title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
[Python3] heap | max-value-of-equation | 0 | 1 | Algo\nSince the target to be optimized is `yi + yj + |xi - xj|` (aka `xj + yj + yi - xi` given `j > i`), the value of interest is `yi - xi`. While we loop through the array with index `j`, we want to keep track of the largest `yi - xi` under the constraint that `xj - xi <= k`. \n\nWe could use a priority queue for this purpose. Since Python provides min-heap, we would stuff `(xj - yj, xj)` to the queue such that top of the queue has points with largest `y-x` and `x` could be used to verify if the constraint is met. \n\n```\nclass Solution:\n def findMaxValueOfEquation(self, points: List[List[int]], k: int) -> int:\n ans = -inf\n hp = [] \n for xj, yj in points:\n while hp and xj - hp[0][1] > k: heappop(hp)\n if hp: \n ans = max(ans, xj + yj - hp[0][0])\n heappush(hp, (xj-yj, xj))\n return ans \n``` | 9 | Write an API that generates fancy sequences using the `append`, `addAll`, and `multAll` operations.
Implement the `Fancy` class:
* `Fancy()` Initializes the object with an empty sequence.
* `void append(val)` Appends an integer `val` to the end of the sequence.
* `void addAll(inc)` Increments all existing values in the sequence by an integer `inc`.
* `void multAll(m)` Multiplies all existing values in the sequence by an integer `m`.
* `int getIndex(idx)` Gets the current value at index `idx` (0-indexed) of the sequence **modulo** `109 + 7`. If the index is greater or equal than the length of the sequence, return `-1`.
**Example 1:**
**Input**
\[ "Fancy ", "append ", "addAll ", "append ", "multAll ", "getIndex ", "addAll ", "append ", "multAll ", "getIndex ", "getIndex ", "getIndex "\]
\[\[\], \[2\], \[3\], \[7\], \[2\], \[0\], \[3\], \[10\], \[2\], \[0\], \[1\], \[2\]\]
**Output**
\[null, null, null, null, null, 10, null, null, null, 26, 34, 20\]
**Explanation**
Fancy fancy = new Fancy();
fancy.append(2); // fancy sequence: \[2\]
fancy.addAll(3); // fancy sequence: \[2+3\] -> \[5\]
fancy.append(7); // fancy sequence: \[5, 7\]
fancy.multAll(2); // fancy sequence: \[5\*2, 7\*2\] -> \[10, 14\]
fancy.getIndex(0); // return 10
fancy.addAll(3); // fancy sequence: \[10+3, 14+3\] -> \[13, 17\]
fancy.append(10); // fancy sequence: \[13, 17, 10\]
fancy.multAll(2); // fancy sequence: \[13\*2, 17\*2, 10\*2\] -> \[26, 34, 20\]
fancy.getIndex(0); // return 26
fancy.getIndex(1); // return 34
fancy.getIndex(2); // return 20
**Constraints:**
* `1 <= val, inc, m <= 100`
* `0 <= idx <= 105`
* At most `105` calls total will be made to `append`, `addAll`, `multAll`, and `getIndex`. | Use a priority queue to store for each point i, the tuple [yi-xi, xi] Loop through the array and pop elements from the heap if the condition xj - xi > k, where j is the current index and i is the point on top the queue. After popping elements from the queue. If the queue is not empty, calculate the equation with the current point and the point on top of the queue and maximize the answer. |
Python Bruteforce and MonoQueue completely Explained | max-value-of-equation | 0 | 1 | This is my first post.Please show some love if you found this helpful.The basic idea is very simple for the bruteforce solution.For every pair of (i,j) caluclate the value of equation and return the maximum answer at the end.The second solution deals with two pointers and monoque structures.If you are unfamiliar with monoque kindly check it out in google.Briefing: A monoque does enq,deq,and findmin/findmax in O(1). Also note that we can only do min or max at a time. For beginners, deque is a better option compared to a list.Always use a collections.deque for faster execution and do not prefer a list over deque for que operations.\nRest the code/solution-2 explains. \n\nSolution-1\n{TLE}\nThis is the brute force solution.\nTime: O(N^2)\nSpace:O(1)\n\n```python\nclass Solution:\n def findMaxValueOfEquation(self, points: List[List[int]], k: int) -> int:\n n = len(points)\n xdif = lambda x,y: abs(x[0]-y[0])\n ysum = lambda x,y: x[1]+y[1]\n \n res = float(\'-inf\')\n for i in range(n):\n for j in range(i+1,n):\n if xdif(points[i],points[j]) <=k :\n res = max(res,ysum(points[i],points[j])+xdif(points[i],points[j]))\n \n return res\n```\n\n\nSolution-2\n{AC}\n\nTopics used: monoque,two-pointers\n\nTime : O(N)\nSpace:O(N),for que\n\nBefore moving on to solution-2, get familiar with `mono-queue`.\n\n`Monoqueue helps in retreiving the max val in O(1)`.\n\n```\nThe front element of the monoque represents the max value in the que.\nfront = [val,count]\nval - max val in the que\ncount - total no of elements pushed before val and including val\nBut,still the order of push is maintained in the que.\n```\n\nThe only disadvantage with monoque is that\nwhen deque operation occurs,it doesnt return the exact deque value,\ninstead returns the max value in the queue.\n\n```python\nclass monoque(collections.deque):\n def enq(self,val):\n count = 1\n \n while self and val > self[-1][0]:\n count += self.pop()[1]\n\n self.append([val,count])\n \n def deq(self):\n ans = self.max()\n self[0][1] -=1\n if self[0][1] <= 0:\n self.popleft()\n \n return ans\n \n def max(self):\n return self[0][0] if self else None\n\n#Now,reframe the equation\n # for a specific j,find imin as explained in the code.Then,\n# max(xj -xi + yi + yj) = xj + yj + max(yi-xi)\n\nclass Solution:\n def findMaxValueOfEquation(self, points: List[List[int]], k: int) -> int:\n n = len(points)\n \n mq = monoque()\n res = float(\'-inf\')\n i = 0\n \n for j in range(n):\n #find the imin such that for all [i,j] imin<=i<j\n #such that xj -xi <=k\n #In this window,find the max(yi-xi)\n while i<j and points[j][0] - points[i][0] > k :\n i+=1\n mq.deq()\n \n mx = mq.max()\n if mx is not None:\n res = max(res, mx + points[j][0] + points[j][1] )\n \n dif = points[j][1] - points[j][0]\n mq.enq(dif)\n \n return res\n```\n | 8 | You are given an array `points` containing the coordinates of points on a 2D plane, sorted by the x-values, where `points[i] = [xi, yi]` such that `xi < xj` for all `1 <= i < j <= points.length`. You are also given an integer `k`.
Return _the maximum value of the equation_ `yi + yj + |xi - xj|` where `|xi - xj| <= k` and `1 <= i < j <= points.length`.
It is guaranteed that there exists at least one pair of points that satisfy the constraint `|xi - xj| <= k`.
**Example 1:**
**Input:** points = \[\[1,3\],\[2,0\],\[5,10\],\[6,-10\]\], k = 1
**Output:** 4
**Explanation:** The first two points satisfy the condition |xi - xj| <= 1 and if we calculate the equation we get 3 + 0 + |1 - 2| = 4. Third and fourth points also satisfy the condition and give a value of 10 + -10 + |5 - 6| = 1.
No other pairs satisfy the condition, so we return the max of 4 and 1.
**Example 2:**
**Input:** points = \[\[0,0\],\[3,0\],\[9,2\]\], k = 3
**Output:** 3
**Explanation:** Only the first two points have an absolute difference of 3 or less in the x-values, and give the value of 0 + 0 + |0 - 3| = 3.
**Constraints:**
* `2 <= points.length <= 105`
* `points[i].length == 2`
* `-108 <= xi, yi <= 108`
* `0 <= k <= 2 * 108`
* `xi < xj` for all `1 <= i < j <= points.length`
* `xi` form a strictly increasing sequence. | Keep track of the engineers by their efficiency in decreasing order. Starting from one engineer, to build a team, it suffices to bring K-1 more engineers who have higher efficiencies as well as high speeds. |
Python Bruteforce and MonoQueue completely Explained | max-value-of-equation | 0 | 1 | This is my first post.Please show some love if you found this helpful.The basic idea is very simple for the bruteforce solution.For every pair of (i,j) caluclate the value of equation and return the maximum answer at the end.The second solution deals with two pointers and monoque structures.If you are unfamiliar with monoque kindly check it out in google.Briefing: A monoque does enq,deq,and findmin/findmax in O(1). Also note that we can only do min or max at a time. For beginners, deque is a better option compared to a list.Always use a collections.deque for faster execution and do not prefer a list over deque for que operations.\nRest the code/solution-2 explains. \n\nSolution-1\n{TLE}\nThis is the brute force solution.\nTime: O(N^2)\nSpace:O(1)\n\n```python\nclass Solution:\n def findMaxValueOfEquation(self, points: List[List[int]], k: int) -> int:\n n = len(points)\n xdif = lambda x,y: abs(x[0]-y[0])\n ysum = lambda x,y: x[1]+y[1]\n \n res = float(\'-inf\')\n for i in range(n):\n for j in range(i+1,n):\n if xdif(points[i],points[j]) <=k :\n res = max(res,ysum(points[i],points[j])+xdif(points[i],points[j]))\n \n return res\n```\n\n\nSolution-2\n{AC}\n\nTopics used: monoque,two-pointers\n\nTime : O(N)\nSpace:O(N),for que\n\nBefore moving on to solution-2, get familiar with `mono-queue`.\n\n`Monoqueue helps in retreiving the max val in O(1)`.\n\n```\nThe front element of the monoque represents the max value in the que.\nfront = [val,count]\nval - max val in the que\ncount - total no of elements pushed before val and including val\nBut,still the order of push is maintained in the que.\n```\n\nThe only disadvantage with monoque is that\nwhen deque operation occurs,it doesnt return the exact deque value,\ninstead returns the max value in the queue.\n\n```python\nclass monoque(collections.deque):\n def enq(self,val):\n count = 1\n \n while self and val > self[-1][0]:\n count += self.pop()[1]\n\n self.append([val,count])\n \n def deq(self):\n ans = self.max()\n self[0][1] -=1\n if self[0][1] <= 0:\n self.popleft()\n \n return ans\n \n def max(self):\n return self[0][0] if self else None\n\n#Now,reframe the equation\n # for a specific j,find imin as explained in the code.Then,\n# max(xj -xi + yi + yj) = xj + yj + max(yi-xi)\n\nclass Solution:\n def findMaxValueOfEquation(self, points: List[List[int]], k: int) -> int:\n n = len(points)\n \n mq = monoque()\n res = float(\'-inf\')\n i = 0\n \n for j in range(n):\n #find the imin such that for all [i,j] imin<=i<j\n #such that xj -xi <=k\n #In this window,find the max(yi-xi)\n while i<j and points[j][0] - points[i][0] > k :\n i+=1\n mq.deq()\n \n mx = mq.max()\n if mx is not None:\n res = max(res, mx + points[j][0] + points[j][1] )\n \n dif = points[j][1] - points[j][0]\n mq.enq(dif)\n \n return res\n```\n | 8 | Write an API that generates fancy sequences using the `append`, `addAll`, and `multAll` operations.
Implement the `Fancy` class:
* `Fancy()` Initializes the object with an empty sequence.
* `void append(val)` Appends an integer `val` to the end of the sequence.
* `void addAll(inc)` Increments all existing values in the sequence by an integer `inc`.
* `void multAll(m)` Multiplies all existing values in the sequence by an integer `m`.
* `int getIndex(idx)` Gets the current value at index `idx` (0-indexed) of the sequence **modulo** `109 + 7`. If the index is greater or equal than the length of the sequence, return `-1`.
**Example 1:**
**Input**
\[ "Fancy ", "append ", "addAll ", "append ", "multAll ", "getIndex ", "addAll ", "append ", "multAll ", "getIndex ", "getIndex ", "getIndex "\]
\[\[\], \[2\], \[3\], \[7\], \[2\], \[0\], \[3\], \[10\], \[2\], \[0\], \[1\], \[2\]\]
**Output**
\[null, null, null, null, null, 10, null, null, null, 26, 34, 20\]
**Explanation**
Fancy fancy = new Fancy();
fancy.append(2); // fancy sequence: \[2\]
fancy.addAll(3); // fancy sequence: \[2+3\] -> \[5\]
fancy.append(7); // fancy sequence: \[5, 7\]
fancy.multAll(2); // fancy sequence: \[5\*2, 7\*2\] -> \[10, 14\]
fancy.getIndex(0); // return 10
fancy.addAll(3); // fancy sequence: \[10+3, 14+3\] -> \[13, 17\]
fancy.append(10); // fancy sequence: \[13, 17, 10\]
fancy.multAll(2); // fancy sequence: \[13\*2, 17\*2, 10\*2\] -> \[26, 34, 20\]
fancy.getIndex(0); // return 26
fancy.getIndex(1); // return 34
fancy.getIndex(2); // return 20
**Constraints:**
* `1 <= val, inc, m <= 100`
* `0 <= idx <= 105`
* At most `105` calls total will be made to `append`, `addAll`, `multAll`, and `getIndex`. | Use a priority queue to store for each point i, the tuple [yi-xi, xi] Loop through the array and pop elements from the heap if the condition xj - xi > k, where j is the current index and i is the point on top the queue. After popping elements from the queue. If the queue is not empty, calculate the equation with the current point and the point on top of the queue and maximize the answer. |
Easiest Deque solution O(n) TC and O(n) SC | max-value-of-equation | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findMaxValueOfEquation(self, points: List[List[int]], k: int) -> int:\n d=deque()\n ans=-1e9\n for x,y in points:\n while d and abs(d[0][0]-x)>k:\n d.popleft()\n if d and abs(d[0][0]-x)<=k:\n ans=max(ans,abs(d[0][0]-x)+d[0][1]+y)\n while d and (x-d[-1][0])+2+d[-1][1]<=2+y:\n d.pop()\n d.append([x,y])\n return ans\n\n``` | 0 | You are given an array `points` containing the coordinates of points on a 2D plane, sorted by the x-values, where `points[i] = [xi, yi]` such that `xi < xj` for all `1 <= i < j <= points.length`. You are also given an integer `k`.
Return _the maximum value of the equation_ `yi + yj + |xi - xj|` where `|xi - xj| <= k` and `1 <= i < j <= points.length`.
It is guaranteed that there exists at least one pair of points that satisfy the constraint `|xi - xj| <= k`.
**Example 1:**
**Input:** points = \[\[1,3\],\[2,0\],\[5,10\],\[6,-10\]\], k = 1
**Output:** 4
**Explanation:** The first two points satisfy the condition |xi - xj| <= 1 and if we calculate the equation we get 3 + 0 + |1 - 2| = 4. Third and fourth points also satisfy the condition and give a value of 10 + -10 + |5 - 6| = 1.
No other pairs satisfy the condition, so we return the max of 4 and 1.
**Example 2:**
**Input:** points = \[\[0,0\],\[3,0\],\[9,2\]\], k = 3
**Output:** 3
**Explanation:** Only the first two points have an absolute difference of 3 or less in the x-values, and give the value of 0 + 0 + |0 - 3| = 3.
**Constraints:**
* `2 <= points.length <= 105`
* `points[i].length == 2`
* `-108 <= xi, yi <= 108`
* `0 <= k <= 2 * 108`
* `xi < xj` for all `1 <= i < j <= points.length`
* `xi` form a strictly increasing sequence. | Keep track of the engineers by their efficiency in decreasing order. Starting from one engineer, to build a team, it suffices to bring K-1 more engineers who have higher efficiencies as well as high speeds. |
Easiest Deque solution O(n) TC and O(n) SC | max-value-of-equation | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def findMaxValueOfEquation(self, points: List[List[int]], k: int) -> int:\n d=deque()\n ans=-1e9\n for x,y in points:\n while d and abs(d[0][0]-x)>k:\n d.popleft()\n if d and abs(d[0][0]-x)<=k:\n ans=max(ans,abs(d[0][0]-x)+d[0][1]+y)\n while d and (x-d[-1][0])+2+d[-1][1]<=2+y:\n d.pop()\n d.append([x,y])\n return ans\n\n``` | 0 | Write an API that generates fancy sequences using the `append`, `addAll`, and `multAll` operations.
Implement the `Fancy` class:
* `Fancy()` Initializes the object with an empty sequence.
* `void append(val)` Appends an integer `val` to the end of the sequence.
* `void addAll(inc)` Increments all existing values in the sequence by an integer `inc`.
* `void multAll(m)` Multiplies all existing values in the sequence by an integer `m`.
* `int getIndex(idx)` Gets the current value at index `idx` (0-indexed) of the sequence **modulo** `109 + 7`. If the index is greater or equal than the length of the sequence, return `-1`.
**Example 1:**
**Input**
\[ "Fancy ", "append ", "addAll ", "append ", "multAll ", "getIndex ", "addAll ", "append ", "multAll ", "getIndex ", "getIndex ", "getIndex "\]
\[\[\], \[2\], \[3\], \[7\], \[2\], \[0\], \[3\], \[10\], \[2\], \[0\], \[1\], \[2\]\]
**Output**
\[null, null, null, null, null, 10, null, null, null, 26, 34, 20\]
**Explanation**
Fancy fancy = new Fancy();
fancy.append(2); // fancy sequence: \[2\]
fancy.addAll(3); // fancy sequence: \[2+3\] -> \[5\]
fancy.append(7); // fancy sequence: \[5, 7\]
fancy.multAll(2); // fancy sequence: \[5\*2, 7\*2\] -> \[10, 14\]
fancy.getIndex(0); // return 10
fancy.addAll(3); // fancy sequence: \[10+3, 14+3\] -> \[13, 17\]
fancy.append(10); // fancy sequence: \[13, 17, 10\]
fancy.multAll(2); // fancy sequence: \[13\*2, 17\*2, 10\*2\] -> \[26, 34, 20\]
fancy.getIndex(0); // return 26
fancy.getIndex(1); // return 34
fancy.getIndex(2); // return 20
**Constraints:**
* `1 <= val, inc, m <= 100`
* `0 <= idx <= 105`
* At most `105` calls total will be made to `append`, `addAll`, `multAll`, and `getIndex`. | Use a priority queue to store for each point i, the tuple [yi-xi, xi] Loop through the array and pop elements from the heap if the condition xj - xi > k, where j is the current index and i is the point on top the queue. After popping elements from the queue. If the queue is not empty, calculate the equation with the current point and the point on top of the queue and maximize the answer. |
Double heap, python | max-value-of-equation | 0 | 1 | # Intuition\n\nThe question is to find\n$$\n \\max (x_j+y_j-\\min\\{(x_i-y_i):i<j,\\,x_j-k\\leq x_i\\})\n$$\nThe `MinSet` class implements the constrained running minimum using two heaps, one for the values seen before $j$, and one for the values that already went out of the window ($x_i<x_j-k$). \n\n# Complexity\n\n- Time complexity: $$O(n\\log n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass MinSet:\n def __init__(self):\n self.active = []\n self.inactive = []\n\n def add(self, value):\n heapq.heappush(self.active, value)\n \n def drop(self, value): \n heapq.heappush(self.inactive, value)\n \n def get(self):\n active, inactive = self.active, self.inactive\n while active and inactive and active[0] >= inactive[0]:\n value = heapq.heappop(inactive)\n if active[0] == value:\n heapq.heappop(active)\n \n return active[0] if active else inf\n \nclass Solution:\n def findMaxValueOfEquation(self, points: List[List[int]], k: int) -> int:\n min_set = MinSet()\n max_val = -inf\n i = 0\n x0, y0 = points[i]\n for x, y in points:\n while x0 < x-k:\n min_set.drop(x0-y0)\n i += 1\n x0, y0 = points[i]\n value = x+y-min_set.get()\n if value > max_val:\n max_val = value\n min_set.add(x-y)\n return max_val\n\n\n``` | 0 | You are given an array `points` containing the coordinates of points on a 2D plane, sorted by the x-values, where `points[i] = [xi, yi]` such that `xi < xj` for all `1 <= i < j <= points.length`. You are also given an integer `k`.
Return _the maximum value of the equation_ `yi + yj + |xi - xj|` where `|xi - xj| <= k` and `1 <= i < j <= points.length`.
It is guaranteed that there exists at least one pair of points that satisfy the constraint `|xi - xj| <= k`.
**Example 1:**
**Input:** points = \[\[1,3\],\[2,0\],\[5,10\],\[6,-10\]\], k = 1
**Output:** 4
**Explanation:** The first two points satisfy the condition |xi - xj| <= 1 and if we calculate the equation we get 3 + 0 + |1 - 2| = 4. Third and fourth points also satisfy the condition and give a value of 10 + -10 + |5 - 6| = 1.
No other pairs satisfy the condition, so we return the max of 4 and 1.
**Example 2:**
**Input:** points = \[\[0,0\],\[3,0\],\[9,2\]\], k = 3
**Output:** 3
**Explanation:** Only the first two points have an absolute difference of 3 or less in the x-values, and give the value of 0 + 0 + |0 - 3| = 3.
**Constraints:**
* `2 <= points.length <= 105`
* `points[i].length == 2`
* `-108 <= xi, yi <= 108`
* `0 <= k <= 2 * 108`
* `xi < xj` for all `1 <= i < j <= points.length`
* `xi` form a strictly increasing sequence. | Keep track of the engineers by their efficiency in decreasing order. Starting from one engineer, to build a team, it suffices to bring K-1 more engineers who have higher efficiencies as well as high speeds. |
Double heap, python | max-value-of-equation | 0 | 1 | # Intuition\n\nThe question is to find\n$$\n \\max (x_j+y_j-\\min\\{(x_i-y_i):i<j,\\,x_j-k\\leq x_i\\})\n$$\nThe `MinSet` class implements the constrained running minimum using two heaps, one for the values seen before $j$, and one for the values that already went out of the window ($x_i<x_j-k$). \n\n# Complexity\n\n- Time complexity: $$O(n\\log n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass MinSet:\n def __init__(self):\n self.active = []\n self.inactive = []\n\n def add(self, value):\n heapq.heappush(self.active, value)\n \n def drop(self, value): \n heapq.heappush(self.inactive, value)\n \n def get(self):\n active, inactive = self.active, self.inactive\n while active and inactive and active[0] >= inactive[0]:\n value = heapq.heappop(inactive)\n if active[0] == value:\n heapq.heappop(active)\n \n return active[0] if active else inf\n \nclass Solution:\n def findMaxValueOfEquation(self, points: List[List[int]], k: int) -> int:\n min_set = MinSet()\n max_val = -inf\n i = 0\n x0, y0 = points[i]\n for x, y in points:\n while x0 < x-k:\n min_set.drop(x0-y0)\n i += 1\n x0, y0 = points[i]\n value = x+y-min_set.get()\n if value > max_val:\n max_val = value\n min_set.add(x-y)\n return max_val\n\n\n``` | 0 | Write an API that generates fancy sequences using the `append`, `addAll`, and `multAll` operations.
Implement the `Fancy` class:
* `Fancy()` Initializes the object with an empty sequence.
* `void append(val)` Appends an integer `val` to the end of the sequence.
* `void addAll(inc)` Increments all existing values in the sequence by an integer `inc`.
* `void multAll(m)` Multiplies all existing values in the sequence by an integer `m`.
* `int getIndex(idx)` Gets the current value at index `idx` (0-indexed) of the sequence **modulo** `109 + 7`. If the index is greater or equal than the length of the sequence, return `-1`.
**Example 1:**
**Input**
\[ "Fancy ", "append ", "addAll ", "append ", "multAll ", "getIndex ", "addAll ", "append ", "multAll ", "getIndex ", "getIndex ", "getIndex "\]
\[\[\], \[2\], \[3\], \[7\], \[2\], \[0\], \[3\], \[10\], \[2\], \[0\], \[1\], \[2\]\]
**Output**
\[null, null, null, null, null, 10, null, null, null, 26, 34, 20\]
**Explanation**
Fancy fancy = new Fancy();
fancy.append(2); // fancy sequence: \[2\]
fancy.addAll(3); // fancy sequence: \[2+3\] -> \[5\]
fancy.append(7); // fancy sequence: \[5, 7\]
fancy.multAll(2); // fancy sequence: \[5\*2, 7\*2\] -> \[10, 14\]
fancy.getIndex(0); // return 10
fancy.addAll(3); // fancy sequence: \[10+3, 14+3\] -> \[13, 17\]
fancy.append(10); // fancy sequence: \[13, 17, 10\]
fancy.multAll(2); // fancy sequence: \[13\*2, 17\*2, 10\*2\] -> \[26, 34, 20\]
fancy.getIndex(0); // return 26
fancy.getIndex(1); // return 34
fancy.getIndex(2); // return 20
**Constraints:**
* `1 <= val, inc, m <= 100`
* `0 <= idx <= 105`
* At most `105` calls total will be made to `append`, `addAll`, `multAll`, and `getIndex`. | Use a priority queue to store for each point i, the tuple [yi-xi, xi] Loop through the array and pop elements from the heap if the condition xj - xi > k, where j is the current index and i is the point on top the queue. After popping elements from the queue. If the queue is not empty, calculate the equation with the current point and the point on top of the queue and maximize the answer. |
Python + Java Solution || Using sorting | can-make-arithmetic-progression-from-sequence | 1 | 1 | # Approach\nSimply sort the array and check the differnce between current element element and previous element , if the diff is same through out the array then it forms arthimetic sequence otherwise it will returns false.\n\n# Complexity\n- Time complexity:\nO(nlogn)\n\n- Space complexity:\nO(1)\n```java []\n public boolean canMakeArithmeticProgression(int[] arr) {\n Arrays.sort(arr);\n int diff= arr[1]-arr[0];\n for(int i=2;i<arr.length;i++){\n if(arr[i]-arr[i-1]!=diff)\n return false;\n }\n return true;\n }\n```\n```python []\ndef canMakeArithmeticProgression(self, arr: List[int]) -> bool:\n arr.sort()\n diff = arr[1]-arr[0]\n for i in range(1,len(arr)):\n if(arr[i]-arr[i-1]!=diff):\n return False\n return True\n```\n\n | 2 | A sequence of numbers is called an **arithmetic progression** if the difference between any two consecutive elements is the same.
Given an array of numbers `arr`, return `true` _if the array can be rearranged to form an **arithmetic progression**. Otherwise, return_ `false`.
**Example 1:**
**Input:** arr = \[3,5,1\]
**Output:** true
**Explanation:** We can reorder the elements as \[1,3,5\] or \[5,3,1\] with differences 2 and -2 respectively, between each consecutive elements.
**Example 2:**
**Input:** arr = \[1,2,4\]
**Output:** false
**Explanation:** There is no way to reorder the elements to obtain an arithmetic progression.
**Constraints:**
* `2 <= arr.length <= 1000`
* `-106 <= arr[i] <= 106` | If the s.length < k we cannot construct k strings from s and answer is false. If the number of characters that have odd counts is > k then the minimum number of palindrome strings we can construct is > k and answer is false. Otherwise you can construct exactly k palindrome strings and answer is true (why ?). |
Python + Java Solution || Using sorting | can-make-arithmetic-progression-from-sequence | 1 | 1 | # Approach\nSimply sort the array and check the differnce between current element element and previous element , if the diff is same through out the array then it forms arthimetic sequence otherwise it will returns false.\n\n# Complexity\n- Time complexity:\nO(nlogn)\n\n- Space complexity:\nO(1)\n```java []\n public boolean canMakeArithmeticProgression(int[] arr) {\n Arrays.sort(arr);\n int diff= arr[1]-arr[0];\n for(int i=2;i<arr.length;i++){\n if(arr[i]-arr[i-1]!=diff)\n return false;\n }\n return true;\n }\n```\n```python []\ndef canMakeArithmeticProgression(self, arr: List[int]) -> bool:\n arr.sort()\n diff = arr[1]-arr[0]\n for i in range(1,len(arr)):\n if(arr[i]-arr[i-1]!=diff):\n return False\n return True\n```\n\n | 2 | You are the manager of a basketball team. For the upcoming tournament, you want to choose the team with the highest overall score. The score of the team is the **sum** of scores of all the players in the team.
However, the basketball team is not allowed to have **conflicts**. A **conflict** exists if a younger player has a **strictly higher** score than an older player. A conflict does **not** occur between players of the same age.
Given two lists, `scores` and `ages`, where each `scores[i]` and `ages[i]` represents the score and age of the `ith` player, respectively, return _the highest overall score of all possible basketball teams_.
**Example 1:**
**Input:** scores = \[1,3,5,10,15\], ages = \[1,2,3,4,5\]
**Output:** 34
**Explanation:** You can choose all the players.
**Example 2:**
**Input:** scores = \[4,5,6,5\], ages = \[2,1,2,1\]
**Output:** 16
**Explanation:** It is best to choose the last 3 players. Notice that you are allowed to choose multiple people of the same age.
**Example 3:**
**Input:** scores = \[1,2,3,5\], ages = \[8,9,10,1\]
**Output:** 6
**Explanation:** It is best to choose the first 3 players.
**Constraints:**
* `1 <= scores.length, ages.length <= 1000`
* `scores.length == ages.length`
* `1 <= scores[i] <= 106`
* `1 <= ages[i] <= 1000` | Consider that any valid arithmetic progression will be in sorted order. Sort the array, then check if the differences of all consecutive elements are equal. |
Simple 2 liner in Python | can-make-arithmetic-progression-from-sequence | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def canMakeArithmeticProgression(self, arr: List[int]) -> bool:\n arr.sort()\n return all(arr[i]-arr[i+1] == arr[0]-arr[1] for i in range(len(arr)-1))\n``` | 1 | A sequence of numbers is called an **arithmetic progression** if the difference between any two consecutive elements is the same.
Given an array of numbers `arr`, return `true` _if the array can be rearranged to form an **arithmetic progression**. Otherwise, return_ `false`.
**Example 1:**
**Input:** arr = \[3,5,1\]
**Output:** true
**Explanation:** We can reorder the elements as \[1,3,5\] or \[5,3,1\] with differences 2 and -2 respectively, between each consecutive elements.
**Example 2:**
**Input:** arr = \[1,2,4\]
**Output:** false
**Explanation:** There is no way to reorder the elements to obtain an arithmetic progression.
**Constraints:**
* `2 <= arr.length <= 1000`
* `-106 <= arr[i] <= 106` | If the s.length < k we cannot construct k strings from s and answer is false. If the number of characters that have odd counts is > k then the minimum number of palindrome strings we can construct is > k and answer is false. Otherwise you can construct exactly k palindrome strings and answer is true (why ?). |
Simple 2 liner in Python | can-make-arithmetic-progression-from-sequence | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def canMakeArithmeticProgression(self, arr: List[int]) -> bool:\n arr.sort()\n return all(arr[i]-arr[i+1] == arr[0]-arr[1] for i in range(len(arr)-1))\n``` | 1 | You are the manager of a basketball team. For the upcoming tournament, you want to choose the team with the highest overall score. The score of the team is the **sum** of scores of all the players in the team.
However, the basketball team is not allowed to have **conflicts**. A **conflict** exists if a younger player has a **strictly higher** score than an older player. A conflict does **not** occur between players of the same age.
Given two lists, `scores` and `ages`, where each `scores[i]` and `ages[i]` represents the score and age of the `ith` player, respectively, return _the highest overall score of all possible basketball teams_.
**Example 1:**
**Input:** scores = \[1,3,5,10,15\], ages = \[1,2,3,4,5\]
**Output:** 34
**Explanation:** You can choose all the players.
**Example 2:**
**Input:** scores = \[4,5,6,5\], ages = \[2,1,2,1\]
**Output:** 16
**Explanation:** It is best to choose the last 3 players. Notice that you are allowed to choose multiple people of the same age.
**Example 3:**
**Input:** scores = \[1,2,3,5\], ages = \[8,9,10,1\]
**Output:** 6
**Explanation:** It is best to choose the first 3 players.
**Constraints:**
* `1 <= scores.length, ages.length <= 1000`
* `scores.length == ages.length`
* `1 <= scores[i] <= 106`
* `1 <= ages[i] <= 1000` | Consider that any valid arithmetic progression will be in sorted order. Sort the array, then check if the differences of all consecutive elements are equal. |
Python3 Solution | can-make-arithmetic-progression-from-sequence | 0 | 1 | \n```\nclass Solution:\n def canMakeArithmeticProgression(self, arr: List[int]) -> bool:\n arr.sort()\n for x,y in zip(arr,arr[1:]):\n if y-x!=arr[1]-arr[0]:\n return False\n\n return True \n``` | 1 | A sequence of numbers is called an **arithmetic progression** if the difference between any two consecutive elements is the same.
Given an array of numbers `arr`, return `true` _if the array can be rearranged to form an **arithmetic progression**. Otherwise, return_ `false`.
**Example 1:**
**Input:** arr = \[3,5,1\]
**Output:** true
**Explanation:** We can reorder the elements as \[1,3,5\] or \[5,3,1\] with differences 2 and -2 respectively, between each consecutive elements.
**Example 2:**
**Input:** arr = \[1,2,4\]
**Output:** false
**Explanation:** There is no way to reorder the elements to obtain an arithmetic progression.
**Constraints:**
* `2 <= arr.length <= 1000`
* `-106 <= arr[i] <= 106` | If the s.length < k we cannot construct k strings from s and answer is false. If the number of characters that have odd counts is > k then the minimum number of palindrome strings we can construct is > k and answer is false. Otherwise you can construct exactly k palindrome strings and answer is true (why ?). |
Python3 Solution | can-make-arithmetic-progression-from-sequence | 0 | 1 | \n```\nclass Solution:\n def canMakeArithmeticProgression(self, arr: List[int]) -> bool:\n arr.sort()\n for x,y in zip(arr,arr[1:]):\n if y-x!=arr[1]-arr[0]:\n return False\n\n return True \n``` | 1 | You are the manager of a basketball team. For the upcoming tournament, you want to choose the team with the highest overall score. The score of the team is the **sum** of scores of all the players in the team.
However, the basketball team is not allowed to have **conflicts**. A **conflict** exists if a younger player has a **strictly higher** score than an older player. A conflict does **not** occur between players of the same age.
Given two lists, `scores` and `ages`, where each `scores[i]` and `ages[i]` represents the score and age of the `ith` player, respectively, return _the highest overall score of all possible basketball teams_.
**Example 1:**
**Input:** scores = \[1,3,5,10,15\], ages = \[1,2,3,4,5\]
**Output:** 34
**Explanation:** You can choose all the players.
**Example 2:**
**Input:** scores = \[4,5,6,5\], ages = \[2,1,2,1\]
**Output:** 16
**Explanation:** It is best to choose the last 3 players. Notice that you are allowed to choose multiple people of the same age.
**Example 3:**
**Input:** scores = \[1,2,3,5\], ages = \[8,9,10,1\]
**Output:** 6
**Explanation:** It is best to choose the first 3 players.
**Constraints:**
* `1 <= scores.length, ages.length <= 1000`
* `scores.length == ages.length`
* `1 <= scores[i] <= 106`
* `1 <= ages[i] <= 1000` | Consider that any valid arithmetic progression will be in sorted order. Sort the array, then check if the differences of all consecutive elements are equal. |
Simple and optimal python3 solution | can-make-arithmetic-progression-from-sequence | 0 | 1 | # Complexity\n- Time complexity: $$O(n \\cdot log(n))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$ (because of TimSort)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n``` python3 []\nimport itertools\n\nclass Solution:\n def canMakeArithmeticProgression(self, arr: List[int]) -> bool:\n arr.sort()\n\n delta = arr[1] - arr[0]\n for a, b in itertools.pairwise(arr):\n if b - a != delta:\n return False\n return True\n\n``` | 1 | A sequence of numbers is called an **arithmetic progression** if the difference between any two consecutive elements is the same.
Given an array of numbers `arr`, return `true` _if the array can be rearranged to form an **arithmetic progression**. Otherwise, return_ `false`.
**Example 1:**
**Input:** arr = \[3,5,1\]
**Output:** true
**Explanation:** We can reorder the elements as \[1,3,5\] or \[5,3,1\] with differences 2 and -2 respectively, between each consecutive elements.
**Example 2:**
**Input:** arr = \[1,2,4\]
**Output:** false
**Explanation:** There is no way to reorder the elements to obtain an arithmetic progression.
**Constraints:**
* `2 <= arr.length <= 1000`
* `-106 <= arr[i] <= 106` | If the s.length < k we cannot construct k strings from s and answer is false. If the number of characters that have odd counts is > k then the minimum number of palindrome strings we can construct is > k and answer is false. Otherwise you can construct exactly k palindrome strings and answer is true (why ?). |
Simple and optimal python3 solution | can-make-arithmetic-progression-from-sequence | 0 | 1 | # Complexity\n- Time complexity: $$O(n \\cdot log(n))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$ (because of TimSort)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n``` python3 []\nimport itertools\n\nclass Solution:\n def canMakeArithmeticProgression(self, arr: List[int]) -> bool:\n arr.sort()\n\n delta = arr[1] - arr[0]\n for a, b in itertools.pairwise(arr):\n if b - a != delta:\n return False\n return True\n\n``` | 1 | You are the manager of a basketball team. For the upcoming tournament, you want to choose the team with the highest overall score. The score of the team is the **sum** of scores of all the players in the team.
However, the basketball team is not allowed to have **conflicts**. A **conflict** exists if a younger player has a **strictly higher** score than an older player. A conflict does **not** occur between players of the same age.
Given two lists, `scores` and `ages`, where each `scores[i]` and `ages[i]` represents the score and age of the `ith` player, respectively, return _the highest overall score of all possible basketball teams_.
**Example 1:**
**Input:** scores = \[1,3,5,10,15\], ages = \[1,2,3,4,5\]
**Output:** 34
**Explanation:** You can choose all the players.
**Example 2:**
**Input:** scores = \[4,5,6,5\], ages = \[2,1,2,1\]
**Output:** 16
**Explanation:** It is best to choose the last 3 players. Notice that you are allowed to choose multiple people of the same age.
**Example 3:**
**Input:** scores = \[1,2,3,5\], ages = \[8,9,10,1\]
**Output:** 6
**Explanation:** It is best to choose the first 3 players.
**Constraints:**
* `1 <= scores.length, ages.length <= 1000`
* `scores.length == ages.length`
* `1 <= scores[i] <= 106`
* `1 <= ages[i] <= 1000` | Consider that any valid arithmetic progression will be in sorted order. Sort the array, then check if the differences of all consecutive elements are equal. |
Python3 29ms very fast solution | can-make-arithmetic-progression-from-sequence | 0 | 1 | # Code\n```\nclass Solution:\n def canMakeArithmeticProgression(self, arr: List[int]) -> bool:\n arr = sorted(arr)\n count, current, apnum = 0, 0, arr[1] - arr[0]\n\n for i in range(len(arr)-1):\n current = apnum + arr[i]\n if current == arr[i+1]:\n count = count + 1\n\n if count+1 == len(arr):\n return True\n else:\n return False\n\n``` | 1 | A sequence of numbers is called an **arithmetic progression** if the difference between any two consecutive elements is the same.
Given an array of numbers `arr`, return `true` _if the array can be rearranged to form an **arithmetic progression**. Otherwise, return_ `false`.
**Example 1:**
**Input:** arr = \[3,5,1\]
**Output:** true
**Explanation:** We can reorder the elements as \[1,3,5\] or \[5,3,1\] with differences 2 and -2 respectively, between each consecutive elements.
**Example 2:**
**Input:** arr = \[1,2,4\]
**Output:** false
**Explanation:** There is no way to reorder the elements to obtain an arithmetic progression.
**Constraints:**
* `2 <= arr.length <= 1000`
* `-106 <= arr[i] <= 106` | If the s.length < k we cannot construct k strings from s and answer is false. If the number of characters that have odd counts is > k then the minimum number of palindrome strings we can construct is > k and answer is false. Otherwise you can construct exactly k palindrome strings and answer is true (why ?). |
Python3 29ms very fast solution | can-make-arithmetic-progression-from-sequence | 0 | 1 | # Code\n```\nclass Solution:\n def canMakeArithmeticProgression(self, arr: List[int]) -> bool:\n arr = sorted(arr)\n count, current, apnum = 0, 0, arr[1] - arr[0]\n\n for i in range(len(arr)-1):\n current = apnum + arr[i]\n if current == arr[i+1]:\n count = count + 1\n\n if count+1 == len(arr):\n return True\n else:\n return False\n\n``` | 1 | You are the manager of a basketball team. For the upcoming tournament, you want to choose the team with the highest overall score. The score of the team is the **sum** of scores of all the players in the team.
However, the basketball team is not allowed to have **conflicts**. A **conflict** exists if a younger player has a **strictly higher** score than an older player. A conflict does **not** occur between players of the same age.
Given two lists, `scores` and `ages`, where each `scores[i]` and `ages[i]` represents the score and age of the `ith` player, respectively, return _the highest overall score of all possible basketball teams_.
**Example 1:**
**Input:** scores = \[1,3,5,10,15\], ages = \[1,2,3,4,5\]
**Output:** 34
**Explanation:** You can choose all the players.
**Example 2:**
**Input:** scores = \[4,5,6,5\], ages = \[2,1,2,1\]
**Output:** 16
**Explanation:** It is best to choose the last 3 players. Notice that you are allowed to choose multiple people of the same age.
