title
stringlengths 1
100
| titleSlug
stringlengths 3
77
| Java
int64 0
1
| Python3
int64 1
1
| content
stringlengths 28
44.4k
| voteCount
int64 0
3.67k
| question_content
stringlengths 65
5k
| question_hints
stringclasses 970
values |
---|---|---|---|---|---|---|---|
✔️[Python3] UNPRECENDENT NUMBER OF COUNTERS o͡͡͡͡͡͡͡͡͡͡͡͡͡͡╮(。❛ᴗ❛。)╭o͡͡͡͡͡͡͡͡͡͡͡͡͡͡, Explained | minimum-domino-rotations-for-equal-row | 0 | 1 | **UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.**\n\nTo be able to make all values the same on the top or bottom, the given dominos should have enough number of same values. So we need to compare the frequency of values on the top and bottom. If there are enough, say `2`s, on the bottom to meet the condition then we can return true. Also, we should account for the dominos with duplicate values. It\'s possible that a frequency of some value shows that we have enough suitable dominos but they may be a part of the duplicates. So we need to count such duplicates. In our case, we create a dedicated counter for every value without duplicates.\n\nTime: **O(n)** - iteration\nSpace: **O(1)** - just 3 lists with constant size\n\nRuntime: 1124 ms, faster than **94.15%** of Python3 online submissions for Minimum Domino Rotations For Equal Row.\nMemory Usage: 15.1 MB, less than **44.98%** of Python3 online submissions for Minimum Domino Rotations For Equal Row.\n\n```\nclass Solution:\n def minDominoRotations(self, tops: List[int], bottoms: List[int]) -> int:\n total = len(tops)\n top_fr, bot_fr, val_total = [0]*7, [0]*7, [total]*7\n for top, bot in zip(tops, bottoms):\n if top == bot:\n val_total[top] -= 1\n else:\n top_fr[top] += 1\n bot_fr[bot] += 1\n \n for val in range(1, 7):\n if (val_total[val] - top_fr[val]) == bot_fr[val]:\n return min(top_fr[val], bot_fr[val])\n \n return -1\n```\n\n**UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.** | 23 | In a row of dominoes, `tops[i]` and `bottoms[i]` represent the top and bottom halves of the `ith` domino. (A domino is a tile with two numbers from 1 to 6 - one on each half of the tile.)
We may rotate the `ith` domino, so that `tops[i]` and `bottoms[i]` swap values.
Return the minimum number of rotations so that all the values in `tops` are the same, or all the values in `bottoms` are the same.
If it cannot be done, return `-1`.
**Example 1:**
**Input:** tops = \[2,1,2,4,2,2\], bottoms = \[5,2,6,2,3,2\]
**Output:** 2
**Explanation:**
The first figure represents the dominoes as given by tops and bottoms: before we do any rotations.
If we rotate the second and fourth dominoes, we can make every value in the top row equal to 2, as indicated by the second figure.
**Example 2:**
**Input:** tops = \[3,5,1,2,3\], bottoms = \[3,6,3,3,4\]
**Output:** -1
**Explanation:**
In this case, it is not possible to rotate the dominoes to make one row of values equal.
**Constraints:**
* `2 <= tops.length <= 2 * 104`
* `bottoms.length == tops.length`
* `1 <= tops[i], bottoms[i] <= 6` | null |
✔️[Python3] UNPRECENDENT NUMBER OF COUNTERS o͡͡͡͡͡͡͡͡͡͡͡͡͡͡╮(。❛ᴗ❛。)╭o͡͡͡͡͡͡͡͡͡͡͡͡͡͡, Explained | minimum-domino-rotations-for-equal-row | 0 | 1 | **UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.**\n\nTo be able to make all values the same on the top or bottom, the given dominos should have enough number of same values. So we need to compare the frequency of values on the top and bottom. If there are enough, say `2`s, on the bottom to meet the condition then we can return true. Also, we should account for the dominos with duplicate values. It\'s possible that a frequency of some value shows that we have enough suitable dominos but they may be a part of the duplicates. So we need to count such duplicates. In our case, we create a dedicated counter for every value without duplicates.\n\nTime: **O(n)** - iteration\nSpace: **O(1)** - just 3 lists with constant size\n\nRuntime: 1124 ms, faster than **94.15%** of Python3 online submissions for Minimum Domino Rotations For Equal Row.\nMemory Usage: 15.1 MB, less than **44.98%** of Python3 online submissions for Minimum Domino Rotations For Equal Row.\n\n```\nclass Solution:\n def minDominoRotations(self, tops: List[int], bottoms: List[int]) -> int:\n total = len(tops)\n top_fr, bot_fr, val_total = [0]*7, [0]*7, [total]*7\n for top, bot in zip(tops, bottoms):\n if top == bot:\n val_total[top] -= 1\n else:\n top_fr[top] += 1\n bot_fr[bot] += 1\n \n for val in range(1, 7):\n if (val_total[val] - top_fr[val]) == bot_fr[val]:\n return min(top_fr[val], bot_fr[val])\n \n return -1\n```\n\n**UPVOTE if you like (\uD83C\uDF38\u25E0\u203F\u25E0), If you have any question, feel free to ask.** | 23 | You are given an array of integers `stones` where `stones[i]` is the weight of the `ith` stone.
We are playing a game with the stones. On each turn, we choose any two stones and smash them together. Suppose the stones have weights `x` and `y` with `x <= y`. The result of this smash is:
* If `x == y`, both stones are destroyed, and
* If `x != y`, the stone of weight `x` is destroyed, and the stone of weight `y` has new weight `y - x`.
At the end of the game, there is **at most one** stone left.
Return _the smallest possible weight of the left stone_. If there are no stones left, return `0`.
**Example 1:**
**Input:** stones = \[2,7,4,1,8,1\]
**Output:** 1
**Explanation:**
We can combine 2 and 4 to get 2, so the array converts to \[2,7,1,8,1\] then,
we can combine 7 and 8 to get 1, so the array converts to \[2,1,1,1\] then,
we can combine 2 and 1 to get 1, so the array converts to \[1,1,1\] then,
we can combine 1 and 1 to get 0, so the array converts to \[1\], then that's the optimal value.
**Example 2:**
**Input:** stones = \[31,26,33,21,40\]
**Output:** 5
**Constraints:**
* `1 <= stones.length <= 30`
* `1 <= stones[i] <= 100` | null |
Simple in Python | minimum-domino-rotations-for-equal-row | 0 | 1 | # Intuition\nThe intuition is that we need to figure out the most common number in both arrays, as that will be the number at either top or bottom after the swaps. \n\nWe also need to make sure that the most common number is in either tops[i] or bottoms[i], because if it is not, then we know we can return -1.\n\nFinally, for the result we can see whether the top or bottom has the higher frequency of the most common number and subtract it from the total length to find the minimum swaps.\n\n# Approach\n1. Find the most common number in both tops and bottoms.\n2. Iterate through length of array, to see if most common number is in either tops[i] or bottoms[i]. If not, then return -1\n3. Return the difference between length and max frequency of most common number at either the top or bottom.\n\n# Code\n```\nclass Solution:\n def minDominoRotations(self, tops: List[int], bottoms: List[int]) -> int:\n \n nlen = len(tops)\n topC = Counter(tops)\n bottomC = Counter(bottoms)\n totalC = topC + bottomC\n\n num, freq = totalC.most_common()[0]\n\n for i in range(nlen):\n if num != tops[i] and num != bottoms[i]:\n return -1\n\n return nlen-max(topC[num], bottomC[num])\n``` | 1 | In a row of dominoes, `tops[i]` and `bottoms[i]` represent the top and bottom halves of the `ith` domino. (A domino is a tile with two numbers from 1 to 6 - one on each half of the tile.)
We may rotate the `ith` domino, so that `tops[i]` and `bottoms[i]` swap values.
Return the minimum number of rotations so that all the values in `tops` are the same, or all the values in `bottoms` are the same.
If it cannot be done, return `-1`.
**Example 1:**
**Input:** tops = \[2,1,2,4,2,2\], bottoms = \[5,2,6,2,3,2\]
**Output:** 2
**Explanation:**
The first figure represents the dominoes as given by tops and bottoms: before we do any rotations.
If we rotate the second and fourth dominoes, we can make every value in the top row equal to 2, as indicated by the second figure.
**Example 2:**
**Input:** tops = \[3,5,1,2,3\], bottoms = \[3,6,3,3,4\]
**Output:** -1
**Explanation:**
In this case, it is not possible to rotate the dominoes to make one row of values equal.
**Constraints:**
* `2 <= tops.length <= 2 * 104`
* `bottoms.length == tops.length`
* `1 <= tops[i], bottoms[i] <= 6` | null |
Simple in Python | minimum-domino-rotations-for-equal-row | 0 | 1 | # Intuition\nThe intuition is that we need to figure out the most common number in both arrays, as that will be the number at either top or bottom after the swaps. \n\nWe also need to make sure that the most common number is in either tops[i] or bottoms[i], because if it is not, then we know we can return -1.\n\nFinally, for the result we can see whether the top or bottom has the higher frequency of the most common number and subtract it from the total length to find the minimum swaps.\n\n# Approach\n1. Find the most common number in both tops and bottoms.\n2. Iterate through length of array, to see if most common number is in either tops[i] or bottoms[i]. If not, then return -1\n3. Return the difference between length and max frequency of most common number at either the top or bottom.\n\n# Code\n```\nclass Solution:\n def minDominoRotations(self, tops: List[int], bottoms: List[int]) -> int:\n \n nlen = len(tops)\n topC = Counter(tops)\n bottomC = Counter(bottoms)\n totalC = topC + bottomC\n\n num, freq = totalC.most_common()[0]\n\n for i in range(nlen):\n if num != tops[i] and num != bottoms[i]:\n return -1\n\n return nlen-max(topC[num], bottomC[num])\n``` | 1 | You are given an array of integers `stones` where `stones[i]` is the weight of the `ith` stone.
We are playing a game with the stones. On each turn, we choose any two stones and smash them together. Suppose the stones have weights `x` and `y` with `x <= y`. The result of this smash is:
* If `x == y`, both stones are destroyed, and
* If `x != y`, the stone of weight `x` is destroyed, and the stone of weight `y` has new weight `y - x`.
At the end of the game, there is **at most one** stone left.
Return _the smallest possible weight of the left stone_. If there are no stones left, return `0`.
**Example 1:**
**Input:** stones = \[2,7,4,1,8,1\]
**Output:** 1
**Explanation:**
We can combine 2 and 4 to get 2, so the array converts to \[2,7,1,8,1\] then,
we can combine 7 and 8 to get 1, so the array converts to \[2,1,1,1\] then,
we can combine 2 and 1 to get 1, so the array converts to \[1,1,1\] then,
we can combine 1 and 1 to get 0, so the array converts to \[1\], then that's the optimal value.
**Example 2:**
**Input:** stones = \[31,26,33,21,40\]
**Output:** 5
**Constraints:**
* `1 <= stones.length <= 30`
* `1 <= stones[i] <= 100` | null |
[ Python ] ✔✔ Simple Python Solution Using Dictionary or Hashmap || O(n) 🔥✌ | minimum-domino-rotations-for-equal-row | 0 | 1 | # If It is Useful to Understand Please Upvote Me \uD83D\uDE4F\uD83D\uDE4F\n# Runtime: 1184 ms, faster than 85.42% of Python3 online submissions for Minimum Domino Rotations For Equal Row.\n# Memory Usage: 15.3 MB, less than 22.57% of Python3 online submissions for Minimum Domino Rotations For Equal Row.\n# Time Complexity : - O(n)\n\tclass Solution:\n\t\tdef minDominoRotations(self, tops: List[int], bottoms: List[int]) -> int:\n\n\t\t\tfrequency_tops = {1:0,2:0,3:0,4:0,5:0,6:0}\n\n\t\t\tfor i in tops:\n\t\t\t\tif i not in frequency_tops:\n\t\t\t\t\tfrequency_tops[i] = 1\n\t\t\t\telse:\n\t\t\t\t\tfrequency_tops[i] = frequency_tops[i] + 1\n\n\t\t\tfrequency_bottoms = {1:0,2:0,3:0,4:0,5:0,6:0}\n\n\t\t\tfor i in bottoms:\n\t\t\t\tif i not in frequency_bottoms:\n\t\t\t\t\tfrequency_bottoms[i] = 1\n\t\t\t\telse:\n\t\t\t\t\tfrequency_bottoms[i] = frequency_bottoms[i] + 1\n\n\t\t\tswap_number = 0\n\n\t\t\tfor i in range(1,7):\n\t\t\t\tif frequency_tops[i] + frequency_bottoms[i] >= len(tops):\n\t\t\t\t\tswap_number = i\n\n\t\t\tif swap_number == 0:\n\t\t\t\treturn -1\n\n\t\t\tmin_num1 = len(tops)-frequency_tops[swap_number]\n\t\t\tmin_num2 = len(bottoms)-frequency_bottoms[swap_number]\n\n\n\t\t\tfor i in range(len(tops)):\n\t\t\t\tif swap_number not in [tops[i],bottoms[i]]:\n\t\t\t\t\treturn -1\n\n\t\t\treturn min(min_num1,min_num2) | 3 | In a row of dominoes, `tops[i]` and `bottoms[i]` represent the top and bottom halves of the `ith` domino. (A domino is a tile with two numbers from 1 to 6 - one on each half of the tile.)
We may rotate the `ith` domino, so that `tops[i]` and `bottoms[i]` swap values.
Return the minimum number of rotations so that all the values in `tops` are the same, or all the values in `bottoms` are the same.
If it cannot be done, return `-1`.
**Example 1:**
**Input:** tops = \[2,1,2,4,2,2\], bottoms = \[5,2,6,2,3,2\]
**Output:** 2
**Explanation:**
The first figure represents the dominoes as given by tops and bottoms: before we do any rotations.
If we rotate the second and fourth dominoes, we can make every value in the top row equal to 2, as indicated by the second figure.
**Example 2:**
**Input:** tops = \[3,5,1,2,3\], bottoms = \[3,6,3,3,4\]
**Output:** -1
**Explanation:**
In this case, it is not possible to rotate the dominoes to make one row of values equal.
**Constraints:**
* `2 <= tops.length <= 2 * 104`
* `bottoms.length == tops.length`
* `1 <= tops[i], bottoms[i] <= 6` | null |
[ Python ] ✔✔ Simple Python Solution Using Dictionary or Hashmap || O(n) 🔥✌ | minimum-domino-rotations-for-equal-row | 0 | 1 | # If It is Useful to Understand Please Upvote Me \uD83D\uDE4F\uD83D\uDE4F\n# Runtime: 1184 ms, faster than 85.42% of Python3 online submissions for Minimum Domino Rotations For Equal Row.\n# Memory Usage: 15.3 MB, less than 22.57% of Python3 online submissions for Minimum Domino Rotations For Equal Row.\n# Time Complexity : - O(n)\n\tclass Solution:\n\t\tdef minDominoRotations(self, tops: List[int], bottoms: List[int]) -> int:\n\n\t\t\tfrequency_tops = {1:0,2:0,3:0,4:0,5:0,6:0}\n\n\t\t\tfor i in tops:\n\t\t\t\tif i not in frequency_tops:\n\t\t\t\t\tfrequency_tops[i] = 1\n\t\t\t\telse:\n\t\t\t\t\tfrequency_tops[i] = frequency_tops[i] + 1\n\n\t\t\tfrequency_bottoms = {1:0,2:0,3:0,4:0,5:0,6:0}\n\n\t\t\tfor i in bottoms:\n\t\t\t\tif i not in frequency_bottoms:\n\t\t\t\t\tfrequency_bottoms[i] = 1\n\t\t\t\telse:\n\t\t\t\t\tfrequency_bottoms[i] = frequency_bottoms[i] + 1\n\n\t\t\tswap_number = 0\n\n\t\t\tfor i in range(1,7):\n\t\t\t\tif frequency_tops[i] + frequency_bottoms[i] >= len(tops):\n\t\t\t\t\tswap_number = i\n\n\t\t\tif swap_number == 0:\n\t\t\t\treturn -1\n\n\t\t\tmin_num1 = len(tops)-frequency_tops[swap_number]\n\t\t\tmin_num2 = len(bottoms)-frequency_bottoms[swap_number]\n\n\n\t\t\tfor i in range(len(tops)):\n\t\t\t\tif swap_number not in [tops[i],bottoms[i]]:\n\t\t\t\t\treturn -1\n\n\t\t\treturn min(min_num1,min_num2) | 3 | You are given an array of integers `stones` where `stones[i]` is the weight of the `ith` stone.
We are playing a game with the stones. On each turn, we choose any two stones and smash them together. Suppose the stones have weights `x` and `y` with `x <= y`. The result of this smash is:
* If `x == y`, both stones are destroyed, and
* If `x != y`, the stone of weight `x` is destroyed, and the stone of weight `y` has new weight `y - x`.
At the end of the game, there is **at most one** stone left.
Return _the smallest possible weight of the left stone_. If there are no stones left, return `0`.
**Example 1:**
**Input:** stones = \[2,7,4,1,8,1\]
**Output:** 1
**Explanation:**
We can combine 2 and 4 to get 2, so the array converts to \[2,7,1,8,1\] then,
we can combine 7 and 8 to get 1, so the array converts to \[2,1,1,1\] then,
we can combine 2 and 1 to get 1, so the array converts to \[1,1,1\] then,
we can combine 1 and 1 to get 0, so the array converts to \[1\], then that's the optimal value.
**Example 2:**
**Input:** stones = \[31,26,33,21,40\]
**Output:** 5
**Constraints:**
* `1 <= stones.length <= 30`
* `1 <= stones[i] <= 100` | null |
Python simple and easy intuitive explained solution | minimum-domino-rotations-for-equal-row | 0 | 1 | ```\nclass Solution:\n def minDominoRotations(self, A: List[int], B: List[int]) -> int:\n def countDifference(A, B, num):\n countA, countB = 0, 0\n \n for i in range(len(A)):\n # if we have a domino which doesn\'t have num at all, num can\'t be the whole row:\n if A[i] != num and B[i] != num:\n return -1\n else:\n if A[i] != num: countA+=1\n if B[i] != num: countB+=1\n \n return min(countA, countB)\n \n # check if A[0] and B[0] can\'t be the number for the whole row:\n if A.count(A[0]) + B.count(A[0]) < len(A) and A.count(B[0]) + B.count(B[0]) < len(A):\n return -1\n \n # first try to make A[0] the whole row, then try B[0]:\n res1 = countDifference(A, B, A[0])\n res2 = countDifference(A, B, B[0])\n \n if min(res1, res2) > 0: return min(res1, res2)\n return max(res1, res2)\n```\n**Like it? please upvote...** | 8 | In a row of dominoes, `tops[i]` and `bottoms[i]` represent the top and bottom halves of the `ith` domino. (A domino is a tile with two numbers from 1 to 6 - one on each half of the tile.)
We may rotate the `ith` domino, so that `tops[i]` and `bottoms[i]` swap values.
Return the minimum number of rotations so that all the values in `tops` are the same, or all the values in `bottoms` are the same.
If it cannot be done, return `-1`.
**Example 1:**
**Input:** tops = \[2,1,2,4,2,2\], bottoms = \[5,2,6,2,3,2\]
**Output:** 2
**Explanation:**
The first figure represents the dominoes as given by tops and bottoms: before we do any rotations.
If we rotate the second and fourth dominoes, we can make every value in the top row equal to 2, as indicated by the second figure.
**Example 2:**
**Input:** tops = \[3,5,1,2,3\], bottoms = \[3,6,3,3,4\]
**Output:** -1
**Explanation:**
In this case, it is not possible to rotate the dominoes to make one row of values equal.
**Constraints:**
* `2 <= tops.length <= 2 * 104`
* `bottoms.length == tops.length`
* `1 <= tops[i], bottoms[i] <= 6` | null |
Python simple and easy intuitive explained solution | minimum-domino-rotations-for-equal-row | 0 | 1 | ```\nclass Solution:\n def minDominoRotations(self, A: List[int], B: List[int]) -> int:\n def countDifference(A, B, num):\n countA, countB = 0, 0\n \n for i in range(len(A)):\n # if we have a domino which doesn\'t have num at all, num can\'t be the whole row:\n if A[i] != num and B[i] != num:\n return -1\n else:\n if A[i] != num: countA+=1\n if B[i] != num: countB+=1\n \n return min(countA, countB)\n \n # check if A[0] and B[0] can\'t be the number for the whole row:\n if A.count(A[0]) + B.count(A[0]) < len(A) and A.count(B[0]) + B.count(B[0]) < len(A):\n return -1\n \n # first try to make A[0] the whole row, then try B[0]:\n res1 = countDifference(A, B, A[0])\n res2 = countDifference(A, B, B[0])\n \n if min(res1, res2) > 0: return min(res1, res2)\n return max(res1, res2)\n```\n**Like it? please upvote...** | 8 | You are given an array of integers `stones` where `stones[i]` is the weight of the `ith` stone.
We are playing a game with the stones. On each turn, we choose any two stones and smash them together. Suppose the stones have weights `x` and `y` with `x <= y`. The result of this smash is:
* If `x == y`, both stones are destroyed, and
* If `x != y`, the stone of weight `x` is destroyed, and the stone of weight `y` has new weight `y - x`.
At the end of the game, there is **at most one** stone left.
Return _the smallest possible weight of the left stone_. If there are no stones left, return `0`.
**Example 1:**
**Input:** stones = \[2,7,4,1,8,1\]
**Output:** 1
**Explanation:**
We can combine 2 and 4 to get 2, so the array converts to \[2,7,1,8,1\] then,
we can combine 7 and 8 to get 1, so the array converts to \[2,1,1,1\] then,
we can combine 2 and 1 to get 1, so the array converts to \[1,1,1\] then,
we can combine 1 and 1 to get 0, so the array converts to \[1\], then that's the optimal value.
**Example 2:**
**Input:** stones = \[31,26,33,21,40\]
**Output:** 5
**Constraints:**
* `1 <= stones.length <= 30`
* `1 <= stones[i] <= 100` | null |
Use Sets for Easily Solving It | minimum-domino-rotations-for-equal-row | 0 | 1 | **Time & Space:**\n\n\n**Intution:**\nThe questions essentially boils down weather their exists a common element b/w all the indices and where to keep that value that is on the top array or the bottom one. Something like this can be easily implemented using sets in python. We just need to find the intersection b/w the current possible intersection set and a set of **{top[i], bottom[i]}**\n\n**Steps:**\n1. Basically maintain a set called inter and initialize it by the values with a set of 1st values of tops and bottom array.\n2. Now loop over all the indices and get the intersection b/w inter and a set of top and bottom values at that index.\n3. Now we can have 3 cases:\n\n**Case 1: `len(inter) == 0`:** No intersection found, so it is not possible to provide the asked arrangement\n**Case 2: `len(inter) == 1`:** Only one value found *(call it **target**)*. But now we have two options that are either to keep target on the top array or to keep target with bottom array. We can simply do it by counting the occurence of target in the tops and bottoms array. We use the array with maximum number of these occurences as it would lead to mininum number of swaps. \n**Case 3: `len(inter) == 2`:** Two values found. Eg Testcase: `top = [1,1,1,2,2]` & `bottom = [2,2,2,1,1]`. Simply count the number of occurence of any in either array *(call it **movements**)*. Return minimum of `movements` or `N - movements`\n**Code:**\n```\nclass Solution:\n def minDominoRotations(self, tops: List[int], bottoms: List[int]) -> int:\n n = len(tops) \n inter = {tops[0], bottoms[0]}\n for i in range(n):\n inter = inter.intersection({tops[i], bottoms[i]})\n\t\t# This gives us the finaly common values b/w all the indices. \n if len(inter) == 0: # Case 1\n return -1\n elif len(inter) == 2: # Case 3\n target = inter.pop()\n m = tops.count(target)\n return min(m, n-m)\n else: # Case 2\n for_tops = for_bottom = 0\n target = inter.pop()\n target_in_tops = tops.count(target)\n target_in_bottoms = bottoms.count(target)\n return n - max(target_in_tops, target_in_bottoms)\n```\n\n\n\n | 2 | In a row of dominoes, `tops[i]` and `bottoms[i]` represent the top and bottom halves of the `ith` domino. (A domino is a tile with two numbers from 1 to 6 - one on each half of the tile.)
We may rotate the `ith` domino, so that `tops[i]` and `bottoms[i]` swap values.
Return the minimum number of rotations so that all the values in `tops` are the same, or all the values in `bottoms` are the same.
If it cannot be done, return `-1`.
**Example 1:**
**Input:** tops = \[2,1,2,4,2,2\], bottoms = \[5,2,6,2,3,2\]
**Output:** 2
**Explanation:**
The first figure represents the dominoes as given by tops and bottoms: before we do any rotations.
If we rotate the second and fourth dominoes, we can make every value in the top row equal to 2, as indicated by the second figure.
**Example 2:**
**Input:** tops = \[3,5,1,2,3\], bottoms = \[3,6,3,3,4\]
**Output:** -1
**Explanation:**
In this case, it is not possible to rotate the dominoes to make one row of values equal.
**Constraints:**
* `2 <= tops.length <= 2 * 104`
* `bottoms.length == tops.length`
* `1 <= tops[i], bottoms[i] <= 6` | null |
Use Sets for Easily Solving It | minimum-domino-rotations-for-equal-row | 0 | 1 | **Time & Space:**\n\n\n**Intution:**\nThe questions essentially boils down weather their exists a common element b/w all the indices and where to keep that value that is on the top array or the bottom one. Something like this can be easily implemented using sets in python. We just need to find the intersection b/w the current possible intersection set and a set of **{top[i], bottom[i]}**\n\n**Steps:**\n1. Basically maintain a set called inter and initialize it by the values with a set of 1st values of tops and bottom array.\n2. Now loop over all the indices and get the intersection b/w inter and a set of top and bottom values at that index.\n3. Now we can have 3 cases:\n\n**Case 1: `len(inter) == 0`:** No intersection found, so it is not possible to provide the asked arrangement\n**Case 2: `len(inter) == 1`:** Only one value found *(call it **target**)*. But now we have two options that are either to keep target on the top array or to keep target with bottom array. We can simply do it by counting the occurence of target in the tops and bottoms array. We use the array with maximum number of these occurences as it would lead to mininum number of swaps. \n**Case 3: `len(inter) == 2`:** Two values found. Eg Testcase: `top = [1,1,1,2,2]` & `bottom = [2,2,2,1,1]`. Simply count the number of occurence of any in either array *(call it **movements**)*. Return minimum of `movements` or `N - movements`\n**Code:**\n```\nclass Solution:\n def minDominoRotations(self, tops: List[int], bottoms: List[int]) -> int:\n n = len(tops) \n inter = {tops[0], bottoms[0]}\n for i in range(n):\n inter = inter.intersection({tops[i], bottoms[i]})\n\t\t# This gives us the finaly common values b/w all the indices. \n if len(inter) == 0: # Case 1\n return -1\n elif len(inter) == 2: # Case 3\n target = inter.pop()\n m = tops.count(target)\n return min(m, n-m)\n else: # Case 2\n for_tops = for_bottom = 0\n target = inter.pop()\n target_in_tops = tops.count(target)\n target_in_bottoms = bottoms.count(target)\n return n - max(target_in_tops, target_in_bottoms)\n```\n\n\n\n | 2 | You are given an array of integers `stones` where `stones[i]` is the weight of the `ith` stone.
We are playing a game with the stones. On each turn, we choose any two stones and smash them together. Suppose the stones have weights `x` and `y` with `x <= y`. The result of this smash is:
* If `x == y`, both stones are destroyed, and
* If `x != y`, the stone of weight `x` is destroyed, and the stone of weight `y` has new weight `y - x`.
At the end of the game, there is **at most one** stone left.
Return _the smallest possible weight of the left stone_. If there are no stones left, return `0`.
**Example 1:**
**Input:** stones = \[2,7,4,1,8,1\]
**Output:** 1
**Explanation:**
We can combine 2 and 4 to get 2, so the array converts to \[2,7,1,8,1\] then,
we can combine 7 and 8 to get 1, so the array converts to \[2,1,1,1\] then,
we can combine 2 and 1 to get 1, so the array converts to \[1,1,1\] then,
we can combine 1 and 1 to get 0, so the array converts to \[1\], then that's the optimal value.
**Example 2:**
**Input:** stones = \[31,26,33,21,40\]
**Output:** 5
**Constraints:**
* `1 <= stones.length <= 30`
* `1 <= stones[i] <= 100` | null |
Superb logic | construct-binary-search-tree-from-preorder-traversal | 0 | 1 | ```\nclass Solution:\n def bstFromPreorder(self, preorder: List[int]) -> Optional[TreeNode]:\n inorder=sorted(preorder)\n def build(preorder,inorder):\n if not preorder or not inorder:\n return \n root=TreeNode(preorder[0])\n mid=inorder.index(preorder[0])\n root.left=build(preorder[1:mid+1],inorder[:mid])\n root.right=build(preorder[mid+1:],inorder[mid+1:])\n return root\n return build(preorder,inorder)\n```\n# please upvote me it would encourage me alot\n | 4 | Given an array of integers preorder, which represents the **preorder traversal** of a BST (i.e., **binary search tree**), construct the tree and return _its root_.
It is **guaranteed** that there is always possible to find a binary search tree with the given requirements for the given test cases.
A **binary search tree** is a binary tree where for every node, any descendant of `Node.left` has a value **strictly less than** `Node.val`, and any descendant of `Node.right` has a value **strictly greater than** `Node.val`.
A **preorder traversal** of a binary tree displays the value of the node first, then traverses `Node.left`, then traverses `Node.right`.
