text
stringlengths 27
775k
|
---|
//
// Author: B4rtik (@b4rtik)
// Project: RedPeanut (https://github.com/b4rtik/RedPeanut)
// License: BSD 3-Clause
//
using System;
using System.Collections.Generic;
using System.Linq;
namespace RedPeanut
{
public class AutoCompletionHandler : IAutoCompleteHandler
{
// new char[] { ' ', '.', '/', '\\', ':' };
public Dictionary<string,string> menu { get; set; }
public char[] Separators { get; set; } = new char[] { };
public string[] GetSuggestions(string text, int index)
{
if (text.Split(' ').Length <= 2)
return menu.Keys.ToArray<string>().Where(f => f.StartsWith(text)).ToArray();
else
return null;
}
}
class AutoCompletionHandlerC2ServerManager : IAutoCompleteHandler
{
public string[] AgentIdList { get; set; }
public Dictionary<string, string> menu { get; set; }
public char[] Separators { get; set; } = new char[] { };
public string[] GetSuggestions(string text, int index)
{
if (!text.StartsWith("interact"))
{
if (text.Split(' ').Length < 2)
return menu.Keys.ToArray<string>().Where(f => f.StartsWith(text)).ToArray();
else
return null;
}
else
{
string[] agl = new string[AgentIdList.Length];
for (int i = 0; i < agl.Length; i++)
agl[i] = "interact " + AgentIdList[i];
if (text.Split(' ').Length <= 2)
return agl.Where(f => f.StartsWith(text)).ToArray();
else
return null;
}
}
}
}
|
#!/bin/bash
project_name=$1
workflow=$2
file_suffix=$3 #extension of input file, does not include .gz if present in input
root_dir=$4
fastq_end1=$5
fastq_end2=$6
input_address=$7 #this is an s3 address e.g. s3://path/to/input/directory
output_address=$8 #this is an s3 address e.g. s3://path/to/output/directory
log_dir=$9
is_zipped=${10} #either "True" or "False", indicates whether input is gzipped
#logging
mkdir -p $log_dir
log_file=$log_dir/'k_diff_cal.log'
exec 1>>$log_file
exec 2>>$log_file
status_file=$log_dir/'status.log'
touch $status_file
#prepare output directories
workspace=$root_dir/$project_name/$workflow
mkdir -p $workspace
echo $workspace
echo "%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%"
date
echo "%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%"
check_step_already_done $JOB_NAME $status_file
# Download group text file
if [ ! -f $workspace/group.txt ] || [ ! -f $workspace/all_gene_counts.txt ]
then
aws s3 cp $input_address/group.txt $workspace/group.txt
aws s3 cp $input_address/all_gene_counts.txt $workspace/all_gene_counts.txt
fi
##END_DOWNLOAD##
# Call R script
check_exit_status "Rscript /shared/workspace/Pipelines/scripts/RNASeq/kallisto/RNA-seq_limma.R \
$workspace/group.txt \
$workspace/all_gene_counts.txt $workspace/" $JOB_NAME $status_file
echo "##########################"
ls $workspace/
echo "##########################"
|
---
layout: post
title: "leet671.second minimum NOde IN a Binary Tree"
author: "yzpwslc"
date: 2018-03-11 23:06
---
<p>题目:在一个特殊二叉树中寻找第二小值</p>
<p>分析:遍历树,将节点值存入列表,排序寻找</p>
<p>代码如下:</p>
{% highlight python %}
class Solution(object):
def findSecondMinimumValue(self, root):
"""
:type root: TreeNode
:rtype: int
"""
treeLst = []
def dfs(node):
if node:
treeLst.append(node.val)
dfs(node.left)
dfs(node.right)
dfs(root)
treeLst = list(set(treeLst))
if len(treeLst) < 2:
return -1
else:
treeLst.sort()
return treeLst[1]
{% endhighlight%}
<p>思考:没有仔细分析这棵树的特点,这棵树是根节点值最小,因此只需寻找一个比根节点值大,比其他值小的值,别人的代码:</p>
{% highlight python %}
class Solution(object):
def findSecondMinimumValue(self, root):
res = [float('inf')]
def traverse(node):
if not node:
return
if root.val < node.val < res[0]:
res[0] = node.val
traverse(node.left)
traverse(node.right)
traverse(root)
return -1 if res[0] == float('inf') else res[0]
{% endhighlight %}
|
---
title: Unity Scriptable Render Pipeline
author: Rito15
date: 2021-08-29 20:50:00 +09:00
categories: [Unity, Unity Study]
tags: [unity, csharp]
math: true
mermaid: true
---
# 목표
---
-
<br>
<!- --------------------------------------------------------------------------- ->
# 개념
---
-
<br>
<!- --------------------------------------------------------------------------- ->
# Source Code
---
- <https://github.com/rito15/UnityStudy2>
<br>
<!- --------------------------------------------------------------------------- ->
# Download
---
-
<br>
<!- --------------------------------------------------------------------------- ->
# References
---
- <https://leehs27.github.io/programming/2021-06-11-Interview-Renderpipeline/>
- <https://cyangamedev.wordpress.com/2020/06/22/urp-post-processing/>
- <https://github.com/Cyanilux/URP_BlitRenderFeature/blob/master/Blit.cs>
- <https://gamedevbill.com/full-screen-shaders-in-unity/>
- <https://blog.naver.com/PostView.naver?blogId=mnpshino&logNo=221633602003>
- <https://www.youtube.com/watch?v=QRlz4-pAtpY>
- <https://www.youtube.com/watch?v=MuzLdCXoJ9I> |
<?php
namespace Packages\System\Observers;
use Packages\System\Models\SystemCounty;
use Uuid;
class SystemCountyObserver
{
public function creating(SystemCounty $systemCounty)
{
$systemCounty->id = $systemCounty->id ?: (string) Uuid::generate(4);
}
/**
* Handle the ChilexpressCounty "created" event.
*
* @param \Packages\System\Models\SystemCounty $chilexpressCounty
* @return void
*/
public function created(SystemCounty $systemCounty)
{
//
}
public function updating(SystemCounty $systemCounty)
{
//
}
/**
* Handle the ChilexpressCounty "updated" event.
*
* @param \Packages\System\Models\SystemCounty $chilexpressCounty
* @return void
*/
public function updated(SystemCounty $systemCounty)
{
//
}
public function saving(SystemCounty $systemCounty)
{
}
public function saved(SystemCounty $systemCounty)
{
}
public function deleting(SystemCounty $systemCounty)
{
}
/**
* Handle the ChilexpressCounty "deleted" event.
*
* @param \Packages\System\Models\SystemCounty $chilexpressCounty
* @return void
*/
public function deleted(SystemCounty $systemCounty)
{
//
}
public function restoring(SystemCounty $systemCounty)
{
}
/**
* Handle the ChilexpressCounty "restored" event.
*
* @param \Packages\System\Models\SystemCounty $chilexpressCounty
* @return void
*/
public function restored(SystemCounty $systemCounty)
{
//
}
/**
* Handle the ChilexpressCounty "force deleted" event.
*
* @param \Packages\System\Models\SystemCounty $chilexpressCounty
* @return void
*/
public function forceDeleted(SystemCounty $systemCounty)
{
//
}
}
|
//*********************************************************
//
// Copyright (c) Microsoft. All rights reserved.
// This code is licensed under the MIT License (MIT).
// THE SOFTWARE IS PROVIDED “AS IS”, WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED,
// INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
// FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT.
// IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM,
// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT,
// TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH
// THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
//
//*********************************************************
using System;
using System.Collections.Generic;
using System.Collections.ObjectModel;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CompositionSampleGallery.Shared
{
public class Thumbnail
{
public Thumbnail()
{
}
public Thumbnail(string name, string url, string description)
{
Name = name;
ImageUrl = url;
Description = description;
}
public string Name
{
get; set;
}
public string ImageUrl
{
get; set;
}
public string Description
{
get; set;
}
}
public class LocalDataSource
{
public LocalDataSource()
{
Items = new ObservableCollection<Thumbnail>();
Items.Add(new Thumbnail("Landscape 1", PREFIX_URL + "Landscape-1.jpg", "24mm f/4.0 1/2500 ISO 200"));
Items.Add(new Thumbnail("Landscape 2", PREFIX_URL + "Landscape-2.jpg", "24mm f/8.0 1/2000 ISO 100"));
Items.Add(new Thumbnail("Landscape 3", PREFIX_URL + "Landscape-3.jpg", "24mm f/8.0 1/640 ISO 100"));
Items.Add(new Thumbnail("Landscape 4", PREFIX_URL + "Landscape-4.jpg", "70mm f/2.8 1/8000 ISO 400"));
Items.Add(new Thumbnail("Landscape 5", PREFIX_URL + "Landscape-5.jpg", "70mm f/2.8 1/5000 ISO 400"));
Items.Add(new Thumbnail("Landscape 6", PREFIX_URL + "Landscape-6.jpg", "70mm f/2.8 1/1250 ISO 100"));
Items.Add(new Thumbnail("Landscape 7", PREFIX_URL + "Landscape-7.jpg", "70mm f/4.0 1/250 ISO 100"));
Items.Add(new Thumbnail("Landscape 8", PREFIX_URL + "Landscape-8.jpg", "70mm f/2.8 1/1250 ISO 100"));
Items.Add(new Thumbnail("Landscape 9", PREFIX_URL + "Landscape-9.jpg", "70mm f/2.8 1/2000 ISO 100"));
Items.Add(new Thumbnail("Landscape 10", PREFIX_URL + "Landscape-10.jpg", "24mm f/4.0 1/1000 ISO 100"));
Items.Add(new Thumbnail("Landscape 11", PREFIX_URL + "Landscape-11.jpg", "50mm f/16 1/125 ISO 100"));
Items.Add(new Thumbnail("Landscape 12", PREFIX_URL + "Landscape-12.jpg", "24mm f/4.0 1/250 ISO 100"));
}
public ObservableCollection<Thumbnail> Items
{
get; set;
}
private static readonly string PREFIX_URL = "ms-appx:///Assets/Landscapes/";
}
}
|
namespace Tilia.Visuals.Tooltip.TextProcessing
{
using UnityEngine;
/// <summary>
/// A basis for text elements that can be set and processed.
/// </summary>
public abstract class BaseTextProcessor : MonoBehaviour
{
/// <summary>
/// Sets the text of the associated text processor.
/// </summary>
/// <param name="text">The text to set.</param>
public abstract void SetText(string text);
/// <summary>
/// Sets the color of the font on the associated text processor.
/// </summary>
/// <param name="color">The color to use.</param>
public abstract void SetColor(Color color);
/// <summary>
/// Sets the size of the font on the associated text processor.
/// </summary>
/// <param name="size">The size to use.</param>
public abstract void SetSize(float size);
}
} |
import * as React from 'react';
export interface IApiConnectorCreatorToggleListProps {
step: number;
i18nDetails: string;
i18nReview: string;
i18nSecurity: string;
i18nSelectMethod: string;
}
export const ApiConnectorCreatorToggleList: React.FunctionComponent<IApiConnectorCreatorToggleListProps> = (
{
i18nDetails,
i18nReview,
i18nSecurity,
i18nSelectMethod,
step,
}) => {
return (
<button
aria-label="Wizard Navigation Toggle"
className="pf-c-wizard__toggle"
aria-expanded="false"
>
<ol className="pf-c-wizard__toggle-list">
{step === 1 && (
<li className="pf-c-wizard__toggle-list-item">
<span className="pf-c-wizard__toggle-num">1</span>
{i18nSelectMethod}
</li>
)}
{step === 2 && (
<li className="pf-c-wizard__toggle-list-item">
<span className="pf-c-wizard__toggle-num">2</span>
{i18nReview}
</li>
)}
{step === 3 && (
<li className="pf-c-wizard__toggle-list-item">
<span className="pf-c-wizard__toggle-num">3</span>
{i18nSecurity}
</li>
)}
{step === 4 && (
<li className="pf-c-wizard__toggle-list-item">
<span className="pf-c-wizard__toggle-num">4</span>
{i18nDetails}
</li>
)}
</ol>
<i
style={{ display: 'none' }}
className="fas fa-caret-down pf-c-wizard__toggle-icon"
aria-hidden="true"
/>
</button>
);
};
|
require "mongoid/publishable/unpublished_object"
module Mongoid
module Publishable
class Queue < Array
# loads the queue from the session
def self.load(session = nil)
# create a new queue
queue = new
# if there was no existing queue, return new
return queue unless session
# create our contents
contents = session.split("\n").map do |data|
UnpublishedObject.deserialize_from_session(data)
end
# add into the queue
queue.replace(contents)
end
# publishes all the objects on the queue to this user
def publish_via(publisher)
remaining = delete_if do |model|
model.publish_via!(publisher)
model.published?
end
# replaces the contents with the remaining models
replace(remaining)
end
# creates a string containing the data of the queue
def dump
map do |model|
model.serialize_for_session
end.join("\n")
end
# adds a new object to the queue
def push(*models)
# map each item to an unpublished object
models = Array(models).map do |model|
UnpublishedObject.new(model: model)
end
# add them to the array
super(*models)
end
alias_method :<<, :push
end
end
end
|
using Entities.Concrete;
using System;
using System.Collections.Generic;
using System.Text;
namespace Business.Constants
{
public static class Messages
{
public static string CarAdded = "Araba eklendi";
public static string CarDailyPriceInvalid = "Araba fiyatı geçersiz";
internal static string MaintenanceTime="Sistem Bakımda";
internal static string CarsListed = "Arabalar listelendi";
public static string CarDeleted = "Araba Silindi.";
public static string CarUpdated = "Araba GÜncellendi.";
}
}
|
import { createStyles, makeStyles } from '@material-ui/core/styles';
import TextField from '@material-ui/core/TextField';
import { Button, CircularProgress } from '@material-ui/core';
import { useEffect, useState } from 'react';
import { useAuthContext } from '../../hooks/UseAuth';
import useRouter from '../../hooks/UseRouter';
/**
* Login Page, redirects to home after
*/
export default function LoginPage({ redirectAfterLogin = '/' }) {
const router = useRouter();
const { user, login } = useAuthContext();
// guarantee that the user will get redirected
useEffect(() => {
if (user) {
// @ts-ignore sadly I haven't found any other way how to get the redirect
const { from } = router.location.state ?? { from: { pathname: redirectAfterLogin } };
router.replace(from);
}
}, [redirectAfterLogin, router, user]);
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const [status, setStatus] = useState<'idle' | 'pending' | 'error'>('idle');
const handleLogin = (e: any) => {
e.preventDefault();
setStatus('pending'); // set pending status to display circle
return login({
credentials: { email, password },
vaccineSerialNumber: '#admin'
}
).catch(() => setStatus('error')); // catch unauthorized
};
const handleTyping = (e: any, change: (value: string) => void) => {
setStatus('idle'); // reset error flag
change(e.target.value);
};
const classes = useStyles();
return (
// we use hidden just as a precaution if the suer already exist
// to not to "blick" with the login window
<div className={classes.page} style={{ visibility: user === null ? 'visible' : 'hidden' }}>
<form className={classes.form} noValidate autoComplete='off'>
<TextField required id='email'
error={status === 'error'}
type='email'
label='e-mail'
value={email}
disabled={status === 'pending'}
onChange={e => handleTyping(e, setEmail)}/>
<TextField required id='password'
error={status === 'error'}
type='password'
label='password'
value={password}
disabled={status === 'pending'}
onChange={e => handleTyping(e, setPassword)}/>
<div>
<Button variant='contained'
type='submit'
fullWidth
onClick={handleLogin}
disabled={!(email && password) || status === 'pending'}>
{status === 'pending'
? <CircularProgress size={'1.5rem'}/>
: <span>Login</span>
}
</Button>
</div>
<div className={classes.infoBox}
style={{ visibility: status === 'error' ? 'visible' : 'hidden' }}>
Unauthorized!
</div>
</form>
</div>
);
}
const useStyles = makeStyles((theme) =>
createStyles({
page: {
display: 'flex',
flexFlow: 'column',
alignContent: 'center',
justifyContent: 'center'
},
form: {
display: 'flex',
flexFlow: 'column',
'& > div': {
margin: '10px',
width: '25ch'
}
},
infoBox: {
color: theme.palette.error.main
}
})
);
|
# Move Panes package
## Move active tab to different panes
Allows moving the active tab to the right, left, up or down. Also supports
cycling the active tab to next/previous panes.
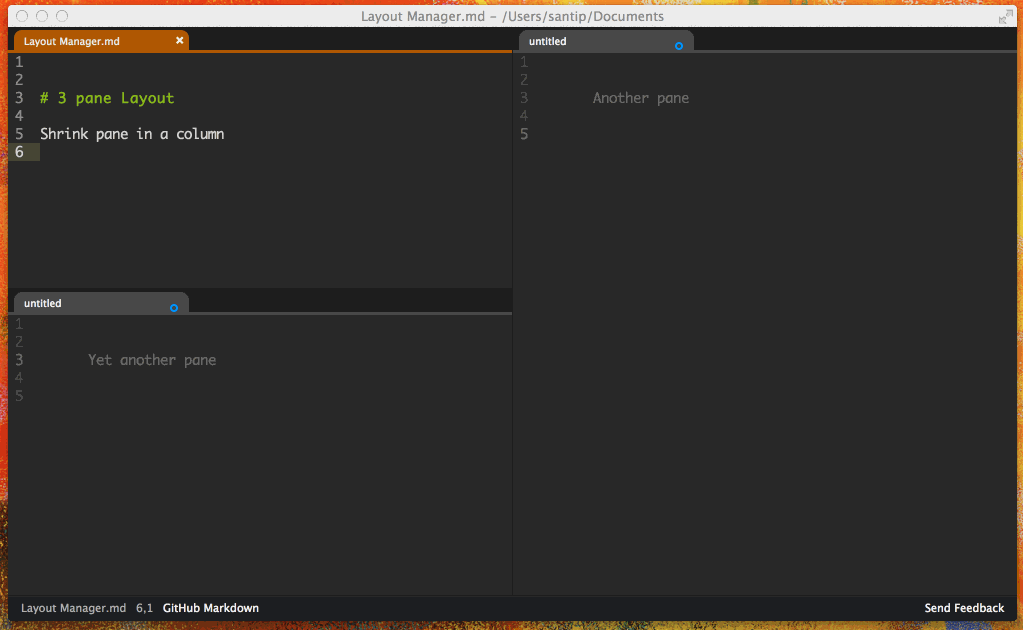
| Command name | Default key binding |
| ----------------------------------- |:----------------------:|
| `move-panes:move-right` | `cmd-ctrl-shift-right` |
| `move-panes:move-left` | `cmd-ctrl-shift-left` |
| `move-panes:move-up` | `cmd-ctrl-shift-up` |
| `move-panes:move-down` | `cmd-ctrl-shift-down` |
| `move-panes:move-next` | None |
| `move-panes:move-previous` | None |
## Related packages
### Other features
- [Maximize Panes](https://atom.io/packages/maximize-panes)
- [Resize panes](https://atom.io/packages/resize-panes)
### All features in a single package
- [Layout Manager](https://atom.io/packages/layout-manager)
# [Feedback](https://github.com/santip/move-panes/issues) and PRs are welcome.
|
#[doc = "Reader of register RAMINFO"]
pub type R = crate::R<u8, super::RAMINFO>;
#[doc = "Reader of field `RAMBITS`"]
pub type RAMBITS_R = crate::R<u8, u8>;
#[doc = "Reader of field `DMACHAN`"]
pub type DMACHAN_R = crate::R<u8, u8>;
impl R {
#[doc = "Bits 0:3 - RAM Address Bus Width"]
#[inline(always)]
pub fn rambits(&self) -> RAMBITS_R {
RAMBITS_R::new((self.bits & 0x0f) as u8)
}
#[doc = "Bits 4:7 - DMA Channels"]
#[inline(always)]
pub fn dmachan(&self) -> DMACHAN_R {
DMACHAN_R::new(((self.bits >> 4) & 0x0f) as u8)
}
}
|
require "pry-byebug/commands/backtrace"
require "pry-byebug/commands/next"
require "pry-byebug/commands/step"
require "pry-byebug/commands/continue"
require "pry-byebug/commands/finish"
require "pry-byebug/commands/up"
require "pry-byebug/commands/down"
require "pry-byebug/commands/frame"
require "pry-byebug/commands/breakpoint"
require "pry-byebug/commands/exit_all"
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Threading.Tasks;
using TCCarShare.Models;
namespace TCCarShare.ViewModels
{
public class WaitingOrder
{
public Order info { get; set; } = new Order();
public ExtensionInfo extension { get; set; } = new ExtensionInfo();
}
public class ExtensionInfo
{
/// <summary>
/// 0-研发,1-运营,2-产品,3-商务,4-客服,5-设计
/// </summary>
public int empStation { get; set; }
public string isSingle { get; set; }
public string passengerName { get; set; }
public string phoneNumber { get; set; }
}
}
|
#!/usr/bin/env bash
OLD_KEY=$(grep APP_KEY= .env)
if [ "$OLD_KEY" != "APP_KEY=" ]; then
read -p "An existing key has been found! Do you want to overwrite it? [y/N] " -n 1 -r
echo
if [[ ! $REPLY =~ ^[Yy]$ ]]; then
echo " > Cancelled."
exit 0
fi
fi
NEW_KEY=$(tr -dc 'a-zA-Z0-9' < /dev/urandom | fold -w 64 | head -n 1)
sed -i.bak "s/^APP_KEY=.*/APP_KEY=$NEW_KEY/" .env
echo " > New key has been set."
|
package ru.tinkoff.deimos.structure.operations
import cats.syntax.flatMap._
import cats.syntax.functor._
import ru.tinkoff.deimos.schema.classes.Element
import ru.tinkoff.deimos.structure.{GeneratedPackage, GlobalName, InvalidSchema, Pure, Tag, XmlCodecInfo}
object ProcessGlobalElement {
def apply(element: Element): XsdMonad[Option[Tag]] = {
def generateTypeByName(name: String): String = name.updated(0, name.head.toUpper)
def name: XsdMonad[String] =
element.name match {
case Some(name) => XsdMonad.pure(name)
case None => XsdMonad.raiseError("Global element name is missing")
}
def namespace: XsdMonad[Option[String]] =
for {
ctx <- XsdMonad.ask
schema = ctx.indices.schemas(ctx.currentPath)
} yield
(schema.targetNamespace, schema.elementFormDefault, element.form) match {
case (Some(uri), _, Some("qualified")) => ctx.indices.namespacePrefixes.get(uri)
case (Some(uri), Some("qualified"), None) => ctx.indices.namespacePrefixes.get(uri)
case _ => None
}
element match {
case _ if element.simpleType.isDefined =>
for {
ctx <- XsdMonad.ask
typ <- ProcessSimpleType(element.simpleType.get)
name <- name
namespace <- namespace
xmlCodec = XmlCodecInfo(typ, name, namespace)
_ <- XsdMonad.addXmlCodec(ctx.currentPath, xmlCodec)
} yield Some(Tag(name, Pure(typ), namespace, None, None))
case _ if element.complexType.isDefined =>
for {
ctx <- XsdMonad.ask
name <- name
realTypeName = generateTypeByName(name) // TODO: Children classes here
globalName = GlobalName(ctx.targetNamespace.getOrElse(""), name)
clazz <- ProcessComplexType(element.complexType.get, realTypeName, Some(globalName))
.local(_.copy(stack = ctx.stack.push(globalName)))
typ = Pure(clazz.name)
namespace <- namespace
xmlCodec = XmlCodecInfo(clazz.name, name, namespace)
_ <- XsdMonad.addXmlCodec(ctx.currentPath, xmlCodec)
} yield Some(Tag(name, typ, namespace, None, None))
case _ if element.`type`.isDefined =>
for {
ctx <- XsdMonad.ask
globalTypeName = element.`type`.map(ctx.toGlobalName).get
typ <- XsdMonad.getSimpleTypeByName(globalTypeName).flatMap {
case Some(simpleType) =>
XsdMonad.pure(Pure(simpleType))
case None =>
XsdMonad.getOrProcessClassName(globalTypeName).map(Pure)
}
name <- name
namespace <- namespace
xmlCodec = XmlCodecInfo(typ.typ, name, namespace)
_ <- XsdMonad.addXmlCodec(ctx.currentPath, xmlCodec)
} yield Some(Tag(name, typ, namespace, None, None))
}
}
}
|
export * from './entry'
export * from './ResponseFixture'
export * from './ResponseBuilder'
export * from './HttpMock'
export * from './HttpMockBuilder'
export * from './HttpMockRepository'
export * from './ResponseDelegate'
|
using Documenter, GeoFormatTypes
makedocs(;
modules = [GeoFormatTypes],
sitename = "GeoFormatTypes.jl",
)
deploydocs(;
repo="github.com/JuliaGeo/GeoFormatTypes.jl",
)
|
package com.banary.base
import org.apache.spark.{SparkConf, SparkContext}
object SparkContextFactory {
def getSparkContext(appName: String): SparkContext = {
val sparkConf = new SparkConf().setMaster("local").setAppName(appName);
return new SparkContext(sparkConf);
}
}
|
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Http\Response;
use App\Models\CustomTeam;
use App\Models\User;
use App\Models\Player;
class CustomTeamController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index(Request $request)
{
try {
$user = \JWTAuth::parseToken()->authenticate();
if (!$user) {
return response('user_not_found', 404);
}
return $user->customTeams()->with('players')->get();
} catch (\Exception $e) {
return response($e->errorInfo, 500);
}
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
try {
$user = \JWTAuth::parseToken()->authenticate();
if (!$user) {
return response('user_not_found', 404);
}
$team = new CustomTeam();
$team->name = $request->name;
$team->user()->associate($user->id);
$team->save();
return $team;
} catch (\Exception $e) {
return response($e->errorInfo, 500);
}
}
/**
* Display the specified resource.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function show($id)
{
try {
$user = \JWTAuth::parseToken()->authenticate();
if (!$user) {
return response('user_not_found', 404);
}
$team = $user->customTeams()->where('id', $id)->first();
if (!$team) {
return response('team_not_found', 404);
}
return $team->setAttribute('players', $team->players()->get());
} catch (\Exception $e) {
return response($e->errorInfo, 500);
}
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param int $id
* @return \Illuminate\Http\Response
*/
public function update(Request $request, $id)
{
//
}
/**
* Remove the specified resource from storage.
