filename
stringlengths 7
140
| content
stringlengths 0
76.7M
|
---|---|
code/cryptography/src/autokey_cipher/autokey.py | # Part of Cosmos by OpenGenus Foundation
# It uses the following Table called Tabula Recta to find the
# ciphertext. The simple intersection of the row and column
# is the ciphertext. Let's say for eg. T is the Key and E is
# the original Plaintext, then the intersection of T column
# and E row is the ciphertext i.e. X. The plaintext and key-
# length must be the same. Autokey Cipher is similar to
# Vigenere Cipher. The only difference lies in how the key is
# chosen. In Vigenere, any random key is chosen which is
# repeated till the length of the plaintext. In Autokey,
# the key chosen contains some part of the plaintext itself.
# A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
# ---------------------------------------------------
# A A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
# B B C D E F G H I J K L M N O P Q R S T U V W X Y Z A
# C C D E F G H I J K L M N O P Q R S T U V W X Y Z A B
# D D E F G H I J K L M N O P Q R S T U V W X Y Z A B C
# E E F G H I J K L M N O P Q R S T U V W X Y Z A B C D
# F F G H I J K L M N O P Q R S T U V W X Y Z A B C D E
# G G H I J K L M N O P Q R S T U V W X Y Z A B C D E F
# H H I J K L M N O P Q R S T U V W X Y Z A B C D E F G
# I I J K L M N O P Q R S T U V W X Y Z A B C D E F G H
# J J K L M N O P Q R S T U V W X Y Z A B C D E F G H I
# K K L M N O P Q R S T U V W X Y Z A B C D E F G H I J
# L L M N O P Q R S T U V W X Y Z A B C D E F G H I J K
# M M N O P Q R S T U V W X Y Z A B C D E F G H I J K L
# N N O P Q R S T U V W X Y Z A B C D E F G H I J K L M
# O O P Q R S T U V W X Y Z A B C D E F G H I J K L M N
# P P Q R S T U V W X Y Z A B C D E F G H I J K L M N O
# Q Q R S T U V W X Y Z A B C D E F G H I J K L M N O P
# R R S T U V W X Y Z A B C D E F G H I J K L M N O P Q
# S S T U V W X Y Z A B C D E F G H I J K L M N O P Q R
# T T U V W X Y Z A B C D E F G H I J K L M N O P Q R S
# U U V W X Y Z A B C D E F G H I J K L M N O P Q R S T
# V V W X Y Z A B C D E F G H I J K L M N O P Q R S T U
# W W X Y Z A B C D E F G H I J K L M N O P Q R S T U V
# X X Y Z A B C D E F G H I J K L M N O P Q R S T U V W
# Y Y Z A B C D E F G H I J K L M N O P Q R S T U V W X
# Z Z A B C D E F G H I J K L M N O P Q R S T U V W X Y
plaintext = "DEFENDTHEEASTWALLOFTHECASTLE"
key = "FORTIFICATIONDEFENDTHEEASTWA"
ciphertext = ""
if __name__ == "__main__":
for index in range(len(plaintext)):
sum_key_plaintext = (ord(key[index]) - 64) + (ord(plaintext[index]) - 64)
if sum_key_plaintext > 27:
ciphertext += chr(sum_key_plaintext - 26 - 1 + 64)
else:
ciphertext += chr(sum_key_plaintext - 1 + 64)
print(ciphertext)
|
code/cryptography/src/autokey_cipher/autokey_cipher.c | #include <stdio.h>
#include <stdlib.h>
#include <string.h>
void flush_stdin()
{
while (fgetc(stdin) != '\n')
;
}
void autokeycipher(char **text, char key, int encrypt)
{
int textsize;
textsize = strlen((*text));
for (int i = 0; i < textsize; ++i)
{
if (isalpha((*text)[i]))
{
(*text)[i] = toupper((*text)[i]);
}
}
int nextkey, keyvalue, result;
nextkey = toupper(key) - 'A';
for (int i = 0; i < textsize; ++i)
{
if (isalpha((*text)[i]))
{
keyvalue = nextkey;
if (encrypt)
{
nextkey = (*text)[i] - 'A';
(*text)[i] = ((*text)[i] - 'A' + keyvalue) % 26 + 'A';
}
else
{
result = ((*text)[i] - 'A' - keyvalue) % 26 + 'A';
(*text)[i] = result < 'A' ? (result + 26) : (result);
nextkey = (*text)[i] - 'A';
}
}
}
}
int main(int argc, char **argv)
{
char key, *plaintext;
printf("Enter the Single Key word: ");
scanf("%c", &key);
int length;
printf("Enter the length of Plain Text: ");
scanf("%d", &length);
plaintext = (char *)malloc((length + 1) * sizeof(char));
flush_stdin();
printf("Enter the Plain Text: ");
fgets(plaintext, length + 1, stdin);
flush_stdin();
printf("\nThe Plain Text is: %s", plaintext);
/* 3rd argument decides whither to do 1-Encryption or 0-Decryption. */
autokeycipher(&plaintext, key, 1);
printf("\nThe Text after Encryption(Cipher text) is: %s", plaintext);
autokeycipher(&plaintext, key, 0);
printf("\nAfter Decryption the Text is: %s", plaintext);
free(plaintext);
return 0;
}
/*
Sample Input:
Enter the Single Key word: L //In A-Z, L will be at 11 index.
Enter the length of Plain Text: 5
Enter the Plain Text: hello
Sample Output:
The Plain Text is: hello
The Text after Encryption(Cipher text) is: slpwz
After Decryption the Text is: hello
*/
|
code/cryptography/src/autokey_cipher/autokey_cipher.cpp | #include <iostream>
std::string autokeycipher(std::string text, char key, int encrypt)
{
for (int i = 0; i < text.length(); ++i)
{
if (isalpha(text[i]))
{
text[i] = toupper(text[i]);
}
}
int nextkey, keyvalue, result;
nextkey = toupper(key) - 'A';
for (int i = 0; i < text.length(); ++i)
{
if (isalpha(text[i]))
{
keyvalue = nextkey;
if (encrypt)
{
nextkey = text[i] - 'A';
text[i] = (text[i] - 'A' + keyvalue) % 26 + 'A';
}
else
{
result = (text[i] - 'A' - keyvalue) % 26 + 'A';
text[i] = result < 'A' ? (result + 26) : (result);
nextkey = text[i] - 'A';
}
}
}
return text;
}
int main(int argc, char **argv)
{
char key;
std::string plaintext;
std::cout << "Enter the Single Key word: ";
std::cin >> key;
std::cout << "Enter the Plain Text: ";
std::cin >> plaintext;
std::cout << std::endl
<< "The Plain Text is: " << plaintext;
/* 3rd argument decides whither to do 1-Encryption or 0-Decryption. */
std::string ciphertext = autokeycipher(plaintext, key, 1);
std::cout << std::endl
<< "The Text after Encryption(Cipher text) is: " << ciphertext;
std::string decryptedtext = autokeycipher(ciphertext, key, 0);
std::cout << std::endl
<< "After Decryption the Text is: " << decryptedtext;
return 0;
}
/*
Sample Input:
Enter the Single Key word: L //In A-Z, L will be at 11 index.
Enter the Plain Text: hello
Sample Output:
The Plain Text is: hello
The Text after Encryption(Cipher text) is: slpwz
After Decryption the Text is: hello
*/
|
code/cryptography/src/autokey_cipher/autokey_cipher.java | import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.HashMap;
public class autokey
{
public static String createkey(String plaintext, int keycount, HashMap<Character, Integer> hashmap1, HashMap<Integer, Character> hashmap2)
{
char keytextarray[] = plaintext.toLowerCase().toCharArray();
int temp = keycount % 26;
for(int i = 0; i < plaintext.length(); ++i)
{
int a = hashmap1.get(plaintext.charAt(i));
if(i == 0)
{
a = (a + temp) % 26;
}
else
{
a = (a + hashmap1.get(plaintext.charAt(i - 1))) % 26;
}
keytextarray[i] = hashmap2.get(a);
}
return new String(keytextarray);
}
public static String encryption(String plaintext, String keytext, HashMap<Character, Integer> hashmap1, HashMap<Integer, Character> hashmap2)
{
char plaintextarray[] = plaintext.toLowerCase().toCharArray();
for(int i = 0; i < plaintext.length(); ++i)
{
int a = hashmap1.get(plaintext.charAt(i));
int b = hashmap1.get(keytext.charAt(i));
a = (a + b) % 26;
plaintextarray[i] = hashmap2.get(a);
}
return new String(plaintextarray);
}
public static String decryption(String encrypted, String keytext, HashMap<Character, Integer> hashmap1, HashMap<Integer, Character> hashmap2)
{
char encryptedarray[] = encrypted.toLowerCase().toCharArray();
for(int i = 0; i < encrypted.length(); ++i)
{
int a = hashmap1.get(encrypted.charAt(i));
int b = hashmap1.get(keytext.charAt(i));
a -= b;
if(a < 0)
{
int x;
x = Math.abs(a) % 26;
a = 26 - x;
}
else
{
a %= 26;
}
encryptedarray[i] = hashmap2.get(a);
}
return new String(encryptedarray);
}
public static void main(String[] args) throws IOException
{
int keycount = 0;
String plaintext = null, keytext = null, encrypted = null, decrypted = null;
HashMap<Character, Integer> hashmap1 = new HashMap<Character, Integer>();
HashMap<Integer, Character> hashmap2 = new HashMap<Integer, Character>();
BufferedReader bufferedreader = new BufferedReader(new InputStreamReader(System.in));
for(int i = 0; i < 26; ++i)
{
hashmap1.put((char)(i + 97), i);
hashmap2.put(i,(char)(i + 97));
}
System.out.print("Enter the Plain Text : ");
plaintext = bufferedreader.readLine();
System.out.print("Enter Key: ");
keycount = Integer.parseInt(bufferedreader.readLine());
System.out.println("Plain Text is: " + plaintext);
keytext = createkey(plaintext, keycount, hashmap1, hashmap2);
encrypted = encryption(plaintext, keytext, hashmap1, hashmap2);
System.out.println("Encrypted Text: " + keytext);
decrypted = decryption(encrypted, keytext, hashmap1, hashmap2);
System.out.println("Decrypted Text: " + decrypted);
bufferedreader.close();
}
}
/*
Sample Input:
Enter the Plain Text : hello
Enter Key: 11(L)
Sample Output:
Plain Text is: hello
Encrypted Text : slpwz
Decrypted Text : hello
*/
|
code/cryptography/src/baconian_cipher/README.md | # Bacon's Cipher
Bacon's cipher (or Baconian cipher) is actually a form of steganography rather than a cipher. This means that it's a method to hide a message in plain sight. It works by utilizing two different typefaces. One would be 'A', the other 'B'. You then write some text using both typefaces mixed together, following a simple pattern corresponding to each letter of the message you want to hide:
| Letter | Code | Letter | Code |
|--------|-------|--------|-------|
| A | aaaaa | N | abbab |
| B | aaaab | O | abbba |
| C | aaaba | P | abbbb |
| D | aaabb | Q | baaaa |
| E | aabaa | R | baaab |
| F | aabab | S | baaba |
| G | aabba | T | baabb |
| H | aabbb | U | babaa |
| I | abaaa | V | babab |
| J | abaab | W | babba |
| K | ababa | X | babbb |
| L | ababb | Y | bbaaa |
| M | abbaa | Z | bbaab |
\* There is also another version of this cipher where I and J, and U and V correspond to the same code. This version, where each letter has it's own distinct code, is more popular and is generally the one used in implementations.
For example, "Hello world" would become "AABBBAABAAABABBABABBABBBABABBAABBBABAAABABABBAAABB" (spaces are ignored). If we wanted to hide this in some Lorem Ipsum, making typeface A normal and typeface B bold, it would look like the following:
Lo**rem** ip**s**um d**o**l**or** s**i**t **am**e**t** **co**n**s**e**ct**et**ur** **a**d**i**pis**c**i**n**g **el**it s**ed**
## Sources and more detailed information:
- https://en.wikipedia.org/wiki/Bacon's_cipher
- http://rumkin.com/tools/cipher/baconian.php
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
code/cryptography/src/baconian_cipher/baconian_cipher.c | #include <stdio.h>
#include <string.h>
#include <stdlib.h>
const char *codes[] = {
"AAAAA", "AAAAB", "AAABA", "AAABB", "AABAA",
"AABAB", "AABBA", "AABBB", "ABAAA", "ABAAB",
"ABABA", "ABABB", "ABBAA", "ABBAB", "ABBBA",
"ABBBB", "BAAAA", "BAAAB", "BAABA", "BAABB",
"BABAA", "BABAB", "BABBA", "BABBB", "BBAAA",
"BBAAB", "BBBAA"
};
/*Function that takes a char and returns its code*/
char*
get_code(const char c)
{
(c >= 97 && c <= 122) ? return (codes[c - 97]) : return (codes[26]);
}
/*Function that takes a code and returns the corresponding char*/
char
get_char(const char *code)
{
int i;
if (!strcmp(codes[26], code)) return ' ';
for (i = 0; i < 26; ++i) {
if (strcmp(codes[i], code) == 0) return 97 + i;
}
printf("\nCode \"%s\" is invalid\n", code);
exit(1);
}
void
str_tolower(char s[])
{
int i;
for (i = 0; i < strlen(s); ++i) s[i] = tolower(s[i]);
}
char*
bacon_encode(char plain_text[], char message[])
{
int i, count;
int plen = strlen(plain_text), mlen = strlen(message);
int elen = 5 * plen;
char c;
char *p, *et = malloc(elen + 1), *mt;
str_tolower(plain_text);
for (i = 0, p = et; i < plen; ++i, p += 5) {
c = plain_text[i];
strncpy(p, get_code(c), 5);
}
*++p = '\0';
/* 'A's to be in lower case, 'B's in upper case */
str_tolower(message);
mt = calloc(mlen + 1, 1);
for (i = 0, count = 0; i < mlen; ++i) {
c = message[i];
if (c >= 'a' && c <= 'z') {
if (et[count] == 'A')
mt[i] = c;
else
mt[i] = c - 32; /* upper case equivalent */
if (++count == elen) break;
}
else mt[i] = c;
}
free(et);
return (mt);
}
char*
bacon_decode(char cipher_text[])
{
int i, count, clen = strlen(cipher_text);
int plen;
char *p, *ct = calloc(clen + 1, 1), *pt;
char c, quintet[6];
for (i = 0, count = 0; i < clen; ++i) {
c = cipher_text[i];
if (c >= 'a' && c <= 'z')
ct[count++] = 'A';
else if (c >= 'A' && c <= 'Z')
ct[count++] = 'B';
}
plen = strlen(ct) / 5;
pt = malloc(plen + 1);
for (i = 0, p = ct; i < plen; ++i, p += 5) {
strncpy(quintet, p, 5);
quintet[5] = '\0';
pt[i] = get_char(quintet);
}
pt[plen] = '\0';
free(ct);
return (pt);
}
int
main()
{
char plain_text[] = "this is plain text";
char message[] = "this is a message";
char *cipher_text, *hidden_text;
cipher_text = bacon_encode(plain_text, message);
printf("Cipher text is\n\n%s\n", cipher_text);
hidden_text = bacon_decode(cipher_text);
printf("\nHidden text is\n\n%s\n", hidden_text);
free(cipher_text);
free(hidden_text);
return (0);
}
|
code/cryptography/src/baconian_cipher/baconian_cipher.cpp | #include <map>
#include <string>
// Part of Cosmos by OpenGenus Foundation
// This function generates a baconian map
std::map<char, std::string> createBaconianMap()
{
std::map<char, std::string> cipher;
std::string static alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
std::string value;
for (std::size_t i = 0; i < alphabet.length(); ++i)
{
for (std::size_t j = 0; j < 5; ++j)
((i & (1 << j)) == 0) ? value = 'A' + value : value = 'B' + value;
char key = alphabet[i];
cipher[key] = value;
value.clear();
}
return cipher;
}
std::string encrypt(std::map<char, std::string>& cipher, std::string message)
{
std::string newMessage;
for (std::size_t i = 0; i < message.length(); ++i)
(message[i] !=
' ') ? newMessage.append(cipher[char(toupper(message[i]))]) : newMessage.append(" ");
return newMessage;
}
std::string decrypt(std::map <char, std::string>& cipher, std::string message)
{
std::string newMessage;
std::string currentString;
int currentStringLength = 0;
for (std::size_t i = 0; i < message.length() + 1; ++i)
{
if (currentStringLength == 5)
{
std::map<char, std::string>::iterator it = cipher.begin();
while (it->second != currentString)
++it;
newMessage += it->first;
currentString.clear();
currentStringLength = 0;
}
if (message[i] == ' ')
newMessage.append(" ");
else
{
currentString += message[i];
currentStringLength++;
}
}
return newMessage;
}
|
code/cryptography/src/baconian_cipher/baconian_cipher.exs | defmodule Cryptography do
@baconian_encryption_table %{
a: "AAAAA",
b: "AAAAB",
c: "AAABA",
d: "AAABB",
e: "AABAA",
f: "AABAB",
g: "AABBA",
h: "AABBB",
i: "ABAAA",
j: "ABAAB",
k: "ABAAB",
l: "ABABB",
m: "ABBAA",
n: "ABBAB",
o: "ABBBA",
p: "ABBBB",
q: "BAAAA",
r: "BAAAB",
s: "BAABA",
t: "BAABB",
u: "BABAA",
v: "BABAB",
w: "BABBA",
x: "BABAB",
y: "BBAAA",
z: "BBAAB"
}
def baconian_encrypt(content) do
content
|> String.downcase()
|> String.split("", trim: true)
|> Enum.map(&Map.get(@baconian_encryption_table, :"#{&1}", ""))
|> Enum.join()
end
def baconian_decrypt(baconian) do
baconian_decryption_table =
Enum.map(@baconian_encryption_table, fn {k, v} -> {v, k} end) |> Enum.into(%{})
baconian
|> String.upcase()
|> String.split("", trim: true)
|> Enum.chunk_every(5)
|> Enum.map(fn x ->
key = Enum.join(x)
Map.get(baconian_decryption_table, key)
|> Atom.to_string()
end)
|> Enum.join()
end
end
msg = "Hello world"
Cryptography.baconian_encrypt(msg)
|> IO.inspect(label: "Encrypting #{msg}")
|> Cryptography.baconian_decrypt()
|> IO.inspect(label: "Decrypting encrypted #{msg}")
|
code/cryptography/src/baconian_cipher/baconian_cipher.java | import java.util.HashMap;
import java.util.Map;
public class Baconian {
private Map<String ,String> cipherMap = new HashMap<>();
public Baconian() {
cipherMap.put("a", "aaaaa");
cipherMap.put("b", "aaaab");
cipherMap.put("c", "aaaba");
cipherMap.put("d", "aaabb");
cipherMap.put("e", "aabaa");
cipherMap.put("f", "aabab");
cipherMap.put("g", "aabba");
cipherMap.put("h", "aabbb");
cipherMap.put("i", "abaaa");
cipherMap.put("j", "abaab");
cipherMap.put("k", "ababa");
cipherMap.put("l", "ababb");
cipherMap.put("m", "abbaa");
cipherMap.put("n", "abbab");
cipherMap.put("o", "abbba");
cipherMap.put("p", "abbbb");
cipherMap.put("q", "baaaa");
cipherMap.put("r", "baaab");
cipherMap.put("s", "baaba");
cipherMap.put("t", "baabb");
cipherMap.put("u", "babaa");
cipherMap.put("v", "babab");
cipherMap.put("w", "babba");
cipherMap.put("x", "babbb");
cipherMap.put("y", "bbaaa");
cipherMap.put("z", "bbaab");
}
public String encode(String word) {
StringBuilder results = new StringBuilder();
word = word.toLowerCase();
for (Character letter : word.toCharArray()) {
results.append(this.cipherMap.get(letter.toString()));
}
return results.toString();
}
public String decode(String word) {
StringBuilder results = new StringBuilder();
word = word.toLowerCase();
for (int start = 0; start < word.length() / 5; ++start) {
StringBuilder code = new StringBuilder();
int startIndex = start * 5;
for (int index = startIndex; index < startIndex + 5; ++index) {
code.append(word.charAt(index));
}
String letter = getKeyFromValue(this.cipherMap, code.toString());
results.append(letter);
}
return results.toString();
}
public static String getKeyFromValue(Map hashmap, Object value) {
for (Object key: hashmap.keySet()) {
if (hashmap.get(key).equals(value)) {
return key.toString();
}
}
return null;
}
} |
code/cryptography/src/baconian_cipher/baconian_cipher.js | function baconianEncrypt(plainText) {
let baconianCodes = {
a: "AAAAA",
b: "AAAAB",
c: "AAABA",
d: "AAABB",
e: "AABAA",
f: "AABAB",
g: "AABBA",
h: "AABBB",
i: "ABAAA",
j: "BBBAA",
k: "ABAAB",
l: "ABABA",
m: "ABABB",
n: "ABBAA",
o: "ABBAB",
p: "ABBBA",
q: "ABBBB",
r: "BAAAA",
s: "BAAAB",
t: "BAABA",
u: "BAABB",
v: "BBBAB",
w: "BABAA",
x: "BABAB",
y: "BABBA",
z: "BABBB"
};
let cipherText = "";
[...plainText].forEach(
char => (cipherText += baconianCodes[char.toLowerCase()])
);
return cipherText;
}
console.log(baconianEncrypt("THISISASECRETTEST"));
console.log(baconianEncrypt("SAVETHECASTLE"));
|
code/cryptography/src/baconian_cipher/baconian_cipher.php | <?php
/**
* Bacon's (aka Baconian) cipher (PHP)
* based on https://en.wikipedia.org/wiki/Bacon%27s_cipher
* implemented by Stefano Amorelli <[email protected]>
* Part of Cosmos by OpenGenus Foundation
*/
class BaconianCipher
{
private $mapping;
function __construct() {
$this->mapping = [];
$this->initMapping();
}
public function encrypt($plainText) {
if (!ctype_alpha($plainText)) {
throw new Exception('Plaintext should be alphabetical strings (no spaces, symbols and numbers).');
}
$plainText = strtoupper($plainText);
$encryptedText = [];
foreach (str_split($plainText) as $character) {
array_push($encryptedText, $this->mapping[$character]);
}
return implode(' ', $encryptedText);
}
public function decrypt($encryptedText) {
$plainText = [];
foreach (explode(' ', $encryptedText) as $character) {
foreach ($this->mapping as $key => $value) {
if ($value === $character) {
$plainCharacter = $key;
continue;
}
}
array_push($plainText, $plainCharacter);
}
return implode('', $plainText);
}
private function initMapping() {
$this->mapping['A'] = 'aaaaa';
$this->mapping['B'] = 'aaaab';
$this->mapping['C'] = 'aaaba';
$this->mapping['D'] = 'aaabb';
$this->mapping['E'] = 'aabaa';
$this->mapping['F'] = 'aabab';
$this->mapping['G'] = 'aabba';
$this->mapping['H'] = 'aabbb';
$this->mapping['I'] = 'abaaa';
$this->mapping['J'] = 'abaab';
$this->mapping['K'] = 'ababa';
$this->mapping['L'] = 'ababb';
$this->mapping['M'] = 'abbaa';
$this->mapping['N'] = 'abbab';
$this->mapping['O'] = 'abbba';
$this->mapping['P'] = 'abbbb';
$this->mapping['Q'] = 'baaaa';
$this->mapping['R'] = 'baaab';
$this->mapping['S'] = 'baaba';
$this->mapping['T'] = 'baabb';
$this->mapping['U'] = 'babaa';
$this->mapping['V'] = 'babab';
$this->mapping['W'] = 'babba';
$this->mapping['X'] = 'babbb';
$this->mapping['Y'] = 'bbaaa';
$this->mapping['Z'] = 'bbaab';
}
}
/* Example usage:
$cipher = new BaconianCipher();
$encrypted = $cipher->encrypt('LOREMIPSUMDOLOR');
$decrypted = $cipher->decrypt($encrypted);
echo $decrypted; // output: LOREMIPSUMDOLOR
*/ |
code/cryptography/src/baconian_cipher/baconian_cipher.py | # Part of Cosmos by OpenGenus Foundation
baconian_codes = {
"a": "AAAAA",
"b": "AAAAB",
"c": "AAABA",
"d": "AAABB",
"e": "AABAA",
"f": "AABAB",
"g": "AABBA",
"h": "AABBB",
"i": "ABAAA",
"j": "BBBAA",
"k": "ABAAB",
"l": "ABABA",
"m": "ABABB",
"n": "ABBAA",
"o": "ABBAB",
"p": "ABBBA",
"q": "ABBBB",
"r": "BAAAA",
"s": "BAAAB",
"t": "BAABA",
"u": "BAABB",
"v": "BBBAB",
"w": "BABAA",
"x": "BABAB",
"y": "BABBA",
"z": "BABBB",
}
if __name__ == "__main__":
plaintext = "Text to be encrypted"
ciphertext = ""
for ch in plaintext:
if ch != " ":
ciphertext += baconian_codes[ch.lower()]
else:
ciphertext += " "
print(ciphertext)
|
code/cryptography/src/baconian_cipher/baconian_cipher.rb | # Written by Michele Riva
BACONIAN_CODE = {
'a' => 'AAAAA', 'b' => 'AAAAB', 'c' => 'AAABA',
'd' => 'AAABB', 'e' => 'AABAA', 'f' => 'AABAB',
'g' => 'AABBA', 'h' => 'AABBB', 'i' => 'ABAAA',
'j' => 'BBBAA', 'k' => 'ABAAB', 'l' => 'ABABA',
'm' => 'ABABB', 'n' => 'ABBAA', 'o' => 'ABBAB',
'p' => 'ABBBA', 'q' => 'ABBBB', 'r' => 'BAAAA',
's' => 'BAAAB', 't' => 'BAABA', 'u' => 'BAABB',
'v' => 'BBBAB', 'w' => 'BABAA', 'x' => 'BABAB',
'y' => 'BABBA', 'z' => 'BABBB'
}.freeze
def encode(string)
text = string.downcase.split('')
encoded_text = []
text.each do |c|
bac_char = BACONIAN_CODE[c]
encoded_text.push(bac_char)
end
encoded_text.join('')
end
# TEST!
puts encode('Hello World') # => AABBBAABAAABABAABABAABBABBABAAABBABBAAAAABABAAAABB
|
code/cryptography/src/caesar_cipher/README.md | # Caesar Cipher
The Caesar Cipher (also known as shift cipher) is a method of encrypting messages. In this cipher, the original message (plaintext) is replaced with a letter corresponding to the original letter shifted by N places according to the English alphabet. It is therefore a Substitution cipher where letter at ‘L th’ position is shifted to ‘L+N th’ position, where **N is the same** for all the letters.
