text
stringlengths 226
34.5k
|
---|
How to parse JSON to two columns in a CSV with Python
Question: For some reason I can't log into the same account on my home computer as my
work computer.
I was able to get Bo10's code to work, but not abernert's and I would really
like to understand why.
Here is my updates to abernert's code:
import csv
import sys
import json
import urllib2
j = urllib2.urlopen('https://citibikenyc.com/stations/json')
js = json.load(j)
citi = js['stationBeanList']
columns = ('stationName', 'totalDocks', 'availableDocks',
'latitude', 'longitude', 'availableBikes')
stations = (operator.itemgetter(columns)(station) for station in citi)
with open('output.csv', 'w') as csv_file:
csv_writer = csv.writer(csv_file)
csv_file.writerows(stations)
I thought adding this line `csv_writer = csv.writer(csv_file)` would fix the object has no attirbute error, but I am still getting it. This is the actual error:
Andrews-MacBook:coding Andrew$ python citibike1.py
Traceback (most recent call last):
File "citibike1.py", line 17, in <module>
csv_file.writerows(stations)
AttributeError: 'file' object has no attribute 'writerows'
* * *
So now I have the changed the code to this and the output is just repeating
the names of the columns 322 times. I changed it on line 14 because i was
getting this error:
Traceback (most recent call last):
File "citibike1.py", line 17, in <module>
csv_writer.writerows(stations)
File "citibike1.py", line 13, in <genexpr>
stations = (operator.itemgetter(columns)(station) for station in citi)
NameError: global name 'operator' is not defined:
import csv
import sys
import json
import urllib2
import operator
j = urllib2.urlopen('https://citibikenyc.com/stations/json')
js = json.load(j)
citi = js['stationBeanList']
columns = ('stationName', 'totalDocks', 'availableDocks',
'latitude', 'longitude', 'availableBikes')
stations = (operator.itemgetter(0,1,2,3,4,5)(columns) for station in citi)
with open('output.csv', 'w') as csv_file:
csv_writer = csv.writer(csv_file)
csv_writer.writerows(stations)
Answer: The problem is that you're not using the `csv` module, you're using the
`pickle` module, and this is what `pickle` output looks like.
To fix it:
csvfile = open('output.csv', 'w')
csv.writer(csvfile).writerows(stationList)
csvfile.close()
* * *
Note that you're going out of your way to build a transposed table, with 6
lists of 322 lists, not 322 lists of 6 lists. So, you're going to get 6 rows
of 322 columns each. If you want the opposite, just don't do that:
stationList = []
for f in citi:
stationList.append((f['stationName'],
f['totalDocks'],
f['availableDocks'],
f['latitude'],
f['longitude'],
f['availableBikes']))
Or, more briefly:
stationlist = map(operator.itemgetter('stationName', 'totalDocks', 'availableDocks',
'latitude', 'longitude', 'availableBikes'),
citi)
* * *
However, instead of building up a huge list, you may want to consider writing
the rows one at a time.
You can do that by putting `csv.writerow` calls into the middle of the for
loop.
But you can also do that just by using `itertools.imap` or a generator
expression instead of `map` or a list comprehension. That will make
`stationlist` into an iterable that creates new values as needed, instead of
creating them all at once.
* * *
Putting that all together, here's how I'd write your program:
import csv
import sys
import json
import urllib2
j = urllib2.urlopen('https://citibikenyc.com/stations/json')
js = json.load(j)
citi = js['stationBeanList']
columns = ('stationName', 'totalDocks', 'availableDocks',
'latitude', 'longitude', 'availableBikes')
stations = (operator.itemgetter(columns)(station) for station in citi)
with open('output.csv', 'w') as csv_file:
csv.writer(csv_file).writerows(stations)
|
python logging with multiple modules does not work
Question: I created some Python files keeping my functions a bit separated to ease
working / fixing. All files are in one directory. The structure may get broken
down to something like:
* a.py (a class A with basic stuff)
* b.py (a class B with basic stuff)
* modA.py (create a class C deriving from A and B)
* modB.py (create a class D deriving from A and B)
* ...
* main_a.py (using class C)
* main_b.py (using class D)
Every module uses the logging stuff from python. An why so ever - only the
root logger messages are written. And I don't see my error.
Here is a minimal example.
`a.py`
import logging
logger = logging.getLogger(__name__)
class A(object):
def __init__(self):
logger.debug("Instance of A")
`b.py`
import logging
logger = logging.getLogger(__name__)
class B(object):
def __init__(self):
logger.debug("Instance of B")
`ab.py`
import a
import b
import logging
logger = logging.getLogger(__name__)
class AB(a.A, b.B):
def __init__(self):
logger.debug("Instance of AB")
a.A.__init__(self)
b.B.__init__(self)
`main_one.py`
import sys
import ab
import logging
import logging.handlers
logger = logging.getLogger(__name__)
logger.setLevel(logging.DEBUG)
handler = logging.StreamHandler(stream=sys.stderr)
handler.setLevel(logging.DEBUG)
handler.setFormatter(logging.Formatter('%(name)s: %(message)s'))
logger.addHandler(handler)
logger.warning("The trouble starts")
ab = ab.AB()
I also tried to use something like `self.logger =
logging.getLogger(type(self).__name__)` to do logging on a per class base, but
the result is the same. So may one of you point out where I went wrong when
reading the python logging manuals?
TIA.
**EDIT 1: My solution**
Thanks to both, @falsetru and @Jakub M., using both answers leads to a working
solution.
First I put everything in a hierarchy.
main_one.py
lib/
__init__.py
ab.py
basic/
__init__.py
a.py
b.py
Second I changed the `logger = logging.getLogger(__name__)` in `main_one.py`
to `logger = logging.getLogger()` (_No name for the root logger!_).
That did the trick.
Very helpful was a code snippet [on
GitHub](https://github.com/mapio/sdt/tree/master/1_observing/active/logging).
Answer: Do `print __name__` for each of your modules and see what you get there
actually. You should put your modules into proper directories so `__name__` is
a ["period separated hierarchical
value"](http://docs.python.org/2/library/logging.html#logger-objects). For
example, if your file hierarchy looked like:
main_a.py
libs/
__init__.py
a.py
modA.py
then `__name__` of your modules (`a.py` and `modA.py`) would be `libs.a` and
`libs.modA`
> The name is potentially a period-separated hierarchical value, like
> foo.bar.baz (though it could also be just plain foo, for example). Loggers
> that are further down in the hierarchical list are children of loggers
> higher up in the list. For example, given a logger with a name of foo,
> loggers with names of foo.bar, foo.bar.baz, and foo.bam are all descendants
> of foo. The logger name hierarchy is analogous to the Python package
> hierarchy, and identical to it if you organise your loggers on a per-module
> basis using the recommended construction logging.getLogger(**name**). That’s
> because in a module, **name** is the module’s name in the Python package
> namespace.
|
How to merge two video parts and get a playable video file using Python?
Question: Here, I actually wants to merge two strings out1 & out2 (which contains the
first and second 30sec long video data) and write that to a file. So that I
will get a 1min long playable video file. But what I am getting is the first
30sec video only. How should I edit this code to achieve that ? Please help
me. Thanks a lot in advance.
import subprocess,os
ffmpeg_command1 = ["ffmpeg", "-i", "PATH/connect.webm", "-vcodec", "copy", "-ss", "00:00:00", "-t", "00:00:30","-f", "webm", "pipe:1"]
p1 = subprocess.Popen(ffmpeg_command1,stdout=subprocess.PIPE)
out1, err = p1.communicate()
ffmpeg_command2 = ["ffmpeg", "-i", "PATH/connect.webm","-vcodec", "copy", "-ss", "00:00:31", "-t", "00:00:30","-f", "webm", "pipe:1"]
p2 = subprocess.Popen(ffmpeg_command2,stdout=subprocess.PIPE)
out2, err1 = p2.communicate()
string = out1 + out2
fname = "PATH/final.webm"
fp = open(fname,'wb')
fp.write(string)
fp.close()
Please help me. I struck.
Answer: If you want to concat two videos with ffmpeg, it works like that:
ffmpeg -vcodec copy -isync -i \
"concat:file1.mp4|file2.mp4|...|fileN.mp4" \
outputfile.mp4
|
python: how to import nested package?
Question: I have a package named myscrapy, the directory structure is:
+ spider-common
--+ myscrapy
----+ basespiders
------+ __init__.py
------+ mod.py
--+ __init__.py
--+ mod.py
And I have an enviroment variable:
export PYTHONPATH=~/spider-common
This is success:
from myscrapy import mod
But it failed:
from myscrapy.basespiders import mod
ImportError: No module named myscrapy.basespiders
why?
Answer: The import command treads every folder only as a package (which is
importable), when a certain structure is present (see [Python
Docs](http://docs.python.org/2/tutorial/modules.html#packages)). You have to
place a `__init__.py` file (could be a blank one) in every subdirectory you
import.
|
How to import a single function to my main.py in python from another module?
Question: In my script I have a function inside a module which I wish to be able to use
in my main module to prevent redundancy. This other module ( not my main, lets
call it `two.py`) contains several classes and to import a class for use in
another module one would use
`from someDirectory.two import ClassA`
Which works fine for importing the entire class, but say I have a function
`myFunction()` in a different class `ClassB` contained in the same `two.py`
module, which I want to be able to use in my `main.py`.
Is there anyway which I can "grab" that function for use in my `main.py` or
other modules, without having to import the entire class or redefine the same
function?
Answer: You need to make sure that the directory you wish to import code from is in
your system path e.g.:
sys.path.insert(0, path_to_your_module_dir)
Then you can go ahead and do
from module import function
**UPDATE**
The following thread has details of how to permanently add the directory to
your pythonpath in either Windows or Unix-like systems:
[Permanently add a directory to
PYTHONPATH](http://stackoverflow.com/questions/3402168/permanently-add-a-
directory-to-pythonpath)
|
Linkedin Api for python not working correctly
Question: Linkedin Documents are confusing like crazy. I just want to get some basic
information. I need to get a company's recent updates, comments for the
update, and how many likes the update got. I tried to follow the documentation
and this is my code:
from linkedin import linkedin
import oauth2 as oauth
import httplib2
api_key = '9puxXXXXXXX'
secret_key = 'brtXoXEkXXXXXXXXX'
auth_token = '75e15760-XXXXXXXXXXXXXXXXXXXXXX'
auth_secret = '10d8caXXXXXXXXXXXXXXXXXXXXXXXXXXXX'
RETURN_URL = 'http://localhost:8000'
cos = oauth.Consumer(api_key,secret_key)
access_token = oauth.Token(key=auth_token, secret=auth_secret)
client = oauth.Client(cos,access_token)
resp,content = client.request("http://api.linkedin.com/v1/companies/1219692/updates?start=0&count=10", "GET", "")
This code is supposed to get the 10 recent updates for apple, but this is what
I get when I
print resp
print content
{'status': '200', 'content-length': '78', 'content-location': u'http://api.linkedin.com/v1/companies/216984/updates?count=10&oauth_body_hash=2jmj7l5rSw0yVb%2FvlWAYkK%2FYBwk%3D&oauth_nonce=87365476&oauth_timestamp=1372347259&oauth_consumer_key=9puxXXXXXXX&oauth_signature_method=HMAC-SHA1&oauth_version=1.0&start=0&oauth_token=75e1576XXXXXXX&oauth_signature=EhcMiQXXXXXXX%3D', 'transfer-encoding': 'chunked', 'vary': '*', 'server': 'Apache-Coyote/1.1', 'connection': 'close', '-content-encoding': 'gzip', 'date': 'Thu, 27 Jun 2013 15:34:18 GMT', 'x-li-request-id': '84BXIU5ZQK', 'x-li-format': 'xml', 'content-type': 'text/xml;charset=UTF-8'}
what am I doing wrong?
Answer: Your code does not make sense, you imported linkedin module, which seems to be
[this module](https://github.com/ozgur/python-linkedin), but I can't see you
are using that API wrapper in your code. If I'm wrong you can still use the
wrapper above and easily handle data back from LinkedIn. Just take a look at
this example:
[Querying updates](https://github.com/ozgur/python-linkedin#company-api)
sample:
from linkedin import server
application = server.quick_api(KEY, SECRET)
application.get_company_updates(1035, params={'count': 2})
where 1035 is the ID of the company you are trying to get updates from.
|
Python Array Rotation
Question: So I am implementing a block swap algorithm in python.
The algorithm I am following is this:
Initialize A = arr[0..d-1] and B = arr[d..n-1] 1) Do following until size of A
is equal to size of B
a) If A is shorter, divide B into Bl and Br such that Br is of same length as
A. Swap A and Br to change ABlBr into BrBlA. Now A is at its final place, so
recur on pieces of B.
b) If A is longer, divide A into Al and Ar such that Al is of same length as B
Swap Al and B to change AlArB into BArAl. Now B is at its final place, so
recur on pieces of A.
2) Finally when A and B are of equal size, block swap them.
The same algorithm has been implemented in C on this website - [Array
Rotation](http://www.geeksforgeeks.org/block-swap-algorithm-for-array-
rotation/)
My python code for the same is
a = [1,2,3,4,5,6,7,8]
x = 2
n = len(a)
def rotate(a,x):
n = len(a)
if x == 0 or x == n:
return a
if x == n -x:
print(a)
for i in range(x):
a[i], a[(i-x+n) % n] = a[(i-x+n) % n], a[i]
print(a)
return a
if x < n-x:
print(a)
for i in range(x):
a[i], a[(i-x+n) % n] = a[(i-x+n) % n], a[i]
print(a)
rotate(a[:n-x],x)
else:
print(a)
for i in range(n-x):
a[i], a[(i-(n-x) + n) % n] = a[(i-(n-x) + n) % n] , a[i]
print(a)
rotate(a[n-x:], n-x)
rotate(a,x)
print(a)
I am getting the right values at each stage but the recursive function call is
not returning the expected result and I cannot seem to understand the cause.
Can someone explain whats wrong with my recursion ? and what can be the
possible alternative.
Answer: You can rotate a list in place in Python by using a
[deque](http://docs.python.org/2/library/collections.html#collections.deque):
>>> from collections import deque
>>> d=deque([1,2,3,4,5])
>>> d
deque([1, 2, 3, 4, 5])
>>> d.rotate(2)
>>> d
deque([4, 5, 1, 2, 3])
>>> d.rotate(-2)
>>> d
deque([1, 2, 3, 4, 5])
Or with list slices:
>>> li=[1,2,3,4,5]
>>> li[2:]+li[:2]
[3, 4, 5, 1, 2]
>>> li[-2:]+li[:-2]
[4, 5, 1, 2, 3]
Note that the sign convention is opposite with deque.rotate vs slices.
If you want a function that has the same sign convention:
def rotate(l, y=1):
if len(l) == 0:
return l
y = -y % len(l) # flip rotation direction
return l[y:] + l[:y]
>>> rotate([1,2,3,4,5],2)
[4, 5, 1, 2, 3]
>>> rotate([1,2,3,4,5],-22)
[3, 4, 5, 1, 2]
>>> rotate('abcdefg',3)
'efgabcd'
|
python pandas time elapsed between dynamic range
Question: I am a new python/pandas user. I am trying to get a time delta (in secs)
between dynamic ranges (based on diff in values) of a time series data frame.
My sample dataframe is:
time price
2013-04-26 09:30:03-04:00 101
2013-04-26 09:30:04-04:00 101
2013-04-26 09:30:05-04:00 102
2013-04-26 09:30:06-04:00 105
2013-04-26 09:30:07-04:00 104
2013-04-26 09:30:08-04:00 105
2013-04-26 09:30:09-04:00 106
2013-04-26 09:30:10-04:00 104
2013-04-26 09:30:11-04:00 110
2013-04-26 09:30:12-04:00 109
2013-04-26 09:30:13-04:00 111
2013-04-26 09:30:14-04:00 108
2013-04-26 09:30:15-04:00 106
2013-04-26 09:30:16-04:00 107
2013-04-26 09:30:17-04:00 107
2013-04-26 09:30:18-04:00 108
2013-04-26 09:30:19-04:00 109
2013-04-26 09:30:20-04:00 109
2013-04-26 09:30:21-04:00 110
I am trying to get the time delta between price diff of 4. Once the diff in
price is reached, that price point become the 'starting point' for the next
calculation and so on. The desired result is something like (time delta in
secs):
time price time delta
2013-04-26 09:30:03-04:00 101
2013-04-26 09:30:04-04:00 101
2013-04-26 09:30:05-04:00 102
2013-04-26 09:30:06-04:00 105 3
2013-04-26 09:30:07-04:00 104
2013-04-26 09:30:08-04:00 105
2013-04-26 09:30:09-04:00 106
2013-04-26 09:30:10-04:00 104
2013-04-26 09:30:11-04:00 110 5
2013-04-26 09:30:12-04:00 109
2013-04-26 09:30:13-04:00 111
2013-04-26 09:30:14-04:00 108
2013-04-26 09:30:15-04:00 106 4
2013-04-26 09:30:16-04:00 107
2013-04-26 09:30:17-04:00 107
2013-04-26 09:30:18-04:00 108
2013-04-26 09:30:19-04:00 109
2013-04-26 09:30:20-04:00 109
2013-04-26 09:30:21-04:00 110 6
Answer: Not sure how great this is performance wise.
import numpy as np
import pandas as pd
gen = df.price.iteritems()
def get_deltas(gen):
time, value = next(gen)
deltas = [np.nan] # initial value
for line in gen:
if np.abs(line[1] - value) >= 4:
deltas.append(np.abs(line[0] - time))
time, value = line
else:
deltas.append(np.nan)
return deltas
deltas = get_deltas(df.price.iteritems())
df['deltas'] = deltas
In [58]: df
Out[58]:
price deltas
time
2013-04-26 13:30:03 101 NaN
2013-04-26 13:30:04 101 NaN
2013-04-26 13:30:05 102 NaN
2013-04-26 13:30:06 105 0:00:03
2013-04-26 13:30:07 104 NaN
2013-04-26 13:30:08 105 NaN
2013-04-26 13:30:09 106 NaN
2013-04-26 13:30:10 104 NaN
2013-04-26 13:30:11 110 0:00:05
2013-04-26 13:30:12 109 NaN
2013-04-26 13:30:13 111 NaN
2013-04-26 13:30:14 108 NaN
2013-04-26 13:30:15 106 0:00:04
2013-04-26 13:30:16 107 NaN
2013-04-26 13:30:17 107 NaN
2013-04-26 13:30:18 108 NaN
2013-04-26 13:30:19 109 NaN
2013-04-26 13:30:20 109 NaN
2013-04-26 13:30:21 110 0:00:06
|
What is acceptable in a python try statement
Question: I have a try statement that roughly follows something like this.
for result in results['matches']:
try:
#runs some functions
except KeyboardInterrupt:
leaveopt = raw_input( 'Would you like to exit or skip the current match? [e/s]:' )
if leaveopt == 'e':
print '\nExiting...'
else:
print '\nSkipping match...'
When I run the program I get no errors but when I press ctrl-c it just skips
the current match rather than asking what I would like to do. I'm wondering if
there is only some content that can be ran in the except portion of a try
statement or if there is another problem.
Answer: There's no restriction on what you can do in an `except` as far as I know
(even if there are some things that you may want to avoid here), and this
works for me (python 2.7.3 / linux mint):
import time
for x in xrange(5000):
try:
print x
time.sleep(5)
except KeyboardInterrupt, e:
leaveopt = raw_input(
'Would you like to exit or skip the current match? [e/s]:'
)
if leaveopt == 'e':
print '\nExiting...'
break
else:
print '\nSkipping match...'
continue
The problem is obviously somewhere in the "run some functions" part.
|
How to build an offline web app using Flask?
Question: I'm prototyping an idea for a website that will use the HTML5 offline
application cache for certain purposes. The website will be built with Python
and Flask and that's where my main problem comes from: I'm working with those
two for the first time, so I'm having a hard time getting the manifest file to
work as expected.
The issue is that I'm getting 404's from the static files included in the
manifest file. The manifest itself seems to be downloaded correctly, but the
files that it points to are not. This is what is spit out in the console when
loading the page:
Creating Application Cache with manifest http://127.0.0.1:5000/static/manifest.appcache offline-app:1
Application Cache Checking event offline-app:1
Application Cache Downloading event offline-app:1
Application Cache Progress event (0 of 2) http://127.0.0.1:5000/style.css offline-app:1
Application Cache Error event: Resource fetch failed (404) http://127.0.0.1:5000/style.css
The error is in the last line.
When the appcache fails even once, it stops the process completely and the
offline cache doesn't work.
This is how my files are structured:
* sandbox
* offline-app
* offline-app.py
* static
* manifest.appcache
* script.js
* style.css
* templates
* offline-app.html
This is the content of **offline-app.py** :
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/offline-app')
def offline_app():
return render_template('offline-app.html')
if __name__ == '__main__':
app.run(host='0.0.0.0', debug=True)
This is what I have in **offline-app.html** :
<!DOCTYPE html>
<html manifest="{{ url_for('static', filename='manifest.appcache') }}">
<head>
<title>Offline App Sandbox - main page</title>
</head>
<body>
<h1>Welcome to the main page for the Offline App Sandbox!</h1>
<p>Some placeholder text</p>
</body>
</html>
This is my **manifest.appcache** file:
CACHE MANIFEST
/style.css
/script.js
I've tried having the manifest file in all different ways I could think of:
CACHE MANIFEST
/static/style.css
/static/script.js
or
CACHE MANIFEST
/offline-app/static/style.css
/offline-app/static/script.js
None of these worked. The same error was returned every time.
I'm certain the issue here is how the server is serving up the files listed in
the manifest. Those files are probably being looked up in the wrong place, I
guess. I either should place them somewhere else or I need something different
in the cache manifest, but I have no idea what. I couldn't find anything
online about having HTML5 offline applications with Flask.
Is anyone able to help me out?
Answer: I would have thought this one would work:
CACHE MANIFEST
/static/style.css
/static/script.js
But in any case, you should not hardcode the URLs for your static files. It's
best to serve the manifest as a template (moved to the "templates" folder) so
that you can use `url_for` to generate the path to the static files, something
like this:
CACHE MANIFEST
{{ url_for('static', filename='style.css') }}
{{ url_for('static', filename='script.js') }}
Then in your HTML template you would have a reference to a route instead of a
static file:
<html manifest="{{ url_for('manifest') }}">
And finally, you would add a new view function that returns the manifest:
from flask import make_response
@app.route('/manifest')
def manifest():
res = make_response(render_template('manifest.appcache'), 200)
res.headers["Content-Type"] = "text/cache-manifest"
return res
|
Accessing the print function from globals()
Question: _Apologies in advance for conflating functions and methods, I don't have time
at the moment to sort out the terminology but I'm aware of the distinction
(generally)._
I'm trying to control what functions are run by my script via command-line
arguments. After a lot of reading here and elsewhere, I'm moving in the
direction of the following example.
# After connecting to a database with MySQLdb and defining a cursor...
cursor.execute(some_query_stored_earlier)
for row in cursor:
for method_name in processing_methods: # ('method1','method2', ...)
globals()[method_name](row)
_(Clarification:`processing_methods` is a tuple of user-defined strings via
command-line argument(s) with `nargs='*'`.)_
However, I'm running into problems with `print` (no surprise there). I would
like `print` to be:
* among the methods that MIGHT be specified from the command line;
* the default method when NO methods are specified from the command line;
* not performed if ONLY OTHER methods are specified from the command line.
Let me acknowledge that I can make things easier on myself by eliminating the
first and third criteria and simply doing:
for row in cursor:
print row
for method_name in processing_methods:
globals[method_name](row)
But I really don't want to ALWAYS print every row in what will sometimes be a
several-million-rows result. I did a future import, hoping that would solve my
problem - no such luck. So I did a little exploring:
>>> from __future__ import print_function
>>> print
<built-in function print>
>>> globals()
{'__builtins__': <module '__builtin__' (built-in)>, '__name__': '__main__', '__doc__': None, 'print_function': _Feature((2, 6, 0, 'alpha', 2), (3, 0, 0, 'alpha', 0), 65536), '__package__': None}
>>> a = "Hello, world!"
>>> print(a)
Hello, world!
>>> globals()['print'](a)
Traceback (most recent call last):
File "<pyshell#33>", line 1, in <module>
globals()['print'](a)
KeyError: 'print' # Okay, no problem, obviously it's...
>>> globals()['print_function'](a)
Traceback (most recent call last):
File "<pyshell#34>", line 1, in <module>
globals()['print_function'](a)
AttributeError: _Feature instance has no __call__ method # ...huh.