*
* @param int $id
* @return \Illuminate\Http\Response
*/
public function destroy($id)
{
try {
$team = CustomTeam::find($id);
if (!$team) {
return response('team_not_found', 404);
}
$team->delete();
return response()->success();
} catch (\Exception $e) {
return response($e->errorInfo, 500);
}
}
public function add_player($teamId, $playerId)
{
try {
$team = CustomTeam::find($teamId);
if (!$team) {
return response('team_not_found', 404);
}
$player = Player::find($playerId);
if (!$player) {
return response('player_not_found', 404);
}
$team->players()->attach($player->id);
return response()->success();
} catch (\Exception $e) {
if ($e->errorInfo[0] == 23000) {
return response('duplicate_player', 500);
}
return response($e->errorInfo, 500);
}
}
public function index_available($pid)
{
try {
$user = \JWTAuth::parseToken()->authenticate();
if (!$user) {
return response('user_not_found', 404);
}
$teams = $user->customTeams()->get();
$ignore_teams = [];
foreach($teams as $team) {
$t = $team->players()->wherePivot('player_id', '=', $pid)->first();
if ($t) {
array_push($ignore_teams, $t->pivot->custom_team_id);
}
}
return $user->customTeams()->whereNotIn('id', $ignore_teams)->get();
} catch (\Exception $e) {
return response($e, 500);
}
}
}
|
package com.force.api.chatter;
import com.force.api.*;
import org.junit.Test;
import java.util.ArrayList;
import static org.junit.Assert.assertEquals;
import static org.junit.Assert.fail;
/**
* Created by jjoergensen on 2/25/17.
*/
public class ChatterTest {
static final String TEST_NAME = "force-rest-api basic chatter test";
@Test
public void chatterCRUDTest() {
ForceApi api = new ForceApi(new ApiConfig()
.setUsername(Fixture.get("username"))
.setPassword(Fixture.get("password"))
.setClientId(Fixture.get("clientId"))
.setClientSecret(Fixture.get("clientSecret")));
// Test simple get
ChatterFeed feed = api.get("/chatter/feeds/user-profile/me/feed-elements").as(ChatterFeed.class);
Identity me = api.getIdentity();
System.out.println(me.getUserId());
assertEquals(feed.elements.get(0).actor.id, me.getUserId());
// Test posting a new feed item
ChatterFeed.FeedItemInput newItem = new ChatterFeed.FeedItemInput();
newItem.subjectId = me.getUserId();
newItem.feedElementType = "FeedItem";
newItem.body = new ChatterFeed.Body();
newItem.body.messageSegments = new ArrayList<ChatterFeed.MessageSegment>();
ChatterFeed.MessageSegment segment = new ChatterFeed.MessageSegment();
segment.type = "text";
segment.text = "Hi from Chatter API";
newItem.body.messageSegments.add(segment);
// Normally you collapse the next two lines into a single line but we need a reference to resrep
// for the test just down below
ResourceRepresentation resrep = api.post("/chatter/feed-elements", newItem);
ChatterFeed.FeedItem resp = resrep.as(ChatterFeed.FeedItem.class);
System.out.println(resp.actor.displayName+" just posted "+resp.body.text);
assertEquals(segment.text, resp.body.text);
// New as of 0.0.37, response code is available in ResourceRepresentation
assertEquals(resrep.getResponseCode(),201);
// Test updating the feed item just posted
ChatterFeed.FeedItemInput updatedItem = new ChatterFeed.FeedItemInput();
updatedItem.body = new ChatterFeed.Body();
updatedItem.body.messageSegments = new ArrayList<ChatterFeed.MessageSegment>();
ChatterFeed.MessageSegment updatedSegment = new ChatterFeed.MessageSegment();
updatedSegment.type = "text";
updatedSegment.text = "I changed my mind";
updatedItem.body.messageSegments.add(updatedSegment);
ChatterFeed.FeedItem updatedresp = api.patch("/chatter/feed-elements/" + resp.id, updatedItem).as(ChatterFeed.FeedItem.class);
System.out.println(resp.actor.displayName+" just updated to "+updatedresp.body.text);
assertEquals(updatedSegment.text, updatedresp.body.text);
// Test deleting the feed item just posted
try {
api.delete("/chatter/feed-elements/" + resp.id);
} catch(ResourceException e){
fail("Delete failed unexpectedly: "+e);
}
}
} |
module Lerk
RSpec.describe HintTag do
describe '::validate' do
it 'ensures case-insensitive uniqueness of the tag' do
create(:hint_tag, tag: 'test')
expect { create(:hint_tag, tag: 'Test') }.to raise_error Sequel::ValidationFailed
end
end
end
end
|
# @author Peter Bell
# Licensed under MIT. See License file in top level directory.
require 'CFSM'
module ConditionOptimisation
describe ConditionsNode do
before(:each) do
@conditions_node =
[ ConditionsNode.new( [1, 2, 3, 4], [:fsm1], [1, 2] ),
ConditionsNode.new( [1, 2, 3, 4], [:fsm1], [1, 2] ),
ConditionsNode.new( [1, 2, 3, 4], [:fsm1], [1] ) ]
end
describe '#=' do
it 'should convert an array of conditions into a set of conditions' do
conditions_node = ConditionsNode.new [], []
conditions_node.conditions = [1, 2, 3, 4]
expect( conditions_node.conditions ).to be_a( Set )
end
end
describe '#similar' do
it 'should find two nodes similar if they agree in every aspect' do
expect( @conditions_node[ 0 ].similar( @conditions_node[ 1 ])).to be_truthy
end
it 'should find two nodes not similar if they differ in the number of edges' do
expect( @conditions_node[ 0 ].similar( @conditions_node[ 2 ])).to be_falsey
end
end
end
end
|
--
-- PostgreSQL database dump
--
-- Dumped from database version 9.5.5
-- Dumped by pg_dump version 9.5.5
SET statement_timeout = 0;
SET lock_timeout = 0;
SET client_encoding = 'UTF8';
SET standard_conforming_strings = on;
SET check_function_bodies = false;
SET client_min_messages = warning;
SET row_security = off;
--
-- Name: plpgsql; Type: EXTENSION; Schema: -; Owner: -
--
CREATE EXTENSION IF NOT EXISTS plpgsql WITH SCHEMA pg_catalog;
--
-- Name: EXTENSION plpgsql; Type: COMMENT; Schema: -; Owner: -
--
COMMENT ON EXTENSION plpgsql IS 'PL/pgSQL procedural language';
SET search_path = public, pg_catalog;
SET default_tablespace = '';
SET default_with_oids = false;
--
-- Name: code_harbor_links; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE code_harbor_links (
id integer NOT NULL,
oauth2token character varying,
created_at timestamp without time zone,
updated_at timestamp without time zone
);
--
-- Name: code_harbor_links_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE code_harbor_links_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: code_harbor_links_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE code_harbor_links_id_seq OWNED BY code_harbor_links.id;
--
-- Name: comments; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE comments (
id integer NOT NULL,
user_id integer,
file_id integer,
user_type character varying,
"row" integer,
"column" integer,
text text,
created_at timestamp without time zone,
updated_at timestamp without time zone
);
--
-- Name: comments_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE comments_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: comments_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE comments_id_seq OWNED BY comments.id;
--
-- Name: consumers; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE consumers (
id integer NOT NULL,
name character varying,
created_at timestamp without time zone,
updated_at timestamp without time zone,
oauth_key character varying,
oauth_secret character varying
);
--
-- Name: consumers_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE consumers_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: consumers_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE consumers_id_seq OWNED BY consumers.id;
--
-- Name: errors; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE errors (
id integer NOT NULL,
execution_environment_id integer,
message text,
created_at timestamp without time zone,
updated_at timestamp without time zone,
submission_id integer
);
--
-- Name: errors_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE errors_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: errors_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE errors_id_seq OWNED BY errors.id;
--
-- Name: execution_environments; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE execution_environments (
id integer NOT NULL,
docker_image character varying,
name character varying,
created_at timestamp without time zone,
updated_at timestamp without time zone,
run_command character varying,
test_command character varying,
testing_framework character varying,
help text,
exposed_ports character varying,
permitted_execution_time integer,
user_id integer,
user_type character varying,
pool_size integer,
file_type_id integer,
memory_limit integer,
network_enabled boolean
);
--
-- Name: execution_environments_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE execution_environments_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: execution_environments_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE execution_environments_id_seq OWNED BY execution_environments.id;
--
-- Name: exercises; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE exercises (
id integer NOT NULL,
description text,
execution_environment_id integer,
title character varying,
created_at timestamp without time zone,
updated_at timestamp without time zone,
user_id integer,
instructions text,
public boolean,
user_type character varying,
token character varying,
hide_file_tree boolean,
allow_file_creation boolean,
allow_auto_completion boolean DEFAULT false
);
--
-- Name: exercises_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE exercises_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: exercises_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE exercises_id_seq OWNED BY exercises.id;
--
-- Name: external_users; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE external_users (
id integer NOT NULL,
consumer_id integer,
email character varying,
external_id character varying,
name character varying,
created_at timestamp without time zone,
updated_at timestamp without time zone
);
--
-- Name: external_users_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE external_users_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: external_users_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE external_users_id_seq OWNED BY external_users.id;
--
-- Name: file_templates; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE file_templates (
id integer NOT NULL,
name character varying,
content text,
file_type_id integer,
created_at timestamp without time zone,
updated_at timestamp without time zone
);
--
-- Name: file_templates_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE file_templates_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: file_templates_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE file_templates_id_seq OWNED BY file_templates.id;
--
-- Name: file_types; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE file_types (
id integer NOT NULL,
editor_mode character varying,
file_extension character varying,
indent_size integer,
name character varying,
user_id integer,
created_at timestamp without time zone,
updated_at timestamp without time zone,
executable boolean,
renderable boolean,
user_type character varying,
"binary" boolean
);
--
-- Name: file_types_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE file_types_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: file_types_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE file_types_id_seq OWNED BY file_types.id;
--
-- Name: files; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE files (
id integer NOT NULL,
content text,
context_id integer,
context_type character varying,
file_id integer,
file_type_id integer,
hidden boolean,
name character varying,
read_only boolean,
created_at timestamp without time zone,
updated_at timestamp without time zone,
native_file character varying,
role character varying,
hashed_content character varying,
feedback_message character varying,
weight double precision,
path character varying,
file_template_id integer
);
--
-- Name: files_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE files_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: files_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE files_id_seq OWNED BY files.id;
--
-- Name: hints; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE hints (
id integer NOT NULL,
execution_environment_id integer,
locale character varying,
message text,
name character varying,
regular_expression character varying,
created_at timestamp without time zone,
updated_at timestamp without time zone
);
--
-- Name: hints_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE hints_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: hints_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE hints_id_seq OWNED BY hints.id;
--
-- Name: internal_users; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE internal_users (
id integer NOT NULL,
consumer_id integer,
email character varying,
name character varying,
role character varying,
created_at timestamp without time zone,
updated_at timestamp without time zone,
crypted_password character varying,
salt character varying,
failed_logins_count integer DEFAULT 0,
lock_expires_at timestamp without time zone,
unlock_token character varying,
remember_me_token character varying,
remember_me_token_expires_at timestamp without time zone,
reset_password_token character varying,
reset_password_token_expires_at timestamp without time zone,
reset_password_email_sent_at timestamp without time zone,
activation_state character varying,
activation_token character varying,
activation_token_expires_at timestamp without time zone
);
--
-- Name: internal_users_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE internal_users_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: internal_users_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE internal_users_id_seq OWNED BY internal_users.id;
--
-- Name: lti_parameters; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE lti_parameters (
id integer NOT NULL,
external_users_id integer,
consumers_id integer,
exercises_id integer,
lti_parameters jsonb DEFAULT '{}'::jsonb NOT NULL,
created_at timestamp without time zone,
updated_at timestamp without time zone
);
--
-- Name: lti_parameters_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE lti_parameters_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: lti_parameters_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE lti_parameters_id_seq OWNED BY lti_parameters.id;
--
-- Name: remote_evaluation_mappings; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE remote_evaluation_mappings (
id integer NOT NULL,
user_id integer NOT NULL,
exercise_id integer NOT NULL,
validation_token character varying NOT NULL,
created_at timestamp without time zone,
updated_at timestamp without time zone
);
--
-- Name: remote_evaluation_mappings_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE remote_evaluation_mappings_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: remote_evaluation_mappings_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE remote_evaluation_mappings_id_seq OWNED BY remote_evaluation_mappings.id;
--
-- Name: request_for_comments; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE request_for_comments (
id integer NOT NULL,
user_id integer NOT NULL,
exercise_id integer NOT NULL,
file_id integer NOT NULL,
created_at timestamp without time zone,
updated_at timestamp without time zone,
user_type character varying,
question text,
solved boolean,
submission_id integer
);
--
-- Name: request_for_comments_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE request_for_comments_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: request_for_comments_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE request_for_comments_id_seq OWNED BY request_for_comments.id;
--
-- Name: schema_migrations; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE schema_migrations (
version character varying NOT NULL
);
--
-- Name: submissions; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE submissions (
id integer NOT NULL,
exercise_id integer,
score double precision,
user_id integer,
created_at timestamp without time zone,
updated_at timestamp without time zone,
cause character varying,
user_type character varying
);
--
-- Name: submissions_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE submissions_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: submissions_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE submissions_id_seq OWNED BY submissions.id;
--
-- Name: testruns; Type: TABLE; Schema: public; Owner: -
--
CREATE TABLE testruns (
id integer NOT NULL,
passed boolean,
output text,
file_id integer,
submission_id integer,
created_at timestamp without time zone,
updated_at timestamp without time zone
);
--
-- Name: testruns_id_seq; Type: SEQUENCE; Schema: public; Owner: -
--
CREATE SEQUENCE testruns_id_seq
START WITH 1
INCREMENT BY 1
NO MINVALUE
NO MAXVALUE
CACHE 1;
--
-- Name: testruns_id_seq; Type: SEQUENCE OWNED BY; Schema: public; Owner: -
--
ALTER SEQUENCE testruns_id_seq OWNED BY testruns.id;
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY code_harbor_links ALTER COLUMN id SET DEFAULT nextval('code_harbor_links_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY comments ALTER COLUMN id SET DEFAULT nextval('comments_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY consumers ALTER COLUMN id SET DEFAULT nextval('consumers_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY errors ALTER COLUMN id SET DEFAULT nextval('errors_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY execution_environments ALTER COLUMN id SET DEFAULT nextval('execution_environments_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY exercises ALTER COLUMN id SET DEFAULT nextval('exercises_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY external_users ALTER COLUMN id SET DEFAULT nextval('external_users_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY file_templates ALTER COLUMN id SET DEFAULT nextval('file_templates_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY file_types ALTER COLUMN id SET DEFAULT nextval('file_types_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY files ALTER COLUMN id SET DEFAULT nextval('files_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY hints ALTER COLUMN id SET DEFAULT nextval('hints_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY internal_users ALTER COLUMN id SET DEFAULT nextval('internal_users_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY lti_parameters ALTER COLUMN id SET DEFAULT nextval('lti_parameters_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY remote_evaluation_mappings ALTER COLUMN id SET DEFAULT nextval('remote_evaluation_mappings_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY request_for_comments ALTER COLUMN id SET DEFAULT nextval('request_for_comments_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY submissions ALTER COLUMN id SET DEFAULT nextval('submissions_id_seq'::regclass);
--
-- Name: id; Type: DEFAULT; Schema: public; Owner: -
--
ALTER TABLE ONLY testruns ALTER COLUMN id SET DEFAULT nextval('testruns_id_seq'::regclass);
--
-- Name: code_harbor_links_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY code_harbor_links
ADD CONSTRAINT code_harbor_links_pkey PRIMARY KEY (id);
--
-- Name: comments_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY comments
ADD CONSTRAINT comments_pkey PRIMARY KEY (id);
--
-- Name: consumers_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY consumers
ADD CONSTRAINT consumers_pkey PRIMARY KEY (id);
--
-- Name: errors_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY errors
ADD CONSTRAINT errors_pkey PRIMARY KEY (id);
--
-- Name: execution_environments_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY execution_environments
ADD CONSTRAINT execution_environments_pkey PRIMARY KEY (id);
--
-- Name: exercises_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY exercises
ADD CONSTRAINT exercises_pkey PRIMARY KEY (id);
--
-- Name: external_users_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY external_users
ADD CONSTRAINT external_users_pkey PRIMARY KEY (id);
--
-- Name: file_templates_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY file_templates
ADD CONSTRAINT file_templates_pkey PRIMARY KEY (id);
--
-- Name: file_types_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY file_types
ADD CONSTRAINT file_types_pkey PRIMARY KEY (id);
--
-- Name: files_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY files
ADD CONSTRAINT files_pkey PRIMARY KEY (id);
--
-- Name: hints_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY hints
ADD CONSTRAINT hints_pkey PRIMARY KEY (id);
--
-- Name: internal_users_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY internal_users
ADD CONSTRAINT internal_users_pkey PRIMARY KEY (id);
--
-- Name: lti_parameters_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY lti_parameters
ADD CONSTRAINT lti_parameters_pkey PRIMARY KEY (id);
--
-- Name: remote_evaluation_mappings_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY remote_evaluation_mappings
ADD CONSTRAINT remote_evaluation_mappings_pkey PRIMARY KEY (id);
--
-- Name: request_for_comments_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY request_for_comments
ADD CONSTRAINT request_for_comments_pkey PRIMARY KEY (id);
--
-- Name: submissions_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY submissions
ADD CONSTRAINT submissions_pkey PRIMARY KEY (id);
--
-- Name: testruns_pkey; Type: CONSTRAINT; Schema: public; Owner: -
--
ALTER TABLE ONLY testruns
ADD CONSTRAINT testruns_pkey PRIMARY KEY (id);
--
-- Name: index_comments_on_file_id; Type: INDEX; Schema: public; Owner: -
--
CREATE INDEX index_comments_on_file_id ON comments USING btree (file_id);
--
-- Name: index_comments_on_user_id; Type: INDEX; Schema: public; Owner: -
--
CREATE INDEX index_comments_on_user_id ON comments USING btree (user_id);
--
-- Name: index_errors_on_submission_id; Type: INDEX; Schema: public; Owner: -
--
CREATE INDEX index_errors_on_submission_id ON errors USING btree (submission_id);
--
-- Name: index_files_on_context_id_and_context_type; Type: INDEX; Schema: public; Owner: -
--
CREATE INDEX index_files_on_context_id_and_context_type ON files USING btree (context_id, context_type);
--
-- Name: index_internal_users_on_activation_token; Type: INDEX; Schema: public; Owner: -
--
CREATE INDEX index_internal_users_on_activation_token ON internal_users USING btree (activation_token);
--
-- Name: index_internal_users_on_email; Type: INDEX; Schema: public; Owner: -
--
CREATE UNIQUE INDEX index_internal_users_on_email ON internal_users USING btree (email);
--
-- Name: index_internal_users_on_remember_me_token; Type: INDEX; Schema: public; Owner: -
--
CREATE INDEX index_internal_users_on_remember_me_token ON internal_users USING btree (remember_me_token);
--
-- Name: index_internal_users_on_reset_password_token; Type: INDEX; Schema: public; Owner: -
--
CREATE INDEX index_internal_users_on_reset_password_token ON internal_users USING btree (reset_password_token);
--
-- Name: unique_schema_migrations; Type: INDEX; Schema: public; Owner: -
--
CREATE UNIQUE INDEX unique_schema_migrations ON schema_migrations USING btree (version);
--
-- PostgreSQL database dump complete
--
SET search_path TO "$user", public;
INSERT INTO schema_migrations (version) VALUES ('20140625134118');
INSERT INTO schema_migrations (version) VALUES ('20140626143132');
INSERT INTO schema_migrations (version) VALUES ('20140626144036');
INSERT INTO schema_migrations (version) VALUES ('20140630093736');
INSERT INTO schema_migrations (version) VALUES ('20140630111215');
INSERT INTO schema_migrations (version) VALUES ('20140701120126');
INSERT INTO schema_migrations (version) VALUES ('20140701122345');
INSERT INTO schema_migrations (version) VALUES ('20140702100130');
INSERT INTO schema_migrations (version) VALUES ('20140703070749');
INSERT INTO schema_migrations (version) VALUES ('20140716153147');
INSERT INTO schema_migrations (version) VALUES ('20140717074902');
INSERT INTO schema_migrations (version) VALUES ('20140722125431');
INSERT INTO schema_migrations (version) VALUES ('20140723135530');
INSERT INTO schema_migrations (version) VALUES ('20140723135747');
INSERT INTO schema_migrations (version) VALUES ('20140724155359');
INSERT INTO schema_migrations (version) VALUES ('20140730114343');
INSERT INTO schema_migrations (version) VALUES ('20140730115010');
INSERT INTO schema_migrations (version) VALUES ('20140805161431');
INSERT INTO schema_migrations (version) VALUES ('20140812102114');
INSERT INTO schema_migrations (version) VALUES ('20140812144733');
INSERT INTO schema_migrations (version) VALUES ('20140812150607');
INSERT INTO schema_migrations (version) VALUES ('20140812150925');
INSERT INTO schema_migrations (version) VALUES ('20140813091722');
INSERT INTO schema_migrations (version) VALUES ('20140820170039');
INSERT INTO schema_migrations (version) VALUES ('20140821064318');
INSERT INTO schema_migrations (version) VALUES ('20140823172643');
INSERT INTO schema_migrations (version) VALUES ('20140823173923');
INSERT INTO schema_migrations (version) VALUES ('20140825121336');
INSERT INTO schema_migrations (version) VALUES ('20140825125801');
INSERT INTO schema_migrations (version) VALUES ('20140825154202');
INSERT INTO schema_migrations (version) VALUES ('20140825161350');
INSERT INTO schema_migrations (version) VALUES ('20140825161358');
INSERT INTO schema_migrations (version) VALUES ('20140825161406');
INSERT INTO schema_migrations (version) VALUES ('20140826073318');
INSERT INTO schema_migrations (version) VALUES ('20140826073319');
INSERT INTO schema_migrations (version) VALUES ('20140826073320');
INSERT INTO schema_migrations (version) VALUES ('20140826073321');
INSERT INTO schema_migrations (version) VALUES ('20140826073322');
INSERT INTO schema_migrations (version) VALUES ('20140827065359');
INSERT INTO schema_migrations (version) VALUES ('20140827083957');
INSERT INTO schema_migrations (version) VALUES ('20140829141913');
INSERT INTO schema_migrations (version) VALUES ('20140903093436');
INSERT INTO schema_migrations (version) VALUES ('20140903165113');
INSERT INTO schema_migrations (version) VALUES ('20140904082810');
INSERT INTO schema_migrations (version) VALUES ('20140909115430');
INSERT INTO schema_migrations (version) VALUES ('20140915095420');
INSERT INTO schema_migrations (version) VALUES ('20140915122846');
INSERT INTO schema_migrations (version) VALUES ('20140918063522');
INSERT INTO schema_migrations (version) VALUES ('20140922161120');
INSERT INTO schema_migrations (version) VALUES ('20140922161226');
INSERT INTO schema_migrations (version) VALUES ('20141003072729');
INSERT INTO schema_migrations (version) VALUES ('20141004114747');
INSERT INTO schema_migrations (version) VALUES ('20141009110434');
INSERT INTO schema_migrations (version) VALUES ('20141011145303');
INSERT INTO schema_migrations (version) VALUES ('20141017110211');
INSERT INTO schema_migrations (version) VALUES ('20141031161603');
INSERT INTO schema_migrations (version) VALUES ('20141119131607');
INSERT INTO schema_migrations (version) VALUES ('20150128083123');
INSERT INTO schema_migrations (version) VALUES ('20150128084834');
INSERT INTO schema_migrations (version) VALUES ('20150128093003');
INSERT INTO schema_migrations (version) VALUES ('20150204080832');
INSERT INTO schema_migrations (version) VALUES ('20150310150712');
INSERT INTO schema_migrations (version) VALUES ('20150317083739');
INSERT INTO schema_migrations (version) VALUES ('20150317115338');
INSERT INTO schema_migrations (version) VALUES ('20150327141740');
INSERT INTO schema_migrations (version) VALUES ('20150408155923');
INSERT INTO schema_migrations (version) VALUES ('20150421074734');
INSERT INTO schema_migrations (version) VALUES ('20150818141554');
INSERT INTO schema_migrations (version) VALUES ('20150818142251');
INSERT INTO schema_migrations (version) VALUES ('20150903152727');
INSERT INTO schema_migrations (version) VALUES ('20150922125415');
INSERT INTO schema_migrations (version) VALUES ('20160204094409');
INSERT INTO schema_migrations (version) VALUES ('20160204111716');
INSERT INTO schema_migrations (version) VALUES ('20160302133540');
INSERT INTO schema_migrations (version) VALUES ('20160426114951');
INSERT INTO schema_migrations (version) VALUES ('20160510145341');
INSERT INTO schema_migrations (version) VALUES ('20160512131539');
INSERT INTO schema_migrations (version) VALUES ('20160609185708');
INSERT INTO schema_migrations (version) VALUES ('20160610111602');
INSERT INTO schema_migrations (version) VALUES ('20160624130951');
INSERT INTO schema_migrations (version) VALUES ('20160630154310');
INSERT INTO schema_migrations (version) VALUES ('20160701092140');
INSERT INTO schema_migrations (version) VALUES ('20160704143402');
INSERT INTO schema_migrations (version) VALUES ('20160907123009');
INSERT INTO schema_migrations (version) VALUES ('20170112151637');
INSERT INTO schema_migrations (version) VALUES ('20170202170437');
|
module Speedup
module Collectors
class Collector
def self.key
self.name.to_s.split('::').last.gsub(/Collector$/, '').underscore.to_sym
end
def initialize(options = {})
@options = options
parse_options
setup_subscribes
end
# Where any subclasses should pick and pull from @options to set any and
# all instance variables they like.
#
# Returns nothing.
def parse_options
# pass
end
# Conditionally enable views based on any gathered data. Helpful
# if you don't want views to show up when they return 0 or are
# touched during the request.
#
# Returns true.
def enabled?
