branch_name
stringclasses 15
values | target
stringlengths 26
10.3M
| directory_id
stringlengths 40
40
| languages
sequencelengths 1
9
| num_files
int64 1
1.47k
| repo_language
stringclasses 34
values | repo_name
stringlengths 6
91
| revision_id
stringlengths 40
40
| snapshot_id
stringlengths 40
40
| input
stringclasses 1
value |
---|---|---|---|---|---|---|---|---|---|
refs/heads/master | <repo_name>jamesanderson9182/StudyBuddy<file_sep>/README.md
# StudyBuddy
>This is an Android App which provides useful tools to students studying.
>>If you are here to look at code its under app/src/main/java/com/example/janderson/studybuddy/
>>>At the time this was last updated the Timer was the only thing that was started.
<file_sep>/app/src/main/java/com/example/janderson/studybuddy/HomeScreenActivity.java
package com.example.janderson.studybuddy;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.LinearLayout;
/**
* @author <NAME>
*/
public class HomeScreenActivity extends Activity {
static Button studyTimerButton;
static Button fillInBlanksButton;
static Button examTrackerButton;
static Button moduleTrackerButton;
static Button flashCardsButton;
static LinearLayout linearLayout;
/**
* Everything that happens when the activity is created
* @param savedInstanceState
* @see Activity
*/
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
//set up layout for activity
this.linearLayout = new LinearLayout(this);
linearLayout.setOrientation(LinearLayout.VERTICAL);
setContentView(linearLayout);
//set up study timer button
this.studyTimerButton = new Button(this);
studyTimerButton.setText("Study Timer");
studyTimerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//Open the study timer button
Intent intent = new Intent(getApplicationContext(), StudyTimerActivity.class);
startActivity(intent);
}
});
//set up fill in the blanks button
this.fillInBlanksButton = new Button(this);
fillInBlanksButton.setText("Fill in the Blanks");
//set up the exam tracker button
this.examTrackerButton = new Button(this);
examTrackerButton.setText("Exam Tracker");
//set up the module tracker button
this.moduleTrackerButton = new Button(this);
moduleTrackerButton.setText("Module Tracker");
//set up the flash cards button
this.flashCardsButton = new Button(this);
flashCardsButton.setText("Flash Cards");
//add everything to the screen
linearLayout.addView(studyTimerButton);
linearLayout.addView(fillInBlanksButton);
linearLayout.addView(examTrackerButton);
linearLayout.addView(moduleTrackerButton);
linearLayout.addView(flashCardsButton);
}
}
| 8525b6cad437e6631b55df9dec7edd06f0d597ce | [
"Markdown",
"Java"
] | 2 | Markdown | jamesanderson9182/StudyBuddy | 713145ebfd2dab6af2a324fb98061958c4179a37 | b14948b065d98c3df9d57818b3081a34f6456619 | |
refs/heads/master | <repo_name>frontweb/vouchers<file_sep>/db/migrate/20100228181210_add_email_to_ad.rb
class AddEmailToAd < ActiveRecord::Migration
def self.up
add_column :ads, :email, :string, :limit => 100
end
def self.down
remove_column :ads, :email
end
end
<file_sep>/app/controllers/admin/comments_controller.rb
class Admin::CommentsController < ApplicationController
before_filter :admin_required
layout 'admin'
# GET /admin/comments
def index
@comments = Comment.paginate :page => params[:page], :order => 'created_at DESC'
end
# DELETE /admin/comments/1
def destroy
Comment.find(params[:id]).destroy
redirect_to admin_comments_url
end
end
<file_sep>/app/models/ad.rb
class Ad < ActiveRecord::Base
apply_simple_captcha :message => "Codul de verificare introdus e gresit"
belongs_to :city
validates_presence_of :city, :message => "Trebuie precizat un oraș"
validates_presence_of :price, :message => "Trebuie precizat prețul (in Euro)"
validates_presence_of :phone_number, :message => "Trebuie introdus un număr de telefon mobil"
validates_numericality_of :price, :message => "Prețul trebuie să fie între 100 și 700 de Euro", :only_integer => true, :greater_than_or_equal_to => 100, :less_than_or_equal_to => 700, :if => Proc.new { |ad| ad.price != nil }
validates_format_of :phone_number, :with => /^07[0-9]{8}$/, :message => "Trebuie un numar de telefon mobil (10 cifre). Introduceți doar cifre (07...)", :if => Proc.new { |ad| ad.price != nil && !ad.phone_number.empty? }
validates_presence_of :email, :message => "Trebuie introdusă o adresa de email"
validates_format_of :email, :with => /\A([^@\s]+)@((?:[-a-z0-9]+\.)+[a-z]{2,})\Z/i, :message => "Adresa de email introdusă nu e corectă", :if => Proc.new { |ad| ad.email != nil && !ad.email.empty? }
def before_create
self.secret_code = random_code
end
def self.per_page
30
end
private
def random_code(length = 6)
#a = ('a'..'z').to_a + (0..9).to_a
a = [:a, :b, :c, :d, :e, :h, :k, :m, :n, :p, :r, :s, :t, :u, :v, :x, :z, 2, 3, 4, 5, 6, 7, 8, 9]
c = ''
1.upto(length) { c += a[rand(a.size)].to_s }
return c
end
end
<file_sep>/app/models/city.rb
class City < ActiveRecord::Base
has_many :ads
validates_presence_of :name
end
<file_sep>/lib/tasks/populate.rake
namespace :db do
desc "Loads initial database models"
task :populate_all => :environment do
Dir[File.join(RAILS_ROOT, 'db', 'fixtures', '*.rb')].sort.each { |fixture| load fixture }
Dir[File.join(RAILS_ROOT, 'db', 'fixtures', RAILS_ENV, '*.rb')].sort.each { |fixture| load fixture }
end
desc "Load a single file"
task :populate => :environment do
unless ENV['file']
puts "usage: rake db:populate file=regions"
exit
end
fixture = File.join(RAILS_ROOT, 'db', 'fixtures', "#{ENV['file']}.rb")
load fixture
end
end
<file_sep>/app/controllers/comments_controller.rb
class CommentsController < ApplicationController
layout 'public'
# GET /article/1/comments/new
def new
@comment = Article.find(params[:article_id]).comments.new
end
# POST /article/1/comments
def create
@article = Article.find(params[:article_id])
@comment = @article.comments.new(params[:comment])
@comment.ip = request.remote_ip
if @comment.save_with_captcha
flash[:success] = "Comentariul a fost adăugat."
redirect_to article_url(@article)
else
render :action => "new"
end
end
end
<file_sep>/app/controllers/application_controller.rb
# Filters added to this controller apply to all controllers in the application.
# Likewise, all the methods added will be available for all controllers.
class ApplicationController < ActionController::Base
include SimpleCaptcha::ControllerHelpers
helper :all # include all helpers, all the time
protect_from_forgery # See ActionController::RequestForgeryProtection for details
# Scrub sensitive parameters from your log
# filter_parameter_logging :password
protected
def admin_required
authenticate_or_request_with_http_basic do |username, password|
username == 'admin' && password == '<PASSWORD>'
end if RAILS_ENV == 'production' || params[:admin_http]
end
end
<file_sep>/app/models/article.rb
class Article < ActiveRecord::Base
has_friendly_id :title, :use_slug => true, :strip_diacritics => true
has_many :comments, :order => 'created_at DESC', :dependent => :destroy
validates_presence_of :title, :message => 'Titlul trebuie completat'
validates_presence_of :content, :message => 'Continutul trebuie completat'
def self.per_page
10
end
end
<file_sep>/app/controllers/page_controller.rb
class PageController < ApplicationController
layout 'public'
def about
end
def contact
end
def cities
@cities = City.all :order => 'name'
end
end
<file_sep>/app/models/notifications.rb
class Notifications < ActionMailer::Base
def new_ad(ad)
subject 'Publicare anunt voucher rabla'
recipients ad.email
from %("<NAME>" <EMAIL>)
body :ad => ad
end
end
<file_sep>/app/helpers/ads_helper.rb
module AdsHelper
def format_phone_number(s)
"#{s[0..3]}.#{s[4..6]}.#{s[7..9]}"
end
end
<file_sep>/db/fixtures/cities.rb
City.create_or_update :id => 1, :name => "Bucuresti"
City.create_or_update :id => 2, :name => "Ilfov"
City.create_or_update :id => 3, :name => "<NAME>"
City.create_or_update :id => 4, :name => "Arad"
City.create_or_update :id => 5, :name => "Pitesti"
City.create_or_update :id => 6, :name => "Bacau"
City.create_or_update :id => 7, :name => "Oradea"
City.create_or_update :id => 8, :name => "Bistrita"
City.create_or_update :id => 9, :name => "Botosani"
City.create_or_update :id => 10, :name => "Braila"
City.create_or_update :id => 11, :name => "Brasov"
City.create_or_update :id => 12, :name => "Buzau"
City.create_or_update :id => 13, :name => "Calarasi"
City.create_or_update :id => 14, :name => "Resita"
City.create_or_update :id => 15, :name => "Cluj"
City.create_or_update :id => 16, :name => "Dej"
City.create_or_update :id => 17, :name => "Constanta"
City.create_or_update :id => 18, :name => "Covasna"
City.create_or_update :id => 19, :name => "Targoviste"
City.create_or_update :id => 20, :name => "Craiova"
City.create_or_update :id => 21, :name => "Galati"
City.create_or_update :id => 22, :name => "Giurgiu"
City.create_or_update :id => 23, :name => "<NAME>"
City.create_or_update :id => 24, :name => "<NAME>"
City.create_or_update :id => 25, :name => "Hunedoara"
City.create_or_update :id => 26, :name => "Slobozia"
City.create_or_update :id => 27, :name => "Iasi"
City.create_or_update :id => 28, :name => "<NAME>"
City.create_or_update :id => 29, :name => "<NAME>"
City.create_or_update :id => 30, :name => "<NAME>"
City.create_or_update :id => 31, :name => "Roman"
City.create_or_update :id => 32, :name => "<NAME>"
City.create_or_update :id => 33, :name => "<NAME>"
City.create_or_update :id => 34, :name => "Slatina"
City.create_or_update :id => 35, :name => "Ploiesti"
City.create_or_update :id => 36, :name => "Zalau"
City.create_or_update :id => 37, :name => "<NAME>"
City.create_or_update :id => 38, :name => "Medias"
City.create_or_update :id => 39, :name => "Sibiu"
City.create_or_update :id => 40, :name => "Suceava"
City.create_or_update :id => 41, :name => "Teleorman"
City.create_or_update :id => 42, :name => "Timisoara"
City.create_or_update :id => 43, :name => "Tulcea"
City.create_or_update :id => 44, :name => "Valcea"
City.create_or_update :id => 45, :name => "Vaslui"
City.create_or_update :id => 46, :name => "Focsani"
<file_sep>/app/controllers/ads_controller.rb
class AdsController < ApplicationController
before_filter :ad_found?, :only => [:edit, :update, :remove, :destroy]
layout 'public'
# GET /ads
def index
# Order
session[:order_column] ||= 'created_at DESC'
order_column = params[:o] ? (params[:o] == 'price' ? 'price' : 'created_at DESC') : session[:order_column]
session[:order_column] = order_column
# Filter by city
@city = nil
if params[:c] && params[:c] == 'all'
session[:city_id] = nil
elsif params[:c]
session[:city_id] = @city.id if @city = City.find(params[:c])
elsif session[:city_id]
@city = City.find(session[:city_id])
end
if @city
@ads = Ad.paginate(:page => params[:page], :conditions => { :city_id => @city.id }, :order => order_column)
else
@ads = Ad.paginate(:page => params[:page], :order => order_column)
end
@articles = Article.all :order => 'created_at DESC', :limit => 3
end
# GET /ads/1
def show
@ad = Ad.find(params[:id])
end
# GET /ads/new
def new
@ad = Ad.new
end
# POST /ads
def create
@ad = Ad.new(params[:ad])
if @ad.save_with_captcha
Notifications.deliver_new_ad(@ad)
flash[:success] = "Anuntul a fost creat. O confirmare a fost trimisa pe email-ul dumneavoastra."
redirect_to ad_url(@ad)
else
render :action => 'new'
end
end
# GET /ads/1/edit?c=secret_code
def edit
end
# PUT /ads/1
def update
if @ad.update_attributes(params[:ad])
flash[:success] = "Modificarile au fost salvate."
redirect_to ad_url(@ad)
else
render :action => 'edit'
end
end
# GET /ads/1/remove?c=secret_code
def remove
end
# DELETE /ads/1?c=secret_code
def destroy
@ad.destroy
flash[:success] = "Anuntul a fost sters."
redirect_to ads_url
end
private
def ad_found?
@ad = Ad.find(:first, :conditions => ["id = ? AND secret_code = ?", params[:id], params[:c]])
unless @ad
flash[:error] = "Anuntul nu a fost gasit."
redirect_to ads_url
return false
end
end
end
<file_sep>/db/migrate/20100228124132_create_ads.rb
class CreateAds < ActiveRecord::Migration
def self.up
create_table :ads do |t|
t.belongs_to :city
t.integer :price
t.string :notes, :phone_number
t.string :secret_code, :limit => 20
t.timestamps
end
add_index(:ads, :city_id)
add_index(:ads, :price)
add_index(:ads, :created_at)
end
def self.down
drop_table :ads
end
end
<file_sep>/app/models/comment.rb
class Comment < ActiveRecord::Base
apply_simple_captcha :message => "Codul de verificare introdus e gresit"
belongs_to :article
validates_presence_of :article_id, :message => "Comentariul trebuie sa apartina unui articol"
validates_presence_of :name, :message => 'Trebuie sa va introduceti numele'
validates_presence_of :email, :message => 'Trebuie sa introduceti o adresa de email'
validates_presence_of :content, :message => 'Comentariul nu poate fi gol'
validates_format_of :email, :with => /\A([^@\s]+)@((?:[-a-z0-9]+\.)+[a-z]{2,})\Z/i, :message => "Adresa de email introdusă nu e corectă", :if => Proc.new { |c| c.email != nil && !c.email.empty? }
def self.per_page
30
end
end
<file_sep>/app/helpers/application_helper.rb
# Methods added to this helper will be available to all templates in the application.
module ApplicationHelper
def error_messages_for(object_name, options = {})
options = options.symbolize_keys
object = instance_variable_get("@#{object_name}")
if object && !object.errors.empty?
txt = ""
object.errors.each { |attr, msg| txt += content_tag("li", msg) }
content_tag("div",
content_tag(options[:header_tag] || "h2", "Corectați erorile următoare:") + content_tag("ul", txt),
:id => options[:id] || "errorExplanation", :class => options[:class] || "errorExplanation"
)
else
""
end
end
# Set title of a page
def title(page_title)
content_for(:title) { page_title }
end
# Set 'description' meta
def meta_description(description)
content_for(:description) { description }
end
# Set 'keywords' meta
def meta_keywords(keywords)
content_for(:keywords) { keywords }
end
end
<file_sep>/test/unit/notifications_test.rb
require 'test_helper'
class NotificationsTest < ActionMailer::TestCase
test "new_ad" do
@expected.subject = 'Notifications#new_ad'
@expected.body = read_fixture('new_ad')
@expected.date = Time.now
assert_equal @expected.encoded, Notifications.create_new_ad(@expected.date).encoded
end
end
<file_sep>/config/deploy.rb
ssh_options[:port] = 383
set :user, "radu"
set :use_sudo, false
set :application, "www.vouchererabla.ro"
set :deploy_to, "/var/apps/#{application}"
set :scm, :git
set :repository, "<EMAIL>:frontweb/vouchers.git"
set :branch, "master"
set :deploy_via, :remote_cache
set :keep_releases, 3
after "deploy:update", "deploy:cleanup"
server "172.16.17.32", :app, :web, :db, :primary => true
namespace :deploy do
desc "Restarting mod_rails with restart.txt"
task :restart, :roles => :app, :except => { :no_release => true } do
run "touch #{current_path}/tmp/restart.txt"
end
[:start, :stop].each do |t|
desc "#{t} task is a no-op with mod_rails"
task t, :roles => :app do ; end
end
end
namespace :my do
desc "Share database"
task :symlink_shares do
run "ln -s #{deploy_to}/#{shared_dir}/database.yml #{release_path}/config/database.yml"
end
end
before "deploy:symlink", "my:symlink_shares"
desc "Tail production log file"
task :tail_log, :roles => :app do
run "tail -f #{shared_path}/log/production.log" do |channel, stream, data|
puts "#{channel[:host]}: #{data}"
break if stream == :err
end
end
desc "Download and load data from production database"
task :db_get, :roles => :app do
require 'yaml'
# Get production database configuration
get "#{current_path}/config/database.yml", "tmp/database.yml"
db = YAML::load_file("tmp/database.yml")
system "rm -f tmp/database.yml"
# Download gzip database dump
run "/usr/bin/mysqldump -u #{db['production']['username']} --password=#{db['production']['password']} --complete-insert #{db['production']['database']} > #{deploy_to}/#{shared_dir}/db.sql"
run "cd #{deploy_to}/#{shared_dir} && tar -czvf db.tar.gz db.sql"
get "#{deploy_to}/#{shared_dir}/db.tar.gz", "tmp/db.tar.gz"
run "rm -f #{deploy_to}/#{shared_dir}/db.sql && rm -f #{deploy_to}/#{shared_dir}/db.tar.gz"
# Untar database
system "cd tmp && tar -xzvf db.tar.gz db.sql"
system "rm -f tmp/db.tar.gz"
# Get development database configuration
db = YAML::load_file("config/database.yml")
# Insert development database name at the begining of the file
File.open("tmp/dump.sql", 'w') do |f|
f.puts "USE '#{db['development']['database']}'"
f.puts File.read("tmp/db.sql")
end
system "rm -f tmp/db.sql"
# Restore dump
system "cat tmp/dump.sql | /usr/local/mysql/bin/mysql -u root"
system "rm -f tmp/dump.sql"
end
<file_sep>/config/initializers/will_paginate.rb
WillPaginate::ViewHelpers.pagination_options[:previous_label] = '« Pagina anterioară'
WillPaginate::ViewHelpers.pagination_options[:next_label] = 'Pagina următoare »'
<file_sep>/app/controllers/articles_controller.rb
class ArticlesController < ApplicationController
layout 'public'
# GET /articles
def index
@articles = Article.paginate :page => params[:page], :order => 'created_at DESC'
end
# GET /articles/1
def show
@article = Article.find(params[:id])
@comment = @article.comments.new
@articles = Article.all :order => 'created_at DESC'
end
end
| ade6af545dea07a679d6b1c81b23355aad522213 | [
"Ruby"
] | 20 | Ruby | frontweb/vouchers | c35428748da2f27f9b9183f685e85d3baa761e69 | 09b959b81cbfa5aa5f7c34494356f7077fa0d6f6 | |
refs/heads/master | <file_sep># Show differences between two playlists.
# Playlists are created using gtkpod with two different ishuffle devices (green and silver)
# In order to sync files use gtkpod instead of the rhythmbox, because dragging files between two devices
# does not works.
# Create a list in gtkpod using Tools->Export Tracks -> export to playlist
require 'rubygems'
class PlayListDifference
def show_diff(master, slave, options)
count_item = 0
slave_name = options.has_key?(:slave_name) ? options[:slave_name] : "slave"
File.open(master, 'r').each_line do |line|
if line =~ /EXTINF/
title = line.split(',')[1].strip.gsub('\n', '')
#puts title
present_in_slave_info = check_title_in_list(slave, title)
present_in_slave = present_in_slave_info[:exact] > 0
partial_in_slave = present_in_slave_info[:partial] > 0
if !present_in_slave
if !partial_in_slave
puts "'#{title}' is missed in #{slave_name}"
elsif options[:print_partial] == true
puts "'#{title}' is partial in #{slave_name}: '#{present_in_slave_info[:partial_titles]}'"
end
end
count_item = count_item + 1
end
end
puts "Found #{count_item} titles"
end
def check_title_in_list(fname, tobe_found)
count_item = 0
partial = 0
partial_arr = []
arr = tobe_found.split('- ')
song_title_only = arr.length > 0 ? arr[arr.length - 1].gsub('.mp3', "") : nil
File.open(fname, 'r').each_line do |line|
if line =~ /EXTINF/
title = line.split(',')[1].strip.gsub('\n', '')
#if title.include?(tobe_found)
if title == tobe_found
#puts "Title '#{tobe_found}' found in '#{title}'"
count_item += 1
else
if song_title_only != nil
if title.include?(song_title_only)
partial += 1
partial_arr << title
end
else
#p tobe_found
end
end
end
end
if partial == 0 and count_item == 0
#p song_title_only
end
return {:exact => count_item, :partial => partial, :partial_titles => partial_arr}
end
end
if $0 == __FILE__
silver_file = 'silver_playlist_2015_08_19'
green_file = 'green_playlist_2015_08_19'
diff = PlayListDifference.new
diff.show_diff(green_file, silver_file, {:print_partial => false, :slave_name => "silver"})
diff.show_diff(silver_file, green_file, {:print_partial => false, :slave_name => "green"})
end
<file_sep># -*- coding: ISO-8859-1 -*-
require 'erb'
fullname = File.dirname(__FILE__) + "/bs_import.rtemp"
file = File.new(fullname, "r")
template = ERB.new(file.read)
file.close
(31..60).each do |ix|
@bsname = "BS"
@bsname += ix < 10 ? "0#{ix}" : "#{ix}"
puts aString = template.result(binding)
end<file_sep>ruby "2.3.1"
source "https://rubygems.org"
gem "pg", '~>0.19.0'
gem "log4r"
gem 'mechanize', '~>2.7.7'<file_sep>ruby_scratch
============
some ruby scripts files
<file_sep>-- statistiche dell'anno
-- Questo script non va lanciato in modo completo, ma vanno selezionate le righe che interressano e poi si preme F5.
-- Uso pgAdmin4 Tools -> Query Tool e qui si carica questo script
-- Il db si aggiorna usando ruby race_picker.rb sotto WLC
-- gare a partire da una data, statistica annuale:
--COPY (
select r.id, r.name, r.title, r.km_length, r.ascending_meter, r.race_date, s.name, r.race_time, r.rank_global, r.rank_class, r.id from race as r
inner join race_subtype s on r.race_subtype_id = s.id
where r.race_date > '2022-01-01' order by r.race_date asc
--) TO 'D:\scratch\postgres\corsa\race-2018-02.csv' With CSV DELIMITER ';' HEADER; -- export in excel con COPY e TO
-- Nota che excel interpreta questo export come ansi. Per l'utf-8, che è lo standard usato dall'export, vuole il BOM.
-- Quindi con notepad++ o converto in ansi o converto in utf8-bom. Poi apro con excel.
-- delete from race where id = 287 (usa questo delete per cancellare una corsa che risulta in conflitto nel db e poi ricaricala di nuovo con race_picker.rb)
-- sommatorie km e Hm percorsi nell'anno
select SUM(r.km_length) as Km_Tot, SUM(r.ascending_meter) as HM_Tot from race as r
inner join race_subtype s on r.race_subtype_id = s.id
where r.race_date >= '2016-01-01' AND r.race_date <= '2016-12-31'
-- mostra tutti i tipi di gara (serve per poi vedere quale filtro usare sul tipo)
SELECT * from race_subtype;
-- Numero di ultra
--select SUM(r.km_length) as Km_Tot, SUM(r.ascending_meter) as HM_Tot from race as r
-- Ultra sopra i cento km
select r.name, r.title, r.km_length, r.ascending_meter as HM, r.race_date, s.name, r.race_time, r.rank_global from race as r
inner join race_subtype s on r.race_subtype_id = s.id
where
(s.id = 5 OR s.id = 6)
--AND r.km_length >= 100
--AND r.race_date > '2017-01-01'
order by r.race_date asc
-- Chilometri totali ultra su asfalto
select SUM(r.km_length) as Km_Tot, SUM(r.ascending_meter) as HM_Tot from race as r
--select r.name, r.title, r.km_length, r.ascending_meter as HM, r.race_date, s.name, r.race_time, r.rank_global from race as r
inner join race_subtype s on r.race_subtype_id = s.id
where
(s.id = 5)
--order by r.race_date asc
-- Chilometri totali ultra su trail
select SUM(r.km_length) as Km_Tot, SUM(r.ascending_meter) as HM_Tot from race as r
--select r.name, r.title, r.km_length, r.ascending_meter as HM, r.race_date, s.name, r.race_time, r.rank_global from race as r
inner join race_subtype s on r.race_subtype_id = s.id
where
(s.id = 6)
--order by r.race_date asc
-- Numero di Maratone (id = 4)
--select SUM(r.km_length) as Km_Tot, SUM(r.ascending_meter) as HM_Tot from race as r
select r.name, r.title, r.km_length, r.ascending_meter as HM, r.race_date, s.name, r.race_time, r.rank_global, r.id from race as r
inner join race_subtype s on r.race_subtype_id = s.id
where
(s.id = 4)
order by r.race_date asc
-- <NAME> (id = 3)
--select SUM(r.km_length) as Km_Tot, SUM(r.ascending_meter) as HM_Tot from race as r
select r.name, r.title, r.km_length, r.ascending_meter as HM, r.race_date, s.name, r.race_time, r.rank_global, r.id from race as r
inner join race_subtype s on r.race_subtype_id = s.id
where
(s.id = 3)
order by r.race_date asc
-- delete from race where id = 145 or id = 259
-- select * from race where id = 191
-- Single Marathon
select r.name, r.title, r.km_length, r.ascending_meter as HM, r.race_date, s.name, r.race_time, r.rank_global, r.id from race as r
inner join race_subtype s on r.race_subtype_id = s.id
where
(s.id = 4)
--AND r.name LIKE '%Welsch%'
AND r.name LIKE '%VCM%'
order by r.race_date asc
-- silverster lauf id = 2 fino ai 10km
--select * from race
select r.name, r.title, r.km_length, r.pace_minkm, r.race_date, s.name, r.race_time, r.rank_global, r.id from race as r
inner join race_subtype s on r.race_subtype_id = s.id
where
(s.id = 2)
AND r.title LIKE '%Silve%'
order by r.race_date asc
select r.title as titolo, r.km_length as distanza, r.race_date as data, r.race_time as tempo, r.rank_global as pos from race as r
inner join race_subtype s on r.race_subtype_id = s.id
where
(s.id = 2)
AND r.title LIKE '%Silve%'
order by r.race_date asc
-- gare da 10km
select r.name, r.title, r.km_length, r.ascending_meter as HM, r.race_date, s.name, r.race_time, r.rank_global, r.id from race as r
inner join race_subtype s on r.race_subtype_id = s.id
where
(s.id = 2) AND r.km_length = 10
--alter table race alter column race_time SET DATA TYPE Time USING race_time::time without time zone
-- Prove con il tempo, per le somme dei tempi si usa interval
SELECT ('13:08:57'::interval + '12:07:48'::interval)
SELECT ('09:30:00'::time - '06:00:00'::time) -- risultato interval
-- SELECT (DATE_PART('hour', '08:56:10'::time - '08:54:55'::time) * 3600 +
-- DATE_PART('minute', '08:56:10'::time - '08:54:55'::time)) * 60 +
-- DATE_PART('second', '08:56:10'::time - '08:54:55'::time);
--- Irdning 2017 dettaglio laps
SELECT rd.lap_number, rd.lap_time, rd.lap_pace_minkm, rd.tot_race_time, rd.tot_km_race, rd.tot_race_minkm,
(rd.tot_km_race / r.km_length * 100) as proc_race_percent, --accumulo della gara
(rd.tot_race_time + r.race_start) as time_in_race -- ora di gara con data
FROM racelap_detail as rd
inner join race as r
on r.id = rd.race_id
where rd.race_id = 249
-- and (CAST (rd.lap_pace_minkm as interval) >= '06:30'::interval)
-- AND (CAST (rd.lap_pace_minkm as interval) < '07:00'::interval)
--AND (CAST (rd.lap_pace_minkm as interval) >= '07:00'::interval)
--and rd.lap_time <= '00:07:18'::interval -- 7:18 come lap è il tempo dei 06:00 min/km
--select 205.156 - 109
-- delete from racelap_detail
select * from race
<file_sep>require 'rubygems'
require 'mechanize'
class EtfEasyChart
def initialize
@agent = Mechanize::Mechanize.new
@agent.user_agent = "Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.1.1) Gecko/20061204 Firefox/2.0.0.1"
@picked_quote = {}
end
def pick_from_easychart(url)
page = @agent.get(url)
data_found = []
#'body//div//div//div//div//table//tbody//tr//td//table//tbody//tr//td//table//tbody//tr//td//table//tbody//tr//td'
#page.search('body//div//div//div//div//td//b').set('class','last').each do |element|
element_with_quote = false
# devo trovare il tr con la classe 'toptitle' e poi vedere il successivo tr. Nei children td ci sono le info che mi servono
page.search('body//div//div//div//div//table').set('class','datatable').search('tr').each do |element|
#p element.inner_html
if element_with_quote
#p element.inner_html
i = 0
# qui una linea separata da td di questo tipo: LU0292106167 XetraETF 18,39 24.02./17 36 -0,05/-0,27%
element.search('td').each do |field|
if i == 0 || i == 1 || i == 4 || i == 5
item = field.inner_html.gsub(' ','')
data_found << item.gsub(/\r\n/,'')
elsif i == 2
data_found << field.search('b')[0].inner_html
end
i = i + 1
#p fields.inner_html
end
break
end
if element.attributes['class'] && (element.attributes['class'].value == 'toptitle')
#p element.inner_html
element_with_quote = true # mark the next item
end
end
#p data_found
data_found << url
@picked_quote[data_found[0]] = data_found
end
def write_quotes(fname)
File.open(fname,'w') do |file|
@picked_quote.each do |k,v|
file.write(v.join(';'))
file.write("\n")
puts "ISIN: #{v[0]}, quote: #{v[2]}"
end
end
puts "File written #{fname}"
#p @picked_quote
end
end
if $0 == __FILE__
#url = 'http://www.finanzen.net/etf/db_x-trackers_DBLCI_-_OY_BALANCED_UCITS_ETF_EUR_1C'
#picker.pick_from_complete_url(url)
puts "get_quote.rb is using hard coded urls..."
urls = []
# 1 LU0290357929 db x-trackers II iBoxx Global Infl.-linked UCITS ETF 1C
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-10904993&menuId=1&pathName=DB%20X-TR.II-IB.GL.IN.-L.1C'
# 2 LU0290355717 db x-trackers II iBoxx Sov. Eurozone UCITS ETF 1C
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-10904984&menuId=1&pathName=DBXTR.II-EUROZ.G.B.(DR)1C'
# 3 LU0292106167 db x-trackers DBLCI - OY Balanced UCITS ETF 1C
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-11057070&menuId=1&pathName=DB%20X-TR.DBLCI-OY%20BAL.%201C'
# 4 LU0489337690 db x-trackers FTSE EPRA/NAREIT Dev. Europe Real Estate
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-23270949&menuId=1&pathName=DB%20X-T.FTSE%20E/N%20DE%20RE%201C'
# 5 IE00B1FZS350 iShares Developed Markets Property Yield UCITS ETF
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-106098910&menuId=1&pathName=ISHSII-DEV.MKT.PR.Y.DLDIS'
# 6 IE00B3VWM098 iShares MSCI USA Small Cap UCITS ETF B
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-20534544&menuId=1&pathName=ISHSVII-MSCI%20USA%20SC%20DL%20AC'
# 7 IE00B2QWDY88 iShares MSCI Japan Small Cap UCITS ETF (Acc) B
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-75100612&menuId=1&pathName=ISHSIII-MSCI%20J.SM.CAP%20DLD'
# 8 IE00B3VWMM18 iShares MSCI EMU Small Cap UCITS ETF B
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-20534543&menuId=1&pathName=ISHSVII-MSCI%20EMU%20SC%20EOACC'
# 9 DE000A0D8Q49 iShares DJ U.S. Select Dividend (DE)
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-3372758&menuId=1&pathName=IS.DJ%20U.S.SELEC.DIV.U.ETF'
#10 LU0292107645 db x-trackers MSCI EM TRN UCITS ETF 1C
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-10980122&menuId=1&pathName=DB%20X-TR.MSCI%20EM%20IDX.1C'
#11 IE00B0M63060 iShares UK Dividend UCITS ETF
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-105446142&menuId=1&pathName=ISHS-UK%20DIVIDEND%20LS%20D'
#12 DE000A0H0744 iShares DJ Asia Pacific Select Dividend 30
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-4076332&menuId=1&pathName=IS.DJ%20AS.PAC.S.D.30%20U.ETF'
#13 DE0002635281 iShares EURO STOXX Select Dividend 30 (DE)
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-2794238&menuId=1&pathName=IS.EO%20ST.SEL.DIV.30%20U.ETF'
#14 IE00BM67HM91 db x-tr.MSCI Wld.Energy I.ETF
urls << 'http://www.easycharts.at/index.asp?action=securities_securityDetails&id=tts-102824320&menuId=1&pathName=DB-XTR.MSCI%20WEIU(PD)%201CDL'
#15 DE000ETF9074 ComStage 1-MDAX UCITS ETF: Inhaber-Anteile IXETRA-Frankfurt
urls << 'http://www.easycharts.at/index.asp?action=securities_chart&id=tts-97685758&menuId=1&pathName=COMSTAGE%201-MDAX%20UCI.ETF%20I'
#16 IE00B1FZS574 ComStage iShsII-MSCI Turkey UCITS ETF: Registered Shs USD (Dist) o.N.
urls << 'http://www.easycharts.at/index.asp?action=securities_chart&id=tts-106098900&menuId=1&pathName=ISHSII-MSCI%20TURKEY%20DLDIS'
picker = EtfEasyChart.new
urls.each do |url|
picker.pick_from_easychart(url)
end
picker.write_quotes('D:\scratch\csharp\PortfolioExcelChecker\PortfolioExcelChecker\bin\Debug\quote.csv')
end<file_sep># file: race_detail_insert.rb
$:.unshift File.dirname(__FILE__)
require 'rubygems'
require 'pg'
require 'db_baseitem'
require 'time'
############################# RaceDetailItem
class RaceDetailItem < DbBaseItem
attr_accessor :lap_number, :lap_time, :lap_meter, :lap_pace_minkm, :tot_meter_race, :tot_race_minkm, :race_id, :tot_race_time, :tot_km_race, :completed
def initialize
@changed_fields = [:lap_number, :lap_time, :lap_meter, :lap_pace_minkm, :tot_meter_race, :tot_race_minkm, :race_id, :tot_race_time, :tot_km_race, :completed]
@field_types = {:lap_number => :int, :lap_meter => :int, :tot_meter_race => :int, :completed => :boolean}
end
end
################################ RaceDetailInsert
class RaceDetailInsert < DbConnectBase
def initialize
@log = Log4r::Logger["RaceDetailInsert"]
super
end
def get_race_reference(title, date)
query = "SELECT id, race_date, title, km_length, lap_meter, tot_race_time_sec FROM race WHERE race_date = '#{date}' AND title = '#{title}' LIMIT 1"
result = exec_query(query)
if result.ntuples == 1
#p result[0]
id_res = result[0]["id"]
km_res = result[0]["km_length"].to_f
lap_meter = result[0]["lap_meter"].to_f
raise "Lap is null (lap_meter), please insert it manually (race id #{id_res})" if lap_meter == 0
tot_race_time_sec = result[0]["tot_race_time_sec"].to_i
raise "Race time is null (tot_race_time_sec), please insert it manually (race id #{id_res})" if tot_race_time_sec == 0
@log.debug "Referenced race is #{title} at #{date} with id #{id_res}, km #{km_res}, lap #{lap_meter}, tot time sec #{format_seconds(tot_race_time_sec)}"
return [id_res, km_res, lap_meter, tot_race_time_sec]
else
raise "Race #{title} at #{date} not found"
end
end
def format_seconds(tot_time_sec)
hh = (tot_time_sec / 3600).floor
min = ((tot_time_sec - (3600 * hh)) / 60).floor
ss = tot_time_sec - (3600 * hh) - (60 * min)
time_str = pad_to_col(hh.to_s, "0", 2) + ':' + pad_to_col(min.to_s, "0", 2) + ':' + pad_to_col(ss.to_s, "0", 2) ;
end
#Provides a pace string. Something like "06:41" for a velocity of 8.98 km/h
def calc_pace_in_min_sec(meter, sec)
dist_km = meter / 1000.0
vel_m_sec = meter / sec
vel_med_in_kmh = vel_m_sec * 3.6
min_part = 1000 / (vel_med_in_kmh / 3.6) / 60 # qualcosa come 4,3 min ma noi interessa tipo 4min 20sec
pace_str = make_min_form(min_part)
end
# Transform the minute velocity fraction in mm:sec format. E.g 4.3 => "04:20"
def make_min_form(min_part)
min_only = min_part.floor;
sec_perc = min_part - min_only;
sec_only = 60 * sec_perc;
if (sec_only > 59.5)
min_only += 1
sec_only = 0
end
mm_formatted = pad_to_col(min_only.to_s, "0", 2) + ':' + pad_to_col(sec_only.round.to_s, "0", 2);
return mm_formatted;
end
def pad_to_col(str, pad, width)
str_res = "" + str;
while (str_res.length < width)
str_res = pad + str_res
end
return str_res.slice(0, width)
end
# lap_meter: lunghezza del lap in metri
# tot_race_time_sec: durata della gara, esempio irdning 3600 * 24
# km_race: km percorsi al termine della gara (finale)
# data_file: file con i tempi parziali di ogni giro percorso
# race_id : referenza della gara (esempio irdning id = 249)
def create_laps(race_id, data_file, lap_meter, km_race, tot_race_time_sec, insert_last_partial)
fname = File.join(File.dirname(__FILE__), data_file)
lap_nr = 1
tot_meter = 0
tot_time_sec = 0
@items = []
File.open(fname, 'r').each_line do |line|
arr_laps_str = line.split(" ")
arr_laps_str.each do |value_s|
#p value_s
t1 = Time.parse(value_s)
#p t1.methods
lap_sec = t1.hour * 3600 + t1.min * 60 + t1.sec
tot_time_sec += lap_sec
tot_meter = lap_meter * lap_nr
item = RaceDetailItem.new
item.lap_number = lap_nr
item.lap_time = value_s
item.lap_meter = lap_meter
item.lap_pace_minkm = calc_pace_in_min_sec(lap_meter, lap_sec)
item.tot_meter_race = tot_meter
item.tot_race_minkm = calc_pace_in_min_sec(tot_meter, tot_time_sec)
item.race_id = race_id
item.tot_race_time = format_seconds(tot_time_sec)
item.tot_km_race = tot_meter / 1000
item.completed = true
#p item
@items << item
lap_nr += 1
#exit if lap_nr == 9
end
#p line
end
@log.debug "Collected #{@items.size} laps. Completed laps km #{@items.last.tot_km_race} in #{@items.last.tot_race_time} "
# last lap is not completed but it is the difference between total km and the last completed sum
if insert_last_partial
t1 = Time.parse(@items.last.tot_race_time)
completed_lap_sec = t1.hour * 3600 + t1.min * 60 + t1.sec
lap_sec = tot_race_time_sec - completed_lap_sec
item = RaceDetailItem.new
item.lap_number = lap_nr
item.lap_time = format_seconds(lap_sec)
item.lap_meter = km_race * 1000 - @items.last.tot_km_race * 1000
item.lap_pace_minkm = calc_pace_in_min_sec(item.lap_meter, lap_sec)
item.tot_meter_race = km_race * 1000
item.tot_race_minkm = calc_pace_in_min_sec(km_race * 1000, tot_race_time_sec)
item.race_id = race_id
item.tot_race_time = format_seconds(tot_race_time_sec)
item.tot_km_race = km_race
item.completed = false
#p item
@items << item
end
end #end create_laps
def store_laps_indb
@log.debug "Store laps into the db."
@items.each do |item|
query = "INSERT INTO racelap_detail (#{item.get_field_title}) VALUES (#{item.get_field_values(@dbpg_conn)})"
exec_query(query)
end
@log.debug "Processed #{@items.size} items, all items are successfuly inserted into the db."
end
end
if $0 == __FILE__
require 'log4r'
include Log4r
@log = Log4r::Logger.new("RaceDetailInsert")
Log4r::Logger['RaceDetailInsert'].outputters << Outputter.stdout
inserter = RaceDetailInsert.new
arr_res = inserter.get_race_reference('24h-Einzel-Bewerb','2017-06-30')
race_id = arr_res[0]
km_race = arr_res[1]
lap_meter = arr_res[2]
tot_race_time_sec = arr_res[3]
#lap_meter = 1217.75
#tot_race_time_sec = 3600 * 24
insert_last_partial = true
inserter.create_laps(race_id, 'data/2017-06-30-24h-irdning.txt', lap_meter, km_race, tot_race_time_sec, insert_last_partial)
#inserter.store_laps_indb # caution, store reinsert previous items
end<file_sep># -*- coding: ISO-8859-1 -*-
require 'rubygems'
require 'fileutils'
require 'filescandir'
class ExtraSPMSLogCreator
def create_structure(low_ix, up_ix, root_dir, src_files_dir)
fscd = FileScanDir.new
fscd.scan_dir(src_files_dir)
(low_ix..up_ix).each do |ix|
bsname = "BS"
bsname += ix < 10 ? "0#{ix}" : "#{ix}"
fullname_bs_dir = File.join(root_dir,bsname)
FileUtils::mkdir_p fullname_bs_dir
FileUtils::mkdir_p File.join(fullname_bs_dir, "Archive")
FileUtils::mkdir_p File.join(fullname_bs_dir, "Error")
target_logs_dir = File.join(fullname_bs_dir, "Logs")
FileUtils::mkdir_p target_logs_dir
fscd.result_list.each do |line|
dst_item = File.join(target_logs_dir, File.basename(line))
FileUtils.cp line, dst_item
end
puts "Created structure in #{fullname_bs_dir}"
end
end
end
if $0 == __FILE__
root_dir = 'D:/tmp/cancme'
src_call_logs_dir = 'C:/temp/SPMS/BS03/Logs'
log_build = ExtraSPMSLogCreator.new
log_build.create_structure(1,3,root_dir, src_call_logs_dir)
end
<file_sep>#file: db_baseitem.rb
class DbBaseItem
def get_field_title
arr = @changed_fields.map{|e| e.to_s}
arr.join(',')
end
def get_field_values(dbpg_conn)
arr = @changed_fields.map{|f| "'" + dbpg_conn.escape_string(serialize_field_value(f,send(f))) + "'"}
arr.join(',')
end
def serialize_field_value(field, value)
if @field_types[field] == :datetime
res = value ? value.strftime("%Y-%m-%d %H:%M:%S") : nil
elsif @field_types[field] == :boolean
res = value ? 1 : 0
elsif @field_types[field] == :int
res = value ? value : 0
elsif @field_types[field] == :numeric
res = value ? value : 0.0
else
res = value.to_s
end
res = res.to_s
end
end #end DbBaseItem
############################################## DbConnectBase
class DbConnectBase
def initialize
@dbpg_conn = nil
@use_debug_sql = true
connect_to_local_db
end
def connect_to_local_db
# su WSL2 localhost non sembra funzionare.
# Si usa su WSL2
# grep -m 1 nameserver /etc/resolv.conf | awk '{print $2}'
# che genera il seguente IP: 172.17.208.1
host_to_connect = `grep -m 1 nameserver /etc/resolv.conf | awk '{print $2}'`
p host_to_connect = host_to_connect.gsub("\n","")
@log.debug "Wanna connect to db on IP #{host_to_connect}"
@dbpg_conn = PG::Connection.open(:dbname => 'corsadb',
:user => 'corsa_user',
:password => '<PASSWORD>',
:host => host_to_connect,
:port => 5432)
@log.debug "Connected to the db"
end
def exec_query(query)
@log.debug query if @use_debug_sql
@dbpg_conn.async_exec(query)
end
end
<file_sep># -*- coding: ISO-8859-1 -*-
require 'rubygems'
require 'log4r'
include Log4r
class WindowsServiesStartOrStop
def initialize
@log = Log4r::Logger.new("WindowsServiesStartOrStop")
@log.outputters << Outputter.stdout
FileOutputter.new('WindowsServiesStartOrStop', :filename=> 'services.log')
Logger['WindowsServiesStartOrStop'].add 'WindowsServiesStartOrStop'
end
def StopServices(serv_arr)
@log.debug "Stopping #{serv_arr}"
submit_net_cmd(:stop, serv_arr)
end
def StartServices(serv_arr)
@log.debug "Starting #{serv_arr}"
submit_net_cmd(:start, serv_arr)
end
private
def submit_net_cmd(cmd_type,serv_arr )
net_cmd = "start"
if cmd_type == :stop
net_cmd = "stop"
end
serv_arr.each do |service|
cmd = "net #{net_cmd} \"#{service}\""
ExecCmd(cmd)
end
@log.debug "All #{net_cmd} requests submitted"
end
def ExecCmd(cmd)
IO.popen(cmd, "r") do |io|
io.each_line do |line|
@log.debug line
end
end
rescue
@log.error "Error: #{$!}"
end
end
if $0 == __FILE__
services = []
services << 'SPMS - Administration Service'
services << 'SPMS - Aggregation Service'
services << 'SPMS - Authorisation Service'
services << 'SPMS - DNS Service'
services << 'SPMS - ETL Service'
services << 'SPMS - LogFile Watcher Service'
services << 'SPMS - Reporting Service'
services << 'SPMS - Scheduler Service'
# SINGLE SERVICE
#services = ['SPMS - Authorisation Service']
#services = ['SPMS - Reporting Service']
#services = ['SPMS - LogFile Watcher Service']
#services = ['SPMS - Aggregation Service']
#services = ['SPMS - ETL Service']
#services = ['SPMS - Administration Service']
#services = ['SPMS - Scheduler Service']
#services = ['SPMS - Reporting Service', 'SPMS - ETL Service']
#services = ['SPMS - LogFile Watcher Service', 'SPMS - ETL Service']
####
starter = WindowsServiesStartOrStop.new
starter.StopServices(services)
#starter.StartServices(services)
end
<file_sep># -*- coding: ISO-8859-1 -*-
require 'rubygems'
require 'rexml/document'
class GPorterConvGpx
def parse_gpx(fname)
p doc_raw = File.open(fname, 'r').read
doc = REXML::Document.new(doc_raw)
titles = []
links = []
p doc
doc.elements.each('trk') do |ele|
titles << ele.name
p ele
end
p titles
end
# Per fare andare il file gpx su garmin connect partendo da un file esportato
# da canway bisogna:
# - Cambiare il nodo gpx, penso che occorra la versione 1.1 anziché la 1.0
# - Usare il nodo metadata
# - Togliere il nodo speed in ogni punto
def remove_speed(fname)
puts "Open file #{fname}"
result = []
line_count = 0
state = :find_gpx
old_time = nil
point_time_insec = nil
space_in_m = nil
accumulate_space = 0
km_tot = 0
File.open(fname, 'r').each_line do |line|
insert_line = true
if state == :insert_metadata
result << "\n<metadata>\n"
state = :find_trk
elsif state == :find_trk and line =~ /trk/
result << "</metadata>\n"
state = :normal
elsif state == :find_gpx and line =~ /gpx/
insert_line = false
result << '<gpx xmlns="http://www.topografix.com/GPX/1/1" xmlns:gpsies="http://www.gpsies.com/GPX/1/0" creator="Igor" version="1.1" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.topografix.com/GPX/1/1 http://www.topografix.com/GPX/1/1/gpx.xsd http://www.gpsies.com/GPX/1/0 http://www.gpsies.com/gpsies.xsd">'
state = :insert_metadata
elsif line =~ /speed/
insert_line = false
var = '<speed>'
var2 = "</speed>\n"
str2 = line.gsub( /#{Regexp.escape(var)}/, '' )
str_speed = str2.gsub( /#{Regexp.escape(var2)}/, '' )
p speed = str_speed.to_f
p point_time_insec
if point_time_insec != nil
p space_in_m = speed * point_time_insec
accumulate_space += space_in_m
if accumulate_space >= 1000
p "Kilometro!!!!!!"
accumulate_space = 0
km_tot += 1
end
end
elsif line =~ /time/
var = '<time>'
var2 = "</time>\n"
str2 = line.gsub( /#{Regexp.escape(var)}/, '' )
str_time = str2.gsub( /#{Regexp.escape(var2)}/, '' )
mytime = DateTime::strptime(str=str_time)
if old_time != nil
diff_time = (mytime - old_time)
point_time_insec = (diff_time * 24 * 60 * 60).to_i
end
old_time = mytime
end
if insert_line
result << line
end
line_count += 1
end
puts "Percorsi km #{km_tot}"
out_fname = File.join(File.dirname(fname), "garmin_#{File.basename(fname)}")
puts "Create a new file without speed item #{out_fname}"
File.open(out_fname, 'w'){|outfile| result.each{ |item| outfile << item } }
puts "File created OK - original count #{line_count}, now #{result.size}"
end
end
if $0 == __FILE__
# NOTA: in questa versione ho provato ad inserire gli split nel file gpx.
# Purtroppo non funziona nell'import in garmin connect, anche se si fa il downlad
# da garmin connect mi da lo stesso file
gp = GPorterConvGpx.new
fname = 'E:\documenti\Tracce_Gps\export_2015-10-18 09-08.gpx'
gp.remove_speed(fname)
#gp.parse_gpx(fname)
end<file_sep>$:.unshift File.dirname(__FILE__)
require 'rubygems'
require 'mechanize'
require 'db_baseitem'
require 'pg'
#Script che uso per fare l'aggiornamento del mio db delle gare memorizzate sul sito pentek
#In questo modo non devo editare le gare due volte, ma solo sul sito Pentek.
#Note: Ho cominciato con arachno e ruby 1.8.6
# il db però usa le stringe in formato utf8 quindi va usato un ruby tipo 2.3.1 quando si va scrivere nel db
class RaceItem < DbBaseItem
attr_accessor :name,:title,:meter_length,:ascending_meter,:descending,:rank_global,:rank_gender,:rank_class,:class_name,:race_date,:sport_type_id,
:race_time,:pace_kmh,:pace_minkm,:comment,:race_subtype_id,:runner_id,:km_length
def initialize
@runner_id = 1
@sport_type_id = 0 # duathlon is 1. Informazione presente solo nel css background del div //*[@id="zeile_zwei_dua_tacho"]
# l'ho messa anche nel titolo
@ascending_meter = 0
@changed_fields = [:name,:title,:meter_length,:ascending_meter,:descending,:rank_global,:rank_gender,:rank_class,:class_name,:race_date,:sport_type_id,
:race_time,:pace_kmh,:pace_minkm,:comment,:race_subtype_id,:runner_id,:km_length]
@field_types = {:race_date => :datetime, :meter_length => :int, :ascending_meter => :int, :descending => :int, :rank_global => :int,
:rank_gender => :int, :rank_class => :int, :sport_type_id => :int, :race_subtype_id => :int, :runner_id => :int, :km_length => :numeric }
end
def title=(tt)
# title format example: "race1 (100HM)"
if tt =~ /\(*.[H,h][m,M]/
arr = tt.split('(')
@title = arr[0].strip
arr[1] = arr[1].downcase
@ascending_meter = arr[1].gsub("(","").gsub(")","").gsub('hm',"").gsub(" ","").to_i
else
@title = tt.strip
end
if @title =~ /Duathlon/
@sport_type_id = 1
end
end
def km_length=(kl)
@km_length = kl.gsub("k","").to_f
@meter_length = (@km_length * 1000).to_i
if @ascending_meter > 300 and @meter_length != 42195
@race_subtype_id = 1
if @meter_length > 10999 and @meter_length < 42195
@race_subtype_id = 7
elsif @meter_length > 42195
@race_subtype_id = 6
end
elsif @meter_length < 10999
@race_subtype_id = 2
elsif @meter_length == 21097
@race_subtype_id = 3
elsif @meter_length == 42195
@race_subtype_id = 4
elsif @meter_length > 42195
@race_subtype_id = 5
else
@race_subtype_id = 8
end
end
def rank_global=(rg)
@rank_global = rg.gsub(".","").to_i
end
def rank_gender=(rg)
rank_gender = rg.gsub(".","").to_i
end
def rank_class=(rg)
@rank_class = rg.gsub(".","").to_i
end
def race_date=(dt)
@race_date = Time.parse(dt)
end
def is_item_recent?(latest_date_in_db)
@race_date > latest_date_in_db
end
end
################################################## RACEPICKER
class RacePicker < DbConnectBase
def initialize
@log = Log4r::Logger["RacePicker"]
@agent = Mechanize.new
@agent.user_agent = "Mozilla/5.0 (Windows; U; Windows NT 5.1; en-US; rv:1.8.1.1) Gecko/20061204 Firefox/2.0.0.1"
@picked_races = {}
if RUBY_VERSION != '1.8.6'
@str_nbs = "\uc2a0".force_encoding('ISO-8859-1')
@str_nbs2 = "\u00A0"
else
@str_nbs = "\240"
@str_nbs2 = "\302\240"
end
super
#@str_nbs = "\240" #http://www.utf8-chartable.de/ non breaking space
end
def get_latest_racedate_indb
query = "SELECT race_date, title FROM race ORDER BY race_date DESC LIMIT 1"
result = exec_query(query)
if result.ntuples == 0
return Time.parse("2000-01-01 00:00:00")
end
#p result[0]
p res = Time.parse(result[0]["race_date"])
return res
end
def is_race_item_indb?(item)
#p item
date_str = item.serialize_field_value(:race_date, item.race_date)
query = "SELECT race_date, title FROM race WHERE race_date = '#{date_str}' AND title = '#{item.title}' LIMIT 1"
result = exec_query(query)
return result.ntuples != 0
end
def insert_indb(race_item)
p race_item
query = "INSERT INTO race (#{race_item.get_field_title}) VALUES (#{race_item.get_field_values(@dbpg_conn)})"
exec_query(query)
end
def pick_races(url, latest_date_in_db)
@log.debug "Url: #{url}, date last race in db #{latest_date_in_db}"
@log.debug "Using parser #{Mechanize.html_parser}" # Nokogiri::HTML
page = @agent.get(url)
#puts page.body
i = 1
@races = []
#per avere l'elemento che si cerca, si usa select che è un nokogiri con attributes e children
# per capire cosa bisogna selezionare, basta usare p link all'interno del block select
page.search("body//div//div//div//div//div").select{|link| link.attributes["id"].value == "ergebnis_container_tacho"}.each do |tacho|
#p link
#p link.inner_html
@log.debug "Found a new item #{i}..."
@race_item = RaceItem.new
tacho.search('div').select{|ldate| ldate.attributes["id"].value == "ergebnis_bewerbdatum"}.each do |date_race|
@race_item.race_date = date_race.inner_html.gsub(@str_nbs, "").gsub(/[[:space:]]+\z/,"").strip #remove all spaces, also   at the end -> "2016-10-29\xA0\xA0"
end
#//*[@id="ergebnis_bewerbname"]
tacho.search('div').select{|litem| litem.attributes["id"].value == "ergebnis_bewerbname"}.each do |item|
name = nil
item.search('a').each do |link_a|
name = link_a.inner_html.gsub(@str_nbs, "")
end
name = item.inner_html if name == nil
@race_item.name = name.gsub(@str_nbs, "")
@race_item.name.strip!
end
#//*[@id="race_tacho"]
tacho.search('div').select{|litem| litem.attributes["id"].value == "race_tacho"}.each do |item_value|
@race_item.title = item_value.inner_html.gsub(@str_nbs, "") #remove non breaking spaces ->  
end
if !@race_item.is_item_recent?(latest_date_in_db)
#p @race_item.race_date
@log.debug "Ignore item #{i} - date: #{@race_item.race_date} - #{@race_item.title}.\nSearch is terminated because now all races should be already into the db, but consider to make a consistency check."
break;
end
#//*[@id="distanz_tacho"]
tacho.search('div').select{|litem| litem.attributes["id"].value == "distanz_tacho"}.each do |item_value|
@race_item.km_length = item_value.children.last.text.strip
end
#//*[@id="zeit_tacho"]
tacho.search('div').select{|litem| litem.attributes["id"].value == "zeit_tacho"}.each do |item_value|
@race_item.race_time = item_value.children.last.text.strip
end
#//*[@id="minprokm_tacho"]
tacho.search('div').select{|litem| litem.attributes["id"].value == "minprokm_tacho"}.each do |item_value|
@race_item.pace_minkm = item_value.children.last.text.strip.gsub(@str_nbs2,"")
end
#//*[@id="kmproh_tacho"]
tacho.search('div').select{|litem| litem.attributes["id"].value == "kmproh_tacho"}.each do |item_value|
@race_item.pace_kmh = item_value.children.last.text.gsub(@str_nbs2, "")
end
#//*[@id="gesamtrang_tacho"]
tacho.search('div').select{|litem| litem.attributes["id"].value == "gesamtrang_tacho"}.each do |item_value|
@race_item.rank_global = item_value.children.first.text
end
#rank_gender
#//*[@id="mwrang_tacho"]
tacho.search('div').select{|litem| litem.attributes["id"].value == "mwrang_tacho"}.each do |item_value|
@race_item.rank_gender = item_value.children.first.text
end
#rank_class
#//*[@id="klassenrang_tacho"]
tacho.search('div').select{|litem| litem.attributes["id"].value == "klassenrang_tacho"}.each do |item_value|
@race_item.rank_class = item_value.children.first.text
@race_item.class_name = item_value.children.last.text.gsub("Kl.","")
end
#//*[@id="container_kommentar_tacho"]
tacho.search('div').select{|litem| litem.attributes["id"].value == "container_kommentar_tacho"}.each do |item_value|
@race_item.comment = item_value.children.last.text
end
#p @race_item
@races << @race_item
@log.debug "#{@race_item.title} - #{@race_item.race_date}"
i += 1
#ergebnis_bewerbdatum
end
@log.debug "Found #{@races.length} items that need to be inserted into the db"
#p @races
end
def insert_races_indb
@races.sort{|a,b| a.race_date <=> b.race_date}.each do |item|
#insert the oldest new item first
#p item
insert_indb(item)
end
if @races.length == 0
@log.debug "Db is already updated, no new races to insert found"
else
@log.debug "Inserted #{@races.length} new races successfully."
end
end
def check_races(opt)
insert_missed = opt[:insert_missed]
@missed_items = []
@inserted = []
@races.sort{|a,b| a.race_date <=> b.race_date}.each do |item|
if is_race_item_indb?(item)
@log.debug "race item #{item.title} - #{item.race_date} is stored OK"
elsif insert_missed
insert_indb(item)
@inserted << item
@log.debug "#{item.title} - #{item.race_date} inserted successfully."
else
@log.debug "race item #{item.title} - #{item.race_date} is not in the db."
@missed_items << item
end
end
@log.debug "Checked #{@races.size} races: #{@missed_items.size} are missed"
if @missed_items.size == 0 && @inserted.size == 0
@log.debug "Race table in Db is OK (race_date and title, if you have changed other fields, please delete the record into the db and run this check again)"
elsif @inserted.size > 0 && @missed_items.size == 0
@log.debug "Inserted #{@inserted.size} races, now db should be sync"
else
@log.debug "Please run again check_races with the insert missed option set to true, or use insert_races_indb"
end
end
end
if $0 == __FILE__
# Nota: non è necessario cambiare questo script, basta usare la linea di comando
# Quello che fa questo script è andare sul sito di Pentenk, prendere le gare e
# importarle nel database. È in grado di importare solo quelle nuove, ma anche tutte le altre e di fare un check tra il db e il sito.
require 'log4r'
include Log4r
@log = Log4r::Logger.new("RacePicker")
Log4r::Logger['RacePicker'].outputters << Outputter.stdout
url = "https://www.membersclub.at/ccmc_showprofile.php?unr=9671&show_tacho=1&pass=008"
picker = RacePicker.new
check_consistency = false
insert_new_races = true
insert_missed = false
if ARGV.size > 0
if ARGV[0] == '/help'
@log.debug 'Advanced Usage : ruby race_picker.rb "{:check_consistency => true, :insert_missed => false, :insert_new_races => false}"'
@log.debug 'Advanced Usage2: ruby race_picker.rb "{:check_consistency => true, :insert_missed => true, :insert_new_races => false}"'
@log.debug "Usual usage: ruby race_picker.rb (default values: check_consistency = false, insert_new_races = true, insert_missed = false)"
exit
else
#p ARGV[0]
opt = eval(ARGV[0])
check_consistency = opt[:check_consistency]
insert_missed = opt[:insert_missed]
insert_new_races = opt[:insert_new_races]
end
end
if insert_missed && !check_consistency
@log.error ":insert_missed works only with :check_consistency"
end
@log.debug "Options are: check_consistency: #{check_consistency} (insert_missed: #{insert_missed}), insert_new_races: #{insert_new_races}"
if check_consistency
picker.pick_races(url, Time.parse("2000-01-01 00:00:00"))
picker.check_races({:insert_missed => insert_missed})
end
if insert_new_races
latest_date_in_db = picker.get_latest_racedate_indb
picker.pick_races(url, latest_date_in_db)
picker.insert_races_indb
end
end
<file_sep># -*- coding: ISO-8859-1 -*-
require 'rubygems'
require 'log4r'
include Log4r
class FileAppenderExtraSim
def initialize
@log = Log4r::Logger.new("FileAppenderExtraSim")
@log.outputters << Outputter.stdout
#FileOutputter.new('FileAppenderExtraSim', :filename=> 'FileAppenderExtraSim.log')
#Logger['FileAppenderExtraSim'].add 'FileAppenderExtraSim'
end
def getnumLinesWritten(target_file)
res = 0
if File.exist?(target_file)
res = File.open(target_file).readlines.size
end
return res
end
def write_some_lines(src_file, target_file, num_to_write)
@log.debug("process #{src_file}")
src_lines = []
num_in_target = getnumLinesWritten(target_file)
@log.debug("Num lines written in target #{num_in_target}")
i = 0
File.open(src_file).each_line do |line|
if i >= num_in_target and src_lines.length < num_to_write
src_lines << line
end
i += 1
end
@log.debug("Source file has #{i} lines, jump the first #{num_in_target} lines and writes #{num_to_write} lines.")
File.open(target_file, 'a'){|f| src_lines.each{|x| f << x}}
@log.debug("Target file #{target_file} has been updated with #{num_to_write} lines")
end
##
# Append lines into the target file using the src_file. Timeout in seconds is used to do the append.
# Lines already written into the target are skipped, so you can restart and stop this function and the append process continue.
def write_lines_after_timeout(src_file, target_file, numrecords, timeout)
@log.debug("process #{src_file}")
src_lines = []
num_in_target = getnumLinesWritten(target_file)
@log.debug("Num lines written in target #{num_in_target}")
i = 0
File.open(src_file).each_line do |line|
if i >= num_in_target
src_lines << line
end
i += 1
end
@log.debug("Start to writes lines after #{timeout} seconds, lines to be written are #{src_lines.size}")
written = 0
arr_num = numrecords.dup
while src_lines.size > 0
sleep timeout
num_to_write = arr_num.pop
curr_slice = src_lines.slice!(0..num_to_write - 1)
File.open(target_file, 'a'){|f| curr_slice.each{|x| f << x}}
written += curr_slice.size
@log.debug("#{curr_slice.size} lines appended, written in this session until now are #{written}")
if arr_num.length == 0
arr_num = numrecords.dup
end
end
end
end
if $0 == __FILE__
app = FileAppenderExtraSim.new
numrec = 5
#src_file = 'C:\temp\SPMS\bs01\Archive\calls_2014.11.03.log'
#target_file = 'C:\temp\KPI\bs01\logs\calls_2014.11.03.log'
#app.write_some_lines(src_file, target_file, numrec)
#change the update file
src_file = 'C:\temp\SPMS\bs01\Archive\calls_2014.11.04.log'
target_file = 'C:\temp\KPI\bs01\logs\xcalls_2015.11.06.log'
app.write_some_lines(src_file, target_file, numrec)
timeout = 1
numrecords = [5,4,3,2,1]
#numrecords = [1]
#app.write_lines_after_timeout(src_file, target_file, numrecords, timeout)
end<file_sep># file: gpx_redux.rb
##
# Used to reduce gpx tracks or split into more files
class GpxReducer
def initialize
@header = []
@footer = []
@all_points = []
@gpx_fname = ''
end
def points_counter(fname)
@gpx_fname = fname
header = []
footer = []
curr_point = []
all_points = []
state = :init_seg
points_count = 0
File.open(fname).each_line do |line|
if line =~ /<trkseg/
header << line
state = :init_point
elsif line =~ /<trkpt/
# inizio punto
if state == :init_point
state = :on_point
points_count += 1
curr_point = []
curr_point << line
end
elsif line =~ /<\/trkpt/
# fine punto
if state == :on_point
curr_point << line
#puts"current point\n#{curr_point}"
all_points << curr_point
state = :init_point
end
elsif line =~ /<\/trkseg/
# fine traccia
footer << line
state = :end_file
else
if state == :init_seg
header << line
elsif state == :end_file
footer << line
elsif state == :on_point
curr_point << line
end
end
end # file open
puts "Header:\n#{header}"
puts "Footer:\n#{footer}"
puts "Points count: #{points_count}(#{all_points.size}) for file #{fname}"
@all_points = all_points
@footer = footer
@header = header
end
def gpx_splitter(num_of_tracks, out_dir)
if @all_points.size == 0
puts "Call firts points_counter() to get points information. No points recognized"
return
end
if num_of_tracks < 2
puts "Nothing to do, use the souce track if you want"
end
if !File.directory?(out_dir)
Dir.mkdir(out_dir)
end
points_per_track = @all_points.size / num_of_tracks
tracks = []
in_track_points = []
@all_points.each do |point|
in_track_points << point
if in_track_points.size >= points_per_track and tracks.size < num_of_tracks - 1
tracks << in_track_points
in_track_points = []
end
end
tracks << in_track_points
ix_track = 1
tracks.each do |track|
trackname = File.join(out_dir, "Track#{ix_track}.gpx")
complete_track = []
complete_track << @header
complete_track << track
complete_track << @footer
#puts "Track #{ix_track}:\n#{complete_track}"
puts "Points in #{trackname} are: #{track.size}"
File.open(trackname, 'w'){|f| complete_track.flatten.each{|x| f << x}}
puts "File written #{trackname}"
ix_track += 1
end
end
def reduce_half(fname, out_dir, out_fname)
arr_res = []
state = :init_point
count_lines = 0
File.open(fname).each_line do |line|
#p line
count_lines += 1
if line =~ /<trkpt/
# inizio punto
if state == :init_point
state = :on_point
arr_res << line
else
p "skip IP (#{state}): #{line}"
end
elsif line =~ /<\/trkpt/
# fine punto
if state == :on_point
arr_res << line
state = :jump__next_point
elsif state == :jump__next_point
p "skip OP (#{state}): #{line}"
state = :init_point
end
elsif line =~ /<\/trkseg/
# fine traccia
state = :end_file
arr_res << line
else
if state != :jump__next_point
arr_res << line
else
p "skip OTHer (#{state}): #{line}"
end
end
end
jumped_points = (count_lines - arr_res.size) / 4
puts "Lines on file now #{arr_res.size}, original lines #{count_lines}, jumped points #{jumped_points}"
res_fname = File.join(out_dir, out_fname)
File.open(res_fname, 'w'){|f| arr_res.each{|x| f << x}}
puts "File created #{res_fname}"
end
end
if $0 == __FILE__
# Usa questo script per ridurre una traccia della metà e poi magari farne uno split.
# Con Canmore è meglio avere dei files con intorno 700 punti massimo, per aprirli e scalarli
# in tempi ragionevoli. I nomi dei files vanno anche cambiati manualmente usando notepad.
# Usa qualcosa tipo 1- desc traccia1 e così via. Basta cambiare il tag <trk><name> (1 punto)
# Usa poi Canway Planner e importa tutte le traccie in un colpo prima di sincronizzare col device.
# Se fai degli errori, chiudi Canway Planner e ricomincia. Le traccie sul device vanno cancellate manulamente
# usando file explorer nel subfolder trips.
# Con il file del Dirndltal Extrem scaricato da gpsies, aveva 1924 punti che ho splitato in 3 files da 641 punti.
gr = GpxReducer.new
#fname = 'D:\Corsa\2020\SpineRace\track\CP5+-+FIN+PADON.gpx'
#fname = 'D:\Corsa\2020\SpineRace\track\reduced\06-cp5-FIN-hpC.gpx'
out_fname = '04-cp3-cp4-hpC.gpx'
out_dir = 'D:\Corsa\2020\SpineRace\track\half-orig\04'
#gr.reduce_half(fname, out_dir, out_fname)
#gr.points_counter(fname)
gr.points_counter(File.join(out_dir, out_fname))
#gr.gpx_splitter(2, out_dir)
end<file_sep># -*- coding: ISO-8859-1 -*-
require 'rubygems'
require 'filescandir'
require 'fileutils'
class JsUIPublisher
def sync_src_with_dest(src_dir, dst_dir, force_dir_arr)
@robocopy_path = 'C:\Windows\SysWOW64\Robocopy.exe'
p "scan src dir"
src_items = scan_path(src_dir)
p "scan dst dir"
dst_items = scan_path(dst_dir)
puts "Src items #{src_items.size}, Dst items #{dst_items.size}"
p "check for missed files in dst"
items_not_in_dst = check_presence(src_items, dst_items)
puts "Items(#{items_not_in_dst.size}) not in destination dir:"
items_not_in_dst.each do |item|
puts item
end
p "check for missed files in src"
items_not_in_src = check_presence(dst_items, src_items)
puts "Items(#{items_not_in_src.size}) not in source dir (delete it manually if you do not need it):"
items_not_in_src.each do |item|
puts item
end
p " - COPY STUFF starts here --"
p "copy src files to dst"
exclude = ['puesraWebUI.csproj','puesraWebUI.csproj.vspscc','app/config.json', 'packages.config']
copy_newfiles_to_dest(dst_dir, exclude, items_not_in_dst, src_dir)
exclude << items_not_in_dst
exclude.flatten!
copy_files_to_dest(dst_dir, exclude, src_items, src_dir, force_dir_arr)
puts "Terminated at #{Time.now}"
end
private
def copy_files_to_dest(dst_dir, filter_items, src_items, src_dir, force_dir_arr)
count = 0
src_items.each do |src_item|
unless filter_items.index(src_item)
dst_fname = File.join(dst_dir, src_item)
src_fname = File.join(src_dir, src_item)
stat_dst = File.stat(dst_fname)
stat_src = File.stat(src_fname)
is_force_item = calc_is_forceitem(src_item, force_dir_arr)
if (stat_dst.mtime != stat_src.mtime and
stat_dst.mtime < stat_src.mtime) or is_force_item
#FileUtils.cp_r(src_fname, dst_fname, :verbose => true)
robocopy(src_dir, dst_dir, src_item)
count += 1
#p stat_dst.mtime
#p stat_src.mtime
p "copy #{src_fname} to #{dst_fname}"
end
end
end
puts "#{count} modified files copied."
end
def robocopy(src_dir, dst_dir, src_item)
arr_names = src_item.split('/')
name = src_item
name_src_dir = src_dir
name_dst_dir = dst_dir
if arr_names.size > 0
last_ix = arr_names.size - 1
name = arr_names[last_ix]
arr_names.delete_at(last_ix)
end
arr_names.each do |nn|
name_src_dir = File.join(name_src_dir, nn)
name_dst_dir = File.join(name_dst_dir, nn)
end
cmd = "#{@robocopy_path} \"#{name_src_dir}\" \"#{name_dst_dir}\" #{name}"
#puts cmd
result_cmd = []
on_error = false
IO.popen(cmd, "r") do |io|
io.each_line do |line|
on_error = true if line =~ /ERROR/
result_cmd << line
end
end
if on_error
puts "ERROR executing #{cmd}"
result_cmd.each do |line|
puts line
end
puts "!!!!!!! Exit because ERROR on robocopy error, FIX this error first please!!!!!"
exit
end
end
def calc_is_forceitem(src_item, force_dir_arr)
item_slash_count = src_item.count('/')
force_dir_arr.each do |dir|
dir.gsub!("\\",'/')
dir_slash_count = dir.count('/') + 1
#p src_item, dir
if src_item.include?(dir) and item_slash_count == dir_slash_count
puts "force #{src_item}"
return true
end
end
return false
end
def copy_newfiles_to_dest(dst_dir, filter_items, items_not_in_dst, src_dir)
count = 0
items_not_in_dst.each do |src_item|
unless filter_items.index(src_item)
dst_fname = File.join(dst_dir, src_item)
src_fname = File.join(src_dir, src_item)
p "new copy #{src_fname} to #{dst_fname}"
#FileUtils.cp_r(src_fname, dst_fname)
robocopy(src_dir, dst_dir, src_item)
count += 1
end
end
puts "#{count} new files copied." if count > 0
end
def scan_path(path_to_scan)
fscd = FileScanDir.new
fscd.is_silent = true
fscd.add_extension_filter(['.cache'])
fscd.scan_dir(path_to_scan)
dir_result = []
fscd.result_list.each do |line|
dir_result << line.gsub(path_to_scan + '/', '')
end
return dir_result
end
def check_presence(src_items, dst_items)
result = []
src_items.each do |item|
is_included = check_included_item(item, dst_items)
if !is_included
result << item
end
end
return result
end
def check_included_item(item_to_find, dst_items)
dst_items.each do |item|
if item == item_to_find
return true
end
end
return false
end
end
if $0 == __FILE__
src_dir = 'D:\ws\SPMS\user_sartorii_001\Application\puesraWebUI'
dst_dir = 'C:\Program Files (x86)\apache-tomcat-7.0.57\webapps\puesraWebUI'
#src_dir = 'D:\ws\openam_custom\12.0.0\war_from_zip\edited'
#dst_dir = 'C:\Program Files (x86)\apache-tomcat-7.0.57\webapps\openam'
#force_dir = ['Scripts']
force_dir = []
sync = JsUIPublisher.new
sync.sync_src_with_dest(src_dir, dst_dir, force_dir)
end | deace84682dd0ba9c19d50b03d790364e88a13f0 | [
"Markdown",
"SQL",
"Ruby"
] | 15 | Ruby | aaaasmile/ruby_scratch | 5751d9edb663034074f95f4309613282284bd3f4 | 20feb1e0e69a1c71798f7bb94a2ad138582f271d | |
refs/heads/master | <file_sep>import Route from '@ember/routing/route';
import { inject as service } from '@ember/service';
export default Route.extend({
init() {
this._super(...arguments);
this.on('routeDidChange', () => {
const page = this.get('router.url');
const title = this.getWithDefault('currentRouteName', 'unknown');
this.get('metrics').trackPage({ page, title });
});
},
metrics: service(),
router: service()
});
<file_sep>{
"name": "ivy-tabs",
"version": "3.3.1",
"description": "WAI-ARIA accessible tabs for Ember.",
"keywords": [
"ember",
"ember-addon",
"tabs"
],
"repository": "https://github.com/IvyApp/ivy-tabs",
"license": "MIT",
"author": "<NAME>",
"directories": {
"doc": "doc",
"test": "tests"
},
"bugs": {
"url": "https://github.com/IvyApp/ivy-tabs/issues"
},
"scripts": {
"build": "ember build",
"deploy": "ember build && ember github-pages:commit --message \"Deploy gh-pages from commit $(git rev-parse HEAD)\" && git push origin gh-pages:gh-pages",
"format": "prettier --single-quote --write './*.js' './{addon,app,config,tests}/{**/,}*.js'",
"lint:hbs": "ember-template-lint .",
"lint:js": "eslint .",
"release": "standard-version",
"start": "ember server",
"test": "ember test",
"test:all": "ember try:each"
},
"dependencies": {
"ember-cli-babel": "^7.7.3",
"ember-cli-htmlbars": "^3.0.1"
},
"devDependencies": {
"@commitlint/config-conventional": "^8.0.0",
"@ember/optional-features": "^1.0.0",
"@types/prettier": "^1.16.1",
"bootstrap": "^4.0.0",
"broccoli-asset-rev": "^3.0.0",
"commitizen": "^3.0.7",
"commitlint": "^8.0.0",
"cz-conventional-changelog": "^2.1.0",
"ember-cli": "~3.14.0",
"ember-cli-app-version": "^3.1.0",
"ember-cli-dependency-checker": "^3.1.0",
"ember-cli-eslint": "^5.1.0",
"ember-cli-github-pages": "^0.2.0",
"ember-cli-htmlbars-inline-precompile": "^3.0.0",
"ember-cli-inject-live-reload": "^2.0.1",
"ember-cli-release": "^0.2.9",
"ember-cli-sri": "^2.1.1",
"ember-cli-template-lint": "^1.0.0-beta.1",
"ember-cli-test-loader": "^2.2.0",
"ember-cli-uglify": "^3.0.0",
"ember-disable-prototype-extensions": "^1.1.3",
"ember-export-application-global": "^2.0.0",
"ember-load-initializers": "^2.0.0",
"ember-maybe-import-regenerator": "^0.1.6",
"ember-metrics": "^0.14.0",
"ember-qunit": "^4.4.1",
"ember-resolver": "^7.0.0",
"ember-source": "~3.15.0",
"ember-source-channel-url": "^2.0.1",
"ember-try": "^1.0.0",
"eslint-plugin-ember": "^6.3.0",
"eslint-plugin-node": "^10.0.0",
"husky": "^3.0.0",
"loader.js": "^4.7.0",
"prettier": "^1.11.1",
"qunit-dom": "^0.9.0",
"standard-version": "^7.0.0"
},
"resolutions": {
"conventional-changelog": "~3.0.6"
},
"engines": {
"node": "8.* || >= 10.*"
},
"ember-addon": {
"configPath": "tests/dummy/config",
"demoURL": "http://ivyapp.github.io/ivy-tabs/"
},
"config": {
"commitizen": {
"path": "cz-conventional-changelog"
}
},
"husky": {
"hooks": {
"commit-msg": "commitlint -E HUSKY_GIT_PARAMS"
}
}
}
| 9cdb6927c97688def83da91eb5e1d2f8cff9f0cc | [
"JavaScript",
"JSON"
] | 2 | JavaScript | jtappa/ivy-tabs | 191ebb6bc1c28fad5276a01dc5f5c25177efc156 | 24eb4bb47d449ee3ed1afe98a51e0d8fb27502e5 | |
refs/heads/master | <repo_name>KairiGG/ENSIIE_S3-CI<file_sep>/src/book.repository.test.js
const BookRepository = require('./book.repository');
describe('Book repository Save', function () {
test('Save a book', () => {
const dbMock = {
get : jest.fn().mockReturnThis(),
push : jest.fn().mockReturnThis(),
write : jest.fn().mockReturnThis()
};
const repository = new BookRepository(dbMock);
repository.save({id: 1, name: "<NAME>"});
expect(dbMock.write.mock.calls.length).toBe(1);
});
});
describe('Book repository GetTotalCount', function () {
test('Get total count of books', () => {
const dbMock = {
get : jest.fn().mockReturnThis(),
size : jest.fn().mockReturnThis(),
value : jest.fn().mockReturnValue(3)
};
const repository = new BookRepository(dbMock);
expect(repository.getTotalCount()).toBe(3);
});
});
describe('Book repository GetTotalPrice', function () {
test('Get total price of books', () => {
const dbMock = {
get : jest.fn().mockReturnThis(),
map : jest.fn().mockReturnThis(),
value : jest.fn().mockReturnValue([5, 5, 5, 5, 5, 5, 5])
};
const repository = new BookRepository(dbMock);
expect(repository.getTotalPrice()).toBe(35);
});
});
describe('Book repository GetBookByName', function () {
test('Get book by name', () => {
const dbMock = {
get : jest.fn().mockReturnThis(),
find : jest.fn().mockReturnThis(),
value : jest.fn().mockReturnValue({ "id": 1, "name": "test", "price": 6.1, "added_at": "2019-01-01" })
};
const repository = new BookRepository(dbMock);
expect(repository.getBookByName('test')).toStrictEqual({ "id": 1, "name": "test", "price": 6.1, "added_at": "2019-01-01" });
});
});
describe('Book repository getCountBookAddedByMonth', function () {
test('Get count book added by month', () => {
const dbMock = {
get : jest.fn().mockReturnThis(),
filter : jest.fn().mockReturnThis(),
map : jest.fn().mockReturnThis(),
value : jest.fn().mockReturnValue([ "2019-06-02", "2021-01-01", "2019-01-01", "2020-03-01", "2019-01-04", "2021-02-01", "2019-01-01", "2032-01-01", "2019-01-01" ])
};
const repository = new BookRepository(dbMock);
expect(repository.getCountBookAddedByMonth('test')).toStrictEqual([ { year: '2019', month: 1, count: 4, count_cumulative: 4 },
{ year: '2019', month: 6, count: 1, count_cumulative: 5 },
{ year: '2020', month: 3, count: 1, count_cumulative: 1 },
{ year: '2021', month: 1, count: 1, count_cumulative: 1 },
{ year: '2021', month: 2, count: 1, count_cumulative: 2 },
{ year: '2032', month: 1, count: 1, count_cumulative: 1 } ]
);
});
});
<file_sep>/src/interval.test.js
const Interval = require('./interval');
describe('overlaps', function () {
test('Test overlapping, if two intervals overlap each other {(1, 3),(2, 4)}', () => {
let interval1 = new Interval(1, 3);
let interval2 = new Interval(2, 4);
expect(interval1.overlaps(interval2)).toBe(true);
});
test('Test overlapping, if two intervals don\'t overlap each other{(1, 2),(3, 4)}', () => {
let interval1 = new Interval(1,2);
let interval2 = new Interval(3,4);
expect(interval1.overlaps(interval2)).toBe(false);
});
test('Test overlapping, if two intervals don\'t overlap each other{(3, 4),(1, 2)}', () => {
let interval1 = new Interval(3,4);
let interval2 = new Interval(1,2);
expect(interval1.overlaps(interval2)).toBe(false);
});
});
describe('includes', function () {
test('Test include function, if the interval contains the argument {(1, 4),(2, 3)}', () => {
let interval1 = new Interval(1, 4);
let interval2 = new Interval(2, 3);
expect(interval1.includes(interval2)).toBe(true);
});
test('Test include function, if the interval start after the argument {(2, 4),(1, 3)}', () => {
let interval1 = new Interval(2, 4);
let interval2 = new Interval(1, 3);
expect(interval1.includes(interval2)).toBe(false);
});
test('Test include function, if the interval end before the argument {(1, 3),(2, 4)}', () => {
let interval1 = new Interval(1,3);
let interval2 = new Interval(2,4);
expect(interval1.includes(interval2)).toBe(false);
});
test('Test include function, if the interval end equals the argument {(1, 4),(1, 4)}', () => {
let interval1 = new Interval(1,4);
let interval2 = new Interval(1,4);
expect(interval1.includes(interval2)).toBe(true);
});
});
describe('union', function () {
test('Test union function, if the interval overlap the argument {(1, 3),(2, 4)}', () => {
let interval1 = new Interval(1, 3);
let interval2 = new Interval(2, 4);
expect(interval1.union(interval2)).toStrictEqual([new Interval(1,4)]);
});
test('Test union function, if the interval doesn\'t overlap the argument {(1, 2),(3, 4)}', () => {
let interval1 = new Interval(1, 2);
let interval2 = new Interval(3, 4);
expect(interval1.union(interval2)).toStrictEqual([interval1, interval2]);
});
});
describe('intersection', function () {
test('Test intersection function, if the interval overlap the argument {(1, 3),(2, 4)}', () => {
let interval1 = new Interval(1, 3);
let interval2 = new Interval(2, 4);
expect(interval1.intersection(interval2)).toStrictEqual(new Interval(2,3));
});
test('Test intersection function, if the interval doesn\'t overlap the argument {(1, 2),(3, 4)}', () => {
let interval1 = new Interval(1, 2);
let interval2 = new Interval(3, 4);
expect(interval1.intersection(interval2)).toStrictEqual(null);
});
});
describe('exclusion', function () {
test('Test exclusion function, if the interval overlap the argument {(1, 3),(2, 4)}', () => {
let interval1 = new Interval(1, 3);
let interval2 = new Interval(2, 4);
expect(interval1.exclusion(interval2)).toStrictEqual([new Interval(1,2), new Interval(3,4)]);
});
test('Test exclusion function, if the interval doesn\'t overlap the argument {(1, 2),(3, 4)}', () => {
let interval1 = new Interval(1, 2);
let interval2 = new Interval(3, 4);
expect(interval1.exclusion(interval2)).toStrictEqual([interval1, interval2]);
});
});
<file_sep>/src/math.js
let Util = {};
Util.factorial = (n) => {
if (n === 0) {
return 1;
}
if (n >= 3000) {
throw 'n too large'
}
if (n < 0) {
throw 'n is negative'
}
return n * Util.factorial(n - 1);
};
/**
* Détermine si n est un nombre premier.
* Util.isPrime(5) => false
* Util.isPrime(6) => true
*
* @param {number} n
* @returns {boolean}
*/
Util.isPrime = function (n) {
if (n === 1 || n === 0) {
return false;
}
if (n < 0) {
throw 'Unable to compute prime for n < 0'
}
for (var i = 2; i < n; i++)
if (n % i === 0) return false;
return true;
};
/**
* Additionne l'ensemble des nombres premiers de 2 à n
*
* Util.sumPrime(6) = 2 + 3 + 5 = 10
* Util.sumPrime(8) = 2 + 3 + 5 + 7 = 17
*
* @param {number} n
* @returns {number}
*/
Util.sumPrime = function(n) {
if(!Util.isPrime(n)) {
throw 'n is not prime'
}
else {
let result = 0;
for(let i = 2; i <= n; i++) {
if(Util.isPrime(i))
result += i;
}
return result;
}
};
/**
* Cette méthode doit retourner un tableau de 1 à n tel que:
* - Pour les nombres multiples de 3, les remplacer par "Fizz"
* - Pour les nombres multiples de 5, les remplacer par "Buzz"
* - Pour les nombres multiples de 3 et 5, les remplacer par "FizzBuzz"
*
* Exp :
* Util.fizzBuzz(15) => [1, 2, "Fizz", 4, "Buzz", "Fizz", 7, 8, "Fizz", "Buzz", 11, "Fizz", 13, 14, "FizzBuzz"]
*
* @param {number} n
* @returns {array}
*/
Util.fizzBuzz = function(n) {
if(n < 1)
throw 'n should be superior to 0'
let result = []
for(let i=1; i < n + 1; i++)
result.push((i%3?'':'Fizz') + (i%5?'':'Buzz') || i)
return result;
};
/**
* Chiffre une phrase selon la règle suivante : Les A deviennent des B, les B des C, etc.
*
* Exp :
* Util.cipher("Test Unitaire") => "Uftu Tojubjsf"
*
* @param phrase
* @returns {string}
*/
Util.cipher = function (phrase) {
let charTransformation = (char) => {
let charValue = char.charCodeAt(0);
if(charValue < 65 || charValue > 122
|| (charValue > 90 && charValue < 97)) {
return char; //special char -> return char
} else {
if(charValue == 90) {
return 'A'; //'Z' case -> return 'A'
} else if(charValue == 122) {
return 'a'; //'z' case -> return 'a'
} else {
return String.fromCharCode(charValue + 1);
}
}
};
let charCollection = phrase.split("");
return charCollection.map( c => charTransformation(c) )
.reduce( (str, char) => str += char );
};
module.exports = Util;
<file_sep>/src/math.test.js
const Util = require('./math');
describe('isPrime', function () {
test('Test factoriel de 0 => 1', () => {
expect(Util.factorial(0)).toBe(1);
});
test('Test factoriel de 2 => 2', () => {
expect(Util.factorial(3)).toBe(6);
});
test('Test factoriel de 3 => 6', () => {
expect(Util.factorial(3)).toBe(6);
});
test('Test factoriel de 3000', () => {
expect(()=> {Util.factorial(3000)}).toThrow();
});
test('Test factoriel -10', () => {
expect(()=> {Util.factorial(-10)}).toThrow(/negative/);
});
});
describe('isPrime', function () {
test('Test prime de 1 => false', () => {
expect(Util.isPrime(1)).toBe(false)
});
test('Test prime de 0 => false', () => {
expect(Util.isPrime(0)).toBe(false)
});
test('Test prime < 0 => throw exception', () => {
expect(() => { Util.isPrime(-10) }).toThrow('Unable to compute prime for n < 0');
});
test.each([
[2, true],
[5, true],
[17, true],
[18, false],
[53, true],
[55, false],
])(
'isPrime %i equals to %i',
(n, expected) => {
expect(Util.isPrime(n)).toBe(expected);
}
);
});
describe('sumPrime', function () {
test('Test somme d\'un nombre non-premier négatif, n=-10', () => {
expect(() => {Util.sumPrime(-10)}).toThrow('Unable to compute prime for n < 0');
});
test('Test somme d\'un nombre non-premier, n=26', () => {
expect(() => {Util.sumPrime(26)}).toThrow('n is not prime');
});
test('Test somme d\'un nombre premier, n=7', () => {
expect(Util.sumPrime(7)).toBe(17);
});
test('Test somme d\'un nombre premier, n=47', () => {
expect(Util.sumPrime(47)).toBe(328);
});
});
describe('fizzBuzz', function () {
test('Test FizzBuzz n < 1, n=0', () => {
expect(() => {Util.fizzBuzz(0)}).toThrow('n should be superior to 0');
});
test('Test FizzBuzz n < 1, n=-63', () => {
expect(() => {Util.fizzBuzz(-63)}).toThrow('n should be superior to 0');
});
test('Test FizzBuzz, n=15', () => {
let expectedResult = [1, 2, "Fizz", 4, "Buzz", "Fizz", 7, 8, "Fizz", "Buzz", 11, "Fizz", 13, 14, "FizzBuzz"];
expect(Util.fizzBuzz(15)).toStrictEqual(expectedResult);
});
test('Test FizzBuzz, n=30', () => {
let expectedResult = [1, 2, "Fizz", 4, "Buzz", "Fizz", 7, 8, "Fizz", "Buzz", 11, "Fizz", 13, 14, "FizzBuzz"
, 16, 17, "Fizz", 19, "Buzz", "Fizz", 22, 23, "Fizz", "Buzz", 26, "Fizz", 28, 29, "FizzBuzz"];
expect(Util.fizzBuzz(30)).toStrictEqual(expectedResult);
});
});
describe('cipher', function () {
test('Test cipher, phrase="Test Unitaire"', () => {
expect(Util.cipher('Test Unitaire')).toBe('Uftu Vojubjsf');
});
test('Test cipher avec phrase contenant "z", phrase="Test Unitaire du z"', () => {
expect(Util.cipher('Test Unitaire du z')).toBe('Uftu Vojubjsf ev a');
});
test('Test cipher avec phrase contenant "Z", phrase="Test Unitaire du Z"', () => {
expect(Util.cipher('Test Unitaire du Z')).toBe('Uftu Vojubjsf ev A');
});
test('Test cipher avec phrase contenant des caractèresspéciaux, phrase="\'"&@=+!§^?,;é"', () => {
expect(Util.cipher('\'"&@=+!§^?,;é')).toBe('\'"&@=+!§^?,;é');
});
});
<file_sep>/src/test-script.js
const BookRepository = require('./book.repository');
const db = require('./db')
const repository = new BookRepository(db);
<file_sep>/src/book.repository.js
class BookRepository {
/**
* @param db
*/
constructor(db) {
this.db = db;
}
save (book) {
this.db.get('books').push(book).write();
}
/**
* Nombre total de livre
*/
getTotalCount() {
return this.db.get('books').size().value();
}
/**
* Somme du prix de tous les livre
*/
getTotalPrice() {
return this.db.get('books').map('price').value()
.reduce( (acc, val) => acc += val );
}
/**
* Retourne un livre
*/
getBookByName(bookName) {
return this.db.get('books')
.find({ name: bookName })
.value();
}
/**
* Nombre de livre ajouté par mois
*
* [
* {
* year: 2017,
* month: 2,
* count: 129,
* count_cumulative: 129
* },
* {
* year: 2017,
* month: 3,
* count: 200,
* count_cumulative: 329
* },
* ....
* ]
*/
getCountBookAddedByMonth(bookName) {
let dates = this.db.get('books')
.filter({ name: bookName})
.map('added_at')
.value()
.map( function(date){ return {year: new Date(date).getYear() + 1900, month: new Date(date).getMonth()}} );
let planning = {};
for(let date of dates) {
if(!(date.year in planning))
planning[date.year] = new Array(12).fill(0);
planning[date.year][date.month] += 1;
}
let cumulativePlanning = JSON.parse(JSON.stringify(planning))
for(let year in cumulativePlanning)
for(let i = 1; i < cumulativePlanning[year].length; i++)
cumulativePlanning[year][i] += cumulativePlanning[year][i - 1];
let accounting = [];
for(let year in planning) {
for(let i = 0; i < planning[year].length; i++)
if(planning[year][i] > 0)
accounting.push(
{year: year, month: i + 1, count: planning[year][i], count_cumulative: cumulativePlanning[year][i]}
);
}
return accounting;
}
}
module.exports = BookRepository;
| 406aca7832eb0295f5d193740b624933c3854256 | [
"JavaScript"
] | 6 | JavaScript | KairiGG/ENSIIE_S3-CI | 38f8a2ddfa4dd7b963c50bf58e19e4eccee39d23 | c7e5f9966729dbadee494bb9665950e39c7d813c | |
refs/heads/master | <repo_name>RyanZhu1024/java_concurrency<file_sep>/src/main/java/producerconsumers/Main.java
package producerconsumers;
/**
* Created by shuxuan on 1/22/15.
*/
public class Main {
public static void main(String[] args) {
FileMock fileMock=new FileMock(100,10);
Buffer buffer=new Buffer(20);
Producer producer=new Producer(fileMock,buffer);
Consumer[] consumers=new Consumer[3];
Thread threadProducer=new Thread(producer, "producer");
Thread[] threadConsumers=new Thread[3];
for(int i=0;i<3;i++){
consumers[i]=new Consumer(buffer);
threadConsumers[i]=new Thread(consumers[i],"consumer"+i);
}
threadProducer.start();
for(int i=0;i<3;i++){
threadConsumers[i].start();
}
}
}
<file_sep>/src/main/java/forkjoin/DocumentTask.java
package forkjoin;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.ForkJoinTask;
import java.util.concurrent.RecursiveTask;
/**
* Created by shuxuan on 1/30/15.
*/
public class DocumentTask extends RecursiveTask<Integer> {
private String[][] document;
private int start;
private int end;
private String word;
public DocumentTask(String[][] document, int start, int end, String word) {
this.document = document;
this.start = start;
this.end = end;
this.word = word;
}
@Override
protected Integer compute() {
int result = 0;
if (end - start < 10) {
result = processLine(document, start, end, word);
} else {
int mid = (end + start) / 2;
DocumentTask task1 = new DocumentTask(document, start, mid, word);
DocumentTask task2 = new DocumentTask(document, mid, end, word);
invokeAll(task1, task2);
try {
result = groupResults(task1.get(), task2.get());
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
}
return result;
}
private int groupResults(Integer number1, Integer number2) {
return number1 + number2;
}
private int processLine(String[][] document, int start, int end, String word) {
List<LineTask> tasks = new ArrayList<LineTask>();
for (int i = start; i < end; i++) {
String[] line = document[i];
LineTask lineTask = new LineTask(0, line.length, word, line);
tasks.add(lineTask);
}
invokeAll(tasks);// synchronous call
int result = 0;
for (int i = 0; i < tasks.size(); i++) {
try {
result += tasks.get(i).get();
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
}
return result;
}
}
<file_sep>/src/main/java/executor/Task.java
package executor;
import java.util.concurrent.TimeUnit;
/**
* Created by shuxuan on 1/27/15.
*/
public class Task implements Runnable {
private String name;
public Task(String name) {
this.name = name;
}
@Override
public void run() {
System.out.println(this.name+" is running");
try {
TimeUnit.SECONDS.sleep(5L);
} catch (InterruptedException e) {
e.printStackTrace();
}
throw new RuntimeException();
}
}
<file_sep>/README.md
# java_concurrency
a java concurrency repo
<file_sep>/src/main/java/phaser/StudentMain.java
package phaser;
/**
* Created by shuxuan on 1/26/15.
*/
public class StudentMain {
public static void main(String[] args) throws InterruptedException {
MyPhaser myPhaser=new MyPhaser();
Student[] students=new Student[5];
for(int i=0;i<5;i++){
students[i]=new Student(myPhaser);
myPhaser.register();
}
Thread[] threads=new Thread[5];
for(int i=0;i<5;i++){
threads[i]=new Thread(students[i]);
threads[i].start();
}
for(int i=0;i<5;i++){
threads[i].join();
}
System.out.println("All threads have been terminated");
}
}
<file_sep>/src/main/java/exchanger/Consumer.java
package exchanger;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.concurrent.Exchanger;
/**
* Created by shuxuan on 1/27/15.
*/
public class Consumer implements Runnable {
private List<String> buffer;
private final Exchanger<List<String>> exchanger;
public Consumer(List<String> buffer, Exchanger<List<String>> exchanger) {
this.exchanger = exchanger;
this.buffer = buffer;
}
@Override
public void run() {
int cycle = 1;
for (int i = 0; i < 10; i++) {
System.out.println("Consumer: " + cycle);
try {
this.buffer = this.exchanger.exchange(buffer);
} catch (InterruptedException e) {
e.printStackTrace();
}
Iterator<String> iterator = this.buffer.iterator();
while (iterator.hasNext()) {
System.out.println("Consumer message: " + iterator.next());
iterator.remove();
}
cycle++;
}
}
}
<file_sep>/src/main/java/exchanger/Main.java
package exchanger;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.Exchanger;
/**
* Created by shuxuan on 1/27/15.
*/
public class Main {
public static void main(String[] args) {
List<String> consumerList=new ArrayList<String>();
List<String> producerList=new ArrayList<String>();
Exchanger<List<String>> exchanger=new Exchanger<List<String>>();
Producer producer=new Producer(producerList,exchanger);
Consumer consumer=new Consumer(consumerList,exchanger);
Thread proTh=new Thread(producer);
Thread conTh=new Thread(consumer);
proTh.start();
conTh.start();
}
}
<file_sep>/src/main/java/executor/FactorialMain.java
package executor;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import java.util.concurrent.*;
/**
* Created by shuxuan on 1/27/15.
*/
public class FactorialMain {
public static void main(String[] args) {
ThreadPoolExecutor threadPoolExecutor = (ThreadPoolExecutor) Executors.newFixedThreadPool(2);
List<Future<Integer>> resultList = new ArrayList();
Random random = new Random();
for (int i = 0; i < 10; i++) {
Integer num = random.nextInt(10);
FactorialCalculator factorialCalculator = new FactorialCalculator(num);
Future<Integer> result = threadPoolExecutor.submit(factorialCalculator);
resultList.add(result);
}
do {
System.out.println("Main: currently done tasks: " + threadPoolExecutor.getCompletedTaskCount());
for (int i = 0; i < resultList.size(); i++) {
Future<Integer> future = resultList.get(i);
System.out.println("Task: " + i + " is done? " + future.isDone());
}
// try {
// TimeUnit.MILLISECONDS.sleep(50);
// } catch (InterruptedException e) {
// e.printStackTrace();
// }
} while (threadPoolExecutor.getCompletedTaskCount() < resultList.size());// similar to the cyclicbarrier
for (int i = 0; i < resultList.size(); i++) {
Future<Integer> future = resultList.get(i);
try {
System.out.println("Task: " + i + " has value: " + future.get());
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
threadPoolExecutor.shutdown();
}
}
}
<file_sep>/src/main/java/executor/InvokeMain.java
package executor;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.*;
/**
* Created by shuxuan on 1/28/15.
*/
public class InvokeMain {
public static void main(String[] args) {
// ScheduledThreadPoolExecutor executorService = (ScheduledThreadPoolExecutor) Executors.newScheduledThreadPool(1000);
ScheduledThreadPoolExecutor executorService = new MyExecutor(1);
List<InvokeTask> taskList = new ArrayList<InvokeTask>();
for (int i = 0; i < 3; i++) {
InvokeTask task = new InvokeTask(String.valueOf(i));
taskList.add(task);
}
List<Future<Result>> futureList = new ArrayList();
Task task = new Task("asd");
// executorService.submit(new FutureTask(task,String.class));
executorService.scheduleAtFixedRate(task, 1, 1, TimeUnit.MILLISECONDS);
// executorService.scheduleWithFixedDelay(task,1,100,TimeUnit.MILLISECONDS);
// futureList = executorService.scheduleAtFixedRate(task,1,1,TimeUnit.SECONDS);
executorService.setContinueExistingPeriodicTasksAfterShutdownPolicy(true);
executorService.shutdown();
System.out.println("Main: printing all results");
for (Future<Result> resultFuture : futureList) {
try {
Result result = resultFuture.get();
System.out.println("Name: " + result.getName() + " and value: " + result.getValue());
// executorService.awaitTermination(1, TimeUnit.DAYS);
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
}
}
public void test() {
ScheduledThreadPoolExecutor executorService = (ScheduledThreadPoolExecutor) Executors.newScheduledThreadPool(1);
executorService.setExecuteExistingDelayedTasksAfterShutdownPolicy(true);
ScheduledExecutorService service = Executors.newScheduledThreadPool(1);
}
}
<file_sep>/src/main/java/completionService/ReportProcessor.java
package completionService;
import java.util.concurrent.CompletionService;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
/**
* Created by shuxuan on 1/29/15.
*/
public class ReportProcessor implements Runnable {
private CompletionService<String> service;
public void setEnd(boolean end) {
this.end = end;
}
private boolean end;
public ReportProcessor(CompletionService<String> service) {
this.service = service;
this.end = false;
}
@Override
public void run() {
while(!end){
Future<String> future=service.poll();
if(future!=null){
try {
String result=future.get();
System.out.println("Report Received: "+result);
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
}
}
}
}
<file_sep>/src/main/java/countDownlatch/Main.java
package countDownlatch;
/**
* Created by shuxuan on 1/23/15.
*/
public class Main {
public static void main(String[] args) {
VideoConference conference=new VideoConference(10);
Thread conferenceThread=new Thread(conference);
conferenceThread.start();
for(int i=0;i<10;i++){
Thread participantTh=new Thread(new Participant(conference,"Participant"+i));
participantTh.start();
}
}
}
<file_sep>/src/main/java/executor/Server.java
package executor;
import java.util.concurrent.Executors;
import java.util.concurrent.ThreadFactory;
import java.util.concurrent.ThreadPoolExecutor;
/**
* Created by shuxuan on 1/27/15.
*/
public class Server {
private ThreadPoolExecutor threadPoolExecutor;
public Server() {
// this.threadPoolExecutor= (ThreadPoolExecutor) Executors.newCachedThreadPool();
this.threadPoolExecutor = (ThreadPoolExecutor) Executors.newFixedThreadPool(5, Executors.defaultThreadFactory());
}
public void executeTask(Task task) {
System.out.println("Server: a new Task arrived");
this.threadPoolExecutor.execute(task);
// System.out.println("Pool size: "+this.threadPoolExecutor.getPoolSize());
// System.out.println("Active count: "+this.threadPoolExecutor.getActiveCount());
// System.out.println("Server: completed tasks: "+this.threadPoolExecutor.getCompletedTaskCount());
}
public void endServer() {
this.threadPoolExecutor.shutdown();
}
}
<file_sep>/src/main/java/semaphoreControlling/Job.java
package semaphoreControlling;
/**
* Created by shuxuan on 1/23/15.
*/
public class Job implements Runnable {
private PrintQueue printQueue;
public Job(PrintQueue queue) {
this.printQueue=queue;
}
@Override
public void run() {
System.out.println("Ready for printing job for thread: "+Thread.currentThread().getName());
this.printQueue.printJob(new Object());
System.out.println("Finish printing job for thread: "+Thread.currentThread().getName());
}
}
<file_sep>/src/main/java/forkjoin/Main3.java
package forkjoin;
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.TimeUnit;
/**
* Created by shuxuan on 1/30/15.
*/
public class Main3 {
public static void main(String[] args) {
ForkJoinPool pool = new ForkJoinPool();
FolderProcessor processor1 = new FolderProcessor("log", "/var/log");
FolderProcessor processor2 = new FolderProcessor("log", "/var/log");
FolderProcessor processor3 = new FolderProcessor("log", "/var/log");
pool.execute(processor2);
pool.execute(processor3);
pool.execute(processor1);
do {
System.out.printf("***************************************** *\n");
System.out.printf("Main: Parallelism: %d\n", pool.
getParallelism());
System.out.printf("Main: Active Threads: %d\n", pool.
getActiveThreadCount());
System.out.printf("Main: Task Count: %d\n", pool.
getQueuedTaskCount());
System.out.printf("Main: Steal Count: %d\n", pool.
getStealCount());
System.out.printf("***************************************** *\n");
try {
TimeUnit.SECONDS.sleep(1);
} catch (InterruptedException e) {
e.printStackTrace();
}
} while (!processor1.isDone() || !processor2.isDone() || !processor3.isDone());
System.out.println("Processor1: "+processor1.join().size());
System.out.println("Processor2: "+processor2.join().size());
System.out.println("Processor3: "+processor3.join().size());
}
}
<file_sep>/src/main/java/exchanger/Producer.java
package exchanger;
import java.util.List;
import java.util.concurrent.Exchanger;
/**
* Created by shuxuan on 1/27/15.
*/
public class Producer implements Runnable {
private List<String> buffer;
private final Exchanger<List<String>> exchanger;
public Producer(List<String> buffer, Exchanger<List<String>> exchanger) {
this.buffer = buffer;
this.exchanger = exchanger;
}
@Override
public void run() {
int cycle=1;
for(int i=0;i<10;i++){
System.out.println("Producer: Cycle "+cycle);
for(int j=0;j<10;j++){
String message="Event: "+((i*10)+j);
System.out.println("Producer: message "+message);
this.buffer.add(message);
}
try {
/*
transfer this buffer to another thread as the parameter
receive another thread's buffer as a return
*/
this.buffer=this.exchanger.exchange(buffer);
} catch (InterruptedException e) {
e.printStackTrace();
}
cycle++;
}
}
}
<file_sep>/src/main/java/cyclicBarrier/Grouper.java
package cyclicBarrier;
/**
* Created by shuxuan on 1/23/15.
*/
public class Grouper implements Runnable{
private Result result;
public Grouper(Result result) {
this.result = result;
}
@Override
public void run() {
int finalResult=0;
System.out.println("processing results---------");
int data[]=result.getData();
for(int number:data){
finalResult+=number;
}
System.out.println("printing result: "+finalResult);
}
}
<file_sep>/src/main/java/forkjoin/ProductGenerator.java
package forkjoin;
import java.util.ArrayList;
import java.util.List;
/**
* Created by shuxuan on 1/29/15.
*/
public class ProductGenerator {
public List<Product> generate(int size){
List<Product> products=new ArrayList<Product>(size);
for(int i=0;i<size;i++){
Product product=new Product();
product.setName("Product"+i);
product.setPrice(10);
products.add(product);
}
return products;
}
}
| 8958fcdbf80526360ee9c0501269dc1cde43c629 | [
"Markdown",
"Java"
] | 17 | Java | RyanZhu1024/java_concurrency | 75b79f4564c81f283fd2acedb3e13e78be599f78 | 7766f75ac970d89d9275347449e378ff3357e160 | |
refs/heads/master | <repo_name>Ivan-Veselov/coding-hw<file_sep>/numbers.cpp
#include "numbers.h"
bool isPrime(Natural number) {
if (number < 2) {
return false;
}
for (Natural divisor = 2; Natural64(divisor) * Natural64(divisor) <= number; ++divisor) {
if (number % divisor == 0) {
return false;
}
}
return true;
}
<file_sep>/main.cpp
#include <iostream>
#include "FinitePrimeFieldElement.h"
#include "Polynomial.h"
void findAllPrimePolynomials() {
auto polynomials = Polynomial<GF<2>>::allPolynomials(3);
for (const auto &polynomial : polynomials) {
if (polynomial == Polynomial<GF<2>>::ZERO || polynomial[polynomial.deg()] != GF<2>::ONE) {
continue;
}
bool isDivisible = false;
for (const auto &other : polynomials) {
if (other.deg() < 1 || other.deg() >= polynomial.deg()) {
continue;
}
if (polynomial % other == Polynomial<GF<2>>::ZERO) {
std::cout << polynomial.formulaString() << " = (" << other.formulaString() << ") * (" << (polynomial / other).formulaString() << ")" << std::endl;
isDivisible = true;
break;
}
}
if (!isDivisible) {
std::cout << polynomial.formulaString() << " is a prime polynomial" << std::endl;
}
}
}
void solve() {
// x^3 + x + 1 for 2**3
// x^2 + x + 2 for 3**2
auto polynomials = Polynomial<GF<3>>::allPolynomials(1);
auto x = Polynomial<GF<3>>::X;
auto prime = x(2) + x(1) + 2;
Natural power = 3 * 3 - 2;
for (const auto &elem : polynomials) {
std::cout << "The inverse of " << elem.formulaString() << " is " << std::endl;
std::cout << "(" << elem.formulaString() << ")**" << power << " = " << std::endl;
auto inverse = elem;
for (int i = 0; i < power - 1; ++i) {
inverse *= elem;
}
inverse %= prime;
std::cout << inverse.formulaString() << std::endl;
std::cout << "The product is " << ((elem * inverse) % prime).formulaString() << "\n" << std::endl;
}
}
int main() {
solve();
/*auto x = Polynomial<GF<2>>::X;
auto prime = x(3) + x(1) + 1;
auto pol = x(1) + 1;
std::cout << ((pol * pol * pol * pol * pol * pol) % prime).prettyString() << std::endl;
std::cout << ((fastPower(pol, 6)) % prime).prettyString() << std::endl;*/
return 0;
}<file_sep>/CMakeLists.txt
cmake_minimum_required(VERSION 3.13)
project(project)
set(CMAKE_CXX_STANDARD 17)
add_executable(project main.cpp FinitePrimeFieldElement.h numbers.h numbers.cpp Polynomial.h)<file_sep>/numbers.h
#pragma once
#include <cstdint>
typedef uint32_t Natural32;
typedef uint64_t Natural64;
typedef Natural32 Natural;
typedef int32_t Whole32;
typedef int64_t Whole64;
typedef Whole32 Whole;
bool isPrime(Natural number);
template<typename Number>
Number multiplicationNeutralElement() {
return Number(1);
}
template <typename Number>
Number fastPower(Number value, Natural power) {
if (power == 0) {
return multiplicationNeutralElement<Number>();
}
if (power == 1) {
return value;
}
Number result = fastPower(value, power / 2);
result *= result;
if (power % 2 == 1) {
result *= value;
}
return result;
}
<file_sep>/Polynomial.h
#pragma once
#include <vector>
#include "numbers.h"
template <typename Coefficient>
class Polynomial;
template <typename Coefficient>
bool operator == (const Polynomial<Coefficient>&, const Polynomial<Coefficient>&);
template <typename Coefficient>
bool operator != (const Polynomial<Coefficient>&, const Polynomial<Coefficient>&);
template <typename Coefficient>
class Polynomial {
friend bool operator == <>(const Polynomial<Coefficient>&, const Polynomial<Coefficient>&);
friend bool operator != <>(const Polynomial<Coefficient>&, const Polynomial<Coefficient>&);
private:
std::vector<Coefficient> coefficients;
// coefficients[i] is a coefficient for x^i
public:
class Variable {
public:
Polynomial<Coefficient> operator() (const Natural &power) const {
return Polynomial<Coefficient>(power);
}
};
static const Variable X;
public:
static const Polynomial ZERO;
static const Polynomial ONE;
public:
Polynomial()
: coefficients({Coefficient::ZERO}) {
}
Polynomial(const Coefficient &scalar)
: coefficients({scalar}) {
}
explicit Polynomial(const Natural &power)
: coefficients(power + 1, Coefficient::ZERO) {
coefficients[power] = Coefficient::ONE;
}
static std::vector<Polynomial> allPolynomials(Natural maxDeg) {
auto allCoefficients = Coefficient::allElements();
std::vector<Polynomial> polynomials;
if (maxDeg == 0) {
for (const auto &coefficient : allCoefficients) {
polynomials.push_back(Polynomial(coefficient));
}
return polynomials;
}
std::vector<Polynomial> lowerDegreePolynomials = allPolynomials(maxDeg - 1);
polynomials.insert(polynomials.end(), lowerDegreePolynomials.begin(), lowerDegreePolynomials.end());
for (const auto &coefficient : allCoefficients) {
if (coefficient == Coefficient::ZERO) {
continue;
}
for (auto lowerPolynomial : lowerDegreePolynomials) {
lowerPolynomial += Polynomial(maxDeg) * coefficient;
polynomials.push_back(lowerPolynomial);
}
}
return polynomials;
}
Natural deg() const {
return coefficients.size() - 1;
}
const Coefficient& operator [] (const Natural °ree) const {
return coefficients[degree];
}
Polynomial& operator += (const Polynomial &other) {
const auto numOfConsideredCoefficients = other.coefficients.size();
addZeroCoefficientsUpTo(numOfConsideredCoefficients);
for (auto i = 0; i < numOfConsideredCoefficients; ++i) {
coefficients[i] += other[i];
}
normalize();
return *this;
}
Polynomial& operator -= (const Polynomial &other) {
const auto numOfConsideredCoefficients = other.coefficients.size();
addZeroCoefficientsUpTo(numOfConsideredCoefficients);
for (auto i = 0; i < numOfConsideredCoefficients; ++i) {
coefficients[i] -= other[i];
}
normalize();
return *this;
}
Polynomial& operator *= (const Coefficient &scalar) {
for (auto &c : coefficients) {
c *= scalar;
}
normalize();
return *this;
}
Polynomial& operator *= (const Polynomial &other) {
const auto numOfConsideredCoefficients = deg() + other.deg() + 1;
addZeroCoefficientsUpTo(numOfConsideredCoefficients);
for (int i = numOfConsideredCoefficients - 1; i >= 0; --i) {
// i is an index of resulting multiplication
for (int j = std::min(i, int(deg())); j >= 0 && i - j <= other.deg(); --j) {
// j is an index of original polynomial
if (j != i) {
coefficients[i] += coefficients[j] * other[i - j];
} else {
coefficients[i] = coefficients[j] * other[i - j];
}
}
}
normalize();
return *this;
}
// this may be passed as a 'remainder' or 'quotient'
void divide(const Polynomial &divisor, Polynomial "ient, Polynomial &remainder) const {
assert(divisor != ZERO);
remainder = *this;
if (deg() < divisor.deg()) {
quotient = ZERO;
return;
}
Natural divisorDeg = divisor.deg();
Natural quotientPower = deg() - divisorDeg + 1;
quotient.coefficients.resize(quotientPower);
Coefficient divisorHighCoefficientInv = divisor.coefficients.back().inv();
do {
--quotientPower;
Coefficient quotientCoefficient = remainder[quotientPower + divisorDeg] * divisorHighCoefficientInv;
quotient[quotientPower] = quotientCoefficient;
for (auto i = 0; i <= divisorDeg; ++i) {
remainder[quotientPower + i] -= divisor[i] * quotientCoefficient;
}
} while (quotientPower != 0);
remainder.normalize();
}
Polynomial& operator /= (const Polynomial &other) {
Polynomial remainder;
divide(other, *this, remainder);
return *this;
}
Polynomial& operator %= (const Polynomial &other) {
Polynomial quotient;
divide(other, quotient, *this);
return *this;
}
std::string formulaString() const {
std::vector<std::string> terms;
for (auto power = coefficients.size() - 1; power > 0; --power) {
auto c = coefficients[power];
if (c == Coefficient::ZERO) {
continue;
}
std::string termStr;
if (c != Coefficient::ONE) {
termStr = c.formulaString() + " * ";
}
termStr += "x";
if (power > 1) {
termStr += "^" + std::to_string(power);
}
terms.push_back(termStr);
}
auto bias = coefficients[0];
if (bias != Coefficient::ZERO || terms.empty()) {
terms.push_back(bias.formulaString());
}
std::string result;
for (auto i = 0; i < terms.size(); ++i) {
if (i > 0) {
result += " + ";
}
result += terms[i];
}
return result;
}
std::string prettyString() const {
return formulaString() + ", a_i is from " + Coefficient::setPrettyString();
}
private:
Coefficient& operator [] (const Natural °ree) {
return coefficients[degree];
}
void normalize() {
while (coefficients.back() == Coefficient::ZERO && coefficients.size() > 1) {
coefficients.pop_back();
}
}
void addZeroCoefficientsUpTo(const Natural numberOfCoefficients) {
if (numberOfCoefficients > coefficients.size()) {
coefficients.resize(numberOfCoefficients, Coefficient::ZERO);
}
}
};
template <typename Coefficient>
const Polynomial<Coefficient> Polynomial<Coefficient>::ZERO = Coefficient::ZERO;
template <typename Coefficient>
const Polynomial<Coefficient> Polynomial<Coefficient>::ONE = Coefficient::ONE;
template <typename Coefficient>
Polynomial<Coefficient> operator + (Polynomial<Coefficient> element1, const Polynomial<Coefficient> &element2) {
return element1 += element2;
}
template <typename Coefficient>
Polynomial<Coefficient> operator - (Polynomial<Coefficient> element1, const Polynomial<Coefficient> &element2) {
return element1 -= element2;
}
template <typename Coefficient, typename Scalar>
Polynomial<Coefficient> operator + (Polynomial<Coefficient> element, const Scalar &scalar) {
return element += Coefficient(scalar);
}
template <typename Coefficient, typename Scalar>
Polynomial<Coefficient> operator + (const Scalar &scalar, Polynomial<Coefficient> element) {
return element += Coefficient(scalar);
}
template <typename Coefficient, typename Scalar>
Polynomial<Coefficient> operator - (Polynomial<Coefficient> element, const Scalar &scalar) {
return element -= Coefficient(scalar);
}
template <typename Coefficient, typename Scalar>
Polynomial<Coefficient> operator - (const Scalar &scalar, Polynomial<Coefficient> element) {
return element -= Coefficient(scalar);
}
template <typename Coefficient, typename Scalar>
Polynomial<Coefficient> operator * (Polynomial<Coefficient> element, const Scalar &scalar) {
return element *= scalar;
}
template <typename Coefficient, typename Scalar>
Polynomial<Coefficient> operator * (const Scalar &scalar, Polynomial<Coefficient> element) {
return element *= scalar;
}
template <typename Coefficient>
Polynomial<Coefficient> operator * (Polynomial<Coefficient> element1, const Polynomial<Coefficient> &element2) {
return element1 *= element2;
}
template <typename Coefficient>
Polynomial<Coefficient> operator / (Polynomial<Coefficient> element1, const Polynomial<Coefficient> &element2) {
return element1 /= element2;
}
template <typename Coefficient>
Polynomial<Coefficient> operator % (Polynomial<Coefficient> element1, const Polynomial<Coefficient> &element2) {
return element1 %= element2;
}
template <typename Coefficient>
bool operator == (const Polynomial<Coefficient> &element1, const Polynomial<Coefficient> &element2) {
return element1.coefficients == element2.coefficients;
}
template <typename Coefficient>
bool operator != (const Polynomial<Coefficient> &element1, const Polynomial<Coefficient> &element2) {
return element1.coefficients != element2.coefficients;
}
namespace Polynomials {
template <typename Coefficient>
Polynomial<Coefficient> X(const Natural &power) {
return Polynomial<Coefficient>(power);
}
}
/*
https://www.fluentcpp.com/2017/08/15/function-templates-partial-specialization-cpp/
template <typename Coefficient>
Polynomial<Coefficient> multiplicationNeutralElement() {
return Polynomial<Coefficient>(Coefficient::ONE);
}
*/
<file_sep>/FinitePrimeFieldElement.h
#pragma once
#include <string>
#include <cassert>
#include <vector>
#include "numbers.h"
template <Natural P>
class FinitePrimeFieldElement;
template <Natural P>
using GF = FinitePrimeFieldElement<P>;
template <Natural P>
bool operator == (const FinitePrimeFieldElement<P>&, const FinitePrimeFieldElement<P>&);
template <Natural P>
bool operator != (const FinitePrimeFieldElement<P>&, const FinitePrimeFieldElement<P>&);
template <Natural P>
class FinitePrimeFieldElement {
friend bool operator == <>(const FinitePrimeFieldElement<P>&, const FinitePrimeFieldElement<P>&);
friend bool operator != <>(const FinitePrimeFieldElement<P>&, const FinitePrimeFieldElement<P>&);
private:
static bool isChecked;
Whole remainder;
public:
static const FinitePrimeFieldElement ZERO;
static const FinitePrimeFieldElement ONE;
public:
FinitePrimeFieldElement()
: remainder(0) {
if (!isChecked) {
check();
}
}
FinitePrimeFieldElement(Whole number)
: remainder(toRemainderClassMainRepresentative(number)) {
if (!isChecked) {
check();
}
}
static std::vector<FinitePrimeFieldElement> allElements() {
std::vector<FinitePrimeFieldElement> elements;
for (Whole remainder = 0; remainder < P; ++remainder) {
elements.push_back(FinitePrimeFieldElement(remainder));
}
return elements;
}
FinitePrimeFieldElement inv() const {
assert(remainder != 0);
return fastPower(*this, P - 2);
}
FinitePrimeFieldElement& operator += (const FinitePrimeFieldElement &other) {
remainder += other.remainder;
if (remainder >= P) {
remainder -= P;
}
return *this;
}
FinitePrimeFieldElement& operator -= (const FinitePrimeFieldElement &other) {
remainder -= other.remainder;
if (remainder < 0) {
remainder += P;
}
return *this;
}
FinitePrimeFieldElement& operator *= (const FinitePrimeFieldElement &other) {
Whole64 tmp = Whole64(remainder) * other.remainder;
remainder = Whole(tmp % P);
return *this;
}
FinitePrimeFieldElement& operator /= (const FinitePrimeFieldElement &other) {
return *this *= other.inv();
}
std::string prettyString() const {
return std::to_string(remainder) + " (mod " + std::to_string(P) + ")";
}
std::string formulaString() const {
return std::to_string(remainder);
}
static std::string setPrettyString() {
return "GF(" + std::to_string(P) + ")";
}
private:
static void check() {
assert(isPrime(P));
}
Whole toRemainderClassMainRepresentative(Whole aRemainder) {
Whole representative = aRemainder % P;
if (representative < 0) {
representative += P;
}
return representative;
}
};
template <Natural P>
bool FinitePrimeFieldElement<P>::isChecked = false;
template <Natural P>
const FinitePrimeFieldElement<P> FinitePrimeFieldElement<P>::ZERO = 0;
template <Natural P>
const FinitePrimeFieldElement<P> FinitePrimeFieldElement<P>::ONE = 1;
template <Natural P>
FinitePrimeFieldElement<P> operator + (FinitePrimeFieldElement<P> element1, const FinitePrimeFieldElement<P> &element2) {
return element1 += element2;
}
template <Natural P>
FinitePrimeFieldElement<P> operator - (FinitePrimeFieldElement<P> element1, const FinitePrimeFieldElement<P> &element2) {
return element1 -= element2;
}
template <Natural P>
FinitePrimeFieldElement<P> operator * (FinitePrimeFieldElement<P> element1, const FinitePrimeFieldElement<P> &element2) {
return element1 *= element2;
}
template <Natural P>
FinitePrimeFieldElement<P> operator / (FinitePrimeFieldElement<P> element1, const FinitePrimeFieldElement<P> &element2) {
return element1 /= element2;
}
template <Natural P>
bool operator == (const FinitePrimeFieldElement<P> &element1, const FinitePrimeFieldElement<P> &element2) {
return element1.remainder == element2.remainder;
}
template <Natural P>
bool operator != (const FinitePrimeFieldElement<P> &element1, const FinitePrimeFieldElement<P> &element2) {
return element1.remainder != element2.remainder;
}
/*
https://www.fluentcpp.com/2017/08/15/function-templates-partial-specialization-cpp/
template <Natural P>
FinitePrimeFieldElement<P> multiplicationNeutralElement() {
return FinitePrimeFieldElement<P>(1);
}
*/ | b0ef6b404836584f74d10445a879a49df5e992fd | [
"CMake",
"C++"
] | 6 | C++ | Ivan-Veselov/coding-hw | a0cdb5b7d78bb37271c8526898e42e552fcadc39 | 579214265a314be71d3e0419bcc2beb1f0a018b8 | |
refs/heads/master | <file_sep>export default function ({context, store}) {
console.log('inside userparam')
store.commit('SET_USER', {'name' : 'APADMIN'})
}<file_sep>const app = require('express')()
const { Nuxt, Builder } = require('nuxt')
const server = require('http').createServer(app)
const io = require('socket.io')(server)
const axios = require('axios')
const host = process.env.HOST || '127.0.0.1' // new code in RC3
const port = process.env.PORT || 3000
const isProd = process.env.NODE_ENV === 'production'
const { isRealString } = require('./utils/validation')
const { getJDEAvailability } = require('./JDE/jde')
const { User } = require('./models/user')
var { mongoose } = require('./db/mongoose')
// // We instantiate Nuxt.js with the options
// let config = require('./nuxt.config.js')
// config.dev = !isProd
// // const nuxt = new Nuxt(config)
// const nuxt = new Nuxt(config)
// Import and set Nuxt.js options . RC3
let config = require('./nuxt.config.js')
config.dev = !(process.env.NODE_ENV === 'production')
const nuxt = new Nuxt(config)
// Start build process in dev mode
if (config.dev) {
const builder = new Builder(nuxt)
builder.build()
}
// let nuxt = new Nuxt(config)
app.use(nuxt.render)
// // Start build process (only in development)
// if (config.dev) {
// new Builder(nuxt).build().then().catch((error) => {
// console.log(error)
// })
// }
// // Build only in dev mode
// if (config.dev) {
// nuxt.build()
// .catch((error) => {
// console.error(error) // eslint-disable-line no-console
// process.exit(1)
// })
// }
var jdeUser = null
User.find().then((users) => {
jdeUser = users[0];
}, (e) => {
console.log(e);
})
// Listen the server
// server.listen(port, '0.0.0.0')
// console.log('Server listening on localhost:' + port) // eslint-disable-line no-console
// RC3
app.listen(port, host)
console.log('Server listening on ' + host + ':' + port)
// Socket.io
io.on('connection', (socket) => {
console.log('New user connected')
console.log('JDE user info to be user', jdeUser)
socket.on('getAvailabilityData', function () {
console.log('getAvailabilityData called by client')
// var itemAvailabilityData = {
// itemNames: ['Item Z', 'Item Y', 'Item X', 'Item W', 'Item V'],
// itemAvailableNos: [getRandomInt(-100, 100), getRandomInt(-100, 100), getRandomInt(-100, 100), getRandomInt(-100, 100), getRandomInt(-100, 100)]
// };
// socket.emit('updateAvailabilityData', itemAvailabilityData);
getJDEAvailability(socket, jdeUser)
});
});
function getRandomInt(min, max) {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min)) + min; //The maximum is exclusive and the minimum is inclusive
}
| 497ffa70dae5f8fc7800ff0fa9e599e23eb91dd6 | [
"JavaScript"
] | 2 | JavaScript | kathirvGitHub/nuxt-chart1 | f387c5b8a1cbda4d69e10ed8399e8242f74e4183 | 60a256b553f6effdf564955df4832c152b748ccf | |
refs/heads/main | <repo_name>jmc18/ReacJS-Course<file_sep>/react-complete-guide/src/components/Expenses/ExpenseList.js
import React, { useState } from 'react';
import ExpenseItem from './ExpenseItem';
import Card from '../UI/Card';
import ExpensesFilter from '../Expenses/ExpensesFilter';
import './ExpenseList.css';
const ExpenseList = (props) => {
const [filterYear, setFilterYear] = useState('2020');
const filterChangeHandler = (selectedYear) => {
setFilterYear(selectedYear);
props.filterYear(filterYear);
};
return (
<Card className="expenses">
<ExpensesFilter
selectedYear={filterYear}
onSelectedYear={filterChangeHandler}
/>
{props.expenses.map((item, index) => (
<ExpenseItem
key={index}
date={item.date}
title={item.title}
amount={item.amount}
/>
))}
</Card>
);
};
export default ExpenseList;
| 5112df3ec3ef80ccac5579645818a51da97b56f2 | [
"JavaScript"
] | 1 | JavaScript | jmc18/ReacJS-Course | f5050408f5cbbf7615efe9a06f76989a573f0503 | 5c58c2fdec1f128214d5a81a7942798ad93ae4fc | |
refs/heads/master | <repo_name>mayank2424/Vue-jS<file_sep>/README.md
# Vue-jS
Todo list project built in vuejs
<file_sep>/app/src/store/modules/todos.js
import axios from 'axios';
//Vuex State
const state = {
todos:[],
reset_todos:[],
showModal:false,
modalMsg:''
};
//actions
const actions = {
//getting todos
async fetchTodos({ commit }){
const response = await axios.get('https://jsonplaceholder.typicode.com/todos/');
// console.log(response.data);
//called or commit mutation
commit('setTodos', response.data)
//Initially setting all todos to different array
commit('resetTodos', response.data)
},
//Adding todos
async addTodo({ commit }, title){
const response = await axios.post('https://jsonplaceholder.typicode.com/todos/',{title, completed:false});
commit('addTodo', response.data)
},
//Delete Todo
async deleteTodo({commit}, delObj){
await axios.delete(`https://jsonplaceholder.typicode.com/todos/${delObj.id}`);
console.log(delObj)
console.log(delObj.showModal, delObj.modalMsg)
commit('deleteTodo',delObj)
},
//filter todo
async filterTodos({commit},filterObj){
console.log(filterObj)
//Getting select option value from targete event
if(filterObj.f_type === 'num'){
console.log('num')
const limit = parseInt(filterObj.e.target.options[filterObj.e.target.options.selectedIndex].innerText);
const response = await axios.get(`https://jsonplaceholder.typicode.com/todos?_limit=${limit}`);
commit('setTodos', response.data)
} else {
const value = filterObj.e.target.options[filterObj.e.target.options.selectedIndex].innerText;
if(value == 'Completed'){
filterObj.completed = true
commit('filter', filterObj)
console.log(filterObj)
} else {
filterObj.completed = false;
commit('filter', filterObj)
console.log(filterObj)
}
console.log(value)
}
},
//update todo
async updateTodo({ commit }, updTodo) {
const response = await axios.put(`https://jsonplaceholder.typicode.com/todos/${updTodo.id}`, updTodo)
console.log(response)
commit('updateTodo',response.data);
},
//close Modal
async closeModal({commit}, modalObj){
console.log(modalObj)
commit('closeModal', modalObj)
}
};
//mutations
const mutations = {
setTodos : (state, todos) => (state.todos = state.reset_todos = todos),
resetTodos:(state,todos) => (state.reset_todos =todos),
addTodo : (state, todo) => state.todos.unshift(todo),
deleteTodo : (state, delObj) => {state.todos = state.todos.filter(todo => todo.id !== delObj.id), state.showModal = delObj.showModal, state.modalMsg = delObj.modalMsg},
updateTodo : (state,updTodo) => {
const index = state.todos.findIndex(todo => todo.id === updTodo.id)
if(index !== -1) {
//replaced selected id with updated id
state.todos.splice(index, 1, updTodo)
}
},
filter:(state, filterObj) => {state.todos = state.reset_todos.filter(todo => todo.completed == filterObj.completed)},
closeModal:(state, modalObj) => {state.showModal = modalObj.showModal, state.modalMsg = modalObj.modalMsg}
};
//getters
const getters = {
allTodos : (state) => state.todos,
modal:(state) => state
};
export default {
state,
getters,
mutations,
actions
} | 7b797d879ec4950b3a40adabbb84338ce244be7a | [
"Markdown",
"JavaScript"
] | 2 | Markdown | mayank2424/Vue-jS | af7dc811094298f413fcb3aad56bab40969030fe | 014de96cb40411ea1552965d725e96787a987d59 | |
refs/heads/main | <file_sep>import React from 'react';
import BottomNavigation from '@material-ui/core/BottomNavigation';
import BottomNavigationAction from '@material-ui/core/BottomNavigationAction';
import fb from '../logo-FB.ico';
import insta from '../logo-IG.ico';
import yt from '../logo-YT.ico';
import { Box } from '@material-ui/core';
import { makeStyles } from '@material-ui/core/styles';
const useStyles = makeStyles((theme) => ({
root: {
minwidth: 200,
backgroundColor: "black"
}
// bot:{
// position:"fixed",
// left:0,
// bottom:0,
// right:0,
// // marginBottom:"1px"
// }
}));
export default function Footer() {
const classes = useStyles();
const [value, setValue] = React.useState(0);
return (
<Box mt={10}>
<BottomNavigation>
value={value}
onChange={(event, newValue) => {
setValue(newValue);
}}
showLabels
className={classes.root}
<BottomNavigationAction label="Facebook" icon={<img src={fb} style={{ height: 25, width: 25 }} />} />
<BottomNavigationAction label="Instagram" icon={<img src={insta} style={{ height: 25, width: 25 }} />} />
<BottomNavigationAction label="Youtube" icon={<img src={yt} style={{ height: 25, width: 25 }} />} />
</BottomNavigation>
</Box>
)
}<file_sep>import React from 'react';
import { useHistory } from 'react-router-dom';
import { Grid, Paper, Typography, TextField, Button } from '@material-ui/core';
import logo from './logo.jpg';
import { Form, Formik, ErrorMessage, Field } from 'formik';
import * as Yup from 'yup';
import axios from 'axios';
const Register = () => {
const paperStyle = { padding: '30px 20px', width: 300, margin: '20px auto', border: "solid black" }
const headStyle = { margin: 0, fontFamily: 'san-serif', color: 'blue' }
const marginTop = { margin: 15 }
const formStyle = { textAlign: 'center' }
const initialValues = {
firstname: '',
lastname: '',
email: '',
password: '',
confirmpassword: ''
}
let history = useHistory();
const onSubmit = (values, props) => {
const user = {
firstname: values.firstname,
lastname: values.lastname,
email: values.email,
password: <PASSWORD>
}
console.log(user)
axios.post("http://localhost:8081/account/register", user)
.then((response) => {
var res = response.status;
console.log(response.data)
console.log(response.status)
if (res === 200) {
alert("Successfully registered")
history.push('/login');
}
})
.catch((error) => {
if (error.response.status === 400) {
console.log(error.response.data.message);
alert("Email already exist")
props.resetForm()
}
else
alert("Something went wrong")
console.log(error)
});
}
const validationSchema = Yup.object().shape({
firstname: Yup.string()
//.min(2, "Its too short")
.required("Required"),
lastname: Yup.string().required("Required"),
email: Yup.string().email("Enter valid email").required("Required"),
password: Yup.string()
.matches(/^.*(?=.{8,})((?=.*[!@#$%^&*()\-_=+{};:,<.>]){1})(?=.*\d)((?=.*[a-z]){1})((?=.*[A-Z]){1}).*$/,
"Password must contain at least 8 characters, one uppercase, one number and one special case character")
.required("Required"),
confirmpassword: Yup.string().required("Please confirm your password")
.when("password", {
is: password => (password && password.length > 0 ? true : false),
then: Yup.string().oneOf([Yup.ref("password")], "Password doesn't match")
})
}
)
return (
<Grid >
<Paper elevation={20} style={paperStyle}>
<Grid align='center'>
<Grid>
<img src={logo} alt="logo" width='180' height='100' />
</Grid>
<h2 style={headStyle} >
Register
</h2>
<Typography variant='caption'>
Please fill this form to create an account!
</Typography>
</Grid>
<Formik initialValues={initialValues} validationSchema={validationSchema} onSubmit={onSubmit}>
{(props) => (
<Form style={formStyle}>
<Field as={TextField} fullWidth label='First Name' name='firstname' value={props.values.firstname}
required error={props.errors.firstname && props.touched.firstname}
onChange={props.handleChange} helperText={<ErrorMessage name='firstname' />} />
<Field as={TextField} fullWidth label='Last Name' name='lastname' required error={props.errors.lastname && props.touched.lastname}
value={props.values.lastname} onChange={props.handleChange} helperText={<ErrorMessage name='lastname' />} />
<Field as={TextField} fullWidth label='Email' type='email' required error={props.errors.email && props.touched.email}
name='email' value={props.values.email} onChange={props.handleChange} helperText={<ErrorMessage name='email' />} />
<Field as={TextField} fullWidth label='Password' type='password' required error={props.errors.password && props.touched.password}
value={props.values.password} onChange={props.handleChange} name='password' helperText={<ErrorMessage name='password' />} />
<Field as={TextField} fullWidth label='Confirm Password' required error={props.errors.confirmpassword && props.touched.confirmpassword}
value={props.values.confirmpassword} onChange={props.handleChange} name='confirmpassword' helperText={<ErrorMessage name='confirmpassword' />} />
<Button type='submit' variant='contained' color='primary' style={marginTop} align='center'>Register</Button>
</Form>
)}
</Formik>
</Paper>
</Grid>
);
}
export default Register;
<file_sep>/*import React from "react";
const home=()=>
{
return(
<div align='center'>
Home<br/><br></br>
<input type="button"
value="Go"
/>
</div>
)
}
export default home;*/
import React, { useEffect } from 'react';
import { Grid, Typography,Button } from '@material-ui/core';
import Card from '@material-ui/core/Card';
import { makeStyles } from '@material-ui/core/styles';
import CardActionArea from '@material-ui/core/CardActionArea';
//import CardActions from '@material-ui/core/CardActions';
import CardContent from '@material-ui/core/CardContent';
//import CardMedia from '@material-ui/core/CardMedia';
import Box from '@material-ui/core/Box';
import logo from './logo.jpeg';
import explore from './explore.jpeg';
import {Component} from 'react';
import opp from './opp.jpeg';
import AppBar from '@material-ui/core/AppBar';
import IconButton from '@material-ui/core/IconButton';
import MenuIcon from '@material-ui/icons/Menu';
import HomeIcon from '@material-ui/icons/Home';
import Toolbar from '@material-ui/core/Toolbar';
import { positions } from '@material-ui/system';
import BottomNavigation from '@material-ui/core/BottomNavigation';
import BottomNavigationAction from '@material-ui/core/BottomNavigationAction';
import InstagramIcon from '@material-ui/icons/Instagram';
import YouTubeIcon from '@material-ui/icons/YouTube';
import FacebookIcon from '@material-ui/icons/Facebook';
import MenuItem from '@material-ui/core/MenuItem';
import Menu from '@material-ui/core/Menu';
import axios from 'axios';
import {useState} from 'react';
import {Route, useHistory } from 'react-router-dom';
import { SettingsCellOutlined } from '@material-ui/icons';
import FormControlLabel from '@material-ui/core/FormControlLabel';
import {Formik, Form} from 'formik';
import moment from 'moment';
import { blue } from '@material-ui/core/colors';
import DashboardIcon from '@material-ui/icons/Dashboard';
const imgstyle = {
margin: '10px 60px'
}
const useStyles = makeStyles((theme) => ({
root: {
minwidth:200,
},
menuButton: {
marginRight: theme.spacing(2),
},
homeButton: {
marginLeft: theme.spacing(143),
},
media: {
height: 140,
/*minwidth:200,
alignItems:'center'*/
}
}));
export default function ButtonAppBar() {
/*const dataInfo=JSON.parse(localStorage.getItem("myInfo"))
const id=dataInfo.userId*/
const [event, setEvent] = useState([]);
let id=11
useEffect(()=>{
{
axios.get(`http://localhost:8081/account/events/getEventParticipated/${id}`)
.then((response) => {
setEvent(response.data.events)
})
};
}, [])
const classes = useStyles();
const [value, setValue] = React.useState(0);
const [anchorEl, setAnchorEl] = React.useState(null);
const handleClick = (event) => {
setAnchorEl(event.currentTarget);
};
const handleClose = () => {
setAnchorEl(null);};
/*
let history = useHistory();
const home = () => {
history.push('/home');
};
let history = useHistory();
const login = () => {
setAnchorEl(null);
history.push('/login');
};
*/
/*const history = useHistory();*/
return (
<Box mr={1}>
<AppBar position="static">
<Toolbar>
<IconButton edge="start" className={classes.menuButton} color="inherit" aria-label="menu">
<DashboardIcon />
</IconButton>
<Typography variant="h6" className={classes.title}>
Dashboard
</Typography>
<IconButton edge="end" padding="20px" className={classes.homeButton} color="inherit" aria-label="menu" >
<HomeIcon />
</IconButton>
<div>
<Button aria-controls="simple-menu" aria-haspopup="true" onClick={handleClick} variant="contained" color="primary">
Hello user,
</Button>
<Menu
id="simple-menu"
anchorEl={anchorEl}
keepMounted
open={Boolean(anchorEl)}
onClose={handleClose}
>
<MenuItem onClick={handleClose}>Profile</MenuItem>
<MenuItem onClick={handleClose}>My account</MenuItem>
<MenuItem onClick={handleClose}>Logout</MenuItem>
</Menu>
</div>
</Toolbar>
</AppBar>
<br/>
<center> <h2 color="primary">Oppurtunities Participated</h2> </center>
<center>
<Grid item xs={12} sm={6} md={3}>
<Card className={useStyles.root}>
<CardActionArea>
<CardContent style={{backgroundColor:"yellow"}}>
<Typography gutterBottom variant="h6" component="h1" color="inherit">
<Formik align='center'>
{(props) => (
event.map(post=>(
<Form key={post.event_id}>
{post.name} <br/>
Event id : {post.event_id}<br/>
Type : {post.event_type} <br/>
Venue : {post.venue} <br/>
</Form>
))
)}
</Formik>
</Typography>
</CardContent>
</CardActionArea>
</Card>
</Grid>
</center>
</Box>
)
}
/*Date : {moment(post.start_time).format('MMMM Do YYYY')}<br></br>
Time : {moment(post.start_time).format('h:mm a')}<br></br>*/<file_sep>package com.targetplatform.model;
import javax.validation.constraints.NotEmpty;
import javax.validation.constraints.Email;
public class User {
@NotEmpty(message="first name is mandatory")
private String firstName;
@NotEmpty
private String lastName;
@NotEmpty(message="email is mandatory")
@Email
private String email;
@NotEmpty(message="new password is mandatory")
@ValidPassword
private String password;
//default constructor
public User()
{
}
//constructor with fields
public User(String firstName,String lastName,String email) {
super();
this.firstName=firstName;
this.lastName=lastName;
this.email=email;
}
//Getters and Setters
public String getFirstname() {
return firstName;
}
public void setFirstname(String firstName) {
this.firstName = firstName;
}
public String getLastname() {
return lastName;
}
public void setLastname(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
}
<file_sep>import React from "react";
consthome=(props)=>{
const handleLogout=()=>{
props.history.push('/login)')
}
return(
<div>
Welcome
<input type="button"
value="Logout"
onClick={handleLogout}
/>
</div>
)
}
export default home;<file_sep>package com.target.VolunteeringPlatform.RequestResponse;
public class LoginResponse {
private int id;
private String email;
private String roles;
public LoginResponse(int id,String email, String roles) {
super();
this.id = id;
this.email = email;
this.roles = roles;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getRoles() {
return roles;
}
public void setRoles(String roles) {
this.roles = roles;
}
}
<file_sep>package com.target.Volunteeringplatform;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class VolunteeringPlatformProfileApplication {
public static void main(String[] args) {
SpringApplication.run(VolunteeringPlatformProfileApplication.class, args);
}
}
<file_sep>plugins {
id 'org.springframework.boot' version '2.5.2'
id 'io.spring.dependency-management' version '1.0.11.RELEASE'
id 'java'
}
group = 'com.target.igniteplus'
version = '0.0.1-SNAPSHOT'
sourceCompatibility = '1.8'
repositories {
mavenCentral()
}
dependencies {
implementation 'org.springframework.boot:spring-boot-starter-web'
implementation group: 'org.springframework', name: 'spring-webmvc'
runtimeOnly 'com.h2database:h2'
implementation group: 'org.apache.tomcat.embed', name: 'tomcat-embed-jasper'
implementation group: 'org.springframework.data', name: 'spring-data-jpa'
implementation group: 'javax.persistence', name: 'javax.persistence-api'
implementation group: 'org.springframework', name: 'spring-jdbc'
testImplementation group: 'com.h2database', name: 'h2'
testImplementation 'org.springframework.boot:spring-boot-starter-test'
}
test {
useJUnitPlatform()
}
<file_sep>import React from 'react';
import {BrowserRouter as Router, Switch, Route} from 'react-router-dom';
import './App.css';
import PastEvents from './Components/PastEvents';
import EditEvents from './Components/EditEvents';
import LeaderRR from './Components/LeaderRR';
//import home from './Components/home';
function App() {
return (
<Router>
<Switch>
<Route exact path = '/pastevents' component = {PastEvents} />
<Route exact path = '/editevents' component = {EditEvents} />
<Route exact path = '/leaderrr' component = {LeaderRR} />
</Switch>
</Router>
);
}
export default App;
<file_sep>rootProject.name = 'VolunteeringPlatform'
<file_sep>CREATE TABLE IF NOT EXISTS user (
user_id bigint(20) NOT NULL,
email varchar(255) DEFAULT NULL,
first_name varchar(255) DEFAULT NULL,
last_name varchar(255) DEFAULT NULL,
password varchar(255) DEFAULT NULL,
active int(10) DEFAULT NULL,
role varchar(255) DEFAULT NULL,
PRIMARY KEY (user_id),
UNIQUE KEY (email)
);<file_sep>import React, {useEffect, useState} from 'react';
import {Grid, Box, Card, CardContent, Button, Typography, Tooltip, makeStyles} from '@material-ui/core';
import logo from './HH-logo.jpg';
import wkndevnt1 from './img1.jpg';
//import wkndevnt2 from './img2.jpg';
import moment from 'moment';
import axios from 'axios';
const useStyles = makeStyles({
card:{
backgroundColor:"#D6EAF8",
'&:hover':{
backgroundColor:"#EBF5FB",
}
},
button:{
color:"primary",
'&:hover':{
backgroundColor:"#2471A3",
},
marginTop:"8px"
}
});
const PastEvents = (props) => {
const gridStyle={margin:'3px auto', padding:'5px auto'}
const headStyle = {margin:'0', fontFamily:'serif', color:'blue'}
const btnStyle = {margin:'8px 0'}
const logoStyle = {height:98, width:128}
//const imgStyle = {height:'100px', width:'180px'}
//const stepperStyle = {}
/*const handleChange = () => {
props.history.push('/home)')
}*/
const [pevent, setPevent] = useState([])
const event = "Past event"
useEffect(() => {
axios.get('http://localhost:8081/account/events/getEventsList/false/Weekend event')
//('http://localhost:8081/account/events/getEvents/'.concat('/isFutureEvent').concat('past'))
//(`http://localhost:8081/account/events/getEventsList/isFutureEvent${past}/eventTypes${event}`)
.then(response => {
console.log(response)
console.log(response.data[0].event_id)
setPevent(response.data)
})
.catch(error => {
if(error.response.status===400 || error.response.status===401) {
console.log(error.response.data.message)
}
else {
console.log("Oop! Something went wrong")
}
})
},[event])
const classes = useStyles();
return(
<Box>
<Box mt={1} mb={3} align="center">
<img src={logo} style={logoStyle} alt="Logo" />
</Box>
<Box align="center">
<Typography variant='h4' style={headStyle}>
<b> Past Events</b>
</Typography>
</Box>
<Box m={5}>
<Grid container spacing={6}>
{pevent.map((post)=>(
<Grid item xs={12} sm={6} md={6}>
<Card style={{minwidth:200}} className={classes.card}>
<CardContent>
<Grid container spacing={5}>
<Grid item xs={6} style={gridStyle}>
<Tooltip title={post.name}>
<img src={wkndevnt1} height='100%' width='100%' alt="Old Age Home" />
</Tooltip>
</Grid>
<Grid item xs={6} style={gridStyle}>
<p align="left">
<br></br>
<b>{post.name}</b>
<br></br>
Venue: {post.venue}
<br></br>
Date: {moment(post.start_time).format('MMMM Do YYYY')}
<br></br>
Start-Time: {moment(post.start_time).format('h:mm a')}
<br></br>
End-Time: {moment(post.end_time).format('h:mm a')}
<br></br>
About: {post.description}
<br></br>
</p>
</Grid>
</Grid>
</CardContent>
</Card>
</Grid>
))}
</Grid>
<br></br>
<br></br>
<Grid align="right">
<Button style={btnStyle} color='primary' variant='contained'>Go To Home Page</Button>
</Grid>
</Box>
</Box>
)
}
export default PastEvents;
<file_sep>package com.targetplatform.controller;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.ModelAttribute;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import com.targetplatform.model.User;
@Controller
@RequestMapping("/registration")
public class UserController {
@ModelAttribute("user")
public User userRegistration()
{
return new User();
}
@GetMapping
public String showRegistrationForm(Model model)
{
return "registration";
}
@PostMapping
public String registerUser(@Valid @ModelAttribute("user") User userRegistration
, BindingResult bindingResult )
/*BindingResult - interface which dictates how the object that stores the result
of validation should store and retrieve the results of the validation */
{
if(bindingResult.hasErrors()) {
return "registration";
}
return "success";
}
}
<file_sep>
import './App.css';
import React, { useState } from 'react';
import Navbar from './components/Navbar';
import { makeStyles } from '@material-ui/core/styles';
import { Carousel } from 'react-bootstrap';
import { blueGrey } from '@material-ui/core/colors';
import Card from '@material-ui/core/Card';
import Grid from '@material-ui/core/Grid';
import CardContent from '@material-ui/core/CardContent';
import Button from '@material-ui/core/Button';
import Footer from './components/Footer';
import Typography from '@material-ui/core/Typography';
import Box from '@material-ui/core/Box';
const useStyles = makeStyles({
card: {
paddingTop: '5px',
height: '300px',
width: '1200px',
margin: 'auto',
// marginLeft : '70px',
backgroundColor: "#E5E7E9",
'&:hover': {
backgroundColor: "#AEB6BF",
}
},
});
function App() {
const classes = useStyles();
const [index, setIndex] = useState(0);
const handleSelect = (selectedIndex, e) => {
setIndex(selectedIndex);
};
return (
<div className="App">
<Navbar />
<br />
<Carousel fade activeIndex={index} onSelect={handleSelect} >
<Carousel.Item interval={2000}>
<img
className="d-block w-100"
src="images/cs1.jpg"
alt="Waste Collection"
/>
<Carousel.Caption>
<h3>Waste Collection Program</h3>
</Carousel.Caption>
</Carousel.Item>
<Carousel.Item interval={2000}>
<img
className="d-block w-100"
src="images/cs3.jpg"
alt="Food Distribution Event"
/>
<Carousel.Caption>
<h3>Food Distribution Event</h3>
</Carousel.Caption>
</Carousel.Item>
<Carousel.Item interval={2000}>
<img
className="d-block w-100"
src="images/cs2.jpg"
alt="Clean Environment"
/>
<Carousel.Caption>
<h3>Clean Environment Program</h3>
</Carousel.Caption>
</Carousel.Item>
</Carousel>
<br />
<br />
<Card style={{ marginTop: '30px' }} className={classes.card}>
<Grid container spacing={2}
direction="column"
alignItems="center"
justify="center"
>
<CardContent>
<Typography coponent="div">
<Box fontFamily="Display" fontSize="h4.fontSize" style={{color:"#11052C"}}>
About Volunteering
</Box>
<Box fontFamily="cursive" style={{color:"orange"}}>
<i>Making differences in lives...</i>
</Box>
<br />
<p>
Offering a variety of volunteer opportunities is one way to ensure that employees or community members find
at least one thing in which they’d feel comfortable participating.
People choose to volunteer for a variety of reasons. For some it offers the chance to give something back to the c
ommunity or make a difference to the people around them. For others it provides an opportunity to develop new skills
or
build on existing experience and knowledge.
<br />
Volunteering allows you to connect to your community and make it a better place. ... And volunteering is a
two-way street: It can benefit you and your family as much as the cause you choose to help. Dedicating your
time as a volunteer helps you make new friends, expand your network, and boost your social skills.
