxWindow;\n}\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***0ms / 7.0MB*** (beats 100.00% / 100.00%).\n* ***C++***\n```\n int characterReplacement(string a, int k) {\n int i=0,j=0, maxRepeating = 0, maxLen = 0;\n unordered_map<char, int> m;\n \n //Character other than maximum repeating character should at most be k, becuase we can make only k changes in the string.\n //(length of substring - number of times of the maximum occurring character in the substring) <= k\n\t\t\n for(j=0; j<a.size(); j++){\n m[a[j]]++;\n maxRepeating = max(maxRepeating, m[a[j]]);\n //When other characters become greater than k, we move window ahead.\n if(j-i+1 - maxRepeating > k){\n m[a[i]]--;\n i++;\n }\n maxLen = max(maxLen, j-i+1);\n }\n return maxLen;\n }\n```\n\n```\n```\n\n```\n```\n\n\nThe best result for the code below is ***26ms / 12.2MB*** (beats 95.42% / 82.32%).\n* ***Python***\n```\n def characterReplacement(self, s: str, k: int) -> int:\n \n l = 0\n c_frequency = {}\n longest_str_len = 0\n for r in range(len(s)):\n \n if not s[r] in c_frequency:\n c_frequency[s[r]] = 0\n c_frequency[s[r]] += 1\n \n # Replacements cost = cells count between left and right - highest frequency\n cells_count = r - l + 1\n if cells_count - max(c_frequency.values()) <= k:\n longest_str_len = max(longest_str_len, cells_count)\n \n else:\n c_frequency[s[l]] -= 1\n if not c_frequency[s[l]]:\n c_frequency.pop(s[l])\n l += 1\n \n return longest_str_len\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***51ms / 34.2MB*** (beats 100.00% / 84.12%).\n* ***JavaScript***\n```\nconst characterReplacement = (s, k) => {\n let left = 0;\n let right = 0;\n let maxCharCount = 0;\n const visited = {};\n\n while (right < s.length) {\n const char = s[right];\n visited[char] = visited[char] ? visited[char] + 1 : 1;\n\n if (visited[char] > maxCharCount) maxCharCount = visited[char];\n\n if (right - left + 1 - maxCharCount > k) {\n visited[s[left]]--;\n left++;\n }\n\n right++;\n }\n\n return right - left;\n};\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***68ms / 44.2MB*** (beats 100.00% / 45.25%).\n* ***Kotlin***\n```\nclass Solution {\n fun characterReplacement(s: String, k: Int): Int {\n var mostFreqCharCount = 0; var start = 0; var max=0\n val map = mutableMapOf<Char, Int>()\n \n for(end in 0 until s.length){\n map.put(s[end], map.getOrDefault(s[end], 0) + 1)\n mostFreqCharCount = Math.max(map.get(s[end])!!, mostFreqCharCount)\n if(end - start + 1 > mostFreqCharCount + k){\n map.put(s[start], map.get(s[start])!! - 1)\n start++ \n }\n max = Math.max(max, end - start + 1)\n }\n return max\n }\n}\n```\n\n```\n```\n\n```\n```\n\nThe best result for the code below is ***12ms / 32.2MB*** (beats 95% / 84%).\n* ***Swift***\n```\nclass Solution {\n \n func characterReplacement(_ s: String, _ k: Int) -> Int {\n var windowStart = 0, mx = 0, maxChar = 0\n var inputString = Array(s)\n var dict = [Character: Int]()\n \n for windowEnd in 0..<inputString.count{\n dict[inputString[windowEnd], default: 0 ] += 1\n maxChar = max(maxChar, dict[inputString[windowEnd], default: 0])\n let replaceCharCount = (windowEnd - windowStart + 1) - maxChar\n if replaceCharCount <= k{\n mx = max(mx, windowEnd - windowStart + 1 )\n }else{\n while( ((windowEnd - windowStart + 1) - maxChar) > k){\n dict[inputString[windowStart], default: 0 ] -= 1\n windowStart += 1\n }\n }\n \n }\n return mx\n }\n}\n```\n\n```\n```\n\n```\n```\n\n***"Open your eyes. Expect us." - \uD835\uDCD0\uD835\uDCF7\uD835\uDCF8\uD835\uDCF7\uD835\uDD02\uD835\uDCF6\uD835\uDCF8\uD835\uDCFE\uD835\uDCFC*** | 6 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
✅ 🔥 O(n) Python3 || ⚡ easy solution | longest-repeating-character-replacement | 0 | 1 | \n```\nclass Solution:\n def characterReplacement(self, s: str, k: int) -> int:\n left = right = 0\n freq = {}\n max_count = 0\n longest = 0\n while right < len(s):\n freq[s[right]] = freq.get(s[right], 0) + 1\n max_count = max(max_count, freq[s[right]])\n if right - left + 1 - max_count > k:\n freq[s[left]] -= 1\n left += 1\n longest = max(longest, right - left + 1)\n right += 1\n return longest \n``` | 3 | You are given a string `s` and an integer `k`. You can choose any character of the string and change it to any other uppercase English character. You can perform this operation at most `k` times.