true
end
# Returns Symbol.
def key
self.class.key
end
alias defer_key key
def render?
enabled?
end
# Returns String.
def context_id
"speedup-context-#{key}"
end
# The wrapper ID for the individual panel in the Speedup bar.
#
# Returns String.
def dom_id
"speedup-panel-#{key}"
end
def subscribe(*args, &block)
ActiveSupport::Notifications.subscribe(*args, &block)
end
def register(*args, &block)
if block_given?
subscribe(*args, &block)
else
subscribe(*args) do |*args|
next unless Speedup.enabled?
evt = ActiveSupport::Notifications::Event.new(*args)
store_event(evt) unless filter_event?(evt)
end
end
end
# Stores en event to request context.
# Uses a event_to_data method.
def store_event(evt, key=nil)
key ||= self.key
Speedup.request.store_event(key, event_to_data(evt) ) if Speedup.request
end
# Transfer the event to actual stored data.
# Default implementation takes full payload, event time and event duration.
def event_to_data(evt)
evt.payload.merge( time: evt.time, duration: evt.duration )
end
def filter_event?(evt)
!Speedup.enabled?
end
protected
def clean_trace
Rails.backtrace_cleaner.clean(caller[2..-1])
end
def setup_subscribes
# pass
end
# Helper method for subscribing to the event that is fired when new
# requests are made.
def before_request(&block)
subscribe 'start_processing.action_controller', &block
end
# Helper method for subscribing to the event that is fired when requests
# are finished.
def after_request(&block)
subscribe 'process_action.action_controller', &block
end
end
end
end
|
package config
import (
"bytes"
"encoding/json"
"fmt"
"log"
"sync"
"github.com/joho/godotenv"
"github.com/kelseyhightower/envconfig"
)
type Config struct {
LogLevel string `envconfig:"LOG_LEVEL"`
PgURL string `envconfig:"PG_URL"`
PgMigrationsPath string `envconfig:"PG_MIGRATIONS_PATH"`
MysqlAddr string `envconfig:"MYSQL_ADDR"`
MysqlUser string `envconfig:"MYSQL_USER"`
MysqlPassword string `envconfig:"MYSQL_PASSWORD"`
MysqlDB string `envconfig:"MYSQL_DB"`
MysqlMigrationsPath string `envconfig:"MYSQL_MIGRATIONS_PATH"`
HTTPAddr string `envconfig:"HTTP_ADDR"`
GCBucket string `envconfig:"GC_BUCKET"`
FilePath string `envconfig:"FILE_PATH"`
}
var (
config Config
once sync.Once
)
func Get() *Config {
once.Do(func() {
if err := godotenv.Load(); err != nil {
log.Fatal(err)
}
err := envconfig.Process("", &config)
if err != nil {
log.Fatal(err)
}
cb, err := json.MarshalIndent(config, "", " ")
cb = bytes.ReplaceAll(cb, []byte(`\u003c`), []byte("<"))
cb = bytes.ReplaceAll(cb, []byte(`\u003e`), []byte(">"))
cb = bytes.ReplaceAll(cb, []byte(`\u0026`), []byte("&"))
if err != nil {
log.Fatal(err)
}
fmt.Println("Configuration:", string(cb))
})
return &config
}
|
// ------------------------------------------------------------
// Copyright (c) Microsoft Corporation. All rights reserved.
// Licensed under the MIT License (MIT). See License.txt in the repo root for license information.
// ------------------------------------------------------------
#pragma once
namespace Management
{
namespace NetworkInventoryManager
{
//--------
// This class represents the SF service that manages (overlay) network definition and pool allocation.
// Network definition defines a network: name, id, subnets, ip and mac pools.
// When a pool allocation is completed, route updates are sent to every node where the network is active.
class NetworkInventoryService :
public Common::ComponentRoot,
public Common::TextTraceComponent<Common::TraceTaskCodes::FM>
{
DENY_COPY(NetworkInventoryService);
public:
NetworkInventoryService(Reliability::FailoverManagerComponent::FailoverManager & fm);
~NetworkInventoryService();
Common::ErrorCode Initialize();
void Close();
__declspec (property(get=get_FM)) Reliability::FailoverManagerComponent::FailoverManager & FM;
Reliability::FailoverManagerComponent::FailoverManager & get_FM() { return fm_; }
__declspec (property(get=get_Id)) std::wstring const& Id;
std::wstring const& get_Id() const { return fm_.Id; }
AsyncOperationSPtr BeginSendPublishNetworkTablesRequestMessage(
PublishNetworkTablesRequestMessage const & params,
const Federation::NodeInstance& nodeInstance,
TimeSpan const timeout,
AsyncCallback const & callback);
ErrorCode EndSendPublishNetworkTablesRequestMessage(AsyncOperationSPtr const & operation,
__out NetworkErrorCodeResponseMessage & reply);
Common::ErrorCode EnumerateNetworks(
ServiceModel::QueryArgumentMap const & queryArgs,
ServiceModel::QueryResult & queryResult,
Common::ActivityId const & activityId);
ErrorCode ProcessNIMQuery(Query::QueryNames::Enum queryName,
ServiceModel::QueryArgumentMap const & queryArgs,
ServiceModel::QueryResult & queryResult,
Common::ActivityId const & activityId);
Common::ErrorCode GetNetworkApplicationList(
ServiceModel::QueryArgumentMap const & queryArgs,
ServiceModel::QueryResult & queryResult,
Common::ActivityId const & activityId);
Common::ErrorCode GetNetworkNodeList(
ServiceModel::QueryArgumentMap const & queryArgs,
ServiceModel::QueryResult & queryResult,
Common::ActivityId const & activityId);
Common::ErrorCode GetApplicationNetworkList(
ServiceModel::QueryArgumentMap const & queryArgs,
ServiceModel::QueryResult & queryResult,
Common::ActivityId const & activityId);
std::vector<ServiceModel::ApplicationNetworkQueryResult> GetApplicationNetworkList(
std::wstring const & applicationName);
Common::ErrorCode GetDeployedNetworkList(
ServiceModel::QueryArgumentMap const & queryArgs,
ServiceModel::QueryResult & queryResult,
Common::ActivityId const & activityId);
Common::AsyncOperationSPtr BeginNetworkCreate(
CreateNetworkMessageBody & requestBody,
Common::TimeSpan const & timeout,
Common::AsyncCallback const & callback,
AsyncOperationSPtr const & state);
Common::ErrorCode EndNetworkCreate(
Common::AsyncOperationSPtr const & operation,
__out std::shared_ptr<NetworkCreateResponseMessage> & reply);
Common::AsyncOperationSPtr BeginNetworkAssociation(
std::wstring const & networkName,
std::wstring const & applicationName,
Common::AsyncCallback const & callback,
AsyncOperationSPtr const & state);
Common::ErrorCode EndNetworkAssociation(
Common::AsyncOperationSPtr const & operation);
Common::AsyncOperationSPtr BeginNetworkDissociation(
std::wstring const & networkName,
std::wstring const & applicationName,
Common::AsyncCallback const & callback,
AsyncOperationSPtr const & state);
Common::ErrorCode EndNetworkDissociation(
Common::AsyncOperationSPtr const & operation);
private:
//--------
// Register to handle federation messages from nodes.
void RegisterRequestHandler();
void UnregisterRequestHandler();
void ProcessMessage(
__in Transport::MessageUPtr & message,
__in Federation::RequestReceiverContextUPtr & context);
void OnNetworkAllocationRequest(
__in Transport::MessageUPtr & message,
__in Federation::RequestReceiverContextUPtr & context);
void OnNetworkCreateRequest(
__in Transport::MessageUPtr & message,
__in Federation::RequestReceiverContextUPtr & context);
void OnNetworkRemoveRequest(
__in Transport::MessageUPtr & message,
__in Federation::RequestReceiverContextUPtr & context);
void OnNetworkValidateRequest(
__in Transport::MessageUPtr & message,
__in Federation::RequestReceiverContextUPtr & context);
void OnNetworkNodeRemoveRequest(
__in Transport::MessageUPtr & message,
__in Federation::RequestReceiverContextUPtr & context);
void OnRequestPublishNetworkTablesRequest(
__in Transport::MessageUPtr & message,
__in Federation::RequestReceiverContextUPtr & context);
void OnNetworkEnumerationRequest(
__in Transport::MessageUPtr & message,
__in Federation::RequestReceiverContextUPtr & context);
ErrorCode InternalStartRefreshProcessing();
void OnTimeout(AsyncOperationSPtr const & operation, bool expectedCompletedSynchronously);
template <class TRequest, class TReply>
class NIMRequestAsyncOperation;
AsyncOperationSPtr periodicAsyncTask_;
Reliability::FailoverManagerComponent::FailoverManager & fm_;
MUTABLE_RWLOCK(FM.NetworkInventoryService, lockObject_);
std::unique_ptr<NetworkInventoryAllocationManager> allocationManagerUPtr_;
MACAllocationPoolSPtr macAddressPool_;
VSIDAllocationPoolSPtr vsidPool_;
};
typedef std::unique_ptr<NetworkInventoryService> NetworkInventoryServiceUPtr;
}
}
|
<?php
namespace Bpost;
use Bpost\BpostApiClient\Bpost\Order\Address;
use Bpost\BpostApiClient\Bpost\Order\Box;
use Bpost\BpostApiClient\Bpost\Order\Box\AtHome;
use Bpost\BpostApiClient\Bpost\Order\Box\International;
use Bpost\BpostApiClient\Bpost\Order\Receiver;
use Bpost\BpostApiClient\Bpost\Order\Sender;
use Bpost\BpostApiClient\Exception\BpostLogicException\BpostInvalidValueException;
class BoxTest extends \PHPUnit_Framework_TestCase
{
/**
* Create a generic DOM Document
*
* @return \DOMDocument
*/
private static function createDomDocument()
{
$document = new \DOMDocument('1.0', 'utf-8');
$document->preserveWhiteSpace = false;
$document->formatOutput = true;
return $document;
}
/**
* Tests Box->toXML
*/
public function testNationalToXML()
{
$data = array(
'sender' => array(
'name' => 'Tijs Verkoyen',
'company' => 'Sumo Coders',
'address' => array(
'streetName' => 'Afrikalaan',
'number' => '289',
'box' => '3',
'postalCode' => '9000',
'locality' => 'Gent',
'countryCode' => 'BE',
),
'emailAddress' => '[email protected]',
'phoneNumber' => '+32 9 395 02 51',
),
'nationalBox' => array(
'atHome' => array(
'product' => 'bpack 24h Pro',
'weight' => 2000,
'receiver' => array(
'name' => 'Tijs Verkoyen',
'company' => 'Sumo Coders',
'address' => array(
'streetName' => 'Kerkstraat',
'number' => '108',
'postalCode' => '9050',
'locality' => 'Gentbrugge',
'countryCode' => 'BE',
),
'emailAddress' => '[email protected]',
'phoneNumber' => '+32 9 395 02 51',
),
),
),
'remark' => 'remark',
'barcode' => 'BARCODE',
);
$expectedDocument = self::createDomDocument();
$box = $expectedDocument->createElement('box');
$expectedDocument->appendChild($box);
$sender = $expectedDocument->createElement('sender');
foreach ($data['sender'] as $key => $value) {
$key = 'common:' . $key;
if ($key == 'common:address') {
$address = $expectedDocument->createElement($key);
foreach ($value as $key2 => $value2) {
$key2 = 'common:' . $key2;
$address->appendChild(
$expectedDocument->createElement($key2, $value2)
);
}
$sender->appendChild($address);
} else {
$sender->appendChild(
$expectedDocument->createElement($key, $value)
);
}
}
$nationalBox = $expectedDocument->createElement('nationalBox');
$atHome = $expectedDocument->createElement('atHome');
$nationalBox->appendChild($atHome);
$atHome->appendChild($expectedDocument->createElement('product', $data['nationalBox']['atHome']['product']));
$atHome->appendChild($expectedDocument->createElement('weight', $data['nationalBox']['atHome']['weight']));
$receiver = $expectedDocument->createElement('receiver');
$atHome->appendChild($receiver);
foreach ($data['nationalBox']['atHome']['receiver'] as $key => $value) {
$key = 'common:' . $key;
if ($key == 'common:address') {
$address = $expectedDocument->createElement($key);
foreach ($value as $key2 => $value2) {
$key2 = 'common:' . $key2;
$address->appendChild(
$expectedDocument->createElement($key2, $value2)
);
}
$receiver->appendChild($address);
} else {
$receiver->appendChild(
$expectedDocument->createElement($key, $value)
);
}
}
$box->appendChild($sender);
$box->appendChild($nationalBox);
$box->appendChild($expectedDocument->createElement('remark', $data['remark']));
$box->appendChild($expectedDocument->createElement('barcode', $data['barcode']));
$actualDocument = self::createDomDocument();
$address = new Address(
$data['sender']['address']['streetName'],
$data['sender']['address']['number'],
$data['sender']['address']['box'],
$data['sender']['address']['postalCode'],
$data['sender']['address']['locality'],
$data['sender']['address']['countryCode']
);
$sender = new Sender();
$sender->setName($data['sender']['name']);
$sender->setCompany($data['sender']['company']);
$sender->setAddress($address);
$sender->setEmailAddress($data['sender']['emailAddress']);
$sender->setPhoneNumber($data['sender']['phoneNumber']);
$address = new Address(
$data['nationalBox']['atHome']['receiver']['address']['streetName'],
$data['nationalBox']['atHome']['receiver']['address']['number'],
null,
$data['nationalBox']['atHome']['receiver']['address']['postalCode'],
$data['nationalBox']['atHome']['receiver']['address']['locality'],
$data['nationalBox']['atHome']['receiver']['address']['countryCode']
);
$receiver = new Receiver();
$receiver->setAddress($address);
$receiver->setName($data['nationalBox']['atHome']['receiver']['name']);
$receiver->setCompany($data['nationalBox']['atHome']['receiver']['company']);
$receiver->setPhoneNumber($data['nationalBox']['atHome']['receiver']['phoneNumber']);
$receiver->setEmailAddress($data['nationalBox']['atHome']['receiver']['emailAddress']);
$atHome = new AtHome();
$atHome->setProduct($data['nationalBox']['atHome']['product']);
$atHome->setWeight($data['nationalBox']['atHome']['weight']);
$atHome->setReceiver($receiver);
$box = new Box();
$box->setSender($sender);
$box->setNationalBox($atHome);
$box->setRemark($data['remark']);
$box->setBarcode($data['barcode']);
$actualDocument->appendChild(
$box->toXML($actualDocument, null)
);
$this->assertSame($expectedDocument->saveXML(), $actualDocument->saveXML());
}
/**
* Tests Box->toXML
*/
public function testInternationalToXML()
{
$data = array(
'sender' => array(
'name' => 'Tijs Verkoyen',
'company' => 'Sumo Coders',
'address' => array(
'streetName' => 'Afrikalaan',
'number' => '289',
'box' => '3',
'postalCode' => '9000',
'locality' => 'Gent',
'countryCode' => 'BE',
),
'emailAddress' => '[email protected]',
'phoneNumber' => '+32 9 395 02 51',
),
'internationalBox' => array(
'international' => array(
'product' => 'bpack World Express Pro',
'receiver' => array(
'name' => 'Tijs Verkoyen',
'company' => 'Sumo Coders',
'address' => array(
'streetName' => 'Kerkstraat',
'number' => '108',
'postalCode' => '9050',
'locality' => 'Gentbrugge',
'countryCode' => 'BE',
),
'emailAddress' => '[email protected]',
'phoneNumber' => '+32 9 395 02 51',
),
),
),
'remark' => 'remark',
'barcode' => 'BARCODE',
);
$expectedDocument = self::createDomDocument();
$box = $expectedDocument->createElement('box');
$expectedDocument->appendChild($box);
$sender = $expectedDocument->createElement('sender');
foreach ($data['sender'] as $key => $value) {
$key = 'common:' . $key;
if ($key == 'common:address') {
$address = $expectedDocument->createElement($key);
foreach ($value as $key2 => $value2) {
$key2 = 'common:' . $key2;
$address->appendChild(
$expectedDocument->createElement($key2, $value2)
);
}
$sender->appendChild($address);
} else {
$sender->appendChild(
$expectedDocument->createElement($key, $value)
);
}
}
$nationalBox = $expectedDocument->createElement('internationalBox');
$atHome = $expectedDocument->createElement('international:international');
$nationalBox->appendChild($atHome);
$atHome->appendChild(
$expectedDocument->createElement(
'international:product',
$data['internationalBox']['international']['product']
)
);
$receiver = $expectedDocument->createElement('international:receiver');
$atHome->appendChild($receiver);
foreach ($data['internationalBox']['international']['receiver'] as $key => $value) {
$key = 'common:' . $key;
if ($key == 'common:address') {
$address = $expectedDocument->createElement($key);
foreach ($value as $key2 => $value2) {
$key2 = 'common:' . $key2;
$address->appendChild(
$expectedDocument->createElement($key2, $value2)
);
}
$receiver->appendChild($address);
} else {
$receiver->appendChild(
$expectedDocument->createElement($key, $value)
);
}
}
$box->appendChild($sender);
$box->appendChild($nationalBox);
$box->appendChild($expectedDocument->createElement('remark', $data['remark']));
$box->appendChild($expectedDocument->createElement('barcode', $data['barcode']));
$actualDocument = self::createDomDocument();
$address = new Address(
$data['sender']['address']['streetName'],
$data['sender']['address']['number'],
$data['sender']['address']['box'],
$data['sender']['address']['postalCode'],
$data['sender']['address']['locality'],
$data['sender']['address']['countryCode']
);
$sender = new Sender();
$sender->setName($data['sender']['name']);
$sender->setCompany($data['sender']['company']);
$sender->setAddress($address);
$sender->setEmailAddress($data['sender']['emailAddress']);
$sender->setPhoneNumber($data['sender']['phoneNumber']);
$address = new Address(
$data['internationalBox']['international']['receiver']['address']['streetName'],
$data['internationalBox']['international']['receiver']['address']['number'],
null,
$data['internationalBox']['international']['receiver']['address']['postalCode'],
$data['internationalBox']['international']['receiver']['address']['locality'],
$data['internationalBox']['international']['receiver']['address']['countryCode']
);
$receiver = new Receiver();
$receiver->setAddress($address);
$receiver->setName($data['internationalBox']['international']['receiver']['name']);
$receiver->setCompany($data['internationalBox']['international']['receiver']['company']);
$receiver->setPhoneNumber($data['internationalBox']['international']['receiver']['phoneNumber']);
$receiver->setEmailAddress($data['internationalBox']['international']['receiver']['emailAddress']);
$international = new International();
$international->setProduct($data['internationalBox']['international']['product']);
$international->setReceiver($receiver);
$box = new Box();
$box->setSender($sender);
$box->setInternationalBox($international);
$box->setRemark($data['remark']);
$box->setBarcode($data['barcode']);
$actualDocument->appendChild(
$box->toXML($actualDocument, null)
);
$this->assertSame($expectedDocument->saveXML(), $actualDocument->saveXML());
}
/**
* Test validation in the setters
*/
public function testFaultyProperties()
{
$box = new Box();
try {
$box->setStatus(str_repeat('a', 10));
$this->fail('BpostInvalidValueException not launched');
} catch (BpostInvalidValueException $e) {
// Nothing, the exception is good
} catch (\Exception $e) {
$this->fail('BpostInvalidValueException not caught');
}
// Exceptions were caught,
$this->assertTrue(true);
}
}
|
package com.mushdap.methodtest.utility;
import com.mushdap.methodtest.model.MethodTest;
import org.junit.Test;
import java.lang.reflect.Method;
import static org.assertj.core.api.Assertions.assertThat;
public class MethodUtilityTest {
@Test
public void getMethodsByName() {
// Act
Method[] methods = MethodUtility.getMethodsByName(MethodTest.class, "create");
Method[] methodsUnknown = MethodUtility.getMethodsByName(MethodTest.class, "UnknownMethod");
// Assert
assertThat(methods.length == 8).isTrue();
assertThat(methodsUnknown.length == 0).isTrue();
}
@Test
public void getMethodByName() {
// Act
Method method = MethodUtility.getMethodByName(MethodTest.class, "create");
Method methodUnknown = MethodUtility.getMethodByName(MethodTest.class, "UnknownMethod");
// Assert
assertThat(method).isNotNull();
assertThat(methodUnknown).isNull();
}
@Test
public void getMethodParametersValues() {
// Act
int totalNumberOfFields = MethodTest.class.getDeclaredFields().length + 1;
Object[] parametersValues =
MethodUtility.getMethodParametersValues(MethodUtility.getMethodByName(MethodTest.class, "setName"));
// Assert
assertThat(TypeUtility.getValue("", String.class, totalNumberOfFields)).isEqualTo(parametersValues[0]);
}
@Test
public void getMethodNullParametersValues() {
// Act
Object[] parametersValues = MethodUtility.getMethodNullParametersValues(MethodUtility.getMethodByName
(MethodTest.class, "setName"));
// Assert
assertThat(parametersValues[0]).isNull();
}
@Test
public void isMethodParametersMatched() {
assertThat((MethodUtility.isMethodParametersMatched(new Class[]{String.class}, new Class[]{String.class})))
.isTrue();
assertThat(MethodUtility.isMethodParametersMatched(new Class[]{String.class}, new Class[]{Integer.class}))
.isFalse();
}
}
|
<?php
/**
* Created by PhpStorm.
* User: gseidel
* Date: 08.12.18
* Time: 13:01
*/
namespace Enhavo\Bundle\AppBundle\Viewer;
use Sylius\Component\Resource\Metadata\MetadataInterface;
class DummyMetadata implements MetadataInterface
{
public function getAlias(): string
{
return '';
}
public function getApplicationName(): string
{
return '';
}
public function getName(): string
{
return '';
}
public function getHumanizedName(): string
{
return '';
}
public function getPluralName(): string
{
return '';
}
public function getDriver(): string
{
return '';
}
public function getTemplatesNamespace(): ?string
{
return '';
}
public function getParameter(string $name)
{
return '';
}
public function getParameters(): array
{
return [];
}
public function hasParameter(string $name): bool
{
return '';
}
public function getClass(string $name): string
{
return '';
}
public function hasClass(string $name): bool
{
return '';
}
public function getServiceId(string $serviceName): string
{
return '';
}
public function getPermissionCode(string $permissionName): string
{
return '';
}
} |
#!/usr/bin/env ruby
# rssdigest.rb - rss to email digest daemon
# non-copyright (c) 2008 rodrigo franco <[email protected]>
$:.unshift(File.dirname(__FILE__) + '/../lib')
require 'ssl'
require 'utils'
# Required Gems
require 'rubygems'
require "simpleconsole"
require 'rubygems'
require 'active_record' #(sqlite3)
require 'action_mailer'
require 'openssl'
require 'simple-rss'
# Non Gems required libs
require 'time'
require 'pathname'
require 'open-uri'
require 'cgi'
require 'htmlentities'
# Configuration Variables
FEED_TITLE = 'AppShopper'
FEED_URL = 'http://appshopper.com/feed/'
SMTP_SERVER = 'smtp.gmail.com'
SMTP_PORT = 587
SMTP_USER = 'foo'
SMTP_PASS = 'bar'
# Application Code
class Controller < SimpleConsole::Controller
params :string => {:e => :exec}
def default
puts "\n"
puts "--------"
puts "rssdigest.rb"
puts "--------"
puts "\n"
puts "rss to email digest daemon. experimental code, no warranty or"
puts "guarantee it will work. it may blow your computer and your house!"
puts "\n"
puts "valid options are:"
puts "\n"
puts "\t * database -e [create|destroy]"
puts "\t * retrieve"
puts "\t * status "
puts "\t * digest -e [preview|send]"
puts "\n"
puts "------"
puts "setup"
puts "------"
puts "\n"
puts "\t* Edit the configuration variables and run '#{$0} setup'"
puts "\t* If everything goes OK, follow the instructions after the setup execution. If not, see manual setup."
puts "\n"
puts "-------------"
puts "manual setup"
puts "-------------"
puts "\n"
puts "\t* Create the database using '#{$0} database -e create'"
puts "\t* Test the retrieval using '#{$0} retrieve'"
puts "\t* Preview the digest using '#{$0} digest preview'"
puts "\t* Test the email sending using '#{$0} digest mail'"
puts "\t* Check your email"
puts "\t* Add the following cronjobs in your server:"
puts "\n"
puts "\t\t 10 * * * * #{$0} retrieve"
puts "\t\t 59 23 * * * #{$0} digest -e send"
puts "\n"
puts "\t - Done :-)"
puts "\n"
end
# deals with database related functionality
# including create and destroy the database.
def database
case params[:exec]
when "create"
# define a migration
CreateEntries.migrate(:up)
when "destroy"
CreateEntries.migrate(:down)
else
puts "valid options: database -e [create | destroy]"
end
end
# retrieve the entries
# and store them in our database
def retrieve
feed = SimpleRSS.parse open(FEED_URL)
feed.items.each do |item|
next unless Entry.find_by_title_and_pubdate(item.title, item.pubDate).empty?
e = Entry.new
e.title = item.title
e.body = item.content
e.body = item.description if item.content.nil?
e.pubdate = item.pubDate
e.author = item.author
e.save
end
end
# prints the total tweets that will be sent
# in the next digest sending
def status
puts "#{Entry.find(:all).size} entries in the queue."
end
# deal with the email, generating and sending the
# digests to the user
def digest
d = String.new
coder = HTMLEntities.new
Entry.find(:all).each do |t|
next if t.created_at.to_date != Date.today
d << "<h1>#{t.title}</h1>#{coder.decode(t.body).strip_html(%w(p br a h1 h2 h3 h4 h5 img)).gsub('h1','h2')}"
end
case params[:exec]
when "preview"
puts DigestMailer.create_message(d)
when "send"
DigestMailer.deliver_message(d)
puts "Digest sent, purging database..."