</p>
</Typography>
</CardContent>
</Grid>
</Card>
<br />
<Footer />
<Button>Learn More</Button>
</div>
);
}
export default App;
<file_sep>import React, {useState, useEffect} from 'react';
import {Grid, Paper, Box, Button, Card, CardContent, Select, NativeSelect, MenuItem, InputLabel, FormControl, FormHelperText, Tooltip, Typography, TextField, AppBar, IconButton, Toolbar , makeStyles} from '@material-ui/core';
import {ArrowBack, Home, Menu } from '@material-ui/icons';
import {Formik, Form, Field, ErrorMesage} from 'formik';
//import logo from './HH-logo.jpg';
import icon from './HH-icon.ico';
import 'date-fns';
import DateFnsUtils from '@date-io/date-fns';
import { MuiPickersUtilsProvider, KeyboardTimePicker, KeyboardDatePicker, } from '@material-ui/pickers';
//import Dropdown from 'react-dropdown';
import es from 'date-fns/locale/es';
import moment from 'moment';
import axios from 'axios';
const EditEvents = (props) => {
const paperStyle = {padding:'10px 25px', height:'auto', width:'auto', margin:'20px 25px', border:' black'}
const gridStyle = {margin:'auto auto', padding:'auto auto'}
const headStyle = {margin:'0', fontFamily:'sans-serif', color:'#8A2BE2'}
const btnStyle = {margin:'15px 15px'}
const logoStyle = {height:98, width:128}
const iconStyle = {height:45, width:45}
const formStyle = {width:'100%'}
const [wevent, setWevent] = useState([])
const event = "Weekend event"
//const future=true
useEffect(() => {
axios.get('http://localhost:8081/account/events/getEventsList/true/Weekend event')
//('http://localhost:8081/account/events/getEvents/'.concat('/isFutureEvent').concat('event'))
//(`http://localhost:8081/account/events/getEventsList/isFutureEvent${future}/eventTypes${event}`)
.then(response => {
console.log(response)
console.log(response.data[0].event_id)
setWevent(response.data)
})
.catch(error => {
if(error.response.status===400 || error.response.status===401) {
console.log(error.response.data.message)
}
else {
console.log("Oop! Something went wrong")
}
})
},[event])
const [selectedDate, setSelectedDate] = React.useState(new Date());
const handleDateChange = (date) => {
setSelectedDate(date);
};
const onSubmit = (values, props) => {
const event = Object.create(values)
axios.post("localhost:8081/")
}
const options = [
{ value: 'weekendevents', label: 'Weekend Events' },
{ value: 'webinarforNGOs', label: 'Webinar for NGOs' },
{ value: 'foodforthought', label: 'Food for Thought' },
{ value: 'artsandcraft', label: 'Arts & Craft' },
];
const useStyles = makeStyles((theme) => ({
root: {
display: 'flex',
'& > *': {
margin: theme.spacing(1),
},
},
homeButton: {
marginRight: theme.spacing(2),
},
backButton: {
marginLeft: theme.spacing(1),
},
button:{
color:"primary",
'&:hover':{
backgroundColor:"#2471A3",
},
marginTop:"8px"
}
}));
const classes = useStyles();
return (
<Box >
<AppBar position="sticky">
<Toolbar>
<IconButton align='center' edge="start" className={classes.homeButton} color="inherit" aria-label="Home">
<img src={icon} style={iconStyle} alt='HH' />
</IconButton>
<Typography>
<b>Helping Hands</b>
</Typography>
</Toolbar>
</AppBar>
<MuiPickersUtilsProvider utils={DateFnsUtils}>
<Grid style={gridStyle} >
<Paper style={paperStyle} elevation={2}>
<Grid item xs={12} align='center'>
<br />
<Typography variant='h4' style={headStyle}>
<b>Edit Events</b>
</Typography>
<br /><br />
</Grid>
<FormControl style={formStyle} variant="outlined" align="center">
<InputLabel>Event Type</InputLabel>
<NativeSelect label="Event Type" >
<option align-items='center' value="" />
<option value="weekendevent">Weekend Event</option>
<option disabled value="webinarforngo">Webinars for NGO</option>
<option disabled value="artsandcraft">Arts & Craft</option>
<option disabled value="foodforthought">Food for Thought</option>
</NativeSelect>
<FormHelperText>Find event by type</FormHelperText>
<br />
</FormControl>
<Grid container spacing={4}>
{wevent.map((post)=>(
<Grid item xs={12} >
<Card style={{minwidth:200}} className={classes.card}>
<CardContent>
<Grid container spacing={2}>
<Grid style={gridStyle}>
<Formik>
{(props) => (
<Form >
<p align="left">
<Field as={TextField} fullWidth label="Event Name" name="eventname" value={post.name} required />
<br /><br />
<Field as={TextField} fullWidth label="Venue" name="venue" value={post.venue} required />
<br /><br />
<TextField
id="standard-multiline-static"
label="Description"
name="description"
multiline
fullWidth
maxRows={4}
defaultValue={post.description}
required
/>
</p>
</Form>
)}
</Formik>
</Grid>
<Grid style={gridStyle}>
<Formik>
{(props) => (
<Form >
<p align="left">
<TextField
id="standard-read-only-input"
label="Event ID"
defaultValue={post.event_id}
inputProps={{readOnly: true,}}
/>
<br />
<KeyboardDatePicker
disableToolbar
disablePast
variant="inline"
format="MMM dd yyyy"
margin="normal"
id="date-picker-inline"
label="Date"
defaultValue={moment(post.start_time)}
required
onChange={handleDateChange}
KeyboardButtonProps = {{
'aria-label': 'change date',
}}
/>
<br /><br />
<Field as={TextField} fullWidth label="Start Time" name="starttime" value={moment(post.start_time).format('h:mm a')} required />
<br /><br />
<Field as={TextField} fullWidth label="End Time" name="endtime" value={moment(post.end_time).format('h:mm a')} required />
<br />
</p>
</Form>
)}
</Formik>
</Grid>
</Grid>
</CardContent>
<Button type='submit' style={btnStyle} color='primary' align='right' variant='contained' >
Save Changes
</Button>
</Card>
</Grid>
))}
</Grid>
</Paper>
</Grid>
</MuiPickersUtilsProvider>
</Box>
)
}
export default EditEvents;
<file_sep>package com.target.VolunteeringPlatform.Controller;
import com.target.VolunteeringPlatform.DAO.UserRepository;
import com.target.VolunteeringPlatform.RequestResponse.LoginRequest;
import com.target.VolunteeringPlatform.RequestResponse.LoginResponse;
import com.target.VolunteeringPlatform.RequestResponse.MessageResponse;
import com.target.VolunteeringPlatform.RequestResponse.SignupRequest;
import com.target.VolunteeringPlatform.Service.UserDetailsImpl;
import com.target.VolunteeringPlatform.Service.UserDetailsServiceImpl;
import com.target.VolunteeringPlatform.model.User;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.Authentication;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.web.bind.annotation.*;
import java.util.List;
import java.util.stream.Collectors;
@RestController
@RequestMapping("/account")
public class UserController {
@Autowired
UserDetailsServiceImpl userService;
@Autowired
UserRepository userRepository;
@Autowired
AuthenticationManager authenticationManager;
@Autowired
PasswordEncoder encoder;
@CrossOrigin("http://localhost:3000")
@RequestMapping(value = "/register", method = RequestMethod.POST)
public ResponseEntity<?> createUser(@RequestBody SignupRequest newUser) {
if (userRepository.findByEmail(newUser.getEmail()) != null) {
return ResponseEntity
.badRequest()
.body(new MessageResponse("Error: Email is already taken!"));
}
User user = new User(newUser.getEmail(),newUser.getFirstname(),newUser.getLastname(), encoder.encode(newUser.getPassword()));
userService.saveUser(user);
return ResponseEntity.ok(new MessageResponse("User registered successfully!"));
}
@CrossOrigin("http://localhost:3000")
@RequestMapping(value = "/login", method = RequestMethod.POST)
public ResponseEntity<?> validateUser(@RequestBody LoginRequest loginRequest) {
Authentication authentication = authenticationManager.authenticate(
new UsernamePasswordAuthenticationToken(loginRequest.getEmail(), loginRequest.getPassword()));
SecurityContextHolder.getContext().setAuthentication(authentication);
UserDetailsImpl userDetails = (UserDetailsImpl) authentication.getPrincipal();
List<String> roles = userDetails.getAuthorities().stream()
.map(GrantedAuthority::getAuthority)
.collect(Collectors.toList());
return ResponseEntity.ok(new LoginResponse(userDetails.getId(),
userDetails.getEmail(), roles.get(0)));
}
}
<file_sep>import React, { useEffect } from 'react';
import { Grid, Typography,Button } from '@material-ui/core';
import Card from '@material-ui/core/Card';
import { makeStyles } from '@material-ui/core/styles';
import CardActionArea from '@material-ui/core/CardActionArea';
//import CardActions from '@material-ui/core/CardActions';
import CardContent from '@material-ui/core/CardContent';
//import CardMedia from '@material-ui/core/CardMedia';
import Box from '@material-ui/core/Box';
import logo from './logo.jpeg';
import explore from './explore.jpeg';
import opp from './opp.jpeg';
import AppBar from '@material-ui/core/AppBar';
import IconButton from '@material-ui/core/IconButton';
import MenuIcon from '@material-ui/icons/Menu';
import HomeIcon from '@material-ui/icons/Home';
import Toolbar from '@material-ui/core/Toolbar';
import { positions } from '@material-ui/system';
import BottomNavigation from '@material-ui/core/BottomNavigation';
import BottomNavigationAction from '@material-ui/core/BottomNavigationAction';
import InstagramIcon from '@material-ui/icons/Instagram';
import YouTubeIcon from '@material-ui/icons/YouTube';
import FacebookIcon from '@material-ui/icons/Facebook';
import MenuItem from '@material-ui/core/MenuItem';
import Menu from '@material-ui/core/Menu';
import axios from 'axios';
import {useState} from 'react';
import {Route, useHistory } from 'react-router-dom';
import DashboardIcon from '@material-ui/icons/Dashboard';
const imgstyle = {
margin: '10px 60px'
}
const useStyles = makeStyles((theme) => ({
root: {
minwidth:200,
},
menuButton: {
marginRight: theme.spacing(2),
},
homeButton: {
marginLeft: theme.spacing(143),
},
media: {
height: 140,
/*minwidth:200,
alignItems:'center'*/
}
}));
export default function ButtonAppBar() {
/*const dataInfo=JSON.parse(localStorage.getItem("myInfo"))
const id=dataInfo.userId*/
const id=11
const [count,setCount]=useState(0);
useEffect(()=>{
{
axios.get(`http://localhost:8081/account/events/getEventParticipated/${id}`)
.then((response) => {
console.log(response.data.events);
var ct = response.data.eventsCount;
setCount(ct);
})
};
})
const classes = useStyles();
const [value, setValue] = React.useState(0);
const [anchorEl, setAnchorEl] = React.useState(null);
const handleClick = (event) => {
setAnchorEl(event.currentTarget);
};
const handleClose = () => {
setAnchorEl(null);};
/*
let history = useHistory();
const home = () => {
history.push('/home');
};
let history = useHistory();
const login = () => {
setAnchorEl(null);
history.push('/login');
};
*/
const history = useHistory();
return (
<Box mr={1}>
<AppBar position="static">
<Toolbar>
<IconButton edge="start" className={classes.menuButton} color="inherit" aria-label="menu">
<DashboardIcon/>
</IconButton>
<Typography variant="h6" className={classes.title}>
Dashboard
</Typography>
<IconButton edge="end" padding="20px" className={classes.homeButton} color="inherit" aria-label="menu" onClick={()=>{history.push("/done");}} >
<HomeIcon />
</IconButton>
<div>
<Button aria-controls="simple-menu" aria-haspopup="true" onClick={handleClick} variant="contained" color="primary">
Hello user,
</Button>
<Menu
id="simple-menu"
anchorEl={anchorEl}
keepMounted
open={Boolean(anchorEl)}
onClose={handleClose}
>
<MenuItem onClick={handleClose}>Profile</MenuItem>
<MenuItem onClick={handleClose}>My account</MenuItem>
<MenuItem onClick={()=>{history.push("/login");}}>Logout</MenuItem>
</Menu>
</div>
</Toolbar>
</AppBar>
<Box mt={3} align="center">
<img src={logo} alt="logo" width='200' height='150' />
</Box>
<br/>
<center><h2>Welcome user,</h2></center>
<center>
<Box m={3}>
<center>
<Grid container spacing={10} direction="row"
justifyContent="center"
alignItems="center">
<Grid item xs={12} sm={6} md={3}>
<Card className={useStyles.root}>
<CardActionArea onClick={()=>{history.push("/home");}} >
<img src={opp} alt="oppurtunities participated" width='40%' height='120' style={imgstyle} />
<CardContent>
<Button variant="contained" color="secondary">
{count}
</Button>
<Typography gutterBottom variant="h6" component="h1" color="primary" >
Oppurtunities Participated
</Typography>
</CardContent>
</CardActionArea>
</Card>
</Grid>
<Grid item xs={12} sm={6} md={3}>
<Card className={useStyles.root}>
<CardActionArea>
<img src={explore} alt="explore" width='40%' height='120' style={imgstyle} />
<CardContent>
<Typography gutterBottom variant="h6" component="h1" color="primary">
<br/>
Explore Oppurtunities
</Typography>
</CardContent>
</CardActionArea>
</Card>
</Grid>
</Grid>
</center>
</Box>
<br/>
<br/>
<br/>
<BottomNavigation
value={value}
onChange={(event, newValue) => {
setValue(newValue);
}}
showLabels
className={classes.root}
>
<BottomNavigationAction label="Facebook" icon={<FacebookIcon />} />
<BottomNavigationAction label="Instagram" icon={<InstagramIcon />} />
<BottomNavigationAction label="YouTube" icon={<YouTubeIcon />} />
</BottomNavigation>
</center>
</Box>
)
}<file_sep>server.port=8081
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
spring.datasource.url=jdbc:h2:mem:targetuserdb;DB_CLOSE_DELAY=-1
spring.datasource.driverClassName=org.h2.Driver
spring.datasource.username=sa
spring.datasource.password=
spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
spring.h2.console.enabled=true
spring.h2.console.path=/account/h2
<file_sep>package com.target.igniteplus.mvcdemo.controller;
public @interface CrossOrigin {
}
<file_sep>rootProject.name = 'VolunteeringPlatformProfile'
<file_sep>import React, {useEffect, useState} from 'react';
import {Grid, Box, AppBar, Toolbar, Typography, IconButton, Paper, Card, CardContent, Avatar, Checkbox, Button, makeStyles, FormGroup, FormControlLabel} from '@material-ui/core';
import {ArrowBack, Home, Menu } from '@material-ui/icons';
import {Formik, Form, Field } from 'formik';
import logo from './HH-logo.jpg';
import icon from './HH-icon.ico';
import axios from 'axios';
const LeaderRR = () => {
const paperStyle = {padding:'10px 25px', height:'auto', width:'auto', margin:'15px 20px', border:' black'}
const gridStyle = {margin:'3px auto', padding:'5px auto'}
const headStyle = {margin:'0', fontFamily:'sans-serif', color:'#8A2BE2'}
const btnStyle = {margin:'8px 0'}
const logoStyle = {height:98, width:128}
const iconStyle = {height:45, width:45}
const formStyle = {textAlign:'center'}
const [eventRR, setEventRR] = useState([])
const event = "Past event RR"
useEffect(() => {
axios.get('http://localhost:8081/account/events/getEventsList/false/Weekend event')
//('http://localhost:8081/account/events/getEvents/'.concat('/isFutureEvent').concat('future'))
//(`http://localhost:8081/account/events/getEventsList/isFutureEvent${future}/eventTypes${event}`)
.then(response => {
console.log(response)
console.log(response.data[0].event_id)
setEventRR(response.data)
})
.catch(error => {
if(error.response.status===400 || error.response.status===401) {
console.log(error.response.data.message)
}
else {
console.log("Oop! Something went wrong")
}
})
},[event])
const useStyles = makeStyles((theme) => ({
root: {
display: 'flex',
'& > *': {
margin: theme.spacing(1),
},
},
large: {
width: theme.spacing(10),
height: theme.spacing(10),
},
homeButton: {
marginRight: theme.spacing(5),
},
button:{
color:"primary",
'&:hover':{
backgroundColor:"#2471A3",
},
marginTop:"8px"
}
}));
const classes = useStyles();
return (
<Box>
<AppBar position="sticky">
<Toolbar>
<IconButton align='center' edge="end" className={classes.homeButton} color="inherit" aria-label="Home">
<img src={icon} style={iconStyle} alt='HH' />
</IconButton>
<Typography>
<b>Helping Hands</b>
</Typography>
</Toolbar>
</AppBar>
<Grid style={gridStyle}>
<Grid align='center'>
<br />
<Typography variant='h4' style={headStyle}>
<b>Rewards & Recognition</b>
</Typography>
</Grid>
{eventRR.map((postOne)=>(
<Formik >
{(props) => (
<Form style={formStyle}>
<Paper style={paperStyle} elevation={15}>
<p align="left">
<Typography style={{textTransform: "uppercase"}} variant='h6' align="center">
<b>{postOne.name}</b>
</Typography>
<br />
<b> Participant(s): </b>
<div className={classes.root}>
<Card >
<CardContent>
<Avatar src="/broken-image.jpg" className={classes.large} />
<Typography>
Participant1
</Typography>
</CardContent>
<Checkbox color='primary' inputProps = {{'aria-label': 'uncontrolled-checkbox'}} />
</Card>
<Card>
<CardContent>
<Avatar src="/broken-image.jpg" className={classes.large} />
<Typography>
Participant2
</Typography>
</CardContent>
<Checkbox color='primary' inputProps = {{'aria-label': 'uncontrolled-checkbox'}} />
</Card>
<Card>
<CardContent>
<Avatar src="/broken-image.jpg" className={classes.large} />
<Typography>
Participant3
</Typography>
</CardContent>
<Checkbox color='primary' inputProps = {{'aria-label': 'uncontrolled-checkbox'}} />
</Card>
</div>
</p>
<Button style={btnStyle} align='right' type='submit' color='primary' variant="contained">Nominate</Button>
</Paper>
</Form>
)}
</Formik>
))}
</Grid>
</Box>
)
}
export default LeaderRR;
<file_sep>import React, {useEffect, useState} from 'react';
import {Grid, Box, AppBar, Toolbar, IconButton, Card, CardContent, Button, Typography, Tooltip, makeStyles} from '@material-ui/core';
import logo from './HH-logo.jpg';
import icon from './HH-icon.ico';
import wkndevnt1 from './img1.jpg';
//import wkndevnt2 from './img2.jpg';
import moment from 'moment';
import axios from 'axios';
const useStyles = makeStyles({
card:{
backgroundColor:"#D6EAF8",
'&:hover':{
backgroundColor:"#EBF5FB",
}
},
button:{
color:"primary",
'&:hover':{
backgroundColor:"#2471A3",
},
marginTop:"8px"
}
});
const PastEvents = (props) => {
const gridStyle={margin:'3px auto', padding:'5px auto'}
const headStyle = {margin:'0', fontFamily:'sans-serif', color:'#8A2BE2'}
const btnStyle = {margin:'8px 0'}
const logoStyle = {height:98, width:128}
const iconStyle = {height:45, width:45}
//const imgStyle = {height:'100px', width:'180px'}
//const stepperStyle = {}
/*const handleChange = () => {
props.history.push('/home)')
}*/
const [pevent, setPevent] = useState([])
const event = "Past event"
useEffect(() => {
axios.get('http://localhost:8081/account/events/getEventsList/false/Weekend event')
//('http://localhost:8081/account/events/getEvents/'.concat('/isFutureEvent').concat('past'))
//(`http://localhost:8081/account/events/getEventsList/isFutureEvent${past}/eventTypes${event}`)
.then(response => {
console.log(response)
console.log(response.data[0].event_id)
setPevent(response.data)
})
.catch(error => {
if(error.response.status===400 || error.response.status===401) {
console.log(error.response.data.message)
}
else {
console.log("Oop! Something went wrong")
}
})
},[event])
const classes = useStyles();
return(
<Box>
<AppBar position="sticky">
<Toolbar>
<IconButton align='center' edge="end" className={classes.homeButton} color="inherit" aria-label="Home">
<img src={icon} style={iconStyle} alt='HH' />
</IconButton>
<Typography>
<b>Helping Hands</b>
</Typography>
</Toolbar>
</AppBar>
<Box align="center">
<br />
<Typography variant='h4' style={headStyle}>
<b>Past Events</b>
</Typography>
</Box>
<Box m={5}>
<Grid container spacing={6}>
{pevent.map((post)=>(
<Grid item xs={12} sm={6} md={6}>
<Card style={{minwidth:200}} className={classes.card}>
<CardContent>
<Grid container spacing={5}>
<Grid item xs={6} style={gridStyle}>
<Tooltip title={post.name}>
<img src={wkndevnt1} height='100%' width='100%' alt="Old Age Home" />
</Tooltip>
</Grid>
<Grid item xs={6} style={gridStyle}>
<Typography style={{textTransform: "uppercase"}} align="center">
<b>{post.name}</b>
</Typography>
<p align="left">
<b>Venue:</b> {post.venue}
<br />
<b>Date:</b> {moment(post.start_time).format('MMMM Do YYYY')}
<br />
<b>Start Time:</b> {moment(post.start_time).format('h:mm a')}
<br />
<b>End Time:</b> {moment(post.end_time).format('h:mm a')}
<br />
<b>Description:</b> {post.description}
<br />
</p>
</Grid>
</Grid>
</CardContent>
</Card>
</Grid>
))}
</Grid>
<br></br>
<br></br>
<Grid align="right">
<Button style={btnStyle} color='primary' variant='contained'>Go To Home Page</Button>
</Grid>
</Box>
</Box>
)
}
export default PastEvents;
| 799999465d25a6bda56038c7546f15494099e84a | [
"SQL",
"JavaScript",
"INI",
"Gradle",
"Java"
] | 22 | JavaScript | ignite-plus-2021/helpinghands | b4b2c09c833d40c779e1bcb9943895e26faabca6 | a91d662d4d82b55b914b5c354330856b63b37f43 | |
refs/heads/main | <repo_name>HighVoltages/MQTT-in-MATLAB<file_sep>/README.md
# MQTT in MATLAB
In this tutorial of MQTT, we will learn how to use MQTT in MATLAB. We will start with basics like making connections with MQTT broker, Publish and subscribe and then we will plot real-time data coming from ESP8266 over MQTT.
Read more at: https://highvoltages.co/iot-internet-of-things/how-to-mqtt/mqtt-in-matlab/
# watch complete tutorial:
[](https://youtu.be/ptdNuqGuf6E "MQTT in MATLAB | Realtime Data Plotting")
<file_sep>/ESP8266/esp8266_mqtt_matlab/esp8266_mqtt_matlab.ino
#include <Adafruit_Sensor.h> //Library for Adafruit sensors , we are using for DHT
#include <DHT_U.h> //DHT library which uses some func from Adafruit Sensor library
#include <ESP8266WiFi.h> //library for using ESP8266 WiFi
#include <PubSubClient.h> //library for MQTT
#include <ArduinoJson.h> //library for Parsing JSON
//defining Pins
#define DHTPIN 5
#define LED D2
//DHT parameters
#define DHTTYPE DHT11 // DHT 11
DHT_Unified dht(DHTPIN, DHTTYPE);
uint32_t delayMS;
//MQTT Credentials
const char* ssid = "SSID";//setting your ap ssid
const char* password = "<PASSWORD>";//setting your ap psk
const char* mqttServer = "iot.reyax.com"; //MQTT URL
const char* mqttUserName = "MQTT username"; // MQTT username
const char* mqttPwd = "<PASSWORD>"; // MQTT password
const char* clientID = "username0001"; // client id username+0001
const char* topic = "ESP8266/data"; //publish topic
//parameters for using non-blocking delay
unsigned long previousMillis = 0;
const long interval = 5000;
String msgStr = ""; // MQTT message buffer
float temp, hum;
//setting up wifi and mqtt client
WiFiClient espClient;
PubSubClient client(espClient);
void setup_wifi() {
delay(10);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.println("");
Serial.println("WiFi connected");
Serial.println("IP address: ");
Serial.println(WiFi.localIP());
}
void reconnect() {
while (!client.connected()) {
if (client.connect(clientID, mqttUserName, mqttPwd)) {
Serial.println("MQTT connected");
client.subscribe("Led1/status");
Serial.println("Topic Subscribed");
}
else {
Serial.print("failed, rc=");
Serial.print(client.state());
Serial.println(" try again in 5 seconds");
delay(5000); // wait 5sec and retry
}
}
}
//subscribe call back
void callback(char*topic, byte* payload, unsigned int length) {
Serial.print("Message arrived in topic: ");
Serial.println(topic);
Serial.print("Message:");
String data = "";
for (int i = 0; i < length; i++) {
Serial.print((char)payload[i]);
data += (char)payload[i];
}
if (data == "1") {
Serial.println("LED");
digitalWrite(LED, HIGH);
}
else {
digitalWrite(LED, LOW);
}
}
void setup() {
Serial.begin(115200);
// Initialize device.
dht.begin();
// get temperature sensor details.
sensor_t sensor;
dht.temperature().getSensor(&sensor);
dht.humidity().getSensor(&sensor);
pinMode(LED, OUTPUT);
digitalWrite(LED, LOW);
setup_wifi();
client.setServer(mqttServer, 1883); //setting MQTT server
client.setCallback(callback); //defining function which will be called when message is recieved.
randomSeed(analogRead(0));
}
void loop() {
if (!client.connected()) { //if client is not connected
reconnect(); //try to reconnect
}
client.loop();
unsigned long currentMillis = millis(); //read current time
if (currentMillis - previousMillis >= interval) { //if current time - last time > 5 sec
previousMillis = currentMillis;
//read temp and humidity
sensors_event_t event;
dht.temperature().getEvent(&event);
if (isnan(event.temperature)) {
Serial.println(F("Error reading temperature!"));
}
else {
Serial.print(F("Temperature: "));
temp = event.temperature;
Serial.print(temp);
Serial.println(F("°C"));
}
// Get humidity event and print its value.
dht.humidity().getEvent(&event);
if (isnan(event.relative_humidity)) {
Serial.println(F("Error reading humidity!"));
}
else {
Serial.print(F("Humidity: "));
hum = event.relative_humidity;
Serial.print(hum);
Serial.println(F("%"));
}
int rand_num = random(90);
msgStr = "{\"parameters\" : { \"temp\" : " + String(temp) + ",\"humidity\": " + String(hum) + ",\"Random\": " + String(rand_num) + "}}";
byte arrSize = msgStr.length() + 1;
char msg[arrSize];
Serial.print("PUBLISH DATA:");
Serial.println(msgStr);
msgStr.toCharArray(msg, arrSize);
client.publish(topic, msg);
msgStr = "";
delay(50);
}
}
| 24a37fa4aac65dea4bee2b3ffb170d2feb669e1e | [
"Markdown",
"C++"
] | 2 | Markdown | HighVoltages/MQTT-in-MATLAB | 080424c4727be7ecb85704fcab1008d27d3780bf | a6715371753f6c9a0f48cb5867790f8398741921 | |
refs/heads/master | <repo_name>zeromile/php-training<file_sep>/arrays.php
<?php
$myIndexArray = array("Katie", "Em", "Molly");
$myAssocArray = array(
"name" => "Katie",
"latinx" => True,
"cohort" => "design"
);
$myOtherAssocArray = array();
$myOtherAssocArray["name"] = "Nikki";
<file_sep>/README.md
# Basic PHP #
## Day 01 ##
[Basic PHP/MySQL Install](basic-php-instructions.md)
- Create ```index.php```
- Comments ```// /* */```
- ```echo``` Command
- Variables
- Strings
- Concatenation
- Arrays
- Indexed
- Associative
- Multi-dimensional
## Day 02 ##
- ```include_once``` vs ```require_once()```
- Control structures
- ```for```
- ```if```
- ```==``` vs ```=```
- Functions
Challenge: Create a 3 page website (```about.php```, ```index.php```, ```contact.php```) whose navigation and page content is fed by arrays; use functions to access the arrays and echo the content. You will need: ```content.php```, ```functions.php```, and ```nav.php``` files
## Day 03 ##
- Make a backup of your Vagrant file ```$ mv Vagrantfile Vagrantfile.log```
- Download [Vagrantfile](Vagrantfile) from the repo to your local ```php-training``` folder (or copy-paste to an empty ```Vagrantfile``` - click on file, view "raw", then file/save)
- Create a basic form on the ```contact.php``` page (firstname, lastname, submit)
- Use ```$_POST/$_GET``` to pull data into the index.php
- Use "Null Coalescing" ```??``` operators to set default value of variable from ```$_POST```
Challenge: Split into teams of 2 and, on the White boards, write out a basic HTML page that uses PHP to set a name variable before the HTML and then echo out that variable in the body of the HTML.
Challenge: Same teams, on the white boards, before your HTML use php to set two names with an array and then use a loop in the body of the HTML to echo each name out in ```<p>``` tags.
Challenge: Use PHP to get the first and last name variables from ```$_POST``` and echo them out as the default content for the two first and last name fields in the form.
## Day 04 ##
- Databases (what are they? how do they even work?)
- MySQL
- Log in to mysql locally
- Show Databases
- Show Tables
- Describe tables
- Select data
- Create a database
- Create a table
- Insert rows
## Day 05 ##
- Enable PHP Error Reporting
- [enable-php-error-reporting](enable-php-error-reporting.md)
- Connect to database in PHP
## Day 06 ##
## Day 07 ##
- Back up database with ```mysqldump```
- Import database using ```mysql```
- Update ```Vagrant``` provisioners to backup and import database
- Begin restructure of database tables
- create navigation table
- update content table
- Update ```index.php``` and ```function-new.php``` to load new navigation
## Day 08 ##
- Enable error checking without editing ```php.ini```
- ```.htaccess``` file directives for error checking
- ```php_flag display_startup_errors on```
- ```php_flag display_errors on```
- PHP file directives for error checking (add to ```connect.php```)
- ```ini_set('display_errors', 1);```
- ```ini_set('display_startup_errors', 1);```
- ```error_reporting(E_ALL);```
- Update tables to remove ```.php``` from filename field
- Create file redirects
- ```.htaccess``` redirects:
```
# Turn rewrite on
Options +FollowSymLinks
RewriteEngine On
# Redirect requests to index.php
RewriteCond %{REQUEST_URI} !=index.php
RewriteCond %{REQUEST_URI} !.*\.png$ [NC]
RewriteCond %{REQUEST_URI} !.*\.jpg$ [NC]
RewriteCond %{REQUEST_URI} !.*\.css$ [NC]
RewriteCond %{REQUEST_URI} !.*\.gif$ [NC]
RewriteCond %{REQUEST_URI} !.*\.js$ [NC]
RewriteRule .* index.php
```
- ```$ sudo a2enmod rewrite```
- ```sudo sed -i '/<Directory \/var\/www\/>/,/<\/Directory>/ s/AllowOverride None/AllowOverride All/' /etc/apache2/apache2.conf```
- ```sudo service apache2 restart```
- edit ```connect.php``` to include redirect code
- ```ini_set('display_errors', 1);```
- ```ini_set('display_startup_errors', 1);```
- ```error_reporting(E_ALL);```
- Add code to grab page name from URL
```
$uriSegments = explode("parse_url($_SERVER['REQUEST_URI'], PHP_URL_PATH));
$thisPage = array_pop($uriSegments);
if($thisPage=="") {
$thisPage="home";
}
$thisPagename = $thisPage;
```
- Updated ```makeNav()```
- Updated ```makeContent()``` to loop through multiple content items
## Day 09 ##
- Add comments to relevant files
- Review new Vagranfile updates for enabling redirects, adding the following to the shell scripts to the APACHE2-RESTART section
```
echo "----------------APACHE2-RESTART--------"
sudo a2enmod rewrite
sudo sed -i '/<Directory \\/var\\/www\\/>/,/<\\/Directory>/ s/AllowOverride None/AllowOverride All/' /etc/apache2/apache2.conf
sudo service apache2 restart
```
- Update ```.htaccess``` to allow php files
- Comment this line
- ```#RewriteCond %{REQUEST_URI} !=index.php```
- And add this line after
- ```RewriteCond %{REQUEST_URI} !.*\.php$ [NC]```
- Create a login link in nav function
- Show how variables do not get passed from page to page
- set $loggedIn
- echo this in index.php
- create login.php to echo
- it fails
- Set session variable in index first
- Echo session variable in login
- Change nav so that login link only shows when not "logged in" is true
- Create ```secret.php``` which will display a special message when logged in but redirect back to index.php if not
```
<?php
session_start();
$loggedIn = $_SESSION['loggedin'];
if ( $loggedIn == "logged in" ) {
echo "Welcome to the secret page. K. That's it. Bye.";
} else {
header("Location: http://192.168.33.10/index");
}
```
## Day 10 ##
Today we will continue adding to our login system.
- Add and commit your changes
```
$ git add .
$ git commit -m "beginning of day 10"
```
- Create and checkout day10 branch
```
$ git checkout -b day10
```
OR
```
$ git branch day10
$ git checkout day10
```
- Remove these files: ```contact.php```, ```content.php```, ```nav.php```, ```about.php```
- remove these two lines from top of ```connect.php```
```
//$loggedIn = "not logged in";
$_SESSION["loggedin"] = "not logged in";
```
- Add logout link in ```function-new.php```
```
if ($loggedIn == "not logged in"){
echo "<li><a href='login.php'>Log In</a></li>";
} else {
echo "<li><a href='logout.php'>Log Out</a></li>";
}
```
- Create ```logout.php```
- ```$ touch logout.php```
- Add this code to ```logout.php```
```
session_start();
$_SESSION = array();
session_destroy();
header("Location: http://192.168.33.10/index");
```
- Update line 5 ```index.php```
```
$loggedIn = $_SESSION['loggedin'] ?? "not logged in";
```
- Revise ```login.php```
```
session_start();
require_once('connect.php');
if (!empty($_POST)){
// if not logged in then check if login was submitted
$userName = $_POST["username"];
$passWord = $_POST["<PASSWORD>"];
$sql = "SELECT username, password, realname FROM test.users WHERE username = '$userName' LIMIT 1";
$result = $conn->query($sql);
$row = $result->fetch_assoc();
if ($userName == $row["username"] &&
md5($passWord) == $row["password"])
{
$_SESSION["loggedin"] = "logged in";
$loggedIn = $_SESSION["loggedin"];
} else {
echo "Try again";
$loggedIn = $_SESSION["loggedin"] ?? "not logged in";
}
} else {
$loggedIn = $_SESSION["loggedin"] ?? "not logged in";
}
if ($loggedIn == "logged in") {
// if "logged in" then redirect to index
header("Location: http://192.168.33.10/index");
} else {
// if not logged in show login form
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<h1>Login Page</h1>
<form action="login.php" method="post">
Username: <input type="text" name="username"> </br>
Password: <input type="<PASSWORD>" name="password"> </br>
<input type="submit" value="login">
</form>
</body>
</html>
<?php }
```
- Create ```users``` table (id, login, password)
- Verify log in using the MD5 Hash function in PHP
## Day 11 ##
- Challenge: Add code to your site that will show the user's "realname" when they are logged in to the site
- Solution: Two lines, yo:
- Add the ```$_SESSION["realname"]``` line to the following code in ```login.php```
```
if ($userName == $row["username"] &&
md5($passWord) == $row["password"])
{
$_SESSION["loggedin"] = "logged in";
$_SESSION["realname"] = $row["realname"]; // add this line
```
- Add the ```$_SESSION["realname"]``` line to the followiung code in ```function-new.php```
```
if ($loggedIn == "not logged in"){
echo "<li><a href='login.php'>Log In</a></li>";
} else {
echo "<li><a href='logout.php'>Log Out</a></li>";
echo "<p> Howdy " . $_SESSION["realname"] . "</p>"; //add this line
}
echo "</ul>";
```
## Day 12 ##
- Challenge: Add CSS to your php-training project that does the following:
- Horizontal navigation w/ background colors:
- ```#BF3F7F``` when logged in
- ```#7FBF3F``` when logged out
- Styles applied to ```<h>``` and ```<p>``` tags (that, at the very least, change the default typefaces)
## Day 13 - Part 1: CSS Challenge Solution##
- Video at [CSS Challenge Solution](https://youtu.be/XTf1NzeU_UY)
- Change name of ```function-new.php``` to ```functions.php```
- Change the reference to ```functions.php``` in ```index.php```
- Add ```<?php echo $_SERVER['PHP_SELF']; ?>``` in the action portion of the form in ```login.php```
- Solution to CSS Challenge: Sharing is caring
- Create ```main.css```
- Add this css:
```
h1, h2, h3, p, a {
font-family: sans-serif;
}
ul {
list-style-type: none;
margin: 0;
padding: 0;
overflow: hidden;
background-color: #333;
}
li {
float: left;
}
li a {
display: block;
color: white;
text-align: center;
padding: 14px 16px;
text-decoration: none;
}
/* Change the link color to #111 (black) on hover */
li a:hover {
background-color: #111;
}
section {
padding: 0 30px 0 30px;
}
```
- In ```index.php``` a
- Add the link to the ```main.css``` file in the head section with:
```
<link rel="stylesheet" href="main.css">
```
- Add the ```$thisPageName``` variable into the ```makeNav()``` function call
```
makeNav($conn, $loggedIn, $thisPagename);
```
- In ```functions.php```:
- Add the ```$thisPagename``` variable into the ```makeNav()``` function parameters to receive the page name
```
function makeNav($conn, $loggedIn, $thisPagename){
```
- Change the background color of the nav by revising the ```makeNav()``` function block to swap a class based on the logged in status of ```$loggedIn``` and echoing out the class in the opening ```<ul>```
```
if($loggedIn == "logged in"){
$loggedInClass = "class='loggedin'";
} else {
$loggedInClass = "class='notloggedin'";
}
echo "<ul " . $loggedInClass . ">";
```
- Add an active class to the navigation link by revising the ```makeNav()``` function block to check if the supplied page name is equal to the active page name and setting the echo'd variable ```$active```.
```
while ( $row = $result->fetch_assoc() ) {
if ( $thisPagename == $row['pagename']) {
$active = "class='active'";
} else {
$active = "";
}
echo "<li " . $active . "><a href='" . $row['pagename'] . "'>" .$row['pagetitle']. "</a></li>";
}
```
- In ```main.css``` add the following classes
```
.active {
background-color: #4CAF50;
}
.loggedin {
background-color: #BF3F7F;
}
.notloggedin {
background-color: #7FBF3F;
}
```
## Day 13 - Part 2: Intro to Classes ##
A class is a created object that is a collection of variables and functions (in a class they are referred to as properties and methods). The advantage of putting your functions and variables into a class is that because a class is scoped to the instantiating object your methods and properties will be protected and also available to each other within the class.
- Create a new file named ```class.php```
- In the ```class.php``` file create the class construct named ```Square```:
```
<?php
class MyClass
{
}
```
- Now let's add a property:
```
class MyClass
{
/*
Create the variable, sets the default
value, and makes the variable available
to the other class member's methods &
properties
*/
public $myname = 'Jason';
}
```
- Notice that we have added the word ```public``` in front of our variable declaration. This allows the variable to be accessed from outside of the instantiated class, which means it can be used in our general program and not just inside the class.
- There are two other variable visibility keywords: ```protected``` and ```private```, which can also be applied to methods. They set the "scope" of the class member they are applied to.
- ```public``` - member will be available everywhere (must still be called by associating it with the class)
- ```protected``` - member will be available only inside the class itself and inheriting and parent classes
- ```private``` - member will be available only within the class that defines the member
- We can see this in action by using the php cli
- ```$ vagrant ssh``` into your running Vagrant box
- ```$ cd /vagrant``` // change into your vagrant folder
- ```$ php -a``` // start the php CLI
- Type each of the following lines into the CLI (at the ```php >``` prompt)
- Load the ```class.php``` file that contains our ```MyClass``` class
- ```php > require('class.php');```
- Instantiate the class in a new variable
- ```php > $myClass = new MyClass();```
- Echo out the ```$myname``` variable
- ```php > echo $myClass->myname;```
- Now lets add a method:
```
...
public $myname = 'Jason';
public function printHello()
{
return $this->myname;
}
...
```
- Notice that there is a new keyword called ```return```. A return will cease execution of the function where the return is called and send any elements after it, in the same statement, back to wherever the function was called from. ```return``` Is not a function/method but a "language construct"
- Call the function named ```printHello()``` and echo it's output
- ```php > echo $myClass->printHello();```
<file_sep>/mysql-cli-database-connection.md
# Connecting to a Database using the MySQL CLI #
This will be a walk-through of how to use the MySQL CLI (command line interface) to connect to MySQL, create a database, create a table, and select data from that table.
We need to start by making sure we have a database selected. We'll be using MySQL and PHP's MySQLi connector. There is another method called PDO (PHP Data Objects) which allows you to connect to 12 different database systems but we will start with the MySQLi for now.
SSH into your running php-training Vagrant box that we set up previously in Day 03. <br/>
```$ vagrant ssh```
Connect to the MySQL CLI
```$ mysql -u root -p```
The password is ```<PASSWORD>```
You will be presented with the MySQLi CLI prompt:
```mysql>```
Command reference:
```SHOW DATABASES;``` - Show a list of all the DATABASES
```SHOW TABLES in {DATABASENAME};``` - Show a list of tables in a particular DATABASE
```USE {DATABASENAME};``` - This chooses which database you want to use by default
```SHOW TABLES;``` - Show a list of tables in the currently selected DATABASE
```DESCRIBE {TABLENAME};``` - Show a list of the columns and column data types for the table name provided
```SELECT * FROM {TABLENAME};``` - Selects all records and all columns from the table name provided
```CREATE DATABASE {DATABASENAME};``` - Create a database
```CREATE TABLE {TABLENAME} ({OPTIONS});``` - Create a table on the chosen database (USE {DATABASENAME})
```INSERT INTO {TABLENAME} ({COLUMNNAME},{COLUMNNAME},{COLUMNNAME}...)```
```VALUES ({VALUE}, {VALUE}, {VALUE}...);``` - Create a record in the provided table
<file_sep>/index.php
<?php
session_start();
require_once("connect.php");
require_once("functions.php");
$loggedIn = $_SESSION['loggedin'] ?? "not logged in";
/*
this pulls the text from after the first / in the
url and sets it to an array
*/
$uriSegments = explode("/", parse_url($_SERVER['REQUEST_URI'],PHP_URL_PATH));
/*
this pulls the last item out of the uri array and sets
$thisPagename variabe to that item
*/
$thisPagename = array_pop($uriSegments);
// this sets the pagename if none is provided in the url
if ($thisPagename == ""){
$thisPagename = "index";
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link rel="stylesheet" href="main.css">
<title><?php // makeTitle($conn, $thisPagename); ?></title>
</head>
<body>
<nav>
<?php
makeNav($conn, $loggedIn, $thisPagename);
echo "<p>" . $loggedIn . "</p>";
?>
</nav>
<section>
<div>
<?php
//echo $thisPagename;
makeContent($conn, $thisPagename);
?>
</div>
</section>
</body>
</html>
<file_sep>/functions.php
<?php
function makeNav($conn, $loggedIn, $thisPagename){
// This creates the navigation from the navigation table
$sql = "SELECT pagename, pagetitle FROM test.navigation";
$result = $conn->query($sql);
if($loggedIn == "logged in"){
$loggedInClass = "loggedin";
} else {
$loggedInClass = "notloggedin";
}
echo "<ul class='" . $loggedInClass . "'>";
// outputs the rows of results from the navigation,
// setting the active variable for the page that is active
while ( $row = $result->fetch_assoc() ) {
if ( $thisPagename == $row['pagename']){
$active = "active";
} else {
$active = "";
}
echo "<li class='" . $active . "''><a href='" . $row['pagename'] . "'>" .$row['pagetitle']. "</a></li>";
}
// Outputs the login links
if ($loggedIn == "not logged in"){
echo "<li><a href='login.php'>Log In</a></li>";
} else {
echo "<li><a href='logout.php'>Log Out " . $_SESSION["realname"] . "</a></li>";
}
echo "</ul>";
} // end of makeNav function
function makeContent($conn, $thisPagename){
/*
this creates the content from the content
table based on the supplied $thisPagename variable.
It will then loop through all the matching content
records and export those individually.
*/
$sql = "SELECT * FROM test.content WHERE pagename = '$thisPagename'";
$result = $conn->query($sql);
while($row = $result->fetch_assoc()){
echo $row['contenttitle'];
echo $row['content'];
}
} // end of makeContent
function makeTitle($conn, $thisPagename){
/*
This will echo out the current page title
from the content table based on the page name
in $thisPageName
*/
$sql = "SELECT * FROM test.content WHERE pagename = '$thisPagename'";
$result = $conn->query($sql);
$row = $result->fetch_assoc();
echo $row['pagename'];
}
<file_sep>/login.php
<?php
session_start();
require_once('connect.php');
require_once('functions.php');
if (!empty($_POST)){
// if not logged in then check if login was submitted
$userName = $_POST["username"];
$passWord = $_POST["password"];
$sql = "SELECT username, password, realname FROM test.users WHERE username = '$userName' LIMIT 1";
$result = $conn->query($sql);
$row = $result->fetch_assoc();
if ($userName == $row["username"] &&
md5($passWord) == $row["password"])
{
$_SESSION["loggedin"] = "logged in";
$_SESSION["realname"] = $row["realname"];
$loggedIn = $_SESSION["loggedin"];
} else {
echo "Try again";
$loggedIn = $_SESSION["loggedin"] ?? "not logged in";
}
} else {
$loggedIn = $_SESSION["loggedin"] ?? "not logged in";
}
if ($loggedIn == "logged in") {
// if "logged in" then redirect to index
header("Location: http://192.168.33.10/index");
} else {
// if not logged in show login form
$thisPage = $_SERVER["PHP_SELF"];
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<h1>Login Page</h1>
<form action='<?php echo $thisPage ?>' method="post">
Username: <input type="text" name="username"> </br>
Password: <input type="<PASSWORD>" name="password"> </br>
<input type="submit" value="login">
</form>
</body>
</html>
<?php
}
<file_sep>/class.php
<?php
class MyClass
{
public $myname = 'Jason';
public function printHello()
{
return ($this->myname);
}
}
<file_sep>/secret.php
<?php
session_start();
$loggedIn = $_SESSION['loggedin'];
if ( $loggedIn == "logged in" ) {
echo "Welcome to the secret page. K. That's it. Bye.";
} else {
header("Location: http://192.168.33.10/index");
}
<file_sep>/basic-php-instructions.md
# Basic PHP/MySQL Install #
Once you have VirtualBox and Vagrant
running you can use the following
instructions to help you stand up a
basic PHP/MySQL virtual machine.
The following instructions are INSECURE...
which means you should not do this on a
production machine that is live on the
Internets. This is only for your local testing.
```
$ mkdir php-training folder
$ cd php-training
$ vagrant init ubuntu/xenial64
```
Edit the Vagrantfile
1. set private network to 192.168.33.10
2. config vm synced folder to:
```
".", "/vagrant", :group => "www-data", :mount_options => ['dmode=775', 'fmode=644']
```
Save and exit Vagrantfile
```
$ vagrant up
$ vagrant ssh
```
Check for and install updates
```
$ sudo apt-get update -y
$ sudo apt-get upgrade -y
```
Install apache2, set up linking for external folder
```
$ sudo apt-get install apache2
$ sudo rm -rf /var/www/html
$ sudo ln -fs /vagrant /var/www/html
```
Install MySQL (sets password as '<PASSWORD>')
```
$ sudo debconf-set-selections <<< 'mysql-server mysql-server/root_password password secret'
$ sudo debconf-set-selections <<< 'mysql-server mysql-server/root_password_again password secret'
$ sudo apt-get -y install mysql-server
```
Install PHP
```
$ sudo apt-get install -y php7.0 libapache2-mod-php7.0 php7.0-curl php7.0-cli php7.0-dev php7.0-gd php7.0-intl php7.0-mcrypt php7.0-json php7.0-mysql php7.0-opcache php7.0-bcmath php7.0-mbstring php7.0-soap php7.0-xml php7.0-zip -y
```
Restart Apache, updates again, then exit
```
$ sudo service apache2 restart
$ sudo apt-get update -y
$ sudo apt-get upgrade -y
$ exit
```
Create index.php
```
$ touch index.php
```
Add some code! (using nano or your editor of preference)
```
$ nano index.php
```
Save and then test in your browser at 192.168.33.10
Now create a database to use in our class (password will be '<PASSWORD>', when asked)
```
echo "create database testdb" | mysql -u root -p
```
<file_sep>/test.sql
-- MySQL dump 10.13 Distrib 5.7.27, for Linux (x86_64)
--
-- Host: localhost Database: test
-- ------------------------------------------------------
-- Server version 5.7.27-0ubuntu0.16.04.1
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8 */;
/*!40103 SET @OLD_TIME_ZONE=@@TIME_ZONE */;
/*!40103 SET TIME_ZONE='+00:00' */;
/*!40014 SET @OLD_UNIQUE_CHECKS=@@UNIQUE_CHECKS, UNIQUE_CHECKS=0 */;
/*!40014 SET @OLD_FOREIGN_KEY_CHECKS=@@FOREIGN_KEY_CHECKS, FOREIGN_KEY_CHECKS=0 */;
/*!40101 SET @OLD_SQL_MODE=@@SQL_MODE, SQL_MODE='NO_AUTO_VALUE_ON_ZERO' */;
/*!40111 SET @OLD_SQL_NOTES=@@SQL_NOTES, SQL_NOTES=0 */;
--
-- Table structure for table `content`
--
DROP TABLE IF EXISTS `content`;
/*!40101 SET @saved_cs_client = @@character_set_client */;
/*!40101 SET character_set_client = utf8 */;
CREATE TABLE `content` (
`id` int(4) unsigned NOT NULL AUTO_INCREMENT,
`pagename` varchar(30) NOT NULL,
`contenttitle` varchar(30) NOT NULL,
`content` mediumtext,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=8 DEFAULT CHARSET=latin1;
/*!40101 SET character_set_client = @saved_cs_client */;
--
-- Dumping data for table `content`
--
LOCK TABLES `content` WRITE;
/*!40000 ALTER TABLE `content` DISABLE KEYS */;
INSERT INTO `content` VALUES (1,'index','<h2>Welcome</h2>','<p>Welcome to our site. This is an example of a snippet of content. We are going to make more.</p>'),(2,'about','<h2>Our Story</h2>','<p>We started with a simple idea: ball bearings. And then we made more ball bearings. The end.</p>'),(3,'contact','<h2>Contact</h2>','<p>Phone:</p>\r\n<p>559-555-1212</p>\r\n<a href=\"mailto:<EMAIL>\">Bob</a>'),(6,'about','<h2>Our Team</h2>','<p>Our team is the best. They have the bigliest hearts. And their brains are so smart, smarter than the other guys for sure.</p>\r\n<h3>Our President</h3>\r\n<img src=\'http://lorempixel.com/400/200/cats/2\' alt=\'picture of our president, a cat\'>'),(7,'index','<h2>What we do</h2>','<p>What we do is nunya! Actually we do a lot of cool stuff. Did we mention ball bearings? No? Well it\'s the future. Fletch knowsit. You know it. Your Grandma is pretty sure she knows it.</p>');
/*!40000 ALTER TABLE `content` ENABLE KEYS */;
UNLOCK TABLES;
--
-- Table structure for table `navigation`
--
DROP TABLE IF EXISTS `navigation`;
/*!40101 SET @saved_cs_client = @@character_set_client */;
/*!40101 SET character_set_client = utf8 */;
CREATE TABLE `navigation` (
`id` int(1) unsigned NOT NULL AUTO_INCREMENT,
`pagename` varchar(30) NOT NULL,
`pagetitle` varchar(30) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=4 DEFAULT CHARSET=latin1;
/*!40101 SET character_set_client = @saved_cs_client */;
--
-- Dumping data for table `navigation`
--
LOCK TABLES `navigation` WRITE;
/*!40000 ALTER TABLE `navigation` DISABLE KEYS */;
INSERT INTO `navigation` VALUES (1,'index','Home'),(2,'contact','Contact Us'),(3,'about','About');
/*!40000 ALTER TABLE `navigation` ENABLE KEYS */;
UNLOCK TABLES;
--
-- Table structure for table `users`
--
DROP TABLE IF EXISTS `users`;
/*!40101 SET @saved_cs_client = @@character_set_client */;
/*!40101 SET character_set_client = utf8 */;
CREATE TABLE `users` (
`id` int(11) unsigned NOT NULL AUTO_INCREMENT,
`username` varchar(255) NOT NULL,
`password` varchar(255) NOT NULL,
`realname` varchar(255) NOT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=2 DEFAULT CHARSET=latin1;
/*!40101 SET character_set_client = @saved_cs_client */;
--
-- Dumping data for table `users`
--
LOCK TABLES `users` WRITE;
/*!40000 ALTER TABLE `users` DISABLE KEYS */;
INSERT INTO `users` VALUES (1,'jason','<PASSWORD>','<NAME>');
/*!40000 ALTER TABLE `users` ENABLE KEYS */;
UNLOCK TABLES;
/*!40103 SET TIME_ZONE=@OLD_TIME_ZONE */;
/*!40101 SET SQL_MODE=@OLD_SQL_MODE */;
/*!40014 SET FOREIGN_KEY_CHECKS=@OLD_FOREIGN_KEY_CHECKS */;
/*!40014 SET UNIQUE_CHECKS=@OLD_UNIQUE_CHECKS */;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
/*!40111 SET SQL_NOTES=@OLD_SQL_NOTES */;
-- Dump completed on 2019-10-16 5:13:42
<file_sep>/connect.php
<?php
// this allows apache to redirect files based on .htaccess
ini_set('display_errors', 1);
ini_set('display_startup_errors', 1);
error_reporting(E_ALL);
$servername = "localhost";
$username = "root";
$password = "<PASSWORD>";
// Create connection
$conn = new mysqli($servername,$username,$password);
// Check connection
if ($conn->connect_error) {
die("No worky " . $conn->connect_error);
} else {
$success = "It worked!";
}
<file_sep>/my-plugin.php
<?php
/*
* Plugin Name: Custom Directory Plugin
* Description: A custom post type
* Author: Me
* Author URI: Https://www.jasonlee.com
* Version: 1.0
*/
function register_custom_post_type() {
$args = [
'labels' => [
'name' => 'Directory',
'singular_name' => 'Directory Listing',
'add_new_item' => 'Add Directory Listing'
],
'public' => true,
'supports' => [
'title', 'thumbnail', 'editor' //these are the options for what shows on the page thing
],
'taxonomies' => []
];
// register our Custom directory using the args above
// the namd of the post type can't be more than 20 characters
register_post_type('dir_custom_posts', $args);
}
//finding the template file\directory
function custom_include_template_function( $template_path ) {
if( get_post_type() == 'my_directory'){
if(is_single()){
if ($theme_file == locate_template(['single-my_directory.php']){
$template_path = $theme_file;
} else {
$template_path = plugin_dir_path(__FILE__) . '/single-my_directory.php');
}
}
}
return $template_path;
}
//add_action ( when, what )
add_action('init', 'register_custom_post_type')
//filters change information
add_filter('template_include', 'custom_include_template_function')
| f0395e1ad2c8469ee456eb88382071e2ebf47413 | [
"Markdown",
"SQL",
"PHP"
] | 12 | PHP | zeromile/php-training | 1a6103d5ef1da713b9e757c634635cbf8c528a47 | c80a2d970c367a57ec604c15ac7710c0ead6242f | |
refs/heads/master | <file_sep>package main
func p1easy(n int) int {
sum := 0
for i:=0; i<=n; i++ {
if i%3==0 || i%5==0 {
sum += i
}
}
return sum
}
<file_sep>package main
import (
"testing"
)
func TestP1easy(t *testing.T) {
pairs := [...][2]int{{9, 23}, {999, 233168}}
for _, pair := range pairs {
in, out := pair[0], pair[1]
if x:=p1easy(in); x!=out {
t.Errorf("p1easy(%d) = %d, should be %d", in, x, out)
} else {
t.Log("p1easy(%d) = %d, should be %d", in, x, out)
}
}
}
func BenchmarkP1easy(b *testing.B) {
in := 1000
b.StartTimer()
for i:=0; i<b.N; i++ {
p1easy(in)
}
b.StopTimer()
}
| 69662b84ff6508a3372228b5fb90d1be06846513 | [
"Go"
] | 2 | Go | ian910297/ProjectEuler | c6bb7a5fb16199815f9a3803945462acfa29c208 | af4ca9d95b122b7d4ea3d07414b07814787da08f | |
refs/heads/master | <file_sep>//
// AppManager.swift
// Mood
//
// Created by Hascats on 2021/6/19.
//
import Foundation
import Combine
struct AppManager {
static let Authenticated = PassthroughSubject<Bool, Never>()
static func IsAuthenticated() -> Bool {
return UserDefaults.standard.string(forKey: "token") != nil
}
}
<file_sep>/root/.virtualenvs/ios/bin/python /root/workspace/iOSproject/crawler.py<file_sep>//
// ContentView.swift
// Mood
//
// Created by Hascats on 2021/6/18.
//
import SwiftUI
struct Result:Codable{
let id : Int
let labels: String
let url : String
}
struct Response:Codable{
let results : [Result]
}
struct ContentView: View {
@State var isAuthenticated = AppManager.IsAuthenticated()
@State var results : [Result] = []
var body: some View {
TabView{
NavigationView{
ScrollView(.vertical){
ForEach(self.results,id:\.id){ item in
LazyVStack{
URLImage(withURL: item.url)
HStack{
Text(item.labels)
}.frame(width: 300, height: 40, alignment: /*@START_MENU_TOKEN@*/.center/*@END_MENU_TOKEN@*/)
.background(Color.gray)
}
}
}.navigationBarTitle(Text("Mood"))
}
.tabItem {
Image(systemName: "photo.on.rectangle")
Text("Mood")
}
Group {
isAuthenticated ? AnyView(HomeView()) : AnyView(LoginView())
}
.onReceive(AppManager.Authenticated, perform: {
isAuthenticated = $0
}).tabItem {
Image(systemName: "person.crop.circle")
Text("Login")
}
}
.onAppear(perform: request_data)
}
func request_data(){
guard let url = URL(string:"https://www.hascats.cn")
else { return }
URLSession.shared.dataTask(with: url){ (data,response,error) in
guard let data = data,
let decodedData = try? JSONDecoder().decode(Response.self,from: data)
else { return }
self.results = decodedData.results
}.resume()
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
<file_sep>//
// LoginView.swift
// Mood
//
// Created by Hascats on 2021/6/19.
//
import SwiftUI
import Combine
struct LoginView: View {
@ObservedObject var vm = LoginViewModel()
@State var showAlert = false
var body: some View {
VStack {
Text("Login")
.font(.largeTitle)
.bold()
.padding(.bottom, 40)
VStack {
TextField("Email", text: $vm.email)
.keyboardType(.emailAddress)
.textContentType(.emailAddress)
.autocapitalization(.none)
.textFieldStyle(RoundedBorderTextFieldStyle())
}
.padding()
VStack {
SecureField("Password", text: $vm.password)
.textContentType(.password)
.textFieldStyle(RoundedBorderTextFieldStyle())
}
.padding()
Button("Login") {
showAlert = !vm.loginUser()
}
.padding()
.frame(width: 100, height: 40, alignment: .center)
.background(Color.green)
.foregroundColor(.white)
.cornerRadius(8)
}
.alert(isPresented: $showAlert, content: {
Alert(title: Text(vm.errorMessage))
})
}
}
struct LoginView_Previews: PreviewProvider {
static var previews: some View {
LoginView()
}
}
<file_sep>//
// HomeViewModel.swift
// Mood
//
// Created by Hascats on 2021/6/19.
//
import Foundation
import Combine
class HomeViewModel: ObservableObject {
func logoutUser() {
UserDefaults.standard.removeObject(forKey: "token")
AppManager.Authenticated.send(false)
}
}
<file_sep># Mood
主要思路:
1. iOS端App通过网络请求,请求服务器
2. 服务器查询云数据库,获得图片的相关信息(id,url)等
3. 服务器以通过HTTP Web应用返回JSON数据,包含以上信息
4. App通过图片的url获取图片,并进行渲染
5. 图片的url信息由服务器上的爬虫程序定时爬取(每天凌晨三点),保证图片的更新
### 路径解释:
- /Mood 包含App源代码
- /server:
- crawer.py 爬虫程序
- server.py web服务程序
- nohup.out 程序输出
- paged.txt 辅助爬虫程序的一个文件
- run_crawler.sh 爬虫程序启动脚本,由服务器定时任务程序cronb执行
<file_sep>import pymysql
from typing import *
from parsel import Selector
import httpx
import asyncio
import random
import pickle
class Spider():
def __init__(self,page) -> None:
self.headers = {"User-Agent":"Mozilla/5.0 (Linux; Android 4.1.1; Nexus 7 Build/JRO03D) AppleWebKit/535.19 (KHTML, like Gecko) Chrome/18.0.1025.166 Safari/535.19"}
self.path = 'spider_downloads'
self.url = 'https://www.wallpapermaiden.com/category/anime?page=' + str(page)
self.download_urls = list(tuple())
self.page = page
def connect_db(self):
conn = pymysql.connect(
host='bj-cynosdbmysql-grp-rjihok1e.sql.tencentcdb.com',
port=21280,
user='root',
password='<PASSWORD>',
db='mood',
charset='utf8',
# autocommit=True, # 如果插入数据,, 是否自动提交? 和conn.commit()功能一致。
)
return conn
async def __get_download_url__(self,url):
async with httpx.AsyncClient() as client:
response = await client.get(url,headers=self.headers)
download_url = Selector(response.text).css('.wpBig > a::attr(href)').get()
self.download_urls.append(download_url)
def get_urls(self):
response = httpx.get(self.url)
selector = Selector(response.text)
wallpaper_list = selector.css('.wallpaperList')
urls = wallpaper_list.css('.wallpaperBgImage > img::attr(src)').getall()
labels = wallpaper_list.css('.wallpaperBgImage > img::attr(alt)').getall()
info = list(zip(labels,map(lambda url:'https://www.wallpapermaiden.com'+url,urls)))
self.download_urls = info
# loop = asyncio.get_event_loop()
# tasks = [
# self.__get_download_url__(url)\
# for url in urls
# ]
# loop.run_until_complete(asyncio.wait(tasks))
# loop.close()
async def parse_single_page(self,url):
async with httpx.AsyncClient() as client:
conn = self.connect_db()
cur = conn.cursor()
insert_sqli = f"insert into images(liked,labels,url) values(0,'{url[0]}','{url[1]}');"
cur.execute(insert_sqli)
conn.commit()
cur.close()
conn.close()
def crawl(self)->None:
try:
print(f"爬取第{self.page}页的图片")
self.get_urls()
loop = asyncio.get_event_loop()
tasks = [
self.parse_single_page(url)\
for url in self.download_urls
]
loop.run_until_complete(asyncio.wait(tasks))
loop.close()
except Exception as e:
print(e)
if __name__ == '__main__':
page = random.randint(2,955)
ls = []
with open("paged.txt","rb") as f:
ls = pickle.load(f)
while(page in ls):
page = random.randint(2,955)
spider = Spider(page)
spider.crawl()
print(f"爬取第{page}页成功!")
ls.append(page)
with open("paged.txt","wb") as f:
pickle.dump(ls,f)
<file_sep># -*- coding: UTF-8 -*-
from flask import Flask
import pymysql
app = Flask(__name__)
@app.get("/")
def read_item():
conn = pymysql.connect(
host='bj-cynosdbmysql-grp-rjihok1e.sql.tencentcdb.com',
port=21280,
user='root',
password='<PASSWORD>',
db='mood',
charset='utf8',
# autocommit=True, # 如果插入数据,, 是否自动提交? 和conn.commit()功能一致。
)
cur = conn.cursor()
sqli = "select id,labels,url from images order by time desc limit 10;"
result = cur.execute(sqli) # 默认不返回查询结果集, 返回数据记录数。
info = cur.fetchall() # 3). 获取所有的查询结果
info = list(map(lambda tup: {"id":tup[0],"labels":tup[1],"url":tup[2]},info))
info = {"results":info}
cur.close()
conn.close()
return info
if __name__ == '__main__':
app.run( host="0.0.0.0", port=443,debug=False,ssl_context=('/root/workspace/lqw/5581010_www.hascats.cn.pem','/root/workspace/lqw/5581010_www.hascats.cn.key'))
<file_sep>//
// HomeView.swift
// Mood
//
// Created by Hascats on 2021/6/19.
//
import SwiftUI
struct HomeView: View {
@ObservedObject var vm = HomeViewModel()
var body: some View {
VStack {
Text("Welcome")
.font(.largeTitle)
Spacer()
.frame(height: 100)
Button("Logout") {
vm.logoutUser()
}
.padding()
.frame(width: 100, height: 40, alignment: .center)
.background(Color.green)
.foregroundColor(.white)
.cornerRadius(8)
}
}
}
struct HomeView_Previews: PreviewProvider {
static var previews: some View {
HomeView()
}
}
<file_sep>//
// LoginViewModel.swift
// Mood
//
// Created by Hascats on 2021/6/19.
//
import SwiftUI
import Combine
class LoginViewModel: ObservableObject {
@Published var email = ""
@Published var password = ""
var errorMessage = ""
}
extension LoginViewModel {
func loginUser() -> Bool {
if email == "<EMAIL>",
password == "<PASSWORD>" {
let token = UUID().uuidString
UserDefaults.standard.set(token, forKey: "token")
AppManager.Authenticated.send(true)
return true
} else {
errorMessage = "Invalid credentials"
return false
}
}
}
| 476622546de458ca70495547d272d029805f27a3 | [
"Swift",
"Python",
"Markdown",
"Shell"
] | 10 | Swift | OMnitrixGit/Mood | cc27348cbfa632ec8fcaccfcbd3ca93d830617f7 | 1c2981918bebd268c26ce5c902d0724c65a1b04f | |
refs/heads/master | <file_sep># double-tracker-canny-filter
double tracker canny filter
<file_sep>#include <math.h>
#include "opencv2/imgproc/imgproc.hpp"
#include "opencv2/highgui/highgui.hpp"
using namespace cv;
using namespace std;
int main()
{
int A[3][3] = { { -1, 0, 1 }, { -2, 0, 2 }, { -1, 0, 1 } };
int B[3][3] = { { 1, 2, 1 }, { 0, 0, 0 }, { -1, -2, -1 } };
int Gx, Gy;
int e,f;
int c = 0;
int d = 0;
Mat var3 = imread("image1.jpg", 1);
Mat var1(var3.rows, var3.cols, CV_8UC1, Scalar(0));
Mat var2(var3.rows, var3.cols, CV_8UC1, Scalar(0));
Mat var4(var3.rows, var3.cols, CV_8UC1, Scalar(0));
Mat var5(var3.rows, var3.cols, CV_8UC1, Scalar(0));
Mat var6(var3.rows, var3.cols, CV_8UC1, Scalar(0));
cvtColor(var3, var1, CV_BGR2GRAY);
for (int i = 0; i < var1.rows; i++)
{
var2.at<uchar>(i, 0) = var1.at<uchar>(i, 0);
var2.at<uchar>(i, var1.cols - 1) = var1.at<uchar>(i, var1.cols - 1);
var5.at<uchar>(i, 0) = var1.at<uchar>(i, 0);
var5.at<uchar>(i, var1.cols - 1) = var1.at<uchar>(i, var1.cols - 1);
var4.at<uchar>(i, 0) = var1.at<uchar>(i, 0);
var4.at<uchar>(i, var1.cols - 1) = var1.at<uchar>(i, var1.cols - 1);
}
for (int i = 0; i < var1.cols; i++)
{
var2.at<uchar>(0, i) = var1.at<uchar>(0, i);
var2.at<uchar>(var1.rows - 1, i) = var1.at<uchar>(var1.rows - 1, i);
}
for (int i = 0; i < var1.rows - 1; i++){
for (int j = 0; j < var1.cols - 1; j++)
{
Gx = 0; Gy = 0;
c = 0;
for (int m = i; m < var1.rows; m++, c++)
{
if (c < 3)
{
d = 0;
for (int n = j; n < var1.cols; n++, d++)
{
if (d < 3)
{
if ((i + 1) < var1.rows && (j + 1) < var1.cols)
{
Gx += var1.at<uchar>(m, n) * A[m - i][n - j];
Gy += var1.at<uchar>(m, n) * B[m - i][n - j];
}
}
}
}
}
var4.at<uchar>(i + 1, j + 1) = atan(Gx / Gy)/3.14*180 + 90;
e = (int)sqrt(Gx*Gx + Gy*Gy);
if (e > 255)
var2.at<uchar>(i + 1, j + 1) = 255;
else
var2.at<uchar>(i + 1, j + 1) = e;
}
}
for (int i = 1; i < var1.rows - 1; i++){
for (int j = 1; j < var1.cols - 1; j++)
{
f = var4.at<uchar>(i, j);
if (f>0 && f < 45/2)
{
if (f>var4.at<uchar>(i, j - 1) && f>var4.at<uchar>(i, j + 1))
var5.at<uchar>(i, j) = var2.at<uchar>(i, j);
else
var5.at<uchar>(i, j) = 0;
}
else if (f>45/2 && f < 135/2)
{
if (f>var4.at<uchar>(i+1, j - 1) && f>var4.at<uchar>(i-1, j + 1))
var5.at<uchar>(i, j) = var2.at<uchar>(i, j);
else
var5.at<uchar>(i, j) = 0;
}
else if (f>135/2 && f < 225/2)
{
if (f>var4.at<uchar>(i - 1, j ) && f>var4.at<uchar>(i + 1, j ))
var5.at<uchar>(i, j) = var2.at<uchar>(i, j);
else
var5.at<uchar>(i, j) = 0;
}
else
{
if (f>var4.at<uchar>(i - 1, j-1) && f>var4.at<uchar>(i + 1, j+1))
var5.at<uchar>(i, j) = var2.at<uchar>(i, j);
else
var5.at<uchar>(i, j) = 0;
}
}
}
int min, max;
min = 150;
max = 250;
namedWindow("windows11", WINDOW_AUTOSIZE);
createTrackbar("track", "windows11", &min, 255);
namedWindow("windows12", WINDOW_AUTOSIZE);
createTrackbar("track", "windows12", &max, 255);
while (1)
{
for (int i = 0; i < var1.rows ; i++){
for (int j = 0; j < var1.cols ; j++)
{
if (var5.at<uchar>(i, j)>min && var5.at<uchar>(i, j) < max)
var6.at<uchar>(i, j) = 255;
else
var6.at<uchar>(i, j) = 0;
}
}
imshow("window6", var6);
imshow("window1", var1);
imshow("window2", var2);
imshow("window3", var3);
//imshow("window4", var4);
imshow("window5", var5);
if(waitKey(10) =='q')
break;
}
/*imshow("window1", var1);
imshow("window2", var2);
imshow("window3", var3);
//imshow("window4", var4);
imshow("window5", var5);
waitKey(0);*/
return(0);
} | 93a86f5107771cb8b8fbb5f3a134f2cbf1e1b627 | [
"Markdown",
"C++"
] | 2 | Markdown | sweta1947/double-tracker-canny-filter | 2c3284b86add0335082eb217f0a625753541636b | 747349fa427ed9f13378e167c2df0ea26afdd3d9 | |
refs/heads/master | <repo_name>tranbaquang/Login<file_sep>/src/FileWriterExample.java
import java.io.FileWriter;
import java.util.Scanner;
public class FileWriterExample {
public static void main(String args[]) {
try {
FileWriter userData = new FileWriter("user.txt");
FileWriter passData = new FileWriter("password.txt");
Scanner scan = new Scanner(System.in);
String user;
String password;
String rePassword;
do {
System.out.println(">>>>>Register an account<<<<<");
System.out.print("User name: ");
user = scan.nextLine();
System.out.print("Password: ");
password = scan.nextLine();
System.out.print("Re-enter Password: ");
rePassword = scan.nextLine();
} while (user.equals("") || !password.equals(rePassword) || password.equals(""));
userData.write(user + "\n");
passData.write(password + "\n");
userData.write(user + "\n");
passData.write(password + "\n");
userData.close();
passData.close();
// if (!user.equals("") && password.equals(rePassword)) {
// userData.write(user);
// passData.write(password);
// userData.close();
// passData.close();
// }
} catch (Exception e) {
System.out.println(e);
}
System.out.println("Successful account registration !");
}
}
| 5ca8e1713b332fc2244fd925ef9825a2ad947783 | [
"Java"
] | 1 | Java | tranbaquang/Login | 9ab3abb01d546a0ba5c96d79141cc7e69503bf0e | dc60e75e5c1a2c349667c42f149feb6831d52ecb | |
refs/heads/master | <repo_name>krissukoco/prac-mongodb<file_sep>/playground/mongodb-delete.js
const MongoClient = require('mongodb').MongoClient;
MongoClient.connect('mongodb://localhost:27017/TodoApp', (err, client) => {
if (err) {
return console.log('Unable to connect to MongoDB server');
} // 'return' will prevent codes below to excecute
console.log('Connection successful');
const db = client.db('TodoApp'); // For MongoDB v3.x.x
db.collection('Todos').findOneAndDelete({text: 'Wash car'}).then((result) => {
console.log(result);
}, (err) => {
console.log(err);
});
client.close();
});
<file_sep>/server/tests/server.test.js
const expect = require('expect');
const request = require('supertest');
const { ObjectID } = require('mongodb');
const { app } = require('./../server');
const { Todo } = require('./../models/todo');
const { User } = require('./../models/user');
const todosDummy = [{
_id: new ObjectID(),
text: 'First todo',
completed: false,
completedAt: 123
}, {
_id: new ObjectID(),
text: 'Second todo',
completed: false,
completedAt: 123
}, {
_id: new ObjectID(),
text: 'Third todo',
completed: false,
completedAt: 123
}];
// Set Todo to be empty, then insert todosDummy
beforeEach((done) => {
Todo.remove({}).then(() => {
Todo.insertMany(todosDummy, function(error, docs) {
console.log('Before Each: ', docs.length);
});
}).then(() => done());
});
// Test POST /todos
describe('POST /todos', () => {
it('should create a new todo', (done) => {
var anyText = 'Changed a bit';
request(app)
.post('/todos')
.send({text: anyText}) // required to send a 'text' field (POST)
.expect(200)
.expect((res) => {
expect(res.body.text).toBe(anyText);
console.log('Res.Body: ', res.body);
})
.end((err, res) => {
if (err) {
return done(err); // print error
// 'return' is merely to stop next lines to execute
}
// console.log(Todo); OPT
// Ensure that MongoDB has stored the data
// With (preset) todos is empty (with beforeEach; line 9)
// Todo.findOne({text: anyText}).then((todos) => { // returns 'todos' as array of documents
// console.log('Todos: ', todos);
// expect(todos.length).toBe(1);
// console.log('Length: ', todos.length);
// expect(todos[0].text).toBe(anyText);
// done();
Todo.find({ text: 'Changed a bit' }, function(err, todos) {
console.log(todos);
done();
});
// console.log(todos[0].text);
// }).catch((e) => done(e));
});
}); //
// No returns for bad data
it('should NOT send a todo with BAD data', (done) => {
request(app)
.post('/todos')
.send({})
.expect(400)
.end((err, res) => {
if(err) {
console.log('Other error occured');
return done(err);
}
Todo.find().then((todos) => {
console.log('3 Todos: ', todos);
expect(todos.length).toBe(3);
done();
}).catch((err) => done(err));
});
});
});
// Test GET /todos
describe('GET /todos', () => {
it('should get all todos', (done) => {
request(app)
.get('/todos')
.expect(200)
.expect((res) => {
expect(res.body.todos.length).toBe(3);
})
.end(done);
});
});
// Todo with ID
describe('GET /todos/:id', () => {
it('should return Todo with correct ID', (done) => {
request(app)
.get(`/todos/${todosDummy[0]._id.toHexString()}`)
.expect(200)
.expect((res) => {
expect(res.body.todo.text).toBe(todosDummy[0].text);
})
.end(done);
});
it('should return 404 if Todo NOT FOUND', (done) => {
// Some Random ID
var hexId = new ObjectID().toHexString();
request(app)
.get(`/todos/${hexId}`)
.expect(404)
.expect((res) => {
expect(res.body).toEqual({});
})
.end(done);
});
it('should return 404 with INVALID ID', (done) => {
request(app)
.get('/todos/123')
.expect(404)
.expect((res) => {
expect(res.body).toEqual({});
})
.end(done);
});
});
// DELETE with ID
describe('DELETE /todos/:id', () => {
it('should remove a todo', (done) => {
var idToRemove = todosDummy[0]._id.toHexString();
request(app)
.delete(`/todos/${idToRemove}`)
.expect(200)
.expect((res) => {
expect(res.body.todo._id).toBe(idToRemove);
})
.end((err, res) => {
if (err) {
return done(err);
}
Todo.findById(idToRemove).then((todo) => {
expect(todo).toNotExist();
done();
}).catch((e) => done(e));
});
});
it('should return 404 if NOT FOUND', (done) => {
var hexId = new ObjectID().toHexString();
request(app)
.delete(`/todos/${hexId}`)
.expect(404)
.expect((res) => {
expect(res.body).toEqual({});
})
.end(done);
});
it('should return 404 if object ID INVALID', (done) => {
request(app)
.get('/todos/123')
.expect(404)
.expect((res) => {
expect(res.body).toEqual({});
})
.end(done);
});
});
/////////////////////
// PATCHING with ID
describe('PATCH /todos/:id', () => {
var idToUpdate = todosDummy[0]._id.toHexString();
var updateReq = {
text: 'I am updated',
completed: true
};
it('should update with correct queries', (done) => {
request(app)
.patch(`/todos/${idToUpdate}`)
.send(updateReq)
.expect(200)
.expect((res) => {
expect(res.body.todo.text).toBe(updateReq.text);
expect(res.body.todo.completed).toBe(updateReq.completed);
// expect(res.body.todo.completedAt).toBe(123); // Why this fails?
}).end(done);
});
it('should remove completedAt when completed is false', (done) => {
request(app)
.patch(`/todos/${idToUpdate}`)
.send({completed: false})
.expect(200)
.expect((res) => {
expect(res.body.todo.completed).toBe(false);
expect(res.body.todo.completedAt).toBe(null);
}).end(done);
});
})
<file_sep>/playground/mongodb-fetch.js
const MongoClient = require('mongodb').MongoClient;
MongoClient.connect('mongodb://localhost:27017/TodoApp', (err, client) => {
if (err) {
return console.log('Unable to connect to MongoDB server');
} // 'return' will prevent codes below to excecute
console.log('Connection successful');
const db = client.db('TodoApp'); // For MongoDB v3.x.x
var searchName = 'Frank'; // Change the searchName
// Fetch users with compatible searchName
db.collection('Users')
.find({name: searchName})
.toArray()
.then((user) => {
console.log('Users found:');
console.log(JSON.stringify(user, undefined, 2));
}, (err) => {
console.log('Unable to find user', err);
});
client.close();
});
<file_sep>/server/models/todo.js
const mongoose = require('mongoose');
// Create a new mongoose model
// Named 'Todo', but create a collection with a name 'todos'
var Todo = mongoose.model('Todo', {
text: {
type: String,
required: true,
minlength: 1,
trim: true
},
completed: {
type: Boolean,
default: false
},
completedAt: {
type: Number,
default: null
}
});
module.exports = { Todo };
// // Create a new Object with the rules of the Todo model
// var newTodo = new Todo({
// text: 'Buy foods'
// });
//
// // Saving to the database
// nweTodo.save().then((doc) => {
// console.log('Todo saved!', doc);
// }, (err) => {
// console.log('Unable to save todo', err);
// })
| 95c177dbd9775df469429117023c9296af4242bf | [
"JavaScript"
] | 4 | JavaScript | krissukoco/prac-mongodb | 74b3e7b6eeb667af949d2460711bf1ab8d61fb0b | f1f60adac613a2507818dd04e8edac5527548baf | |
refs/heads/master | <repo_name>valeriaedw/Examen2-Comp<file_sep>/src/main/java/com/cenfotec/examen/service/workshop/WorkShopServiceImpl.java
package com.cenfotec.examen.service.workshop;
import com.cenfotec.examen.domain.WorkShop;
import com.cenfotec.examen.repo.WorkShopRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.Optional;
@Service
public class WorkShopServiceImpl implements WorkShopService{
@Autowired
WorkShopRepository repo;
@Override
public void save(WorkShop workshop) {
repo.save(workshop);
}
@Override
public List<WorkShop> getAll() {
return repo.findAll();
}
@Override
public Optional<WorkShop> get(Long id) {
return repo.findById(id);
}
@Override
public List<WorkShop> find(String name) {
return repo.findByNameContaining(name);
}
@Override
public List<WorkShop> findByCategory(String category) {
return repo.findByCategory(category);
}
@Override
public List<WorkShop> findByAutor(String autor) {
return repo.findByAutor(autor);
}
@Override
public List<WorkShop> findByKeyword(String keyword) {
return repo.findByKeyword(keyword);
}
}
<file_sep>/src/main/java/com/cenfotec/examen/service/workshop/WorkShopService.java
package com.cenfotec.examen.service.workshop;
import com.cenfotec.examen.domain.WorkShop;
import java.util.List;
import java.util.Optional;
public interface WorkShopService {
public void save(WorkShop workshop);
public List<WorkShop> getAll();
public Optional<WorkShop> get(Long id);
public List<WorkShop> find(String name);
public List<WorkShop> findByCategory(String category);
public List<WorkShop> findByAutor(String autor);
public List<WorkShop> findByKeyword( String keyword);
}
<file_sep>/src/main/java/com/cenfotec/examen/controller/WordController.java
package com.cenfotec.examen.controller;
import com.cenfotec.examen.domain.WorkShop;
import com.cenfotec.examen.service.workshop.WorkShopService;
import org.apache.poi.xwpf.usermodel.XWPFDocument;
import org.apache.poi.xwpf.usermodel.XWPFParagraph;
import org.apache.poi.xwpf.usermodel.XWPFRun;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.util.Optional;
@RestController
@RequestMapping("/report/{id}")
public class WordController{
@Autowired
WorkShopService workShopService;
@GetMapping
public void getGeneratedDocument(HttpServletResponse response, @PathVariable long id) throws IOException {
response.setHeader("Content-disposition","attachment; filename=test.docx");
Optional<WorkShop> workShopData = workShopService.get(id);
if (workShopData.isPresent()){
WorkShop workshop = workShopData.get();
XWPFDocument document = new XWPFDocument();
XWPFParagraph body = document.createParagraph();
XWPFRun bodyRun = body.createRun();
bodyRun.setText("Taller: "+ workshop.getName()+ "\n"+"Autor: "+ workshop.getAutor()+"\nObjetivo: "+workshop.getObjetivo()+
"\nCategoria: "+ workshop.getCategory().getName()+"\nPalabras clave: "+workshop.getKeyword1() + " " +workshop.getKeyword2()+
" "+workshop.getKeyword3() + "\nActividades: " + workshop.getActivity().toString());
document.write(response.getOutputStream());
}
}
}
<file_sep>/src/main/java/com/cenfotec/examen/domain/WorkShop.java
package com.cenfotec.examen.domain;
import javax.persistence.*;
import java.time.LocalTime;
import java.util.Set;
@Entity
public class WorkShop {
@Id
@GeneratedValue(strategy = GenerationType.AUTO)
private long id;
private String name;
private String autor;
private String objetivo;
private String keyword1;
private String keyword2;
private String keyword3;
private LocalTime duracionTotal = LocalTime.of(0,0);
@ManyToOne
@JoinColumn(name="cart_id", nullable = false)
private Category category;
@OneToMany(fetch=FetchType.LAZY, mappedBy="workshop")
private Set<Activity> activity;
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAutor() {
return autor;
}
public void setAutor(String autor) {
this.autor = autor;
}
public String getObjetivo() {
return objetivo;
}
public void setObjetivo(String objetivo) {
this.objetivo = objetivo;
}
public String getKeyword1() {
return keyword1;
}
public void setKeyword1(String keyword1) {
this.keyword1 = keyword1;
}
public String getKeyword2() {
return keyword2;
}
public void setKeyword2(String keyword2) {
this.keyword2 = keyword2;
}
public String getKeyword3() {
return keyword3;
}
public void setKeyword3(String keyword3) {
this.keyword3 = keyword3;
}
public Category getCategory() {
return category;
}
public void setCategory(Category category) {
this.category = category;
}
public Set<Activity> getActivity() {
return activity;
}
public void setActivity(Set<Activity> activity) {
this.activity = activity;
}
public LocalTime getDuracionTotal() {
return duracionTotal;
}
public void setDuracionTotal(LocalTime duracionTotal) {
this.duracionTotal = duracionTotal;
}
}
<file_sep>/src/main/java/com/cenfotec/examen/service/activity/ActivityServiceImpl.java
package com.cenfotec.examen.service.activity;
import com.cenfotec.examen.domain.Activity;
import com.cenfotec.examen.repo.ActivityRepository;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.Optional;
@Service
public class ActivityServiceImpl implements ActivityService {
@Autowired
ActivityRepository repo;
@Override
public void save(Activity activity) {
repo.save(activity);
}
@Override
public List<Activity> getAll() {
return repo.findAll();
}
@Override
public Optional<Activity> get(Long id) {
return repo.findById(id);
}
@Override
public List<Activity> find(String name) {
return repo.findByNameContaining(name);
}
}
<file_sep>/src/main/java/com/cenfotec/examen/controller/ActivityController.java
package com.cenfotec.examen.controller;
import com.cenfotec.examen.domain.Activity;
import com.cenfotec.examen.service.activity.ActivityService;
import com.cenfotec.examen.service.workshop.WorkShopService;
import com.cenfotec.examen.domain.WorkShop;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.PathVariable;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import java.time.LocalTime;
import java.util.Optional;
@Controller
public class ActivityController {
@Autowired
ActivityService activityService;
@Autowired
WorkShopService workShopService;
@RequestMapping(value="/registra_actividades/{id}")
public String recoverForAddActivity(Model model, @PathVariable long id) {
Optional<WorkShop> workShop = workShopService.get(id);
Activity newActivity = new Activity();
if (workShop.isPresent()) {
newActivity.setWorkshop(workShop.get());
model.addAttribute("workshop",workShop.get());
model.addAttribute("activity",newActivity);
return "registra_actividades";
}
return "notfound";
}
@RequestMapping(value="/registra_actividades/{id}", method = RequestMethod.POST)
public String saveActivity(Activity activity, Model model, @PathVariable long id) {
Optional<WorkShop> workShop = workShopService.get(id);
if (workShop.isPresent()) {
LocalTime total = sumaDuracion(workShop.get().getDuracionTotal(), activity.getHour());
workShop.get().setDuracionTotal(total);
workShopService.save(workShop.get());
activity.setWorkshop(workShop.get());
activityService.save(activity);
return "index";
}
return "notfound";
}
private LocalTime sumaDuracion(LocalTime duracionActual, LocalTime newTime){
Integer actualHour = duracionActual.getHour();
Integer actualMinute = duracionActual.getMinute();
Integer newHour = newTime.getHour();
Integer newMinute = newTime.getMinute();
Integer hour = actualHour += newHour;
Integer minute = actualMinute += newMinute;
return LocalTime.of(hour,minute);
}
}
| 82e8569e8a24948c191a28a7a64a1d15b80ce471 | [
"Java"
] | 6 | Java | valeriaedw/Examen2-Comp | bbd4dea9c483e485d645ad13913ce72f05315e07 | 0c47c213e23b1d9b36662230d9a5cb5c0daa3a1b | |
refs/heads/master | <repo_name>JuandaGarcia/aplicacion-multas<file_sep>/paginas/EditarPersona.js
import React, { useState, useEffect } from 'react'
import {
Text,
SafeAreaView,
ScrollView,
View,
ActivityIndicator,
TextInput,
TouchableHighlight,
Alert
} from 'react-native'
import {
handleAndroidBackButton,
removeAndroidBackButtonHandler
} from '../modulos/androidBackButton'
import styles from '../modulos/styles'
import axios from 'axios'
import { useHistory } from 'react-router-native'
export default function EditarPersona(props) {
const [nombre, setNombre] = useState('')
const [identificacion, setIdentifiacion] = useState('')
const [telefono, setTelefono] = useState('')
const [direccion, setDireccion] = useState('')
const [ciudad, setCiudad] = useState('')
const [persona, setPersona] = useState('')
const [loading, setLoading] = useState('')
const [error, setError] = useState('')
const id_parametros = props.match.params.identificacion
const history = useHistory()
useEffect(() => {
fetchData()
handleAndroidBackButton(() => history.push('/personas'))
return () => {
removeAndroidBackButtonHandler()
}
}, [])
useEffect(() => {
setNombre(persona.nombre)
setTelefono(persona.telefono)
setDireccion(persona.direccion)
setCiudad(persona.ciudad)
}, [persona])
const AlertaPUT = id => {
Alert.alert(
'Se actualizo correctamente',
'Oprima Aceptar para continuar',
[
{
text: 'Aceptar',
onPress: () => {
history.push('/personas')
}
}
],
{ cancelable: false }
)
}
const fetchData = async () => {
setLoading(true)
setError(null)
try {
await axios
.get(`http://157.245.245.214/personas/${id_parametros}`)
.then(res => {
setPersona(res.data)
})
setLoading(false)
} catch (error) {
setError(error)
}
}
const PutData = async () => {
setLoading(true)
setError(null)
try {
const data = {
identificacion: id_parametros,
nombre: nombre,
telefono: telefono,
direccion: direccion,
ciudad: ciudad
}
const headers = {
'Content-Type': 'application/json'
}
axios
.put(`http://192.168.127.12/personas/${id_parametros}`, data, {
headers: headers
})
.then(response => {
AlertaPUT()
})
.catch(error => {})
setLoading(false)
} catch (error) {
setError(error)
}
}
if (loading) {
return (
<SafeAreaView>
<Text style={styles.text_HeaderList}>Editar Persona</Text>
<ScrollView style={styles.scrollArea}>
<View style={styles.contenedorFormulario}>
<Text style={styles.textoidentificacion}>
Identificación: {id_parametros}
</Text>
<ActivityIndicator size="large" color="#0000ff" />
</View>
</ScrollView>
</SafeAreaView>
)
}
if (error) {
return (
<SafeAreaView>
<Text style={styles.text_HeaderList}>Editar Persona</Text>
<ScrollView style={styles.scrollArea}>
<View style={styles.contenedorFormulario}>
<Text style={styles.textoidentificacion}>
Identificación: {id_parametros}
</Text>
<Text style={styles.textoidentificacion}>Error</Text>
</View>
</ScrollView>
</SafeAreaView>
)
}
return (
<SafeAreaView>
<Text style={styles.text_HeaderList}>Editar Persona</Text>
<ScrollView style={styles.scrollArea}>
<View style={styles.contenedorFormulario}>
<Text style={styles.textoidentificacion}>
Identificación: {id_parametros}
</Text>
<Text>Nombre</Text>
<TextInput
style={styles.inputText}
onChangeText={text => setNombre(text)}
value={nombre}
placeholder="ingresa el nombre"
maxLength={40}
/>
<Text>Teléfono</Text>
<TextInput
style={styles.inputText}
onChangeText={text => setTelefono(text)}
value={telefono}
maxLength={10}
placeholder="ingresa el teléfono"
keyboardType="numeric"
/>
<Text>Dirección</Text>
<TextInput
style={styles.inputText}
onChangeText={text => setDireccion(text)}
value={direccion}
placeholder="ingresa la dirección"
maxLength={40}
/>
<Text>Ciudad</Text>
<TextInput
style={styles.inputText}
onChangeText={text => setCiudad(text)}
value={ciudad}
placeholder="ingresa la ciudad"
maxLength={40}
/>
<View style={styles.contenedorBotonNPersona}>
<TouchableHighlight
underlayColor="rgba(0,0,0,0.1)"
style={styles.buttonAñadirPersona}
onPress={() => {
PutData()
}}
>
<Text style={styles.textVerMas}>Guarda cambios</Text>
</TouchableHighlight>
</View>
</View>
</ScrollView>
</SafeAreaView>
)
}
<file_sep>/paginas/Vehiculos.js
import React, { useState, useEffect } from 'react'
import {
Text,
SafeAreaView,
ScrollView,
View,
ActivityIndicator
} from 'react-native'
import {
handleAndroidBackButton,
removeAndroidBackButtonHandler
} from '../modulos/androidBackButton'
import styles from '../modulos/styles'
import axios from 'axios'
export default ({ history }) => {
const [vehiculos, setVehiculos] = useState([])
const [loading, setLoading] = useState('')
const [error, setError] = useState('')
useEffect(() => {
handleAndroidBackButton(() => history.push('/'))
fetchData()
return () => {
removeAndroidBackButtonHandler()
}
}, [])
const fetchData = async () => {
setLoading(true)
setError(null)
try {
await axios.get(`http://172.16.58.3/vehiculos`).then(res => {
setVehiculos(res.data)
})
setLoading(false)
} catch (error) {
setError(error)
}
}
if (loading) {
return (
<SafeAreaView>
<Text style={styles.text_HeaderList}>Vehículos</Text>
<Text style={styles.texto_info_vehiculos}>
Lista de vehículos registrados en la región
</Text>
<ScrollView style={styles.scrollArea}>
<View style={styles.contenedorPersonas}>
<ActivityIndicator size="large" color="#0000ff" />
</View>
</ScrollView>
</SafeAreaView>
)
}
if (error) {
return (
<SafeAreaView>
<Text style={styles.text_HeaderList}>Vehículos</Text>
<Text style={styles.texto_info_vehiculos}>
Lista de vehículos registrados en la región
</Text>
<ScrollView style={styles.scrollArea}>
<View style={styles.contenedorPersonas}>
<Text style={styles.textError}>Algo salió mal!</Text>
</View>
</ScrollView>
</SafeAreaView>
)
}
return (
<SafeAreaView>
<Text style={styles.text_HeaderList}>Vehículos</Text>
<Text style={styles.texto_info_vehiculos}>
Lista de vehículos registrados en la región
</Text>
<ScrollView style={styles.scrollArea}>
<View style={styles.contenedorPersonas}>
{vehiculos.map(vehiculo => {
return (
<View
key={vehiculo.placa}
style={[
styles.Marco,
styles.container_Personas,
styles.horizontal
]}
>
<View style={styles.contenedo_info_vehiculo}>
<Text style={styles.nombreVehiculo}>{vehiculo.modelo}</Text>
<Text>Placa: {vehiculo.placa}</Text>
</View>
</View>
)
})}
</View>
</ScrollView>
</SafeAreaView>
)
}
<file_sep>/componets/HeaderList.js
import React from 'react'
import { Text, TouchableHighlight, StyleSheet, View } from 'react-native'
import styles from '../modulos/styles'
export default function HeaderList(props) {
return (
<View style={styles.contenedorTop_HeaderList}>
<Text style={styles.text_HeaderList}>{props.titulo}</Text>
<TouchableHighlight
underlayColor="rgba(0,0,0,0.1)"
style={styles.buttonAñadir}
onPress={() => props.history.push(`/${props.url}`)}
>
<Text> Añadir {props.textoBoton} </Text>
</TouchableHighlight>
</View>
)
}
<file_sep>/paginas/AñadirPersona.js
import React, { useState, useEffect } from 'react'
import {
Text,
SafeAreaView,
ScrollView,
View,
ActivityIndicator,
TextInput,
TouchableHighlight,
Alert
} from 'react-native'
import {
handleAndroidBackButton,
removeAndroidBackButtonHandler
} from '../modulos/androidBackButton'
import styles from '../modulos/styles'
import axios from 'axios'
export default ({ history }) => {
const [nombre, setNombre] = useState('')
const [identificacion, setIdentifiacion] = useState('')
const [telefono, setTelefono] = useState('')
const [direccion, setDireccion] = useState('')
const [ciudad, setCiudad] = useState('')
const [loading, setLoading] = useState('')
const [error, setError] = useState('')
useEffect(() => {
handleAndroidBackButton(() => history.push('/personas'))
return () => {
removeAndroidBackButtonHandler()
}
}, [])
const PostData = async () => {
setLoading(true)
setError(null)
try {
const data = {
identificacion: identificacion,
nombre: nombre,
telefono: telefono,
direccion: direccion,
ciudad: ciudad
}
const headers = {
'Content-Type': 'application/json'
}
axios
.post(`http://157.245.245.214/personas/`, data, {
headers: headers
})
.then(response => {
AlertaPOST()
/* dispatch({
type: FOUND_USER,
data: response.data[0]
}) */
})
.catch(error => {
/* dispatch({
type: ERROR_FINDING_USER
}) */
})
setLoading(false)
} catch (error) {
setError(error)
}
}
const AlertaPOST = () => {
Alert.alert(
'Se agrego correctamente',
'Oprima Aceptar para continuar',
[
{
text: 'Aceptar',
onPress: () => {
history.push('/personas')
}
}
],
{ cancelable: false }
)
}
return (
<SafeAreaView>
<Text style={styles.text_HeaderList}>Añadir Persona</Text>
<ScrollView style={styles.scrollArea}>
<View style={styles.contenedorFormulario}>
<Text>Nombre</Text>
<TextInput
style={styles.inputText}
onChangeText={text => setNombre(text)}
value={nombre}
placeholder="ingresa el nombre"
maxLength={40}
/>
<Text>Identificación</Text>
<TextInput
style={styles.inputText}
onChangeText={text => setIdentifiacion(text)}
value={identificacion}
placeholder="ingresa la identificación"
keyboardType="numeric"
maxLength={15}
/>
<Text>Teléfono</Text>
<TextInput
style={styles.inputText}
onChangeText={text => setTelefono(text)}
value={telefono}
maxLength={10}
placeholder="ingresa el teléfono"
keyboardType="numeric"
/>
<Text>Dirección</Text>
<TextInput
style={styles.inputText}
onChangeText={text => setDireccion(text)}
value={direccion}
placeholder="ingresa la dirección"
maxLength={40}
/>
<Text>Ciudad</Text>
<TextInput
style={styles.inputText}
onChangeText={text => setCiudad(text)}
value={ciudad}
placeholder="ingresa la ciudad"
maxLength={40}
/>
<View style={styles.contenedorBotonNPersona}>
<TouchableHighlight
underlayColor="rgba(0,0,0,0.1)"
style={styles.buttonAñadirPersona}
onPress={() => PostData()}
>
<Text style={styles.textVerMas}>Añadir persona</Text>
</TouchableHighlight>
</View>
</View>
</ScrollView>
</SafeAreaView>
)
}
<file_sep>/paginas/EditarMulta.js
import React, { useState, useEffect } from 'react'
import {
Text,
SafeAreaView,
ScrollView,
View,
ActivityIndicator,
TextInput,
TouchableHighlight,
Alert,
Picker
} from 'react-native'
import {
handleAndroidBackButton,
removeAndroidBackButtonHandler
} from '../modulos/androidBackButton'
import styles from '../modulos/styles'
import axios from 'axios'
import { useHistory } from 'react-router-native'
export default props => {
const [fecha, setFecha] = useState('')
const [observacion, setObservacion] = useState('')
const [total_pagar, setTotal_pagar] = useState('')
const [id_persona, setId_persona] = useState('')
const [placa_carro, setPlaca_carro] = useState('')
const [personas, setPersonas] = useState([])
const [vehiculos, setVehiculos] = useState([])
const [multa, setMulta] = useState([])
const [loading, setLoading] = useState('')
const [error, setError] = useState('')
const codigo_parametros = props.match.params.codigo
const history = useHistory()
useEffect(() => {
handleAndroidBackButton(() => history.push('/multas'))
fetchData()
return () => {
removeAndroidBackButtonHandler()
}
}, [])
useEffect(() => {
setFecha(multa.fecha)
setObservacion(multa.observacion)
setTotal_pagar(multa.total_pagar)
setId_persona(multa.id_persona)
setPlaca_carro(multa.placa_carro)
}, [multa])
const fetchData = async () => {
setLoading(true)
setError(null)
try {
await axios.get(`http://172.16.31.10/personas/`).then(res => {
setPersonas(res.data)
})
await axios.get(`http://157.245.245.214/vehiculos/`).then(res => {
setVehiculos(res.data)
})
await axios
.get(`http://157.245.245.214/multas/${codigo_parametros}`)
.then(res => {
setMulta(res.data)
})
setLoading(false)
} catch (error) {
setError(error)
}
}
const PostData = async () => {
setLoading(true)
setError(null)
try {
const data = {
fecha: fecha,
observacion: observacion,
total_pagar: total_pagar,
id_persona: id_persona,
placa_carro: placa_carro
}
const headers = {
'Content-Type': 'application/json'
}
axios
.post(`http://157.245.245.214/multas/`, data, {
headers: headers
})
.then(response => {
AlertaPOST()
})
.catch(error => {})
setLoading(false)
} catch (error) {
setError(error)
}
}
const AlertaPUT = id => {
Alert.alert(
'Se actualizo correctamente',
'Oprima Aceptar para continuar',
[
{
text: 'Aceptar',
onPress: () => {
history.push('/multas')
}
}
],
{ cancelable: false }
)
}
const PutData = async () => {
setLoading(true)
setError(null)
try {
const data = {
fecha: fecha,
observacion: observacion,
total_pagar: total_pagar,
id_persona: id_persona,
placa_carro: placa_carro
}
const headers = {
'Content-Type': 'application/json'
}
axios
.put(`http://157.245.245.214/multas/${codigo_parametros}`, data, {
headers: headers
})
.then(response => {
AlertaPUT()
})
.catch(error => {})
setLoading(false)
} catch (error) {
setError(error)
}
}
if (loading) {
return (
<SafeAreaView>
<Text style={styles.text_HeaderList}>Editar multa</Text>
<ScrollView style={styles.scrollArea}>
<View style={styles.contenedorFormulario}>
<Text style={styles.textoidentificacion}>
Codigo: {codigo_parametros}
</Text>
<ActivityIndicator size="large" color="#0000ff" />
</View>
</ScrollView>
</SafeAreaView>
)
}
if (error) {
return (
<SafeAreaView>
<Text style={styles.text_HeaderList}>Editar multa</Text>
<ScrollView style={styles.scrollArea}>
<View style={styles.contenedorFormulario}>
<Text style={styles.textoidentificacion}>
Codigo: {codigo_parametros}
</Text>
<Text style={styles.textError}>Algo salió mal!</Text>
</View>
</ScrollView>
</SafeAreaView>
)
}
return (
<SafeAreaView>
<Text style={styles.text_HeaderList}>Editar multa</Text>
<ScrollView style={styles.scrollArea}>
<View style={styles.contenedorFormulario}>
<Text style={styles.textoidentificacion}>
Codigo: {codigo_parametros}
</Text>
<Text>Fecha</Text>
<TextInput
style={styles.inputText}
onChangeText={text => setFecha(text)}
value={fecha}
placeholder="AAAA-MM-DD"
maxLength={40}
/>
<Text>Observación</Text>
<TextInput
style={styles.inputText}
onChangeText={text => setObservacion(text)}
value={observacion}
placeholder="ingresa la observación"
maxLength={200}
/>
<Text>Total a pagar {`(Actual: ${total_pagar})`}</Text>
<TextInput
style={styles.inputText}
onChangeText={text => setTotal_pagar(text)}
value={total_pagar}
placeholder="ingresa el total a pagar"
keyboardType="numeric"
maxLength={15}
/>
<Text>Identificacion de la persona</Text>
<Text>{`(Actual: ${id_persona})`}</Text>
<Picker
selectedValue={id_persona}
style={{ height: 50, width: 300 }}
onValueChange={text => setId_persona(text)}
>
{personas.map(persona => {
return (
<Picker.Item
key={persona.identificacion}
label={`${persona.identificacion} - ${persona.nombre}`}
value={persona.identificacion}
/>
)
})}
</Picker>
<Text>Placa del carro</Text>
<Text>{`(Actual: ${placa_carro})`}</Text>
<Picker
selectedValue={placa_carro}
style={{ height: 50, width: 300 }}
onValueChange={text => setPlaca_carro(text)}
>
{vehiculos.map(vehiculo => {
return (
<Picker.Item
key={vehiculo.placa}
label={`${vehiculo.placa} - ${vehiculo.modelo}`}
value={vehiculo.placa}
/>
)
})}
</Picker>
<View style={styles.contenedorBotonNPersona}>
<TouchableHighlight
underlayColor="rgba(0,0,0,0.1)"
style={styles.buttonAñadirPersona}
onPress={() => {
PutData()
}}
>
<Text style={styles.textVerMas}>Editar multa</Text>
</TouchableHighlight>
</View>
</View>
</ScrollView>
</SafeAreaView>
)
}
<file_sep>/App.js
import React from 'react'
import { NativeRouter, Switch, Route } from 'react-router-native'
import Home from './paginas/Home'
import Vehiculos from './paginas/Vehiculos'
import Personas from './paginas/Personas'
import Multas from './paginas/Multas'
import EditarPersona from './paginas/EditarPersona'
import AñadirPersona from './paginas/AñadirPersona'
import AñadirMultas from './paginas/AñadirMultas'
import EditarMulta from './paginas/EditarMulta'
export default function App() {
return (
<NativeRouter>
<Switch>
<Route exact path="/" component={Home} />
<Route exact path="/personas" component={Personas} />
<Route
exact
path="/editar-persona/:identificacion"
component={EditarPersona}
/>
<Route exact path="/añadir-personas" component={AñadirPersona} />
<Route exact path="/multas" component={Multas} />
<Route exact path="/añadir-multas" component={AñadirMultas} />
<Route exact path="/editar-multa/:codigo" component={EditarMulta} />
<Route exact path="/vehiculos" component={Vehiculos} />
</Switch>
</NativeRouter>
)
}
<file_sep>/paginas/Multas.js
import React, { useState, useEffect } from 'react'
import {
Text,
TouchableHighlight,
SafeAreaView,
ScrollView,
View,
ActivityIndicator,
Alert
} from 'react-native'
import {
handleAndroidBackButton,
removeAndroidBackButtonHandler
} from '../modulos/androidBackButton'
import HeaderList from '../componets/HeaderList'
import axios from 'axios'
import styles from '../modulos/styles'
export default ({ history }) => {
const [multas, setMultas] = useState([])
const [loading, setLoading] = useState('')
const [error, setError] = useState('')
useEffect(() => {
handleAndroidBackButton(() => history.push('/'))
fetchData()
return () => {
removeAndroidBackButtonHandler()
}
}, [])
const fetchData = async () => {
setLoading(true)
setError(null)
try {
await axios.get(`http://172.16.58.3/multas/`).then(res => {
setMultas(res.data)
})
setLoading(false)
} catch (error) {
setError(error)
}
}
const AlertaEliminar = id => {
Alert.alert(
'Eliminar',
'¿Está seguro que desea eliminar esta multa?',
[
{
text: 'Cancelar',
onPress: () => console.log('Cancel Pressed'),
style: 'cancel'
},
{
text: 'Eliminar',
onPress: () => {
DeleteMulta(id)
}
}
],
{ cancelable: false }
)
}
const DeleteMulta = async id => {
setLoading(true)
setError(null)
try {
await axios.delete(`http://192.168.127.124/multas/${id}`).then(res => {})
await axios.get(`http://157.245.245.214/multas/`).then(res => {
setMultas(res.data)
})
setLoading(false)
} catch (error) {
setError(error)
}
}
if (loading) {
return (
<SafeAreaView>
<HeaderList
history={history}
titulo={'Multas'}
textoBoton={'multa'}
url={'añadir-multas'}
/>
<ScrollView style={styles.scrollArea}>
<ActivityIndicator size="large" color="#0000ff" />
</ScrollView>
</SafeAreaView>
)
}
if (error) {
return (
<SafeAreaView>
<HeaderList
history={history}
titulo={'Multas'}
textoBoton={'multa'}
url={'añadir-multas'}
/>
<ScrollView style={styles.scrollArea}>
<Text style={styles.textError}>Algo salió mal!</Text>
</ScrollView>
</SafeAreaView>
)
}
return (
<SafeAreaView style={{ flex: 1 }}>
<HeaderList
history={history}
titulo={'Multas'}
textoBoton={'multa'}
url={'añadir-multas'}
/>
<ScrollView style={styles.scrollArea}>
<View style={styles.contenedorPersonas}>
{multas.map(multa => {
return (
<View
key={multa.codigo}
style={[
styles.ItemMulta,
styles.container_Personas,
styles.horizontal
]}
>
<View style={styles.contenedo_info_vehiculo}>
<Text style={styles.nombrePersona}>
Codigo: {multa.codigo}
</Text>
<Text style={styles.nombrePersona}>
Persona: {multa.id_persona}
</Text>
<Text>Fecha: {multa.fecha}</Text>
<Text style={styles.containerObservacion}>
Observacion: {multa.observacion}
</Text>
<Text>Placa del carro: {multa.placa_carro}</Text>
<Text style={styles.nombrePersona}>
Total a pagar: {multa.total_pagar}
</Text>
</View>
<View style={styles.contenedor_botones_persona}>
<TouchableHighlight
underlayColor="rgba(0,0,0,0.1)"
style={styles.verMas}
onPress={() => {
history.push(`/editar-multa/${multa.codigo}`)
}}
>
<Text style={styles.textVerMas}>Editar</Text>
</TouchableHighlight>
<TouchableHighlight
underlayColor="rgba(0,0,0,0.1)"
style={styles.Eliminar_persona}
onPress={() => {
AlertaEliminar(multa.codigo)
}}
>
<Text style={styles.textVerMas}>Eliminar</Text>
</TouchableHighlight>
</View>
</View>
)
})}
</View>
</ScrollView>
</SafeAreaView>
)
}
<file_sep>/modulos/styles.js
import { StyleSheet, Dimensions } from 'react-native'
const styles = StyleSheet.create({
container_Home: {
flex: 1,
backgroundColor: '#fff',
alignItems: 'center',
justifyContent: 'center'
},
button_Home: {
alignItems: 'center',
justifyContent: 'center',
borderWidth: 2,
borderColor: '#292b98',
backgroundColor: 'transparent',
borderRadius: 25,
height: 50,
width: 230,
marginBottom: 20
},
MarcoPersona_Personas: {
alignItems: 'center',
justifyContent: 'center',
borderWidth: 2,
borderColor: '#292b98',
backgroundColor: 'transparent',
borderRadius: 5,
height: 150,
width: 350,
marginBottom: 20
},
Marco: {
alignItems: 'center',
justifyContent: 'center',
borderWidth: 2,
borderColor: '#292b98',
backgroundColor: 'transparent',
borderRadius: 5,
height: 70,
width: 310,
marginBottom: 20
},
scrollArea: {
width: Dimensions.get('window').width
},
contenedorPersonas: {
alignItems: 'center'
},
contenedorTitulo: {
display: 'flex'
},
goBack: {
width: 40
},
textError: {
textAlign: 'center',
fontSize: 20,
color: '#FFA9A9'
},
container_Personas: {
flex: 1,
justifyContent: 'center'
},
horizontal: {
flexDirection: 'row',
justifyContent: 'space-around',
padding: 10
},
contenedor_botones_persona: {
position: 'absolute',
right: 15
},
verMas: {
alignItems: 'center',
justifyContent: 'center',
height: 50,
width: 100,
backgroundColor: '#68B1FF',
borderRadius: 5,
margin: 10
},
Eliminar_persona: {
alignItems: 'center',
justifyContent: 'center',
height: 50,
width: 100,
backgroundColor: '#FF8D8D',
borderRadius: 5,
margin: 10
},
textVerMas: {
color: 'white',
fontWeight: 'bold'
},
nombrePersona: {
fontWeight: 'bold'
},
nombreVehiculo: {
fontWeight: 'bold'
},
contenedorTop_HeaderList: {
alignItems: 'center'
},
buttonAñadir: {
alignItems: 'center',
justifyContent: 'center',
borderWidth: 2,
borderColor: '#292b98',
backgroundColor: 'transparent',
borderRadius: 25,
height: 50,
width: 230,
marginBottom: 35
},
text_HeaderList: {
fontSize: 40,
fontWeight: 'bold',
paddingTop: 40,
paddingBottom: 10,
textAlign: 'center'
},
texto_info_vehiculos: {
textAlign: 'center',
marginBottom: 40
},
contenedo_info_vehiculo: {
position: 'absolute',
left: 15
},
inputText: {
height: 50,
borderColor: 'gray',
borderWidth: 2,
borderRadius: 5,
marginBottom: 15,
marginTop: 5,
padding: 10
},
contenedorFormulario: {
padding: 40,
paddingTop: 0,
marginTop: 20
},
buttonAñadirPersona: {
borderRadius: 5,
height: 50,
width: 200,
backgroundColor: '#8DFFAE',
justifyContent: 'center',
alignItems: 'center',
marginTop: 15
},
contenedorBotonNPersona: {
alignItems: 'center'
},
textoidentificacion: {
fontWeight: 'bold',
marginBottom: 10
},
containerObservacion: {
width: 200,
marginBottom: 10,
marginTop: 10
},
ItemMulta: {
alignItems: 'center',
justifyContent: 'center',
borderWidth: 2,
borderColor: '#292b98',
backgroundColor: 'transparent',
borderRadius: 5,
height: 230,
width: 350,
marginBottom: 20
}
})
export default styles
<file_sep>/paginas/Personas.js
import React, { useState, useEffect } from 'react'
import {
Text,
TouchableHighlight,
SafeAreaView,
ScrollView,
View,
ActivityIndicator,
Alert
} from 'react-native'
import {
handleAndroidBackButton,
removeAndroidBackButtonHandler
} from '../modulos/androidBackButton'
import HeaderList from '../componets/HeaderList'
import axios from 'axios'
import styles from '../modulos/styles'
export default ({ history }) => {
const [personas, setPersonas] = useState([])
const [loading, setLoading] = useState('')
const [error, setError] = useState('')
useEffect(() => {
handleAndroidBackButton(() => history.push('/'))
fetchData()
return () => {
removeAndroidBackButtonHandler()
}
}, [])
const fetchData = async () => {
setLoading(true)
setError(null)
try {
await axios.get(`http://172.16.31.10/personas/`).then(res => {
setPersonas(res.data)
})
setLoading(false)
} catch (error) {
setError(error)
}
}
const AlertaEliminar = id => {
Alert.alert(
'Eliminar',
'¿Está seguro que desea eliminar esta persona?',
[
{
text: 'Cancelar',
onPress: () => console.log('Cancel Pressed'),
style: 'cancel'
},
{
text: 'Eliminar',
onPress: () => {
DeletePerson(id)
}
}
],
{ cancelable: false }
)
}
const DeletePerson = async id => {
setLoading(true)
setError(null)
try {
await axios
.delete(`http://192.168.127.124/personas/${id}`)
.then(res => {})
await axios.get(`http://157.245.245.214/personas/`).then(res => {
setPersonas(res.data)
})
setLoading(false)
} catch (error) {
setError(error)
}
}
if (loading) {
return (
<SafeAreaView>
<HeaderList
history={history}
titulo={'Personas'}
textoBoton={'Persona'}
url={'añadir-personas'}
/>
<ScrollView style={styles.scrollArea}>
<ActivityIndicator size="large" color="#0000ff" />
</ScrollView>
</SafeAreaView>
)
}
if (error) {
return (
<SafeAreaView>
<HeaderList
history={history}
titulo={'Personas'}
textoBoton={'Persona'}
url={'añadir-personas'}
/>
<ScrollView style={styles.scrollArea}>
<Text style={styles.textError}>Algo salió mal!</Text>
</ScrollView>
</SafeAreaView>
)
}
return (
<SafeAreaView style={{ flex: 1 }}>
<HeaderList
history={history}
titulo={'Personas'}
textoBoton={'Persona'}
url={'añadir-personas'}
/>
<ScrollView style={styles.scrollArea}>
<View style={styles.contenedorPersonas}>
{personas.map(persona => {
return (
<View
key={persona.identificacion}
style={[
styles.MarcoPersona_Personas,
styles.container_Personas,
styles.horizontal
]}
>
<View style={styles.contenedo_info_vehiculo}>
<Text style={styles.nombrePersona}>{persona.nombre}</Text>
<Text>{persona.identificacion}</Text>
<Text>Teléfono: {persona.telefono}</Text>
<Text>Dirección: {persona.direccion}</Text>
<Text>Ciudad: {persona.ciudad}</Text>
</View>
<View style={styles.contenedor_botones_persona}>
<TouchableHighlight
underlayColor="rgba(0,0,0,0.1)"
style={styles.verMas}
onPress={() =>
history.push(`/editar-persona/${persona.identificacion}`)
}
>
<Text style={styles.textVerMas}>Editar</Text>
</TouchableHighlight>
<TouchableHighlight
underlayColor="rgba(0,0,0,0.1)"
style={styles.Eliminar_persona}
onPress={() => {
AlertaEliminar(persona.identificacion)
}}
>
<Text style={styles.textVerMas}>Eliminar</Text>
</TouchableHighlight>
</View>
</View>
)
})}
</View>
</ScrollView>
</SafeAreaView>
)
}
| d452bd59b7c87352ab0f96591b3ba4dd9a7f2a71 | [
"JavaScript"
] | 9 | JavaScript | JuandaGarcia/aplicacion-multas | 02bf01b0a34afaf3e8b11eb4dec226b80161024e | 9b5f57a07cb531f3e0f1f0e5ce88650ab43f5bc0 | |
refs/heads/master | <repo_name>dhagerty9009/watch-the-corners<file_sep>/Watch The Corners/ViewController.swift
//
// ViewController.swift
// Watch The Corners
//
// Created by <NAME> on 2/13/15.
// Copyright (c) 2015 TwistSix, LLC. All rights reserved.
//
import UIKit
class ViewController: UIViewController {
@IBOutlet weak var playGameButton: UIButton!
@IBOutlet weak var highScoreButton: UIButton!
override func viewDidLoad() {
super.viewDidLoad()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func goToHighScores(sender: UIButton) {
showViewController(scoresViewController(), sender: self)
}
@IBAction func playGamePressed(sender: UIButton) {
showViewController(gameViewController(), sender: self)
}
@IBAction func goToRules(sender: UIButton) {
showViewController(rulesViewController(), sender: self)
}
@IBAction func goToGameCenter(sender: UIButton) {
GCHelper.showGameCenter(self, viewState: .Leaderboards)
}
}
<file_sep>/fastlane/Deliverfile
########################################
# App Metadata
########################################
# This folder has to include one folder for each language
# More information about automatic screenshot upload:
# https://github.com/KrauseFx/deliver#upload-screenshots-to-itunes-connect
screenshots_path './screenshots/'
app_identifier 'TS.Watch-The-Corners'
apple_id '978732428' # App ID in iTunes Connect
email '<EMAIL>'
########################################
# Building and Testing
########################################
ipa do
'./build/ipa/WatchTheCorners.ipa'
success do
system("say 'Successfully deployed a new version!'")
end
end
beta_ipa do
'.build/ipa/WatchTheCorners.ipa'
success do
system("say 'Deployed a new beta!'")
end
end
<file_sep>/Watch The Corners WatchKit Extension/resultController.swift
//
// resultController.swift
// Watch The Corners
//
// Created by <NAME> on 3/4/15.
// Copyright (c) 2015 TwistSix, LLC. All rights reserved.
//
import WatchKit
import Foundation
class resultController: WKInterfaceController {
let storage: NSUserDefaults = NSUserDefaults.standardUserDefaults()
let score = Score.sharedInstance
// The final score from the game.
@IBOutlet weak var finalScore: WKInterfaceLabel!
@IBOutlet weak var thirdScore: WKInterfaceLabel!
@IBOutlet weak var secondScore: WKInterfaceLabel!
@IBOutlet weak var highestScore: WKInterfaceLabel!
@IBAction func startNewGame() {
pushControllerWithName("gameController", context: nil)
}
func loadDefaults() {
storage.synchronize()
if storage.arrayForKey("watchHighScores") != nil {
score.highScores = storage.arrayForKey("watchHighScores") as! [Int]
}
}
func saveDefaults() {
storage.setObject(score.highScores, forKey: "watchHighScores")
}
override func awakeWithContext(context: AnyObject?) {
super.awakeWithContext(context)
loadDefaults()
score.addScoreToHighScores(score.currentScore)
saveDefaults()
finalScore.setText("\(score.currentScore)")
highestScore.setText("\(score.highScores[0])")
secondScore.setText("\(score.highScores[1])")
thirdScore.setText("\(score.highScores[2])")
}
}
<file_sep>/Watch The Corners/gameViewController.swift
//
// gameViewController.swift
// Watch The Corners
//
// Created by <NAME> on 3/23/15.
// Copyright (c) 2015 TwistSix, LLC. All rights reserved.
//
import UIKit
import Foundation
import AVFoundation
class gameViewController: UIViewController {
//Colors
let WHITE: UIColor = UIColor.init(red: 0.94, green: 0.94, blue: 0.94, alpha: 1)
let BLACK: UIColor = UIColor.blackColor()
let GREEN: UIColor = UIColor.init(red: 0.64, green: 0.83, blue: 0.63, alpha: 1)
let GRAY: UIColor = UIColor.init(red: 0.58, green: 0.59, blue: 0.6, alpha: 1)
let RED: UIColor = UIColor.init(red: 0.93, green: 0.34, blue: 0.34, alpha: 1)
let TRUE_WHITE: UIColor = UIColor.whiteColor()
var buttonSound = NSURL.fileURLWithPath(NSBundle.mainBundle().pathForResource("blip2", ofType: "wav")!)
var deathSound = NSURL.fileURLWithPath(NSBundle.mainBundle().pathForResource("endgame", ofType: "wav")!)
var buttonPlayer = AVAudioPlayer()
var deathPlayer = AVAudioPlayer()
// Game variables and constants
var board: GameBoard!
var gameTimer: NSTimer!
var secondTimer: NSTimer!
var timeLeft: Int!
var scoreLabel: UILabel = UILabel()
var gameTimerLabel: UILabel = UILabel()
var timeLabel: UILabel = UILabel()
var buttonOne: GameButton!
var buttonTwo: GameButton!
var buttonThree: GameButton!
var buttonFour: GameButton!
let score = Score.sharedInstance
let labelFont = UIFont.systemFontOfSize(50)
let ONE_SECOND: NSTimeInterval = 1
let TIME_INTERVAL: NSTimeInterval = 10
let ADDITIONAL_TIME: NSTimeInterval = 2
let GOAL: Int = 8
let WIDTH = UIScreen.mainScreen().bounds.width
let HEIGHT = UIScreen.mainScreen().bounds.height
let HALF_WIDTH = UIScreen.mainScreen().bounds.width/2
enum EndGameReason {
case TimeOut
case WrongButton
}
var endGameReason: EndGameReason = .TimeOut
func makeButtons() {
buttonOne = GameButton.buttonWithType(UIButtonType.Custom) as! GameButton
buttonOne.frame = CGRectMake(0, 20, HALF_WIDTH, HALF_WIDTH)
buttonOne.backgroundColor = GRAY
buttonOne.tag = 1
buttonTwo = GameButton.buttonWithType(UIButtonType.Custom) as! GameButton
buttonTwo.frame = CGRectMake(HALF_WIDTH, 20, HALF_WIDTH, HALF_WIDTH)
buttonTwo.backgroundColor = GRAY
buttonTwo.tag = 2
buttonThree = GameButton.buttonWithType(UIButtonType.Custom) as! GameButton
buttonThree.frame = CGRectMake(0, HEIGHT-HALF_WIDTH, HALF_WIDTH, HALF_WIDTH)
buttonThree.backgroundColor = GRAY
buttonThree.tag = 3
buttonFour = GameButton.buttonWithType(UIButtonType.Custom) as! GameButton
buttonFour.frame = CGRectMake(HALF_WIDTH, HEIGHT - HALF_WIDTH, HALF_WIDTH, HALF_WIDTH)
buttonFour.backgroundColor = GRAY
buttonFour.tag = 4
buttonOne.addTarget(self, action: Selector("buttonTapped:"), forControlEvents: .TouchUpInside)
buttonTwo.addTarget(self, action: Selector("buttonTapped:"), forControlEvents: .TouchUpInside)
buttonThree.addTarget(self, action: Selector("buttonTapped:"), forControlEvents: .TouchUpInside)
buttonFour.addTarget(self, action: Selector("buttonTapped:"), forControlEvents: .TouchUpInside)
self.view.addSubview(buttonOne)
self.view.addSubview(buttonTwo)
self.view.addSubview(buttonThree)
self.view.addSubview(buttonFour)
}
func makeLabels() {
scoreLabel.frame = CGRectMake(10, HEIGHT/2, HALF_WIDTH - 10, 60)
scoreLabel.textColor = GREEN
scoreLabel.textAlignment = NSTextAlignment.Left
scoreLabel.font = labelFont
scoreLabel.text = "0"
gameTimerLabel.frame = CGRectMake(HALF_WIDTH, HEIGHT/2, HALF_WIDTH - 10, 60)
gameTimerLabel.textColor = RED
gameTimerLabel.textAlignment = NSTextAlignment.Right
gameTimerLabel.font = labelFont
gameTimerLabel.text = "10"
timeLabel.frame = CGRectMake(0, HEIGHT/2, WIDTH, 60)
timeLabel.textColor = GRAY
timeLabel.textAlignment = NSTextAlignment.Center
timeLabel.font = labelFont
timeLabel.text = ""
self.view.addSubview(scoreLabel)
self.view.addSubview(gameTimerLabel)
self.view.addSubview(timeLabel)
}
func updateLabels() {
timeLabel.text = ""
gameTimerLabel.text = "\(timeLeft - 1)"
timeLeft = timeLeft - 1
}
func buttonTapped(sender: UIButton) {
var button = sender.tag
var active = board.activeButton
if button == active {
buttonPlayer.play()
score.currentScore = score.currentScore + 1
if score.currentScore % GOAL == 0 {
timeLabel.text = "+2"
timeLeft = timeLeft + Int(ADDITIONAL_TIME)
invalidateTimers()
setTimers()
}
scoreLabel.text = ("\(score.currentScore)")
board.makeRandomButtonActive()
activateButton(board.activeButton)
} else {
timeLabel.text = ""
endGameReason = .WrongButton
sender.backgroundColor = RED
gameOver()
}
}
func activateButton(button: Int) {
buttonOne.backgroundColor = GRAY
buttonTwo.backgroundColor = GRAY
buttonThree.backgroundColor = GRAY
buttonFour.backgroundColor = GRAY
var pressed = button
switch pressed {
case 1:
buttonOne.backgroundColor = GREEN
case 2:
buttonTwo.backgroundColor = GREEN
case 3:
buttonThree.backgroundColor = GREEN
case 4:
buttonFour.backgroundColor = GREEN
default:
break
}
}
func setTimers() {
let TIME = NSTimeInterval(timeLeft)
gameTimer = NSTimer.scheduledTimerWithTimeInterval(TIME, target: self, selector: Selector("gameOver"), userInfo: nil, repeats: false)
secondTimer = NSTimer.scheduledTimerWithTimeInterval(ONE_SECOND, target: self, selector: Selector("updateLabels"), userInfo: nil, repeats: true)
}
func invalidateTimers() {
gameTimer.invalidate()
secondTimer.invalidate()
}
func gameOver() {
// disable all interaction with buttons
buttonOne.enabled = false
buttonTwo.enabled = false
buttonThree.enabled = false
buttonFour.enabled = false
deathPlayer.play()
gameTimerLabel.text = "0"
invalidateTimers()
switch endGameReason {
case .WrongButton:
NSLog("Wrong button tapped")
case .TimeOut:
NSLog("Timer timed out")
buttonOne.backgroundColor = RED
buttonTwo.backgroundColor = RED
buttonThree.backgroundColor = RED
buttonFour.backgroundColor = RED
default:
NSLog("Something Went Wrong")
}
var waitTimer = NSTimer.scheduledTimerWithTimeInterval(ONE_SECOND*2, target: self, selector: Selector("toScoreScreen"), userInfo: nil, repeats: false)
}
func toScoreScreen() {
showViewController(resultViewController(), sender: self)
}
func startGame() {
score.currentScore = 0
timeLeft = Int(TIME_INTERVAL)
setTimers()
board = GameBoard()
activateButton(board.activeButton)
}
override func viewDidLoad() {
super.viewDidLoad()
self.view.backgroundColor = WHITE
buttonPlayer = AVAudioPlayer(contentsOfURL: buttonSound, error: nil)
deathPlayer = AVAudioPlayer(contentsOfURL: deathSound, error: nil)
buttonPlayer.prepareToPlay()
deathPlayer.prepareToPlay()
makeButtons()
makeLabels()
startGame()
}
}
<file_sep>/Watch The Corners/GameButton.swift
//
// GameButton.swift
// Watch The Corners
//
// Created by <NAME> on 4/22/15.
// Copyright (c) 2015 TwistSix, LLC. All rights reserved.
//
import UIKit
class GameButton: UIButton {
let WHITE: UIColor = UIColor.init(red: 0.94, green: 0.94, blue: 0.94, alpha: 1)
let BLACK: UIColor = UIColor.blackColor()
let GREEN: UIColor = UIColor.init(red: 0.64, green: 0.83, blue: 0.63, alpha: 1)
let GRAY: UIColor = UIColor.init(red: 0.58, green: 0.59, blue: 0.6, alpha: 1)
let RED: UIColor = UIColor.init(red: 0.93, green: 0.34, blue: 0.34, alpha: 1)
let TRUE_WHITE: UIColor = UIColor.whiteColor()
let WIDTH = UIScreen.mainScreen().bounds.width
let HEIGHT = UIScreen.mainScreen().bounds.height
let HALF_WIDTH = UIScreen.mainScreen().bounds.width/2
required init(coder aDecoder: NSCoder) {
super.init(coder: aDecoder)
self.backgroundColor = GRAY
}
override init(frame: CGRect) {
super.init(frame: frame)
}
override var highlighted: Bool {
get {
return super.highlighted
}
set {
if newValue {
backgroundColor = TRUE_WHITE
} else {
backgroundColor = backgroundColor
}
super.highlighted = newValue
}
}
}
<file_sep>/Watch The Corners/Score.swift
//
// Score.swift
// Watch The Corners
//
// Created by <NAME> on 3/25/15.
// Copyright (c) 2015 TwistSix, LLC. All rights reserved.
//
import Foundation
class Score {
class var sharedInstance : Score {
struct Static {
static var onceToken : dispatch_once_t = 0
static var instance : Score? = nil
}
dispatch_once(&Static.onceToken) {
Static.instance = Score()
}
return Static.instance!
}
var currentScore: Int!
var mostRecentScore: Int!
var highScores = [0, 0, 0, 0, 0]
var duplicateScore: Bool!
func addScoreToHighScores(score: Int) {
mostRecentScore = highScores[0]
println("\(mostRecentScore), \(currentScore)")
if currentScore != mostRecentScore {
duplicateScore = false
for (index, storedScore) in enumerate(highScores) {
if score > storedScore {
var saved = storedScore
highScores.removeAtIndex(index)
highScores.insert(score, atIndex: index)
if index + 1 != 5 {
highScores.insert(saved, atIndex: index + 1)
}
highScores.removeLast()
break
}
}
} else if currentScore == mostRecentScore {
duplicateScore = true
}
}
func getHighScore() -> Int {
return highScores[0]
}
func currentScoreIsHighScore() -> Bool {
if currentScore == highScores[0] {
return true
} else {
return false
}
}
func reportToGameCenter() {
GCHelper.reportLeaderboardIdentifier("1", score: currentScore)
}
}
<file_sep>/Watch The Corners WatchKit Extension/Score.swift
//
// Score.swift
// Watch The Corners
//
// Created by <NAME> on 4/3/15.
// Copyright (c) 2015 TwistSix, LLC. All rights reserved.
//
import Foundation
class Score {
class var sharedInstance : Score {
struct Static {
static var onceToken : dispatch_once_t = 0
static var instance : Score? = nil
}
dispatch_once(&Static.onceToken) {
Static.instance = Score()
}
return Static.instance!
}
var currentScore: Int!
var highScores = [0, 0, 0]
func addScoreToHighScores(score: Int) {
for (index, storedScore) in enumerate(highScores) {
if score > storedScore {
var saved = storedScore
highScores.removeAtIndex(index)
highScores.insert(score, atIndex: index)
if index + 1 != 5 {
highScores.insert(saved, atIndex: index + 1)
}
highScores.removeLast()
break
}
}
}
func currentScoreIsHighScore() -> Bool {
if currentScore == highScores[0] {
return true
} else {
return false
}
}
}
<file_sep>/Watch The Corners WatchKit Extension/gameController.swift
//
// InterfaceController.swift
// Watch The Corners WatchKit Extension
//
// Created by <NAME> on 2/13/15.
// Copyright (c) 2015 TwistSix, LLC. All rights reserved.
//
import WatchKit
import Foundation
class gameController: WKInterfaceController {
// Color constants
let WHITE: UIColor = UIColor.init(red: 0.94, green: 0.94, blue: 0.94, alpha: 1)
let BLACK: UIColor = UIColor.blackColor()
let GREEN: UIColor = UIColor.init(red: 0.64, green: 0.83, blue: 0.63, alpha: 1)
let GRAY: UIColor = UIColor.init(red: 0.58, green: 0.59, blue: 0.6, alpha: 1)
let RED : UIColor = UIColor.init(red: 0.93, green: 0.34, blue: 0.34, alpha: 1)
// Time Constants
let TIME_INTERVAL: NSTimeInterval = 10
let ONE_SECOND: NSTimeInterval = 1
let ADDITIONAL_TIME: NSTimeInterval = 3
let GOAL: Int = 5
// Game data
let score = Score.sharedInstance
var minuteTimer: NSTimer!
var secondTimer: NSTimer!
var board:GameBoard!
var gameTimeLeft: Int!
// Game data outlets
@IBOutlet weak var scoreLabel: WKInterfaceLabel!
@IBOutlet weak var gameTimer: WKInterfaceLabel!
// The game's buttons
@IBOutlet weak var buttonOne: WKInterfaceButton!
@IBOutlet weak var buttonTwo: WKInterfaceButton!
@IBOutlet weak var buttonThree: WKInterfaceButton!
@IBOutlet weak var buttonFour: WKInterfaceButton!
// End Game Enum
enum EndGameReason {
case TimeOut
case WrongButton
}
var endGameReason = EndGameReason.TimeOut
// Button Tapped
var tapped = 5
// 5 = error
// Event handlers for all the buttons.
@IBAction func TapOne() {
tappedButton(1)
}
@IBAction func TapTwo() {
tappedButton(2)
}
@IBAction func TapThree() {
tappedButton(3)
}
@IBAction func TapFour() {
tappedButton(4)
}
func tappedButton(button: Int) {
var button = button
var active = board.activeButton
if button == active {
score.currentScore = score.currentScore + 1
if score.currentScore % GOAL == 0 {
gameTimeLeft = gameTimeLeft + Int(ADDITIONAL_TIME)
secondTimer.invalidate()
minuteTimer.invalidate()
setTimer()
}
scoreLabel.setText("\(score.currentScore)")
board.makeRandomButtonActive()
activateButton(board.activeButton)
} else {
endGameReason = EndGameReason.WrongButton
gameOver(button)
}
}
func setTimer() {
let TIME = NSTimeInterval(gameTimeLeft)
minuteTimer = NSTimer.scheduledTimerWithTimeInterval(TIME, target: self, selector: Selector("gameOver:"), userInfo: 0, repeats: false)
secondTimer = NSTimer.scheduledTimerWithTimeInterval(ONE_SECOND, target: self, selector: Selector("updateLabel"), userInfo: nil, repeats: true)
}
// This function changes the color of a button depending on whether it is the active button or not.
func activateButton(button: Int) {
buttonOne.setBackgroundColor(GRAY)
buttonTwo.setBackgroundColor(GRAY)
buttonThree.setBackgroundColor(GRAY)
buttonFour.setBackgroundColor(GRAY)
var newButton = button
switch newButton {
case 1:
buttonOne.setBackgroundColor(GREEN)
case 2:
buttonTwo.setBackgroundColor(GREEN)
case 3:
buttonThree.setBackgroundColor(GREEN)
case 4:
buttonFour.setBackgroundColor(GREEN)
default:
break
}
}
func updateLabel() {
gameTimer.setText("\(gameTimeLeft - 1)")
gameTimeLeft = gameTimeLeft - 1
}
// This is the game timer, started when the player pushes the start button.
func startGame() {
score.currentScore = 0
gameTimeLeft = Int(TIME_INTERVAL)
setTimer()
board = GameBoard()
activateButton(board.activeButton)
}
// A game over function to return the app to the initial state
func gameOver(button: Int) {
var tapped = button
gameTimer.setText("0")
var endGameText: String = ""
secondTimer.invalidate()
minuteTimer.invalidate()
// What to do for each reason the game was ended
switch endGameReason{
case .WrongButton:
endGameText = "wrong button"
switch tapped{
case 1:
buttonOne.setBackgroundColor(RED)
case 2:
buttonTwo.setBackgroundColor(RED)
case 3:
buttonThree.setBackgroundColor(RED)
case 4:
buttonFour.setBackgroundColor(RED)
default:
break
}
case .TimeOut:
endGameText = "time out"
buttonOne.setBackgroundColor(RED)
buttonTwo.setBackgroundColor(RED)
buttonThree.setBackgroundColor(RED)
buttonFour.setBackgroundColor(RED)
}
NSLog("End Game Reason : \(endGameText)")
NSLog("Game over: score was \(score.currentScore)")
var waitTimer: NSTimer = NSTimer.scheduledTimerWithTimeInterval(2, target: self, selector: Selector("toResults"), userInfo: nil, repeats: false)
}
func toResults() {
pushControllerWithName("resultController", context: nil)
}
// The default initialization function
override func awakeWithContext(context: AnyObject?) {
super.awakeWithContext(context)
startGame()
}
}
<file_sep>/Watch The Corners WatchKit Extension/mainController.swift
//
// mainController.swift
// Watch The Corners
//
// Created by <NAME> on 3/2/15.
// Copyright (c) 2015 TwistSix, LLC. All rights reserved.
//
import WatchKit
import Foundation
class mainController: WKInterfaceController {
override func awakeWithContext(context: AnyObject?) {
super.awakeWithContext(context)
}
@IBAction func startGame() {
pushControllerWithName("gameController", context: nil)
}
}
<file_sep>/README.md
# Watch the Corners
An Apple Watch game
<file_sep>/Watch The Corners/scoresViewController.swift
//
// scoresViewController.swift
// Watch The Corners
//
// Created by <NAME> on 4/13/15.
// Copyright (c) 2015 TwistSix, LLC. All rights reserved.
//
import UIKit
import iAd
class scoresViewController: UIViewController {
let storage = NSUserDefaults.standardUserDefaults()
let score = Score.sharedInstance
let WHITE: UIColor = UIColor.init(red: 0.94, green: 0.94, blue: 0.94, alpha: 1)
let BLACK: UIColor = UIColor.blackColor()
let GREEN: UIColor = UIColor.init(red: 0.64, green: 0.83, blue: 0.63, alpha: 1)
let DARK_GRAY: UIColor = UIColor.init(red: 0.18, green: 0.19, blue: 0.2, alpha: 1)
let LIGHT_GRAY: UIColor = UIColor.lightGrayColor()
let RED : UIColor = UIColor.init(red: 0.93, green: 0.34, blue: 0.34, alpha: 1)
let WIDTH = UIScreen.mainScreen().bounds.width
let HEIGHT = UIScreen.mainScreen().bounds.height
let labelFont = UIFont.boldSystemFontOfSize(30)
let buttonFont = UIFont.systemFontOfSize(50)
let scoreFont = UIFont.boldSystemFontOfSize(150)
let scoreListFont = UIFont.systemFontOfSize(40)
var scoresAsText = ""
var numberOfScores = 0
var highScore: Int!
var newScoreText: UILabel = UILabel()
var highScoresLabel: UILabel = UILabel()
var scoreLabel: UILabel = UILabel()
var highScoreText: UIButton = UIButton.buttonWithType(.System) as! UIButton
var button: UIButton = UIButton.buttonWithType(UIButtonType.System) as! UIButton
func backToGame() {
storage.synchronize()
showViewController(gameViewController(), sender: self)
}
func goToGameCenter() {
GCHelper.showGameCenter(self, viewState: .Leaderboards)
}
func displayScores() {
showLogo()
highScoreLabels()
playAgainButton()
}
func showLogo() {
let imageView = UIImageView()
imageView.frame = CGRectMake(WIDTH/8, 30, WIDTH*3/4, WIDTH*3/4)
imageView.image = UIImage(named: "logo")
self.view.addSubview(imageView)
}
func highScoreLabels() {
// high score labels
highScoresLabel.frame = CGRectMake(0, HEIGHT/2 + 20, WIDTH, 50)
highScoresLabel.text = scoresAsText + "|"
highScoresLabel.textAlignment = NSTextAlignment.Center
highScoresLabel.textColor = DARK_GRAY
highScoresLabel.font = scoreListFont
highScoresLabel.adjustsFontSizeToFitWidth = true
// A label to describe the high scores
highScoreText.frame = CGRectMake(0, HEIGHT/2 + 70, WIDTH, 30)
highScoreText.titleLabel?.font = UIFont.systemFontOfSize(20)
highScoreText.titleLabel?.textAlignment = NSTextAlignment.Center
highScoreText.setTitle("High Scores", forState: .Normal)
highScoreText.setTitleColor(DARK_GRAY, forState: .Normal)
highScoreText.addTarget(self, action: Selector("goToGameCenter"), forControlEvents: .TouchUpInside)
self.view.addSubview(highScoresLabel)
self.view.addSubview(highScoreText)
}
func playAgainButton() {
// The button to allow the player to start a new game
button.frame = CGRectMake(5, HEIGHT*2/3 + 30, WIDTH-10, 60)
button.layer.cornerRadius = 10
button.backgroundColor = GREEN
button.titleLabel?.font = buttonFont
button.titleLabel?.textAlignment = NSTextAlignment.Center
button.setTitle("Play Game", forState: UIControlState.Normal)
button.setTitleColor(DARK_GRAY, forState: UIControlState.Normal)
button.addTarget(self, action: Selector("backToGame"), forControlEvents: UIControlEvents.TouchUpInside)
self.view.addSubview(button)
}
func formatHighScores() {
for value in score.highScores {
if value > 0 {
scoresAsText = scoresAsText + "| \(value) "
} else if value == 0 {
scoresAsText = scoresAsText + "| 0 "
}
}
}
func loadDefaults() {
storage.synchronize()
if storage.arrayForKey("highScores") != nil {
score.highScores = storage.arrayForKey("highScores") as! [Int]
}
}
override func viewDidLoad() {
super.viewDidLoad()
loadDefaults()
self.interstitialPresentationPolicy = ADInterstitialPresentationPolicy.Automatic
self.canDisplayBannerAds = true
self.view.backgroundColor = WHITE
formatHighScores()
displayScores()
}
}
<file_sep>/fastlane/Snapfile
# Uncomment the lines below you want to change by removing the # in the beginning
# A list of devices you want to take the screenshots from
devices([
'iPhone 6',
'iPhone 6 Plus',
'iPhone 5s'
])
languages([
'en-US'
])
# Where should the resulting screenshots be stored?
screenshots_path './screenshots'
clear_previous_screenshots # remove the '#'' to clear all previously generated screenshots before creating new ones
# JavaScript UIAutomation file
js_file './snapshot.js'
# The name of the project's scheme
scheme 'Watch The Corners'
# Where is your project (or workspace)? Provide the full path here
project_path '../Watch The Corners.xcworkspace'
<file_sep>/Watch The Corners/resultViewController.swift
//
// resultViewController.swift
// Watch The Corners
//
// Created by <NAME> on 3/23/15.
// Copyright (c) 2015 TwistSix, LLC. All rights reserved.
//
import UIKit
import iAd
import Social
class resultViewController: UIViewController {
let storage = NSUserDefaults.standardUserDefaults()
let score = Score.sharedInstance
let WHITE: UIColor = UIColor.init(red: 0.94, green: 0.94, blue: 0.94, alpha: 1)
let BLACK: UIColor = UIColor.blackColor()
let GREEN: UIColor = UIColor.init(red: 0.64, green: 0.83, blue: 0.63, alpha: 1)
let DARK_GRAY: UIColor = UIColor.init(red: 0.18, green: 0.19, blue: 0.2, alpha: 1)
let LIGHT_GRAY: UIColor = UIColor.lightGrayColor()
let RED : UIColor = UIColor.init(red: 0.93, green: 0.34, blue: 0.34, alpha: 1)
let WIDTH = UIScreen.mainScreen().bounds.width
let HEIGHT = UIScreen.mainScreen().bounds.height - 50
let labelFont = UIFont.boldSystemFontOfSize(30)
let buttonFont = UIFont.systemFontOfSize(30)
let scoreFont = UIFont.boldSystemFontOfSize(150)
let scoreListFont = UIFont.systemFontOfSize(40)
let highScoreFont = UIFont.boldSystemFontOfSize(30)
let FACEBOOK_TEXT = "I scored big in Watch the Corners! Try and beat me!"
let TWITTER_TEXT = "I scored big in #watchthecorners! Try and beat me!"
let TWITTER_APP_URL: NSURL = NSURL(string: "https://itunes.apple.com/app/apple-store/id978732428?pt=96139182&ct=Twitter&mt=8")!
let FACEBOOK_APP_URL: NSURL = NSURL(string: "https://itunes.apple.com/app/apple-store/id978732428?pt=96139182&ct=Facebook&mt=8")!
var scoresAsText = ""
var numberOfScores = 0
var highScore: Int!
var newScoreText: UILabel = UILabel()
var scoreLabel: UILabel = UILabel()
var highScoreText: UIButton = UIButton.buttonWithType(.System) as! UIButton
var gameScoreTextFrame: CGRect!
var highScoreTextFrame: CGRect!
var gameScoreFrame: CGRect!
var buttonFrame: CGRect!
var logoFrame: CGRect!
var twitterFrame: CGRect!
var facebookFrame: CGRect!
func backToGame() {
storage.synchronize()
showViewController(gameViewController(), sender: self)
}
func goToScores() {
storage.synchronize()
showViewController(scoresViewController(), sender: self)
}
func makeFrames() {
logoFrame = CGRectMake(10, 30, WIDTH, WIDTH/4)
gameScoreTextFrame = CGRectMake(0, WIDTH/4 + 30, WIDTH, 60)
gameScoreFrame = CGRectMake(0, WIDTH/2, WIDTH, 160)
highScoreTextFrame = CGRectMake(0, HEIGHT/2 + 90, WIDTH, 35)
buttonFrame = CGRectMake(80, HEIGHT*2/3 + 65, WIDTH - 160, 70)
twitterFrame = CGRectMake(WIDTH - 65, HEIGHT*2/3 + 75, 50, 50)
facebookFrame = CGRectMake(15, HEIGHT*2/3 + 75, 50, 50)
}
func displayScores() {
gameScoreLabel()
gameScoreText()
highScoreLabels()
playAgainButton()
scoreLogicHandler()
showLogo()
}
func showLogo() {
let imageView = UIImageView()
imageView.frame = logoFrame
imageView.image = UIImage(named: "text-logo")
self.view.addSubview(imageView)
}
func scoreLogicHandler() {
highScore = score.getHighScore()
var topTenPercent = Int(floor(0.9 * Float(highScore)))
var bottomTenPercent = Int(floor(0.1 * Float(highScore)))
if !score.duplicateScore {
if score.currentScoreIsHighScore() && score.currentScore != 0 {
score.reportToGameCenter()
newScoreText.text = "New High Score!"
newScoreText.textColor = GREEN
scoreLabel.textColor = GREEN
} else if score.currentScore == 0 {
newScoreText.text = "Better Luck Next Time!"
newScoreText.textColor = RED
scoreLabel.textColor = RED
} else if (score.currentScore >= topTenPercent && highScore > score.currentScore) {
newScoreText.text = "So Close!"
newScoreText.textColor = DARK_GRAY
scoreLabel.textColor = DARK_GRAY
} else if (bottomTenPercent >= score.currentScore && score.currentScore > 0) {
newScoreText.text = "Almost There..."
newScoreText.textColor = DARK_GRAY
scoreLabel.textColor = DARK_GRAY
} else {
newScoreText.text = "Good Job!"
newScoreText.textColor = LIGHT_GRAY
scoreLabel.textColor = LIGHT_GRAY
}
} else if (score.duplicateScore == true) {
newScoreText.text = "Better Luck Next Time!"
newScoreText.textColor = DARK_GRAY
scoreLabel.textColor = DARK_GRAY
}
}
func gameScoreText() {
newScoreText.frame = gameScoreTextFrame
newScoreText.textAlignment = .Center
newScoreText.font = labelFont
newScoreText.adjustsFontSizeToFitWidth = true
self.view.addSubview(newScoreText)
}
func highScoreLabels() {
// A label to describe the high scores
highScoreText.frame = highScoreTextFrame
highScoreText.titleLabel?.font = highScoreFont
highScoreText.titleLabel?.textAlignment = .Center
highScoreText.setTitle("High Score: \(score.getHighScore())", forState: .Normal)
highScoreText.setTitleColor(DARK_GRAY, forState: .Normal)
highScoreText.addTarget(self, action: Selector("goToScores"), forControlEvents: .TouchUpInside)
self.view.addSubview(highScoreText)
}
func gameScoreLabel() {
// The player's score from the completed game
scoreLabel.text = "\(score.currentScore)"
scoreLabel.frame = gameScoreFrame
scoreLabel.textAlignment = .Center
scoreLabel.font = scoreFont
self.view.addSubview(scoreLabel)
}
func playAgainButton() {
// The button to allow the player to start a new game
let button = UIButton.buttonWithType(.System) as! UIButton
button.frame = buttonFrame
button.layer.cornerRadius = 10
button.backgroundColor = GREEN
button.titleLabel?.font = buttonFont
button.titleLabel?.textAlignment = .Center
button.setTitle("Play Again", forState: UIControlState.Normal)
button.setTitleColor(DARK_GRAY, forState: UIControlState.Normal)
button.addTarget(self, action: Selector("backToGame"), forControlEvents: UIControlEvents.TouchUpInside)
// The button that shares to Facebook
let facebookShare = UIButton.buttonWithType(.Custom) as! UIButton
facebookShare.frame = facebookFrame
facebookShare.setImage(UIImage(named: "facebook-logo"), forState: UIControlState.Normal)
facebookShare.addTarget(self, action: Selector("shareToFacebook"), forControlEvents: .TouchUpInside)
// The button that shares to Twitter
let twitterShare = UIButton.buttonWithType(.Custom) as! UIButton
twitterShare.frame = twitterFrame
twitterShare.layer.cornerRadius = 5
twitterShare.setImage(UIImage(named: "twitter-logo-white"), forState: UIControlState.Normal)
twitterShare.addTarget(self, action: Selector("shareToTwitter"), forControlEvents: .TouchUpInside)
self.view.addSubview(button)
self.view.addSubview(facebookShare)
self.view.addSubview(twitterShare)
}
func loadDefaults() {
storage.synchronize()
if storage.arrayForKey("highScores") != nil {
score.highScores = storage.arrayForKey("highScores") as! [Int]
}
}
func saveDefaults() {
storage.setObject(score.highScores, forKey: "highScores")
}
func saveScreenshot(view: UIView) -> UIImage {
var frame: CGRect = CGRectMake(0, 0, WIDTH, (WIDTH/2) + 162)
UIGraphicsBeginImageContext(frame.size)
let context: CGContextRef = UIGraphicsGetCurrentContext()
view.layer.renderInContext(context)
var screenshot: UIImage = UIGraphicsGetImageFromCurrentImageContext()
UIGraphicsEndImageContext()
return screenshot
}
func shareToFacebook() {
if SLComposeViewController.isAvailableForServiceType(SLServiceTypeFacebook) {
var sharesheet = SLComposeViewController(forServiceType: SLServiceTypeFacebook)
sharesheet.setInitialText(FACEBOOK_TEXT)
sharesheet.addImage(saveScreenshot(view))
sharesheet.addURL(FACEBOOK_APP_URL)
self.presentViewController(sharesheet, animated: true, completion: nil)
}
}
func shareToTwitter() {
if SLComposeViewController.isAvailableForServiceType(SLServiceTypeTwitter) {
var sharesheet = SLComposeViewController(forServiceType: SLServiceTypeTwitter)
sharesheet.setInitialText(TWITTER_TEXT)
sharesheet.addImage(saveScreenshot(view))
sharesheet.addURL(TWITTER_APP_URL)
self.presentViewController(sharesheet, animated: true, completion: nil)
}
}
override func viewDidLoad() {
super.viewDidLoad()
loadDefaults()
score.addScoreToHighScores(score.currentScore)
saveDefaults()
self.interstitialPresentationPolicy = ADInterstitialPresentationPolicy.Automatic
self.canDisplayBannerAds = true
self.view.backgroundColor = WHITE
makeFrames()
displayScores()
}
}
<file_sep>/Watch The Corners/rulesViewController.swift
//
// rulesViewController.swift
// Watch The Corners
//
// Created by <NAME> on 4/13/15.
// Copyright (c) 2015 TwistSix, LLC. All rights reserved.
//
import UIKit
let WHITE: UIColor = UIColor.init(red: 0.94, green: 0.94, blue: 0.94, alpha: 1)
let BLACK: UIColor = UIColor.blackColor()
let GREEN: UIColor = UIColor.init(red: 0.64, green: 0.83, blue: 0.63, alpha: 1)
let DARK_GRAY: UIColor = UIColor.init(red: 0.18, green: 0.19, blue: 0.2, alpha: 1)
let LIGHT_GRAY: UIColor = UIColor.init(red: 0.58, green: 0.59, blue: 0.6, alpha: 1)
let RED : UIColor = UIColor.init(red: 0.93, green: 0.34, blue: 0.34, alpha: 1)
let WIDTH = UIScreen.mainScreen().bounds.width
let HALF_WIDTH = UIScreen.mainScreen().bounds.width/2
let HEIGHT = UIScreen.mainScreen().bounds.height
let HALF_HEIGHT = UIScreen.mainScreen().bounds.height/2
let labelFont = UIFont.boldSystemFontOfSize(25)
class rulesViewController: UIViewController {
var testButton: UIButton = UIButton.buttonWithType(UIButtonType.Custom) as! UIButton
var rulesLabel: UILabel = UILabel()
var scoreRulesLabel: UILabel = UILabel()
var extraTimeLabel: UILabel = UILabel()
func makeButtons() {
testButton.frame = CGRectMake(WIDTH/4, 30, WIDTH/2, WIDTH/2)
testButton.backgroundColor = GREEN
testButton.addTarget(self, action: Selector("testTapped"), forControlEvents: UIControlEvents.TouchUpInside)
self.view.addSubview(testButton)
rulesLabel.frame = CGRectMake(0, HALF_WIDTH + 40, WIDTH, 30)
rulesLabel.font = labelFont
rulesLabel.text = "Tap the GREEN button."
rulesLabel.textAlignment = NSTextAlignment.Center
rulesLabel.textColor = DARK_GRAY
self.view.addSubview(rulesLabel)
}
func testTapped() {
rulesLabel.text = "Good Job!"
testButton.enabled = false
testButton.backgroundColor = LIGHT_GRAY
var waitTimer = NSTimer.scheduledTimerWithTimeInterval(2, target: self, selector: Selector("scoreRules"), userInfo: nil, repeats: false)
}
func scoreRules() {
scoreRulesLabel.text = "Try to get as many points as you can in TEN seconds."
scoreRulesLabel.font = labelFont
scoreRulesLabel.frame = CGRectMake(10, HALF_HEIGHT-75, WIDTH-20, 180)
scoreRulesLabel.textAlignment = NSTextAlignment.Center
scoreRulesLabel.textColor = DARK_GRAY
scoreRulesLabel.numberOfLines = 3
scoreRulesLabel.lineBreakMode = NSLineBreakMode.ByWordWrapping
self.view.addSubview(scoreRulesLabel)
var waitTimer = NSTimer.scheduledTimerWithTimeInterval(5, target: self, selector: Selector("extraTimeRules"), userInfo: nil, repeats: false)
}
func extraTimeRules() {
extraTimeLabel.text = "Every TEN points, you get TWO extra seconds."
extraTimeLabel.font = labelFont
extraTimeLabel.frame = CGRectMake(10, HALF_HEIGHT + 20, WIDTH - 20, 180)
extraTimeLabel.textAlignment = NSTextAlignment.Center
extraTimeLabel.textColor = DARK_GRAY
extraTimeLabel.numberOfLines = 3
extraTimeLabel.lineBreakMode = NSLineBreakMode.ByWordWrapping
self.view.addSubview(extraTimeLabel)
var waitTimer = NSTimer.scheduledTimerWithTimeInterval(5, target: self, selector: Selector("playGame"), userInfo: nil, repeats: false)
}
func playGame() {
rulesLabel.text = "Tap the button to play."
rulesLabel.textColor = RED
testButton.backgroundColor = GREEN
testButton.enabled = true
testButton.addTarget(self, action: Selector("goToGame"), forControlEvents: UIControlEvents.TouchUpInside)
}
func goToGame() {
showViewController(gameViewController(), sender: self)
}
override func viewDidLoad() {
super.viewDidLoad()
self.view.backgroundColor = WHITE
makeButtons()
}
}
<file_sep>/Watch The Corners/GameBoard.swift
//
// GameBoard.swift
// Watch The Corners
//
// Created by <NAME> on 3/23/15.
// Copyright (c) 2015 TwistSix, LLC. All rights reserved.