**Example 1:**
**Input:** preorder = \[8,5,1,7,10,12\]
**Output:** \[8,5,10,1,7,null,12\]
**Example 2:**
**Input:** preorder = \[1,3\]
**Output:** \[1,null,3\]
**Constraints:**
* `1 <= preorder.length <= 100`
* `1 <= preorder[i] <= 1000`
* All the values of `preorder` are **unique**. | null |
Python Easy Solution | construct-binary-search-tree-from-preorder-traversal | 0 | 1 | # Python3 Solution\n```\nclass TreeNode:\n def __init__(self, val=0, left=None, right=None):\n self.val = val\n self.left = left\n self.right = right\n\nclass Solution:\n def bstFromPreorder(self, preorder: List[int]) -> Optional[TreeNode]:\n if len(preorder) == 0:\n return None\n root = TreeNode(preorder[0])\n\n def insertNode(root, newVal):\n if root.val > newVal:\n if root.left is None:\n root.left = TreeNode(newVal)\n return\n insertNode(root.left, newVal)\n if root.val < newVal:\n if root.right is None:\n root.right = TreeNode(newVal)\n return\n insertNode(root.right, newVal)\n \n for val in preorder[1:]:\n insertNode(root, val)\n return root\n``` | 1 | Given an array of integers preorder, which represents the **preorder traversal** of a BST (i.e., **binary search tree**), construct the tree and return _its root_.
It is **guaranteed** that there is always possible to find a binary search tree with the given requirements for the given test cases.
A **binary search tree** is a binary tree where for every node, any descendant of `Node.left` has a value **strictly less than** `Node.val`, and any descendant of `Node.right` has a value **strictly greater than** `Node.val`.
A **preorder traversal** of a binary tree displays the value of the node first, then traverses `Node.left`, then traverses `Node.right`.
**Example 1:**
**Input:** preorder = \[8,5,1,7,10,12\]
**Output:** \[8,5,10,1,7,null,12\]
**Example 2:**
**Input:** preorder = \[1,3\]
**Output:** \[1,null,3\]
**Constraints:**
* `1 <= preorder.length <= 100`
* `1 <= preorder[i] <= 1000`
* All the values of `preorder` are **unique**. | null |
Python || Easy || Recursive Solution | construct-binary-search-tree-from-preorder-traversal | 0 | 1 | ```\ndef bstFromPreorder(self, preorder: List[int]) -> Optional[TreeNode]:\n i=[0]\n def construct(preorder,i,bound):\n if i[0]==len(preorder) or preorder[i[0]]>bound:\n return None\n root=TreeNode(preorder[i[0]])\n i[0]+=1\n root.left=construct(preorder,i,root.val)\n root.right=construct(preorder,i,bound)\n return root \n return construct(preorder,i,float(\'inf\'))\n```\n**An upvote will be encouraging** | 2 | Given an array of integers preorder, which represents the **preorder traversal** of a BST (i.e., **binary search tree**), construct the tree and return _its root_.
It is **guaranteed** that there is always possible to find a binary search tree with the given requirements for the given test cases.
A **binary search tree** is a binary tree where for every node, any descendant of `Node.left` has a value **strictly less than** `Node.val`, and any descendant of `Node.right` has a value **strictly greater than** `Node.val`.
A **preorder traversal** of a binary tree displays the value of the node first, then traverses `Node.left`, then traverses `Node.right`.
**Example 1:**
**Input:** preorder = \[8,5,1,7,10,12\]
**Output:** \[8,5,10,1,7,null,12\]
**Example 2:**
**Input:** preorder = \[1,3\]
**Output:** \[1,null,3\]
**Constraints:**
* `1 <= preorder.length <= 100`
* `1 <= preorder[i] <= 1000`
* All the values of `preorder` are **unique**. | null |
Beginner-friendly || Construct a TreeNode with using list comprehension in Python3 | construct-binary-search-tree-from-preorder-traversal | 0 | 1 | # Intuition\nThe problem description is the following:\n- there\'s a list of nodes, that\'re placed by **preorder traversal**\n- we need to construct a **Binary Search Tree** from this list\n\n```py\n# Example\nnodes = [2, 1, 3]\n# 1. The list of nodes will represent the following BST:\n# (2)\n# / \\\n# (1) (3)\n\n# 2. At each step we need to split a given list of nodes for those,\n# whose value GREATER, and for those, whose value FEWER, than \n# the FIRST in that list. \nnode = TreeNode(nodes[0])\nnode.left = TreeNode(nodes[1])\nnode.right = TreeNode(nodes[2])\n\n# The real example could have at least 10, 100 or more nodes,\n# so it\'ll be exhausted to build this tree like the step above.\n\n# 3. So, according to p.2 we should SPLIT and recursively build\n# the tree by providing a list of corresponding nodes due \n# to BST implementation. \n```\n\n---\n\nIf you haven\'t familiar with [BST](https://en.wikipedia.org/wiki/Binary_search_tree), follow the links (preorder traversal is described in that article too + the other kinds, respectively).\n\n# Approach\n1. check, if we don\'t have any nodes in `preorder` and return `None`\n2. otherwise create a `TreeNode` with `val=preorder[0]`\n3. recursively create `left` and `right` children by filtering values `preorder[i]` by `x` in both sides **(less or greater)**\n4. return the `node`\n\n# Complexity\n- Time complexity: **O(n^2)**, because of iterating over `preorder` at each recursive call\n\n- Space complexity: **O(n)**, because of recursive call stack\n# Code\n```\n# Definition for a binary tree node.\n# class TreeNode:\n# def __init__(self, val=0, left=None, right=None):\n# self.val = val\n# self.left = left\n# self.right = right\nclass Solution:\n def bstFromPreorder(self, preorder: List[int]) -> Optional[TreeNode]:\n if not preorder:\n return None\n \n node = TreeNode(preorder[0])\n node.left = self.bstFromPreorder([x for x in preorder if x < preorder[0]])\n node.right = self.bstFromPreorder([x for x in preorder if x > preorder[0]])\n\n return node\n``` | 1 | Given an array of integers preorder, which represents the **preorder traversal** of a BST (i.e., **binary search tree**), construct the tree and return _its root_.
It is **guaranteed** that there is always possible to find a binary search tree with the given requirements for the given test cases.
A **binary search tree** is a binary tree where for every node, any descendant of `Node.left` has a value **strictly less than** `Node.val`, and any descendant of `Node.right` has a value **strictly greater than** `Node.val`.
A **preorder traversal** of a binary tree displays the value of the node first, then traverses `Node.left`, then traverses `Node.right`.
**Example 1:**
**Input:** preorder = \[8,5,1,7,10,12\]
**Output:** \[8,5,10,1,7,null,12\]
**Example 2:**
**Input:** preorder = \[1,3\]
**Output:** \[1,null,3\]
**Constraints:**
* `1 <= preorder.length <= 100`
* `1 <= preorder[i] <= 1000`
* All the values of `preorder` are **unique**. | null |
Python 3, Recursive, Easy to understand | construct-binary-search-tree-from-preorder-traversal | 0 | 1 | ```\nclass Solution:\n def bstFromPreorder(self, preorder: List[int]) -> TreeNode:\n if not preorder:\n return None\n root = TreeNode(preorder[0])\n i = 1\n while i<len(preorder) and preorder[i] < root.val:\n i+=1\n root.left = self.bstFromPreorder(preorder[1:i])\n root.right = self.bstFromPreorder(preorder[i:])\n return root\n```\n\nWork through with example `preorder = [8,5,1,7,10,12]`\n- root = preorder[0] = 8\n- root.left = [5,1,7]\n\t- root.left = 5\n\t- root.left.left = [1]\n\t\t- root.left.left = 1\n\t- root.left.right = [7]\n\t\t- root.left.right = 7\n- root.right = [10,12]\n\t- root.right = 10\n\t- root.right.left = None\n\t- root.right.right = [12]\n\t\t- root.right.right = 12\n\n\n***\nCheck on my [repo](https://github.com/zengtian006/LeetCode) to get Leetcode solution(Python) with classification: https://github.com/zengtian006/LeetCode\n***\n\n | 77 | Given an array of integers preorder, which represents the **preorder traversal** of a BST (i.e., **binary search tree**), construct the tree and return _its root_.
It is **guaranteed** that there is always possible to find a binary search tree with the given requirements for the given test cases.
A **binary search tree** is a binary tree where for every node, any descendant of `Node.left` has a value **strictly less than** `Node.val`, and any descendant of `Node.right` has a value **strictly greater than** `Node.val`.
A **preorder traversal** of a binary tree displays the value of the node first, then traverses `Node.left`, then traverses `Node.right`.
**Example 1:**
**Input:** preorder = \[8,5,1,7,10,12\]
**Output:** \[8,5,10,1,7,null,12\]
**Example 2:**
**Input:** preorder = \[1,3\]
**Output:** \[1,null,3\]
**Constraints:**
* `1 <= preorder.length <= 100`
* `1 <= preorder[i] <= 1000`
* All the values of `preorder` are **unique**. | null |
Solution | construct-binary-search-tree-from-preorder-traversal | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n void BST(TreeNode* root,int x){\n while(1){\n if(root->val<x){\n if(root->right==NULL){\n TreeNode* new_node=new TreeNode(x);\n root->right=new_node;\n return;\n }\n else{\n root=root->right;\n }\n }\n else{\n if(root->left==NULL){\n TreeNode* new_node=new TreeNode(x);\n root->left=new_node;\n return;\n }\n else{\n root=root->left;\n }\n }\n }\n }\n TreeNode* bstFromPreorder(vector<int> &preorder) {\n TreeNode* root=new TreeNode(preorder[0]);\n int n=preorder.size();\n for(int i=1;i<n;i++){\n BST(root,preorder[i]);\n }\n return root;\n }\n};\n```\n\n```Python3 []\nclass Solution:\n preorder_index = 0\n def bstFromPreorder(self, preorder: List[int]) -> Optional[TreeNode]:\n root = TreeNode(preorder[0])\n for index, val in enumerate(preorder):\n if index == 0:\n continue\n\n self.insert(root, val)\n return root\n\n def insert(self, root, val):\n curr = root\n while curr:\n if curr.val > val:\n if curr.left is None:\n curr.left = TreeNode(val)\n break\n curr = curr.left\n if curr.val < val :\n if curr.right is None:\n curr.right = TreeNode(val)\n break\n curr = curr.right\n```\n\n```Java []\nclass Solution {\n public TreeNode bstFromPreorder(int[] preorder) {\n return helper(preorder, 0, preorder.length - 1); \n }\n private TreeNode helper(int[] preorder, int start, int end) {\n if(start > end) return null;\n \n TreeNode node = new TreeNode(preorder[start]);\n int i;\n for(i=start;i<=end;i++) {\n if(preorder[i] > node.val)\n break;\n }\n node.left = helper(preorder, start+1, i-1);\n node.right = helper(preorder, i, end);\n return node; \n }\n}\n``` | 2 | Given an array of integers preorder, which represents the **preorder traversal** of a BST (i.e., **binary search tree**), construct the tree and return _its root_.
It is **guaranteed** that there is always possible to find a binary search tree with the given requirements for the given test cases.
A **binary search tree** is a binary tree where for every node, any descendant of `Node.left` has a value **strictly less than** `Node.val`, and any descendant of `Node.right` has a value **strictly greater than** `Node.val`.
A **preorder traversal** of a binary tree displays the value of the node first, then traverses `Node.left`, then traverses `Node.right`.
**Example 1:**
**Input:** preorder = \[8,5,1,7,10,12\]
**Output:** \[8,5,10,1,7,null,12\]
**Example 2:**
**Input:** preorder = \[1,3\]
**Output:** \[1,null,3\]
**Constraints:**
* `1 <= preorder.length <= 100`
* `1 <= preorder[i] <= 1000`
* All the values of `preorder` are **unique**. | null |
Solution | complement-of-base-10-integer | 1 | 1 | ```C++ []\nclass Solution {\n public:\n int bitwiseComplement(int N) {\n int mask = 1;\n\n while (mask < N)\n mask = (mask << 1) + 1;\n\n return mask ^ N;\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def bitwiseComplement(self, n: int) -> int:\n cnt=0\n ans=0\n if n==0:\n return 1\n while n>0:\n if n&1:\n cnt+=1\n else:\n ans =ans +(2**cnt)\n cnt+=1\n n=n>>1\n return ans\n```\n\n```Java []\nclass Solution {\n public int bitwiseComplement(int n) {\n if(n == 0) return 1;\n int res = 0;\n int fac = 1;\n \n while(n != 0){\n res += fac * (n % 2 == 0 ? 1 : 0);\n fac *= 2;\n n /= 2;\n }\n return res;\n }\n}\n``` | 456 | The **complement** of an integer is the integer you get when you flip all the `0`'s to `1`'s and all the `1`'s to `0`'s in its binary representation.
* For example, The integer `5` is `"101 "` in binary and its **complement** is `"010 "` which is the integer `2`.
Given an integer `n`, return _its complement_.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation:** 5 is "101 " in binary, with complement "010 " in binary, which is 2 in base-10.
**Example 2:**
**Input:** n = 7
**Output:** 0
**Explanation:** 7 is "111 " in binary, with complement "000 " in binary, which is 0 in base-10.
**Example 3:**
**Input:** n = 10
**Output:** 5
**Explanation:** 10 is "1010 " in binary, with complement "0101 " in binary, which is 5 in base-10.
**Constraints:**
* `0 <= n < 109`
**Note:** This question is the same as 476: [https://leetcode.com/problems/number-complement/](https://leetcode.com/problems/number-complement/) | null |
Solution | complement-of-base-10-integer | 1 | 1 | ```C++ []\nclass Solution {\n public:\n int bitwiseComplement(int N) {\n int mask = 1;\n\n while (mask < N)\n mask = (mask << 1) + 1;\n\n return mask ^ N;\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def bitwiseComplement(self, n: int) -> int:\n cnt=0\n ans=0\n if n==0:\n return 1\n while n>0:\n if n&1:\n cnt+=1\n else:\n ans =ans +(2**cnt)\n cnt+=1\n n=n>>1\n return ans\n```\n\n```Java []\nclass Solution {\n public int bitwiseComplement(int n) {\n if(n == 0) return 1;\n int res = 0;\n int fac = 1;\n \n while(n != 0){\n res += fac * (n % 2 == 0 ? 1 : 0);\n fac *= 2;\n n /= 2;\n }\n return res;\n }\n}\n``` | 456 | In a warehouse, there is a row of barcodes, where the `ith` barcode is `barcodes[i]`.
Rearrange the barcodes so that no two adjacent barcodes are equal. You may return any answer, and it is guaranteed an answer exists.
**Example 1:**
**Input:** barcodes = \[1,1,1,2,2,2\]
**Output:** \[2,1,2,1,2,1\]
**Example 2:**
**Input:** barcodes = \[1,1,1,1,2,2,3,3\]
**Output:** \[1,3,1,3,1,2,1,2\]
**Constraints:**
* `1 <= barcodes.length <= 10000`
* `1 <= barcodes[i] <= 10000` | A binary number plus its complement will equal 111....111 in binary. Also, N = 0 is a corner case. |
2 Solutions one-liner beat 90.93% 26ms Python3 | complement-of-base-10-integer | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def bitwiseComplement(self, n: int) -> int:\n return (int((bin(n)[2:]).replace(\'1\', \'2\').replace(\'0\',\'1\').replace(\'2\',\'0\'),2))\n\n return (int((bin(n)[2:]).translate(str.maketrans("01", "10")),2))\n``` | 1 | The **complement** of an integer is the integer you get when you flip all the `0`'s to `1`'s and all the `1`'s to `0`'s in its binary representation.
* For example, The integer `5` is `"101 "` in binary and its **complement** is `"010 "` which is the integer `2`.
Given an integer `n`, return _its complement_.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation:** 5 is "101 " in binary, with complement "010 " in binary, which is 2 in base-10.
**Example 2:**
**Input:** n = 7
**Output:** 0
**Explanation:** 7 is "111 " in binary, with complement "000 " in binary, which is 0 in base-10.
**Example 3:**
**Input:** n = 10
**Output:** 5
**Explanation:** 10 is "1010 " in binary, with complement "0101 " in binary, which is 5 in base-10.
**Constraints:**
* `0 <= n < 109`
**Note:** This question is the same as 476: [https://leetcode.com/problems/number-complement/](https://leetcode.com/problems/number-complement/) | null |
2 Solutions one-liner beat 90.93% 26ms Python3 | complement-of-base-10-integer | 0 | 1 | \n\n# Code\n```\nclass Solution:\n def bitwiseComplement(self, n: int) -> int:\n return (int((bin(n)[2:]).replace(\'1\', \'2\').replace(\'0\',\'1\').replace(\'2\',\'0\'),2))\n\n return (int((bin(n)[2:]).translate(str.maketrans("01", "10")),2))\n``` | 1 | In a warehouse, there is a row of barcodes, where the `ith` barcode is `barcodes[i]`.
Rearrange the barcodes so that no two adjacent barcodes are equal. You may return any answer, and it is guaranteed an answer exists.
**Example 1:**
**Input:** barcodes = \[1,1,1,2,2,2\]
**Output:** \[2,1,2,1,2,1\]
**Example 2:**
**Input:** barcodes = \[1,1,1,1,2,2,3,3\]
**Output:** \[1,3,1,3,1,2,1,2\]
**Constraints:**
* `1 <= barcodes.length <= 10000`
* `1 <= barcodes[i] <= 10000` | A binary number plus its complement will equal 111....111 in binary. Also, N = 0 is a corner case. |
Python 2 Approach 97.96% beats | complement-of-base-10-integer | 0 | 1 | **If you got help from this,... Plz Upvote .. it encourage me**\n# EXAMPLE , \n # N = 101 (5)\n # MASK WILL BE 111 (7)\n # COMPLEMENT MASK - N = 7-5 = 2 (010)\n# Code\n```\n<!-- 1ST APPROACH -->\nclass Solution:\n def bitwiseComplement(self, n: int) -> int:\n \n if n == 0:\n return 1\n\n mask = 0\n m = n\n while m != 0:\n mask = mask << 1 | 1\n m = m >> 1\n \n return mask - n\n # OR\n #return (~n) & mask \n\n<!-- ============================================================ -->\n<!-- 2ND APPROACH -->\nclass Solution:\n def bitwiseComplement(self, n: int) -> int:\n x = 1\n while n > x:\n x = x * 2 + 1\n return x - n\n``` | 2 | The **complement** of an integer is the integer you get when you flip all the `0`'s to `1`'s and all the `1`'s to `0`'s in its binary representation.
* For example, The integer `5` is `"101 "` in binary and its **complement** is `"010 "` which is the integer `2`.
Given an integer `n`, return _its complement_.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation:** 5 is "101 " in binary, with complement "010 " in binary, which is 2 in base-10.
**Example 2:**
**Input:** n = 7
**Output:** 0
**Explanation:** 7 is "111 " in binary, with complement "000 " in binary, which is 0 in base-10.
**Example 3:**
**Input:** n = 10
**Output:** 5
**Explanation:** 10 is "1010 " in binary, with complement "0101 " in binary, which is 5 in base-10.
**Constraints:**
* `0 <= n < 109`
**Note:** This question is the same as 476: [https://leetcode.com/problems/number-complement/](https://leetcode.com/problems/number-complement/) | null |
Python 2 Approach 97.96% beats | complement-of-base-10-integer | 0 | 1 | **If you got help from this,... Plz Upvote .. it encourage me**\n# EXAMPLE , \n # N = 101 (5)\n # MASK WILL BE 111 (7)\n # COMPLEMENT MASK - N = 7-5 = 2 (010)\n# Code\n```\n<!-- 1ST APPROACH -->\nclass Solution:\n def bitwiseComplement(self, n: int) -> int:\n \n if n == 0:\n return 1\n\n mask = 0\n m = n\n while m != 0:\n mask = mask << 1 | 1\n m = m >> 1\n \n return mask - n\n # OR\n #return (~n) & mask \n\n<!-- ============================================================ -->\n<!-- 2ND APPROACH -->\nclass Solution:\n def bitwiseComplement(self, n: int) -> int:\n x = 1\n while n > x:\n x = x * 2 + 1\n return x - n\n``` | 2 | In a warehouse, there is a row of barcodes, where the `ith` barcode is `barcodes[i]`.
Rearrange the barcodes so that no two adjacent barcodes are equal. You may return any answer, and it is guaranteed an answer exists.
**Example 1:**
**Input:** barcodes = \[1,1,1,2,2,2\]
**Output:** \[2,1,2,1,2,1\]
**Example 2:**
**Input:** barcodes = \[1,1,1,1,2,2,3,3\]
**Output:** \[1,3,1,3,1,2,1,2\]
**Constraints:**
* `1 <= barcodes.length <= 10000`
* `1 <= barcodes[i] <= 10000` | A binary number plus its complement will equal 111....111 in binary. Also, N = 0 is a corner case. |
Beginner Friendly Python || Beats 91% | complement-of-base-10-integer | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nLet\'s assume a number, say 10. \n\n```\nBinary version = 1010\nComplement = 0101\nInteger compelement = 5\n```\n\n```\nmask is initially 0,\nTill m = 10 (1010) is not 0,\n1. add a 1 (left shift 0 at the end + OR with 1), mask = 1\n2. divide 10 by 2 or right shift by 1\n3. now m = 5(101)\n- Iteration 2: mask = 11, m = 2 (10)\n- Iteration 3: mask = 111, m = 1 (1)\n- Iteration 4: mask = 1111, m = 0\n```\n\n\n\nTo do this using code, we follow these steps:\n\n1. First, we check if the given number is 0. If it is, the complement is 1, because flipping 0 gives us 1.\n```\nif n == 0:\n return 1\n```\n\n2. To find the complement for any other number, we need to figure out how many bits it has. \n\n\n3. Now, we create a "mask," which is a pattern of bits. We set as many bits as our number has to 1. So, for 10, our mask is \'1111\' (4 ones in binary).\n\n```\nmask is basically all ones. \nfor example: \nmask for 10 (1010) = 1111\nmask for 5 (101) = 111\nmask for 11 (1011) = 1111\n```\n```\nmask is initially 0,\nTill m = 10 (1010) is not 0,\n- add a 1 (left shift 0 at the end + OR with 1), mask = 1\n- divide 10 by 2 or right shift by 1\n- now m = 5(101)\n- Iteration 2: mask = 11, m = 2 (10)\n- Iteration 3: mask = 111, m = 1 (1)\n- Iteration 4: mask = 1111, m = 0\n\n```\n\n4. Take the complement of the original number (flipping the bits using \'~\' in code) and apply the mask to it (using \'&\' in code). This gives us the complement of the number.\n\n# Complexity\n- Time complexity: O(1)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def bitwiseComplement(self, n: int) -> int:\n #generate a new number\n if n == 0:\n return 1\n m = n\n mask = 0\n while (m!=0):\n #generate a mask\n mask = (mask << 1) | 1\n m = m >> 1\n answer = (~n) & mask\n \n return answer\n \n \n\n \n \n``` | 2 | The **complement** of an integer is the integer you get when you flip all the `0`'s to `1`'s and all the `1`'s to `0`'s in its binary representation.
* For example, The integer `5` is `"101 "` in binary and its **complement** is `"010 "` which is the integer `2`.
Given an integer `n`, return _its complement_.
**Example 1:**
**Input:** n = 5
**Output:** 2
**Explanation:** 5 is "101 " in binary, with complement "010 " in binary, which is 2 in base-10.
**Example 2:**
**Input:** n = 7
**Output:** 0
**Explanation:** 7 is "111 " in binary, with complement "000 " in binary, which is 0 in base-10.
**Example 3:**
**Input:** n = 10
**Output:** 5
**Explanation:** 10 is "1010 " in binary, with complement "0101 " in binary, which is 5 in base-10.
**Constraints:**
* `0 <= n < 109`
**Note:** This question is the same as 476: [https://leetcode.com/problems/number-complement/](https://leetcode.com/problems/number-complement/) | null |
Beginner Friendly Python || Beats 91% | complement-of-base-10-integer | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\nLet\'s assume a number, say 10. \n\n```\nBinary version = 1010\nComplement = 0101\nInteger compelement = 5\n```\n\n```\nmask is initially 0,\nTill m = 10 (1010) is not 0,\n1. add a 1 (left shift 0 at the end + OR with 1), mask = 1\n2. divide 10 by 2 or right shift by 1\n3. now m = 5(101)\n- Iteration 2: mask = 11, m = 2 (10)\n- Iteration 3: mask = 111, m = 1 (1)\n- Iteration 4: mask = 1111, m = 0\n```\n\n\n\nTo do this using code, we follow these steps:\n\n1. First, we check if the given number is 0. If it is, the complement is 1, because flipping 0 gives us 1.\n```\nif n == 0:\n return 1\n```\n\n2. To find the complement for any other number, we need to figure out how many bits it has. \n\n\n3. Now, we create a "mask," which is a pattern of bits. We set as many bits as our number has to 1. So, for 10, our mask is \'1111\' (4 ones in binary).\n\n```\nmask is basically all ones. \nfor example: \nmask for 10 (1010) = 1111\nmask for 5 (101) = 111\nmask for 11 (1011) = 1111\n```\n```\nmask is initially 0,\nTill m = 10 (1010) is not 0,\n- add a 1 (left shift 0 at the end + OR with 1), mask = 1\n- divide 10 by 2 or right shift by 1\n- now m = 5(101)\n- Iteration 2: mask = 11, m = 2 (10)\n- Iteration 3: mask = 111, m = 1 (1)\n- Iteration 4: mask = 1111, m = 0\n\n```\n\n4. Take the complement of the original number (flipping the bits using \'~\' in code) and apply the mask to it (using \'&\' in code). This gives us the complement of the number.\n\n# Complexity\n- Time complexity: O(1)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: O(1)\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def bitwiseComplement(self, n: int) -> int:\n #generate a new number\n if n == 0:\n return 1\n m = n\n mask = 0\n while (m!=0):\n #generate a mask\n mask = (mask << 1) | 1\n m = m >> 1\n answer = (~n) & mask\n \n return answer\n \n \n\n \n \n``` | 2 | In a warehouse, there is a row of barcodes, where the `ith` barcode is `barcodes[i]`.
Rearrange the barcodes so that no two adjacent barcodes are equal. You may return any answer, and it is guaranteed an answer exists.
**Example 1:**
**Input:** barcodes = \[1,1,1,2,2,2\]
**Output:** \[2,1,2,1,2,1\]
**Example 2:**
**Input:** barcodes = \[1,1,1,1,2,2,3,3\]
**Output:** \[1,3,1,3,1,2,1,2\]
**Constraints:**
* `1 <= barcodes.length <= 10000`
* `1 <= barcodes[i] <= 10000` | A binary number plus its complement will equal 111....111 in binary. Also, N = 0 is a corner case. |
Solution | pairs-of-songs-with-total-durations-divisible-by-60 | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n int numPairsDivisibleBy60(vector<int>& time) {\n vector<int> remainders(60);\n int count = 0;\n for(int t : time) {\n if(t % 60 == 0) count += remainders[0];\n\n else count += remainders[60 - t % 60]; \n\n remainders[t % 60]++;\n }\n return count;\n }\n};\n```\n\n```Python3 []\nfrom collections import Counter\n\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n L = [t % 60 for t in time]\n L = Counter(L)\n res = 0\n for t in L:\n if t < 30 :\n res += L[t] * L[60 - t]\n return res + (L[0] * (L[0] - 1) // 2) + (L[30] * (L[30] - 1) // 2)\n```\n\n```Java []\nclass Solution {\n public int numPairsDivisibleBy60(int[] time) {\n int[] freq = new int[60];\n int res=0;\n\n for(int t: time){\n int val = t%60;\n if(val==0){\n res += freq[0];\n }\n else{\n res += freq[60-val];\n }\n freq[val]++;\n }\n return res;\n }\n}\n``` | 1 | You are given a list of songs where the `ith` song has a duration of `time[i]` seconds.
Return _the number of pairs of songs for which their total duration in seconds is divisible by_ `60`. Formally, we want the number of indices `i`, `j` such that `i < j` with `(time[i] + time[j]) % 60 == 0`.
**Example 1:**
**Input:** time = \[30,20,150,100,40\]
**Output:** 3
**Explanation:** Three pairs have a total duration divisible by 60:
(time\[0\] = 30, time\[2\] = 150): total duration 180
(time\[1\] = 20, time\[3\] = 100): total duration 120
(time\[1\] = 20, time\[4\] = 40): total duration 60
**Example 2:**
**Input:** time = \[60,60,60\]
**Output:** 3
**Explanation:** All three pairs have a total duration of 120, which is divisible by 60.
**Constraints:**
* `1 <= time.length <= 6 * 104`
* `1 <= time[i] <= 500` | null |
Solution | pairs-of-songs-with-total-durations-divisible-by-60 | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n int numPairsDivisibleBy60(vector<int>& time) {\n vector<int> remainders(60);\n int count = 0;\n for(int t : time) {\n if(t % 60 == 0) count += remainders[0];\n\n else count += remainders[60 - t % 60]; \n\n remainders[t % 60]++;\n }\n return count;\n }\n};\n```\n\n```Python3 []\nfrom collections import Counter\n\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n L = [t % 60 for t in time]\n L = Counter(L)\n res = 0\n for t in L:\n if t < 30 :\n res += L[t] * L[60 - t]\n return res + (L[0] * (L[0] - 1) // 2) + (L[30] * (L[30] - 1) // 2)\n```\n\n```Java []\nclass Solution {\n public int numPairsDivisibleBy60(int[] time) {\n int[] freq = new int[60];\n int res=0;\n\n for(int t: time){\n int val = t%60;\n if(val==0){\n res += freq[0];\n }\n else{\n res += freq[60-val];\n }\n freq[val]++;\n }\n return res;\n }\n}\n``` | 1 | A **subsequence** of a string is a new string that is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (i.e., `"ace "` is a subsequence of `"abcde "` while `"aec "` is not).