Entry.delete(:all)
else
puts "valid options: digest -e [preview | send]"
end
end
# execute all required actions to setup the application
# make sure there's no database created before run it.
def setup
actions = ["database -e create", "retrieve", "digest -e preview", "digest -e send" ]
actions.each do |a|
puts "[#{Time.now}]#{$0} #{a}..."
Kernel.system("#{$0} #{a}")
exit if $? != 0
end
puts "setup done, now add the following cronjobs in your server:"
puts "\n"
puts "\t\t 10 * * * * #{$0} retrieve"
puts "\t\t 59 23 * * * #{$0} digest -e mail"
puts "\n"
end
end
class View < SimpleConsole::View; end
# Database Migration
class CreateEntries< ActiveRecord::Migration
def self.up
create_table :entries do |t|
t.column :title, :text
t.column :author, :string
t.column :body, :text
t.column :created_at, :datetime
t.column :pubdate, :datetime
end
end
def self.down
drop_table :entries
end
end
# Connect to the database (sqlite in this case)
ActiveRecord::Base.establish_connection({
:adapter => "sqlite3",
:dbfile => "#{Pathname.new(File.open($0).path).dirname}/entries.sqlite"
})
# Setup actionmailer
ActionMailer::Base.smtp_settings = {
:address => SMTP_SERVER,
:port => SMTP_PORT,
:user_name => SMTP_USER,
:password => SMTP_PASS,
:authentication => :plain
}
# Tweet AR Class
class Entry < ActiveRecord::Base
def self.find_by_title_and_pubdate(title, pubdate)
find(:all, :conditions => ["title = ? and pubdate = ?", title, pubdate.to_time])
end
end
# DigestMailer AM Class
class DigestMailer < ActionMailer::Base
def message(digest)
from '[email protected]'
recipients '[email protected]'
subject "#{FEED_TITLE} Digest - #{Date.today}"
content_type 'text/html'
body digest
end
end
# Run the app
SimpleConsole::Application.run(ARGV, Controller, View) |
package org.firstinspires.ftc.teamcode.ftc16072;
import com.qualcomm.robotcore.eventloop.opmode.OpMode;
import com.qualcomm.robotcore.eventloop.opmode.TeleOp;
import org.firstinspires.ftc.robotcore.external.navigation.AngleUnit;
import org.firstinspires.ftc.robotcore.external.navigation.DistanceUnit;
/**
* Autonomous Parking OpMode contributed by broncobots - ftc
* For red alliance: start with robot in square next to the blue depot, facing toward the building zone.
* For blue alliance: start with robot in square next to the blue building site, facing toward the loading zone.
*/
@TeleOp(name = "auto park opmode", group = "ftc16072")
public class AutoParkOpMode extends OpMode {
private Robot robot = new Robot();
int state = 0;
// Code to run ONCE when the driver hits INIT
@Override
public void init() {
robot.init(hardwareMap);
}
@Override
public void start() {
robot.nav.setPosition(60, -36, DistanceUnit.INCH);
}
// Code to run REPEATEDLY after the driver hits PLAY but before they hit STOP
@Override
public void loop() {
telemetry.addData("X", robot.nav.getEstimatedPosition().getX(DistanceUnit.INCH));
telemetry.addData("Y", robot.nav.getEstimatedPosition().getY(DistanceUnit.INCH));
telemetry.addData("state", state);
switch (state) {
case 0:
if (robot.nav.driveTo(32, -36, DistanceUnit.INCH)) {
state = 1;
}
break;
case 1:
if (robot.nav.driveTo(32, -0, DistanceUnit.INCH)) {
state = 2;
}
break;
}
}
}
|
<?php
namespace App\Http\Controllers;
use App\Models\Categories;
class CategoriesController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$category = Categories::orderBy('name')->get();
if ($category) {
return success($category);
} else {
return error(400, 'Failed to Get Categories');
}
}
}
|
Evema.Modules[ "Grid" ] = ( function() {
const Grid = {
'Instance': null,
'Context': null
};
Grid.Init = function() {
const that = Grid;
const instance = document.getElementById( 'e-grid' );
if ( instance === null ) {
console.error( 'Evema.Core.Grid.Init error' );
console.error( 'Can\'t find element "e-grid" in document' );
return;
}
that.Instance = instance;
that.Context = instance.getContext( '2d' );
Evema.SetLocal( that, 'Instance', that.Instance );
Evema.SetLocal( that, 'Context', that.Context );
that.Rebuild();
};
Grid.Rebuild = function() {
const that = Grid;
const instance = that.Instance;
if ( instance === null ) {
console.warn( 'Can\'t rebuild grid, the grid instance is null!' );
return;
}
const width = Evema.GetLocal( that, 'Width' );
const height = Evema.GetLocal( that, 'Height' );
const cellWidth = Evema.GetLocal( that, 'CellWidth' );
const cellHeight = Evema.GetLocal( that, 'CellHeight' );
instance.width = width * cellWidth;
instance.height = height * cellHeight;
that.Redraw();
}
Grid.Redraw = function() {
const that = Grid;
that.Clear();
that.Draw();
}
Grid.Clear = function() {
const that = Grid;
const instance = that.Instance;
if ( instance === null ) {
console.warn( 'Can\'t clear grid, the grid instance is null!' );
return;
}
const context = that.Context;
const backgroundColor = Evema.GetLocal( that, 'BackgroundColor' );
context.clearRect( 0, 0, instance.width, instance.height );
document.documentElement.style.setProperty( '--grid-background-color', backgroundColor );
}
Grid.Draw = function() {
const that = Grid;
const instance = that.Instance;
if ( instance === null ) {
console.warn( 'Can\'t draw grid, the grid instance is null!' );
return;
}
const context = that.Context;
const type = Evema.GetLocal( that, 'Type' );
const lineColor = Evema.GetLocal( that, 'LineColor' );
const cw = Evema.GetLocal( that, 'CellWidth' );
const ch = Evema.GetLocal( that, 'CellHeight' );
const width = Evema.GetLocal( that, 'Width' );
const height = Evema.GetLocal( that, 'Height' );
const ox = Evema.GetLocal( that, 'OffsetX' ) % cw;
const oy = Evema.GetLocal( that, 'OffsetY' ) % ch;
context.fillStyle = lineColor;
if ( type === 'pixel' ) {
// Draw one pixel on each cell in left-top corner
for ( let y = 0; y < height; y++ ) {
for ( let x = 0; x < width; x++ ) {
context.fillRect(
cw * x + ox,
ch * y + oy,
1, 1
);
}
}
} else {
console.warn( `Unknown draw type "${type}"` );
}
}
Grid.Options = {
Standard: {
Instance: null,
Context: null,
BackgroundColor: '#D3D1BB',
LineColor: '#000000',
CellWidth: 8,
CellHeight: 8,
Width: 100,
Height: 60,
Type: 'pixel',
OffsetX: 0,
OffsetY: 0
},
Current: {}
};
Grid.Actions = {
'Init' : Grid.Init,
'Rebuild' : Grid.Rebuild,
'Redraw' : Grid.Redraw,
'Clear' : Grid.Clear,
'Draw' : Grid.Draw
};
return Grid; } )();
|
// Code generated by sqlc. DO NOT EDIT.
package harddrive
import (
"time"
)
type HDD struct {
ID int64
MakeID int64
Name string
SizeBytes int64
Rpm int64
CreatedAt time.Time
UpdatedAt time.Time
}
|
#!/bin/sh
# Delete comments if needed
alg=9 #Run with SAMA-DiEGO-A (PV + MI-ES), if run with B, C, D please set to 10, 11, 12, accordingly
func=2 # Function id, the second choice of 'Func_ids' in IOHPBO_config.json
dim=1 # The first choice of 'Dims' in IOHPBO_config.json, the dimensionality
# run: stands for count of repetition
# Example exclusively experiment on the eighth problem in IOHPBO_config.json with 11 independent runs
for run in 1 3 5 7 9 11
do
if [ $run -eq 11 ]
then
python run_IOHPBO_test.py -F $func -A $alg -R $run -D $dim
else
next_r=$(( run + 1 ))
python run_IOHPBO_test.py -F $func -A $alg -R $run -D $dim &
python run_IOHPBO_test.py -F $func -A $alg -R $next_r -D $dim
fi
done
# Run multiple problems together by specifying the function id here
for func in 8 9 10 11 12
do
for run in 1 3 5 7 9 11 # Number of runs
do
if [ $run -eq 11 ]
then
python run_IOHPBO_test.py -F $func -A $alg -R $run -D $dim
else
next_r=$(( run + 1 ))
python run_IOHPBO_test.py -F $func -A $alg -R $run -D $dim &
python run_IOHPBO_test.py -F $func -A $alg -R $next_r -D $dim
fi
done
done
|
import pyramid_handlers
from blueyellow_pycharm_app.controllers.base_controller import BaseController
from blueyellow_pycharm_app.infrastructure.suppressor import suppress
class HomeController(BaseController):
@pyramid_handlers.action(renderer = 'templates/home/index.pt')
def index(self):
return {'value' : 'HOME'}
@pyramid_handlers.action(renderer = 'templates/home/about.pt')
def about(self):
return {'value' : 'ABOUT'}
@pyramid_handlers.action(renderer = 'templates/home/contact.pt')
def contact(self):
return {'value' : 'CONTACT'}
@suppress
def dont_expose_as_web_action(self):
print("Called dont_expose...") |
# ====================================================================
# MultiModel
#
mutable struct MultiModel <: AbstractMultiModel
models::Vector{Model}
patchfuncts::Vector{ExprFunction}
patchcomps::Vector{OrderedDict{Symbol, PatchComp}}
function MultiModel(v::Vararg{Model})
multi = new([v...], Vector{ExprFunction}(),
Vector{OrderedDict{Symbol, PatchComp}}())
for m in v
m.parent = multi
end
evaluate!(multi)
return multi
end
end
Base.getindex(m::MultiModel, id::Int) = m.models[id]
Base.length(m::MultiModel) = length(m.models)
function evaluate!(multi::MultiModel)
eval1!(multi)
eval2!(multi)
eval3!(multi)
eval4!(multi)
end
function eval1!(multi::MultiModel)
for id in 1:length(multi.models)
eval1!(multi.models[id])
end
empty!(multi.patchcomps)
for id in 1:length(multi.models)
push!(multi.patchcomps, multi.models[id].meval.patchcomps)
end
end
function eval2!(multi::MultiModel)
for id in 1:length(multi.models)
eval2!(multi.models[id], fromparent=true)
end
for pf in multi.patchfuncts
pf.funct(multi.patchcomps)
end
nothing
end
function eval3!(multi::MultiModel)
for id in 1:length(multi.models)
eval3!(multi.models[id])
end
end
function eval4!(multi::MultiModel)
for id in 1:length(multi.models)
eval4!(multi.models[id])
end
end
function push!(multi::MultiModel, model::Model)
push!(multi.models, model)
model.parent = multi
evaluate!(multi)
end
function patch!(multi::MultiModel, exfunc::ExprFunction)
evaluate!(multi) # ensure sub models are evaluated before adding
# new patch functions
push!(multi.patchfuncts, exfunc)
evaluate!(multi)
return multi
end
|
package com.escodro.domain.usecase.task
import com.escodro.domain.model.Task
import com.escodro.domain.usecase.fake.AlarmInteractorFake
import com.escodro.domain.usecase.fake.CalendarProviderFake
import com.escodro.domain.usecase.fake.NotificationInteractorFake
import com.escodro.domain.usecase.fake.TaskRepositoryFake
import com.escodro.domain.usecase.task.implementation.AddTaskImpl
import com.escodro.domain.usecase.task.implementation.LoadTaskImpl
import com.escodro.domain.usecase.task.implementation.UpdateTaskStatusImpl
import kotlinx.coroutines.ExperimentalCoroutinesApi
import kotlinx.coroutines.test.runBlockingTest
import org.junit.Assert
import org.junit.Before
import org.junit.Test
@ExperimentalCoroutinesApi
internal class CompleteTaskTest {
private val taskRepository = TaskRepositoryFake()
private val alarmInteractor = AlarmInteractorFake()
private val notificationInteractor = NotificationInteractorFake()
private val calendarProvider = CalendarProviderFake()
private val addTaskUseCase = AddTaskImpl(taskRepository)
private val completeTaskUseCase =
CompleteTask(taskRepository, alarmInteractor, notificationInteractor, calendarProvider)
private val uncompleteTaskUseCase = UncompleteTask(taskRepository)
private val updateTaskStatusUseCase =
UpdateTaskStatusImpl(taskRepository, completeTaskUseCase, uncompleteTaskUseCase)
private val getTaskUseCase = LoadTaskImpl(taskRepository)
@Before
fun setup() = runBlockingTest {
taskRepository.cleanTable()
alarmInteractor.clear()
notificationInteractor.clear()
}
@Test
fun `test if a task is updated as completed`() = runBlockingTest {
val task = Task(id = 18, title = "buy soy milk", completed = false)
addTaskUseCase(task)
completeTaskUseCase(task)
val result = getTaskUseCase(task.id)
Assert.assertNotNull(result)
Assert.assertTrue(result?.completed == true)
}
@Test
fun `test if a task is updated as completed by id`() = runBlockingTest {
val task = Task(id = 17, title = "change smartphone", completed = false)
addTaskUseCase(task)
completeTaskUseCase(task.id)
val result = getTaskUseCase(task.id)
require(result != null)
Assert.assertTrue(result.completed)
}
@Test
fun `test if a task is updated as uncompleted`() = runBlockingTest {
val task = Task(id = 18, title = "buy soy milk", completed = false)
addTaskUseCase(task)
uncompleteTaskUseCase(task)
val result = getTaskUseCase(task.id)
require(result != null)
Assert.assertFalse(result.completed)
}
@Test
fun `test if the completed status is inverted`() = runBlockingTest {
val task1 = Task(id = 99, title = "watch tech talk", completed = true)
val task2 = Task(id = 88, title = "write paper", completed = false)
addTaskUseCase(task1)
addTaskUseCase(task2)
updateTaskStatusUseCase(task1.id)
updateTaskStatusUseCase(task2.id)
val loadedTask1 = getTaskUseCase(task1.id)
val loadedTask2 = getTaskUseCase(task2.id)
require(loadedTask1 != null)
require(loadedTask2 != null)
Assert.assertFalse(loadedTask1.completed)
Assert.assertTrue(loadedTask2.completed)
}
@Test
fun `test if the alarm is canceled when the task is completed`() = runBlockingTest {
val task = Task(id = 19, title = "sato's meeting", completed = false)
addTaskUseCase(task)
completeTaskUseCase(task)
Assert.assertFalse(alarmInteractor.isAlarmScheduled(task.id))
}
@Test
fun `test if the notification is dismissed when the task is completed`() = runBlockingTest {
val task = Task(id = 20, title = "scrum master dog", completed = false)
addTaskUseCase(task)
completeTaskUseCase(task)
Assert.assertFalse(notificationInteractor.isNotificationShown(task.id))
}
}
|
package net.chigita.savepoint.ui.fragment
import android.os.Bundle
import android.view.LayoutInflater
import android.view.Menu
import android.view.MenuInflater
import android.view.MenuItem
import android.view.View
import android.view.ViewGroup
import androidx.databinding.DataBindingUtil
import androidx.fragment.app.Fragment
import androidx.lifecycle.ViewModelProvider
import androidx.lifecycle.ViewModelProviders
import androidx.navigation.fragment.findNavController
import androidx.navigation.fragment.navArgs
import com.xwray.groupie.GroupAdapter
import com.xwray.groupie.ViewHolder
import net.chigita.savepoint.R
import net.chigita.savepoint.databinding.FragmentRegisterBinding
import net.chigita.savepoint.di.Injectable
import net.chigita.savepoint.ui.adapter.AnglesSection
import net.chigita.savepoint.util.changed
import net.chigita.savepoint.viewmodel.AngleViewModel
import net.chigita.savepoint.viewmodel.ThingViewModel
import javax.inject.Inject
/**
* Created by chigichan24 on 2019-05-14.
*/
class RegisterFragment : Fragment(), Injectable {
private lateinit var binding: FragmentRegisterBinding
@Inject lateinit var viewModelFactory: ViewModelProvider.Factory
lateinit var thingViewModel: ThingViewModel
lateinit var angleViewModel: AngleViewModel
private val args by navArgs<RegisterFragmentArgs>()
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setHasOptionsMenu(true)
}
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View? {
binding = DataBindingUtil.inflate(
inflater,
R.layout.fragment_register,
container,
false
)
return binding.root
}
override fun onActivityCreated(savedInstanceState: Bundle?) {
super.onActivityCreated(savedInstanceState)
val args = RegisterFragmentArgs.fromBundle(arguments!!)
binding.rootConstraint.setOnTouchListener { v, _ ->
v.requestFocus()
}
thingViewModel = ViewModelProviders.of(this, viewModelFactory)
.get(ThingViewModel::class.java)
angleViewModel = ViewModelProviders.of(this, viewModelFactory)
.get(AngleViewModel::class.java)
thingViewModel.thingLiveData.changed(viewLifecycleOwner) {
binding.thingEditText.setText(it.name)
}
angleViewModel.registedAnglesLiveData.changed(viewLifecycleOwner) {
binding.recyclerView.adapter = GroupAdapter<ViewHolder>().apply {
add(AnglesSection(it, viewLifecycleOwner))
}
}
binding.fab.setOnClickListener {
(args.uuid)?.let {
navigateToAngleRegister(it)
}
}
(args.uuid)?.let {
thingViewModel.loadThing(it)
angleViewModel.loadRegistedAngles(it)
}
}
override fun onCreateOptionsMenu(menu: Menu, inflater: MenuInflater) {
inflater.inflate(R.menu.menu_fragment_register, menu)
}
override fun onOptionsItemSelected(item: MenuItem): Boolean {
if (item.itemId == R.id.menu_completed) {
if (args.uuid == null) {
thingViewModel.registerThing(binding.thingEditText.text.toString())
navigateToHome()
}
return false
}
return true
}
fun navigateToAngleRegister(uuid: String) {
val bundle = AngleRegisterFragmentArgs.Builder()
.setThingUuid(uuid)
.build()
.toBundle()
findNavController().navigate(R.id.action_register_to_angle_register, bundle)
}
fun navigateToHome() = findNavController().popBackStack()
}
|
<?php
namespace Accompli\Deployment;
use Accompli\Deployment\Connection\ConnectionAdapterInterface;
use UnexpectedValueException;
/**
* Host.
*
* @author Niels Nijens <[email protected]>
*/
class Host
{
/**
* The constant to identify a host in the test stage.
*
* @var string
*/
const STAGE_TEST = 'test';
/**
* The constant to identify a host in the acceptance stage.
*
* @var string
*/
const STAGE_ACCEPTANCE = 'acceptance';
/**
* The constant to identify a host in the production stage.
*
* @var string
*/
const STAGE_PRODUCTION = 'production';
/**
* The stage (test, acceptance, production) of this host.
*
* @var string
*/
private $stage;
/**
* The connection type for this host.
*
* @var string
*/
private $connectionType;
/**
* The hostname of this host.
*
* @var string|null
*/
private $hostname;
/**
* The base workspace path on this host.
*
* @var string
*/
private $path;
/**
* The array with connection options.
*
* @var array
*/
private $connectionOptions;
/**
* The connection instance used to connect to and communicate with this Host.
*
* @var ConnectionAdapterInterface
*/
private $connection;
/**
* Constructs a new Host instance.
*
* @param string $stage
* @param string $connectionType
* @param string $hostname
* @param string $path
* @param array $connectionOptions
*
* @throws UnexpectedValueException when $stage is not a valid type
*/
public function __construct($stage, $connectionType, $hostname, $path, array $connectionOptions = array())
{
if (self::isValidStage($stage) === false) {
throw new UnexpectedValueException(sprintf("'%s' is not a valid stage.", $stage));
}
$this->stage = $stage;
$this->connectionType = $connectionType;
$this->hostname = $hostname;
$this->path = $path;
$this->connectionOptions = $connectionOptions;
}
/**
* Returns true if this Host has a connection instance.
*
* @return ConnectionAdapterInterface
*/
public function hasConnection()
{
return ($this->connection instanceof ConnectionAdapterInterface);
}
/**
* Returns the stage of this host.
*
* @return string
*/
public function getStage()
{
return $this->stage;
}
/**
* Returns the connection type of this host.
*
* @return string
*/
public function getConnectionType()
{
return $this->connectionType;
}
/**
* Returns the hostname of this host.
*
* @return string
*/
public function getHostname()
{
return $this->hostname;
}
/**
* Returns the connection instance.
*
* @return ConnectionAdapterInterface
*/
public function getConnection()
{
return $this->connection;
}
/**
* Returns the base workspace path.
*
* @return string
*/
public function getPath()
{
return $this->path;
}
/**
* Returns the connection options.
*
* @return array
*/
public function getConnectionOptions()
{
return $this->connectionOptions;
}
/**
* Sets the connection instance.
*
* @param ConnectionAdapterInterface $connection
*/
public function setConnection(ConnectionAdapterInterface $connection)
{
$this->connection = $connection;
}
/**
* Returns true if $stage is a valid stage type.
