status
stringclasses 1
value | repo_name
stringclasses 13
values | repo_url
stringclasses 13
values | issue_id
int64 1
104k
| updated_files
stringlengths 11
1.76k
| title
stringlengths 4
369
| body
stringlengths 0
254k
⌀ | issue_url
stringlengths 38
55
| pull_url
stringlengths 38
53
| before_fix_sha
stringlengths 40
40
| after_fix_sha
stringlengths 40
40
| report_datetime
unknown | language
stringclasses 5
values | commit_datetime
unknown |
---|---|---|---|---|---|---|---|---|---|---|---|---|---|
closed | dagger/dagger | https://github.com/dagger/dagger | 5,205 | ["cmd/dagger/listen.go"] | 🐞 `dagger listen` stopped working in v0.6.0 release | ### What is the issue?
`dagger listen` doesn't work anymore since starting from v0.6.0, it starts the TUI instead of executing the actual `listen` command
### Log output

### Steps to reproduce
run `dagger listen`
### SDK version
Engine v0.6.0
### OS version
N/A | https://github.com/dagger/dagger/issues/5205 | https://github.com/dagger/dagger/pull/5219 | 2dd8717ee0c09230dbc2d9bee5d3f6343e8ccd36 | 7a48b9b25a612d3568f198e17fb3e3889219b276 | "2023-05-26T02:20:22Z" | go | "2023-05-31T21:54:07Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,196 | ["cmd/dagger/engine.go", "cmd/dagger/run.go"] | ✨ `dagger run` does not print the running program's stdout | ### What are you trying to do?
Weird use-case I'm sure but I have a Dagger pipeline ran using 'go run ./cmd', which prints some data to stdout, which we are writing to a file.
```
go run ./cmd > out.txt
```
When we use `dagger run` with this, it doesn't print anything.
### Why is this important to you?
There's probably a lot of weird cases where one would want your porgram's stdout to be piped to another program or to be written to a file. We are using it to write down the file paths of objects that are created by the pipeline where other processes will be used to copy them.
### How are you currently working around this?
We're just not using 'dagger run' for this at the moment.
---
I don't necessarily think this is a bug. | https://github.com/dagger/dagger/issues/5196 | https://github.com/dagger/dagger/pull/5231 | dfc9063a109d00b5831862e7e549e25718d08815 | e4bbec135098db82515c8b1033b820b888d2e125 | "2023-05-25T16:10:02Z" | go | "2023-06-01T13:21:27Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,192 | ["sdk/python/src/dagger/connection.py", "sdk/python/tests/api/test_integration_sync.py"] | Deprecate sync Python | ## Summary
Raise a deprecation warning when using the synchronous version of the Python SDK in favor of only the asynchronous one.
## Motivation
It’s simple to move from sync to async, but the opposite isn’t true.
There’s multiple disadvantages for having these two versions:
- more complicated codebase leading to a higher **maintenance** burden
- adds **fragmentation** to the ecosystem since you can’t share `async` functions in sync code
- *sync* is **rarely used** from what we can see
But there’s another even more compelling reason.
### Concurrency
It doesn’t make much sense to use the synchronous API with Dagger.
Due to the nature of our client/server architecture, we need to make concurrent requests for things that can run in parallel by the engine. If you’re using the synchronous API you’ll have to use threads, deal with the GIL and generally jump through hoops when we already have an asynchronous API that makes this easy.
Let’s take the example of running tests against a code base for multiple Python versions. This is a good candidate for running concurrent requests since they can run in parallel. Otherwise you need to test in sequence, taking much longer:
- [helderco/dagger-examples/test_multiple.py](https://github.com/helderco/dagger-examples/blob/main/test_multiple.py) → ~3m
- [helderco/dagger-examples/test_concurrency.py](https://github.com/helderco/dagger-examples/blob/main/test_concurrency.py) → ~1m
```mermaid
gantt
title Testing the FastAPI library with and without concurrency
dateFormat x
axisFormat %M:%S
section sync
Python 3.7 : 68, 37831
Python 3.8 : 37873, 63971
Python 3.9 : 64010, 97783
Python 3.10 : 97824, 128072
Python 3.11 : 128109, 162619
section concurrent
Python 3.7: 48, 51079
Python 3.8: 58, 50979
Python 3.9: 52, 51978
Python 3.10: 55, 52633
Python 3.11: 56, 59450
```
You can learn more about this in:
- https://github.com/dagger/dagger/issues/4766
### Confusion
The two versions (sync vs async) can also lead to more confusion.
#### `await` allows sequencial execution
It’s a possible misconception by users unfamiliar with asynchronous code that by using an `await` it makes an asynchronous call without waiting for it to finish.
> We use the sync code because we have to execute it in the order. Build the image and then deploy it. In the build image part we create a base image with rust and everything we need to cross-compile the application. So here we are free to do that async because we build the image for the different platforms/architecture.
\- From [Discord](https://discord.com/channels/707636530424053791/1088575225026846822/1090224327816908962)
That’s incorrect. Just by using `async`/`await` is not asynchronous on it’s own (not concurrent to be exact). The `await` name should imply that it will wait for the asynchronous task to finish before proceeding, but this may not be obvious to some.
You need to use concurrency primitives like a *task group* to run multiple asynchronous functions concurrently.
#### Not concurrent
```python
async def test(client: dagger.Client):
await testX(client)
await testY(client)
await testZ(client)
```
#### Concurrent
```python
async def test(client: dagger.Client):
async with anyio.create_task_group() as tg:
tg.start_soon(testX, client)
tg.start_soon(testY, client)
tg.start_soon(testZ, client)
```
See [But aren’t I using “async” with `await`?](https://github.com/dagger/dagger/issues/4766#python-sdk) for more information.
#### Wrong context manager!
Since `dagger.Connection` can be used with both a synchronous context manager and an asynchronous one, you don't get an error if you use the wrong one, not directly. What you get is confusing behavior and side effects.
For example, if you're using Dagger with [asyncclick](https://pypi.org/project/asyncclick/), it can be useful to let it manage the dagger connection as [a resource](https://click.palletsprojects.com/en/8.1.x/advanced/#managing-resources) to make available to subcommands:
```python
@click.pass_context
def cli(ctx):
ctx.client = ctx.with_resource(dagger.Connection())
```
Except that that's wrong! `with_resource` is sync, so it will use the `SyncClient` instead of the async `Client`. You need to use the async manager instead:
```python
@click.pass_context
async def cli(ctx):
ctx.client = await ctx.with_async_resource(dagger.Connection())
```
Removing `SyncClient` will fix this footgun.
## <a name="async-migration"></a>How to migrate to async?
Here’s the **TL;DR**:
- Add `async` to the connection context manager;
- Add an `await` before methods that make requests to the API;
- If a function has `await` or `async` statements, add `async` to the function as well;
- Wrap the call to the first `async` function in `anyio.run()`.
```diff
+import anyio
import dagger
-def main():
+async def main():
- with dagger.Connection() as client:
+ async with dagger.Connection() as client:
ctr = client.container().from_("alpine").with_exec(["echo", "hello"])
- output = ctr.stdout()
+ output = await ctr.stdout()
print(output)
-main()
+anyio.run(main)
```
If you’re importing types from the synchronous API, replace with the one defined in `dagger` instead:
```diff
import dagger
-from dagger.api.gen_sync import BuildArg
-BuildArg("version", "2")
+dagger.BuildArg("version", "2")
```
### Running from a synchronous function
If your code base is fully “sync”, you can still call asynchronous Dagger from any synchronous scope. Just pass the first asynchronous function (coroutine) to `anyio.run()`:
```python
import anyio
import dagger
async def my_dagger_func():
async with dagger.Connection() as client:
...
def my_sync_func():
anyio.run(my_dagger_func)
# do more after that's done
```
`anyio.run` kicks off the chain of coroutines in an event loop and waits until it’s all done.
### Blocking code
In fully synchronous code bases, there’s no need to worry about calling blocking code inside asynchronous functions, at least at the start.
It only matters if you’re running multiple tasks concurrently. Even If you’re not doing that yourself the SDK does it when it can (for example when converting objects to IDs before sending a request), but if you’re already using synchronous Dagger you already have that performance bottleneck.
At least after the initial quick migration, you’ll be able to **gradually migrate** more code to be non-blocking when you can invest the time to do it, including learning about it more.
Then you’ll see performance improvements where you couldn’t otherwise.
## <a name="resources"></a>Resources
For more information:
- [FastAPI: Concurrency and async / await](https://fastapi.tiangolo.com/async)
- https://github.com/dagger/dagger/issues/4766
- https://github.com/dagger/dagger/issues/4705
| https://github.com/dagger/dagger/issues/5192 | https://github.com/dagger/dagger/pull/5193 | 6f171dca6d2456ad5602dba4e1348b1762bd2ec8 | ddac9322b7a6fe0ae561f5f06aafa87f7c56e9e5 | "2023-05-25T12:09:24Z" | go | "2023-05-25T14:22:23Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,191 | ["cmd/dagger/engine.go", "cmd/dagger/run.go", "internal/tui/item.go", "internal/tui/tree.go"] | 🐞 TUI display incorrect when sdk crash with exception | ### What is the issue?
From https://github.com/wingyplus/kaogeek-discord-bot/tree/dagger/deployment
I accidentally set export to `docker.io/kaogeek` and found crash when running in auto provisioning mode. When running with TUI feature I see all pass at first but it hide the crash log in the first step and step export not show in the TUI. I expected to see the step is a failure at least and may show the export step failure, this could spot the problems more easier.
### Log output
Auto provisioning mode:
```
file:///Users/thanabodee/src/github.com/creatorsgarten/kaogeek-discord-bot/deployment/node_modules/.pnpm/@[email protected]/node_modules/@dagger.io/dagger/dist/api/utils.js:144
throw new GraphQLRequestError("Error message", {
^
GraphQLRequestError: Error message
at file:///Users/thanabodee/src/github.com/creatorsgarten/kaogeek-discord-bot/deployment/node_modules/.pnpm/@[email protected]/node_modules/@dagger.io/dagger/dist/api/utils.js:144:23
at Generator.throw (<anonymous>)
at rejected (file:///Users/thanabodee/src/github.com/creatorsgarten/kaogeek-discord-bot/deployment/node_modules/.pnpm/@[email protected]/node_modules/@dagger.io/dagger/dist/api/utils.js:5:65)
at process.processTicksAndRejections (node:internal/process/task_queues:95:5) {
cause: ClientError: open /Users/thanabodee/src/github.com/creatorsgarten/kaogeek-discord-bot/deployment/docker.io/kaogeek: no such file or directory: {"response":{"data":null,"errors":[{"message":"open /Users/thanabodee/src/github.com/creatorsgarten/kaogeek-discord-bot/deployment/docker.io/kaogeek: no such file or directory","locations":[{"line":2,"column":58719}],"path":....
```
TUI:
<img width="1915" alt="Screenshot 2566-05-25 at 09 57 48" src="https://github.com/dagger/dagger/assets/484530/47d5de01-a842-41cc-81a0-40d833afffd0">
### Steps to reproduce
Provisioning mode run by `pnpm build`. And TUI mode run with `_EXPERIMENT_DAGGER_TUI=1 dagger run pnpm run build`.
### SDK version
nodjs sdk 0.5.3
### OS version
macOS 13.1 (22C65) | https://github.com/dagger/dagger/issues/5191 | https://github.com/dagger/dagger/pull/5232 | b267d5999a927ba73e7978f0938fc827eb642c9c | 6d75e9b34a4bf70f454e201849998f34713352db | "2023-05-25T03:17:54Z" | go | "2023-06-01T18:17:32Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,171 | ["core/schema/secret.go", "router/schema.go"] | 🐞 Running TUI with `--debug` flag exposes secret values | ### What is the issue?
Running the TUI with `dagger run --debug` exposes the secrets otherwise masked in the logs. I can observe the `withSecretVariable` being exposed in the listing of stages.
### Log output
_No response_
### Steps to reproduce
Run any pipeline that has a `withSecretVar` with `dagger run --debug go run pipeline.go`
### SDK version
Go SDK 0.6.3
### OS version
Mac OS 12.6.2 | https://github.com/dagger/dagger/issues/5171 | https://github.com/dagger/dagger/pull/5186 | e171dc4252e464031737b51fee4c54531b4b537d | 6f171dca6d2456ad5602dba4e1348b1762bd2ec8 | "2023-05-23T04:41:10Z" | go | "2023-05-25T14:21:41Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,140 | ["core/integration/container_test.go", "core/schema/container.go", "core/schema/container.graphqls", "core/util.go", "sdk/go/api.gen.go", "sdk/nodejs/api/client.gen.ts", "sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py"] | Expand environment variables in `withEnvVariable` values | ### Discussed in https://github.com/dagger/dagger/discussions/5001
<div type='discussions-op-text'>
<sup>Originally posted by **wingyplus** April 21, 2023</sup>
I want to install something and append it to `PATH` to make it accessible. So how to prepend the new directory into the env variable as we do with `PATH`?</div>
Solution proposed by @vito :
> I agree; extending $PATH seems like a common use case. it would be nice to have parity with Dockerfile ENV which supports interpolation.
>
> It might be a surprising default, since who knows what values people might try to set in env vars. An expand: true param would be simple enough to implement by using [os.Expand](https://pkg.go.dev/os#Expand) and fetching values from the current image config.
>
> ```
> container {
> withEnvVariable(name: "PATH", value: "/opt/venv/bin:${PATH}", expand: true) {
> withExec(args: []string{"sh", "-c", "echo $PATH"}) {
> stdout
> }
> }
> }
> ```
> (Interpolate might be a more obvious name.) | https://github.com/dagger/dagger/issues/5140 | https://github.com/dagger/dagger/pull/5160 | c2fcf8390801585188042d12ee8190b436cc9c2f | e171dc4252e464031737b51fee4c54531b4b537d | "2023-05-16T15:18:49Z" | go | "2023-05-25T09:29:47Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,124 | [".changes/unreleased/Deprecated-20230718-173905.yaml", "core/schema/container.graphqls", "sdk/go/api.gen.go", "sdk/nodejs/api/client.gen.ts", "sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py"] | Deprecate `exitCode` | ## Summary
I’m proposing we deprecate `exitCode` in favor of [`sync`](https://github.com/dagger/dagger/pull/5071) and [`ExecError`](https://github.com/dagger/dagger/pull/5184) as an alternative solution to https://github.com/dagger/dagger/issues/3192.
## Motivation
The `Container.exitCode` field doesn’t make sense because you always get zero on success and you can’t get the non-zero value when the command fails due to a bug:
- https://github.com/dagger/dagger/issues/3192
So since the result on success is useless, just replace with `sync`:
- https://github.com/dagger/dagger/pull/5071
But if you do need the non-zero exit code value, you can get it from `ExecError`:
- https://github.com/dagger/dagger/pull/5184
## Examples
**Don't care about the result**
```diff
- _, err := ctr.ExitCode(ctx)
+ _, err := ctr.Sync(ctx)
if err != nil {
return err
}
```
**Need the exit code**
```go
func GetExitCode(ctr *dagger.Container) (int, error) {
_, err := ctr.Sync(ctx)
if e, ok := err.(*dagger.ExecError); ok {
return e.ExitCode, nil
}
return 0, err
}
```
## Specification
Just add the deprecation in the API:
```diff
type Container {
"""
Exit code of the last executed command. Zero means success.
Will execute default command if none is set, or error if there's no default.
"""
- exitCode: Int!
+ exitCode: Int! @deprecated(reason: "Use `sync` instead.")
}
```
## Caveat
There is one big difference between `ExitCode(ctx)` and `Sync(ctx)`. While the former triggers command execution, defaulting to the entrypoint and default args if no `WithExec` is defined in the pipeline, the latter does not.
Just “renaming” to a new `.Run(ctx)` that behaves the same doesn’t seem worth it. And some users don’t actually want to run the command (e.g., just do a `Dockerfile` build), so it pays to understand what’s going on.
We’ll just have to make this clear in documentation and examples:
- https://github.com/dagger/dagger/issues/3617 | https://github.com/dagger/dagger/issues/5124 | https://github.com/dagger/dagger/pull/5481 | 9d87f496046ccfc5ec82d86a7c0aea71933df3a1 | 9aff03b6150c1ab45189523a63f2a97f1ddac283 | "2023-05-10T15:14:10Z" | go | "2023-07-18T18:03:07Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,084 | [".changes/unreleased/Added-20230815-154334.yaml", "core/directory.go", "core/file.go"] | TUI: show directory export target | ## Problem
In the new (and awesome) TUI:
* directory imports (reading from host filesystem) are shown with the source path ✅
* but directory exports (writing from host filesystem) don't 😢
Example:

## Solution
Change how directory exports are displayed in the TUI, so that the target path is visible. | https://github.com/dagger/dagger/issues/5084 | https://github.com/dagger/dagger/pull/5632 | ece3f3691ac5d241a2e8d3bf047c2ea8cf952547 | badf974d4e5d4592602c4ed876a0da50c9d74e5c | "2023-05-04T05:59:34Z" | go | "2023-08-15T16:22:20Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,077 | ["cmd/shim/main.go", "core/gateway.go", "core/integration/container_test.go"] | Add `(truncated output)` to stdout/stderr errors | @helderco mentioned:
> Can we append some notice like "truncated output"? Multiple users have been confused that the output is truncated, reporting it as a bug. | https://github.com/dagger/dagger/issues/5077 | https://github.com/dagger/dagger/pull/5163 | 7d8738d64889572fd5ac7d9930c2f16b4c3629d2 | e0fecd2682e63b5c0fb18a18e77f4ea001bf6219 | "2023-05-02T21:11:43Z" | go | "2023-05-22T21:21:17Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,069 | [".changes/unreleased/Added-20230727-114058.yaml", "core/integration/host_test.go", "core/schema/host.go", "core/schema/host.graphqls", "core/schema/secret.go", "sdk/go/api.gen.go", "sdk/nodejs/api/client.gen.ts", "sdk/python/src/dagger/api/gen.py", "secret/store.go"] | Handling Binary Data | ## Background
While trying to implement examples for issue [Allow directories to be mounted as secrets](https://github.com/dagger/dagger/issues/4896), it became apparent that there are limitations when dealing with binary data in GraphQL using strings. This is relevant for the purpose of mounting directories as secrets, where binary data needs to be handled.
I encountered a problem when attempting to handle GPG files. The goal was to create and GPG-sign a built binary with Dagger by mounting the `~/.gnupg` directory into the container and running `gpg --detach-sign --armor binary-name`.
This required the following files:
- ~/.gnupg/pubring.kbx
- ~/.gnupg/trustdb.gpg
- ~/.gnupg/private-keys-v1.d/*
Handling binary data like GPG files in GraphQL led to errors due to improper escaping:
```shell
go run .
Connected to engine c01f0fd17590
panic: input:1: Syntax Error GraphQL request (1:49) Invalid character escape sequence: \\x.
```
The initial idea was to implement a union type `SecretData = String | Bytes` in the `setSecret` query. However, according to the [discussion](https://github.com/dagger/dagger/issues/5000#issuecomment-1518352932), using unions as input values in GraphQL is not feasible.
## Issue
The main issue is finding a solution to handle both string and binary data when setting secrets in GraphQL, as the initial proposal of using a union type is not possible. This is important for mounting directories as secrets and handling binary data efficiently.
## Proposed Solution
Based on the comments and limitations mentioned in the discussion, the best solution seems to be to create a new function that accepts binary data as Base64 encoded string as input. This function would be a variation of withSecretVariable and handle binary data separately.
### Advantages
- No character encoding issues: By using Base64 encoding, we can ensure that all binary data is properly serialized and deserialized without any issues related to character encoding.
- Efficient data transfer: Base64-encoded binary data is more compact than a string representation, reducing the overhead associated with transferring binary data in GraphQL queries or mutations.
- Clear type information: Defining a custom type allows us to be explicit regarding the expected encoded format
#### Steps to implement the solution:
1. Create a new custom type `Base64` in the GraphQL schema to handle binary data.
```graphql
type Base64 string
```
2. Define a new function in the client SDK called WithSecretBytes that accepts an environment variable name and Base64 data as input.
```go
func (c *Container) setSecretBytes(name string, data Base64) *Container
```
3. Update the GraphQL schema to include the new function setSecretBytes.
```graphql
extend type Query {
"Sets a secret given a user-defined name to its binary data and returns the secret."
setSecretBytes(
"""
The user-defined name for this secret
"""
name: String!