//
import Foundation
class GameBoard {
let BOARD_SIZE = 4
var activeButton:Int!
init() {
makeRandomButtonActive()
}
// makes a random 'button' active
func makeRandomButtonActive() {
let number = Int(arc4random_uniform(4)+1)
self.activeButton = number
}
}
<file_sep>/fastlane/Fastfile
# Fastfile for Watch the Corners. This will speed up deployment of the game.
fastlane_version '0.3.0'
before_all do
update_fastlane
ensure_git_status_clean
increment_build_number
xcclean
end
lane :major do
increment_version_number(
bump_type: 'major'
)
commit_version_bump
end
lane :minor do
increment_version_number(
bump_type: 'minor'
)
commit_version_bump
end
lane :patch do
increment_version_number
commit_version_bump
end
lane :deploy do
add_git_tag(
grouping: 'releases',
prefix: 'v'
)
ipa({
workspace: 'Watch The Corners.xcworkspace',
configuration: 'Release',
scheme: 'Watch The Corners',
clean: true,
destination: './build/ipa',
ipa: 'WatchTheCorners.ipa'
})
sigh
deliver(
skip_deploy: true,
force: true
)
end
lane :crashlytics do
ipa({
workspace: 'Watch The Corners.xcworkspace',
configuration: 'Release',
scheme: 'Watch The Corners',
clean: true,
destination: './build/ipa',
ipa: 'WatchTheCorners.ipa'
})
sigh
crashlytics({
crashlytics_path: './Crashlytics.framework',
ipa_path: './build/ipa/WatchTheCorners.ipa'
})
end
lane :testflight do
ipa({
workspace: 'Watch The Corners.xcworkspace',
configuration: 'Release',
scheme: 'Watch The Corners',
clean: true,
destination: './build/ipa',
ipa: 'WatchTheCorners.ipa'
})
sigh
deliver(
beta: true,
force: true
)
end
after_all do |lane|
clean_build_artifacts
slack(
message: "Deployed a #{lane} update for Watch The Corners.",
success: true
)
notify "Finished running #{lane}!"
end
error do |lane, exception|
slack(
message: "Error deploying a #{lane} update for Watch the Corners, caused by #{exception}",
success: false
)
notify "Error running #{lane}."
end
| bdb2f8728433740e8a9d56f25311768e9c32fd54 | [
"Swift",
"Ruby",
"Markdown"
] | 16 | Swift | dhagerty9009/watch-the-corners | 6576cf7dca2a3ba4ef34d71ae23201f5e48c5f2f | 21aa37bc3fba5716a7f1b58298365aa5c0c16976 | |
refs/heads/main | <repo_name>StudentLifeMarketingAndDesign/annual-report-2013<file_sep>/Gruntfile.js
module.exports = function(grunt) {
var globalConfig = {
themeDir: 'themes/annual-report'
};
// Project configuration.
grunt.initConfig({
globalConfig: globalConfig,
pkg: grunt.file.readJSON('package.json'),
sass: {
dist: {
files: {
'<%=globalConfig.themeDir %>/css/master.css' : '<%=globalConfig.themeDir %>/scss/master.scss',
'<%=globalConfig.themeDir %>/css/editor.css' : 'annual-report-project/scss/editor.scss'
}, // Target
options: { // Target options
style: 'compressed'
}
}
},
//concat all the files into the build folder
concat: {
js:{
src: [
'division-bar/js/division-bar.js',
'annual-report-project/bower_components/bootstrap/js/affix.js',
'annual-report-project/bower_components/bootstrap/js/transition.js',
'annual-report-project/bower_components/bootstrap/js/collapse.js',
'annual-report-project/bower_components/isotope/dist/isotope.pkgd.js',
'annual-report-project/js/plugins/jquery-scrollto.js',
'annual-report-project/bower_components/history.js/scripts/bundled/html4+html5/jquery.history.js',
'annual-report-project/js/plugins/socialcount.min.js',
'annual-report-project/js/plugins/ajaxify-html5.js',
'annual-report-project/js/plugins/jquery.fitvids.js',
'annual-report-project/js/main.js',
'<%=globalConfig.themeDir %>/js/app.js'
],
dest: '<%=globalConfig.themeDir %>/build/build.src.js'
}
},
//minify those concated files
//toggle mangle to leave variable names intact
uglify: {
my_target:{
files:{
'<%=globalConfig.themeDir %>/build/build.js': ['<%=globalConfig.themeDir %>/build/build.src.js'],
}
}
},
watch: {
scripts: {
files: ['<%=globalConfig.themeDir %>/js/*.js',
'<%=globalConfig.themeDir %>/js/**/*.js',
'annual-report-project/js/*.js',
'annual-report-project/js/**/*.js',
],
tasks: ['concat', 'uglify'],
options: {
spawn: true,
}
},
css: {
files: ['<%=globalConfig.themeDir %>/scss/*.scss',
'<%=globalConfig.themeDir %>/scss/**/*.scss',
'<%=globalConfig.themeDir %>/scss/**/**/*.scss',
'annual-report-project/scss/*.scss',
'annual-report-project/scss/**/*.scss',
'annual-report-project/scss/**/**/*.scss',
],
tasks: ['sass'],
options: {
spawn: true,
}
}
},
});
// Load the plugin that provides the "uglify" task.
grunt.loadNpmTasks('grunt-contrib-concat');
grunt.loadNpmTasks('grunt-contrib-uglify');
grunt.loadNpmTasks('grunt-contrib-sass');
//grunt.loadNpmTasks('grunt-contrib-imagemin');
grunt.loadNpmTasks('grunt-contrib-watch');
// Default task(s).
// Note: order of tasks is very important
grunt.registerTask('default', ['sass', 'concat', 'uglify', 'watch']);
};
<file_sep>/mysite/_config.php
<?php
global $project;
$project = 'mysite';
global $database;
$database = 'annualreport13';
// Use _ss_environment.php file for configuration
require_once("conf/ConfigureFromEnv.php");
MySQLDatabase::set_connection_charset('utf8');
// add a button to remove formatting
HtmlEditorConfig::get('cms')->insertButtonsBefore(
'styleselect',
'removeformat'
);
// tell the button which tags it may remove
HtmlEditorConfig::get('cms')->setOption(
'removeformat_selector',
'b,strong,em,i,span,ins'
);
//remove font->span conversion
HtmlEditorConfig::get('cms')->setOption(
'convert_fonts_to_spans', 'false,'
);
HtmlEditorConfig::get('cms')->setOptions(array(
'extended_valid_elements' => "img[class|src|alt|title|hspace|vspace|width|height|align|onmouseover|onmouseout|name|usemap],#iframe[src|name|width|height|align|frameborder|marginwidth|marginheight|scrolling],object[width|height|data|type],param[name|value],map[class|name|id],area[shape|coords|href|target|alt],script[language|type|src]",
));
// Set the site locale
i18n::set_locale('en_US');
if(Director::isLive()) {
Director::forceSSL();
}
| 61c27ac1eabc815e2753816dc616668cde26cfd6 | [
"JavaScript",
"PHP"
] | 2 | JavaScript | StudentLifeMarketingAndDesign/annual-report-2013 | 9946b15f088646e2fb4d9d5a4c66de0da3a02b97 | 37886ab2605d1815d1f8b910e95feb2ffdbb3d87 | |
refs/heads/master | <repo_name>StaffanJ/PizzaChallenge<file_sep>/Pizza.Domain/CustomerCheckValidationException.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Pizza.Domain
{
public class CustomerCheckValidationException : Exception
{
public string Message;
public CustomerCheckValidationException(string Message)
{
this.Message = Message;
}
}
}
<file_sep>/Pizza.Presentation/Default.aspx.cs
using System;
using Pizza.Domain;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Diagnostics;
using System.Data.Entity.Validation;
namespace Pizza.Presentation
{
public partial class Default : System.Web.UI.Page
{
PizzaManager pizzaManager = new PizzaManager();
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
displayInformation();
displayCustomers();
}
resultLabel.Text = gatherInformation();
}
private void displayCustomers()
{
var customers = pizzaManager.GetCustomers();
foreach (var customer in customers)
{
customerDropDown.Items.Add(new ListItem(customer.Name, customer.customerID.ToString()));
}
}
private void displayInformation()
{
var crusts = pizzaManager.GetCrusts();
var ingredients = pizzaManager.GetIngredients();
var sizes = pizzaManager.GetSizes();
foreach (var crust in crusts)
{
crustDropDownList.Items.Add(new ListItem(crust.Name, crust.Price.ToString()));
}
foreach (var size in sizes)
{
sizesDropDownList.Items.Add(new ListItem(size.Name, size.Price.ToString()));
}
foreach (var ingredient in ingredients)
{
ingredientsCheckBox.Items.Add(new ListItem(ingredient.Name, ingredient.Price.ToString()));
}
}
private string gatherInformation()
{
string result = "";
decimal totalPrice = calculatePrice();
return result = string.Format("Total cost of the pizza is: {0:C}", totalPrice);
}
private decimal calculatePrice()
{
decimal crustPrice = 0, sizePrice = 0, ingredientPrice = 0, totalPrice = 0;
crustPrice = Decimal.Parse(crustDropDownList.SelectedValue);
sizePrice = Decimal.Parse(sizesDropDownList.SelectedValue);
for (int i = 0; i < ingredientsCheckBox.Items.Count; i++)
{
if (ingredientsCheckBox.Items[i].Selected)
{
ingredientPrice += Decimal.Parse(ingredientsCheckBox.Items[i].Value.ToString());
}
}
return totalPrice = crustPrice + sizePrice + ingredientPrice;
}
private DTO.Customer createCustomer()
{
var customer = new DTO.Customer()
{
customerID = Guid.NewGuid(),
Name = nameTextBox.Text,
PostalCode = postalTextBox.Text,
Phone = phoneTextBox.Text,
Address = addressTextBox.Text,
};
return customer;
}
private void AddCustomer()
{
var customer = createCustomer();
string errorMessage = "";
try
{
if (customer.Name == "")
{
errorMessage = "Name cannot be empty";
throw new CustomerCheckValidationException(errorMessage);
}
if (customer.Address == "")
{
errorMessage = "Address cannot be empty";
throw new CustomerCheckValidationException(errorMessage);
}
if (customer.PostalCode == "")
{
errorMessage = "Postal code cannot be empty";
throw new CustomerCheckValidationException(errorMessage);
}
if (customer.Phone == "")
{
errorMessage = "Phonenumber cannot be empty";
throw new CustomerCheckValidationException(errorMessage);
}
pizzaManager.AddCustomer(customer);
customerLabel.Text = string.Format("{0} have been added!", customer.Name);
}
catch (DbEntityValidationException ex)
{
foreach (var validations in ex.EntityValidationErrors)
{
foreach (var validation in validations.ValidationErrors)
{
customerLabel.Text += string.Format("There was an issue with: {0}", validation.PropertyName);
}
}
}
catch (CustomerCheckValidationException ex)
{
customerLabel.Text = ex.Message;
}
catch (Exception ex)
{
customerLabel.Text = string.Format("There was an issue with: {0}", ex.Message);
}
}
private DTO.Pizzas createPizza()
{
decimal totalCost = calculatePrice();
var createPizza = new DTO.Pizzas() {
pizzaID = Guid.NewGuid(),
customerID = Guid.Parse(customerDropDown.SelectedValue),
Price = totalCost,
Completed = 1
};
return createPizza;
}
private void AddPizza()
{
var pizza = createPizza();
string errorMessage = "";
try
{
if (pizza.Price.ToString() == "")
{
errorMessage = "There must be a price";
throw new PizzaCheckValidationException(errorMessage);
}
pizzaManager.AddPizza(pizza);
resultLabel.Text = "Your order have been added";
}
catch (DbEntityValidationException ex)
{
foreach (var validations in ex.EntityValidationErrors)
{
foreach (var validation in validations.ValidationErrors)
{
customerLabel.Text += string.Format("There was an issue with: {0}", validation.PropertyName);
}
}
}
catch (PizzaCheckValidationException ex)
{
customerLabel.Text = ex.Message;
}
catch (Exception ex)
{
customerLabel.Text = string.Format("There was an issue with: {0}", ex.Message);
}
}
//Event logic
protected void addCustomer_Click(object sender, EventArgs e)
{
AddCustomer();
}
protected void orderButton_Click(object sender, EventArgs e)
{
AddPizza();
}
}
}<file_sep>/Pizza.Presentation/Pizzas.aspx.cs
using System;
using Pizza.Domain;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace Pizza.Presentation
{
public partial class Pizzas : System.Web.UI.Page
{
PizzaManager pizzaManager = new PizzaManager();
protected void Page_Load(object sender, EventArgs e)
{
if(!IsPostBack)
getPizzas();
}
private void getPizzas()
{
var pizzas = pizzaManager.GetPizzas();
var uncompletedOrder = new List<DTO.Pizzas>();
foreach (var pizza in pizzas)
{
if(pizza.Completed == 0)
{
uncompletedOrder.Add(pizza);
}
}
pizzaGridView.DataSource = uncompletedOrder;
pizzaGridView.DataBind();
}
protected void pizzaGridView_RowCommand(object sender, GridViewCommandEventArgs e)
{
int index = Convert.ToInt32(e.CommandArgument);
GridViewRow row = pizzaGridView.Rows[index];
var value = row.Cells[1].Text.ToString();
Guid pizzaId = Guid.Parse(row.Cells[1].Text.ToString());
pizzaManager.UpdatePizza(pizzaId);
getPizzas();
}
}
}<file_sep>/Pizza.DTO/Pizzas.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Pizza.DTO
{
public class Pizzas
{
public System.Guid pizzaID { get; set; }
public System.Guid customerID { get; set; }
public decimal Price { get; set; }
public Nullable<int> Completed { get; set; }
}
}
<file_sep>/Pizza.DTO/Customer.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Pizza.DTO
{
public class Customer
{
public System.Guid customerID { get; set; }
public string Name { get; set; }
public string Address { get; set; }
public string PostalCode { get; set; }
public string Phone { get; set; }
}
}
<file_sep>/Pizza.DTO/Ingredients.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Pizza.DTO
{
public class Ingredients
{
public System.Guid ingredientID { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
}
<file_sep>/Pizza.Persistence/PizzaRepository.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Pizza.Persistence
{
public class PizzaRepository
{
PizzaEntities db = new PizzaEntities();
//Get information from the database and sends it to the domain level.
public List<DTO.Customer> GetCustomers()
{
var customers = db.Customer;
var dtoCustomers = new List<DTO.Customer>();
foreach (var customer in customers)
{
var dtoCustomer = new DTO.Customer() {
customerID = customer.customerID,
Name = customer.Name,
Address = customer.Address,
Phone = customer.Phone,
PostalCode = customer.PostalCode
};
dtoCustomers.Add(dtoCustomer);
}
return dtoCustomers;
}
public List<DTO.Crusts> GetCrusts()
{
var crusts = db.Crusts;
var dtoCrusts = new List<DTO.Crusts>();
foreach (var crust in crusts)
{
var dtoCrust = new DTO.Crusts() {
Name = crust.Name,
Price = crust.Price
};
dtoCrusts.Add(dtoCrust);
}
return dtoCrusts;
}
public List<DTO.Ingredients> GetIngredients()
{
var ingredients = db.Ingredients;
var dtoIngredients = new List<DTO.Ingredients>();
foreach (var ingridient in ingredients)
{
var dtoIngridient = new DTO.Ingredients() {
Name = ingridient.Name,
Price = ingridient.Price
};
dtoIngredients.Add(dtoIngridient);
}
return dtoIngredients;
}
public List<DTO.Size> GetSize()
{
var sizes = db.Size;
var dtoSizes = new List<DTO.Size>();
foreach (var size in sizes)
{
var dtoSize = new DTO.Size() {
Name = size.Name,
Price = size.Price
};
dtoSizes.Add(dtoSize);
}
return dtoSizes;
}
public List<DTO.Pizzas> GetPizzas()
{
var pizzas = db.Pizzas;
var dtoPizzas = new List<DTO.Pizzas>();
foreach (var pizza in pizzas)
{
var dtoPizza = new DTO.Pizzas()
{
pizzaID = pizza.pizzaID,
customerID = pizza.customerID,
Price = pizza.Price,
Completed = pizza.Completed
};
dtoPizzas.Add(dtoPizza);
}
return dtoPizzas;
}
//Add information to the database
public void AddCustomer(DTO.Customer newCustomer)
{
var customer = new Customer()
{
customerID = newCustomer.customerID,
Name = newCustomer.Name,
Address = newCustomer.Address,
PostalCode = newCustomer.PostalCode,
Phone = newCustomer.Phone
};
var dbCustomer = db.Customer;
dbCustomer.Add(customer);
db.SaveChanges();
}
public void AddPizza(DTO.Pizzas pizza)
{
var pizzas = new Pizzas() {
pizzaID = pizza.pizzaID,
customerID = pizza.customerID,
Price = pizza.Price,
Completed = pizza.Completed
};
var dbPizza = db.Pizzas;
dbPizza.Add(pizzas);
db.SaveChanges();
}
//Update information to the dabase
public void UpdatePizza(Guid pizza)
{
var pizzas = db.Pizzas.Find(pizza);
pizzas.Completed = 1;
db.SaveChanges();
}
}
}
<file_sep>/Pizza.Domain/PizzaManager.cs
using System;
using Pizza.DTO;
using Pizza.Persistence;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Pizza.Domain
{
public class PizzaManager
{
PizzaRepository pizzaRepository = new PizzaRepository();
//Get information from the persistence layer and sends it to the presentation layer
public List<DTO.Customer> GetCustomers()
{
var customers = pizzaRepository.GetCustomers();
return customers;
}
public List<DTO.Crusts> GetCrusts() {
List<DTO.Crusts> crusts = pizzaRepository.GetCrusts();
return crusts;
}
public List<DTO.Ingredients> GetIngredients()
{
List<DTO.Ingredients> ingredients = pizzaRepository.GetIngredients();
return ingredients;
}
public List<DTO.Size> GetSizes()
{
List<DTO.Size> sizes = pizzaRepository.GetSize();
return sizes;
}
public List<DTO.Pizzas> GetPizzas()
{
var pizzas = pizzaRepository.GetPizzas();
return pizzas;
}
//Add information to the persistence layer.
public void AddCustomer(DTO.Customer customer)
{
pizzaRepository.AddCustomer(customer);
}
public void AddPizza(DTO.Pizzas pizza)
{
pizzaRepository.AddPizza(pizza);
}
public void UpdatePizza(Guid pizza)
{
pizzaRepository.UpdatePizza(pizza);
}
}
}
<file_sep>/Pizza.DTO/Size.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Pizza.DTO
{
public class Size
{
public System.Guid sizeID { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
}
| 5864081e2ab6831dd27253f887bcf27f028973a3 | [
"C#"
] | 9 | C# | StaffanJ/PizzaChallenge | 570d355c5ac51064c0c5ce703bfb4997a9327861 | 3f1957d2ce0b458b0a6981f76c539e8df8eead6a | |
refs/heads/master | <repo_name>bstansberry/wildflysite<file_sep>/_docs/docs.md
---
layout: docs
title: Wildfly Documentation
permalink: /docs/
---
<file_sep>/_docs/13/_testsuite/Pre-requisites_-_test_quality_standards.adoc
[[Pre-requisites_-_test_quality_standards]]
== Before you add a test
Every added test, whether ported or new should follow the same
guidelines:
== Verify the test belongs in WildFly
AS6 has a lot of tests for things that are discontinued. For example
the +
legacy JBoss Transaction Manager which was replaced by Arjuna. Also we +
had tests for SPIs that no longer exist. None of these things should
be +
migrated.
== Only add CORRECT and UNDERSTANDABLE tests
If you don't understand what a test is doing (perhaps too complex), or +
it's going about things in a strange way that might not be correct,
THEN +
DO NOT PORT IT. Instead we should have a simpler, understandable, and +
correct test. Write a new one, ping the author, or skip it altogether.
== Do not add duplicate tests
Always check that the test you are adding doesn't have coverage +
elsewhere (try using "git grep"). As mentioned above we have some +
overlap between 6 and 7. The 7 test version will likely be better.
== Don't name tests after JIRAs
A JIRA number is useless without an internet connection, and they are +
hard to read. If I get a test failure thats XDGR-843534578 I have to
dig +
to figure out the context. It's perfectly fine though to link to a
JIRA +
number in the comments of the test. Also the commit log is always
available.
== Tests should contain javadoc that explains what is being tested
This is especially critical if the test is non-trivial
== Prefer expanding an EXISTING test over a new test class
If you are looking at migrating or creating a test with similar +
functionality to an exiting test, it is better to +
expand upon the existing one by adding more test methods, rather than +
creating a whole new test. In general each +
new test class adds at least 300ms to execution time, so as long as it +
makes sense it is better to add it to an +
existing test case.
== Organize tests by subsystem
Integration tests should be packaged in subpackages under the relevant +
subsystem (e.g org.jboss.as.test.integration.ejb.async). When a test +
impacts multiple subsystems this is a bit of a judgement call, but in +
general the tests should go into the package of +
the spec that defines the functionality (e.g. CDI based constructor +
injection into an EJB, even though this involves CDI and EJB, +
the CDI spec defines this behaviour)
== Explain non-obvious spec behavior in comments
The EE spec is full of odd requirements. If the test is covering +
behavior that is not obvious then please add something like "Verifies
EE +
X.T.Z - The widget can't have a foobar if it is declared like blah"
== Put integration test resources in the source directory of the test
At the moment there is not real organization of these files. It makes +
sense for most apps to have this separation, however the testsuite is +
different. e.g. most apps will have a single deployment descriptor of
a +
given type, for the testsuite will have hundreds, and maintaining
mirroring +
package structures is error prone. +
This also makes the tests easier to understand, as all the artifacts
in +
the deployment are in one place, and that place tends to be small
(only +
a handful of files).
== Do not hard-code values likely to need configuration (URLs, ports, ...)
URLs hardcoded to certain address (localhost) or port (like the default
8080 for web) prevent running the test against different address or with
IPv6 adress. +
Always use the configurable values provided by Arquillian or as a system
property. +
If you come across a value which is not configurable but you think it
should be, file an WildFly {wildflyVersion} jira issue with component "Test suite". +
See
https://github.com/arquillian/arquillian/blob/master/examples/junit/src/test/java/com/acme/web/LocalRunServletTestCase.java[@ArquillianResourrce
usage example].
== Follow best committing practices
* Only do changes related to the topic of the jira/pull request.
* Do not clutter your pull request with e.g. reformatting, fixing typos
spotted along the way - do another pull request for such.
* Prefer smaller changes in more pull request over one big pull request
which are difficult to merge.
* Keep the code consistent across commits - e.g. when renaming
something, be sure to update all references to it.
* Describe your commits properly as they will appear in master's linear
history.
* If you're working on a jira issue, include it's ID in the commit
message(s).
== Do not use blind timeouts
Do not use Thread.sleep() without checking for the actual condition you
need to be fulfilled. +
You may use active waiting with a timeout, but prefer using timeouts of
the API/SPI you test where available.
Make the timeouts configurable: For a group of similar test, use a
configurable timeout value with a default if not set.
== Provide messages in assert*() and fail() calls
Definitely, it's better to see "File x/y/z.xml not found" instead of:
[source, java]
----
junit.framework.AssertionFailedError
at junit.framework.Assert.fail(Assert.java:48) [arquillian-service:]
at junit.framework.Assert.assertTrue(Assert.java:20) [arquillian-service:]
at junit.framework.Assert.assertTrue(Assert.java:27) [arquillian-service:]
at org.jboss.as.test.smoke.embedded.parse.ParseAndMarshalModelsTestCase.getOriginalStandaloneXml(ParseAndMarshalModelsTestCase.java:554) [bogus.jar:]
----
== Provide configuration properties hints in exceptions
If your test uses some configuration property and it fails possibly due
to misconfiguration, note the property and it's value in the exception:
[source, java]
----
File jdbcJar = new File( System.getProperty("jbossas.ts.dir", "."),
"integration/src/test/resources/mysql-connector-java-5.1.15.jar");
if( !jdbcJar.exists() )
throw new IllegalStateException("Can't find " + jdbcJar + " using $\{jbossas.ts.dir} == " + System.getProperty("jbossas.ts.dir") );
----
== Clean up
* Close sockets, connections, file descriptors;
* Don't put much data to static fields, or clean them in a finaly {...} block.
* Don't alter AS config (unless you are absolutely sure that it will
reload in a final \{...} block or an @After* method)
== Keep the tests configurable
Keep these things in properties, set them at the beginning of the test:
* Timeouts
* Paths
* URLs
* Numbers (of whatever)
They either will be or already are provided in form of system
properties, or a simple testsuite until API (soon to come).
<file_sep>/_docs/13/_high-availability/subsystem-support/JGroups_Subsystem.adoc
[[JGroups_Subsystem]]
== JGroups Subsystem
[[jgroups-purpose]]
== Purpose
The JGroups subsystem provides group communication support for HA
services in the form of JGroups channels.
Named channel instances permit application peers in a cluster to
communicate as a group and in such a way that the communication
satisfies defined properties (e.g. reliable, ordered,
failure-sensitive). Communication properties are configurable for each
channel and are defined by the protocol stack used to create the
channel. Protocol stacks consist of a base transport layer (used to
transport messages around the cluster) together with a user-defined,
ordered stack of protocol layers, where each protocol layer supports a
given communication property.
The JGroups subsystem provides the following features:
* allows definition of named protocol stacks
* view run-time metrics associated with channels
* specify a default stack for general use
In the following sections, we describe the JGroups subsystem.
[IMPORTANT]
JGroups channels are created transparently as part of the clustering
functionality (e.g. on clustered application deployment, channels will
be created behind the scenes to support clustered features such as
session replication or transmission of SSO contexts around the cluster).
[[jgroups-configuration-example]]
== Configuration example
What follows is a sample JGroups subsystem configuration showing all of
the possible elements and attributes which may be configured. We shall
use this example to explain the meaning of the various elements and
attributes.
[IMPORTANT]
The schema for the subsystem, describing all valid elements and
attributes, can be found in the WildFly distribution, in the `docs/schema`
directory.
[source, xml]
----
<subsystem xmlns="urn:jboss:domain:jgroups:6.0">
<channels default="ee">
<channel name="ee" stack="udp" cluster="ejb"/>
</channels>
<stacks>
<stack name="udp">
<transport type="UDP" socket-binding="jgroups-udp"/>
<protocol type="PING"/>
<protocol type="MERGE3"/>
<protocol type="FD_SOCK"/>
<protocol type="FD_ALL"/>
<protocol type="VERIFY_SUSPECT"/>
<protocol type="pbcast.NAKACK2"/>
<protocol type="UNICAST3"/>
<protocol type="pbcast.STABLE"/>
<protocol type="pbcast.GMS"/>
<protocol type="UFC"/>
<protocol type="MFC"/>
<protocol type="FRAG3"/>
</stack>
<stack name="tcp">
<transport type="TCP" socket-binding="jgroups-tcp"/>
<socket-protocol type="MPING" socket-binding="jgroups-mping"/>
<protocol type="MERGE3"/>
<protocol type="FD_SOCK"/>
<protocol type="FD_ALL"/>
<protocol type="VERIFY_SUSPECT"/>
<protocol type="pbcast.NAKACK2"/>
<protocol type="UNICAST3"/>
<protocol type="pbcast.STABLE"/>
<protocol type="pbcast.GMS"/>
<protocol type="MFC"/>
<protocol type="FRAG3"/>
</stack>
</stacks>
</subsystem>
----
[[subsystem]]
=== <subsystem>
This element is used to configure the subsystem within a WildFly system
profile.
* `xmlns` This attribute specifies the XML namespace of the JGroups
subsystem and, in particular, its version.
* `default-stack` This attribute is used to specify a default stack for
the JGroups subsystem. This default stack will be used whenever a stack
is required but no stack is specified.
[[stack]]
=== <stack>
This element is used to configure a JGroups protocol stack.
* `name` This attribute is used to specify the name of the stack.
[[jgroups-transport]]
=== <transport>
This element is used to configure the transport layer (required) of the
protocol stack.
* `type` This attribute specifies the transport type (e.g. UDP, TCP,
TCPGOSSIP)
* `socket-binding` This attribute references a defined socket binding in
the server profile. It is used when JGroups needs to create general
sockets internally.
* `diagnostics-socket-binding` This attribute references a defined
socket binding in the server profile. It is used when JGroups needs to
create sockets for use with the diagnostics program. For more about the
use of diagnostics, see the JGroups documentation for probe.sh.
* `default-executor` This attribute references a defined thread pool
executor in the threads subsystem. It governs the allocation and
execution of runnable tasks to handle incoming JGroups messages.
* `oob-executor` This attribute references a defined thread pool
executor in the threads subsystem. It governs the allocation and
execution of runnable tasks to handle incoming JGroups OOB
(out-of-bound) messages.
* `timer-executor` This attribute references a defined thread pool
executor in the threads subsystem. It governs the allocation and
execution of runnable timer-related tasks.
* `shared` This attribute indicates whether or not this transport is
shared amongst several JGroups stacks or not.
* `thread-factory` This attribute references a defined thread factory in
the threads subsystem. It governs the allocation of threads for running
tasks which are not handled by the executors above.
* `site` This attribute defines a site (data centre) id for this node.
* `rack` This attribute defines a rack (server rack) id for this node.
* `machine` This attribute defines a machine (host) is for this node.
[IMPORTANT]
site, rack and machine ids are used by the Infinispan topology-aware
consistent hash function, which when using dist mode, prevents dist mode
replicas from being stored on the same host, rack or site
.
[[property]]
==== <property>
This element is used to configure a transport property.
* `name` This attribute specifies the name of the protocol property. The
value is provided as text for the property element.
[[protocol]]
=== <protocol>
This element is used to configure a (non-transport) protocol layer in
the JGroups stack. Protocol layers are ordered within the stack.
* `type` This attribute specifies the name of the JGroups protocol
implementation (e.g. MPING, pbcast.GMS), with the package prefix
org.jgroups.protocols removed.
* `socket-binding` This attribute references a defined socket binding in
the server profile. It is used when JGroups needs to create general
sockets internally for this protocol instance.
[[relay]]
=== <relay>
This element is used to configure the RELAY protocol for a JGroups
stack. RELAY is a protocol which provides cross-site replication between
defined sites (data centres). In the RELAY protocol, defined sites
specify the names of remote sites (backup sites) to which their data
should be backed up. Channels are defined between sites to permit the
RELAY protocol to transport the data from the current site to a backup
site.
* `site` This attribute specifies the name of the current site. Site
names can be referenced elsewhere (e.g. in the JGroups remote-site
configuration elements, as well as backup configuration elements in the
Infinispan subsystem)
[[remote-site]]
==== <remote-site>
This element is used to configure a remote site for the RELAY protocol.
* `name` This attribute specifies the name of the remote site to which
this configuration applies.
* `stack` This attribute specifies a JGroups protocol stack to use for
communication between this site and the remote site.
* `cluster` This attribute specifies the name of the JGroups channel to
use for communication between this site and the remote site.
[[jgroups-use-cases]]
== Use Cases
In many cases, channels will be configured via XML as in the example
above, so that the channels will be available upon server startup.
However, channels may also be added, removed or have their
configurations changed in a running server by making use of the WildFly
management API command-line interface (CLI). In this section, we present
some key use cases for the JGroups management API.
The key use cases covered are:
* adding a stack
* adding a protocol to an existing stack
* adding a property to a protocol
[IMPORTANT]
The WildFly management API command-line interface (CLI) itself can be
used to provide extensive information on the attributes and commands
available in the JGroups subsystem interface used in these examples.
[[add-a-stack]]
=== Add a stack
[source]
----
/subsystem=jgroups/stack=mystack:add(transport={}, protocols={})
----
[[add-a-protocol-to-a-stack]]
=== Add a protocol to a stack
[source]
----
/subsystem=jgroups/stack=mystack/transport=TRANSPORT:add(type=<type>, socket-binding=<socketbinding>)
----
[source]
----
/subsystem=jgroups/stack=mystack:add-protocol(type=<type>, socket-binding=<socketbinding>)
----
[[add-a-property-to-a-protocol]]
=== Add a property to a protocol
[source]
----
/subsystem=jgroups/stack=mystack/transport=TRANSPORT/property=<property>:add(value=<value>)
----
<file_sep>/_docs/14/JavaEE_Tutorial.adoc
[[JavaEE_Tutorial]]
= JavaEE 7 Tutorial
WildFly team;
:revnumber: {version}
:revdate: {localdate}
:toc: macro
:toclevels: 3
:toc-title: JavaEE 7 Tutorial
:doctype: book
:icons: font
:source-highlighter: coderay
:wildflyVersion: 14
:leveloffset: +1
ifndef::ebook-format[:leveloffset: 1]
(C) 2017 The original authors.
:numbered:
Coming Soon
[IMPORTANT]
This guide is still under development, check back soon!
include::_javaee-guide/Java_API_for_RESTful_Web_Services_(JAX-RS).adoc[]
include::_javaee-guide/Java_Servlet_Technology.adoc[]
include::_javaee-guide/Java_Server_Faces_Technology_(JSF).adoc[]
include::_javaee-guide/Java_Persistence_API_(JPA).adoc[]
include::_javaee-guide/Java_Transaction_API_(JTA).adoc[]
include::_javaee-guide/Managed_Beans.adoc[]
include::_javaee-guide/Contexts_and_Dependency_Injection_(CDI).adoc[]
include::_javaee-guide/Bean_Validation.adoc[]
include::_javaee-guide/Java_Message_Service_API_(JMS).adoc[]
include::_javaee-guide/JavaEE_Connector_Architecture_(JCA).adoc[]
include::_javaee-guide/JavaMail_API.adoc[]
include::_javaee-guide/Java_Authorization_Contract_for_Containers_(JACC).adoc[]
include::_javaee-guide/Java_Authentication_Service_Provider_Interface_for_Containers_(JASPIC).adoc[]
include::_javaee-guide/Enterprise_JavaBeans_Technology_(EJB).adoc[]
include::_javaee-guide/Java_API_for_XML_Web_Services_(JAX-WS).adoc[]
<file_sep>/_docs/13/Testsuite.adoc
[[Testsuite]]
= WildFly Testsuite
WildFly team;
:revnumber: {version}
:revdate: {localdate}
:toc: macro
:toclevels: 3
:toc-title: WildFly testsuite
:doctype: book
:icons: font
:source-highlighter: coderay
:wildflyVersion: 13
:leveloffset: +1
ifndef::ebook-format[:leveloffset: 1]
(C) 2017 The original authors.
:numbered:
include::_testsuite/WildFly_Testsuite_Overview.adoc[]
include::_testsuite/WildFly_Integration_Testsuite_User_Guide.adoc[]
include::_testsuite/WildFly_Testsuite_Harness_Developer_Guide.adoc[]
include::_testsuite/WildFly_Testsuite_Test_Developer_Guide.adoc[]<file_sep>/_docs/13/_high-availability/subsystem-support/Infinispan_Subsystem.adoc
[[Infinispan_Subsystem]]
== Infinispan Subsystem
The Infinispan subsystem configures a set of Infinispan cache containers and cache configurations for use by WildFly clustering services.
== Cache container
A cache container manages a set of cache configurations that share the same transport and marshalling configuration.
Cache containers returned by the Infinispan subsystem are auto-configured with the following customizations:
* A custom xref:transport[transport] capable of sharing a JGroups channel defined by the JGroups subsystem.
* Uses WildFly's mbean server, if the org.jboss.as.jmx extension is present.
* Marshaller configured to resolve classes using JBoss Modules.
* Marshaller configured with a set of marshalling optimizations for common JDK classes
* Marshaller configured with additional Externalizers loadable from the configured module attribute.
e.g. To create a new cache container that loads marshallers from the "org.bar" module:
[source]
----
/subsystem=infinispan/cache-container=foo:add(module=org.foo)
----
A cache container may designate a specific cache as its default cache, i.e. the cache returned via http://docs.jboss.org/infinispan/9.2/apidocs/org/infinispan/manager/CacheContainer.html#getCache--[CacheContainer.getCache()]:
e.g. To set "bar" as the default cache of the "foo" container:
[source]
----
/subsystem=infinispan/cache-container=foo:write-attribute(name=default-cache, value=bar)
----
[[transport]]
=== Transport
Configures the mechanism used by clustered caches to communicate with each other.
It is only necessary to define a transport if the cache container contains clustered caches.
To create a JGroups transport using the default channel of the server:
[source]
----
/subsystem=infinispan/cache-container=foo/transport=jgroups:add()
----
To create a JGroups transport using a distinct "alpha" channel, that uses the "tcp" stack:
[source]
----
/subsystem=jgroups/channel=alpha:add(stack=tcp)
/subsystem=infinispan/cache-container=foo/transport=jgroups:add(channel=alpha)
----
For a complete list of transport attributes, refer to the https://wildscribe.github.io/[WildFly management model documentation]
To remove an existing JGroups transport, you can either use the standard remove resource operation:
[source]
----
/subsystem=infinispan/cache-container=foo/transport=jgroups:remove()
----
{empty}... or by adding the "none" transport (which will auto-remove any existing transport):
[source]
----
/subsystem=infinispan/cache-container=foo/transport=none:add(){allow-resource-service-restart=true}
----
=== Cache types
Infinispan supports a number of cache types for use in both HA and non-HA server profiles.
==== Local
A local cache stores a given cache entry only on the local node.
A local cache does not require a transport, as cache reads and writes are always local.
For more information about this cache type, refer to the http://infinispan.org/docs/stable/user_guide/user_guide.html#local_mode[the Infinispan documentation].
To create a local cache:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar:add()
----
For a complete list of local-cache attributes, refer to the https://wildscribe.github.io/[WildFly management model documentation]
==== Replicated
A replicated cache stores a given cache entry on every node in the cluster.
A replicated cache requires a transport, as cache writes are replicated to all nodes in the cluster on which the associated cache is running.
For more information about this cache type, refer to the http://infinispan.org/docs/stable/user_guide/user_guide.html#replicated_mode[the Infinispan documentation].
To create a replicated cache:
[source]
----
/subsystem=infinispan/cache-container=foo/replicated-cache=bar:add()
----
For a complete list of replicated-cache attributes, refer to the https://wildscribe.github.io/[WildFly management model documentation]
==== Distributed
A distributed cache stores a given cache entry on a configurable number of nodes in the cluster, assigned via an algorithm based on consistent hashing.
A distributed cache requires a transport, as cache writes need to forward to each owner, and cache reads from a non-owner require a remote request.
For more information about this cache type, refer to the http://infinispan.org/docs/stable/user_guide/user_guide.html#distribution_mode[the Infinispan documentation].
To create a distributed cache where a given entry is stored on 3 nodes:
[source]
----
/subsystem=infinispan/cache-container=foo/distributed-cache=bar:add(owners=3)
----
For a complete list of distributed-cache attributes, refer to the https://wildscribe.github.io/[WildFly management model documentation]
==== Scattered
A scattered cache is a variation of a distributed cache that maintains 2 copies of a particular cache entry.
Consequently, it can only tolerate failure of a single node at a time.
Primary ownership of a cache entry is determined by the same mechanism used by a distributed cache,
while the backup copy is the node that last updated the entry.
This design means that a scattered cache only requires 1 remote invocation to write a given cache entry, regardless of which node initiated the cache operation.
By comparison, a distributed cache (with 2 owners) uses 1 remote invocation to write a cache entry if and only if the primary owner initiated the cache operation, and otherwise requires 2 remote invocations.
For more information about this cache type, refer to the http://infinispan.org/docs/stable/user_guide/user_guide.html#scattered_mode[the Infinispan documentation].
To create a scattered cache:
[source]
----
/subsystem=infinispan/cache-container=foo/scattered-cache=bar:add()
----
For a complete list of scattered-cache attributes, refer to the https://wildscribe.github.io/[WildFly management model documentation]
==== Invalidation
An invalidation cache is a special type of clustered cache that does not share state, but instead ensures that remote state is invalidated any time a given entry is updated locally.
An invalidation cache requires a transport, as cache writes trigger invalidation on remote nodes on which the associated cache is running.
For more information about this cache type, refer to the http://infinispan.org/docs/stable/user_guide/user_guide.html#invalidation_mode[the Infinispan documentation].
To create an invalidation cache:
[source]
----
/subsystem=infinispan/cache-container=foo/invalidation-cache=bar:add()
----
For a complete list of invalidation-cache attributes, refer to the https://wildscribe.github.io/[WildFly management model documentation]
=== Cache features
The configuration of a cache is divided into several components, each defining a specific cache feature.
Because a given cache configuration requires each component relevant to its cache type, cache add operations and cache component add operations are typically batched.
Any undefined components are auto-created using their defaults.
e.g. The following cache add operation:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar:add()
----
{empty}... is actually equivalent to the following sequence of operations:
[source]
----
batch
/subsystem=infinispan/cache-container=foo/local-cache=bar:add()
/subsystem=infinispan/cache-container=foo/local-cache=bar/component=expiration:add()
/subsystem=infinispan/cache-container=foo/local-cache=bar/component=locking:add()
/subsystem=infinispan/cache-container=foo/local-cache=bar/component=transaction:add()
/subsystem=infinispan/cache-container=foo/local-cache=bar/memory=object:add()
/subsystem=infinispan/cache-container=foo/local-cache=bar/store=none:add()
run-batch
----
Similarly, you can reset all the attributes of a component by simply removing the component.
e.g.
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar:component=expiration:remove(){allow-resource-service-restart=true}
----
{empty}... is equivalent to:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar:component=expiration:remove(){allow-resource-service-restart=true}
/subsystem=infinispan/cache-container=foo/local-cache=bar:component=expiration:add(){allow-resource-service-restart=true}
----
==== Memory
An Infinispan cache can be configured to store cache entries as Java objects or as binary data (i.e. byte[]), either on or off the JVM heap.
The type of storage used has semantic implications for the user of the cache.
When using object storage, the cache has store-as-reference semantics, whereas when using binary storage the cache has call-by-value semantics.
Consider the following logic:
[source,java]
----
List<String> list = new ArrayList<>();
cache.startBatch();
cache.put("a", list);
list.add("test");
cache.endBatch(true);
List<String> result = cache.get("a");
System.out.println(result.size());
----
How many elements are in the "result" list? The answer depends on how the cache is configured.
When the cache is configured to use object memory, our result list has 1 element.
When the cache is configured to use binary (or off-heap) memory, our result list is empty.
When using binary memory, the cache value must be marshalled to a byte[] on write and unmarshalled on read, thus any mutations of the cache value in the interim are not reflected in the cache.
===== Object storage
When using object storage, cache keys and values are stored as Java object references.
Object storage may be configured with a maximum size.
When the number of entries in the cache exceeds this threshold, the least recently used entries are evicted from memory.
e.g. To store a maximum of 100 objects in the Java heap:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar/memory=object:add(size=100)
----
For a complete list of memory=object attributes, refer to the https://wildscribe.github.io/[WildFly management model documentation]
===== Binary storage (on-heap)
When using binary storage, each cache entry is stored as a byte[] within the JVM heap.
Binary storage may also be configured with a maximum size.
This size can be specified either as a maximum number of entries (i.e. COUNT), or as a maximum number of bytes (i.e. MEMORY).
When the number of entries in the cache exceeds this threshold, the least recently used entries are evicted from memory.
e.g. To store a maximum of 1 MB of binary data in the Java heap:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar/memory=binary:add(size=1048576, eviction-type=MEMORY)
----
For a complete list of memory=binary attributes, refer to the https://wildscribe.github.io/[WildFly management model documentation]
===== Off-heap binary storage
When using off-heap storage, each cache entry is stored as a byte[] in native memory allocated via sun.misc.Unsafe.
Off-heap memory storage may also be configured with a maximum size, specified either as a maximum number of entries (i.e. COUNT), or as a maximum number of bytes (i.e. MEMORY).
When the number of entries in the cache exceeds this threshold, the least recently used entries are evicted from memory.
e.g. To store a maximum of 1 GB of binary data in native memory outside of the Java heap:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar/memory=off-heap:add(size=1073741824)
----
For a complete list of memory=off-heap attributes, refer to the https://wildscribe.github.io/[WildFly management model documentation]
==== Transactions
An Infinispan cache can be configured as transactional or non-transactional.
This behavior is determined by the mode attribute, which supports the following values:
NONE::
Non-transactional cache (the default behavior).
BATCH::
Transactional cache using a local Infinispan transaction manager.
Infinispan transactions are started/committed/rolled-back using http://docs.jboss.org/infinispan/9.2/apidocs/org/infinispan/commons/api/BatchingCache.html[Infinispan's batching API].
NON_XA::
Transactional cache configured to use the server's transaction manager, registering as a Synchronization to the current transaction.
Cache commit/rollback happens after the associated transaction completes.
NON_DURABLE_XA::
Transactional cache configured to use the server's transaction manager, enlisting as an XAResource to the current transaction, but without transaction recovery support.
FULL_XA::
Transactional cache configured to use the server's transaction manager, with full transaction recovery support.
Within the context of a transaction, cache write operations must obtain a lock on the affected keys.
Locks may be acquired either pessimistically (the default), i.e. before invoking the operation, or optimistically, i.e. before transaction commit.
e.g. To configure a transactional cache using local Infinispan transactions with OPTIMISTIC locking:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar/component=transaction(mode=BATCH, locking=OPTIMISTIC)
----
For a complete list of transaction attributes, refer to the https://wildscribe.github.io/[WildFly management model documentation]
==== Locking
Within the context of a transaction, entries read from the cache are isolated from other concurrent transactions according to the configured isolation level.
Infinispan supports the following transaction isolation levels:
READ_COMMITTED::
A cache read may return a different value than a previous read within the same transaction, even if a concurrent transaction updated the entry.
This is the default isolation level.
REPEATABLE_READ::
A cache read will return the same value as a previous read within the same transaction, even if a concurrent transaction updated the entry.
IMPORTANT: Cache reads are always lock-free unless invoked using Flag.FORCE_WRITE_LOCK.
e.g. To configure a cache using REPEATABLE_READ isolation:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar/component=locking(isolation=REPEATABLE_READ)
----
For a complete list of locking attributes, refer to the https://wildscribe.github.io/[WildFly management model documentation]
==== Expiration
The expiration component configures expiration defaults for cache entries.
Cache entries may be configured to expire after some duration since creation (i.e. lifespan) or since last accessed (i.e. max-idle).
e.g. To configure expiration of entries older than 1 day, or that have not been accessed within the past hour:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar/component=expiration(lifespan=86400000, max-idle=3600000)
----
CAUTION: max-idle based expiration is not generally safe for use with clustered caches, as the meta data of a cache entry is not replicated by cache read operations
For a complete list of expiration attributes, refer to the https://wildscribe.github.io/[WildFly management model documentation]
==== Persistence
An Infinispan cache can optionally load/store cache entries from an external storage.
All cache stores support the following attributes:
fetch-state::
Indicates whether to refresh persistent state from cluster members on cache start.
Does not apply to a local or invalidation cache, nor a shared store.
Default is true.
passivation::
Indicates whether cache entries should only be persisted upon eviction from memory.
Default is true.
preload::
Indicates whether cache entries should be loaded into memory on cache start.
Default is false.
purge::
Indicates whether the cache store should be purged on cache start.
Purge should never be enabled on a shared store.
Default is true.
shared::
Indicates that the same cache store endpoint (e.g. database, data grid, etc.) is used by all members of the cluster.
When using a shared cache store, cache entries are only persisted by the primary owner of a given cache entry.
Default is false.
To remove an existing cache store, you can either use the standard resource remove operation:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar/store=file:remove()
----
{empty}... or by adding the "none" store (which auto-removes any existing store):
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar/store=none:add(){allow-resource-service-restart=true}
----
===== File store
A file store persists cache entries to the local filesystem.
By default, files are stored in a file named "_cache-name_.dat" within a subdirectory named "infinispan/_container-name_" relative to the server's data directory.
e.g. To persist cache entries to $HOME/foo/bar.dat:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar/store=file:add(path=foo, relative-to=user.home)
----
===== JDBC store
A JDBC store persists cache entries to a database.
e.g. To persist cache entries to an H2 database via the ExampleDS data-source:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar/store=jdbc:add(data-source=ExampleDS, dialect=H2)
----
===== Remote cache store
A remote store persists cache entries to a set of remote Infinispan server instances via the HotRod protocol.
[source]
----
/socket-binding-group=standard-sockets/remote-destination-outbound-socket-binding=node1:add(host=server1, port=1000)
/socket-binding-group=standard-sockets/remote-destination-outbound-socket-binding=node2:add(host=server2, port=1000)
/subsystem=infinispan/cache-container=foo/local-cache=bar/store=remote:add(remote-servers=[node1,node2])
----
==== State transfer
The state transfer component defines the behavior for the initial transfer of state from remote caches on cache start.
State transfer is only applicable to distributed and replicated caches.
When configured with a timeout, a cache is only available after its initial state transfer completes.
If state transfer does not complete within the configured timeout, the cache will fail to start.
e.g. To configure a state-transfer timeout of 1 minute:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar/component=state-transfer:add(timeout=60000)
----
Alternatively, state transfer can be configured to be non-blocking, by configuring a timeout of 0.
While this prevents timeouts due to large state transfers, cache operations on the new node will require remote invocations to retrieve the requisite state until state transfer is complete.
e.g. To configure a non-blocking state transfer:
[source]
----
/subsystem=infinispan/cache-container=foo/local-cache=bar/component=state-transfer:add(timeout=0)
----
For a complete list of state-transfer attributes, refer to the https://wildscribe.github.io/[WildFly management model documentation]
<file_sep>/_docs/13/_testsuite/How_to_Add_a_Test_Case.adoc
[[How_to_Add_a_Test_Case]]
== How to Add a Test Case
_(Please don't (re)move - this is a landing page from a Jira link.)_
*Thank you for finding time to contribute to WildFly {wildflyVersion} quality.* +
Covering corner cases found by community users with tests is very
important to increase stability. +
If you're providing a test case to support your bug report, it's very
likely that your bug will be fixed much sooner.
[[create-a-test-case.]]
== 1) Create a test case.
It's quite easy - a simple use case may even consist of one short .java
file.
Check WildFly {wildflyVersion}
https://github.com/wildfly/wildfly/tree/master/testsuite/integration/basic/src/test/java/org/jboss/as/test/integration[test
suite test cases] for examples.
For more information, see <<WildFly_Testsuite_Test_Developer_Guide,WildFly Testsuite Test
Developer Guide>>. Check the requirements for a test to be included in
the testsuite.
Ask for help at WildFly {wildflyVersion} forum or at IRC - #wildfly @ FreeNode.
[[push-your-test-case-to-github-and-create-a-pull-request.]]
== 2) Push your test case to GitHub and create a pull request.
For information on how to create a GitHub account and push your code
therein, see https://community.jboss.org/wiki/HackingOnWildFly[Hacking
on WildFly].
If you're not into Git, send a diff file to JBoss forums, someone might
pick it up.
[[wait-for-the-outcome.]]
== 3) Wait for the outcome.
Your test case will be reviewed and eventually added. It may take few
days.
When something happens, you'll receive a notification e-mail.
<file_sep>/_docs/14/Getting_Started_Guide.adoc
[[Getting_Started_Guide]]
= Getting Started Guide
WildFly team;
:revnumber: {version}
:revdate: {localdate}
:toc: macro
:toclevels: 3
:toc-title: Getting Started Guide
:doctype: book
:icons: font
:source-highlighter: coderay
ifndef::ebook-format[:leveloffset: 1]
(C) 2017 The original authors.
:numbered:
[[getting-started-with-wildfly]]
== Getting Started with WildFly {wildflyVersion}
WildFly {wildflyVersion} is the latest release in a series of JBoss open-source
application server offerings. WildFly {wildflyVersion} is an exceptionally fast,
lightweight and powerful implementation of the Java Enterprise Edition {javaee_version}
Platform specifications. The state-of-the-art architecture built on the
Modular Service Container enables services on-demand when your
application requires them. The table below lists the Java Enterprise
Edition {javaee_version} technologies and the technologies available in WildFly {wildflyVersion}
server configuration profiles.
[cols=",,,,",options="header"]
|=======================================================================
|Java EE {javaee_version} Platform Technology |Java EE {javaee_version} Full Profile |Java EE {javaee_version} Web
Profile |WildFly {wildflyVersion} Full Profile |WildFly {wildflyVersion} Web Profile
|JSR-356: Java API for Web Socket |X |X |X |X
|JSR-374: Java API for JSON Processing 1.1 |X |X |X |X
|JSR-367: Java API for JSON Binding 1.0 |X |X |X |X
|JSR-369: Java Servlet 4.0 |X |X |X |X
|JSR-372: JavaServer Faces 2.3 |X |X |X |X
|JSR-341: Expression Language 3.0 |X |X |X |X
|JSR-245: JavaServer Pages 2.3 |X |X |X |X
|JSR-52: Standard Tag Library for JavaServer Pages (JSTL) 1.2 |X |X |X
|X
|JSR-352: Batch Applications for the Java Platform 1.0 |X |-- |X |--
|JSR-236: Concurrency Utilities for Java EE 1.0 |X |X |X |X
|JSR-365: Contexts and Dependency Injection for Java 2.0 |X |X |X |X
|JSR-330: Dependency Injection for Java 1.0 |X |X |X |X
|JSR-380: Bean Validation 2.0 |X |X |X |X
|JSR-345: Enterprise JavaBeans 3.2 |XCMP 2.0Optional |X(Lite) |XCMP
2.0Not Available |X(Lite)
|JSR-318: Interceptors 1.2 |X |X |X |X
|JSR-322: Java EE Connector Architecture 1.7 |X |-- |X |X
|JSR-338: Java Persistence 2.2 |X |X |X |X
|JSR-250: Common Annotations for the Java Platform 1.3 |X |X |X |X
|JSR-343: Java Message Service API 2.0 |X |-- |X |--
|JSR-907: Java Transaction API 1.2 |X |X |X |X
|JSR-919: JavaMail 1.6 |X |-- |X |X
|JSR-370: Java API for RESTFul Web Services 2.1 |X |X |X |X
|JSR-109: Implementing Enterprise Web Services 1.3 |X |-- |X |--
|JSR-224: Java API for XML-Based Web Services 2.3 |X |X |X |X
|JSR-181: Web Services Metadata for the Java Platform |X |-- |X |--
|JSR-101: Java API for XML-Based RPC 1.1 |Optional |-- |-- |--
|JSR-67: Java APIs for XML Messaging 1.3 |X |-- |X |--
|JSR-93: Java API for XML Registries |Optional |-- |-- |--
|JSR-222: Java Architecture for XML Binding (JAXB) 2.3 |X |X |X |X
|JSR-196: Java Authentication Service Provider Interface for Containers
1.1 |X |-- |X |--
|JSR-115: Java Authorization Contract for Containers 1.5 |X |-- |X |--
|JSR-88: Java EE Application Deployment 1.2 |Optional |-- |-- |--
|JSR-77: J2EE Management 1.1 |X | |X |
|JSR-45: Debugging Support for Other Languages 1.0 |X |X |X |X
|JSR-375: Java EE Security API 1.0 |X |X |X |X
|=======================================================================
Missing HornetQ and JMS?
[WARNING]
The WildFly Web Profile doesn't include JMS (provided by HornetQ) by
default. If you want to use messaging, make sure you start the server
using the "Full Profile" configuration.
This document provides a quick overview on how to download and get
started using WildFly {wildflyVersion} for your application development. For in-depth
content on administrative features, refer to the WildFly {wildflyVersion} Admin Guide.
[[download]]
=== Download
WildFly {wildflyVersion} distributions can be obtained from:
http://www.wildfly.org/downloads/[wildfly.org/downloads]
WildFly {wildflyVersion} provides a single distribution available in zip or tar file
formats.
* *wildfly-{wildflyVersion}.0.0.Final.zip*
* *wildfly-{wildflyVersion}.0.0.Final.tar.gz*
[[requirements]]
=== Requirements
* Java SE 8 or later (we recommend that you use the latest update
available)
[[installation]]
=== Installation
Simply extract your chosen download to the directory of your choice. You
can install WildFly {wildflyVersion} on any operating system that supports the zip or
tar formats. Refer to the Release Notes for additional information
related to the release.
[[wildfly---a-quick-tour]]
=== Wild**Fly** - A Quick Tour
Now that you've downloaded WildFly {wildflyVersion}, the next thing to discuss is the
layout of the distribution and explore the server directory structure,
key configuration files, log files, user deployments and so on. It's
worth familiarizing yourself with the layout so that you'll be able to
find your way around when it comes to deploying your own applications.
[[wildfly-directory-structure]]
==== Wild**Fly** Directory Structure
[cols=",",options="header"]
|=======================================================================
|DIRECTORY |DESCRIPTION
|appclient |Configuration files, deployment content, and writable areas
used by the application client container run from this installation.
|bin |Start up scripts, start up configuration files and various command
line utilities like Vault, add-user and Java diagnostic reportavailable
for Unix and Windows environments
|bin/client |Contains a client jar for use by non-maven based clients.
|docs/schema |XML schema definition files
|docs/examples/configs |Example configuration files representing
specific use cases
|domain |Configuration files, deployment content, and writable areas
used by the domain mode processes run from this installation.
|modules |WildFly is based on a modular classloading architecture.
The various modules used in the server are stored here.
|standalone |Configuration files, deployment content, and writable areas
used by the single standalone server run from this installation.
|welcome-content |Default Welcome Page content
|=======================================================================
[[standalone-directory-structure]]
===== Standalone Directory Structure
In " *_standalone_* " mode each WildFly {wildflyVersion} server instance is an
independent process (similar to previous JBoss AS versions; e.g., 3, 4,
5, or 6). The configuration files, deployment content and writable areas
used by the single standalone server run from a WildFly installation are
found in the following subdirectories under the top level "standalone"
directory:
[cols=",",options="header"]
|=======================================================================
|DIRECTORY |DESCRIPTION
|configuration |Configuration files for the standalone server that runs
off of this installation. All configuration information for the running
server is located here and is the single place for configuration
modifications for the standalone server.
|data |Persistent information written by the server to survive a restart
of the server
|deployments |End user deployment content can be placed in this
directory for automatic detection and deployment of that content into
the server's runtime.NOTE: The server's management API is recommended
for installing deployment content. File system based deployment scanning
capabilities remain for developer convenience.
|lib/ext |Location for installed library jars referenced by applications
using the Extension-List mechanism
|log |standalone server log files
|tmp |location for temporary files written by the server
|tmp/auth |Special location used to exchange authentication tokens with
local clients so they can confirm that they are local to the running AS
process.
|=======================================================================
[[domain-directory-structure]]
===== Domain Directory Structure
A key feature of WildFly {wildflyVersion} is the managing multiple servers from a
single control point. A collection of multiple servers are referred to
as a " *_domain_* ". Domains can span multiple physical (or virtual)
machines with all WildFly instances on a given host under the control of
a Host Controller process. The Host Controllers interact with the Domain
Controller to control the lifecycle of the WildFly instances running on
that host and to assist the Domain Controller in managing them. The
configuration files, deployment content and writeable areas used by
domain mode processes run from a WildFly installation are found in the
following subdirectories under the top level "domain" directory:
[cols=",",options="header"]
|=======================================================================
|DIRECTORY |DESCRIPTION
|configuration |Configuration files for the domain and for the Host
Controller and any servers running off of this installation. All
configuration information for the servers managed wtihin the domain is
located here and is the single place for configuration information.
|content |an internal working area for the Host Controller that controls
this installation. This is where it internally stores deployment
content. This directory is not meant to be manipulated by end users.Note
that "domain" mode does not support deploying content based on scanning
a file system.
|lib/ext |Location for installed library jars referenced by applications
using the Extension-List mechanism
|log |Location where the Host Controller process writes its logs. The
Process Controller, a small lightweight process that actually spawns the
other Host Controller process and any Application Server processes also
writes a log here.
|servers |Writable area used by each Application Server instance that
runs from this installation. Each Application Server instance will have
its own subdirectory, created when the server is first started. In each
server's subdirectory there will be the following subdirectories:data --
information written by the server that needs to survive a restart of the
serverlog -- the server's log filestmp -- location for temporary files
written by the server
|tmp |location for temporary files written by the server
|tmp/auth |Special location used to exchange authentication tokens with
local clients so they can confirm that they are local to the running AS
process.
|=======================================================================
[[wildfly-10-configurations]]
==== Wild**Fly** {wildflyVersion} Configurations
[[standalone-server-configurations]]
===== Standalone Server Configurations
* standalone.xml (_default_)
** Java Enterprise Edition {javaee_version} web profile certified configuration with
the required technologies plus those noted in the table above.
* standalone-ha.xml
** Java Enterprise Edition {javaee_version} web profile certified configuration with
high availability
* standalone-full.xml
** Java Enterprise Edition {javaee_version} full profile certified configuration
including all the required EE {javaee_version} technologies
* standalone-full-ha.xml
** Java Enterprise Edition {javaee_version} full profile certified configuration with
high availability
[[domain-server-configurations]]
===== Domain Server Configurations
* domain.xml
** Java Enterprise Edition {javaee_version} full and web profiles available with or
without high availability
Important to note is that the *_domain_* and *_standalone_* modes
determine how the servers are managed not what capabilities they
provide.
[[starting-wildfly-10]]
==== Starting WildFly {wildflyVersion}
To start WildFly {wildflyVersion} using the default web profile configuration in "
_standalone_" mode, change directory to $JBOSS_HOME/bin.
[source]
----
./standalone.sh
----
To start the default web profile configuration using domain management
capabilities,
[source]
----
./domain.sh
----
[[starting-wildfly-with-an-alternate-configuration]]
==== Starting WildFly with an Alternate Configuration
If you choose to start your server with one of the other provided
configurations, they can be accessed by passing the --server-config
argument with the server-config file to be used.
To use the full profile with clustering capabilities, use the following
syntax from $JBOSS_HOME/bin:
[source, bash]
----
./standalone.sh --server-config=standalone-full-ha.xml
----
Similarly to start an alternate configuration in _domain_ mode:
[source, bash]
----
./domain.sh --domain-config=my-domain-configuration.xml
----
Alternatively, you can create your own selecting the additional
subsystems you want to add, remove, or modify.
[[test-your-installation]]
===== Test Your Installation
After executing one of the above commands, you should see output similar
to what's shown below.
[source, bash]
----
=========================================================================
JBoss Bootstrap Environment
JBOSS_HOME: /opt/wildfly-10.0.0.Final
JAVA: java
JAVA_OPTS: -server -Xms64m -Xmx512m -XX:MetaspaceSize=96M -XX:MaxMetaspaceSize=256m -Djava.net.preferIPv4Stack=true -Djboss.modules.system.pkgs=com.yourkit,org.jboss.byteman -Djava.awt.headless=true
=========================================================================
11:46:11,161 INFO [org.jboss.modules] (main) JBoss Modules version 1.5.1.Final
11:46:11,331 INFO [org.jboss.msc] (main) JBoss MSC version 1.2.6.Final
11:46:11,391 INFO [org.jboss.as] (MSC service thread 1-6) WFLYSRV0049: WildFly Full 10.0.0.Final (WildFly Core 2.0.10.Final) starting
<snip>
11:46:14,300 INFO [org.jboss.as] (Controller Boot Thread) WFLYSRV0025: WildFly Full 10.0.0.Final (WildFly Core 2.0.10.Final) started in 1909ms - Started 267 of 553 services (371 services are lazy, passive or on-demand)
----
As with previous WildFly releases, you can point your browser to
*_http://localhost:8080_* (if using the default configured http port)
which brings you to the Welcome Screen:
image:images/wildfly.png[wildfly.png]
From here you can access links to the WildFly community documentation
set, stay up-to-date on the latest project information, have a
discussion in the user forum and access the enhanced web-based
Administration Console. Or, if you uncover a defect while using WildFly,
report an issue to inform us (attached patches will be reviewed). This
landing page is recommended for convenient access to information about
WildFly {wildflyVersion} but can easily be replaced with your own if desired.
[[managing-your-wildfly-10]]
==== Managing your WildFly {wildflyVersion}
WildFly {wildflyVersion} offers two administrative mechanisms for managing your
running instance:
* web-based Administration Console
* command-line interface
===== Authentication
By default WildFly {wildflyVersion} is now distributed with security enabled for the
management interfaces, this means that before you connect using the
administration console or remotely using the CLI you will need to add a
new user, this can be achieved simply by using the _add-user.sh_ script
in the bin folder.
After starting the script you will be guided through the process to add
a new user: -
[source, java]
----
./add-user.sh
What type of user do you wish to add?
a) Management User (mgmt-users.properties)
b) Application User (application-users.properties)
(a):
----
In this case a new user is being added for the purpose of managing the
servers so select option a.
You will then be prompted to enter the details of the new user being
added: -
[source, bash]
----
Enter the details of the new user to add.
Realm (ManagementRealm) :
Username :
Password :
Re-enter Password :
----
It is important to leave the name of the realm as 'ManagementRealm' as
this needs to match the name used in the server's configuration, for the
remaining fields enter the new username, password and password
confirmation.
Provided there are no errors in the values entered you will then be
asked to confirm that you want to add the user, the user will be written
to the properties files used for authentication and a confirmation
message will be displayed.
The modified time of the properties files are inspected at the time of
authentication and the files reloaded if they have changed, for this
reason you do not need to re-start the server after adding a new user.
[[administration-console]]
===== Administration Console
To access the web-based Administration Console, simply follow the link
from the Welcome Screen. To directly access the Management Console,
point your browser at:
*_http://localhost:9990/console_*
NOTE: port 9990 is the default port configured.
[source, xml]
----
<management-interfaces>
<native-interface security-realm="ManagementRealm">
<socket-binding native="management-native"/>
</native-interface>
<http-interface security-realm="ManagementRealm">
<socket-binding http="management-http"/>
</http-interface>
</management-interfaces>
----
If you modify the _management-http_ socket binding in your running
configuration: adjust the above command accordingly. If such
modifications are made, then the link from the Welcome Screen will also
be inaccessible.
If you have not yet added at least one management user an error page
will be displayed asking you to add a new user, after a user has been
added you can click on the 'Try Again' link at the bottom of the error
page to try connecting to the administration console again.
[[command-line-interface]]
===== Command-Line Interface
If you prefer to manage your server from the command line (or batching),
the _jboss-cli.sh_ script provides the same capabilities available via
the web-based UI. This script is accessed from $JBOSS_HOME/bin
directory; e.g.,
[source, bash]
----
$JBOSS_HOME/bin/jboss-cli.sh --connect
Connected to standalone controller at localhost:9990
----
Notice if no host or port information provided, it will default to
localhost:9990.
When running locally to the WildFly process the CLI will silently
authenticate against the server by exchanging tokens on the file system,
the purpose of this exchange is to verify that the client does have
access to the local file system. If the CLI is connecting to a remote
WildFly installation then you will be prompted to enter the username and
password of a user already added to the realm.
Once connected you can add, modify, remove resources and deploy or
undeploy applications. For a complete list of commands and command
syntax, type *_help_* once connected.
[[modifying-the-example-datasource]]
==== Modifying the Example DataSource
As with previous JBoss application server releases, a default data
source, *_ExampleDS_* , is configured using the embedded H2 database for
developer convenience. There are two ways to define datasource
configurations:
1. as a module
2. as a deployment
In the provided configurations, H2 is configured as a module. The module
is located in the $JBOSS_HOME/modules/com/h2database/h2 directory. The
H2 datasource configuration is shown below.
[source, xml]
----
<subsystem xmlns="urn:jboss:domain:datasources:1.0">
<datasources>
<datasource jndi-name="java:jboss/datasources/ExampleDS" pool-name="ExampleDS">
<connection-url>jdbc:h2:mem:test;DB_CLOSE_DELAY=-1</connection-url>
<driver>h2</driver>
<pool>
<min-pool-size>10</min-pool-size>
<max-pool-size>20</max-pool-size>
<prefill>true</prefill>
</pool>
<security>
<user-name>sa</user-name>
<password>sa</password>
</security>
</datasource>
<xa-datasource jndi-name="java:jboss/datasources/ExampleXADS" pool-name="ExampleXADS">
<driver>h2</driver>
<xa-datasource-property name="URL">jdbc:h2:mem:test</xa-datasource-property>
<xa-pool>
<min-pool-size>10</min-pool-size>
<max-pool-size>20</max-pool-size>
<prefill>true</prefill>
</xa-pool>
<security>
<user-name>sa</user-name>
<password><PASSWORD>>
</security>
</xa-datasource>
<drivers>
<driver name="h2" module="com.h2database.h2">
<xa-datasource-class>org.h2.jdbcx.JdbcDataSource</xa-datasource-class>
</driver>
</drivers>
</datasources>
</subsystem>
----
The datasource subsystem is provided by the
http://www.jboss.org/ironjacamar[IronJacamar] project. For a detailed
description of the available configuration properties, please consult
the project documentation.
* IronJacamar homepage: http://www.jboss.org/ironjacamar
* Project Documentation: http://www.jboss.org/ironjacamar/docs
* Schema description:
http://docs.jboss.org/ironjacamar/userguide/1.0/en-US/html/deployment.html#deployingds_descriptor
[[configure-logging-in-wildfly]]
===== Configure Logging in WildFly
WildFly logging can be configured with the web console or the command
line interface. You can get more detail on the link:Admin_Guide{outfilesuffix}#Logging[Logging
Configuration] page.
Turn on debugging for a specific category with CLI:
[source, ruby]
----
/subsystem=logging/logger=org.jboss.as:add(level=DEBUG)
----
By default the `server.log` is configured to include all levels in it's
log output. In the above example we changed the console to also display
debug messages.
<file_sep>/_docs/13/_developer-guide/Migrate_Seam_2_Booking_EAR_Binaries_-_Step_by_Step.adoc
[[Migrate_Seam_2_Booking_EAR_Binaries_-_Step_by_Step]]
== Seam 2 Booking Application - Migration of Binaries from EAP5.1 to
WildFly
=========================================================================
This is a step-by-step how-to guide on porting the Seam Booking
application binaries from EAP5.1 to WildFly {wildflyVersion}. Although there are better
approaches for migrating applications, the purpose of this document is
to show the types of issues you might encounter when migrating an
application and how to debug and resolve those issues.
For this example, the application EAR is deployed to the
JBOSS_HOME/standalone/deployments directory with no changes other than
extracting the archives so we can modify the XML files contained within
them.
[[step-1-build-and-deploy-the-eap5.1-version-of-the-seam-booking-application]]
== Step 1: Build and deploy the EAP5.1 version of the Seam Booking
application
1. Build the EAR
+
[source, java]
----
cd /EAP5_HOME/jboss-eap5.1/seam/examples/booking
~/tools/apache-ant-1.8.2/bin/ant explode
----
* Copy the EAR to the JBOSS_HOME deployments directory:
+
[source, java]
----
cp -r EAP5_HOME/jboss-eap-5.1/seam/examples/booking/exploded-archives/jboss-seam-booking.ear AS7_HOME/standalone/deployments/
cp -r EAP5_HOME/jboss-eap-5.1/seam/examples/booking/exploded-archives/jboss-seam-booking.war AS7_HOME/standalone/deployments/jboss-seam.ear
cp -r EAP5_HOME/jboss-eap-5.1/seam/examples/booking/exploded-archives/jboss-seam-booking.jar AS7_HOME/standalone/deployments/jboss-seam.ear
----
* Start the WildFly server and check the log. You will see:
+
[source, java]
----
INFO [org.jboss.as.deployment] (DeploymentScanner-threads - 1) Found jboss-seam-booking.ear in deployment directory. To trigger deployment create a file called jboss-seam-booking.ear.dodeploy
----
* Create an empty file with the name `jboss-seam-booking.ear.dodeploy`
and copy it into the deployments directory. In the log, you will now see
the following, indicating that it is deploying:
+
[source, java]
----
INFO [org.jboss.as.server.deployment] (MSC service thread 1-1) Starting deployment of "jboss-seam-booking.ear"
INFO [org.jboss.as.server.deployment] (MSC service thread 1-3) Starting deployment of "jboss-seam-booking.jar"
INFO [org.jboss.as.server.deployment] (MSC service thread 1-6) Starting deployment of "jboss-seam.jar"
INFO [org.jboss.as.server.deployment] (MSC service thread 1-2) Starting deployment of "jboss-seam-booking.war"
----
* At this point, you will first encounter your first deployment error.
In the next section, we will step through each issue and how to debug
and resolve it.
+
[[step-2-debug-and-resolve-deployment-errors-and-exceptions]]
== Step 2: Debug and resolve deployment errors and exceptions
[[first-issue-java.lang.classnotfoundexception-javax.faces.facesexception]]
=== First Issue: java.lang.ClassNotFoundException:
javax.faces.FacesException
When you deploy the application, the log contains the following error:
[source, java]
----
ERROR \[org.jboss.msc.service.fail\] (MSC service thread 1-1) MSC00001: Failed to start service jboss.deployment.subunit."jboss-seam-booking.ear"."jboss-seam-booking.war".POST_MODULE:
org.jboss.msc.service.StartException in service jboss.deployment.subunit."jboss-seam-booking.ear"."jboss-seam-booking.war".POST_MODULE:
Failed to process phase POST_MODULE of subdeployment "jboss-seam-booking.war" of deployment "jboss-seam-booking.ear"
(.. additional logs removed ...)
Caused by: java.lang.ClassNotFoundException: javax.faces.FacesException from \[Module "deployment.jboss-seam-booking.ear:main" from Service Module Loader\]
at org.jboss.modules.ModuleClassLoader.findClass(ModuleClassLoader.java:191)
----
[[what-it-means]]
==== What it means:
The ClassNotFoundException indicates a missing dependency. In this case,
it can not find the class `javax.faces.FacesException` and you need to
explicitly add the dependency.
[[how-to-resolve-it]]
==== How to resolve it:
Find the module name for that class in the `AS7_HOME/modules` directory
by looking for a path that matches the missing class. In this case, you
will find 2 modules that match:
[source, java]
----
javax/faces/api/main
javax/faces/api/1.2
----
Both modules have the same module name: "javax.faces.api" but one in the
main directory is for JSF 2.0 and the one located in the 1.2 directory
is for JSF 1.2. If there was only one module available, we could simply
create a `MANIFEST.MF` file and added the module dependency. But in this
case, we want to use the JSF 1.2 version and not the 2.0 version in
main, so we need to be able to specify one and exclude the other. To do
this, we create a `jboss-deployment-structure.xml` file in the EAR
`META-INF/` directory that contains the following data:
[source, java]
----
<jboss-deployment-structure xmlns="urn:jboss:deployment-structure:1.0">
<deployment>
<dependencies>
<module name="javax.faces.api" slot="1.2" export="true"/>
</dependencies>
</deployment>
<sub-deployment name="jboss-seam-booking.war">
<exclusions>
<module name="javax.faces.api" slot="main"/>
</exclusions>
<dependencies>
<module name="javax.faces.api" slot="1.2"/>
</dependencies>
</sub-deployment>
</jboss-deployment-structure>
----
+
In the "deployment" section, we add the dependency for the
`javax.faces.api` for the JSF 1.2 module. We also add the dependency for
the JSF 1.2 module in the sub-deployment section for the WAR and exclude
the module for JSF 2.0.
+
Redeploy the application by deleting the
`standalone/deployments/jboss-seam-booking.ear.failed` file and creating
a blank `jboss-seam-booking.ear.dodeploy` file in the same directory.
+
[[next-issue-java.lang.classnotfoundexception-org.apache.commons.logging.log]]
=== Next Issue: java.lang.ClassNotFoundException:
org.apache.commons.logging.Log
When you deploy the application, the log contains the following error:
[source, java]
----
ERROR [org.jboss.msc.service.fail] (MSC service thread 1-8) MSC00001: Failed to start service jboss.deployment.unit."jboss-seam-booking.ear".INSTALL:
org.jboss.msc.service.StartException in service jboss.deployment.unit."jboss-seam-booking.ear".INSTALL:
Failed to process phase INSTALL of deployment "jboss-seam-booking.ear"
(.. additional logs removed ...)
Caused by: java.lang.ClassNotFoundException: org.apache.commons.logging.Log from [Module "deployment.jboss-seam-booking.ear.jboss-seam-booking.war:main" from Service Module Loader]
----
+
[[what-it-means-1]]
==== What it means:
The ClassNotFoundException indicates a missing dependency. In this case,
it can not find the class `org.apache.commons.logging.Log` and you need
to explicitly add the dependency.
+
[[how-to-resolve-it-1]]
==== How to resolve it:
Find the module name for that class in the `JBOSS_HOME/modules/`
directory by looking for a path that matches the missing class. In this
case, you will find one module that matches the path
`org/apache/commons/logging/`. The module name is
"org.apache.commons.logging".
Modify the `jboss-deployment-structure.xml` to add the module dependency
to the deployment section of the file.
[source, java]
----
<module name="org.apache.commons.logging" export="true"/>
----
+
The `jboss-deployment-structure.xml` should now look like this:
+
[source, java]
----
<jboss-deployment-structure xmlns="urn:jboss:deployment-structure:1.0">
<deployment>
<dependencies>
<module name="javax.faces.api" slot="1.2" export="true"/>
<module name="org.apache.commons.logging" export="true"/>
</dependencies>
</deployment>
<sub-deployment name="jboss-seam-booking.war">
<exclusions>
<module name="javax.faces.api" slot="main"/>
</exclusions>
<dependencies>
<module name="javax.faces.api" slot="1.2"/>
</dependencies>
</sub-deployment>
</jboss-deployment-structure>
----
+
Redeploy the application by deleting the
`standalone/deployments/jboss-seam-booking.ear.failed` file and creating
a blank `jboss-seam-booking.ear.dodeploy file` in the same directory.
+
[[next-issue-java.lang.classnotfoundexception-org.dom4j.documentexception]]
=== Next Issue: java.lang.ClassNotFoundException:
org.dom4j.DocumentException
When you deploy the application, the log contains the following error:
[source, java]
----
ERROR [org.apache.catalina.core.ContainerBase.[jboss.web].[default-host].[/seam-booking]] (MSC service thread 1-3) Exception sending context initialized event to listener instance of class org.jboss.seam.servlet.SeamListener: java.lang.NoClassDefFoundError: org/dom4j/DocumentException
(... additional logs removed ...)
Caused by: java.lang.ClassNotFoundException: org.dom4j.DocumentException from [Module "deployment.jboss-seam-booking.ear.jboss-seam.jar:main" from Service Module Loader]
----
+
[[what-it-means-2]]
==== What it means:
Again, the ClassNotFoundException indicates a missing dependency. In
this case, it can not find the class `org.dom4j.DocumentException`.
+
[[how-to-resolve-it-2]]
==== How to resolve it:
Find the module name in the `JBOSS_HOME/modules/` directory by looking
for the `org/dom4j/DocumentException`. The module name is "org.dom4j".
Modify the `jboss-deployment-structure.xml` to add the module dependency
to the deployment section of the file.
[source, java]
----
<module name="org.dom4j" export="true"/>
----
+
The `jboss-deployment-structure.xml` file should now look like this:
+
[source, java]
----
<jboss-deployment-structure xmlns="urn:jboss:deployment-structure:1.0">
<deployment>
<dependencies>
<module name="javax.faces.api" slot="1.2" export="true"/>
<module name="org.apache.commons.logging" export="true"/>
<module name="org.dom4j" export="true"/>
</dependencies>
</deployment>
<sub-deployment name="jboss-seam-booking.war">
<exclusions>
<module name="javax.faces.api" slot="main"/>
</exclusions>
<dependencies>
<module name="javax.faces.api" slot="1.2"/>
</dependencies>
</sub-deployment>
</jboss-deployment-structure>
----
+
Redeploy the application by deleting the
`standalone/deployments/jboss-seam-booking.ear.failed` file and creating
a blank `jboss-seam-booking.ear.dodeploy file` in the same directory.
+
[[next-issue-java.lang.classnotfoundexception-org.hibernate.validator.invalidvalue]]
=== Next Issue: java.lang.ClassNotFoundException:
org.hibernate.validator.InvalidValue
When you deploy the application, the log contains the following error:
[source, java]
----
ERROR [org.apache.catalina.core.ContainerBase.[jboss.web].[default-host].[/seam-booking]] (MSC service thread 1-6) Exception sending context initialized event to listener instance of class org.jboss.seam.servlet.SeamListener: java.lang.RuntimeException: Could not create Component: org.jboss.seam.international.statusMessages
(... additional logs removed ...)
Caused by: java.lang.ClassNotFoundException: org.hibernate.validator.InvalidValue from [Module "deployment.jboss-seam-booking.ear.jboss-seam.jar:main" from Service Module Loader]
----
+
[[what-it-means-3]]
==== What it means:
Again, the ClassNotFoundException indicates a missing dependency. In
this case, it can not find the class
`org.hibernate.validator.InvalidValue`.
+
[[how-to-resolve-it-3]]
==== How to resolve it:
There is a module "org.hibernate.validator", but the JAR does not
contain the `org.hibernate.validator.InvalidValue` class, so adding the
module dependency will not resolve this issue.
In this case, the JAR containing the class was part of the EAP 5.1
deployment. We will look for the JAR that contains the missing class in
the `EAP5_HOME/jboss-eap-5.1/seam/lib/` directory. To do this, open a
console and type the following:
[source, java]
----
cd EAP5_HOME/jboss-eap-5.1/seam/lib
grep 'org.hibernate.validator.InvalidValue' `find . -name '*.jar'`
----
+
The result shows:
+
[source, java]
----
Binary file ./hibernate-validator.jar matches
Binary file ./test/hibernate-all.jar matches
----
+
In this case, we need to copy the `hibernate-validator.jar` to the
`jboss-seam-booking.ear/lib/` directory:
+
[source, java]
----
cp EAP5_HOME/jboss-eap-5.1/seam/lib/hibernate-validator.jar jboss-seam-booking.ear/lib
----
+
Redeploy the application by deleting the
`standalone/deployments/jboss-seam-booking.ear.failed` file and creating
a blank `jboss-seam-booking.ear.dodeploy file` in the same directory.
+
[[next-issue-java.lang.instantiationexception-org.jboss.seam.jsf.seamapplicationfactory]]
=== Next Issue: java.lang.InstantiationException:
org.jboss.seam.jsf.SeamApplicationFactory
When you deploy the application, the log contains the following error:
[source, java]
----
INFO [javax.enterprise.resource.webcontainer.jsf.config] (MSC service thread 1-7) Unsanitized stacktrace from failed start...: com.sun.faces.config.ConfigurationException: Factory 'javax.faces.application.ApplicationFactory' was not configured properly.
at com.sun.faces.config.processor.FactoryConfigProcessor.verifyFactoriesExist(FactoryConfigProcessor.java:296) [jsf-impl-2.0.4-b09-jbossorg-4.jar:2.0.4-b09-jbossorg-4]
(... additional logs removed ...)
Caused by: javax.faces.FacesException: org.jboss.seam.jsf.SeamApplicationFactory
at javax.faces.FactoryFinder.getImplGivenPreviousImpl(FactoryFinder.java:606) [jsf-api-1.2_13.jar:1.2_13-b01-FCS]
(... additional logs removed ...)
at com.sun.faces.config.processor.FactoryConfigProcessor.verifyFactoriesExist(FactoryConfigProcessor.java:294) [jsf-impl-2.0.4-b09-jbossorg-4.jar:2.0.4-b09-jbossorg-4]
... 11 more
Caused by: java.lang.InstantiationException: org.jboss.seam.jsf.SeamApplicationFactory
at java.lang.Class.newInstance0(Class.java:340) [:1.6.0_25]
at java.lang.Class.newInstance(Class.java:308) [:1.6.0_25]
at javax.faces.FactoryFinder.getImplGivenPreviousImpl(FactoryFinder.java:604) [jsf-api-1.2_13.jar:1.2_13-b01-FCS]
... 16 more
----
+
[[what-it-means-4]]
==== What it means:
The com.sun.faces.config.ConfigurationException and
java.lang.InstantiationException indicate a dependency issue. In this
case, it is not as obvious.
+
[[how-to-resolve-it-4]]
==== How to resolve it:
We need to find the module that contains the com.sun.faces classes.
While there is no com.sun.faces module, there are are two
com.sun.jsf-impl modules. A quick check of the jsf-impl-1.2_13.jar in
the 1.2 directory shows it contains the com.sun.faces classes.
As we did with the javax.faces.FacesException ClassNotFoundException, we
want to use the JSF 1.2 version and not the JSF 2.0 version in main, so
we need to be able to specify one and exclude the other. We need to
modify the jboss-deployment-structure.xml to add the module dependency
to the deployment section of the file. We also need to add it to the WAR
subdeployment and exclude the JSF 2.0 module. The file should now look
like this:
[source, java]
----
<jboss-deployment-structure xmlns="urn:jboss:deployment-structure:1.0">
<deployment>
<dependencies>
<module name="javax.faces.api" slot="1.2" export="true"/>
<module name="com.sun.jsf-impl" slot="1.2" export="true"/>
<module name="org.apache.commons.logging" export="true"/>
<module name="org.dom4j" export="true"/>
</dependencies>
</deployment>
<sub-deployment name="jboss-seam-booking.war">
<exclusions>
<module name="javax.faces.api" slot="main"/>
<module name="com.sun.jsf-impl" slot="main"/>
</exclusions>
<dependencies>
<module name="javax.faces.api" slot="1.2"/>
<module name="com.sun.jsf-impl" slot="1.2"/>
</dependencies>
</sub-deployment>
</jboss-deployment-structure>
----
+
Redeploy the application by deleting the
`standalone/deployments/jboss-seam-booking.ear.failed` file and creating
a blank `jboss-seam-booking.ear.dodeploy file` in the same directory.
+
[[next-issue-java.lang.classnotfoundexception-org.apache.commons.collections.arraystack]]
=== Next Issue: java.lang.ClassNotFoundException:
org.apache.commons.collections.ArrayStack
When you deploy the application, the log contains the following error:
[source, java]
----
ERROR [org.apache.catalina.core.ContainerBase.[jboss.web].[default-host].[/seam-booking]] (MSC service thread 1-1) Exception sending context initialized event to listener instance of class com.sun.faces.config.ConfigureListener: java.lang.RuntimeException: com.sun.faces.config.ConfigurationException: CONFIGURATION FAILED! org.apache.commons.collections.ArrayStack from [Module "deployment.jboss-seam-booking.ear:main" from Service Module Loader]
(... additional logs removed ...)
Caused by: java.lang.ClassNotFoundException: org.apache.commons.collections.ArrayStack from [Module "deployment.jboss-seam-booking.ear:main" from Service Module Loader]
----
+
[[what-it-means-5]]
==== What it means:
Again, the ClassNotFoundException indicates a missing dependency. In
this case, it can not find the class
`org.apache.commons.collections.ArrayStack`.
+
[[how-to-resolve-it-5]]
==== How to resolve it:
Find the module name in the `JBOSS_HOME/modules/` directory by looking
for the `org/apache/commons/collections` path. The module name is
"org.apache.commons.collections".
Modify the `jboss-deployment-structure.xml` to add the module dependency
to the deployment section of the file.
[source, java]
----
<module name="org.apache.commons.collections" export="true"/>
----
+
The `jboss-deployment-structure.xml` file should now look like this:
+
[source, java]
----
<jboss-deployment-structure xmlns="urn:jboss:deployment-structure:1.0">
<deployment>
<dependencies>
<module name="javax.faces.api" slot="1.2" export="true"/>
<module name="com.sun.jsf-impl" slot="1.2" export="true"/>
<module name="org.apache.commons.logging" export="true"/>
<module name="org.dom4j" export="true"/>
<module name="org.apache.commons.collections" export="true"/>
</dependencies>
</deployment>
<sub-deployment name="jboss-seam-booking.war">
<exclusions>
<module name="javax.faces.api" slot="main"/>
<module name="com.sun.jsf-impl" slot="main"/>
</exclusions>
<dependencies>
<module name="javax.faces.api" slot="1.2"/>
<module name="com.sun.jsf-impl" slot="1.2"/>
</dependencies>
</sub-deployment>
</jboss-deployment-structure>
----
+
Redeploy the application by deleting the
`standalone/deployments/jboss-seam-booking.ear.failed` file and creating
a blank `jboss-seam-booking.ear.dodeploy file` in the same directory.
+
[[next-issue-services-with-missingunavailable-dependencies]]
=== Next Issue: Services with missing/unavailable dependencies
When you deploy the application, the log contains the following error:
[source, java]
----
ERROR [org.jboss.as.deployment] (DeploymentScanner-threads - 2) {"Composite operation failed and was rolled back. Steps that failed:" => {"Operation step-2" => {"Services with missing/unavailable dependencies" => ["jboss.deployment.subunit.\"jboss-seam-booking.ear\".\"jboss-seam-booking.jar\".component.AuthenticatorAction.START missing [ jboss.naming.context.java.comp.jboss-seam-booking.\"jboss-seam-booking.jar\".AuthenticatorAction.\"env/org.jboss.seam.example.booking.AuthenticatorAction/em\" ]","jboss.deployment.subunit.\"jboss-seam-booking.ear\".\"jboss-seam-booking.jar\".component.HotelSearchingAction.START missing [ jboss.naming.context.java.comp.jboss-seam-booking.\"jboss-seam-booking.jar\".HotelSearchingAction.\"env/org.jboss.seam.example.booking.HotelSearchingAction/em\" ]","
<... additional logs removed ...>
"jboss.deployment.subunit.\"jboss-seam-booking.ear\".\"jboss-seam-booking.jar\".component.BookingListAction.START missing [ jboss.naming.context.java.comp.jboss-seam-booking.\"jboss-seam-booking.jar\".BookingListAction.\"env/org.jboss.seam.example.booking.BookingListAction/em\" ]","jboss.persistenceunit.\"jboss-seam-booking.ear/jboss-seam-booking.jar#bookingDatabase\" missing [ jboss.naming.context.java.bookingDatasource ]"]}}}
----
+
[[what-it-means-6]]
==== What it means:
When you get a "Services with missing/unavailable dependencies" error,
look that the text within the brackets after "missing".
In this case you see:
[source, java]
----
missing [ jboss.naming.context.java.comp.jboss-seam-booking.\"jboss-seam-booking.jar\".AuthenticatorAction.\"env/org.jboss.seam.example.booking.AuthenticatorAction/em\" ]
----
+
The "/em" indicates an Entity Manager and datasource issue.
+
[[how-to-resolve-it-6]]
==== How to resolve it:
In WildFly {wildflyVersion}, datasource configuration has changed and needs to be
defined in the `standalone/configuration/standalone.xml` file. Since
WildFly ships with an example database that is already defined in the
standalone.xml file, we will modify the `persistence.xml` file to use
that example database. Looking in the `standalone.xml` file, you can see
that the jndi-name for the example database is
"java:jboss/datasources/ExampleDS".
Modify the `jboss-seam-booking.jar/META-INF/persistence.xml` file to
comment the existing jta-data-source element and replace it as follows:
[source, java]
----
<!-- <jta-data-source>java:/bookingDatasource</jta-data-source> -->
<jta-data-source>java:jboss/datasources/ExampleDS</jta-data-source>
----
+
Redeploy the application by deleting the
`standalone/deployments/jboss-seam-booking.ear.failed` file and creating
a blank `jboss-seam-booking.ear.dodeploy file` in the same directory.
+
[[next-issue-java.lang.classnotfoundexception-org.hibernate.cache.hashtablecacheprovider]]
=== Next Issue: java.lang.ClassNotFoundException:
org.hibernate.cache.HashtableCacheProvider
When you deploy the application, the log contains the following error:
[source, java]
----
ERROR [org.jboss.msc.service.fail] (MSC service thread 1-4) MSC00001: Failed to start service jboss.persistenceunit."jboss-seam-booking.ear/jboss-seam-booking.jar#bookingDatabase": org.jboss.msc.service.StartException in service jboss.persistenceunit."jboss-seam-booking.ear/jboss-seam-booking.jar#bookingDatabase": Failed to start service
at org.jboss.msc.service.ServiceControllerImpl$StartTask.run(ServiceControllerImpl.java:1786)
(... log messages removed ...)
Caused by: javax.persistence.PersistenceException: [PersistenceUnit: bookingDatabase] Unable to build EntityManagerFactory
at org.hibernate.ejb.Ejb3Configuration.buildEntityManagerFactory(Ejb3Configuration.java:903)
{... log messages removed ...)
Caused by: org.hibernate.HibernateException: could not instantiate RegionFactory [org.hibernate.cache.internal.bridge.RegionFactoryCacheProviderBridge]
at org.hibernate.cfg.SettingsFactory.createRegionFactory(SettingsFactory.java:355)
(... log messages removed ...)
Caused by: java.lang.reflect.InvocationTargetException
at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method) [:1.6.0_25]
(... log messages removed ...)
Caused by: org.hibernate.cache.CacheException: could not instantiate CacheProvider [org.hibernate.cache.HashtableCacheProvider]
at org.hibernate.cache.internal.bridge.RegionFactoryCacheProviderBridge.<init>(RegionFactoryCacheProviderBridge.java:68)
... 20 more
Caused by: java.lang.ClassNotFoundException: org.hibernate.cache.HashtableCacheProvider from [Module "org.hibernate:main" from local module loader @12a3793 (roots: /home/sgilda/tools/jboss7/modules)]
at org.jboss.modules.ModuleClassLoader.findClass(ModuleClassLoader.java:191)
(... log messages removed ...)
----
+
[[what-it-means-7]]
==== What it means:
The ClassNotFoundException indicates a missing dependency. In this case,
it can not find the class org.hibernate.cache.HashtableCacheProvider.
+
[[how-to-resolve-it-7]]
==== How to resolve it:
There is no module for "org.hibernate.cache". In this case, the JAR
containing the class was part of the EAP 5.1 deployment. We will look
for the JAR that contains the missing class in the
`EAP5_HOME/jboss-eap-5.1/seam/lib/` directory.
To do this, open a console and type the following:
[source, java]
----
cd EAP5_HOME/jboss-eap-5.1/seam/lib
grep 'org.hibernate.validator.InvalidValue' `find . -name '*.jar'`
----
+
The result shows:
+
[source, java]
----
Binary file ./hibernate-core.jar matches
Binary file ./test/hibernate-all.jar matches
----
+
In this case, we need to copy the `hibernate-core.jar` to the
`jboss-seam-booking.ear/lib/` directory:
+
[source, java]
----
cp EAP5_HOME/jboss-eap-5.1/seam/lib/hibernate-core.jar jboss-seam-booking.ear/lib
----
+
Redeploy the application by deleting the
`standalone/deployments/jboss-seam-booking.ear.failed` file and creating
a blank `jboss-seam-booking.ear.dodeploy file` in the same directory.
+
[[next-issue-java.lang.classcastexception-org.hibernate.cache.hashtablecacheprovider]]
=== Next Issue: java.lang.ClassCastException:
org.hibernate.cache.HashtableCacheProvider
When you deploy the application, the log contains the following error:
[source, java]
----
ERROR [org.jboss.msc.service.fail] (MSC service thread 1-2) MSC00001: Failed to start service jboss.persistenceunit."jboss-seam-booking.ear/jboss-seam-booking.jar#bookingDatabase": org.jboss.msc.service.StartException in service jboss.persistenceunit."jboss-seam-booking.ear/jboss-seam-booking.jar#bookingDatabase": Failed to start service
at org.jboss.msc.service.ServiceControllerImpl$StartTask.run(ServiceControllerImpl.java:1786)
(... log messages removed ...)
Caused by: javax.persistence.PersistenceException: [PersistenceUnit: bookingDatabase] Unable to build EntityManagerFactory
at org.hibernate.ejb.Ejb3Configuration.buildEntityManagerFactory(Ejb3Configuration.java:903)
(... log messages removed ...)
Caused by: org.hibernate.HibernateException: could not instantiate RegionFactory [org.hibernate.cache.internal.bridge.RegionFactoryCacheProviderBridge]
at org.hibernate.cfg.SettingsFactory.createRegionFactory(SettingsFactory.java:355)
(... log messages removed ...)
Caused by: java.lang.reflect.InvocationTargetException
at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method) [:1.6.0_25]
(... log messages removed ...)
Caused by: org.hibernate.cache.CacheException: could not instantiate CacheProvider [org.hibernate.cache.HashtableCacheProvider]
at org.hibernate.cache.internal.bridge.RegionFactoryCacheProviderBridge.<init>(RegionFactoryCacheProviderBridge.java:68)
... 20 more
Caused by: java.lang.ClassCastException: org.hibernate.cache.HashtableCacheProvider cannot be cast to org.hibernate.cache.spi.CacheProvider
at org.hibernate.cache.internal.bridge.RegionFactoryCacheProviderBridge.<init>(RegionFactoryCacheProviderBridge.java:65)
... 20 more
----
+
[[what-it-means-8]]
==== What it means:
A ClassCastException can be a result of many problems. If you look at
this exception in the log, it appears the class
org.hibernate.cache.HashtableCacheProvider extends
org.hibernate.cache.spi.CacheProvider and is being loaded by a different
class loader than the class it extends. The
org.hibernate.cache.HashtableCacheProvider class is in in the
hibernate-core.jar and is being loaded by the application class loader.
The class it extends, org.hibernate.cache.spi.CacheProvider, is in the
org/hibernate/main/hibernate-core-4.0.0.Beta1.jar and is implicitly
loaded by that module.
This is not obvious, but due to changes in Hibernate 4, this problem is
caused by a backward compatibility issue due moving the
HashtableCacheProvider class into another package. This class was moved
from the org.hibernate.cache package to the org.hibernate.cache.internal
package. If you don't remove the hibernate.cache.provider_class property
from the persistence.xml file, it will force the Seam application to
bundle the old Hibernate libraries, resulting in ClassCastExceptions, In
WildFly, you should move away from using HashtableCacheProvider and use
Infinispan instead.
+
[[how-to-resolve-it-8]]
==== How to resolve it:
In WildFly, you need to comment out the hibernate.cache.provider_class
property in the `jboss-seam-booking.jar/META-INF persistence.xml` file
as follows:
[source, java]
----
<!-- <property name="hibernate.cache.provider_class" value="org.hibernate.cache.HashtableCacheProvider"/> -->
----
+
Redeploy the application by deleting the
`standalone/deployments/jboss-seam-booking.ear.failed` file and creating
a blank `jboss-seam-booking.ear.dodeploy file` in the same directory.
+
[[no-more-issues-deployment-errors-should-be-resolved]]
=== No more issues: Deployment errors should be resolved
At this point, the application should deploy without errors, but when
you access the URL " http://localhost:8080/seam-booking/" in a browser
and attempt "Account Login", you will get a runtime error "The page
isn't redirecting properly". In the next section, we will step through
each runtime issue and how to debug and resolve it.
+
[[step-3-debug-and-resolve-runtime-errors-and-exceptions]]
== Step 3: Debug and resolve runtime errors and exceptions
[[first-issue-javax.naming.namenotfoundexception-name-jboss-seam-booking-not-found-in-context]]
=== First Issue: javax.naming.NameNotFoundException: Name
'jboss-seam-booking' not found in context ''
The application deploys successfully, but when you access the URL "
http://localhost:8080/seam-booking/" in a browser, you get "The page
isn't redirecting properly" and the log contains the following error:
[source, java]
----
SEVERE [org.jboss.seam.jsf.SeamPhaseListener] (http--127.0.0.1-8080-1) swallowing exception: java.lang.IllegalStateException: Could not start transaction
at org.jboss.seam.jsf.SeamPhaseListener.begin(SeamPhaseListener.java:598) [jboss-seam.jar:]
(... log messages removed ...)
Caused by: org.jboss.seam.InstantiationException: Could not instantiate Seam component: org.jboss.seam.transaction.synchronizations
at org.jboss.seam.Component.newInstance(Component.java:2170) [jboss-seam.jar:]
(... log messages removed ...)
Caused by: javax.naming.NameNotFoundException: Name 'jboss-seam-booking' not found in context ''
at org.jboss.as.naming.util.NamingUtils.nameNotFoundException(NamingUtils.java:109)
(... log messages removed ...)
----
[[what-it-means-9]]
==== What it means:
A NameNotFoundException indications a JNDI naming issue. JNDI naming
rules have changed in WildFly and we need to modify the lookup names to
follow the new rules.
[[how-to-resolve-it-9]]
==== How to resolve it:
To debug this, look earlier in the server log trace to what JNDI binding
were used. Looking at the server log we see this:
[source, java]
----
15:01:16,138 INFO [org.jboss.as.ejb3.deployment.processors.EjbJndiBindingsDeploymentUnitProcessor] (MSC service thread 1-1) JNDI bindings for session bean named RegisterAction in deployment unit subdeployment "jboss-seam-booking.jar" of deployment "jboss-seam-booking.ear" are as follows:
java:global/jboss-seam-booking/jboss-seam-booking.jar/RegisterAction!org.jboss.seam.example.booking.Register
java:app/jboss-seam-booking.jar/RegisterAction!org.jboss.seam.example.booking.Register
java:module/RegisterAction!org.jboss.seam.example.booking.Register
java:global/jboss-seam-booking/jboss-seam-booking.jar/RegisterAction
java:app/jboss-seam-booking.jar/RegisterAction
java:module/RegisterAction
15:01:16,138 INFO [org.jboss.as.ejb3.deployment.processors.EjbJndiBindingsDeploymentUnitProcessor] (MSC service thread 1-1) JNDI bindings for session bean named BookingListAction in deployment unit subdeployment "jboss-seam-booking.jar" of deployment "jboss-seam-booking.ear" are as follows:
java:global/jboss-seam-booking/jboss-seam-booking.jar/BookingListAction!org.jboss.seam.example.booking.BookingList
java:app/jboss-seam-booking.jar/BookingListAction!org.jboss.seam.example.booking.BookingList
java:module/BookingListAction!org.jboss.seam.example.booking.BookingList
java:global/jboss-seam-booking/jboss-seam-booking.jar/BookingListAction
java:app/jboss-seam-booking.jar/BookingListAction
java:module/BookingListAction
15:01:16,138 INFO [org.jboss.as.ejb3.deployment.processors.EjbJndiBindingsDeploymentUnitProcessor] (MSC service thread 1-1) JNDI bindings for session bean named HotelBookingAction in deployment unit subdeployment "jboss-seam-booking.jar" of deployment "jboss-seam-booking.ear" are as follows:
java:global/jboss-seam-booking/jboss-seam-booking.jar/HotelBookingAction!org.jboss.seam.example.booking.HotelBooking
java:app/jboss-seam-booking.jar/HotelBookingAction!org.jboss.seam.example.booking.HotelBooking
java:module/HotelBookingAction!org.jboss.seam.example.booking.HotelBooking
java:global/jboss-seam-booking/jboss-seam-booking.jar/HotelBookingAction
java:app/jboss-seam-booking.jar/HotelBookingAction
java:module/HotelBookingAction
15:01:16,138 INFO [org.jboss.as.ejb3.deployment.processors.EjbJndiBindingsDeploymentUnitProcessor] (MSC service thread 1-1) JNDI bindings for session bean named AuthenticatorAction in deployment unit subdeployment "jboss-seam-booking.jar" of deployment "jboss-seam-booking.ear" are as follows:
java:global/jboss-seam-booking/jboss-seam-booking.jar/AuthenticatorAction!org.jboss.seam.example.booking.Authenticator
java:app/jboss-seam-booking.jar/AuthenticatorAction!org.jboss.seam.example.booking.Authenticator
java:module/AuthenticatorAction!org.jboss.seam.example.booking.Authenticator
java:global/jboss-seam-booking/jboss-seam-booking.jar/AuthenticatorAction
java:app/jboss-seam-booking.jar/AuthenticatorAction
java:module/AuthenticatorAction
15:01:16,139 INFO [org.jboss.as.ejb3.deployment.processors.EjbJndiBindingsDeploymentUnitProcessor] (MSC service thread 1-1) JNDI bindings for session bean named ChangePasswordAction in deployment unit subdeployment "jboss-seam-booking.jar" of deployment "jboss-seam-booking.ear" are as follows:
java:global/jboss-seam-booking/jboss-seam-booking.jar/ChangePasswordAction!org.jboss.seam.example.booking.ChangePassword
java:app/jboss-seam-booking.jar/ChangePasswordAction!org.jboss.seam.example.booking.ChangePassword
java:module/ChangePasswordAction!org.jboss.seam.example.booking.ChangePassword
java:global/jboss-seam-booking/jboss-seam-booking.jar/ChangePasswordAction
java:app/jboss-seam-booking.jar/ChangePasswordAction
java:module/ChangePasswordAction
15:01:16,139 INFO [org.jboss.as.ejb3.deployment.processors.EjbJndiBindingsDeploymentUnitProcessor] (MSC service thread 1-1) JNDI bindings for session bean named HotelSearchingAction in deployment unit subdeployment "jboss-seam-booking.jar" of deployment "jboss-seam-booking.ear" are as follows:
java:global/jboss-seam-booking/jboss-seam-booking.jar/HotelSearchingAction!org.jboss.seam.example.booking.HotelSearching
java:app/jboss-seam-booking.jar/HotelSearchingAction!org.jboss.seam.example.booking.HotelSearching
java:module/HotelSearchingAction!org.jboss.seam.example.booking.HotelSearching
java:global/jboss-seam-booking/jboss-seam-booking.jar/HotelSearchingAction
java:app/jboss-seam-booking.jar/HotelSearchingAction
java:module/HotelSearchingAction
15:01:16,140 INFO [org.jboss.as.ejb3.deployment.processors.EjbJndiBindingsDeploymentUnitProcessor] (MSC service thread 1-6) JNDI bindings for session bean named EjbSynchronizations in deployment unit subdeployment "jboss-seam.jar" of deployment "jboss-seam-booking.ear" are as follows:
java:global/jboss-seam-booking/jboss-seam/EjbSynchronizations!org.jboss.seam.transaction.LocalEjbSynchronizations
java:app/jboss-seam/EjbSynchronizations!org.jboss.seam.transaction.LocalEjbSynchronizations
java:module/EjbSynchronizations!org.jboss.seam.transaction.LocalEjbSynchronizations
java:global/jboss-seam-booking/jboss-seam/EjbSynchronizations
java:app/jboss-seam/EjbSynchronizations
java:module/EjbSynchronizations
15:01:16,140 INFO [org.jboss.as.ejb3.deployment.processors.EjbJndiBindingsDeploymentUnitProcessor] (MSC service thread 1-6) JNDI bindings for session bean named TimerServiceDispatcher in deployment unit subdeployment "jboss-seam.jar" of deployment "jboss-seam-booking.ear" are as follows:
java:global/jboss-seam-booking/jboss-seam/TimerServiceDispatcher!org.jboss.seam.async.LocalTimerServiceDispatcher
java:app/jboss-seam/TimerServiceDispatcher!org.jboss.seam.async.LocalTimerServiceDispatcher
java:module/TimerServiceDispatcher!org.jboss.seam.async.LocalTimerServiceDispatcher
java:global/jboss-seam-booking/jboss-seam/TimerServiceDispatcher
java:app/jboss-seam/TimerServiceDispatcher
java:module/TimerServiceDispatcher
----
We need to modify the WAR's lib/components.xml file to use the new JNDI
bindings. In the log, note the EJB JNDI bindings all start with
"java:app/jboss-seam-booking.jar"
Replace the <core:init> element as follows:
[source, java]
----
<!-- <core:init jndi-pattern="jboss-seam-booking/#{ejbName}/local" debug="true" distributable="false"/> -->
<core:init jndi-pattern="java:app/jboss-seam-booking.jar/#{ejbName}" debug="true" distributable="false"/>
----
+
Next, we need to add the EjbSynchronizations and TimerServiceDispatcher
JNDI bindings. Add the following component elements to the file:
+
[source, java]
----
<component class="org.jboss.seam.transaction.EjbSynchronizations" jndi-name="java:app/jboss-seam/EjbSynchronizations"/>
<component class="org.jboss.seam.async.TimerServiceDispatcher" jndi-name="java:app/jboss-seam/TimerServiceDispatcher"/>
----
+
The components.xml file should now look like this:
+
[source, java]
----
<?xml version="1.0" encoding="UTF-8"?>
<components xmlns="http://jboss.com/products/seam/components"
xmlns:core="http://jboss.com/products/seam/core"
xmlns:security="http://jboss.com/products/seam/security"
xmlns:transaction="http://jboss.com/products/seam/transaction"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation=
"http://jboss.com/products/seam/core http://jboss.com/products/seam/core-2.2.xsd
http://jboss.com/products/seam/transaction http://jboss.com/products/seam/transaction-2.2.xsd
http://jboss.com/products/seam/security http://jboss.com/products/seam/security-2.2.xsd
http://jboss.com/products/seam/components http://jboss.com/products/seam/components-2.2.xsd">
<!-- <core:init jndi-pattern="jboss-seam-booking/#{ejbName}/local" debug="true" distributable="false"/> -->
<core:init jndi-pattern="java:app/jboss-seam-booking.jar/#{ejbName}" debug="true" distributable="false"/>
<core:manager conversation-timeout="120000"
concurrent-request-timeout="500"
conversation-id-parameter="cid"/>
<transaction:ejb-transaction/>
<security:identity authenticate-method="#{authenticator.authenticate}"/>
<component class="org.jboss.seam.transaction.EjbSynchronizations"
jndi-name="java:app/jboss-seam/EjbSynchronizations"/>
<component class="org.jboss.seam.async.TimerServiceDispatcher"
jndi-name="java:app/jboss-seam/TimerServiceDispatcher"/>
</components>
----
+
Redeploy the application by deleting the
`standalone/deployments/jboss-seam-booking.ear.failed` file and creating
a blank `jboss-seam-booking.ear.dodeploy` file in the same directory.
+
At this point, the application should deploy and run without error. When
you access the URL " http://localhost:8080/seam-booking/" in a browser,
you will be able to login successfully.
+
[[step-4-access-the-application]]
== Step 4: Access the application
Access the URL " http://localhost:8080/seam-booking/" in a browser and
login with demo/demo. You should the Booking welcome page.
+
[[summary-of-changes]]
== Summary of Changes
Although it would be much more efficient to determine dependencies in
advance and add the implicit dependencies in one step, this exercise
shows how problems appear in the log and provides some information on
how to debug and resolve them.
The following is a summary of changes made to the application when
migrating it to WildFly:
1. We created a `jboss-deployment-structure.xml` file in the EAR's
`META-INF/` directory. We added "dependencies" and "exclusions" to
resolve ClassNotFoundExceptions. This file contains the following data:
+
[source, java]
----
<jboss-deployment-structure xmlns="urn:jboss:deployment-structure:1.0">
<deployment>
<dependencies>
<module name="javax.faces.api" slot="1.2" export="true"/>
<module name="com.sun.jsf-impl" slot="1.2" export="true"/>
<module name="org.apache.commons.logging" export="true"/>
<module name="org.dom4j" export="true"/>
<module name="org.apache.commons.collections" export="true"/>
</dependencies>
</deployment>
<sub-deployment name="jboss-seam-booking.war">
<exclusions>
<module name="javax.faces.api" slot="main"/>
<module name="com.sun.jsf-impl" slot="main"/>
</exclusions>
<dependencies>
<module name="javax.faces.api" slot="1.2"/>
<module name="com.sun.jsf-impl" slot="1.2"/>
</dependencies>
</sub-deployment>
</jboss-deployment-structure>
----
* We copied the following JARs from the
`EAP5_HOME/jboss-eap-5.1/seam/lib/` directory to the
`jboss-seam-booking.ear/lib/` directory to resolve
ClassNotFoundExceptions:
+
[source, java]
----
hibernate-core.jar
hibernate-validator.jar
----
* We modified the \{\{jboss-seam-booking.jar/META-INF/persistence.xml}
file as follows.
1. First, we changed the jta-data-source element to use the Example
database that ships with AS7:
+
[source, java]
----
<!-- <jta-data-source>java:/bookingDatasource</jta-data-source> -->
<jta-data-source>java:jboss/datasources/ExampleDS</jta-data-source>
----
2. Next, we commented out the hibernate.cache.provider_class property:
+
[source, java]
----
<!-- <property name="hibernate.cache.provider_class" value="org.hibernate.cache.HashtableCacheProvider"/> -->
----
* We modified the WAR's lib/components.xml file to use the new JNDI
bindings
1. We replaced the <core:init> existing element as follows:
+
[source, java]
----
<!-- <core:init jndi-pattern="jboss-seam-booking/#{ejbName}/local" debug="true" distributable="false"/> -->
<core:init jndi-pattern="java:app/jboss-seam-booking.jar/#{ejbName}" debug="true" distributable="false"/>
----
2. We added component elements for the "EjbSynchronizations" and
"TimerServiceDispatcher" JNDI bindings
+
[source, java]
----
<component class="org.jboss.seam.transaction.EjbSynchronizations" jndi-name="java:app/jboss-seam/EjbSynchronizations"/>
<component class="org.jboss.seam.async.TimerServiceDispatcher" jndi-name="java:app/jboss-seam/TimerServiceDispatcher"/>
----
* The unmodified EAR from EAP 5.1 (jboss-seam-booking-eap51.ear.tar.gz)
and the EAR as modified to run on AS7
(jboss-seam-booking-as7.ear.tar.gz) are attached to this document.
<file_sep>/_docs/13/_testsuite/How_the_server_is_built_and_configured_for_testsuite_modules.adoc
[[How_the_server_is_built_and_configured_for_testsuite_modules]]
== How the WildFly is built and configured for testsuite modules.
Refer to <<Shortened_Maven_Run_Overview,Shortened Maven Run Overview>> to see the
mentioned build steps.
\1) AS instance is copied from `${jboss.dist`} to
`testsuite/target/jbossas`. +
Defaults to AS which is built by the project (
`build/target/jboss-as-*`).
2)
*testsuite/pom.xml:*
from $\{jboss.home} to $\{basedir}/target/jbossas +
phase generate-test-resources: resource-plugin, goal copy-resources
*testsuite/integration/pom.xml:*
phase process-test-resources: antrun-plugin:
[source, xml]
----
<ant antfile="$\{basedir}/src/test/scripts/basic-integration-build.xml">
<target name="build-basic-integration"/>
<target name="build-basic-integration-jts"/>
</ant>
----
Which invokes
[source, xml]
----
<target name="build-basic-integration" description="Builds server configuration for basic-integration tests">
<build-server-config name="jbossas"/>
----
Which invokes
[source, xml]
----
<!-- Copy the base distribution. -->
<!-- We exclude modules and bundles as they are read-only and we locate the via sys props. -->
<copy todir="@{output.dir}/@{name}">
<fileset dir="@{jboss.dist}">
<exclude name="**/modules/**"/>
<exclude name="**/bundles/**"/>
</fileset>
</copy>
<!-- overwrite with configs from test-configs and apply property filtering -->
<copy todir="@{output.dir}/@{name}" overwrite="true" failonerror="false">
<fileset dir="@{test.configs.dir}/@{name}"/>
<filterset begintoken="${" endtoken="}">
<filter token="node0" value="${node0}"/>
<filter token="node1" value="${node1}"/>
<filter token="udpGroup" value="${udpGroup}"/>
<filter-elements/>
</filterset>
</copy>
----
[[arquillian-config-file-location]]
== Arquillian config file location
[source, bash]
----
-Darquillian.xml=some-file-or-classpath-resource.xml
----
<file_sep>/_docs/13/common_attributes.adoc
:oracle-javadoc: https://docs.oracle.com/javase/8/docs/api/java
:appservername: WildFly<file_sep>/_docs/14/_admin-guide/add-user-utility.adoc
[[add-user-utility]]
= add-user utility
ifdef::env-github[:imagesdir: ../]
For use with the default configuration we supply a utility `add-user`
which can be used to manage the properties files for the default realms
used to store the users and their roles.
The add-user utility can be used to manage both the users in the
`ManagementRealm` and the users in the `ApplicationRealm`, changes made
apply to the properties file used both for domain mode and standalone
mode.
[TIP]
After you have installed your application server and decided if you are
going to run in standalone mode or domain mode you can delete the parent
folder for the mode you are not using, the add-user utility will then
only be managing the properties file for the mode in use.
The add-user utility is a command line utility however it can be run in
both interactive and non-interactive mode. Depending on your platform
the script to run the add-user utility is either `add-user.sh` or
`add-user.bat` which can be found in \{ `jboss.home}/bin`.
This guide now contains a couple of examples of this utility in use to
accomplish the most common tasks.
[[adding-a-user]]
== Adding a User
Adding users to the properties files is the primary purpose of this
utility. Usernames can only contain the following characters in any
number and in any order:
* Alphanumeric characters (a-z, A-Z, 0-9)
* Dashes (-), periods (.), commas (,), at (@)
* Escaped backslash ( \\ )
* Escaped equals (\=)
[NOTE]
The server caches the contents of the properties files in memory,
however the server does check the modified time of the properties files
on each authentication request and re-load if the time has been updated
- this means all changes made by this utility are immediately applied to
any running server.
[[a-management-user]]
=== A Management User
[NOTE]
The default name of the realm for management users is `ManagementRealm`,
when the utility prompts for the realm name just accept the default
unless you have switched to a different realm.
[[interactive-mode]]
==== Interactive Mode
image:images/add-mgmt-user-interactive.png[Add mgmt user interactive]
Here we have added a new Management User called `adminUser`, as you can
see some of the questions offer default responses so you can just press
enter without repeating the default value.
For now just answer `n` or `no` to the final question, adding users to
be used by processes is described in more detail in the domain
management chapter.
[[add-user-non-interactive-mode]]
==== Non-Interactive Mode
To add a user in non-interactive mode the command
`./add-user.sh {username} {password`} can be used.
image:images/add-mgmt-user-non-interactive.png[add-mgmt-user-non-interactive.png]
[WARNING]
If you add users using this approach there is a risk that any other user
that can view the list of running process may see the arguments
including the password of the user being added, there is also the risk
that the username / password combination will be cached in the history
file of the shell you are currently using.
[[an-application-user]]
=== An Application User
When adding application users in addition to adding the user with their
pre-hashed password it is also now possible to define the roles of the
user.
[[interactive-mode-1]]
==== Interactive Mode
image:images/add-app-user-interactive.png[add-app-user-interactive.png]
Here a new user called `appUser` has been added, in this case a comma
separated list of roles has also been specified.
As with adding a management user just answer `n` or `no` to the final
question until you know you are adding a user that will be establishing
a connection from one server to another.
[[non-interactive-mode-1]]
==== Non-Interactive Mode
To add an application user non-interactively use the command
`./add-user.sh -a {username} {password`}.
image:images/add-app-user-non-interactive.png[add-app-user-non-interactive.png]
[NOTE]
Non-interactive mode does not support defining a list of users, to
associate a user with a set of roles you will need to manually edit the
`application-roles.properties` file by hand.
[[updating-a-user]]
== Updating a User
Within the add-user utility it is also possible to update existing
users, in interactive mode you will be prompted to confirm if this is
your intention.
[[a-management-user-1]]
=== A Management User
[[interactive-mode-2]]
==== Interactive Mode
image:images/update-mgmt-user-interactive.png[update-mgmt-user-interactive.png]
[[non-interactive-mode-2]]
==== Non-Interactive Mode
In non-interactive mode if a user already exists the update is automatic
with no confirmation prompt.
[[an-application-user-1]]
=== An Application User
==== Interactive Mode
image:images/update-app-user-interactive.png[images/update-app-user-interactive.png]
[NOTE]
On updating a user with roles you will need to re-enter the list of
roles assigned to the user.
==== Non-Interactive Mode
In non-interactive mode if a user already exists the update is automatic
with no confirmation prompt.
[[community-contributions]]
== Community Contributions
There are still a few features to add to the add-user utility such as
removing users or adding application users with roles in non-interactive
mode, if you are interested in contributing to Wild**Fly** development the
add-user utility is a good place to start as it is a stand alone
utility, however it is a part of the AS build so you can become familiar
with the AS development processes without needing to delve straight into
the internals of the application server.
<file_sep>/_docs/13/_testsuite/WildFly_Testsuite_Harness_Developer_Guide.adoc
[[WildFly_Testsuite_Harness_Developer_Guide]]
== WildFly Testsuite Harness Developer Guide
*Audience:* Whoever wants to change the testsuite harness
*JIRA*: https://issues.jboss.org/browse/WFLY-576[WFLY-576]
[[testsuite-requirements]]
== Testsuite requirements
http://community.jboss.org/wiki/ASTestsuiteRequirements will probably be
merged here later.
[[adding-a-new-maven-plugin]]
== Adding a new maven plugin
The plugin version needs to be specified in jboss-parent. See
https://github.com/jboss/jboss-parent-pom/blob/master/pom.xml#L65 .
[[shortened-maven-run-overview]]
== Shortened Maven run overview
See <<Shortened_Maven_Run_Overview,Shortened Maven Run Overview>>.
[[how-the-as-instance-is-built]]
== How the AS instance is built
See <<How_the_server_is_built_and_configured_for_testsuite_modules,How the JBoss AS instance is built and configured
for testsuite modules.>>
[[properties-and-their-propagation]]
== Properties and their propagation
Propagated to tests through arquillian.xml: +
`<property name="javaVmArguments">${server.jvm.args}</property>` +
TBD: https://issues.jboss.org/browse/ARQ-647
[[jboss-as-instance-dir]]
=== JBoss AS instance dir
*integration/pom.xml*
(currently nothing)
**-arquillian.xml*
[source, xml]
----
<container qualifier="jboss" default="true">
<configuration>
<property name="jbossHome">${basedir}/target/jbossas</property>
----
[[server-jvm-arguments]]
=== Server JVM arguments
[source, xml]
----
<surefire.memory.args>-Xmx512m -XX:MaxPermSize=256m</surefire.memory.args>
<surefire.jpda.args></surefire.jpda.args>
<surefire.system.args>${surefire.memory.args} ${surefire.jpda.args}</surefire.system.args>
----
[[ip-settings]]
=== IP settings
* `${ip.server.stack` *}* - used in
`<systemPropertyVariables> / <server.jvm.args>` which is used in
`*-arquillian.xml`.
[[testsuite-directories]]
=== Testsuite directories
* `${jbossas.ts.integ.dir`}
* `${jbossas.ts.dir`}
* `${jbossas.project.dir`}
[[clustering-properties]]
=== Clustering properties
* node0
* node1
== Debug parameters propagation
[source, xml]
----
<surefire.jpda.args></surefire.jpda.args> - default
<surefire.jpda.args>-Xrunjdwp:transport=dt_socket,address=${as.debug.port},server=y,suspend=y</surefire.jpda.args> - activated by -Ddebug or -Djpda
testsuite/pom.xml: <surefire.system.args>... ${surefire.jpda.args} ...</surefire.system.args>
testsuite/pom.xml: <jboss.options>${surefire.system.args}</jboss.options>
testsuite/integration/pom.xml: <server.jvm.args>${surefire.system.args} ${jvm.args.ip.server} ${jvm.args.security} ${jvm.args.timeouts} -Dnode0=${node0} -Dnode1=
integration/pom.xml:
<server.jvm.args>${surefire.system.args} ${jvm.args.ip.server} ${jvm.args.security} ${jvm.args.timeouts} -Dnode0=${node0} -Dnode1=${node1} -DudpGroup=${udpGroup} ${jvm.args.dirs}</server.jvm.args>
arquillian.xml:
<property name="javaVmArguments">${server.jvm.args} -Djboss.inst=${basedir}/target/jbossas</property>
----
include::How_the_server_is_built_and_configured_for_testsuite_modules.adoc[]
include::Plugin_executions_matrix.adoc[]
include::Shortened_Maven_Run_Overview.adoc[]
<file_sep>/assets/javascript/random-color-picker.js
$(document).ready(function(){
var listClasses = ["red", "orange", "yellow", "teal", "blue"];
var blockClasses = ["red", "orange", "yellow", "teal"];
// Random color class for blog feed on /blog
$(".blog-list-item").each(function(){
$(this).addClass(listClasses[~~(Math.random()*listClasses.length)]);
});
// Random color class for blog feed in blocks format on homepage
$(".blog-block-item").each(function(){
$(this).addClass(blockClasses[~~(Math.random()*blockClasses.length)]);
});
});
<file_sep>/_docs/14/_testsuite/WildFly_Testsuite_Overview.adoc
[[WildFly_Testsuite_Overview]]
= Wild**Fly** Testsuite Overview
This document will detail the implementation of the testsuite
Integration submodule as it guides you on adding your own test cases.
The Wild**Fly** integration test suite has been designed with the following
goals:
* support execution of all identified test case use cases
* employ a design/organization which is scalable and maintainable
* provide support for the automated measurement of test suite quality
(generation of feature coverage reports, code coverage reports)
In addition, these requirements were considered:
* identifying distinct test case runs of the same test case with a
different set of client side parameters and server side parameters
* separately maintaining server side execution results (e.g. logs, the
original server configuration) for post-execution debugging
* running the testsuite in conjunction with a debugger
* the execution of a single test (for debugging purposes)
* running test cases against different container modes (managed in the
main, but also remote and embedded)
* configuring client and server JVMs separately (e.g., IPv6 testing)
[[test-suite-organization]]
== Test Suite Organization
The testsuite module has a small number of submodules:
* *benchmark* - holds all benchmark tests intended to assess relative
performance of specific feature
* *domain* - holds all domain management tests
* *integration* - holds all integration tests
* *stress* - holds all stress tests
It is expected that test contributions fit into one of these categories.
The pom.xml file located in the testsuite module is inherited by all
submodules and is used to do the following:
* set defaults for common testsuite system properties (which can then be
overridden on the command line)
* define dependencies common to all tests (Arquillian, junit or testng,
and container type)
* provide a workaround for @Resource(lookup=...) which requires
libraries in jbossas/endorsed
It should not:
* define module-specific server configuration build steps
* define module-specific surefire executions
These elements should be defined in logical profiles associated with
each logical grouping of tests; e.g., in the pom for the module which
contains the tests. The submodule poms contain additional details of
their function and purpose as well as expanded information as shown in
this document.
[[profiles]]
== Profiles
You should not activate the abovementioned profiles by -P, because that
disables other profiles which are activated by default.
Instead, you should always use activating properties, which are in
parenthesis in the lists below.
*Testsuite profiles* are used to group tests into logical groups.
* all-modules.module.profile (all-modules)
* integration.module.profile (integration.module)
* compat.module.profile (compat.module)
* domain.module.profile (domain.module)
* benchmark.module.profile (benchmark.module)
* stress.module.profile (stress.module)
They also pprepare WildFly instances and resources for respective
testsuite submodules.
* jpda.profile - sets surefire.jpda.args (debug)
* ds.profile -sets database properties and prepares the datasource
(ds=<db id>)
** Has related database-specific profiles, like mysql51.profile etc.
*Integration testsuite profiles* configure surefire executions.
* smoke.integration.tests.profile
* basic.integration.tests.profile
* clustering.integration.tests.profile
== Integration tests
[[smoke--dts.smoke--dts.nosmoke]]
=== Smoke -Dts.smoke, -Dts.noSmoke
Contains smoke tests.
Runs by default; use -Dts.noSmoke to prevent running.
Tests should execute quickly.
Divided into two Surefire executions:
* One with full profile
* Second with web profile (majority of tests).
[[basic--dts.basic]]
=== Basic -Dts.basic
Basic integration tests - those which do not need special configuration
like cluster.
Divided into three Surefire executions:
* One with full profile,
* Second with web profile (majority of tests).
* Third with web profile, but needs to be run after server restart to
check whether persistent data are really persisted.
[[cluster--dts.clust]]
=== Cluster -Dts.clust
Sets up a cluster of two nodes.
[[iiop--dts.iiop]]
=== IIOP -Dts.iiop
[[xts--dts.xts]]
== XTS -Dts.XTS
[[multinode--dts.multinode]]
=== Multinode -Dts.multinode
<file_sep>/_docs/14/_admin-guide/Subsystem_configuration.adoc
[[Subsystem_configuration]]
= Subsystem configuration
Subsystem configuration reference
:author: <EMAIL>
:icons: font
:source-highlighter: coderay
:toc: macro
:toclevels: 2
The following chapters will focus on the high level management use cases
that are available through the CLI and the web interface. For a detailed
description of each subsystem configuration property, please consult the
respective component reference.
Schema Location
TIP: The configuration schemas can found in `$JBOSS_HOME/docs/schema`.
:leveloffset: +1
include::subsystem-configuration/EE.adoc[]
include::subsystem-configuration/Naming.adoc[]
include::subsystem-configuration/DataSource.adoc[]
include::subsystem-configuration/Datasources_Agroal.adoc[]
include::subsystem-configuration/Logging.adoc[]
include::subsystem-configuration/EJB3.adoc[]
include::subsystem-configuration/Undertow.adoc[]
include::subsystem-configuration/Messaging.adoc[]
include::subsystem-configuration/MicroProfile_Config_SmallRye.adoc[]
include::subsystem-configuration/MicroProfile_OpenTracing_SmallRye.adoc[]
include::subsystem-configuration/Security.adoc[]
include::subsystem-configuration/Web_services.adoc[]
include::subsystem-configuration/Resource_adapters.adoc[]
include::subsystem-configuration/Batch_(JSR-352).adoc[]
include::subsystem-configuration/JSF.adoc[]
include::subsystem-configuration/JMX.adoc[]
include::subsystem-configuration/Deployment_Scanner.adoc[]
include::subsystem-configuration/Core_Management.adoc[]
include::subsystem-configuration/Simple_configuration_subsystems.adoc[]
:leveloffset: -1
<file_sep>/_docs/13/_high-availability/ha-singleton/Singleton_MSC_services.adoc
[[Singleton_MSC_services]]
== Singleton MSC services
The singleton service facility exposes a mechanism for installing an MSC service such that the service only starts on a single member of a cluster at a time.
If the member providing the singleton service is shutdown or crashes, the facility automatically elects a new primary provider and starts the service on that node.
In general, a singleton election happens in response to any change of membership, where the membership is defined as the set of cluster nodes on which the given service was installed.
[[installing-an-msc-service-using-an-existing-singleton-policy]]
== Installing an MSC service using an existing singleton policy
While singleton MSC services have been around since AS7, WildFly adds the ability to leverage the singleton subsystem to create singleton MSC services from existing singleton policies.
The singleton subsystem exposes capabilities for each singleton policy it defines.
These policies, encapsulated by the `org.wildfly.clustering.singleton.service.SingletonPolicy` interface, can be referenced via the following capability name:
"org.wildfly.clustering.singleton.policy" + _policy-name_
You can reference the default singleton policy of the server via the name:
"org.wildfly.clustering.singleton.default-policy"
e.g.
[source, java]
----
public class MyServiceActivator implements ServiceActivator {
@Override
public void activate(ServiceActivatorContext context) {
ServiceName name = ServiceName.parse("my.service.name");
// Use default singleton policy
Supplier<SingletonPolicy> policy = new ActiveServiceSupplier<>(context.getServiceTarget(), ServiceName.parse(SingletonDefaultRequirement.SINGLETON_POLICY.getName()));
ServiceBuilder<?> builder = policy.get().createSingletonServiceConfigurator(name).build(context.getServiceTarget());
Service service = new MyService();
builder.setInstance(service).install();
}
}
----
[[installing-an-msc-service-using-dynamic-singleton-policy]]
== Installing an MSC service using dynamic singleton policy
Alternatively, you can configure a singleton policy dynamically, which is particularly useful if you want to use a custom singleton election policy.
`org.wildfly.clustering.singleton.service.SingletonPolicy` is a generalization of the `org.wildfly.clustering.singleton.service.SingletonServiceConfiguratorFactory` interface,
which includes support for specifying an election policy and, optionally, a quorum.
The 'SingletonServiceConfiguratorFactory' capability may be references using the following capability name:
"org.wildfly.clustering.cache.singleton-service-configurator-factory" + _container-name_ + "." + _cache-name_
You can reference a 'SingletonServiceConfiguratorFactory' using the default cache of a given cache container via the name:
"org.wildfly.clustering.cache.default-singleton-service-configurator-factory" + _container-name_
e.g.
[source, java]
----
public class MyServiceActivator implements ServiceActivator {
@Override
public void activate(ServiceActivatorContext context) {
String containerName = "foo";
ElectionPolicy policy = new MySingletonElectionPolicy();
int quorum = 3;
ServiceName name = ServiceName.parse("my.service.name");
// Use a SingletonServiceConfiguratorFactory backed by default cache of "foo" container
Supplier<SingletonServiceConfiguratorFactory> factory = new ActiveServiceSupplier<>(context.getServiceTarget(), ServiceName.parse(SingletonDefaultCacheRequirement.SINGLETON_SERVICE_CONFIGURATOR_FACTORY.resolve(containerName).getName()));
ServiceBuilder<?> builder = factory.get().createSingletonServiceConfigurator(name)
.electionPolicy(policy)
.requireQuorum(quorum)
.build(context.getServiceTarget());
Service service = new MyService();
builder.setInstance(service).install();
}
}
----
<file_sep>/_docs/13/Admin_Guide.adoc
[[Admin_Guide]]
= Wild**Fly** Admin Guide
Wild**Fly** developer team;
:revnumber: {version}
:revdate: {localdate}
:toc: macro
:toclevels: 2
:toc-title: WildFly Admin guide
:doctype: book
:icons: font
:source-highlighter: coderay
:wildflyVersion: 13
:sectlinks:
:idseparator: -
:include-depth: 2
ifdef::env-github[:outfilesuffix: .adoc]
ifndef::ebook-format[:leveloffset: 1]
(C) 2017 The original authors.
:numbered:
////
:leveloffset: 1
////
include::_admin-guide/Target_Audience.adoc[]
include::_admin-guide/Core_management_concepts.adoc[]
include::_admin-guide/Management_Clients.adoc[]
include::_admin-guide/Interfaces_and_ports.adoc[leveloffset=1]
= Administrative security
include::_admin-guide/Security_Realms.adoc[]
include::_admin-guide/RBAC.adoc[]
include::_admin-guide/Application_deployment.adoc[]
include::_admin-guide/Subsystem_configuration.adoc[]
include::_admin-guide/Domain_Setup.adoc[]
include::_admin-guide/Management_tasks.adoc[]
include::_admin-guide/Management_API_reference.adoc[]
include::_admin-guide/CLI_Recipes.adoc[]
<file_sep>/_docs/13/_testsuite/Shared_Test_Classes_and_Resources.adoc
[[Shared_Test_Classes_and_Resources]]
== Shared Test Classes and Resources
[[among-testsuite-modules]]
== Among Testsuite Modules
Use the testsuite/shared module.
Classes and resources in this module are available in all testsuite
modules - i.e. in testsuite/* .
Only use it if necessary - don't put things "for future use" in there.
*Don't split packages across modules.* *Make sure the java package is
unique in the WildFly project.*
*Document your util classes* (javadoc) so they can be easily found and
reused! A generated list will be put here.
[[between-components-and-testsuite-modules]]
== Between Components and Testsuite Modules
To share component's test classes with some module in testsuite, you
don't need to split to submodules.
You can create a jar with classifier using this:
[source, xml]
----
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-jar-plugin</artifactId>
<executions>
<execution>
<goals>
<goal>test-jar</goal>
</goals>
</execution>
</executions>
</plugin>
----
This creates a jar with classifier "tests", so you can add it as
dependency to a testsuite module:
[source, xml]
----
<dependency>
<groupId>org.wildfly</groupId>
<artifactId>wildfly-clustering-common</artifactId>
<classifier>tests</classifier>
<version>${project.version}</version>
<scope>test</scope>
</dependency>
----<file_sep>/_docs/14/_extending-wildfly/WildFly_JNDI_Implementation.adoc
[[WildFly_JNDI_Implementation]]
= Wild**Fly** JNDI Implementation
[[introduction]]
== Introduction
This page proposes a reworked WildFly JNDI implementation, and
new/updated APIs for WildFly subsystem and EE deployment processors
developers to bind new resources easier.
To support discussion in the community, the content includes a big focus
on comparing WildFly {wildflyVersion} JNDI implementation with the new proposal, and
should later evolve to the prime guide for WildFly developers needing to
interact with JNDI at subsystem level.
[[architecture]]
== Architecture
WildFly relies on MSC to provide the data source for the JNDI tree. Each
resource bound in JNDI is stored in a MSC service (BinderService), and
such services are installed as children of subsystem/deployment
services, for an automatically unbound as consequence of uninstall of
the parent services.
Since there is the need to know what entries are bound, and MSC does not
provides that, there is also the (ServiceBased)NamingStore concept,
which internally manage the set of service names bound. There are
multiple naming stores in every WildFly instance, serving different JNDI
namespaces:
* java:comp - the standard EE namespace for entries scoped to a specific
component, such as an EJB
* java:module - the standard EE namespace for entries scoped to specific
module, such as an EJB jar, and shared by all components in it
* java:app - the standard EE namespace for entries scoped to a specific
application, i.e. EAR, and shared by all modules in it
* java:global - the standard EE namespace for entries shared by all
deployments
* java:jboss - a proprietary namespace "global" namespace
* java:jboss/exported - a proprietary "global" namespace which entries
are exposed to remote JNDI
* java: - any entries not in the other namespaces
One particular implementation choice, to save resources, is that JNDI
contexts by default are not bound, the naming stores will search for any
entry bound with a name that is a child of the context name, if found
then its assumed the context exists.
The reworked implementation introduces shared/global java:comp,
java:module and java:app namespaces. Any entry bound on these will
automatically be available to every EE deployment scoped instance of
these namespaces, what should result in a significant reduction of
binder services, and also of EE deployment processors. Also, the Naming
subsystem may now configure bind on these shared contexts, and these
contexts will be available when there is no EE component in the
invocation, which means that entries such as java:comp/DefaultDatasource
will always be available.
[[binding-apis]]
== Binding APIs
WildFly Naming subsystem exposes high level APIs to bind new JNDI
resources, there is no need to deal with the low level BinderService
type anymore.
[[subsystem]]
=== Subsystem
At the lowest level a JNDI entry is bound by installing a BinderService
to a ServiceTarget:
[source, java]
----
/**
* Binds a new entry to JNDI.
* @param serviceTarget the binder service's target
* @param name the new JNDI entry's name
* @param value the new JNDI entry's value
*/
private ServiceController<?> bind(ServiceTarget serviceTarget, String name, Object value) {
// the bind info object provides MSC service names to use when creating the binder service
final ContextNames.BindInfo bindInfo = ContextNames.bindInfoFor(name);
final BinderService binderService = new BinderService(bindInfo.getBindName());
// the entry's value is provided by a managed reference factory,
// since the value may need to be obtained on lookup (e.g. EJB reference)
final ManagedReferenceFactory managedReferenceFactory = new ImmediateManagedReferenceFactory(value);
return serviceTarget
// add binder service to specified target
.addService(bindInfo.getBinderServiceName(), binderService)
// when started the service will be injected with the factory
.addInjection(binderService.getManagedObjectInjector(), managedReferenceFactory)
// the binder service depends on the related naming store service,
// and on start/stop will add/remove its service name
.addDependency(bindInfo.getParentContextServiceName(),
ServiceBasedNamingStore.class,
binderService.getNamingStoreInjector())
.install();
}
----
But the example above is the simplest usage possible, it may become
quite complicated if the entry's value is not immediately available, for
instance it is a value in another MSC service, or is a value in another
JNDI entry. It's also quite easy to introduce bugs when working with the
service names, or incorrectly assume that other MSC functionality, such
as alias names, may be used.
Using the new high level API, it's as simple as:
[source, java]
----
// bind an immediate value
ContextNames.bindInfoFor("java:comp/ORB").bind(serviceTarget, this.orb);
// bind value from another JNDI entry (an alias/linkref)
ContextNames.bindInfoFor("java:global/x").bind(serviceTarget, new JndiName("java:jboss/x"));
// bind value obtained from a MSC service
ContextNames.bindInfoFor("java:global/z").bind(serviceTarget, serviceName);
----
If there is the need to access the binder's service builder, perhaps to
add a service verification handler or simply not install the binder
service right away:
[source, java]
----
ContextNames.bindInfoFor("java:comp/ORB").builder(serviceTarget, verificationHandler, ServiceController.Mode.ON_DEMAND).installService(this.orb);
----
[[ee-deployment]]
=== EE Deployment
With respect to EE deployments, the subsystem API should not be used,
since bindings may need to be discarded/overridden, thus a EE deployment
processor should add a new binding in the form of a
BindingConfiguration, to the EeModuleDescription or
ComponentDescription, depending if the bind is specific to a component
or not. An example of a deployment processor adding a binding:
[source, java]
----
public class ModuleNameBindingProcessor implements DeploymentUnitProcessor {
// jndi name objects are immutable
private static final JndiName JNDI_NAME_java_module_ModuleName = new JndiName("java:module/ModuleName");
@Override
public void deploy(DeploymentPhaseContext phaseContext) throws DeploymentUnitProcessingException {
final DeploymentUnit deploymentUnit = phaseContext.getDeploymentUnit();
// skip deployment unit if it's the top level EAR
if (DeploymentTypeMarker.isType(DeploymentType.EAR, deploymentUnit)) {
return;
}
// the module's description is in the DUs attachments
final EEModuleDescription moduleDescription = deploymentUnit
.getAttachment(org.jboss.as.ee.component.Attachments.EE_MODULE_DESCRIPTION);
if (moduleDescription == null) {
return;
}
// add the java:module/ModuleName binding
// the value's injection source for an immediate available value
final InjectionSource injectionSource = new ImmediateInjectionSource(moduleDescription.getModuleName());
// add the binding configuration to the module's description bindings configurations
moduleDescription.getBindingConfigurations()
.addDeploymentBinding(new BindingConfiguration(JNDI_NAME_java_module_ModuleName, injectionSource));
}
//...
}
----
[IMPORTANT]
When adding the binding configuration use:
* addDeploymentBinding() for a binding that may not be overriden, such
as the ones found in xml descriptors
* addPlatformBinding() for a binding which may be overriden by a
deployment descriptor bind or annotation, for instance
java:comp/DefaultDatasource
A deployment processor may now also add a binding configuration to all
components in a module:
[source, java]
----
moduleDescription.getBindingConfigurations().addPlatformBindingToAllComponents(bindingConfiguration);
----
[IMPORTANT]
In the reworked implementation there is now no need to behave
differently considering the deployment type, for instance if deployment
is a WAR or app client, the Module/Component BindingConfigurations
objects handle all of that. The processor should simply go for the 3 use
cases: module binding, component binding or binding shared by all
components.
[IMPORTANT]
All deployment binding configurations MUST be added before INSTALL
phase, this is needed because on such phase, when the bindings are
actually done, there must be a final set of deployment binding names
known, such information is need to understand if a resource injection
targets entries in the global or scoped EE namespaces.
Most cases for adding bindings to EE deployments are in the context of a
processor deploying a XML descriptor, or scanning deployment classes for
annotations, and there abstract types, such as the
AbstractDeploymentDescriptorBindingsProcessor, which simplifies greatly
the processor code for such use cases.
One particular use case is the parsing of EE Resource Definitions, and
the reworked implementation provides high level abstract deployment
processors for both XML descriptor and annotations, an example for each:
[source, java]
----
/**
* Deployment processor responsible for processing administered-object deployment descriptor elements
*
* @author <NAME>
*/
public class AdministeredObjectDefinitionDescriptorProcessor extends ResourceDefinitionDescriptorProcessor {
@Override
protected void processEnvironment(RemoteEnvironment environment, ResourceDefinitionInjectionSources injectionSources) throws DeploymentUnitProcessingException {
final AdministeredObjectsMetaData metaDatas = environment.getAdministeredObjects();
if (metaDatas != null) {
for(AdministeredObjectMetaData metaData : metaDatas) {
injectionSources.addResourceDefinitionInjectionSource(getResourceDefinitionInjectionSource(metaData));
}
}
}
private ResourceDefinitionInjectionSource getResourceDefinitionInjectionSource(final AdministeredObjectMetaData metaData) {
final String name = metaData.getName();
final String className = metaData.getClassName();
final String resourceAdapter = metaData.getResourceAdapter();
final AdministeredObjectDefinitionInjectionSource resourceDefinitionInjectionSource = new AdministeredObjectDefinitionInjectionSource(name, className, resourceAdapter);
resourceDefinitionInjectionSource.setInterface(metaData.getInterfaceName());
if (metaData.getDescriptions() != null) {
resourceDefinitionInjectionSource.setDescription(metaData.getDescriptions().toString());
}
resourceDefinitionInjectionSource.addProperties(metaData.getProperties());
return resourceDefinitionInjectionSource;
}
}
----
and
[source, java]
----
/**
* Deployment processor responsible for processing {@link javax.resource.AdministeredObjectDefinition} and {@link javax.resource.AdministeredObjectDefinitions}.
*
* @author <NAME>
* @author <NAME>
*/
public class AdministeredObjectDefinitionAnnotationProcessor extends ResourceDefinitionAnnotationProcessor {
private static final DotName ANNOTATION_NAME = DotName.createSimple(AdministeredObjectDefinition.class.getName());
private static final DotName COLLECTION_ANNOTATION_NAME = DotName.createSimple(AdministeredObjectDefinitions.class.getName());
@Override
protected DotName getAnnotationDotName() {
return ANNOTATION_NAME;
}
@Override
protected DotName getAnnotationCollectionDotName() {
return COLLECTION_ANNOTATION_NAME;
}
@Override
protected ResourceDefinitionInjectionSource processAnnotation(AnnotationInstance annotationInstance) throws DeploymentUnitProcessingException {
final String name = AnnotationElement.asRequiredString(annotationInstance, AnnotationElement.NAME);
final String className = AnnotationElement.asRequiredString(annotationInstance, "className");
final String ra = AnnotationElement.asRequiredString(annotationInstance, "resourceAdapter");
final AdministeredObjectDefinitionInjectionSource directAdministeredObjectInjectionSource =
new AdministeredObjectDefinitionInjectionSource(name, className, ra);
directAdministeredObjectInjectionSource.setDescription(AnnotationElement.asOptionalString(annotationInstance,
AdministeredObjectDefinitionInjectionSource.DESCRIPTION));
directAdministeredObjectInjectionSource.setInterface(AnnotationElement.asOptionalString(annotationInstance,
AdministeredObjectDefinitionInjectionSource.INTERFACE));
directAdministeredObjectInjectionSource.addProperties(AnnotationElement.asOptionalStringArray(annotationInstance,
AdministeredObjectDefinitionInjectionSource.PROPERTIES));
return directAdministeredObjectInjectionSource;
}
}
----
[IMPORTANT]
The abstract processors with respect to Resource Definitions are already
submitted through WFLY-3292's PR.
[[resource-ref-processing]]
== Resource Ref Processing
TODO for now no changes on this in the reworked WildFly Naming.
<file_sep>/_docs/14/_extending-wildfly/Key_Interfaces_and_Classes_Relevant_to_Extension_Developers.adoc
[[Key_Interfaces_and_Classes_Relevant_to_Extension_Developers]]
= Key Interfaces and Classes Relevant to Extension Developers
In the first major section of this guide, we provided an example of how
to implement an extension to the AS. The emphasis there was learning by
doing. In this section, we'll focus a bit more on the major WildFly
interfaces and classes that most are relevant to extension developers.
The best way to learn about these interfaces and classes in detail is to
look at their javadoc. What we'll try to do here is provide a brief
introduction of the key items and how they relate to each other.
Before digging into this section, readers are encouraged to read the
"Core Management Concepts" section of the Admin Guide.
[[extension-interface]]
== Extension Interface
The `org.jboss.as.controller.Extension` interface is the hook by which
your extension to the AS kernel is able to integrate with the AS. During
boot of the AS, when the `<extension>` element in the AS's xml
configuration file naming your extension is parsed, the JBoss Modules
module named in the element's name attribute is loaded. The standard JDK
`java.lang.ServiceLoader` mechanism is then used to load your module's
implementation of this interface.
The function of an `Extension` implementation is to register with the
core AS the management API, xml parsers and xml marshallers associated
with the extension module's subsystems. An `Extension` can register
multiple subsystems, although the usual practice is to register just one
per extension.
Once the `Extension` is loaded, the core AS will make two invocations
upon it:
* `void initializeParsers(ExtensionParsingContext context)`
When this is invoked, it is the `Extension` implementation's
responsibility to initialize the XML parsers for this extension's
subsystems and register them with the given `ExtensionParsingContext`.
The parser's job when it is later called is to create
`org.jboss.dmr.ModelNode` objects representing WildFly management API
operations needed make the AS's running configuration match what is
described in the xml. Those management operation `ModelNode` s are added
to a list passed in to the parser.
A parser for each version of the xml schema used by a subsystem should
be registered. A well behaved subsystem should be able to parse any
version of its schema that it has ever published in a final release.
* `void initialize(ExtensionContext context)`
When this is invoked, it is the `Extension` implementation's
responsibility to register with the core AS the management API for its
subsystems, and to register the object that is capable of marshalling
the subsystem's in-memory configuration back to XML. Only one XML
marshaller is registered per subsystem, even though multiple XML parsers
can be registered. The subsystem should always write documents that
conform to the latest version of its XML schema.
The registration of a subsystem's management API is done via the
`ManagementResourceRegistration` interface. Before discussing that
interface in detail, let's describe how it (and the related `Resource`
interface) relate to the notion of managed resources in the AS.
[[wildfly-managed-resources]]
== Wild**Fly** Managed Resources
Each subsystem is responsible for managing one or more management
resources. The conceptual characteristics of a management resource are
covered in some detail in the link:Admin_Guide{outfilesuffix}#Management_resources[Admin
Guide]; here we'll just summarize the main points. A management resource
has
* An *_address_* consisting of a list of key/value pairs that uniquely
identifies a resource
* Zero or more *_attributes_* , the value of which is some sort of
`org.jboss.dmr.ModelNode`
* Zero or more supported *_operations_* . An operation has a string name
and zero or more parameters, each of which is a key/value pair where the
key is a string naming the parameter and the value is some sort of
`ModelNode`
* Zero or more *_children_* , each of which in turn is a managed
resource
The implementation of a managed resource is somewhat analogous to the
implementation of a Java object. A managed resource will have a "type",
which encapsulates API information about that resource and logic used to
implement that API. And then there are actual instances of the resource,
which primarily store data representing the current state of a
particular resource. This is somewhat analogous to the "class" and
"object" notions in Java.
A managed resource's type is encapsulated by the
`org.jboss.as.controller.registry.ManagementResourceRegistration` the
core AS creates when the type is registered. The data for a particular
instance is encapsulated in an implementation of the
`org.jboss.as.controller.registry.Resource` interface.
[[managementresourceregistration-interface]]
== ManagementResourceRegistration Interface
In the Java analogy used above, the `ManagementResourceRegistration` is
analogous to the "class", while the `Resource` discussed below is
analogous to an instance of that class.
A `ManagementResourceRegistration` represents the specification for a
particular managed resource type. All resources whose address matches
the same pattern will be of the same type, specified by the type's
`ManagementResourceRegistration`. The MRR encapsulates:
* A `PathAddress` showing the address pattern that matches resources of
that type. This `PathAddress` can and typically does involve wildcards
in the value of one or more elements of the address. In this case there
can be more than one instance of the type, i.e. different `Resource`
instances.
* Definition of the various attributes exposed by resources of this
type, including the `OperationStepHandler` implementations used for
reading and writing the attribute values.
* Definition of the various operations exposed by resources of this
type, including the `OperationStepHandler` implementations used for
handling user invocations of those operations.
* Definition of child resource types. `ManagementResourceRegistration`
instances form a tree.
* Definition of management notifications emitted by resources of this
type.
* Definition of
<<Working_with_WildFly_Capabilities,capabilities>> provided by
resources of this type.
* Definition of link:Admin_Guide{outfilesuffix}#RBAC[RBAC] access constraints that should be
applied by the management kernel when authorizing operations against
resources of this type.
* Whether the resource type is an alias to another resource type, and if
so information about that relationship. Aliases are primarily used to
preserve backwards compatibility of the management API when the location
of a given type of resources is moved in a newer release.
The `ManagementResourceRegistration` interface is a subinterface of
`ImmutableManagementResourceRegistration`, which provides a read-only
view of the information encapsulated by the MRR. The MRR subinterface
adds the methods needed for registering the attributes, operations,
children, etc.
Extension developers do not directly instantiate an MRR. Instead they
create a `ResourceDefinition` for the root resource type for each
subsystem, and register it with the `ExtensionContext` passed in to
their `Extension` implementation's `initialize` method:
[source, java]
----
public void initialize(ExtensionContext context) {
SubsystemRegistration subsystem = context.registerSubsystem(SUBSYSTEM_NAME, CURRENT_VERSION);
subsystem.registerXMLElementWriter(getOurXmlWriter());
ResourceDefinition rd = getOurSubsystemDefinition();
ManagementResourceRegistration mrr = subsystem.registerSubsystemModel(rd));
}
----
The kernel uses the provided `ResourceDefinition` to construct a
`ManagementResourceRegistration` and then passes that MRR to the various
`registerXXX` methods implemented by the `ResourceDefinition`, giving it
the change to record the resource type's attributes, operations and
children.
[[resourcedefinition-interface]]
== ResourceDefinition Interface
An implementation of `ResourceDefinition` is the primary class used by
an extension developer when defining a managed resource type. It
provides basic information about the type, exposes a
`DescriptionProvider` used to generate a DMR description of the type,
and implements callbacks the kernel can invoke when building up the
`ManagementResourceRegistration` to ask for registration of definitions
of attributes, operations, children, notifications and capabilities.
Almost always an extension author will create their `ResourceDefinition`
by creating a subclass of the
`org.jboss.as.controller.SimpleResourceDefinition` class or of its
`PersistentResourceDefinition` subclass. Both of these classes have
constructors that take a `Parameters` object, which is a simple builder
class to use to provide most of the key information about the resource
type. The extension-specific subclass would then take responsibility for
any additional behavior needed by overriding the `registerAttributes`,
`registerOperations`, `registerNotifications` and `registerChildren`
callbacks to do whatever is needed beyond what is provided by the
superclasses.
For example, to add a writable attribute:
[source, java]
----
@Override
public void registerAttributes(ManagementResourceRegistration resourceRegistration) {
super.registerAttributes(resourceRegistration);
// Now we register the 'foo' attribute
AttributeDefinition ad = FOO; // constant declared elsewhere
OperationStepHandler writeHandler = new FooWriteAttributeHandler();
resourceRegistration.registerReadWriteHandler(ad, null, writeHandler); // null read handler means use default read handling
}
----
To register a custom operation:
[source, java]
----
@Override
public void registerOperations(ManagementResourceRegistration resourceRegistration) {
super.registerOperations(resourceRegistration);
// Now we register the 'foo-bar' custom operation
OperationDefinition od = FooBarOperationStepHandler.getDefinition();
OperationStepHandler osh = new FooBarOperationStepHandler();
resourceRegistration.registerOperationHandler(od, osh);
}
----
To register a child resource type:
[source, java]
----
@Override
public void registerChildren(ManagementResourceRegistration resourceRegistration) {
super.registerChildren(resourceRegistration);
// Now we register the 'baz=*' child type
ResourceDefinition rd = new BazResourceDefinition();
resourceRegistration.registerSubmodel(rd);
}
----
[[resourcedescriptionresolver]]
=== ResourceDescriptionResolver
One of the things a `ResourceDefinition` must be able to do is provide a
`DescriptionProvider` that provides a proper DMR description of the
resource to use as the output for the standard
`read-resource-description` management operation. Since you are almost
certainly going to be using one of the standard `ResourceDefinition`
implementations like `SimpleResourceDefinition`, the creation of this
`DescriptionProvider` is largely handled for you. The one thing that is
not handled for you is providing the localized free form text
descriptions of the various attributes, operations, operation
parameters, child types, etc used in creating the resource description.
For this you must provide an implementation of the
`ResourceDescriptionResolver` interface, typically passed to the
`Parameters` object provided to the `SimpleResourceDefinition`
constructor. This interface has various methods that are invoked when a
piece of localized text description is needed.
Almost certainly you'll satisfy this requirement by providing an
instance of the `StandardResourceDescriptionResolver` class.
`StandardResourceDescriptionResolver` uses a `ResourceBundle` to load
text from a properties file available on the classpath. The keys in the
properties file must follow patterns expected by
`StandardResourceDescriptionResolver`. See the
`StandardResourceDescriptionResolver` javadoc for further details.
The biggest task here is to create the properties file and add the text
descriptions. A text description must be provided for everything. The
typical thing to do is to store this properties file in the same package
as your `Extension` implementation, in a file named
`LocalDescriptions.properties`.
[[attributedefinition-class]]
== AttributeDefinition Class
The `AttributeDefinition` class is used to create the static definition
of one of a managed resource's attributes. It's a bit poorly named
though, because the same interface is used to define the details of
parameters to operations, and to define fields in the result of of
operations.
The definition includes all the static information about the
attribute/operation parameter/result field, e.g. the DMR `ModelType` of
its value, whether its presence is required, whether it supports
expressions, etc. See
link:Admin_Guide{outfilesuffix}#Description_of_the_Management_Model[Description of the
Management Model] for a description of the metadata available. Almost
all of this comes from the `AttributeDefinition`.