So then I did a little more reading, and [this
Q&A](http://stackoverflow.com/questions/15771393/python-global-function-like-
print) prompted some more exploring:
>>> dir()
['__builtins__', '__doc__', '__name__', '__package__']
>>> __builtins__
<module '__builtin__' (built-in)>
>>> 'print' in dir(__builtins__)
True # now we're getting somewhere!
>>> __builtins__.print something
SyntaxError: invalid syntax # fair enough.
>>> __builtins__.print('something')
SyntaxError: invalid syntax # wait, what?
>>> dir(__builtins__.print)
SyntaxError: invalid syntax # -_-
Something is going on here that I just don't understand, and [this other
Q&A](http://stackoverflow.com/questions/11181519/python-whats-the-difference-
between-builtin-and-builtins) hasn't made it any clearer. I think the easy
solution to my particular issue is going to be a mildly awkward wrapper like:
def printrows(row):
print row # assuming no future import, of course
But it's driving me crazy: Why can't I access `print` via the globals
dictionary? Am I doing it wrong, or is it just something you can't do with
built-in functions?
Answer: Did you forget to repeat `from __future__ import print_function` when you
opened a new shell for your second try (where you got all those syntax
errors)? It works for me: <https://ideone.com/JOBAAk>
|
Creating a Terminal Program with Python
Question: I recently started learning python. I have created some basic webapps with
Django and wrote some simple scripts. After using VIM as a Python IDE I really
fell I love with "Terminal programs" (is there an official term for this?).
Right now I am capable of doing simple things like asking someones age and
printing it to the screen. However this comes down to running a .py script and
after this script is done the normal bash return. I would like create a
program that I can run from the command line and that would allow the same
user experience as VIM (one that you open and close). For example I created a
simple script to import RSS feeds. It would be cool if I could open my
terminal type the name of my program -> program would open -> Then I would
like to use commands like :findsomething. Basically have real interaction with
my program.
To conclude:
* How would I go about creating such a program?
* What kinds of modules, books or site would you recommend
Answer: A true command-line program is something in the vein of `ls` or `grep`; it is
started from the command-line, but it's non-interactive and can be used in
pipelines and combined with other programs. A typical command-line program has
no interactive user experience, instead relying on shell's history and init
file for customization.
What you want to create is a _curses_ application, that uses the full
capabilities of the TTY as an interactive platform, for better or worse. To do
that, look up [curses](http://docs.python.org/2/library/curses.html).
|
Facebook python api documentation on all commands
Question: I am using the facebook api but currently their code only teaches how to get
your friends. I played with the graph api tool on their site and now I know
what I need to extract, but is there a document out there that tells you all
the commands for:
* gettings posts are there from a user
* how many likes a user's/company's page has
* what is the talking_about_count
* all posts that have a user's name tagged
this is all I have so far:
import facebook
token = "XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX"
graph = facebook.GraphAPI(token)
profile = graph.get_object("travelguard")
friends = graph.get_connections("me", "friends")
friend_list = [friend['name'] for friend in friends['data']]
print profile['talking_about_count']
print profile['likes']
print friends
Answer: Man, no one here seems to know anything on facebook or linkedin apis. I
figured it out myself...
from facepy import GraphAPI
graph = GraphAPI("CAAErZAZAHDByABAHtXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX")
graph.get('me/posts')
#if you want to get someone else's post: graph.get('{id}/posts')
|
Python regex example
Question: If I want to replace a pattern in the following statement structure:
cat&345;
bat &#hut;
I want to replace elements starting from `&` and ending before (not including
`;`). What is the best way to do so?
Answer: Here is a good regex
`import re
result = re.sub("(?<=\\&).*(?=;)", replacementstr, searchText)`
Basically this will put the replacement in between the `&` and the `;`
|
Implementing an algorithm to determine if a string has all unique characters
Question: Context: I'm a CS n00b working my way through "Cracking the Coding Interview."
The first problem asks to "implement an algorithm to determine if a string has
all unique characters." My (likely naive) implementation is as follows:
def isUniqueChars2(string):
uchars = []
for c in string:
if c in uchars:
return False
else:
uchars.append(c)
return True
The author suggests the following implementation:
def isUniqueChars(string):
checker = 0
for c in string:
val = ord(c) - ord('a')
if (checker & (1 << val) > 0):
return False
else:
checker |= (1 << val)
return True
What makes the author's implementation better than mine (FWIW, the author's
solution was in Java and I converted it to Python -- is my solution one that
is not possible to implement in Java)? Or, more generally, what is desirable
in a solution to this problem? What is wrong with the approach I've taken? I'm
assuming there are some fundamental CS concepts (that I'm not familiar with)
that are important and help inform the choice of which approach to take to
this problem.
Answer: Here is how I would write this:
def unique(s):
return len(set(s)) == len(s)
Strings are iterable so you can pass your argument directly to `set()` to get
a set of the characters from the string (which by definition will not contain
any duplicates). If the length of that set is the same as the length of the
original string then you have entirely unique characters.
Your current approach is fine and in my opinion it is much more Pythonic and
readable than the version proposed by the author, but you should change
`uchars` to be a set instead of a list. Sets have O(1) membership test so `c
in uchars` will be considerably faster on average if `uchars` is a set rather
than a list. So your code could be written as follows:
def unique(s):
uchars = set()
for c in s:
if c in uchars:
return False
uchars.add(c)
return True
This will actually be more efficient than my version if the string is large
and there are duplicates early, because it will short-circuit (exit as soon as
the first duplicate is found).
|
Django unicode error when removing ImageField from Admin interface
Question: When using the Clear checkbox to remove an uploaded image and clicking Save in
the Django Admin interface, I get the following result:
> TypeError at /admin/foo/bar/1/
>
> coercing to Unicode: need string or buffer, ImageFieldFile found
>
> Exception Value: coercing to Unicode: need string or buffer, ImageFieldFile
> found Exception Location: /home/ec2-user/ve/Project/lib/python2.6/site-
> packages/django/core/files/images.py in get_image_dimensions, line 47
I am aware that this is probably to do with the unicode type of the return so
have changed from `return image` to `return image.name` in the relevant
`ImageField` code but this has not solved the problem. This is the debug log:
/home/user/ve/Project/lib/python2.6/site-packages/django/db/transaction.py
in inner
return func(*args, **kwargs) ...
/home/user/ve/Project/lib/python2.6/site-packages/django/contrib/admin/options.py
in change_view
if form.is_valid(): ...
/home/user/ve/Project/lib/python2.6/site-packages/django/forms/forms.py
in is_valid
return self.is_bound and not bool(self.errors) ...
/home/user/ve/Project/lib/python2.6/site-packages/django/forms/forms.py
in _get_errors
self.full_clean() ...
/home/user/ve/Project/lib/python2.6/site-packages/django/forms/forms.py
in full_clean
self._clean_fields() ...
/home/user/ve/Project/lib/python2.6/site-packages/django/forms/forms.py
in _clean_fields
value = field.clean(value, initial) ...
/home/user/Devel/Project/project-cms/project/forms.py in clean
w, h = get_image_dimensions(image) ...
/home/user/ve/Project/lib/python2.6/site-packages/django/core/files/images.py
in get_image_dimensions
file = open(file_or_path, 'rb') ...
The get_image_dimensions method is imported as such: from
django.core.files.images import get_image_dimensions
This is the body of the clean function:
def clean(self, value, initial=None):
image = super(TheImageField, self).clean(value, initial)
if image is None: return
w, h = get_image_dimensions(image)
if w != TheImageField.required_width or h != TheImageField.required_height:
error_message = self.error_messages['incorrect_size'] % (w,h,)
raise util.ValidationError(error_message)
return image
Answer: [The signature for function
`get_image_dimensions`](https://github.com/disqus/django/blob/master/django/core/files/images.py)
is this one:
def get_image_dimensions(file_or_path, close=False)
`file_or_path` should be a string to a file in the disk, not an `ImageField`,
hence the error.
You can do it like this:
def clean(self, value, initial=None):
image = super(TheImageField, self).clean(value, initial)
if image is None: return
# this is where I changed the code to add image.path
w, h = get_image_dimensions(image.path)
if w != TheImageField.required_width or h != TheImageField.required_height:
error_message = self.error_messages['incorrect_size'] % (w,h,)
raise util.ValidationError(error_message)
return image
Hope this helps!
|
add new elements to a dictionary
Question: I would like to convert stuff from my csv.reader to a dictionary. I
implemented the instructions from this post [Add to a dictionary in
Python?](http://stackoverflow.com/questions/1024847/add-to-a-dictionary-in-
python) but I keep getting `IndexError: list index out of range`. How can I
fix this?
import wx
import csv
info = csv.reader(open('report.csv', 'rb'),delimiter=',')
length = 0
info_list = []
for row in info: #search each row in the report
info_list.append([length,row[1],row[4]])
length = length + 1
print length
dict_info = {}
rows = 0
counter = 0
while counter < length:
for item in info_list:
dict_info[item[rows]] = [item[rows + 1], item[rows + 2]]
rows = rows + 3
counter = counter + 1
print dict_info
Answer:
for index, row in enumerate(info):
dict_info[index] = [row[1], row[4]]
|
Inspecting data descriptor attributes in python
Question: I'm attempting to use a data descriptor to provide some custom get/set
functionality to attributes of a class. I'd like to be able to inspect the
class at runtime and get a list of data descriptors on that class, and maybe
even determine what the type of the descriptor is.
The problem is that when I look at the members I get using
`inspect.getmembers` my data descriptor attributes are resolved (their
`__get__` method is already called and that result is set as the value of the
object).
I'm using the example from: <http://docs.python.org/2/howto/descriptor.html>
import inspect
class RevealAccess(object):
"""A data descriptor that sets and returns values
normally and prints a message logging their access.
"""
def __init__(self, initval=None, name='var'):
self.val = initval
self.name = name
def __get__(self, obj, objtype):
print 'Retrieving', self.name
return self.val
def __set__(self, obj, val):
print 'Updating', self.name
self.val = val
class MyClass(object):
x = RevealAccess(10, 'var "x"')
y = 5
if __name__ == '__main__':
for x in inspect.getmembers(MyClass, inspect.isdatadescriptor):
print x
When I run this, I get:
Retrieving var "x"
('__weakref__', <attribute '__weakref__' of 'MyClass' objects>)
What I _expect_ is more like:
('x', <attribute 'x' of 'MyClass' objects>)
('__weakref__', <attribute '__weakref__' of 'MyClass' objects>)
I know I'm missing something I just can't put my finger on it. Any help
appreciated.
Answer: To get the descriptor itself, you can look into class `__dict__`:
MyClass.__dict__['x']
But the better way is to modify the getter:
def __get__(self, obj, objtype):
print 'Retrieving', self.name
if obj is None: # accessed as class attribute
return self # return the descriptor itself
else: # accessed as instance attribute
return self.val # return a value
Which gives:
Retrieving var "x"
('__weakref__', <attribute '__weakref__' of 'MyClass' objects>)
('x', <__main__.RevealAccess object at 0x7f32ef989890>)
|
gunicorn_django -b 0.0.0.0 project_name/settings/production.py
Question: $ python manage.py run_gunicorn 0.0.0.0:80
--settings=project_name.settings.production
<\- It's run, OK.
but, $ gunicorn_django -b 0.0.0.0:80 project_name/settings/production.py
* * *
Traceback (most recent call last):
File "/usr/local/lib/python2.7/dist-packages/gunicorn/arbiter.py", line 473, in spawn_worker
worker.init_process()
File "/usr/local/lib/python2.7/dist-packages/gunicorn/workers/base.py", line 100, in init_process
self.wsgi = self.app.wsgi()
File "/usr/local/lib/python2.7/dist-packages/gunicorn/app/base.py", line 106, in wsgi
self.callable = self.load()
File "/usr/local/lib/python2.7/dist-packages/gunicorn/app/djangoapp.py", line 102, in load
return mod.make_wsgi_application()
File "/usr/local/lib/python2.7/dist-packages/gunicorn/app/django_wsgi.py", line 36, in make_wsgi_application
if get_validation_errors(s):
File "/usr/local/lib/python2.7/dist-packages/django/core/management/validation.py", line 35, in get_validation_errors
for (app_name, error) in get_app_errors().items():
File "/usr/local/lib/python2.7/dist-packages/django/db/models/loading.py", line 166, in get_app_errors
self._populate()
File "/usr/local/lib/python2.7/dist-packages/django/db/models/loading.py", line 72, in _populate
self.load_app(app_name, True)
File "/usr/local/lib/python2.7/dist-packages/django/db/models/loading.py", line 94, in load_app
app_module = import_module(app_name)
File "/usr/local/lib/python2.7/dist-packages/django/utils/importlib.py", line 35, in import_module
__import__(name)
> ImportError: No module named accounts
2013-06-29 01:56:53 [30859] [INFO] Worker exiting (pid: 30859)
2013-06-29 01:56:53 [30854] [INFO] Shutting down: Master
2013-06-29 01:56:53 [30854] [INFO] Reason: Worker failed to boot.
* * *
How can I solve this problem? I want to run on daemon.
Thanks.
Answer: This looks like an issue related to your `PYTHONPATH`. Try adding the path
that contains the `accounts` module to your python path, using gunicorn's
[`--pythonpath`](http://docs.gunicorn.org/en/latest/configure.html#pythonpath).
If you have newer gunicorn/Django versions using gunicorn via [management
command](http://docs.gunicorn.org/en/latest/run.html#django-manage-py) should
be the preffered way - and it should be more safe regarding issues like this.
|
Python Slice Notation with Comma/List
Question: I have come across some python code with slice notation that I am having
trouble figuring out. It looks like slice notation but uses a comma and a
list:
list[:, [1, 2, 3]]
Is this syntax valid? If so what does it do?
**edit** looks like it is a 2D numpy array
Answer: Assuming that the object is really a `numpy` array, this is known as [advanced
indexing](http://docs.scipy.org/doc/numpy/reference/arrays.indexing.html#advanced-
indexing), and picks out the specified columns:
>>> import numpy as np
>>> a = np.arange(12).reshape(3,4)
>>> a
array([[ 0, 1, 2, 3],
[ 4, 5, 6, 7],
[ 8, 9, 10, 11]])
>>> a[:, [1,2,3]]
array([[ 1, 2, 3],
[ 5, 6, 7],
[ 9, 10, 11]])
>>> a[:, [1,3]]
array([[ 1, 3],
[ 5, 7],
[ 9, 11]])
Note that this won't work with the standard Python list:
>>> a.tolist()
[[0, 1, 2, 3], [4, 5, 6, 7], [8, 9, 10, 11]]
>>> a.tolist()[:,[1,2,3]]
Traceback (most recent call last):
File "<ipython-input-17-7d77de02047a>", line 1, in <module>
a.tolist()[:,[1,2,3]]
TypeError: list indices must be integers, not tuple
|
Send keystrokes to vnc server without GUI
Question: I want to make a program that connects to a VNC server and then sends a
sequence of key presses, then disconnects. And all without ever showing a GUI.
Example use:
vnckeysender SERVER KEYPRESSES
Where SERVER would be something like "10.0.0.1" and KEYPRESSES would be
something like "The quick brown fox".
I would like to write it in python, but I am not sure where to start. Any help
would be appreciated.
Answer: There is a command line utility written in python called
[vncdotool](https://github.com/sibson/vncdotool). It can also be used as a
library and imported into existing python scripts to connect and send keyboard
and mouse input to a VNC server.
|
Could not import settings in Django over OpenShift
Question: I'm testing OpenShift with Django/Python 2.7 and I'm getting this:
127.5.232.1 - - [2013-06-28 17:01:37] "GET / HTTP/1.1" 500 161 0.013770
Traceback (most recent call last):
File "build/bdist.linux-x86_64/egg/gevent/pywsgi.py", line 438, in handle_one_response
self.run_application()
File "build/bdist.linux-x86_64/egg/gevent/pywsgi.py", line 424, in run_application
self.result = self.application(self.environ, self.start_response)
File "/var/lib/openshift/51cd757de0b8cd31130000c1/python/virtenv/lib/python2.7/site-packages/Django-1.5.1-py2.7.egg/django/core/handlers/wsgi.py", line 236, in __call__
self.load_middleware()
File "/var/lib/openshift/51cd757de0b8cd31130000c1/python/virtenv/lib/python2.7/site-packages/Django-1.5.1-py2.7.egg/django/core/handlers/base.py", line 45, in load_middleware
for middleware_path in settings.MIDDLEWARE_CLASSES:
File "/var/lib/openshift/51cd757de0b8cd31130000c1/python/virtenv/lib/python2.7/site-packages/Django-1.5.1-py2.7.egg/django/conf/__init__.py", line 53, in __getattr__
self._setup(name)
File "/var/lib/openshift/51cd757de0b8cd31130000c1/python/virtenv/lib/python2.7/site-packages/Django-1.5.1-py2.7.egg/django/conf/__init__.py", line 48, in _setup
self._wrapped = Settings(settings_module)
File "/var/lib/openshift/51cd757de0b8cd31130000c1/python/virtenv/lib/python2.7/site-packages/Django-1.5.1-py2.7.egg/django/conf/__init__.py", line 134, in __init__
raise ImportError("Could not import settings '%s' (Is it on sys.path?): %s" % (self.SETTINGS_MODULE, e))
ImportError: Could not import settings 'www_project.settings' (Is it on sys.path?): No module named openshiftlibs
<WSGIServer fileno=6 address=127.5.232.1:8080>: Failed to handle request:
request = GET /favicon.ico HTTP/1.1 from ('127.5.232.1', 22073)
application = <django.core.handlers.wsgi.WSGIHandler object at 0x7f65c45dc510>
What I did?:
1. Created an application using rhc command line tool
2. Created an "isolated Django application" and copied into the wsgi directory
3. Replaced the application and settings file for the official django example
4. Pushed and tried
This is my application file:
#!/usr/bin/env python
import os
import sys
os.environ['DJANGO_SETTINGS_MODULE'] = 'www_project.settings'
sys.path.append(os.path.join(os.environ['OPENSHIFT_REPO_DIR'], 'wsgi', 'www_project'))
virtenv = os.environ['OPENSHIFT_REPO_DIR'] + '/virtenv/'
os.environ['PYTHON_EGG_CACHE'] = os.path.join(virtenv, 'lib/python2.7/site-packages')
virtualenv = os.path.join(virtenv, 'bin/activate_this.py')
try:
execfile(virtualenv, dict(__file__=virtualenv))
except:
pass
import django.core.handlers.wsgi
application = django.core.handlers.wsgi.WSGIHandler()
**My question:**
What am I missing?, if you need something else please let me know. Thanks in
advance!
Answer: I was missing the openshiftlibs.py file
|
Seemingly strange behavior with python counter program
Question: A portion of a recent assignment was to design a program that counts days
between dates. I have yet to finish, and I know this could be improved
drastically. However, my question is: when I run this program with **date2**
(below), an error occurs, but this runs as planned with **date1** (again,
below). I find this behavior strange. These dates are only one day apart and
yet one fails and one doesn't. I've tried this with multiple dates, but each
fails after 994 days regardless of month, year, day, whatever. **Why?**
clarification: I define "fail" as
File "first.py", line 35, in counter
return counter(date_array, x+1)
.
def date_test(date_array):
first = (date_array[0], date_array[1], date_array[2])
second = (date_array[3], date_array[4], date_array[5])
if first > second:
return False
elif first != second:
return True
elif first == second:
return False
else:
return "Error: This shouldn't happen."
def counter(date_array, x = 0):
months = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]
while(date_test(date_array)):
if(date_array[2] == 31 and date_array[1] == 12):
date_array[0] += 1; date_array[1], date_array[2] = 1, 1
return counter(date_array, x+1)
else:
if date_array[2] < months[date_array[1]-1]:
date_array[2] += 1
return counter(date_array, x+1)
else:
date_array[1] += 1; date_array[2] = 1;
return counter(date_array, x+1);
return x
def tuple_test(date):
date_array = []
for x in date:
date_array.append(x)
if not date_test(date_array):
return "The first date is before the second. Swap them."
return counter(date_array)
date1 = (1900,1,1,1902,9,22)
date2 = (1900,1,1,1902,9,23)
print tuple_test(date1)
print tuple_test(date2)
Answer: you should use the tools python provides
>>> import datetime
>>> date1 = (1900,1,1,1902,9,22) #your weird list with 2 dates
>>> dt1 = datetime.date(*date1[:3]) #create a date object == datetime.date(1900,1,1)
>>> dt2 = datetime.date(*date1[3:]) #create a date object == datetime.date(1902,9,22)
>>> if dt1 < dt2: dt1,dt2 = dt2,dt1 #if dt1 is smaller than dt2, swap them
...
>>> print (dt1 - dt2).days #subtract them and print their days
994
>>>
|
Decode JSON in Bash using python mjson.tool
Question: I need to get a `key` from JSON in standard bash, and found the following:
echo '{"first_key": "value", "second_key": "value2"}' | python -mjson.tool | grep 'first_key'
But this returns:
"first_key": "value",
How can I just return `value`, i.e. not the key, and remove the quotes and
comma.
Thanks.
Answer:
$ echo '{"first_key": "value", "second_key": "value2"}' | python -c 'import sys, json; print json.load(sys.stdin)[sys.argv[1]]' first_key
value
|
python plotting overrides data
Question: I have lot of binary and ascii files in one folder. I am reading them using
glob module. Doing processing of the binary data so that I can plot them. And
finally, I am trying to plot simplified binary data in one subplot and normal
ascii file in another subplot. The problem I am facing is that it can generate
plots for the corresponding binary files. But for the ascii files it just
simply override the previous files and always generates the same plot. Here is
the simplied version of the code for an example-
import glob
import numpy as np
from struct import unpack
import matplotlib.pyplot as plt
chi = sorted(glob.glob('C:/Users/Desktop/bin/*.chi'))
for index,fh in enumerate(chi):
data = np.genfromtxt(fh, dtype = float)
x = [row[0] for row in data]
y = [row[1] for row in data]
binary = sorted(glob.glob('C:/Users/Desktop/bin/*.bin'))
for count,FILE in enumerate(binary):
F = open(FILE,'rb')
B = unpack('f'*1023183, F.read(4*1023183))
A = np.array(B).reshape(1043, 981)
F.close()
#a = something column 1 # some further processing
#b = something column 2 # and generates 1D data
fig = plt.figure(figsize=(11, 8.0))
ax1 =fig.add_subplot(211,axisbg='w')
ax1.plot(a,b)
ax2 =fig.add_subplot(212, axisbg ='w')
ax2.plot(x,y)
plt.show()
Can somebody please explain why the files are replacing each other during
plotting only for one set of data where the other set is plotting correctly?
Answer: the structures of the loops is not correct in your example, you must have the
plot command inside the loop over the ascii file, else only the last one is
plotted. This should work:
try it like this:
import glob
import numpy as np
from struct import unpack
import matplotlib.pyplot as plt
fig = plt.figure(figsize=(11, 8.0))
chi = sorted(glob.glob('C:/Users/Desktop/bin/*.chi'))
for index,fh in enumerate(chi):
data = np.genfromtxt(fh, dtype = float)
x = [row[0] for row in data]
y = [row[1] for row in data]
ax1 =fig.add_subplot(211, axisbg ='w')
ax1.plot(x,y)
binary = sorted(glob.glob('C:/Users/Desktop/bin/*.bin'))
for count,FILE in enumerate(binary):
F = open(FILE,'rb')
B = unpack('f'*1023183, F.read(4*1023183))
A = np.array(B).reshape(1043, 981)
F.close()
#a = something column 1 # some further processing
#b = something column 2 # and generates 1D data
ax2 =fig.add_subplot(212,axisbg='w')
ax2.plot(a,b)
plt.show()
|
Updating a clock on a notepad tab
Question: I'm making an application through Python and one of the tabs I'm creating is
to show the current times: local and GMT. When I start the program, it gives
me the time at which the program was started. I was wondering if there was a
way to update the times so that I can have the actual time on the application.
I tried this type of code for my clocks:
<http://ygchan.blogspot.com/2012/05/python-how-to-make-clock-timer-in.html>
This is what I have for my application so far:
from Tkinter import *
from ttk import *
import time
root = Tk()
root.geometry('1000x900')
root.title("Astronomical Observing Recording Program")
frame1 = Frame(root, name='frame1')
frame1.pack(fill=BOTH)
localtime = time.asctime(time.localtime(time.time()))
gmttime = time.asctime(time.gmtime(time.time()))
welcome_message = "Hi" #shortened
current_time = "The current time is: " + str(localtime)
gmt_time = "The current GMT time is: " + str(gmttime)
root.protocol("WM_DELETE_WINDOW", frame1.quit)
nb = Notebook(frame1, name='nb')
nb.pack(fill=BOTH, padx=2, pady=3)
welcome_screen = Frame(nb, name='welcome_screen')
Label(welcome_screen, text=welcome_message).pack(side=LEFT)
nb.add(welcome_screen, text='Welcome')
time_screen = Frame(frame1, name='time_screen')
Label(time_screen, text=current_time).pack(side=TOP)
Label(time_screen, text=gmt_time).pack(side=TOP)
nb.add(time_screen, text='Time Bar')
frame1.mainloop()
It would be appreciated if someone could help me, or even set me in the right
direction so I could try to fix it myself.