Using this method, the original message that is understandable to any English speaking user, needs to be deciphered before arriving at context.
## Example
This example uses a right shift of 3.
```text
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
| | | | | | | | | | | | | | | | | | | | | | | | | |
V V V V V V V V V V V V V V V V V V V V V V V V V V
D E F G H I J K L M N O P Q R S T U V W X Y Z A B C
```
#### Plain text:
I LOVE WORKING ON GITHUB
#### Cipher text:
L ORYH ZRUNLQJ RQ JLWKXE
## Further Reading
[Caesar Cipher](https://en.wikipedia.org/wiki/Caesar_cipher)
#### Fun Trivia:
This cipher is named after Julius Caesar, who used this in his private correspondence.
|
code/cryptography/src/caesar_cipher/caesar_cipher.c | // A C program to illustrate Caesar Cipher Technique
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h>
//argc gives the number of arguments and argv[1] gives the key of the cipher
int main(int argc, char* argv[])
{
if(argc == 2)
{
int r = atoi(argv[1]); //Converts key from string type to integer type and stores in r
printf("plaintext: ");
char s[100]; //Stores plaintext
scanf("%s", s);
printf("ciphertext: \n");
//Traverses through all characters of plaintext
for(int i = 0; i < strlen(s); i++)
{
if(isalpha(s[i])) //Checks if the character is an alphabet
{
if(isupper(s[i])) //Checks if the character is an uppercase alphabet
{
printf("%c",((s[i] - 65 + r) % 26) + 65); //Performs encryption
}
if(islower(s[i])) //Checks if the character is an lowercase alphabet
{
printf("%c",((s[i] - 97 + r) % 26) + 97); //Performs encryption
}
}
else
printf("%c",s[i]); //Simply prints the character if it is not an alphabet
}
printf("\n");
return 0;
}
else //If key is not given as argument argv[1]
{
printf("Invalid key Entered.\n");
return 1;
}
}
|
code/cryptography/src/caesar_cipher/caesar_cipher.cpp | // Part of Cosmos by OpenGenus Foundation
// A C++ program to illustrate Caesar Cipher Technique
#include <iostream>
using namespace std;
// returns the encrypted text
string caesarcipher(string text, int s)
{
string result = "";
// traverse text
for (size_t i = 0; i < text.length(); i++)
{
//Takes care of spaces
if (int(text[i]) == 32)
{
result += char(32);
continue;
}
// Encrypt Uppercase letters
if (isupper(text[i]))
result += char(int(text[i] + s - 65) % 26 + 65);
// Encrypt Lowercase letters
else
result += char(int(text[i] + s - 97) % 26 + 97);
}
// Return the resulting string
return result;
}
int main()
{
string text;
cout << "\nEnter string to encrypt\n";
getline(cin, text);
int s;
cout << "\nEnter no of shifts:\n";
cin >> s;
cout << "\nText : " << text;
cout << "\nShift: " << s;
text = caesarcipher(text, s);
cout << "\nEncrypted: " << text;
cout << "\nDecrypted: " << caesarcipher(text, 26 - s);
return 0;
}
|
code/cryptography/src/caesar_cipher/caesar_cipher.cs | // CAESAR CIPHER USING C#
// Sample Input:
// qwertyuiopasdfghjklzxcvbnm@QWERTYUIOPASDFGHJKLZXCVBNM
// 3
// Sample Output:
// Encrypted value is: rxfsuzvjpqbteghiklmaydwcon?RXFSUZVJPQBTEGHIKLMAYDWCON
// Decrypted value is: qwertyuiopasdfghjklzxcvbnm?QWERTYUIOPASDFGHJKLZXCVBNM
// Part of Cosmos by OpenGenus Foundation
using System.IO;
using System;
class Program
{
const int NUM_ALPHABETS = 26;
static char addValue(char character, int valueToShiftBy){
if(character >= 'a' && character <= 'z'){
return Convert.ToChar((((character - 'a') + valueToShiftBy) % NUM_ALPHABETS) + 'a');
}
else if(character >= 'A' && character <= 'Z'){
return Convert.ToChar((((character - 'A') + valueToShiftBy) % NUM_ALPHABETS) + 'A');
}
// Not sure if Caesar's happy with this :)
return '?';
}
static char subtractValue(char character, int valueToShiftBy){
return addValue(character, NUM_ALPHABETS-(valueToShiftBy % NUM_ALPHABETS));
}
static string DecryptByCaesarCipher(string stringToEncrypt, int keyToShiftBy){
string result = string.Empty;
foreach(var character in stringToEncrypt){
result += subtractValue(character, keyToShiftBy);
}
return result;
}
static string EncryptByCaesarCipher(string stringToEncrypt, int keyToShiftBy){
string result = string.Empty;
foreach(var character in stringToEncrypt){
result += addValue(character, keyToShiftBy);
}
return result;
}
static void Main()
{
Console.WriteLine("Enter string and key!");
var stringToEncrypt = Console.ReadLine();
var keyToShiftBy = int.Parse(Console.ReadLine());
var encryptedValue = EncryptByCaesarCipher(stringToEncrypt, keyToShiftBy);
Console.WriteLine($"Encrypted value is: {encryptedValue}");
var decryptedValue = DecryptByCaesarCipher(encryptedValue, keyToShiftBy);
Console.WriteLine($"Decrypted value is: {decryptedValue}");
}
}
|
code/cryptography/src/caesar_cipher/caesar_cipher.go | package main
import (
"fmt"
"strings"
)
func Encrypt(str string, key int) string {
if err := checkKey(key); err != nil {
fmt.Println("Error: ", err.Error())
return ""
}
shift := rune(key)
dec := strings.Map(func(r rune) rune {
if r >= 'a' && r <= 'z'-shift || r >= 'A' && r <= 'Z'-shift {
return r + shift
} else if r > 'z'-shift && r <= 'z' || r > 'Z'-shift && r <= 'Z' {
return r + shift - 26
}
return r
}, str)
return dec
}
func Decrypt(str string, key int) string {
if err := checkKey(key); err != nil {
fmt.Println("Error: ", err.Error())
return ""
}
shift := rune(key)
dec := strings.Map(func(r rune) rune {
if r >= 'a'+shift && r <= 'z' || r >= 'A'+shift && r <= 'Z' {
return r - shift
} else if r >= 'a' && r < 'a'+shift || r >= 'A' && r < 'A'+shift {
return r - shift + 26
}
return r
}, str)
return dec
}
func checkKey(k int) error {
if k <= 26 {
return nil
}
return fmt.Errorf("Invalid key\n 0 <= Key <= 26")
}
func main() {
var msg string
var key int
var mode rune
fmt.Println("Enter text to encrypt\\decrypt")
fmt.Scanf("%s\n", &msg)
fmt.Println("Enter key")
fmt.Scanf("%d\n", &key)
fmt.Println("Enter 'e' to encrypt or 'd' to decrypt")
fmt.Scanf("%c", &mode)
if mode == rune('e') {
fmt.Println("Encrypted Message: ", Encrypt(msg, key))
} else if mode == rune('d') {
fmt.Println("Deccrypted Message: ", Decrypt(msg, key))
} else {
fmt.Println("Unknown mode")
}
}
|
code/cryptography/src/caesar_cipher/caesar_cipher.hs | import Data.Char (ord, chr, isUpper, isAlpha)
caesar, uncaesar :: Int -> String -> String
caesar = (<$>) . tr
uncaesar = caesar . negate
tr :: Int -> Char -> Char
tr offset c
| isAlpha c = chr $ intAlpha + mod ((ord c - intAlpha) + offset) 26
| otherwise = c
where
intAlpha =
ord
(if isUpper c
then 'A'
else 'a')
main :: IO ()
main = mapM_ print [encoded, decoded]
where
encoded = caesar (-114) "Veni, vidi, vici"
decoded = uncaesar (-114) encoded |
code/cryptography/src/caesar_cipher/caesar_cipher.java | import java.util.Scanner;
public final class CaesarCipher {
private final static int MIN_KEY = 1;
private final static int MAX_KEY = 26;
public static void main(String[] args){
final Scanner scanner = new Scanner(System.in);
String ans = "";
System.out.print("Enter a message: ");
String message = scanner.nextLine();
System.out.print("Encrypt or Decrypt: ");
char type = scanner.next().charAt(0);
int key = 0;
do {
System.out.print("Enter a key: ");
key = scanner.nextInt();
} while (key < MIN_KEY || key > MAX_KEY);
key = (type == 'd' || type == 'D') ? -key : key;
for (char c : message.toCharArray()) {
int num = (int) c;
if (Character.isAlphabetic(c)) {
num += key;
if (Character.isUpperCase(c)) {
if (num > 'Z') {
num -= MAX_KEY;
}
else if (num < 'A') {
num += MAX_KEY;
}
}
else if (Character.isLowerCase(c)) {
if (num > 'z') {
num -= MAX_KEY;
}
else if (num < 'a') {
num += MAX_KEY;
}
}
}
ans += (char) num;
}
System.out.println(ans);
}
}
|
code/cryptography/src/caesar_cipher/caesar_cipher.js | /* Part of Cosmos by OpenGenus Foundation */
// Caesar Cipher in javascript
//Use the 'Ref' string to set the range of characters that can be encrypted. Characters not in 'Ref' will not be encoded
const Ref = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789";
// text = the string to be encrypted/decrypted
// shift = the amount of shift for encryption/decryption
function caesar_cipher(text, shift) {
shift %= Ref.length;
if (shift < 0) {
shift += Ref.length;
}
return text
.split("")
.map(cv => {
let loc = Ref.indexOf(cv);
return loc !== -1
? Ref.charAt(
loc + shift < Ref.length ? loc + shift : loc + shift - Ref.length
)
: cv;
})
.join("");
}
/*
-Sample use:
let encrypted = caesar_cipher( "Hello World", 10 );
-Then to decrypt
let decrypted = caesar_cipher( encrypted, -10 );
*/
|
code/cryptography/src/caesar_cipher/caesar_cipher.php | <?php
/* Part of Cosmos by OpenGenus Foundation */
const REF = 'AaBbCcDdEeFfGgHhIiJjKkLlMmNnOoPpQqRrSsTtUuVvWwXxYyZz';
function caesar_cipher(string $message, int $shift): string {
$shift %= 26;
$ref_shifted = substr(REF, $shift * 2) . substr(REF, 0, $shift * 2);
return strtr($message, REF, $ref_shifted);
}
echo 'Encoding "Hello World", using a right shift of 10:' . PHP_EOL;
echo caesar_cipher('Hello World', 10) . PHP_EOL . PHP_EOL;
echo 'Decoding "byFFI qILFx", using a left shift of 6:' . PHP_EOL;
echo caesar_cipher('byFFI qILFx', -6); |
code/cryptography/src/caesar_cipher/caesar_cipher.py | MAX_KEY_SIZE = 26
# Mode to select (e)ncryption or (d)ecryption
def get_mode():
while True:
print("Do you wish to encrypt or decrypt a message?")
mode = input().lower()
if mode in "encrypt e decrypt d".split():
return mode
else:
print('Enter either "encrypt" or "e" or "decrypt" or "d".')
# Gets message from the user
def get_message():
print("Enter your message:")
return input()
# Gets key from the user
def get_key():
key = 0
while key < 1 or key > MAX_KEY_SIZE:
print("Enter the key number (1-%s)" % (MAX_KEY_SIZE))
key = int(input())
return key
# Translation logic here
def get_translated_message(mode, message, key):
if mode[0] == "d":
key = -key
translated = ""
for symbol in message:
if symbol.isalpha():
num = ord(symbol)
num += key
if symbol.isupper():
if num > ord("Z"):
num -= 26
elif num < ord("A"):
num += 26
elif symbol.islower():
if num > ord("z"):
num -= 26
elif num < ord("a"):
num += 26
translated += chr(num)
else:
translated += symbol
return translated
mode = get_mode()
message = get_message()
key = get_key()
print("Your translated text is:")
print(get_translated_message(mode, message, key))
|
code/cryptography/src/caesar_cipher/caesar_cipher.rb | # Part of Cosmos by OpenGenus Foundation
# Written by Michele Riva
CHARS_HASH = Hash[('a'..'z').to_a.zip((0..25).to_a)]
def caesar(string, shift)
shift = 25 if shift > 25
text = string.downcase.split('')
encoded = []
text.each do |c|
orig_num = CHARS_HASH[c]
if orig_num - shift > 0
encoded_num = orig_num - shift
else
pre = shift - orig_num
encoded_num = 26 - pre
end
encoded_char = CHARS_HASH.key(encoded_num)
encoded.push(encoded_char)
end
encoded.join('')
end
# Test!
# Input your string as first argument and the shift as second argument.
puts caesar('cosmos', 5) # => xjnhjn
|
code/cryptography/src/caesar_cipher/decryption.cpp | #include <iostream>
using namespace std;
int main()
{
char message[100], ch;
int i, key;
cout << "Enter a message to decrypt: ";
cin.getline(message, 100);
cout << "Enter key: ";
cin >> key;
//Traverses the ciphertext
for (i = 0; message[i] != '\0'; ++i)
{
ch = message[i];
if (ch >= 'a' && ch <= 'z') //Checks if the character of ciphertext is a lowercase alphabet
{
ch = ch - key; //Performs decryption
if (ch < 'a') //If character goes below lowercase a
ch = ch + 'z' - 'a' + 1; //then we bring it within the range of lowercase a to z
message[i] = ch;
}
else if (ch >= 'A' && ch <= 'Z') //Checks if the character of ciphertext is a uppercase alphabet
{
ch = ch - key; //Performs decryption
if (ch > 'a') //If character goes above lowercase a
ch = ch + 'Z' - 'A' + 1; //then we bring it within the range of uppercase A to Z
message[i] = ch;
}
}
cout << "Decrypted message: " << message;
return 0;
}
|
code/cryptography/src/caesar_cipher/encryption.cpp | #include <iostream>
using namespace std;
int main()
{
char message[100], ch;
int i, key;
cout << "Enter a message to encrypt: ";
cin.getline(message, 100);
cout << "Enter key: ";
cin >> key;
for (i = 0; message[i] != '\0'; ++i)
{
ch = message[i];
if (ch >= 'a' && ch <= 'z')
{
ch = ch + key;
if (ch > 'z')
ch = ch - 'z' + 'a' - 1;
message[i] = ch;
}
else if (ch >= 'A' && ch <= 'Z')
{
ch = ch + key;
if (ch > 'Z')
ch = ch - 'Z' + 'A' - 1;
message[i] = ch;
}
}
cout << "Encrypted message: " << message;
return 0;
}
|
code/cryptography/src/columnar_transposition_cipher/columnar_transposition_cipher.cpp | // CPP program for
// Columnar Transposition Cipher
#include <string>
#include <iostream>
#include <map>
using namespace std;
map<int, int> keyMap;
void setPermutationOrder(string key)
{
// Add the permutation
for (size_t i = 0; i < key.length(); i++)
keyMap[key[i]] = i;
}
// Encryption code for cipher
string encrypt(string object, string key)
{
int row, col, j;
string cipher = "";
/* calculate column of the matrix*/
col = key.length();
/* calculate Maximum row of the matrix*/
row = object.length() / col;
if (object.length() % col)
row += 1;
char matrix[row][col];
for (int i = 0, k = 0; i < row; i++)
for (int j = 0; j < col;)
{
if (object[k] == '\0')
{
/* Adding the padding character '_' */
matrix[i][j] = '_';
j++;
}
if (isalpha(object[k]) || object[k] == ' ')
{
/* Adding only space and alphabet into matrix*/
matrix[i][j] = object[k];
j++;
}
k++;
}
for (map<int, int>::iterator ii = keyMap.begin(); ii != keyMap.end(); ++ii)
{
j = ii->second;
// getting cipher text from matrix column wise using permuted key
for (int i = 0; i < row; i++)
if (isalpha(matrix[i][j]) || matrix[i][j] == ' ' || matrix[i][j] == '_')
cipher += matrix[i][j];
}
return cipher;
}
// Decryption code for cipher
string decrypt(string cipher, string key)
{
/* calculate row and column for cipher Matrix */
int col = key.length();
int row = cipher.length() / col;
char cipherMat[row][col];
/* add character into matrix column wise */
for (int j = 0, k = 0; j < col; j++)
for (int i = 0; i < row; i++)
cipherMat[i][j] = cipher[k++];
/* update the order of key for decryption */
int index = 0;
for (map<int, int>::iterator ii = keyMap.begin(); ii != keyMap.end(); ++ii)
ii->second = index++;
/* Arrange the matrix column wise according
* to permutation order by adding into new matrix */
char decCipher[row][col];
int k = 0;
for (int l = 0, j; key[l] != '\0'; k++)
{
j = keyMap[key[l++]];
for (int i = 0; i < row; i++)
decCipher[i][k] = cipherMat[i][j];
}
/* getting Message using matrix */
string msg = "";
for (int i = 0; i < row; i++)
for (int j = 0; j < col; j++)
if (decCipher[i][j] != '_')
msg += decCipher[i][j];
return msg;
}
// Driver Program for columnar transposition
int main()
{
/* message */
string msg = "defend the east wall of the castle";
string key = "GERMAN";
setPermutationOrder(key);
string cipher = encrypt(msg, key);
cout << "Encrypted Message: " << cipher << endl;
cout << "Decrypted Message: " << decrypt(cipher, key) << endl;
return 0;
}
|
code/cryptography/src/des_cipher/des_java/.gitignore | *.class |
code/cryptography/src/des_cipher/des_java/DES.java | // Java code for the above approach
import java.util.*;
public class DES {
// CONSTANTS
// Initial Permutation Table
int[] IP = {58, 50, 42, 34, 26, 18, 10, 2, 60, 52, 44, 36, 28, 20, 12, 4, 62, 54, 46, 38, 30,
22, 14, 6, 64, 56, 48, 40, 32, 24, 16, 8, 57, 49, 41, 33, 25, 17, 9, 1, 59, 51, 43, 35,
27, 19, 11, 3, 61, 53, 45, 37, 29, 21, 13, 5, 63, 55, 47, 39, 31, 23, 15, 7};
// Inverse Initial Permutation Table
int[] IP1 = {40, 8, 48, 16, 56, 24, 64, 32, 39, 7, 47, 15, 55, 23, 63, 31, 38, 6, 46, 14, 54,
22, 62, 30, 37, 5, 45, 13, 53, 21, 61, 29, 36, 4, 44, 12, 52, 20, 60, 28, 35, 3, 43, 11,
51, 19, 59, 27, 34, 2, 42, 10, 50, 18, 58, 26, 33, 1, 41, 9, 49, 17, 57, 25};
// first key-hePermutation Table
int[] PC1 = {57, 49, 41, 33, 25, 17, 9, 1, 58, 50, 42, 34, 26, 18, 10, 2, 59, 51, 43, 35, 27,
19, 11, 3, 60, 52, 44, 36, 63, 55, 47, 39, 31, 23, 15, 7, 62, 54, 46, 38, 30, 22, 14, 6,
61, 53, 45, 37, 29, 21, 13, 5, 28, 20, 12, 4};
// second key-Permutation Table
int[] PC2 = {14, 17, 11, 24, 1, 5, 3, 28, 15, 6, 21, 10, 23, 19, 12, 4, 26, 8, 16, 7, 27, 20,
13, 2, 41, 52, 31, 37, 47, 55, 30, 40, 51, 45, 33, 48, 44, 49, 39, 56, 34, 53, 46, 42,
50, 36, 29, 32};
// Expansion D-box Table
int[] EP = {32, 1, 2, 3, 4, 5, 4, 5, 6, 7, 8, 9, 8, 9, 10, 11, 12, 13, 12, 13, 14, 15, 16, 17,
16, 17, 18, 19, 20, 21, 20, 21, 22, 23, 24, 25, 24, 25, 26, 27, 28, 29, 28, 29, 30, 31,
32, 1};
// Straight Permutation Table
int[] P = {16, 7, 20, 21, 29, 12, 28, 17, 1, 15, 23, 26, 5, 18, 31, 10, 2, 8, 24, 14, 32, 27, 3,
9, 19, 13, 30, 6, 22, 11, 4, 25};
// S-box Table
int[][][] sbox = {
{{14, 4, 13, 1, 2, 15, 11, 8, 3, 10, 6, 12, 5, 9, 0, 7},
{0, 15, 7, 4, 14, 2, 13, 1, 10, 6, 12, 11, 9, 5, 3, 8},
{4, 1, 14, 8, 13, 6, 2, 11, 15, 12, 9, 7, 3, 10, 5, 0},
{15, 12, 8, 2, 4, 9, 1, 7, 5, 11, 3, 14, 10, 0, 6, 13}},
{{15, 1, 8, 14, 6, 11, 3, 4, 9, 7, 2, 13, 12, 0, 5, 10},
{3, 13, 4, 7, 15, 2, 8, 14, 12, 0, 1, 10, 6, 9, 11, 5},
{0, 14, 7, 11, 10, 4, 13, 1, 5, 8, 12, 6, 9, 3, 2, 15},
{13, 8, 10, 1, 3, 15, 4, 2, 11, 6, 7, 12, 0, 5, 14, 9}},
{{10, 0, 9, 14, 6, 3, 15, 5, 1, 13, 12, 7, 11, 4, 2, 8},
{13, 7, 0, 9, 3, 4, 6, 10, 2, 8, 5, 14, 12, 11, 15, 1},
{13, 6, 4, 9, 8, 15, 3, 0, 11, 1, 2, 12, 5, 10, 14, 7},
{1, 10, 13, 0, 6, 9, 8, 7, 4, 15, 14, 3, 11, 5, 2, 12}},
{{7, 13, 14, 3, 0, 6, 9, 10, 1, 2, 8, 5, 11, 12, 4, 15},
{13, 8, 11, 5, 6, 15, 0, 3, 4, 7, 2, 12, 1, 10, 14, 9},
{10, 6, 9, 0, 12, 11, 7, 13, 15, 1, 3, 14, 5, 2, 8, 4},
{3, 15, 0, 6, 10, 1, 13, 8, 9, 4, 5, 11, 12, 7, 2, 14}},
{{2, 12, 4, 1, 7, 10, 11, 6, 8, 5, 3, 15, 13, 0, 14, 9},
{14, 11, 2, 12, 4, 7, 13, 1, 5, 0, 15, 10, 3, 9, 8, 6},
{4, 2, 1, 11, 10, 13, 7, 8, 15, 9, 12, 5, 6, 3, 0, 14},
{11, 8, 12, 7, 1, 14, 2, 13, 6, 15, 0, 9, 10, 4, 5, 3}},
{{12, 1, 10, 15, 9, 2, 6, 8, 0, 13, 3, 4, 14, 7, 5, 11},
{10, 15, 4, 2, 7, 12, 9, 5, 6, 1, 13, 14, 0, 11, 3, 8},
{9, 14, 15, 5, 2, 8, 12, 3, 7, 0, 4, 10, 1, 13, 11, 6},
{4, 3, 2, 12, 9, 5, 15, 10, 11, 14, 1, 7, 6, 0, 8, 13}},
{{4, 11, 2, 14, 15, 0, 8, 13, 3, 12, 9, 7, 5, 10, 6, 1},
{13, 0, 11, 7, 4, 9, 1, 10, 14, 3, 5, 12, 2, 15, 8, 6},
{1, 4, 11, 13, 12, 3, 7, 14, 10, 15, 6, 8, 0, 5, 9, 2},
{6, 11, 13, 8, 1, 4, 10, 7, 9, 5, 0, 15, 14, 2, 3, 12}},
{{13, 2, 8, 4, 6, 15, 11, 1, 10, 9, 3, 14, 5, 0, 12, 7},
{1, 15, 13, 8, 10, 3, 7, 4, 12, 5, 6, 11, 0, 14, 9, 2},
{7, 11, 4, 1, 9, 12, 14, 2, 0, 6, 10, 13, 15, 3, 5, 8},
{2, 1, 14, 7, 4, 10, 8, 13, 15, 12, 9, 0, 3, 5, 6, 11}}};
int[] shiftBits = {1, 1, 2, 2, 2, 2, 2, 2, 1, 2, 2, 2, 2, 2, 2, 1};
// hexadecimal to binary conversion
String hextoBin(String input) {
int n = input.length() * 4;
input = Long.toBinaryString(Long.parseUnsignedLong(input, 16));
while (input.length() < n)
input = "0" + input;
return input;
}
// binary to hexadecimal conversion
String binToHex(String input) {
int n = (int) input.length() / 4;
input = Long.toHexString(Long.parseUnsignedLong(input, 2));
while (input.length() < n)
input = "0" + input;
return input;
}
/***
* per-mutate input hexadecimal according to specified sequence
*
* @param sequence permutation table
* @param input
* @return
*/
String permutation(int[] sequence, String input) {
String output = "";
input = hextoBin(input);
for (int i = 0; i < sequence.length; i++)
output += input.charAt(sequence[i] - 1);
output = binToHex(output);
return output;
}
// xor 2 hexadecimal strings
String xor(String a, String b) {
// hexadecimal to decimal(base 10)
long t_a = Long.parseUnsignedLong(a, 16);
// hexadecimal to decimal(base 10)
long t_b = Long.parseUnsignedLong(b, 16);
// xor
t_a = t_a ^ t_b;
// decimal to hexadecimal
a = Long.toHexString(t_a);
// prepend 0's to maintain length
while (a.length() < b.length())
a = "0" + a;
return a;
}
// left Circular Shifting bits
String leftCircularShift(String input, int numBits) {
int n = input.length() * 4;
int perm[] = new int[n];
for (int i = 0; i < n - 1; i++)
perm[i] = (i + 2);
perm[n - 1] = 1;
while (numBits-- > 0)
input = permutation(perm, input);
return input;
}
// preparing 16 keys for 16 rounds
String[] getKeys(String key) {
String keys[] = new String[16];
// first key permutation
key = permutation(PC1, key);
for (int i = 0; i < 16; i++) {
key = leftCircularShift(key.substring(0, 7), shiftBits[i])
+ leftCircularShift(key.substring(7, 14), shiftBits[i]);
// second key permutation
keys[i] = permutation(PC2, key);
}
return keys;
}
// s-box lookup
String sBox(String input) {
String output = "";
input = hextoBin(input);
for (int i = 0; i < 48; i += 6) {
String temp = input.substring(i, i + 6);
int num = i / 6;
int row = Integer.parseInt(temp.charAt(0) + "" + temp.charAt(5), 2);
int col = Integer.parseInt(temp.substring(1, 5), 2);
output += Integer.toHexString(sbox[num][row][col]);
}
return output;
}
/***
* Round function that takes 64-bit and 48-bit key Gives a 64-bit output
*
* There will be 16 rounds in DES
*
* @param input 64-bit
* @param key 48-bit
* @param num Round Number
* @return String 64-bit
*/
String round(String input, String key, int num) {
// fk
String left = input.substring(0, 8);
String temp = input.substring(8, 16);
String right = temp;
// Expansion permutation
temp = permutation(EP, temp);
// xor temp and round key
temp = xor(temp, key);
// lookup in s-box table
temp = sBox(temp);
// Straight D-box
temp = permutation(P, temp);
// xor
left = xor(left, temp);
// System.out.println("Round " + (num + 1) + " " + right.toUpperCase() + " "
// + left.toUpperCase() + " " + key.toUpperCase());
// swapper
return right + left;
}
/***
* Whole DES encryption process.