Return _the length of the longest substring containing the same letter you can get after performing the above operations_.
**Example 1:**
**Input:** s = "ABAB ", k = 2
**Output:** 4
**Explanation:** Replace the two 'A's with two 'B's or vice versa.
**Example 2:**
**Input:** s = "AABABBA ", k = 1
**Output:** 4
**Explanation:** Replace the one 'A' in the middle with 'B' and form "AABBBBA ".
The substring "BBBB " has the longest repeating letters, which is 4.
**Constraints:**
* `1 <= s.length <= 105`
* `s` consists of only uppercase English letters.
* `0 <= k <= s.length` | null |
[Python] Recursion; Explained | construct-quad-tree | 0 | 1 | This is a simple recursion problem.\n\nWe can go from top to bottom (i.e., 2 ^ n --> 1) and build the tree based on the return value of the four children.\n\n(1) if the number of element in the grid region is 1, this is a leaf node, we build a new node and return it;\n(2) check the four children, if they all are leaf nodes and have the same value, we can merge the four nodes by create a new leaf node;\n(3) otherwise, just build a new node from the four children.\n\n\n```\nclass Solution:\n def construct(self, grid: List[List[int]]) -> \'Node\':\n level = len(grid)\n root = None\n if level >= 1:\n root = self.buildTree(grid, 0, level, 0, level, level)\n return root\n \n \n def buildTree(self, grid, rs, re, cs, ce, level):\n if level == 1:\n # this is a leaf node:\n return Node(grid[rs][cs], True, None, None, None, None)\n \n next_level = level // 2\n tl = self.buildTree(grid, rs, re - next_level, cs, ce - next_level, next_level)\n tr = self.buildTree(grid, rs, re - next_level, ce - next_level, ce, next_level)\n bl = self.buildTree(grid, re - next_level, re, cs, ce - next_level, next_level)\n br = self.buildTree(grid, re - next_level, re, ce - next_level, ce, next_level)\n \n if tl.isLeaf and tr.isLeaf and bl.isLeaf and br.isLeaf:\n if tl.val == tr.val == bl.val == br.val:\n new_node = Node(tl.val, True, None, None, None, None)\n else:\n new_node = Node(tl.val, False, tl, tr, bl, br)\n else:\n new_node = Node(tl.val, False, tl, tr, bl, br)\n \n return new_node\n``` | 2 | Given a `n * n` matrix `grid` of `0's` and `1's` only. We want to represent `grid` with a Quad-Tree.
Return _the root of the Quad-Tree representing_ `grid`.
A Quad-Tree is a tree data structure in which each internal node has exactly four children. Besides, each node has two attributes:
* `val`: True if the node represents a grid of 1's or False if the node represents a grid of 0's. Notice that you can assign the `val` to True or False when `isLeaf` is False, and both are accepted in the answer.
* `isLeaf`: True if the node is a leaf node on the tree or False if the node has four children.
class Node {
public boolean val;
public boolean isLeaf;
public Node topLeft;
public Node topRight;
public Node bottomLeft;
public Node bottomRight;
}
We can construct a Quad-Tree from a two-dimensional area using the following steps:
1. If the current grid has the same value (i.e all `1's` or all `0's`) set `isLeaf` True and set `val` to the value of the grid and set the four children to Null and stop.