Besides basic metadata, the `AttributeDefinition` can also hold custom
logic the kernel should use when dealing with the attribute/operation
parameter/result field. For example, a `ParameterValidator` to use to
perform special validation of values (beyond basic things like DMR type
checks and defined/undefined checks), or an `AttributeParser` or
`AttributeMarshaller` to use to perform customized parsing from and
marshaling to XML.
WildFly Core's `controller` module provides a number of subclasses of
`AttributeDefinition` used for the usual kinds of attributes. For each
there is an associated builder class which you should use to build the
`AttributeDefinition`. Most commonly used are
`SimpleAttributeDefinition`, built by the associated
`SimpleAttributeDefinitionBuilder`. This is used for attributes whose
values are analogous to java primitives, `String` or byte[]. For
collections, there are various subclasses of `ListAttributeDefinition`
and `MapAttributeDefinition`. All have a `Builder` inner class. For
complex attributes, i.e. those with a fixed set of fully defined fields,
use `ObjectTypeAttributeDefinition`. (Each field in the complex type is
itself specified by an `AttributeDefinition`.) Finally there's
`ObjectListAttributeDefinition` and `ObjectMapAttributeDefinition` for
lists whose elements are complex types and maps whose values are complex
types respectively.
Here's an example of creating a simple attribute definition with extra
validation of the range of allowed values:
[source, java]
----
static final AttributeDefinition QUEUE_LENGTH = new SimpleAttributeDefinitionBuilder("queue-length", ModelType.INT)
.setRequired(true)
.setAllowExpression(true)
.setValidator(new IntRangeValidator(1, Integer.MAX_VALUE))
.setRestartAllServices() // means modification after resource add puts the server in reload-required
.build();
----
Via a bit of dark magic, the kernel knows that the `IntRangeValidator`
defined here is a reliable source of information on min and max values
for the attribute, so when creating the `read-resource-description`
output for the attribute it will use it and output `min` and `max`
metadata. For STRING attributes, `StringLengthValidator` can also be
used, and the kernel will see this and provide `min-length` and
`max-length` metadata. In both cases the kernel is checking for the
presence of a `MinMaxValidator` and if found it provides the appropriate
metadata based on the type of the attribute.
Use `EnumValidator` to restrict a STRING attribute's values to a set of
legal values:
[source, java]
----
static final SimpleAttributeDefinition TIME_UNIT = new SimpleAttributeDefinitionBuilder("unit", ModelType.STRING)
.setRequired(true)
.setAllowExpression(true)
.setValidator(new EnumValidator<TimeUnit>(TimeUnit.class))
.build();
----
`EnumValidator` is an implementation of `AllowedValuesValidator` that
works with Java enums. You can use other implementations or write your
own to do other types of restriction to certain values.
Via a bit of dark magic similar to what is done with `MinMaxValidator`,
the kernel recognizes the presence of an `AllowedValuesValidator` and
uses it to seed the `allowed-values` metadata in
`read-resource-description` output.
[[key-uses-of-attributedefinition]]
=== Key Uses of AttributeDefinition
Your `AttributeDefinition` instances will be some of the most commonly
used objects in your extension code. Following are the most typical
uses. In each of these examples assume there is a
`SimpleAttributeDefinition` stored in a constant FOO_AD that is
available to the code. Typically FOO_AD would be a constant in the
relevant `ResourceDefinition` implementation class. Assume FOO_AD
represents an INT attribute.
Note that for all of these cases except for "Use in Extracting Data from
the Configuration Model for Use in Runtime Services" there may be
utility code that handles this for you. For example
`PersistentResourceXMLParser` can handle the XML cases, and
`AbstractAddStepHandler` can handle the "Use in Storing Data Provided by
the User to the Configuration Model" case.
[[use-in-xml-parsing]]
==== Use in XML Parsing
Here we have your extension's implementation of
`XMLElementReader<List<ModelNode>>` that is being used to parse the xml
for your subsystem and add `ModelNode` operations to the list that will
be used to boot the server.
[source, java]
----
@Override
public void readElement(final XMLExtendedStreamReader reader, final List<ModelNode> operationList) throws XMLStreamException {
// Create a node for the op to add our subsystem
ModelNode addOp = new ModelNode();
addOp.get("address").add("subsystem", "mysubsystem");
addOp.get("operation").set("add");
operationList.add(addOp);
for (int i = 0; i < reader.getAttributeCount(); i++) {
final String value = reader.getAttributeValue(i);
final String attribute = reader.getAttributeLocalName(i);
if (FOO_AD.getXmlName().equals(attribute) {
FOO_AD.parseAndSetParameter(value, addOp, reader);
} else ....
}
... more parsing
}
----
Note that the parsing code has deliberately been abbreviated. The key
point is the `parseAndSetParameter` call. FOO_AD will validate the
`value` read from XML, throwing an XMLStreamException with a useful
message if invalid, including a reference to the current location of the
`reader`. If valid, `value` will be converted to a DMR `ModelNode` of
the appropriate type and stored as a parameter field of `addOp`. The
name of the parameter will be what `FOO_AD.getName()` returns.
If you use `PersistentResourceXMLParser` this parsing logic is handled
for you and you don't need to write it yourself.
[[use-in-storing-data-provided-by-the-user-to-the-configuration-model]]
==== Use in Storing Data Provided by the User to the Configuration Model
Here we illustrate code in an `OperationStepHandler` that extracts a
value from a user-provided `operation` and stores it in the internal
model:
[source, java]
----
@Override
public void execute(OperationContext context, ModelNode operation) throws OperationFailedException {
// Get the Resource targeted by this operation
Resource resource = context.readResourceForUpdate(PathAddress.EMPTY_ADDRESS);
ModelNode model = resource.getModel();
// Store the value of any 'foo' param to the model's 'foo' attribute
FOO_AD.validateAndSet(operation, model);
... do other stuff
}
----
As the name implies `validateAndSet` will validate the value in
`operation` before setting it. A validation failure will result in an
`OperationFailedException` with an appropriate message, which the kernel
will use to provide a failure response to the user.
Note that `validateAndSet` will not perform expression resolution.
Expression resolution is not appropriate at this stage, when we are just
trying to store data to the persistent configuration model. However, it
will check for expressions and fail validation if found and FOO_AD
wasn't built with `setAllowExpressions(true)`.
This work of storing data to the configuration model is usually done in
handlers for the `add` and `write-attribute` operations. If you base
your handler implementations on the standard classes provided by WildFly
Core, this part of the work will be handled for you.
[[use-in-extracting-data-from-the-configuration-model-for-use-in-runtime-services]]
==== Use in Extracting Data from the Configuration Model for Use in
Runtime Services
This is the example you are most likely to use in your code, as this is
where data needs to be extracted from the configuration model and passed
to your runtime services. What your services need is custom, so there's
no utility code we provide.
Assume as part of `... do other stuff` in the last example that your
handler adds a step to do further work once operation execution proceeds
to RUNTIME state (see Operation Execution and the `OperationContext` for
more on what this means):
[source, java]
----
context.addStep(new OperationStepHandler() {
@Override
public void execute(OperationContext context, ModelNode operation) throws OperationFailedException {
// Get the Resource targetted by this operation
Resource resource = context.readResource(PathAddress.EMPTY_ADDRESS);
ModelNode model = resource.getModel();
// Extract the value of the 'foo' attribute from the model
int foo = FOO_AD.resolveModelAttribute(context, model).asInt();
Service<XyZ> service = new MyService(foo);
... do other stuff, like install 'service' with MSC
}
}, Stage.RUNTIME);
----
Use `resolveModelAttribute` to extract data from the model. It does a
number of things:
* reads the value from the model
* if it's an expression and expressions are supported, resolves it
* if it's undefined and undefined is allowed but FOO_AD was configured
with a default value, uses the default value
* validates the result of that (which is how we check that expressions
resolve to legal values), throwing OperationFailedException with a
useful message if invalid
* returns that as a `ModelNode`
If when you built FOO_AD you configured it such that the user must
provide a value, or if you configured it with a default value, then you
know the return value of `resolveModelAttribute` will be a defined
`ModelNode`. Hence you can safely perform type conversions with it, as
we do in the example above with the call to `asInt()`. If FOO_AD was
configured such that it's possible that the attribute won't have a
defined value, you need to guard against that, e.g.:
[source, java]
----
ModelNode node = FOO_AD.resolveModelAttribute(context, model);
Integer foo = node.isDefined() ? node.asInt() : null;
----
[[use-in-marshaling-configuration-model-data-to-xml]]
==== Use in Marshaling Configuration Model Data to XML
Your `Extension` must register an
`XMLElementWriter<SubsystemMarshallingContext>` for each subsystem. This
is used to marshal the subsystem's configuration to XML. If you don't
use `PersistentResourceXMLParser` for this you'll need to write your own
marshaling code, and `AttributeDefinition` will be used.
[source, java]
----
@Override
public void writeContent(XMLExtendedStreamWriter writer, SubsystemMarshallingContext context) throws XMLStreamException {
context.startSubsystemElement(Namespace.CURRENT.getUriString(), false);
ModelNode subsystemModel = context.getModelNode();
// we persist foo as an xml attribute
FOO_AD.marshalAsAttribute(subsystemModel, writer);
// We also have a different attribute that we marshal as an element
BAR_AD.marshalAsElement(subsystemModel, writer);
}
----
The `SubsystemMarshallingContext` provides a `ModelNode` that represents
the entire resource tree for the subsystem (including child resources).
Your `XMLElementWriter` should walk through that model, using
`marshalAsAttribute` or `marshalAsElement` to write the attributes in
each resource. If the model includes child node trees that represent
child resources, create child xml elements for those and continue down
the tree.
[[operationdefinition-and-operationstephandler-interfaces]]
== OperationDefinition and OperationStepHandler Interfaces
`OperationDefinition` defines an operation, particularly its name, its
parameters and the details of any result value, with
`AttributeDefinition` instances used to define the parameters and result
details. The `OperationDefinition` is used to generate the
`read-operation-description` output for the operation, and in some cases
is also used by the kernel to decide details as to how to execute the
operation.
Typically `SimpleOperationDefinitionBuilder` is used to create an
`OperationDefinition`. Usually you only need to create an
`OperationDefinition` for custom operations. For the common `add` and
`remove` operations, if you provide minimal information about your
handlers to your `SimpleResourceDefinition` implementation via the
`Parameters` object passed to its constructor, then
`SimpleResourceDefinition` can generate a correct `OperationDefinition`
for those operations.
The `OperationStepHandler` is what contains the actual logic for doing
what the user requests when they invoke an operation. As its name
implies, each OSH is responsible for doing one step in the overall
sequence of things necessary to give effect to what the user requested.
One of the things an OSH can do is add other steps, with the result that
an overall operation can involve a great number of OSHs executing. (See
Operation Execution and the `OperationContext` for more on this.)
Each OSH is provided in its `execute` method with a reference to the
`OperationContext` that is controlling the overall operation, plus an
`operation` `ModelNode` that represents the operation that particular
OSH is being asked to deal with. The `operation` node will be of
`ModelType.OBJECT` with the following key/value pairs:
* a key named `operation` with a value of `ModelType.STRING` that
represents the name of the operation. Typically an OSH doesn't care
about this information as it is written for an operation with a
particular name and will only be invoked for that operation.
* a key named `address` with a value of `ModelType.LIST` with list
elements of `ModelType.PROPERTY`. This value represents the address of
the resource the operation targets. If this key is not present or the
value is undefined or an empty list, the target is the root resource.
Typically an OSH doesn't care about this information as it can more
efficiently get the address from the `OperationContext` via its
`getCurrentAddress()` method.
* other key/value pairs that represent parameters to the operation, with
the key the name of the parameter. This is the main information an OSH
would want from the `operation` node.
There are a variety of situations where extension code will instantiate
an `OperationStepHandler`
* When registering a writable attribute with a
`ManagementResourceRegistration` (typically in an implementation of
`ResourceDefinition.registerAttributes`), an OSH must be provided to
handle the `write-attribute` operation.
* When registering a read-only or read-write attribute that needs
special handling of the `read-attribute` operation, an OSH must be
provided.
* When registering a metric attribute, an OSH must be provided to handle
the `read-attribute` operation.
* Most resources need OSHs created for the `add` and `remove`
operations. These are passed to the `Parameters` object given to the
`SimpleResourceDefinition` constructor, for use by the
`SimpleResourceDefinition` in its implementation of the
`registerOperations` method.
* If your resource has custom operations, you will instantiate them to
register with a `ManagementResourceRegistration`, typically in an
implementation of `ResourceDefinition.registerOperations`
* If an OSH needs to tell the `OperationContext` to add additional steps
to do further handling, the OSH will create another OSH to execute that
step. This second OSH is typically an inner class of the first OSH.
[[operation-execution-and-the-operationcontext]]
== Operation Execution and the OperationContext
When the `ModelController` at the heart of the WildFly Core management
layer handles a request to execute an operation, it instantiates an
implementation of the `OperationContext` interface to do the work. The
`OperationContext` is configured with an initial list of operation steps
it must execute. This is done in one of two ways:
* During boot, multiple steps are configured, one for each operation in
the list generated by the parser of the xml configuration file. For each
operation, the `ModelController` finds the
`ManagementResourceRegistration` that matches the address of the
operation and finds the `OperationStepHandler` registered with that MRR
for the operation's name. A step is added to the `OperationContext` for
each operation by providing the operation `ModelNode` itself, plus the
`OperationStepHandler`.
* After boot, any management request involves only a single operation,
so only a single step is added. (Note that a `composite` operation is
still a single operation; it's just one that internally executes via
multiple steps.)
The `ModelController` then asks the `OperationContext` to execute the
operation.
The `OperationContext` acts as both the engine for operation execution,
and as the interface provided to `OperationStepHandler` implementations
to let them interact with the rest of the system.
[[execution-process]]
=== Execution Process
Operation execution proceeds via execution by the `OperationContext` of
a series of "steps" with an `OperationStepHandler` doing the key work
for each step. As mentioned above, during boot the OC is initially
configured with a number of steps, but post boot operations involve only
a single step initially. But even a post-boot operation can end up
involving numerous steps before completion. In the case of a
`/:read-resource(recursive=true)` operation, thousands of steps might
execute. This is possible because one of the key things an
`OperationStepHandler` can do is ask the `OperationContext` to add
additional steps to execute later.
Execution proceeds via a series of "stages", with a queue of steps
maintained for each stage. An `OperationStepHandler` can tell the
`OperationContext` to add a step for any stage equal to or later than
the currently executing stage. The instruction can either be to add the
step to the head of the queue for the stage or to place it at the end of
the stage's queue.
Execution of a stage continues until there are no longer any steps in
the stage's queue. Then an internal transition task can execute, and the
processing of the next stage's steps begins.
Here is some brief information about each stage:
[[stage.model]]
==== Stage.MODEL
This stage is concerned with interacting with the persistent
configuration model, either making changes to it or reading information
from it. Handlers for this stage should not make changes to the runtime,
and handlers running after this stage should not make changes to the
persistent configuration model.
If any step fails during this stage, the operation will automatically
roll back. Rollback of MODEL stage failures cannot be turned off.
Rollback during boot results in abort of the process start.
The initial step or steps added to the `OperationContext` by the
`ModelController` all execute in Stage.MODEL. This means that all
`OperationStepHandler` instances your extension registers with a
`ManagementResourceRegistration` must be designed for execution in
`Stage.MODEL`. If you need work done in later stages your `Stage.MODEL`
handler must add a step for that work.
When this stage completes, the `OperationContext` internally performs
model validation work before proceeding on to the next stage. Validation
failures will result in rollback.
[[stage.runtime]]
==== Stage.RUNTIME
This stage is concerned with interacting with the server runtime, either
reading from it or modifying it (e.g. installing or removing services or
updating their configuration.) By the time this stage begins, all model
changes are complete and model validity has been checked. So typically
handlers in this stage read their inputs from the model, not from the
original `operation` `ModelNode` provided by the user.
Most `OperationStepHandler` logic written by extension authors will be
for Stage.RUNTIME. The vast majority of Stage.MODEL handling can best be
performed by the base handler classes WildFly Core provides in its
`controller` module. (See below for more on those.)
During boot failures in `Stage.RUNTIME` will not trigger rollback and
abort of the server boot. After boot, by default failures here will
trigger rollback, but users can prevent that by using the
`rollback-on-runtime-failure` header. However, a RuntimeException thrown
by a handler will trigger rollback.
At the end of `Stage.RUNTIME`, the `OperationContext` blocks waiting for
the MSC service container to stabilize (i.e. for all services to have
reached a rest state) before moving on to the next stage.
[[stage.verify]]
==== Stage.VERIFY
Service container verification work is performed in this stage, checking
that any MSC changes made in `Stage.RUNTIME` had the expected effect.
Typically extension authors do not add any steps in this stage, as the
steps automatically added by the `OperationContext` itself are all that
are needed. You can add a step here though if you have an unusual use
case where you need to verify something after MSC has stabilized.
Handlers in this stage should not make any further runtime changes;
their purpose is simply to do verification work and fail the operation
if verification is unsuccessful.
During boot failures in `Stage.VERIFY` will not trigger rollback and
abort of the server boot. After boot, by default failures here will
trigger rollback, but users can prevent that by using the
`rollback-on-runtime-failure` header. However, a RuntimeException thrown
by a handler will trigger rollback.
There is no special transition work at the end of this stage.
[[stage.domain]]
==== Stage.DOMAIN
Extension authors should not add steps in this stage; it is only for use
by the kernel.
Steps needed to execute rollout across the domain of an operation that
affects multiple processes in a managed domain run here. This stage is
only run on Host Contoller processes, never on servers.
[[stage.done-and-resulthandler-rollbackhandler-execution]]
==== Stage.DONE and ResultHandler / RollbackHandler Execution
This stage doesn't maintain a queue of steps; no `OperationStepHandler`
executes here. What does happen here is persistence of any configuration
changes to the xml file and commit or rollback of changes affecting
multiple processes in a managed domain.
While no `OperationStepHandler` executes in this stage, following
persistence and transaction commit all `ResultHandler` or
`RollbackHandler` callbacks registered with the `OperationContext` by
the steps that executed are invoked. This is done in the reverse order
of step execution, so the callback for the last step to run is the first
to be executed. The most common thing for a callback to do is to respond
to a rollback by doing whatever is necessary to reverse changes made in
`Stage.RUNTIME`. (No reversal of `Stage.MODEL` changes is needed,
because if an operation rolls back the updated model produced by the
operation is simply never published and is discarded.)
[[tips-about-adding-steps]]
==== Tips About Adding Steps
Here are some useful tips about how to add steps:
* Add a step to the head of the current stage's queue if you want it to
execute next, prior to any other steps. Typically you would use this
technique if you are trying to decompose some complex work into pieces,
with reusable logic handling each piece. There would be an
`OperationStepHandler` for each part of the work, added to the head of
the queue in the correct sequence. This would be a pretty advanced use
case for an extension author but is quite common in the handlers
provided by the kernel.
* Add a step to the end of the queue if either you don't care when it
executes or if you do care and want to be sure it executes after any
already registered steps.
** A very common example of this is a `Stage.MODEL` handler adding a
step for its associated `Stage.RUNTIME` work. If there are multiple
model steps that will execute (e.g. at boot or as part of handling a
`composite`), each will want to add a runtime step, and likely the best
order for those runtime steps is the same as the order of the model
steps. So if each adds its runtime step at the end, the desired result
will be achieved.
** A more sophisticated but important scenario is when a step may or may
not be executing as part of a larger set of steps, i.e. it may be one
step in a `composite` or it may not. There is no way for the handler to
know. But it can assume that if it is part of a composite, the steps for
the other operations in the composite *are already registered in the
queue*. (The handler for the `composite` op guarantees this.) So, if it
wants to do some work (say validation of the relationship between
different attributes or resources) the input to which may be affected by
possible other already registered steps, instead of doing that work
itself, it should register a different step at the *end* of the queue
and have that step do the work. This will ensure that when the
validation step runs, the other steps in the `composite` will have had a
chance to do their work. *Rule of thumb: always doing any extra
validation work in an added step.*
[[passing-data-to-an-added-step]]
===== Passing Data to an Added Step
Often a handler author will want to share state between the handler for
a step it adds and the handler that added it. There are a number of ways
this can be done:
* Very often the `OperationStepHandler` for the added class is an inner
class of the handler that adds it. So here sharing state is easily done
using final variables in the outer class.
* The handler for the added step can accept values passed to its
constructor which can serve as shared state.
* The `OperationContext` includes an Attachment API which allows
arbitary data to be attached to the context and retrieved by any handler
that has access to the attachment key.
* The `OperationContext.addStep` methods include overloaded variants
where the caller can pass in an `operation` `ModelNode` that will in
turn be passed to the `execute` method of the handler for the added
step. So, state can be passed via this `ModelNode`. It's important to
remember though that the `address` field of the `operation` will govern
what the `OperationContext` sees as the target of operation when that
added step's handler executes.
[[controlling-output-from-an-added-step]]
===== Controlling Output from an Added Step
When an `OperationStepHandler` wants to report an operation result, it
calls the `OperationContext.getResult()` method and manipulates the
returned `ModelNode`. Similarly for failure messages it can call
`OperationContext.getFailureDescription()`. The usual assumption when
such a call is made is that the result or failure description being
modified is the one at the root of the response to the end user. But
this is not necessarily the case.
When an `OperationStepHandler` adds a step it can use one of the
overloaded `OperationContext.addStep` variants that takes a `response`
`ModelNode` parameter. If it does, whatever `ModelNode` it passes in
will be what is updated as a result of `OperationContext.getResult()`
and `OperationContext.getFailureDescription()` calls by the step's
handler. This node does not need to be one that is directly associated
with the response to the user.
How then does the handler that adds a step in this manner make use of
whatever results the added step produces, since the added step will not
run until the adding step completes execution? There are a couple of
ways this can be done.
The first is to add yet another step, and provide it a reference to the
`response` node used by the second step. It will execute after the
second step and can read its response and use it in formulating its own
response.
The second way involves using a `ResultHandler`. The `ResultHandler` for
a step will execute *after* any step that it adds executes. And, it is
legal for a `ResultHandler` to manipulate the "result" value for an
operation, or its "failure-description" in case of failure. So, the
handler that adds a step can provide to its `ResultHandler` a reference
to the `response` node it passed to `addStep`, and the `ResultHandler`
can in turn and use its contents to manipulate its own response.
This kind of handling wouldn't commonly be done by extension authors and
great care needs to be taken if it is done. It is often done in some of
the kernel handlers.
[[operationstephandler-use-of-the-operationcontext]]
=== OperationStepHandler use of the OperationContext
All useful work an `OperationStepHandler` performs is done by invoking
methods on the `OperationContext`. The `OperationContext` interface is
extensively javadoced, so this section will just provide a brief partial
overview. The OSH can use the `OperationContext` to:
* Learn about the environment in which it is executing (
`getProcessType`, `getRunningMode`, `isBooting`, `getCurrentStage`,
`getCallEnvironment`, `getSecurityIdentity`, `isDefaultRequiresRuntime`,
`isNormalServer`)
* Learn about the operation ( `getCurrentAddress`,
`getCurrentAddressValue`, `getAttachmentStream`,
`getAttachmentStreamCount`)
* Read the `Resource` tree ( `readResource`, `readResourceFromRoot`,
`getOriginalRootResource`)
* Manipulate the `Resource` tree ( `createResource`, `addResource`,
`readResourceForUpdate`, `removeResource`)
* Read the resource type information ( `getResourceRegistration`,
`getRootResourceRegistration`)
* Manipulate the resource type information (
`getResourceRegistrationForUpdate`)
* Read the MSC service container ( `getServiceRegistry(false)`)
* Manipulate the MSC service container ( `getServiceTarget`,
`getServiceRegistry(true)`, `removeService`)
* Manipulate the process state ( `reloadRequired`,
`revertReloadRequired`, `restartRequired`, `revertRestartRequired`
* Resolve expressions ( `resolveExpressions`)
* Manipulate the operation response ( `getResult`,
`getFailureDescription`, `attachResultStream`, `runtimeUpdateSkipped`)
* Force operation rollback ( `setRollbackOnly`)
* Add other steps ( `addStep`)
* Share data with other steps ( `attach`, `attachIfAbsent`,
`getAttachment`, `detach`)
* Work with capabilities (numerous methods)
* Emit notifications ( `emit`)
* Request a callback to a `ResultHandler` or `RollbackHandler` (
`completeStep`)
[[locking-and-change-visibility]]
=== Locking and Change Visibility
The `ModelController` and `OperationContext` work together to ensure
that only one operation at a time is modifying the state of the system.
This is done via an exclusive lock maintained by the `ModelController`.
Any operation that does not need to write never requests the lock and is
able to proceed without being blocked by an operation that holds the
lock (i.e. writes do not block reads.) If two operations wish to
concurrently write, one or the other will get the lock and the loser
will block waiting for the winner to complete and release the lock.
The `OperationContext` requests the exclusive lock the first time any of
the following occur:
* A step calls one of its methods that indicates a wish to modify the
resource tree ( `createResource`, `addResource`,
`readResourceForUpdate`, `removeResource`)
* A step calls one of its methods that indicates a wish to modify the
`ManagementResourceRegistration` tree (
`getResourceRegistrationForUpdate`)
* A step calls one of its methods that indicates a desire to change MSC
services ( `getServiceTarget`, `removeService` or `getServiceRegistry`
with the `modify` param set to `true`)
* A step calls one of its methods that manipulates the capability
registry (various)
* A step explicitly requests the lock by calling the
`acquireControllerLock` method (doing this is discouraged)
The step that acquired the lock is tracked, and the lock is released
when the `ResultHandler` added by that step has executed. (If the step
doesn't add a result handler, a default no-op one is automatically
added).
When an operation first expresses a desire to manipulate the `Resource`
tree or the capability registry, a private copy of the tree or registry
is created and thereafter the `OperationContext` works with that copy.
The copy is published back to the `ModelController` in `Stage.DONE` if
the operation commits. Until that happens any changes to the tree or
capability registry made by the operation are invisible to other
threads. If the operation does not commit, the private copies are simply
discarded.
However, the `OperationContext` does not make a private copy of the
`ManagementResourceRegistration` tree before manipulating it, nor is
there a private copy of the MSC service container. So, any changes made
by an operation to either of those are immediately visible to other
threads.
[[resource-interface]]
== Resource Interface
An instance of the `Resource` interface holds the state for a particular
instance of a type defined by a `ManagementResourceRegistration`.
Referring back to the analogy mentioned earlier the
`ManagementResourceRegistration` is analogous to a Java class while the
`Resource` is analogous to an instance of that class.
The `Resource` makes available state information, primarily
* Some descriptive metadata, such as its address, whether it is
runtime-only and whether it represents a proxy to a another primary
resource that resides on another process in a managed domain
* A `ModelNode` of `ModelType.OBJECT` whose keys are the resource's
attributes and whose values are the attribute values
* Links to child resources such that the resources form a tree
[[creating-resources]]
=== Creating Resources
Typically extensions create resources via `OperationStepHandler` calls
to the `OperationContext.createResource` method. However it is allowed
for handlers to use their own `Resource` implementations by
instantiating the resource and invoking `OperationContext.addResource`.
The `AbstractModelResource` class can be used as a base class.
[[runtime-only-and-synthetic-resources-and-the-placeholderresourceentry-class]]
=== Runtime-Only and Synthetic Resources and the PlaceholderResourceEntry Class
A runtime-only resource is one whose state is not persisted to the xml
configuration file. Many runtime-only resources are also "synthetic"
meaning they are not added or removed as a result of user initiated
management operations. Rather these resources are "synthesized" in order
to allow users to use the management API to examine some aspect of the
internal state of the process. A good example of synthetic resources are
the resources in the `/core-service=platform-mbeans` branch of the
resource tree. There are resources there that represent various aspects
of the JVM (classloaders, memory pools, etc) but which resources are
present entirely depends on what the JVM is doing, not on any management
action. Another example are resources representing "core queues" in the
WildFly messaging and messaging-artemismq subsystems. Queues are created
as a result of activity in the message broker which may not involve
calls to the management API. But for each such queue a management
resource is available to allow management users to perform management
operations against the queue.
It is a requirement of execution of a management operation that the
`OperationContext` can navigate through the resource tree to a
`Resource` object located at the address specified. This requirement
holds true even for synthetic resources. How can this be handled, given
the fact these resources are not created in response to management
operations?
The trick involves using special implementations of `Resource`. Let's
imagine a simple case where we have a parent resource which is fairly
normal (i.e. it holds persistent configuration and is added via a user's
`add` operation) except for the fact that one of its child types
represents synthetic resources (e.g. message queues). How would this be
handled?
First, the parent resource would require a custom implementation of the
`Resource` interface. The `OperationStepHandler` for the `add` operation
would instantiate it, providing it with access to whatever API is needed
for it to work out what items exist for which a synthetic resource
should be made available (e.g. an API provided by the message broker
that provides access to its queues). The `add` handler would use the
`OperationContext.addResource` method to tie this custom resource into
the overall resource tree.
The custom `Resource` implementation would use special implementations
of the various methods that relate to accessing children. For all calls
that relate to the synthetic child type (e.g. core-queue) the custom
implementation would use whatever API call is needed to provide the
correct data for that child type (e.g. ask the message broker for the
names of queues).
A nice strategy for creating such a custom resource is to use
delegation. Use `Resource.Factory.create}()` to create a standard
resource. Then pass it to the constructor of your custom resource type
for use as a delegate. The custom resource type's logic is focused on
the synthetic children; all other work it passes on to the delegate.
What about the synthetic resources themselves, i.e. the leaf nodes in
this part of the tree? These are created on the fly by the parent
resource in response to `getChild`, `requireChild`, `getChildren` and
`navigate` calls that target the synthetic resource type. These
created-on-the-fly resources can be very lightweight, since they store
no configuration model and have no children. The
`PlaceholderResourceEntry` class is perfect for this. It's a very
lightweight `Resource` implementation with minimal logic that only
stores the final element of the resource's address as state.
See `LoggingResource` in the WildFly Core logging subsystem for an
example of this kind of thing. Searching for other uses of
`PlaceholderResourceEntry` will show other examples.
[[deploymentunitprocessor-interface]]
== DeploymentUnitProcessor Interface
TODO
[[useful-classes-for-implementing-operationstephandler]]
== Useful classes for implementing OperationStepHandler
The WildFly Core `controller` module includes a number of
`OperationStepHandler` implementations that in some cases you can use
directly, and that in other cases can serve as the base class for your
own handler implementation. In all of these a general goal is to
eliminate the need for your code to do anything in `Stage.MODEL` while
providing support for whatever is appropriate for `Stage.RUNTIME`.
[[add-handlers]]
=== Add Handlers
`AbstractAddStepHandler` is a base class for handlers for `add`
operations. There are a number of ways you can configure its behavior,
the most commonly used of which are to:
* Configure its behavior in `Stage.MODEL` by passing to its constructor
`AttributeDefinition` and `RuntimeCapability` instances for the
attributes and capabilities provided by the resource. The handler will
automatically validate the operation parameters whose names match the
provided attributes and store their values in the model of the newly
added `Resource`. It will also record the presence of the given
capabilities.
* Control whether a `Stage.RUNTIME` step for the operation needs to be
added, by overriding the
`protected boolean requiresRuntime(OperationContext context)` method.
Doing this is atypical; the standard behavior in the base class is
appropriate for most cases.
* Implement the primary logic of the `Stage.RUNTIME` step by overriding
the
`protected void performRuntime(final OperationContext context, final ModelNode operation, final Resource resource)`
method. This is typically the bulk of the code in an
`AbstractAddStepHandler` subclass. This is where you read data from the
`Resource` model and use it to do things like configure and install MSC
services.
* Handle any unusual needs of any rollback of the `Stage.RUNTIME` step
by overriding
`protected void rollbackRuntime(OperationContext context, final ModelNode operation, final Resource resource)`.
Doing this is not typically needed, since if the rollback behavior
needed is simply to remove any MSC services installed in
`performRuntime`, the `OperationContext` will do this for you
automatically.
`AbstractBoottimeAddStepHandler` is a subclass of
`AbstractAddStepHandler` meant for use by `add` operations that should
only do their normal `Stage.RUNTIME` work in server, boot, with the
server being put in `reload-required` if executed later. Primarily this
is used for `add` operations that register `DeploymentUnitProcessor`
implementations, as this can only be done at boot.
Usage of `AbstractBoottimeAddStepHandler` is the same as for
`AbstractAddStepHandler` except that instead of overriding
`performRuntime` you override
`protected void performBoottime(OperationContext context, ModelNode operation, Resource resource)`.
A typical thing to do in `performBoottime` is to add a special step that
registers one or more `DeploymentUnitProcessor` s.
[source, java]
----
@Override
public void performBoottime(OperationContext context, ModelNode operation, final Resource resource)
throws OperationFailedException {
context.addStep(new AbstractDeploymentChainStep() {
@Override
protected void execute(DeploymentProcessorTarget processorTarget) {
processorTarget.addDeploymentProcessor(RequestControllerExtension.SUBSYSTEM_NAME, Phase.STRUCTURE, Phase.STRUCTURE_GLOBAL_REQUEST_CONTROLLER, new RequestControllerDeploymentUnitProcessor());
}
}, OperationContext.Stage.RUNTIME);
... do other things
----
[[remove-handlers]]
=== Remove Handlers
TODO `AbstractRemoveStepHandler` `ServiceRemoveStepHandler`
[[write-attribute-handlers]]
=== Write attribute handlers
TODO `AbstractWriteAttributeHandler`
[[reload-required-handlers]]
=== Reload-required handlers
`ReloadRequiredAddStepHandler` `ReloadRequiredRemoveStepHandler`
`ReloadRequiredWriteAttributeHandler`
Use these for cases where, post-boot, the change to the configuration
model made by the operation cannot be reflected in the runtime until the
process is reloaded. These handle the mechanics of recording the need
for reload and reverting it if the operation rolls back.
[[restart-parent-resource-handlers]]
=== Restart Parent Resource Handlers
`RestartParentResourceAddHandler` `RestartParentResourceRemoveHandler`
`RestartParentWriteAttributeHandler`
Use these in cases where a management resource doesn't directly control
any runtime services, but instead simply represents a chunk of
configuration that a parent resource uses to configure services it
installs. (Really, this kind of situation is now considered to be a poor
management API design and is discouraged. Instead of using child
resources for configuration chunks, complex attributes on the parent
resource should be used.)
These handlers help you deal with the mechanics of the fact that,
post-boot, any change to the child resource likely requires a restart of
the service provided by the parent.
[[model-only-handlers]]
=== Model Only Handlers
`ModelOnlyAddStepHandler` `ModelOnlyRemoveStepHandler`
`ModelOnlyWriteAttributeHandler`
Use these for cases where the operation never affects the runtime, even
at boot. All it does is update the configuration model. In most cases
such a thing would be odd. These are primarily useful for legacy
subsystems that are no longer usable on current version servers and thus
will never do anything in the runtime. However, current version Domain
Controllers must be able to understand the subsystem's configuration
model to allow them to manage older Host Controllers running previous
versions where the subsystem is still usable by servers. So these
handlers allow the DC to maintain the configuration model for the
subsystem.
[[misc]]
=== Misc
`AbstractRuntimeOnlyHandler` is used for custom operations that don't
involve the configuration model. Create a subclass and implement the
`protected abstract void executeRuntimeStep(OperationContext context, ModelNode operation)`
method. The superclass takes care of adding a `Stage.RUNTIME` step that
calls your method.
`ReadResourceNameOperationStepHandler` is for cases where a resource
type includes a 'name' attribute whose value is simply the value of the
last element in the resource's address. There is no need to store the
value of such an attribute in the resource's model, since it can always
be determined from the resource address. But, if the value is not stored
in the resource model, when the attribute is registered with
`ManagementResourceRegistration.registerReadAttribute` an
`OperationStepHandler` to handle the `read-attribute` operation must be
provided. Use `ReadResourceNameOperationStepHandler` for this. (Note
that including such an attribute in your management API is considered to
be poor practice as it's just redundant data.)
<file_sep>/_docs/13/_high-availability/ha-singleton/Singleton_subsystem.adoc
[[Singleton_subsystem]]
== Singleton subsystem
WildFly 10 introduced a "singleton" subsystem, which defines a set of
policies that define how an HA singleton should behave. A singleton
policy can be used to instrument singleton deployments or to create
singleton MSC services.
[[singleton-configuration]]
== Configuration
The
https://github.com/wildfly/wildfly/blob/10.0.0.Final/clustering/singleton/extension/src/main/resources/schema/wildfly-singleton_1_0.xsd[default
subsystem configuration] from WildFly's ha and full-ha profile looks
like:
[source, xml]
----
<subsystem xmlns="urn:jboss:domain:singleton:1.0">
<singleton-policies default="default">
<singleton-policy name="default" cache-container="server">
<simple-election-policy/>
</singleton-policy>
</singleton-policies>
</subsystem>
----
A singleton policy defines:
1. A unique name
2. A cache container and cache with which to register singleton provider candidates
3. An election policy
4. A quorum (optional)
One can add a new singleton policy via the following management
operation:
[source]
----
/subsystem=singleton/singleton-policy=foo:add(cache-container=server)
----
[[cache-configuration]]
=== Cache configuration
The cache-container and cache attributes of a singleton policy must
reference a valid cache from the Infinispan subsystem. If no specific
cache is defined, the default cache of the cache container is assumed.
This cache is used as a registry of which nodes can provide a given
service and will typically use a replicated-cache configuration.
[[election-policies]]
=== Election policies
WildFly includes two singleton election policy implementations:
* *simple* +
Elects the provider (a.k.a. master) of a singleton service based on a
specified position in a circular linked list of eligible nodes sorted by
descending age. Position=0, the default value, refers to the oldest
node, 1 is second oldest, etc. ; while position=-1 refers to the
youngest node, -2 to the second youngest, etc. +
e.g.
+
[source]
----
/subsystem=singleton/singleton-policy=foo/election-policy=simple:add(position=-1)
----
* *random* +
Elects a random member to be the provider of a singleton service +
e.g.
+
[source]
----
/subsystem=singleton/singleton-policy=foo/election-policy=random:add()
----
[[preferences]]
==== Preferences
Additionally, any singleton election policy may indicate a preference
for one or more members of a cluster. Preferences may be defined either
via node name or via outbound socket binding name. Node preferences
always take precedent over the results of an election policy. +
e.g.
[source]
----
/subsystem=singleton/singleton-policy=foo/election-policy=simple:list-add(name=name-preferences, value=nodeA)
/subsystem=singleton/singleton-policy=bar/election-policy=random:list-add(name=socket-binding-preferences, value=nodeA)
----
[[quorum]]
=== Quorum
Network partitions are particularly problematic for singleton services,
since they can trigger multiple singleton providers for the same service
to run at the same time. To defend against this scenario, a singleton
policy may define a quorum that requires a minimum number of nodes to be
present before a singleton provider election can take place. A typical
deployment scenario uses a quorum of N/2 + 1, where N is the anticipated
cluster size. This value can be updated at runtime, and will immediately
affect any active singleton services. +
e.g.
[source]
----
/subsystem=singleton/singleton-policy=foo:write-attribute(name=quorum, value=3)
----
[[non-ha-environments]]
== Non-HA environments
The singleton subsystem can be used in a non-HA profile, so long as the
cache that it references uses a local-cache configuration. In this
manner, an application leveraging singleton functionality (via the
singleton API or using a singleton deployment descriptor) will continue
function as if the server was a sole member of a cluster. For obvious
reasons, the use of a quorum does not make sense in such a
configuration.
<file_sep>/_docs/14/_testsuite/WildFly_Testsuite_Test_Developer_Guide.adoc
[[WildFly_Testsuite_Test_Developer_Guide]]
= Wild**Fly** Testsuite Test Developer Guide
*See also:* <<WildFly_Integration_Testsuite_User_Guide,WildFly
Integration Testsuite User Guide>>
[[pre-requisites]]
== Pre-requisites
Please be sure to read <<Pre-requisites_-_test_quality_standards,Pre-requisites - test quality
standards>> and follow those guidelines.
[[arquillian-container-configuration]]
== Arquillian container configuration
See
https://docs.jboss.org/author/display/ARQ/JBoss+AS+7.1%2C+JBoss+EAP+6.0+-+Managed[AS
7.1 managed container adapter refrence].
[[managementclient-and-modelnode-usage-example]]
== ManagementClient and ModelNode usage example
[source, java]
----
final ModelNode operation = new ModelNode();
operation.get(ModelDescriptionConstants.OP).set(ModelDescriptionConstants.READ_RESOURCE_OPERATION);
operation.get(ModelDescriptionConstants.OP_ADDR).set(address);
operation.get(ModelDescriptionConstants.RECURSIVE).set(true);
final ModelNode result = managementClient.getControllerClient().execute(operation);
Assert.assertEquals(ModelDescriptionConstants.SUCCESS, result.get(ModelDescriptionConstants.OUTCOME).asString());
----
ManagementClient can be obtained as described below.
[[arquillian-features-available-in-tests]]
== Arquillian features available in tests
@ServerSetup
TBD
[source, java]
----
@ContainerResource private ManagementClient managementClient;
final ModelNode result = managementClient.getControllerClient().execute(operation);
----
TBD
[source, java]
----
@ArquillianResource private ManagementClient managementClient;
ModelControllerClient client = managementClient.getControllerClient();
----
[source, java]
----
@ArquillianResource ContainerController cc;
@Test
public void test() {
cc.setup("test", ...properties..)
cc.start("test")
}
----
[source, xml]
----
<arquillian>
<container qualifier="test" mode="manual" />
</arquillian>
----
[source, java]
----
// Targeted containers HTTP context.
@ArquillianResource URL url;
----
[source, java]
----
// Targeted containers HTTP context where servlet is located.
@ArquillianResource(SomeServlet.class) URL url;
----
[source, java]
----
// Targeted containers initial context.
@ArquillianResource InitialContext|Context context;
----
[source, java]
----
// The manual deployer.
@ArquillianResource Deployer deployer;
----
See
https://docs.jboss.org/author/display/ARQ/Resource+injection[Arquillian's
Resource Injection docs] for more info,
https://github.com/arquillian/arquillian-examples for examples.
See also
https://docs.jboss.org/author/display/ARQ/Reference+Guide[Arquillian
Reference].
Note to @ServerSetup annotation: It works as expected only on non-manual
containers. In case of manual mode containers it calls setup() method
after each server start up which is right (or actually before
deployment), but the tearDown() method is called only at AfterClass
event, i.e. usually after your manual shutdown of the server. Which
limits you on the ability to revert some configuration changes on the
server and so on. I cloned the annotation and changed it to fit the
manual mode, but it is still in my github branch :)
[[properties-available-in-tests]]
== Properties available in tests
[[directories]]
=== Directories
* jbosssa.project.dir - Project's root dir (where ./build.sh is).
* jbossas.ts.dir - Testsuite dir.
* jbossas.ts.integ.dir - Testsuite's integration module dir.
* jboss.dist - Path to AS distribution, either built
(build/target/jboss-as-...) or user-provided via -Djboss.dist
* jboss.inst - (Arquillian in-container only) Path to the AS instance in
which the test is running (until ARQ-650 is possibly done)
* [line-through]*jboss.home* - Deprecated as it's name is unclear and
confusing. Use jboss.dist or jboss.inst.
[[networking]]
=== Networking
* node0
* node1
* 172.16.31.10
[[time-related-coefficients-ratios]]
=== Time-related coefficients (ratios)
In case some of the following causes timeouts, you may prolong the
timeouts by setting value >= 100:
100 = leave as is, +
150 = 50 % longer, etc.
* timeout.ratio.gen - General ratio - can be used to adjust all
timeouts.When this and specific are defined, both apply.
* timeout.ratio.fs- Filesystem IO
* timeout.ratio.net - Network IO
* timeout.ratio.mem - Memory IO
* timeout.ratio.cpu - Processor
* timeout.ratio.db - Database
Time ratios will soon be provided by
`org.jboss.as.test.shared.time.TimeRatio.for*()` methods.
[[negative-tests]]
== Negative tests
To test invalid deployment handling: @ShouldThrowException
Currently doesn't work due to
https://issues.jboss.org/browse/WFLY-673[WFLY-673].
optionally you might be able to catch it using the manual deployer
[source, java]
----
@Deployment(name = "X", managed = false) ...
@Test
public void shouldFail(@ArquillianResource Deployer deployer) throws Exception {
try {
deployer.deploy("X")
}
catch(Exception e) {
// do something
}
}
----
[[clustering-tests-wfly-616]]
== Clustering tests (WFLY-616)
You need to deploy the same thing twice, so two deployment methods that
just return the same thing. +
And then you have tests that run against each.
[source, java]
----
@Deployment(name = "deplA", testable = false)
@TargetsContainer("serverB")
public static Archive<?> deployment()
@Deployment(name = "deplB", testable = false)
@TargetsContainer("serverA")
public static Archive<?> deployment(){ ... }
@Test
@OperateOnDeployment("deplA")
public void testA(){ ... }
@Test
@OperateOnDeployment("deplA")
public void testA() {...}
----
[[how-to-get-the-tests-to-master]]
== How to get the tests to master
* First of all, *be sure to read the "Before you add a test" section*.
* *Fetch* the newest mater:
`git fetch upstream # Provided you have the jbossas/jbossas GitHub repo`
`as a remote called 'upstream'.`
* *Rebase* your branch: git checkout WFLY-1234-your-branch; git rebase
upstream/master
* *Run* *_whole_* *testsuite* (integration-tests -DallTests). You may
use
https://jenkins.mw.lab.eng.bos.redhat.com/hudson/job/wildfly-as-testsuite-RHEL-matrix-openJDK7/lastCompletedBuild/testReport/.
** If any tests fail and they do not fail in master, fix it and go back
to the "Fetch" step.
* *Push* to a new branch in your GitHub repo:
`git push origin WFLY-1234-new-XY-tests`
* *Create a pull-request* on GitHub. Go to your branch and click on
"Pull Request".
** If you have a jira, start the title with it, like - WFLY-1234 New
tests for XYZ.
** If you don't, write some apposite title. In the description, describe
in detail what was done and why should it be merged. Keep in mind that
the diff will be visible under your description.
* *Keep the branch rebased daily* until it's merged (see the Fetch
step). If you don't, you're dramatically decreasing chance to get it
merged.
* There's a mailing list, jbossas-pull-requests, which is notified of
every pull-request.
* You might have someone with merge privileges to cooperate with you, so
they know what you're doing, and expect your pull request.
* When your pull request is reviewed and merged, you'll be notified by
mail from GitHub.
* You may also check if it was merged by the following:
`git fetch upstream; git cherry` `<branch> ## Or` git branch
--contains\{\{<branch> - see}} `here`
* Your commits will appear in master. They will have the same hash as in
your branch.
** You are now safe to delete both your local and remote branches:
`git branch -D WFLY-1234-your-branch; git push origin :WFLY-1234-your-branch`
include::How_to_Add_a_Test_Case.adoc[]
include::Pre-requisites_-_test_quality_standards.adoc[]
include::Shared_Test_Classes_and_Resources.adoc[]
<file_sep>/_docs/14/_developer-guide/JNDI_Local_Reference.adoc
[[JNDI_Local_Reference]]
= Local JNDI
The Java EE platform specification defines the following JNDI contexts:
* `java:comp` - The namespace is scoped to the current component (i.e.
EJB)
* `java:module` - Scoped to the current module
* `java:app` - Scoped to the current application
* `java:global` - Scoped to the application server
In addition to the standard namespaces, Wild**Fly** also provides the
following two global namespaces:
* java:jboss
* java:/
[IMPORTANT]
Only entries within the `java:jboss/exported` context are accessible
over remote JNDI.
[IMPORTANT]
For web deployments `java:comp` is aliased to `java:module`, so EJB's
deployed in a war do not have their own comp namespace.
[[binding-entries-to-jndi]]
== Binding entries to JNDI
There are several methods that can be used to bind entries into JNDI in
Wild**Fly**.
[[using-a-deployment-descriptor]]
=== Using a deployment descriptor
For Java EE applications the recommended way is to use a
<<Deployment_Descriptors_used_In_WildFly,deployment descriptor>> to create the binding. For
example the following `web.xml` binds the string `"Hello World"` to
`java:global/mystring` and the string `"Hello Module"` to
`java:comp/env/hello` (any non absolute JNDI name is relative to
`java:comp/env` context).
[source, java]
----
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd"
version="3.1">
<env-entry>
<env-entry-name>java:global/mystring</env-entry-name>
<env-entry-type>java.lang.String</env-entry-type>
<env-entry-value>Hello World</env-entry-value>
</env-entry>
<env-entry>
<env-entry-name>hello</env-entry-name>
<env-entry-type>java.lang.String</env-entry-type>
<env-entry-value>Hello Module</env-entry-value>
</env-entry>
</web-app>
----
For more details, see the http://jcp.org/en/jsr/detail?id=342[Java EE
Platform Specification].
[[programatically]]
=== Programatically
[[java-ee-applications]]
==== Java EE Applications
Standard Java EE applications may use the standard JNDI API, included
with Java SE, to bind entries in the global namespaces (the standard
`java:comp`, `java:module` and `java:app` namespaces are read-only, as
mandated by the Java EE Platform Specification).
[source, java]
----
InitialContext initialContext = new InitialContext();
initialContext.bind("java:global/a", 100);
----
[IMPORTANT]
There is no need to unbind entries created programatically, since
Wild**Fly** tracks which bindings belong to a deployment, and the bindings
are automatically removed when the deployment is undeployed.
[[wildfly-modules-and-extensions]]
==== Wild**Fly** Modules and Extensions
With respect to code in Wild**Fly** Modules/Extensions, which is executed
out of a Java EE application context, using the standard JNDI API may
result in a UnsupportedOperationException if the target namespace uses a
WritableServiceBasedNamingStore. To work around that, the bind()
invocation needs to be wrapped using Wild**Fly** proprietary APIs:
[source, java]
----
InitialContext initialContext = new InitialContext();
WritableServiceBasedNamingStore.pushOwner(serviceTarget);
try {
initialContext.bind("java:global/a", 100);
} finally {
WritableServiceBasedNamingStore.popOwner();
}
----
[IMPORTANT]
The ServiceTarget removes the bind when uninstalled, thus using one out
of the module/extension domain usage should be avoided, unless entries
are removed using unbind().
[[naming-subsystem-configuration]]
=== Naming Subsystem Configuration
It is also possible to bind to one of the three global namespaces using
configuration in the naming subsystem. This can be done by either
editing the `standalone.xml/domain.xml` file directly, or through the
management API.
Four different types of bindings are supported:
* Simple - A primitive or java.net.URL entry (default is
`java.lang.String`).
* Object Factory - This allows to to specify the
`javax.naming.spi.ObjectFactory` that is used to create the looked up
value.
* External Context - An external context to federate, such as an LDAP
Directory Service
* Lookup - The allows to create JNDI aliases, when this entry is looked
up it will lookup the target and return the result.
An example standalone.xml might look like:
[source, java]
----
<subsystem xmlns="urn:jboss:domain:naming:2.0" >
<bindings>
<simple name="java:global/a" value="100" type="int" />
<simple name="java:global/jbossDocs" value="https://docs.jboss.org" type="java.net.URL" />
<object-factory name="java:global/b" module="com.acme" class="org.acme.MyObjectFactory" />
<external-context name="java:global/federation/ldap/example" class="javax.naming.directory.InitialDirContext" cache="true">
<environment>
<property name="java.naming.factory.initial" value="com.sun.jndi.ldap.LdapCtxFactory" />
<property name="java.naming.provider.url" value="ldap://ldap.example.com:389" />
<property name="java.naming.security.authentication" value="simple" />
<property name="java.naming.security.principal" value="uid=admin,ou=system" />
<property name="java.naming.security.credentials" value="secret" />
</environment>
</external-context>
<lookup name="java:global/c" lookup="java:global/b" />
</bindings>
</subsystem>
----
The CLI may also be used to bind an entry. As an example:
[source, java]
----
/subsystem=naming/binding=java\:global\/mybinding:add(binding-type=simple, type=long, value=1000)
----
[IMPORTANT]
Wild**Fly**'s Administrator Guide includes a section describing in detail
the Naming subsystem configuration.
[[retrieving-entries-from-jndi]]
== Retrieving entries from JNDI
[[resource-injection]]
=== Resource Injection
For Java EE applications the recommended way to lookup a JNDI entry is
to use `@Resource` injection:
[source, java]
----
@Resource(lookup = "java:global/mystring")
private String myString;
@Resource(name = "hello")
private String hello;
@Resource
ManagedExecutorService executor;
----
Note that `@Resource` is more than a JNDI lookup, it also binds an entry
in the component's JNDI environment. The new bind JNDI name is defined
by `@Resource`'s `name` attribute, which value, if unspecified, is the
Java type concatenated with `/` and the field's name, for instance
`java.lang.String/myString`. More, similar to when using deployment
descriptors to bind JNDI entries. unless the name is an absolute JNDI
name, it is considered relative to `java:comp/env`. For instance, with
respect to the field named `myString` above, the `@Resource`'s `lookup`
attribute instructs Wild**Fly** to lookup the value in
`java:global/mystring`, bind it in
`java:comp/env/java.lang.String/myString`, and then inject such value
into the field.
With respect to the field named `hello`, there is no `lookup` attribute
value defined, so the responsibility to provide the entry's value is
delegated to the deployment descriptor. Considering that the deployment
descriptor was the `web.xml` previously shown, which defines an
environment entry with same `hello` name, then Wild**Fly** inject the valued
defined in the deployment descriptor into the field.
The `executor` field has no attributes specified, so the bind's name
would default to
`java:comp/env/javax.enterprise.concurrent.ManagedExecutorService/executor`,
but there is no such entry in the deployment descriptor, and when that
happens it's up to Wild**Fly** to provide a default value or null, depending
on the field's Java type. In this particular case Wild**Fly** would inject
the default instance of a managed executor service, the value in
`java:comp/DefaultManagedExecutorService`, as mandated by the EE
Concurrency Utilities 1.0 Specification (JSR 236).
[[standard-java-se-jndi-api]]
=== Standard Java SE JNDI API
Java EE applications may use, without any additional configuration
needed, the standard JNDI API to lookup an entry from JNDI:
[source, java]
----
String myString = (String) new InitialContext().lookup("java:global/mystring");
----
or simply
[source, java]
----
String myString = InitialContext.doLookup("java:global/mystring");
----
<file_sep>/_docs/13/_developer-guide/wsconsume.adoc
[[wsconsume]]
== wsconsume
_wsconsume_ is a command line tool and ant task that "consumes" the
abstract contract (WSDL file) and produces portable JAX-WS service and
client artifacts.
[[command-line-tool]]
== Command Line Tool
The command line tool has the following usage:
....
usage: wsconsume [options] <wsdl-url>
options:
-h, --help Show this help message
-b, --binding=<file> One or more JAX-WS or JAXB binding files
-k, --keep Keep/Generate Java source
-c --catalog=<file> Oasis XML Catalog file for entity resolution
-j --clientjar=<name> Create a jar file of the generated artifacts for calling the webservice
-p --package=<name> The target package for generated source
-w --wsdlLocation=<loc> Value to use for @WebServiceClient.wsdlLocation
-o, --output=<directory> The directory to put generated artifacts
-s, --source=<directory> The directory to put Java source
-t, --target=<2.0|2.1|2.2> The JAX-WS specification target
-q, --quiet Be somewhat more quiet
-v, --verbose Show full exception stack traces
-l, --load-consumer Load the consumer and exit (debug utility)
-e, --extension Enable SOAP 1.2 binding extension
-a, --additionalHeaders Enables processing of implicit SOAP headers
-n, --nocompile Do not compile generated sources
....
[IMPORTANT]
The wsdlLocation is used when creating the Service to be used by clients
and will be added to the @WebServiceClient annotation, for an endpoint
implementation based on the generated service endpoint interface you
will need to manually add the wsdlLocation to the @WebService annotation
on your web service implementation and not the service endpoint
interface.
[[examples]]
=== Examples
Generate artifacts in Java class form only:
....
wsconsume Example.wsdl
....
Generate source and class files:
....
wsconsume -k Example.wsdl
....
Generate source and class files in a custom directory:
....
wsconsume -k -o custom Example.wsdl
....
Generate source and class files in the org.foo package:
....
wsconsume -k -p org.foo Example.wsdl
....
Generate source and class files using multiple binding files:
....
wsconsume -k -b wsdl-binding.xml -b schema1-binding.xml -b schema2-binding.xml
....
[[maven-plugin]]
== Maven Plugin
The wsconsume tools is included in the
*org.jboss.ws.plugins:jaxws-tools-maven-plugin* plugin. The plugin has
two goals for running the tool, _wsconsume_ and _wsconsume-test_, which
basically do the same during different maven build phases (the former
triggers the sources generation during _generate-sources_ phase, the
latter during the _generate-test-sources_ one).
The _wsconsume_ plugin has the following parameters:
[cols=",,",options="header"]
|=======================================================================
|Attribute |Description |Default
|bindingFiles |JAXWS or JAXB binding file |true
|classpathElements |Each classpathElement provides alibrary file to be
added to classpath
|$\{project.compileClasspathElements}or$\{project.testClasspathElements}
|catalog |Oasis XML Catalog file for entity resolution |none
|targetPackage |The target Java package for generated code. |generated
|bindingFiles |One or more JAX-WS or JAXB binding file |none
|wsdlLocation |Value to use for @WebServiceClient.wsdlLocation
|generated
|outputDirectory |The output directory for generated artifacts.
|$\{project.build.outputDirectory}or$\{project.build.testOutputDirectory}
|sourceDirectory |The output directory for Java source.
|$\{project.build.directory}/wsconsume/java
|verbose |Enables more informational output about command progress.
|false
|wsdls |The WSDL files or URLs to consume |n/a
|extension |Enable SOAP 1.2 binding extension. |false
|encoding |The charset encoding to use for generated sources.
|$\{project.build.sourceEncoding}
|argLine |An optional additional argline to be used when running in fork
mode;can be used to set endorse dir, enable debugging,
etc.Example<argLine>-Djava.endorsed.dirs=...</argLine> |none
|fork |Whether or not to run the generation task in a separate VM.
|false
|target |A preference for the JAX-WS specification target |Depends on
the underlying stack and endorsed dirs if any
|=======================================================================
[[examples-1]]
=== Examples
You can use _wsconsume_ in your own project build simply referencing the
_jaxws-tools-maven-plugin_ in the configured plugins in your pom.xml
file.
The following example makes the plugin consume the test.wsdl file and
generate SEI and wrappers' java sources. The generated sources are then
compiled together with the other project classes.
[source,xml]
----
<build>
<plugins>
<plugin>
<groupId>org.jboss.ws.plugins</groupId>
<artifactId>jaxws-tools-maven-plugin</artifactId>
<version>1.2.0.Beta1</version>
<configuration>
<wsdls>
<wsdl>${basedir}/test.wsdl</wsdl>
</wsdls>
</configuration>
<executions>
<execution>
<goals>
<goal>wsconsume</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
----
You can also specify multiple wsdl files, as well as force the target
package, enable SOAP 1.2 binding and turn the tool's verbose mode on:
[source,xml]
----
<build>
<plugins>
<plugin>
<groupId>org.jboss.ws.plugins</groupId>
<artifactId>jaxws-tools-maven-plugin</artifactId>
<version>1.2.0.Beta1</version>
<configuration>
<wsdls>
<wsdl>${basedir}/test.wsdl</wsdl>
<wsdl>${basedir}/test2.wsdl</wsdl>
</wsdls>
<targetPackage>foo.bar</targetPackage>
<extension>true</extension>
<verbose>true</verbose>
</configuration>
<executions>
<execution>
<goals>
<goal>wsconsume</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
----
Finally, if the wsconsume invocation is required for consuming a wsdl to
be used in your testsuite only, you might want to use the
_wsconsume-test_ goal as follows:
[source,xml]
----
<build>
<plugins>
<plugin>
<groupId>org.jboss.ws.plugins</groupId>
<artifactId>jaxws-tools-maven-plugin</artifactId>
<version>1.2.0.Beta1</version>
<configuration>
<wsdls>
<wsdl>${basedir}/test.wsdl</wsdl>
</wsdls>
</configuration>
<executions>
<execution>
<goals>
<goal>wsconsume-test</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
----
Plugin stack dependencyThe plugin itself does not have an explicit
dependency to a JBossWS stack, as it's meant for being used with
implementations of any supported version of the _JBossWS SPI_. So the
user is expected to set a dependency in his own `pom.xml` to the desired
_JBossWS_ stack version. The plugin will rely on the that for using the
proper tooling.
[source,xml]
----
<dependencies>
<dependency>
<groupId>org.jboss.ws.cxf</groupId>
<artifactId>jbossws-cxf-client</artifactId>
<version>4.0.0.GA</version>
</dependency>
</dependencies>
----
[TIP]
Be careful when using this plugin with the Maven War Plugin as that
include any project dependency into the generated application war
archive. You might want to set `<scope>provided</scope>` for the
_JBossWS_ stack dependency to avoid that.
[IMPORTANT]
Up to version 1.1.2.Final, the _artifactId_ of the plugin was
*maven-jaxws-tools-plugin*.
[[ant-task]]
== Ant Task
The _wsconsume_ Ant task ( _org.jboss.ws.tools.ant.WSConsumeTask_) has
the following attributes:
[cols=",,",options="header"]
|=======================================================================
|Attribute |Description |Default
|fork |Whether or not to run the generation task in a separate VM. |true
|keep |Keep/Enable Java source code generation. |false
|catalog |Oasis XML Catalog file for entity resolution |none
|package |The target Java package for generated code. |generated
|binding |A JAX-WS or JAXB binding file |none
|wsdlLocation |Value to use for @WebServiceClient.wsdlLocation
|generated
|encoding |The charset encoding to use for generated sources |n/a
|destdir |The output directory for generated artifacts. |"output"
|sourcedestdir |The output directory for Java source. |value of destdir
|target |The JAX-WS specification target. Allowed values are 2.0, 2.1
and 2.2 |
|verbose |Enables more informational output about command progress.
|false
|wsdl |The WSDL file or URL |n/a
|extension |Enable SOAP 1.2 binding extension. |false
|additionalHeaders |Enables processing of implicit SOAP headers |false
|=======================================================================
[NOTE]
Users also need to put streamBuffer.jar and stax-ex.jar to the classpath
of the ant task to generate the appropriate artefacts.
[NOTE]
The wsdlLocation is used when creating the Service to be used by clients
and will be added to the @WebServiceClient annotation, for an endpoint
implementation based on the generated service endpoint interface you
will need to manually add the wsdlLocation to the @WebService annotation
on your web service implementation and not the service endpoint
interface.
Also, the following nested elements are supported:
[cols=",,",options="header"]
|=================================================
|Element |Description |Default
|binding |A JAXWS or JAXB binding file |none
|jvmarg |Allows setting of custom jvm arguments |
|=================================================
[[examples-2]]
=== Examples
Generate JAX-WS source and classes in a separate JVM with separate
directories, a custom wsdl location attribute, and a list of binding
files from foo.wsdl:
[source,xml]
----
<wsconsume
fork="true"
verbose="true"
destdir="output"
sourcedestdir="gen-src"
keep="true"
wsdllocation="handEdited.wsdl"
wsdl="foo.wsdl">
<binding dir="binding-files" includes="*.xml" excludes="bad.xml"/>
</wsconsume>
----
<file_sep>/_docs/13/_high-availability/Subsystem_Support.adoc
[[Subsystem_Support]]
== Subsystem Support
////
todo look into moving this into admin guide!
////
include::subsystem-support/Infinispan_Subsystem.adoc[]
include::subsystem-support/Offloading_session_state_to_remote_Infinispan_server_cluster.adoc[]
include::subsystem-support/JGroups_Subsystem.adoc[]
include::subsystem-support/Kubernetes.adoc[]
include::subsystem-support/mod_cluster_Subsystem.adoc[]<file_sep>/_docs/13/_high-availability/Clustering_and_Domain_Setup_Walkthrough.adoc
[[Clustering_and_Domain_Setup_Walkthrough]]
= Clustering and Domain Setup Walkthrough
ifdef::env-github[:imagesdir: ../]
In this article, I'd like to show you how to setup WildFly in domain
mode and enable clustering so we could get HA and session replication
among the nodes. It's a step to step guide so you can follow the
instructions in this article and build the sandbox by yourself.
[[preparation-scenario]]
== Preparation & Scenario
[[preparation]]
=== Preparation
We need to prepare two hosts (or virtual hosts) to do the experiment. We
will use these two hosts as following:
* Install Fedora on them (Other linux version may also fine but I'll
use Fedora in this article)
* Make sure that they are in same local network
* Make sure that they can access each other via different TCP/UDP
ports (better turn off firewall and disable SELinux during the experiment
or they will cause network problems).
[[scenario]]
=== Scenario
Here are some details on what we are going to do:
* Let's call one host as 'master', the other one as 'slave'.
* Both master and slave will run WildFly, and master will run as
domain controller, slave will under the domain management of master.
* Apache httpd will be run on master, and in httpd we will enable the
mod_cluster module. The WildFly on master and slave will form a
cluster and discovered by httpd.
image:images/clustering/Clustering.jpg[images/clustering/Clustering.jpg]
* We will deploy a demo project into domain, and verify that the project
is deployed into both master and slave by domain controller. Thus we
could see that domain management provide us a single point to manage the
deployments across multiple hosts in a single domain.
* We will access the cluster URL and verify that httpd has distributed
the request to one of the WildFly host. So we could see the cluster is
working properly.
* We will try to make a request on cluster, and if the request is
forwarded to master, we then kill the WildFly process on master. After
that we will go on requesting cluster and we should see the request is
forwarded to slave, but the session is not lost. Our goal is to verify
the HA is working and sessions are replicated.
* After previous step finished, we reconnect the master by restarting
it. We should see the master is registered back into cluster, also we
should see slave sees master as domain controller again and connect to
it.
image:images/clustering/test_scenario.jpg[images/clustering/test_scenario.jpg]
Please don't worry if you cannot digest so many details currently. Let's
move on and you will get the points step by step.
[[download-wildfly]]
== Download WildFly
First we should download WildFly from the http://wildfly.org/downloads/[WildFly website].
Then I unzipped the package to master and try to make a test run:
[source, bash, subs="verbatim,attributes"]
----
unzip wildfly-{wildflyVersion}.0.0.Final.zip
cd wildfly-{wildflyVersion}.0.0.Final/bin
./domain.sh
----
If everything ok we should see WildFly successfully startup in domain
mode:
[source, bash, subs="verbatim,attributes"]
----
wildfly-{wildflyVersion}.0.0.Final/bin$ ./domain.sh
=========================================================================
JBoss Bootstrap Environment
JBOSS_HOME: /Users/weli/Downloads/wildfly-{wildflyVersion}.0.0.Final
JAVA: /Library/Java/Home/bin/java
JAVA_OPTS: -Xms64m -Xmx512m -XX:MaxPermSize=256m -Djava.net.preferIPv4Stack=true -Dorg.jboss.resolver.warning=true -Dsun.rmi.dgc.client.gcInterval=3600000 -Dsun.rmi.dgc.server.gcInterval=3600000 -Djboss.modules.system.pkgs=org.jboss.byteman -Djava.awt.headless=true
=========================================================================
...
[Server:server-two] 14:46:12,375 INFO [org.jboss.as] (Controller Boot Thread) JBAS015874: WildFly {wildflyVersion}.0.0.Final "Kenny" started in 8860ms - Started 210 of 258 services (8{wildflyVersion} services are lazy, passive or on-demand)
----
Now exit master and let's repeat the same steps on slave host. Finally
we get WildFly run on both master and slave, then we could move on to
next step.
[[domain-configuration]]
== Domain Configuration
[[interface-config-on-master]]
=== Interface config on master
In this section we'll setup both master and slave for them to run in
domain mode. And we will configure master to be the domain controller.
First open the host.xml in master for editing:
[source, bash]
----
vi domain/configuration/host.xml
----
The default settings for interface in this file is like:
[source, xml]
----
<interfaces>
<interface name="management">
<inet-address value="${jboss.bind.address.management:127.0.0.1}"/>
</interface>
<interface name="public">
<inet-address value="${jboss.bind.address:127.0.0.1}"/>
</interface>
<interface name="unsecured">
<inet-address value="127.0.0.1" />
</interface>
</interfaces>
----
We need to change the address to the management interface so slave could
connect to master. The public interface allows the application to be
accessed by non-local HTTP, and the unsecured interface allows remote
RMI access. My master's ip address is 10.211.55.7, so I change the
config to:
[source, xml]
----
<interfaces>
<interface name="management"
<inet-address value="${jboss.bind.address.management:10.211.55.7}"/>
</interface>
<interface name="public">
<inet-address value="${jboss.bind.address:10.211.55.7}"/>
</interface>
<interface name="unsecured">
<inet-address value="10.211.55.7" />
</interface>
</interfaces>
----
[[interface-config-on-slave]]
=== Interface config on slave
Now we will setup interfaces on slave. Let's edit host.xml. Similar to
the steps on master, open host.xml first:
[source, bash]
----
vi domain/configuration/host.xml
----
The configuration we'll use on slave is a little bit different, because
we need to let slave connect to master. First we need to set the
hostname. We change the name property from:
[source, xml]
----
<host name="master" xmlns="urn:jboss:domain:3.0">
----
to:
[source, xml]
----
<host name="slave" xmlns="urn:jboss:domain:3.0">
----
Then we need to modify domain-controller section so slave can connect to
master's management port:
[source, xml]
----
<domain-controller>
<remote protocol="remote" host="10.211.55.7" port="9999" />
</domain-controller>
----
As we know, 10.211.55.7 is the ip address of master.
You may use discovery options to define multiple mechanisms to connect
to the remote domain controller :
[source, xml]
----
<domain-controller>
<remote security-realm="ManagementRealm" >
<discovery-options>
<static-discovery name="master-native" protocol="remote" host="10.211.55.7" port=9999" />
<static-discovery name="master-https" protocol="https-remoting" host="10.211.55.7" port="9993" security-realm="ManagementRealm"/>
<static-discovery name="master-http" protocol="http-remoting" host="10.211.55.7" port="9990" />
</discovery-options>
</remote>
</domain-controller>
----
Finally, we also need to configure interfaces section and expose the
management ports to public address:
[source, xml]
----
<interfaces>
<interface name="management">
<inet-address value="${jboss.bind.address.management:10.211.55.2}"/>
</interface>
<interface name="public">
<inet-address value="${jboss.bind.address:10.211.55.2}"/>
</interface>
<interface name="unsecured">
<inet-address value="10.211.55.2" />
</interface>
</interfaces>
----
10.211.55.2 is the ip address of the slave. Refer to the domain
controller configuration above for an explanation of the management,
public, and unsecured interfaces.
[TIP]
It is easier to turn off all firewalls for testing, but in production,
you need to enable the firewall and allow access to the following ports:
9999.
[[security-configuration]]
=== Security Configuration
If you start WildFly on both master and slave now, you will see the
slave cannot be started with following error:
[source]
----
[Host Controller] 20:31:24,575 ERROR [org.jboss.remoting.remote] (Remoting "endpoint" read-1) JBREM000200: Remote connection failed: javax.security.sasl.SaslException: Authentication failed: all available authentication mechanisms failed
[Host Controller] 20:31:24,579 WARN [org.jboss.as.host.controller] (Controller Boot Thread) JBAS010900: Could not connect to remote domain controller 10.211.55.7:9999
[Host Controller] 20:31:24,582 ERROR [org.jboss.as.host.controller] (Controller Boot Thread) JBAS010901: Could not connect to master. Aborting. Error was: java.lang.IllegalStateException: JBAS010942: Unable to connect due to authentication failure.
----
Because we haven't properly set up the authentication between master and
slave. Now let's work on it:
[[master]]
==== Master
In bin directory there is a script called add-user.sh, we'll use it to
add new users to the properties file used for domain management
authentication:
[source, bash, subs="verbatim,attributes"]
----
./add-user.sh
Enter the details of the new user to add.
Realm (ManagementRealm) :
Username : admin
Password recommendations are listed below. To modify these restrictions edit the add-user.properties configuration file.
- The password should not be one of the following restricted values {root, admin, administrator}
- The password should contain at least 8 characters, 1 alphabetic character(s), 1 digit(s), 1 non-alphanumeric symbol(s)
- The password should be different from the username
Password : <PASSWORD>!
Re-enter Password : <PASSWORD>!
The username 'admin' is easy to guess
Are you sure you want to add user 'admin' yes/no? yes
About to add user 'admin' for realm 'ManagementRealm'
Is this correct yes/no? yes
Added user 'admin' to file '/home/weli/projs/wildfly-{wildflyVersion}.0.0.Final/standalone/configuration/mgmt-users.properties'
Added user 'admin' to file '/home/weli/projs/wildfly-{wildflyVersion}.0.0.Final/domain/configuration/mgmt-users.properties'
----
As shown above, we have created a user named 'admin' and its password is
'<PASSWORD>!'. Then we add another user called 'slave':
[source, bash, subs="verbatim,attributes"]
----
./add-user.sh
Enter the details of the new user to add.
Realm (ManagementRealm) :
Username : slave
Password recommendations are listed below. To modify these restrictions edit the add-user.properties configuration file.
- The password should not be one of the following restricted values {root, admin, administrator}
- The password should contain at least 8 characters, 1 alphabetic character(s), 1 digit(s), 1 non-alphanumeric symbol(s)
- The password should be different from the username
Password : <PASSWORD>!
Re-enter Password : <PASSWORD>!
What groups do you want this user to belong to? (Please enter a comma separated list, or leave blank for none)[ ]:
About to add user 'slave' for realm 'ManagementRealm'
Is this correct yes/no? yes
Added user 'slave' to file '/home/weli/projs/wildfly-{wildflyVersion}.0.0.Final/standalone/configuration/mgmt-users.properties'
Added user 'slave' to file '/home/weli/projs/wildfly-{wildflyVersion}.0.0.Final/domain/configuration/mgmt-users.properties'
Added user 'slave' with groups to file '/home/weli/projs/wildfly-{wildflyVersion}.0.0.Final/standalone/configuration/mgmt-groups.properties'
Added user 'slave' with groups to file '/home/weli/projs/wildfly-{wildflyVersion}.0.0.Final/domain/configuration/mgmt-groups.properties'
Is this new user going to be used for one AS process to connect to another AS process?
e.g. for a slave host controller connecting to the master or for a Remoting connection for server to server EJB calls.
yes/no? yes
To represent the user add the following to the server-identities definition <secret value="cGFzc3cwcmQh" />
----
We will use this user for slave host to connect to master. The
add-user.sh will let you choose the type of the user. Here we need to
choose 'Management User' type for both 'admin' and 'slave' account.
[[slave]]
==== Slave
In slave we need to configure host.xml for authentication. We should
change the security-realms section as following:
[source, java]
----
<security-realms>
<security-realm name="ManagementRealm">
<server-identities>
<secret value="cGFzc3cwcmQh" />
<!-- This is required for SSL remoting -->
<ssl>
<keystore path="server.keystore" relative-to="jboss.domain.config.dir" keystore-password="<PASSWORD>" alias="jboss" key-password="<PASSWORD>"/>
</ssl>
</server-identities>
<authentication>
<properties path="mgmt-users.properties" relative-to="jboss.domain.config.dir"/>
</authentication>
</security-realm>
</security-realms>
----
We've added server-identities into security-realm, which is used for
authentication host when slave tries to connect to master. In secret
value property we have 'cGFzc3cwcmQh', which is the base64 code for
'passw0rd!'. You can generate this value by using a base64 calculator
such as the one at http://www.webutils.pl/index.php?idx=base64.
Then in domain controller section we also need to add security-realm
property:
[source, xml]
----
<domain-controller>
<remote protocol="remote" host="10.211.55.7" port="9999" username="slave" security-realm="ManagementRealm" />
</domain-controller>
----
So the slave host could use the authentication information we provided
in 'ManagementRealm'.
[[dry-run]]
==== Dry Run
Now everything is set for the two hosts to run in domain mode. Let's
start them by running domain.sh on both hosts. If everything goes fine,
we could see from the log on master:
[source, java]
----
[Host Controller] 21:30:52,042 INFO [org.jboss.as.domain] (management-handler-threads - 1) JBAS010918: Registered remote slave host slave
----
That means all the configurations are correct and two hosts are run in
domain mode now as expected. Hurrah!
[[deployment]]
== Deployment
Now we can deploy a demo project into the domain. I have created a
simple project located at:
[source, java]
----
https://github.com/liweinan/cluster-demo
----
We can use git command to fetch a copy of the demo:
[source, java]
----
git clone git://github.com/liweinan/cluster-demo.git
----
In this demo project we have a very simple web application. In web.xml
we've enabled session replication by adding following entry:
[source, xml]
----
<distributable/>
----
And it contains a jsp page called put.jsp which will put current time to
a session entry called 'current.time':
[source, java]
----
<%
session.setAttribute("current.time", new java.util.Date());
%>
----
Then we could fetch this value from get.jsp:
[source, java]
----
The time is <%= session.getAttribute("current.time") %>
----
It's an extremely simple project but it could help us to test the
cluster later: We will access put.jsp from cluster and see the request
are distributed to master, then we disconnect master and access get.jsp.
We should see the request is forwarded to slave but the 'current.time'
value is held by session replication. We'll cover more details on this
one later.
Let's go back to this demo project. Now we need to create a war from it.
In the project directory, run the following command to get the war:
[source]
----
mvn package
----
It will generate cluster-demo.war. Then we need to deploy the war into
domain. First we should access the http management console on
master(Because master is acting as domain controller):
[source]
----
http://10.211.55.7:9990
----
It will popup a windows ask you to input account name and password, we
can use the 'admin' account we've added just now. After logging in we
could see the 'Server Instances' window. By default there are three
servers listed, which are:
* server-one
* server-two
* server-three
We could see server-one and server-two are in running status and they
belong to main-server-group; server-three is in idle status, and it
belongs to other-server-group.
All these servers and server groups are set in domain.xml on master as7.
What we are interested in is the 'other-server-group' in domain.xml:
[source, xml]
----
<server-group name="other-server-group" profile="ha">
<jvm name="default">
<heap size="64m" max-size="512m"/>
</jvm>
<socket-binding-group ref="ha-sockets"/>
</server-group>
----
We could see this server-group is using 'ha' profile, which then uses
'ha-sockets' socket binding group. It enable all the modules we need to
establish cluster later(including infinispan, jgroup and mod_cluster
modules). So we will deploy our demo project into a server that belongs
to 'other-server-group', so 'server-three' is our choice.
[IMPORTANT]
In newer version of WildFly, the profile 'ha' changes to 'full-ha':
[source, xml]
----
<server-group name="other-server-group" profile="full-ha">
----
Let's go back to domain controller's management console:
[source]
----
http://10.211.55.7:9990
----
Now server-three is not running, so let's click on 'server-three' and
then click the 'start' button at bottom right of the server list. Wait a
moment and server-three should start now.
Now we should also enable 'server-three' on slave: From the top of menu
list on left side of the page, we could see now we are managing master
currently. Click on the list, and click 'slave', then choose
'server-three', and we are in slave host management page now.
Then repeat the steps we've done on master to start 'server-three' on
slave.
[TIP]
server-three on master and slave are two different hosts, their names
can be different.
After server-three on both master and slave are started, we will add our
cluster-demo.war for deployment. Click on the 'Manage Deployments' link
at the bottom of left menu list.
image:images/clustering/JBoss_Management.png[images/clustering/JBoss_Management.png] +
(We should ensure the server-three should be started on both master and
slave)
After enter 'Manage Deployments' page, click 'Add Content' at top right
corner. Then we should choose our cluster-demo.war, and follow the
instruction to add it into our content repository.
Now we can see cluster-demo.war is added. Next we click 'Add to Groups'
button and add the war to 'other-server-group' and then click 'save'.
Wait a few seconds, management console will tell you that the project is
deployed into 'other-server-group'.:
image:images/clustering/JBoss_Management_2.png[images/clustering/JBoss_Management_2.png]
Please note we have two hosts participate in this server group, so the
project should be deployed in both master and slave now - that's the
power of domain management.
Now let's verify this, trying to access cluster-demo from both master
and slave, and they should all work now:
[source]
----
http://10.211.55.7:8330/cluster-demo/
----
image:images/clustering/http---10.211.55.7-8330-cluster-demo-.png[images/clustering/http---10.211.55.7-8330-cluster-demo-.png]
[source]
----
http://10.211.55.2:8330/cluster-demo/
----
image:images/clustering/http---10.211.55.2-8330-cluster-demo-.png[images/clustering/http---10.211.55.2-8330-cluster-demo-.png]
Now that we have finished the project deployment and see the usages of
domain controller, we will then head up for using these two hosts to
establish a cluster icon:smile-o[role="yellow"]
[IMPORTANT]
Why is the port number 8330 instead of 8080? Please check the settings
in host.xml on both master and slave:
[source, xml]
----
<server name="server-three" group="other-server-group" auto-start="false">
<!-- server-three avoids port conflicts by incrementing the ports in
the default socket-group declared in the server-group -->
<socket-bindings port-offset="250"/>
</server>
----
The port-offset is set to 250, so 8080 + 250 = 8330
Now we quit the WildFly process on both master and slave. We have some
work left on host.xml configurations. Open the host.xml of master, and
do some modifications the servers section from:
[source, xml]
----
<server name="server-three" group="other-server-group" auto-start="false">
<!-- server-three avoids port conflicts by incrementing the ports in
the default socket-group declared in the server-group -->
<socket-bindings port-offset="250"/>
</server>
----
to:
[source, xml]
----
<server name="server-three" group="other-server-group" auto-start="true">
<!-- server-three avoids port conflicts by incrementing the ports in
the default socket-group declared in the server-group -->
<socket-bindings port-offset="250"/>
</server>
----
We've set auto-start to true so we don't need to enable it in management
console each time WildFly restart. Now open slave's host.xml, and modify
the server-three section:
[source, xml]
----
<server name="server-three-slave" group="other-server-group" auto-start="true">
<!-- server-three avoids port conflicts by incrementing the ports in
the default socket-group declared in the server-group -->
<socket-bindings port-offset="250"/>
</server>
----
Besides setting auto-start to true, we've renamed the 'server-three' to
'server-three-slave'. We need to do this because mod_cluster will fail
to register the hosts with same name in a single server group. It will
cause name conflict.
After finishing the above configuration, let's restart two as7 hosts and
go on cluster configuration.
[[cluster-configuration]]
== Cluster Configuration
We will use mod_cluster + apache httpd on master as our cluster
controller here. Because WildFly has been configured to support
mod_cluster out of box so it's the easiest way.
[IMPORTANT]
The WildFly domain controller and httpd are not necessary to be on
same host. But in this article I just install them all on master for
convenience.
First we need to ensure that httpd is installed:
[source, bash]
----
sudo yum install httpd
----
And then we need to download newer version of mod_cluster from its
website:
[source]
----
http://www.jboss.org/mod_cluster/downloads
----
The version I downloaded is:
[source]
----
http://downloads.jboss.org/mod_cluster/1.1.3.Final/mod_cluster-1.1.3.Final-linux2-x86-so.tar.gz
----
[TIP]
Jean-Frederic has suggested to use mod_cluster 1.2.x. Because 1.1.x it
is affected by CVE-2011-4608
With mod_cluster-1.2.0 you need to add EnableMCPMReceive in the
VirtualHost.
Then we extract it into:
[source]
----
/etc/httpd/modules
----
Then we edit httpd.conf:
[source, bash]
----
sudo vi /etc/httpd/conf/httpd.conf
----
We should add the modules:
[source]
----
LoadModule slotmem_module modules/mod_slotmem.so
LoadModule manager_module modules/mod_manager.so
LoadModule proxy_cluster_module modules/mod_proxy_cluster.so
LoadModule advertise_module modules/mod_advertise.so
----
Please note we should comment out:
[source, java]
----
#LoadModule proxy_balancer_module modules/mod_proxy_balancer.so
----
This is conflicted with cluster module. And then we need to make httpd
to listen to public address so we could do the testing. Because we
installed httpd on master host so we know the ip address of it:
[source]
----
Listen 10.211.55.7:80
----
Then we do the necessary configuration at the bottom of httpd.conf:
[source]
----
# This Listen port is for the mod_cluster-manager, where you can see the status of mod_cluster.
# Port 10001 is not a reserved port, so this prevents problems with SELinux.
Listen 10.211.55.7:10001
# This directive only applies to Red Hat Enterprise Linux. It prevents the temmporary
# files from being written to /etc/httpd/logs/ which is not an appropriate location.
MemManagerFile /var/cache/httpd
<VirtualHost 10.211.55.7:10001>
<Directory />
Order deny,allow
Deny from all
Allow from 10.211.55.
</Directory>
# This directive allows you to view mod_cluster status at URL http://10.211.55.4:10001/mod_cluster-manager
<Location /mod_cluster-manager>
SetHandler mod_cluster-manager
Order deny,allow
Deny from all
Allow from 10.211.55.
</Location>
KeepAliveTimeout 60
MaxKeepAliveRequests 0
ManagerBalancerName other-server-group
AdvertiseFrequency 5
</VirtualHost>
----
[IMPORTANT]
For more details on mod_cluster configurations please see this document:
[source, java]
----
http://docs.jboss.org/mod_cluster/1.1.0/html/Quick_Start_Guide.html
----
[[testing]]
== Testing
If everything goes fine we can start httpd service now:
[source, bash]
----
service httpd start
----
Now we access the cluster:
[source]
----
http://10.211.55.7/cluster-demo/put.jsp
----
image:images/clustering/http---10.211.55.7-cluster-demo-put.jsp.png[images/clustering/http---10.211.55.7-cluster-demo-put.jsp.png]
We should see the request is distributed to one of the hosts(master or
slave) from the WildFly log. For me the request is sent to master:
[source]
----
[Server:server-three] 16:06:22,256 INFO [stdout] (http-10.211.55.7-10.211.55.7-8330-4) Putting date now
----
Now I disconnect master by using the management interface. Select
'runtime' and the server 'master' in the upper corners.
Select 'server-three' and kick the stop button, the active-icon should
change.
Killing the server by using system commands will have the effect that
the Host-Controller restart the instance immediately!
Then wait for a few seconds and access cluster:
[source]
----
http://10.211.55.7/cluster-demo/get.jsp
----
image:images/clustering/http---10.211.55.7-cluster-demo-get.jsp.png[images/clustering/http---10.211.55.7-cluster-demo-get.jsp.png]
Now the request should be served by slave and we should see the log from
slave:
[source, bash]
----
[Server:server-three-slave] 16:08:29,860 INFO [stdout] (http-10.211.55.2-10.211.55.2-8330-1) Getting date now
----
And from the get.jsp we should see that the time we get is the same
we've put by 'put.jsp'. Thus it's proven that the session is correctly
replicated to slave.
Now we restart master and should see the host is registered back to
cluster.
[TIP]
It doesn't matter if you found the request is distributed to slave at
first time. Then just disconnect slave and do the testing, the request
should be sent to master instead. The point is we should see the request
is redirect from one host to another and the session is held.
[[special-thanks]]
== Special Thanks
https://community.jboss.org/people/wdfink[<NAME>] has
contributed the updated add-user.sh usages and configs in host.xml from
7.1.0.Final. +
https://community.jboss.org/people/jfclere[<NAME>] provided
the mod_cluster 1.2.0 usages. +
<NAME> has given a lot of suggestions and helps to make
this document readable.
<file_sep>/_docs/13/_high-availability/subsystem-support/mod_cluster_Subsystem.adoc
[[mod_cluster_Subsystem]]
== mod_cluster Subsystem
The mod_cluster integration is done via the `mod_cluster` subsystem.
[[configuration]]
== Configuration
[[instance-id-or-jvmroute]]
=== Instance ID or JVMRoute
The instance-id or JVMRoute defaults to `jboss.node.name` property passed
on server startup (e.g. via `-Djboss.node.name=XYZ`).
[source]
----
[standalone@localhost:9990 /] /subsystem=undertow/:read-attribute(name=instance-id)
{
"outcome" => "success",
"result" => expression "${jboss.node.name}"
}
----
To configure instance-id statically, configure the corresponding
property in Undertow subsystem:
[source]
----
[standalone@localhost:9990 /] /subsystem=undertow/:write-attribute(name=instance-id,value=myroute)
{
"outcome" => "success",
"response-headers" => {
"operation-requires-reload" => true,
"process-state" => "reload-required"
}
}
----
[[proxies]]
=== Proxies
By default, mod_cluster is configured for multicast-based discovery. To
specify a static list of proxies, create a remote-socket-binding for
each proxy and then reference them in the 'proxies' attribute. See the
following example for configuration in the domain mode:
[source]
----
[domain@localhost:9990 /] /socket-binding-group=ha-sockets/remote-destination-outbound-socket-binding=proxy1:add(host=10.21.152.86, port=6666)
{
"outcome" => "success",
"result" => undefined,
"server-groups" => undefined
}
[domain@localhost:9990 /] /socket-binding-group=ha-sockets/remote-destination-outbound-socket-binding=proxy2:add(host=10.21.152.87, port=6666)
{
"outcome" => "success",
"result" => undefined,
"server-groups" => undefined
}
[domain@localhost:9990 /] /profile=ha/subsystem=modcluster/mod-cluster-config=configuration/:write-attribute(name=proxies, value=[proxy1, proxy2])
{
"outcome" => "success",
"result" => undefined,
"server-groups" => undefined
}
[domain@localhost:9990 /] :reload-servers
{
"outcome" => "success",
"result" => undefined,
"server-groups" => undefined
}
----
[[runtime-operations]]
== Runtime Operations
The modcluster subsystem supports several operations:
[source]
----
[standalone@localhost:9999 subsystem=modcluster] :read-operation-names
{
"outcome" => "success",
"result" => [
"add",
"add-custom-metric",
"add-metric",
"add-proxy",
"disable",
"disable-context",
"enable",
"enable-context",
"list-proxies",
"read-attribute",
"read-children-names",
"read-children-resources",
"read-children-types",
"read-operation-description",
"read-operation-names",
"read-proxies-configuration",
"read-proxies-info",
"read-resource",
"read-resource-description",
"refresh",
"remove-custom-metric",
"remove-metric",
"remove-proxy",
"reset",
"stop",
"stop-context",
"validate-address",
"write-attribute"
]
}
----
The operations specific to the modcluster subsystem are divided in 3
categories the ones that affects the configuration and require a restart
of the subsystem, the one that just modify the behaviour temporarily and
the ones that display information from the httpd part.
[[operations-displaying-httpd-informations]]
=== operations displaying httpd information
There are 2 operations that display how Apache httpd sees the node:
[[read-proxies-configuration]]
==== read-proxies-configuration
Send a DUMP message to all Apache httpd the node is connected to and
display the message received from Apache httpd.
[source]
----
[standalone@localhost:9999 subsystem=modcluster] :read-proxies-configuration
{
"outcome" => "success",
"result" => [
"neo3:6666",
"balancer: [1] Name: mycluster Sticky: 1 [JSESSIONID]/[jsessionid] remove: 0 force: 1 Timeout: 0 Maxtry: 1
node: [1:1],Balancer: mycluster,JVMRoute: 498bb1f0-00d9-3436-a341-7f012bc2e7ec,Domain: [],Host: 127.0.0.1,Port: 8080,Type: http,flushpackets: 0,flushwait: 10,ping: 10,smax: 26,ttl: 60,timeout: 0
host: 1 [example.com] vhost: 1 node: 1
host: 2 [localhost] vhost: 1 node: 1
host: 3 [default-host] vhost: 1 node: 1
context: 1 [/myapp] vhost: 1 node: 1 status: 1
context: 2 [/] vhost: 1 node: 1 status: 1
",
"jfcpc:6666",
"balancer: [1] Name: mycluster Sticky: 1 [JSESSIONID]/[jsessionid] remove: 0 force: 1 Timeout: 0 maxAttempts: 1
node: [1:1],Balancer: mycluster,JVMRoute: 498bb1f0-00d9-3436-a341-7f012bc2e7ec,LBGroup: [],Host: 127.0.0.1,Port: 8080,Type: http,flushpackets: 0,flushwait: 10,ping: 10,smax: 26,ttl: 60,timeout: 0
host: 1 [default-host] vhost: 1 node: 1
host: 2 [localhost] vhost: 1 node: 1
host: 3 [example.com] vhost: 1 node: 1
context: 1 [/] vhost: 1 node: 1 status: 1
context: 2 [/myapp] vhost: 1 node: 1 status: 1
"
]
}
----
[[read-proxies-info]]
==== read-proxies-info
Send a INFO message to all Apache httpd the node is connected to and
display the message received from Apache httpd.
[source]
----
[standalone@localhost:9999 subsystem=modcluster] :read-proxies-info
{
"outcome" => "success",
"result" => [
"neo3:6666",
"Node: [1],Name: 498bb1f0-00d9-3436-a341-7f012bc2e7ec,Balancer: mycluster,Domain: ,Host: 127.0.0.1,Port: 8080,Type: http,Flushpackets: Off,Flushwait: 10000,Ping: 10000000,Smax: 26,Ttl: 60000000,Elected: 0,Read: 0,Transfered: 0,Connected: 0,Load: -1
Vhost: [1:1:1], Alias: example.com
Vhost: [1:1:2], Alias: localhost
Vhost: [1:1:3], Alias: default-host
Context: [1:1:1], Context: /myapp, Status: ENABLED
Context: [1:1:2], Context: /, Status: ENABLED
",
"jfcpc:6666",
"Node: [1],Name: 498bb1f0-00d9-3436-a341-7f012bc2e7ec,Balancer: mycluster,LBGroup: ,Host: 127.0.0.1,Port: 8080,Type: http,Flushpackets: Off,Flushwait: 10,Ping: 10,Smax: 26,Ttl: 60,Elected: 0,Read: 0,Transfered: 0,Connected: 0,Load: 1
Vhost: [1:1:1], Alias: default-host
Vhost: [1:1:2], Alias: localhost
Vhost: [1:1:3], Alias: example.com
Context: [1:1:1], Context: /, Status: ENABLED
Context: [1:1:2], Context: /myapp, Status: ENABLED
"
]
}
----
[[operations-that-handle-the-proxies-the-node-is-connected-too]]
==== operations that handle the proxies the node is connected too
There are 3 operation that could be used to manipulate the list of
Apache httpd the node is connected to.
[[list-proxies]]
==== list-proxies
Displays the httpd that are connected to the node. The httpd could be
discovered via the Advertise protocol or via the proxy-list attribute.
[source]
----
[standalone@localhost:9999 subsystem=modcluster] :list-proxies
{
"outcome" => "success",
"result" => [
"proxy1:6666",
"proxy2:6666"
]
}
----
[[remove-proxy]]
==== remove-proxy
Remove a proxy from the discovered proxies or temporarily from the
proxy-list attribute.
[source]
----
[standalone@localhost:9999 subsystem=modcluster] :remove-proxy(host=jfcpc, port=6666)
{"outcome" => "success"}
----
[[add-proxy]]
==== add-proxy
Add a proxy to the discovered proxies or temporarily to the proxy-list
attribute.
[source]
----
[standalone@localhost:9999 subsystem=modcluster] :add-proxy(host=jfcpc, port=6666)
{"outcome" => "success"}
----
[[context-related-operations]]
=== Context related operations
Those operations allow to send context related commands to Apache httpd.
They are send automatically when deploying or undeploying webapps.
[[enable-context]]
==== enable-context
Tell Apache httpd that the context is ready receive requests.
[source]
----
[standalone@localhost:9999 subsystem=modcluster] :enable-context(context=/myapp, virtualhost=default-host)
{"outcome" => "success"}
----
[[disable-context]]
==== disable-context
Tell Apache httpd that it shouldn't send new session requests to the
context of the virtualhost.
[source]
----
[standalone@localhost:9999 subsystem=modcluster] :disable-context(context=/myapp, virtualhost=default-host)
{"outcome" => "success"}
----
[[stop-context]]
==== stop-context
Tell Apache httpd that it shouldn't send requests to the context of the
virtualhost.
[source]
----
[standalone@localhost:9999 subsystem=modcluster] :stop-context(context=/myapp, virtualhost=default-host, waittime=50)
{"outcome" => "success"}
----
[[node-related-operations]]
=== Node related operations
Those operations are like the context operation but they apply to all
webapps running on the node and operation that affect the whole node.
[[refresh]]
==== refresh
Refresh the node by sending a new CONFIG message to Apache httpd.
[[reset]]
==== reset
Reset the connection between Apache httpd and the node.
[[configuration-1]]
=== Configuration
[[metric-configuration]]
==== Metric configuration
There are 4 metric operations corresponding to add and remove load
metrics to the dynamic-load-provider. Note that when nothing is defined
a simple-load-provider is use with a fixed load factor of one.
[source]
----
[standalone@localhost:9999 subsystem=modcluster] :read-resource(name=mod-cluster-config)
{
"outcome" => "success",
"result" => {"simple-load-provider" => {"factor" => "1"}}
}
----
that corresponds to the following configuration:
[source, xml]
----
<subsystem xmlns="urn:jboss:domain:modcluster:1.0">
<mod-cluster-config>
<simple-load-provider factor="1"/>
</mod-cluster-config>
</subsystem>
----
[[add-metric]]
===== add-metric
Add a metric to the dynamic-load-provider, the dynamic-load-provider in
configuration is created if needed.
[source, ruby]
----
[standalone@localhost:9999 subsystem=modcluster] :add-metric(type=cpu)
{"outcome" => "success"}
[standalone@localhost:9999 subsystem=modcluster] :read-resource(name=mod-cluster-config)
{
"outcome" => "success",
"result" => {
"dynamic-load-provider" => {
"history" => 9,
"decay" => 2,
"load-metric" => [{
"type" => "cpu"
}]
}
}
}
----
[[remove-metric]]
===== remove-metric
Remove a metric from the dynamic-load-provider.
[source]
----
[standalone@localhost:9999 subsystem=modcluster] :remove-metric(type=cpu)
{"outcome" => "success"}
----
[[add-custom-metric-remove-custom-metric]]
===== add-custom-metric / remove-custom-metric
like the add-metric and remove-metric except they require a class
parameter instead the type. Usually they needed additional properties
which can be specified
[source]
----
[standalone@localhost:9999 subsystem=modcluster] :add-custom-metric(class=myclass, property=[("pro1" => "value1"), ("pro2" => "value2")]
{"outcome" => "success"}
----
which corresponds the following in the xml configuration file:
[source, xml]
----
<subsystem xmlns="urn:jboss:domain:modcluster:1.0">
<mod-cluster-config>
<dynamic-load-provider history="9" decay="2">
<custom-load-metric class="myclass">
<property name="pro1" value="value1"/>
<property name="pro2" value="value2"/>
</custom-load-metric>
</dynamic-load-provider>
</mod-cluster-config>
</subsystem>
----
include::SSL_Configuration_using_Elytron_Subsystem.adoc[leveloffset=+1]<file_sep>/_docs/13/_high-availability/subsystem-support/SSL_Configuration_using_Elytron_Subsystem.adoc
[[SSL_Configuration_using_Elytron_Subsystem]]
== SSL Configuration using Elytron Subsystem
[abstract]
This document provides information how to configure mod_cluster
subsystem to protect communication between mod_cluster and load balancer
using SSL/TLS using link:WildFly_Elytron_Security{outfilesuffix}#Elytron_Subsystem[Elytron Subsystem].
== Overview
Elytron subsystem provides a powerful and flexible model to configure
different security aspects for applications and the application server
itself. At its core, Elytron subsystem exposes different capabilities to
the application server in order centralize security related
configuration in a single place and to allow other subsystems to consume
these capabilities. One of the security capabilities exposed by Elytron
subsystem is a Client `ssl-context` that can be used to configure
mod_cluster subsystem to communicate with a load balancer using SSL/TLS.
When protecting the communication between the application server and the
load balancer, you need do define a Client `ssl-context` in order to:
* Define a trust store holding the certificate chain that will be used
to validate load balancer's certificate
* Define a trust manager to perform validations against the load
balancer's certificate
[[defining-a-trust-store-with-the-trusted-certificates]]
== Defining a Trust Store with the Trusted Certificates
To define a trust store in Elytron you can execute the following CLI
command:
[source]
----
[standalone@localhost:9990 /] /subsystem=elytron/key-store=default-trust-store:add(type=JKS, relative-to=jboss.server.config.dir, path=application.truststore, credential-reference={clear-text=password})
----
In order to successfully execute the command above you must have a
*application.truststore* file inside your
*JBOSS_HOME/standalone/configuration* directory. Where the trust store
is protected by a password with a value *password*. The trust store must
contain the certificates associated with the load balancer or a
certificate chain in case the load balancer's certificate is signed by a
CA.
We strongly recommend you to avoid using self-signed certificates with
your load balancer. Ideally, certificates should be signed by a CA and
your trust store should contain a certificate chain representing your
ROOT and Intermediary CAs.
[[defining-a-trust-manager-to-validate-certificates]]
== Defining a Trust Manager To Validate Certificates
To define a trust manager in Elytron you can execute the following CLI
command:
[source]
----
[standalone@localhost:9990 /] /subsystem=elytron/trust-managers=default-trust-manager:add(algorithm=PKIX, key-store=default-trust-store)
----
Here we are setting the *default-trust-store* as the source of the
certificates that the application server trusts.
[[defining-a-client-ssl-context-and-configuring-mod_cluster-subsystem]]
== Defining a Client SSL Context and Configuring mod_cluster Subsystem
Finally, you can create the Client SSL Context that is going to be used
by the mod_cluster subsystem when connecting to the load balancer using
SSL/TLS:
[source]
----
[standalone@localhost:9990 /] /subsystem=elytron/client-ssl-context=modcluster-client-ssl-context:add(trust-managers=default-trust-manager)
----
Now that the Client `ssl-context` is defined you can configure
mod_cluster subsystem as follows:
[source]
----
[standalone@localhost:9990 /] /subsystem=modcluster/mod-cluster-config=configuration:write-attribute(name=ssl-context, value=modcluster-client-ssl-context)
----
Once you execute the last command above, reload the server:
[source]
----
[standalone@localhost:9990 /] reload
----
[[using-a-certificate-revocation-list]]
== Using a Certificate Revocation List
In case you want to validate the load balancer certificate against a
Certificate Revocation List (CRL), you can configure the `trust-manager`
in Elytron subsystem as follows:
[source]
----
[standalone@localhost:9990 /] /subsystem=elytron/trust-managers=default-trust-manager:write-attribute(name=certificate-revocation-list.path, value=intermediate.crl.pem)
----
To use a CRL your trust store must contain the certificate chain in
order to check validity of both CRL list and the load balancer`s
certificate.
A different way to configure a CRL is using the _Distribution Points_
embedded in your certificates. For that, you need to configure a
`certificate-revocation-list` as follows:
[source]
----
/subsystem=elytron/trust-managers=default-trust-manager:write-attribute(name=certificate-revocation-list)
----
<file_sep>/_docs/14/Developer_Guide.adoc
[[Developer_Guide]]
= Developer Guide
WildFly developer team;
:revnumber: {version}
:revdate: {localdate}
:toc: macro
:toclevels: 2
:toc-title: WildFly Developer guide
:doctype: book
:icons: font
:source-highlighter: coderay
:wildflyVersion: 14
ifndef::ebook-format[:leveloffset: 1]
(C) 2017 The original authors.
:numbered:
.Target Audience
Java Developers
:leveloffset: 1
include::_developer-guide/Class_Loading_in_WildFly.adoc[]
include::_developer-guide/Implicit_module_dependencies_for_deployments.adoc[]
include::_developer-guide/Deployment_Descriptors_used_In_WildFly.adoc[]
include::_developer-guide/Development_Guidelines_and_Recommended_Practices.adoc[]
include::_developer-guide/Application_Client_Reference.adoc[]
include::_developer-guide/Embedded_API.adoc[]
include::_developer-guide/CDI_Reference.adoc[]
include::_developer-guide/EE_Concurrency_Utilities.adoc[]
include::_developer-guide/EJB3_Reference_Guide.adoc[]
include::_developer-guide/JPA_Reference_Guide.adoc[]
include::_developer-guide/JNDI_Reference.adoc[]
include::_developer-guide/JAX-RS_Reference_Guide.adoc[]
include::_developer-guide/Web_(Undertow)_Reference_Guide.adoc[]
include::_developer-guide/JAX-WS_Reference_guide.adoc[]
include::_developer-guide/Scoped_EJB_client_contexts.adoc[]
include::_developer-guide/EJB_invocations_from_a_remote_client_using_JNDI.adoc[]
include::_developer-guide/EJB_invocations_from_a_remote_server_instance.adoc[]
include::_developer-guide/Remote_EJB_invocations_via_JNDI_-_EJB_client_API_or_remote-naming_project.adoc[]
include::_developer-guide/Migrated_to_WildFly_Example_Applications.adoc[]
include::_developer-guide/Spring_applications_development_and_migration_guide.adoc[]
include::_developer-guide/How_do_I_migrate_my_application_from_AS7_to_WildFly.adoc[]
include::_developer-guide/How_do_I_migrate_my_application_from_WebLogic_to_WildFly.adoc[]
include::_developer-guide/How_do_I_migrate_my_application_from_WebSphere_to_WildFly.adoc[]
<file_sep>/_docs/13/_developer-guide/JNDI_Remote_Reference.adoc
[[JNDI_Remote_Reference]]
== Remote JNDI Access
WildFly supports two different types of remote JNDI.
[[http-remoting]]
== http-remoting:
The `http-remoting:` protocol implementation is provided by JBoss Remote
Naming project, and uses http upgrade to lookup items from the servers
local JNDI. To use it, you must have the appropriate jars on the class
path, if you are maven user can be done simply by adding the following
to your `pom.xml` dependencies:
[source, xml]
----
<dependency>
<groupId>org.wildfly</groupId>
<artifactId>wildfly-ejb-client-bom</artifactId>
<version>11.0.0.Final</version>
<type>pom</type>
</dependency>
----
If you are not using maven a shaded jar that contains all required
classes +
can be found in the `bin/client` directory of WildFly's distribution.
[source, java]
----
final Properties env = new Properties();
env.put(Context.INITIAL_CONTEXT_FACTORY, "org.jboss.naming.remote.client.InitialContextFactory");
env.put(Context.PROVIDER_URL, "http-remoting://localhost:8080");
// the property below is required ONLY if there is no ejb client configuration loaded (such as a
// jboss-ejb-client.properties in the class path) and the context will be used to lookup EJBs
env.put("jboss.naming.client.ejb.context", true);
InitialContext remoteContext = new InitialContext(env);
RemoteCalculator ejb = (RemoteCalculator) remoteContext.lookup("wildfly-http-remoting-ejb/CalculatorBean!"
+ RemoteCalculator.class.getName());
----
[IMPORTANT]
The http-remoting client assumes JNDI names in remote lookups are
relative to java:jboss/exported namespace, a lookup of an absolute JNDI
name will fail.
[[ejb]]
== ejb:
The ejb: namespace implementation is provided by the jboss-ejb-client
library, and allows the lookup of EJB's using their application name,
module name, ejb name and interface type. To use it, you must have the
appropriate jars on the class path, if you are maven user can be done
simply by adding the following to your `pom.xml` dependencies:
[source, xml]
----
<dependency>
<groupId>org.wildfly</groupId>
<artifactId>wildfly-ejb-client-bom</artifactId>
<version>11.0.0.Final</version>
<type>pom</type>
</dependency>
----
If you are not using maven a shaded jar that contains all required
classes +
can be found in the `bin/client` directory of WildFly's distribution.
This is a client side JNDI implementation. Instead of looking up an EJB
on the server the lookup name contains enough information for the client
side library to generate a proxy with the EJB information. When you
invoke a method on this proxy it will use the current EJB client context
to perform the invocation. If the current context does not have a
connection to a server with the specified EJB deployed then an error
will occur. Using this protocol it is possible to look up EJB's that do
not actually exist, and no error will be thrown until the proxy is
actually used. The exception to this is stateful session beans, which
need to connect to a server when they are created in order to create the
session bean instance on the server.
[source, java]
----
final Properties env = new Properties();
env.put(Context.URL_PKG_PREFIXES, "org.jboss.ejb.client.naming");
InitialContext remoteContext = new InitialContext(env);
MyRemoteInterface myRemote = (MyRemoteInterface) remoteContext.lookup("ejb:myapp/myejbjar/MyEjbName\!com.test.MyRemoteInterface");
MyStatefulRemoteInterface myStatefulRemote = (MyStatefulRemoteInterface) remoteContext.lookup("ejb:myapp/myejbjar/MyStatefulName\!comp.test.MyStatefulRemoteInterface?stateful");
----
The first example is a lookup of a singleton, stateless or EJB 2.x home
interface. This lookup will not hit the server, instead a proxy will be
generated for the remote interface specified in the name. The second
example is for a stateful session bean, in this case the JNDI lookup
will hit the server, in order to tell the server to create the SFSB
session.
[IMPORTANT]
For more details on how the server connections are configured, including
the *required* jboss ejb client setup, please see
<<EJB_invocations_from_a_remote_client_using_JNDI,EJB invocations from a remote client using JNDI>>.
<file_sep>/_docs/13/_high-availability/ha-singleton/Singleton_deployments.adoc
[[Singleton_deployments]]
== Singleton deployments
WildFly 10 resurrected the ability to start a given deployment on a
single node in the cluster at any given time. If that node shuts down,
or fails, the application will automatically start on another node on
which the given deployment exists. Long time users of JBoss AS will
recognize this functionality as being akin to the
https://docs.jboss.org/jbossclustering/cluster_guide/5.1/html/deployment.chapt.html#d0e1220[HASingletonDeployer],
a.k.a. "
https://docs.jboss.org/jbossclustering/cluster_guide/5.1/html/deployment.chapt.html#d0e1220[deploy-hasingleton]",
feature of AS6 and earlier.
[[usage]]
== Usage
A deployment indicates that it should be deployed as a singleton via a
deployment descriptor. This can either be a standalone
`/META-INF/singleton-deployment.xml` file or embedded within an existing
jboss-all.xml descriptor. This descriptor may be applied to any
deployment type, e.g. JAR, WAR, EAR, etc., with the exception of a
subdeployment within an EAR. +
e.g.
[source, xml]
----
<singleton-deployment xmlns="urn:jboss:singleton-deployment:1.0" policy="foo"/>
----
The singleton deployment descriptor defines which
<<Singleton_subsystem,singleton policy>> should be used to deploy the
application. If undefined, the default singleton policy is used, as
defined by the singleton subsystem.
Using a standalone descriptor is often preferable, since it may be
overlaid onto an existing deployment archive. +
e.g.
[source]
----
deployment-overlay add --name=singleton-policy-foo --content=/META-INF/singleton-deployment.xml=/path/to/singleton-deployment.xml --deployments=my-app.jar --redeploy-affected
----
<file_sep>/_docs/13/_admin-guide/Core_management_concepts.adoc
[[Core_management_concepts]]
= Core management concepts
:toclevels: 2
:toc-title: Core management concepts
:toc: macro
:leveloffset: +1
:numbered:
include::Operating_modes.adoc[]
include::General_configuration_concepts.adoc[]
include::Management_resources.adoc[]
:leveloffset: -1
<file_sep>/_docs/13/_testsuite/Shortened_Maven_Run_Overview.adoc
[[Shortened_Maven_Run_Overview]]
== Shortened Maven Run Overview
[[how-to-get-it]]
== How to get it
[source, bash]
----
./integration-tests.sh clean install -DallTests | tee TS.txt | testsuite/tools/runSummary.sh
----
[[how-its-done]]
== How it's done
Run this script on the output of the AS7 testsuite run:
[source, bash]
----
## Cat the file or stdin if no args,
## filter only interesting lines - plugin executions and modules separators,
## plus Test runs summaries,
## and remove the boring plugins like enforcer etc.
cat $1 \
| egrep ' --- |Building| ---------|Tests run: | T E S T S' \
| grep -v 'Time elapsed'
| sed 's|Tests run:| Tests run:|' \
| grep -v maven-clean-plugin \
| grep -v maven-enforcer-plugin \
| grep -v buildnumber-maven-plugin \
| grep -v maven-help-plugin \
| grep -v properties-maven-plugin:.*:write-project-properties \
;
----
You'll get an overview of the run.
[[example-output-with-comments.]]
== Example output with comments.
[source, bash]
----
ondra@ondra-redhat: ~/work/AS7/ozizka-as7 $ ./integration-tests.sh clean install -DallTests | tee TS.txt | testsuite/tools/runSummary.sh
[INFO] ------------------------------------------------------------------------
[INFO] ------------------------------------------------------------------------
[INFO] Building JBoss Application Server Test Suite: Aggregator 7.1.0.CR1-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] --- maven-dependency-plugin:2.3:copy (copy-annotations-endorsed) @ jboss-as-testsuite ---
Copies org.jboss.spec.javax.annotation:jboss-annotations-api_1.1_spec to ${project.build.directory}/endorsed .
Inherited - needed for compilation of all submodules.
[INFO] --- maven-resources-plugin:2.5:copy-resources (build-jbossas.server) @ jboss-as-testsuite ---
Copies ${jboss.home} to target/jbossas . TODO: Should be jboss.dist.
[INFO] --- xml-maven-plugin:1.0:transform (update-ip-addresses-jbossas.server) @ jboss-as-testsuite ---
Changes IP addresses used in server config files -
applies ${xslt.scripts.dir}/changeIPAddresses.xsl on ${basedir}/target/jbossas/standalone/configuration/standalone-*.xml
Currently inherited, IMO should not be.
[INFO] --- maven-source-plugin:2.1.2:jar-no-fork (attach-sources) @ jboss-as-testsuite ---
TODO: Remove
[INFO] --- maven-install-plugin:2.3.1:install (default-install) @ jboss-as-testsuite ---
[INFO] ------------------------------------------------------------------------
[INFO] Building JBoss Application Server Test Suite: Integration Aggregator 7.1.0.CR1-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] --- maven-dependency-plugin:2.3:copy (copy-annotations-endorsed) @ jboss-as-testsuite-integration-agg ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (build-jbossas.server) @ jboss-as-testsuite-integration-agg ---
TODO: Remove
[INFO] --- maven-resources-plugin:2.5:copy-resources (ts.copy-jbossas) @ jboss-as-testsuite-integration-agg ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (ts.copy-jbossas.groups) @ jboss-as-testsuite-integration-agg ---
[INFO] --- xml-maven-plugin:1.0:transform (update-ip-addresses-jbossas.server) @ jboss-as-testsuite-integration-agg ---
TODO: Remove
[INFO] --- maven-source-plugin:2.1.2:jar-no-fork (attach-sources) @ jboss-as-testsuite-integration-agg ---
TODO: Remove
[INFO] --- maven-install-plugin:2.3.1:install (default-install) @ jboss-as-testsuite-integration-agg ---
[INFO] ------------------------------------------------------------------------
[INFO] Building JBoss AS Test Suite: Integration - Smoke 7.1.0.CR1-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] --- maven-dependency-plugin:2.3:copy (copy-annotations-endorsed) @ jboss-as-testsuite-integration-smoke ---
[INFO] --- maven-resources-plugin:2.5:resources (default-resources) @ jboss-as-testsuite-integration-smoke ---
TODO: Remove
[INFO] --- maven-compiler-plugin:2.3.2:compile (default-compile) @ jboss-as-testsuite-integration-smoke ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (build-jbossas.server) @ jboss-as-testsuite-integration-smoke ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (ts.copy-jbossas.groups) @ jboss-as-testsuite-integration-smoke ---
[INFO] --- maven-resources-plugin:2.5:testResources (default-testResources) @ jboss-as-testsuite-integration-smoke ---
[INFO] --- xml-maven-plugin:1.0:transform (update-ip-addresses-jbossas.server) @ jboss-as-testsuite-integration-smoke ---
TODO: Remove
[INFO] --- maven-antrun-plugin:1.6:run (build-smoke.server) @ jboss-as-testsuite-integration-smoke ---
[echo] Building AS instance "smoke" from /home/ondra/work/EAP/EAP6-DR9 to /home/ondra/work/AS7/ozizka-as7/testsuite/integration/smoke/target
TODO: Should be running one level above!
[INFO] --- maven-compiler-plugin:2.3.2:testCompile (default-testCompile) @ jboss-as-testsuite-integration-smoke ---
[INFO] --- maven-surefire-plugin:2.10:test (smoke-full.surefire) @ jboss-as-testsuite-integration-smoke ---
T E S T S
Tests run: 4, Failures: 0, Errors: 4, Skipped: 0
----
[[example-output-unchanged]]
== Example output, unchanged
[source, bash]
----
ondra@lenovo:~/work/AS7/ozizka-git$ ./integration-tests.sh clean install -DallTests | tee TS.txt | testsuite/tools/runSummary.sh
SSCmeetingWestfordJan [copy] Warning: /home/ondra/work/AS7/ozizka-git/testsuite/integration/src/test/resources/test-configs/smoke does not exist.
[copy] Warning: /home/ondra/work/AS7/ozizka-git/testsuite/integration/src/test/resources/test-configs/clustering-udp-0 does not exist.
[copy] Warning: /home/ondra/work/AS7/ozizka-git/testsuite/integration/src/test/resources/test-configs/clustering-udp-1 does not exist.
[copy] Warning: /home/ondra/work/AS7/ozizka-git/testsuite/integration/src/test/resources/test-configs/iiop-client does not exist.
[copy] Warning: /home/ondra/work/AS7/ozizka-git/testsuite/integration/src/test/resources/test-configs/iiop-server does not exist.
[INFO] ------------------------------------------------------------------------
[INFO] ------------------------------------------------------------------------
[INFO] Building JBoss Application Server Test Suite: Aggregator 7.1.0.Final-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] --- maven-antrun-plugin:1.6:run (banner) @ jboss-as-testsuite ---
[INFO] --- maven-dependency-plugin:2.3:copy (copy-annotations-endorsed) @ jboss-as-testsuite ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (build-jbossas.server) @ jboss-as-testsuite ---
[INFO] --- xml-maven-plugin:1.0:transform (update-ip-addresses-jbossas.server) @ jboss-as-testsuite ---
[INFO] --- maven-install-plugin:2.3.1:install (default-install) @ jboss-as-testsuite ---
[INFO] ------------------------------------------------------------------------
[INFO] Building JBoss Application Server Test Suite: Integration 7.1.0.Final-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] --- maven-dependency-plugin:2.3:copy (copy-annotations-endorsed) @ jboss-as-testsuite-integration-agg ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (build-jbossas.server) @ jboss-as-testsuite-integration-agg ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (ts.copy-jbossas) @ jboss-as-testsuite-integration-agg ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (ts.copy-jbossas.groups) @ jboss-as-testsuite-integration-agg ---
[INFO] --- maven-install-plugin:2.3.1:install (default-install) @ jboss-as-testsuite-integration-agg ---
[INFO] ------------------------------------------------------------------------
[INFO] Building JBoss Application Server Test Suite: Integration - Smoke 7.1.0.Final-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] --- maven-dependency-plugin:2.3:copy (copy-annotations-endorsed) @ jboss-as-testsuite-integration-smoke ---
[INFO] --- maven-resources-plugin:2.5:resources (default-resources) @ jboss-as-testsuite-integration-smoke ---
[INFO] --- maven-compiler-plugin:2.3.2:compile (default-compile) @ jboss-as-testsuite-integration-smoke ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (build-jbossas.server) @ jboss-as-testsuite-integration-smoke ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (ts.copy-jbossas.groups) @ jboss-as-testsuite-integration-smoke ---
[INFO] --- maven-resources-plugin:2.5:testResources (default-testResources) @ jboss-as-testsuite-integration-smoke ---
[INFO] --- maven-antrun-plugin:1.6:run (build-smoke.server) @ jboss-as-testsuite-integration-smoke ---
[echo] Building AS instance "smoke" from /home/ondra/work/AS7/ozizka-git/testsuite/integration/smoke/../../../build/target/jboss-as-7.1.0.Final-SNAPSHOT to /home/ondra/work/AS7/ozizka-git/testsuite/integration/smoke/target
[INFO] --- maven-compiler-plugin:2.3.2:testCompile (default-testCompile) @ jboss-as-testsuite-integration-smoke ---
[INFO] --- maven-surefire-plugin:2.10:test (smoke-full.surefire) @ jboss-as-testsuite-integration-smoke ---
T E S T S
Tests run: 4, Failures: 0, Errors: 0, Skipped: 0
[INFO] --- maven-surefire-plugin:2.10:test (smoke-web.surefire) @ jboss-as-testsuite-integration-smoke ---
T E S T S
Tests run: 116, Failures: 0, Errors: 0, Skipped: 6
[INFO] --- maven-jar-plugin:2.3.1:jar (default-jar) @ jboss-as-testsuite-integration-smoke ---
[INFO] Building jar: /home/ondra/work/AS7/ozizka-git/testsuite/integration/smoke/target/jboss-as-testsuite-integration-smoke-7.1.0.Final-SNAPSHOT.jar
[INFO] --- maven-install-plugin:2.3.1:install (default-install) @ jboss-as-testsuite-integration-smoke ---
[INFO] ------------------------------------------------------------------------
[INFO] Building JBoss Application Server Test Suite: Integration - Basic 7.1.0.Final-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] --- maven-dependency-plugin:2.3:copy (copy-annotations-endorsed) @ jboss-as-testsuite-integration-basic ---
[INFO] --- maven-resources-plugin:2.5:resources (default-resources) @ jboss-as-testsuite-integration-basic ---
[INFO] --- maven-compiler-plugin:2.3.2:compile (default-compile) @ jboss-as-testsuite-integration-basic ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (build-jbossas.server) @ jboss-as-testsuite-integration-basic ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (ts.copy-jbossas.groups) @ jboss-as-testsuite-integration-basic ---
[INFO] --- maven-resources-plugin:2.5:testResources (default-testResources) @ jboss-as-testsuite-integration-basic ---
[INFO] --- maven-antrun-plugin:1.6:run (prepare-jars-basic-integration.server) @ jboss-as-testsuite-integration-basic ---
[INFO] --- maven-compiler-plugin:2.3.2:testCompile (default-testCompile) @ jboss-as-testsuite-integration-basic ---
[INFO] --- maven-surefire-plugin:2.10:test (basic-integration-default-full.surefire) @ jboss-as-testsuite-integration-basic ---
T E S T S
Tests run: 323, Failures: 0, Errors: 4, Skipped: 30
[INFO] ------------------------------------------------------------------------
[INFO] Building JBoss Application Server Test Suite: Integration - Clustering 7.1.0.Final-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] --- maven-dependency-plugin:2.3:copy (copy-annotations-endorsed) @ jboss-as-testsuite-integration-clust ---
[INFO] --- maven-resources-plugin:2.5:resources (default-resources) @ jboss-as-testsuite-integration-clust ---
[INFO] --- maven-compiler-plugin:2.3.2:compile (default-compile) @ jboss-as-testsuite-integration-clust ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (build-jbossas.server) @ jboss-as-testsuite-integration-clust ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (ts.copy-jbossas.groups) @ jboss-as-testsuite-integration-clust ---
[INFO] --- maven-resources-plugin:2.5:testResources (default-testResources) @ jboss-as-testsuite-integration-clust ---
[INFO] --- maven-antrun-plugin:1.6:run (build-clustering.server) @ jboss-as-testsuite-integration-clust ---
[echo] Building config clustering-udp-0
[echo] Building AS instance "clustering-udp-0" from /home/ondra/work/AS7/ozizka-git/testsuite/integration/clust/../../../build/target/jboss-as-7.1.0.Final-SNAPSHOT to /home/ondra/work/AS7/ozizka-git/testsuite/integration/clust/target
[echo] Building config clustering-udp-1
[echo] Building AS instance "clustering-udp-1" from /home/ondra/work/AS7/ozizka-git/testsuite/integration/clust/../../../build/target/jboss-as-7.1.0.Final-SNAPSHOT to /home/ondra/work/AS7/ozizka-git/testsuite/integration/clust/target
[INFO] --- maven-compiler-plugin:2.3.2:testCompile (default-testCompile) @ jboss-as-testsuite-integration-clust ---
[INFO] --- maven-surefire-plugin:2.10:test (tests-clustering-multi-node-unmanaged.surefire) @ jboss-as-testsuite-integration-clust ---
T E S T S
Tests run: 9, Failures: 0, Errors: 0, Skipped: 0
[INFO] --- maven-surefire-plugin:2.10:test (tests-clustering-single-node.surefire) @ jboss-as-testsuite-integration-clust ---
T E S T S
Tests run: 1, Failures: 0, Errors: 0, Skipped: 0
[INFO] --- maven-surefire-plugin:2.10:test (tests-clustering-multi-node.surefire) @ jboss-as-testsuite-integration-clust ---
T E S T S
Tests run: 8, Failures: 0, Errors: 0, Skipped: 0
[INFO] --- maven-jar-plugin:2.3.1:jar (default-jar) @ jboss-as-testsuite-integration-clust ---
[INFO] Building jar: /home/ondra/work/AS7/ozizka-git/testsuite/integration/clust/target/jboss-as-testsuite-integration-clust-7.1.0.Final-SNAPSHOT.jar
[INFO] --- maven-install-plugin:2.3.1:install (default-install) @ jboss-as-testsuite-integration-clust ---
[INFO] ------------------------------------------------------------------------
[INFO] Building JBoss Application Server Test Suite: Integration - IIOP 7.1.0.Final-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] --- maven-dependency-plugin:2.3:copy (copy-annotations-endorsed) @ jboss-as-testsuite-integration-iiop ---
[INFO] --- maven-resources-plugin:2.5:resources (default-resources) @ jboss-as-testsuite-integration-iiop ---
[INFO] --- maven-compiler-plugin:2.3.2:compile (default-compile) @ jboss-as-testsuite-integration-iiop ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (build-jbossas.server) @ jboss-as-testsuite-integration-iiop ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (ts.copy-jbossas.groups) @ jboss-as-testsuite-integration-iiop ---
[INFO] --- maven-resources-plugin:2.5:testResources (default-testResources) @ jboss-as-testsuite-integration-iiop ---
[INFO] --- maven-antrun-plugin:1.6:run (build-clustering.server) @ jboss-as-testsuite-integration-iiop ---
[echo] Building config iiop-client
[echo] Building AS instance "iiop-client" from /home/ondra/work/AS7/ozizka-git/testsuite/integration/iiop/../../../build/target/jboss-as-7.1.0.Final-SNAPSHOT to /home/ondra/work/AS7/ozizka-git/testsuite/integration/iiop/target
[echo] Building config iiop-server
[echo] Building AS instance "iiop-server" from /home/ondra/work/AS7/ozizka-git/testsuite/integration/iiop/../../../build/target/jboss-as-7.1.0.Final-SNAPSHOT to /home/ondra/work/AS7/ozizka-git/testsuite/integration/iiop/target
[INFO] --- maven-compiler-plugin:2.3.2:testCompile (default-testCompile) @ jboss-as-testsuite-integration-iiop ---
[INFO] --- maven-surefire-plugin:2.10:test (tests-iiop-multi-node.surefire) @ jboss-as-testsuite-integration-iiop ---
T E S T S
Tests run: 12, Failures: 0, Errors: 0, Skipped: 0
[INFO] --- maven-jar-plugin:2.3.1:jar (default-jar) @ jboss-as-testsuite-integration-iiop ---
[INFO] Building jar: /home/ondra/work/AS7/ozizka-git/testsuite/integration/iiop/target/jboss-as-testsuite-integration-iiop-7.1.0.Final-SNAPSHOT.jar
[INFO] --- maven-install-plugin:2.3.1:install (default-install) @ jboss-as-testsuite-integration-iiop ---
[INFO] ------------------------------------------------------------------------
[INFO] Building JBoss Application Server Test Suite: Compatibility Tests 7.1.0.Final-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] --- maven-dependency-plugin:2.3:copy (copy-annotations-endorsed) @ jboss-as-testsuite-integration-compat ---
[INFO] --- maven-resources-plugin:2.5:resources (default-resources) @ jboss-as-testsuite-integration-compat ---
[INFO] --- maven-compiler-plugin:2.3.2:compile (default-compile) @ jboss-as-testsuite-integration-compat ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (build-jbossas.server) @ jboss-as-testsuite-integration-compat ---
[INFO] --- maven-resources-plugin:2.5:testResources (default-testResources) @ jboss-as-testsuite-integration-compat ---
[INFO] --- maven-compiler-plugin:2.3.2:testCompile (default-testCompile) @ jboss-as-testsuite-integration-compat ---
[INFO] --- maven-antrun-plugin:1.6:run (build-jars) @ jboss-as-testsuite-integration-compat ---
[INFO] --- maven-surefire-plugin:2.10:test (default-test) @ jboss-as-testsuite-integration-compat ---
T E S T S
Tests run: 7, Failures: 0, Errors: 4, Skipped: 3
[INFO] ------------------------------------------------------------------------
[INFO] Building JBoss Application Server Test Suite: Domain Mode Integration Tests 7.1.0.Final-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] --- maven-dependency-plugin:2.3:copy (copy-annotations-endorsed) @ jboss-as-testsuite-integration-domain ---
[INFO] --- maven-resources-plugin:2.5:resources (default-resources) @ jboss-as-testsuite-integration-domain ---
[INFO] --- maven-compiler-plugin:2.3.2:compile (default-compile) @ jboss-as-testsuite-integration-domain ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (build-jbossas.server) @ jboss-as-testsuite-integration-domain ---
[INFO] --- maven-resources-plugin:2.5:testResources (default-testResources) @ jboss-as-testsuite-integration-domain ---
[INFO] --- maven-compiler-plugin:2.3.2:testCompile (default-testCompile) @ jboss-as-testsuite-integration-domain ---
[INFO] --- maven-surefire-plugin:2.10:test (default-test) @ jboss-as-testsuite-integration-domain ---
T E S T S
Tests run: 89, Failures: 0, Errors: 0, Skipped: 4
[INFO] --- maven-jar-plugin:2.3.1:jar (default-jar) @ jboss-as-testsuite-integration-domain ---
[INFO] Building jar: /home/ondra/work/AS7/ozizka-git/testsuite/domain/target/jboss-as-testsuite-integration-domain-7.1.0.Final-SNAPSHOT.jar
[INFO] --- maven-install-plugin:2.3.1:install (default-install) @ jboss-as-testsuite-integration-domain ---
[INFO] ------------------------------------------------------------------------
[INFO] Building JBoss Application Server Test Suite: Benchmark Tests 7.1.0.Final-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] --- maven-dependency-plugin:2.3:copy (copy-annotations-endorsed) @ jboss-as-testsuite-benchmark ---
[INFO] --- maven-resources-plugin:2.5:resources (default-resources) @ jboss-as-testsuite-benchmark ---
[INFO] --- maven-compiler-plugin:2.3.2:compile (default-compile) @ jboss-as-testsuite-benchmark ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (build-jbossas.server) @ jboss-as-testsuite-benchmark ---
[INFO] --- maven-resources-plugin:2.5:testResources (default-testResources) @ jboss-as-testsuite-benchmark ---
[INFO] --- maven-compiler-plugin:2.3.2:testCompile (default-testCompile) @ jboss-as-testsuite-benchmark ---
[INFO] --- maven-surefire-plugin:2.10:test (default-test) @ jboss-as-testsuite-benchmark ---
T E S T S
Tests run: 0, Failures: 0, Errors: 0, Skipped: 0
[INFO] --- maven-jar-plugin:2.3.1:jar (default-jar) @ jboss-as-testsuite-benchmark ---
[INFO] Building jar: /home/ondra/work/AS7/ozizka-git/testsuite/benchmark/target/jboss-as-testsuite-benchmark-7.1.0.Final-SNAPSHOT.jar
[INFO] --- maven-install-plugin:2.3.1:install (default-install) @ jboss-as-testsuite-benchmark ---
[INFO] ------------------------------------------------------------------------
[INFO] Building JBoss Application Server Test Suite: Stress Tests 7.1.0.Final-SNAPSHOT
[INFO] ------------------------------------------------------------------------
[INFO] --- maven-dependency-plugin:2.3:copy (copy-annotations-endorsed) @ jboss-as-testsuite-stress ---
[INFO] --- maven-resources-plugin:2.5:resources (default-resources) @ jboss-as-testsuite-stress ---
[INFO] --- maven-compiler-plugin:2.3.2:compile (default-compile) @ jboss-as-testsuite-stress ---
[INFO] --- maven-resources-plugin:2.5:copy-resources (build-jbossas.server) @ jboss-as-testsuite-stress ---
[INFO] --- maven-resources-plugin:2.5:testResources (default-testResources) @ jboss-as-testsuite-stress ---
[INFO] --- maven-compiler-plugin:2.3.2:testCompile (default-testCompile) @ jboss-as-testsuite-stress ---
[INFO] --- maven-surefire-plugin:2.10:test (default-test) @ jboss-as-testsuite-stress ---
T E S T S
Tests run: 0, Failures: 0, Errors: 0, Skipped: 0
[INFO] --- maven-jar-plugin:2.3.1:jar (default-jar) @ jboss-as-testsuite-stress ---
[INFO] Building jar: /home/ondra/work/AS7/ozizka-git/testsuite/stress/target/jboss-as-testsuite-stress-7.1.0.Final-SNAPSHOT.jar
[INFO] --- maven-install-plugin:2.3.1:install (default-install) @ jboss-as-testsuite-stress ---
[INFO] ------------------------------------------------------------------------
[INFO] ------------------------------------------------------------------------
[INFO] ------------------------------------------------------------------------
[INFO] ------------------------------------------------------------------------
----
<file_sep>/_docs/13/_developer-guide/wsprovide.adoc
[[wsprovide]]
== wsprovide
_wsprovide_ is a command line tool, Maven plugin and Ant task that
generates portable JAX-WS artifacts for a service endpoint
implementation. It also has the option to "provide" the abstract
contract for offline usage.
[[command-line-tool]]
== Command Line Tool
The command line tool has the following usage:
....
usage: wsprovide [options] <endpoint class name>
options:
-h, --help Show this help message
-k, --keep Keep/Generate Java source
-w, --wsdl Enable WSDL file generation
-a, --address The generated port soap:address in wsdl
-c. --classpath=<path> The classpath that contains the endpoint
-o, --output=<directory> The directory to put generated artifacts
-r, --resource=<directory> The directory to put resource artifacts
-s, --source=<directory> The directory to put Java source
-e, --extension Enable SOAP 1.2 binding extension
-q, --quiet Be somewhat more quiet
-t, --show-traces Show full exception stack traces
....
[[examples]]
=== Examples
Generating wrapper classes for portable artifacts in the "generated"
directory:
....
wsprovide -o generated foo.Endpoint
....
Generating wrapper classes and WSDL in the "generated" directory
....
wsprovide -o generated -w foo.Endpoint
....
Using an endpoint that references other jars
....
wsprovide -o generated -c application1.jar:application2.jar foo.Endpoint
....
[[maven-plugin]]
== Maven Plugin
The _wsprovide_ tools is included in the
*org.jboss.ws.plugins:jaxws-tools-* *maven-* *plugin* plugin. The plugin
has two goals for running the tool, _wsprovide_ and _wsprovide-test_,
which basically do the same during different Maven build phases (the
former triggers the sources generation during _process-classes_ phase,
the latter during the _process-test-classes_ one).
The _wsprovide_ plugin has the following parameters:
[cols=",,",options="header"]
|=======================================================================
|Attribute |Description |Default
|testClasspathElements |Each classpathElement provides alibrary file to
be added to classpath
|$\{project.compileClasspathElements}or$\{project.testClasspathElements}
|outputDirectory |The output directory for generated artifacts.
|$\{project.build.outputDirectory}or$\{project.build.testOutputDirectory}
|resourceDirectory |The output directory for resource artifacts
(WSDL/XSD). |$\{project.build.directory}/wsprovide/resources
|sourceDirectory |The output directory for Java source.
|$\{project.build.directory}/wsprovide/java
|extension |Enable SOAP 1.2 binding extension. |false
|generateWsdl |Whether or not to generate WSDL. |false
|verbose |Enables more informational output about command progress.
|false
|portSoapAddress |The generated port soap:address in the WSDL |
|endpointClass |Service Endpoint Implementation. |
|=======================================================================
[[examples-1]]
=== Examples
You can use _wsprovide_ in your own project build simply referencing the
_maven-jaxws-tools-plugin_ in the configured plugins in your _pom.xml_
file.
The following example makes the plugin provide the wsdl file and
artifact sources for the specified endpoint class:
[source,xml]
----
<build>
<plugins>
<plugin>
<groupId>org.jboss.ws.plugins</groupId>
<artifactId>jaxws-tools-maven-plugin</artifactId>
<version>1.2.0.Beta1</version>
<configuration>
<verbose>true</verbose>
<endpointClass>org.jboss.test.ws.plugins.tools.wsprovide.TestEndpoint</endpointClass>
<generateWsdl>true</generateWsdl>
</configuration>
<executions>
<execution>
<goals>
<goal>wsprovide</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
----
The following example does the same, but is meant for use in your own
testsuite:
[source,xml]
----
<build>
<plugins>
<plugin>
<groupId>org.jboss.ws.plugins</groupId>
<artifactId>jaxws-tools-maven-plugin</artifactId>
<version>1.2.0.Beta1</version>
<configuration>
<verbose>true</verbose>
<endpointClass>org.jboss.test.ws.plugins.tools.wsprovide.TestEndpoint2</endpointClass>
<generateWsdl>true</generateWsdl>
</configuration>
<executions>
<execution>
<goals>
<goal>wsprovide-test</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
----
Plugin stack dependencyThe plugin itself does not have an explicit
dependency to a JBossWS stack, as it's meant for being used with
implementations of any supported version of the _JBossWS SPI_. So the
user is expected to set a dependency in his own `pom.xml` to the desired
_JBossWS_ stack version. The plugin will rely on the that for using the
proper tooling.
[source,xml]
----
<dependencies>
<dependency>
<groupId>org.jboss.ws.cxf</groupId>
<artifactId>jbossws-cxf-client</artifactId>
<version>5.0.0.CR1</version>
</dependency>
</dependencies>
----
[TIP]
Be careful when using this plugin with the Maven War Plugin as that
include any project dependency into the generated application war
archive. You might want to set `<scope>provided</scope>` for the
_JBossWS_ stack dependency to avoid that.
[IMPORTANT]
Up to version 1.1.2.Final, the _artifactId_ of the plugin was
*maven-jaxws-tools-plugin*.
[[ant-task]]
== Ant Task
The wsprovide ant task ( _org.jboss.ws.tools.ant.WSProvideTask_) has the
following attributes:
[cols=",,",options="header"]
|=======================================================================
|Attribute |Description |Default
|fork |Whether or not to run the generation task in a separate VM. |true
|keep |Keep/Enable Java source code generation. |false
|destdir |The output directory for generated artifacts. |"output"
|resourcedestdir |The output directory for resource artifacts
(WSDL/XSD). |value of destdir
|sourcedestdir |The output directory for Java source. |value of destdir
|extension |Enable SOAP 1.2 binding extension. |false
|genwsdl |Whether or not to generate WSDL. |false
|address |The generated port soap:address in wsdl. |
|verbose |Enables more informational output about command progress.
|false
|sei |Service Endpoint Implementation. |
|classpath |The classpath that contains the service endpoint
implementation. |"."
|=======================================================================
[[examples-2]]
=== Examples
Executing wsprovide in verbose mode with separate output directories for
source, resources, and classes:
[source,xml]
----
<target name="test-wsproivde" depends="init">
<taskdef name="wsprovide" classname="org.jboss.ws.tools.ant.WSProvideTask">
<classpath refid="core.classpath"/>
</taskdef>
<wsprovide
fork="false"
keep="true"
destdir="out"
resourcedestdir="out-resource"
sourcedestdir="out-source"
genwsdl="true"
verbose="true"
sei="org.jboss.test.ws.jaxws.jsr181.soapbinding.DocWrappedServiceImpl">
<classpath>
<pathelement path="${tests.output.dir}/classes"/>
</classpath>
</wsprovide>
</target>
----
<file_sep>/_docs/14/_testsuite/WildFly_Integration_Testsuite_User_Guide.adoc
[[WildFly_Integration_Testsuite_User_Guide]]
= Wild**Fly** Integration Testsuite User Guide
*See also:* <<WildFly_Testsuite_Test_Developer_Guide,WildFly
Testsuite Test Developer Guide>>
*Target Audience:* Those interested in running the testsuite or a subset
thereof, with various configuration options.
[[running-the-testsuite]]
== Running the testsuite
The tests can be run using:
* `build.sh` or `build.bat`, as a part of WildFly build.
** By default, only smoke tests are run. To run all tests, run build.sh
install -DallTests.
* `integration-tests.sh` or `integration-tests.bat`, a convenience
script which uses bundled Maven (currently 3.0.3), and runs all parent
testsuite modules (which configure the AS server).
* pure maven run, using `mvn install`.
The scripts are wrappers around Maven-based build. Their arguments are
passed to Maven (with few exceptions described below). This means you
can use:
* build.sh (defaults to install)
* build.sh install
* build.sh clean install
* integration-tests.sh install
* ...etc.
[[supported-maven-phases]]
=== Supported Maven phases
Testsuite actions are bounds to various Maven phases up to `verify`.
Running the build with earlier phases may fail in the submodules due to
missed configuration steps. Therefore, the only Maven phases you may
safely run, are:
* clean
* install
* site
The `test` phase is not recommended to be used for scripted jobs as we
are planning to switch to the `failsafe` plugin bound to the
`integration-test` and `verify` phases. See
https://issues.jboss.org/browse/WFLY-625[WFLY-625] and
https://issues.jboss.org/browse/WFLY-228[WFLY-228].
[[testsuite-structure]]
=== Testsuite structure
testsuite +
integration +
smoke +
basic +
clust +
iiop +
multinode +
xts +
compat +
domain +
mixed-domain +
stress +
benchmark
[[test-groups]]
=== Test groups
To define groups of tests to be run, these properties are available:
* `-DallTests` - Runs all subgroups.
* `-DallInteg` - Runs all integration tests. Same as
`cd testsuite/integration; mvn clean install -DallTests`
* `-Dts.integ` - Basic integration + clustering tests.
* `-Dts.clust` - Clustering tests.
* `-Dts.iiop` - IIOP tests.
* `-Dts.multinode `- Tests with many nodes.
* `-Dts.manualmode` - Tests with manual mode Arquillian containers.
* `-Dts.bench` - Benchmark tests.
* `-Dts.stress` - Stress tests.
* `-Dts.domain` - Domain mode tests.
* `-Dts.compat` - Compatibility tests.
[[examples]]
== Examples
* `integration-tests.sh [install] ` `` `-- Runs smoke tests.`
* `integration-tests.sh clean install -- Cleans the target directory, then runs smoke tests.`
* `integration-tests.sh install -Dts.smoke ` `` `-- Same as above.`
* `integration-tests.sh install -DallTests ` ``
`-- Runs all testsuite tests.`
* `integration-tests.sh install -Dts.stress -- Runs smoke tests and stress tests.`
* `integration-tests.sh install -Dts.stress -Dts.noSmoke -- Runs stress tests only.`
*Pure maven* - if you prefer not to use scripts, you may achieve the
same result with:
* `mvn ... -rf testsuite`
The `-rf ...` parameter stands for "resume from" and causes Maven to run
the specified module _and all successive_.
It's possible to run only a single module (provided the ancestor modules
were already run to create the AS copies) :
* `mvn ... -pl testsuite/integration/cluster`
The `-pl ...` parameter stands for "project list" and causes Maven to
run the specified module _only_.
[[output-to-console]]
=== Output to console
[source, java]
----
-DtestLogToFile
----
[[other-options]]
=== Other options
`-DnoWebProfile` - Run all tests with the _full_ profile (
`standalone-full.xml`). By default, most tests are run under _web_
profile ( `standalone.xml`).
`-Dts.skipTests` - Skip testsuite's tests. Defaults to the value of
`-DskipTests`, which defaults to `false`. To build AS, skip unit tests
and run testsuite, use `-DskipTests -Dts.skipTests=false`.
[[timeouts]]
=== Timeouts
[[surefire-execution-timeout]]
==== Surefire execution timeout
Unfortunatelly, no math can be done in Maven, so instead of applying a
timeout ratio, you need to specify timeout manually for Surefire.
[source, java]
----
-Dsurefire.forked.process.timeout=900
----
[[in-test-timeout-ratios]]
==== In-test timeout ratios
Ratio in prercent - 100 = default, 200 = two times longer timeouts for
given category.
Currently we have five different ratios. Later, it could be replaced
with just one generic, one for database and one for deployment
operations.
[source, java]
----
-Dtimeout.ratio.fsio=100
-Dtimeout.ratio.netio=100
-Dtimeout.ratio.memio=100
-Dtimeout.ratio.proc=100
-Dtimeout.ratio.db=100
----
[[running-a-single-test-or-specified-tests]]
=== Running a single test (or specified tests)
Single test is run using *-Dtest=...* . Examples:
* `./integration-tests.sh install -Dtest='` `*` `Clustered` `*`
`' -Dintegration.module` `-Dts.clust`
* `./integration-tests.sh clean install -Dtest=org` `/` `jboss` `/` `as`
`/` `test` `/` `integration` `/` `ejb/async/*TestCase.java`
`-Dintegration.module` `-Dts.basic`
* `cd testsuite; mvn install` `-Dtest='` `*Clustered` `*` `'`
`-Dts.basic #`
`No need for -Dintegration.module - integration module is active by default.`
The same shortcuts listed in "Test groups" may be used to activate the
module and group profile.
Note that `-Dtest=` overrides `<includes>` and `<exludes>` defined in
pom.xml, so do not rely on them when using wildcards - all compiled test
classes matching the wildcard will be run.
*Which Surefire execution is used?*
Due to Surefire's design flaw, tests run multiple times if there are
multiple surefire executions. +
To prevent this, if `-Dtest=`... is specified, non-default executions
are disabled, and standalone-full is used for all tests. +
If you need it other way, you can overcome that need:
* `basic-integration-web.surefire with standalone.xml - Configure standalone.xml to be used as server config.`
* `basic-integration-non-web.surefire - For tests included here, technically nothing changes.`
* `basic-integration-2nd.surefire - Simply run the second test in another invocation of Maven.`
[[running-against-existing-as-copy-not-the-one-from-buildtargetjboss-as-]]
=== Running against existing AS copy (not the one from
build/target/jboss-as-*)
*-Djboss.dist=<path/to/jboss-as>* will tell the testsuite to copy that
AS into submodules to run the tests against.
For example, you might want to run the testsuite against AS located in
`/opt/wildfly-8` :
[source, java]
----
./integration-tests.sh -DallTests -Djboss.dist=/opt/wildfly-8
----
The difference between jboss.dist and jboss.home:
jboss.dist is the location of the tested binaries. It gets copied to
testsuite submodules.
jboss.home is internally used and points to those copied AS instances
(for multinode tests, may be even different for each AS started by
Arquillian).
[[running-against-a-running-jboss-as-instance]]
==== Running against a running JBoss AS instance
Arquillian's WildFly {wildflyVersion} container adapter allows specifying
`allowConnectingToRunningServer` in `arquillian.xml`, which makes it
check whether AS is listening at `managementAddress:managementPort`, and
if so, it uses that server instead of launching a new one, and doesn't
shut it down at the end.
All arquillian.xml's in the testsuite specify this parameter. Thus, if
you have a server already running, it will be re-used.
[[running-against-jboss-enterprise-application-platform-eap-6.0]]
==== Running against JBoss Enterprise Application Platform (EAP) 6.0
To run the testsuite against AS included JBoss Enterprise Application
Platform 6.x (EAP), special steps are needed.
Assuming you already have the sources available, and the distributed EAP
maven repository unzipped in e.g. `/opt/jboss/eap6-maven-repo/` :
1) Configure maven in settings.xml to use only the EAP repository. This
repo contains all artifacts necessary for building EAP, including maven
plugins. +
The build (unlike running testsuite) may be done offline. +
The recommended way of configuring is to use special settings.xml, not
your local one (typically in .m2/settings.xml).
[source, xml]
----
<mirror>
<id>eap6-mirror-setting</id>
<mirrorOf>
*,!central-eap6,!central-eap6-plugins,!jboss-public-eap6,!jboss-public-eap6-plugins
</mirrorOf>
<name>Mirror Settings for EAP 6 build</name>
<url>file:///opt/jboss/eap6-maven-repo</url>
</mirror>
</mirrors>
----
\2) Build EAP. You won't use the resulting EAP build, though. The
purpose is to get the artifacts which the testsuite depends on.
[source, java]
----
mvn clean install -s settings.xml -Dmaven.repo.local=local-repo-eap
----
\3) Run the testsuite. Assuming that EAP is located in `/opt/eap6`, you
would run:
[source, java]
----
./integration-tests.sh -DallTests -Djboss.dist=/opt/eap6
----
For further information on building EAP and running the testsuite
against it, see the official EAP documentation (link to be added)
https://docspace.corp.redhat.com/docs/DOC-86875[.]
How-to for EAP QA can be found
https://docspace.corp.redhat.com/docs/DOC-89200[here] (Red Hat internal
only).
[[running-with-a-debugger]]
=== Running with a debugger
[cols=",,,",options="header"]
|=======================================================================
|Argument |What will start with debugger |Default port |Port change arg.
|-Ddebug |AS instances run by Arquillian |8787 |-Das.debug.port=...
|-Djpda |alias for -Ddebug | |
|-DdebugClient |Test JVMs (currently Surefire) |5050
|-Ddebug.port.surefire=...
|-DdebugCLI |AS CLI |5051 |-Ddebug.port.cli=...
|=======================================================================
[[examples-1]]
==== Examples
[source, java]
----
./integration-tests.sh install -DdebugClient -Ddebug.port.surefire=4040
...
-------------------------------------------------------
T E S T S
-------------------------------------------------------
Listening for transport dt_socket at address: 4040
----
[source, bash]
----
./integration-tests.sh install -DdebugClient -Ddebug.port.surefire
...
-------------------------------------------------------
T E S T S
-------------------------------------------------------
Listening for transport dt_socket at address: 5050
----
[source, java]
----
./integration-tests.sh install -Ddebug
----
[source, java]
----
./integration-tests.sh install -Ddebug -Das.debug.port=5005
----
[IMPORTANT]
JBoss AS is started by Arquillian, when the first test which requires
given instance is run. Unless you pass *-DtestLogToFile=false,* *there's
(currently) no challenge text in the console*; it will look like the
first test is stuck. This is being solved in
http://jira.codehaus.org/browse/SUREFIRE-781.
[IMPORTANT]
Depending on which test group(s) you run, multiple AS instances may be
started. In that case, you need to attach the debugger multiple times.
[[running-tests-with-custom-database]]
=== Running tests with custom database
To run with different database, specify the `-Dds` and use these
properties (with the following defaults):
[source, java]
----
-Dds.jdbc.driver=
-Dds.jdbc.driver.version=
-Dds.jdbc.url=
-Dds.jdbc.user=test
-Dds.jdbc.pass=<PASSWORD>
-Dds.jdbc.driver.jar=${ds.db}-jdbc-driver.jar
----
`driver` is JDBC driver class. JDBC `url`, `user` and `pass` is as
expected.
`driver.version` is used for automated JDBC driver downloading. Users
can set up internal Maven repository hosting JDBC drivers, with
artifacts with
GAV = `jdbcdrivers:${ds.db}:${ds.jdbc.driver.version`}
Internally, JBoss has such repo at
http://nexus.qa.jboss.com:8081/nexus/content/repositories/thirdparty/jdbcdrivers/
.
The `ds.db` value is set depending on ds. E.g. `-Dds=mssql2005` sets
`ds.db=mssql` (since they have the same driver). `-Dds.db` may be
overriden to use different driver.
[line-through]*In case you don't want to use such driver, set just
-Dds.db= (empty) and provide the driver to the AS manually.* +
_Not supported; work in progress on parameter to provide JDBC Driver
jar._
[[default-values]]
==== Default values
For WildFly continuous integration, there are some predefined values for
some of databases, which can be set using:
[source, java]
----
-Dds.db=<database-identifier>
----
Where database-identifier is one of: `h2`, `mysql51`
[[running-tests-with-ipv6]]
=== Running tests with IPv6
`-Dipv6` - Runs AS with
`-Djava.net.preferIPv4Stack=false -Djava.net.preferIPv6Addresses=true`
and the following defaults, overridable by respective parameter:
[cols=",,,",options="header"]
|=======================================================================
|Parameter |IPv4 default |IPv6 default |
|-Dnode0 |127.0.0.1 |::1 |Single-node tests.
|-Dnode1 |127.0.0.1 |::1 |Two-node tests (e.g. cluster) use this for the
2nd node.
|-Dmcast |192.168.3.11 |fd00:c2b6:b24b:be67:2827:688d:e6a1:6a3b |fd00:c2b6:b24b:be67:2827:688d:e6a1:6a3b is IPv6 Node-Local scope mcast
addr.
|-Dmcast.jgroupsDiag |192.168.3.11 |fdf8:f53e:61e4::18 |JGroups diagnostics
multicast address.
|-Dmcast.modcluster |172.16.31.10 |fc00:e968:6179::de52:7100 |mod_cluster multicast
address.
|=======================================================================
Values are set in AS configuration XML, replaced in resources (like
ejb-jar.xml) and used in tests.
[[running-tests-with-security-manager-custom-security-policy]]
=== Running tests with security manager / custom security policy
`-Dsecurity.manager` - Run with default policy.
`-Dsecurity.policy=<path>` - Run with the given policy.
`-Dsecurity.manager.other=<set of Java properties>` - Run with the given
properties. Whole set is included in all server startup parameters.
Example:
[source, java]
----
./integration-tests.sh clean install -Dintegration.module -DallTests \
\"-Dsecurity.manager.other=-Djava.security.manager \
-Djava.security.policy==$(pwd)/testsuite/shared/src/main/resources/secman/permitt_all.policy \
-Djava.security.debug=access:failure \"
----
Notice the \" quotes delimiting the whole `-Dsecurity.manager.other`
property.
[[creating-test-reports]]
=== Creating test reports
Test reports are created in the form known from EAP 5. To create them,
simply run the testsuite, which will create Surefire XML files.
Creation of the reports is bound to the `site` Maven phase, so it must
be run separatedly afterwards. Use one of these:
[source, bash]
----
./integration-tests.sh site
cd testsuite; mvn site
mvn -pl testsuite site
----
Note that it will take all test results under `testsuite/integration/` -
the pattern is `**/*TestCase.xml`, without need to specify `-DallTests`.
[[creating-coverage-reports]]
=== Creating coverage reports
*Jira:* https://issues.jboss.org/browse/WFLY-585
Coverage reports are created by
http://www.eclemma.org/jacoco/trunk/index.html[JaCoCo].
During the integration tests, Arquillian is passed a JVM argument which
makes it run with JaCoCo agent, which records the executions into
`${basedir}/target/jacoco` .
In the `site` phase, a HTML, XML and CSV reports are generated. That is
done using `jacoco:report` Ant task in `maven-ant-plugin` since JaCoCo's
maven report goal doesn't support getting classes outside
target/classes.
[[usage]]
==== Usage
[source, bash]
----
./build.sh clean install -DskipTests
./integration-tests.sh clean install -DallTests -Dcoverage
./integration-tests.sh site -DallTests -Dcoverage ## Must run in separatedly.