**Example 3:**
**Input:** scores = \[1,2,3,5\], ages = \[8,9,10,1\]
**Output:** 6
**Explanation:** It is best to choose the first 3 players.
**Constraints:**
* `1 <= scores.length, ages.length <= 1000`
* `scores.length == ages.length`
* `1 <= scores[i] <= 106`
* `1 <= ages[i] <= 1000` | Consider that any valid arithmetic progression will be in sorted order. Sort the array, then check if the differences of all consecutive elements are equal. |
C++ 3 lines & python 1 line|| physics | last-moment-before-all-ants-fall-out-of-a-plank | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nI don\'t like this description. If you use moving balls instead, it just reflects the physics.(I am just borrowing concepts from physics and have no intention of discussing the size of the moving ball and its possible physical properties. )\n\nFind the times for last ants for the right & left. The time they need is exactly the distance.\n\nEach ant has its "task".\n\nThis trick is the the fundamental technique to solve a much hard problem [Leetcode 2731. Movement of Robots](https://leetcode.com/problems/movement-of-robots/solutions/4248711/c-sort-sum-88ms-beats-95-92/)\n# Approach\n<!-- Describe your approach to solving the problem. -->\n[Please turn English subtitles if necessary]\n[https://youtu.be/odbN3KooN8s?si=fvLjz2a0rGpmFZXM](https://youtu.be/odbN3KooN8s?si=fvLjz2a0rGpmFZXM)\nWhen 2 ants meet, they both change the directions and also interchange their "tasks". This is much like the collision of balls, transferring energy to the opponent. One ant may meet several ants, and they interchange their "tasks" when they meet. \n\nThe "tasks" must be done and do not matter which ants finish them.\n\nPython code can be written in line. C code uses loops!\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n# Code\n```\nclass Solution {\npublic:\n int getLastMoment(int n, vector<int>& left, vector<int>& right) {\n int l=right.empty()?0:n-*min_element(right.begin(), right.end());\n int r=left.empty()?0:*max_element(left.begin(), left.end());\n return max(l, r);\n }\n};\n\n```\n# Python code\n\n```\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n return max(n-min(right+[n]), max(left+[0]))\n \n```\n# C code\n```\nint getLastMoment(int n, int* left, int leftSize, int* right, int rightSize) \n{\n int l=0, r=0;\n for(register i=0; i<rightSize; i++) if (n-l>right[i]) l=n-right[i];\n for(register i=0; i<leftSize; i++) if (r<left[i]) r=left[i];\n return (r>l)?r:l;\n}\n``` | 10 | We have a wooden plank of the length `n` **units**. Some ants are walking on the plank, each ant moves with a speed of **1 unit per second**. Some of the ants move to the **left**, the other move to the **right**.
When two ants moving in two **different** directions meet at some point, they change their directions and continue moving again. Assume changing directions does not take any additional time.
When an ant reaches **one end** of the plank at a time `t`, it falls out of the plank immediately.
Given an integer `n` and two integer arrays `left` and `right`, the positions of the ants moving to the left and the right, return _the moment when the last ant(s) fall out of the plank_.
**Example 1:**
**Input:** n = 4, left = \[4,3\], right = \[0,1\]
**Output:** 4
**Explanation:** In the image above:
-The ant at index 0 is named A and going to the right.
-The ant at index 1 is named B and going to the right.
-The ant at index 3 is named C and going to the left.
-The ant at index 4 is named D and going to the left.
The last moment when an ant was on the plank is t = 4 seconds. After that, it falls immediately out of the plank. (i.e., We can say that at t = 4.0000000001, there are no ants on the plank).
**Example 2:**
**Input:** n = 7, left = \[\], right = \[0,1,2,3,4,5,6,7\]
**Output:** 7
**Explanation:** All ants are going to the right, the ant at index 0 needs 7 seconds to fall.
**Example 3:**
**Input:** n = 7, left = \[0,1,2,3,4,5,6,7\], right = \[\]
**Output:** 7
**Explanation:** All ants are going to the left, the ant at index 7 needs 7 seconds to fall.
**Constraints:**
* `1 <= n <= 104`
* `0 <= left.length <= n + 1`
* `0 <= left[i] <= n`
* `0 <= right.length <= n + 1`
* `0 <= right[i] <= n`
* `1 <= left.length + right.length <= n + 1`
* All values of `left` and `right` are unique, and each value can appear **only in one** of the two arrays. | Use dynamic programming to find the optimal solution by saving the previous best like-time coefficient and its corresponding element sum. If adding the current element to the previous best like-time coefficient and its corresponding element sum would increase the best like-time coefficient, then go ahead and add it. Otherwise, keep the previous best like-time coefficient. |
C++ 3 lines & python 1 line|| physics | last-moment-before-all-ants-fall-out-of-a-plank | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nI don\'t like this description. If you use moving balls instead, it just reflects the physics.(I am just borrowing concepts from physics and have no intention of discussing the size of the moving ball and its possible physical properties. )\n\nFind the times for last ants for the right & left. The time they need is exactly the distance.\n\nEach ant has its "task".\n\nThis trick is the the fundamental technique to solve a much hard problem [Leetcode 2731. Movement of Robots](https://leetcode.com/problems/movement-of-robots/solutions/4248711/c-sort-sum-88ms-beats-95-92/)\n# Approach\n<!-- Describe your approach to solving the problem. -->\n[Please turn English subtitles if necessary]\n[https://youtu.be/odbN3KooN8s?si=fvLjz2a0rGpmFZXM](https://youtu.be/odbN3KooN8s?si=fvLjz2a0rGpmFZXM)\nWhen 2 ants meet, they both change the directions and also interchange their "tasks". This is much like the collision of balls, transferring energy to the opponent. One ant may meet several ants, and they interchange their "tasks" when they meet. \n\nThe "tasks" must be done and do not matter which ants finish them.\n\nPython code can be written in line. C code uses loops!\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n$$O(n)$$\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n$$O(1)$$\n# Code\n```\nclass Solution {\npublic:\n int getLastMoment(int n, vector<int>& left, vector<int>& right) {\n int l=right.empty()?0:n-*min_element(right.begin(), right.end());\n int r=left.empty()?0:*max_element(left.begin(), left.end());\n return max(l, r);\n }\n};\n\n```\n# Python code\n\n```\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n return max(n-min(right+[n]), max(left+[0]))\n \n```\n# C code\n```\nint getLastMoment(int n, int* left, int leftSize, int* right, int rightSize) \n{\n int l=0, r=0;\n for(register i=0; i<rightSize; i++) if (n-l>right[i]) l=n-right[i];\n for(register i=0; i<leftSize; i++) if (r<left[i]) r=left[i];\n return (r>l)?r:l;\n}\n``` | 10 | We have `n` cities labeled from `1` to `n`. Two different cities with labels `x` and `y` are directly connected by a bidirectional road if and only if `x` and `y` share a common divisor **strictly greater** than some `threshold`. More formally, cities with labels `x` and `y` have a road between them if there exists an integer `z` such that all of the following are true:
* `x % z == 0`,
* `y % z == 0`, and
* `z > threshold`.
Given the two integers, `n` and `threshold`, and an array of `queries`, you must determine for each `queries[i] = [ai, bi]` if cities `ai` and `bi` are connected directly or indirectly. (i.e. there is some path between them).
Return _an array_ `answer`_, where_ `answer.length == queries.length` _and_ `answer[i]` _is_ `true` _if for the_ `ith` _query, there is a path between_ `ai` _and_ `bi`_, or_ `answer[i]` _is_ `false` _if there is no path._
**Example 1:**
**Input:** n = 6, threshold = 2, queries = \[\[1,4\],\[2,5\],\[3,6\]\]
**Output:** \[false,false,true\]
**Explanation:** The divisors for each number:
1: 1
2: 1, 2
3: 1, 3
4: 1, 2, 4
5: 1, 5
6: 1, 2, 3, 6
Using the underlined divisors above the threshold, only cities 3 and 6 share a common divisor, so they are the
only ones directly connected. The result of each query:
\[1,4\] 1 is not connected to 4
\[2,5\] 2 is not connected to 5
\[3,6\] 3 is connected to 6 through path 3--6
**Example 2:**
**Input:** n = 6, threshold = 0, queries = \[\[4,5\],\[3,4\],\[3,2\],\[2,6\],\[1,3\]\]
**Output:** \[true,true,true,true,true\]
**Explanation:** The divisors for each number are the same as the previous example. However, since the threshold is 0,
all divisors can be used. Since all numbers share 1 as a divisor, all cities are connected.
**Example 3:**
**Input:** n = 5, threshold = 1, queries = \[\[4,5\],\[4,5\],\[3,2\],\[2,3\],\[3,4\]\]
**Output:** \[false,false,false,false,false\]
**Explanation:** Only cities 2 and 4 share a common divisor 2 which is strictly greater than the threshold 1, so they are the only ones directly connected.
Please notice that there can be multiple queries for the same pair of nodes \[x, y\], and that the query \[x, y\] is equivalent to the query \[y, x\].
**Constraints:**
* `2 <= n <= 104`
* `0 <= threshold <= n`
* `1 <= queries.length <= 105`
* `queries[i].length == 2`
* `1 <= ai, bi <= cities`
* `ai != bi` | The ants change their way when they meet is equivalent to continue moving without changing their direction. Answer is the max distance for one ant to reach the end of the plank in the facing direction. |
✅For Beginners ✅II O(n) II just for loop✅ | last-moment-before-all-ants-fall-out-of-a-plank | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe given code defines a class Solution with a method getLastMoment that calculates the last moment when a particle at position n will hit a wall. The particle is initially located at position 0 and can move either to the left or the right. There are two arrays, left and right, which represent the positions of walls to the left and right of the particle\'s initial position.\n\nThe goal is to determine the time it takes for the particle to hit either the leftmost or the rightmost wall and return the maximum of these two times.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n1. Initialize a variable `ans` to keep track of the maximum time it takes for the particle to hit a wall. Set it to 0 initially.\n\n2. Iterate through the `left` array to consider the walls to the left of the particle\'s initial position. For each wall position `left[i]`, calculate the time it takes for the particle to hit this wall by finding the absolute difference between the initial position (0) and the wall position. Update `ans` with the maximum of its current value and this calculated time.\n\n3. Similarly, iterate through the `right` array to consider the walls to the right of the particle\'s initial position. For each wall position `right[i]`, calculate the time it takes for the particle to hit this wall by finding the absolute difference between the wall position and the maximum position `n`. Update `ans` with the maximum of its current value and this calculated time.\n\n4. After processing both the `left` and `right` arrays, `ans` will contain the maximum time it takes for the particle to hit a wall, whether on the left or the right side.\n\n5. Return `ans` as the result, which represents the last moment when the particle hits a wall.\n\nThe code\'s approach efficiently calculates the last moment for the particle to hit a wall by considering both the walls to the left and the walls to the right and taking the maximum of these two times.\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n int getLastMoment(int n, vector<int>& left, vector<int>& right) {\n int ans=0;\n for(int i=0;i<left.size();i++) // you can also use for-each loop like " for(int i : left) "\n ans=max(ans,(abs(0-left[i]))); \n for(int i=0;i<right.size();i++)\n ans=max(ans,abs(n-right[i])); \n return ans; \n }\n};\n```\n```python []\nclass Solution:\n def getLastMoment(self, n, left, right):\n ans = 0\n \n for i in left:\n ans = max(ans, abs(0 - i))\n \n for i in right:\n ans = max(ans, abs(n - i))\n \n return ans\n\n```\n```java []\nclass Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n int totalDistanceLeft;\n int totalDistanceRight;\n if (left.length == 0)\n totalDistanceLeft = 0;\n else \n totalDistanceLeft = Arrays.stream(left).max().getAsInt();\n if (right.length == 0)\n totalDistanceRight = n;\n else \n totalDistanceRight = Arrays.stream(right).min().getAsInt();\n return Math.max(totalDistanceLeft, n-totalDistanceRight);\n \n }\n}\n\n```\n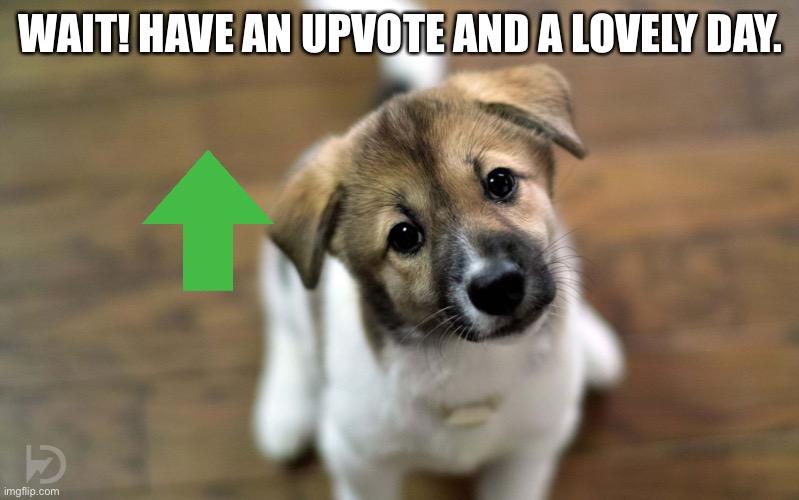\n | 46 | We have a wooden plank of the length `n` **units**. Some ants are walking on the plank, each ant moves with a speed of **1 unit per second**. Some of the ants move to the **left**, the other move to the **right**.
When two ants moving in two **different** directions meet at some point, they change their directions and continue moving again. Assume changing directions does not take any additional time.
When an ant reaches **one end** of the plank at a time `t`, it falls out of the plank immediately.
Given an integer `n` and two integer arrays `left` and `right`, the positions of the ants moving to the left and the right, return _the moment when the last ant(s) fall out of the plank_.
**Example 1:**
**Input:** n = 4, left = \[4,3\], right = \[0,1\]
**Output:** 4
**Explanation:** In the image above:
-The ant at index 0 is named A and going to the right.
-The ant at index 1 is named B and going to the right.
-The ant at index 3 is named C and going to the left.
-The ant at index 4 is named D and going to the left.
The last moment when an ant was on the plank is t = 4 seconds. After that, it falls immediately out of the plank. (i.e., We can say that at t = 4.0000000001, there are no ants on the plank).
**Example 2:**
**Input:** n = 7, left = \[\], right = \[0,1,2,3,4,5,6,7\]
**Output:** 7
**Explanation:** All ants are going to the right, the ant at index 0 needs 7 seconds to fall.
**Example 3:**
**Input:** n = 7, left = \[0,1,2,3,4,5,6,7\], right = \[\]
**Output:** 7
**Explanation:** All ants are going to the left, the ant at index 7 needs 7 seconds to fall.
**Constraints:**
* `1 <= n <= 104`
* `0 <= left.length <= n + 1`
* `0 <= left[i] <= n`
* `0 <= right.length <= n + 1`
* `0 <= right[i] <= n`
* `1 <= left.length + right.length <= n + 1`
* All values of `left` and `right` are unique, and each value can appear **only in one** of the two arrays. | Use dynamic programming to find the optimal solution by saving the previous best like-time coefficient and its corresponding element sum. If adding the current element to the previous best like-time coefficient and its corresponding element sum would increase the best like-time coefficient, then go ahead and add it. Otherwise, keep the previous best like-time coefficient. |
✅For Beginners ✅II O(n) II just for loop✅ | last-moment-before-all-ants-fall-out-of-a-plank | 1 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nThe given code defines a class Solution with a method getLastMoment that calculates the last moment when a particle at position n will hit a wall. The particle is initially located at position 0 and can move either to the left or the right. There are two arrays, left and right, which represent the positions of walls to the left and right of the particle\'s initial position.\n\nThe goal is to determine the time it takes for the particle to hit either the leftmost or the rightmost wall and return the maximum of these two times.\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n1. Initialize a variable `ans` to keep track of the maximum time it takes for the particle to hit a wall. Set it to 0 initially.\n\n2. Iterate through the `left` array to consider the walls to the left of the particle\'s initial position. For each wall position `left[i]`, calculate the time it takes for the particle to hit this wall by finding the absolute difference between the initial position (0) and the wall position. Update `ans` with the maximum of its current value and this calculated time.\n\n3. Similarly, iterate through the `right` array to consider the walls to the right of the particle\'s initial position. For each wall position `right[i]`, calculate the time it takes for the particle to hit this wall by finding the absolute difference between the wall position and the maximum position `n`. Update `ans` with the maximum of its current value and this calculated time.\n\n4. After processing both the `left` and `right` arrays, `ans` will contain the maximum time it takes for the particle to hit a wall, whether on the left or the right side.\n\n5. Return `ans` as the result, which represents the last moment when the particle hits a wall.\n\nThe code\'s approach efficiently calculates the last moment for the particle to hit a wall by considering both the walls to the left and the walls to the right and taking the maximum of these two times.\n# Complexity\n- Time complexity: O(n)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```cpp []\nclass Solution {\npublic:\n int getLastMoment(int n, vector<int>& left, vector<int>& right) {\n int ans=0;\n for(int i=0;i<left.size();i++) // you can also use for-each loop like " for(int i : left) "\n ans=max(ans,(abs(0-left[i]))); \n for(int i=0;i<right.size();i++)\n ans=max(ans,abs(n-right[i])); \n return ans; \n }\n};\n```\n```python []\nclass Solution:\n def getLastMoment(self, n, left, right):\n ans = 0\n \n for i in left:\n ans = max(ans, abs(0 - i))\n \n for i in right:\n ans = max(ans, abs(n - i))\n \n return ans\n\n```\n```java []\nclass Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n int totalDistanceLeft;\n int totalDistanceRight;\n if (left.length == 0)\n totalDistanceLeft = 0;\n else \n totalDistanceLeft = Arrays.stream(left).max().getAsInt();\n if (right.length == 0)\n totalDistanceRight = n;\n else \n totalDistanceRight = Arrays.stream(right).min().getAsInt();\n return Math.max(totalDistanceLeft, n-totalDistanceRight);\n \n }\n}\n\n```\n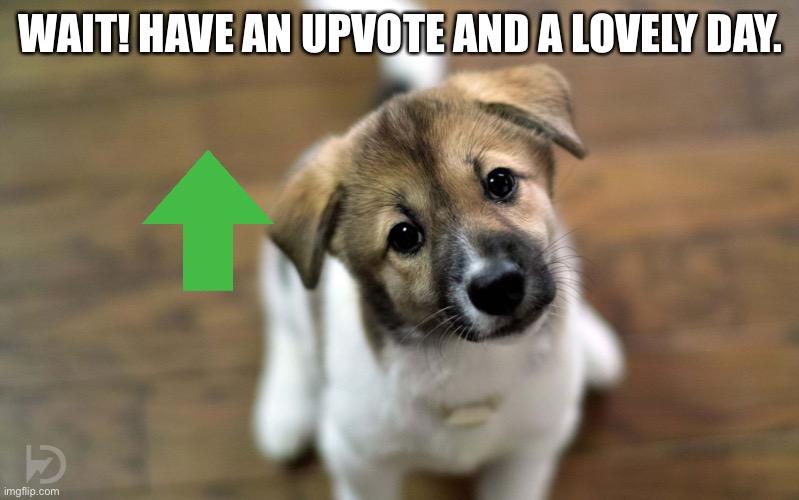\n | 46 | We have `n` cities labeled from `1` to `n`. Two different cities with labels `x` and `y` are directly connected by a bidirectional road if and only if `x` and `y` share a common divisor **strictly greater** than some `threshold`. More formally, cities with labels `x` and `y` have a road between them if there exists an integer `z` such that all of the following are true:
* `x % z == 0`,
* `y % z == 0`, and
* `z > threshold`.
Given the two integers, `n` and `threshold`, and an array of `queries`, you must determine for each `queries[i] = [ai, bi]` if cities `ai` and `bi` are connected directly or indirectly. (i.e. there is some path between them).
Return _an array_ `answer`_, where_ `answer.length == queries.length` _and_ `answer[i]` _is_ `true` _if for the_ `ith` _query, there is a path between_ `ai` _and_ `bi`_, or_ `answer[i]` _is_ `false` _if there is no path._
**Example 1:**
**Input:** n = 6, threshold = 2, queries = \[\[1,4\],\[2,5\],\[3,6\]\]
**Output:** \[false,false,true\]
**Explanation:** The divisors for each number:
1: 1
2: 1, 2
3: 1, 3
4: 1, 2, 4
5: 1, 5
6: 1, 2, 3, 6
Using the underlined divisors above the threshold, only cities 3 and 6 share a common divisor, so they are the
only ones directly connected. The result of each query:
\[1,4\] 1 is not connected to 4
\[2,5\] 2 is not connected to 5
\[3,6\] 3 is connected to 6 through path 3--6
**Example 2:**
**Input:** n = 6, threshold = 0, queries = \[\[4,5\],\[3,4\],\[3,2\],\[2,6\],\[1,3\]\]
**Output:** \[true,true,true,true,true\]
**Explanation:** The divisors for each number are the same as the previous example. However, since the threshold is 0,
all divisors can be used. Since all numbers share 1 as a divisor, all cities are connected.
**Example 3:**
**Input:** n = 5, threshold = 1, queries = \[\[4,5\],\[4,5\],\[3,2\],\[2,3\],\[3,4\]\]
**Output:** \[false,false,false,false,false\]
**Explanation:** Only cities 2 and 4 share a common divisor 2 which is strictly greater than the threshold 1, so they are the only ones directly connected.
Please notice that there can be multiple queries for the same pair of nodes \[x, y\], and that the query \[x, y\] is equivalent to the query \[y, x\].
**Constraints:**
* `2 <= n <= 104`
* `0 <= threshold <= n`
* `1 <= queries.length <= 105`
* `queries[i].length == 2`
* `1 <= ai, bi <= cities`
* `ai != bi` | The ants change their way when they meet is equivalent to continue moving without changing their direction. Answer is the max distance for one ant to reach the end of the plank in the facing direction. |
🔥More than one way |🔥Detailed Explanations | Java | C++ | Python | JavaScript | C# | | last-moment-before-all-ants-fall-out-of-a-plank | 1 | 1 | ### The first method is the best, while the second method is just for your curiosity.\n\n>If you want to understand How to think for this solution ? \nAfter explaining 2nd mehtod i had explained below at last\n\n# 1st Method :- Two Passes\uD83D\uDD25\n\n## Intuition \uD83D\uDE80\nThe problem involves ants moving on a wooden plank. The goal is to find the time when the last ant(s) fall off the plank. Ants can be moving in either direction, and they change direction when they meet another ant. To solve this problem, we need to determine the moment in time when the last ant(s) fall off the plank.\n\n## Approach \uD83D\uDE80\n1. **Variable Initialization**: We start by initializing a variable `time` to keep track of the maximum time it takes for the last ant(s) to fall off the plank. We set it to 0 initially.\n\n2. **Handling Ants Moving to the Left**:\n - We iterate through the positions of ants moving to the left (specified by the `left` array).\n - For each left-moving ant, we check its position.\n - If the current left-moving ant\'s position is greater than the previously recorded maximum time (`time`), we update `time` with the current ant\'s position.\n - This step ensures that we keep track of the ant moving to the left that falls last.\n\n3. **Handling Ants Moving to the Right**:\n - Next, we iterate through the positions of ants moving to the right (specified by the `right` array).\n - For each right-moving ant, we calculate its position relative to the right end of the plank by subtracting its position from the total length of the plank, which is `n`.\n - We compare this calculated position with the previously recorded maximum time (`time`) and update `time` if the calculated position is greater.\n - This step ensures that we account for the ant moving to the right that falls last.\n\n4. **Return Result**:\n - Finally, the `time` variable contains the maximum time, which represents when the last ant(s) fall off the plank, whether they are moving to the left or the right.\n - We return this maximum time as the result of the function.\n\nThe key insight here is that the last ant(s) to fall off the plank will either be the one farthest to the left among left-moving ants or the one farthest to the right among right-moving ants. By keeping track of the maximum position reached by ants from both directions, we can determine the moment when the last ant(s) fall off the plank, and this approach simplifies the problem considerably.\n\n## \u2712\uFE0FCode\n``` Java []\nclass Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n // Initialize a variable to keep track of the maximum time\n int time = 0;\n\n // Iterate through the positions of ants moving to the left\n for (int pos : left) {\n // Update the maximum time if the current left-moving ant\'s position is greater\n // than the previously recorded maximum time\n time = Math.max(time, pos);\n }\n\n // Iterate through the positions of ants moving to the right\n for (int pos : right) {\n // Update the maximum time if the current right-moving ant\'s position (relative to\n // the right end of the plank) is greater than the previously recorded maximum time\n time = Math.max(time, n - pos);\n }\n\n // The final \'time\' variable contains the maximum time, which is when the last ant(s)\n // fall off the plank, so return it as the result.\n return time;\n }\n\n}\n```\n``` C++ []\nclass Solution {\npublic:\n int getLastMoment(int n, vector<int>& left, vector<int>& right) {\n // Initialize a variable to keep track of the maximum time\n int time = 0;\n\n // Iterate through the positions of ants moving to the left\n for (int pos : left) {\n // Update the maximum time if the current left-moving ant\'s position is greater\n // than the previously recorded maximum time\n time = max(time, pos);\n }\n\n // Iterate through the positions of ants moving to the right\n for (int pos : right) {\n // Update the maximum time if the current right-moving ant\'s position (relative to\n // the right end of the plank) is greater than the previously recorded maximum time\n time = max(time, n - pos);\n }\n\n // The final \'time\' variable contains the maximum time, which is when the last ant(s)\n // fall off the plank, so return it as the result.\n return time;\n }\n};\n```\n``` Python []\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n # Initialize a variable to keep track of the maximum time\n time = 0\n\n # Iterate through the positions of ants moving to the left\n for pos in left:\n # Update the maximum time if the current left-moving ant\'s position is greater\n # than the previously recorded maximum time\n time = max(time, pos)\n\n # Iterate through the positions of ants moving to the right\n for pos in right:\n # Update the maximum time if the current right-moving ant\'s position (relative to\n # the right end of the plank) is greater than the previously recorded maximum time\n time = max(time, n - pos)\n\n # The final \'time\' variable contains the maximum time, which is when the last ant(s)\n # fall off the plank, so return it as the result.\n return time\n\n```\n``` JavaScript []\nvar getLastMoment = function(n, left, right) {\n // Initialize a variable to keep track of the maximum time\n let time = 0;\n\n // Iterate through the positions of ants moving to the left\n for (let pos of left) {\n // Update the maximum time if the current left-moving ant\'s position is greater\n // than the previously recorded maximum time\n time = Math.max(time, pos);\n }\n\n // Iterate through the positions of ants moving to the right\n for (let pos of right) {\n // Update the maximum time if the current right-moving ant\'s position (relative to\n // the right end of the plank) is greater than the previously recorded maximum time\n time = Math.max(time, n - pos);\n }\n\n // The final \'time\' variable contains the maximum time, which is when the last ant(s)\n // fall off the plank, so return it as the result.\n return time;\n}\n\n```\n``` C# []\npublic class Solution {\n public int GetLastMoment(int n, int[] left, int[] right) {\n // Initialize a variable to keep track of the maximum time\n int time = 0;\n\n // Iterate through the positions of ants moving to the left\n foreach (int pos in left) {\n // Update the maximum time if the current left-moving ant\'s position is greater\n // than the previously recorded maximum time\n time = Math.Max(time, pos);\n }\n\n // Iterate through the positions of ants moving to the right\n foreach (int pos in right) {\n // Update the maximum time if the current right-moving ant\'s position (relative to\n // the right end of the plank) is greater than the previously recorded maximum time\n time = Math.Max(time, n - pos);\n }\n\n // The final \'time\' variable contains the maximum time, which is when the last ant(s)\n // fall off the plank, so return it as the result.\n return time;\n }\n\n}\n```\n\n## Complexity \uD83D\uDE81\n### \uD83C\uDFF9Time complexity: O(N)\n- The time complexity of this code is O(N), where N is the length of the plank. This is because we iterate through the positions of ants moving to the left and right once, comparing each ant\'s position with the current maximum time. Both loops iterate through the left and right ant positions separately, but they do not depend on each other or nest within each other, so the time complexity remains linear.\n\n### \uD83C\uDFF9Space complexity: O(1)\n- The space complexity of this code is O(1). It uses a constant amount of additional space regardless of the input size. The only extra space used is for the `time` variable and the loop control variables (`pos`), which do not depend on the input size. The input arrays `left` and `right` are not creating additional space in proportion to the input size, so they do not contribute to the space complexity.\n\n\n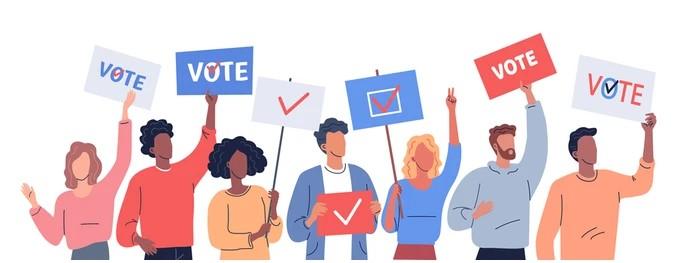\n\n---\n\n# 2nd Method :- Two Passes with Stream and Math Operations\uD83D\uDD25\n\n## Approach \uD83D\uDE80\nThe approach used in this code is based on the "Two Passes" approach.\n\n1. **Finding the Farthest Position Among Ants Moving to the Left**:\n - In the first step, the code calculates the farthest position that any ant moving to the left can reach before falling off the plank.\n - It does this by finding the maximum value in the `left` array using `Arrays.stream(left).max()`. This operation finds the ant with the highest position among all ants moving to the left.\n - The `orElse(0)` part is used as a default value in case the `left` array is empty, ensuring that the code returns 0 if there are no ants moving to the left.\n\n2. **Finding the Nearest Position Among Ants Moving to the Right**:\n - In the second step, the code calculates the nearest position that any ant moving to the right can reach before falling off the plank.\n - It does this by finding the minimum value in the `right` array using `Arrays.stream(right).min()`. This operation finds the ant with the lowest position among all ants moving to the right.\n - The code then calculates the distance from the right end of the plank (`n`) to the nearest ant moving to the right by subtracting the minimum position from `n`.\n - The `orElse(n)` part is used as a default value in case the `right` array is empty, ensuring that the code returns the full length of the plank (`n`) if there are no ants moving to the right.\n\n3. **Calculating the Time When the Last Ant(s) Fall**:\n - Finally, the code calculates the time when the last ant(s) fall by taking the maximum of two values:\n - `maxLeft`: The farthest position among ants moving to the left.\n - `minRight`: The distance from the right end to the nearest ant moving to the right.\n - This maximum value represents the time it takes for the last ant(s) to fall out of the plank.\n\nThe "Two Passes" approach is used here because it makes two passes through the ant positions, first to find the farthest left position and second to find the nearest right position. It efficiently determines the time when the last ant(s) fall without the need for simulating the motion of individual ants or sorting the positions. This code leverages Java\'s stream and mathematical functions to achieve an elegant and efficient solution to the problem.\n\n\n## \u2712\uFE0FCode\n``` Java []\nclass Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n // Find the farthest position among ants moving to the left.\n int maxLeft = Arrays.stream(left).max().orElse(0);\n \n // Find the nearest position among ants moving to the right.\n int minRight = n - Arrays.stream(right).min().orElse(n);\n \n // Calculate the time when the last ant(s) fall.\n return Math.max(maxLeft, minRight);\n }\n}\n\n```\n``` C++ []\nclass Solution {\npublic:\n int getLastMoment(int n, std::vector<int>& left, std::vector<int>& right) {\n // Find the farthest position among ants moving to the left.\n // If \'left\' is empty, set maxLeft to 0.\n int maxLeft = left.empty() ? 0 : *std::max_element(left.begin(), left.end());\n\n // Find the nearest position among ants moving to the right.\n // If \'right\' is empty, set minRight to the right end of the plank (n).\n int minRight = right.empty() ? n : *std::min_element(right.begin(), right.end());\n\n // Calculate the time when the last ant(s) fall.\n // The last ant(s) to fall will be at the maximum position from the left or\n // the maximum position from the right end of the plank.\n return std::max(maxLeft, n - minRight);\n }\n};\n\n```\n``` Python []\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n # Find the farthest position among ants moving to the left.\n maxLeft = max(left, default=0)\n\n # Find the nearest position among ants moving to the right.\n minRight = n - min(right, default=n)\n\n # Calculate the time when the last ant(s) fall.\n return max(maxLeft, minRight)\n```\n``` JavaScript []\nvar getLastMoment = function(n, left, right) {\n // Find the farthest position among ants moving to the left.\n const maxLeft = Math.max(...left, 0);\n \n // Find the nearest position among ants moving to the right.\n const minRight = n - Math.min(...right, n);\n \n // Calculate the time when the last ant(s) fall.\n return Math.max(maxLeft, minRight);\n}\n\n```\n``` C# []\npublic class Solution {\n public int GetLastMoment(int n, int[] left, int[] right) {\n // Find the farthest position among ants moving to the left.\n int maxLeft = left.Length > 0 ? left.Max() : 0;\n \n // Find the nearest position among ants moving to the right.\n int minRight = right.Length > 0 ? right.Min() : n;\n \n // Calculate the time when the last ant(s) fall.\n return Math.Max(maxLeft, n - minRight); // Corrected this line\n }\n\n}\n```\n\n## Complexity \uD83D\uDE81\n### \uD83C\uDFF9Time complexity: O(n_left + n_right)\n- Finding the maximum position among ants moving to the left using `Arrays.stream(left).max()` takes O(n_left) time, where `n_left` is the number of ants moving to the left.\n- Finding the minimum position among ants moving to the right using `Arrays.stream(right).min()` takes O(n_right) time, where `n_right` is the number of ants moving to the right.\n- The time complexity of the `Math.max()` operation is O(1), and the same applies to the subtraction and addition operations.\n- The overall time complexity of the code is O(n_left + n_right), where `n_left` and `n_right` are the sizes of the `left` and `right` arrays, respectively.\n\n### \uD83C\uDFF9Space complexity: O(n_left + n_right)\n- The space complexity is primarily determined by the additional space used for the streams and variables.\n- The space required for the stream created from the `left` and `right` arrays is O(n_left + n_right), as it effectively stores a copy of these arrays.\n- The space used for the `maxLeft` and `minRight` variables is O(1) because they are single integers.\n- Therefore, the overall space complexity is O(n_left + n_right).\n\n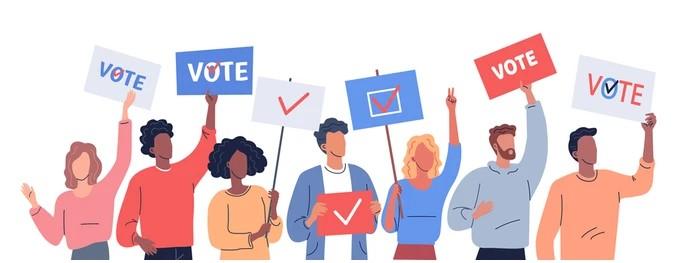\n\n---\n---\n---\n### UnderStand How to think for this solution\n**Problem Description:** You are given an integer n, representing the length of a wooden plank, and an array of integers representing the positions of ants on the plank. Ants can move to the left or right. The goal is to find the last moment when all ants have fallen off the plank. You need to return the earliest possible time (in seconds) when this occurs.\n\n>2nd & 4th step is important to understand\nbelow i had explaned with an example\n\n**Steps to Solve:**\n\n1. **Understand the Problem:** Carefully read and understand the problem statement. Note that the ants can move in both directions, and you need to determine the earliest time when all ants have fallen off the plank.\n\n2. **Encode Ant Directions:** First, you need to understand the importance of ant directions. If two ants collide, they will pass through each other without changing direction. This means that you can treat each ant as if it\'s facing a specific direction (left or right) and calculate the time it takes for that ant to fall off the plank.\n\n3. **Determine Maximum and Minimum Times:** To find the earliest possible time when all ants have fallen off, you can determine the maximum and minimum times for each ant. For an ant at position `x`, the maximum time is the maximum of `x` and `n - x`, and the minimum time is the minimum of `x` and `n - x`.\n\n4. **Find the Maximum of Minimums:** To get the earliest time when all ants have fallen off, you need to find the maximum of the minimum times for all ants. This is because the ant that takes the longest to fall off will determine the time for all ants.\n\n5. **Write the Code:** Implement the above steps in code. Iterate through the ant positions, calculate the maximum and minimum times for each ant, and keep track of the maximum of the minimum times.\n\n6. **Return the Result:** Finally, return the maximum of the minimum times as the earliest time when all ants have fallen off.\n\n# Example:\n\nSuppose you have a plank of length `n = 10`, and you have two ants with positions:\n\n- Ant 1 at position 2 (moving to the right).\n- Ant 2 at position 6 (moving to the left).\n\nHere\'s how you can calculate the earliest moment when all ants have fallen off:\n\n1. **Encode Ant Directions:** Since ant 1 is moving to the right and ant 2 is moving to the left, we can treat them as if they are both moving in one direction. We\'ll choose a common direction, which in this case will be to the right. We\'ll calculate the time it takes for each ant to fall off the plank while moving to the right.\n\n2. **Calculate Maximum and Minimum Times:**\n\n - For Ant 1 at position 2:\n - Maximum time = max(2, 10 - 2) = max(2, 8) = 8\n - Minimum time = min(2, 10 - 2) = min(2, 8) = 2\n\n - For Ant 2 at position 6:\n - Maximum time = max(6, 10 - 6) = max(6, 4) = 6\n - Minimum time = min(6, 10 - 6) = min(6, 4) = 4\n\n3. **Find the Maximum of Minimums:**\n - The maximum of the minimum times is max(2, 4) = 4.\n\nSo, in this example, the earliest moment when all ants have fallen off the plank while moving in one direction (to the right) is 4 seconds.\n\nsee The 1st Method\'s code\n\n\n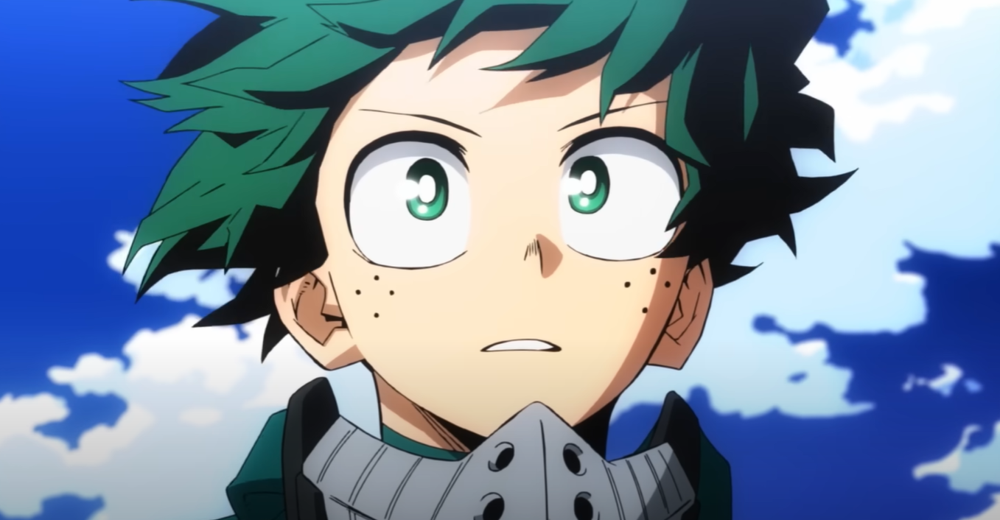\n# Up Vote Guys\n | 77 | We have a wooden plank of the length `n` **units**. Some ants are walking on the plank, each ant moves with a speed of **1 unit per second**. Some of the ants move to the **left**, the other move to the **right**.
When two ants moving in two **different** directions meet at some point, they change their directions and continue moving again. Assume changing directions does not take any additional time.
When an ant reaches **one end** of the plank at a time `t`, it falls out of the plank immediately.
Given an integer `n` and two integer arrays `left` and `right`, the positions of the ants moving to the left and the right, return _the moment when the last ant(s) fall out of the plank_.
**Example 1:**
**Input:** n = 4, left = \[4,3\], right = \[0,1\]
**Output:** 4
**Explanation:** In the image above:
-The ant at index 0 is named A and going to the right.
-The ant at index 1 is named B and going to the right.
-The ant at index 3 is named C and going to the left.
-The ant at index 4 is named D and going to the left.
The last moment when an ant was on the plank is t = 4 seconds. After that, it falls immediately out of the plank. (i.e., We can say that at t = 4.0000000001, there are no ants on the plank).