Thanks
Answer: Use [after](http://effbot.org/tkinterbook/widget.htm#Tkinter.Widget.after-
method) which will call the function after given time.
from Tkinter import *
from ttk import *
import time
root = Tk()
root.geometry('1000x900')
root.title("Astronomical Observing Recording Program")
frame1 = Frame(root, name='frame1')
frame1.pack(fill=BOTH)
welcome_message = "Hi" #shortened
root.protocol("WM_DELETE_WINDOW", frame1.quit)
nb = Notebook(frame1, name='nb')
nb.pack(fill=BOTH, padx=2, pady=3)
welcome_screen = Frame(nb, name='welcome_screen')
Label(welcome_screen, text=welcome_message).pack(side=LEFT)
nb.add(welcome_screen, text='Welcome')
time_screen = Frame(frame1, name='time_screen')
lb_current_time = Label(time_screen)
lb_current_time.pack(side=TOP)
lb_gmt_time = Label(time_screen)
lb_gmt_time.pack(side=TOP)
nb.add(time_screen, text='Time Bar')
def update_time():
localtime = time.asctime(time.localtime(time.time()))
gmttime = time.asctime(time.gmtime(time.time()))
current_time = "The current time is: " + localtime
gmt_time = "The current GMT time is: " + gmttime
lb_current_time['text'] = current_time
lb_gmt_time['text'] = gmt_time
root.after(1000, update_time)
update_time()
frame1.mainloop()
|
How can I parse a formatted file into variables using Python?
Question: I have a pre-formatted text file with some variables in it, like this:
header one
name = "this is my name"
last_name = "this is my last name"
addr = "somewhere"
addr_no = 35
header
header two
first_var = 1.002E-3
second_var = -2.002E-8
header
As you can see, each score starts with the string `header` followed by the
name of the scope (one, two, etc.).
I can't figure out how to programmatically parse those options using Python so
that they would be accesible to my script in this manner:
one.name = "this is my name"
one.last_name = "this is my last name"
two.first_var = 1.002E-3
Can anyone point me to a tutorial or a library or to a specific part of the
docs that would help me achieve my goal?
Answer: I'd parse that with a generator, yielding sections as you parse the file.
`ast.literal_eval()` takes care of interpreting the value as a Python literal:
import ast
def load_sections(filename):
with open(filename, 'r') as infile:
for line in infile:
if not line.startswith('header'):
continue # skip to the next line until we find a header
sectionname = line.split(None, 1)[-1].strip()
section = {}
for line in infile:
if line.startswith('header'):
break # end of section
line = line.strip()
key, value = line.split(' = ', 1)
section[key] = ast.literal_eval(value)
yield sectionname, section
Loop over the above function to receive `(name, section_dict)` tuples:
for name, section in load_sections(somefilename):
print name, section
For your sample input data, that results in:
>>> for name, section in load_sections('/tmp/example'):
... print name, section
...
one {'last_name': 'this is my last name', 'name': 'this is my name', 'addr_no': 35, 'addr': 'somewhere'}
two {'first_var': 0.001002, 'second_var': -2.002e-08}
|
Python: Unicode encoding of returned String from parsed Query (MeCab)
Question: I am trying to use a program called MeCab, which does syntax analysis on
Japanese text. The problem I am having is that it returns a byte string and if
I try to print it, it prints question marks for almost all characters.
However, if I try to use `.decode`, it throws an error. Here is my code:
#!/usr/bin/python
# -*- coding:utf-8 -*-
import MeCab
tagger = MeCab.Tagger("-Owakati")
text = 'MeCabで遊んでみよう!'
print text
result = tagger.parse(text)
print result
result = unicode(result, 'utf-8')
print result
This is my output:
MeCabで遊んでみよう!
MeCab �� �� ��んで�� �� ��う!
Traceback (most recent call last):
File "test.py", line 12, in <module>
result = unicode(result, 'utf-8')
UnicodeDecodeError: 'utf8' codec can't decode bytes in position 6-7: invalid continuation byte
------------------
(program exited with code: 1)
Press return to continue
Also, my terminal is able to display Japanese characters properly. For example
`print '日本語'` works perfectly fine.
Any ideas?
Answer: MeCab doesn't return UTF8 by default. Below is a quote from the following link
(via Google Translate):
<http://mecab.googlecode.com/svn/trunk/mecab/doc/index.html#charset>
> Unless otherwise specified, euc is used. If you would like to use the utf8
> or shift-jis, change the charset with configure options dictionary, please
> rebuild the dictionary. Now, and shift-jis, dictionary of utf8 is created.
Try `result = tagger.parse(text).decode('euc-jp')`.
|
Python mongolab REST api
Question: I am trying to access the mongolab REST api through python. Is the correct way
to do this via pythons urllib2? I have tried the following:
import urllib2
p = urllib2.urlopen("https://api.mongolab.com/api/1/databases/mydb/collections/mycollection?apiKey=XXXXXXXXXXXXXXXX")
But this gives me an error:
urllib2.URLError: <urlopen error unknown url type: https>
What is the correct way of doing this? After connecting, how do I go on to
POST a document to my collection? If someone could post up a code example, I
would be very grateful. Thanks all for the help!
EDIT:
I've recompiled python with ssl support. How do I POST insert a document to a
collection using mongolab REST API? Here is the code I have atm:
import urllib
import urllib2
url = "https://api.mongolab.com/api/1/databases/mydb/collections/mycollection?apiKey=XXXXXXXXXXXXXXXX"
data = {"x" : "1"}
request = urllib2.Request(url, data)
p = urllib2.urlopen(request)
Now, when I run this, I get the error
urllib2.HTTPError: HTTP Error 415: Unsupported Media Type
How do I insert documents using HTTP POST? Thanks!
Answer: That error is raised if you version of python does not include ssl support.
What version are you using? Did you compile it yourself?
That said, when you get a version including ssl, using
[requests](https://requests.readthedocs.org/en/v1.1.0/) is a lot easier than
urllib2, especially when POSTing data.
|
Python re.sub - replacing character when context does not match
Question: I am trying to clean up some corrupted csv-Files. One problem is that they
contain line feeds within data fields thus splitting one data set in two. I am
looking for a piece of python-code that eliminates line feeds should they not
be followed by 8 digits. My code so far:
filetoparse = open('test.csv', encoding='utf-8')
data = filetoparse.read()
data = re.sub(r'\n(\d{8})',r'§§§\1',data)
data = re.sub(r'\n',r'',data)
data = re.sub(r'§§§','\n',data)
Basically I am using the §§§ as a placeholder for correct line feeds,
eliminate all line feeds in the data and then replace the placeholders with
line feeds again.
It does work, but is there a way to do this more elegantly?
Answer: Use a [negative lookahead
pattern](http://docs.python.org/2/library/re.html#regular-expression-syntax):
data = re.sub(r'\n(?!\d{8})', '', data)
For example,
import re
data = '''
12345678 foo
bar
baz
12345678 foo
'''
data = re.sub(r'\n(?!\d{8})', '', data)
print(data)
yields
12345678 foobarbaz
12345678 foo
|
Argv - String into Integer
Question: I'm pretty new at python and I've been playing with argv. I wrote this simple
program here and getting an error that says :
> TypeError: %d format: a number is required, not str
from sys import argv
file_name, num1, num2 = argv
int(argv[1])
int(argv[2])
def addfunc(num1, num2):
print "This function adds %d and %d" % (num1, num2)
return num1 + num2
addsum = addfunc(num1, num2)
print "The final sum of addfunc is: " + str(addsum)
When I run filename.py 2 2, does argv put 2 2 into strings? If so, how do I
convert these into integers?
Thanks for your help.
Answer: `sys.argv` is indeed a list of strings. Use the `int()` function to turn a
string to a number, provided the string _can_ be converted.
You need to _assign_ the result, however:
num1 = int(argv[1])
num2 = int(argv[2])
or simply use:
num1, num2 = int(num1), int(num2)
You did call `int()` but ignored the return value.
|
python run in powershell with script give 'non-utf' error
Question: I am a python beginner, trying to run from power shell on vista.
when trying to call a simple script with:
> python vc.py
gives error: "File "vcpy", line 1 syntaxError: Non-UTF-8 code starting with
'\xff'
... where vc.py is: import sys print sys.version
It does work when I invoke instead:
> cat vc.py | python
The problem with this latter approach is that it is giving us problems with
the raw input function.
Answer: It seems your file is started with Unicode BOM. Try to save your file in Utf-8
without BOM.
|
FIlling in randomized form with Python and Mechanize
Question: I'm trying to use mechanize to automatically log into a website and check some
figures. I'm pretty sure I've got past the first page with the usual username
password form but the second login page asks for specific characters from an
answer to a security question you pick during account creation.
Like if your favorite pet was called garfield and it asked for the 2nd, 4th
and 5th characters you would have to fill out 3 inputs with a, f, i and post
that form.
Im not sure what the best way of having mechanize search for which characters
it wants each time it logs in is. The start of the source code for the form
contains this.
<label for="frmentermemorableinformation1:strEnterMemorableInformation_memInfo1">Character 5  </label>
It has another 2 of those labels for the other 2 characters to be filled in on
the form, I thought it would be a good idea to get mechanize to read through
the source searching for the first 3 occurances of "character " then reading
the character right after each occurance as the first 3 times that text comes
up is for those labels.
How would i go about doing this and is there an easier way to do this rather
than reading through the entire source code, can mechanize specifically search
for labels within that form or some other shortcut? Also I'm not very
experienced with programming so any extra detail or explanations would be
great. Thank you.
Answer: > How would i go about doing this and is there an easier way to do this rather
> than reading through the entire source code, can mechanize specifically
> search for labels within that form or some other shortcut?
I'm not that familiar with mechanize in python, but with mechanize in ruby for
instance, you use an html parser to search through the html. python's html
parser is BeautifulSoup or libxml. BeautifulSoup is easier to install--libxml
has lots of dependencies and can be a bear to install. Here is a BeautifulSoup
example:
from BeautifulSoup import BeautifulSoup as bs
import re
soup = bs(open('html.html'))
form = soup.find(id="form1")
labels = form.findAll('label', text=re.compile("Character \d+") )
labels = labels[:3]
for label in labels:
print(label.string)
--output:--
Character 5  
Character 6  
Character 7  
|
How do I wait for a certain number of buttons to be clicked in Tkinter/Python?
Question: I'm trying to write a simple 'Simon' game, but I have hit a road block here,
and honestly have no idea how to get around it.
So here, I made a class for the four buttons in the GUI:
class button:
def buttonclicked(self):
self.butclicked= True
def checkIfClicked(self):
if self.butclicked== True:
global pressed
pressed.append(self.color)
self.butclicked= False
def __init__(self, color1):
self.color= color1
self.button= tk.Button(root, text=' '*10, bg= self.color, command= self.buttonclicked)
self.button.pack(side='left')
self.butclicked=False
I then created four instances of this class in `blue, red, yellow, and green
as bb, rb, yb,` and `gb`.
Once everything is packed into the Tk() module, it enters a while loop that
appends a random color to a list activecolors. I try to use the following loop
to wait until the list pressed is at least as long as the list activecolors
before comparing the two to see if the user was correct:
while len(pressed)<len(activecolors):
sleep(.25)
print('In the check loop')
bb.checkIfClicked()
rb.checkIfClicked()
yb.checkIfClicked()
gb.checkIfClicked()
However, since it is stuck inside the while loop, the program can't tell that
the button has been clicked. I thought adding the sleep method into the loop
would allow the code to have time to do other things (such as process button
clicks), but this is not the case. Any help is appreciated.
[Here is the
link](https://docs.google.com/document/d/16zQlQk_mFoSro7iq_8NDYWen2QwfhGNUgcs7JSkFwvU/edit?usp=sharing)
to the full code, if you would like to see it. A warning though: it's not
pretty.
Edit: I ended up just changing the code to check the list only after a new
button was clicked, telling the computer the code was ready. I've updated the
Google Document if you'd like to see it.
Answer: You are making it too complicated. This program uses partial from
functiontools to allow a variable to be passed to the function so one function
handles all clicks (Python 2.7).
from Tkinter import *
from functools import partial
class ButtonsTest:
def __init__(self):
self.top = Tk()
self.top.title('Buttons Test')
self.top_frame = Frame(self.top, width =400, height=400)
self.colors = ("red", "green", "blue", "yellow")
self.colors_selected = []
self.num_clicks = 0
self.wait_for_number = 5
self.buttons()
self.top_frame.grid(row=0, column=1)
Button(self.top_frame, text='Exit',
command=self.top.quit).grid(row=2,column=1, columnspan=5)
self.top.mainloop()
##-------------------------------------------------------------------
def buttons(self):
for but_num in range(4):
b = Button(self.top_frame, text = str(but_num+1),
command=partial(self.cb_handler, but_num))
b.grid(row=1, column=but_num+1)
##----------------------------------------------------------------
def cb_handler( self, cb_number ):
print "\ncb_handler", cb_number
self.num_clicks += 1
this_color = self.colors[cb_number]
if (self.num_clicks > self.wait_for_number) \
and (this_color in self.colors_selected):
print "%s already selected" % (this_color)
self.colors_selected.append(this_color)
##===================================================================
BT=ButtonsTest()
|
Generating permutation in Python with specific rule
Question: Let say a=[A, B, C, D], each element has a weight w, and is set to 1 if
selected, 0 if otherwise. I'd like to generate permutation in the below order
1,1,1,1
1,1,1,0
1,1,0,1
1,1,0,0
1,0,1,1
1,0,1,0
1,0,0,1
1,0,0,0
0,1,1,1
0,1,1,0
0,1,0,1
0,1,0,0
0,0,1,1
0,0,1,0
0,0,0,1
0,0,0,0
Let's w=[1,2,3,4] for item A,B,C,D ... and max_weight = 4. For each
permutation, if the accum weight has exceeded max_weight, stop calculation for
that permutation, move to next permutation. For eg.
1,1,1 --> 6 > 4, exceeded, stop, move to next
1,1,1 --> 6 > 4, exceeded, stop, move to next
1,1,0,1 --> 7 > 4 finished, move to next
1,1,0,0 --> 3 finished, move to next
1,0,1,1 --> 8 > 4, finished, move to next
1,0,1,0 --> 4 finished, move to next
1,0,0,1 --> 5 > 4 finished, move to next
1,0,0,0 --> 1 finished, move to next
etc calculation continue
So far, [1,0,1,0] is the best combination which does not exceeded max_weight 4
My questions are
1. What's the algorithm which generate the required permutation? Or any suggestion I could generate the permutation?
2. As the number of element can be up to 10000, and the calculation stop if the accum weight for the branch exceeds max_weight, it is not necessary to generate all permutation first before the calculation. How can the algo in (1) generate permutation on the fly?
Answer: Use
[itertools.product](http://docs.python.org/2/library/itertools.html#itertools.compress)
function to generate permutation.
from itertools import *
w = [1,2,3,4]
max_weight = 4
for selection in product([1,0], repeat=len(w)):
accum = sum(compress(w, selection))
if accum > 4:
print '{} --> {} > {}, exceeded, stop, move to next'.format(selection, accum, max_weight)
else:
print '{} --> {} , finished, move to next'.format(selection, accum)
Use
[itertools.compress](http://docs.python.org/2/library/itertools.html#itertools.compress)
to filter weights by selection.
>>> from itertools import *
>>> compress([1,2,3,4], [1,0,1,1])
<itertools.compress object at 0x00000000027A07F0>
>>> list(compress([1,2,3,4], [1,0,1,1]))
[1, 3, 4]
|
python: How to remove punctuations from file
Question: I have an input file, each line of which is in the formar of a list in python.
It looks something like this:
['people', 'desert', 'snow']
['people', 'flower', 'garden', 'goat']
I want to process this file and remove all the punctuations from it, i.e. "[",
"]", "," and "'"
I am using the following code:
import string
import re
openfile=open('jcn','r')
writefile=open('jcnout','w')
punctuation=["[","]",",","'"]
for line in openfile:
line.translate(None, string.punctuation)
writefile.write(line)
writefile.flush()
writefile.close()
openfile.close()
But it doesnt seem to work, i.e. punctuation are retaind in the output file.
Could someone please tell me where i am wrong
Answer: You need to change
line.translate(None, string.punctuation)
to
line = line.translate(None, string.punctuation)
In Python, strings are immutable. Correspondingly, `translate()` doesn't
change the string in place, but rather _returns_ the translated string (which
you're ignoring).
|
freebase not working in python
Question: Im trying to run freebase using python on Ubuntu 12.10 the first time. here's
what i did
import freebase
query = {
"id" : "/en/the_beatles",
"type" : "/music/artist",
"album" : [{
"name" : None,
"release_date" : None,
"track": {
"return" : "count"
},
"sort" : "release_date"
}]
}
freebase.mqlread(query)
Here's that error i got
Traceback (most recent call last): File "", line 1, in File
"/usr/local/lib/python2.7/dist-
packages/freebase-1.0.8-py2.7.egg/freebase/api/session.py", line 597, in
mqlread r = self._httpreq_json(service, 'POST', form=dict(query=qstr)) File
"/usr/local/lib/python2.7/dist-
packages/freebase-1.0.8-py2.7.egg/freebase/api/session.py", line 420, in
_httpreq_json resp, body = self._httpreq(*args, **kws) File
"/usr/local/lib/python2.7/dist-
packages/freebase-1.0.8-py2.7.egg/freebase/api/session.py", line 406, in
_httpreq return self._http_request(url, method, body, headers) File
"/usr/local/lib/python2.7/dist-
packages/freebase-1.0.8-py2.7.egg/freebase/api/httpclients.py", line 66, in
**call** self.log.error('SOCKET FAILURE: %s', e.fp.read()) AttributeError:
'error' object has no attribute 'fp'
Could anyone help me resolve this?
Thansk in advance
Answer: If you're using the old Python client library it won't work because Google
never migrated it to work with the new APIs. You'll need to use the standard
Google APIs Python library and the discovery interface.
<https://developers.google.com/api-client-library/python/start/get_started>
|
CvtColor Error at higher resolutions
Question: I have a Logitech Pro 9000 webcam and right now I try to learn OpenCV. I use
OpenCV 2.4.5 together with Python 2.7. I'm having problems with the CvtColor
function at higher resolutions. The following script is working in 640x480,
but not with higher resolutions (800X600 and up).
import cv
cv.NamedWindow("Video", cv.CV_WINDOW_AUTOSIZE)
camera_index = 1
capture = cv.CaptureFromCAM(camera_index)
cv.SetCaptureProperty(capture,cv.CV_CAP_PROP_FRAME_WIDTH,1200)
cv.SetCaptureProperty(capture,cv.CV_CAP_PROP_FRAME_HEIGHT,800)
grey_frame = cv.CreateImage((1200,800),8,1)
while True:
input_frame = cv.QueryFrame(capture)
cv.CvtColor(input_frame,grey_frame,cv.CV_BGR2GRAY)
cv.WaitKey(5)
cv.ShowImage("Video", grey_frame)
I get the following error
cv2.error: dst.data == dst0.data
EDIT: SOLVED After saving the captured input_frame. I noticed that the
resolution of the saved image is not matching my set values. I set 1200x800 as
capture properties and got a 1280x800 image. After using this resolution,
everything worked fine.
Answer: After saving the captured input_frame. I noticed that the resolution of the
saved image is not matching my set values. I set 1200x800 as capture
properties and got a 1280x800 image. After using this resolution, everything
worked fine.
|
How to join a string to a URL in Python?
Question: I am trying to join a string in a URL, but the problem is that since it's
spaced the other part does not get recognized as part of the URL.
Here would be an example:
import urllib
import urllib2
website = "http://example.php?id=1 order by 1--"
request = urllib2.Request(website)
response = urllib2.urlopen(request)
html = response.read()
The "`order by 1--`" part is not recognized as part of the URL.
Answer: You should better use
[`urllib.urlencode`](http://docs.python.org/2/library/urllib.html#urllib.urlencode)
or
[`urllib.quote`](http://docs.python.org/2/library/urllib.html#urllib.quote):
website = "http://example.com/?" + urllib.quote("?id=1 order by 1--")
or
website = "http://example.com/?" + urllib.urlencode({"id": "1 order by 1 --"})
and about the query you're trying to achieve:
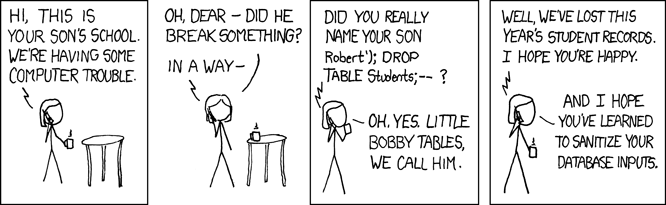
I think you're forgetting a `;` to end the first query.
|
IPython on Emacs 24.2 doesn't work
Question: I'm a beginner in emacs and I'm trying to extend so it acts like a comfortable
python IDE. Trouble is I can't seem to integrate Ipython to emacs. I'm running
emacs 24.2 with Ipython 0.13.2 on Xubuntu 13.04.
I tried adding this to my .emacs file:
(setq
python-shell-interpreter "ipython"
python-shell-interpreter-args ""
python-shell-prompt-regexp "In \\[[0-9]+\\]: "
python-shell-prompt-output-regexp "Out\\[[0-9]+\\]: "
python-shell-completion-setup-code
"from IPython.core.completerlib import module_completion"
python-shell-completion-module-string-code
"';'.join(module_completion('''%s'''))\n"
python-shell-completion-string-code
"';'.join(get_ipython().Completer.all_completions('''%s'''))\n")
But running python console from emacs (Python > Start Interpreter) just brings
"Inferior Python Mode". I also tried "emacs-ipython-notebook" but that doesn't
seem to be what I'm looking for...
Thanks!
Answer: Emacs 24.3 comes with a different python.el than 24.2. Your install points at
symbols of 24.3, 24.2 python.el will ignore this.
|
Multiple-choice answers not working
Question: I'm creating a menu that is meant to look like this
1-quit
2-multiplication
3-division
I cannot seem to fix this error it has something to do with `random.randint`
What I've done so far:
iFirst= random.randint(1,10)
iSecond = random.randint (1,10)
import random
print(" Made by: Ahmed\n --------------------")
print("1 - quit")
print("2 - Multiplication")
print("3 - Division")
while 1:
choose=input("\n\t:")
if choose=="1":
break # we basically break the while loop so it stops
if choose=="2":
a=iFirst*iSecond
print(str(iFirst) + "*" + str(iSecond))
print("a") + (random.randint()1,10)
print("a") + (random.randint()1,10)
print("b") + (random.randint()1,10)
print("c") + (str(a)
if choose =="3":
I'm trying to make it so that if I choose '2' it would randomly generate a
multiplication question with random answers, all different all the time, and
the real answer wouldn't stay at a certain letter. eg. If answer was 'b' the
next question will be different.
I want it to go a little bit like this but I cannot because it's too
challenging for me.
5*4 = ?
a)10
b)32
c)20
d)42
I'm trying to make it so that it randomizes the numbers each time I push 2 or
I get an incorrect answer. From there I can do the rest but at the moment it
gives me an error that I cannot figure out because i'm a beginner in Python. I
need help. I don't want you guys to do all of it for me because I know you're
not meant to, you're only here to help people like me.
Thanks in advance!
Answer: You can put the answers into a `list` and then use `random.shuffle()`
>>> answers = [10, 32, 20, 42]
>>> import random
>>> random.shuffle(answers)
>>> for c, item in zip('abcd', answers):
... print("{}) {}".format(c, item))
...
a) 20
b) 32
c) 10
d) 42
shuffle again gives a different order
>>> random.shuffle(answers)
>>> for c, item in zip('abcd', answers):
... print("{}) {}".format(c, item))
...
a) 32
b) 20
c) 42
d) 10
|
Send email in python using subprocess module
Question: I wrote a script to retrieve weather report from a website and send it to my
girfriend in the morning.
Using Gmail. Of course I can send it using my Postfix server. Here is the
script.
What **I'm not sure is how to use Popen() function in the situation with so
many arguments.**
I can send the mail using the command.