----
Alternative:
[source, bash]
----
mvn clean install -DskipTests
mvn -rf testsuite clean install -DallTests -Dcoverage
mvn -rf testsuite site -DallTests -Dcoverage
----
[[cleaning-the-project]]
=== Cleaning the project
To have most stable build process, it should start with:
* clean target directories
* only central Maven repo configured
* clean local repository or at least:
** free of artefacts to be built
** free of dependencies to be used (especially snapshots)
To use , you may use these commands:
[source, java]
----
mvn clean install -DskipTests -DallTests ## ...to clean all testsuite modules.
mvn dependency:purge-local-repository build-helper:remove-project-artifact -Dbuildhelper.removeAll
----
In case the build happens in a shared environment (e.g. network disk),
it's recommended to use local repository:
[source, java]
----
cp /home/hudson/.m2/settings.xml .
sed "s|<settings>|<settings><localRepository>/home/ozizka/hudson-repos/$JOBNAME</localRepository>|" -i settings.xml
----
Or:
[source, java]
----
mvn clean install ... -Dmaven.repo.local=localrepo
----
See also https://issues.jboss.org/browse/WFLY-628.
<file_sep>/_docs/13/Client_Guide.adoc
[[Client_Guide]]
= Wild**Fly** Client Configuration
:revnumber: {version}
:revdate: {localdate}
:toc: macro
:toclevels: 2
:toc-title: WildFly Client Configuration Guide
:doctype: book
:icons: font
:source-highlighter: coderay
:wildflyVersion: 13
:numbered:
ifndef::ebook-format[:leveloffset: 1]
:leveloffset: 0
== Introduction
As of Wild**Fly** 11 a common configuration framework has been introduced for use by the client libraries to define configuration, this allows for the configuration to be shared across multiple clients rather than relying on their own configuration files. As an example the configuration used by an EJB client can be shared with the JBoss CLI, if both of these required SSL configuration this can now be defined once and re-used.
Programmatic APIs are also available for many of the options however this document is focusing on the configuration available within the common `wildfly-config.xml` configuration file.
== wildfly-config.xml Discovery
At the time a client requires access to its configuration, the class path is scanned for a `wildfly-config.xml` or `META-INF/wildfly-config.xml` file. Once the file is located the configuration will be parsed to be made available for that client.
Alternatively, the `wildfly.config.url` system property can also be specified to identify the location of the configuration that should be used.
== Configuration Sections
:leveloffset: +2
include::_client-guide/authentication-client.adoc[]
include::_client-guide/jboss-ejb-client.adoc[]
include::_client-guide/Remoting_Client_Configuration.adoc[]
include::_client-guide/XNIO_Client_Configuration.adoc[]
:leveloffset: -2
<file_sep>/_docs/13/_developer-guide/Migrate_Order_Application_from_EAP5.1_to_WildFly.adoc
[[Migrate_Order_Application_from_EAP5]]
== Porting the Order Application from EAP 5.1 to WildFly {wildflyVersion}
<NAME> ported an example Order application that was used for
performance testing from EAP 5.1 to WildFly {wildflyVersion}. These are the notes he
made during the migration process.
[[overview-of-the-application]]
== Overview of the application
The application is relatively simple. it contains three servlets, some
stateless session beans, a stateful session bean, and some entities.
In addition to application code changes, modifications were made to the
way the EAR was packaged. This is because WildFly removed support of
some proprietary features that were available in EAP 5.1.
[[summary-of-changes]]
== Summary of changes
[[code-changes]]
=== Code Changes
[[modify-jndi-lookup-code]]
==== Modify JNDI lookup code
Since this application was first written for EAP 4.2/4.3, which did not
support EJB reference injection, the servlets were using pre-EE 5
methods for looking up stateless and stateful session bean interfaces.
While migrating to WildFly, it seemed a good time to change the code to
use the @EJB annotation, although this was not a required change.
The real difference is in the lookup name. WildFly only supports the new
EE 6 portable JNDI names rather than the old EAR structure based names.
The JNDI lookup code changed as follows:
Example of code in the EAP 5.1 version:
[source, java]
----
try {
context = new InitialContext();
distributionCenterManager = (DistributionCenterManager) context.lookup("OrderManagerApp/DistributionCenterManagerBean/local");
} catch(Exception lookupError) {
throw new ServletException("Couldn't find DistributionCenterManager bean", lookupError);
}
try {
customerManager = (CustomerManager) context.lookup("OrderManagerApp/CustomerManagerBean/local");
} catch(Exception lookupError) {
throw new ServletException("Couldn't find CustomerManager bean", lookupError);
}
try {
productManager = (ProductManager) context.lookup("OrderManagerApp/ProductManagerBean/local");
} catch(Exception lookupError) {
throw new ServletException("Couldn't find the ProductManager bean", lookupError);
}
----
Example of how this is now coded in WildFly:
[source, java]
----
@EJB(lookup="java:app/OrderManagerEJB/DistributionCenterManagerBean!services.ejb.DistributionCenterManager")
private DistributionCenterManager distributionCenterManager;
@EJB(lookup="java:app/OrderManagerEJB/CustomerManagerBean!services.ejb.CustomerManager")
private CustomerManager customerManager;
@EJB(lookup="java:app/OrderManagerEJB/ProductManagerBean!services.ejb.ProductManager")
private ProductManager productManager;
----
In addition to the change to injection, which was supported in EAP
5.1.0, the lookup name changed from:
[source, java]
----
OrderManagerApp/DistributionCenterManagerBean/local
----
to:
[source, java]
----
java:app/OrderManagerEJB/DistributionCenterManagerBean!services.ejb.DistributionCenterManager
----
All the other beans were changed in a similar manner. They are now based
on the portable JNDI names described in EE 6.
[[modify-logging-code]]
=== Modify logging code
The next major change was to logging within the application. The old
version was using the commons logging infrastructure and Log4J that is
bundled in the application server. Rather than bundling third-party
logging, the application was modified to use the new WildFly Logging
infrastructure.
The code changes themselves are rather trivial, as this example
illustrates:
Old JBoss Commons Logging/Log4J:
[source, java]
----
private static Log log = LogFactory.getLog(CustomerManagerBean.class);
----
New WildFly Logging
[source, java]
----
private static Logger logger = Logger.getLogger(CustomerManagerBean.class.toString());
----
Old JBoss Commons Logging/Log4J:
[source, java]
----
if(log.isTraceEnabled()) {
log.trace("Just flushed " + batchSize + " rows to the database.");
log.trace("Total rows flushed is " + (i+1));
}
----
New WildFly Logging:
[source, java]
----
if(logger.isLoggable(Level.TRACE)) {
logger.log(Level.TRACE, "Just flushed " + batchSize + " rows to the database.");
logger.log(Level.TRACE, "Total rows flushed is " + (i+1));
}
----
In addition to the code changes made to use the new AS7 JBoss log
manager module, you must add this dependency to the `MANIFEST.MF` file
as follows:
[source, java]
----
Manifest-Version: 1.0
Dependencies: org.jboss.logmanager
----
[[modify-the-code-to-use-infinispan-for-2nd-level-cache]]
=== Modify the code to use Infinispan for 2nd level cache
Jboss Cache has been replaced by Infinispan for 2nd level cache. This
requires modification of the `persistence.xml` file.
This is what the file looked like in EAP 5.1:
[source, java]
----
<properties>
<property name="hibernate.cache.region.factory_class" value="org.hibernate.cache.jbc2.JndiMultiplexedJBossCacheRegionFactory"/>
<property name="hibernate.cache.region.jbc2.cachefactory" value="java:CacheManager"/>
<property name="hibernate.cache.use_second_level_cache" value="true"/>
<property name="hibernate.cache.use_query_cache" value="false"/>
<property name="hibernate.cache.use_minimal_puts" value="true"/>
<property name="hibernate.cache.region.jbc2.cfg.entity" value="mvcc-entity"/>
<property name="hibernate.cache.region_prefix" value="services"/>
</properties>
----
This is how it was modified to use Infinispan for the same
configuration:
[source, java]
----
<properties>
<property name="hibernate.cache.use_second_level_cache" value="true"/>
<property name="hibernate.cache.use_minimal_puts" value="true"/>
</properties>
<shared-cache-mode>ENABLE_SELECTIVE</shared-cache-mode>
----
Most of the properties are removed since they will default to the
correct values for the second level cache. See
https://docs.jboss.org/author/display/AS71/JPA+Reference+Guide#JPAReferenceGuide-UsingtheInfinispansecondlevelcache["Using
the Infinispan second level cache"] for more details.
That was the extent of the code changes required to migrate the
application to AS7.
[[ear-packaging-changes]]
=== EAR Packaging Changes
Due to modular class loading changes, the structure of the existing EAR
failed to deploy successfully in WildFly.
The old structure of the EAR was as follows:
[source, java]
----
$ jar tf OrderManagerApp.ear
META-INF/MANIFEST.MF
META-INF/application.xml
OrderManagerWeb.war
OrderManagerEntities.jar
OrderManagerEJB.jar
META-INF/
----
In this structure, the entities and the `persistence.xml` were in one
jar file, `OrderManagerEntities.jar`, and the stateless and stateful
session beans were in another jar file, `OrderManagerEJB.jar`. This did
not work due to modular class loading changes in WildFly. There are a
couple of ways to resolve this issue:
1. Modify the class path in the `MANIFEST.MF`
2. Flatten the code and put all the beans in one JAR file.
The second approach was selected because it simplified the EAR
structure:
[source, java]
----
$ jar tf OrderManagerApp.ear
META-INF/application.xml
OrderManagerWeb.war
OrderManagerEJB.jar
META-INF/
----
Since there is no longer an `OrderManagerEntities.jar` file, the
`applcation.xml` file was modified to remove the entry.
An entry was added to the `MANIFEST.MF` file in the
`OrderManagerWeb.war` to resolve another class loading issue resulting
from the modification to use EJB reference injection in the servlets.
[source, java]
----
Manifest-Version: 1.0
Dependencies: org.jboss.logmanager
Class-Path: OrderManagerEJB.jar
----
The `Class-Path` entry tells the application to look in the
`OrderManagerEJB.jar` file for the injected beans.
[[summary]]
=== Summary
Although the existing EAR structure could have worked with additional
modifications to the `MANIFEST.MF` file, this approach seemed more
appealing because it simplified the structure while maintaining the web
tier in its own WAR.
The source files for both versions is attached so you can view the
changes that were made to the application.
| 3c7b7cb4b5f1c274c74c0ab50d426f101b848b6d | [
"Markdown",
"JavaScript",
"AsciiDoc"
] | 38 | Markdown | bstansberry/wildflysite | cf32390538befe8db6c832e07681a18dda332f69 | c31749390b9b344a077c0b3c1edbfeb4114d55b6 | |
refs/heads/main | <repo_name>rignellj/Full-Stack-Open-2021<file_sep>/Part_4/blogilista/utils/middleware.js
const { SECRET } = require('./config');
const jwt = require('jsonwebtoken');
const User = require('../models/user');
const errorHandler = (error, request, response, next) => {
const { message, name } = error;
if (name === 'ValidationError') {
return response.status(400).send({ error: message });
} else if (name === 'JsonWebTokenError') {
return response.status(401).json({
error: 'invalid token'
});
} else if (name === 'TokenExpiredError') {
return response.status(401).json({
error: 'token expired'
});
}
next(error);
};
const tokenExtractor = (req, res, next) => {
const getTokenFrom = req => {
const auth = req.get('authorization');
if (auth && auth.toLowerCase().startsWith('bearer ')) {
return auth.substring(7);
}
return null;
};
req.token = getTokenFrom(req);
next();
};
const userExtractor = async (req, res, next) => {
const getUser = async (req) => {
const { token } = req;
const { id } = jwt.verify(token, SECRET);
if (!token || !id) {
return res.status(401).json({ error: 'token missing or invalid' });
}
return await User.findById(id);
};
req.user = await getUser(req);
next();
};
module.exports = { errorHandler, tokenExtractor, userExtractor };
<file_sep>/Part_3/puhelinluettelo/models/contact.js
const mongoose = require('mongoose');
const url = process.env.MONGODB_URI;
mongoose.connect(url, {
useNewUrlParser: true,
useUnifiedTopology: true,
useFindAndModify: false,
useCreateIndex: true
})
.then(() => {
console.log('connected to MongoDB');
})
.catch(err => {
console.log('error connecting to MongoDB: ', err.message);
});
const contactSchema = new mongoose.Schema({
name: {
type: String,
minlength: 3,
required: true
},
number: {
type: String,
minlength: 10,
required: true
}
});
contactSchema.set('toJSON', {
transform: (document, returnedObject) => {
returnedObject.id = returnedObject._id.toString();
delete returnedObject._id;
delete returnedObject.__v;
}
});
module.exports = mongoose.model('Contact', contactSchema);
<file_sep>/Part_2/puhelinluettelo_front/src/App.js
import React, { useState, useEffect } from 'react';
import service from './services/address';
import PersonForm from './components/PersonForm';
import Persons from './components/Persons';
import Filter from './components/Filter';
import Notification from './components/Notification';
import './App.css';
const App = () => {
const [ persons, setPersons ] = useState(undefined);
const [ newName, setNewName ] = useState('');
const [ newNumber, setNewNumber ] = useState('');
const [ filterName, setFilterName ] = useState('');
const [ notification, setNotification ] = useState([null, null]);
const onSubmitHandler = (event) => {
event.preventDefault();
const filteredPersons = persons.filter(person => person.name === newName.trim());
const { length } = filteredPersons;
if (length === 0) {
const newAddress = {
name: newName,
number: newNumber
};
service
.create(newAddress)
.then(res => {
setPersons(persons.concat(res.data));
setNotification([`Added ${newAddress.name}`, 'added']);
})
.catch(err => {
setNotification([`${err.response.data.error}`, null]);
})
} else {
const confirm = window.confirm(`${newName.trim()} is already added to phonebook, replace the old with a new one?`);
const person = filteredPersons[0];
if (confirm) {
const updatedAddress = {
name: newName.trim(),
number: newNumber
};
service
.update(person.id, updatedAddress)
.then(res => {
console.log(res.data);
setNotification([`Number was changed for ${updatedAddress.name}`, 'changed']);
})
.catch(err => {
console.log(err);
setNotification([`Address ${updatedAddress.name} was already deleted from server`, null]);
});
}
}
setTimeout(() => {
setNotification([null, null])
}, 5000)
setNewName('');
setNewNumber('');
};
const onNameChangeHandler = (event) => setNewName(event.target.value);
const onNumberChangeHandler = (event) => setNewNumber(event.target.value);
const filterCHangeHandler = (event) => setFilterName(event.target.value);
useEffect(() => {
service
.getAll()
.then(res => {
console.log(res);
setPersons(res.data);
})
.catch(err => {
console.log(err);
});
}, []);
return (
<React.Fragment>
<h2>Phonebook</h2>
<Notification notification={notification} />
<Filter
filterCHangeHandler={filterCHangeHandler}
filterName={filterName}
/>
<h3>Add a new</h3>
<PersonForm
onSubmitHandler={onSubmitHandler}
onNameChangeHandler={onNameChangeHandler}
onNumberChangeHandler={onNumberChangeHandler}
newName={newName}
newNumber={newNumber}
/>
<h2>Numbers</h2>
<Persons
setNotification={setNotification}
filterName={filterName}
persons={persons}
setPersons={setPersons}
/>
</React.Fragment>
);
}
export default App;
<file_sep>/Part_2/Kurssitiedot/src/components/Course.js
import React from 'react';
const Header = ({ name }) => <h1>{name}</h1>;
const Part = ({ name, exercises }) => <p>{name} {exercises}</p>;
const Total = ({ total }) => <p>Number of exercises {total}</p>;
const Content = ({ parts }) => {
return (
<React.Fragment>
{parts.map(part =>
<Part
key={part.id}
name={part.name}
exercises={part.exercises}
/>
)}
</React.Fragment>
);
};
const Course = ({ course }) => {
const reducer = (accumulator, currentValue) => {
return {
exercises: accumulator.exercises + currentValue.exercises
};
};
const { name, parts } = course;
const { exercises } = parts.reduce(reducer);
return (
<React.Fragment>
<Header name={name} />
<Content parts={parts} />
<Total total={exercises}/>
</React.Fragment>
);
};
export default Course;
<file_sep>/Part_2/maiden_tiedot/src/App.js
import React, { useEffect, useState } from 'react';
import axios from 'axios';
import Countries from './components/Countries';
import './App.css';
const App = () => {
const [ countries, setCountries ] = useState([]);
const [ countryName, setCountryName ] = useState([]);
const countriesURL = 'https://restcountries.eu/rest/v2/all';
const inputChangeHandler = (event) => setCountryName(event.target.value);
useEffect(() => {
axios.get(countriesURL)
.then(res => {
setCountries(res.data)
});
}, []);
return (
<React.Fragment>
<h1>Find Countries</h1>
<input value={countryName} onChange={inputChangeHandler} />
<Countries countries={countries} countryName={countryName} setCountryName={setCountryName} />
</React.Fragment>
);
}
export default App;
<file_sep>/Part_4/blogilista/tests/blog_api.test.js
const mongoose = require('mongoose');
const supertest = require('supertest');
const app = require('../app');
const api = supertest(app);
const Blog = require('../models/blog');
const {
initialBlogs,
newBlog,
newBlogWithoutLikes,
blogWithoutUrl,
blogWithoutTitle,
blogWithoutTitleOrUrl,
blogToBeDeleted,
apiUrl
} = require('./blog_helper.test');
beforeEach(async () => {
await Blog.deleteMany({});
const blogObjects = initialBlogs.map(blog => new Blog(blog));
const promiseArray = blogObjects.map(blog => blog.save());
await Promise.all(promiseArray);
});
describe('form', () => {
test('blogs are returned as JSON', async () => {
await api
.get(apiUrl)
.expect(200)
.expect('Content-Type', /application\/json/);
});
});
describe('blogs based tests', () => {
test('all blogs are returned', async () => {
const { body } = await api.get(apiUrl);
expect(body).toHaveLength(initialBlogs.length);
});
test('a spesific url is within returned blogs', async () => {
const { body } = await api.get(apiUrl);
const contents = body.map(item => item.url);
expect(contents).toContain('www.com.www');
});
test('id property check', async () => {
const { body } = await api.get(apiUrl);
const ids = body.map(item => item.id);
expect(ids[0]).toBeDefined();
});
});
describe('routes', () => {
test('new blog can be added', async () => {
await api
.post(apiUrl)
.send(newBlog)
.expect(201)
.expect('Content-Type', /application\/json/);
const { body } = await api.get(apiUrl);
const titles = body.map(item => item.title);
expect(body).toHaveLength(initialBlogs.length + 1);
expect(titles).toContain('new blog can be added');
});
test('likes are initialized to 0 if not specified', async () => {
const { body } = await api
.post(apiUrl)
.send(newBlogWithoutLikes)
.expect(201);
expect(body.likes).toBe(0);
});
test('post request without url or title fails with 400 status code', async () => {
await api
.post(apiUrl)
.send(blogWithoutUrl)
.expect(400);
await api
.post(apiUrl)
.send(blogWithoutTitle)
.expect(400);
await api
.post(apiUrl)
.send(blogWithoutTitleOrUrl)
.expect(400);
});
test('delete request returns 204 status code', async () => {
const { body } = await api
.post(apiUrl)
.send(blogToBeDeleted)
.expect(201);
const { id } = body;
await api
.delete(apiUrl + '/' + id)
.expect(204);
});
test('likes increase by one if blog is updated', async () => {
const { body } = await api
.post(apiUrl)
.send(newBlogWithoutLikes)
.expect(201);
const { id } = body;
const response = await api
.put(apiUrl + '/' + id)
.send(body);
expect(response.body.likes).toBe(1);
});
});
afterAll(() => {
mongoose.connection.close();
});
<file_sep>/Part_4/blogilista/tests/blog_helper.test.js
const initialBlogs = [
{
title: '1',
author: '1',
url: 'www.com'
},
{
title: '2',
author: '2',
url: 'www.com'
},
{
title: '3',
author: '3',
url: 'www.com.www'
},
];
const newBlog = {
title: 'new blog can be added',
author: '4',
url: 'www.fi',
likes: 7
};
const newBlogWithoutLikes = {
title: 'blog without likes',
author: '5',
url: 'www.fi.www'
};
const blogWithoutUrl = {
title: 'blog without likes',
author: '6',
likes: 1
};
const blogWithoutTitle = {
author: '7',
url: 'www.fi.www',
likes: 1
};
const blogWithoutTitleOrUrl = {
author: '8',
likes: 1
};
const blogToBeDeleted = {
title: 'blog to be deleted',
author: '5',
url: 'www.fi.www',
likes: 2
};
const apiUrl = '/api/blogs';
module.exports = {
initialBlogs,
newBlog,
newBlogWithoutLikes,
blogWithoutUrl,
blogWithoutTitle,
blogWithoutTitleOrUrl,
blogToBeDeleted,
apiUrl
};
<file_sep>/Part_3/puhelinluettelo/README.md
My Heroku deploy
https://phonebook-fso2021.herokuapp.com/
<file_sep>/Part_4/blogilista/utils/list_helper.js
const dummy = () => {
return 1;
};
const totalLikes = (arrayOfBlogs) => {
const reducer = (sum, item) => {
return sum + item.likes;
};
if (arrayOfBlogs.length === 0) {
return 0;
}
return arrayOfBlogs.reduce(reducer, 0);
};
const favoriteBlog = (arrayOfBlogs) => {
const reducer = (favorite, item) => {
return favorite.likes > item.likes ? favorite : item;
};
return arrayOfBlogs.reduce(reducer);
};
module.exports = { dummy, totalLikes, favoriteBlog };
<file_sep>/Part_4/blogilista/tests/user_api.test.js
const mongoose = require('mongoose');
const supertest = require('supertest');
const app = require('../app');
const api = supertest(app);
const User = require('../models/user');
const {
initialUsers,
apiURL,
invalidUser,
invalidUser2
} = require('./user_helper.test');
beforeEach(async () => {
await User.deleteMany({});
const userObjects = initialUsers.map(user => new User(user));
const promiseArray = userObjects.map(user => user.save());
await Promise.all(promiseArray);
});
describe('user creation', () => {
test('creating a user that alreaydy exists', async () => {
await api
.post(apiURL)
.send(initialUsers[0])
.expect(400);
});
test('creating two invalid users', async () => {
await api
.post(apiURL)
.send(invalidUser)
.expect(400);
await api
.post(apiURL)
.send(invalidUser2)
.expect(400);
});
});
afterAll(() => {
mongoose.connection.close();
});
<file_sep>/Part_4/blogilista/tests/user_helper.test.js
const initialUsers = [
{
username: 'jrignell',
password: '<PASSWORD>',
name: '<NAME>'
},
{
username: 'hellas',
password: '<PASSWORD>',
name: '<NAME>'
},
{
username: 'mluukkai',
password: '<PASSWORD>',
name: '<NAME>'
}
];
const invalidUser = {
name: 'Martta'
}
const invalidUser2 = {
name: 'Martta',
username: 'marttaOnHirmuinenKartta',
password: '0'
}
const apiURL = '/api/users';
module.exports = { initialUsers, apiURL, invalidUser, invalidUser2 };<file_sep>/Part_3/puhelinluettelo/index.js
require('dotenv').config();
const express = require('express');
const morgan = require('morgan');
const cors = require('cors');
const app = express();
const Contact = require('./models/contact');
app.use(express.json());
app.use(express.static('build'));
app.use(cors());
const errorHandler = (err, req, res, next) => {
const { message, name } = err;
console.log(message);
if (name === 'CastError') {
return res.status(400).send({ error: 'malformatted id' });
} else if (name === 'ValidationError') {
return res.status(400).send({ error: message });
}
next(err);
};
morgan.token('post_content', function getContent (req) {
return JSON.stringify(req.body);
});
app.use(morgan(':method :url :status :res[content-length] - :response-time ms :post_content', {
skip: (req) => {
return req.method !== 'POST';
}
}));
app.get('/info', (req, res, next) => {
Contact.find({})
.then(result => {
const content = `<p>Phone book has info for ${result.length} people</p><p>${new Date()}</p>`;
res.send(content);
})
.catch(err => next(err));
});
app.get('/api/persons', (req, res, next) => {
Contact.find({})
.then(contact => res.json(contact))
.catch(err => next(err));
});
app.get('/api/persons/:id', (req, res, next) => {
Contact.findById(req.params.id)
.then(contact => {
if (contact) {
res.json(contact);
} else {
res.status(404).end();
}
})
.catch(err => next(err));
});
app.post('/api/persons', (req, res, next) => {
const { body } = req;
const { name, number } = body;
Contact.find({})
.then(result => {
const foundContact = result.filter(person => person.name === name);
if (foundContact.length > 0) {
return res.status(400).json({ error: 'Person is already in the PhoneBook' });
}
})
.catch(err => next(err));
const person = new Contact({ name, number });
person.save()
.then(savedPerson => res.json(savedPerson))
.catch(err => next(err));
});
app.delete('/api/persons/:id', (req, res, next) => {
Contact.findByIdAndRemove(req.params.id)
.then(() => res.status(204).end())
.catch(err => next(err));
});
app.put('/api/persons/:id', (req, res, next) => {
const { body, params } = req;
const { name, number } = body;
const contact = { name, number };
Contact.findByIdAndUpdate(params.id, contact, { new: true })
.then(updatedContact => res.json(updatedContact))
.catch(err => next(err));
});
const unknowEndPoint = (req, res) => {
res.status(404).send({ error: 'Unknown error' });
};
app.use(unknowEndPoint);
app.use(errorHandler);
const PORT = process.env.PORT;
app.listen(PORT || 3001, () => {
console.log(`Server running on port ${PORT}`);
});
<file_sep>/Part_1/unicafe/src/App.js
import React, { useState } from 'react';
import './App.css';
const Header = () => {
return <h1>Give feedback</h1>
};
const Button = ({ option, clickHandler }) => {
const handleClick = (event) => {
clickHandler(prevValue => prevValue + 1);
};
return (
<button
value={option}
type="submit"
onClick={handleClick}
>
{option}
</button>
);
};
const StatisticsLine = ({ text, value, percent }) => {
return (
<tr>
<td>{text}</td>
<td>{value} {percent ? '%' : ''}</td>
</tr>
);
};
const Statistics = ({ goods, neutrals, bads }) => {
const all = goods + neutrals + bads;
const positive = all === 0 ? 0 : goods / all * 100;
let content;
if (all === 0) {
content = <p>No feedback given</p>
} else {
content = (
<React.Fragment>
<h1>Statistics</h1>
<table>
<tbody>
<StatisticsLine text="good" value={goods} />
<StatisticsLine text="neutral" value={neutrals} />
<StatisticsLine text="bad" value={bads} />
<StatisticsLine text="all" value={all} />
<StatisticsLine text="positive" percent={true} value={positive} />
</tbody>
</table>
</React.Fragment>
)
}
return (
<React.Fragment>
{content}
</React.Fragment>
);
};
const App = () => {
const [good, setGood] = useState(0);
const [neutral, setNeutral] = useState(0);
const [bad, setBad] = useState(0);
return (
<React.Fragment>
<Header />
<Button
option="Good"
clickHandler={setGood}
value={good}
/>
<Button
option="Neutral"
clickHandler={setNeutral}
value={neutral}
/>
<Button
option="Bad"
clickHandler={setBad}
value={bad}
/>
<Statistics goods={good} neutrals={neutral} bads={bad} />
</React.Fragment>
);
}
export default App;
<file_sep>/Part_2/puhelinluettelo_front/src/components/Person.js
import React from 'react';
import service from '../services/address';
const Person = ({ name, number, id, setPersons, setNotification }) => {
const deleteHandler = (id) => {
const confirm = window.confirm(`Delete ${name}?`);
if (confirm) {
service
.deleteAddress(id)
.then(res => {
console.log(res, 'delete address');
})
setNotification([`Deleted ${name}`, 'deleted'])
setTimeout(() => {
setNotification([null, null])
}, 5000)
service
.getAll()
.then(res => {
setPersons(res.data);
});
}
};
return (
<React.Fragment>
<tr>
<td>{name}</td>
<td>{number}</td>
<td><button onClick={() => deleteHandler(id)}>Delete</button></td>
</tr>
</React.Fragment>
);
};
export default Person;
<file_sep>/Part_4/blogilista/controllers/blogs.js
const blogsRouter = require('express').Router();
const Blog = require('../models/blog');
blogsRouter.get('/', async (req, res) => {
const blogs = await Blog
.find({})
.populate('userId', { username: 1, name: 1, id: 1 });
res.json(blogs);
});
blogsRouter.post('/', async (req, res, next) => {
const { body, user } = req;
let { title, author, url, likes } = body;
likes = !likes ? 0 : likes;
if (!title || !url) {
return res.status(400).end();
}
try {
const blog = new Blog({ title, author, url, likes, user: user._id });
const result = await blog.save();
user.blogs = user.blogs.concat(result._id);
await user.save();
res.status(201).json(result);
} catch (error) {
next(error);
}
});
blogsRouter.delete('/:id', async (req, res) => {
const { params, user } = req;
const { userId } = await Blog.findById(params.id).populate('userId');
const { _id: blogId } = userId;
if (user._id.toString() === blogId.toString()) {
await Blog.findOneAndDelete(params.id);
}
res.status(204).end();
});
blogsRouter.put('/:id', async (req, res) => {
const { body, params } = req;
const { likes, title, author, url } = body;
const blog = {
likes: likes + 1,
title,
author,
url
};
const updatedBlog = await Blog.findByIdAndUpdate(params.id, blog, { new: true });
res.json(updatedBlog);
});
module.exports = blogsRouter;
<file_sep>/Part_2/puhelinluettelo_front/src/components/Filter.js
import React from 'react';
const Filter = ({ filterName, filterCHangeHandler }) => {
return (
<div>
filter: <input onChange={filterCHangeHandler} value={filterName} />
</div>
);
};
export default Filter;
<file_sep>/Part_2/puhelinluettelo_front/src/components/PersonForm.js
import React from 'react';
const PersonForm = ({
onSubmitHandler,
onNameChangeHandler,
newName,
onNumberChangeHandler,
newNumber
}) => {
return (
<form onSubmit={onSubmitHandler}>
<div>
name: <input onChange={onNameChangeHandler} value={newName} />
</div>
<div>
number: <input onChange={onNumberChangeHandler} value={newNumber} />
</div>
<div>
<button type="submit">Add</button>
</div>
</form>
);
};
export default PersonForm;
<file_sep>/Part_2/puhelinluettelo_front/src/components/Notification.js
import React from 'react';
import styles from './Notification.module.css';
const Notification = ({ notification }) => {
const [ message, event ] = notification;
if (message === null) {
return null
}
if (event) {
return (
<div className={`${styles.notification} ${styles.ok}`}>
{message}
</div>
);
}
return (
<div className={`${styles.notification} ${styles.error}`}>
{message}
</div>
);
};
export default Notification;
<file_sep>/Part_3/tietokanta_komentorivilta/mongo.js
const mongoose = require('mongoose');
let name = null;
let number = null;
if (process.argv.length == 5) {
name = process.argv[3];
number = process.argv[4];
} else if (process.argv.length == 3) {
} else {
console.log('Give password, name and number as argument');
console.log('OR');
console.log('Give just password to get all records from database');
console.log('Exiting with 1');
process.exit(1);
}
const password = process.argv[2];
const url = `mongodb+srv://tunnus:${password}@<EMAIL>.<EMAIL>/phonebook?retryWrites=true&w=majority`
mongoose.connect(url, {
useNewUrlParser: true,
useUnifiedTopology: true,
useFindAndModify: false,
useCreateIndex: true
});
const contactSchema = new mongoose.Schema({
name: String,
number: String
});
const Contact = mongoose.model('Contact', contactSchema);
if (name && number) {
const contact = new Contact({
name: name,
number: number
});
contact.save()
.then(res => {
console.log(`Contact name: ${name}, number: ${number} saved to phonebook!`);
console.log(res);
mongoose.connection.close();
});
} else {
Contact.find({})
.then(res => {
console.log('Phonebook:');
res.forEach(contact => console.log(`${contact.name} ${contact.number}`));
mongoose.connection.close();
});
}
<file_sep>/Part_2/puhelinluettelo_front/src/components/Persons.js
import React from 'react';
import Person from './Person';
const Persons = ({ persons, filterName, setPersons, setNotification }) => {
let content;
if (persons === undefined) {
return <p>Loading...</p>;
}
const filteredPersons = persons.filter(person => person.name.includes(filterName));
if (filteredPersons.length || filterName) {
content = (
filteredPersons.map(person => (
<Person
setNotification={setNotification}
setPersons={setPersons}
id={person.id}
key={person.name}
name={person.name}
number={person.number}
/>
)
));
} else {
content = (
persons.map(person => (
<Person
setNotification={setNotification}
setPersons={setPersons}
id={person.id}
key={person.name}
name={person.name}
number={person.number}
/>
)
));
}
return (
<table>
<tbody>
{content}
</tbody>
</table>
);
};
export default Persons;
<file_sep>/Part_2/maiden_tiedot/src/components/Country.js
import React, { useEffect, useState } from 'react';
import axios from 'axios';
import Weather from './Weather';
const Country = ({ country }) => {
const { name, capital, languages, population, flag } = country;
const api_key = process.env.REACT_APP_API_KEY;
const [ weather, setWeather ] = useState(undefined);
const weatherURL = `http://api.weatherstack.com/current?access_key=${api_key}&query=${capital}`
useEffect(() => {
axios.get(weatherURL)
.then(res => {
setWeather(res.data);
});
}, [weatherURL]);
return (
<React.Fragment>
<h2>{name}</h2>
<p>Capital: {capital}</p>
<p>Population: {population}</p>
<h3>Languages</h3>
<ul>
{languages.map(language => <li key={language.name}>{language.name}</li>)}
</ul>
<img src={flag} style={{width:"5rem", height:"auto"}} alt="flag" />
<Weather capital={capital} weather={weather} />
</React.Fragment>
);
};
export default Country;
<file_sep>/Part_2/maiden_tiedot/src/components/Weather.js
import React from 'react';
const Weather = ({ weather, capital }) => {
let content;
if (weather === undefined) {
content = <p></p>
} else {
const { temperature, weather_icons } = weather.current
content = (
<React.Fragment>
<p>Temperature: {temperature} °C</p>
<img src={weather_icons} style={{width:"5rem", height:"auto"}} alt="weather" />
</React.Fragment>
)
}
return (
<React.Fragment>
<h3>Weather in {capital}</h3>
{content}
</React.Fragment>
);
};
export default Weather;
| e1bd8d7e20a8d5e703764c5a59167698df622b85 | [
"JavaScript",
"Markdown"
] | 22 | JavaScript | rignellj/Full-Stack-Open-2021 | 0ed8fc150bf2e2c6bf9ac4c72e3f4dc94c711b0a | facf3595bccaa9d3a4f649fc246ab3974becd1cd | |
refs/heads/main | <file_sep>const audioContext = new AudioContext();
const $one = document.querySelector("#one");
const $two = document.querySelector("#two");
const audioOne = new Audio();
const audioTwo = new Audio();
$one.addEventListener("click", () => {
// Play voice
audioOne.src = "./voice.mp3";
audioOne.play();
audioTwo.volume = 0.2;
audioOne.onended = () => {
audioTwo.volume = 1;
};
});
$two.addEventListener("click", () => {
audioTwo.src = "./music.mp3";
audioTwo.play();
});
<file_sep># Audio Ducking example
## Working
1. Players `music.mp3`
2. When `voice.mp3` is played, `music.mp3`'s volume is temporarily reduced until done
3. `music.mp3`'s volume goes up again
## Limitations
- Need to pre-download all music and load to the website (could be solved with youtube-dl)
| 7c1719c3f07085c8864b607d4a8b51662be2b86b | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | HavenOfExcellence/audio-ducker | d953b81827a088ccc3475103ecba9b49921f9161 | 469610267e61d3b5e5e2cb69b0246648dbb29e7c | |
refs/heads/master | <file_sep>
document.addEventListener('DOMContentLoaded', function() {
var notify = document.getElementById("notify");
notify.addEventListener('click', function() {
chrome.runtime.sendMessage({update: true}, function(response) {
});
window.close();
});
});
<file_sep># PongOS-Chrome-Extension
**PongOS alerts employees of Solstice Mobile to the current state of the Employee Lounge through the color of the ping pong paddle**
**Firebase is also required for this application to run correctly**
##Setup
- manifest.json controls the entire chrome extension.
- The popup template (yes.html) is initialized here
- The background process (background.html) is also initialized here
- The entension has permission to access the users current open tab on Chrome
- In order to package and distribute this chrome extension you will need to either create or get ahold of a .pem file
## Functionality
- When the ping pong paddle is red, the no.html file will be displayed when the paddle is clicked
- The user has the option to click 'Notify me when open', and when the room is unoccupied, a popup will appear on screen
notifying the user that room is now available.
- When the ping pong paddle is green, the yes.html file will be displayed when the paddle is clicked
- This template has no additional functionality
- The background.html controls most of the functionality of the application
- This file initializes the background.js file
- background.js connects to Firebase and is alerted whenever a sensor detects movement in the Employee lounge
- If the sensor detects movement, the ping pong paddle will turn red, otherwise it's green
| d286fc2ddc112a0bfa78388e218c82daf64785ab | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | Spacewolf21/PongOS-Chrome-Extension | 32cc06b3f5954181ed1b275bc8af79a135170c05 | 582f765c27a38cce038ec9555315900821dfca48 | |
refs/heads/master | <repo_name>fkaaa568/Rust-Mysql<file_sep>/src/main.rs
#[macro_use]
extern crate mysql;
use std::io;
use mysql as my;
#[derive(Debug, PartialEq, Eq)]
struct Student {
student_id: i32,
student_name: Option<String>,
student_email: Option<String>,
student_age: i32,
student_status: Option<String>,
}
fn main() {
println!("Enter your Student_id");
let mut student_id = String::new();
io::stdin().read_line(&mut student_id);
let student_id:i32 = student_id.trim().parse().unwrap();
println!("Enter your Name");
let mut student_name = String::new();
io::stdin().read_line(&mut student_name);
let student_name = student_name.trim().parse().unwrap();
println!("Enter your email");
let mut student_email = String::new();
io::stdin().read_line(&mut student_email);
let student_email = student_email.trim().parse().unwrap();
println!("Enter your age");
let mut student_age = String::new();
io::stdin().read_line(&mut student_age);
let student_age = student_age.trim().parse().unwrap();
println!("Enter your Status");
let mut student_status = String::new();
io::stdin().read_line(&mut student_status);
let student_status = student_status.trim().parse().unwrap();
let student = Student{student_id:student_id,student_name:Some(student_name),student_email:Some(student_email),student_age:student_age,student_status:Some(student_status)};
insert(student);
fetch();
}
fn insert(student: Student){
let pool = my::Pool::new("mysql://root:@localhost:3309/test").unwrap();
// // Let's create payment table.
// // Unwrap just to make sure no error happened.
let students = vec![
student
];
// Let's insert payments to the database
// We will use into_iter() because we do not need to map Stmt to anything else.
// Also we assume that no error happened in `prepare`.
for mut stmt in pool.prepare(r"INSERT INTO tblStudent
(student_id, student_name, student_email, student_age,student_status)
VALUES
(:student_id, :student_name, :student_email, :student_age, :student_status)").into_iter() {
for s in students.iter() {
// `execute` takes ownership of `params` so we pass account name by reference.
// Unwrap each result just to make sure no errors happened.
stmt.execute(params!{
"student_id" => s.student_id,
"student_name" => &s.student_name,
"student_email" => &s.student_email,
"student_age" => &s.student_age,
"student_status" => &s.student_status,
}).unwrap();
}
}
}
fn update(student:Student){
let pool = my::Pool::new("mysql://root:@localhost:3309/test").unwrap();
// // Let's create payment table.
// // Unwrap just to make sure no error happened.
let students = vec![
student
];
// Let's insert payments to the database
// We will use into_iter() because we do not need to map Stmt to anything else.
// Also we assume that no error happened in `prepare`.
for mut stmt in pool.prepare(r"Update tblStudent
set student_name=:student_name,
student_email=:student_email,
student_age=:student_age,
student_status=:student_status
where student_id=:student_id
").into_iter() {
for s in students.iter() {
// `execute` takes ownership of `params` so we pass account name by reference.
// Unwrap each result just to make sure no errors happened.
stmt.execute(params!{
"student_id" => s.student_id,
"student_name" => &s.student_name,
"student_email" => &s.student_email,
"student_age" => &s.student_age,
"student_status" => &s.student_status,
}).unwrap();
}
}
}
fn delete(student: Student){
let pool = my::Pool::new("mysql://root:@localhost:3309/test").unwrap();
// // Let's create payment table.
// // Unwrap just to make sure no error happened.
let students = vec![
student
];
// Let's insert payments to the database
// We will use into_iter() because we do not need to map Stmt to anything else.
// Also we assume that no error happened in `prepare`.
for mut stmt in pool.prepare(r"delete from tblStudent
where sid=:sid
").into_iter() {
for s in students.iter() {
// `execute` takes ownership of `params` so we pass account name by reference.
// Unwrap each result just to make sure no errors happened.
stmt.execute(params!{
"student_id" => s.student_id,
"student_name" => &s.student_name,
"student_email" => &s.student_email,
"student_age" => &s.student_age,
"student_status" => &s.student_status,
}).unwrap();
}
}
}
fn fetch(){
let pool = my::Pool::new("mysql://root:@localhost:3309/test").unwrap();
// Let's select payments from database
let selected_students: Vec<Student> =
pool.prep_exec("SELECT student_id, student_name, student_email, student_age, student_status from tblStudent", ())
.map(|result| { // In this closure we will map `QueryResult` to `Vec<Payment>`
// `QueryResult` is iterator over `MyResult<row, err>` so first call to `map`
// will map each `MyResult` to contained `row` (no proper error handling)
// and second call to `map` will map each `row` to `Payment`
result.map(|x| x.unwrap()).map(|row| {
// ⚠️ Note that from_row will panic if you don't follow your schema
let (student_id, student_name, student_email, student_age, student_status) = my::from_row(row);
Student {
student_id: student_id,
student_name: student_name,
student_email: student_email,
student_age: student_age,
student_status: student_status,
}
}).collect() // Collect payments so now `QueryResult` is mapped to `Vec<Payment>`
}).unwrap(); // Unwrap `Vec<Payment>`
for s in 0..selected_students.len(){
println!("ID: {} Name: {:?} Email: {:?} Age: {:?} Status: {:?}",selected_students[s].student_id,selected_students[s].student_name,
selected_students[s].student_email,selected_students[s].student_age,selected_students[s].student_status);
}
} | 40783cb7183d2315c3f01aab1e3f89a4615ccfee | [
"Rust"
] | 1 | Rust | fkaaa568/Rust-Mysql | 08bcdd384c890fa12801e3917ec057d955014196 | 9608c61f073d861c8cea606ffd94d8ecccf89cab | |
refs/heads/master | <file_sep>#include <interrupt/idt.h>
#include <lib/stdtypes.h>
#include <lib/x86.h>
#include <lib/string.h>
#include <drivers/screen/textmode.h>
extern void idt_flush(uint32_t);
static void idt_set_gate(uint8_t, uint32_t, uint16_t, uint8_t);
idt_entry_t idt_entries[256];
idt_ptr_t idt_ptr;
interrupt_handler_t interrupt_handlers[256];
void idt_init(void)
{
// Zero all interrupt handlers
memset(&interrupt_handlers, 0, sizeof(interrupt_handler_t) * 256);
// Just like the GDT, the IDT has a "limit" field that is set to the last valid byte in the IDT,
// after adding in the start position (i.e. size-1).
idt_ptr.limit = sizeof(idt_entry_t) * 256 - 1;
idt_ptr.base = (uint32_t) &idt_entries;
// Zero the IDT
memset(&idt_entries, 0, sizeof(idt_entry_t) * 255);
// Remap IRQs
outportb(0x20, 0x11);
outportb(0xA0, 0x11);
outportb(0x21, 0x20);
outportb(0xA1, 0x28);
outportb(0x21, 0x04);
outportb(0xA1, 0x02);
outportb(0x21, 0x01);
outportb(0xA1, 0x01);
outportb(0x21, 0x0);
outportb(0xA1, 0x0);
// Set each gate in the IDT that we care about - that is:
// 0-32: Used by the CPU to report conditions, both normal and error.
// 255: Will be used later as a way to execute system calls.
idt_set_gate(0, (uint32_t)isr0 , 0x08, 0x8E);
idt_set_gate(1, (uint32_t)isr1 , 0x08, 0x8E);
idt_set_gate(2, (uint32_t)isr2 , 0x08, 0x8E);
idt_set_gate(3, (uint32_t)isr3 , 0x08, 0x8E);
idt_set_gate(4, (uint32_t)isr4 , 0x08, 0x8E);
idt_set_gate(5, (uint32_t)isr5 , 0x08, 0x8E);
idt_set_gate(6, (uint32_t)isr6 , 0x08, 0x8E);
idt_set_gate(7, (uint32_t)isr7 , 0x08, 0x8E);
idt_set_gate(8, (uint32_t)isr8 , 0x08, 0x8E);
idt_set_gate(9, (uint32_t)isr9 , 0x08, 0x8E);
idt_set_gate(10, (uint32_t)isr10, 0x08, 0x8E);
idt_set_gate(11, (uint32_t)isr11, 0x08, 0x8E);
idt_set_gate(12, (uint32_t)isr12, 0x08, 0x8E);
idt_set_gate(13, (uint32_t)isr13, 0x08, 0x8E);
idt_set_gate(14, (uint32_t)isr14, 0x08, 0x8E);
idt_set_gate(15, (uint32_t)isr15, 0x08, 0x8E);
idt_set_gate(16, (uint32_t)isr16, 0x08, 0x8E);
idt_set_gate(17, (uint32_t)isr17, 0x08, 0x8E);
idt_set_gate(18, (uint32_t)isr18, 0x08, 0x8E);
idt_set_gate(19, (uint32_t)isr19, 0x08, 0x8E);
idt_set_gate(20, (uint32_t)isr20, 0x08, 0x8E);
idt_set_gate(21, (uint32_t)isr21, 0x08, 0x8E);
idt_set_gate(22, (uint32_t)isr22, 0x08, 0x8E);
idt_set_gate(23, (uint32_t)isr23, 0x08, 0x8E);
idt_set_gate(24, (uint32_t)isr24, 0x08, 0x8E);
idt_set_gate(25, (uint32_t)isr25, 0x08, 0x8E);
idt_set_gate(26, (uint32_t)isr26, 0x08, 0x8E);
idt_set_gate(27, (uint32_t)isr27, 0x08, 0x8E);
idt_set_gate(28, (uint32_t)isr28, 0x08, 0x8E);
idt_set_gate(29, (uint32_t)isr29, 0x08, 0x8E);
idt_set_gate(30, (uint32_t)isr30, 0x08, 0x8E);
idt_set_gate(31, (uint32_t)isr31, 0x08, 0x8E);
idt_set_gate(32, (uint32_t)irq0, 0x08, 0x8E);
idt_set_gate(33, (uint32_t)irq1, 0x08, 0x8E);
idt_set_gate(34, (uint32_t)irq2, 0x08, 0x8E);
idt_set_gate(35, (uint32_t)irq3, 0x08, 0x8E);
idt_set_gate(36, (uint32_t)irq4, 0x08, 0x8E);
idt_set_gate(37, (uint32_t)irq5, 0x08, 0x8E);
idt_set_gate(38, (uint32_t)irq6, 0x08, 0x8E);
idt_set_gate(39, (uint32_t)irq7, 0x08, 0x8E);
idt_set_gate(40, (uint32_t)irq8, 0x08, 0x8E);
idt_set_gate(41, (uint32_t)irq9, 0x08, 0x8E);
idt_set_gate(42, (uint32_t)irq10, 0x08, 0x8E);
idt_set_gate(43, (uint32_t)irq11, 0x08, 0x8E);
idt_set_gate(44, (uint32_t)irq12, 0x08, 0x8E);
idt_set_gate(45, (uint32_t)irq13, 0x08, 0x8E);
idt_set_gate(46, (uint32_t)irq14, 0x08, 0x8E);
idt_set_gate(47, (uint32_t)irq15, 0x08, 0x8E);
idt_set_gate(255, (uint32_t)isr255, 0x08, 0x8E);
idt_flush((uint32_t)&idt_ptr);
}
static void idt_set_gate(uint8_t num, uint32_t base, uint16_t sel, uint8_t flags)
{
idt_entries[num].base_lo = base & 0xFFFF;
idt_entries[num].base_hi = (base >> 16) & 0xFFFF;
idt_entries[num].sel = sel;
idt_entries[num].always0 = 0;
// We must uncomment the OR below when we get to using user-mode
// It sets the interrupt gate's privilege level to 3
idt_entries[num].flags = flags /* | 0x60 */;
}
<file_sep>#include <cpu/fpu.h>
#include <lib/stdtypes.h>
#include <drivers/screen/textmode.h>
void fpu_init(void) // TODO: Check FPU is present
{
size_t cr4;
__asm __volatile__("mov %%cr4, %0" : "=r"(cr4));
cr4 |= 0x200;
__asm __volatile__("mov %0, %%cr4" :: "r"(cr4));
fpu_set_cw(0x37F);
}
void fpu_set_cw(const uint16_t cw)
{
__asm __volatile__("fldcw %0" :: "m"(cw));
}
<file_sep>#ifndef LIB_STRING_H
#define LIB_STRING_H
#include <lib/stdtypes.h>
#include <lib/stddef.h>
void *memcpy(void *dest, const void *src, size_t count);
void *memset(void *dest, int8_t value, size_t count);
void *memsetw(void *dest, uint16_t value, size_t count);
int32_t memcmp(const void *s1, const void *s2, size_t n);
int8_t *itoa(int32_t value, int8_t *result, int32_t base);
int32_t strcmp(const int8_t *str1, const int8_t *str2);
int32_t strncmp(const int8_t *str1, const int8_t *str2, int32_t count);
int8_t *strcpy(int8_t *dest, const int8_t *src);
int8_t *strncpy(int8_t *dest, const int8_t *src, int32_t count);
int8_t *strcat(int8_t *dest, const int8_t *src);
int32_t strlen(const int8_t *src);
int32_t isspace(int8_t c);
int32_t isupper(int8_t c);
int32_t islower(int8_t c);
int32_t isdigit(int8_t c);
int32_t isalpha(int8_t c);
int8_t toupper(int8_t c);
int8_t tolower(int8_t c);
#endif
<file_sep>#ifndef DRIVERS_SERIAL_H
#define DRIVERS_SERIAL_H
#include <lib/stdtypes.h>
#include <interrupt/isr.h>
#define SERIAL_IRQ 4
#define SERIAL_PORT_A 0x3F8
#define SERIAL_PORT_B 0x2F8
#define SERIAL_PORT_C 0x3E8
#define SERIAL_PORT_D 0x2E8
void serial_handler_a(registers_t *regs);
void serial_handler_b(registers_t *regs);
void serial_enable(int32_t device);
void serial_init(void);
void serial_write(int32_t device, const int8_t *out);
#endif
<file_sep>#ifndef DRIVERS_PCI_PCI_H
#define DRIVERS_PCI_PCI_H
void pci_scan(void);
#endif
<file_sep># SimpleOS #
## Simple, yet ingenious. ##
**SimpleOS** is a hobby x86 kernel and operating system loosely based on POSIX, written in C with ASM where needed.
**SimpleOS** aims to keep things simple, small and efficient.
<file_sep>#ifndef DRIVERS_PCI_REGISTRY_H
#define DRIVERS_PCI_REGISTRY_H
#include <lib/stdtypes.h>
const int8_t *pci_device_name(uint32_t vendorId, uint32_t deviceId);
const int8_t *pci_class_name(uint32_t classCode, uint32_t subclass, uint32_t progIntf);
// PCI Vendors
#define VENDOR_INTEL 0x8086
// PCI Classes
#define PCI_CLASS_LEGACY 0x00
#define PCI_CLASS_STORAGE 0x01
#define PCI_CLASS_NETWORK 0x02
#define PCI_CLASS_DISPLAY 0x03
#define PCI_CLASS_MULTIMEDIA 0x04
#define PCI_CLASS_MEMORY 0x05
#define PCI_CLASS_BRIDGE_DEVICE 0x06
#define PCI_CLASS_COMMUNICATION 0x07
#define PCI_CLASS_PERIHPERALS 0x08
#define PCI_CLASS_INPUT_DEVICES 0x09
#define PCI_CLASS_DOCKING_STATION 0x0a
#define PCI_CLASS_PROCESSOR 0x0b
#define PCI_CLASS_SERIAL_BUS 0x0c
#define PCI_CLASS_WIRELESS 0x0d
#define PCI_CLASS_INTELLIGENT_IO 0x0e
#define PCI_CLASS_SATELLITE 0x0f
#define PCI_CLASS_CRYPT 0x10
#define PCI_CLASS_SIGNAL_PROCESSING 0x11
#define PCI_CLASS_UNDEFINED 0xff
// Undefined Class
#define PCI_UNCLASSIFIED 0x0000
#define PCI_VGA_COMPATIBLE 0x0001
// Mass Storage Controller
#define PCI_STORAGE_SCSI 0x0100
#define PCI_STORAGE_IDE 0x0101
#define PCI_STORAGE_FLOPPY 0x0102
#define PCI_STORAGE_IPI 0x0103
#define PCI_STORAGE_RAID 0x0104
#define PCI_STORAGE_ATA 0x0105
#define PCI_STORAGE_SATA 0x0106
#define PCI_STORAGE_OTHER 0x0180
// Network Controller
#define PCI_NETWORK_ETHERNET 0x0200
#define PCI_NETWORK_TOKEN_RING 0x0201
#define PCI_NETWORK_FDDI 0x0202
#define PCI_NETWORK_ATM 0x0203
#define PCI_NETWORK_ISDN 0x0204
#define PCI_NETWORK_WORLDFIP 0x0205
#define PCI_NETWORK_PICGMG 0x0206
#define PCI_NETWORK_OTHER 0x0280
// Display Controller
#define PCI_DISPLAY_VGA 0x0300
#define PCI_DISPLAY_XGA 0x0301
#define PCI_DISPLAY_3D 0x0302
#define PCI_DISPLAY_OTHER 0x0380
// Multimedia Controller
#define PCI_MULTIMEDIA_VIDEO 0x0400
#define PCI_MULTIMEDIA_AUDIO 0x0401
#define PCI_MULTIMEDIA_PHONE 0x0402
#define PCI_MULTIMEDIA_AUDIO_DEVICE 0x0403
#define PCI_MULTIMEDIA_OTHER 0x0480
// Memory Controller
#define PCI_MEMORY_RAM 0x0500
#define PCI_MEMORY_FLASH 0x0501
#define PCI_MEMORY_OTHER 0x0580
// Bridge Device
#define PCI_BRIDGE_HOST 0x0600
#define PCI_BRIDGE_ISA 0x0601
#define PCI_BRIDGE_EISA 0x0602
#define PCI_BRIDGE_MCA 0x0603
#define PCI_BRIDGE_PCI 0x0604
#define PCI_BRIDGE_PCMCIA 0x0605
#define PCI_BRIDGE_NUBUS 0x0606
#define PCI_BRIDGE_CARDBUS 0x0607
#define PCI_BRIDGE_RACEWAY 0x0608
#define PCI_BRIDGE_OTHER 0x0680
// Simple Communication Controller
#define PCI_COMM_SERIAL 0x0700
#define PCI_COMM_PARALLEL 0x0701
#define PCI_COMM_MULTIPORT 0x0702
#define PCI_COMM_MODEM 0x0703
#define PCI_COMM_GPIB 0x0704
#define PCI_COMM_SMARTCARD 0x0705
#define PCI_COMM_OTHER 0x0780
// Base System Peripherals
#define PCI_SYSTEM_PIC 0x0800
#define PCI_SYSTEM_DMA 0x0801
#define PCI_SYSTEM_TIMER 0x0802
#define PCI_SYSTEM_RTC 0x0803
#define PCI_SYSTEM_PCI_HOTPLUG 0x0804
#define PCI_SYSTEM_SD 0x0805
#define PCI_SYSTEM_OTHER 0x0880
// Input Devices
#define PCI_INPUT_KEYBOARD 0x0900
#define PCI_INPUT_PEN 0x0901
#define PCI_INPUT_MOUSE 0x0902
#define PCI_INPUT_SCANNER 0x0903
#define PCI_INPUT_GAMEPORT 0x0904
#define PCI_INPUT_OTHER 0x0980
// Docking Stations
#define PCI_DOCKING_GENERIC 0x0a00
#define PCI_DOCKING_OTHER 0x0a80
// Processors
#define PCI_PROCESSOR_386 0x0b00
#define PCI_PROCESSOR_486 0x0b01
#define PCI_PROCESSOR_PENTIUM 0x0b02
#define PCI_PROCESSOR_ALPHA 0x0b10
#define PCI_PROCESSOR_POWERPC 0x0b20
#define PCI_PROCESSOR_MIPS 0x0b30
#define PCI_PROCESSOR_CO 0x0b40
// Serial Bus Controllers
#define PCI_SERIAL_FIREWIRE 0x0c00
#define PCI_SERIAL_ACCESS 0x0c01
#define PCI_SERIAL_SSA 0x0c02
#define PCI_SERIAL_USB 0x0c03
#define PCI_SERIAL_FIBER 0x0c04
#define PCI_SERIAL_SMBUS 0x0c05
#define PCI_SERIAL_USB_UHCI 0x00
#define PCI_SERIAL_USB_OHCI 0x10
#define PCI_SERIAL_USB_EHCI 0x20
#define PCI_SERIAL_USB_XHCI 0x30
#define PCI_SERIAL_USB_OTHER 0x80
// Wireless Controllers
#define PCI_WIRELESS_IRDA 0x0d00
#define PCI_WIRLESSS_IR 0x0d01
#define PCI_WIRLESSS_RF 0x0d10
#define PCI_WIRLESSS_BLUETOOTH 0x0d11
#define PCI_WIRLESSS_BROADBAND 0x0d12
#define PCI_WIRLESSS_ETHERNET_A 0x0d20
#define PCI_WIRLESSS_ETHERNET_B 0x0d21
#define PCI_WIRELESS_OTHER 0x0d80
// Intelligent I/O Controllers
#define PCI_INTELLIGENT_I2O 0x0e00
// Satellite Communication Controllers
#define PCI_SATELLITE_TV 0x0f00
#define PCI_SATELLITE_AUDIO 0x0f01
#define PCI_SATELLITE_VOICE 0x0f03
#define PCI_SATELLITE_DATA 0x0f04
// Encryption/Decryption Controllers
#define PCI_CRYPT_NETWORK 0x1000
#define PCI_CRYPT_ENTERTAINMENT 0x1001
#define PCI_CRYPT_OTHER 0x1080
// Data Acquisition and Signal Processing Controllers
#define PCI_SP_DPIO 0x1100
#define PCI_SP_OTHER 0x1180
#endif
<file_sep>#include <drivers/screen/textmode.h>
#include <lib/stdtypes.h>
#include <lib/x86.h>
#include <lib/string.h>
#include <lib/vsprintf.h>
// VGA framebuffer location
uint16_t *screen_memory = (uint16_t *)0xB8000;
// Cursor position
uint8_t cursor_x = 0;
uint8_t cursor_y = 0;
// Cursor attributes
int32_t attrib;
static void put_cursor(void);
static void scroll(void);
static void textmode_put(int8_t c);
static void textmode_write(int8_t *c);
void textmode_init(void)
{
textmode_set_colors(COLOR_LTGRAY, COLOR_BLACK); // Default colors
if (((*(volatile uint16_t *)0x410) & 0x30) == 0x30) {
screen_memory = (uint16_t *)0xB0000; // Monochrome display
// TODO: Panic
}
textmode_clear_screen();
}
void textmode_set_colors(uint8_t forecolor, uint8_t backcolor)
{
attrib = (backcolor << 4) | (forecolor & 0x0F);
}
void textmode_move_cursor(int32_t x, int32_t y)
{
cursor_x = x;
cursor_y = y;
put_cursor();
}
static void put_cursor(void)
{
uint16_t position = (cursor_y * SCREEN_WIDTH) + cursor_x;
outportb(0x3D4, 14);
outportb(0x3D5, position >> 8);
outportb(0x3D4, 15);
outportb(0x3D5, position);
}
void textmode_clear_screen(void)
{
int32_t i;
for (i = 0; i < SCREEN_HEIGHT; i++) {
memsetw(screen_memory + i * SCREEN_WIDTH, 0, SCREEN_WIDTH);
}
textmode_move_cursor(0, 0);
}
static void scroll(void)
{
if (cursor_y >= SCREEN_HEIGHT) {
uint32_t tmp = (cursor_y - SCREEN_HEIGHT) + 1;
memcpy(screen_memory, screen_memory + tmp * SCREEN_WIDTH, (SCREEN_HEIGHT - tmp) * SCREEN_WIDTH * 2);
memsetw(screen_memory + (SCREEN_HEIGHT - tmp) * SCREEN_WIDTH, 0, SCREEN_WIDTH);
cursor_y = (SCREEN_HEIGHT - 1);
put_cursor();
}
}
static void textmode_put(int8_t c)
{
scroll();
int8_t *memory = (int8_t *)screen_memory;
int32_t i = ((cursor_y * SCREEN_WIDTH) + cursor_x) * 2;
if (c == '\b' && cursor_x) {
cursor_x--;
} else if (c == '\t') {
cursor_x = (cursor_x + 8) & ~(8 - 1);
} else if (c == '\r') {
cursor_x = 0;
} else if (c == '\n') {
cursor_x = 0;
cursor_y++;
} else if (c >= ' ') {
memory[i] = c;
i++;
memory[i] = attrib;
i++;
cursor_x++;
}
if (cursor_x >= SCREEN_WIDTH) {
cursor_x = 0;
cursor_y++;
}
put_cursor();
}
static void textmode_write(int8_t *c)
{
while (*c) {
textmode_put(*c++);
}
}
void printf(const int8_t *fmt, ...)
{
static int8_t buf[1920];
va_list args;
int32_t i;
va_start(args, fmt);
i = vsprintf(buf, fmt, args);
va_end(args);
buf[i] = '\0';
textmode_write(buf);
}
<file_sep>APP = kernel
OBJDIR = build
AUXFILES = Makefile link.ld floppy.img cd.iso
# Pick output [floppy|iso|both]
OUTPUT = both
# Location to mount floppy.img
FLOPPYDIR = /mnt/floppy
VERSION_MAJOR = 0
VERSION_MINOR = 2
VERSION_BUILD := $(shell cat .build 2>/dev/null || (echo 1 > .build && echo 1))
VERSION_DATE := $(shell date +'%Y-%m-%d')
AS = nasm
#CC = gcc # Stock compiler may work, but not recommended
CC = gcc-4.8.2 # Cross compiler
LD = ld
ASFLAGS = -f elf
CFLAGS = -std=c99 -m32 -nodefaultlibs -nostdlib -nostdinc -fno-builtin -ffreestanding -I src/include \
-Wall -Wextra -Wshadow -Wpointer-arith -Wcast-align -Wwrite-strings -Wmissing-prototypes -Wmissing-declarations -Wredundant-decls -Wnested-externs -Winline -Wno-long-long -Wstrict-prototypes \
-Wno-unused-function -Wno-unused-parameter -Wno-unused-variable -Wno-unused-but-set-variable \
-DVERSION_MAJOR=$(VERSION_MAJOR) -DVERSION_MINOR=$(VERSION_MINOR) -DVERSION_BUILD=$(VERSION_BUILD) -DVERSION_DATE=\"$(VERSION_DATE)\"
# -Wpedantic -Wconversion
LDFLAGS = -m elf_i386 -T link.ld
AFILES := $(shell find src/ -name '*.s' | sed 's/src\///')
CFILES := $(shell find src/ -name '*.c' | sed 's/src\///')
FILES = $(AFILES) $(CFILES)
ADIRS := $(shell find src/ -name '*.s' -exec dirname {} \; | sed 's/src//' | uniq)
CDIRS := $(shell find src/ -name '*.c' -exec dirname {} \; | sed 's/src//' | uniq)
DIRS = $(ADIRS) $(CDIRS)
AOBJS := $(patsubst %.s, $(OBJDIR)/%.o, $(AFILES))
COBJS := $(patsubst %.c, $(OBJDIR)/%.o, $(CFILES))
OBJS = $(AOBJS) $(COBJS)
.PHONY: all clean clean-floppy build-floppy build-iso build-both todo dist backup cloc $(OBJDIR)
all: $(OBJDIR) $(OBJDIR)/$(APP) build-$(OUTPUT)
@echo Done
$(OBJDIR):
@$(shell for dir in $(DIRS); do mkdir -p $(OBJDIR)/$$dir; done)
$(OBJDIR)/$(APP): $(OBJS)
@echo $$(($$(cat .build) + 1)) > .build
@echo Linking $(APP)
@$(LD) $(LDFLAGS) -o $(OBJDIR)/$(APP) $(OBJS)
$(OBJDIR)/%.o: src/%.s
@echo Assembling $<
@$(AS) $(ASFLAGS) $< -o $@
$(OBJDIR)/%.o: src/%.c
@echo Compiling $<
@$(CC) $(CFLAGS) -c $< -o $@
clean:
@echo Cleaning
-@-rm -rf $(OBJDIR)/* simpleos.tgz
clean-floppy:
@echo Cleaning floppy.img
@losetup /dev/loop0 floppy.img
@mkdir -p $(FLOPPYDIR)
@mount /dev/loop0 $(FLOPPYDIR)
@rm -f $(FLOPPYDIR)/$(APP)
@sync
@umount /dev/loop0
@losetup -d /dev/loop0
build-floppy:
@echo Updating floppy.img
@losetup /dev/loop0 floppy.img
@mkdir -p $(FLOPPYDIR)
@mount /dev/loop0 $(FLOPPYDIR)
@cp $(OBJDIR)/$(APP) $(FLOPPYDIR)/$(APP)
@sync
@umount /dev/loop0
@losetup -d /dev/loop0
build-iso:
@echo Creating cd.iso
@rm -f cd.iso
@mkdir -p iso/boot/grub
@cp /usr/share/grub/x86_64-redhat/stage2_eltorito iso/boot/grub/
@printf "timeout 0\n\ntitle SimpleOS\nroot (cd)\nkernel /kernel\n" > iso/boot/grub/grub.conf
@cp $(OBJDIR)/$(APP) iso/$(APP)
@mkisofs -quiet -R -l -b boot/grub/stage2_eltorito -no-emul-boot -boot-load-size 4 -boot-info-table -V SimpleOS -o cd.iso iso
@rm -rf iso/
build-both: build-floppy build-iso
todo:
@for file in $(FILES); do fgrep -H -e TODO -e FIXME src/$$file; done; true
dist: clean all build-both
@echo Building simpleos.tgz
@tar czf simpleos.tgz --exclude='*.suo' --exclude='*.user' build/ src/ $(AUXFILES)
backup: dist
@echo Saving to backup/simpleos-$(shell date +%Y-%m-%d).tgz
@mkdir -p backup/
@mv simpleos.tgz backup/simpleos-$(shell date +%Y-%m-%d).tgz
cloc:
@cloc --quiet src/ $(AUXFILES) | tail -n +3
<file_sep>#ifndef DRIVERS_PCI_DRIVER_H
#define DRIVERS_PCI_DRIVER_H
#include <lib/stdtypes.h>
#define PCI_MAKE_ID(bus, dev, func) ((bus) << 16) | ((dev) << 11) | ((func) << 8)
// I/O Ports
#define PCI_CONFIG_ADDR 0xcf8
#define PCI_CONFIG_DATA 0xcfC
// Header Type
#define PCI_TYPE_MULTIFUNC 0x80
#define PCI_TYPE_GENERIC 0x00
#define PCI_TYPE_PCI_BRIDGE 0x01
#define PCI_TYPE_CARDBUS_BRIDGE 0x02
// PCI Configuration Registers
#define PCI_CONFIG_VENDOR_ID 0x00
#define PCI_CONFIG_DEVICE_ID 0x02
#define PCI_CONFIG_COMMAND 0x04
#define PCI_CONFIG_STATUS 0x06
#define PCI_CONFIG_REVISION_ID 0x08
#define PCI_CONFIG_PROG_INTF 0x09
#define PCI_CONFIG_SUBCLASS 0x0a
#define PCI_CONFIG_CLASS_CODE 0x0b
#define PCI_CONFIG_CACHELINE_SIZE 0x0c
#define PCI_CONFIG_LATENCY 0x0d
#define PCI_CONFIG_HEADER_TYPE 0x0e
#define PCI_CONFIG_BIST 0x0f
// Type 0x00 (Generic) Configuration Registers
#define PCI_CONFIG_BAR0 0x10
#define PCI_CONFIG_BAR1 0x14
#define PCI_CONFIG_BAR2 0x18
#define PCI_CONFIG_BAR3 0x1c
#define PCI_CONFIG_BAR4 0x20
#define PCI_CONFIG_BAR5 0x24
#define PCI_CONFIG_CARDBUS_CIS 0x28
#define PCI_CONFIG_SUBSYSTEM_VENDOR_ID 0x2c
#define PCI_CONFIG_SUBSYSTEM_DEVICE_ID 0x2e
#define PCI_CONFIG_EXPANSION_ROM 0x30
#define PCI_CONFIG_CAPABILITIES 0x34
#define PCI_CONFIG_INTERRUPT_LINE 0x3c
#define PCI_CONFIG_INTERRUPT_PIN 0x3d
#define PCI_CONFIG_MIN_GRANT 0x3e
#define PCI_CONFIG_MAX_LATENCY 0x3f
// PCI BAR
#define PCI_BAR_IO 0x01
#define PCI_BAR_LOWMEM 0x02
#define PCI_BAR_64 0x04
#define PCI_BAR_PREFETCH 0x08
typedef struct {
union {
void *address;
uint16_t port;
} u;
uint64_t size;
uint32_t flags;
} pci_bar_t;
typedef struct {
uint16_t vendorId;
uint16_t deviceId;
uint8_t classCode;
uint8_t subclass;
uint8_t progIntf;
} pci_device_info_t;
typedef struct {
void (*init)(uint32_t id, pci_device_info_t *info);
} pci_driver_t;
uint8_t pci_read8(uint32_t id, uint32_t reg);
uint16_t pci_read16(uint32_t id, uint32_t reg);
uint32_t pci_read32(uint32_t id, uint32_t reg);
void pci_write8(uint32_t id, uint32_t reg, uint8_t data);
void pci_write16(uint32_t id, uint32_t reg, uint16_t data);
void pci_write32(uint32_t id, uint32_t reg, uint32_t data);
void pci_get_bar(pci_bar_t *bar, uint32_t id, uint32_t index);
#endif
<file_sep>#ifndef LIB_VSPRINTF_H
#define LIB_VSPRINTF_H
#include <lib/stdtypes.h>
#include <lib/stdarg.h>
int32_t vsprintf(int8_t *buf, const int8_t *fmt, va_list args);
int32_t sprintf(int8_t *buf, const int8_t *fmt, ...);
#endif
<file_sep>#ifndef LIB_STDBOOL_H
#define LIB_STDBOOL_H
#define bool _Bool
#define true 1
#define false 0
#endif
<file_sep>#ifndef INTERRUPT_ISR_H
#define INTERRUPT_ISR_H
#include <lib/stdtypes.h>
#define IRQ0 32
#define IRQ1 33
#define IRQ2 34
#define IRQ3 35
#define IRQ4 36
#define IRQ5 37
#define IRQ6 38
#define IRQ7 39
#define IRQ8 40
#define IRQ9 41
#define IRQ10 42
#define IRQ11 43
#define IRQ12 44
#define IRQ13 45
#define IRQ14 46
#define IRQ15 47
typedef struct {
uint32_t ds; // Data segment selector
uint32_t edi, esi, ebp, esp, ebx, edx, ecx, eax; // Pushed by pusha
uint32_t int_no, err_code; // Interrupt number and error code (if applicable)
uint32_t eip, cs, eflags, useresp, ss; // Pushed by the processor automatically
} registers_t;
typedef void (*interrupt_handler_t)(registers_t *);
void register_interrupt_handler(uint8_t n, interrupt_handler_t h);
void irq_handler(registers_t *regs);
void isr_handler(registers_t *regs);
void irq_ack(int32_t irq);
#endif
<file_sep>#include <lib/x86.h>
#include <lib/stdtypes.h>
uint8_t inportb(uint16_t port)
{
uint8_t rv;
__asm __volatile__("in %%dx,%%al" : "=a"(rv) : "d"(port));
return rv;
}
uint16_t inportw(uint16_t port)
{
uint16_t rv;
__asm __volatile__("in %%dx, %%eax" : "=a"(rv) : "d"(port));
return rv;
}
uint32_t inportl(uint16_t port)
{
uint32_t rv;
__asm __volatile__("in %%dx,%%eax" : "=a"(rv) : "d"(port));
return rv;
}
void outportb(uint16_t port, uint8_t value)
{
__asm __volatile__("outb %%al,%%dx" : : "a"(value), "d"(port));
}
void outportw(uint16_t port, uint16_t value)
{
__asm __volatile__("outw %%ax,%%dx" : : "a"(value), "d"(port));
}
void outportl(uint16_t port, uint32_t value)
{
__asm __volatile__("outl %%eax,%%dx" : : "a"(value), "d"(port));
}
<file_sep>#include <cpu/cpuid.h>
#include <lib/stdtypes.h>
#include <lib/string.h>
#include <drivers/screen/textmode.h>
void cpuid(uint32_t reg, uint32_t *eax, uint32_t *ebx, uint32_t *ecx, uint32_t *edx)
{
__asm __volatile__("cpuid" : "=a"(*eax), "=b"(*ebx), "=c"(*ecx), "=d"(*edx) : "a"(reg));
}
void cpu_detect(void)
{
printf("Scanning CPU...\n");
uint32_t eax, ebx, ecx, edx;
uint32_t largestStandardFunc;
// Function 0x00 - Vendor-ID and Largest Standard Function
int8_t vendor[13];
cpuid(0, &largestStandardFunc, (uint32_t *)(vendor + 0), (uint32_t *)(vendor + 8), (uint32_t *)(vendor + 4));
vendor[12] = '\0';
const int8_t *vendorName = "Unknown";
if (strcmp(vendor, "AuthenticAMD") == 0 || strcmp(vendor, "AMDisbetter") == 0) {
vendorName = "AMD";
} else if (strcmp(vendor, "GenuineIntel") == 0) {
vendorName = "Intel";
} else if (strcmp(vendor, "CentaurHauls") == 0) {
vendorName = "Centaur";
} else if (strcmp(vendor, "CyrixInstead") == 0) {
vendorName = "Cyrix";
} else if (strcmp(vendor, "TransmetaCPU") == 0 || strcmp(vendor, "GenuineTMx86") == 0) {
vendorName = "Transmeta";
} else if (strcmp(vendor, "Geode by NSC") == 0) {
vendorName = "National Semiconductor";
} else if (strcmp(vendor, "NexGenDriven") == 0) {
vendorName = "NexGen";
} else if (strcmp(vendor, "RiseRiseRise") == 0) {
vendorName = "Rise";
} else if (strcmp(vendor, "SiS SiS SiS ") == 0) {
vendorName = "SiS";
} else if (strcmp(vendor, "UMC UMC UMC ") == 0) {
vendorName = "UMC";
} else if (strcmp(vendor, "VIA VIA VIA ") == 0) {
vendorName = "VIA";
} else if (strcmp(vendor, "Vortex86 SoC") == 0) {
vendorName = "Vortex";
} else if (strcmp(vendor, "KVMKVMKVMKVM") == 0) {
vendorName = "KVM";
}
printf("Vendor: %s\n", vendorName);
// Function 0x01 - Feature Information
if (largestStandardFunc >= 0x01) {
cpuid(0x01, &eax, &ebx, &ecx, &edx);
//struct cpu_signature_s *cpu = (struct cpu_signature_s *)&eax;
uint32_t stepping = eax & 0x0F;
uint32_t model = (eax >> 4) & 0x0F;
uint32_t family = (eax >> 8) & 0x0F;
if (strcmp(vendor, "GenuineIntel") == 0) {
uint32_t exmodel = (eax >> 16) & 0x0F;
uint32_t exfamily = (eax >> 20) & 0xFF;
if (family == 0x0F) {
family += exfamily;
model += (exmodel << 4);
} else if (family == 0x06) {
model += (exmodel << 4);
}
}
printf("Family: %X, Model: %X, Stepping: %X\n", family, model, stepping);
printf("Features:");
if (edx & EDX_PSE) {
printf(" PSE");
}
if (edx & EDX_PAE) {
printf(" PAE");
}
if (edx & EDX_APIC) {
printf(" APIC");
}
if (edx & EDX_MTRR) {
printf(" MTRR");
}
printf("\nInstructions:");
if (edx & EDX_HTT) {
printf(" HTT");
}
if (edx & EDX_TSC) {
printf(" TSC");
}
if (edx & EDX_MSR) {
printf(" MSR");
}
if (edx & EDX_SSE) {
printf(" SSE");
}
if (edx & EDX_SSE2) {
printf(" SSE2");
}
if (ecx & ECX_SSE3) {
printf(" SSE3");
}
if (ecx & ECX_SSSE3) {
printf(" SSSE3");
}
if (ecx & ECX_SSE41) {
printf(" SSE41");
}
if (ecx & ECX_SSE42) {
printf(" SSE42");
}
if (ecx & ECX_AVX) {
printf(" AVX");
}
if (ecx & ECX_F16C) {
printf(" F16C");
}
if (ecx & ECX_RDRAND) {
printf(" RDRAND");
}
printf("\n");
}
// Extended Function 0x00 - Largest Extended Function
uint32_t largestExtendedFunc;
cpuid(0x80000000, &largestExtendedFunc, &ebx, &ecx, &edx);
// Extended Function 0x01 - Extended Feature Bits
int32_t bits = 32;
if (largestExtendedFunc >= 0x80000001) {
cpuid(0x80000001, &eax, &ebx, &ecx, &edx);
if (edx & EDX_64_BIT) {
bits = 64;
}
}
printf("Architecture: %i-bit\n", bits);
// Extended Function 0x02-0x04 - Processor Name / Brand String
if (largestExtendedFunc >= 0x80000004) {
int8_t name[48];
cpuid(0x80000002, (uint32_t *)(name + 0), (uint32_t *)(name + 4), (uint32_t *)(name + 8), (uint32_t *)(name + 12));
cpuid(0x80000003, (uint32_t *)(name + 16), (uint32_t *)(name + 20), (uint32_t *)(name + 24), (uint32_t *)(name + 28));
cpuid(0x80000004, (uint32_t *)(name + 32), (uint32_t *)(name + 36), (uint32_t *)(name + 40), (uint32_t *)(name + 44));
const int8_t *p = name;
while (*p == ' ') {
++p;
}
printf("CPU Name: %s\n", p);
}
}
<file_sep>#ifndef LIB_STDDEF_H
#define LIB_STDDEF_H
#if defined(_MSC_VER) // Visual Studio
#define PACKED( __Declaration__ ) __pragma( pack(push, 1) ) __Declaration__ __pragma( pack(pop) )
#else
#define PACKED( __Declaration__ ) __Declaration__ __attribute__((__packed__)) // GCC
#endif
#define NULL ((void *)0)
typedef unsigned long size_t;
typedef long intptr_t;
typedef unsigned long uintptr_t;
#endif
<file_sep>#ifndef DRIVERS_PIT_H
#define DRIVERS_PIT_H
#include <lib/stdtypes.h>
#define PIT_FREQUENCY 1193182
void pit_init(void);
void pit_wait(uint32_t ms);
#endif
<file_sep>#ifndef LIB_STDARG_H
#define LIB_STDARG_H
#include <lib/stdtypes.h>
#if defined(_MSC_VER) // Visual Studio
typedef int8_t *va_list;
#define intsizeof(n) ((sizeof(n) + sizeof(int32_t) - 1) &~(sizeof(int32_t) - 1))
#define va_start(ap, v) (ap = (va_list)&(v) + intsizeof(v))
#define va_arg(ap, t) (*(t *) ((ap += intsizeof(t)) - intsizeof(t)))
#define va_end(ap) (ap = (va_list)0)
#else // GCC
typedef __builtin_va_list va_list;
#define va_start(v,l) __builtin_va_start(v,l)
#define va_arg(v,l) __builtin_va_arg(v,l)
#define va_end(v) __builtin_va_end(v)
#define va_copy(d,s) __builtin_va_copy(d,s)
#endif
#endif
<file_sep>#ifndef LIB_TIME_H
#define LIB_TIME_H
#include <lib/stdtypes.h>
#define LOCAL_TIME_ZONE 0 * 60; // Time zone offset in minutes
typedef uint32_t time_t; // Expiry: 2038
typedef struct datetime {
int sec; // [0, 59]
int min; // [0, 59]
int hour; // [0, 23]
int day; // [1, 31]
int month; // [1, 12]
int year; // [1970, 2038]
int week_day; // [0, 6] Sunday = 0
int year_day; // [0, 365]
int tz_offset; // offset in minutes
} datetime_t;
#endif
<file_sep>#ifndef LIB_STDTYPES_H
#define LIB_STDTYPES_H
// 32-bit x86
typedef unsigned long long uint64_t;
typedef long long int64_t;
typedef unsigned int uint32_t;
typedef int int32_t;
typedef unsigned short uint16_t;
typedef short int16_t;
typedef unsigned char uint8_t;
typedef char int8_t;
#endif
<file_sep>#include <drivers/pit.h>
#include <lib/stdtypes.h>
#include <lib/x86.h>
#include <interrupt/isr.h>
#include <drivers/screen/textmode.h>
volatile uint32_t ticks = 0;
static void pit_callback(registers_t *regs)
{
ticks++;
}
void pit_init(void)
{
register_interrupt_handler(IRQ0, &pit_callback);
int16_t divisor = PIT_FREQUENCY / 1000;
outportb(0x43, 0x36);
outportb(0x40, (uint8_t)(divisor & 0xFF));
outportb(0x40, (uint8_t)((divisor >> 8) & 0xFF));
}
void pit_wait(uint32_t ms)
{
uint32_t now = ticks;
++ms;
while (ticks - now < ms) {
;
}
}
<file_sep>#include <interrupt/isr.h>
#include <lib/stdtypes.h>
#include <lib/x86.h>
#include <drivers/screen/textmode.h>
const int8_t *exception_messages[] = {
"Division By Zero",
"Debug",
"Non Maskable Interrupt",
"Breakpoint",
"Into Detected Overflow",
"Out of Bounds",
"Invalid Opcode",
"No Coprocessor",
"Double Fault",
"Coprocessor Segment Overrun",
"Bad TSS",
"Segment Not Present",
"Stack Fault",
"General Protection Fault",
"Page Fault",
"Unknown Interrupt",
"Coprocessor Fault",
"Alignment Check",
"Machine Check",
"Reserved",
"Reserved",
"Reserved",
"Reserved",
"Reserved",
"Reserved",
"Reserved",
"Reserved",
"Reserved",
"Reserved",
"Reserved",
"Reserved",
"Reserved"
};
interrupt_handler_t interrupt_handlers[256];
void register_interrupt_handler(uint8_t n, interrupt_handler_t h)
{
interrupt_handlers[n] = h;
}
void isr_handler(registers_t *regs)
{
if (interrupt_handlers[regs->int_no]) {
interrupt_handlers[regs->int_no](regs);
} else {
printf("Unhandled interrupt: %i (%s Exception)\n", regs->int_no, exception_messages[regs->int_no]);
// TODO: Panic
}
}
void irq_handler(registers_t *regs)
{
// Send an EOI (end of interrupt) signal to the PICs
// If this interrupt involved the slave
if (regs->int_no >= 40) {
// Send reset signal to slave.
outportb(0xA0, 0x20);
}
// Send reset signal to master. (As well as slave, if necessary)
outportb(0x20, 0x20);
if (interrupt_handlers[regs->int_no] != 0) {
interrupt_handlers[regs->int_no](regs);
}
}
void irq_ack(int32_t irq)
{
if (irq >= 12) {
outportb(0xA0, 0x20);
}
outportb(0x20, 0x20);
}
<file_sep>#!/bin/bash
echo Creating floppy.img
dd if=/dev/zero of=floppy.img bs=1k count=1440 > /dev/null 2>&1
losetup /dev/loop0 floppy.img
mke2fs /dev/loop0 > /dev/null 2>&1
echo "(fd0) /dev/loop0" > floppy.map
mount /dev/loop0 /mnt/floppy
mkdir -p /mnt/floppy/boot/grub
cp /usr/share/grub/x86_64-redhat/stage1 /mnt/floppy/boot/grub/stage1
cp /usr/share/grub/x86_64-redhat/stage2 /mnt/floppy/boot/grub/stage2
grub --batch --device-map=floppy.map /dev/loop0 <<EOT > /dev/null 2>&1
root (fd0)
setup (fd0)
quit
EOT
rm -f floppy.map
if [ -f grub.conf ]; then
cp grub.conf /mnt/floppy/boot/grub/grub.conf
else
cat << EOF > /mnt/floppy/boot/grub/grub.conf
timeout 0
title SimpleOS
root (fd0)
kernel /kernel
EOF
fi
umount /dev/loop0
losetup -d /dev/loop0
echo Done
<file_sep>#include <lib/rand.h>
#include <lib/stdtypes.h>
static uint32_t next = 1;
int32_t rand(void)
{
next = next * 1103515245 + 12345;
return (uint32_t)(next / 65536) % 32768;
}
void srand(uint32_t seed)
{
next = seed;
}
<file_sep>#include <drivers/rtc.h>
#include <lib/stdtypes.h>
#include <lib/x86.h>
#include <lib/time.h>
#include <drivers/pit.h>
#include <drivers/screen/textmode.h>
static uint8_t rtc_read(uint8_t addr)
{
outportb(IO_RTC_INDEX, addr);
return inportb(IO_RTC_TARGET);
}
static void rtc_write(uint8_t addr, uint8_t val)
{
outportb(IO_RTC_INDEX, addr);
outportb(IO_RTC_TARGET, val);
}
static uint8_t bcd_to_bin(uint8_t val)
{
return (val & 0xf) + (val >> 4) * 10;
}
static uint8_t bin_to_bcd(uint8_t val)
{
return ((val / 10) << 4) + (val % 10);
}
void rtc_get(datetime_t *dt)
{
// Wait if update is in progress
if (rtc_read(RTC_A) & RTC_A_UIP) {
pit_wait(3);
}
// Read Registers
uint8_t sec = rtc_read(RTC_SEC);
uint8_t min = rtc_read(RTC_MIN);
uint8_t hour = rtc_read(RTC_HOUR);
uint8_t weekDay = rtc_read(RTC_WEEK_DAY);
uint8_t day = rtc_read(RTC_DAY);
uint8_t month = rtc_read(RTC_MONTH);
uint16_t year = rtc_read(RTC_YEAR);
// Get Data configuration
uint8_t regb = rtc_read(RTC_B);
// BCD conversion
if (~regb & RTC_B_DM) {
sec = bcd_to_bin(sec);
min = bcd_to_bin(min);
hour = bcd_to_bin(hour);
day = bcd_to_bin(day);
month = bcd_to_bin(month);
year = bcd_to_bin(year);
}
// Century support
year += 2000;
// Week day conversion: Sunday as the first day of the week (0-6)
weekDay--;
// Write results
dt->sec = sec;
dt->min = min;
dt->hour = hour;
dt->day = day;
dt->month = month;
dt->year = year;
dt->week_day = weekDay;
dt->tz_offset = LOCAL_TIME_ZONE;
}
void rtc_set(const datetime_t *dt)
{
uint8_t sec = dt->sec;
uint8_t min = dt->min;
uint8_t hour = dt->hour;
uint8_t day = dt->day;
uint8_t month = dt->month;
uint8_t year = dt->year - 2000;
uint8_t week_day = dt->week_day + 1;
// Validate data
if (sec >= 60 || min >= 60 || hour >= 24 || day > 31 || month > 12 || year >= 100 || week_day > 7) {
printf("rtc_set: bad data\n");
return;
}
// Get data configuration
uint8_t regb = rtc_read(RTC_B);
// BCD conversion
if (~regb & RTC_B_DM) {
sec = bin_to_bcd(sec);
min = bin_to_bcd(min);
hour = bin_to_bcd(hour);
day = bin_to_bcd(day);
month = bin_to_bcd(month);
year = bin_to_bcd(year);
}
// Wait if update is in progress
if (rtc_read(RTC_A) & RTC_A_UIP) {
pit_wait(3);
}
// Write registers
rtc_write(RTC_SEC, sec);
rtc_write(RTC_MIN, min);
rtc_write(RTC_HOUR, hour);
rtc_write(RTC_WEEK_DAY, week_day);
rtc_write(RTC_DAY, day);
rtc_write(RTC_MONTH, month);
rtc_write(RTC_YEAR, year);
}<file_sep>#include <drivers/serial.h>
#include <interrupt/isr.h>
#include <lib/stdtypes.h>
#include <lib/x86.h>
#include <lib/string.h>
#include <drivers/screen/textmode.h>
static int32_t serial_rcvd(int32_t device);
static int8_t serial_recv(int32_t device);
static int8_t serial_recv_async(int32_t device);
static int32_t serial_send_empty(int32_t device);
static void serial_send(int32_t device, int8_t out);
void serial_handler_a(registers_t *regs)
{
int8_t serial = serial_recv(SERIAL_PORT_A);
irq_ack(SERIAL_IRQ);
if (serial == 13) {
serial = '\n';
}
printf("%c", serial);
serial_send(SERIAL_PORT_B, serial);
}
void serial_handler_b(registers_t *regs)
{
int8_t serial = serial_recv(SERIAL_PORT_B);
irq_ack(SERIAL_IRQ - 1);
serial_send(SERIAL_PORT_A, serial);
}
void serial_enable(int32_t device)
{
outportb(device + 1, 0x00);
outportb(device + 3, 0x80); /* Enable divisor mode */
outportb(device + 0, 0x03); /* Div Low: 03 Set the port to 38400 bps */
outportb(device + 1, 0x00); /* Div High: 00 */
outportb(device + 3, 0x03);
outportb(device + 2, 0xC7);
outportb(device + 4, 0x0B);
}
void serial_init(void)
{
serial_enable(SERIAL_PORT_A);
serial_enable(SERIAL_PORT_B);
register_interrupt_handler(SERIAL_IRQ, serial_handler_a); /* Install the serial input handler */
register_interrupt_handler(SERIAL_IRQ - 1, serial_handler_b); /* Install the serial input handler */
outportb(SERIAL_PORT_A + 1, 0x01); /* Enable interrupts on receive */
outportb(SERIAL_PORT_B + 1, 0x01); /* Enable interrupts on receive */
}
static int32_t serial_rcvd(int32_t device)
{
return inportb(device + 5) & 1;
}
static int8_t serial_recv(int32_t device)
{
while (serial_rcvd(device) == 0);
return inportb(device);
}
static int8_t serial_recv_async(int32_t device)
{
return inportb(device);
}
static int32_t serial_send_empty(int32_t device)
{
return inportb(device + 5) & 0x20;
}
static void serial_send(int32_t device, int8_t out)
{
while (serial_send_empty(device) == 0);
outportb(device, out);
}
void serial_write(int32_t device, const int8_t *out)
{
for (int32_t i = 0; i < strlen(out); ++i) {
serial_send(device, out[i]);
}
}
<file_sep>#ifndef CPU_FPU_H
#define CPU_FPU_H
#include <lib/stdtypes.h>
void fpu_init(void);
void fpu_set_cw(const uint16_t cw);
#endif
<file_sep>#ifndef INTERRUPT_IDT_H
#define INTERRUPT_IDT_H
#include <lib/stdtypes.h>
#include <lib/stddef.h>
#include <interrupt/isr.h>
typedef PACKED(struct {
uint16_t base_lo; // The lower 16 bits of the address to jump to
uint16_t sel; // Kernel segment selector
uint8_t always0; // This must always be zero
uint8_t flags; // More flags. See documentation
uint16_t base_hi; // The upper 16 bits of the address to jump to
}) idt_entry_t;
typedef PACKED(struct {
uint16_t limit;
uint32_t base; // The address of the first element in our idt_entry_t array
}) idt_ptr_t;
void idt_init(void);
extern void isr0(void);
extern void isr1(void);
extern void isr2(void);
extern void isr3(void);
extern void isr4(void);
extern void isr5(void);
extern void isr6(void);
extern void isr7(void);
extern void isr8(void);
extern void isr9(void);
extern void isr10(void);
extern void isr11(void);
extern void isr12(void);
extern void isr13(void);
extern void isr14(void);
extern void isr15(void);
extern void isr16(void);
extern void isr17(void);
extern void isr18(void);
extern void isr19(void);
extern void isr20(void);
extern void isr21(void);
extern void isr22(void);
extern void isr23(void);
extern void isr24(void);
extern void isr25(void);
extern void isr26(void);
extern void isr27(void);
extern void isr28(void);
extern void isr29(void);
extern void isr30(void);
extern void isr31(void);
extern void isr255(void);
extern void irq0(void);
extern void irq1(void);
extern void irq2(void);
extern void irq3(void);
extern void irq4(void);
extern void irq5(void);
extern void irq6(void);
extern void irq7(void);
extern void irq8(void);
extern void irq9(void);
extern void irq10(void);
extern void irq11(void);
extern void irq12(void);
extern void irq13(void);
extern void irq14(void);
extern void irq15(void);
#endif
<file_sep>#ifndef LIB_RAND_H
#define LIB_RAND_H
#include <lib/stdtypes.h>
int32_t rand(void);
void srand(uint32_t seed);
#endif
<file_sep>#ifndef INTERRUPT_GDT_H
#define INTERRUPT_GDT_H
#include <lib/stdtypes.h>
#include <lib/stddef.h>
void gdt_init(void);
typedef PACKED(struct {
uint16_t limit_low; // The lower 16 bits of the limit
uint16_t base_low; // The lower 16 bits of the base
uint8_t base_middle; // The next 8 bits of the base
uint8_t access; // Access flags, determines what ring this segment can be used in
uint8_t granularity;
uint8_t base_high; // The last 8 bits of the base
}) gdt_entry_t;
typedef PACKED(struct {
uint16_t limit; // The Global Descriptor Table limit
uint32_t base; // The address of the first gdt_entry_t struct
}) gdt_ptr_t;
#endif
<file_sep>#ifndef DRIVERS_RTC_H
#define DRIVERS_RTC_H
#include <lib/stdtypes.h>
#include <lib/time.h>
// I/O Ports
#define IO_RTC_INDEX 0x70
#define IO_RTC_TARGET 0x71
// Indexed Registers
#define RTC_SEC 0x00
#define RTC_SEC_ALARM 0x01
#define RTC_MIN 0x02
#define RTC_MIN_ALARM 0x03
#define RTC_HOUR 0x04
#define RTC_HOUR_ALARM 0x05
#define RTC_WEEK_DAY 0x06
#define RTC_DAY 0x07
#define RTC_MONTH 0x08
#define RTC_YEAR 0x09
#define RTC_A 0x0a
#define RTC_B 0x0b
#define RTC_C 0x0c
#define RTC_D 0x0d
// General Configuration Registers
#define RTC_A_UIP (1 << 7) // Update In Progress
#define RTC_B_HOURFORM (1 << 1) // Hour Format (0 = 12hr, 1 = 24hr)
#define RTC_B_DM (1 << 2) // Data Mode (0 = BCD, 1 = Binary)
void rtc_get(datetime_t *dt);
void rtc_set(const datetime_t *dt);
#endif
<file_sep>#ifndef DRIVERS_SCREEN_TEXTMODE_H
#define DRIVERS_SCREEN_TEXTMODE_H
#include <lib/stdtypes.h>
#include <lib/stddef.h>
#define SCREEN_WIDTH 80
#define SCREEN_HEIGHT 25
#define COLOR_BLACK 0
#define COLOR_BLUE 1
#define COLOR_GREEN 2
#define COLOR_CYAN 3
#define COLOR_RED 4
#define COLOR_MAGENTA 5
#define COLOR_BROWN 6
#define COLOR_LTGRAY 7
#define COLOR_GRAY 8
#define COLOR_LTBLUE 9
#define COLOR_LTGREEN 10
#define COLOR_LTCYAN 11
#define COLOR_LTRED 12
#define COLOR_LTMAGENTA 13
#define COLOR_YELLOW 14
#define COLOR_WHITE 15
void textmode_init(void);
void textmode_set_colors(uint8_t forecolor, uint8_t backcolor);
void textmode_move_cursor(int32_t x, int32_t y);
void textmode_clear_screen(void);
void printf(const int8_t *fmt, ...);
#endif
<file_sep>#include <lib/string.h>
#include <lib/stdtypes.h>
void *memcpy(void *dest, const void *src, size_t count)
{
const int8_t *sp = (const int8_t *)src;
int8_t *dp = (int8_t *)dest;
for (; count != 0; count--) {
*dp++ = *sp++;
}
return dest;
}
void *memset(void *dest, int8_t value, size_t count)
{
int8_t *temp = (int8_t *)dest;
for (; count != 0; count--) {
*temp++ = value;
}
return dest;
}
void *memsetw(void *dest, uint16_t value, size_t count)
{
uint16_t *temp = (uint16_t *)dest;
for (; count != 0; count--) {
*temp++ = value;
}
return dest;
}
int32_t memcmp(const void *s1, const void *s2, size_t n)
{
if (n) {
register const uint8_t *p1 = s1, *p2 = s2;
do {
if (*p1++ != *p2++) {
return (*--p1 - *--p2);
}
} while (--n);
}
return 0;
}
int8_t *itoa(int32_t value, int8_t *result, int32_t base)
{
if (base < 2 || base > 36) {
*result = '\0';
return result;
}
int8_t *ptr = result;
int8_t *ptr1 = result;
int8_t tmp_char;
int32_t tmp_value;
do {
tmp_value = value;
value /= base;
*ptr++ = "zyxwvutsrqp<KEY>"[35 + (tmp_value - value * base)];
} while (value);
if (tmp_value < 0) {
*ptr++ = '-';
}
*ptr-- = '\0';
while (ptr1 < ptr) {
tmp_char = *ptr;
*ptr-- = *ptr1;
*ptr1++ = tmp_char;
}
return result;
}
int32_t strcmp(const int8_t *str1, const int8_t *str2)
{
int32_t i = 0;
int32_t failed = 0;
while (str1[i] != '\0' && str2[i] != '\0') {
if (str1[i] != str2[i]) {
failed = 1;
break;
}
i++;
}
if ((str1[i] == '\0' && str2[i] != '\0') || (str1[i] != '\0' && str2[i] == '\0')) {
failed = 1;
}
return failed;
}
int32_t strncmp(const int8_t *str1, const int8_t *str2, int32_t count)
{
int32_t i = 0;
int32_t failed = 0;
while (str1[i] != '\0' && str2[i] != '\0') {
if (str1[i] != str2[i]) {
failed = 1;
break;
}
i++;
count--;
}
if (count && ((str1[i] == '\0' && str2[i] != '\0') || (str1[i] != '\0' && str2[i] == '\0'))) {
failed = 1;
}
return failed;
}
int8_t *strcpy(int8_t *dest, const int8_t *src)
{
while (*src) {
*dest++ = *src++;
}
*dest = '\0';
return dest;
}
int8_t *strncpy(int8_t *dest, const int8_t *src, int32_t count)
{
while (*src && count) {
*dest++ = *src++;
count--;
}
*dest = '\0';
return dest;
}
int8_t *strcat(int8_t *dest, const int8_t *src)
{
strcpy(dest + strlen(dest), src);
return dest;
}
int32_t strlen(const int8_t *src)
{
int32_t i = 0;
while (*src++) {
i++;
}
return i;
}
int32_t isspace(int8_t c)
{
return (c == ' ' || c == '\n' || c == '\r' || c == '\t');
}
int32_t isupper(int8_t c)
{
return (c >= 'A' && c <= 'Z');
}
int32_t islower(int8_t c)
{
return (c >= 'a' && c <= 'z');
}
int32_t isdigit(int8_t c)
{
return (c >= '0' && c <= '9');
}
int32_t isalpha(int8_t c)
{
return isupper(c) || islower(c) || isdigit(c);
}
int8_t toupper(int8_t c)
{
if (islower(c)) {
return 'A' + (c - 'a');
} else {
return c;
}
}
int8_t tolower(int8_t c)
{
if (isupper(c)) {
return 'a' + (c - 'A');
} else {
return c;
}
}
<file_sep>#ifndef MAIN_H
#define MAIN_H
#include <lib/stdtypes.h>
#include <lib/multiboot.h>
int32_t kernel_main(multiboot_t *mboot_ptr);
#endif
<file_sep>#ifndef LIB_IO_H
#define LIB_IO_H
#include <lib/stdtypes.h>
uint8_t inportb(uint16_t port);
uint16_t inportw(uint16_t port);
uint32_t inportl(uint16_t port);
void outportb(uint16_t port, uint8_t value);
void outportw(uint16_t port, uint16_t value);
void outportl(uint16_t port, uint32_t value);
#endif
<file_sep>#include <drivers/pci/pci.h>
#include <drivers/pci/driver.h>
#include <drivers/pci/registry.h>
#include <lib/stdtypes.h>
#include <lib/string.h>
#include <drivers/screen/textmode.h>
static void pci_check(uint32_t bus, uint32_t dev, uint32_t func)
{
uint32_t id = PCI_MAKE_ID(bus, dev, func);
pci_device_info_t info;
info.vendorId = pci_read16(id, PCI_CONFIG_VENDOR_ID);
if (info.vendorId == 0xFFFF) {
return;
}
info.deviceId = pci_read16(id, PCI_CONFIG_DEVICE_ID);
info.progIntf = pci_read8(id, PCI_CONFIG_PROG_INTF);
info.subclass = pci_read8(id, PCI_CONFIG_SUBCLASS);
info.classCode = pci_read8(id, PCI_CONFIG_CLASS_CODE);
uint32_t result = pci_read32(id, PCI_CONFIG_VENDOR_ID);
uint16_t dev_id = result >> 0x10;
uint16_t ven_id = result & 0xFFFF;
printf("%02x:%02x.%d %04x:%04x %s\n", bus, dev, func, info.vendorId, info.deviceId, pci_class_name(info.classCode, info.subclass, info.progIntf));
}
void pci_scan(void)
{
printf("Scanning PCI bus...\n");
for (uint32_t bus = 0; bus < 256; ++bus) {
for (uint32_t dev = 0; dev < 32; ++dev) {
uint32_t baseId = PCI_MAKE_ID(bus, dev, 0);
uint8_t headerType = pci_read8(baseId, PCI_CONFIG_HEADER_TYPE);
uint32_t funcCount = headerType & PCI_TYPE_MULTIFUNC ? 8 : 1;
for (uint32_t func = 0; func < funcCount; ++func) {
pci_check(bus, dev, func);
}
}
}
}<file_sep>#include <main.h>
#include <lib/stdtypes.h>
#include <lib/multiboot.h>
#include <lib/vsprintf.h>
#include <cpu/cpuid.h>
#include <cpu/fpu.h>
#include <interrupt/gdt.h>
#include <interrupt/idt.h>
#include <drivers/pit.h>
#include <drivers/rtc.h>
#include <drivers/serial.h>
#include <drivers/pci/pci.h>
#include <drivers/screen/textmode.h>
int32_t kernel_main(multiboot_t *mboot_ptr)
{
textmode_init();
printf(" _____ _ _ ____ _____ \n / ____(_) | | / __ \\ / ____|\n | (___ _ _ __ ___ _ __ | | ___| | | | (___ \n \\___ \\| | '_ ` _ \\| '_ \\| |/ _ \\ | | |\\___ \\ \n ____) | | | | | | | |_) | | __/ |__| |____) |\n |_____/|_|_| |_| |_| .__/|_|\\___|\\____/|_____/ \n | | \n |_| \n");
textmode_set_colors(COLOR_RED, COLOR_BLACK);
textmode_move_cursor(19, 6);
printf("Version %i.%i.%i", VERSION_MAJOR, VERSION_MINOR, VERSION_BUILD);
textmode_move_cursor(19, 7);
printf("Built %s", VERSION_DATE);
textmode_set_colors(COLOR_GREEN, COLOR_BLACK);
textmode_move_cursor(41, 6);
printf("Written by <NAME>");
textmode_set_colors(COLOR_LTGRAY, COLOR_BLACK);
textmode_move_cursor(0, 9);
fpu_init();
gdt_init();
idt_init();
__asm __volatile__("sti"); // Start interrupts
pit_init();
serial_init();
// Test serial
int8_t buffer[50];
sprintf(buffer, "SimpleOS %i.%i.%i (%s)\n", VERSION_MAJOR, VERSION_MINOR, VERSION_BUILD, VERSION_DATE);
serial_write(SERIAL_PORT_A, buffer);
// Test RTC
datetime_t *dt = 0;
rtc_get(dt);
printf("Started at %02i-%02i-%02i %02i:%02i:%02i\n\n", dt->year, dt->month, dt->day, dt->hour, dt->min, dt->sec);
// Test CPU
cpu_detect();
printf("\n");
// Test PCI
pci_scan();
for (;;);
return 0x12345678;
}
<file_sep>#ifndef CPU_CPUID_H
#define CPU_CPUID_H
#include <lib/stdtypes.h>
// Function 0x01
#define ECX_SSE3 (1 << 0) // Streaming SIMD Extensions 3
#define ECX_PCLMULQDQ (1 << 1) // PCLMULQDQ Instruction
#define ECX_DTES64 (1 << 2) // 64-Bit Debug Store Area
#define ECX_MONITOR (1 << 3) // MONITOR/MWAIT
#define ECX_DS_CPL (1 << 4) // CPL Qualified Debug Store
#define ECX_VMX (1 << 5) // Virtual Machine Extensions
#define ECX_SMX (1 << 6) // Safer Mode Extensions
#define ECX_EST (1 << 7) // Enhanced SpeedStep Technology
#define ECX_TM2 (1 << 8) // Thermal Monitor 2
#define ECX_SSSE3 (1 << 9) // Supplemental Streaming SIMD Extensions 3
#define ECX_CNXT_ID (1 << 10) // L1 Context ID
#define ECX_FMA (1 << 12) // Fused Multiply Add
#define ECX_CX16 (1 << 13) // CMPXCHG16B Instruction
#define ECX_XTPR (1 << 14) // xTPR Update Control
#define ECX_PDCM (1 << 15) // Perf/Debug Capability MSR
#define ECX_PCID (1 << 17) // Process-context Identifiers
#define ECX_DCA (1 << 18) // Direct Cache Access
#define ECX_SSE41 (1 << 19) // Streaming SIMD Extensions 4.1
#define ECX_SSE42 (1 << 20) // Streaming SIMD Extensions 4.2
#define ECX_X2APIC (1 << 21) // Extended xAPIC Support
#define ECX_MOVBE (1 << 22) // MOVBE Instruction
#define ECX_POPCNT (1 << 23) // POPCNT Instruction
#define ECX_TSC (1 << 24) // Local APIC supports TSC Deadline
#define ECX_AESNI (1 << 25) // AESNI Instruction
#define ECX_XSAVE (1 << 26) // XSAVE/XSTOR States
#define ECX_OSXSAVE (1 << 27) // OS Enabled Extended State Management
#define ECX_AVX (1 << 28) // AVX Instructions
#define ECX_F16C (1 << 29) // 16-bit Floating Point Instructions
#define ECX_RDRAND (1 << 30) // RDRAND Instruction
#define EDX_FPU (1 << 0) // Floating-Point Unit On-Chip
#define EDX_VME (1 << 1) // Virtual 8086 Mode Extensions
#define EDX_DE (1 << 2) // Debugging Extensions
#define EDX_PSE (1 << 3) // Page Size Extension
#define EDX_TSC (1 << 4) // Time Stamp Counter
#define EDX_MSR (1 << 5) // Model Specific Registers
#define EDX_PAE (1 << 6) // Physical Address Extension
#define EDX_MCE (1 << 7) // Machine-Check Exception
#define EDX_CX8 (1 << 8) // CMPXCHG8 Instruction
#define EDX_APIC (1 << 9) // APIC On-Chip
#define EDX_SEP (1 << 11) // SYSENTER/SYSEXIT instructions
#define EDX_MTRR (1 << 12) // Memory Type Range Registers
#define EDX_PGE (1 << 13) // Page Global Bit
#define EDX_MCA (1 << 14) // Machine-Check Architecture
#define EDX_CMOV (1 << 15) // Conditional Move Instruction
#define EDX_PAT (1 << 16) // Page Attribute Table
#define EDX_PSE36 (1 << 17) // 36-bit Page Size Extension
#define EDX_PSN (1 << 18) // Processor Serial Number
#define EDX_CLFLUSH (1 << 19) // CLFLUSH Instruction
#define EDX_DS (1 << 21) // Debug Store
#define EDX_ACPI (1 << 22) // Thermal Monitor and Software Clock Facilities
#define EDX_MMX (1 << 23) // MMX Technology
#define EDX_FXSR (1 << 24) // FXSAVE and FXSTOR Instructions
#define EDX_SSE (1 << 25) // Streaming SIMD Extensions
#define EDX_SSE2 (1 << 26) // Streaming SIMD Extensions 2
#define EDX_SS (1 << 27) // Self Snoop
#define EDX_HTT (1 << 28) // Multi-Threading
#define EDX_TM (1 << 29) // Thermal Monitor
#define EDX_PBE (1 << 31) // Pending Break Enable
// Extended Function 0x01
#define EDX_SYSCALL (1 << 11) // SYSCALL/SYSRET
#define EDX_XD (1 << 20) // Execute Disable Bit
#define EDX_1GB_PAGE (1 << 26) // 1 GB Pages
#define EDX_RDTSCP (1 << 27) // RDTSCP and IA32_TSC_AUX
#define EDX_64_BIT (1 << 29) // 64-bit Architecture
void cpu_detect(void);
void cpuid(uint32_t reg, uint32_t *eax, uint32_t *ebx, uint32_t *ecx, uint32_t *edx);
#endif
<file_sep>#include <drivers/pci/driver.h>
#include <lib/stdtypes.h>
#include <lib/x86.h>
uint8_t pci_read8(uint32_t id, uint32_t reg)
{
uint32_t addr = 0x80000000 | id | (reg & 0xfc);
outportl(PCI_CONFIG_ADDR, addr);
return inportb(PCI_CONFIG_DATA + (reg & 0x03));
}
uint16_t pci_read16(uint32_t id, uint32_t reg)
{
uint32_t addr = 0x80000000 | id | (reg & 0xfc);
outportl(PCI_CONFIG_ADDR, addr);
return inportw(PCI_CONFIG_DATA + (reg & 0x02));
}
uint32_t pci_read32(uint32_t id, uint32_t reg)
{
uint32_t addr = 0x80000000 | id | (reg & 0xfc);
outportl(PCI_CONFIG_ADDR, addr);
return inportl(PCI_CONFIG_DATA);
}
void pci_write8(uint32_t id, uint32_t reg, uint8_t data)
{
uint32_t address = 0x80000000 | id | (reg & 0xfc);
outportl(PCI_CONFIG_ADDR, address);
outportb(PCI_CONFIG_DATA + (reg & 0x03), data);
}
void pci_write16(uint32_t id, uint32_t reg, uint16_t data)
{
uint32_t address = 0x80000000 | id | (reg & 0xfc);
outportl(PCI_CONFIG_ADDR, address);
outportw(PCI_CONFIG_DATA + (reg & 0x02), data);
}
void pci_write32(uint32_t id, uint32_t reg, uint32_t data)
{
uint32_t address = 0x80000000 | id | (reg & 0xfc);
outportl(PCI_CONFIG_ADDR, address);
outportl(PCI_CONFIG_DATA, data);
}
static void pci_read_bar(uint32_t id, uint32_t index, uint32_t *address, uint32_t *mask)
{
uint32_t reg = PCI_CONFIG_BAR0 + index * sizeof(uint32_t);
// Get address
*address = pci_read32(id, reg);
// Find size of the bar
pci_write32(id, reg, 0xffffffff);
*mask = pci_read32(id, reg);
// Restore adddress
pci_write32(id, reg, *address);
}
void pci_get_bar(pci_bar_t *bar, uint32_t id, uint32_t index)
{
// Read PCI bar register
uint32_t addressLow;
uint32_t maskLow;
pci_read_bar(id, index, &addressLow, &maskLow);
if (addressLow & PCI_BAR_64) {
// 64-bit MMIO
uint32_t addressHigh;
uint32_t maskHigh;
pci_read_bar(id, index + 1, &addressHigh, &maskHigh);
bar->u.address = (void *)(((uint32_t)addressHigh << 16) | (addressLow & ~0xf));
bar->size = ~(((uint32_t)maskHigh << 16) | (maskLow & ~0xf)) + 1;
bar->flags = addressLow & 0xf;
} else if (addressLow & PCI_BAR_IO) {
// I/O register
bar->u.port = (uint16_t)(addressLow & ~0x3);
bar->size = (uint16_t)(~(maskLow & ~0x3) + 1);
bar->flags = addressLow & 0x3;
} else {
// 32-bit MMIO
bar->u.address = (void *)(uint32_t)(addressLow & ~0xf);
bar->size = ~(maskLow & ~0xf) + 1;
bar->flags = addressLow & 0xf;
}
}<file_sep>#include <interrupt/gdt.h>
#include <lib/stdtypes.h>
extern void gdt_flush(uint32_t);
static void gdt_set_gate(int32_t, uint32_t, uint32_t, uint8_t, uint8_t);
gdt_entry_t gdt_entries[3];
gdt_ptr_t gdt_ptr;
void gdt_init(void)
{
// The limit is the last valid byte from the start of the GDT - i.e. the size of the GDT - 1
gdt_ptr.limit = sizeof(gdt_entry_t) * 3 - 1;
gdt_ptr.base = (uint32_t) &gdt_entries;
gdt_set_gate(0, 0, 0, 0, 0); // Null segment
gdt_set_gate(1, 0, 0xFFFFF, 0x9A, 0xCF); // Code segment
gdt_set_gate(2, 0, 0xFFFFF, 0x92, 0xCF); // Data segment
gdt_flush((uint32_t) &gdt_ptr);
}
static void gdt_set_gate(int32_t num, uint32_t base, uint32_t limit, uint8_t access, uint8_t gran)
{
gdt_entries[num].base_low = (base & 0xFFFF);
gdt_entries[num].base_middle = (base >> 16) & 0xFF;
gdt_entries[num].base_high = (base >> 24) & 0xFF;
gdt_entries[num].limit_low = (limit & 0xFFFF);
gdt_entries[num].granularity = (limit >> 16) & 0x0F;
gdt_entries[num].granularity |= gran & 0xF0;
gdt_entries[num].access = access;
}
| cb2971409b0a8cabf9f1fc8d73068581416c7067 | [
"Markdown",
"C",
"Makefile",
"Shell"
] | 40 | C | BackupGGCode/simpleos | 7f4f73f019e8b8e894b5480489833e10a7bcccb6 | e73f5222f888ceca04a40ccdb5628931be888213 | |
refs/heads/master | <file_sep>// JavaScript Document
function UpdateGrupo() {
var form = document.frmGrupoPopUp;
for (var i=0; i < parseInt(form.hiddenIntGrupoCliente.value); i++) {
if (form.radioThisGroup[i].checked) {
window.location.href="frmGrupoPopUp.asp?cnpj="+form.cnpj.value+"&idgrupo="+form.radioThisGroup[i].value+"&action=alterar"
}
}
}
<file_sep>$(function(){
$('#language').change(function() {
var selectLanguage = $('#language').val();
var currentUrl = window.location.href;
var langParam = currentUrl.match(/lang=[0-9a-zA-Z]*/);
if (langParam == null){
if (currentUrl.indexOf('?') > 0) {
window.location.href = currentUrl + '&lang=' + selectLanguage;
} else {
window.location.href = currentUrl + '?lang=' + selectLanguage;
}
} else {
window.location.href = currentUrl.replace(langParam, 'lang=' + selectLanguage);
}
});
$('input[name="supportManualsTab"]').change(function() {
var downloadManualUrl = $("input[name='supportManualsTab']:checked").val();
$('#supportManualDownload a').attr('href', downloadManualUrl);
});
$('#softwareOs').change(function() {
softwareDropDownListReload();
});
$('#softwareLanguage').change(function() {
softwareDropDownListReload();
});
function softwareDropDownListReload() {
var selectOs = $('#softwareOs').val();
var selectLanguage = $('#softwareLanguage').val();
var currentUrl = window.location.href;
var langParam = currentUrl.match(/lang=[0-9a-zA-Z]*/);
var osParam = currentUrl.match(/os=[0-9a-zA-Z]*/);
if (langParam == null && osParam == null) {
if (currentUrl.indexOf('?') > 0) {
if (selectOs != "") {
window.location.href = currentUrl + '&os=' + selectOs + '&lang=' + selectLanguage;
} else {
window.location.href = currentUrl + '&lang=' + selectLanguage;
}
} else {
if (selectOs != "") {
window.location.href = currentUrl + '?os=' + selectOs + '&lang=' + selectLanguage;
} else {
window.location.href = currentUrl + '?lang=' + selectLanguage;
}
}
} else if (langParam != null && osParam == null) {
if (selectOs != "") {
window.location.href = currentUrl.replace(langParam, 'os=' + selectOs + '&lang=' + selectLanguage);
} else {
window.location.href = currentUrl.replace(langParam, 'lang=' + selectLanguage);
}
} else if (langParam == null && osParam != null) {
if (selectOs != "") {
window.location.href = currentUrl.replace(osParam, 'os=' + selectOs + '&lang=' + selectLanguage);
} else {
window.location.href = currentUrl.replace(osParam, 'lang=' + selectLanguage);
}
} else if (langParam != null && osParam != null) {
if (selectOs != "") {
window.location.href = currentUrl.replace(osParam, 'os=' + selectOs).replace(langParam, 'lang=' + selectLanguage);
} else {
if (currentUrl.match(/&os=[0-9a-zA-Z]*/) != null) {
window.location.href = currentUrl.replace('&' + osParam, '').replace(langParam, 'lang=' + selectLanguage);
} else {
window.location.href = currentUrl.replace(osParam + '&', '').replace(langParam, 'lang=' + selectLanguage);
}
}
}
}
});
<file_sep>// JavaScript Document
function updateTransp() {
var error = 0;
// alert(parseInt(document.frmTransportadoraLc.hiddenIntTransp.value + 1));
for (var i=0; i < parseInt(document.frmTransportadoraLc.hiddenIntTransp.value); i++) {
if (!document.frmTransportadoraLc.transp[i].checked) {
error++;
}
}
if (error == parseInt(document.frmTransportadoraLc.hiddenIntTransp.value)) {
alert("Por favor escolha uma transportadora");
return
} else {
document.frmTransportadoraLc.submit();
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Web;
using System.Configuration;
using System.Data;
using System.Data.SqlClient;
/// <summary>
/// Summary description for dbclass
/// </summary>
///
namespace dbclass
{
public class dbclass
{
public dbclass()
{
//
// TODO: Add constructor logic here
//
}
private static SqlConnection connection()
{
try
{
SqlConnection sqlconnection = new SqlConnection(ConfigurationSettings.AppSettings["ConnectionString"].ToString());
//Verifica se a conexão esta fechada.
if (sqlconnection.State == ConnectionState.Closed)
{
//Abri a conexão.
sqlconnection.Open();
}
//Retorna o sqlconnection.
return sqlconnection;
}
catch (Exception)
{
throw;
}
}
/// <summary>
/// Método que retorna um datareader com o resultado da query.
/// </summary>
/// <param name="query"></param>
/// <returns></returns>
public SqlDataReader retornaQuery(string query)
{
try
{
//Instância o sqlcommand com a query sql que será executada e a conexão.
SqlCommand comando = new SqlCommand(query, connection());
//Executa a query sql.
SqlDataReader retornaQuery = comando.ExecuteReader();
//Fecha a conexão.
connection().Close();
//Retorna o dataReader com o resultado
return retornaQuery;
}
catch (SqlException ex)
{
throw ex;
}
}
public DataSet retornaQueryDataSet(string query)
{
try
{
//Instância o sqlcommand com a query sql que será executada e a conexão.
SqlCommand comando = new SqlCommand(query, connection());
//Instância o sqldataAdapter.
SqlDataAdapter adapter = new SqlDataAdapter(comando);
//Instância o dataSet de retorno.
DataSet dataSet = new DataSet();
//Atualiza o dataSet
adapter.Fill(dataSet);
//Retorna o dataSet com o resultado da query sql.
return dataSet;
}
catch (Exception ex)
{
throw ex;
}
}
}
}<file_sep>// JavaScript Document
function Ajax() {
var ajax = null;
if (window.ActiveXObject) {
try {
ajax = new ActiveXObject("Msxml2.XMLHTTP");
} catch (ex) {
try {
ajax = new ActiveXObject("Microsoft.XMLHTTP");
} catch(ex2) {
alert("Seu browser não suporta Ajax.");
}
}
} else {
if (window.XMLHttpRequest) {
try {
ajax = new XMLHttpRequest();
} catch(ex3) {
alert("Seu browser não suporta Ajax.");
}
}
}
return ajax;
}
function date(campo) {
var string = campo.value;
var _char = "/";
for (var i=0;i<string.length;i++) {
if (i == 2 || i == 5) {
continue;
}
}
switch (string.length) {
case 2:
string += _char;
break;
case 5:
string += _char;
break;
}
campo.value = string;
}
function aprovar(ID) {
_data();
document.frmEditSolicitacaoColetaPontoColetaAdm.cbStatusSolColeta.value = 2;
if (document.frmEditSolicitacaoColetaPontoColetaAdm.cbStatusSolColeta.value == 2) {
if (confirm("Deseja realmente aprovar essa solicitação?")) {
try {
var oAjax = Ajax();
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
document.getElementById("msgret").innerHTML = oAjax.responseText;
document.getElementById("btnprove").style.display = 'none';
document.getElementById("btnatualizar").style.display = 'block';
}
}
oAjax.open('GET','ajax/Ajax.asp?sub=aprovarsolicitacao&id='+ID,true);
oAjax.setRequestHeader("Content-Type","application/x-form-www-urlencoded; charset=iso-8859-1");
oAjax.send(null);
} catch (exception) {
alert(exception);
}
window.opener.location.reload();
window.close();
}
}
}
function reprovar(ID) {
var change = document.frmEditSolicitacaoColetaPontoColetaAdm.cbStatusSolColeta.value;
document.frmEditSolicitacaoColetaPontoColetaAdm.cbStatusSolColeta.value = 3;
if (confirm("Deseja realmente rejeitar essa Solicitação?")) {
document.frmEditSolicitacaoColetaPontoColetaAdm.submit();
} else {
document.frmEditSolicitacaoColetaPontoColetaAdm.cbStatusSolColeta.value = change;
return;
}
}
function cancelar(ID) {
document.frmEditSolicitacaoColetaPontoColetaAdm.cbStatusSolColeta.value = 4;
if (confirm("Deseja realmente cancelar essa Solicitação?")) {
document.frmEditSolicitacaoColetaPontoColetaAdm.submit();
}
}
function _data() {
var data = new Date();
var _dia = data.getDate();
var _mes = parseInt(data.getMonth()) + 1;
var _ano = data.getFullYear();
if (_dia < 10) {
_dia = "0" + _dia;
}
if (_mes < 10) {
_mes = "0" + _mes;
}
return _dia + "/" + _mes + "/" + _ano;
}
function validateStandByColect() {
var form = document.frmEditSolicitacaoColetaPontoColetaAdm;
if (form.cbStatusSolColeta.value == 5) {
if (form.hiddenReqColetaDomiciliar.value == 1) {
if (form.txtDataEnvioTransportadora.value == "") {
alert("O status escolhido necessita que o campo Data Envio para Transportadora esteja preenchido!");
return false;
}
}
}
return true;
}
function validateInTransit() {
var form = document.frmEditSolicitacaoColetaPontoColetaAdm;
if (form.cbStatusSolColeta.value == 7) {
if (form.txtDataEntregaPontoColeta.value == "") {
alert("O status escolhido necessita que o campo Data Entrega no Ponto de Coleta esteja preenchido!");
return false;
}
}
return true;
}
function validateFinish() {
var form = document.frmEditSolicitacaoColetaPontoColetaAdm;
if (form.cbStatusSolColeta.value == 6) {
if (form.txtDataRecebimento.value == "") {
alert("O status escolhido necessita que o campo Data recebimento pelo Operador Logístico esteja preenchido!");
return false;
}
}
return true;
}
function validateForm() {
var error = 0;
if (!validateStandByColect()) {
error++;
}
if (!validateInTransit()) {
error++;
} else {
if (!validaDataEnvPontoColeta()) {
error++;
}
}
if (!validateFinish()) {
error++;
}
if (error == 0) {
document.frmEditSolicitacaoColetaPontoColetaAdm.submit();
}
}
function getData(value) {
var _date = new Date();
var _arrData = value.split("/");
var _dia = _arrData[0];
var _mes = _arrData[1];
var _ano = _arrData[2];
//alert(_dia + "/" + _mes + "/" + _ano);
//alert(_dia);
//alert(_mes);
_date.setFullYear(_ano, _mes, _dia);
//_date = _dia + "/" + _mes + "/" + _ano
return _date;
}
function validaDataEnvPontoColeta() {
var form = document.frmEditSolicitacaoColetaPontoColetaAdm;
if (form.txtDataAprovacao.value == "") {
alert("Data de Aprovação tem que estar preenchido!");
return false;
} else {
if (form.txtDataEntregaPontoColeta.value == "") {
alert("Preencha o campo Data Entrega no Ponto Coleta corretamente!");
return false;
} else {
if (!validateGetDate(form.txtDataAprovacao.value, form.txtDataEntregaPontoColeta.value)) {
alert("Preencha o campo Data Entrega no Ponto Coleta corretamente!");
return false;
} else {
form.cbStatusSolColeta.value = 8;
return true;
}
}
}
}
function validateGetDate(dataDefault, date)
{
//alert(date);
//alert(dataDefault);
var arrData1 = dataDefault.split("/");
var arrData2 = date.split("/");
var dia1 = arrData1[0];
var mes1 = arrData1[1];
var ano1 = arrData1[2];
var dia2 = arrData2[0];
var mes2 = arrData2[1];
var ano2 = arrData2[2];
var data1 = ano1+mes1+dia1
var data2 = ano2+mes2+dia2
//alert(data2);
//alert(data1);
if (parseInt(data2) >= parseInt(data1))
{
//alert("maior ou igual");
return true;
}
else
{
//alert("menor");
return false;
}
}
function validateGetDate1(dataDefault, date) {
// alert(dataDefault.getDate() + "/" + dataDefault.getMonth() + "/" + dataDefault.getFullYear());
// alert(date.getDate() + "/" + date.getMonth() + "/" + date.getFullYear());
if (parseInt(date.getFullYear()) < parseInt(dataDefault.getFullYear())) {
return false;
} else {
if (parseInt(date.getMonth()) < parseInt(dataDefault.getMonth())) {
return false;
} else {
switch(parseInt(date.getMonth() + 1)) {
case 1: // Janeiro
if (parseInt(date.getDate()) > 31 || parseInt(date.getDate()) < 1) {
return false
}
break;
case 2: // Fevereiro
if (parseInt(date.getDate()) > 28 || parseInt(date.getDate()) < 1) {
return false;
}
break;
case 3: // Março
if (parseInt(date.getDate()) > 31 || parseInt(date.getDate()) < 1) {
return false;
}
break;
case 4: // Abril
if (parseInt(date.getDate()) > 30 || parseInt(date.getDate()) < 1) {
return false;
}
break;
case 5: // Maio
if (parseInt(date.getDate()) > 31 || parseInt(date.getDate()) < 1) {
return false;
}
break;
case 6: // Junho
if (parseInt(date.getDate()) > 31 || parseInt(date.getDate()) < 1) {
return false;
}
break;
case 7: // Julho
if (parseInt(date.getDate()) > 31 || parseInt(date.getDate()) < 1) {
return false;
}
break;
case 8: // Agosto
if (parseInt(date.getDate()) > 31 || parseInt(date.getDate()) < 1) {
return false;
}
break;
case 9: // Setembro
if (parseInt(date.getDate()) > 30 || parseInt(date.getDate()) < 1) {
return false;
}
break;
case 10: // Outubro
if (parseInt(date.getDate()) > 31 || parseInt(date.getDate()) < 1) {
return false;
}
break;
case 11: // Novembro
if (parseInt(date.getDate()) > 30 || parseInt(date.getDate()) < 1) {
return false;
}
break;
case 12: // Dezembro
if (parseInt(date.getDate()) > 31 || parseInt(date.getDate()) < 1) {
return false;
}
break;
default:
return false;
break;
}
if (parseInt(date.getDate()) < parseInt(dataDefault.getDate())) {
return false;
}
return true;
}
}
}
// JavaScript Document<file_sep>
//Peterson 5-5-2014
//Função para retornar o id do contato a ser editado.
//
function lcEditContato(element) {
var sid = element;
window.location.href="frmAddContato.asp?Query=UPDATE&ID="+sid
}
<file_sep>$(function(){
$('.bxslider').bxSlider({
buildPager: function(slideIndex){
/*$.each($(".slideImg").data('images'), function(i, img){
if (slideIndex === i) {
//console.log('<img src="' + img + '">')
//return '<img src="' + img + '">';
console.log(slideIndex + $(".slideImg").data("images")[i])
}
});*/
switch(slideIndex){
case 0:
return '<img src="' + $(".slideImg").data("images")[0] + '">';
case 1:
return '<img src="' + $(".slideImg").data("images")[1] + '">';
case 2:
return '<img src="' + $(".slideImg").data("images")[2] + '">';
case 3:
return '<img src="' + $(".slideImg").data("images")[3] + '">';
case 4:
return '<img src="' + $(".slideImg").data("images")[4] + '">';
}
}
});
});<file_sep>// JavaScript Document
function updateTransp() {
var error = 0;
// alert(parseInt(document.frmSearchTranspSol.hiddenIntTransp.value + 1));
for (var i=0; i < parseInt(document.frmSearchTranspSol.hiddenIntTransp.value); i++) {
if (parseInt(document.frmSearchTranspSol.hiddenIntTransp.value) == 1) {
if (!document.frmSearchTranspSol.transp.checked) {
error++;
}
} else {
if (!document.frmSearchTranspSol.transp[i].checked) {
error++;
}
}
}
if (error == parseInt(document.frmSearchTranspSol.hiddenIntTransp.value)) {
alert("Por favor escolha uma transportadora");
return
} else {
document.frmSearchTranspSol.submit();
}
}<file_sep>// JavaScript Document
/*
'|--------------------------------------------------------------------
'| Arquivo: frmCadCliente.js
'| Autor: <NAME> (<EMAIL>)
'| Data Criação: 13/04/2007
'| Data Modificação : 15/04/2007
'| Descrição: Arquivo de Formulário para cadastro de Cliente (Javascript)
'|--------------------------------------------------------------------
*/
var errForm = false;
var msgErrForm = "Os seguintes campos foram preenchidos incorretamente!\n";
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Verifica se a coleta tem que ser domiciliar ou de ponto de coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function ckeckColeta() {
var form = document.frmCadCliente;
if (form.cbCategorias.value == -1) {
alert("Escolha uma Categoria!");
return false;
} else {
if (isNaN(form.txtQtdCartuchos.value)) {
alert("Digite somente números na quantidade de cartuchos!");
return false;
}
if (form.txtQtdCartuchos.value == 0 || form.txtQtdCartuchos.value == "") {
alert("Digite a quantidade de cartuchos a ser coletada!");
return false;
} else {
if (form.hiddenControleColeta.value == 0) {
form.hiddenTypeColeta.value = 0;
} else {
if (parseInt(form.txtQtdCartuchos.value) < parseInt(form.hiddenMinCartuchos.value)) {
form.hiddenTypeColeta.value = 0;
} else {
form.hiddenTypeColeta.value = 1;
}
}
if (form.hiddenTypeColeta.value == 0) {
document.getElementById("tableCadClienteCategoria").style.display = 'none';
document.getElementById("tableCadClientePontoColeta").style.display = 'block';
document.frmCadCliente.btnNextToCadEmpresaPontoColeta.disabled = true;
} else {
document.getElementById("tableCadClienteCategoria").style.display = 'none';
document.getElementById("tableCadCliente").style.display = 'block';
}
}
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Conforme a escolha do usuário
// Verifica se o usuario é coleta Domiciliar ou coleta em Ponto de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function setTypeColeta() {
var oAjax = Ajax();
var form = document.frmCadCliente;
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
form.hiddenControleColeta.value = oAjax.responseText;
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=gettypecoleta&id="+form.cbCategorias.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Atualiza o valor do campo hidden para seja guardado o valor do mínimo de cartuchos
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function updateMinimo() {
var oAjax = Ajax();
var form = document.frmCadCliente;
if (form.cbCategorias.value != -1) {
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
form.hiddenMinCartuchos.value = oAjax.responseText;
setTypeColeta();
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getminimo&id="+form.cbCategorias.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Retorno do Usuario quando o mesmo é Coleta Domiciliar
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function returnCadCategoriaDomiciliar() {
document.getElementById("tableCadCliente").style.display = 'none';
if (!checkTypeColeta()) {
document.getElementById("tableCadClientePontoColeta").style.display = 'block';
} else {
document.getElementById("tableCadClienteCategoria").style.display = 'block';
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Retorno do Usuario quando o mesmo é Coleta Ponto de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function returnCadCategoriaPontoColeta() {
document.getElementById("tableCadClientePontoColeta").style.display = 'none';
document.getElementById("tableCadClienteCategoria").style.display = 'block';
}
//=========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Preenchimento do Endereço da Empresa
// É validado os campos referente ao Cadastro da Empresa
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function showClienteEndereco() {
var form = document.frmCadCliente;
if (form.radioPessoa[0].checked) {
if (form.txtNome.value == "") {
msgErrForm += "Campo: Nome\n";
errForm = true;
}
validateCPF();
validaDDD();
validaTelefone();
if (!errForm) {
document.getElementById('tableCadClienteEndereco').style.display = 'block';
document.getElementById('tableCadCliente').style.display = 'none';
document.getElementById('tableCadClienteCategoria').style.display = 'none';
form.txtNumero.value = "";
form.chkMesmoEndereco.checked = false;
form.txtCepColeta.value = "";
form.txtLogradouroColeta.value = "";
form.txtCompLogradouroColeta.value = "";
form.txtNumeroColeta.value = "";
form.txtBairroColeta.value = "";
form.txtMunicipioColeta.value = "";
form.txtEstadoColeta.value = "";
} else {
alert(msgErrForm);
msgErrForm = "Os seguintes campos foram preenchidos incorretamente!\n";
}
errForm = false;
} else {
if (form.radioPessoa[1].checked) {
if (form.txtRazaoSocial.value == "") {
msgErrForm += "Campo: Razão Social\n";
errForm = true;
}
if (form.txtNomeFantasia.value == "") {
msgErrForm += "Campo: Nome Fantasia\n";
errForm = true;
}
validaCnpj();
if (updateDisplayIE()) {
if (form.txtInscricaoEstadual.value == "") {
msgErrForm += "Campo: Inscrição Estadual\n";
errForm = true;
}
if (form.txtInscricaoEstadual.value.length < 15) {
msgErrForm += "Campo: Inscrição Estadual\n";
errForm = true;
}
}
validaDDD();
validaTelefone();
if (!errForm) {
document.getElementById('tableCadClienteEndereco').style.display = 'block';
document.getElementById('tableCadCliente').style.display = 'none';
document.getElementById('tableCadClienteCategoria').style.display = 'none';
form.txtNumero.value = "";
form.chkMesmoEndereco.checked = false;
form.txtCepColeta.value = "";
form.txtLogradouroColeta.value = "";
form.txtCompLogradouroColeta.value = "";
form.txtNumeroColeta.value = "";
form.txtBairroColeta.value = "";
form.txtMunicipioColeta.value = "";
form.txtEstadoColeta.value = "";
} else {
alert(msgErrForm);
msgErrForm = "Os seguintes campos foram preenchidos incorretamente!\n";
}
errForm = false;
}
}
}
//=========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Direciona para o cadastro da empresa
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function showCadCliente() {
if (document.frmCadCliente.txtCepConsultaPonto.value == "") {
alert("Por favor preencha o campo de Cep para Busca dos Pontos de Coleta!");
return false;
}
if (checkChangePontoColeta()) {
document.getElementById('tableCadClientePontoColeta').style.display = 'none';
document.getElementById('tableCadCliente').style.display = 'block';
} else {
alert("Escolha um Ponto de Coleta para que seja feita a Solicitação!");
}
}
//=========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Check se foi selecionado algum ponto de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function checkChangePontoColeta() {
var bErr = false;
var bSelected = false;
for (var i=0;i <= parseInt(document.frmCadCliente.hiddenIntPontoColeta.value);i++) {
if (!document.getElementById("radioCheckPonto"+i).checked) {
bErr = true;
} else {
bSelected = true;
document.frmCadCliente.hiddenIntChangePontoColeta.value = document.getElementById("radioCheckPonto"+i).value;
}
}
if (bErr && !bSelected) {
return false
} else {
return true;
}
}
//=========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Check se foi selecionado algum ponto de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function checkTypeContinue() {
if (document.frmCadCliente.txtNumero.value == "") {
alert("Preencha o número da Localização da Empresa");
document.frmCadCliente.txtNumero.focus();
return false;
}
if (document.frmCadCliente.txtCep.value == "") {
alert("Preencha o campo Cep para Busca do Endereço de Coleta!");
return false;
}
if (isNaN(document.frmCadCliente.txtCep.value)) {
alert("Preencha o Cep somente com números!");
return false;
}
if (document.frmCadCliente.txtCep.value.length < 8) {
alert("Preencha corretamente o Campo Cep!");
return false;
} else {
document.getElementById("tableCadClienteEndereco").style.display = 'none';
if (!checkTypeColeta()) {
document.getElementById("tableCadClienteContato").style.display = 'block';
} else {
document.getElementById("tableCadClienteEnderecoColeta").style.display = 'block';
}
}
}
//=========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Check qual tipo selecionado de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function checkTypeColeta() {
if (document.frmCadCliente.hiddenTypeColeta.value == 0) {
return false;
} else {
return true;
}
}
//=========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Validação do CNPJ da Empresa
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function validaCnpj() {
var form = document.frmCadCliente;
var numeros1Dig = new Array(5,4,3,2,9,8,7,6,5,4,3,2);
var soma1Dig = 0;
var resto1Dig = 0;
var digVer1 = 0;
var numeros2Dig = new Array(6,5,4,3,2,9,8,7,6,5,4,3,2);
var soma2Dig = 0;
var resto2Dig = 0;
var digVer2 = 0;
var i = 0;
var j = 0;
var cnpj = "";
cnpj = form.txtCNPJ.value;
digVer2 = cnpj.charAt(cnpj.length - 1);
digVer1 = cnpj.charAt(cnpj.length - 2);
if (form.txtCNPJ.value == "") {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
if (form.txtCNPJ.value.indexOf('/') == -1) {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
if (form.txtCNPJ.value.length < 18) {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
cnpj = cnpj.replace('/','');
cnpj = cnpj.replace('-','');
cnpj = cnpj.replace('.','');
cnpj = cnpj.replace('.','');
for(i = 0; i < cnpj.length - 2; i++) {
if (!isNaN(cnpj.charAt(i)) && !isNaN(numeros1Dig[i])) {
soma1Dig += cnpj.charAt(i) * numeros1Dig[i];
}
}
resto1Dig = soma1Dig % 11;
if (resto1Dig < 2) {
if (!(digVer1 == 0)) {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
} else {
resto1Dig = 11 - resto1Dig;
if (!(resto1Dig == digVer1)) {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
}
for(j = 0; j < cnpj.length - 1; j++) {
soma2Dig += cnpj.charAt(j) * numeros2Dig[j];
}
resto2Dig = soma2Dig % 11;
if (resto2Dig < 2) {
if (!(digVer2 == 0)) {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
} else {
resto2Dig = 11 - resto2Dig;
if (!(resto2Dig == digVer2)) {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Validação DDD e auto preenchimento
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function validaDDD() {
var form = document.frmCadCliente;
if (form.txtDDD.value == "") {
msgErrForm += "Campo: DDD\n";
errForm = true;
return false;
}
if (isNaN(form.txtDDD.value)) {
msgErrForm += "Campo: DDD\n";
errForm = true;
return false;
}
if (form.txtDDD.value.length == 1) {
msgErrForm += "Campo: DDD\n";
errForm = true;
return false;
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Busca De Pontos de Coleta do cliente
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function showClientePostoColeta() {
var form = document.frmCadCliente;
var oAjax = Ajax();
var strRet = "";
document.body.style.cursor='wait';
//form.btnBuscarCepUser.disabled = true;
if (form.txtCepConsultaPonto.value.length < 8) {
alert("Preencha corretamente o Cep para Busca!");
form.txtCepConsultaPonto.focus();
return false;
}
if (isNaN(form.txtCepConsultaPonto.value)) {
alert("Preencha somente números no Cep para Busca!");
form.txtCepConsultaPonto.focus();
return false;
}
if (form.txtCepConsultaPonto.value == "") {
alert("Preencha o campo de Cep para busca dos Pontos de Coleta!");
form.txtCepConsultaPonto.focus();
return false;
} else {
document.frmCadCliente.btnBuscarCepUser.disabled = 'true';
document.frmCadCliente.btnNextToCadEmpresaPontoColeta.disabled = '';
document.frmCadCliente.txtCepConsultaPonto.value;
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
// alert(oAjax.responseText);
document.frmCadCliente.btnBuscarCepUser.disabled = false;
strRet = oAjax.responseText.split(";");
document.getElementById("titTableListPontoColeta").style.display = 'block';
document.getElementById("tableListPontoColeta").innerHTML = strRet[0];
document.frmCadCliente.hiddenIntPontoColeta.value = strRet[1];
if (document.frmCadCliente.hiddenIntPontoColeta.value == -1) {
alert("Não foi encontrado nenhum ponto de coleta no CEP digitado, favor tente outro CEP!");
document.frmCadCliente.btnNextToCadEmpresaPontoColeta.disabled = 'true';
document.frmCadCliente.txtCepConsultaPonto.value = "";
document.frmCadCliente.txtCepConsultaPonto.focus();
return false;
} else {
document.frmCadCliente.btnNextToCadEmpresaPontoColeta.disabled = '';
if (!confirm("Este é o endereço do(s) ponto(s) de coleta mais próximo(s), onde você deverá entregar os cartuchos vazios.\nDeseja prosseguir com a solicitação?")) {
alert("Grato pelo contato!");
window.location.href="index.asp";
} else {
document.body.style.cursor='default';
}
}
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getlistpontocoleta&id="+document.frmCadCliente.txtCepConsultaPonto.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Busca do Endereço para Preenchimento automático do Endereço de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function loadCepColeta() {
var oAjax = Ajax();
var strRet = "";
if (document.frmCadCliente.txtCepColeta.value == "") {
alert("Preencha o campo Cep para Busca do Endereço de Coleta!");
return false;
}
if (isNaN(document.frmCadCliente.txtCepColeta.value)) {
alert("Preencha o Cep somente com números!");
return false;
}
if (document.frmCadCliente.txtCepColeta.value.length < 8) {
alert("Preencha corretamente o Campo Cep!");
return false;
}
//====================================================================
// Bloqueio dos campos de consulta
//====================================================================
document.frmCadCliente.chkMesmoEndereco.checked = false;
document.frmCadCliente.btnBuscarCepComum.disabled = true;
document.frmCadCliente.txtLogradouroColeta.disabled = true;
document.frmCadCliente.txtLogradouroColeta.value = "Carregando...";
document.frmCadCliente.txtCompLogradouroColeta.disabled = true;
document.frmCadCliente.txtNumeroColeta.disabled = true;
document.frmCadCliente.txtBairroColeta.disabled = true;
document.frmCadCliente.txtBairroColeta.value = "Carregando...";
document.frmCadCliente.txtMunicipioColeta.disabled = true;
document.frmCadCliente.txtMunicipioColeta.value = "Carregando...";
document.frmCadCliente.txtEstadoColeta.disabled = true;
document.frmCadCliente.txtEstadoColeta.value = "Carregando...";
document.frmCadCliente.txtCompLogradouroColeta.value = "";
document.frmCadCliente.txtNumeroColeta.value = "";
document.getElementById("btnBuscarCepComum").style.cursor = 'wait';
document.frmCadCliente.btnNextToContatoMaster.style.cursor = 'wait';
document.frmCadCliente.btnBackCadClienteColeta.style.cursor = 'wait';
document.body.style.cursor = 'wait';
//====================================================================
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
strRet = oAjax.responseText.split(";");
document.frmCadCliente.btnBuscarCepComum.disabled = false;
document.frmCadCliente.hiddenIntEnderecoCepColeta.value = strRet[0];
document.frmCadCliente.txtLogradouroColeta.value = strRet[2];
document.frmCadCliente.txtBairroColeta.value = strRet[3];
document.frmCadCliente.txtMunicipioColeta.value = strRet[4];
document.frmCadCliente.txtEstadoColeta.value = strRet[5];
document.frmCadCliente.txtLogradouroColeta.disabled = false;
document.frmCadCliente.txtCompLogradouroColeta.disabled = false;
document.frmCadCliente.txtNumeroColeta.disabled = false;
document.frmCadCliente.txtBairroColeta.disabled = false;
document.frmCadCliente.txtMunicipioColeta.disabled = false;
document.frmCadCliente.txtEstadoColeta.disabled = false;
document.getElementById("btnBuscarCepComum").style.cursor = 'pointer';
document.frmCadCliente.btnNextToContatoMaster.style.cursor = 'default';
document.frmCadCliente.btnBackCadClienteColeta.style.cursor = 'default';
document.body.style.cursor = 'default';
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getcependereco&id="+document.frmCadCliente.txtCepColeta.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Busca do Endereço para Preenchimento automático do Endereço de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function loadCepComum() {
var oAjax = Ajax();
var strRet = "";
if (document.frmCadCliente.txtCep.value == "") {
alert("Preencha o campo Cep para Busca do Endereço de Coleta!");
return false;
}
if (isNaN(document.frmCadCliente.txtCep.value)) {
alert("Preencha o Cep somente com números!");
return false;
}
if (document.frmCadCliente.txtCep.value.length < 8) {
alert("Preencha corretamente o Campo Cep!");
return false;
}
//====================================================================
// Bloqueio dos campos de consulta
//====================================================================
// document.frmCadCliente.chkMesmoEndereco.checked = false;
document.frmCadCliente.btnBuscarCepColeta.disabled = true;
document.frmCadCliente.txtLogradouro.disabled = true;
document.frmCadCliente.txtLogradouro.value = "Carregando...";
document.frmCadCliente.txtCompLogradouro.disabled = true;
document.frmCadCliente.txtNumero.disabled = true;
document.frmCadCliente.txtBairro.disabled = true;
document.frmCadCliente.txtBairro.value = "Carregando...";
document.frmCadCliente.txtMunicipio.disabled = true;
document.frmCadCliente.txtMunicipio.value = "Carregando...";
document.frmCadCliente.txtEstado.disabled = true;
document.frmCadCliente.txtEstado.value = "Carregando...";
document.frmCadCliente.txtCompLogradouro.value = "";
document.frmCadCliente.txtNumero.value = "";
// document.getElementById("btnBuscarCepColeta").style.cursor = 'wait';
document.frmCadCliente.btnNextToEnderecoColeta.style.cursor = 'wait';
document.frmCadCliente.btnBackCadCliente.style.cursor = 'wait';
document.body.style.cursor = 'wait';
//====================================================================
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
strRet = oAjax.responseText.split(";");
document.frmCadCliente.btnBuscarCepColeta.disabled = false;
document.frmCadCliente.hiddenIntEnderecoCep.value = strRet[0];
document.frmCadCliente.txtLogradouro.value = strRet[2];
document.frmCadCliente.txtBairro.value = strRet[3];
document.frmCadCliente.txtMunicipio.value = strRet[4];
document.frmCadCliente.txtEstado.value = strRet[5];
document.frmCadCliente.txtLogradouro.disabled = false;
document.frmCadCliente.txtCompLogradouro.disabled = false;
document.frmCadCliente.txtNumero.disabled = false;
document.frmCadCliente.txtBairro.disabled = false;
document.frmCadCliente.txtMunicipio.disabled = false;
document.frmCadCliente.txtEstado.disabled = false;
// document.getElementById("btnBuscarCepColeta").style.cursor = 'pointer';
document.frmCadCliente.btnNextToEnderecoColeta.style.cursor = 'default';
document.frmCadCliente.btnBackCadCliente.style.cursor = 'default';
document.body.style.cursor = 'default';
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getcependereco&id="+document.frmCadCliente.txtCep.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Busca do Endereço para Preenchimento automático do Endereço de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function preencheEnderecoToCep() {
var oAjax = Ajax();
var form = document.frmCadCliente;
var strRet = "";
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
strRet = oAjax.responseText.split(";");
strRet[6] = strRet[6].replace(" ",'');
form.hiddenIntEnderecoCep.value = strRet[0];
form.txtLogradouro.value = strRet[6] + ". " + strRet[2];
form.txtBairro.value = strRet[3];
form.txtMunicipio.value = strRet[4];
form.txtEstado.value = strRet[5];
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getcependereco&id="+form.txtCep.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Preenchimento Automático do mesmo Endereço
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function preencheMesmoEndereco() {
var form = document.frmCadCliente;
if (form.chkMesmoEndereco.checked) {
form.txtCepColeta.value = form.txtCep.value;
form.txtLogradouroColeta.value = form.txtLogradouro.value;
form.txtCompLogradouroColeta.value = form.txtCompLogradouro.value;
form.txtNumeroColeta.value = form.txtNumero.value;
form.txtBairroColeta.value = form.txtBairro.value;
form.txtMunicipioColeta.value = form.txtMunicipio.value;
form.txtEstadoColeta.value = form.txtEstado.value;
} else {
form.txtCepColeta.value = "";
form.txtLogradouroColeta.value = "";
form.txtCompLogradouroColeta.value = "";
form.txtNumeroColeta.value = "";
form.txtBairroColeta.value = "";
form.txtMunicipioColeta.value = "";
form.txtEstadoColeta.value = "";
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Validação do Ambiente de Contato com o cliente
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function validaCadClienteContato() {
var form = document.frmCadCliente;
if (form.txtContatoColeta.value == "") {
alert("Preencha o campo Contato!");
return false;
}
if (form.txtUsuario.value == "") {
alert("Preencha o campo Usuario!");
return false;
}
if (form.txtUsuario.value.length < 6) {
alert("Preencha o campo Usuário com no mínimo 6 caracteres!");
return false;
}
if (form.txtSenha.value == "") {
alert("Preencha o campo Senha!");
return false;
}
if (form.txtSenha.value.length < 6) {
alert("Preencha o campo Senha com no mínimo 6 caracteres!");
return false;
}
if (form.txtEmail.value == "") {
alert("Preencha o campo Email!");
return false;
}
if (form.txtEmail.value.indexOf('@') == -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('@.') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('.@') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('.') == -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('com') == -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('[') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf(']') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('(') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf(')') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('/') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('\\') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('..') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('com.com') != -1) {
alert("Email inválido!");
return false;
}
checkUserContato();
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Validação do Telefone da Empresa
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function validaTelefone() {
var form = document.frmCadCliente;
if (form.txtTelefone.value == "") {
msgErrForm += "Campo: Telefone\n";
errForm = true;
return false;
}
if (isNaN(form.txtTelefone.value)) {
msgErrForm += "Campo: Telefone\n";
errForm = true;
return false;
}
if (form.txtTelefone.value.length < 8) {
msgErrForm += "Campo: Telefone\n";
errForm = true;
return false;
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Criação do Objeto Ajax
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function Ajax() {
var ajax = null;
if (window.ActiveXObject) {
try {
ajax = new ActiveXObject("Msxml2.XMLHTTP");
} catch (ex) {
try {
ajax = new ActiveXObject("Microsoft.XMLHTTP");
} catch(ex2) {
alert("Seu browser não suporta Ajax.");
}
}
} else {
if (window.XMLHttpRequest) {
try {
ajax = new XMLHttpRequest();
} catch(ex3) {
alert("Seu browser não suporta Ajax.");
}
}
}
return ajax;
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Retorno do Contato corretamente de acordo com o tipo de Cliente
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function checkReturnEndCorrespondente() {
var form = document.frmCadCliente;
if (form.hiddenTypeColeta.value == 0) {
document.getElementById("tableCadClienteContato").style.display = 'none';
document.getElementById("tableCadClienteEndereco").style.display = 'block';
} else {
document.getElementById("tableCadClienteContato").style.display = 'none';
document.getElementById("tableCadClienteEnderecoColeta").style.display = 'block';
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Retorno do Endereço do Cliente para o Cadastro da Empresa
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function returnCadCliente() {
document.getElementById("tableCadClienteEndereco").style.display = 'none';
document.getElementById("tableCadCliente").style.display = 'block';
}
function returnCadClienteColeta() {
document.getElementById("tableCadClienteEnderecoColeta").style.display = 'none';
document.getElementById("tableCadClienteEndereco").style.display = 'block';
}
function returnClienteEnderecoPonto() {
document.getElementById("tableCadClientePontoColeta").style.display = 'none';
document.getElementById("tableCadClienteEndereco").style.display = 'block';
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Prossegue para o cadastro do Contato no Tipo Coleta Domiciliar
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function showCadClienteContato() {
var form = document.frmCadCliente;
if (form.txtCepColeta.value == "") {
alert("Preencha o campo de Cep do Endereço de Coleta!");
form.txtCepColeta.focus();
return false;
}
if (form.txtNumeroColeta.value == "") {
alert("Preecha o campo número do Endereço de Coleta!");
form.txtNumeroColeta.focus();
return false;
}
if (form.txtContatoRespColeta.value == "") {
alert("Preencha o campo do Contato responsável pela Coleta!");
form.txtContatoRespColeta.focus();
return false;
}
if (form.txtDDDContatoRespColeta.value == "") {
alert("Preencha o campo do DDD do responsável pela Coleta!");
form.txtDDDContatoRespColeta.focus();
return false;
}
if (isNaN(form.txtDDDContatoRespColeta.value)) {
alert("Preencha o campo DDD do Contato somente com dados numéricos!");
form.txtDDDContatoRespColeta.focus();
return false;
}
if (form.txtDDDContatoRespColeta.value.length < 2) {
alert("Preencha o campo do DDD do Contato com no mínimo 2 caracteres válidos!");
form.txtDDDContatoRespColeta.focus();
return false;
}
if (form.txtTelefoneContatoRespColeta.value == "") {
alert("Preencha o campo do Telefone do responsável pela Coleta!");
form.txtTelefoneContatoRespColeta.focus();
return false;
}
if (isNaN(form.txtTelefoneContatoRespColeta.value)) {
alert("Preencha o campo Telefone do Contato somente com dados numéricos!");
form.txtTelefoneContatoRespColeta.focus();
return false;
}
if (form.txtTelefoneContatoRespColeta.value.length < 8) {
alert("Preencha o campo do Telefone do Contato com 8 caracteres válidos!");
form.txtTelefoneContatoRespColeta.focus();
return false;
}
document.getElementById("tableCadClienteEnderecoColeta").style.display = 'none';
document.getElementById("tableCadClienteContato").style.display = 'block';
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Verifica se o usuário já está cadastrado
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function checkUserContato() {
var oAjax = Ajax();
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
if (oAjax.responseText == "true") {
alert("Usuário ou Senha já cadastrado!");
} else {
document.frmCadCliente.submit();
}
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getcheckusercontato&id=0&user="+document.frmCadCliente.txtUsuario.value+"&senha="+document.frmCadCliente.txtSenha.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
function checkUsuario() {
var oAjaxUsuario = Ajax();
oAjaxUsuario.onreadystatechange = function() {
if (oAjaxUsuario.readyState == 4 && oAjaxUsuario.status == 200) {
if (oAjaxUsuario.responseText == "true") {
alert("Usuário já cadastrado. Favor cadastre outro usuário");
document.frmCadCliente.txtUsuario.focus();
return;
}
}
}
oAjaxUsuario.open("GET", "ajax/frmCadCliente.asp?sub=getcheckusuario&id=0&user="+document.frmCadCliente.txtUsuario.value, true);
oAjaxUsuario.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjaxUsuario.send(null);
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Verifica se o o CNPJ se já está cadastrado
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function checkCNPJEmpresa() {
var oAjax = Ajax();
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
if (oAjax.responseText == "true") {
alert("CNPJ já cadastrado. Favor cadastrar outro CNPJ!");
document.frmCadCliente.txtCNPJ.focus();
return false;
} else {
return true;
}
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getcheckcnpjempresa&id="+document.frmCadCliente.txtCNPJ.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
function checkCPF() {
var oAjax = Ajax();
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
if (oAjax.responseText == "true") {
alert("CPF já cadastrado. Favor cadastrar outro CPF!");
document.frmCadCliente.txtCPF.focus();
return false;
} else {
return true;
}
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getcheckcpf&id="+document.frmCadCliente.txtCPF.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
function checkPessoa() {
var form = document.frmCadCliente;
if (form.radioPessoa[0].checked) {
document.getElementById("razaosocial").style.display = 'none';
document.getElementById("nomefantasia").style.display = 'none';
document.getElementById("cnpj").style.display = 'none';
document.getElementById("inscestadual").style.display = 'none';
document.getElementById("nome").style.display = 'block';
document.getElementById("cpf").style.display = 'block';
document.getElementById("possuiinscestadual").style.display = 'none';
} else {
if (form.radioPessoa[1].checked) {
document.getElementById("razaosocial").style.display = 'block';
document.getElementById("nomefantasia").style.display = 'block';
document.getElementById("cnpj").style.display = 'block';
document.getElementById("inscestadual").style.display = 'block';
document.getElementById("nome").style.display = 'none';
document.getElementById("cpf").style.display = 'none';
document.getElementById("possuiinscestadual").style.display = 'block';
}
}
}
function validateCPF() {
var form = document.frmCadCliente;
var numeros1Dig = new Array(10,9,8,7,6,5,4,3,2);
var soma1Dig = 0;
var resto1Dig = 0;
var digVer1 = 0;
var numeros2Dig = new Array(11,10,9,8,7,6,5,4,3,2);
var soma2Dig = 0;
var resto2Dig = 0;
var digVer2 = 0;
var i = 0;
var j = 0;
var cnpj = "";
cnpj = form.txtCPF.value;
digVer2 = cnpj.charAt(cnpj.length - 1);
digVer1 = cnpj.charAt(cnpj.length - 2);
if (form.txtCPF.value == "") {
msgErrForm += "Campo: CPF\n";
errForm = true;
return false;
}
if (form.txtCPF.value.length < 14) {
msgErrForm += "Campo: CPF\n";
errForm = true;
return false;
}
cnpj = cnpj.replace('-','');
cnpj = cnpj.replace('.','');
cnpj = cnpj.replace('.','');
for(i = 0; i < cnpj.length - 2; i++) {
if (!isNaN(cnpj.charAt(i)) && !isNaN(numeros1Dig[i])) {
soma1Dig += cnpj.charAt(i) * numeros1Dig[i];
}
}
resto1Dig = soma1Dig % 11;
if (resto1Dig < 2) {
if (!(digVer1 == 0)) {
msgErrForm += "Campo: CPF\n";
errForm = true;
return false;
}
} else {
resto1Dig = 11 - resto1Dig;
if (!(resto1Dig == digVer1)) {
msgErrForm += "Campo: CPF\n";
errForm = true;
return false;
}
}
for(j = 0; j < cnpj.length - 1; j++) {
soma2Dig += cnpj.charAt(j) * numeros2Dig[j];
}
resto2Dig = soma2Dig % 11;
if (resto2Dig < 2) {
if (!(digVer2 == 0)) {
msgErrForm += "Campo: CPF\n";
errForm = true;
return false;
}
} else {
resto2Dig = 11 - resto2Dig;
if (!(resto2Dig == digVer2)) {
msgErrForm += "Campo: CPF\n";
errForm = true;
return false;
}
}
}
function updateDisplayIE() {
var form = document.frmCadCliente;
if (form.hasEstadual[0].checked) {
document.getElementById("inscestadual").style.display = 'block';
return true;
}
if (form.hasEstadual[1].checked) {
document.getElementById("inscestadual").style.display = 'none';
return false;
}
}
function cnpj_format(cnpj) {
var form = document.frmCadCliente;
if (cnpj.value.length == 2 || cnpj.value.length == 6) {
form.txtCNPJ.value += ".";
}
if (cnpj.value.length == 10) {
form.txtCNPJ.value += "/";
}
if (cnpj.value.length == 15) {
form.txtCNPJ.value += "-";
}
}
function cpf_format(cpf) {
var form = document.frmCadCliente;
if (cpf.value.length == 3 || cpf.value.length == 7) {
form.txtCPF.value += ".";
}
if (cpf.value.length == 11) {
form.txtCPF.value += "-";
}
}
function keypressIE(value) {
var form = document.frmCadCliente;
if (value.length == 3 || value.length == 7 || value.length == 11) {
form.txtInscricaoEstadual.value += ".";
}
}
<file_sep>// JavaScript Document
function updateTransp() {
var error = 0;
var valor = null;
// alert(parseInt(document.frmSearchTranspSol.hiddenIntTransp.value + 1));
for (var i=0; i < parseInt(document.frmSearchTranspPontoColeta.hiddenIntTransp.value); i++) {
if (parseInt(document.frmSearchTranspPontoColeta.hiddenIntTransp.value) == 1) {
if (!document.frmSearchTranspPontoColeta.transp.checked) {
error++;
} else {
valor = document.frmSearchTranspPontoColeta.transp.value;
}
} else {
if (!document.frmSearchTranspPontoColeta.transp[i].checked) {
error++;
} else {
valor = document.frmSearchTranspPontoColeta.transp[i].value;
}
}
}
if (error == parseInt(document.frmSearchTranspPontoColeta.hiddenIntTransp.value)) {
alert("Por favor escolha uma transportadora");
return
} else {
window.opener.frmPontoColetaAdm.cbTransp.value = valor;
window.close();
}
}<file_sep>// JavaScript Document
/*
'|--------------------------------------------------------------------
'| Arquivo: frmAddContato.js
'| Autor: <NAME> (<EMAIL>)
'| Data Criação: 13/04/2007
'| Data Modificação : 15/04/2007
'| Descrição: Arquivo de Formulário para cadastro de Contato (Javascript)
'|--------------------------------------------------------------------
*/
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Criação do Objeto Ajax
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function Ajax() {
var ajax = null;
if (window.ActiveXObject) {
try {
ajax = new ActiveXObject("Msxml2.XMLHTTP");
} catch (ex) {
try {
ajax = new ActiveXObject("Microsoft.XMLHTTP");
} catch(ex2) {
alert("Seu browser não suporta Ajax.");
}
}
} else {
if (window.XMLHttpRequest) {
try {
ajax = new XMLHttpRequest();
} catch(ex3) {
alert("Seu browser não suporta Ajax.");
}
}
}
return ajax;
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Validação do Ambiente de Contato com o cliente
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function validaCadClienteContato() {
var form = document.frmAddContato;
if (form.txtContatoColeta.value == "") {
alert("Preencha o campo Contato!");
return false;
}
if (form.txtUsuario.value == "") {
alert("Preencha o campo Usuario!");
return false;
}
if (form.txtUsuario.value.length < 6) {
alert("Preencha o campo Usuário com no mínimo 6 caracteres!");
return false;
}
if (form.txtSenha.value == "") {
alert("Preencha o campo Senha!");
return false;
}
if (form.txtSenha.value.length < 6) {
alert("Preencha o campo Senha com no mínimo 6 caracteres!");
return false;
}
if (!is_email(form.txtEmail.value))
{
alert("Email inválido!");
return false;
}
if (form.txtTelefone.value == "") {
alert("Preenchar o campo telefone!");
return false;
}
if ( isNaN(form.txtTelefone.value) ) {
alert("Preencher o campo telefone somente com números");
return false;
}
if (form.txtRamal.value == "") {
alert("Preenchar o campo Ramal!");
return false;
}
if (form.txtDDD.value == "") {
alert("Preenchar o campo DDD!");
return false;
}
if (isNaN(form.txtDDD.value)) {
alert("Preencher o campo DDD somente com números");
return false;
}
/*
if (form.txtEmail.value.indexOf('@') == -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('@.') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('.@') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('.') == -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('com') == -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('[') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf(']') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('(') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf(')') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('/') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('\\') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('..') != -1) {
alert("Email inválido!");
return false;
}
if (form.txtEmail.value.indexOf('com.com') != -1) {
alert("Email inválido!");
return false;
}
*/
if (!form.radioIsMaster[0].checked && !form.radioIsMaster[1].checked) {
alert("Escolha o Tipo de Usuário (Master)!");
return false;
}
document.frmAddContato.submit();
}
function is_email(email)
{
er = /^[a-zA-Z0-9][a-zA-Z0-9\._-]+@([a-zA-Z0-9\._-]+\.)[a-zA-Z-0-9]{2}/;
if(er.exec(email))
{
return true;
} else {
return false;
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Verifica se o usuário já está cadastrado
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//function checkUserContato() {
// var oAjax = Ajax();
// oAjax.onreadystatechange = function() {
// if (oAjax.readyState == 4 && oAjax.status == 200) {
// if (oAjax.responseText == "true") {
// alert("Usuário já cadastrado. Favor cadastre outro Usuário!");
// } else {
// document.frmAddContato.submit();
// }
// }
// }
//
// oAjax.open("GET", "ajax/frmAddContato.asp?sub=getcheckusercontato&id=0&user="+document.frmAddContato.txtUsuario.value+"&senha="+document.frmAddContato.txtSenha.value, true);
// oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
// oAjax.send(null);
//}
//==========================================================================================================
function redirActionContato() {
var contCheckFalse = 0;
var elemento;
for (var cont=0; cont < document.frmAddContato.hiddenIntContatos.value; cont++) {
if (!document.getElementById("checkContato"+cont).checked) {
contCheckFalse++;
} else {
elemento = document.getElementById("checkContato"+cont);
}
}
if (contCheckFalse == document.frmAddContato.hiddenIntContatos.value && !document.getElementById("checkContato"+document.frmAddContato.hiddenIntContatos.value).checked) {
alert("Escolha um Contato para executar a Ação escolhida!");
return false;
} else {
if (document.getElementById("checkContato"+document.frmAddContato.hiddenIntContatos.value).checked) {
elemento = document.getElementById("checkContato"+document.frmAddContato.hiddenIntContatos.value);
}
if (document.frmAddContato.cbActionContatos.value == 1) {
window.location.href="frmAddContato.asp?Query=UPDATE&ID="+elemento.value
} else if(document.frmAddContato.cbActionContatos.value == 2) {
window.location.href="frmAddContato.asp?Query=DELETE&ID="+elemento.value
}
}
}
<file_sep>$(function() {
// carousel play pause //
$('#main_carousel').carousel({
interval : 4000,
pause : "false"
});
$('#carouselButton').on('click', function() {
if ($(this).hasClass("pause")) {
$('#main_carousel').carousel('pause');
$(this).removeClass("pause");
$(this).addClass("play");
} else {
$('#main_carousel').carousel('cycle');
$(this).removeClass("play");
$(this).addClass("pause");
}
});
// campanyText //
$("[class^=companyImg]").on('mouseenter', function(){
var $discription = $(this).find("[class^=contentsDescription]")
if (touchDevice()) {
setTimeout(function(){
$discription.show();
}, 300);
} else {
$discription.show();
}
});
$("[class^=companyImg]").on('mouseleave', function(){
var $discription = $(this).find("[class^=contentsDescription]")
$discription.hide();
});
var touchDevice = function(){
var agent = navigator.userAgent;
if(agent.search(/iPhone/) != -1 || agent.search(/iPad/) != -1 || agent.search(/iPod/) != -1 || agent.search(/Android/) != -1){
return true;
}
};
});
<file_sep>using System;
using System.Collections.Generic;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
using System.IO;
public partial class rptcoletas : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
String sid = Request.QueryString["id"];
String sgrupo = Request.QueryString["grupo"];
String sdataini = Request.QueryString["dataini"];
String sdatafinal = Request.QueryString["datafinal"];
String sstatus = Request.QueryString["status"];
this.ExportToExcel(sid, sgrupo, sdataini, sdatafinal, sstatus);
}
/// <summary>
/// faz a conexão com o banco de dados sql serve conforme script wen.config.