**Example 2:**
**Input:** n = 7, left = \[\], right = \[0,1,2,3,4,5,6,7\]
**Output:** 7
**Explanation:** All ants are going to the right, the ant at index 0 needs 7 seconds to fall.
**Example 3:**
**Input:** n = 7, left = \[0,1,2,3,4,5,6,7\], right = \[\]
**Output:** 7
**Explanation:** All ants are going to the left, the ant at index 7 needs 7 seconds to fall.
**Constraints:**
* `1 <= n <= 104`
* `0 <= left.length <= n + 1`
* `0 <= left[i] <= n`
* `0 <= right.length <= n + 1`
* `0 <= right[i] <= n`
* `1 <= left.length + right.length <= n + 1`
* All values of `left` and `right` are unique, and each value can appear **only in one** of the two arrays. | Use dynamic programming to find the optimal solution by saving the previous best like-time coefficient and its corresponding element sum. If adding the current element to the previous best like-time coefficient and its corresponding element sum would increase the best like-time coefficient, then go ahead and add it. Otherwise, keep the previous best like-time coefficient. |
🔥More than one way |🔥Detailed Explanations | Java | C++ | Python | JavaScript | C# | | last-moment-before-all-ants-fall-out-of-a-plank | 1 | 1 | ### The first method is the best, while the second method is just for your curiosity.\n\n>If you want to understand How to think for this solution ? \nAfter explaining 2nd mehtod i had explained below at last\n\n# 1st Method :- Two Passes\uD83D\uDD25\n\n## Intuition \uD83D\uDE80\nThe problem involves ants moving on a wooden plank. The goal is to find the time when the last ant(s) fall off the plank. Ants can be moving in either direction, and they change direction when they meet another ant. To solve this problem, we need to determine the moment in time when the last ant(s) fall off the plank.\n\n## Approach \uD83D\uDE80\n1. **Variable Initialization**: We start by initializing a variable `time` to keep track of the maximum time it takes for the last ant(s) to fall off the plank. We set it to 0 initially.\n\n2. **Handling Ants Moving to the Left**:\n - We iterate through the positions of ants moving to the left (specified by the `left` array).\n - For each left-moving ant, we check its position.\n - If the current left-moving ant\'s position is greater than the previously recorded maximum time (`time`), we update `time` with the current ant\'s position.\n - This step ensures that we keep track of the ant moving to the left that falls last.\n\n3. **Handling Ants Moving to the Right**:\n - Next, we iterate through the positions of ants moving to the right (specified by the `right` array).\n - For each right-moving ant, we calculate its position relative to the right end of the plank by subtracting its position from the total length of the plank, which is `n`.\n - We compare this calculated position with the previously recorded maximum time (`time`) and update `time` if the calculated position is greater.\n - This step ensures that we account for the ant moving to the right that falls last.\n\n4. **Return Result**:\n - Finally, the `time` variable contains the maximum time, which represents when the last ant(s) fall off the plank, whether they are moving to the left or the right.\n - We return this maximum time as the result of the function.\n\nThe key insight here is that the last ant(s) to fall off the plank will either be the one farthest to the left among left-moving ants or the one farthest to the right among right-moving ants. By keeping track of the maximum position reached by ants from both directions, we can determine the moment when the last ant(s) fall off the plank, and this approach simplifies the problem considerably.\n\n## \u2712\uFE0FCode\n``` Java []\nclass Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n // Initialize a variable to keep track of the maximum time\n int time = 0;\n\n // Iterate through the positions of ants moving to the left\n for (int pos : left) {\n // Update the maximum time if the current left-moving ant\'s position is greater\n // than the previously recorded maximum time\n time = Math.max(time, pos);\n }\n\n // Iterate through the positions of ants moving to the right\n for (int pos : right) {\n // Update the maximum time if the current right-moving ant\'s position (relative to\n // the right end of the plank) is greater than the previously recorded maximum time\n time = Math.max(time, n - pos);\n }\n\n // The final \'time\' variable contains the maximum time, which is when the last ant(s)\n // fall off the plank, so return it as the result.\n return time;\n }\n\n}\n```\n``` C++ []\nclass Solution {\npublic:\n int getLastMoment(int n, vector<int>& left, vector<int>& right) {\n // Initialize a variable to keep track of the maximum time\n int time = 0;\n\n // Iterate through the positions of ants moving to the left\n for (int pos : left) {\n // Update the maximum time if the current left-moving ant\'s position is greater\n // than the previously recorded maximum time\n time = max(time, pos);\n }\n\n // Iterate through the positions of ants moving to the right\n for (int pos : right) {\n // Update the maximum time if the current right-moving ant\'s position (relative to\n // the right end of the plank) is greater than the previously recorded maximum time\n time = max(time, n - pos);\n }\n\n // The final \'time\' variable contains the maximum time, which is when the last ant(s)\n // fall off the plank, so return it as the result.\n return time;\n }\n};\n```\n``` Python []\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n # Initialize a variable to keep track of the maximum time\n time = 0\n\n # Iterate through the positions of ants moving to the left\n for pos in left:\n # Update the maximum time if the current left-moving ant\'s position is greater\n # than the previously recorded maximum time\n time = max(time, pos)\n\n # Iterate through the positions of ants moving to the right\n for pos in right:\n # Update the maximum time if the current right-moving ant\'s position (relative to\n # the right end of the plank) is greater than the previously recorded maximum time\n time = max(time, n - pos)\n\n # The final \'time\' variable contains the maximum time, which is when the last ant(s)\n # fall off the plank, so return it as the result.\n return time\n\n```\n``` JavaScript []\nvar getLastMoment = function(n, left, right) {\n // Initialize a variable to keep track of the maximum time\n let time = 0;\n\n // Iterate through the positions of ants moving to the left\n for (let pos of left) {\n // Update the maximum time if the current left-moving ant\'s position is greater\n // than the previously recorded maximum time\n time = Math.max(time, pos);\n }\n\n // Iterate through the positions of ants moving to the right\n for (let pos of right) {\n // Update the maximum time if the current right-moving ant\'s position (relative to\n // the right end of the plank) is greater than the previously recorded maximum time\n time = Math.max(time, n - pos);\n }\n\n // The final \'time\' variable contains the maximum time, which is when the last ant(s)\n // fall off the plank, so return it as the result.\n return time;\n}\n\n```\n``` C# []\npublic class Solution {\n public int GetLastMoment(int n, int[] left, int[] right) {\n // Initialize a variable to keep track of the maximum time\n int time = 0;\n\n // Iterate through the positions of ants moving to the left\n foreach (int pos in left) {\n // Update the maximum time if the current left-moving ant\'s position is greater\n // than the previously recorded maximum time\n time = Math.Max(time, pos);\n }\n\n // Iterate through the positions of ants moving to the right\n foreach (int pos in right) {\n // Update the maximum time if the current right-moving ant\'s position (relative to\n // the right end of the plank) is greater than the previously recorded maximum time\n time = Math.Max(time, n - pos);\n }\n\n // The final \'time\' variable contains the maximum time, which is when the last ant(s)\n // fall off the plank, so return it as the result.\n return time;\n }\n\n}\n```\n\n## Complexity \uD83D\uDE81\n### \uD83C\uDFF9Time complexity: O(N)\n- The time complexity of this code is O(N), where N is the length of the plank. This is because we iterate through the positions of ants moving to the left and right once, comparing each ant\'s position with the current maximum time. Both loops iterate through the left and right ant positions separately, but they do not depend on each other or nest within each other, so the time complexity remains linear.\n\n### \uD83C\uDFF9Space complexity: O(1)\n- The space complexity of this code is O(1). It uses a constant amount of additional space regardless of the input size. The only extra space used is for the `time` variable and the loop control variables (`pos`), which do not depend on the input size. The input arrays `left` and `right` are not creating additional space in proportion to the input size, so they do not contribute to the space complexity.\n\n\n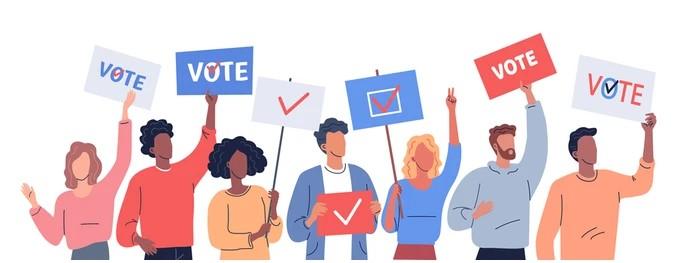\n\n---\n\n# 2nd Method :- Two Passes with Stream and Math Operations\uD83D\uDD25\n\n## Approach \uD83D\uDE80\nThe approach used in this code is based on the "Two Passes" approach.\n\n1. **Finding the Farthest Position Among Ants Moving to the Left**:\n - In the first step, the code calculates the farthest position that any ant moving to the left can reach before falling off the plank.\n - It does this by finding the maximum value in the `left` array using `Arrays.stream(left).max()`. This operation finds the ant with the highest position among all ants moving to the left.\n - The `orElse(0)` part is used as a default value in case the `left` array is empty, ensuring that the code returns 0 if there are no ants moving to the left.\n\n2. **Finding the Nearest Position Among Ants Moving to the Right**:\n - In the second step, the code calculates the nearest position that any ant moving to the right can reach before falling off the plank.\n - It does this by finding the minimum value in the `right` array using `Arrays.stream(right).min()`. This operation finds the ant with the lowest position among all ants moving to the right.\n - The code then calculates the distance from the right end of the plank (`n`) to the nearest ant moving to the right by subtracting the minimum position from `n`.\n - The `orElse(n)` part is used as a default value in case the `right` array is empty, ensuring that the code returns the full length of the plank (`n`) if there are no ants moving to the right.\n\n3. **Calculating the Time When the Last Ant(s) Fall**:\n - Finally, the code calculates the time when the last ant(s) fall by taking the maximum of two values:\n - `maxLeft`: The farthest position among ants moving to the left.\n - `minRight`: The distance from the right end to the nearest ant moving to the right.\n - This maximum value represents the time it takes for the last ant(s) to fall out of the plank.\n\nThe "Two Passes" approach is used here because it makes two passes through the ant positions, first to find the farthest left position and second to find the nearest right position. It efficiently determines the time when the last ant(s) fall without the need for simulating the motion of individual ants or sorting the positions. This code leverages Java\'s stream and mathematical functions to achieve an elegant and efficient solution to the problem.\n\n\n## \u2712\uFE0FCode\n``` Java []\nclass Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n // Find the farthest position among ants moving to the left.\n int maxLeft = Arrays.stream(left).max().orElse(0);\n \n // Find the nearest position among ants moving to the right.\n int minRight = n - Arrays.stream(right).min().orElse(n);\n \n // Calculate the time when the last ant(s) fall.\n return Math.max(maxLeft, minRight);\n }\n}\n\n```\n``` C++ []\nclass Solution {\npublic:\n int getLastMoment(int n, std::vector<int>& left, std::vector<int>& right) {\n // Find the farthest position among ants moving to the left.\n // If \'left\' is empty, set maxLeft to 0.\n int maxLeft = left.empty() ? 0 : *std::max_element(left.begin(), left.end());\n\n // Find the nearest position among ants moving to the right.\n // If \'right\' is empty, set minRight to the right end of the plank (n).\n int minRight = right.empty() ? n : *std::min_element(right.begin(), right.end());\n\n // Calculate the time when the last ant(s) fall.\n // The last ant(s) to fall will be at the maximum position from the left or\n // the maximum position from the right end of the plank.\n return std::max(maxLeft, n - minRight);\n }\n};\n\n```\n``` Python []\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n # Find the farthest position among ants moving to the left.\n maxLeft = max(left, default=0)\n\n # Find the nearest position among ants moving to the right.\n minRight = n - min(right, default=n)\n\n # Calculate the time when the last ant(s) fall.\n return max(maxLeft, minRight)\n```\n``` JavaScript []\nvar getLastMoment = function(n, left, right) {\n // Find the farthest position among ants moving to the left.\n const maxLeft = Math.max(...left, 0);\n \n // Find the nearest position among ants moving to the right.\n const minRight = n - Math.min(...right, n);\n \n // Calculate the time when the last ant(s) fall.\n return Math.max(maxLeft, minRight);\n}\n\n```\n``` C# []\npublic class Solution {\n public int GetLastMoment(int n, int[] left, int[] right) {\n // Find the farthest position among ants moving to the left.\n int maxLeft = left.Length > 0 ? left.Max() : 0;\n \n // Find the nearest position among ants moving to the right.\n int minRight = right.Length > 0 ? right.Min() : n;\n \n // Calculate the time when the last ant(s) fall.\n return Math.Max(maxLeft, n - minRight); // Corrected this line\n }\n\n}\n```\n\n## Complexity \uD83D\uDE81\n### \uD83C\uDFF9Time complexity: O(n_left + n_right)\n- Finding the maximum position among ants moving to the left using `Arrays.stream(left).max()` takes O(n_left) time, where `n_left` is the number of ants moving to the left.\n- Finding the minimum position among ants moving to the right using `Arrays.stream(right).min()` takes O(n_right) time, where `n_right` is the number of ants moving to the right.\n- The time complexity of the `Math.max()` operation is O(1), and the same applies to the subtraction and addition operations.\n- The overall time complexity of the code is O(n_left + n_right), where `n_left` and `n_right` are the sizes of the `left` and `right` arrays, respectively.\n\n### \uD83C\uDFF9Space complexity: O(n_left + n_right)\n- The space complexity is primarily determined by the additional space used for the streams and variables.\n- The space required for the stream created from the `left` and `right` arrays is O(n_left + n_right), as it effectively stores a copy of these arrays.\n- The space used for the `maxLeft` and `minRight` variables is O(1) because they are single integers.\n- Therefore, the overall space complexity is O(n_left + n_right).\n\n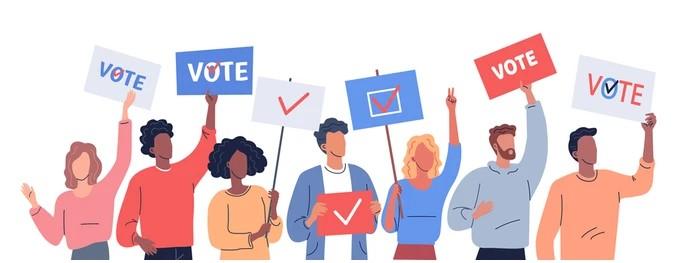\n\n---\n---\n---\n### UnderStand How to think for this solution\n**Problem Description:** You are given an integer n, representing the length of a wooden plank, and an array of integers representing the positions of ants on the plank. Ants can move to the left or right. The goal is to find the last moment when all ants have fallen off the plank. You need to return the earliest possible time (in seconds) when this occurs.\n\n>2nd & 4th step is important to understand\nbelow i had explaned with an example\n\n**Steps to Solve:**\n\n1. **Understand the Problem:** Carefully read and understand the problem statement. Note that the ants can move in both directions, and you need to determine the earliest time when all ants have fallen off the plank.\n\n2. **Encode Ant Directions:** First, you need to understand the importance of ant directions. If two ants collide, they will pass through each other without changing direction. This means that you can treat each ant as if it\'s facing a specific direction (left or right) and calculate the time it takes for that ant to fall off the plank.\n\n3. **Determine Maximum and Minimum Times:** To find the earliest possible time when all ants have fallen off, you can determine the maximum and minimum times for each ant. For an ant at position `x`, the maximum time is the maximum of `x` and `n - x`, and the minimum time is the minimum of `x` and `n - x`.\n\n4. **Find the Maximum of Minimums:** To get the earliest time when all ants have fallen off, you need to find the maximum of the minimum times for all ants. This is because the ant that takes the longest to fall off will determine the time for all ants.\n\n5. **Write the Code:** Implement the above steps in code. Iterate through the ant positions, calculate the maximum and minimum times for each ant, and keep track of the maximum of the minimum times.\n\n6. **Return the Result:** Finally, return the maximum of the minimum times as the earliest time when all ants have fallen off.\n\n# Example:\n\nSuppose you have a plank of length `n = 10`, and you have two ants with positions:\n\n- Ant 1 at position 2 (moving to the right).\n- Ant 2 at position 6 (moving to the left).\n\nHere\'s how you can calculate the earliest moment when all ants have fallen off:\n\n1. **Encode Ant Directions:** Since ant 1 is moving to the right and ant 2 is moving to the left, we can treat them as if they are both moving in one direction. We\'ll choose a common direction, which in this case will be to the right. We\'ll calculate the time it takes for each ant to fall off the plank while moving to the right.\n\n2. **Calculate Maximum and Minimum Times:**\n\n - For Ant 1 at position 2:\n - Maximum time = max(2, 10 - 2) = max(2, 8) = 8\n - Minimum time = min(2, 10 - 2) = min(2, 8) = 2\n\n - For Ant 2 at position 6:\n - Maximum time = max(6, 10 - 6) = max(6, 4) = 6\n - Minimum time = min(6, 10 - 6) = min(6, 4) = 4\n\n3. **Find the Maximum of Minimums:**\n - The maximum of the minimum times is max(2, 4) = 4.\n\nSo, in this example, the earliest moment when all ants have fallen off the plank while moving in one direction (to the right) is 4 seconds.\n\nsee The 1st Method\'s code\n\n\n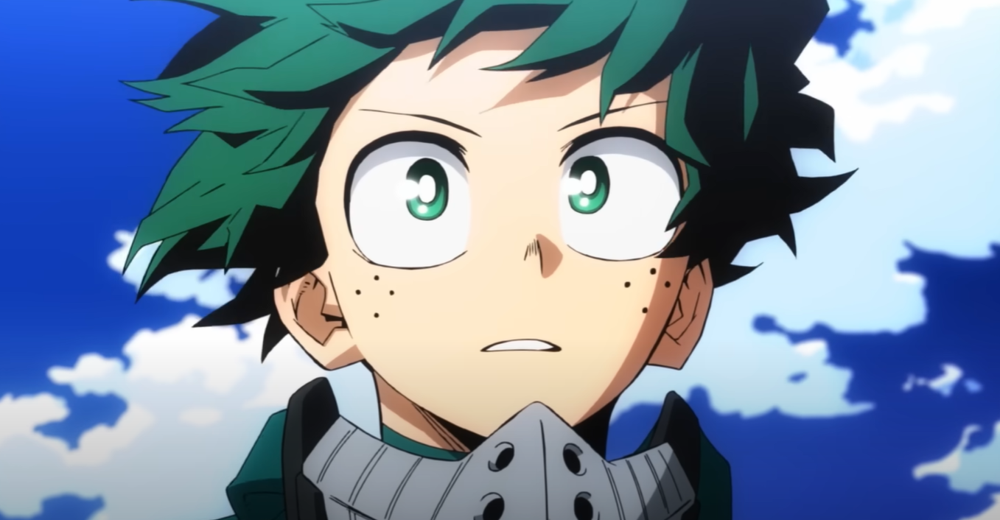\n# Up Vote Guys\n | 77 | We have `n` cities labeled from `1` to `n`. Two different cities with labels `x` and `y` are directly connected by a bidirectional road if and only if `x` and `y` share a common divisor **strictly greater** than some `threshold`. More formally, cities with labels `x` and `y` have a road between them if there exists an integer `z` such that all of the following are true:
* `x % z == 0`,
* `y % z == 0`, and
* `z > threshold`.
Given the two integers, `n` and `threshold`, and an array of `queries`, you must determine for each `queries[i] = [ai, bi]` if cities `ai` and `bi` are connected directly or indirectly. (i.e. there is some path between them).
Return _an array_ `answer`_, where_ `answer.length == queries.length` _and_ `answer[i]` _is_ `true` _if for the_ `ith` _query, there is a path between_ `ai` _and_ `bi`_, or_ `answer[i]` _is_ `false` _if there is no path._
**Example 1:**
**Input:** n = 6, threshold = 2, queries = \[\[1,4\],\[2,5\],\[3,6\]\]
**Output:** \[false,false,true\]
**Explanation:** The divisors for each number:
1: 1
2: 1, 2
3: 1, 3
4: 1, 2, 4
5: 1, 5
6: 1, 2, 3, 6
Using the underlined divisors above the threshold, only cities 3 and 6 share a common divisor, so they are the
only ones directly connected. The result of each query:
\[1,4\] 1 is not connected to 4
\[2,5\] 2 is not connected to 5
\[3,6\] 3 is connected to 6 through path 3--6
**Example 2:**
**Input:** n = 6, threshold = 0, queries = \[\[4,5\],\[3,4\],\[3,2\],\[2,6\],\[1,3\]\]
**Output:** \[true,true,true,true,true\]
**Explanation:** The divisors for each number are the same as the previous example. However, since the threshold is 0,
all divisors can be used. Since all numbers share 1 as a divisor, all cities are connected.
**Example 3:**
**Input:** n = 5, threshold = 1, queries = \[\[4,5\],\[4,5\],\[3,2\],\[2,3\],\[3,4\]\]
**Output:** \[false,false,false,false,false\]
**Explanation:** Only cities 2 and 4 share a common divisor 2 which is strictly greater than the threshold 1, so they are the only ones directly connected.
Please notice that there can be multiple queries for the same pair of nodes \[x, y\], and that the query \[x, y\] is equivalent to the query \[y, x\].
**Constraints:**
* `2 <= n <= 104`
* `0 <= threshold <= n`
* `1 <= queries.length <= 105`
* `queries[i].length == 2`
* `1 <= ai, bi <= cities`
* `ai != bi` | The ants change their way when they meet is equivalent to continue moving without changing their direction. Answer is the max distance for one ant to reach the end of the plank in the facing direction. |
Python3 Solution | last-moment-before-all-ants-fall-out-of-a-plank | 0 | 1 | \n```\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n ans=0\n for i in left:\n ans=max(i,ans)\n for i in right:\n ans=max(n-i,ans)\n return ans \n``` | 4 | We have a wooden plank of the length `n` **units**. Some ants are walking on the plank, each ant moves with a speed of **1 unit per second**. Some of the ants move to the **left**, the other move to the **right**.
When two ants moving in two **different** directions meet at some point, they change their directions and continue moving again. Assume changing directions does not take any additional time.
When an ant reaches **one end** of the plank at a time `t`, it falls out of the plank immediately.
Given an integer `n` and two integer arrays `left` and `right`, the positions of the ants moving to the left and the right, return _the moment when the last ant(s) fall out of the plank_.
**Example 1:**
**Input:** n = 4, left = \[4,3\], right = \[0,1\]
**Output:** 4
**Explanation:** In the image above:
-The ant at index 0 is named A and going to the right.
-The ant at index 1 is named B and going to the right.
-The ant at index 3 is named C and going to the left.
-The ant at index 4 is named D and going to the left.
The last moment when an ant was on the plank is t = 4 seconds. After that, it falls immediately out of the plank. (i.e., We can say that at t = 4.0000000001, there are no ants on the plank).
**Example 2:**
**Input:** n = 7, left = \[\], right = \[0,1,2,3,4,5,6,7\]
**Output:** 7
**Explanation:** All ants are going to the right, the ant at index 0 needs 7 seconds to fall.
**Example 3:**
**Input:** n = 7, left = \[0,1,2,3,4,5,6,7\], right = \[\]
**Output:** 7
**Explanation:** All ants are going to the left, the ant at index 7 needs 7 seconds to fall.
**Constraints:**
* `1 <= n <= 104`
* `0 <= left.length <= n + 1`
* `0 <= left[i] <= n`
* `0 <= right.length <= n + 1`
* `0 <= right[i] <= n`
* `1 <= left.length + right.length <= n + 1`
* All values of `left` and `right` are unique, and each value can appear **only in one** of the two arrays. | Use dynamic programming to find the optimal solution by saving the previous best like-time coefficient and its corresponding element sum. If adding the current element to the previous best like-time coefficient and its corresponding element sum would increase the best like-time coefficient, then go ahead and add it. Otherwise, keep the previous best like-time coefficient. |
Python3 Solution | last-moment-before-all-ants-fall-out-of-a-plank | 0 | 1 | \n```\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n ans=0\n for i in left:\n ans=max(i,ans)\n for i in right:\n ans=max(n-i,ans)\n return ans \n``` | 4 | We have `n` cities labeled from `1` to `n`. Two different cities with labels `x` and `y` are directly connected by a bidirectional road if and only if `x` and `y` share a common divisor **strictly greater** than some `threshold`. More formally, cities with labels `x` and `y` have a road between them if there exists an integer `z` such that all of the following are true:
* `x % z == 0`,
* `y % z == 0`, and
* `z > threshold`.
Given the two integers, `n` and `threshold`, and an array of `queries`, you must determine for each `queries[i] = [ai, bi]` if cities `ai` and `bi` are connected directly or indirectly. (i.e. there is some path between them).
Return _an array_ `answer`_, where_ `answer.length == queries.length` _and_ `answer[i]` _is_ `true` _if for the_ `ith` _query, there is a path between_ `ai` _and_ `bi`_, or_ `answer[i]` _is_ `false` _if there is no path._
**Example 1:**
**Input:** n = 6, threshold = 2, queries = \[\[1,4\],\[2,5\],\[3,6\]\]
**Output:** \[false,false,true\]
**Explanation:** The divisors for each number:
1: 1
2: 1, 2
3: 1, 3
4: 1, 2, 4
5: 1, 5
6: 1, 2, 3, 6
Using the underlined divisors above the threshold, only cities 3 and 6 share a common divisor, so they are the
only ones directly connected. The result of each query:
\[1,4\] 1 is not connected to 4
\[2,5\] 2 is not connected to 5
\[3,6\] 3 is connected to 6 through path 3--6
**Example 2:**
**Input:** n = 6, threshold = 0, queries = \[\[4,5\],\[3,4\],\[3,2\],\[2,6\],\[1,3\]\]
**Output:** \[true,true,true,true,true\]
**Explanation:** The divisors for each number are the same as the previous example. However, since the threshold is 0,
all divisors can be used. Since all numbers share 1 as a divisor, all cities are connected.
**Example 3:**
**Input:** n = 5, threshold = 1, queries = \[\[4,5\],\[4,5\],\[3,2\],\[2,3\],\[3,4\]\]
**Output:** \[false,false,false,false,false\]
**Explanation:** Only cities 2 and 4 share a common divisor 2 which is strictly greater than the threshold 1, so they are the only ones directly connected.
Please notice that there can be multiple queries for the same pair of nodes \[x, y\], and that the query \[x, y\] is equivalent to the query \[y, x\].
**Constraints:**
* `2 <= n <= 104`
* `0 <= threshold <= n`
* `1 <= queries.length <= 105`
* `queries[i].length == 2`
* `1 <= ai, bi <= cities`
* `ai != bi` | The ants change their way when they meet is equivalent to continue moving without changing their direction. Answer is the max distance for one ant to reach the end of the plank in the facing direction. |
faster than 95.7% of solutions greedy | last-moment-before-all-ants-fall-out-of-a-plank | 0 | 1 | \n# Intuition\nThe first thought I had on how to solve this problem was to find the maximum time taken by the ants to fall off the plank. Since the ants move with a speed of 1 unit per second, the maximum time taken by the ants to fall off the plank is the maximum distance between the ants and the ends of the plank.\n\n# Approach\nThe approach I took to solve this problem was to first calculate the maximum distance between the ants and the ends of the plank. If there are no ants moving in one direction, then the maximum distance is the distance between the ant closest to the end of the plank and the end of the plank. If there are ants moving in both directions, then the maximum distance is the maximum of the distance between the ant closest to the left end of the plank and the left end of the plank and the distance between the ant closest to the right end of the plank and the right end of the plank.\n\n# Complexity\nTime complexity: O(n)\nSpace complexity: O(1)\n\n# **Code**\n\n```python\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n if len(right) == 0:\n return n - (n - max(left))\n if len(left) == 0:\n return n - min(right)\n return max(n - (n - max(left)), n - min(right))\n```\n\nThis code is efficient because it only needs to iterate over the left and right lists once. The space complexity is also efficient because it only needs to store the maximum and minimum values of the left and right lists.\n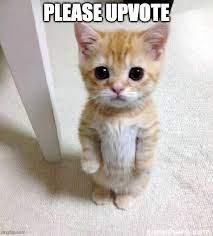\n\n | 3 | We have a wooden plank of the length `n` **units**. Some ants are walking on the plank, each ant moves with a speed of **1 unit per second**. Some of the ants move to the **left**, the other move to the **right**.
When two ants moving in two **different** directions meet at some point, they change their directions and continue moving again. Assume changing directions does not take any additional time.
When an ant reaches **one end** of the plank at a time `t`, it falls out of the plank immediately.
Given an integer `n` and two integer arrays `left` and `right`, the positions of the ants moving to the left and the right, return _the moment when the last ant(s) fall out of the plank_.
**Example 1:**
**Input:** n = 4, left = \[4,3\], right = \[0,1\]
**Output:** 4
**Explanation:** In the image above:
-The ant at index 0 is named A and going to the right.
-The ant at index 1 is named B and going to the right.
-The ant at index 3 is named C and going to the left.
-The ant at index 4 is named D and going to the left.
The last moment when an ant was on the plank is t = 4 seconds. After that, it falls immediately out of the plank. (i.e., We can say that at t = 4.0000000001, there are no ants on the plank).
**Example 2:**
**Input:** n = 7, left = \[\], right = \[0,1,2,3,4,5,6,7\]
**Output:** 7
**Explanation:** All ants are going to the right, the ant at index 0 needs 7 seconds to fall.
**Example 3:**
**Input:** n = 7, left = \[0,1,2,3,4,5,6,7\], right = \[\]
**Output:** 7
**Explanation:** All ants are going to the left, the ant at index 7 needs 7 seconds to fall.
**Constraints:**
* `1 <= n <= 104`
* `0 <= left.length <= n + 1`
* `0 <= left[i] <= n`
* `0 <= right.length <= n + 1`
* `0 <= right[i] <= n`
* `1 <= left.length + right.length <= n + 1`
* All values of `left` and `right` are unique, and each value can appear **only in one** of the two arrays. | Use dynamic programming to find the optimal solution by saving the previous best like-time coefficient and its corresponding element sum. If adding the current element to the previous best like-time coefficient and its corresponding element sum would increase the best like-time coefficient, then go ahead and add it. Otherwise, keep the previous best like-time coefficient. |
faster than 95.7% of solutions greedy | last-moment-before-all-ants-fall-out-of-a-plank | 0 | 1 | \n# Intuition\nThe first thought I had on how to solve this problem was to find the maximum time taken by the ants to fall off the plank. Since the ants move with a speed of 1 unit per second, the maximum time taken by the ants to fall off the plank is the maximum distance between the ants and the ends of the plank.\n\n# Approach\nThe approach I took to solve this problem was to first calculate the maximum distance between the ants and the ends of the plank. If there are no ants moving in one direction, then the maximum distance is the distance between the ant closest to the end of the plank and the end of the plank. If there are ants moving in both directions, then the maximum distance is the maximum of the distance between the ant closest to the left end of the plank and the left end of the plank and the distance between the ant closest to the right end of the plank and the right end of the plank.\n\n# Complexity\nTime complexity: O(n)\nSpace complexity: O(1)\n\n# **Code**\n\n```python\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n if len(right) == 0:\n return n - (n - max(left))\n if len(left) == 0:\n return n - min(right)\n return max(n - (n - max(left)), n - min(right))\n```\n\nThis code is efficient because it only needs to iterate over the left and right lists once. The space complexity is also efficient because it only needs to store the maximum and minimum values of the left and right lists.\n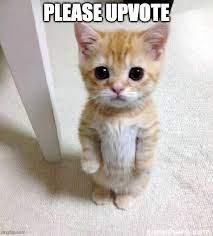\n\n | 3 | We have `n` cities labeled from `1` to `n`. Two different cities with labels `x` and `y` are directly connected by a bidirectional road if and only if `x` and `y` share a common divisor **strictly greater** than some `threshold`. More formally, cities with labels `x` and `y` have a road between them if there exists an integer `z` such that all of the following are true:
* `x % z == 0`,
* `y % z == 0`, and
* `z > threshold`.
Given the two integers, `n` and `threshold`, and an array of `queries`, you must determine for each `queries[i] = [ai, bi]` if cities `ai` and `bi` are connected directly or indirectly. (i.e. there is some path between them).
Return _an array_ `answer`_, where_ `answer.length == queries.length` _and_ `answer[i]` _is_ `true` _if for the_ `ith` _query, there is a path between_ `ai` _and_ `bi`_, or_ `answer[i]` _is_ `false` _if there is no path._
**Example 1:**
**Input:** n = 6, threshold = 2, queries = \[\[1,4\],\[2,5\],\[3,6\]\]
**Output:** \[false,false,true\]
**Explanation:** The divisors for each number:
1: 1
2: 1, 2
3: 1, 3
4: 1, 2, 4
5: 1, 5
6: 1, 2, 3, 6
Using the underlined divisors above the threshold, only cities 3 and 6 share a common divisor, so they are the
only ones directly connected. The result of each query:
\[1,4\] 1 is not connected to 4
\[2,5\] 2 is not connected to 5
\[3,6\] 3 is connected to 6 through path 3--6
**Example 2:**
**Input:** n = 6, threshold = 0, queries = \[\[4,5\],\[3,4\],\[3,2\],\[2,6\],\[1,3\]\]
**Output:** \[true,true,true,true,true\]
**Explanation:** The divisors for each number are the same as the previous example. However, since the threshold is 0,
all divisors can be used. Since all numbers share 1 as a divisor, all cities are connected.
**Example 3:**
**Input:** n = 5, threshold = 1, queries = \[\[4,5\],\[4,5\],\[3,2\],\[2,3\],\[3,4\]\]
**Output:** \[false,false,false,false,false\]
**Explanation:** Only cities 2 and 4 share a common divisor 2 which is strictly greater than the threshold 1, so they are the only ones directly connected.
Please notice that there can be multiple queries for the same pair of nodes \[x, y\], and that the query \[x, y\] is equivalent to the query \[y, x\].
**Constraints:**
* `2 <= n <= 104`
* `0 <= threshold <= n`
* `1 <= queries.length <= 105`
* `queries[i].length == 2`
* `1 <= ai, bi <= cities`
* `ai != bi` | The ants change their way when they meet is equivalent to continue moving without changing their direction. Answer is the max distance for one ant to reach the end of the plank in the facing direction. |
✅☑[C++/Java/Python/JavaScript] || 2 Approaches || EXPLAINED🔥 | last-moment-before-all-ants-fall-out-of-a-plank | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n**(Also explained in the code)**\n\n#### ***Approach 1 (Easy Traverse)***\n1. We initialize the `ans` variable to 0, which will be used to keep track of the maximum moment when an ant falls off the plank.\n1. We loop through the `left` vector to find the maximum position of ants moving to the left.\n1. We loop through the `right` vector and use `n - num` to find the equivalent position on the left side for ants moving to the right, and update `ans` with the maximum of these positions.\n1. Finally, we return `ans`, which represents the last moment when an ant falls off the plank.\n\n# Complexity\n- *Time complexity:*\n $$O(n+m)$$\n \n\n- *Space complexity:*\n $$O(1)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int getLastMoment(int n, vector<int>& left, vector<int>& right) {\n int ans = 0; // Initialize a variable \'ans\' to store the maximum time when an ant falls off.\n\n // Loop through the \'left\' vector, which contains positions of ants moving to the left.\n for (int num : left) {\n ans = max(ans, num); // Update \'ans\' with the maximum position from the \'left\' vector.\n }\n \n // Loop through the \'right\' vector, which contains positions of ants moving to the right.\n for (int num : right) {\n ans = max(ans, n - num); // Update \'ans\' with the maximum position from the \'right\' vector (using n - num to account for ants moving right).\n }\n \n return ans; // Return the final value of \'ans\', which represents the moment when the last ant falls off.\n }\n};\n\n\n\n```\n```C []\n\nint getLastMoment(int n, int* left, int leftSize, int* right, int rightSize) {\n int ans = 0; // Initialize a variable \'ans\' to store the maximum time when an ant falls off.\n\n // Loop through the \'left\' array, which contains positions of ants moving to the left.\n for (int i = 0; i < leftSize; i++) {\n int num = left[i];\n ans = (ans > num) ? ans : num; // Update \'ans\' with the maximum position from the \'left\' array.\n }\n\n // Loop through the \'right\' array, which contains positions of ants moving to the right.\n for (int i = 0; i < rightSize; i++) {\n int num = right[i];\n ans = (ans > n - num) ? ans : n - num; // Update \'ans\' with the maximum position from the \'right\' array (using n - num to account for ants moving right).\n }\n\n return ans; // Return the final value of \'ans\', which represents the moment when the last ant falls off.\n}\n\n\n```\n```Java []\nclass Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n int ans = 0;\n for (int num : left) {\n ans = Math.max(ans, num);\n }\n \n for (int num : right) {\n ans = Math.max(ans, n - num);\n }\n \n return ans;\n }\n}\n\n```\n\n```python3 []\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n ans = 0\n for num in left:\n ans = max(ans, num)\n \n for num in right:\n ans = max(ans, n - num)\n \n return ans\n\n\n```\n```javascript []\nvar getLastMoment = function(n, left, right) {\n let ans = 0; // Initialize a variable \'ans\' to store the maximum time when an ant falls off.\n\n // Loop through the \'left\' array, which contains positions of ants moving to the left.\n for (let num of left) {\n ans = Math.max(ans, num); // Update \'ans\' with the maximum position from the \'left\' array.\n }\n\n // Loop through the \'right\' array, which contains positions of ants moving to the right.\n for (let num of right) {\n ans = Math.max(ans, n - num); // Update \'ans\' with the maximum position from the \'right\' array (using n - num to account for ants moving right).\n }\n\n return ans; // Return the final value of \'ans\', which represents the moment when the last ant falls off.\n};\n\n```\n\n\n---\n#### ***Approach 2 (Math Operation)***\n1. The `getLastMoment` function takes three parameters: the total length of the wooden plank `n`, a vector `left` containing positions of ants moving to the left, and another vector `right` containing positions of ants moving to the right.\n\n1. The code first checks if the `left` vector is empty. If it is empty, it means there are no ants moving to the left, so `maxLeft` is set to 0. Otherwise, it finds the farthest position among ants moving to the left by using `*std::max_element(left.begin(), left.end())`.\n\n1. Similarly, it checks if the `right` vector is empty. If it\'s empty, it means there are no ants moving to the right, so `minRight` is set to `n`, which represents the right end of the plank. Otherwise, it finds the nearest position among ants moving to the right using `*std::min_element(right.begin(), right.end())`.\n\n1. The code then calculates the time when the last ant(s) will fall off the plank. The last ant(s) to fall will be at the maximum position from the left (`maxLeft`) or the maximum position from the right end of the plank (`n - minRight`).\n\n1. The maximum of these two positions is returned as the result, representing the moment when the last ant(s) fall off the plank.\n\n# Complexity\n- *Time complexity:*\n $$O(nleft + nright)$$\n(where `nleft` is the number of ants moving to the left.\nwhere `nright` is the number of ants moving to the right.)\n \n\n- *Space complexity:*\n $$ O(nleft + nright)$$\nwhere `nleft` is the number of ants moving to the left.\nwhere `nright` is the number of ants moving to the right.\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int getLastMoment(int n, std::vector<int>& left, std::vector<int>& right) {\n // Find the farthest position among ants moving to the left.\n // If \'left\' is empty, set maxLeft to 0.\n int maxLeft = left.empty() ? 0 : *std::max_element(left.begin(), left.end());\n\n // Find the nearest position among ants moving to the right.\n // If \'right\' is empty, set minRight to the right end of the plank (n).\n int minRight = right.empty() ? n : *std::min_element(right.begin(), right.end());\n\n // Calculate the time when the last ant(s) fall.\n // The last ant(s) to fall will be at the maximum position from the left or\n // the maximum position from the right end of the plank.\n return std::max(maxLeft, n - minRight);\n }\n};\n\n\n```\n```C []\n\n\nint getLastMoment(int n, int* left, int leftSize, int* right, int rightSize) {\n // Find the farthest position among ants moving to the left.\n int maxLeft = (leftSize == 0) ? 0 : left[0]; // Initialize maxLeft to 0 if \'left\' is empty.\n\n for (int i = 1; i < leftSize; i++) {\n if (left[i] > maxLeft) {\n maxLeft = left[i]; // Update maxLeft if a larger position is found.\n }\n }\n\n // Find the nearest position among ants moving to the right.\n int minRight = (rightSize == 0) ? n : right[0]; // Initialize minRight to n if \'right\' is empty.\n\n for (int i = 1; i < rightSize; i++) {\n if (right[i] < minRight) {\n minRight = right[i]; // Update minRight if a smaller position is found.\n }\n }\n\n // Calculate the time when the last ant(s) fall.\n // The last ant(s) to fall will be at the maximum position from the left or\n // the maximum position from the right end of the plank.\n int lastFall = (maxLeft > n - minRight) ? maxLeft : n - minRight;\n\n return lastFall;\n}\n\n\n\n\n\n\n```\n```Java []\nclass Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n // Find the farthest position among ants moving to the left.\n int maxLeft = Arrays.stream(left).max().orElse(0);\n \n // Find the nearest position among ants moving to the right.\n int minRight = n - Arrays.stream(right).min().orElse(n);\n \n // Calculate the time when the last ant(s) fall.\n return Math.max(maxLeft, minRight);\n }\n}\n\n```\n\n```python []\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n # Find the farthest position among ants moving to the left.\n maxLeft = max(left, default=0)\n\n # Find the nearest position among ants moving to the right.\n minRight = n - min(right, default=n)\n\n # Calculate the time when the last ant(s) fall.\n return max(maxLeft, minRight)\n\n\n```\n```javascript []\nvar getLastMoment = function(n, left, right) {\n // Find the farthest position among ants moving to the left.\n const maxLeft = Math.max(...left, 0);\n \n // Find the nearest position among ants moving to the right.\n const minRight = n - Math.min(...right, n);\n \n // Calculate the time when the last ant(s) fall.\n return Math.max(maxLeft, minRight);\n}\n```\n\n\n---\n\n\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n--- | 2 | We have a wooden plank of the length `n` **units**. Some ants are walking on the plank, each ant moves with a speed of **1 unit per second**. Some of the ants move to the **left**, the other move to the **right**.
When two ants moving in two **different** directions meet at some point, they change their directions and continue moving again. Assume changing directions does not take any additional time.
When an ant reaches **one end** of the plank at a time `t`, it falls out of the plank immediately.
Given an integer `n` and two integer arrays `left` and `right`, the positions of the ants moving to the left and the right, return _the moment when the last ant(s) fall out of the plank_.
**Example 1:**
**Input:** n = 4, left = \[4,3\], right = \[0,1\]
**Output:** 4
**Explanation:** In the image above:
-The ant at index 0 is named A and going to the right.
-The ant at index 1 is named B and going to the right.
-The ant at index 3 is named C and going to the left.
-The ant at index 4 is named D and going to the left.
The last moment when an ant was on the plank is t = 4 seconds. After that, it falls immediately out of the plank. (i.e., We can say that at t = 4.0000000001, there are no ants on the plank).