2. If the current grid has different values, set `isLeaf` to False and set `val` to any value and divide the current grid into four sub-grids as shown in the photo.
3. Recurse for each of the children with the proper sub-grid.
If you want to know more about the Quad-Tree, you can refer to the [wiki](https://en.wikipedia.org/wiki/Quadtree).
**Quad-Tree format:**
You don't need to read this section for solving the problem. This is only if you want to understand the output format here. The output represents the serialized format of a Quad-Tree using level order traversal, where `null` signifies a path terminator where no node exists below.
It is very similar to the serialization of the binary tree. The only difference is that the node is represented as a list `[isLeaf, val]`.
If the value of `isLeaf` or `val` is True we represent it as **1** in the list `[isLeaf, val]` and if the value of `isLeaf` or `val` is False we represent it as **0**.
**Example 1:**
**Input:** grid = \[\[0,1\],\[1,0\]\]
**Output:** \[\[0,1\],\[1,0\],\[1,1\],\[1,1\],\[1,0\]\]
**Explanation:** The explanation of this example is shown below:
Notice that 0 represnts False and 1 represents True in the photo representing the Quad-Tree.
**Example 2:**
**Input:** grid = \[\[1,1,1,1,0,0,0,0\],\[1,1,1,1,0,0,0,0\],\[1,1,1,1,1,1,1,1\],\[1,1,1,1,1,1,1,1\],\[1,1,1,1,0,0,0,0\],\[1,1,1,1,0,0,0,0\],\[1,1,1,1,0,0,0,0\],\[1,1,1,1,0,0,0,0\]\]
**Output:** \[\[0,1\],\[1,1\],\[0,1\],\[1,1\],\[1,0\],null,null,null,null,\[1,0\],\[1,0\],\[1,1\],\[1,1\]\]
**Explanation:** All values in the grid are not the same. We divide the grid into four sub-grids.
The topLeft, bottomLeft and bottomRight each has the same value.
The topRight have different values so we divide it into 4 sub-grids where each has the same value.
Explanation is shown in the photo below:
**Constraints:**
* `n == grid.length == grid[i].length`
* `n == 2x` where `0 <= x <= 6` | null |
[Python] Recursion; Explained | construct-quad-tree | 0 | 1 | This is a simple recursion problem.\n\nWe can go from top to bottom (i.e., 2 ^ n --> 1) and build the tree based on the return value of the four children.\n\n(1) if the number of element in the grid region is 1, this is a leaf node, we build a new node and return it;\n(2) check the four children, if they all are leaf nodes and have the same value, we can merge the four nodes by create a new leaf node;\n(3) otherwise, just build a new node from the four children.\n\n\n```\nclass Solution:\n def construct(self, grid: List[List[int]]) -> \'Node\':\n level = len(grid)\n root = None\n if level >= 1:\n root = self.buildTree(grid, 0, level, 0, level, level)\n return root\n \n \n def buildTree(self, grid, rs, re, cs, ce, level):\n if level == 1:\n # this is a leaf node:\n return Node(grid[rs][cs], True, None, None, None, None)\n \n next_level = level // 2\n tl = self.buildTree(grid, rs, re - next_level, cs, ce - next_level, next_level)\n tr = self.buildTree(grid, rs, re - next_level, ce - next_level, ce, next_level)\n bl = self.buildTree(grid, re - next_level, re, cs, ce - next_level, next_level)\n br = self.buildTree(grid, re - next_level, re, ce - next_level, ce, next_level)\n \n if tl.isLeaf and tr.isLeaf and bl.isLeaf and br.isLeaf:\n if tl.val == tr.val == bl.val == br.val:\n new_node = Node(tl.val, True, None, None, None, None)\n else:\n new_node = Node(tl.val, False, tl, tr, bl, br)\n else:\n new_node = Node(tl.val, False, tl, tr, bl, br)\n \n return new_node\n``` | 2 | Implement a basic calculator to evaluate a simple expression string.