"""
The binary data of the secret
"""
data: Base64!
): Secret!
}
```
4. Implement deserialization functions for the Base64 `setSecretBytes`, handling the decoding of binary from Base64.
5. Update the SDK example to show how to convert binary data to Base64-encoded strings before sending them as secret arguments.
By implementing this new function, we can effectively handle binary data in GraphQL, making it possible to mount directories as secrets in a more convenient and efficient manner.
cc @vikram-dagger @helderco @vito | https://github.com/dagger/dagger/issues/5069 | https://github.com/dagger/dagger/pull/5500 | 99a054bc0bc14baf91783871d8364b5be5d10177 | 09677c0311acb73891b7798d65777957f1a5d513 | "2023-05-02T14:47:43Z" | go | "2023-07-28T00:04:29Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,055 | ["core/docs/d7yxc-operator_manual.md", "docs/current/faq.md"] | 🖐️ How do we specify registry mirrors? | ### What happened? What did you expect to happen?
Some people (e.g: #4217), including myself, are trying to use a private registry as a mirror for the Docker Hub registry.
This, [according to the Buildkit documentation](https://docs.docker.com/build/buildkit/configure/) is achieved with the following `/etc/buildkit.toml` file:
```toml
debug = true
[registry."docker.io"]
mirrors = ["mirror.gcr.io"]
```
How does one do that for Dagger?
As an example, the following method should pull from `mirror.gcr.io/golang:latest`:
```golang
func build(ctx context.Context) error {
fmt.Println("Building with Dagger")
// initialize Dagger client
client, err := dagger.Connect(ctx, dagger.WithLogOutput(os.Stdout))
if err != nil {
return err
}
defer client.Close()
// get reference to the local project
src := client.Host().Directory(".")
// get `golang` image
golang := client.Container().From("golang:latest")
// mount cloned repository into `golang` image
golang = golang.WithDirectory("/src", src).WithWorkdir("/src")
// define the application build command
path := "build/"
golang = golang.WithExec([]string{"go", "build", "-o", path})
// get reference to build output directory in container
output := golang.Directory(path)
// write contents of container build/ directory to the host
_, err = output.Export(ctx, path)
if err != nil {
return err
}
return nil
}
```
Any tips? | https://github.com/dagger/dagger/issues/5055 | https://github.com/dagger/dagger/pull/5828 | 33cdd129e5395e538df38aaca8a34baa38b90c5f | 74e6393e816af380e410f7582e37de5c52ea02e8 | "2023-04-28T16:28:41Z" | go | "2023-10-19T06:11:07Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,045 | ["docs/current/guides/235290-troubleshooting.md"] | Create Dagger troubleshooting guide | ### What is the issue?
Create a guide to troubleshoot Dagger.
Potentially this could also be a guide to troubleshoot Dagger pipelines.
Inspired by https://discord.com/channels/707636530424053791/1101085612750143568 | https://github.com/dagger/dagger/issues/5045 | https://github.com/dagger/dagger/pull/5046 | 98cccd79262962d7081e9048da41a03a3d0ebb50 | 08dcc156b8b0724a9f1c2cf4e5c68020334bebfb | "2023-04-27T14:07:41Z" | go | "2023-05-04T13:43:48Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,027 | ["docs/current/sdk/python/628797-get-started.md"] | Clarify Python SDK installation instructions | ### What is the issue?
The Python SDK installation page at https://docs.dagger.io/sdk/python/628797/get-started does not specify how to create a virtual environment. This should be included for users unfamiliar with Python.
From Discord: "Hi, I'm trying the basic example in the tutorial at https://docs.dagger.io/sdk/python/628797/get-started. I've installed dagger-io and copied the test.py script into the fastapi dir, verbatim. When i run py test.py, i get no output and the process just hangs. Is there a bug in the sample script?"
https://discord.com/channels/707636530424053791/1100107534951514283
| https://github.com/dagger/dagger/issues/5027 | https://github.com/dagger/dagger/pull/5029 | 793f5073eda34be29092c88ca48fa9e699ed946e | cdcb1df5f2eeaf8cdbd3035cf0b5987e5e4c6c0a | "2023-04-25T14:45:04Z" | go | "2023-04-27T09:20:41Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,017 | ["docs/current/guides/541047-alternative-runtimes.md"] | Create guide for substituting Docker with podman and others | ### What is the issue?
This task is to create a guide to setup Dagger in order to use podman instead of Docker.
This has been requested multiple times:
https://discord.com/channels/707636530424053791/1100004042207416404
https://discord.com/channels/707636530424053791/1100004042207416404/1100081213760340029
https://discord.com/channels/707636530424053791/1073859480560865360 | https://github.com/dagger/dagger/issues/5017 | https://github.com/dagger/dagger/pull/5053 | 08dcc156b8b0724a9f1c2cf4e5c68020334bebfb | 08cbce9c4e1b0345f31bbfb2ca9f17dff3aa4bc4 | "2023-04-24T18:54:06Z" | go | "2023-05-04T13:48:30Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 5,010 | ["package.json", "yarn.lock"] | 🐞 Dagger stop running when use docker dind as a service binding | ### What is the issue?
I want to use Docker as a service in Dagger. But when binding docker with service binding, the container stop running without executing any command.
I expected at least the container (Elixir container in the example) should execute `elixir --version` and return the result. Or if there's anything wrong, it may log something that indicate the error.
### Log output
From `go run .`:
```
#1 resolve image config for docker.io/library/docker:23.0.4-dind-rootless
#1 DONE 2.2s
#2 resolve image config for docker.io/hexpm/elixir:1.14.0-erlang-24.3.4-alpine-3.16.0
#2 DONE 0.7s
```
From Dagger container (registry.dagger.io/engine:v0.5.0):
```
time="2023-04-24T02:40:54Z" level=debug msg="session started" spanID=1c305bea8789cca0 traceID=7f366793e8b5dc8ce00251ece0ec17d9
time="2023-04-24T02:40:54Z" level=debug msg="unsupported cache type , defaulting export off" spanID=75f5f9e5452a0361 traceID=08b31aa4bc15a904a73711048d9b9106
time="2023-04-24T02:40:54Z" level=debug msg="cache exporter resolver for dagger returned nil, skipping exporter" spanID=75f5f9e5452a0361 traceID=08b31aa4bc15a904a73711048d9b9106
time="2023-04-24T02:40:56Z" level=debug msg=resolving host=registry-1.docker.io spanID=0db54ab81774c660 traceID=f622981b876d3fe3a174069c9db24006
time="2023-04-24T02:40:56Z" level=debug msg="do request" host=registry-1.docker.io request.header.accept="application/vnd.docker.distribution.manifest.v2+json, application/vnd.docker.distribution.manifest.list.v2+json, application/vnd.oci.image.manifest.v1+json, application/vnd.oci.image.index.v1+json, */*" request.header.user-agent=buildkit/v0.0-dev request.method=HEAD spanID=0db54ab81774c660 traceID=f622981b876d3fe3a174069c9db24006 url="https://registry-1.docker.io/v2/library/docker/manifests/23.0.4-dind-rootless"
time="2023-04-24T02:40:56Z" level=debug msg="healthcheck completed" actualDuration="805.208µs" spanID=bd5a4f1c3e10427d timeout=30s traceID=aff580b3237f87444c0460531b52a609
time="2023-04-24T02:40:59Z" level=debug msg="fetch response received" host=registry-1.docker.io response.header.content-length=549 response.header.content-type=application/vnd.docker.distribution.manifest.list.v2+json response.header.date="Mon, 24 Apr 2023 02:40:59 GMT" response.header.docker-content-digest="sha256:d9ad5130fa11a3dc1abe42bec5cbf255b1ce02bdf710a088a1ce8b617e49870a" response.header.docker-distribution-api-version=registry/2.0 response.header.docker-ratelimit-source=97d94f38-74c0-11e4-bea4-0242ac11001b response.header.etag="\"sha256:d9ad5130fa11a3dc1abe42bec5cbf255b1ce02bdf710a088a1ce8b617e49870a\"" response.header.ratelimit-limit="200;w=21600" response.header.ratelimit-remaining="198;w=21600" response.header.strict-transport-security="max-age=31536000" response.status="200 OK" spanID=0db54ab81774c660 traceID=f622981b876d3fe3a174069c9db24006 url="https://registry-1.docker.io/v2/library/docker/manifests/23.0.4-dind-rootless"
time="2023-04-24T02:40:59Z" level=debug msg=resolved desc.digest="sha256:d9ad5130fa11a3dc1abe42bec5cbf255b1ce02bdf710a088a1ce8b617e49870a" host=registry-1.docker.io spanID=0db54ab81774c660 traceID=f622981b876d3fe3a174069c9db24006
time="2023-04-24T02:40:59Z" level=debug msg=fetch digest="sha256:d9ad5130fa11a3dc1abe42bec5cbf255b1ce02bdf710a088a1ce8b617e49870a" mediatype=application/vnd.docker.distribution.manifest.list.v2+json size=549 spanID=0db54ab81774c660 traceID=f622981b876d3fe3a174069c9db24006
time="2023-04-24T02:40:59Z" level=debug msg=fetch digest="sha256:2538ea1aec9be5b0e93fb9d2088a5e3af5d2ad3d7ab280d0a779fab15a541ad7" mediatype=application/vnd.docker.distribution.manifest.v2+json size=4296 spanID=0db54ab81774c660 traceID=f622981b876d3fe3a174069c9db24006
time="2023-04-24T02:40:59Z" level=debug msg=fetch digest="sha256:da5f146df7948b500b2001573b8471329eb8044c0595b1aee105b1433961919c" mediatype=application/vnd.docker.container.image.v1+json size=13711 spanID=0db54ab81774c660 traceID=f622981b876d3fe3a174069c9db24006
time="2023-04-24T02:40:59Z" level=debug msg=resolving host=registry-1.docker.io spanID=2d43bf3dc310aad8 traceID=3238d7b42125c07be12936e1c8c67430
time="2023-04-24T02:40:59Z" level=debug msg="do request" host=registry-1.docker.io request.header.accept="application/vnd.docker.distribution.manifest.v2+json, application/vnd.docker.distribution.manifest.list.v2+json, application/vnd.oci.image.manifest.v1+json, application/vnd.oci.image.index.v1+json, */*" request.header.user-agent=buildkit/v0.0-dev request.method=HEAD spanID=2d43bf3dc310aad8 traceID=3238d7b42125c07be12936e1c8c67430 url="https://registry-1.docker.io/v2/library/docker/manifests/23.0.4-dind-rootless"
time="2023-04-24T02:40:59Z" level=debug msg="fetch response received" host=registry-1.docker.io response.header.content-length=549 response.header.content-type=application/vnd.docker.distribution.manifest.list.v2+json response.header.date="Mon, 24 Apr 2023 02:41:00 GMT" response.header.docker-content-digest="sha256:d9ad5130fa11a3dc1abe42bec5cbf255b1ce02bdf710a088a1ce8b617e49870a" response.header.docker-distribution-api-version=registry/2.0 response.header.docker-ratelimit-source=97d94f38-74c0-11e4-bea4-0242ac11001b response.header.etag="\"sha256:d9ad5130fa11a3dc1abe42bec5cbf255b1ce02bdf710a088a1ce8b617e49870a\"" response.header.ratelimit-limit="200;w=21600" response.header.ratelimit-remaining="198;w=21600" response.header.strict-transport-security="max-age=31536000" response.status="200 OK" spanID=2d43bf3dc310aad8 traceID=3238d7b42125c07be12936e1c8c67430 url="https://registry-1.docker.io/v2/library/docker/manifests/23.0.4-dind-rootless"
time="2023-04-24T02:40:59Z" level=debug msg=resolved desc.digest="sha256:d9ad5130fa11a3dc1abe42bec5cbf255b1ce02bdf710a088a1ce8b617e49870a" host=registry-1.docker.io spanID=2d43bf3dc310aad8 traceID=3238d7b42125c07be12936e1c8c67430
time="2023-04-24T02:40:59Z" level=debug msg=fetch digest="sha256:d9ad5130fa11a3dc1abe42bec5cbf255b1ce02bdf710a088a1ce8b617e49870a" mediatype=application/vnd.docker.distribution.manifest.list.v2+json size=549 spanID=2d43bf3dc310aad8 traceID=3238d7b42125c07be12936e1c8c67430
time="2023-04-24T02:40:59Z" level=debug msg=fetch digest="sha256:2538ea1aec9be5b0e93fb9d2088a5e3af5d2ad3d7ab280d0a779fab15a541ad7" mediatype=application/vnd.docker.distribution.manifest.v2+json size=4296 spanID=2d43bf3dc310aad8 traceID=3238d7b42125c07be12936e1c8c67430
time="2023-04-24T02:40:59Z" level=debug msg=fetch digest="sha256:da5f146df7948b500b2001573b8471329eb8044c0595b1aee105b1433961919c" mediatype=application/vnd.docker.container.image.v1+json size=13711 spanID=2d43bf3dc310aad8 traceID=3238d7b42125c07be12936e1c8c67430
time="2023-04-24T02:40:59Z" level=debug msg=resolving host=registry-1.docker.io spanID=44f82957783fd7ee traceID=d2b1c05e9a874e9c16d8c3fa8cdcf1e3
time="2023-04-24T02:40:59Z" level=debug msg="do request" host=registry-1.docker.io request.header.accept="application/vnd.docker.distribution.manifest.v2+json, application/vnd.docker.distribution.manifest.list.v2+json, application/vnd.oci.image.manifest.v1+json, application/vnd.oci.image.index.v1+json, */*" request.header.user-agent=buildkit/v0.0-dev request.method=HEAD spanID=44f82957783fd7ee traceID=d2b1c05e9a874e9c16d8c3fa8cdcf1e3 url="https://registry-1.docker.io/v2/hexpm/elixir/manifests/1.14.0-erlang-24.3.4-alpine-3.16.0"
time="2023-04-24T02:40:59Z" level=debug msg="healthcheck completed" actualDuration=3.728458ms spanID=1c305bea8789cca0 timeout=30s traceID=7f366793e8b5dc8ce00251ece0ec17d9
time="2023-04-24T02:41:00Z" level=debug msg="fetch response received" host=registry-1.docker.io response.header.content-length=772 response.header.content-type=application/vnd.docker.distribution.manifest.list.v2+json response.header.date="Mon, 24 Apr 2023 02:41:00 GMT" response.header.docker-content-digest="sha256:bfcd284f327e98adf231cad592f9629fc7adf78d7f1a5511cd451085a528eeab" response.header.docker-distribution-api-version=registry/2.0 response.header.docker-ratelimit-source=97d94f38-74c0-11e4-bea4-0242ac11001b response.header.etag="\"sha256:bfcd284f327e98adf231cad592f9629fc7adf78d7f1a5511cd451085a528eeab\"" response.header.ratelimit-limit="200;w=21600" response.header.ratelimit-remaining="198;w=21600" response.header.strict-transport-security="max-age=31536000" response.status="200 OK" spanID=44f82957783fd7ee traceID=d2b1c05e9a874e9c16d8c3fa8cdcf1e3 url="https://registry-1.docker.io/v2/hexpm/elixir/manifests/1.14.0-erlang-24.3.4-alpine-3.16.0"
time="2023-04-24T02:41:00Z" level=debug msg=resolved desc.digest="sha256:bfcd284f327e98adf231cad592f9629fc7adf78d7f1a5511cd451085a528eeab" host=registry-1.docker.io spanID=44f82957783fd7ee traceID=d2b1c05e9a874e9c16d8c3fa8cdcf1e3
time="2023-04-24T02:41:00Z" level=debug msg=fetch digest="sha256:bfcd284f327e98adf231cad592f9629fc7adf78d7f1a5511cd451085a528eeab" mediatype=application/vnd.docker.distribution.manifest.list.v2+json size=772 spanID=44f82957783fd7ee traceID=d2b1c05e9a874e9c16d8c3fa8cdcf1e3
time="2023-04-24T02:41:00Z" level=debug msg=fetch digest="sha256:161437f2e1fe49f354abab2a91b4cff7fab1f405550770f4cbc350ce315c603d" mediatype=application/vnd.docker.distribution.manifest.v2+json size=1162 spanID=44f82957783fd7ee traceID=d2b1c05e9a874e9c16d8c3fa8cdcf1e3
time="2023-04-24T02:41:00Z" level=debug msg=fetch digest="sha256:2bb75ba5041cb2f11c0da587f998a98730cd6cc276d1ceb93f965846770bea91" mediatype=application/vnd.docker.container.image.v1+json size=2383 spanID=44f82957783fd7ee traceID=d2b1c05e9a874e9c16d8c3fa8cdcf1e3
time="2023-04-24T02:41:00Z" level=debug msg=resolving host=registry-1.docker.io spanID=50a0ba61b4381a15 traceID=f5622e8770e9332a9506075e6fd48dcd
time="2023-04-24T02:41:00Z" level=debug msg="do request" host=registry-1.docker.io request.header.accept="application/vnd.docker.distribution.manifest.v2+json, application/vnd.docker.distribution.manifest.list.v2+json, application/vnd.oci.image.manifest.v1+json, application/vnd.oci.image.index.v1+json, */*" request.header.user-agent=buildkit/v0.0-dev request.method=HEAD spanID=50a0ba61b4381a15 traceID=f5622e8770e9332a9506075e6fd48dcd url="https://registry-1.docker.io/v2/hexpm/elixir/manifests/1.14.0-erlang-24.3.4-alpine-3.16.0"
time="2023-04-24T02:41:00Z" level=debug msg="fetch response received" host=registry-1.docker.io response.header.content-length=772 response.header.content-type=application/vnd.docker.distribution.manifest.list.v2+json response.header.date="Mon, 24 Apr 2023 02:41:01 GMT" response.header.docker-content-digest="sha256:bfcd284f327e98adf231cad592f9629fc7adf78d7f1a5511cd451085a528eeab" response.header.docker-distribution-api-version=registry/2.0 response.header.docker-ratelimit-source=97d94f38-74c0-11e4-bea4-0242ac11001b response.header.etag="\"sha256:bfcd284f327e98adf231cad592f9629fc7adf78d7f1a5511cd451085a528eeab\"" response.header.ratelimit-limit="200;w=21600" response.header.ratelimit-remaining="198;w=21600" response.header.strict-transport-security="max-age=31536000" response.status="200 OK" spanID=50a0ba61b4381a15 traceID=f5622e8770e9332a9506075e6fd48dcd url="https://registry-1.docker.io/v2/hexpm/elixir/manifests/1.14.0-erlang-24.3.4-alpine-3.16.0"
time="2023-04-24T02:41:00Z" level=debug msg=resolved desc.digest="sha256:bfcd284f327e98adf231cad592f9629fc7adf78d7f1a5511cd451085a528eeab" host=registry-1.docker.io spanID=50a0ba61b4381a15 traceID=f5622e8770e9332a9506075e6fd48dcd
time="2023-04-24T02:41:00Z" level=debug msg=fetch digest="sha256:bfcd284f327e98adf231cad592f9629fc7adf78d7f1a5511cd451085a528eeab" mediatype=application/vnd.docker.distribution.manifest.list.v2+json size=772 spanID=50a0ba61b4381a15 traceID=f5622e8770e9332a9506075e6fd48dcd
time="2023-04-24T02:41:00Z" level=debug msg=fetch digest="sha256:161437f2e1fe49f354abab2a91b4cff7fab1f405550770f4cbc350ce315c603d" mediatype=application/vnd.docker.distribution.manifest.v2+json size=1162 spanID=50a0ba61b4381a15 traceID=f5622e8770e9332a9506075e6fd48dcd
time="2023-04-24T02:41:00Z" level=debug msg=fetch digest="sha256:2bb75ba5041cb2f11c0da587f998a98730cd6cc276d1ceb93f965846770bea91" mediatype=application/vnd.docker.container.image.v1+json size=2383 spanID=50a0ba61b4381a15 traceID=f5622e8770e9332a9506075e6fd48dcd
time="2023-04-24T02:41:00Z" level=debug msg="session finished: <nil>" spanID=1c305bea8789cca0 traceID=7f366793e8b5dc8ce00251ece0ec17d9
time="2023-04-24T02:41:01Z" level=debug msg="healthcheck completed" actualDuration=1.358334ms spanID=bd5a4f1c3e10427d timeout=30s traceID=aff580b3237f87444c0460531b52a609
```
### Steps to reproduce
Create new directory, run `go mod init` and create go source with content below:
```go
package main
import (
"context"
"os"
"dagger.io/dagger"
)
func main() {
ctx := context.Background()
client, err := dagger.Connect(ctx, dagger.WithLogOutput(os.Stderr))
if err != nil {
panic(err)
}
defer client.Close()
dockerCertsCA := client.CacheVolume("docker-certs-ca")
dockerCertsClient := client.CacheVolume("docker-certs-client")
dockerDind := client.Container().
