repo_id
stringlengths 21
168
| file_path
stringlengths 36
210
| content
stringlengths 1
9.98M
| __index_level_0__
int64 0
0
|
---|---|---|---|
mirrored_repositories/project_lw/lib | mirrored_repositories/project_lw/lib/utils/spf_keys.dart | class SpfKeys {
static const String LAST_WALLPAPER = 'LAST_WALLPAPER';
static const String FIRST = 'FIRST';
}
| 0 |
mirrored_repositories/project_lw/lib | mirrored_repositories/project_lw/lib/utils/wallpaper_file_utils.dart | import 'dart:convert';
import 'dart:io';
import 'package:archive/archive.dart';
import 'package:archive/archive_io.dart';
import 'package:es_compression/brotli.dart';
import 'package:file_utils/file_utils.dart';
import 'package:project_lw/entity/wallpaper.dart';
class WallpaperFileUtil {
static const WALLPAPER_INFO_FILE_NAME = 'wallpaper.json';
static const VIEW_INFO_FILE_NAME = 'view_config.json';
static const PACK_NAME = 'wallpaper.$SUPPORTED_WALLPAPER_FORMAT';
static const TEMP_TAR_NAME = 'content.tar';
static const TEMP_FOLDER_NAME = '.temp';
static const SUPPORTED_WALLPAPER_FORMAT = 'lwpak';
static const SUPPORTED_IMAGE_FORMAT = 'webp';
static const SUPPORTED_VIDEO_FORMAT = 'mp4';
static const SUPPORTED_HTML_FORMAT = 'html';
/// 解包 wallpaper pack
/// [wallpaperFile] 传入的 wallpaper 包
/// [unpackDir] 解包路径
static Future<void> unpackWallpaper(final File wallpaperFile, final Directory unpackDir) async {
if (wallpaperFile == null || unpackDir == null) throw ArgumentError.notNull('wallpaperFile');
if (!wallpaperFile.path.endsWith(SUPPORTED_WALLPAPER_FORMAT)) throw ArgumentError('!wallpaperFile.path.endsWith(SUPPORTED_WALLPAPER_FORMAT)');
if (!wallpaperFile.existsSync() || !unpackDir.existsSync()) throw ArgumentError('!wallpaperFile.existsSync() || !unpackPath.existsSync()');
final bytes = await wallpaperFile.readAsBytes();
final result = brotli.decode(bytes);
final tarDecoder = TarDecoder();
final archive = tarDecoder.decodeBytes(result);
await for (final file in Stream.fromIterable(archive.files)) {
final targetPath = unpackDir.path + Platform.pathSeparator + file.name;
if (file.isFile) {
final data = file.content as List<int>;
final f = File(targetPath);
await f.create(recursive: true);
await f.writeAsBytes(data);
} else {
final dir = Directory(targetPath);
await dir.create(recursive: true);
}
}
}
static Wallpaper? parseWallpaperPack(final Directory directory) {
if (!directory.existsSync()) return null;
final jsonFile = File(directory.path + Platform.pathSeparator + WALLPAPER_INFO_FILE_NAME);
if (!jsonFile.existsSync()) return null;
final Wallpaper wallpaper = Wallpaper.fromJson(json.decode(jsonFile.readAsStringSync()) as Map<String, dynamic>);
return wallpaper;
}
/// 打包 wallpaper pack
/// [wallpaperSource] 资源路径
static Future<File> packWallpaper(final Directory wallpaperSource) async {
if (!wallpaperSource.existsSync()) throw ArgumentError('!wallpaperSource.existsSync()');
final fileList = wallpaperSource.listSync(recursive: true);
final tempDir = Directory(wallpaperSource.path + Platform.pathSeparator + TEMP_FOLDER_NAME);
await tempDir.create();
await for (final element in Stream.fromIterable(fileList)) {
await element.rename(tempDir.path + Platform.pathSeparator + FileUtils.basename(element.path));
}
final tarEncoder = TarFileEncoder();
tarEncoder.create(wallpaperSource.path + Platform.pathSeparator + TEMP_TAR_NAME);
tarEncoder.addDirectory(tempDir);
final fileListNew = tempDir.listSync(recursive: true);
await for (final element in Stream.fromIterable(fileListNew)) {
await element.rename(wallpaperSource.path + Platform.pathSeparator + FileUtils.basename(element.path));
}
await tempDir.delete();
final tarFile = File(tarEncoder.tarPath);
tarEncoder.close();
final result = brotli.encode(await tarFile.readAsBytes());
final pak = File(wallpaperSource.path + Platform.pathSeparator + PACK_NAME);
if (pak.existsSync()) pak.deleteSync();
pak.writeAsBytesSync(result);
tarFile.deleteSync();
return pak;
}
static bool checkWallpaperConfigFile(File? file) {
if (file == null) return false;
if (!file.existsSync()) return false;
if (!file.path.contains(WALLPAPER_INFO_FILE_NAME)) return false;
Wallpaper wallpaper;
try {
wallpaper = Wallpaper.fromJson(json.decode(file.readAsStringSync()) as Map<String, dynamic>);
} catch (e, s) {
print(e);
print(s);
return false;
}
for (final value in wallpaper.toMap().values) {
if (value == null) return false;
}
if (wallpaper.id.trim().isEmpty) return false;
if (wallpaper.mainFilepath.trim().isEmpty) return false;
return true;
}
}
| 0 |
mirrored_repositories/project_lw/lib | mirrored_repositories/project_lw/lib/utils/native_tools.dart | import 'package:project_lw/entity/wallpaper.dart';
import 'package:project_lw/main.dart';
class NativeTool {
static void setWallpaper(final Wallpaper wallpaper) {
methodChannel.invokeMethod('SET_WALLPAPER', {
'wallpaper': wallpaper.toMap(),
});
}
static void clearWallpaper() {
methodChannel.invokeMethod('CLEAR_WALLPAPER');
}
static void gotoLiveWallpaperSettings() {
methodChannel.invokeMethod('GOTO_WALLPAPER_CHOOSER');
}
}
| 0 |
mirrored_repositories/project_lw/lib | mirrored_repositories/project_lw/lib/utils/wallpaper_tools.dart | import 'dart:async';
import 'dart:convert';
import 'dart:io';
import 'package:file_utils/file_utils.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_ffmpeg/flutter_ffmpeg.dart';
import 'package:path_provider/path_provider.dart';
import 'package:project_lw/entity/center/data_center.dart';
import 'package:project_lw/entity/wallpaper.dart';
import 'package:project_lw/entity/wallpaper_info.dart';
import 'package:project_lw/main_cmd.dart';
import 'package:project_lw/misc/const.dart';
import 'package:project_lw/utils/wallpaper_file_utils.dart';
import 'package:share_plus/share_plus.dart';
class WallpaperTools {
static WallpaperTools instance = WallpaperTools._();
WallpaperTools._();
/// 指壁纸存储位置
late Directory wallpaperPlaceDir;
Future<void> init() async {
wallpaperPlaceDir = await getApplicationSupportDirectory();
}
/// 初始化预设壁纸
Future<void> initPresetWallpaper(BuildContext context) async {
final dir = WallpaperTools.instance.wallpaperPlaceDir;
await for (final path in Stream.fromIterable(PRESET_WALLPAPER_PATH)) {
final wallpaperId = uuid.v1();
final dest = Directory(dir.path + Platform.pathSeparator + wallpaperId);
dest.createSync();
final data = await rootBundle.load(path);
final tempPakFile = File(dir.path + Platform.pathSeparator + wallpaperId + '.' + WallpaperFileUtil.SUPPORTED_WALLPAPER_FORMAT);
await tempPakFile.writeAsBytes(data.buffer.asUint8List(data.offsetInBytes, data.lengthInBytes));
await WallpaperFileUtil.unpackWallpaper(tempPakFile, dest);
tempPakFile.deleteSync();
final wallpaper = WallpaperFileUtil.parseWallpaperPack(dest);
await DataCenter.get(context).addWallpaper(wallpaper?.copyWith(id: wallpaperId));
}
}
/// 导入壁纸包
/// [wallpaperPack] 壁纸包文件
Future<void> importWallpaper(final BuildContext context, final File? wallpaperPack) async {
if (wallpaperPack == null || !wallpaperPack.existsSync()) return;
final tempDir = Directory(
wallpaperPlaceDir.path + Platform.pathSeparator + 'temp-${DateTime.now().millisecondsSinceEpoch}',
);
tempDir.createSync();
Future<void> clean() async {
await tempDir.delete(recursive: true);
}
await WallpaperFileUtil.unpackWallpaper(wallpaperPack, tempDir);
final config = File(tempDir.path + Platform.pathSeparator + WallpaperFileUtil.WALLPAPER_INFO_FILE_NAME);
if (!config.existsSync()) {
await clean();
return;
}
final wallpaper = Wallpaper.fromJson(json.decode(config.readAsStringSync()) as Map<String, dynamic>);
if (wallpaper == null && !WallpaperFileUtil.checkWallpaperConfigFile(config)) {
await clean();
return;
}
final newDir = Directory(wallpaperPlaceDir.path + Platform.pathSeparator + wallpaper.id);
if (newDir.existsSync()) {
await clean();
return;
}
newDir.createSync();
await for (final element in Stream.fromIterable(tempDir.listSync(recursive: true))) {
await element.rename(newDir.path + Platform.pathSeparator + FileUtils.basename(element.path));
}
print('------new-------');
print(newDir.listSync(recursive: true).map((e) => e.path));
await DataCenter.get(context).addWallpaper(wallpaper);
}
/// 分享壁纸包
/// [wallpaper] 指定的壁纸包
Future<void> shareWallpaper(Wallpaper wallpaper) async {
final dir = Directory(wallpaper.getDirPath());
final pak = await WallpaperFileUtil.packWallpaper(dir);
print('----------------------------------');
print(pak.path);
print(pak.existsSync());
final newPath = (await getTemporaryDirectory()).path + Platform.pathSeparator + FileUtils.basename(pak.path);
pak.renameSync(newPath);
print('----------------------------------');
print(newPath);
print(File(newPath));
if (pak != null && pak.existsSync()) {
Share.shareFiles([newPath], text: '${wallpaper.name}\n${wallpaper.description}');
}
}
void removeWallpaper(BuildContext context, Wallpaper wallpaper) {
if (wallpaper == null) return;
final dir = Directory(wallpaper.getDirPath());
dir.deleteSync(recursive: true);
DataCenter.get(context).removeWallpaper(wallpaper);
}
Future<void> importVideo(BuildContext context, List<String?>? files) async {
if (files == null || files.isEmpty) return;
final target = <String>[];
for (final element in files) {
if (element == null) continue;
if (element.endsWith(WallpaperFileUtil.SUPPORTED_VIDEO_FORMAT)) target.add(element);
}
final FlutterFFmpeg _flutterFFmpeg = FlutterFFmpeg();
await for (final videoPath in Stream.fromIterable(target)) {
final item = File(videoPath);
final fileName = FileUtils.basename(videoPath);
final id = uuid.v1();
final tempName = item.parent.path + Platform.pathSeparator + '${DateTime.now().millisecondsSinceEpoch}.png';
final result = await _flutterFFmpeg.executeWithArguments([
// '-ss',
// '00:01:00',
// '-i',
// path,
// '-vframes',
// '1',
// '-q:v',
// '2',
'-i',
'$videoPath',
'-ss',
'4.500',
'-vframes',
'1',
'-q:v',
'2',
tempName,
]);
if (result != 0 || !File(tempName).existsSync()) continue;
final wallpaperConfig = Wallpaper(
id: id,
name: fileName,
author: 'Unknown',
description: 'None',
mainFilepath: fileName,
thumbnails: ['thumbnail1.png'],
tags: ['NONE'],
versionCode: 1,
versionName: '1.0',
wallpaperType: WallpaperType.VIDEO);
final targetDir = Directory(WallpaperTools.instance.wallpaperPlaceDir.path + Platform.pathSeparator + wallpaperConfig.id);
targetDir.createSync();
final configFile = File(targetDir.path + Platform.pathSeparator + WallpaperFileUtil.WALLPAPER_INFO_FILE_NAME);
configFile.writeAsStringSync(json.encode(wallpaperConfig));
final thumbnailFile = File(tempName);
await thumbnailFile.copy(targetDir.path + Platform.pathSeparator + 'thumbnail1.png');
try {
await thumbnailFile.delete();
} catch (e, s) {
print(e);
print(s);
}
// thumbnailFile.renameSync(targetDir.path +
// Platform.pathSeparator +
// FileUtils.basename(thumbnailFile.path));
final videoFile = File(videoPath);
await videoFile.copy(targetDir.path + Platform.pathSeparator + FileUtils.basename(videoFile.path));
await DataCenter.get(context).addWallpaper(wallpaperConfig);
}
}
Future<void> importImage(BuildContext context, List<String?>? files) async {
if (files == null || files.isEmpty) return;
final target = <String>[];
for (final element in files) {
if (element == null) continue;
if (element.endsWith('.png') || element.endsWith('.jpg')) target.add(element);
}
await for (final itemPath in Stream.fromIterable(target)) {
print('importImageFromDir: $itemPath');
final item = File(itemPath);
final fileName = FileUtils.basename(itemPath);
final id = uuid.v1();
final wallpaperConfig = Wallpaper(
id: id,
name: fileName,
author: 'Unknown',
description: 'None',
mainFilepath: fileName,
thumbnails: [fileName],
versionCode: 1,
versionName: '1.0',
wallpaperType: WallpaperType.IMAGE,
);
final targetDir = Directory(
WallpaperTools.instance.wallpaperPlaceDir.path + Platform.pathSeparator + wallpaperConfig.id,
);
targetDir.createSync();
item.copySync(wallpaperPlaceDir.path + Platform.pathSeparator + '$id' + Platform.pathSeparator + fileName);
final configFile = File(targetDir.path + Platform.pathSeparator + WallpaperFileUtil.WALLPAPER_INFO_FILE_NAME);
configFile.writeAsStringSync(json.encode(wallpaperConfig));
await DataCenter.get(context).addWallpaper(wallpaperConfig);
}
}
Future<void> importUrl(BuildContext context, String url) async {
if (url == null || url.trim().isEmpty) return;
print('importUrl--------------$url');
final id = uuid.v1();
final wallpaperConfig = Wallpaper(
id: id,
name: url,
author: 'Unknown',
description: 'None',
mainFilepath: url,
thumbnails: [],
versionCode: 1,
versionName: '1.0',
wallpaperType: WallpaperType.HTML,
tags: []);
final targetDir = Directory(
WallpaperTools.instance.wallpaperPlaceDir.path + Platform.pathSeparator + wallpaperConfig.id,
);
targetDir.createSync();
final configFile = File(targetDir.path + Platform.pathSeparator + WallpaperFileUtil.WALLPAPER_INFO_FILE_NAME);
configFile.writeAsStringSync(json.encode(wallpaperConfig));
await DataCenter.get(context).addWallpaper(wallpaperConfig);
print(DataCenter.get(context).wallpapers);
}
// /// 导入指定目录下的所有视频图片(层级1)
// Future<void> importImageFromDir(BuildContext context,
// Directory directory) async {
// if (directory == null || !directory.existsSync()) return;
//
// final files = directory.listSync();
// final target = <String>[];
//
// files.forEach((element) {
// if (element.path.endsWith('png') || element.path.endsWith('jpg'))
// target.add(element.path);
// });
//
// await for (final itemPath in Stream.fromIterable(target)) {
// print('importImageFromDir: $itemPath');
// final item = File(itemPath);
//
// item.copySync(directory.path +
// Platform.pathSeparator +
// FileUtils.basename(itemPath));
//
// final fileName = FileUtils.basename(itemPath);
//
// final wallpaperConfig = Wallpaper(
// id: uuid.v1(),
// name: fileName,
// author: 'Unknown',
// description: 'None',
// mainFilepath: fileName,
// thumbnails: [fileName],
// versionCode: 1,
// versionName: '1.0',
// wallpaperType: WallpaperType.IMAGE,
// );
//
// final targetDir = Directory(
// WallpaperTools.instance.wallpaperPlaceDir.path +
// Platform.pathSeparator +
// wallpaperConfig.id,
// );
//
// targetDir.createSync();
//
// final configFile = File(targetDir.path +
// Platform.pathSeparator +
// WallpaperFileUtil.WALLPAPER_INFO_FILE_NAME);
//
// configFile.writeAsStringSync(json.encode(wallpaperConfig));
//
// await DataCenter.get(context).addWallpaper(wallpaperConfig);
// }
// }
}
extension WallpaperExt on Wallpaper {
String? getMainThumbnailPath() {
if (thumbnails == null || thumbnails!.isEmpty) return null;
return WallpaperTools.instance.wallpaperPlaceDir.path + Platform.pathSeparator + id + Platform.pathSeparator + thumbnails!.first;
}
List<String>? getAllThumbnailPath() {
return thumbnails?.map((e) => WallpaperTools.instance.wallpaperPlaceDir.path + Platform.pathSeparator + id + Platform.pathSeparator + e).toList();
}
String getDirPath() => WallpaperTools.instance.wallpaperPlaceDir.path + Platform.pathSeparator + id;
Future<WallpaperExtInfo> extInfo() async {
List<String> getAllFilePath(Directory? directory) {
if (directory == null) return [];
final data = <String>[];
for (final element in directory.listSync()) {
final temp = Directory(element.path);
if (temp.existsSync()) {
return getAllFilePath(temp);
} else {
data.add(element.path);
}
}
return data;
}
final dirPath = this.getDirPath();
final allPath = Directory(dirPath).listSync(recursive: true).map((e) => e.path).toList();
int len = 0;
final all = getAllFilePath(Directory(dirPath));
all.forEach((element) {
len += File(element).lengthSync();
});
return WallpaperExtInfo(dirPath, allPath, len, false);
}
}
| 0 |
mirrored_repositories/project_lw/lib | mirrored_repositories/project_lw/lib/utils/sheet_utils.dart | import 'package:flutter/material.dart';
class SheetUtils {
static Future<T?> showSheetNoAPNoBlurCommon<T>(
BuildContext context,
final Widget child, {
bool cancelable = true,
}) {
return showModalBottomSheet<T>(
context: context,
isDismissible: cancelable,
isScrollControlled: true,
clipBehavior: Clip.antiAlias,
shape: RoundedRectangleBorder(borderRadius: BorderRadius.vertical(top: Radius.circular(24.0))),
builder: (ctx) {
return Material(color: Theme.of(ctx).scaffoldBackgroundColor, child: child);
});
}
}
| 0 |
mirrored_repositories/project_lw/lib | mirrored_repositories/project_lw/lib/utils/data_base_helper.dart | import 'package:project_lw/entity/wallpaper.dart';
import 'package:sqflite/sqflite.dart';
class DataBaseHelper {
static DataBaseHelper instance = DataBaseHelper._();
DataBaseHelper._();
late Database db;
Future<void> init() async {
db = await openDatabase('database.db', version: 1, onCreate: (database, version) async {
var batch = database.batch();
batch.execute(Wallpaper.CREATE_TABLE);
await batch.commit();
}, onUpgrade: (database, oldVersion, newVersion) async {
var batch = database.batch();
for (var i = oldVersion; i < newVersion; i++) {
print('========================== DB VERSION ==========================');
print(i);
// switch (i) {
// case 1:
// batch.execute('ALTER TABLE ${EventData.TABLE} ADD COLUMN isComplete INTEGER');
// break;
// }
}
await batch.commit();
});
}
}
| 0 |
mirrored_repositories/project_lw/lib | mirrored_repositories/project_lw/lib/utils/lw_theme_utils.dart | import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
@Deprecated('抽出')
class LWThemeUtil {
static const double pageTitleTopPadding = 50;
static const EdgeInsets pageTitlePadding = const EdgeInsets.only(left: 20, right: 20, top: 50, bottom: 30);
static const double navBarHeight = 65;
static SystemUiOverlayStyle getSystemStyle(BuildContext context) {
return SystemUiOverlayStyle.dark.copyWith(
systemNavigationBarColor: Theme.of(context).backgroundColor,
systemNavigationBarIconBrightness: Theme.of(context).brightness == Brightness.dark ? Brightness.light : Brightness.dark,
statusBarIconBrightness: Theme.of(context).brightness == Brightness.dark ? Brightness.light : Brightness.dark,
statusBarColor: Colors.transparent,
/* statusBarBrightness: Theme.of(context).brightness == Brightness.dark
? Brightness.light
: Brightness.dark*/
);
}
static TextStyle pageTitleStyle(BuildContext context) => Theme.of(context).textTheme.headline4!.copyWith(
color: Theme.of(context).brightness == Brightness.light ? Color.fromARGB(255, 49, 55, 63) : Colors.white, fontWeight: FontWeight.w500);
}
| 0 |
mirrored_repositories/MyFlutterLearn/drawer | mirrored_repositories/MyFlutterLearn/drawer/lib/main.dart | import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyDrawer(),
);
}
}
class MyDrawer extends StatelessWidget{
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Drawer'),
),
drawer: Drawer(
child: _listDrawer(context),
),
);
}
Widget _myDrawer(){
return UserAccountsDrawerHeader(
accountName: Text('Rangga Dika'),
accountEmail: Text('[email protected]'),
currentAccountPicture: CircleAvatar(
backgroundColor: Colors.white,
child: Text('R', style: TextStyle(fontSize: 40.0)),
),
);
}
Widget _listDrawer(BuildContext context){
return ListView(
children: <Widget>[
_myDrawer(),
ListTile(
title: Text('Hello World'),
onTap: () => Navigator.push(context, MaterialPageRoute(builder: (context) => PageHelloWorld())),
),
ListTile(
title: Text('Item 2'),
onTap: () {},
),
],
);
}
}
class PageHelloWorld extends StatelessWidget{
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Hello World'),
),
body: Center(
child: Text('Hello World'),
),
);
}
} | 0 |
mirrored_repositories/MyFlutterLearn/drawer | mirrored_repositories/MyFlutterLearn/drawer/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:drawer/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/MyFlutterLearn/load_image | mirrored_repositories/MyFlutterLearn/load_image/lib/main.dart | import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyLoadImage(),
);
}
}
class MyLoadImage extends StatelessWidget{
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Load Image"),
),
body: Container(
decoration: BoxDecoration(
image: DecorationImage(
image: AssetImage('images/img.jpg')
)
),
),
);
}
}
| 0 |
mirrored_repositories/MyFlutterLearn/load_image | mirrored_repositories/MyFlutterLearn/load_image/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:load_image/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/MyFlutterLearn/form | mirrored_repositories/MyFlutterLearn/form/lib/main.dart | import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Form',
theme: ThemeData(primaryColor: Colors.green),
home: FormLogin(),
);
}
}
class FormLogin extends StatefulWidget {
@override
_FormLoginState createState() => _FormLoginState();
}
class _FormLoginState extends State<FormLogin> {
TextStyle styleText = TextStyle(fontFamily: 'Montserrat', fontSize: 20.0);
@override
Widget build(BuildContext context) {
final email = TextField(
obscureText: false,
style: TextStyle(
fontSize: 20.0,
),
decoration: InputDecoration(
contentPadding: EdgeInsets.fromLTRB(20.0, 15.0, 20.0, 15.0),
hintText: "Email",
border: OutlineInputBorder(borderRadius: BorderRadius.circular(32.0)),
),
);
final password = TextField(
obscureText: false,
style: TextStyle(
fontSize: 20.0,
),
decoration: InputDecoration(
contentPadding: EdgeInsets.fromLTRB(20.0, 15.0, 20.0, 15.0),
hintText: "Password",
border: OutlineInputBorder(borderRadius: BorderRadius.circular(32.0)),
),
);
final buttonLogin = Material(
borderRadius: BorderRadius.circular(32.0),
color: Colors.deepOrange,
child: MaterialButton(
onPressed: () {},
child: Text(
"Login",
textAlign: TextAlign.center,
style: TextStyle(
fontWeight: FontWeight.bold,
color: Colors.white,
),
),
),
);
return Scaffold(
appBar: AppBar(
title: Text("Form"),
),
body: Center(
child: Container(
child: Padding(
padding: EdgeInsets.all(30.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
email,
SizedBox(
height: 15.0,
),
password,
SizedBox(
height: 30.0,
),
buttonLogin,
],
),
),
),
));
}
}
| 0 |
mirrored_repositories/MyFlutterLearn/form | mirrored_repositories/MyFlutterLearn/form/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:form/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/MyFlutterLearn/timer | mirrored_repositories/MyFlutterLearn/timer/lib/main.dart | import 'package:flutter/material.dart';
import 'dart:async';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Timer',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyTimer(),
);
}
}
class MyTimer extends StatefulWidget {
@override
_MyTimerState createState() => _MyTimerState();
}
class _MyTimerState extends State<MyTimer>{
Timer _timer;
int start = 1;
void startTimer() {
start = 0;
const oneSec = const Duration(seconds: 1);
_timer = new Timer.periodic(
oneSec,
(Timer timer) => setState(
() {
if (start > 9) {
timer.cancel();
} else {
start = start + 1;
}
},
),
);
}
@override
void dispose() {
_timer.cancel();
super.dispose();
}
Widget build(BuildContext context) {
return new Scaffold(
appBar: AppBar(
title: Text("Timer")
),
body: Center(
child: Padding(
padding: EdgeInsets.all(16.0),
child: Column(
children: <Widget>[
Material(
borderRadius: BorderRadius.circular(16.0),
color: Colors.deepOrange,
child: MaterialButton(
onPressed: () {
startTimer();
},
child: Text(
"Start",
textAlign: TextAlign.center,
style: TextStyle(
fontWeight: FontWeight.bold,
color: Colors.white,
),
),
),
),
SizedBox(
height: 30.0,
),
Text(
"$start",
style: TextStyle(
fontSize: 50.0,
fontWeight: FontWeight.bold,
fontFamily: 'Montserrat',
),
),
],
),
),
)
);
}
}
| 0 |
mirrored_repositories/MyFlutterLearn/timer | mirrored_repositories/MyFlutterLearn/timer/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:timer/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/MyFlutterLearn/edittext | mirrored_repositories/MyFlutterLearn/edittext/lib/main.dart | import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: "EditText",
home: MyEditText(),
);
}
}
class MyEditText extends StatefulWidget {
@override
_MyEditTextState createState() => _MyEditTextState();
}
class _MyEditTextState extends State<MyEditText> {
final myController = TextEditingController();
@override
void dispose() {
myController.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Center(
child: Text("My Edit Text"),
),
),
body: Padding(
padding: EdgeInsets.all(16.0),
child: TextField(
controller: myController,
),
),
floatingActionButton: FloatingActionButton.extended(
onPressed: () {
return showDialog(
context: context,
builder: (context) {
return AlertDialog(
title: Center(
child: Text('The value of text!'),
),
content: Text(myController.text),
);
});
},
label: Text('Show the value'),
backgroundColor: Colors.deepOrange,
icon: Icon(Icons.done_all),
),
);
}
}
| 0 |
mirrored_repositories/MyFlutterLearn/edittext | mirrored_repositories/MyFlutterLearn/edittext/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:editText/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/MyFlutterLearn/sharedpref | mirrored_repositories/MyFlutterLearn/sharedpref/lib/main.dart | import 'package:flutter/material.dart';
import 'package:shared_preferences/shared_preferences.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyHomePage(),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
final textController = TextEditingController();
String text = "";
saveValue() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
await prefs.setString('key', textController.text);
}
void getValue() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
//Return String
setState(() {
text = prefs.getString("key");
});
_dialog();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text("MyPref"),),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
_edittext(),
_row(),
],
),
),
);
}
Widget _edittext(){
return Container(
padding: EdgeInsets.all(8.0),
child: TextField(
controller: textController,
decoration: InputDecoration(
hintText: 'Type text'
),
),
);
}
Widget _row(){
return Row(
mainAxisAlignment: MainAxisAlignment.spaceAround,
mainAxisSize: MainAxisSize.max,
children: <Widget>[
Container(
width: MediaQuery.of(context).size.width/3,
child: RaisedButton(
hoverColor: Colors.grey,
padding: EdgeInsets.all(16.0),
onPressed: saveValue,
elevation: 8,
child: Text("Save"),
color: Colors.green,
textColor: Colors.white,
),
),
Container(
width: MediaQuery.of(context).size.width/3,
child: RaisedButton(
hoverColor: Colors.grey,
padding: EdgeInsets.all(16.0),
onPressed: getValue,
elevation: 8,
child: Text("Load"),
color: Colors.blue,
textColor: Colors.white,
),
),
],
);
}
void _dialog(){
showDialog(
context: context,
builder: (_) => new AlertDialog(
content: Text('$text'),
));
}
}
| 0 |
mirrored_repositories/MyFlutterLearn/sharedpref | mirrored_repositories/MyFlutterLearn/sharedpref/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:sharedpref/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/MyFlutterLearn/route | mirrored_repositories/MyFlutterLearn/route/lib/secondpage.dart | import 'package:flutter/material.dart';
class SecondPage extends StatelessWidget{
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text("Route"),),
body: Center(
child: RaisedButton(
onPressed: (){
Navigator.pop(context);
},
elevation: 8.0,
child: Text("Back To First Page"),
),
),
backgroundColor: Colors.lightBlue,
);
}
} | 0 |
mirrored_repositories/MyFlutterLearn/route | mirrored_repositories/MyFlutterLearn/route/lib/firstpage.dart | import 'package:flutter/material.dart';
import 'package:route/secondpage.dart';
class FirstPage extends StatelessWidget{
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text("Route"),),
body: Center(
child: RaisedButton(
onPressed: (){
Navigator.push(
context,
MaterialPageRoute(builder: (context) => SecondPage()),
);
},
elevation: 8.0,
child: Text("Go To Second Page"),
),
),
backgroundColor: Colors.green,
);
}
} | 0 |
mirrored_repositories/MyFlutterLearn/route | mirrored_repositories/MyFlutterLearn/route/lib/main.dart | import 'package:flutter/material.dart';
import 'firstpage.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Route',
theme: ThemeData(
primarySwatch: Colors.blue,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: FirstPage(),
);
}
}
| 0 |
mirrored_repositories/MyFlutterLearn/route | mirrored_repositories/MyFlutterLearn/route/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:route/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/MyFlutterLearn/bottom_tab | mirrored_repositories/MyFlutterLearn/bottom_tab/lib/tab2.dart | import 'package:flutter/material.dart';
class Tab2 extends StatefulWidget{
@override
_Tab2State createState() => _Tab2State();
}
class _Tab2State extends State<Tab2> {
@override
void initState() {
super.initState();
print('initState Tab2');
}
@override
Widget build(BuildContext context) {
print('build Tab2');
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Tab2'),
),
body: Center(
child: Text(
'This is content of Tab2',
style: TextStyle(fontSize: 30),
),
),
backgroundColor: Colors.deepOrange,
),
theme: ThemeData(
textTheme: TextTheme(bodyText2: TextStyle(color: Colors.white)),
),
);
}
} | 0 |
mirrored_repositories/MyFlutterLearn/bottom_tab | mirrored_repositories/MyFlutterLearn/bottom_tab/lib/tab1.dart | import 'package:flutter/material.dart';
class Tab1 extends StatefulWidget{
@override
_Tab1State createState() => _Tab1State();
}
class _Tab1State extends State<Tab1> {
@override
void initState() {
super.initState();
print('initState Tab1');
}
@override
Widget build(BuildContext context) {
print('build Tab1');
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Tab1'),
),
body: Center(
child: Text(
'This is content of Tab1',
style: TextStyle(fontSize: 30),
),
),
backgroundColor: Colors.purple,
),
theme: ThemeData(
textTheme: TextTheme(bodyText2: TextStyle(color: Colors.white)),
),
);
}
} | 0 |
mirrored_repositories/MyFlutterLearn/bottom_tab | mirrored_repositories/MyFlutterLearn/bottom_tab/lib/main.dart | /// Flutter code sample for BottomNavigationBar
// This example shows a [BottomNavigationBar] as it is used within a [Scaffold]
// widget. The [BottomNavigationBar] has three [BottomNavigationBarItem]
// widgets and the [currentIndex] is set to index 0. The selected item is
// amber. The `_onItemTapped` function changes the selected item's index
// and displays a corresponding message in the center of the [Scaffold].