`$ mail -s "おお様からの天気予報" [email protected] < foo`
#!/usr/bin/env python
# -*- coding: utf-8 -*-
from bs4 import BeautifulSoup
import urllib2
import subprocess
weather_url = "http://www.weather.com.cn/weather/101020100.shtml"
f=urllib2.urlopen(weather_url)
html = f.read()
soup = BeautifulSoup(html)
content = soup.title.string
with open("foo","w") as mail:
mail.write(content.encode('utf-8'))
command_line = 'mail -s "おお様からの天気予報" [email protected] < foo'
li = command_line.split()
process = subprocess.Popen(li, shell=True)
returncode = process.wait()
The content of the weather report is in the `foo` file. Can somebody tell me
how to use `Popen()` with so many arguments?
I tried a lot.
This script just doesn't seem to work.
Answer: You don't need to pass an argument list when you use `shell=True` you can just
pass an argument string...
command_line = 'mail -s "おお様からの天気予報" [email protected] < foo'
process = subprocess.Popen(command_line, shell=True)
Or.. you can not use the shell to interpret your arguments and pass a list...
command_line = 'mail -s "おお様からの天気予報" [email protected] < foo'
li = command_line.split()
process = subprocess.Popen(li)
But you can't pass an argument list _and_ use the shell to interpret it.
Based on the nature of your command, I would recommend passing a string to the
shell to interpret. (the first option)
|
Get a datastore object by getattr without knowing its class
Question: I want to get a entity through a ReferencePropery in this way.
getattr(model, refrence)
where model is a `db.Model` and reference is `db.ReferenceProperty`. But I get
a KindError, which I can avoid by adding import of following sort.
from foomodule import ReferedKind
Where `ReferedKind` is the class of the entity I want to get by above
`getattr`.
But my problem is in my case because of the nature of the function I want to
call this in, the `model` can belong to any Model class of mine. So I don't
know what the `ReferedKind` will be.
How can I call `getattr` and get the referred entity without importing every
possible model class?
Is there a way I can know the class of a entity referred by a
`ReferanceProperty` in advance so I can dynamically import it?
**Aditional info**
I am writing a function that would return a json serializable python dict.
Because in some cases dictionary returned by db.to_dict() is not json
serializable. So the function doesn't know in advance what kind of a model it
will have to put into a dict.
Answer: Normally you need to import all potential models that you could retrieve
before doing anything.
Your making things hard for yourself.
The only way you do it would be to work at a lower level than db.Model or
ndb.Model to fetch the raw data then examine it then you will still need to
import the model before dereferencing a referenceproperty or fetching a key.
If you decide to do this ndm will be easier as it doesn't have a reference
property, but a KeyProperty, you can use getattr, check if you have a key,
then fetch the object referred to be the key.
If you want to stick with db. it will be messy because you need to use
`get_value_for_datastore`
Which would involve,
getting the class of the entity, getting the property of the class and then
calling get_value_for_datastore passing in the instance then assuming you have
imported the you can get the key.
so if you have an instance from the datastore called foo you would
prop = getattr(foo.__class__,"the_property_name")
obj_key = prop.get_value_for_datastore(foo)
Then you will need to get the kind from the key, then work out how to import
the model for that kind, before you get() the key, other wise you will still
get the `KindError`
I am not sure all the effort is worth it, you will find it easier to just
import the models. You ultimately need to import any model before you use it.
The only thing you are might be gaining is a lazy import potentially saving
some startup time.
|
Python: How to store output of ls -lrt
Question: Problem : In python, how to store the o/p of 'ls -lrt' in a string so that we
can search for files of a particular pattern
This is inorder to find all files in a directory that has been modified
between date1 and date2
Thanks in advance
Answer:
>>> import subprocess
>>> op = subprocess.check_output(["ls","-lrt"])
>>> print(op)
total 0
drwxr-xr-x+ 4 henry staff 136 16 Jun 11:20 Public
drwx------+ 3 henry staff 102 16 Jun 11:20 Movies
drwx------+ 4 henry staff 136 16 Jun 12:54 Pictures
drwx------@ 48 henry staff 1632 25 Jun 21:35 Library
drwx------+ 39 henry staff 1326 28 Jun 09:15 Desktop
drwx------+ 8 henry staff 272 28 Jun 12:24 Documents
drwx------+ 19 henry staff 646 29 Jun 15:15 Music
drwx------+ 146 henry staff 4964 30 Jun 17:49 Downloads
|
Python - Pandas: Get a group of mean values of a daily range in a longer range period
Question: For each day, I want to get the mean value of values between a range of 8am to
5pm. With those daily mean-values I want to make a new mean value for a range-
period of for example a month or a year or a custom chosen range. How can I do
that in Pandas?
for example the mean value for a period of aug-2011 to nov-2011 for a daily-
range between 8am and 5pm.
Time T_Sanyo_Gesloten
2010-08-31 12:30:00 33.910
2010-08-31 12:40:00 33.250
2010-08-31 12:50:00 30.500
2010-08-31 13:00:00 27.065
2010-08-31 13:10:00 25.610
...
2013-06-07 02:10:00 16.970
2013-06-07 02:20:00 16.955
2013-06-07 02:30:00 17.000
2013-06-07 02:40:00 17.015
2013-06-07 02:50:00 16.910
Answer:
import datetime as DT
import numpy as np
import pandas as pd
np.random.seed(2013)
N = 10**4
df = pd.DataFrame(
np.cumsum(np.random.random(N) - 0.5),
index=pd.date_range('2010-8-31', freq='10T', periods=N))
# 0
# 2010-08-31 00:00:00 0.175448
# 2010-08-31 00:10:00 0.631796
# 2010-08-31 00:20:00 0.399373
# 2010-08-31 00:30:00 0.499184
# 2010-08-31 00:40:00 0.631005
# ...
# 2010-11-08 09:50:00 -3.474801
# 2010-11-08 10:00:00 -3.172819
# 2010-11-08 10:10:00 -2.988451
# 2010-11-08 10:20:00 -3.101262
# 2010-11-08 10:30:00 -3.477685
eight_to_five = df.ix[df.index.indexer_between_time(DT.time(8), DT.time(17))]
# 0
# 2010-08-31 08:00:00 1.440543
# 2010-08-31 08:10:00 1.450957
# 2010-08-31 08:20:00 1.746454
# 2010-08-31 08:30:00 1.443941
# 2010-08-31 08:40:00 1.845446
# ...
# 2010-11-08 09:50:00 -3.474801
# 2010-11-08 10:00:00 -3.172819
# 2010-11-08 10:10:00 -2.988451
# 2010-11-08 10:20:00 -3.101262
# 2010-11-08 10:30:00 -3.477685
# daily_mean = eight_to_five.groupby()
daily_mean = eight_to_five.resample('D', how='mean')
# 0
# 2010-08-31 0.754004
# 2010-09-01 0.203610
# 2010-09-02 5.219528
# 2010-09-03 6.337688
# 2010-09-04 2.765504
monthly_mean = daily_mean.resample('M', how='mean')
# 0
# 2010-08-31 0.754004
# 2010-09-30 -0.437582
# 2010-10-31 3.533525
# 2010-11-30 4.356728
yearly_mean = daily_mean.groupby(daily_mean.index.year).mean()
# 0
# 2010 1.885995
To get a custom mean you'd change the argument passed to `groupby`.
|
running python-daemon as non-priviliged user and keeping group-memberships
Question: i'm writing a daemon in python, using the [python-
daemon](https://pypi.python.org/pypi/python-daemon/) package. the daemon is
started at boot-time (init.d) and needs to access various devices. the daemon
is to run on an embedded sysytem ([beaglebone](http://beagleboard.org/bone))
running ubuntu.
now my problem is that i want to run the daemon as an unpriviliged user rather
(e.g. `mydaemon`) than `root`.
in order to allow the daemon to access the devices i added that user to the
required groups. in the python code i use
`daemon.DaemonContext(uid=uidofmydamon)`.
the process started by `root` daemonizes nicely and is owned by the correct
user, but i get _permission denied_ errors when trying to access the devices.
i wrote a small test application, and it seems that the process does not
inherit the group-memberships of the user.
#!/usr/bin/python
import logging, daemon, os
if __name__ == '__main__':
lh=logging.StreamHandler()
logger = logging.getLogger()
logger.setLevel(logging.INFO)
logger.addHandler(lh)
uid=1001 ## UID of the daemon user
with daemon.DaemonContext(uid=uid,
files_preserve=[lh.stream],
stderr=lh.stream):
logger.warn("UID : %s" % str(os.getuid()))
logger.warn("groups: %s" % str(os.getgroups()))
when i run the above code as the user with uid=1001 i get something like
$ ./testdaemon.py
UID: 1001
groups: [29,107,1001]
wheras when i run the above code as root (or `su`), i get:
$ sudo ./testdaemon.py
UID: 1001
groups: [0]
how can i create a daemon-process started by root but with a different
effective uid _and_ intact group memberships?
Answer: my current solution involves dropping root priviliges before starting the
actual daemon, using the `chuid` argument for `start-stop-daemon`:
start-stop-daemon \
--start \
--chuid daemonuser \
--name testdaemon \
--pidfile /var/run/testdaemon/test.pid \
--startas /tmp/testdaemon.py \
-- \
--pidfile /var/run/testdaemon/test.pid \
--logfile=/var/log/testdaemon/testdaemon.log
the drawback of this solution is, that i need to create all directories, where
the daemon ought to write to (noteably `/var/run/testdaemon` and
`/var/log/testdaemon`), _before_ starting the actual daemon (with the proper
file permissions).
i would have preferred to write that logic in python rather than bash.
for now that works, but me thinketh that this should be solveable in a more
elegant fashion.
|
(Python) How to XOR two hex strings so that each byte is XORed separately?
Question: I have been posting similar questions here for a couple of days now, but it
seems like I was not asking the right thing, so excuse me if I have exhausted
you with my XOR questions :D.
To the point - I have two hex strings and I want to XOR these strings such
that each byte is XORed separately (i.e. each pair of numbers is XORed
separately). And I want to do this in python, and I want to be able to have
strings of different lengths. I will do an example manually to illustrate my
point (I used the code environment because it allows me to put in spaces where
I want them to be):
Input:
s1 = "48656c6c6f"
s2 = "61736b"
Encoding in binary:
48 65 6c 6c 6f = 01001000 01100101 01101100 01101100 01101111
61 73 6b = 01100001 01110011 01101011
XORing the strings:
01001000 01100101 01101100 01101100 01101111
01100001 01110011 01101011
00001101 00011111 00000100
Converting the result to hex:
00001101 00011111 00000100 = 0d 1f 04
Output:
0d1f04
So, to summarize, I want to be able to input two hex strings (these will
usually be ASCII letters encoded in hex) of different or equal length, and get
their XOR such that each byte is XORed separately.
Answer: Use
[`binascii.unhexlify()`](http://docs.python.org/2/library/binascii.html#binascii.unhexlify)
to turn your hex strings to binary data, then XOR that, going back to hex with
[`binascii.hexlify()`](http://docs.python.org/2/library/binascii.html#binascii.hexlify):
>>> from binascii import unhexlify, hexlify
>>> s1 = "48656c6c6f"
>>> s2 = "61736b"
>>> hexlify(''.join(chr(ord(c1) ^ ord(c2)) for c1, c2 in zip(unhexlify(s1[-len(s2):]), unhexlify(s2))))
'0d1f04'
The actual XOR is applied per byte of the decoded data (using `ord()` and
`chr()` to go to and from integers).
Note that like in your example, I truncated `s1` to be the same length as `s2`
(ignoring characters from the start of `s1`). You can encode _all_ of `s1`
with a shorter key `s2` by cycling the bytes:
>>> from itertools import cycle
>>> hexlify(''.join(chr(ord(c1) ^ ord(c2)) for c1, c2 in zip(unhexlify(s1), cycle(unhexlify(s2)))))
'2916070d1c'
You don't _have_ to use `unhexlify()`, but it is a lot easier than looping
over `s1` and `s2` 2 characters at a time and using `int(twocharacters, 16)`
to turn that into integer values for XOR operations.
|
Parse data from html page to table
Question: I would like make table of chosen physical properties of elements (for example
atomization enthalpy, vaporization enthalpy, heat of vaporization, boiling
point), which are accessible on [this
page](http://environmentalchemistry.com/yogi/periodic/W.html).
It is a huge pain to do it by hand, and I didn't find any other machine-
processing-friendly source of such data on the internet.
I was trying to learn how to to do it in Python (because I want to use this
data for my other code written in Python / NumPy / Pandas).
I was able to download the webpage HTML code with urllib2, and I was trying to
learn how to use some HTML/XML parser like ElementTree or MiniDom. However I
have no experience with web programing and HTML/XML processing.
Answer: Using lxml's xpath support, you can parse the data easily. Here's an example
parsing the atomization enthalpy
import lxml.html
import urllib2
html = urllib2.urlopen("http://http://environmentalchemistry.com/yogi/periodic/W.html").read()
doc = lxml.html.document_fromstring(html)
result = doc.xpath("/html/body/div[2]/div[2]/div[1]/div[1]/ul[7]/li[8]")
You could dynamically generate the xpath string for the different elements,
and use a dict to parse the require fields.
|
Elementtree, check if element has certain parent?
Question: I am parsing an xml file: <http://pastebin.com/fw151jQN> I wish to read it in
copy a lot of it and write it to a new file, some of it modified, a lot of it
unmodified, and a lot of it ignored. As an initial pass I want to find certain
xml, and write it to a new file unchanged.
Here is the section of the xml that is of interest at first:
<COMMAND name="shutdown"
help="Shutdown the selected interface">
<CONFIG priority="0x7F01" />
<ACTION>
/klas/klish-scripts/interfaces.py conf -i ${iface} --enable 0
</ACTION>
</COMMAND>
<COMMAND name="no shutdown"
help="Enable the selected interface">
<CONFIG operation="unset" pattern="shutdown"/>
<ACTION>
/klas/klish-scripts/interfaces.py conf -i ${iface} --enable 1
</ACTION>
</COMMAND>
My code is below
#!/usr/bin/python -tt
import xml.etree.ElementTree as ET
tree = ET.parse('interface_range_test.xml')
root = tree.getroot()
namespaces = {'command': 'http://clish.sourceforge.net/XMLSchema}COMMAND','config': 'http://clish.sourceforge.net/XMLSchema}CONFIG'}
all_links = tree.findall('.//')
for i in all_links:
if namespaces['command'] in i.tag:
if i.attrib['name'] == "shutdown":
print i.attrib
if namespaces['config'] in i.tag:
print i.attrib
Output:
{'name': 'shutdown', 'help': 'Shutdown the selected interface'}
{'priority': '0x7F01'}
{'pattern': 'shutdown', 'operation': 'unset'}
This reads in the file and I can find the shutdown information, now I want to
find the `CONFIG` information, and then the `action` information and it's
text, but when I search there is `CONFIG` information for both `shutdown` and
`no shutdown`. This case will occur in a lot of the xml, a lot of it has the
same format.
Shutdown: {'priority': '0x7F01'} no shutdown: {'pattern': 'shutdown',
'operation': 'unset'}
How can I specify which to look at, can I check the parent of this
information? Or can I check the children of the super element above it
(`http://clish.sourceforge.net/XMLSchema}COMMAND`)?
Answer: You can search all COMMANDS as node(element), and getting CONFIG info from
there, e.g.
import xml.etree.ElementTree as ET
tree = ET.parse('interface_range_test.xml')
root = tree.getroot()
for command in root.iter("{http://clish.sourceforge.net/XMLSchema}COMMAND"):
subs = list(command.iter('{http://clish.sourceforge.net/XMLSchema}CONFIG'))
if len(subs) > 0: #we found CONFIG
print command.tag, command.attrib, subs[0].tag, subs[0].attrib
And you will get:
{http://clish.sourceforge.net/XMLSchema}COMMAND {'name': 'shutdown', 'help': 'Shutdown the selected interface'} {http://clish.sourceforge.net/XMLSchema}CONFIG {'priority': '0x7F01'}
{http://clish.sourceforge.net/XMLSchema}COMMAND {'name': 'no shutdown', 'help': 'Enable the selected interface'} {http://clish.sourceforge.net/XMLSchema}CONFIG {'pattern': 'shutdown', 'operation': 'unset'}
BTW, if you need to handling big xml files, I would recommend to use
[lxml](http://lxml.de), which also has [ElementTree compatible
interface](http://lxml.de/tutorial.html) but much faster than python's
standard xml lib.
|
AppEngine (Python): can I know programmatically how much memory is used by the current instance?
Question: In the AppEngine control panel I can see the active instances and how much
memory they are using.
Is it possible to get the same information programmatically?
I mean, when a request is processed, is there a function I can call that will
return the number of bytes currently used by the instance that is handling the
request?
Thanks
Answer:
from google.appengine.api import runtime
runtime.memory_usage().current()
[docs](https://developers.google.com/appengine/docs/python/backends/runtimeapi)
|
Python scattered chart legend
Question: I'm plotting a scattered chart which look like this :
from pylab import *
n = 1024
X = np.random.normal(0,1,n)
Y = np.random.normal(0,1,n)
T = np.arctan2(Y,X)
axes([0.025,0.025,0.95,0.95])
scatter(X,Y, s=75, c=T, alpha=.5)
xlim(-1.5,1.5), xticks([])
ylim(-1.5,1.5), yticks([])
# savefig('../figures/scatter_ex.png',dpi=48)
show()

I would like to add a legend to explain the color scale :
Like yellow is 0 red is 10 etc...
I cannot find a way to do it , it works for imshow like this one :
from pylab import *
def f(x,y): return (1-x/2+x**5+y**3)*np.exp(-x**2-y**2)
n = 10
x = np.linspace(-3,3,3.5*n)
y = np.linspace(-3,3,3.0*n)
X,Y = np.meshgrid(x,y)
Z = f(X,Y)
axes([0.025,0.025,0.95,0.95])
imshow(Z,interpolation='nearest', cmap='bone', origin='lower')
colorbar(shrink=.92)
xticks([]), yticks([])
# savefig('../figures/imshow_ex.png', dpi=48)
show()

BUt I cannot find a way to add it on scattered charts.
DOes anyone already had to deal with this issue ?
Thanks for your help.
Answer: Change the last few lines to:
>>> s = scatter(X,Y, s=75, c=T, alpha=.5)
>>> colorbar(s)
>>> show()

|
how to create a file and throw exception if already exists
Question: In my program, many processes can try to create a file if the file doesnt
exist currently. Now I want to ensure that only one of the processes is able
to create the file and the rest get an exception if its already been
created(kind of process safe and thread safe open() implementation). How can I
achieve this in python.
Just for clarity, what I want is that the file is created if it doesnt exist.
But if it already exists then throw an exception. And this all should happen
atomicly.
Answer: In Python 2.x:
import os
fd = os.open('filename', os.O_CREAT|os.O_EXCL)
with os.fdopen(fd, 'w') as f:
....
In Python 3.3+:
with open('filename', 'x') as f:
....
|
Python sending and receiving HTTP POST
Question: I am in the process of learning Python and I am trying to do something really
simple: send an HTTP POST from one application and receive it in the other,
not only I can't get it to work, I can't get it to work with what would seem
reasonable, using def post(self). This is the code I have, which doesn't give
errors, but doesn't do the task either: Sender Application:
import cgi
import webapp2
import urllib
import urllib2
import json
from google.appengine.api import urlfetch
from google.appengine.ext import webapp
senddata = {}
senddata["message"] = 'Testing the Sender'
class MainPagePost(webapp2.RequestHandler):
def get(self):
txt_url_values = urllib.urlencode(senddata)
txturl = 'http://localhost:10080'
result = urllib.urlopen(txturl, txt_url_values)
self.redirect('http://localhost:10080')
application = webapp2.WSGIApplication([
('/', MainPagePost),
], debug=True)
Recieving Application:
import cgi
import webapp2
import urllib
import urllib2
import json
from google.appengine.api import urlfetch
from google.appengine.ext import webapp
class MainPageGet(webapp2.RequestHandler):
def get(self):
self.response.write('you sent:')
con = self.request.get("message")
self.response.write(con)
application = webapp2.WSGIApplication([
('/', MainPageGet),
], debug=True)
All I get on the localhost is "you sent:" :( Worst of all I don't understand
why both defs need to be "get(self)" so that I don't get 405 error... Thanks
all :)
This is the "new" code, for the sender no change:
import cgi
import webapp2
import urllib
import urllib2
import json
from google.appengine.api import urlfetch
from google.appengine.ext import webapp
senddata = {}
senddata["message"] = 'Testing Tester'
class MainPagePost(webapp2.RequestHandler):
def get(self):
txt_url_values = urllib.urlencode(senddata)
txturl = 'http://localhost:10080'
result = urllib.urlopen(txturl, txt_url_values)
self.redirect('http://localhost:10080')
application = webapp2.WSGIApplication([
('/', MainPagePost),
], debug=True)
The receiver I changed to post, as Sam suggested, but I am getting 405:
# -*- coding: utf-8 -*-
import cgi
import webapp2
import urllib
import urllib2
import json
from google.appengine.api import urlfetch
from google.appengine.ext import webapp
class MainPageGet(webapp2.RequestHandler):
def post(self):
# self.response.write('you sent:')
con = self.request.get("message")
self.response.write('you sent: ' + con)
application = webapp2.WSGIApplication([
('/', MainPageGet),
], debug=True)
Thanks :)
Answer: Check this [example](http://webapp-improved.appspot.com/guide/response.html):
self.response.write("<html><body><p>Hi there!</p></body></html>")
> The response buffers all output in memory, then sends the final output when
> the handler exits. webapp2 does not support streaming data to the client.
so basically, response.write must be the last thing you call:
def get(self):
con = self.request.get("message")
self.response.write("you sent: " + con )
Also, I suggest you check this
[link](https://developers.google.com/appengine/docs/python/gettingstartedpython27/handlingforms)
to read more about POST and GET requests with forms on Appengine. I don't
understand what you're trying to do with those two views, but they clash with
each other
|
Sorting represented data from a dictionary
Question: I am trying to sort my data, something similar to sorting example in here:
<http://www.blog.pythonlibrary.org/2011/01/04/wxpython-wx-listctrl-tips-and-
tricks/>
But for some reason when my data is represented in the table, things are all
over the place. For example, the computer name does not match the owner. At
first I though that the dictionary was not create correctly. But then I tried
printing it and the dictionary looks perfectly fine. So, the error must be
somewhere in lines with list_ctrl. Do you see where my mistake is?
#!/usr/bin/env python
import wx
import wx.lib.mixins.listctrl as listmix
import csv
from wxPython.wx import *
key_index = 4
##########################################################################
info = csv.reader(open(report.csv', 'rb'),delimiter=',')
length = 0
info_list = []
for row in info: #search each row in the report
info_list.append([length,row[1],row[4]])
length = length + 1
dict_info = {}
rows = 0
for item in info_list:
dict_info[item[rows]] = (item[rows + 1], item[rows + 2])
####################################################################
class TestListCtrl(wx.ListCtrl):
def __init__(self, parent, ID=wx.ID_ANY, pos=wx.DefaultPosition,
size=wx.DefaultSize, style=0):
wx.ListCtrl.__init__(self, parent, ID, pos, size, style)
########################################################################
class TestListCtrlPanel(wx.Panel, listmix.ColumnSorterMixin):
def __init__(self, parent):
wx.Panel.__init__(self, parent, -1, style=wx.WANTS_CHARS)
self.index = 0
self.list_ctrl = TestListCtrl(self, size=(-1,100),style=wx.LC_REPORT
|wx.BORDER_SUNKEN|wx.LC_SORT_ASCENDING)
self.list_ctrl.InsertColumn(0, "Computer Name")
self.list_ctrl.InsertColumn(1, "Owner")
items = dict_info.items()
index = 0
for key, data in items:
self.list_ctrl.InsertStringItem(self.index, data[0])
self.list_ctrl.SetStringItem(self.index, 1, data[1])
self.list_ctrl.SetItemData(self.index, key)
index += 1
# Now that the list exists we can init the other base class,
# see wx/lib/mixins/listctrl.py
self.itemDataMap = dict_info
listmix.ColumnSorterMixin.__init__(self, 2)
self.Bind(wx.EVT_LIST_COL_CLICK, self.OnColClick, self.list_ctrl)
sizer = wx.BoxSizer(wx.VERTICAL)
sizer.Add(self.list_ctrl, 0, wx.ALL|wx.EXPAND, 5)
self.SetSizer(sizer)
#----------------------------------------------------------------------
# Used by the ColumnSorterMixin, see wx/lib/mixins/listctrl.py
def GetListCtrl(self):
return self.list_ctrl
#----------------------------------------------------------------------
def OnColClick(self, event):
print "column clicked"
event.Skip()
########################################################################
###################################################################
class MyFrame(wx.Frame):
def __init__(self):
wx.Frame.__init__(self, None, wx.ID_ANY,
"Search")
panel = TestListCtrlPanel(self) #create a panel
#run code
if __name__ == '__main__':
app = wx.App(False)
frame = MyFrame()
frame.Show()
app.MainLoop()
Answer: Looks like you copied and pasted my code but changed a critical piece. You
need to change the for loop in your **init** to look like this:
for key, data in items:
print "%s: %s - %s" % (key, data[0], data[1])
print
self.list_ctrl.InsertStringItem(index, data[0])
self.list_ctrl.SetStringItem(index, 1, data[1])
self.list_ctrl.SetItemData(index, key)
index += 1
The "self.index" that I left in my example is set to zero, but I never use it
in that loop. Instead I create a local variable called **index** and use that
instead. I have fixed my article by removing the **self.index** bit since it
is unneeded in that particular example.