From("docker:23.0.4-dind-rootless").
WithMountedCache("/certs/ca", dockerCertsCA).
WithMountedCache("/certs/client", dockerCertsClient).
WithEnvVariable("DOCKER_TLS_CERTDIR", "/certs")
client.Container().
From("hexpm/elixir:1.14.0-erlang-24.3.4-alpine-3.16.0").
WithServiceBinding("docker-dind", dockerDind).
WithMountedCache("/certs/client", dockerCertsClient).
WithExec([]string{"elixir", "--version"}).
Stdout(ctx)
}
```
And then run `go run .`.
### SDK version
Go SDK 0.6.0
### OS version
macOS 13.1 (22C65) | https://github.com/dagger/dagger/issues/5010 | https://github.com/dagger/dagger/pull/3381 | 5b1ad4de0aaab70e4d936ad4cd29628b42e27765 | e8f5d405a3d0f236181b23c18a9ab811a5b577e3 | "2023-04-23T15:53:19Z" | go | "2022-10-15T00:57:56Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,958 | ["docs/current/162770-faq.md"] | Update Dagger FAQ | ### What is the issue?
The answer to the question in https://docs.dagger.io/162770/faq#how-do-i-log-in-to-a-container-registry-using-a-dagger-sdk is outdated, as we also now have SDK methods for registry authentication. This issue is to update the answer in the FAQ to reflect the above. | https://github.com/dagger/dagger/issues/4958 | https://github.com/dagger/dagger/pull/4959 | 19447fd4cbc0069e408b6fdf08f924a0106b4a2b | 71054e36398e60f7c3f493e5e9d72ba6fe0f7bbc | "2023-04-14T06:35:50Z" | go | "2023-04-21T14:15:37Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,954 | ["docs/current/guides/757394-use-service-containers.md"] | Improve the service containers guide | ### What is the issue?
From https://discord.com/channels/707636530424053791/1095626134919594084:
> include a note that service containers should be configured to listen on 0.0.0.0 instead of 127.0.0.1...
> yep - the issue with 127.0.0.1 is it'll only be reachable within the container itself, so other services (including our healthcheck) won't be able to connect to it. 0.0.0.0 on the other hand will allow connections to any destination IP, i.e. the container's 10.87.xx.xx IP in the Dagger network | https://github.com/dagger/dagger/issues/4954 | https://github.com/dagger/dagger/pull/4955 | 07e7939f5fac71082f0a22025f6b83c5accebafd | 0b377e03d99b257c6ade55581056f7422a3ea39e | "2023-04-13T11:41:14Z" | go | "2023-04-13T12:47:48Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,953 | ["docs/current/guides/723462-use-secrets.md"] | Improve the secrets guide | ### What is the issue?
From https://github.com/dagger/dagger/pull/4822#issuecomment-1494400399:
> A couple of thoughts:
> We should emphasise that WithSecretVariable doesn't end in the container image history, or the final layer. WithEnvVariable does, and sometimes a regular env variable that should not stick around (maybe because that container will be published) should be declared via WithSecretVariable instead. I hit this problem a few weeks ago: https://github.com/thechangelog/changelog.com/commit/0aa5e6d38d9abac78f0d6d2b4bdf7deff803677a
> We should caution users against using WithEnvVariable for secrets. I mention this because it is the common way of using secrets in GitHub Actions
| https://github.com/dagger/dagger/issues/4953 | https://github.com/dagger/dagger/pull/4956 | 0b377e03d99b257c6ade55581056f7422a3ea39e | 65649cb838d8a9a7ed83477e1b2fa840cf52919d | "2023-04-13T11:40:51Z" | go | "2023-04-13T14:50:20Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,943 | ["docs/current/guides/145912-ci.md", "docs/current/guides/snippets/ci/go/actions.yml", "docs/current/guides/snippets/ci/python/Jenkinsfile", "docs/current/guides/snippets/ci/python/actions.yml", "docs/current/guides/snippets/ci/python/circle.yml", "docs/current/guides/snippets/ci/python/gitlab.yml"] | docs: Simplify CI guide | > The python dependencies install could be simplified and the go version I think is 1.20, but that can be in a follow up.
_Originally posted by @helderco in https://github.com/dagger/dagger/pull/4937#pullrequestreview-1380990279_
| https://github.com/dagger/dagger/issues/4943 | https://github.com/dagger/dagger/pull/4952 | 321d5e54079d3b4d4e88b45c43a6c81eb8f40cf8 | f0a4a3264751e93906bd07f9e10ac38b377e81a8 | "2023-04-12T16:51:18Z" | go | "2023-04-22T10:03:05Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,935 | ["docs/current/quickstart/349011-quickstart-build.mdx", "docs/current/quickstart/429462-quickstart-build-dockerfile.mdx", "docs/current/quickstart/472910-quickstart-build-multi.mdx", "docs/current/quickstart/593914-quickstart-hello.mdx", "docs/current/quickstart/635927-quickstart-caching.mdx", "docs/current/quickstart/730264-quickstart-publish.mdx", "docs/current/quickstart/947391-quickstart-test.mdx"] | Selecting a specific language in code snippets does not persist consistently across pages | ### What is the issue?
1. Selecting a specific language tab in a code snippet is not persistent across pages.
Reproduction:
- https://docs.dagger.io/472910/quickstart-build-multi and select Node
- https://docs.dagger.io/252029/load-images-local-docker-engine - Node is not pre-selected
2. Selecting a specific language tab in the quickstart is not consistent across quickstart pages.
Reproduction:
- https://docs.dagger.io/947391/quickstart-test and select Node
- https://docs.dagger.io/349011/quickstart-build - Node is pre-selected
- https://docs.dagger.io/628381/quickstart-sdk - Node is not pre-selected
Reported by @gerhard on 11/04/2023 | https://github.com/dagger/dagger/issues/4935 | https://github.com/dagger/dagger/pull/5018 | f5376628bc10ebcdf80807c809a5c8546bebe5d8 | 793f5073eda34be29092c88ca48fa9e699ed946e | "2023-04-12T06:40:25Z" | go | "2023-04-26T07:06:08Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,934 | ["website/sidebars.js", "website/src/css/custom.scss"] | Documentation left sidebar menu is too long | ### What is the issue?
Reported by @gerhard on 11/04/23: Currently, the docs navigation menu (left menu) has sub-pages expanded. This creates a very long menu. The items at the bottom are not visible immediately unless you scroll down (eg. a user may not realize we have an FAQ since it's at the bottom of the list). Also, when browsing the docs, it's easy to lose your place in the navigation menu due to its length.

| https://github.com/dagger/dagger/issues/4934 | https://github.com/dagger/dagger/pull/5120 | 7327f3381fe914f0acc754a024176dcc506a703f | 406efacb52ab3b2c898b327d4209ff1e9cc07400 | "2023-04-12T06:17:10Z" | go | "2023-05-11T06:32:34Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,896 | ["docs/current/742989-cookbook.md", "docs/current/cookbook/snippets/mount-directories-as-secrets/index.ts", "docs/current/cookbook/snippets/mount-directories-as-secrets/main.go", "docs/current/cookbook/snippets/mount-directories-as-secrets/main.py"] | ✨Allow directories to be mounted as secrets | ### What are you trying to do?
I'm trying to create and GPG-sign a built binary with Dagger.
To do this, I need to mount my ~/.gnupg directory into my container and run `gpg --detach-sign --armor binary-name`.
The above command requires the following files (keyrings and trustdb) to create the signature:
- `~/.gnupg/pubring.kbx`
- `~/.gnupg/trustdb.gpg`
- `~/.gnupg/private-keys-v1.d/*`
Currently Dagger only permits mounting files as secrets. It's not possible to mount an entire directory hierarchy as a secret; each file has to be mounted manually.
Can Dagger allow directories to be mounted as secrets, as it does with files?
### Why is this important to you?
It will make it simpler to sign binary builds with GPG.
### How are you currently working around this?
_No response_ | https://github.com/dagger/dagger/issues/4896 | https://github.com/dagger/dagger/pull/5520 | 4322a3f514514fbf18351d57b3433beca61714e7 | 1b09e476ea9b598f70eb8ecdc5f25e0d711b4f15 | "2023-04-06T13:06:11Z" | go | "2023-08-03T17:22:16Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,890 | ["go.mod", "go.sum"] | 🖐️ Execute multiple commands in the same 'WithExec' sentence | ### What happened? What did you expect to happen?
Hi,
I whould like to know how can i build a container with golang making a:
[...]
WithExec([]string{"apt-get", "update"}).
WithExec([]string{"rm", "-rf", "/var/lib/apt/lists/*"}).
[...]
if i try using something like this:
WithExec([]string{"apt-get", "update","&&","rm", "-rf", "/var/lib/apt/lists/*"}).
The contaner build complains saying that the command "apt-get update" only takes one argument.
I dont fully underestand how the commands are interpreted in order to fail in this case.
Using the tool "dive" https://github.com/wagoodman/dive i can see that multiple layers are generated for an apt update and i whould like to optimize as much as possible the number of layers. Run this kind of creation and eliminations at once whould prevent from creating aditional layers, making the container bigger.
I am missing something?
Sorry in advance if it's a naive question, i really like this project and i whould like to build my pipelines with it. | https://github.com/dagger/dagger/issues/4890 | https://github.com/dagger/dagger/pull/1092 | 164e6e3eb8d3776de95a77fc810475c18d0a6aff | b4b472390c0087836c0b0c56843888ff2316a122 | "2023-04-03T21:18:28Z" | go | "2021-11-03T22:08:13Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,878 | ["website/src/css/custom.scss"] | Change highlighting of code in Getting Started etc in docs. Hard to read with light-colored boxes on top of code | Change highlighting of code in Getting Started etc in docs. Hard to read with large light-colored boxes on top of code. Maybe some annotations on side? Like a heavy vertical line?
Looks great
<img width="200" alt="image" src="https://user-images.githubusercontent.com/3187222/229232155-3299838d-8a62-40ea-8a75-b89e33b285e2.png">
Harder to read
<img width="200" alt="image" src="https://user-images.githubusercontent.com/3187222/229232249-a72310cc-a98a-40f4-9153-b39a1649137a.png">
| https://github.com/dagger/dagger/issues/4878 | https://github.com/dagger/dagger/pull/4882 | 72d64d2d6426be1d62d2f0a8374046f0c51a37cb | 8183343df314a40281e5175b92d9a4af8e691444 | "2023-03-31T21:14:29Z" | go | "2023-04-04T23:06:06Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,866 | ["docs/current/guides/757394-use-service-containers.md", "docs/current/guides/snippets/use-services/use-db-service/index.ts", "docs/current/guides/snippets/use-services/use-db-service/main.go", "docs/current/guides/snippets/use-services/use-db-service/main.py"] | Add a full-fledged example to the service containers guide | ### What is the issue?
Add an example of standing up a database service for application testing to the guide at https://docs.dagger.io/757394/use-service-containers
This is a pending task from when the guide was originally created by @vito | https://github.com/dagger/dagger/issues/4866 | https://github.com/dagger/dagger/pull/4867 | 8a6c8e200cc33536edf5cb1746d265f068f40758 | ec917dc02128089f55d35c0d3450a900caf4fbe7 | "2023-03-30T09:02:15Z" | go | "2023-03-30T18:48:11Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,865 | ["docs/current/sdk/nodejs/783645-get-started.md"] | Update Node get started to specify the TS configuration file if not present | ### What is the issue?
The Typescript code fails if the user doesn't have a `tsconfig.json` file already. This is not explicitly stated anywhere, so should be added. The command to create the file if not already present is `tsc --init --module esnext --moduleResolution nodenext` | https://github.com/dagger/dagger/issues/4865 | https://github.com/dagger/dagger/pull/4868 | b56aee2fe0875c1e3b5b47372e91afbee158c9ce | 522e4b82710927a4826f1a904cc1414a73d3a2be | "2023-03-30T08:55:53Z" | go | "2023-03-30T14:11:49Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,856 | ["sdk/python/pyproject.toml", "sdk/python/src/dagger/api/base.py"] | ✨ Python reference: coroutines aren't clearly shown | ### What are you trying to do?
The Python reference documentation doesn't clearly show a method as being a coroutine.
<img width="720" alt="Screenshot 2023-03-29 at 17 12 08" src="https://user-images.githubusercontent.com/174525/228616311-f72cbc39-692a-4b85-9afd-6a0f60701a6f.png">
### Why is this important to you?
We used to have a note in the description clarifying that it needs to be `await`ed, but it was removed for simplicity. Making it clearer again can be helpful to users.
### How are you currently working around this?
The IDE's autocomplete should show the return type as being a coroutine:
<img width="820" alt="Screenshot 2023-02-22 at 12 25 06" src="https://user-images.githubusercontent.com/174525/228614792-374a7a8b-61b4-43f7-be2d-99a617d45fae.png">
In the reference you can notice that if it raises errors, then it's a coroutine because coroutines are only the methods that make a request to the API, which can return an error. It's also only when a simple value is returned (e.g., `str` primitive).
<img width="727" alt="Screenshot 2023-03-29 at 16 58 33" src="https://user-images.githubusercontent.com/174525/228613390-87509deb-efb3-4cea-9816-96c92e301eb0.png">
| https://github.com/dagger/dagger/issues/4856 | https://github.com/dagger/dagger/pull/5477 | d9249d675855405598247977bac98a616649e3ed | e0b1b8e4b32fb3048ec5caf1ab631b10bced8cdf | "2023-03-29T17:08:54Z" | go | "2023-07-18T12:47:21Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,823 | ["sdk/nodejs/provisioning/bin.ts"] | 🐞 Dagger doesn't respect SIGINT (ctrl-c) | ### What is the issue?
From @RonanQuigley
> Dagger does not respect SIGINT (ctrl + c). Able to replicate on Mac and Linux.
> We would request prioritising getting the SIGINT issue fixed ASAP. We found that it's a productivity killer (we made a lot of mistakes when making our pipelines, and having to kill the process manually each time gets tedious very quickly).
### Log output
n/a
### Steps to reproduce
run a pipeline and hit ctrl-c. It seems like SIGINT is ignored while the Dagger pipeline is running.
### SDK version
Node.js SDK
### OS version
mac, linux | https://github.com/dagger/dagger/issues/4823 | https://github.com/dagger/dagger/pull/4911 | 0433b11cd2fc894a40a8c9f6ea94c6a87cd6fe78 | 4be9d6ee11cb5d3a66ec3e0f846436535009fd5e | "2023-03-23T17:12:40Z" | go | "2023-04-09T13:40:31Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,821 | ["sdk/python/src/dagger/api/base.py", "sdk/python/tests/api/test_inputs.py", "sdk/python/tests/api/test_integration.py"] | 🐞 Env Variable as empty string blows up | ### What is the issue?
🐛 If you have an env var that is an empty string, Graphql explodes as it's converted to null or undefined. To fix it you have to add whitespace
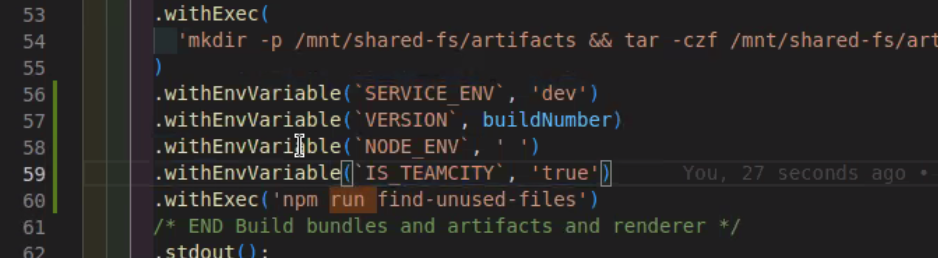
### Log output
```console
Field "withEnvVariable" argument "value" of type "String!" is required but not provided.
```
### Steps to reproduce
```javascript
import * as dagger from '@dagger.io/dagger';
dagger.connect(async (client) => {
const out = await client
.container()
.from('alpine')
.withEnvVariable('FOO', '')
.withExec(['sh', '-c', 'env | grep FOO'])
.stdout();
console.log(out)
})
```
This produces the following query, which is missing the value:
<pre>
{
container {
from (address: "alpine") {
withEnvVariable (<b>name: "FOO"</b>) {
withExec (args: ["sh","-c","env | grep FOO"]) {
stdout
}
}
}
}
}
</pre>
### SDK version
NodeJS v0.4.2
| https://github.com/dagger/dagger/issues/4821 | https://github.com/dagger/dagger/pull/4884 | d272752757543708fa602748674934cdf9f49321 | 928ecb215844247b65d29146ce6c23f6c0bdce8a | "2023-03-23T15:54:41Z" | go | "2023-04-03T16:55:03Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,812 | ["docs/current/guides/252029-load-images-local-docker-engine.md", "docs/current/guides/snippets/load-images-local-docker-engine/export/index.mjs", "docs/current/guides/snippets/load-images-local-docker-engine/export/main.go", "docs/current/guides/snippets/load-images-local-docker-engine/export/main.py", "docs/current/guides/snippets/load-images-local-docker-engine/push/index.mjs", "docs/current/guides/snippets/load-images-local-docker-engine/push/main.go", "docs/current/guides/snippets/load-images-local-docker-engine/push/main.py"] | Create a guide about building a Docker container for local use with Dagger | There are quite a few questions in Discord about building an image locally without publishing to a remote registry:
https://discord.com/channels/707636530424053791/1068148348240019498
https://discord.com/channels/707636530424053791/1046817210695417906/1046817210695417906
https://discord.com/channels/707636530424053791/1081963303921799269/1081963303921799269
https://discord.com/channels/707636530424053791/1047912353469059165
https://discord.com/channels/707636530424053791/1080194783953305661
https://github.com/dagger/dagger/issues/4579
https://github.com/dagger/dagger/issues/4089
This issue is to create a guide outlining the options to do this with Dagger. | https://github.com/dagger/dagger/issues/4812 | https://github.com/dagger/dagger/pull/4814 | 70268101d7e661cc856a31b63918d16eeccaece2 | b758ad7ae59471f2fbb94c1f3b61c47381dc349c | "2023-03-22T08:36:20Z" | go | "2023-03-31T18:28:47Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,801 | ["core/integration/container_test.go", "core/schema/container.go"] | Using a secret from `setSecret` with `withRegistryAuth` fails | @vikram-dagger tried to use a new version of secrets in the registry authentication and it failed:
Here is a repro
```go
package main
import (
"context"
"fmt"
"os"
"dagger.io/dagger"
)
func main() {
ctx := context.Background()
c, err := dagger.Connect(ctx, dagger.WithLogOutput(os.Stderr))
if err != nil {
panic(err)
}
sec := c.SetSecret("my-secret-id", "yolo")
stdout, err := c.Container().WithRegistryAuth("localhost:8888", "YOLO", sec).Publish(ctx, "localhost:8888/myorg/myapp")
if err != nil {
panic(err)
}
fmt.Println(stdout)
}
```
Result:
```console
panic: input:1: container.withRegistryAuth plaintext: empty secret?