//
// 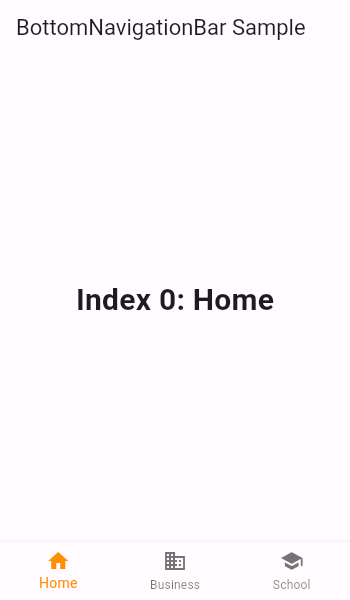
import 'package:flutter/material.dart';
import 'tab1.dart';
import 'tab2.dart';
import 'tab3.dart';
void main() => runApp(MyApp());
/// This is the main application widget.
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: TabContainerBottom(),
// TabContainerBottom(),
// TabContainerLoad1Time(),
// TabContainerIndexedStack(),
// TabContainer(),
);
}
}
class TabContainerBottom extends StatefulWidget{
@override
_TabContainerBottomState createState() => _TabContainerBottomState();
}
class _TabContainerBottomState extends State<TabContainerBottom> {
int tabIndex = 0;
List<Widget> listScreens;
@override
void initState() {
super.initState();
listScreens = [
Tab1(),
Tab2(),
Tab3(),
];
}
@override
Widget build(BuildContext context) {
return MaterialApp(
color: Colors.yellow,
home: Scaffold(
body: listScreens[tabIndex],
bottomNavigationBar: BottomNavigationBar(
selectedItemColor: Colors.white,
unselectedItemColor: Colors.grey[400],
backgroundColor: Theme.of(context).primaryColor,
currentIndex: tabIndex,
onTap: (int index) {
setState(() {
tabIndex = index;
});
},
items: [
BottomNavigationBarItem(
icon: Icon(Icons.home),
title: Text('Tab1'),
),
BottomNavigationBarItem(
icon: Icon(Icons.assignment),
title: Text('Tab2'),
),
BottomNavigationBarItem(
icon: Icon(Icons.settings),
title: Text('Tab3'),
),
]),
backgroundColor: Theme.of(context).primaryColor,
),
);
}
}
// class Tab1 extends StatefulWidget{
// @override
// _Tab1State createState() => _Tab1State();
// }
//
// class _Tab1State extends State<Tab1> {
// @override
// void initState() {
// super.initState();
// print('initState Tab1');
// }
// @override
// Widget build(BuildContext context) {
// print('build Tab1');
// return Scaffold(
// appBar: AppBar(
// title: Text('Tab1'),
// ),
// body: Center(
// child: Text(
// 'This is content of Tab1',
// style: TextStyle(fontSize: 30),
// ),
// ),
// );
// }
// }
| 0 |
mirrored_repositories/MyFlutterLearn/bottom_tab | mirrored_repositories/MyFlutterLearn/bottom_tab/lib/tab3.dart | import 'package:flutter/material.dart';
class Tab3 extends StatefulWidget{
@override
_Tab3State createState() => _Tab3State();
}
class _Tab3State extends State<Tab3> {
@override
void initState() {
super.initState();
print('initState Tab3');
}
@override
Widget build(BuildContext context) {
print('build Tab3');
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Tab3'),
),
body: Center(
child: Text(
'This is content of Tab3',
style: TextStyle(fontSize: 30),
),
),
backgroundColor: Colors.green,
),
theme: ThemeData(
textTheme: TextTheme(bodyText2: TextStyle(color: Colors.white)),
),
);
}
} | 0 |
mirrored_repositories/MyFlutterLearn/bottom_tab | mirrored_repositories/MyFlutterLearn/bottom_tab/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:bottom_tab/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/MyFlutterLearn/gridview | mirrored_repositories/MyFlutterLearn/gridview/lib/main.dart | import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
// This is the theme of your application.
//
// Try running your application with "flutter run". You'll see the
// application has a blue toolbar. Then, without quitting the app, try
// changing the primarySwatch below to Colors.green and then invoke
// "hot reload" (press "r" in the console where you ran "flutter run",
// or simply save your changes to "hot reload" in a Flutter IDE).
// Notice that the counter didn't reset back to zero; the application
// is not restarted.
primarySwatch: Colors.blue,
// This makes the visual density adapt to the platform that you run
// the app on. For desktop platforms, the controls will be smaller and
// closer together (more dense) than on mobile platforms.
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MyGridView(),
);
}
}
class MyGridView extends StatelessWidget {
final TextStyle style = TextStyle(color: Colors.white);
@override
Widget build(BuildContext context) {
const desc = ['computer', 'desktop mac', 'desktop windows', 'gamepad',
'headset', 'keyboard', 'laptop', 'laptop chromebook', 'laptop mac',
'laptop windows', 'tablet android', 'tablet mac', 'router',
'scanner', 'mouse', 'watch', 'tv'];
const icons = [Icons.computer, Icons.desktop_mac,
Icons.desktop_windows, Icons.gamepad, Icons.headset,
Icons.keyboard, Icons.laptop, Icons.laptop_chromebook,
Icons.laptop_mac, Icons.laptop_windows, Icons.tablet_android,
Icons.tablet_mac, Icons.router, Icons.scanner,
Icons.mouse, Icons.watch, Icons.tv];
const colors = [Color(0xFFFFAB91), Color(0xFFFF8A65),
Color(0xFFFFECB3), Color(0xFFFFE082), Color(0xFFFFD54F),
Color(0xFFF0F4C3), Color(0xFFE6EE9C), Color(0xFFDCE775),
Color(0xFFB3E5FC), Color(0xFF81D4FA), Color(0xFF4FC3F7),
Color(0xFFD1C4E9), Color(0xFFB39DDB), Color(0xFF9575CD),
Color(0xFFF8BBD0), Color(0xFFF48FB1), Color(0xFFF06292)];
return Scaffold(
appBar: AppBar(
title: Text("Gridview", style: style),
),
body: GridView.count(
crossAxisCount: 2,
crossAxisSpacing: 4.0,
mainAxisSpacing: 8.0,
children: List.generate(desc.length, (_index) {
return Container(
child: Card(
color: colors[_index],
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
children: <Widget>[
Expanded(child: Icon(icons[_index], size:50.0,)),
Text(desc[_index]),
],
),
),
);
}),
)
);
}
} | 0 |
mirrored_repositories/MyFlutterLearn/gridview | mirrored_repositories/MyFlutterLearn/gridview/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:gridview/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/MyFlutterLearn/tab | mirrored_repositories/MyFlutterLearn/tab/lib/tab2.dart | import 'package:flutter/material.dart';
class Tab2 extends StatelessWidget {
@override
Widget build(BuildContext context) {
print('build Tab2');
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
body: Center(
child: Text(
'This is content of Tab2',
style: TextStyle(fontSize: 30),
),
),
backgroundColor: Colors.deepOrange,
),
theme: ThemeData(
textTheme: TextTheme(bodyText2: TextStyle(color: Colors.white)),
),
);
}
} | 0 |
mirrored_repositories/MyFlutterLearn/tab | mirrored_repositories/MyFlutterLearn/tab/lib/tab1.dart | import 'package:flutter/material.dart';
class Tab1 extends StatelessWidget {
@override
Widget build(BuildContext context) {
print('build Tab1');
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
body: Center(
child: Text(
'This is content of Tab1',
style: TextStyle(fontSize: 30),
),
),
backgroundColor: Colors.purple,
),
theme: ThemeData(
textTheme: TextTheme(bodyText2: TextStyle(color: Colors.white)),
),
);
}
} | 0 |
mirrored_repositories/MyFlutterLearn/tab | mirrored_repositories/MyFlutterLearn/tab/lib/main.dart | import 'package:flutter/material.dart';
import 'tab1.dart';
import 'tab2.dart';
import 'tab3.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyTab(),
);
}
}
class MyTab extends StatelessWidget {
final _tabPages = <Widget>[
Tab1(),
Tab2(),
Tab3()
];
final _tab = <Tab>[
Tab(text: 'Tab1'),
Tab(text: 'Tab2'),
Tab(text: 'Tab3')
];
@override
Widget build(BuildContext context) {
return DefaultTabController(
length: _tab.length,
child: Scaffold(
appBar: AppBar(
title: Text("Tab"),
backgroundColor: Colors.cyan,
bottom: TabBar(
tabs: _tab,
),
),
body: TabBarView(
children: _tabPages,
),
),
);
}
}
| 0 |
mirrored_repositories/MyFlutterLearn/tab | mirrored_repositories/MyFlutterLearn/tab/lib/tab3.dart | import 'package:flutter/material.dart';
class Tab3 extends StatelessWidget {
@override
Widget build(BuildContext context) {
print('build Tab3');
return MaterialApp(
debugShowCheckedModeBanner: false,
home: Scaffold(
body: Center(
child: Text(
'This is content of Tab3',
style: TextStyle(fontSize: 30),
),
),
backgroundColor: Colors.green,
),
theme: ThemeData(
textTheme: TextTheme(bodyText2: TextStyle(color: Colors.white)),
),
);
}
} | 0 |
mirrored_repositories/MyFlutterLearn/tab | mirrored_repositories/MyFlutterLearn/tab/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:tab/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/MyFlutterLearn/splash_screen | mirrored_repositories/MyFlutterLearn/splash_screen/lib/home_page.dart | import 'package:flutter/material.dart';
class MyHomePage extends StatelessWidget{
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("My Home"),
),
body: Center(
child: Text("This is my home page"),
),
);
}
} | 0 |
mirrored_repositories/MyFlutterLearn/splash_screen | mirrored_repositories/MyFlutterLearn/splash_screen/lib/screen_page.dart | import 'package:flutter/material.dart';
import 'dart:async';
import 'home_page.dart';
class MySplashScreenPage extends StatefulWidget{
@override
_MySplashScreenPageState createState() => _MySplashScreenPageState();
}
class _MySplashScreenPageState extends State<MySplashScreenPage>{
@override
void initState(){
super.initState();
startSplashScreen();
}
startSplashScreen() async{
var duration = Duration(seconds: 5);
return Timer(duration, (){
Navigator.of(context).pushReplacement(MaterialPageRoute(
builder: (_) {
return MyHomePage();
}
));
});
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Center(
child: Text("My Splash Screen"),
),
backgroundColor: Colors.deepOrange,
),
theme: ThemeData(
primaryColor: Colors.deepOrange,
accentColor: Color.fromARGB(255, 255, 0, 0),
textTheme: TextTheme(bodyText2: TextStyle(color: Colors.white, fontSize: 20.0, fontWeight: FontWeight.bold))),
);
}
} | 0 |
mirrored_repositories/MyFlutterLearn/splash_screen | mirrored_repositories/MyFlutterLearn/splash_screen/lib/main.dart | import 'package:flutter/material.dart';
import 'screen_page.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
title: 'Splash Screen',
theme: ThemeData(
primarySwatch: Colors.deepOrange,
visualDensity: VisualDensity.adaptivePlatformDensity,
),
home: MySplashScreenPage(),
);
}
} | 0 |
mirrored_repositories/MyFlutterLearn/splash_screen | mirrored_repositories/MyFlutterLearn/splash_screen/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:splash_screen/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/MyFlutterLearn/helloworld | mirrored_repositories/MyFlutterLearn/helloworld/lib/main.dart | import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'First App',
home: Scaffold(
appBar: AppBar(
title: Center(
child: Text("First App"),
),
),
body: Center(
child: Text("Hello, World!!"),
),
),
theme: ThemeData(
primaryColor: Color.fromARGB(255, 0, 255, 0),
accentColor: Color.fromARGB(255, 255, 0, 0),
textTheme: TextTheme(bodyText2: TextStyle(color: Colors.purple))),
);
}
}
| 0 |
mirrored_repositories/MyFlutterLearn/helloworld | mirrored_repositories/MyFlutterLearn/helloworld/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:hello_world/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/MyFlutterLearn/listview | mirrored_repositories/MyFlutterLearn/listview/lib/main.dart | import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'List View',
theme: ThemeData(
primarySwatch: Colors.deepOrange,
),
home: MyListView(),
);
}
}
class MyListView extends StatelessWidget{
@override
Widget build(BuildContext context){
const desc = ['computer', 'desktop mac', 'desktop windows', 'gamepad',
'headset', 'keyboard', 'laptop', 'laptop chromebook', 'laptop mac',
'laptop windows', 'tablet android', 'tablet mac', 'router',
'scanner', 'mouse', 'watch', 'tv'];
const icons = [Icons.computer, Icons.desktop_mac,
Icons.desktop_windows, Icons.gamepad, Icons.headset,
Icons.keyboard, Icons.laptop, Icons.laptop_chromebook,
Icons.laptop_mac, Icons.laptop_windows, Icons.tablet_android,
Icons.tablet_mac, Icons.router, Icons.scanner,
Icons.mouse, Icons.watch, Icons.tv];
const colors = [Color(0xFFFFAB91), Color(0xFFFF8A65),
Color(0xFFFFECB3), Color(0xFFFFE082), Color(0xFFFFD54F),
Color(0xFFF0F4C3), Color(0xFFE6EE9C), Color(0xFFDCE775),
Color(0xFFB3E5FC), Color(0xFF81D4FA), Color(0xFF4FC3F7),
Color(0xFFD1C4E9), Color(0xFFB39DDB), Color(0xFF9575CD),
Color(0xFFF8BBD0), Color(0xFFF48FB1), Color(0xFFF06292)];
return Scaffold(
appBar: AppBar(
title: Text("List View"),
),
body: ListView.builder(
itemCount: desc.length,
itemBuilder: (context, index) {
return Card( // <-- Card widget
child: ListTile(
leading: Icon(icons[index]),
title: Text(desc[index]),
tileColor: colors[index],
),
);
},
),
);
}
}
| 0 |
mirrored_repositories/MyFlutterLearn/listview | mirrored_repositories/MyFlutterLearn/listview/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:listview/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/flutter_ui_package | mirrored_repositories/flutter_ui_package/lib/flutteruipackage.dart | library flutteruipackage;
export 'src/dialogboxes.dart';
export 'src/buttons.dart';
export 'src/inputfields.dart';
export 'src/dropdowns.dart'; | 0 |
mirrored_repositories/flutter_ui_package/lib | mirrored_repositories/flutter_ui_package/lib/src/customdropdown.dart | import 'dart:math' as math;
import 'package:flutter/material.dart';
// source of the code : https://stackoverflow.com/a/59859741/12802591
const Duration _kDropdownMenuDuration = Duration(milliseconds: 300);
const double _kMenuItemHeight = 48.0;
const double _kDenseButtonHeight = 24.0;
const EdgeInsets _kMenuItemPadding = EdgeInsets.symmetric(horizontal: 16.0);
const EdgeInsetsGeometry _kAlignedButtonPadding =
EdgeInsetsDirectional.only(start: 16.0, end: 4.0);
const EdgeInsets _kUnalignedButtonPadding = EdgeInsets.zero;
const EdgeInsets _kAlignedMenuMargin = EdgeInsets.zero;
const EdgeInsetsGeometry _kUnalignedMenuMargin =
EdgeInsetsDirectional.only(start: 16.0, end: 24.0);
class _DropdownMenuPainter extends CustomPainter {
_DropdownMenuPainter({
this.color,
this.elevation,
this.selectedIndex,
this.resize,
}) : _painter = new BoxDecoration(
// If you add an image here, you must provide a real
// configuration in the paint() function and you must provide some sort
// of onChanged callback here.
color: color,
borderRadius: new BorderRadius.circular(2.0),
boxShadow: kElevationToShadow[elevation])
.createBoxPainter(),
super(repaint: resize);
final Color color;
final int elevation;
final int selectedIndex;
final Animation<double> resize;
final BoxPainter _painter;
@override
void paint(Canvas canvas, Size size) {
final double selectedItemOffset =
selectedIndex * _kMenuItemHeight + kMaterialListPadding.top;
final Tween<double> top = new Tween<double>(
begin: selectedItemOffset.clamp(0.0, size.height - _kMenuItemHeight),
end: 0.0,
);
final Tween<double> bottom = new Tween<double>(
begin:
(top.begin + _kMenuItemHeight).clamp(_kMenuItemHeight, size.height),
end: size.height,
);
final Rect rect = new Rect.fromLTRB(
0.0, top.evaluate(resize), size.width, bottom.evaluate(resize));
_painter.paint(
canvas, rect.topLeft, new ImageConfiguration(size: rect.size));
}
@override
bool shouldRepaint(_DropdownMenuPainter oldPainter) {
return oldPainter.color != color ||
oldPainter.elevation != elevation ||
oldPainter.selectedIndex != selectedIndex ||
oldPainter.resize != resize;
}
}
// Do not use the platform-specific default scroll configuration.
// Dropdown menus should never overscroll or display an overscroll indicator.
class _DropdownScrollBehavior extends ScrollBehavior {
const _DropdownScrollBehavior();
@override
TargetPlatform getPlatform(BuildContext context) =>
Theme.of(context).platform;
@override
Widget buildViewportChrome(
BuildContext context, Widget child, AxisDirection axisDirection) =>
child;
@override
ScrollPhysics getScrollPhysics(BuildContext context) =>
const ClampingScrollPhysics();
}
class _DropdownMenu<T> extends StatefulWidget {
const _DropdownMenu({
Key key,
this.padding,
this.route,
}) : super(key: key);
final _DropdownRoute<T> route;
final EdgeInsets padding;
@override
_DropdownMenuState<T> createState() => new _DropdownMenuState<T>();
}
class _DropdownMenuState<T> extends State<_DropdownMenu<T>> {
CurvedAnimation _fadeOpacity;
CurvedAnimation _resize;
@override
void initState() {
super.initState();
// We need to hold these animations as state because of their curve
// direction. When the route's animation reverses, if we were to recreate
// the CurvedAnimation objects in build, we'd lose
// CurvedAnimation._curveDirection.
_fadeOpacity = new CurvedAnimation(
parent: widget.route.animation,
curve: const Interval(0.0, 0.25),
reverseCurve: const Interval(0.75, 1.0),
);
_resize = new CurvedAnimation(
parent: widget.route.animation,
curve: const Interval(0.25, 0.5),
reverseCurve: const Threshold(0.0),
);
}
@override
Widget build(BuildContext context) {
// The menu is shown in three stages (unit timing in brackets):
// [0s - 0.25s] - Fade in a rect-sized menu container with the selected item.
// [0.25s - 0.5s] - Grow the otherwise empty menu container from the center
// until it's big enough for as many items as we're going to show.
// [0.5s - 1.0s] Fade in the remaining visible items from top to bottom.
//
// When the menu is dismissed we just fade the entire thing out
// in the first 0.25s.
final MaterialLocalizations localizations =
MaterialLocalizations.of(context);
final _DropdownRoute<T> route = widget.route;
final double unit = 0.5 / (route.items.length + 1.5);
final List<Widget> children = <Widget>[];
for (int itemIndex = 0; itemIndex < route.items.length; ++itemIndex) {
CurvedAnimation opacity;
if (itemIndex == route.selectedIndex) {
opacity = new CurvedAnimation(
parent: route.animation, curve: const Threshold(0.0));
} else {
final double start = (0.5 + (itemIndex + 1) * unit).clamp(0.0, 1.0);
final double end = (start + 1.5 * unit).clamp(0.0, 1.0);
opacity = new CurvedAnimation(
parent: route.animation, curve: new Interval(start, end));
}
children.add(new FadeTransition(
opacity: opacity,
child: new InkWell(
child: new Container(
padding: widget.padding,
child: route.items[itemIndex],
),
onTap: () => Navigator.pop(
context,
new _DropdownRouteResult<T>(route.items[itemIndex].value),
),
),
));
}
return new FadeTransition(
opacity: _fadeOpacity,
child: new CustomPaint(
painter: new _DropdownMenuPainter(
color: Theme.of(context).canvasColor,
elevation: route.elevation,
selectedIndex: route.selectedIndex,
resize: _resize,
),
child: new Semantics(
scopesRoute: true,
namesRoute: true,
explicitChildNodes: true,
label: localizations.popupMenuLabel,
child: new Material(
type: MaterialType.transparency,
textStyle: route.style,
child: new ScrollConfiguration(
behavior: const _DropdownScrollBehavior(),
child: new Scrollbar(
child: new ListView(
controller: widget.route.scrollController,
padding: kMaterialListPadding,
itemExtent: _kMenuItemHeight,
shrinkWrap: true,
children: children,
),
),
),
),
),
),
);
}
}
class _DropdownMenuRouteLayout<T> extends SingleChildLayoutDelegate {
_DropdownMenuRouteLayout({
@required this.buttonRect,
@required this.menuTop,
@required this.menuHeight,
@required this.textDirection,
});
final Rect buttonRect;
final double menuTop;
final double menuHeight;
final TextDirection textDirection;
@override
BoxConstraints getConstraintsForChild(BoxConstraints constraints) {
// The maximum height of a simple menu should be one or more rows less than
// the view height. This ensures a tappable area outside of the simple menu
// with which to dismiss the menu.
// -- https://material.google.com/components/menus.html#menus-simple-menus
final double maxHeight =
math.max(0.0, constraints.maxHeight - 2 * _kMenuItemHeight);
// The width of a menu should be at most the view width. This ensures that
// the menu does not extend past the left and right edges of the screen.
final double width = math.min(constraints.maxWidth, buttonRect.width);
return new BoxConstraints(
minWidth: width,
maxWidth: width,
minHeight: 0.0,
maxHeight: maxHeight,
);
}
@override
Offset getPositionForChild(Size size, Size childSize) {
assert(() {
final Rect container = Offset.zero & size;
if (container.intersect(buttonRect) == buttonRect) {
// If the button was entirely on-screen, then verify
// that the menu is also on-screen.
// If the button was a bit off-screen, then, oh well.
assert(menuTop >= 0.0);
assert(menuTop + menuHeight <= size.height);
}
return true;
}());
assert(textDirection != null);
double left;
switch (textDirection) {
case TextDirection.rtl:
left = buttonRect.right.clamp(0.0, size.width) - childSize.width;
break;
case TextDirection.ltr:
left = buttonRect.left.clamp(0.0, size.width - childSize.width);
break;
}
return new Offset(left, menuTop);
}
@override
bool shouldRelayout(_DropdownMenuRouteLayout<T> oldDelegate) {
return buttonRect != oldDelegate.buttonRect ||
menuTop != oldDelegate.menuTop ||
menuHeight != oldDelegate.menuHeight ||
textDirection != oldDelegate.textDirection;
}
}
class _DropdownRouteResult<T> {
const _DropdownRouteResult(this.result);
final T result;
@override
bool operator ==(dynamic other) {
if (other is! _DropdownRouteResult<T>) return false;
final _DropdownRouteResult<T> typedOther = other;
return result == typedOther.result;
}
@override
int get hashCode => result.hashCode;
}
class _DropdownRoute<T> extends PopupRoute<_DropdownRouteResult<T>> {
_DropdownRoute({
this.items,
this.padding,
this.buttonRect,
this.selectedIndex,
this.elevation = 8,
this.theme,
@required this.style,
this.barrierLabel,
}) : assert(style != null);
final List<DropdownMenuItem<T>> items;
final EdgeInsetsGeometry padding;
final Rect buttonRect;
final int selectedIndex;
final int elevation;
final ThemeData theme;
final TextStyle style;
ScrollController scrollController;
@override
Duration get transitionDuration => _kDropdownMenuDuration;
@override
bool get barrierDismissible => true;
@override
Color get barrierColor => null;
@override
final String barrierLabel;
@override
Widget buildPage(BuildContext context, Animation<double> animation,
Animation<double> secondaryAnimation) {
assert(debugCheckHasDirectionality(context));
final double screenHeight = MediaQuery.of(context).size.height;
final double maxMenuHeight = screenHeight - 2.0 * _kMenuItemHeight;
final double preferredMenuHeight =
(items.length * _kMenuItemHeight) + kMaterialListPadding.vertical;
final double menuHeight = math.min(maxMenuHeight, preferredMenuHeight);
final double buttonTop = buttonRect.top;
final double selectedItemOffset =
selectedIndex * _kMenuItemHeight + kMaterialListPadding.top;
double menuTop = (buttonTop - selectedItemOffset) -
(_kMenuItemHeight - buttonRect.height) / 2.0;
const double topPreferredLimit = _kMenuItemHeight;
if (menuTop < topPreferredLimit)
menuTop = math.min(buttonTop, topPreferredLimit);
double bottom = menuTop + menuHeight;
final double bottomPreferredLimit = screenHeight - _kMenuItemHeight;
if (bottom > bottomPreferredLimit) {
bottom = math.max(buttonTop + _kMenuItemHeight, bottomPreferredLimit);
menuTop = bottom - menuHeight;
}
if (scrollController == null) {
double scrollOffset = 0.0;
if (preferredMenuHeight > maxMenuHeight)
scrollOffset = selectedItemOffset - (buttonTop - menuTop);
scrollController =
new ScrollController(initialScrollOffset: scrollOffset);
}
final TextDirection textDirection = Directionality.of(context);
Widget menu = new _DropdownMenu<T>(
route: this,
padding: padding.resolve(textDirection),
);
if (theme != null) menu = new Theme(data: theme, child: menu);
return new MediaQuery.removePadding(
context: context,
removeTop: true,
removeBottom: true,
removeLeft: true,
removeRight: true,
child: new Builder(
builder: (BuildContext context) {
return new CustomSingleChildLayout(
delegate: new _DropdownMenuRouteLayout<T>(
buttonRect: buttonRect,
menuTop: menuTop,
menuHeight: menuHeight,
textDirection: textDirection,
),
child: menu,
);
},
),
);
}
void _dismiss() {
navigator?.removeRoute(this);
}
}
class CustomDropdownButton<T> extends StatefulWidget {
/// Creates a dropdown button.