In your code, you increment index, but use self.index in all your
self.list_ctrl.*() methods.
|
Interpret list of integers as a float in Python
Question: I am attempting to convert a C code snippet into python.
The purpose of said function is to take 4, 8-bit readings from a PLC, and
decode them into a single float.
float conv_float_s7_pc(char * plc_real)
{
char tmp[4];
tmp[0] = * (plc_real + 3);
tmp[1] = * (plc_real + 2);
tmp[2] = * (plc_real + 1);
tmp[3] = * (plc_real + 0);
return (* (float *) tmp) ;
}
Is there some Python magic that can perform this function cleanly?
While I am attempting to convert the above function, the more general question
is, how would you perform memory "reinterpretations" such as this in python?
## Edit
This got me what I needed:
import struct
def conv_to_float(plc):
temp = struct.pack("BBBB", plc[0], plc[1], plc[2], plc[3])
output = struct.unpack(">f", temp)[0]
return output
Answer: Use the [struct module](http://docs.python.org/2/library/struct.html#format-
characters) with the `f` format character
>>> import struct
>>> plc_real = "1234"
>>> struct.unpack("f", plc_real)[0]
1.6688933612840628e-07
Make sure you use `<` or `>` to set the endianness you need
|
Python Requests encoding POST data
Question: Version: Python 2.7.3
Other libraries: Python-Requests 1.2.3, jinja2 (2.6)
I have a script that submits data to a forum and the problem is that non-ascii
characters appear as garbage. For instance a name like André Téchiné comes out
as André Téchiné.
Here's how the data is submitted:
1) Data is initially loaded from a UTF-8 encoded CSV file like so:
entries = []
with codecs.open(filename, 'r', 'utf-8') as f:
for row in unicode_csv_reader(f.readlines()[1:]):
entries.append(dict(zip(csv_header, row)))
unicode_csv_reader is from the bottom of Python CSV documentation page:
<http://docs.python.org/2/library/csv.html>
When I type the entries name in the interpreter, I see the name as `u'Andr\xe9
T\xe9chin\xe9'`.
2) Next I render the data through jinja2:
tpl = tpl_env.get_template(u'forumpost.html')
rendered = tpl.render(entries=entries)
When I type the name rendered in the interpreter I see again the same:
`u'Andr\xe9 T\xe9chin\xe9'`
Now, if I write the rendered variable to a filename like this, it displays
correctly:
with codecs.open('out.txt', 'a', 'utf-8') as f:
f.write(rendered)
But I must send it to the forum:
3) In the POST request code I have:
params = {u'post': rendered}
headers = {u'content-type': u'application/x-www-form-urlencoded'}
session.post(posturl, data=params, headers=headers, cookies=session.cookies)
session is a Requests session.
And the name is displayed broken in the forum post. I have tried the
following:
* Leave out headers
* Encode rendered as rendered.encode('utf-8') (same result)
* rendered = urllib.quote_plus(rendered) (comes out as all %XY)
If I type rendered.encode('utf-8') I see the following:
'Andr\xc3\xa9 T\xc3\xa9chin\xc3\xa9'
How could I fix the issue? Thanks.
Answer: Your client behaves as it should e.g. running `nc -l 8888` as a server and
making a request:
import requests
requests.post('http://localhost:8888', data={u'post': u'Andr\xe9 T\xe9chin\xe9'})
shows:
POST / HTTP/1.1
Host: localhost:8888
Content-Length: 33
Content-Type: application/x-www-form-urlencoded
Accept-Encoding: gzip, deflate, compress
Accept: */*
User-Agent: python-requests/1.2.3 CPython/2.7.3
post=Andr%C3%A9+T%C3%A9chin%C3%A9
You can check that it is correct:
>>> import urllib
>>> urllib.unquote_plus(b"Andr%C3%A9+T%C3%A9chin%C3%A9").decode('utf-8')
u'Andr\xe9 T\xe9chin\xe9'
* check the server decodes the request correctly. You could try to specify the charset:
headers = {"Content-Type": "application/x-www-form-urlencoded; charset=UTF-8"}
the body contains only ascii characters so it shouldn't hurt and the correct
server would ignore any parameters for `x-www-form-urlencoded` type anyway.
Look for gory details in [URL-encoded form
data](http://www.whatwg.org/specs/web-apps/current-work/multipage/association-
of-controls-and-forms.html#url-encoded-form-data)
* check the issue is not a display artefact i.e., the value is correct but it displays incorrectly
|
Hadoop: Sending Files or File paths to a map reduce job
Question: supposed I had N files to process using hadoop map-reduce, let's assume they
are large, well beyond the block size and there are only a few hundred of
them. Now I would like to process each of these files, let's assume the word
counting example.
My question is: What is the difference between creating a map-reduce job whose
input is a text file with the paths to each of the files as opposed to sending
each of the files directly to the map function i.e. concatenating all the
files and pushing **them into different mappers [EDIT]**.
Are these both valid approaches? Are there any drawbacks to them?
**Thanks for the prompt answers I've included a detailed description of my
problem since my abstraction may have missed a few important topics:**
I have N small files on Hadoop HDFS in my application and I just need to
process each file. So I am using a map function to apply a python script to
each file (actually image [I've already looked at all the hadoop image
processing links out there]), I am aware of the small file problem and the
typical recommendation is to group the smaller files so we avoid the overhead
of moving files around (the basic recommendation using sequence files or
creating your own data structures as in the case of the HIPI).
_**This makes me wonder can't we just tell each mapper to look for files that
are local to him and operate on those?_**
I haven't found a solution to that issue which is why I was looking at either
sending a path to the files to each mapper or the file it self.
Creating a list of path names for each of the collection of images seems to be
ok, but as mentioned in the comments I loose the data locality property.
Now when I looked at the hadoop streaming interface it mentions that the
different pieces communicate based on stdin and stdout typically used for text
files. That's where I get confused, if I am just sending a list of path names
this shouldn't be an issue since each mapper would just try to find the
collection of images it is assigned. But when I look at the word count example
the input is the file which then gets split up across the mappers and **so
that's when I was confused as to if I should concatenate images into groups
and then send these concatenated groups just like the text document to the
different mappers or if I should instead concatenate the images leave them in
hadoop HDFS and then just pass the path to them to the mapper**... I hope this
makes sense... maybe I'm completely off here...
Thanks again!
Answer: Both are valid. But latter would incur extra overhead and performance will go
down because you are talking about concatenating all the files into one and
feeding it to just 1 mapper. And by doing that you would go against one of the
most basic principles of Hadoop, `parallelism`. Parallelism is what makes
Hadoop so efficient.
FYI, if you really need to do that you have to set `isSplittable` to false in
your `InputFormat` class, otherwise the framework will split the file(based on
your InputFormat).
And as far as input path is considered, you just need to give the path of the
input directory. Each file inside this directory will be processed without
human intervention.
HTH
* * *
In response to your edit :
I think you have misunderstood this a bit. You don't have to worry about
localization. Hadoop takes care of that. You just have to run your job and the
data will be processed on the node where it is present. Size of the file has
nothing to with it. You don't have to tell anything to mappers. Process goes
like this :
You submit your job to JT. JT directs the TT running on the node which has the
block of data required by the job to start start the mappers. If the slots are
occupied by some other process, then same thing takes place on some other node
having the data block.
|
Objective C JSON object null but responseData not empty
Question: I am sending an HTTP request to a web service and I should get a JSON as
response. In Objective C the responseData is not empty, but the serialization
of it as JSON is null. This is my code:
- (IBAction)getProfileInfo:(id)sender
{
NSString *code = @"oanure!1";
//Start request
NSURL *url = [NSURL URLWithString:@"http://192.168.1.8:5000/login?json"];
ASIFormDataRequest *request = [ASIFormDataRequest requestWithURL:url];
[request setPostValue:code forKey:@"logincode"];
[request setDelegate:self];
[request startAsynchronous];
}
- (void)requestFinished: (ASIHTTPRequest *) request{
if (request.responseStatusCode == 400){
self.profileInfo.text = @"Invalid code";
} else if (request.responseStatusCode == 403) {
self.profileInfo.text = @"Code alredy used";
} else if (request.responseStatusCode == 200) {
NSData *responseData = [request responseData];
NSLog(@"%@", responseData);
NSError *error;
//I tried with NSDictionary as well
NSArray *json = (NSArray *)
[NSJSONSerialization JSONObjectWithData:responseData
options:kNilOptions
error:&error];
NSLog(@"%@", json);
}
else {
self.profileInfo.text = @"Unexpected error";
}
}
The console prints out:
2013-07-02 16:30:16.047 AppName[1396:c07] <3c68746d 6c3e0a0a 3c686561 643e0a3c
7469746c 653e5072 6f66696c 65206f66 206f616e 7572653c 2f746974 6c653e0a 0a3c7363
72697074 20747970 653d2274 6578742f 6a617661 53637269 70742220 7372633d 22737461
7469632f 6a717565 7279312e 392e6a73 223e3c2f 73637269 70743e0a 0a0a3c2f 68656164
3e0a0a3c 626f6479 3e0a3c68 313e4865 6c6c6f2c 206f616e 75726521 3c2f6831 3e0a3c74
61626c65 3e0a2020 3c74723e 3c746820 636f6c73 70616e3d 323e4661 6365626f 6f6b3c2f
74683e3c 2f74723e 0a20203c 74723e0a 20202020 3c74643e 75736572 6e616d65 3c2f7464
3e3c7464 3e6f616e 7572653c 2f74643e 0a20203c 2f74723e 0a3c2f74 61626c65 3e0a3c2f
626f6479 3e0a0a3c 2f68746d 6c3e0a>
2013-07-02 16:30:16.048 AppName[1396:c07] (null)
I suppose I am not doing the serialization right...
Just so to prove that the web service works, I wrote a small script in Python:
import httplib, urllib
params = urllib.urlencode({
'logincode' : 'oanure!1',
})
conn = httplib.HTTPConnection("192.168.1.8:5000")
conn.request("POST", "/login?json",
params)
response = conn.getresponse()
print response.status, response.reason
data = response.read()
print data
conn.close()
In this case the console printed out: 200 OK {"loggedin": 0, "error": "Not
logged in"}
I would like to get the same thing in Objective C.
Please help!
Answer: I have tried to convert the responseData using hex to string online converter
and it is in HTML (not JSON format). Please check in server side whether is it
sending data correctly or not. Here is the converted responseData:
<html>
<head>
<?title>Profile of oanure</title>
<sc?ript type="text/javaScript" src="sta?tic/jquery1.9.js"></script>
</head?>
<body>
<h1>Hello, oanure!</h1>
<t?able>
<tr><th colspan=2>Facebook</?th></tr>
<tr>
<td>username</td?><td>oanure</td>
</tr>
</table>
</?body>
</html>
Hex converter:
<http://www.string-functions.com/hex-string.aspx>
Secondly, probably you should print out the errMessage as well to check the
detailed error. Once JSON couldn't convert the format, it will always return
nil (null) to json object.
|
How to: Pass Arguments to Python Script via Powershell
Question: I am attempting to pass 2 arguments to a python script via Powershell.
CODE:
$env:PATHEXT += ";.py"
[Environment]::SetEnvironmentVariable("Path", "$env:Path;c:\Program Files\lcpython15\", "User")
$args1 = "Test1"
$args2 = "Test2"
$Python_SetAttrib = "c:\ProgramData\set_cust_attr.py "
python $Python_SetAttrib $args1 $args2
USAGE FROM CMD.exe:
c:\ProgramData\set_cust_attr.py <custom attribute name> <custom attribute value>
ERROR:
PS C:\ProgramData> python $Python_SetAttrib + $args1 + $args2
usage: c:\ProgramData\set_cust_attr.sh <custom attribute name> <custom attribute value>
OR
usage: c:\ProgramData\Opsware\set_cust_attr.sh --valuefile <path to file with value in it> <custom attribute name>
python.exe : Got more than one custom attribute name.
At line:1 char:7
+ python <<<< $Python_SetAttrib + $args1 + $args2
+ CategoryInfo : NotSpecified: (Got more than one custom attribute name.:String) [], RemoteException
+ FullyQualifiedErrorId : NativeCommandError
Unhandled exception in thread started by
Traceback (most recent call last):
File ".\client\__init__.py", line 88, in pumpthread
File "C:\Program Files\lcpython15\lib\site-packages\pythoncom.py", line 3, in ?
pywintypes.__import_pywin32_system_module__("pythoncom", globals())
File "C:\Program Files\lcpython15\Lib\site-packages\win32\lib\pywintypes.py", line 68, in __import_pywin32_system_module__
import _win32sysloader
ImportError: No module named _win32sysloader
Answer: Got it! Darn single quotes.....
$env:Path += ";c:\Program Files\lcpython15";
$env:PATHEXT += ";.py";
$arg1 = "Test3"
$arg2 = "Testing"
$arg3 = 'c:\ProgramData\set_cust_attr.py'
python $arg3 $arg1 $arg2
|
Contour plotting orbitals in pyquante2 using matplotlib
Question: I'm currently writing line and contour plotting functions for my
[PyQuante](https://github.com/rpmuller/pyquante2) quantum chemistry package
using matplotlib. I have some great functions that evaluate basis sets along a
(npts,3) array of points, e.g.
from somewhere import basisset, line
bfs = basisset(h2) # Generate a basis set
points = line((0,0,-5),(0,0,5)) # Create a line in 3d space
bfmesh = bfs.mesh(points)
for i in range(bfmesh.shape[1]):
plot(bfmesh[:,i])
This is fast because it evaluates all of the basis functions at once, and I
got some great help from stackoverflow
[here](http://stackoverflow.com/questions/17396164/numpythonic-way-to-
make-3d-meshes-for-line-plotting) and
[here](http://stackoverflow.com/questions/17391052/compute-square-distances-
from-numpy-array) to make them extra-nice.
I would now like to update this to do contour plotting as well. The slow way
I've done this in the past is to create two one-d vectors using linspace(),
mesh these into a 2D grid using meshgrid(), and then iterating over all xyz
points and evaluating each one:
f = np.empty((50,50),dtype=float)
xvals = np.linspace(0,10)
yvals = np.linspace(0,20)
z = 0
for x in xvals:
for y in yvals:
f = bf(x,y,z)
X,Y = np.meshgrid(xvals,yvals)
contourplot(X,Y,f)
(this isn't real code -- may have done something dumb)
What I would like to do is to generate the mesh in more or less the same way I
do in the contour plot example, "unravel" it to a (npts,3) list of points,
evaluate the basis functions using my new fast routines, then "re-ravel" it
back to X,Y matrices for plotting with contourplot.
The problem is that I don't have anything that I can simply call .ravel() on:
I either have 1d meshes of xvals and yvals, the 2D versions X,Y, and the
single z value.
Can anyone think of a nice, pythonic way to do this?
Answer: If you can express `f` as a function of `X` and `Y`, you could avoid the
Python `for-loop`s this way:
import matplotlib.pyplot as plt
import numpy as np
def bf(x, y):
return np.sin(np.sqrt(x**2+y**2))
xvals = np.linspace(0,10)
yvals = np.linspace(0,20)
X, Y = np.meshgrid(xvals,yvals)
f = bf(X,Y)
plt.contour(X,Y,f)
plt.show()
yields

|
How to make a summation under a condition in Biopython
Question: I have a FASTA file with three defined elements in the "description" line.
The first element, defined as `dato[0]`, is the one that has to carry out with
the condition and the third element, defined as `dato[2]`, is the one that I
want to sum. The FASTA description line is like this:
PIN4 HOIAQKS02C4SWQ 1761
PIN1 HOIAQKS02D3JZ3 572
And I want to sum the values (`dato[2]`) that carry out the condition `dato[0]
== PIN1` in one row and the condition `dato[0] == PIN4` in another.
I am using the following code:
from Bio import SeqIO
secuencias=SeqIO.parse("/Users/imac/Desktop/Pruebas_UniFrac/otu1_alpin1+4.fasta", "fasta")
PIN_records=list(SeqIO.parse("/Users/imac/Desktop/Pruebas_UniFrac/otu1_alpin1+4.fasta", "fasta")
archivo1=open("/Users/imac/Desktop/Pruebas_UniFrac/pruebaalpin1+4_fin.fasta", "w")
archivo2=open("/Users/imac/Desktop/Pruebas_UniFrac/pruebaalpin1+4_seqsotus.fasta", "w")
archivo3=open("/Users/imac/Desktop/Pruebas_UniFrac/pruebaalpin1+4_sumas.fasta", "w")
x = 0
y = x+1
for linea in secuencias:
dato = linea.description.split(" ")
seqs = str(linea.seq)
if dato[0] != "PIN1":
if dato[0] != "PIN4":
if dato[0] == "consensus":
archivo1.write("hacia arriba OTU" + str(y) + "\n" + "x" + "\n" + "x" + "\n")
archivo2.write(">" + "OTU" + str(y) + "\n" + seqs + "\n")
archivo3.write("fin del OTU" + "\n")
y = y+1
else:
archivo1.write(str(dato[0]) + "," + str(dato[2]) + "\n")
#num = int(dato[2])
#archivo3.write("PIN4=" + str(sum(dato[2])) + "\n")
#archivo3.write("PIN4=%d\n" % sum(dato[2]))
archivo3.write("PIN4={}\n".format(sum(dato[2])))
else:
archivo1.write(str(dato[0]) + "," + str(dato[2]) + "\n")
#num = int(dato[2])
#archivo3.write("PIN1=" + str(sum(dato[2])) + "\n")
#archivo3.write("PIN1=%d\n" % sum(dato[2]))
archivo3.write("PIN1={}\n".format(sum(dato[2])))
archivo1.close()
archivo2.close()
archivo3.close()
And when I do that, I get this error message:
TypeError: unsupported operand type(s) for +: 'int' and 'str'
## How can I do that?
After following posterior comments, I have introduced changes in my code, but
I can't get it working properly and I do not know how to fix it.
Thanks a lot
With this code, I get the following error:
File "./lectura_msaout_pruebaalpin1+4_final.py", line 16
archivo1=open("/Users/imac/Desktop/Pruebas_UniFrac/pruebaalpin1+4_fin.fasta", "w")
^
SyntaxError: invalid syntax
Answer: Your code has two main issues.
1. You're trying to call `sum()` on string data.
2. You're trying to format a numeric value as a string.
## Fixing summation
You want to sum an iterable of numeric values, as summing is undefined for
string values. You can convert string values to an integer by calling `int()`
on each value (use the `map()` function to do this).
### Example:
>>> sum(["1", "2", "3"])
TypeError: unsupported operand type(s) for +: 'int' and 'str'
>>> sum([1, 2, 3])
6
map(int, ["1", "2", "3"])
[1, 2, 3]
>>> sum(map(int, ["1", "2", "3"]))
6
### Application to your code
Do you really want to sum the single digits of `dato[2]`? It'd look like this:
>>> dato = ['PIN4', 'HOIAQKS02C4SWQ', '1761']
>>> sum(map(int, dato[2])) # 1 + 7 + 6 + 1
15
## Fixing the string formatting
You can't append an integer to a string (see [String and integer
concatenation](http://stackoverflow.com/questions/2847386/string-and-integer-
concatenation)).
The solution is to either convert the integer to a string before
concatenating, or to format the integer within a string. In your case, the
solutions look like this:
1. Convert to string:
archivo3.write("PIN1=" + str(dato_2_sum) + "\n")
2. Use string formatting:
archivo3.write("PIN1=%d\n" % dato_2_sum)
3. Use newstyle formatting:
archivo3.write("PIN1={}\n".format(dato_2_sum)
|
Matplotlib figures not working after Tkinter file dialog
Question: I'm using the following function which I found as a reply to [this
question](http://stackoverflow.com/questions/9319317/quick-and-easy-file-
dialog-in-python) to show a dialog window for file selection.
[ Edit: Turns out the distro differences here are merely because Matplotlib is
using gtk3agg on Fedora and TkAgg for drawing windows on each system ]
**On Fedora 18:** Everything works fine.
**On Ubuntu 12.10:** Matplotlib hangs after closing any figure displayed after
the file dialog. For example, in the code below, on Ubuntu I can never get to
the "made it" line. I am still able to type in the terminal, though nothing
happens. If I remove the file dialog, Matplotlib figures work as expected.
import Tkinter, tkFileDialog
import pylab
def ask_for_config_file():
print "Please provide location of configuration file."
root = Tkinter.Tk()
root.withdraw()
file_path = tkFileDialog.askopenfilename()
return file_path
def main():
config_file_path = ask_for_config_file()
pylab.figure()
pylab.show()
print "Made it."
Any suggestions?
Answer: I just needed a `root.destroy()` at the end of the dialog function!
|
Import class in same file in Python
Question: I'm new to python and I have a file with several classes. In a method in the
class "class1" I want to use a method from another class "class2". How do I do
the import and how do I call the method from class1? I have tried several
different things but nothing seems to work.
Answer: you dont need to import them becuase they are already in the same file
do something like this:
class1 = Class1() #assigns class1 to youre first class
then call a method of Class1 like this:
Class2():
def method2(self):
class1.method1() #call youre method from class2
basically you are taking `Class2()` and giving it the instance `class2` then
you are calling a method of that class by doing `class2.method2()` its just
like calling a function but you need the class name in front of it
here is an example:
class Class1():
def method1(self):
print "hello"
class Class2():
def method2(self)
class1 = Class1()
class1.method1()
then when you call `Class2()` it will print 'hello'
|
fifo - reading in a loop
Question: I want to use [os.mkfifo](http://docs.python.org/2/library/os.html#os.mkfifo)
for simple communication between programs. I have a problem with reading from
the fifo in a loop.
Consider this toy example, where I have a reader and a writer working with the
fifo. I want to be able to run the reader in a loop to read everything that
enters the fifo.
# reader.py
import os
import atexit
FIFO = 'json.fifo'
@atexit.register
def cleanup():
try:
os.unlink(FIFO)
except:
pass
def main():
os.mkfifo(FIFO)
with open(FIFO) as fifo:
# for line in fifo: # closes after single reading
# for line in fifo.readlines(): # closes after single reading
while True:
line = fifo.read() # will return empty lines (non-blocking)
print repr(line)
main()
And the writer:
# writer.py
import sys
FIFO = 'json.fifo'
def main():
with open(FIFO, 'a') as fifo:
fifo.write(sys.argv[1])
main()
If I run `python reader.py` and later `python writer.py foo`, "foo" will be
printed but the fifo will be closed and the reader will exit (or spin inside
the `while` loop). I want reader to stay in the loop, so I can execute the
writer many times.
**Edit**
I use this snippet to handle the issue:
def read_fifo(filename):
while True:
with open(filename) as fifo:
yield fifo.read()
but maybe there is some neater way to handle it, instead of repetitively
opening the file...
**Related**
* [Getting readline to block on a FIFO](http://stackoverflow.com/questions/2406365/getting-readline-to-block-on-a-fifo)
Answer: A FIFO works (on the reader side) exactly this way: it can be read from, until
all writers are gone. Then it signals EOF to the reader.
If you want the reader to continue reading, you'll have to open again and read
from there. So your snippet is exactly the way to go.
If you have mutliple writers, you'll have to ensure that each data portion
written by them is smaller than `PIPE_BUF` on order not to mix up the
messages.
|
New regex module fuzzy function error value. Python
Question: im trying out the fuzzy function of the new regex module. in this case, i want
there to find a match for all strings with <= 1 errors, but i'm having trouble
with it
import regex
statement = 'eol the dark elf'
test_1 = 'the dark'
test_2 = 'the darc'
test_3 = 'the black'
print regex.search('{}'.format(test_1),statement).group(0) #works
>>> 'the dark'
print regex.search('{}'.format(test_1){e<=1},statement).group(0)
>>> print regex.search('{}'.format(test_1){e<=1},statement).group(0) #doesn't work
^
SyntaxError: invalid syntax
i have also tried
print regex.search('(?:drk){e<=1}',statement).group(0) #works
>>> 'dark'
but this . . .
print regex.search(('(?:{}){e<=1}'.format(test_1)),statement).group(0) #doesn't work
>>> SyntaxError: invalid syntax
Answer: In your first snippet, you forgot to put the `{e<=1}` in a string. In your
final snippet, I think the problem is, that `format` tries to deal with the
`{e<=1}` itself. So either you use concatenation:
print regex.search(test_1 + '{e<=1}', statement).group(0)
or you escape the literal braces, by doubling them:
print regex.search('{}{{e<=1}}'.format(test_1), statement).group(0)
This can then easily be extended to
print regex.search('{}{{e<={}}}'.format(test_1, num_of_errors), statement).group(0)
|
Does python's multiprocessing leak memory?