Please visit https://dagger.io/help#go for troubleshooting guidance.
goroutine 1 [running]:
main.main()
/home/dolanor/src/daggerr/vikram-setsecret-empty/main.go:21 +0x1b1
exit status 2
``` | https://github.com/dagger/dagger/issues/4801 | https://github.com/dagger/dagger/pull/4809 | 0fa00a1f4905be1eb6fb017f3c87e0a09112c586 | aaba659eccbc858a0f330c5178cb7ea20f997c94 | "2023-03-21T15:16:35Z" | go | "2023-03-22T08:14:34Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,790 | ["sdk/nodejs/api/test/api.spec.ts", "sdk/nodejs/api/utils.ts"] | 🐞 Using containers in `platformVariants` seems to give too much data (queryTree) | ### What is the issue?
reference: https://discord.com/channels/707636530424053791/1086025025947193444/1086025025947193444
It seems reusing a container to put in a `platformVariants` in Node SDK transform it as the Query Object, and not the container itself
### Log output
```graphql
{ container { publish (address: "registry.gitlab.com/jobilla/backend-services/migrations/migrated-8:latest",platformVariants: [{"_queryTree":[{operation:"container",args:{platform:"linux/amd64"}},{operation:"from",args:{address:"mysql:8.0"}},{operation:"withEnvVariable",args:{name:"MYSQL_USER",value:"jobilla"}},{operation:"withEnvVariable",args:{name:"MYSQL_PASSWORD",value:"jobilla"}},{operation:"withEnvVariable",args:{name:"MYSQL_DATABASE",value:"jobilla"}},{operation:"withEnvVariable",args:{name:"MYSQL_ROOT_PASSWORD",value:"password"}},{operation:"withExposedPort",args:{port:3306}},{operation:"withExec",args:{args:[]}}],clientHost:"127.0.0.1:56726",sessionToken:"f74916b3-f236-47e4-bd1f-58e367035a32",client:{url:"http://127.0.0.1:56726/query",options:{headers:{Authorization:"Basic Zjc0OTE2YjMtZjIzNi00N2U0LWJkMWYtNThlMzY3MDM1YTMyOg=="}}}},{"_queryTree":[{operation:"container",args:{platform:"linux/arm64"}},{operation:"from",args:{address:"mysql:8.0"}},{operation:"withEnvVariable",args:{name:"MYSQL_USER",value:"jobilla"}},..."}}}}]) } }
```
### Steps to reproduce
```typescript
import Client, { connect } from "@dagger.io/dagger";
import { ClientContainerOpts, ContainerPublishOpts } from "@dagger.io/dagger/dist/api/client.gen";
connect(async (client: Client) => {
let migratedPlatformVariants = [];
let seededPlatformVariants = [];
for (let platform of ["linux/amd64", "linux/arm64"]) {
const db = client
.container({ platform } as ClientContainerOpts)
.from("mysql:8.0")
.withEnvVariable("MYSQL_USER", "jobilla")
.withEnvVariable("MYSQL_PASSWORD", "jobilla")
.withEnvVariable("MYSQL_DATABASE", "jobilla")
.withEnvVariable("MYSQL_ROOT_PASSWORD", "password")
.withExposedPort(3306)
.withExec(["ls"]);
await db.exitCode();
seededPlatformVariants.push(db);
}
await client.container().publish(`docker.io/marcosnils/migrated-8:latest`, {
platformVariants: seededPlatformVariants
});
}, { LogOutput: process.stderr });
```
### SDK version
Node.js SDK v0.4.1
### OS version
Ubuntu 20.04 | https://github.com/dagger/dagger/issues/4790 | https://github.com/dagger/dagger/pull/4800 | 7215711bac3ff9442d0bb59b8d2cc6b2bee70867 | b8051d3fa73d932050d16863bd1205d68a85c663 | "2023-03-18T09:19:02Z" | go | "2023-03-23T02:32:42Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,783 | ["website/src/css/custom.scss"] | [Docs] Codeblock size inside guides is too narrow | The codeblocks inside the guides is a bit more narrow than it should.
The UX when looking at code snippets is not the best, as it requires constant horizontal scrolling to read large lines.
<img src="https://user-images.githubusercontent.com/70334844/225992687-8c613d89-be19-441d-8e47-4d453cb41a1a.png" height="700px"><img> | https://github.com/dagger/dagger/issues/4783 | https://github.com/dagger/dagger/pull/4784 | bd9b551b9cae4c22708014c9a5c8e3ade92d29c5 | d2c645a81535e19d2fddd1727817b5fdb5bc7fbc | "2023-03-17T18:51:58Z" | go | "2023-03-21T10:49:11Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,773 | ["sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py", "sdk/python/src/dagger/codegen.py", "sdk/python/tests/api/test_inputs.py"] | 🐞 Can't instantiate BuildArg() | You can't instantiate input objects like [BuildArg](https://dagger-io.readthedocs.io/en/sdk-python-v0.4.0/api.html#dagger.api.gen.BuildArg) in [Container.build](https://dagger-io.readthedocs.io/en/sdk-python-v0.4.0/api.html#dagger.api.gen.Container.build) with Python SDK v0.4.0:
```python
import dagger
arg = dagger.BuildArg("NAME", "value") # TypeError: BuildArg() takes no arguments
```
Current workaround:
```python
import dagger
arg = dagger.BuildArg()
arg.name = "NAME"
arg.value = "value
```
Reported by a [discord](https://discord.com/channels/707636530424053791/1085912251627745431/1085912953464815616) user. | https://github.com/dagger/dagger/issues/4773 | https://github.com/dagger/dagger/pull/4774 | 7439aeb3350ef1f3de10520208dc80341483d09d | 5ca5adad5a9fe23f67ea956f08aec16f47f6f6f5 | "2023-03-16T13:58:36Z" | go | "2023-03-16T15:35:08Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,770 | ["package.json", "yarn.lock"] | Docs: guides URL | For our docs URL schema, we've been using the "knowledge base" model, where each article has a unique ID, allowing decoupling between its content and the overall information architecture. This has proven useful overall.
But I think we have applied this model to certain pages where it doesn't make sense. One example is [our guides section](https://docs.dagger.io/278912/guides). A list of guides is not a knowledge base article, and therefore should not have an article ID embedded in its URL. It would make more sense for that page to be at `https://docs.dagger.io/guides`. This is part of the decoupling I mention above.
There may be other pages where the same observation applies. I haven't checked thoroughly.
| https://github.com/dagger/dagger/issues/4770 | https://github.com/dagger/dagger/pull/3412 | 6c873dce63f70d381d7d9f4e1ecede44897c6c03 | 1a054f0e84665492c850e043355c5c409d1b881c | "2023-03-15T20:25:30Z" | go | "2022-10-21T08:50:02Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,761 | ["website/src/theme/DocTagDocListPage/index.js", "website/src/theme/DocTagsListPage/index.js"] | [Docs] Tags page should be hidden | This discussion arose from #4759
> Docusaurus has a default tags page, which https://github.com/facebook/docusaurus/discussions/5542.
> Currently it lives [here](https://docs.dagger.io/tags/).
> It also leads to [these pages being rendered](https://docs.dagger.io/tags/circle-ci/).
@vikram-dagger LMK if we should hide these pages.
[The tags are also present at the end of the guides.](https://docs.dagger.io/648384/multi-builds/) | https://github.com/dagger/dagger/issues/4761 | https://github.com/dagger/dagger/pull/4794 | d2c645a81535e19d2fddd1727817b5fdb5bc7fbc | 7fd75993168d1632cd2b30570881b7d1ef204270 | "2023-03-14T19:10:55Z" | go | "2023-03-21T10:49:34Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,748 | ["cmd/engine/main.go", "engine/remotecache/cache.go", "engine/remotecache/s3.go"] | remotecache: error exporting manifest during concurrent garbage collection | Found while debugging a large job that was running on a small (20 GB) disk. The small disk size caused buildkit to very aggressively prune almost the whole local cache, but that happened in parallel with the export which caused a layer to not be found and failed the export.
The very temporary workaround is to use a larger disk size, but that will just make the issue more rare.
The fix on our end is to grab a lease on the content in the content store in the time between the client passing off the export request and when the s3 cache manager actually pushes it. Should be able to just pass the content store and the lease manager to the s3 manager since that's all available in our main func. | https://github.com/dagger/dagger/issues/4748 | https://github.com/dagger/dagger/pull/4758 | 076b60be9a01c1e42a47ccf23f81405c2c640c9b | 67c7e7635cf4ea0e446e2fed522a3e314c960f6a | "2023-03-10T21:02:05Z" | go | "2023-03-14T16:43:00Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,745 | ["core/host.go", "core/integration/host_test.go", "core/schema/host.go", "core/schema/host.graphqls", "sdk/go/api.gen.go", "sdk/nodejs/api/client.gen.ts", "sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py"] | ✨ `(*dagger.Client).Host().Directory(...)` equivalent for a `*dagger.File` | ### What are you trying to do?
I am using `container.WithMountedFile` but `Host()` only lets me retrieve a `*dagger.Directory`, and there is no equivalent for a `*dagger.File`
### Why is this important to you?
It would be nice to have and would eliminate some boilerplate :)
### How are you currently working around this?
Right now I'm doing
```go
path := "/path/to/my/file.txt"
file := client.Host().Directory(filepath.Dir(path)).File(filepath.Base(path))
client.Container().From("alpine").WithFile("/file.txt", file)
``` | https://github.com/dagger/dagger/issues/4745 | https://github.com/dagger/dagger/pull/5317 | 3df28c2ba50452ed4df7ed2693ae97611428a007 | a89a7c3cc34bdb4c15cbdbe415b491157717d14d | "2023-03-09T21:56:42Z" | go | "2023-06-15T19:10:03Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,742 | ["docs/current/guides/205271-replace-dockerfile.md", "docs/current/guides/406009-multiplatform-support.md", "docs/current/guides/421437-work-with-host-filesystem.md", "docs/current/guides/544174-multistage-build.md", "docs/current/partials/_install-sdk-go.md", "docs/current/quickstart/628381-quickstart-sdk.mdx", "docs/current/sdk/go/959738-get-started.md", "website/static/guides.json"] | As of v0.5.0, Go SDK requires Go v1.20 | ### What is the issue?
Most of our Go SDK docs - like this page https://docs.dagger.io/sdk/go/371491/install - mention that the Dagger Go SDK requires Go 1.15 or later.
As of https://github.com/dagger/dagger/releases/tag/sdk%2Fgo%2Fv0.5.0, we require Go 1.20 or later. This is what happens when we run Go SDK v0.5.0 with Go v1.19: https://github.com/dagger/registry-redirect/actions/runs/4377426015/jobs/7660890220#step:4:17
<img width="975" alt="image" src="https://user-images.githubusercontent.com/3342/224125782-44e496d4-32b2-42eb-8787-0e73da525c3b.png">
We should update our docs to reflect this.
My suggestion would be to require everywhere Go 1.20 or newer.
| https://github.com/dagger/dagger/issues/4742 | https://github.com/dagger/dagger/pull/4743 | 479c3d3b4dd2933711317f87990e8d632e10a76e | 92d23111368d9d480107f2980b7adfba0ddfb496 | "2023-03-09T18:50:53Z" | go | "2023-03-10T16:43:19Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,708 | ["sdk/python/src/dagger/engine/cli.py"] | Python: Don't buffer output from `dagger session` | Especially when using `log_output=sys.stderr`, it'll help get the output lines from the engine in a better order ([context](https://discord.com/channels/707636530424053791/1080527877688275015/1080874047325339708)). | https://github.com/dagger/dagger/issues/4708 | https://github.com/dagger/dagger/pull/4719 | fd61da9d094ef8c31c0d54bfd2d47e592ac49c9b | 71b45ad788cae77b89016d47ddbb16444f8cad26 | "2023-03-06T14:29:27Z" | go | "2023-03-08T18:36:50Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,670 | ["website/plugins/docusaurus-plugin-guides/index.js", "website/src/components/molecules/guideIndex.js", "website/src/css/molecules/guideIndex.module.scss", "website/src/css/molecules/tag.module.scss"] | Add filtering method to guide index page | When visiting [the current guide index](https://docs.dagger.io/278912/guides/), we can only filter the guides by clicking in the tags made visible by the first few guides. We should give the user an option to see all available filters when visiting the page so they can search freely across guides.
I'll look into the best implementation for this. I've already mentioned [this showcase](https://docusaurus.io/showcase) as inspiration for tag filtering, but agree with @vikram-dagger that we'll have a hard time once we have more tags to display. | https://github.com/dagger/dagger/issues/4670 | https://github.com/dagger/dagger/pull/4686 | 71b45ad788cae77b89016d47ddbb16444f8cad26 | dd953f3982a4fd9cd3cb2140b876e0910bce144f | "2023-02-28T20:52:34Z" | go | "2023-03-08T18:54:12Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,668 | ["core/container.go", "core/errors.go", "core/integration/container_test.go", "core/integration/services_test.go", "core/schema/container.go", "core/schema/container.graphqls", "sdk/go/api.gen.go", "sdk/nodejs/api/client.gen.ts", "sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py"] | Lazy executions are confusing to understand and sometimes don't work as expected | ## Summary
Developers are often confused by a property of the Dagger engine called "laziness": pipelines are executed at the latest possible moment, to maximize performance. There are several dimensions to this problem; below is an overview of different dimensions, and status of possible solutions.
| Issues | Proposals |
| ------------------------------------------- | ------------------------ |
| [No `withExec`](#with-exec) | <li>#4833</li> |
| [`Dockerfile` build (without exec)](#build) | <li>#5065</li> |
| [Implicit query execution](#implicit) | <li>#5065</li> |
| [Multiple ways to execute](#multiple) | “Pipeline builder” model |
| [Documentation](#docs) | <li>#3617</li> |
## <a name="with-exec"></a>Issue: no `withExec`
We had some users report that part of their pipeline wasn't being executed, for which they had to add a `WithExec(nil)` statement for it to work:
```go
_, err := c.Container().Build(src).ExitCode(ctx) // doesn't work
_, err := c.Container().From("alpine").ExitCode(ctx) // same thing
```
### Explanation
Users may assume that since they know there’s an `Entrypoint`/`Cmd` in the docker image it should work, but it’s just updating the dagger container metadata. There’s nothing to run, it’s equivalent to the following:
```go
_, err := ctr.
WithEntrypoint([]string{}).
WithDefaultArgs(dagger.ContainerWithDefaultArgsOpts{
Args: []string{"/bin/sh"},
})
ExitCode(ctx) // nothing to execute!
```
`ExitCode` and `Stdout` only return something for the **last executed command**. That means the equivalent of a `RUN` instruction in a `Dockerfile` or running a container with `docker run`.
### Workaround
Add a `WithExec()` to tell dagger to execute the container:
```diff
_, err := client.Container().
Build(src).
+ WithExec(nil).
ExitCode(ctx)
```
The empty (`nil`) argument to `WithExec` will execute the **entrypoint and default args** configured in the dagger container.
> **Note**
> If you replace the `.ExitCode()` with a `Publish()`, you see that `Build()` is called and the image is published, because `Publish` doesn’t depend on execution but `Build` is still a dependency.
The same is true for a bound service:
```diff
db := client.Container().From("postgres").
WithExposedPort(5432).
+ WithExec(nil)
ctr := app.WithServiceBinding("db", db)
```
Here, `WithServiceBinding` clearly needs to execute/run the *postgres* container so that *app* can connect to it, so we need the `WithExec` here too (with `nil` for default entrypoint and arguments).
### Proposals
To avoid astonishment, a fix was added (#4716) to raise an error when fields like `.ExitCode` or `.WithServiceBinding` (that depend on `WithExec`) are used on a container that hasn’t been executed.
However, perhaps a better solution is to implicitly execute the entrypoint and default arguments because if you’re using a field that depends on an execution, we can assume that you mean to execute the container.
This is what #4833 proposes, meaning the following would now work as expected by users:
```go
// ExitCode → needs execution so use default exec
_, err := c.Container().From("alpine").ExitCode(ctx)
// WithServiceBinding → needs execution so use default exec
db := client.Container().From("postgres").WithExposedPort(5432)
ctr := app.WithServiceBinding("db", db)
```
```[tasklist]
### No `withExec`
- [x] #4716
- [ ] #4833
```
## <a name="build"></a>Issue: `Dockerfile` build (without exec)
Some users just want to test if a `Dockerfile` build succeeds or not, and **don’t want to execute the entrypoint** (e.g., long running executable):
```go
_, err = client.Container().Build(src).ExitCode(ctx)
```
In this case users are just using `ExitCode` as a way to trigger the build when they also don’t want to `Publish`. It’s the same problem as above, but the intent is different.
### Workarounds
With #4919, you’ll be able to skip the entrypoint:
```go
_, err = client.Container().
Build(src).
WithExec([]string{"/bin/true"}, dagger.ContainerWithExecOpts{
SkipEntrypoint: true,
}).
ExitCode(ctx)
```
But executing the container isn’t even needed to build, so `ExitCode` isn’t a good choice here. It’s just simpler to use another field such as:
```diff
- _, err = client.Container().Build(src).ExitCode(ctx)
+ _, err = client.Container().Build(src).Rootfs().Entries(ctx)
```
However this isn’t intuitive and is clearly a workaround (not meant for this).
### Proposal
Perhaps the best solution is to use a general synchronization primitive (#5065) that simply forces resolving the laziness in the pipeline, especially since the result is discarded in the above workarounds:
```diff
- _, err = client.Container().Build(src).ExitCode(ctx)
+ _, err = client.Container().Build(src).Sync(ctx)
```
```[tasklist]
### `Dockerfile` build (without exec)
- [x] #4919
- [ ] #5065
```
## <a name="implicit"></a>Issue: Implicit query execution
Some functions are “lazy” and don’t result in a [query execution](http://spec.graphql.org/October2021/#sec-Execution) (e.g., `From`, `Build`, `WithXXX`), while others execute (e.g., `ExitCode`, `Stdout`, `Publish`).
It’s not clear to some users which is which.
### Explanation
The model is implicit, with a “rule of thumb” in each language to hint which ones execute:
- **Go:** functions taking a context and returning an error
- **Python** and **Node.js:** `async` functions that need an `await`
Essentially, each SDK’s codegen (the feature that introspects the API and builds a dagger client that is idiomatic in each language) **transforms [leaf fields](http://spec.graphql.org/October2021/#sec-Leaf-Field-Selections) into an implicit API request** when called, and return the **value from the response**.
So the “rule of thumb” is based on the need to make a request to the GraphQL server, the problem is that it may not be immediately clear and the syntax can vary depending on the language so there’s different “rules” to understand.
This was discussed in:
- #3555
- #3558
### Proposal
The same [Pipeline Synchronization](https://github.com/dagger/dagger/issues/5065) proposal from the previous issue helps make this a bit more explicit:
```go
_, err := ctr.Sync(ctx)
```
```[tasklist]
### Implicit query execution
- [x] #3555
- [x] #3558
- [ ] #5065
```
## <a name="multiple"></a>Issue: Multiple ways to execute
“Execution” sometimes mean different things:
- **Container execution** (i.e., `Container.withExec`)
- **[Query execution](http://spec.graphql.org/October2021/#sec-Execution)** (i.e., making a request to the GraphQL API)
- **”Engine” execution** (i.e., doing actual work in BuildKit)
The *ID* fields like `Container.ID` for example, make a request to the API, but don’t do any actual work building the container. We reduced the scope of the issue in the SDKs by avoiding passing IDs around (#3558), and keeping the pipeline as lazy as possible until an output is needed (see [Implicit query execution](#implicit) above).
More importantly, users have been using `.ExitCode(ctx)` as the goto solution to “synchronize” the laziness, but as we’ve seen in the above issues, it triggers the container to execute and there’s cases where you don’t want to do that.
However, adding the general `.Sync()` (#4205) to fix that may make people shift to using it as the goto solution to “resolve” the laziness instead (“synchronize”), which actually makes sense. The problem is that we now go back to needing `WithExec(nil)` because `.Sync()` can’t assume you want to execute the container.
That’s a catch 22 situation! **There’s no single execute function** to “rule them all”.
It requires the user to have a good enough grasp on these concepts and the Dagger model to chose the right function for each purpose:
```go
// exec the container (build since it's a dependency)
c.Container().Build(src).ExitCode(ctx)
// just build (don't exec)
c.Container().Build(src).Sync(ctx)
```
### Proposal
During the “implicit vs explicit” discussions, the proposal for the most explicit solution was for a “pipeline builder” model (https://github.com/dagger/dagger/issues/3555#issuecomment-1301327344).
The idea was to make a clear separation between **building** the lazy pipeline and **executing** the query:
```go
// ExitCode doesn't implicitly execute query here! Still lazy.
// Just setting expected output, and adding exec as a dependency.
// Build is a dependency for exec so it also runs.
q := c.Container().Build(src).ExitCode()
// Same as above but don't care about output, just exec.
q := c.Container().Build(src).WithExec(nil)
// Same as above but don't want to exec, just build.
q := c.Container().Build(src)
// Only one way to execute query!
client.Query(q)
```
### Downsides
- It’s a big **breaking change** so it’s not seen as a viable solution now
- No great solution to grab output values
- More boilerplate for simple things
### Solution
Embrace the laziness!
## Issue: <a name="docs"></a>Documentation
We have a guide on [Lazy Evaluation](https://docs.dagger.io/api/975146/concepts#lazy-evaluation) but it’s focused on the GraphQL API and isn’t enough to explain the above issues.