The expression string contains only non-negative integers, `'+'`, `'-'`, `'*'`, `'/'` operators, and open `'('` and closing parentheses `')'`. The integer division should **truncate toward zero**.
You may assume that the given expression is always valid. All intermediate results will be in the range of `[-231, 231 - 1]`.
**Note:** You are not allowed to use any built-in function which evaluates strings as mathematical expressions, such as `eval()`.
**Example 1:**
**Input:** s = "1+1 "
**Output:** 2
**Example 2:**
**Input:** s = "6-4/2 "
**Output:** 4
**Example 3:**
**Input:** s = "2\*(5+5\*2)/3+(6/2+8) "
**Output:** 21
**Constraints:**
* `1 <= s <= 104`
* `s` consists of digits, `'+'`, `'-'`, `'*'`, `'/'`, `'('`, and `')'`.
* `s` is a **valid** expression. | null |
Solution | construct-quad-tree | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n bool sameVal(vector<vector<int>>& grid, int x, int y, int size)\n {\n for(auto i{x}; i<x+size; ++i)\n {\n for(auto j{y}; j<y+size; ++j)\n {\n if(grid[i][j] != grid[x][y])\n {\n return false;\n }\n }\n }\n return true;\n }\n void rec(Node* root, vector<vector<int>>& grid, int x, int y, int size)\n {\n if(sameVal(grid, x, y, size))\n {\n root->val = grid[x][y];\n root->isLeaf=true;\n return;\n }\n const auto new_size{size/2};\n \n root->topLeft = new Node();\n rec(root->topLeft, grid, x, y, new_size);\n \n root->topRight = new Node();\n rec(root->topRight, grid, x, y+new_size, new_size);\n \n root->bottomLeft = new Node();\n rec(root->bottomLeft, grid, x+new_size, y, new_size);\n\n root->bottomRight = new Node();\n rec(root->bottomRight, grid, x+new_size, y+new_size, new_size);\n }\n Node* construct(vector<vector<int>>& grid) \n {\n const auto n{grid.size()};\n auto root = new Node();\n rec(root, grid, 0, 0, n);\n return root;\n }\n};\n```\n\n```Python3 []\nclass Node:\n def __init__(self, val, isLeaf, topLeft, topRight, bottomLeft, bottomRight):\n self.val = val\n self.isLeaf = isLeaf\n self.topLeft = topLeft\n self.topRight = topRight\n self.bottomLeft = bottomLeft\n self.bottomRight = bottomRight\n\nclass Solution:\n def construct(self, grid: List[List[int]]) -> Node:\n \n def helper(grid, i, j, w):\n if allSame(grid, i, j, w):\n return Node(grid[i][j] == 1, True)\n\n node = Node(True, False)\n node.topLeft = helper(grid, i, j, w // 2)\n node.topRight = helper(grid, i, j + w // 2, w // 2)\n node.bottomLeft = helper(grid, i + w // 2, j, w // 2)\n node.bottomRight = helper(grid, i + w // 2, j + w // 2, w // 2)\n return node\n\n def allSame(grid, i, j, w):\n for x in range(i, i + w):\n for y in range(j, j + w):\n if grid[x][y] != grid[i][j]:\n return False\n return True\n\n return helper(grid, 0, 0, len(grid))\n```\n\n```Java []\nclass Solution {\n public Node construct(int[][] grid) {\n return build(grid, 0, 0, grid.length);\n }\n public Node build(int[][] grid, int r, int c, int size) {\n if (size == 1 || checkSame(grid, r, c, size)) {\n Node leaf = new Node(grid[r][c] == 1, true);\n return leaf;\n } else {\n int new_size = size/2;\n Node curr = new Node(true, false, \n build(grid, r, c, new_size), \n build(grid, r, c + new_size, new_size), \n build(grid, r + new_size, c, new_size), \n build(grid, r + new_size, c + new_size, new_size));\n return curr;\n }\n }\n public boolean checkSame(int[][] grid, int r, int c, int size) {\n int first = grid[r][c];\n for (int i = r; i < r+size; i++) {\n for (int j = c; j < c+size; j++) {\n if (grid[i][j] != first) {\n return false;\n }\n }\n }\n return true;\n }\n}\n```\n | 1 | Given a `n * n` matrix `grid` of `0's` and `1's` only. We want to represent `grid` with a Quad-Tree.