///
/// The [items] must have distinct values and if [value] isn't null it must be among them.
///
/// The [elevation] and [iconSize] arguments must not be null (they both have
/// defaults, so do not need to be specified).
CustomDropdownButton({
Key key,
@required this.items,
this.value,
this.hint,
@required this.onChanged,
this.elevation = 8,
this.style,
this.iconSize = 24.0,
this.icon = const Icon(
Icons.keyboard_arrow_down,
),
this.isDense = false,
}) : assert(items != null),
assert(value == null ||
items
.where((DropdownMenuItem<T> item) => item.value == value)
.length ==
1),
super(key: key);
/// The list of possible items to select among.
final List<DropdownMenuItem<T>> items;
/// The currently selected item, or null if no item has been selected. If
/// value is null then the menu is popped up as if the first item was
/// selected.
final T value;
/// Displayed if [value] is null.
final Widget hint;
/// Called when the user selects an item.
final ValueChanged<T> onChanged;
/// The z-coordinate at which to place the menu when open.
///
/// The following elevations have defined shadows: 1, 2, 3, 4, 6, 8, 9, 12, 16, 24
///
/// Defaults to 8, the appropriate elevation for dropdown buttons.
final int elevation;
/// The text style to use for text in the dropdown button and the dropdown
/// menu that appears when you tap the button.
///
/// Defaults to the [TextTheme.subhead] value of the current
/// [ThemeData.textTheme] of the current [Theme].
final TextStyle style;
/// Icon on the right of the hint
Widget icon;
/// The size to use for the drop-down button's down arrow icon button.
///
/// Defaults to 24.0.
final double iconSize;
/// Reduce the button's height.
///
/// By default this button's height is the same as its menu items' heights.
/// If isDense is true, the button's height is reduced by about half. This
/// can be useful when the button is embedded in a container that adds
/// its own decorations, like [InputDecorator].
final bool isDense;
@override
_DropdownButtonState<T> createState() => new _DropdownButtonState<T>();
}
class _DropdownButtonState<T> extends State<CustomDropdownButton<T>>
with WidgetsBindingObserver {
int _selectedIndex;
_DropdownRoute<T> _dropdownRoute;
@override
void initState() {
super.initState();
// _updateSelectedIndex();
WidgetsBinding.instance.addObserver(this);
}
@override
void dispose() {
WidgetsBinding.instance.removeObserver(this);
_removeDropdownRoute();
super.dispose();
}
// Typically called because the device's orientation has changed.
// Defined by WidgetsBindingObserver
@override
void didChangeMetrics() {
_removeDropdownRoute();
}
void _removeDropdownRoute() {
_dropdownRoute?._dismiss();
_dropdownRoute = null;
}
@override
void didUpdateWidget(CustomDropdownButton<T> oldWidget) {
super.didUpdateWidget(oldWidget);
_updateSelectedIndex();
}
void _updateSelectedIndex() {
assert(widget.value == null ||
widget.items
.where((DropdownMenuItem<T> item) => item.value == widget.value)
.length ==
1);
_selectedIndex = null;
for (int itemIndex = 0; itemIndex < widget.items.length; itemIndex++) {
if (widget.items[itemIndex].value == widget.value) {
_selectedIndex = itemIndex;
return;
}
}
}
TextStyle get _textStyle =>
widget.style ?? Theme.of(context).textTheme.subhead;
void _handleTap() {
final RenderBox itemBox = context.findRenderObject();
final Rect itemRect = itemBox.localToGlobal(Offset.zero) & itemBox.size;
final TextDirection textDirection = Directionality.of(context);
final EdgeInsetsGeometry menuMargin =
ButtonTheme.of(context).alignedDropdown
? _kAlignedMenuMargin
: _kUnalignedMenuMargin;
assert(_dropdownRoute == null);
_dropdownRoute = new _DropdownRoute<T>(
items: widget.items,
buttonRect: menuMargin.resolve(textDirection).inflateRect(itemRect),
padding: _kMenuItemPadding.resolve(textDirection),
selectedIndex: -1,
elevation: widget.elevation,
theme: Theme.of(context, shadowThemeOnly: true),
style: _textStyle,
barrierLabel: MaterialLocalizations.of(context).modalBarrierDismissLabel,
);
Navigator.push(context, _dropdownRoute)
.then<void>((_DropdownRouteResult<T> newValue) {
_dropdownRoute = null;
if (!mounted || newValue == null) return;
if (widget.onChanged != null) widget.onChanged(newValue.result);
});
}
// When isDense is true, reduce the height of this button from _kMenuItemHeight to
// _kDenseButtonHeight, but don't make it smaller than the text that it contains.
// Similarly, we don't reduce the height of the button so much that its icon
// would be clipped.
double get _denseButtonHeight {
return math.max(
_textStyle.fontSize, math.max(widget.iconSize, _kDenseButtonHeight));
}
@override
Widget build(BuildContext context) {
assert(debugCheckHasMaterial(context));
// The width of the button and the menu are defined by the widest
// item and the width of the hint.
final List<Widget> items = new List<Widget>.from(widget.items);
int hintIndex;
if (widget.hint != null) {
hintIndex = items.length;
items.add(new DefaultTextStyle(
style: _textStyle.copyWith(color: Theme.of(context).hintColor),
child: new IgnorePointer(
child: widget.hint,
ignoringSemantics: false,
),
));
}
final EdgeInsetsGeometry padding = ButtonTheme.of(context).alignedDropdown
? _kAlignedButtonPadding
: _kUnalignedButtonPadding;
Widget result = new DefaultTextStyle(
style: _textStyle,
child: new Container(
padding: padding.resolve(Directionality.of(context)),
height: widget.isDense ? _denseButtonHeight : null,
child: new Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
// If value is null (then _selectedIndex is null) then we display
// the hint or nothing at all.
Expanded(
child: new IndexedStack(
index: _selectedIndex ?? hintIndex,
alignment: AlignmentDirectional.centerStart,
children: items,
),
),
widget.icon,
],
),
),
);
return new Semantics(
button: true,
child: new GestureDetector(
onTap: _handleTap, behavior: HitTestBehavior.opaque, child: result),
);
}
}
| 0 |
mirrored_repositories/flutter_ui_package/lib | mirrored_repositories/flutter_ui_package/lib/src/dialogboxes.dart | import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'buttons.dart';
enum ButtonsPosition{
HORIZONTAL,
VERTICAL,
}
enum ButtonsAlignement{
LEFT,
RIGHT,
CENTER,
}
// return a Widget array of buttons according to buttons (success, dismiss) input texts
List<Widget> AddButtons(BuildContext context, ButtonsPosition buttonsPosition, double buttonsWidth, String successText, VoidCallback onSuccessPressed, String dismissText, VoidCallback onDismissPressed, Color backgroundColor, Color themeColor){
TextStyle successtextstyle= new TextStyle(fontSize: 17 , fontWeight: FontWeight.w800, color: backgroundColor);
TextStyle dismisstextstyle= new TextStyle(fontSize: 17 , fontWeight: FontWeight.w800, color: themeColor);
if (successText.isEmpty && dismissText.isEmpty){
return [];
}else{
if (successText.isNotEmpty && dismissText.isNotEmpty){
return [
BOutlined(
width: buttonsWidth,
height: 45,
text: dismissText,
textStyle: dismisstextstyle,
outlineColor: themeColor,
backgroundColor: backgroundColor,
onPressed: onDismissPressed,
),
SizedBox(
width: 12,
height: 10,
),
BFilled(
width: buttonsWidth,
height: 45,
text: successText,
textStyle: successtextstyle,
backgroundColor: themeColor,
onPressed: onSuccessPressed,
)
];
}else{
if (successText.isNotEmpty) return [
BFilled(
width: buttonsWidth,
height: 45,
text: successText,
textStyle: successtextstyle,
backgroundColor: themeColor,
onPressed: onSuccessPressed,
)
];
else return [
BOutlined(
width: buttonsWidth,
height: 45,
text: dismissText,
textStyle: dismisstextstyle,
outlineColor: themeColor,
backgroundColor: backgroundColor,
onPressed: onDismissPressed,
),
];
}
}
}
// place buttons on a row or colum according to buttonsPosition value (HORIZONTAL, VERTICAL)
Widget PlaceButtons(ButtonsPosition position, List<Widget> array, ButtonsAlignement alignement){
MainAxisAlignment mainAxisAlignment;
CrossAxisAlignment crossAxisAlignment;
switch (alignement){
case ButtonsAlignement.CENTER:
mainAxisAlignment= MainAxisAlignment.center;
crossAxisAlignment = CrossAxisAlignment.center;
break;
case ButtonsAlignement.LEFT:
mainAxisAlignment= MainAxisAlignment.start;
crossAxisAlignment = CrossAxisAlignment.start;
break;
case ButtonsAlignement.RIGHT:
mainAxisAlignment= MainAxisAlignment.end;
crossAxisAlignment= CrossAxisAlignment.end;
break;
}
if (array.isEmpty){
return Container(width: 0, height: 0, color: Colors.transparent,);
}
switch (position){
case ButtonsPosition.HORIZONTAL:
return Padding(
padding: const EdgeInsets.only(top: 20),
child: Row(
mainAxisAlignment: mainAxisAlignment,
children: array,
),
);
case ButtonsPosition.VERTICAL :
return Padding(
padding: const EdgeInsets.only(top: 20),
child: Column(
crossAxisAlignment: crossAxisAlignment,
//mainAxisAlignment: mainAxisAlignment,
children: array,
),
);
}
}
// return buttons width, full width for vertical alignement, and custom width for horizontal
double getButtonsWidth(BuildContext context, ButtonsPosition position){
double width = MediaQuery.of(context).size.width;
switch (position){
case ButtonsPosition.VERTICAL :
return width;
case ButtonsPosition.HORIZONTAL :
return width/3.5;
}
}
// Dialog Box with customizable widget between title and description
class BasicAlertBox {
double dialogRadius;
double buttonsWidth;
Color themeColor, backgroundColor;
String title, description;
String successText, dismissText;
Widget imageWidget;
ButtonsPosition buttonsPosition;
ButtonsAlignement buttonsAlignement;
VoidCallback onSuccessPressed, onDismissPressed;
BasicAlertBox({
this.dialogRadius= 7,
this.buttonsWidth,
this.themeColor= Colors.blue,
this.backgroundColor = Colors.white,
this.title = "Alert Dialog",
this.description= "This is an alert dialog !",
this.imageWidget= const Card(color: Colors.transparent,),
this.successText = "",
this.onSuccessPressed,
this.dismissText= "",
this.onDismissPressed,
this.buttonsAlignement = ButtonsAlignement.CENTER,
this.buttonsPosition = ButtonsPosition.HORIZONTAL
});
Future show({
@required BuildContext context,
}) {
assert(context != null, "context is null!");
if (buttonsWidth==null) buttonsWidth=getButtonsWidth(context, buttonsPosition);
TextStyle titletext= new TextStyle(fontSize: 26 , fontWeight: FontWeight.w700, color: themeColor);
TextStyle descriptiontext= new TextStyle(fontSize: 14 , fontWeight: FontWeight.w400, color: Colors.black);
return showDialog(
context: context,
builder: (context) {
return AlertDialog(
backgroundColor: backgroundColor,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(dialogRadius)),
),
content: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Text(title, style: titletext, textAlign: TextAlign.center,),
SizedBox(height: 5,),
imageWidget,
SizedBox(height: 5,),
Text(description, style: descriptiontext, textAlign: TextAlign.center,),
PlaceButtons(
buttonsPosition,
AddButtons(context, buttonsPosition, buttonsWidth, successText, onSuccessPressed, dismissText, onDismissPressed, backgroundColor, themeColor),
buttonsAlignement
),
],
),
elevation: 10,
);
});
}
}
// Dialog with Widget half in half out the box
class StackAlertBox {
double dialogRadius;
double buttonsWidth;
Color themeColor, backgroundColor, circleColor;
String title, description;
String successText, dismissText;
Widget imageWidget;
ButtonsPosition buttonsPosition;
ButtonsAlignement buttonsAlignement;
VoidCallback onSuccessPressed, onDismissPressed;
StackAlertBox({
this.dialogRadius = 7,
this.buttonsWidth,
this.themeColor= Colors.blue,
this.backgroundColor = Colors.white,
this.title = "Alert Dialog",
this.description= "This is an alert dialog !",
this.circleColor = Colors.transparent,
this.imageWidget= const Card(color: Colors.transparent,),
this.successText = "",
this.onSuccessPressed,
this.dismissText = "",
this.onDismissPressed,
this.buttonsAlignement = ButtonsAlignement.CENTER,
this.buttonsPosition=ButtonsPosition.HORIZONTAL
});
Future show({
@required BuildContext context,
}) {
assert(context != null, "context is null!");
TextStyle titletext= new TextStyle(fontSize: 26 , fontWeight: FontWeight.w700, color: themeColor);
TextStyle descriptiontext= new TextStyle(fontSize: 14 , fontWeight: FontWeight.w400, color: Colors.black);
if (buttonsWidth==null) buttonsWidth=getButtonsWidth(context, buttonsPosition);
return showDialog(
context: context,
builder: (context) {
return Dialog(
backgroundColor: Colors.transparent,
elevation: 0,
child: Stack(children: <Widget>[
Container(
margin: EdgeInsets.only(top: 50),
decoration: BoxDecoration(
color: backgroundColor,
borderRadius: BorderRadius.circular(dialogRadius),
),
padding: EdgeInsets.only(top: 60, left: 20, right: 20, bottom: 20),
child: Column(
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Text(title, style: titletext, textAlign: TextAlign.center,),
SizedBox(height: 10,width: 0,),
Text(description, style: descriptiontext, textAlign: TextAlign.center,),
PlaceButtons(
buttonsPosition,
AddButtons(context, buttonsPosition, buttonsWidth, successText, onSuccessPressed, dismissText, onDismissPressed, backgroundColor, themeColor),
buttonsAlignement
),
],
),
),
Positioned(
left: 16,
right: 16,
child: CircleAvatar(
child: imageWidget,
backgroundColor: circleColor,
radius: 50,
),
),
],),
);
});
}
} | 0 |
mirrored_repositories/flutter_ui_package/lib | mirrored_repositories/flutter_ui_package/lib/src/dropdowns.dart | import 'package:flutter/material.dart';
import 'customdropdown.dart';
enum Dots{
HORIZONTAL,
VERTICAL
}
// ---------------- Classic Dropdown from material
// using customdropdown.dart (from stackoverflow)
// to persist the dropdown list under button -----------
// doesn't work when the list get longer
class ClassicDropdown extends StatefulWidget {
List<Item> list;
String hint;
Color outlineColor;
Color backgroundColor, boxColor;
double width, borderRadius, borderThickness, iconSize;
TextStyle style;
Widget icon;
ClassicDropdown({
this.list,
this.borderThickness = 2,
this.borderRadius = 10,
this.iconSize = 40,
this.icon ,
this.width = 200,
this.boxColor = Colors.white,
this.outlineColor = Colors.white,
this.hint = "Select",
this.backgroundColor = Colors.white,
this.style = const TextStyle(
fontSize: 14, fontWeight: FontWeight.w600, color: Colors.grey),
});
@override
_CdropState createState() => _CdropState();
}
class _CdropState extends State<ClassicDropdown> {
Item selectedUser;
@override
Widget build(BuildContext context) {
return new Container(
width: widget.width,
//padding: EdgeInsets.only(left: 10),
decoration: BoxDecoration(
color: widget.boxColor,
border: Border.all(
color: widget.outlineColor, width: widget.borderThickness),
borderRadius: BorderRadius.circular(widget.borderRadius)),
child: new Theme(
data: Theme.of(context).copyWith(
canvasColor: widget.backgroundColor,
),
child :ButtonTheme(
alignedDropdown: true,
child : CustomDropdownButton<Item>(
hint: Text(
widget.hint.toString(),
style: widget.style,
),
icon: widget.icon,
iconSize: widget.iconSize,
style: widget.style,
// underline: SizedBox(),
value: selectedUser,
onChanged: (Item Value) {
setState(() {
selectedUser = Value;
});
},
items: widget.list.map((Item user) {
return DropdownMenuItem<Item>(
value: user,
child: Row(
children: <Widget>[
user.icon,
SizedBox(
width: 10,
),
Text(
user.name,
style: widget.style,
),
],
),
);
}).toList(),
),
),
),
);
}
}
// ----------------------------------------------
//----------- dropdown from Material----------------
class MaterialDropdown extends StatefulWidget {
List<Item> list;
String hint;
Color outlineColor;
Color backgroundColor, boxColor;
double borderRadius, borderThickness, iconSize;
TextStyle style;
Widget icon;
MaterialDropdown({
this.list,
this.borderThickness = 2,
this.borderRadius = 10,
this.iconSize = 40,
this.icon = const Icon(
Icons.keyboard_arrow_down,
),
this.boxColor = Colors.white,
this.outlineColor = Colors.white,
this.hint = "Select",
this.backgroundColor = Colors.white,
this.style = const TextStyle(
fontSize: 14, fontWeight: FontWeight.w600, color: Colors.grey),
});
@override
_MdropState createState() => _MdropState();
}
class _MdropState extends State<MaterialDropdown> {
Item selectedUser;
@override
Widget build(BuildContext context) {
return new Container(
//padding: EdgeInsets.only(left: 10),
decoration: BoxDecoration(
color: widget.boxColor,
border: Border.all(
color: widget.outlineColor, width: widget.borderThickness),
borderRadius: BorderRadius.circular(widget.borderRadius)),
child: new Theme(
data: Theme.of(context).copyWith(
canvasColor: widget.backgroundColor,
),
child :ButtonTheme(
alignedDropdown: true,
child : DropdownButton<Item>(
hint: Text(
widget.hint.toString(),
style: widget.style,
),
icon: widget.icon,
iconSize: widget.iconSize,
style: widget.style,
underline: SizedBox(),
value: selectedUser,
onChanged: (Item Value) {
setState(() {
selectedUser = Value;
});
},
items: widget.list.map((Item user) {
return DropdownMenuItem<Item>(
value: user,
child: Row(
children: <Widget>[
user.icon,
SizedBox(
width: 10,
),
Text(
user.name,
style: widget.style,
),
],
),
);
}).toList(),
),
),
),
);
}
}
//-----------------------------------------
//------------ a class to store dropdown items,
// it contains a text and a widget which can be an icon, image ... ----------
class Item {
const Item({this.name="", this.icon = const SizedBox()});
final String name;
final Widget icon;
}
//-----------------------------------------
//-----------Scrollable PopupMenu with dots-------------------
class DotsMenu extends StatefulWidget {
Dots dots ;
List<Item> items;
double width, height, borderRadius, dotsSize;
Color backgroundColor, outlineColor;
Color iconColor, dotsColor;
ValueChanged<int> onChange;
TextStyle style;
DotsMenu({
Key key,
this.items,
this.dotsSize=30,
this.dots= Dots.HORIZONTAL,
this.width = 100,
this.height = 300,
this.style = const TextStyle(
fontSize: 14, fontWeight: FontWeight.w600, color: Colors.grey),
this.dotsColor= Colors.black,
this.borderRadius = 4,
this.backgroundColor = const Color(0xFFFFFFFFF),
this.outlineColor = Colors.transparent,
this.iconColor = Colors.black,
this.onChange,
});
@override
_DotsMenuState createState() => _DotsMenuState();
}
class _DotsMenuState extends State<DotsMenu> {
GlobalKey _key;
bool isMenuOpen = false;
Offset buttonPosition;
Size buttonSize;
OverlayEntry _overlayEntry;
@override
void initState() {
_key = LabeledGlobalKey("button");
super.initState();
}
findButton() {
RenderBox renderBox = _key.currentContext.findRenderObject();
buttonSize = renderBox.size;
buttonPosition = renderBox.localToGlobal(Offset.zero);
}
void closeMenu() {
_overlayEntry.remove();
isMenuOpen = !isMenuOpen;
}
void openMenu() {
findButton();
_overlayEntry = _overlayEntryBuilder();
Overlay.of(context).insert(_overlayEntry);
isMenuOpen = !isMenuOpen;
}
@override
Widget build(BuildContext context) {
Icon dotsIcon;
switch(widget.dots) {
case Dots.HORIZONTAL:
dotsIcon = Icon(Icons.more_horiz, size: widget.dotsSize,);
break;
case Dots.VERTICAL:
dotsIcon= Icon(Icons.more_vert, size: widget.dotsSize,);
break;
}
return Container(
key: _key,
child: IconButton(
icon: dotsIcon,
color: widget.dotsColor,
onPressed: () {
if (isMenuOpen) {
closeMenu();
} else {
openMenu();
}
},
),
);
}
OverlayEntry _overlayEntryBuilder() {
return OverlayEntry(
builder: (context) {
return Stack (children :[
Positioned.fill(
child: GestureDetector(
onTap:closeMenu,
child: Container(
color: Colors.transparent,
),
)
),
Positioned(
top: buttonPosition.dy + buttonSize.height/1.5,
right: buttonPosition.dx + buttonSize.height/3.5,
width: widget.width,
child: Material(
color: Colors.transparent,
child: Padding(
padding: const EdgeInsets.only(top: 5.0),
child: ConstrainedBox(
constraints: new BoxConstraints(
maxHeight: widget.height,
maxWidth: widget.width,
),
child: Container(
padding: const EdgeInsets.only(left: 5.0, right: 5.0),
height: widget.items.length * buttonSize.height,
decoration: BoxDecoration(
color: widget.backgroundColor,
border: Border.all(color: widget.outlineColor ,width: 2.0),
borderRadius: BorderRadius.circular(widget.borderRadius),
),
child: Theme(
data: ThemeData(
iconTheme: IconThemeData(
color: widget.iconColor,
),
),
child: ListView(
padding: EdgeInsets.zero,
children:[ Column(
mainAxisSize: MainAxisSize.min,
children: List.generate(widget.items.length, (index) {
return GestureDetector(
onTap: () {
widget.onChange(index);
closeMenu();
},
child: Container(
width: widget.width,
height: buttonSize.height,
child: Row (
children : [
widget.items[index].icon,
SizedBox(width: 10,),
Text(widget.items[index].name, style: widget.style,)
],
)
),
);
}),
),] ,
),
),
),
),
),
),
),],);
},
);
}
}
//-----------------------------------------
// --------A notch for our AwesomeMenu-------------
class ArrowClipper extends CustomClipper<Path> {
@override
Path getClip(Size size) {
Path path = Path();
path.moveTo(0, size.height);
path.lineTo(size.width / 2, size.height / 2);
path.lineTo(size.width, size.height);
return path;
}
@override
bool shouldReclip(CustomClipper<Path> oldClipper) {
return true;
}
}
//-----------------------------------------
// ---------A PopupMenu with a triangle arrow-------------
class AwesomeMenu extends StatefulWidget {
List<Item> items;
double width, height, borderRadius, iconSize;
Icon icon;
Color backgroundColor;
Color iconColor;
ValueChanged<int> onChange;
TextStyle style;
AwesomeMenu({
Key key,
this.items,
this.icon= const Icon(Icons.add_alert, size: 38,),
this.width = 100,
this.height = 300,
this.style = const TextStyle(
fontSize: 14, fontWeight: FontWeight.w600, color: Colors.grey),
this.borderRadius = 4,
this.backgroundColor = Colors.red,
this.iconColor = Colors.black,
this.onChange,
});
@override
_AwesomeMenuState createState() => _AwesomeMenuState();
}
class _AwesomeMenuState extends State<AwesomeMenu> {
GlobalKey _key;
bool isMenuOpen = false;
Offset buttonPosition;
Size buttonSize;
OverlayEntry _overlayEntry;
@override
void initState() {
_key = LabeledGlobalKey("button_icon");
super.initState();
}
findButton() {
RenderBox renderBox = _key.currentContext.findRenderObject();
buttonSize = renderBox.size;
buttonPosition = renderBox.localToGlobal(Offset.zero);
}
void closeMenu() {
_overlayEntry.remove();
isMenuOpen = !isMenuOpen;
}
void openMenu() {
findButton();
_overlayEntry = _overlayEntryBuilder();
Overlay.of(context).insert(_overlayEntry);
isMenuOpen = !isMenuOpen;
}
@override
Widget build(BuildContext context) {
return Container(
key: _key,
child: IconButton(
icon: widget.icon,
color: widget.iconColor,
onPressed: () {
if (isMenuOpen) {
closeMenu();
} else {
openMenu();
}
},
),
);
}
OverlayEntry _overlayEntryBuilder() {
return OverlayEntry(
builder: (context) {
return Stack (children :[
Positioned.fill(
child: GestureDetector(
onTap:closeMenu,
child: Container(
color: Colors.transparent,
),
)
),
Positioned(
top: buttonPosition.dy + buttonSize.height/1.5,
left: buttonPosition.dx - buttonSize.height/2,
width: widget.width,
child: Material(
color: Colors.transparent,
child: Stack(
children: <Widget>[
Align(
alignment: Alignment.topCenter,
child: ClipPath(
clipper: ArrowClipper(),
child: Container(
width: 20,
height: 20,
color: widget.backgroundColor ?? Color(0xFFF),
),
),
),
Padding(
padding: const EdgeInsets.only(top: 19.0),
child: ConstrainedBox(
constraints: new BoxConstraints(
maxHeight: widget.height,
maxWidth: widget.width,
),
child: Container(
padding: const EdgeInsets.only(left: 5.0, right: 5.0),
height: widget.items.length * buttonSize.height,
decoration: BoxDecoration(
color: widget.backgroundColor,
borderRadius: BorderRadius.circular(widget.borderRadius),
),
child: Theme(
data: ThemeData(
iconTheme: IconThemeData(
color: widget.iconColor,
),
),
child: ListView(
padding: EdgeInsets.zero,
children:[ Column(
mainAxisSize: MainAxisSize.min,
children: List.generate(widget.items.length, (index) {
return GestureDetector(
onTap: () {
widget.onChange(index);
closeMenu();
},
child: Container(
width: widget.width,
height: buttonSize.height,
child: Row (
children : [
widget.items[index].icon,
SizedBox(width: 10,),
Text(widget.items[index].name, style: widget.style,)
],
)
),
);
}),
),] ,
),
),
),
),
),
],
),
),
),
],);
},
);
}
}
// ----------- a function to show popup menu anywhere -------------
void showPopupMenu(BuildContext context, Offset offset) async {
double left = offset.dx;
double top = offset.dy;
await showMenu(
context: context,
position: RelativeRect.fromLTRB(left, top, 100, 100),
items: [
PopupMenuItem<String>(
child: const Text('One'), value: 'One'),
PopupMenuItem<String>(
child: const Text('Two'), value: 'Two'),
],
elevation: 8.0,
);
}
//-----------------------------------------
// ----------- Material PopupMenu -------------
class MaterialPopup extends StatefulWidget{
Offset offset ;
Color backgroundColor, outlineColor;
String text;
double width, height;
List<String> items ;
TextStyle style;
double borderRadius;
MaterialPopup({
this.offset= const Offset (50,50),
this.backgroundColor= Colors.blue,
this.outlineColor = Colors.transparent,
this.text= 'Select',
this.items,
this.width = 100,
this.height = 50,
this.style = const TextStyle( fontSize: 18, fontWeight: FontWeight.w600, color: Colors.white),
this.borderRadius = 10
});
@override
_MaterialPopupState createState() => _MaterialPopupState();
}
class _MaterialPopupState extends State<MaterialPopup> {
String _selection;
@override
Widget build(BuildContext context) {
List <PopupMenuItem<String>> list = widget.items.map((String text){
return PopupMenuItem<String>(
value: text,
child: Text(text),
); }).toList();
return PopupMenuButton<String>(
onSelected: (String value) {
setState(() {
_selection = value;
});
},
offset: widget.offset,
child: Container(
width: widget.width,
height: widget.height,
padding: EdgeInsets.all(10),
decoration: BoxDecoration(
color: widget.backgroundColor,
borderRadius: BorderRadius.circular(widget.borderRadius),
border: Border.all(color: widget.outlineColor, width: 2),
),