*
* @param string $stage
*
* @return bool
*/
public static function isValidStage($stage)
{
return in_array($stage, array(self::STAGE_TEST, self::STAGE_ACCEPTANCE, self::STAGE_PRODUCTION));
}
}
|
class MainActivity < Android::App::Activity
def onCreate(savedInstanceState)
super
layout = Android::Widget::LinearLayout.new(self)
layout.orientation = Android::Widget::LinearLayout::VERTICAL
@paintView = PaintView.new(self)
layout.addView(@paintView, Android::Widget::LinearLayout::LayoutParams.new(Android::View::ViewGroup::LayoutParams::MATCH_PARENT, 0.0, 1.0))
button = Android::Widget::Button.new(self)
button.text = 'Clear'
button.onClickListener = self
layout.addView(button)
self.contentView = layout
end
def onClick(view)
@paintView.clearPaths
end
end
|
package nl.dyonb.discordfabriclink.util;
import net.minecraft.text.Text;
import nl.dyonb.chathistory.ChatHistory;
import nl.dyonb.chathistory.util.ChatMessage;
import java.util.UUID;
public class ChatHistoryInteraction {
public static void addMessage(Text text, UUID uuid) {
ChatHistory.CHAT_HISTORY.add(new ChatMessage(text, uuid));
}
}
|
using Distributions
using ArrayViews
using Base.LinAlg.BLAS
import Base.length
abstract RBM
typealias Mat{T} AbstractArray{T, 2}
typealias Vec{T} AbstractArray{T, 1}
const UNIT_CLASSES = [:bernoulli, :gaussian]
type Units
bias::Vector{Float64}
class::Symbol
function Units(num::Int, class::Symbol=:bernoulli)
#@assert class in UNIT_CLASSES
new(zeros(num), class)
end
function Units(params::Dict)
@assert haskey(params, "num")
if haskey(params, "class")
return new(zeros(params["num"]), symbol(params["class"]))
else
return new(zeros(params["num"]), :bernoulli)
end
end
function Base.show(io::IO, units::Units)
num = length(units)
print(io, "Units(class=$(units.class), num=$num)")
end
end
length(units::Units) = length(units.bias)
insert!(units::Units, index::Int, item::Any) = insert!(units.bias, index, item)
deleteat!(units::Units, index::Int) = deleteat!(units.bias, index)
type BaseRBM <: RBM
W::Matrix{Float64}
visible::Units
hidden::Units
dW_prev::Matrix{Float64}
persistent_chain::Matrix{Float64}
momentum::Float64
directory
function BaseRBM(visible::Units, hidden::Units; sigma=0.001, momentum=0.9, directory="./")
n_hid = length(hidden)
n_vis = length(visible)
new(
rand(Normal(0, sigma), (n_hid, n_vis)),
visible, hidden,
zeros(n_hid, n_vis),
Array(Float64, 0, 0),
momentum,
directory
)
end
function BaseRBM(params::Dict)
@assert haskey(params, "visible")
@assert haskey(params, "hidden")
visible = Units(params["visible"])
hidden = Units(params["hidden"])
sigma = 0.001
momentum = 0.9
directory="./"
sigma = haskey(params, "sigma") ? params["sigma"] : sigma
momentum = haskey(params, "momentum") ? params["momentum"] : momentum
directory = haskey(params, "directory") ? params["directory"] : directory
n_vis = length(visible)
n_hid = length(hidden)
new(
rand(Normal(0, sigma), (n_hid, n_vis)),
visible, hidden,
zeros(n_hid, n_vis),
Array(Float64, 0, 0),
momentum,
directory
)
end
function Base.show(io::IO, rbm::BaseRBM)
n_vis = length(rbm.visible)
n_hid = length(rbm.hidden)
print(io, "BaseRBM($n_vis, $n_hid, momentum=$(rbm.momentum))")
end
end
function logistic(x)
return 1 ./ (1 + exp(-x))
end
function mean_hiddens(rbm::RBM, vis::Mat{Float64})
p = rbm.W * vis .+ rbm.hidden.bias
return logistic(p)
end
function sample_hiddens(rbm::RBM, vis::Mat{Float64})
p = mean_hiddens(rbm, vis)
return float(rand(size(p)) .< p)
end
function sample_visibles(rbm::RBM, hid::Mat{Float64})
if rbm.visible.class == :bernoulli
p = rbm.W' * hid .+ rbm.visible.bias
p = logistic(p)
return float(rand(size(p)) .< p)
elseif rbm.visible.class == :gaussian
mu = logistic(rbm.W' * hid .+ rbm.visible.bias)
sigma2 = 0.01 # using fixed standard diviation
samples = zeros(size(mu))
for j=1:size(mu, 2), i=1:size(mu, 1)
samples[i, j] = rand(Normal(mu[i, j], sigma2))
end
return samples
end
end
function gibbs(rbm::RBM, vis::Mat{Float64}; n_times=1)
v_pos = vis
h_pos = sample_hiddens(rbm, v_pos)
v_neg = sample_visibles(rbm, h_pos)
h_neg = sample_hiddens(rbm, v_neg)
for i=1:n_times-1
v_neg = sample_visibles(rbm, h_neg)
h_neg = sample_hiddens(rbm, v_neg)
end
return v_pos, h_pos, v_neg, h_neg
end
function free_energy(rbm::RBM, vis::Mat{Float64})
vb = sum(vis .* rbm.visible.bias, 1)
Wx_b_log = sum(log(1 + exp(rbm.W * vis .+ rbm.hidden.bias)), 1)
return - vb - Wx_b_log
end
# This function is very memory intensive. Force subsampling of the input
# space with vectors should allow people to trade of itereations of memory consumption
function score_samples(rbm::RBM, X::Mat{Float64}, scoring_ratio)
# Randomly select the scoring sample view
sample_size = int(size(X, 2) * scoring_ratio)
start = rand(1:size(X,2)-sample_size)
vis = view(X, :, start:start+sample_size)
# Still have to do a copy
n_feat, n_samples = size(vis)
vis_corrupted = copy(vis)
idxs = rand(1:n_feat, n_samples)
for (i, j) in zip(idxs, 1:n_samples)
vis_corrupted[i, j] = 1 - vis_corrupted[i, j]
end
# These are expensive, but necessary for now.
fe = free_energy(rbm, vis)
fe_corrupted = free_energy(rbm, vis_corrupted)
return n_feat * log(logistic(fe_corrupted - fe))
end
function update_weights!(rbm::RBM, h_pos, v_pos, h_neg, v_neg, lr, buf)
dW = buf
# dW = (h_pos * v_pos') - (h_neg * v_neg')
gemm!('N', 'T', 1.0, h_neg, v_neg, 0.0, dW)
gemm!('N', 'T', 1.0, h_pos, v_pos, -1.0, dW)
# rbm.W += lr * dW
axpy!(lr, dW, rbm.W)
# rbm.W += rbm.momentum * rbm.dW_prev
axpy!(lr * rbm.momentum, rbm.dW_prev, rbm.W)
# save current dW
copy!(rbm.dW_prev, dW)
end
function contdiv(rbm::RBM, vis::Mat{Float64}, n_gibbs::Int)
v_pos, h_pos, v_neg, h_neg = gibbs(rbm, vis, n_times=n_gibbs)
return v_pos, h_pos, v_neg, h_neg
end
function persistent_contdiv(rbm::RBM, vis::Mat{Float64}, n_gibbs::Int)
if size(rbm.persistent_chain) != size(vis)
# persistent_chain not initialized or batch size changed, re-initialize
rbm.persistent_chain = vis
end
# take positive samples from real data
v_pos, h_pos, _, _ = gibbs(rbm, vis)
# take negative samples from "fantasy particles"
rbm.persistent_chain, _, v_neg, h_neg = gibbs(rbm, vis, n_times=n_gibbs)
return v_pos, h_pos, v_neg, h_neg
end
function fit_batch!(rbm::RBM, vis::Mat{Float64};
persistent=true, buf=None, lr=0.1, n_gibbs=1)
buf = buf == None ? zeros(size(rbm.W)) : buf
# v_pos, h_pos, v_neg, h_neg = gibbs(rbm, vis, n_times=n_gibbs)
sampler = persistent ? persistent_contdiv : contdiv
v_pos, h_pos, v_neg, h_neg = sampler(rbm, vis, n_gibbs)
lr = lr / size(v_pos, 1)
update_weights!(rbm, h_pos, v_pos, h_neg, v_neg, lr, buf)
rbm.hidden.bias += vec(lr * (sum(h_pos, 2) - sum(h_neg, 2)))
rbm.visible.bias += vec(lr * (sum(v_pos, 2) - sum(v_neg, 2)))
return rbm
end
function fit(rbm::RBM, X::Mat{Float64};
persistent=true, lr=0.1, n_iter=10, batch_size=100, n_gibbs=1, scoring_ratio=0.1)
@assert minimum(X) >= 0 && maximum(X) <= 1
n_samples = size(X, 2)
n_batches = int(ceil(n_samples / batch_size))
w_buf = zeros(size(rbm.W))
batch = Array(Float64, size(X, 1), batch_size) # pre-allocate batch
for itr=1:n_iter
tic()
for i=1:n_batches
# println("fitting $(i)th batch")
batch = X[:, ((i-1)*batch_size + 1):min(i*batch_size, size(X, 2))]
batch = full(batch)
fit_batch!(rbm, batch, persistent=persistent,
buf=w_buf, n_gibbs=n_gibbs)
end
toc()
pseudo_likelihood = mean(score_samples(rbm, X, scoring_ratio))
info("Iteration #$itr, pseudo-likelihood = $pseudo_likelihood")
end
return rbm
end
function transform(rbm::RBM, X::Mat{Float64})
return mean_hiddens(rbm, X)
end
function generate(rbm::RBM, vis::Vec{Float64}; n_gibbs=1)
return gibbs(rbm, reshape(vis, length(vis), 1); n_times=n_gibbs)[3]
end
function generate(rbm::RBM, X::Mat{Float64}; n_gibbs=1)
return gibbs(rbm, X; n_times=n_gibbs)[3]
end
function features(rbm::RBM; transpose=true)
return if transpose rbm.W' else rbm.W end
end
# synonym for backward compatibility
components(rbm::RBM; transpose=true) = features(rbm, transpose)
|
import { CanvasViewer } from './canvasViewer';
import { floor } from 'suf-utils';
export class CanvasViewerRect extends CanvasViewer {
constructor(public rect: { width: number, height: number }, scale = 1, cssScale = 1) {
super(rect.width, scale, cssScale)
this.canvas.style.width = `${rect.width * scale * cssScale}px`
this.canvas.style.height = `${rect.height * scale * cssScale}px`
this.canvas.width = rect.width * scale
this.canvas.height = rect.height * scale
}
drawWaveArray(arr: number[], skip = 1) {
this.ctx.fillStyle = '#fff'
let i = 0
for (let x = 0; x < this.rect.width; x++) {
const y = arr[i];
const middlePoint = (this.rect.height / 2);
this.ctx.fillRect(x, middlePoint, this.scale, floor(middlePoint * y))
i += skip;
}
}
} |
// Copyright 2017 David Conran
#include "IRsend.h"
#include "IRsend_test.h"
#include "gtest/gtest.h"
// Tests for sendGlobalCache().
// Test sending a typical command wihtout a repeat.
TEST(TestSendGlobalCache, NonRepeatingCode) {
IRsendTest irsend(4);
IRrecv irrecv(4);
irsend.begin();
irsend.reset();
// Modified NEC TV "Power On" from Global Cache with no repeats
uint16_t gc_test[71] = {38000, 1, 1, 342, 172, 21, 22, 21, 21, 21, 65, 21,
21, 21, 22, 21, 22, 21, 21, 21, 22, 21, 65, 21,
65, 21, 22, 21, 65, 21, 65, 21, 65, 21, 65, 21,
65, 21, 65, 21, 22, 21, 22, 21, 21, 21, 22, 21,
22, 21, 65, 21, 22, 21, 21, 21, 65, 21, 65, 21,
65, 21, 64, 22, 65, 21, 22, 21, 65, 21, 1519};
irsend.sendGC(gc_test, 71);
irsend.makeDecodeResult();
EXPECT_TRUE(irrecv.decodeNEC(&irsend.capture));
EXPECT_EQ(NEC, irsend.capture.decode_type);
EXPECT_EQ(kNECBits, irsend.capture.bits);
EXPECT_EQ(0x20DF827D, irsend.capture.value);
EXPECT_EQ(0x4, irsend.capture.address);
EXPECT_EQ(0x41, irsend.capture.command);
EXPECT_EQ(
"m8892s4472m546s572m546s546m546s1690m546s546m546s572m546s572"
"m546s546m546s572m546s1690m546s1690m546s572m546s1690m546s1690"
"m546s1690m546s1690m546s1690m546s1690m546s572m546s572m546s546"
"m546s572m546s572m546s1690m546s572m546s546m546s1690m546s1690"
"m546s1690m546s1664m572s1690m546s572m546s1690m546s39494",
irsend.outputStr());
}
// Test sending typical command with repeats.
TEST(TestSendGlobalCache, RepeatCode) {
IRsendTest irsend(4);
IRrecv irrecv(4);
irsend.begin();
irsend.reset();
// Sherwood (NEC-like) "Power On" from Global Cache with 2 repeats
uint16_t gc_test[75] = {
38000, 2, 69, 341, 171, 21, 64, 21, 64, 21, 21, 21, 21, 21, 21,
21, 21, 21, 21, 21, 64, 21, 64, 21, 21, 21, 64, 21, 21, 21,
21, 21, 21, 21, 64, 21, 21, 21, 64, 21, 21, 21, 21, 21, 21,
21, 64, 21, 21, 21, 21, 21, 21, 21, 21, 21, 64, 21, 64, 21,
64, 21, 21, 21, 64, 21, 64, 21, 64, 21, 1600, 341, 85, 21, 3647};
irsend.sendGC(gc_test, 75);
irsend.makeDecodeResult();
EXPECT_TRUE(irrecv.decodeNEC(&irsend.capture));
EXPECT_EQ(NEC, irsend.capture.decode_type);
EXPECT_EQ(kNECBits, irsend.capture.bits);
EXPECT_EQ(0xC1A28877, irsend.capture.value);
EXPECT_EQ(0x4583, irsend.capture.address);
EXPECT_EQ(0x11, irsend.capture.command);
EXPECT_EQ(
"m8866s4446m546s1664m546s1664m546s546m546s546m546s546m546s546"
"m546s546m546s1664m546s1664m546s546m546s1664m546s546m546s546"
"m546s546m546s1664m546s546m546s1664m546s546m546s546m546s546"
"m546s1664m546s546m546s546m546s546m546s546m546s1664m546s1664"
"m546s1664m546s546m546s1664m546s1664m546s1664m546s41600"
"m8866s2210m546s94822"
"m8866s2210m546s94822",
irsend.outputStr());
}
|
<?php
namespace files;
use atomar\Atomar;
use model\File;
/**
* Stores files on the local disk
* Class LocalDataStore
* @package files\controller
*/
class LocalDataStore implements DataStore {
public function generateUpload(File $file, int $ttl) {
$upload = \R::dispense('fileupload');
$upload->token = md5($file->file_path . $file->id . time());
$upload->file = $file;
$upload->ttl = $ttl;
$upload->created_at = db_date();
if (store($upload)) {
return $upload;
} else {
return null;
}
}
public function postProcessUpload(File $file) {
$meta = $this->getMeta($file);
$file->size = $meta['content_length'];
$file->type = $meta['content_type'];
return store($file);
}
public function getMeta(File $file) {
$path = $this->absolutePath($file);
if(!file_exists($path)) return null;
$info = pathinfo($path);
$meta = array();
$meta['content_length'] = filesize($path);
$meta['content_size'] = $info['extension'];
if (function_exists('mime_content_type')) {
$meta['content_type'] = mime_content_type($path);
}
$meta['path'] = $path;
return $meta;
}
/**
* Returns the fully qualified path to the file
*
* @param File $file
* @return string the path to the file
*/
public function absolutePath(File $file) {
$path = Atomar::$config['files'] . $file->file_path;
return realpath($path);
}
public function download(File $file, bool $view_in_browser = false) {
if ($file->id) {
$path = $this->absolutePath($file);
if (file_exists($path)) {
if ($view_in_browser) {
$finfo = finfo_open(FILEINFO_MIME_TYPE);
$mime = finfo_file($finfo, $path);
header('Content-Type: ' . $mime);
header('Content-Disposition: inline; filename=' . basename($path));
readfile($path);
} else {
stream_file($path, array(
'name' => $file->name,
'content_type' => $file->type,
'download' => true
));
}
} else {
header('HTTP/1.1 404 Not Found');
echo 'Missing file data';
}
} else {
header('HTTP/1.1 404 Not Found');
}
exit;
}
/**
* Performs any initialization required by the data store
*/
public function init() {
}
/**
* returns a download url for the file
*
* @param File $file the file to be downloaded
* @param int $ttl
* @return string the download link
*/
public function getDownloadURL(File $file, int $ttl) {
return '';
}
} |
package frame.styles
import javafx.scene.paint.Color
import tornadofx.Stylesheet
import tornadofx.box
import tornadofx.cssclass
import tornadofx.px
class NoteStyles: Stylesheet() {
companion object {
val notesPane_ by cssclass()
val paneToolbar_ by cssclass()
val editor_ by cssclass()
}
init {
notesPane_ {
padding = box(2.px)
}
paneToolbar_ {
backgroundColor += Color.TRANSPARENT
padding = box(0.px, 0.px, 0.px, 20.px)
}
editor_ {
maxHeight = 300.px
}
}
}
|
package MetaCPAN::TestApp;
use strict;
use warnings;
use LWP::ConsoleLogger::Easy qw( debug_ua );
use MetaCPAN::Server::Test qw( app );
use Moose;
use Plack::Test::Agent;
has _test_agent => (
is => 'ro',
isa => 'Plack::Test::Agent',
handles => ['get'],
lazy => 1,
default => sub {
my $self = shift;
return Plack::Test::Agent->new(
app => app(),
ua => $self->_user_agent,
# server => 'HTTP::Server::Simple',
);
},
);
# set a server value above if you want to see debugging info
has _user_agent => (
is => 'ro',
isa => 'LWP::UserAgent',
lazy => 1,
default => sub {
my $ua = LWP::UserAgent->new;
debug_ua($ua);
return $ua;
},
);
__PACKAGE__->meta->make_immutable();
1;
|
OVPN_DATA=$1
CLIENT_NAME=$2
# Generate client configuration
docker run --volumes-from $OVPN_DATA --rm kylemanna/openvpn easyrsa build-client-full $CLIENT_NAME nopass
# Export client configuration
docker run --volumes-from $OVPN_DATA --rm kylemanna/openvpn ovpn_getclient $CLIENT_NAME > config/$CLIENT_NAME.ovpn
|
/*---------------------------------------------------------------------------------------------
* Copyright (c) Microsoft Corporation. All rights reserved.
* Licensed under the MIT License. See License.txt in the project root for license information.
*--------------------------------------------------------------------------------------------*/
import { URI } from 'vs/base/common/uri';
import { Disposable, toDisposable } from 'vs/base/common/lifecycle';
import { Schemas } from 'vs/base/common/network';
import { localize } from 'vs/nls';
import { IEnvironmentService } from 'vs/platform/environment/common/environment';
import { IFileService } from 'vs/platform/files/common/files';
import { ILabelService } from 'vs/platform/label/common/label';
import { ILogService } from 'vs/platform/log/common/log';
import { IWorkbenchContribution } from 'vs/workbench/common/contributions';
import { ITerminalEditorService, ITerminalGroupService, ITerminalService, terminalEditorId } from 'vs/workbench/contrib/terminal/browser/terminal';
import { terminalStrings } from 'vs/workbench/contrib/terminal/common/terminalStrings';
import { IEditorResolverService, RegisteredEditorPriority } from 'vs/workbench/services/editor/common/editorResolverService';
import { registerLogChannel } from 'vs/workbench/services/output/common/output';
import { join } from 'vs/base/common/path';
import { TerminalLogConstants } from 'vs/platform/terminal/common/terminal';
/**
* The main contribution for the terminal contrib. This contains calls to other components necessary
* to set up the terminal but don't need to be tracked in the long term (where TerminalService would
* be more relevant).
*/
export class TerminalMainContribution extends Disposable implements IWorkbenchContribution {
constructor(
@IEditorResolverService editorResolverService: IEditorResolverService,
@IEnvironmentService environmentService: IEnvironmentService,
@IFileService private readonly _fileService: IFileService,
@ILabelService labelService: ILabelService,
@ILogService private readonly _logService: ILogService,
@ITerminalService terminalService: ITerminalService,
@ITerminalEditorService terminalEditorService: ITerminalEditorService,
@ITerminalGroupService terminalGroupService: ITerminalGroupService
) {
super();
// Register terminal editors
editorResolverService.registerEditor(
`${Schemas.vscodeTerminal}:/**`,
{
id: terminalEditorId,
label: terminalStrings.terminal,
priority: RegisteredEditorPriority.exclusive
},
{
canHandleDiff: false,
canSupportResource: uri => uri.scheme === Schemas.vscodeTerminal,
singlePerResource: true
},
({ resource, options }) => {
const instance = terminalService.getInstanceFromResource(resource);
if (instance) {
const sourceGroup = terminalGroupService.getGroupForInstance(instance);
sourceGroup?.removeInstance(instance);
}
const resolvedResource = terminalEditorService.resolveResource(instance || resource);
const editor = terminalEditorService.getInputFromResource(resolvedResource) || { editor: resolvedResource };
return {
editor,
options: {
...options,
pinned: true,
forceReload: true,
override: terminalEditorId
}
};
}
);
// Register a resource formatter for terminal URIs
labelService.registerFormatter({
scheme: Schemas.vscodeTerminal,
formatting: {
label: '${path}',
separator: ''
}
});
// Register log channel
this._registerLogChannel('ptyHostLog', localize('ptyHost', "Pty Host"), URI.file(join(environmentService.logsPath, `${TerminalLogConstants.FileName}.log`)));
}
private _registerLogChannel(id: string, label: string, file: URI): void {
const promise = registerLogChannel(id, label, file, this._fileService, this._logService);
this._register(toDisposable(() => promise.cancel()));
}
}
|
@file:Suppress("unused", "MemberVisibilityCanBePrivate")
package bulma
import org.w3c.dom.HTMLElement
interface BulmaElement {
val root: HTMLElement
var text: String
get() = root.innerText
set(value) { root.innerText = value }
var hidden: Boolean
get() = root.classList.contains("is-hidden")
set(value) { root.classList.toggle("is-hidden", value) }
var invisible: Boolean
get() = root.classList.contains("is-invisible")
set(value) { root.classList.toggle("is-invisible", value)}
}
interface HasClassName {
val className: String
}
enum class Size(override val className: String) : HasClassName {
None(""), Small("is-small"), Normal("is-normal"),
Medium("is-medium"), Large("is-large");
fun toFas() = when (this) {
None -> FasSize.None
Small -> FasSize.Small
Normal -> FasSize.None
Medium -> FasSize.Medium
Large -> FasSize.Large
}
}
enum class ButtonsSize(override val className: String) : HasClassName {
None(""), Small("are-small"),
Medium("are-medium"), Large("are-large");
}
enum class TagsSize(override val className: String) : HasClassName {
None(""), Normal("are-normal"),
Medium("are-medium"), Large("are-large");
}
enum class FasSize(override val className: String) : HasClassName {
None(""), Small("fa-sm"),
Medium("fa-2x"), Large("fa-3x")
}
enum class FaRotate(override val className: String) : HasClassName {
None(""), R90("fa-rotate-90"), R180("fa-rotate-180"), R270("fa-rotate-270")
}
enum class FaFlip(override val className: String) : HasClassName {
None(""), Horizontal("fa-flip-horizontal"), Vertical("fa-flip-vertical"), Both("fa-flip-both")
}
enum class ElementColor(override val className: String) : HasClassName {
None(""),
White("is-white"), Black("is-black"),
Light("is-light"), Dark("is-dark"),
Primary("is-primary"), Info("is-info"),
Link("is-link"), Success("is-success"),
Warning("is-warning"), Danger("is-danger");
fun next() = values().let { it[(ordinal + 1) % it.count()] }
}
enum class TextSize(override val className: String) : HasClassName {
None(""),
S1("is-1"), S2("is-2"), S3("is-3"),
S4("is-4"), S5("is-5"), S6("is-6");
}
enum class TextColor(override val className: String) : HasClassName {
None(""), White("has-text-white"),
Black("has-text-black"), Light("has-text-light"),
Dark("has-text-dark"), Primary("has-text-primary"),
Info("has-text-info"), Link("has-text-link"),
Success("has-text-success"), Warning("has-text-warning"), Danger("has-text-danger"),
BlackBis("has-text-black-bis"), BlackBer("has-text-black-ter"),
GreyDarker("has-text-grey-darker"), GreyDark("has-text-grey-dark"),
Grey("has-text-grey"),
GreyLight("has-text-grey-light"), GreyLighter("has-text-grey-lighter"),
WhiteTer("has-text-white-ter"), WhiteBis("has-text-white-bis");
fun next() = values().let { it[(ordinal + 1) % it.count()] }
}
enum class Alignment(override val className: String) : HasClassName {
Left(""), Centered("is-centered"), Right("is-right")
}
enum class Spacing(override val className: String) : HasClassName {
None(""),
M("m"), M0("m-0"), M1("m-1"), M2("m-2"), M3("m-3"), M4("m-4"), M5("m-5"), M6("m-6"),
Mt("mt"), Mt0("mt-0"), Mt1("mt-1"), Mt2("mt-2"), Mt3("mt-3"), Mt4("mt-4"), Mt5("mt-5"), Mt6("mt-6"),
Mr("mr"), Mr0("mr-0"), Mr1("mr-1"), Mr2("mr-2"), Mr3("mr-3"), Mr4("mr-4"), Mr5("mr-5"), Mr6("mr-6"),
Mb("mb"), Mb0("mb-0"), Mb1("mb-1"), Mb2("mb-2"), Mb3("mb-3"), Mb4("mb-4"), Mb5("mb-5"), Mb6("mb-6"),
Ml("ml"), Ml0("ml-0"), Ml1("ml-1"), Ml2("ml-2"), Ml3("ml-3"), Ml4("ml-4"), Ml5("ml-5"), Ml6("ml-6"),
Mx("mx"), Mx0("mx-0"), Mx1("mx-1"), Mx2("mx-2"), Mx3("mx-3"), Mx4("mx-4"), Mx5("mx-5"), Mx6("mx-6"),
My("my"), My0("my-0"), My1("my-1"), My2("my-2"), My3("my-3"), My4("my-4"), My5("my-5"), My6("my-6"),
P("p"), P0("p-0"), P1("p-1"), P2("p-2"), P3("p-3"), P4("p-4"), P5("p-5"), P6("p-6"),
Pt("pt"), Pt0("pt-0"), Pt1("pt-1"), Pt2("pt-2"), Pt3("pt-3"), Pt4("pt-4"), Pt5("pt-5"), Pt6("pt-6"),
Pr("pr"), Pr0("pr-0"), Pr1("pr-1"), Pr2("pr-2"), Pr3("pr-3"), Pr4("pr-4"), Pr5("pr-5"), Pr6("pr-6"),
Pb("pb"), Pb0("pb-0"), Pb1("pb-1"), Pb2("pb-2"), Pb3("pb-3"), Pb4("pb-4"), Pb5("pb-5"), Pb6("pb-6"),
Pl("pl"), Pl0("pl-0"), Pl1("pl-1"), Pl2("pl-2"), Pl3("pl-3"), Pl4("pl-4"), Pl5("pl-5"), Pl6("pl-6"),
Px("px"), Px0("px-0"), Px1("px-1"), Px2("px-2"), Px3("px-3"), Px4("px-4"), Px5("px-5"), Px6("px-6"),
Py("py"), Py0("py-0"), Py1("py-1"), Py2("py-2"), Py3("py-3"), Py4("py-4"), Py5("py-5"), Py6("py-6"),
} |
/** @file AnimationsMixer.cpp
@author Philip Abbet
Implementation of the class 'Athena::Entities::AnimationsMixer'
*/
#include <Athena-Entities/AnimationsMixer.h>
#include <Athena-Entities/Animation.h>
using namespace Athena::Entities;
using namespace Athena::Utils;
using namespace std;
/***************************** CONSTRUCTION / DESTRUCTION *******************************/
AnimationsMixer::AnimationsMixer()
: m_pCurrentAnimation(0), m_pPreviousAnimation(0)
{
}
//-----------------------------------------------------------------------
AnimationsMixer::~AnimationsMixer()
{
tAnimationsIterator iter = getAnimationsIterator();
while (iter.hasMoreElements())
delete iter.getNext();
m_animations.clear();
}
/************************************** METHODS *****************************************/
void AnimationsMixer::update(float fSecondsElapsed)
{