Return _the root of the Quad-Tree representing_ `grid`.
A Quad-Tree is a tree data structure in which each internal node has exactly four children. Besides, each node has two attributes:
* `val`: True if the node represents a grid of 1's or False if the node represents a grid of 0's. Notice that you can assign the `val` to True or False when `isLeaf` is False, and both are accepted in the answer.
* `isLeaf`: True if the node is a leaf node on the tree or False if the node has four children.
class Node {
public boolean val;
public boolean isLeaf;
public Node topLeft;
public Node topRight;
public Node bottomLeft;
public Node bottomRight;
}
We can construct a Quad-Tree from a two-dimensional area using the following steps:
1. If the current grid has the same value (i.e all `1's` or all `0's`) set `isLeaf` True and set `val` to the value of the grid and set the four children to Null and stop.
2. If the current grid has different values, set `isLeaf` to False and set `val` to any value and divide the current grid into four sub-grids as shown in the photo.
3. Recurse for each of the children with the proper sub-grid.
If you want to know more about the Quad-Tree, you can refer to the [wiki](https://en.wikipedia.org/wiki/Quadtree).
**Quad-Tree format:**
You don't need to read this section for solving the problem. This is only if you want to understand the output format here. The output represents the serialized format of a Quad-Tree using level order traversal, where `null` signifies a path terminator where no node exists below.
It is very similar to the serialization of the binary tree. The only difference is that the node is represented as a list `[isLeaf, val]`.
If the value of `isLeaf` or `val` is True we represent it as **1** in the list `[isLeaf, val]` and if the value of `isLeaf` or `val` is False we represent it as **0**.
**Example 1:**
**Input:** grid = \[\[0,1\],\[1,0\]\]
**Output:** \[\[0,1\],\[1,0\],\[1,1\],\[1,1\],\[1,0\]\]
**Explanation:** The explanation of this example is shown below:
Notice that 0 represnts False and 1 represents True in the photo representing the Quad-Tree.
**Example 2:**
**Input:** grid = \[\[1,1,1,1,0,0,0,0\],\[1,1,1,1,0,0,0,0\],\[1,1,1,1,1,1,1,1\],\[1,1,1,1,1,1,1,1\],\[1,1,1,1,0,0,0,0\],\[1,1,1,1,0,0,0,0\],\[1,1,1,1,0,0,0,0\],\[1,1,1,1,0,0,0,0\]\]
**Output:** \[\[0,1\],\[1,1\],\[0,1\],\[1,1\],\[1,0\],null,null,null,null,\[1,0\],\[1,0\],\[1,1\],\[1,1\]\]
**Explanation:** All values in the grid are not the same. We divide the grid into four sub-grids.
The topLeft, bottomLeft and bottomRight each has the same value.
The topRight have different values so we divide it into 4 sub-grids where each has the same value.