*
* @param plainText String 64-bit
* @param key String 64-bit
* @return String 64-bit encrypted cipher text
*/
public String encrypt(String plainText, String key) {
int i;
// get round keys
String keys[] = getKeys(key);
// Initial permutation
plainText = permutation(IP, plainText);
// System.out.println("After initial permutation: " + plainText.toUpperCase());
// System.out.println("After splitting: L0=" + plainText.substring(0, 8).toUpperCase() +
// "R0="
// + plainText.substring(8, 16).toUpperCase() + "\n");
// 16 rounds
for (i = 0; i < 16; i++) {
plainText = round(plainText, keys[i], i);
}
// 32-bit swap
plainText = plainText.substring(8, 16) + plainText.substring(0, 8);
// Final permutation
plainText = permutation(IP1, plainText);
return plainText;
}
/***
* Whole DES decryption process.
*
* @param cipherText String 64-bit
* @param key String 64-bit
* @return String 64-bit encrypted cipher text
*/
String decrypt(String cipherText, String key) {
int i;
// get round keys
String keys[] = getKeys(key);
// Initial permutation
cipherText = permutation(IP, cipherText);
// System.out.println("After initial permutation: " + cipherText.toUpperCase());
// System.out.println("After splitting: L0=" + cipherText.substring(0, 8).toUpperCase()
// + " R0=" + cipherText.substring(8, 16).toUpperCase() + "\n");
// 16-rounds
for (i = 15; i > -1; i--) {
cipherText = round(cipherText, keys[i], 15 - i);
}
// 32-bit swap
cipherText = cipherText.substring(8, 16) + cipherText.substring(0, 8);
// Final permutation
cipherText = permutation(IP1, cipherText);
// System.out.println("After final permutation: " + cipherText.toUpperCase());
return cipherText;
}
}
|
code/cryptography/src/des_cipher/des_java/Main.java | import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Arrays;
import java.util.Scanner;
public class Main {
public static void writeToCipherFile(String file_path, String cipher_text) {
try {
FileWriter myWriter = new FileWriter(file_path);
myWriter.write(cipher_text);
myWriter.close();
} catch (IOException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
}
/***
* Parsing the text from the file Converting it to byte arrays Converting it ot 64-bit blocks
*
* @param file_path
* @param block_size
* @return
*/
public String[] desPlaintextBlock(String file_path, int block_size) {
String plain_text = "";
try {
File myObj = new File(file_path);
Scanner myReader = new Scanner(myObj);
while (myReader.hasNextLine()) {
plain_text += myReader.nextLine().toUpperCase();
}
myReader.close();
} catch (FileNotFoundException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
byte[] byte_array = plain_text.getBytes();
plain_text = "";
for (byte value : byte_array) {
plain_text += value;
}
// System.out.println(plain_text);
return split(plain_text, block_size);
}
/***
* Converting blocks of decrypted text back into byte array then into String
*
* @param blocks
* @return String
*/
public static String blocksToPlainText(String[] blocks) {
String decrypted_blocks = String.join("", blocks);
writeToCipherFile("files/cipher.txt", decrypted_blocks);
String[] decrypted_blocks_array = decrypted_blocks.split("(?<=\\G.{2})");
byte[] byte_array = new byte[decrypted_blocks_array.length];
for (int i = 0; i < byte_array.length; i++) {
byte_array[i] = (byte) Integer.parseInt(decrypted_blocks_array[i]);
}
return new String(byte_array);
}
/***
* If a block size is less than 64-bit padding it with zeros
*
* @param inputString
* @param length
* @return
*/
public String padLeftZeros(String inputString, int length) {
if (inputString.length() >= length) {
return inputString;
}
StringBuilder sb = new StringBuilder();
while (sb.length() < length - inputString.length()) {
sb.append('0');
}
sb.append(inputString);
return sb.toString();
}
/**
* Splitting the string into desired blocks
*/
public String[] split(String src, int len) {
String[] result = new String[(int) Math.ceil((double) src.length() / (double) len)];
for (int i = 0; i < result.length; i++)
result[i] = src.substring(i * len, Math.min(src.length(), (i + 1) * len));
result[result.length - 1] = padLeftZeros(result[result.length - 1], len);
return result;
}
// Driver code
public static void main(String args[]) {
// READING KEY
String key = "";
try {
File myObj = new File("files/key.txt");
Scanner myReader = new Scanner(myObj);
while (myReader.hasNextLine()) {
key += myReader.nextLine().toUpperCase();
}
myReader.close();
} catch (FileNotFoundException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
Main driver = new Main();
// PARSING TEXT
String[] blocks = driver.desPlaintextBlock("files/msg.txt", 16);
String[] cipher_blocks = blocks;
System.out.println("\n== ENCRYPTION ==");
System.out.println("\n== 64-bit blocks of file ==\n");
System.out.println(Arrays.toString(blocks));
System.out.println();
// ENCRYPTING
DES cipher = new DES();
for (int i = 0; i < blocks.length; i++) {
cipher_blocks[i] = cipher.encrypt(blocks[i], key);
}
System.out.println("\n== CIPHERED BLOCKS ==\n");
System.out.println(Arrays.toString(cipher_blocks));
System.out.println("\n== DECRYPTION ==");
// DECRYPTING
for (int i = 0; i < cipher_blocks.length; i++) {
blocks[i] = cipher.decrypt(blocks[i], key);
}
System.out.println("\n== DECRYPTED BLOCKS ==\n");
System.out.println(Arrays.toString(blocks));
System.out.println();
// BYTE ARR TO STRING
System.out.println("\n== PLAIN TEXT ==\n");
System.out.println(blocksToPlainText(blocks));
}
}
|
code/cryptography/src/des_cipher/des_java/README.MD | # Explanation
- KeyFile = _files:/key.txt_
- MsgFile = _files:/msg.txt_
- CphFile = _files:/cipher.txt_
**1. `desPlaintextBlock()` that takes the plaintext as input and splits it up into 64 bit blocks. If the last block is less than 64 bits, pad it with 0s.**
```
public String[] desPlaintextBlock(String file_path, int block_size) {
String plain_text = "";
try {
File myObj = new File(file_path);
Scanner myReader = new Scanner(myObj);
while (myReader.hasNextLine()) {
plain_text += myReader.nextLine().toUpperCase();
}
myReader.close();
} catch (FileNotFoundException e) {
System.out.println("An error occurred.");
e.printStackTrace();
}
return split(plain_text, block_size);
}
public String padLeftZeros(String inputString, int length) {
if (inputString.length() >= length) {
return inputString;
}
StringBuilder sb = new StringBuilder();
while (sb.length() < length - inputString.length()) {
sb.append('0');
}
sb.append(inputString);
return sb.toString();
}
public String[] split(String src, int len) {
String[] result = new String[(int) Math.ceil((double) src.length() / (double) len)];
for (int i = 0; i < result.length; i++)
result[i] = src.substring(i * len, Math.min(src.length(), (i + 1) * len));
result[result.length - 1] = padLeftZeros(result[result.length - 1], len);
return result;
}
```
**2. `permutation()` that takes a 64-bit block, performs permutation according to the 8 x 8 IP table and returns the permuted block.**
CONSTANTS:
```
// CONSTANTS
// Initial Permutation Table
int[] IP = {58, 50, 42, 34, 26, 18, 10, 2, 60, 52, 44, 36, 28, 20, 12, 4, 62, 54, 46, 38, 30,
22, 14, 6, 64, 56, 48, 40, 32, 24, 16, 8, 57, 49, 41, 33, 25, 17, 9, 1, 59, 51, 43, 35,
27, 19, 11, 3, 61, 53, 45, 37, 29, 21, 13, 5, 63, 55, 47, 39, 31, 23, 15, 7};
```
METHOD:
```
/***
* per-mutate input hexadecimal
* according to specified sequence
*
* @param sequence permutation table
* @param input
* @return
*/
String permutation(int[] sequence, String input) {
String output = "";
input = hextoBin(input);
for (int i = 0; i < sequence.length; i++)
output += input.charAt(sequence[i] - 1);
output = binToHex(output);
return output;
}
```
USAGE:
```
// Initial permutation
plainText = permutation(IP, plainText);
```
**3. FinalPermutation that takes a 64-bit block, performs permutation according to the 8 x 8 FP table and returns the permuted block.**
```
// Inverse Initial Permutation Table
int[] IP1 = {40, 8, 48, 16, 56, 24, 64, 32, 39, 7, 47, 15, 55, 23, 63, 31, 38, 6, 46, 14, 54,
22, 62, 30, 37, 5, 45, 13, 53, 21, 61, 29, 36, 4, 44, 12, 52, 20, 60, 28, 35, 3, 43, 11,
51, 19, 59, 27, 34, 2, 42, 10, 50, 18, 58, 26, 33, 1, 41, 9, 49, 17, 57, 25};
USAGE:
// Final permutation
plainText = permutation(IP1, plainText);
```
|
code/cryptography/src/des_cipher/des_java/files/cipher.txt | 84727383327383328385808079836968328479326669326978678289808469683287697676326578683265768379326869678289808469680000003287697676 |
code/cryptography/src/des_cipher/des_java/files/key.txt | AABB09182736CCDD |
code/cryptography/src/des_cipher/des_java/files/msg.txt | this is supposed to be encrypted well and also decrypted well |
code/cryptography/src/elgamal/README.md | # ElGamal encryption
The ElGamal encryption system is an asymmetric key encryption algorithm for public-key cryptography .
ElGamal encryption can be defined over any cyclic group $G$, like multiplicative group of integers modulo n. Its security depends upon the difficulty of a certain problem in $G$ related to computing discrete logarithms.
## Sources and more detailed information:
https://en.wikipedia.org/wiki/ElGamal_encryption
|
code/cryptography/src/elgamal/elgamal.py | # ElGamal encryption
# pip install pycryptodome
from Crypto.Util import number
# Key generation algorithm
def elgamal_gen_key(bits):
# Prime number p
while True:
q = number.getPrime(bits - 1)
p = 2 * q + 1
if number.isPrime(p):
break
# Primitive element g
while True:
g = number.getRandomRange(3, p)
# Primitive element judgment
if pow(g, 2, p) == 1:
continue
if pow(g, q, p) == 1:
continue
break
# Secret value x
x = number.getRandomRange(2, p - 1)
# Public value y
y = pow(g, x, p)
return (p, g, y), x
# Encryption algorithm
def elgamal_encrypt(m, pk):
p, g, y = pk
assert 0 <= m < p
r = number.getRandomRange(2, p - 1)
c1 = pow(g, r, p)
c2 = (m * pow(y, r, p)) % p
return (c1, c2)
# Decryption algorithm
def elgamal_decrypt(c, pk, sk):
p, g, y = pk
c1, c2 = c
return (c2 * pow(c1, p - 1 - sk, p)) % p
pk, sk = elgamal_gen_key(bits=20)
print("pk:", pk) # Public key
print("sk:", sk) # Private key
print()
m = 314159 # Plain text
print("m:", m)
c = elgamal_encrypt(m, pk) # Encryption
print("c:", c)
d = elgamal_decrypt(c, pk, sk) # Decryption
print("d:", d)
|
code/cryptography/src/hill_cipher/hill_cipher.py | # Python Hill Cipher
keyMatrix = [[0] * 3 for i in range(3)]
messageVector = [[0] for i in range(3)]
cipherMatrix = [[0] for i in range(3)]
def getKeyMatrix(key):
k = 0
for i in range(3):
for j in range(3):
keyMatrix[i][j] = ord(key[k]) % 65
k += 1
def encrypt(messageVector):
for i in range(3):
for j in range(1):
cipherMatrix[i][j] = 0
for x in range(3):
cipherMatrix[i][j] += (keyMatrix[i][x] *
messageVector[x][j])
cipherMatrix[i][j] = cipherMatrix[i][j] % 26
def HillCipher(message, key):
getKeyMatrix(key)
for i in range(3):
messageVector[i][0] = ord(message[i]) % 65
encrypt(messageVector)
CipherText = []
for i in range(3):
CipherText.append(chr(cipherMatrix[i][0] + 65))
print("Ciphertext: ", "".join(CipherText))
# Driver Code
def main():
message = "ACT"
key = "GYBNQKURP"
HillCipher(message, key)
if __name__ == "__main__":
main()
|
code/cryptography/src/huffman_encoding/README.md | # Huffman Encoding
Huffman Encoding is a famous greedy algorithm that is used for the loseless compression of file/data. It uses variable length encoding where variable length codes are assigned to all the characters depending on how frequently they occur in the given text. The character which occurs most frequently gets the smallest code and the character which occurs least frequently gets the largest code.