child: Align(
alignment: Alignment.center,
child: Text(
_selection == null ? widget.text : _selection.toString(),
// textAlign: TextAlign.center,
style: widget.style,
),
),
),
itemBuilder: (BuildContext context) {
return list;
}
);
}
}
//-----------------------------------------
| 0 |
mirrored_repositories/flutter_ui_package/lib | mirrored_repositories/flutter_ui_package/lib/src/buttons.dart | import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
enum Position{
CENTER,
LEFT,
RIGHT
}
MainAxisAlignment getPosition(Position position){
switch(position){
case Position.CENTER :
return MainAxisAlignment.center;
case Position.LEFT :
return MainAxisAlignment.start;
case Position.RIGHT :
return MainAxisAlignment.end;
}
}
// get a Widget List of the Button Content
List<Widget> ButtonContent(IconData icon, Color iconColor, double iconSize, double icontextSpace, String text, TextStyle textStyle){
if (icon != null){
return [
Icon(
icon,
color: iconColor,
size: iconSize,
),
SizedBox(
width: icontextSpace,
),
Text(
text,
style: textStyle,
),
];
}else return [
Text(
text,
style: textStyle,
),
];
}
class BFilled extends StatelessWidget {
String text;
Color iconColor, backgroundColor;
double width, height, radius, iconSize, icontextSpace;
IconData icon;
VoidCallback onPressed;
TextStyle textStyle;
Position alignment;
BFilled({
this.width =100,
this.height= 50,
this.radius = 7,
this.text = "",
this.textStyle= const TextStyle(fontSize: 18 , fontWeight: FontWeight.w700, color: Colors.white),
this.backgroundColor= Colors.blue,
this.icon,
this.iconColor= Colors.white,
this.icontextSpace =10,
this.iconSize =32,
this.alignment = Position.CENTER,
this.onPressed
});
@override
Widget build(BuildContext context) {
return Container(
width: width,
height: height,
decoration: BoxDecoration(
color: backgroundColor,
borderRadius: BorderRadius.all(Radius.circular(radius)),
),
child: SizedBox.expand(
child : FlatButton(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(radius)),
),
padding: EdgeInsets.all(10),
onPressed: onPressed,
child: Row(
mainAxisAlignment: getPosition(alignment),
children: ButtonContent(icon, iconColor, iconSize, icontextSpace, text, textStyle),
),
),
)
);
}
}
class BOutlined extends StatelessWidget {
String text;
Color iconColor, backgroundColor, outlineColor;
double width, height, radius, iconSize, icontextSpace;
IconData icon;
VoidCallback onPressed;
TextStyle textStyle;
Position alignment;
BOutlined({
this.width =100,
this.height= 50,
this.radius = 7,
this.text = "",
this.textStyle= const TextStyle(fontSize: 18 , fontWeight: FontWeight.w700, color: Colors.black),
this.backgroundColor= Colors.transparent,
this.icon,
this.iconColor= Colors.blue,
this.icontextSpace =10,
this.iconSize =32,
this.alignment = Position.CENTER,
this.outlineColor= Colors.blue,
this.onPressed
});
@override
Widget build(BuildContext context) {
return Container(
width: width,
height: height,
decoration: BoxDecoration(
color: backgroundColor,
border: Border.all(color: outlineColor ,width: 2.0),
borderRadius: BorderRadius.all(Radius.circular(radius)),
),
child: SizedBox.expand(
child : FlatButton(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(radius)),
),
padding: EdgeInsets.all(10),
onPressed: onPressed,
child: Row(
mainAxisAlignment: getPosition(alignment),
children: ButtonContent(icon, iconColor, iconSize, icontextSpace, text, textStyle),
),
),
),
);
}
}
class BRaised extends StatelessWidget {
String text;
Color backgroundColor, iconColor, shadowColor;
double width, height, radius, iconSize, icontextSpace, spreadRadius, blurRadius;
Offset shadowOffset;
VoidCallback onPressed;
TextStyle textStyle;
IconData icon;
Position alignment;
BRaised ({
this.width =100,
this.height= 50,
this.radius = 7,
this.text = "",
this.textStyle= const TextStyle(fontSize: 18 , fontWeight: FontWeight.w700, color: Colors.white),
this.backgroundColor= Colors.blue,
this.icon,
this.iconColor= Colors.white,
this.icontextSpace =10,
this.iconSize =32,
this.alignment = Position.CENTER,
this.shadowColor= const Color(0x80303030),
this.spreadRadius = 2,
this.blurRadius = 4,
this.shadowOffset = const Offset(0, 2),
this.onPressed
});
@override
Widget build(BuildContext context) {
return Container(
width: width,
height: height,
decoration: BoxDecoration(
color: backgroundColor,
boxShadow: [BoxShadow(color: shadowColor, spreadRadius: spreadRadius, blurRadius: blurRadius, offset: shadowOffset)],
borderRadius: BorderRadius.all(Radius.circular(radius)),
),
child: SizedBox.expand(
child: FlatButton(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(radius)),
),
padding: EdgeInsets.all(10),
onPressed: onPressed,
child: Row(
mainAxisAlignment: getPosition(alignment),
children: ButtonContent(icon, iconColor, iconSize, icontextSpace, text, textStyle),
),
),
),
);
}
} | 0 |
mirrored_repositories/flutter_ui_package/lib | mirrored_repositories/flutter_ui_package/lib/src/inputfields.dart | import 'package:flutter/material.dart';
class Entry extends StatelessWidget {
String title, hint;
Color outlineColor, cursorColor;
double radius, outlineThikness;
bool isPassword, showCursor;
TextStyle hintStyle, inputStyle;
Entry({
this.title = "Field",
this.hint= "hint",
this.outlineColor = Colors.blue,
this.radius = 10,
this.outlineThikness=2,
this.isPassword = false,
this.showCursor = true,
this.cursorColor = Colors.blue,
this.hintStyle= const TextStyle(fontWeight: FontWeight.bold, fontSize: 15),
this.inputStyle= const TextStyle(fontSize: 18),
});
@override
Widget build(BuildContext context) {
return Container(
padding: EdgeInsets.all(10),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Text(
title,
style: hintStyle,
),
SizedBox(
height: 10,
),
TextField(
showCursor: showCursor,
style: inputStyle,
cursorColor: cursorColor,
obscureText: isPassword,
decoration: InputDecoration(
contentPadding: EdgeInsets.only(right: 10, left: 10),
enabledBorder: OutlineInputBorder(
borderSide: BorderSide(color: outlineColor, width: outlineThikness),
borderRadius: BorderRadius.all(Radius.circular(radius)),
),
focusedBorder: OutlineInputBorder(
borderSide: BorderSide(color: outlineColor, width: outlineThikness),
borderRadius: BorderRadius.all(Radius.circular(radius)),
),
hintText: hint,
),
)
],
),
);
}
} | 0 |
mirrored_repositories/flutter_ui_package | mirrored_repositories/flutter_ui_package/test/flutteruipackage_test.dart | import 'package:flutter_test/flutter_test.dart';
import 'package:flutteruipackage/flutteruipackage.dart';
void main() {
}
| 0 |
mirrored_repositories/flutter_ui_package/example/flutter_app | mirrored_repositories/flutter_ui_package/example/flutter_app/lib/dialogs_usecases.dart | import 'package:flutter/material.dart';
import 'package:flutteruipackage/flutteruipackage.dart';
class MyDialogs extends StatefulWidget {
MyDialogs({Key key}) : super(key: key);
@override
_MyDialogsState createState() => _MyDialogsState();
}
class _MyDialogsState extends State<MyDialogs> {
@override
Widget build(BuildContext context) {
Widget iconwidget = new Icon(
Icons.warning,
color: Colors.white,
size: 70,
);
return Scaffold(
backgroundColor: Colors.white,
appBar: AppBar(
title: Text("Alert Dialogs"),
),
body: Center(
child: Column(
children: <Widget>[
SizedBox(
height: 10,
),
BFilled(
width: 200,
height: 50,
icon: Icons.done,
iconSize: 20,
text: "No Button Dialog",
backgroundColor: Colors.green,
onPressed: () { //a dialog with no button, no image
BasicAlertBox(
themeColor: Colors.indigo,
backgroundColor: Colors.tealAccent[100],
title: "Alert Dialog",
description: "This is a dialog with no button",
dialogRadius: 10,
buttonsWidth: 50,
).show(context: context);
},
),
SizedBox(
height: 10,
),
BFilled(
width: 200,
height: 50,
text: "Horizontal Buttons",
backgroundColor: Colors.redAccent,
onPressed: () { //shows a dialog with two Horizantal buttons + network gif image
BasicAlertBox(
imageWidget: Image.network(
"https://cdn.dribbble.com/users/146798/screenshots/2933118/rocket.gif",
width: 150,
height: 150,
),
dialogRadius: 7,
title: "An Alert Box",
description: "Example of a dialog with two horizontal buttons and a widget between title and description.",
successText: "OK",
dismissText: "CANCEL",
buttonsWidth: 100,
onSuccessPressed: () {
Navigator.of(context).pop();
},
onDismissPressed: () {
Navigator.of(context).pop();
},
).show(context: context);
},
),
SizedBox(
height: 10,
),
BFilled(
width: 200,
height: 50,
text: "Vertical Buttons",
backgroundColor: Colors.redAccent,
onPressed: () { //a dialog with two Vertical buttons
BasicAlertBox(
dialogRadius: 7,
buttonsWidth: 200,
themeColor: Colors.teal,
title: "An Alert Box",
description: "Example of a dialog with two vertical buttons.",
successText: "OK",
dismissText: "CANCEL",
onSuccessPressed: () {
Navigator.of(context).pop();
},
onDismissPressed: () {
Navigator.of(context).pop();
},
buttonsPosition: ButtonsPosition.VERTICAL,
).show(context: context);
},
),
SizedBox(
height: 10,
),
BFilled(
width: 200,
height: 50,
text: "Stack Dialog 1",
backgroundColor: Colors.blue,
onPressed: () { //shows a dialog with popup icon in a blue circle
StackAlertBox(
dialogRadius: 15,
buttonsWidth: 150,
themeColor: Colors.blue,
backgroundColor: Colors.white,
circleColor: Colors.blue,
title: "Alert Box",
description: "A Stack Dialog with a custom widget inside the circle above.",
imageWidget: iconwidget,
successText: "OK",
dismissText: "CANCEL",
buttonsPosition: ButtonsPosition.VERTICAL,
).show(context: context);
},
),
SizedBox(
height: 10,
),
BFilled(
width: 200,
height: 50,
text: "Stack Dialog 2",
backgroundColor: Colors.blue,
onPressed: () {
StackAlertBox( //a dialog with popup png image from network
imageWidget: Image.network(
"https://www.freepngimg.com/thumb/technology/35452-1-robot-hd.png",
width: 170,
height: 190,
),
title: "An Alert Dialog",
description: "An other stack dialog with a png image, check your network connection to appear",
buttonsWidth: 100,
successText: "OK",
themeColor: Colors.blueGrey,
buttonsAlignement: ButtonsAlignement.RIGHT
).show(context: context);
},
),
],
),
),
);
}
}
| 0 |
mirrored_repositories/flutter_ui_package/example/flutter_app | mirrored_repositories/flutter_ui_package/example/flutter_app/lib/buttons_usecases.dart | import 'package:flutter/material.dart';
import 'package:flutteruipackage/flutteruipackage.dart';
class MyButtons extends StatefulWidget {
MyButtons({Key key}) : super(key: key);
@override
_MyButtonsState createState() => _MyButtonsState();
}
class _MyButtonsState extends State<MyButtons> {
@override
Widget build (BuildContext context) {
TextStyle text = new TextStyle(
fontSize: 16, fontWeight: FontWeight.w500, color: Colors.white);
TextStyle textstyle = new TextStyle(
fontSize: 16, fontWeight: FontWeight.w500, color: Colors.green);
return Scaffold(
backgroundColor: Colors.white,
appBar: AppBar(
title: Text("Buttons"),
),
body: Center(
child: Column(
children: <Widget>[
SizedBox(
height: 10,
),
BFilled(
width: 200,
height: 50,
text: "LOGIN WITH FACEBOOK",
textStyle: text,
backgroundColor: Color(0xff2872ba),
),
SizedBox(
height: 10,
),
BFilled(
width: 200,
height: 50,
text: "Button",
radius: 30,
alignment: Position.LEFT,
textStyle: text,
backgroundColor: Colors.blue,
),
SizedBox(
height: 10,
),
BFilled(
width: 200,
height: 50,
icon: Icons.subscriptions,
iconSize: 20,
icontextSpace: 30,
text: "Subscribe Now",
backgroundColor: Colors.red,
onPressed: () {},
),
SizedBox(
height: 10,
),
BOutlined(
width: 200,
height: 50,
text: "SAVE TO PLAYLIST",
backgroundColor: Colors.blueGrey[50],
outlineColor: Colors.red,
onPressed: () {},
),
SizedBox(
height: 10,
),
BOutlined(
width: 200,
height: 50,
icon: Icons.done,
iconSize: 20,
iconColor: Colors.green,
text: "ADD TO FAVORITES",
textStyle: textstyle,
outlineColor: Colors.green,
onPressed: () {},
),
SizedBox(
height: 10,
),
BRaised(
width: 210,
height: 50,
text: "LOGIN",
backgroundColor: Colors.orange,
shadowColor: Colors.lightBlue[200],
shadowOffset: Offset(2,2),
spreadRadius: 1,
blurRadius: 1,
onPressed: () {},
),
SizedBox(
height: 10,
),
BRaised(
width: 210,
height: 50,
text: "CREATE ACCOUNT",
icon: Icons.person,
iconColor: Colors.white,
iconSize: 25,
icontextSpace: 5,
backgroundColor: Colors.lightBlue,
shadowColor: Colors.orange[200],
shadowOffset: Offset(0,2),
spreadRadius: 1,
blurRadius: 7,
onPressed: () {},
),
],
),
),
);
}
}
| 0 |
mirrored_repositories/flutter_ui_package/example/flutter_app | mirrored_repositories/flutter_ui_package/example/flutter_app/lib/main.dart | import 'package:flutter/material.dart';
import 'package:flutteruipackage/flutteruipackage.dart';
import 'buttons_usecases.dart';
import 'dialogs_usecases.dart';
import 'dropdown_usecases.dart';
import 'inputs_usecases.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Demo',
home: MyHomePage(title: 'Flutter UI Demo'),
);
}
}
class MyHomePage extends StatefulWidget {
MyHomePage({Key key, this.title}) : super(key: key);
final String title;
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
TextStyle textstyle = new TextStyle(
fontSize: 18, fontWeight: FontWeight.w300, color: Colors.white);
return Scaffold(
backgroundColor: Colors.white,
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
children: <Widget>[
Text(
'A demo for Custom UI widgets',
),
SizedBox(
height: 30,
),
BFilled(
width: 200,
height: 50,
text: "Buttons",
textStyle: textstyle,
backgroundColor: Colors.blue,
onPressed: () {
Navigator.push(context,
MaterialPageRoute(builder: (context) => MyButtons()));
},
),
SizedBox(
height: 10,
),
BFilled(
width: 200,
height: 50,
text: "Alert Dialogs",
textStyle: textstyle,
backgroundColor: Colors.blue,
onPressed: () {
Navigator.push(context,
MaterialPageRoute(builder: (context) => MyDialogs()));
},
),
SizedBox(
height: 10,
),
BFilled(
width: 200,
height: 50,
text: "Input Fields",
textStyle: textstyle,
backgroundColor: Colors.blue,
onPressed: () {
Navigator.push(context,
MaterialPageRoute(builder: (context) => MyInputs()));
},
),
SizedBox(
height: 10,
),
BFilled(
width: 200,
height: 50,
text: "Dropdowns",
textStyle: textstyle,
backgroundColor: Colors.blue,
onPressed: () {
Navigator.push(context,
MaterialPageRoute(builder: (context) => MyDrops()));
},
),
SizedBox(
height: 10,
),
],
),
),
);
}
}
| 0 |
mirrored_repositories/flutter_ui_package/example/flutter_app | mirrored_repositories/flutter_ui_package/example/flutter_app/lib/inputs_usecases.dart | import 'package:flutter/material.dart';
import 'package:flutteruipackage/flutteruipackage.dart';
class MyInputs extends StatefulWidget {
MyInputs({Key key}) : super(key: key);
@override
_MyInputsState createState() => _MyInputsState();
}
class _MyInputsState extends State<MyInputs> {
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.white,
appBar: AppBar(
title: Text("Input Fields"),
),
body: SingleChildScrollView(
child: Column(
children: <Widget>[
Entry(
title: "Username",
hint: "username",
isPassword: false,
outlineColor: Colors.blue,
radius: 0,
outlineThikness: 1,
cursorColor: Colors.orange,
inputStyle: TextStyle(fontWeight: FontWeight.bold, fontSize: 25),
),
Entry(
title: "Password",
hint: "********",
isPassword: true,
outlineColor: Colors.blueGrey,
radius: 10,
outlineThikness: 2,
),
],
),
),
);
}
}
| 0 |
mirrored_repositories/flutter_ui_package/example/flutter_app | mirrored_repositories/flutter_ui_package/example/flutter_app/lib/dropdown_usecases.dart | import 'package:flutter/material.dart';
import 'package:flutteruipackage/flutteruipackage.dart';
class MyDrops extends StatefulWidget {
MyDrops({Key key}) : super(key: key);
@override
_MyDropState createState() => _MyDropState();
}
class _MyDropState extends State<MyDrops> {
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.white,
appBar: AppBar(
title: Text("Dropdowns"),
),
body: Center(
child: Column(
children: <Widget>[
// 3 dots popup menu
DotsMenu(
items: [
Item(name : 'Profil', icon: Icon(Icons.person)),
Item(name : 'Phone', icon: Icon(Icons.phone)),
Item(name : 'Email', icon: Icon(Icons.email)),
Item(name : 'Adress', icon: Icon(Icons.home)),
Item(name : 'Job', icon: Icon(Icons.work)),
],
height: 200,
width: 100,
dots: Dots.HORIZONTAL,
borderRadius: 5,
style: TextStyle(fontSize: 14, fontWeight: FontWeight.w600, color: Colors.white),
iconColor: Colors.white,
outlineColor: Colors.black,
backgroundColor: Colors.red,
onChange: (index) {
print(index); //action setted when an item is chosen
},
),
SizedBox(height: 20,),
// the classic dropdown from material
MaterialDropdown(
list: [
Item(name : 'Android', icon: Icon(Icons.android, color: Colors.teal,)),
Item(name : 'Flutter',),
Item(name : 'iOS',icon: Icon(Icons.mobile_screen_share,color: Colors.teal)),
Item(name : 'ReactNative',),
],
outlineColor: Colors.blue,
icon: Icon(Icons.keyboard_arrow_down, color: Colors.blue,),
iconSize: 30,
borderRadius: 5,
hint: "Select Language",
style: TextStyle(fontSize: 16, fontWeight: FontWeight.w600, color: Colors.black),
),
SizedBox(height: 20,),
// the same material dropdown but it persists opening the menu under the button, isn't working for a big list
ClassicDropdown(
width: 250,
list: [
Item(name : 'Android', icon: Icon(Icons.android, color: Colors.teal,)),
Item(name : 'Flutter',),
Item(name : 'iOS',icon: Icon(Icons.mobile_screen_share,color: Colors.teal)),
Item(name : 'ReactNative',),
],
outlineColor: Colors.teal,
icon: Icon(Icons.keyboard_arrow_up, color: Colors.teal,),
iconSize: 30,
borderRadius: 5,
hint: "Select Language",
style: TextStyle(fontSize: 16, fontWeight: FontWeight.w600, color: Colors.black),
),
SizedBox(height: 20,),
// Popup Menu of Material
MaterialPopup(
text: 'Choose a number',
width: 160,
height: 50,
offset: Offset(50,50),
borderRadius: 5,
backgroundColor: Colors.blueGrey[50],
outlineColor: Colors.blue,
style: TextStyle(fontSize: 16, fontWeight: FontWeight.w700, color: Colors.blue) ,
items: ["One","Two", "Three"],
),
SizedBox(height: 20,),
AwesomeMenu(
icon: Icon(Icons.notification_important, color: Colors.black, size: 35,),
iconColor: Colors.black,
items: [
Item(name : 'Profil', icon: Icon(Icons.person)),
Item(name : 'Phone', icon: Icon(Icons.phone)),
Item(name : 'Email', icon: Icon(Icons.email)),
],
borderRadius: 4,
backgroundColor: Colors.red,
style: TextStyle(fontSize: 14, fontWeight: FontWeight.w700, color: Colors.white),
),
],
),
),
);
}
}
| 0 |
mirrored_repositories/flutter_ui_package/example/flutter_app | mirrored_repositories/flutter_ui_package/example/flutter_app/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
void main() {
}
| 0 |
mirrored_repositories/travee-flutter-app | mirrored_repositories/travee-flutter-app/lib/demo_file.dart | import 'package:flutter/material.dart';
import 'dart:ui';
class DemoApp extends StatelessWidget {
const DemoApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
theme: ThemeData(
primarySwatch: Colors.pink,
),
home: const HomePage(),
);
}
}
class HomePage extends StatelessWidget {
const HomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
double listContainerHeight = MediaQuery.of(context).size.height - 150;
return PageWrapper(
containerHeight: listContainerHeight,
body: SafeArea(
child: SingleChildScrollView(
clipBehavior: Clip.none,
padding: const EdgeInsets.symmetric(horizontal: 30),
child: Column(
children: [
const SizedBox(height: 20),
BlurContainer(
containerHeight: listContainerHeight,
child: ItemPicker(containerHeight: listContainerHeight),
),
const SizedBox(height: 20),
const BlurSlider(),
const SizedBox(height: 20),
const ExplicitAnimations(),
const SizedBox(height: 20),
const AnimatedBuilderExample(),
const SizedBox(height: 20),
const AnimatedWidgetExample(),
const SizedBox(height: 20),
],
),
),
),
);
}
}
class ExplicitAnimations extends StatefulWidget {
const ExplicitAnimations({Key? key}) : super(key: key);
@override
State<ExplicitAnimations> createState() => _ExplicitAnimationsState();
}
class _ExplicitAnimationsState extends State<ExplicitAnimations> with SingleTickerProviderStateMixin {
late AnimationController _controller;
late final Animation<AlignmentGeometry> _alignAnimation;
late final Animation<double> _rotationAnimation;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: const Duration(milliseconds: 800),
vsync: this,
)..repeat(reverse: true);
_alignAnimation = Tween<AlignmentGeometry>(
begin: Alignment.centerLeft,
end: Alignment.centerRight,
).animate(
CurvedAnimation(
parent: _controller,
curve: Curves.easeInOutCubic,
),
);
_rotationAnimation = Tween<double>(begin: 0, end: 2).animate(
CurvedAnimation(
parent: _controller,
curve: Curves.easeInOutCubic,
),
);
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return BlurContainer(
containerHeight: 200,
child: AlignTransition(
alignment: _alignAnimation,
child: RotationTransition(
turns: _rotationAnimation,
child: const Rectangle(
color1: pink,
color2: pinkDark,
width: 50,
height: 50,
),
),
),
);
}
}
class Rectangle extends StatelessWidget {
const Rectangle({
Key? key,
this.width = 60,
this.height = 40,
required this.color1,
required this.color2,
this.top,
this.bottom,
this.left,
this.right,
}) : super(key: key);
final double width;
final double height;
final Color color1;
final Color color2;
final double? top;
final double? bottom;
final double? left;
final double? right;
@override
Widget build(BuildContext context) {
return Container(
width: width,
height: height,
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [color1, color2],
begin: Alignment.topLeft,
end: Alignment.bottomRight,
),
border: Border.all(width: 1, color: Colors.white),
borderRadius: BorderRadius.circular(12),
),
);
}
}
class BlurSlider extends StatefulWidget {
const BlurSlider({Key? key}) : super(key: key);
@override
State<BlurSlider> createState() => _BlurSliderState();
}
class _BlurSliderState extends State<BlurSlider> {
double _sliderValue = 0.01;
@override
Widget build(BuildContext context) {
return TweenAnimationBuilder(
duration: const Duration(milliseconds: 200),
tween: Tween<double>(begin: 0.01, end: _sliderValue),
child: Container(
height: 300,
decoration: BoxDecoration(
color: Colors.white.withOpacity(0.2),
border: Border.all(
color: Colors.white.withOpacity(0.5),
width: 1.5,
),
borderRadius: BorderRadius.circular(15),
),
alignment: Alignment.center,
child: Slider(
value: _sliderValue,
min: 0.01,
onChanged: (value) {
setState(() => _sliderValue = value);
},
),
),
builder: (BuildContext context, double? value, Widget? child) {
return ClipRect(
child: BackdropFilter(
filter: ImageFilter.blur(
sigmaX: 40 * (value ?? 0.01),
sigmaY: 40 * (value ?? 0.01),
),
child: child,
),
);
},
);
}
}
class ItemPicker extends StatefulWidget {
const ItemPicker({
Key? key,
required this.containerHeight,
}) : super(key: key);
final double containerHeight;
@override
State<ItemPicker> createState() => _ItemPickerState();
}
class _ItemPickerState extends State<ItemPicker> {
int selectedItemIndex = 0;
@override
Widget build(BuildContext context) {
const double listContainerBorderWidth = 1.5;
const int itemsCount = 8;
double itemHeight = (widget.containerHeight - listContainerBorderWidth * 2) / itemsCount;
return SizedBox(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
child: Stack(
children: [
AnimatedPositioned(
top: selectedItemIndex * itemHeight,
left: 0,
right: 0,
height: itemHeight,
duration: const Duration(milliseconds: 200),
curve: Curves.easeInOut,
child: Container(
margin: const EdgeInsets.symmetric(horizontal: 10, vertical: 10),
decoration: BoxDecoration(
color: Colors.white.withOpacity(0.3),
borderRadius: BorderRadius.circular(10),
),
),
),
Positioned.fill(
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: List.generate(
8,
(i) => Expanded(
child: InkWell(
onTap: () => setState(() => selectedItemIndex = i),
child: Padding(
padding: const EdgeInsets.symmetric(horizontal: 30),
child: Center(
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
Text(
'List Item ${i + 1}',
style: const TextStyle(
fontSize: 18,
color: Colors.white,
),
),
if (i == 3)
AnimatedContainer(
duration: const Duration(milliseconds: 200),
curve: Curves.easeInOut,
padding: const EdgeInsets.all(8),
decoration: BoxDecoration(
color: selectedItemIndex == i ? yellow : pink,
borderRadius: BorderRadius.circular(8),
border: Border.all(
color: selectedItemIndex == i ? Colors.white : Colors.transparent,
width: 2,
),
),
child: AnimatedDefaultTextStyle(
duration: const Duration(milliseconds: 200),
style: TextStyle(
fontSize: 14,
color: selectedItemIndex == i ? Colors.black : Colors.white,
),
child: const Text('Featured!'),
),
),
],
),
),
),
),
),
),
),
),
],
),
);
}
}
class BlurContainer extends StatelessWidget {
const BlurContainer({
Key? key,
this.containerHeight = 120,
this.child,
}) : super(key: key);
final double containerHeight;
final Widget? child;
@override
Widget build(BuildContext context) {
return ClipRRect(
borderRadius: BorderRadius.circular(15),
child: BackdropFilter(
filter: ImageFilter.blur(sigmaX: 40, sigmaY: 40),
child: Container(
clipBehavior: Clip.none,
height: containerHeight,
decoration: BoxDecoration(
color: Colors.white.withOpacity(0.2),
border: Border.all(
color: Colors.white.withOpacity(0.5),
width: 1.5,
),
borderRadius: BorderRadius.circular(15),
),
alignment: Alignment.center,
child: child,
),
),
);
}
}
class AnimatedBuilderExample extends StatefulWidget {
const AnimatedBuilderExample({Key? key}) : super(key: key);
@override
State<AnimatedBuilderExample> createState() => _AnimatedBuilderExampleState();
}
class _AnimatedBuilderExampleState extends State<AnimatedBuilderExample> with SingleTickerProviderStateMixin {
late final AnimationController _controller;
@override
void initState() {
_controller = AnimationController(
duration: const Duration(milliseconds: 500),
vsync: this,
)..repeat(reverse: true);
super.initState();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return AnimatedBuilder(
animation: _controller,
builder: (BuildContext context, Widget? child) {
return Container(
height: 100,
decoration: BoxDecoration(