We need better documentation to help users understand the “lazy DAG” model (https://github.com/dagger/dagger/issues/3617). It’s even more important if the “pipeline builder” model above isn’t viable.
```[tasklist]
### Documentation
- [x] #3622
- [ ] #3617
```
## Affected users
These are only some examples of users that were affected by this:
- from @RonanQuigley
> DX or a Bug: In order to have a dockerfile's entrypoint executed, why did we need to use a dummy withExec? There was a unamious 😩 in our team call after we figured this out.
- https://discord.com/channels/707636530424053791/708371226174685314/1079926439064911972
- https://discord.com/channels/707636530424053791/1080160708123185264/1080174051965812766
- https://discord.com/channels/707636530424053791/1080160708123185264/1080174051965812766
- #5010
| https://github.com/dagger/dagger/issues/4668 | https://github.com/dagger/dagger/pull/4716 | f2a62f276d36918b0e453389dc7c63cad195da59 | ad722627391f3e7d5bf51534f846913afc95d555 | "2023-02-28T17:37:30Z" | go | "2023-03-07T23:54:56Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,652 | ["cmd/engine/config.go", "cmd/engine/main.go", "go.mod", "go.sum", "internal/mage/engine.go", "network/network.go"] | 🐞 network issue with [email protected] in k8s pod | ### What is the issue?
May cause by https://github.com/dagger/dagger/pull/4505
Here a new search in `/etc/resolv.conf` added before k8s searches
```conf
search dns.dagger
search gitlab.svc.cluster.local. svc.cluster.local. cluster.local. openstacklocal
options ndots:5
```
Then network because very slow to fetch anything.
### Log output
_No response_
### Steps to reproduce
_No response_
### SDK version
Go SDK v0.4.6
### OS version
Unbuntu | https://github.com/dagger/dagger/issues/4652 | https://github.com/dagger/dagger/pull/4666 | 253e3b227216468202a5b512838a77bd4315ed06 | 318cb775bed51438f6eb45fb4c96db1c2a2d39cd | "2023-02-27T08:40:03Z" | go | "2023-03-01T01:16:55Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,648 | ["cmd/engine/main.go", "core/integration/remotecache_test.go", "engine/remotecache/cache.go", "engine/remotecache/combined.go", "engine/remotecache/s3.go"] | Fix cache export running when image/local exports run | We currently have to open separate sessions when doing an image/local export. This causes cache export to run in these subsessions, which is confusing because it means the manifest will be written for the subsession and then later the main session. Additionally, if the refs being tracked only end up in these subsessions, then if the manifest written by the main session at the end overwrites those refs might not be part of the export 😵💫
If you use the pooling support this is less of an issue since manifests are additive, but even then it's still not ideal. | https://github.com/dagger/dagger/issues/4648 | https://github.com/dagger/dagger/pull/4715 | 2c04a71b0cdcbbe0e9712743a383f652a9f1211e | 2b4831aff2fddbf68a5941b873e03ceb1e48f185 | "2023-02-24T21:53:07Z" | go | "2023-03-07T18:19:57Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,633 | ["go.mod", "go.sum"] | API Playground embed throws errors | ### What is the issue?
To reproduce:
- Visit https://docs.dagger.io/593914/quickstart-hello
- Click the Play button in the API playground embed (any language)
- Login if prompted
- Click the Play button
- An error message appears like `forwarding ResolveImageConfig: no such job 7lnkxo8kly8o9exjzsue7qgv0`
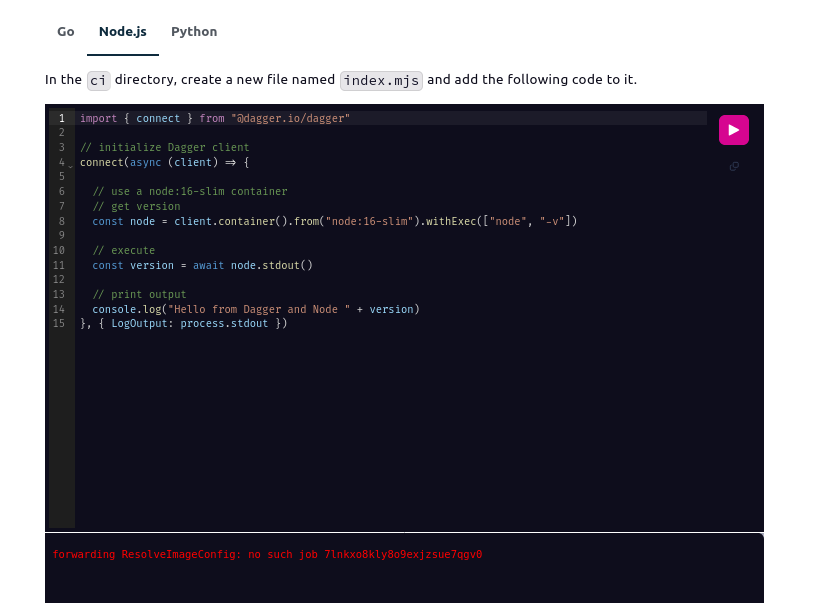
| https://github.com/dagger/dagger/issues/4633 | https://github.com/dagger/dagger/pull/1021 | 6a663c5c0ac4287395384f359a150bdb00fb5991 | 61c783773463d05d36863ff8042721d1778e5564 | "2023-02-22T09:18:14Z" | go | "2021-09-27T21:54:18Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,620 | ["core/integration/container_test.go", "go.mod", "go.sum", "internal/mage/engine.go"] | Engine fails, hangs or becomes really slow when several operations are chained | Some users (cc @cpuguy83 ) have observed that when chaining several `WithMountedDirectory` calls, sometimes the engine becomes extremely slow or completely unresponsive.
Discord thread: https://discord.com/channels/707636530424053791/1075544944388882522
@cpuguy83 managed to come up with a simple repro here.
Repro snippet: https://gist.github.com/cpuguy83/b61f22d05fd05a6407008d944f5858f4
cc @sipsma | https://github.com/dagger/dagger/issues/4620 | https://github.com/dagger/dagger/pull/4817 | aaba659eccbc858a0f330c5178cb7ea20f997c94 | 7215711bac3ff9442d0bb59b8d2cc6b2bee70867 | "2023-02-17T23:17:51Z" | go | "2023-03-22T23:13:15Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,588 | ["core/container.go", "core/integration/container_test.go", "core/util.go"] | DX: Container.From() and Container.Build() creates fresh container | Discord context: https://discord.com/channels/707636530424053791/1074736111131304057/1074747695765405696
Summary:
Container.From() and Container.Build() create a new container. Chaining this with `.client.Container()` instead of directly `client.From()`/`container.Build()`, or `client.ContainerFrom()`/`client.ContainerBuild()` introduces opportunity for potential footguns.
For example:
```js
const foo = await client
.container()
.withEnvVariable('BUST_CACHE', Math.random().toString())
.build(node)
.withExec(['node', '--version'])
.stdout();
```
The cache busting env variable will never actually make it to the engine since the environment is replaced (correct me if this is wrong) | https://github.com/dagger/dagger/issues/4588 | https://github.com/dagger/dagger/pull/5052 | f5f76fcbda4b772a54aecc43812bd03e51c0d62c | 7d8738d64889572fd5ac7d9930c2f16b4c3629d2 | "2023-02-13T18:00:22Z" | go | "2023-05-19T18:05:02Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,570 | ["go.mod", "go.sum"] | API Reference Unification | As discussed in #4291, it would be great to present all references of all different SDKs in the same page.
Right now, every SDK has their own specific generation, with a tool of their own language.
We should find a possible implementation and try to estimate how much engineering time it would take to implement something like this.
Dagger might be a possible answer, as we could create different containers, one for each SDK, and export the type/interface/functions.
Context:
- [Python SDK](https://dagger-io.readthedocs.io/en/sdk-python-v0.3.2/)
- [GO SDK](https://pkg.go.dev/dagger.io/dagger)
- [Node SDK](https://deploy-preview-4537--devel-docs-dagger-io.netlify.app/current/sdk/nodejs/reference/modules)
- [GraphQL API](https://docs.dagger.io/api/reference/) | https://github.com/dagger/dagger/issues/4570 | https://github.com/dagger/dagger/pull/900 | 922802185fb0c7351741fe7e9e7206f626c00c0e | 4468898f4bb209c08576810d47f6c39d3b4e5ae0 | "2023-02-09T22:27:13Z" | go | "2021-08-20T15:09:01Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,538 | ["sdk/go/internal/engineconn/session.go"] | 🐞 Sometimes pipelines fail with EOF randomly | ### What is the issue?
Some users have reported that seems like after bumping go to 1.20, some pipelines have randomly started to fail with an `EOF` error:
- https://discord.com/channels/707636530424053791/1070997951503151104
- https://discord.com/channels/707636530424053791/1071076724458131610
### Log output
```
time="2023-02-03T16:10:48Z" level=debug msg="session finished: <nil>"
time="2023-02-03T16:10:49Z" level=debug msg="remove snapshot" key=a3pnf2678hnh2qw8fsy4dyuws snapshotter=overlayfs
time="2023-02-03T16:10:49Z" level=debug msg="remove snapshot" key=ky13d6x635szkhqgvp2u076q9 snapshotter=overlayfs
time="2023-02-03T16:10:49Z" level=debug msg="remove snapshot" key=m4ifraund99pseap731l6ubyw snapshotter=overlayfs
time="2023-02-03T16:10:49Z" level=debug msg="remove snapshot" key=w5ogr03j22gzyqg5wzkg0fh35 snapshotter=overlayfs
time="2023-02-03T16:10:49Z" level=debug msg="remove snapshot" key=x7qr5jqyi5jht905l8idi9vzd snapshotter=overlayfs
time="2023-02-03T16:10:49Z" level=debug msg="schedule snapshotter cleanup" snapshotter=overlayfs
time="2023-02-03T16:10:49Z" level=debug msg="removed snapshot" key=buildkit/26/m4ifraund99pseap731l6ubyw snapshotter=overlayfs
time="2023-02-03T16:10:49Z" level=debug msg="removed snapshot" key=buildkit/31/ky13d6x635szkhqgvp2u076q9 snapshotter=overlayfs
time="2023-02-03T16:10:49Z" level=debug msg="removed snapshot" key=buildkit/34/x7qr5jqyi5jht905l8idi9vzd snapshotter=overlayfs
time="2023-02-03T16:10:49Z" level=debug msg="removed snapshot" key=buildkit/45/a3pnf2678hnh2qw8fsy4dyuws snapshotter=overlayfs
time="2023-02-03T16:10:49Z" level=debug msg="removed snapshot" key=buildkit/41/w5ogr03j22gzyqg5wzkg0fh35 snapshotter=overlayfs
time="2023-02-03T16:10:49Z" level=debug msg="snapshot garbage collected" d=102.083581ms snapshotter=overlayfs
time="2023-02-03T16:10:50Z" level=error msg="/moby.buildkit.v1.frontend.LLBBridge/ReadFile returned error: rpc error: code = Canceled desc = context canceled\n"
```
```
› go version
go version go1.20 linux/amd64
› mage -v test:unit
Running target: Test:Unit
...
#1 DONE 2.0s
#2 copy / /
#2 DONE 0.3s
#3 copy /go.mod /
#3 DONE 0.1s
#4 copy /go.sum /
#4 DONE 0.6s
Error: Post "http://dagger/query": EOF
Please visit https://dagger.io/help#go for troubleshooting guidance.
```
```
"error": "Post \"http://dagger/query\": EOF\nPlease visit https://dagger.io/help#go for troubleshooting guidance."
```
### Steps to reproduce
I haven't been able to reproduce locally since users don't know what triggers it. However, some users reported that they can make it fail every time on their machines. We're continuing to troubleshoot on this thread: https://discord.com/channels/707636530424053791/1070997951503151104
### SDK version
0.4.2 to 0.4.4
### OS version
N/A | https://github.com/dagger/dagger/issues/4538 | https://github.com/dagger/dagger/pull/4551 | 18744003b6fd6865f7c518f6f8801ac52c25d5d2 | 5455656a4128f569b2d8f664d777120aa79d715d | "2023-02-06T18:17:01Z" | go | "2023-02-08T13:47:37Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,524 | ["docs/current/sdk/nodejs/guides/620941-github-google-cloud.md", "docs/current/sdk/nodejs/snippets/github-google-cloud/main.mjs"] | Add architecture/platform notes in Github + Google Cloud guide | ### What is the issue?
From @d3rp3tt3 :
I am stuck on step 4, "Test the Dagger pipeline on the local host." Specifically, when I run the pipeline, it mostly succeeds, but the container fails to start. Here are the relevant snippets from the Google Cloud logs for the service. The key message looks to be: "terminated: Application failed to start: Failed to create init process: failed to load /usr/local/bin/npm: exec format error"
Solution from @marcosnils confirmed by @d3rp3tt3
I had to add linux as a prefix to get it to work for that example. I looked at the GraphQL reference to see the options.
```
// get Node image
const node = daggerClient.container({ platform: "linux/amd64" }).from("node:16")
```
Add a note regarding this in the guide. | https://github.com/dagger/dagger/issues/4524 | https://github.com/dagger/dagger/pull/4526 | a7c74a4745a6d3d3797e46876bef56eab1277d20 | ff04bc261d8e6fece592526c70d84023d33647b0 | "2023-02-03T05:59:58Z" | go | "2023-02-03T14:47:27Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,523 | ["docs/current/sdk/nodejs/guides/620941-github-google-cloud.md"] | Update command in Github + Google Cloud guide | ### What is the issue?
From @d3rp3tt3 : I ran into an error when I tried to follow one step of the Google Cloud example: https://docs.dagger.io/sdk/nodejs/620941/github-google-cloud#appendix-a-create-a-github-repository-with-an-example-express-application. I had to add the -e flag before the expression so that sed would take the command.
Updated command: sed -i -e 's/Express/Dagger/g' routes/index.js
Discord: https://discord.com/channels/707636530424053791/708371226174685314/1070485752321953843 | https://github.com/dagger/dagger/issues/4523 | https://github.com/dagger/dagger/pull/4525 | ff04bc261d8e6fece592526c70d84023d33647b0 | 8c95aebd534c40d478c88d4279ad83c2db0da741 | "2023-02-03T05:57:05Z" | go | "2023-02-03T14:48:00Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,479 | ["docs/current/quickstart/120918-quickstart-setup.mdx"] | Transfer quickstart example code to Dagger org repo | ### What is the issue?
This task is to transfer the repository from https://github.com/vikram-dagger/hello-dagger to https://github.com/dagger/hello-dagger so that it resides in the organization's location rather than a personal one. | https://github.com/dagger/dagger/issues/4479 | https://github.com/dagger/dagger/pull/4527 | 856c790691c940f45019f7812aec0105fef49b53 | a7c74a4745a6d3d3797e46876bef56eab1277d20 | "2023-01-30T15:02:39Z" | go | "2023-02-03T14:42:07Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,470 | ["docs/current/quickstart/628381-quickstart-sdk.mdx", "docs/current/sdk/python/866944-install.md", "sdk/python/README.md"] | Python: Update documentation for Conda usage | ### What is the issue?
Now that we're on conda we need to update docs to use it (e.g., links, installation, badge).
- https://github.com/dagger/dagger/issues/3942 | https://github.com/dagger/dagger/issues/4470 | https://github.com/dagger/dagger/pull/4651 | 90f49ec1cc14f01c93fdb7c2662d1bedc87f7582 | 73231802715618fb88f3972e0738cb8fd5c15a4a | "2023-01-27T17:41:20Z" | go | "2023-02-27T11:39:37Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,469 | ["sdk/python/src/dagger/api/base.py", "sdk/python/tests/api/test_integration.py", "sdk/python/tests/api/test_integration_sync.py"] | 🐞 Python SDK: `platform_variants` argument to `Contaner.export` and `Container.publish` is not resolved to `ContainerID`s. | ### What is the issue?
When `platform_variants` of the type `Optional[Sequence[Container]]` is passed to `Container.export` and `Container.publish` the the following error is raised:
```
TypeError: Cannot convert value to AST: <Container instance>.
```
More information in Discord: https://discord.com/channels/707636530424053791/1037455051821682718/1068415400146108447
### Steps to reproduce
```python
import asyncio
import dagger
async def main():
cfg = dagger.Config()
async with dagger.Connection(cfg) as client:
# images = await build(client)
images = (client.container().from_("python:alpine"),)
# Export
await client.container().export(
path="./export.tar.gz", platform_variants=images
)
# Publish
await client.container().publish(
address="example.com/foo/bar:test", platform_variants=images
)
if __name__ == "__main__":
asyncio.run(main())
```
### SDK version
`d92f4db7` (current main branch)
### OS version
Linux 6.1.8-arch1-1 | https://github.com/dagger/dagger/issues/4469 | https://github.com/dagger/dagger/pull/4471 | 9c817bf968c3afa6f47c798956dc945fe4597b6f | e9f95e0f6b52b8f59500824f2b2c1e8b6f0ced0a | "2023-01-27T17:00:22Z" | go | "2023-01-30T15:48:49Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,458 | ["core/host.go"] | 🐞 Error with export function | ### What is the issue?
I was trying dagger with the [Get Started](https://docs.dagger.io/sdk/go/959738/get-started#step-4-create-a-single-build-pipeline)
page. The failing function is the Export function.
### Log output
input:1: container.from.withMountedDirectory.withWorkdir.withExec.directory.export destination "C:\\Users\\***\\GolandProjects\\FilesManagement\\build" escapes workdir
### Steps to reproduce
code:
```go
func daggerBuild() error {
ctx := context.Background()
client, err := dagger.Connect(ctx, dagger.WithLogOutput(os.Stdout))
if err != nil {
fmt.Printf("Error connecting to Dagger Engine: %s\n", err)
os.Exit(1)
}
defer client.Close()
src := client.Host().Directory(".")
golang := client.Container().From("golang:latest")
golang = golang.WithMountedDirectory("/src", src).WithWorkdir("/src")
path := "build/"
golang = golang.WithExec([]string{"go", "build", "-o", path})
output := golang.Directory(path)
_, err = output.Export(ctx, path)
if err != nil {
fmt.Printf("Error on export: %s", err)
}
return nil
}
```
### SDK version
Go SDK 0.4.3
### OS version
Windows 11 | https://github.com/dagger/dagger/issues/4458 | https://github.com/dagger/dagger/pull/4486 | 0c415b96f3fc86e9087a05fab868b98b3f775f45 | c257683ca9ea81f75de8c3c9c07804d286ab9087 | "2023-01-26T18:10:41Z" | go | "2023-01-30T22:46:16Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,398 | ["go.mod", "go.sum"] | SDKs fail to return leaf arrays of structs (GraphQL) | ### Problem
When implementing https://github.com/dagger/dagger/pull/4387 tests using the Go SDK, we realized that SDKs fail to execute some endpoints that do work as graphQL queries.
After talking with @vito, it turns out that this touches all schemas that return arrays of custom structs:
- [envVariables](https://github.com/dagger/dagger/blob/1e54736caeaf9d1f851371178d4f04a93acb545c/core/schema/container.graphqls#L83)
- [labels](https://github.com/dagger/dagger/pull/4387) (future implementation)
### Repro
#### Go SDK
Given this Go SDK example:
```go
package main
import (
"context"
"fmt"
"log"
"dagger.io/dagger"
)
func main() {
ctx := context.Background()
c, err := dagger.Connect(ctx)
if err != nil {
log.Fatalf("debug: %s", err)
}
defer c.Close()
labels, err := c.Container().From("nginx").EnvVariables(ctx)
if err != nil {
log.Fatalf("debug2: %s", err)
}
fmt.Println("good", labels)
}
```
We get this error:
```shell
$ go run .
debug2 input:1: Field "envVariables" of type "[EnvVariable!]!" must have a sub selection.
Please visit https://dagger.io/help#go for troubleshooting guidance.