## Sources and more detailed information:
- https://iq.opengenus.org/huffman-encoding/
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
code/cryptography/src/huffman_encoding/huffman.java | import java.io.File;
import java.io.FileNotFoundException;
import java.util.PriorityQueue;
import java.util.Scanner;
import java.util.TreeMap;
/* Huffman coding , decoding */
public class Huffman {
static final boolean readFromFile = false;
static final boolean newTextBasedOnOldOne = false;
static PriorityQueue<Node> nodes = new PriorityQueue<>((o1, o2) -> (o1.value < o2.value) ? -1 : 1);
static TreeMap<Character, String> codes = new TreeMap<>();
static String text = "";
static String encoded = "";
static String decoded = "";
static int ASCII[] = new int[128];
public static void main(String[] args) throws FileNotFoundException {
Scanner scanner = (readFromFile) ? new Scanner(new File("input.txt")) : new Scanner(System.in);
int decision = 1;
while (decision != -1) {
if (handlingDecision(scanner, decision)) continue;
decision = consoleMenu(scanner);
}
}
private static int consoleMenu(Scanner scanner) {
int decision;
System.out.println("\n---- Menu ----\n" +
"-- [-1] to exit \n" +
"-- [1] to enter new text\n" +
"-- [2] to encode a text\n" +
"-- [3] to decode a text");
decision = Integer.parseInt(scanner.nextLine());
if (readFromFile)
System.out.println("Decision: " + decision + "\n---- End of Menu ----\n");
return decision;
}
private static boolean handlingDecision(Scanner scanner, int decision) {
if (decision == 1) {
if (handleNewText(scanner)) return true;
} else if (decision == 2) {
if (handleEncodingNewText(scanner)) return true;
} else if (decision == 3) {
handleDecodingNewText(scanner);
}
return false;
}
private static void handleDecodingNewText(Scanner scanner) {
System.out.println("Enter the text to decode:");
encoded = scanner.nextLine();
System.out.println("Text to Decode: " + encoded);
decodeText();
}
private static boolean handleEncodingNewText(Scanner scanner) {
System.out.println("Enter the text to encode:");
text = scanner.nextLine();
System.out.println("Text to Encode: " + text);
if (!IsSameCharacterSet()) {
System.out.println("Not Valid input");
text = "";
return true;
}
encodeText();
return false;
}
private static boolean handleNewText(Scanner scanner) {
int oldTextLength = text.length();
System.out.println("Enter the text:");
text = scanner.nextLine();
if (newTextBasedOnOldOne && (oldTextLength != 0 && !IsSameCharacterSet())) {
System.out.println("Not Valid input");
text = "";
return true;
}
ASCII = new int[128];
nodes.clear();
codes.clear();
encoded = "";
decoded = "";
System.out.println("Text: " + text);
calculateCharIntervals(nodes, true);
buildTree(nodes);
generateCodes(nodes.peek(), "");
printCodes();
System.out.println("-- Encoding/Decoding --");
encodeText();
decodeText();
return false;
}
private static boolean IsSameCharacterSet() {
boolean flag = true;
for (int i = 0; i < text.length(); i++)
if (ASCII[text.charAt(i)] == 0) {
flag = false;
break;
}
return flag;
}
private static void decodeText() {
decoded = "";
Node node = nodes.peek();
for (int i = 0; i < encoded.length(); ) {
Node tmpNode = node;
while (tmpNode.left != null && tmpNode.right != null && i < encoded.length()) {
if (encoded.charAt(i) == '1')
tmpNode = tmpNode.right;
else tmpNode = tmpNode.left;
i++;
}
if (tmpNode != null)
if (tmpNode.character.length() == 1)
decoded += tmpNode.character;
else
System.out.println("Input not Valid");
}
System.out.println("Decoded Text: " + decoded);
}
private static void encodeText() {
encoded = "";
for (int i = 0; i < text.length(); i++)
encoded += codes.get(text.charAt(i));
System.out.println("Encoded Text: " + encoded);
}
private static void buildTree(PriorityQueue<Node> vector) {
while (vector.size() > 1)
vector.add(new Node(vector.poll(), vector.poll()));
}
private static void printCodes() {
System.out.println("--- Printing Codes ---");
codes.forEach((k, v) -> System.out.println("'" + k + "' : " + v));
}
private static void calculateCharIntervals(PriorityQueue<Node> vector, boolean printIntervals) {
if (printIntervals) System.out.println("-- intervals --");
for (int i = 0; i < text.length(); i++)
ASCII[text.charAt(i)]++;
for (int i = 0; i < ASCII.length; i++)
if (ASCII[i] > 0) {
vector.add(new Node(ASCII[i] / (text.length() * 1.0), ((char) i) + ""));
if (printIntervals)
System.out.println("'" + ((char) i) + "' : " + ASCII[i] / (text.length() * 1.0));
}
}
private static void generateCodes(Node node, String s) {
if (node != null) {
if (node.right != null)
generateCodes(node.right, s + "1");
if (node.left != null)
generateCodes(node.left, s + "0");
if (node.left == null && node.right == null)
codes.put(node.character.charAt(0), s);
}
}
}
class Node {
Node left, right;
double value;
String character;
public Node(double value, String character) {
this.value = value;
this.character = character;
left = null;
right = null;
}
public Node(Node left, Node right) {
this.value = left.value + right.value;
character = left.character + right.character;
if (left.value < right.value) {
this.right = right;
this.left = left;
} else {
this.right = left;
this.left = right;
}
}
} |
code/cryptography/src/huffman_encoding/huffman.py | # A Huffman Tree Node
class Node:
def __init__(self, prob, symbol, left=None, right=None):
# probability of symbol
self.prob = prob
# symbol
self.symbol = symbol
# left node
self.left = left
# right node
self.right = right
# tree direction (0/1)
self.code = ''
""" A helper function to print the codes of symbols by traveling Huffman Tree"""
codes = dict()
def Calculate_Codes(node, val=''):
# huffman code for current node
newVal = val + str(node.code)
if(node.left):
Calculate_Codes(node.left, newVal)
if(node.right):
Calculate_Codes(node.right, newVal)
if(not node.left and not node.right):
codes[node.symbol] = newVal
return codes
""" A helper function to calculate the probabilities of symbols in given data"""
def Calculate_Probability(data):
symbols = dict()
for element in data:
if symbols.get(element) == None:
symbols[element] = 1
else:
symbols[element] += 1
return symbols
""" A helper function to obtain the encoded output"""
def Output_Encoded(data, coding):
encoding_output = []
for c in data:
# print(coding[c], end = '')
encoding_output.append(coding[c])
string = ''.join([str(item) for item in encoding_output])
return string
""" A helper function to calculate the space difference between compressed and non-compressed data"""
def Total_Gain(data, coding):
before_compression = len(data) * 8 # total bit space to stor the data before compression
after_compression = 0
symbols = coding.keys()
for symbol in symbols:
count = data.count(symbol)
after_compression += count * len(coding[symbol]) # calculate how many bit is required for that symbol in total
print("Space usage before compression (in bits):", before_compression)
print("Space usage after compression (in bits):", after_compression)
def Huffman_Encoding(data):
symbol_with_probs = Calculate_Probability(data)
symbols = symbol_with_probs.keys()
probabilities = symbol_with_probs.values()
print("symbols: ", symbols)
print("probabilities: ", probabilities)
nodes = []
# converting symbols and probabilities into huffman tree nodes
for symbol in symbols:
nodes.append(Node(symbol_with_probs.get(symbol), symbol))
while len(nodes) > 1:
# sort all the nodes in ascending order based on their probability
nodes = sorted(nodes, key=lambda x: x.prob)
# pick 2 smallest nodes
right = nodes[0]
left = nodes[1]
left.code = 0
right.code = 1
# combine the 2 smallest nodes to create new node
newNode = Node(left.prob+right.prob, left.symbol+right.symbol, left, right)
nodes.remove(left)
nodes.remove(right)
nodes.append(newNode)
huffman_encoding = Calculate_Codes(nodes[0])
print("symbols with codes", huffman_encoding)
Total_Gain(data, huffman_encoding)
encoded_output = Output_Encoded(data,huffman_encoding)
return encoded_output, nodes[0]
def Huffman_Decoding(encoded_data, huffman_tree):
tree_head = huffman_tree
decoded_output = []
for x in encoded_data:
if x == '1':
huffman_tree = huffman_tree.right
elif x == '0':
huffman_tree = huffman_tree.left
try:
if huffman_tree.left.symbol == None and huffman_tree.right.symbol == None:
pass
except AttributeError:
decoded_output.append(huffman_tree.symbol)
huffman_tree = tree_head
string = ''.join([str(item) for item in decoded_output])
return string |
code/cryptography/src/huffman_encoding/huffman_encoding.c | #include <stdio.h>
#include <stdlib.h>
typedef struct node{
int freq;
char alpha[26];
struct node *next;
struct node *left;
struct node *right;
struct node *parent;
}node;
node* getnode(){
node* newnode = (node*)malloc(sizeof(node));
newnode->freq = -1;
int i;
for(i=0;i<26;i++)newnode->alpha[i] = '$';
newnode->next = NULL;
newnode->left = NULL;
newnode->right = NULL;
newnode->parent = NULL;
return newnode;
}
node* adds(node* headl,node* temp){
if(headl == NULL){
headl = temp;
return headl;
}
else{
temp->next = headl;
headl = temp;
return headl;
}
}
node* addl(node* taill,node* temp){
if(taill == NULL){
taill = temp;
return taill;
}
else{
taill->next = temp;
taill = temp;
return taill;
}
}
node* addbw(node* smaller,node* temp,node* greater){
temp->next = greater;
smaller->next = temp;
return temp;
}
node* addafterp(node* p, node* temp){
node *te = p->next;
temp = addbw(p,temp,te);
return temp;
}
void printl(node* headl){
while(headl != NULL){
printf("%c%d ",headl->alpha[0],headl->freq);
headl = headl->next;
}
}
node* search(node* headl,node *temp){
node* t1 = headl;
node* t2 = headl;
if(t2->next != NULL){
t2 = t2->next;
}
if(t2 == headl){
if(t2->freq > temp->freq){
headl = adds(t2,temp);
return headl;
}
else if(t2->freq < temp->freq){
temp = addl(t2,temp);
return headl;
}
else if(t2->freq == temp->freq){
if(t2->alpha[0] > temp->alpha[0]){
temp = addl(t2,temp);
return headl;
}
else if(t2->alpha[0] < temp->alpha[0]){
temp = adds(t2,temp);
return headl;
}
}
}
while(t1 != t2){
if(temp->freq < t1->freq){
temp->next = t1;
headl = temp;
return headl;
}
else if(temp->freq >t2->freq){
if(t2->next == NULL){
temp = addl(t2,temp);
return headl;
}
else if(temp->freq > t2->freq && temp->freq < (t2->next)->freq){
temp = addafterp(t2,temp);
return headl;
}
}
else if(t1->freq < temp->freq && t2->freq > temp->freq){
temp = addbw(t1,temp,t2);
return headl;
}
else if(t1->freq < temp->freq && t2->freq == temp->freq){
if(temp->alpha[0] < t2->alpha[0]){
temp = addbw(t1,temp,t2);
return headl;
}
if(t2->next == NULL){
temp = addl(t2,temp);
return headl;
}
}
else if(t1->freq == temp->freq && t2->freq == temp->freq){
if(temp->alpha[0] < t2->alpha[0] && temp->alpha[0] > t1->alpha[0]){
temp = addbw(t1,temp,t2);
return headl;
}
if(t2->next == NULL){
temp = addl(t2,temp);
return headl;
}
}
else if(t1->freq >temp->freq && t2->next == NULL){
headl = adds(t1,temp);
return headl;
}
else if(t1->freq == temp->freq && t2->freq > temp->freq){
if(temp->alpha[0] > t1->alpha[0]){
temp = addbw(t1,temp,t2);
return headl;
}
else{
headl = adds(t1,temp);
return headl;
}
}
if(t1->next != NULL){
t1 = t1->next;
}
if(t2->next != NULL){
t2 = t2->next;
}
}
}
node* pop(node *headl){
node* temp = headl;
headl = headl->next;
temp->next = NULL;
return temp;
}
node* buildtree(node* headl){
while(headl->next != NULL){
node* t2 = headl;
headl = headl->next;
t2->next = NULL;
node* t1 = headl;
headl = headl->next;
t1->next = NULL;
node* par = getnode();
par->left = t2;
par->right = t1;
par->alpha[0] = (par->left)->alpha[0];
par->freq = t1->freq + t2->freq;
t1->parent = par;
t2->parent = par;
if(headl == NULL){
headl = par;
}
else{
headl = search(headl,par);
}
}
return headl;
}
void printleaf(node *headl){
if(headl == NULL)return;
else
{
if(headl->left == NULL && headl->right == NULL)printf("%c ",headl->alpha[0]);
else{
printleaf(headl->left);
printleaf(headl->right);
}
}
}
void printarr(int *arr, int n)
{
int i;
for (i = 0; i < n; ++i)printf("%d", arr[i]);
printf("\n");
}
void printcodes(node* headl, int *arr, int top)
{
if (headl->left)
{
arr[top] = 0;
printcodes(headl->left, arr, top + 1);
}
if (headl->right)
{
arr[top] = 1;
printcodes(headl->right, arr, top + 1);
}
if (headl->left == NULL && headl->right == NULL)
{
printf("%c: ", headl->alpha[0]);
printarr(arr, top);
}
}
int main(){
node *head = getnode();
char input[100];
int top =0,result[20];
printf("Enter the string to be coded: ");
scanf("%s",input);
int frequency[26] = {0};
int d,i;
for(i=0;input[i] != '\0';i++){
d = input[i];
d -= 97;
frequency[d]++;
}
int f=0;
for(i=0;i<26;i++){
if(frequency[i] != 0){
if(f==0){
head->alpha[0] = i+97;
head->freq = frequency[i];
f=1;
}
else
{
node *t1 = getnode();
t1->alpha[0] = i+97;
t1->freq = frequency[i];
head = search(head,t1);
}
}
}
head = buildtree(head);
printf("List of codes: \n");
printcodes(head,result,top);
return 0;
}
|
code/cryptography/src/monoalphabetic_cipher/monoalphabetic.py | import random
alpha = "abcdefghijklmnopqrstuvwxyz"
#Encrypts the plain text message
def encrypt(original, key=None):
if key is None:
l = list(alpha)
random.shuffle(l)
key = "".join(l)
new = []
for letter in original:
new.append(key[alpha.index(letter)])
return ["".join(new), key]
#Decrypts the encrypted message
def decrypt(cipher, key=None):
if key is not None:
new = []
for letter in cipher:
new.append(alpha[key.index(letter)])
return "".join(new)
e = encrypt('monoalphabetic', None)
print(e) #Prints encrypted message
print(decrypt(e[0], e[1])) #Decodes the message and prints it |
code/cryptography/src/monoalphabetic_cipher/monoalphabeticCipher.py | keyDict = {}
# Enter a custom map for the monoalphabetic encryption mapping
def create_Map():
print("Enter the monoalphabetic map as a string(lenght 26)")
inputMap = input()
cur = "a"
curIndex = 0
while curIndex < 26:
keyDict[cur] = inputMap[curIndex]
cur = chr(ord(cur) + 1)
curIndex += 1
# Return the corresponding decrypted char for a given input char
def get_key(value):
for key, val in keyDict.items():
if val == value:
return key
# Encrypt the input text
def monoalphabetic_encrypt():
plainText = input("Enter plain text-")
wordList = plainText.split(" ")
cipherText = ""
for word in wordList:
c = ""
for i in word:
i = keyDict[i]
c += i
cipherText += c + " "
cipherText = cipherText[:-1]
return cipherText
# De-crypt the input text
def monoalphabetic_decrypt():
cipherText = input("Enter cipher text-")
wordList = cipherText.split(" ")
plainText = ""
for word in wordList:
c = ""
for i in word:
i = get_key(i)
c += i
plainText += c + " "
plainText = plainText[:-1]
return plainText
create_Map()
print(monoalphabetic_encrypt())
print(monoalphabetic_decrypt())
|
code/cryptography/src/morse_cipher/README.md | # cosmos
Your personal library of every algorithm and data structure code that you will ever encounter
|
code/cryptography/src/morse_cipher/morse_code.java | // Part of Cosmos by OpenGenus Foundation
import java.util.HashMap;
import java.util.Map;
public class MorseCode {
private Map<String, String> engToMorse;
private Map<String, String> morseToEng;
private static final String rawMorseCodes = "A:.-|B:-...|C:-.-.|D:-..|E:.|F:..-.|G:--.|H:....|I:..|J:.---|K:-.-|L:.-..|M:--|N:-.|O:---|P:.--.|Q:--.-|R:.-.|S:...|T:-|U:..-|V:...-|W:.--|X:-..-|Y:-.--|Z:--..|1:.----|2:..---|3:...--|4:....-|5:.....|6:-....|7:--...|8:---..|9:----.|0:-----|,:--..--|.:.-.-.-|?:..--..|/:-..-.|-:-....-|(:-.--.|):-.--.-";
public MorseCode() {
engToMorse = new HashMap<String, String>();
morseToEng = new HashMap<String, String>();
String[] morseCodes = rawMorseCodes.split("\\|");
for (String morseCode: morseCodes) {
String[] keyValue = morseCode.split(":");
String english = keyValue[0];
String morse = keyValue[1];
engToMorse.put(english, morse);
morseToEng.put(morse, english);
}
}
public String encrypt(String english) {
String cipherText = "";
english = english.toUpperCase();
for (int i = 0; i < english.length(); i++) {
if (english.charAt(i) != ' ') {
cipherText += engToMorse.get(String.valueOf(english.charAt(i)));
cipherText += ' ';
}
else {
cipherText += ' ';
}
}
return cipherText;
}
public String decrypt(String morse) {
String plainText = "";
String[] words = morse.split("\\s{2}");
for (String word : words) {
String[] letters = word.split("\\s");
for (String letter : letters) {
plainText += morseToEng.get(letter);
}
plainText += " ";
}
return plainText;
}
public static void main(String[] args) {
String msg = "alice killed bob";
String morseMsg = ".- .-.. .. -.-. . -.- .. .-.. .-.. . -.. -... --- -...";
MorseCode mCode = new MorseCode();
System.out.println(mCode.encrypt(msg));
System.out.println(mCode.decrypt(morseMsg));
}
}
|
code/cryptography/src/morse_cipher/morse_code.sh | #!/bin/bash
#get the file and be ready to decrypt one time internet file download is required
pwd=$PWD
#check if file doesnt exist
if ! [[ -f "$pwd/morse_code.txt" ]]
then
curl http://www.qsl.net/we6w/text/morse.txt >> morse.txt 2>/dev/null
sed -i '17,367d' morse.txt
cat morse.txt|grep -vi morse > morse_code.txt
rm -f morse.txt
fi
#function to encrypt the msg
function encrypt(){
col1=`cat morse_code.txt|awk '{print $1,$2}'`
col2=`cat morse_code.txt|awk '{print $3,$4}'`
col3=`cat morse_code.txt|awk '{print $5,$6}'`
col4=`cat morse_code.txt|awk '{print $7,$8}'`
while read -n1 char
do
echo "$col1"|grep -i $char 2>/dev/null
echo "$col2"|grep -i $char 2>/dev/null
echo "$col3"|grep -i $char 2> /dev/null
echo "$col4"|grep -i $char 2> /dev/null
done<$pwd/msg.txt
}
#Program begins
read -p "Enter The Message " MS
echo "$MS" > $pwd/msg.txt
encrypt
rm -f $pwd/msg.txt
|
code/cryptography/src/morse_cipher/morse_code_generator.bf | Morse Code Generator
store slash
++++++[>+++++++<-]>+++++>
store space
++++[>++++++++<-]>>
store dash & dot
+++++[>+++++++++>+++++++++<<-]>+>>
, Prime loop
Main loop
[
--------------------------------
space [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<<< <<.>> .>>>>> [-]]
<
---------------- Shift so that 48 (char '0') is now 0
0 [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.....>> <<<<<.>>>>> [-]]
<-
1 [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>....>> <<<<<.>>>>> [-]]
<-
2 [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<..>...>> <<<<<.>>>>> [-]]
<-
3 [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<...>..>> <<<<<.>>>>> [-]]
<-
4 [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<....>.>> <<<<<.>>>>> [-]]
<-
5 [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.....>>> <<<<<.>>>>> [-]]
<-
6 [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<....>>> <<<<<.>>>>> [-]]
<-
7 [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<..<...>>> <<<<<.>>>>> [-]]
<-
8 [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<...<..>>> <<<<<.>>>>> [-]]
<-
9 [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<....<.>>> <<<<<.>>>>> [-]]
<
-------- Uppercase letters
a [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>.>> <<<<<.>>>>> [-]]
<-
b [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<...>>> <<<<<.>>>>> [-]]
<-
c [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<.>.<.>>> <<<<<.>>>>> [-]]
<-
d [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<..>>> <<<<<.>>>>> [-]]
<-
e [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>>> <<<<<.>>>>> [-]]
<-
f [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<..>.<.>>> <<<<<.>>>>> [-]]
<-
g [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<..<.>>> <<<<<.>>>>> [-]]
<-
h [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<....>>> <<<<<.>>>>> [-]]
<-
i [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<..>>> <<<<<.>>>>> [-]]
<-
j [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>...>> <<<<<.>>>>> [-]]
<-
k [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<.>.>> <<<<<.>>>>> [-]]
<-
l [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>.<..>>> <<<<<.>>>>> [-]]
<-
m [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<..>> <<<<<.>>>>> [-]]
<-
n [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<.>>> <<<<<.>>>>> [-]]
<-
o [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<...>> <<<<<.>>>>> [-]]
<-
p [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>..<.>>> <<<<<.>>>>> [-]]
<-
q [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<..<.>.>> <<<<<.>>>>> [-]]
<-
r [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>.<.>>> <<<<<.>>>>> [-]]
<-
s [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<...>>> <<<<<.>>>>> [-]]
<-
t [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.>> <<<<<.>>>>> [-]]
<-
u [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<..>.>> <<<<<.>>>>> [-]]
<-
v [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<...>.>> <<<<<.>>>>> [-]]
<-
w [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>..>> <<<<<.>>>>> [-]]
<-
x [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<..>.>> <<<<<.>>>>> [-]]
<-
y [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<.>..>> <<<<<.>>>>> [-]]
<-
z [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<..<..>>> <<<<<.>>>>> [-]]
<
-------
a [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>.>> <<<<<.>>>>> [-]]
<-
b [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<...>>> <<<<<.>>>>> [-]]
<-
c [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<.>.<.>>> <<<<<.>>>>> [-]]
<-
d [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<..>>> <<<<<.>>>>> [-]]
<-
#
e [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>>> <<<<<.>>>>> [-]]
<-
f [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<..>.<.>>> <<<<<.>>>>> [-]]
<-
g [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<..<.>>> <<<<<.>>>>> [-]]
<-
h [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<....>>> <<<<<.>>>>> [-]]
<-
i [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<..>>> <<<<<.>>>>> [-]]
<-
j [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>...>> <<<<<.>>>>> [-]]
<-
k [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<.>.>> <<<<<.>>>>> [-]]
<-
l [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>.<..>>> <<<<<.>>>>> [-]]
<-
m [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<..>> <<<<<.>>>>> [-]]
<-
n [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<.>>> <<<<<.>>>>> [-]]
<-
o [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<...>> <<<<<.>>>>> [-]]
<-
p [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>..<.>>> <<<<<.>>>>> [-]]
<-
q [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<..<.>.>> <<<<<.>>>>> [-]]
<-
r [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>.<.>>> <<<<<.>>>>> [-]]
<-
s [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<...>>> <<<<<.>>>>> [-]]
<-
t [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.>> <<<<<.>>>>> [-]]
<-
u [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<..>.>> <<<<<.>>>>> [-]]
<-
v [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<...>.>> <<<<<.>>>>> [-]]
<-
w [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<<.>..>> <<<<<.>>>>> [-]]
<-
x [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<..>.>> <<<<<.>>>>> [-]]
<-
y [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<.<.>..>> <<<<<.>>>>> [-]]
<-
z [->+>+<<]>>[-<<+>>]<<
>
[->[-]+<]+>[-<->]<
[ <<..<..>>> <<<<<.>>>>> [-]]
<-
[-]>[-]>[-]<<, Take input
]
|
code/cryptography/src/morse_cipher/morse_code_generator.c | /* Part of Cosmos by OpenGenus Foundation */
//C implementation of morse code generator
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void
encrypt(char* msg)
{
int size = strlen(msg);
char * morse_code = (char*) malloc(size * 5);
// Morse Code for the numbers [0-10]
char* num[] = {"-----", ".----", "..---", "...--", "....-", ".....", "-....", "--...", "---..", "----."};
// Morse Code for the alphabet
char* letters[] = {".-", "-...", "-.-.", "-..", ".", "..-.", "--.", "....", "..", ".---", "-.-", ".-..",
"--", "-.", "---", ".--.", "--.-", ".-.", "...", "-", "..-", "...-", ".--", "-..-", "-.--", "--.."};
int i;
for (i = 0;i < size; i++)
if (msg[i] != ' ')
if (msg[i] >= '0' && msg[i] <= '9')
strcat(morse_code, num[msg[i] - 48]);
else
strcat(morse_code, letters[msg[i] - 65]);
else
strcat(morse_code, " ");
printf("%s\n", morse_code);
free(morse_code);
}
int
main()
{
char* msg = "CONVERT THIS MORSE CODE";
encrypt(msg);
return (0);
}
|
code/cryptography/src/morse_cipher/morse_code_generator.cpp | //C++ implemention of morse code generator
#include <iostream>
using namespace std;
#include <map> //For map data structure
// Encryption Function
void encryption(string msg)
{
// Decalaring map structure and Defination of map
map<char, string> encrypt;
encrypt['A'] = ".-"; encrypt['B'] = "-..."; encrypt['C'] = "-.-."; encrypt['D'] = "-..";
encrypt['E'] = "."; encrypt['F'] = "..-."; encrypt['G'] = "--."; encrypt['H'] = "....";
encrypt['I'] = ".."; encrypt['J'] = ".---"; encrypt['K'] = "-.-"; encrypt['L'] = ".-..";
encrypt['M'] = "--"; encrypt['N'] = "-."; encrypt['O'] = "---"; encrypt['P'] = ".--.";
encrypt['Q'] = "--.-"; encrypt['R'] = ".-."; encrypt['S'] = "..."; encrypt['T'] = "-";
encrypt['U'] = "..-"; encrypt['V'] = "...-"; encrypt['W'] = ".--"; encrypt['X'] = "-..-";
encrypt['Y'] = "-.--"; encrypt['Z'] = "--..";
encrypt['1'] = ".----"; encrypt['2'] = "..---"; encrypt['3'] = "...--"; encrypt['4'] = "....-";
encrypt['5'] = "....."; encrypt['6'] = "-...."; encrypt['7'] = "--...";
encrypt['8'] = "---.."; encrypt['9'] = "----."; encrypt['0'] = "-----";
string cipher;
for (size_t i = 0; i < msg.size(); i++)
{
if (msg[i] != ' ')
cipher = cipher + encrypt[msg[i]] + " ";
else
cipher = cipher + " ";
}
cout << cipher;
}
//Driver program
int main()
{
string key = "CONVERT THIS TO MORSE CODE";
//Calling Function
encryption(key);
return 0;
}
|
code/cryptography/src/morse_cipher/morse_code_generator.rb | MORSE_CODES = {
'a' => '.-', 'b' => '-...', 'c' => '-.-.',
'd' => '-..', 'e' => '.', 'f' => '..-.',
'g' => '--.', 'h' => '....', 'i' => '..',
'j' => '.---', 'k' => '-.-', 'l' => '.-..',
'm' => '--', 'n' => '-.', 'o' => '---',
'p' => '.--.', 'q' => '--.-', 'r' => '.-.',
's' => '...', 't' => '-', 'u' => '..-',
'v' => '...-', 'w' => '.--', 'x' => '-..-',
'y' => '-.--', 'z' => '--..', ' ' => ' ',
'1' => '.----', '2' => '..---', '3' => '...--',
'4' => '....-', '5' => '.....', '6' => '-....',
'7' => '--...', '8' => '---..', '9' => '----.',
'0' => '-----'
}.freeze
def encode(string)
text = string.downcase.split('')
encoded_text = []
text.each do |c|
morse_char = MORSE_CODES[c]
encoded_text.push(morse_char)
end
encoded_text.join('')
end
# TEST!
puts encode('Hello World') # => ......-...-..--- .-----.-..-..-..
|
code/cryptography/src/morse_cipher/morse_code_translator.cpp | //
// Created by miszk on 10/4/2017.