**Example 2:**
**Input:** n = 7, left = \[\], right = \[0,1,2,3,4,5,6,7\]
**Output:** 7
**Explanation:** All ants are going to the right, the ant at index 0 needs 7 seconds to fall.
**Example 3:**
**Input:** n = 7, left = \[0,1,2,3,4,5,6,7\], right = \[\]
**Output:** 7
**Explanation:** All ants are going to the left, the ant at index 7 needs 7 seconds to fall.
**Constraints:**
* `1 <= n <= 104`
* `0 <= left.length <= n + 1`
* `0 <= left[i] <= n`
* `0 <= right.length <= n + 1`
* `0 <= right[i] <= n`
* `1 <= left.length + right.length <= n + 1`
* All values of `left` and `right` are unique, and each value can appear **only in one** of the two arrays. | Use dynamic programming to find the optimal solution by saving the previous best like-time coefficient and its corresponding element sum. If adding the current element to the previous best like-time coefficient and its corresponding element sum would increase the best like-time coefficient, then go ahead and add it. Otherwise, keep the previous best like-time coefficient. |
✅☑[C++/Java/Python/JavaScript] || 2 Approaches || EXPLAINED🔥 | last-moment-before-all-ants-fall-out-of-a-plank | 1 | 1 | # PLEASE UPVOTE IF IT HELPED\n\n---\n\n\n# Approaches\n**(Also explained in the code)**\n\n#### ***Approach 1 (Easy Traverse)***\n1. We initialize the `ans` variable to 0, which will be used to keep track of the maximum moment when an ant falls off the plank.\n1. We loop through the `left` vector to find the maximum position of ants moving to the left.\n1. We loop through the `right` vector and use `n - num` to find the equivalent position on the left side for ants moving to the right, and update `ans` with the maximum of these positions.\n1. Finally, we return `ans`, which represents the last moment when an ant falls off the plank.\n\n# Complexity\n- *Time complexity:*\n $$O(n+m)$$\n \n\n- *Space complexity:*\n $$O(1)$$\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int getLastMoment(int n, vector<int>& left, vector<int>& right) {\n int ans = 0; // Initialize a variable \'ans\' to store the maximum time when an ant falls off.\n\n // Loop through the \'left\' vector, which contains positions of ants moving to the left.\n for (int num : left) {\n ans = max(ans, num); // Update \'ans\' with the maximum position from the \'left\' vector.\n }\n \n // Loop through the \'right\' vector, which contains positions of ants moving to the right.\n for (int num : right) {\n ans = max(ans, n - num); // Update \'ans\' with the maximum position from the \'right\' vector (using n - num to account for ants moving right).\n }\n \n return ans; // Return the final value of \'ans\', which represents the moment when the last ant falls off.\n }\n};\n\n\n\n```\n```C []\n\nint getLastMoment(int n, int* left, int leftSize, int* right, int rightSize) {\n int ans = 0; // Initialize a variable \'ans\' to store the maximum time when an ant falls off.\n\n // Loop through the \'left\' array, which contains positions of ants moving to the left.\n for (int i = 0; i < leftSize; i++) {\n int num = left[i];\n ans = (ans > num) ? ans : num; // Update \'ans\' with the maximum position from the \'left\' array.\n }\n\n // Loop through the \'right\' array, which contains positions of ants moving to the right.\n for (int i = 0; i < rightSize; i++) {\n int num = right[i];\n ans = (ans > n - num) ? ans : n - num; // Update \'ans\' with the maximum position from the \'right\' array (using n - num to account for ants moving right).\n }\n\n return ans; // Return the final value of \'ans\', which represents the moment when the last ant falls off.\n}\n\n\n```\n```Java []\nclass Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n int ans = 0;\n for (int num : left) {\n ans = Math.max(ans, num);\n }\n \n for (int num : right) {\n ans = Math.max(ans, n - num);\n }\n \n return ans;\n }\n}\n\n```\n\n```python3 []\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n ans = 0\n for num in left:\n ans = max(ans, num)\n \n for num in right:\n ans = max(ans, n - num)\n \n return ans\n\n\n```\n```javascript []\nvar getLastMoment = function(n, left, right) {\n let ans = 0; // Initialize a variable \'ans\' to store the maximum time when an ant falls off.\n\n // Loop through the \'left\' array, which contains positions of ants moving to the left.\n for (let num of left) {\n ans = Math.max(ans, num); // Update \'ans\' with the maximum position from the \'left\' array.\n }\n\n // Loop through the \'right\' array, which contains positions of ants moving to the right.\n for (let num of right) {\n ans = Math.max(ans, n - num); // Update \'ans\' with the maximum position from the \'right\' array (using n - num to account for ants moving right).\n }\n\n return ans; // Return the final value of \'ans\', which represents the moment when the last ant falls off.\n};\n\n```\n\n\n---\n#### ***Approach 2 (Math Operation)***\n1. The `getLastMoment` function takes three parameters: the total length of the wooden plank `n`, a vector `left` containing positions of ants moving to the left, and another vector `right` containing positions of ants moving to the right.\n\n1. The code first checks if the `left` vector is empty. If it is empty, it means there are no ants moving to the left, so `maxLeft` is set to 0. Otherwise, it finds the farthest position among ants moving to the left by using `*std::max_element(left.begin(), left.end())`.\n\n1. Similarly, it checks if the `right` vector is empty. If it\'s empty, it means there are no ants moving to the right, so `minRight` is set to `n`, which represents the right end of the plank. Otherwise, it finds the nearest position among ants moving to the right using `*std::min_element(right.begin(), right.end())`.\n\n1. The code then calculates the time when the last ant(s) will fall off the plank. The last ant(s) to fall will be at the maximum position from the left (`maxLeft`) or the maximum position from the right end of the plank (`n - minRight`).\n\n1. The maximum of these two positions is returned as the result, representing the moment when the last ant(s) fall off the plank.\n\n# Complexity\n- *Time complexity:*\n $$O(nleft + nright)$$\n(where `nleft` is the number of ants moving to the left.\nwhere `nright` is the number of ants moving to the right.)\n \n\n- *Space complexity:*\n $$ O(nleft + nright)$$\nwhere `nleft` is the number of ants moving to the left.\nwhere `nright` is the number of ants moving to the right.\n \n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n int getLastMoment(int n, std::vector<int>& left, std::vector<int>& right) {\n // Find the farthest position among ants moving to the left.\n // If \'left\' is empty, set maxLeft to 0.\n int maxLeft = left.empty() ? 0 : *std::max_element(left.begin(), left.end());\n\n // Find the nearest position among ants moving to the right.\n // If \'right\' is empty, set minRight to the right end of the plank (n).\n int minRight = right.empty() ? n : *std::min_element(right.begin(), right.end());\n\n // Calculate the time when the last ant(s) fall.\n // The last ant(s) to fall will be at the maximum position from the left or\n // the maximum position from the right end of the plank.\n return std::max(maxLeft, n - minRight);\n }\n};\n\n\n```\n```C []\n\n\nint getLastMoment(int n, int* left, int leftSize, int* right, int rightSize) {\n // Find the farthest position among ants moving to the left.\n int maxLeft = (leftSize == 0) ? 0 : left[0]; // Initialize maxLeft to 0 if \'left\' is empty.\n\n for (int i = 1; i < leftSize; i++) {\n if (left[i] > maxLeft) {\n maxLeft = left[i]; // Update maxLeft if a larger position is found.\n }\n }\n\n // Find the nearest position among ants moving to the right.\n int minRight = (rightSize == 0) ? n : right[0]; // Initialize minRight to n if \'right\' is empty.\n\n for (int i = 1; i < rightSize; i++) {\n if (right[i] < minRight) {\n minRight = right[i]; // Update minRight if a smaller position is found.\n }\n }\n\n // Calculate the time when the last ant(s) fall.\n // The last ant(s) to fall will be at the maximum position from the left or\n // the maximum position from the right end of the plank.\n int lastFall = (maxLeft > n - minRight) ? maxLeft : n - minRight;\n\n return lastFall;\n}\n\n\n\n\n\n\n```\n```Java []\nclass Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n // Find the farthest position among ants moving to the left.\n int maxLeft = Arrays.stream(left).max().orElse(0);\n \n // Find the nearest position among ants moving to the right.\n int minRight = n - Arrays.stream(right).min().orElse(n);\n \n // Calculate the time when the last ant(s) fall.\n return Math.max(maxLeft, minRight);\n }\n}\n\n```\n\n```python []\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n # Find the farthest position among ants moving to the left.\n maxLeft = max(left, default=0)\n\n # Find the nearest position among ants moving to the right.\n minRight = n - min(right, default=n)\n\n # Calculate the time when the last ant(s) fall.\n return max(maxLeft, minRight)\n\n\n```\n```javascript []\nvar getLastMoment = function(n, left, right) {\n // Find the farthest position among ants moving to the left.\n const maxLeft = Math.max(...left, 0);\n \n // Find the nearest position among ants moving to the right.\n const minRight = n - Math.min(...right, n);\n \n // Calculate the time when the last ant(s) fall.\n return Math.max(maxLeft, minRight);\n}\n```\n\n\n---\n\n\n# PLEASE UPVOTE IF IT HELPED\n\n---\n---\n\n\n--- | 2 | We have `n` cities labeled from `1` to `n`. Two different cities with labels `x` and `y` are directly connected by a bidirectional road if and only if `x` and `y` share a common divisor **strictly greater** than some `threshold`. More formally, cities with labels `x` and `y` have a road between them if there exists an integer `z` such that all of the following are true:
* `x % z == 0`,
* `y % z == 0`, and
* `z > threshold`.
Given the two integers, `n` and `threshold`, and an array of `queries`, you must determine for each `queries[i] = [ai, bi]` if cities `ai` and `bi` are connected directly or indirectly. (i.e. there is some path between them).
Return _an array_ `answer`_, where_ `answer.length == queries.length` _and_ `answer[i]` _is_ `true` _if for the_ `ith` _query, there is a path between_ `ai` _and_ `bi`_, or_ `answer[i]` _is_ `false` _if there is no path._
**Example 1:**
**Input:** n = 6, threshold = 2, queries = \[\[1,4\],\[2,5\],\[3,6\]\]
**Output:** \[false,false,true\]
**Explanation:** The divisors for each number:
1: 1
2: 1, 2
3: 1, 3
4: 1, 2, 4
5: 1, 5
6: 1, 2, 3, 6
Using the underlined divisors above the threshold, only cities 3 and 6 share a common divisor, so they are the
only ones directly connected. The result of each query:
\[1,4\] 1 is not connected to 4
\[2,5\] 2 is not connected to 5
\[3,6\] 3 is connected to 6 through path 3--6
**Example 2:**
**Input:** n = 6, threshold = 0, queries = \[\[4,5\],\[3,4\],\[3,2\],\[2,6\],\[1,3\]\]
**Output:** \[true,true,true,true,true\]
**Explanation:** The divisors for each number are the same as the previous example. However, since the threshold is 0,
all divisors can be used. Since all numbers share 1 as a divisor, all cities are connected.
**Example 3:**
**Input:** n = 5, threshold = 1, queries = \[\[4,5\],\[4,5\],\[3,2\],\[2,3\],\[3,4\]\]
**Output:** \[false,false,false,false,false\]
**Explanation:** Only cities 2 and 4 share a common divisor 2 which is strictly greater than the threshold 1, so they are the only ones directly connected.
Please notice that there can be multiple queries for the same pair of nodes \[x, y\], and that the query \[x, y\] is equivalent to the query \[y, x\].
**Constraints:**
* `2 <= n <= 104`
* `0 <= threshold <= n`
* `1 <= queries.length <= 105`
* `queries[i].length == 2`
* `1 <= ai, bi <= cities`
* `ai != bi` | The ants change their way when they meet is equivalent to continue moving without changing their direction. Answer is the max distance for one ant to reach the end of the plank in the facing direction. |
✅ 91.37% Ants' Behavior & One Line | last-moment-before-all-ants-fall-out-of-a-plank | 1 | 1 | # Intuition\nWhen faced with this problem, the first visualization that comes to mind is ants moving along a line, bouncing back when they meet each other, and eventually falling off the plank. This seems like a dynamic system with multiple interactions. However, upon deeper contemplation, one realizes that the interaction (changing directions) of two ants doesn\'t affect the outcome of when they fall off. It\'s as if they just passed through one another. This simplifies our problem and makes it tractable.\n\n# Live Coding & More\nhttps://youtu.be/E-ygoOBUpOs?si=RaH5wXUjXc9MdB3K\n\n# Approach\nOur approach leverages the key observation that two ants meeting and changing direction is functionally equivalent to them passing straight through each other without interaction. This means that we can simply calculate the time it takes for each ant to fall off the plank if it were the only ant on the plank.\n\n1. For ants moving to the left: An ant at position `p` will take exactly `p` seconds to fall off.\n2. For ants moving to the right: An ant at position `p` will take exactly `n - p` seconds to fall off.\n\nThe last moment when an ant falls off the plank is the maximum of these times. Thus, we need to find:\n- The maximum position of ants moving to the left (this gives the longest time for ants moving left).\n- `n` minus the minimum position of ants moving to the right (this gives the longest time for ants moving right).\n\nFinally, our answer is the maximum of the two times computed above.\n\n# Why is our primary approach optimal?\nOur chosen approach requires a single pass through each list (`left` and `right`) to find the max/min values, giving a time complexity of $O(n)$. This is optimal because we have to look at every ant at least once to determine the solution. The space complexity is $O(1)$ because we only store a constant amount of information (max and min values).\n\nThe alternative simulation approach would be much less efficient, with a time complexity in the worst case of $O(n^2)$ since we\'d need to check each ant\'s position in each second. The collapsing plank approach, once fully understood, leads back to our primary approach, but it\'s a useful mental exercise to visualize the problem.\n\n# Complexity\n- **Time complexity**: $O(n)$ - We make a single pass through the `left` and `right` arrays.\n- **Space complexity**: $O(1)$ - We only store the maximum and minimum values, which requires constant space.\n\n# Code\n``` Python []\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n return max(max(left, default=0), n - min(right, default=n))\n```\n``` C++ []\nclass Solution {\npublic:\n int getLastMoment(int n, vector<int>& left, vector<int>& right) {\n int maxLeft = left.empty() ? 0 : *max_element(left.begin(), left.end());\n int minRight = right.empty() ? n : *min_element(right.begin(), right.end());\n return max(maxLeft, n - minRight);\n }\n};\n```\n``` Java []\npublic class Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n int maxLeft = left.length == 0 ? 0 : Arrays.stream(left).max().getAsInt();\n int minRight = right.length == 0 ? n : Arrays.stream(right).min().getAsInt();\n return Math.max(maxLeft, n - minRight);\n }\n}\n```\n``` Go []\nfunc getLastMoment(n int, left []int, right []int) int {\n maxLeft := 0\n for _, val := range left {\n if val > maxLeft {\n maxLeft = val\n }\n }\n \n minRight := n\n for _, val := range right {\n if val < minRight {\n minRight = val\n }\n }\n \n return int(math.Max(float64(maxLeft), float64(n - minRight)))\n}\n```\n``` Rust []\nimpl Solution {\n pub fn get_last_moment(n: i32, left: Vec<i32>, right: Vec<i32>) -> i32 {\n let max_left = match left.iter().max() {\n Some(&max_val) => max_val,\n None => 0,\n };\n \n let min_right = match right.iter().min() {\n Some(&min_val) => min_val,\n None => n,\n };\n \n std::cmp::max(max_left, n - min_right)\n }\n}\n```\n``` C# []\npublic class Solution {\n public int GetLastMoment(int n, int[] left, int[] right) {\n int maxLeft = left.Length == 0 ? 0 : left.Max();\n int minRight = right.Length == 0 ? n : right.Min();\n return Math.Max(maxLeft, n - minRight);\n }\n}\n```\n``` JavaScript []\nvar getLastMoment = function(n, left, right) {\n const maxLeft = left.length === 0 ? 0 : Math.max(...left);\n const minRight = right.length === 0 ? n : Math.min(...right);\n return Math.max(maxLeft, n - minRight);\n};\n```\n``` PHP []\nclass Solution {\n function getLastMoment($n, $left, $right) {\n $maxLeft = empty($left) ? 0 : max($left);\n $minRight = empty($right) ? $n : min($right);\n return max($maxLeft, $n - $minRight);\n }\n}\n```\n\nIn this code, the use of the `default` argument in the `max` and `min` functions ensures that we handle cases where the `left` or `right` lists might be empty, providing a default value to be used in such cases. This makes the code more concise and readable.\n\n# Performance\n\n| Language | Execution Time (ms) | Memory Used |\n|------------|---------------------|--------------|\n| Rust | 3 | 2.4 MB |\n| Java | 3 | 43.9 MB |\n| C++ | 11 | 23.1 MB |\n| Go | 16 | 6.4 MB |\n| PHP | 45 | 20.4 MB |\n| JavaScript | 55 | 44.5 MB |\n| C# | 98 | 46.1 MB |\n| Python3 | 140 | 17.2 MB |\n\n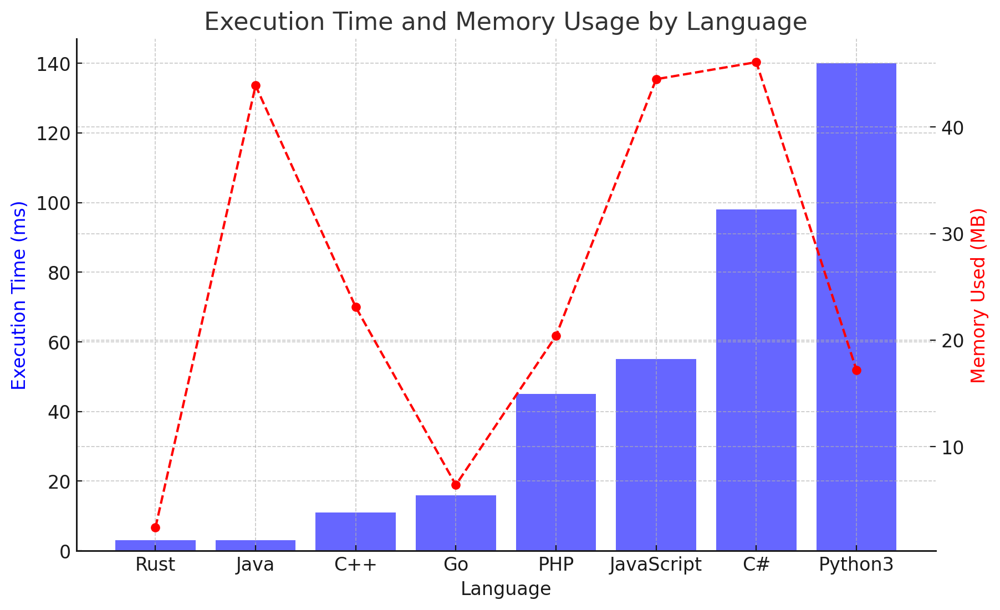\n\n\n---\n\n# Alternative Approach - Collapsing the Plank Approach\n\nThis approach involves a mental model of shrinking the plank at a speed of 1 unit/second from both ends. This makes the ants seem like they are moving faster towards the end. We can visualize the problem as if the plank is collapsing from both ends, and the ants are stationary. The ant that\'s last to hit the shrinking end is the one that falls off last.\n\n## Steps:\n\n1. For each ant moving to the left, calculate how long it will take for the left end of the plank to collapse and reach the ant.\n2. For each ant moving to the right, calculate how long it will take for the right end of the plank to collapse and reach the ant.\n3. The maximum of all these times will be the time the last ant falls off.\n\n``` Python []\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n left_times = [pos for pos in left]\n right_times = [n - pos for pos in right]\n return max(left_times + right_times)\n```\n\n# Complexity Analysis for the Collapsing the Plank Approach:\n\n- **Time Complexity**: $O(n)$ as we go through the list of ants once.\n- **Space Complexity**: $O(n)$ to store the times for all ants.\n\n### Why does the Collapsing the Plank Approach work?\nThis approach works because of our key observation that ants changing direction upon meeting each other is functionally equivalent to them passing straight through each other without interaction. By imagining the plank collapsing, we\'re simply reframing the problem to view the "endpoint" (or time to fall off) for each ant without having to consider their interactions.\n\n### Optimality:\nWhile this approach provides a unique perspective, its computational complexity is the same as our primary approach. However, it may be more intuitive for some people, as it provides a clear visualization of the problem. | 34 | We have a wooden plank of the length `n` **units**. Some ants are walking on the plank, each ant moves with a speed of **1 unit per second**. Some of the ants move to the **left**, the other move to the **right**.
When two ants moving in two **different** directions meet at some point, they change their directions and continue moving again. Assume changing directions does not take any additional time.
When an ant reaches **one end** of the plank at a time `t`, it falls out of the plank immediately.
Given an integer `n` and two integer arrays `left` and `right`, the positions of the ants moving to the left and the right, return _the moment when the last ant(s) fall out of the plank_.
**Example 1:**
**Input:** n = 4, left = \[4,3\], right = \[0,1\]
**Output:** 4
**Explanation:** In the image above:
-The ant at index 0 is named A and going to the right.
-The ant at index 1 is named B and going to the right.
-The ant at index 3 is named C and going to the left.
-The ant at index 4 is named D and going to the left.
The last moment when an ant was on the plank is t = 4 seconds. After that, it falls immediately out of the plank. (i.e., We can say that at t = 4.0000000001, there are no ants on the plank).
**Example 2:**
**Input:** n = 7, left = \[\], right = \[0,1,2,3,4,5,6,7\]
**Output:** 7
**Explanation:** All ants are going to the right, the ant at index 0 needs 7 seconds to fall.
**Example 3:**
**Input:** n = 7, left = \[0,1,2,3,4,5,6,7\], right = \[\]
**Output:** 7
**Explanation:** All ants are going to the left, the ant at index 7 needs 7 seconds to fall.
**Constraints:**
* `1 <= n <= 104`
* `0 <= left.length <= n + 1`
* `0 <= left[i] <= n`
* `0 <= right.length <= n + 1`
* `0 <= right[i] <= n`
* `1 <= left.length + right.length <= n + 1`
* All values of `left` and `right` are unique, and each value can appear **only in one** of the two arrays. | Use dynamic programming to find the optimal solution by saving the previous best like-time coefficient and its corresponding element sum. If adding the current element to the previous best like-time coefficient and its corresponding element sum would increase the best like-time coefficient, then go ahead and add it. Otherwise, keep the previous best like-time coefficient. |
✅ 91.37% Ants' Behavior & One Line | last-moment-before-all-ants-fall-out-of-a-plank | 1 | 1 | # Intuition\nWhen faced with this problem, the first visualization that comes to mind is ants moving along a line, bouncing back when they meet each other, and eventually falling off the plank. This seems like a dynamic system with multiple interactions. However, upon deeper contemplation, one realizes that the interaction (changing directions) of two ants doesn\'t affect the outcome of when they fall off. It\'s as if they just passed through one another. This simplifies our problem and makes it tractable.\n\n# Live Coding & More\nhttps://youtu.be/E-ygoOBUpOs?si=RaH5wXUjXc9MdB3K\n\n# Approach\nOur approach leverages the key observation that two ants meeting and changing direction is functionally equivalent to them passing straight through each other without interaction. This means that we can simply calculate the time it takes for each ant to fall off the plank if it were the only ant on the plank.\n\n1. For ants moving to the left: An ant at position `p` will take exactly `p` seconds to fall off.\n2. For ants moving to the right: An ant at position `p` will take exactly `n - p` seconds to fall off.\n\nThe last moment when an ant falls off the plank is the maximum of these times. Thus, we need to find:\n- The maximum position of ants moving to the left (this gives the longest time for ants moving left).\n- `n` minus the minimum position of ants moving to the right (this gives the longest time for ants moving right).\n\nFinally, our answer is the maximum of the two times computed above.\n\n# Why is our primary approach optimal?\nOur chosen approach requires a single pass through each list (`left` and `right`) to find the max/min values, giving a time complexity of $O(n)$. This is optimal because we have to look at every ant at least once to determine the solution. The space complexity is $O(1)$ because we only store a constant amount of information (max and min values).\n\nThe alternative simulation approach would be much less efficient, with a time complexity in the worst case of $O(n^2)$ since we\'d need to check each ant\'s position in each second. The collapsing plank approach, once fully understood, leads back to our primary approach, but it\'s a useful mental exercise to visualize the problem.\n\n# Complexity\n- **Time complexity**: $O(n)$ - We make a single pass through the `left` and `right` arrays.\n- **Space complexity**: $O(1)$ - We only store the maximum and minimum values, which requires constant space.\n\n# Code\n``` Python []\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n return max(max(left, default=0), n - min(right, default=n))\n```\n``` C++ []\nclass Solution {\npublic:\n int getLastMoment(int n, vector<int>& left, vector<int>& right) {\n int maxLeft = left.empty() ? 0 : *max_element(left.begin(), left.end());\n int minRight = right.empty() ? n : *min_element(right.begin(), right.end());\n return max(maxLeft, n - minRight);\n }\n};\n```\n``` Java []\npublic class Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n int maxLeft = left.length == 0 ? 0 : Arrays.stream(left).max().getAsInt();\n int minRight = right.length == 0 ? n : Arrays.stream(right).min().getAsInt();\n return Math.max(maxLeft, n - minRight);\n }\n}\n```\n``` Go []\nfunc getLastMoment(n int, left []int, right []int) int {\n maxLeft := 0\n for _, val := range left {\n if val > maxLeft {\n maxLeft = val\n }\n }\n \n minRight := n\n for _, val := range right {\n if val < minRight {\n minRight = val\n }\n }\n \n return int(math.Max(float64(maxLeft), float64(n - minRight)))\n}\n```\n``` Rust []\nimpl Solution {\n pub fn get_last_moment(n: i32, left: Vec<i32>, right: Vec<i32>) -> i32 {\n let max_left = match left.iter().max() {\n Some(&max_val) => max_val,\n None => 0,\n };\n \n let min_right = match right.iter().min() {\n Some(&min_val) => min_val,\n None => n,\n };\n \n std::cmp::max(max_left, n - min_right)\n }\n}\n```\n``` C# []\npublic class Solution {\n public int GetLastMoment(int n, int[] left, int[] right) {\n int maxLeft = left.Length == 0 ? 0 : left.Max();\n int minRight = right.Length == 0 ? n : right.Min();\n return Math.Max(maxLeft, n - minRight);\n }\n}\n```\n``` JavaScript []\nvar getLastMoment = function(n, left, right) {\n const maxLeft = left.length === 0 ? 0 : Math.max(...left);\n const minRight = right.length === 0 ? n : Math.min(...right);\n return Math.max(maxLeft, n - minRight);\n};\n```\n``` PHP []\nclass Solution {\n function getLastMoment($n, $left, $right) {\n $maxLeft = empty($left) ? 0 : max($left);\n $minRight = empty($right) ? $n : min($right);\n return max($maxLeft, $n - $minRight);\n }\n}\n```\n\nIn this code, the use of the `default` argument in the `max` and `min` functions ensures that we handle cases where the `left` or `right` lists might be empty, providing a default value to be used in such cases. This makes the code more concise and readable.\n\n# Performance\n\n| Language | Execution Time (ms) | Memory Used |\n|------------|---------------------|--------------|\n| Rust | 3 | 2.4 MB |\n| Java | 3 | 43.9 MB |\n| C++ | 11 | 23.1 MB |\n| Go | 16 | 6.4 MB |\n| PHP | 45 | 20.4 MB |\n| JavaScript | 55 | 44.5 MB |\n| C# | 98 | 46.1 MB |\n| Python3 | 140 | 17.2 MB |\n\n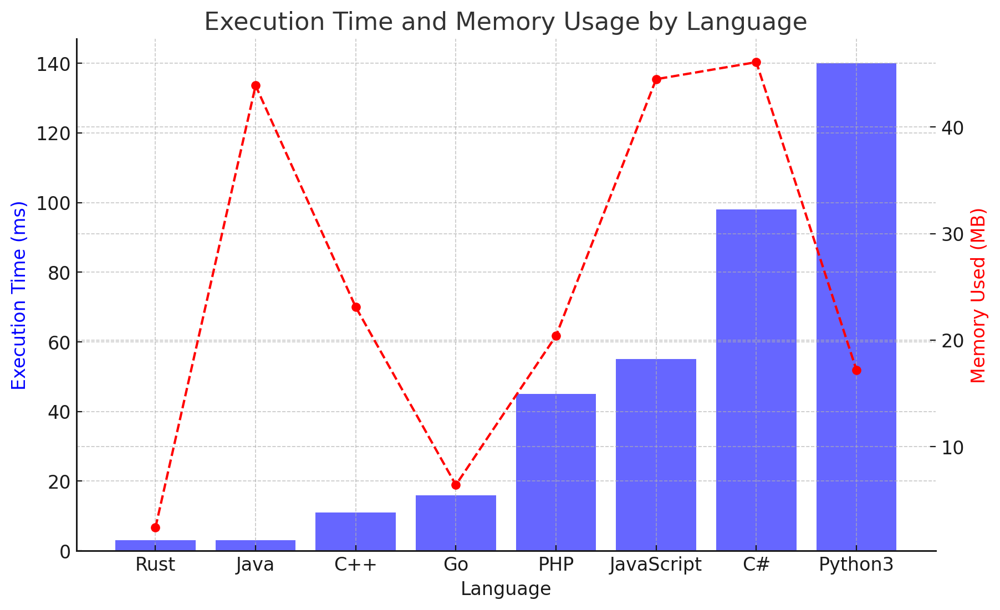\n\n\n---\n\n# Alternative Approach - Collapsing the Plank Approach\n\nThis approach involves a mental model of shrinking the plank at a speed of 1 unit/second from both ends. This makes the ants seem like they are moving faster towards the end. We can visualize the problem as if the plank is collapsing from both ends, and the ants are stationary. The ant that\'s last to hit the shrinking end is the one that falls off last.\n\n## Steps:\n\n1. For each ant moving to the left, calculate how long it will take for the left end of the plank to collapse and reach the ant.\n2. For each ant moving to the right, calculate how long it will take for the right end of the plank to collapse and reach the ant.\n3. The maximum of all these times will be the time the last ant falls off.\n\n``` Python []\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n left_times = [pos for pos in left]\n right_times = [n - pos for pos in right]\n return max(left_times + right_times)\n```\n\n# Complexity Analysis for the Collapsing the Plank Approach:\n\n- **Time Complexity**: $O(n)$ as we go through the list of ants once.\n- **Space Complexity**: $O(n)$ to store the times for all ants.\n\n### Why does the Collapsing the Plank Approach work?\nThis approach works because of our key observation that ants changing direction upon meeting each other is functionally equivalent to them passing straight through each other without interaction. By imagining the plank collapsing, we\'re simply reframing the problem to view the "endpoint" (or time to fall off) for each ant without having to consider their interactions.\n\n### Optimality:\nWhile this approach provides a unique perspective, its computational complexity is the same as our primary approach. However, it may be more intuitive for some people, as it provides a clear visualization of the problem. | 34 | We have `n` cities labeled from `1` to `n`. Two different cities with labels `x` and `y` are directly connected by a bidirectional road if and only if `x` and `y` share a common divisor **strictly greater** than some `threshold`. More formally, cities with labels `x` and `y` have a road between them if there exists an integer `z` such that all of the following are true:
* `x % z == 0`,
* `y % z == 0`, and
* `z > threshold`.
Given the two integers, `n` and `threshold`, and an array of `queries`, you must determine for each `queries[i] = [ai, bi]` if cities `ai` and `bi` are connected directly or indirectly. (i.e. there is some path between them).
Return _an array_ `answer`_, where_ `answer.length == queries.length` _and_ `answer[i]` _is_ `true` _if for the_ `ith` _query, there is a path between_ `ai` _and_ `bi`_, or_ `answer[i]` _is_ `false` _if there is no path._
**Example 1:**
**Input:** n = 6, threshold = 2, queries = \[\[1,4\],\[2,5\],\[3,6\]\]
**Output:** \[false,false,true\]
**Explanation:** The divisors for each number:
1: 1
2: 1, 2
3: 1, 3
4: 1, 2, 4
5: 1, 5
6: 1, 2, 3, 6
Using the underlined divisors above the threshold, only cities 3 and 6 share a common divisor, so they are the
only ones directly connected. The result of each query:
\[1,4\] 1 is not connected to 4
\[2,5\] 2 is not connected to 5
\[3,6\] 3 is connected to 6 through path 3--6
**Example 2:**
**Input:** n = 6, threshold = 0, queries = \[\[4,5\],\[3,4\],\[3,2\],\[2,6\],\[1,3\]\]
**Output:** \[true,true,true,true,true\]
**Explanation:** The divisors for each number are the same as the previous example. However, since the threshold is 0,
all divisors can be used. Since all numbers share 1 as a divisor, all cities are connected.
**Example 3:**
**Input:** n = 5, threshold = 1, queries = \[\[4,5\],\[4,5\],\[3,2\],\[2,3\],\[3,4\]\]
**Output:** \[false,false,false,false,false\]
**Explanation:** Only cities 2 and 4 share a common divisor 2 which is strictly greater than the threshold 1, so they are the only ones directly connected.
Please notice that there can be multiple queries for the same pair of nodes \[x, y\], and that the query \[x, y\] is equivalent to the query \[y, x\].
**Constraints:**
* `2 <= n <= 104`
* `0 <= threshold <= n`
* `1 <= queries.length <= 105`
* `queries[i].length == 2`
* `1 <= ai, bi <= cities`
* `ai != bi` | The ants change their way when they meet is equivalent to continue moving without changing their direction. Answer is the max distance for one ant to reach the end of the plank in the facing direction. |
🔥The only shortest 1 line🔥 | last-moment-before-all-ants-fall-out-of-a-plank | 0 | 1 | # Code\n```\nclass Solution:\n def getLastMoment(self, n: int, l: List[int], r: List[int]) -> int:\n return max(l + [n - x for x in r])\n```\nplease upvote :D | 1 | We have a wooden plank of the length `n` **units**. Some ants are walking on the plank, each ant moves with a speed of **1 unit per second**. Some of the ants move to the **left**, the other move to the **right**.
When two ants moving in two **different** directions meet at some point, they change their directions and continue moving again. Assume changing directions does not take any additional time.
When an ant reaches **one end** of the plank at a time `t`, it falls out of the plank immediately.
Given an integer `n` and two integer arrays `left` and `right`, the positions of the ants moving to the left and the right, return _the moment when the last ant(s) fall out of the plank_.
**Example 1:**
**Input:** n = 4, left = \[4,3\], right = \[0,1\]
**Output:** 4
**Explanation:** In the image above:
-The ant at index 0 is named A and going to the right.
-The ant at index 1 is named B and going to the right.
-The ant at index 3 is named C and going to the left.
-The ant at index 4 is named D and going to the left.
The last moment when an ant was on the plank is t = 4 seconds. After that, it falls immediately out of the plank. (i.e., We can say that at t = 4.0000000001, there are no ants on the plank).
**Example 2:**
**Input:** n = 7, left = \[\], right = \[0,1,2,3,4,5,6,7\]
**Output:** 7
**Explanation:** All ants are going to the right, the ant at index 0 needs 7 seconds to fall.
**Example 3:**
**Input:** n = 7, left = \[0,1,2,3,4,5,6,7\], right = \[\]
**Output:** 7
**Explanation:** All ants are going to the left, the ant at index 7 needs 7 seconds to fall.
**Constraints:**
* `1 <= n <= 104`
* `0 <= left.length <= n + 1`
* `0 <= left[i] <= n`
* `0 <= right.length <= n + 1`
* `0 <= right[i] <= n`
* `1 <= left.length + right.length <= n + 1`
* All values of `left` and `right` are unique, and each value can appear **only in one** of the two arrays. | Use dynamic programming to find the optimal solution by saving the previous best like-time coefficient and its corresponding element sum. If adding the current element to the previous best like-time coefficient and its corresponding element sum would increase the best like-time coefficient, then go ahead and add it. Otherwise, keep the previous best like-time coefficient. |
🔥The only shortest 1 line🔥 | last-moment-before-all-ants-fall-out-of-a-plank | 0 | 1 | # Code\n```\nclass Solution:\n def getLastMoment(self, n: int, l: List[int], r: List[int]) -> int:\n return max(l + [n - x for x in r])\n```\nplease upvote :D | 1 | We have `n` cities labeled from `1` to `n`. Two different cities with labels `x` and `y` are directly connected by a bidirectional road if and only if `x` and `y` share a common divisor **strictly greater** than some `threshold`. More formally, cities with labels `x` and `y` have a road between them if there exists an integer `z` such that all of the following are true:
* `x % z == 0`,
* `y % z == 0`, and
* `z > threshold`.
Given the two integers, `n` and `threshold`, and an array of `queries`, you must determine for each `queries[i] = [ai, bi]` if cities `ai` and `bi` are connected directly or indirectly. (i.e. there is some path between them).
Return _an array_ `answer`_, where_ `answer.length == queries.length` _and_ `answer[i]` _is_ `true` _if for the_ `ith` _query, there is a path between_ `ai` _and_ `bi`_, or_ `answer[i]` _is_ `false` _if there is no path._
**Example 1:**
**Input:** n = 6, threshold = 2, queries = \[\[1,4\],\[2,5\],\[3,6\]\]
**Output:** \[false,false,true\]
**Explanation:** The divisors for each number:
1: 1
2: 1, 2
3: 1, 3
4: 1, 2, 4
5: 1, 5
6: 1, 2, 3, 6
Using the underlined divisors above the threshold, only cities 3 and 6 share a common divisor, so they are the
only ones directly connected. The result of each query:
\[1,4\] 1 is not connected to 4
\[2,5\] 2 is not connected to 5
\[3,6\] 3 is connected to 6 through path 3--6
**Example 2:**
**Input:** n = 6, threshold = 0, queries = \[\[4,5\],\[3,4\],\[3,2\],\[2,6\],\[1,3\]\]
**Output:** \[true,true,true,true,true\]
**Explanation:** The divisors for each number are the same as the previous example. However, since the threshold is 0,
all divisors can be used. Since all numbers share 1 as a divisor, all cities are connected.
**Example 3:**
**Input:** n = 5, threshold = 1, queries = \[\[4,5\],\[4,5\],\[3,2\],\[2,3\],\[3,4\]\]
**Output:** \[false,false,false,false,false\]
**Explanation:** Only cities 2 and 4 share a common divisor 2 which is strictly greater than the threshold 1, so they are the only ones directly connected.
Please notice that there can be multiple queries for the same pair of nodes \[x, y\], and that the query \[x, y\] is equivalent to the query \[y, x\].
**Constraints:**
* `2 <= n <= 104`
* `0 <= threshold <= n`
* `1 <= queries.length <= 105`
* `queries[i].length == 2`
* `1 <= ai, bi <= cities`
* `ai != bi` | The ants change their way when they meet is equivalent to continue moving without changing their direction. Answer is the max distance for one ant to reach the end of the plank in the facing direction. |
🚀 100% || Easy Iterative Approach || Explained Intuition 🚀 | last-moment-before-all-ants-fall-out-of-a-plank | 1 | 1 | # Problem Description\nYou have a wooden plank of a **specific** length, measured in **units**. On this plank, there are a group of ants moving in **two opposite** directions. Each ant moves at a constant speed of `1 unit per second`. When ants traveling in opposite directions **meet**, they instantaneously **reverse** their direction and continue moving `collide`.\n\nThe following information are provided:\n1. The **length** of the wooden plank, denoted as `n` units.\n2. Two integer arrays:\n - `left`: An array containing the initial positions of ants moving to the left.\n - `right`: An array containing the initial positions of ants moving to the right.\n \nThe task is to determine the exact moment at which the last ant falls off the plank.\n\n- Constraints:\n - $1 <= n <= 10^4$\n - $0 <= left.length <= n + 1$\n - $0 <= left[i] <= n$\n - $0 <= right.length <= n + 1$\n - $0 <= right[i] <= n$\n - $1 <= left.length + right.length <= n + 1$\n - All values of left and right are **unique**, and each value can appear only **in one** of the two arrays.\n\n---\n\n# Intuition\n\nHi there, \uD83D\uDE04\n\nLet\'s dive\uD83E\uDD3F deep into our today\'s problem.\n\nThe task today is to **examine** the behaviour of a group of friendly ants. Given that they are on a wooden board and each ant is going **left** or **right**, we want to calculate the time it takes them to fell of the board.\nBut what happens when two ants hit eachother ?\uD83E\uDD14\nin this case, they reverse their direction in **no time** -they collide-.\nNoting that collision doesn\'t take time.\n\nLet\'s take a look at these examples \nGiven wooden board of length 10 :\nAt t = 0\n\nAt t = 1\n\n\nAt t = 2\n\n\nAt t = 9\n\n\n\n\n---\n\n\n\nLet\'s take a look at this example also \nAt t = 0\n\n\nAt t = 1\n\n\nAt t = 2\n\n\nAt t = 9\n\n\n\n\n---\n\n\nLet\'s take a look at this complex example also \nAt t = 0\n\n\nAt t = 1\n\n\nAt t = 2\n\n\nAt t = 3\n\n\nAt t = 8\n\n\n\nDid you noticed something ?\uD83E\uDD14\nYES, from the **first** and **second** examples, the collision **doesn\'t matter** since it doesn\'t affect the total time.\n\nBut why ?\uD83E\uDD14 ......\uD83E\uDD28\nBecause it is like **swapping** places. When two ants are about to collide they act like they are **swapping** places.\nAnd if there are **many** collisions like the example in the description of the problem they will swap places **many** times.\n\nSo the final solution is pretty **easy**.\uD83E\uDD29\nOnly see the position of each ant and take the **maximum** of the time for any ant to reach the end of the board if it was **alone** on the board.\n\nAnd this is the solution for our today\'S problem I hope that you understood it\uD83D\uDE80\uD83D\uDE80\n\n\n\n---\n\n\n# Approach\n1. Initialize `lastFallTime` to `0`, which will store the time when the **last** ant falls off the plank.\n2. **Iterate** through the positions of ants moving to the **left** (`left` vector) and update `lastFallTime` to the **maximum** value of the current `leftPosition` and the current `lastFallTime`. This step finds the farthest position reached by ants moving **left**.\n3. Next, **iterate** through the positions of ants moving to the **right** (`right` vector). Calculate the effective position by subtracting `rightPosition` from the total length of the plank (`n`), which accounts for ants starting from the right end. Update `lastFallTime` to the **maximum** value of this calculated position and the current `lastFallTime`. This step finds the farthest position reached by ants moving **right**.\n4. **Return** the value of `lastFallTime` as the result.\n\n\n# Complexity\n- **Time complexity:** $O(N)$\nSince we are only using two **for** **loops** to traverse over the arrays and the lengths of the two arrays are maximum `N` then time complexity is `O(N)`.\n- **Space complexity:** $O(1)$\nSince we are using **constant** variables.\n\n\n\n---\n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n \n int getLastMoment(int n, vector<int>& left, vector<int>& right) {\n int lastFallTime = 0;\n \n // Determine the farthest position reached by ants moving to the left.\n for (int leftPosition : left) {\n lastFallTime = max(lastFallTime, leftPosition);\n }\n \n // Determine the farthest position reached by ants moving to the right,\n // considering they start from the right end of the plank (n - position).\n for (int rightPosition : right) {\n lastFallTime = max(lastFallTime, n - rightPosition);\n }\n \n return lastFallTime;\n }\n};\n```\n```Java []\n\nclass Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n int lastFallTime = 0;\n \n // Determine the farthest position reached by ants moving to the left.\n for (int leftPosition : left) {\n lastFallTime = Math.max(lastFallTime, leftPosition);\n }\n \n // Determine the farthest position reached by ants moving to the right,\n // considering they start from the right end of the plank (n - position).\n for (int rightPosition : right) {\n lastFallTime = Math.max(lastFallTime, n - rightPosition);\n }\n \n return lastFallTime;\n }\n}\n```\n```Python []\nclass Solution:\n def getLastMoment(self, n, left, right):\n last_fall_time = 0\n\n # Determine the farthest position reached by ants moving to the left.\n for left_position in left:\n last_fall_time = max(last_fall_time, left_position)\n\n # Determine the farthest position reached by ants moving to the right,\n # considering they start from the right end of the plank (n - position).\n for right_position in right:\n last_fall_time = max(last_fall_time, n - right_position)\n\n return last_fall_time\n```\n```C []\nint getLastMoment(int n, int* left, int leftSize, int* right, int rightSize) {\n int lastFallTime = 0;\n\n // Determine the farthest position reached by ants moving to the left.\n for (int i = 0; i < leftSize; i++) {\n int leftPosition = left[i];\n lastFallTime = (lastFallTime > leftPosition) ? lastFallTime : leftPosition;\n }\n\n // Determine the farthest position reached by ants moving to the right,\n // considering they start from the right end of the plank (n - position).\n for (int i = 0; i < rightSize; i++) {\n int rightPosition = right[i];\n lastFallTime = (lastFallTime > n - rightPosition) ? lastFallTime : n - rightPosition;\n }\n\n return lastFallTime;\n}\n```\n\n\n\n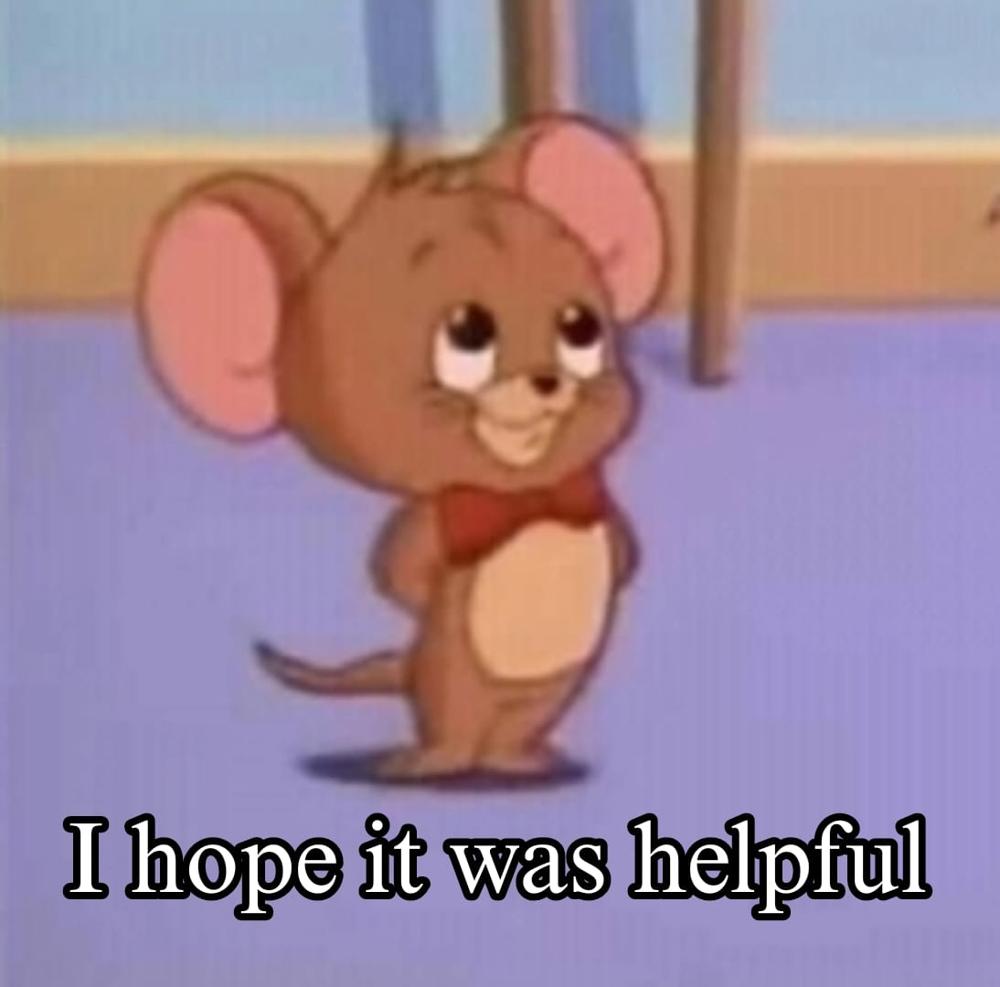\n | 27 | We have a wooden plank of the length `n` **units**. Some ants are walking on the plank, each ant moves with a speed of **1 unit per second**. Some of the ants move to the **left**, the other move to the **right**.