```
> Error is consistent with the one encountered on current implementation of `labels`
#### GraphQL equivalent
However, the graphQL equivalent:
```graphQL
{
container {
from(address: "nginx") {
envVariables {
name
value
}
}
}
}
```
We get:
```json
{
"data": {
"container": {
"from": {
"envVariables": [
{
"name": "PATH",
"value": "/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin"
}
]
}
}
}
}
```
### Why didn't we see it ?
Most of container tests have been implemented as graphQL queries, thus making us unaware of this problem.
cc @vito @aluzzardi | https://github.com/dagger/dagger/issues/4398 | https://github.com/dagger/dagger/pull/461 | a79f10a9bb62552daaae493fee91dfcf93494894 | 1927ebbe1da1964d833a4eff4234c06083081aa5 | "2023-01-17T23:30:37Z" | go | "2021-05-13T18:51:13Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,386 | ["sdk/nodejs/api/utils.ts", "sdk/nodejs/common/errors/NotAwaitedRequestError.ts", "sdk/nodejs/common/errors/errors-codes.ts", "sdk/nodejs/common/errors/index.ts"] | Node.js SDK - check for await | ## Problem
Many users get confused with their async pipeline.
When should I use await ? Is my pipeline executing ?
The error doesn't give any clue of what's happening.
```shell
throw new UnknownDaggerError("Encountered an unknown error while requesting data via graphql", { cause: e });
```
## A better approach
Display an understandable error message if users omit to await the promise. | https://github.com/dagger/dagger/issues/4386 | https://github.com/dagger/dagger/pull/4485 | 2e478c522374c4621872a1f0bc697488545dc061 | a80a11ea7b3fcb6f42f2cedb793fa62b64d404f6 | "2023-01-16T14:30:10Z" | go | "2023-02-09T12:31:14Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,373 | ["docs/current/sdk/nodejs/783645-get-started.md", "docs/current/sdk/nodejs/835948-install.md", "docs/current/sdk/nodejs/guides/620941-github-google-cloud.md"] | Update Installation for Node.js SDK | ### What is the issue?
To make sure user get the latest version of the SDK, we should update the installation command :
From:
```shell
npm install @dagger.io/dagger --save-dev
```
To:
```shell
npm install @dagger.io/dagger@latest --save-dev
```
This command can also be run inside an existing project to update dagger SDK version.
cc @vikram-dagger | https://github.com/dagger/dagger/issues/4373 | https://github.com/dagger/dagger/pull/4374 | babbbe9953eb790bcad393fb2261d31812fe45e5 | 8c1f0e87e8fd9b00057fa397a63e8b0a2c4170ea | "2023-01-12T16:00:29Z" | go | "2023-01-16T09:32:09Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,322 | ["sdk/nodejs/common/errors/EngineSessionErrorOptions.ts", "sdk/nodejs/common/errors/errors-codes.ts", "sdk/nodejs/common/errors/index.ts", "sdk/nodejs/provisioning/bin.ts"] | feat: Node SDK: handle errors from establishing session | Similar to #4321
Whenever a dagger session connection fails, the output error message is not forwarded by the Node SDK.
Below is an example with a modified version of our dagger session where it should retrieve the stderr of buildkit.
Instead, we get the stack trace error with a hardcoded string `"No line was found to parse the engine connect params"`:
```
➜ typescript_sdk node --loader ts-node/esm copy.ts
(node:83510) ExperimentalWarning: Custom ESM Loaders is an experimental feature. This feature could change at any time
(Use `node --trace-warnings ...` to show where the warning was created)
file:///private/tmp/dagger/sdk/nodejs/dist/provisioning/bin.js:166
throw new EngineSessionEOFError("No line was found to parse the engine connect params");
^
EngineSessionEOFError: No line was found to parse the engine connect params
at Bin.<anonymous> (file:///private/tmp/dagger/sdk/nodejs/dist/provisioning/bin.js:166:19)
at Generator.next (<anonymous>)
at fulfilled (file:///private/tmp/dagger/sdk/nodejs/dist/provisioning/bin.js:4:58)
at processTicksAndRejections (node:internal/process/task_queues:95:5) {
cause: undefined,
code: 'D105'
}
```
cc @slumbering | https://github.com/dagger/dagger/issues/4322 | https://github.com/dagger/dagger/pull/4421 | e9f95e0f6b52b8f59500824f2b2c1e8b6f0ced0a | 0c415b96f3fc86e9087a05fab868b98b3f775f45 | "2023-01-06T10:29:55Z" | go | "2023-01-30T15:59:17Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,311 | ["docs/current/sdk/nodejs/783645-get-started.md", "docs/current/sdk/nodejs/snippets/get-started/step2/build.js", "docs/current/sdk/nodejs/snippets/get-started/step2/build.mjs", "docs/current/sdk/nodejs/snippets/get-started/step2/build.mts", "docs/current/sdk/nodejs/snippets/get-started/step3/build.js", "docs/current/sdk/nodejs/snippets/get-started/step3/build.mjs", "docs/current/sdk/nodejs/snippets/get-started/step3/build.mts", "docs/current/sdk/nodejs/snippets/get-started/step4/build.js", "docs/current/sdk/nodejs/snippets/get-started/step4/build.mjs", "docs/current/sdk/nodejs/snippets/get-started/step4/build.mts"] | Node.js SDK - Document how to use it with a commonjs app | @marcosnils pointed here how to use Node.js SDK with a cjs app: https://discord.com/channels/707636530424053791/708371226174685314/1060221923180691496
Here is the code snippet:
```ts
(async function() {
// initialize Dagger client
let connect = (await import('@dagger.io/dagger')).connect;
connect(async (client) => {
// get Node image
// get Node version
const node = client.container().from("node:16").withExec(["node", "-v"])
// execute
const version = await node.stdout()
// print output
console.log("Hello from Dagger and Node " + version)
}, { LogOutput: process.stdout });
})();
```
This should be documented as some old users projects are still using common Javascript.
@vikram-dagger what do you think of:
- Add a warning here to tell users that step 3 (Define the package type as a module) is required if their project is ESM only https://docs.dagger.io/sdk/nodejs/783645/get-started#step-1-create-a-react-application
- Having two tabs called `Javascript ESM` and `Javascript CJS` here:
- https://docs.dagger.io/sdk/nodejs/783645/get-started#step-1-create-a-react-application
- https://docs.dagger.io/sdk/nodejs/783645/get-started#step-4-test-against-a-single-nodejs-version
- https://docs.dagger.io/sdk/nodejs/783645/get-started#step-5-test-against-multiple-nodejs-versions
These points are suggestions, let me know if I'm missing something. Thanks 🙏
| https://github.com/dagger/dagger/issues/4311 | https://github.com/dagger/dagger/pull/4377 | 937323182e02fe6b02b601f77d134aaec3305435 | babbbe9953eb790bcad393fb2261d31812fe45e5 | "2023-01-05T09:05:40Z" | go | "2023-01-16T09:31:43Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,305 | ["website/docs-graphql/custom-theme/data/index.js", "website/docs-graphql/custom-theme/helpers/spanWrap.js", "website/docs-graphql/custom-theme/views/partials/graphql/kinds/_fields.hbs", "website/docs-graphql/custom-theme/views/partials/graphql/name-and-type.hbs"] | Individual GraphQL field reference is not linkable | ## Problem
When using the [GraphQL API reference documentation](https://docs.dagger.io/api/reference/), I often want to link to a specific field or query. This is currently not possible.
Note: types are linkable, but not fields. Example of a link to the [Container type](https://docs.dagger.io/api/reference/#definition-Container)
## Solution
Make fields linkable in the same way as types.
| https://github.com/dagger/dagger/issues/4305 | https://github.com/dagger/dagger/pull/4410 | 5cd27322a2a2ab4a6692088248b86ff9411ab5a6 | 76bcf891570b839a8780076fffc247712edfc32e | "2023-01-04T18:57:08Z" | go | "2023-01-19T17:23:40Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,270 | ["go.mod", "go.sum"] | 🐞 Unable to run Dockerfile with "yarn install" `dagger-cue` | ### What is the issue?
I am unable to build my Next-App with dagger-cue, even the simplest solution with an inline Dockerfile did not work out:
**ERROR**: process "**/bin/sh -c yarn install --frozen-lockfile**" did not complete successfully: exit code: 1
### Log output
[✔] client.filesystem.".".read 0.2s
[✗] actions.build 1.3s
dependency detected dependency=client.filesystem.".".read
Create inline Dockerfile
sha256:e666fd2924a849f401ea2295df44a2a13117a4ca25e1451f16d93abe50cc1371
DONE 0.0s
[1/4] FROM docker.io/library/node:19.0-alpine@sha256:2241dcb089d4d46d9321cc69f67769f741a04b08c9ead1530e8054d7eaac97a5
sha256:603e1cfa5c1694acd93e6e78c2c2f061488cbea93050dbd0183aee0e62dd7834
resolve docker.io/library/node:19.0-alpine@sha256:2241dcb089d4d46d9321cc69f67769f741a04b08c9ead1530e8054d7eaac97a5 0.0s done
DONE 0.0s
[2/4] WORKDIR /app
sha256:52d970ce883a47808ce9d68208b07f15c84b3d4a91726619fbc323a6967b7f7a
DONE 0.0s
[3/4] COPY package.json yarn.lock ./
sha256:99951fd58d8949b6b6046ce47ba7ca7aea4c0f3740095ed314898edd3dbcb085
DONE 0.0s
[4/4] RUN yarn install --frozen-lockfile
sha256:c6a8dadfa8055dc659ebce71afec4587fda43564c167a8949992e6ee5ce146ef 0.166 Error while loading init: No such file or directory
0.198 container_linux.go:380: starting container process caused: process_linux.go:393: copying bootstrap data to pipe caused: write init-p: broken pipe
**ERROR**: process "**/bin/sh -c yarn install --frozen-lockfile**" did not complete successfully: exit code: 1
------
> [4/4] **RUN yarn install --frozen-lockfile**:
------
[internal] load metadata for docker.io/library/node:19.0-alpine
sha256:8d6aedba791302256a8bfd1f3994441176793042d0c00298cf5327f109a16a1f
DONE 1.0s
12:59PM FATAL failed to execute plan: task failed: actions.build._build: process "/bin/sh -c yarn install --frozen-lockfile" did not complete successfully: exit code: 1
### Install simple Next-App
```bash
npx create-next-app@latest
or
yarn create next-app
or
pnpm create next-app
```
### Steps to reproduce
```cue
dagger.#Plan & {
client: {
filesystem: {
".": read: {
contents: dagger.#FS
exclude: [".next", "node_modules", "cue.mod", "README.md"]
}
}
}
actions: {
build: docker.#Dockerfile & {
source: client.filesystem.".".read.contents
dockerfile: contents: #"""
FROM node:19.0-alpine AS deps
WORKDIR /app
COPY package.json yarn.lock ./
RUN yarn install --frozen-lockfile
"""#
}
}
}
```
### SDK version
CUE-SDK: dagger 0.2.232
### OS version
macOS 13.1 | https://github.com/dagger/dagger/issues/4270 | https://github.com/dagger/dagger/pull/478 | 8c511a8dc3b57446ec0608d69162fa0a61d0c9d0 | f498a9bb846fa7071e2b175f333b9bf2f4b9b228 | "2022-12-30T12:43:58Z" | go | "2021-05-24T18:18:30Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,258 | ["codegen/generator/nodejs/templates/functions.go", "codegen/generator/nodejs/templates/functions_test.go", "codegen/generator/nodejs/templates/src/type.ts.tmpl"] | NodeJS SDK has input type order generated randomly (which makes CI fail) | https://github.com/dagger/dagger/actions/runs/3765190410/jobs/6400412139
We need to order it alphabetically. | https://github.com/dagger/dagger/issues/4258 | https://github.com/dagger/dagger/pull/4259 | 9680b708c649db8cc1527411c5787ab2816223d7 | 22b205cdd9bd303148ec751af89a02cd30001339 | "2022-12-23T15:40:52Z" | go | "2022-12-23T18:11:34Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,257 | ["sdk/nodejs/api/test/api.spec.ts", "sdk/nodejs/api/utils.ts"] | 🐞 NodeJS .build() fail with a simple Dockerfile | ### What is the issue?
reference: https://discord.com/channels/707636530424053791/1055501264387063899/1055501264387063899
Using `build()` with a Dockerfile containing only a `FROM alpine` instruction fails but it works if we call a `container().from("alpine")`.
In Go it works.
### Log output
```console
errors: [
{
message: 'Syntax Error GraphQL request (5:26) Expected Name, found String "_queryTree"\n' +
'\n' +
'4: \n' +
'5: build (context: {"_queryTree":[{operation:"directory","args":{}},{operation:"withNewFile","args":{path:"Dockerfile","contents":"\\n FROM alpine \\n "}}],"clientHost":"127.0.0.1:45369","client":{url:"http://127.0.0.1:45369/query","options":{}}}) {\n' +
' ^\n' +
'6: \n',
locations: [ [Object] ]
}
],
```
### Steps to reproduce
## Doesn't work
```typescript
const image = client.directory().withNewFile(
"Dockerfile",
`
FROM alpine
`
);
const builder = client
.container()
.build(image)
.withWorkdir("/")
.withEntrypoint(["sh", "-c"])
.withExec(["echo htrshtrhrthrts > file.txt"])
.withExec(["cat file.txt"]);
const anotherImage = client
.container()
.from("alpine")
.withWorkdir("/")
.withFile("/copied-file.txt", builder.file("/file.txt"))
.withEntrypoint(["sh", "-c"])
.withExec(["cat copied-file.txt"]);
```
## Works
```typescript
const builder = client
.container()
.from("alpine") // from instead of build
.withWorkdir("/")
.withEntrypoint(["sh", "-c"])
.withExec(["echo htrshtrhrthrts > file.txt"])
.withExec(["cat file.txt"]);
const anotherImage = client
.container()
.from("alpine")
.withWorkdir("/")
.withFile("/copied-file.txt", builder.file("/file.txt"))
.withEntrypoint(["sh", "-c"])
.withExec(["cat copied-file.txt"]);
```
Or in Go
```go
// Build our app
builder := client.
Container().
Build(image). // works fine
WithWorkdir("/").
WithEntrypoint([]string{"sh", "-c"}).
WithExec([]string{"echo htrshtrhrthrts > file.txt"}).
WithExec([]string{"cat file.txt"})
prodImage := client.
Container().
From("alpine").
WithWorkdir("/").
WithFile("/copied-file.txt", builder.File("/file.txt")).
WithEntrypoint([]string{"sh", "-c"}).
WithExec([]string{"cat copied-file.txt"})
out, err := prodImage.Stdout(ctx)
```
Also, using secret like this breaks
```typescript
const builder = client
.container()
.from("alpine")
.withSecretVariable("TOKEN", client.host().envVariable("FOOBAR").secret()) // adding this line borks it
.withWorkdir("/")
.withEntrypoint(["sh", "-c"])
.withExec(["echo htrshtrhrthrts > file.txt"])
.withExec(["cat file.txt"]);
const anotherImage = client
.container()
.from("alpine")
.withWorkdir("/")
.withFile("/copied-file.txt", builder.file("/file.txt"))
.withEntrypoint(["sh", "-c"])
.withExec(["cat copied-file.txt"]);
const out = await anotherImage.stdout();
```
### SDK version
Go SDK v0.4.2, NodeJS SDK v0.3.0
### OS version
?? | https://github.com/dagger/dagger/issues/4257 | https://github.com/dagger/dagger/pull/4266 | b5d37ab067065d08679a07cad0cd9e40288f3acb | 74af2ec1c6f2491d8536b70afa49ee9e8d857606 | "2022-12-23T11:31:23Z" | go | "2023-01-02T22:36:26Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,245 | ["core/integration/container_test.go", "core/schema/container.go", "core/schema/container.graphqls", "sdk/go/api.gen.go", "sdk/nodejs/api/client.gen.ts", "sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py"] | Implement labels for Dockerfile build | This allows a user to add labels the Dockerfile build
```graphql
input Label {
key: String!
value: String!
}
type Directory {
dockerBuild(dockerfile: String, platform: Platform, labels: [Label!]): Container!
}
``` | https://github.com/dagger/dagger/issues/4245 | https://github.com/dagger/dagger/pull/4387 | c5c5e0e3a51b6a80eee69f07b24aec36ae99cc13 | e24ce91f30cec5ad7f35f975c46f34969ad3bae3 | "2022-12-21T20:35:16Z" | go | "2023-01-19T18:15:20Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,216 | ["codegen/generator/nodejs/templates/src/type.ts.tmpl", "codegen/generator/nodejs/templates/src/type_test.go"] | 🐞 NodeJS: Support GraphQL `input` types | ### What is the issue?
As evidenced in https://github.com/dagger/dagger/pull/4207, the NodeJS/Typescript codegen doesn't currently support [GraphQL input types](https://graphql.org/learn/schema/#input-types).
### Log output
```
#26 docker-entrypoint.sh yarn test
#26 0.304 yarn run v1.22.19
#26 0.354 $ mocha
#26 0.643 (node:39) ExperimentalWarning: Custom ESM Loaders is an experimental feature. This feature could change at any time
#26 0.643 (Use `node --trace-warnings ...` to show where the warning was created)
#26 4.956
#26 4.956 TSError: ⨯ Unable to compile TypeScript:
#26 4.956 api/client.gen.ts(62,15): error TS2304: Cannot find name 'BuildArg'.
#26 4.956 api/client.gen.ts(156,15): error TS2304: Cannot find name 'BuildArg'.
#26 4.956
#26 4.956 at createTSError (/workdir/node_modules/ts-node/src/index.ts:859:12)
#26 4.956 at reportTSError (/workdir/node_modules/ts-node/src/index.ts:863:19)
#26 4.956 at getOutput (/workdir/node_modules/ts-node/src/index.ts:1077:36)
#26 4.956 at Object.compile (/workdir/node_modules/ts-node/src/index.ts:1433:41)
#26 4.956 at transformSource (/workdir/node_modules/ts-node/src/esm.ts:400:37)
#26 4.956 at /workdir/node_modules/ts-node/src/esm.ts:278:53
#26 4.956 at async addShortCircuitFlag (/workdir/node_modules/ts-node/src/esm.ts:409:15)
#26 4.956 at async nextLoad (node:internal/modules/esm/loader:163:22)
#26 4.956 at async ESMLoader.load (node:internal/modules/esm/loader:605:20)
#26 4.956 at async ESMLoader.moduleProvider (node:internal/modules/esm/loader:457:11)
#26 5.003 error Command failed with exit code 1.
#26 5.003 info Visit https://yarnpkg.com/en/docs/cli/run for documentation about this command.
#26 ERROR: process "docker-entrypoint.sh yarn test" did not complete successfully: exit code: 1
Error: input:1: container.from.withWorkdir.withRootfs.withExec.withRootfs.withUnixSocket.withMountedFile.withMountedFile.withEnvVariable.withEnvVariable.withMountedDirectory.withExec.exitCode process "docker-entrypoint.sh yarn test" did not complete successfully: exit code: 1
```
### Steps to reproduce
_No response_
### SDK version
Latest NodeJS SDK
### OS version
All of them. :) | https://github.com/dagger/dagger/issues/4216 | https://github.com/dagger/dagger/pull/4247 | 7725948fedd64364e87d55404dfa0416b55c637b | 385d9a9fabb838b783b13a107f0488c0d67f1721 | "2022-12-16T23:03:21Z" | go | "2022-12-22T14:49:15Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,215 | ["RELEASING.md", "core/docs/d7yxc-operator_manual.md"] | Document new interface for using custom provisioned engine | Splitting this out from the task list [here](https://github.com/dagger/dagger/issues/3830) so it can be tracked by users and discussed separately.
Given how common a question and enormous a pain point this is, I think we should document:
1. The new `_EXPERIMENTAL_*` env knobs for connecting an SDK to an already provisioned engine
2. Where our engine image is located (so users can set it up themselves)
3. How to obtain a CLI corresponding to a given engine image
We need to decide where this documentation should live given its unstable, experimental status. Personally, given how common a question it is, I'd be okay with putting this somewhere on our website alongside big red warnings about it being experimental and subject to change. But if there's concerns from others about that I'm also not opposed to just putting it in a markdown doc on our repo and pointing power users to that.
The docs should be pretty minimal to start I think, just enough to get users who are okay with some DIY going. Obviously the goal would then be to expand and clean these docs up as we finalize+flesh out the actual desired end OX. | https://github.com/dagger/dagger/issues/4215 | https://github.com/dagger/dagger/pull/4232 | 4fb99b422cc1a8fe13e55fcf4534b225928bfd2c | 031c50c95f8f76144c998c706f7bf57ce2186a2f | "2022-12-16T21:55:35Z" | go | "2023-01-09T17:17:06Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,212 | ["sdk/python/.flake8", "sdk/python/src/dagger/api/base.py", "sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py", "sdk/python/src/dagger/codegen.py", "sdk/python/tests/api/test_codegen.py", "sdk/python/tests/api/test_inputs.py", "sdk/python/tests/api/test_response.py"] | Python: Improve error message in runtime type checking | ## Overview
Follow up to:
- https://github.com/dagger/dagger/pull/4195
Initial implementation had the actual type in the error message, but it’s not always simple to get a good string representation of a type, so it was left out of the change.