//
#include <map>
#include <iostream>
using std::string;
using std::cin;
using std::cout;
using std::endl;
std::map<std::string,
string> dictionary =
{ {"A", ".-"}, { "B", "-..."}, { "C", "-.-."}, { "D", "-.."}, { "E", "."}, { "F", "..-."},
{ "G", "--."}, { "H", "...."}, { "I", ".."}, { "J", ".---"}, { "K", "-.-"}, { "L", ".-.."},
{ "M", "--"}, { "N", "-."}, { "O", "---"}, { "P", ".--."}, { "Q", "--.-"}, { "R", ".-."},
{ "S", "..."}, { "T", "-"}, { "U", "..-"}, { "V", "...-"}, { "W", ".--"}, { "X", "-..-"},
{ "Y", "-.--"}, { "Z", "--.."}, { "1", ".----"}, { "2", "..---"}, { "3", "...--"},
{ "4", "....-"}, { "5", "....."}, { "6", "-...."}, { "7", "--..."}, { "8", "---.."},
{ "9", "----."}, { "0", "-----"}, { "}, {", "--..--"}, { ".", ".-.-.-"}, { "?", "..--.."},
{ "/", "-..-."}, { "-", "-....-"}, { "(", "-.--."}, { ")", "-.--.-"}};
// Function to encrypt the string according to the morse code chart
string encrytp(string message)
{
string encrypted = "";
string to_string;
for (char c : message)
{
//check if char is a letter
if (c != ' ')
{
//add morse letter (first make it uppercase letter 'cause we'll search for it in our dictionary)
to_string = toupper(c);
encrypted += dictionary.find(string(to_string))->second;
//add a space to separate morse letters
encrypted += " ";
}
else
encrypted += " ";
}
return encrypted;
}
// Function to decrypt the string from morse to english
string decrypt (string message)
{
string morse_letter = "";
string decrypted = "";
for (char i : message)
{
// check for space or the end of current morse letter
if (i == ' ')
{
if (morse_letter.length() != 0)
{
// it's the end of morse letter
//search for key in dictionary
for (auto o : dictionary)
if (o.second == morse_letter)
{
decrypted += o.first;
break;
}
morse_letter = "";
}
else
//it's just a space -- we copy it
decrypted += " ";
}
else
morse_letter += i;
}
return decrypted;
}
int main()
{
string msg = "alice killed bob";
string morse_msg = ".- .-.. .. -.-. . -.- .. .-.. .-.. . -.. -... --- -... ";
cout << encrytp(msg) << endl << decrypt(morse_msg) << endl;
return 0;
}
|
code/cryptography/src/morse_cipher/morse_code_translator.exs | defmodule Cryptography do
@morse_encryption_table %{
A: ".-",
B: "-...",
C: "-.-.",
D: "-..",
E: ".",
F: "..-.",
G: "--.",
H: "....",
I: "..",
J: ".---",
K: "-.-",
L: ".-..",
M: "--",
N: "-.",
O: "---",
P: ".--.",
Q: "--.-",
R: ".-.",
S: "...",
T: "-",
U: "..-",
V: "...-",
W: ".--",
X: "-..-",
Y: "-.--",
Z: "--..",
"1": ".----",
"2": "..---",
"3": "...--",
"4": "....-",
"5": ".....",
"6": "-....",
"7": "--...",
"8": "---..",
"9": "----.",
"0": "-----",
",": "--..--",
".": ".-.-.-",
"?": "..--..",
/: "-..-.",
-: "-....-",
"(": "-.--.",
")": "-.--.-"
}
def morse_encrypt(content) do
content
|> String.upcase()
|> String.split("", trim: true)
|> Enum.map(fn x ->
@morse_encryption_table[:"#{x}"]
end)
|> Enum.join(" ")
end
def morse_decrypt(morse) do
translation_table =
Enum.map(@morse_encryption_table, fn {k, v} -> {v, k} end)
|> Enum.into(%{})
morse
|> String.split(" ")
|> Enum.map(fn
"" ->
" "
x ->
"#{Map.get(translation_table, x)}"
end)
|> Enum.join("")
end
end
msg = "Hello world"
Cryptography.morse_encrypt(msg)
|> IO.inspect(label: "Encrypting #{msg} in morse")
|> Cryptography.morse_decrypt()
|> IO.inspect(label: "Decrypting #{msg}")
|
code/cryptography/src/morse_cipher/morse_code_translator.js | // Javascript program to implement morse code translator
/*
* VARIABLE KEY
* 'cipher' -> 'stores the morse translated form of the english string'
* 'decipher' -> 'stores the english translated form of the morse string'
* 'citext' -> 'stores morse code of a single character'
* 'i' -> 'keeps count of the spaces between morse characters'
* 'message' -> 'stores the string to be encoded or decoded'
*/
// Object representing the morse code chart
const MORSE_CODES = {
A: ".-",
B: "-...",
C: "-.-.",
D: "-..",
E: ".",
F: "..-.",
G: "--.",
H: "....",
I: "..",
J: ".---",
K: "-.-",
L: ".-..",
M: "--",
N: "-.",
O: "---",
P: ".--.",
Q: "--.-",
R: ".-.",
S: "...",
T: "-",
U: "..-",
V: "...-",
W: ".--",
X: "-..-",
Y: "-.--",
Z: "--..",
"1": ".----",
"2": "..---",
"3": "...--",
"4": "....-",
"5": ".....",
"6": "-....",
"7": "--...",
"8": "---..",
"9": "----.",
"0": "-----",
",": "--..--",
".": ".-.-.-",
"?": "..--..",
"/": "-..-.",
"-": "-....-",
"(": "-.--.",
")": "-.--.-"
};
// Array of values from above
const CODE_VALS = Object.keys(MORSE_CODES).map(key => MORSE_CODES[key]);
// Function to encrypt the string according to the morse code chart
function encrypt(message) {
var cipher = "";
message.split("").forEach(letter => {
if (letter !== " ") {
// looks up the dictionary and adds the correspponding morse code
// along with a space to separate morse codes for different characters
cipher += MORSE_CODES[letter] + " ";
} else {
// 1 space indicates different characters & 2 indicates different words
cipher += " ";
}
});
return cipher;
}
// Function to decrypt the string from morse to english
function decrypt(message) {
message += " "; // extra space added at the end to access the last morse code
var decipher = "";
var citext = "";
var i = 0; // counter to keep track of space
message.split("").forEach(letter => {
// checks for space
if (letter !== " ") {
i = 0;
citext += letter; // storing morse code of a single character
}
// in case of space
else {
// if i = 1 that indicates a new character
i += 1;
// if i = 2 that indicates a new word
if (i === 2) {
decipher += " "; // adding space to separate words
} else {
// accessing the keys using their values (reverse of encryption via array of values)
decipher += Object.keys(MORSE_CODES)[CODE_VALS.indexOf(citext)];
citext = "";
}
}
});
return decipher;
}
//===================RUN IT====================
var message1 = "ALICE KILED BOB";
var result1 = encrypt(message1.toUpperCase());
console.log(result1);
var message2 =
"-.- . ...- .. -. ... .--. .- -.-. . -.-- .. ... -.- . -.-- ... . .-. ... --- --.. .";
var result2 = decrypt(message2);
console.log(result2);
|
code/cryptography/src/morse_cipher/morse_code_translator.lua | -- Lua code to convert text to morse code // tested on Lua 5.3.3
function enc(message)
-- Lua dictionary containing the morse alphabets
change = {
A = ".-", B = "-...", C = "-.-.", D = "-..", E = ".",
F = "..-.", G = "--.", H = "....", I = "..", J = ".---",
K = "-.-", L = ".-..", M = "--", N = "-.", O = "---",
P = ".--.", Q = "--.-", R = ".-.", S = "...", T = "-",
U = "..-", V = "...-", W = ".--", X = "-..-", Y = "-.--",
Z = "--..", [1]=".----", [2]="..---", [3]="...--",
[4]="....-", [5]=".....", [6]="-....", [7]="--...",
[8]="---..", [9]="----.", [0]="-----"
}
cipherText = ""
for i=1, string.len(message) do
-- (s.sub(s, i, i))
str = string.sub(message, i, i)
if str ~= " " then
-- one space after every letter
cipherText = cipherText .. change[str] .. " "
else
-- two spaces after every word
cipherText = cipherText .. " "
end
end
-- print the final cipher text
print(cipherText)
end
-- user input from STDIN
str = io.read()
-- calling main translation function
enc(str)
|
code/cryptography/src/morse_cipher/morse_code_translator.php | <?php
/* Part of Cosmos by OpenGenus Foundation */
const LATIN_TO_MORSE_DICTIONARY = [
'A' => '.-',
'B' => '-...',
'C' => '-.-.',
'D' => '-..',
'E' => '.',
'F' => '..-.',
'G' => '--.',
'H' => '....',
'I' => '..',
'J' => '.---',
'K' => '-.-',
'L' => '.-..',
'M' => '--',
'N' => '-.',
'O' => '---',
'P' => '.--.',
'Q' => '--.-',
'R' => '.-.',
'S' => '...',
'T' => '-',
'U' => '..-',
'V' => '...-',
'W' => '.--',
'X' => '-..-',
'Y' => '-.--',
'Z' => '--..',
'0' => '-----',
'1' => '.----',
'2' => '..---',
'3' => '...--',
'4' => '....-',
'5' => '.....',
'6' => '-....',
'7' => '--...',
'8' => '---..',
'9' => '----.',
',' => '--..--',
'.' => '.-.-.-',
'?' => '..--..',
'/' => '-..-.',
'-' => '-....-',
'(' => '-.--.',
')' => '-.--.-',
' ' => ' ',
];
// Converts $message to morse code
function encrypt(string $message): string {
$chars = str_split(strtoupper(trim($message)));
$morse = '';
foreach ($chars AS $char) {
if (array_key_exists($char, LATIN_TO_MORSE_DICTIONARY)) {
$morse .= LATIN_TO_MORSE_DICTIONARY[$char];
}
$morse .= ' ';
}
return rtrim($morse);
}
// Decrypts morse code
function decrypt(string $morse): string {
$tokens = explode(' ', $morse);
$message = '';
$morse_to_latin_dictionary = array_flip(LATIN_TO_MORSE_DICTIONARY);
foreach ($tokens AS $token) {
if (array_key_exists($token, $morse_to_latin_dictionary)) {
$message .= $morse_to_latin_dictionary[$token];
}
else if ('' === $token){
$message .= ' ';
}
}
return rtrim($message);
}
echo 'Encoding "Alice":' . PHP_EOL;
echo encrypt('Alice') . PHP_EOL . PHP_EOL;
echo 'Decoding ".... .. -... --- -...":' . PHP_EOL;
echo decrypt('.... .. -... --- -...'); |
code/cryptography/src/morse_cipher/morse_code_translator.py | # Python program to implement Morse Code Translator
"""
VARIABLE KEY
'cipher' -> 'stores the morse translated form of the english string'
'decipher' -> 'stores the english translated form of the morse string'
'citext' -> 'stores morse code of a single character'
'i' -> 'keeps count of the spaces between morse characters'
'message' -> 'stores the string to be encoded or decoded'
"""
# Dictionary representing the morse code chart
MORSE_CODE_DICT = {
"A": ".-",
"B": "-...",
"C": "-.-.",
"D": "-..",
"E": ".",
"F": "..-.",
"G": "--.",
"H": "....",
"I": "..",
"J": ".---",
"K": "-.-",
"L": ".-..",
"M": "--",
"N": "-.",
"O": "---",
"P": ".--.",
"Q": "--.-",
"R": ".-.",
"S": "...",
"T": "-",
"U": "..-",
"V": "...-",
"W": ".--",
"X": "-..-",
"Y": "-.--",
"Z": "--..",
"1": ".----",
"2": "..---",
"3": "...--",
"4": "....-",
"5": ".....",
"6": "-....",
"7": "--...",
"8": "---..",
"9": "----.",
"0": "-----",
",": "--..--",
".": ".-.-.-",
"?": "..--..",
"/": "-..-.",
"-": "-....-",
"(": "-.--.",
")": "-.--.-",
}
# Function to encrypt the string according to the morse code chart
def encrypt(message):
cipher = ""
for letter in message:
if letter != " ":
# looks up the dictionary and adds the correspponding morse code
# along with a space to separate morse codes for different characters
cipher += MORSE_CODE_DICT[letter] + " "
else:
# 1 space indicates different characters & 2 indicates different words
cipher += " "
return cipher
# Function to decrypt the string from morse to english
def decrypt(message):
message += " " # extra space added at the end to access the last morse code
decipher = ""
citext = ""
for letter in message:
# checks for space
if letter != " ":
i = 0 # couunter to keep track of space
citext += letter # storing morse code of a single character
# in case of space
else:
# if i = 1 that indicates a new character
i += 1
# if i = 2 that indicates a new word
if i == 2:
decipher += " " # adding space to separate words
else:
# accessing the keys using their values (reverse of encryption)
decipher += list(MORSE_CODE_DICT.keys())[
list(MORSE_CODE_DICT.values()).index(citext)
]
citext = ""
return decipher
# Hard-coded driver function to run the program
def main():
message = "ALICE KILED BOB"
result = encrypt(message.upper())
print(result)
message = "-.- . ...- .. -. ... .--. .- -.-. . -.-- .. ... -.- . -.-- ... . .-. ... --- --.. ."
result = decrypt(message)
print(result)
# Executes the main function
if __name__ == "__main__":
main()
|
code/cryptography/src/morse_cipher/morse_code_translator.ts | // Program to convert morse code to ASCII and vice-versa
const MORSE_MAP = {
A: '.-',
B: '-...',
C: '-.-.',
D: '-..',
E: '.',
F: '..-.',
G: '--.',
H: '....',
I: '..',
J: '.---',
K: '-.-',
L: '.-..',
M: '--',
N: '-.',
O: '---',
P: '.--.',
Q: '--.-',
R: '.-.',
S: '...',
T: '-',
U: '..-',
V: '...-',
W: '.--',
X: '-..-',
Y: '-.--',
Z: '--..',
'1': '.----',
'2': '..---',
'3': '...--',
'4': '....-',
'5': '.....',
'6': '-....',
'7': '--...',
'8': '---..',
'9': '----.',
'0': '-----',
',': '--..--',
'.': '.-.-.-',
'?': '..--..',
'/': '-..-.',
'-': '-....-',
'(': '-.--.',
')': '-.--.-',
}
/**
* Encrypt a text string into morse code. One space implies
* a different character, while two spaces implies a different word.
*
* @param str The ASCII input string
*/
export function encrypt(str: string): string {
let encrypted = '';
str.toUpperCase().split('').forEach((char: string) => {
if (char !== ' ') {
encrypted += MORSE_MAP[char] + ' ';
} else {
encrypted += ' ';
}
})
return encrypted;
}
/**
* Convert a morse code string back into regular text.
*
* @param str The Morse code input string
*/
export function decrypt(str: string): string {
let decrypted = '';
str.split(' ').forEach((word: string) => {
word.split(' ').forEach((letter: string) => {
decrypted += Object.keys(MORSE_MAP).filter(key => MORSE_MAP[key] === letter)[0];
})
// Add a space to split words
decrypted += ' ';
})
return decrypted;
}
const input1 = 'Hello world.';
const input2 = '.... . .-.. .-.. --- .-- --- .-. .-.. -.. .-.-.-';
const result1 = encrypt(input1);
const result2 = decrypt(input2);
console.log(`Encrypted text: ${result1}`);
console.log(`Decrypted text: ${result2}`);
|
code/cryptography/src/password_generator/strongpasswordgenerator.py | # This program will generate a strong password using cryptography principles
# Import secrets and string module
# The string module defines the alphabet, and the secret module generates cryptographically sound random numbers
import secrets
import string
# Define the alphabet to be digits, letters, and special characters
letters = string.ascii_letters
digits = string.digits
special = string.punctuation
alphabet = letters + digits + special
# Set the password length
while True:
try:
password_length = int(input("Please enter the length of your password: "))
break
except ValueError:
print("Please enter an integer length.")
# Generates strong password with at least one special character and one digit
while True:
password = ''
for i in range(password_length):
password += ''.join(secrets.choice(alphabet))
if (any(char in special for char in password) and any(char in digits for char in password)):
break
print("--------Your Password Has Been Generated---------")
print(password)
print("--------Make Sure To Keep Your Password Safe---------")
|
code/cryptography/src/playfair_cipher/playfair_cipher.c | // C program to implement Playfair Cipher
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SIZE 30
// function to convert the string to lowercase
void toLowerCase(char arr[], int ps)
{
int i;
for (i = 0; i < ps; i++) {
if (arr[i] > 64 && arr[i] < 91)
arr[i] += 32;
}
}
// function to remove all spaces in a string
int removeSpaces(char* arr, int ps)
{
int i, count = 0;
for (i = 0; i < ps; i++)
if (arr[i] != ' ')
arr[count++] = arr[i];
arr[count] = '\0';
return count;
}
// function to generate the 5x5 square matrix key
void generateKeyTable(char arr[], int ks, char key_M[5][5])
{
int i, j, k, flag = 0, *arr1;
// hashmap to store count of the alphabet
arr1 = (int*)calloc(26, sizeof(int));
for (i = 0; i < ks; i++) {
if (arr[i] != 'j')
arr1[arr[i] - 97] = 2;
}
arr1['j' - 97] = 1;
i = 0;
j = 0;
for (k = 0; k < ks; k++) {
if (arr1[arr[k] - 97] == 2) {
arr1[arr[k] - 97] -= 1;
key_M[i][j] = arr[k];
j++;
if (j == 5) {
i++;
j = 0;
}
}
}
for (k = 0; k < 26; k++) {
if (arr1[k] == 0) {
key_M[i][j] = (char)(k + 97);
j++;
if (j == 5) {
i++;
j = 0;
}
}
}
}
// Function to search for the characters of a digraph
// in the key square and return their position
void search(char key_M[5][5], char a, char b, int arr[])
{
int i, j;
if (a == 'j')
a = 'i';
else if (b == 'j')
b = 'i';
for (i = 0; i < 5; i++) {
for (j = 0; j < 5; j++) {
if (key_M[i][j] == a) {
arr[0] = i;
arr[1] = j;
}
else if (key_M[i][j] == b) {
arr[2] = i;
arr[3] = j;
}
}
}
}
// function to find the modulus with 5
int mod5(int a)
{
return (a % 5);
}
// function to make the plain text length to be even
int prepare(char arr[], int n)
{
if (n % 2 != 0) {
arr[n++] = 'z';
arr[n] = '\0';
}
return n;
}
// function for assistance in encryption of the data
void encrypt(char arr[], char key_M[5][5], int ps)
{
int i, arr1[4];
for (i = 0; i < ps; i += 2) {
search(key_M, arr[i], arr[i + 1], arr1);
if (arr1[0] == arr1[2]) {
arr[i] = key_M[arr1[0]][mod5(arr1[1] + 1)];
arr[i + 1] = key_M[arr1[0]][mod5(arr1[3] + 1)];
}
else if (arr1[1] == arr1[3]) {
arr[i] = key_M[mod5(arr1[0] + 1)][arr1[1]];
arr[i + 1] = key_M[mod5(arr1[2] + 1)][arr1[1]];
}
else {
arr[i] = key_M[arr1[0]][arr1[3]];
arr[i + 1] = key_M[arr1[2]][arr1[1]];
}
}
}
// function to encrypt using Playfair Cipher
void encryptByPlayfairCipher(char arr[], char key[])
{
char ps, ks, key_M[5][5];
// Key
ks = strlen(key);
ks = removeSpaces(key, ks);
toLowerCase(key, ks);
// Plaintext
ps = strlen(arr);
toLowerCase(arr, ps);
ps = removeSpaces(arr, ps);
ps = prepare(arr, ps);
generateKeyTable(key, ks, key_M);
encrypt(arr, key_M, ps);
}
// Main function that gets executed first
int main()
{
char arr[SIZE], key[SIZE];
// Key to be encrypted
strcpy(key, "Germany");
printf("Key: %s\n", key);
// Plaintext to be encrypted
strcpy(arr, "Dusseldorf");
printf("Text to be encrypted: %s\n", arr);
// encrypt using Playfair Cipher
encryptByPlayfairCipher(arr, key);
printf("Cipher: %s\n", arr);
return 0;
}
// C program to implement Playfair Cipher
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define SIZE 30
// function to convert the string to lowercase
void toLowerCase(char arr[], int ps)
{
int i;
for (i = 0; i < ps; i++) {
if (arr[i] > 64 && arr[i] < 91)
arr[i] += 32;
}
}
// function to remove all spaces in a string
int removeSpaces(char* arr, int ps)
{
int i, count = 0;
for (i = 0; i < ps; i++)
if (arr[i] != ' ')
arr[count++] = arr[i];
arr[count] = '\0';
return count;
}
// function to generate the 5x5 square matrix key
void generateKeyTable(char arr[], int ks, char key_M[5][5])
{
int i, j, k, flag = 0, *arr1;
// hashmap to store count of the alphabet
arr1 = (int*)calloc(26, sizeof(int));
for (i = 0; i < ks; i++) {
if (arr[i] != 'j')
arr1[arr[i] - 97] = 2;
}
arr1['j' - 97] = 1;
i = 0;
j = 0;
for (k = 0; k < ks; k++) {
if (arr1[arr[k] - 97] == 2) {
arr1[arr[k] - 97] -= 1;
key_M[i][j] = arr[k];
j++;
if (j == 5) {
i++;
j = 0;
}
}
}
for (k = 0; k < 26; k++) {
if (arr1[k] == 0) {
key_M[i][j] = (char)(k + 97);
j++;
if (j == 5) {
i++;
j = 0;
}
}
}
}
// Function to search for the characters of a digraph
// in the key square and return their position
void search(char key_M[5][5], char a, char b, int arr[])
{
int i, j;
if (a == 'j')
a = 'i';
else if (b == 'j')
b = 'i';
for (i = 0; i < 5; i++) {
for (j = 0; j < 5; j++) {
if (key_M[i][j] == a) {
arr[0] = i;
arr[1] = j;
}
else if (key_M[i][j] == b) {
arr[2] = i;
arr[3] = j;
}
}
}
}
// function to find the modulus with 5
int mod5(int a)
{
return (a % 5);
}
// function to make the plain text length to be even
int prepare(char arr[], int n)
{
if (n % 2 != 0) {
arr[n++] = 'z';
arr[n] = '\0';
}
return n;
}
// function for assistance in encryption of the data
void encrypt(char arr[], char key_M[5][5], int ps)
{
int i, arr1[4];
for (i = 0; i < ps; i += 2) {
search(key_M, arr[i], arr[i + 1], arr1);
if (arr1[0] == arr1[2]) {
arr[i] = key_M[arr1[0]][mod5(arr1[1] + 1)];
arr[i + 1] = key_M[arr1[0]][mod5(arr1[3] + 1)];
}
else if (arr1[1] == arr1[3]) {
arr[i] = key_M[mod5(arr1[0] + 1)][arr1[1]];
arr[i + 1] = key_M[mod5(arr1[2] + 1)][arr1[1]];
}
else {
arr[i] = key_M[arr1[0]][arr1[3]];
arr[i + 1] = key_M[arr1[2]][arr1[1]];
}
}
}
// function to encrypt using Playfair Cipher
void encryptByPlayfairCipher(char arr[], char key[])
{
char ps, ks, key_M[5][5];
// Key
ks = strlen(key);
ks = removeSpaces(key, ks);
toLowerCase(key, ks);
// Plaintext
ps = strlen(arr);
toLowerCase(arr, ps);
ps = removeSpaces(arr, ps);
ps = prepare(arr, ps);
generateKeyTable(key, ks, key_M);
encrypt(arr, key_M, ps);
}
// Main function that gets executed first
int main()
{
char arr[SIZE], key[SIZE];
// Key to be encrypted
strcpy(key, "Germany");
printf("Key: %s\n", key);
// Plaintext to be encrypted
strcpy(arr, "Dusseldorf");
printf("Text to be encrypted: %s\n", arr);
// encrypt using Playfair Cipher
encryptByPlayfairCipher(arr, key);
printf("Cipher: %s\n", arr);
return 0;
}
// OUTPUT
// Key: Germany
// Text to be encrypted: Dusseldorf
// Cipher: nzoxahntgi
// Code curated by Subhadeep Das(username:- Raven1233) |
code/cryptography/src/polybius_cipher/polybius_cipher.py | from pycipher.base import Cipher
import re
####################################################################################
class PolybiusSquare(Cipher):
"""The Polybius square is a simple substitution cipher that outputs 2 characters of ciphertext for each character of plaintext. It has a key consisting
which depends on 'size'. By default 'size' is 5, and the key is 25 letters (5^2). For a size of 6 a 36 letter key required etc.
For a more detailed look at how it works see http://www.practicalcryptography.com/ciphers pycipher.polybius-square-cipher/.
:param key: The keysquare, each row one after the other. The key must by size^2 characters in length.
:param size: The size of the keysquare, if size=5, the keysquare uses 5^2 or 25 characters.
:param chars: the set of characters to use. By default ABCDE are used, this parameter should have the same length as size.
"""
def __init__(self, key="phqgiumeaylnofdxkrcvstzwb", size=5, chars=None):
self.key = "".join([k.upper() for k in key])
self.chars = chars or "ABCDEFGHIJKLMNOPQRSTUVWXYZ"[:size]
self.size = size
assert (
len(self.key) == size * size
), "invalid key in init: must have length size*size, has length " + str(
len(key)
)
assert (
len(self.chars) == size
), "invalid chars in init: must have length=size, has length " + str(len(chars))
def encipher_char(self, ch):
row = (int)(self.key.index(ch) / self.size)
col = self.key.index(ch) % self.size
return self.chars[row] + self.chars[col]
def decipher_pair(self, pair):
row = self.chars.index(pair[0])
col = self.chars.index(pair[1])
return self.key[row * self.size + col]
def encipher(self, string):
"""Encipher string using Polybius square cipher according to initialised key.
Example::
ciphertext = Polybius('APCZWRLFBDKOTYUQGENHXMIVS',5,'MKSBU').encipher(plaintext)
:param string: The string to encipher.
:returns: The enciphered string. The ciphertext will be twice the length of the plaintext.
"""
string = self.remove_punctuation(string) # ,filter='[^'+self.key+']')
ret = ""
for c in range(0, len(string)):
ret += self.encipher_char(string[c])
return ret
def decipher(self, string):
"""Decipher string using Polybius square cipher according to initialised key.
Example::
plaintext = Polybius('APCZWRLFBDKOTYUQGENHXMIVS',5,'MKSBU').decipher(ciphertext)
:param string: The string to decipher.
:returns: The deciphered string. The plaintext will be half the length of the ciphertext.
"""
string = self.remove_punctuation(string) # ,filter='[^'+self.chars+']')
ret = ""
for i in range(0, len(string), 2):
ret += self.decipher_pair(string[i : i + 2])
return ret
if __name__ == "__main__":
print('use "import pycipher" to access functions')
|
code/cryptography/src/porta_cipher/porta_cipher.py | from pycipher.base import Cipher
####################################################################################
class Porta(Cipher):
"""The Porta Cipher is a polyalphabetic substitution cipher, and has a key consisting of a word e.g. 'FORTIFICATION'.
:param key: The keyword, any word or phrase will do. Must consist of alphabetical characters only, no punctuation of numbers.
"""
def __init__(self, key="FORTIFICATION"):
self.key = [k.upper() for k in key]
def encipher(self, string):
"""Encipher string using Porta cipher according to initialised key. Punctuation and whitespace
are removed from the input.