When two ants moving in two **different** directions meet at some point, they change their directions and continue moving again. Assume changing directions does not take any additional time.
When an ant reaches **one end** of the plank at a time `t`, it falls out of the plank immediately.
Given an integer `n` and two integer arrays `left` and `right`, the positions of the ants moving to the left and the right, return _the moment when the last ant(s) fall out of the plank_.
**Example 1:**
**Input:** n = 4, left = \[4,3\], right = \[0,1\]
**Output:** 4
**Explanation:** In the image above:
-The ant at index 0 is named A and going to the right.
-The ant at index 1 is named B and going to the right.
-The ant at index 3 is named C and going to the left.
-The ant at index 4 is named D and going to the left.
The last moment when an ant was on the plank is t = 4 seconds. After that, it falls immediately out of the plank. (i.e., We can say that at t = 4.0000000001, there are no ants on the plank).
**Example 2:**
**Input:** n = 7, left = \[\], right = \[0,1,2,3,4,5,6,7\]
**Output:** 7
**Explanation:** All ants are going to the right, the ant at index 0 needs 7 seconds to fall.
**Example 3:**
**Input:** n = 7, left = \[0,1,2,3,4,5,6,7\], right = \[\]
**Output:** 7
**Explanation:** All ants are going to the left, the ant at index 7 needs 7 seconds to fall.
**Constraints:**
* `1 <= n <= 104`
* `0 <= left.length <= n + 1`
* `0 <= left[i] <= n`
* `0 <= right.length <= n + 1`
* `0 <= right[i] <= n`
* `1 <= left.length + right.length <= n + 1`
* All values of `left` and `right` are unique, and each value can appear **only in one** of the two arrays. | Use dynamic programming to find the optimal solution by saving the previous best like-time coefficient and its corresponding element sum. If adding the current element to the previous best like-time coefficient and its corresponding element sum would increase the best like-time coefficient, then go ahead and add it. Otherwise, keep the previous best like-time coefficient. |
🚀 100% || Easy Iterative Approach || Explained Intuition 🚀 | last-moment-before-all-ants-fall-out-of-a-plank | 1 | 1 | # Problem Description\nYou have a wooden plank of a **specific** length, measured in **units**. On this plank, there are a group of ants moving in **two opposite** directions. Each ant moves at a constant speed of `1 unit per second`. When ants traveling in opposite directions **meet**, they instantaneously **reverse** their direction and continue moving `collide`.\n\nThe following information are provided:\n1. The **length** of the wooden plank, denoted as `n` units.\n2. Two integer arrays:\n - `left`: An array containing the initial positions of ants moving to the left.\n - `right`: An array containing the initial positions of ants moving to the right.\n \nThe task is to determine the exact moment at which the last ant falls off the plank.\n\n- Constraints:\n - $1 <= n <= 10^4$\n - $0 <= left.length <= n + 1$\n - $0 <= left[i] <= n$\n - $0 <= right.length <= n + 1$\n - $0 <= right[i] <= n$\n - $1 <= left.length + right.length <= n + 1$\n - All values of left and right are **unique**, and each value can appear only **in one** of the two arrays.\n\n---\n\n# Intuition\n\nHi there, \uD83D\uDE04\n\nLet\'s dive\uD83E\uDD3F deep into our today\'s problem.\n\nThe task today is to **examine** the behaviour of a group of friendly ants. Given that they are on a wooden board and each ant is going **left** or **right**, we want to calculate the time it takes them to fell of the board.\nBut what happens when two ants hit eachother ?\uD83E\uDD14\nin this case, they reverse their direction in **no time** -they collide-.\nNoting that collision doesn\'t take time.\n\nLet\'s take a look at these examples \nGiven wooden board of length 10 :\nAt t = 0\n\nAt t = 1\n\n\nAt t = 2\n\n\nAt t = 9\n\n\n\n\n---\n\n\n\nLet\'s take a look at this example also \nAt t = 0\n\n\nAt t = 1\n\n\nAt t = 2\n\n\nAt t = 9\n\n\n\n\n---\n\n\nLet\'s take a look at this complex example also \nAt t = 0\n\n\nAt t = 1\n\n\nAt t = 2\n\n\nAt t = 3\n\n\nAt t = 8\n\n\n\nDid you noticed something ?\uD83E\uDD14\nYES, from the **first** and **second** examples, the collision **doesn\'t matter** since it doesn\'t affect the total time.\n\nBut why ?\uD83E\uDD14 ......\uD83E\uDD28\nBecause it is like **swapping** places. When two ants are about to collide they act like they are **swapping** places.\nAnd if there are **many** collisions like the example in the description of the problem they will swap places **many** times.\n\nSo the final solution is pretty **easy**.\uD83E\uDD29\nOnly see the position of each ant and take the **maximum** of the time for any ant to reach the end of the board if it was **alone** on the board.\n\nAnd this is the solution for our today\'S problem I hope that you understood it\uD83D\uDE80\uD83D\uDE80\n\n\n\n---\n\n\n# Approach\n1. Initialize `lastFallTime` to `0`, which will store the time when the **last** ant falls off the plank.\n2. **Iterate** through the positions of ants moving to the **left** (`left` vector) and update `lastFallTime` to the **maximum** value of the current `leftPosition` and the current `lastFallTime`. This step finds the farthest position reached by ants moving **left**.\n3. Next, **iterate** through the positions of ants moving to the **right** (`right` vector). Calculate the effective position by subtracting `rightPosition` from the total length of the plank (`n`), which accounts for ants starting from the right end. Update `lastFallTime` to the **maximum** value of this calculated position and the current `lastFallTime`. This step finds the farthest position reached by ants moving **right**.\n4. **Return** the value of `lastFallTime` as the result.\n\n\n# Complexity\n- **Time complexity:** $O(N)$\nSince we are only using two **for** **loops** to traverse over the arrays and the lengths of the two arrays are maximum `N` then time complexity is `O(N)`.\n- **Space complexity:** $O(1)$\nSince we are using **constant** variables.\n\n\n\n---\n\n\n# Code\n```C++ []\nclass Solution {\npublic:\n \n int getLastMoment(int n, vector<int>& left, vector<int>& right) {\n int lastFallTime = 0;\n \n // Determine the farthest position reached by ants moving to the left.\n for (int leftPosition : left) {\n lastFallTime = max(lastFallTime, leftPosition);\n }\n \n // Determine the farthest position reached by ants moving to the right,\n // considering they start from the right end of the plank (n - position).\n for (int rightPosition : right) {\n lastFallTime = max(lastFallTime, n - rightPosition);\n }\n \n return lastFallTime;\n }\n};\n```\n```Java []\n\nclass Solution {\n public int getLastMoment(int n, int[] left, int[] right) {\n int lastFallTime = 0;\n \n // Determine the farthest position reached by ants moving to the left.\n for (int leftPosition : left) {\n lastFallTime = Math.max(lastFallTime, leftPosition);\n }\n \n // Determine the farthest position reached by ants moving to the right,\n // considering they start from the right end of the plank (n - position).\n for (int rightPosition : right) {\n lastFallTime = Math.max(lastFallTime, n - rightPosition);\n }\n \n return lastFallTime;\n }\n}\n```\n```Python []\nclass Solution:\n def getLastMoment(self, n, left, right):\n last_fall_time = 0\n\n # Determine the farthest position reached by ants moving to the left.\n for left_position in left:\n last_fall_time = max(last_fall_time, left_position)\n\n # Determine the farthest position reached by ants moving to the right,\n # considering they start from the right end of the plank (n - position).\n for right_position in right:\n last_fall_time = max(last_fall_time, n - right_position)\n\n return last_fall_time\n```\n```C []\nint getLastMoment(int n, int* left, int leftSize, int* right, int rightSize) {\n int lastFallTime = 0;\n\n // Determine the farthest position reached by ants moving to the left.\n for (int i = 0; i < leftSize; i++) {\n int leftPosition = left[i];\n lastFallTime = (lastFallTime > leftPosition) ? lastFallTime : leftPosition;\n }\n\n // Determine the farthest position reached by ants moving to the right,\n // considering they start from the right end of the plank (n - position).\n for (int i = 0; i < rightSize; i++) {\n int rightPosition = right[i];\n lastFallTime = (lastFallTime > n - rightPosition) ? lastFallTime : n - rightPosition;\n }\n\n return lastFallTime;\n}\n```\n\n\n\n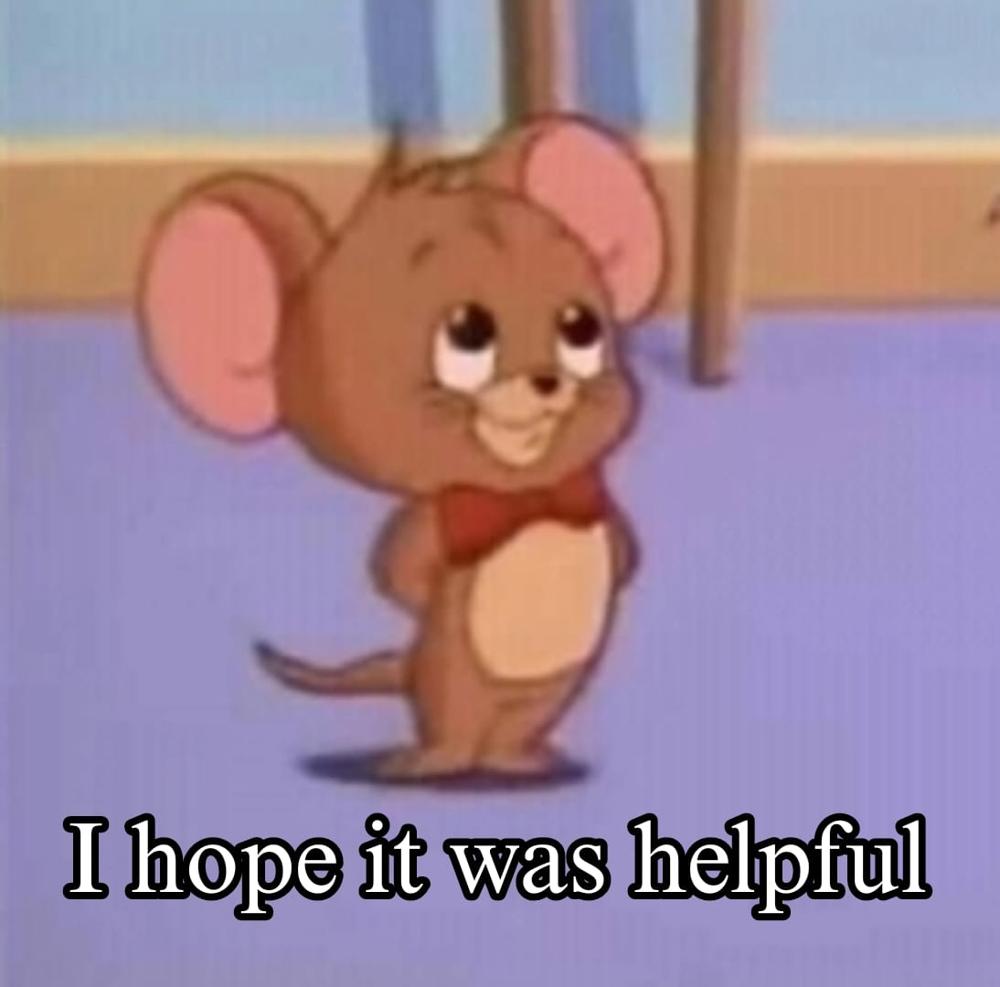\n | 27 | We have `n` cities labeled from `1` to `n`. Two different cities with labels `x` and `y` are directly connected by a bidirectional road if and only if `x` and `y` share a common divisor **strictly greater** than some `threshold`. More formally, cities with labels `x` and `y` have a road between them if there exists an integer `z` such that all of the following are true:
* `x % z == 0`,
* `y % z == 0`, and
* `z > threshold`.
Given the two integers, `n` and `threshold`, and an array of `queries`, you must determine for each `queries[i] = [ai, bi]` if cities `ai` and `bi` are connected directly or indirectly. (i.e. there is some path between them).
Return _an array_ `answer`_, where_ `answer.length == queries.length` _and_ `answer[i]` _is_ `true` _if for the_ `ith` _query, there is a path between_ `ai` _and_ `bi`_, or_ `answer[i]` _is_ `false` _if there is no path._
**Example 1:**
**Input:** n = 6, threshold = 2, queries = \[\[1,4\],\[2,5\],\[3,6\]\]
**Output:** \[false,false,true\]
**Explanation:** The divisors for each number:
1: 1
2: 1, 2
3: 1, 3
4: 1, 2, 4
5: 1, 5
6: 1, 2, 3, 6
Using the underlined divisors above the threshold, only cities 3 and 6 share a common divisor, so they are the
only ones directly connected. The result of each query:
\[1,4\] 1 is not connected to 4
\[2,5\] 2 is not connected to 5
\[3,6\] 3 is connected to 6 through path 3--6
**Example 2:**
**Input:** n = 6, threshold = 0, queries = \[\[4,5\],\[3,4\],\[3,2\],\[2,6\],\[1,3\]\]
**Output:** \[true,true,true,true,true\]
**Explanation:** The divisors for each number are the same as the previous example. However, since the threshold is 0,
all divisors can be used. Since all numbers share 1 as a divisor, all cities are connected.
**Example 3:**
**Input:** n = 5, threshold = 1, queries = \[\[4,5\],\[4,5\],\[3,2\],\[2,3\],\[3,4\]\]
**Output:** \[false,false,false,false,false\]
**Explanation:** Only cities 2 and 4 share a common divisor 2 which is strictly greater than the threshold 1, so they are the only ones directly connected.
Please notice that there can be multiple queries for the same pair of nodes \[x, y\], and that the query \[x, y\] is equivalent to the query \[y, x\].
**Constraints:**
* `2 <= n <= 104`
* `0 <= threshold <= n`
* `1 <= queries.length <= 105`
* `queries[i].length == 2`
* `1 <= ai, bi <= cities`
* `ai != bi` | The ants change their way when they meet is equivalent to continue moving without changing their direction. Answer is the max distance for one ant to reach the end of the plank in the facing direction. |
Easy peezy lemon squeezy | last-moment-before-all-ants-fall-out-of-a-plank | 0 | 1 | # Intuition\nThe bumping of two ants is a catch here. Actually it doesn\'t matter. When two ants \'collide\' is like they\'re just swapping places. So the ant that is the furthest from the edge is going to be the last to bump and it\'s distance from the edge is the time `t`.\n\n# Approach\nBasically calculate max from the left and min for the right. \nSubtract min from the right array from n to get the longest distance for ants moving right and get the max from left to get the longest distance for ants moving to the left. Find maximum of these two values.\n\n# Complexity\n- Time complexity:\n`O(N)` to find the `max` of the `left` and `min` of the `right` arrays\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n farthest_left = max(left) if len(left) != 0 else 0\n farthest_right = n - min(right) if len(right) != 0 else 0\n return max(farthest_left, farthest_right)\n``` | 1 | We have a wooden plank of the length `n` **units**. Some ants are walking on the plank, each ant moves with a speed of **1 unit per second**. Some of the ants move to the **left**, the other move to the **right**.
When two ants moving in two **different** directions meet at some point, they change their directions and continue moving again. Assume changing directions does not take any additional time.
When an ant reaches **one end** of the plank at a time `t`, it falls out of the plank immediately.
Given an integer `n` and two integer arrays `left` and `right`, the positions of the ants moving to the left and the right, return _the moment when the last ant(s) fall out of the plank_.
**Example 1:**
**Input:** n = 4, left = \[4,3\], right = \[0,1\]
**Output:** 4
**Explanation:** In the image above:
-The ant at index 0 is named A and going to the right.
-The ant at index 1 is named B and going to the right.
-The ant at index 3 is named C and going to the left.
-The ant at index 4 is named D and going to the left.
The last moment when an ant was on the plank is t = 4 seconds. After that, it falls immediately out of the plank. (i.e., We can say that at t = 4.0000000001, there are no ants on the plank).
**Example 2:**
**Input:** n = 7, left = \[\], right = \[0,1,2,3,4,5,6,7\]
**Output:** 7
**Explanation:** All ants are going to the right, the ant at index 0 needs 7 seconds to fall.
**Example 3:**
**Input:** n = 7, left = \[0,1,2,3,4,5,6,7\], right = \[\]
**Output:** 7
**Explanation:** All ants are going to the left, the ant at index 7 needs 7 seconds to fall.
**Constraints:**
* `1 <= n <= 104`
* `0 <= left.length <= n + 1`
* `0 <= left[i] <= n`
* `0 <= right.length <= n + 1`
* `0 <= right[i] <= n`
* `1 <= left.length + right.length <= n + 1`
* All values of `left` and `right` are unique, and each value can appear **only in one** of the two arrays. | Use dynamic programming to find the optimal solution by saving the previous best like-time coefficient and its corresponding element sum. If adding the current element to the previous best like-time coefficient and its corresponding element sum would increase the best like-time coefficient, then go ahead and add it. Otherwise, keep the previous best like-time coefficient. |
Easy peezy lemon squeezy | last-moment-before-all-ants-fall-out-of-a-plank | 0 | 1 | # Intuition\nThe bumping of two ants is a catch here. Actually it doesn\'t matter. When two ants \'collide\' is like they\'re just swapping places. So the ant that is the furthest from the edge is going to be the last to bump and it\'s distance from the edge is the time `t`.\n\n# Approach\nBasically calculate max from the left and min for the right. \nSubtract min from the right array from n to get the longest distance for ants moving right and get the max from left to get the longest distance for ants moving to the left. Find maximum of these two values.\n\n# Complexity\n- Time complexity:\n`O(N)` to find the `max` of the `left` and `min` of the `right` arrays\n\n- Space complexity:\nO(1)\n\n# Code\n```\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n farthest_left = max(left) if len(left) != 0 else 0\n farthest_right = n - min(right) if len(right) != 0 else 0\n return max(farthest_left, farthest_right)\n``` | 1 | We have `n` cities labeled from `1` to `n`. Two different cities with labels `x` and `y` are directly connected by a bidirectional road if and only if `x` and `y` share a common divisor **strictly greater** than some `threshold`. More formally, cities with labels `x` and `y` have a road between them if there exists an integer `z` such that all of the following are true:
* `x % z == 0`,
* `y % z == 0`, and
* `z > threshold`.
Given the two integers, `n` and `threshold`, and an array of `queries`, you must determine for each `queries[i] = [ai, bi]` if cities `ai` and `bi` are connected directly or indirectly. (i.e. there is some path between them).
Return _an array_ `answer`_, where_ `answer.length == queries.length` _and_ `answer[i]` _is_ `true` _if for the_ `ith` _query, there is a path between_ `ai` _and_ `bi`_, or_ `answer[i]` _is_ `false` _if there is no path._
**Example 1:**
**Input:** n = 6, threshold = 2, queries = \[\[1,4\],\[2,5\],\[3,6\]\]
**Output:** \[false,false,true\]
**Explanation:** The divisors for each number:
1: 1
2: 1, 2
3: 1, 3
4: 1, 2, 4
5: 1, 5
6: 1, 2, 3, 6
Using the underlined divisors above the threshold, only cities 3 and 6 share a common divisor, so they are the
only ones directly connected. The result of each query:
\[1,4\] 1 is not connected to 4
\[2,5\] 2 is not connected to 5
\[3,6\] 3 is connected to 6 through path 3--6
**Example 2:**
**Input:** n = 6, threshold = 0, queries = \[\[4,5\],\[3,4\],\[3,2\],\[2,6\],\[1,3\]\]
**Output:** \[true,true,true,true,true\]
**Explanation:** The divisors for each number are the same as the previous example. However, since the threshold is 0,
all divisors can be used. Since all numbers share 1 as a divisor, all cities are connected.
**Example 3:**
**Input:** n = 5, threshold = 1, queries = \[\[4,5\],\[4,5\],\[3,2\],\[2,3\],\[3,4\]\]
**Output:** \[false,false,false,false,false\]
**Explanation:** Only cities 2 and 4 share a common divisor 2 which is strictly greater than the threshold 1, so they are the only ones directly connected.
Please notice that there can be multiple queries for the same pair of nodes \[x, y\], and that the query \[x, y\] is equivalent to the query \[y, x\].
**Constraints:**
* `2 <= n <= 104`
* `0 <= threshold <= n`
* `1 <= queries.length <= 105`
* `queries[i].length == 2`
* `1 <= ai, bi <= cities`
* `ai != bi` | The ants change their way when they meet is equivalent to continue moving without changing their direction. Answer is the max distance for one ant to reach the end of the plank in the facing direction. |
simple solution just by using max() | last-moment-before-all-ants-fall-out-of-a-plank | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFirst of all even if they do turn around when they meet it doesn\'t matter because the total number of ants going that way remais the same.\n\nNo position change, no distance changig in between any two ants\nso it doesn\'t matter.\n\nThe second thing that you need to keep in mind is that it\'s only when the last ant crosses the edge then only it can be said that all the ants have passed through.\n\nso when the ants the first ant of the keft train crosses the mark of n and the last ant of the right crosses the mark of 0 it is only then that all ants have fallen down.\n\nThus the answer is max(time take by ant on the earlist index of \'left\', time take by the ant on the last index \'right\' )\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\nO(len(left)+len(right)) \n\nsince time complexity of max is O(n) for list\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n# Code\n```\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n max_left = max(left) if left else 0\n max_right = n - min(right) if right else 0\n return max(max_left, max_right)\n\n``` | 1 | We have a wooden plank of the length `n` **units**. Some ants are walking on the plank, each ant moves with a speed of **1 unit per second**. Some of the ants move to the **left**, the other move to the **right**.
When two ants moving in two **different** directions meet at some point, they change their directions and continue moving again. Assume changing directions does not take any additional time.
When an ant reaches **one end** of the plank at a time `t`, it falls out of the plank immediately.
Given an integer `n` and two integer arrays `left` and `right`, the positions of the ants moving to the left and the right, return _the moment when the last ant(s) fall out of the plank_.
**Example 1:**
**Input:** n = 4, left = \[4,3\], right = \[0,1\]
**Output:** 4
**Explanation:** In the image above:
-The ant at index 0 is named A and going to the right.
-The ant at index 1 is named B and going to the right.
-The ant at index 3 is named C and going to the left.
-The ant at index 4 is named D and going to the left.
The last moment when an ant was on the plank is t = 4 seconds. After that, it falls immediately out of the plank. (i.e., We can say that at t = 4.0000000001, there are no ants on the plank).
**Example 2:**
**Input:** n = 7, left = \[\], right = \[0,1,2,3,4,5,6,7\]
**Output:** 7
**Explanation:** All ants are going to the right, the ant at index 0 needs 7 seconds to fall.
**Example 3:**
**Input:** n = 7, left = \[0,1,2,3,4,5,6,7\], right = \[\]
**Output:** 7
**Explanation:** All ants are going to the left, the ant at index 7 needs 7 seconds to fall.
**Constraints:**
* `1 <= n <= 104`
* `0 <= left.length <= n + 1`
* `0 <= left[i] <= n`
* `0 <= right.length <= n + 1`
* `0 <= right[i] <= n`
* `1 <= left.length + right.length <= n + 1`
* All values of `left` and `right` are unique, and each value can appear **only in one** of the two arrays. | Use dynamic programming to find the optimal solution by saving the previous best like-time coefficient and its corresponding element sum. If adding the current element to the previous best like-time coefficient and its corresponding element sum would increase the best like-time coefficient, then go ahead and add it. Otherwise, keep the previous best like-time coefficient. |
simple solution just by using max() | last-moment-before-all-ants-fall-out-of-a-plank | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nFirst of all even if they do turn around when they meet it doesn\'t matter because the total number of ants going that way remais the same.\n\nNo position change, no distance changig in between any two ants\nso it doesn\'t matter.\n\nThe second thing that you need to keep in mind is that it\'s only when the last ant crosses the edge then only it can be said that all the ants have passed through.\n\nso when the ants the first ant of the keft train crosses the mark of n and the last ant of the right crosses the mark of 0 it is only then that all ants have fallen down.\n\nThus the answer is max(time take by ant on the earlist index of \'left\', time take by the ant on the last index \'right\' )\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\nO(len(left)+len(right)) \n\nsince time complexity of max is O(n) for list\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nO(1)\n# Code\n```\nclass Solution:\n def getLastMoment(self, n: int, left: List[int], right: List[int]) -> int:\n max_left = max(left) if left else 0\n max_right = n - min(right) if right else 0\n return max(max_left, max_right)\n\n``` | 1 | We have `n` cities labeled from `1` to `n`. Two different cities with labels `x` and `y` are directly connected by a bidirectional road if and only if `x` and `y` share a common divisor **strictly greater** than some `threshold`. More formally, cities with labels `x` and `y` have a road between them if there exists an integer `z` such that all of the following are true:
* `x % z == 0`,
* `y % z == 0`, and
* `z > threshold`.
Given the two integers, `n` and `threshold`, and an array of `queries`, you must determine for each `queries[i] = [ai, bi]` if cities `ai` and `bi` are connected directly or indirectly. (i.e. there is some path between them).
Return _an array_ `answer`_, where_ `answer.length == queries.length` _and_ `answer[i]` _is_ `true` _if for the_ `ith` _query, there is a path between_ `ai` _and_ `bi`_, or_ `answer[i]` _is_ `false` _if there is no path._
**Example 1:**
**Input:** n = 6, threshold = 2, queries = \[\[1,4\],\[2,5\],\[3,6\]\]
**Output:** \[false,false,true\]
**Explanation:** The divisors for each number:
1: 1
2: 1, 2
3: 1, 3
4: 1, 2, 4
5: 1, 5
6: 1, 2, 3, 6
Using the underlined divisors above the threshold, only cities 3 and 6 share a common divisor, so they are the
only ones directly connected. The result of each query:
\[1,4\] 1 is not connected to 4
\[2,5\] 2 is not connected to 5
\[3,6\] 3 is connected to 6 through path 3--6
**Example 2:**
**Input:** n = 6, threshold = 0, queries = \[\[4,5\],\[3,4\],\[3,2\],\[2,6\],\[1,3\]\]
**Output:** \[true,true,true,true,true\]
**Explanation:** The divisors for each number are the same as the previous example. However, since the threshold is 0,
all divisors can be used. Since all numbers share 1 as a divisor, all cities are connected.
**Example 3:**
**Input:** n = 5, threshold = 1, queries = \[\[4,5\],\[4,5\],\[3,2\],\[2,3\],\[3,4\]\]
**Output:** \[false,false,false,false,false\]
**Explanation:** Only cities 2 and 4 share a common divisor 2 which is strictly greater than the threshold 1, so they are the only ones directly connected.
Please notice that there can be multiple queries for the same pair of nodes \[x, y\], and that the query \[x, y\] is equivalent to the query \[y, x\].
**Constraints:**
* `2 <= n <= 104`
* `0 <= threshold <= n`
* `1 <= queries.length <= 105`
* `queries[i].length == 2`
* `1 <= ai, bi <= cities`
* `ai != bi` | The ants change their way when they meet is equivalent to continue moving without changing their direction. Answer is the max distance for one ant to reach the end of the plank in the facing direction. |
[Python3] O(MN) histogram model | count-submatrices-with-all-ones | 0 | 1 | Algo \nIn the first step, stack `mat` row by row to get the "histogram model". For example, \n```\nmat = [[1,0,1],\n [1,1,0],\n [1,1,0]]\n```\nbecomes \n```\nmat = [[1,0,1],\n [2,1,0],\n [3,2,0]]\n```\nIn the second step, traverse the stacked matrix row by row. At each position `i, j`, compute the number of all-1 submatrices like below. \n\nDefine a `stack` to store indices of non-decreasing height, and a variable `cnt` for the number of all-1 submatrices at given position (`i, j`). Take the height of row `i` as an example, say `h = mat[i]`. At column `j`, if `h[j-1] <= h[j]`, it is apparent that `cnt[j] = cnt[j-1] + h[j]`, since every case that contributes to `cnt[j-1]` could be added a new column of 1\'s from the `j`th column to contribute to `cnt[j]`. \n\nThe tricky part is when `h[j-1] > h[j]`. In this case, we need to "hypothetically" lower `h[j-1]` to `h[j]` to get an updated `cnt*[j-1]` before adding `h[j]` to get `cnt[j]`. Suppose that the histogram is like below to reflect `3,3,3,2`. To compute `cnt[3]`, we have to adjust `cnt[2]` to a hypothetical height of 2 by removing top row before adding the new column to get `cnt[3]`. The specific operation is done using a mono-stack which stores indices of non-decreasing height. Whenever a new height comes in, pop out the heights in the stack that are higher than the new height while removing the quota contributed by the extra height (between poped height and new height). \n\n```\n* * * \n* * * * \n* * * *\n```\n\n```\nclass Solution:\n def numSubmat(self, mat: List[List[int]]) -> int:\n m, n = len(mat), len(mat[0])\n \n #precipitate mat to histogram \n for i in range(m):\n for j in range(n):\n if mat[i][j] and i > 0: \n mat[i][j] += mat[i-1][j] #histogram \n \n ans = 0\n for i in range(m):\n stack = [] #mono-stack of indices of non-decreasing height\n cnt = 0\n for j in range(n):\n while stack and mat[i][stack[-1]] > mat[i][j]: \n jj = stack.pop() #start\n kk = stack[-1] if stack else -1 #end\n cnt -= (mat[i][jj] - mat[i][j])*(jj - kk) #adjust to reflect lower height\n\n cnt += mat[i][j] #count submatrices bottom-right at (i, j)\n ans += cnt\n stack.append(j)\n\n return ans\n``` | 124 | Given an `m x n` binary matrix `mat`, _return the number of **submatrices** that have all ones_.
**Example 1:**
**Input:** mat = \[\[1,0,1\],\[1,1,0\],\[1,1,0\]\]
**Output:** 13
**Explanation:**
There are 6 rectangles of side 1x1.
There are 2 rectangles of side 1x2.
There are 3 rectangles of side 2x1.
There is 1 rectangle of side 2x2.
There is 1 rectangle of side 3x1.
Total number of rectangles = 6 + 2 + 3 + 1 + 1 = 13.
**Example 2:**
**Input:** mat = \[\[0,1,1,0\],\[0,1,1,1\],\[1,1,1,0\]\]
**Output:** 24
**Explanation:**
There are 8 rectangles of side 1x1.
There are 5 rectangles of side 1x2.
There are 2 rectangles of side 1x3.
There are 4 rectangles of side 2x1.
There are 2 rectangles of side 2x2.
There are 2 rectangles of side 3x1.
There is 1 rectangle of side 3x2.
Total number of rectangles = 8 + 5 + 2 + 4 + 2 + 2 + 1 = 24.
**Constraints:**
* `1 <= m, n <= 150`
* `mat[i][j]` is either `0` or `1`. | null |
Without dp, 80% TC and 65% SC easy python solution | count-submatrices-with-all-ones | 0 | 1 | Without dp\n```\ndef numSubmat(self, mat: List[List[int]]) -> int:\n\tm, n = len(mat), len(mat[0])\n\tpref = [[0] for _ in range(m)]\n\tfor i in range(m):\n\t\tfor j in range(n):\n\t\t\tpref[i].append(pref[i][-1] + mat[i][j])\n\tans = 0\n\tfor i in range(n+1):\n\t\tfor j in range(i+1, n+1):\n\t\t\tdiff = j-i\n\t\t\tc = 0\n\t\t\tfor k in range(m):\n\t\t\t\tif(diff == pref[k][j] - pref[k][i]):\n\t\t\t\t\tc += 1\n\t\t\t\telse:\n\t\t\t\t\tans += ((c+1)*c)//2\n\t\t\t\t\tc = 0\n\t\t\tans += ((c+1)*c)//2\n\treturn ans\n``` | 1 | Given an `m x n` binary matrix `mat`, _return the number of **submatrices** that have all ones_.
**Example 1:**
**Input:** mat = \[\[1,0,1\],\[1,1,0\],\[1,1,0\]\]
**Output:** 13
**Explanation:**
There are 6 rectangles of side 1x1.
There are 2 rectangles of side 1x2.
There are 3 rectangles of side 2x1.
There is 1 rectangle of side 2x2.
There is 1 rectangle of side 3x1.
Total number of rectangles = 6 + 2 + 3 + 1 + 1 = 13.
**Example 2:**
**Input:** mat = \[\[0,1,1,0\],\[0,1,1,1\],\[1,1,1,0\]\]
**Output:** 24
**Explanation:**
There are 8 rectangles of side 1x1.
There are 5 rectangles of side 1x2.
There are 2 rectangles of side 1x3.
There are 4 rectangles of side 2x1.
There are 2 rectangles of side 2x2.
There are 2 rectangles of side 3x1.
There is 1 rectangle of side 3x2.
Total number of rectangles = 8 + 5 + 2 + 4 + 2 + 2 + 1 = 24.
**Constraints:**
* `1 <= m, n <= 150`
* `mat[i][j]` is either `0` or `1`. | null |
Python solution | count-submatrices-with-all-ones | 0 | 1 | loop through each row. use a list to record the height of each column, and a stack to record the height and the # of additional submatrices created by column for each row\n\nif the height of the cur column< that of the previous column in the stack, pop the record of the previous column from the stack until either the stack is empty or the previous column has a lower height\n\nthe \uFF03of additional submatrices created by the cur column equals (the index of the cur column - the index of the previous column with lower height)* the height of cur column, plus the submatries owing to the previous column with lower height\n\n\n\'\'\'\n\n\t def numSubmat(self, mat: List[List[int]]) -> int:\n col=len(mat[0])\n h,res=[0]*col,0\n\n\n for row in mat:\n\n memo=[] \n\n for i in range(col):\n\n h[i]=(row[i]==1)*(h[i]+1)\n\n while memo and h[i]<h[memo[-1][0]]:\n memo.pop()\n\n\n ct=(i-memo[-1][0])*h[i]+memo[-1][1] if memo else h[i]*(i+1)\n\n res+=ct \n memo.append((i,ct)) #(index, additonal submatrices)\n \n \n return res\n\'\'\' | 9 | Given an `m x n` binary matrix `mat`, _return the number of **submatrices** that have all ones_.
**Example 1:**
**Input:** mat = \[\[1,0,1\],\[1,1,0\],\[1,1,0\]\]
**Output:** 13
**Explanation:**
There are 6 rectangles of side 1x1.
There are 2 rectangles of side 1x2.
There are 3 rectangles of side 2x1.
There is 1 rectangle of side 2x2.
There is 1 rectangle of side 3x1.
Total number of rectangles = 6 + 2 + 3 + 1 + 1 = 13.
**Example 2:**
**Input:** mat = \[\[0,1,1,0\],\[0,1,1,1\],\[1,1,1,0\]\]
**Output:** 24
**Explanation:**
There are 8 rectangles of side 1x1.
There are 5 rectangles of side 1x2.
There are 2 rectangles of side 1x3.
There are 4 rectangles of side 2x1.
There are 2 rectangles of side 2x2.
There are 2 rectangles of side 3x1.
There is 1 rectangle of side 3x2.
Total number of rectangles = 8 + 5 + 2 + 4 + 2 + 2 + 1 = 24.
**Constraints:**
* `1 <= m, n <= 150`
* `mat[i][j]` is either `0` or `1`. | null |
Python3 - O(mn) time complexity, using stack | count-submatrices-with-all-ones | 0 | 1 | # Intuition\nThis problem is advanced problem of https://leetcode.com/problems/sum-of-subarray-minimums/\n\n# Approach\n\n\n# Complexity\n- Time complexity: O(mn)\n- Space complexity: O(n)\n\n# Code\n```\nclass Solution:\n def numSubmat(self, matrix: List[List[int]]) -> int:\n m = len(matrix)\n n = len(matrix[0])\n result = 0\n heights = [0] * n\n for i in range(m):\n stack = []\n lastZeroIndex = -1\n for j in range(n):\n value = matrix[i][j]\n heights[j] = heights[j] + 1 if value == 1 else 0\n while len(stack) and (heights[stack[-1][0]] >= heights[j] or value == 0):\n stack.pop()\n if value == 0:\n lastZeroIndex = j\n continue\n k, countOfK = stack[-1] if len(stack) > 0 else (lastZeroIndex, 0)\n count = (j - k) * heights[j] + countOfK\n result += count\n stack.append((j, count))\n return result\n``` | 0 | Given an `m x n` binary matrix `mat`, _return the number of **submatrices** that have all ones_.
**Example 1:**
**Input:** mat = \[\[1,0,1\],\[1,1,0\],\[1,1,0\]\]
**Output:** 13
**Explanation:**
There are 6 rectangles of side 1x1.
There are 2 rectangles of side 1x2.
There are 3 rectangles of side 2x1.
There is 1 rectangle of side 2x2.
There is 1 rectangle of side 3x1.
Total number of rectangles = 6 + 2 + 3 + 1 + 1 = 13.
**Example 2:**
**Input:** mat = \[\[0,1,1,0\],\[0,1,1,1\],\[1,1,1,0\]\]
**Output:** 24
**Explanation:**
There are 8 rectangles of side 1x1.
There are 5 rectangles of side 1x2.
There are 2 rectangles of side 1x3.
There are 4 rectangles of side 2x1.
There are 2 rectangles of side 2x2.
There are 2 rectangles of side 3x1.
There is 1 rectangle of side 3x2.
Total number of rectangles = 8 + 5 + 2 + 4 + 2 + 2 + 1 = 24.
**Constraints:**
* `1 <= m, n <= 150`
* `mat[i][j]` is either `0` or `1`. | null |
Power of Monotonic Stack . . . | count-submatrices-with-all-ones | 0 | 1 | \n# Time complexity: $$O(n^3)$$\n\n# Code\n```\nclass Solution:\n def numSubmat(self, mat) -> int:\n res = 0\n for col_limit in range(1, len(mat[0]) + 1):\n for c in range(len(mat[0]) - col_limit + 1):\n length = 1\n for r in range(len(mat)):\n sub_mat = mat[r][c : c + col_limit]\n if 0 not in sub_mat:\n res += length\n length += 1\n else:\n stack = []\n length = 1\n \n \n return res\n``` | 0 | Given an `m x n` binary matrix `mat`, _return the number of **submatrices** that have all ones_.
**Example 1:**
**Input:** mat = \[\[1,0,1\],\[1,1,0\],\[1,1,0\]\]
**Output:** 13
**Explanation:**
There are 6 rectangles of side 1x1.
There are 2 rectangles of side 1x2.
There are 3 rectangles of side 2x1.
There is 1 rectangle of side 2x2.
There is 1 rectangle of side 3x1.
Total number of rectangles = 6 + 2 + 3 + 1 + 1 = 13.
**Example 2:**
**Input:** mat = \[\[0,1,1,0\],\[0,1,1,1\],\[1,1,1,0\]\]
**Output:** 24
**Explanation:**
There are 8 rectangles of side 1x1.
There are 5 rectangles of side 1x2.
There are 2 rectangles of side 1x3.
There are 4 rectangles of side 2x1.
There are 2 rectangles of side 2x2.
There are 2 rectangles of side 3x1.
There is 1 rectangle of side 3x2.
Total number of rectangles = 8 + 5 + 2 + 4 + 2 + 2 + 1 = 24.