However, it really helps in debugging to show it:
```diff
File "test.py", line 21, in test
.with_directory("/spam", dir_id)
- TypeError: Wrong type for 'directory' parameter. Expected a 'Directory' instead.
+ TypeError: Wrong type 'DirectoryID' for 'directory' parameter. Expected a 'Directory' instead.
```
## Generics
When we get to generics it's a bit more tricky. Helpful if we can do better than the naive implementation:
```diff
File "test.py", line 23, in test
.with_exec(["sleep", 1])
- TypeError: Wrong type 'list' for 'args' parameter. Expected a 'list[str]' instead.
+ TypeError: Wrong type 'list[str | int]' for 'directory' parameter. Expected a 'list[str]' instead.
```
## Coroutines
Specifically for coroutines Python warns if one isn’t awaited, but not if an exception is raised first which is what happens with the runtime type checking. Instead of just showing the general error message though, we can show a helpful hint.
```diff
File "test.py", line 18, in test
.directory(src.id())
- TypeError: Wrong type 'Coroutine' for 'id' parameter. Expected a 'DirectoryID' instead.
+ TypeError: Unexpected coroutine in 'id' parameter. Did you forget to await?
```
| https://github.com/dagger/dagger/issues/4212 | https://github.com/dagger/dagger/pull/4333 | fbee5bdcf840513b7817436f1699e36292d542f9 | c541313b7b3648b1d45e67253c14e27272f9d68d | "2022-12-16T18:13:56Z" | go | "2023-01-09T15:07:31Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,200 | ["docs/current/cli/698277-index.md"] | docs: Ensure users looking at Dagger CLI docs can discover `dagger run` capabilities/examples | Right now the CLI docs focus on `dagger query` and the `dagger run` examples live under the GraphQL section. There should be links or some other way to make sure all of these capabilities are discovered by users.
cc @marcosnils | https://github.com/dagger/dagger/issues/4200 | https://github.com/dagger/dagger/pull/4202 | 6f89d303ee49502902703ca577580ff3cd9d7806 | 8e4c8e459c8440af1278a71c4f3bfb0d79c141ae | "2022-12-15T17:28:11Z" | go | "2022-12-19T18:19:16Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,192 | [".changes/unreleased/Breaking-20230727-115352.yaml", "core/schema/container.go", "core/schema/container.graphqls", "sdk/go/api.gen.go", "sdk/nodejs/api/client.gen.ts", "sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py", "sdk/python/src/dagger/codegen.py"] | Rename `id` argument name in fields that don’t return its type | Some top-level fields return an object if you have their ID:
```graphql
type Query {
container(id: ContainerID, platform: Platform): Container!
directory(id: DirectoryID): Directory!
file(id: FileID!): File
secret(id: SecretID!): Secret!
socket(id: SocketID): Socket!
}
```
There’s one exception to this “rule”. I think it makes sense for us to rename this parameter to make it more consistent:
```diff
type Container {
"Initializes this container from this DirectoryID."
- withRootfs(id: DirectoryID!): Container!
+ withRootfs(directory: DirectoryID!): Container!
}
```
### Notes
- `directory` is consistent with `withDirectory(path: String!, directory: DirectoryID!)`. `withRootfs` is similar just with `path` set to `/`;
- `Container.withFS` doesn’t matter as it’s deprecated and is going to be removed;
- Downside is the breaking change but for codegen clients, `withRootfs`‘s `id` being a required argument, the name is hidden from usage so it shouldn’t actually break most users;
- This was surfaced in https://github.com/dagger/dagger/issues/4191.
| https://github.com/dagger/dagger/issues/4192 | https://github.com/dagger/dagger/pull/5513 | 18f57d142656400fd6318d1d6ed486101f3c14d6 | cf4c4689391d801fbfee00a56134ba03417eb8c2 | "2022-12-13T23:59:19Z" | go | "2023-07-27T18:08:17Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,191 | ["sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py", "sdk/python/src/dagger/codegen.py"] | Python: Don’t accept an object for parameters named `id` (and the return is of the same type) | ## Overview
Some top-level fields return an object if you have an ID:
```python
def directory(self, id: DirectoryID | Directory | None = None) -> Directory: ...
def file(self, id: FileID | File) -> File: ...
```
If you already have an object it doesn’t make sense to use these fields to get the same object. So these fields only make sense if you have an *ID* somehow, and need to get the object they represent.
Note: It’s not clear when you’d need these fields in a codegen client. IDs are an implementation detail.
## Solution
Replace all ID inputs with object references, unless the argument name is `id` and the field’s return type is that object type.
### Rename outliers
That’s a good rule of thumb that is future proof, because there’s two outliers with `id` param names that I think should be renamed, but there can be other cases in the future.
```diff
type Git {
- commit(id: string)
+ commit(hash: string)
}
type Container {
- withRootfs(id: DirectoryID!): Container!
+ withRootfs(source: DirectoryID!): Container!
}
```
Note: `Git.commit` is harmless since it’s not asking for an *ID* type, but I still think it should be renamed.
## Explanation
This is a follow up to the following changes:
- https://github.com/dagger/dagger/pull/3598
- https://github.com/dagger/dagger/pull/3870
- https://github.com/dagger/dagger/pull/3936
Somehow I got confused with the top-level selectors:
> Top-level selectors (e.g. `File(id FileID)`) become weird (`File(id *File)`) -- if you already have the file, what's the point of having a function to look it up? I've kept them as `File(id FileID)`
\- *Originally posted in https://github.com/dagger/dagger/pull/3598*
In #3870 I just replaced every ID scalar with an object. Later, in #3936, I realized these fields with an `id` parameter looked weird by passing an object, so I added a union:
```python
# before
def file(self, id: FileID) -> File: ...
# after hiding IDs
def file(self, id: File) -> File: ...
# follow-up to bring back ID here
def file(self, id: FileID | File) -> File: ...
```
Now I realize these should have been left alone:
```python
def file(self, id: FileID) -> File: ...
```
| https://github.com/dagger/dagger/issues/4191 | https://github.com/dagger/dagger/pull/4195 | 91accd96689ffb183915495fe6898d34c8210a26 | 5bba71ad79cdb787f1852f882d377b069df4a5f3 | "2022-12-13T23:24:20Z" | go | "2022-12-15T14:49:06Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,190 | ["core/directory.go", "core/integration/directory_test.go"] | Enforce size limit on paths in engine | If you provide a path that exceeds a certain length you will get an error from buildkit like
```
ClientError: lstat /var/lib/buildkit/runc-overlayfs/cachemounts/buildkit1109656980/app/eyJsbGIiOnsiZGVmIjpbIkdvSUNDa2xzYjJOaGJEb3ZMeTlWYzJWeWN5OXNaVzl6YW05aVpYSm5MME52WkdVdmFtOWlhV3hzWVM5aVlXTnJaVzVrTDI5dGJtbGhMMkoxYVd4a0wyRnlaMjh0YldGdWFXWmxjM1J6RWwwS0UyeHZZMkZzTG5Ob1lYSmxaR3RsZVdocGJuUVNSbWh2YzNRNkwxVnpaWEp6TDJ4bGIzTnFiMkpsY21jdlEyOWtaUzlxYjJKcGJHeGhMMkpoWTJ0bGJtUXZiMjF1YVdFdlluVnBiR1F2WVhKbmJ5MXRZVzVwWm1WemRITVNWZ29NYkc5allXd3VkVzVwY1hWbEVrWm9iM04wT2k5VmMyVnljeTlzWlc5emFtOWlaWEpuTDBOdlpHVXZhbTlpYVd4c1lTOWlZV05yWlc1a0wyOXRibWxoTDJKMWFXeGtMMkZ5WjI4dGJXRnVhV1psYzNSeldnQT0iLCJDa2tLUjNOb1lUSTFOam96TlRaaE16azVNakprTXpsak4yVmpOVEkzWVRnNE5ERmtZMlkwWkRFek9UTTVNRGsyTnpGaFpHUTFPRFZtWXpJNFpqa3hNRGMyTm1Kak5XTmtNemt3SWlzU0tRai8vLy8vLy8vLy8vOEJJaHdLQVM4U0FTOGcvLy8vLy8vLy8vLy9BVmovLy8vLy8vLy8vLzhCVWc0S0JXRnliVFkwRWdWc2FXNTFlRm9BIiwiQ2trS1IzTm9ZVEkxTmpwaU9ERTVZVFEyWkRjek16ZzVPV00zWXpjMllqWmtZamRrWmpBeU5qZzVNekEyWTJSaU1UZzFNV0ZqWldNMlpERTNZVEF5WVRRMk1XUTFZVGMxTmpFeCJdLCJtZXRhZGF0YSI6eyJzaGEyNTY6MzU2YTM5OTIyZDM5YzdlYzUyN2E4ODQxZGNmNGQxMzkzOTA5NjcxYWRkNTg1ZmMyOGY5MTA3NjZiYzVjZDM5MCI6eyJjYXBzIjp7InNvdXJjZS5sb2NhbCI6dHJ1ZSwic291cmNlLmxvY2FsLnNoYXJlZGtleWhpbnQiOnRydWUsInNvdXJjZS5sb2NhbC51bmlxdWUiOnRydWV9fSwic2hhMjU2OmI4MTlhNDZkNzMzODk5YzdjNzZiNmRiN2RmMDI2ODkzMDZjZGIxODUxYWNlYzZkMTdhMDJhNDYxZDVhNzU2MTEiOnsiY2FwcyI6eyJmaWxlLmJhc2UiOnRydWV9fSwic2hhMjU2OmUxODE1ZjUyYzk1MzllMzNmODY1ZDU0OTQ4YTk4MDYxNmM1ZjExZjM0MjZlNzc5YTFlZDgzOThiMmY0NWI4M2MiOnsiY2FwcyI6eyJjb25zdHJhaW50cyI6dHJ1ZSwicGxhdGZvcm0iOnRydWV9fX0sIlNvdXJjZSI6eyJsb2NhdGlvbnMiOnsic2hhMjU2OjM1NmEzOTkyMmQzOWM3ZWM1MjdhODg0MWRjZjRkMTM5MzkwOTY3MWFkZDU4NWZjMjhmOTEwNzY2YmM1Y2QzOTAiOnt9LCJzaGEyNTY6YjgxOWE0NmQ3MzM4OTljN2M3NmI2ZGI3ZGYwMjY4OTMwNmNkYjE4NTFhY2VjNmQxN2EwMmE0NjFkNWE3NTYxMSI6e319fX0sImRpciI6IiIsInBsYXRmb3JtIjp7ImFyY2hpdGVjdHVyZSI6ImFybTY0Iiwib3MiOiJsaW51eCJ9fQ==: file name too long
```
This is not very clear to end users though since it is a path that buildkit itself is trying to mount, not the exact path that the user specified.
We should instead enforce the size limit in our engine code and return a more clear error message. | https://github.com/dagger/dagger/issues/4190 | https://github.com/dagger/dagger/pull/4654 | 78f8c1050bed0ec349dbf3be27398c4955136439 | 253e3b227216468202a5b512838a77bd4315ed06 | "2022-12-13T22:23:18Z" | go | "2023-02-28T23:42:33Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,182 | ["docs/current/guides/snippets/load-images-local-docker-engine/export/main.py", "docs/current/guides/snippets/load-images-local-docker-engine/push/main.py", "docs/current/guides/snippets/use-secrets/sdk/main.py", "docs/current/quickstart/snippets/caching/main.py", "sdk/python/.changes/unreleased/Changed-20230726-105626.yaml", "sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py", "sdk/python/src/dagger/codegen.py", "sdk/python/tests/api/test_integration.py"] | Python: force keyword arguments for optional parameters | ## Overview
Even though you can easily reach for a named parameter in Python, I think we should make it required for optional parameters.
This is to **avoid** someone actually using positional params even though it's obvious that named is better here.
## Example
```python
# ❌ don't allow this
ctr.with_exec(["wat"], None, None, "/out/stderrr")
# ✅ force this instead
ctr.with_exec(["wat"], redirect_stderr="/out/stderr")
```
## Motivation
- Clearer when reading code
- Order of params can change if a new one is added | https://github.com/dagger/dagger/issues/4182 | https://github.com/dagger/dagger/pull/5508 | 22d1ca63bcf7e0b3d05b9c491b74e12c8de45688 | 62ab7216d8b6f01fe3348778aa909b57dc68d420 | "2022-12-13T17:09:54Z" | go | "2023-07-26T12:18:27Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,179 | ["sdk/python/poetry.lock", "sdk/python/pyproject.toml", "sdk/python/src/dagger/engine/download.py"] | Implement correct user cache detection in Python SDK | null | https://github.com/dagger/dagger/issues/4179 | https://github.com/dagger/dagger/pull/4281 | 23c2dc2b261eb5c2682017fe86340af12a8d0e72 | c1b6c9e3bb9a48215ce4b80fc726af52e6521590 | "2022-12-13T14:27:08Z" | go | "2023-01-04T00:31:08Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,174 | ["website/docs-graphql/data/examples/queries/socket/gql.md"] | docs: Missing example for socket API | ### What is the issue?
When building the GraphQL API reference documentation, the build process throws an error on account of a missing example for the `socket` API. This should be added.

| https://github.com/dagger/dagger/issues/4174 | https://github.com/dagger/dagger/pull/4187 | ff331f30c03ff7117f029272e45fd45b11bdf42b | 91accd96689ffb183915495fe6898d34c8210a26 | "2022-12-13T10:38:18Z" | go | "2022-12-14T14:58:57Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,152 | ["go.mod", "go.sum"] | Cannot get size of file > 2.14GB (max int32) | ### What is the issue?
If I try to get the `size` of a file that's larger than max int32 (2147483647 bytes or ~2.14GB), I get an error.
The [GraphQL spec](https://graphql.org/learn/schema/#scalar-types) has this to say:
> `Int`: A signed 32‐bit integer.
So we might need a different representation here.
### Log output
```
Cannot return null for non-nullable field File.size.
```
### Steps to reproduce
https://play.dagger.cloud/playground/sNxo3mQis2M
```graphql
query {
container {
from(address: "alpine") {
withExec(args: ["dd", "if=/dev/zero", "of=./foo", "bs=1000", "count=2147484"]) {
file(path:"foo"){
size
}
}
}
}
}
```
### Dagger version
24c0ac804d5c9c3ecdd2c6c565ee9799653c3313 (`master`, but all versions)
### OS version
Linux | https://github.com/dagger/dagger/issues/4152 | https://github.com/dagger/dagger/pull/5227 | 679b27fdf996beef5031ce80a61be78facb7a9d9 | 9d55c22d1fb94a96d2be464823cbca27e8df9302 | "2022-12-09T17:42:01Z" | go | "2023-06-01T12:04:40Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,131 | [".goreleaser.yml", "cmd/client-gen/main.go", "cmd/dagger/engine.go", "cmd/dagger/version.go", "cmd/engine-session/main.go", "codegen/generator/generator.go", "codegen/generator/nodejs/templates/functions_test.go", "internal/engine/version.go"] | 🐞 ./cmd/client-gen doesn't work out of the box | ### What is the issue?
I wanted to regenerate locally some SDK code.
It failed inside the engine of a `./hack/make sdk:nodejs:generate` and I couldn't get more details.
I wanted to see on my host FS what was failing, so I decided to do a:
```
$ go run ./cmd/client-gen/ --lang nodejs -o sdk/nodejs/api/client.gen.ts
```
### Log output
```
Error: failed to start engine session bin: EOF: Error: unknown engine provider:
Please visit https://dagger.io/help#go for troubleshooting guidance.
Usage:
client-gen [flags]
Flags:
-h, --help help for client-gen
--lang string language to generate in
-o, --output string output file
--package string package name
-p, --project string project config file
--workdir string The host workdir loaded into dagger
Error: failed to start engine session bin: EOF: Error: unknown engine provider:
Please visit https://dagger.io/help#go for troubleshooting guidance.
exit status 1
```
### Steps to reproduce
get the latest main (5219a37e)
and run
```
go run ./cmd/client-gen/ --lang nodejs -o sdk/nodejs/api/client.gen.ts
```
### Dagger version
5219a37e
### OS version
Ubuntu 20.04 | https://github.com/dagger/dagger/issues/4131 | https://github.com/dagger/dagger/pull/4143 | 1d14ca04b9aa13589649c7a441a587d1e2b18e30 | 2844748a9db7c4851e5f7acc87c01651f07bb598 | "2022-12-08T09:21:34Z" | go | "2022-12-09T21:36:44Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,111 | ["sdk/python/poetry.lock", "sdk/python/src/dagger/engine/cli.py"] | Python: increase execution timeout | The default timeout for executing a query in the Python SDK is 5 min. This may be too short a default for dagger.
What are the timeouts in other SDKs?
- Go?
- Nodejs?
Is 30 mins good, or too much? What should we converge on @sipsma?
This was hit by a user doing a build. | https://github.com/dagger/dagger/issues/4111 | https://github.com/dagger/dagger/pull/5074 | c77f63e96ea77ac0af22ce9d98f0de4cd4315802 | 4506a1f0bb437d1696d4008426d3159e556d68ef | "2022-12-06T14:21:45Z" | go | "2023-05-05T00:14:54Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,095 | ["docs/current/sdk/go/guides/421437-work-with-host-filesystem.md", "docs/current/sdk/go/snippets/work-with-host-filesystem/list-dir-exclude-include/main.go"] | Document include / exclude behavior | The Dagger API supports "including" or "excluding" files in some [directory operations](https://docs.dagger.io/api/reference/#definition-Directory). But the behavior of these fields is not explained in detail.
For example @maxdeviant [asked on discord](https://discord.com/channels/707636530424053791/1040027260155088956/1048370602219683970):
> how does the exclude parameter for .directory work? Does it support globs?
| https://github.com/dagger/dagger/issues/4095 | https://github.com/dagger/dagger/pull/4110 | 047d49318b0db7ffa83a209aea7a353d941fcc3e | 9eb15124f2a719b2a0f5d45bd1fc27c7fda4d7b5 | "2022-12-05T18:29:46Z" | go | "2022-12-07T10:28:13Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,093 | ["docs/current/sdk/cue/getting-started/470907-get-started.md"] | Fix CUE Get started with GitLab CI | ### What is the issue?
The getting started for Dagger CUE on GitLab CI doesn't work.
In the `before_script` the dagger CLI is installed instead of dagger-cue CLI:
```yaml
before_script:
- |
# install dagger
cd /usr/local
wget -O - https://dl.dagger.io/dagger/install.sh | sh
cd -
dagger-cue version
```
To fix it, I'm going to open a PR where :
- I replaced `https://dl.dagger.io/dagger/install.sh` by `https://dl.dagger.io/dagger-cue/install.sh`
- I specify a variable `VERSION` to dagger-cue installation script because it doesn't support `DAGGER_VERSION` variable
But, I think it is better to update the dagger-cue install script to use variable `DAGGER_VERSION` in order to have consistent variables naming in installation scripts. Additionally, the script should fetch the last version if I don't specify `VERSION` variable but it doesn't work. I'm going to open another PR dedicated to this update. | https://github.com/dagger/dagger/issues/4093 | https://github.com/dagger/dagger/pull/4108 | 302902b8110188c6458295d25be0ac802dba3bdd | 29362b1e1530e4d24bdcd581c5d60657b8372ad6 | "2022-12-05T16:23:58Z" | go | "2022-12-06T13:09:19Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,085 | ["core/container.go"] | Container From removes existing env vars | Example: https://play.dagger.cloud/playground/JBT8x7KCicC
```graphql
{
container {
withEnvVariable(name:"FOO", value:"BAR") {
from(address:"alpine:latest") {
withExec(args:["env"]) {
stdout
}
}
}
}
}
```
That will not show `FOO` in the output.
---
I suppose the correct behavior is not totally obvious, but I'd argue that we should not remove existing env vars because:
1. It can be nice for certain use cases. I was trying to set some env vars that would be shared across all our sdk base containers but I couldn't set them in a common base container because each SDK calls `From`.