/// </summary>
/// <returns></returns>
private static SqlConnection connection()
{
try
{
string sconexao = ConfigurationSettings.AppSettings["conexaoSQL"];
SqlConnection sqlconnection = new SqlConnection(sconexao);
//Verifica se a conexão esta fechada.
if (sqlconnection.State == ConnectionState.Closed)
{
//Abri a conexão.
sqlconnection.Open();
}
//Retorna o sqlconnection.
return sqlconnection;
}
catch (SqlException ex)
{
throw ex;
}
}
/// <summary>
/// GetData abre a conexão com o SQlServer, faz o Select e retorna um Dt
/// </summary>
/// <param name="cmd"></param>
/// <returns></returns>
private DataTable GetData(SqlCommand cmd)
{
DataTable dt = new DataTable();
String strConnString = ConfigurationSettings.AppSettings["conexaoSQL"];
SqlConnection con = new SqlConnection(strConnString);
SqlDataAdapter sda = new SqlDataAdapter();
cmd.CommandType = CommandType.Text;
cmd.Connection = con;
try
{
con.Open();
sda.SelectCommand = cmd;
sda.Fill(dt);
return dt;
}
catch (Exception ex)
{
throw ex;
}
finally
{
con.Close();
sda.Dispose();
con.Dispose();
}
}
/// <summary>
/// Exporta os dados da query para o excel
/// </summary>
private void ExportToExcel(string sid, string sgrupo, string sdataini, string sdatafinal, string sstatus)
{
string strQuery = "SELECT ";
strQuery += " convert( nvarchar(10), A.[data_solicitacao], 103), A.[numero_solicitacao_coleta], C.[idClientes], C.[razao_social], C.[nome_fantasia], A.[qtd_cartuchos], convert( nvarchar(10), A.[data_programada],103) , ";
strQuery += " D.[status_coleta] ";
strQuery += "FROM [marketingoki2].[dbo].[Solicitacao_coleta] AS A ";
strQuery += "LEFT JOIN [marketingoki2].[dbo].[Status_coleta] as D on D.[idStatus_coleta] = A.[Status_coleta_idStatus_coleta] ";
strQuery += "LEFT JOIN [marketingoki2].[dbo].[Solicitacao_coleta_has_Clientes] AS B ";
strQuery += "ON A.[idSolicitacao_coleta] = B.[Solicitacao_coleta_idSolicitacao_coleta] ";
strQuery += "LEFT JOIN [marketingoki2].[dbo].[Clientes] AS C ";
strQuery += "ON B.[Clientes_idClientes] = C.[idClientes] where A.[isMaster] = 0 ";
strQuery += " AND C.[idClientes] = " + sid+ "";
if ( !String.IsNullOrEmpty(sstatus.ToString()) && Convert.ToInt16(sstatus.ToString()) != 0 )
{
strQuery += " AND A.[Status_coleta_idStatus_coleta] = " + sstatus + "";
}
if (!String.IsNullOrEmpty(sdataini.ToString()) && String.IsNullOrEmpty(sdatafinal.ToString()))
{
strQuery += " AND A.[data_solicitacao] between convert(datetime, '" + sdataini + " 00:01') and convert(datetime, '" + sdataini + " 23:59')";
}
else if (!String.IsNullOrEmpty(sdataini.ToString()) && !String.IsNullOrEmpty(sdatafinal.ToString()))
{
strQuery += " AND A.[data_solicitacao] between convert(datetime, '" + sdataini + " 00:01') and convert(datetime, '" + sdatafinal + " 23:59')";
}
else if (String.IsNullOrEmpty(sdataini.ToString()) && !String.IsNullOrEmpty(sdatafinal.ToString()))
{
strQuery += " AND A.[data_solicitacao] between convert(datetime, '" + sdatafinal + " 00:01') and convert(datetime, '" + sdatafinal + " 23:59')";
}
/*
strQuery += " A.[idSolicitacao_coleta], A.[Status_coleta_idStatus_coleta], A.[numero_solicitacao_coleta], A.[qtd_cartuchos], A.[qtd_cartuchos_recebidos], A.[data_solicitacao] ";
strQuery += ",A.[data_aprovacao], A.[data_envio_transportadora], A.[data_entrega_pontocoleta], A.[data_recebimento], A.[motivo_status], A.[isMaster], B.[Solicitacao_coleta_idSolicitacao_coleta] ";
strQuery += ",B.[typeColect], B.[Pontos_coleta_idPontos_coleta], B.[Contatos_idContatos], B.[Clientes_idClientes], B.[cep_coleta], B.[logradouro_coleta] ";
strQuery += ",B.[bairro_coleta], B.[numero_endereco_coleta], B.[comp_endereco_coleta], B.[municipio_coleta], B.[estado_coleta], B.[ddd_resp_coleta] ";
strQuery += ",B.[telefone_resp_coleta], B.[contato_coleta], C.[idClientes], C.[Grupos_idGrupos], C.[Categorias_idCategorias], C.[razao_social] ";
strQuery += ",C.[nome_fantasia], C.[cnpj], C.[inscricao_estadual], C.[ddd], C.[telefone], C.[compl_endereco], C.[compl_endereco_coleta], C.[numero_endereco] ";
strQuery += ",C.[numero_endereco_coleta], C.[contato_respcoleta], C.[ddd_respcoleta], C.[telefone_respcoleta], C.[numero_sequencial], C.[data_atualizacao_sequencial] ";
strQuery += ",C.[minCartuchos], C.[typeColect], C.[status_cliente], C.[motivo_status], C.[bonus_type], C.[Transportadoras_idTransportadoras] ";
strQuery += ",C.[tipopessoa], C.[cod_cli_consolidador], C.[cod_bonus_cli], A.[data_programada] ";
strQuery += "FROM [marketingoki2].[dbo].[Solicitacao_coleta] AS A ";
strQuery += "LEFT JOIN [marketingoki2].[dbo].[Solicitacao_coleta_has_Clientes] AS B ";
strQuery += "ON A.[idSolicitacao_coleta] = B.[Solicitacao_coleta_idSolicitacao_coleta] ";
strQuery += "LEFT JOIN [marketingoki2].[dbo].[Clientes] AS C ";
strQuery += "ON B.[Clientes_idClientes] = C.[idClientes] where A.[isMaster] = 0 ";
*/
SqlCommand cmd = new SqlCommand(strQuery);
DataTable dt = GetData(cmd);
//Create a dummy GridView
GridView GridView1 = new GridView();
GridView1.AllowPaging = false;
GridView1.DataSource = dt;
GridView1.DataBind();
Response.Clear();
Response.Buffer = true;
Response.AddHeader("content-disposition","attachment;filename=DataTable.xls");
Response.Charset = "";
Response.ContentType = "application/vnd.ms-excel";
StringWriter sw = new StringWriter();
HtmlTextWriter hw = new HtmlTextWriter(sw);
for (int i = 0; i < GridView1.Rows.Count; i++)
{
//Apply text style to each Row
GridView1.Rows[i].Attributes.Add("class", "textmode");
}
GridView1.RenderControl(hw);
//style to format numbers to string
string style = @"<style> .textmode { mso-number-format:\@; } </style>";
Response.Write(style);
Response.Output.Write(sw.ToString());
Response.Flush();
Response.End();
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
using System.IO;
public partial class rptcoletascli : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
String sid = Request.QueryString["id"];
String sgrupo = Request.QueryString["grupo"];
String sdataini = Request.QueryString["dataini"];
String sdatafinal = Request.QueryString["datafinal"];
String sstatus = Request.QueryString["status"];
String sql = Request.QueryString["query"];
this.ExportToExcel(sid, sgrupo, sdataini, sdatafinal, sstatus, sql);
}
/// <summary>
/// faz a conexão com o banco de dados sql serve conforme script wen.config.
/// </summary>
/// <returns></returns>
private static SqlConnection connection()
{
try
{
string sconexao = ConfigurationSettings.AppSettings["conexaoSQL"];
SqlConnection sqlconnection = new SqlConnection(sconexao);
//Verifica se a conexão esta fechada.
if (sqlconnection.State == ConnectionState.Closed)
{
//Abri a conexão.
sqlconnection.Open();
}
//Retorna o sqlconnection.
return sqlconnection;
}
catch (SqlException ex)
{
throw ex;
}
}
/// <summary>
/// GetData abre a conexão com o SQlServer, faz o Select e retorna um Dt
/// </summary>
/// <param name="cmd"></param>
/// <returns></returns>
private DataTable GetData(SqlCommand cmd)
{
DataTable dt = new DataTable();
String strConnString = ConfigurationSettings.AppSettings["conexaoSQL"];
SqlConnection con = new SqlConnection(strConnString);
SqlDataAdapter sda = new SqlDataAdapter();
cmd.CommandType = CommandType.Text;
cmd.Connection = con;
try
{
con.Open();
sda.SelectCommand = cmd;
sda.Fill(dt);
return dt;
}
catch (Exception ex)
{
Response.Write(ex);
}
finally
{
con.Close();
sda.Dispose();
con.Dispose();
}
return dt;
}
/// <summary>
/// Exporta os dados da query para o excel
/// </summary>
private void ExportToExcel(string sid, string sgrupo, string sdataini, string sdatafinal, string sstatus,string sql)
{
string strQuery = sql;
SqlCommand cmd = new SqlCommand(strQuery);
DataTable dt = GetData(cmd);
//Create a dummy GridView
GridView GridView1 = new GridView();
GridView1.AllowPaging = false;
GridView1.DataSource = dt;
GridView1.DataBind();
Response.Clear();
Response.Buffer = true;
Response.AddHeader("content-disposition","attachment;filename=DataTable.xls");
Response.Charset = "";
Response.ContentType = "application/vnd.ms-excel";
StringWriter sw = new StringWriter();
HtmlTextWriter hw = new HtmlTextWriter(sw);
for (int i = 0; i < GridView1.Rows.Count; i++)
{
//Apply text style to each Row
GridView1.Rows[i].Attributes.Add("class", "textmode");
}
GridView1.RenderControl(hw);
//style to format numbers to string
string style = @"<style> .textmode { mso-number-format:\@; } </style>";
Response.Write(style);
Response.Output.Write(sw.ToString());
Response.Flush();
Response.End();
}
}<file_sep>// JavaScript Document
function validate() {
var form = document.frmCategoriasAdm;
if (form.txtDescricao.value == "") {
alert("Preencha o campo Descrição da Categoria!");
return;
}
if (form.txtQtdMinima.value == "") {
alert("Preencha o campo Quantidade Mínima!");
return;
}
if (isNaN(form.txtQtdMinima.value)) {
alert("O campo Quantidade Mínima só aceita dados numéricos!");
return;
}
form.submit();
}<file_sep>function tipopessoa() {
return;
}
function windowLocationFind(url) {
var find = true;
var busca = document.getElementsByName("txtFindCliente")[0].value;
var por = document.getElementsByName("typeFindCliente")[0].value;
var status = document.getElementsByName("cbStatusFindCliente")[0].value;
var categoria = document.getElementsByName("cbCategoriasFindCliente")[0].value;
//var grupos = document.frmEditCadastroClienteLc.grupos.value;
//window.location.href = url + "frmEditCadastroClienteLc.asp?find=true&search="+busca+"&changetype="+por+"&status="+status+"&categoria="+categoria;
window.location.href = url + "../adm/frmCadastroClienteAdm.asp?find=true&search="+busca+"&changetype="+por+"&status="+status+"&categoria="+categoria;
}
function aprovar() {
if (document.frmEditCadastroClienteLc.hiddenActionIsColetaDomiciliar.value == 1)
{
//if (document.frmEditCadastroClienteLc.cbTransp.value == "-1")
//{
// alert("Selecione uma Transportadora!");
// return;
//}
}
document.frmEditCadastroClienteLc.hiddenActionManagerProve.value = 'true';
document.frmEditCadastroClienteLc.hiddenActionForm.value = "APROVAR";
document.frmEditCadastroClienteLc.submit();
}
function reprovar() {
document.frmEditCadastroClienteLc.hiddenActionManagerProve.value = 'false';
document.frmEditCadastroClienteLc.hiddenActionForm.value = "REPROVAR";
document.frmEditCadastroClienteLc.submit();
}
function validar() {
document.getElementsByName("hiddenActionForm")[0].value = "SALVAR";
document.frmEditCadastroClienteLc.submit();
}
function validaCnpj() {
var numeros1Dig = new Array(5,4,3,2,9,8,7,6,5,4,3,2);
var soma1Dig = 0;
var resto1Dig = 0;
var digVer1 = 0;
var numeros2Dig = new Array(6,5,4,3,2,9,8,7,6,5,4,3,2);
var soma2Dig = 0;
var resto2Dig = 0;
var digVer2 = 0;
var i = 0;
var j = 0;
var cnpj = "";
cnpj = document.frmEditCadastroClienteLc.txtCNPJCliente.value;
digVer2 = cnpj.charAt(cnpj.length - 1);
digVer1 = cnpj.charAt(cnpj.length - 2);
if (document.frmEditCadastroClienteLc.txtCNPJCliente.value.indexOf('/') == -1) {
alert("Preencha corretamente o campo CNPJ erro:215");
return;
}
if (document.frmEditCadastroClienteLc.txtCNPJCliente.value.length < 18) {
alert("Preencha corretamente o campo CNPJ erro:219");
return;
}
cnpj = cnpj.replace('/','');
cnpj = cnpj.replace('-','');
cnpj = cnpj.replace('.','');
cnpj = cnpj.replace('.','');
for(i = 0; i < cnpj.length - 2; i++) {
if (!isNaN(cnpj.charAt(i)) && !isNaN(numeros1Dig[i])) {
soma1Dig += cnpj.charAt(i) * numeros1Dig[i];
}
}
resto1Dig = soma1Dig % 11;
if (resto1Dig < 2) {
if (!(digVer1 == 0)) {
alert("Preencha corretamente o campo CNPJ erro:234");
return;
}
} else {
resto1Dig = 11 - resto1Dig;
if (!(resto1Dig == digVer1)) {
alert("Preencha corretamente o campo CNPJ erro:240");
return;
}
}
for(j = 0; j < cnpj.length - 1; j++) {
soma2Dig += cnpj.charAt(j) * numeros2Dig[j];
}
resto2Dig = soma2Dig % 11;
if (resto2Dig < 2) {
if (!(digVer2 == 0)) {
alert("Preencha corretamente o campo CNPJ erro:250");
return;
}
} else {
resto2Dig = 11 - resto2Dig;
if (!(resto2Dig == digVer2)) {
alert("Preencha corretamente o campo CNPJ eroo:256");
return;
}
}
}
function Ajax() {
var ajax = null;
if (window.ActiveXObject) {
try {
ajax = new ActiveXObject("Msxml2.XMLHTTP");
} catch (ex) {
try {
ajax = new ActiveXObject("Microsoft.XMLHTTP");
} catch(ex2) {
alert("Seu browser não suporta Ajax.");
}
}
} else {
if (window.XMLHttpRequest) {
try {
ajax = new XMLHttpRequest();
} catch(ex3) {
alert("Seu browser não suporta Ajax.");
}
}
}
return ajax;
}
function getEndereco() {
var oAjax = Ajax();
var form = document.frmEditCadastroClienteLc;
var strRet = "";
form.txtLogradouro.value = "Carregando...";
form.txtBairro.value = "Carregando...";
form.txtMunicipio.value = "Carregando...";
form.txtEstado.value = "Carregando...";
form.txtCompLogradouro.value = "";
form.txtNumero.value = "";
document.body.style.cursor = 'wait';
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
strRet = oAjax.responseText.split(";");
strRet[6] = strRet[6].replace(" ", '');
form.txtLogradouro.value = strRet[6] + "" + strRet[2];
form.txtBairro.value = strRet[3];
form.txtMunicipio.value = strRet[4];
form.txtEstado.value = strRet[5];
form.txtLogradouro.value = strRet[2];
document.body.style.cursor = 'default';
}
}
oAjax.open("GET", "../ajax/frmCadCliente.asp?sub=getcependereco&id="+form.txtCEPEnderecoComumCliente.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
return;
}
function getEnderecoColeta() {
var oAjax = Ajax();
var form = document.frmEditCadastroClienteLc;
var strRet = "";
form.txtLogradouroColeta.value = "Carregando...";
form.txtBairroColeta.value = "Carregando...";
form.txtMunicipioColeta.value = "Carregando...";
form.txtEstadoColeta.value = "Carregando...";
form.txtCompLogradouroColeta.value = "";
form.txtNumeroColeta.value = "";
document.body.style.cursor = 'wait';
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
strRet = oAjax.responseText.split(";");
strRet[6] = strRet[6].replace(" ",'');
form.txtLogradouroColeta.value = strRet[6] + ". " + strRet[2];
form.txtBairroColeta.value = strRet[3];
form.txtMunicipioColeta.value = strRet[4];
form.txtEstadoColeta.value = strRet[5];
document.body.style.cursor = 'default';
}
}
oAjax.open("GET", "../ajax/frmCadCliente.asp?sub=getcependereco&id="+form.txtCEPEnderecoComumClienteColeta.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
function validaCPF() {
var numeros1Dig = new Array(10,9,8,7,6,5,4,3,2);
var soma1Dig = 0;
var resto1Dig = 0;
var digVer1 = 0;
var numeros2Dig = new Array(11,10,9,8,7,6,5,4,3,2);
var soma2Dig = 0;
var resto2Dig = 0;
var digVer2 = 0;
var i = 0;
var j = 0;
var cnpj = "";
cnpj = document.frmEditCadastroClienteLc.txtCPFCliente.value;
digVer2 = cnpj.charAt(cnpj.length - 1);
digVer1 = cnpj.charAt(cnpj.length - 2);
if (document.frmEditCadastroClienteLc.txtCPFCliente.value == "") {
alert("Preencha o campo CPF!");
return;
}
if (document.frmEditCadastroClienteLc.txtCPFCliente.value.length < 14) {
alert("Preencha o campo CPF corretamente!");
return;
}
cnpj = cnpj.replace('-','');
cnpj = cnpj.replace('.','');
cnpj = cnpj.replace('.','');
for(i = 0; i < cnpj.length - 2; i++) {
if (!isNaN(cnpj.charAt(i)) && !isNaN(numeros1Dig[i])) {
soma1Dig += cnpj.charAt(i) * numeros1Dig[i];
}
}
resto1Dig = soma1Dig % 11;
if (resto1Dig < 2) {
if (!(digVer1 == 0)) {
alert("Preencha o campo CPF corretamente!");
return;
}
} else {
resto1Dig = 11 - resto1Dig;
if (!(resto1Dig == digVer1)) {
alert("Preencha o campo CPF corretamente!");
return;
}
}
for(j = 0; j < cnpj.length - 1; j++) {
soma2Dig += cnpj.charAt(j) * numeros2Dig[j];
}
resto2Dig = soma2Dig % 11;
if (resto2Dig < 2) {
if (!(digVer2 == 0)) {
alert("Preencha o campo CPF corretamente!");
return;
}
} else {
resto2Dig = 11 - resto2Dig;
if (!(resto2Dig == digVer2)) {
alert("Preencha o campo CPF corretamente!");
return;
}
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
using System.IO;
using System.Text;
using System.Text.RegularExpressions;
public partial class rpttoexcel : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
String sid = Request.QueryString["id"];
String sgrupo = Request.QueryString["grupo"];
String sdataini = Request.QueryString["dataini"];
String sdatafinal = Request.QueryString["datafinal"];
String sstatus = Request.QueryString["status"];
String sql = Request.QueryString["query"];
//this.ExportToExcel(sid, sgrupo, sdataini, sdatafinal, sstatus, sql);
ExportToExcel2(sid, sgrupo, sdataini, sdatafinal, sstatus, sql);
}
/// <summary>
/// faz a conexão com o banco de dados sql serve conforme script wen.config.
/// </summary>
/// <returns></returns>
private static SqlConnection connection()
{
try
{
string sconexao = ConfigurationSettings.AppSettings["conexaoSQL"];
SqlConnection sqlconnection = new SqlConnection(sconexao);
//Verifica se a conexão esta fechada.
if (sqlconnection.State == ConnectionState.Closed)
{
//Abri a conexão.
sqlconnection.Open();
}
//Retorna o sqlconnection.
return sqlconnection;
}
catch (SqlException ex)
{
throw ex;
}
}
/// <summary>
/// GetData abre a conexão com o SQlServer, faz o Select e retorna um Dt
/// </summary>
/// <param name="cmd"></param>
/// <returns></returns>
private DataTable GetData(SqlCommand cmd)
{
DataTable dt = new DataTable();
String strConnString = ConfigurationSettings.AppSettings["conexaoSQL"];
SqlConnection con = new SqlConnection(strConnString);
SqlDataAdapter sda = new SqlDataAdapter();
cmd.CommandType = CommandType.Text;
cmd.Connection = con;
try
{
con.Open();
sda.SelectCommand = cmd;
sda.Fill(dt);
return dt;
}
catch (Exception ex)
{
Response.Write(ex);
}
finally
{
con.Close();
sda.Dispose();
con.Dispose();
}
return dt;
}
/// <summary>
/// Exporta os dados da query para o excel
/// </summary>
private void ExportToExcel(string sid, string sgrupo, string sdataini, string sdatafinal, string sstatus,string sql)
{
string strQuery = sql;
SqlCommand cmd = new SqlCommand(strQuery);
DataTable dt = GetData(cmd);
//Create a dummy GridView
GridView GridView1 = new GridView();
GridView1.AllowPaging = false;
GridView1.DataSource = dt;
GridView1.DataBind();
Response.Clear();
Response.Buffer = true;
Response.AddHeader("content-disposition", "attachment;filename=DataTable.xls");
Response.Charset = "";
Response.ContentType = "application/vnd.ms-excel";
StringWriter sw = new StringWriter();
HtmlTextWriter hw = new HtmlTextWriter(sw);
for (int i = 0; i < GridView1.Rows.Count; i++)
{
//Apply text style to each Row
GridView1.Rows[i].Attributes.Add("class", "textmode");
}
GridView1.RenderControl(hw);
//style to format numbers to string
string style = @"<style> .textmode { mso-number-format:\@; } </style>";
Response.Write(style);
Response.Output.Write(sw.ToString());
Response.Flush();
Response.End();
}
public void ExportToExcel2(string sid, string sgrupo, string sdataini, string sdatafinal, string sstatus, string sql)
{
string strQuery = sql;
SqlCommand cmd = new SqlCommand(strQuery);
DataTable dt = GetData(cmd);
string str = GetTableContent(dt);
byte[] bytes = new byte[str.Length * sizeof(char)];
System.Buffer.BlockCopy(str.ToCharArray(), 0, bytes, 0, bytes.Length);
try
{
Response.Clear();
Response.AddHeader("Content-Disposition", "attachment;filename=\"DataTable.csv\"");
Response.AddHeader("Content-Length", bytes.Length.ToString());
Response.ContentType = "application/octet-stream";
Response.BinaryWrite(bytes);
Response.Flush();
}
catch (Exception ex)
{
Response.ContentType = "application/vnd.ms-excel";
Response.Write(ex.Message);
}
finally
{
Response.End();
}
}
private string GetTableContent (DataTable dt)
{
string rw = "";
StringBuilder builder = new StringBuilder();
for (int a = 0; a < dt.Columns.Count; a++)
{
rw = GetStringNoAccents(dt.Columns[a].ToString());
if (rw.Contains(";")) rw = "\"" + rw + "\"";
builder.Append(rw + ";");
}
builder.Append(Environment.NewLine);
foreach(DataRow dr in dt.Rows)
{
for(int i = 0; i < dt.Columns.Count; i++)
{
rw = dr[i].ToString();
if (rw.Contains(";")) rw = "\"" + rw + "\"";
builder.Append(rw + ";");
}
builder.Append(Environment.NewLine);
}
return builder.ToString();
}
public static string GetStringNoAccents(string str)
{
/** Troca os caracteres acentuados por não acentuados **/
string[] acentos = new string[] { "ç", "Ç", "á", "é", "í", "ó", "ú", "ý", "Á", "É", "Í", "Ó", "Ú", "Ý", "à", "è", "ì", "ò", "ù", "À", "È", "Ì", "Ò", "Ù", "ã", "õ", "ñ", "ä", "ë", "ï", "ö", "ü", "ÿ", "Ä", "Ë", "Ï", "Ö", "Ü", "Ã", "Õ", "Ñ", "â", "ê", "î", "ô", "û", "Â", "Ê", "Î", "Ô", "Û" };
string[] semAcento = new string[] { "c", "C", "a", "e", "i", "o", "u", "y", "A", "E", "I", "O", "U", "Y", "a", "e", "i", "o", "u", "A", "E", "I", "O", "U", "a", "o", "n", "a", "e", "i", "o", "u", "y", "A", "E", "I", "O", "U", "A", "O", "N", "a", "e", "i", "o", "u", "A", "E", "I", "O", "U" };
for (int i = 0; i < acentos.Length; i++)
{
str = str.Replace(acentos[i], semAcento[i]);
}
return str;
//string pattern = @"(?i)[^0-9a-záéíóúàèìòùâêîôûãõç\s]";
//string replacement = "";
//Regex rgx = new Regex(pattern);
//return rgx.Replace(str, replacement);
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Net.Mail;
using System.Net;
using System.Text;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
public partial class _Default : System.Web.UI.Page
{
private static SqlConnection connection()
{
try
{
string sconexao = ConfigurationSettings.AppSettings["conexaoSQL"];
SqlConnection sqlconnection = new SqlConnection(sconexao);
//Verifica se a conexão esta fechada.
if (sqlconnection.State == ConnectionState.Closed)
{
//Abri a conexão.
sqlconnection.Open();
}
//Retorna o sqlconnection.
return sqlconnection;
}
catch (SqlException ex)
{
throw ex;
}
}
/// <summary>
/// Método que retorna um datareader com o resultado da query.
/// </summary>
/// <param name="query"></param>
/// <returns></returns>
public string retornaQuery(string query)
{
try
{
//Instância o sqlcommand com a query sql que será executada e a conexão.
SqlCommand comando = new SqlCommand(query, connection());
//Executa a query sql.
SqlDataReader retornaQuery = comando.ExecuteReader();
if (retornaQuery.Read())
{
connection().Close();
string sretorno = retornaQuery[0].ToString();
return sretorno;
}
else
{
connection().Close();
return "conteudo_embranco";
}
}
catch (SqlException ex)
{
throw ex;
}
}
public string retornaQuery2(string query)
{
try
{
//Instância o sqlcommand com a query sql que será executada e a conexão.
SqlCommand comando = new SqlCommand(query, connection());
//Executa a query sql.
SqlDataReader retornaQuery2 = comando.ExecuteReader();
if (retornaQuery2.Read())
{
connection().Close();
string sretorno2 = retornaQuery2[1].ToString();
return sretorno2;
}
else
{
connection().Close();
return "conteudo_embranco";
}
}
catch (SqlException ex)
{
throw ex;
}
}
public DataSet retornaQueryDataSet(string query)
{
try
{
//Instância o sqlcommand com a query sql que será executada e a conexão.
SqlCommand comando = new SqlCommand(query, connection());
//Instância o sqldataAdapter.
SqlDataAdapter adapter = new SqlDataAdapter(comando);
//Instância o dataSet de retorno.
DataSet dataSet = new DataSet();
//Atualiza o dataSet
adapter.Fill(dataSet);
//Retorna o dataSet com o resultado da query sql.
return dataSet;
}
catch (Exception ex)
{
throw ex;
}
}
protected void Page_Load(object sender, EventArgs e)
{
if (Page.IsPostBack)
{
return;
}
}
protected void Button1_Click(object sender, EventArgs e)
{
string suser;
string ssenha;
string squery = "select usuario,senha from [marketingoki2].[dbo].[Contatos] where email = '" + txtBemail.Text.ToLower() + "' and status_contato = 1 ";
suser = retornaQuery(squery);
ssenha = retornaQuery2(squery);
if (IsPostBack && !string.IsNullOrEmpty(txtBemail.Text))
{
//crio objeto responsável pela mensagem de email
MailMessage objEmail = new MailMessage();
objEmail.From = new MailAddress("<EMAIL>");
objEmail.To.Add(txtBemail.Text.ToLower());
//objEmail.CC.Add("<EMAIL>");
objEmail.IsBodyHtml = true;
objEmail.Subject = "OKIDATA - Recuperamos sua senha";
String cBody = "";
cBody += "<!DOCTYPE html>";
cBody += "<html>";
cBody += "<head>";
cBody += "<link rel='stylesheet' type='text/css' href='css/geral.css'>";
cBody += "<title>Recuperar Senha</title>";
cBody += "<meta http-equiv='Content-Type' content='text/html; charset=iso-8859-1'>";
cBody += "</head>";
cBody += "<table cellspacing='0' cellpadding='0' width='775'>";
cBody += " <tr>";
cBody += " <td width='11' background='img/Bg_LatEsq.gif'> </td>";
cBody += "";
cBody += " <td id='conteudo'>";
cBody += " <table width='100%' cellspacing='0' cellpadding='0'>";
cBody += " <tr>";
cBody += " <td width='100%'>";
cBody += " <img src='http://www.sustentabilidadeoki.com.br/banner_oki.png' />";
cBody += " </p>";
cBody += " <font face='Verdana, Arial' color='black' size='4'>";
cBody += " Solicitação de Recuperação de senha</p></Font>";
cBody += " <tr><td>";
cBody += " <font face='Verdana, Arial' color='black' size='2'> Estamos reenviando sua senha do portal Sustentabilidade OKI:<br></td></tr>";
cBody += " </td>";
cBody += " </tr>";
cBody += " <tr>";
cBody += " <td width='100%'> <font face='Verdana, Arial' color='black' size='1'><b>Usuário: " + suser + "<b></font><br>";
cBody += " </td>";
cBody += " </tr>";
cBody += " <tr>";
cBody += " <td width='100%'> <font face='Verdana, Arial' color='black' size='1'><b>Senha: " + ssenha + "<b></font><br>";
cBody += " </td>";
cBody += " </tr>";
cBody += " <tr>";
cBody += " <t;d width='200'> <br>";
cBody += " </td>";
cBody += " </tr> ";
cBody += " </table>";
cBody += " </td>";
cBody += " <td width='11' background='img/Bg_LatDir.gif'> </td>";
cBody += " </tr>";
cBody += " </table>";
cBody += "</html>";
objEmail.Body = cBody;
objEmail.BodyEncoding = Encoding.GetEncoding("ISO-8859-1");
SmtpClient objSmtp = new SmtpClient();
objSmtp.Host = "smtp.sustentabilidadeoki.com.br"; //"mail.okidata.com.br";
objSmtp.Credentials = new NetworkCredential("<EMAIL>", "Oki!321!"); //("<EMAIL>", "!nfe321!");
//objSmtp.Credentials = new NetworkCredential("<EMAIL>", "Oki!321!");
objSmtp.Port = 587;
objSmtp.Send(objEmail);
Response.Redirect("frmlogincliente.asp");
}
else
{
if (string.IsNullOrEmpty(txtBemail.Text))
{
}
}
}
protected void TextBox1_TextChanged(object sender, EventArgs e)
{
}
}<file_sep>// JavaScript Document
function validate() {
var form = document.frmGrupoProdutosAdm;
if (form.txtDesc.value == "") {
alert("Preencha o campo Descrição!");
return;
}
form.submit();
}
function showOnClick() {
var form = document.frmGrupoProdutosAdm;
var bChecked = false;
var cont = 0;
for (var i=0; i < parseInt(form.hiddenIntProdutos.value); i++) {
if (parseInt(form.hiddenIntProdutos.value) == 1) {
if (form.radioIntProduto.checked) {cont = i;bChecked = true;}
} else {
if (form.radioIntProduto[i].checked) {cont = i;bChecked = true;}
}
}
(bChecked)?document.getElementById("cbgrupos").style.display = "block":document.getElementById("cbgrupos").style.display = "none";
}
function validateChangeListener() {
document.frmGrupoProdutosAdm.action.value = "updategroup";
document.frmGrupoProdutosAdm.submit();
}<file_sep>// JavaScript Document
/*
'|--------------------------------------------------------------------
'| Arquivo: frmEditaCadastroCliente.js
'| Autor: <NAME> (<EMAIL>)
'| Data Criação: 13/04/2007
'| Data Modificação: 15/04/2007
'| Descrição: Arquivo de Formulário para UPDATE do Cliente (Javascript)
'|--------------------------------------------------------------------
*/
var errForm = false;
var msgErrForm = "Os seguintes campos foram preenchidos incorretamente!\n";
function showClienteEndColeta() {
var form = document.frmEditaCadastroCliente;
if (form.txtColetaCep.value == "") {
msgErrForm += "CEP Coleta inválido\n";
errForm = true;
}
if (isNaN(form.txtColetaCep.value)) {
msgErrForm += "CEP de Coleta deve ter somente números\n";
errForm = true;
}
if (form.txtColetaCep.value.length < 8) {
msgErrForm += "CEP de Coleta deve ter 8 digitos\n";
errForm = true;
}
if (!errForm) {
preencheEndColetaToCep();
} else {
alert(msgErrForm);
msgErrForm = "Os seguintes campos foram preenchidos incorretamente!\n";
errForm = false;
return false;
}
errForm = false;
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Busca do Endereço para Preenchimento automático do Endereço de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function preencheEndColetaToCep() {
var oAjax = Ajax();
var form = document.frmEditaCadastroCliente;
var strRet = "";
document.getElementById("loadingdisplay").style.display = 'block';
form.txtLogradouroColeta.value = "Loading...";
form.txtBairroColeta.value = "Loading...";
form.txtMunicipioColeta.value = "Loading...";
form.txtEstadoColeta.value = "Loading...";
form.txtCompLogradouroColeta.value = "";
form.txtNumeroColeta.value = "";
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
document.getElementById("loadingdisplay").style.display = 'none';
strRet = oAjax.responseText.split(";");
strRet[6] = strRet[6].replace(" ",'');
form.txtLogradouroColeta.value = strRet[6] + ". " + strRet[2];
form.txtBairroColeta.value = strRet[3];
form.txtMunicipioColeta.value = strRet[4];
form.txtEstadoColeta.value = strRet[5];
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getcependereco&id="+form.txtColetaCep.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
function usaMesmoEndereco() {
var form = document.frmEditaCadastroCliente;
var bReadOnly = true;
if (document.frmEditaCadastroCliente.chkMesmoEndereco.checked == 1){
bReadOnly = true;
}
else {
bReadOnly = false;
}
document.frmEditaCadastroCliente.txtColetaCep.readOnly = bReadOnly;
document.frmEditaCadastroCliente.txtLogradouroColeta.readOnly = bReadOnly;
document.frmEditaCadastroCliente.txtCompLogradouroColeta.readOnly = bReadOnly;
document.frmEditaCadastroCliente.txtNumeroColeta.readOnly = bReadOnly;
document.frmEditaCadastroCliente.txtBairroColeta.readOnly = bReadOnly;
document.frmEditaCadastroCliente.txtMunicipioColeta.readOnly = bReadOnly;
document.frmEditaCadastroCliente.txtEstadoColeta.readOnly = bReadOnly;
if (bReadOnly == true) {
document.frmEditaCadastroCliente.txtColetaCep.value = document.frmEditaCadastroCliente.txtCep.value;
document.frmEditaCadastroCliente.txtLogradouroColeta.value = document.frmEditaCadastroCliente.txtLogradouro.value;
document.frmEditaCadastroCliente.txtCompLogradouroColeta.value = document.frmEditaCadastroCliente.txtCompLogradouro.value;
document.frmEditaCadastroCliente.txtNumeroColeta.value = document.frmEditaCadastroCliente.txtNumero.value;
document.frmEditaCadastroCliente.txtBairroColeta.value = document.frmEditaCadastroCliente.txtBairro.value;
document.frmEditaCadastroCliente.txtMunicipioColeta.value = document.frmEditaCadastroCliente.txtMunicipio.value;
document.frmEditaCadastroCliente.txtEstadoColeta.value = document.frmEditaCadastroCliente.txtEstado.value;
}
else {
document.frmEditaCadastroCliente.txtColetaCep.value = "";
document.frmEditaCadastroCliente.txtLogradouroColeta.value = "";
document.frmEditaCadastroCliente.txtCompLogradouroColeta.value = "";
document.frmEditaCadastroCliente.txtNumeroColeta.value = "";
document.frmEditaCadastroCliente.txtBairroColeta.value = "";
document.frmEditaCadastroCliente.txtMunicipioColeta.value = "";
document.frmEditaCadastroCliente.txtEstadoColeta.value = "";
}
}<file_sep>using System;
using System.Collections.Generic;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.Data.SqlClient;
using System.Configuration;
using System.IO;
public partial class Adm_rptcoletasaprovadas : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
String sfiltro = Request.QueryString["filtra"];
this.ExportToExcel(sfiltro);
}
/// <summary>
/// faz a conexão com o banco de dados sql serve conforme script wen.config.
/// </summary>
/// <returns></returns>
private static SqlConnection connection()
{
try
{
string sconexao = ConfigurationSettings.AppSettings["conexaoSQL"];
SqlConnection sqlconnection = new SqlConnection(sconexao);
//Verifica se a conexão esta fechada.
if (sqlconnection.State == ConnectionState.Closed)
{
//Abri a conexão.
sqlconnection.Open();
}
//Retorna o sqlconnection.
return sqlconnection;
}
catch (SqlException ex)
{
throw ex;
}
}
/// <summary>
/// GetData abre a conexão com o SQlServer, faz o Select e retorna um Dt
/// </summary>
/// <param name="cmd"></param>
/// <returns></returns>
private DataTable GetData(SqlCommand cmd)
{
DataTable dt = new DataTable();
String strConnString = ConfigurationSettings.AppSettings["conexaoSQL"];
SqlConnection con = new SqlConnection(strConnString);
SqlDataAdapter sda = new SqlDataAdapter();
cmd.CommandType = CommandType.Text;
cmd.Connection = con;
try
{
con.Open();
sda.SelectCommand = cmd;
sda.Fill(dt);
return dt;
}
catch (Exception ex)
{
throw ex;
}
finally
{
con.Close();
sda.Dispose();
con.Dispose();
}
}
/// <summary>
/// Exporta os dados da query para o excel
/// </summary>
private void ExportToExcel(string sfiltro)
{
string strQuery = "SELECT ";
strQuery += "a.idSolicitacao_coleta as 'Id',a.numero_solicitacao_coleta as 'Numero', ";
strQuery += "c.Clientes_idClientes as 'Cod.Cliente', ";
strQuery += "e.razao_social as 'Nome', ";
strQuery += "c.cep_coleta as 'CEP', ";
strQuery += "c.logradouro_coleta as 'Endereço', ";
strQuery += "c.comp_endereco_coleta as 'Complemento', ";
strQuery += "c.bairro_coleta as 'Bairro', ";
strQuery += "c.municipio_coleta as 'Município', ";
strQuery += "c.estado_coleta as 'Estado', ";
strQuery += "c.contato_coleta as 'Contato', c.ddd_resp_coleta as 'DDD', c.telefone_resp_coleta as 'Telefone', ";
strQuery += "c.ramal_resp_coleta as 'Ramal', ";
strQuery += "c.depto_resp_coleta as 'Departamento' ";
strQuery += "from dbo.Solicitacao_coleta as a ";
strQuery += "left outer join solicitacao_coleta_has_clientes as c ";
strQuery += "on c.Solicitacao_coleta_idSolicitacao_coleta = a.idSolicitacao_coleta ";
strQuery += "left outer join Solicitacao_coleta as d on a.idSolicitacao_coleta = d.idSolicitacao_coleta ";
strQuery += "left outer join Clientes as e on e.idClientes = c.Clientes_idClientes ";
strQuery += "left outer join Transportadoras as f on f.idTransportadoras = e.Transportadoras_idTransportadoras ";
strQuery += "where d.Status_coleta_idStatus_coleta = 2 ";
strQuery += sfiltro;
strQuery += " order by a.idSolicitacao_coleta ";
SqlCommand cmd = new SqlCommand(strQuery);
DataTable dt = GetData(cmd);
//Create a dummy GridView
GridView GridView1 = new GridView();
GridView1.AllowPaging = false;
GridView1.DataSource = dt;
GridView1.DataBind();
Response.Clear();
Response.Buffer = true;
Response.AddHeader("content-disposition","attachment;filename=DataTable.xls");
Response.Charset = "";
Response.ContentType = "application/vnd.ms-excel";
StringWriter sw = new StringWriter();
HtmlTextWriter hw = new HtmlTextWriter(sw);
for (int i = 0; i < GridView1.Rows.Count; i++)
{
//Apply text style to each Row
GridView1.Rows[i].Attributes.Add("class", "textmode");
}
GridView1.RenderControl(hw);
//style to format numbers to string
string style = @"<style> .textmode { mso-number-format:\@; } </style>";
Response.Write(style);
Response.Output.Write(sw.ToString());
Response.Flush();
Response.End();
}
}<file_sep>// JavaScript Document
function windowLocationFind(url) {
var find = true;
var busca = document.frmCepEndAdm.search.value;
var por = document.frmCepEndAdm.cbBusca.value;
window.location.href = url + "/adm/frmCepEndAdm.asp?find=true&search="+busca+"&changetype="+por;
}
<file_sep> // JavaScript Document
//
//<NAME> - 4/5/2014
//Preenche com os dados do endereço padrão de coleta
//
function preencheEndColeta() {
var form = document.frmAddSolicitacao;
var oAjax = Ajax();
var strRet;
//if (form.chkMesmoEndereco.checked) {
document.frmAddSolicitacao.txtLogradouroColeta.disabled = true;
document.frmAddSolicitacao.txtLogradouroColeta.value = "Loading...";
document.frmAddSolicitacao.txtCompLogradouroColeta.disabled = true;
document.frmAddSolicitacao.txtNumeroColeta.disabled = true;
document.frmAddSolicitacao.txtBairroColeta.disabled = true;
document.frmAddSolicitacao.txtBairroColeta.value = "Loading...";
document.frmAddSolicitacao.txtMunicipioColeta.disabled = true;
document.frmAddSolicitacao.txtMunicipioColeta.value = "Loading...";
document.frmAddSolicitacao.txtEstadoColeta.disabled = true;
document.frmAddSolicitacao.txtEstadoColeta.value = "Loading...";
document.getElementById("btnBuscarCepColeta").style.cursor = 'wait';
document.body.style.cursor = 'wait';
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
strRet = oAjax.responseText.split(";");
document.frmAddSolicitacao.hiddenIntEnderecoCepColeta.value = strRet[0];
document.frmAddSolicitacao.txtCepColeta.value = document.frmAddSolicitacao.hiddenCEPCol.value;
document.frmAddSolicitacao.txtLogradouroColeta.value = document.frmAddSolicitacao.hiddenLogrCol.value;;
document.frmAddSolicitacao.txtCompLogradouroColeta.value = document.frmAddSolicitacao.hiddenComplCol.value;
document.frmAddSolicitacao.txtNumeroColeta.value = document.frmAddSolicitacao.hiddenNumCol.value;
document.frmAddSolicitacao.txtBairroColeta.value = document.frmAddSolicitacao.hiddenBaiCol.value;
document.frmAddSolicitacao.txtMunicipioColeta.value = document.frmAddSolicitacao.hiddenMunCol.value;
document.frmAddSolicitacao.txtEstadoColeta.value = document.frmAddSolicitacao.hiddenEstCol.value;
document.frmAddSolicitacao.txtLogradouroColeta.disabled = false;
document.frmAddSolicitacao.txtCompLogradouroColeta.disabled = false;
document.frmAddSolicitacao.txtNumeroColeta.disabled = false;
document.frmAddSolicitacao.txtBairroColeta.disabled = false;
document.frmAddSolicitacao.txtMunicipioColeta.disabled = false;
document.frmAddSolicitacao.txtEstadoColeta.disabled = false;
document.getElementById("btnBuscarCepColeta").style.cursor = 'pointer';
document.body.style.cursor = 'default';
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getcependereco&id="+document.frmAddSolicitacao.hiddenGetCepEnderecoComum.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
/*
} else {
form.txtCepColeta.value = "";
form.txtLogradouroColeta.value = "";
form.txtCompLogradouroColeta.value = "";
form.txtNumeroColeta.value = "";
form.txtBairroColeta.value = "";
form.txtMunicipioColeta.value = "";
form.txtEstadoColeta.value = "";
}*/
//lock texts
document.frmAddSolicitacao.txtLogradouroColeta.readOnly = true;
document.frmAddSolicitacao.txtContatoRespColeta.readOnly = true;
document.frmAddSolicitacao.txtLogradouroColeta.readOnly = true;
document.frmAddSolicitacao.txtCepColeta.readOnly = true;
document.frmAddSolicitacao.txtCompLogradouroColeta.readOnly = true;
document.frmAddSolicitacao.txtNumeroColeta.readOnly = true;
document.frmAddSolicitacao.txtBairroColeta.readOnly = true;
document.frmAddSolicitacao.txtMunicipioColeta.readOnly = true;
document.frmAddSolicitacao.txtEstadoColeta.readOnly = true;
document.frmAddSolicitacao.txtContatoRespColeta.readOnly = false;
document.frmAddSolicitacao.txtDDDContatoRespColeta.readOnly = false;
document.frmAddSolicitacao.txtTelefoneContatoRespColeta.readOnly = false;
document.frmAddSolicitacao.txtRamalContatoRespColeta.readOnly = false;
document.frmAddSolicitacao.txtDepContatoRespColeta.readOnly = false;
}<file_sep>// JavaScript Document
/*
'|--------------------------------------------------------------------
'| Arquivo: frmEditaCadastroCliente.js
'| Autor: <NAME> (<EMAIL>)
'| Data Criação: 13/04/2007
'| Data Modificação: 15/04/2007
'| Descrição: Arquivo de Formulário para UPDATE do Cliente (Javascript)
'|--------------------------------------------------------------------
*/
var errForm = false;
var msgErrForm = "Os seguintes campos foram preenchidos incorretamente!\n";
function showClienteEndereco() {
var form = document.frmEditaCadastroCliente;
if (form.txtNomeFantasia.value == "") {
msgErrForm += "Campo: Nome Fantasia\n";
errForm = true;
}
if (form.txtCep.value == "") {
msgErrForm += "Campo: Cep\n";
errForm = true;
}
if (isNaN(form.txtCep.value)) {
msgErrForm += "Campo: Somente números no Cep\n";
errForm = true;
}
if (form.txtCep.value.length < 8) {
msgErrForm += "Campo: Cep\n";
errForm = true;
}
if (parseInt(form.tipopessoa.value) == 1) {
validaCnpj();
} else {
validaDDD();
}
validaTelefone();
if (!errForm) {
preencheEnderecoToCep();
} else {
alert(msgErrForm);
msgErrForm = "Os seguintes campos foram preenchidos incorretamente!\n";
errForm = false;
return false;
}
errForm = false;
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Validação do CNPJ da Empresa
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function validaCnpj() {
var form = document.frmEditaCadastroCliente;
var numeros1Dig = new Array(5,4,3,2,9,8,7,6,5,4,3,2);
var soma1Dig = 0;
var resto1Dig = 0;
var digVer1 = 0;
var numeros2Dig = new Array(6,5,4,3,2,9,8,7,6,5,4,3,2);
var soma2Dig = 0;
var resto2Dig = 0;
var digVer2 = 0;
var i = 0;
var j = 0;
var cnpj = "";
cnpj = form.txtCNPJ.value;
digVer2 = cnpj.charAt(cnpj.length - 1);
digVer1 = cnpj.charAt(cnpj.length - 2);
if (form.txtCNPJ.value == "") {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
if (form.txtCNPJ.value.indexOf('/') == -1) {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
if (form.txtCNPJ.value.length < 18) {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
cnpj = cnpj.replace('/','');
cnpj = cnpj.replace('-','');
cnpj = cnpj.replace('.','');
cnpj = cnpj.replace('.','');
for(i = 0; i < cnpj.length - 2; i++) {
if (!isNaN(cnpj.charAt(i)) && !isNaN(numeros1Dig[i])) {
soma1Dig += cnpj.charAt(i) * numeros1Dig[i];
}
}
resto1Dig = soma1Dig % 11;
if (resto1Dig < 2) {
if (!(digVer1 == 0)) {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
} else {
resto1Dig = 11 - resto1Dig;
if (!(resto1Dig == digVer1)) {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
}
for(j = 0; j < cnpj.length - 1; j++) {
soma2Dig += cnpj.charAt(j) * numeros2Dig[j];
}
resto2Dig = soma2Dig % 11;
if (resto2Dig < 2) {
if (!(digVer2 == 0)) {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
} else {
resto2Dig = 11 - resto2Dig;
if (!(resto2Dig == digVer2)) {
msgErrForm += "Campo: CNPJ\n";
errForm = true;
return false;
}
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Validação DDD e auto preenchimento
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function validaDDD() {
var form = document.frmEditaCadastroCliente;
if (form.txtDDD.value == "") {
msgErrForm += "Campo: DDD\n";
errForm = true;
return false;
}
if (isNaN(form.txtDDD.value)) {
msgErrForm += "Campo: DDD\n";
errForm = true;
return false;
}
if (form.txtDDD.value.length == 1) {
msgErrForm += "Campo: DDD\n";
errForm = true;
return false;
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Validação do Telefone da Empresa
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function validaTelefone() {
var form = document.frmEditaCadastroCliente;
if (form.txtTelefone.value == "") {
msgErrForm += "Campo: Telefone\n";
errForm = true;
return false;
}
if (isNaN(form.txtTelefone.value)) {
msgErrForm += "Campo: Telefone\n";
errForm = true;
return false;
}
if (form.txtTelefone.value.length < 8) {
msgErrForm += "Campo: Telefone\n";
errForm = true;
return false;
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Busca do Endereço para Preenchimento automático do Endereço de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function preencheEnderecoToCep() {
var oAjax = Ajax();
var form = document.frmEditaCadastroCliente;
var strRet = "";
document.getElementById("loadingdisplay").style.display = 'block';
form.txtLogradouro.value = "Carregando...";
form.txtBairro.value = "Carregando...";
form.txtMunicipio.value = "Carregando...";
form.txtEstado.value = "Carregando...";
form.txtCompLogradouro.value = "";
form.txtNumero.value = "";
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
document.getElementById("loadingdisplay").style.display = 'none';
strRet = oAjax.responseText.split(";");
strRet[6] = strRet[6].replace(" ",'');
form.txtLogradouro.value = strRet[6] + ". " + strRet[2];
form.txtBairro.value = strRet[3];
form.txtMunicipio.value = strRet[4];
form.txtEstado.value = strRet[5];
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getcependereco&id="+form.txtCep.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Criação do Objeto Ajax
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function Ajax() {
var ajax = null;
if (window.ActiveXObject) {
try {
ajax = new ActiveXObject("Msxml2.XMLHTTP");
} catch (ex) {
try {
ajax = new ActiveXObject("Microsoft.XMLHTTP");
} catch(ex2) {
alert("Seu browser não suporta Ajax.");
}
}
} else {
if (window.XMLHttpRequest) {
try {
ajax = new XMLHttpRequest();
} catch(ex3) {
alert("Seu browser não suporta Ajax.");
}
}
}
return ajax;
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Verifica se o o CNPJ se já está cadastrado
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function checkCNPJEmpresa() {
var oAjax = Ajax();
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
if (oAjax.responseText == "true") {
alert("CNPJ já cadastrado. Favor cadastrar outro CNPJ!");
document.frmEditaCadastroCliente.txtCNPJ.focus();
return false;
} else {
return true;
}
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getcheckcnpjempresa&id="+document.frmEditaCadastroCliente.txtCNPJ.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Busca De Pontos de Coleta do cliente
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function showClientePostoColeta() {
var form = document.frmEditaCadastroCliente;
var oAjax = Ajax();
var strRet = "";
if (form.txtCepConsultaPonto.value.length < 8) {
alert("Preencha corretamente o Cep para Busca!");
form.txtCepConsultaPonto.focus();
return false;
}
if (isNaN(form.txtCepConsultaPonto.value)) {
alert("Preencha somente números no Cep para Busca!");
form.txtCepConsultaPonto.focus();
return false;
}
if (form.txtCepConsultaPonto.value == "") {
alert("Preencha o campo de Cep para busca dos Pontos de Coleta!");
form.txtCepConsultaPonto.focus();
return false;
} else {
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
strRet = oAjax.responseText.split(";");
document.getElementById("titTableListPontoColeta").style.display = 'block';
document.getElementById("tableListPontoColeta").innerHTML = strRet[0];
document.frmEditaCadastroCliente.hiddenIntPontoColeta.value = strRet[1];
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getlistpontocoleta&id="+document.frmEditaCadastroCliente.txtCepConsultaPonto.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
}
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Busca do Endereço para Preenchimento automático do Endereço de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function loadCepColeta() {
var oAjax = Ajax();
var strRet = "";
if (document.frmEditaCadastroCliente.txtCepColeta.value == "") {
alert("Preencha o campo Cep para Busca do Endereço de Coleta!");
return false;
}
if (isNaN(document.frmEditaCadastroCliente.txtCepColeta.value)) {
alert("Preencha o Cep somente com números!");
return false;
}
if (document.frmEditaCadastroCliente.txtCepColeta.value.length < 8) {
alert("Preencha corretamente o Campo Cep!");
return false;
}
//====================================================================
// Bloqueio dos campos de consulta
//====================================================================
document.frmEditaCadastroCliente.chkMesmoEndereco.checked = false;
document.frmEditaCadastroCliente.txtLogradouroColeta.disabled = true;
document.frmEditaCadastroCliente.txtLogradouroColeta.value = "Carregando...";
document.frmEditaCadastroCliente.txtCompLogradouroColeta.disabled = true;
document.frmEditaCadastroCliente.txtNumeroColeta.disabled = true;
document.frmEditaCadastroCliente.txtBairroColeta.disabled = true;
document.frmEditaCadastroCliente.txtBairroColeta.value = "Carregando...";
document.frmEditaCadastroCliente.txtMunicipioColeta.disabled = true;
document.frmEditaCadastroCliente.txtMunicipioColeta.value = "Carregando...";
document.frmEditaCadastroCliente.txtEstadoColeta.disabled = true;
document.frmEditaCadastroCliente.txtEstadoColeta.value = "Carregando...";
document.getElementById("btnBuscarCepColeta").style.cursor = 'wait';
document.body.style.cursor = 'wait';
//====================================================================
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
strRet = oAjax.responseText.split(";");
document.frmEditaCadastroCliente.hiddenIntEnderecoCepColeta.value = strRet[0];
document.frmEditaCadastroCliente.txtLogradouroColeta.value = strRet[2];
document.frmEditaCadastroCliente.txtBairroColeta.value = strRet[3];
document.frmEditaCadastroCliente.txtMunicipioColeta.value = strRet[4];
document.frmEditaCadastroCliente.txtEstadoColeta.value = strRet[5];
document.frmEditaCadastroCliente.txtLogradouroColeta.disabled = false;
document.frmEditaCadastroCliente.txtCompLogradouroColeta.disabled = false;
document.frmEditaCadastroCliente.txtNumeroColeta.disabled = false;
document.frmEditaCadastroCliente.txtBairroColeta.disabled = false;
document.frmEditaCadastroCliente.txtMunicipioColeta.disabled = false;
document.frmEditaCadastroCliente.txtEstadoColeta.disabled = false;
document.getElementById("btnBuscarCepColeta").style.cursor = 'pointer';
document.body.style.cursor = 'default';
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getcependereco&id="+document.frmEditaCadastroCliente.txtCepColeta.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Preenchimento Automático do mesmo Endereço
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function preencheMesmoEndereco() {
var form = document.frmEditaCadastroCliente;
if (form.chkMesmoEndereco.checked) {
form.txtCepColeta.value = form.txtCep.value;
form.txtLogradouroColeta.value = form.txtLogradouro.value;
form.txtCompLogradouroColeta.value = form.txtCompLogradouro.value;
form.txtNumeroColeta.value = form.txtNumero.value;
form.txtBairroColeta.value = form.txtBairro.value;
form.txtMunicipioColeta.value = form.txtMunicipio.value;
form.txtEstadoColeta.value = form.txtEstado.value;
} else {
form.txtCepColeta.value = "";
form.txtLogradouroColeta.value = "";
form.txtCompLogradouroColeta.value = "";
form.txtNumeroColeta.value = "";
form.txtBairroColeta.value = "";
form.txtMunicipioColeta.value = "";
form.txtEstadoColeta.value = "";
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Check se foi selecionado algum ponto de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function checkChangePontoColeta() {
var bErr = false;
var bSelected = false;
for (var i=0;i <= parseInt(document.frmEditaCadastroCliente.hiddenIntPontoColeta.value);i++) {
if (!document.getElementById("radioCheckPonto"+i).checked) {
bErr = true;
} else {
bSelected = true;
document.frmEditaCadastroCliente.hiddenIntChangePontoColeta.value = document.getElementById("radioCheckPonto"+i).value;
}
}
if (bErr && !bSelected) {
return false
} else {
return true;
}
}
//=========================================================================================================
function validate() {
var form = document.frmEditaCadastroCliente;
if (form.txtNomeFantasia.value == "") {
msgErrForm += "Campo: Nome Fantasia\n";
errForm = true;
}
if (form.txtCep.value == "") {
msgErrForm += "Campo: Cep\n";
errForm = true;
}
if (isNaN(form.txtCep.value)) {
msgErrForm += "Campo: Somente números no Cep\n";
errForm = true;
}
if (form.txtCep.value.length < 8) {
msgErrForm += "Campo: Cep\n";
errForm = true;
}
if (parseInt(form.tipopessoa.value) == 1) {
validaCnpj();
} else {
validateCPF();
}
validaDDD();
validaTelefone();
if (form.txtNumero.value == "") {
msgErrForm += "Campo: Numero\n";
errForm = true;
}
if (!errForm) {
form.submit();
} else {
alert(msgErrForm);
msgErrForm = "Os seguintes campos foram preenchidos incorretamente!\n";
errForm = false;
return false;
}
errForm = false;
}
function validateCPF() {
var form = document.frmEditaCadastroCliente;
var numeros1Dig = new Array(10,9,8,7,6,5,4,3,2);
var soma1Dig = 0;
var resto1Dig = 0;
var digVer1 = 0;
var numeros2Dig = new Array(11,10,9,8,7,6,5,4,3,2);
var soma2Dig = 0;
var resto2Dig = 0;
var digVer2 = 0;
var i = 0;
var j = 0;
var cnpj = "";
cnpj = form.txtCPF.value;
digVer2 = cnpj.charAt(cnpj.length - 1);
digVer1 = cnpj.charAt(cnpj.length - 2);
if (form.txtCPF.value == "") {
msgErrForm += "Campo: CPF\n";
errForm = true;
return false;
}
if (form.txtCPF.value.length < 14) {
msgErrForm += "Campo: CPF\n";
errForm = true;
return false;
}
cnpj = cnpj.replace('-','');
cnpj = cnpj.replace('.','');
cnpj = cnpj.replace('.','');
for(i = 0; i < cnpj.length - 2; i++) {
if (!isNaN(cnpj.charAt(i)) && !isNaN(numeros1Dig[i])) {
soma1Dig += cnpj.charAt(i) * numeros1Dig[i];
}
}
resto1Dig = soma1Dig % 11;
if (resto1Dig < 2) {
if (!(digVer1 == 0)) {
msgErrForm += "Campo: CPF\n";
errForm = true;
return false;
}
} else {
resto1Dig = 11 - resto1Dig;
if (!(resto1Dig == digVer1)) {
msgErrForm += "Campo: CPF\n";
errForm = true;
return false;
}
}
for(j = 0; j < cnpj.length - 1; j++) {
soma2Dig += cnpj.charAt(j) * numeros2Dig[j];
}
resto2Dig = soma2Dig % 11;
if (resto2Dig < 2) {
if (!(digVer2 == 0)) {
msgErrForm += "Campo: CPF\n";
errForm = true;
return false;
}
} else {
resto2Dig = 11 - resto2Dig;
if (!(resto2Dig == digVer2)) {
msgErrForm += "Campo: CPF\n";
errForm = true;
return false;
}
}
}
<file_sep>// JavaScript Document
/*
'|--------------------------------------------------------------------
'| Arquivo: frmAddSolicitacao.js
'| Autor: <NAME> (<EMAIL>)
'| Data Criação: 13/04/2007
'| Data Modificação: 15/04/2007
'| Descrição: Arquivo de Formulário para Nova Solicitacao (Javascript)
'|--------------------------------------------------------------------
*/
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Criação do Objeto Ajax
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function Ajax() {
var ajax = null;
if (window.ActiveXObject) {
try {
ajax = new ActiveXObject("Msxml2.XMLHTTP");
} catch (ex) {
try {
ajax = new ActiveXObject("Microsoft.XMLHTTP");
} catch(ex2) {
alert("Seu browser não suporta Ajax.");
}
}
} else {
if (window.XMLHttpRequest) {
try {
ajax = new XMLHttpRequest();
} catch(ex3) {
alert("Seu browser não suporta Ajax.");
}
}
}
return ajax;
}
//libera digitação do endereço novo
function checkEndereco(clicked_id) {
var elThisElement = clicked_id;
var elToggled = document.getElementById("tagendnovo");
var elToggledmesmo = document.getElementById("tagendmesmo");
var oPreenche = preencheMesmoEndereco();
if (elThisElement == "radioendmesmo") {
elToggled.style.display = "none";
elToggledmesmo.style.display = "block";
}
if (elThisElement == "radioendnovo") {
elToggled.style.display = "block";
elToggledmesmo.style.display = "none";
}
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Check se foi selecionado algum ponto de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function checkChangePontoColeta() {
try {
var bErr = false;
var bSelected = false;
for (var i=0;i <= parseInt(document.frmAddSolicitacao.hiddenIntPontoColeta.value);i++) {
if (!document.getElementById("radioCheckPonto"+i).checked) {
bErr = true;
} else {
bSelected = true;
document.frmAddSolicitacao.hiddenIntChangePontoColeta.value = document.getElementById("radioCheckPonto"+i).value;
}
}
if (bErr && !bSelected) {
return false
} else {
return true;
}
} catch (ex) {
return true;
}
}
//=========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Valida Formulário de Nova Solicitação
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function validaFormulario() {
if (document.frmAddSolicitacao.txtQtdCartuchos.value == "") {
alert("Preencha o campo Quantidade de Cartuchos");
return false;
}
if (isNaN(document.frmAddSolicitacao.txtQtdCartuchos.value)) {
alert("O Campo Quantidade de Cartuchos só aceita dados numéricos");
return false;
}
//if (parseInt(document.frmAddSolicitacao.txtQtdCartuchos.value) < parseInt(document.frmAddSolicitacao.hiddenMinCartuchos.value)) {
// alert("O mínimo de cartuchos para esta categoria é de " + document.frmAddSolicitacao.hiddenMinCartuchos.value + " cartuchos. Por favor preencha com um valor maior de cartuchos a serem entregues!");
// return false;
//}
//if (!checkChangePontoColeta()) {
// if (!confirm("Deseja usar o mesmo Ponto de Coleta da Solicitação anterior?")) {
// alert("Selecione um Ponto de Coleta");
// return false;
// }
//}
if (document.frmAddSolicitacao.hiddenSessionisColetaDomiciliar.value == 1) {
if (isNaN(document.frmAddSolicitacao.txtCepColeta.value)) {
alert("Preencha o campo CEP apenas com números");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
return false;
}
if (document.frmAddSolicitacao.txtCepColeta.value.length < 8) {
alert("Preencha o campo CEP com 8 números!");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
return false;
}
if (document.frmAddSolicitacao.txtCepColeta.value == "") {
alert("Preencha o campo CEP de coleta");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
return false;
}
if (document.frmAddSolicitacao.txtLogradouroColeta.value == "") {
alert("Preencha o campo Endereço");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
return false;
}
if (document.frmAddSolicitacao.txtNumeroColeta.value == "") {
alert("Preencha o campo Numero do Endereço");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
return false;
}
if (document.frmAddSolicitacao.txtBairroColeta.value == "") {
alert("Preencha o campo Bairro");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
return false;
}
if (document.frmAddSolicitacao.txtMunicipioColeta.value == "") {
alert("Preencha o campo Município");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
return false;
}
if (document.frmAddSolicitacao.txtEstadoColeta.value == "") {
alert("Preencha o campo Estado");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
return false;
}
if (document.frmAddSolicitacao.txtRespColContato.value == "") {
alert("Preencha o campo do Contato responsável pela Coleta!");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
form.txtRespColContato.focus();
return false;
}
if (document.frmAddSolicitacao.txtDDDContatoRespColeta.value == "") {
alert("Preencha o campo do DDD do responsável pela Coleta!");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
form.txtDDDContatoRespColeta.focus();
return false;
}
if (isNaN(document.frmAddSolicitacao.txtDDDContatoRespColeta.value)) {
alert("Preencha o campo DDD do Contato somente com dados numéricos!");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
form.txtDDDContatoRespColeta.focus();
return false;
}
if (document.frmAddSolicitacao.txtDDDContatoRespColeta.value.length < 2) {
alert("Preencha o campo do DDD do Contato com no mínimo 2 caracteres válidos!");
form.txtDDDContatoRespColeta.focus();
return false;
}
if (document.frmAddSolicitacao.txtTelefoneContatoRespColeta.value == "") {
alert("Preencha o campo do Telefone do responsável pela Coleta!");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
form.txtTelefoneContatoRespColeta.focus();
return false;
}
if (isNaN(document.frmAddSolicitacao.txtTelefoneContatoRespColeta.value)) {
alert("Preencha o campo Telefone do Contato somente com dados numéricos!");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
form.txtTelefoneContatoRespColeta.focus();
return false;
}
if (document.frmAddSolicitacao.txtTelefoneContatoRespColeta.value.length < 8) {
alert("Preencha o campo do Telefone do Contato com no mínimo 8 caracteres válidos!");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
form.txtTelefoneContatoRespColeta.focus();
return false;
}
if (document.frmAddSolicitacao.txtDepContatoRespColeta.value == "") {
alert("Preencha o campo Departamento do contato!");
document.frmAddSolicitacao.hiddenActionForm.value = "3";
form.txtDepContatoRespColeta.focus();
return false;
}
}
document.frmAddSolicitacao.submit();
}
//=========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Busca De Pontos de Coleta do cliente
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function showClientePostoColeta() {
var form = document.frmAddSolicitacao;
var oAjax = Ajax();
var strRet = "";
if (form.txtCepConsultaPonto.value.length < 8) {
alert("Preencha corretamente o Cep para Busca!");
form.txtCepConsultaPonto.focus();
return false;
}
if (isNaN(form.txtCepConsultaPonto.value)) {
alert("Preencha somente números no Cep para Busca!");
form.txtCepConsultaPonto.focus();
return false;
}
if (form.txtCepConsultaPonto.value == "") {
alert("Preencha o campo de Cep para busca dos Pontos de Coleta!");
form.txtCepConsultaPonto.focus();
return false;
} else {
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
strRet = oAjax.responseText.split(";");
document.getElementById("titTableListPontoColeta").style.display = 'block';
document.getElementById("tableListPontoColeta").innerHTML = strRet[0];
document.frmAddSolicitacao.hiddenIntPontoColeta.value = strRet[1];
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getlistpontocoleta&id="+form.txtCepConsultaPonto.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
}
//=========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Busca do Endereço para Preenchimento automático do Endereço de Coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function loadCepColeta() {
var oAjax = Ajax();
var strRet = "";
if (document.frmAddSolicitacao.txtCepColeta.value == "") {
alert("Preencha o campo Cep para Busca do Endereço de Coleta!");
return false;
}
if (isNaN(document.frmAddSolicitacao.txtCepColeta.value)) {
alert("Preencha o Cep somente com números!");
return false;
}
if (document.frmAddSolicitacao.txtCepColeta.value.length < 8) {
alert("Preencha corretamente o Campo Cep!");
return false;
}
//====================================================================
// Bloqueio dos campos de consulta
//====================================================================
document.frmAddSolicitacao.chkMesmoEndereco.checked = false;
document.frmAddSolicitacao.chkNovoEndereco.checked = true;
document.frmAddSolicitacao.txtLogradouroColeta.disabled = true;
document.frmAddSolicitacao.txtLogradouroColeta.value = "Loading...";
document.frmAddSolicitacao.txtCompLogradouroColeta.value = "";
document.frmAddSolicitacao.txtNumeroColeta.value = "";
document.frmAddSolicitacao.txtBairroColeta.disabled = true;
document.frmAddSolicitacao.txtBairroColeta.value = "Loading...";
document.frmAddSolicitacao.txtMunicipioColeta.disabled = true;
document.frmAddSolicitacao.txtMunicipioColeta.value = "Loading...";
document.frmAddSolicitacao.txtEstadoColeta.disabled = true;
document.frmAddSolicitacao.txtEstadoColeta.value = "Loading...";
document.getElementById("btnBuscarCepColeta").style.cursor = 'wait';
document.body.style.cursor = 'wait';
//====================================================================
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
strRet = oAjax.responseText.split(";");
document.frmAddSolicitacao.hiddenIntEnderecoCepColeta.value = strRet[0];
document.frmAddSolicitacao.txtLogradouroColeta.value = strRet[6] + " "+ strRet[2];
document.frmAddSolicitacao.txtBairroColeta.value = strRet[3];
document.frmAddSolicitacao.txtMunicipioColeta.value = strRet[4];
document.frmAddSolicitacao.txtEstadoColeta.value = strRet[5];
document.frmAddSolicitacao.txtLogradouroColeta.disabled = false;
document.frmAddSolicitacao.txtCompLogradouroColeta.disabled = false;
document.frmAddSolicitacao.txtNumeroColeta.disabled = false;
document.frmAddSolicitacao.txtBairroColeta.disabled = false;
document.frmAddSolicitacao.txtMunicipioColeta.disabled = false;
document.frmAddSolicitacao.txtEstadoColeta.disabled = false;
document.getElementById("btnBuscarCepColeta").style.cursor = 'pointer';
document.body.style.cursor = 'default';
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getcependereco&id="+document.frmAddSolicitacao.txtCepColeta.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
}
function preencheNovoEndereco(){
var form = document.frmAddSolicitacao;
var oAjax = Ajax();
var strRet;
//if (form.chkNovoEndereco.checked) {
form.txtCepColeta.value = "";
form.txtLogradouroColeta.value = "";
form.txtCompLogradouroColeta.value = "";
form.txtNumeroColeta.value = "";
form.txtBairroColeta.value = "";
form.txtMunicipioColeta.value = "";
form.txtEstadoColeta.value = "";
//lock texts
//lock texts
document.frmAddSolicitacao.txtLogradouroColeta.readOnly = false;
document.frmAddSolicitacao.txtRespColContato.readOnly = false;
document.frmAddSolicitacao.txtLogradouroColeta.readOnly = false;
document.frmAddSolicitacao.txtCepColeta.readOnly = false;
document.frmAddSolicitacao.txtCompLogradouroColeta.readOnly = false;
document.frmAddSolicitacao.txtNumeroColeta.readOnly = false;
document.frmAddSolicitacao.txtBairroColeta.readOnly = false;
document.frmAddSolicitacao.txtMunicipioColeta.readOnly = false;
document.frmAddSolicitacao.txtEstadoColeta.readOnly = false;
document.frmAddSolicitacao.txtDDDContatoRespColeta.readOnly = false;
document.frmAddSolicitacao.txtTelefoneContatoRespColeta.readOnly = false;
document.frmAddSolicitacao.txtRamalContatoRespColeta.readOnly = false;
document.frmAddSolicitacao.txtDepContatoRespColeta.readOnly = false;
//}
}
//
//<NAME> - 4/5/2014
//Preenche com os dados do endereço padrão de coleta
//
function preencheEndColeta() {
var form = document.frmAddSolicitacao;
var oAjax = Ajax();
var strRet;
//if (form.chkMesmoEndereco.checked) {
document.frmAddSolicitacao.txtLogradouroColeta.disabled = true;
document.frmAddSolicitacao.txtLogradouroColeta.value = "Loading...";
document.frmAddSolicitacao.txtCompLogradouroColeta.disabled = true;
document.frmAddSolicitacao.txtNumeroColeta.disabled = true;
document.frmAddSolicitacao.txtNumeroColeta.value = "Loading...";
document.frmAddSolicitacao.txtBairroColeta.disabled = true;
document.frmAddSolicitacao.txtBairroColeta.value = "Loading...";
document.frmAddSolicitacao.txtMunicipioColeta.disabled = true;
document.frmAddSolicitacao.txtMunicipioColeta.value = "Loading...";
document.frmAddSolicitacao.txtEstadoColeta.disabled = true;
document.frmAddSolicitacao.txtEstadoColeta.value = "Loading...";
document.getElementById("btnBuscarCepColeta").style.cursor = 'wait';
document.body.style.cursor = 'wait';
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
strRet = oAjax.responseText.split(";");
document.frmAddSolicitacao.hiddenIntEnderecoCepColeta.value = strRet[0];
document.frmAddSolicitacao.txtCepColeta.value = strRet[1];
document.frmAddSolicitacao.txtLogradouroColeta.value = strRet[2];
document.frmAddSolicitacao.txtCompLogradouroColeta.value = strRet[7]
document.frmAddSolicitacao.txtNumeroColeta.value = strRet[6];
document.frmAddSolicitacao.txtBairroColeta.value = strRet[3];
document.frmAddSolicitacao.txtMunicipioColeta.value = strRet[4];
document.frmAddSolicitacao.txtEstadoColeta.value = strRet[5];
document.frmAddSolicitacao.txtLogradouroColeta.disabled = false;
document.frmAddSolicitacao.txtCompLogradouroColeta.disabled = false;
document.frmAddSolicitacao.txtNumeroColeta.disabled = false;
document.frmAddSolicitacao.txtBairroColeta.disabled = false;
document.frmAddSolicitacao.txtMunicipioColeta.disabled = false;
document.frmAddSolicitacao.txtEstadoColeta.disabled = false;
document.getElementById("btnBuscarCepColeta").style.cursor = 'pointer';
document.body.style.cursor = 'default';
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getEndColeta&id="+document.frmAddSolicitacao.hiddenClienteId.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
/*
} else {
form.txtCepColeta.value = "";
form.txtLogradouroColeta.value = "";
form.txtCompLogradouroColeta.value = "";
form.txtNumeroColeta.value = "";
form.txtBairroColeta.value = "";
form.txtMunicipioColeta.value = "";
form.txtEstadoColeta.value = "";
}*/
//lock texts
document.frmAddSolicitacao.txtLogradouroColeta.readOnly = true;
document.frmAddSolicitacao.txtCepColeta.readOnly = true;
document.frmAddSolicitacao.txtCompLogradouroColeta.readOnly = true;
document.frmAddSolicitacao.txtNumeroColeta.readOnly = true;
document.frmAddSolicitacao.txtBairroColeta.readOnly = true;
document.frmAddSolicitacao.txtMunicipioColeta.readOnly = true;
document.frmAddSolicitacao.txtEstadoColeta.readOnly = true;
document.frmAddSolicitacao.txtRespColContato.readOnly = false;
document.frmAddSolicitacao.txtDDDContatoRespColeta.readOnly = false;
document.frmAddSolicitacao.txtTelefoneContatoRespColeta.readOnly = false;
document.frmAddSolicitacao.txtRamalContatoRespColeta.readOnly = false;
document.frmAddSolicitacao.txtDepContatoRespColeta.readOnly = false;
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Preenchimento Automático do mesmo Endereço
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function preencheMesmoEndereco() {
var form = document.frmAddSolicitacao;
var oAjax = Ajax();
var strRet;
//if (form.chkMesmoEndereco.checked) {
document.frmAddSolicitacao.txtLogradouroColeta.disabled = true;
document.frmAddSolicitacao.txtLogradouroColeta.value = "Loading...";
document.frmAddSolicitacao.txtCompLogradouroColeta.disabled = true;
document.frmAddSolicitacao.txtNumeroColeta.disabled = true;
document.frmAddSolicitacao.txtBairroColeta.disabled = true;
document.frmAddSolicitacao.txtBairroColeta.value = "Loading...";
document.frmAddSolicitacao.txtMunicipioColeta.disabled = true;
document.frmAddSolicitacao.txtMunicipioColeta.value = "Loading...";
document.frmAddSolicitacao.txtEstadoColeta.disabled = true;
document.frmAddSolicitacao.txtEstadoColeta.value = "Loading...";
document.getElementById("btnBuscarCepColeta").style.cursor = 'wait';
document.body.style.cursor = 'wait';
oAjax.onreadystatechange = function() {
if (oAjax.readyState == 4 && oAjax.status == 200) {
strRet = oAjax.responseText.split(";");
document.frmAddSolicitacao.hiddenIntEnderecoCepColeta.value = strRet[0];
document.frmAddSolicitacao.txtCepColeta.value = document.frmAddSolicitacao.hiddenGetCepEnderecoComum.value;
document.frmAddSolicitacao.txtLogradouroColeta.value = strRet[6] + " " +strRet[2];
document.frmAddSolicitacao.txtCompLogradouroColeta.value = document.frmAddSolicitacao.hiddenGetCompLogradouroEnderecoCliente.value;
document.frmAddSolicitacao.txtNumeroColeta.value = document.frmAddSolicitacao.hiddenGetNumeroEnderecoCliente.value;
document.frmAddSolicitacao.txtBairroColeta.value = strRet[3];
document.frmAddSolicitacao.txtMunicipioColeta.value = strRet[4];
document.frmAddSolicitacao.txtEstadoColeta.value = strRet[5];
document.frmAddSolicitacao.txtLogradouroColeta.disabled = false;
document.frmAddSolicitacao.txtCompLogradouroColeta.disabled = false;
document.frmAddSolicitacao.txtNumeroColeta.disabled = false;
document.frmAddSolicitacao.txtBairroColeta.disabled = false;
document.frmAddSolicitacao.txtMunicipioColeta.disabled = false;
document.frmAddSolicitacao.txtEstadoColeta.disabled = false;
document.getElementById("btnBuscarCepColeta").style.cursor = 'pointer';
document.body.style.cursor = 'default';
}
}
oAjax.open("GET", "ajax/frmCadCliente.asp?sub=getcependereco&id="+document.frmAddSolicitacao.hiddenGetCepEnderecoComum.value, true);
oAjax.setRequestHeader("Content-Type","application/x-www-form-urlencoded; charset=iso-8859-1");
oAjax.send(null);
/*
} else {
form.txtCepColeta.value = "";
form.txtLogradouroColeta.value = "";
form.txtCompLogradouroColeta.value = "";
form.txtNumeroColeta.value = "";
form.txtBairroColeta.value = "";
form.txtMunicipioColeta.value = "";
form.txtEstadoColeta.value = "";
}*/
//lock texts
document.frmAddSolicitacao.txtLogradouroColeta.readOnly = true;
document.frmAddSolicitacao.txtCepColeta.readOnly = true;
document.frmAddSolicitacao.txtCompLogradouroColeta.readOnly = true;
document.frmAddSolicitacao.txtNumeroColeta.readOnly = true;
document.frmAddSolicitacao.txtBairroColeta.readOnly = true;
document.frmAddSolicitacao.txtMunicipioColeta.readOnly = true;
document.frmAddSolicitacao.txtEstadoColeta.readOnly = true;
document.frmAddSolicitacao.txtRespColContato.readOnly = false;
document.frmAddSolicitacao.txtDDDContatoRespColeta.readOnly = false;
document.frmAddSolicitacao.txtTelefoneContatoRespColeta.readOnly = false;
document.frmAddSolicitacao.txtRamalContatoRespColeta.readOnly = false;
document.frmAddSolicitacao.txtDepContatoRespColeta.readOnly = false;
}
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Autentica a edição do endereço de Coleta do Cliente
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function authenticateUpdateAdress() {
if (document.frmAddSolicitacao.txtCepColeta.value == "") {
alert("Preencha o campo CEP de Coleta");
return false;
}
if (document.frmAddSolicitacao.txtLogradouroColeta.value == "") {
alert("Preencha o campo Logradouro");
return false;
}
if (document.frmAddSolicitacao.txtNumeroColeta.value == "") {
alert("Preencha o campo Numero do Endereço");
return false;
}
if (document.frmAddSolicitacao.txtBairroColeta.value == "") {
alert("Preencha o campo Bairro");
return false;
}
if (document.frmAddSolicitacao.txtMunicipioColeta.value == "") {
alert("Preencha o campo Município");
return false;
}
if (document.frmAddSolicitacao.txtEstadoColeta.value == "") {
alert("Preencha o campo Estado");
return false;
}
if (document.frmAddSolicitacao.txtRespColContato.value == "") {
alert("Preencha o campo do Contato responsável pela Coleta!");
form.txtRespColContato.focus();
return false;
}
if (document.frmAddSolicitacao.txtDDDContatoRespColeta.value == "") {
alert("Preencha o campo do DDD do responsável pela Coleta!");
form.txtDDDContatoRespColeta.focus();
return false;
}
if (isNaN(document.frmAddSolicitacao.txtDDDContatoRespColeta.value)) {
alert("Preencha o campo DDD do Contato somente com dados numéricos!");
form.txtDDDContatoRespColeta.focus();
return false;
}
if (document.frmAddSolicitacao.txtDDDContatoRespColeta.value.length < 2) {
alert("Preencha o campo do DDD do Contato com no mínimo 2 caracteres válidos!");
form.txtDDDContatoRespColeta.focus();
return false;
}
if (document.frmAddSolicitacao.txtTelefoneContatoRespColeta.value == "") {
alert("Preencha o campo do Telefone do responsável pela Coleta!");
form.txtTelefoneContatoRespColeta.focus();
return false;
}
if (isNaN(document.frmAddSolicitacao.txtTelefoneContatoRespColeta.value)) {
alert("Preencha o campo Telefone do Contato somente com dados numéricos!");
form.txtTelefoneContatoRespColeta.focus();
return false;
}
if (document.frmAddSolicitacao.txtTelefoneContatoRespColeta.value.length < 8) {
alert("Preencha o campo do Telefone do Contato com 8 caracteres válidos!");
form.txtTelefoneContatoRespColeta.focus();
return false;
}
document.frmAddSolicitacao.hiddenActionForm.value = "1";
document.frmAddSolicitacao.submit();
}
////////////////////////////////////////////////////////////////////////////////////////////////////////////
//==========================================================================================================
// Carrega as informações se for o mesmo endereço para coleta
//==========================================================================================================
////////////////////////////////////////////////////////////////////////////////////////////////////////////
function loadInfoSameAdress() {
if (document.frmAddSolicitacao.txtCepColeta.value == document.frmAddSolicitacao.hiddenGetCepEnderecoComum.value) {
document.frmAddSolicitacao.chkMesmoEndereco.checked = true;
document.frmAddSolicitacao.txtCompLogradouroColeta.value = document.frmAddSolicitacao.hiddenGetCompLogradouroEnderecoCliente.value;
}
}
function loadClear() {
var form = document.frmAddSolicitacao;
var oAjax = Ajax();
var strRet;
form.txtCepColeta.value = "";
form.txtLogradouroColeta.value = "";
form.txtCompLogradouroColeta.value = "";
form.txtNumeroColeta.value = "";
form.txtBairroColeta.value = "";
form.txtMunicipioColeta.value = "";
form.txtEstadoColeta.value = "";
form.txtRespColContato.value = "";
form.txtDDDContatoRespColeta.value = "";
form.txtTelefoneContatoRespColeta.value = "";
document.getElementsByName('txtRespColContato').readOnly = true;
document.getElementsByName('txtLogradouroColeta').readOnly = true;
document.getElementsByName('txtCepColeta').readOnly = true;
document.getElementsByName('txtCompLogradouroColeta').readOnly = true;
document.getElementsByName('txtNumeroColeta').readOnly = true;
document.getElementsByName('txtBairroColeta').readOnly = true;
document.getElementsByName('txtMunicipioColeta').readOnly = true;
document.getElementsByName('txtEstadoColeta').readOnly = true;
document.getElementsByName('txtDDDContatoRespColeta').readOnly = true;
document.getElementsByName('txtTelefoneContatoRespColeta').readOnly = true;
document.getElementsByName('txtRamalContatoRespColeta').readOnly = true;
document.getElementsByName('txtDepContatoRespColeta').readOnly = true;
}
<file_sep>$(function(){
$('.simple-table').stacktable();
});<file_sep>// JavaScript Document
function validate() {
var form = document.frmCadProdutosAdm;
if (form.cbGrupos.value == -1) {
alert("Escolha um Grupo!");
return;
}
if (form.txtDesc.value == "") {
alert("Preencha o campo Descrição!");
return;
}
form.submit();
} | 0a6c5854a768ba35512bec27d52a7a9ef52a373a | [
"JavaScript",
"C#"
] | 27 | JavaScript | brunobritoapps/.NET-oki | b4c22195a98e77bec3db762e89b4698b7289d3f8 | 063895989cf8f3c4b64857e733ef02ccda45f1ff | |
refs/heads/master | <file_sep># Object Recognition Drone
This project is an ongoing work under <NAME>, Data Scientist Sr. at Microsoft. Anusua is a mentor to <NAME> & <NAME>, who are the main contributors to this project.
The Object Recognition Drone is a platform that allows one to perform object recognition using a drone. This software implements the Microsoft Computer Vision API and the Microsoft Bing Speech API and combines it with the Node Javascript file to access a Web Interface for full control of the drone functionalities. By invoking the server.js, you can fly your drone and have it recognize objects in real-time.
For more information on Microsoft Computer Vision APIs and the Microsoft Bing Speech APIs, see the Microsoft Computer Vision API Documentation and Microsoft Bing Speech APIs on docs.microsoft.com.
Inspiration for this project came from <NAME>’s article at https://www.oreilly.com/ideas/how-to-build-an-autonomous-voice-controlled-face-recognizing-drone-for-200.
If you run into any problems, feel free to contact <NAME> at <EMAIL>, <NAME> at <EMAIL> or <NAME> at <EMAIL>
LinkedIns:
https://www.linkedin.com/in/anusua/
https://www.linkedin.com/in/ishachakraborty/
https://www.linkedin.com/in/neelagreevgriddalur/
# Source Code
Clone the sources: git clone https://github.com/Object_Recognition_Drone
# The Project
This is a Node.js and Python application to demonstrate the uses and integrations of the Computer Vision APIs and Bing Speech APIs.
# Dependencies
This repository contains all of the necessary files and dependencies excluding node.js and Python needed to build this project and use it yourself. A drone is needed for recreating this project, a Parrot AR Drone 2.0 Power Edition Quadricopter is recommended for best compatibility.
To install Node.js, https://nodejs.org/en/
To install node-ar drone libraries, run “npm install git://github.com/felixge/node-ar-drone.git”
Run “pip install git+https://github.com/westparkcom/Python-Bing-TTS.git”
Install ffmpeg
# Running the Project
Note: This specific drone only supports 2.4 GHz networks.
Turn the Drone on and connect to its network.
Run the command, “./start.sh” to run the shell file and follow the instructions present on your terminal window by entering the credentials of your desired network and reconnect to it after completion.
In the same terminal window, run “node server.js” and navigate to “localhost:3001” in your browser window.
Open a second terminal window In the window, run “./object_detect.sh” to take the picture. This will run the ComputerVision.py file to identify the object.
If you want to run the facial recognition, run "./startfacerec.sh" to take the picture. This will run the OpenCV code and the bat files to identify the person. This can only be done once you "enroll" the subject, details of how to do that can be found in the README.md file in the facerec folder.
<file_sep>#!/bin/bash
cd /Users/neelgriddalur/Documents/Microsoft\ AI\ Project/2\ -\ Face\ Recog\ Drone/FaceRecognitionTesting/facerec/
brew tap homebrew/science
brew install opencv
export PYTHONPATH=$PYTHONPATH:/usr/local/lib/python2.7/site-packages
pip install numpy
pip install pillow
pip install argparse
curl https://raw.githubusercontent.com/shhavel/facerec/master/facerec>/usr/local/bin/facerec;chmod +x /usr/local/bin/facerec
sed -i '' s,/usr/share/opencv/haarcascades/haarcascade_frontalface_alt2.xml,/usr/local/opt/opencv/share/OpenCV/haarcascades/haarcascade_frontalface_alt2.xml, /usr/local/bin/facerec
| 93bff9096443d8c8c727e428888d2e592c0aadb5 | [
"Markdown",
"Shell"
] | 2 | Markdown | merico34/Object_Recognition_Drone | efe9cdc71da3e173d96b4d8ce755febd6d863bfc | 09e717e4aa1b4acf6a5d07599c847131fe6f1158 | |
refs/heads/main | <repo_name>bytesun/non-fungible-token<file_sep>/example.sh
#!/usr/bin/env sh
RED='\033[0;31m'
GREEN='\033[0;32m'
NC='\033[0m'
bold() {
tput bold
echo $1
tput sgr0
}
check() {
if [ "$1" = "$2" ]; then
echo "${GREEN}OK${NC}"
else
echo "${RED}NOK${NC}: expected ${2}, got ${1}"
dfx -q stop > /dev/null 2>&1
exit 1
fi
}
bold "| Starting replica."
dfx -q start --background --clean > /dev/null 2>&1
bold "| Deploying canisters (this can take a while): \c"
dfx -q identity new "admin" > /dev/null 2>&1
dfx -q identity use "admin"
adminID="$(dfx identity get-principal)"
dfx -q deploy --no-wallet
dfx canister id nft > /dev/null 2>&1
hubID="$(dfx canister id nft)"
echo "${GREEN}DONE${NC}"
bold "| Initializing hub: \c"
check "$(dfx canister call nft init "(
vec{},
record{ name = \"aviate\"; symbol = \"av8\" }
)")" "()"
bold "| Checking contract metadata: \c"
check "$(dfx canister call nft getMetadata)" "(record { name = \"aviate\"; symbol = \"av8\" })"
bold "| Mint first NFT: \c"
check "$(dfx canister call nft mint "(record{
contentType = \"\";
payload = variant{ Payload = vec{ 0x00 } };
owner = null;
properties = vec{};
isPrivate = false;
})")" "(variant { ok = \"0\" })"
bold "| Check owner (hub) of new NFT: \c"
check "$(dfx canister call nft ownerOf "(\"0\")")" "(variant { ok = principal \"${hubID}\" })"
bold "| Transfer NFT to admin: \c"
check "$(dfx canister call nft transfer "(
principal \"${adminID}\", \"0\"
)")" "(variant { ok })"
bold "| Check balance of admin: \c"
check "$(dfx canister call nft balanceOf "(
principal \"${adminID}\"
)")" "(vec { \"0\" })"
dfx -q stop > /dev/null 2>&1
| c3cf7ddad796e23b535eca6dc31766a382929bb0 | [
"Shell"
] | 1 | Shell | bytesun/non-fungible-token | 44ccbc28372d2059d20b963cc9572fa9bb004cbb | 3b6b2c20e43429ae559313a942ae2cb1c7026954 | |
refs/heads/master | <repo_name>LamGiang1912/Lamgiang1<file_sep>/Dropbox/nnlt2/ConsoleApplication2/ConsoleApplication2/Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ConsoleApplication1
{
class Program
{
static int[] arr = new int[100];
static int n;
static void Khoitao()
{
Console.OutputEncoding = Encoding.UTF8;
Console.WriteLine("nhap day so, Enter de bat dau nhap!!");
int i = 0;
int j = 0;
int err = 0;
int lt = 0;
bool bl = true;
bool[] kt = { true, true, true };
while ((lt != 3))
{
Console.Write("sai {0}\tarr[{1}]= ", err, i);
bl = int.TryParse(Console.ReadLine(), out arr[i]);
if (bl == true)
{
i++;
kt[j % 3] = true;
}
else
{
err++;
kt[j % 3] = false;
if ((kt[0] == kt[1]) && (kt[1] == kt[2]) && (kt[2] == kt[0]))
{
Console.WriteLine("nhap sai 3 lan lien tiep!!!!");
lt = 3;
}
if (err == 5)
{
Console.WriteLine("nhap sai 5 lan!!!!");
break;
}
}
j++;
}
n = i;
Console.WriteLine();
}
static void try1()
{
int sum = 0;
for (int i = 0; i < n; i++)
{
sum += arr[i];
}
Console.WriteLine("Tong day so: " + sum);
Console.WriteLine("Da nhap vao {0} so", n);
int am = 0;
int duong = am;
for (int i = 0; i < n; i++)
{
if (arr[i] < 0) am++;
else if (arr[i] > 0) duong++;
}
Console.WriteLine("\tTrong do co {0} so am, {1} so duong", am, duong);
}
static void Main(string[] args)
{
try
{
Khoitao();
try1();
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
Console.ReadKey();
}
}
}
| c33595eeb64b742983e1b4c47234468676b5492e | [
"C#"
] | 1 | C# | LamGiang1912/Lamgiang1 | 280a3f488efeec49b2ef6eeb69a314ea019b62c3 | 9c3c7a12b89871fb78e897a2ce189c7c1e390ab4 | |
refs/heads/master | <repo_name>aldefouw/redcap-bulk-assign-users-hook<file_sep>/README.md
# redcap-bulk-assign-users-hook
PHP, HTML, and JavaScript code that allows you to bulk assign a User Role to several different usernames.
<file_sep>/user_rights/bulkUserModuleClass.php
<?php
class bulkUserModuleClass {
private $add_bulk_user_path;
private $project_id;
function __construct($project_id) {
$this->setProjectID($project_id);
$this->setAddBulkUsersPath();
}
function setProjectID($project_id){
$this->project_id = $project_id;
}
function getProjectID(){
return $this->project_id;
}
function setAddBulkUsersPath(){
$this->add_bulk_user_path = dirname(__FILE__).'/add_bulk_users.php';
}
function getAddBulkUsersPath(){
return $this->add_bulk_user_path;
}
function getProjectRoles(){
$sql = "SELECT role_id, role_name FROM redcap_user_roles WHERE project_ID = ".$this->getProjectID();
$q = db_query($sql);
$select_menu = '<select id="bulk_add_roles">';
while ($row = db_fetch_assoc($q)) {
$select_menu .= '<option value="'.$row['role_id'].'">'.$row['role_name'].'</option>';
}
$select_menu .= '</select>';
if(db_num_rows($q)) return $select_menu;
}
function getHTMLOutput(){
if( is_file( $this->getAddBulkUsersPath() ) && strlen($this->getProjectRoles()) ) {
//Output buffer will fetch the output of this PHP file
ob_start();
require($this->getAddBulkUsersPath());
$output = ob_get_contents();
ob_end_clean();
return $output;
}
}
}
?>
<file_sep>/user_rights/add_bulk_users.php
<div id="addBulkUserRole" style="margin:20px 0;font-size:12px;font-weight:normal;padding:10px;border:1px solid #ccc;background-color:#eee;max-width:630px;">
<p><strong>Bulk Add Users to Role</strong>: Separate users with a line break.</p>
<textarea style="width:95%;height:100px;" id="addUsersToRole"></textarea>
<p><strong>Role:</strong> <?php echo $this->getProjectRoles(); ?></p>
<input type="checkbox" id="notify_all_with_email" value="1"> Notify ALL Users With Email?
<p><strong>Double check your list before submitting.</strong></p>
<p><button class="jqbuttonmed ui-button ui-widget ui-state-default ui-corner-all ui-button-text-only" id="bulkUserAddBtn" role="button"><span class="ui-button-text">Assign Users to Role</span></button></p>
</div>
<script type='text/javascript'>
var messages = '';
var loaded = [];
// Assign user to role (via ajax)
function bulkAssignUserRole(username,role_id,index) {
showProgress(1);
checkIfuserRights(username, role_id, function(data){
if(data == 1){
// Ajax request
$.post(app_path_webroot+'UserRights/assign_user.php?pid='+pid, { username: username, role_id: role_id, notify_email_role: sendEmail() }, function(data){
if (data == '') { alert(woops); return; }
$('#user_rights_roles_table_parent').html(data);
messages += $('#user_rights_roles_table_parent div.userSaveMsg')[0].innerHTML + '<br />';
}).done(function() {
loaded[index] = true;
}).fail(function() {
loaded[index] = true;
messages = "An error occurred for " + username + '.';
});
} else {
loaded[index] = true;
messages = "Please note that the role you are trying to assign yourself to does not have User Rights permissions, and thus it would prevent you from accessing the User Rights page. Try selecting another role, or modify the one you are trying to assign.";
}
});
}
function checkIfLoaded(load){
return load == true;
}
function stopLoading() {
if(loaded.every(checkIfLoaded)){
showProgress(0,0);
simpleDialogAlt(messages, 3);
enablePageJS();
messages = '';
$('textarea#addUsersToRole').val("");
} else {
setTimeout(function(){ stopLoading(); },500);
}
}
function sendEmail(){
if ( $('#notify_all_with_email').prop("checked") ){
return 1;
} else {
return 0;
}
}
$(document).ready(function(){
$("button#bulkUserAddBtn").click(function(e) {
e.preventDefault();
var users_to_add = $('textarea#addUsersToRole').val().split("\n");
//Trim whitespace and blank lines
users_to_add = $.map(users_to_add, $.trim);
for (var i in users_to_add) {
loaded[i] = false;
bulkAssignUserRole(users_to_add[i], $("select#bulk_add_roles").val(), i);
}
stopLoading();
});
});
</script><file_sep>/hooks.php
<?php
/*
ANY OTHER FILES YOU NEED TO REQUIRE UP HERE
*/
require dirname(__FILE__) . '/hooks/user_rights/bulkUserModuleClass.php';
function redcap_user_rights($project_id){
//ONLY AVAILABLE FOR SUPER ADMINS!
if (SUPER_USER) {
$add_bulk_users = new bulkUserModuleClass($project_id);
echo $add_bulk_users->getHTMLOutput();
}
}
?> | c07d2de8ae8a27bf551ec45b62a5f3c97d78e444 | [
"Markdown",
"PHP"
] | 4 | Markdown | aldefouw/redcap-bulk-assign-users-hook | d03485b3991027afb8112c8f90c4ea05f7028e81 | 6810fbd568f7e60c71714a672e92c4112eb11422 | |
refs/heads/master | <file_sep><h1>Редактировать задачу</h1>
<form method='post' action='#'>
<div class="form-group">
<label for="description">Описание</label>
<textarea rows = "5" cols = "50" class="form-control" id="description" placeholder="Введите описание" name="description" >
<?php if (isset($task["description"])) echo $task["description"];?>
</textarea>
</div>
<button type="submit" class="btn btn-primary">Сохранить</button>
</form><file_sep><h1>Создать задачу</h1>
<form method='post' action='#'>
<div class="form-group row">
<label for="title" class="col-sm-2 col-form-label">Имя пользователя</label>
<div class="col-sm-7">
<input type="text" class="form-control" id="username" placeholder="Введите имя" name="username" value="<?= $username?>" >
</div>
<?if(!empty($errors["username_error"])):?>
<div class="col-sm-3">
<small class="text-danger">
<?= $errors["username_error"]?>
</small>
</div>
<?endif;?>
</div>
<div class="form-group row">
<label for="title" class="col-sm-2 col-form-label">Email</label>
<div class="col-sm-7">
<input type="email" class="form-control" id="email" placeholder="Введите ваш email" name="email" value="<?=$email?>" >
</div>
<?if(!empty($errors["email_error"])):?>
<div class="col-sm-3">
<small class="text-danger">
<?= $errors["email_error"]?>
</small>
</div>
<?endif;?>
</div>
<div class="form-group row">
<label for="description" class="col-sm-2 col-form-label">Описание</label>
<div class="col-sm-7">
<textarea rows = "5" cols = "50" class="form-control" id="description" placeholder="Введите описание" name="description" ><?=$description?></textarea>
</div>
<?if(!empty($errors["description_error"])):?>
<div class="col-sm-3">
<small class="text-danger">
<?= $errors["description_error"]?>
</small>
</div>
<?endif;?>
</div>
<button type="submit" class="btn btn-primary">Отправить</button>
</form><file_sep><?php
class Router
{
static public function parse($url, $request)
{
$url = trim($url);
$explode_url = explode('/', $url);
$explode_url = array_slice($explode_url, 1);
$request->controller = $explode_url[0];
$action = explode("?",$explode_url[1]);
$request->action = $action[0];
$request->params = $_REQUEST;
if(!isset($request->controller) || empty($request->controller)){
header("Location: /tasks/index");
}
}
}
?><file_sep><h1>Вход</h1>
<form method='post' action='#'>
<div class="form-group row">
<label for="login" class="col-sm-2 col-form-label">Логин</label>
<div class="col-sm-7">
<input type="text" class="form-control" id="login" placeholder="Введите логин" name="login" value="<?= $login?>" >
</div>
<?if(!empty($errors["username_error"])):?>
<div class="col-sm-3">
<small class="text-danger">
<?= $errors["username_error"]?>
</small>
</div>
<?endif;?>
</div>
<div class="form-group row">
<label for="password" class="col-sm-2 col-form-label">Пароль</label>
<div class="col-sm-7">
<input type="password" class="form-control" id="password" placeholder="Введите пароль" name="password" value="<?=$password?>" >
</div>
<?if(!empty($errors["password_error"])):?>
<div class="col-sm-3">
<small class="text-danger">
<?= $errors["password_error"]?>
</small>
</div>
<?endif;?>
</div>
<button type="submit" class="btn btn-primary">Отправить</button>
</form><file_sep><?php
class Task extends Model
{
public function create($username, $email, $description)
{
$sql = "INSERT INTO tasks (username,email, description, created_at, updated_at) VALUES (:username,:email, :description, :created_at, :updated_at)";
$req = Database::getBdd()->prepare($sql);
return $req->execute([
'username' => $username,
'email' => $email,
'description' => $description,
'created_at' => date('Y-m-d H:i:s'),
'updated_at' => date('Y-m-d H:i:s')
]);
}
public function showTask($id)
{
$sql = "SELECT * FROM tasks WHERE id =" . $id;
$req = Database::getBdd()->prepare($sql);
$req->execute();
return $req->fetch();
}
public function showAllTasks($offset, $limit, $column, $sort)
{
$sql = "SELECT * FROM tasks" . " ORDER BY " . "$column $sort" . " LIMIT " . $limit . " OFFSET " . $offset;
$req = Database::getBdd()->prepare($sql);
$req->execute();
return $req->fetchAll();
}
public function edit($id, $description)
{
$sql = "UPDATE tasks SET description = :description, updated_at = :updated_at WHERE id = :id";
$req = Database::getBdd()->prepare($sql);
return $req->execute([
'id' => $id,
'description' => $description,
'updated_at' => date('Y-m-d H:i:s')
]);
}
public function setStatus($id)
{
$sql = 'UPDATE tasks SET status = 1 WHERE id = :id';
$req = Database::getBdd()->prepare($sql);
return $req->execute(["id" => $id]);
}
public function getTotalCount()
{
$sql = 'SELECT count(*) FROM tasks';
$req = Database::getBdd()->query($sql)->fetchColumn();
return $req;
}
}
?><file_sep><?php
/**
* Created by PhpStorm.
* User: Lexz
* Date: 02.06.2019
* Time: 10:06
*/
class User
{
public function login($login, $password){
$sql = "SELECT access_token FROM admin WHERE login =\"" . $login . "\" AND password=\"" . $password . "\"";
$req = Database::getBdd()->prepare($sql);
$req->execute();
return $req->fetchColumn();
}
public function logout(){
}
public function authentificated($access_token){
$sql = "SELECT access_token FROM admin WHERE access_token =\"" . $access_token . "\"";
$req = Database::getBdd()->prepare($sql);
$req->execute();
return $req->fetchColumn();
}
}<file_sep><!doctype html>
<head>
<meta charset="utf-8">
<title>Tracker Ara</title>
<link href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-beta.2/css/bootstrap.min.css" rel="stylesheet">
<link href="https://pagination.js.org/dist/2.1.4/pagination.css" rel="stylesheet">
<link href="/css/style.css" rel="stylesheet">
<style>
body {
padding-top: 5rem;
}
.starter-template {
padding: 3rem 1.5rem;
text-align: center;
}
</style>
</head>
<body>
<nav class="navbar navbar-expand-md navbar-dark bg-dark fixed-top">
<a class="navbar-brand" href="#">Tracker Ara</a>
<? if (!$isAdmin): ?>
<a class="navbar-brand" href="/auth/login">Вход</a>
<? else: ?>
<a class="navbar-brand" href="/auth/logout">Выход</a>
<? endif; ?>
</nav>
<main role="main" class="container">
<div>
<?php
echo $content_for_layout;
?>
</div>
</main>
<script src="https://code.jquery.com/jquery-3.4.1.min.js"></script>
<script src="https://kit.fontawesome.com/afa0f3ed32.js"></script>
<script src="https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script>
<script src="/js/script.js"></script>
</body>
</html>
<file_sep><?php
/**
* Created by PhpStorm.
* User: Lexz
* Date: 02.06.2019
* Time: 10:06
*/
class AuthController extends Controller
{
public function login(){
if(!$this->isAdmin()) {
require_once(ROOT . 'Models/User.php');
if (!empty($_POST["login"] && !empty($_POST["password"]))) {
$user = new User();
$login = $_POST["login"];
$password = hash("<PASSWORD>", $_POST["password"]);
if ($access = $user->login($login, $password)) {
$_SESSION["access_token"] = $access;
header("Location: /tasks/index");
} else {
$data["error"] = "Пользователь с таким логином\паролем не найден";
$this->set($data);
$this->render("login");
}
}
$this->render("login");
}else{
header("Location: /tasks/index");
}
}
public function logout(){
unset($_SESSION['access_token']);
header("Location: /tasks/index");
}
}<file_sep><?php
/**
* Created by PhpStorm.
* User: Lexz
* Date: 26.05.2019
* Time: 14:31
*/
class errorsController extends Controller
{
function index()
{
$this->render("404");
}
}<file_sep><?php
class tasksController extends Controller
{
private $limit = 3;
public function index()
{
$this->render("index");
}
public function getAllTasks($page = 1, $column = "id", $sort = "asc")
{
require(ROOT . 'Models/Task.php');
$tasks = new Task();
$offset = ($page - 1) * $this->limit;
$res_tasks = $tasks->showAllTasks($offset, $this->limit, $column, $sort);
$res_count = $tasks->getTotalCount();
$res = "";
foreach ($res_tasks as $task) {
$res .= '<tr>';
$res .= "<td>" . $task['id'] . "</td>";
$res .= "<td>" . $task['username'] . "</td>";
$res .= "<td>" . $task['email'] . "</td>";
if($task["status"] == 1){
$res .= "<td><i class=\"far fa-check-circle\"></i></td>";
}else{
$res .= "<td><i class=\"fas fa-star-of-life\"></i></td>";
}
$res .= "<td>";
if($this->isAdmin()) {
$res .= "<a class='btn btn-info btn-xs' href='/tasks/edit/?id=" . $task["id"] . "' > Редактировать</a>";
if ($task["status"] != 1) {
$res .= "<a href='/tasks/agree/?id=" . $task["id"] . "' class='btn btn-info btn-xs'></span>Завершить задачу</a>";
}
}
$res .= "</td>";
$res .= "</tr>";
}
$data["tasks"] = $res;
$data["count"] = $res_count;
$data["limit"] = $this->limit;
echo json_encode($data);
}
public function create()
{
if ($_SERVER['REQUEST_METHOD'] == "POST") {
$data = $this->validate($_POST);
if (empty($data["errors"])) {
require(ROOT . 'Models/Task.php');
$task = new Task();
if ($task->create($data["username"], $data["email"], $data["description"])) {
header("Location: /tasks/index");
}
$this->render("create");
} else {
$this->set($data);
$this->render("create");
}
} else {
$this->render("create");
}
}
public function edit($id)
{
if($this->isAdmin()) {
require(ROOT . 'Models/Task.php');
$task = new Task();
$d["task"] = $task->showTask($id);
if (isset($_POST["description"])) {
if ($task->edit($id, $_POST["description"])) {
header("Location: /tasks/index");
}
}
$this->set($d);
$this->render("edit");
}else{
header("Location: /tasks/index");
}
}
public function agree($id)
{
if($this->isAdmin()) {
require(ROOT . 'Models/Task.php');
$task = new Task();
if ($task->setStatus($id)) {
header("Location: tasks/index");
}
}else{
header("Location: /tasks/index");
}
}
private function validate($post)
{
$username = $post["username"];
$email = $post["email"];
$description = $post["description"];
$username = $this->clean($username);
$email = $this->clean($email);
$description = $this->clean($description);
$data = [];
$data["username"] = $username;
$data["email"] = $email;
$data["description"] = $description;
if (empty($username)) {
$data["errors"]["username_error"] = "Заполните имя";
}
if (empty($email)) {
$data["errors"]["email_error"] = "Заполните email";
}
if (empty($description)) {
$data["errors"]["description_error"] = "Заполните описание задачи";
}
if (!empty($data["errors"])) {
return $data;
}
$email_validate = filter_var($email, FILTER_VALIDATE_EMAIL);
if (!$email_validate) {
$data["errors"]["email_error"] = "Неправильно заполнен email";
}
return $data;
}
private function clean($value = "")
{
$value = trim($value);
$value = stripslashes($value);
$value = strip_tags($value);
$value = htmlspecialchars($value);
return $value;
}
}
?><file_sep>var current_page = 1;
var total_count = 0;
var records_per_page = 0;
var sort = "asc";
var field = 'id';
$(document).ready(function () {
load_data(current_page,field,sort);
});
function load_data(page,field,sort) {
$.ajax({
url: "/tasks/getAllTasks",
method: "POST",
data: {page: page,field:field,sort:sort},
dataType: 'json',
success: function (data) {
total_count = data.count;
records_per_page = data.limit;
$("#tasks tbody").html('');
$("#tasks tbody").append(data.tasks);
}
})
};
$("#btn_prev").on("click", function () {
if (current_page > 1) {
current_page--;
load_data(current_page,field,sort);
}
});
$("#btn_next").on("click", function () {
if (current_page < numPages()) {
current_page++;
load_data(current_page,field,sort);
}
});
$("#tasks thead th").on("click", function(){
if(typeof $(this).attr("id") == "undefined"){
return;
}
field = $(this).attr("id");
if(sort === "asc"){
sort = "desc";
}else{
sort = "asc";
}
load_data(current_page,field,sort);
});
function numPages() {
return Math.ceil(total_count / records_per_page);
}
| 3019237e8b6073d90cd853460093e57eea218d6c | [
"JavaScript",
"PHP"
] | 11 | PHP | borlano/tracker | a3e462f5e93cf39257fd27386a13ea799451e3b5 | 5e21c76d0deb7b60e89e7cff60c442f872de3046 | |
refs/heads/main | <file_sep>#!/bin/bash
# ### const value Start ###
WARNING="WARNING:"
ERROR="ERROR:"
# ### const value End ###
# venv 作成パス
VENVPATH="`cd $(dirname ${0}) && pwd`/venv"
# venv スクリプトパス
SCRIPTSPATH="$VENVPATH/bin"
echo "### Start Setup. ###"
# venv が作成されていれば venv は作成しない
echo -n "create venv ... "
if [ ! -e $VENVPATH ]; then
if [ "$(uname)" == "Darwin" ]; then
python3 -m venv $VENVPATH
elif [ "$(expr substr $(uname -s) 1 5)" == "MINGW" ]; then
python -m venv $VENVPATH
elif [ "$(expr substr $(uname -s) 1 5)" == "Linux" ]; then
python3 -m venv $VENVPATH
else
"Unknown OS"
exit 0
fi
echo "ok"
else
echo "ok"
echo "$WARNING Already exist venv! Please remove $VENVPATH if you want to clean setup."
fi
# venv を有効化
if [ "$(uname)" == "Darwin" ]; then
source "$VENVPATH/bin/activate"
elif [ "$(expr substr $(uname -s) 1 5)" == "MINGW" ]; then
. "$VENVPATH/Scripts/activate"
elif [ "$(expr substr $(uname -s) 1 5)" == "Linux" ]; then
source "$VENVPATH/bin/activate"
fi
echo -n "install library ... "
# ライブラリインストール
pip --disable-pip-version-check --quiet install -r "`cd $(dirname ${0}) && pwd`/requirements.txt"
echo "ok"
echo -e
# インストール後の環境
pip --disable-pip-version-check list
# venv を非アクティブ化
if [ "$(uname)" == "Darwin" ]; then
source "$VENVPATH/bin/deactivate"
elif [ "$(expr substr $(uname -s) 1 5)" == "MINGW" ]; then
deactivate
elif [ "$(expr substr $(uname -s) 1 5)" == "Linux" ]; then
source "$VENVPATH/bin/deactivate"
fi
echo -e
echo "### Finished Setup. ###"
exit 0<file_sep># venv_setup
| 0eb611b070f6e981dda1948306940e3c3e350e8d | [
"Markdown",
"Shell"
] | 2 | Shell | shindy-dev/venv_setup | 259aef787f0bb58a5839490304b4d13b886393dd | 5c753b21f09f0638064d14c0dbbb39da9daabe70 | |
refs/heads/master | <file_sep>-- sd-up
ALTER TABLE table2 ADD col5 text;
-- ALTER TABLE table2 ADD col6 int;
<file_sep>-- sd-up
CREATE TABLE IF NOT EXISTS table2 (
col1 uuid,
col2 boolean,
col3 int,
col4 timestamp,
PRIMARY KEY (col1)
);
<file_sep>-- sd-up
CREATE TABLE IF NOT EXISTS schemadeploy.table1 (
col1 date,
col2 counter,
PRIMARY KEY (col1)
);
-- sd-down
DROP TABLE IF EXISTS schemadeploy.table1;
| 5b624b9d851275ff97e0fb002091c86ea9e4afbd | [
"SQL"
] | 3 | SQL | cloudboys/cassandraSchemaDeploy | 7343b01f41c3afa97d3466f7537fd570cdff6da8 | 1bf5ba650512b78568bfe3fdb83aacdb19d46ac7 | |
refs/heads/master | <file_sep>#-*- coding:utf-8 -*-
import os
import hou
hip_dir = os.path.expandvars("$HIP")
log_dir = "/script/sim_log/"
density_log = hip_dir + log_dir + "density.txt"
temperature_log = hip_dir + log_dir + "temperature.txt"
heat_log = hip_dir + log_dir + "heat.txt"
padding = 4
density_max = str(hou.hscriptExpression('volumemax(0,0)'))
density_max = density_max.split('.')
density_max = density_max[0].zfill(padding) + '.' + density_max[1][:2]
temperature_max = str(hou.hscriptExpression('volumemax(0,1)'))
temperature_max = temperature_max.split('.')
temperature_max = temperature_max[0].zfill(padding) + '.' + temperature_max[1][:2]
heat_max = str(hou.hscriptExpression('volumemax(0,2)'))
heat_max = heat_max.split('.')
heat_max = heat_max[0].zfill(padding) + '.' + heat_max[1][:2]
with open(density_log, 'a') as density_log_file:
density_log_file.write(density_max + "\n")
with open(temperature_log, 'a') as temperature_log_file:
temperature_log_file.write(temperature_max + "\n")
with open(heat_log, 'a') as heat_log_file:
heat_log_file.write(heat_max + "\n")
<file_sep>
hou.hipFile.load(file_name='/home/gulliver/Desktop/Job/Study/command_line/test_v01.hip')
hip_dir = hou.hscriptExpression('$HIP')
new_geo = hou.node('/obj').createNode('geo')
font_node = new_geo.createNode('font')
font_node.parm('text').set(hip_dir)
print(hou.isUIAvailable(1))
#print(hou.ui.paneTabs())
hou.hipFile.save(file_name='/home/gulliver/Desktop/Job/Study/command_line/test_v02.hip')
#
# print(hip_dir)
# #ver = 'v' + str(hou.hscriptExpression('$VER').zfill(2))
# ver = 'v01'
# #wipver = str(hou.hscriptExpression('$WIPVER').zfill(2))
# wipver = 'w01'
# #justin = hou.hscriptFloatExpression('$JUSTIN')
# #justout = hou.hscriptFloatExpression('$JUSTOUT')
#
# panes = hou.ui.paneTabs()
#
# sceneview = None
#
# for i in panes:
# if 'Scene' in str(i.type()):
# sceneview = i
# else:
# pass
#
# flipbook_setup = sceneview.flipbookSettings()
#
# flipbook_setup.frameRange((1, 50))
# # flipbook_setup.resolution((1920,1080))
# flipbook_setup.useResolution(0)
#
# flipbook_setup.output(hip_dir + '/flipbook/' + ver + '_' + wipver + '/' + 'test_$F4.png')
# flipbook_setup.outputToMPlay(0)
#
# sceneview.flipbook()
<file_sep># Name: Oncreated.py
manager_color = (0, 0.533, 0) # green
generator_color = (1, 0.9, 0.65) # ivory
# Common node type colors. These colors affect these types in all contexts.
# Node names are matched identically with keys.
common_type_colors = {
"null": (0, 0, 0), # black
"switch": (1, 0.4, 1), # pink
"attribvop": (0.188, 0.529, 0.459), # blue green
"volumevop": (0.188, 0.529, 0.459), # blue green
"attribwrangle": (0.1, 0.37, 0.7), # blue
"volumewrangle": (0.1, 0.37, 0.7), # blue
}
# Specific/general node type colors based on context. By default, node names are matched
# by a search result. "hlight", "indirectlight", etc will all be matched to "light". To
# match identically, change the last argument to the checkDicionaryForTypeName() call
# to False
node_type_colors = {
"object": {
"null": (0, 0.4, 1), # blue
"geo": (0.8, 0.8, 0.8), # gray
"light": (1, 0.8, 0), # yellow
"ambient": (1, 0.8, 0), # yellow
"cam": (0.6, 0.8, 1), # skyblue
"bone": (0.867, 0, 0), # red
},
"dop": {
},
}
# Specific tab menu folders based on context. For example, all the nodes who
# ars located in the "Forces" tab in a DOP context will be colored orange.
tool_menu_colors = {
"dop": {
"Solvers": (0, 0.3, 1), # blue
"Objects": (0.4, 1, 0.4), # green
"Forces": (1, 0.4, 0), # orange
},
"sop": {
"Manipulate": (1, 0.8, 0), # yellow
"Import": (0.573, 0.353, 0), # brown
},
"vop": {
"Geometry": (0.4, 1, 0.4), # green
"Utility": (1, 0.4, 0), # orange
},
}
def getNodeTypeTool(node_type):
""" Get the hou.Tool entry for a particular node type. """
tools = hou.shelves.tools()
tool_name = "%s_%s" % (node_type.category().name().lower(), node_type.name())
return tools.get(tool_name)
def checkDicionaryForTypeName(type_dictionary, type_name, soft_check=True):
""" Check a dictionary for a specific node type name.
soft_check: Use a soft check by searching for the specified type
name in the string rather than an absolute string
match. This allows generic names like 'light' to
encompass several nodes at once.
"""
if soft_check:
for key, color in type_dictionary.iteritems():
if type_name.find(key) != -1:
return color
return None
else:
# Try for a direct match of the type name.
return type_dictionary.get(type_name)
# Node type information
node = kwargs["node"]
node_type = node.type()
node_type_name = node_type.name()
type_category_name = node_type.category().name().lower()
# Start with no node color:
node_color = None
# Manager nodes (sopnet, chopnet, ropnet, etc).
if node_type.isManager():
node_color = manager_color
# Common node types (nulls, switches, etc) are colored the same across
# different contexts.
elif node_type_name in common_type_colors:
node_color = common_type_colors[node_type_name]
else:
# Get the color mappings for this context.
type_colors = node_type_colors.get(type_category_name)
if type_colors is not None:
# Check to see if there ars any mappings for this type.
node_color = checkDicionaryForTypeName(type_colors, node_type_name, True)
# If there were no mappings for the specific type, check for the tab
# menu.
if node_color is None:
# Get the tool for the node type.
tool = getNodeTypeTool(node_type)
# Get a tuple of possible tab menu locations this tool will appear.
try:
menu_locations = tool.toolMenuLocations()
except AttributeError:
pass
# Get any possible colored menu locations for this context.
menu_colors = tool_menu_colors.get(type_category_name)
if menu_colors is not None:
# Iterate over all the possible locations for the tool.
try:
for location in menu_locations:
# Try and get the color of the location.
node_color = menu_colors.get(location)
# If we got a color then use it.
if node_color is not None:
break
except NameError:
pass
# If there have been no previous assignments, color generator nodes.
if node_color is None and node_type.isGenerator():
node_color = generator_color
# If we found a color mapping, set the color of the node.
if node_color is not None:
node.setColor(hou.Color(node_color))<file_sep>import hou
import os
import json
from utility_scripts import main as util
reload(util)
proj_list = 'D:\Job\Study\Rebelway\Python\Week_6\project_list.json'
def read_json():
try:
with open(proj_list, 'r') as f:
data = json.load(f)
except ValueError:
data = {}
return data
# def fix_path(old_path, new_sep='/'):
# _path = old_path.replace('\\', '/')
# _path = old_path.replace('\\\\', '/')
# _path = _path.replace('//', '/')
#
# if _path.endswith('/'):
# _path = _path[:-1]
#
# _path = _path.replace('/', new_sep)
#
# new_path = _path
# return new_path
#
#
# def error(message, severity=hou.severityType.Error):
# print('{severity}: {message}'.format(severity=severity.name(), message=message))
# hou.ui.displayMessage(message, severity=severity)
#
#
# def print_report(header, *body):
# print('\n{header:-^50}'.format(header=header))
#
# for b in body:
# print('\t{0}'.format(b))
#
# print('-'*50)
def manage_proj():
sel = hou.ui.displayMessage('Select an action', buttons=('Add', 'Remove', 'Details', 'Cancel'))
if sel == 0:
add_proj()
elif sel == 1:
remove_proj()
elif sel == 2:
details_proj()
else:
return
def add_proj():
data = read_json()
# Init
_proj_name = None
path = None
path_name = None
path_code = None
path_fps = None
# Select Proejct Path
repeat = True
while repeat:
repeat = False
_path = hou.ui.selectFile(title='Select Project Directory', file_type=hou.fileType.Directory)
path = os.path.dirname(_path)
if path == '':
return
for k, v in data.items():
if util.fix_path(path) == util.fix_path(v['PATH']):
util.error('Project path is already used by another project {0}\nPlease select a different path'.format(k))
repeat = True
break
if repeat:
continue
_proj_name = os.path.split(path)[-1]
# Select Project Name, Code and FPS
repeat = True
while repeat:
repeat = False
inputs = hou.ui.readMultiInput(message='Enter Project Data', input_labels=('Project Name', 'Project Code', 'FPS'),
buttons=('OK', 'Cancel'), initial_contents=(_proj_name, '', '24'))
if inputs[0] == 1:
return
proj_name = inputs[1][0]
proj_code = inputs[1][1]
proj_fps = inputs[1][2]
if proj_name == '' or proj_code == '' or proj_fps == '':
util.error('Please fill in all fields')
repeat = True
continue
try:
proj_fps = int(proj_fps)
except ValueError:
util.error('FPS not set to a number. \n Please enter a whole number')
repeat = True
continue
for k, v in data.items():
if proj_name == k:
util.error('Project name is already in use\nPlease select a different name')
repeat = True
break
if proj_code == v['CODE']:
util.error('Project code is already in use\nPlease select a different code')
repeat = True
break
if repeat:
continue
if proj_name and proj_code and proj_fps:
proj_data = {
'CODE' : proj_code,
'PATH' : path,
'FPS' : proj_fps
}
data[proj_name] = proj_data
if data:
with open(proj_list, 'w') as f:
json.dump(data, f, sort_keys=True, indent=4)
def remove_proj():
data = read_json()
projects = data.keys()
sel = hou.ui.selectFromList(projects, message='Select Project to Remove', title='Remove Project',
column_header='Projects', clear_on_cancel=True)
if len(sel) == 0:
return
for i in sel:
key = projects[i]
del data[key]
with open(proj_list, 'w') as f:
json.dump(data, f, sort_keys=True, indent=4)
def details_proj():
data = read_json()
projects = data.keys()
sel = hou.ui.selectFromList(projects, exclusive=True, message='Select Project to view details',
title='Project Details', column_header='Projects', clear_on_cancel=True)
if len(sel) == 0:
return
proj = projects[sel[0]]
proj_data = data[proj]
util.print_report('Project Details',
'Name: {0}'.format(proj),
'Code: {0}'.format(proj_data['CODE']),
'Path: {0}'.format(proj_data['PATH']),
'FPS: {0}'.format(proj_data['FPS'])
)
<file_sep>#-*- coding:utf-8 -*-
import hou
import os
import math
from pprint import pprint
from itertools import product
from decimal import Decimal
menu_list = []
# 파라미터에 입력된 Wedge 값들을 얻어오는 함수
def get():
global menu_list
node = hou.pwd()
parm = 'wedge_parm'
node.parm('wedge_select').set(0)
wedge_parms = node.parm(parm).multiParmInstances()
value_list = []
for i in wedge_parms:
value_list.append(i.eval())
dl = divide_list(value_list, 0, len(value_list), 6)
wedge_val_list = []
for j in dl:
# Use Set Value가 켜져있는지 체크
if j[4] == 0:
# 상승값이 0일 경우 첫번째 Parm의 값으로 리스트 구성
if j[3] == 0:
wedge_val_list.append([j[1], j[1], j[1]])
else:
wedge_val_list.append(increase(j[1], j[2], j[3]))
else:
custom_list = j[-1].split(' ')
set_val = []
for c in custom_list:
set_val.append(float(c))
wedge_val_list.append(set_val)
noc_list = list(product(*wedge_val_list))
for i, n in enumerate(noc_list):
noc_list[i] = list(n)
wirte_wedge_val(node, len(noc_list), noc_list)
menu_list = wedge_select_list(len(noc_list))
# 최종적으로 현재씬에 적용할 Wedge 값을 불러오는 함수
def import_btn():
node = hou.pwd()
parm = node.parm('wedge_select')
parm_val = parm.eval()
load_value = node.parm('wedge_list').eval().split('\n')
value_list = []
wedge_parms = node.parm('wedge_parm').multiParmInstances()
for i in wedge_parms:
if 'chs' in i.rawValue():
value_list.append(i.rawValue()[6:-3])
else:
pass
import_value_list = []
for l in load_value:
import_value_list.append(l.split(' - ')[-1])
import_value = import_value_list[parm_val][1:-1]
import_parm = node.parm('import_value')
import_parm.lock(False)
import_parm.set(import_value)
import_parm.lock(True)
set_value = import_value_list[parm_val][1:-1].split(', ')
for v, s in zip(value_list, set_value):
hou.parm(v).set(float(s))
# Wedge에 대한 값들을 Text로 출력해주는 함수
def wirte_wedge_val(node, wedge_count, noc):
text = ''
for i, n in zip(range(wedge_count), noc):
text = text + 'WEDGE ' + str(i) + ' - ' + str(n) + '\n'
list_parm = node.parm('wedge_list')
list_parm.lock(False)
list_parm.set(text)
list_parm.lock(True)
total_parm = node.parm('total_count')
total_parm.lock(False)
total_parm.set(str(wedge_count))
total_parm.lock(True)
def wedge_select_list(wedge_count):
result_list = []
for i in range(wedge_count):
result_list.append('{}'.format(i))
result_list.append('WEDGE {}'.format(i))
return result_list
# 기능 구현만 해두고 사용하지 않은 함수임
# Wedge 경우의 수에 따른 파라미터 생성
def add_parm(node):
ptg = node.parmTemplateGroup()
if ptg.parmTemplates()[-1].name() == 'wedge_list':
for i in ptg.parmTemplates()[-1].parmTemplates():
node.parm(i.name()).set(10)
else:
parm_folder = hou.FolderParmTemplate('wedge_list', 'Wedge List')
parm_folder.setFolderType(hou.folderType.Simple)
parm_folder.addParmTemplate(hou.FloatParmTemplate('first', 'First', 1))
parm_folder.addParmTemplate(hou.FloatParmTemplate('last', 'Last', 1))
ptg.append(parm_folder)
node.setParmTemplateGroup(ptg)
# 리스트를 특정 갯수로 나누는 함수
def divide_list(source_list, start_pos, end_pos, div):
div_list = []
for i in range(start_pos, end_pos + div, div):
out = source_list[start_pos:start_pos + div]
if out != []:
div_list.append(out)
start_pos = start_pos + div
return div_list
# 주어진 숫자가 상승되는 리스트
def increase(val0, val1, val2):
list = [val0, val1, val2]
icnt = list[0]
val_list = []
while (icnt <= list[1]):
icnt += list[-1]
val_list.append(float(str(icnt - list[-1]))) # 부동소수점 제거를 위해 float을 str로 변환하고 다시 float으로 변환
return val_list
<file_sep>import datetime
import hou
import os
import re
import json
import shutil
from save_scene import main as save_scene
reload(save_scene)
from utility_scripts import main as util
reload(util)
def cache_dir():
root = hou.getenv('OUTPUT_PATH')
if not root:
root = hou.getenv('HIP')
root = os.path.join(root, 'Output')
root = util.fix_path(root)
sub_folders = ['cache']
path = util.fix_path(os.sep.join(root.split('/') + sub_folders))
return path
def cache_path(mode='path'):
node = hou.pwd()
name = node.evalParm('cache_name')
if node.evalParm('enable_version'):
ver = '_v{0:0>3}'.format(node.evalParm('version'))
else:
ver = ''
if node.evalParm('trange') > 0:
frame = '.{0:0>4}'.format(int(hou.frame()))
else:
frame = ''
ext = node.evalParm('ext')
full_name = '{name}{ver}{frame}{ext}'.format(name=name, ver=ver, frame=frame, ext=ext)
path = util.fix_path(os.path.join(node.evalParm('cache_dir'), full_name))
if mode == 'path':
return path
elif mode == 'name':
return full_name
else:
return
def auto_version(node, forced=False):
if node.evalParm('enable_version') and (node.evalParm('auto_version') or forced):
cache_dir = node.evalParm('cache_dir')
if not os.access(cache_dir, os.F_OK):
node.parm('version').set(1)
return
files = os.listdir(cache_dir)
versions_list = []
for f in files:
if not os.path.isdir(os.path.join(cache_dir, f)):
regex = '(?P<name>{0})_v(?P<ver>\\d+)\\..+'.format(node.evalParm('cache_name'))
match = re.match(regex, f)
if match:
versions_list.append(int(match.group('ver')))
if len(versions_list) == 0:
node.parm('version').set(1)
return
else:
versions_list = list(set(versions_list))
versions_list.sort()
latest_version = versions_list[-1]
if forced:
node.parm('version').set(latest_version)
else:
node.parm('version').set(latest_version+1)
return
def latest_version(kwargs):
node = kwargs['node']
auto_version(node, forced=True)
node.parm('reload').pressButton()
return
def watchlist_val_parm(node_num, parm_num):
node = hou.parm('watch_node_{0}'.format(node_num)).evalAsNode()
parm_name = hou.evalParm('watch_parm_{0}_{1}'.format(node_num, parm_num))
watch_parm = node.parm(parm_name)
if not watch_parm:
return
has_keyframe = len(watch_parm.keyframes()) > 0
try:
watch_parm.expression()
is_expr = True
except hou.OperationFailed:
is_expr = False
if has_keyframe and not is_expr:
return '<< Animated Parameter >>'
elif has_keyframe and is_expr:
return '<< Expression >>'
else:
return watch_parm.evalAsString()
def watchlist_action(kwargs):
node = kwargs['node']
parent_num = kwargs['script_multiparm_index2']
parent_parm = node.parm('watch_node_{0}'.format(parent_num))
parent_node = parent_parm.evalAsNode()
if not parent_node:
util.error('Invalid node selected')
return
parm_list = []
for p in parent_node.parms():
template = p.parmTemplate()
parm_type = template.type()
if parm_type == hou.parmTemplateType.Folder or parm_type == hou.parmTemplateType.FolderSet:
continue
parm_list.append(p)
tree_list = []
for p in parm_list:
hierarchy = list(p.containingFolders())
hierarchy.append('{0} ({1})'.format(p.parmTemplate().label(), p.name()))
tree_list.append('/'.join(hierarchy))
choice = hou.ui.selectFromTree(tree_list, exclusive=True, message='Select a parameter to watch',
title='Watchlist Select', clear_on_cancel=True)
if len(choice) > 0:
idx = tree_list.index(choice[0])
parm = parm_list[idx]
val_parm = kwargs['parmtuple'][0]
name_parm = node.parm(val_parm.name()[:-4])
name_parm.set(parm.name())
return
def watchlist_write():
def set_string_attrib(attrib_name, attrib_val):
if not geo.findGlobalAttrib(attrib_name):
geo.addAttrib(hou.attribType.Global, attrib_name, '', create_local_variable=False)
geo.setGlobalAttribValue(attrib_name, attrib_val)
node = hou.pwd()
parent = node.parent()
geo = node.geometry()
set_string_attrib('hipfile_path', parent.evalParm('hipfile_path'))
set_string_attrib('hipfile_date', parent.evalParm('hipfile_date'))
set_string_attrib('hipfile_project', parent.evalParm('hipfile_project'))
set_string_attrib('hipfile_source', parent.evalParm('hipfile_source'))
set_string_attrib('hipfile_error', parent.evalParm('hipfile_error'))
watch_metadata = {}
num_watch_nodes = parent.evalParm('watchlist_parms')
for n in range(num_watch_nodes):
watch_node = parent.parm('watch_node_{0}'.format(n+1)).evalAsNode()
if not watch_node:
continue
watch_node_path = watch_node.path()
watch_metadata[watch_node_path] = {}
num_watch_parms = parent.evalParm('watch_parms_{0}'.format(n+1))
for p in range(num_watch_parms):
key = parent.evalParm('watch_parm_{0}_{1}'.format(n+1, p+1))
watch_parm = hou.parm('{0}/{1}'.format(watch_node_path, key))
if not watch_parm:
continue
has_keyframe = len(watch_parm.keyframes()) > 0
try:
watch_parm.expression()
is_expr = True
except hou.OperationFailed:
is_expr = False
if has_keyframe and not is_expr:
val = ('<< Animated Parameter >>', '<< Animated Parameter >>')
else:
val = (watch_parm.eval(), watch_parm.evalAsString())
watch_metadata[watch_node_path][key] = val
_watch_metadata = json.dumps(watch_metadata)
set_string_attrib('watchlist', _watch_metadata)
return
def watchlist_read(kwargs):
def lock_parms(val):
for p in node.parms():
if p.name().startswith('read_') and p.name().endswith('_val'):
p.lock(val)
node = kwargs['node']
read_node = node.node('READ_METADATA')
geo = read_node.geometry()
if not geo or not node.evalParm('load'):
watchlist_dict = {}
else:
try:
watchlist = geo.attribValue('watchlist')
watchlist_dict = json.loads(watchlist)
except hou.OperationFailed:
watchlist_dict = {}
lock_parms(False)
num_keys = len(watchlist_dict.keys())
node.parm('read_watchlist_parms').set(num_keys)
for i, (k, v) in enumerate(watchlist_dict.items()):
node.parm('read_watch_node_{0}'.format(i+1)).set(k)
num_parm_keys = len(v.keys())
node.parm('read_watch_parms_{0}'.format(i+1)).set(num_parm_keys)
for i2, (k2, v2) in enumerate(v.items()):
node.parm('read_watch_parm_{0}_{1}'.format(i+1, i2+1)).set(k2)
node.parm('read_watch_parm_{0}_{1}_val'.format(i+1, i2+1)).set(v2[1])
lock_parms(True)
def watchlist_restore_single(kwargs):
node = kwargs['node']
read_node = node.node('READ_METADATA')
geo = read_node.geometry()
watchlist = geo.attribValue('watchlist')
watchlist_dict = json.loads(watchlist)
parent_num = kwargs['script_multiparm_index2']
parent_parm = node.parm('read_watch_node_{0}'.format(parent_num))
parent_node = parent_parm.evalAsNode()
if not parent_node:
util.error('Node to restore to [{0}] does not exist'.format(parent_parm.evalAsString()))
return
parm_num = kwargs['script_multiparm_index']
parm = node.parm('read_watch_parm_{0}_{1}'.format(parent_num, parm_num))
parm_name = parm.eval()
parm_to_restore = parent_node.parm(parm_name)
if not parm_to_restore:
util.error('Parm to restore [{0}/{1}] does not exist'.format(parent_node.path(), parm_name))
return
vals = watchlist_dict[parent_node.path()][parm_name]
if vals[0] == '<< Animated Parameter >>':
util.error('This parm [{0}] is an Animated Parameter and cannot be restored this way'.format(parm_name),
severity=hou.severityType.Warning)
return
try:
parm_to_restore.set(vals[0])
print('Parm [{0}/{1}] restored to [{2}]'.format(parent_node.path(), parm_name, vals[1]))
except TypeError:
parm_to_restore.set(vals[1])
print('Parm [{0}/{1}] restored to [{2}]'.format(parent_node.path(), parm_name, vals[1]))
return
def save_backup_hip(node):
def generateReport(success, **kwargs):
parent.parm('hipfile_path').set('None')
parent.parm('hipfile_date').set('None')
parent.parm('hipfile_error').set('None')
parent.parm('hipfile_project').set('None')
parent.parm('hipfile_source').set('None')
if success:
util.print_report(
'Scene Backup Report',
'Status: Successful',
'Scene File: {0}'.format(kwargs['scenefile']),
'Date Created: {0}'.format(kwargs['date'])
)
parent.parm('hipfile_path').set(kwargs['scenefile'])
parent.parm('hipfile_date').set(kwargs['date'])
parent.parm('hipfile_project').set(hou.getenv('PROJECT'))
parent.parm('hipfile_source').set(hou.hipFile.basename())
else:
util.print_report(
'Scene Backup Report',
'Status Failed',
'Error Log: {0}'.format(kwargs['error'])
)
parent.parm('hipfile_error').set(kwargs['error'])
def error_report(message, severity=hou.severityType.Error):
util.error(message, severity=severity)
generateReport(False, error=message)
### Notes ###
# file = name of file
# dir = path to folder
# path = dir/file
parent = node.parent()
backup_dir = hou.getenv('HOUDINI_BACKUP_DIR')
if not backup_dir:
hip = hou.getenv('HIP')
backup_dir = util.fix_path(os.path.join(hip, 'backup'))
# hou.putenv('HOUDINI_BACKUP_DIR', backup_dir)
if not os.access(backup_dir, os.F_OK):
try:
os.makedirs(backup_dir)
except:
error_report('Unable to create backup directory')
return
old_files = os.listdir(backup_dir)
hou.hipFile.save()
hou.hipFile.saveAsBackup()
new_files = os.listdir(backup_dir)
# delta = []
# for f in new_files:
# if f not in old_files:
# delta.append(f)
delta = [f for f in new_files if f not in old_files]
if len(delta) > 1:
error_report('Unable to link backup file')
return
new_backup_file = delta[0]
if not new_backup_file:
error_report('Unable to link backup file')
return
new_backup_path = util.fix_path(os.path.join(backup_dir, new_backup_file))
cache_path = node.evalParm('sopoutput')
_split = os.path.split(cache_path)
cache_dir = util.fix_path(_split[0])
cache_file = _split[1]
cache_name = cache_file.split('.')[0]
cache_hip_dir = os.path.join(cache_dir, 'cache_hips')
if not os.access(cache_hip_dir, os.F_OK):
try:
os.makedirs(cache_hip_dir)
except:
error_report('Unable to create a directory for cache .hip files')
return
cache_hip_file = '{name}{ext}'.format(name=cache_name, ext=os.path.splitext(new_backup_file)[-1])
cache_hip_path = util.fix_path(os.path.join(cache_hip_dir, cache_hip_file))
try:
shutil.copy(new_backup_path, cache_hip_path)
except:
error_report('Unable to copy backup file to cache .hip file directory')
return
date = datetime.datetime.now()
generateReport(True, scenefile=cache_hip_path, date=str(date))
def hip_actions(action):
node = hou.pwd()
metadata_node = node.node('READ_METADATA')
hip_file = metadata_node.geometry().attribValue('hipfile_path')
if hip_file == '' or not hip_file:
return
if not os.access(hip_file, os.F_OK):
return
if action == 'open':
hou.hipFile.load(hip_file)
if action == 'explore':
util.open_explorer(hip_file)
pass
return
def restore(kwargs):
node = kwargs['node']
scene_data = None
for n in hou.node('/obj').children():
if n.type().nameComponents()[2] == 'scene_data':
scene_data = n
break
if not scene_data:
util.error('Scene Data node not found. Scene cannot be correctly restored')
return
if os.path.dirname(hou.hipFile.path()) == hou.getenv('SAVE_PATH'):
message = 'Current .hip already seems to be saved to \"working_files\" directory\n'\
'Are you sure you want to perform this operation?'
choice = hou.ui.displayMessage(message, buttons=('Yes', 'No'), severity=hou.severityType.Warning)
if choice == 1:
return
try:
metadata = node.node('READ_METADATA').geometry().attribValue('hipfile_source')
except hou.OperationFailed:
util.error('Unable to find source file in metadata')
return
regex = r'(?P<scene>^\w+)-(?P<task>\w+)-(?P<ver>v\d{3,})(-(?P<desc>\w+))?-(?P<user>\w+)\.(?P<ext>hip\w*$)'
match = re.match(regex, metadata)
if not match:
util.error('Source does not align with correct naming convertion. \n'
'Unable to restore file correctly')
return
task = match.group('task')
if not task or task == '':
util.error('Unable to find task name from metadata.\n'
'Reverting to "restore" task name', severity=hou.severityType.Warning)
task = 'restore'
desc = 'restore'
save_scene.save_scene(None, task, desc)
def prerender(node):
auto_version(node.parent())
save_backup_hip(node)
return
def preframe(node):
# print('preframe')
return
def postframe(node):
# print('postframe')
return
def postwrite(node):
# print('postwrite')
return
def postrender(node):
# print('postrender')
return<file_sep>#-*- coding:utf-8 -*-
import os
import hou
hip_dir = hou.hscriptExpression('$HIP')
log_dir = '/script/sim_log/'
node_name = hou.hscriptExpression('opname("..")')
ver = hou.hscriptExpression('$VER')
wipver = hou.hscriptExpression('$WIPVER')
density_log = open(hip_dir + log_dir + 'density.txt', 'r')
density_lead = density_log.readlines()
# 개행문자 제거
density_lead = list(map(lambda s: s.strip(), density_lead))
density_max = max(density_lead)
temperature_log = open(hip_dir + log_dir + 'temperature.txt', 'r')
temperature_lead = temperature_log.readlines()
# 개행문자 제거
temperature_lead = list(map(lambda s: s.strip(), temperature_lead))
temperature_max = max(temperature_lead)
heat_log = open(hip_dir + log_dir + 'heat.txt', 'r')
heat_lead = heat_log.readlines()
# 개행문자 제거
heat_lead = list(map(lambda s: s.strip(), heat_lead))
heat_max = max(heat_lead)
# 정수 패딩 제거
density_max = density_max.replace('0', ' ').lstrip().replace(' ', '0')
temperature_max = temperature_max.replace('0', ' ').lstrip().replace(' ', '0')
heat_max = heat_max.replace('0', ' ').lstrip().replace(' ', '0')
max_log_dir = hip_dir + "/script/" + node_name + '_v' + ver.zfill(2) + '_w' + wipver.zfill(2) + '_' +"max_log.txt"
with open(max_log_dir, 'w') as max_log:
max_log.write('density: ' + str(density_max) + "\n")
max_log.write('temperature: ' + str(temperature_max) + "\n")
max_log.write('heat: ' + str(heat_max) + "\n")
<file_sep>import hou
import json
import os
import sys
from utility_scripts import main as util
proj_list = 'D:\Job\Study\Rebelway\Python\Week_6\project_list.json'
def read_json():
try:
with open(proj_list, 'r') as f:
data = json.load(f)
except ValueError:
data = {}
return data
def create_folder_and_var(var, path):
path = util.fix_path(path)
hou.putenv(var, path)
if not os.access(path, os.F_OK):
os.makedirs(path)
print('Creating variable {0} and folder [{1}]'.format(var, path))
return
def unset_all_scene_vars():
variables = [
'SCENE',
'SCENE_PATH',
'SAVE_PATH',
'OUTPUT_PATH',
'CACHE_PATH',
'RENDER_PATH'
]
for v in variables:
hou.unsetenv(v)
return
def project_menu(kwargs):
data = read_json()
menu = ['-', '-']
for k in data.keys():
menu.append(k)
menu.append(k)
return menu
def scene_menu(kwargs):
menu = []
scenes_path = hou.getenv('SCENES_PATH')
if scenes_path and os.access(scenes_path, os.F_OK):
for f in os.listdir(scenes_path):
if os.path.isdir(util.fix_path(os.path.join(scenes_path, f))):
menu.append(f)
menu.append(f)
return menu
def set_project(kwargs):
node = kwargs['node']
project_name = node.evalParm('proj')
# project_name = kwargs['script_value']
node.parm('scene').set('')
unset_all_scene_vars()
data = read_json()
if project_name == '-':
hou.unsetenv('PROJECT')
hou.unsetenv('CODE')
hou.unsetenv('PROJ_FPS')
hou.setFps(24)
hou.unsetenv('PROJECT_PATH')
hou.unsetenv('SCENES_PATH')
hou.unsetenv('HDA_PATH')
hou.unsetenv('SCRIPTS_PATH')
node.cook(True)
return
try:
project_data = data[project_name]
except KeyError:
util.error('Project Data not found')
return
hou.putenv('PROJECT', project_name)
hou.putenv('CODE', project_data['CODE'])
fps = project_data['FPS']
hou.putenv('PROJ_FPS', str(fps))
hou.setFps(fps)
project_path = project_data['PATH']
hou.putenv('PROJECT_PATH', project_data['PATH'])
create_folder_and_var('SCENES_PATH', util.fix_path(os.path.join(project_path, 'scenes')))
hda_path = util.fix_path(os.path.join(project_path, 'hda'))
create_folder_and_var('HDA_PATH', hda_path)
scripts_path = util.fix_path(os.path.join(project_path, 'scripts'))
create_folder_and_var('SCRIPTS_PATH', scripts_path)
hda_paths = [hda_path, ]
scan_path = hou.getenv('HOUDINI_OTLSCAN_PATH')
if scan_path:
hda_paths += scan_path.split(';')
hda_paths = list(set(hda_paths))
hou.putenv('HOUDINI_OTLSCAN_PATH', ';'.join(hda_paths))
hou.hda.reloadAllFiles()
sys.path.append(scripts_path)
node.cook(True)
def set_scene(kwargs):
node = kwargs['node']
scene_name = node.evalParm('scene')
# If null scene > unset all
if scene_name == '':
unset_all_scene_vars()
node.cook(True)
return
# If no PROJECT_PATH var
project_path = hou.getenv('PROJECT_PATH')
if not project_path or not os.access(project_path, os.F_OK):
util.error('Project path is invalid')
return
# If no SCENES_PATH var
scenes_path = hou.getenv('SCENES_PATH')
if not scenes_path or not os.access(scenes_path, os.F_OK):
util.error('Scenes path is invalid')
return
# If SCENES_PATH var but no folder
if not os.access(scenes_path, os.F_OK):
os.makedirs(scenes_path)
scene_path = util.fix_path(os.path.join(scenes_path, scene_name))
if not os.access(scene_path, os.F_OK):
message = 'Scene folder "{0}" does not exist\n'\
'Create this folder now?'.format(scene_name)
choice = hou.ui.displayMessage(message, severity=hou.severityType.ImportantMessage, buttons=('Yes', 'No'))
if choice == 0:
os.makedirs(scene_path)
else:
unset_all_scene_vars()
node.cook(True)
return
hou.putenv('SCENE', scene_name)
hou.putenv('SCENE_PATH', scene_path)
create_folder_and_var('SAVE_PATH', os.path.join(scene_path, 'working_files'))
output_path = os.path.join(scene_path, 'output')
create_folder_and_var('OUTPUT_PATH', output_path)
create_folder_and_var('CACHE_PATH', os.path.join(output_path, 'cache'))
create_folder_and_var('RENDER_PATH', os.path.join(output_path, 'render'))
node.cook(True)
def onLoaded(kwargs):
node = kwargs['node']
curr_scene = node.evalParm('scene')
# print(curr_scene)
node.parm('proj').pressButton()
if not curr_scene == '':
node.parm('scene').set(curr_scene)
if curr_scene in scene_menu({}):
node.parm('scene').pressButton()
def onCreated(kwargs):
node = kwargs['node']
node.setName('SCENE_DATA')
# node.setUserData('nodeshape', 'circle')
node.setColor(hou.Color(0.58, 0.2, 0.48))
node.setGenericFlag(hou.nodeFlag.Display, False)
node.setGenericFlag(hou.nodeFlag.Selectable, False)
node.parm('proj').set(hou.getenv('PROJECT'))
node.parm('scene').set(hou.getenv('SCENE'))
<file_sep>#-*- coding:utf-8 -*-
import hou
import os
import json
import time
reload(time)
from collections import OrderedDict
# Slate에 작성될 GLOBAL 변수
hip_dir = hou.hscriptExpression("$HIP")
hip_name = hou.hscriptExpression("$HIPNAME")
# username = hou.hscriptExpression("$FXUSER")
username = 'nosung'
# Simulation Log에 대한 json 데이터를 저장할 경로
log_dir = hip_dir + "/simulation_log"
hip_dict = {'hip_dir':hip_dir, 'hip_name':hip_name, 'username':username, 'log_dir':log_dir}
houdini_os = hou.hscriptExpression("$HOUDINI_OS")
def convert_path(path):
if 'window' in houdini_os.lower():
repath = path.replace('/', '\\\\')
else:
repath = path
return repath
def read_info():
node = hou.pwd()
today = time.strftime('%Y-%m-%d', time.localtime(time.time()))
nowtime = time.strftime('%H-%M-%S', time.localtime(time.time()))
path_list = []
parm_path_list = hou.pwd().parms()
for i in list(parm_path_list):
if 'parm_path' in i.name():
path = i.rawValue().split('"')[1]
path = path
path_list.append(path)
lv_list = []
for j in path_list:
parm = hou.parm(j)
parm_label = parm.description() + ' (' + parm.name() + ')'
parm_value = parm.eval()
lv_list.append([parm_label, str(parm_value)])
parm_dict = dict(zip(range(len(lv_list)), lv_list))
for i, v in enumerate(parm_dict.items()):
parm_dict[i] = dict([parm_dict[i]])
write_dict = dict(Hip_information=hip_dict, Parameter=parm_dict)
write_json(write_dict, today, nowtime)
load_list = load_json(today, nowtime)
node.parm('title').set(hip_name)
node.parm('user').set(username)
node.parm('time').set(today + ' ' + nowtime)
text = ''
for l in load_list:
text = text + str(l)
node.parm('text').set(text + '\n')
def write_json(dict_data, today, nowtime):
json_data = OrderedDict()
json_data = dict_data
json_save_dir = log_dir + "/json"
if os.path.isdir(log_dir) == False:
os.mkdir(log_dir)
if os.path.isdir(json_save_dir) == False:
os.mkdir(json_save_dir)
json_file = json_save_dir + '/' + hip_name + '_' + today + '_' + nowtime + ".json"
with open(convert_path(json_file), 'w') as make_file:
json.dump(json_data, make_file, ensure_ascii=False, indent=4)
def load_json(today, nowtime):
json_save_dir = log_dir + "/json"
json_file = json_save_dir + '/' + hip_name + '_' + today + '_' + nowtime + ".json"
out_list = []
with open(convert_path(json_file)) as load_file:
json_data = json.load(load_file)
parm_data = json_data['Parameter']
parm_data = sorted(parm_data.items())
for data in parm_data:
k_encode = data[0].encode('utf-8')
v = data[1].items()[0]
v_encode = str(v[0]).encode('utf-8') + ': ' + str(v[1]).encode('utf-8') + '\n'
convert_json = k_encode + ' ' + v_encode
out_list.append(convert_json)
out_list.sort()
write_list = [i[2:] for i in out_list]
return write_list
<file_sep>import hou
hou.node('/obj').createNode('geo')
hou.hipFile.save(file_name='/home/gulliver/Desktop/Job/Study/command_line/test_v01.hip')
<file_sep># -*- coding:utf-8 -*-
import sys
sys.path.append("/core/inhouse/rez/packages/python_library/2.7/python/site-packages")
import pyperclip
import os
import hou
nodes = hou.selectedNodes()
cam_path_list = []
out_path_list = []
start_f_list = []
end_f_list = []
resx_list = []
resy_list = []
name_list = []
for node in nodes:
cam_path_list.append(node.parm('camera').eval())
start_f_list.append(node.parm('f1').eval())
end_f_list.append(node.parm('f2').eval())
name_list.append(node.name())
if node.parm('override_camerares').eval() == 0:
resx_list.append(hou.parm(node.parm('camera').eval() + '/resx').eval())
resy_list.append(hou.parm(node.parm('camera').eval() + '/resy').eval())
else:
res_mult = node.parm('res_fraction').eval()
resx = hou.parm(node.parm('camera').eval() + '/resx').eval()
resy = hou.parm(node.parm('camera').eval() + '/resy').eval()
resx_list.append(int(round(float(resx) * float(res_mult))))
resy_list.append(int(round(float(resy) * float(res_mult))))
node_name = node.type().name()
if 'Arnold' in node_name:
out_path_split = node.parm('ar_picture').eval().split('.')
elif 'Mantra' in node_name:
out_path_split = node.parm('vm_picture').eval().split('.')
else:
pass
out_path_split[-2] = '####'
new_path = '.'.join(out_path_split)
out_path_list.append(new_path)
def write_script(path, resx, resy, start_f, end_f, name, xpos, ypos):
head = 'set cut_paste_input [stack 0]\nversion 12.0 v3\n'
read_head = 'Read {\n inputs 0\n'
file_type = ' file_type exr\n'
file_path = ' file {}\n'.format(path)
img_format = ' format "{0} {1}"\n'.format(resx, resy)
first = ' first ' + str(int(start_f)) + '\n'
last = ' last ' + str(int(end_f)) + '\n'
origfirst = ' origfirst ' + str(int(start_f)) + '\n'
origlast = ' origlast ' + str(int(end_f)) + '\n'
origset = ' origset true\n'
version = ' version 0\n'
name = ' name {}\n'.format(name)
selected = ' selected true\n'
xpos = ' xpos {}\n'.format(xpos)
ypos = ' ypos {}\n'.format(ypos)
end = '}'
read_list = [
head,
read_head,
file_type,
file_path,
img_format,
first,
last,
origfirst,
origlast,
version,
name,
selected,
xpos,
ypos,
end
]
read_script = ''
for j in read_list:
read_script += j
return read_script
result = ''
for i, (out, resx, resy, start_f, end_f) in enumerate(zip(out_path_list, resx_list, resy_list, start_f_list, end_f_list)):
result += write_script(out_path_list[i], resx_list[i], resy_list[i], start_f_list[i], end_f_list[i], name_list[i], i*100, 0) + '\n'
pyperclip.copy(result)<file_sep>import subprocess
import os
import hou
def fix_path(old_path, new_sep='/'):
_path = old_path.replace('\\', '/')
# _path = old_path.replace('\\\\', '/')
_path = _path.replace('//', '/')
if _path.endswith('/'):
_path = _path[:-1]
_path = _path.replace('/', new_sep)
new_path = _path
return new_path
def error(message, severity=hou.severityType.Error):
print('{severity}: {message}'.format(severity=severity.name(), message=message))
hou.ui.displayMessage(message, severity=severity)
def print_report(header, *body):
print('\n{header:-^50}'.format(header=header))
for b in body:
print('\t{0}'.format(b))
print('-'*50)
def open_explorer(path):
path = os.path.dirname(path)
path = fix_path(path, os.path.sep)
open_path = 'explorer {0}'.format(path)
subprocess.Popen(open_path, shell=True)
return<file_sep>print('importing the Project Tools Module')<file_sep>import hou
import os
import re
from utility_scripts import main as util
reload(util)
def clean_name(name):
new_name = name.lower().strip()
regex = r'\W+'
new_name = re.sub(regex, '_', new_name)
return new_name
def save_scene(kwargs=None, task=None, desc=None):
if kwargs:
ctrl = kwargs['ctrlclick']
else:
ctrl = False
# Find scene_data node
scene_data = None
scene_data_nodes = []
for n in hou.node('/obj').children():
if n.type().nameComponents()[2].startswith('scene_data'):
scene_data_nodes.append(n)
if len(scene_data_nodes) > 1:
util.error('Multiple SCENE DATA nodes found\nAborting Operation')
elif len(scene_data_nodes) == 1:
scene_data = scene_data_nodes[0]
else:
util.error('SCENE DATA node not found\nPlease create one and select a scene before saving')
return
# Gather variables
save_path = hou.getenv('SAVE_PATH')
scene = hou.getenv('SCENE')
if not save_path or not scene:
util.error('Local variables not defined\nPlease use SCENE DATA node to set these correctly')
return
# Set task and desc from curr scene or initialize
curr_name = hou.hipFile.basename()
regex = r'^(?P<scene>\w+)-(?P<task>\w+)-(?P<full_ver>v(?P<ver>\d{3,}))(-(?P<desc>\w+))?-(?P<user>\w+)' \
r'(\.(?P<ext>\w+))$'
match = re.match(regex, curr_name, flags=re.IGNORECASE)
if match:
if not task:
task = match.group('task')
if not desc:
desc = match.group('desc')
if not task:
task = ''
if not desc:
desc = ''
ask_input = True
if ctrl and task != '':
ask_input = False
# Input Task and Description
if ask_input:
repeat = True
while repeat:
repeat = False
user_input = hou.ui.readMultiInput('Select Task and Description', ('Task', 'Description (optional'),
buttons=('OK', 'Cancel'), initial_contents=(task, desc))
if user_input[0] == 1:
return
task = user_input[1][0]
desc = user_input[1][1]
if task == '':
util.error('Task cannot be left blank', hou.severityType.Warning)
repeat = True
continue
# Set version
regex = '(?P<scene>{scene})-(?P<task>{task})-v(?P<ver_num>\\d{{3,}})'.format(scene=scene, task=task)
ver_nums = []
for f in os.listdir(save_path):
if not os.path.isdir(f):
match = re.match(regex, f, flags=re.IGNORECASE)
if match:
ver_nums.append(int(match.group('ver_num')))
if len(ver_nums) > 0:
high_ver = sorted(ver_nums)[-1]
ver = 'v{0:>03}'.format(high_ver+1)
else:
ver = 'v001'
# Set User
user = hou.getenv('USER').lower()
components = [scene, task, ver, desc, user]
for i, c in enumerate(components):
if c and c != '':
components[i] = clean_name(c)
else:
del components[i]
# Set extension
lic_dict = {
'Commercial': 'hip',
'Indie': 'hiplc',
'Apprentice': 'hipnc',
'ApprenticeHD': 'hipnc',
'Education': 'hipnc'
}
lic = hou.licenseCategory().name()
ext = lic_dict[lic]
# Build Filename
name = '-'.join(components)
filename = '{path}/{name}.{ext}'.format(path=save_path, name=name, ext=ext)
# Save
hou.hipFile.save(filename)<file_sep>#-*- coding:utf-8 -*-
import os
import hou
hip_dir = hou.hscriptExpression('$HIP')
log_dir = hip_dir + '/script/sim_log/'
# log_dir 경로가 없을 경우 경로를 생성하고, 있을 경우 파일을 제거
if os.path.isdir(log_dir) == False:
os.mkdir(log_dir)
else:
if os.path.isfile(log_dir + 'density.txt'):
os.remove(log_dir + 'density.txt')
if os.path.isfile(log_dir + 'temperature.txt'):
os.remove(log_dir + 'temperature.txt')
if os.path.isfile(log_dir + 'heat.txt'):
os.remove(log_dir + 'heat.txt')
| d206312343623f8584b6e718e546d99440ebcdab | [
"Python"
] | 15 | Python | zmfovlr/Houdini | b47cfd72f5183c9e6ab5243a52fb5c6b897bed65 | 0a8f188836a46650d519ea2e0704eb7f89f3b829 | |
refs/heads/master | <repo_name>UQuark0/ADS-Labs<file_sep>/src/me/uquark/univ/ads/labs/lab7/Tree.java
package me.uquark.univ.ads.labs.lab7;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Consumer;
public class Tree<T> {
public static class Optional<K> {
public final boolean isEmpty;
public final K value;
public static final Optional empty = new Optional(null, true);
public Optional(K value, boolean isEmpty) {
this.value = value;
this.isEmpty = isEmpty;
}
public Optional(K value) {
this(value, false);
}
}
public interface Searcher<T> {
boolean accepts(T value);
}
public T value;
public Tree<T> left, right;
public Tree(T value) {
this.value = value;
}
public void add(T value) {
if (this.value == null) {
this.value = value;
return;
}
if (left == null) {
left = new Tree<>(value);
return;
}
if (right == null) {
right = new Tree<>(value);
return;
}
if (left.getNodesCount(false) <= right.getNodesCount(false))
left.add(value);
else
right.add(value);
}
public static Tree fromArray(Object... array) {
if (array.length == 0)
return null;
Tree tree = new Tree(array[0]);
for (int i=1; i < array.length; i++)
tree.add(array[i]);
return tree;
}
public Optional<Tree<T>> delete(T value) {
if (this.value.equals(value)) {
delete(left, right);
if (left != null)
return new Optional<>(left);
if (right != null)
return new Optional<>(right);
return new Optional<>(null);
}
Optional<Tree<T>> newTree;
if (left != null) {
newTree = left.delete(value);
if (!newTree.isEmpty) {
left = newTree.value;
return Optional.empty;
}
} else {
if (right != null) {
newTree = right.delete(value);
if (!newTree.isEmpty) {
right = newTree.value;
return Optional.empty;
}
}
return Optional.empty;
}
if (right != null) {
newTree = right.delete(value);
if (!newTree.isEmpty) {
right = newTree.value;
return Optional.empty;
}
}
return Optional.empty;
}
public void delete(Tree<T> newLeft, Tree<T> newRight) {
if (left != null) {
left.delete(null, right);
} else if (right != null) {
right.delete(left, null);
}
if (newLeft != null)
left = newLeft;
if (newRight != null)
right = newRight;
}
public int getNodesCount(boolean root) {
int nodes = 0;
if (root)
nodes = 1;
if (left != null)
nodes += left.getNodesCount(false) + 1;
if (right != null)
nodes += right.getNodesCount(false) + 1;
return nodes;
}
public List<Tree<T>> getNodes(boolean root) {
List<Tree<T>> list = new ArrayList<>();
if (root)
list.add(this);
list.add(left);
list.add(right);
if (left != null) {
list.addAll(left.getNodes(false));
}
if (right != null) {
list.addAll(right.getNodes(false));
}
return list;
}
public void forEachNode(Consumer<Tree<T>> consumer) {
List<Tree<T>> nodes = getNodes(true);
for (Tree<T> node : nodes)
consumer.accept(node);
}
public Tree<T> find(Searcher<T> searcher) {
if (searcher.accepts(value))
return this;
Tree<T> result = null;
if (left != null)
result = left.find(searcher);
if (result != null)
return result;
if (right != null)
result = right.find(searcher);
return result;
}
public List<Tree<T>> findAll(Searcher<T> searcher) {
List<Tree<T>> result = new ArrayList<>();
if (searcher.accepts(value))
result.add(this);
if (left != null)
result.addAll(left.findAll(searcher));
if (right != null)
result.addAll(right.findAll(searcher));
return result;
}
}
<file_sep>/src/me/uquark/univ/ads/labs/Lab6.java
package me.uquark.univ.ads.labs;
import me.uquark.univ.ads.labs.lab4.Task1;
public class Lab6 {
private static class Queue<T extends Task1.Printable> extends Task1.ForwardLinkedList<T> {
public void push(T object) {
addToEnd(object);
}
public T pop() {
if (begin == null)
return null;
T result = begin.object;
begin = begin.next;
return result;
}
}
private static class PrintableFloat implements Task1.Printable {
public final float value;
public PrintableFloat(float value) {
this.value = value;
}
@Override
public void print() {
System.out.print(value);
}
}
public static void main(String[] args) {
Queue<PrintableFloat> queue = new Queue<>();
queue.push(new PrintableFloat(-2.2f));
queue.push(new PrintableFloat(2.3f));
queue.push(new PrintableFloat(2.2f));
queue.push(new PrintableFloat(5.1f));
queue.push(new PrintableFloat(6.7f));
queue.printAll(" ");
System.out.println();
queue.pop();
queue.pop();
queue.pop();
queue.push(new PrintableFloat(1.9f));
queue.printAll(" ");
}
}
/*
Контрольні запитання
1. Що таке черга?