gradient: LinearGradient(
colors: const [purple, pink, yellow],
stops: [0, _controller.value, 1],
),
borderRadius: BorderRadius.circular(15),
border: Border.all(color: Colors.white),
),
);
},
);
}
}
class AnimatedWidgetExample extends StatefulWidget {
const AnimatedWidgetExample({Key? key}) : super(key: key);
@override
State<AnimatedWidgetExample> createState() => _AnimatedWidgetExampleState();
}
class _AnimatedWidgetExampleState extends State<AnimatedWidgetExample> with SingleTickerProviderStateMixin {
late final AnimationController _controller;
@override
void initState() {
_controller = AnimationController(
duration: const Duration(milliseconds: 500),
vsync: this,
)..repeat(reverse: true);
super.initState();
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Container(
decoration: BoxDecoration(
gradient: LinearGradient(
colors: const [purple, pink, yellow],
stops: [0, _controller.value, 1],
),
borderRadius: BorderRadius.circular(15),
border: Border.all(color: Colors.white),
),
child: ClipRRect(
borderRadius: BorderRadius.circular(16),
child: GradientTransition(gradientEnd: _controller),
),
);
}
}
class GradientTransition extends AnimatedWidget {
final Animation<double> gradientEnd;
const GradientTransition({
Key? key,
required this.gradientEnd,
}) : super(key: key, listenable: gradientEnd);
@override
Widget build(BuildContext context) {
return Container(
height: 100,
decoration: BoxDecoration(
gradient: LinearGradient(
colors: const [purple, pink, yellow],
stops: [0, gradientEnd.value, 1],
),
),
);
}
}
const Color pink = Color(0xffEC6283);
const Color pinkDark = Color(0xffD64265);
const Color yellow = Color(0xffE7F18F);
const Color yellowDark = Color(0xffB8C72B);
const Color purple = Color(0xff323AE9);
class PageWrapper extends StatelessWidget {
const PageWrapper({
Key? key,
this.body,
this.containerHeight = 500,
this.hasContainer = true,
}) : super(key: key);
final Widget? body;
final double containerHeight;
final bool hasContainer;
@override
Widget build(BuildContext context) {
return Scaffold(
body: Container(
decoration: const BoxDecoration(
gradient: LinearGradient(
colors: [Color(0xff323AE9), Color(0xff4D59D1)],
begin: Alignment.topLeft,
end: Alignment.bottomRight,
),
),
child: Stack(
children: [
..._backgroundCircles,
Positioned.fill(
child: Center(
child: body,
),
),
],
),
),
);
}
List<Widget> get _backgroundCircles => const [
Circle(
color1: pink,
color2: pinkDark,
radius: 350,
left: -120,
top: -100,
),
Circle(
color1: pink,
color2: pinkDark,
radius: 280,
right: -120,
bottom: 200,
),
Circle(
color1: yellow,
color2: yellowDark,
radius: 180,
right: -60,
top: -40,
),
Circle(
color1: yellow,
color2: yellowDark,
radius: 400,
left: -160,
bottom: -120,
),
];
}
class Circle extends StatelessWidget {
final Color color1;
final Color color2;
final double? left;
final double? right;
final double? top;
final double? bottom;
final double? radius;
final bool isPositioned;
const Circle({
required this.color1,
required this.color2,
this.left,
this.right,
this.top,
this.bottom,
this.radius,
this.isPositioned = true,
});
@override
Widget build(BuildContext context) {
Widget circle = Container(
height: radius,
width: radius,
decoration: BoxDecoration(
shape: BoxShape.circle,
gradient: LinearGradient(
colors: [color1, color2],
end: Alignment.topLeft,
begin: Alignment.bottomRight,
),
),
);
return isPositioned
? Positioned(
bottom: bottom,
right: right,
top: top,
left: left,
height: radius,
width: radius,
child: circle,
)
: circle;
}
}
| 0 |
mirrored_repositories/travee-flutter-app | mirrored_repositories/travee-flutter-app/lib/main.dart | import 'package:dart_openai/dart_openai.dart';
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'package:travee/screens/homescreen/bottom_nav_bar.dart';
void main() {
String gptToken = "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";
String gptApiKey = "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";
// String orgId = "org-3EMmsvASfykyFOG55GAadAch";
OpenAI.apiKey = gptApiKey;
// OpenAI.organization = orgId;
runApp(const MyApp()); // travee app
//runApp(const DemoApp()); // animation class
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
@override
Widget build(BuildContext context) {
return GetMaterialApp(
title: 'travee',
theme: ThemeData(
primarySwatch: Colors.blue,
),
debugShowCheckedModeBanner: false,
home: const BottomNavBar(),
);
}
}
| 0 |
mirrored_repositories/travee-flutter-app/lib | mirrored_repositories/travee-flutter-app/lib/resources/color.dart | import 'package:flutter/material.dart';
//Color kPinkColor = const Color(0xFFF273E6);
Color kPrimaryColor = const Color(0xFF7149C6);
Color kWhiteColor = const Color(0xFFFFFFFF);
Color kBlackColor = const Color(0xFF000000);
Color kGreyColor = const Color(0xFF808080);
Color kLightGreyColor = const Color(0xFFEAEAEA);
Color kBackgroundColor = const Color(0xFFEFF1F3);
// Color kColor1 = const Color(0xFFF1F4FA);
MaterialColor mPinkColor = const MaterialColor(
0xFFE5068B,
<int, Color>{
50: Color(0xFFEFE8FF),
100: Color(0xFFE6DBFF),
200: Color(0xFFD9CAFF),
300: Color(0xFFD1BFFF),
400: Color(0xFFCCB6FF),
500: Color(0xFFCAAFFF),
600: Color(0xFFB898FF),
700: Color(0xFF9871FF),
800: Color(0xFF895EFF),
900: Color(0xFF6C39FF),
},
);
| 0 |
mirrored_repositories/travee-flutter-app/lib | mirrored_repositories/travee-flutter-app/lib/data/tour_category_data.dart | import 'package:travee/model/itinerary_model.dart';
import 'package:travee/model/tour_categories_model.dart';
import 'package:travee/model/transport_model.dart';
import '../model/tour_package_model.dart';
class TourCategoryData {
List<Package> trekkingPackageList = [
Package(
name: "Saputara",
photo: "assets/images/tour_images/saputara1.jpg",
price: "3500",
duration: "3D-2N",
transport: Transport(
by: "Bus",
toSource: "Ahmedabad",
fromDestination: "Saputara",
pickupTime: "6:30 am",
dropTime: "9:30 pm",
),
fromDate: "August 23,2024",
toDate: "August 26,2024",
included: "meals, transportation, sightseeing, accommodation, activities",
itinerary: [
Itinerary(
day: "1",
place: Place(
name: "Saputara Lake",
details: " Lake & Backwaters\n"
"Duration of visit: 30 Mins - 1 Hr\n"
"Timings: Boating: 8.30 AM - 6.30 PM\n"
"Entry Fee: Boating: Rs. 20 for Pedal Boat & Rs. 5 for Row Boat (For 30 min)\n"
"At a distance of 1 km from Saputara Bus Station, Saputara Lake is a beautiful lake situated at the heart of Saputara hill station in Gujarat. It is considered one of the most famous picnic spots for the locals and among the most scenic",
),
),
Itinerary(
day: "2",
place: Place(
name: "Lake garden",
details: "Park : Duration of visit: 30 Mins\n"
"Timings: 9 AM - 8 PM\n"
"Entry Fee: Free\n"
"At a distance of 1 km from Saputara Bus Station and 1.5 km from Saputara Lake Boating Point, Lake Garden is a beautiful garden in Saputara, the lone hill station of Gujarat. Also called as Lake View Garden, it is an ideal picnic spot and also one of the prime places to visit in Saputara\n",
),
),
Itinerary(
day: "3",
place: Place(
name: "Step garden",
details: " Park : Duration of visit: 30 Mins\n"
"Timings: 8 AM - 12 PM & 3 PM - 7 PM, Closed on Tuesday\n"
"Entry Fee: Rs. 10 for Person\n"
"At a distance of 1 km from Saputara Bus Station, Step Garden is a laid out garden located in Saputara, only hill station of Gujarat. Situated on the Table Land Road, it is one of the best maintained gardens in Saputara and among the peaceful places to visit in Saputara.",
),
),
],
), // saputara
Package(
name: "Manali",
photo: "assets/images/tour_images/manali1.jpg",
price: "22824",
duration: "5D-4N",
transport: Transport(
by: "Plane",
toSource: "Ahmedabad",
fromDestination: "Manali",
pickupTime: "8:30 am",
dropTime: "11:30 pm",
),
fromDate: "January 14,2024",
toDate: "January 19,2024",
included: "meals, transportation, sightseeing, accommodation, activities",
itinerary: [
Itinerary(
day: "1",
place: Place(
name: "Delhi to Manali",
details: "Arrival, transfer, stay included\n"
"As per your itinerary, your trip starts with a road-trip from Delhi to Manali. On your way, you will be delighted to see the various sightseeing places such as Sundernagar Lake, Mini Vaishno Devi Temple, Pandoh Dam, etc. Upon arrival in Manali, our driver will drive you to the hotel and complete your check-in formalities. After check-in, have dinner and relax for an overnight stay at the hotel.",
),
),
Itinerary(
day: "2",
place: Place(
name: "Manali: Sightseeing",
details: "local sightseeing, manali market\n"
"After breakfast, get ready for your half day city tour of Manali. The city tour starts with the visit to holy Hadimba Temple, a wooden temple located in the middle of Van Vihar",
),
),
Itinerary(
day: "3",
place: Place(
name: "Solang Valley, Rohtang Pass, Day Tour",
details:
"After breakfast, get ready for a drive to Rohtang Pass. Rohtang Pass connects Manali to Lahaul and Spiti district and is known to be a high mountain pass usually covered with snow. Enjoy snow activities at the pass such as snow scooter ride, skiing, and horse riding",
),
),
],
), // manali
Package(
name: "Mussoorie",
photo: "assets/images/tour_images/masoorie1.jpg",
price: "14999",
duration: "5D-4N",
transport: Transport(
by: "Train",
toSource: "Ahmedabad",
fromDestination: "Mussoorie",
pickupTime: "10:30 am",
dropTime: "12:30 pm",
),
fromDate: "December 07,2024",
toDate: "December 12,2024",
included: "meals, transportation, sightseeing, accommodation, activities",
itinerary: [
Itinerary(
day: "1",
place: Place(
name: "Train from Ahmedabad to Corbett",
details:
"Corbett, in the recent times, has become a favourite tourist haunt for its rich biodiversity. Established in 1936, it is known for being the oldest national park in the mainland Asia and first Tiger Reserve in the country. The park has varied landscapes – plain and mountainous, wet and dry, gentle and rugged, which is home to numerous animal and plant species. Among the most popular of Corbett’s wild residents are the Asiatic elephant and Bengal tiger. However, the area is equally famous for over 600 species of avifauna that makes Corbett one of the richest bird regions in the country",
),
),
Itinerary(
day: "2",
place: Place(
name: "Safari in Corbett National Park",
details:
"Mussoorie, located in the Indian state of Uttarakhand, gives a stunning view of the spectacular Himalayas. Renowned as the ‘Queen of Hills’, Mussoorie was the summer getaway for the British during their colonial rule in India. A climb to Lal Tibba, the highest point in town, enriches the soul with inner peace one misses in busy city lives. Be spellbound by the beauty of the gushing Kempty Falls and enjoy various activities. The Happy Valley is a place where HH Dalai Lama settled with fellow Tibetans and started first Tibetan school in 1960. Saint Mary’s church, is the oldest church in the Himalayan region, which is currently under renovation. Company Gardens, Gun Hill, Lake Misty, Jwala Devi Temple, Jawahar Aquarium, Jhari Pani and Bhatta falls are some of the other attractions in Mussoorie",
),
),
Itinerary(
day: "3",
place: Place(
name: "Drive from Corbett to Mussoorie",
details: "Check-out from the hotel and embark on a road journey to the spectacular town of Mussoorie"
"A lovely hill resort, Mussoorie is located on the foothills of the Garhwal Himalayan ranges in Uttarakhand. Blessed with mighty hills, pleasing climate and varied flora and fauna, this place is rightly known as 'Queen of Hill Stations'. A paradise for nature walkers and adventure lovers, Mussoorie offers a fantastic view of the Doon Valley"
"Upon arrival in Mussoorie, complete the check-in formalities at the hotel. Enjoy a comfortable overnight stay and planning about the next day",
),
),
],
), // mussoorie
Package(
name: "Saputara",
photo: "assets/images/tour_images/saputara1.jpg",
price: "3500",
duration: "3D-2N",
transport: Transport(
by: "Bus",
toSource: "Ahmedabad",
fromDestination: "Saputara",
pickupTime: "6:30 am",
dropTime: "9:30 pm",
),
fromDate: "August 23,2024",
toDate: "August 26,2024",
included: "meals, transportation, sightseeing, accommodation, activities",
itinerary: [
Itinerary(
day: "1",
place: Place(
name: "Saputara Lake",
details: " Lake & Backwaters\n"
"Duration of visit: 30 Mins - 1 Hr\n"
"Timings: Boating: 8.30 AM - 6.30 PM\n"
"Entry Fee: Boating: Rs. 20 for Pedal Boat & Rs. 5 for Row Boat (For 30 min)\n"
"At a distance of 1 km from Saputara Bus Station, Saputara Lake is a beautiful lake situated at the heart of Saputara hill station in Gujarat. It is considered one of the most famous picnic spots for the locals and among the most scenic",
),
),
Itinerary(
day: "2",
place: Place(
name: "Lake garden",
details: "Park : Duration of visit: 30 Mins\n"
"Timings: 9 AM - 8 PM\n"
"Entry Fee: Free\n"
"At a distance of 1 km from Saputara Bus Station and 1.5 km from Saputara Lake Boating Point, Lake Garden is a beautiful garden in Saputara, the lone hill station of Gujarat. Also called as Lake View Garden, it is an ideal picnic spot and also one of the prime places to visit in Saputara\n",
),
),
Itinerary(
day: "3",
place: Place(
name: "Step garden",
details: " Park : Duration of visit: 30 Mins\n"
"Timings: 8 AM - 12 PM & 3 PM - 7 PM, Closed on Tuesday\n"
"Entry Fee: Rs. 10 for Person\n"
"At a distance of 1 km from Saputara Bus Station, Step Garden is a laid out garden located in Saputara, only hill station of Gujarat. Situated on the Table Land Road, it is one of the best maintained gardens in Saputara and among the peaceful places to visit in Saputara.",
),
),
],
), // saputara
Package(
name: "Manali",
photo: "assets/images/tour_images/manali1.jpg",
price: "22824",
duration: "5D-4N",
transport: Transport(
by: "Plane",
toSource: "Ahmedabad",
fromDestination: "Manali",
pickupTime: "8:30 am",
dropTime: "11:30 pm",
),
fromDate: "January 14,2024",
toDate: "January 19,2024",
included: "meals, transportation, sightseeing, accommodation, activities",
itinerary: [
Itinerary(
day: "1",
place: Place(
name: "Delhi to Manali",
details: "Arrival, transfer, stay included\n"
"As per your itinerary, your trip starts with a road-trip from Delhi to Manali. On your way, you will be delighted to see the various sightseeing places such as Sundernagar Lake, Mini Vaishno Devi Temple, Pandoh Dam, etc. Upon arrival in Manali, our driver will drive you to the hotel and complete your check-in formalities. After check-in, have dinner and relax for an overnight stay at the hotel.",
),
),
Itinerary(
day: "2",
place: Place(
name: "Manali: Sightseeing",
details: "local sightseeing, manali market\n"
"After breakfast, get ready for your half day city tour of Manali. The city tour starts with the visit to holy Hadimba Temple, a wooden temple located in the middle of Van Vihar",
),
),
Itinerary(
day: "3",
place: Place(
name: "Solang Valley, Rohtang Pass, Day Tour",
details:
"After breakfast, get ready for a drive to Rohtang Pass. Rohtang Pass connects Manali to Lahaul and Spiti district and is known to be a high mountain pass usually covered with snow. Enjoy snow activities at the pass such as snow scooter ride, skiing, and horse riding",
),
),
],
), // manali
Package(
name: "Mussoorie",
photo: "assets/images/tour_images/masoorie1.jpg",
price: "14999",
duration: "5D-4N",
transport: Transport(
by: "Train",
toSource: "Ahmedabad",
fromDestination: "Mussoorie",
pickupTime: "10:30 am",
dropTime: "12:30 pm",
),
fromDate: "December 07,2024",
toDate: "December 12,2024",
included: "meals, transportation, sightseeing, accommodation, activities",
itinerary: [
Itinerary(
day: "1",
place: Place(
name: "Train from Ahmedabad to Corbett",
details:
"Corbett, in the recent times, has become a favourite tourist haunt for its rich biodiversity. Established in 1936, it is known for being the oldest national park in the mainland Asia and first Tiger Reserve in the country. The park has varied landscapes – plain and mountainous, wet and dry, gentle and rugged, which is home to numerous animal and plant species. Among the most popular of Corbett’s wild residents are the Asiatic elephant and Bengal tiger. However, the area is equally famous for over 600 species of avifauna that makes Corbett one of the richest bird regions in the country",
),
),
Itinerary(
day: "2",
place: Place(
name: "Safari in Corbett National Park",
details:
"Mussoorie, located in the Indian state of Uttarakhand, gives a stunning view of the spectacular Himalayas. Renowned as the ‘Queen of Hills’, Mussoorie was the summer getaway for the British during their colonial rule in India. A climb to Lal Tibba, the highest point in town, enriches the soul with inner peace one misses in busy city lives. Be spellbound by the beauty of the gushing Kempty Falls and enjoy various activities. The Happy Valley is a place where HH Dalai Lama settled with fellow Tibetans and started first Tibetan school in 1960. Saint Mary’s church, is the oldest church in the Himalayan region, which is currently under renovation. Company Gardens, Gun Hill, Lake Misty, Jwala Devi Temple, Jawahar Aquarium, Jhari Pani and Bhatta falls are some of the other attractions in Mussoorie",
),
),
Itinerary(
day: "3",
place: Place(
name: "Drive from Corbett to Mussoorie",
details: "Check-out from the hotel and embark on a road journey to the spectacular town of Mussoorie"
"A lovely hill resort, Mussoorie is located on the foothills of the Garhwal Himalayan ranges in Uttarakhand. Blessed with mighty hills, pleasing climate and varied flora and fauna, this place is rightly known as 'Queen of Hill Stations'. A paradise for nature walkers and adventure lovers, Mussoorie offers a fantastic view of the Doon Valley"
"Upon arrival in Mussoorie, complete the check-in formalities at the hotel. Enjoy a comfortable overnight stay and planning about the next day",
),
),
],
), // mussoorie
Package(
name: "Saputara",
photo: "assets/images/tour_images/saputara1.jpg",
price: "3500",
duration: "3D-2N",
transport: Transport(
by: "Bus",
toSource: "Ahmedabad",
fromDestination: "Saputara",
pickupTime: "6:30 am",
dropTime: "9:30 pm",
),
fromDate: "August 23,2024",
toDate: "August 26,2024",
included: "meals, transportation, sightseeing, accommodation, activities",
itinerary: [
Itinerary(
day: "1",
place: Place(
name: "Saputara Lake",
details: " Lake & Backwaters\n"
"Duration of visit: 30 Mins - 1 Hr\n"
"Timings: Boating: 8.30 AM - 6.30 PM\n"
"Entry Fee: Boating: Rs. 20 for Pedal Boat & Rs. 5 for Row Boat (For 30 min)\n"
"At a distance of 1 km from Saputara Bus Station, Saputara Lake is a beautiful lake situated at the heart of Saputara hill station in Gujarat. It is considered one of the most famous picnic spots for the locals and among the most scenic",
),
),
Itinerary(
day: "2",
place: Place(
name: "Lake garden",
details: "Park : Duration of visit: 30 Mins\n"
"Timings: 9 AM - 8 PM\n"
"Entry Fee: Free\n"
"At a distance of 1 km from Saputara Bus Station and 1.5 km from Saputara Lake Boating Point, Lake Garden is a beautiful garden in Saputara, the lone hill station of Gujarat. Also called as Lake View Garden, it is an ideal picnic spot and also one of the prime places to visit in Saputara\n",
),
),
Itinerary(
day: "3",
place: Place(
name: "Step garden",
details: " Park : Duration of visit: 30 Mins\n"
"Timings: 8 AM - 12 PM & 3 PM - 7 PM, Closed on Tuesday\n"
"Entry Fee: Rs. 10 for Person\n"
"At a distance of 1 km from Saputara Bus Station, Step Garden is a laid out garden located in Saputara, only hill station of Gujarat. Situated on the Table Land Road, it is one of the best maintained gardens in Saputara and among the peaceful places to visit in Saputara.",
),
),
],
), // saputara
Package(
name: "Manali",
photo: "assets/images/tour_images/manali1.jpg",
price: "22824",
duration: "5D-4N",
transport: Transport(
by: "Plane",
toSource: "Ahmedabad",
fromDestination: "Manali",
pickupTime: "8:30 am",
dropTime: "11:30 pm",
),
fromDate: "January 14,2024",
toDate: "January 19,2024",
included: "meals, transportation, sightseeing, accommodation, activities",
itinerary: [
Itinerary(
day: "1",
place: Place(
name: "Delhi to Manali",
details: "Arrival, transfer, stay included\n"
"As per your itinerary, your trip starts with a road-trip from Delhi to Manali. On your way, you will be delighted to see the various sightseeing places such as Sundernagar Lake, Mini Vaishno Devi Temple, Pandoh Dam, etc. Upon arrival in Manali, our driver will drive you to the hotel and complete your check-in formalities. After check-in, have dinner and relax for an overnight stay at the hotel.",
),
),
Itinerary(
day: "2",
place: Place(
name: "Manali: Sightseeing",
details: "local sightseeing, manali market\n"
"After breakfast, get ready for your half day city tour of Manali. The city tour starts with the visit to holy Hadimba Temple, a wooden temple located in the middle of Van Vihar",
),
),
Itinerary(
day: "3",
place: Place(
name: "Solang Valley, Rohtang Pass, Day Tour",
details:
"After breakfast, get ready for a drive to Rohtang Pass. Rohtang Pass connects Manali to Lahaul and Spiti district and is known to be a high mountain pass usually covered with snow. Enjoy snow activities at the pass such as snow scooter ride, skiing, and horse riding",
),
),
],
), // manali
Package(
name: "Mussoorie",
photo: "assets/images/tour_images/masoorie1.jpg",
price: "14999",
duration: "5D-4N",
transport: Transport(
by: "Train",
toSource: "Ahmedabad",
fromDestination: "Mussoorie",
pickupTime: "10:30 am",
dropTime: "12:30 pm",
),
fromDate: "December 07,2024",
toDate: "December 12,2024",
included: "meals, transportation, sightseeing, accommodation, activities",
itinerary: [
Itinerary(
day: "1",
place: Place(
name: "Train from Ahmedabad to Corbett",
details:
"Corbett, in the recent times, has become a favourite tourist haunt for its rich biodiversity. Established in 1936, it is known for being the oldest national park in the mainland Asia and first Tiger Reserve in the country. The park has varied landscapes – plain and mountainous, wet and dry, gentle and rugged, which is home to numerous animal and plant species. Among the most popular of Corbett’s wild residents are the Asiatic elephant and Bengal tiger. However, the area is equally famous for over 600 species of avifauna that makes Corbett one of the richest bird regions in the country",
),
),
Itinerary(
day: "2",
place: Place(
name: "Safari in Corbett National Park",
details:
"Mussoorie, located in the Indian state of Uttarakhand, gives a stunning view of the spectacular Himalayas. Renowned as the ‘Queen of Hills’, Mussoorie was the summer getaway for the British during their colonial rule in India. A climb to Lal Tibba, the highest point in town, enriches the soul with inner peace one misses in busy city lives. Be spellbound by the beauty of the gushing Kempty Falls and enjoy various activities. The Happy Valley is a place where HH Dalai Lama settled with fellow Tibetans and started first Tibetan school in 1960. Saint Mary’s church, is the oldest church in the Himalayan region, which is currently under renovation. Company Gardens, Gun Hill, Lake Misty, Jwala Devi Temple, Jawahar Aquarium, Jhari Pani and Bhatta falls are some of the other attractions in Mussoorie",
),
),
Itinerary(
day: "3",
place: Place(
name: "Drive from Corbett to Mussoorie",
details: "Check-out from the hotel and embark on a road journey to the spectacular town of Mussoorie"
"A lovely hill resort, Mussoorie is located on the foothills of the Garhwal Himalayan ranges in Uttarakhand. Blessed with mighty hills, pleasing climate and varied flora and fauna, this place is rightly known as 'Queen of Hill Stations'. A paradise for nature walkers and adventure lovers, Mussoorie offers a fantastic view of the Doon Valley"
"Upon arrival in Mussoorie, complete the check-in formalities at the hotel. Enjoy a comfortable overnight stay and planning about the next day",
),
),
],
), // mussoorie
];
late List<TourCategoriesModel> tourCategoriesList = [
TourCategoriesModel(
name: "Weekends",
photo: "assets/images/tour_images/weekend.png",
packageList: trekkingPackageList,
),
TourCategoriesModel(
name: "Trekking",
photo: "assets/images/tour_images/trekking.png",
packageList: trekkingPackageList,
),
TourCategoriesModel(
name: "Beach",
photo: "assets/images/tour_images/beach.png",
packageList: trekkingPackageList,
),
TourCategoriesModel(
name: "Road Trip",
photo: "assets/images/tour_images/bike.png",
packageList: trekkingPackageList,
),
TourCategoriesModel(
name: "Holidays",
photo: "assets/images/tour_images/holidays.png",
packageList: trekkingPackageList,
),
];
}
| 0 |
mirrored_repositories/travee-flutter-app/lib | mirrored_repositories/travee-flutter-app/lib/model/itinerary_model.dart | class Itinerary {
String day;
Place place;
Itinerary({
required this.day,
required this.place,
});
}
class Place {
final String name;
final String details;
Place({
required this.name,
required this.details,
});
}
| 0 |
mirrored_repositories/travee-flutter-app/lib | mirrored_repositories/travee-flutter-app/lib/model/tour_categories_model.dart | import 'dart:core';
import 'package:travee/model/tour_package_model.dart';
class TourCategoriesModel {
final String name;
final String photo;
List<Package> packageList;
TourCategoriesModel({
required this.name,
required this.photo,
required this.packageList,
});
}
// Transportation: Airfare or ground transportation to/from the destination and for excursions.
// Accommodations: Hotel or lodging arrangements.
// Meals: Some or all meals depending on the package.
// Activities: Sightseeing tours, cultural experiences, and/or adventure activities.
// Tour guide: A guide to provide information and assistance throughout the trip.
// Entrance fees: Admission fees to museums, parks, or other attractions.
// Travel insurance: Optional insurance coverage for unexpected events.
// Itinerary: A detailed schedule of the trip, including dates, times, and locations.