Explanation is shown in the photo below:
**Constraints:**
* `n == grid.length == grid[i].length`
* `n == 2x` where `0 <= x <= 6` | null |
Solution | construct-quad-tree | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n bool sameVal(vector<vector<int>>& grid, int x, int y, int size)\n {\n for(auto i{x}; i<x+size; ++i)\n {\n for(auto j{y}; j<y+size; ++j)\n {\n if(grid[i][j] != grid[x][y])\n {\n return false;\n }\n }\n }\n return true;\n }\n void rec(Node* root, vector<vector<int>>& grid, int x, int y, int size)\n {\n if(sameVal(grid, x, y, size))\n {\n root->val = grid[x][y];\n root->isLeaf=true;\n return;\n }\n const auto new_size{size/2};\n \n root->topLeft = new Node();\n rec(root->topLeft, grid, x, y, new_size);\n \n root->topRight = new Node();\n rec(root->topRight, grid, x, y+new_size, new_size);\n \n root->bottomLeft = new Node();\n rec(root->bottomLeft, grid, x+new_size, y, new_size);\n\n root->bottomRight = new Node();\n rec(root->bottomRight, grid, x+new_size, y+new_size, new_size);\n }\n Node* construct(vector<vector<int>>& grid) \n {\n const auto n{grid.size()};\n auto root = new Node();\n rec(root, grid, 0, 0, n);\n return root;\n }\n};\n```\n\n```Python3 []\nclass Node:\n def __init__(self, val, isLeaf, topLeft, topRight, bottomLeft, bottomRight):\n self.val = val\n self.isLeaf = isLeaf\n self.topLeft = topLeft\n self.topRight = topRight\n self.bottomLeft = bottomLeft\n self.bottomRight = bottomRight\n\nclass Solution:\n def construct(self, grid: List[List[int]]) -> Node:\n \n def helper(grid, i, j, w):\n if allSame(grid, i, j, w):\n return Node(grid[i][j] == 1, True)\n\n node = Node(True, False)\n node.topLeft = helper(grid, i, j, w // 2)\n node.topRight = helper(grid, i, j + w // 2, w // 2)\n node.bottomLeft = helper(grid, i + w // 2, j, w // 2)\n node.bottomRight = helper(grid, i + w // 2, j + w // 2, w // 2)\n return node\n\n def allSame(grid, i, j, w):\n for x in range(i, i + w):\n for y in range(j, j + w):\n if grid[x][y] != grid[i][j]:\n return False\n return True\n\n return helper(grid, 0, 0, len(grid))\n```\n\n```Java []\nclass Solution {\n public Node construct(int[][] grid) {\n return build(grid, 0, 0, grid.length);\n }\n public Node build(int[][] grid, int r, int c, int size) {\n if (size == 1 || checkSame(grid, r, c, size)) {\n Node leaf = new Node(grid[r][c] == 1, true);\n return leaf;\n } else {\n int new_size = size/2;\n Node curr = new Node(true, false, \n build(grid, r, c, new_size), \n build(grid, r, c + new_size, new_size), \n build(grid, r + new_size, c, new_size), \n build(grid, r + new_size, c + new_size, new_size));\n return curr;\n }\n }\n public boolean checkSame(int[][] grid, int r, int c, int size) {\n int first = grid[r][c];\n for (int i = r; i < r+size; i++) {\n for (int j = c; j < c+size; j++) {\n if (grid[i][j] != first) {\n return false;\n }\n }\n }\n return true;\n }\n}\n```\n | 1 | Implement a basic calculator to evaluate a simple expression string.
The expression string contains only non-negative integers, `'+'`, `'-'`, `'*'`, `'/'` operators, and open `'('` and closing parentheses `')'`. The integer division should **truncate toward zero**.
You may assume that the given expression is always valid. All intermediate results will be in the range of `[-231, 231 - 1]`.
**Note:** You are not allowed to use any built-in function which evaluates strings as mathematical expressions, such as `eval()`.
**Example 1:**
**Input:** s = "1+1 "
**Output:** 2
**Example 2:**
**Input:** s = "6-4/2 "
**Output:** 4
**Example 3:**
**Input:** s = "2\*(5+5\*2)/3+(6/2+8) "
**Output:** 21
**Constraints:**
* `1 <= s <= 104`
* `s` consists of digits, `'+'`, `'-'`, `'*'`, `'/'`, `'('`, and `')'`.