2. Опишіть порядок роботи з чергою.
3. Які ви знаєте операції над чергами?
4. Що таке стек?
5. Опишіть порядок роботи зі стеком.
1. Очередь - это структура данных, реализующая модель FIFO (First In, First Out)
2. В очередь можно добавлять элементы (push) и удалять элементы (pop)
3. См. п. 2
4. Стек - это структура данных, реализующая модель LIFO (Last In, First Out)
5. В стек можно добалять элементы (push) и удалять элементы (pop)
*/<file_sep>/README.md
# ADS-Labs
## <NAME>.
### Вариант 2
Ответы на контрольные вопросы находятся в .java файлах как комментарии.<file_sep>/src/me/uquark/univ/ads/labs/lab4/Task1.java
package me.uquark.univ.ads.labs.lab4;
public class Task1 {
public interface Printable {
void print();
}
public static class Element<T> {
public final T object;
public Element<T> next, previous;
public Element(T object) {
this.object = object;
}
}
public static class ForwardLinkedList<T extends Printable> {
public interface Searcher<K> {
boolean compare(K object);
}
public Element<T> begin = null, end = null;
public void addToEnd(T object) {
if (begin == null || end == null) {
begin = new Element<>(object);
end = begin;
return;
}
Element<T> newElement = new Element<>(object);
newElement.previous = end;
end.next = newElement;
end = newElement;
}
public void delete(int index) {
Element<T> current = begin;
for (int i=0; i < index; i++)
if (current == null || current.next == null)
return;
else
current = current.next;
current.previous.next = current.next;
current.next.previous = current.previous;
}
public void add(int index, T object) {
Element<T> current = begin;
if (current == null) {
addToEnd(object);
return;
}
for (int i=0; i < index; i++)
if (current.next == null)
return;
else
current = current.next;
Element<T> newElement = new Element<>(object);
if (current.next == null)
addToEnd(object);
else
current.next = newElement;
}
public T find(Searcher<T> searcher) {
Element<T> current = begin;
while (current != null) {
if (searcher.compare(current.object))
return current.object;
current = current.next;
}
return null;
}
public void printAll(String separator) {
Element<T> current = begin;
while (current != null) {
current.object.print();
System.out.print(separator);
current = current.next;
}
}
public int getCount() {
int counter = 0;
Element<T> current = begin;
while (current != null) {
counter++;
current = current.next;
}
return counter;
}
}
public static class LoopedForwardLinkedList<T extends Printable> extends ForwardLinkedList<T> {
@Override
public void addToEnd(T object) {
super.addToEnd(object);
end.next = begin;
}
@Override
public void printAll(String separator) {
Element<T> current = begin;
while (current != null) {
current.object.print();
System.out.print(separator);
current = current.next;
if (current == begin)
break;
}
}
@Override
public T find(Searcher<T> searcher) {
Element<T> current = begin;
while (current != null) {
if (searcher.compare(current.object))
return current.object;
current = current.next;
if (current == begin)
break;
}
return null;
}
@Override
public int getCount() {
int counter = 0;
Element<T> current = begin;
while (current != null) {
counter++;
current = current.next;
if (current == begin)
break;
}
return counter;
}
}
}
<file_sep>/LICENSE.md
Copyright (C) 2020 <NAME>
| b24d5ae9e115566014b32e8baf53d7670ee9701c | [
"Markdown",
"Java"
] | 5 | Java | UQuark0/ADS-Labs | 997b0d064b831f9927da9fd7f4b824e44a2fd130 | bed5761a28ebea2b420db1beec8d9a398b89f28b | |
refs/heads/main | <repo_name>sargull-1998/Mybrary<file_sep>/routes/books.js
const express= require('express')
const router= express.Router()
// const path = require('path')
// const fs = require('fs')
const Book= require('../models/book')
const Author= require('../models/author')
// const uploadPath = path.join('public',Book.coverImageBasePath)
const imageMimeTypes = ['image/jpeg','image/png','image/gif','image/jpg']
//const upload = multer({
// dest: uploadPath,
//fileFilter: (req,file,callback) => {
//callback(null,imageMimeTypes.includes(file.mimetype))
//}
// })
// all books route
router.get('/', async (req,res) =>{
let query = Book.find()
if(req.query.title != null && req.query.title != ''){
query = query.regex('title', new RegExp( req.query.title, 'i'))
}
if(req.query.publishedBefore != null && req.query.publishedBefore != ''){
query = query.lte('publishDate',req.query.publishedBefore)
}
if(req.query.publishedAfter != null && req.query.publishedAfter != ''){
query = query.gte('publishDate',req.query.publishedAftere)
}
try{
const books = await query.exec()
res.render('books/index',{
books: books,
searchOptions: req.query
})
}catch{
res.redirect('/')
}
})
// new book route
router.get('/new', async (req,res) =>{
renderNewPage(res, new Book())
})
//create book route
router.post('/', async (req,res)=>{
// const fileName = req.file !=null ? req.file.filename : null
const book = new Book({
title: req.body.title,
author: req.body.author.trim(),
publishDate: new Date (req.body.publishDate),
pageCount: parseInt(req.body.pageCount),
description: req.body.description,
//coverImageName: fileName
})
saveCover(book ,req.body.cover)
try {
const newBook = await book.save()
//res.redirect(`books/${newBook.id}`)
res.redirect(`books`)
} catch {
// if(book.coverImageName != null) {
//removeBookCover(book.coverImageName)
//}
renderNewPage(res, book(), true)
}
})
//function removeBookCover(fileName){
//fs.unlink(path.join(uploadPath,fileName), err =>{
//if(err) console.error(err)
//})
//}
async function renderNewPage(res,book, hasError = false){
try {
const authors = await Author.find({})
const params = {
authors: authors,
book: book
}
if (hasError) params.errorMessage = 'Error Creating Book'
res.render('books/new',params)
} catch {
res.redirect('/books')
}
}
function saveCover (book , coverEncoded) {
if(coverEncoded == null) return
const cover = JSON.parse(coverEncoded)
if(cover != null && imageMimeTypes.includes(cover.type)){
book.coverImage = new Buffer.from(cover.data,'base64')
book.coverImageType = cover.type
}
}
module.exports = router
| ce3c9ee76c12ff625c46d03fdf6ea30e52f59c14 | [
"JavaScript"
] | 1 | JavaScript | sargull-1998/Mybrary | b693062c32dae615de95c448e33ab064e57ebd77 | 157c085c8ba2ab07ce9039f75dbc58e9a831caae | |
refs/heads/master | <repo_name>ocadotechnology/newrelic-alerts-configurator<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/exception/NewRelicSyncException.java
package com.ocadotechnology.newrelic.alertsconfigurator.exception;
public class NewRelicSyncException extends RuntimeException {
public NewRelicSyncException(String message) {
super(message);
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/model/transactions/KeyTransaction.java
package com.ocadotechnology.newrelic.apiclient.model.transactions;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.ocadotechnology.newrelic.apiclient.model.applications.ApplicationSummary;
import com.ocadotechnology.newrelic.apiclient.model.applications.EndUserSummary;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Value;
import java.time.OffsetDateTime;
/**
* See <a href="https://rpm.newrelic.com/api/explore/key_transactions/list">Doc</a>
*/
@Value
@Builder
@AllArgsConstructor
public class KeyTransaction {
@JsonProperty
Integer id;
@JsonProperty
String name;
@JsonProperty("transaction_name")
String transactionName;
@JsonProperty("health_status")
String healthStatus;
@JsonProperty
Boolean reporting;
@JsonProperty("last_reported_at")
OffsetDateTime lastReportedAt;
@JsonProperty("application_summary")
ApplicationSummary applicationSummary;
@JsonProperty("end_user_summary")
EndUserSummary endUserSummary;
@JsonProperty
KeyTransactionLinks links;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/graphql/apiclient/internal/GraphqlAlertsPoliciesApi.java
package com.ocadotechnology.newrelic.graphql.apiclient.internal;
import com.ocadotechnology.newrelic.apiclient.AlertsPoliciesApi;
import com.ocadotechnology.newrelic.apiclient.model.policies.AlertsPolicy;
import com.ocadotechnology.newrelic.apiclient.model.policies.AlertsPolicyChannels;
import com.ocadotechnology.newrelic.graphql.apiclient.internal.transport.JavaLikeEnum;
import com.ocadotechnology.newrelic.graphql.apiclient.internal.transport.Query;
import com.ocadotechnology.newrelic.graphql.apiclient.internal.transport.Result;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
import static com.github.k0kubun.builder.query.graphql.GraphQLQueryBuilder.query;
import static com.ocadotechnology.newrelic.graphql.apiclient.internal.util.Preconditions.checkArgument;
import static java.util.Objects.nonNull;
public class GraphqlAlertsPoliciesApi extends ApiBase implements AlertsPoliciesApi {
private final long accountId;
public GraphqlAlertsPoliciesApi(QueryExecutor executor, long accountId) {
super(executor);
this.accountId = accountId;
}
@Override
public Optional<AlertsPolicy> getByName(String alertsPolicyName) {
String query = query()
.object("actor",
object(actor -> actor.object("account", param("id", accountId),
object(account -> account.object("alerts",
object(alerts -> alerts.object(
"policiesSearch",
param("searchCriteria", complexParamValue(param("name", alertsPolicyName))),
object(policiesSearch -> policiesSearch.object("policies", object(policies -> policies
.field("id")
.field("name")
.field("incidentPreference"))))))))))).build();
List<AlertsPolicy> policies = execute(Query.wrapped(query)).getPolicies();
return policies.isEmpty() ? Optional.empty() : Optional.ofNullable(policies.get(0));
}
public Optional<AlertsPolicy> getById(int policyId) {
return getPolicy(doGetById(policyId));
}
private Result doGetById(int policyId) {
String query = query()
.object("actor",
object(actor -> actor.object("account", param("id", accountId),
object(account -> account.object("alerts",
object(alerts -> alerts.object("policy", param("id", policyId),
object(policy -> policy
.field("id")
.field("name")
.field("incidentPreference"))))))))).build();
return execute(Query.wrapped(query));
}
private Optional<AlertsPolicy> getPolicy(Result result) {
return result.notFound() ? Optional.empty() : throwOnError(result).getAlertsPolicy();
}
@Override
public AlertsPolicy create(AlertsPolicy policy) {
checkArgument(nonNull(policy), "policy must not be null");
checkArgument(nonNull(policy.getName()), "name must not be null");
checkArgument(nonNull(policy.getIncidentPreference()), "incident preference must not be null");
String query = query()
.object("mutation",
object(mutation -> mutation.object(
"alertsPolicyCreate",
params(
"accountId", accountId,
"policy", complexParamValue(params(
"name", policy.getName(),
"incidentPreference", new JavaLikeEnum(policy.getIncidentPreference())))),
object(alertsPolicyCreate -> alertsPolicyCreate
.field("id")
.field("name")
.field("incidentPreference"))))).build();
return throwOnError(execute(Query.of(query))).getAlertsPolicyCreate().get();
}
@Override
public AlertsPolicy delete(int policyId) {
AlertsPolicy policy = throwOnError(doGetById(policyId)).getAlertsPolicy().get();
String query = query()
.object("mutation",
object(mutation -> mutation.object(
"alertsPolicyDelete",
params(
"accountId", accountId,
"id", "" + policyId),
object(alertsPolicyDelete -> alertsPolicyDelete.field("id")))))
.build();
throwOnError(execute(Query.of(query)));
return policy;
}
@Override
public AlertsPolicyChannels updateChannels(AlertsPolicyChannels channels) {
checkArgument(nonNull(channels), "channels must not be null");
checkArgument(nonNull(channels.getPolicyId()), "policy id must not be null");
String query = query()
.object("mutation",
object(mutation -> mutation.object(
"alertsNotificationChannelsAddToPolicy",
params(
"accountId", accountId,
"policyId", channels.getPolicyId(),
"notificationChannelIds", Optional.ofNullable(channels.getChannelIds()).orElseGet(ArrayList::new)),
object(alertsNotificationChannelsAddToPolicy -> alertsNotificationChannelsAddToPolicy
.object("notificationChannels", object(notificationChannels -> notificationChannels
.field("id")))
.object("errors", object(errors -> errors
.field("description")
.field("errorType")
.field("notificationChannelId")))))))
.build();
List<Integer> notificationChannelIds = throwOnError(execute(Query.of(query)), Result::getAlertsNotificationChannelsAddToPolicy)
.orElseGet(ArrayList::new);
return AlertsPolicyChannels.builder().policyId(channels.getPolicyId()).channelIds(notificationChannelIds).build();
}
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/condition/NrqlConditionDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.NrqlCondition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal.NrqlSignalConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.NrqlTermsConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition.terms.NrqlTermConfigurations
@NewRelicConfigurationMarker
class NrqlConditionDsl {
var conditionName: String? = null
var enabled: Boolean = true
var runBookUrl: String? = null
var valueFunction: NrqlCondition.ValueFunction? = null
var query: String? = null
internal val terms: MutableList<NrqlTermsConfiguration> = mutableListOf()
var nrqlSignalConfiguration: NrqlSignalConfiguration? = null
fun terms(block: NrqlTermConfigurations.() -> Unit) = terms.addAll(NrqlTermConfigurations().apply(block).terms)
fun signal(block: NrqlSignalConfigurationDsl.() -> Unit) {
nrqlSignalConfiguration = nrqlSignalConfiguration(block)
}
}
fun nrqlCondition(block: NrqlConditionDsl.() -> Unit): NrqlCondition {
val dsl = NrqlConditionDsl()
dsl.block()
return NrqlCondition.builder()
.conditionName(requireNotNull(dsl.conditionName) { "Nrql condition name cannot be null" })
.enabled(dsl.enabled)
.runBookUrl(dsl.runBookUrl)
.terms(dsl.terms)
.valueFunction(requireNotNull(dsl.valueFunction) { "Nrql condition value cannot be null" })
.query(requireNotNull(dsl.query) { "Nrql condition query cannot be null" })
.signal(dsl.nrqlSignalConfiguration)
.build()
}<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/model/synthetics/Monitor.java
package com.ocadotechnology.newrelic.apiclient.model.synthetics;
import java.util.Collection;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Singular;
import lombok.Value;
/**
* See <a href="https://docs.newrelic.com/docs/apis/synthetics-rest-api/monitor-examples/manage-synthetics-monitors-rest-api">Doc</a>
*/
@Value
@Builder
@AllArgsConstructor
public class Monitor {
@JsonProperty("id")
String uuid;
@JsonProperty
String name;
@JsonProperty
String type;
@JsonProperty
Integer frequency;
@JsonProperty
@Singular
Collection<String> locations;
@JsonProperty
String uri;
@JsonProperty
String status;
@JsonProperty
Double slaThreshold;
@JsonProperty
Integer userId;
@JsonProperty
String apiVersion;
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/violationclosetimer/ViolationCloseTimer.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.violationclosetimer;
import lombok.AccessLevel;
import lombok.AllArgsConstructor;
import lombok.Getter;
@Getter
@AllArgsConstructor(access = AccessLevel.PACKAGE)
public enum ViolationCloseTimer {
DURATION_1("1"),
DURATION_2("2"),
DURATION_4("4"),
DURATION_8("8"),
DURATION_12("12"),
DURATION_24("24");
String duration;
}
<file_sep>/settings.gradle
rootProject.name = 'newrelic-alerts-configurator'
include 'newrelic-api-client', 'newrelic-alerts-configurator', 'newrelic-alerts-configurator-examples', 'newrelic-alerts-configurator-dsl'
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/ApiBase.java
package com.ocadotechnology.newrelic.apiclient.internal;
import com.ocadotechnology.newrelic.apiclient.internal.client.NewRelicClient;
import com.ocadotechnology.newrelic.apiclient.internal.model.ObjectList;
import javax.ws.rs.client.Invocation.Builder;
import javax.ws.rs.core.Link;
import javax.ws.rs.core.Response;
import static javax.ws.rs.core.MediaType.APPLICATION_JSON_TYPE;
abstract class ApiBase {
protected final NewRelicClient client;
ApiBase(NewRelicClient client) {
this.client = client;
}
<T, LT extends ObjectList<T, LT>> LT getPageable(Builder request, Class<LT> responseType) {
Response response = request.get();
LT responseBody = response.readEntity(responseType);
Link next = response.getLink("next");
return followPageable(next, responseBody, responseType);
}
private <T, LT extends ObjectList<T, LT>> LT followPageable(Link next, LT responseBody, Class<LT> responseType) {
while (next != null) {
Response nextResponse = client.target(next).request(APPLICATION_JSON_TYPE).get();
LT nextResponseBody = nextResponse.readEntity(responseType);
responseBody = responseBody.merge(nextResponseBody);
next = nextResponse.getLink("next");
}
return responseBody;
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/model/servers/ServerLinks.java
package com.ocadotechnology.newrelic.apiclient.model.servers;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.Value;
@Value
public class ServerLinks {
@JsonProperty("alert_policy")
Integer alertPolicy;
}
<file_sep>/MIGRATION.md
# Migrating from version 2 to 3
## New Conditions and Channels management policy
In version 3 we changed the way condition and channel synchronization works. In version 3 all conditions and channels are managed
in the same way.
1. If you don't define any conditions in the policy, synchronization process will not modify already existing conditions.
Example:
```java
PolicyConfiguration configuration = PolicyConfiguration.builder()
.policyName("Policy name")
.incidentPreference(PolicyConfiguration.IncidentPreference.PER_POLICY)
.build()
```
This configuration will not remove, create or update any existing condition.
1. If you define some conditions in the policy, then:
- conditions which are defined in configuration, but are missing will be created.
- conditions which are defined in configuration and already exist will be updated.
- conditions which are not defined in configuration, but already exist will be removed.
Example:
```java
PolicyConfiguration configuration = PolicyConfiguration.builder()
.policyName("Policy name")
.incidentPreference(PolicyConfiguration.IncidentPreference.PER_POLICY)
.condition(condition1)
.condition(condition2)
.build()
```
This configuration will create/update condition1 and condition2, and will remove all other conditions.
1. If you define empty conditions list in the policy, then synchronization process will remove all existing conditions.
Example:
```java
PolicyConfiguration configuration = PolicyConfiguration.builder()
.policyName("Policy name")
.incidentPreference(PolicyConfiguration.IncidentPreference.PER_POLICY)
.conditions(Collections.emptyList())
.build()
```
This configuration will not create nor update any conditions, but will remove all existing ones.
In version 2 there was no way to preserve already existing conditions. Existing conditions were removed in both cases:
when you
provided empty conditions list and when you didn't provide anything at all
## Changed default condition state
In version 2 all conditions were by default disabled. In version 3 it has been changed and conditions are by default enabled<file_sep>/newrelic-alerts-configurator/src/test/java/com/ocadotechnology/newrelic/alertsconfigurator/ApmUserDefinedConditionConfiguratorTest.java
package com.ocadotechnology.newrelic.alertsconfigurator;
import com.google.common.collect.ImmutableList;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.PolicyConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ApmAppCondition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.Condition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.UserDefinedConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.DurationTerm;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.OperatorTerm;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.PriorityTerm;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.TermsConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.TimeFunctionTerm;
import com.ocadotechnology.newrelic.alertsconfigurator.internal.entities.EntityResolver;
import com.ocadotechnology.newrelic.apiclient.model.conditions.AlertsCondition;
import com.ocadotechnology.newrelic.apiclient.model.policies.AlertsPolicy;
import org.assertj.core.groups.Tuple;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.junit.rules.ExpectedException;
import org.mockito.ArgumentCaptor;
import org.mockito.Captor;
import org.mockito.InjectMocks;
import org.mockito.Mock;
import java.util.Collections;
import java.util.Optional;
import static org.assertj.core.api.Assertions.assertThat;
import static org.mockito.Matchers.any;
import static org.mockito.Matchers.eq;
import static org.mockito.Mockito.verify;
import static org.mockito.Mockito.when;
public class ApmUserDefinedConditionConfiguratorTest extends AbstractConfiguratorTest {
@Rule
public final ExpectedException expectedException = ExpectedException.none();
private static final String POLICY_NAME = "policyName";
private static final String METRIC = "userDefined";
private static final String CONDITION_NAME = "conditionName";
private static final boolean ENABLED = true;
private static final String APPLICATION_NAME = "applicationName";
private static final int APPLICATION_ENTITY_ID = 1;
private static final ApmAppCondition.ConditionScope CONDITION_SCOPE = ApmAppCondition.ConditionScope.APPLICATION;
private static final AlertsPolicy POLICY = AlertsPolicy.builder().id(42).name(POLICY_NAME).build();
private static final TermsConfiguration TERMS_CONFIGURATION = createTermsConfiguration().build();
private static final UserDefinedConfiguration USER_DEFINED_CONFIGURATION = createUserDefinedConfiguration();
private static final Condition APP_CONDITION = createAppCondition(CONDITION_NAME);
private static final PolicyConfiguration CONFIGURATION = createConfiguration();
@Captor
private ArgumentCaptor<AlertsCondition> alertsConditionCaptor;
@Mock
private EntityResolver entityResolverMock;
@InjectMocks
private ConditionConfigurator testee;
@Before
public void setUp() {
when(alertsPoliciesApiMock.getByName(POLICY_NAME)).thenReturn(Optional.of(POLICY));
when(entityResolverMock.resolveEntities(apiMock, APP_CONDITION)).thenReturn(Collections.singletonList(APPLICATION_ENTITY_ID));
}
@Test
public void shouldCorrectlyCreateUserDefinedCondition() throws Exception {
//given
when(alertsConditionsApiMock.list(POLICY.getId())).thenReturn(ImmutableList.of());
when(alertsConditionsApiMock.create(eq(POLICY.getId()), any(AlertsCondition.class))).thenReturn(AlertsCondition.builder().build());
//when
testee.sync(CONFIGURATION);
//then
verify(alertsConditionsApiMock).create(eq(POLICY.getId()), alertsConditionCaptor.capture());
AlertsCondition result = alertsConditionCaptor.getValue();
assertThat(result.getConditionScope()).isEqualTo("application");
assertThat(result.getType()).isEqualTo("apm_app_metric");
assertThat(result.getName()).isEqualTo(CONDITION_NAME);
assertThat(result.getEnabled()).isEqualTo(true);
assertThat(result.getEntities()).containsExactly(APPLICATION_ENTITY_ID);
assertThat(result.getMetric()).isEqualTo("user_defined");
assertThat(result.getTerms())
.extracting("duration", "operator", "priority", "threshold", "timeFunction")
.containsExactly(new Tuple("5", "above", "critical", "0.5", "all"));
assertThat(result.getUserDefined().getMetric()).isEqualTo(METRIC);
assertThat(result.getUserDefined().getValueFunction()).isEqualTo("average");
}
private static PolicyConfiguration createConfiguration() {
return PolicyConfiguration.builder()
.policyName(POLICY_NAME)
.incidentPreference(PolicyConfiguration.IncidentPreference.PER_POLICY)
.condition(APP_CONDITION)
.build();
}
private static TermsConfiguration.TermsConfigurationBuilder createTermsConfiguration() {
return TermsConfiguration.builder()
.durationTerm(DurationTerm.DURATION_5)
.operatorTerm(OperatorTerm.ABOVE)
.priorityTerm(PriorityTerm.CRITICAL)
.thresholdTerm(0.5f)
.timeFunctionTerm(TimeFunctionTerm.ALL);
}
private static ApmAppCondition createAppCondition(String conditionName) {
return ApmAppCondition.builder()
.conditionName(conditionName)
.enabled(ENABLED)
.application(APPLICATION_NAME)
.metric(ApmAppCondition.Metric.USER_DEFINED)
.conditionScope(CONDITION_SCOPE)
.term(TERMS_CONFIGURATION)
.userDefinedConfiguration(USER_DEFINED_CONFIGURATION)
.build();
}
private static UserDefinedConfiguration createUserDefinedConfiguration() {
return UserDefinedConfiguration.builder()
.metric(METRIC)
.valueFunction(UserDefinedConfiguration.ValueFunction.AVERAGE).build();
}
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/channel/SlackChannel.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.internal.SlackChannelTypeSupport;
import lombok.Builder;
import lombok.Getter;
import lombok.NonNull;
/**
* Slack channel configuration.
* Configuration parameters:
* <ul>
* <li>{@link #channelName}</li>
* <li>{@link #slackUrl}</li>
* <li>{@link #teamChannel} (optional)</li>
* </ul>
*/
@Builder
@Getter
public class SlackChannel implements Channel {
private final ChannelType type = ChannelType.SLACK;
/**
* Name of your alerts channel
*/
@NonNull
private String channelName;
/**
* Slack url to your channel
*/
@NonNull
private String slackUrl;
/**
* Name of your team channel
*/
private String teamChannel;
private final ChannelTypeSupport channelTypeSupport = new SlackChannelTypeSupport(this);
@Override
public ChannelTypeSupport getChannelTypeSupport() {
return channelTypeSupport;
}
}
<file_sep>/CONTRIBUTING.md
## How to contribute to NewRelic Alerts Configurator
#### Did you find a bug?
- Ensure the bug was not already reported by searching on GitHub under [Issues](https://github.com/ocadotechnology/newrelic-alerts-configurator/issues).
- Open new issue. Include a title and description with as much information as possible.
Code samples and test cases demonstrating the problem or steps to reproduce would be a great help.
#### Did you write a patch that fixes a bug?
- Make sure an issue describing the bug exists.
- Open a new GitHub [pull request](https://help.github.com/categories/collaborating-with-issues-and-pull-requests/) with the
patch.
#### Do you intend to add a new feature or change an existing one?
- Open a new GitHub [pull request](https://help.github.com/categories/collaborating-with-issues-and-pull-requests/) with the
patch. Describe introduced feature in details. Remember to provide unit tests and documentation.
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/internal/entities/IdProvider.java
package com.ocadotechnology.newrelic.alertsconfigurator.internal.entities;
import com.ocadotechnology.newrelic.apiclient.NewRelicApi;
public interface IdProvider {
Integer getId(NewRelicApi api, String name);
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/terms/TermsUtils.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms;
import com.ocadotechnology.newrelic.apiclient.model.conditions.Terms;
import java.util.Collection;
import java.util.stream.Collectors;
public final class TermsUtils {
private TermsUtils() {
}
public static Collection<Terms> createTerms(Collection<TermsConfiguration> termsConfigurations) {
return termsConfigurations.stream().map(TermsUtils::mapTerms).collect(Collectors.toList());
}
private static Terms mapTerms(TermsConfiguration termsConfiguration) {
return Terms.builder()
.duration(String.valueOf(termsConfiguration.getDurationTerm().getDuration()))
.operator(termsConfiguration.getOperatorTerm().name().toLowerCase())
.priority(termsConfiguration.getPriorityTerm().name().toLowerCase())
.threshold(String.valueOf(termsConfiguration.getThresholdTerm()))
.timeFunction(termsConfiguration.getTimeFunctionTerm().name().toLowerCase())
.build();
}
public static Collection<Terms> createNrqlTerms(Collection<NrqlTermsConfiguration> termsConfigurations) {
return termsConfigurations.stream().map(TermsUtils::mapNrqlTerms).collect(Collectors.toList());
}
private static Terms mapNrqlTerms(NrqlTermsConfiguration termsConfiguration) {
return Terms.builder()
.duration(String.valueOf(termsConfiguration.getDurationTerm().getDuration()))
.operator(termsConfiguration.getOperatorTerm().name().toLowerCase())
.priority(termsConfiguration.getPriorityTerm().name().toLowerCase())
.threshold(String.valueOf(termsConfiguration.getThresholdTerm()))
.timeFunction(termsConfiguration.getTimeFunctionTerm().name().toLowerCase())
.build();
}
}
<file_sep>/newrelic-alerts-configurator-dsl/README.md
# Table of contents
- [Configurator DSL](#configurator-dsl)
- [Application configuration DSL](#application-configuration-dsl)
- [Policy configuration DSL](#policy-configuration-dsl)
- Alerts condition
- [APM application metric condition DSL](#apm-application-metric-condition-dsl)
- [APM JVM metric condition DSL](#apm-jvm-metric-condition-dsl)
- [APM key transaction metric condition DSL](#apm-key-transaction-metric-condition-dsl)
- [Server metric condition DSL](#server-metric-condition-dsl)
- [New Relic Browser metric condition DSL](#new-relic-browser-metric-condition-dsl)
- Alerts external service condition
- [APM external service condition DSL](#apm-external-service-condition-dsl)
- [Alerts NRQL condition DSL](#alerts-nrql-condition-dsl)
- [Synthetics Monitor Failure condition DSL](#synthetics-monitor-failure-condition-dsl)
- [User defined configuration DSL](#user-defined-configuration-dsl)
- [Term DSL](#term-configurations-dsl)
- [NRQL Term DSL](#nrql-term-configuration-dsl)
- Notification channel
- [Email channel DSL](#email-channel-dsl)
- [Slack channel DSL](#slack-channel-dsl)
- [Webhook channel DSL](#webhook-channel-dsl)
- [PagerDuty channel DSL](#pagerduty-channel-dsl)
- [NewRelic user channel DSL](#newrelic-user-channel-dsl)
## Configurator DSL
To create and run [Configurator](../newrelic-alerts-configurator#configurator) use `configuration` dsl.
```kotlin
val appConfig: ApplicationConfiguration
val policyConfig: PolicyConfiguration
configuration(yourApiKey) {
applications {
application { /* configuration described in Application configuration DSL section */ }
application { /* configuration described in Application configuration DSL section */ }
+appConfig // if you already have ApplicationConfiguration defined, you can add it by using unary plus operator
}
policies {
policy { /* configuration described in Policy configuration DSL section */ }
policy { /* configuration described in Policy configuration DSL section */ }
policy { /* configuration described in Policy configuration DSL section */ }
+policyConfig // if you already have PolicyConfiguration defined, you can add it by using unary plus operator
}
}
```
This will create `Configurator`, set applications and policies, and *run* synchronization process
# Application configuration DSL
To create [ApplicationConfiguration](../newrelic-alerts-configurator#application-configuration) use `applicationConfiguration` dsl.
```kotlin
val config: ApplicationConfiguration = applicationConfiguration {
applicationName = "test"
appApdexThreshold = 0.5f
endUserApdexThreshold = 0.7f
enableRealUserMonitoring = true
}
```
## Policy configuration DSL
To create [PolicyConfiguration](../newrelic-alerts-configurator#policy-configuration) use `policyConfiguration` dsl.
```kotlin
val conditionConfig: ApmJvmCondition
val channelConfig: EmailChannel
val config: PolicyConfiguration = policyConfiguration {
policyName = "Application Policy"
incidentPreference = PolicyConfiguration.IncidentPreference.PER_POLICY
conditions {
nrql { /* configuration described in NRQL condition DSL section */ }
apmApp { /* configuration described in APM application metric condition DSL section */ }
apmJvm { /* configuration described in Apm JVM condition DSL section */ }
browser { /* configuration described in New Relic browser metric condition DSL section */ }
synthetics { /* configuration described in Synthetics Monitor Failure condition DSL section */ }
serversMetric { /* configuration described in Servers metric condition DSL section */ }
apmKeyTransation { /* configuration described in Apm key transaction condition DSL section */ }
apmExternalService { /* configuration described in APM external service condition DSL section */ }
+conditionConfig // if you already have condition defined, you can add it by using unary plus operator
}
channels {
user { /* configuration described in User channel DSL section */ }
email { /* configuration described in Email channel DSL section */ }
slack { /* configuration described in Slack channel DSL section */ }
webhook { /* configuration described in Webhook channel DSL section */ }
pagerDuty { /* configuration described in PagerDuty channel DSL section */ }
+channelConfig // if you already have channel defined, you can add it by using unary plus operator
}
}
```
## APM application metric condition DSL
To create [ApmAppCondition](../newrelic-alerts-configurator#apm-application-metric-condition) use `apmAppCondition` dsl.
```kotlin
val termConfig: TermsConfiguration
val userDefinedConfig: UserDefinedConfiguration
val config: ApmAppCondition = apmAppCondition {
conditionName = "Condition name"
enabled = true
applications = listOf("app1", "app2")
metric = ApmAppCondition.Metric.APDEX
conditionScope = ApmAppCondition.ConditionScope.APPLICATION
runBookUrl = "runBookUrl"
violationCloseTimer = ViolationCloseTimer.DURATION_24
userDefined { /* configuration described in User defined configuration DSL section */ }
// if you already have user defined configuration, you can add it:
// userDefinedConfiguration = userDefinedConfig
terms {
term { /* configuration described in Term configuration DSL section */ }
term { /* configuration described in Term configuration DSL section */ }
+termConfig // if you already have term defined, you can add it by using unary plus operator
// you can also use special fluent syntax to define term
PriorityTerm.CRITICAL given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.BELOW value 0.7f
}
}
```
## APM JVM metric condition DSL
To create [ApmJVMCondition](../newrelic-alerts-configurator#apm-jvm-metric-condition) use `apmJvmCondition` dsl.
```kotlin
val termConfig: TermsConfiguration
val config: ApmJVMCondition = apmJvmCondition {
conditionName = "Condition name"
enabled = true
applications = listOf("app1", "app2")
metric = ApmJvmCondition.Metric.GC_CPU_TIME
gcMetric = ApmJvmCondition.GcMetric.GC_MARK_SWEEP
runBookUrl = "runBookUrl"
violationCloseTimer = ViolationCloseTimer.DURATION_24
terms {
term { /* configuration described in Term configuration DSL section */ }
term { /* configuration described in Term configuration DSL section */ }
+termConfig // if you already have term defined, you can add it by using unary plus operator
// you can also use special fluent syntax to define term
PriorityTerm.CRITICAL given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.BELOW value 0.7f
}
}
```
## APM key transaction metric condition DSL
To create [ApmKeyTransactionCondition](../newrelic-alerts-configurator#apm-key-transaction-metric-condition) use `apmKeyTransactionCondition` dsl.
```kotlin
val termConfig: TermsConfiguration
val config: ApmKeyTransactionCondition = apmKeyTransactionCondition {
conditionName = "Condition name"
enabled = true
keyTransaction = "Key Transaction name"
metric = ApmKeyTransactionCondition.Metric.APDEX
runBookUrl = "runBookUrl"
terms {
term { /* configuration described in Term configuration DSL section */ }
term { /* configuration described in Term configuration DSL section */ }
+termConfig // if you already have term defined, you can add it by using unary plus operator
// you can also use special fluent syntax to define term
PriorityTerm.CRITICAL given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.BELOW value 0.7f
}
}
```
## Server metric condition DSL
To create [ServersMetricCondition](../newrelic-alerts-configurator#server-metric-condition) use `serverMetricCondition` dsl.
```kotlin
val termConfig: TermsConfiguration
val userDefinedConfig: UserDefinedConfiguration
val config: ServersMetricCondition = serverMetricCondition {
conditionName = "Condition name"
enabled = true
servers = listOf("some-host", "some-host-2")
metric = ServersMetricCondition.Metric.CPU_PERCENTAGE
runBookUrl = "runBookUrl"
userDefined { /* configuration described in User defined configuration DSL section */ }
// if you already have user defined configuration, you can add it:
// userDefinedConfiguration = userDefinedConfig
terms {
term { /* configuration described in Term configuration DSL section */ }
term { /* configuration described in Term configuration DSL section */ }
+termConfig // if you already have term defined, you can add it by using unary plus operator
// you can also use special fluent syntax to define term
PriorityTerm.CRITICAL given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.BELOW value 0.7f
}
}
```
## New Relic browser metric condition DSL
To create [BrowserCondition](../newrelic-alerts-configurator#new-relic-browser-metric-condition) use `browserCondition` dsl.
```kotlin
val termConfig: TermsConfiguration
val userDefinedConfig: UserDefinedConfiguration
val config: BrowserCondition = browserCondition {
conditionName = "Condition name"
enabled = true
applications = listOf("application 1", "application 2")
metric = BrowserCondition.Metric.PAGE_VIEWS_WITH_JS_ERRORS
runBookUrl = "runBookUrl"
userDefined { /* configuration described in User defined configuration DSL section */ }
// if you already have user defined configuration, you can add it:
// userDefinedConfiguration = userDefinedConfig
terms {
term { /* configuration described in Term configuration DSL section */ }
term { /* configuration described in Term configuration DSL section */ }
+termConfig // if you already have term defined, you can add it by using unary plus operator
// you can also use special fluent syntax to define term
PriorityTerm.CRITICAL given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.BELOW value 0.7f
}
}
```
## APM external service condition DSL
To create [ApmExternalServiceCondition](../newrelic-alerts-configurator#apm-external-service-condition) use `apmExternalServiceCondition` dsl.
```kotlin
val termConfig: TermsConfiguration
val config: ApmExternalServiceCondition = apmExternalServiceCondition {
conditionName = "Condition name"
enabled = true
applications = listOf("application 1", "application 2")
externalServiceUrl = "externalServiceUrl"
metric = ApmExternalServiceCondition.Metric.RESPONSE_TIME_AVERAGE
runBookUrl = "runBookUrl"
terms {
term { /* configuration described in Term configuration DSL section */ }
term { /* configuration described in Term configuration DSL section */ }
+termConfig // if you already have term defined, you can add it by using unary plus operator
// you can also use special fluent syntax to define term
PriorityTerm.CRITICAL given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.BELOW value 0.7f
}
}
```
## Alerts NRQL condition DSL
To create [NrqlCondition](../newrelic-alerts-configurator#alerts-nrql-condition) use `nrqlCondition` dsl.
```kotlin
val termConfig: NrqlTermsConfiguration
val config: NrqlCondition = nrqlCondition {
conditionName = "Condition name"
enabled = true
valueFunction = NrqlCondition.ValueFunction.SINGLE_VALUE
query = "SELECT count(*) FROM `myApp:HealthStatus` WHERE healthy IS false"
runBookUrl = "runBookUrl"
terms {
term { /* configuration described in NRQL term configuration DSL section */ }
term { /* configuration described in NRQL term configuration DSL section */ }
+termConfig // if you already have term defined, you can add it by using unary plus operator
// you can also use special fluent syntax to define term
PriorityTerm.CRITICAL given TimeFunctionTerm.ALL inLast NrqlDurationTerm.DURATION_5 minutes OperatorTerm.BELOW value 0.7f
}
signal {
aggregationWindow = 1
evaluationWindows = 15
signalFillOption = SignalFillOption.LAST_KNOWN_VALUE
signalLost {
signalIsLostAfter = 22
openNewViolationOnSignalLost = true
closeCurrentViolationsOnSignalLost = false
}
}
}
```
## Synthetics Monitor Failure condition DSL
To create [SyntheticsCondition](../newrelic-alerts-configurator#synthetics-monitor-failure-condition) use `syntheticsCondition` dsl.
```kotlin
val config: SyntheticsCondition = syntheticsCondition {
conditionName = "MyMonitor failed"
enabled = true
monitorName = "MyMonitor"
runBookUrl = "runBookUrl"
}
```
## User defined configuration DSL
To create [UserDefinedConfiguration](../newrelic-alerts-configurator#user-defined-configuration) use `userDefinedConfiguration` dsl.
```kotlin
val config: UserDefinedConfiguration = userDefinedConfiguration {
metric = "MY_CUSTOM_METRIC"
valueFunction = UserDefinedConfiguration.ValueFunction.MAX
}
```
## Term configurations DSL
To create [TermsConfiguration](../newrelic-alerts-configurator#term) use `termsConfiguration` dsl.
```kotlin
val config: TermsConfiguration = termsConfiguration {
durationTerm = DurationTerm.DURATION_5
operatorTerm = OperatorTerm.BELOW
thresholdTerm = 0.8f
priorityTerm = PriorityTerm.CRITICAL
timeFunctionTerm = TimeFunctionTerm.ALL
}
```
## NRQL term configuration DSL
To create [NrqlTermsConfiguration](../newrelic-alerts-configurator#nrql-term) use `nrqlTermsConfiguration` dsl.
```kotlin
val config: NrqlTerms = nrqlTermsConfiguration {
durationTerm = NrqlDurationTerm.DURATION_5
operatorTerm = OperatorTerm.BELOW
thresholdTerm = 0.8f
priorityTerm = PriorityTerm.CRITICAL
timeFunctionTerm = TimeFunctionTerm.ALL
}
```
## Email channel DSL
To create [EmailChannel](../newrelic-alerts-configurator#email-channel) use `emailChannel` dsl.
```kotlin
val config: EmailChannel = emailChannel {
channelName = "Channel name"
emailAddress = "<EMAIL>"
includeJsonAttachment = true
}
```
## Slack channel DSL
To create [SlackChannel](../newrelic-alerts-configurator#slack-channel) use `slackChannel` dsl.
```kotlin
val config: SlackChannel = slackChannel {
channelName = "Channel name"
slackUrl = "https://hooks.slack.com/services/..."
teamName = "My team slack name"
}
```
## Webhook channel DSL
To create [WebhookChannel](../newrelic-alerts-configurator#webhook-channel) use `webhookChannel` dsl.
```kotlin
val config: WebhookChannel = webhookChannel {
channelName = "Channel name"
baseUrl = "https://example.com/webhook/abcd123"
authUsername = "john.doea"
authPassword = "<PASSWORD>"
}
```
## PagerDuty channel DSL
To create [PagerDutyChannel](../newrelic-alerts-configurator#pagerduty-channel) use `pagerDutyChannel` dsl.
```kotlin
val config: PagerDutyChannel = pagerDutyChannel {
channelName = "Channel name"
serviceKey = "<KEY>"
}
```
## NewRelic user channel DSL
To create [UserChannel](../newrelic-alerts-configurator#newrelic-user-channel) use `userChannel` dsl.
```kotlin
val config: UserChannel = userChannel {
emailAddress = "<EMAIL>"
}
```<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/internal/entities/ApplicationIdProvider.java
package com.ocadotechnology.newrelic.alertsconfigurator.internal.entities;
import com.ocadotechnology.newrelic.alertsconfigurator.exception.NewRelicSyncException;
import com.ocadotechnology.newrelic.apiclient.NewRelicApi;
import com.ocadotechnology.newrelic.apiclient.model.applications.Application;
import java.util.Optional;
import static java.lang.String.format;
class ApplicationIdProvider implements IdProvider {
@Override
public Integer getId(NewRelicApi api, String name) {
Optional<Application> applicationOptional = api.getApplicationsApi().getByName(name);
Application application = applicationOptional.orElseThrow(
() -> new NewRelicSyncException(format("Application %s does not exist", name)));
return application.getId();
}
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/channel/UserChannelDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.channel
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.UserChannel
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class UserChannelDsl {
var userEmail: String? = null
}
fun userChannel(block: UserChannelDsl.() -> Unit): UserChannel {
val dsl = UserChannelDsl()
dsl.block()
return UserChannel.builder()
.userEmail(requireNotNull(dsl.userEmail) { "User channel name cannot be null" })
.build()
}<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/ExternalServiceCondition.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.TermsConfiguration;
import java.util.Collection;
/**
* Alerts external service condition configuration.
* Implementations:
* <ul>
* <li>{@link ApmExternalServiceCondition}</li>
* </ul>
*/
public interface ExternalServiceCondition {
/**
* Returns condition type. Each ExternalServiceCondition implementation should have unique type.
*
* @return condition type
*/
ExternalServiceConditionType getType();
/**
* Returns condition type in string format.
*
* @return condition type
*/
String getTypeString();
/**
* Returns name of the condition.
*
* @return condition name
*/
String getConditionName();
/**
* Returns if condition is enabled.
*
* @return {@code true} if condition is enabled, {@code false} otherwise
*/
boolean isEnabled();
/**
* Returns collection of entities for which this condition is applied.
* The type of the entity depends on concrete condition implementation. This can be an application
* name or host name for example.
* <p>
* If entity with given name does not exist an exception will be thrown.
*
* @return entity names
*/
Collection<String> getEntities();
/**
* Returns URL of the external service to be monitored. This string must not include the protocol.
*
* @return external service URL
*/
String getExternalServiceUrl();
/**
* Returns metric used in given condition.
*
* @return metric
*/
String getMetric();
/**
* Returns the runbook URL to display in notifications.
*
* @return runbook URL
*/
String getRunBookUrl();
/**
* Returns collection of terms used for alerts condition.
*
* @return terms
*/
Collection<TermsConfiguration> getTerms();
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/signal/ExpirationUtils.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal;
import com.ocadotechnology.newrelic.apiclient.model.conditions.Expiration;
public final class ExpirationUtils {
private ExpirationUtils() {
}
public static Expiration createExpiration(SignalLostConfiguration signalLostConfiguration) {
if (signalLostConfiguration == null) {
return null;
}
return Expiration.builder()
.expirationDuration(String.valueOf(signalLostConfiguration.getSignalIsLostAfter()))
.closeViolationsOnExpiration(signalLostConfiguration.isCloseCurrentViolationsOnSignalLost())
.openViolationOnExpiration(signalLostConfiguration.isOpenNewViolationOnSignalLost())
.build();
}
}
<file_sep>/newrelic-api-client/src/test/java/com/ocadotechnology/newrelic/apiclient/NewRelicApiIntegrationTest.java
package com.ocadotechnology.newrelic.apiclient;
import com.github.tomakehurst.wiremock.junit.WireMockClassRule;
import com.ocadotechnology.newrelic.apiclient.model.applications.Application;
import com.ocadotechnology.newrelic.apiclient.model.channels.AlertsChannel;
import org.apache.commons.io.IOUtils;
import org.apache.http.HttpHeaders;
import org.junit.Before;
import org.junit.ClassRule;
import org.junit.Test;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.nio.charset.Charset;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
import static com.github.tomakehurst.wiremock.client.WireMock.*;
import static org.apache.http.HttpStatus.SC_OK;
import static org.assertj.core.api.Assertions.assertThat;
public class NewRelicApiIntegrationTest {
@ClassRule
public static final WireMockClassRule WIRE_MOCK = new WireMockClassRule(6766);
private static final String APPLICATION_JSON = "application/json";
private static final Charset UTF_8 = Charset.forName("UTF-8");
private NewRelicApi testee;
private String applications;
private String channels1;
private String channels2;
@Before
public void setUp() throws IOException {
WIRE_MOCK.resetMappings();
testee = new NewRelicRestApi("http://localhost:6766", "http://localhost:6766", "secret");
applications = IOUtils.toString(NewRelicApiIntegrationTest.class.getResource("/applications.json"), UTF_8);
channels1 = IOUtils.toString(NewRelicApiIntegrationTest.class.getResource("/channels1.json"), UTF_8);
channels2 = IOUtils.toString(NewRelicApiIntegrationTest.class.getResource("/channels2.json"), UTF_8);
}
@Test
public void shouldGetApplicationByNameCorrectly() throws IOException {
// given
newRelicReturnsApplications();
// when
Optional<Application> app = testee.getApplicationsApi().getByName("application_name");
// then
assertThat(app).isNotEmpty();
}
@Test
public void shouldGetPaginatedChannelsCorrectly() {
// given
newRelicReturnsPaginatedChannels();
// when
List<AlertsChannel> channels = testee.getAlertsChannelsApi().list();
// then
Set<Integer> channelsIds = channels.stream().map(AlertsChannel::getId).collect(Collectors.toSet());
assertThat(channelsIds).containsExactlyInAnyOrder(1, 2);
}
private void newRelicReturnsApplications() throws UnsupportedEncodingException {
String queryParam = URLEncoder.encode("filter[name]", UTF_8.name());
WIRE_MOCK.addStubMapping(
get(urlPathEqualTo("/v2/applications.json"))
.withQueryParam(queryParam, equalTo("application_name"))
.withHeader("X-Api-Key", equalTo("secret"))
.willReturn(aResponse()
.withStatus(SC_OK)
.withHeader(HttpHeaders.CONTENT_TYPE, APPLICATION_JSON)
.withBody(applications)
).build());
}
private void newRelicReturnsPaginatedChannels() {
WIRE_MOCK.addStubMapping(
get(urlPathEqualTo("/v2/alerts_channels.json"))
.withHeader("X-Api-Key", equalTo("secret"))
.willReturn(aResponse()
.withStatus(SC_OK)
.withHeader(HttpHeaders.CONTENT_TYPE, APPLICATION_JSON)
.withHeader("Link",
"<http://localhost:6766/v2/alerts_channels.json?page=2>; rel=\"next\", " +
"<http://localhost:6766/v2/alerts_channels.json?page=2>; rel=\"last\"")
.withBody(channels1)
).build());
WIRE_MOCK.addStubMapping(
get(urlPathEqualTo("/v2/alerts_channels.json"))
.withQueryParam("page", equalTo(String.valueOf(2)))
.withHeader("X-Api-Key", equalTo("secret"))
.willReturn(aResponse()
.withStatus(SC_OK)
.withHeader(HttpHeaders.CONTENT_TYPE, APPLICATION_JSON)
.withHeader("Link",
"<http://localhost:6766/v2/alerts_channels.json?page=2>; rel=\"last\"")
.withBody(channels2)
).build());
}
}
<file_sep>/newrelic-api-client/src/test/java/com/ocadotechnology/newrelic/graphql/apiclient/internal/testutil/TestResources.java
package com.ocadotechnology.newrelic.graphql.apiclient.internal.testutil;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.*;
public class TestResources {
private static final ObjectMapper OBJECT_MAPPER = new ObjectMapper();
public static InputStream inputStream(String fileName) {
return TestResources.class.getResourceAsStream(fileName);
}
public static <T> T fromJson(String fileName, Class<T> clazz) {
try {
return OBJECT_MAPPER.readValue(new InputStreamReader(inputStream(fileName)), clazz);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
public static String fromJson(String fileName) {
try (BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream(fileName)))) {
StringBuilder builder = new StringBuilder();
while (reader.ready()) {
builder.append(reader.readLine()).append("\n");
}
return builder.toString();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/condition/UserDefinedConfigurationDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.UserDefinedConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class UserDefinedConfigurationDsl {
var metric: String? = null
var valueFunction: UserDefinedConfiguration.ValueFunction? = null
}
fun userDefinedConfiguration(block: UserDefinedConfigurationDsl.() -> Unit): UserDefinedConfiguration {
val dsl = UserDefinedConfigurationDsl()
dsl.block()
return UserDefinedConfiguration.builder()
.metric(requireNotNull(dsl.metric) { "User defined configuration metric cannot be null" })
.valueFunction(requireNotNull(dsl.valueFunction) { "User defined configuration value cannot be null" })
.build()
}<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/ApplicationsApi.java
package com.ocadotechnology.newrelic.apiclient;
import com.ocadotechnology.newrelic.apiclient.model.applications.Application;
import java.util.Optional;
public interface ApplicationsApi {
/**
* Gets {@link Application} object using its name.
*
* @param applicationName name of the application registered in NewRelic
* @return Optional containing {@link Application} object, or empty if application not found
*/
Optional<Application> getByName(String applicationName);
/**
* Updates {@link Application} object.
*
* @param applicationId id of the application to be updated
* @param application application definition to be updated
* @return updated {@link Application}
*/
Application update(int applicationId, Application application);
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/NrqlCondition.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal.NrqlSignalConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.NrqlTermsConfiguration;
import java.util.Collection;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Getter;
import lombok.NonNull;
import lombok.Singular;
/**
* NRQL condition.
* Configuration parameters:
* <ul>
* <li>{@link #conditionName}</li>
* <li>{@link #enabled} (optional)</li>
* <li>{@link #runBookUrl} (optional)</li>
* <li>{@link #terms}</li>
* <li>{@link #valueFunction}</li>
* <li>{@link #query}</li>
* <li>{@link #signal}</li>
* </ul>
*/
@Getter
@Builder
public class NrqlCondition {
public static final int DEFAULT_VIOLATION_TIME_LIMIT_SECONDS = 259_200;
/**
* Name of your NRQL condition
*/
@NonNull
private String conditionName;
/**
* If your NRQL condition is enabled. Default is true
*/
@Builder.Default
private boolean enabled = true;
/**
* Used to automatically close instance-based incidents after the number of seconds specified.
* <br>
* Default is {@link #DEFAULT_VIOLATION_TIME_LIMIT_SECONDS}. Maximum is 30 days.
* <br>
* Used for:
* <li>Location conditions</li>
* <li>NRQL conditions</li>
* @see <a href="https://docs.newrelic.com/docs/alerts-applied-intelligence/new-relic-alerts/advanced-alerts/rest-api-alerts/alerts-conditions-api-field-names/#violation_time_limit_seconds">Alerts conditions API field names - violation_time_limit_seconds</a>
*/
@Builder.Default
private Integer violationTimeLimitSeconds = DEFAULT_VIOLATION_TIME_LIMIT_SECONDS;
/**
* The runbook URL to display in notifications
*/
private String runBookUrl;
/**
* Collection of terms used for alerts condition
*/
@NonNull
@Singular
private Collection<NrqlTermsConfiguration> terms;
/**
* Value function
*/
@NonNull
private ValueFunction valueFunction;
/**
* This is NRQL query that will be executed by the condition.
*/
@NonNull
private String query;
/**
* NRQL Signal configuration.
*/
private NrqlSignalConfiguration signal;
@Getter
@AllArgsConstructor
public enum ValueFunction {
SINGLE_VALUE("single_value"),
SUM("sum");
private final String valueString;
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/model/AlertsPolicyWrapper.java
package com.ocadotechnology.newrelic.apiclient.internal.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.ocadotechnology.newrelic.apiclient.model.policies.AlertsPolicy;
import lombok.Value;
@Value
public class AlertsPolicyWrapper {
@JsonProperty
AlertsPolicy policy;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/graphql/apiclient/internal/transport/NotificationChannels.java
package com.ocadotechnology.newrelic.graphql.apiclient.internal.transport;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import lombok.Value;
import java.util.List;
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
public class NotificationChannels implements Page<List<NotificationChannel>> {
List<NotificationChannel> channels;
String nextCursor;
@Override
public List<NotificationChannel> getContent() {
return getChannels();
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/model/policies/AlertsPolicyChannels.java
package com.ocadotechnology.newrelic.apiclient.model.policies;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Value;
import java.util.Collection;
@Value
@Builder
@AllArgsConstructor
public class AlertsPolicyChannels {
@JsonProperty("id")
Integer policyId;
@JsonProperty("channel_ids")
Collection<Integer> channelIds;
}
<file_sep>/newrelic-alerts-configurator/src/test/java/com/ocadotechnology/newrelic/alertsconfigurator/ExternalServiceConditionConfiguratorTest.java
package com.ocadotechnology.newrelic.alertsconfigurator;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.PolicyConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ApmExternalServiceCondition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ExternalServiceCondition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ExternalServiceConditionType;
import com.ocadotechnology.newrelic.apiclient.PolicyItemApi;
import com.ocadotechnology.newrelic.apiclient.model.conditions.Terms;
import com.ocadotechnology.newrelic.apiclient.model.conditions.external.AlertsExternalServiceCondition;
import org.junit.Before;
import java.util.Collections;
import static org.mockito.Mockito.when;
public class ExternalServiceConditionConfiguratorTest extends AbstractPolicyItemConfiguratorTest<ExternalServiceConditionConfigurator, AlertsExternalServiceCondition> {
private static final ApmExternalServiceCondition.Metric METRIC = ApmExternalServiceCondition.Metric.RESPONSE_TIME_AVERAGE;
private static final String EXTERNAL_SERVICE_URL = "externalServiceUrl";
private static final ExternalServiceCondition EXTERNAL_SERVICE_CONDITION = createCondition(CONDITION_NAME);
private static final AlertsExternalServiceCondition ALERTS_CONDITION_SAME = createConditionBuilder().id(1).build();
private static final AlertsExternalServiceCondition ALERTS_CONDITION_FROM_CONFIG = createConditionBuilder().build();
private static final AlertsExternalServiceCondition ALERTS_CONDITION_UPDATED = createConditionBuilder().id(2).enabled(!ENABLED).build();
private static final AlertsExternalServiceCondition ALERTS_CONDITION_DIFFERENT = createConditionBuilder().id(3).name("different").build();
private static final PolicyConfiguration CONFIGURATION = createConfiguration();
@Before
public void setUp() {
super.setUp();
when(entityResolverMock.resolveEntities(apiMock, EXTERNAL_SERVICE_CONDITION)).thenReturn(Collections.singletonList(APPLICATION_ENTITY_ID));
testee = new ExternalServiceConditionConfigurator(apiMock, entityResolverMock);
}
@Override
protected PolicyConfiguration getPolicyConfiguration() {
return CONFIGURATION;
}
@Override
protected PolicyItemApi<AlertsExternalServiceCondition> getPolicyItemApiMock() {
return alertsExternalServiceConditionsApiMock;
}
@Override
protected AlertsExternalServiceCondition getItemFromConfig() {
return ALERTS_CONDITION_FROM_CONFIG;
}
@Override
protected AlertsExternalServiceCondition getItemSame() {
return ALERTS_CONDITION_SAME;
}
@Override
protected AlertsExternalServiceCondition getItemUpdated() {
return ALERTS_CONDITION_UPDATED;
}
@Override
protected AlertsExternalServiceCondition getItemDifferent() {
return ALERTS_CONDITION_DIFFERENT;
}
private static PolicyConfiguration createConfiguration() {
return PolicyConfiguration.builder()
.policyName(POLICY_NAME)
.incidentPreference(INCIDENT_PREFERENCE)
.externalServiceCondition(EXTERNAL_SERVICE_CONDITION)
.build();
}
private static ApmExternalServiceCondition createCondition(String conditionName) {
return ApmExternalServiceCondition.builder()
.conditionName(conditionName)
.enabled(ENABLED)
.application(APPLICATION_NAME)
.metric(METRIC)
.externalServiceUrl(EXTERNAL_SERVICE_URL)
.term(TERMS_CONFIGURATION)
.build();
}
private static AlertsExternalServiceCondition.AlertsExternalServiceConditionBuilder createConditionBuilder() {
return AlertsExternalServiceCondition.builder()
.type(ExternalServiceConditionType.APM.getTypeString())
.name(CONDITION_NAME)
.enabled(ENABLED)
.entity(APPLICATION_ENTITY_ID)
.metric(METRIC.name().toLowerCase())
.externalServiceUrl(EXTERNAL_SERVICE_URL)
.term(Terms.builder()
.duration(String.valueOf(TERMS_CONFIGURATION.getDurationTerm().getDuration()))
.operator(TERMS_CONFIGURATION.getOperatorTerm().name().toLowerCase())
.priority(TERMS_CONFIGURATION.getPriorityTerm().name().toLowerCase())
.threshold(String.valueOf(TERMS_CONFIGURATION.getThresholdTerm()))
.timeFunction(TERMS_CONFIGURATION.getTimeFunctionTerm().name().toLowerCase())
.build()
);
}
}
<file_sep>/newrelic-alerts-configurator-examples/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/examples/Defaults.kt
package com.ocadotechnology.newrelic.alertsconfigurator.examples
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.ApplicationConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.Channel
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.*
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.*
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.violationclosetimer.ViolationCloseTimer
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.applicationConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.channel.emailChannel
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.channel.slackChannel
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition.*
fun applicationConfiguration(name: String): ApplicationConfiguration {
return applicationConfiguration {
applicationName = name
appApdexThreshold = 0.5f
endUserApdexThreshold = 0.7f
enableRealUserMonitoring = true
}
}
fun apdexCondition(applicationName: String): Condition {
return apmAppCondition {
conditionName = "Apdex"
enabled = true
applications = listOf(applicationName)
metric = ApmAppCondition.Metric.APDEX
conditionScope = ApmAppCondition.ConditionScope.APPLICATION
terms {
PriorityTerm.CRITICAL given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.BELOW value 0.7f
PriorityTerm.WARNING given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.BELOW value 0.85f
}
}
}
fun diskSpaceCondition(vararg serverNames: String): Condition {
return serversMetricCondition {
conditionName = "Fullest Disk %"
enabled = true
servers = listOf(*serverNames)
metric = ServersMetricCondition.Metric.FULLEST_DISK_PERCENTAGE
terms {
PriorityTerm.CRITICAL given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.ABOVE value 80.0f
PriorityTerm.WARNING given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.ABOVE value 65.0f
}
}
}
fun cpuUsageCondition(vararg serverNames: String): Condition {
return serversMetricCondition {
conditionName = "CPU Usage %"
enabled = true
servers = listOf(*serverNames)
metric = ServersMetricCondition.Metric.CPU_PERCENTAGE
terms {
PriorityTerm.CRITICAL given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.ABOVE value 90.0f
PriorityTerm.WARNING given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.ABOVE value 70.0f
}
}
}
fun heapUsageCondition(applicationName: String): Condition {
return apmJvmCondition {
conditionName = "Heap usage"
enabled = true
applications = listOf(applicationName)
metric = ApmJvmCondition.Metric.HEAP_MEMORY_USAGE
violationCloseTimer = ViolationCloseTimer.DURATION_24
terms {
PriorityTerm.CRITICAL given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.ABOVE value 85.0f
PriorityTerm.WARNING given TimeFunctionTerm.ALL inLast DurationTerm.DURATION_5 minutes OperatorTerm.ABOVE value 65.0f
}
}
}
fun healthCheckCondition(applicationName: String): NrqlCondition {
return nrqlCondition {
conditionName = "Health Check"
enabled = true
valueFunction = NrqlCondition.ValueFunction.SINGLE_VALUE
query = "SELECT count(*) FROM `$applicationName:HealthStatus` WHERE healthy IS false"
terms {
term {
priorityTerm = PriorityTerm.CRITICAL
durationTerm = NrqlDurationTerm.DURATION_1
timeFunctionTerm = TimeFunctionTerm.ANY
operatorTerm = OperatorTerm.ABOVE
thresholdTerm = 0.0f
}
}
}
}
fun jsErrorsCondition(applicationName: String): BrowserCondition {
return browserCondition {
conditionName = "Page views with JS errors"
enabled = true
applications = listOf(applicationName)
metric = BrowserCondition.Metric.PAGE_VIEWS_WITH_JS_ERRORS
terms {
term {
priorityTerm = PriorityTerm.WARNING
durationTerm = DurationTerm.DURATION_5
timeFunctionTerm = TimeFunctionTerm.ANY
operatorTerm = OperatorTerm.ABOVE
thresholdTerm = 1.0f
}
}
}
}
fun teamEmail(): Channel {
return emailChannel {
channelName = "My Team - email"
emailAddress = "<EMAIL>"
includeJsonAttachment = true
}
}
fun slack(): Channel {
return slackChannel {
channelName = "My Team - slack"
slackUrl = "https://hooks.slack.com/services/aaaaaaaaa/bbbbbbbbb/cccccccccccccccccccccccc"
teamChannel = "newrelic-alerts"
}
}<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/condition/NrqlConditionConfigurationDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal.NrqlSignalConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal.SignalAggregationMethod
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal.SignalFillOption
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal.SignalLostConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class NrqlSignalConfigurationDsl {
var aggregationWindow: Int? = null
var aggregationMethod: SignalAggregationMethod? = null
var aggregationDelay: Int? = null
var aggregationTimer: Int? = null
var signalFillOption: SignalFillOption? = null
var signalFillValue: String? = null
var signalLostConfiguration: SignalLostConfiguration? = null
fun signalLost(block: SignalLostConfigurationDsl.() -> Unit) {
signalLostConfiguration = signalLostConfiguration(block)
}
}
fun nrqlSignalConfiguration(block: NrqlSignalConfigurationDsl.() -> Unit): NrqlSignalConfiguration {
val dsl = NrqlSignalConfigurationDsl()
dsl.block()
val signalConfigurationBuilder = NrqlSignalConfiguration.builder()
.aggregationMethod(requireNotNull(dsl.aggregationMethod) { "Aggregation method cannot be null" })
.aggregationWindow(requireNotNull(dsl.aggregationWindow) { "Aggregation window cannot be null" })
.signalFillOption(requireNotNull(dsl.signalFillOption) { "Signal fill option cannot be null" })
.signalLostConfiguration(dsl.signalLostConfiguration)
if (dsl.aggregationMethod == SignalAggregationMethod.EVENT_FLOW || dsl.aggregationMethod == SignalAggregationMethod.CADENCE) {
signalConfigurationBuilder.aggregationDelay(requireNotNull(dsl.aggregationDelay) { "Aggregation delay cannot be null for selected aggregation method." })
} else if (dsl.aggregationMethod == SignalAggregationMethod.EVENT_TIMER) {
signalConfigurationBuilder.aggregationTimer(requireNotNull(dsl.aggregationTimer) { "Aggregation timer cannot be null for selected aggregation method." })
}
if (dsl.signalFillOption == SignalFillOption.STATIC) {
signalConfigurationBuilder.signalFillValue(requireNotNull(dsl.signalFillValue) {"Signal fill value is required when signal fill is STATIC"})
}
return signalConfigurationBuilder
.build()
}
<file_sep>/newrelic-api-client/src/test/java/com/ocadotechnology/newrelic/apiclient/internal/DefaultSyntheticsMonitorsApiTest.java
package com.ocadotechnology.newrelic.apiclient.internal;
import com.ocadotechnology.newrelic.apiclient.internal.client.NewRelicClient;
import com.ocadotechnology.newrelic.apiclient.internal.model.MonitorList;
import com.ocadotechnology.newrelic.apiclient.model.synthetics.Monitor;
import static java.util.Collections.singletonList;
import static javax.ws.rs.core.MediaType.APPLICATION_JSON_TYPE;
import static org.assertj.core.api.Assertions.assertThat;
import static org.assertj.core.api.Assertions.assertThatThrownBy;
import org.junit.Before;
import org.junit.Test;
import org.junit.runner.RunWith;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.when;
import org.mockito.junit.MockitoJUnitRunner;
import javax.ws.rs.client.Entity;
import javax.ws.rs.client.Invocation;
import javax.ws.rs.client.WebTarget;
import javax.ws.rs.core.Response;
import java.util.Collections;
import java.util.UUID;
@RunWith(MockitoJUnitRunner.class)
public class DefaultSyntheticsMonitorsApiTest {
private Response postResponseMock;
private Response getResponseMock;
private NewRelicClient clientMock;
private WebTarget webTargetMock;
private Invocation.Builder builderMock;
private DefaultSyntheticsMonitorsApi syntheticsMonitorsApi;
@Before
public void setUp() {
postResponseMock = mock(Response.class);
getResponseMock = mock(Response.class);
clientMock = mock(NewRelicClient.class);
webTargetMock = mock(WebTarget.class);
builderMock = mock(Invocation.Builder.class);
syntheticsMonitorsApi = new DefaultSyntheticsMonitorsApi(clientMock);
}
@Test
public void create_shouldCreateANewMonitorAndReturnDetails() throws Exception {
final Monitor expectedMonitorObject = new Monitor(UUID.randomUUID().toString(), "test-monitor", "SIMPLE", 1, singletonList("AWS_US_WEST_1"), "http://newrelic-url", "ENABLED", 1.0, null, null);;
final Monitor actualMonitorToBeCreated = new Monitor("", "test-monitor", "SIMPLE", 1, singletonList("AWS_US_WEST_1"), "http://newrelic-url", "ENABLED", 1.0, null, null);
final MonitorList monitorList = new MonitorList(Collections.singletonList(expectedMonitorObject));
when(postResponseMock.getStatus()).thenReturn(201);
when(builderMock.post(Entity.entity(actualMonitorToBeCreated, APPLICATION_JSON_TYPE))).thenReturn(postResponseMock);
when(getResponseMock.readEntity(MonitorList.class)).thenReturn(monitorList);
when(builderMock.get()).thenReturn(getResponseMock);
when(webTargetMock.request(APPLICATION_JSON_TYPE)).thenReturn(builderMock);
when(webTargetMock.request()).thenReturn(builderMock);
when(clientMock.target("/v3/monitors")).thenReturn(webTargetMock).thenReturn(webTargetMock);
final Monitor monitor = syntheticsMonitorsApi.create(actualMonitorToBeCreated);
assertThat(monitor).isEqualTo(expectedMonitorObject);
}
@Test
public void create_shouldThrowErrorIfFailedToCreateMonitor() {
final Monitor actualMonitorToBeCreated = new Monitor("", "test-monitor", "SIMPLE", 1, singletonList("AWS_US_WEST_1"), "http://newrelic-url", "ENABLED", 1.0, null, null);
when(postResponseMock.getStatus()).thenReturn(400);
when(builderMock.post(Entity.entity(actualMonitorToBeCreated, APPLICATION_JSON_TYPE))).thenReturn(postResponseMock);
when(webTargetMock.request(APPLICATION_JSON_TYPE)).thenReturn(builderMock);
when(clientMock.target("/v3/monitors")).thenReturn(webTargetMock);
assertThatThrownBy(() -> syntheticsMonitorsApi.create(actualMonitorToBeCreated)).isInstanceOf(Exception.class);
}
@Test
public void delete_shouldDeleteTheMonitor() {
final Monitor actualMonitorToBeDeleted = new Monitor(UUID.randomUUID().toString(), "test-monitor", "SIMPLE", 1, singletonList("AWS_US_WEST_1"), "http://newrelic-url", "ENABLED", 1.0, null, null);
when(builderMock.delete()).thenReturn(postResponseMock);
when(webTargetMock.request(APPLICATION_JSON_TYPE)).thenReturn(builderMock);
when(clientMock.target("/v3/monitors/"+actualMonitorToBeDeleted.getUuid())).thenReturn(webTargetMock);
final Monitor monitor = syntheticsMonitorsApi.delete(actualMonitorToBeDeleted);
assertThat(monitor).isEqualTo(actualMonitorToBeDeleted);
}
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/ConditionType.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition;
import lombok.AllArgsConstructor;
import lombok.Getter;
@Getter
@AllArgsConstructor
public enum ConditionType {
APM_APP("apm_app_metric"),
APM_KEY_TRANSACTION("apm_kt_metric"),
SERVERS_METRIC("servers_metric"),
APM_JVM("apm_jvm_metric"),
BROWSER_METRIC("browser_metric");
private final String typeString;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/model/ObjectList.java
package com.ocadotechnology.newrelic.apiclient.internal.model;
import lombok.EqualsAndHashCode;
import java.util.List;
import java.util.Optional;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.stream.Collectors;
import java.util.stream.Stream;
@EqualsAndHashCode(of = "items")
public abstract class ObjectList<T, LT extends ObjectList<T, LT>> {
private final List<T> items;
private final Function<List<T>, LT> constructor;
protected ObjectList(List<T> items, Function<List<T>, LT> constructor) {
this.items = items;
this.constructor = constructor;
}
public final List<T> getList() {
return items;
}
public final Optional<T> getSingle() {
List<T> list = getList();
if (list.isEmpty()) {
return Optional.empty();
}
if (list.size() == 1) {
return Optional.of(list.get(0));
}
throw new IllegalStateException("Expected single element in the list but found: " + list.size());
}
public final LT merge(ObjectList<T, LT> list) {
List<T> mergedItems = Stream.concat(
items.stream(),
list.items.stream())
.collect(Collectors.toList());
return constructor.apply(mergedItems);
}
public final LT filter(Predicate<? super T> predicate) {
List<T> filteredItems = items.stream().filter(predicate).collect(Collectors.toList());
return constructor.apply(filteredItems);
}
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/channel/internal/UserChannelTypeSupport.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.internal;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.Channel;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.ChannelTypeSupport;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.UserChannel;
import com.ocadotechnology.newrelic.alertsconfigurator.exception.NewRelicSyncException;
import com.ocadotechnology.newrelic.apiclient.NewRelicApi;
import com.ocadotechnology.newrelic.apiclient.model.channels.AlertsChannelConfiguration;
import com.ocadotechnology.newrelic.apiclient.model.users.User;
import lombok.AllArgsConstructor;
import static java.lang.String.format;
@AllArgsConstructor
public class UserChannelTypeSupport implements ChannelTypeSupport {
private Channel channel;
@Override
public AlertsChannelConfiguration generateAlertsChannelConfiguration(NewRelicApi api) {
UserChannel userChannel = (UserChannel) channel;
int userId = getUserId(api, userChannel.getUserEmail());
AlertsChannelConfiguration.AlertsChannelConfigurationBuilder builder = AlertsChannelConfiguration.builder();
builder.userId(userId);
return builder.build();
}
private static int getUserId(NewRelicApi api, String userEmail) {
return api.getUsersApi().getByEmail(userEmail)
.map(User::getId)
.orElseThrow(() -> new NewRelicSyncException(format("NewRelic user with email %s does not exist", userEmail)));
}
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/signal/SignalAggregationMethod.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal;
import lombok.AllArgsConstructor;
import lombok.Getter;
@Getter
@AllArgsConstructor
public enum SignalAggregationMethod {
EVENT_FLOW("EVENT_FLOW"), EVENT_TIMER("EVENT_TIMER"), CADENCE("CADENCE");
private final String value;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/client/NewRelicClient.java
package com.ocadotechnology.newrelic.apiclient.internal.client;
import javax.ws.rs.client.WebTarget;
import javax.ws.rs.core.Link;
public interface NewRelicClient {
WebTarget target(String path);
WebTarget target(Link link);
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/NrqlConditionConfigurator.java
package com.ocadotechnology.newrelic.alertsconfigurator;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.PolicyConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.NrqlCondition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal.ExpirationUtils;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal.NrqlSignalConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal.SignalUtils;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.TermsUtils;
import com.ocadotechnology.newrelic.apiclient.NewRelicApi;
import com.ocadotechnology.newrelic.apiclient.PolicyItemApi;
import com.ocadotechnology.newrelic.apiclient.model.conditions.nrql.AlertsNrqlCondition;
import com.ocadotechnology.newrelic.apiclient.model.conditions.nrql.Nrql;
import java.util.Collection;
import java.util.Optional;
import lombok.NonNull;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
@Slf4j
class NrqlConditionConfigurator extends AbstractPolicyItemConfigurator<AlertsNrqlCondition, NrqlCondition> {
NrqlConditionConfigurator(@NonNull NewRelicApi api) {
super(api);
}
@Override
protected Optional<Collection<NrqlCondition>> getConfigItems(PolicyConfiguration config) {
return config.getNrqlConditions();
}
@Override
protected PolicyItemApi<AlertsNrqlCondition> getItemsApi() {
return api.getAlertsNrqlConditionsApi();
}
@Override
protected AlertsNrqlCondition convertFromConfigItem(NrqlCondition condition) {
AlertsNrqlCondition.AlertsNrqlConditionBuilder nrqlConditionBuilder = AlertsNrqlCondition.builder()
.name(condition.getConditionName())
.enabled(condition.isEnabled())
.runbookUrl(condition.getRunBookUrl())
.terms(TermsUtils.createNrqlTerms(condition.getTerms()))
.valueFunction(condition.getValueFunction().getValueString())
.violationTimeLimitSeconds(condition.getViolationTimeLimitSeconds())
.nrql(Nrql.builder()
.query(condition.getQuery())
.build());
NrqlSignalConfiguration nrqlSignalConfiguration = condition.getSignal();
if (nrqlSignalConfiguration != null) {
nrqlConditionBuilder
.signal(SignalUtils.createSignal(nrqlSignalConfiguration))
.expiration(ExpirationUtils.createExpiration(nrqlSignalConfiguration.getSignalLostConfiguration()));
}
return nrqlConditionBuilder.build();
}
@Override
protected boolean sameInstance(AlertsNrqlCondition alertsNrqlCondition1, AlertsNrqlCondition alertsNrqlCondition2) {
return StringUtils.equals(alertsNrqlCondition1.getName(), alertsNrqlCondition2.getName());
}
}
<file_sep>/newrelic-alerts-configurator/src/test/java/com/ocadotechnology/newrelic/alertsconfigurator/internal/entities/EntityResolverTest.java
package com.ocadotechnology.newrelic.alertsconfigurator.internal.entities;
import static org.assertj.core.api.Assertions.assertThat;
import static org.junit.Assert.assertNotNull;
import static org.mockito.Mockito.mock;
import static org.mockito.Mockito.verify;
import static org.mockito.Mockito.when;
import java.util.Arrays;
import java.util.Collection;
import org.junit.Rule;
import org.junit.Test;
import org.mockito.Mock;
import org.mockito.Spy;
import org.mockito.junit.MockitoJUnit;
import org.mockito.junit.MockitoRule;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.Condition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ConditionType;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ExternalServiceCondition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ExternalServiceConditionType;
import com.ocadotechnology.newrelic.apiclient.NewRelicApi;
public class EntityResolverTest {
@Rule
public final MockitoRule mockito = MockitoJUnit.rule();
@Mock
private NewRelicApi apiMock;
@Mock
private IdProvider idProviderMock;
@Spy
private final EntityResolver testee = new EntityResolver() {
@Override
protected IdProvider getIdProvider(String conditionType) {
return idProviderMock;
}
@Override
protected UuidProvider getUuidProvider(String conditionType) {
return null;
}
};
@Test
public void resolveEntities_shouldResolveConditionEntities() {
// given
Condition condition = mock(Condition.class);
when(condition.getType()).thenReturn(ConditionType.APM_KEY_TRANSACTION);
when(condition.getEntities()).thenReturn(Arrays.asList("e1", "e2"));
when(idProviderMock.getId(apiMock, "e1")).thenReturn(1);
when(idProviderMock.getId(apiMock, "e2")).thenReturn(2);
// when
Collection<Integer> ids = testee.resolveEntities(apiMock, condition);
// then
assertThat(ids).containsExactly(1, 2);
verify(testee).getIdProvider(ConditionType.APM_KEY_TRANSACTION.getTypeString());
}
@Test
public void resolveEntities_shouldResolveExternalServiceConditionEntities() {
// given
ExternalServiceCondition condition = mock(ExternalServiceCondition.class);
when(condition.getType()).thenReturn(ExternalServiceConditionType.APM);
when(condition.getEntities()).thenReturn(Arrays.asList("e1", "e2"));
when(idProviderMock.getId(apiMock, "e1")).thenReturn(1);
when(idProviderMock.getId(apiMock, "e2")).thenReturn(2);
// when
Collection<Integer> ids = testee.resolveEntities(apiMock, condition);
// then
assertThat(ids).containsExactly(1, 2);
verify(testee).getIdProvider(ExternalServiceConditionType.APM.getTypeString());
}
@Test
public void defaultInstance_shouldSupportAllConditionEntities() {
for (ConditionType type : ConditionType.values()) {
assertCanResolveType(type.getTypeString());
}
}
@Test
public void defaultInstance_shouldSupportAllExternalServiceCondtionEntities() {
for (ExternalServiceConditionType type : ExternalServiceConditionType.values()) {
assertCanResolveType(type.getTypeString());
}
}
private void assertCanResolveType(String conditionType) {
IdProvider provider = EntityResolver.defaultInstance().getIdProvider(conditionType);
assertNotNull("Should resolve id for " + conditionType, provider);
}
}
<file_sep>/newrelic-alerts-configurator-examples/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/examples/ApplicationConfigurator.java
package com.ocadotechnology.newrelic.alertsconfigurator.examples;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.ApplicationConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.PolicyConfiguration;
/**
* Returns configuration for one application in NewRelic. In this sample, each application will have one application
* configuration and one policy configuration
*/
public interface ApplicationConfigurator {
ApplicationConfiguration getApplicationConfiguration();
PolicyConfiguration getPolicyConfigurations();
}
<file_sep>/newrelic-api-client/src/test/java/com/ocadotechnology/newrelic/graphql/apiclient/internal/testutil/QueryExecutorMock.java
package com.ocadotechnology.newrelic.graphql.apiclient.internal.testutil;
import com.ocadotechnology.newrelic.graphql.apiclient.internal.QueryExecutor;
import com.ocadotechnology.newrelic.graphql.apiclient.internal.transport.Query;
import com.ocadotechnology.newrelic.graphql.apiclient.internal.transport.Result;
import java.util.HashMap;
import java.util.Map;
import java.util.Optional;
import static java.lang.String.format;
public class QueryExecutorMock implements QueryExecutor {
private final Map<Query, Result> resultsByQuery = new HashMap<>();
@Override
public Result execute(Query query) {
return Optional.ofNullable(resultsByQuery.get(query))
.orElseThrow(() -> new UnsupportedOperationException(format("No scenario found for query:'\n%s\n'", query)));
}
public QueryExecutorMock addScenario(Query query, Result result) {
resultsByQuery.put(query, result);
return this;
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/DefaultDeploymentsApi.java
package com.ocadotechnology.newrelic.apiclient.internal;
import com.ocadotechnology.newrelic.apiclient.DeploymentsApi;
import com.ocadotechnology.newrelic.apiclient.internal.client.NewRelicClient;
import com.ocadotechnology.newrelic.apiclient.internal.model.DeploymentWrapper;
import com.ocadotechnology.newrelic.apiclient.internal.model.DeploymentsList;
import com.ocadotechnology.newrelic.apiclient.model.deployments.Deployment;
import javax.ws.rs.client.Entity;
import java.util.List;
import static javax.ws.rs.core.MediaType.APPLICATION_JSON_TYPE;
public class DefaultDeploymentsApi extends ApiBase implements DeploymentsApi {
private static final String DEPLOYMENTS_URL = "/v2/applications/{application_id}/deployments.json";
private static final String DEPLOYMENT_URL = "/v2/applications/{application_id}/deployments/{deployment_id}.json";
public DefaultDeploymentsApi(NewRelicClient client) {
super(client);
}
@Override
public List<Deployment> list(int applicationId) {
return getPageable(
client.target(DEPLOYMENTS_URL)
.resolveTemplate("application_id", applicationId)
.request(APPLICATION_JSON_TYPE),
DeploymentsList.class)
.getList();
}
@Override
public Deployment create(int applicationId, Deployment deployment) {
return client
.target(DEPLOYMENTS_URL)
.resolveTemplate("application_id", applicationId)
.request(APPLICATION_JSON_TYPE)
.post(Entity.entity(new DeploymentWrapper(deployment), APPLICATION_JSON_TYPE), DeploymentWrapper.class)
.getDeployment();
}
@Override
public Deployment delete(int applicationId, int deploymentId) {
return client
.target(DEPLOYMENT_URL)
.resolveTemplate("application_id", applicationId)
.resolveTemplate("deployment_id", deploymentId)
.request(APPLICATION_JSON_TYPE)
.delete(DeploymentWrapper.class)
.getDeployment();
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/graphql/apiclient/internal/transport/AssociatedPolicies.java
package com.ocadotechnology.newrelic.graphql.apiclient.internal.transport;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import lombok.Value;
import java.util.List;
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
public class AssociatedPolicies {
List<Identifiable> policies;
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/condition/SyntheticsConditionDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.SyntheticsCondition
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class SyntheticsConditionDsl {
var conditionName: String? = null
var monitorName: String? = null
var enabled: Boolean = true
var runBookUrl: String? = null
}
fun syntheticsCondition(block: SyntheticsConditionDsl.() -> Unit): SyntheticsCondition {
val dsl = SyntheticsConditionDsl()
dsl.block()
return SyntheticsCondition.builder()
.conditionName(requireNotNull(dsl.conditionName) { "Synthetics condition name cannot be null" })
.monitorName(requireNotNull(dsl.monitorName) { "Synthetics condition monitor name cannot be null" })
.enabled(dsl.enabled)
.runBookUrl(dsl.runBookUrl)
.build()
}<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/model/AlertsConditionList.java
package com.ocadotechnology.newrelic.apiclient.internal.model;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.ocadotechnology.newrelic.apiclient.model.conditions.AlertsCondition;
import lombok.EqualsAndHashCode;
import java.util.List;
@EqualsAndHashCode(callSuper = true)
public class AlertsConditionList extends ObjectList<AlertsCondition, AlertsConditionList> {
@JsonCreator
public AlertsConditionList(@JsonProperty("conditions") List<AlertsCondition> items) {
super(items, AlertsConditionList::new);
}
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/condition/SignalLostConfigurationDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal.SignalLostConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class SignalLostConfigurationDsl {
var signalIsLostAfter: Int? = null
var openNewViolationOnSignalLost: Boolean = false
var closeCurrentViolationsOnSignalLost: Boolean = false
}
fun signalLostConfiguration(block: SignalLostConfigurationDsl.() -> Unit): SignalLostConfiguration {
val dsl = SignalLostConfigurationDsl()
dsl.block()
return SignalLostConfiguration.builder()
.signalIsLostAfter(requireNotNull(dsl.signalIsLostAfter, { "Signal is lost after cannot be null" }))
.openNewViolationOnSignalLost(dsl.openNewViolationOnSignalLost)
.closeCurrentViolationsOnSignalLost(dsl.closeCurrentViolationsOnSignalLost)
.build()
}<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/model/dashboards/widget/WidgetLayout.java
package com.ocadotechnology.newrelic.apiclient.model.dashboards.widget;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Value;
@Value
@Builder(toBuilder = true)
@AllArgsConstructor
public class WidgetLayout {
@JsonProperty
Integer width;
@JsonProperty
Integer height;
@JsonProperty
Integer row;
@JsonProperty
Integer column;
}
<file_sep>/build.gradle
plugins {
id 'org.jetbrains.kotlin.jvm' version '1.3.72' apply false
}
allprojects {
group = 'com.ocadotechnology.newrelicalertsconfigurator'
apply plugin: 'java-library'
apply plugin: 'maven'
apply plugin: 'signing'
sourceCompatibility = 1.8
targetCompatibility = 1.8
compileJava.options.encoding = 'UTF-8'
}
subprojects {
repositories {
mavenCentral()
}
dependencies {
api 'org.slf4j:slf4j-api:1.7.30'
compileOnly 'org.projectlombok:lombok:1.18.12'
annotationProcessor 'org.projectlombok:lombok:1.18.12'
testImplementation 'junit:junit:4.13'
testImplementation 'com.google.guava:guava:29.0-jre'
testImplementation 'org.mockito:mockito-core:3.4.6'
testImplementation 'com.github.tomakehurst:wiremock:2.27.1'
testImplementation 'org.assertj:assertj-core:3.16.1'
}
}
configure(subprojects - project(':newrelic-alerts-configurator-examples')) {
task sourcesJar(type: Jar, dependsOn: classes) {
archiveClassifier.set('sources')
from sourceSets.main.allSource
}
task javadocJar(type: Jar, dependsOn: javadoc) {
archiveClassifier.set('javadoc')
from javadoc.destinationDir
}
artifacts {
archives javadocJar, sourcesJar
}
if (project.hasProperty('release')) {
signing {
required { !version.endsWith("-SNAPSHOT") && gradle.taskGraph.hasTask("uploadArchives") }
useInMemoryPgpKeys(findProperty("signingKey").toString().split("(?<=\\G.{64})").join("\n"), findProperty("signingPassword").toString())
sign configurations.archives
}
uploadArchives {
doFirst {
println "Uploading archive ${project.name} in version ${version}"
}
repositories {
mavenDeployer {
beforeDeployment { MavenDeployment deployment -> signing.signPom(deployment) }
repository(url: "https://oss.sonatype.org/service/local/staging/deploy/maven2/") {
authentication(userName: ossrhUsername, password: <PASSWORD>)
}
snapshotRepository(url: "https://oss.sonatype.org/content/repositories/snapshots/") {
authentication(userName: ossrhUsername, password: <PASSWORD>)
}
pom.project {
name 'NewRelic Alerts Configurator'
packaging 'jar'
// optionally artifactId can be defined here
description 'NewRelic Alerts Configurator can be used to configure NewRelic alerts for your application. Instead of defining alerts through UI you can define them in code. It allows you to automatize alerts configuration, easily recover them in case of wipe out and have full history of changes in your version control system.'
url 'https://github.com/ocadotechnology/newrelic-alerts-configurator'
scm {
connection 'scm:git:git://github.com/ocadotechnology/newrelic-alerts-configurator.git'
developerConnection 'scm:git:[email protected]:ocadotechnology/newrelic-alerts-configurator.git'
url 'https://github.com/ocadotechnology/newrelic-alerts-configurator'
}
licenses {
license {
name 'The Apache License, Version 2.0'
url 'http://www.apache.org/licenses/LICENSE-2.0.txt'
}
}
organization {
name 'Ocado Technology'
url 'http://www.ocadotechnology.com/'
}
developers {
developer {
id 'panda.team'
name '<NAME>'
email '<EMAIL>'
}
}
}
}
}
}
}
}
project(':newrelic-api-client') {
dependencies {
api 'commons-io:commons-io:2.7'
api 'org.apache.commons:commons-lang3:3.11'
api 'org.glassfish.jersey.core:jersey-client:2.24.1'
api 'org.glassfish.jersey.media:jersey-media-json-jackson:2.24.1'
api 'org.glassfish.jersey.connectors:jersey-apache-connector:2.24.1'
api 'com.fasterxml.jackson.core:jackson-databind:2.11.1'
api 'com.fasterxml.jackson.datatype:jackson-datatype-jdk8:2.11.1'
api 'com.fasterxml.jackson.datatype:jackson-datatype-jsr310:2.11.1'
implementation 'org.apache.commons:commons-text:1.10.0'
implementation('com.github.k0kubun:graphql-query-builder:0.4.2') {
exclude group: 'com.google.guava', module: 'guava'
}
}
}
project(':newrelic-alerts-configurator') {
dependencies {
api project(':newrelic-api-client')
}
}
project(':newrelic-alerts-configurator-examples') {
apply plugin: 'kotlin'
dependencies {
api project(':newrelic-alerts-configurator')
api project(':newrelic-alerts-configurator-dsl')
}
}
project(':newrelic-alerts-configurator-dsl') {
apply plugin: 'kotlin'
dependencies {
api project(':newrelic-alerts-configurator')
api 'org.jetbrains.kotlin:kotlin-stdlib'
}
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/channel/Channels.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.channel
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.Channel
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class Channels {
internal val channels: MutableList<Channel> = mutableListOf()
fun email(block: EmailChannelDsl.() -> Unit) = channels.add(emailChannel(block))
fun pagerDuty(block: PagerDutyChannelDsl.() -> Unit) = channels.add(pagerDutyChannel(block))
fun slack(block: SlackChannelDsl.() -> Unit) = channels.add(slackChannel(block))
fun user(block: UserChannelDsl.() -> Unit) = channels.add(userChannel(block))
fun webhook(block: WebhookChannelDsl.() -> Unit) = channels.add(webhookChannel(block))
operator fun Channel.unaryPlus() = channels.add(this)
}<file_sep>/newrelic-alerts-configurator/src/test/java/com/ocadotechnology/newrelic/alertsconfigurator/ConfiguratorTest.java
package com.ocadotechnology.newrelic.alertsconfigurator;
import static org.mockito.Mockito.inOrder;
import static org.mockito.Mockito.mock;
import com.ocadotechnology.newrelic.apiclient.NewRelicApi;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.junit.rules.ExpectedException;
import org.junit.runner.RunWith;
import org.mockito.InOrder;
import org.mockito.Mock;
import org.mockito.junit.MockitoJUnitRunner;
import com.google.common.collect.ImmutableList;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.ApplicationConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.PolicyConfiguration;
@RunWith(MockitoJUnitRunner.class)
public class ConfiguratorTest {
@Rule
public final ExpectedException expectedException = ExpectedException.none();
@Mock
private ApplicationConfigurator applicationConfiguratorMock;
@Mock
private PolicyConfigurator policyConfiguratorMock;
@Mock
private ConditionConfigurator conditionConfiguratorMock;
@Mock
private ExternalServiceConditionConfigurator externalServiceConditionConfiguratorMock;
@Mock
private NrqlConditionConfigurator nrqlConditionConfiguratorMock;
@Mock
private SyntheticsConditionConfigurator syntheticsConditionConfiguratorMock;
@Mock
private ChannelConfigurator channelConfiguratorMock;
@Mock
private ApplicationConfiguration applicationConfigurationMock;
@Mock
private PolicyConfiguration policyConfigurationMock;
private Configurator testee;
@Before
public void setUp() {
testee = new Configurator(applicationConfiguratorMock,
policyConfiguratorMock,
conditionConfiguratorMock,
externalServiceConditionConfiguratorMock,
nrqlConditionConfiguratorMock,
syntheticsConditionConfiguratorMock,
channelConfiguratorMock);
}
@Test
public void shouldThrowException_whenNoApiKey() {
// given
// then - exception
expectedException.expect(NullPointerException.class);
expectedException.expectMessage("apiKey");
// when
new Configurator((String) null);
}
@Test
public void shouldThrowException_whenNoApi() {
// given
// then - exception
expectedException.expect(NullPointerException.class);
expectedException.expectMessage("api");
// when
new Configurator((NewRelicApi) null);
}
@Test
public void shouldNotSynchronizeAnything_whenNoConfigurationsSet() {
// given
// when
testee.sync();
// then
InOrder order = inOrder(applicationConfiguratorMock,
policyConfiguratorMock,
conditionConfiguratorMock,
externalServiceConditionConfiguratorMock,
nrqlConditionConfiguratorMock,
syntheticsConditionConfiguratorMock,
channelConfiguratorMock);
order.verifyNoMoreInteractions();
}
@Test
public void shouldSynchronizeMoreThanOneConfigurations_whenMoreThanOneConfigurationsSet() {
// given
ApplicationConfiguration applicationConfigurationMock2 = mock(ApplicationConfiguration.class);
PolicyConfiguration policyConfigurationMock2 = mock(PolicyConfiguration.class);
testee.setApplicationConfigurations(ImmutableList.of(applicationConfigurationMock, applicationConfigurationMock2));
testee.setPolicyConfigurations(ImmutableList.of(policyConfigurationMock, policyConfigurationMock2));
// when
testee.sync();
// then
InOrder order = inOrder(applicationConfiguratorMock,
policyConfiguratorMock,
conditionConfiguratorMock,
externalServiceConditionConfiguratorMock,
nrqlConditionConfiguratorMock,
syntheticsConditionConfiguratorMock,
channelConfiguratorMock);
order.verify(applicationConfiguratorMock).sync(applicationConfigurationMock);
order.verify(applicationConfiguratorMock).sync(applicationConfigurationMock2);
order.verify(policyConfiguratorMock).sync(policyConfigurationMock);
order.verify(policyConfiguratorMock).sync(policyConfigurationMock2);
}
@Test
public void shouldSynchronizeAllConfigurationsForPolicy_whenFullPolicyConfiguration() {
// given
testee.setPolicyConfigurations(ImmutableList.of(policyConfigurationMock));
// when
testee.sync();
// then
InOrder order = inOrder(applicationConfiguratorMock,
policyConfiguratorMock,
conditionConfiguratorMock,
externalServiceConditionConfiguratorMock,
nrqlConditionConfiguratorMock,
syntheticsConditionConfiguratorMock,
channelConfiguratorMock);
order.verify(policyConfiguratorMock).sync(policyConfigurationMock);
order.verify(conditionConfiguratorMock).sync(policyConfigurationMock);
order.verify(externalServiceConditionConfiguratorMock).sync(policyConfigurationMock);
order.verify(nrqlConditionConfiguratorMock).sync(policyConfigurationMock);
order.verify(syntheticsConditionConfiguratorMock).sync(policyConfigurationMock);
order.verify(channelConfiguratorMock).sync(policyConfigurationMock);
order.verifyNoMoreInteractions();
}
}
<file_sep>/newrelic-api-client/README.md
New Relic API wrapper.
See https://rpm.newrelic.com/api/explore<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/channel/internal/EmailChannelTypeSupport.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.internal;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.Channel;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.ChannelTypeSupport;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.EmailChannel;
import com.ocadotechnology.newrelic.apiclient.NewRelicApi;
import com.ocadotechnology.newrelic.apiclient.model.channels.AlertsChannelConfiguration;
import lombok.AllArgsConstructor;
import org.apache.commons.lang3.BooleanUtils;
@AllArgsConstructor
public class EmailChannelTypeSupport implements ChannelTypeSupport {
private Channel channel;
@Override
public AlertsChannelConfiguration generateAlertsChannelConfiguration(NewRelicApi api) {
EmailChannel emailChannel = (EmailChannel) channel;
AlertsChannelConfiguration.AlertsChannelConfigurationBuilder builder = AlertsChannelConfiguration.builder();
builder.recipients(emailChannel.getEmailAddress());
if (BooleanUtils.isTrue(emailChannel.getIncludeJsonAttachment())) {
builder.includeJsonAttachment(emailChannel.getIncludeJsonAttachment());
}
return builder.build();
}
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/internal/entities/SyntheticsMonitorUuidProvider.java
package com.ocadotechnology.newrelic.alertsconfigurator.internal.entities;
import static java.lang.String.format;
import java.util.Optional;
import com.ocadotechnology.newrelic.alertsconfigurator.exception.NewRelicSyncException;
import com.ocadotechnology.newrelic.apiclient.NewRelicApi;
import com.ocadotechnology.newrelic.apiclient.model.synthetics.Monitor;
class SyntheticsMonitorUuidProvider implements UuidProvider {
@Override
public String getUuid(NewRelicApi api, String name) {
Optional<Monitor> monitorOptional = api.getSyntheticsMonitorsApi().getByName(name);
Monitor monitor = monitorOptional.orElseThrow(
() -> new NewRelicSyncException(format("Synthetics Monitor %s does not exist", name)));
return monitor.getUuid();
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/model/transactions/KeyTransactionLinks.java
package com.ocadotechnology.newrelic.apiclient.model.transactions;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.Value;
@Value
public class KeyTransactionLinks {
@JsonProperty
Integer application;
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/condition/terms/TermsConfigurationDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition.terms
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.*
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class TermsConfigurationDsl {
var durationTerm: DurationTerm? = null
var operatorTerm: OperatorTerm? = null
var priorityTerm: PriorityTerm? = null
var thresholdTerm: Float? = null
var timeFunctionTerm: TimeFunctionTerm? = null
}
fun termsConfiguration(block: TermsConfigurationDsl.() -> Unit): TermsConfiguration {
val dsl = TermsConfigurationDsl()
dsl.block()
return TermsConfiguration.builder()
.durationTerm(requireNotNull(dsl.durationTerm) { "Terms duration cannot be null" })
.operatorTerm(requireNotNull(dsl.operatorTerm) { "Terms operator cannot be null" })
.priorityTerm(requireNotNull(dsl.priorityTerm) { "Terms priority cannot be null" })
.thresholdTerm(requireNotNull(dsl.thresholdTerm) { "Terms threshold cannot be null" })
.timeFunctionTerm(requireNotNull(dsl.timeFunctionTerm) { "Terms time cannot be null" })
.build()
}
@NewRelicConfigurationMarker
class TermConfigurations {
internal val terms: MutableList<TermsConfiguration> = mutableListOf()
fun term(block: TermsConfigurationDsl.() -> Unit) = terms.add(com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition.terms.termsConfiguration(block))
operator fun TermsConfiguration.unaryPlus() = terms.add(this)
infix fun PriorityTerm.given(timeFunctionTerm: TimeFunctionTerm) : AfterGiven {
return AfterGiven(TermsConfiguration.builder().priorityTerm(this).timeFunctionTerm(timeFunctionTerm))
}
infix fun AfterGiven.inLast(durationTerm: DurationTerm) : AfterInLast {
return AfterInLast(this.builder.durationTerm(durationTerm))
}
infix fun AfterInLast.minutes(operatorTerm: OperatorTerm) : AfterMinutes {
return AfterMinutes(this.builder.operatorTerm(operatorTerm))
}
infix fun AfterMinutes.value(thresholdTerm: Float) {
terms.add(this.builder.thresholdTerm(thresholdTerm).build())
}
class AfterGiven(internal val builder: TermsConfiguration.TermsConfigurationBuilder)
class AfterInLast(internal val builder: TermsConfiguration.TermsConfigurationBuilder)
class AfterMinutes(internal val builder: TermsConfiguration.TermsConfigurationBuilder)
}<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/DashboardsApi.java
package com.ocadotechnology.newrelic.apiclient;
import com.ocadotechnology.newrelic.apiclient.model.dashboards.Dashboard;
import java.util.List;
/**
* See <a href="https://docs.newrelic.com/docs/insights/insights-api/manage-dashboards/insights-dashboard-api">Doc</a>
*/
public interface DashboardsApi {
/**
* View a list of {@link Dashboard}. The list shows dashboard metadata only; no widgets will appear in the list.
*
* @param dashboardTitle name of the dashboard(s) registered in NewRelic. This may be a partial name.
* @return List containing {@link Dashboard} objects, or empty if no dashboard including dashboardTitle exist.
* This
*/
List<Dashboard> getByTitle(String dashboardTitle);
/**
* View an existing {@link Dashboard} and all accessible widgets for the dashboard id.
*
* @param dashboardId id of the dashboard registered in NewRelic
* @return found {@link Dashboard}
*/
Dashboard getById(int dashboardId);
/**
* Create a new {@link Dashboard}.
* <p>
* The API permits a maximum of 300 widgets when creating or updating a dashboard. Attempting to POST more than 300 widgets will produce an error.
*
* @param dashboard to be created in NewRelic
* @return created {@link Dashboard}
*/
Dashboard create(Dashboard dashboard);
/**
* Update an existing {@link Dashboard} for the dashboard id.
* <p>
* The API permits a maximum of 300 widgets when creating or updating a dashboard. Attempting to PUT more than 300 widgets will produce an error.
*
* @param dashboard to be updated in NewRelic
* @return updated {@link Dashboard}
*/
Dashboard update(Dashboard dashboard);
/**
* Delete an existing {@link Dashboard} indicated by the dashboard id.
*
* @param dashboardId of the dashboard to be deleted
* @return deleted {@link Dashboard}
*/
Dashboard delete(int dashboardId);
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/channel/internal/WebhookChannelTypeSupport.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.internal;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.Channel;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.ChannelTypeSupport;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.WebhookChannel;
import com.ocadotechnology.newrelic.apiclient.NewRelicApi;
import com.ocadotechnology.newrelic.apiclient.model.channels.AlertsChannelConfiguration;
import lombok.AllArgsConstructor;
@AllArgsConstructor
public class WebhookChannelTypeSupport implements ChannelTypeSupport {
private Channel channel;
@Override
public AlertsChannelConfiguration generateAlertsChannelConfiguration(NewRelicApi api) {
WebhookChannel webhookChannel = (WebhookChannel) channel;
return AlertsChannelConfiguration.builder()
.baseUrl(webhookChannel.getBaseUrl())
.authUsername(webhookChannel.getAuthUsername())
.authPassword(webhookChannel.getAuthPassword())
.build();
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/graphql/apiclient/internal/transport/mutation/MutationResult.java
package com.ocadotechnology.newrelic.graphql.apiclient.internal.transport.mutation;
import java.util.List;
import java.util.Map;
import static java.util.Objects.nonNull;
public interface MutationResult<T> {
T get();
List<Map<String, Object>> getErrors();
default boolean hasErrors() {
return nonNull(getErrors()) && !getErrors().isEmpty();
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/KeyTransactionsApi.java
package com.ocadotechnology.newrelic.apiclient;
import com.ocadotechnology.newrelic.apiclient.model.transactions.KeyTransaction;
import java.util.Optional;
public interface KeyTransactionsApi {
/**
* Gets {@link KeyTransaction} object using its name.
*
* @param keyTransactionName name of the key transaction registered in NewRelic
* @return Optional containing {@link KeyTransaction} object, or empty if transaction not found
*/
Optional<KeyTransaction> getByName(String keyTransactionName);
/**
* Gets {@link KeyTransaction} object using its name.
*
* @param keyTransactionId id of the key transaction registered in NewRelic
* @return found {@link KeyTransaction}
*/
KeyTransaction getById(int keyTransactionId);
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/Configurator.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl
import com.ocadotechnology.newrelic.alertsconfigurator.Configurator
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.ApplicationConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.PolicyConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.ApplicationConfigurations
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.PolicyConfigurations
@NewRelicConfigurationMarker
class ConfiguratorDsl {
internal val applicationConfigurations: MutableList<ApplicationConfiguration> = mutableListOf()
internal val policyConfigurations: MutableList<PolicyConfiguration> = mutableListOf()
fun applications(block: ApplicationConfigurations.() -> Unit) = applicationConfigurations.addAll(ApplicationConfigurations().apply(block).applications)
fun policies(block: PolicyConfigurations.() -> Unit) = policyConfigurations.addAll(PolicyConfigurations().apply(block).policies)
}
fun configuration(apiKey: String, block: ConfiguratorDsl.() -> Unit) {
val dsl = ConfiguratorDsl()
dsl.apply(block)
val configurator = Configurator(apiKey)
configurator.setApplicationConfigurations(dsl.applicationConfigurations)
configurator.setPolicyConfigurations(dsl.policyConfigurations)
configurator.sync()
}<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/model/applications/ApplicationLinks.java
package com.ocadotechnology.newrelic.apiclient.model.applications;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.Value;
import java.util.List;
@Value
public class ApplicationLinks {
@JsonProperty("alert_policy")
Integer alertPolicy;
@JsonProperty
List<Integer> servers;
@JsonProperty("application_hosts")
List<Integer> hosts;
@JsonProperty("application_instances")
List<Integer> instances;
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/condition/ApmExternalServiceConditionDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ApmExternalServiceCondition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.TermsConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition.terms.TermConfigurations
@NewRelicConfigurationMarker
class ApmExternalServiceConditionDsl {
var conditionName: String? = null
var enabled: Boolean = true
var applications: Collection<String> = mutableListOf()
var externalServiceUrl: String? = null
var metric: ApmExternalServiceCondition.Metric? = null
var runBookUrl: String? = null
internal val terms: MutableList<TermsConfiguration> = mutableListOf()
fun terms(block: TermConfigurations.() -> Unit) = terms.addAll(TermConfigurations().apply(block).terms)
}
fun apmExternalServiceCondition(block: ApmExternalServiceConditionDsl.() -> Unit): ApmExternalServiceCondition {
val dsl = ApmExternalServiceConditionDsl()
dsl.block()
return ApmExternalServiceCondition.builder()
.conditionName(requireNotNull(dsl.conditionName) { "Apm external service condition name cannot be null" })
.enabled(dsl.enabled)
.applications(dsl.applications)
.externalServiceUrl(requireNotNull(dsl.externalServiceUrl) { "Apm external service condition url cannot be null" })
.metric(requireNotNull(dsl.metric) { "Apm external service condition metric cannot be null" })
.runBookUrl(dsl.runBookUrl)
.terms(dsl.terms)
.build()
}<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/channel/WebhookChannelDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.channel
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.WebhookChannel
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class WebhookChannelDsl {
var channelName: String? = null
var baseUrl: String? = null
val authUsername: String? = null
val authPassword: String? = null
}
fun webhookChannel(block: WebhookChannelDsl.() -> Unit): WebhookChannel {
val dsl = WebhookChannelDsl()
dsl.block()
return WebhookChannel.builder()
.channelName(requireNotNull(dsl.channelName) { "Webhook channel name cannot be null" })
.baseUrl(requireNotNull(dsl.baseUrl) { "Webhook channel url cannot be null" })
.authUsername(dsl.authUsername)
.authPassword(<PASSWORD>)
.build()
}<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/graphql/apiclient/internal/transport/mutation/EmptyMutationResult.java
package com.ocadotechnology.newrelic.graphql.apiclient.internal.transport.mutation;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import lombok.Getter;
import lombok.Value;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import static java.util.Collections.singletonList;
import static lombok.AccessLevel.NONE;
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
@Getter(NONE)
public class EmptyMutationResult implements MutationResult<Void> {
Map<String, Object> error;
@Override
public Void get() {
return null;
}
@Override
public List<Map<String, Object>> getErrors() {
return Optional.ofNullable(error).map(e -> singletonList(error)).orElse(null);
}
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/channel/EmailChannelDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.channel
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.EmailChannel
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class EmailChannelDsl {
var channelName: String? = null
var emailAddress: String? = null
var includeJsonAttachment: Boolean = false
}
fun emailChannel(block: EmailChannelDsl.() -> Unit): EmailChannel {
val dsl = EmailChannelDsl()
dsl.block()
return EmailChannel.builder()
.channelName(requireNotNull(dsl.channelName) { "Email channel name cannot be null" })
.emailAddress(requireNotNull(dsl.emailAddress) { "Email channel address cannot be null" })
.includeJsonAttachment(dsl.includeJsonAttachment)
.build()
}<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/graphql/apiclient/internal/transport/PageIterator.java
package com.ocadotechnology.newrelic.graphql.apiclient.internal.transport;
import lombok.RequiredArgsConstructor;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Optional;
import java.util.function.Function;
import static java.util.Objects.isNull;
import static java.util.Objects.nonNull;
@RequiredArgsConstructor
public class PageIterator<C, W extends Page<List<C>>> implements Iterator<List<C>> {
private W current;
private final Function<String, Optional<W>> fetch;
@Override
public boolean hasNext() {
return isNull(current) || nonNull(current.getNextCursor());
}
@Override
public List<C> next() {
if (hasNext()) {
current = fetch.apply(Optional.ofNullable(current).map(Page::getNextCursor).orElse("")).get();
return Optional.ofNullable(current.getContent()).orElseGet(ArrayList::new);
}
throw new IllegalStateException("Cannot get next");
}
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/PolicyConfigurationDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.PolicyConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.Channel
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.Condition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ExternalServiceCondition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.NrqlCondition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.SyntheticsCondition
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.channel.Channels
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition.Conditions
@NewRelicConfigurationMarker
class PolicyConfigurationDsl {
var policyName: String? = null
var incidentPreference: PolicyConfiguration.IncidentPreference? = null
internal val channels: MutableList<Channel> = mutableListOf()
internal val conditions: MutableList<Condition> = mutableListOf()
internal val externalServiceConditions: MutableList<ExternalServiceCondition> = mutableListOf()
internal val nrqlConditions: MutableList<NrqlCondition> = mutableListOf()
internal val syntheticsConditions: MutableList<SyntheticsCondition> = mutableListOf()
fun channels(block: Channels.() -> Unit) = channels.addAll(Channels().apply(block).channels)
fun conditions(block: Conditions.() -> Unit) {
val conds = Conditions().apply(block)
conditions.addAll(conds.conditions)
externalServiceConditions.addAll(conds.externalServiceConditions)
nrqlConditions.addAll(conds.nrqlConditions)
syntheticsConditions.addAll(conds.syntheticsConditions)
}
}
fun policyConfiguration(block: PolicyConfigurationDsl.() -> Unit): PolicyConfiguration {
val dsl = PolicyConfigurationDsl()
dsl.block()
return PolicyConfiguration.builder()
.policyName(requireNotNull(dsl.policyName) { "Policy name cannot be null" })
.incidentPreference(requireNotNull(dsl.incidentPreference) { "Policy incident preference cannot be null" })
.channels(dsl.channels)
.conditions(dsl.conditions)
.externalServiceConditions(dsl.externalServiceConditions)
.nrqlConditions(dsl.nrqlConditions)
.syntheticsConditions(dsl.syntheticsConditions)
.build()
}
@NewRelicConfigurationMarker
class PolicyConfigurations {
internal val policies: MutableList<PolicyConfiguration> = mutableListOf()
fun policy(block: PolicyConfigurationDsl.() -> Unit) = policies.add(policyConfiguration(block))
operator fun PolicyConfiguration.unaryPlus() = policies.add(this)
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/ServersMetricCondition.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.TermsConfiguration;
import lombok.Builder;
import lombok.Getter;
import lombok.NonNull;
import lombok.Singular;
import java.util.Collection;
/**
* Servers metric configuration.
* Configuration parameters:
* <ul>
* <li>{@link #conditionName}</li>
* <li>{@link #enabled}</li>
* <li>{@link #servers}</li>
* <li>{@link #metric}</li>
* <li>{@link #runBookUrl}</li>
* <li>{@link #terms}</li>
* <li>{@link #userDefinedConfiguration}</li>
* </ul>
*/
@Getter
@Builder
public class ServersMetricCondition implements Condition {
private final ConditionType type = ConditionType.SERVERS_METRIC;
/**
* Name of your servers metric condition.
*/
@NonNull
private String conditionName;
/**
* If your servers metric condition is enabled. Default is true.
*/
@Builder.Default
private boolean enabled = true;
/**
* Collection of server names for which this condition is applied.
* If server with given name does not exist exception will be thrown.
*/
@NonNull
@Singular
private Collection<String> servers;
/**
* Metric used in given condition.
*/
@NonNull
private Metric metric;
/**
* The runbook URL to display in notifications.
*/
private String runBookUrl;
/**
* Collection of terms used for alerts condition.
*/
@NonNull
@Singular
private Collection<TermsConfiguration> terms;
/**
* Configuration for user defined metric. Should be provided when {@link #metric} is set to USER_DEFINED
*/
private UserDefinedConfiguration userDefinedConfiguration;
@Override
public Collection<String> getEntities() {
return getServers();
}
@Override
public String getMetricAsString() {
return metric.name().toLowerCase();
}
@Override
public UserDefinedConfiguration getUserDefinedMetric() {
return userDefinedConfiguration;
}
public enum Metric {
CPU_PERCENTAGE, DISK_IO_PERCENTAGE, MEMORY_PERCENTAGE, FULLEST_DISK_PERCENTAGE,
LOAD_AVERAGE_ONE_MINUTE, USER_DEFINED
}
}
<file_sep>/CHANGELOG.md
# 5.0.1 (13-07-2023)
- Added support for `violationTimeLimitSeconds`
# 5.0.0 (05-06-2023)
- Added support for new NR API
# 4.3.0 (07-03-2023)
- Added redirect following
# 4.2.0 (19-07-2022)
- Added possibility to define custom duration
# 4.1.0 (06-07-2022)
- Removed deprecated `sinceValue` from Nrql Condition
# 4.0.1 (03-06-2022)
- Allow usage of aggregationTimer when EVENT_TIMER streaming method is used
# 4.0.0 (19-05-2022)
- Adapt to Signal having aggregationMethod
# 3.6.1 (13-10-2021)
- Added support for signal configuration without expiration
# 3.6.0 (09-09-2021)
- Added support for `signalFillValue` in NRQL Signaling
- Updated conditions REST API url
# 3.5.3 (25-08-2021)
- Added SyntheticsMonitorsApi
# 3.5.2 (08-03-2021)
- Added NRQL signal configuration to Kotlin DSL
# 3.5.1 (14-01-2021)
- Fixed setting explicit null value for field 'fill_value' in Signal configuration
# 3.5.0 (02-12-2020)
- Added Signal configuration
# 3.4.1 (26-02-2020)
- Added new GcMetric `GC/MarkSweepCompact` and `GC/Copy`
# 3.4.0 (06-06-2019)
- Added newrelic-alerts-configurator-dsl module with kotlin dsl
# 3.3.1 (03-10-2018)
- Added more "Since" values to NRQL Condition
# 3.3.0 (07-08-2018)
- Added support for Synthetics Monitor Failure conditions
- Removed unused AlertsNrqlConditionsApi interface
# 3.2.0 (09.04.2018)
- Added new GcMetric `GC/ParNew` and `GC/ConcurrentMarkSweep`
# 3.1.0 (23.02.2018)
- Added support for New Relic Browser conditions
# 3.0.0 (17.01.2018)
- Added support for NRQL conditions
- Changed conditions and channels management logic. Changed default conditions state. Those are breaking changes - see details in
[Migration guide](MIGRATION.md)
# 2.0.3 (06.12.2017)
- Added support for JVM metrics conditions
# 2.0.2 (20.09.2017)
- Add violation_close_timer
- Make incident preference required in policy configuration
# 2.0.0 (15.02.2017)
- Changed groupdId and artifactId
- Changed packages to `com.ocadotechnology.newrelic`
- Changed `AlertsChannelConfiguration` field `payload` to type `Object`
- JSON deserialization configured to convert empty string into null POJO reference
# 1.4.0 (06.02.2017)
- Added support for user notification channel
- Simplified channel synchronization logic
# 1.3.0 (24.01.2017)
- Added UserDefined condition type support for `ApmAppCondition` and `ServersMetricCondition`
# 1.2.0 (19.01.2017)
- Added PagerDuty channel support
# 1.1.2
- Added Webhook channel support
# 1.1.1
- fixed Maven publication problems
# 1.1.0
- added support for server metric conditions
- fixed type of `links` property in `KeyTransaction`
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/graphql/apiclient/internal/ModelTranslator.java
package com.ocadotechnology.newrelic.graphql.apiclient.internal;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.ocadotechnology.newrelic.apiclient.model.channels.AlertsChannel;
import com.ocadotechnology.newrelic.apiclient.model.channels.AlertsChannelConfiguration;
import com.ocadotechnology.newrelic.apiclient.model.channels.AlertsChannelLinks;
import com.ocadotechnology.newrelic.apiclient.model.conditions.Expiration;
import com.ocadotechnology.newrelic.apiclient.model.conditions.Signal;
import com.ocadotechnology.newrelic.apiclient.model.conditions.Terms;
import com.ocadotechnology.newrelic.apiclient.model.conditions.nrql.AlertsNrqlCondition;
import com.ocadotechnology.newrelic.apiclient.model.conditions.nrql.Nrql;
import com.ocadotechnology.newrelic.graphql.apiclient.internal.transport.*;
import lombok.RequiredArgsConstructor;
import lombok.SneakyThrows;
import org.apache.commons.text.StringEscapeUtils;
import java.io.StringReader;
import java.io.StringWriter;
import java.util.*;
import java.util.function.Consumer;
import static com.fasterxml.jackson.databind.DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES;
import static com.ocadotechnology.newrelic.graphql.apiclient.internal.util.Preconditions.checkArgument;
import static java.lang.String.format;
import static java.util.Collections.emptyList;
import static java.util.Objects.isNull;
import static java.util.Objects.nonNull;
import static java.util.stream.Collectors.toList;
import static java.util.stream.Collectors.toMap;
@RequiredArgsConstructor
public class ModelTranslator {
private static final ObjectMapper DEFAULT_OBJECT_MAPPER = new ObjectMapper()
.disable(FAIL_ON_UNKNOWN_PROPERTIES);
private final ObjectMapper objectMapper;
public ModelTranslator() {
this.objectMapper = DEFAULT_OBJECT_MAPPER;
}
public AlertsChannel toModel(NotificationChannel notificationChannel) {
return Optional.ofNullable(notificationChannel)
.map(channel -> AlertsChannel.builder()
.id(Optional.ofNullable(channel.getId()).map(Integer::parseInt).orElse(null))
.name(channel.getName())
.type(Optional.ofNullable(channel.getType()).map(String::toLowerCase).orElse(null))
.links(new AlertsChannelLinks(Optional.ofNullable(channel.getAssociatedPolicies())
.map(AssociatedPolicies::getPolicies)
.orElse(Collections.emptyList())
.stream().map(Identifiable::getId)
.collect(toList())))
.configuration(toModel(channel.getConfig()))
.build())
.orElse(null);
}
public AlertsNrqlCondition toModel(NrqlCondition dto) {
return AlertsNrqlCondition.builder()
.id(Optional.ofNullable(dto.getId()).map(Integer::parseInt).orElse(null))
.name(dto.getName())
.enabled(dto.getEnabled())
.runbookUrl(dto.getRunbookUrl())
.nrql(Optional.ofNullable(dto.getNrql())
.map(nrql -> new Nrql(nrql.getQuery()))
.orElse(null))
.expiration(Optional.ofNullable(dto.getExpiration())
.map(expiration -> Expiration.builder()
.closeViolationsOnExpiration(expiration.isCloseViolationsOnExpiration())
.expirationDuration(expiration.getExpirationDuration())
.openViolationOnExpiration(expiration.isOpenViolationOnExpiration())
.build())
.orElse(null))
.signal(Optional.ofNullable(dto.getSignal())
.map(signal -> Signal.builder()
.aggregationDelay(signal.getAggregationDelay())
.aggregationMethod(Optional.ofNullable(signal.getAggregationMethod()).map(String::toUpperCase).orElse(null))
.aggregationTimer(signal.getAggregationTimer())
.aggregationWindow(signal.getAggregationWindow())
.fillOption(Optional.ofNullable(signal.getFillOption()).map(String::toLowerCase).orElse(null))
.fillValue(signal.getFillValue())
.build())
.orElse(null))
.terms(Optional.ofNullable(dto.getTerms())
.map(terms -> terms.stream()
.map(term -> Terms.builder()
.operator(Optional.ofNullable(term.getOperator()).map(String::toLowerCase).orElse(null))
.priority(Optional.ofNullable(term.getPriority()).map(String::toLowerCase).orElse(null))
.threshold(term.getThreshold())
.duration(Optional.ofNullable(term.getThresholdDuration())
.map(Long::parseLong)
.map(durationSeconds -> "" + (long) (durationSeconds / 60))
.orElse(null))
.timeFunction(Optional.ofNullable(term.getThresholdOccurrences())
.map(this::thresholdOccurrencesToTimeFunctionTo)
.map(String::toLowerCase)
.orElse(null))
.build())
.collect(toList()))
.orElse(null))
.valueFunction(Optional.ofNullable(dto.getValueFunction()).map(String::toLowerCase).orElse(null))
.build();
}
private AlertsChannelConfiguration toModel(NotificationChannelConfig dto) {
return Optional.ofNullable(dto)
.filter(config -> !config.isEmpty())
.map(config -> AlertsChannelConfiguration.builder()
.recipients(Optional.ofNullable(config.getEmails()).orElse(emptyList())
.stream().reduce(null, (acc, email) -> (isNull(acc) ? "" : acc + ",") + email))
.includeJsonAttachment(config.getIncludeJson())
.userId(Optional.ofNullable(config.getUserId())
.map(Integer::parseInt).orElse(null))
.channel(config.getTeamChannel())
.baseUrl(config.getBaseUrl())
.headers(Optional.ofNullable(config.getCustomHttpHeaders())
.map(httpHeaders -> httpHeaders.stream().collect(toMap(HttpHeader::getName, HttpHeader::getValue)))
.filter(httpHeaders -> !httpHeaders.isEmpty())
.orElse(null))
.authUsername(Optional.ofNullable(config.getBasicAuth()).map(NotificationChannelConfig.BasicAuth::getUsername).orElse(null))
.payloadType(payloadTypeToModel(config.getCustomPayloadType()))
.payload(payloadToModel(config.getCustomPayloadType(), config.getCustomPayloadBody()))
.build()
).orElse(null);
}
@SuppressWarnings("unchecked")
public Map<String, Object> toChannelConfig(String type, String name, AlertsChannelConfiguration configuration) {
checkArgument(nonNull(type), "channel type cannot be null");
checkArgument(nonNull(name), "channel name cannot be null");
checkArgument(nonNull(configuration), "configuration name cannot be null");
Map<String, Object> config = map();
config.put("name", name);
String wrapperName = type;
switch (type) {
case "email":
config.put("emails", Optional.ofNullable(configuration.getRecipients())
.map(recipients -> Arrays.stream(recipients.split(",")).map(String::trim).collect(toList())).orElse(null));
config.put("includeJson", Optional.ofNullable(configuration.getIncludeJsonAttachment()).orElse(false));
break;
case "pagerduty":
config.put("apiKey", configuration.getServiceKey());
wrapperName = "pagerDuty";
break;
case "slack":
config.put("teamChannel", configuration.getChannel());
config.put("url", configuration.getUrl());
break;
case "webhook":
AlertsWebhookCustomPayloadType payloadType = Optional.ofNullable(configuration.getPayloadType())
.map(AlertsWebhookCustomPayloadType::byType).orElse(null);
config.put("baseUrl", configuration.getBaseUrl());
config.put("basicAuth", Optional.ofNullable(configuration.getAuthUsername())
.map(__ -> {
Map<String, Object> basicAuth = map();
basicAuth.put("username", configuration.getAuthUsername());
basicAuth.put("password", configuration.getAuthPassword());
return basicAuth;
}).orElse(null));
config.put("customPayloadType", Optional.ofNullable(payloadType)
.map(t -> new JavaLikeEnum(t.toString())).orElse(null));
config.put("customHttpHeaders", Optional.ofNullable(configuration.getHeaders())
.map(headers -> headers.entrySet().stream().map(header -> {
Map<String, String> headerObject = map();
headerObject.put("name", header.getKey());
headerObject.put("value", header.getValue());
return headerObject;
}).collect(toList())).orElse(null));
config.put("customPayloadBody", Optional.ofNullable(configuration.getPayload())
.flatMap(__ -> Optional.ofNullable(payloadType))
.map(pt -> {
switch (pt) {
case JSON:
return writeJson(configuration.getPayload());
case FORM:
return configuration.getPayload() instanceof String
? configuration.getPayload()
: writeProperties((Map<String, Object>) configuration.getPayload());
}
return null;
}).orElse(null)
);
break;
default:
throw new IllegalArgumentException(format("Unsupported channel type: '%s'", type));
}
Map<String, Object> wrapper = new HashMap<>();
wrapper.put(wrapperName, config);
return wrapper;
}
public Map<String, Object> toNrqlCondition(AlertsNrqlCondition condition) {
Map<String, Object> cond = map();
cond.put("enabled", condition.getEnabled());
cond.put("name", condition.getName());
onPresent(condition.getRunbookUrl(), runbookUrl -> cond.put("runbookUrl", runbookUrl));
Map<String, Object> nrql = map();
nrql.put("query", Optional.ofNullable(condition.getNrql()).map(Nrql::getQuery).orElse(null));
cond.put("nrql", nrql);
onPresent(condition.getExpiration(), expiration -> {
Map<String, Object> result = map();
result.put("closeViolationsOnExpiration", expiration.isCloseViolationsOnExpiration());
result.put("openViolationOnExpiration", expiration.isOpenViolationOnExpiration());
onPresent(expiration.getExpirationDuration(),
expirationDuration -> result.put("expirationDuration", toLong(expiration.getExpirationDuration())));
cond.put("expiration", result);
});
onPresent(condition.getSignal(), signal -> {
Map<String, Object> result = map();
onPresent(signal.getAggregationDelay(), aggregationDelay -> result.put("aggregationDelay", toLong(aggregationDelay)));
onPresent(signal.getAggregationMethod(), aggregationMethod -> result.put("aggregationMethod", toEnum(aggregationMethod)));
onPresent(signal.getAggregationTimer(), aggregationTimer -> result.put("aggregationTimer", toLong(aggregationTimer)));
onPresent(signal.getAggregationWindow(), aggregationWindow -> result.put("aggregationWindow", toLong(aggregationWindow)));
onPresent(signal.getFillOption(), fillOption -> result.put("fillOption", toEnum(fillOption)));
onPresent(signal.getFillValue(), fillValue -> result.put("fillValue", fillValue));
cond.put("signal", result);
});
cond.put("terms", Optional.ofNullable(condition.getTerms()).orElseGet(ArrayList::new).stream()
.map(term -> {
LinkedHashMap<String, Object> result = map();
onPresent(term.getOperator(), operator -> result.put("operator", toEnum(operator)));
onPresent(term.getPriority(), priority -> result.put("priority", toEnum(priority)));
onPresent(term.getThreshold(), threshold -> result.put("threshold", toFloat(threshold)));
onPresent(term.getDuration(), duration -> result.put("thresholdDuration", toLong(duration) * 60));
onPresent(term.getTimeFunction(), timeFunction -> result.put("thresholdOccurrences", toEnum(timeFunctionToThresholdOccurrences(timeFunction))));
return result;
})
.collect(toList()));
onPresent(condition.getValueFunction(), valueFunction -> cond.put("valueFunction", toEnum(valueFunction)));
return cond;
}
private String timeFunctionToThresholdOccurrences(String input) {
return input.equalsIgnoreCase("any") ? "at_least_once" : input;
}
private String thresholdOccurrencesToTimeFunctionTo(String input) {
return input.equalsIgnoreCase("at_least_once") ? "any" : input;
}
private static <T1, T2> LinkedHashMap<T1, T2> map() {
return new LinkedHashMap<>();
}
private JavaLikeEnum toEnum(String fillOption) {
return new JavaLikeEnum(fillOption.toUpperCase());
}
private long toLong(String value) {
return Long.parseLong(value);
}
private float toFloat(String value) {
return Float.parseFloat(value);
}
private <T> void onPresent(T object, Consumer<T> consumer) {
if (Objects.nonNull(object)) {
consumer.accept(object);
}
}
private String payloadTypeToModel(String customPayloadType) {
return Optional.ofNullable(customPayloadType)
.map(AlertsWebhookCustomPayloadType::valueOf)
.map(AlertsWebhookCustomPayloadType::getType)
.orElse(null);
}
private Map<String, Object> payloadToModel(String customPayloadType, String customPayloadBody) {
return Optional.ofNullable(customPayloadBody)
.map(__ -> customPayloadType)
.map(AlertsWebhookCustomPayloadType::valueOf)
.map(type -> {
switch (type) {
case JSON:
return readJson(StringEscapeUtils.unescapeJson(customPayloadBody));
case FORM:
return readProperties(customPayloadBody);
default:
return null;
}
})
.orElse(null);
}
@SneakyThrows
private String writeJson(Object value) {
return objectMapper.writeValueAsString(value);
}
@SuppressWarnings("unchecked")
@SneakyThrows
private Map<String, Object> readJson(String value) {
return objectMapper.readValue(value, Map.class);
}
@SuppressWarnings({"unchecked", "rawtypes"})
@SneakyThrows
private Map<String, Object> readProperties(String value) {
Properties properties = new Properties();
properties.load(new StringReader(value));
return (Map<String, Object>) (Map) properties;
}
@SneakyThrows
public static String writeProperties(Map<String, Object> value) {
Properties properties = new Properties();
properties.putAll(value);
StringWriter writer = new StringWriter();
properties.store(writer, null);
String output = writer.toString();
String sep = System.getProperty("line.separator");
return output.substring(output.indexOf(sep) + sep.length()).trim().replaceAll(sep, "\n");
}
}
<file_sep>/gradle.properties
version=5.0.2-SNAPSHOT<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/ApmAppCondition.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.TermsConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.violationclosetimer.ViolationCloseTimer;
import lombok.Builder;
import lombok.Getter;
import lombok.NonNull;
import lombok.Singular;
import java.util.Collection;
/**
* APM application configuration.
* Configuration parameters:
* <ul>
* <li>{@link #conditionName}</li>
* <li>{@link #enabled}</li>
* <li>{@link #applications}</li>
* <li>{@link #metric}</li>
* <li>{@link #conditionScope}</li>
* <li>{@link #runBookUrl}</li>
* <li>{@link #terms}</li>
* <li>{@link #violationCloseTimer}</li>
* <li>{@link #userDefinedConfiguration}</li>
* </ul>
*/
@Getter
@Builder
public class ApmAppCondition implements Condition {
private final ConditionType type = ConditionType.APM_APP;
/**
* Name of your APM application metric condition
*/
@NonNull
private String conditionName;
/**
* If your APM application metric condition is enabled. Default is true
*/
private boolean enabled;
/**
* Collection of application names for which this condition is applied.
* If application with given name does not exist exception will be thrown
*/
@NonNull
@Singular
private Collection<String> applications;
/**
* Metric used in given condition
*/
@NonNull
private Metric metric;
/**
* Condition scope used in given condition
*/
@NonNull
private ConditionScope conditionScope;
/**
* The runbook URL to display in notifications
*/
private String runBookUrl;
/**
* Collection of terms used for alerts condition
*/
@NonNull
@Singular
private Collection<TermsConfiguration> terms;
/**
* Duration (in hours) after which instance-based violations will automatically close.
* Required when {@link #conditionScope} is set to {@link ConditionScope#INSTANCE}
*/
private ViolationCloseTimer violationCloseTimer;
/**
* Configuration for user defined metric. Should be provided when {@link #metric} is set to USER_DEFINED
*/
private UserDefinedConfiguration userDefinedConfiguration;
@Override
public Collection<String> getEntities() {
return getApplications();
}
@Override
public String getMetricAsString() {
return metric.name().toLowerCase();
}
@Override
public String getConditionScopeAsString() {
return conditionScope.name().toLowerCase();
}
@Override
public String getViolationCloseTimerAsString() {
return violationCloseTimer == null ? null : violationCloseTimer.getDuration();
}
@Override
public UserDefinedConfiguration getUserDefinedMetric() {
return userDefinedConfiguration;
}
public enum Metric {
APDEX, ERROR_PERCENTAGE, RESPONSE_TIME_WEB, RESPONSE_TIME_BACKGROUND, THROUGHPUT_WEB,
THROUGHPUT_BACKGROUND, USER_DEFINED
}
public enum ConditionScope {
APPLICATION, INSTANCE
}
public static class ApmAppConditionBuilder {
private boolean enabled = true;
public ApmAppCondition build() {
validateViolationCloseTimer();
return new ApmAppCondition(conditionName, enabled, applications, metric, conditionScope,
runBookUrl, terms, violationCloseTimer, userDefinedConfiguration);
}
private void validateViolationCloseTimer() {
if (conditionScope == ConditionScope.INSTANCE && violationCloseTimer == null) {
throw new NullPointerException("violationCloseTimer");
}
}
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/AlertsPoliciesApi.java
package com.ocadotechnology.newrelic.apiclient;
import com.ocadotechnology.newrelic.apiclient.model.policies.AlertsPolicy;
import com.ocadotechnology.newrelic.apiclient.model.policies.AlertsPolicyChannels;
import java.util.Optional;
public interface AlertsPoliciesApi {
/**
* Gets {@link AlertsPolicy} object using its name.
*
* @param alertsPolicyName name of the alert policy registered in NewRelic
* @return Optional containing {@link AlertsPolicy} object, or empty if alert policy not found
*/
Optional<AlertsPolicy> getByName(String alertsPolicyName);
/**
* Creates Alerts Policy instance.
*
* @param policy policy definition to be created
* @return created {@link AlertsPolicy}
*/
AlertsPolicy create(AlertsPolicy policy);
/**
* Deletes Alerts Policy instance.
*
* @param policyId id of the policy to be removed
* @return deleted {@link AlertsPolicy}
*/
AlertsPolicy delete(int policyId);
/**
* Associates given channels to the policy. This method does not remove previously linked channel but not provided in the list.