Given two strings `source` and `target`, return _the minimum number of **subsequences** of_ `source` _such that their concatenation equals_ `target`. If the task is impossible, return `-1`.
**Example 1:**
**Input:** source = "abc ", target = "abcbc "
**Output:** 2
**Explanation:** The target "abcbc " can be formed by "abc " and "bc ", which are subsequences of source "abc ".
**Example 2:**
**Input:** source = "abc ", target = "acdbc "
**Output:** -1
**Explanation:** The target string cannot be constructed from the subsequences of source string due to the character "d " in target string.
**Example 3:**
**Input:** source = "xyz ", target = "xzyxz "
**Output:** 3
**Explanation:** The target string can be constructed as follows "xz " + "y " + "xz ".
**Constraints:**
* `1 <= source.length, target.length <= 1000`
* `source` and `target` consist of lowercase English letters. | We only need to consider each song length modulo 60. We can count the number of songs with (length % 60) equal to r, and store that in an array of size 60. |
[LC-1010-M | Python3] A Plain Solution | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | Note that `i > j` is symmetric with `i < j` for calculation.\nTC: $\\Omicron(n)$ | SC: $\\Omicron(n)$, where `n = len(time)`.\n \n```Python3 []\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n cnter = defaultdict(int)\n res = 0\n for t in time:\n t %= 60\n res += cnter[(60-t) % 60]\n cnter[t] += 1\n \n return res\n``` | 1 | You are given a list of songs where the `ith` song has a duration of `time[i]` seconds.
Return _the number of pairs of songs for which their total duration in seconds is divisible by_ `60`. Formally, we want the number of indices `i`, `j` such that `i < j` with `(time[i] + time[j]) % 60 == 0`.
**Example 1:**
**Input:** time = \[30,20,150,100,40\]
**Output:** 3
**Explanation:** Three pairs have a total duration divisible by 60:
(time\[0\] = 30, time\[2\] = 150): total duration 180
(time\[1\] = 20, time\[3\] = 100): total duration 120
(time\[1\] = 20, time\[4\] = 40): total duration 60
**Example 2:**
**Input:** time = \[60,60,60\]
**Output:** 3
**Explanation:** All three pairs have a total duration of 120, which is divisible by 60.
**Constraints:**
* `1 <= time.length <= 6 * 104`
* `1 <= time[i] <= 500` | null |
[LC-1010-M | Python3] A Plain Solution | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | Note that `i > j` is symmetric with `i < j` for calculation.\nTC: $\\Omicron(n)$ | SC: $\\Omicron(n)$, where `n = len(time)`.\n \n```Python3 []\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n cnter = defaultdict(int)\n res = 0\n for t in time:\n t %= 60\n res += cnter[(60-t) % 60]\n cnter[t] += 1\n \n return res\n``` | 1 | A **subsequence** of a string is a new string that is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (i.e., `"ace "` is a subsequence of `"abcde "` while `"aec "` is not).
Given two strings `source` and `target`, return _the minimum number of **subsequences** of_ `source` _such that their concatenation equals_ `target`. If the task is impossible, return `-1`.
**Example 1:**
**Input:** source = "abc ", target = "abcbc "
**Output:** 2
**Explanation:** The target "abcbc " can be formed by "abc " and "bc ", which are subsequences of source "abc ".
**Example 2:**
**Input:** source = "abc ", target = "acdbc "
**Output:** -1
**Explanation:** The target string cannot be constructed from the subsequences of source string due to the character "d " in target string.
**Example 3:**
**Input:** source = "xyz ", target = "xzyxz "
**Output:** 3
**Explanation:** The target string can be constructed as follows "xz " + "y " + "xz ".
**Constraints:**
* `1 <= source.length, target.length <= 1000`
* `source` and `target` consist of lowercase English letters. | We only need to consider each song length modulo 60. We can count the number of songs with (length % 60) equal to r, and store that in an array of size 60. |
99% Faster || Python3 || | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | class Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int: \n\t \n\t\trem={}\n count=0\n for x in time:\n r=x%60\n if r in rem:\n rem[r]=rem[r]+1\n else:\n rem[r]=1 \n if 0 in rem:\n n=rem[0]\n count=count+(n*(n-1))//2\n del rem[0]\n if 30 in rem:\n n=rem[30]\n count=count+(n*(n-1))//2\n del rem[30]\n for i in range(1,30):\n if(60-i) in rem and i in rem:\n p=rem[i]\n q=rem[60-i]\n count=count+(p*q)\n return(count)\n | 1 | You are given a list of songs where the `ith` song has a duration of `time[i]` seconds.
Return _the number of pairs of songs for which their total duration in seconds is divisible by_ `60`. Formally, we want the number of indices `i`, `j` such that `i < j` with `(time[i] + time[j]) % 60 == 0`.
**Example 1:**
**Input:** time = \[30,20,150,100,40\]
**Output:** 3
**Explanation:** Three pairs have a total duration divisible by 60:
(time\[0\] = 30, time\[2\] = 150): total duration 180
(time\[1\] = 20, time\[3\] = 100): total duration 120
(time\[1\] = 20, time\[4\] = 40): total duration 60
**Example 2:**
**Input:** time = \[60,60,60\]
**Output:** 3
**Explanation:** All three pairs have a total duration of 120, which is divisible by 60.
**Constraints:**
* `1 <= time.length <= 6 * 104`
* `1 <= time[i] <= 500` | null |
99% Faster || Python3 || | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | class Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int: \n\t \n\t\trem={}\n count=0\n for x in time:\n r=x%60\n if r in rem:\n rem[r]=rem[r]+1\n else:\n rem[r]=1 \n if 0 in rem:\n n=rem[0]\n count=count+(n*(n-1))//2\n del rem[0]\n if 30 in rem:\n n=rem[30]\n count=count+(n*(n-1))//2\n del rem[30]\n for i in range(1,30):\n if(60-i) in rem and i in rem:\n p=rem[i]\n q=rem[60-i]\n count=count+(p*q)\n return(count)\n | 1 | A **subsequence** of a string is a new string that is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (i.e., `"ace "` is a subsequence of `"abcde "` while `"aec "` is not).
Given two strings `source` and `target`, return _the minimum number of **subsequences** of_ `source` _such that their concatenation equals_ `target`. If the task is impossible, return `-1`.
**Example 1:**
**Input:** source = "abc ", target = "abcbc "
**Output:** 2
**Explanation:** The target "abcbc " can be formed by "abc " and "bc ", which are subsequences of source "abc ".
**Example 2:**
**Input:** source = "abc ", target = "acdbc "
**Output:** -1
**Explanation:** The target string cannot be constructed from the subsequences of source string due to the character "d " in target string.
**Example 3:**
**Input:** source = "xyz ", target = "xzyxz "
**Output:** 3
**Explanation:** The target string can be constructed as follows "xz " + "y " + "xz ".
**Constraints:**
* `1 <= source.length, target.length <= 1000`
* `source` and `target` consist of lowercase English letters. | We only need to consider each song length modulo 60. We can count the number of songs with (length % 60) equal to r, and store that in an array of size 60. |
Python : HashMap/Dictionary | Complexity O(n) | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | ```\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n HashMap = {}\n pairs = 0\n \n for t in time:\n numMod = t % 60\n \n if numMod == 0:\n if 0 in HashMap:\n pairs += HashMap[0]\n elif (60 - numMod) in HashMap:\n pairs += HashMap[60 - numMod]\n \n if numMod in HashMap:\n HashMap[numMod] += 1\n else:\n HashMap[numMod] = 1\n \n return pairs\n \n``` | 23 | You are given a list of songs where the `ith` song has a duration of `time[i]` seconds.
Return _the number of pairs of songs for which their total duration in seconds is divisible by_ `60`. Formally, we want the number of indices `i`, `j` such that `i < j` with `(time[i] + time[j]) % 60 == 0`.
**Example 1:**
**Input:** time = \[30,20,150,100,40\]
**Output:** 3
**Explanation:** Three pairs have a total duration divisible by 60:
(time\[0\] = 30, time\[2\] = 150): total duration 180
(time\[1\] = 20, time\[3\] = 100): total duration 120
(time\[1\] = 20, time\[4\] = 40): total duration 60
**Example 2:**
**Input:** time = \[60,60,60\]
**Output:** 3
**Explanation:** All three pairs have a total duration of 120, which is divisible by 60.
**Constraints:**
* `1 <= time.length <= 6 * 104`
* `1 <= time[i] <= 500` | null |
Python : HashMap/Dictionary | Complexity O(n) | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | ```\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n HashMap = {}\n pairs = 0\n \n for t in time:\n numMod = t % 60\n \n if numMod == 0:\n if 0 in HashMap:\n pairs += HashMap[0]\n elif (60 - numMod) in HashMap:\n pairs += HashMap[60 - numMod]\n \n if numMod in HashMap:\n HashMap[numMod] += 1\n else:\n HashMap[numMod] = 1\n \n return pairs\n \n``` | 23 | A **subsequence** of a string is a new string that is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (i.e., `"ace "` is a subsequence of `"abcde "` while `"aec "` is not).
Given two strings `source` and `target`, return _the minimum number of **subsequences** of_ `source` _such that their concatenation equals_ `target`. If the task is impossible, return `-1`.
**Example 1:**
**Input:** source = "abc ", target = "abcbc "
**Output:** 2
**Explanation:** The target "abcbc " can be formed by "abc " and "bc ", which are subsequences of source "abc ".
**Example 2:**
**Input:** source = "abc ", target = "acdbc "
**Output:** -1
**Explanation:** The target string cannot be constructed from the subsequences of source string due to the character "d " in target string.
**Example 3:**
**Input:** source = "xyz ", target = "xzyxz "
**Output:** 3
**Explanation:** The target string can be constructed as follows "xz " + "y " + "xz ".
**Constraints:**
* `1 <= source.length, target.length <= 1000`
* `source` and `target` consist of lowercase English letters. | We only need to consider each song length modulo 60. We can count the number of songs with (length % 60) equal to r, and store that in an array of size 60. |
Python | HashMap | O(n) Time and Space | 80% T 75% S | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | We only need to consider song length modulo 60. \n\nFor every pair(a, b) we know a + b should = 60 to count as valid. \nSolving for a, we get 60 - b which we can hash and use to check against the rest of the array to generate the number of valid pairs.\n\nTo handle the case where pair(a % 60 = 0, b % 60 = 0) ex. (60,120), we add up the # of sixty\'s or numbers perfectly divisible and \nperform an n choose k operation where k = 2 and n = # of sixty\'s or numbers perfectly divisible.\n\n*n choose k = (C(n,k)) ways to choose an (unordered) subset of k elements from a fixed set of n elements.\n\n```\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n reverseMap = {}\n count = 0\n sixtyCount = 0\n \n for song in time:\n index = 60 - song % 60\n\n if(song % 60 == 0):\n sixtyCount += 1\n continue\n \n if(song % 60 in reverseMap):\n count += reverseMap[song % 60]\n \n if(index in reverseMap):\n reverseMap[index] += 1\n else:\n reverseMap[index] = 1\n \n # n choose 2 where n = # songs perfectly divisible by 60\n return count + comb(sixtyCount, 2) | 3 | You are given a list of songs where the `ith` song has a duration of `time[i]` seconds.
Return _the number of pairs of songs for which their total duration in seconds is divisible by_ `60`. Formally, we want the number of indices `i`, `j` such that `i < j` with `(time[i] + time[j]) % 60 == 0`.
**Example 1:**
**Input:** time = \[30,20,150,100,40\]
**Output:** 3
**Explanation:** Three pairs have a total duration divisible by 60:
(time\[0\] = 30, time\[2\] = 150): total duration 180
(time\[1\] = 20, time\[3\] = 100): total duration 120
(time\[1\] = 20, time\[4\] = 40): total duration 60
**Example 2:**
**Input:** time = \[60,60,60\]
**Output:** 3
**Explanation:** All three pairs have a total duration of 120, which is divisible by 60.
**Constraints:**
* `1 <= time.length <= 6 * 104`
* `1 <= time[i] <= 500` | null |
Python | HashMap | O(n) Time and Space | 80% T 75% S | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | We only need to consider song length modulo 60. \n\nFor every pair(a, b) we know a + b should = 60 to count as valid. \nSolving for a, we get 60 - b which we can hash and use to check against the rest of the array to generate the number of valid pairs.\n\nTo handle the case where pair(a % 60 = 0, b % 60 = 0) ex. (60,120), we add up the # of sixty\'s or numbers perfectly divisible and \nperform an n choose k operation where k = 2 and n = # of sixty\'s or numbers perfectly divisible.\n\n*n choose k = (C(n,k)) ways to choose an (unordered) subset of k elements from a fixed set of n elements.\n\n```\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n reverseMap = {}\n count = 0\n sixtyCount = 0\n \n for song in time:\n index = 60 - song % 60\n\n if(song % 60 == 0):\n sixtyCount += 1\n continue\n \n if(song % 60 in reverseMap):\n count += reverseMap[song % 60]\n \n if(index in reverseMap):\n reverseMap[index] += 1\n else:\n reverseMap[index] = 1\n \n # n choose 2 where n = # songs perfectly divisible by 60\n return count + comb(sixtyCount, 2) | 3 | A **subsequence** of a string is a new string that is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (i.e., `"ace "` is a subsequence of `"abcde "` while `"aec "` is not).
Given two strings `source` and `target`, return _the minimum number of **subsequences** of_ `source` _such that their concatenation equals_ `target`. If the task is impossible, return `-1`.
**Example 1:**
**Input:** source = "abc ", target = "abcbc "
**Output:** 2
**Explanation:** The target "abcbc " can be formed by "abc " and "bc ", which are subsequences of source "abc ".
**Example 2:**
**Input:** source = "abc ", target = "acdbc "
**Output:** -1
**Explanation:** The target string cannot be constructed from the subsequences of source string due to the character "d " in target string.
**Example 3:**
**Input:** source = "xyz ", target = "xzyxz "
**Output:** 3
**Explanation:** The target string can be constructed as follows "xz " + "y " + "xz ".
**Constraints:**
* `1 <= source.length, target.length <= 1000`
* `source` and `target` consist of lowercase English letters. | We only need to consider each song length modulo 60. We can count the number of songs with (length % 60) equal to r, and store that in an array of size 60. |
Python Solution | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | ```\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n arr=[0]*60\n count=0\n for i in range(len(time)):\n temp=time[i]%60\n count+=arr[-temp%60]\n arr[temp]+=1\n return count\n``` | 1 | You are given a list of songs where the `ith` song has a duration of `time[i]` seconds.
Return _the number of pairs of songs for which their total duration in seconds is divisible by_ `60`. Formally, we want the number of indices `i`, `j` such that `i < j` with `(time[i] + time[j]) % 60 == 0`.
**Example 1:**
**Input:** time = \[30,20,150,100,40\]
**Output:** 3
**Explanation:** Three pairs have a total duration divisible by 60:
(time\[0\] = 30, time\[2\] = 150): total duration 180
(time\[1\] = 20, time\[3\] = 100): total duration 120
(time\[1\] = 20, time\[4\] = 40): total duration 60
**Example 2:**
**Input:** time = \[60,60,60\]
**Output:** 3
**Explanation:** All three pairs have a total duration of 120, which is divisible by 60.
**Constraints:**
* `1 <= time.length <= 6 * 104`
* `1 <= time[i] <= 500` | null |
Python Solution | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | ```\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n arr=[0]*60\n count=0\n for i in range(len(time)):\n temp=time[i]%60\n count+=arr[-temp%60]\n arr[temp]+=1\n return count\n``` | 1 | A **subsequence** of a string is a new string that is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (i.e., `"ace "` is a subsequence of `"abcde "` while `"aec "` is not).
Given two strings `source` and `target`, return _the minimum number of **subsequences** of_ `source` _such that their concatenation equals_ `target`. If the task is impossible, return `-1`.
**Example 1:**
**Input:** source = "abc ", target = "abcbc "
**Output:** 2
**Explanation:** The target "abcbc " can be formed by "abc " and "bc ", which are subsequences of source "abc ".
**Example 2:**
**Input:** source = "abc ", target = "acdbc "
**Output:** -1
**Explanation:** The target string cannot be constructed from the subsequences of source string due to the character "d " in target string.
**Example 3:**
**Input:** source = "xyz ", target = "xzyxz "
**Output:** 3
**Explanation:** The target string can be constructed as follows "xz " + "y " + "xz ".
**Constraints:**
* `1 <= source.length, target.length <= 1000`
* `source` and `target` consist of lowercase English letters. | We only need to consider each song length modulo 60. We can count the number of songs with (length % 60) equal to r, and store that in an array of size 60. |
Python O(n) 6 Lines beats 86% time | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | ```python\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n res , count = 0, [0] * 60\n for one in range(len(time)):\n index = time[one] % 60\n res += count[(60 - index)%60] # %60 is for index==0\n count[index] += 1\n return res\n```\n\n**If it helps you ,Please give me a star.\nThanks** | 30 | You are given a list of songs where the `ith` song has a duration of `time[i]` seconds.
Return _the number of pairs of songs for which their total duration in seconds is divisible by_ `60`. Formally, we want the number of indices `i`, `j` such that `i < j` with `(time[i] + time[j]) % 60 == 0`.
**Example 1:**
**Input:** time = \[30,20,150,100,40\]
**Output:** 3
**Explanation:** Three pairs have a total duration divisible by 60:
(time\[0\] = 30, time\[2\] = 150): total duration 180
(time\[1\] = 20, time\[3\] = 100): total duration 120
(time\[1\] = 20, time\[4\] = 40): total duration 60
**Example 2:**
**Input:** time = \[60,60,60\]
**Output:** 3
**Explanation:** All three pairs have a total duration of 120, which is divisible by 60.
**Constraints:**
* `1 <= time.length <= 6 * 104`
* `1 <= time[i] <= 500` | null |
Python O(n) 6 Lines beats 86% time | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | ```python\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n res , count = 0, [0] * 60\n for one in range(len(time)):\n index = time[one] % 60\n res += count[(60 - index)%60] # %60 is for index==0\n count[index] += 1\n return res\n```\n\n**If it helps you ,Please give me a star.\nThanks** | 30 | A **subsequence** of a string is a new string that is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (i.e., `"ace "` is a subsequence of `"abcde "` while `"aec "` is not).
Given two strings `source` and `target`, return _the minimum number of **subsequences** of_ `source` _such that their concatenation equals_ `target`. If the task is impossible, return `-1`.
**Example 1:**
**Input:** source = "abc ", target = "abcbc "
**Output:** 2
**Explanation:** The target "abcbc " can be formed by "abc " and "bc ", which are subsequences of source "abc ".
**Example 2:**
**Input:** source = "abc ", target = "acdbc "
**Output:** -1
**Explanation:** The target string cannot be constructed from the subsequences of source string due to the character "d " in target string.
**Example 3:**
**Input:** source = "xyz ", target = "xzyxz "
**Output:** 3
**Explanation:** The target string can be constructed as follows "xz " + "y " + "xz ".
**Constraints:**
* `1 <= source.length, target.length <= 1000`
* `source` and `target` consist of lowercase English letters. | We only need to consider each song length modulo 60. We can count the number of songs with (length % 60) equal to r, and store that in an array of size 60. |
✔️[Python3] HASHMAP, °‿‿° Explained | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | We can notice that to find a pair that is divisible by 60 we need to find two songs that have the sum of their modulo equal to 60 or 0. For example for 20 and 100 we have `20%60=20` and `100%60=40`, i.e. their modulo add up to 60, thus thees pair satisfy the condition. So we can create a hashmap where a key is `time[i]%60` and a value is the count of songs with the same reminder. For example, 40 and 100 have the same reminder 40, thus `hashmap[40] = 2`. In sum, we simply iterate over the `time`, and for every time look into the hashmap to determine how many pairs we can form.\n\nTime: **O(n)** - for scan\nSpace: **O(1)** - maximum 60 key-value pairs in the hashmap\n\nRuntime: 236 ms, faster than **55.71%** of Python3 online submissions for Pairs of Songs With Total Durations Divisible by 60.\nMemory Usage: 17.5 MB, less than **93.55%** of Python3 online submissions for Pairs of Songs With Total Durations Divisible by 60.\n\n```\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n hashmap = defaultdict(int)\n for t in time:\n hashmap[t%60] += 1\n \n res = 0\n for t in time:\n reminder = t%60\n hashmap[reminder] -= 1\n \n res += hashmap[0 if reminder == 0 else 60 - reminder]\n \n return res\n``` | 8 | You are given a list of songs where the `ith` song has a duration of `time[i]` seconds.
Return _the number of pairs of songs for which their total duration in seconds is divisible by_ `60`. Formally, we want the number of indices `i`, `j` such that `i < j` with `(time[i] + time[j]) % 60 == 0`.
**Example 1:**
**Input:** time = \[30,20,150,100,40\]
**Output:** 3
**Explanation:** Three pairs have a total duration divisible by 60:
(time\[0\] = 30, time\[2\] = 150): total duration 180
(time\[1\] = 20, time\[3\] = 100): total duration 120
(time\[1\] = 20, time\[4\] = 40): total duration 60
**Example 2:**
**Input:** time = \[60,60,60\]
**Output:** 3
**Explanation:** All three pairs have a total duration of 120, which is divisible by 60.
**Constraints:**
* `1 <= time.length <= 6 * 104`
* `1 <= time[i] <= 500` | null |
✔️[Python3] HASHMAP, °‿‿° Explained | pairs-of-songs-with-total-durations-divisible-by-60 | 0 | 1 | We can notice that to find a pair that is divisible by 60 we need to find two songs that have the sum of their modulo equal to 60 or 0. For example for 20 and 100 we have `20%60=20` and `100%60=40`, i.e. their modulo add up to 60, thus thees pair satisfy the condition. So we can create a hashmap where a key is `time[i]%60` and a value is the count of songs with the same reminder. For example, 40 and 100 have the same reminder 40, thus `hashmap[40] = 2`. In sum, we simply iterate over the `time`, and for every time look into the hashmap to determine how many pairs we can form.\n\nTime: **O(n)** - for scan\nSpace: **O(1)** - maximum 60 key-value pairs in the hashmap\n\nRuntime: 236 ms, faster than **55.71%** of Python3 online submissions for Pairs of Songs With Total Durations Divisible by 60.\nMemory Usage: 17.5 MB, less than **93.55%** of Python3 online submissions for Pairs of Songs With Total Durations Divisible by 60.\n\n```\nclass Solution:\n def numPairsDivisibleBy60(self, time: List[int]) -> int:\n hashmap = defaultdict(int)\n for t in time:\n hashmap[t%60] += 1\n \n res = 0\n for t in time:\n reminder = t%60\n hashmap[reminder] -= 1\n \n res += hashmap[0 if reminder == 0 else 60 - reminder]\n \n return res\n``` | 8 | A **subsequence** of a string is a new string that is formed from the original string by deleting some (can be none) of the characters without disturbing the relative positions of the remaining characters. (i.e., `"ace "` is a subsequence of `"abcde "` while `"aec "` is not).
Given two strings `source` and `target`, return _the minimum number of **subsequences** of_ `source` _such that their concatenation equals_ `target`. If the task is impossible, return `-1`.
**Example 1:**
**Input:** source = "abc ", target = "abcbc "
**Output:** 2
**Explanation:** The target "abcbc " can be formed by "abc " and "bc ", which are subsequences of source "abc ".
**Example 2:**
**Input:** source = "abc ", target = "acdbc "
**Output:** -1
**Explanation:** The target string cannot be constructed from the subsequences of source string due to the character "d " in target string.
**Example 3:**
**Input:** source = "xyz ", target = "xzyxz "
**Output:** 3
**Explanation:** The target string can be constructed as follows "xz " + "y " + "xz ".
**Constraints:**
* `1 <= source.length, target.length <= 1000`
* `source` and `target` consist of lowercase English letters. | We only need to consider each song length modulo 60. We can count the number of songs with (length % 60) equal to r, and store that in an array of size 60. |
🔥Easy Binary Search Explanation🔥 | capacity-to-ship-packages-within-d-days | 0 | 1 | # Intuition\r\nSuppose we know capacity `x` doesn\'t work, then we know that we should try a capacity greater than `x`. We can stop checking capacities less than `x`.\r\n\r\nIf we know capacity `y` works, we need to check capacities less than `y` to see if there\'s a smaller capacity that also works. We no longer need to check capacities greater than `y`, as that would only make our answer worse.\r\n\r\n# Approach\r\nWe\'ll use binary search. Set the lower bound to `max(weights)` since the capacity needs to be at least as big as the largest weight, otherwise the ship will never be able to carry that weight. Set the upper bound to `sum(weights)` since the largest the capacity needs to be is the sum of all weights - we could just load all the weights onto the ship in a single day.\r\n\r\nTo check if a capacity works, we add as many weights as possible without exceeding the capacity and keep track of how many days we need to load all the weights. If the number of `days_used` is at most the `days` limit, then we know the capacity is large enough.\r\n\r\n# Complexity\r\n- Time complexity: O(nlogC) where `n` is the number of `weights` and `C` is the sum of all `weights`. Each check takes O(n) time, and we perform at most O(logC) checks in the binary search.\r\n\r\n- Space complexity: O(1). Only constant memory is used.\r\n\r\n# Code\r\n```python\r\nclass Solution:\r\n def shipWithinDays(self, weights: List[int], days: int) -> int:\r\n def check(capacity):\r\n days_used = 0\r\n capacity_used = capacity\r\n for weight in weights:\r\n if capacity_used + weight > capacity:\r\n days_used += 1\r\n capacity_used = 0\r\n capacity_used += weight\r\n return days_used <= days\r\n\r\n l, r = max(weights), sum(weights)\r\n min_capacity = r\r\n while l <= r:\r\n capacity = (l + r) // 2\r\n if check(capacity):\r\n min_capacity = capacity\r\n r = capacity - 1\r\n else:\r\n l = capacity + 1\r\n return min_capacity\r\n``` | 4 | A conveyor belt has packages that must be shipped from one port to another within `days` days.
The `ith` package on the conveyor belt has a weight of `weights[i]`. Each day, we load the ship with packages on the conveyor belt (in the order given by `weights`). We may not load more weight than the maximum weight capacity of the ship.
Return the least weight capacity of the ship that will result in all the packages on the conveyor belt being shipped within `days` days.
**Example 1:**
**Input:** weights = \[1,2,3,4,5,6,7,8,9,10\], days = 5
**Output:** 15
**Explanation:** A ship capacity of 15 is the minimum to ship all the packages in 5 days like this:
1st day: 1, 2, 3, 4, 5
2nd day: 6, 7
3rd day: 8
4th day: 9
5th day: 10
Note that the cargo must be shipped in the order given, so using a ship of capacity 14 and splitting the packages into parts like (2, 3, 4, 5), (1, 6, 7), (8), (9), (10) is not allowed.
**Example 2:**
**Input:** weights = \[3,2,2,4,1,4\], days = 3
**Output:** 6
**Explanation:** A ship capacity of 6 is the minimum to ship all the packages in 3 days like this:
1st day: 3, 2
2nd day: 2, 4
3rd day: 1, 4
**Example 3:**
**Input:** weights = \[1,2,3,1,1\], days = 4
**Output:** 3
**Explanation:**
1st day: 1
2nd day: 2
3rd day: 3
4th day: 1, 1
**Constraints:**
* `1 <= days <= weights.length <= 5 * 104`
* `1 <= weights[i] <= 500` | null |
🔥Easy Binary Search Explanation🔥 | capacity-to-ship-packages-within-d-days | 0 | 1 | # Intuition\r\nSuppose we know capacity `x` doesn\'t work, then we know that we should try a capacity greater than `x`. We can stop checking capacities less than `x`.\r\n\r\nIf we know capacity `y` works, we need to check capacities less than `y` to see if there\'s a smaller capacity that also works. We no longer need to check capacities greater than `y`, as that would only make our answer worse.\r\n\r\n# Approach\r\nWe\'ll use binary search. Set the lower bound to `max(weights)` since the capacity needs to be at least as big as the largest weight, otherwise the ship will never be able to carry that weight. Set the upper bound to `sum(weights)` since the largest the capacity needs to be is the sum of all weights - we could just load all the weights onto the ship in a single day.\r\n\r\nTo check if a capacity works, we add as many weights as possible without exceeding the capacity and keep track of how many days we need to load all the weights. If the number of `days_used` is at most the `days` limit, then we know the capacity is large enough.\r\n\r\n# Complexity\r\n- Time complexity: O(nlogC) where `n` is the number of `weights` and `C` is the sum of all `weights`. Each check takes O(n) time, and we perform at most O(logC) checks in the binary search.\r\n\r\n- Space complexity: O(1). Only constant memory is used.\r\n\r\n# Code\r\n```python\r\nclass Solution:\r\n def shipWithinDays(self, weights: List[int], days: int) -> int:\r\n def check(capacity):\r\n days_used = 0\r\n capacity_used = capacity\r\n for weight in weights:\r\n if capacity_used + weight > capacity:\r\n days_used += 1\r\n capacity_used = 0\r\n capacity_used += weight\r\n return days_used <= days\r\n\r\n l, r = max(weights), sum(weights)\r\n min_capacity = r\r\n while l <= r:\r\n capacity = (l + r) // 2\r\n if check(capacity):\r\n min_capacity = capacity\r\n r = capacity - 1\r\n else:\r\n l = capacity + 1\r\n return min_capacity\r\n``` | 4 | A **confusing number** is a number that when rotated `180` degrees becomes a different number with **each digit valid**.