Question: I've narrowed down a piece of code to the following minimal (working?)
example:
import multiprocessing
def f(x): return x**2
for n in xrange(2000):
P = multiprocessing.Pool()
sol = list(P.imap(f, range(20)))
When I run this on my computer (Ubuntu 12.04, 8-core), python proceeds to
consume all available memory and eventually it hangs the system due to an
unresponsive swap. I've heard that Python doesn't release memory until it's
finished, but I think it's a bit silly that this program should consume over
8Gb of RAM. If I want to create `multiprocessing.Pool` objects over and over,
how can I do so without reallocating new memory each time?
Answer: You're creating a new multiprocessing pool every loop iteration - don't you
only want one pool to which to feed your processing jobs?
import multiprocessing
def f(x): return x**2
P = multiprocessing.Pool()
for n in xrange(2000):
sol = list(P.imap(f, range(20)))
|
Python: randomly assign one of two values
Question:
if losttwice <= 2:
bet = _________ # <- Here
elif losttwice <= 5:
bet = bet * 2
else:
bet = startingbet
Can anyone help me to add one more thing to this? I would like to do a random
50% chance when `losttwice <= 2` (when I lost 1-2 times) for it to be `bet =
startingbet` or `bet = bet * 2` based on 50% chance.
Answer: `if random.random() > 0.5:` (and `import random` on the top) might be useful.
You should be able to figure it out based on that.
|
Converting a serial task to parallel to map inputs and outputs
Question: I have tens of thousands of simulations to run on a system with several cores.
Currently, it is done in serial, where I know my input parameters, and store
my results in a dict.
# Serial version
import time
import random
class MyModel(object):
input = None
output = None
def run(self):
time.sleep(random.random()) # simulate a complex task
self.output = self.input * 10
# Run serial tasks and store results for each parameter
parameters = range(10)
results = {}
for p in parameters:
m = MyModel()
m.input = p
m.run()
results[p] = m.output
print('results: ' + str(results))
Which takes <10 seconds, and displays correct results:
results: {0: 0, 1: 10, 2: 20, 3: 30, 4: 40, 5: 50, 6: 60, 7: 70, 8: 80, 9: 90}
# Parallel version
My attempts to parallelize this procedure are based on the example in the
`multiprocessing` module near the text ["An example showing how to use queues
to feed tasks to a collection of worker processes and collect the
results"](http://docs.python.org/2/library/multiprocessing.html#examples)
(sorry, no URL anchor available).
The following builds on the top half of the serial version:
from multiprocessing import Process, Queue
NUMBER_OF_PROCESSES = 4
def worker(input, output):
for args in iter(input.get, 'STOP'):
m = MyModel()
m.input = args[0]
m.run()
output.put(m.output)
# Run parallel tasks and store results for each parameter
parameters = range(10)
results = {}
# Create queues
task_queue = Queue()
done_queue = Queue()
# Submit tasks
tasks = [(t,) for t in parameters]
for task in tasks:
task_queue.put(task)
# Start worker processes
for i in range(NUMBER_OF_PROCESSES):
Process(target=worker, args=(task_queue, done_queue)).start()
# Get unordered results
for i in range(len(tasks)):
results[i] = done_queue.get()
# Tell child processes to stop
for i in range(NUMBER_OF_PROCESSES):
task_queue.put('STOP')
print('results: ' + str(results))
Takes only a few seconds now, but the mapping orders between inputs and
results are mixed up.
results: {0: 10, 1: 0, 2: 60, 3: 40, 4: 20, 5: 80, 6: 30, 7: 90, 8: 70, 9: 50}
I realise that I'm populating the `results` based on an unordered
`done_queue.get()`, but I'm not sure how to get the correct mapping to
`task_queue`. Any ideas? Any other way to make this somehow cleaner?
Answer: A-ha! The worker needs to embed some kind of ID, such as the input
parameter(s) used to return to the output queue, which can be used to identify
the returned process. Here are the required modifications:
def worker(input, output):
for args in iter(input.get, 'STOP'):
m = MyModel()
m.input = args[0]
m.run()
# Return a tuple of an ID (the input parameter), and the model output
return_obj = (m.input, m.output)
output.put(return_obj)
and
# Get unordered results
for i in range(len(tasks)):
# Unravel output tuple, which has the input parameter 'p' used as an ID
p, result = done_queue.get()
results[p] = result
|
Match the folder list by using regex in python
Question: How to match the following case in python regex,
str = "https://10.0.4.3/myrepos/Projects/ID87_070_138"
I need to match "ID87_070_138" this type of folders from the list of folders.
The pattern is "ID<number>_<number>_<Number>".
Thanks in advance.
Answer:
ID\d+_\d+_\d+
Matches the `ID` followed by three groups of _one_ or _more_ digits, separated
by underscore.
And the Python code:
> import re
> str = "https://10.0.4.3/myrepos/Projects/ID87_070_138"
> print re.findall(r"ID\d+_\d+_\d+", str)
With the result:
['ID87_070_138']
|
cannot use document[0] (type uint8) as type []byte in function argument
Question: I'm trying to get a JSON string pulled from a document and into SimpleJson in
GOlang, though I've run into a problem with the types (again..)
I get the following error:
> cannot use document[0] (type uint8) as type []byte in function argument
and the line which bugs up is:
js, err := simplejson.NewJson(document[0])
Could anyone please help me fix this, and also is there a good place I can
read up about types and conversions? Having come from php which has no types
and python where the conversions are simple, GO is a bit confusing on this
front.
Thanks :-)
Answer: First of all, `uint8` is simply an alias for `byte`. Therefor `[]uint8` is the
same as `[]byte`.
What the error message tells you is that document[0] is of type `uint8` and
not `[]byte`
From your comment it is clear that `document` is a `string`. You should just
convert it to []byte. If the JSON data in itself contains an array, you must
_first_ parse the document (pass it to NewJson) before you can retrieve the
first value of the JSON array.
So, instead change your code to:
js, err := simplejson.NewJson([]byte(document))
// error testing
jsonArray, err := js.Array()
// more error testing
fmt.Println(jsonArray[0])
(I assume you have imported the `github.com/xiocode/toolkit/simplejson`
package. The only one I could find with simplejson.NewJson)
|
Error when assigning tempfile.TemporaryFile to a variable and executing
Question: So let's say that my class looks like the below, with all relevant imports
already done:
class LargeRequest(server.Request):
memory_limit = 1024*1024*25
temp_type = tempfile.TemporaryFile
def parse_multipart(self, fp, pdict):
while loop_condition:
if self.temp_type.__name__ == 'SpooledTemporaryFile':
data = self.temp_type(max_size=self.memory_limit)
else:
data = self.temp_type()
...
data.write('stuff')
When I run this I get an error:
File "/home/user/workspace/twisted/server.py", line 226, in parse_multipart
data = self.temp_type()
File "/usr/lib/python2.7/tempfile.py", line 484, in TemporaryFile
if 'b' in mode:
exceptions.TypeError: argument of type 'instance' is not iterable
Which refers to [this line in
tempfile.py](http://hg.python.org/cpython/file/2.7/Lib/tempfile.py#l448).
Also, this error does not occur when I simply do:
data = tempfile.TemporaryFile()
But I would like a bit more flexibility. What am I doing wrong?
Answer: `temp_type` become instance method. `self.temp_type()` call become
`TemporaryFile(self)`.
Try following:
temp_type = staticmethod(tempfile.TemporaryFile)
|
import error while using python and Django
Question: I am making a website using the django api. Problem is I am getting a weird
import error. I have a function in a file which calls another function in
another file which in turn calls back a third function in the first file.
Problem is during that third function. When I try to import it I get an error
cannot import deletefromS3.
A full stack trace is given below
<http://dpaste.com/1288190/>
Here are the snippets of the two modules:
topichandler.py:
from sdbhandler.mediahandler import deleteMediaParent
def deletefromS3(itemid,folder):
itemid=folder+itemid
bucket = connect_s3()
for key in bucket:
fname=key.split(".")[0]
if(fname==itemid):
bucket.delete_key(key)
return []
def deleteTopic(itemid,parentId='NULL'):
sdb=connect()
domain= sdb.get_domain(DOMAIN)
rootitem = domain.get_item(itemid)
if(parentId=='NULL'):
query= 'select * from ' + DOMAIN + ' where itemName()="'+itemid+'"'
rs = domain.select(query)
else:
rs = [rootitem]
for item in rs:
deleteMediaParent(item.name)
deletefromS3(item.name,'topicsK2/')
domain.delete_attributes(item.name)
deleteMediaParent(rootitem.name)
deletefromS3(rootitem.name,'topicsK2/')
domain.delete_attributes(rootitem.name)
mediahandler.py:
from sdbhandler.topichandler import deletefromS3
def deleteMediaParent(parentid):
sdb=connect()
domain = sdb.get_domain(DOMAIN)
query = 'select * from '+ DOMAIN + 'where ' +FIELD_TopicID + ' = "' + parentid + '"'
rs = domain.select(query)
for item in rs:
deleteQuestionParent(item.name)
deletefromS3(item.name,'mediaK2/')
domain.delete_attributes(item.name)
There are more dependencies but I cannot post my whole code that would be way
too much. Can I not import from the file from which a method was called?
Answer: The import is impossible because a module has to finish loading before things
can be imported from it. When the `topichandler` module loads, it tries to
import `deleteMediaParent` from `mediahandler`. But `mediahandler` tries to
import `deletefromS3` from `topichandler`, which triggers another attempt to
load `topichandler`. Python catches the infinite loop that’s about to happen
and raises an error instead.
Let’s look at this with a simpler example. Here is `foo.py`:
#!/usr/bin/env python2.7
x = 3
from bar import y
print x, y
and here is `bar.py`:
from foo import x
y = x
This gives the same error you got, for the same reason.
Although the best solution is probably to restructure your code into more
coherent standalone modules, there is a workaround. You can delay the import
by moving it into a function, as in:
#!/usr/bin/env python2.7
x = 3
from bar import y
print x, y()
`bar.py`:
def y():
from foo import x
return x
Note that if you run this the `print` will actually be imported twice… again,
you probably are better off moving related functions into the same module.
|
Avoid storing passwords in plaintext for IMAP access via Python
Question: I'm trying to write a script that helps to clear IMAP inboxes, and I'm running
into a problem with passwords; namely, that to get access to the server I need
to have access to the plaintext. I've checked, and my mailserver isn't showing
MD5 as an available method (otherwise I could use `IMAP4.login_cram_md5`). How
do I go about accessing the server without plaintext passwords?
Answer: You can check the authentication methods available like this (I'm using
`IMAP4_SSL`, use `IMAP4` if you want an insecure connection, but I don't
recommend ever using non-SSL connections if you have the choice).
import imaplib
imap_server = imaplib.IMAP4_SSL("imap.server.com")
print "\n".join(i for i in imap_server.capabilities if i.startswith("AUTH="))
You can then write an authentication object that can be passed to
`imap_server.authenticate()` for your chosen method. [This bug
report](http://bugs.python.org/issue13700) happens to have some examples of
its use and [this SO
question](http://stackoverflow.com/questions/7963820/gmail-imap-
authentication-imaplib-authenticate-takes-exactly-3-args-4-given) shows
someone authenticating via OAuth to Gmail.
If the server doesn't provide any authentication methods other than plaintext
passwords, or you can't / don't wish to support them, then storing the
password in a form where you can recover the plaintext is unfortunately
unavoidable. You could encrypt it in some form (e.g. AES using
[PyCrypto](https://www.dlitz.net/software/pycrypto/), example
[here](http://stackoverflow.com/questions/5778304/simple-example-
aes256-crypt)), but the encryption key is still going to be stored somewhere
unless you want to prompt the user each time (in which case you might as well
just prompt for the password anyway). I would suggest at least some trivial
obfuscation, such as Base64 encoding, just to prevent someone idly glancing at
the code or config seeing the password in the clear.
**EDIT:** One other point which might be obvious is to make sure that if you
do need to store plaintext passwords, you never store them in a file which
needs to be world-readable. For example, don't ever hard-code them in scripts
even if it's just a quick script which has all its other settings as global
variables or similar. Instead, try to make sure the password is in a separate
file which can have OS-specific permissions restricted as required.
|
Python date string manipulation based on timezone - DST
Question: My objective is to take string(containg UTC date and time) as the input and
convert it to local timezone based on Timezone difference. I have come up with
the following code
**Code**
import time
print "Timezone Diff", time.timezone/3600
def convertTime(string):
print "Before Conversion"
print "year",string[0:4],"month",string[5:7],"day",string[8:10]
print "hour",string[11:13],"min",string[14:16]
print "After Conversion"
print "newhour",int(string[11:13])-(time.timezone/3600)
newhour = int(string[11:13])-(time.timezone/3600)
if newhour>=24:
print "year",string[0:4],"month",string[5:7],"newday",int(string[8:10])+1
print "hour",newhour-24,"min",string[14:16]
convertTime('2013:07:04:14:00')
**Output:**
Timezone Diff -10
Before Conversion
year 2013 month 07 day 04
hour 14 min 00
After Conversion
newhour 24
year 2013 month 07 newday 5
hour 0 min 00
This code is very basic , and clearly wouldn't work for month /year changes
and not consider leap years. Can anyone suggest me a better approach to this
issue.
Answer: Here's a solution with the
[`datetime`](http://docs.python.org/2/library/datetime.html) and
[`pytz`](http://pytz.sourceforge.net/) modules, using my timezone as an
example:
import pytz
import datetime
s = '2013:07:04:14:00'
mydate = datetime.datetime.strptime(s, '%Y:%m:%d:%H:%M')
mydate = mydate.replace(tzinfo=timezone('Australia/Sydney'))
print mydate
Prints:
2013-07-04 14:00:00+10:00
You may have to "reshape" the code to work for your exact output, but I hope
this helps in any way!
|
greater than 'date' python 3
Question: I would like to be able to do greater than and less than against dates. How
would I go about doing that? For example:
date1 = "20/06/2013"
date2 = "25/06/2013"
date3 = "01/07/2013"
date4 = "07/07/2013"
datelist = [date1, date2, date3]
for j in datelist:
if j <= date4:
print j
If I run the above, I get date3 back and not date1 or date2. I think I need I
need to get the system to realise it's a date and I don't know how to do that.
Can someone lend a hand?
Thanks
Answer: You can use the [`datetime`](http://docs.python.org/2/library/datetime.html)
module to convert them all to datetime objects. You are comparing strings in
your example:
>>> from datetime import datetime
>>> date1 = datetime.strptime(date1, "%d/%m/%Y")
>>> date2 = datetime.strptime(date2, "%d/%m/%Y")
>>> date3 = datetime.strptime(date3, "%d/%m/%Y")
>>> date4 = datetime.strptime(date4, "%d/%m/%Y")
>>> datelist = [date1, date2, date3]
>>> for j in datelist:
... if j <= date4:
... print(j.strftime('%d/%m/%Y'))
...
20/06/2013
25/06/2013
01/07/2013
|
Python - creating a histogram
Question: I'm using data of the form: `[num1,num2,..., numk]` (an array of integers).
I would like to plot a histogram of a particular form, which I will use an
example to describe.
Suppose `data = [0,5,7,2,3]`. I want a histogram with:
* Bins of width 1.
* x-axis ticks at 0,1,2,...,4 (one for each element of the array, e.g. if the array had 10 elements the ticks would run from 0 to 9)
* For the bin between tick i and i+1, we have frequency (height) equal to `data[i] + data[i+1]`, e.g. between 1 and 2 we have a rectangle of height 12.
How do I create such a histogram using matplotlib? Or numpy, if you prefer.
Answer: histogram usage is e.g. here:
<http://matplotlib.org/examples/api/histogram_demo.html>
<http://matplotlib.org/examples/pylab_examples/histogram_demo_extended.html>
I'd create this special data structure you want beforehand, then feed it into
the histogram:
map(int.__add__, data[1:], data[0:-1])
> [5, 12, 9, 5]
If you already have numpy imported, you can also do
a=numpy.array(data[0:-1])
b=numpy.array(data[1:])
a+b
> array([ 5, 12, 9, 5])
|
Adding values resulting from input() Python3
Question: i know this is a completely noob question, but how can i add the result from a
input(), add it and print it? this is the code:
import time
#valores de definicao da cortina
cortinas_cm = 100
cortina_preco = 5
#nome e apelido
nome = input("1º Nome" "\n")
apelido = input("Apelido" "\n")
#teste de listagem de cliente
inventario = []
inventario.append(nome + " " + apelido)
#inputs de utilizador para calcular cortinas
quantidade_cm = input("Quantos cm pretende? em cm!" "\n")
inventario.append(quantidade_cm)
print("------------------\n")
print(inventario)
print("\n------------------\n")
#converter o input em string
quantidade = float(quantidade_cm)
#valor total com taxa de comerciante
preco = quantidade * cortina_preco * 1.05
print("preço total e: ")
#converte se em string para que se possa imprimir com texto depois
print (str(preco) + " eur!")
#print de restante de tecido
quantidade_restante = cortinas_cm - quantidade
print("quantidade restante: ")
print(str(quantidade_restante)+ " cm!")
#teste de if
tecla = input("Comprar mais: y or n? \n")
if tecla == "n":
hora = time.strftime('%X %x %Z')
print (hora)
else:
mais_mais = input("Quanto mais deseja? \n")
total_float = float(mais_mais)
total_total = quantidade_restante + mais_mais
print(total_total, total_float)
#loop teste
if quantidade_restante <= 10:
print("Quase no limite de stock")
else:
print("Obrigado pela sua compra")
#para manter a janela aberta
input()
Every time i run the program when i enter in the "if" and add other value, it
gives an error regarding cannot add str with float. I have already transformed
the input() in float! Can you help me out?! The comments are in Portuguese, so
any questions regarding any statement just ask!
Answer: You assigned the conversion to `float` to the _wrong name_ :
mais_mais = input("Quanto mais deseja? \n")
total_float = float(mais_mais)
total_total = quantidade_restante + mais_mais
`mais_mais` is still a string. Assign the conversion to `mais_mais` instead:
mais_mais = input("Quanto mais deseja? \n")
mais_mais = float(mais_mais)
total_total = quantidade_restante + mais_mais
|
How to read an attribute in another class?
Question: still a beginner in Python, so be kind :) FYI : Python 2.7.5, PySide 1.1.2,
OSX 10.8 Simple question. I have this function :
def openFileDialog(self):
import os
path, _ = QtGui.QFileDialog.getOpenFileName(self, "Open File", os.getcwd())
self.label.setText(path)
print(path)
And I want to use this "path" in another function which does not belong to the
same class... I tried different things to display this path in my other
function, for example, I tried
print(testWindow.openFileDialog.path)
but it didn't work. How would you do this...? Thank you!
Answer: You want to
[return](http://docs.python.org/2/reference/simple_stmts.html#grammar-token-
return_stmt) the path.
def openFileDialog(self):
import os
path, _ = QtGui.QFileDialog.getOpenFileName(self, "Open File", os.getcwd())
self.label.setText(path)
return path
This way you can call it as follows
path = testWindow.openFileDialog()
and store it in a local variable. Then do whatever you want with it.
print path
If `testWindow` is the class containing `openFileDialog` you will have to
create an instance of the
[class](http://docs.python.org/2/tutorial/classes.html) first, then call the
method on that.
x = testWindow()
path = x.openFileDialog()
|
Python, handle persistent http connection in web application
Question: I'm in a bit over my head as a beginner with python, but I've managed to setup
a connection to the twitter streaming api via a Django application and
[tweetstream](https://pypi.python.org/pypi/tweetstream).
Within the application I can do the following and get a constant stream of
tweets outputted to the console via the django test web server.
with tweetstream.FilterStream(arg, arg, arg, arg, arg) as stream:
for tweet in stream:
print tweet
I can also do something like this so I can query the stream for information.
my_tweetstream = tweetstream.FilterStream(arg, arg, arg, arg, arg)
print my_tweetstream.variable
Ideally, I'd like to start tweetstream so that it's able to log tweets, but
also be able to visit an admin page which on refresh would query the
connection and return data on how long it's been connected, how many tweets
have been returned etc
The problem is I have no idea how this can be done with the code I have got so
far. For instance, how can i 'store' the connection so i can query it?
Please would someone mind explaining the right approach to going about this,
and which resources might help me understand the problem better?
Thanks in advance,
Answer: I did this recently for a project. You'll need to run the stream consumer as a
separate python process. It doesn't need to be part of your Django application
at all.
Basically I had:
from tweepy import OAuthHandler
from tweepy import Stream
from tweepy.streaming import StreamListener
from myproject.myapp.utils import do_something_with_tweet
class StdOutListener(StreamListener):
def on_data(self, data):
do_something_with_tweet(data)
return True
def main():
listener = StdOutListener()
auth = OAuthHandler(
TWITTER_CONSUMER_KEY,
TWITTER_CONSUMER_SECRET)
auth.set_access_token(
TWITTER_ACCESS_TOKEN,
TWITTER_ACCESS_SECRET)
try:
stream = Stream(auth, listener)
stream.filter(track=['#something', ])
except (KeyboardInterrupt, SystemExit):
print 'Stopping Twitter Streaming Client'
if __name__ == '__main__':
main()
This way you can run this as a separate process and pass the tweet data to
some function to save it or whatever and Django can run happily elsewhere.
Plus points would be to use celery to process your tweet data in asynchronous
tasks: <https://celery.readthedocs.org>
|
Python ctype - How to pass data between C functions
Question: I have a self-made C library that I want to access using python. The problem
is that the code consists essentially of two parts, an initialization to read
in data from a number of files and a few calculations that need to be done
only once. The other part is called in a loop and uses the data generated
before repeatedly. To this function I want to pass parameters from python.
My idea was to write two C wrapper functions, "init" and "loop" - "init" reads
the data and returns a void pointer to a structure that "loop" can use
together with additional parameters that I can pass on from python. Something
like
void *init() {
struct *mystruct ret = (mystruct *)malloc(sizeof(mystruct));
/* Fill ret with data */
return ret;
}
float loop(void *data, float par1, float par2) {
/* do stuff with data, par1, par2, return result */
}
I tried calling "init" from python as a c_void_p, but since "loop" changes
some of the contents of "data" and ctypes' void pointers are immutable, this
did not work.
Other solutions to similar problems I saw seem to require knowledge of how
much memory "init" would use, and I do not know that.
Is there a way to pass data from one C function to another through python
without telling python exactly what or how much it is? Or is there another way
to solve my problem?
* * *
I tried (and failed) to write a minimum crashing example, and after some
debugging it turned out there was a bug in my C code. Thanks to everyone who
replied! Hoping that this might help other people, here is a sort-of-minimal
working version (still without separate 'free' - sorry):
pybug.c:
#include <stdio.h>
#include <stdlib.h>
typedef struct inner_struct_s {
int length;
float *array;
} inner_struct_t;
typedef struct mystruct_S {
int id;
float start;
float end;
inner_struct_t *inner;
} mystruct_t;
void init(void **data) {
int i;
mystruct_t *mystruct = (mystruct_t *)malloc(sizeof(mystruct_t));
inner_struct_t *inner = (inner_struct_t *)malloc(sizeof(inner_struct_t));
inner->length = 10;
inner->array = calloc(inner->length, sizeof(float));
for (i=0; i<inner->length; i++)
inner->array[i] = 2*i;
mystruct->id = 0;
mystruct->start = 0;
mystruct->end = inner->length;
mystruct->inner = inner;
*data = mystruct;
}
float loop(void *data, float par1, float par2, int newsize) {
mystruct_t *str = data;
inner_struct_t *inner = str->inner;
int i;
inner->length = newsize;
inner->array = realloc(inner->array, newsize * sizeof(float));
for (i=0; i<inner->length; i++)
inner->array[i] = par1 + i * par2;
return inner->array[inner->length-1];
}
compile as
cc -c -fPIC pybug.c
cc -shared -o libbug.so pybug.o
Run in python:
from ctypes import *
sl = CDLL('libbug.so')
# What arguments do functions take / return?
sl.init.argtype = c_void_p
sl.loop.restype = c_float
sl.loop.argtypes = [c_void_p, c_float, c_float, c_int]
# Init takes a pointer to a pointer
px = c_void_p()
sl.init(byref(px))
# Call the loop a couple of times
for i in range(10):
print sl.loop(px, i, 5, 10*i+5)
Answer: You should have a corresponding function to `free` the data buffer when the
caller is done. Otherwise I don't see the issue. Just pass the pointer to
`loop` that you get from `init`.
init.restype = c_void_p
loop.argtypes = [c_void_p, c_float, c_float]
loop.restype = c_float
I'm not sure what you mean by "ctypes' void pointers are immutable", unless
you're talking about `c_char_p` and `c_wchar_p`. The issue there is if you
pass a Python string as an argument it uses Python's private pointer to the
string buffer. If a function can change the string, you should first copy it
to a `c_char` or `c_wchar` array.