**Constraints:**
* `1 <= m, n <= 150`
* `mat[i][j]` is either `0` or `1`. | null |
Python (Simple BIT) | minimum-possible-integer-after-at-most-k-adjacent-swaps-on-digits | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass BIT:\n def __init__(self,n):\n self.ans = [0]*(n+1)\n\n def query(self,i):\n res = 0\n while i > 0:\n res += self.ans[i]\n i -= i&-i\n return res\n\n def update(self,i,val):\n while i < len(self.ans):\n self.ans[i] += val\n i += i&-i\n\nclass Solution:\n def minInteger(self, num, k):\n n, dict1, res = len(num), defaultdict(deque), ""\n\n for i,x in enumerate(num):\n dict1[x].append(i)\n\n result = BIT(n)\n\n for i in range(n):\n result.update(i+1,1)\n\n for i in range(n):\n for v in "0123456789":\n if dict1[v]:\n idx = dict1[v][0]\n cnt = result.query(idx)\n if cnt <= k:\n dict1[v].popleft()\n k -= cnt\n res += v\n result.update(idx+1,-1)\n break\n\n return res\n\n\n \n\n\n \n``` | 0 | You are given a string `num` representing **the digits** of a very large integer and an integer `k`. You are allowed to swap any two adjacent digits of the integer **at most** `k` times.
Return _the minimum integer you can obtain also as a string_.
**Example 1:**
**Input:** num = "4321 ", k = 4
**Output:** "1342 "
**Explanation:** The steps to obtain the minimum integer from 4321 with 4 adjacent swaps are shown.
**Example 2:**
**Input:** num = "100 ", k = 1
**Output:** "010 "
**Explanation:** It's ok for the output to have leading zeros, but the input is guaranteed not to have any leading zeros.
**Example 3:**
**Input:** num = "36789 ", k = 1000
**Output:** "36789 "
**Explanation:** We can keep the number without any swaps.
**Constraints:**
* `1 <= num.length <= 3 * 104`
* `num` consists of only **digits** and does not contain **leading zeros**.
* `1 <= k <= 109` | Simulate the process and fill corresponding numbers in the designated spots. |
Python (Simple BIT) | minimum-possible-integer-after-at-most-k-adjacent-swaps-on-digits | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass BIT:\n def __init__(self,n):\n self.ans = [0]*(n+1)\n\n def query(self,i):\n res = 0\n while i > 0:\n res += self.ans[i]\n i -= i&-i\n return res\n\n def update(self,i,val):\n while i < len(self.ans):\n self.ans[i] += val\n i += i&-i\n\nclass Solution:\n def minInteger(self, num, k):\n n, dict1, res = len(num), defaultdict(deque), ""\n\n for i,x in enumerate(num):\n dict1[x].append(i)\n\n result = BIT(n)\n\n for i in range(n):\n result.update(i+1,1)\n\n for i in range(n):\n for v in "0123456789":\n if dict1[v]:\n idx = dict1[v][0]\n cnt = result.query(idx)\n if cnt <= k:\n dict1[v].popleft()\n k -= cnt\n res += v\n result.update(idx+1,-1)\n break\n\n return res\n\n\n \n\n\n \n``` | 0 | A newly designed keypad was tested, where a tester pressed a sequence of `n` keys, one at a time.
You are given a string `keysPressed` of length `n`, where `keysPressed[i]` was the `ith` key pressed in the testing sequence, and a sorted list `releaseTimes`, where `releaseTimes[i]` was the time the `ith` key was released. Both arrays are **0-indexed**. The `0th` key was pressed at the time `0`, and every subsequent key was pressed at the **exact** time the previous key was released.
The tester wants to know the key of the keypress that had the **longest duration**. The `ith` keypress had a **duration** of `releaseTimes[i] - releaseTimes[i - 1]`, and the `0th` keypress had a duration of `releaseTimes[0]`.
Note that the same key could have been pressed multiple times during the test, and these multiple presses of the same key **may not** have had the same **duration**.
_Return the key of the keypress that had the **longest duration**. If there are multiple such keypresses, return the lexicographically largest key of the keypresses._
**Example 1:**
**Input:** releaseTimes = \[9,29,49,50\], keysPressed = "cbcd "
**Output:** "c "
**Explanation:** The keypresses were as follows:
Keypress for 'c' had a duration of 9 (pressed at time 0 and released at time 9).
Keypress for 'b' had a duration of 29 - 9 = 20 (pressed at time 9 right after the release of the previous character and released at time 29).
Keypress for 'c' had a duration of 49 - 29 = 20 (pressed at time 29 right after the release of the previous character and released at time 49).
Keypress for 'd' had a duration of 50 - 49 = 1 (pressed at time 49 right after the release of the previous character and released at time 50).
The longest of these was the keypress for 'b' and the second keypress for 'c', both with duration 20.
'c' is lexicographically larger than 'b', so the answer is 'c'.
**Example 2:**
**Input:** releaseTimes = \[12,23,36,46,62\], keysPressed = "spuda "
**Output:** "a "
**Explanation:** The keypresses were as follows:
Keypress for 's' had a duration of 12.
Keypress for 'p' had a duration of 23 - 12 = 11.
Keypress for 'u' had a duration of 36 - 23 = 13.
Keypress for 'd' had a duration of 46 - 36 = 10.
Keypress for 'a' had a duration of 62 - 46 = 16.
The longest of these was the keypress for 'a' with duration 16.
**Constraints:**
* `releaseTimes.length == n`
* `keysPressed.length == n`
* `2 <= n <= 1000`
* `1 <= releaseTimes[i] <= 109`
* `releaseTimes[i] < releaseTimes[i+1]`
* `keysPressed` contains only lowercase English letters. | We want to make the smaller digits the most significant digits in the number. For each index i, check the smallest digit in a window of size k and append it to the answer. Update the indices of all digits in this range accordingly. |
O(NlogN), a SortedList and 10 queues | minimum-possible-integer-after-at-most-k-adjacent-swaps-on-digits | 0 | 1 | # Intuition\nIterating from the left, we want to find the smallest reacheable digit and put it on each place.\n\n# Approach\nWe keep 10 queues, containing sequences of indices for each digit. At each step we pick the smallest digit that is not further than `k` steps away. After that we remove it from its queue, and reduce `k` by the number of steps used.\n\nThe question, then, rises, how to recalculate the correct indices and distances after a digit is removed from its place. Of course, we don\'t want to modify the queues, that would be too slow. But, it can be seen that the correction to every index is simply the number of used indices to the left of it, which we can calculate efficiently with `SortedList`.\n\n# Complexity\n- Time complexity: $$O(nlogn)$$, dominated by `SortedList` operations, at most `n` inserts and `n` bisections. Technically, `SortedList` has larger [time complexity](https://grantjenks.com/docs/sortedcontainers/performance-scale.html), but in practice it is fast.\n\n- Space complexity: $$O(n)$$, one `SortedList` and 10 queues each\n\n# Code\n```\nfrom collections import deque\nfrom sortedcontainers import SortedList\n\nclass Solution:\n def minInteger(self, num: str, k: int) -> str:\n N = len(num)\n lists = [deque() for _ in range(10)]\n for i,c in enumerate(num):\n lists[int(c)].append(i)\n res = []\n used = SortedList()\n unused = set(range(N))\n while k and len(res) < N:\n for d in range(10):\n if not lists[d]:\n continue\n i = lists[d][0]\n i -= used.bisect_left(i)\n if i <= k:\n k -= i\n i = lists[d].popleft()\n used.add(i)\n unused.remove(i)\n res.append(i)\n break\n res.extend(unused)\n return \'\'.join(num[x] for x in res)\n\n``` | 0 | You are given a string `num` representing **the digits** of a very large integer and an integer `k`. You are allowed to swap any two adjacent digits of the integer **at most** `k` times.
Return _the minimum integer you can obtain also as a string_.
**Example 1:**
**Input:** num = "4321 ", k = 4
**Output:** "1342 "
**Explanation:** The steps to obtain the minimum integer from 4321 with 4 adjacent swaps are shown.
**Example 2:**
**Input:** num = "100 ", k = 1
**Output:** "010 "
**Explanation:** It's ok for the output to have leading zeros, but the input is guaranteed not to have any leading zeros.
**Example 3:**
**Input:** num = "36789 ", k = 1000
**Output:** "36789 "
**Explanation:** We can keep the number without any swaps.
**Constraints:**
* `1 <= num.length <= 3 * 104`
* `num` consists of only **digits** and does not contain **leading zeros**.
* `1 <= k <= 109` | Simulate the process and fill corresponding numbers in the designated spots. |
O(NlogN), a SortedList and 10 queues | minimum-possible-integer-after-at-most-k-adjacent-swaps-on-digits | 0 | 1 | # Intuition\nIterating from the left, we want to find the smallest reacheable digit and put it on each place.\n\n# Approach\nWe keep 10 queues, containing sequences of indices for each digit. At each step we pick the smallest digit that is not further than `k` steps away. After that we remove it from its queue, and reduce `k` by the number of steps used.\n\nThe question, then, rises, how to recalculate the correct indices and distances after a digit is removed from its place. Of course, we don\'t want to modify the queues, that would be too slow. But, it can be seen that the correction to every index is simply the number of used indices to the left of it, which we can calculate efficiently with `SortedList`.\n\n# Complexity\n- Time complexity: $$O(nlogn)$$, dominated by `SortedList` operations, at most `n` inserts and `n` bisections. Technically, `SortedList` has larger [time complexity](https://grantjenks.com/docs/sortedcontainers/performance-scale.html), but in practice it is fast.\n\n- Space complexity: $$O(n)$$, one `SortedList` and 10 queues each\n\n# Code\n```\nfrom collections import deque\nfrom sortedcontainers import SortedList\n\nclass Solution:\n def minInteger(self, num: str, k: int) -> str:\n N = len(num)\n lists = [deque() for _ in range(10)]\n for i,c in enumerate(num):\n lists[int(c)].append(i)\n res = []\n used = SortedList()\n unused = set(range(N))\n while k and len(res) < N:\n for d in range(10):\n if not lists[d]:\n continue\n i = lists[d][0]\n i -= used.bisect_left(i)\n if i <= k:\n k -= i\n i = lists[d].popleft()\n used.add(i)\n unused.remove(i)\n res.append(i)\n break\n res.extend(unused)\n return \'\'.join(num[x] for x in res)\n\n``` | 0 | A newly designed keypad was tested, where a tester pressed a sequence of `n` keys, one at a time.
You are given a string `keysPressed` of length `n`, where `keysPressed[i]` was the `ith` key pressed in the testing sequence, and a sorted list `releaseTimes`, where `releaseTimes[i]` was the time the `ith` key was released. Both arrays are **0-indexed**. The `0th` key was pressed at the time `0`, and every subsequent key was pressed at the **exact** time the previous key was released.
The tester wants to know the key of the keypress that had the **longest duration**. The `ith` keypress had a **duration** of `releaseTimes[i] - releaseTimes[i - 1]`, and the `0th` keypress had a duration of `releaseTimes[0]`.
Note that the same key could have been pressed multiple times during the test, and these multiple presses of the same key **may not** have had the same **duration**.
_Return the key of the keypress that had the **longest duration**. If there are multiple such keypresses, return the lexicographically largest key of the keypresses._
**Example 1:**
**Input:** releaseTimes = \[9,29,49,50\], keysPressed = "cbcd "
**Output:** "c "
**Explanation:** The keypresses were as follows:
Keypress for 'c' had a duration of 9 (pressed at time 0 and released at time 9).
Keypress for 'b' had a duration of 29 - 9 = 20 (pressed at time 9 right after the release of the previous character and released at time 29).
Keypress for 'c' had a duration of 49 - 29 = 20 (pressed at time 29 right after the release of the previous character and released at time 49).
Keypress for 'd' had a duration of 50 - 49 = 1 (pressed at time 49 right after the release of the previous character and released at time 50).
The longest of these was the keypress for 'b' and the second keypress for 'c', both with duration 20.
'c' is lexicographically larger than 'b', so the answer is 'c'.
**Example 2:**
**Input:** releaseTimes = \[12,23,36,46,62\], keysPressed = "spuda "
**Output:** "a "
**Explanation:** The keypresses were as follows:
Keypress for 's' had a duration of 12.
Keypress for 'p' had a duration of 23 - 12 = 11.
Keypress for 'u' had a duration of 36 - 23 = 13.
Keypress for 'd' had a duration of 46 - 36 = 10.
Keypress for 'a' had a duration of 62 - 46 = 16.
The longest of these was the keypress for 'a' with duration 16.
**Constraints:**
* `releaseTimes.length == n`
* `keysPressed.length == n`
* `2 <= n <= 1000`
* `1 <= releaseTimes[i] <= 109`
* `releaseTimes[i] < releaseTimes[i+1]`
* `keysPressed` contains only lowercase English letters. | We want to make the smaller digits the most significant digits in the number. For each index i, check the smallest digit in a window of size k and append it to the answer. Update the indices of all digits in this range accordingly. |
Python Sliding Window + AVL Tree Solution | Easy to Understand | minimum-possible-integer-after-at-most-k-adjacent-swaps-on-digits | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nMaintain a window of size ```k``` in a AVL Tree, pop out the minimum element in the index in $$O(log(n))$$ time, find its index, decrement ```k``` accordingly until ```k``` becomes ```0```.\n\n# Complexity\n- Time complexity: $$O(n * log(n))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python []\nfrom sortedcontainers import SortedList\n\nclass Solution:\n def minInteger(self, num: str, k: int) -> str:\n sz, window = len(num), SortedList()\n remainedIndices, poppedIndices = SortedList(range(sz)), []\n while k > 0:\n while len(window) < k + 1 and len(window) < len(remainedIndices):\n idx = remainedIndices[len(window)]\n window.add((num[idx], idx))\n if not window:\n break\n index = window.pop(0)[1]\n k -= remainedIndices.bisect_left(index)\n remainedIndices.remove(index)\n poppedIndices.append(index)\n for idx in remainedIndices[k + 1: len(window)]:\n window.remove((num[idx], idx))\n poppedSet = set(poppedIndices)\n return "".join(num[idx] for idx in poppedIndices) + "".join(num[idx] for idx in range(sz) if idx not in poppedSet)\n``` | 0 | You are given a string `num` representing **the digits** of a very large integer and an integer `k`. You are allowed to swap any two adjacent digits of the integer **at most** `k` times.
Return _the minimum integer you can obtain also as a string_.
**Example 1:**
**Input:** num = "4321 ", k = 4
**Output:** "1342 "
**Explanation:** The steps to obtain the minimum integer from 4321 with 4 adjacent swaps are shown.
**Example 2:**
**Input:** num = "100 ", k = 1
**Output:** "010 "
**Explanation:** It's ok for the output to have leading zeros, but the input is guaranteed not to have any leading zeros.
**Example 3:**
**Input:** num = "36789 ", k = 1000
**Output:** "36789 "
**Explanation:** We can keep the number without any swaps.
**Constraints:**
* `1 <= num.length <= 3 * 104`
* `num` consists of only **digits** and does not contain **leading zeros**.
* `1 <= k <= 109` | Simulate the process and fill corresponding numbers in the designated spots. |
Python Sliding Window + AVL Tree Solution | Easy to Understand | minimum-possible-integer-after-at-most-k-adjacent-swaps-on-digits | 0 | 1 | # Approach\n<!-- Describe your approach to solving the problem. -->\nMaintain a window of size ```k``` in a AVL Tree, pop out the minimum element in the index in $$O(log(n))$$ time, find its index, decrement ```k``` accordingly until ```k``` becomes ```0```.\n\n# Complexity\n- Time complexity: $$O(n * log(n))$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python []\nfrom sortedcontainers import SortedList\n\nclass Solution:\n def minInteger(self, num: str, k: int) -> str:\n sz, window = len(num), SortedList()\n remainedIndices, poppedIndices = SortedList(range(sz)), []\n while k > 0:\n while len(window) < k + 1 and len(window) < len(remainedIndices):\n idx = remainedIndices[len(window)]\n window.add((num[idx], idx))\n if not window:\n break\n index = window.pop(0)[1]\n k -= remainedIndices.bisect_left(index)\n remainedIndices.remove(index)\n poppedIndices.append(index)\n for idx in remainedIndices[k + 1: len(window)]:\n window.remove((num[idx], idx))\n poppedSet = set(poppedIndices)\n return "".join(num[idx] for idx in poppedIndices) + "".join(num[idx] for idx in range(sz) if idx not in poppedSet)\n``` | 0 | A newly designed keypad was tested, where a tester pressed a sequence of `n` keys, one at a time.
You are given a string `keysPressed` of length `n`, where `keysPressed[i]` was the `ith` key pressed in the testing sequence, and a sorted list `releaseTimes`, where `releaseTimes[i]` was the time the `ith` key was released. Both arrays are **0-indexed**. The `0th` key was pressed at the time `0`, and every subsequent key was pressed at the **exact** time the previous key was released.
The tester wants to know the key of the keypress that had the **longest duration**. The `ith` keypress had a **duration** of `releaseTimes[i] - releaseTimes[i - 1]`, and the `0th` keypress had a duration of `releaseTimes[0]`.
Note that the same key could have been pressed multiple times during the test, and these multiple presses of the same key **may not** have had the same **duration**.
_Return the key of the keypress that had the **longest duration**. If there are multiple such keypresses, return the lexicographically largest key of the keypresses._
**Example 1:**
**Input:** releaseTimes = \[9,29,49,50\], keysPressed = "cbcd "
**Output:** "c "
**Explanation:** The keypresses were as follows:
Keypress for 'c' had a duration of 9 (pressed at time 0 and released at time 9).
Keypress for 'b' had a duration of 29 - 9 = 20 (pressed at time 9 right after the release of the previous character and released at time 29).
Keypress for 'c' had a duration of 49 - 29 = 20 (pressed at time 29 right after the release of the previous character and released at time 49).
Keypress for 'd' had a duration of 50 - 49 = 1 (pressed at time 49 right after the release of the previous character and released at time 50).
The longest of these was the keypress for 'b' and the second keypress for 'c', both with duration 20.
'c' is lexicographically larger than 'b', so the answer is 'c'.
**Example 2:**
**Input:** releaseTimes = \[12,23,36,46,62\], keysPressed = "spuda "
**Output:** "a "
**Explanation:** The keypresses were as follows:
Keypress for 's' had a duration of 12.
Keypress for 'p' had a duration of 23 - 12 = 11.
Keypress for 'u' had a duration of 36 - 23 = 13.
Keypress for 'd' had a duration of 46 - 36 = 10.
Keypress for 'a' had a duration of 62 - 46 = 16.
The longest of these was the keypress for 'a' with duration 16.
**Constraints:**
* `releaseTimes.length == n`
* `keysPressed.length == n`
* `2 <= n <= 1000`
* `1 <= releaseTimes[i] <= 109`
* `releaseTimes[i] < releaseTimes[i+1]`
* `keysPressed` contains only lowercase English letters. | We want to make the smaller digits the most significant digits in the number. For each index i, check the smallest digit in a window of size k and append it to the answer. Update the indices of all digits in this range accordingly. |
[Python3] brute force | minimum-possible-integer-after-at-most-k-adjacent-swaps-on-digits | 0 | 1 | Algo\nScan through the string. At each index, look for the smallest element behind it within k swaps. Upon finding such minimum, swap it to replece the current element, and reduce k to reflect the swaps. Do this for all element until k becomes 0. \n\n`O(N^2)` time & `O(N)` space \n```\nclass Solution:\n def minInteger(self, num: str, k: int) -> str:\n n = len(num)\n if k >= n*(n-1)//2: return "".join(sorted(num)) #special case\n \n #find smallest elements within k swaps \n #and swap it to current position \n num = list(num)\n for i in range(n):\n if not k: break \n #find minimum within k swaps\n ii = i\n for j in range(i+1, min(n, i+k+1)): \n if num[ii] > num[j]: ii = j \n #swap the min to current position \n if ii != i: \n k -= ii-i\n for j in range(ii, i, -1):\n num[j-1], num[j] = num[j], num[j-1]\n return "".join(num)\n``` | 2 | You are given a string `num` representing **the digits** of a very large integer and an integer `k`. You are allowed to swap any two adjacent digits of the integer **at most** `k` times.
Return _the minimum integer you can obtain also as a string_.
**Example 1:**
**Input:** num = "4321 ", k = 4
**Output:** "1342 "
**Explanation:** The steps to obtain the minimum integer from 4321 with 4 adjacent swaps are shown.
**Example 2:**
**Input:** num = "100 ", k = 1
**Output:** "010 "
**Explanation:** It's ok for the output to have leading zeros, but the input is guaranteed not to have any leading zeros.
**Example 3:**
**Input:** num = "36789 ", k = 1000
**Output:** "36789 "
**Explanation:** We can keep the number without any swaps.
**Constraints:**
* `1 <= num.length <= 3 * 104`
* `num` consists of only **digits** and does not contain **leading zeros**.
* `1 <= k <= 109` | Simulate the process and fill corresponding numbers in the designated spots. |
[Python3] brute force | minimum-possible-integer-after-at-most-k-adjacent-swaps-on-digits | 0 | 1 | Algo\nScan through the string. At each index, look for the smallest element behind it within k swaps. Upon finding such minimum, swap it to replece the current element, and reduce k to reflect the swaps. Do this for all element until k becomes 0. \n\n`O(N^2)` time & `O(N)` space \n```\nclass Solution:\n def minInteger(self, num: str, k: int) -> str:\n n = len(num)\n if k >= n*(n-1)//2: return "".join(sorted(num)) #special case\n \n #find smallest elements within k swaps \n #and swap it to current position \n num = list(num)\n for i in range(n):\n if not k: break \n #find minimum within k swaps\n ii = i\n for j in range(i+1, min(n, i+k+1)): \n if num[ii] > num[j]: ii = j \n #swap the min to current position \n if ii != i: \n k -= ii-i\n for j in range(ii, i, -1):\n num[j-1], num[j] = num[j], num[j-1]\n return "".join(num)\n``` | 2 | A newly designed keypad was tested, where a tester pressed a sequence of `n` keys, one at a time.
You are given a string `keysPressed` of length `n`, where `keysPressed[i]` was the `ith` key pressed in the testing sequence, and a sorted list `releaseTimes`, where `releaseTimes[i]` was the time the `ith` key was released. Both arrays are **0-indexed**. The `0th` key was pressed at the time `0`, and every subsequent key was pressed at the **exact** time the previous key was released.
The tester wants to know the key of the keypress that had the **longest duration**. The `ith` keypress had a **duration** of `releaseTimes[i] - releaseTimes[i - 1]`, and the `0th` keypress had a duration of `releaseTimes[0]`.
Note that the same key could have been pressed multiple times during the test, and these multiple presses of the same key **may not** have had the same **duration**.
_Return the key of the keypress that had the **longest duration**. If there are multiple such keypresses, return the lexicographically largest key of the keypresses._
**Example 1:**
**Input:** releaseTimes = \[9,29,49,50\], keysPressed = "cbcd "
**Output:** "c "
**Explanation:** The keypresses were as follows:
Keypress for 'c' had a duration of 9 (pressed at time 0 and released at time 9).
Keypress for 'b' had a duration of 29 - 9 = 20 (pressed at time 9 right after the release of the previous character and released at time 29).
Keypress for 'c' had a duration of 49 - 29 = 20 (pressed at time 29 right after the release of the previous character and released at time 49).
Keypress for 'd' had a duration of 50 - 49 = 1 (pressed at time 49 right after the release of the previous character and released at time 50).
The longest of these was the keypress for 'b' and the second keypress for 'c', both with duration 20.
'c' is lexicographically larger than 'b', so the answer is 'c'.
**Example 2:**
**Input:** releaseTimes = \[12,23,36,46,62\], keysPressed = "spuda "
**Output:** "a "
**Explanation:** The keypresses were as follows:
Keypress for 's' had a duration of 12.
Keypress for 'p' had a duration of 23 - 12 = 11.
Keypress for 'u' had a duration of 36 - 23 = 13.
Keypress for 'd' had a duration of 46 - 36 = 10.
Keypress for 'a' had a duration of 62 - 46 = 16.
The longest of these was the keypress for 'a' with duration 16.
**Constraints:**
* `releaseTimes.length == n`
* `keysPressed.length == n`
* `2 <= n <= 1000`
* `1 <= releaseTimes[i] <= 109`
* `releaseTimes[i] < releaseTimes[i+1]`
* `keysPressed` contains only lowercase English letters. | We want to make the smaller digits the most significant digits in the number. For each index i, check the smallest digit in a window of size k and append it to the answer. Update the indices of all digits in this range accordingly. |
Simple solution. Beats 97% in Python | reformat-date | 0 | 1 | # Steps:\n1. Split the given string and unpack it into variables.\n2. Cut off the suffix from the day\'s string as long as it will not be needed in the final answer.\n3. If the day value is less than 10, add a zero to the beginning of the string. To do so:\n a. Append zero to the end of the string.\n b. Reverse the string.\n4. To manage months correctly, use a hash map.\n5. Form the final answer and return it.\n# Complexity\n- Time complexity:$$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python []\nclass Solution:\n months = {\n \'Jan\': \'01\', "Feb": \'02\', "Mar": \'03\', \n "Apr": \'04\', "May": \'05\', "Jun": \'06\', \n "Jul": \'07\', "Aug": \'08\', "Sep": \'09\', \n "Oct": \'10\', "Nov": \'11\', "Dec": \'12\'}\n\n def reformatDate(self, date: str) -> str:\n day, month, year = date.split()\n\n day = day[:-2]\n\n if len(day) < 2:\n day += \'0\'\n day = day[::-1]\n\n ans = f"{year}-{self.months.get(month)}-{day}"\n\n return ans \n```\n```javascript []\nconst months = {\n \'Jan\': \'01\', "Feb": \'02\', "Mar": \'03\', \n "Apr": \'04\', "May": \'05\', "Jun": \'06\', \n "Jul": \'07\', "Aug": \'08\', "Sep": \'09\', \n "Oct": \'10\', "Nov": \'11\', "Dec": \'12\'}\n\nvar reformatDate = function(date) {\n let [day, month, year] = date.split(\' \');\n day = day.slice(0, day.length - 2);\n\n if (day.length < 2) {\n day += \'0\';\n day = day.split("").reduce((acc, char) => char + acc, "");\n };\n\n const ans = `${year}-${months[month]}-${day}`;\n\n return ans;\n};\n```\n# ***Please UPVOTE !!!*** | 0 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
Simple solution. Beats 97% in Python | reformat-date | 0 | 1 | # Steps:\n1. Split the given string and unpack it into variables.\n2. Cut off the suffix from the day\'s string as long as it will not be needed in the final answer.\n3. If the day value is less than 10, add a zero to the beginning of the string. To do so:\n a. Append zero to the end of the string.\n b. Reverse the string.\n4. To manage months correctly, use a hash map.\n5. Form the final answer and return it.\n# Complexity\n- Time complexity:$$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:$$O(n)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```python []\nclass Solution:\n months = {\n \'Jan\': \'01\', "Feb": \'02\', "Mar": \'03\', \n "Apr": \'04\', "May": \'05\', "Jun": \'06\', \n "Jul": \'07\', "Aug": \'08\', "Sep": \'09\', \n "Oct": \'10\', "Nov": \'11\', "Dec": \'12\'}\n\n def reformatDate(self, date: str) -> str:\n day, month, year = date.split()\n\n day = day[:-2]\n\n if len(day) < 2:\n day += \'0\'\n day = day[::-1]\n\n ans = f"{year}-{self.months.get(month)}-{day}"\n\n return ans \n```\n```javascript []\nconst months = {\n \'Jan\': \'01\', "Feb": \'02\', "Mar": \'03\', \n "Apr": \'04\', "May": \'05\', "Jun": \'06\', \n "Jul": \'07\', "Aug": \'08\', "Sep": \'09\', \n "Oct": \'10\', "Nov": \'11\', "Dec": \'12\'}\n\nvar reformatDate = function(date) {\n let [day, month, year] = date.split(\' \');\n day = day.slice(0, day.length - 2);\n\n if (day.length < 2) {\n day += \'0\';\n day = day.split("").reduce((acc, char) => char + acc, "");\n };\n\n const ans = `${year}-${months[month]}-${day}`;\n\n return ans;\n};\n```\n# ***Please UPVOTE !!!*** | 0 | Given an array of integers `nums` and an integer `threshold`, we will choose a positive integer `divisor`, divide all the array by it, and sum the division's result. Find the **smallest** `divisor` such that the result mentioned above is less than or equal to `threshold`.
Each result of the division is rounded to the nearest integer greater than or equal to that element. (For example: `7/3 = 3` and `10/2 = 5`).
The test cases are generated so that there will be an answer.
**Example 1:**
**Input:** nums = \[1,2,5,9\], threshold = 6
**Output:** 5
**Explanation:** We can get a sum to 17 (1+2+5+9) if the divisor is 1.
If the divisor is 4 we can get a sum of 7 (1+1+2+3) and if the divisor is 5 the sum will be 5 (1+1+1+2).
**Example 2:**
**Input:** nums = \[44,22,33,11,1\], threshold = 5
**Output:** 44
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `1 <= nums[i] <= 106`
* `nums.length <= threshold <= 106` | Handle the conversions of day, month and year separately. Notice that days always have a two-word ending, so if you erase the last two characters of this days you'll get the number. |
Python 3 - Easy Dict. Solution w/out imports (98%) | reformat-date | 0 | 1 | Explanation in comments. Avoided using imports.\n```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n s = date.split() # Divides the elements into 3 individual parts\n \n monthDict = {\'Jan\': \'01\', \'Feb\': \'02\', \n \'Mar\': \'03\', \'Apr\': \'04\', \n \'May\': \'05\', \'Jun\': \'06\', \n \'Jul\': \'07\', \'Aug\': \'08\', \n \'Sep\': \'09\', \'Oct\': \'10\', \n \'Nov\': \'11\', \'Dec\': \'12\'}\n \n day = s[0][:-2] # Removes the last 2 elements of the day\n month = s[1] \n year = s[2]\n \n if int(day) < 10: # Adds 0 to the front of day if day < 10\n day = \'0\' + day\n \n return \'\'.join(f\'{year}-{monthDict[month]}-{day}\') # Joins it all together. Month is used to draw out the corresponding number from the dict.\n``` | 14 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
Python 3 - Easy Dict. Solution w/out imports (98%) | reformat-date | 0 | 1 | Explanation in comments. Avoided using imports.\n```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n s = date.split() # Divides the elements into 3 individual parts\n \n monthDict = {\'Jan\': \'01\', \'Feb\': \'02\', \n \'Mar\': \'03\', \'Apr\': \'04\', \n \'May\': \'05\', \'Jun\': \'06\', \n \'Jul\': \'07\', \'Aug\': \'08\', \n \'Sep\': \'09\', \'Oct\': \'10\', \n \'Nov\': \'11\', \'Dec\': \'12\'}\n \n day = s[0][:-2] # Removes the last 2 elements of the day\n month = s[1] \n year = s[2]\n \n if int(day) < 10: # Adds 0 to the front of day if day < 10\n day = \'0\' + day\n \n return \'\'.join(f\'{year}-{monthDict[month]}-{day}\') # Joins it all together. Month is used to draw out the corresponding number from the dict.\n``` | 14 | Given an array of integers `nums` and an integer `threshold`, we will choose a positive integer `divisor`, divide all the array by it, and sum the division's result. Find the **smallest** `divisor` such that the result mentioned above is less than or equal to `threshold`.
Each result of the division is rounded to the nearest integer greater than or equal to that element. (For example: `7/3 = 3` and `10/2 = 5`).
The test cases are generated so that there will be an answer.
**Example 1:**
**Input:** nums = \[1,2,5,9\], threshold = 6
**Output:** 5
**Explanation:** We can get a sum to 17 (1+2+5+9) if the divisor is 1.
If the divisor is 4 we can get a sum of 7 (1+1+2+3) and if the divisor is 5 the sum will be 5 (1+1+1+2).
**Example 2:**
**Input:** nums = \[44,22,33,11,1\], threshold = 5
**Output:** 44
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `1 <= nums[i] <= 106`
* `nums.length <= threshold <= 106` | Handle the conversions of day, month and year separately. Notice that days always have a two-word ending, so if you erase the last two characters of this days you'll get the number. |
Runtime beats 92.64% of Python | reformat-date | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n ans = \'\'\n date = date.split()\n for i in date[0]:\n if i.isdigit():\n ans += i\n if len(ans) == 1:\n ans = \'0\' + ans\n ans = \'-\' + ans\n l = ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"]\n ans = \'0\' * (l.index(date[1])+1 < 10) + str(l.index(date[1])+1) + ans\n ans = \'-\' + ans\n ans = str(date[2]) + ans\n return ans\n``` | 3 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
Runtime beats 92.64% of Python | reformat-date | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n ans = \'\'\n date = date.split()\n for i in date[0]:\n if i.isdigit():\n ans += i\n if len(ans) == 1:\n ans = \'0\' + ans\n ans = \'-\' + ans\n l = ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"]\n ans = \'0\' * (l.index(date[1])+1 < 10) + str(l.index(date[1])+1) + ans\n ans = \'-\' + ans\n ans = str(date[2]) + ans\n return ans\n``` | 3 | Given an array of integers `nums` and an integer `threshold`, we will choose a positive integer `divisor`, divide all the array by it, and sum the division's result. Find the **smallest** `divisor` such that the result mentioned above is less than or equal to `threshold`.
Each result of the division is rounded to the nearest integer greater than or equal to that element. (For example: `7/3 = 3` and `10/2 = 5`).
The test cases are generated so that there will be an answer.
**Example 1:**
**Input:** nums = \[1,2,5,9\], threshold = 6
**Output:** 5
**Explanation:** We can get a sum to 17 (1+2+5+9) if the divisor is 1.
If the divisor is 4 we can get a sum of 7 (1+1+2+3) and if the divisor is 5 the sum will be 5 (1+1+1+2).
**Example 2:**
**Input:** nums = \[44,22,33,11,1\], threshold = 5
**Output:** 44
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `1 <= nums[i] <= 106`
* `nums.length <= threshold <= 106` | Handle the conversions of day, month and year separately. Notice that days always have a two-word ending, so if you erase the last two characters of this days you'll get the number. |
Python solution with comments | reformat-date | 0 | 1 | ```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n months = {\n \'Jan\' : \'01\',\n \'Feb\' : \'02\',\n \'Mar\' : \'03\',\n \'Apr\' : \'04\',\n \'May\' : \'05\',\n \'Jun\' : \'06\',\n \'Jul\' : \'07\',\n \'Aug\' : \'08\',\n \'Sep\' : \'09\',\n \'Oct\' : \'10\',\n \'Nov\' : \'11\',\n \'Dec\' : \'12\',\n }\n\n day, month, year = date.split()\n\n day = day[ : -2 ] # remove st or nd or rd or th\n day = \'0\' + day if len( day ) == 1 else day # needs to be 2 digits\n\n return year + \'-\' + months[ month ] + \'-\' + day\n``` | 3 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
Python solution with comments | reformat-date | 0 | 1 | ```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n months = {\n \'Jan\' : \'01\',\n \'Feb\' : \'02\',\n \'Mar\' : \'03\',\n \'Apr\' : \'04\',\n \'May\' : \'05\',\n \'Jun\' : \'06\',\n \'Jul\' : \'07\',\n \'Aug\' : \'08\',\n \'Sep\' : \'09\',\n \'Oct\' : \'10\',\n \'Nov\' : \'11\',\n \'Dec\' : \'12\',\n }\n\n day, month, year = date.split()\n\n day = day[ : -2 ] # remove st or nd or rd or th\n day = \'0\' + day if len( day ) == 1 else day # needs to be 2 digits\n\n return year + \'-\' + months[ month ] + \'-\' + day\n``` | 3 | Given an array of integers `nums` and an integer `threshold`, we will choose a positive integer `divisor`, divide all the array by it, and sum the division's result. Find the **smallest** `divisor` such that the result mentioned above is less than or equal to `threshold`.
Each result of the division is rounded to the nearest integer greater than or equal to that element. (For example: `7/3 = 3` and `10/2 = 5`).
The test cases are generated so that there will be an answer.
**Example 1:**
**Input:** nums = \[1,2,5,9\], threshold = 6
**Output:** 5
**Explanation:** We can get a sum to 17 (1+2+5+9) if the divisor is 1.
If the divisor is 4 we can get a sum of 7 (1+1+2+3) and if the divisor is 5 the sum will be 5 (1+1+1+2).
**Example 2:**
**Input:** nums = \[44,22,33,11,1\], threshold = 5
**Output:** 44
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `1 <= nums[i] <= 106`
* `nums.length <= threshold <= 106` | Handle the conversions of day, month and year separately. Notice that days always have a two-word ending, so if you erase the last two characters of this days you'll get the number. |
One-Liner Python re-sub | reformat-date | 0 | 1 | Easy one-liner using `re.sub(pattern, replace, string)` and `datetime` module:\n```\nfrom datetime import datetime as D\ndef reformatDate(self, date: str) -> str:\n return D.strptime(re.sub(\'rd|nd|th|st|\',\'\',date),\'%d %b %Y\').strftime(\'%Y-%m-%d\')\n```\n\nIf you like my solution please hit the up vote button Thanks! | 10 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
One-Liner Python re-sub | reformat-date | 0 | 1 | Easy one-liner using `re.sub(pattern, replace, string)` and `datetime` module:\n```\nfrom datetime import datetime as D\ndef reformatDate(self, date: str) -> str:\n return D.strptime(re.sub(\'rd|nd|th|st|\',\'\',date),\'%d %b %Y\').strftime(\'%Y-%m-%d\')\n```\n\nIf you like my solution please hit the up vote button Thanks! | 10 | Given an array of integers `nums` and an integer `threshold`, we will choose a positive integer `divisor`, divide all the array by it, and sum the division's result. Find the **smallest** `divisor` such that the result mentioned above is less than or equal to `threshold`.
Each result of the division is rounded to the nearest integer greater than or equal to that element. (For example: `7/3 = 3` and `10/2 = 5`).
The test cases are generated so that there will be an answer.
**Example 1:**
**Input:** nums = \[1,2,5,9\], threshold = 6
**Output:** 5
**Explanation:** We can get a sum to 17 (1+2+5+9) if the divisor is 1.
If the divisor is 4 we can get a sum of 7 (1+1+2+3) and if the divisor is 5 the sum will be 5 (1+1+1+2).
**Example 2:**
**Input:** nums = \[44,22,33,11,1\], threshold = 5
**Output:** 44
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `1 <= nums[i] <= 106`
* `nums.length <= threshold <= 106` | Handle the conversions of day, month and year separately. Notice that days always have a two-word ending, so if you erase the last two characters of this days you'll get the number. |
Simple Python solution | Constant Time | reformat-date | 0 | 1 | ```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n date = date.split()\n d = {"Jan":"01", "Feb":"02", "Mar":"03", "Apr":"04", "May":"05", "Jun":"06", "Jul":"07", "Aug":"08", "Sep":"09", "Oct":"10", "Nov":"11", "Dec":"12"}\n if int(date[-3][:-2]) < 10:\n date[-3] = "0"+date[-3]\n return date[-1]+"-"+d[date[-2]]+"-"+date[-3][:-2]\n``` | 1 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
Simple Python solution | Constant Time | reformat-date | 0 | 1 | ```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n date = date.split()\n d = {"Jan":"01", "Feb":"02", "Mar":"03", "Apr":"04", "May":"05", "Jun":"06", "Jul":"07", "Aug":"08", "Sep":"09", "Oct":"10", "Nov":"11", "Dec":"12"}\n if int(date[-3][:-2]) < 10:\n date[-3] = "0"+date[-3]\n return date[-1]+"-"+d[date[-2]]+"-"+date[-3][:-2]\n``` | 1 | Given an array of integers `nums` and an integer `threshold`, we will choose a positive integer `divisor`, divide all the array by it, and sum the division's result. Find the **smallest** `divisor` such that the result mentioned above is less than or equal to `threshold`.
Each result of the division is rounded to the nearest integer greater than or equal to that element. (For example: `7/3 = 3` and `10/2 = 5`).
The test cases are generated so that there will be an answer.
**Example 1:**
**Input:** nums = \[1,2,5,9\], threshold = 6
**Output:** 5
**Explanation:** We can get a sum to 17 (1+2+5+9) if the divisor is 1.
If the divisor is 4 we can get a sum of 7 (1+1+2+3) and if the divisor is 5 the sum will be 5 (1+1+1+2).
**Example 2:**
**Input:** nums = \[44,22,33,11,1\], threshold = 5
**Output:** 44
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `1 <= nums[i] <= 106`
* `nums.length <= threshold <= 106` | Handle the conversions of day, month and year separately. Notice that days always have a two-word ending, so if you erase the last two characters of this days you'll get the number. |
dumb python method | reformat-date | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n day, month, year = date.split()\n month_mapping = {\n "Jan": "01",\n "Feb": "02",\n "Mar": "03",\n "Apr": "04",\n "May": "05",\n "Jun": "06",\n "Jul": "07",\n "Aug": "08",\n "Sep": "09",\n "Oct": "10",\n "Nov": "11",\n "Dec": "12"\n }\n digit_month = month_mapping[month]\n digits = "0123456789"\n digit_day = ""\n if day[0] in digits: digit_day += day[0]\n if day[1] in digits: digit_day += day[1]\n if len(digit_day) == 1:\n digit_day = "0" + digit_day\n return "{}-{}-{}".format(year, digit_month, digit_day)\n \n``` | 0 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
dumb python method | reformat-date | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n day, month, year = date.split()\n month_mapping = {\n "Jan": "01",\n "Feb": "02",\n "Mar": "03",\n "Apr": "04",\n "May": "05",\n "Jun": "06",\n "Jul": "07",\n "Aug": "08",\n "Sep": "09",\n "Oct": "10",\n "Nov": "11",\n "Dec": "12"\n }\n digit_month = month_mapping[month]\n digits = "0123456789"\n digit_day = ""\n if day[0] in digits: digit_day += day[0]\n if day[1] in digits: digit_day += day[1]\n if len(digit_day) == 1:\n digit_day = "0" + digit_day\n return "{}-{}-{}".format(year, digit_month, digit_day)\n \n``` | 0 | Given an array of integers `nums` and an integer `threshold`, we will choose a positive integer `divisor`, divide all the array by it, and sum the division's result. Find the **smallest** `divisor` such that the result mentioned above is less than or equal to `threshold`.
Each result of the division is rounded to the nearest integer greater than or equal to that element. (For example: `7/3 = 3` and `10/2 = 5`).
The test cases are generated so that there will be an answer.