// If the previous animation isn't finished yet (because it's blended with
// the current one), update it
if (m_pPreviousAnimation)
{
// Compute the new weight of the previous animation
float fWeight = m_pPreviousAnimation->getWeight();
fWeight -= fSecondsElapsed * 10.0f;
// If the weight goes to zero, disable the previous animation
if (fWeight <= 0.0f)
{
m_pPreviousAnimation->setEnabled(false);
m_pCurrentAnimation->setWeight(1.0f);
m_pPreviousAnimation = 0;
}
// Otherwise, continue to blend the 2 animations with the new weight
else
{
m_pPreviousAnimation->setWeight(fWeight);
m_pCurrentAnimation->setWeight(1.0f - fWeight);
}
}
// Add the elapsed time to the animations
if (m_pCurrentAnimation)
m_pCurrentAnimation->update(fSecondsElapsed);
if (m_pPreviousAnimation)
m_pPreviousAnimation->update(fSecondsElapsed);
}
/*************************** MANAGEMENT OF THE ANIMATIONS *******************************/
bool AnimationsMixer::addAnimation(tAnimation animation, Animation* pAnimation)
{
// Assertions
assert(pAnimation);
assert(animation > 0);
// Check that the animation ID doesn't already exists in the list
tAnimationsList::iterator iter = m_animations.find(animation);
if (iter != m_animations.end())
return false;
// Add the animation in the list
m_animations[animation] = pAnimation;
return true;
}
//-----------------------------------------------------------------------
tAnimation AnimationsMixer::addAnimation(Animation* pAnimation)
{
// Assertions
assert(pAnimation);
// Create an unique ID for the animation
tAnimation animation = m_animations.rbegin()->first + 1;
// Add the animation in the list
m_animations[animation] = pAnimation;
return animation;
}
//-----------------------------------------------------------------------
void AnimationsMixer::removeAnimation(tAnimation animation)
{
assert(animation > 0);
// Check that the animation ID exists in the list
tAnimationsList::iterator iter = m_animations.find(animation);
if (iter != m_animations.end())
{
delete iter->second;
m_animations.erase(iter);
}
}
void AnimationsMixer::removeAnimation(const std::string& strName)
{
assert(!strName.empty());
tAnimationsList::iterator iter, iterEnd;
for (iter = m_animations.begin(), iterEnd = m_animations.end(); iter != iterEnd; ++iter)
{
if (iter->second->getName() == strName)
{
delete iter->second;
m_animations.erase(iter);
break;
}
}
}
//-----------------------------------------------------------------------
void AnimationsMixer::removeAnimation(Animation* pAnimation)
{
assert(pAnimation);
tAnimationsList::iterator iter, iterEnd;
for (iter = m_animations.begin(), iterEnd = m_animations.end(); iter != iterEnd; ++iter)
{
if (iter->second == pAnimation)
{
delete iter->second;
m_animations.erase(iter);
break;
}
}
}
//-----------------------------------------------------------------------
Animation* AnimationsMixer::getAnimation(tAnimation animation)
{
assert(animation > 0);
tAnimationsList::iterator iter = m_animations.find(animation);
if (iter != m_animations.end())
return iter->second;
return 0;
}
//-----------------------------------------------------------------------
Animation* AnimationsMixer::getAnimation(const std::string& strName)
{
tAnimationsIterator iter = getAnimationsIterator();
while (iter.hasMoreElements())
{
if (iter.peekNextValue()->getName() == strName)
return iter.peekNextValue();
iter.moveNext();
}
return 0;
}
//-----------------------------------------------------------------------
void AnimationsMixer::startAnimation(tAnimation animation, bool bReset)
{
Animation* pNewAnimation = getAnimation(animation);
if (pNewAnimation)
startAnimation(pNewAnimation, bReset);
}
//-----------------------------------------------------------------------
void AnimationsMixer::startAnimation(const std::string& strName, bool bReset)
{
Animation* pNewAnimation = getAnimation(strName);
if (pNewAnimation)
startAnimation(pNewAnimation, bReset);
}
//-----------------------------------------------------------------------
void AnimationsMixer::startAnimation(Animation* pNewAnimation, bool bReset)
{
assert(pNewAnimation);
if (bReset)
stopAnimation();
// Test if there is currently no animation played
if (!m_pCurrentAnimation)
{
m_pCurrentAnimation = pNewAnimation;
m_pCurrentAnimation->setTimePosition(0.0f);
m_pCurrentAnimation->setWeight(1.0f);
m_pCurrentAnimation->setEnabled(true);
}
// Test if the animation currently played is the same than the new one
else if (m_pCurrentAnimation == pNewAnimation)
{
if (m_pPreviousAnimation)
{
m_pPreviousAnimation->setEnabled(false);
m_pPreviousAnimation = 0;
}
m_pCurrentAnimation->setTimePosition(0.0f);
m_pCurrentAnimation->setWeight(1.0f);
m_pCurrentAnimation->setEnabled(true);
}
// We must blend the new animation with the previous one
else
{
if (m_pPreviousAnimation)
{
m_pPreviousAnimation->setEnabled(false);
m_pPreviousAnimation = 0;
}
m_pPreviousAnimation = m_pCurrentAnimation;
m_pCurrentAnimation = pNewAnimation;
m_pPreviousAnimation->setWeight(1.0f);
m_pCurrentAnimation->setTimePosition(0.0f);
m_pCurrentAnimation->setWeight(0.0f);
m_pCurrentAnimation->setEnabled(true);
}
}
//-----------------------------------------------------------------------
void AnimationsMixer::stopAnimation()
{
if (m_pPreviousAnimation)
{
m_pPreviousAnimation->setEnabled(false);
m_pPreviousAnimation = 0;
}
if (m_pCurrentAnimation)
{
m_pCurrentAnimation->setEnabled(false);
m_pCurrentAnimation = 0;
}
}
//-----------------------------------------------------------------------
bool AnimationsMixer::isCurrentAnimationDone()
{
if (m_pCurrentAnimation && !m_pCurrentAnimation->isLooping())
return (m_pCurrentAnimation->getTimePosition() >= m_pCurrentAnimation->getLength());
return false;
}
//-----------------------------------------------------------------------
float AnimationsMixer::getCurrentAnimationTime()
{
if (m_pCurrentAnimation)
return m_pCurrentAnimation->getTimePosition();
return 0.0f;
}
//-----------------------------------------------------------------------
float AnimationsMixer::getCurrentAnimationLength()
{
if (m_pCurrentAnimation)
return m_pCurrentAnimation->getTimePosition();
return 0.0f;
}
//-----------------------------------------------------------------------
tAnimation AnimationsMixer::getCurrentAnimationID()
{
tAnimationsIterator iter = getAnimationsIterator();
while (iter.hasMoreElements())
{
if (iter.peekNextValue() == m_pCurrentAnimation)
return iter.peekNextKey();
iter.moveNext();
}
return 0;
}
|
module ServiceNow
class Client
Dir[File.expand_path('../client/*.rb', __FILE__)].each { |f| require f }
attr_reader :connection
class << self
def authenticate(instance_id, client_id, client_secret, username, password)
connection_options = {
url: "https://#{instance_id}.service-now.com/"
}
conn = Faraday.new(connection_options) do |faraday|
faraday.request :url_encoded
faraday.response :json
faraday.response :raise_error
faraday.adapter Faraday.default_adapter
end
params = {
grant_type: "password",
client_id: client_id,
client_secret: client_secret,
username: username,
password: password
}
response = conn.post('oauth_token.do', params)
connection = Connection.new(instance_id, response.body["access_token"])
Client.new(connection)
end
end
def initialize(connection)
@connection = connection
end
def incidents
@incidents ||= Client::Tables.new(@connection, "incident")
end
def users
@users ||= Client::Tables.new(@connection, "sys_user")
end
def tables(table_name)
Client::Tables.new(@connection, table_name)
end
def cmdb_instance(instance_class)
Client::CMDB.new(@connection, instance_class)
end
end
end |
const path = require('path');
const share = require('./share');
module.exports = share;
|
package com.example
interface HelloSayer {
fun sayHello(): String
} |
package thu.brainmatrix.visualization
import thu.brainmatrix.synapse_symbol._
import thu.brainmatrix.Shape
import scala.util.parsing.json._
import thu.brainmatrix.Symbol
import thu.brainmatrix.Visualization
object SynapseVis {
def main(args: Array[String]): Unit = {
val leis = new ExampleVis
leis.net
val ctx = Config.CTX
val xpreinput1 = new Input("input1")(ctx);
xpreinput1.initial(3)
// create an axon
val xaxon1 = new Axon(ctx,"axon1");
xaxon1.addSpikeInput(xpreinput1);
// create a dendrite
val xdendrite1 = new Dendrite(ctx,"Dendrite1");
// create an synapse
val xsynapse1 = new Synapse(ctx,"Synapse1");
xaxon1.addSynapse(xsynapse1);
xdendrite1.addSynapse(xsynapse1);
// input with higher input rates
val xpreinput2 = new Input("input2")(ctx);
xpreinput2.initial(5)
val xaxon2 = new Axon(ctx,"axon2");
xaxon2.addSpikeInput(xpreinput2);
val xdendrite2 = new Dendrite(ctx,"Dendrite2");
val xsynapse2 = new Synapse(ctx,"Synapse2");
xaxon2.addSynapse(xsynapse2);
xdendrite2.addSynapse(xsynapse2);
// create an model
val model = new Model(ctx);
//
model.addModule(xaxon1);
model.addModule(xaxon2);
model.addModule(xsynapse1);
model.addModule(xsynapse2);
model.addModule(xdendrite1);
model.addModule(xdendrite2);
val (sym, shape) = (model.update(),Shape(1, 1, 28, 28))
val dot = Visualization.plotNetwork(symbol = sym,
title = leis.net, shape = Map("data" -> shape),
nodeAttrs = Map("shape" -> "rect", "fixedsize" -> "false"))
dot.render(engine = "dot", format = "pdf", fileName = leis.net, path = leis.outDir)
}
} |
#!/bin/bash
export KONG_ADMIN_ENDPOINT="http://localhost:8001"
export KONG_PROXY_ENDPOINT="https://localhost:8443"
export API_PATH="/myapi"
export PROVISION_KEY="uKRXEw1RyKdHlZ6S7q6edY97zHZpZnro"
export DEMO_CLIENT_ID="y9FTvz0ovdczj3oxZf4NKkKUm0MMu4ii"
go run main.go |
#
# Licensed to the Apache Software Foundation (ASF) under one or more
# contributor license agreements. See the NOTICE file distributed with
# this work for additional information regarding copyright ownership.
# The ASF licenses this file to You under the Apache License, Version 2.0
# (the "License"); you may not use this file except in compliance with
# the License. You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#
from __future__ import print_function
class TaskContext(object):
"""
.. note:: Experimental
Contextual information about a task which can be read or mutated during
execution. To access the TaskContext for a running task, use:
L{TaskContext.get()}.
"""
_taskContext = None
_attemptNumber = None
_partitionId = None
_stageId = None
_taskAttemptId = None
def __new__(cls):
"""Even if users construct TaskContext instead of using get, give them the singleton."""
taskContext = cls._taskContext
if taskContext is not None:
return taskContext
cls._taskContext = taskContext = object.__new__(cls)
return taskContext
def __init__(self):
"""Construct a TaskContext, use get instead"""
pass
@classmethod
def _getOrCreate(cls):
"""Internal function to get or create global TaskContext."""
if cls._taskContext is None:
cls._taskContext = TaskContext()
return cls._taskContext
@classmethod
def get(cls):
"""
Return the currently active TaskContext. This can be called inside of
user functions to access contextual information about running tasks.
.. note:: Must be called on the worker, not the driver. Returns None if not initialized.
"""
return cls._taskContext
def stageId(self):
"""The ID of the stage that this task belong to."""
return self._stageId
def partitionId(self):
"""
The ID of the RDD partition that is computed by this task.
"""
return self._partitionId
def attemptNumber(self):
""""
How many times this task has been attempted. The first task attempt will be assigned
attemptNumber = 0, and subsequent attempts will have increasing attempt numbers.
"""
return self._attemptNumber
def taskAttemptId(self):
"""
An ID that is unique to this task attempt (within the same SparkContext, no two task
attempts will share the same attempt ID). This is roughly equivalent to Hadoop's
TaskAttemptID.
"""
return self._taskAttemptId
|
<?php
use Faker\Generator as Faker;
use App\Models\KeyResult;
$factory->define(KeyResult::class, function (Faker $faker) {
static $i = 0;
$i++;
return [
'organization_id' => $i % 2 ? 1 : 2,
'objective_id' => rand(1, 4),
'user_id' => rand(1, 10),
'title' => $faker->words(3, true),
'description' => $faker->sentence,
'type' => $i % 2 ? KeyResult::TYPES['boolean'] : KeyResult::TYPES['number'],
'criteria' => KeyResult::GTE,
'initial' => rand(1, 50),
'current' => rand(51, 90),
'target' => 100,
'done' => false,
'format' => $i % 2 ? KeyResult::FORMATS['currency'] : KeyResult::FORMATS['percentage'],
];
});
|
type Model
ws::Vector
end
function (m::Model)(s::State)
tokens = s.tokens
s0 = tokens[s.top]
s1 = isnull(s.left) ? tokens[s.left.top] : nulltoken
b0 = s.right <= length(tokens) ? tokens[s.right] : nulltoken
sl = isnull(s.lch) ? tokens[s.lch.top] : nulltoken
sr = isnull(s.rch) ? tokens[s.rch.top] : nulltoken
end
function train()
opt = SGD()
for epoch = 1:nepochs
println("epoch: $(epoch)")
opt.rate = 0.0075 / epoch
loss = fit(train_x, train_y, model, crossentropy, opt)
println("loss: $(loss)")
test_z = map(x -> predict(model,x), test_x)
acc = accuracy(test_y, test_z)
println("test acc.: $(acc)")
println("")
end
end
|
import '~/android/RtpService'
import * as Application from '@nativescript/core/application'
import { DefineProperty } from '~/utils/decorators'
Application.android.on('activityCreated', function activityCreated(args) {
android.os.StrictMode.setThreadPolicy(
new android.os.StrictMode.ThreadPolicy.Builder().permitAll().build(),
)
})
Object.assign(global, { ad: {} })
DefineProperty(global.ad, 'context', () => Application.android.context)
DefineProperty(global.ad, 'foregroundActivity', () => Application.android.foregroundActivity)
DefineProperty(global.ad, 'nativeApp', () => Application.android.nativeApp)
DefineProperty(global.ad, 'startActivity', () => Application.android.startActivity)
declare global {
export module ad {
var context: android.content.Context
var foregroundActivity: androidx.appcompat.app.AppCompatActivity
var nativeApp: android.app.Application
var startActivity: androidx.appcompat.app.AppCompatActivity
}
}
// Application.android.on('activityNewIntent', function activityNewIntent(args) {
// console.log('activityNewIntent ->', args)
// })
|
use std::sync::mpsc::{channel, Sender};
use std::thread;
use std::vec::Vec;
#[derive(Default)]
pub struct Service {
web_sockets: Vec<ws::Sender>,
}
pub type ServiceSender = Sender<Message>;
pub enum Message {
AddWS(ws::Sender),
RemoveWS(ws::Sender),
SendEvent(String),
}
impl Service {
pub fn run_thread() -> ServiceSender {
let (service_sender, rx) = channel();
let mut service = Service::default();
thread::Builder::new()
.name("frontend service".to_string())
.spawn(move || {
for message in rx {
match message {
Message::SendEvent(jsonrpc_data) => {
service.send_event(jsonrpc_data);
}
Message::AddWS(web_socket) => {
service.add_ws(web_socket);
}
Message::RemoveWS(web_socket) => {
service.remove_ws(web_socket);
}
}
}
})
.expect("Should success running client service thread");
service_sender
}
pub fn send_event(&mut self, data: String) {
for web_socket in &self.web_sockets {
if let Err(err) = web_socket.send(data.clone()) {
cwarn!("Error when sending event to frontend {}", err);
}
}
}
pub fn add_ws(&mut self, web_socket: ws::Sender) {
debug_assert_eq!(false, self.web_sockets.contains(&web_socket));
self.web_sockets.push(web_socket);
}
pub fn remove_ws(&mut self, web_socket: ws::Sender) {
debug_assert_eq!(true, self.web_sockets.contains(&web_socket));
let index = self.web_sockets.iter().position(|web_socket_iter| *web_socket_iter == web_socket);
match index {
None => cerror!("Cannot find websocket to delete, {:?}", web_socket.token()),
Some(index) => {
self.web_sockets.remove(index);
}
}
}
}
|
package org.decembrist.domain.content.classes
import org.decembrist.domain.content.IContent
import org.decembrist.domain.content.IAnnotated
import org.decembrist.domain.content.IVisibilityModified
interface IEntityContent: IAnnotated, IContent, IVisibilityModified {
val name: String
fun isAbstract(): Boolean
fun isFinal(): Boolean
fun isOpen(): Boolean
} |
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use DB;
use App\Http\Requests;
use App\Http\Controllers\Controller;
use App\Models\Products;
class CheckoutController extends Controller{
public function checkout(){
// $product = new Products(); //make an instance of the class products
return view('checkout');
}
} |
# quickly edit the bash_profile no matter wherever you are
#
alias bprofile='nano ~/.bash_profile'
# free to make an error when typing clear
# any other common mistakes will be added here
#
alias clera='clear'
alias dir='ls -al'
alias terminal='gnome-terminal'
# Make a directory and immediately change into it
# usage:
# user:~$ mkdircd folder_name
# user:~/folder_name$
function mkdircd() {
command mkdir $1 && cd $1
}
# easier way to go up N levels when deep in a path
# usage:
# user:~/a/b/c/d/e/f/g/h/i$ up 2
# user:~/a/b/c/d/e/f/g$
function up() {
LIMIT=$1
P=$PWD
for ((i=1; i <= LIMIT; i++))
do
P=$P/..
done
cd $P
export MPWD=$P
}
# did you overshoot using up? Quickly correct your mistake
# usage:
# user:~/a/b/c/d/e/f/g$ back 1
# user:~/a/b/c/d/e/f/g/h$
function back() {
LIMIT=$1
P=$MPWD
for ((i=1; i <= LIMIT; i++))
do
P=${P%/..}
done
cd $P
export MPWD=$P
}
|
// Copyright 2018-2019 @paritytech/substrate-light-ui authors & contributors
// This software may be modified and distributed under the terms
// of the Apache-2.0 license. See the LICENSE file for details.
import SUIContainer from 'semantic-ui-react/dist/commonjs/elements/Container';
import SUIInput from 'semantic-ui-react/dist/commonjs/elements/Input';
import styled from 'styled-components';
import { FONT_SIZES, MARGIN_SIZES } from './constants';
import { polkadotOfficialTheme } from './globalStyle';
import {
DynamicSizeTextProps,
FlexItemProps,
HeaderProps,
StackProps,
StyledNavButtonProps,
StyledNavLinkProps,
SubHeaderProps,
WithSpaceAroundProps,
WithSpaceBetweenProps,
WrapperDivProps,
} from './StyleProps';
// eslint-disable-next-line @typescript-eslint/no-explicit-any
export const Input = styled<any>(SUIInput)`
width: ${(props): string => props.width || '100%'};
`;
export const Container = styled(SUIContainer)`
padding: ${MARGIN_SIZES.large};
`;
/**
* Fixed-width container
*/
export const FixedWidthContainer = styled(Container)`
@media only screen and (min-width: 320px) and (max-width: 479px) {
width: 300px;
}
@media only screen and (min-width: 480px) and (max-width: 767px) {
width: 400px;
}
@media only screen and (min-width: 768px) and (max-width: 991px) {
width: 700px;
}
@media only screen and (min-width: 992px) {
width: 950px;
}
`;
export const BoldText = styled.b`
color: ${polkadotOfficialTheme.black};
`;
export const FadedText = styled.p`
color: ${polkadotOfficialTheme.black};
opacity: 0.5;
text-align: center;
`;
export const FlexItem = styled.div<FlexItemProps>`
flex: ${(props): number => props.flex || 1};
`;
export const ErrorText = styled.p`
color: red;
text-align: center;
font-weight: 500;
`;
export const SuccessText = styled.p`
color: green;
text-align: center;
font-weight: 500;
`;
export const WithSpace = styled.div`
margin: ${MARGIN_SIZES.medium} auto;
`;
export const WithSpaceAround = styled.div<WithSpaceAroundProps>`
margin: ${(props): string => MARGIN_SIZES[props.margin || 'medium']};
padding: ${(props): string => MARGIN_SIZES[props.padding || 'medium']};
`;
export const WithSpaceBetween = styled.div<WithSpaceBetweenProps>`
display: flex ${(props): string => props.flexDirection || 'row'};
justify-content: space-between;
align-items: space-between;
`;
export const WithPadding = styled.div`
padding: ${MARGIN_SIZES.medium} auto;
`;
export const Header = styled.h2<HeaderProps>`
color: ${(props): string =>
props.color
? polkadotOfficialTheme[props.color]
: polkadotOfficialTheme.grey};
font-weight: 300;
font-size: ${FONT_SIZES.big};
margin: ${(props): string =>
props.margin ? MARGIN_SIZES[props.margin] : `${MARGIN_SIZES.big} 0`};
padding: ${MARGIN_SIZES.small} ${MARGIN_SIZES.medium};
text-align: ${(props): string => props.textAlign || 'center'};
`;
export const DynamicSizeText = styled.p<DynamicSizeTextProps>`
font-size: ${(props): string => FONT_SIZES[props.fontSize || 'medium']};
font-weight: ${(props): string => props.fontWeight || 'light'};
margin: 0 0;
text-align: center;
`;
export const RefreshButton = styled.button`
border: none;
background-color: inherit;
color: ${polkadotOfficialTheme.hotPink};
:hover {
cursor: pointer;
color: ${polkadotOfficialTheme.maroon};
}
`;
export const StyledNavLink = styled.span<StyledNavLinkProps>`
background: none;
border: none;
color: ${(props): string =>
props.inverted
? polkadotOfficialTheme.white
: polkadotOfficialTheme.hotPink};
font-size: ${FONT_SIZES.medium};
font-weight: 300;
:hover {
cursor: pointer;
}
`;
export const StyledLinkButton = styled.button`
align-items: space-between;
background: none;
border: none;
color: ${(props): string => props.color || polkadotOfficialTheme.hotPink};
display: flex;
font-size: ${FONT_SIZES.medium};
font-weight: 300;
justify-content: space-between;
:hover {
cursor: pointer;
}
`;
export const StyledNavButton = styled.button<StyledNavButtonProps>`
background-image: linear-gradient(
107deg,
${(props): string =>
props.disabled
? polkadotOfficialTheme.grey
: props.negative
? polkadotOfficialTheme.grey
: polkadotOfficialTheme.white},
${(props): string =>
props.disabled
? polkadotOfficialTheme.grey
: props.negative
? polkadotOfficialTheme.maroon
: polkadotOfficialTheme.hotPink}
);
border: none;
border-radius: 15px;
box-shadow: 0 4px 6px 0 rgba(${polkadotOfficialTheme.black}, 0.3);
color: ${polkadotOfficialTheme.white};
fontsize: ${FONT_SIZES.large};
height: 42px;
width: 134px;
:hover {
cursor: ${(props): string => (props.disabled ? 'not-allowed' : 'pointer')};
}
`;
export const VoteNayButton = styled.button`
background-image: linear-gradient(
107deg,
${polkadotOfficialTheme.lightBlue1},
${polkadotOfficialTheme.neonBlue}
);
border: none;
border-radius: 8px;
box-shadow: 0 4px 6px 0 rgba(${polkadotOfficialTheme.black}, 0.3);
color: ${polkadotOfficialTheme.white};
fontsize: ${FONT_SIZES.large};
height: 21px;
width: 51px;
:hover {
cursor: pointer;
}
`;
export const VoteYayButton = styled.button`
background-image: linear-gradient(
107deg,
${polkadotOfficialTheme.lightBlue1},
${polkadotOfficialTheme.lightBlue2}
);
border: none;
border-radius: 8px;
box-shadow: 0 4px 6px 0 rgba(${polkadotOfficialTheme.black}, 0.3);
color: ${polkadotOfficialTheme.white};
fontsize: ${FONT_SIZES.large};
height: 21px;
width: 51px;
:hover {
cursor: pointer;
}
`;
export const Stacked = styled.div<StackProps>`
align-items: ${(props): string => props.alignItems || 'center'};
display: flex;
flex: 1;
flex-direction: column;
justify-content: ${(props): string => props.justifyContent || 'center'};
text-align: ${(props): string => props.textAlign || 'center'};
`;
export const StackedHorizontal = styled.div<StackProps>`
align-items: ${(props): string => props.alignItems || 'center'};
display: flex;
flex: 1;
flex-direction: row;
flex-grow: 1;
flex-wrap: wrap;
margin: ${(props): string | number => props.margin || 0}
justify-content: ${(props): string => props.justifyContent || 'center'};
text-align: ${(props): string => props.textAlign || 'center'};
`;
export const SubHeader = styled.h3<SubHeaderProps>`
color: ${polkadotOfficialTheme.black};
font-weight: 200;
font-size: ${FONT_SIZES.medium};
margin: ${(props): string =>
props.noMargin ? '0 0' : '1rem auto 0.3rem auto'};
text-align: ${(props): string => props.textAlign || 'left'};
`;
export const InlineSubHeader = styled(SubHeader)`
display: inline;
`;
export const WrapperDiv = styled.div<WrapperDivProps>`
margin: ${(props): string => props.margin || '1rem'};
padding: ${(props): string => props.padding || '1rem'};
width: ${(props): string => props.width || '30rem'};
height: ${(props): string => props.height || '100%'};
`;
|
module Operations
def sparsity
assert valid?