We can rotate digits of a number by `180` degrees to form new digits.
* When `0`, `1`, `6`, `8`, and `9` are rotated `180` degrees, they become `0`, `1`, `9`, `8`, and `6` respectively.
* When `2`, `3`, `4`, `5`, and `7` are rotated `180` degrees, they become **invalid**.
Note that after rotating a number, we can ignore leading zeros.
* For example, after rotating `8000`, we have `0008` which is considered as just `8`.
Given an integer `n`, return `true` _if it is a **confusing number**, or_ `false` _otherwise_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** We get 9 after rotating 6, 9 is a valid number, and 9 != 6.
**Example 2:**
**Input:** n = 89
**Output:** true
**Explanation:** We get 68 after rotating 89, 68 is a valid number and 68 != 89.
**Example 3:**
**Input:** n = 11
**Output:** false
**Explanation:** We get 11 after rotating 11, 11 is a valid number but the value remains the same, thus 11 is not a confusing number
**Constraints:**
* `0 <= n <= 109` | Binary search on the answer. We need a function possible(capacity) which returns true if and only if we can do the task in D days. |
Solution | capacity-to-ship-packages-within-d-days | 1 | 1 | ```C++ []\r\nclass Solution {\r\npublic:\r\n int shipWithinDays(vector<int>& weights, int days) {\r\n if (weights.size() == 0)\r\n\t\t\treturn 0;\r\n\r\n\t\tif (weights.size() < days)\r\n\t\t\treturn 0;\r\n\r\n\t\tint minVal = weights[0], maxVal = 0;\r\n\t\tfor (auto& elem : weights)\r\n\t\t{\r\n\t\t\tif (elem > minVal)\r\n\t\t\t\tminVal = elem;\r\n\t\t\tmaxVal += elem;\r\n\t\t}\r\n\t\tint start = minVal, end = maxVal, mid;\r\n\t\twhile (start < end)\r\n\t\t{\r\n\t\t\tint pieces = 1, sum = 0;\r\n\t\t\tmid = start + (end - start) / 2;\r\n\t\t\tfor (auto& elem : weights)\r\n\t\t\t{\r\n\t\t\t\tsum += elem;\r\n\t\t\t\tif (sum > mid)\r\n\t\t\t\t{\r\n\t\t\t\t\t++pieces;\r\n\t\t\t\t\tsum = elem;\r\n\t\t\t\t}\r\n\t\t\t}\r\n\t\t\tif (pieces > days)\r\n\t\t\t\tstart = mid + 1;\r\n\t\t\telse\r\n\t\t\t\tend = mid;\r\n\t\t}\r\n\t\treturn start;\r\n }\r\n};\r\n```\r\n\r\n```Python3 []\r\nclass Solution:\r\n def shipWithinDays(self, weights: List[int], days: int) -> int:\r\n def is_enough(max_load):\r\n total = 1\r\n curr_load = 0\r\n for w in weights:\r\n if curr_load + w <= max_load:\r\n curr_load += w\r\n else:\r\n total += 1\r\n curr_load = w\r\n return total <= days\r\n\r\n max_load = max(weights)\r\n daily = (len(weights)-1) // days + 1\r\n h = max_load * daily\r\n l = max(max_load, min(weights)*daily)\r\n print(l, h)\r\n while l <= h:\r\n m = (l + h) // 2\r\n if is_enough(m):\r\n h = m - 1\r\n else:\r\n l = m + 1\r\n return l\r\n```\r\n\r\n```Java []\r\nclass Solution {\r\n public int shipWithinDays(int[] weights, int days) {\r\n int lo = -1, hi = -1, sum = 0;\r\n for (int w : weights){\r\n sum += w;\r\n lo = Math.max(lo, w);\r\n }\r\n hi = lo * (weights.length / days + Integer.signum(weights.length % days));\r\n lo = Math.max(lo, sum / days);\r\n while(lo < hi){ \r\n int mid = lo + (hi - lo) / 2;\r\n if (isOK(mid, weights, days)) hi = mid;\r\n else lo = mid + 1;\r\n }\r\n return lo;\r\n }\r\n private boolean isOK(int guess, int[] arr, int d){ \r\n int sum = 0;\r\n for (int n : arr){\r\n if (sum + n > guess){\r\n sum = 0;\r\n d--;\r\n }\r\n sum += n;\r\n }\r\n return d > 0;\r\n }\r\n}\r\n``` | 1 | A conveyor belt has packages that must be shipped from one port to another within `days` days.
The `ith` package on the conveyor belt has a weight of `weights[i]`. Each day, we load the ship with packages on the conveyor belt (in the order given by `weights`). We may not load more weight than the maximum weight capacity of the ship.
Return the least weight capacity of the ship that will result in all the packages on the conveyor belt being shipped within `days` days.
**Example 1:**
**Input:** weights = \[1,2,3,4,5,6,7,8,9,10\], days = 5
**Output:** 15
**Explanation:** A ship capacity of 15 is the minimum to ship all the packages in 5 days like this:
1st day: 1, 2, 3, 4, 5
2nd day: 6, 7
3rd day: 8
4th day: 9
5th day: 10
Note that the cargo must be shipped in the order given, so using a ship of capacity 14 and splitting the packages into parts like (2, 3, 4, 5), (1, 6, 7), (8), (9), (10) is not allowed.
**Example 2:**
**Input:** weights = \[3,2,2,4,1,4\], days = 3
**Output:** 6
**Explanation:** A ship capacity of 6 is the minimum to ship all the packages in 3 days like this:
1st day: 3, 2
2nd day: 2, 4
3rd day: 1, 4
**Example 3:**
**Input:** weights = \[1,2,3,1,1\], days = 4
**Output:** 3
**Explanation:**
1st day: 1
2nd day: 2
3rd day: 3
4th day: 1, 1
**Constraints:**
* `1 <= days <= weights.length <= 5 * 104`
* `1 <= weights[i] <= 500` | null |
Solution | capacity-to-ship-packages-within-d-days | 1 | 1 | ```C++ []\r\nclass Solution {\r\npublic:\r\n int shipWithinDays(vector<int>& weights, int days) {\r\n if (weights.size() == 0)\r\n\t\t\treturn 0;\r\n\r\n\t\tif (weights.size() < days)\r\n\t\t\treturn 0;\r\n\r\n\t\tint minVal = weights[0], maxVal = 0;\r\n\t\tfor (auto& elem : weights)\r\n\t\t{\r\n\t\t\tif (elem > minVal)\r\n\t\t\t\tminVal = elem;\r\n\t\t\tmaxVal += elem;\r\n\t\t}\r\n\t\tint start = minVal, end = maxVal, mid;\r\n\t\twhile (start < end)\r\n\t\t{\r\n\t\t\tint pieces = 1, sum = 0;\r\n\t\t\tmid = start + (end - start) / 2;\r\n\t\t\tfor (auto& elem : weights)\r\n\t\t\t{\r\n\t\t\t\tsum += elem;\r\n\t\t\t\tif (sum > mid)\r\n\t\t\t\t{\r\n\t\t\t\t\t++pieces;\r\n\t\t\t\t\tsum = elem;\r\n\t\t\t\t}\r\n\t\t\t}\r\n\t\t\tif (pieces > days)\r\n\t\t\t\tstart = mid + 1;\r\n\t\t\telse\r\n\t\t\t\tend = mid;\r\n\t\t}\r\n\t\treturn start;\r\n }\r\n};\r\n```\r\n\r\n```Python3 []\r\nclass Solution:\r\n def shipWithinDays(self, weights: List[int], days: int) -> int:\r\n def is_enough(max_load):\r\n total = 1\r\n curr_load = 0\r\n for w in weights:\r\n if curr_load + w <= max_load:\r\n curr_load += w\r\n else:\r\n total += 1\r\n curr_load = w\r\n return total <= days\r\n\r\n max_load = max(weights)\r\n daily = (len(weights)-1) // days + 1\r\n h = max_load * daily\r\n l = max(max_load, min(weights)*daily)\r\n print(l, h)\r\n while l <= h:\r\n m = (l + h) // 2\r\n if is_enough(m):\r\n h = m - 1\r\n else:\r\n l = m + 1\r\n return l\r\n```\r\n\r\n```Java []\r\nclass Solution {\r\n public int shipWithinDays(int[] weights, int days) {\r\n int lo = -1, hi = -1, sum = 0;\r\n for (int w : weights){\r\n sum += w;\r\n lo = Math.max(lo, w);\r\n }\r\n hi = lo * (weights.length / days + Integer.signum(weights.length % days));\r\n lo = Math.max(lo, sum / days);\r\n while(lo < hi){ \r\n int mid = lo + (hi - lo) / 2;\r\n if (isOK(mid, weights, days)) hi = mid;\r\n else lo = mid + 1;\r\n }\r\n return lo;\r\n }\r\n private boolean isOK(int guess, int[] arr, int d){ \r\n int sum = 0;\r\n for (int n : arr){\r\n if (sum + n > guess){\r\n sum = 0;\r\n d--;\r\n }\r\n sum += n;\r\n }\r\n return d > 0;\r\n }\r\n}\r\n``` | 1 | A **confusing number** is a number that when rotated `180` degrees becomes a different number with **each digit valid**.
We can rotate digits of a number by `180` degrees to form new digits.
* When `0`, `1`, `6`, `8`, and `9` are rotated `180` degrees, they become `0`, `1`, `9`, `8`, and `6` respectively.
* When `2`, `3`, `4`, `5`, and `7` are rotated `180` degrees, they become **invalid**.
Note that after rotating a number, we can ignore leading zeros.
* For example, after rotating `8000`, we have `0008` which is considered as just `8`.
Given an integer `n`, return `true` _if it is a **confusing number**, or_ `false` _otherwise_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** We get 9 after rotating 6, 9 is a valid number, and 9 != 6.
**Example 2:**
**Input:** n = 89
**Output:** true
**Explanation:** We get 68 after rotating 89, 68 is a valid number and 68 != 89.
**Example 3:**
**Input:** n = 11
**Output:** false
**Explanation:** We get 11 after rotating 11, 11 is a valid number but the value remains the same, thus 11 is not a confusing number
**Constraints:**
* `0 <= n <= 109` | Binary search on the answer. We need a function possible(capacity) which returns true if and only if we can do the task in D days. |
Easy solution on python3 | capacity-to-ship-packages-within-d-days | 0 | 1 | # Intuition\r\n<!-- Describe your first thoughts on how to solve this problem. -->\r\n\r\n# Approach\r\n<!-- Describe your approach to solving the problem. -->\r\n\r\n# Complexity\r\n- Time complexity:\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\n\r\n- Space complexity:\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\n\r\n# Code\r\n```\r\nimport functools\r\nclass Solution:\r\n def shipWithinDays(self, weights: List[int], days: int) -> int:\r\n N = len(weights)\r\n l, r = max(weights), sum(weights)\r\n m = None\r\n @functools.cache\r\n def _days(capacity:int) -> int:\r\n i = 0\r\n d = 0\r\n while i < N:\r\n d+=1\r\n tank = capacity\r\n while i < N and tank >= weights[i]:\r\n tank-=weights[i]\r\n i+=1\r\n return d\r\n while True:\r\n m = (l+r)//2\r\n if _days(m)==days and _days(m-1)<days:\r\n return m\r\n elif _days(m) <= days:\r\n r = m\r\n elif _days(m) > days:\r\n l = m\r\n if r-l<=1:\r\n if _days(l)<=days:\r\n return l\r\n return r\r\n \r\n``` | 1 | A conveyor belt has packages that must be shipped from one port to another within `days` days.
The `ith` package on the conveyor belt has a weight of `weights[i]`. Each day, we load the ship with packages on the conveyor belt (in the order given by `weights`). We may not load more weight than the maximum weight capacity of the ship.
Return the least weight capacity of the ship that will result in all the packages on the conveyor belt being shipped within `days` days.
**Example 1:**
**Input:** weights = \[1,2,3,4,5,6,7,8,9,10\], days = 5
**Output:** 15
**Explanation:** A ship capacity of 15 is the minimum to ship all the packages in 5 days like this:
1st day: 1, 2, 3, 4, 5
2nd day: 6, 7
3rd day: 8
4th day: 9
5th day: 10
Note that the cargo must be shipped in the order given, so using a ship of capacity 14 and splitting the packages into parts like (2, 3, 4, 5), (1, 6, 7), (8), (9), (10) is not allowed.
**Example 2:**
**Input:** weights = \[3,2,2,4,1,4\], days = 3
**Output:** 6
**Explanation:** A ship capacity of 6 is the minimum to ship all the packages in 3 days like this:
1st day: 3, 2
2nd day: 2, 4
3rd day: 1, 4
**Example 3:**
**Input:** weights = \[1,2,3,1,1\], days = 4
**Output:** 3
**Explanation:**
1st day: 1
2nd day: 2
3rd day: 3
4th day: 1, 1
**Constraints:**
* `1 <= days <= weights.length <= 5 * 104`
* `1 <= weights[i] <= 500` | null |
Easy solution on python3 | capacity-to-ship-packages-within-d-days | 0 | 1 | # Intuition\r\n<!-- Describe your first thoughts on how to solve this problem. -->\r\n\r\n# Approach\r\n<!-- Describe your approach to solving the problem. -->\r\n\r\n# Complexity\r\n- Time complexity:\r\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\r\n\r\n- Space complexity:\r\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\r\n\r\n# Code\r\n```\r\nimport functools\r\nclass Solution:\r\n def shipWithinDays(self, weights: List[int], days: int) -> int:\r\n N = len(weights)\r\n l, r = max(weights), sum(weights)\r\n m = None\r\n @functools.cache\r\n def _days(capacity:int) -> int:\r\n i = 0\r\n d = 0\r\n while i < N:\r\n d+=1\r\n tank = capacity\r\n while i < N and tank >= weights[i]:\r\n tank-=weights[i]\r\n i+=1\r\n return d\r\n while True:\r\n m = (l+r)//2\r\n if _days(m)==days and _days(m-1)<days:\r\n return m\r\n elif _days(m) <= days:\r\n r = m\r\n elif _days(m) > days:\r\n l = m\r\n if r-l<=1:\r\n if _days(l)<=days:\r\n return l\r\n return r\r\n \r\n``` | 1 | A **confusing number** is a number that when rotated `180` degrees becomes a different number with **each digit valid**.
We can rotate digits of a number by `180` degrees to form new digits.
* When `0`, `1`, `6`, `8`, and `9` are rotated `180` degrees, they become `0`, `1`, `9`, `8`, and `6` respectively.
* When `2`, `3`, `4`, `5`, and `7` are rotated `180` degrees, they become **invalid**.
Note that after rotating a number, we can ignore leading zeros.
* For example, after rotating `8000`, we have `0008` which is considered as just `8`.
Given an integer `n`, return `true` _if it is a **confusing number**, or_ `false` _otherwise_.
**Example 1:**
**Input:** n = 6
**Output:** true
**Explanation:** We get 9 after rotating 6, 9 is a valid number, and 9 != 6.
**Example 2:**
**Input:** n = 89
**Output:** true
**Explanation:** We get 68 after rotating 89, 68 is a valid number and 68 != 89.
**Example 3:**
**Input:** n = 11
**Output:** false
**Explanation:** We get 11 after rotating 11, 11 is a valid number but the value remains the same, thus 11 is not a confusing number
**Constraints:**
* `0 <= n <= 109` | Binary search on the answer. We need a function possible(capacity) which returns true if and only if we can do the task in D days. |
Solution | numbers-with-repeated-digits | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n int numDupDigitsAtMostN(int n) {\n vector<int> digits{};\n int temp = n + 1;\n while (temp > 0) {\n digits.emplace_back(temp % 10);\n temp /= 10;\n }\n int result = 0;\n int len = digits.size();\n int curr = 9;\n for (int i = 0; i < len - 1; i++) {\n result += curr;\n curr *= 9 - i;\n }\n curr /= 9;\n vector<bool> seen(10, false);\n for (int i = 0; i < len; i++) {\n int d = digits[len - i - 1];\n\n for (int j = i == 0 ? 1 : 0; j < d; j++) if (!seen[j]) result += curr;\n curr /= 9 - i;\n\n if (seen[d]) break;\n seen[d] = true;\n }\n return n - result;\n }\n};\n```\n\n```Python3 []\nfrom math import perm\n\nclass Solution:\n def numDupDigitsAtMostN(self, n: int) -> int:\n digits = list(map(int, str(n + 1)))\n nl = len(digits)\n res = sum(9 * perm(9, i) for i in range(nl - 1))\n s = set()\n for i, x in enumerate(digits):\n for y in range(i == 0, x):\n if y not in s:\n res += perm(9 - i, nl - i - 1)\n if x in s:\n break\n s.add(x)\n return n - res\n```\n\n```Java []\nclass Solution {\n public int numDupDigitsAtMostN(int N) {\n ArrayList<Integer> L = new ArrayList<Integer>();\n for (int x = N + 1; x > 0; x /= 10)\n L.add(0, x % 10);\n\n int res = 0, n = L.size();\n for (int i = 1; i < n; ++i)\n res += 9 * A(9, i - 1);\n\n HashSet<Integer> seen = new HashSet<>();\n for (int i = 0; i < n; ++i) {\n for (int j = i > 0 ? 0 : 1; j < L.get(i); ++j)\n if (!seen.contains(j))\n res += A(9 - i, n - i - 1);\n if (seen.contains(L.get(i))) break;\n seen.add(L.get(i));\n }\n return N - res;\n }\n public int A(int m, int n) {\n return n == 0 ? 1 : A(m, n - 1) * (m - n + 1);\n }\n}\n``` | 442 | Given an integer `n`, return _the number of positive integers in the range_ `[1, n]` _that have **at least one** repeated digit_.
**Example 1:**
**Input:** n = 20
**Output:** 1
**Explanation:** The only positive number (<= 20) with at least 1 repeated digit is 11.
**Example 2:**
**Input:** n = 100
**Output:** 10
**Explanation:** The positive numbers (<= 100) with atleast 1 repeated digit are 11, 22, 33, 44, 55, 66, 77, 88, 99, and 100.
**Example 3:**
**Input:** n = 1000
**Output:** 262
**Constraints:**
* `1 <= n <= 109` | null |
Solution | numbers-with-repeated-digits | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n int numDupDigitsAtMostN(int n) {\n vector<int> digits{};\n int temp = n + 1;\n while (temp > 0) {\n digits.emplace_back(temp % 10);\n temp /= 10;\n }\n int result = 0;\n int len = digits.size();\n int curr = 9;\n for (int i = 0; i < len - 1; i++) {\n result += curr;\n curr *= 9 - i;\n }\n curr /= 9;\n vector<bool> seen(10, false);\n for (int i = 0; i < len; i++) {\n int d = digits[len - i - 1];\n\n for (int j = i == 0 ? 1 : 0; j < d; j++) if (!seen[j]) result += curr;\n curr /= 9 - i;\n\n if (seen[d]) break;\n seen[d] = true;\n }\n return n - result;\n }\n};\n```\n\n```Python3 []\nfrom math import perm\n\nclass Solution:\n def numDupDigitsAtMostN(self, n: int) -> int:\n digits = list(map(int, str(n + 1)))\n nl = len(digits)\n res = sum(9 * perm(9, i) for i in range(nl - 1))\n s = set()\n for i, x in enumerate(digits):\n for y in range(i == 0, x):\n if y not in s:\n res += perm(9 - i, nl - i - 1)\n if x in s:\n break\n s.add(x)\n return n - res\n```\n\n```Java []\nclass Solution {\n public int numDupDigitsAtMostN(int N) {\n ArrayList<Integer> L = new ArrayList<Integer>();\n for (int x = N + 1; x > 0; x /= 10)\n L.add(0, x % 10);\n\n int res = 0, n = L.size();\n for (int i = 1; i < n; ++i)\n res += 9 * A(9, i - 1);\n\n HashSet<Integer> seen = new HashSet<>();\n for (int i = 0; i < n; ++i) {\n for (int j = i > 0 ? 0 : 1; j < L.get(i); ++j)\n if (!seen.contains(j))\n res += A(9 - i, n - i - 1);\n if (seen.contains(L.get(i))) break;\n seen.add(L.get(i));\n }\n return N - res;\n }\n public int A(int m, int n) {\n return n == 0 ? 1 : A(m, n - 1) * (m - n + 1);\n }\n}\n``` | 442 | On a campus represented on the X-Y plane, there are `n` workers and `m` bikes, with `n <= m`.
You are given an array `workers` of length `n` where `workers[i] = [xi, yi]` is the position of the `ith` worker. You are also given an array `bikes` of length `m` where `bikes[j] = [xj, yj]` is the position of the `jth` bike. All the given positions are **unique**.
Assign a bike to each worker. Among the available bikes and workers, we choose the `(workeri, bikej)` pair with the shortest **Manhattan distance** between each other and assign the bike to that worker.
If there are multiple `(workeri, bikej)` pairs with the same shortest **Manhattan distance**, we choose the pair with **the smallest worker index**. If there are multiple ways to do that, we choose the pair with **the smallest bike index**. Repeat this process until there are no available workers.
Return _an array_ `answer` _of length_ `n`_, where_ `answer[i]` _is the index (**0-indexed**) of the bike that the_ `ith` _worker is assigned to_.
The **Manhattan distance** between two points `p1` and `p2` is `Manhattan(p1, p2) = |p1.x - p2.x| + |p1.y - p2.y|`.
**Example 1:**
**Input:** workers = \[\[0,0\],\[2,1\]\], bikes = \[\[1,2\],\[3,3\]\]
**Output:** \[1,0\]
**Explanation:** Worker 1 grabs Bike 0 as they are closest (without ties), and Worker 0 is assigned Bike 1. So the output is \[1, 0\].
**Example 2:**
**Input:** workers = \[\[0,0\],\[1,1\],\[2,0\]\], bikes = \[\[1,0\],\[2,2\],\[2,1\]\]
**Output:** \[0,2,1\]
**Explanation:** Worker 0 grabs Bike 0 at first. Worker 1 and Worker 2 share the same distance to Bike 2, thus Worker 1 is assigned to Bike 2, and Worker 2 will take Bike 1. So the output is \[0,2,1\].
**Constraints:**
* `n == workers.length`
* `m == bikes.length`
* `1 <= n <= m <= 1000`
* `workers[i].length == bikes[j].length == 2`
* `0 <= xi, yi < 1000`
* `0 <= xj, yj < 1000`
* All worker and bike locations are **unique**. | How many numbers with no duplicate digits? How many numbers with K digits and no duplicates? How many numbers with same length as N? How many numbers with same prefix as N? |
I spent 4 hours on this shit I hate my life | numbers-with-repeated-digits | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIts basically just math, Im gonna die \n# Approach\n<!-- Describe your approach to solving the problem. -->\nlet n be a K digit integer\nWe will count the total number of non-duplicated integers\nWe split them up in 2 cases by counting <K digits and K digits\n\nFor < K digits\nI did abit of trial and error for this but the general form that you would get would be for each k number of digits\n$$9\\bullet\\sum^{K-3}_{i=0}\\Pi^9_{j=9-i}j + 9$$\n\nSo how i visualised it was for e.g. we wamt let K = 5 so we want to find all possible non-duplicated integers <10 000 i.e. [1,9 999]\n\nFrom 1000 to 9999 we have 10000 - 1000 = 9000 integers \nwe fix the 1st digit so we have 1XXX then for the 2nd digit we have 9 remaining unique digits so on and so forth. Resulting in 9x8x7 integers\nNow we permutate the first digit to be between 1 to 9\nHence from 1000 - 9999 we have 9x9x8x7 non-duplicated integers\n\nSimilarly, from 100 - 999 we have 9x9x8\nfrom 10 - 99 we have 9x9\nfrom 1 - 9 we have 9\n\nNow if we take the sum we get \n9x9x8x7 9x9x8 + 9x9 + 9\n\n\nFor a fixed length of K digits\nWe store each leading digit in seen\nThen we build the integer up by iterating from 0 (if not first digit) and 1(if first digit) up to the leading digit. If any of the digit was seen before then we skip it. We then permutate the remaining digits left. If the leading digit was already seen we can stop as we know it will no longer give us any non-duplicated integers.\n\nE.g. if we let n = 13579\nWe will calculate this in parts as well specifically from \n1XXXX - 1XXXX\n10XXX - 12XXX\n130XX - 134XX\n1350X - 1356X\n1357X - 13578\n(remember last number not inclusive, pretty sure you can tweak the code to include)\n\nAt the start we have 10 000, since the leading digit is the first digit and is 1 we skip it. We then store 1 inside seen. Now we have fixed the first digit.\n\nFor the second digit, we start building from 0 to 2 so we have 10XXX, 11XXX and 12XXX but since 11XXX is already a duplicate we will skip it. Giving us 2x8x7x6 non-duplicated integers. Now we fix the second digit to be 3\n\nFor the third digit we will consider 130XX, 131XX, 132XX, 133XX, 134XX, w can see that 131XX and 133XX already contains duplicates and hence we will skip them. Giving us 3x7x6. Then we fix the third digit to be 5\n\nSo on and so forth, resulting in a total of 2x8x7x6 + 3x7x6 + 4x6 + 5\n\nNow we take the sum \n9x9x8x7 9x9x8 + 9x9 + 9 + \n2x8x7x6 + 3x7x6 + 4x6 + 5 + 1 (to include n=13579) = 6102\nWe return 13579 - 6102 = 7477 which is the ans\n \n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nI believe it is $$O(log_{10}n)$$ as we are counting the number of digits\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nI think it is $$O(log_{10}n)$$ as well? as we only store the number of digits in both seen and numList\n# Code\n```\nclass Solution:\n def numDupDigitsAtMostN(self, n: int) -> int:\n temp = n\n numList = []\n while temp > 0:\n numList.insert(0, temp%10)\n temp //=10\n digits = len(numList)\n totalNum = 0\n res = 0\n # less than K digits\n for j in range(digits-1, 0, -1):\n res = 9\n for k in range(9, 9-j+1,-1):\n res *= k\n totalNum += res\n #K digits\n seen = {}\n for i in range(0,digits):\n leadingDigit = numList[i]\n remainingDigits = len(numList) - i - 1\n for j in range(1 if i==0 else 0, leadingDigit):\n if j in seen:\n continue\n res = 1\n for k in range(9-i, 9-i-remainingDigits,-1):\n res *= k\n totalNum += res\n if leadingDigit in seen:\n break\n seen[leadingDigit] = 1\n seen = {}\n for num in numList:\n if num in seen:\n return n-totalNum\n seen[num] = 1\n return n-totalNum - 1\n``` | 2 | Given an integer `n`, return _the number of positive integers in the range_ `[1, n]` _that have **at least one** repeated digit_.
**Example 1:**
**Input:** n = 20
**Output:** 1
**Explanation:** The only positive number (<= 20) with at least 1 repeated digit is 11.
**Example 2:**
**Input:** n = 100
**Output:** 10
**Explanation:** The positive numbers (<= 100) with atleast 1 repeated digit are 11, 22, 33, 44, 55, 66, 77, 88, 99, and 100.
**Example 3:**
**Input:** n = 1000
**Output:** 262
**Constraints:**
* `1 <= n <= 109` | null |
I spent 4 hours on this shit I hate my life | numbers-with-repeated-digits | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nIts basically just math, Im gonna die \n# Approach\n<!-- Describe your approach to solving the problem. -->\nlet n be a K digit integer\nWe will count the total number of non-duplicated integers\nWe split them up in 2 cases by counting <K digits and K digits\n\nFor < K digits\nI did abit of trial and error for this but the general form that you would get would be for each k number of digits\n$$9\\bullet\\sum^{K-3}_{i=0}\\Pi^9_{j=9-i}j + 9$$\n\nSo how i visualised it was for e.g. we wamt let K = 5 so we want to find all possible non-duplicated integers <10 000 i.e. [1,9 999]\n\nFrom 1000 to 9999 we have 10000 - 1000 = 9000 integers \nwe fix the 1st digit so we have 1XXX then for the 2nd digit we have 9 remaining unique digits so on and so forth. Resulting in 9x8x7 integers\nNow we permutate the first digit to be between 1 to 9\nHence from 1000 - 9999 we have 9x9x8x7 non-duplicated integers\n\nSimilarly, from 100 - 999 we have 9x9x8\nfrom 10 - 99 we have 9x9\nfrom 1 - 9 we have 9\n\nNow if we take the sum we get \n9x9x8x7 9x9x8 + 9x9 + 9\n\n\nFor a fixed length of K digits\nWe store each leading digit in seen\nThen we build the integer up by iterating from 0 (if not first digit) and 1(if first digit) up to the leading digit. If any of the digit was seen before then we skip it. We then permutate the remaining digits left. If the leading digit was already seen we can stop as we know it will no longer give us any non-duplicated integers.\n\nE.g. if we let n = 13579\nWe will calculate this in parts as well specifically from \n1XXXX - 1XXXX\n10XXX - 12XXX\n130XX - 134XX\n1350X - 1356X\n1357X - 13578\n(remember last number not inclusive, pretty sure you can tweak the code to include)\n\nAt the start we have 10 000, since the leading digit is the first digit and is 1 we skip it. We then store 1 inside seen. Now we have fixed the first digit.\n\nFor the second digit, we start building from 0 to 2 so we have 10XXX, 11XXX and 12XXX but since 11XXX is already a duplicate we will skip it. Giving us 2x8x7x6 non-duplicated integers. Now we fix the second digit to be 3\n\nFor the third digit we will consider 130XX, 131XX, 132XX, 133XX, 134XX, w can see that 131XX and 133XX already contains duplicates and hence we will skip them. Giving us 3x7x6. Then we fix the third digit to be 5\n\nSo on and so forth, resulting in a total of 2x8x7x6 + 3x7x6 + 4x6 + 5\n\nNow we take the sum \n9x9x8x7 9x9x8 + 9x9 + 9 + \n2x8x7x6 + 3x7x6 + 4x6 + 5 + 1 (to include n=13579) = 6102\nWe return 13579 - 6102 = 7477 which is the ans\n \n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\nI believe it is $$O(log_{10}n)$$ as we are counting the number of digits\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\nI think it is $$O(log_{10}n)$$ as well? as we only store the number of digits in both seen and numList\n# Code\n```\nclass Solution:\n def numDupDigitsAtMostN(self, n: int) -> int:\n temp = n\n numList = []\n while temp > 0:\n numList.insert(0, temp%10)\n temp //=10\n digits = len(numList)\n totalNum = 0\n res = 0\n # less than K digits\n for j in range(digits-1, 0, -1):\n res = 9\n for k in range(9, 9-j+1,-1):\n res *= k\n totalNum += res\n #K digits\n seen = {}\n for i in range(0,digits):\n leadingDigit = numList[i]\n remainingDigits = len(numList) - i - 1\n for j in range(1 if i==0 else 0, leadingDigit):\n if j in seen:\n continue\n res = 1\n for k in range(9-i, 9-i-remainingDigits,-1):\n res *= k\n totalNum += res\n if leadingDigit in seen:\n break\n seen[leadingDigit] = 1\n seen = {}\n for num in numList:\n if num in seen:\n return n-totalNum\n seen[num] = 1\n return n-totalNum - 1\n``` | 2 | On a campus represented on the X-Y plane, there are `n` workers and `m` bikes, with `n <= m`.