// Other services: Additional services such as visa assistance, airport transfers, or language translation.
| 0 |
mirrored_repositories/travee-flutter-app/lib | mirrored_repositories/travee-flutter-app/lib/model/transport_model.dart | class Transport {
String by; // road, train or plan
String toSource; // source = pickup point
String fromDestination; // destination = drop point
String pickupTime;
String dropTime;
Transport({
required this.by,
required this.toSource,
required this.fromDestination,
required this.pickupTime,
required this.dropTime,
});
}
| 0 |
mirrored_repositories/travee-flutter-app/lib | mirrored_repositories/travee-flutter-app/lib/model/tour_package_model.dart | import 'package:travee/model/itinerary_model.dart';
import 'transport_model.dart';
class Package {
String name;
String photo;
String price;
String duration; // like 7 Days, 6 Nights
Transport transport;
String fromDate;
String toDate;
String included; // like meals, flight, Accommodations, activities,
List<Itinerary> itinerary; //A detailed schedule of the trip, including dates, times, and locations
Package({
required this.name,
required this.photo,
required this.price,
required this.duration,
required this.transport,
required this.fromDate,
required this.toDate,
required this.included,
required this.itinerary,
});
}
| 0 |
mirrored_repositories/travee-flutter-app/lib/screens | mirrored_repositories/travee-flutter-app/lib/screens/package_list_screen/package_list_screen.dart | import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_staggered_grid_view/flutter_staggered_grid_view.dart';
import 'package:get/get.dart';
import 'package:travee/resources/color.dart';
import 'package:travee/screens/package_list_screen/package_detail_screen.dart';
import '../../data/tour_category_data.dart';
import '../../model/tour_package_model.dart';
class PackageListScreen extends StatefulWidget {
final String packageName;
const PackageListScreen({
Key? key,
required this.packageName,
}) : super(key: key);
@override
State<PackageListScreen> createState() => _PackageListScreenState();
}
class _PackageListScreenState extends State<PackageListScreen> {
TourCategoryData tourCategoryData = TourCategoryData();
late List<Package> packageList;
@override
void initState() {
// SystemChrome.setEnabledSystemUIMode(SystemUiMode.manual, overlays: [SystemUiOverlay.top]);
// SystemChrome.setSystemUIOverlayStyle(
// SystemUiOverlayStyle(
// statusBarColor: kWhiteColor,
// statusBarIconBrightness: Brightness.dark,
// statusBarBrightness: Brightness.dark,
// systemNavigationBarDividerColor: Colors.transparent,
// systemNavigationBarColor: Colors.transparent,
// ),
// );
packageList = tourCategoryData.tourCategoriesList.first.packageList;
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
backgroundColor: kWhiteColor,
elevation: 0,
centerTitle: true,
titleSpacing: 0,
title: Text(
"Travee",
style: TextStyle(color: kBlackColor, fontSize: 22, fontWeight: FontWeight.bold),
),
leading: InkWell(
onTap: () => Get.back(),
child: Icon(
Icons.arrow_back_ios,
size: 22,
color: kBlackColor,
),
),
),
backgroundColor: kWhiteColor,
body: Container(
height: Get.height,
width: Get.width,
margin: const EdgeInsets.all(16),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
/// package name
Text(
widget.packageName,
style: TextStyle(color: kBlackColor, fontSize: 24, fontWeight: FontWeight.bold),
),
const SizedBox(height: 20),
/// search bar
Container(
padding: const EdgeInsets.only(top: 5, bottom: 5, right: 5),
decoration: BoxDecoration(
color: kWhiteColor,
borderRadius: BorderRadius.circular(14),
),
child: Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Expanded(
child: TextFormField(
keyboardType: TextInputType.text,
obscureText: false,
cursorColor: kPrimaryColor,
style: TextStyle(color: kBlackColor),
decoration: InputDecoration(
enabledBorder: OutlineInputBorder(
borderRadius: const BorderRadius.all(Radius.circular(20)),
borderSide: BorderSide(color: kGreyColor),
),
focusedBorder: OutlineInputBorder(
borderRadius: const BorderRadius.all(Radius.circular(20)),
borderSide: BorderSide(color: kGreyColor),
),
border: OutlineInputBorder(
borderRadius: const BorderRadius.all(Radius.circular(20)),
borderSide: BorderSide(color: kGreyColor),
),
labelStyle: TextStyle(color: kGreyColor),
labelText: "Search packages",
contentPadding: const EdgeInsets.symmetric(vertical: 10.0, horizontal: 10.0),
),
onFieldSubmitted: (value) {},
),
),
const SizedBox(width: 10),
Container(
padding: const EdgeInsets.all(10),
decoration: BoxDecoration(color: kPrimaryColor, borderRadius: BorderRadius.circular(14)),
child: Icon(
Icons.search,
color: kWhiteColor,
size: 26,
))
],
),
),
const SizedBox(height: 20),
/// package list view
Expanded(
child: StaggeredGridView.countBuilder(
padding: EdgeInsets.zero,
shrinkWrap: true,
physics: const AlwaysScrollableScrollPhysics(),
crossAxisCount: 2,
crossAxisSpacing: 10,
mainAxisSpacing: 10,
itemCount: packageList.length,
itemBuilder: (context, index) {
return GestureDetector(
onTap: () {
Get.to(PackageDetailScreen(
package: packageList[index],
heroTag: '',
));
},
child: Stack(
fit: StackFit.expand,
children: [
Container(
decoration: const BoxDecoration(
color: Colors.transparent, borderRadius: BorderRadius.all(Radius.circular(15))),
child: ClipRRect(
borderRadius: const BorderRadius.all(Radius.circular(15)),
child: Image.asset(packageList[index].photo, fit: BoxFit.cover),
),
),
Container(
padding: const EdgeInsets.all(10),
decoration: BoxDecoration(
color: kBlackColor.withOpacity(0.25),
borderRadius: const BorderRadius.all(Radius.circular(15))),
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
/// dummy row
Row(
children: const [Text(" ")],
),
/// package name
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
packageList[index].name,
style: TextStyle(color: kWhiteColor, fontSize: 20, fontWeight: FontWeight.bold),
)
],
),
/// dummy row
Row(
children: const [Text(" ")],
),
],
),
)
],
),
);
},
staggeredTileBuilder: (index) {
return StaggeredTile.count(1, index.isEven ? 1.2 : 1.8);
},
),
),
],
),
),
);
}
}
| 0 |
mirrored_repositories/travee-flutter-app/lib/screens | mirrored_repositories/travee-flutter-app/lib/screens/package_list_screen/package_detail_screen.dart | import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'package:travee/model/tour_package_model.dart';
import 'package:travee/resources/color.dart';
class PackageDetailScreen extends StatefulWidget {
final Package package;
final String heroTag;
const PackageDetailScreen({Key? key, required this.package, required this.heroTag}) : super(key: key);
@override
State<PackageDetailScreen> createState() => _PackageDetailScreenState();
}
class _PackageDetailScreenState extends State<PackageDetailScreen> {
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: kBackgroundColor,
body: Column(
children: [
/// package name price & duration
Hero(
tag: widget.heroTag,
child: Container(
margin: const EdgeInsets.only(bottom: 10),
decoration: BoxDecoration(
image: DecorationImage(image: AssetImage(widget.package.photo), fit: BoxFit.cover),
borderRadius: BorderRadius.circular(30),
),
child: Container(
height: Get.height / 3.5,
width: Get.width,
padding: const EdgeInsets.all(20),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(30),
gradient: LinearGradient(
begin: Alignment.topCenter,
end: Alignment.bottomCenter,
colors: [
Colors.transparent,
Colors.transparent,
kBlackColor,
],
)),
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
GestureDetector(
onTap: () => Get.back(),
child: Container(
height: 48,
width: 48,
margin: const EdgeInsets.symmetric(vertical: 30),
padding: const EdgeInsets.only(left: 5),
decoration:
BoxDecoration(color: kBlackColor.withOpacity(0.5), borderRadius: BorderRadius.circular(48)),
child: Icon(
Icons.arrow_back_ios,
color: kWhiteColor,
size: 20,
),
),
),
Flexible(
flex: 5,
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.end,
children: [
Column(
mainAxisAlignment: MainAxisAlignment.end,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
widget.package.name,
style: TextStyle(color: kWhiteColor, fontSize: 20, fontWeight: FontWeight.bold),
),
const SizedBox(height: 10),
Text(
"\u{20B9} ${widget.package.price}",
style: TextStyle(color: kWhiteColor, fontSize: 16),
)
],
),
Text(
widget.package.duration,
style: TextStyle(color: kWhiteColor, fontSize: 16),
)
],
),
),
],
),
),
),
),
/// package transportation detail
Expanded(
child: SingleChildScrollView(
child: Column(
children: [
/// transportation
Container(
margin: const EdgeInsets.all(16),
padding: const EdgeInsets.all(16),
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(20),
color: kWhiteColor,
),
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
//from source
Column(
children: [
Text(
widget.package.transport.toSource,
style: TextStyle(color: kBlackColor, fontSize: 18),
),
const SizedBox(height: 5),
Text(
widget.package.transport.pickupTime,
style: TextStyle(color: kGreyColor, fontSize: 14),
),
],
),
// vehicle image
Image.asset(
widget.package.transport.by == "Bus"
? "assets/images/bus.png"
: widget.package.transport.by == "Plane"
? "assets/images/plane.png"
: "assets/images/train.png",
color: widget.package.transport.by == "Bus"
? Colors.red
: widget.package.transport.by == "Plane"
? Colors.blueAccent
: Colors.purpleAccent,
height: 26,
width: 26,
),
//to destination
Column(
children: [
Text(
widget.package.transport.fromDestination,
style: TextStyle(color: kBlackColor, fontSize: 18),
),
const SizedBox(height: 5),
Text(
widget.package.transport.dropTime,
style: TextStyle(color: kGreyColor, fontSize: 14),
),
],
)
],
),
const SizedBox(height: 30),
Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
//from date
Text(
widget.package.fromDate,
style: TextStyle(color: kGreyColor, fontSize: 14),
),
//to date
Text(
widget.package.toDate,
style: TextStyle(color: kGreyColor, fontSize: 14),
)
],
),
const SizedBox(height: 20),
Text(
"Included : ${widget.package.included}",
style: TextStyle(color: kGreyColor, fontSize: 14),
),
],
),
),
/// itinerary
Container(
margin: const EdgeInsets.all(16),
padding: const EdgeInsets.all(16),
width: Get.width,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(20),
color: kWhiteColor,
),
child: Column(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
"Itinerary",
style: TextStyle(color: kBlackColor, fontSize: 18, fontWeight: FontWeight.bold),
),
const SizedBox(height: 20),
ListView.builder(
padding: EdgeInsets.zero,
shrinkWrap: true,
physics: const NeverScrollableScrollPhysics(),
itemCount: widget.package.itinerary.length,
itemBuilder: (context, index) {
return Container(
padding: const EdgeInsets.all(10),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
"Day ${widget.package.itinerary[index].day}",
style: TextStyle(color: kBlackColor, fontSize: 14, fontWeight: FontWeight.bold),
),
const SizedBox(height: 10),
Text(
widget.package.itinerary[index].place.name,
style: TextStyle(
color: kBlackColor,
fontSize: 14,
),
),
const SizedBox(height: 10),
Text(
widget.package.itinerary[index].place.details,
style: TextStyle(
color: kBlackColor,
fontSize: 14,
),
),
],
),
);
},
),
],
),
),
],
),
),
),
InkWell(
//onTap: () => Get.to(BlurSlider()),
child: Container(
width: Get.width,
height: 48,
margin: const EdgeInsets.only(left: 16, right: 16, top: 20, bottom: 30),
decoration: BoxDecoration(color: kPrimaryColor, borderRadius: BorderRadius.circular(14)),
child: Center(
child: Text(
"Book Now",
textAlign: TextAlign.center,
style: TextStyle(color: kWhiteColor, fontSize: 18, fontWeight: FontWeight.bold),
),
),
),
)
],
),
);
}
}
| 0 |
mirrored_repositories/travee-flutter-app/lib/screens | mirrored_repositories/travee-flutter-app/lib/screens/mainscreen/main_screen1.dart | import 'package:action_slider/action_slider.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:get/get.dart';
import 'package:travee/resources/color.dart';
import 'main_screen2.dart';
class MainScreen1 extends StatefulWidget {
const MainScreen1({super.key});
@override
State<MainScreen1> createState() => _MainScreen1State();
}
class _MainScreen1State extends State<MainScreen1> {
@override
void initState() {
SystemChrome.setEnabledSystemUIMode(SystemUiMode.manual, overlays: [SystemUiOverlay.top]);
SystemChrome.setSystemUIOverlayStyle(
const SystemUiOverlayStyle(
statusBarColor: Colors.transparent,
statusBarIconBrightness: Brightness.dark,
statusBarBrightness: Brightness.dark,
systemNavigationBarDividerColor: Colors.transparent,
systemNavigationBarColor: Colors.transparent,
),
);
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
extendBody: true,
body: Stack(
children: [
///background image
Container(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
decoration: const BoxDecoration(
image: DecorationImage(
fit: BoxFit.cover,
image: AssetImage("assets/images/road.jpg"),
),
),
),
/// content on image
Container(
padding: const EdgeInsets.symmetric(horizontal: 20, vertical: 70),
child: Column(
children: [
Expanded(child: Container()),
/// title
const Text(
"Explore the world",
style: TextStyle(color: Colors.white, fontSize: 30, fontWeight: FontWeight.bold),
),
Expanded(child: Container()),
ActionSlider.standard(
width: Get.width / 2,
toggleColor: kPrimaryColor,
icon: const Icon(
Icons.navigate_next,
color: Colors.white,
size: 25,
),
loadingIcon: const CircularProgressIndicator(color: Colors.white, strokeWidth: 1),
successIcon: const Icon(
Icons.check,
color: Colors.white,
size: 25,
),
backgroundColor: Colors.black.withOpacity(.4),
action: (controller) async {
controller.loading(); //starts loading animation
await Future.delayed(const Duration(seconds: 1));
controller.success(); //starts success animation
await Future.delayed(const Duration(seconds: 1));
Get.offAll(
const MainScreen2(),
transition: Transition.zoom,
duration: const Duration(milliseconds: 500),
);
},
child: const Padding(
padding: EdgeInsets.only(left: 20),
child: Text(
'Explore',
textAlign: TextAlign.end,
style: TextStyle(color: Colors.white, fontSize: 16, fontWeight: FontWeight.bold),
),
),
),
],
),
)
],
),
);
}
}
| 0 |
mirrored_repositories/travee-flutter-app/lib/screens | mirrored_repositories/travee-flutter-app/lib/screens/mainscreen/main_screen2.dart | import 'package:action_slider/action_slider.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:get/get.dart';
import 'package:travee/resources/color.dart';
import 'main_screen3.dart';
class MainScreen2 extends StatefulWidget {
const MainScreen2({super.key});
@override
State<MainScreen2> createState() => _MainScreen2State();
}
class _MainScreen2State extends State<MainScreen2> {
@override
void initState() {
SystemChrome.setEnabledSystemUIMode(SystemUiMode.manual, overlays: [SystemUiOverlay.top]);
SystemChrome.setSystemUIOverlayStyle(
const SystemUiOverlayStyle(
statusBarColor: Colors.transparent,
statusBarIconBrightness: Brightness.light,
statusBarBrightness: Brightness.light,
systemNavigationBarDividerColor: Colors.transparent,
systemNavigationBarColor: Colors.transparent,
),
);
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Stack(
children: [
///background image
Container(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
decoration: const BoxDecoration(
image: DecorationImage(
fit: BoxFit.cover,
image: AssetImage("assets/images/boy_girl_travel.jpg"),
),
),
),
/// content on image
Container(
padding: const EdgeInsets.symmetric(horizontal: 20, vertical: 70),
child: Column(
children: [
/// travel map
Image.asset(
"assets/images/travel_map.png",
width: Get.width,
height: Get.width / 3,
),
Expanded(child: Container()),
/// title
const Text(
"Find your travel mate",
//textAlign: TextAlign.center,
style: TextStyle(color: Colors.white, fontSize: 30, fontWeight: FontWeight.bold),
),
const SizedBox(height: 30),
FloatingActionButton(
onPressed: () {
Get.offAll(
const MainScreen3(),
transition: Transition.rightToLeft,
duration: const Duration(milliseconds: 500),
);
},
backgroundColor: kPrimaryColor,
elevation: 8,
child: const Icon(Icons.navigate_next),
),
],
),
)
],
),
);
}
}
| 0 |
mirrored_repositories/travee-flutter-app/lib/screens | mirrored_repositories/travee-flutter-app/lib/screens/mainscreen/main_screen3.dart | import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:get/get.dart';
import 'package:travee/resources/color.dart';
import '../homescreen/home_screen.dart';
class MainScreen3 extends StatefulWidget {
const MainScreen3({super.key});
@override
State<MainScreen3> createState() => _MainScreen3State();
}
class _MainScreen3State extends State<MainScreen3> {
@override
void initState() {
SystemChrome.setEnabledSystemUIMode(SystemUiMode.manual, overlays: [SystemUiOverlay.top]);
SystemChrome.setSystemUIOverlayStyle(
const SystemUiOverlayStyle(
statusBarColor: Colors.transparent,
statusBarIconBrightness: Brightness.light,
statusBarBrightness: Brightness.light,
systemNavigationBarDividerColor: Colors.transparent,
systemNavigationBarColor: Colors.transparent,
),
);
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Stack(
children: [
///background image
Container(
height: MediaQuery.of(context).size.height,
width: MediaQuery.of(context).size.width,
decoration: const BoxDecoration(
image: DecorationImage(
fit: BoxFit.cover,
image: AssetImage("assets/images/boat.jpg"),
),
),
),
/// content on image
Container(
padding: const EdgeInsets.symmetric(horizontal: 20, vertical: 70),
child: Column(
children: [
Expanded(child: Container()),
/// title
const Text(
"Plan your trip",
//textAlign: TextAlign.center,
style: TextStyle(color: Colors.white, fontSize: 30, fontWeight: FontWeight.bold),
),
const SizedBox(height: 50),
FloatingActionButton(
onPressed: () {
Get.offAll(
const HomeScreen(),
transition: Transition.rightToLeft,
duration: const Duration(milliseconds: 500),
);
},
backgroundColor: kPrimaryColor,
elevation: 8,
child: const Icon(Icons.navigate_next),
),
],
),
)
],
),
);
}
}
| 0 |
mirrored_repositories/travee-flutter-app/lib/screens | mirrored_repositories/travee-flutter-app/lib/screens/chatbot/chat_bot_screen.dart | import 'package:chat_gpt_sdk/chat_gpt_sdk.dart';
import 'package:flutter/material.dart';
import 'package:flutter_chat_bubble/chat_bubble.dart';
import 'package:get/get.dart';
import 'package:lottie/lottie.dart';
import '../../resources/color.dart';
class ChatModel {
String type; //question or answer
String text;
ChatModel({
required this.type,
required this.text,
});
}
class ChatBotScreen extends StatefulWidget {
const ChatBotScreen({Key? key}) : super(key: key);
@override
State<ChatBotScreen> createState() => _ChatBotScreenState();
}
class _ChatBotScreenState extends State<ChatBotScreen> {
List<ChatModel> chatList = [];
var textController = TextEditingController();
bool isChatLoading = false;
@override
void initState() {
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
child: Container(
height: Get.height,
width: Get.width,
padding: const EdgeInsets.all(10),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
/// back arrow & title
Row(
children: [
IconButton(
onPressed: () => Get.back(),
icon: const Icon(Icons.arrow_back_ios),
),
const Text(
"Search your Query",
style: TextStyle(fontSize: 24, fontWeight: FontWeight.bold, color: Colors.black),
)
],
),
/// chat container
Expanded(
child: Container(
width: Get.width,
margin: const EdgeInsets.symmetric(vertical: 20),
child: ListView.builder(
padding: EdgeInsets.zero,
reverse: true,
shrinkWrap: true,
controller: ScrollController(keepScrollOffset: true),
physics: const AlwaysScrollableScrollPhysics(),
itemCount: chatList.length,
itemBuilder: (context, index) {
final reversedIndex = chatList.length - 1 - index;
final item = chatList[reversedIndex];
return Align(
alignment: item.type == "a" ? Alignment.centerLeft : Alignment.centerRight,
child: item.type == "q"
? ChatBubble(
clipper: ChatBubbleClipper2(type: BubbleType.sendBubble),
alignment: Alignment.topRight,
margin: const EdgeInsets.only(top: 20),
backGroundColor: kPrimaryColor,
child: Container(
constraints: BoxConstraints(
maxWidth: MediaQuery.of(context).size.width * 0.7,
),
child: Text(
item.text,
style: const TextStyle(color: Colors.white, fontSize: 16),
),
),
)
: item.text.isEmpty
? Container(
margin: const EdgeInsets.only(top: 20),
child: Lottie.asset(
"assets/images/chat_anim.json",
width: 38,
height: 38,
fit: BoxFit.cover,
),
)
: ChatBubble(
clipper: ChatBubbleClipper2(type: BubbleType.receiverBubble),
backGroundColor: const Color(0xffE7E7ED),
margin: const EdgeInsets.only(top: 20),
child: Container(
constraints: BoxConstraints(
maxWidth: MediaQuery.of(context).size.width * 0.7,
),
child: Text(
item.text,
style: const TextStyle(color: Colors.black, fontSize: 16),
),
),
),
);
},
),
),
),
/// search bar
Container(
padding: const EdgeInsets.only(top: 5, bottom: 5, right: 5),
decoration: BoxDecoration(
color: kWhiteColor,
borderRadius: BorderRadius.circular(14),
),
child: Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Expanded(
child: TextFormField(
controller: textController,
keyboardType: TextInputType.text,
obscureText: false,
cursorColor: kPrimaryColor,
style: TextStyle(color: kBlackColor),
decoration: InputDecoration(
enabledBorder: OutlineInputBorder(
borderRadius: const BorderRadius.all(Radius.circular(20)),
borderSide: BorderSide(color: kGreyColor),
),
focusedBorder: OutlineInputBorder(
borderRadius: const BorderRadius.all(Radius.circular(20)),
borderSide: BorderSide(color: kGreyColor),
),
border: OutlineInputBorder(
borderRadius: const BorderRadius.all(Radius.circular(20)),
borderSide: BorderSide(color: kGreyColor),
),
labelStyle: TextStyle(color: kGreyColor),
labelText: "Search places",
contentPadding: const EdgeInsets.symmetric(vertical: 10.0, horizontal: 10.0),
),
onFieldSubmitted: (value) {},
),
),
const SizedBox(width: 10),
InkWell(
onTap: () {
setState(() {
chatList.add(ChatModel(type: "q", text: textController.text));
chatGptModel(textController.text);
// getChats(textController.text);
//getAnswer(textController.text);
});
},
child: Container(
padding: const EdgeInsets.all(10),
decoration: BoxDecoration(color: kPrimaryColor, borderRadius: BorderRadius.circular(14)),
child: Icon(
Icons.search,
color: kWhiteColor,
size: 26,
)),
)
],
),
)
],
),
),
),
);
}
void chatGptModel(String question) async {
chatList.add(ChatModel(type: "a", text: ""));
setState(() {});
String answer = "";
String gptApiKey = "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";
final openAI = OpenAI.instance
.build(token: gptApiKey, baseOption: HttpSetup(receiveTimeout: const Duration(minutes: 30)), enableLog: true);
final request = ChatCompleteText(messages: [
Map.of({"role": "user", "content": question})
], maxToken: 200, model: GptTurboChatModel());
final response = await openAI.onChatCompletion(request: request);
for (var element in response!.choices) {
answer += element.message!.content;
print("data -> ${element.message?.content}");
}
chatList.removeLast();
chatList.add(ChatModel(type: "a", text: answer));
setState(() {});
}
Future<void> getChats(String question) async {
String answer = "";
String gptApiKey = "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";
final openAI = OpenAI.instance
.build(token: gptApiKey, baseOption: HttpSetup(receiveTimeout: const Duration(minutes: 30)), enableLog: true);
final request = CompleteText(prompt: question, maxTokens: 200, model: TextDavinci3Model());
openAI.onCompletionSSE(request: request).listen((it) {
debugPrint("complete text model================>${it.choices.last.text}");
});
}
/* void getAnswer(String question) async {
String answer = "";
List<OpenAIModelModel> models = await OpenAI.instance.model.list();
OpenAIModelModel firstModel = models.first;
*/ /*models.forEach((element) {
print("model ----> ${element.id}");
});
*/ /*
OpenAICompletionModel completionModel = await OpenAI.instance.completion.create(
//model: "text-davinci-003", // use ada model if this limit is over
model: "gpt-3.5-turbo-0301",
prompt: question,
maxTokens: 100,
temperature: 0.5,
topP: 1,
);
completionModel.choices.forEach((element) {
answer += element.text;
});
log("chat model :::::::::::::: $answer");
chatList.add(ChatModel(type: "a", text: answer));
setState(() {});
// completionModel.listen((event) {
// final firstCompletionChoice = event.choices.first;
// answer += firstCompletionChoice.text;
// });
}*/
}
| 0 |
mirrored_repositories/travee-flutter-app/lib/screens | mirrored_repositories/travee-flutter-app/lib/screens/homescreen/user_screen.dart | import 'package:flutter/material.dart';
class UserPackagesScreen extends StatefulWidget {
const UserPackagesScreen({Key? key}) : super(key: key);
@override
State<UserPackagesScreen> createState() => _UserPackagesScreenState();
}
class _UserPackagesScreenState extends State<UserPackagesScreen> {
@override
Widget build(BuildContext context) {
return const Center(
child: Text("user package screen"),
);
}
}
| 0 |
mirrored_repositories/travee-flutter-app/lib/screens | mirrored_repositories/travee-flutter-app/lib/screens/homescreen/home_screen.dart | import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:get/get.dart';
import 'package:travee/data/tour_category_data.dart';
import 'package:travee/screens/chatbot/chat_bot_screen.dart';
import 'package:travee/screens/package_list_screen/package_detail_screen.dart';
import 'package:travee/screens/package_list_screen/package_list_screen.dart';
import '../../model/tour_package_model.dart';
import '../../resources/color.dart';
class HomeScreen extends StatefulWidget {
const HomeScreen({Key? key}) : super(key: key);
@override
State<HomeScreen> createState() => _HomeScreenState();
}
class _HomeScreenState extends State<HomeScreen> {
TourCategoryData tourCategoryData = TourCategoryData();
late List<Package> packageList;
List<String> imageList = [
'https://cdn.pixabay.com/photo/2019/03/15/09/49/girl-4056684_960_720.jpg',
'https://cdn.pixabay.com/photo/2020/12/15/16/25/clock-5834193__340.jpg',
'https://cdn.pixabay.com/photo/2020/09/18/19/31/laptop-5582775_960_720.jpg',
'https://media.istockphoto.com/photos/woman-kayaking-in-fjord-in-norway-picture-id1059380230?b=1&k=6&m=1059380230&s=170667a&w=0&h=kA_A_XrhZJjw2bo5jIJ7089-VktFK0h0I4OWDqaac0c=',
'https://cdn.pixabay.com/photo/2019/11/05/00/53/cellular-4602489_960_720.jpg',
'https://cdn.pixabay.com/photo/2017/02/12/10/29/christmas-2059698_960_720.jpg',
'https://cdn.pixabay.com/photo/2020/01/29/17/09/snowboard-4803050_960_720.jpg',
'https://cdn.pixabay.com/photo/2020/02/06/20/01/university-library-4825366_960_720.jpg',
'https://cdn.pixabay.com/photo/2020/11/22/17/28/cat-5767334_960_720.jpg',
'https://cdn.pixabay.com/photo/2020/12/13/16/22/snow-5828736_960_720.jpg',
'https://cdn.pixabay.com/photo/2020/12/09/09/27/women-5816861_960_720.jpg',
];
@override
void initState() {
SystemChrome.setEnabledSystemUIMode(SystemUiMode.manual, overlays: [SystemUiOverlay.top]);
SystemChrome.setSystemUIOverlayStyle(
const SystemUiOverlayStyle(
statusBarColor: Colors.transparent,
statusBarIconBrightness: Brightness.dark,
statusBarBrightness: Brightness.dark,
systemNavigationBarDividerColor: Colors.transparent,
systemNavigationBarColor: Colors.transparent,
),
);
packageList = tourCategoryData.tourCategoriesList.first.packageList;
super.initState();
}
@override
Widget build(BuildContext context) {
return SafeArea(
child: Scaffold(
resizeToAvoidBottomInset: false,
backgroundColor: kWhiteColor,
body: Container(
height: Get.height,
width: Get.width,
padding: const EdgeInsets.symmetric(horizontal: 16),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
/// app bar
SizedBox(
height: 68,
width: Get.width,
child: Row(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
/// user icon
Container(
height: 48,
width: 48,
padding: const EdgeInsets.all(5),
decoration: BoxDecoration(borderRadius: BorderRadius.circular(50), color: kBackgroundColor),
child: Image.asset("assets/images/man.png", fit: BoxFit.cover),
),
const SizedBox(width: 10),
/// username
const Text(
"Hi, John",
style: TextStyle(fontSize: 20, fontWeight: FontWeight.w500, color: Colors.black),
),
Expanded(child: Container()),
/// notification icon
Container(
height: 48,
width: 48,
padding: const EdgeInsets.all(15),
decoration: BoxDecoration(borderRadius: BorderRadius.circular(50), color: kBackgroundColor),