@storage.sparsity
end
def trace
#pre
assert valid?
assert @storage.rows == @storage.columns
result = @storage.trace
assert valid?
result
end
def rank
assert valid?
return self.to_matrix.rank
end
def row_sum(rowNum)
assert valid?
assert rowNum.is_a? Integer
assert rowNum >= 0
assert rowNum < @storage.rows
result = @storage.row_sum(rowNum)
assert valid?
result
end
def col_sum(colNum)
assert valid?
assert colNum.is_a? Integer
assert colNum >= 0
assert colNum < @storage.columns
result = @storage.col_sum(colNum)
assert valid?
result
end
def total_sum
assert valid?
result = @storage.total_sum
assert valid?
result
end
def transpose
assert valid?
#pre
#matrix to be transposed has been initialized
result = SMatrix.new(@storage.transpose, self.ftype)
#post
assert valid?
assert result.shape[0] == @storage.shape[1]
assert result.shape[1] == @storage.shape[0]
result
end
# TODO:
# NMatrix only implements this for dense matrices
# so we must convert types then calculate then convert back.
# Inefficient but necessary for now.
def inverse
assert valid?
#pre
assert self.determinant != 0
assert square?
assert regular?, "Not a regular matrix (thus not invertible)."
#post
assert square?
assert valid?
SMatrix.new(self.to_matrix.inverse, self.ftype)
end
def determinant
assert valid?
#pre
assert square? #square
#post
return self.to_matrix.determinant
end
# in a SMatrix.
def cholesky
raise NotImplementedError
end
def eigensystem
assert valid?
v, d, v_inv = self.to_matrix.eigensystem
v = SMatrix.new(v, self.ftype)
d = SMatrix.new(d, self.ftype)
v_inv = SMatrix.new(v_inv, self.ftype)
assert d.diagonal?
assert ~v == v_inv
return [v, d, v_inv]
end
def lu_factorization
# matrices in SMatrix so conversions MUST be done.
#pre
assert valid?
assert square?
l, u, perm = self.to_matrix.lup
l = SMatrix.new(l, self.ftype)
u = SMatrix.new(u, self.ftype)
perm = SMatrix.new(perm, self.ftype)
assert l.lower_triangular?
assert u.upper_triangular?
assert perm.permutation?
return [l, u, perm]
end
def conjugate
# Pre-conditions
yFactory = YaleFactory.new
assert valid?
# Post
# We need to wrap returned matrix as an SMatrix
return yFactory.create(@storage.complex_conjugate)
end
def partition(rows = [0, self.rows], columns = [0, self.columns])
# returns a partion of the current matrix
assert valid?
assert (rows.is_a? Array) and (columns.is_a? Array)
assert (rows[0] < rows[1]) and (columns[0] < columns[1])
assert (rows[1] <= self.rows) and (columns[1] <= self.columns)
row_count = rows[1] - rows[0]
column_count = columns[1] - columns[0]
matrix = Matrix.zero(row_count, column_count)
part = SMatrix.new(matrix)
part.each_index do |i, j|
part[i, j] = self[i + rows[0], j + columns[0]]
end
part
end
def ~
assert valid?
return self.inverse
end
def t
assert valid?
return self.transpose
end
end
|
class Curso {
String addressedTo;
DateTime created;
String creator;
DateTime date;
String description;
List<DownloadableContent> downloadableContent;
Duration duration;
DateTime edited;
String img;
List<Instructor> instructors;
String module;
String name;
String place;
Postulation postulation;
String schedule;
String id;
Curso.fromJSON({Map<String, dynamic> data, String id}) {
this.id = id;
this.addressedTo = data['addressedTo'];
this.created = data['created'];
this.creator = data['creator'];
this.date = data['date'];
this.description = data['description'];
this.edited = data['edited'];
this.img = data['img'];
this.module = data['module'];
this.name = data['name'];
this.place = data['place'];
this.schedule = data['schedule'];
this.duration = Duration.fromJSON(data: data['duration']);
this.downloadableContent = (data['downloadableContent'] as List<dynamic>)
.map((item) => DownloadableContent.fromJSON(data: item))
.toList();
this.instructors = (data['instructors'] as List<dynamic>)
.map((item) => Instructor.fromJSON(data: item))
.toList();
this.postulation = Postulation.fromJSON(data: data['postulation']);
}
}
class DownloadableContent {
String url;
DownloadableContent.fromJSON({
Map<dynamic, dynamic> data,
}) {
this.url = data['url'];
}
}
class Duration {
int days;
int hours;
int weeks;
Duration.fromJSON({
Map<dynamic, dynamic> data,
}) {
this.days = data['days'];
this.hours = data['hours'];
this.weeks = data['weeks'];
}
}
class Instructor {
String about;
String name;
Instructor.fromJSON({
Map<dynamic, dynamic> data,
}) {
this.about = data['about'];
this.name = data['name'];
}
}
class Postulation {
DateTime date;
String link;
String message;
Postulation.fromJSON({
Map<dynamic, dynamic> data,
}) {
this.date = data['date'];
this.link = data['link'];
this.message = data['message'];
}
}
|
import matplotlib.pyplot as plt
import pandas as pd
def read_data():
'''
:return: the climate data with some pre-processing
'''
with open('./climate.data', 'r') as f:
data = pd.read_csv(f)
return data.rename(columns={name: name[1:] for name in data.columns})
def plot_part_line():
data = read_data()
date = ['{}/{}'.format(year, month) for year, month in zip(data['Year'], data['Mo'])]
data.insert(2, "Date", date)
data = data.loc[200:300, ]
plt.figure(figsize=(12, 10))
data = data.drop(columns=['Year', 'Mo'])
x = data.loc[::2, 'Date']
plt.plot(x, data.loc[::2, 'Globe'], '-k', label='Globe')
plt.plot(x, data.loc[::2, 'Land'], ':g', label='Land')
plt.plot(x, data.loc[::2, 'Ocean'], ':b', label='Ocean')
plt.xticks(x[::5], rotation='vertical')
plt.xlabel('Date')
plt.ylabel('Average degree')
plt.legend()
plt.title('Average degree change from {} to {}'.format(x.iloc[0], x.iloc[-1]))
plt.savefig('./question_a.png', dpi=1000)
def plot_year():
data = read_data()
data = data.iloc[1:-1, :]
plt.figure(figsize=(12, 10))
x = data.loc[::4, 'Year']
plt.plot(x, data.loc[::4, 'Globe'], '-k', label='Globe')
plt.plot(x, data.loc[::4, 'Land'], ':g', label='Land')
plt.plot(x, data.loc[::4, 'Ocean'], ':b', label='Ocean')
plt.xticks(x[::12], rotation='vertical')
plt.xlabel('Date')
plt.ylabel('Average degree')
plt.legend()
plt.title('Average degree change from {} to {}'.format(x.iloc[0], x.iloc[-1]))
plt.savefig('question_b.png', dpi=1000)
plot_year()
|
package org.firezenk.goldenbleetle.features.common
class Store {
private val states: MutableList<State> = mutableListOf()
val frozenStates: List<State>
get() = states.toList()
internal fun add(function: () -> State) {
states.add(function())
}
internal fun clear() = states.clear()
inline fun <reified S> provideLast(): S? = frozenStates.findLast { it is S } as S?
} |
#!/usr/bin/env bash
mkdir -p /var/www/data/stats-data
ln -s /var/www/data/stats-data stats-data |
import * as React from 'react';
import defaultOptions from '../../defaultOptions';
import { SortingMode } from '../../enums';
import { Column } from '../../Models/Column';
import { DispatchFunc } from '../../types';
import HeadCellContent from '../HeadCellContent/HeadCellContent';
export interface IHeadCellProps {
areAllRowsSelected: boolean;
column: Column;
dispatch: DispatchFunc;
sortingMode: SortingMode;
}
const HeadCell: React.FunctionComponent<IHeadCellProps> = (props) => {
const {
column: { style },
} = props;
return (
<th scope='col' style={style} className={defaultOptions.css.theadCell}>
<HeadCellContent {...props}/>
</th>
);
};
export default HeadCell;
|
/*
* =====================================================================================
*
* Filename: aer_backend.hpp
*
* Description:
*
* Version: 1.0
* Created: 09/28/2021 04:38:06 PM
* Revision: none
* Compiler: gcc
*
* Author: YOUR NAME (),
* Organization:
*
* =====================================================================================
*/
#ifndef AER_BACKEND_HPP
#define AER_BACKEND_HPP
#if defined( AER_THRUST_CUDA ) || defined( AER_THRUST_ROCm)
#define AER_THRUST_GPU
#endif
#ifdef AER_THRUST_CUDA
#include <cuda.h>
#include <cuda_runtime.h>
#endif
#ifdef AER_THRUST_ROCm
// Types
#define cudaStream_t hipStream_t
#define cudaSuccess hipSuccess
#define cudaStreamNonBlocking hipStreamNonBlocking
// Functions
#define cudaMemGetInfo hipMemGetInfo
#define cudaSetDevice hipSetDevice
#define cudaStreamSynchronize hipStreamSynchronize
#define cudaGetDeviceCount hipGetDeviceCount
#define cudaDeviceCanAccessPeer hipDeviceCanAccessPeer
#define cudaDeviceEnablePeerAccess hipDeviceEnablePeerAccess
#define cudaGetLastError hipGetLastError
#define cudaStreamCreateWithFlags hipStreamCreateWithFlags
#define cudaMemcpyAsync hipMemcpyAsync
#define cudaMemcpyHostToDevice hipMemcpyHostToDevice
#define cudaMemcpyDeviceToHost hipMemcpyDeviceToHost
#endif // AER_THRUST_ROCm
#endif // AER_BACKEND_HPP
|
class V8::Object
def to_hash
to_hash0(self)
end
def to_hash0(obj)
case obj
when V8::Array
obj.map {|v| to_hash0(v) }
when V8::Object
h = {}
obj.each do |k, v|
h[to_hash0(k)] = to_hash0(v)
end
h
else
obj
end
end
end
|
package com.example.calculator.presentation.settings
import androidx.lifecycle.LiveData
import androidx.lifecycle.MutableLiveData
import androidx.lifecycle.ViewModel
import androidx.lifecycle.viewModelScope
import com.example.calculator.domain.SettingsDao
import com.example.calculator.domain.entity.ForceVibrationTypeEnum
import com.example.calculator.domain.entity.FormatResultEnum
import com.example.calculator.domain.entity.ResultPanelType
import kotlinx.coroutines.launch
class SettingsViewModel(
private val settingsDao: SettingsDao
) : ViewModel() {
private val _resultPanelState = MutableLiveData<ResultPanelType>(ResultPanelType.LEFT)
val resultPanelState: LiveData<ResultPanelType> = _resultPanelState
private val _openResultPanelAction = SingleLiveEvent<ResultPanelType>()
val openResultPanelAction: LiveData<ResultPanelType> = _openResultPanelAction
private val _formatResultState = MutableLiveData<FormatResultEnum>(FormatResultEnum.MANY)
val formatResultState: LiveData<FormatResultEnum> = _formatResultState
private val _openFormatResultAction = SingleLiveEvent<FormatResultEnum>()
val openFormatResultAction: LiveData<FormatResultEnum> = _openFormatResultAction
private val _forceVibrationState = MutableLiveData<ForceVibrationTypeEnum>(
ForceVibrationTypeEnum.SMALL
)
val forceVibrationState: LiveData<ForceVibrationTypeEnum> = _forceVibrationState
private val _openForceVibrationAction = SingleLiveEvent<ForceVibrationTypeEnum>()
val openForceVibrationAction: LiveData<ForceVibrationTypeEnum> = _openForceVibrationAction
init {
viewModelScope.launch {
_resultPanelState.value = settingsDao.getResultPanelType()
_formatResultState.value = settingsDao.getFormatResultType()
_forceVibrationState.value = settingsDao.getForceVibrationType()
}
}
fun onFormatResultChanged(formatResultType: FormatResultEnum) {
_formatResultState.value = formatResultType
viewModelScope.launch {
settingsDao.setFormatResultType(formatResultType)
}
}
fun onFormatResultPanelTypeClicked() {
_openFormatResultAction.value = _formatResultState.value
}
fun onResultPanelTypeChanged(resultPanelType: ResultPanelType) {
_resultPanelState.value = resultPanelType
viewModelScope.launch {
settingsDao.setResultPanelType(resultPanelType)
}
}
fun onResultPanelTypeClicked() {
_openResultPanelAction.value = _resultPanelState.value
}
fun onForceVibrationChanged(forceVibrationType: ForceVibrationTypeEnum) {
_forceVibrationState.value = forceVibrationType
viewModelScope.launch {
settingsDao.setForceVibrationType(forceVibrationType)
}
}
fun onForceVibrationPanelTypeClicked() {
_openForceVibrationAction.value = _forceVibrationState.value
}
}
|
package cn.edu.cug.cs.gtl.geom;
import cn.edu.cug.cs.gtl.io.Serializable;
import java.io.DataInput;
import java.io.DataOutput;
import java.io.IOException;
/**
* Created by hadoop on 17-3-27.
*/
public class Track implements Serializable, Comparable<Track> {
private static final long serialVersionUID = 1L;
Vector origin;
Vector velocity;
public Track() {
}
public Track(Vector origin, Vector velocity) {
this.origin = (Vector) origin.clone();
this.velocity = (Vector) velocity.clone();
}
public Vector getOrigin() {
return origin;
}
public void setOrigin(Vector origin) {
this.origin = (Vector) origin.clone();
}
public Vector getVelocity() {
return velocity;
}
public void setVelocity(Vector velocity) {
this.velocity = (Vector) velocity.clone();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Track track = (Track) o;
if (!origin.equals(track.origin)) return false;
return velocity.equals(track.velocity);
}
@Override
public int hashCode() {
int result = origin.hashCode();
result = 31 * result + velocity.hashCode();
return result;
}
@Override
public String toString() {
return "Track{" +
"origin=" + origin +
", velocity=" + velocity +
'}';
}
@Override
public Object clone() {
return null;
}
@Override
public void copyFrom(Object i) {
}
@Override
public boolean load(DataInput in) throws IOException {
return false;
}
@Override
public boolean store(DataOutput out) throws IOException {
return false;
}
@Override
public long getByteArraySize() {
return 0;
}
@Override
public int compareTo(Track o) {
return 0;
}
}
|
const fs = require('fs')
const data = require('./query_results.json')
const metrics = data.other_metrics;
handleErr = err => {
if(err){
console.log('error while writing:' , err);
}
}
var headers = "timestamp"
var dict = {}
metrics.forEach(element => {
headers = `${headers},${element.metric_name}` ;
element.data_points.forEach(dp => {
dict[dp[0]] = dict[dp[0]] == undefined ? `${dp[1]}` : `${dict[dp[0]]},${dp[1]}`;
});
});
fs.appendFile('parsed.csv', `${headers}\n`, handleErr);
Object.keys(dict).forEach( key => {
fs.appendFile('parsed.csv', `${key},${dict[key]}\n`, handleErr)
})
|
const nuke = require("./../../build/system/fs/nuke");
const perf = require("./index").perf;
module.exports = function (dir) {
return perf(function () {
nuke.nukeSync(dir);
});
};
|
#ifndef __clang__
unsigned short __builtin_subcs(unsigned short, unsigned short, unsigned short, unsigned short *);
#endif
int main(int argc, const char **argv) {
unsigned short carryout, res;
res = __builtin_subcs((unsigned short)0x0, (unsigned short)0x0, 0, &carryout);
if (res != 0x0 || carryout != 0) {
return -1;
}
res = __builtin_subcs((unsigned short)0xFFFF, (unsigned short)0x0, 0, &carryout);
if (res != 0xFFFF || carryout != 0) {
return -1;
}
res = __builtin_subcs((unsigned short)0x0, (unsigned short)0xFFFF, 0, &carryout);
if (res != 0x01 || carryout != 1) {
return -1;
}
res = __builtin_subcs((unsigned short)0xFFFF, (unsigned short)0x1, 0, &carryout);
if (res != 0xFFFE || carryout != 0) {
return -1;
}
res = __builtin_subcs((unsigned short)0x1, (unsigned short)0xFFFF, 0, &carryout);
if (res != 0x2 || carryout != 1) {
return -1;
}
res = __builtin_subcs((unsigned short)0xFFFF, (unsigned short)0xFFFF, 0, &carryout);
if (res != 0x0 || carryout != 0) {
return -1;
}
res = __builtin_subcs((unsigned short)0x8FFF, (unsigned short)0x0FFF, 0, &carryout);
if (res != 0x8000 || carryout != 0) {
return res;
}
res = __builtin_subcs((unsigned short)0x0, (unsigned short)0xFFFE, 1, &carryout);
if (res != 0x1 || carryout != 1) {
return -1;
}
res = __builtin_subcs((unsigned short)0x0, (unsigned short)0xFFFF, 1, &carryout);
if (res != 0x0 || carryout != 1) {
return -1;
}
res = __builtin_subcs((unsigned short)0xFFFE, (unsigned short)0x0, 1, &carryout);
if (res != 0xFFFD || carryout != 0) {
return -1;
}
res = __builtin_subcs((unsigned short)0xFFFE, (unsigned short)0xFFFE, 1, &carryout);
if (res != 0xFFFF || carryout != 1) {
return res;
}
res = __builtin_subcs((unsigned short)0xFFFE, (unsigned short)0xFFFF, 0, &carryout);
if (res != 0xFFFF || carryout != 1) {
return res;
}
res = __builtin_subcs((unsigned short)0xFFFE, (unsigned short)0xFFFF, 1, &carryout);
if (res != 0xFFFE || carryout != 1) {
return res;
}
res = __builtin_subcs((unsigned short)0xFFFF, (unsigned short)0x0, 1, &carryout);
if (res != 0xFFFE || carryout != 0) {
return -1;
}
res = __builtin_subcs((unsigned short)0xFFFF, (unsigned short)0xFFFF, 1, &carryout);
if (res != 0xFFFF || carryout != 1) {
return -1;
}
res = __builtin_subcs((unsigned short)0x0F, (unsigned short)0x1, 0, &carryout);
if (res != 0x0E || carryout != 0) {
return -1;
}
res = __builtin_subcs((unsigned short)0x0F, (unsigned short)0x1, 1, &carryout);
if (res != 0x0D || carryout != 0) {
return -1;
}
return 0;
}
|
module DeployGate
module Config
class Credential < Base
class << self
# @return [String]
def file_path
File.join(ENV["HOME"], '.dg/credentials')
end
end
end
end
end
|
# frozen_string_literal: true
RSpec.describe ActiveCampaignWrapper::Core::EmailActivityGateway, :vcr do
let(:email_activity_gateway) do
described_class.new(ActiveCampaignWrapper::Client.new)
end
describe '#all' do
subject(:response) { email_activity_gateway.all }
it 'returns email activities hash' do
expect(response).to include_json(email_activities: [])
end
end
end
|
#!/data/data/com.termux/files/usr/bin/bash -e
## Setup Colors
reset='\033[0m'
red='\033[1;31m'
blue='\033[1;34m'
yellow='\033[1;33m'
FS="$HOME/.mytermux/linux/kali/linux-fs"
## if file .setup-linux.sh exists, run with ash shell
setup="./.setup-linux.sh"
echo "[ -s $setup ] && $setup" > $FS/root/.profile
## change to main repo
repo="http://http.kali.org/kali kali-rolling main non-free contrib"
echo "deb $repo" > $FS/etc/apt/sources.list
echo "deb-src $repo" >> $FS/etc/apt/sources.list
## create `.setup-linux.sh` for initial setup
github="https://raw.githubusercontent.com"
ohMyZsh="$github/robbyrussell/oh-my-zsh/master/tools/install.sh"
linuxFS="$FS/root/.setup-linux.sh"
echo "apt-get -y update" > $linuxFS
echo "apt-get -y upgrade" >> $linuxFS
echo "apt-get -y install vim git zsh curl" >> $linuxFS
echo "$(curl -fsSL $ohMyZsh)" >> $linuxFS
sed -i 's/ +chsh -s.*//' $linuxFS
echo "rm ~/.setup-linux.sh" >> $linuxFS
echo "exit" >> $linuxFS
chmod +x $linuxFS
## Start Linux
#printf "$yellow [*] Start Linux$reset\n"
#sed -i 's/zsh --/bash --/g' ./start.sh
#./start.sh
#sed -i 's/bash --/zsh --/g' ./start.sh
#exit
|
<?php
namespace app\models;
use yii\base\Model;
class Users extends model {
function Get_userAll() {
$sql = "SELECT * FROM masuser";
$result = \Yii::$app->db->createCommand($sql)->queryAll();
return $result;
}
}
|
;;; Purpose: Read a file of kif into a hashtable indexed by the line number at which
;;; each toplevel form appears.
(in-package :kif)
(defparameter *kif-readtable* (copy-readtable nil))
(defvar *kif-std-readtable* (copy-readtable nil))
(defvar *kif-line* 1 "Line at which we are reading")
(defparameter *kif-forms-ht* (make-hash-table)) ; set to the ht in a kif-forms object, usually.
(defun set-variable-property (x)
(cond ((atom x)
(cl:and (symbolp x)
(cl:or (when (char= #\? (char (symbol-name x) 0))
(setf (get x 'kvar) t))
(when (char= #\@ (char (symbol-name x) 0))
(setf (get x 'row-variable) t)))))
((consp x)
(set-variable-property (car x))
(set-variable-property (cdr x))))
x)
(let ((paren-cnt 0))
(defun clear-paren-cnt () (setf paren-cnt 0))
(defun kif-lparen (stream char)
(incf paren-cnt)
(let ((save-line *kif-line*)
(result (funcall (get-macro-character #\( *kif-std-readtable*) stream char)))
;; everything else in this file just counts lines.
(when (= paren-cnt 1) (setf (gethash save-line *kif-forms-ht*) result))
(decf paren-cnt)
result))
)
(defun kif-newline (stream char)
(declare (ignore stream char))
(incf *kif-line*)
(values))
(defun kif-comment (stream char)
(funcall (get-macro-character #\; *kif-std-readtable*) stream char)
(incf *kif-line*)
(values))
(defun kif-string (stream char)
(let ((str (funcall (get-macro-character #\" *kif-std-readtable*) stream char)))
(incf *kif-line* (count #\Newline str))
str))
(defun kif-word-token (stream char)
(unread-char char stream)
(loop with string = (make-array 100 :element-type 'character :adjustable t)
and strlen = 100 and stridx = 0
for ch = (read-char stream t nil t)
while (cl:or (digit-char-p ch)
(char<= #\a ch #\z)
(char<= #\A ch #\Z)
(char= ch #\?)
(char= ch #\@)
(char= ch #\-)
(char= ch #\_)
(char= ch #\+))
finally (progn (adjust-array string stridx)
(unread-char ch stream)
(cl:return (intern string)))
do (setf (aref string stridx) ch)
(incf stridx)
(when (= stridx strlen)
(incf strlen 40)
(setf string (adjust-array string strlen :element-type 'character)))))
(defun kif-ignore (stream char)
(declare (ignore stream char))
(values))
(let ((*readtable* *kif-readtable*))
(set-macro-character #\( #'kif-lparen)
(set-macro-character #\Newline #'kif-newline)
(set-macro-character #\; #'kif-comment)
(set-macro-character #\" #'kif-string)
(loop for ch in '(#\a #\b #\c #\d #\e #\f #\g #\h #\i #\j #\k #\l #\m
#\n #\o #\p #\q #\r #\s #\t #\u #\v #\w #\x #\y #\z
#\A #\B #\C #\D #\E #\F #\G #\H #\I #\J #\K #\L #\M
#\N #\O #\P #\Q #\R #\S #\T #\U #\V #\W #\X #\Y #\Z
#\? #\@ #\-)
do (set-macro-character ch #'kif-word-token))
(loop for code from 160 to 255
do (set-macro-character (code-char code) #'kif-ignore)))
(defun kif-read (&rest args)
(let ((*readtable* *kif-readtable*)
(*package* (find-package :ku)))
(set-variable-property (apply #'read args))))
(defmethod kif-readfile (filename (kif-forms kif-forms) &key continue)
(with-slots (forms-ht files-read lines-read) kif-forms
(unless continue
(setf lines-read 1) ; actually current line ???
(clrhash forms-ht))
(setf *kif-forms-ht* forms-ht)
(setf *kif-line* lines-read)
(pushnew filename files-read :test #'string=)
(clear-paren-cnt)
(with-open-file (stream filename :direction :input)
(loop for form = (kif-read stream nil :eof)
when (eql form :eof) return *kif-line*))
(setf lines-read *kif-line*)))
(defun kif-read-from-string (str)
(setf str (strcat str " ")) ; POD get around a bug...
(clear-paren-cnt)
(with-input-from-string (stream str)
(kif-read stream nil :eof)))
(defun kif-n2form (n &key ht)
(gethash n ht))
(defun kif-form2n (form &key ht)
(loop for f being the hash-value of ht using (hash-key key)
when (eql f form) return key))
(defun kif-export-safe-symbols ()
" 'Safe' means it has lowercase and it isn't a variable."