You are given an array `workers` of length `n` where `workers[i] = [xi, yi]` is the position of the `ith` worker. You are also given an array `bikes` of length `m` where `bikes[j] = [xj, yj]` is the position of the `jth` bike. All the given positions are **unique**.
Assign a bike to each worker. Among the available bikes and workers, we choose the `(workeri, bikej)` pair with the shortest **Manhattan distance** between each other and assign the bike to that worker.
If there are multiple `(workeri, bikej)` pairs with the same shortest **Manhattan distance**, we choose the pair with **the smallest worker index**. If there are multiple ways to do that, we choose the pair with **the smallest bike index**. Repeat this process until there are no available workers.
Return _an array_ `answer` _of length_ `n`_, where_ `answer[i]` _is the index (**0-indexed**) of the bike that the_ `ith` _worker is assigned to_.
The **Manhattan distance** between two points `p1` and `p2` is `Manhattan(p1, p2) = |p1.x - p2.x| + |p1.y - p2.y|`.
**Example 1:**
**Input:** workers = \[\[0,0\],\[2,1\]\], bikes = \[\[1,2\],\[3,3\]\]
**Output:** \[1,0\]
**Explanation:** Worker 1 grabs Bike 0 as they are closest (without ties), and Worker 0 is assigned Bike 1. So the output is \[1, 0\].
**Example 2:**
**Input:** workers = \[\[0,0\],\[1,1\],\[2,0\]\], bikes = \[\[1,0\],\[2,2\],\[2,1\]\]
**Output:** \[0,2,1\]
**Explanation:** Worker 0 grabs Bike 0 at first. Worker 1 and Worker 2 share the same distance to Bike 2, thus Worker 1 is assigned to Bike 2, and Worker 2 will take Bike 1. So the output is \[0,2,1\].
**Constraints:**
* `n == workers.length`
* `m == bikes.length`
* `1 <= n <= m <= 1000`
* `workers[i].length == bikes[j].length == 2`
* `0 <= xi, yi < 1000`
* `0 <= xj, yj < 1000`
* All worker and bike locations are **unique**. | How many numbers with no duplicate digits? How many numbers with K digits and no duplicates? How many numbers with same length as N? How many numbers with same prefix as N? |
Math solution | numbers-with-repeated-digits | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(logn)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numDupDigitsAtMostN(self, n: int) -> int:\n num_list = []\n original_n = n\n while n > 0:\n num_list.append(n % 10)\n n = n // 10\n num_list.reverse()\n\n digit_num = len(num_list)\n # str_n = str(n)\n o = len(num_list) - 1\n \n ans_o = 0\n while o > 0:\n o_a = 9\n for i in range(o-1):\n o_a *= (9-i)\n ans_o += o_a\n o -= 1\n \n ans = 0\n should_break = 0\n\n for i in range(digit_num):\n print(i)\n # if num_list[i] != 0:\n minus_num = 0\n\n for j in range(i):\n if num_list[j] < num_list[i]:\n minus_num += 1\n elif num_list[j] == num_list[i]:\n should_break = 1\n\n p = 1\n for q in range(10-i-1, 10 - digit_num, -1):\n p *= q\n \n if i == 0:\n ans += (num_list[i] - 1) * p + ans_o\n print(\'ti\', ans)\n\n elif i != 0:\n ans += (num_list[i] - minus_num) * p\n print(\'to\', ans)\n\n if should_break:\n break\n\n print(ans)\n\n # last_digit + 1 & first_digit zero cases\n return original_n - ans - 1 if len(set(num_list))==digit_num else original_n - ans\n \n\n \n``` | 0 | Given an integer `n`, return _the number of positive integers in the range_ `[1, n]` _that have **at least one** repeated digit_.
**Example 1:**
**Input:** n = 20
**Output:** 1
**Explanation:** The only positive number (<= 20) with at least 1 repeated digit is 11.
**Example 2:**
**Input:** n = 100
**Output:** 10
**Explanation:** The positive numbers (<= 100) with atleast 1 repeated digit are 11, 22, 33, 44, 55, 66, 77, 88, 99, and 100.
**Example 3:**
**Input:** n = 1000
**Output:** 262
**Constraints:**
* `1 <= n <= 109` | null |
Math solution | numbers-with-repeated-digits | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity: O(logn)\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numDupDigitsAtMostN(self, n: int) -> int:\n num_list = []\n original_n = n\n while n > 0:\n num_list.append(n % 10)\n n = n // 10\n num_list.reverse()\n\n digit_num = len(num_list)\n # str_n = str(n)\n o = len(num_list) - 1\n \n ans_o = 0\n while o > 0:\n o_a = 9\n for i in range(o-1):\n o_a *= (9-i)\n ans_o += o_a\n o -= 1\n \n ans = 0\n should_break = 0\n\n for i in range(digit_num):\n print(i)\n # if num_list[i] != 0:\n minus_num = 0\n\n for j in range(i):\n if num_list[j] < num_list[i]:\n minus_num += 1\n elif num_list[j] == num_list[i]:\n should_break = 1\n\n p = 1\n for q in range(10-i-1, 10 - digit_num, -1):\n p *= q\n \n if i == 0:\n ans += (num_list[i] - 1) * p + ans_o\n print(\'ti\', ans)\n\n elif i != 0:\n ans += (num_list[i] - minus_num) * p\n print(\'to\', ans)\n\n if should_break:\n break\n\n print(ans)\n\n # last_digit + 1 & first_digit zero cases\n return original_n - ans - 1 if len(set(num_list))==digit_num else original_n - ans\n \n\n \n``` | 0 | On a campus represented on the X-Y plane, there are `n` workers and `m` bikes, with `n <= m`.
You are given an array `workers` of length `n` where `workers[i] = [xi, yi]` is the position of the `ith` worker. You are also given an array `bikes` of length `m` where `bikes[j] = [xj, yj]` is the position of the `jth` bike. All the given positions are **unique**.
Assign a bike to each worker. Among the available bikes and workers, we choose the `(workeri, bikej)` pair with the shortest **Manhattan distance** between each other and assign the bike to that worker.
If there are multiple `(workeri, bikej)` pairs with the same shortest **Manhattan distance**, we choose the pair with **the smallest worker index**. If there are multiple ways to do that, we choose the pair with **the smallest bike index**. Repeat this process until there are no available workers.
Return _an array_ `answer` _of length_ `n`_, where_ `answer[i]` _is the index (**0-indexed**) of the bike that the_ `ith` _worker is assigned to_.
The **Manhattan distance** between two points `p1` and `p2` is `Manhattan(p1, p2) = |p1.x - p2.x| + |p1.y - p2.y|`.
**Example 1:**
**Input:** workers = \[\[0,0\],\[2,1\]\], bikes = \[\[1,2\],\[3,3\]\]
**Output:** \[1,0\]
**Explanation:** Worker 1 grabs Bike 0 as they are closest (without ties), and Worker 0 is assigned Bike 1. So the output is \[1, 0\].
**Example 2:**
**Input:** workers = \[\[0,0\],\[1,1\],\[2,0\]\], bikes = \[\[1,0\],\[2,2\],\[2,1\]\]
**Output:** \[0,2,1\]
**Explanation:** Worker 0 grabs Bike 0 at first. Worker 1 and Worker 2 share the same distance to Bike 2, thus Worker 1 is assigned to Bike 2, and Worker 2 will take Bike 1. So the output is \[0,2,1\].
**Constraints:**
* `n == workers.length`
* `m == bikes.length`
* `1 <= n <= m <= 1000`
* `workers[i].length == bikes[j].length == 2`
* `0 <= xi, yi < 1000`
* `0 <= xj, yj < 1000`
* All worker and bike locations are **unique**. | How many numbers with no duplicate digits? How many numbers with K digits and no duplicates? How many numbers with same length as N? How many numbers with same prefix as N? |
easy dp python digit dp | numbers-with-repeated-digits | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numDupDigitsAtMostN(self, n: int) -> int:\n s=str(n)\n @cache\n def rec(ind,done,h,exists,z):\n nonlocal s\n if ind==len(s):\n if done:\n return 1\n return 0\n top=int(s[ind]) if h else 9\n ans=0\n for i in range(top+1):\n hh=h and (i==int(s[ind]))\n existsnew=exists\n donenew=done\n if not( i==0 and z) and (exists & 1<<i)!=0:\n donenew=True\n elif not (i==0 and z):\n existsnew =exists | 1<<i\n zz=z and i==0\n ans+=rec(ind+1,donenew,hh,existsnew,zz)\n return ans\n return rec(0,False,True,0,True)\n \n \n``` | 0 | Given an integer `n`, return _the number of positive integers in the range_ `[1, n]` _that have **at least one** repeated digit_.
**Example 1:**
**Input:** n = 20
**Output:** 1
**Explanation:** The only positive number (<= 20) with at least 1 repeated digit is 11.
**Example 2:**
**Input:** n = 100
**Output:** 10
**Explanation:** The positive numbers (<= 100) with atleast 1 repeated digit are 11, 22, 33, 44, 55, 66, 77, 88, 99, and 100.
**Example 3:**
**Input:** n = 1000
**Output:** 262
**Constraints:**
* `1 <= n <= 109` | null |
easy dp python digit dp | numbers-with-repeated-digits | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def numDupDigitsAtMostN(self, n: int) -> int:\n s=str(n)\n @cache\n def rec(ind,done,h,exists,z):\n nonlocal s\n if ind==len(s):\n if done:\n return 1\n return 0\n top=int(s[ind]) if h else 9\n ans=0\n for i in range(top+1):\n hh=h and (i==int(s[ind]))\n existsnew=exists\n donenew=done\n if not( i==0 and z) and (exists & 1<<i)!=0:\n donenew=True\n elif not (i==0 and z):\n existsnew =exists | 1<<i\n zz=z and i==0\n ans+=rec(ind+1,donenew,hh,existsnew,zz)\n return ans\n return rec(0,False,True,0,True)\n \n \n``` | 0 | On a campus represented on the X-Y plane, there are `n` workers and `m` bikes, with `n <= m`.
You are given an array `workers` of length `n` where `workers[i] = [xi, yi]` is the position of the `ith` worker. You are also given an array `bikes` of length `m` where `bikes[j] = [xj, yj]` is the position of the `jth` bike. All the given positions are **unique**.
Assign a bike to each worker. Among the available bikes and workers, we choose the `(workeri, bikej)` pair with the shortest **Manhattan distance** between each other and assign the bike to that worker.
If there are multiple `(workeri, bikej)` pairs with the same shortest **Manhattan distance**, we choose the pair with **the smallest worker index**. If there are multiple ways to do that, we choose the pair with **the smallest bike index**. Repeat this process until there are no available workers.
Return _an array_ `answer` _of length_ `n`_, where_ `answer[i]` _is the index (**0-indexed**) of the bike that the_ `ith` _worker is assigned to_.
The **Manhattan distance** between two points `p1` and `p2` is `Manhattan(p1, p2) = |p1.x - p2.x| + |p1.y - p2.y|`.
**Example 1:**
**Input:** workers = \[\[0,0\],\[2,1\]\], bikes = \[\[1,2\],\[3,3\]\]
**Output:** \[1,0\]
**Explanation:** Worker 1 grabs Bike 0 as they are closest (without ties), and Worker 0 is assigned Bike 1. So the output is \[1, 0\].
**Example 2:**
**Input:** workers = \[\[0,0\],\[1,1\],\[2,0\]\], bikes = \[\[1,0\],\[2,2\],\[2,1\]\]
**Output:** \[0,2,1\]
**Explanation:** Worker 0 grabs Bike 0 at first. Worker 1 and Worker 2 share the same distance to Bike 2, thus Worker 1 is assigned to Bike 2, and Worker 2 will take Bike 1. So the output is \[0,2,1\].
**Constraints:**
* `n == workers.length`
* `m == bikes.length`
* `1 <= n <= m <= 1000`
* `workers[i].length == bikes[j].length == 2`
* `0 <= xi, yi < 1000`
* `0 <= xj, yj < 1000`
* All worker and bike locations are **unique**. | How many numbers with no duplicate digits? How many numbers with K digits and no duplicates? How many numbers with same length as N? How many numbers with same prefix as N? |
80%faster Easy and optimal solution | partition-array-into-three-parts-with-equal-sum | 0 | 1 | ```\n def canThreePartsEqualSum(self, arr: List[int]) -> bool:\n \n res=sum(arr)\n if(res%3!=0):\n return False\n res=res//3\n li=0\n sm=0\n for i in arr:\n sm+=i\n if(sm==res):\n li+=1\n sm=0\n if(li==3):\n return True\n return li==3\n``` | 1 | Given an array of integers `arr`, return `true` if we can partition the array into three **non-empty** parts with equal sums.
Formally, we can partition the array if we can find indexes `i + 1 < j` with `(arr[0] + arr[1] + ... + arr[i] == arr[i + 1] + arr[i + 2] + ... + arr[j - 1] == arr[j] + arr[j + 1] + ... + arr[arr.length - 1])`
**Example 1:**
**Input:** arr = \[0,2,1,-6,6,-7,9,1,2,0,1\]
**Output:** true
**Explanation:** 0 + 2 + 1 = -6 + 6 - 7 + 9 + 1 = 2 + 0 + 1
**Example 2:**
**Input:** arr = \[0,2,1,-6,6,7,9,-1,2,0,1\]
**Output:** false
**Example 3:**
**Input:** arr = \[3,3,6,5,-2,2,5,1,-9,4\]
**Output:** true
**Explanation:** 3 + 3 = 6 = 5 - 2 + 2 + 5 + 1 - 9 + 4
**Constraints:**
* `3 <= arr.length <= 5 * 104`
* `-104 <= arr[i] <= 104` | null |
80%faster Easy and optimal solution | partition-array-into-three-parts-with-equal-sum | 0 | 1 | ```\n def canThreePartsEqualSum(self, arr: List[int]) -> bool:\n \n res=sum(arr)\n if(res%3!=0):\n return False\n res=res//3\n li=0\n sm=0\n for i in arr:\n sm+=i\n if(sm==res):\n li+=1\n sm=0\n if(li==3):\n return True\n return li==3\n``` | 1 | Given a string `s`, return _the length of the longest repeating substrings_. If no repeating substring exists, return `0`.
**Example 1:**
**Input:** s = "abcd "
**Output:** 0
**Explanation:** There is no repeating substring.
**Example 2:**
**Input:** s = "abbaba "
**Output:** 2
**Explanation:** The longest repeating substrings are "ab " and "ba ", each of which occurs twice.
**Example 3:**
**Input:** s = "aabcaabdaab "
**Output:** 3
**Explanation:** The longest repeating substring is "aab ", which occurs `3` times.
**Constraints:**
* `1 <= s.length <= 2000`
* `s` consists of lowercase English letters. | If we have three parts with the same sum, what is the sum of each?
If you can find the first part, can you find the second part? |
Solution in Python 3 (beats ~99%) (With Detailed Explanation) ( O(n) time ) ( O(1) space ) | partition-array-into-three-parts-with-equal-sum | 0 | 1 | _Explanation:_\n\nWe are asked to determine if the array can be partioned into three _adjacent_ regions each of which have the same sum. Since we must use all of the elements in the array the sum of each of the three regions must be sum(A)//3. If sum(A) is not divisible by 3, one can immediately return False because a number which is not a multiple of 3 cannot be divided evenly into three equal numbers. The program starts by setting _g_ equal to the sum that each region must be. The variable _C_ is initialized to zero and will hold the cumulative sum as we iterate from left to right. The variable _p_ is initialized to zero and is the count of the number of successful partition points we have found thus far. Since the iteration is yet to begin, we have not yet found any partition points so _p_ is equal to zero. The goal of the program is to successfully find two partition points or partition indices such that the sum of the values within each region is equal. Note that the second partition point cannot be at the last index of A because then there would be no room for the third partition.\n\nThe for loop iterates through each element of A from left to right, adding the element to the the cumulative sum _C_. If the variable _C_ eventually equals _g_ then that means that we\'ve found a continuous group of numbers starting from the beginning of the list that add up to our goal sum for each of the three regions. So if _C_ == _g_, we can set _p_ equal to one because we have now found the first partition point. We reset the cumulative sum _C_ to zero to being accumulating the numbers in the second region. Note that if _C_ never reaches the goal sum _g_ then there can be no first partition and the for loop will eventually finish and the program will return False.\n\nNow that _p_ equals one and _C_ has been reset to zero we continue on with the loop, adding the elements to _C_ one by one. Note that we are now currently in the middle (or the second) of the three partitions that we hope to find. Once again as _C_ increases (or decreases if the numbers are negative) we check to see if _C_ has reached our goal sum of _g_ yet. Again, if _C_ never reaches the goal sum _g_ then there can be no second partition and the for loop will eventually finish and the program will return False. If we do successfully reach the goal sum _g_ (i.e. _C_ == _g_) for the second time, we have successfully found the second partition point.\n\nNote that the for loop does not continue onto the last index of A. This is because if the second partition point is not found by the time the last index is reached, there cannot be three partitions. Thus, if two partition points can be found before the last index of A is reached, the program can end and return True. The reason that we don\'t have to search any further or add any more numbers is because if the first two regions each have a sum of _g_ then the third region must also add to _g_ since _g_ was defined to be one third of the total sum of array A.\n\nI\'ve also included a one line version just for fun!\n\n\n```\n```\n_Detailed Version (with explanation above):_\n```\nclass Solution:\n def canThreePartsEqualSum(self, A: List[int]) -> bool:\n \tS = sum(A)\n \tif S % 3 != 0: return False\n \tg, C, p = S//3, 0, 0\n \tfor a in A[:-1]:\n \t\tC += a\n \t\tif C == g:\n \t\t\tif p == 1: return True\n \t\t\tC, p = 0, 1\n \treturn False\n\n```\t\t\t\t\n_One-Line Version:_ (beats ~100%)\n```\nclass Solution:\n def canThreePartsEqualSum(self, A: List[int]) -> bool:\n \treturn (lambda x,y: x in y and 2*x in y and 3*x in y)(sum(A)//3,itertools.accumulate(A))\n\t\t\t\t\n\t\t\t\t\n\t\t\t\t\nJunaid Mansuri\nChicago, IL | 23 | Given an array of integers `arr`, return `true` if we can partition the array into three **non-empty** parts with equal sums.
Formally, we can partition the array if we can find indexes `i + 1 < j` with `(arr[0] + arr[1] + ... + arr[i] == arr[i + 1] + arr[i + 2] + ... + arr[j - 1] == arr[j] + arr[j + 1] + ... + arr[arr.length - 1])`
**Example 1:**
**Input:** arr = \[0,2,1,-6,6,-7,9,1,2,0,1\]
**Output:** true
**Explanation:** 0 + 2 + 1 = -6 + 6 - 7 + 9 + 1 = 2 + 0 + 1
**Example 2:**
**Input:** arr = \[0,2,1,-6,6,7,9,-1,2,0,1\]
**Output:** false
**Example 3:**
**Input:** arr = \[3,3,6,5,-2,2,5,1,-9,4\]
**Output:** true
**Explanation:** 3 + 3 = 6 = 5 - 2 + 2 + 5 + 1 - 9 + 4
**Constraints:**
* `3 <= arr.length <= 5 * 104`
* `-104 <= arr[i] <= 104` | null |
Solution in Python 3 (beats ~99%) (With Detailed Explanation) ( O(n) time ) ( O(1) space ) | partition-array-into-three-parts-with-equal-sum | 0 | 1 | _Explanation:_\n\nWe are asked to determine if the array can be partioned into three _adjacent_ regions each of which have the same sum. Since we must use all of the elements in the array the sum of each of the three regions must be sum(A)//3. If sum(A) is not divisible by 3, one can immediately return False because a number which is not a multiple of 3 cannot be divided evenly into three equal numbers. The program starts by setting _g_ equal to the sum that each region must be. The variable _C_ is initialized to zero and will hold the cumulative sum as we iterate from left to right. The variable _p_ is initialized to zero and is the count of the number of successful partition points we have found thus far. Since the iteration is yet to begin, we have not yet found any partition points so _p_ is equal to zero. The goal of the program is to successfully find two partition points or partition indices such that the sum of the values within each region is equal. Note that the second partition point cannot be at the last index of A because then there would be no room for the third partition.\n\nThe for loop iterates through each element of A from left to right, adding the element to the the cumulative sum _C_. If the variable _C_ eventually equals _g_ then that means that we\'ve found a continuous group of numbers starting from the beginning of the list that add up to our goal sum for each of the three regions. So if _C_ == _g_, we can set _p_ equal to one because we have now found the first partition point. We reset the cumulative sum _C_ to zero to being accumulating the numbers in the second region. Note that if _C_ never reaches the goal sum _g_ then there can be no first partition and the for loop will eventually finish and the program will return False.\n\nNow that _p_ equals one and _C_ has been reset to zero we continue on with the loop, adding the elements to _C_ one by one. Note that we are now currently in the middle (or the second) of the three partitions that we hope to find. Once again as _C_ increases (or decreases if the numbers are negative) we check to see if _C_ has reached our goal sum of _g_ yet. Again, if _C_ never reaches the goal sum _g_ then there can be no second partition and the for loop will eventually finish and the program will return False. If we do successfully reach the goal sum _g_ (i.e. _C_ == _g_) for the second time, we have successfully found the second partition point.\n\nNote that the for loop does not continue onto the last index of A. This is because if the second partition point is not found by the time the last index is reached, there cannot be three partitions. Thus, if two partition points can be found before the last index of A is reached, the program can end and return True. The reason that we don\'t have to search any further or add any more numbers is because if the first two regions each have a sum of _g_ then the third region must also add to _g_ since _g_ was defined to be one third of the total sum of array A.\n\nI\'ve also included a one line version just for fun!\n\n\n```\n```\n_Detailed Version (with explanation above):_\n```\nclass Solution:\n def canThreePartsEqualSum(self, A: List[int]) -> bool:\n \tS = sum(A)\n \tif S % 3 != 0: return False\n \tg, C, p = S//3, 0, 0\n \tfor a in A[:-1]:\n \t\tC += a\n \t\tif C == g:\n \t\t\tif p == 1: return True\n \t\t\tC, p = 0, 1\n \treturn False\n\n```\t\t\t\t\n_One-Line Version:_ (beats ~100%)\n```\nclass Solution:\n def canThreePartsEqualSum(self, A: List[int]) -> bool:\n \treturn (lambda x,y: x in y and 2*x in y and 3*x in y)(sum(A)//3,itertools.accumulate(A))\n\t\t\t\t\n\t\t\t\t\n\t\t\t\t\nJunaid Mansuri\nChicago, IL | 23 | Given a string `s`, return _the length of the longest repeating substrings_. If no repeating substring exists, return `0`.
**Example 1:**
**Input:** s = "abcd "
**Output:** 0
**Explanation:** There is no repeating substring.
**Example 2:**
**Input:** s = "abbaba "
**Output:** 2
**Explanation:** The longest repeating substrings are "ab " and "ba ", each of which occurs twice.
**Example 3:**
**Input:** s = "aabcaabdaab "
**Output:** 3
**Explanation:** The longest repeating substring is "aab ", which occurs `3` times.
**Constraints:**
* `1 <= s.length <= 2000`
* `s` consists of lowercase English letters. | If we have three parts with the same sum, what is the sum of each?
If you can find the first part, can you find the second part? |
Solution | partition-array-into-three-parts-with-equal-sum | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n bool canThreePartsEqualSum(vector<int>&v) {\n ios::sync_with_stdio(0);\n int ts=accumulate(v.begin(),v.end(),0),c=0,s=0,n=v.size()-1,sum;\n if(ts%3 == 0) sum=ts/3;\n else return 0;\n for(int i=0;i<=n;i++)\n {\n s+=v[i];\n if(s==sum) {s=0;c++;}\n }\n return (c>=3);\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def canThreePartsEqualSum(self, A: List[int]) -> bool:\n \tS = sum(A)\n \tif S % 3 != 0: return False\n \tg, C, p = S//3, 0, 0\n \tfor a in A[:-1]:\n \t\tC += a\n \t\tif C == g:\n \t\t\tif p == 1: return True\n \t\t\tC, p = 0, 1\n \treturn False\n```\n\n```Java []\nclass Solution {\n public boolean canThreePartsEqualSum(int[] arr) {\n int sum = 0;\n for(int i : arr){\n sum += i;\n }\n int average = sum / 3;\n int total = 0;\n int count = 0;\n\n for(int i : arr){\n total += i;\n if(total == average){\n count++;\n total = 0;\n }\n }\n return count >= 3 && sum % 3 == 0;\n }\n}\n``` | 2 | Given an array of integers `arr`, return `true` if we can partition the array into three **non-empty** parts with equal sums.
Formally, we can partition the array if we can find indexes `i + 1 < j` with `(arr[0] + arr[1] + ... + arr[i] == arr[i + 1] + arr[i + 2] + ... + arr[j - 1] == arr[j] + arr[j + 1] + ... + arr[arr.length - 1])`
**Example 1:**
**Input:** arr = \[0,2,1,-6,6,-7,9,1,2,0,1\]
**Output:** true
**Explanation:** 0 + 2 + 1 = -6 + 6 - 7 + 9 + 1 = 2 + 0 + 1
**Example 2:**
**Input:** arr = \[0,2,1,-6,6,7,9,-1,2,0,1\]
**Output:** false
**Example 3:**
**Input:** arr = \[3,3,6,5,-2,2,5,1,-9,4\]
**Output:** true
**Explanation:** 3 + 3 = 6 = 5 - 2 + 2 + 5 + 1 - 9 + 4
**Constraints:**
* `3 <= arr.length <= 5 * 104`
* `-104 <= arr[i] <= 104` | null |
Solution | partition-array-into-three-parts-with-equal-sum | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n bool canThreePartsEqualSum(vector<int>&v) {\n ios::sync_with_stdio(0);\n int ts=accumulate(v.begin(),v.end(),0),c=0,s=0,n=v.size()-1,sum;\n if(ts%3 == 0) sum=ts/3;\n else return 0;\n for(int i=0;i<=n;i++)\n {\n s+=v[i];\n if(s==sum) {s=0;c++;}\n }\n return (c>=3);\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def canThreePartsEqualSum(self, A: List[int]) -> bool:\n \tS = sum(A)\n \tif S % 3 != 0: return False\n \tg, C, p = S//3, 0, 0\n \tfor a in A[:-1]:\n \t\tC += a\n \t\tif C == g:\n \t\t\tif p == 1: return True\n \t\t\tC, p = 0, 1\n \treturn False\n```\n\n```Java []\nclass Solution {\n public boolean canThreePartsEqualSum(int[] arr) {\n int sum = 0;\n for(int i : arr){\n sum += i;\n }\n int average = sum / 3;\n int total = 0;\n int count = 0;\n\n for(int i : arr){\n total += i;\n if(total == average){\n count++;\n total = 0;\n }\n }\n return count >= 3 && sum % 3 == 0;\n }\n}\n``` | 2 | Given a string `s`, return _the length of the longest repeating substrings_. If no repeating substring exists, return `0`.
**Example 1:**
**Input:** s = "abcd "
**Output:** 0
**Explanation:** There is no repeating substring.
**Example 2:**
**Input:** s = "abbaba "
**Output:** 2
**Explanation:** The longest repeating substrings are "ab " and "ba ", each of which occurs twice.
**Example 3:**
**Input:** s = "aabcaabdaab "
**Output:** 3
**Explanation:** The longest repeating substring is "aab ", which occurs `3` times.
**Constraints:**
* `1 <= s.length <= 2000`
* `s` consists of lowercase English letters. | If we have three parts with the same sum, what is the sum of each?