Here's a simple example showing the problem of passing a Python string (2.x
byte string) as an argument to a function that modifies it. In this case it
changes index 0 to '\x00':
>>> import os
>>> from ctypes import *
>>> open('tmp.c', 'w').write("void f(char *s) {s[0] = 0;}")
>>> os.system('gcc -shared -fPIC -o tmp.so tmp.c')
0
>>> tmp = CDLL('./tmp.so')
>>> tmp.f.argtypes = [c_void_p]
>>> tmp.f.restype = None
>>> tmp.f('a')
>>> 'a'
'\x00'
>>> s = 'abc'
>>> tmp.f(s)
>>> s
'\x00bc'
This is specific to passing Python strings as arguments. It isn't a problem to
pass pointers to data structures that are intended to be mutable, either
ctypes data objects such as a `Structure`, or pointers returned by libraries.
|
Convert timestamps of "yyyy-MM-dd'T'HH:mm:ss.SSSZ" format in Python
Question: I have a log file with timestamps like "2012-05-12T13:04:35.347-07:00". I want
to convert each timestamp into a number so that I sort them by ascending order
based on time.
How can I do this in Python? In Java I found out that I can convert timestamps
for such format with `SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss.SSSZ")` but for
Python I couldn't find anything.
Answer: As py2.x has issues with the `%z` directive you've to do something like this:
from datetime import timedelta,datetime
strs = "2012-05-12T13:04:35.347-07:00"
#replace the last ':' with an empty string, as python UTC offset format is +HHMM
strs = strs[::-1].replace(':','',1)[::-1]
As `datetime.striptime` doesn't supports `%z`(UTC offset)(at least not in
py2.x), so you need a work around:
#Snippet taken from http://stackoverflow.com/a/526450/846892
try:
offset = int(strs[-5:])
except:
print "Error"
delta = timedelta(hours = offset / 100)
Now apply formatting to : `'2012-05-12T13:04:35.347'`
time = datetime.strptime(strs[:-5], "%Y-%m-%dT%H:%M:%S.%f")
time -= delta #reduce the delta from this time object
print time
#2012-05-12 20:04:35.347000
|
Python i18n using pygettext.py
Question: I am starting on localisation, but I get stuck.
1. The program
#example.py
import gettext
t = gettext.translation('cn', 'C:\locale', fallback=True)
_ = t.ugettext
print _('Hello!')
does work.
2. But when try to use pygettext
python pygettext.py -d cn -o cn.pot example.py
I get the message `"invalid syntax:<string>, line 1, pos 18`
I tried even the most simple pygettest command :
python pygettext.py example.py
which returns the same error. I have tried with different other settings and
changes in the program, but this error keeps returning.
The complete example I used the code from is <http://achilles-keep-
moving.blogspot.nl/2011/07/minimal-tutorial-for-python.html>
What I am doing wrong?
Answer: You should escape the `\` character in the string like `'C:\\foo.bar'` or
`r'C:\foo.bar'`.
|
scrape google resultstats with python
Question: I would like to get the estimated results number from google for a keyword. Im
using Python3.3 and try to accomplish this task with BeautifulSoup and
urllib.request. This is my simple code so far
def numResults():
try:
page_google = '''http://www.google.de/#output=search&sclient=psy-ab&q=pokerbonus&oq=pokerbonus&gs_l=hp.3..0i10l2j0i10i30l2.16503.18949.0.20819.10.9.0.1.1.0.413.2110.2-6j1j1.8.0....0...1c.1.19.psy-ab.FEBvxrgi0KU&pbx=1&bav=on.2,or.r_qf.&bvm=bv.48705608,d.Yms&'''
req_google = Request(page_google)
req_google.add_header('User Agent', 'Mozilla/5.0 (Windows NT 6.1; WOW64; rv:15.0) Gecko/20120427 Firefox/15.0a1')
html_google = urlopen(req_google).read()
soup = BeautifulSoup(html_google)
scounttext = soup.find('div', id='resultStats')
except URLError as e:
print(e)
return scounttext
My problem is that my soup variable is somehow encoded and that i cant get any
information out of it. So i get back a None because soup.find doesnt work.
What am i doing wrong and how can i extract the wanted resultstats? Many
thanks!
Answer: If you haven't solved this problem yet, it looks like the reason BeautifulSoup
is failing to find anything is that the resultStats never appear in the soup -
your Request(page_google) is only returning JavaScript, not any search results
that the JavaScript is dynamically loading in. You can verify this by adding a
print(soup)
command to your code and you will see that the resultStats div doesn't appear.
The following code:
import sys
from urllib2 import Request, urlopen
import urllib
from bs4 import BeautifulSoup
query = 'pokerbonus'
url = "http://www.google.de/search?q=%s" % urllib.quote_plus(query)
req_google = Request(url)
req_google.add_header('User-Agent', 'Mozilla/5.0 (Windows; U; Windows NT 5.1; en-GB; rv:1.9.0.3) Gecko/2008092417 Firefox/3.0.3')
html_google = urlopen(req_google).read()
soup = BeautifulSoup(html_google)
scounttext = soup.find('div', id='resultStats')
print(scounttext)
Will print
<div class="sd" id="resultStats">Ungefähr 1.060.000 Ergebnisse</div>
Lastly, using a tool like Selenium Webdriver might be a better way to go about
solving this, as Google does not allow bots to scrape search results.
|
python scrapy - output csv file empty
Question: My main Spider code:
from scrapy.spider import BaseSpider
from scrapy.selector import HtmlXPathSelector
from Belray_oil.items import BelrayOilItem
class BelraySpider(BaseSpider):
name = "Belray_oil"
allowed_domains = ["mxdirtrider.com/"]
start_urls = ["http://www.mxdirtrider.com/h-products/bel-ray/2011-02/pr-bel-ray-accessories-lubricant-oil-2-stroke-2t-mineral-engine.htm?ref=search"]
def parse(self, response):
hxs = HtmlXPathSelector(response)
name = hxs.select("//div[@id='product-title']/h1/span/text()").extract()
MSRP = hxs.select("//div[@id='price']/span[1]/text()").extract()
Sale = hxs.select("//div[@id='price']/span[2]/strong/text()").extract()
print name, MSRP, Sale
my items file:
from scrapy.item import Item, Field
class BelrayOilItem(Item):
name = Field()
MSRP = Field()
Sale = Field()
my terminal log output whene I run : scrapy crawl Belray_oil -o items.csv -t
csv
2013-07-05 18:03:25-0400 [scrapy] INFO: Scrapy 0.14.4 started (bot: Belray_oil)
2013-07-05 18:03:25-0400 [scrapy] DEBUG: Enabled extensions: FeedExporter, LogStats, TelnetConsole, CloseSpider, WebService, CoreStats, MemoryUsage, SpiderState
2013-07-05 18:03:25-0400 [scrapy] DEBUG: Enabled downloader middlewares: HttpAuthMiddleware, DownloadTimeoutMiddleware, UserAgentMiddleware, RetryMiddleware, DefaultHeadersMiddleware, RedirectMiddleware, CookiesMiddleware, HttpCompressionMiddleware, ChunkedTransferMiddleware, DownloaderStats
2013-07-05 18:03:25-0400 [scrapy] DEBUG: Enabled spider middlewares: HttpErrorMiddleware, OffsiteMiddleware, RefererMiddleware, UrlLengthMiddleware, DepthMiddleware
2013-07-05 18:03:25-0400 [scrapy] DEBUG: Enabled item pipelines:
2013-07-05 18:03:25-0400 [Belray_oil] INFO: Spider opened
2013-07-05 18:03:25-0400 [Belray_oil] INFO: Crawled 0 pages (at 0 pages/min), scraped 0 items (at 0 items/min)
2013-07-05 18:03:25-0400 [scrapy] DEBUG: Telnet console listening on 0.0.0.0:6023
2013-07-05 18:03:25-0400 [scrapy] DEBUG: Web service listening on 0.0.0.0:6080
2013-07-05 18:03:26-0400 [Belray_oil] DEBUG: Crawled (200) <GET http://www.mxdirtrider.com/h-products/bel-ray/2011-02/pr-bel-ray-accessories-lubricant-oil-2-stroke-2t-mineral-engine.htm?ref=search> (referer: None)
2013-07-05 18:03:26-0400 [Belray_oil] ERROR: Spider error processing <GET http://www.mxdirtrider.com/h-products/bel-ray/2011-02/pr-bel-ray-accessories-lubricant-oil-2-stroke-2t-mineral-engine.htm?ref=search>
Traceback (most recent call last):
File "/usr/lib/python2.7/dist-packages/twisted/internet/base.py", line 1182, in mainLoop
self.runUntilCurrent()
File "/usr/lib/python2.7/dist-packages/twisted/internet/base.py", line 805, in runUntilCurrent
call.func(*call.args, **call.kw)
File "/usr/lib/python2.7/dist-packages/twisted/internet/defer.py", line 381, in callback
self._startRunCallbacks(result)
File "/usr/lib/python2.7/dist-packages/twisted/internet/defer.py", line 489, in _startRunCallbacks
self._runCallbacks()
--- <exception caught here> ---
File "/usr/lib/python2.7/dist-packages/twisted/internet/defer.py", line 576, in _runCallbacks
current.result = callback(current.result, *args, **kw)
File "/usr/lib/python2.7/dist-packages/scrapy/spider.py", line 62, in parse
raise NotImplementedError
exceptions.NotImplementedError:
2013-07-05 18:03:26-0400 [Belray_oil] INFO: Closing spider (finished)
2013-07-05 18:03:26-0400 [Belray_oil] INFO: Dumping spider stats:
{'downloader/request_bytes': 310,
'downloader/request_count': 1,
'downloader/request_method_count/GET': 1,
'downloader/response_bytes': 13379,
'downloader/response_count': 1,
'downloader/response_status_count/200': 1,
'finish_reason': 'finished',
'finish_time': datetime.datetime(2013, 7, 5, 22, 3, 26, 204316),
'scheduler/memory_enqueued': 1,
'spider_exceptions/NotImplementedError': 1,
'start_time': datetime.datetime(2013, 7, 5, 22, 3, 25, 970550)}
2013-07-05 18:03:26-0400 [Belray_oil] INFO: Spider closed (finished)
2013-07-05 18:03:26-0400 [scrapy] INFO: Dumping global stats:
{'memusage/max': 116150272, 'memusage/startup': 116150272}
The CSV in the output is always empty, and I couldn't figure out what's the
problem exactly. Please little Help!
Answer: You should return an `Item` in your `parse` method:
from scrapy.spider import BaseSpider
from scrapy.selector import HtmlXPathSelector
from Belray_oil.items import BelrayOilItem
class BelraySpider(BaseSpider):
name = "Belray_oil"
allowed_domains = ["mxdirtrider.com"]
start_urls = [
"http://www.mxdirtrider.com/h-products/bel-ray/2011-02/pr-bel-ray-accessories-lubricant-oil-2-stroke-2t-mineral-engine.htm?ref=search"]
def parse(self, response):
hxs = HtmlXPathSelector(response)
item = BelrayOilItem()
item['name'] = hxs.select("//div[@id='product-title']/h1/span/text()").extract()
item['MSRP'] = hxs.select("//div[@id='price']/span[1]/text()").extract()
item['Sale'] = hxs.select("//div[@id='price']/span[2]/strong/text()").extract()
return item
Then, in `items.csv` you'll have:
name,msrp,sale
Bel-Ray 2T Mineral Engine 2-Stroke ,MSRP $9.75,$8.13
Hope that helps.
|
python program for fetching link from a page
Question: I am trying to download an entire play list for Android development tutorial
from Youtube. So I used [savefrom](http://en.savefrom.net/) for generating
playlist for download. But the problem is that I have so many videos in that
playlist. So, I decided to write a python script for making this work simpler.
But the problem is it uses Java Script to generate the link so I cant able to
fetch generated link using javascript()
Example: <http://ssyoutube.com/watch?v=AfleuRtrJoA> It takes 5 sec to generate
download links.
I want to get page source only after 5 sec **from the browse**.
For this kind of work I found a good package named
[selenium](https://pypi.python.org/pypi/selenium).
import time
from selenium import webdriver
def savefromnotnet(url):
browser = webdriver.Firefox() # Get local session of firefox
browser.get(url) # Load page
time.sleep(5) # Let the page load, will be added to the API
return browser.page_source()
source = savefromnotnet("http://ssyoutube.com/watch?v=AfleuRtrJoA")
The `savefromnotnet` function open's Firefox and it will request the url, up
to this every thing works fine. But when I want to get page source
`browser.page_source()` it shows the following error.
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
File "C:\Python27\lib\site-packages\spyderlib\widgets\externalshell\sitecustomize.py", line 523, in runfile
execfile(filename, namespace)
File "C:\Users\BK\Desktop\Working Folder\Python Temp\temp.py", line 10, in <module>
source = savefromnotnet("http://ssyoutube.com/watch?v=AfleuRtrJoA")
File "C:\Users\BK\Desktop\Working Folder\Python Temp\temp.py", line 8, in savefromnotnet
return browser.page_source()
TypeError: 'unicode' object is not callable
Answer: Error occured on following line.
return browser.page_source()
I think brackets did not need.
return browser.page_source
|
Pictures not attached in file
Question: I am using python win32 to drive excel 2010. I want to insert pictures into
certain cells
import win32com.client as win32
from win32com.client import constants as constants
excel = win32.gencache.EnsureDispatch('Excel.Application')
excel.Visible = True
wb = excel.Workbooks.Add()
ws = wb.Worksheets('Sheet1')
ws.Name = 'PicDemo' ## rename worksheet
## select the active sheet
excel.Sheets('PicDemo').Select()
ws = excel.ActiveSheet
ws.Cells(9,9).Select()
## insert a png pic into this cell
ws.Pictures().Insert(pic file path)
I can see the pictures inserted on my PC, but when I send the excel to my
friend, they couldn't see the picture but red cross with 'invalid link'.
I search the web and a lot of people complained about this glitch in excel
2010. I just want the pics saved absolutely without any dependent linking.
Is there a python win32 workaround?
Answer: Microsoft changed the behavior in Excel 2010 and 2013. Try this:
xlApp = win32.Dispatch(r'Excel.Application')
xlBook = xlApp.Workbooks.Open(filename)
xlSheet = xlBook.Sheets(sheet_name)
rng = xlSheet.Range("B2:F8")
height = rng.Offset(rng.Rows.Count, 0).Top - rng.Top
width = rng.Offset(0, rng.Columns.Count).Left - rng.Left
xlSheet.Shapes.AddPicture(image_file_name, False, True, rng.Left, rng.Top, height, width)
[AddPicture](https://msdn.microsoft.com/en-us/library/office/ff198302.aspx)
has an option to save the image with the document.
Height and Width are required. In the code above I am using the Range to size
the image.
|
Using regex in python call to API to clean up return
Question: The following Python Script:
def lookup(guildname):
try:
guildname = gw2api.get_guild_details(guildid)
return guildname
except:
return''
Returns results that look like this: (each are single line)
{u'emblem': {u'foreground_secondary_color_id': 443, u'foreground_primary_color_id': 584, u'foreground_id': 107, u'background_id': 27, u'flags': [], u'background_color_id': 11}, u'guild_id': u'4FAEB34C-BA01-49C8-AD19-C651D69F9981', u'tag': u'RAWK', u'guild_name': u'Ready And Willing Knights'}
{u'emblem': {u'foreground_secondary_color_id': 443, u'foreground_primary_color_id': 11, u'foreground_id': 144, u'background_id': 22, u'flags': [u'FlipBackgroundHorizontal'], u'background_color_id': 4}, u'guild_id': u'DDE74A26-FC28-4514-926D-9BB590E6BDD2', u'tag': u'AI', u'guild_name': u'Alchemy Incorporated'}
{u'emblem': {u'foreground_secondary_color_id': 64, u'foreground_primary_color_id': 146, u'foreground_id': 148, u'background_id': 21, u'flags': [], u'background_color_id': 617}, u'guild_id': u'190E573E-8970-440E-8EA1-653098296EDB', u'tag': u'RoMS', u'guild_name': u'Roses Of The Moonlight Sigil'}
Using Regex I think I can get the guild name
([A-Z0-9a-z-]*\b [A-Z0-9a-z-]*)
But how do I apply that to the above python script? It needs to return just
the unique guild name like `Roses Of The Moonlight Sigil` and `Alchemy
Incorporated` instead of that huge big block. Not really sure if I have the
right expression
Edit with proper function:
import json, gw2api
getguild = gw2api.get_servers()
guildapi = json.dumps(getguild)
apiresult = json.loads(guildapi)
print apiresult['name']
Answer: Use [`json.loads()`](http://docs.python.org/2/library/json.html#json.loads),
then access it as a normal dictionary. Regex is not needed:
import json
...
return json.loads(guildname)
thedict = the_one_returned
print thedict['guild_name']
# Prints Ready And Willing Knights
|
How to load custom configuration file with twisted?
Question: I'm creating a simple server with twisted. I want to store config values in a
yaml file. I can't find examples of configuring twisted services or
applications with app-specific config.
Since the actual Resource object I'm serving will be created for each request,
obviously this isn't the right place to read a config file.
Would I read the config file in my factory perhaps, and then subclass Site to
pass it to my resource? I just can't seem to find the pattern documented
anywhere.
Here's my code:
#!/usr/bin/env python
from twisted.internet import reactor
from twisted.web.server import Site
from twisted.web.resource import Resource
import yaml
def load_config():
return yaml.load(file('./test/config_file.yaml', 'r'))
# how can I make this resource have access to my config?
class ScaledImage(Resource):
isLeaf = True
def render_POST(self, request):
return """
<h1>image scaled</h1>
"""
factory = Site(ScaledImage())
reactor.listenTCP(8000, factory)
reactor.run()
Answer: How about just changing this:
factory = Site(ScaledImage(load_config(...)))
Then make your `ScaledImage` initializer accept the configuration.
As a general point, you probably _shouldn't_ pass your _entire_ configuration
around. Configuration files usually end up as big confusing balls of random
stuff. You don't want to push this big mess through your APIs. Instead, pick
out the piece of configuration that `ScaledImage` is interested in and pass
_that_ in:
config = load_config(...)
scaleFactor = getScaleFactorFromConfig(config)
factory = Site(ScaledImage(scaleFactor))
|
list indices must be integers python nested dictionaries
Question: In python 3, I need a function to dynamically return a value from a nested
key.
nesteddict = {'a':'a1','b':'b1','c':{'cn':'cn1'}}
print(nesteddict['c']['cn']) #gives cn1
def nestedvalueget(keys):
print(nesteddict[keys])
nestedvalueget(['n']['cn'])
How should nestedvalueget be written?
I'm not sure the title is properly phrased, but I'm not sure how else to best
describe this.
Answer: If you want to traverse dictionaries, use a loop:
def nestedvalueget(*keys):
ob = nesteddict
for key in keys:
ob = ob[key]
return ob
or use
[`functools.reduce()`](http://docs.python.org/3/library/functools.html#functools.reduce):
from functools import reduce
from operator import getitem
def nestedvalueget(*keys):
return reduce(getitem, keys, nesteddict)
then use either version as:
nestedvalueget('c', 'cn')
Note that either version takes a variable number of arguments to let you pas 0
or more keys as positional arguments.
Demos:
>>> nesteddict = {'a':'a1','b':'b1','c':{'cn':'cn1'}}
>>> def nestedvalueget(*keys):
... ob = nesteddict
... for key in keys:
... ob = ob[key]
... return ob
...
>>> nestedvalueget('c', 'cn')
'cn1'
>>> from functools import reduce
>>> from operator import getitem
>>> def nestedvalueget(*keys):
... return reduce(getitem, keys, nesteddict)
...
>>> nestedvalueget('c', 'cn')
'cn1'
And to clarify your error message: You passed the expression `['n']['cn']` to
your function call, which defines a list with one element (`['n']`), which you
then try to index with `'cn'`, a string. List indices can only be integers:
>>> ['n']['cn']
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
TypeError: list indices must be integers, not str
>>> ['n'][0]
'n'
|
Creating simply image gallery in Python, Tkinter & PIL
Question: So, I'm on simple project for a online course to make an image gallery using
python. The thing is to create 3 buttons one Next, Previous and Quit. So far
the quit button works and the next loads a new image but in a different
window, I'm quite new to python and GUI-programming with Tkinter so this is a
big part of the begineers course.
So far my code looks like this and everything works. But I need help in HOW to
make a previous and a next button, I've used the NEW statement so far but it
opens in a different window. I simply want to display 1 image then click next
image with some simple text.
import Image
import ImageTk
import Tkinter
root = Tkinter.Tk();
text = Tkinter.Text(root, width=50, height=15);
myImage = ImageTk.PhotoImage(file='nesta.png');
def new():
wind = Tkinter.Toplevel()
wind.geometry('600x600')
imageFile2 = Image.open("signori.png")
image2 = ImageTk.PhotoImage(imageFile2)
panel2 = Tkinter.Label(wind , image=image2)
panel2.place(relx=0.0, rely=0.0)
wind.mainloop()
master = Tkinter.Tk()
master.geometry('600x600')
B = Tkinter.Button(master, text = 'Previous picture', command = new).pack()
B = Tkinter.Button(master, text = 'Quit', command = quit).pack()
B = Tkinter.Button(master, text = 'Next picture', command = new).pack()
master.mainloop()
Answer: Change image by setting image item: `Label['image'] = photoimage_obj`
import Image
import ImageTk
import Tkinter
image_list = ['1.jpg', '2.jpg', '5.jpg']
text_list = ['apple', 'bird', 'cat']
current = 0
def move(delta):
global current, image_list
if not (0 <= current + delta < len(image_list)):
tkMessageBox.showinfo('End', 'No more image.')
return
current += delta
image = Image.open(image_list[current])
photo = ImageTk.PhotoImage(image)
label['text'] = text_list[current]
label['image'] = photo
label.photo = photo
root = Tkinter.Tk()
label = Tkinter.Label(root, compound=Tkinter.TOP)
label.pack()
frame = Tkinter.Frame(root)
frame.pack()
Tkinter.Button(frame, text='Previous picture', command=lambda: move(-1)).pack(side=Tkinter.LEFT)
Tkinter.Button(frame, text='Next picture', command=lambda: move(+1)).pack(side=Tkinter.LEFT)
Tkinter.Button(frame, text='Quit', command=root.quit).pack(side=Tkinter.LEFT)
move(0)
root.mainloop()
|
Copying lists in python
Question: I am relatively new to Python. I am trying to come up with a function that
takes a list, adds +1 to a value at random in that list, and then places a new
list with the modified value as a new index.
For instance, if I have
blank=[]
list_one=[1,2,3,4,5] #
The possible outcome might be
blank=[ [1,2,3,4,5], [1,3,3,4,5],[1,3,3,4,6],[2,3,3,4,6],[2,4,3,4,6] ]
So the function takes blank[0], copies and modifies it, and returns it as
blank[1]. Similarly, the function takes blank[1], copies it and modifies it,
and returns it as blank[2]
This is my code:
import random
list_one=[1,2,3,4,5]
def new_function(value):
for i in range(1,value):
list_copy=list_one[:]
blank.append(list_copy)
x=random.choice(blank[i])
y=blank[i].index(x)
blank[i][y]=x+1
return blank
print new_function(4)
"""Yields [ [1,2,3,4,5],[1,3,3,4,5],[2,2,3,4,5],[1,2,4,4,5] ]
(this is the list, blank)"""
my problem is that blank[1], blank[2], and blank[3] are all modifications of
blank[0], when each indexed list should be a modification of the previous
indexed list.
What am I doing wrong here???