Example::
ciphertext = Porta('HELLO').encipher(plaintext)
:param string: The string to encipher.
:returns: The enciphered string.
"""
string = self.remove_punctuation(string)
ret = ""
for (i, c) in enumerate(string):
i = i % len(self.key)
if self.key[i] in "AB":
ret += "NOPQRSTUVWXYZABCDEFGHIJKLM"[self.a2i(c)]
elif self.key[i] in "YZ":
ret += "ZNOPQRSTUVWXYBCDEFGHIJKLMA"[self.a2i(c)]
elif self.key[i] in "WX":
ret += "YZNOPQRSTUVWXCDEFGHIJKLMAB"[self.a2i(c)]
elif self.key[i] in "UV":
ret += "XYZNOPQRSTUVWDEFGHIJKLMABC"[self.a2i(c)]
elif self.key[i] in "ST":
ret += "WXYZNOPQRSTUVEFGHIJKLMABCD"[self.a2i(c)]
elif self.key[i] in "QR":
ret += "VWXYZNOPQRSTUFGHIJKLMABCDE"[self.a2i(c)]
elif self.key[i] in "OP":
ret += "UVWXYZNOPQRSTGHIJKLMABCDEF"[self.a2i(c)]
elif self.key[i] in "MN":
ret += "TUVWXYZNOPQRSHIJKLMABCDEFG"[self.a2i(c)]
elif self.key[i] in "KL":
ret += "STUVWXYZNOPQRIJKLMABCDEFGH"[self.a2i(c)]
elif self.key[i] in "IJ":
ret += "RSTUVWXYZNOPQJKLMABCDEFGHI"[self.a2i(c)]
elif self.key[i] in "GH":
ret += "QRSTUVWXYZNOPKLMABCDEFGHIJ"[self.a2i(c)]
elif self.key[i] in "EF":
ret += "PQRSTUVWXYZNOLMABCDEFGHIJK"[self.a2i(c)]
elif self.key[i] in "CD":
ret += "OPQRSTUVWXYZNMABCDEFGHIJKL"[self.a2i(c)]
return ret
def decipher(self, string):
"""Decipher string using Porta cipher according to initialised key. Punctuation and whitespace
are removed from the input. For the Porta cipher, enciphering and deciphering are the same operation.
Example::
plaintext = Porta('HELLO').decipher(ciphertext)
:param string: The string to decipher.
:returns: The deciphered string.
"""
return self.encipher(string)
if __name__ == "__main__":
print('use "import pycipher" to access functions')
|
code/cryptography/src/rail_fence_cipher/rail_fence_cipher.cpp | #include <iostream>
#include <cstring>
#include <string>
// Part of Cosmos by OpenGenus Foundation
// Rails fence cipher - Cryptography
using namespace std;
string Encryptor(string msg, int key)
{
int msgLen = msg.size();
bool direction = true;
char enc[key][msgLen];
for (int i = 0; i < key; i++)
for (int j = 0; j < msgLen; j++)
enc[i][j] = '\n';
for (int i = 0, row = 0, col = 0; i < msgLen; i++)
{
if (row == 0 || row == key - 1)
direction = !direction;
enc[row][col++] = msg[i];
direction ? row-- : row++;
}
string cipher;
for (int i = 0; i < key; i++)
for (int j = 0; j < msgLen; j++)
if (enc[i][j] != '\n')
cipher.push_back(enc[i][j]);
return cipher;
}
string Decryptor(string cipher, int key)
{
int cipLen = cipher.size();
bool direction;
char dec[key][cipLen];
for (int i = 0; i < key; i++)
for (int j = 0; j < cipLen; j++)
dec[i][j] = '\n';
for (int i = 0, row = 0, col = 0; i < cipLen; i++)
{
if (row == 0)
direction = false;
else if (row == key - 1)
direction = true;
dec[row][col++] = '*';
direction ? row-- : row++;
}
int index = 0;
for (int i = 0; i < key; i++)
for (int j = 0; j < cipLen; j++)
if (dec[i][j] == '*' && index < cipLen)
dec[i][j] = cipher[index++];
string msg;
for (int i = 0, row = 0, col = 0; i < cipLen; i++)
{
if (row == 0)
direction = false;
else if (row == key - 1)
direction = true;
if (dec[row][col] != '*')
msg.push_back(dec[row][col++]);
direction ? row-- : row++;
}
return msg;
}
int main()
{
int key, choice;
std::string cip, msg;
cout << "1. Encrypt \n2. Decrypt \n>>";
cin >> choice;
if (choice == 1)
{
cout << "Enter Message\n>>";
cin.ignore();
std::getline(std::cin, msg);
cout << "Enter Key\n>>";
cin >> key;
cout << "Encrypted Message\n>>" << Encryptor(msg, key);
}
else if (choice == 2)
{
cout << "Enter Encrypted Message\n>>";
cin.ignore();
std::getline(std::cin, cip);
cout << "Enter Key\n>>";
cin >> key;
cout << "Original Message\n>>" << Decryptor(cip, key);
}
return 0;
}
|
code/cryptography/src/rail_fence_cipher/rail_fence_cipher.py | string = "Aditya Krishnakumar"
key = 2
mode = 0
def cipher(s, k, m):
w = len(s)
h = k
matrix = [[0 for x in range(w)] for y in range(h)]
output = []
replace = []
def c(string):
cur_x, cur_y, not_0 = 0, 0, 0
for char in string:
matrix[cur_y][cur_x] = char
cur_x += 1
if cur_y < h - 1 and not_0 == 0:
cur_y += 1
else:
if cur_y > 0:
cur_y -= 1
not_0 = 1
else:
cur_y += 1
not_0 = 0
return matrix
def d(string):
pointer = 0
for i in range(len(string)):
replace.append("r")
for row in c("".join(replace)):
for pos, char in enumerate(row):
if char == "r":
row[pos] = list(string)[pointer]
pointer += 1
return matrix
if m == 0:
for row in c(string):
print(row)
for char in row:
if char is not 0:
output.append(char)
return "".join(output)
if m == 1:
cur_x, cur_y, not_0 = 0, 0, 0
matrix = d(string)
for row in matrix:
print(row)
while len(output) < len(list(string)):
output.append(matrix[cur_y][cur_x])
cur_x += 1
if cur_y < h - 1 and not_0 == 0:
cur_y += 1
else:
if cur_y > 0:
cur_y -= 1
not_0 = 1
else:
cur_y += 1
not_0 = 0
return "".join(output)
print(string, "->", cipher(string, key, mode))
|
code/cryptography/src/rail_fence_cipher/rail_fence_cipher.rb | # Part of Cosmos by OpenGenus Foundation
require 'optparse'
options = {}
OptionParser.new do |opt|
opt.banner = 'Usage: rail_fence.rb [options]'
opt.on('-e', '--encipher KEY') do |o|
options[:encipher] = o
end
opt.on('-d', '--decipher KEY') do |o|
options[:decipher] = o
end
end.parse!
##
# A class for enciphering and deciphering messages using the Rail-fence cipher.
#
# See: http://practicalcryptography.com/ciphers/rail-fence-cipher/
class RailFence
##
# Encipher a +message+ with the Rail-fence result.
#
# Params:
# +message+::Message to encipher.
# +key+::Key to encipher message with.
def self.encipher(message, key)
message.tr! ' ', '_'
# Handle case where key = 1
return message if key == 1
# Initialize a matrix of characters
matrix = Array.new(key.to_i) do
Array.new(message.length, '.')
end
down = false
row = 0
col = 0
# Iterate over characters in message to create matrix
message.split('').each do |char|
# Swap direction at the top or bottom row
down = !down if (row == 0) || (row == (key - 1))
# Fill corresponding letter in matrix
matrix[row][col] = char
col += 1
# Increment or decrement row
down ? row += 1 : row -= 1
end
# Construct result from matrix
cipher = []
matrix.each do |array|
array.each do |char|
cipher.push(char) unless char == '.'
end
end
cipher.join('')
end
##
# Decipher a +message+ with the Rail-fence result.
#
# Params:
# +message+::Message to decipher.
# +key+::Key to decipher message.
def self.decipher(message, key)
message.tr! ' ', '_'
# Handle case where key = 1
return message if key == 1
# Initialize a matrix of characters
matrix = Array.new(key.to_i) do
Array.new(message.length, '.')
end
down = false
row = 0
col = 0
# Iterate over characters in message to create matrix
message.split('').each do |_char|
# Determine direction
down = true if row == 0
down = false if row == key - 1
# Fill corresponding position with marker
matrix[row][col] = '*'
col += 1
# Increment or decrement row
down ? row += 1 : row -= 1
end
index = 0
characters = message.split('')
# Fill the matrix with the message
matrix.each_with_index do |array, i|
array.each_with_index do |char, j|
if char == '*'
matrix[i][j] = characters[index]
index += 1
end
end
end
# Construct result from matrix
result = []
down = false
row = 0
col = 0
while col < message.length
# Determine which direction to move in
down = true if row == 0
down = false if row == key - 1
result.push matrix[row][col]
col += 1
# Increment or decrement row
down ? row += 1 : row -= 1
end
result.join('')
end
end
if options.key? :encipher
key = options[:encipher]
puts RailFence.encipher ARGV.join(' '), key.to_i
end
if options.key? :decipher
key = options[:decipher]
puts RailFence.decipher ARGV.join(' '), key.to_i
end
|
code/cryptography/src/rot13_cipher/README.md | # ROT-13 Cipher
ROT-13 is a simple substitution cipher in which each letter is shifted by 13 letters in the alphabet, wrapping around when neccesary. An example is shown below:
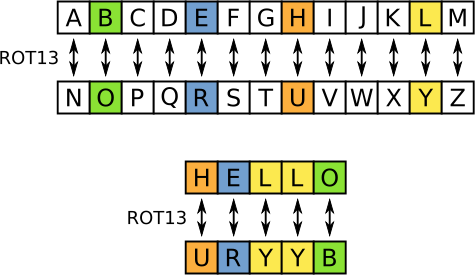
Image credit: `By Benjamin D. Esham (bdesham) - Based upon ROT13.png by en:User:Matt Crypto. This version created by bdesham in Inkscape.This vector image was created with Inkscape., Public Domain, https://commons.wikimedia.org/w/index.php?curid=2707244`
## ROT-N
ROT-N, more commonly known as a Caesar cipher, is similar to ROT-13, although letters can be shifted by any number of spaces. This makes ROT-13 a subset of a Caesar cipher where letters are shifted by 13 spaces.
## Sources and more detailed information:
- https://en.wikipedia.org/wiki/ROT13
- https://en.wikipedia.org/wiki/Caesar_cipher
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
code/cryptography/src/rot13_cipher/rot13_cipher.c | #include <stdio.h>
#include <string.h>
#include <ctype.h>
// Run rotation cipher on a string
// The string will be modified
void rotN(char * string, int n) {
char *p;
char sub;
// Loop through input text
for(p = string ; *p != 0 ; p++) {
// Check if character is a letter
if (isalpha(*p)) {
// Subtract 'A' if uppercase, 'a' if lowercase
sub = (*p >= 65 && *p <= 90) ? 'A' : 'a';
// Convert character to alphabet index starting from zero (0 - 25)
*p -= sub;
// Shift
*p += n;
// Wrap around
*p %= 26;
// Convert back into ASCII
*p += sub;
}
}
}
int main() {
// Example
char str[] = "Hello, world!";
rotN(str, 13);
puts(str); // Outputs "Uryyb, jbeyq!"
return 0;
}
|
code/cryptography/src/rot13_cipher/rot13_cipher.cpp | #include <string>
#include <iostream>
#include <cctype>
std::string rot13(const std::string &text)
{
std::string output;
// Loop through input text
for (auto c : text)
{
// Check if character is a letter
if (isalpha(c))
{
// Subtract 'A' if uppercase, 'a' if lowercase
char sub = (c >= 65 && c <= 90) ? 'A' : 'a';
// Convert character to alphabet index starting from zero (0 - 25)
c -= sub;
// Shift
c += 13;
// Wrap around
c %= 26;
// Convert back into ASCII and add to output
output += (c + sub);
}
else
output += c;
}
return output;
}
int main()
{
// Example
std::cout << rot13("Hello, world!") << "\n"; // Outputs "Uryyb, jbeyq!"
std::cout << rot13("Uryyb, jbeyq!") << "\n"; // Output "Hello, world!"
return 0;
}
|
code/cryptography/src/rot13_cipher/rot13_cipher.java | // Part of Cosmos by OpenGenus Foundation
public class RotN {
public static void main(String[] args) {
String test = "test";
System.out.println(rot(test, 13)); // prints 'grfg'
System.out.println(rot(test, 26)); // prints 'test'
}
public static String rot(String str, int n) {
n = Math.max(0, n);
String result = "";
for (char c : str.toCharArray()) {
if (!Character.isLetter(c))
result += c;
else {
int b = c - 'a';
int m = (b + n) % 26;
result += (char) (m + 'a');
}
}
return result;
}
}
|
code/cryptography/src/rot13_cipher/rot13_cipher.js | function rot13(text) {
return text.replace(/[a-z]/gi, function(char) {
return String.fromCharCode(
(char <= "Z" ? 90 : 122) >= (char = char.charCodeAt(0) + 13)
? char
: char - 26
);
});
}
|
code/cryptography/src/rot13_cipher/rot13_cipher.py | # Part of Cosmos by OpenGenus Foundation
if __name__ == "__main__":
plaintext = "This is unencrypted sample text"
ciphertext = ""
for ch in plaintext:
ascii_value = ord(ch)
if ch == " ":
ciphertext += " "
elif ascii_value >= 65 and ascii_value <= 90:
if ascii_value + 13 > 90:
ciphertext += chr(ascii_value - 13)
else:
ciphertext += chr(ascii_value + 13)
elif ascii_value >= 97 and ascii_value <= 122:
if ascii_value + 13 > 122:
ciphertext += chr(ascii_value - 13)
else:
ciphertext += chr(ascii_value + 13)
print(ciphertext)
|
code/cryptography/src/rot13_cipher/rot13_cipher.rb | # Part of Cosmos by OpenGenus Foundation
puts 'Enter text you want to encrypt: '
text = gets.chomp
puts text.tr('A-Za-z', 'N-ZA-Mn-za-m')
|
code/cryptography/src/rot13_cipher/rot13_cipher.sh | #!/bin/sh
# Run rot13 on a string
rot13()
{
str=$1
result=$(echo -n "$str" | tr '[A-Za-z]' '[N-ZA-Mn-za-m]')
echo $result
}
# Example
# $ ./rot13.sh "Hello world!"
# $ Uryyb jbeyq!
if [ "$#" -ne 1 ] ; then
echo "Usage: $0 <string>"
exit 1
fi
rot13 "$1"
|
code/cryptography/src/rot13_cipher/rot13_cipher2.cpp | #include <iostream>
#include <string>
#include <cctype>
using namespace std;
/// @brief Run rotation cipher on a string
/// @param text Text to encrypt
/// @param n Amount to shift by
/// @brief Encoded text
std::string rotN(const std::string &text, int n)
{
std::string output;
// Loop through input text
for (auto c : text)
{
// Check if character is a letter
if (isalpha(c))
{
// Subtract 'A' if uppercase, 'a' if lowercase
char sub = (c >= 65 && c <= 90) ? 'A' : 'a';
// Convert character to alphabet index starting from zero (0 - 25)
c -= sub;
// Shift
c += n;
// Wrap around
c %= 26;
// Convert back into ASCII and add to output
output += (c + sub);
}
else
output += c;
}
return output;
}
int main()
{
// Example
cout << rotN("Hello, world!", 13) << endl; // Outputs "Uryyb, jbeyq!"
return 0;
}
|
code/cryptography/src/rot13_cipher/rot13_cipher2.js | /**
* Run rotation cipher on a string
* @param {string} text - Text to encrypt
* @param {number} n - Amount to shift by
* @return {string} Encoded string
*/
const rotN = function rot(text, n) {
let output = "";
// Loop through input
for (let char of text) {
// Get ASCII code
let ascii = char.charCodeAt(0);
// Check if character is not a letter
if (ascii < 65 || ascii > 122) {
output += String(char);
} else {
// Subtract 'A' if uppercase, 'a' if lowercase
let sub = ascii >= 65 && ascii <= 90 ? "A" : "a";
// Convert to alphabet index starting from zero (0 - 25)
ascii -= sub.charCodeAt(0);
// Shift
ascii += n;
// Wrap around
ascii = ascii % 26;
// Convert back to ASCII code and add to output
output += String.fromCharCode(ascii + sub.charCodeAt(0));
}
}
return output;
};
// Examples
console.log(rotN("Hello, world!", 13)); // Outputs "Uryyb, jbeyq!"
|
code/cryptography/src/rot13_cipher/rotn_cipher.exs | defmodule Cryptography do
# Its rotn, but defaults to rot13
def rotn(input, n \\ 13) do
alphabet = "abcdefghijklmnopqrstuvwxyz"
input
|> String.downcase()
|> String.split("", trim: true)
|> Enum.map(fn
" " ->
" "
a ->
alphabet_ind =
String.split(alphabet, "", trim: true) |> Enum.find_index(fn x -> x == a end)
if !is_nil(alphabet_ind) do
rot = alphabet_ind + n
rot_ind =
cond do
rot < 0 -> rem(rot, 26) * -1
true -> rem(rot, 26)
end
String.at(alphabet, rot_ind)
else
a
end
end)
|> Enum.join("")
end
end
msg = "Hello world"
n = 13
Cryptography.rotn(msg, n)
|> IO.inspect(label: "Encrypting '#{msg}' in rot#{n}") # uryyb jbeyq
|
code/cryptography/src/rsa/rsa.c | /* Part of Cosmos by OpenGenus Foundation
* RSA(Rivest–Shamir–Adleman) Public Key Cryptosystem
*/
#include <stdio.h>
#include <math.h>
#include <stdlib.h>
#include<stdbool.h>
long long p, q, n, t, d, e, m[100], en[100];
/*
p: prime 1
q: prime 2
n: p*q
t: euler totient function phi(n)
e: encryption key
d: decryption key
Public Key: n, e
Private Key: n, d (or p, q, t)
*/
char msg[100];
bool prime(int n)
{
if ( n == 1 )
return 0;
int sq = sqrt(n);
for ( int i = 2 ; i <= sq ; i++ )
{
if ( n % i == 0 )
return 0;
}
return 1;
}
void chooseED()
{
for ( int i = 2 ; i < t ; i++ )
{
if ( t % i == 0 )
continue;
if ( !prime(i) )
continue;
int res = 1;
while ( 1 )
{
res += t;
if ( res % i == 0 )
{
e = i;
d = res / i;
return;
}
}
}
}
// fast modular exponentiation, O(log n)
long long modular_pow(long long base, long long exponent, long long modulus)
{
long long result = 1;
while (exponent > 0)
{
if (exponent % 2 == 1)
result = (result * base) % modulus;
exponent = exponent >> 1;
base = (base * base) % modulus;
}
return result;
}
int main()
{
freopen("rsa_input.in", "r", stdin);
printf("Enter first prime number:\n");
scanf("%lld", &p);
if ( !prime(p) )
{
printf("Error: \'p\' is not prime\n");
exit(0);
}
printf("Enter second prime number:\n");
scanf("%lld", &q);
if ( !prime(q) )
{
printf("Error: \'q\' is not prime\n");
exit(0);
}
n = p * q;
t = (p-1) * (q-1);
chooseED();
printf("Enter your secret message:\n");
scanf("%s", msg);
for ( int i = 0 ; msg[i] != '\0' ; i++ )
{
m[i] = msg[i];
en[i] = modular_pow(m[i], e, n);
en[i+1] = -1;
}
printf("Encrypted Message:\n");
for ( int i = 0 ; en[i] != -1 ; i++ )
{
printf("%lld ", en[i]);
}
printf("\n");
long long ans;
printf("Decrypted Message:\n");
for ( int i = 0 ; en[i] != -1 ; i++ )
{
ans = modular_pow(en[i], d, n);
printf("%c", (char)ans);
}
}
|
code/cryptography/src/rsa/rsa.cs | /*
* Bailey Thompson
* github.com/bkthomps
*
* Simple implementation of RSA Encryption.
*/
using System;
using System.Numerics;
using System.Collections.Generic;
namespace rsa
{
/*
* Entry point of the program, display information to user on console.
*/
internal static class Program
{
private static void Main()
{
var primeGen = new Primes();
var key = primeGen.GetKey();
const string message = "Hello World!";
Console.WriteLine("Original Text: \"" + message + "\"");
var cypherText = key.Encrypt(message);
Console.Write("Cypher Text: ");
var isFirstLetter = true;
foreach (var place in cypherText)
{
if (isFirstLetter)
{
isFirstLetter = false;
Console.Write(place);
continue;
}
Console.Write(", " + place);
}
Console.WriteLine();
var decryptedText = key.Decrypt(cypherText);
Console.WriteLine("Decrypted Text: \"" + decryptedText + "\"");
}
}
/*
* Create a list of primes, with ability to create keys using the list.
*/
internal class Primes
{
private const int MaxValue = 25000;
private readonly bool[] isPrime = new bool[MaxValue + 1];
private readonly List<int> primes = new List<int>();
internal Primes()
{
for (var i = 2; i <= MaxValue; i++)
{
if (!isPrime[i])
{
primes.Add(i);
for (var j = i * i; j <= MaxValue; j += i)
{
isPrime[j] = true;
}
}
}
}
internal Key GetKey()
{
var end = primes.Count - 1;
var start = end / 4;
var random = new Random();
var primeOne = primes[random.Next(start, end)];
var primeTwo = primes[random.Next(start, end)];
while (primeTwo == primeOne)
{
primeTwo = primes[random.Next(start, end)];
}
return new Key(primeOne, primeTwo, primes);
}
}
/*
* Create a key using two primes. The variable n is both primes multiplied, phi is each prime minus one multiplied
* together. The variable e has to satisfy gcd(e, phi) = 1, so just another prime. The variable d has to satisfy
* ed = 1 mod phi. The public key is (e, n) and the private key is (d, n).