If you can find the first part, can you find the second part? |
Python 3 faster than 90% | partition-array-into-three-parts-with-equal-sum | 0 | 1 | ```\nclass Solution:\n def canThreePartsEqualSum(self, arr: List[int]) -> bool:\n s = sum(arr)\n if s % 3:\n return False\n \n part_sum = s / 3\n \n cur_sum = parts = 0\n for num in arr:\n cur_sum += num\n if cur_sum == part_sum:\n if parts == 2:\n return True\n cur_sum = 0\n parts += 1\n \n return False | 3 | Given an array of integers `arr`, return `true` if we can partition the array into three **non-empty** parts with equal sums.
Formally, we can partition the array if we can find indexes `i + 1 < j` with `(arr[0] + arr[1] + ... + arr[i] == arr[i + 1] + arr[i + 2] + ... + arr[j - 1] == arr[j] + arr[j + 1] + ... + arr[arr.length - 1])`
**Example 1:**
**Input:** arr = \[0,2,1,-6,6,-7,9,1,2,0,1\]
**Output:** true
**Explanation:** 0 + 2 + 1 = -6 + 6 - 7 + 9 + 1 = 2 + 0 + 1
**Example 2:**
**Input:** arr = \[0,2,1,-6,6,7,9,-1,2,0,1\]
**Output:** false
**Example 3:**
**Input:** arr = \[3,3,6,5,-2,2,5,1,-9,4\]
**Output:** true
**Explanation:** 3 + 3 = 6 = 5 - 2 + 2 + 5 + 1 - 9 + 4
**Constraints:**
* `3 <= arr.length <= 5 * 104`
* `-104 <= arr[i] <= 104` | null |
Python 3 faster than 90% | partition-array-into-three-parts-with-equal-sum | 0 | 1 | ```\nclass Solution:\n def canThreePartsEqualSum(self, arr: List[int]) -> bool:\n s = sum(arr)\n if s % 3:\n return False\n \n part_sum = s / 3\n \n cur_sum = parts = 0\n for num in arr:\n cur_sum += num\n if cur_sum == part_sum:\n if parts == 2:\n return True\n cur_sum = 0\n parts += 1\n \n return False | 3 | Given a string `s`, return _the length of the longest repeating substrings_. If no repeating substring exists, return `0`.
**Example 1:**
**Input:** s = "abcd "
**Output:** 0
**Explanation:** There is no repeating substring.
**Example 2:**
**Input:** s = "abbaba "
**Output:** 2
**Explanation:** The longest repeating substrings are "ab " and "ba ", each of which occurs twice.
**Example 3:**
**Input:** s = "aabcaabdaab "
**Output:** 3
**Explanation:** The longest repeating substring is "aab ", which occurs `3` times.
**Constraints:**
* `1 <= s.length <= 2000`
* `s` consists of lowercase English letters. | If we have three parts with the same sum, what is the sum of each?
If you can find the first part, can you find the second part? |
Solution | best-sightseeing-pair | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n int maxScoreSightseeingPair(vector<int>& values) {\n int maxval = values[0];\n int minval = INT_MAX;\n int res = 0;\n for (int i = 1; i < values.size(); i++)\n {\n minval = values[i] - i;\n res = max(res, maxval + minval);\n maxval = max(maxval, values[i] + i);\n }\n return res;\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def maxScoreSightseeingPair(self, values: List[int]) -> int:\n best = ans = 0\n for n in values:\n if n+best > ans:\n ans = n+best\n if n > best:\n best = n-1\n else:\n best -= 1\n return ans\n```\n\n```Java []\nclass Solution {\n public int maxScoreSightseeingPair(int[] A) {\n int i=0;\n\t\tint max = A[i] + i;\n for(int j=1;j<A.length;j++){\n int curr = A[i] + A[j] + i - j;\n max = curr > max ? curr : max;\n \n if(A[i] + i < A[j] + j){\n i=j;\n }\n }\n return max;\n }\n}\n``` | 1 | You are given an integer array `values` where values\[i\] represents the value of the `ith` sightseeing spot. Two sightseeing spots `i` and `j` have a **distance** `j - i` between them.
The score of a pair (`i < j`) of sightseeing spots is `values[i] + values[j] + i - j`: the sum of the values of the sightseeing spots, minus the distance between them.
Return _the maximum score of a pair of sightseeing spots_.
**Example 1:**
**Input:** values = \[8,1,5,2,6\]
**Output:** 11
**Explanation:** i = 0, j = 2, values\[i\] + values\[j\] + i - j = 8 + 5 + 0 - 2 = 11
**Example 2:**
**Input:** values = \[1,2\]
**Output:** 2
**Constraints:**
* `2 <= values.length <= 5 * 104`
* `1 <= values[i] <= 1000` | null |
Solution | best-sightseeing-pair | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n int maxScoreSightseeingPair(vector<int>& values) {\n int maxval = values[0];\n int minval = INT_MAX;\n int res = 0;\n for (int i = 1; i < values.size(); i++)\n {\n minval = values[i] - i;\n res = max(res, maxval + minval);\n maxval = max(maxval, values[i] + i);\n }\n return res;\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def maxScoreSightseeingPair(self, values: List[int]) -> int:\n best = ans = 0\n for n in values:\n if n+best > ans:\n ans = n+best\n if n > best:\n best = n-1\n else:\n best -= 1\n return ans\n```\n\n```Java []\nclass Solution {\n public int maxScoreSightseeingPair(int[] A) {\n int i=0;\n\t\tint max = A[i] + i;\n for(int j=1;j<A.length;j++){\n int curr = A[i] + A[j] + i - j;\n max = curr > max ? curr : max;\n \n if(A[i] + i < A[j] + j){\n i=j;\n }\n }\n return max;\n }\n}\n``` | 1 | Given an integer array `nums`, return _the number of non-empty **subarrays** with the leftmost element of the subarray not larger than other elements in the subarray_.
A **subarray** is a **contiguous** part of an array.
**Example 1:**
**Input:** nums = \[1,4,2,5,3\]
**Output:** 11
**Explanation:** There are 11 valid subarrays: \[1\],\[4\],\[2\],\[5\],\[3\],\[1,4\],\[2,5\],\[1,4,2\],\[2,5,3\],\[1,4,2,5\],\[1,4,2,5,3\].
**Example 2:**
**Input:** nums = \[3,2,1\]
**Output:** 3
**Explanation:** The 3 valid subarrays are: \[3\],\[2\],\[1\].
**Example 3:**
**Input:** nums = \[2,2,2\]
**Output:** 6
**Explanation:** There are 6 valid subarrays: \[2\],\[2\],\[2\],\[2,2\],\[2,2\],\[2,2,2\].
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `0 <= nums[i] <= 105` | Can you tell the best sightseeing spot in one pass (ie. as you iterate over the input?) What should we store or keep track of as we iterate to do this? |
✅ || Very easy explanation || DP || Complexity Analysis || Python | best-sightseeing-pair | 0 | 1 | ## **Solution**\n\n\n#### **LOGIC**\n\nSuppose we have a = ```[8,1,5,2,6]```\ncreate a dp = ```[0, 0, 0, 0]``` where dp[i] represents the best possible answer if we consider all pairs forming with a[i] (like i = 3 then it will be (0, 3),(1, 3), (2, 3))\n\n* For d[0] it will be 0\n* For d[1] it will be a[0] + a[1] + 0 - 1\n* For d[2] it will be max((a[0] + a[2] + 0 - 2), (a[1] + a[2] + 1 - 2))\n* For d[3] it will be max((a[0] + a[3] + 0 - 3), (a[1] + a[3] + 1 - 3), (a[2] + a[3] + 2 - 3))\n* For d[4] it will be max(a[0] + a[4] + 0 - 4), (a[1] + a[4] + 1 - 4), (a[2] + a[4] + 2 - 4), (a[3] + a[4] + 3 - 4))\n\nRewriting above by taking about common term out of max() function\n* For d[0] it will be 0\n* For d[1] it will be a[0] + 0 + a[1] - 1\n* For d[2] it will be max((a[0] + 0), (a[1] + 1)) + a[2] - 2\n* For d[3] it will be max((a[0] + 0 ), (a[1] + 1), (a[2] + 2)) + a[3] - 3\n* For d[4] it will be max(a[0] + 0 ), (a[1] + 1), (a[2] + 2), (a[3] + 3 )) + a[4] - 4\n\nFor a minute lets ignore the part outside the max function and drop it for now, So make a new series and call it dp\n* For dp[0] it will be 0\n* For dp[1] it will be a[0] + 0\n* For dp[2] it will be max((a[0] + 0), (a[1] + 1))\n* For dp[3] it will be max((a[0] + 0 ), (a[1] + 1), (a[2] + 2))\n* For dp[4] it will be max(a[0] + 0 ), (a[1] + 1), (a[2] + 2), (a[3] + 3 ))\n\n**Here is the catch\ndp[i] = max(dp[i-1], a[i-1] + i - 1)\nYou can Clearly see this pattern in above dp series**\n\nCombining this to d series we can get:\n* For d[0] it will be 0\n* For d[1] it will be dp[0]+ a[1] - 1\n* For d[2] it will be max(dp[1], (a[1] + 1)) + a[2] - 2\n* For d[3] it will be max(dp[2], (a[2] + 2)) + a[3] - 3\n* For d[4] it will be max(dp[3], (a[3] + 3 )) + a[4] - 4\n\n**Now our answer can simply the maximum of d that is max(d), but for improving space complexity i used maxVal to keep track of maximum value**\n\n#### **Code**\n\n##### Apporach : 1 \n```cpp\nclass Solution:\n def maxScoreSightseeingPair(self, values: List[int]) -> int: \n dp = [0]*(len(values))\n dp[0] = values[0]\n maxVal = 0\n \n for i in range(1, len(values)):\n dp[i] = max(dp[i-1], values[i-1]+i-1)\n maxVal = max(maxVal, dp[i]+values[i]-i)\n \n return maxVal\n```\n\n##### Apporach : 2[Memory Efficient]\n```cpp\nclass Solution:\n\n def maxScoreSightseeingPair(self, values: List[int]) -> int: \n maxVal = 0\n cur = 0 \n for i in range(1, len(values)):\n cur = max(cur, values[i-1]+i-1)\n maxVal = max(maxVal, cur+values[i]-i)\n return maxVal\n\n```\n## **Complexity**\n\n##### __Apporach : 1__ \n##### Time Complexity: **O(n)**.\n\n##### Space Complexity: **O(n)**\n\n##### __Apporach : 2__ \n##### Time Complexity: **O(n)**.\n\n##### Space Complexity: **O(1)**\n<br>\n\n __Check out all [my](https://leetcode.com/siddp6/) recent solutions [here](https://github.com/sidd6p/LeetCode)__\n\n \n __Feel Free to Ask Doubts\nAnd Please Share Some Suggestions\nHAPPY CODING :)__\n\n\n | 50 | You are given an integer array `values` where values\[i\] represents the value of the `ith` sightseeing spot. Two sightseeing spots `i` and `j` have a **distance** `j - i` between them.
The score of a pair (`i < j`) of sightseeing spots is `values[i] + values[j] + i - j`: the sum of the values of the sightseeing spots, minus the distance between them.
Return _the maximum score of a pair of sightseeing spots_.
**Example 1:**
**Input:** values = \[8,1,5,2,6\]
**Output:** 11
**Explanation:** i = 0, j = 2, values\[i\] + values\[j\] + i - j = 8 + 5 + 0 - 2 = 11
**Example 2:**
**Input:** values = \[1,2\]
**Output:** 2
**Constraints:**
* `2 <= values.length <= 5 * 104`
* `1 <= values[i] <= 1000` | null |
✅ || Very easy explanation || DP || Complexity Analysis || Python | best-sightseeing-pair | 0 | 1 | ## **Solution**\n\n\n#### **LOGIC**\n\nSuppose we have a = ```[8,1,5,2,6]```\ncreate a dp = ```[0, 0, 0, 0]``` where dp[i] represents the best possible answer if we consider all pairs forming with a[i] (like i = 3 then it will be (0, 3),(1, 3), (2, 3))\n\n* For d[0] it will be 0\n* For d[1] it will be a[0] + a[1] + 0 - 1\n* For d[2] it will be max((a[0] + a[2] + 0 - 2), (a[1] + a[2] + 1 - 2))\n* For d[3] it will be max((a[0] + a[3] + 0 - 3), (a[1] + a[3] + 1 - 3), (a[2] + a[3] + 2 - 3))\n* For d[4] it will be max(a[0] + a[4] + 0 - 4), (a[1] + a[4] + 1 - 4), (a[2] + a[4] + 2 - 4), (a[3] + a[4] + 3 - 4))\n\nRewriting above by taking about common term out of max() function\n* For d[0] it will be 0\n* For d[1] it will be a[0] + 0 + a[1] - 1\n* For d[2] it will be max((a[0] + 0), (a[1] + 1)) + a[2] - 2\n* For d[3] it will be max((a[0] + 0 ), (a[1] + 1), (a[2] + 2)) + a[3] - 3\n* For d[4] it will be max(a[0] + 0 ), (a[1] + 1), (a[2] + 2), (a[3] + 3 )) + a[4] - 4\n\nFor a minute lets ignore the part outside the max function and drop it for now, So make a new series and call it dp\n* For dp[0] it will be 0\n* For dp[1] it will be a[0] + 0\n* For dp[2] it will be max((a[0] + 0), (a[1] + 1))\n* For dp[3] it will be max((a[0] + 0 ), (a[1] + 1), (a[2] + 2))\n* For dp[4] it will be max(a[0] + 0 ), (a[1] + 1), (a[2] + 2), (a[3] + 3 ))\n\n**Here is the catch\ndp[i] = max(dp[i-1], a[i-1] + i - 1)\nYou can Clearly see this pattern in above dp series**\n\nCombining this to d series we can get:\n* For d[0] it will be 0\n* For d[1] it will be dp[0]+ a[1] - 1\n* For d[2] it will be max(dp[1], (a[1] + 1)) + a[2] - 2\n* For d[3] it will be max(dp[2], (a[2] + 2)) + a[3] - 3\n* For d[4] it will be max(dp[3], (a[3] + 3 )) + a[4] - 4\n\n**Now our answer can simply the maximum of d that is max(d), but for improving space complexity i used maxVal to keep track of maximum value**\n\n#### **Code**\n\n##### Apporach : 1 \n```cpp\nclass Solution:\n def maxScoreSightseeingPair(self, values: List[int]) -> int: \n dp = [0]*(len(values))\n dp[0] = values[0]\n maxVal = 0\n \n for i in range(1, len(values)):\n dp[i] = max(dp[i-1], values[i-1]+i-1)\n maxVal = max(maxVal, dp[i]+values[i]-i)\n \n return maxVal\n```\n\n##### Apporach : 2[Memory Efficient]\n```cpp\nclass Solution:\n\n def maxScoreSightseeingPair(self, values: List[int]) -> int: \n maxVal = 0\n cur = 0 \n for i in range(1, len(values)):\n cur = max(cur, values[i-1]+i-1)\n maxVal = max(maxVal, cur+values[i]-i)\n return maxVal\n\n```\n## **Complexity**\n\n##### __Apporach : 1__ \n##### Time Complexity: **O(n)**.\n\n##### Space Complexity: **O(n)**\n\n##### __Apporach : 2__ \n##### Time Complexity: **O(n)**.\n\n##### Space Complexity: **O(1)**\n<br>\n\n __Check out all [my](https://leetcode.com/siddp6/) recent solutions [here](https://github.com/sidd6p/LeetCode)__\n\n \n __Feel Free to Ask Doubts\nAnd Please Share Some Suggestions\nHAPPY CODING :)__\n\n\n | 50 | Given an integer array `nums`, return _the number of non-empty **subarrays** with the leftmost element of the subarray not larger than other elements in the subarray_.
A **subarray** is a **contiguous** part of an array.
**Example 1:**
**Input:** nums = \[1,4,2,5,3\]
**Output:** 11
**Explanation:** There are 11 valid subarrays: \[1\],\[4\],\[2\],\[5\],\[3\],\[1,4\],\[2,5\],\[1,4,2\],\[2,5,3\],\[1,4,2,5\],\[1,4,2,5,3\].
**Example 2:**
**Input:** nums = \[3,2,1\]
**Output:** 3
**Explanation:** The 3 valid subarrays are: \[3\],\[2\],\[1\].
**Example 3:**
**Input:** nums = \[2,2,2\]
**Output:** 6
**Explanation:** There are 6 valid subarrays: \[2\],\[2\],\[2\],\[2,2\],\[2,2\],\[2,2,2\].
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `0 <= nums[i] <= 105` | Can you tell the best sightseeing spot in one pass (ie. as you iterate over the input?) What should we store or keep track of as we iterate to do this? |
✅Python 3 | O(n) | Very clearly explained! | best-sightseeing-pair | 0 | 1 | ***Solution 1: Brute Force [Time Limit Exceeded]***\nThe most naive solution is that we can have a brute force solution by simply getting all possible combinations of index `i` and `j`, and then finding the maximum of `values[i] + values[j] + i - j` when `i < j` for all combinations and that will be our answer. We can do this by using a 2 nested for loops keeping track of index `i` and index `j`, while skipping to the next iteration if `i >= j`. We initialize a variable `maximum` and each iteration we check to see if the current value is higher than maximum, if so, we set maximum to this new value. Returning `maximum` in the end will give us our answer.\n```\nclass Solution:\n def maxScoreSightseeingPair(self, values: List[int]) -> int:\n n = len(values)\n maximum = 0\n for i in range(n):\n for j in range(i+1, n):\n if i >= j: continue\n maximum = max(maximum, values[i] + values[j] + i - j)\n return maximum\n```\nTime Complexity: O(n^2)\nSpace Complexity: O(1)\n\n***Solution 2: One Pass***\n\n**Intuition**\nNotice how in the brute force solution, we skip to the next iteration if index `i >= j`. Although we skip to the next iteration using `continue`, we still iterated through many unnecessary iterations giving us a slow O(n^2) time complexity which exceeded the time limit. Thus, we can simply skip all the unnecessary iterations where `i >= j` altogether using this one pass algorithm.\n\n**Algorithm**\nWe can initialize `i` to be 0, and then this time we only need to start 1 for loop tracking the index `j`, same thing as the brute force solution, we keep track of a variable `maximum` and in the iteration if `values[i] + values[j] + i - j` is bigger than the current `maximum` we replace it with that. However, one thing we are doing differently is that instead of starting a nested for loop we write an if statement saying that `values[j] + j - i > values[i]` then we set `i=j`. Basically, you can treat `if values[j] + j - i > values[i]: i = j` the same thing as `if i >= j: continue` in the brute force solution, except we are not iterating unnecessarily.\n\n**Implementation**\n```\nclass Solution:\n def maxScoreSightseeingPair(self, values: List[int]) -> int:\n n = len(values)\n i = maximum = 0\n for j in range(i+1, n):\n maximum = max(maximum, values[i] + values[j] + i - j)\n if values[j] + j - i > values[i]: i = j\n return maximum\n```\nTime Complexity: O(n)\nSpace Complexity: O(1)\n\n**Please consider upvoting if this solution helped you. Good luck!**\n\n | 4 | You are given an integer array `values` where values\[i\] represents the value of the `ith` sightseeing spot. Two sightseeing spots `i` and `j` have a **distance** `j - i` between them.
The score of a pair (`i < j`) of sightseeing spots is `values[i] + values[j] + i - j`: the sum of the values of the sightseeing spots, minus the distance between them.
Return _the maximum score of a pair of sightseeing spots_.
**Example 1:**
**Input:** values = \[8,1,5,2,6\]
**Output:** 11
**Explanation:** i = 0, j = 2, values\[i\] + values\[j\] + i - j = 8 + 5 + 0 - 2 = 11
**Example 2:**
**Input:** values = \[1,2\]
**Output:** 2
**Constraints:**
* `2 <= values.length <= 5 * 104`
* `1 <= values[i] <= 1000` | null |
✅Python 3 | O(n) | Very clearly explained! | best-sightseeing-pair | 0 | 1 | ***Solution 1: Brute Force [Time Limit Exceeded]***\nThe most naive solution is that we can have a brute force solution by simply getting all possible combinations of index `i` and `j`, and then finding the maximum of `values[i] + values[j] + i - j` when `i < j` for all combinations and that will be our answer. We can do this by using a 2 nested for loops keeping track of index `i` and index `j`, while skipping to the next iteration if `i >= j`. We initialize a variable `maximum` and each iteration we check to see if the current value is higher than maximum, if so, we set maximum to this new value. Returning `maximum` in the end will give us our answer.\n```\nclass Solution:\n def maxScoreSightseeingPair(self, values: List[int]) -> int:\n n = len(values)\n maximum = 0\n for i in range(n):\n for j in range(i+1, n):\n if i >= j: continue\n maximum = max(maximum, values[i] + values[j] + i - j)\n return maximum\n```\nTime Complexity: O(n^2)\nSpace Complexity: O(1)\n\n***Solution 2: One Pass***\n\n**Intuition**\nNotice how in the brute force solution, we skip to the next iteration if index `i >= j`. Although we skip to the next iteration using `continue`, we still iterated through many unnecessary iterations giving us a slow O(n^2) time complexity which exceeded the time limit. Thus, we can simply skip all the unnecessary iterations where `i >= j` altogether using this one pass algorithm.\n\n**Algorithm**\nWe can initialize `i` to be 0, and then this time we only need to start 1 for loop tracking the index `j`, same thing as the brute force solution, we keep track of a variable `maximum` and in the iteration if `values[i] + values[j] + i - j` is bigger than the current `maximum` we replace it with that. However, one thing we are doing differently is that instead of starting a nested for loop we write an if statement saying that `values[j] + j - i > values[i]` then we set `i=j`. Basically, you can treat `if values[j] + j - i > values[i]: i = j` the same thing as `if i >= j: continue` in the brute force solution, except we are not iterating unnecessarily.\n\n**Implementation**\n```\nclass Solution:\n def maxScoreSightseeingPair(self, values: List[int]) -> int:\n n = len(values)\n i = maximum = 0\n for j in range(i+1, n):\n maximum = max(maximum, values[i] + values[j] + i - j)\n if values[j] + j - i > values[i]: i = j\n return maximum\n```\nTime Complexity: O(n)\nSpace Complexity: O(1)\n\n**Please consider upvoting if this solution helped you. Good luck!**\n\n | 4 | Given an integer array `nums`, return _the number of non-empty **subarrays** with the leftmost element of the subarray not larger than other elements in the subarray_.
A **subarray** is a **contiguous** part of an array.
**Example 1:**
**Input:** nums = \[1,4,2,5,3\]
**Output:** 11
**Explanation:** There are 11 valid subarrays: \[1\],\[4\],\[2\],\[5\],\[3\],\[1,4\],\[2,5\],\[1,4,2\],\[2,5,3\],\[1,4,2,5\],\[1,4,2,5,3\].
**Example 2:**
**Input:** nums = \[3,2,1\]
**Output:** 3
**Explanation:** The 3 valid subarrays are: \[3\],\[2\],\[1\].
**Example 3:**
**Input:** nums = \[2,2,2\]
**Output:** 6
**Explanation:** There are 6 valid subarrays: \[2\],\[2\],\[2\],\[2,2\],\[2,2\],\[2,2,2\].
**Constraints:**
* `1 <= nums.length <= 5 * 104`
* `0 <= nums[i] <= 105` | Can you tell the best sightseeing spot in one pass (ie. as you iterate over the input?) What should we store or keep track of as we iterate to do this? |
Solution | smallest-integer-divisible-by-k | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n int smallestRepunitDivByK(int k) {\n for (int r=0,N=1;N<=k;N++) {\n if ((r=(r*10+1)%k)==0) {\n return N;\n }\n }\n return -1;\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def smallestRepunitDivByK(self, k: int) -> int:\n if k%2==0 or k%5==0:\n return -1\n else:\n cur = 1\n res = 1\n while cur%k!=0:\n cur = (10*cur+1)%k\n res += 1\n return res\n```\n\n```Java []\nclass Solution {\n public int smallestRepunitDivByK(int k) {\n if (k % 2 == 0 || k % 5 == 0) return -1;\n \n int phi = get_euler(k * 9);\n int res = phi;\n for (int i = 1; i <= phi / i; i++) {\n if (phi % i == 0) {\n if (qmi(10, i, 9 * k) == 1) return i;\n if (qmi(10, phi / i, 9 * k) == 1) res = Math.min(res, phi / i);\n }\n }\n return res;\n }\n private int get_euler(int x) {\n int res = x;\n for (int i = 2; i <= x / i; i++) {\n if (x % i == 0) {\n res = res / i * (i - 1);\n while (x % i == 0) x /= i;\n }\n }\n if (x > 1) res = res / x * (x - 1);\n return res;\n }\n private long qmi(long a, long k, long p) {\n long res = 1;\n while (k > 0) {\n if ((k & 1) == 1) res = res * a % p;\n a = a * a % p;\n k >>= 1;\n }\n return res;\n }\n}\n``` | 1 | Given a positive integer `k`, you need to find the **length** of the **smallest** positive integer `n` such that `n` is divisible by `k`, and `n` only contains the digit `1`.
Return _the **length** of_ `n`. If there is no such `n`, return -1.
**Note:** `n` may not fit in a 64-bit signed integer.
**Example 1:**
**Input:** k = 1
**Output:** 1
**Explanation:** The smallest answer is n = 1, which has length 1.
**Example 2:**
**Input:** k = 2
**Output:** -1
**Explanation:** There is no such positive integer n divisible by 2.
**Example 3:**
**Input:** k = 3
**Output:** 3
**Explanation:** The smallest answer is n = 111, which has length 3.
**Constraints:**
* `1 <= k <= 105` | null |
Solution | smallest-integer-divisible-by-k | 1 | 1 | ```C++ []\nclass Solution {\npublic:\n int smallestRepunitDivByK(int k) {\n for (int r=0,N=1;N<=k;N++) {\n if ((r=(r*10+1)%k)==0) {\n return N;\n }\n }\n return -1;\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def smallestRepunitDivByK(self, k: int) -> int:\n if k%2==0 or k%5==0:\n return -1\n else:\n cur = 1\n res = 1\n while cur%k!=0:\n cur = (10*cur+1)%k\n res += 1\n return res\n```\n\n```Java []\nclass Solution {\n public int smallestRepunitDivByK(int k) {\n if (k % 2 == 0 || k % 5 == 0) return -1;\n \n int phi = get_euler(k * 9);\n int res = phi;\n for (int i = 1; i <= phi / i; i++) {\n if (phi % i == 0) {\n if (qmi(10, i, 9 * k) == 1) return i;\n if (qmi(10, phi / i, 9 * k) == 1) res = Math.min(res, phi / i);\n }\n }\n return res;\n }\n private int get_euler(int x) {\n int res = x;\n for (int i = 2; i <= x / i; i++) {\n if (x % i == 0) {\n res = res / i * (i - 1);\n while (x % i == 0) x /= i;\n }\n }\n if (x > 1) res = res / x * (x - 1);\n return res;\n }\n private long qmi(long a, long k, long p) {\n long res = 1;\n while (k > 0) {\n if ((k & 1) == 1) res = res * a % p;\n a = a * a % p;\n k >>= 1;\n }\n return res;\n }\n}\n``` | 1 | Given an array of distinct integers `arr`, where `arr` is sorted in **ascending order**, return the smallest index `i` that satisfies `arr[i] == i`. If there is no such index, return `-1`.
**Example 1:**
**Input:** arr = \[-10,-5,0,3,7\]
**Output:** 3
**Explanation:** For the given array, `arr[0] = -10, arr[1] = -5, arr[2] = 0, arr[3] = 3`, thus the output is 3.
**Example 2:**
**Input:** arr = \[0,2,5,8,17\]
**Output:** 0
**Explanation:** `arr[0] = 0`, thus the output is 0.
**Example 3:**
**Input:** arr = \[-10,-5,3,4,7,9\]
**Output:** -1
**Explanation:** There is no such `i` that `arr[i] == i`, thus the output is -1.
**Constraints:**
* `1 <= arr.length < 104`
* `-109 <= arr[i] <= 109`
**Follow up:** The `O(n)` solution is very straightforward. Can we do better? | 11111 = 1111 * 10 + 1
We only need to store remainders modulo K. If we never get a remainder of 0, why would that happen, and how would we know that? |
[Python3] ✔️ Less Math, More Intuition ✔️ | 2 Accepted Solutions | Intuitive | smallest-integer-divisible-by-k | 0 | 1 | **Important**: A number containing only `1`s cannot be divisible by `2` or `5`. You can easily convince yourself that this is true since:\n* A number divisble by 2 can only end with `0`, `2`, `4`, `6`, or `8`.\n* A number divisble by 5 can only end with `0`, or `5`.\n\n\u2714\uFE0F **[Accepted] First Approach:** *Making use of Python Big Integer*\nHere, we solve the problem as if overflow does not exist. In fact, Python supports arbitrarily large numbers. However, this comes at the cost of speed/memory, but anyways this is the naive way of doing it:\n```\nclass Solution:\n def smallestRepunitDivByK(self, k: int) -> int:\n if not k % 2 or not k % 5: return -1\n n = length = 1\n while True:\n if not n % k: return length\n length += 1\n n = 10*n + 1\n```\n\n\u2714\uFE0F **[Accepted] Second Approach:** *Using some math*\nTo improve that, the trick to avoid overflow is that we only need to store the remainder. A small proof for that can be found below:\n\n1. At every iteration, `n = kq + r` for some quotient `q` and remainder `r`.\n2. Therefore, `10*n + 1` = `10(kq + r) + 1` = `10kq + 10r + 1`.\n3. `10kq` is divisible by `k`, so for `10*n + 1` to be divisible by `k`, it all depends on if `10r + 1` is divisible by `k`.\n4. Therefore, we only have to keep track of `r`!\n\n```\nclass Solution:\n def smallestRepunitDivByK(self, k: int) -> int:\n if not k % 2 or not k % 5: return -1\n r = length = 1\n while True:\n r = r % k\n if not r: return length\n length += 1\n r = 10*r + 1\n``` | 27 | Given a positive integer `k`, you need to find the **length** of the **smallest** positive integer `n` such that `n` is divisible by `k`, and `n` only contains the digit `1`.