(let ((package (find-package :ku)))
(do-symbols (s package)
(when (cl:and (cl:not (kvar-p s))
(some #'lower-case-p (symbol-name s)))
; (format t " |~A|" (symbol-name s))
(export s package)))))
|
package tfimportables
import (
"testing"
"github.com/onelogin/onelogin/clients"
"github.com/stretchr/testify/assert"
)
func TestGetImportable(t *testing.T) {
clientList := &clients.Clients{
ClientConfigs: clients.ClientConfigs{
OneLoginClientID: "test",
OneLoginClientSecret: "test",
OneLoginURL: "test.com",
OktaOrgName: "test",
OktaBaseURL: "test.com",
OktaAPIToken: "test",
},
}
importableNames := [7]string{
"onelogin_apps",
"onelogin_users",
"onelogin_apps",
"onelogin_user_mappings",
"onelogin_roles",
"okta_apps",
"aws_iam_user",
}
tests := map[string]struct {
Importables *ImportableList
}{
"It creates and returns importables": {
Importables: New(clientList),
},
}
for name, test := range tests {
t.Run(name, func(t *testing.T) {
for _, name := range importableNames {
importable := test.Importables.GetImportable(name)
memoizedImportable := test.Importables.GetImportable(name)
assert.Equal(t, test.Importables.importables[name], importable)
assert.Equal(t, test.Importables.importables[name], memoizedImportable)
}
})
}
}
|
using RobotDynamics
using ForwardDiff
using LinearAlgebra
using RobotDynamics: dynamics, discrete_dynamics
function check_jacobians(model, z)
t,dt, = z.t, z.dt
xn = zeros(RD.state_dim(model))
J0 = ForwardDiff.jacobian(z->RD.discrete_dynamics(model, z[1:4], z[5:5], t, dt), z.z)
J = similar(J0)
for sig in (RD.StaticReturn(), RD.InPlace()), diff in (RD.ForwardAD(), RD.FiniteDifference(), RD.UserDefined())
atol = diff == RD.FiniteDifference() ? 1e-6 : 1e-10
RD.jacobian!(sig, diff, model, J, xn, z)
@test J ≈ J0 atol=atol
end
end
cmodel = Cartpole()
x,u = rand(cmodel)
n,m = RD.dims(cmodel)
dt = 0.01
t = 0.0
z = RD.KnotPoint(x,u,t,dt)
# Check the Cartpole analytical Jacobian
J = zeros(n, n+m)
J0 = zero(J)
xdot = zeros(n)
RD.jacobian!(RD.StaticReturn(), RD.ForwardAD(), cmodel, J, xdot, z)
RD.jacobian!(RD.StaticReturn(), RD.UserDefined(), cmodel, J0, xdot, z)
@test J0 ≈ J
# Test Euler
model = RD.DiscretizedDynamics{RD.Euler}(cmodel)
xdot = RD.dynamics(cmodel, z)
@test discrete_dynamics(model, z) ≈ x + xdot * dt
check_jacobians(model, z)
# # Test RK2
# _k1 = dynamics(cmodel, x, u)*dt
# _k2 = dynamics(cmodel, x + _k1/2, u)*dt
# @test discrete_dynamics(model, z) ≈ x + _k2
# Test RK4
model = RD.DiscretizedDynamics{RD.RK4}(cmodel)
_k1 = dynamics(cmodel, x, u)*dt
_k2 = dynamics(cmodel, x + _k1/2, u)*dt
_k3 = dynamics(cmodel, x + _k2/2, u)*dt
_k4 = dynamics(cmodel, x + _k3, u)*dt
@test discrete_dynamics(model, z) ≈ x + (_k1 + 2*_k2 + 2*_k3 + _k4)/6
xn = zeros(n)
RD.discrete_dynamics!(model, xn, z)
@test xn ≈ x + (_k1 + 2*_k2 + 2*_k3 + _k4)/6
RD.discrete_dynamics!(model, xn, x, u, t, dt)
@test xn ≈ x + (_k1 + 2*_k2 + 2*_k3 + _k4)/6
check_jacobians(model, z)
J0 = ForwardDiff.jacobian(z->RD.discrete_dynamics(model, z[1:4], z[5:5], t, dt), z.z)
J = similar(J0)
RD.jacobian!(RD.InPlace(), RD.ForwardAD(), model, J, xn, z)
@test J ≈ J0
RD.jacobian!(RD.StaticReturn(), RD.UserDefined(), model, J, xn, z)
@test J ≈ J0
RD.jacobian!(RD.StaticReturn(), RD.UserDefined(), model, J, xn, z)
RD.jacobian!(RD.InPlace(), RD.UserDefined(), model, J, xn, z)
@test J ≈ J0
int1 = RD.RK4(cmodel)
int2 = copy(int1)
@test int1.k1 !== int2.k1
@test int1.k2 !== int2.k2
@test int1.A !== int2.A
# Test RK3 and jacobian
model = RD.DiscretizedDynamics{RD.RK3}(cmodel)
k1(x) = dynamics(cmodel, x, u, t )*dt;
k2(x) = dynamics(cmodel, x + k1(x)/2, u, t + dt/2)*dt;
k3(x) = dynamics(cmodel, x - k1(x) + 2*k2(x), u, t + dt )*dt;
xnext(x) = x + (k1(x) + 4*k2(x) + k3(x))/6
k1_(u) = dynamics(cmodel, x, u, t )*dt;
k2_(u) = dynamics(cmodel, x + k1_(u)/2, u, t + dt/2)*dt;
k3_(u) = dynamics(cmodel, x - k1_(u) + 2*k2_(u), u, t + dt )*dt;
xnext_(u) = x + (k1_(u) + 4*k2_(u) + k3_(u))/6
# Make sure the test functions match
@test k1(x) == k1_(u)
@test k2(x) == k2_(u)
@test k3(x) == k3_(u)
@test xnext(x) == xnext_(u)
# Check actual implementation
@test xnext(x) ≈ RD.discrete_dynamics(model, z)
@test xnext_(u) ≈ RD.discrete_dynamics(model, z)
@test xnext(x) ≈ RD.discrete_dynamics(model, x, u, t, dt)
# Check Jacobian
A = ForwardDiff.jacobian(xnext, x)
B = ForwardDiff.jacobian(xnext_, u)
J0 = [A B]
J = zeros(n,n+m)
RD.jacobian!(RD.StaticReturn(), RD.UserDefined(), model, J, xn, z)
@test J ≈ J0
RD.jacobian!(RD.InPlace(), RD.UserDefined(), model, J, xn, z)
@test J ≈ J0
check_jacobians(model, z) |
package util
import (
"os"
"go.uber.org/zap"
"go.uber.org/zap/zapcore"
)
func NewLogger(json bool) *zap.Logger {
econf := zapcore.EncoderConfig{
MessageKey: "msg",
LevelKey: "level",
NameKey: "logger",
EncodeLevel: zapcore.LowercaseLevelEncoder,
EncodeTime: zapcore.ISO8601TimeEncoder,
EncodeDuration: zapcore.StringDurationEncoder,
}
var core zapcore.Core
if json {
core = zapcore.NewCore(zapcore.NewJSONEncoder(econf), os.Stdout, zap.DebugLevel)
} else {
econf.EncodeLevel = zapcore.CapitalColorLevelEncoder
core = zapcore.NewCore(zapcore.NewConsoleEncoder(econf), os.Stdout, zap.DebugLevel)
}
return zap.New(core)
}
|
package chapter16
import (
"testing"
"github.com/stretchr/testify/assert"
)
func TestMaxNumWithoutComparison(t *testing.T) {
testData := []struct {
name string
a, b, max int
}{
{"comparing possitive numbers", 1, 2, 2},
{"comparing zero values", 0, 0, 0},
{"comparing negative numbers", -123, -1212, -123},
}
for _, data := range testData {
t.Run(data.name, func(t *testing.T) {
assert.Equal(t, data.max, MaxNumWithoutComparison(data.a, data.b))
})
}
}
|
# Copyright (c) 2019 Herbert Shen <[email protected]> All Rights Reserved.
# Released under the terms of the MIT License.
bacon_export prompt
declare -ga BACON_PROMPT_PS1_LAYOUT=()
declare -ga BACON_PROMPT_COUNTERS=()
declare -gA BACON_PROMPT_COLOR=()
declare -gA BACON_PROMPT_CHARS=()
bacon_promptc() {
local color
while bacon_has_map BACON_COLOR "$1"; do
color+="${BACON_COLOR[$1]}"; shift
done
color="${color:-${BACON_COLOR[default]}}"
local IFS=' '
printf '\[%s\]%s\[\033[00m\]\n' "$color" "$*"
}
bacon_prompt_last_status() {
local color="${BACON_PROMPT_COLOR[last_fail]:-red}"
[[ $LAST_STATUS -eq 0 ]] && color="${BACON_PROMPT_COLOR[last_ok]:-green}"
bacon_promptc "$color" "${BACON_PROMPT_CHARS[last_status]:-&}"
}
bacon_prompt_time() {
bacon_promptc "${BACON_PROMPT_COLOR[time]:-green}" "${BACON_PROMPT_CHARS[time]:-[\A]}"
}
bacon_prompt_location() {
bacon_promptc "${BACON_PROMPT_COLOR[location]:-blue}" "${BACON_PROMPT_CHARS[location]:-[\u@\h:\W]}"
}
bacon_prompt_counter() {
local -A counters
local str='' cnt
for cnt in "${BACON_PROMPT_COUNTERS[@]}"; do
# shellcheck disable=SC2155
local nr="$(eval "$cnt")"
[[ $nr =~ ^[[:digit:]]+$ && $nr != 0 ]] || continue
cnt="${cnt// /}"
for ((len = 1; len <= ${#cnt}; len++)); do
[[ -z ${counters[${cnt:0:$len}]} ]] || continue
str+="${cnt:0:$len}$nr"
counters[${cnt:0:$len}]="$nr"
break
done
done
[[ -n $str ]] && bacon_promptc "${BACON_PROMPT_COLOR[counter]:-yellow}" "[$str]"
}
bacon_prompt_dollar() {
bacon_promptc "${BACON_PROMPT_COLOR[dollar]:-blue}" "${BACON_PROMPT_CHARS[dollar]:-\$ }"
}
bacon_prompt_ps1() {
local ps1 layout
for layout in "${BACON_PROMPT_PS1_LAYOUT[@]}"; do
bacon_definedf "$layout" && ps1+="$($layout)"
done
ps1+="$(bacon_prompt_dollar)"
echo "$ps1"
}
# vim:set ft=sh ts=4 sw=4:
|
# Headers
# h1
## h2
### h3
#### h4
##### h5
###### h6
# h1
## h2
### h3
#### h4
##### h5
###### h6
# header *italic*
## header _italic text_
### header **bold text**
#### header __bold text__
##### header *italic*
###### header *italic*
# header `code`
## header ```code```
### header `code`
#### header ```code```
##### header `code`
###### header ```code```
# header ~~strikethrough~~
## header ~~strikethrough~~
### header ~~strikethrough~~
#### header ~~strikethrough~~
##### header ~~strikethrough~~
###### header ~~strikethrough~~
# header [~~url~~](uefasdfs)
## header `code` **~~[**opuscapita**](https://www.opuscapita.com/)~~
### header [*url*](uefasdfs)
#### header ~~[opuscapita](https://www.opuscapita.com/)~~
##### header [url](uefasdfs)
###### header [*url*](uefasdfs)
# Inline code
text `inline code` text
text ```inline code``` text
`inline code`
```inline code```
# URL
[opuscapita](https://www.opuscapita.com/)
**[opuscapita](https://www.opuscapita.com/)**
[**opuscapita**](https://www.opuscapita.com/)
[**opuscapita*](https://www.opuscapita.com/)
*[**opuscapita**](https://www.opuscapita.com/)*
~~[**opuscapita**](https://www.opuscapita.com/)~~
**~~[**opuscapita**](https://www.opuscapita.com/)~~
# Blockquotes
> blockquotes **bold**
>> nested blockquotes **bold _italic_**
>>> nested blockquotes ***italic bold***
# Horisontal rules
---
***
___
# Ordered lists
1. first line
1) first first line
2) first second line
2. second line
3. third line
1) third first line
1. third first first line
# Unordered list
+ Create a list by starting a line with `+`, `-`, or `*`
+ Sub-lists are made by indenting 2 spaces:
- Marker character change forces new list start:
* Ac tristique libero volutpat at
+ Facilisis in pretium nisl aliquet
- Nulla volutpat aliquam velit
+ Very easy!
# Emphasis
_~~_test2_~~
~~_test2_~~_
~~*test2*~~*
*~~*test2*~~
***test** (value should be bold);
_ _**test**_ (value should be bold and italic);
**__test__** (value should be bold);
~~test1~~ testing ~~test2~~
__bold text_bold__
__bold_text bold__
_**bold**
**bold**_
**bold***
**bold**bold
bold**bold**
**bold**bold**bold**
**bold bold**bold**
**bold**bold bold**
***bold**bold bold**
***bold**bold**bold**
**bold**bold bold***
**bold**bold**bold***
**bold**bold***bold**
**bold***bold**bold**
_italic_italic
italic_italic_
_italic_italic_italic_
_italic italic_italic_
_italic_italic italic_
__italic_italic italic_
__italic_italic_italic_
_italic_italic italic__
_italic_italic_italic__
_italic_italic__italic_
_italic__italic_italic_
just a text
**bold text**
*italic text*
***bold italic text***
*italic* simple *italic*
**bold** simple **bold**
***bold italic text** italic*
***bold italic text* bold**
**_bold italic text_ bold**
__*bold italic text* bold__
***italic bold* bold *italic bold***
***italic bold** italic **italic bold***
***italic bold*** simple ***italic bold***
*italic **bold italic** italic*
*italic __bold italic__ italic*
**bold *italic bold* bold**
**bold _italic bold_ bold**
__bold text__
_italic text_
___bold italic text___
_italic_ simple _italic_
__bold__ simple __bold__
___italic bold_ bold _italic bold___
___italic bold__ italic __italic bold___
___italic bold___ simple ___italic bold___
_italic __bold italic__ italic_
_italic **bold italic** italic_
__bold *italic bold* bold__
__bold _italic bold_ bold__
___bold italic text__ italic_
___bold italic text_ bold__
~~strike-through text~~
*~~strike-through text inside italic~~*
~~*italic inside strike-through text*~~
**~~strike-through text inside bold~~**
~~**bold inside strike-through text**~~
# Other
## Code blocks
```
code block
code block
code block
```
## Tables
| c1 | c2 | c3 | c4 |
|--|--|--|--|
| a | b | c | d |
| 1 | 2 | 3 | 4 |
|
-- --------------------------------------------------------
-- Host: 127.0.0.1
-- Server version: 5.1.63-community - MySQL Community Server (GPL)
-- Server OS: Win64
-- HeidiSQL version: 7.0.0.4053
-- Date/time: 2012-10-14 17:59:16
-- --------------------------------------------------------
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET NAMES utf8 */;
/*!40014 SET FOREIGN_KEY_CHECKS=0 */;
-- Dumping structure for table swganh_static.conversation_animations
DROP TABLE IF EXISTS `conversation_animations`;
CREATE TABLE IF NOT EXISTS `conversation_animations` (
`id` int(11) unsigned NOT NULL AUTO_INCREMENT COMMENT 'Conversation Animation ID',
`name` varchar(255) NOT NULL DEFAULT 'none' COMMENT 'Conversation Animation Name',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=47 DEFAULT CHARSET=utf8 COMMENT='Conversation Animations (NPC)';
-- Dumping data for table swganh_static.conversation_animations: ~46 rows (approximately)
/*!40000 ALTER TABLE `conversation_animations` DISABLE KEYS */;
INSERT INTO `conversation_animations` (`id`, `name`) VALUES
(1, 'bow'),
(2, 'beckon'),
(3, 'adn'),
(4, 'apologize'),
(5, 'ayt'),
(6, 'backhand'),
(7, 'blame'),
(8, 'bow2'),
(9, 'bow3'),
(10, 'bow4'),
(11, 'claw'),
(12, 'cuckoo'),
(13, 'curtsey'),
(14, 'dream'),
(15, 'rose'),
(16, 'giveup'),
(17, 'helpme'),
(18, 'huge'),
(19, 'medium'),
(20, 'small'),
(21, 'tiny'),
(22, 'jam'),
(23, 'loser'),
(24, 'mock'),
(25, 'model'),
(26, 'nod'),
(27, 'poke'),
(28, 'rude'),
(29, 'scare'),
(30, 'scared'),
(31, 'scream'),
(32, 'shiver'),
(33, 'shoo'),
(34, 'snap'),
(35, 'spin'),
(36, 'squirm'),
(37, 'strut'),
(38, 'sweat'),
(39, 'thank'),
(40, 'throwdown'),
(41, 'tiphat'),
(42, 'twitch'),
(43, 'worship'),
(44, 'yawn'),
(45, 'yes'),
(46, 'none');
/*!40000 ALTER TABLE `conversation_animations` ENABLE KEYS */;
/*!40014 SET FOREIGN_KEY_CHECKS=1 */;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
|
package com.jeffpdavidson.kotwords.formats
import com.jeffpdavidson.kotwords.formats.AcrossLite.Companion.asAcrossLiteBinary
import com.jeffpdavidson.kotwords.readBinaryResource
import com.jeffpdavidson.kotwords.readStringResource
import com.jeffpdavidson.kotwords.runTest
import kotlin.test.Test
import kotlin.test.assertTrue
class CrosshareTest {
@Test
fun asCrossword() = runTest {
assertTrue(
readBinaryResource(CrosshareTest::class, "puz/test.puz").contentEquals(
Crosshare(readStringResource(CrosshareTest::class, "crosshare.json"))
.asPuzzle().asAcrossLiteBinary()
)
)
}
} |
---
Title: ソースからビルド
nav: ja
---
# ソースからビルド
Content App は [Angular CLI](https://cli.angular.io) をベースとしており、CLI でサポートされているすべてのコマンド、ジェネレーター、およびブループリントを使用できます。
## ビルドの前提条件
- [Node.js](https://nodejs.org/ja/) LTS
- (オプション) [Angular CLI](https://cli.angular.io/) 7.3.4 以降
> Angular CLI ライブラリはすでにセットアップの一部です。
> CLI コマンドを個別に使用する場合にのみ、グローバル (推奨) ツールとしてインストールすることをお勧めします。
## クローンと実行
次のコマンドを使用してプロジェクトのクローンを作成し、依存関係をインストールして実行します。
```sh
git clone https://github.com/Alfresco/alfresco-content-app.git
cd alfresco-content-app
npm install
npm start
```
アプリケーションはデフォルトで `4200` ポートで実行され、プロジェクトがコンパイルされるとデフォルトのブラウザで自動的に開きます。
## 環境変数の設定
ローカルの開発サーバーを実行できるようにするために、一部の環境変数を設定する必要があるかもしれません。プロジェクトのルートフォルダに、次のデータを含む `.env` ファイル (これは gitignored です) を作成してください。
```bash
API_CONTENT_HOST="http://your-url-here"
```
## プロキシの設定
Content App は、ローカル開発サーバーのプロキシ設定を提供します。これにより、CORS とネイティブの認証ダイアログを使用して特定のシナリオに対処できます。
設定は、プロジェクトの `src` ディレクトリにある `proxy.conf.js` ファイルに記述されています。デフォルトでは、環境変数からの設定が優先されます。
**注:** プロキシの設定は、`npm start` スクリプトでアプリケーションを実行するたびに自動的に適用されます。
設定を変更するたびに、アプリケーションを再起動する必要があります。
## 単体テストの実行
[Karma](https://karma-runner.github.io) を介して単体テストを実行するには、`npm test` を実行します。
|
## pin map
|引脚 |功能 |片上外设 |功能模块 |
|:----------|:----------|:--------------|:--------------|
|P0.03 |ADC_IN1 |ADC |battery |
|P0.06 |BAT_CHRG |GPIOTE |battery |
|P0.07 |BAT_STDBY |GPIOTE |battery |
| | | | |
|P0.04 |VIBRATION |GPIOTE |vibration motor|
| | | | |
|P0.12 |IIC_CLK |IIC0 |MPU6050 |
|P0.11 |IIC_SDA |IIC0 |MPU6050 |
|P0.13 |INT |GPIOTE |MPU6050 |
| | | | |
|P0.24 |SPI_CLK |SPI1 |SPI FLASH |
|P0.25 |SPI_CS |SPI1 |SPI FLASH |
|P0.26 |SPI_MISO |SPI1 |SPI FLASH |
|P0.27 |SPI_MOSI |SPI1 |SPI FLASH |
| | | | |
|P0.14 |SPI_CLK |SPI2 |TFT_LCD |
|P0.15 |SPI_MOSI |SPI2 |TFT_LCD |
|P0.16 |D/C sel |GPIO |TFT_LCD |
|P0.17 |RESET |GPIO |TFT_LCD |
|P0.18 |SPI_CS |GPIO |TFT_LCD |
|P0.19 |LEDA |PWM |TFT_LCD |
| | | | |
|P0.12 |IIC_CLK |IIC0 |HRS3300 |
|P0.11 |IIC_SDA |IIC0 |HRS3300 |
|
import { NO_OP } from "@thi.ng/api";
import { Reducer } from "../api";
import { reduce, reducer } from "../reduce";
export function last<T>(): Reducer<T, T>;
export function last<T>(xs: Iterable<T>): T;
export function last<T>(xs?: Iterable<T>): any {
return xs ? reduce(last(), xs) : reducer<T, T>(<any>NO_OP, (_, x) => x);
}
|
import numpy as np
from pypex.poly2d import polygon
def main():
poly1 = polygon.Polygon([[0.0, 0.0], [1.0, 0.0], [0.0, 1.0], [1.0, 1.0]])
poly2 = polygon.Polygon([[0.5, 0.3], [0.0, -1.0], [1.0, -1.0]])
print("Polygon with hull defined by {} \n is automaticaly sorted to clokwise corners as {}\n"
"".format([[0.0, 0.0], [1.0, 0.0], [0.0, 1.0], [1.0, 1.0]], poly1.hull))
try:
import matplotlib
import matplotlib.pyplot as plt
from matplotlib.patches import Polygon as polyg
from matplotlib.collections import PatchCollection
# mpl rcParams
params = {'legend.fontsize': 8, 'legend.handlelength': 0.5, "font.size": 8}
matplotlib.rcParams.update(params)
fig, ax = plt.subplots()
patches = [polyg(poly1.hull, True), polyg(poly2.hull, True)]
p = PatchCollection(patches, cmap=matplotlib.cm.jet, alpha=0.4)
colors = 100 * np.random.rand(len(patches))
p.set_array(np.array(colors))
ax.add_collection(p)
plt.xlim(-2, 2)
plt.ylim(-2, 2)
plt.show()
except ImportError:
pass
print("\n {} has following edges".format(poly1))
for edge in poly1.edges():
print("edge {}".format(edge))
print("\n")
intersects = poly1.intersects(poly2)
print("{} intersects {}: {}".format(poly1, poly2, intersects))
intersection = poly1.intersection(poly2)
print("Intersection of {} and {} is following polygon: \n"
" {}".format(poly1, poly2, intersection))
try:
import matplotlib
import matplotlib.pyplot as plt
from matplotlib.patches import Polygon as polyg
from matplotlib.collections import PatchCollection
# mpl rcParams
params = {'legend.fontsize': 8, 'legend.handlelength': 0.5, "font.size": 8}
matplotlib.rcParams.update(params)
fig, ax = plt.subplots()
patches = [polyg(poly1.hull, True), polyg(poly2.hull, True)]
p = PatchCollection(patches, cmap=matplotlib.cm.jet, alpha=0.4, edgecolors="k")
colors = 100 * np.random.rand(len(patches))
p.set_array(np.array(colors))
ax.add_collection(p)
plt.scatter(intersection.hull.T[0], intersection.hull.T[1])
plt.xlim(-0.5, 1.5)
plt.ylim(-1.5, 1.5)
plt.show()
except ImportError:
pass
# inpolygon
_polygon = np.array([[0.0, 0.0], [0.3, 0.0], [0.4, 1.1], [0.1, 0.5]])
poly = polygon.Polygon(_polygon)
inpolygon = poly.inpolygon()
try:
import matplotlib
import matplotlib.pyplot as plt
from matplotlib.patches import Polygon as polyg
from matplotlib.collections import PatchCollection
# mpl rcParams
params = {'legend.fontsize': 8, 'legend.handlelength': 0.5, "font.size": 8}
matplotlib.rcParams.update(params)
fig, ax = plt.subplots()
patches = [polyg(poly.hull, True), polyg(inpolygon.hull, True)]
p = PatchCollection(patches, cmap=matplotlib.cm.jet, alpha=0.4, edgecolors="k")
colors = 100 * np.random.rand(len(patches))
p.set_array(np.array(colors))
ax.add_collection(p)
plt.xlim(-0.25, 0.6)
plt.ylim(-0.25, 1.2)
plt.show()
except ImportError:
pass
if __name__ == "__main__":
main()
|
#ifndef _LINUX_TIMEKEEPING_WRAPPER_H
#define _LINUX_TIMEKEEPING_WRAPPER_H
#ifndef HAVE_KTIME_GET_TS64
#define ktime_get_ts64 ktime_get_ts
#define timespec64 timespec
#else
#include_next <linux/timekeeping.h>
#endif
#endif
|
<?php
namespace App;
use Illuminate\Database\Eloquent\Model;
use Illuminate\Support\Facades\Hash;
use Illuminate\Foundation\Auth\User as Authenticatable;
class Mahasiswa extends Authenticatable
{
protected $table = "mahasiswa";
protected $fillable = [
'nim',
'username',
'nama_mahasiswa',
'kode_jurusan',
'email',
'password',
'alamat',
'nama_sekolah',
'kode_dosen',
'tanggal_lahir',
'tempat_lahir', //
'jenis_kelamin', //
'agama', //
'kelas', //
'tahun_masuk',
'semester_aktif', //
// 'sekolah_asal', //
// 'nisn',
'status',
'nama_ayah', //
'nama_ibu', //
'alama_ortu', //
'kerja_ortu', //
'no_hp_ortu' //
];
protected $primaryKey = 'nim';
public $incrementing = false;
function setPasswordAttribute($value)
{
$this->attributes['password'] = Hash::make($value);
}
}
|
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.