*/
internal class Key
{
private readonly int n;
private readonly int e;
private int d;
internal Key(int primeOne, int primeTwo, List<int> primes)
{
n = primeOne * primeTwo;
var phi = (primeOne - 1) * (primeTwo - 1);
var end = primes.Count - 1;
var start = end / 4;
var random = new Random();
do
{
do
{
e = primes[random.Next(start, end)];
} while (e == primeOne || e == primeTwo);
} while (!IsFoundD(phi));
Console.WriteLine("Public Key: (e, n) = (" + e + ", " + n + ")");
}
private bool IsFoundD(int phi)
{
for (var i = phi - 1; i > 1; i--)
{
var mul = BigInteger.Multiply(e, i);
var result = BigInteger.Remainder(mul, phi);
if (result.Equals(1))
{
d = i;
Console.WriteLine("Private Key: (d, n) = (" + d + ", " + n + ")");
return true;
}
}
return false;
}
internal int[] Encrypt(string message)
{
var charArray = message.ToCharArray();
var array = new int[charArray.Length];
for (var i = 0; i < array.Length; i++)
{
array[i] = (int) BigInteger.ModPow(charArray[i], e, n);
}
return array;
}
internal string Decrypt(int[] cyphertext)
{
var array = new char[cyphertext.Length];
for (var i = 0; i < array.Length; i++)
{
array[i] = (char) BigInteger.ModPow(cyphertext[i], d, n);
}
return new string(array);
}
}
}
|
code/cryptography/src/rsa/rsa.java | import java.math.BigInteger;
import java.util.Random;
import java.util.Scanner;
/**
*
* @author thompson naidu
*/
public class RSA {
private BigInteger p,q,N,phi,e,d;
private int bitlength=1024;
private Random rand;
public RSA(){
rand=new Random();
p=BigInteger.probablePrime(bitlength, rand);
q=BigInteger.probablePrime(bitlength, rand);
N=p.multiply(q);
phi= p.subtract(BigInteger.ONE).multiply(q.subtract(BigInteger.ONE));
e=BigInteger.probablePrime(bitlength/2, rand);
while(phi.gcd(e).compareTo(BigInteger.ONE)>0 && e.compareTo(phi)< 0){
e.add(BigInteger.ONE);
}
d=e.modInverse(phi);
}
public static String bytesToString(byte[] bytes){
String text="";
for(byte b:bytes){
text+=Byte.toString(b);
}
return text;
}
//encrypt the message
public byte[] encrypt(byte[] message){
return (new BigInteger(message)).modPow(e,N).toByteArray();
}
//decrypt the message
public byte[] decrypt(byte[] message){
return (new BigInteger(message)).modPow(d,N).toByteArray();
}
public static void main(String[] args) {
RSA rsa=new RSA();
Scanner sc=new Scanner(System.in);
System.out.println("Enter the plain text: ");
String plaintext=sc.nextLine();
System.out.println("Encryption in progress..");
System.out.println("String in bytes : "+bytesToString(plaintext.getBytes()));
// encrypt
byte[] encrypted = rsa.encrypt(plaintext.getBytes());
// decrypt
byte[] decrypted = rsa.decrypt(encrypted);
System.out.println("Decrypting Bytes: " + bytesToString(decrypted));
System.out.println("Decrypted String: " + new String(decrypted));
}
}
|
code/cryptography/src/rsa/rsa.py | #!/usr/bin/env python
from Crypto.PublicKey import RSA
# Convert string to number
def strToNum(s):
return int(s.encode("hex"), 16)
# Convert number to string
def numToStr(n):
s = "%x" % n
if len(s) % 2 != 0:
s = "0" + s
return s.decode("hex")
# Encrypt s using public key (e,n)
def encrypt(s, e, n):
return numToStr(pow(strToNum(s), e, n))
# Decrypt s using private key (d,n)
def decrypt(s, d, n):
return numToStr(pow(strToNum(s), d, n))
# Example
# Generate random key
key = RSA.generate(2048)
n = key.n
d = key.d
e = key.e
# decrypt(encrypt(m)) = m
# Output: Hello world!
print(decrypt(encrypt("Hello world!", e, n), d, n))
|
code/cryptography/src/rsa/rsa_input.in | 103 347
hellohowareyou |
code/cryptography/src/rsa_digital_signature/rsa_digital_signature.ipynb | {
"cells": [
{
"cell_type": "markdown",
"metadata": {
"deletable": true,
"editable": true
},
"source": [
"# RSA Digital Signature"
]
},
{
"cell_type": "code",
"execution_count": 59,
"metadata": {
"collapsed": true
},
"outputs": [],
"source": [
"from math import sqrt"
]
},
{
"cell_type": "code",
"execution_count": 1,
"metadata": {
"collapsed": true,
"deletable": true,
"editable": true
},
"outputs": [],
"source": [
"def exp_mod(a, b, n):\n",
" \"\"\"\n",
" a to the power of b, mod n\n",
" >>> exp_mod(2, 3, 6)\n",
" 2\n",
" \"\"\"\n",
" return (a**b) % n"
]
},
{
"cell_type": "code",
"execution_count": 2,
"metadata": {
"collapsed": true,
"deletable": true,
"editable": true
},
"outputs": [],
"source": [
"def gcd(a, b):\n",
" \"\"\"\n",
" Greatest common divisor\n",
" >>> gcd(72, 5)\n",
" 1\n",
" \"\"\"\n",
" if b > a:\n",
" return gcd(b, a)\n",
" if a % b == 0:\n",
" return b\n",
" return gcd(b, a % b)"
]
},
{
"cell_type": "code",
"execution_count": 3,
"metadata": {
"collapsed": false,
"deletable": true,
"editable": true
},
"outputs": [],
"source": [
"def gcd_qs(a, b):\n",
" \"\"\"\n",
" List of q values from gcd(a, b)\n",
" >>> gcd(72, 5)\n",
" [14, 2]\n",
" \"\"\"\n",
" if b > a:\n",
" a, b = b, a\n",
" assert a > b\n",
" assert gcd(a, b) == 1\n",
" \n",
" rem = None\n",
" while rem != 1:\n",
" q = a // b # 72 // 5 = 14 5 // 2 = 2\n",
" rem = a - q * b # 72 - 5*14 = 2 5 - 2*2 = 1\n",
" a = b # a is now 5 a is now 2\n",
" b = rem # b is now 2 b is now 1\n",
" yield q"
]
},
{
"cell_type": "code",
"execution_count": 4,
"metadata": {
"collapsed": true,
"deletable": true,
"editable": true
},
"outputs": [],
"source": [
"def mod_inv(a, n):\n",
" \"\"\" \n",
" Modular inverse\n",
" >>> inv_mod(72, 5)\n",
" 29\n",
" \"\"\"\n",
" t0, t1 = 0, 1\n",
" for q in gcd_qs(a, n):\n",
" t = t0 - q * t1\n",
" t0, t1 = t1, t # move them all back one space\n",
" return t"
]
},
{
"cell_type": "code",
"execution_count": 63,
"metadata": {
"collapsed": true,
"deletable": true,
"editable": true
},
"outputs": [],
"source": [
"def is_prime(n):\n",
" \"\"\"\n",
" Check whether a number is prime\n",
" >>> is_prime(5)\n",
" True\n",
" \"\"\"\n",
" return all(n % i != 0 \n",
" for i in range(2, int(sqrt(n))))"
]
},
{
"cell_type": "markdown",
"metadata": {
"deletable": true,
"editable": true
},
"source": [
"## 1) Generate Keys"
]
},
{
"cell_type": "code",
"execution_count": 47,
"metadata": {
"collapsed": true
},
"outputs": [],
"source": [
"from random import shuffle"
]
},
{
"cell_type": "code",
"execution_count": 68,
"metadata": {
"collapsed": false,
"deletable": true,
"editable": true
},
"outputs": [],
"source": [
"# pick two big prime numbers\n",
"p = 101\n",
"q = 499\n",
"\n",
"assert is_prime(p)\n",
"assert is_prime(q)\n",
"\n",
"n = p * q # first part of public key\n",
"phi_n = (p-1)*(q-1)"
]
},
{
"cell_type": "code",
"execution_count": 55,
"metadata": {
"collapsed": false,
"deletable": true,
"editable": true,
"scrolled": true
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"v = 167\n"
]
}
],
"source": [
"# pick v, second part of public key\n",
"valid_range = list(range(p+1, q))\n",
"shuffle(valid_range) # shuffles in place\n",
"\n",
"for v in valid_range: # search for a prime in (p, q)\n",
" if not is_prime(v): # need v to be prime\n",
" continue\n",
" if gcd(v, phi_n) == 1: # found one that fits\n",
" break\n",
" \n",
"print(f'v = {v}')"
]
},
{
"cell_type": "markdown",
"metadata": {
"deletable": true,
"editable": true
},
"source": [
"## 2) Sign Message"
]
},
{
"cell_type": "code",
"execution_count": 56,
"metadata": {
"collapsed": false,
"deletable": true,
"editable": true,
"scrolled": true
},
"outputs": [],
"source": [
"# compute s\n",
"s = mod_inv(v, phi_n) # private key"
]
},
{
"cell_type": "code",
"execution_count": 57,
"metadata": {
"collapsed": false,
"deletable": true,
"editable": true
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"signed message = 16437\n"
]
}
],
"source": [
"# desired message to send\n",
"msg = 321 \n",
"assert 1 < msg < n\n",
"\n",
"signed_msg = exp_mod(msg, s, n)\n",
"print(f'signed message = {signed_msg}')"
]
},
{
"cell_type": "markdown",
"metadata": {
"deletable": true,
"editable": true
},
"source": [
"## 3) Verify Authenticity"
]
},
{
"cell_type": "code",
"execution_count": 69,
"metadata": {
"collapsed": false,
"deletable": true,
"editable": true
},
"outputs": [
{
"name": "stdout",
"output_type": "stream",
"text": [
"decrypted message = 321\n",
"same as original message: True\n"
]
}
],
"source": [
"decrypted = exp_mod(signed_msg, v, n)\n",
"print(f'decrypted message = {decrypted}')\n",
"print(f'same as original message: {decrypted == msg}')"
]
}
],
"metadata": {
"kernelspec": {
"display_name": "Python 3",
"language": "python",
"name": "python3"
},
"language_info": {
"codemirror_mode": {
"name": "ipython",
"version": 3
},
"file_extension": ".py",
"mimetype": "text/x-python",
"name": "python",
"nbconvert_exporter": "python",
"pygments_lexer": "ipython3",
"version": "3.6.0"
}
},
"nbformat": 4,
"nbformat_minor": 2
}
|
code/cryptography/src/runningkey_cipher/README.md | # cosmos
> Your personal library of every algorithm and data structure code that you will ever encounter
Part of Cosmos by OpenGenus Foundation
It uses the following Table called Tabula Recta to find the ciphertext. The simple intersection of the row and column is the ciphertext. Let's say for eg. T is the Key and E is the original Plaintext, then the intersection of T column and E row is the ciphertext i.e. X. The plaintext and key-length must be the same. Running Key Cipher is similar to Vigenere Cipher. The difference lies in how the key is chosen; the Vigenere cipher uses a short key that repeats, whereas the running key cipher uses a long key such as an excerpt from a book. This means the key does not repeat, making cryptanalysis more difficult.
A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
---------------------------------------------------
A A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
B B C D E F G H I J K L M N O P Q R S T U V W X Y Z A
C C D E F G H I J K L M N O P Q R S T U V W X Y Z A B
D D E F G H I J K L M N O P Q R S T U V W X Y Z A B C
E E F G H I J K L M N O P Q R S T U V W X Y Z A B C D
F F G H I J K L M N O P Q R S T U V W X Y Z A B C D E
G G H I J K L M N O P Q R S T U V W X Y Z A B C D E F
H H I J K L M N O P Q R S T U V W X Y Z A B C D E F G
I I J K L M N O P Q R S T U V W X Y Z A B C D E F G H
J J K L M N O P Q R S T U V W X Y Z A B C D E F G H I
K K L M N O P Q R S T U V W X Y Z A B C D E F G H I J
L L M N O P Q R S T U V W X Y Z A B C D E F G H I J K
M M N O P Q R S T U V W X Y Z A B C D E F G H I J K L
N N O P Q R S T U V W X Y Z A B C D E F G H I J K L M
O O P Q R S T U V W X Y Z A B C D E F G H I J K L M N
P P Q R S T U V W X Y Z A B C D E F G H I J K L M N O
Q Q R S T U V W X Y Z A B C D E F G H I J K L M N O P
R R S T U V W X Y Z A B C D E F G H I J K L M N O P Q
S S T U V W X Y Z A B C D E F G H I J K L M N O P Q R
T T U V W X Y Z A B C D E F G H I J K L M N O P Q R S
U U V W X Y Z A B C D E F G H I J K L M N O P Q R S T
V V W X Y Z A B C D E F G H I J K L M N O P Q R S T U
W W X Y Z A B C D E F G H I J K L M N O P Q R S T U V
X X Y Z A B C D E F G H I J K L M N O P Q R S T U V W
Y Y Z A B C D E F G H I J K L M N O P Q R S T U V W X
Z Z A B C D E F G H I J K L M N O P Q R S T U V W X Y
---
<p align="center">
A massive collaborative effort by <a href="https://github.com/OpenGenus/cosmos">OpenGenus Foundation</a>
</p>
---
|
code/cryptography/src/runningkey_cipher/runningkey_cipher.py | # Part of Cosmos by OpenGenus Foundation
#
# It uses the following Table called Tabula Recta to find the
# ciphertext. The simple intersection of the row and column
# is the ciphertext. Let's say for eg. T is the Key and E is
# the original Plaintext, then the intersection of T column
# and E row is the ciphertext i.e. X. The plaintext and key-
# length must be the same. Running Key Cipher is similar to
# Vigenere Cipher. The difference lies in how the key is chosen;
# the Vigenere cipher uses a short key that repeats, whereas the
# running key cipher uses a long key such as an excerpt from
# a book. This means the key does not repeat, making cryptanalysis
# more difficult.
# A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
# ---------------------------------------------------
# A A B C D E F G H I J K L M N O P Q R S T U V W X Y Z
# B B C D E F G H I J K L M N O P Q R S T U V W X Y Z A
# C C D E F G H I J K L M N O P Q R S T U V W X Y Z A B
# D D E F G H I J K L M N O P Q R S T U V W X Y Z A B C
# E E F G H I J K L M N O P Q R S T U V W X Y Z A B C D
# F F G H I J K L M N O P Q R S T U V W X Y Z A B C D E
# G G H I J K L M N O P Q R S T U V W X Y Z A B C D E F
# H H I J K L M N O P Q R S T U V W X Y Z A B C D E F G
# I I J K L M N O P Q R S T U V W X Y Z A B C D E F G H
# J J K L M N O P Q R S T U V W X Y Z A B C D E F G H I
# K K L M N O P Q R S T U V W X Y Z A B C D E F G H I J
# L L M N O P Q R S T U V W X Y Z A B C D E F G H I J K
# M M N O P Q R S T U V W X Y Z A B C D E F G H I J K L
# N N O P Q R S T U V W X Y Z A B C D E F G H I J K L M
# O O P Q R S T U V W X Y Z A B C D E F G H I J K L M N
# P P Q R S T U V W X Y Z A B C D E F G H I J K L M N O
# Q Q R S T U V W X Y Z A B C D E F G H I J K L M N O P
# R R S T U V W X Y Z A B C D E F G H I J K L M N O P Q
# S S T U V W X Y Z A B C D E F G H I J K L M N O P Q R
# T T U V W X Y Z A B C D E F G H I J K L M N O P Q R S
# U U V W X Y Z A B C D E F G H I J K L M N O P Q R S T
# V V W X Y Z A B C D E F G H I J K L M N O P Q R S T U
# W W X Y Z A B C D E F G H I J K L M N O P Q R S T U V
# X X Y Z A B C D E F G H I J K L M N O P Q R S T U V W
# Y Y Z A B C D E F G H I J K L M N O P Q R S T U V W X
# Z Z A B C D E F G H I J K L M N O P Q R S T U V W X Y
plaintext = "DEFENDTHEEASTWALLOFTHECASTLE"
key = "HOWDOESTHEDUCKKNOWTHATSAIDVI"
ciphertext = ""
if __name__ == "__main__":
for index in range(len(plaintext)):
sum_key_plaintext = (ord(key[index]) - 64) + (ord(plaintext[index]) - 64)
if sum_key_plaintext > 27:
ciphertext += chr(sum_key_plaintext - 26 - 1 + 64)
else:
ciphertext += chr(sum_key_plaintext - 1 + 64)
print(ciphertext)
|
code/cryptography/src/sha/sha_256/sha_256.py | initial_hash_values = [
"6a09e667",
"bb67ae85",
"3c6ef372",
"a54ff53a",
"510e527f",
"9b05688c",
"1f83d9ab",
"5be0cd19",
]
sha_256_constants = [
"428a2f98",
"71374491",
"b5c0fbcf",
"e9b5dba5",
"3956c25b",
"59f111f1",
"923f82a4",
"ab1c5ed5",
"d807aa98",
"12835b01",
"243185be",
"550c7dc3",
"72be5d74",
"80deb1fe",
"9bdc06a7",
"c19bf174",
"e49b69c1",
"efbe4786",
"0fc19dc6",
"240ca1cc",
"2de92c6f",
"4a7484aa",
"5cb0a9dc",
"76f988da",
"983e5152",
"a831c66d",
"b00327c8",
"bf597fc7",
"c6e00bf3",
"d5a79147",
"06ca6351",
"14292967",
"27b70a85",
"2e1b2138",
"4d2c6dfc",
"53380d13",
"650a7354",
"766a0abb",
"81c2c92e",
"92722c85",
"a2bfe8a1",
"a81a664b",
"c24b8b70",
"c76c51a3",
"d192e819",
"d6990624",
"f40e3585",
"106aa070",
"19a4c116",
"1e376c08",
"2748774c",
"34b0bcb5",
"391c0cb3",
"4ed8aa4a",
"5b9cca4f",
"682e6ff3",
"748f82ee",
"78a5636f",
"84c87814",
"8cc70208",
"90befffa",
"a4506ceb",
"bef9a3f7",
"c67178f2",
]
def dec_to_bin(dec):
return str(format(dec, "b"))
def dec_to_bin_8_bit(dec):
return str(format(dec, "08b"))
def dec_to_bin_32_bit(dec):
return str(format(dec, "032b"))
def dec_to_bin_64_bit(dec):
return str(format(dec, "064b"))
def dec_to_hex(dec):
return str(format(dec, "x"))
def bin_to_dec(bin_string):
return int(bin_string, 2)
def hex_to_dec(hex_string):
return int(hex_string, 16)
def L_P(SET, n):
to_return = []
j = 0
k = n
while k < len(SET) + 1:
to_return.append(SET[j:k])
j = k
k += n
return to_return
def string_to_list(bit_string):
bit_list = []
for i in range(len(bit_string)):
bit_list.append(bit_string[i])
return bit_list
def list_to_string(bit_list):
bit_string = ""
for i in range(len(bit_list)):
bit_string += bit_list[i]
return bit_string
def rotate_right(bit_string, n):
bit_list = string_to_list(bit_string)
for i in range(n):
last_elem = list(bit_list.pop(-1))
bit_list = last_elem + bit_list
return list_to_string(bit_list)
def shift_right(bit_string, n):
len_bit_string = len(bit_string)
bit_string = bit_string[: len_bit_string - n :]
front = "0" * n
return front + bit_string
def mod_32_addition(input_set):
return sum(input_set) % 4294967296
def xor_2str(string_1, string_2):
xor_string = ""
for i in range(len(string_1)):
if string_1[i] == string_2[i]:
xor_string += "0"
else:
xor_string += "1"
return xor_string
def and_2str(string_1, string_2):
and_list = []
for i in range(len(string_1)):
if string_1[i] == "1" and string_2[i] == "1":
and_list.append("1")
else:
and_list.append("0")
return list_to_string(and_list)
def or_2str(string_1, string_2):
or_list = []
for i in range(len(string_1)):
if string_1[i] == "0" and string_2[i] == "0":
or_list.append("0")
else:
or_list.append("1")
return list_to_string(or_list)
def not_str(bit_string):
not_string = ""
for i in range(len(bit_string)):
if bit_string[i] == "0":
not_string += "1"
else:
not_string += "0"
return not_string
"""
SHA-256 Specific Functions:
"""
def Ch(x, y, z):
return xor_2str(and_2str(x, y), and_2str(not_str(x), z))
def Maj(x, y, z):
return xor_2str(xor_2str(and_2str(x, y), and_2str(x, z)), and_2str(y, z))
def e_0(x):
return xor_2str(
xor_2str(rotate_right(x, 2), rotate_right(x, 13)), rotate_right(x, 22)
)
def e_1(x):
return xor_2str(
xor_2str(rotate_right(x, 6), rotate_right(x, 11)), rotate_right(x, 25)
)
def s_0(x):
return xor_2str(
xor_2str(rotate_right(x, 7), rotate_right(x, 18)), shift_right(x, 3)
)
def s_1(x):
return xor_2str(
xor_2str(rotate_right(x, 17), rotate_right(x, 19)), shift_right(x, 10)
)
def message_pad(bit_list):
pad_one = bit_list + "1"
pad_len = len(pad_one)
k = 0
while ((pad_len + k) - 448) % 512 != 0:
k += 1
back_append_0 = "0" * k
back_append_1 = dec_to_bin_64_bit(len(bit_list))
return pad_one + back_append_0 + back_append_1
def message_bit_return(string_input):
bit_list = []
for i in range(len(string_input)):
bit_list.append(dec_to_bin_8_bit(ord(string_input[i])))
return list_to_string(bit_list)
def message_pre_pro(input_string):
bit_main = message_bit_return(input_string)
return message_pad(bit_main)
def message_parsing(input_string):
return L_P(message_pre_pro(input_string), 32)
def message_schedule(index, w_t):
new_word = dec_to_bin_32_bit(
mod_32_addition(
[
bin_to_dec(s_1(w_t[index - 2])),
bin_to_dec(w_t[index - 7]),
bin_to_dec(s_0(w_t[index - 15])),
bin_to_dec(w_t[index - 16]),
]
)
)
return new_word
def sha_256(input_string):
w_t = message_parsing(input_string)
a = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[0]))
b = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[1]))
c = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[2]))
d = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[3]))
e = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[4]))
f = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[5]))
g = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[6]))
h = dec_to_bin_32_bit(hex_to_dec(initial_hash_values[7]))
for i in range(0, 64):
if i <= 15:
t_1 = mod_32_addition(
[
bin_to_dec(h),
bin_to_dec(e_1(e)),
bin_to_dec(Ch(e, f, g)),
hex_to_dec(sha_256_constants[i]),
bin_to_dec(w_t[i]),
]
)
t_2 = mod_32_addition([bin_to_dec(e_0(a)), bin_to_dec(Maj(a, b, c))])
h = g
g = f
f = e
e = mod_32_addition([bin_to_dec(d), t_1])
d = c
c = b
b = a
a = mod_32_addition([t_1, t_2])
a = dec_to_bin_32_bit(a)
e = dec_to_bin_32_bit(e)
else:
w_t.append(message_schedule(i, w_t))
t_1 = mod_32_addition(
[
bin_to_dec(h),
bin_to_dec(e_1(e)),
bin_to_dec(Ch(e, f, g)),
hex_to_dec(sha_256_constants[i]),
bin_to_dec(w_t[i]),
]
)
t_2 = mod_32_addition([bin_to_dec(e_0(a)), bin_to_dec(Maj(a, b, c))])
h = g
g = f
f = e
e = mod_32_addition([bin_to_dec(d), t_1])
d = c
c = b
b = a
a = mod_32_addition([t_1, t_2])
a = dec_to_bin_32_bit(a)
e = dec_to_bin_32_bit(e)
hash_0 = mod_32_addition([hex_to_dec(initial_hash_values[0]), bin_to_dec(a)])
hash_1 = mod_32_addition([hex_to_dec(initial_hash_values[1]), bin_to_dec(b)])
hash_2 = mod_32_addition([hex_to_dec(initial_hash_values[2]), bin_to_dec(c)])
hash_3 = mod_32_addition([hex_to_dec(initial_hash_values[3]), bin_to_dec(d)])
hash_4 = mod_32_addition([hex_to_dec(initial_hash_values[4]), bin_to_dec(e)])
hash_5 = mod_32_addition([hex_to_dec(initial_hash_values[5]), bin_to_dec(f)])
hash_6 = mod_32_addition([hex_to_dec(initial_hash_values[6]), bin_to_dec(g)])
hash_7 = mod_32_addition([hex_to_dec(initial_hash_values[7]), bin_to_dec(h)])
final_hash = [
dec_to_hex(hash_0),
dec_to_hex(hash_1),
dec_to_hex(hash_2),
dec_to_hex(hash_3),
dec_to_hex(hash_4),
dec_to_hex(hash_5),
dec_to_hex(hash_6),
dec_to_hex(hash_7),
]
return "".join(final_hash)
assert (
sha_256("cosmos")
) == "4cbe19716b1aa73a67dc4b28c34391879b503259fc76852082b4dafcf0de85b2"
|
code/cryptography/src/simple_des/sdes.py | def p_10(k):
p_list=[2,4,1,6,3,9,0,8,7,5]
for i in range(len(k)):
p_list[i]=k[p_list[i]]
return p_list
def p_8(k):
p_list=[5,2,6,3,7,4,9,8]
for i in range(len(p_list)):
p_list[i]=k[p_list[i]]
return p_list
def p_4(k):
permutation_list=[1,3,2,0]
for i in range(len(permutation_list)):
permutation_list[i]=k[permutation_list[i]]
return permutation_list
def left_shift_by_1(k):
k.append(k[0])
k.pop(0)
return k
def left_shift_by_2(k):
k.append(k[0])
k.append(k[1])
k.pop(0)
k.pop(0)
return k
def generate_keys(key):
p_10_list=p_10(key)
l=p_10_list[:5]
r=p_10_list[5:]
l1=left_shift_by_1(l)
r1=left_shift_by_1(r)
key1=l1+r1
key1=p_8(key1)
l2=left_shift_by_2(l)
r2=left_shift_by_2(r)
key2=l2+r2
key2=p_8(key2)
print("Key1 Generated : ","".join(key1))
print("Key2 Generated : ","".join(key2))
keys=[]
keys.append(key1)
keys.append(key2)
return keys
def i_p(pt):
p_list=[1,5,2,0,3,7,4,6]
for i in range(len(p_list)):
p_list[i]=pt[p_list[i]]
return p_list
def inverse_i_p(k):
i_p_list=[3,0,2,4,6,1,7,5]
for i in range(len(i_p_list)):
i_p_list[i]=k[i_p_list[i]]
return i_p_list
def e_p(k):
e_list=[3,0,1,2,1,2,3,0]
for i in range(len(e_list)):
e_list[i]=k[e_list[i]]
return e_list
def xor(l1,l2):
xor_list=[]
for i in range(len(l1)):
xor_list.append(str(int(l1[i])^int(l2[i])))
return xor_list
def Part_1(pt):
return i_p(pt)
def Part_2(pt,key,sbox1,sbox2):
l=pt[:4]
r=pt[4:]
expanded_right_half=e_p(r)
xor_out=xor(expanded_right_half,key)
xor_left_half=xor_out[:4]
xor_right_half=xor_out[4:]
left_row_value=2*(int(xor_left_half[0]))+int(xor_left_half[3])
left_column_value=2*(int(xor_left_half[1]))+int(xor_left_half[2])
right_row_value=2*(int(xor_right_half[0]))+int(xor_right_half[3])
right_column_value=2*(int(xor_right_half[1]))+int(xor_right_half[2])
s0b=sbox1[left_row_value][left_column_value]
s1b=sbox2[right_row_value][right_column_value]
s0b=bin(s0b).replace("0b","")
s1b=bin(s1b).replace("0b","")
if(len(s0b)<2):
s0b="0"+s0b
if(len(s1b)<2):
s1b="0"+s1b
combined_s_value=s0b+s1b
combined_s_value = p_4(combined_s_value)
xcs=xor(l,combined_s_value)
xcs = xcs+r
return xcs
def encrypt(pt,keys,sbox1,sbox2):
initial_permutation_list=Part_1(pt)
xcs_r1 = Part_2(initial_permutation_list ,keys[0],sbox1,sbox2)
xcs_r2 = Part_2(xcs_r1,keys[1],sbox1,sbox2)
xcs_r2=xcs_r2[4:]+xcs_r2[:4]
inverse_initial_permutation_value=inverse_i_p(xcs_r2)
encrypted_text=""
for i in range(len(inverse_initial_permutation_value)):
encrypted_text=encrypted_text+inverse_initial_permutation_value[i]
return encrypted_text
def decrypt(dt,keys,sbox1,sbox2):
initial_permutation_list=Part_1(dt)
initial_permutation_list = initial_permutation_list[4:]+initial_permutation_list[:4]
xcs_r1 = Part_2(initial_permutation_list,keys[1],sbox1,sbox2)
xcs_r2 = Part_2(xcs_r1,keys[0],sbox1,sbox2)
inverse_initial_permutation_value=inverse_i_p(xcs_r2)
decrypted_text=""
for i in range(len(inverse_initial_permutation_value)):
decrypted_text=decrypted_text+inverse_initial_permutation_value[i]
return decrypted_text
# key = list(input("Enter the 10-bit Key Value : "))
# pt = list(input("Enter the 8-bit Plain Text : "))
key = list("1010000010")
pt = list("10010101")
sbox1=[[1,0,3,2],
[3,2,1,0],
[0,2,1,3],
[3,1,3,2]]
sbox2=[[0,1,2,3],
[2,0,1,3],
[3,0,1,0],
[2,1,0,3]]
print("Entered Plain text : ","".join(pt))
keys=generate_keys(key)
encrypted_text=encrypt(pt,keys,sbox1,sbox2)
print("After Encryption, Cipher Text : ",encrypted_text)
decrypted_text = decrypt(encrypted_text,keys,sbox1,sbox2)
print("After Decryption, Original Plain Text : ",decrypted_text)
|
code/cryptography/src/vernam_cipher/vernam_cipher.py | mappingsDict = {}
def vernamEncryption(plaintext, key):
ciphertext = ''
for i in range(len(plaintext)):
ptLetter = plaintext[i]
keyLetter = key[i]
sum = mappingsDict[ptLetter] + mappingsDict[keyLetter]
if sum >= 26:
sum -= 26
ciphertext += chr(sum + 65)
return ciphertext
def vernamDecryption(ciphertext, key):
plaintext = ''
for i in range(len(ciphertext)):
ctLetter = ciphertext[i]
keyLetter = key[i]
diff = mappingsDict[ctLetter] - mappingsDict[keyLetter]
if diff < 0:
diff += 26
plaintext += chr(diff + 65)
return plaintext
plaintext = input("Enter the plaintext : ")
key = input("Enter the key : ")
alphabets = "abcdefghijklmnopqrstuvwxyz".upper()
for alphabet in alphabets:
mappingsDict[alphabet] = ord(alphabet) - 65
plaintext = plaintext.upper()
key = key.upper()
if len(key) < len(plaintext):
print("Length of Key Must be >= Length of Plaintext")
else:
ciphertext = vernamEncryption(plaintext, key)
plaintext = vernamDecryption(ciphertext, key)
print("Encrypted ciphertext is : ", ciphertext)
print("Decrypted plaintext is : ", plaintext)
|
code/cryptography/src/vigenere_cipher/README.md | # cosmos
Your personal library of every algorithm and data structure code that you will ever encounter
|
code/cryptography/src/vigenere_cipher/vigenere_cipher.c | // Vigenere Cipher
// Author: Rishav Pandey
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <ctype.h>
int main(int argc, char* argv[])
{
//check if user give more or less than two arguments.