* `s` is a **valid** expression. | null |
Python Solution O(n^2) Solution Beats 94% | construct-quad-tree | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThink of how you would do divide and conquer on an array if we had to divide the array in half everytime we do a recursion.\n\nWe will typically have a start index and number of elements from the start. \nFor example if our array is like this - \n\n[0,1,2,3,4,5,6,7]\n\nIn our first stack our recursive calls will look like -\nstart = 0, num_items = 4 # [0,1,2,3]\nstart = 4, num_items = 4 # [4,5,6,7]\n\nOur next recursive call for [4,5,6,7] will look like -\nstart = 4, num_items = 2 # [4,5]\nstart = 6, num_items = 2 # [6,7]\n\nOur base case will be when num_items == 1.\n\nUsing this understanding above, how can we equally divide a 2D array.\nFrom the above example we see that we need a `start` point and `num_items` to describe a 1D array.\n\nTherefore to describe a 2D array we will need a start point in the form of a tuple `(start_row, start_col)` and `size` of our grid, since we will always have `nxn` grid.\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nInorder to keep track of the grid that we are evalulating we will keep 4 variables - \n`pos` - This will be our starting point (described above). For simplicity we will unpack our tuple `r, c = pos` and use `r` as our starting row and `c` as starting column.\n`grid` - This is our original grid\n`size` - number of rows and columns we want to evaluate (described above)\n\nLet\'s understand our base cases here -\n- We will stop recursing if the grid size that we are evaluating is 1x1. Since each grid that we divide has equal number of rows and columns, we will use `abs(c-num_cols) == 1`\n - Create a node and return it `return Node(grid[r][c], True, None, None, None, None)`. Our leaf is set to `True` and we use the first element of the grid as the marker.\n- The second scenario is when all nodes in our current grid is same.\n - We start from `r` (our starting row) and loop until `r+size`\n - For the column we start from `c` and loop until `c+size`\n - If at any point our current element doesn\'t match with the first element of our current grid `grid[row][col] != grid[r][c]:` We break out of the loop and recurse further (See below)\n - Otherwise return same as above `return Node(grid[r][c], True, None, None, None, None)`\n```\nif abs(c-size) == 1:\n return Node(grid[r][c], True, None, None, None, None)\nfor row in range(r, r+size):\n is_break = False\n for col in range(c, c+size):\n if grid[row][col] != grid[r][c]:\n is_break = True\n break\n if is_break:\n break\nelse:\n return Node(grid[r][c], True, None, None, None, None)\n```\nAlternatively you can merge these 2 cases, but I left it there for understanding sake.\n\nLet\'s go over how we are going to recursive if the elements of our current grid are not the same.\n\nWe have 4 cases for recursion\n- Top left `top_left = helper((r,c), grid, size//2)`\n\n ```\n [x o - -]\n [o o - -]\n [- - - -]\n [- - - -]\n ``` \n - Here our `x` represets our starting point, and squares marked with `o` represet our grid that we wil evaluate in next recursion which is of size `n // 2`\n - In our next recursive stack our `(r,c)` is (0,0) our `size` drops from 4 - > 2\n\n\n- Top right `top_right = helper((r,c+size//2), grid, size//2)`\n ```\n [- - x o]\n [- - o o]\n [- - - -]\n [- - - -]\n ``` \n - Here `x` x starts from our original column `c` + half the size of the grid `size//2` our `size` stays the same as before\n - In our next recursive stack our `(r,c)` is (0,2) our `size` drops from 4 - > 2\n\n\n- Bottom Left `bottom_left = helper((r+size//2,c), grid, size//2)`\n ```\n [- - - -]\n [- - - -]\n [x o - -]\n [o o - -]\n ``` \n - Here `x` x starts from our original row `r` + half the size of the grid `size//2` our `size` stays the same as before.\n - In our next recursive stack our `(r,c)` is (2,0) our `size` drops from 4 - > 2\n\n\n- Bottom Right `bottom_right = helper((r+size//2,c+size//2), grid, size//2)`\n ```\n [- - - -]\n [- - - -]\n [- - x o]\n [- - o o]\n ``` \n - Here `x` x starts from our original row `r` and original column `c` + half the size of the grid `size//2` our `size` stays the same as before.\n - In our next recursive stack our `(r,c)` is (2,2) our `size` drops from 4 - > 2\n\nFinally we create a `Node` and populate it with the variables `above`. Our leaf will be set to `False` and since we are allowed to pick any value for `val` we will pick our current grid\'s starting cell value - `grid[r][c]`.\n```\n top_left = helper((r,c), grid, size//2)\n top_right = helper((r,c+size//2), grid, size//2)\n bottom_left = helper((r+size//2,c), grid, size//2)\n bottom_right = helper((r+size//2,c+size//2), grid, size//2)\n return Node(grid[r][c], False, top_left, top_right, bottom_left, bottom_right)\n```\n\nNote: Our first call will have a starting point `(0,0)` and `size` will be `len(grid)`\n```\n return helper((0,0), grid, len(grid))\n```\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nO(n^2) \n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1) \n\n# Code\n```\nclass Solution:\n def construct(self, grid: List[List[int]]) -> \'Node\':\n\n def helper(pos, grid, size):\n r, c = pos\n if abs(c-size) == 1:\n return Node(grid[r][c], True, None, None, None, None)\n for row in range(r, r+size):\n is_break = False\n for col in range(c, c+size):\n if grid[row][col] != grid[r][c]:\n is_break = True\n break\n if is_break:\n break\n else:\n return Node(grid[r][c], True, None, None, None, None)\n top_left = helper((r,c), grid, size//2)\n top_right = helper((r,c+size//2), grid, size//2)\n bottom_left = helper((r+size//2,c), grid, size//2)\n bottom_right = helper((r+size//2,c+size//2), grid, size//2)\n return Node(grid[r][c], False, top_left, top_right, bottom_left, bottom_right)\n return helper((0,0), grid, len(grid))\n``` | 1 | Given a `n * n` matrix `grid` of `0's` and `1's` only. We want to represent `grid` with a Quad-Tree.