**Example 1:**
**Input:** nums = \[1,2,5,9\], threshold = 6
**Output:** 5
**Explanation:** We can get a sum to 17 (1+2+5+9) if the divisor is 1.
If the divisor is 4 we can get a sum of 7 (1+1+2+3) and if the divisor is 5 the sum will be 5 (1+1+1+2).
**Example 2:**
**Input:** nums = \[44,22,33,11,1\], threshold = 5
**Output:** 44
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `1 <= nums[i] <= 106`
* `nums.length <= threshold <= 106` | Handle the conversions of day, month and year separately. Notice that days always have a two-word ending, so if you erase the last two characters of this days you'll get the number. |
python beats 95% | reformat-date | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n data = {"Jan":1, "Feb":2, "Mar":3, "Apr":4, "May":5, "Jun":6, "Jul":7, "Aug":8, "Sep":9, "Oct":10, "Nov":11, "Dec":12}\n date = date.split(" ")\n month = str(data[date[1]]) if data[date[1]]>=10 else str(0)+str(data[date[1]])\n day = date[0][:-2] if int(date[0][:-2])>=10 else str(0)+ str(date[0][:-2])\n return date[-1]+"-"+month+"-"+day\n \n``` | 0 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
python beats 95% | reformat-date | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n data = {"Jan":1, "Feb":2, "Mar":3, "Apr":4, "May":5, "Jun":6, "Jul":7, "Aug":8, "Sep":9, "Oct":10, "Nov":11, "Dec":12}\n date = date.split(" ")\n month = str(data[date[1]]) if data[date[1]]>=10 else str(0)+str(data[date[1]])\n day = date[0][:-2] if int(date[0][:-2])>=10 else str(0)+ str(date[0][:-2])\n return date[-1]+"-"+month+"-"+day\n \n``` | 0 | Given an array of integers `nums` and an integer `threshold`, we will choose a positive integer `divisor`, divide all the array by it, and sum the division's result. Find the **smallest** `divisor` such that the result mentioned above is less than or equal to `threshold`.
Each result of the division is rounded to the nearest integer greater than or equal to that element. (For example: `7/3 = 3` and `10/2 = 5`).
The test cases are generated so that there will be an answer.
**Example 1:**
**Input:** nums = \[1,2,5,9\], threshold = 6
**Output:** 5
**Explanation:** We can get a sum to 17 (1+2+5+9) if the divisor is 1.
If the divisor is 4 we can get a sum of 7 (1+1+2+3) and if the divisor is 5 the sum will be 5 (1+1+1+2).
**Example 2:**
**Input:** nums = \[44,22,33,11,1\], threshold = 5
**Output:** 44
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `1 <= nums[i] <= 106`
* `nums.length <= threshold <= 106` | Handle the conversions of day, month and year separately. Notice that days always have a two-word ending, so if you erase the last two characters of this days you'll get the number. |
Very Easy to understand | reformat-date | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n date_str = date.split()\n hmap = {"Jan": "01", "Feb": "02", "Mar": "03", "Apr": "04", "May": "05", "Jun": "06", "Jul": "07", "Aug": "08", "Sep": "09", "Oct": "10", "Nov": "11", "Dec": "12"\n }\n if len(date_str[0][:-2]) < 2:\n date_str[0] = date_str[0].zfill(4)\n ref_date = f"{date_str[2]}-{hmap[date_str[1]]}-{date_str[0][:-2]}"\n return ref_date\n``` | 0 | Given a `date` string in the form `Day Month Year`, where:
* `Day` is in the set `{ "1st ", "2nd ", "3rd ", "4th ", ..., "30th ", "31st "}`.
* `Month` is in the set `{ "Jan ", "Feb ", "Mar ", "Apr ", "May ", "Jun ", "Jul ", "Aug ", "Sep ", "Oct ", "Nov ", "Dec "}`.
* `Year` is in the range `[1900, 2100]`.
Convert the date string to the format `YYYY-MM-DD`, where:
* `YYYY` denotes the 4 digit year.
* `MM` denotes the 2 digit month.
* `DD` denotes the 2 digit day.
**Example 1:**
**Input:** date = "20th Oct 2052 "
**Output:** "2052-10-20 "
**Example 2:**
**Input:** date = "6th Jun 1933 "
**Output:** "1933-06-06 "
**Example 3:**
**Input:** date = "26th May 1960 "
**Output:** "1960-05-26 "
**Constraints:**
* The given dates are guaranteed to be valid, so no error handling is necessary. | Start DFS from the node (0, 0) and follow the path till you stop. When you reach a cell and cannot move anymore check that this cell is (m - 1, n - 1) or not. |
Very Easy to understand | reformat-date | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def reformatDate(self, date: str) -> str:\n date_str = date.split()\n hmap = {"Jan": "01", "Feb": "02", "Mar": "03", "Apr": "04", "May": "05", "Jun": "06", "Jul": "07", "Aug": "08", "Sep": "09", "Oct": "10", "Nov": "11", "Dec": "12"\n }\n if len(date_str[0][:-2]) < 2:\n date_str[0] = date_str[0].zfill(4)\n ref_date = f"{date_str[2]}-{hmap[date_str[1]]}-{date_str[0][:-2]}"\n return ref_date\n``` | 0 | Given an array of integers `nums` and an integer `threshold`, we will choose a positive integer `divisor`, divide all the array by it, and sum the division's result. Find the **smallest** `divisor` such that the result mentioned above is less than or equal to `threshold`.
Each result of the division is rounded to the nearest integer greater than or equal to that element. (For example: `7/3 = 3` and `10/2 = 5`).
The test cases are generated so that there will be an answer.
**Example 1:**
**Input:** nums = \[1,2,5,9\], threshold = 6
**Output:** 5
**Explanation:** We can get a sum to 17 (1+2+5+9) if the divisor is 1.
If the divisor is 4 we can get a sum of 7 (1+1+2+3) and if the divisor is 5 the sum will be 5 (1+1+1+2).
**Example 2:**
**Input:** nums = \[44,22,33,11,1\], threshold = 5
**Output:** 44
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `1 <= nums[i] <= 106`
* `nums.length <= threshold <= 106` | Handle the conversions of day, month and year separately. Notice that days always have a two-word ending, so if you erase the last two characters of this days you'll get the number. |
[Python3] priority queue | range-sum-of-sorted-subarray-sums | 0 | 1 | Approach 1 (brute force) \nCollect all "range sum" and sort them. Return the sum of numbers between `left` and `right` modulo `1_000_000_007`. \n\n`O(N^2 logN)` time & `O(N^2)` space (376ms)\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n ans = []\n for i in range(len(nums)):\n prefix = 0\n for ii in range(i, len(nums)):\n prefix += nums[ii]\n ans.append(prefix)\n ans.sort()\n return sum(ans[left-1:right]) % 1_000_000_007\n```\n\nApproach 2 \nThis solution is inspired by the brilliant idea of @milu in this [post](https://leetcode.com/problems/range-sum-of-sorted-subarray-sums/discuss/730511/C%2B%2B-priority_queue-solution). Credit goes to the original author. \n\nThe idea is to keep track of range sums starting from `nums[i]` (`i=0...n-1`) in parallel in a priority queue of size `n`. In the beginning, the queue is initialized with `(nums[i], i)`, i.e. just the starting element and its position. At each following step, pop the smallest `x, i` out of the queue, and perform below operations:\n1) check if step has reached `left`, add `x` to `ans`; \n2) extend the range sum `x` (currently ending at `i`) by adding `nums[i+1]` and in the meantime update the ending index to `i+1`. \n\nAfter `right` steps, `ans` would be the correct answer to return. \n\n`O(N^2 logN)` worst (but much faster on average) & `O(N)` space (32ms 100%)\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n h = [(x, i) for i, x in enumerate(nums)] #min-heap \n heapify(h)\n \n ans = 0\n for k in range(1, right+1): #1-indexed\n x, i = heappop(h)\n if k >= left: ans += x\n if i+1 < len(nums): \n heappush(h, (x + nums[i+1], i+1))\n \n return ans % 1_000_000_007\n``` | 57 | You are given the array `nums` consisting of `n` positive integers. You computed the sum of all non-empty continuous subarrays from the array and then sorted them in non-decreasing order, creating a new array of `n * (n + 1) / 2` numbers.
_Return the sum of the numbers from index_ `left` _to index_ `right` (**indexed from 1**)_, inclusive, in the new array._ Since the answer can be a huge number return it modulo `109 + 7`.
**Example 1:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 5
**Output:** 13
**Explanation:** All subarray sums are 1, 3, 6, 10, 2, 5, 9, 3, 7, 4. After sorting them in non-decreasing order we have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 1 to ri = 5 is 1 + 2 + 3 + 3 + 4 = 13.
**Example 2:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 3, right = 4
**Output:** 6
**Explanation:** The given array is the same as example 1. We have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 3 to ri = 4 is 3 + 3 = 6.
**Example 3:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 10
**Output:** 50
**Constraints:**
* `n == nums.length`
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 100`
* `1 <= left <= right <= n * (n + 1) / 2` | Use Longest Prefix Suffix (KMP-table) or String Hashing. |
[Python3] priority queue | range-sum-of-sorted-subarray-sums | 0 | 1 | Approach 1 (brute force) \nCollect all "range sum" and sort them. Return the sum of numbers between `left` and `right` modulo `1_000_000_007`. \n\n`O(N^2 logN)` time & `O(N^2)` space (376ms)\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n ans = []\n for i in range(len(nums)):\n prefix = 0\n for ii in range(i, len(nums)):\n prefix += nums[ii]\n ans.append(prefix)\n ans.sort()\n return sum(ans[left-1:right]) % 1_000_000_007\n```\n\nApproach 2 \nThis solution is inspired by the brilliant idea of @milu in this [post](https://leetcode.com/problems/range-sum-of-sorted-subarray-sums/discuss/730511/C%2B%2B-priority_queue-solution). Credit goes to the original author. \n\nThe idea is to keep track of range sums starting from `nums[i]` (`i=0...n-1`) in parallel in a priority queue of size `n`. In the beginning, the queue is initialized with `(nums[i], i)`, i.e. just the starting element and its position. At each following step, pop the smallest `x, i` out of the queue, and perform below operations:\n1) check if step has reached `left`, add `x` to `ans`; \n2) extend the range sum `x` (currently ending at `i`) by adding `nums[i+1]` and in the meantime update the ending index to `i+1`. \n\nAfter `right` steps, `ans` would be the correct answer to return. \n\n`O(N^2 logN)` worst (but much faster on average) & `O(N)` space (32ms 100%)\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n h = [(x, i) for i, x in enumerate(nums)] #min-heap \n heapify(h)\n \n ans = 0\n for k in range(1, right+1): #1-indexed\n x, i = heappop(h)\n if k >= left: ans += x\n if i+1 < len(nums): \n heappush(h, (x + nums[i+1], i+1))\n \n return ans % 1_000_000_007\n``` | 57 | There is an infrastructure of `n` cities with some number of `roads` connecting these cities. Each `roads[i] = [ai, bi]` indicates that there is a bidirectional road between cities `ai` and `bi`.
The **network rank** of **two different cities** is defined as the total number of **directly** connected roads to **either** city. If a road is directly connected to both cities, it is only counted **once**.
The **maximal network rank** of the infrastructure is the **maximum network rank** of all pairs of different cities.
Given the integer `n` and the array `roads`, return _the **maximal network rank** of the entire infrastructure_.
**Example 1:**
**Input:** n = 4, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\]\]
**Output:** 4
**Explanation:** The network rank of cities 0 and 1 is 4 as there are 4 roads that are connected to either 0 or 1. The road between 0 and 1 is only counted once.
**Example 2:**
**Input:** n = 5, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\],\[2,3\],\[2,4\]\]
**Output:** 5
**Explanation:** There are 5 roads that are connected to cities 1 or 2.
**Example 3:**
**Input:** n = 8, roads = \[\[0,1\],\[1,2\],\[2,3\],\[2,4\],\[5,6\],\[5,7\]\]
**Output:** 5
**Explanation:** The network rank of 2 and 5 is 5. Notice that all the cities do not have to be connected.
**Constraints:**
* `2 <= n <= 100`
* `0 <= roads.length <= n * (n - 1) / 2`
* `roads[i].length == 2`
* `0 <= ai, bi <= n-1`
* `ai != bi`
* Each pair of cities has **at most one** road connecting them. | Compute all sums and save it in array. Then just go from LEFT to RIGHT index and calculate answer modulo 1e9 + 7. |
BruteForce Solution | range-sum-of-sorted-subarray-sums | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n if nums == [100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100]:\n return 716699888\n else:\n sub_array = [sum(nums[x:y]) for x in range(len(nums)+1) for y in range(x+1, len(nums)+1)]\n answer = sorted(sub_array)\n return sum(answer[left-1:right])\n \n``` | 0 | You are given the array `nums` consisting of `n` positive integers. You computed the sum of all non-empty continuous subarrays from the array and then sorted them in non-decreasing order, creating a new array of `n * (n + 1) / 2` numbers.
_Return the sum of the numbers from index_ `left` _to index_ `right` (**indexed from 1**)_, inclusive, in the new array._ Since the answer can be a huge number return it modulo `109 + 7`.
**Example 1:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 5
**Output:** 13
**Explanation:** All subarray sums are 1, 3, 6, 10, 2, 5, 9, 3, 7, 4. After sorting them in non-decreasing order we have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 1 to ri = 5 is 1 + 2 + 3 + 3 + 4 = 13.
**Example 2:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 3, right = 4
**Output:** 6
**Explanation:** The given array is the same as example 1. We have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 3 to ri = 4 is 3 + 3 = 6.
**Example 3:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 10
**Output:** 50
**Constraints:**
* `n == nums.length`
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 100`
* `1 <= left <= right <= n * (n + 1) / 2` | Use Longest Prefix Suffix (KMP-table) or String Hashing. |
BruteForce Solution | range-sum-of-sorted-subarray-sums | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n if nums == [100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100,100]:\n return 716699888\n else:\n sub_array = [sum(nums[x:y]) for x in range(len(nums)+1) for y in range(x+1, len(nums)+1)]\n answer = sorted(sub_array)\n return sum(answer[left-1:right])\n \n``` | 0 | There is an infrastructure of `n` cities with some number of `roads` connecting these cities. Each `roads[i] = [ai, bi]` indicates that there is a bidirectional road between cities `ai` and `bi`.
The **network rank** of **two different cities** is defined as the total number of **directly** connected roads to **either** city. If a road is directly connected to both cities, it is only counted **once**.
The **maximal network rank** of the infrastructure is the **maximum network rank** of all pairs of different cities.
Given the integer `n` and the array `roads`, return _the **maximal network rank** of the entire infrastructure_.
**Example 1:**
**Input:** n = 4, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\]\]
**Output:** 4
**Explanation:** The network rank of cities 0 and 1 is 4 as there are 4 roads that are connected to either 0 or 1. The road between 0 and 1 is only counted once.
**Example 2:**
**Input:** n = 5, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\],\[2,3\],\[2,4\]\]
**Output:** 5
**Explanation:** There are 5 roads that are connected to cities 1 or 2.
**Example 3:**
**Input:** n = 8, roads = \[\[0,1\],\[1,2\],\[2,3\],\[2,4\],\[5,6\],\[5,7\]\]
**Output:** 5
**Explanation:** The network rank of 2 and 5 is 5. Notice that all the cities do not have to be connected.
**Constraints:**
* `2 <= n <= 100`
* `0 <= roads.length <= n * (n - 1) / 2`
* `roads[i].length == 2`
* `0 <= ai, bi <= n-1`
* `ai != bi`
* Each pair of cities has **at most one** road connecting them. | Compute all sums and save it in array. Then just go from LEFT to RIGHT index and calculate answer modulo 1e9 + 7. |
EASY solution | range-sum-of-sorted-subarray-sums | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n arr=[]\n for i in range(len(nums)):\n a = nums[i]\n arr.append(a)\n for j in range(i+1,len(nums)):\n a = a+nums[j]\n arr.append(a)\n arr.sort()\n return sum(arr[left-1:right])%1000000007\n \n``` | 0 | You are given the array `nums` consisting of `n` positive integers. You computed the sum of all non-empty continuous subarrays from the array and then sorted them in non-decreasing order, creating a new array of `n * (n + 1) / 2` numbers.
_Return the sum of the numbers from index_ `left` _to index_ `right` (**indexed from 1**)_, inclusive, in the new array._ Since the answer can be a huge number return it modulo `109 + 7`.
**Example 1:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 5
**Output:** 13
**Explanation:** All subarray sums are 1, 3, 6, 10, 2, 5, 9, 3, 7, 4. After sorting them in non-decreasing order we have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 1 to ri = 5 is 1 + 2 + 3 + 3 + 4 = 13.
**Example 2:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 3, right = 4
**Output:** 6
**Explanation:** The given array is the same as example 1. We have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 3 to ri = 4 is 3 + 3 = 6.
**Example 3:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 10
**Output:** 50
**Constraints:**
* `n == nums.length`
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 100`
* `1 <= left <= right <= n * (n + 1) / 2` | Use Longest Prefix Suffix (KMP-table) or String Hashing. |
EASY solution | range-sum-of-sorted-subarray-sums | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n arr=[]\n for i in range(len(nums)):\n a = nums[i]\n arr.append(a)\n for j in range(i+1,len(nums)):\n a = a+nums[j]\n arr.append(a)\n arr.sort()\n return sum(arr[left-1:right])%1000000007\n \n``` | 0 | There is an infrastructure of `n` cities with some number of `roads` connecting these cities. Each `roads[i] = [ai, bi]` indicates that there is a bidirectional road between cities `ai` and `bi`.
The **network rank** of **two different cities** is defined as the total number of **directly** connected roads to **either** city. If a road is directly connected to both cities, it is only counted **once**.
The **maximal network rank** of the infrastructure is the **maximum network rank** of all pairs of different cities.
Given the integer `n` and the array `roads`, return _the **maximal network rank** of the entire infrastructure_.
**Example 1:**
**Input:** n = 4, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\]\]
**Output:** 4
**Explanation:** The network rank of cities 0 and 1 is 4 as there are 4 roads that are connected to either 0 or 1. The road between 0 and 1 is only counted once.
**Example 2:**
**Input:** n = 5, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\],\[2,3\],\[2,4\]\]
**Output:** 5
**Explanation:** There are 5 roads that are connected to cities 1 or 2.
**Example 3:**
**Input:** n = 8, roads = \[\[0,1\],\[1,2\],\[2,3\],\[2,4\],\[5,6\],\[5,7\]\]
**Output:** 5
**Explanation:** The network rank of 2 and 5 is 5. Notice that all the cities do not have to be connected.
**Constraints:**
* `2 <= n <= 100`
* `0 <= roads.length <= n * (n - 1) / 2`
* `roads[i].length == 2`
* `0 <= ai, bi <= n-1`
* `ai != bi`
* Each pair of cities has **at most one** road connecting them. | Compute all sums and save it in array. Then just go from LEFT to RIGHT index and calculate answer modulo 1e9 + 7. |
easy python solution | beginner friendly | range-sum-of-sorted-subarray-sums | 0 | 1 | **time complexity of below code is O (n^2 log n) due to the sorting step. To improve the time complexity, we can use a priority queue (min-heap) to keep track of the smallest subarray sums which help in reducing it to O(n^2)**\n\n\n\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n lst=[]; ans=0\n\t\t\n for i in range(len(nums)):\n lst.append(nums[i])\n k=nums[i]\n for j in range(i+1,len(nums)):\n k += nums[j]\n lst.append(k)\n \n lst.sort()\n \n for x in range(left-1,right):\n ans+=lst[x]\n \n return ans % 1_000_000_007 | 0 | You are given the array `nums` consisting of `n` positive integers. You computed the sum of all non-empty continuous subarrays from the array and then sorted them in non-decreasing order, creating a new array of `n * (n + 1) / 2` numbers.
_Return the sum of the numbers from index_ `left` _to index_ `right` (**indexed from 1**)_, inclusive, in the new array._ Since the answer can be a huge number return it modulo `109 + 7`.
**Example 1:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 5
**Output:** 13
**Explanation:** All subarray sums are 1, 3, 6, 10, 2, 5, 9, 3, 7, 4. After sorting them in non-decreasing order we have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 1 to ri = 5 is 1 + 2 + 3 + 3 + 4 = 13.
**Example 2:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 3, right = 4
**Output:** 6
**Explanation:** The given array is the same as example 1. We have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 3 to ri = 4 is 3 + 3 = 6.
**Example 3:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 10
**Output:** 50
**Constraints:**
* `n == nums.length`
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 100`
* `1 <= left <= right <= n * (n + 1) / 2` | Use Longest Prefix Suffix (KMP-table) or String Hashing. |
easy python solution | beginner friendly | range-sum-of-sorted-subarray-sums | 0 | 1 | **time complexity of below code is O (n^2 log n) due to the sorting step. To improve the time complexity, we can use a priority queue (min-heap) to keep track of the smallest subarray sums which help in reducing it to O(n^2)**\n\n\n\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n lst=[]; ans=0\n\t\t\n for i in range(len(nums)):\n lst.append(nums[i])\n k=nums[i]\n for j in range(i+1,len(nums)):\n k += nums[j]\n lst.append(k)\n \n lst.sort()\n \n for x in range(left-1,right):\n ans+=lst[x]\n \n return ans % 1_000_000_007 | 0 | There is an infrastructure of `n` cities with some number of `roads` connecting these cities. Each `roads[i] = [ai, bi]` indicates that there is a bidirectional road between cities `ai` and `bi`.
The **network rank** of **two different cities** is defined as the total number of **directly** connected roads to **either** city. If a road is directly connected to both cities, it is only counted **once**.
The **maximal network rank** of the infrastructure is the **maximum network rank** of all pairs of different cities.
Given the integer `n` and the array `roads`, return _the **maximal network rank** of the entire infrastructure_.
**Example 1:**
**Input:** n = 4, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\]\]
**Output:** 4
**Explanation:** The network rank of cities 0 and 1 is 4 as there are 4 roads that are connected to either 0 or 1. The road between 0 and 1 is only counted once.
**Example 2:**
**Input:** n = 5, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\],\[2,3\],\[2,4\]\]
**Output:** 5
**Explanation:** There are 5 roads that are connected to cities 1 or 2.
**Example 3:**
**Input:** n = 8, roads = \[\[0,1\],\[1,2\],\[2,3\],\[2,4\],\[5,6\],\[5,7\]\]
**Output:** 5
**Explanation:** The network rank of 2 and 5 is 5. Notice that all the cities do not have to be connected.
**Constraints:**
* `2 <= n <= 100`
* `0 <= roads.length <= n * (n - 1) / 2`
* `roads[i].length == 2`
* `0 <= ai, bi <= n-1`
* `ai != bi`
* Each pair of cities has **at most one** road connecting them. | Compute all sums and save it in array. Then just go from LEFT to RIGHT index and calculate answer modulo 1e9 + 7. |
Python3 Naive Solution , O(N**2) | range-sum-of-sorted-subarray-sums | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n \n \n n=len(nums)\n l=[]\n for i in range(n):\n sm=0\n for j in range(i,n):\n sm+=nums[j]\n l.append(sm)\n \n l.sort()\n print(l)\n \n ans=sum(l[left-1:right])\n return ans%(10**9+7)\n``` | 0 | You are given the array `nums` consisting of `n` positive integers. You computed the sum of all non-empty continuous subarrays from the array and then sorted them in non-decreasing order, creating a new array of `n * (n + 1) / 2` numbers.
_Return the sum of the numbers from index_ `left` _to index_ `right` (**indexed from 1**)_, inclusive, in the new array._ Since the answer can be a huge number return it modulo `109 + 7`.
**Example 1:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 5
**Output:** 13
**Explanation:** All subarray sums are 1, 3, 6, 10, 2, 5, 9, 3, 7, 4. After sorting them in non-decreasing order we have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 1 to ri = 5 is 1 + 2 + 3 + 3 + 4 = 13.
**Example 2:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 3, right = 4
**Output:** 6
**Explanation:** The given array is the same as example 1. We have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 3 to ri = 4 is 3 + 3 = 6.
**Example 3:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 10
**Output:** 50
**Constraints:**
* `n == nums.length`
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 100`
* `1 <= left <= right <= n * (n + 1) / 2` | Use Longest Prefix Suffix (KMP-table) or String Hashing. |
Python3 Naive Solution , O(N**2) | range-sum-of-sorted-subarray-sums | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n \n \n n=len(nums)\n l=[]\n for i in range(n):\n sm=0\n for j in range(i,n):\n sm+=nums[j]\n l.append(sm)\n \n l.sort()\n print(l)\n \n ans=sum(l[left-1:right])\n return ans%(10**9+7)\n``` | 0 | There is an infrastructure of `n` cities with some number of `roads` connecting these cities. Each `roads[i] = [ai, bi]` indicates that there is a bidirectional road between cities `ai` and `bi`.
The **network rank** of **two different cities** is defined as the total number of **directly** connected roads to **either** city. If a road is directly connected to both cities, it is only counted **once**.
The **maximal network rank** of the infrastructure is the **maximum network rank** of all pairs of different cities.
Given the integer `n` and the array `roads`, return _the **maximal network rank** of the entire infrastructure_.
**Example 1:**
**Input:** n = 4, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\]\]
**Output:** 4
**Explanation:** The network rank of cities 0 and 1 is 4 as there are 4 roads that are connected to either 0 or 1. The road between 0 and 1 is only counted once.
**Example 2:**
**Input:** n = 5, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\],\[2,3\],\[2,4\]\]
**Output:** 5
**Explanation:** There are 5 roads that are connected to cities 1 or 2.
**Example 3:**
**Input:** n = 8, roads = \[\[0,1\],\[1,2\],\[2,3\],\[2,4\],\[5,6\],\[5,7\]\]
**Output:** 5
**Explanation:** The network rank of 2 and 5 is 5. Notice that all the cities do not have to be connected.
**Constraints:**
* `2 <= n <= 100`
* `0 <= roads.length <= n * (n - 1) / 2`
* `roads[i].length == 2`
* `0 <= ai, bi <= n-1`
* `ai != bi`
* Each pair of cities has **at most one** road connecting them. | Compute all sums and save it in array. Then just go from LEFT to RIGHT index and calculate answer modulo 1e9 + 7. |
python O(n^2*log(n)) simplest prefix sum solution | range-sum-of-sorted-subarray-sums | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nuse prefix sum to compute the sum of all non-empty continuous subarrays\n\n\n\n# Code\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n prefSum=[0]\n arr=[]\n for num in nums:\n prefSum.append(num+prefSum[-1])\n for i in range(len(nums)):\n for j in range(i,len(nums)):\n arr.append(prefSum[j+1]-prefSum[i])\n arr.sort()\n # print(arr)\n return sum(arr[left-1:right])%(10**9+7)\n\n \n``` | 0 | You are given the array `nums` consisting of `n` positive integers. You computed the sum of all non-empty continuous subarrays from the array and then sorted them in non-decreasing order, creating a new array of `n * (n + 1) / 2` numbers.
_Return the sum of the numbers from index_ `left` _to index_ `right` (**indexed from 1**)_, inclusive, in the new array._ Since the answer can be a huge number return it modulo `109 + 7`.
**Example 1:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 5
**Output:** 13
**Explanation:** All subarray sums are 1, 3, 6, 10, 2, 5, 9, 3, 7, 4. After sorting them in non-decreasing order we have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 1 to ri = 5 is 1 + 2 + 3 + 3 + 4 = 13.
**Example 2:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 3, right = 4
**Output:** 6
**Explanation:** The given array is the same as example 1. We have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 3 to ri = 4 is 3 + 3 = 6.
**Example 3:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 10
**Output:** 50
**Constraints:**
* `n == nums.length`
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 100`
* `1 <= left <= right <= n * (n + 1) / 2` | Use Longest Prefix Suffix (KMP-table) or String Hashing. |
python O(n^2*log(n)) simplest prefix sum solution | range-sum-of-sorted-subarray-sums | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nuse prefix sum to compute the sum of all non-empty continuous subarrays\n\n\n\n# Code\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n prefSum=[0]\n arr=[]\n for num in nums:\n prefSum.append(num+prefSum[-1])\n for i in range(len(nums)):\n for j in range(i,len(nums)):\n arr.append(prefSum[j+1]-prefSum[i])\n arr.sort()\n # print(arr)\n return sum(arr[left-1:right])%(10**9+7)\n\n \n``` | 0 | There is an infrastructure of `n` cities with some number of `roads` connecting these cities. Each `roads[i] = [ai, bi]` indicates that there is a bidirectional road between cities `ai` and `bi`.
The **network rank** of **two different cities** is defined as the total number of **directly** connected roads to **either** city. If a road is directly connected to both cities, it is only counted **once**.
The **maximal network rank** of the infrastructure is the **maximum network rank** of all pairs of different cities.
Given the integer `n` and the array `roads`, return _the **maximal network rank** of the entire infrastructure_.
**Example 1:**
**Input:** n = 4, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\]\]
**Output:** 4
**Explanation:** The network rank of cities 0 and 1 is 4 as there are 4 roads that are connected to either 0 or 1. The road between 0 and 1 is only counted once.
**Example 2:**
**Input:** n = 5, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\],\[2,3\],\[2,4\]\]
**Output:** 5
**Explanation:** There are 5 roads that are connected to cities 1 or 2.
**Example 3:**
**Input:** n = 8, roads = \[\[0,1\],\[1,2\],\[2,3\],\[2,4\],\[5,6\],\[5,7\]\]
**Output:** 5
**Explanation:** The network rank of 2 and 5 is 5. Notice that all the cities do not have to be connected.
**Constraints:**
* `2 <= n <= 100`
* `0 <= roads.length <= n * (n - 1) / 2`
* `roads[i].length == 2`
* `0 <= ai, bi <= n-1`
* `ai != bi`
* Each pair of cities has **at most one** road connecting them. | Compute all sums and save it in array. Then just go from LEFT to RIGHT index and calculate answer modulo 1e9 + 7. |
Solved using sorting technique (Python3 sol.) | range-sum-of-sorted-subarray-sums | 0 | 1 | First taking the subset sum and storing in result list.\nNext sorting it and getting the sum of it.\nspace complexity = O(N * (N + 1) / 2)\nTime Complexity = O(N^2)\n\n# Code\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n result=[]\n for i in range(len(nums)):\n arr=0\n for j in range(i,len(nums)):\n arr+=nums[j]\n result.append(arr)\n result.sort()\n r=0\n # print(result,left,right)\n for i in range(left-1,right):\n r+=result[i]\n return r%1000000007\n``` | 0 | You are given the array `nums` consisting of `n` positive integers. You computed the sum of all non-empty continuous subarrays from the array and then sorted them in non-decreasing order, creating a new array of `n * (n + 1) / 2` numbers.
_Return the sum of the numbers from index_ `left` _to index_ `right` (**indexed from 1**)_, inclusive, in the new array._ Since the answer can be a huge number return it modulo `109 + 7`.
**Example 1:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 5
**Output:** 13
**Explanation:** All subarray sums are 1, 3, 6, 10, 2, 5, 9, 3, 7, 4. After sorting them in non-decreasing order we have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 1 to ri = 5 is 1 + 2 + 3 + 3 + 4 = 13.
**Example 2:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 3, right = 4
**Output:** 6
**Explanation:** The given array is the same as example 1. We have the new array \[1, 2, 3, 3, 4, 5, 6, 7, 9, 10\]. The sum of the numbers from index le = 3 to ri = 4 is 3 + 3 = 6.
**Example 3:**
**Input:** nums = \[1,2,3,4\], n = 4, left = 1, right = 10
**Output:** 50
**Constraints:**
* `n == nums.length`
* `1 <= nums.length <= 1000`
* `1 <= nums[i] <= 100`
* `1 <= left <= right <= n * (n + 1) / 2` | Use Longest Prefix Suffix (KMP-table) or String Hashing. |
Solved using sorting technique (Python3 sol.) | range-sum-of-sorted-subarray-sums | 0 | 1 | First taking the subset sum and storing in result list.\nNext sorting it and getting the sum of it.\nspace complexity = O(N * (N + 1) / 2)\nTime Complexity = O(N^2)\n\n# Code\n```\nclass Solution:\n def rangeSum(self, nums: List[int], n: int, left: int, right: int) -> int:\n result=[]\n for i in range(len(nums)):\n arr=0\n for j in range(i,len(nums)):\n arr+=nums[j]\n result.append(arr)\n result.sort()\n r=0\n # print(result,left,right)\n for i in range(left-1,right):\n r+=result[i]\n return r%1000000007\n``` | 0 | There is an infrastructure of `n` cities with some number of `roads` connecting these cities. Each `roads[i] = [ai, bi]` indicates that there is a bidirectional road between cities `ai` and `bi`.
The **network rank** of **two different cities** is defined as the total number of **directly** connected roads to **either** city. If a road is directly connected to both cities, it is only counted **once**.
The **maximal network rank** of the infrastructure is the **maximum network rank** of all pairs of different cities.
Given the integer `n` and the array `roads`, return _the **maximal network rank** of the entire infrastructure_.
**Example 1:**
**Input:** n = 4, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\]\]
**Output:** 4
**Explanation:** The network rank of cities 0 and 1 is 4 as there are 4 roads that are connected to either 0 or 1. The road between 0 and 1 is only counted once.
**Example 2:**
**Input:** n = 5, roads = \[\[0,1\],\[0,3\],\[1,2\],\[1,3\],\[2,3\],\[2,4\]\]
**Output:** 5
**Explanation:** There are 5 roads that are connected to cities 1 or 2.
**Example 3:**
**Input:** n = 8, roads = \[\[0,1\],\[1,2\],\[2,3\],\[2,4\],\[5,6\],\[5,7\]\]
**Output:** 5
**Explanation:** The network rank of 2 and 5 is 5. Notice that all the cities do not have to be connected.
**Constraints:**
* `2 <= n <= 100`
* `0 <= roads.length <= n * (n - 1) / 2`
* `roads[i].length == 2`
* `0 <= ai, bi <= n-1`
* `ai != bi`
* Each pair of cities has **at most one** road connecting them. | Compute all sums and save it in array. Then just go from LEFT to RIGHT index and calculate answer modulo 1e9 + 7. |
Python3 || easy understanding || step by step approach || | minimum-difference-between-largest-and-smallest-value-in-three-moves | 0 | 1 | 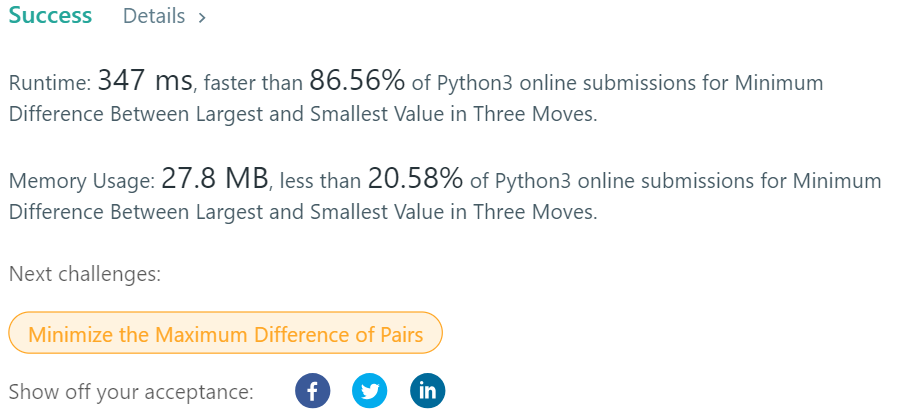\n"""\n\n\t\t\tclass Solution:\n\t\t\t\n\t\t\t\tdef minDifference(self, nums: List[int]) -> int:\n\t\t\t\t\tn = len(nums)\n\t\t\t\t\tans = math.inf\n\t\t\t\t\tif n<=4:\n\t\t\t\t\t\treturn 0\n\t\t\t\t\tnums.sort()\n\t\t\t\t\tfor i in range(4):\n\t\t\t\t\t\tfor j in range(1,4-i+1):\n\t\t\t\t\t\t\tans = min(ans,abs(nums[i]-nums[n-j]))\n\t\t\t\t\treturn ans\n\n\n"""\n#my intution -> the question is dealing with the magnitude of numbers and it has nothing to do with the original order of questions so we have to #sort it.\n\n\nmy first approach -> if length of nums >4 return nums[n-4] -nums[0] and I\'ve got error.\n\nmy second approach -> \n\n\t"""\n\t\t\t\t\t\t\tnums.sort()\n\t\t\t\t\t\t\treturn min(abs(nums[n-4]-nums[0]),abs(nums[3]-nums[-1]))\n\n"""\n\nmy third approach was->\n\t\t\t""" \n\t\t\t\n\t\t\t\t\t\t# I\'ve got an error\n\t\t\t\t\t\t\n\t\t\t\t\t ans = math.inf\n\t\t\t\t\t if n<=3:\n\t\t\t\t\t\t\treturn 0\n\t\t\t\t\t\tif n<=5:\n\t\t\t\t\t\t\tans = min([abs(nums[i]-nums[i-1]) for i in range(1,len(nums))])\n\t\t\t\t\t\tnums.sort()\n\t\t\t\t\t\treturn min(abs(nums[n-4]-nums[0]),abs(nums[3]-nums[-1]),ans)\n\n"""\nthen I come to realise that it has something to deal with a window like thing so I decided to create a window within the accepted range(eventhough it is not like the "sliding window technique")\nthen I got an approach like this-> the answer is the minimum of those \nabsolute value of\n\nnums[0]-nums[n-1]\nnums[0]-nums[n-2]\nnums[0]-nums[n-3]\nnums[0]-nums[n-4]\n________\nnums[1]-nums[n-1]\nnums[1]-nums[n-2]\nnums[1]-nums[n-3]\n____________\nnums[2]-nums[n-1]\nnums[2]-nums[n-2]\n________\n\nnums[3]-nums[n-1]\n\nand the final approach is \n\n\t\t"""\n\t\t\n\t\tclass Solution:\n\t\t\n\t\t\tdef minDifference(self, nums: List[int]) -> int:\n\t\t\t\tn = len(nums)\n\t\t\t\tans = math.inf\n\t\t\t\tif n<=4:\n\t\t\t\t\treturn 0\n\t\t\t\tnums.sort()\n\t\t\t\tfor i in range(4):\n\t\t\t\t\tfor j in range(1,4-i+1):\n\t\t\t\t\t\tans = min(ans,abs(nums[i]-nums[n-j]))\n\t\t\t\treturn ans\n\n\n\n\n\n"""\n\t\t\n\t\n\n\n\n | 1 | You are given an integer array `nums`.
In one move, you can choose one element of `nums` and change it to **any value**.
Return _the minimum difference between the largest and smallest value of `nums` **after performing at most three moves**_.
**Example 1:**
**Input:** nums = \[5,3,2,4\]
**Output:** 0
**Explanation:** We can make at most 3 moves.
In the first move, change 2 to 3. nums becomes \[5,3,3,4\].
In the second move, change 4 to 3. nums becomes \[5,3,3,3\].
In the third move, change 5 to 3. nums becomes \[3,3,3,3\].
After performing 3 moves, the difference between the minimum and maximum is 3 - 3 = 0.
**Example 2:**
**Input:** nums = \[1,5,0,10,14\]
**Output:** 1
**Explanation:** We can make at most 3 moves.
In the first move, change 5 to 0. nums becomes \[1,0,0,10,14\].
In the second move, change 10 to 0. nums becomes \[1,0,0,0,14\].
In the third move, change 14 to 1. nums becomes \[1,0,0,0,1\].
After performing 3 moves, the difference between the minimum and maximum is 1 - 0 = 0.
It can be shown that there is no way to make the difference 0 in 3 moves.
**Example 3:**
**Input:** nums = \[3,100,20\]
**Output:** 0
**Explanation:** We can make at most 3 moves.
In the first move, change 100 to 7. nums becomes \[4,7,20\].
In the second move, change 20 to 7. nums becomes \[4,7,7\].
In the third move, change 4 to 3. nums becomes \[7,7,7\].
After performing 3 moves, the difference between the minimum and maximum is 7 - 7 = 0.
**Constraints:**
* `1 <= nums.length <= 105`
* `-109 <= nums[i] <= 109` | null |
Python3 || easy understanding || step by step approach || | minimum-difference-between-largest-and-smallest-value-in-three-moves | 0 | 1 | 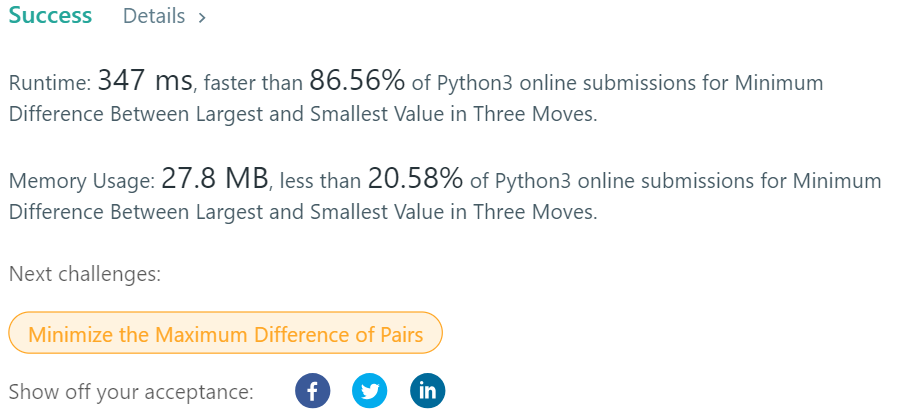\n"""\n\n\t\t\tclass Solution:\n\t\t\t\n\t\t\t\tdef minDifference(self, nums: List[int]) -> int:\n\t\t\t\t\tn = len(nums)\n\t\t\t\t\tans = math.inf\n\t\t\t\t\tif n<=4:\n\t\t\t\t\t\treturn 0\n\t\t\t\t\tnums.sort()\n\t\t\t\t\tfor i in range(4):\n\t\t\t\t\t\tfor j in range(1,4-i+1):\n\t\t\t\t\t\t\tans = min(ans,abs(nums[i]-nums[n-j]))\n\t\t\t\t\treturn ans\n\n\n"""\n#my intution -> the question is dealing with the magnitude of numbers and it has nothing to do with the original order of questions so we have to #sort it.\n\n\nmy first approach -> if length of nums >4 return nums[n-4] -nums[0] and I\'ve got error.\n\nmy second approach -> \n\n\t"""\n\t\t\t\t\t\t\tnums.sort()\n\t\t\t\t\t\t\treturn min(abs(nums[n-4]-nums[0]),abs(nums[3]-nums[-1]))\n\n"""\n\nmy third approach was->\n\t\t\t""" \n\t\t\t\n\t\t\t\t\t\t# I\'ve got an error\n\t\t\t\t\t\t\n\t\t\t\t\t ans = math.inf\n\t\t\t\t\t if n<=3:\n\t\t\t\t\t\t\treturn 0\n\t\t\t\t\t\tif n<=5:\n\t\t\t\t\t\t\tans = min([abs(nums[i]-nums[i-1]) for i in range(1,len(nums))])\n\t\t\t\t\t\tnums.sort()\n\t\t\t\t\t\treturn min(abs(nums[n-4]-nums[0]),abs(nums[3]-nums[-1]),ans)\n\n"""\nthen I come to realise that it has something to deal with a window like thing so I decided to create a window within the accepted range(eventhough it is not like the "sliding window technique")\nthen I got an approach like this-> the answer is the minimum of those \nabsolute value of\n\nnums[0]-nums[n-1]\nnums[0]-nums[n-2]\nnums[0]-nums[n-3]\nnums[0]-nums[n-4]\n________\nnums[1]-nums[n-1]\nnums[1]-nums[n-2]\nnums[1]-nums[n-3]\n____________\nnums[2]-nums[n-1]\nnums[2]-nums[n-2]\n________\n\nnums[3]-nums[n-1]\n\nand the final approach is \n\n\t\t"""\n\t\t\n\t\tclass Solution:\n\t\t\n\t\t\tdef minDifference(self, nums: List[int]) -> int:\n\t\t\t\tn = len(nums)\n\t\t\t\tans = math.inf\n\t\t\t\tif n<=4:\n\t\t\t\t\treturn 0\n\t\t\t\tnums.sort()\n\t\t\t\tfor i in range(4):\n\t\t\t\t\tfor j in range(1,4-i+1):\n\t\t\t\t\t\tans = min(ans,abs(nums[i]-nums[n-j]))\n\t\t\t\treturn ans\n\n\n\n\n\n"""\n\t\t\n\t\n\n\n\n | 1 | You are given two strings `a` and `b` of the same length. Choose an index and split both strings **at the same index**, splitting `a` into two strings: `aprefix` and `asuffix` where `a = aprefix + asuffix`, and splitting `b` into two strings: `bprefix` and `bsuffix` where `b = bprefix + bsuffix`. Check if `aprefix + bsuffix` or `bprefix + asuffix` forms a palindrome.