2. Other APIs like `WithMountedFile` change the container state but don't get removed by calling `From`, so we should be consistent one way or the other at least | https://github.com/dagger/dagger/issues/4085 | https://github.com/dagger/dagger/pull/4455 | 3c0192e2b9c644ee4a5aad285df94f07a107ccd8 | b43d267a7f7657017188bec57d7826059475a2f3 | "2022-12-02T23:30:33Z" | go | "2023-01-31T18:49:32Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,054 | ["website/docs-graphql/config.yml", "website/docs-graphql/custom-theme/views/partials/layout/page.hbs"] | Add ability to get back to Dagger docs from API reference docs | Right now, there is no way to get back to the Dagger docs from the API reference doc. It would be great if we could make the Dagger logo clickable and add a link back to the main docs homepage or maybe just the API section. | https://github.com/dagger/dagger/issues/4054 | https://github.com/dagger/dagger/pull/4056 | f86ff05ff72523a345995bae9b02334cdc87505c | e11fff5ba0e8676526ff6f923710c41909ba6218 | "2022-11-30T21:31:16Z" | go | "2022-12-01T15:42:50Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,051 | ["internal/mage/sdk/nodejs.go"] | Fix lint errors on nodejs engine bump | The automatic engine bump PR seems to generate code that the nodejs linter disagrees with, requiring manual updates to the PR whenever it's opened, e.g. https://github.com/dagger/dagger/actions/runs/3586757292/jobs/6036336215 | https://github.com/dagger/dagger/issues/4051 | https://github.com/dagger/dagger/pull/4072 | ec51da155e85ecda92ba6c14812d567f37269f8a | bec73f147a6901d77aca780b5386434b27863cdf | "2022-11-30T20:20:05Z" | go | "2022-12-01T23:00:13Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,050 | [".github/workflows/lint.yml", ".github/workflows/publish.yml", ".github/workflows/test.yml", "RELEASING.md"] | Test+Lint workflows don't run automatically in engine bump PRs | See for example here: https://github.com/dagger/dagger/pull/4049#issuecomment-1332689127
Really not sure why this is. The yamls seem to configure the workflows such that they should run. cc @gerhard in case you have any ideas what would cause this | https://github.com/dagger/dagger/issues/4050 | https://github.com/dagger/dagger/pull/4560 | 9f1e65e0213e1f2290263349d9bc7fd27c360514 | e4e5fa65ac5ebc0ed58b92f6cd9d29156a63e132 | "2022-11-30T20:17:20Z" | go | "2023-02-08T23:16:02Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,020 | ["cmd/dagger/do.go", "cmd/dagger/main.go"] | cli: `dagger do` with warning message | ## Problem
The new `dagger` CLI (0.3) works very differently from the old one (0.2). This can confuse some users who upgrade, run the typical `dagger do` command from 0.2, and get a "command not found".
## Solution
Implement a hidden `dagger do` command in 0.3, which does nothing but prints a message explaining the change and where to go to navigate it. | https://github.com/dagger/dagger/issues/4020 | https://github.com/dagger/dagger/pull/4071 | f378cabc6254b69fc1f4765d3c83e57e12057841 | c8e90811f74d0ea13508e4bfaede11046b3fa522 | "2022-11-29T18:01:17Z" | go | "2022-12-02T05:38:57Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,015 | ["core/integration/directory_test.go", "core/schema/directory.go", "core/schema/directory.graphqls", "sdk/go/api.gen.go", "sdk/nodejs/api/client.gen.ts", "sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py"] | API: directory { dockerBuild } | ## Problem
The Dagger API already supports native docker build with `container { build }`. This makes for a perfectly fine DX in native SDKs, I have no complaints. **But** it is less pleasant in the context of a raw GraphQL query, because of limitations in the GraphQL language: stitching must be done manually by querying an ID in one query and copy-pasting it into the other. This is not a problem when developing "real" pipelines, since stitching is trivially available. But it is problem when using the API playground to evaluate what the Dagger API can do. Chaining a docker build from a directory would allow for much cooler demos, for example `git { directory { dockerBuild } }` for a trivial monorepo build example; etc.
## Solution
Implement `directory { dockerBuild }` as an alias to `container { build }`.
Yes, I am aware that I am contradicting my own argument for cutting `container { build }`. My worry at the time was crossing a one-way door and setting a precedent for extensions. Now that our understanding of the extension design has evolved, I am no longer worried about that (each set of extensions results in a unique graphql schema which in turn will produce a unique set of generated native clients. Whatever namespacing / extension points issues we have, they will be caught at generation and will be specific to each code generation project, which is acceptable).
TLDR: @aluzzardi you were right ;) | https://github.com/dagger/dagger/issues/4015 | https://github.com/dagger/dagger/pull/4016 | 39327ce003e55b79ca55a5d84aef97e5036a5924 | c0e8bb1511524f1a372b3aa3def5621e6f4eb9d1 | "2022-11-29T08:34:10Z" | go | "2022-12-01T07:39:20Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 4,002 | [".github/workflows/publish.yml", ".goreleaser.yml", "internal/mage/cli.go", "internal/mage/engine.go", "internal/mage/util/util.go"] | CLI release test | Issue to track progress on the CLI release.
- [x] `curl -L https://dl.dagger.io/dagger/install.sh DAGGER_VERSION=? | sh`
- [x] `brew install dagger/tap/dagger` - for [our tap formula](https://github.com/dagger/homebrew-tap)
- Move the existing `dagger.rb` to `[email protected]`
- [x] `brew install dagger` - for [bottled formula](https://github.com/Homebrew/homebrew-core/blob/master/Formula/dagger.rb)
- Move the existing `dagger.rb` to `[email protected]` | https://github.com/dagger/dagger/issues/4002 | https://github.com/dagger/dagger/pull/4012 | 064879cb6b4fcaa50bf28bbcdb6d40d60dd25cba | 5d18d9f5e56d704f95242304661ae9656ae85780 | "2022-11-28T14:30:21Z" | go | "2022-11-29T21:52:29Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 3,990 | ["sdk/nodejs/api/test/api.spec.ts", "sdk/nodejs/api/utils.ts"] | 🐞 [Node SDK] `withExec` removes whitespace of arguments | ### What is the issue?
When calling `withExec` and passing a single argument that contains whitespace, all whitespace is stripped.
For example, `.withExec(['echo', 'foo bar'])` will print `foobar`.
The expected output is `foo bar`.
### Log output
N/A
### Steps to reproduce
1. Run the following
```ts
import Client, { connect } from "@dagger.io/dagger";
connect(async (client: Client) => {
await client.container().from('alpine:latest')
.withExec(['echo', 'foo bar'])
}, { LogOutput: process.stdout })
```
2. observe that the output is `foobar` rather than the expected output
### Dagger version
dagger v0.2.36 (ea275a3ba) darwin/arm64
### OS version
macOS 12.1 | https://github.com/dagger/dagger/issues/3990 | https://github.com/dagger/dagger/pull/3992 | 58c782e6d5de4992046f2b1ebeea52948ae793fd | 925d95069da66f425019e5140201c37929293cd1 | "2022-11-25T17:33:27Z" | go | "2022-11-29T09:51:03Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 3,966 | ["sdk/python/experimental/hello/main.py", "sdk/python/experimental/hello/requirements.txt", "sdk/python/poetry.lock", "sdk/python/pyproject.toml", "sdk/python/src/dagger/__init__.py"] | Python: Make strawberry dependency an extra | The Python SDK started out with good extension support via _strawberry_. We then put a pause on extension support to release clients. The released Python SDK is only a client right now, but we still have _strawberry_ as a dependency. We should make it an extra:
```shell
$ pip install dagger-io[server]
```
Even after extensions are released, there's a high chance you'll want to run client code in an extension's resolver so the client part of the SDK should stay required and extensions optional. | https://github.com/dagger/dagger/issues/3966 | https://github.com/dagger/dagger/pull/3967 | 9d7ac01dd8d636c20acbbfd72db9f4bfe924f0c5 | 64a40a222640842b2f57c2829fd905c1db416aa3 | "2022-11-23T09:18:01Z" | go | "2022-11-23T12:22:14Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 3,950 | ["internal/mage/util/util.go"] | 🐞 Can't run mage on macos | ### What is the issue?
Running mage tasks locally in macos fails because, I suppose, the `test-dagger-engine` container has a binary for my host (`dagger-engine-session-darwin-arm64`) but not for running inside a container (linux in dagger-in-dagger).
Examples:
```shell
$ ./hack/make sdk:python:test
$ ./hack/make sdk:go:lint
```
### Log output
```
#0 0.056 container_linux.go:380: starting container process caused: process_linux.go:545: container init caused: rootfs_linux.go:76: mounting "/usr/bin/dagger-engine-session-linux-arm64" to rootfs at "/.dagger_engine_session" caused: stat /usr/bin/dagger-engine-session-linux-arm64: no such file or directory
```
\cc @sipsma | https://github.com/dagger/dagger/issues/3950 | https://github.com/dagger/dagger/pull/3954 | 9e4aac59a0e6e99c58c046ccc0c61ea6e2e82b95 | eb9ef56fc4958bf5e1ac781533cdf0cb92da4401 | "2022-11-22T13:20:15Z" | go | "2022-11-22T17:13:25Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 3,894 | ["sdk/python/.flake8", "sdk/python/pyproject.toml", "sdk/python/src/dagger/__init__.py", "sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py", "sdk/python/src/dagger/codegen.py"] | Python: Import API types in `dagger` root | ## Motivation
```diff
import dagger
-from dagger.api.gen import Container
-def base(client: dagger.Client) -> Container:
+def base(client: dagger.Client) -> dagger.Container:
....
``` | https://github.com/dagger/dagger/issues/3894 | https://github.com/dagger/dagger/pull/3940 | 1b50af0be38a350e238b6a47c4ea79766250f1f8 | 67b02cdafce581a8c863e75769adc28f9c7db2c4 | "2022-11-17T12:45:23Z" | go | "2022-11-21T20:50:23Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 3,887 | ["core/integration/container_test.go", "core/schema/container.go", "core/schema/container.graphqls", "sdk/go/api.gen.go", "sdk/nodejs/api/client.gen.ts", "sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py"] | ✨ Ability to add LABELs to Docker images published via SDK | ### What are you trying to do?
Generate and publish a docker image which has LABELs.
Related discussion : https://discord.com/channels/707636530424053791/1042501877906022481
### Why is this important to you?
We have corporate policies that mandate certain labels on all docker images
### How are you currently working around this?
The workaround is to manually create a dockerfile with the LABELs added. But this is inconvenient and bypasses the features provided by the API and SDK
For broader set of OCI configs: #3886 | https://github.com/dagger/dagger/issues/3887 | https://github.com/dagger/dagger/pull/4387 | c5c5e0e3a51b6a80eee69f07b24aec36ae99cc13 | e24ce91f30cec5ad7f35f975c46f34969ad3bae3 | "2022-11-17T05:20:38Z" | go | "2023-01-19T18:15:20Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 3,871 | ["docs/current/sdk/python/628797-get-started.md", "docs/current/sdk/python/guides/648384-multi-builds.md", "docs/current/sdk/python/snippets/get-started/step3/test.py", "docs/current/sdk/python/snippets/get-started/step4a/test.py", "docs/current/sdk/python/snippets/get-started/step4b/test.py", "docs/current/sdk/python/snippets/multi-builds/build.py"] | Update docs with recent DX changes | ### What is the issue?
The next Python release will include a breaking change[^1]:
- https://github.com/dagger/dagger/pull/3870
We need to update the docs examples but only after releasing to PyPI.
[^1]: Actually shouldn't break in runtime, just warnings in the IDE. | https://github.com/dagger/dagger/issues/3871 | https://github.com/dagger/dagger/pull/3941 | 0cb9ae22bb95eb3682e9a50304eee46e7b136523 | 1b50af0be38a350e238b6a47c4ea79766250f1f8 | "2022-11-16T11:28:24Z" | go | "2022-11-21T20:05:57Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 3,836 | ["sdk/nodejs/connect.ts", "sdk/nodejs/provisioning/docker-provision/image.ts", "sdk/nodejs/provisioning/engineconn.ts"] | NodeJS SDK: replace execa library with nodejs Stream | Another follow up fix, we should probably not use an `execa` type in our public interface since we may want to switch to a different library in the future. I think the nodejs `Stream` type might be all we need.
_Originally posted by @sipsma in https://github.com/dagger/dagger/pull/3820#discussion_r1022255634_
| https://github.com/dagger/dagger/issues/3836 | https://github.com/dagger/dagger/pull/3861 | 325ac37b14ca763856e757d2aa92d80e3f044416 | dfb4b8b4979ca9c2f46b818fb9c899b3a11e2b89 | "2022-11-15T10:34:18Z" | go | "2022-11-16T16:13:06Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 3,772 | ["sdk/python/poetry.lock", "sdk/python/pyproject.toml", "sdk/python/src/dagger/api/base.py", "sdk/python/src/dagger/api/gen.py", "sdk/python/src/dagger/api/gen_sync.py", "sdk/python/src/dagger/codegen.py", "sdk/python/tests/api/test_codegen.py", "sdk/python/tests/api/test_inputs.py"] | sdk: python: Codegen - check types on runtime | Python's typing system doesn't work on runtime. You should get warnings from the IDE but nothing's stopping you from using the wrong value types while building the query.
If you do run a script like this you'll get a more cryptic error during serialization of the GraphQL query string.
We can do better and add runtime checks for this.
Thanks @charliermarsh for reporting! | https://github.com/dagger/dagger/issues/3772 | https://github.com/dagger/dagger/pull/4034 | fe43fe207d38ecb2c614bc5dcac6340b2c9293e1 | ff331f30c03ff7117f029272e45fd45b11bdf42b | "2022-11-10T13:36:12Z" | go | "2022-12-14T13:57:58Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 3,737 | ["go.mod", "go.sum", "sdk/go/go.mod", "sdk/go/go.sum", "sdk/go/internal/engineconn/dockerprovision/container.go"] | Errors getting credentials on macos when using dagger-in-dagger | A few of us running `mage sdk:python:test` are getting very cryptic errors on the first step that runs dagger-in-dagger:
```
#1 resolve image config for docker.io/library/golang:1.19-alpine
Error: input:1: container.from error getting credentials - err: signal: killed, out: ``
Please visit https://dagger.io/help#go for troubleshooting guidance.
#1 ERROR: error getting credentials - err: signal: killed, out: ``
```
But only on macos.
I did some digging and eventually found the line where it comes from, forked and added a little extra debug information: https://github.com/sipsma/docker-credential-helpers/blob/9706447df656d46a595e2e2788637eddcf962a4c/client/client.go#L68
With that change it shows the error:
```
error getting credentials - err: signal: killed, prog: docker-credential-desktop get, out: `
```
When I try to manually invoke docker-credential-desktop get from my terminal, it hangs indefinitely, ignoring even SIGTERM and I have to SIGKILL it.
I have no idea why this *seems* to only happen once we get to the dagger-in-dagger step. It is worth confirming that understanding and worth confirming that the credential provider is indeed trying to run `docker-credential-desktop` on macos directly as opposed to inside the Linux container.
It's actually not even obvious that it should be trying the macos client auth provider since the buildkit client is technically inside dagger and thus linux. However, buildkit has a lot of logic where it fallsback to trying any connected session, so *maybe* it is trying the macos client that is also connected to buildkit? Overall, kind of mysterious for a number of reasons, needs more investigation. | https://github.com/dagger/dagger/issues/3737 | https://github.com/dagger/dagger/pull/3758 | 15a897278460408ccc4f832fd7e91dd2ddfaa90a | 4a797801ef3bb6148d7186dac5d9d544cc913960 | "2022-11-08T21:58:43Z" | go | "2022-11-10T00:30:26Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 3,733 | ["cmd/shim/main.go", "core/container.go", "core/shim/cmd/Dockerfile", "core/shim/cmd/main.go", "core/shim/shim.go", "go.mod", "go.sum", "internal/mage/util/util.go"] | Building shim can cause rate limit errors from dockerhub | We build our shim binary on the fly, which requires we pull an image. We currently pull from dockerhub, which can result in 429 throttling errors, which is surprising to users since they never even requested that image be pulled.
Two options:
1. Pull the image from a different registry that doesn't have the same ratelimiting
2. Don't build the shim like that anymore; maybe pre-build it and include it in our engine image now that that's an option
Current preference is 2. That approach would:
1. save us from managing another image.
2. make it easier for users to customize the engine image in the future or to mirror it on their own registries (i.e. an internal company one)
3. be more performant (pull everything at once)
---
Option 2 is easier said than done unfortunately. There's not really a built-in way to just mount a binary from the **buildkitd's host filesystem** into arbitrary execs. Execs are supposed to be fully specified by LLB and LLB alone, and LLB can only include references to the **client's** host filesystem (which is not the same buildkitd's).
There are bunch of possible approaches, but there's one that sticks out to me as being not that hacky and also *relatively* easy: hook into the runc executor.
Right now, our buildkitd uses `runc` to start containers. We can use oci runtime hooks to modify the spec of those containers to include a mount of our shim binary into each container.
* I know of [this existing tool](https://github.com/awslabs/oci-add-hooks) for accomplishing this pretty easily, but it's also not even that hard to roll our own if a compelling need arises.
In the future if we decide to switch to the containerd backend then that same approach will probably still work because the default containerd runtime uses runc. But at that point we could also have our own runtime (containerd coincidentally also uses the terminology "shim"...) too, so there will be lots of options.
Pros:
1. Fairly easy
2. Robust enough - just reuses existing well defined mechanisms for hooking into container runtimes
3. Doesn't require any upstream changes to buildkitd afaict
4. A nice re-usable mechanism in the future in case we ever need to mount anything else from the buildkitd host direct into the container
Cons:
1. The shim binary will no longer be part of LLB and thus won't count towards the cache key. Changing the shim won't invalidate cache
* Actually, as I write this, that might just make sense... the shim is a component of the runner, so just like changing buildkitd doesn't invalidate cache, neither should the shim? Sort of a gray area, but this isn't actually an obvious con I suppose. | https://github.com/dagger/dagger/issues/3733 | https://github.com/dagger/dagger/pull/3913 | 9443adaa8f5bafe062eb757ac596c198391c9b61 | 32c1d82fa2c715a32e7d7d0c95d6a50e96c09fae | "2022-11-08T19:17:23Z" | go | "2022-11-18T03:37:21Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 3,727 | [".github/dependabot.yml"] | Use dependabot with Python | ~May depend on #3702~ | https://github.com/dagger/dagger/issues/3727 | https://github.com/dagger/dagger/pull/3815 | 76d28eb66f86e7d447c8aa4d98ac6bff055dc1b4 | 32e16359c17ff34cd22aa1328b9b8d7489562c9d | "2022-11-08T09:26:24Z" | go | "2022-11-16T09:45:16Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 3,723 | ["internal/buildkitd/buildkitd.go"] | Fix spurious `WARN[0000] commandConn.CloseWrite: commandconn: failed to wait: signal: terminated` | Caused by our use of the buildkit connhelper more often now (we always wait to check if it's running first) | https://github.com/dagger/dagger/issues/3723 | https://github.com/dagger/dagger/pull/3731 | 9bd33ed56ab5e64de7ec5759b9416cd05baec5ee | d0e08e48a909fc14647b8be5047597f8243d48a4 | "2022-11-08T01:47:22Z" | go | "2022-11-08T18:33:42Z" |
closed | dagger/dagger | https://github.com/dagger/dagger | 3,719 | [".github/workflows/publish-sdk-nodejs.yml", ".gitignore", "internal/mage/sdk/all.go", "internal/mage/sdk/nodejs.go", "sdk/nodejs/package.json"] | NodeJS SDK : Publish NodeJS SDK to npm | Publishes the NodeJS SDK to the registry so that it can be installed by name.
ex: `npm publish @dagger.io/nodejs-sdk`
https://www.npmjs.com is the npm Registry that has a public collection of packages of open-source code for Node.js.
In order to publish, follow the developer guide: https://docs.npmjs.com/cli/v9/using-npm/developers#the-packagejson-file
Need to discuss everything related to the CI with github action (Test, build and publish to NPM). cc @gerhard
Tasks:
- [x] add a README.md
- [x] LICENSE
Npm automatically handles node_modules ignore. Do not add node_modules to .npmignore.
| https://github.com/dagger/dagger/issues/3719 | https://github.com/dagger/dagger/pull/3809 | 88e795da2c1b78e4b2b79f542a3233c57dd1fbed | dea51153c37566831d1075479f708d7d51bbcf5d | "2022-11-07T20:47:22Z" | go | "2022-11-15T20:39:32Z" |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.