Return _the root of the Quad-Tree representing_ `grid`.
A Quad-Tree is a tree data structure in which each internal node has exactly four children. Besides, each node has two attributes:
* `val`: True if the node represents a grid of 1's or False if the node represents a grid of 0's. Notice that you can assign the `val` to True or False when `isLeaf` is False, and both are accepted in the answer.
* `isLeaf`: True if the node is a leaf node on the tree or False if the node has four children.
class Node {
public boolean val;
public boolean isLeaf;
public Node topLeft;
public Node topRight;
public Node bottomLeft;
public Node bottomRight;
}
We can construct a Quad-Tree from a two-dimensional area using the following steps:
1. If the current grid has the same value (i.e all `1's` or all `0's`) set `isLeaf` True and set `val` to the value of the grid and set the four children to Null and stop.
2. If the current grid has different values, set `isLeaf` to False and set `val` to any value and divide the current grid into four sub-grids as shown in the photo.
3. Recurse for each of the children with the proper sub-grid.
If you want to know more about the Quad-Tree, you can refer to the [wiki](https://en.wikipedia.org/wiki/Quadtree).
**Quad-Tree format:**
You don't need to read this section for solving the problem. This is only if you want to understand the output format here. The output represents the serialized format of a Quad-Tree using level order traversal, where `null` signifies a path terminator where no node exists below.
It is very similar to the serialization of the binary tree. The only difference is that the node is represented as a list `[isLeaf, val]`.
If the value of `isLeaf` or `val` is True we represent it as **1** in the list `[isLeaf, val]` and if the value of `isLeaf` or `val` is False we represent it as **0**.
**Example 1:**
**Input:** grid = \[\[0,1\],\[1,0\]\]
**Output:** \[\[0,1\],\[1,0\],\[1,1\],\[1,1\],\[1,0\]\]
**Explanation:** The explanation of this example is shown below:
Notice that 0 represnts False and 1 represents True in the photo representing the Quad-Tree.
**Example 2:**
**Input:** grid = \[\[1,1,1,1,0,0,0,0\],\[1,1,1,1,0,0,0,0\],\[1,1,1,1,1,1,1,1\],\[1,1,1,1,1,1,1,1\],\[1,1,1,1,0,0,0,0\],\[1,1,1,1,0,0,0,0\],\[1,1,1,1,0,0,0,0\],\[1,1,1,1,0,0,0,0\]\]
**Output:** \[\[0,1\],\[1,1\],\[0,1\],\[1,1\],\[1,0\],null,null,null,null,\[1,0\],\[1,0\],\[1,1\],\[1,1\]\]
**Explanation:** All values in the grid are not the same. We divide the grid into four sub-grids.
The topLeft, bottomLeft and bottomRight each has the same value.
The topRight have different values so we divide it into 4 sub-grids where each has the same value.
Explanation is shown in the photo below:
**Constraints:**
* `n == grid.length == grid[i].length`
* `n == 2x` where `0 <= x <= 6` | null |
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.