Return _the **length** of_ `n`. If there is no such `n`, return -1.
**Note:** `n` may not fit in a 64-bit signed integer.
**Example 1:**
**Input:** k = 1
**Output:** 1
**Explanation:** The smallest answer is n = 1, which has length 1.
**Example 2:**
**Input:** k = 2
**Output:** -1
**Explanation:** There is no such positive integer n divisible by 2.
**Example 3:**
**Input:** k = 3
**Output:** 3
**Explanation:** The smallest answer is n = 111, which has length 3.
**Constraints:**
* `1 <= k <= 105` | null |
[Python3] ✔️ Less Math, More Intuition ✔️ | 2 Accepted Solutions | Intuitive | smallest-integer-divisible-by-k | 0 | 1 | **Important**: A number containing only `1`s cannot be divisible by `2` or `5`. You can easily convince yourself that this is true since:\n* A number divisble by 2 can only end with `0`, `2`, `4`, `6`, or `8`.\n* A number divisble by 5 can only end with `0`, or `5`.\n\n\u2714\uFE0F **[Accepted] First Approach:** *Making use of Python Big Integer*\nHere, we solve the problem as if overflow does not exist. In fact, Python supports arbitrarily large numbers. However, this comes at the cost of speed/memory, but anyways this is the naive way of doing it:\n```\nclass Solution:\n def smallestRepunitDivByK(self, k: int) -> int:\n if not k % 2 or not k % 5: return -1\n n = length = 1\n while True:\n if not n % k: return length\n length += 1\n n = 10*n + 1\n```\n\n\u2714\uFE0F **[Accepted] Second Approach:** *Using some math*\nTo improve that, the trick to avoid overflow is that we only need to store the remainder. A small proof for that can be found below:\n\n1. At every iteration, `n = kq + r` for some quotient `q` and remainder `r`.\n2. Therefore, `10*n + 1` = `10(kq + r) + 1` = `10kq + 10r + 1`.\n3. `10kq` is divisible by `k`, so for `10*n + 1` to be divisible by `k`, it all depends on if `10r + 1` is divisible by `k`.\n4. Therefore, we only have to keep track of `r`!\n\n```\nclass Solution:\n def smallestRepunitDivByK(self, k: int) -> int:\n if not k % 2 or not k % 5: return -1\n r = length = 1\n while True:\n r = r % k\n if not r: return length\n length += 1\n r = 10*r + 1\n``` | 27 | Given an array of distinct integers `arr`, where `arr` is sorted in **ascending order**, return the smallest index `i` that satisfies `arr[i] == i`. If there is no such index, return `-1`.
**Example 1:**
**Input:** arr = \[-10,-5,0,3,7\]
**Output:** 3
**Explanation:** For the given array, `arr[0] = -10, arr[1] = -5, arr[2] = 0, arr[3] = 3`, thus the output is 3.
**Example 2:**
**Input:** arr = \[0,2,5,8,17\]
**Output:** 0
**Explanation:** `arr[0] = 0`, thus the output is 0.
**Example 3:**
**Input:** arr = \[-10,-5,3,4,7,9\]
**Output:** -1
**Explanation:** There is no such `i` that `arr[i] == i`, thus the output is -1.
**Constraints:**
* `1 <= arr.length < 104`
* `-109 <= arr[i] <= 109`
**Follow up:** The `O(n)` solution is very straightforward. Can we do better? | 11111 = 1111 * 10 + 1
We only need to store remainders modulo K. If we never get a remainder of 0, why would that happen, and how would we know that? |
Smallest Integer Divisible by K | smallest-integer-divisible-by-k | 0 | 1 | Given a positive integer `K`, we must find the *length* of the smallest positive integer `N` such that `N` is divisible by `K`, and `N` only contains the digit `1`. If there is no such `N`, return `-1`. Therefore the following constraints must be met.\n\n* `N % K == 0`\n* length of `N` is minimized\n* `N` only contains the digit `1`\n\nThe first important item to note is that `N` may not fit into a 64-bit signed integer. This means that we can not simply iterate though the possible values of `N` and mod `K`. \n\nTo find a way to reduce the problem, lets take the example of `K=3`. The value `3` has the following possible remainders when dividing.\n\n| 0 | 1 | 2 |\n|:-:|:-:|:-:|\n\nNow, lets find the value of `N` by brute-force. In the table below, we can see that the condition `N % K == 0` is first met with the value of `111`. More interestingly, we can see that the pattern `1 -> 2 -> 0` repeats. \n\n| N | 1 | 11 | 111 | 1111 | 11111 | 111111\n|:---:|:-:|:--:|:---:|:----:|:-----:|:-----:|\n| N%K | 1 | 2 | 0 | 1 | 2 | 0 |\n\nNow, lets take a look at the example `K=6`. We can see that there are a finite number of possible remainders for any value mod `K` and that we only need to iterate thought the sequence of remainders *once* to find that `N` is not divisible by `K`.\n\n| 0 | 1 | 2 | 3 | 4 | 5 |\n|:-:|:-:|:-:|:-:|:-:|:-:|\n\n| N | 1 | 11 | 111 | 1111 | 11111 | 111111 | 1111111 |\n|:---:|:-:|:--:|:---:|:----:|:-----:|:------:|:-------:|\n| N%K | 1 | 5 | 3 | 1 | 5 | 3 | 1 |\n\nThe final piece that we must solve is how to calculate the `N%K` sequence: how to find the next remainder. We can\'t go though the values of `N` because it might not fit in a 64-bit integer. Let take a look at how we would calculate the next value of `N` and go from there. \n\n\n`N next = (N * 10) + 1` : the next value of `N` `1 -> 11 -> 111 ...`\n`remainder = N%K` : the remainder\n\n`(N next)%K = ((N * 10) + 1)%K` : we can mod both sides of our next remainder equation \n`(N next)%K = ((N * 10)%K + 1%K)%K` : the equation can then be rearranged. "It is useful to know that modulos can be taken anywhere in the calculation if it involves only addition and multiplication." *[- duke-cps102](http://db.cs.duke.edu/courses/cps102/spring10/Lectures/L-04.pdf)*\n`(N next)%K = ((N * 10)%K + 1)%K` : `1` mod anything is `1`\n`(N next)%K = ((N * 10)%K + 1)%K` : `1` mod anything is `1`\n**`remainder next = (remainder*10 + 1) %K`** : knowing that `N%K` is our remainder, we have now have a general equation that can find our next remainder value without knowing the value of `N`.\n\n```\ndef smallestRepunitDivByK(self, K: int) -> int:\n r = 0\n for c in range(1, K+1):\n r = (r*10 + 1)%K\n if r == 0: return c\n return -1\n```\n\nThe runtime of this solution is **`O(K)`**. \nThe space complexity is **`O(1)`**. | 20 | Given a positive integer `k`, you need to find the **length** of the **smallest** positive integer `n` such that `n` is divisible by `k`, and `n` only contains the digit `1`.
Return _the **length** of_ `n`. If there is no such `n`, return -1.
**Note:** `n` may not fit in a 64-bit signed integer.
**Example 1:**
**Input:** k = 1
**Output:** 1
**Explanation:** The smallest answer is n = 1, which has length 1.
**Example 2:**
**Input:** k = 2
**Output:** -1
**Explanation:** There is no such positive integer n divisible by 2.
**Example 3:**
**Input:** k = 3
**Output:** 3
**Explanation:** The smallest answer is n = 111, which has length 3.
**Constraints:**
* `1 <= k <= 105` | null |
Smallest Integer Divisible by K | smallest-integer-divisible-by-k | 0 | 1 | Given a positive integer `K`, we must find the *length* of the smallest positive integer `N` such that `N` is divisible by `K`, and `N` only contains the digit `1`. If there is no such `N`, return `-1`. Therefore the following constraints must be met.\n\n* `N % K == 0`\n* length of `N` is minimized\n* `N` only contains the digit `1`\n\nThe first important item to note is that `N` may not fit into a 64-bit signed integer. This means that we can not simply iterate though the possible values of `N` and mod `K`. \n\nTo find a way to reduce the problem, lets take the example of `K=3`. The value `3` has the following possible remainders when dividing.\n\n| 0 | 1 | 2 |\n|:-:|:-:|:-:|\n\nNow, lets find the value of `N` by brute-force. In the table below, we can see that the condition `N % K == 0` is first met with the value of `111`. More interestingly, we can see that the pattern `1 -> 2 -> 0` repeats. \n\n| N | 1 | 11 | 111 | 1111 | 11111 | 111111\n|:---:|:-:|:--:|:---:|:----:|:-----:|:-----:|\n| N%K | 1 | 2 | 0 | 1 | 2 | 0 |\n\nNow, lets take a look at the example `K=6`. We can see that there are a finite number of possible remainders for any value mod `K` and that we only need to iterate thought the sequence of remainders *once* to find that `N` is not divisible by `K`.\n\n| 0 | 1 | 2 | 3 | 4 | 5 |\n|:-:|:-:|:-:|:-:|:-:|:-:|\n\n| N | 1 | 11 | 111 | 1111 | 11111 | 111111 | 1111111 |\n|:---:|:-:|:--:|:---:|:----:|:-----:|:------:|:-------:|\n| N%K | 1 | 5 | 3 | 1 | 5 | 3 | 1 |\n\nThe final piece that we must solve is how to calculate the `N%K` sequence: how to find the next remainder. We can\'t go though the values of `N` because it might not fit in a 64-bit integer. Let take a look at how we would calculate the next value of `N` and go from there. \n\n\n`N next = (N * 10) + 1` : the next value of `N` `1 -> 11 -> 111 ...`\n`remainder = N%K` : the remainder\n\n`(N next)%K = ((N * 10) + 1)%K` : we can mod both sides of our next remainder equation \n`(N next)%K = ((N * 10)%K + 1%K)%K` : the equation can then be rearranged. "It is useful to know that modulos can be taken anywhere in the calculation if it involves only addition and multiplication." *[- duke-cps102](http://db.cs.duke.edu/courses/cps102/spring10/Lectures/L-04.pdf)*\n`(N next)%K = ((N * 10)%K + 1)%K` : `1` mod anything is `1`\n`(N next)%K = ((N * 10)%K + 1)%K` : `1` mod anything is `1`\n**`remainder next = (remainder*10 + 1) %K`** : knowing that `N%K` is our remainder, we have now have a general equation that can find our next remainder value without knowing the value of `N`.\n\n```\ndef smallestRepunitDivByK(self, K: int) -> int:\n r = 0\n for c in range(1, K+1):\n r = (r*10 + 1)%K\n if r == 0: return c\n return -1\n```\n\nThe runtime of this solution is **`O(K)`**. \nThe space complexity is **`O(1)`**. | 20 | Given an array of distinct integers `arr`, where `arr` is sorted in **ascending order**, return the smallest index `i` that satisfies `arr[i] == i`. If there is no such index, return `-1`.
**Example 1:**
**Input:** arr = \[-10,-5,0,3,7\]
**Output:** 3
**Explanation:** For the given array, `arr[0] = -10, arr[1] = -5, arr[2] = 0, arr[3] = 3`, thus the output is 3.
**Example 2:**
**Input:** arr = \[0,2,5,8,17\]
**Output:** 0
**Explanation:** `arr[0] = 0`, thus the output is 0.
**Example 3:**
**Input:** arr = \[-10,-5,3,4,7,9\]
**Output:** -1
**Explanation:** There is no such `i` that `arr[i] == i`, thus the output is -1.
**Constraints:**
* `1 <= arr.length < 104`
* `-109 <= arr[i] <= 109`
**Follow up:** The `O(n)` solution is very straightforward. Can we do better? | 11111 = 1111 * 10 + 1
We only need to store remainders modulo K. If we never get a remainder of 0, why would that happen, and how would we know that? |
Farhad Zada -> Beats 100% Python3 | smallest-integer-divisible-by-k | 0 | 1 | # Intuition\nThe point is that you need to find the remnants of something. Let me explain what is it. \nExample when you `100 % 7` you get `2`, let\'s see why. \n`1 % 7 = 1` this is obvious. \n`10 % 7 = 3` \n`30 % 7 = 2` because if you get 3 when you divide 10 by 7, 100 is just 10 times 10, you will get 10 3s as remnants. And when you sum these all up it makes 30 and now you do not need to divide big numbers into 7, but only this ones. And any next power of then is just ten times of the remnant. Get it? \n\n# Approach\nIf it is even number it is imposible to have a number that is dvisible and no remnants, because the n itself is an odd number and any odd number divided by even number will have a remnant. \n\n# Complexity\n- Time complexity:\n$$O(idk)$$\n\n- Space complexity:\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def smallestRepunitDivByK(self, k: int) -> int:\n if not k % 2 or not k % 5: return -1\n remnant, remnants, length = (1, 1, 1)\n while remnants % k:\n remnant *= 10\n remnant %= k\n remnants += remnant\n length += 1\n return length\n```\n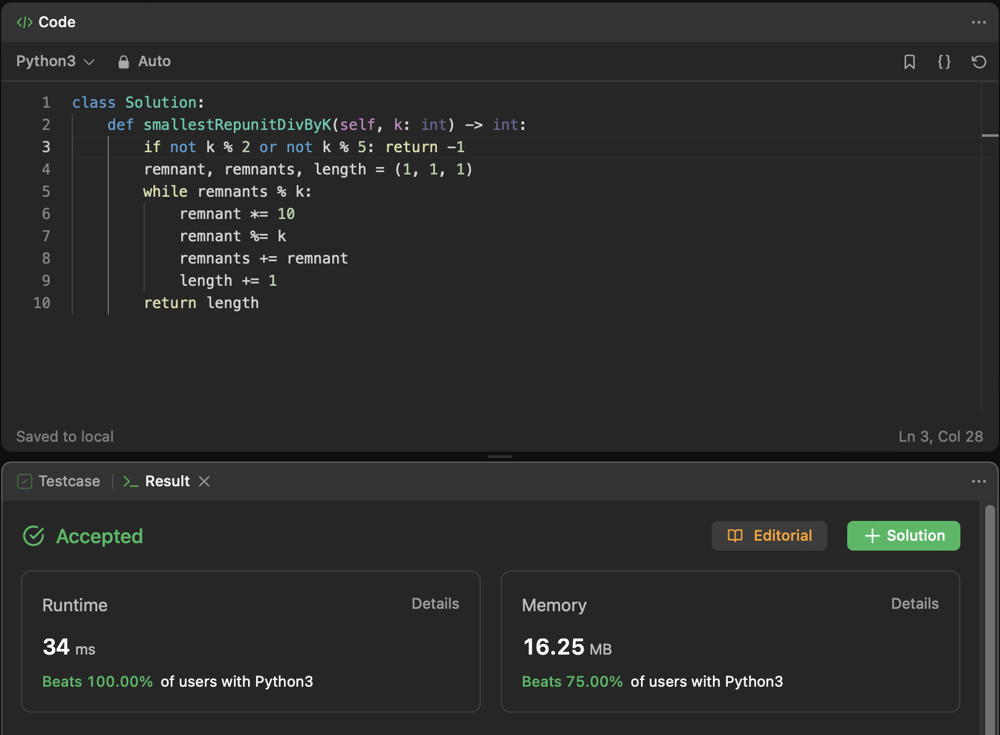\n\n\n# If liked pleae upvote | 0 | Given a positive integer `k`, you need to find the **length** of the **smallest** positive integer `n` such that `n` is divisible by `k`, and `n` only contains the digit `1`.
Return _the **length** of_ `n`. If there is no such `n`, return -1.
**Note:** `n` may not fit in a 64-bit signed integer.
**Example 1:**
**Input:** k = 1
**Output:** 1
**Explanation:** The smallest answer is n = 1, which has length 1.
**Example 2:**
**Input:** k = 2
**Output:** -1
**Explanation:** There is no such positive integer n divisible by 2.
**Example 3:**
**Input:** k = 3
**Output:** 3
**Explanation:** The smallest answer is n = 111, which has length 3.
**Constraints:**
* `1 <= k <= 105` | null |
Farhad Zada -> Beats 100% Python3 | smallest-integer-divisible-by-k | 0 | 1 | # Intuition\nThe point is that you need to find the remnants of something. Let me explain what is it. \nExample when you `100 % 7` you get `2`, let\'s see why. \n`1 % 7 = 1` this is obvious. \n`10 % 7 = 3` \n`30 % 7 = 2` because if you get 3 when you divide 10 by 7, 100 is just 10 times 10, you will get 10 3s as remnants. And when you sum these all up it makes 30 and now you do not need to divide big numbers into 7, but only this ones. And any next power of then is just ten times of the remnant. Get it? \n\n# Approach\nIf it is even number it is imposible to have a number that is dvisible and no remnants, because the n itself is an odd number and any odd number divided by even number will have a remnant. \n\n# Complexity\n- Time complexity:\n$$O(idk)$$\n\n- Space complexity:\n$$O(1)$$\n\n# Code\n```\nclass Solution:\n def smallestRepunitDivByK(self, k: int) -> int:\n if not k % 2 or not k % 5: return -1\n remnant, remnants, length = (1, 1, 1)\n while remnants % k:\n remnant *= 10\n remnant %= k\n remnants += remnant\n length += 1\n return length\n```\n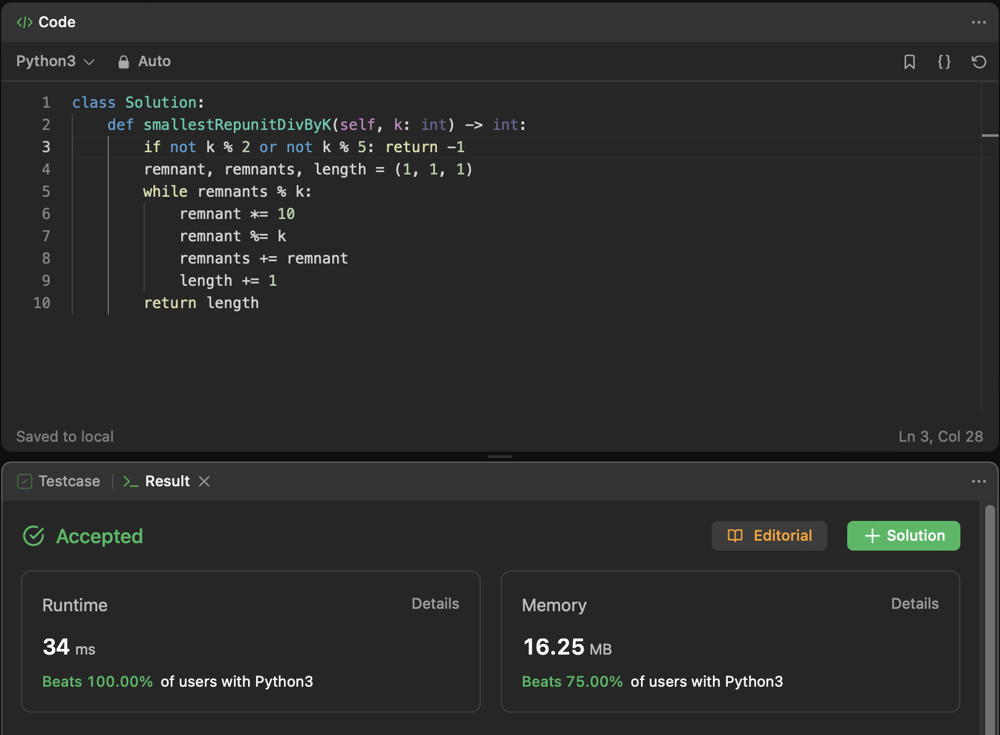\n\n\n# If liked pleae upvote | 0 | Given an array of distinct integers `arr`, where `arr` is sorted in **ascending order**, return the smallest index `i` that satisfies `arr[i] == i`. If there is no such index, return `-1`.
**Example 1:**
**Input:** arr = \[-10,-5,0,3,7\]
**Output:** 3
**Explanation:** For the given array, `arr[0] = -10, arr[1] = -5, arr[2] = 0, arr[3] = 3`, thus the output is 3.
**Example 2:**
**Input:** arr = \[0,2,5,8,17\]
**Output:** 0
**Explanation:** `arr[0] = 0`, thus the output is 0.
**Example 3:**
**Input:** arr = \[-10,-5,3,4,7,9\]
**Output:** -1
**Explanation:** There is no such `i` that `arr[i] == i`, thus the output is -1.
**Constraints:**
* `1 <= arr.length < 104`
* `-109 <= arr[i] <= 109`
**Follow up:** The `O(n)` solution is very straightforward. Can we do better? | 11111 = 1111 * 10 + 1
We only need to store remainders modulo K. If we never get a remainder of 0, why would that happen, and how would we know that? |
python3 solution | smallest-integer-divisible-by-k | 0 | 1 | \n# Approach\ninitialize the remainder with zero and make a set to store all the remainders.\nBy looping k times we have to update the remainder by (remainder*10+1)%k.\nif the remainder will be zero return i+1 as i is zero base and for returning the length will be 1 base so i+1 instead of i.\nif remainder is in the set, return -1;\nthen add the remainder to the set.\nwe will get the length of n.\n\n\n# Code\n```\nclass Solution:\n def smallestRepunitDivByK(self, k: int) -> int:\n remainder=0\n allremain=set()\n for i in range(k):\n remainder=(remainder*10+1)%k\n\n if remainder==0:\n return i+1\n if remainder in allremain:\n return -1\n allremain.add(remainder)\n``` | 0 | Given a positive integer `k`, you need to find the **length** of the **smallest** positive integer `n` such that `n` is divisible by `k`, and `n` only contains the digit `1`.
Return _the **length** of_ `n`. If there is no such `n`, return -1.
**Note:** `n` may not fit in a 64-bit signed integer.
**Example 1:**
**Input:** k = 1
**Output:** 1
**Explanation:** The smallest answer is n = 1, which has length 1.
**Example 2:**
**Input:** k = 2
**Output:** -1
**Explanation:** There is no such positive integer n divisible by 2.
**Example 3:**
**Input:** k = 3
**Output:** 3
**Explanation:** The smallest answer is n = 111, which has length 3.
**Constraints:**
* `1 <= k <= 105` | null |
python3 solution | smallest-integer-divisible-by-k | 0 | 1 | \n# Approach\ninitialize the remainder with zero and make a set to store all the remainders.\nBy looping k times we have to update the remainder by (remainder*10+1)%k.\nif the remainder will be zero return i+1 as i is zero base and for returning the length will be 1 base so i+1 instead of i.\nif remainder is in the set, return -1;\nthen add the remainder to the set.\nwe will get the length of n.\n\n\n# Code\n```\nclass Solution:\n def smallestRepunitDivByK(self, k: int) -> int:\n remainder=0\n allremain=set()\n for i in range(k):\n remainder=(remainder*10+1)%k\n\n if remainder==0:\n return i+1\n if remainder in allremain:\n return -1\n allremain.add(remainder)\n``` | 0 | Given an array of distinct integers `arr`, where `arr` is sorted in **ascending order**, return the smallest index `i` that satisfies `arr[i] == i`. If there is no such index, return `-1`.
**Example 1:**
**Input:** arr = \[-10,-5,0,3,7\]
**Output:** 3
**Explanation:** For the given array, `arr[0] = -10, arr[1] = -5, arr[2] = 0, arr[3] = 3`, thus the output is 3.
**Example 2:**
**Input:** arr = \[0,2,5,8,17\]
**Output:** 0
**Explanation:** `arr[0] = 0`, thus the output is 0.
**Example 3:**
**Input:** arr = \[-10,-5,3,4,7,9\]
**Output:** -1
**Explanation:** There is no such `i` that `arr[i] == i`, thus the output is -1.
**Constraints:**
* `1 <= arr.length < 104`
* `-109 <= arr[i] <= 109`
**Follow up:** The `O(n)` solution is very straightforward. Can we do better? | 11111 = 1111 * 10 + 1
We only need to store remainders modulo K. If we never get a remainder of 0, why would that happen, and how would we know that? |
Solution | binary-string-with-substrings-representing-1-to-n | 1 | 1 | ```C++ []\nclass Solution {\n public:\n bool queryString(string S, int N) {\n if (N > 1511)\n return false;\n\n for (int i = N; i > N / 2; --i) {\n string binary = bitset<32>(i).to_string();\n binary = binary.substr(binary.find("1"));\n if (S.find(binary) == string::npos)\n return false;\n }\n return true;\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n for i in range(1, n+1):\n if bin(i)[2:] not in s:\n return False\n return True\n```\n\n```Java []\nclass Solution {\n public boolean queryString(String s, int n) {\n for(int i=1;i<=n;i++){\n if(!s.contains(Integer.toBinaryString(i))){\n return false;\n }\n }\n return true;\n }\n}\n``` | 1 | Given a binary string `s` and a positive integer `n`, return `true` _if the binary representation of all the integers in the range_ `[1, n]` _are **substrings** of_ `s`_, or_ `false` _otherwise_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "0110", n = 3
**Output:** true
**Example 2:**
**Input:** s = "0110", n = 4
**Output:** false
**Constraints:**
* `1 <= s.length <= 1000`
* `s[i]` is either `'0'` or `'1'`.
* `1 <= n <= 109` | null |
Solution | binary-string-with-substrings-representing-1-to-n | 1 | 1 | ```C++ []\nclass Solution {\n public:\n bool queryString(string S, int N) {\n if (N > 1511)\n return false;\n\n for (int i = N; i > N / 2; --i) {\n string binary = bitset<32>(i).to_string();\n binary = binary.substr(binary.find("1"));\n if (S.find(binary) == string::npos)\n return false;\n }\n return true;\n }\n};\n```\n\n```Python3 []\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n for i in range(1, n+1):\n if bin(i)[2:] not in s:\n return False\n return True\n```\n\n```Java []\nclass Solution {\n public boolean queryString(String s, int n) {\n for(int i=1;i<=n;i++){\n if(!s.contains(Integer.toBinaryString(i))){\n return false;\n }\n }\n return true;\n }\n}\n``` | 1 | Given a string `text` and an array of strings `words`, return _an array of all index pairs_ `[i, j]` _so that the substring_ `text[i...j]` _is in `words`_.
Return the pairs `[i, j]` in sorted order (i.e., sort them by their first coordinate, and in case of ties sort them by their second coordinate).
**Example 1:**
**Input:** text = "thestoryofleetcodeandme ", words = \[ "story ", "fleet ", "leetcode "\]
**Output:** \[\[3,7\],\[9,13\],\[10,17\]\]
**Example 2:**
**Input:** text = "ababa ", words = \[ "aba ", "ab "\]
**Output:** \[\[0,1\],\[0,2\],\[2,3\],\[2,4\]\]
**Explanation:** Notice that matches can overlap, see "aba " is found in \[0,2\] and \[2,4\].
**Constraints:**
* `1 <= text.length <= 100`
* `1 <= words.length <= 20`
* `1 <= words[i].length <= 50`
* `text` and `words[i]` consist of lowercase English letters.
* All the strings of `words` are **unique**. | We only need to check substrings of length at most 30, because 10^9 has 30 bits. |
2 LINES ||| PYTHON EASY SOLUTION|| USING STRING | binary-string-with-substrings-representing-1-to-n | 0 | 1 | ```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n for i in range(1,n+1):\n if bin(i)[2:] not in s:return 0\n return 1 | 1 | Given a binary string `s` and a positive integer `n`, return `true` _if the binary representation of all the integers in the range_ `[1, n]` _are **substrings** of_ `s`_, or_ `false` _otherwise_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "0110", n = 3
**Output:** true
**Example 2:**
**Input:** s = "0110", n = 4
**Output:** false
**Constraints:**
* `1 <= s.length <= 1000`
* `s[i]` is either `'0'` or `'1'`.
* `1 <= n <= 109` | null |
2 LINES ||| PYTHON EASY SOLUTION|| USING STRING | binary-string-with-substrings-representing-1-to-n | 0 | 1 | ```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n for i in range(1,n+1):\n if bin(i)[2:] not in s:return 0\n return 1 | 1 | Given a string `text` and an array of strings `words`, return _an array of all index pairs_ `[i, j]` _so that the substring_ `text[i...j]` _is in `words`_.
Return the pairs `[i, j]` in sorted order (i.e., sort them by their first coordinate, and in case of ties sort them by their second coordinate).
**Example 1:**
**Input:** text = "thestoryofleetcodeandme ", words = \[ "story ", "fleet ", "leetcode "\]
**Output:** \[\[3,7\],\[9,13\],\[10,17\]\]
**Example 2:**
**Input:** text = "ababa ", words = \[ "aba ", "ab "\]
**Output:** \[\[0,1\],\[0,2\],\[2,3\],\[2,4\]\]
**Explanation:** Notice that matches can overlap, see "aba " is found in \[0,2\] and \[2,4\].
**Constraints:**
* `1 <= text.length <= 100`
* `1 <= words.length <= 20`
* `1 <= words[i].length <= 50`
* `text` and `words[i]` consist of lowercase English letters.