Answer: The issue is that you always copy the original list, not the new one. This is
easily solved by using the same name for the new list, thus, when the loop
comes round again, the new list is copied:
import random
def randomly_increment(seq, n):
for _ in range(n):
x = random.randrange(len(seq))
seq = list(seq)
seq[x] += 1
yield seq
Which I believe works as intended:
>>> list(randomly_increment([1, 2, 3, 4, 5], 4))
[[1, 2, 4, 4, 5], [1, 2, 4, 5, 5], [1, 2, 4, 5, 6], [1, 2, 4, 6, 6]]
Other changes:
* Rather than building a list, this is a generator, which is generally more flexible and easier to read/write. If you need a list, then wrap the call with `list()` as above.
* Rather than making a random choice, then finding it's place to change it, it makes more sense to pick a place, and change it. This also avoids the issue where `seq.index()` will return the first value matching the given one, not necessarily the 'picked' one.
* Using `list(seq)` to copy over `seq[:]`, as noted in my comment. This is more readable.
|
Entire JSON into One SQLite Field with Python
Question: I have what is likely an easy question. I'm trying to pull a JSON from an
online source, and store it in a SQLite table. In addition to storing the data
in a rich table, corresponding to the many fields in the JSON, I would like to
also just dump the entire JSON into a table every time it is pulled.
The table looks like:
CREATE TABLE Raw_JSONs (ID INTEGER PRIMARY KEY ASC, T DATE DEFAULT (datetime('now','localtime')), JSON text);
I've pulled a JSON from some URL using the following python code:
from pyquery import PyQuery
from lxml import etree
import urllib
x = PyQuery(url='json')
y = x('p').text()
Now, I'd like to execute the following INSERT command:
import sqlite3
db = sqlite3.connect('a.db')
c = db.cursor()
c.execute("insert into Raw_JSONs values(NULL,DATETIME('now'),?)", y)
But I'm told that I've supplied the incorrect number bindings (i.e. thousands,
instead of just 1). I gather it's reading the y variable as all the different
elements of the JSON.
Can someone help me store just the JSON, in it's entirety?
Also, as I'm obviously new to this JSON game, any online resources to
recommend would be amazing.
Thanks!
Answer: `.execute()` expects a _sequence_ , better give it a one-element tuple:
c.execute("insert into Raw_JSONs values(NULL,DATETIME('now'),?)", (y,))
A Python string is a sequence too, one of individual characters. So the
`.execute()` call tried to treat each separate character as a parameter for
your query, and unless your string is one character short that means it'll not
provide the right number of parameters.
Don't forget to commit your inserts:
db.commit()
or use the database connection as a context manager:
with db:
# inserts executed here will automatically commit if no exceptions are raised.
|
How do I modify the file upload handlers in a class based View with CSRF middleware?
Question: In my Django project I will have to modify the tuple of file upload handlers
"on the fly" [as
documented](https://docs.djangoproject.com/en/dev/topics/http/file-
uploads/#modifying-upload-handlers-on-the-fly), to have the ability to modify
the file stream as it is being uploaded. I need this "on the fly", because I
have to provide the handler some data from the View (see `setup()` method in
the code below).
The documentation also mentions how to take care of doing this if you use CSRF
protection. This is special because the CSRF protection middleware accesses
the POST data in the request resulting in the file upload process will be
fired at the time before my View gets called. However, this is only documented
for old-style Views, but I want to accomplish the same using a Class Based
View.
Here's a minimal code example of my View:
from django.views.decorators.csrf import csrf_exempt, csrf_protect
class MyView(TemplateResponseMixin, ContextMixin, View):
template_name = 'mytemplate.html'
def __init__(self, *args, **kwargs):
self.fileuploadhandler = MyUploadHandler()
super(MyView, self).__init__(*args, **kwargs)
def get(self, request, *args, **kwargs):
return self.render_to_response(
self.get_context_data(form=MyForm()))
#@csrf_protect # this gives the error below
def post(self, request, *args, **kwargs):
# Set up the FileUploadHandler
# SNIP - some data is being gathered here
self.fileuploadhandler.setup(mydata)
# Process the POST data by loading the ModelForm
form = MyForm(request.POST, request.FILES)
if form.is_valid():
# SNIP processing Form
else:
return self.render_to_response(self.get_context_data(form=form))
def get_context_data(self, **kwargs):
context = super(MyView, self).get_context_data(**kwargs)
return context
@csrf_exempt # I have to do this
def dispatch(self, *args, **kwargs):
self.request.upload_handlers.insert(0, self.fileuploadhandler)
return super(MyView, self).dispatch(*args, **kwargs)
The error I get when using `@csrf_protect` on the `post` method is:
Traceback (most recent call last):
File "/some/path/to/Envs/someenv/local/lib/python2.7/site-packages/django/core/handlers/base.py", line 115, in get_response
response = callback(request, *callback_args, **callback_kwargs)
File "/some/path/to/Envs/someenv/local/lib/python2.7/site-packages/django/views/generic/base.py", line 68, in view
return self.dispatch(request, *args, **kwargs)
File "/some/path/to/Envs/someenv/local/lib/python2.7/site-packages/django/utils/decorators.py", line 25, in _wrapper
return bound_func(*args, **kwargs)
File "/some/path/to/Envs/someenv/local/lib/python2.7/site-packages/django/contrib/auth/decorators.py", line 25, in _wrapped_view
return view_func(request, *args, **kwargs)
File "/some/path/to/Envs/someenv/local/lib/python2.7/site-packages/django/utils/decorators.py", line 21, in bound_func
return func(self, *args2, **kwargs2)
File "/some/path/to/Envs/someenv/local/lib/python2.7/site-packages/django/utils/decorators.py", line 25, in _wrapper
return bound_func(*args, **kwargs)
File "/some/path/to/Envs/someenv/local/lib/python2.7/site-packages/django/contrib/auth/decorators.py", line 25, in _wrapped_view
return view_func(request, *args, **kwargs)
File "/some/path/to/Envs/someenv/local/lib/python2.7/site-packages/django/utils/decorators.py", line 21, in bound_func
return func(self, *args2, **kwargs2)
File "/some/path/to/Envs/someenv/local/lib/python2.7/site-packages/django/views/decorators/csrf.py", line 77, in wrapped_view
return view_func(*args, **kwargs)
File "/some/path/to/project/myapp/views.py", line 01234, in dispatch
return super(MyView, self).dispatch(*args, **kwargs)
File "/some/path/to/Envs/someenv/local/lib/python2.7/site-packages/django/views/generic/base.py", line 86, in dispatch
return handler(request, *args, **kwargs)
File "/some/path/to/Envs/someenv/local/lib/python2.7/site-packages/django/utils/decorators.py", line 87, in _wrapped_view
result = middleware.process_view(request, view_func, args, kwargs)
File "/some/path/to/Envs/someenv/local/lib/python2.7/site-packages/django/middleware/csrf.py", line 95, in process_view
request.COOKIES[settings.CSRF_COOKIE_NAME])
AttributeError: 'MyView' object has no attribute 'COOKIES'
So, how can I have the combination of the following three properties of my
View?
* the use of Class Based Views
* ability to modify the file upload handler "on the fly"
* proper CSRF protection on the View
Django version used: 1.5.1, Python 2.7.3.
Answer: With the help of a colleague I've found a bit of an ugly way of using the CSRF
middleware to check the token manually within the View. Here's the recipe:
from django.views.decorators.csrf import csrf_exempt, csrf_protect
from django.middleware.csrf import CsrfViewMiddleware
class MyView(TemplateResponseMixin, ContextMixin, View):
template_name = 'mytemplate.html'
def __init__(self, *args, **kwargs):
self.fileuploadhandler = MyUploadHandler()
super(MyView, self).__init__(*args, **kwargs)
def post(self, request, *args, **kwargs):
# Set up the FileUploadHandler
# SNIP - some data is being gathered here
self.fileuploadhandler.setup(mydata)
# Check CSRF manually *after* initializing the file upload handlers.
csrf_checker = CsrfViewMiddleware()
csrf_error = csrf_checker.process_view(request, None, None, None)
if csrf_error is not None:
return csrf_error # csrf_error is the regular CSRF error View
# Process the POST data by loading the ModelForm
form = MyForm(request.POST, request.FILES)
if form.is_valid():
# SNIP processing Form
else:
return self.render_to_response(self.get_context_data(form=form))
@csrf_exempt # Important to skip CSRF checking here.
def dispatch(self, *args, **kwargs):
self.request.upload_handlers.insert(0, self.fileuploadhandler)
return super(MyView, self).dispatch(*args, **kwargs)
I think here's some room for improvement in Django - the CSRF middleware
should provide a separate `check_token` method wrapped in `process_view`, in
my opinion.
|
Why doesn't opencv give this rectangle a colour using python's cv2
Question: The following code draws a white rectangle. However it is not supposed to do
that. Considering opencv uses BGR colorspace, it should look like this
<http://www.colorpicker.com/?colorcode=9F635F>
import cv2
import numpy as np
drawing = np.zeros([500, 500, 3])
cv2.rectangle(drawing, (0, 0), (250, 250), (95, 99, 159), -1)
cv2.imshow("drawing", drawing)
cv2.waitKey()
Answer: It will draw fine if you change
drawing = np.zeros([500, 500, 3])
to
drawing = np.zeros([500, 500, 3], np.uint8)
Otherwise, the image will be of 32F type.

|
How do I monitor the change of my gtalk status message?
Question: I want to write a program (preferably in python) which could monitor my gtalk
status messages, and whenever I post a new gtalk status message, this program
will get the content of this message and post it somewhere else.
Is there a way for me to register for notification of my gtalk status change?
Or do I have to poll my status constantly? Where can I find the API to do it?
Answer: I'd suggest you to use sleekxmpp. You can register a callback like this:
self.add_event_handler("changed_status", self.my_callback_function)
Where self is your instance of a class that inherits from
`sleekxmpp.ClientXMPP`.
**Edit** : I've just made this code for you (free to use as you wish)
import sleekxmpp
from ConfigParser import ConfigParser
class StatusWatcher(sleekxmpp.ClientXMPP):
def __init__(self, jid_to_watch):
self._jid_to_watch = jid_to_watch
config = ConfigParser()
config.read("config.ini")
jid = config.get("general", "jid")
resource = config.get("general", "resource")
password = config.get("general", "password")
sleekxmpp.ClientXMPP.__init__(self, jid + "/" + resource, password)
self.add_event_handler("session_start", self.handle_XMPP_connected)
self.add_event_handler("changed_status", self.handle_changed_status)
def handle_XMPP_connected(self, event):
print "connected"
self.sendPresence(pstatus="I'm just a Bot.")
self.get_roster()
def handle_changed_status(self, pres):
if pres['from'].bare == self._jid_to_watch:
print pres['status']
xmpp = StatusWatcher("[email protected]") # The account to monitor
xmpp.register_plugin('xep_0030')
xmpp.register_plugin('xep_0199')
if xmpp.connect():
xmpp.process(threaded=False)
You need to create a file `config.ini` with your credentials:
[general]
[email protected]
resource=presence_watcher
password=yourpwd
|
Python: Threads stopping without any reason
Question: I am trying to make a hash breaking application that will check all the lines
of one file with all the lines in the rockyou dictionary. While with pre-
hashing the rock you i got the time of checking one hash down to a few seconds
its still not enough. This is why i am moving my program to multithreading.
But my threads stop without rising any exceptions.
import threading
import datetime
class ThreadClass(threading.Thread):
hash_list=0
def file_len(fname):
with open(fname) as f:
for i, l in enumerate(f):
pass
return i + 1
list_len=file_len("list.txt")
def run(self):
while ThreadClass.list_len>0:
ThreadClass.list_len=ThreadClass.list_len-1
print str(threading.current_thread())+":"+str(ThreadClass.list_len)
for i in range(20):
try:
t = ThreadClass()
t.start()
except:
raise
Here is output:  When i run it after some time
there is only one thread reporting. Why? Thanks for all help
EDIT: One of the threads rises a key error.I dont know what that is
Answer: As calculating hashes is a CPU bound problems, using multithreading won't help
you in cPython [because of the
GIL](http://docs.python.org/2/c-api/init.html#threads).
If anything, you need to use
[`multiprocessing`](http://docs.python.org/3/library/multiprocessing). Using a
[`Pool`](http://docs.python.org/3/library/multiprocessing#multiprocessing.pool.Pool),
your whole code could be reduced to something like:
import multiprocessing
def calculate(line):
# ... calculate the hash ...
return (line, 'calculated_result')
pool = multiprocessing.Pool(multiprocessing.cpu_count())
with open('input.txt') as inputfile:
result = pool.map(calculate, inputfile)
print(result)
# compare results
* * *
As to your problem with the threads: You're concurrently accessing
`ThreadClass.list_len` from multiple theads. First you access it and compare
it to 0. Then you access it again, decrease it and store it back, which is
[not thread safe](http://effbot.org/pyfaq/what-kinds-of-global-value-mutation-
are-thread-safe.htm) And then you access it again when you print it. Between
any of these operations, another thread could modify the value.
To show this, I've modified your code a little:
import threading
import datetime
lns = []
class ThreadClass(threading.Thread):
hash_list=0
list_len= 10000
def run(self):
while ThreadClass.list_len>0:
ThreadClass.list_len=ThreadClass.list_len-1
ln = ThreadClass.list_len # copy for later use ...
lns.append(ln)
threads = []
for i in range(20):
t = ThreadClass()
t.start()
threads.append(t)
for t in threads:
t.join()
print len(lns), len(set(lns)), min(lns)
When I run this 10 times, what i get is:
13473 9999 -1
10000 10000 0
10000 10000 0
12778 10002 -2
10140 10000 0
10000 10000 0
15579 10000 -1
10866 9996 0
10000 10000 0
10164 9999 -1
So sometimes it seems to run ok, but others there are a lot of values that
have been added multiple times, and list_len even manages to get negative.
If you disassemble the run method, you'll see this:
>>> dis.dis(ThreadClass.run)
11 0 SETUP_LOOP 57 (to 60)
>> 3 LOAD_GLOBAL 0 (ThreadClass)
6 LOAD_ATTR 1 (list_len)
9 LOAD_CONST 1 (0)
12 COMPARE_OP 4 (>)
15 POP_JUMP_IF_FALSE 59
12 18 LOAD_GLOBAL 0 (ThreadClass)
21 LOAD_ATTR 1 (list_len)
24 LOAD_CONST 2 (1)
27 BINARY_SUBTRACT
28 LOAD_GLOBAL 0 (ThreadClass)
31 STORE_ATTR 1 (list_len)
13 34 LOAD_GLOBAL 0 (ThreadClass)
37 LOAD_ATTR 1 (list_len)
40 STORE_FAST 1 (ln)
14 43 LOAD_GLOBAL 2 (lns)
46 LOAD_ATTR 3 (append)
49 LOAD_FAST 1 (ln)
52 CALL_FUNCTION 1
55 POP_TOP
56 JUMP_ABSOLUTE 3
>> 59 POP_BLOCK
>> 60 LOAD_CONST 0 (None)
63 RETURN_VALUE
Simplified you can say, between any of these lines another thread could run
and modify something. To safely access a value from multiple threads, you need
to synchronize the access.
For example using `threading.Lock` the code could be modified like this:
class ThreadClass(threading.Thread):
# ...
lock = threading.Lock()
def run(self):
while True:
with self.lock:
# code accessing shared variables inside lock
if ThreadClass.list_len <= 0:
return
ThreadClass.list_len -= 1
list_len = ThreadClass.list_len # store for later use...
# not accessing shared state, outside of lock
I'm not entirely sure that this is the cause of your problem, but it may be,
specially if you're also reading from an input file in your run method.
|
Get sourcecode for urls
Question: I have following codes:
import urllib2
from itertools import product
with open('urllist.txt') as urllist:
urls=[line.strip() for line in urllist]
for url in product(urls):
usock = urllib2.urlopen(url)
data = usock.read()
usock.close()
sourcecode=open('./sourcecode', 'w+')
sourcecode.write(data)
When I ran it, it gave:
Traceback (most recent call last):
File "12.py", line 8, in <module>
usock = urllib2.urlopen(url)
File "/opt/python2.7.1/lib/python2.7/urllib2.py", line 126, in urlopen
return _opener.open(url, data, timeout)
File "/opt/python2.7.1/lib/python2.7/urllib2.py", line 383, in open
req.timeout = timeout
AttributeError: 'tuple' object has no attribute 'timeout'
Any idea how to fix it? Many thanks!
Answer: [`itertools.product`](http://docs.python.org/2/library/itertools.html#itertools.product)
returns a tuple not the item itself.:
>>> from itertools import product
>>> lis = ['a','b','c']
>>> for p in product(lis):
... print p
...
('a',)
('b',)
('c',)
Use a simple loop over urls:
for url in urls:
usock = urllib2.urlopen(url)
|
Django ImportError on Heroku
Question: I tried several things to get my app working on heroku, but now I'm out of
ideas. I can install my project on heroku's rep, but I get a 500 error code.
My application works very well using virtualenv on my machine after I followed
the steps described on heroku documentation for django.
When I do my "git push heroku master" and try in browser, I get the following
error:
2013-07-07T15:39:11.170514+00:00 app[web.1]: ImportError: No module named apps.base
2013-07-07T15:39:11.170059+00:00 app[web.1]: File "/app/.heroku/python/lib/python2.7/site-packages/django/utils/dateformat.py", line 35, in format
2013-07-07T15:39:11.170202+00:00 app[web.1]: app = import_module(appname)
2013-07-07T15:39:11.170202+00:00 app[web.1]: default_translation = _fetch(settings.LANGUAGE_CODE)
2013-07-07T15:39:11.170202+00:00 app[web.1]: _default = translation(settings.LANGUAGE_CODE)
I tought it was caused by a directory structure that wasn't supported on
heroku, so I adjusted it from the default one that was created with django
startproject command.
Here is my new file structure. I adjusted the import reference everywhere and
as I said, I works pefctly in local:
manage.py
Procfile
requirements.txt
vielfaltig
|____apps
| |____base
| | |____models.py
| | |____templates
| | |____tests.py
| | |____urls.py
| | |____views.py
| |____projects
| | |____admin.py
| | |____models.py
| | |____templates
| | |____templatetags
| | |____tests.py
| | |____translation.py
| | |____urls.py
| | |____views.py
|____locale
|____media
|____settings.py
|____static
|____urls.py
|____vielfaltig.db
|____wsgi.py
As you notice, I have 2 apps (base and projects). In the code, I import them
using "vielfaltig.apps.base" for example. I changed this everywhere. I had
this error before and I changed the directory structure according to what I
read when I googled the error. I also tried to put everything in the root
directory (along with the requirements.txt and procfile). I don't know why it
keeps telling me an ImportError for "apps.base" while I reference the app
using "vielfaltif.apps.base" everywhere... ?
Does anyone have an idea? I will paste my settings.py if needed. For now I
think it would just take a lot of space.
Thank you very much for any help !
Answer: I guess it will work with `import vielfaltig.apps.base` instead of
`apps.base`. It can be fixed if you insert path `vielfaltig` to python system
path in your `settings.py`
import sys
sys.path.append('vielfaltig')
|
Fast 2 dimensional array of floats for python (access/write)
Question: For my project use, I need to store certain amount (~100x100) of floats in two
dimensional array. And during the function calculation I need to read and
write to the array and since the function is really the bottleneck (consuming
98% of time) I really would need it to be fast.
I did some experiments with numpy and cython:
import numpy
import time
cimport numpy
cimport cython
cdef int col, row
DTYPE = numpy.int
ctypedef numpy.int_t DTYPE_t
cdef numpy.ndarray[DTYPE_t, ndim=2] matrix_c = numpy.zeros([100 + 1, 100 + 1], dtype=DTYPE)
time_ = time.time()
for l in xrange(5000):
for col in xrange(100):
for row in xrange(100):
matrix_c[<unsigned int>row + 1][<unsigned int>col + 1] = matrix_c[<unsigned int>row][<unsigned int>col]
print "Numpy + cython time: {0}".format(time.time() - time_)
but I found out that in spite of all my attempts, the version using python
lists, is still significantly faster.
Code using lists:
matrix = []
for i in xrange(100 + 1):
matrix.append([])
for j in xrange(100 + 1):
matrix[i].append(0)
time_ = time.time()
for l in xrange(5000):
for col in xrange(100):
for row in xrange(100):
matrix[row + 1][col + 1] = matrix[row][col]
print "list time: {0}".format(time.time() - time_)
And results:
list time: 0.0141758918762
Numpy + cython time: 0.484772920609
Have I done something wrong? If not, is there anything that would help me to
improve the results?
Answer: Here's my version of the code you have. There are three functions, dealing
with integer arrays, 32 bit floating point arrays and double precision
floating point arrays, respectively.
from numpy cimport ndarray as ar
cimport numpy as np
import numpy as np
cimport cython
import time
@cython.boundscheck(False)
@cython.wraparound(False)
def access_write_int(ar[int,ndim=2] c, int n):
cdef int l, col, row, h=c.shape[0], w=c.shape[1]
time_ = time.time()
for l in range(n):
for row in range(h-1):
for col in range(w-1):
c[row+1,col+1] = c[row,col]
print "Numpy + cython time: {0}".format(time.time() - time_)
@cython.boundscheck(False)
@cython.wraparound(False)
def access_write_float(ar[np.float32_t,ndim=2] c, int n):
cdef int l, col, row, h=c.shape[0], w=c.shape[1]
time_ = time.time()
for l in range(n):
for row in range(h-1):
for col in range(w-1):
c[row+1,col+1] = c[row,col]
print "Numpy + cython time: {0}".format(time.time() - time_)
@cython.boundscheck(False)
@cython.wraparound(False)
def access_write_double(ar[double,ndim=2] c, int n):
cdef int l, col, row, h=c.shape[0], w=c.shape[1]
time_ = time.time()
for l in range(n):
for row in range(h-1):
for col in range(w-1):
c[row+1,col+1] = c[row,col]
print "Numpy + cython time: {0}".format(time.time() - time_)
To call these functions from Python I run this
import numpy as np
from numpy.random import rand, randint
print "integers"
c = randint(0, high=20, size=(101,101))
access_write_int(c, 5000)
print "32 bit float"
c = rand(101, 101).astype(np.float32)
access_write_float(c, 5000)
print "double precision"
c = rand(101, 101)
access_write_double(c, 5000)
The following changes are important:
1. Avoid slicing the array by accessing it using indices of the form `[i,j]` instead of `[i][j]`
2. Define the variables `l`, `col`, and `row`, as integers so that the for loops run in C.
3. Use the function decorators `@cython.boundscheck(False)` and '@cython.wraparound(False)` to turn off boundschecking and wraparound indexing for the key portion of the program. This allows for out of bounds memory accesses, so you should do this _only_ when you are certain that your indices are what they should be.
4. Swap the two innermost `for` loops so that you access your array according to how it is arranged in memory. This makes a much bigger difference for larger arrays. The arrays given by `np.zeros` `np.random.rand`, etc. are usually C contiguous, so rows are stored in contiguous blocks and it is faster to vary the index along the rows in the outer `for` loop and not the inner one. If you want to keep the for loops as they are, consider taking the transpose of your array before you run the function on it so that the columns are in contiguous blocks instead.
|
Python 2.7.3 Math Flaw( 40 million is less then six hundred thousand )
Question: **SOLVED**
In my program, it thinks that 40 million is less then 600,000.
Here is the code: (Stop it after it loops 20 times)
import re
import urllib2
x = 0
d = 1
c = 1
highestmemberid = 1
highestmembercash = 4301848
while (d==1):
x = float(x + 1)
if (x==14 or x==3 or x==11 or x==13 or x==15):
x = x + 1
print x,
url = "http://www.marapets.com/profile.php?id=" + str(x)
home = opener.open(url)
matchpoint = re.compile("<td align='left' valign=middle><B style='color:#(......);'>(.*?)</B></td>")
home = home.read()
home = home
points = re.findall(matchpoint,home)
if ("http://images.marapets.com/stuff/banned.gif" in home or "This user does not exist" in home):
print "banned/dosen't exist"
else:
mp = points[0][1]
mp = mp.replace(" MP","")
mpcheck= mp.replace(",","")
mp = float(mpcheck)
if (mpcheck > highestmembercash):
highestmembercash = mpcheck
highestmemberid = x
print "richer"
else:
print "Not richer!"
print mp
print "The richest player in marapets is player id #: " + str(highestmemberid) + "Who has: " + str(highestmembercash) + " MP."
if(x == 5368561):
print "The richest player in marapets is player id #: " + str(highestmemberid) + "Who has: " + str(highestmembercash) + " MP."
What the program does is grab cash amounts from the page, and then sees if
this is the highest amount. It loops about 5 million times.
Answer: `mpcheck` is a string, you want to check that `mp > highestmembercash` and
assign `highestmembercash = mp`.
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.