child: Image.asset("assets/images/notification.png", height: 20, width: 20, fit: BoxFit.cover),
)
],
),
),
const SizedBox(height: 20),
/// search places
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
const Text(
"Leading you\nFrom here to beyond",
style: TextStyle(fontSize: 24, fontWeight: FontWeight.bold, color: Colors.black),
),
const SizedBox(height: 20),
//search bar
Container(
padding: const EdgeInsets.only(top: 5, bottom: 5, right: 5),
decoration: BoxDecoration(
color: kWhiteColor,
borderRadius: BorderRadius.circular(14),
),
child: Row(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Expanded(
child: TextFormField(
keyboardType: TextInputType.text,
obscureText: false,
cursorColor: kPrimaryColor,
style: TextStyle(color: kBlackColor),
decoration: InputDecoration(
enabledBorder: OutlineInputBorder(
borderRadius: const BorderRadius.all(Radius.circular(20)),
borderSide: BorderSide(color: kGreyColor),
),
focusedBorder: OutlineInputBorder(
borderRadius: const BorderRadius.all(Radius.circular(20)),
borderSide: BorderSide(color: kGreyColor),
),
border: OutlineInputBorder(
borderRadius: const BorderRadius.all(Radius.circular(20)),
borderSide: BorderSide(color: kGreyColor),
),
labelStyle: TextStyle(color: kGreyColor),
labelText: "Search places",
contentPadding: const EdgeInsets.symmetric(vertical: 10.0, horizontal: 10.0),
),
onFieldSubmitted: (value) {},
),
),
const SizedBox(width: 10),
Container(
padding: const EdgeInsets.all(10),
decoration: BoxDecoration(color: kPrimaryColor, borderRadius: BorderRadius.circular(14)),
child: Icon(
Icons.search,
color: kWhiteColor,
size: 26,
))
],
),
),
],
),
/// tour categories
Container(
width: Get.width,
margin: const EdgeInsets.symmetric(vertical: 20),
padding: const EdgeInsets.symmetric(vertical: 8),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
"Categories",
style: TextStyle(color: kBlackColor, fontSize: 20, fontWeight: FontWeight.bold),
),
const SizedBox(height: 10),
SizedBox(
height: 58,
child: ListView.builder(
padding: EdgeInsets.zero,
shrinkWrap: true,
physics: const AlwaysScrollableScrollPhysics(),
scrollDirection: Axis.horizontal,
itemCount: tourCategoryData.tourCategoriesList.length,
itemBuilder: (context, index) {
return GestureDetector(
onTap: () {
Get.to(PackageListScreen(
packageName: tourCategoryData.tourCategoriesList[index].name,
));
},
child: Container(
margin: const EdgeInsets.only(right: 10),
padding: const EdgeInsets.all(8),
decoration: BoxDecoration(
color: kBackgroundColor,
borderRadius: BorderRadius.circular(10),
),
//color: Colors.redAccent,
child: Row(
children: [
Container(
padding: const EdgeInsets.all(5),
decoration: BoxDecoration(
color: kWhiteColor,
borderRadius: BorderRadius.circular(10),
),
child: Image.asset(
tourCategoryData.tourCategoriesList[index].photo,
height: 38,
width: 38,
fit: BoxFit.cover,
),
),
const SizedBox(width: 10),
Text(
tourCategoryData.tourCategoriesList[index].name,
style: TextStyle(color: kBlackColor, fontSize: 14, fontWeight: FontWeight.bold),
),
],
),
),
);
},
),
),
],
),
),
///top picks
Flexible(
child: Container(
margin: const EdgeInsets.only(bottom: 20),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
"Top picks",
style: TextStyle(color: kBlackColor, fontSize: 20, fontWeight: FontWeight.bold),
),
const SizedBox(height: 20),
Flexible(
child: SizedBox(
height: Get.height / 3,
child: ListView.builder(
padding: EdgeInsets.zero,
shrinkWrap: true,
physics: const AlwaysScrollableScrollPhysics(),
scrollDirection: Axis.horizontal,
itemCount: packageList.length,
itemBuilder: (context, index) {
return Hero(
tag: "packageImage$index",
transitionOnUserGestures: true,
child: GestureDetector(
onTap: () => Get.to(
PackageDetailScreen(
package: packageList[index],
heroTag: 'packageImage$index',
),
transition: Transition.native),
child: Stack(
// alignment: Alignment.center,
children: [
Container(
width: Get.width / 2.5,
margin: const EdgeInsets.only(right: 10),
padding: const EdgeInsets.all(5),
decoration: BoxDecoration(
color: kWhiteColor,
image: DecorationImage(
image: AssetImage(
packageList[index].photo,
),
fit: BoxFit.cover,
),
borderRadius: BorderRadius.circular(10),
),
),
Container(
width: Get.width / 2.5,
margin: const EdgeInsets.only(right: 10),
padding: const EdgeInsets.all(5),
decoration: BoxDecoration(
color: kBlackColor.withOpacity(0.25),
borderRadius: BorderRadius.circular(10),
),
child: Center(
child: Text(
packageList[index].name,
style: TextStyle(
color: kWhiteColor, fontSize: 20, fontWeight: FontWeight.bold),
),
),
),
],
),
),
);
},
),
),
),
],
),
),
),
],
),
),
floatingActionButton: FloatingActionButton(
elevation: 3,
backgroundColor: kPrimaryColor,
onPressed: () => Get.to(const ChatBotScreen()),
child: const Icon(Icons.chat),
),
floatingActionButtonLocation: FloatingActionButtonLocation.endFloat,
),
);
}
}
| 0 |
mirrored_repositories/travee-flutter-app/lib/screens | mirrored_repositories/travee-flutter-app/lib/screens/homescreen/trending_packages_screen.dart | import 'package:flutter/material.dart';
class TrendingPackagesScreen extends StatefulWidget {
const TrendingPackagesScreen({Key? key}) : super(key: key);
@override
State<TrendingPackagesScreen> createState() => _TrendingPackagesScreenState();
}
class _TrendingPackagesScreenState extends State<TrendingPackagesScreen> {
@override
Widget build(BuildContext context) {
return const Center(
child: Text("trending package screen"),
);
}
}
| 0 |
mirrored_repositories/travee-flutter-app/lib/screens | mirrored_repositories/travee-flutter-app/lib/screens/homescreen/bottom_nav_bar.dart | import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:persistent_bottom_nav_bar/persistent_tab_view.dart';
import 'package:travee/resources/color.dart';
import 'package:travee/screens/homescreen/home_screen.dart';
import 'package:travee/screens/homescreen/liked_packages_screen.dart';
import 'package:travee/screens/homescreen/trending_packages_screen.dart';
import 'package:travee/screens/homescreen/user_screen.dart';
class BottomNavBar extends StatefulWidget {
const BottomNavBar({Key? key}) : super(key: key);
@override
State<BottomNavBar> createState() => _BottomNavBarState();
}
class _BottomNavBarState extends State<BottomNavBar> {
late PersistentTabController _controller;
List<Widget> _buildScreens() {
return [
const HomeScreen(),
const TrendingPackagesScreen(),
const LikedPackagesScreen(),
const UserPackagesScreen(),
];
}
List<PersistentBottomNavBarItem> _navBarsItems() {
return [
PersistentBottomNavBarItem(
icon: Image.asset("assets/images/nav_bar_images/home.png", height: 26, width: 26, color: kWhiteColor),
inactiveIcon: Image.asset("assets/images/nav_bar_images/home.png", height: 26, width: 26, color: kPrimaryColor),
activeColorSecondary: kWhiteColor,
title: ("Home"),
activeColorPrimary: kPrimaryColor,
inactiveColorPrimary: CupertinoColors.systemGrey,
),
PersistentBottomNavBarItem(
icon: Image.asset("assets/images/nav_bar_images/trending.png", height: 26, width: 26, color: kWhiteColor),
inactiveIcon:
Image.asset("assets/images/nav_bar_images/trending.png", height: 26, width: 26, color: kPrimaryColor),
activeColorSecondary: kWhiteColor,
title: ("Trending"),
activeColorPrimary: kPrimaryColor,
inactiveColorPrimary: CupertinoColors.systemGrey,
),
PersistentBottomNavBarItem(
icon: Image.asset("assets/images/nav_bar_images/like.png", height: 26, width: 26, color: kWhiteColor),
inactiveIcon: Image.asset("assets/images/nav_bar_images/like.png", height: 26, width: 26, color: kPrimaryColor),
activeColorSecondary: kWhiteColor,
title: ("Like"),
activeColorPrimary: kPrimaryColor,
inactiveColorPrimary: CupertinoColors.systemGrey,
),
PersistentBottomNavBarItem(
icon: Image.asset("assets/images/nav_bar_images/user.png", height: 26, width: 26, color: kWhiteColor),
inactiveIcon: Image.asset("assets/images/nav_bar_images/user.png", height: 26, width: 26, color: kPrimaryColor),
activeColorSecondary: kWhiteColor,
title: ("User"),
activeColorPrimary: kPrimaryColor,
inactiveColorPrimary: CupertinoColors.systemGrey,
),
];
}
@override
void initState() {
SystemChrome.setEnabledSystemUIMode(SystemUiMode.manual, overlays: [SystemUiOverlay.top]);
SystemChrome.setSystemUIOverlayStyle(
SystemUiOverlayStyle(
statusBarColor: kBackgroundColor,
statusBarIconBrightness: Brightness.dark,
statusBarBrightness: Brightness.dark,
systemNavigationBarDividerColor: kBackgroundColor,
systemNavigationBarColor: kBackgroundColor,
),
);
_controller = PersistentTabController(initialIndex: 0);
super.initState();
}
@override
Widget build(BuildContext context) {
return Expanded(
child: PersistentTabView(
context,
controller: _controller,
screens: _buildScreens(),
items: _navBarsItems(),
margin: const EdgeInsets.only(bottom: 0),
padding: const NavBarPadding.all(0),
confineInSafeArea: true,
backgroundColor: kWhiteColor,
handleAndroidBackButtonPress: true,
resizeToAvoidBottomInset: true,
stateManagement: true,
hideNavigationBarWhenKeyboardShows: true,
decoration: NavBarDecoration(
borderRadius: BorderRadius.circular(10.0),
colorBehindNavBar: Colors.white,
),
popAllScreensOnTapOfSelectedTab: true,
popActionScreens: PopActionScreensType.all,
itemAnimationProperties: const ItemAnimationProperties(
// Navigation Bar's items animation properties.
duration: Duration(milliseconds: 200),
curve: Curves.ease,
),
screenTransitionAnimation: const ScreenTransitionAnimation(
// Screen transition animation on change of selected tab.
animateTabTransition: true,
curve: Curves.ease,
duration: Duration(milliseconds: 200),
),
navBarStyle: NavBarStyle.style10, // Choose the nav bar style with this property.
),
);
}
}
| 0 |
mirrored_repositories/travee-flutter-app/lib/screens | mirrored_repositories/travee-flutter-app/lib/screens/homescreen/liked_packages_screen.dart | import 'package:flutter/material.dart';
class LikedPackagesScreen extends StatefulWidget {
const LikedPackagesScreen({Key? key}) : super(key: key);
@override
State<LikedPackagesScreen> createState() => _LikedPackagesScreenState();
}
class _LikedPackagesScreenState extends State<LikedPackagesScreen> {
@override
Widget build(BuildContext context) {
return const Center(
child: Text("liked package screen"),
);
}
}
| 0 |
mirrored_repositories/travee-flutter-app | mirrored_repositories/travee-flutter-app/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility in the flutter_test package. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:travee/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(const MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/fluttering | mirrored_repositories/fluttering/lib/WelcomeScreen.dart | import "package:flutter/material.dart";
class WelcomeScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Text(
"Welcome Screen",
style: TextStyle(fontSize: 20),
),
),
);
}
}
| 0 |
mirrored_repositories/fluttering | mirrored_repositories/fluttering/lib/HomeScreen.dart | import "package:flutter/material.dart";
class HomeScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Center(
child: Text(
"Home Screen",
style: TextStyle(fontSize: 20),
),
),
);
}
}
| 0 |
mirrored_repositories/fluttering | mirrored_repositories/fluttering/lib/main.dart | import 'package:flutter/material.dart';
import 'package:fluttering/WelcomeScreen.dart';
import 'package:shared_preferences/shared_preferences.dart';
import 'HomeScreen.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: "Provider Basic",
theme: ThemeData(primarySwatch: Colors.blue),
home: MainScreen());
}
}
class MainScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Shared Preferences"),
),
body: Center(
child: FlatButton(
child: Text(
"Proceed",
style: TextStyle(color: Colors.white),
),
color: Colors.deepPurple,
onPressed: () async {
bool visitingFlag = await getVisitedFlag();
setVisitedFlag();
if (visitingFlag) {
Navigator.of(context)
.push(MaterialPageRoute(builder: (context) => HomeScreen()));
} else {
Navigator.of(context).push(
MaterialPageRoute(builder: (context) => WelcomeScreen()));
}
},
),
),
);
}
}
setVisitedFlag() async {
SharedPreferences preferences = await SharedPreferences.getInstance();
preferences.setBool("already_visited", true);
}
getVisitedFlag() async {
SharedPreferences preferences = await SharedPreferences.getInstance();
bool alradyVisited = preferences.getBool('already_visited') ?? false;
return alradyVisited;
}
| 0 |
mirrored_repositories/fluttering | mirrored_repositories/fluttering/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:fluttering/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/Crop-Care | mirrored_repositories/Crop-Care/lib/plant_details.dart | // ignore_for_file: constant_identifier_names
import 'package:crop_capture/model/plant.dart';
const PLANT_DETAILS = [
Plant(
index: 1,
name: "Corn",
disease: "Cercospora Leaf Spot",
type: "Fungal Disease",
pathogen: "Cercospora zeae-maydis",
symptoms: [
"Small, circular to elongated grayish-green lesions on the lower leaves of the plant",
"Lesions can range in size from 1 to 2 mm and can be surrounded by a yellow halo",
"Lesions will become larger and turn brown or gray with a tan center",
"Lesions may merge together, causing significant damage to the foliage and leading to premature leaf death",
"Lesions can also appear on the husks of the ears, reducing grain quality and yield",
"Stunted growth, reduced yield, and an increased susceptibility to other diseases and pests",
],
remedies: [
"Crop Rotation: Cercospora zeae-maydis can survive in infected plant debris in the soil, so rotating crops can help break the disease cycle.",
"Fungicides: Applying fungicides can help control the disease, but they should be used as a last resort and only when necessary.",
"Resistant Varieties: Planting resistant varieties of corn can be an effective way to prevent Cercospora leaf spot disease.",
"Proper Crop Management: Good crop management practices such as proper irrigation, nutrient management, and weed control can help reduce the severity of the disease.",
"Sanitation: Removing infected plant debris and destroying it can help reduce the spread of the disease.",
"Early Detection: Regular scouting and monitoring can help detect the disease early, which can make it easier to manage,It's also important to remove and destroy infected plant debris to prevent the spread of the disease to healthy plants.",
],
),
Plant(
index: 3,
name: "Corn",
disease: "Northern Leaf Blight",
type: "Fungal Disease",
pathogen: "Setosphaeria turcica",
symptoms: [
"Cigar-shaped, gray-green lesions on leaves with yellow or brown borders",
"Lesions tend to start on lower leaves and move up the plant",
"In severe cases, lesions can merge together and kill entire leaves",
"Yield loss and reduced grain quality can occur",
],
remedies: [
"Plant Resistant Varieties: Plant corn varieties that are resistant to northern leaf blight.",
"Crop Rotation: Rotate corn crops with non-host crops to break the disease cycle and reduce the amount of inoculum in the soil.",
"Fungicides: Apply fungicides preventively, especially during periods of high humidity and rainfall.",
"Tillage and Sanitation: Till infected crop debris into the soil and avoid planting corn in the same field for at least one year. Remove and destroy crop debris at the end of the season to reduce the amount of inoculum for the next season.",
"Monitoring: Regularly monitor corn crops for signs of northern leaf blight, especially during periods of high humidity and rainfall. Early detection can help prevent the disease from spreading and causing significant damage.",
"Balanced Nutrition: Providing balanced nutrition to the corn plants can help improve their overall health and resilience against diseases.",
],
),
Plant(
index: 4,
name: "Cotton",
disease: "Aphids",
type: "Biotic disease",
pathogen: "Pear-shaped Insects",
symptoms: [
"Stunted plant growth.",
"Yellowing of leaves.",
"Curling or distortion of leaves.",
"Presence of sticky, sugary substance (honeydew) on leaves, which can lead to the growth of sooty mold.",
"Presence of the aphids themselves on the undersides of leaves.",
],
remedies: [
"Biological control: Encourage natural enemies of cotton aphids, such as lady beetles, lacewings, and parasitic wasps, by planting companion plants and avoiding broad-spectrum insecticides that can harm beneficial insects.",
"Insecticidal soap: Apply insecticidal soap to the undersides of leaves to kill aphids. Be sure to follow label instructions and use appropriate safety precautions.",
"Neem oil: Apply neem oil to the undersides of leaves to kill aphids. Be sure to follow label instructions and use appropriate safety precautions.",
"Horticultural oil: Apply horticultural oil to the undersides of leaves to suffocate aphids. Be sure to follow label instructions and use appropriate safety precautions.",
"Cultural practices: Avoid over-fertilizing with nitrogen, as this can promote aphid populations. Use drip irrigation instead of overhead irrigation to avoid creating humid conditions that can promote aphid populations.",
],
),
Plant(
index: 5,
name: "Cotton",
disease: "Army Worm",
type: "Biotic disease",
pathogen: "Moth Species",
symptoms: [
"Holes in leaves and buds.",
"Defoliation of plants.",
"Presence of small greenish-brown caterpillars with light stripes on the sides of the body.",
"Presence of adult moths, which are brown or gray with a wingspan of about 1-1.5 inches.",
],
remedies: [
"Biological control: Encourage natural enemies of cotton armyworms, such as parasitic wasps, predatory bugs, and birds, by planting companion plants and avoiding broad-spectrum insecticides that can harm beneficial insects.",
"Bacillus thuringiensis (Bt): Apply Bt to the undersides of leaves to kill caterpillars. Bt is a naturally occurring soil bacterium that produces a protein toxic to caterpillars. Be sure to follow label instructions and use appropriate safety precautions.",
"Insecticides: Apply insecticides to control caterpillar populations. Be sure to follow label instructions and use appropriate safety precautions.",
"Cultural practices: Rotate crops to reduce the likelihood of armyworm infestations. Maintain good weed control to eliminate alternative hosts for armyworms. Avoid over-fertilizing with nitrogen, which can promote armyworm populations",
],
),
Plant(
index: 6,
name: "Cotton",
disease: "Bacterial Blight",
type: "Bacterial Disease",
pathogen: "bacterium Xanthomonas citri subsp.malvacearum",
symptoms: [
"Water-soaked lesions on the leaves that turn brown or black and become angular in shape.",
"Lesions may be surrounded by a yellow halo.",
"Lesions may merge to form larger areas of necrosis.",
"Infection can spread to other parts of the plant, causing wilt and death of the plant.",
"The disease is more severe in warm, humid weather.",
],
remedies: [
"Plant resistant varieties: There are some cotton varieties that have been bred for resistance to bacterial blight.",
"Crop rotation: Rotate cotton with non-host crops to reduce the buildup of bacteria in the soil.",
"Sanitation: Remove and destroy infected plants to prevent the spread of the disease.",
"Chemical control: Copper-based bactericides can be used to control bacterial blight, but they must be applied preventively and may have negative impacts on beneficial bacteria in the soil.",
"Cultural practices: Avoid overhead irrigation, as this can promote the spread of the disease. Use drip irrigation instead. Also, avoid working in the field when plants are wet to prevent spreading the disease.",
],
),
Plant(
index: 8,
name: "Cotton",
disease: "Powdery Mildew",
type: "Fungal Disease",
pathogen: "Species of the genus Erysiphe",
symptoms: [
"A white, powdery growth on the leaves, stems, and flowers of the plant.",
"Infected leaves may become distorted and turn yellow or brown.",
"Infected leaves may curl or distort, which can lead to reduced photosynthesis and lower yields.",
"As the disease progresses, infected leaves may develop necrotic areas or brown spots, which can eventually lead to leaf drop.",
"Severe infections can cause stunted growth and reduced yield, as the plant's ability to produce energy through photosynthesis is reduced.",
"Powdery mildew can also affect the quality of the cotton fibers, leading to lower prices and reduced profits for growers.",
],
remedies: [
"Plant resistant varieties: There are some cotton varieties that have been bred for resistance to powdery mildew.",
"Crop rotation: Rotate cotton with non-host crops to reduce the buildup of fungal spores in the soil.",
"Sanitation: Remove and destroy infected plant material to prevent the spread of the disease.",
"Fungicides: Apply fungicides early in the season to prevent the disease from becoming established. Be sure to follow label instructions and use appropriate safety precautions.",
"Cultural practices: Avoid overhead irrigation, as this can promote the spread of the disease. Use drip irrigation instead. Also, avoid working in the field when plants are wet to prevent spreading the disease.",
],
),
Plant(
index: 9,
name: "Grape",
disease: "Black Rot",
type: "Fungal Disease",
pathogen: "Guignardia bidwellii",
symptoms: [
"Small, circular lesions on the leaves, stems, and fruit of the grapevine.",
"As the disease progresses, the lesions enlarge and turn brown or black.",
"Infected fruit may become mummified and fall from the vine.",
"The disease is most common in warm, humid weather.",
],
remedies: [
"Pruning: Prune out infected plant material and remove fallen fruit from the ground to reduce the spread of the disease.",
"Sanitation: Remove and destroy infected plant material to prevent the spread of the disease.",
"Fungicides: Apply fungicides preventatively to control the disease. Be sure to follow label instructions and use appropriate safety precautions.",
"Cultural practices: Avoid overhead irrigation, as this can promote the spread of the disease. Use drip irrigation instead. Also, avoid working in the vineyard when plants are wet to prevent spreading the disease.",
],
),
Plant(
index: 11,
name: "Grape",
disease: "Leaf Blight",
type: "Fungal Disease",
pathogen: "Isariopsis griseola",
symptoms: [
"Small, circular to angular spots on the leaves, which can be gray or brown in color.",
"The spots may be surrounded by a yellow halo.",
"As the disease progresses, the spots may coalesce and cover larger areas of the leaf.",
"Severe infections can cause premature defoliation.",
],
remedies: [
"Sanitation: Remove and destroy infected plant material to prevent the spread of the disease.",
"Fungicides: Apply fungicides preventatively to control the disease. Be sure to follow label instructions and use appropriate safety precautions.",
"Cultural practices: Promote good air circulation around the vines by pruning and trellising, as this can help to reduce humidity and slow the spread of the disease. Also, avoid overhead irrigation, as this can promote the spread of the disease.",
"Resistant cultivars: Some grape varieties are more resistant to leaf blight than others. Choosing resistant varieties can be an effective long-term strategy for managing the disease.",
],
),
Plant(
index: 12,
name: "Potato",
disease: "Early Blight",
type: "Fungal Disease",
pathogen: "Alternaria solani",
symptoms: [
"Small, brown lesions with concentric rings develop on older leaves first.",
"Lesions may coalesce and cause the leaves to turn yellow and die.",
"Black, sunken spots may appear on stems.",
"Yield reduction due to premature defoliation.",
],
remedies: [
"Sanitation: Remove and destroy infected plant material to prevent the spread of the disease.",
"Fungicides: Apply fungicides preventatively to control the disease. Be sure to follow label instructions and use appropriate safety precautions.",
"Crop rotation: Rotate potatoes with non-solanaceous crops (e.g. legumes, cereals, grasses) to reduce the build-up of pathogen inoculum in the soil.",
"Cultural practices: Avoid overhead irrigation, as this can promote the spread of the disease. Prune lower leaves to improve air circulation and reduce humidity, which can slow the spread of the disease. Avoid over-fertilizing with nitrogen, which can make plants more susceptible to the disease.",
"Resistant varieties: Some potato varieties are more resistant to early blight than others. Choosing resistant varieties can be an effective long-term strategy for managing the disease.",
],
),
Plant(
index: 14,
name: "Potato",
disease: "Late Blight",
type: "Fungal Disease",
pathogen: "Phytophthora infestan",
symptoms: [
"Dark brown to black lesions with irregular margins develop on leaves.",
"Lesions can rapidly spread to cover entire leaves and stems.",
"A white, fuzzy mold may be visible on the undersides of leaves during periods of high humidity.",
"Infected tubers may have a reddish-brown discoloration under the skin and a granular texture.",
],
remedies: [
"Sanitation: Remove and destroy infected plant material to prevent the spread of the disease.",
"Fungicides: Apply fungicides preventatively to control the disease. Be sure to follow label instructions and use appropriate safety precautions. Fungicides may need to be applied at shorter intervals during periods of high disease pressure.",
"Cultural practices: Avoid overhead irrigation, as this can promote the spread of the disease. Prune lower leaves to improve air circulation and reduce humidity, which can slow the spread of the disease. Avoid over-fertilizing with nitrogen, which can make plants more susceptible to the disease.",
"Resistant varieties: Some potato varieties are more resistant to late blight than others. Choosing resistant varieties can be an effective long-term strategy for managing the disease.",
"It is important to note that late blight can be difficult to control once it becomes established in a field. Early detection and prompt action are essential for preventing the disease from spreading. Integrated management strategies that combine multiple approaches are most likely to be successful in controlling the disease.",
],
),
];
| 0 |
mirrored_repositories/Crop-Care | mirrored_repositories/Crop-Care/lib/main.dart | import 'package:flutter/material.dart';
import './pages/history_page.dart';
import './pages/plant_details_screen.dart';
import './pages/tflite_model.dart';
import './pages/home_page.dart';
import './pages/splash_screen.dart';
import './constants/constants.dart';
import './pages/profile_screen.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Crop Care',
theme: ThemeData(
primaryColor: primaryColor,
primarySwatch: primaryColorSwatch,
useMaterial3: true,
),
routes: {
'/': (context) => const SplashScreen(),
HomePage.routeName: (context) => const HomePage(),
TfliteModel.routeName: (context) => TfliteModel(),
HistoryPage.routeName: (context) => const HistoryPage(),
PlantDetailsScreen.routeName: (context) => PlantDetailsScreen(),
ProfilePage.routeName: (context) => ProfilePage(),
},
debugShowCheckedModeBanner: false,
);
}
}
| 0 |
mirrored_repositories/Crop-Care/lib | mirrored_repositories/Crop-Care/lib/constants/constants.dart | import 'package:flutter/material.dart';
const Color primaryColor = Color(0xFF87E4D5);
const Color secondaryColor = Color(0xFF00BFA5);
const Color onPrimaryColor = Color(0xFFFFFFFF);
const Color textColor = Color(0xFFFFFFFF);
const Color textColor2 = Color(0xFF000000);
const Color textColor2Lite = Color(0xFF696868);
const Color iconColor = Color(0xFF242222);
MaterialColor primaryColorSwatch = MaterialColor(
primaryColor.value,
const <int, Color>{
50: Color(0xFFE0F2F1),
100: Color(0xFFB2DFDB),
200: Color(0xFF80CBC4),
300: Color(0xFF4DB6AC),
400: Color(0xFF26A69A),
500: Color(0xFF009688),
600: Color(0xFF00897B),
700: Color(0xFF00796B),
800: Color(0xFF00695C),
900: Color(0xFF004D40),
},
);
| 0 |
mirrored_repositories/Crop-Care/lib | mirrored_repositories/Crop-Care/lib/widgets/plant_details_widget.dart | import 'dart:io';
import 'package:crop_capture/constants/constants.dart';
import 'package:crop_capture/pages/plant_details_screen.dart';
import 'package:flutter/material.dart';
class PlantWidget extends StatelessWidget {
const PlantWidget({
required this.name,
required this.disease,
required this.imageFile,
});
final String name;
final String disease;
final File imageFile;
void selectPlant(BuildContext context) {