if(argc != 2)
{
printf("Enter the Valid Key");
return 1;
}
else
{
for(int i =0, n = strlen(argv[1]); i<n ;i++)
{
//check weather the key is only alphabet or not
if(!isalpha(argv[1][i]))
{
printf("Enter only Alphabets");
return 1;
}
}
}
printf("plaintext: ");
char s[100];
scanf("%s", s);
char* k = argv[1];
int klen = strlen(k);
printf("ciphertext: ");
for(int i = 0, j = 0, n = strlen(s); i<n ; i++)
{
//wrap key for plaintext.
int key = tolower(k[j % klen]) - 97;
if(isalpha(s[i]))
{
//for upper case letters.
if(isupper(s[i]))
{
printf("%c",((s[i]-65+key)%26)+65);
//increase key for next char.
j++;
}
//for lower case letters.
else if(islower(s[i]))
{
printf("%c",((s[i]-97+key)%26)+97);
//for lower case letters.
j++;
//increase key for next char.
}
}
//for non alphabetical values.
else
{
printf("%c",s[i]);
}
}
printf("\n");
return 0;
} |
code/cryptography/src/vigenere_cipher/vigenere_cipher.cpp | //C++ code for Vigenere Ciphere
#include <iostream>
#include <string>
class Vigenere
{
public:
Vigenere(const std::string& key)
{ //Traverses through the key
for (size_t i = 0; i < key.size(); ++i)
{
if (key[i] >= 'A' && key[i] <= 'Z') //Check if the character of key is an uppercase alphabet
this->key += key[i];
else if (key[i] >= 'a' && key[i] <= 'z') //Check if the character of key is a lowercase alphabet
this->key += key[i] + 'A' - 'a';
}
}
//A function to perform encryption
std::string encrypt(const std::string& text)
{
std::string result;
//Traverses the plaintext
for (size_t i = 0, j = 0; i < text.length(); ++i)
{
char c = text[i];
if (c >= 'a' && c <= 'z') //Check if the character is a lowercase alphabet
c += 'A' - 'a'; //Converts lowercase to uppercase characters
else if (c < 'A' || c > 'Z') //Check if the character is not an alphabet
continue;
result += (c + key[j] - 2 * 'A') % 26 + 'A'; //Encryption of character is put into result by adding key to plaintext
j = (j + 1) % key.length(); //Increment j within length of key
}
return result;
}
//A function to perform decryption
std::string decrypt(const std::string& text)
{
std::string result;
//Traverses the ciphertext
for (size_t i = 0, j = 0; i < text.length(); ++i)
{
char c = text[i];
if (c >= 'a' && c <= 'z') //Check if the character is a lowercase alphabet
c += 'A' - 'a'; //Converts lowercase to uppercase characters
else if (c < 'A' || c > 'Z') //Check if the character is not an alphabet
continue;
result += (c - key[j] + 26) % 26 + 'A'; //Decryption of character is put into result by Subtracting key from ciphertext
j = (j + 1) % key.length(); //Increment j within length of key
}
return result;
}
private:
std::string key;
};
int main()
{
Vigenere cipher{"VIGENERECIPHER"}; //key is VIGENERECIPHER of length 14
std::string original =
"Beware the Jabberwock, my son! The jaws that bite, the claws that catch!"; //Plaintext
std::string encrypted = cipher.encrypt(original);
std::string decrypted = cipher.decrypt(encrypted);
std::cout << original << "\n";
std::cout << "Encrypted: " << encrypted << "\n";
std::cout << "Decrypted: " << decrypted << "\n";
}
|
code/cryptography/src/vigenere_cipher/vigenere_cipher.go | package main
import (
"fmt"
)
func Encrypt(text string, key string) string {
if err := checkKey(key); err != nil {
fmt.Println("Error: ", err.Error())
return "Err"
}
j := 0
n := len(text)
res := ""
for i := 0; i < n; i++ {
r := text[i]
if r >= 'a' && r <= 'z' {
res += string((r+key[j]-2*'a')%26 + 'a')
} else if r >= 'A' && r <= 'Z' {
res += string((r+key[j]-2*'A')%26 + 'A')
} else {
res += string(r)
}
j = (j + 1) % len(key)
}
return res
}
func Decrypt(text string, key string) string {
if err := checkKey(key); err != nil {
fmt.Println("Error: ", err.Error())
return "Err"
}
res := ""
j := 0
n := len(text)
for i := 0; i < n; i++ {
r := text[i]
if r >= 'a' && r <= 'z' {
res += string((r-key[j]+26)%26 + 'a')
} else if r >= 'A' && r <= 'Z' {
res += string((r-key[j]+26)%26 + 'A')
} else {
res += string(r)
}
j = (j + 1) % len(key)
}
return res
}
func checkKey(key string) error {
if len(key) == 0 {
return fmt.Errorf("Key length is 0")
}
for _, r := range key {
if !(r >= 'a' && r <= 'z' || r >= 'A' && r <= 'Z') {
return fmt.Errorf("Invalid Key")
}
}
return nil
}
func main() {
var (
msg, key string
mode rune
)
fmt.Println("Enter text to encrypt\\decrypt")
fmt.Scanf("%s\n", &msg)
fmt.Println("Enter key")
fmt.Scanf("%s\n", &key)
fmt.Println("Enter 'e' to encrypt or 'd' to decrypt")
fmt.Scanf("%c", &mode)
if mode == rune('e') {
fmt.Println("Encrypted Message: ", Encrypt(msg, key))
} else if mode == rune('d') {
fmt.Println("Deccrypted Message: ", Decrypt(msg, key))
} else {
fmt.Println("Unknown mode")
}
}
|
code/cryptography/src/vigenere_cipher/vigenere_cipher.hs | --Vigenere cipher
module Vigenere where
import Data.Char
chrRg :: Int
chrRg = length ['A'..'Z']
encode :: Char -> Int
encode = subtract (ord 'A') . ord . toUpper
decode :: Int -> Char
decode = chr . (ord 'A' +)
shiftr :: Char -> Char -> Char
shiftr c k = decode $ mod (encode c + encode k) chrRg
shiftl :: Char -> Char -> Char
shiftl c k = decode $ mod (encode c - encode k) chrRg
vigenere :: String -> String -> String
vigenere = vigProc shiftr
unVigenere :: String -> String -> String
unVigenere = vigProc shiftl
vigProc :: (Char -> Char -> Char) -> (String -> String -> String)
vigProc s cs = vigProc' s cs . cycle
vigProc' :: (Char -> Char -> Char) -> (String -> String -> String)
vigProc' _ [] _ = []
vigProc' s (c:cs) (k:ks)
| isLetter c = s c k : vigProc' s cs ks
| otherwise = c : vigProc' s cs (k:ks)
encrypt :: IO ()
encrypt = do
putStrLn "Enter message to be encrypted: "
mes <- getLine
putStrLn "Enter key: "
key <- getLine
putStrLn "Encrypted message: "
putStrLn $ vigenere mes key
{- Ex.
encrypt
Enter message to be encrypted:
"Hello, World!"
Enter key:
"World"
Encrypted message:
"CAZCZ, ZJMHR!" -}
decrypt :: IO ()
decrypt = do
putStrLn "Enter message to be decrypted: "
mes <- getLine
putStrLn "Enter key: "
key <- getLine
putStrLn "Decrypted message: "
putStrLn $ unVigenere mes key
{- Ex.
decrypt
Enter message to be decrypted:
"CAZCZ, ZJMHR!"
Enter key:
"World"
Decrypted message:
"HELLO, WORLD!" -}
|
code/cryptography/src/vigenere_cipher/vigenere_cipher.java | /**
* Modified code from: https://rosettacode.org/wiki/Vigen%C3%A8re_cipher#Java
* Part of Cosmos by Open Genus Foundation
*/
import java.util.Scanner;
public class vigenere_cipher{
public static void main(String[] args) {
System.out.println("Enter key: ");
Scanner scanner = new Scanner(System.in);
String key = scanner.nextLine();
System.out.println("Enter text to encrypt: ");
String text = scanner.nextLine();
String enc = encrypt(text, key);
System.out.println(enc);
System.out.println(decrypt(enc, key));
}
static String encrypt(String text, final String key) {
String res = "";
for (int i = 0, j = 0; i < text.length(); i++) {
char c = text.charAt(i);
if (Character.isUpperCase(c)){
res += (char)((c + key.charAt(j) - 2 * 'A') % 26 + 'A');
j = ++j % key.length();
}
if (Character.isLowerCase(c)){
res += (char)((c + Character.toLowerCase(key.charAt(j)) - 2 * 'a') % 26 + 'a');
j = ++j % key.length();
}
}
return res;
}
static String decrypt(String text, final String key) {
String res = "";
for (int i = 0, j = 0; i < text.length(); i++) {
char c = text.charAt(i);
if (Character.isUpperCase(c)) {
res += (char)((c - key.charAt(j) + 26) % 26 + 'A');
j = ++j % key.length();
}
if (Character.isLowerCase(c)) {
res += (char)((c - Character.toLowerCase(key.charAt(j)) + 26) % 26 + 'a');
j = ++j % key.length();
}
}
return res;
}
} |
code/cryptography/src/vigenere_cipher/vigenere_cipher.jl | ###!/* Part of Cosmos by OpenGenus Foundation */
###Vigenere cipher implementation in Julia
function encrypt(msg::AbstractString, key::AbstractString)
msg = uppercase(join(filter(isalpha, collect(msg))))
key = uppercase(join(filter(isalpha, collect(key))))
msglen = length(msg)
keylen = length(key)
if keylen < msglen
key = repeat(key, div(msglen - keylen, keylen) + 2)[1:msglen]
end
enc = Vector{Char}(msglen)
@inbounds for i in 1:length(msg)
enc[i] = Char((Int(msg[i]) + Int(key[i]) - 130) % 26 + 65)
end
return join(enc)
end
function decrypt(enc::AbstractString, key::AbstractString)
enc = uppercase(join(filter(isalpha, collect(enc))))
key = uppercase(join(filter(isalpha, collect(key))))
msglen = length(enc)
keylen = length(key)
if keylen < msglen
key = repeat(key, div(msglen - keylen, keylen) + 2)[1:msglen]
end
msg = Vector{Char}(msglen)
@inbounds for i in 1:length(enc)
msg[i] = Char((Int(enc[i]) - Int(key[i]) + 26) % 26 + 65)
end
return join(msg)
end
const messages = ("Attack at dawn.", "Don't attack.", "The war is over.", "Happy new year!")
const key = "LEMON"
for msg in messages
enc = encrypt(msg, key)
dec = decrypt(enc, key)
println("Original: $msg\n -> encrypted: $enc\n -> decrypted: $dec")
end
|
code/cryptography/src/vigenere_cipher/vigenere_cipher.js | "use strict";
class Viegenere {
static alphabetLen_() {
return Viegenere.ALPHABET.length;
}
static encript(text, key) {
let result = "";
const preparedText = text.replace(new RegExp("\\s", "g"), "").toLowerCase();
const preparedKey = key.toLowerCase();
const alphabetLen = Viegenere.alphabetLen_();
for (let i = 0; i < preparedText.length; i++) {
const char = preparedText[i];
const keyChar = preparedKey[i % preparedKey.length];
const shift = Viegenere.ALPHABET.indexOf(keyChar) % alphabetLen;
const indexInAlphabet = Viegenere.ALPHABET.indexOf(char);
result += Viegenere.ALPHABET[(indexInAlphabet + shift) % alphabetLen];
}
return result;
}
static decript(encripted, key) {
let result = "";
const preparedKey = key.toLowerCase();
const alphabetLen = Viegenere.alphabetLen_();
for (let i = 0; i < encripted.length; i++) {
const char = encripted[i];
const keyChar = preparedKey[i % preparedKey.length];
let shift =
Viegenere.ALPHABET.indexOf(char) - Viegenere.ALPHABET.indexOf(keyChar);
shift = shift >= 0 ? shift : alphabetLen + shift;
result += Viegenere.ALPHABET[shift];
}
return result;
}
}
Viegenere.ALPHABET = "abcdefghijklmnopqrstuvwxyz";
const key = "LEMONLEMONLE";
const text = "Lorem ipsum dolor sit amet";
const encripted = Viegenere.encript(text, key);
const decripted = Viegenere.decript(encripted, key);
console.log(encripted);
console.log(decripted);
|
code/cryptography/src/vigenere_cipher/vigenere_cipher.php | <?php
/**
* Vigenère cipher (PHP)
* based on https://en.wikipedia.org/wiki/Vigen%C3%A8re_cipher
* implemented by Stefano Amorelli <[email protected]>
* Part of Cosmos by OpenGenus Foundation
*/
class VigenereCipher
{
public function encrypt($plainText, $key) {
if (!ctype_alpha($plainText) || !ctype_alpha($key)) {
throw new Exception('Plaintext and key should be alphabetical strings (no spaces, symbols and numbers).');
}
$plainText = strtolower($plainText);
$key = strtolower($key);
$encryptedText = [];
$i = 0;
foreach (str_split($plainText) as $character) {
array_push($encryptedText, $this->encryptCharacter($character, $key[$i]));
$i++;
$i = $i % strlen($key);
}
return implode('', $encryptedText);
}
public function decrypt($encryptedText, $key) {
if (!ctype_alpha($encryptedText) || !ctype_alpha($key)) {
throw new Exception('Encrypted text and key should be alphabetical strings (no spaces, symbols and numbers).');
}
$encryptedText = strtolower($encryptedText);
$key = strtolower($key);
$plainText = [];
$i = 0;
foreach (str_split($encryptedText) as $character) {
array_push($plainText, $this->decryptCharacter($character, $key[$i]));
$i++;
$i = $i % strlen($key);
}
return implode('', $plainText);
}
private function normalizeAscii($character) {
return ord($character) - ord('a');
}
private function returnAscii($normalizedAscii) {
return chr($normalizedAscii + ord('a'));
}
private function encryptCharacter($plainCharacter, $keyCharacter) {
return $this->returnAscii(($this->normalizeAscii($plainCharacter) + $this->normalizeAscii($keyCharacter)) % 26);
}
private function decryptCharacter($encryptedCharacter, $keyCharacter) {
return $this->returnAscii(($this->normalizeAscii($encryptedCharacter) - $this->normalizeAscii($keyCharacter) + 26) % 26);
}
}
/* Example usage:
$cipher = new VigenereCipher();
$secret = 'mysecret';
$encrypted = $cipher->encrypt('loremipsumdolor', $secret);
$plain = $cipher->decrypt($encrypted, $secret);
echo $plain;
*/
|
code/cryptography/src/vigenere_cipher/vigenere_cipher.py | #Method for encryption that takes key and plaintext as arguments
def encrypt(key, text):
alfa = "abcdefghijklmnopqrstuvwxyz"
msg_to_be_decrypted = text.replace(" ", "") #Removes spaces from plaintext
msg_to_be_decrypted = msg_to_be_decrypted.lower() #Converts all characters to lowercase
encrypted = ""
#Traverses the plaintext
for i in range(len(msg_to_be_decrypted)):
letter_in_key = key[i % len(key)] #Puts the required character of the key in variable letter_in_key
shift = (alfa.find(letter_in_key) + 1) % 26 #Puts the amount to be shifted in variable shift
index_of_letter = alfa.find(msg_to_be_decrypted[i]) #Finds the character of plaintext to be encrypted
encrypted += alfa[(index_of_letter + shift) % 26]
return encrypted
#Method for encryption that takes key and ciphertext as arguments
def decrypt(key, encrypted):
alfa = "abcdefghijklmnopqrstuvwxyz"
decrypted = ""
#Traverses the ciphertext
for i in range(len(encrypted)):
letter_in_key = key[i % len(key)] #Puts the required character of the key in variable letter_in_key
shift = (alfa.find(encrypted[i]) - alfa.find(letter_in_key) - 1) % 26 #Puts the amount to be shifted in variable shift
decrypted += alfa[shift] #Performs decryption
return decrypted
key = "abc"
text = "ZZAla ma kota"
cipher = encrypt(key, text)
print(cipher)
msg = decrypt(key, cipher)
print(msg)
|
code/cryptography/src/vigenere_cipher/vigenere_cipher.rb | class Vigenere
@@alphabet = 'abcdefghijklmnopqrstuvwxyz'
def initialize(key)
@key = key.downcase
end
def encrypt(message)
message = message.downcase
result = ''
for i in 0..message.length - 1
letterNum = @@alphabet.index(message[i])
keyNum = @@alphabet.index(@key[i % @key.length]) + 1
resultNum = (letterNum + keyNum) % @@alphabet.length
result << @@alphabet[resultNum]
end
result
end
def decrypt(cipher)
cipher = cipher.downcase
result = ''
for i in 0..cipher.length - 1
letterNum = @@alphabet.index(cipher[i])
keyNum = @@alphabet.index(@key[i % @key.length]) + 1
resultNum = (letterNum - keyNum) % @@alphabet.length
result << @@alphabet[resultNum]
end
result
end
def self.encryptMessage(key, message)
message = message.downcase
result = ''
for i in 0..message.length - 1
letterNum = @@alphabet.index(message[i])
keyNum = @@alphabet.index(key[i % key.length]) + 1
resultNum = (letterNum + keyNum) % @@alphabet.length
result << @@alphabet[resultNum]
end
result
end
def self.decryptMessage(key, cipher)
cipher = cipher.downcase
result = ''
for i in 0..cipher.length - 1
letterNum = @@alphabet.index(cipher[i])
keyNum = @@alphabet.index(key[i % key.length]) + 1
resultNum = (letterNum - keyNum) % @@alphabet.length
result << @@alphabet[resultNum]
end
result
end
end
# vig = Vigenere.new("abc")
# cipher = vig.encrypt("hello")
# puts cipher
# puts vig.decrypt(cipher)
# cipher2 = Vigenere.encryptMessage("abc", "hello")
# puts cipher2
# puts Vigenere.decryptMessage("abc", cipher2)
|
code/cryptography/test/README.md | # cosmos
Your personal library of every algorithm and data structure code that you will ever encounter
|
code/cryptography/test/baconian_cipher/baconian_test.cpp | #include <assert.h>
#include <iostream>
#include "./../../src/baconian_cipher/baconian.cpp"
// Part of Cosmos by OpenGenus Foundation
int main()
{
std::string baconianString = "AABBBAABAAABABBABABBABBBA BABBAABBBABAAABABABBAAABB";
std::string englishString = "HELLO WORLD";
std::map<char, std::string> generatedCipher = createBaconianMap();
assert(encrypt(generatedCipher, englishString) == baconianString);
assert(decrypt(generatedCipher, baconianString) == englishString);
std::cout << "Testing Complete\n";
}
|
code/data_structures/src/2d_array/SpiralMatrix.cpp | #include <iostream>
#include <vector>
using namespace std;
vector<vector<int>> generateSpiralMatrix(int n) {
vector<vector<int>> matrix(n, vector<int>(n));
int left = 0;
int right = n - 1;
int top = 0;
int bottom = n - 1;
int direction = 0;
int value = 1;
while (left <= right && top <= bottom) {
if (direction == 0) {
for (int i = left; i <= right; i++) {
matrix[top][i] = value++;
}
top++;
} else if (direction == 1) {
for (int j = top; j <= bottom; j++) {
matrix[j][right] = value++;
}
right--;
} else if (direction == 2) {
for (int i = right; i >= left; i--) {
matrix[bottom][i] = value++;
}
bottom--;
} else {
for (int j = bottom; j >= top; j--) {
matrix[j][left] = value++;
}
left++;
}
direction = (direction + 1) % 4;
}
return matrix;
}
int main() {
int n;
cin >> n;
vector<vector<int>> spiralMatrix = generateSpiralMatrix(n);
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
cout << spiralMatrix[i][j] << " ";
}
cout << endl;
}
return 0;
}
|
Subsets and Splits
No saved queries yet
Save your SQL queries to embed, download, and access them later. Queries will appear here once saved.