* All the strings of `words` are **unique**. | We only need to check substrings of length at most 30, because 10^9 has 30 bits. |
Python solution beats 100% | binary-string-with-substrings-representing-1-to-n | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nMy thought is to loop through numbers from `n` to `n//2` and check if their binary representation is in the string `s`.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe can loop through numbers from `n` to `n//2` and check if their binary representation is in the string `s`. If the binary representation is not found in the string, we can immediately return `False`. If we loop through all numbers and each one is found in `s`, then we can return `True`.\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n for i in range(n, n//2, -1):\n if bin(i)[2:] not in s:\n return False\n return True\n``` | 1 | Given a binary string `s` and a positive integer `n`, return `true` _if the binary representation of all the integers in the range_ `[1, n]` _are **substrings** of_ `s`_, or_ `false` _otherwise_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "0110", n = 3
**Output:** true
**Example 2:**
**Input:** s = "0110", n = 4
**Output:** false
**Constraints:**
* `1 <= s.length <= 1000`
* `s[i]` is either `'0'` or `'1'`.
* `1 <= n <= 109` | null |
Python solution beats 100% | binary-string-with-substrings-representing-1-to-n | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\nMy thought is to loop through numbers from `n` to `n//2` and check if their binary representation is in the string `s`.\n# Approach\n<!-- Describe your approach to solving the problem. -->\nWe can loop through numbers from `n` to `n//2` and check if their binary representation is in the string `s`. If the binary representation is not found in the string, we can immediately return `False`. If we loop through all numbers and each one is found in `s`, then we can return `True`.\n# Complexity\n- Time complexity: $$O(n)$$\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity: $$O(1)$$\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n for i in range(n, n//2, -1):\n if bin(i)[2:] not in s:\n return False\n return True\n``` | 1 | Given a string `text` and an array of strings `words`, return _an array of all index pairs_ `[i, j]` _so that the substring_ `text[i...j]` _is in `words`_.
Return the pairs `[i, j]` in sorted order (i.e., sort them by their first coordinate, and in case of ties sort them by their second coordinate).
**Example 1:**
**Input:** text = "thestoryofleetcodeandme ", words = \[ "story ", "fleet ", "leetcode "\]
**Output:** \[\[3,7\],\[9,13\],\[10,17\]\]
**Example 2:**
**Input:** text = "ababa ", words = \[ "aba ", "ab "\]
**Output:** \[\[0,1\],\[0,2\],\[2,3\],\[2,4\]\]
**Explanation:** Notice that matches can overlap, see "aba " is found in \[0,2\] and \[2,4\].
**Constraints:**
* `1 <= text.length <= 100`
* `1 <= words.length <= 20`
* `1 <= words[i].length <= 50`
* `text` and `words[i]` consist of lowercase English letters.
* All the strings of `words` are **unique**. | We only need to check substrings of length at most 30, because 10^9 has 30 bits. |
Easy Python solution || 80%runtime | binary-string-with-substrings-representing-1-to-n | 0 | 1 | \n# Code\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n for i in range(1,n+1):\n if bin(i)[2:] not in s:return False\n return True\n``` | 2 | Given a binary string `s` and a positive integer `n`, return `true` _if the binary representation of all the integers in the range_ `[1, n]` _are **substrings** of_ `s`_, or_ `false` _otherwise_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "0110", n = 3
**Output:** true
**Example 2:**
**Input:** s = "0110", n = 4
**Output:** false
**Constraints:**
* `1 <= s.length <= 1000`
* `s[i]` is either `'0'` or `'1'`.
* `1 <= n <= 109` | null |
Easy Python solution || 80%runtime | binary-string-with-substrings-representing-1-to-n | 0 | 1 | \n# Code\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n for i in range(1,n+1):\n if bin(i)[2:] not in s:return False\n return True\n``` | 2 | Given a string `text` and an array of strings `words`, return _an array of all index pairs_ `[i, j]` _so that the substring_ `text[i...j]` _is in `words`_.
Return the pairs `[i, j]` in sorted order (i.e., sort them by their first coordinate, and in case of ties sort them by their second coordinate).
**Example 1:**
**Input:** text = "thestoryofleetcodeandme ", words = \[ "story ", "fleet ", "leetcode "\]
**Output:** \[\[3,7\],\[9,13\],\[10,17\]\]
**Example 2:**
**Input:** text = "ababa ", words = \[ "aba ", "ab "\]
**Output:** \[\[0,1\],\[0,2\],\[2,3\],\[2,4\]\]
**Explanation:** Notice that matches can overlap, see "aba " is found in \[0,2\] and \[2,4\].
**Constraints:**
* `1 <= text.length <= 100`
* `1 <= words.length <= 20`
* `1 <= words[i].length <= 50`
* `text` and `words[i]` consist of lowercase English letters.
* All the strings of `words` are **unique**. | We only need to check substrings of length at most 30, because 10^9 has 30 bits. |
✅ Explained - Simple and Clear Python3 Code✅ | binary-string-with-substrings-representing-1-to-n | 0 | 1 | # Intuition\nTo determine if the binary representation of all integers in the range [1, n] are substrings of a given binary string s, we can iterate through the range and check if each binary representation exists as a substring in s. If we find any missing substring, we return False; otherwise, we return True.\n\n# Approach:\nWe initialize a boolean variable test as True, which will be used to keep track of whether all substrings are present or not. We start iterating from 1 to n using a while loop. For each iteration, we convert the current integer i into its binary representation using the format(i, "b") function. We then use the find() method on the binary string s to check if the binary representation exists as a substring. If it does not, we update test to False and break out of the loop. Finally, we increment i by 1.\n\nAfter the loop completes, we return the value of test, which will be True if all substrings are present, or False if any substring is missing.\n\n# Time complexity:\nThe time complexity of this approach is O(n * m), where n is the given integer n and m is the average length of the binary representations of the integers from 1 to n. This is because we iterate through the range [1, n] and, for each iteration, perform a string search operation using the find() method, which takes O(m) time.\n\n# Space complexity:\nThe space complexity of this approach is O(1) because we only use a constant amount of extra space to store the boolean variable test, the integer variable i, and the temporary binary representation of each integer. The input string s is not counted in the space complexity as it is given and does not require any additional space.\n\n# Code\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n test=True\n i=1\n while i<=n:\n if s.find(format(i,"b"))==-1:\n test=False\n break\n i+=1\n return test\n``` | 5 | Given a binary string `s` and a positive integer `n`, return `true` _if the binary representation of all the integers in the range_ `[1, n]` _are **substrings** of_ `s`_, or_ `false` _otherwise_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "0110", n = 3
**Output:** true
**Example 2:**
**Input:** s = "0110", n = 4
**Output:** false
**Constraints:**
* `1 <= s.length <= 1000`
* `s[i]` is either `'0'` or `'1'`.
* `1 <= n <= 109` | null |
✅ Explained - Simple and Clear Python3 Code✅ | binary-string-with-substrings-representing-1-to-n | 0 | 1 | # Intuition\nTo determine if the binary representation of all integers in the range [1, n] are substrings of a given binary string s, we can iterate through the range and check if each binary representation exists as a substring in s. If we find any missing substring, we return False; otherwise, we return True.\n\n# Approach:\nWe initialize a boolean variable test as True, which will be used to keep track of whether all substrings are present or not. We start iterating from 1 to n using a while loop. For each iteration, we convert the current integer i into its binary representation using the format(i, "b") function. We then use the find() method on the binary string s to check if the binary representation exists as a substring. If it does not, we update test to False and break out of the loop. Finally, we increment i by 1.\n\nAfter the loop completes, we return the value of test, which will be True if all substrings are present, or False if any substring is missing.\n\n# Time complexity:\nThe time complexity of this approach is O(n * m), where n is the given integer n and m is the average length of the binary representations of the integers from 1 to n. This is because we iterate through the range [1, n] and, for each iteration, perform a string search operation using the find() method, which takes O(m) time.\n\n# Space complexity:\nThe space complexity of this approach is O(1) because we only use a constant amount of extra space to store the boolean variable test, the integer variable i, and the temporary binary representation of each integer. The input string s is not counted in the space complexity as it is given and does not require any additional space.\n\n# Code\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n test=True\n i=1\n while i<=n:\n if s.find(format(i,"b"))==-1:\n test=False\n break\n i+=1\n return test\n``` | 5 | Given a string `text` and an array of strings `words`, return _an array of all index pairs_ `[i, j]` _so that the substring_ `text[i...j]` _is in `words`_.
Return the pairs `[i, j]` in sorted order (i.e., sort them by their first coordinate, and in case of ties sort them by their second coordinate).
**Example 1:**
**Input:** text = "thestoryofleetcodeandme ", words = \[ "story ", "fleet ", "leetcode "\]
**Output:** \[\[3,7\],\[9,13\],\[10,17\]\]
**Example 2:**
**Input:** text = "ababa ", words = \[ "aba ", "ab "\]
**Output:** \[\[0,1\],\[0,2\],\[2,3\],\[2,4\]\]
**Explanation:** Notice that matches can overlap, see "aba " is found in \[0,2\] and \[2,4\].
**Constraints:**
* `1 <= text.length <= 100`
* `1 <= words.length <= 20`
* `1 <= words[i].length <= 50`
* `text` and `words[i]` consist of lowercase English letters.
* All the strings of `words` are **unique**. | We only need to check substrings of length at most 30, because 10^9 has 30 bits. |
Python + C# solutions: another bluffing question... | binary-string-with-substrings-representing-1-to-n | 0 | 1 | List of bluffing Leetcode questions:\n1. [1016. Binary String With Substrings Representing 1 To N](https://leetcode.com/problems/binary-string-with-substrings-representing-1-to-n/)\n2. [2311. Longest Binary Subsequence Less Than or Equal to K](https://leetcode.com/problems/longest-binary-subsequence-less-than-or-equal-to-k/)\n\n**Python**\n```\nclass Solution:\n # First impression of the question suggests the check priorities should be from high n to low.\n # 1 <= n <= 10^9\n # Think about it a little more, how long s need to be to cover [1 10^9]?\n # It would be enormous, on the order of 10^8\n # but in our case, 1 <= s.length <= 1000\n # Therefore if we can just keep scanning from n downwards and report false if cannot find\n # generating binary string of n is easy\n def queryString(self, s: str, n: int) -> bool:\n for i in range(n, 0,-1):\n if bin(i)[2:] not in s:\n return False\n return True\n```\n**C#**\n```\nusing System;\npublic class Solution \n{\n public bool QueryString(string s, int n) \n {\n for (int i = n; i > 0; i--)\n {\n if (!s.Contains(Convert.ToString(i, 2)))\n {\n return false;\n }\n }\n return true;\n }\n}\n``` | 2 | Given a binary string `s` and a positive integer `n`, return `true` _if the binary representation of all the integers in the range_ `[1, n]` _are **substrings** of_ `s`_, or_ `false` _otherwise_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "0110", n = 3
**Output:** true
**Example 2:**
**Input:** s = "0110", n = 4
**Output:** false
**Constraints:**
* `1 <= s.length <= 1000`
* `s[i]` is either `'0'` or `'1'`.
* `1 <= n <= 109` | null |
Python + C# solutions: another bluffing question... | binary-string-with-substrings-representing-1-to-n | 0 | 1 | List of bluffing Leetcode questions:\n1. [1016. Binary String With Substrings Representing 1 To N](https://leetcode.com/problems/binary-string-with-substrings-representing-1-to-n/)\n2. [2311. Longest Binary Subsequence Less Than or Equal to K](https://leetcode.com/problems/longest-binary-subsequence-less-than-or-equal-to-k/)\n\n**Python**\n```\nclass Solution:\n # First impression of the question suggests the check priorities should be from high n to low.\n # 1 <= n <= 10^9\n # Think about it a little more, how long s need to be to cover [1 10^9]?\n # It would be enormous, on the order of 10^8\n # but in our case, 1 <= s.length <= 1000\n # Therefore if we can just keep scanning from n downwards and report false if cannot find\n # generating binary string of n is easy\n def queryString(self, s: str, n: int) -> bool:\n for i in range(n, 0,-1):\n if bin(i)[2:] not in s:\n return False\n return True\n```\n**C#**\n```\nusing System;\npublic class Solution \n{\n public bool QueryString(string s, int n) \n {\n for (int i = n; i > 0; i--)\n {\n if (!s.Contains(Convert.ToString(i, 2)))\n {\n return false;\n }\n }\n return true;\n }\n}\n``` | 2 | Given a string `text` and an array of strings `words`, return _an array of all index pairs_ `[i, j]` _so that the substring_ `text[i...j]` _is in `words`_.
Return the pairs `[i, j]` in sorted order (i.e., sort them by their first coordinate, and in case of ties sort them by their second coordinate).
**Example 1:**
**Input:** text = "thestoryofleetcodeandme ", words = \[ "story ", "fleet ", "leetcode "\]
**Output:** \[\[3,7\],\[9,13\],\[10,17\]\]
**Example 2:**
**Input:** text = "ababa ", words = \[ "aba ", "ab "\]
**Output:** \[\[0,1\],\[0,2\],\[2,3\],\[2,4\]\]
**Explanation:** Notice that matches can overlap, see "aba " is found in \[0,2\] and \[2,4\].
**Constraints:**
* `1 <= text.length <= 100`
* `1 <= words.length <= 20`
* `1 <= words[i].length <= 50`
* `text` and `words[i]` consist of lowercase English letters.
* All the strings of `words` are **unique**. | We only need to check substrings of length at most 30, because 10^9 has 30 bits. |
python3 solution beats 100% in runtime(24ms) & 100% in memory(16.1MB) | binary-string-with-substrings-representing-1-to-n | 0 | 1 | \nI deserve Upvotes\n\n\n\n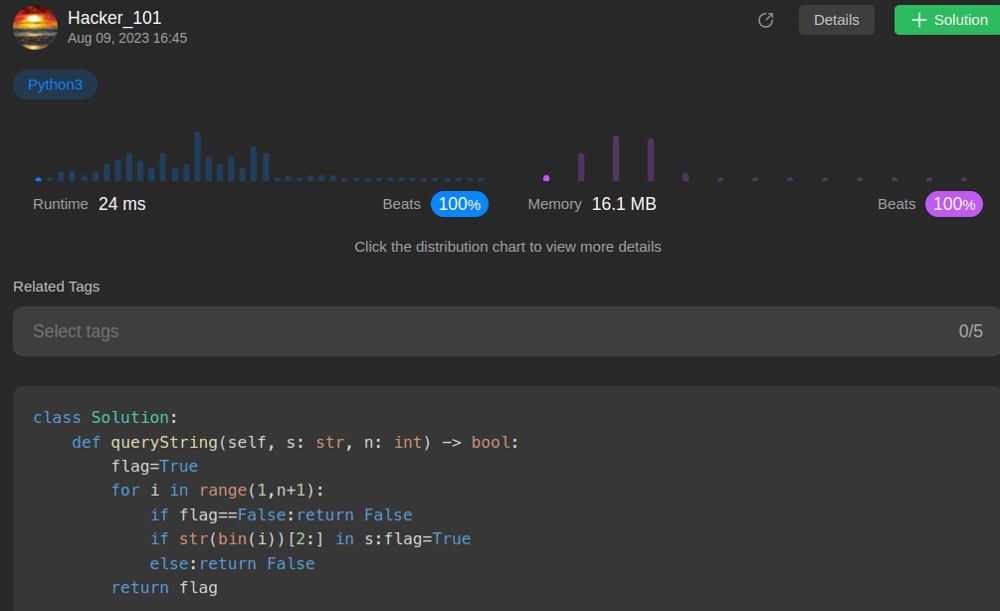\n\n# Code\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n flag=True\n for i in range(1,n+1):\n if flag==False:return False\n if str(bin(i))[2:] in s:flag=True\n else:return False\n return flag\n``` | 3 | Given a binary string `s` and a positive integer `n`, return `true` _if the binary representation of all the integers in the range_ `[1, n]` _are **substrings** of_ `s`_, or_ `false` _otherwise_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "0110", n = 3
**Output:** true
**Example 2:**
**Input:** s = "0110", n = 4
**Output:** false
**Constraints:**
* `1 <= s.length <= 1000`
* `s[i]` is either `'0'` or `'1'`.
* `1 <= n <= 109` | null |
python3 solution beats 100% in runtime(24ms) & 100% in memory(16.1MB) | binary-string-with-substrings-representing-1-to-n | 0 | 1 | \nI deserve Upvotes\n\n\n\n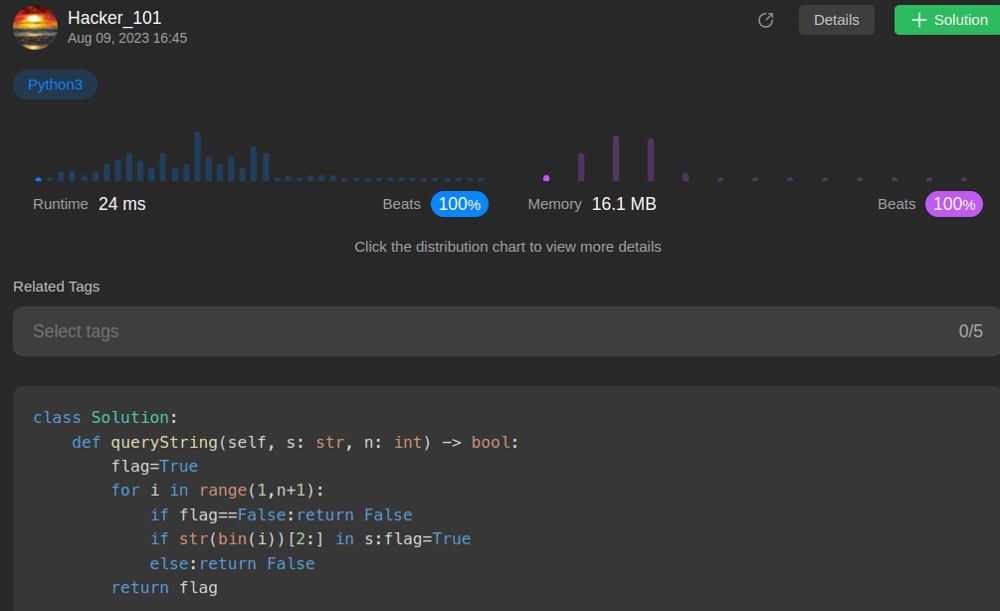\n\n# Code\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n flag=True\n for i in range(1,n+1):\n if flag==False:return False\n if str(bin(i))[2:] in s:flag=True\n else:return False\n return flag\n``` | 3 | Given a string `text` and an array of strings `words`, return _an array of all index pairs_ `[i, j]` _so that the substring_ `text[i...j]` _is in `words`_.
Return the pairs `[i, j]` in sorted order (i.e., sort them by their first coordinate, and in case of ties sort them by their second coordinate).
**Example 1:**
**Input:** text = "thestoryofleetcodeandme ", words = \[ "story ", "fleet ", "leetcode "\]
**Output:** \[\[3,7\],\[9,13\],\[10,17\]\]
**Example 2:**
**Input:** text = "ababa ", words = \[ "aba ", "ab "\]
**Output:** \[\[0,1\],\[0,2\],\[2,3\],\[2,4\]\]
**Explanation:** Notice that matches can overlap, see "aba " is found in \[0,2\] and \[2,4\].
**Constraints:**
* `1 <= text.length <= 100`
* `1 <= words.length <= 20`
* `1 <= words[i].length <= 50`
* `text` and `words[i]` consist of lowercase English letters.
* All the strings of `words` are **unique**. | We only need to check substrings of length at most 30, because 10^9 has 30 bits. |
Python 3 || 3 lines, w/ comment || T/M: 93% / 98% | binary-string-with-substrings-representing-1-to-n | 0 | 1 | Pretty much the same as the other brute force solutions, with one enhancement: *If`num`is in s then so is`num`//2.* For example, 13 = "1101" and 13//2= 6 = "110." \n\nIt follows then we only need to verify`num`over `range(n//2+1, n+1)`.\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n\n for num in range(n//2+1, n+1):\n if bin(num)[2:] not in s: return False\n\n return True\n```\n[https://leetcode.com/problems/binary-string-with-substrings-representing-1-to-n/submissions/872267745/](http://)\n\nI could be wrong, but I think that time complexity is *O*(*N*) and space complexity is *O*(*N*). | 4 | Given a binary string `s` and a positive integer `n`, return `true` _if the binary representation of all the integers in the range_ `[1, n]` _are **substrings** of_ `s`_, or_ `false` _otherwise_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "0110", n = 3
**Output:** true
**Example 2:**
**Input:** s = "0110", n = 4
**Output:** false
**Constraints:**
* `1 <= s.length <= 1000`
* `s[i]` is either `'0'` or `'1'`.
* `1 <= n <= 109` | null |
Python 3 || 3 lines, w/ comment || T/M: 93% / 98% | binary-string-with-substrings-representing-1-to-n | 0 | 1 | Pretty much the same as the other brute force solutions, with one enhancement: *If`num`is in s then so is`num`//2.* For example, 13 = "1101" and 13//2= 6 = "110." \n\nIt follows then we only need to verify`num`over `range(n//2+1, n+1)`.\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n\n for num in range(n//2+1, n+1):\n if bin(num)[2:] not in s: return False\n\n return True\n```\n[https://leetcode.com/problems/binary-string-with-substrings-representing-1-to-n/submissions/872267745/](http://)\n\nI could be wrong, but I think that time complexity is *O*(*N*) and space complexity is *O*(*N*). | 4 | Given a string `text` and an array of strings `words`, return _an array of all index pairs_ `[i, j]` _so that the substring_ `text[i...j]` _is in `words`_.
Return the pairs `[i, j]` in sorted order (i.e., sort them by their first coordinate, and in case of ties sort them by their second coordinate).
**Example 1:**
**Input:** text = "thestoryofleetcodeandme ", words = \[ "story ", "fleet ", "leetcode "\]
**Output:** \[\[3,7\],\[9,13\],\[10,17\]\]
**Example 2:**
**Input:** text = "ababa ", words = \[ "aba ", "ab "\]
**Output:** \[\[0,1\],\[0,2\],\[2,3\],\[2,4\]\]
**Explanation:** Notice that matches can overlap, see "aba " is found in \[0,2\] and \[2,4\].
**Constraints:**
* `1 <= text.length <= 100`
* `1 <= words.length <= 20`
* `1 <= words[i].length <= 50`
* `text` and `words[i]` consist of lowercase English letters.
* All the strings of `words` are **unique**. | We only need to check substrings of length at most 30, because 10^9 has 30 bits. |
[Python3] 2 approaches | binary-string-with-substrings-representing-1-to-n | 0 | 1 | Approach 1 - check if binary of number is in `S`\n```\nclass Solution:\n def queryString(self, S: str, N: int) -> bool:\n for x in range(N, 0, -1):\n if bin(x)[2:] not in S: return False \n return True \n```\n\nApproach 2 - collect all numbers in `S` and check if they fill `1` to `N`\n```\nclass Solution:\n def queryString(self, S: str, N: int) -> bool:\n ans = set()\n for i in range(len(S)):\n for ii in range(i, i + N.bit_length()): \n x = int(S[i:ii+1], 2)\n if 1 <= x <= N: ans.add(x)\n return len(ans) == N\n``` | 7 | Given a binary string `s` and a positive integer `n`, return `true` _if the binary representation of all the integers in the range_ `[1, n]` _are **substrings** of_ `s`_, or_ `false` _otherwise_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "0110", n = 3
**Output:** true
**Example 2:**
**Input:** s = "0110", n = 4
**Output:** false
**Constraints:**
* `1 <= s.length <= 1000`
* `s[i]` is either `'0'` or `'1'`.
* `1 <= n <= 109` | null |
[Python3] 2 approaches | binary-string-with-substrings-representing-1-to-n | 0 | 1 | Approach 1 - check if binary of number is in `S`\n```\nclass Solution:\n def queryString(self, S: str, N: int) -> bool:\n for x in range(N, 0, -1):\n if bin(x)[2:] not in S: return False \n return True \n```\n\nApproach 2 - collect all numbers in `S` and check if they fill `1` to `N`\n```\nclass Solution:\n def queryString(self, S: str, N: int) -> bool:\n ans = set()\n for i in range(len(S)):\n for ii in range(i, i + N.bit_length()): \n x = int(S[i:ii+1], 2)\n if 1 <= x <= N: ans.add(x)\n return len(ans) == N\n``` | 7 | Given a string `text` and an array of strings `words`, return _an array of all index pairs_ `[i, j]` _so that the substring_ `text[i...j]` _is in `words`_.
Return the pairs `[i, j]` in sorted order (i.e., sort them by their first coordinate, and in case of ties sort them by their second coordinate).
**Example 1:**
**Input:** text = "thestoryofleetcodeandme ", words = \[ "story ", "fleet ", "leetcode "\]
**Output:** \[\[3,7\],\[9,13\],\[10,17\]\]
**Example 2:**
**Input:** text = "ababa ", words = \[ "aba ", "ab "\]
**Output:** \[\[0,1\],\[0,2\],\[2,3\],\[2,4\]\]
**Explanation:** Notice that matches can overlap, see "aba " is found in \[0,2\] and \[2,4\].
**Constraints:**
* `1 <= text.length <= 100`
* `1 <= words.length <= 20`
* `1 <= words[i].length <= 50`
* `text` and `words[i]` consist of lowercase English letters.
* All the strings of `words` are **unique**. | We only need to check substrings of length at most 30, because 10^9 has 30 bits. |
simple easy to understand beats 80% | binary-string-with-substrings-representing-1-to-n | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n for i in range(1,n+1):\n b=bin(i)[2:]\n if b not in s:\n return False\n else:\n return True\n \n``` | 0 | Given a binary string `s` and a positive integer `n`, return `true` _if the binary representation of all the integers in the range_ `[1, n]` _are **substrings** of_ `s`_, or_ `false` _otherwise_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "0110", n = 3
**Output:** true
**Example 2:**
**Input:** s = "0110", n = 4
**Output:** false
**Constraints:**
* `1 <= s.length <= 1000`
* `s[i]` is either `'0'` or `'1'`.
* `1 <= n <= 109` | null |
simple easy to understand beats 80% | binary-string-with-substrings-representing-1-to-n | 0 | 1 | # Intuition\n<!-- Describe your first thoughts on how to solve this problem. -->\n\n# Approach\n<!-- Describe your approach to solving the problem. -->\n\n# Complexity\n- Time complexity:\n<!-- Add your time complexity here, e.g. $$O(n)$$ -->\n\n- Space complexity:\n<!-- Add your space complexity here, e.g. $$O(n)$$ -->\n\n# Code\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n for i in range(1,n+1):\n b=bin(i)[2:]\n if b not in s:\n return False\n else:\n return True\n \n``` | 0 | Given a string `text` and an array of strings `words`, return _an array of all index pairs_ `[i, j]` _so that the substring_ `text[i...j]` _is in `words`_.
Return the pairs `[i, j]` in sorted order (i.e., sort them by their first coordinate, and in case of ties sort them by their second coordinate).
**Example 1:**
**Input:** text = "thestoryofleetcodeandme ", words = \[ "story ", "fleet ", "leetcode "\]
**Output:** \[\[3,7\],\[9,13\],\[10,17\]\]
**Example 2:**
**Input:** text = "ababa ", words = \[ "aba ", "ab "\]
**Output:** \[\[0,1\],\[0,2\],\[2,3\],\[2,4\]\]
**Explanation:** Notice that matches can overlap, see "aba " is found in \[0,2\] and \[2,4\].
**Constraints:**
* `1 <= text.length <= 100`
* `1 <= words.length <= 20`
* `1 <= words[i].length <= 50`
* `text` and `words[i]` consist of lowercase English letters.
* All the strings of `words` are **unique**. | We only need to check substrings of length at most 30, because 10^9 has 30 bits. |
Python Solution | binary-string-with-substrings-representing-1-to-n | 0 | 1 | # Code\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n for i in range(1,n+1):\n st=""\n no=i\n while(no>0):\n st=str(no&1)+st\n no=no>>1\n if(st not in s):\n return False\n return True\n``` | 0 | Given a binary string `s` and a positive integer `n`, return `true` _if the binary representation of all the integers in the range_ `[1, n]` _are **substrings** of_ `s`_, or_ `false` _otherwise_.
A **substring** is a contiguous sequence of characters within a string.
**Example 1:**
**Input:** s = "0110", n = 3
**Output:** true
**Example 2:**
**Input:** s = "0110", n = 4
**Output:** false
**Constraints:**
* `1 <= s.length <= 1000`
* `s[i]` is either `'0'` or `'1'`.
* `1 <= n <= 109` | null |
Python Solution | binary-string-with-substrings-representing-1-to-n | 0 | 1 | # Code\n```\nclass Solution:\n def queryString(self, s: str, n: int) -> bool:\n for i in range(1,n+1):\n st=""\n no=i\n while(no>0):\n st=str(no&1)+st\n no=no>>1\n if(st not in s):\n return False\n return True\n``` | 0 | Given a string `text` and an array of strings `words`, return _an array of all index pairs_ `[i, j]` _so that the substring_ `text[i...j]` _is in `words`_.
Return the pairs `[i, j]` in sorted order (i.e., sort them by their first coordinate, and in case of ties sort them by their second coordinate).
**Example 1:**
**Input:** text = "thestoryofleetcodeandme ", words = \[ "story ", "fleet ", "leetcode "\]
**Output:** \[\[3,7\],\[9,13\],\[10,17\]\]
**Example 2:**
**Input:** text = "ababa ", words = \[ "aba ", "ab "\]
**Output:** \[\[0,1\],\[0,2\],\[2,3\],\[2,4\]\]
**Explanation:** Notice that matches can overlap, see "aba " is found in \[0,2\] and \[2,4\].
**Constraints:**
* `1 <= text.length <= 100`
* `1 <= words.length <= 20`
* `1 <= words[i].length <= 50`
* `text` and `words[i]` consist of lowercase English letters.
* All the strings of `words` are **unique**. | We only need to check substrings of length at most 30, because 10^9 has 30 bits. |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.