Navigator.of(context).pushNamed(PlantDetailsScreen.routeName, arguments: {
'name': name,
'disease': disease,
'imageFile': imageFile,
});
}
@override
Widget build(BuildContext context) {
return InkWell(
onTap: () => selectPlant(context),
child: Card(
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(15),
),
elevation: 4,
margin: const EdgeInsets.all(10),
child: Column(
children: [
Stack(
children: [
ClipRRect(
borderRadius: const BorderRadius.all(
Radius.circular(15),
),
child: SizedBox(
width: double.infinity,
height: 200,
child: Hero(
tag: imageFile.path,
child: Image.file(
imageFile,
width: double.infinity,
height: 200,
fit: BoxFit.cover,
),
),
),
),
],
),
Padding(
padding: const EdgeInsets.all(10),
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: [
SizedBox(
height: 60,
child: Container(
width: 300,
padding: const EdgeInsets.symmetric(
vertical: 2,
horizontal: 20,
),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
name,
style: const TextStyle(
fontSize: 20,
color: textColor2,
),
softWrap: true,
overflow: TextOverflow.fade,
),
Text(
disease,
style: const TextStyle(
fontSize: 15,
color: textColor2Lite,
),
softWrap: true,
overflow: TextOverflow.fade,
),
],
),
),
),
const Icon(
Icons.arrow_forward_ios_outlined,
size: 15,
color: iconColor,
),
],
),
),
],
),
),
);
}
}
| 0 |
mirrored_repositories/Crop-Care/lib | mirrored_repositories/Crop-Care/lib/widgets/text_animator.dart | import 'package:flutter/material.dart';
import 'package:crop_capture/constants/constants.dart';
class SplashTextAnimation extends StatefulWidget {
const SplashTextAnimation({Key? key}) : super(key: key);
@override
State<SplashTextAnimation> createState() => _SplashTextAnimationState();
}
class _SplashTextAnimationState extends State<SplashTextAnimation> {
final String title = 'Crop Care';
@override
Widget build(BuildContext context) {
return TweenAnimationBuilder<int>(
duration: const Duration(seconds: 2),
builder: (BuildContext context, int value, Widget? child) {
return Text(
title.substring(0, value),
style: const TextStyle(
color: secondaryColor,
fontSize: 40,
),
);
},
tween: IntTween(
begin: 0,
end: title.length,
),
);
}
}
| 0 |
mirrored_repositories/Crop-Care/lib | mirrored_repositories/Crop-Care/lib/pages/home_page.dart | import 'package:flutter/material.dart';
import 'package:permission_handler/permission_handler.dart';
import '../constants/constants.dart';
import './tflite_model.dart';
import './history_page.dart';
import './profile_screen.dart';
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
static const String routeName = '/home-page';
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
void initState() {
super.initState();
requestStoragePermission();
}
Future<void> requestStoragePermission() async {
final PermissionStatus status = await Permission.storage.request();
if (status != PermissionStatus.granted) {
throw Exception('Permission denied');
}
}
int _selectedIndex = 1;
final List<Widget> _pages = [
const HistoryPage(),
TfliteModel(),
ProfilePage(),
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Crop Care'),
),
backgroundColor: onPrimaryColor,
body: _pages[_selectedIndex],
bottomNavigationBar: NavigationBar(
backgroundColor: primaryColor,
destinations: const [
NavigationDestination(
icon: Icon(Icons.home),
label: 'Home',
),
NavigationDestination(
icon: Icon(Icons.camera_alt),
label: 'Camera',
),
NavigationDestination(
icon: Icon(Icons.person),
label: 'Profile',
),
],
animationDuration: const Duration(milliseconds: 300),
selectedIndex: _selectedIndex,
onDestinationSelected: (index) {
setState(() {
_selectedIndex = index;
});
},
),
);
}
}
| 0 |
mirrored_repositories/Crop-Care/lib | mirrored_repositories/Crop-Care/lib/pages/history_page.dart | import 'dart:io';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:lottie/lottie.dart';
import 'package:path_provider/path_provider.dart';
import 'package:path/path.dart' as pth;
import '../constants/constants.dart';
import '../plant_details.dart';
import '../widgets/plant_details_widget.dart';
class HistoryPage extends StatefulWidget {
const HistoryPage({Key? key}) : super(key: key);
static const routeName = '/history-page';
@override
State<HistoryPage> createState() => _HistoryPageState();
}
class _HistoryPageState extends State<HistoryPage>
with AutomaticKeepAliveClientMixin {
List<File> _imageFiles = [];
@override
void initState() {
super.initState();
_loadSavedImages();
}
Future<void> _loadSavedImages() async {
try {
final directory = await getExternalStorageDirectory();
final files = await directory?.list().toList();
final imageFiles = files
?.where((file) => file.path.endsWith('.png'))
.map((file) => File(file.path))
.toList();
setState(() {
_imageFiles = imageFiles ?? [];
});
} catch (e) {
if (kDebugMode) {
print('Failed to load saved images: $e');
}
}
}
List<String> getDetails(String path) {
final fileName = pth.basename(path);
final nameAndDisease = fileName.split('____');
final details = nameAndDisease[0].split('_');
final name = details[0];
final disease = details.sublist(1, details.length).join(' ');
if (kDebugMode) {
int? index = 0;
for (var i = 0; i < PLANT_DETAILS.length; i++) {
if (PLANT_DETAILS[i].name == name &&
PLANT_DETAILS[i].disease == disease) {
index = PLANT_DETAILS[i].index;
break;
}
}
print('Index : $index \nPlant : $name\nDisease : $disease\n\n');
}
return [name, disease];
}
@override
Widget build(BuildContext context) {
super.build(context);
return Scaffold(
backgroundColor: onPrimaryColor,
body: _imageFiles.isNotEmpty
? Column(
mainAxisSize: MainAxisSize.max,
children: [
Expanded(
child: ListView.builder(
padding: EdgeInsets.zero,
scrollDirection: Axis.vertical,
itemCount: _imageFiles.length,
itemBuilder: (BuildContext context, int index) {
final file = _imageFiles[index];
final details = getDetails(file.path);
return Padding(
padding: const EdgeInsets.symmetric(
vertical: 10,
horizontal: 5,
),
child: PlantWidget(
name: details[0],
disease: details[1],
imageFile: file,
),
);
},
),
),
],
)
: Center(
child: Lottie.asset(
'assets/icons/no_images.json',
fit: BoxFit.cover,
repeat: false,
height: 250.0,
width: 250.0,
),
),
);
}
@override
bool get wantKeepAlive => true;
}
| 0 |
mirrored_repositories/Crop-Care/lib | mirrored_repositories/Crop-Care/lib/pages/profile_screen.dart | import 'package:flutter/material.dart';
class ProfilePage extends StatefulWidget {
static const routeName = '/profile-page';
@override
State<ProfilePage> createState() => _ProfilePageState();
}
class _ProfilePageState extends State<ProfilePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
body: Padding(
padding: const EdgeInsets.only(
top: 30,
),
child: Column(
children: <Widget>[
Container(
padding: const EdgeInsets.all(16.0),
child: Column(
children: const [
CircleAvatar(
backgroundImage: AssetImage('assets/images/profile.png'),
radius: 50.0,
),
SizedBox(height: 16.0),
Text(
'John Doe',
style: TextStyle(
fontSize: 24.0,
fontWeight: FontWeight.bold,
),
),
],
),
),
const Divider(),
ListTile(
leading: const Icon(Icons.settings),
title: const Text('Settings'),
onTap: () {},
),
const Divider(),
ListTile(
leading: const Icon(Icons.logout),
title: const Text('Logout'),
onTap: () {},
),
],
),
),
);
}
}
| 0 |
mirrored_repositories/Crop-Care/lib | mirrored_repositories/Crop-Care/lib/pages/plant_details_screen.dart | import 'dart:io';
import 'package:flutter/material.dart';
import 'package:lottie/lottie.dart';
import '../plant_details.dart';
class PlantDetailsScreen extends StatelessWidget {
static const String routeName = '/plant-details-screen';
@override
Widget build(BuildContext context) {
final Map<String, dynamic>? routeArgs =
ModalRoute.of(context)?.settings.arguments as Map<String, dynamic>?;
final name = routeArgs!['name'];
final disease = routeArgs['disease'];
final imageFile = routeArgs['imageFile'].path;
final plantDetailsList = PLANT_DETAILS.where((plant) {
return plant.name == name && plant.disease == disease;
}).toList();
final plantDetails =
plantDetailsList.isNotEmpty ? plantDetailsList.first : null;
return Scaffold(
body: CustomScrollView(
slivers: [
SliverAppBar(
expandedHeight: 300.0,
pinned: true,
flexibleSpace: FlexibleSpaceBar(
title: Text(
plantDetails != null ? plantDetails.disease : name,
textAlign: TextAlign.start,
),
background: SizedBox(
height: MediaQuery.of(context).size.height * 0.3,
child: Hero(
tag: imageFile,
child: Image.file(
File(imageFile),
fit: BoxFit.cover,
),
),
),
),
),
SliverList(
delegate: SliverChildListDelegate(
[
Padding(
padding: const EdgeInsets.all(16.0),
child: plantDetails == null
? Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Lottie.asset(
'assets/icons/happy_plant.json',
height: 250.0,
width: 250.0,
fit: BoxFit.cover,
repeat: false,
),
const SizedBox(height: 16.0),
const Text(
'Your plant is healthy!',
style: TextStyle(
fontSize: 24.0,
fontWeight: FontWeight.bold,
),
),
],
)
: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
plantDetails.name,
style: const TextStyle(
fontSize: 24.0,
fontWeight: FontWeight.bold,
),
),
const SizedBox(height: 8.0),
Text(
plantDetails.type,
style: const TextStyle(
fontSize: 16.0,
fontStyle: FontStyle.italic,
),
),
const SizedBox(height: 8.0),
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
...plantDetails.symptoms
.asMap()
.entries
.map((entry) {
final index = entry.key + 1;
final symptom = entry.value;
return Padding(
padding: const EdgeInsets.only(bottom: 8.0),
child: Row(
crossAxisAlignment:
CrossAxisAlignment.start,
children: [
Text(
'$index. ',
style: const TextStyle(
fontWeight: FontWeight.bold,
fontSize: 16.0,
),
),
Expanded(
child: Text(
symptom,
style:
const TextStyle(fontSize: 16.0),
),
),
],
),
);
}).toList(),
],
),
const SizedBox(height: 16.0),
const Text(
'Remedies:',
style: TextStyle(
fontWeight: FontWeight.bold,
),
),
const SizedBox(height: 8.0),
Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
...plantDetails.remedies.map((remedy) {
final remedyParts = remedy.split(':');
return Padding(
padding: const EdgeInsets.only(bottom: 8.0),
child: RichText(
text: TextSpan(
style: const TextStyle(
color: Colors.black,
fontSize: 16.0,
),
children: [
TextSpan(
text: remedyParts[0],
style: const TextStyle(
fontWeight: FontWeight.bold,
fontSize: 16.0,
),
),
TextSpan(text: ": ${remedyParts[1]}"),
],
),
),
);
}).toList(),
],
),
],
),
),
const SizedBox(height: 300.0),
],
),
),
],
),
);
}
}
| 0 |
mirrored_repositories/Crop-Care/lib | mirrored_repositories/Crop-Care/lib/pages/tflite_model.dart | import 'dart:io';
import 'package:flutter/foundation.dart';
import 'package:flutter/material.dart';
import 'package:image_picker/image_picker.dart';
import 'package:path_provider/path_provider.dart';
import 'package:tflite/tflite.dart';
import '../constants/constants.dart';
class TfliteModel extends StatefulWidget {
static const String routeName = '/tflite-model';
@override
_TfliteModelState createState() => _TfliteModelState();
}
class _TfliteModelState extends State<TfliteModel> {
late File _image;
bool imageSelect = false;
bool isLoading = false;
late String imageURL;
late String label = '';
@override
void initState() {
super.initState();
loadModel();
}
Future pickImage(ImageSource source) async {
final ImagePicker picker = ImagePicker();
final XFile? pickedFile = await picker.pickImage(
source: source,
);
setState(() {
isLoading = true;
imageSelect = false;
});
File image = File(pickedFile!.path);
Map<String, String> results = await imageClassification(image);
setState(() {
label = 'Plant: ${results['plant']}\nDisease: ${results['disease']}';
isLoading = false;
});
}
Future loadModel() async {
String res;
res = (await Tflite.loadModel(
model: "assets/model/SequentialModel_v3.tflite",
labels: "assets/model/labels.txt"))!;
if (kDebugMode) {
print("Models loading status: $res");
}
}
@override
void dispose() {
super.dispose();
Tflite.close();
}
Future<void> saveImage(File imageFile, List<String> labelParts) async {
final imageName =
'${labelParts[0]}_${labelParts[1]}____${DateTime.now().millisecondsSinceEpoch}.png';
final externalDirectory = await getExternalStorageDirectory();
final publicImagePath = '${externalDirectory?.path}/$imageName';
await imageFile.copy(publicImagePath);
if (kDebugMode) {
print('Public image saved at $publicImagePath');
}
}
Future<Map<String, String>> imageClassification(File image) async {
final List? recognitions = await Tflite.runModelOnImage(
path: image.path,
numResults: 15,
threshold: 0.1,
imageMean: 0.0,
imageStd: 255.0,
);
final String label = recognitions![0]['label'];
final List<String> labelParts = label.split(" ");
final String plant = labelParts[0];
String disease = "no disease";
if (labelParts.length > 1) {
disease = labelParts[1];
}
if (kDebugMode) {
print('Plant: $plant, Disease: $disease');
}
await saveImage(image, labelParts);
setState(() {
_image = image;
imageSelect = true;
isLoading = false;
});
return {'plant': plant, 'disease': disease};
}
Widget customButton(String textOnButton, ImageSource source) {
return ElevatedButton(
style: ButtonStyle(
shape: MaterialStateProperty.all<RoundedRectangleBorder>(
RoundedRectangleBorder(
borderRadius: BorderRadius.circular(18.0),
),
),
backgroundColor: MaterialStateProperty.all<Color>(primaryColor),
padding: MaterialStateProperty.all<EdgeInsetsGeometry>(
const EdgeInsets.symmetric(vertical: 16.0, horizontal: 40.0),
),
textStyle: MaterialStateProperty.all<TextStyle>(
const TextStyle(fontSize: 16),
),
),
child: Text(
textOnButton,
style: const TextStyle(
color: textColor,
fontWeight: FontWeight.bold,
),
),
onPressed: () {
pickImage(source);
},
);
}
@override
Widget build(BuildContext context) {
final double height = MediaQuery.of(context).size.height;
final double width = MediaQuery.of(context).size.width;
return Scaffold(
backgroundColor: onPrimaryColor,
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
const SizedBox(
height: 10,
),
(imageSelect)
? Container(
margin: const EdgeInsets.all(10),
child: Image.file(
_image,
fit: BoxFit.cover,
width: height * 0.4,
height: width * 0.9,
),
)
: Container(
margin: const EdgeInsets.all(10),
child: const Opacity(
opacity: 0.8,
child: Center(
child: Text("No image selected"),
),
),
),
const SizedBox(height: 10),
Text(
label,
textAlign: TextAlign.center,
style: const TextStyle(
color: textColor2,
fontSize: 20,
),
),
const SizedBox(height: 10),
customButton("Pick Image from Gallery", ImageSource.gallery),
const SizedBox(height: 10),
customButton("Pick Image from Camera", ImageSource.camera),
],
),
),
);
}
}
| 0 |
mirrored_repositories/Crop-Care/lib | mirrored_repositories/Crop-Care/lib/pages/splash_screen.dart | import 'dart:async';
import 'package:crop_capture/widgets/text_animator.dart';
import 'package:flutter/material.dart';
import 'package:lottie/lottie.dart';
import 'package:crop_capture/pages/home_page.dart';
class SplashScreen extends StatefulWidget {
const SplashScreen({super.key});
@override
SplashScreenState createState() => SplashScreenState();
}
class SplashScreenState extends State<SplashScreen> {
@override
void initState() {
super.initState();
Timer(
const Duration(seconds: 3),
() => Navigator.pushReplacement(
context,
PageRouteBuilder(
pageBuilder: (context, animation, secondaryAnimation) {
return const HomePage();
},
transitionDuration: const Duration(milliseconds: 600),
transitionsBuilder: (context, animation, secondaryAnimation, child) {
return FadeTransition(
opacity: animation,
child: child,
);
},
),
),
);
}
@override
Widget build(BuildContext context) {
double size = MediaQuery.of(context).size.height;
return Scaffold(
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
SizedBox(
height: size * 0.3,
width: size * 0.3,
child: Lottie.asset(
'assets/icons/leaf_icon.json',
fit: BoxFit.fill,
),
),
const SplashTextAnimation(),
],
),
));
}
}
| 0 |
mirrored_repositories/Crop-Care/lib | mirrored_repositories/Crop-Care/lib/model/details_model.dart | import 'dart:io';
class PlantImageDetailsCard {
final int index;
final String name;
final String disease;
final File image;
PlantImageDetailsCard({
required this.index,
required this.name,
required this.disease,
required this.image,
});
}
| 0 |
mirrored_repositories/Crop-Care/lib | mirrored_repositories/Crop-Care/lib/model/plant.dart | class Plant {
final int index;
final String name;
final String disease;
final String type;
final String pathogen;
final List<String> symptoms;
final List<String> remedies;
const Plant({
required this.index,
required this.name,
required this.disease,
required this.type,
required this.pathogen,
required this.symptoms,
required this.remedies,
});
}
| 0 |
mirrored_repositories/Crop-Care | mirrored_repositories/Crop-Care/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility in the flutter_test package. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:crop_capture/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(const MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/Flutter_Firebase-Storage | mirrored_repositories/Flutter_Firebase-Storage/lib/add_image.dart | import 'dart:io';
import 'package:cloud_firestore/cloud_firestore.dart';
import 'package:flutter/material.dart';
import 'package:image_picker/image_picker.dart';
import 'package:firebase_storage/firebase_storage.dart' as firebase_storage;
import 'package:path/path.dart' as Path;
class AddImage extends StatefulWidget {
@override
_AddImageState createState() => _AddImageState();
}
class _AddImageState extends State<AddImage> {
bool uploading = false;
double val = 0;
CollectionReference imgRef;
firebase_storage.Reference ref;
List<File> _image = [];
final picker = ImagePicker();
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Add Image'),
actions: [
FlatButton(
onPressed: () {
setState(() {
uploading = true;
});
uploadFile().whenComplete(() => Navigator.of(context).pop());
},
child: Text(
'upload',
style: TextStyle(color: Colors.white),
))
],
),
body: Stack(
children: [
Container(
padding: EdgeInsets.all(4),
child: GridView.builder(
itemCount: _image.length + 1,
gridDelegate: SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 3),
itemBuilder: (context, index) {
return index == 0
? Center(
child: IconButton(
icon: Icon(Icons.add),
onPressed: () =>
!uploading ? chooseImage() : null),
)
: Container(
margin: EdgeInsets.all(3),
decoration: BoxDecoration(
image: DecorationImage(
image: FileImage(_image[index - 1]),
fit: BoxFit.cover)),
);
}),
),
uploading
? Center(
child: Column(
mainAxisSize: MainAxisSize.min,
children: [
Container(
child: Text(
'uploading...',
style: TextStyle(fontSize: 20),
),
),
SizedBox(
height: 10,
),
CircularProgressIndicator(
value: val,
valueColor: AlwaysStoppedAnimation<Color>(Colors.green),
)
],
))
: Container(),
],
));
}
chooseImage() async {
final pickedFile = await picker.getImage(source: ImageSource.gallery);
setState(() {
_image.add(File(pickedFile?.path));
});
if (pickedFile.path == null) retrieveLostData();
}
Future<void> retrieveLostData() async {
final LostData response = await picker.getLostData();
if (response.isEmpty) {
return;
}
if (response.file != null) {
setState(() {
_image.add(File(response.file.path));
});
} else {
print(response.file);
}
}
Future uploadFile() async {
int i = 1;
for (var img in _image) {
setState(() {
val = i / _image.length;
});
ref = firebase_storage.FirebaseStorage.instance
.ref()
.child('images/${Path.basename(img.path)}');
await ref.putFile(img).whenComplete(() async {
await ref.getDownloadURL().then((value) {
imgRef.add({'url': value});
i++;
});
});
}
}
@override
void initState() {
super.initState();
imgRef = FirebaseFirestore.instance.collection('imageURLs');
}
}
| 0 |
mirrored_repositories/Flutter_Firebase-Storage | mirrored_repositories/Flutter_Firebase-Storage/lib/main.dart | import 'package:firebase_core/firebase_core.dart';
import 'package:flutter/material.dart';
import 'home.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
await Firebase.initializeApp();
runApp(MaterialApp(
home: MyApp(),
debugShowCheckedModeBanner: false,
));
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
Widget build(BuildContext context) {
return HomePage();
}
}
| 0 |
mirrored_repositories/Flutter_Firebase-Storage | mirrored_repositories/Flutter_Firebase-Storage/lib/home.dart | import 'package:cloud_firestore/cloud_firestore.dart';
import 'package:flutter/material.dart';
import 'package:transparent_image/transparent_image.dart';
import 'add_image.dart';
class HomePage extends StatefulWidget {
@override
_HomePageState createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Home Page')),
floatingActionButton: FloatingActionButton(
child: Icon(Icons.add),
onPressed: () {
Navigator.of(context)
.push(MaterialPageRoute(builder: (context) => AddImage()));
},
),
body: StreamBuilder(
stream: FirebaseFirestore.instance.collection('imageURLs').snapshots(),
builder: (context, snapshot) {
return !snapshot.hasData
? Center(
child: CircularProgressIndicator(),
)
: Container(
padding: EdgeInsets.all(4),
child: GridView.builder(
itemCount: snapshot.data.documents.length,
gridDelegate: SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 3),
itemBuilder: (context, index) {
return Container(
margin: EdgeInsets.all(3),
child: FadeInImage.memoryNetwork(
fit: BoxFit.cover,
placeholder: kTransparentImage,
image: snapshot.data.documents[index].get('url')),
);
}),
);
},
),
);
}
}
| 0 |
mirrored_repositories/Flutter_Firebase-Storage | mirrored_repositories/Flutter_Firebase-Storage/test/widget_test.dart | // This is a basic Flutter widget test.
//
// To perform an interaction with a widget in your test, use the WidgetTester
// utility that Flutter provides. For example, you can send tap and scroll
// gestures. You can also use WidgetTester to find child widgets in the widget
// tree, read text, and verify that the values of widget properties are correct.
import 'package:flutter/material.dart';
import 'package:flutter_test/flutter_test.dart';
import 'package:firebase_storage_project/main.dart';
void main() {
testWidgets('Counter increments smoke test', (WidgetTester tester) async {
// Build our app and trigger a frame.
await tester.pumpWidget(MyApp());
// Verify that our counter starts at 0.
expect(find.text('0'), findsOneWidget);
expect(find.text('1'), findsNothing);
// Tap the '+' icon and trigger a frame.
await tester.tap(find.byIcon(Icons.add));
await tester.pump();
// Verify that our counter has incremented.
expect(find.text('0'), findsNothing);
expect(find.text('1'), findsOneWidget);
});
}
| 0 |
mirrored_repositories/drag_drop_listview_example | mirrored_repositories/drag_drop_listview_example/lib/main.dart | import 'package:drag_and_drop_lists/drag_and_drop_item.dart';
import 'package:drag_and_drop_lists/drag_and_drop_list.dart';
import 'package:drag_and_drop_lists/drag_and_drop_lists.dart';
import 'package:drag_drop_listview_example/data/draggable_lists.dart';
import 'package:flutter/material.dart';
import 'package:flutter/rendering.dart';
import 'package:flutter/widgets.dart';
import 'package:flutter/services.dart';
import 'model/draggable_list.dart';
Future main() async {
WidgetsFlutterBinding.ensureInitialized();
await SystemChrome.setPreferredOrientations([
DeviceOrientation.portraitUp,
DeviceOrientation.portraitDown,
]);
runApp(MyApp());
}
class MyApp extends StatelessWidget {
static final String title = 'Drag & Drop ListView';
@override
Widget build(BuildContext context) => MaterialApp(
debugShowCheckedModeBanner: false,
title: title,
theme: ThemeData(primarySwatch: Colors.red),
home: MainPage(),
);
}
class MainPage extends StatefulWidget {
@override
_MainPage createState() => _MainPage();
}
class _MainPage extends State<MainPage> {
late List<DragAndDropList> lists;
@override
void initState() {
super.initState();
lists = allLists.map(buildList).toList();
}
@override
Widget build(BuildContext context) {
final backgroundColor = Color.fromARGB(255, 243, 242, 248);
return Scaffold(
backgroundColor: backgroundColor,
appBar: AppBar(
title: Text(MyApp.title),
centerTitle: true,
),
body: DragAndDropLists(
// lastItemTargetHeight: 50,
// addLastItemTargetHeightToTop: true,
// lastListTargetSize: 30,
listPadding: EdgeInsets.all(16),
listInnerDecoration: BoxDecoration(
color: Theme.of(context).canvasColor,
borderRadius: BorderRadius.circular(10),
),
children: lists,
itemDivider: Divider(thickness: 2, height: 2, color: backgroundColor),
itemDecorationWhileDragging: BoxDecoration(
color: Colors.white,
boxShadow: [BoxShadow(color: Colors.black12, blurRadius: 4)],
),
listDragHandle: buildDragHandle(isList: true),
itemDragHandle: buildDragHandle(),
onItemReorder: onReorderListItem,
onListReorder: onReorderList,
),
);
}
DragHandle buildDragHandle({bool isList = false}) {
final verticalAlignment = isList
? DragHandleVerticalAlignment.top
: DragHandleVerticalAlignment.center;
final color = isList ? Colors.blueGrey : Colors.black26;
return DragHandle(
verticalAlignment: verticalAlignment,
child: Container(
padding: EdgeInsets.only(right: 10),
child: Icon(Icons.menu, color: color),
),
);
}
DragAndDropList buildList(DraggableList list) => DragAndDropList(
header: Container(
padding: EdgeInsets.all(8),
child: Text(
list.header,
style: TextStyle(fontWeight: FontWeight.bold, fontSize: 16),
),
),
children: list.items
.map((item) => DragAndDropItem(
child: ListTile(
leading: Image.network(
item.urlImage,
width: 40,
height: 40,
fit: BoxFit.cover,
),
title: Text(item.title),
),
))
.toList(),
);
void onReorderListItem(
int oldItemIndex,
int oldListIndex,
int newItemIndex,
int newListIndex,
) {
setState(() {
final oldListItems = lists[oldListIndex].children;
final newListItems = lists[newListIndex].children;
final movedItem = oldListItems.removeAt(oldItemIndex);
newListItems.insert(newItemIndex, movedItem);
});
}
void onReorderList(
int oldListIndex,
int newListIndex,
) {
setState(() {
final movedList = lists.removeAt(oldListIndex);
lists.insert(newListIndex, movedList);
});
}
}
| 0 |
mirrored_repositories/drag_drop_listview_example/lib | mirrored_repositories/drag_drop_listview_example/lib/data/draggable_lists.dart | import 'package:drag_drop_listview_example/model/draggable_list.dart';
List<DraggableList> allLists = [
DraggableList(
header: 'Best Fruits',
items: [
DraggableListItem(
title: 'Orange',
urlImage:
'https://images.unsplash.com/photo-1582979512210-99b6a53386f9?ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&ixlib=rb-1.2.1&auto=format&fit=crop&w=934&q=80',
),
DraggableListItem(
title: 'Apple',
urlImage:
'https://images.unsplash.com/photo-1560806887-1e4cd0b6cbd6?ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&ixlib=rb-1.2.1&auto=format&fit=crop&w=3367&q=80',
),
DraggableListItem(
title: 'Blueberries',
urlImage:
'https://images.unsplash.com/photo-1595231776515-ddffb1f4eb73?ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&ixlib=rb-1.2.1&auto=format&fit=crop&w=1950&q=80',
),
],
),
DraggableList(
header: 'Good Fruits',
items: [
DraggableListItem(
title: 'Lemon',
urlImage:
'https://images.unsplash.com/photo-1590502593747-42a996133562?ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&ixlib=rb-1.2.1&auto=format&fit=crop&w=975&q=80',
),
DraggableListItem(
title: 'Melon',
urlImage:
'https://images.unsplash.com/photo-1571575173700-afb9492e6a50?ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&ixlib=rb-1.2.1&auto=format&fit=crop&w=976&q=80',
),
DraggableListItem(
title: 'Papaya',
urlImage:
'https://images.unsplash.com/photo-1617112848923-cc2234396a8d?ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&ixlib=rb-1.2.1&auto=format&fit=crop&w=1567&q=80',
),
],
),
DraggableList(
header: 'Disliked Fruits',
items: [
DraggableListItem(
title: 'Banana',
urlImage:
'https://images.unsplash.com/photo-1543218024-57a70143c369?ixlib=rb-1.2.1&ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&auto=format&fit=crop&w=975&q=80',
),
DraggableListItem(
title: 'Strawberries',
urlImage:
'https://images.unsplash.com/photo-1464965911861-746a04b4bca6?ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&ixlib=rb-1.2.1&auto=format&fit=crop&w=1950&q=80',
),
DraggableListItem(
title: 'Grapefruit',
urlImage:
'https://images.unsplash.com/photo-1577234286642-fc512a5f8f11?ixid=MnwxMjA3fDB8MHxwaG90by1wYWdlfHx8fGVufDB8fHx8&ixlib=rb-1.2.1&auto=format&fit=crop&w=975&q=80',
),
],
),
];
| 0 |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.