When you split a string `s` into `sprefix` and `ssuffix`, either `ssuffix` or `sprefix` is allowed to be empty. For example, if `s = "abc "`, then `" " + "abc "`, `"a " + "bc "`, `"ab " + "c "` , and `"abc " + " "` are valid splits.
Return `true` _if it is possible to form_ _a palindrome string, otherwise return_ `false`.
**Notice** that `x + y` denotes the concatenation of strings `x` and `y`.
**Example 1:**
**Input:** a = "x ", b = "y "
**Output:** true
**Explaination:** If either a or b are palindromes the answer is true since you can split in the following way:
aprefix = " ", asuffix = "x "
bprefix = " ", bsuffix = "y "
Then, aprefix + bsuffix = " " + "y " = "y ", which is a palindrome.
**Example 2:**
**Input:** a = "xbdef ", b = "xecab "
**Output:** false
**Example 3:**
**Input:** a = "ulacfd ", b = "jizalu "
**Output:** true
**Explaination:** Split them at index 3:
aprefix = "ula ", asuffix = "cfd "
bprefix = "jiz ", bsuffix = "alu "
Then, aprefix + bsuffix = "ula " + "alu " = "ulaalu ", which is a palindrome.
**Constraints:**
* `1 <= a.length, b.length <= 105`
* `a.length == b.length`
* `a` and `b` consist of lowercase English letters | The minimum difference possible is is obtained by removing 3 elements between the 3 smallest and 3 largest values in the array. |
Python, 3 lines, well explained, Greedy O(nlogn) | minimum-difference-between-largest-and-smallest-value-in-three-moves | 0 | 1 | If the size <= 4, then just delete all the elements and either no or one element would be left giving the answer 0.\nelse sort the array\nthere are 4 possibilities now:-\n1. delete 3 elements from left and none from right\n2. delete 2 elements from left and one from right\nand so on.. now just print the minima.\n```\nclass Solution:\n def minDifference(self, nums: List[int]) -> int:\n \n if len(nums) <= 4: return 0\n nums.sort()\n return min(nums[-1] - nums[3], nums[-2] - nums[2], nums[-3] - nums[1], nums[-4] - nums[0])\n``` | 77 | You are given an integer array `nums`.
In one move, you can choose one element of `nums` and change it to **any value**.
Return _the minimum difference between the largest and smallest value of `nums` **after performing at most three moves**_.
**Example 1:**
**Input:** nums = \[5,3,2,4\]
**Output:** 0
**Explanation:** We can make at most 3 moves.
In the first move, change 2 to 3. nums becomes \[5,3,3,4\].
In the second move, change 4 to 3. nums becomes \[5,3,3,3\].
In the third move, change 5 to 3. nums becomes \[3,3,3,3\].
After performing 3 moves, the difference between the minimum and maximum is 3 - 3 = 0.
**Example 2:**
**Input:** nums = \[1,5,0,10,14\]
**Output:** 1
**Explanation:** We can make at most 3 moves.
In the first move, change 5 to 0. nums becomes \[1,0,0,10,14\].
In the second move, change 10 to 0. nums becomes \[1,0,0,0,14\].
In the third move, change 14 to 1. nums becomes \[1,0,0,0,1\].
After performing 3 moves, the difference between the minimum and maximum is 1 - 0 = 0.
It can be shown that there is no way to make the difference 0 in 3 moves.
**Example 3:**
**Input:** nums = \[3,100,20\]
**Output:** 0
**Explanation:** We can make at most 3 moves.
In the first move, change 100 to 7. nums becomes \[4,7,20\].
In the second move, change 20 to 7. nums becomes \[4,7,7\].
In the third move, change 4 to 3. nums becomes \[7,7,7\].
After performing 3 moves, the difference between the minimum and maximum is 7 - 7 = 0.
**Constraints:**
* `1 <= nums.length <= 105`
* `-109 <= nums[i] <= 109` | null |
Python, 3 lines, well explained, Greedy O(nlogn) | minimum-difference-between-largest-and-smallest-value-in-three-moves | 0 | 1 | If the size <= 4, then just delete all the elements and either no or one element would be left giving the answer 0.\nelse sort the array\nthere are 4 possibilities now:-\n1. delete 3 elements from left and none from right\n2. delete 2 elements from left and one from right\nand so on.. now just print the minima.\n```\nclass Solution:\n def minDifference(self, nums: List[int]) -> int:\n \n if len(nums) <= 4: return 0\n nums.sort()\n return min(nums[-1] - nums[3], nums[-2] - nums[2], nums[-3] - nums[1], nums[-4] - nums[0])\n``` | 77 | You are given two strings `a` and `b` of the same length. Choose an index and split both strings **at the same index**, splitting `a` into two strings: `aprefix` and `asuffix` where `a = aprefix + asuffix`, and splitting `b` into two strings: `bprefix` and `bsuffix` where `b = bprefix + bsuffix`. Check if `aprefix + bsuffix` or `bprefix + asuffix` forms a palindrome.
When you split a string `s` into `sprefix` and `ssuffix`, either `ssuffix` or `sprefix` is allowed to be empty. For example, if `s = "abc "`, then `" " + "abc "`, `"a " + "bc "`, `"ab " + "c "` , and `"abc " + " "` are valid splits.
Return `true` _if it is possible to form_ _a palindrome string, otherwise return_ `false`.
**Notice** that `x + y` denotes the concatenation of strings `x` and `y`.
**Example 1:**
**Input:** a = "x ", b = "y "
**Output:** true
**Explaination:** If either a or b are palindromes the answer is true since you can split in the following way:
aprefix = " ", asuffix = "x "
bprefix = " ", bsuffix = "y "
Then, aprefix + bsuffix = " " + "y " = "y ", which is a palindrome.
**Example 2:**
**Input:** a = "xbdef ", b = "xecab "
**Output:** false
**Example 3:**
**Input:** a = "ulacfd ", b = "jizalu "
**Output:** true
**Explaination:** Split them at index 3:
aprefix = "ula ", asuffix = "cfd "
bprefix = "jiz ", bsuffix = "alu "
Then, aprefix + bsuffix = "ula " + "alu " = "ulaalu ", which is a palindrome.
**Constraints:**
* `1 <= a.length, b.length <= 105`
* `a.length == b.length`
* `a` and `b` consist of lowercase English letters | The minimum difference possible is is obtained by removing 3 elements between the 3 smallest and 3 largest values in the array. |
[Python3/Python] Easy readable solution with comments. | minimum-difference-between-largest-and-smallest-value-in-three-moves | 0 | 1 | ```\nclass Solution:\n \n def minDifference(self, nums: List[int]) -> int:\n \n n = len(nums)\n # If nums are less than 3 all can be replace,\n # so min diff will be 0, which is default condition\n if n > 3:\n \n # Init min difference\n min_diff = float("inf")\n \n # sort the array\n nums = sorted(nums)\n \n # Get the window size, this indicates, if we\n # remove 3 element in an array how many element\n # are left, consider 0 as the index, window\n # size should be (n-3), but for array starting\n # with 0 it should be ((n-1)-3)\n window = (n-1)-3\n \n # Run through the entire array slinding the\n # window and calculating minimum difference\n # between the first and the last element of\n # that window\n for i in range(n):\n if i+window >= n:\n break\n else:\n min_diff = min(nums[i+window]-nums[i], min_diff)\n \n # return calculated minimum difference\n return min_diff\n \n return 0 # default condition\n``` | 10 | You are given an integer array `nums`.
In one move, you can choose one element of `nums` and change it to **any value**.
Return _the minimum difference between the largest and smallest value of `nums` **after performing at most three moves**_.
**Example 1:**
**Input:** nums = \[5,3,2,4\]
**Output:** 0
**Explanation:** We can make at most 3 moves.
In the first move, change 2 to 3. nums becomes \[5,3,3,4\].
In the second move, change 4 to 3. nums becomes \[5,3,3,3\].
In the third move, change 5 to 3. nums becomes \[3,3,3,3\].
After performing 3 moves, the difference between the minimum and maximum is 3 - 3 = 0.
**Example 2:**
**Input:** nums = \[1,5,0,10,14\]
**Output:** 1
**Explanation:** We can make at most 3 moves.
In the first move, change 5 to 0. nums becomes \[1,0,0,10,14\].
In the second move, change 10 to 0. nums becomes \[1,0,0,0,14\].
In the third move, change 14 to 1. nums becomes \[1,0,0,0,1\].
After performing 3 moves, the difference between the minimum and maximum is 1 - 0 = 0.
It can be shown that there is no way to make the difference 0 in 3 moves.
**Example 3:**
**Input:** nums = \[3,100,20\]
**Output:** 0
**Explanation:** We can make at most 3 moves.
In the first move, change 100 to 7. nums becomes \[4,7,20\].
In the second move, change 20 to 7. nums becomes \[4,7,7\].
In the third move, change 4 to 3. nums becomes \[7,7,7\].
After performing 3 moves, the difference between the minimum and maximum is 7 - 7 = 0.
**Constraints:**
* `1 <= nums.length <= 105`
* `-109 <= nums[i] <= 109` | null |
[Python3/Python] Easy readable solution with comments. | minimum-difference-between-largest-and-smallest-value-in-three-moves | 0 | 1 | ```\nclass Solution:\n \n def minDifference(self, nums: List[int]) -> int:\n \n n = len(nums)\n # If nums are less than 3 all can be replace,\n # so min diff will be 0, which is default condition\n if n > 3:\n \n # Init min difference\n min_diff = float("inf")\n \n # sort the array\n nums = sorted(nums)\n \n # Get the window size, this indicates, if we\n # remove 3 element in an array how many element\n # are left, consider 0 as the index, window\n # size should be (n-3), but for array starting\n # with 0 it should be ((n-1)-3)\n window = (n-1)-3\n \n # Run through the entire array slinding the\n # window and calculating minimum difference\n # between the first and the last element of\n # that window\n for i in range(n):\n if i+window >= n:\n break\n else:\n min_diff = min(nums[i+window]-nums[i], min_diff)\n \n # return calculated minimum difference\n return min_diff\n \n return 0 # default condition\n``` | 10 | You are given two strings `a` and `b` of the same length. Choose an index and split both strings **at the same index**, splitting `a` into two strings: `aprefix` and `asuffix` where `a = aprefix + asuffix`, and splitting `b` into two strings: `bprefix` and `bsuffix` where `b = bprefix + bsuffix`. Check if `aprefix + bsuffix` or `bprefix + asuffix` forms a palindrome.
When you split a string `s` into `sprefix` and `ssuffix`, either `ssuffix` or `sprefix` is allowed to be empty. For example, if `s = "abc "`, then `" " + "abc "`, `"a " + "bc "`, `"ab " + "c "` , and `"abc " + " "` are valid splits.
Return `true` _if it is possible to form_ _a palindrome string, otherwise return_ `false`.
**Notice** that `x + y` denotes the concatenation of strings `x` and `y`.
**Example 1:**
**Input:** a = "x ", b = "y "
**Output:** true
**Explaination:** If either a or b are palindromes the answer is true since you can split in the following way:
aprefix = " ", asuffix = "x "
bprefix = " ", bsuffix = "y "
Then, aprefix + bsuffix = " " + "y " = "y ", which is a palindrome.
**Example 2:**
**Input:** a = "xbdef ", b = "xecab "
**Output:** false
**Example 3:**
**Input:** a = "ulacfd ", b = "jizalu "
**Output:** true
**Explaination:** Split them at index 3:
aprefix = "ula ", asuffix = "cfd "
bprefix = "jiz ", bsuffix = "alu "
Then, aprefix + bsuffix = "ula " + "alu " = "ulaalu ", which is a palindrome.
**Constraints:**
* `1 <= a.length, b.length <= 105`
* `a.length == b.length`
* `a` and `b` consist of lowercase English letters | The minimum difference possible is is obtained by removing 3 elements between the 3 smallest and 3 largest values in the array. |
[Python3] 1-line O(N) | minimum-difference-between-largest-and-smallest-value-in-three-moves | 0 | 1 | Algo\nHere, at most 8 numbers are relevant, i.e. the 4 smallest and the 4 largest. Suppose they are labeled as \n\n`m1, m2, m3, m4, ... M4, M3, M2, M1` \n\nthen `min(M4-m1, M3-m2, M2-m3, M1-m4)` gives the solution. It is linear `O(N)` to find `m1-m4` and `M1-M4` like below. \n\n`O(N)` time & `O(1)` space leveraging on the `heapq` module \n\n```\nclass Solution:\n def minDifference(self, nums: List[int]) -> int:\n return min(x-y for x, y in zip(nlargest(4, nums), reversed(nsmallest(4, nums))))\n```\n\nA more readable version is below \n```\nclass Solution:\n def minDifference(self, nums: List[int]) -> int:\n small = nsmallest(4, nums)\n large = nlargest(4, nums)\n return min(x-y for x, y in zip(large, reversed(small)))\n``` | 9 | You are given an integer array `nums`.
In one move, you can choose one element of `nums` and change it to **any value**.
Return _the minimum difference between the largest and smallest value of `nums` **after performing at most three moves**_.
**Example 1:**
**Input:** nums = \[5,3,2,4\]
**Output:** 0
**Explanation:** We can make at most 3 moves.
In the first move, change 2 to 3. nums becomes \[5,3,3,4\].
In the second move, change 4 to 3. nums becomes \[5,3,3,3\].
In the third move, change 5 to 3. nums becomes \[3,3,3,3\].
After performing 3 moves, the difference between the minimum and maximum is 3 - 3 = 0.
**Example 2:**
**Input:** nums = \[1,5,0,10,14\]
**Output:** 1
**Explanation:** We can make at most 3 moves.
In the first move, change 5 to 0. nums becomes \[1,0,0,10,14\].
In the second move, change 10 to 0. nums becomes \[1,0,0,0,14\].
In the third move, change 14 to 1. nums becomes \[1,0,0,0,1\].
After performing 3 moves, the difference between the minimum and maximum is 1 - 0 = 0.
It can be shown that there is no way to make the difference 0 in 3 moves.
**Example 3:**
**Input:** nums = \[3,100,20\]
**Output:** 0
**Explanation:** We can make at most 3 moves.
In the first move, change 100 to 7. nums becomes \[4,7,20\].
In the second move, change 20 to 7. nums becomes \[4,7,7\].
In the third move, change 4 to 3. nums becomes \[7,7,7\].
After performing 3 moves, the difference between the minimum and maximum is 7 - 7 = 0.
**Constraints:**
* `1 <= nums.length <= 105`
* `-109 <= nums[i] <= 109` | null |
[Python3] 1-line O(N) | minimum-difference-between-largest-and-smallest-value-in-three-moves | 0 | 1 | Algo\nHere, at most 8 numbers are relevant, i.e. the 4 smallest and the 4 largest. Suppose they are labeled as \n\n`m1, m2, m3, m4, ... M4, M3, M2, M1` \n\nthen `min(M4-m1, M3-m2, M2-m3, M1-m4)` gives the solution. It is linear `O(N)` to find `m1-m4` and `M1-M4` like below. \n\n`O(N)` time & `O(1)` space leveraging on the `heapq` module \n\n```\nclass Solution:\n def minDifference(self, nums: List[int]) -> int:\n return min(x-y for x, y in zip(nlargest(4, nums), reversed(nsmallest(4, nums))))\n```\n\nA more readable version is below \n```\nclass Solution:\n def minDifference(self, nums: List[int]) -> int:\n small = nsmallest(4, nums)\n large = nlargest(4, nums)\n return min(x-y for x, y in zip(large, reversed(small)))\n``` | 9 | You are given two strings `a` and `b` of the same length. Choose an index and split both strings **at the same index**, splitting `a` into two strings: `aprefix` and `asuffix` where `a = aprefix + asuffix`, and splitting `b` into two strings: `bprefix` and `bsuffix` where `b = bprefix + bsuffix`. Check if `aprefix + bsuffix` or `bprefix + asuffix` forms a palindrome.
When you split a string `s` into `sprefix` and `ssuffix`, either `ssuffix` or `sprefix` is allowed to be empty. For example, if `s = "abc "`, then `" " + "abc "`, `"a " + "bc "`, `"ab " + "c "` , and `"abc " + " "` are valid splits.
Return `true` _if it is possible to form_ _a palindrome string, otherwise return_ `false`.
**Notice** that `x + y` denotes the concatenation of strings `x` and `y`.
**Example 1:**
**Input:** a = "x ", b = "y "
**Output:** true
**Explaination:** If either a or b are palindromes the answer is true since you can split in the following way:
aprefix = " ", asuffix = "x "
bprefix = " ", bsuffix = "y "
Then, aprefix + bsuffix = " " + "y " = "y ", which is a palindrome.
**Example 2:**
**Input:** a = "xbdef ", b = "xecab "
**Output:** false
**Example 3:**
**Input:** a = "ulacfd ", b = "jizalu "
**Output:** true
**Explaination:** Split them at index 3:
aprefix = "ula ", asuffix = "cfd "
bprefix = "jiz ", bsuffix = "alu "
Then, aprefix + bsuffix = "ula " + "alu " = "ulaalu ", which is a palindrome.
**Constraints:**
* `1 <= a.length, b.length <= 105`
* `a.length == b.length`
* `a` and `b` consist of lowercase English letters | The minimum difference possible is is obtained by removing 3 elements between the 3 smallest and 3 largest values in the array. |
Python short and clean 1-liners. Multiple solutions. Functional programming. | stone-game-iv | 0 | 1 | # Approach 1: Top-Down DP\n\n# Complexity\n- Time complexity: $$O(n \\cdot \\sqrt{n})$$\n\n- Space complexity: $$O(n)$$\n\n# Code\nMultiline Recursive:\n```python\nclass Solution:\n def winnerSquareGame(self, n: int) -> bool:\n squares = lambda x: (i * i for i in range(isqrt(x), 0, -1))\n \n @cache\n def can_win(n: int) -> bool:\n return n and not all(can_win(n - s) for s in squares(n))\n \n return can_win(n)\n\n\n```\n\nFunctional. Can be restructured into a 1-liner:\n```python\nclass Solution:\n def winnerSquareGame(self, n: int) -> bool:\n squares = lambda x: (i * i for i in range(isqrt(x), 0, -1))\n can_win = cache(lambda n: n and not all(can_win(n - s) for s in squares(n)))\n return can_win(n)\n\n\n```\n\n---\n\n# Approach 2: Bottom-Up DP\n\n# Complexity\n- Time complexity: $$O(n \\cdot \\sqrt{n})$$\n\n- Space complexity: $$O(n)$$\n\n# Code\nMultiline Iterative:\n```python\nclass Solution:\n def winnerSquareGame(self, n: int) -> bool:\n squares = lambda x: (i * i for i in range(isqrt(x), 0, -1))\n\n dp = [False] * (n + 1)\n for i in range(1, n + 1):\n dp[i] = not all(dp[i - s] for s in squares(i))\n return dp[-1]\n\n\n```\n\nFunctional. Can be restructured into a 1-liner:\n# Code\n```python\nclass Solution:\n def winnerSquareGame(self, n: int) -> bool:\n squares = lambda x: (i * i for i in range(isqrt(x), 0, -1))\n next_wins = lambda dp, i: setitem(dp, i, not all(dp[i - s] for s in squares(i))) or dp\n return reduce(next_wins, range(n + 1), [False] * (n + 1))[-1]\n\n\n``` | 1 | Alice and Bob take turns playing a game, with Alice starting first.
Initially, there are `n` stones in a pile. On each player's turn, that player makes a _move_ consisting of removing **any** non-zero **square number** of stones in the pile.
Also, if a player cannot make a move, he/she loses the game.
Given a positive integer `n`, return `true` if and only if Alice wins the game otherwise return `false`, assuming both players play optimally.
**Example 1:**
**Input:** n = 1
**Output:** true
**Explanation:** Alice can remove 1 stone winning the game because Bob doesn't have any moves.
**Example 2:**
**Input:** n = 2
**Output:** false
**Explanation:** Alice can only remove 1 stone, after that Bob removes the last one winning the game (2 -> 1 -> 0).
**Example 3:**
**Input:** n = 4
**Output:** true
**Explanation:** n is already a perfect square, Alice can win with one move, removing 4 stones (4 -> 0).
**Constraints:**
* `1 <= n <= 105` | Count the frequency of each integer in the array. Get all lucky numbers and return the largest of them. |
Python short and clean 1-liners. Multiple solutions. Functional programming. | stone-game-iv | 0 | 1 | # Approach 1: Top-Down DP\n\n# Complexity\n- Time complexity: $$O(n \\cdot \\sqrt{n})$$\n\n- Space complexity: $$O(n)$$\n\n# Code\nMultiline Recursive:\n```python\nclass Solution:\n def winnerSquareGame(self, n: int) -> bool:\n squares = lambda x: (i * i for i in range(isqrt(x), 0, -1))\n \n @cache\n def can_win(n: int) -> bool:\n return n and not all(can_win(n - s) for s in squares(n))\n \n return can_win(n)\n\n\n```\n\nFunctional. Can be restructured into a 1-liner:\n```python\nclass Solution:\n def winnerSquareGame(self, n: int) -> bool:\n squares = lambda x: (i * i for i in range(isqrt(x), 0, -1))\n can_win = cache(lambda n: n and not all(can_win(n - s) for s in squares(n)))\n return can_win(n)\n\n\n```\n\n---\n\n# Approach 2: Bottom-Up DP\n\n# Complexity\n- Time complexity: $$O(n \\cdot \\sqrt{n})$$\n\n- Space complexity: $$O(n)$$\n\n# Code\nMultiline Iterative:\n```python\nclass Solution:\n def winnerSquareGame(self, n: int) -> bool:\n squares = lambda x: (i * i for i in range(isqrt(x), 0, -1))\n\n dp = [False] * (n + 1)\n for i in range(1, n + 1):\n dp[i] = not all(dp[i - s] for s in squares(i))\n return dp[-1]\n\n\n```\n\nFunctional. Can be restructured into a 1-liner:\n# Code\n```python\nclass Solution:\n def winnerSquareGame(self, n: int) -> bool:\n squares = lambda x: (i * i for i in range(isqrt(x), 0, -1))\n next_wins = lambda dp, i: setitem(dp, i, not all(dp[i - s] for s in squares(i))) or dp\n return reduce(next_wins, range(n + 1), [False] * (n + 1))[-1]\n\n\n``` | 1 | There are `n` cities numbered from `1` to `n`. You are given an array `edges` of size `n-1`, where `edges[i] = [ui, vi]` represents a bidirectional edge between cities `ui` and `vi`. There exists a unique path between each pair of cities. In other words, the cities form a **tree**.
A **subtree** is a subset of cities where every city is reachable from every other city in the subset, where the path between each pair passes through only the cities from the subset. Two subtrees are different if there is a city in one subtree that is not present in the other.
For each `d` from `1` to `n-1`, find the number of subtrees in which the **maximum distance** between any two cities in the subtree is equal to `d`.
Return _an array of size_ `n-1` _where the_ `dth` _element **(1-indexed)** is the number of subtrees in which the **maximum distance** between any two cities is equal to_ `d`.
**Notice** that the **distance** between the two cities is the number of edges in the path between them.
**Example 1:**
**Input:** n = 4, edges = \[\[1,2\],\[2,3\],\[2,4\]\]
**Output:** \[3,4,0\]
**Explanation:**
The subtrees with subsets {1,2}, {2,3} and {2,4} have a max distance of 1.
The subtrees with subsets {1,2,3}, {1,2,4}, {2,3,4} and {1,2,3,4} have a max distance of 2.
No subtree has two nodes where the max distance between them is 3.
**Example 2:**
**Input:** n = 2, edges = \[\[1,2\]\]
**Output:** \[1\]
**Example 3:**
**Input:** n = 3, edges = \[\[1,2\],\[2,3\]\]
**Output:** \[2,1\]
**Constraints:**
* `2 <= n <= 15`
* `edges.length == n-1`
* `edges[i].length == 2`
* `1 <= ui, vi <= n`
* All pairs `(ui, vi)` are distinct. | Use dynamic programming to keep track of winning and losing states. Given some number of stones, Alice can win if she can force Bob onto a losing state. |
✔️ [Python3] JUST 4-LINES, (ノ゚0゚)ノ~ OMG!!!, Explained | stone-game-iv | 0 | 1 | **UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.**\n\nIn the beginning, it may seem that we would need to do a huge amount of scans, recursive calls and take lots of space doing backtracking since the input `n` is in the range `1..10e5`. But the thing is that a player can remove only **squared number** of stones from the pile. That is, if we got `100000` as an input, the number of possible moves is in the more modest range `1..floor(sqrt(100000))` = `1 .. 316`. So it\'s perfectly doable to recursively verify all possible moves of Alice and Bob using memoization.\n\nWe iterate over the range `floor(sqrt(n)) .. 1`, square the index, and recursively call the function asking the question: Can the next player win if he/she starts with the pile of stones `n - i^2`?. If yes that means the current player loses, and we need to check the remaining options.\n\nWhy do we scan in the reversed range? Try to answer this question yourself as an exercise in the comments (\u0E07\u30C4)\u0E27\n\nTime: **O(N*sqrt(N))** - scan\nSpace: **O(N)** - memo and recursive stack\n\nRuntime: 404 ms, faster than **82.63%** of Python3 online submissions for Stone Game IV.\nMemory Usage: 22.7 MB, less than **40.72%** of Python3 online submissions for Stone Game IV.\n\n```\n @cache\n def winnerSquareGame(self, n: int) -> bool:\n if not n: return False\n\t\t\n for i in reversed(range(1, floor(sqrt(n)) + 1)):\n if not self.winnerSquareGame(n - i**2): return True\n\n return False\n```\n\n**UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.** | 11 | Alice and Bob take turns playing a game, with Alice starting first.
Initially, there are `n` stones in a pile. On each player's turn, that player makes a _move_ consisting of removing **any** non-zero **square number** of stones in the pile.
Also, if a player cannot make a move, he/she loses the game.
Given a positive integer `n`, return `true` if and only if Alice wins the game otherwise return `false`, assuming both players play optimally.
**Example 1:**
**Input:** n = 1
**Output:** true
**Explanation:** Alice can remove 1 stone winning the game because Bob doesn't have any moves.
**Example 2:**
**Input:** n = 2
**Output:** false
**Explanation:** Alice can only remove 1 stone, after that Bob removes the last one winning the game (2 -> 1 -> 0).
**Example 3:**
**Input:** n = 4
**Output:** true
**Explanation:** n is already a perfect square, Alice can win with one move, removing 4 stones (4 -> 0).
**Constraints:**
* `1 <= n <= 105` | Count the frequency of each integer in the array. Get all lucky numbers and return the largest of them. |
✔️ [Python3] JUST 4-LINES, (ノ゚0゚)ノ~ OMG!!!, Explained | stone-game-iv | 0 | 1 | **UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.**\n\nIn the beginning, it may seem that we would need to do a huge amount of scans, recursive calls and take lots of space doing backtracking since the input `n` is in the range `1..10e5`. But the thing is that a player can remove only **squared number** of stones from the pile. That is, if we got `100000` as an input, the number of possible moves is in the more modest range `1..floor(sqrt(100000))` = `1 .. 316`. So it\'s perfectly doable to recursively verify all possible moves of Alice and Bob using memoization.\n\nWe iterate over the range `floor(sqrt(n)) .. 1`, square the index, and recursively call the function asking the question: Can the next player win if he/she starts with the pile of stones `n - i^2`?. If yes that means the current player loses, and we need to check the remaining options.\n\nWhy do we scan in the reversed range? Try to answer this question yourself as an exercise in the comments (\u0E07\u30C4)\u0E27\n\nTime: **O(N*sqrt(N))** - scan\nSpace: **O(N)** - memo and recursive stack\n\nRuntime: 404 ms, faster than **82.63%** of Python3 online submissions for Stone Game IV.\nMemory Usage: 22.7 MB, less than **40.72%** of Python3 online submissions for Stone Game IV.\n\n```\n @cache\n def winnerSquareGame(self, n: int) -> bool:\n if not n: return False\n\t\t\n for i in reversed(range(1, floor(sqrt(n)) + 1)):\n if not self.winnerSquareGame(n - i**2): return True\n\n return False\n```\n\n**UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.** | 11 | There are `n` cities numbered from `1` to `n`. You are given an array `edges` of size `n-1`, where `edges[i] = [ui, vi]` represents a bidirectional edge between cities `ui` and `vi`. There exists a unique path between each pair of cities. In other words, the cities form a **tree**.
A **subtree** is a subset of cities where every city is reachable from every other city in the subset, where the path between each pair passes through only the cities from the subset. Two subtrees are different if there is a city in one subtree that is not present in the other.
For each `d` from `1` to `n-1`, find the number of subtrees in which the **maximum distance** between any two cities in the subtree is equal to `d`.
Return _an array of size_ `n-1` _where the_ `dth` _element **(1-indexed)** is the number of subtrees in which the **maximum distance** between any two cities is equal to_ `d`.
**Notice** that the **distance** between the two cities is the number of edges in the path between them.
**Example 1:**
**Input:** n = 4, edges = \[\[1,2\],\[2,3\],\[2,4\]\]
**Output:** \[3,4,0\]
**Explanation:**
The subtrees with subsets {1,2}, {2,3} and {2,4} have a max distance of 1.
The subtrees with subsets {1,2,3}, {1,2,4}, {2,3,4} and {1,2,3,4} have a max distance of 2.
No subtree has two nodes where the max distance between them is 3.
**Example 2:**
**Input:** n = 2, edges = \[\[1,2\]\]
**Output:** \[1\]
**Example 3:**
**Input:** n = 3, edges = \[\[1,2\],\[2,3\]\]
**Output:** \[2,1\]
**Constraints:**
* `2 <= n <= 15`
* `edges.length == n-1`
* `edges[i].length == 2`
* `1 <= ui, vi <= n`
* All pairs `(ui, vi)` are distinct. | Use dynamic programming to keep track of winning and losing states. Given some number of stones, Alice can win if she can force Bob onto a losing state. |
[Python3] DP | stone-game-iv | 0 | 1 | Below is the code, please let me know if you have any questions!\n```\nclass Solution:\n def winnerSquareGame(self, n: int) -> bool:\n dp = [False] * (n + 1)\n squares = []\n curSquare = 1\n for i in range(1, n + 1):\n if i == curSquare * curSquare:\n squares.append(i)\n curSquare += 1\n dp[i] = True\n else:\n for square in squares:\n if not dp[i - square]:\n dp[i] = True\n break\n return dp[n]\n```\n\nEdit: For a clear explanation of the algorithm, check the comment of @Cavalier_Poet below! | 8 | Alice and Bob take turns playing a game, with Alice starting first.
Initially, there are `n` stones in a pile. On each player's turn, that player makes a _move_ consisting of removing **any** non-zero **square number** of stones in the pile.
Also, if a player cannot make a move, he/she loses the game.
Given a positive integer `n`, return `true` if and only if Alice wins the game otherwise return `false`, assuming both players play optimally.
**Example 1:**
**Input:** n = 1
**Output:** true
**Explanation:** Alice can remove 1 stone winning the game because Bob doesn't have any moves.
**Example 2:**
**Input:** n = 2
**Output:** false
**Explanation:** Alice can only remove 1 stone, after that Bob removes the last one winning the game (2 -> 1 -> 0).
**Example 3:**
**Input:** n = 4
**Output:** true
**Explanation:** n is already a perfect square, Alice can win with one move, removing 4 stones (4 -> 0).
**Constraints:**
* `1 <= n <= 105` | Count the frequency of each integer in the array. Get all lucky numbers and return the largest of them. |
[Python3] DP | stone-game-iv | 0 | 1 | Below is the code, please let me know if you have any questions!\n```\nclass Solution:\n def winnerSquareGame(self, n: int) -> bool:\n dp = [False] * (n + 1)\n squares = []\n curSquare = 1\n for i in range(1, n + 1):\n if i == curSquare * curSquare:\n squares.append(i)\n curSquare += 1\n dp[i] = True\n else:\n for square in squares:\n if not dp[i - square]:\n dp[i] = True\n break\n return dp[n]\n```\n\nEdit: For a clear explanation of the algorithm, check the comment of @Cavalier_Poet below! | 8 | There are `n` cities numbered from `1` to `n`. You are given an array `edges` of size `n-1`, where `edges[i] = [ui, vi]` represents a bidirectional edge between cities `ui` and `vi`. There exists a unique path between each pair of cities. In other words, the cities form a **tree**.
A **subtree** is a subset of cities where every city is reachable from every other city in the subset, where the path between each pair passes through only the cities from the subset. Two subtrees are different if there is a city in one subtree that is not present in the other.
For each `d` from `1` to `n-1`, find the number of subtrees in which the **maximum distance** between any two cities in the subtree is equal to `d`.
Return _an array of size_ `n-1` _where the_ `dth` _element **(1-indexed)** is the number of subtrees in which the **maximum distance** between any two cities is equal to_ `d`.
**Notice** that the **distance** between the two cities is the number of edges in the path between them.
**Example 1:**
**Input:** n = 4, edges = \[\[1,2\],\[2,3\],\[2,4\]\]
**Output:** \[3,4,0\]
**Explanation:**
The subtrees with subsets {1,2}, {2,3} and {2,4} have a max distance of 1.
The subtrees with subsets {1,2,3}, {1,2,4}, {2,3,4} and {1,2,3,4} have a max distance of 2.
No subtree has two nodes where the max distance between them is 3.
**Example 2:**
**Input:** n = 2, edges = \[\[1,2\]\]
**Output:** \[1\]
**Example 3:**
**Input:** n = 3, edges = \[\[1,2\],\[2,3\]\]
**Output:** \[2,1\]
**Constraints:**
* `2 <= n <= 15`
* `edges.length == n-1`
* `edges[i].length == 2`
* `1 <= ui, vi <= n`
* All pairs `(ui, vi)` are distinct. | Use dynamic programming to keep track of winning and losing states. Given some number of stones, Alice can win if she can force Bob onto a losing state. |
Standard DP question Explained BEST - ALL you need to know | stone-game-iv | 0 | 1 | We are asked to find the solution for an input of n. So,we find solution for all numbers from [0,n] and we do this because the previous answers help us in finding the solution for a potentially larger number. We have n states of dp,each state represents the solution of that specific state. states = [0,n]. let dp[i] be the state representation.\n\nAnd we have to find the optimal answer.Even if in one possibility alice wins,its a True.\n```\nFor each state i:\n\tj = find all the perfect squares less than i.\n\tfor each j:\n\t\tAssume that the perfectsquare j is taken by alice.\n\t\tThen the game continues as` dp[i-j*j]` for bob as start player.\n\t\tSince we calculated all answers for such states with alice as start player,its the same even if bob becomes the start player because both play optimally.\n\t\tIf bob could wins ,alice loses i.e ` dp[i-j*j]` = True\n\t\telse if bob loses alice wins i.e ` dp[i-j*j]` = False\n\t\tSo,we choose the case where alice wins with alice as start player(In essence we find if atleast one j returns True for dp[i]). or dp[i] |= ! dp[i-j*j]\n```\t\n\t\t\n`Time:O(n^1.5) | Space:O(n) `\nApproach`:DynamicProgramming` Bottom-up\n\n\tclass Solution:\n\t\t\tdef winnerSquareGame(self, n: int) -> bool:\n\t\t\t\tdp = [False]*(n+1)\n\t\t\t\tfor i in range(1,n+1):\n\t\t\t\t\tj = 1\n\t\t\t\t\twhile j*j <= i:\n\t\t\t\t\t\tdp[i] |= not dp[i-j*j]\n\t\t\t\t\t\tj+=1\n\t\t\t\treturn dp[n]\n\nplease leave an upvote if you find it helpful :)\n | 8 | Alice and Bob take turns playing a game, with Alice starting first.
Initially, there are `n` stones in a pile. On each player's turn, that player makes a _move_ consisting of removing **any** non-zero **square number** of stones in the pile.
Also, if a player cannot make a move, he/she loses the game.
Given a positive integer `n`, return `true` if and only if Alice wins the game otherwise return `false`, assuming both players play optimally.
**Example 1:**
**Input:** n = 1
**Output:** true
**Explanation:** Alice can remove 1 stone winning the game because Bob doesn't have any moves.
**Example 2:**
**Input:** n = 2
**Output:** false
**Explanation:** Alice can only remove 1 stone, after that Bob removes the last one winning the game (2 -> 1 -> 0).
**Example 3:**
**Input:** n = 4
**Output:** true
**Explanation:** n is already a perfect square, Alice can win with one move, removing 4 stones (4 -> 0).
**Constraints:**
* `1 <= n <= 105` | Count the frequency of each integer in the array. Get all lucky numbers and return the largest of them. |
Standard DP question Explained BEST - ALL you need to know | stone-game-iv | 0 | 1 | We are asked to find the solution for an input of n. So,we find solution for all numbers from [0,n] and we do this because the previous answers help us in finding the solution for a potentially larger number. We have n states of dp,each state represents the solution of that specific state. states = [0,n]. let dp[i] be the state representation.\n\nAnd we have to find the optimal answer.Even if in one possibility alice wins,its a True.\n```\nFor each state i:\n\tj = find all the perfect squares less than i.\n\tfor each j:\n\t\tAssume that the perfectsquare j is taken by alice.\n\t\tThen the game continues as` dp[i-j*j]` for bob as start player.\n\t\tSince we calculated all answers for such states with alice as start player,its the same even if bob becomes the start player because both play optimally.\n\t\tIf bob could wins ,alice loses i.e ` dp[i-j*j]` = True\n\t\telse if bob loses alice wins i.e ` dp[i-j*j]` = False\n\t\tSo,we choose the case where alice wins with alice as start player(In essence we find if atleast one j returns True for dp[i]). or dp[i] |= ! dp[i-j*j]\n```\t\n\t\t\n`Time:O(n^1.5) | Space:O(n) `\nApproach`:DynamicProgramming` Bottom-up\n\n\tclass Solution:\n\t\t\tdef winnerSquareGame(self, n: int) -> bool:\n\t\t\t\tdp = [False]*(n+1)\n\t\t\t\tfor i in range(1,n+1):\n\t\t\t\t\tj = 1\n\t\t\t\t\twhile j*j <= i:\n\t\t\t\t\t\tdp[i] |= not dp[i-j*j]\n\t\t\t\t\t\tj+=1\n\t\t\t\treturn dp[n]\n\nplease leave an upvote if you find it helpful :)\n | 8 | There are `n` cities numbered from `1` to `n`. You are given an array `edges` of size `n-1`, where `edges[i] = [ui, vi]` represents a bidirectional edge between cities `ui` and `vi`. There exists a unique path between each pair of cities. In other words, the cities form a **tree**.
A **subtree** is a subset of cities where every city is reachable from every other city in the subset, where the path between each pair passes through only the cities from the subset. Two subtrees are different if there is a city in one subtree that is not present in the other.
For each `d` from `1` to `n-1`, find the number of subtrees in which the **maximum distance** between any two cities in the subtree is equal to `d`.
Return _an array of size_ `n-1` _where the_ `dth` _element **(1-indexed)** is the number of subtrees in which the **maximum distance** between any two cities is equal to_ `d`.
**Notice** that the **distance** between the two cities is the number of edges in the path between them.
**Example 1:**
**Input:** n = 4, edges = \[\[1,2\],\[2,3\],\[2,4\]\]
**Output:** \[3,4,0\]
**Explanation:**
The subtrees with subsets {1,2}, {2,3} and {2,4} have a max distance of 1.
The subtrees with subsets {1,2,3}, {1,2,4}, {2,3,4} and {1,2,3,4} have a max distance of 2.
No subtree has two nodes where the max distance between them is 3.
**Example 2:**
**Input:** n = 2, edges = \[\[1,2\]\]
**Output:** \[1\]
**Example 3:**
**Input:** n = 3, edges = \[\[1,2\],\[2,3\]\]
**Output:** \[2,1\]
**Constraints:**
* `2 <= n <= 15`
* `edges.length == n-1`
* `edges[i].length == 2`
* `1 <= ui, vi <= n`
* All pairs `(ui, vi)` are distinct. | Use dynamic programming to keep track of winning and losing states. Given some number of stones, Alice can win if she can force Bob onto a losing state. |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.