*
* @param channels {@link AlertsPolicyChannels} object mapping policy id to the list of channels ids
* @return {@link AlertsPolicyChannels} when successfully set
*/
AlertsPolicyChannels updateChannels(AlertsPolicyChannels channels);
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/model/DashboardList.java
package com.ocadotechnology.newrelic.apiclient.internal.model;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.ocadotechnology.newrelic.apiclient.model.dashboards.Dashboard;
import lombok.EqualsAndHashCode;
import java.util.List;
@EqualsAndHashCode(callSuper = true)
public class DashboardList extends ObjectList<Dashboard, DashboardList> {
@JsonCreator
public DashboardList(@JsonProperty("dashboards") List<Dashboard> items) {
super(items, DashboardList::new);
}
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/channel/ChannelType.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel;
public enum ChannelType {
EMAIL, SLACK, WEBHOOK, PAGERDUTY, USER
}
<file_sep>/newrelic-alerts-configurator/README.md
# Table of contents
- [Configurator](#configurator)
- [Application configuration](#application-configuration)
- [Policy configuration](#policy-configuration)
- [Alerts condition](#alerts-condition)
- [APM application metric condition](#apm-application-metric-condition)
- [APM JVM metric condition](#apm-jvm-metric-condition)
- [APM key transaction metric condition](#apm-key-transaction-metric-condition)
- [Server metric condition](#server-metric-condition)
- [New Relic Browser metric condition](#new-relic-browser-metric-condition)
- [Alerts external service condition](#alerts-external-service-condition)
- [APM external service condition](#apm-external-service-condition)
- [Alerts NRQL condition](#alerts-nrql-condition)
- [Synthetics Monitor Failure condition](#synthetics-monitor-failure-condition)
- [User defined configuration](#user-defined-configuration)
- [Term](#term)
- [NRQL Term](#nrql-term)
- [Notification channel](#notification-channel)
- [Email channel](#email-channel)
- [Slack channel](#slack-channel)
- [Webhook channel](#webhook-channel)
- [PagerDuty channel](#pagerduty-channel)
- [NewRelic user channel](#newrelic-user-channel)
- [Condition and Channel management policy](#condition-and-channel-management-policy)
## Configurator
`Configurator` is the main class used to synchronize all defined configurations with NewRelic. Example use of Configurator:
```java
Configurator configurator = new Configurator(yourApiKey);
configurator.setApplicationConfigurations(yourApplicationConfigurationsCollection);
configurator.setPolicyConfigurations(yourPolicyConfigurationsCollection);
configurator.sync();
```
Both `setApplicationConfigurations` and `setPolicyConfigurations` accept Java Collections of configurations.
## Application configuration
To configure your application in NewRelic you just need to create and pass your application configuration to NewRelic Alerts configurator.
Application configuration can be created using simple builder:
```java
ApplicationConfiguration.builder()
```
What you can set in your configuration:
- application name - Your application name.
- application apdex threshold (optional) - Set apdex threshold for application.
- end user apdex threshold (optional) - Set apdex threshold for end user.
- real user monitoring enabled (optional) - If real user monitoring is enabled. Default value is false.
If application with given application name does not exist exception will be thrown.
Example application configuration:
```java
ApplicationConfiguration configuration = ApplicationConfiguration.builder()
.applicationName("Application name")
.appApdexThreshold(0.5f)
.endUserApdexThreshold(7.0f)
.enableRealUserMonitoring(true)
.build();
```
## Policy configuration
To configure your alerts policy in NewRelic you just need to create and pass your policy configuration to NewRelic Alerts configurator.
Policy configuration can be created using simple builder:
```java
PolicyConfiguration.builder()
```
What you can set in your configuration:
- policy name - Name of your alerts policy.
- incident preference - Rollup strategy options for your alerts policy. Possible values are:
- Per policy
- Per condition
- Per condition and target
- conditions (optional) - Collection of [alerts conditions](#alerts-condition) which needs to be configured for your alerts policy.
- external service conditions (optional) - Collection of [alerts external service conditions](#alerts-external-service-condition)
which needs to be configured for your alerts policy.
- nrql conditions (optional) - Collection of [alerts NRQL conditions](#alerts-nrql-condition) which needs to be configured for
your alerts policy.
- synthetics conditions (optional) - Collection of [Synthetics Monitor Failure conditions](#synthetics-monitor-failure-condition)
which need to be configured for your alerts policy.
- channels (optional) - Collection of [alerts channels](#notification-channel) which needs to be configured for your alerts policy.
If policy with given policy name exists - it will be updated.
If policy with given policy name does not exist - it will be created.
Example alerts policy configuration:
```java
PolicyConfiguration configuration = PolicyConfiguration.builder()
.policyName("Policy name")
.incidentPreference(PolicyConfiguration.IncidentPreference.PER_POLICY)
.condition(apmAppCondition)
.condition(apmKeyTransactionCondition)
.externalServiceCondition(apmExternalServiceCondition)
.nrqlCondition(nrqlCondition)
.channel(emailChannel)
.channel(slackChannel)
.build()
```
### Alerts condition
Currently supported types of alerts policy conditions are:
- [APM application metric condition](#apm-application-metric-condition)
- [APM JVM metric condition](#apm-jvm-metric-condition)
- [APM key transaction metric condition](#apm-key-transaction-metric-condition)
- [Server metric condition](#server-metric-condition)
- [New Relic Browser metric condition](#new-relic-browser-metric-condition)
Condition creation/update/removal policy is described in [Condition and Channel management policy](#condition-and-channel-management-policy)
#### APM application metric condition
To create APM application metric condition for your alerts policy use simple builder:
```java
ApmAppCondition.builder()
```
What you can set for APM application metric condition:
- condition name - Name of your APM application metric condition.
- enabled (optional) - If your APM application metric condition is enabled. Default is true.
- applications - Collection of application names for which this condition is applied. If application with given name does not exist exception will be thrown.
- metric - Metric used in given condition. Possible values are:
- Apdex
- Error percentage
- Response time (web)
- Response time (background)
- Throughput (web)
- Throughput (background)
- User defined
- condition scope - Possible values are:
- Application - The average of all application instances' data is used during evaluation.
- Instance - Each application instance's data is evaluated individually
- run book url (optional) - The runbook URL to display in notifications.
- terms - Collection of [terms](#term) used for alerts condition.
- violation close timer - Duration (in hours) after which instance-based violations will automatically close.
This field is only required when condition scope is set to 'Instance'. Possible values are:
- 1
- 2
- 4
- 8
- 12
- 24
- user defined configuration - configuration for [user defined metric](#user-defined-configuration).
Example APM application metric condition configuration:
```java
Condition apmAppCondition = ApmAppCondition.builder()
.conditionName("Condition name")
.enabled(true)
.application("Application name")
.metric(ApmAppCondition.Metric.APDEX)
.conditionScope(ApmAppCondition.ConditionScope.APPLICATION)
.term(term)
.build();
```
#### APM JVM metric condition
To create APM JVM metric condition for your alerts policy use simple builder:
```java
ApmJvmCondition.builder()
```
What you can set for APM JVM metric condition:
- condition name - Name of your APM application metric condition.
- enabled (optional) - If your APM application metric condition is enabled. Default is true.
- applications - Collection of application names for which this condition is applied. If application with given name does not exist exception will be thrown.
- metric - Metric used in given condition. Possible values are:
- Deadlocked threads
- Heap memory usage
- CPU utilization time
- Garbage collection CPU time
- gc metric - Metric used to get GC CPU time. To use it metric has to be set to measure garbage collection CPU time. Possible values are:
- PS MarkSweep
- PS Scavenge
- ParNew
- ConcurrentMarkSweep
- ###### run book url (optional) - The runbook URL to display in notifications.
- terms - Collection of [terms](#term) used for alerts condition.
- violation close timer - Duration (in hours) after which instance-based violations will automatically close.
Possible values are:
- 1
- 2
- 4
- 8
- 12
- 24
Example APM JVM metric condition configuration:
```java
Condition apmJvmCondition = ApmJvmCondition.builder()
.conditionName("Condition name")
.enabled(true)
.application("Application name")
.metric(ApmJvmCondition.Metric.GC_CPU_TIME)
.gcMetric(ApmJvmCondition.GcMetric.GC_MARK_SWEEP)
.term(term)
.violationCloseTimer(ViolationCloseTimer.DURATION_24)
.build();
```
#### APM key transaction metric condition
To create APM key transaction metric condition for your alerts policy use simple builder:
```java
ApmKeyTransactionCondition.builder()
```
What you can set for APM key transaction metric condition:
- condition name - Name of your APM key transaction metric condition.
- enabled (optional) - If your APM key transaction metric condition is enabled. Default is true.
- keyTransactions - Collection of key transaction names for which this condition is applied. If key transaction with given name does not exist exception will be thrown.
- metric - Metric used in given condition. Possible values are:
- Apdex
- Error percentage
- Error count
- Response time
- Throughput
- run book url (optional) - The runbook URL to display in notifications.
- terms - Collection of [terms](#term) used for alerts condition.
Example APM key transaction metric condition configuration:
```java
Condition apmKeyTransactionCondition = ApmKeyTransactionCondition.builder()
.conditionName("Condition name")
.enabled(true)
.keyTransastion("Key Transaction name")
.metric(ApmKeyTransactionCondition.Metric.APDEX)
.term(term)
.build();
```
**Note that key transaction cannot be created via New Relic API. Before configuration of condition, key transaction needs to be manually created in New Relic.**
#### Server metric condition
To create server metric condition for your alerts policy use simple builder:
```java
ServersMetricCondition.builder()
```
What you can set for server metric condition:
- condition name - Name of your server metric condition.
- enabled (optional) - If your server metric condition is enabled. Default is true.
- servers - Collection of server names for which this condition is applied. If server with given name does not exist exception will be thrown.
- metric - Metric used in given condition. Possible values are:
- CPU percentage
- Disk I/O percentage
- Memory percentage
- Fullest disk percentage
- Load average one minute
- User defined
- run book url (optional) - The runbook URL to display in notifications.
- terms - Collection of [terms](#term) used for alerts condition.
- user defined configuration - configuration for [user defined metric](#user-defined-configuration).
Example server metric condition configuration:
```java
Condition serverMetricCondition = ServerMetricCondition.builder()
.conditionName("Condition name")
.enabled(true)
.server("some-host")
.metric(ServersMetricCondition.Metric.CPU_PERCENTAGE)
.term(term)
.build();
```
#### New Relic Browser metric condition
To create New Relic Browser metric condition for your alerts policy use simple builder:
```java
BrowserCondition.builder()
```
What you can set for New Relic Browser metric condition:
- condition name - Name of your New Relic Browser metric condition
- enabled (optional) - If your New Relic Browser metric condition is enabled. Default is true.
- applications - Collection of application names for which this condition is applied. If application with given name does not exist exception will be thrown.
- metric - Metric used in given condition. Possible values are:
- End user Apdex
- Total page load
- Page rendering
- Web application
- Network
- DOM processing
- Request queuing
- AJAX response time
- Page views with JS errors
- Page view throughput
- AJAX throughput
- User defined
- run book url (optional) - The runbook URL to display in notifications.
- terms - Collection of [terms](#term) used for alerts condition.
- user defined configuration - configuration for [user defined metric](#user-defined-configuration).
Example New Relic Browser metric condition configuration:
```java
Condition browserCondition = BrowserCondition.builder()
.conditionName("Page views with JS errors")
.enabled(true)
.application("Application name")
.metric(BrowserCondition.Metric.PAGE_VIEWS_WITH_JS_ERRORS)
.term(term)
.build();
```
**Please note New Relic Browser agent has to be installed.**
### Alerts external service condition
Currently supported types of alerts policy external service conditions are:
- [APM external service condition](#apm-external-service-condition)
External Service Condition creation/update/removal policy is described in [Condition and Channel management policy](#condition-and-channel-management-policy)
#### APM external service condition
To create APM external service condition for your alerts policy use simple builder:
```java
ApmExternalServiceCondition.builder()
```
What you can set for APM external service condition:
- condition name - Name of your APM external service condition.
- enabled (optional) - If your APM external service condition is enabled. Default is true.
- applications - Collection of application names for which this condition is applied. If application with given name does not exist exception will be thrown.
- metric - Metric used in given condition. Possible values are:
- Response time (average)
- Response time (minimum)
- Response time (maximum)
- Throughput
- external service url - URL of the external service to be monitored. This string must not include the protocol.
- run book url (optional) - The runbook URL to display in notifications.
- terms - Collection of [terms](#term) used for alerts condition.
Example APM external service condition configuration:
```java
ExternalServiceCondition apmExternalServiceCondition = ApmExternalServiceCondition.builder()
.conditionName("Condition name")
.enabled(true)
.application("Application name")
.externalServiceUrl("externalServiceUrl")
.metric(ApmExternalServiceCondition.Metric.RESPONSE_TIME_AVERAGE)
.term(term)
.build();
```
### Alerts NRQL condition
NRQL Condition creation/update/removal policy is described in [Condition and Channel management policy](#condition-and-channel-management-policy)
To create NRQL condition for your alerts policy use simple builder:
```java
NrqlCondition.builder()
```
What you can set for NRQL condition:
- condition name - Name of your NRQL condition.
- enabled (optional) - If your NRQL condition is enabled. Default is true.
- run book url (optional) - The runbook URL to display in notifications.
- terms - Collection of [NRQL terms](#nrql-term) used for alerts condition.
- value function - How condition should be evaluated. Possible values are:
- single_value - the condition will be evaluated by the raw value returned.
- sum - the condition will evaluate on the sum of the query results.
- query - Query in NRQL.
- signal configuration - Determines how data should be collected and what should happened when there is lack of data:
- aggregation window - Time (in seconds) for how long NewRelic collects data before running the NRQL query.
- evaluation windows - Number of windows to evaluate data.
- signal fill option - Configuration of filling data gaps/signal lost. Possible values are:
- NONE - do not fill any data
- LAST_KNOWN_VALUE - fill with last known value
- signal lost configuration:
- signal is lost after - Time (in seconds) for the signal treated as lost.
- open new violation on signal lost - Opens a loss of signal violation when no signal.
- closeCurrentViolationsOnSignalLost - cCoses all currently open violations when no signal is heard.
Example NRQL condition configuration:
```java
NrqlCondition.builder()
.conditionName("Condition name")
.enabled(true)
.valueFunction(NrqlCondition.ValueFunction.SINGLE_VALUE)
.term(term)
.query("SELECT count(*) FROM `myApp:HealthStatus` WHERE healthy IS false")
.build();
```
### Synthetics Monitor Failure condition
Synthetics Monitor Failure condition creation/update/removal policy is described in [Condition and Channel management policy](#condition-and-channel-management-policy)
To create Synthetics Monitor Failure condition for your alerts policy use simple builder:
```java
SyntheticsCondition.builder()
```
What you can set for Synthetics Monitor Failure condition:
- condition name - Name of your Synthetics Monitor Failure condition.
- monitorName - The name of the Synthetics Monitor you want to alert on.
- enabled (optional) - If your Synthetics Monitor Failure condition is enabled. Default is true.
- run book url (optional) - The runbook URL to display in notifications.
Example Synthetics Monitor Failure condition configuration:
```java
SyntheticsCondition.builder()
.conditionName("MyMonitor failed")
.enabled(true)
.monitorName("MyMonitor")
.build();
```
### Synthetic Monitor Create
Synthetic monitor can be created as follows.
```java
new NewRelicApi("api-key").getSyntheticsMonitorsApi().create(new Monitor(...))
```
### Synthetic Monitor Delete
Synthetic monitor can be delete as follows.
```java
new NewRelicApi("api-key").getSyntheticsMonitorsApi().delete(new Monitor(...))
```
### User defined configuration
User defined configuration allows to use custom (user defined) metric in alert definition.
Example user defined configuration:
```java
UserDefinedConfiguration config = UserDefinedConfiguration.builder()
.metric("MY_CUSTOM_METRIC")
.valueFunction(UserDefinedConfiguration.ValueFunction.MAX)
.build();
```
### Term
Terms are used both in alerts conditions and alerts external service conditions.
To create term configuration for condition use simple builder:
```java
TermsConfiguration.builder()
```
What you can set in term configuration:
- duration - Time (in minutes) for the condition to persist before triggering an event. Possible values are:
- 5
- 10
- 15
- 30
- 60
- 120
- operator - Determines what comparison will be used between the monitored value and the threshold term value to trigger an event. Possible values are:
- Above
- Below
- Equal
- priority - Severity level for given term in condition. Possible values are:
- Critical
- Warning
- time function - Time function in which threshold term have to be reached in duration term to trigger an event. Possible values are:
- All (for whole time)
- Any (at least once in)
- threshold - Threshold that the monitored value must be compared to using the operator term for an event to be triggered.
Example term configuration:
```java
TermsConfiguration term = TermsConfiguration.builder()
.durationTerm(DurationTerm.DURATION_5)
.operatorTerm(OperatorTerm.BELOW)
.thresholdTerm(0.8f)
.priorityTerm(PriorityTerm.CRITICAL)
.timeFunctionTerm(TimeFunctionTerm.ALL)
.build();
```
### NRQL Term
NRQL Terms are used in NRQL conditions.
To create term configuration for condition use simple builder:
```java
NrqlTermsConfiguration.builder()
```
What you can set in term configuration:
- duration - Time (in minutes) for the condition to persist before triggering an event. Possible values are:
- 1
- 2
- 3
- 4
- 5
- 10
- 15
- 30
- 60
- 120
- operator - Determines what comparison will be used between the monitored value and the threshold term value to trigger an event. Possible values are:
- Above
- Below
- Equal
- priority - Severity level for given term in condition. Possible values are:
- Critical
- Warning
- time function - Time function in which threshold term have to be reached in duration term to trigger an event. Possible values are:
- All (for whole time)
- Any (at least once in)
- threshold - Threshold that the monitored value must be compared to using the operator term for an event to be triggered.
Example term configuration:
```java
NrqlTermsConfiguration term = NrqlTermsConfiguration.builder()
.durationTerm(NrqlDurationTerm.DURATION_1)
.operatorTerm(OperatorTerm.BELOW)
.thresholdTerm(0.8f)
.priorityTerm(PriorityTerm.CRITICAL)
.timeFunctionTerm(TimeFunctionTerm.ALL)
.build();
```
### Notification channel
Currently supported types of alerts notification channels are:
- [Email channel](#email-channel)
- [Slack channel](#slack-channel)
- [Webhook channel](#webhook-channel)
- [PagerDuty channel](#pagerduty-channel)
- [NewRelic user channel](#newrelic-user-channel)
Channels creation/update/removal policy is described in [Condition and Channel management policy](#condition-and-channel-management-policy)
Only exception is NewRelic user channel - we will not delete it.
#### Email channel
To create email channel use simple builder:
```java
EmailChannel.builder()
```
What you can set in email channel configuration:
- channel name - Name of your alerts channel.
- email address - Email address to which alert event should be sent.
- include json attachment (optional) - If json attachment should be included in alert event email. Default value is false.
Example email channel configuration:
```java
Channel emailChannel = EmailChannel.builder()
.channelName("Channel name")
.emailAddress("<EMAIL>")
.includeJsonAttachment(true)
.build();
```
#### Slack channel
To create slack channel use simple builder:
```java
SlackChannel.builder()
```
What you can set in slack channel configuration:
- channel name - Name of your alerts channel.
- slack url - Slack url to your channel.
- team channel (optional) - Name of your team channel.
Example slack channel configuration:
```java
Channel slackChannel = SlackChannel.builder()
.channelName("Channel name")
.slackUrl("https://hooks.slack.com/services/...")
.teamName("My team slack name")
.build();
```
#### Webhook channel
To create webhook channel use simple builder:
```java
WebhookChannel.builder()
```
What you can set in webhook channel configuration:
- channel name - Name of your alerts channel.
- base URL - URL webhook address to which alert event should be sent.
- auth username - User name for BASIC authorization (optional)
- auth password - User password for BASIC authorization (optional)
Example webhook channel configuration:
```java
Channel webhookChannel = WebhookChannel.builder()
.channelName("Channel name")
.baseUrl("https://example.com/webhook/abcd123")
.authUsername("john.doea")
.authPassword("<PASSWORD>")
.build();
```
#### PagerDuty channel
To create [PagerDuty](https://www.pagerduty.com/) channel use simple builder:
```java
PagerDutyChannel.builder()
```
What you can set in PagerDUty channel configuration:
- channel name - Name of your alerts channel.
- serviceKey - Service key integration ID defined in PagerDuty.
Example PagerDuty channel configuration:
```java
Channel pagerDutyChannel = PagerDutyChannel.builder()
.channelName("Channel name")
.serviceKey("<KEY>")
.build();
```
#### NewRelic user channel
To create NewRelic user channel use simple builder:
```java
UserChannel.builder()
```
What you can set in NewRelic user channel:
- email address - NewRelic user's email address
Example email channel configuration:
```java
Channel userChannel = UserChannel.builder()
.userEmail("<EMAIL>")
.build();
```
#### Condition and Channel management policy
All conditions and channels are managed in the same way.
1. If you don't define any conditions in the policy, synchronization process will not modify already existing conditions.
Example:
```java
PolicyConfiguration configuration = PolicyConfiguration.builder()
.policyName("Policy name")
.incidentPreference(PolicyConfiguration.IncidentPreference.PER_POLICY)
.build()
```
This configuration will not remove, create or update any existing condition.
1. If you define some conditions in the policy, then:
- conditions which are defined in configuration, but are missing will be created.
- conditions which are defined in configuration and already exist will be updated.
- conditions which are not defined in configuration, but already exist will be removed.
Example:
```java
PolicyConfiguration configuration = PolicyConfiguration.builder()
.policyName("Policy name")
.incidentPreference(PolicyConfiguration.IncidentPreference.PER_POLICY)
.condition(condition1)
.condition(condition2)
.build()
```
This configuration will create/update condition1 and condition2, and will remove all other conditions.
1. If you define empty conditions list in the policy, then synchronization process will remove all existing conditions.
Example:
```java
PolicyConfiguration configuration = PolicyConfiguration.builder()
.policyName("Policy name")
.incidentPreference(PolicyConfiguration.IncidentPreference.PER_POLICY)
.conditions(Collections.emptyList())
.build()
```
This configuration will not create nor update any conditions, but will remove all existing ones.
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/channel/SlackChannelDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.channel
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.SlackChannel
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class SlackChannelDsl {
var channelName: String? = null
var slackUrl: String? = null
var teamChannel: String? = null
}
fun slackChannel(block: SlackChannelDsl.() -> Unit): SlackChannel {
val dsl = SlackChannelDsl()
dsl.block()
return SlackChannel.builder()
.channelName(requireNotNull(dsl.channelName) { "Slack channel name cannot be null" })
.slackUrl(requireNotNull(dsl.slackUrl) {"Slack channel url cannot be null"})
.teamChannel(dsl.teamChannel)
.build()
}<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/AlertsChannelsApi.java
package com.ocadotechnology.newrelic.apiclient;
import com.ocadotechnology.newrelic.apiclient.model.channels.AlertsChannel;
import java.util.List;
public interface AlertsChannelsApi {
/**
* Lists all existing Alerts Channels.
* This method supports pagination - which means it returns list of entries combined from all pages.
*
* @return list of all existing {@link AlertsChannel} from NewRelic
*/
List<AlertsChannel> list();
/**
* Creates Alerts Channel.
* <p>
* Although New Relic returns list of channels in its response this method assumes there will be exactly one channel returned.
*
* @param channel channel definition to be created
* @return created {@link AlertsChannel}
*/
AlertsChannel create(AlertsChannel channel);
/**
* Deletes Alerts Channel.
*
* @param channelId id of the channel to be deleted
* @return deleted {@link AlertsChannel}
*/
AlertsChannel delete(int channelId);
/**
* Removes Alerts Channel from Policy definition.
*
* @param policyId id of the policy to be updated
* @param channelId id of the channel to be removed from the given policy
* @return {@link AlertsChannel} for the given channel id regardless of being part of the specified policy
*/
AlertsChannel deleteFromPolicy(int policyId, int channelId);
}
<file_sep>/newrelic-alerts-configurator-examples/README.md
# Sample
This is the sample project that uses NewRelic Alerts Configurator library to configure NewRelic alerts for few applications.
It contains configuration in both Java and Kotlin DSL
## Goals
We have **3** applications:
- `Application1` deployed on `app-1-host`
- `Application2` deployed on `app-2-host`
- `Application3` deployed on `app-3-host`
For **each** application we need to:
- Raise warning alert if **apdex** falls below `0.85`
- Raise critical alert if **apdex** falls below `0.7`
- Send alert notification on **slack** to `newrelic-alerts` channel
- In application configuration **application apdex** threshold should be configured to `0.5f`
- In application configuration **end user apdex** threshold should be configured to `0.7f`
- Real user monitoring have to be enabled
- Raise critical alert if **heap memory usage** is above `85%` for longer than 5 minutes
- Raise warning alert if **heap memory usage** is above `65%` for longer than 5 minutes
For **Application1** we need to additionally:
- Raise warning alert if **disk space** on application host raises above `65%`
- Raise critical alert if **disk space** on application host raises above `80%`
- Send alert notifications to **email** `<EMAIL>`
For **Application2** we need to additionally:
- Raise critical alert if **HealthStatus** returns false. Health statuses are available in **Application2:HealthStatus** transactions.
- Raise warning alert if there are above 1% page views with JS error within 5 min
For **Application3** we need to additionally:
- Raise warning alert if **cpu usage** on application host raised above `70%`
- Raise critical alert if **cpu usage** on application host raised above `90%`
- Send alert notifications to **email** `<EMAIL>`
Moreover, described configuration should be deployed on **two** different NewRelic account:
- one account monitoring staging environment
- one account monitoring production environment<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/PolicyItemConfigurator.java
package com.ocadotechnology.newrelic.alertsconfigurator;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.PolicyConfiguration;
import lombok.NonNull;
interface PolicyItemConfigurator {
void sync(@NonNull PolicyConfiguration config);
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/signal/SignalFillOption.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal;
import lombok.AllArgsConstructor;
import lombok.Getter;
@Getter
@AllArgsConstructor
public enum SignalFillOption {
NONE("none"), LAST_KNOWN_VALUE("last_value"), STATIC("static");
private final String value;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/ServersApi.java
package com.ocadotechnology.newrelic.apiclient;
import com.ocadotechnology.newrelic.apiclient.model.servers.Server;
import java.util.Optional;
public interface ServersApi {
/**
* Gets {@link Server} object using its name.
*
* @param serverName name of the server registered in NewRelic
* @return Optional containing {@link Server} object, or empty if server not found
*/
Optional<Server> getByName(String serverName);
/**
* Gets {@link Server} object using its id.
*
* @param serverId id of the server registered in NewRelic
* @return found {@link Server}
*/
Server getById(int serverId);
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/model/AlertsNrqlConditionWrapper.java
package com.ocadotechnology.newrelic.apiclient.internal.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.ocadotechnology.newrelic.apiclient.model.conditions.nrql.AlertsNrqlCondition;
import lombok.Value;
@Value
public class AlertsNrqlConditionWrapper {
@JsonProperty("nrql_condition")
AlertsNrqlCondition nrqlCondition;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/model/applications/ApplicationSummary.java
package com.ocadotechnology.newrelic.apiclient.model.applications;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.Value;
@Value
public class ApplicationSummary {
@JsonProperty("response_time")
Float responseTime;
@JsonProperty
Float throughput;
@JsonProperty("error_rate")
Float errorRate;
@JsonProperty("apdex_target")
Float apdexTarget;
@JsonProperty("apdex_score")
Float apdexScore;
@JsonProperty("host_count")
Integer hostCount;
@JsonProperty("instance_count")
Integer instanceCount;
@JsonProperty("concurrent_instance_count")
Integer concurrentInstanceCount;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/NewRelicInternalApi.java
package com.ocadotechnology.newrelic.apiclient.internal;
import com.ocadotechnology.newrelic.apiclient.*;
import com.ocadotechnology.newrelic.apiclient.internal.client.NewRelicClient;
import com.ocadotechnology.newrelic.apiclient.internal.client.NewRelicClientFactory;
import com.ocadotechnology.newrelic.apiclient.model.conditions.AlertsCondition;
import com.ocadotechnology.newrelic.apiclient.model.conditions.external.AlertsExternalServiceCondition;
import com.ocadotechnology.newrelic.apiclient.model.conditions.nrql.AlertsNrqlCondition;
import com.ocadotechnology.newrelic.apiclient.model.conditions.synthetics.AlertsSyntheticsCondition;
import lombok.Getter;
/**
* API facade - object exposing NewRelic API endpoints as Java methods. Requires API key.
*/
@Getter
public class NewRelicInternalApi {
private final ApplicationsApi applicationsApi;
private final AlertsChannelsApi alertsChannelsApi;
private final AlertsPoliciesApi alertsPoliciesApi;
private final PolicyItemApi<AlertsCondition> alertsConditionsApi;
private final PolicyItemApi<AlertsNrqlCondition> alertsNrqlConditionsApi;
private final PolicyItemApi<AlertsExternalServiceCondition> alertsExternalServiceConditionsApi;
private final PolicyItemApi<AlertsSyntheticsCondition> alertsSyntheticsConditionApi;
private final KeyTransactionsApi keyTransactionsApi;
private final DeploymentsApi deploymentsApi;
private final ServersApi serversApi;
private final UsersApi usersApi;
private final SyntheticsMonitorsApi syntheticsMonitorsApi;
private final DashboardsApi dashboardsApi;
/**
* NewRelic API constructor.
*
* @param restApiUrl NewRelic REST API URL, for example https://api.newrelic.com
* @param syntheticsApiUrl NewRelic Synthetics API URL
* @param clientFactory NewRelic client factory
*/
public NewRelicInternalApi(String restApiUrl, String syntheticsApiUrl, NewRelicClientFactory clientFactory) {
NewRelicClient client = clientFactory.create(restApiUrl);
applicationsApi = new DefaultApplicationsApi(client);
alertsChannelsApi = new DefaultAlertsChannelsApi(client);
alertsPoliciesApi = new DefaultAlertsPoliciesApi(client);
alertsConditionsApi = new DefaultAlertsConditionsApi(client);
alertsExternalServiceConditionsApi = new DefaultAlertsExternalServiceConditionsApi(client);
alertsNrqlConditionsApi = new DefaultAlertsNrqlConditionsApi(client);
alertsSyntheticsConditionApi = new DefaultAlertsSyntheticsConditionsApi(client);
keyTransactionsApi = new DefaultKeyTransactionsApi(client);
deploymentsApi = new DefaultDeploymentsApi(client);
serversApi = new DefaultServersApi(client);
usersApi = new DefaultUsersApi(client);
dashboardsApi = new DefaultDashboardsApi(client);
NewRelicClient syntheticsClient = clientFactory.create(syntheticsApiUrl);
syntheticsMonitorsApi = new DefaultSyntheticsMonitorsApi(syntheticsClient);
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/DefaultKeyTransactionsApi.java
package com.ocadotechnology.newrelic.apiclient.internal;
import com.ocadotechnology.newrelic.apiclient.KeyTransactionsApi;
import com.ocadotechnology.newrelic.apiclient.internal.client.NewRelicClient;
import com.ocadotechnology.newrelic.apiclient.internal.model.KeyTransactionList;
import com.ocadotechnology.newrelic.apiclient.internal.model.KeyTransactionWrapper;
import com.ocadotechnology.newrelic.apiclient.model.transactions.KeyTransaction;
import org.glassfish.jersey.uri.UriComponent;
import java.util.Optional;
import static javax.ws.rs.core.MediaType.APPLICATION_JSON_TYPE;
import static org.glassfish.jersey.uri.UriComponent.Type.QUERY_PARAM_SPACE_ENCODED;
public class DefaultKeyTransactionsApi extends ApiBase implements KeyTransactionsApi {
private static final String KEY_TRANSACTIONS_URL = "/v2/key_transactions.json";
private static final String KEY_TRANSACTION_URL = "/v2/key_transactions/{key_transaction_id}.json";
public DefaultKeyTransactionsApi(NewRelicClient client) {
super(client);
}
@Override
public Optional<KeyTransaction> getByName(String keyTransactionName) {
String keyTransactionNameEncoded = UriComponent.encode(keyTransactionName, QUERY_PARAM_SPACE_ENCODED);
return client
.target(KEY_TRANSACTIONS_URL)
.queryParam("filter[name]", keyTransactionNameEncoded)
.request(APPLICATION_JSON_TYPE)
.get(KeyTransactionList.class)
.getSingle();
}
@Override
public KeyTransaction getById(int keyTransactionId) {
return client
.target(KEY_TRANSACTION_URL)
.resolveTemplate("key_transaction_id", keyTransactionId)
.request(APPLICATION_JSON_TYPE)
.get(KeyTransactionWrapper.class)
.getKeyTransaction();
}
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/SyntheticsCondition.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition;
import lombok.Builder;
import lombok.Getter;
import lombok.NonNull;
/**
* Synthetics condition.
* Configuration parameters:
* <ul>
* <li>{@link #conditionName}</li>
* <li>{@link #monitorName}</li>
* <li>{@link #enabled} (optional)</li>
* <li>{@link #runBookUrl} (optional)</li>
* </ul>
*/
@Getter
@Builder
public class SyntheticsCondition {
/**
* Name of your Synthetics condition
*/
@NonNull
private String conditionName;
/**
* Name of Synthetics monitor
*/
@NonNull
private String monitorName;
/**
* If your Synthetics condition is enabled. Default is true
*/
@Builder.Default
private boolean enabled = true;
/**
* The runbook URL to display in notifications
*/
private String runBookUrl;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/DefaultDashboardsApi.java
package com.ocadotechnology.newrelic.apiclient.internal;
import com.ocadotechnology.newrelic.apiclient.DashboardsApi;
import com.ocadotechnology.newrelic.apiclient.internal.client.NewRelicClient;
import com.ocadotechnology.newrelic.apiclient.internal.model.DashboardList;
import com.ocadotechnology.newrelic.apiclient.internal.model.DashboardWrapper;
import com.ocadotechnology.newrelic.apiclient.model.dashboards.Dashboard;
import org.glassfish.jersey.uri.UriComponent;
import javax.ws.rs.client.Entity;
import javax.ws.rs.client.Invocation;
import java.util.List;
import static javax.ws.rs.core.MediaType.APPLICATION_JSON_TYPE;
import static org.glassfish.jersey.uri.UriComponent.Type.QUERY_PARAM_SPACE_ENCODED;
public class DefaultDashboardsApi extends ApiBase implements DashboardsApi {
private static final String DASHBOARDS_URL = "/v2/dashboards.json";
private static final String DASHBOARD_URL = "/v2/dashboards/{dashboard_id}.json";
public DefaultDashboardsApi(NewRelicClient client) {
super(client);
}
@Override
public Dashboard getById(int dashboardId) {
return client
.target(DASHBOARD_URL)
.resolveTemplate("dashboard_id", dashboardId)
.request(APPLICATION_JSON_TYPE)
.get(DashboardWrapper.class)
.getDashboard();
}
@Override
public List<Dashboard> getByTitle(String dashboardTitle) {
String dashboardTitleEncoded = UriComponent.encode(dashboardTitle, QUERY_PARAM_SPACE_ENCODED);
Invocation.Builder builder = client
.target(DASHBOARDS_URL)
.queryParam("filter[title]", dashboardTitleEncoded)
.request(APPLICATION_JSON_TYPE);
return getPageable(builder, DashboardList.class)
.getList();
}
@Override
public Dashboard create(Dashboard dashboard) {
return client
.target(DASHBOARDS_URL)
.request(APPLICATION_JSON_TYPE)
.post(Entity.entity(new DashboardWrapper(dashboard), APPLICATION_JSON_TYPE), DashboardWrapper.class)
.getDashboard();
}
@Override
public Dashboard update(Dashboard dashboard) {
return client
.target(DASHBOARD_URL)
.resolveTemplate("dashboard_id", dashboard.getId())
.request(APPLICATION_JSON_TYPE)
.put(Entity.entity(new DashboardWrapper(dashboard), APPLICATION_JSON_TYPE), DashboardWrapper.class)
.getDashboard();
}
@Override
public Dashboard delete(int dashboardId) {
return client
.target(DASHBOARD_URL)
.resolveTemplate("dashboard_id", dashboardId)
.request(APPLICATION_JSON_TYPE)
.delete(DashboardWrapper.class)
.getDashboard();
}
}
<file_sep>/README.md
# NewRelic Alerts Configurator
NewRelic Alerts Configurator can be used to configure NewRelic alerts for your application. Instead of defining alerts
through UI you can define them in code.
It allows you to automatize alerts configuration, easily recover them in case of wipe out and have full history of changes in
your version control system.
## How to use NewRelic Alerts Configurator
First step is to obtain API key for given NewRelic account. Then You can create configuration. For example:
```java
// Create alert condition
TermsConfiguration above90Percent = TermsConfiguration.builder()
.operatorTerm(OperatorTerm.ABOVE)
.thresholdTerm(90f)
.durationTerm(DurationTerm.DURATION_5)
.timeFunctionTerm(TimeFunctionTerm.ALL)
.priorityTerm(PriorityTerm.CRITICAL)
.build();
Condition cpuCondition = ServersMetricCondition.builder()
.conditionName("CPU Usage")
.enabled(true)
.server("some-host")
.metric(ServersMetricCondition.Metric.CPU_PERCENTAGE)
.term(above90Percent)
.build();
// Create email channel for notifications
Channel emailChannel = EmailChannel.builder()
.channelName("Team email")
.emailAddress("<EMAIL>")
.build();
// Create policy
PolicyConfiguration policy = PolicyConfiguration.builder()
.policyName("My application policy")
.condition(cpuCondition)
.channel(emailChannel)
.build();
// Synchronize changes
Configurator configurator = new Configurator("MY_REST_API_KEY");
configurator.setPolicyConfigurations(Collections.singletonList(policy));
configurator.sync();
```
That's all!
This code creates alert policy that will raise critical alert whenever some-host's cpu usage will raise above 90% in last 5
minutes. Information about alert will be emailed to <EMAIL>
More examples can be found in [newrelic-alerts-configurator-examples](newrelic-alerts-configurator-examples) module.
### Experimental configurator
NewRelic actively deprecates parts of it's REST API encouraging a shift towards GraphQL API.
There is therefore an alternative configurator that uses [NewRelic GraphQL API](https://docs.newrelic.com/docs/apis/nerdgraph/get-started/introduction-new-relic-nerdgraph/) where possible.
At this point it's experimental.
```java
NewRelicApi api = new NewRelicHybridApi("MY_REST_API_KEY", MY_ACCOUNT_ID);
Configurator configurator = new Configurator(api);
```
## How to obtain New Relic REST API key and account ID
In above examples we used **MY_REST_API_KEY** and **MY_ACCOUNT_ID**.
Details on how to obtain API key can be found in
[NewRelic's REST API docs](https://docs.newrelic.com/docs/apis/rest-api-v2/getting-started/api-keys)
Details on how to obtain account ID can be found in
[NewRelic's account docs](https://docs.newrelic.com/docs/accounts/accounts-billing/account-structure/account-id/)
**Note that for some configurations you will need Admin User's New Relic API key!**
## Binaries
Library is available in Maven Central
```xml
<dependency>
<groupId>com.ocadotechnology.newrelicalertsconfigurator</groupId>
<artifactId>newrelic-alerts-configurator</artifactId>
<version>5.0.1</version>
</dependency>
```
## Features
Detailed list of supported features can be found in [newrelic-alerts-configurator](newrelic-alerts-configurator/) module.
## NewRelic API Client
This project contains following library useful for developers that monitor their application using New Relic:
- [NewRelic API client](newrelic-api-client/)
Client for New Relic rest API
## Testing
To run tests call:
```bash
./gradlew test
```
## Build
To build call:
```bash
./gradlew clean build
```
## Contributing
We encourage you to contribute to NewRelic Alerts Configurator. Please check out the [Contributing guide](CONTRIBUTING.md) for
guidelines about how to proceed.
## License
This product is licensed under Apache License 2.0. For details see [license file](LICENSE)<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/NewRelicConfigurationMarker.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl
@DslMarker
annotation class NewRelicConfigurationMarker<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/DefaultAlertsNrqlConditionsApi.java
package com.ocadotechnology.newrelic.apiclient.internal;
import com.ocadotechnology.newrelic.apiclient.PolicyItemApi;
import com.ocadotechnology.newrelic.apiclient.internal.client.NewRelicClient;
import com.ocadotechnology.newrelic.apiclient.internal.model.AlertsNrqlConditionList;
import com.ocadotechnology.newrelic.apiclient.internal.model.AlertsNrqlConditionWrapper;
import com.ocadotechnology.newrelic.apiclient.model.conditions.nrql.AlertsNrqlCondition;
import javax.ws.rs.client.Entity;
import java.util.List;
import static javax.ws.rs.core.MediaType.APPLICATION_JSON_TYPE;
public class DefaultAlertsNrqlConditionsApi extends ApiBase implements PolicyItemApi<AlertsNrqlCondition> {
private static final String CONDITIONS_URL = "/v2/alerts_nrql_conditions.json";
private static final String CONDITION_URL = "/v2/alerts_nrql_conditions/{condition_id}.json";
private static final String CONDITION_POLICY_URL = "/v2/alerts_nrql_conditions/policies/{policy_id}.json";
public DefaultAlertsNrqlConditionsApi(NewRelicClient client) {
super(client);
}
@Override
public List<AlertsNrqlCondition> list(int policyId) {
return getPageable(
client.target(CONDITIONS_URL).queryParam("policy_id", policyId).request(APPLICATION_JSON_TYPE),
AlertsNrqlConditionList.class)
.getList();
}
@Override
public AlertsNrqlCondition create(int policyId, AlertsNrqlCondition nrqlCondition) {
return client
.target(CONDITION_POLICY_URL)
.resolveTemplate("policy_id", policyId)
.request(APPLICATION_JSON_TYPE)
.post(Entity.entity(new AlertsNrqlConditionWrapper(nrqlCondition), APPLICATION_JSON_TYPE),
AlertsNrqlConditionWrapper.class)
.getNrqlCondition();
}
@Override
public AlertsNrqlCondition update(int conditionId, AlertsNrqlCondition nrqlCondition) {
return client
.target(CONDITION_URL)
.resolveTemplate("condition_id", conditionId)
.request(APPLICATION_JSON_TYPE)
.put(Entity.entity(new AlertsNrqlConditionWrapper(nrqlCondition), APPLICATION_JSON_TYPE),
AlertsNrqlConditionWrapper.class)
.getNrqlCondition();
}
@Override
public AlertsNrqlCondition delete(int policyId, int conditionId) {
return client
.target(CONDITION_URL)
.resolveTemplate("condition_id", conditionId)
.request(APPLICATION_JSON_TYPE)
.delete(AlertsNrqlConditionWrapper.class)
.getNrqlCondition();
}
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/condition/Conditions.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.Condition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ExternalServiceCondition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.NrqlCondition
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.SyntheticsCondition
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class Conditions {
internal val conditions: MutableList<Condition> = mutableListOf()
internal val externalServiceConditions: MutableList<ExternalServiceCondition> = mutableListOf()
internal val nrqlConditions: MutableList<NrqlCondition> = mutableListOf()
internal val syntheticsConditions: MutableList<SyntheticsCondition> = mutableListOf()
fun apmApp(block: ApmAppConditionDsl.() -> Unit) = conditions.add(apmAppCondition(block))
fun apmExternalService(block: ApmExternalServiceConditionDsl.() -> Unit) = externalServiceConditions.add(apmExternalServiceCondition(block))
fun apmJvm(block: ApmJvmConditionDsl.() -> Unit) = conditions.add(apmJvmCondition(block))
fun apmKeyTransation(block: ApmKeyTransactionConditionDsl.() -> Unit) = conditions.add(apmKeyTransactionCondition(block))
fun browser(block: BrowserConditionDsl.() -> Unit) = conditions.add(browserCondition(block))
fun nrql(block: NrqlConditionDsl.() -> Unit) = nrqlConditions.add(nrqlCondition(block))
fun serversMetric(block: ServersMetricConditionDsl.() -> Unit) = conditions.add(serversMetricCondition(block))
fun synthetics(block: SyntheticsConditionDsl.() -> Unit) = syntheticsConditions.add(syntheticsCondition(block))
operator fun Condition.unaryPlus() = conditions.add(this)
operator fun ExternalServiceCondition.unaryPlus() = externalServiceConditions.add(this)
operator fun NrqlCondition.unaryPlus() = nrqlConditions.add(this)
operator fun SyntheticsCondition.unaryPlus() = syntheticsConditions.add(this)
}<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/UserDefinedConfiguration.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition;
import lombok.Builder;
import lombok.Getter;
import lombok.NonNull;
/**
* User defined metric configuration.
* Configuration parameters:
* <ul>
* <li>{@link #metric}</li>
* <li>{@link #valueFunction}</li>
* </ul>
*/
@Builder
@Getter
public class UserDefinedConfiguration {
/**
* This is the name of a user defined custom metric to be used to determine if an event should be triggered.
*/
@NonNull
private String metric;
/**
* This is the numeric value obtained from the custom metric specified by {@link #metric}
*/
@NonNull
private ValueFunction valueFunction;
public enum ValueFunction {
AVERAGE, MIN, MAX, TOTAL, SAMPLE_SIZE;
public String getAsString() {
return this.name().toLowerCase();
}
}
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/channel/PagerDutyChannelDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.channel
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.PagerDutyChannel
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class PagerDutyChannelDsl {
var channelName: String? = null
var serviceKey: String? = null
}
fun pagerDutyChannel(block: PagerDutyChannelDsl.() -> Unit): PagerDutyChannel {
val dsl = PagerDutyChannelDsl()
dsl.block()
return PagerDutyChannel.builder()
.channelName(requireNotNull(dsl.channelName) { "PagerDuty channel name cannot be null" })
.serviceKey(requireNotNull(dsl.serviceKey) { "PagerDuty channel key cannot be null" })
.build()
}<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/ApplicationConfigurationDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.ApplicationConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class ApplicationConfigurationDsl {
var applicationName: String = ""
var appApdexThreshold: Float = 0.0f
var endUserApdexThreshold: Float = 0.0f
var enableRealUserMonitoring: Boolean = false
}
fun applicationConfiguration(block: ApplicationConfigurationDsl.() -> Unit): ApplicationConfiguration {
val dsl = ApplicationConfigurationDsl()
dsl.block()
return ApplicationConfiguration.builder()
.applicationName(dsl.applicationName)
.appApdexThreshold(dsl.appApdexThreshold)
.endUserApdexThreshold(dsl.endUserApdexThreshold)
.enableRealUserMonitoring(dsl.enableRealUserMonitoring)
.build()
}
@NewRelicConfigurationMarker
class ApplicationConfigurations {
internal val applications: MutableList<ApplicationConfiguration> = mutableListOf()
fun application(block: ApplicationConfigurationDsl.() -> Unit) = applications.add(applicationConfiguration(block))
operator fun ApplicationConfiguration.unaryPlus() = applications.add(this)
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/model/ApplicationWrapper.java
package com.ocadotechnology.newrelic.apiclient.internal.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.ocadotechnology.newrelic.apiclient.model.applications.Application;
import lombok.Value;
@Value
public class ApplicationWrapper {
@JsonProperty
Application application;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/model/conditions/nrql/AlertsNrqlCondition.java
package com.ocadotechnology.newrelic.apiclient.model.conditions.nrql;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.ocadotechnology.newrelic.apiclient.model.PolicyItem;
import com.ocadotechnology.newrelic.apiclient.model.conditions.Expiration;
import com.ocadotechnology.newrelic.apiclient.model.conditions.Signal;
import com.ocadotechnology.newrelic.apiclient.model.conditions.Terms;
import java.util.Collection;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Singular;
import lombok.Value;
import lombok.experimental.NonFinal;
/**
* See <a href="https://docs.newrelic.com/docs/alerts-applied-intelligence/new-relic-alerts/advanced-alerts/rest-api-alerts/rest-api-calls-alerts/#conditions-list">Doc</a>
*/
@Value
@Builder
@AllArgsConstructor
@NonFinal
public class AlertsNrqlCondition implements PolicyItem {
@JsonProperty
Integer id;
@JsonProperty
String name;
@JsonProperty
Boolean enabled;
@JsonProperty("runbook_url")
String runbookUrl;
@JsonProperty
@Singular
Collection<Terms> terms;
@JsonProperty("value_function")
String valueFunction;
@JsonProperty
Nrql nrql;
@JsonProperty
Signal signal;
@JsonProperty
Expiration expiration;
@JsonProperty("violation_time_limit_seconds")
Integer violationTimeLimitSeconds;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/model/AlertsChannelWrapper.java
package com.ocadotechnology.newrelic.apiclient.internal.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.ocadotechnology.newrelic.apiclient.model.channels.AlertsChannel;
import lombok.Value;
@Value
public class AlertsChannelWrapper {
@JsonProperty
AlertsChannel channel;
}
<file_sep>/newrelic-alerts-configurator-examples/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/examples/Main.kt
package com.ocadotechnology.newrelic.alertsconfigurator.examples
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.PolicyConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.policyConfiguration
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration
object APP1 {
private const val NAME = "Application1"
val applicationConfig = applicationConfiguration(NAME)
val policyConfig = policyConfiguration {
policyName = "Application1 Policy"
incidentPreference = PolicyConfiguration.IncidentPreference.PER_POLICY
conditions {
+apdexCondition(NAME)
+diskSpaceCondition("app-1-host")
+heapUsageCondition(NAME)
}
channels {
+teamEmail()
+slack()
}
}
}
object APP2 {
private const val NAME = "Application2"
val applicationConfig = applicationConfiguration(NAME)
val policyConfig = policyConfiguration {
policyName = "Application2 Policy"
incidentPreference = PolicyConfiguration.IncidentPreference.PER_POLICY
conditions {
+apdexCondition(NAME)
+heapUsageCondition(NAME)
+jsErrorsCondition(NAME)
+healthCheckCondition(NAME)
}
channels {
+slack()
}
}
}
object APP3 {
private const val NAME = "Application3"
val applicationConfig = applicationConfiguration(NAME)
val policyConfig = policyConfiguration {
policyName = "Application3 Policy"
incidentPreference = PolicyConfiguration.IncidentPreference.PER_POLICY
conditions {
+apdexCondition(NAME)
+cpuUsageCondition("app-3-host")
+heapUsageCondition(NAME)
}
channels {
+teamEmail()
+slack()
}
}
}
fun main() {
val stagingEnvironmentNewRelicKey = "KEY1"
val productionEnvironmentNewRelicKey = "KEY2"
configure(stagingEnvironmentNewRelicKey)
configure(productionEnvironmentNewRelicKey)
}
fun configure(newRelicKey: String) {
configuration(newRelicKey) {
applications {
+APP1.applicationConfig
+APP2.applicationConfig
+APP3.applicationConfig
}
policies {
+APP1.policyConfig
+APP2.policyConfig
+APP3.policyConfig
}
}
}<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/channel/ChannelTypeSupport.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel;
import com.ocadotechnology.newrelic.apiclient.NewRelicApi;
import com.ocadotechnology.newrelic.apiclient.model.channels.AlertsChannelConfiguration;
/**
* This interface represents support object, that is used to transform implementation of {@link Channel} to
* {@link AlertsChannelConfiguration}, thet will be used by api client.
* Each implementation of {@link Channel} should provide it's own implementation of this interface, that will build correct
* object of {@link AlertsChannelConfiguration}.
*/
public interface ChannelTypeSupport {
/**
* Builds alerts channel configuration.
*
* @param api NewRelic api client. Can be used to obtain additional paremeters required to build alerts channel
* configuration, like {@code userId}
* @return generated alerts channel configuration
*/
AlertsChannelConfiguration generateAlertsChannelConfiguration(NewRelicApi api);
}
<file_sep>/newrelic-alerts-configurator-dsl/src/main/kotlin/com/ocadotechnology/newrelic/alertsconfigurator/dsl/configuration/condition/terms/NrqlTermsConfigurationDsl.kt
package com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition.terms
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.*
import com.ocadotechnology.newrelic.alertsconfigurator.dsl.NewRelicConfigurationMarker
@NewRelicConfigurationMarker
class NrqlTermsConfigurationDsl {
var durationTerm: NrqlDurationTerm? = null
var operatorTerm: OperatorTerm? = null
var priorityTerm: PriorityTerm? = null
var thresholdTerm: Float? = null
var timeFunctionTerm: TimeFunctionTerm? = null
}
fun nrqlTermsConfiguration(block: NrqlTermsConfigurationDsl.() -> Unit): NrqlTermsConfiguration {
val dsl = NrqlTermsConfigurationDsl()
dsl.block()
return NrqlTermsConfiguration.builder()
.durationTerm(requireNotNull(dsl.durationTerm) { "Nrql terms duration cannot be null" })
.operatorTerm(requireNotNull(dsl.operatorTerm) { "Nrql terms operator cannot be null" })
.priorityTerm(requireNotNull(dsl.priorityTerm) { "Nrql terms priority cannot be null" })
.thresholdTerm(requireNotNull(dsl.thresholdTerm) { "Nrql terms threshold cannot be null" })
.timeFunctionTerm(requireNotNull(dsl.timeFunctionTerm) { "Nrql terms time function cannot be null" })
.build()
}
@NewRelicConfigurationMarker
class NrqlTermConfigurations {
internal val terms: MutableList<NrqlTermsConfiguration> = mutableListOf()
fun term(block: NrqlTermsConfigurationDsl.() -> Unit) = terms.add(com.ocadotechnology.newrelic.alertsconfigurator.dsl.configuration.condition.terms.nrqlTermsConfiguration(block))
operator fun NrqlTermsConfiguration.unaryPlus() = terms.add(this)
infix fun PriorityTerm.given(timeFunctionTerm: TimeFunctionTerm) : AfterGiven {
return AfterGiven(NrqlTermsConfiguration.builder().priorityTerm(this).timeFunctionTerm(timeFunctionTerm))
}
infix fun AfterGiven.inLast(durationTerm: NrqlDurationTerm) : AfterInLast {
return AfterInLast(this.builder.durationTerm(durationTerm))
}
infix fun AfterInLast.minutes(operatorTerm: OperatorTerm) : AfterMinutes {
return AfterMinutes(this.builder.operatorTerm(operatorTerm))
}
infix fun AfterMinutes.value(thresholdTerm: Float) {
terms.add(this.builder.thresholdTerm(thresholdTerm).build())
}
class AfterGiven(internal val builder: NrqlTermsConfiguration.NrqlTermsConfigurationBuilder)
class AfterInLast(internal val builder: NrqlTermsConfiguration.NrqlTermsConfigurationBuilder)
class AfterMinutes(internal val builder: NrqlTermsConfiguration.NrqlTermsConfigurationBuilder)
}<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/model/deployments/Deployment.java
package com.ocadotechnology.newrelic.apiclient.model.deployments;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Value;
import java.time.OffsetDateTime;
/**
* See <a href="https://rpm.newrelic.com/api/explore/application_deployments/list">Doc</a>
*/
@Value
@Builder
@AllArgsConstructor
public class Deployment {
@JsonProperty
Integer id;
@JsonProperty
String revision;
@JsonProperty
String changelog;
@JsonProperty
String description;
@JsonProperty
String user;
@JsonProperty
OffsetDateTime timestamp;
@JsonProperty
DeploymentLinks links;
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/Condition.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.TermsConfiguration;
import java.util.Collection;
/**
* Alerts condition configuration.
* Implementations:
* <ul>
* <li>{@link ApmAppCondition}</li>
* <li>{@link ApmKeyTransactionCondition}</li>
* <li>{@link ServersMetricCondition}</li>
* </ul>
*/
public interface Condition {
/**
* Returns condition type. Each Condition implementation should have unique type.
*
* @return condition type
*/
ConditionType getType();
/**
* Returns name of the condition.
*
* @return condition name
*/
String getConditionName();
/**
* Returns if condition is enabled.
*
* @return {@code true} if condition is enabled, {@code false} otherwise
*/
boolean isEnabled();
/**
* Returns collection of entities for which this condition is applied.
* The type of the entity depends on concrete condition implementation. This can be an application
* name or host name for example.
* <p>
* If entity with given name does not exist an exception will be thrown.
*
* @return entity names
*/
Collection<String> getEntities();
/**
* Returns metric in string format.
*
* @return metric
*/
String getMetricAsString();
/**
* Returns the runbook URL to display in notifications.
*
* @return runbook URL
*/
String getRunBookUrl();
/**
* Returns collection of terms used for alerts condition.
*
* @return terms
*/
Collection<TermsConfiguration> getTerms();
/**
* Returns condition scope in string format.
*
* @return condition scope
*/
default String getConditionScopeAsString() {
return null;
}
/**
* Returns time (in hours) after which instance-based violations will automatically close.
* @return violation close timer
*/
default String getViolationCloseTimerAsString() {
return null;
}
/**
* Returns configuration for user defined metric. Should be provided when metric is set to USER_DEFINED.
*
* @return user defined metric
*/
default UserDefinedConfiguration getUserDefinedMetric() {
return null;
}
/**
* Returns type of GC metric for jvm condition if metric set to GC_CPU_TIME.
*
* @return type of GC metric
*/
default String getGcMetricAsString() {
return null;
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/PolicyItemApi.java
package com.ocadotechnology.newrelic.apiclient;
import java.util.List;
public interface PolicyItemApi<T> {
/**
* Lists items for the given policy.
*
* @param policyId id of the policy containing items
* @return list of all existing {@link T} from the given policy
*/
List<T> list(int policyId);
/**
* Creates item instance within specified policy.
*
* @param policyId id of the policy to be updated
* @param item item definition to be created
* @return created {@link T}
*/
T create(int policyId, T item);
/**
* Updates item definition.
*
* @param id id of the item to be updated
* @param item item definition to be updated
* @return created {@link T}
*/
T update(int id, T item);
/**
* Deletes item.
*
* @param policyId policy id
* @param id id of the item to be deleted
* @return deleted {@link T}
*/
T delete(int policyId, int id);
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/terms/NrqlDurationTerm.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms;
import lombok.Getter;
@Getter
public final class NrqlDurationTerm {
// defaults as used in original, enum-based implementation
public static final NrqlDurationTerm DURATION_1 = new NrqlDurationTerm(1);
public static final NrqlDurationTerm DURATION_2 = new NrqlDurationTerm(2);
public static final NrqlDurationTerm DURATION_3 = new NrqlDurationTerm(3);
public static final NrqlDurationTerm DURATION_4 = new NrqlDurationTerm(4);
public static final NrqlDurationTerm DURATION_5 = new NrqlDurationTerm(5);
public static final NrqlDurationTerm DURATION_10 = new NrqlDurationTerm(10);
public static final NrqlDurationTerm DURATION_15 = new NrqlDurationTerm(15);
public static final NrqlDurationTerm DURATION_30 = new NrqlDurationTerm(30);
public static final NrqlDurationTerm DURATION_60 = new NrqlDurationTerm(60);
public static final NrqlDurationTerm DURATION_120 = new NrqlDurationTerm(120);
private final int duration;
public NrqlDurationTerm(int duration) {
assertMoreThanZero(duration);
this.duration = duration;
}
private static void assertMoreThanZero(int duration) {
if (duration <= 0) {
throw new IllegalArgumentException("Duration Term for alert should not be 0 nor negative");
}
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/graphql/apiclient/internal/transport/NotificationChannel.java
package com.ocadotechnology.newrelic.graphql.apiclient.internal.transport;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import lombok.Value;
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
public class NotificationChannel {
String id;
String name;
String type;
AssociatedPolicies associatedPolicies;
NotificationChannelConfig config;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/model/policies/AlertsPolicy.java
package com.ocadotechnology.newrelic.apiclient.model.policies;
import com.fasterxml.jackson.annotation.JsonAlias;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Value;
/**
* See <a href="https://rpm.newrelic.com/api/explore/alerts_policies/list">Doc</a>
*/
@Value
@Builder
@AllArgsConstructor
public class AlertsPolicy {
@JsonProperty
Integer id;
@JsonProperty("incident_preference")
@JsonAlias({"incidentPreference"})
String incidentPreference;
@JsonProperty
String name;
@JsonProperty("created_at")
Long createdAt;
@JsonProperty("updated_at")
Long updatedAt;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/model/deployments/DeploymentLinks.java
package com.ocadotechnology.newrelic.apiclient.model.deployments;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.Value;
@Value
public class DeploymentLinks {
@JsonProperty
Integer application;
}
<file_sep>/newrelic-alerts-configurator-examples/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/examples/Defaults.java
package com.ocadotechnology.newrelic.alertsconfigurator.examples;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.ApplicationConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.Channel;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.EmailChannel;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.channel.SlackChannel;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ApmAppCondition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.BrowserCondition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ApmJvmCondition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.Condition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.NrqlCondition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ServersMetricCondition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.DurationTerm;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.NrqlDurationTerm;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.NrqlTermsConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.OperatorTerm;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.PriorityTerm;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.TermsConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.TimeFunctionTerm;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.violationclosetimer.ViolationCloseTimer;
import java.util.Arrays;
/**
* Utility class used to obtain {@link Channel}, {@link Condition} or {@link ApplicationConfiguration} predefined
* with default values.
*/
public final class Defaults {
private Defaults() {
}
public static ApplicationConfiguration applicationConfiguration(String applicationName) {
return ApplicationConfiguration.builder()
.applicationName(applicationName)
.appApdexThreshold(0.5f)
.endUserApdexThreshold(0.7f)
.enableRealUserMonitoring(true)
.build();
}
public static Condition apdexCondition(String applicationName) {
return ApmAppCondition.builder()
.conditionName("Apdex")
.enabled(true)
.application(applicationName)
.metric(ApmAppCondition.Metric.APDEX)
.conditionScope(ApmAppCondition.ConditionScope.APPLICATION)
// Raise critical if in the last 5 minutes apdex was below 0.7
.term(TermsConfiguration.builder()
.durationTerm(DurationTerm.DURATION_5)
.operatorTerm(OperatorTerm.BELOW)
.priorityTerm(PriorityTerm.CRITICAL)
.timeFunctionTerm(TimeFunctionTerm.ALL)
.thresholdTerm(0.7f)
.build()
)
// Raise warning if in the last 5 minutes apdex was below 0.85
.term(TermsConfiguration.builder()
.durationTerm(DurationTerm.DURATION_5)
.operatorTerm(OperatorTerm.BELOW)
.priorityTerm(PriorityTerm.WARNING)
.timeFunctionTerm(TimeFunctionTerm.ALL)
.thresholdTerm(0.85f)
.build()
)
.build();
}
public static Condition diskSpaceCondition(String... serverNames) {
return ServersMetricCondition.builder()
.conditionName("Fullest Disk %")
.enabled(true)
.servers(Arrays.asList(serverNames))
.metric(ServersMetricCondition.Metric.FULLEST_DISK_PERCENTAGE)
// Raise critical if in the last 5 minutes disk was over 80% full
.term(TermsConfiguration.builder()
.priorityTerm(PriorityTerm.CRITICAL)
.durationTerm(DurationTerm.DURATION_5)
.operatorTerm(OperatorTerm.ABOVE)
.timeFunctionTerm(TimeFunctionTerm.ALL)
.thresholdTerm(80.0f)
.build()
)
// Raise warning if in the last 5 minutes disk was over 65% full
.term(TermsConfiguration.builder()
.priorityTerm(PriorityTerm.WARNING)
.durationTerm(DurationTerm.DURATION_5)
.operatorTerm(OperatorTerm.ABOVE)
.timeFunctionTerm(TimeFunctionTerm.ALL)
.thresholdTerm(65.0f)
.build()
)
.build();
}
public static Condition cpuUsageCondition(String... serverNames) {
return ServersMetricCondition.builder()
.conditionName("CPU Usage %")
.enabled(true)
.servers(Arrays.asList(serverNames))
.metric(ServersMetricCondition.Metric.CPU_PERCENTAGE)
// Raise critical if in the last 5 minutes cpu was over 90%
.term(TermsConfiguration.builder()
.priorityTerm(PriorityTerm.CRITICAL)
.durationTerm(DurationTerm.DURATION_5)
.operatorTerm(OperatorTerm.ABOVE)
.timeFunctionTerm(TimeFunctionTerm.ALL)
.thresholdTerm(90.0f)
.build()
)
// Raise warning if in the last 5 minutes cpu was over 70%
.term(TermsConfiguration.builder()
.priorityTerm(PriorityTerm.WARNING)
.durationTerm(DurationTerm.DURATION_5)
.operatorTerm(OperatorTerm.ABOVE)
.timeFunctionTerm(TimeFunctionTerm.ALL)
.thresholdTerm(70.0f)
.build()
)
.build();
}
public static Condition heapUsageCondition(String applicationName) {
return ApmJvmCondition.builder()
.conditionName("Heap usage")
.enabled(true)
.application(applicationName)
.metric(ApmJvmCondition.Metric.HEAP_MEMORY_USAGE)
// Raise critical if in the last 5 minutes heap memory usage was above 85%
.term(TermsConfiguration.builder()
.durationTerm(DurationTerm.DURATION_5)
.operatorTerm(OperatorTerm.ABOVE)
.priorityTerm(PriorityTerm.CRITICAL)
.timeFunctionTerm(TimeFunctionTerm.ALL)
.thresholdTerm(85f)
.build()
)
// Raise warning if in the last 5 minutes heap memory usage was above 65%
.term(TermsConfiguration.builder()
.durationTerm(DurationTerm.DURATION_5)
.operatorTerm(OperatorTerm.ABOVE)
.priorityTerm(PriorityTerm.WARNING)
.timeFunctionTerm(TimeFunctionTerm.ALL)
.thresholdTerm(65f)
.build()
)
.violationCloseTimer(ViolationCloseTimer.DURATION_24)
.build();
}
public static NrqlCondition healthCheckCondition(String applicationName) {
return NrqlCondition.builder()
.conditionName("Health Check")
.enabled(true)
.valueFunction(NrqlCondition.ValueFunction.SINGLE_VALUE)
.term(NrqlTermsConfiguration.builder()
.priorityTerm(PriorityTerm.CRITICAL)
.durationTerm(NrqlDurationTerm.DURATION_1)
.timeFunctionTerm(TimeFunctionTerm.ANY)
.operatorTerm(OperatorTerm.ABOVE)
.thresholdTerm(0f)
.build())
.query("SELECT count(*) FROM `" + applicationName + ":HealthStatus` WHERE healthy IS false")
.build();
}
public static BrowserCondition jsErrorsCondition(String applicationName) {
return BrowserCondition.builder()
.conditionName("Page views with JS errors")
.enabled(true)
.application(applicationName)
.metric(BrowserCondition.Metric.PAGE_VIEWS_WITH_JS_ERRORS)
.term(TermsConfiguration.builder()
.durationTerm(DurationTerm.DURATION_5)
.operatorTerm(OperatorTerm.ABOVE)
.priorityTerm(PriorityTerm.WARNING)
.thresholdTerm(1f)
.timeFunctionTerm(TimeFunctionTerm.ANY)
.build()
)
.build();
}
public static Channel teamEmailChannel() {
return EmailChannel.builder()
.channelName("My Team - email")
.emailAddress("<EMAIL>")
.includeJsonAttachment(true)
.build();
}
public static Channel slackChannel() {
return SlackChannel.builder()
.channelName("My Team - slack")
.slackUrl("https://hooks.slack.com/services/aaaaaaaaa/bbbbbbbbb/cccccccccccccccccccccccc")
.teamChannel("newrelic-alerts")
.build();
}
}
<file_sep>/newrelic-alerts-configurator/src/main/java/com/ocadotechnology/newrelic/alertsconfigurator/configuration/condition/signal/NrqlSignalConfiguration.java
package com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.signal;
import lombok.Builder;
import lombok.Getter;
import lombok.NonNull;
/**
* NRQL Signal configuration.
* Configuration parameters:
* <ul>
* <li>{@link #aggregationMethod}</li>
* <li>{@link #aggregationWindow}</li>
* <li>{@link #signalFillOption}</li>
* <li>{@link #signalFillValue}</li>
* <li>{@link #signalLostConfiguration}</li>
* </ul>
*/
@Builder
@Getter
public class NrqlSignalConfiguration {
/**
* Configuration of signal aggregation method.
* For reference see: <a href="https://docs.newrelic.com/docs/alerts-applied-intelligence/new-relic-alerts/advanced-alerts/understand-technical-concepts/streaming-alerts-key-terms-concepts/#aggregation-methods">NR Docs</a>
*/
@NonNull
private SignalAggregationMethod aggregationMethod;
/**
* Delay is how long we wait for events that belong in each aggregation window.
* Depending on your data a longer delay may increase accuracy but delay notifications.
* This value is used for EVENT_FLOW and CADENCE aggregation methods.
*/
private Integer aggregationDelay;
/**
* The timer tells us how long to wait after each data point to make sure we've processed the whole batch.
* This value should match data frequency, and preferably be at least equal to aggregationWindow.
* See <a href="https://docs.newrelic.com/docs/alerts-applied-intelligence/new-relic-alerts/advanced-alerts/understand-technical-concepts/streaming-alerts-key-terms-concepts/#aggregation-methods">NR Docs</a> for more information.
* This value is used for EVENT_TIMER aggregation method.
*/
private Integer aggregationTimer;
/**
* Time (in seconds) for how long NewRelic collects data before running the NRQL query.
*/
@NonNull
private Integer aggregationWindow;
/**
* Configuration of filling data gaps/signal lost.
*/
@NonNull
private SignalFillOption signalFillOption;
/**
* Value for filling data gaps/signal lost
*/
private String signalFillValue;
/**
* Configuration of signal lost behaviour.
*/
private SignalLostConfiguration signalLostConfiguration;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/graphql/apiclient/internal/transport/NrqlCondition.java
package com.ocadotechnology.newrelic.graphql.apiclient.internal.transport;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import lombok.Value;
import java.util.List;
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
public class NrqlCondition {
Boolean enabled;
String id;
String name;
String type;
String runbookUrl;
Nrql nrql;
Expiration expiration;
Signal signal;
List<Term> terms;
String valueFunction;
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
public static class Nrql {
String query;
}
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
public static class Expiration {
String expirationDuration;
boolean openViolationOnExpiration;
boolean closeViolationsOnExpiration;
}
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
public static class Signal {
String aggregationMethod;
String aggregationDelay;
String aggregationTimer;
String aggregationWindow;
String fillOption;
String fillValue;
}
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
public static class Term {
String operator;
String priority;
String threshold;
String thresholdDuration;
String thresholdOccurrences;
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/model/dashboards/widget/WidgetData.java
package com.ocadotechnology.newrelic.apiclient.model.dashboards.widget;
import com.fasterxml.jackson.annotation.JsonProperty;
import lombok.AllArgsConstructor;
import lombok.Builder;
import lombok.Value;
@Value
@Builder(toBuilder = true)
@AllArgsConstructor
public class WidgetData {
@JsonProperty
String nrql;
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/graphql/apiclient/internal/transport/Result.java
package com.ocadotechnology.newrelic.graphql.apiclient.internal.transport;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.ocadotechnology.newrelic.apiclient.model.policies.AlertsPolicy;
import com.ocadotechnology.newrelic.graphql.apiclient.internal.transport.mutation.EmptyMutationResult;
import com.ocadotechnology.newrelic.graphql.apiclient.internal.transport.mutation.MutationResult;
import com.ocadotechnology.newrelic.graphql.apiclient.internal.transport.mutation.NotificationChannelResult;
import com.ocadotechnology.newrelic.graphql.apiclient.internal.transport.mutation.NotificationChannelsResult;
import lombok.AllArgsConstructor;
import lombok.ToString;
import lombok.Value;
import java.util.*;
@JsonIgnoreProperties(ignoreUnknown = true)
@ToString
@AllArgsConstructor
public class Result {
private final Data data;
private final List<Error> errors;
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
static class Data {
Actor actor;
AlertsPolicyCreate alertsPolicyCreate;
EmptyMutationResult alertsNotificationChannelDelete;
NotificationChannelsResult alertsNotificationChannelsAddToPolicy;
NotificationChannelsResult alertsNotificationChannelsRemoveFromPolicy;
NotificationChannelResult alertsNotificationChannelCreate;
NrqlCondition alertsNrqlConditionStaticCreate;
NrqlCondition alertsNrqlConditionStaticUpdate;
}
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
static class Actor {
EntitySearch entitySearch;
Account account;
}
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
static class AlertsPolicyCreate {
String id;
String name;
String incidentPreference;
}
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
static class Account {
Alerts alerts;
}
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
static class Alerts {
AlertsPolicy policy;
NotificationChannel notificationChannel;
NotificationChannels notificationChannels;
PoliciesSearch policiesSearch;
NrqlConditionsSearch nrqlConditionsSearch;
}
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
static class PoliciesSearch {
List<AlertsPolicy> policies;
}
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
static class EntitySearch {
Results results;
}
@JsonIgnoreProperties(ignoreUnknown = true)
@Value
static class Results {
List<Map<Object, Object>> entities;
}
public boolean hasErrors() {
return Objects.nonNull(errors);
}
public boolean notFound() {
return Optional.ofNullable(errors)
.orElseGet(ArrayList::new).stream()
.anyMatch(error -> "Not Found".equalsIgnoreCase(error.getMessage()));
}
public List<Error> getErrors() {
return Optional.ofNullable(errors).orElseGet(ArrayList::new);
}
public List<AlertsPolicy> getPolicies() {
return getAlerts()
.map(alerts -> alerts.policiesSearch)
.map(policiesSearch -> policiesSearch.policies).orElseGet(ArrayList::new);
}
public Optional<AlertsPolicy> getAlertsPolicyCreate() {
return getData()
.map(data -> data.alertsPolicyCreate)
.map(alertsPolicyCreate -> AlertsPolicy.builder()
.id(Optional.ofNullable(alertsPolicyCreate.id).map(Integer::parseInt).orElse(null))
.name(alertsPolicyCreate.name)
.incidentPreference(alertsPolicyCreate.incidentPreference)
.build());
}
public Optional<MutationResult<List<Integer>>> getAlertsNotificationChannelsAddToPolicy() {
return getData().map(data -> data.alertsNotificationChannelsAddToPolicy);
}
public Optional<MutationResult<List<Integer>>> getAlertsNotificationChannelsRemoveFromPolicy() {
return getData().map(data -> data.alertsNotificationChannelsRemoveFromPolicy);
}
public Optional<NotificationChannelResult> getAlertsNotificationChannelCreate() {
return getData().map(data -> data.alertsNotificationChannelCreate);
}
public Optional<MutationResult<Void>> getAlertsNotificationChannelDelete() {
return getData().map(data -> data.alertsNotificationChannelDelete);
}
public Optional<AlertsPolicy> getAlertsPolicy() {
return getAlerts().map(alerts -> alerts.policy);
}
public Optional<NotificationChannel> getNotificationChannel() {
return getAlerts().map(alerts -> alerts.notificationChannel);
}
public Optional<NotificationChannels> getNotificationChannels() {
return getAlerts().map(alerts -> alerts.notificationChannels);
}
public Optional<NrqlConditionsSearch> getNrqlConditionsSearch() {
return getAlerts().map(alerts -> alerts.nrqlConditionsSearch);
}
public Optional<NrqlCondition> getAlertsNrqlConditionStaticCreate() {
return getData().map(data -> data.alertsNrqlConditionStaticCreate);
}
public Optional<NrqlCondition> getAlertsNrqlConditionStaticUpdate() {
return getData().map(data -> data.alertsNrqlConditionStaticUpdate);
}
private Optional<Data> getData() {
return Optional.ofNullable(data);
}
private Optional<Alerts> getAlerts() {
return getData()
.map(data -> data.actor)
.map(actor -> actor.account)
.map(account -> account.alerts);
}
}
<file_sep>/newrelic-alerts-configurator/src/test/java/com/ocadotechnology/newrelic/alertsconfigurator/AbstractPolicyItemConfiguratorTest.java
package com.ocadotechnology.newrelic.alertsconfigurator;
import com.google.common.collect.ImmutableList;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.PolicyConfiguration;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.ApmAppCondition;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.terms.*;
import com.ocadotechnology.newrelic.alertsconfigurator.configuration.condition.violationclosetimer.ViolationCloseTimer;
import com.ocadotechnology.newrelic.alertsconfigurator.exception.NewRelicSyncException;
import com.ocadotechnology.newrelic.alertsconfigurator.internal.entities.EntityResolver;
import com.ocadotechnology.newrelic.apiclient.PolicyItemApi;
import com.ocadotechnology.newrelic.apiclient.model.PolicyItem;
import com.ocadotechnology.newrelic.apiclient.model.policies.AlertsPolicy;
import org.junit.Before;
import org.junit.Rule;
import org.junit.Test;
import org.junit.rules.ExpectedException;
import org.mockito.InOrder;
import org.mockito.Mock;
import java.util.Collections;
import java.util.Optional;
import static java.lang.String.format;
import static org.mockito.Mockito.*;
public abstract class AbstractPolicyItemConfiguratorTest<T extends PolicyItemConfigurator, U extends PolicyItem> extends AbstractConfiguratorTest {
@Rule
public final ExpectedException expectedException = ExpectedException.none();
protected static final ApmAppCondition.Metric APP_METRIC = ApmAppCondition.Metric.APDEX;
protected static final String POLICY_NAME = "policyName";
protected static final PolicyConfiguration.IncidentPreference INCIDENT_PREFERENCE = PolicyConfiguration.IncidentPreference.PER_POLICY;
protected static final AlertsPolicy POLICY = AlertsPolicy.builder()
.id(42)
.name(POLICY_NAME)
.incidentPreference(INCIDENT_PREFERENCE.name())
.build();
protected static final String CONDITION_NAME = "conditionName";
protected static final ApmAppCondition.ConditionScope CONDITION_SCOPE = ApmAppCondition.ConditionScope.INSTANCE;
protected static final boolean ENABLED = true;
protected static final String APPLICATION_NAME = "applicationName";
protected static final int APPLICATION_ENTITY_ID = 1;
protected static final ViolationCloseTimer VIOLATION_CLOSE_TIMER = ViolationCloseTimer.DURATION_1;
protected static final TermsConfiguration TERMS_CONFIGURATION = createTermsConfiguration().build();
@Mock
EntityResolver entityResolverMock;
protected T testee;
@Before
public void setUp() {
when(alertsPoliciesApiMock.getByName(POLICY_NAME)).thenReturn(Optional.of(POLICY));
}
@Test
public void shouldThrowException_whenPolicyDoesNotExist() {
// given
when(alertsPoliciesApiMock.getByName(POLICY_NAME)).thenReturn(Optional.empty());
// then - exception
expectedException.expect(NewRelicSyncException.class);
expectedException.expectMessage(format("Policy %s does not exist", POLICY_NAME));
// when
testee.sync(getPolicyConfiguration());
}
@Test
public void shouldDoNothing_whenNullConditionsInConfiguration() {
PolicyItemApi<U> itemApiMock = getPolicyItemApiMock();
// given
PolicyConfiguration config = PolicyConfiguration.builder()
.policyName(POLICY_NAME)
.incidentPreference(PolicyConfiguration.IncidentPreference.PER_POLICY)
.build();
// when
testee.sync(config);
// then
verifyZeroInteractions(itemApiMock);
}
@Test
public void shouldDoNothing_whenEmptyConditionsInConfiguration() {
PolicyItemApi<U> itemApiMock = getPolicyItemApiMock();
// given
PolicyConfiguration config = PolicyConfiguration.builder()
.policyName(POLICY_NAME)
.incidentPreference(PolicyConfiguration.IncidentPreference.PER_POLICY)
.conditions(Collections.emptyList())
.nrqlConditions(Collections.emptyList())
.externalServiceConditions(Collections.emptyList())
.build();
// when
testee.sync(config);
// then
InOrder order = inOrder(itemApiMock);
order.verify(itemApiMock).list(POLICY.getId());
order.verifyNoMoreInteractions();
}
@Test
public void shouldThrowException_whenConditionScopeInstanceButViolationCloseTimerIsNotSet() throws Exception {
// given
// then - exception
expectedException.expect(NullPointerException.class);
expectedException.expectMessage("violationCloseTimer");
// when
ApmAppCondition.builder()
.conditionName("condition name")
.enabled(ENABLED)
.application(APPLICATION_NAME)
.metric(APP_METRIC)
.conditionScope(ApmAppCondition.ConditionScope.INSTANCE)
.term(TERMS_CONFIGURATION)
.build();
}
@Test
public void shouldCreateCondition() {
U itemFromConfig = getItemFromConfig();
U itemSame = getItemSame();
PolicyConfiguration policyConfiguration = getPolicyConfiguration();
PolicyItemApi<U> itemApiMock = getPolicyItemApiMock();
// given
when(itemApiMock.list(POLICY.getId())).thenReturn(ImmutableList.of());
when(itemApiMock.create(POLICY.getId(), itemFromConfig)).thenReturn(itemSame);
// when
testee.sync(policyConfiguration);
// then
InOrder order = inOrder(itemApiMock);
order.verify(itemApiMock).list(POLICY.getId());
order.verify(itemApiMock).create(POLICY.getId(), itemFromConfig);
order.verifyNoMoreInteractions();
}
@Test
public void shouldUpdateCondition() {
U itemFromConfig = getItemFromConfig();
U itemUpdated = getItemUpdated();
PolicyConfiguration policyConfiguration = getPolicyConfiguration();
PolicyItemApi<U> itemApiMock = getPolicyItemApiMock();
// given
when(itemApiMock.list(POLICY.getId())).thenReturn(ImmutableList.of(itemUpdated));
when(itemApiMock.update(itemUpdated.getId(), itemFromConfig)).thenReturn(itemUpdated);
// when
testee.sync(policyConfiguration);
// then
InOrder order = inOrder(itemApiMock);
order.verify(itemApiMock).list(POLICY.getId());
order.verify(itemApiMock).update(itemUpdated.getId(), itemFromConfig);
order.verifyNoMoreInteractions();
}
@Test
public void shouldRemoveOldCondition() {
U itemFromConfig = getItemFromConfig();
U itemSame = getItemSame();
U itemDifferent = getItemDifferent();
PolicyConfiguration policyConfiguration = getPolicyConfiguration();
PolicyItemApi<U> itemApiMock = getPolicyItemApiMock();
// given
when(itemApiMock.list(POLICY.getId())).thenReturn(ImmutableList.of(itemDifferent));
when(itemApiMock.create(POLICY.getId(), itemFromConfig)).thenReturn(itemSame);
// when
testee.sync(policyConfiguration);
// then
InOrder order = inOrder(itemApiMock);
order.verify(itemApiMock).list(POLICY.getId());
order.verify(itemApiMock).create(POLICY.getId(), itemFromConfig);
order.verify(itemApiMock).delete(POLICY.getId(), itemDifferent.getId());
order.verifyNoMoreInteractions();
}
protected abstract PolicyConfiguration getPolicyConfiguration();
protected abstract PolicyItemApi<U> getPolicyItemApiMock();
protected abstract U getItemFromConfig();
protected abstract U getItemSame();
protected abstract U getItemUpdated();
protected abstract U getItemDifferent();
private static TermsConfiguration.TermsConfigurationBuilder createTermsConfiguration() {
return TermsConfiguration.builder()
.durationTerm(DurationTerm.DURATION_5)
.operatorTerm(OperatorTerm.ABOVE)
.priorityTerm(PriorityTerm.CRITICAL)
.thresholdTerm(0.5f)
.timeFunctionTerm(TimeFunctionTerm.ALL);
}
static ApmAppCondition createAppCondition(String conditionName) {
return ApmAppCondition.builder()
.conditionName(conditionName)
.enabled(ENABLED)
.application(APPLICATION_NAME)
.metric(APP_METRIC)
.conditionScope(CONDITION_SCOPE)
.violationCloseTimer(VIOLATION_CLOSE_TIMER)
.term(TERMS_CONFIGURATION)
.build();
}
}
<file_sep>/newrelic-api-client/src/main/java/com/ocadotechnology/newrelic/apiclient/internal/DefaultAlertsChannelsApi.java
package com.ocadotechnology.newrelic.apiclient.internal;
import com.ocadotechnology.newrelic.apiclient.AlertsChannelsApi;
import com.ocadotechnology.newrelic.apiclient.internal.client.NewRelicClient;
import com.ocadotechnology.newrelic.apiclient.internal.model.AlertsChannelList;
import com.ocadotechnology.newrelic.apiclient.internal.model.AlertsChannelWrapper;
import com.ocadotechnology.newrelic.apiclient.model.channels.AlertsChannel;
import javax.ws.rs.client.Entity;
import java.util.List;
import static javax.ws.rs.core.MediaType.APPLICATION_JSON_TYPE;
public class DefaultAlertsChannelsApi extends ApiBase implements AlertsChannelsApi {
private static final String CHANNELS_URL = "/v2/alerts_channels.json";
private static final String CHANNEL_URL = "/v2/alerts_channels/{channel_id}.json";
private static final String POLICY_CHANNELS_URL = "/v2/alerts_policy_channels.json";
public DefaultAlertsChannelsApi(NewRelicClient client) {
super(client);
}
@Override
public List<AlertsChannel> list() {
return getPageable(
client.target(CHANNELS_URL).request(APPLICATION_JSON_TYPE),
AlertsChannelList.class)
.getList();
}
@Override
public AlertsChannel create(AlertsChannel channel) {
return client
.target(CHANNELS_URL)
.request(APPLICATION_JSON_TYPE)
.post(Entity.entity(new AlertsChannelWrapper(channel), APPLICATION_JSON_TYPE), AlertsChannelList.class)
.getSingle()
.orElseThrow(() -> new IllegalStateException("Failed to create channel: empty list returned"));
}
@Override
public AlertsChannel delete(int channelId) {
return client
.target(CHANNEL_URL)
.resolveTemplate("channel_id", channelId)
.request(APPLICATION_JSON_TYPE)
.delete(AlertsChannelWrapper.class)
.getChannel();
}
@Override
public AlertsChannel deleteFromPolicy(int policyId, int channelId) {
return client
.target(POLICY_CHANNELS_URL)
.queryParam("policy_id", policyId)
.queryParam("channel_id", channelId)
.request(APPLICATION_JSON_TYPE)
.delete(AlertsChannelWrapper.class)
.getChannel();
}
}
| 2430711117ce9bb01a5e32756fdcb5e0c8409c82 | [
"Markdown",
"INI",
"Gradle",
"Java",
"Kotlin"
] | 115 | Java | ocadotechnology/newrelic-alerts-configurator | a1066a0ffcc59f3458f39d139c010df6968e9fe8 | e27b1ae0aece8b68441cf91b9199ddbe0bf5721f | |
refs/heads/master | <file_sep>#Write a function named 'pollutantmean' that calculates the mean of a pollutant (sulfate or nitrate) across a
#specified list of monitors. The function 'pollutantmean' takes three arguments: 'directory', 'pollutant', and 'id'.
#Given a vector monitor ID numbers, 'pollutantmean' reads that monitors' particulate matter data from the directory specified
#in the 'directory' argument and returns the mean of the pollutant across all of the monitors, ignoring any missing values coded as NA.
#A prototype of the function is as follows:
pollutantmean <- function (directory, pollutant, id = 1:332){
files <- list.files(directory, full.names = 1)
data <- data.frame()
for (i in id){
data <- rbind(data, read.csv(files[i]))
}
mean(data[,pollutant], na.rm =1 )
}<file_sep># takes a directory of data files and a threshold for complete cases and calculates the correlation
#between sulfate and nitrate for monitor locations where the number of completely observed cases (on all variables)
#is greater than the threshold. The function should return a vector of correlations for the monitors that meet the threshold requirement.
#If no monitors meet the threshold requirement, then the function should return a numeric vector of length 0.
corr <- function(directory, threshold = 0) {
FL<- list.files(path = directory, pattern = ".csv", full.names = TRUE)
cors <- numeric()
for (i in 1:332) {
dat <- read.csv(FL[i])
if (sum(complete.cases(dat)) > threshold) {
cors <- c(cors, cor(dat[["sulfate"]], dat[["nitrate"]], use = "complete.obs"))
}
}
cors
}<file_sep>#Reads a directory full of files and reports the number of completely observed cases in each data file.
#The function should return a data frame where the first column is the name of the file and the second
#column is the number of complete cases.
observation <- function (directory, id = 1:332){
files <- list.files(directory, full.names =1)
data <- data.frame(id = integer(), nobs = integer())
for (i in 1:length(id)){
data[i,1]<- id[i]
data[i,2]<- sum(complete.cases(read.csv(files[i])))
}
data
} | 58bffff88053ead44a06756d7878920962a9ffd7 | [
"R"
] | 3 | R | Dcroix/Programming-Assign-One | 0563313610bbf5f1f7626445b9c2ef3d6537ea10 | ec07d6ab0545a8c8b1ac5f4ec76c7d7a77a76660 | |
refs/heads/master | <file_sep>module Alignment
# An Entry represents a single row or column of an alignment. It is generally
# recommended that instead of generating these objects directly they are
# instead accessed through the corresponding methods in Alignment::Instance.
module Entry
module ClassMethods
# This is the table in the SQL database to store the entry in.
attr_accessor :sql_table
alias :table :sql_table=
# This is the key in the database to use with alignment_id for identifying
attr_accessor :sql_key
alias :key :sql_key=
# This is the prefix to use when adding to the sequence database.
attr_accessor :tt_prefix
alias :prefix :tt_prefix=
def sequence_of(instance, index)
return Sequence.new(instance, self.tt_prefix, index).sequence
end
# Get the dataset for this entry.
#
# @return [Sequel::Dataset] The dataset.
def dataset(instance)
return instance.sql[@sql_table].filter(:alignment_id => instance[:id])
end
end
module InstanceMethods
# The instance this entry is a part of.
attr_reader :instance
# Create a new Entry.
#
# @param [Alignmnet::Instance] sql The instance this entry belongs to.
# @param [String] entry_id The id of this Entry.
def initialize(instance, entry_id)
alignment_id = instance[:id]
query = {
:alignment_id => alignment_id,
self.class.sql_key => entry_id
}
@instance = instance
sql = instance.sql
@meta = Alignment::MetaData.new(sql[self.class.sql_table], query)
prefix = self.class.tt_prefix
@sequence = Alignment::Sequence.new(instance, prefix, @meta[:index])
end
# Get the value of some key in this entry.
#
# @param [String] key The key.
# @return [String, nil] The value.
def [](key)
return (key == :sequence ? @sequence.sequence : @meta[key])
end
# Set the value of some key.
#
# @param [String] key The key to set.
# @param [String] value The value.
# @return [Alignment::Entry] This entry.
def []=(key, value)
(key == :sequence ? @sequence.sequence = value : @meta[key] = value)
return self
end
# Test if this entry is new. Something is new if there is no saved meta
# data about it.
#
# @return [Boolean] true if this is new.
def new?
return @meta.new?
end
# Save this entry. This will throw an error if the entry already exists or
# if some problem occurs while saving.
#
# @return [Alignment::Entry] The entry.
def save!
@meta.save!
@sequence.index = @meta[:index]
@sequence.save!
return self
end
# Delete all information associated with a specific entry.
#
# @return [Alignment::Entry] This entry.
def delete!
if new?
raise ArgumentError, "Cannot delete new Entry"
end
@meta.delete!
@sequence.delete!
return self
end
end
def self.included(receiver)
receiver.extend ClassMethods
receiver.send :include, InstanceMethods
end
end
end
<file_sep>module Alignment
class Import
attr_accessor :parser
def initialize(instance)
@alignment = instance
@alignment.save!
@rows = []
@columns = []
end
def save!
@rows.each_slice(100) do |rows|
@alignment.sql[:alignment_rows].multi_insert(rows)
end
@columns.each_slice(100) do |columns|
@alignment.sql[:alignment_columns].multi_insert(columns)
end
end
def rows(row_file)
load_file(row_file, :row) do |header, index|
@rows << header.merge(:index => index, :alignment_id => @alignment[:id])
end
end
def columns(column_file)
load_file(column_file, :column) do |header, index|
@columns << header.merge(:index => index, :alignment_id => @alignment[:id])
end
end
private
def load_file(name, type)
Fasta.open(name) do |file|
index = 0
prefix = type.to_s
file.each do |header, sequence|
header_data = @parser.send(type, header)
yield header_data,index
sequence.gsub!('t', 'u')
sequence.gsub!('.', '-')
seq = Alignment::Sequence.new(@alignment, prefix, index)
seq.sequence = sequence
seq.save!
index += 1
end
end
end
end
end
<file_sep>require 'set'
module Alignment
# An instance is one specific alignment. This provides a easy way to access
# and manipulate the metadata as well as sequence data for the alignment. This
# can also find sub alignments efficiently.
class Instance
extend Forwardable
# Options for aggregating in some methods.
OPTIONS = { :aggregate => true }.freeze
# The SQL database we connect to.
attr_reader :sql
# The Tokyo Tyrant DB to connect to.
attr_reader :tt
# Force the method for accessing by either row or sequence.
attr_reader :access_method
# Method use to access by either row or column.
attr_reader :method_used
def_delegators :@data, :new?, :[], :[]=
# Create a new Instance.
#
# @param [Rufus::Tokyo::Tyrant] db The db to connect to.
# @param [String] id The ID of this alignment
def initialize(database, query)
@sql = database.sql
@tt = database.tt
@data = Alignment::MetaData.new(@sql[:alignments], query)
end
# Create a new Alignment::Import object for this class.
#
# @return [Alignment::Import] An importer for this class.
def importer
return Alignment::Import.new(self)
end
# Get the row count.
#
# @return [Sequel::Dataset] The dataset for each row.
def rows
return Alignment::Row.dataset(self)
end
# Get the column count.
#
# @return [Integer] The count of all columns.
def columns
return Alignment::Column.dataset(self)
end
# Get the data for a row with the given column id.
#
# @param [String] row_id The row id.
# @return [Hash] A Hash with the information for the given row. Empty if no
# such row exists.
def row(row_id)
return Alignment::Row.new(self, row_id)
end
# Get the data for a column with the given column id.
#
# @param [String] column_id The column id.
# @return [Hash] A Hash with the information for the given column. Empty if
# no such column exists.
def column(column_id)
return Alignment::Column.new(self, column_id)
end
# Save the metadata for this Instance.
#
# @return [Alignment::Instance] This instance.
def save!
@data.save!
return self
end
# Delete all metadata for this Instance.
#
# @return [Alignment::Instance] This instance.
def delete!
@data.delete!
return self
end
# Compute the unique sequences and their ounts for some row, column slice of
# this alignment.
#
# @param [Array<String>] row_ids The ids of each row.
# @param [Array<Array<String>>] column_ids The ids of each column.
# @return [Hash<String, Integer>] Hash of each unique seqeunce and it's
# counts.
def variations(rows, columns, options = {})
opts = OPTIONS.merge(options)
vars = Hash.new { |h, k| h[k] = (opts[:aggregate] ? 0 : []) }
each_subsequence(rows, columns) do |r, c, sequence|
if opts[:aggregate]
vars[sequence] += 1
else
vars[sequence] << r
vars[sequence].uniq!
end
end
return vars
end
# Find all unique subsequences for the given row, column slice.
#
# @param [Array<String>] row_ids The row ids.
# @param [Array<Array<String>>] column_ids The column ids.
# @return [Array<String>] THe unique subsequences.
def unique_sequences(row_ids, column_ids)
unique = Set.new
each_subsequence(row_ids, column_ids) do |_, _, sequence|
unique << sequence
end
return unique.to_a
end
# Find all subsequences for the given row, column slice.
#
# @param [Array<String>] row_ids The row ids.
# @param [Array<Array<String>>] column_ids The column ids.
# @return [Array<String>] THe subsequences.
def subsequences(row_ids, column_ids)
subsequences = []
each_subsequence(row_ids, column_ids) do |_, _, sequence|
subsequences << sequence
end
return subsequences
end
# Iterate over the given rows or all if none are given.
#
# @param [Array<String>] row_ids The ids.
# @yeilds row, sequence
def each_row(row_ids = nil)
row_ids ||= self.rows.select_map(:row_id)
row_ids.each do |id|
yield self.row(id)
end
end
# Iterate over the given columns or all if none are given.
#
# @param [Array<String>] column_ids The ids to iterate over, if none is
# given all columns are used.
# @yeilds column A Column object.
def each_column(column_ids = nil)
column_ids ||= self.columns.select_map(:column_id)
column_ids.each do |id|
yield self.column(id)
end
end
# Iterate over each subsequence in the given row and column set.
def each_subsequence(rows, column_set)
if (@access_method && @access_method == :row) ||
(!@access_method && row_cost(rows, column_set) < column_cost(rows, column_set))
@method_used = :row
each_sub_by_row(rows, column_set) { |r, c, s| yield r, c, s }
elsif (@access_method && @access_method == :column) || !@access_method
@method_used = :column
each_sub_by_col(rows, column_set) { |r, c, s| yield r, c, s }
end
@access_method = nil
end
private
def query(term, ids)
return (ids ? { term => ids} : {})
end
def each_sub_by_row(rows, column_set)
columns = column_set.map do |cols|
cols.map { |c| column(c)[:index] }
end
query = (rows == :all || rows == 'all' ? {} : { :row_id => rows })
all = self.rows.filter(query).all
all.each do |row|
row_id = row[:row_id]
seq = Row.sequence_of(self, row[:index])
columns.each do |cols|
yield row_id, cols, cols.map { |c| seq[c] }.join
end
end
end
def each_sub_by_col(row_ids, column_set)
query = (rows == :all || rows == 'all' ? {} : { :row_id => row_ids })
rows = self.rows.filter(query).select_map(:index)
sequences = Array.new(rows.size) { |i| '' }
column_set.each do |columns|
cols = columns.map { |c| column(c) }
cols.each do |col|
seq = Column.sequence_of(self, col[:index])
rows.each_with_index do |seq_index, array_index|
sequences[array_index] << seq[seq_index]
end
end
sequences.each_with_index do |sequence, index|
yield row_ids[index], columns, sequence
end
sequences = Array.new(rows.size) { |i| '' }
end
end
def row_cost(rows, columns)
return (rows == :all || rows == 'all' ? self.rows.count : rows.size)
end
def column_cost(rows, columns)
return columns.flatten.uniq.size
end
end
end
<file_sep>require 'forwardable'
module Alignment
# Class to manage sequences in the database.
class Sequence
extend Forwardable
# Access and set the sequence. This only manipulates the data in memory.
attr_accessor :sequence
# The index to use for saving
attr_accessor :index
def_delegator :@sequence, :[]
# Create a new sequence.
#
# @param [Rufus::Tokyo::Tyrant] tt The database to store the sequence in.
# @param [String] key The key to access.
def initialize(instance, prefix, index)
@tt = instance.tt
@id = instance[:id]
@prefix = prefix
@index = index
if @index
update_key
@sequence = @tt[@key]
end
end
# Remove the sequence from the database.
#
# @return [Alignment::Sequence] The sequence.
def delete!
update_key
raise ArgumentError, "Must specify index to delete" if !@key
@sequence = nil
@tt.delete(@key)
return self
end
# Update the sequence in the database.
#
# @return [Alignment::Sequence] The sequence.
def save!
update_key
raise ArgumentError, "Must specify index to save" if !@key
@tt[@key] = @sequence
return self
end
private
def update_key
@key = (@index ? "%s-%s:%s" % [@id, @prefix, @index] : nil)
end
end
end
<file_sep>require 'tempfile'
require "alignment"
require "minitest/pride"
require "minitest/autorun"
# TokyoTyrant DB to connect to
temp_file = Tempfile.new(["alignment-test", ".tch"])
TT = Rufus::Tokyo::Cabinet.new(temp_file.path)
# SQL database to connect to
SQL = Sequel.sqlite
Sequel.extension :migration
Sequel::Migrator.apply(SQL, 'spec/migrations')
<file_sep>require "spec_helper"
describe 'Alignment::Import' do
before do
class Parser
def row(l)
return { :row_id => l.to_i }
end
def column(l)
return { :column_id => l.to_i }
end
end
conn = Alignment::Database.new(SQL, TT)
alignment = conn.instance(:source => 'gg', :version => 1)
@id = alignment[:id]
@import = alignment.importer
@import.parser = Parser.new
end
describe 'importing rows' do
before do
@dataset = SQL[:alignment_rows]
@dataset.delete
@import.rows('spec/files/simple_rows.fasta')
@import.save!
end
it 'can import all meta data' do
val = @dataset.select_order_map(:row_id)
val.must_equal %w{10 3}
end
it 'can import all sequences' do
val = TT["#{@id}-row:0"]
val.must_equal '--a'
end
end
describe 'importing columns' do
before do
@dataset = SQL[:alignment_columns]
@dataset.delete
@import.columns('spec/files/simple_columns')
@import.save!
end
it 'can import all meta data' do
val = @dataset.select_order_map(:column_id)
val.must_equal %w{1 2 3}
end
it 'can imoprt all sequences' do
val = TT["#{@id}-column:1"]
val.must_equal '-u'
end
end
end
<file_sep>module Alignment
# An Alignment Database is a way of managing both the sequence information as
# well as the meta data associated with the sequence.
class Database
# The connection the SQL database.
attr_reader :sql
# The connection to the tokyo tyrant.
attr_accessor :tt
# Create a new connection to the alignment.
#
# @param [Hash] sequel_db Hash specifying which SQL database to connect to.
# @param [String] tt_db The socket to connect to.
def initialize(sequel, tt)
@sql = sequel
@tt = tt
end
# Get an instance using a specific query.
#
# @param [Hash] query The query to find the instance.
# @return [Alignment::Instance] The instance.
def instance(query)
return Alignment::Instance.new(self, query)
end
# Close the connection to the database.
#
# @return [Alignment::Database] This database.
def close
@sql.close
@tt.close
return self
end
end
end
<file_sep>Alignment DB
============
This is a tool for accessing both the rows and columns of large alignments
rapidly. This also provides some simple analysis of the columns such as unique
sequences and counts of unique sequences. It is primarly geared towards rapid
analysis of small sections of large alignments but may be useful for other
things.
<file_sep>require "spec_helper"
describe Alignment::Sequence do
before do
TT['1-ex:0'] = 'bob'
conn = Alignment::Database.new(SQL, TT)
@instance = conn.instance(:source => 'test', :version => 1)
@instance.save!
@sequence = Alignment::Sequence.new(@instance, 'ex', 0)
end
after do
TT.delete('1-ex:0')
end
describe 'building' do
describe 'with a null index' do
before do
@sequence = Alignment::Sequence.new(@instance, 'ex', nil)
end
it 'does not load any sequence' do
@sequence.sequence.must_equal nil
end
it 'cannot save' do
lambda do
@sequence.save!
end.must_raise ArgumentError
end
it 'cannot delete' do
lambda do
@sequence.delete!
end.must_raise ArgumentError
end
end
end
describe 'properties' do
it 'has a sequence property as in the database' do
val = @sequence.sequence
val.must_equal 'bob'
end
it 'can access a sequence index using []' do
val = @sequence[1]
val.must_equal 'o'
end
it 'can access a sequence range using []' do
val = @sequence[1..2]
val.must_equal 'ob'
end
end
describe 'editing the sequence' do
before do
@sequence.sequence = 'jones'
end
describe 'without saving' do
it 'changes the in memory sequence' do
@sequence.sequence.must_equal 'jones'
end
it 'does not change the sequence in the database' do
TT['1-ex:0'].must_equal 'bob'
end
end
describe 'with saving' do
before do
@sequence.save!
end
it 'changes the in memory sequence' do
@sequence.sequence.must_equal 'jones'
end
it 'changes the sequence in the database' do
TT['1-ex:0'].must_equal 'jones'
end
end
end
describe 'deleting a sequence' do
before do
@sequence.delete!
end
it 'sets the database sequence to nil' do
TT['1-ex:0'].must_equal nil
end
it 'sets the in memory sequence to nil' do
@sequence.sequence.must_equal nil
end
end
end
<file_sep>require "spec_helper"
describe 'Alignment::Instance' do
def add(type, id, seq, index)
entry = @alignment.send(type, id)
entry[:sequence] = seq
entry[:index] = index
entry.save!
end
before do
@conn = Alignment::Database.new(SQL, TT)
@alignment = @conn.instance(:source => 'test', :version => 1)
add(:row, '10', 'aa', 0)
add(:row, '11', 'ag', 1)
add(:row, '12', 'ac', 2)
add(:row, '13', 'aa', 3)
add(:column, '1', 'aaaa', 0)
add(:column, '2', 'agca', 1)
@alignment.save!
# Run importer to import some data for testing
end
describe 'creating' do
before do
@alignment = @conn.instance(:source => 'a', :version => 2)
end
describe 'before saving' do
it 'knows it is new' do
@alignment.new?.must_equal true
end
end
end
describe 'editing the metadata' do
describe 'after saving' do
before do
@alignment = @conn.instance(:source => 'test2', :version => 1)
@alignment.save!
end
it 'can get the id' do
@alignment[:id].wont_equal nil
end
end
end
describe 'accessing' do
it 'can get a row' do
row = @alignment.row('10')
row[:row_id].must_equal '10'
row[:sequence].must_equal 'aa'
row[:index].must_equal(0)
end
it 'can get a column' do
col = @alignment.column('1')
col[:sequence].must_equal 'aaaa'
col[:index].must_equal 0
end
end
describe 'iterating' do
describe 'over all rows' do
it 'can get meta data' do
ans = %w{10 11 12 13}
val = []
@alignment.each_row { |row| val << row[:row_id] }
val.must_equal ans
end
it 'can get sequence' do
ans = %w{aa ag ac aa}
val = []
@alignment.each_row { |row| val << row[:sequence] }
val.must_equal ans
end
end
describe 'over some rows' do
it 'can get the meta data' do
ids = %w{11 12}
val = []
@alignment.each_row(ids) { |row| val << row[:row_id] }
val.must_equal ids
end
it 'can get the sequence' do
ids = %w{11 12}
ans = %w{ag ac}
val = []
@alignment.each_row(ids) { |row| val << row[:sequence] }
val.must_equal ans
end
end
describe 'over all columns' do
it 'can get meta data' do
ans = %w{1 2}
val = []
@alignment.each_column { |col| val << col[:column_id] }
val.must_equal ans
end
it 'can get sequences' do
ans = %w{aaaa agca}
val = []
@alignment.each_column { |col| val << col[:sequence] }
val.must_equal ans
end
end
describe 'over some columns' do
before do
@ids = %w{1}
@val_ids = []
@val_seqs = []
@alignment.each_column(@ids) do |col|
@val_ids << col[:column_id]
@val_seqs << col[:sequence]
end
end
it 'can get meta data' do
@val_ids.must_equal %w{1}
end
it 'can get sequences' do
@val_seqs.must_equal %w{aaaa}
end
end
end
describe 'analyzing' do
describe 'generic analysis' do
it 'can get all subsequences with counts' do
rows = %w{10 11 13}
cols = [%w{1 2}]
ans = %w{aa ag aa}
val = @alignment.subsequences(rows, cols)
val.must_equal ans
end
it 'can get all unique subsequences with rows' do
rows = %w{10 11 13}
cols = [%w{1 2}]
ans = %w{aa ag}
val = @alignment.unique_sequences(rows, cols)
val.must_equal ans
end
end
describe 'when not aggregating' do
it 'gets all subsequences and the rows' do
rows = %w{10 11 13}
cols = [%w{1 2}, %w{2 1}]
ans = { 'aa' => %w{10 13}, 'ag' => %w{11}, 'ga' => %w{11} }
val = @alignment.variations(rows, cols, :aggregate => false)
val.must_equal ans
end
end
describe 'when aggregating' do
it 'can get all subsequences and counts' do
rows = %w{10 11 13}
cols = [%w{1 2}, %w{2 1}]
ans = { 'aa' => 4, 'ag' => 1, 'ga' => 1 }
val = @alignment.variations(rows, cols)
val.must_equal ans
end
end
end
end
<file_sep>$:.unshift("./lib")
require "alignment"
require "benchmark"
# Read only database
MAX_THREAD_COUNT = 10
connection = Alignment::Connection.new('/tmp/alignment_socket')
alignment = connection['greengenes', 'current']
id_count = 100
ids = alignment.info.keys.shuffle[0 .. id_count]
ids.delete('rows')
max_col = 300
col_count = 900
columns = []
col_count.times do |t|
c1 = rand(max_col) + 1
c2 = rand(max_col) + 1
columns << [c1, c2]
end
Benchmark.bmbm(5) do |x|
x.report('100') do
cols = columns[0..100]
alignment.count_subsequences(ids, cols)
end
x.report('400') do
cols = columns[0..200]
alignment.count_subsequences(ids, cols)
end
x.report('900') do
alignment.count_subsequences(ids, columns)
end
x.report('all') do
cols = columns[0..20]
rows = alignment.info.keys
rows.delete('rows')
alignment.count_subsequences(rows, cols)
end
end
<file_sep>module Alignment
class Column
include Alignment::Entry
table :alignment_columns
prefix 'column'
key :column_id
end
end
<file_sep>Sequel.migration do
change do
create_table :entry_test do
primary_key :id
Integer :alignment_id
Integer :entry_id
Integer :index
String :type
end
end
end
<file_sep>class Parser
def parse(header)
header << "AWESOME"
end
end
<file_sep>Sequel.migration do
change do
create_table :alignment_rows do
primary_key :id
String :row_id, :length => 10
Integer :index
foreign_key :alignment_id, :alignments
end
create_table :alignment_columns do
primary_key :id
String :column_id, :length => 10
Integer :index
foreign_key :alignment_id, :alignments
end
end
end
<file_sep>module Alignment
# MetaData represents data about sequences stored in an SQL database. This
# manages accessing and manipulating the data.
class MetaData
extend Forwardable
# Delegate accessing and setting methods
def_delegators :@data, :[], :[]=
# Create new MetaData.
#
# @param [Sequel::Dataset] dataset The dataset to use.
# @param [Hash] search A search which should specify one row in the dataset.
# If more than one row is found an error is raised. If no rows are found a
# new in memory entry is created using the query.
def initialize(dataset, search)
@dataset = dataset.filter(search)
count = @dataset.count
if count > 1
throw ArgumentError.new("Metadata may specify at most 1 row")
end
@data = (count == 0 ? search : @dataset.first)
end
# Check if this meta data is new. New means this is not yet stored in the
# database. This does not insure that the database and in memory data are
# synced.
#
# @return [Boolean] true if the meta data is new.
def new?
return @dataset.count == 0
end
# Save the meta data. If the data already exists it will be updated,
# otherwise it is inserted to the database.
#
# @return [Alignment::MetaData] Returns itself
def save!
method = (new? ? :insert : :update)
@dataset.send(method, @data)
@data = @dataset.first
return self
end
# Delete this meta data. If it does not exist nothing is done, otherwise the
# data is removed both from the database and memory.
#
# @return [Alignment::MetaData] Returns itself
def delete!
@dataset.delete
@data = {}
return self
end
end
end
<file_sep>module Alignment
class Row
include Alignment::Entry
table :alignment_rows
prefix 'row'
key :row_id
end
end
<file_sep>require "rufus/tokyo/tyrant"
require "sequel"
require "fasta"
require "alignment/database"
require "alignment/sequence"
require "alignment/meta_data"
require "alignment/entry"
require "alignment/row"
require "alignment/column"
require "alignment/instance"
require "alignment/import"
# This alignment class is intended to make it easy to store and manipulate large
# alignments rapidly. Alignments are a combination of Sequence information in a
# key value store and meta data in an SQL store. This manages both of them. The
# basic assumptions are that specific alignments are identified by source and
# version.
module Alignment
end
<file_sep>require "spec_helper"
describe Alignment::MetaData do
before do
@dataset = SQL[:meta_data]
@dataset.delete
@raw = [ { :bob => 'jones', :type => 'A', :id => 1 } ]
@dataset.multi_insert(@raw)
@data = Alignment::MetaData.new(@dataset, :id => 1)
end
after do
@dataset.delete
end
describe 'properties' do
describe 'for meta data that already exists' do
it 'can access properties from the search using []' do
@data[:id].must_equal 1
end
it 'can access properites from the db using []' do
@data[:type] = 'A'
end
end
describe 'for a new meta data' do
before do
@data = Alignment::MetaData.new(@dataset, :id => 2)
end
it 'can access properties from the search' do
@data[:id].must_equal 2
end
it 'knows it is new' do
@data.new?.must_equal true
end
it 'does not add things to the db' do
@dataset.all.must_equal @raw
end
end
end
describe 'editing the meta data' do
before do
@data[:bob] = 'test'
end
describe 'without saving' do
it 'changes the in memory data' do
@data[:bob].must_equal 'test'
end
it 'does not change the data in SQL' do
@dataset.all.must_equal @raw
end
end
describe 'with saving' do
before do
@data.save!
end
it 'changes the data in memory' do
@data[:bob].must_equal 'test'
end
it 'does not insert a new row' do
@dataset.count.must_equal 1
end
it 'changes the data in SQL' do
ans = @raw.first.merge(:bob => 'test')
@dataset.first.must_equal ans
end
end
end
describe 'deleting' do
describe 'an entry that does not exist' do
before do
@data = Alignment::MetaData.new(@dataset, :id => 2)
@data.delete!
end
it 'does not change the database' do
@dataset.all.must_equal @raw
end
it 'erases the data in memory' do
@data[:bob].must_equal nil
end
end
describe 'an entry that does exist' do
before do
@data.delete!
end
it 'erases in memory data' do
@data['bob'].must_equal nil
end
it 'erases data in SQL' do
@dataset.all.must_equal []
end
end
end
end
<file_sep>require "spec_helper"
describe Alignment::Entry do
before do
connection = Alignment::Database.new(SQL, TT)
@instance = connection.instance(:source => 'test', :version => 1)
@instance.save!
@klass = Class.new
@klass.send(:include, Alignment::Entry)
@klass.table :entry_test
@klass.prefix 'ex'
@klass.key :entry_id
end
after do
@instance.sql[:entry_test].delete
end
describe 'class methods' do
it 'can get the dataset for the entry' do
ans = @instance.sql[:entry_test].filter(:alignment_id => 1)
@klass.dataset(@instance).must_equal ans
end
end
describe 'a new entry' do
before do
@data = @klass.new(@instance, '10')
end
describe 'deleting' do
it 'cannot delete' do
lambda { @data.delete! }.must_raise ArgumentError
end
end
describe 'accessing' do
it 'has no sequence' do
@data[:sequence].must_equal nil
end
it 'has no id' do
@data[:id].must_equal nil
end
it 'has no metadata' do
@data[:type].must_equal nil
end
end
describe 'editing the data' do
before do
@data[:type] = 'A'
@data[:sequence] = 'some'
@data[:index] = 4
end
describe 'after saving' do
before do
@data.save!
end
it 'updates meta data in SQL' do
val = @instance.sql[:entry_test].filter(:entry_id => 10).first
val[:type].must_equal 'A'
end
it 'updates the sequence in TT' do
TT['1-ex:4'].must_equal 'some'
end
end
end
end
describe 'an existing entry' do
before do
dataset = @instance.sql[:entry_test]
dataset.delete
@raw = [
{ :alignment_id => 1, :entry_id => 0, :index => 0, :type => 'A', :id => 1 }
]
TT['1-ex:0'] = 'bob'
dataset.multi_insert(@raw)
@data = @klass.new(@instance, 0)
end
describe 'accessing' do
it 'can get the associated sequence' do
@data[:sequence].must_equal 'bob'
end
it 'can get the associated meta data' do
@data[:type].must_equal 'A'
end
end
describe 'editing the data' do
before do
@data[:type] = 'c'
@data[:sequence] = 'something'
end
describe 'after saving' do
before do
@data.save!
end
it 'changes the meta data in memory' do
@data[:type].must_equal 'c'
end
it 'changes the meta data in sql' do
dataset = @instance.sql[:entry_test]
val = dataset.filter(:id => 1).first
val[:type].must_equal 'c'
end
it 'changes the sequence in memory' do
@data[:sequence].must_equal 'something'
end
it 'changes the sequence on disk' do
TT['1-ex:0'].must_equal 'something'
end
it 'knows the entry is not new' do
@data.new?.must_equal false
end
end
describe 'after deleting' do
before do
@data.delete!
end
it 'removes the meta data in memory' do
@data[:type].must_equal nil
end
it 'removes the meta data in sql' do
dataset = @instance.sql[:entry_test]
val = dataset.count.must_equal 0
end
it 'removes the sequence in memory' do
@data[:sequence].must_equal nil
end
it 'removes the sequence on disk' do
TT['1-ex:0'].must_equal nil
end
end
end
describe 'editing the data' do
before do
@data[:type] = 'c'
@data[:sequence] = 'something'
end
describe 'without saving' do
it 'changes the meta data in memory' do
@data[:type].must_equal 'c'
end
it 'does not change the meta data in sql' do
dataset = @instance.sql[:entry_test]
val = dataset.filter(:id => 1).first
val[:type].must_equal 'A'
end
it 'changes the sequence in memory' do
@data[:sequence].must_equal 'something'
end
it 'does not change the sequence on disk' do
TT['1-ex:0'].must_equal 'bob'
end
end
end
end
end
<file_sep>$:.unshift("./lib")
require "alignment"
#alignment = Alignment.new(:main_port => 1979)
connection = Alignment::Connection.new("/tmp/alignment_test_socket")
alignment = connection['test', 'generate']
rows = []
15_000.times do |count|
seq = ''
400.times { |t| seq << 'acgun-'[rand(6)] }
rows << seq
end
columns = Array.new(400) { |i| '' }
rows.each_with_index do |row, index|
alignment.add_row(index, row)
row.each_char.with_index do |char, index|
columns[index] << char
end
end
columns.each_with_index do |column, index|
alignment.add_column(index + 1, column)
end
| 12a2f7e35a06035023b406258370a74b56fb15e3 | [
"Markdown",
"Ruby"
] | 21 | Ruby | blakesweeney/Alignment | d2f18157f841aa83446637fbea8c4a8242853d99 | 6c2643b6570b2aa59a9775594efe5193710f0d7d | |
refs/heads/master | <repo_name>munifgebara/contatosnanuvemAndroid<file_sep>/src/com/example/contatosnanuvem/MainActivity.java
package com.example.contatosnanuvem;
import java.net.URLEncoder;
import java.util.Stack;
import org.apache.http.HttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.util.EntityUtils;
import com.google.gson.Gson;
import com.google.gson.JsonArray;
import com.google.gson.JsonParser;
import android.app.Activity;
import android.app.ActionBar;
import android.app.Fragment;
import android.content.ContentResolver;
import android.content.ContentValues;
import android.database.Cursor;
import android.net.Uri;
import android.os.AsyncTask;
import android.os.Bundle;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.view.ViewGroup;
import android.widget.EditText;
import android.widget.TextView;
import android.os.Build;
import android.provider.ContactsContract;
import android.provider.ContactsContract.Contacts;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
if (savedInstanceState == null) {
getFragmentManager().beginTransaction().add(R.id.container, new PlaceholderFragment()).commit();
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
public void acessaContatos(View v) {
EnviaContatos ec = new EnviaContatos();
EditText etPin=(EditText)findViewById(R.id.etPin);
ec.execute(etPin.getText().toString());
}
public void obtemContatos() {
TextView tv = (TextView) findViewById(R.id.textView1);
String phoneNumber = null;
String email = null;
Uri CONTENT_URI = ContactsContract.Contacts.CONTENT_URI;
String _ID = ContactsContract.Contacts._ID;
String DISPLAY_NAME = ContactsContract.Contacts.DISPLAY_NAME;
String HAS_PHONE_NUMBER = ContactsContract.Contacts.HAS_PHONE_NUMBER;
Uri PhoneCONTENT_URI = ContactsContract.CommonDataKinds.Phone.CONTENT_URI;
String Phone_CONTACT_ID = ContactsContract.CommonDataKinds.Phone.CONTACT_ID;
String NUMBER = ContactsContract.CommonDataKinds.Phone.NUMBER;
Uri EmailCONTENT_URI = ContactsContract.CommonDataKinds.Email.CONTENT_URI;
String EmailCONTACT_ID = ContactsContract.CommonDataKinds.Email.CONTACT_ID;
String DATA = ContactsContract.CommonDataKinds.Email.DATA;
StringBuffer output = new StringBuffer();
ContentResolver contentResolver = getContentResolver();
Cursor cursor = contentResolver.query(CONTENT_URI, null, null, null, null);
// Loop for every contact in the phone
int n = cursor.getCount();
Log.v("contatos", "Inicio da listagem de contatos " + n);
tv.setText("Contatos " + n);
if (n > 0) {
while (cursor.moveToNext()) {
ContatoAndroid contatoAndroid = new ContatoAndroid();
String contact_id = cursor.getString(cursor.getColumnIndex(_ID));
String name = cursor.getString(cursor.getColumnIndex(DISPLAY_NAME));
contatoAndroid.setNome(name);
int hasPhoneNumber = Integer.parseInt(cursor.getString(cursor.getColumnIndex(HAS_PHONE_NUMBER)));
if (hasPhoneNumber > 0) {
// Query and loop for every phone number of the contact
Cursor phoneCursor = contentResolver.query(PhoneCONTENT_URI, null, Phone_CONTACT_ID + " = ?", new String[] { contact_id }, null);
contatoAndroid.setTelefones(new String[phoneCursor.getCount()]);
int i = 0;
while (phoneCursor.moveToNext()) {
phoneNumber = phoneCursor.getString(phoneCursor.getColumnIndex(NUMBER));
contatoAndroid.getTelefones()[i] = phoneNumber;
i++;
}
phoneCursor.close();
// Query and loop for every email of the contact
Cursor emailCursor = contentResolver.query(EmailCONTENT_URI, null, EmailCONTACT_ID + " = ?", new String[] { contact_id }, null);
i = 0;
if (emailCursor.getCount() > 0) {
contatoAndroid.setEmails(new String[emailCursor.getCount()]);
while (emailCursor.moveToNext()) {
email = emailCursor.getString(emailCursor.getColumnIndex(DATA));
contatoAndroid.getEmails()[i] = email;
i++;
}
}
emailCursor.close();
}
if (contatoAndroid.getTelefones() != null || contatoAndroid.getEmails() != null) {
Log.v("contatos", contatoAndroid.toString());
}
}
}
}
/**
* A placeholder fragment containing a simple view.
*/
public static class PlaceholderFragment extends Fragment {
public PlaceholderFragment() {
}
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View rootView = inflater.inflate(R.layout.fragment_main, container, false);
return rootView;
}
}
class EnviaContatos extends AsyncTask<String, String, String> {
@Override
protected String doInBackground(String... params) {
String phoneNumber = null;
String email = null;
Uri CONTENT_URI = ContactsContract.Contacts.CONTENT_URI;
String _ID = ContactsContract.Contacts._ID;
String DISPLAY_NAME = ContactsContract.Contacts.DISPLAY_NAME;
String HAS_PHONE_NUMBER = ContactsContract.Contacts.HAS_PHONE_NUMBER;
Uri PhoneCONTENT_URI = ContactsContract.CommonDataKinds.Phone.CONTENT_URI;
String Phone_CONTACT_ID = ContactsContract.CommonDataKinds.Phone.CONTACT_ID;
String NUMBER = ContactsContract.CommonDataKinds.Phone.NUMBER;
Uri EmailCONTENT_URI = ContactsContract.CommonDataKinds.Email.CONTENT_URI;
String EmailCONTACT_ID = ContactsContract.CommonDataKinds.Email.CONTACT_ID;
String DATA = ContactsContract.CommonDataKinds.Email.DATA;
StringBuffer output = new StringBuffer();
ContentResolver contentResolver = getContentResolver();
Cursor cursor = contentResolver.query(CONTENT_URI, null, null, null, null);
int n = cursor.getCount();
int contador=0;
if (n > 0) {
while (cursor.moveToNext()) {
ContatoAndroid contatoAndroid = new ContatoAndroid();
String contact_id = cursor.getString(cursor.getColumnIndex(_ID));
String name = cursor.getString(cursor.getColumnIndex(DISPLAY_NAME));
contatoAndroid.setNome(name);
int hasPhoneNumber = Integer.parseInt(cursor.getString(cursor.getColumnIndex(HAS_PHONE_NUMBER)));
if (hasPhoneNumber > 0) {
// Query and loop for every phone number of the contact
Cursor phoneCursor = contentResolver.query(PhoneCONTENT_URI, null, Phone_CONTACT_ID + " = ?", new String[] { contact_id }, null);
contatoAndroid.setTelefones(new String[phoneCursor.getCount()]);
int i = 0;
while (phoneCursor.moveToNext()) {
phoneNumber = phoneCursor.getString(phoneCursor.getColumnIndex(NUMBER));
contatoAndroid.getTelefones()[i] = phoneNumber;
i++;
}
phoneCursor.close();
Cursor emailCursor = contentResolver.query(EmailCONTENT_URI, null, EmailCONTACT_ID + " = ?", new String[] { contact_id }, null);
i = 0;
if (emailCursor.getCount() > 0) {
contatoAndroid.setEmails(new String[emailCursor.getCount()]);
while (emailCursor.moveToNext()) {
email = emailCursor.getString(emailCursor.getColumnIndex(DATA));
contatoAndroid.getEmails()[i] = email;
i++;
}
}
emailCursor.close();
}
if (contatoAndroid.getTelefones() != null || contatoAndroid.getEmails() != null) {
contatoAndroid.setPin(params[0]);
enviaContato(contatoAndroid);
}
contador++;
publishProgress(contador+"/"+n);
}
}
return "Enviados";
}
@Override
protected void onPostExecute(String result) {
super.onPostExecute(result);
TextView tv = (TextView) findViewById(R.id.textView1);
tv.setText(result);
}
@Override
protected void onProgressUpdate(String... values) {
super.onProgressUpdate(values);
TextView tv = (TextView) findViewById(R.id.textView1);
tv.setText(values[0]);
}
private void enviaContato(ContatoAndroid contatoAndroid) {
//String servidor="munifgebara.servehttp.com";
String servidor="10.12.15.229:8080";
try {
DefaultHttpClient dhc = new DefaultHttpClient();
String contatoJson=new Gson().toJson(contatoAndroid);
contatoJson=URLEncoder.encode(contatoJson,"UTF-8");
Log.v("contatos",contatoJson);
HttpGet httpGet = new HttpGet("http://"+servidor+"/contatonanuvem/rest/contatos/insere/"+contatoJson);
HttpResponse resposta = dhc.execute(httpGet);
String res = EntityUtils.toString(resposta.getEntity());
}
catch(Exception ex){
Log.v("contatos", ex.toString());
}
}
}
}
| 55f120c93c0884e2a343ca295abc40d4f8ed499d | [
"Java"
] | 1 | Java | munifgebara/contatosnanuvemAndroid | a1982db27c75050ad6841919fb2fae052629d5a8 | deebb893b2199106a40475043abdbf65e97ed194 | |
refs/heads/master | <repo_name>ctg1819/practicaGIT<file_sep>/Ejercicio2_Persona/Ejercicio2_Persona/Program.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Ejercicio2_Persona
{
class Program
{
static void Main(string[] args)
{
/*
Persona per1 = new Persona();
Persona per2 = new Persona();
per1.SetNombre("María");
per2.SetNombre("Elena");
per1.Saludar();
Console.WriteLine("\n");
per2.Saludar();
Console.WriteLine("\n");
*/
/* Añadimos PersonaInglesa
Persona per = new Persona();
PersonaInglesa peringlesa = new PersonaInglesa();
per.SetNombre("Carmen");
peringlesa.SetNombre("Elisabeth");
per.Saludar();
Console.WriteLine("\n");
peringlesa.Saludar();
Console.WriteLine("\n");
peringlesa.TomarTe();
*/
Persona per = new Persona();
PersonaInglesa peringlesa = new PersonaInglesa();
PersonaItaliana peritaliana = new PersonaItaliana();
per.Saludar();
peringlesa.Saludar();
peritaliana.Saludar();
PersonaInglesa peringlesa2 = new PersonaInglesa("Catherine");
peringlesa2.Saludar();
per.SetNombre("Carmen");
peritaliana.SetNombre("Donatella");
per.Saludar();
peritaliana.Saludar();
per.Saludar("Soy una persona...");
peringlesa.Saludar("Soy un/a inglés/a...");
Console.WriteLine();
peritaliana.Saludar("Soy un/a italiano/a...");
Console.WriteLine();
/*
per.SetNombre("Carmen");
peringlesa.SetNombre("Elisabeth");
peritaliana.SetNombre("Donatella");
per.Saludar();
Console.WriteLine();
peringlesa.Saludar();
Console.WriteLine();
peritaliana.Saludar();
Console.WriteLine();
peringlesa.TomarTe();
*/
}
}
}
<file_sep>/Ejercicio2_Persona/Ejercicio2_Persona/PersonaInglesa.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Ejercicio2_Persona
{
class PersonaInglesa:Persona
{
public PersonaInglesa()
{
nombre = "John";
}
public PersonaInglesa(string NuevoValor)
{
nombre = NuevoValor;
}
public void TomarTe()
{
Console.WriteLine("Estoy tomando té");
}
public new void Saludar()
{
Console.WriteLine("Hi, I am: " + nombre);
}
}
}
<file_sep>/Ejercicio2_Persona/Ejercicio2_Persona/Persona.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Ejercicio2_Persona
{
class Persona
{
protected string nombre;
public Persona()
{
nombre = "";
}
public void SetNombre(string nuevoValor)
{
nombre = nuevoValor;
}
public void Saludar()
{
Console.WriteLine("Hola, soy "+ nombre);
}
public new void Saludar(string nuevoSaludo)
{
Console.WriteLine(nuevoSaludo);
}
}
}
<file_sep>/Ejercicio2_Persona/Ejercicio2_Persona/PersonaItaliana.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Ejercicio2_Persona
{
class PersonaItaliana: Persona
{
public PersonaItaliana()
{
nombre = "";
}
public new void Saludar()
{
Console.WriteLine("Ciao: "+nombre);
}
}
}
| 17a872ab7af94c95fd5229c3c88678fa4e48bf27 | [
"C#"
] | 4 | C# | ctg1819/practicaGIT | f5c2143b98644a0cfe32cd95fde50bbb28aca986 | 84b5c0ae2ef5aa9b73d2bf8926cd81f17a932427 | |
refs/heads/master | <repo_name>kirubiel/learn_ruby<file_sep>/03_simon_says/simon_says.rb
#write your code here
def echo(simon_said)
simon_said
end
def shout(simon_said)
simon_said.upcase
end
def repeat(simon_said, times = 2)
((simon_said + ' ') * times).strip
end
def start_of_word(simon_said, no_of_chars = 1)
simon_said[0, no_of_chars]
end
def first_word(simon_said)
simon_said.split.first
end
def titleize(simon_said)
split_word = simon_said.split
split_word.each_with_index do |word, i|
if(not((split_word[i] == 'and' or
word == 'over' or
word == 'the') and
split_word.index(word) > 0))
split_word[i] = word.capitalize
end
end
split_word.join(' ')
end<file_sep>/01_temperature/temperature.rb
#write your code here
def ftoc (fahrenheit)
(fahrenheit - 32) * (5.0/9.0)
end
def ctof (celsius)
(celsius * (9.0 / 5.0)) + 32
end<file_sep>/05_book_titles/book.rb
class Book
# write your code here
def title
@title
end
def title=(title)
conjs_and_prepos = ["and", "in", "the", "of", "a", "an"]
split_title = title.split
split_title.map! {|word|
if conjs_and_prepos.index(word) and word != split_title[0]
word = word
else
word = word.capitalize
end
}
split_title = split_title.join(' ')
@title = split_title
end
end<file_sep>/04_pig_latin/pig_latin.rb
#write your code here
def translate(word)
split_word = word.split
vowels_regex = /^[aieou]/
two_consonants = /\A[^aieou]{2}/
three_consonants = /\A[^aieou]{3}/
split_word.map! {|word|
if vowels_regex.match(word)
word << "ay"
elsif not(vowels_regex.match(word)) and word[1] == "u"
consonant_letter = word[0,2]
word = word.sub(word[0,2], "")
word << consonant_letter + "ay"
elsif three_consonants.match(word)
consonant_letter = word[0,3]
word = word.sub(word[0,3], "")
word << consonant_letter + "ay"
elsif two_consonants.match(word)
if word[2] == "u"
consonant_letter = word[0,3]
word = word.sub(word[0,3], "")
word << consonant_letter + "ay"
else
consonant_letter = word[0,2]
word = word.sub(word[0,2], "")
word << consonant_letter + "ay"
end
else
consonant_letter = word.slice(0)
word = word.sub(word[0], "")
word << consonant_letter + "ay"
end
}
split_word.join(" ")
end<file_sep>/02_calculator/calculator.rb
#write your code here
def add(a, b)
a + b
end
def subtract(a, b)
a - b
end
def sum(arr)
total = 0
arr.each do |item|
total += item
end
total
end | 9b982962f67935bfef12286bd43dab95e20d2cf9 | [
"Ruby"
] | 5 | Ruby | kirubiel/learn_ruby | 462697d89e72f863ea5ad5b71056c6f0c07ca78a | c5d39e1019552654068744b0453767652a423675 | |
refs/heads/master | <file_sep>require 'httparty'
class RecipeSearchWrapper
@app_id = ENV["APPLICATION_ID"]
@app_key = ENV["APPLICATION_KEY"]
@base_url = "https://api.edamam.com/search?"
class RecipeError < StandardError; end
def self.search_recipes(query)
url = @base_url + "app_id=#{@app_id}&app_key=#{@app_key}" + "&q=#{query}" + "&to=100"
encoded_uri = URI.encode(url)
response = HTTParty.get(encoded_uri).parsed_response
# raise_on_error(response)
recipes = []
if response["hits"]
response["hits"].each do |hit|
label = hit["recipe"]["label"]
uri = hit["recipe"]["uri"]
opts = {}
opts["image"] = hit["recipe"]["image"]
recipes << Recipe.new(uri, label, opts)
end
end
return recipes
end
def self.find_recipe(uri)
encoded_uri = URI.encode("https://api.edamam.com/search?app_id=#{@app_id}&app_key=#{@app_key}&r=" + uri)
response = HTTParty.get(encoded_uri).parsed_response
# raise_on_error(response)
unless response.empty?
uri = response[0]["uri"]
label = response[0]["label"]
opts = {}
opts["image"] = response[0]["image"]
opts["url"] = response[0]["url"]
opts["servings"] = response[0]["yield"]
opts["ingredients"] = response[0]["ingredientLines"]
opts["health_labels"] = response[0]["healthLabels"]
opts["calories"] = response[0]["calories"]
opts["fat"] = response[0]["totalNutrients"]["FAT"]["quantity"]
opts["saturated_fat"] = response[0]["totalNutrients"]["FASAT"]["quantity"]
opts["mono_fat"] = response[0]["totalNutrients"]["FAMS"]["quantity"]
opts["carbs"] = response[0]["totalNutrients"]["CHOCDF"]["quantity"]
opts["protein"] = response[0]["totalNutrients"]["PROCNT"]["quantity"]
opts["sodium"] = response[0]["totalNutrients"]["NA"]["quantity"]
opts["fiber"] = response[0]["totalNutrients"]["FIBTG"]["quantity"]
opts["cholesterol"] = response[0]["totalNutrients"]["CHOLE"]["quantity"]
@recipe = Recipe.new(uri, label, opts)
return @recipe
else
RecipeError.new("We couldn't find that recipe. Maybe you could write it?")
end
end
private
def self.raise_on_error(response)
unless response["ok"]
raise RecipeError.new(response["error"])
end
end
end
<file_sep>require 'test_helper'
describe "RecipeApiWrapper" do
describe "search_recipes" do
it "gives a list of recipes" do
VCR.use_cassette("recipes") do
query = "rabbit"
recipes = RecipeSearchWrapper.search_recipes(query)
recipes.each do |recipe|
recipe.must_be_kind_of Recipe
end
end
end
end
describe "find_recipe" do
it "returns a recipe" do
VCR.use_cassette("find") do
rabbits = RecipeSearchWrapper.search_recipes("rabbit")
recipe = rabbits.last
rabbit_recipe = RecipeSearchWrapper.find_recipe(recipe.uri)
rabbit_recipe.must_be_kind_of Recipe
end
end
end
end
<file_sep>class Recipe
attr_reader :uri, :label, :image, :url, :servings, :ingredients, :health_labels, :calories, :fat, :saturated_fat, :mono_fat, :carbs, :protein, :sodium, :fiber, :cholesterol
def initialize(uri, label, opts = {})
if label.nil? || label.empty?
raise ArgumentError.new("The recipe needs a label")
end
if uri.nil? || uri.empty?
raise ArgumentError.new("The recipe needs a URI")
end
@uri = uri
@label = label
@image = opts["image"]
@url = opts["url"]
@servings = opts["servings"]
@ingredients = opts["ingredients"]
@health_labels = opts["health_labels"]
@calories = opts["calories"]
@fat = opts["fat"]
@saturated_fat = opts["saturated_fat"]
@mono_fat = opts["mono_fat"]
@carbs = opts["carbs"]
@protein = opts["protein"]
@sodium = opts["sodium"]
@fiber = opts["fiber"]
@cholesterol = opts["cholesterol"]
end
def self.from_api(raw_recipe)
self.new(
raw_recipe["uri"],
raw_recipe["label"],
opts = {}
)
opts["image"] = raw_recipe["image"],
opts["url"] = raw_recipe["url"],
opts["yield"] = raw_recipe["yield"],
opts["ingredientLines"] = raw_recipe["ingredientLines"],
opts["healthLabels"] = raw_recipe["healthLabels"],
opts["calories"] = raw_recipe["calories"],
opts["fat"] = raw_recipe["totalNutrients"]["FAT"]["quantity"],
opts["saturated_fat"] = raw_recipe["totalNutrients"]["FASAT"]["quantity"],
opts["mono_fat"] = raw_recipe["totalNutrients"]["FAMS"]["quantity"],
opts["carbs"] = raw_recipe["totalNutrients"]["CHOCDF"]["quantity"],
opts["protein"] = raw_recipe["totalNutrients"]["PROCNT"]["quantity"],
opts["sodium"] = raw_recipe["totalNutrients"]["NA"]["quantity"],
opts["fiber"] = raw_recipe["totalNutrients"]["FIBTG"]["quantity"],
opts["cholesterol"] = raw_recipe["totalNutrients"]["CHOLE"]["quantity"]
end
end
<file_sep>require 'test_helper'
describe "recipe" do
describe "initialize" do
it "can be created with required values" do
uri = "test value"
label = "test recipe label"
opts = {}
opts["servings"] = 8
recipe = Recipe.new(uri, label, opts)
recipe.label.must_equal label
recipe.uri.must_equal uri
end
it "raises an error if not enough arguments are provided" do
label = "chicken dinner"
proc { Recipe.new(label)}.must_raise ArgumentError
end
it "accepts optional arguments" do
label = "Best Label"
uri = "Uridiculous"
opts = {
url: "http:://homestarrunner.com",
servings: "200"
}
recipe = Recipe.new(uri, label, opts)
recipe.url.must_equal opts["url"]
recipe.servings.must_equal opts["servings"]
end
end
end
<file_sep>require "test_helper"
describe "RecipesController" do
describe "root" do
it "shows the root page" do
get root_path
must_respond_with :success
end
end
describe "index" do
it "returns a list of search recipes results" do
query = "rabbit"
VCR.use_cassette("recipes") do
RecipeSearchWrapper.search_recipes(query)
get query_recipe_path(query: query)
must_respond_with :success
end
end
it "doesn't return results for a nonsense search term" do
query = "zzzzzzadsfasdfasdfasdf"
VCR.use_cassette("recipes") do
RecipeSearchWrapper.search_recipes(query)
get query_recipe_path(query: query)
must_redirect_to root_path
end
end
end
describe "show" do
it "displays the recipe page for the selected recipe" do
VCR.use_cassette("show") do
uri = "http://www.edamam.com/ontologies/edamam.owl#recipe_b79327d05b8e5b838ad6cfd9576b30b6"
label = "Chicken Vesuvio"
recipe = RecipeSearchWrapper.find_recipe(uri)
recipe.must_be_kind_of Recipe
get recipe_path(uri: uri, label: label)
must_respond_with :success
end
end
it "it returns a RecipeError if the recipe uri does not exist" do
VCR.use_cassette("show") do
uri = "http://www.edamam.com/ontologies/edamam.owl#recipe_b79327d05b8e5b838ad6cfd9576b30b69999999"
result = RecipeSearchWrapper.find_recipe(uri)
result.must_be_kind_of RecipeSearchWrapper::RecipeError
end
end
end
end
<file_sep>
class RecipesController < ApplicationController
around_action :catch_api_error
def root
end
def index
@query = params[:query]
@recipes = RecipeSearchWrapper.search_recipes(@query)
if @recipes.empty?
flash[:status] = :failure
flash[:result_text] = "There are no recipes for #{params[:query]}. Maybe you could write one?"
redirect_to root_path
else
@recipes = @recipes.paginate(:page => params[:page], :per_page => 10)
end
end
def show
@recipe = RecipeSearchWrapper.find_recipe(params[:uri])
unless @recipe
head :not_found
end
end
private
def catch_api_error
begin
# This will run the actual controller action
# Actually the same yield keyword as in
# application.html.erb
yield
rescue RecipeSearchWrapper::RecipeError => error
flash[:status] = :failure
flash[:result_text] = "API call failed: #{error}"
redirect_back fallback_location: root_path
end
end
end
| 3ac2f09b1613ea451bc6dcb878f5c5ed22662da0 | [
"Ruby"
] | 6 | Ruby | annehwatson/api-muncher | 42864aebf62f21a8a5c36c20376f762e6e5a14db | 95cd93de8f9edd3041d092e64bfc9b5d2d696740 | |
refs/heads/master | <repo_name>boikedamhuis/Arduino-soil-moisture-relay-controller<file_sep>/README.md
# Arduino Soil Moisture relay controller
Arduino example to control a relay based on moisture values. The basis for a smart garden irrigation system.
# Wiring relay
- Connect VCC to 3.3v
- Connect ground to ground
- Connect data pin to pin D4/GPIO2
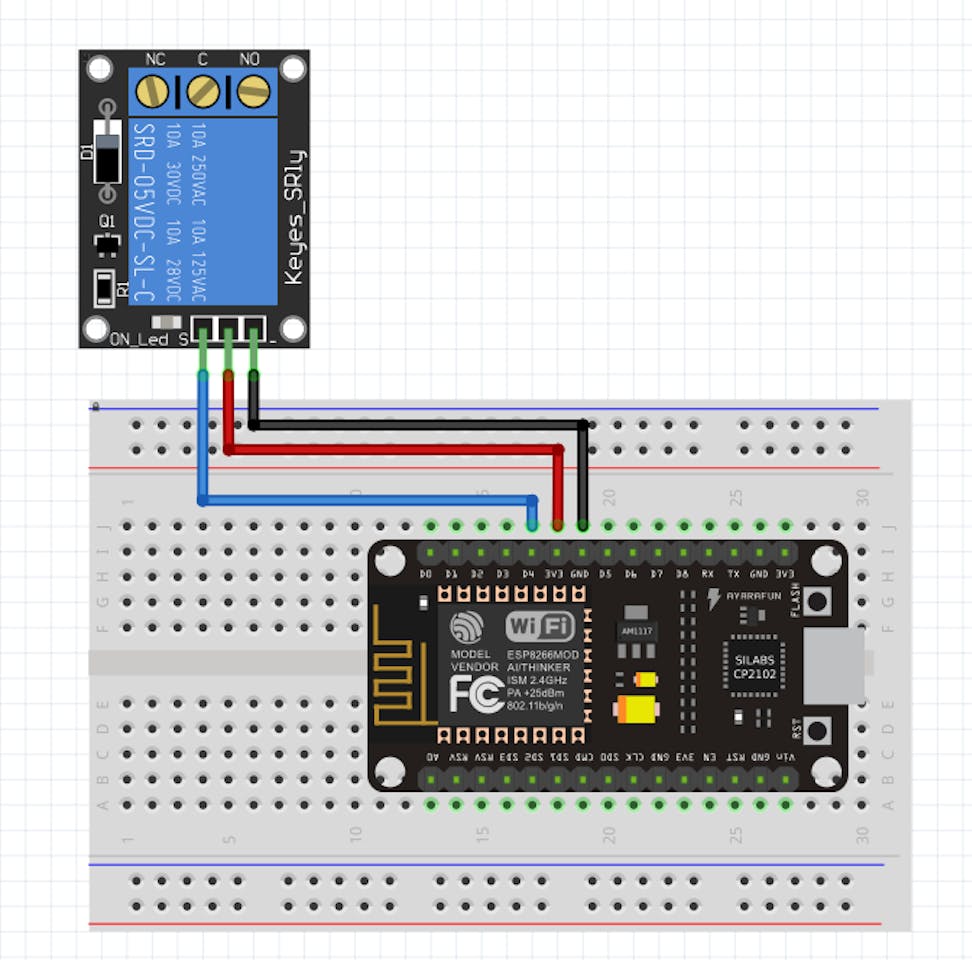
# Wiring soil moisture sensor
- Connect VCC to 3.3v
- Connect ground to ground
- Connect data pin to AO analog pin.

Wire your pump to the relay. The pump will start pumping water as soon as the soil moisture sensor detects too less water.
<file_sep>/Arduino-soil-moisture-relay-controller.ino
//-------------------------------------------------------------------
// Arduino example to control a relay based on moisture values
//-------------------------------------------------------------------
int moisture_pin = A0;
int relay_pin = 2;
int moisture_value;
//Chamge the minimum percentage here!
int minimum_percentage = 50;
void setup() {
//Start serial connection
Serial.begin(9600);
pinMode(relay_pin, OUTPUT);
Serial.println("Getting data from the sensor ...");
//Delay so the sensor can initialize
delay(2000);
}
void loop() {
moisture_value= analogRead(moisture_pin);
moisture_value = map(moisture_value,550,0,0,100);
Serial.print("Mositure value : ");
Serial.print(moisture_value);
Serial.println(" %");
if (moisture_value < minimum_percentage) {
digitalWrite(relay_pin, HIGH);
} else {
digitalWrite(relay_pin, LOW);
}
delay(1000);
}
| ff2f081153dce4186236708b37910ee149e0feab | [
"Markdown",
"C++"
] | 2 | Markdown | boikedamhuis/Arduino-soil-moisture-relay-controller | 76b506edd83f2aa63364b0a782203a18236f6017 | a9c61598bf5c425d63d9e18a0a8b4ea8a15885ac | |
refs/heads/master | <file_sep>import asyncio
import click
from utils.db import db as dbs
@click.group()
def db():
pass
@db.command()
def seed():
asyncio.run(dbs.seed())
cli = click.CommandCollection(sources=[db])
if __name__ == '__main__':
cli()
<file_sep>import asyncio
import itertools
import logging
from lib import telegram as tglib
from conf import config
from utils.functions import commands as bot_commands
from utils.serialization import serialize_response as serialize
from utils import command_parser
tg_app = tglib.TelegramBot(token=config.TELEGRAM_BOT_TOKEN)
logging.basicConfig(level=logging.INFO)
async def send_response(command: str, message: str, function, chat_id: int):
"""
Dynamic choose function for send response to user (result of functions)
"""
response = {
'chat_id': chat_id,
'text': 'Command not found'
}
if not function:
return await tg_app.send_message(response)
send_type = {
'photo': tg_app.send_photo,
'text': tg_app.send_message
}
# do some work
result = function(message)
result_type = result['result_type']
response[result['result_type']] = result['result']
if function.__name__.endswith('cmd'):
response['parse_mode'] = 'MarkdownV2'
# Send typed response
await send_type[result_type](params=response)
async def main():
offset = 0 # last update id
for i in itertools.count(1):
data: dict = await tg_app.get_updates(offset=offset)
# Serialize data
tg_resp = serialize(data.get('result', []))
ready = bool(data['ok'])
if tg_resp.results and ready:
result = tg_resp.results[0]
offset = result.update_id
offset += 1
result = result.dict()
last_chat_text = result['message']['text']
last_chat_id = result['message']['chat']['id']
last_chat_name = result['message']['chat']['username']
command, message = command_parser.parse_user_message(last_chat_text)
if not message:
continue
await send_response(command, message, bot_commands.get(command), last_chat_id)
logging.info('[%s]: [%s]', last_chat_name, last_chat_text)
await asyncio.sleep(1)
if __name__ == '__main__':
asyncio.run(main())
<file_sep>import typing
from typing import AnyStr, Tuple, Union, List
def parse_user_message(user_message: AnyStr = None) -> Union[Tuple[AnyStr, AnyStr], Tuple[AnyStr, None]]:
"""Parsing string with message and split
:user_message: string like '/echo text'
@return: tuple with command/message
"""
command, text = None, ' '
if not user_message:
return command, text
user_message: List[AnyStr, AnyStr] = user_message.split(' ')
try:
# Cut first symbol '/' from command
if len(user_message) == 1:
command = user_message[0][1:] if user_message[0].startswith('/') else user_message[0]
return command, text
command, text = user_message.pop(0)[1:], ' '.join(user_message)
except Exception as e:
# TODO: add logging
pass
return command, text
<file_sep>import aiohttp
import typing
class TelegramBot:
func_result: dict = {}
def __init__(self, token):
self.token: str = token
self.API_URL: str = f'https://api.telegram.org/bot{self.token}/'
async def do_request(self, query: str, params: dict = None) -> dict:
if not params:
params = {}
async with aiohttp.ClientSession() as session:
async with session.get(query, params=params) as resp:
return await resp.json()
async def run_function(self, func, *args, **kwargs):
# TODO: standartize in future functions
self.func_result = func(*args, **kwargs)
return self.func_result
async def get_updates(self, timeout: int = 30, offset: int = 0) -> dict:
"""Pull latest events from bot"""
params = {'timeout': timeout}
if offset:
params['offset'] = offset
query: str = self.API_URL + 'getUpdates'
return await self.do_request(query, params)
async def send_message(self, params: dict) -> dict:
"""Send answer for messages
chat_id (from): int
text: str
"""
query = self.API_URL + 'sendMessage'
return await self.do_request(query, params)
async def send_photo(self, params: dict) -> dict:
"""Send photo for messages
chat_id (from): int
text: str
"""
query = self.API_URL + 'sendPhoto'
return await self.do_request(query, params)
<file_sep>aiodns==2.0.0
aiohttp==3.6.2
aiosqlite==0.15.0
async-timeout==3.0.1
attrs==20.2.0
brotlipy==0.7.0
cchardet==2.1.6
certifi==2020.6.20
cffi==1.14.3
chardet==3.0.4
click==7.1.2
databases==0.3.2
idna==2.10
Mako==1.1.3
markovify==0.8.3
MarkupSafe==1.1.1
multidict==4.7.6
pycares==3.1.1
pycparser==2.20
requests==2.24.0
typing-extensions==3.7.4.3
ujson==4.0.1
Unidecode==1.1.1
urllib3==1.25.10
yarl==1.6.0
<file_sep>from enum import Enum
from dataclasses import dataclass, asdict, field
from typing import List, Dict
ResultTypes = Enum('ResultTypes', ((s, s) for s in ('text', 'photo')))
@dataclass
class Base:
def dict(self):
return asdict(self)
def __getattr__(self, name):
if name.endswith('_'):
return getattr(self, name[:-1])
@dataclass
class BaseResponse(Base):
result_type: str = ResultTypes.text.value
result: str = None
@dataclass
class TelegramMessageFrom(Base):
id: int = 0
is_bot: bool = False
first_name: str = None
last_name: str = None
username: str = None
language_code: str = None
@dataclass
class TelegramMessageChat(Base):
id: int = 0
first_name: str = None
last_name: str = None
username: str = None
type: str = None
@dataclass
class TelegramMessage(Base):
date: int = 0
text: str = None
message_id: int = 0
entities: list = None # [{"offset":0,"length":5,"type":"bot_command"}]
from_: TelegramMessageFrom = field(default_factory=TelegramMessageFrom)
chat: TelegramMessageChat = field(default_factory=TelegramMessageChat)
@dataclass
class TelegramEntity(Base):
update_id: int = None
message: TelegramMessage = field(default_factory=TelegramMessage)
edited_message: TelegramMessage = field(default_factory=TelegramMessage)
@dataclass
class TelegramResponse(Base):
results: List[TelegramEntity] = field(default_factory=TelegramEntity)
<file_sep># This file include function by name
import os
import subprocess
import requests
import itertools
from random import randint
from conf import config
from models.response import BaseResponse, ResultTypes
commands = {}
def command(command_name):
def wrap(f):
commands[command_name[1:]] = f
return f
return wrap
# @command('/cats')
# def command_random_cats(search_query: str = 'cats') -> dict:
# """Get random cats"""
# resp = BaseResponse()
#
# headers = {'Authorization': config.PEXELS_IMAGE_API_TOKEN}
# params = {'query': search_query or 'cats', 'page': randint(1,2852), 'per_page': 1}
#
# r = requests.get(url=config.PEXELS_IMAGE_API_URL, params=params, headers=headers)
# data: dict = r.json()
# if r.status_code == 200 and data:
# resp.result_type = ResultTypes.photo.value
# resp.result = data['photos'][-1]['src']['large']
#
# return resp.dict()
@command('/echo')
def command_echo(text: str) -> dict:
"""Test echo function"""
resp = BaseResponse()
resp.result = text
return resp.dict()
@command('/start')
def command_start(*args) -> dict:
resp = BaseResponse()
text = ''
for name, f in commands.items():
if name == 'start':
continue
text += f"""/{name}: {f.__doc__};
"""
resp.result = text
return resp.dict()
@command('/public_domain')
def command_domain(*args, **kwargs) -> dict:
""" Show public domain for server"""
resp = BaseResponse()
try:
r = requests.get('http://127.0.0.1:4040/api/tunnels')
resp.result = str(r.json()['tunnels'][0]['public_url'])
except Exception as e:
resp.result = str(e)
return resp.dict()
@command('/cmd')
def command_cmd(*args):
""" Server console"""
resp = BaseResponse()
params = list(args)[0].split(' ')
auth = params.pop(0)
if auth == config.AUTH:
try:
r = subprocess.check_output(params)
resp.result = f"""```{r.decode('utf-8')}```"""
except Exception as e:
resp.result = str(e)
return resp.dict()
resp.result = 'Wrong auth'
return resp.dict()
<file_sep>from models.response import TelegramResponse, TelegramEntity, TelegramMessage, TelegramMessageChat
def serialize_response(results: []) -> TelegramResponse:
"""
Serializing response from telegram API to Dataclass objects
"""
telegram_response = TelegramResponse(results=[TelegramEntity(**row) for row in results])
for i, row in enumerate(telegram_response.results):
if row.edited_message:
continue
if row and row.message and row.message.get('from'):
row.message['from_'] = row.message.pop('from')
message = TelegramMessage(**row.message)
message.chat = TelegramMessageChat(**message.chat)
telegram_response.results[i].message = message
return telegram_response
<file_sep>DSN = ''
TELEGRAM_BOT_TOKEN = ''
PEXELS_IMAGE_API_TOKEN = '' # pexels.com
PEXELS_IMAGE_API_URL = 'https://api.pexels.com/v1/search'<file_sep>import asyncio
from databases import Database
from conf import config
class DatabaseTG(Database):
async def seed(self):
await self.connect()
# SQL query for sqlite
queries = ["""
CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY,
tg_id INTEGER,
tg_username TEXT,
created_at TEXT,
about TEXT
);""",
"""
CREATE TABLE IF NOT EXISTS message_log (
id INTEGER PRIMARY KEY,
user_id INTEGER,
message_id INTEGER,
message TEXT,
FOREIGN KEY (user_id) REFERENCES users (id) ON DELETE CASCADE
);"""
]
for q in queries:
await self.execute(q)
db = DatabaseTG(config.DSN)
if __name__ == '__main__':
asyncio.run(db.seed())
| 4a70a4fed1d16930ffd7e8bed9841245d86d353f | [
"Python",
"Text"
] | 10 | Python | infnetdanpro/tg-helper-bot | 904ffc3220777484b1ec730066897d5593ec4fd8 | 40cb1e682ff084bea22d7518f230d94b5f773bf7 | |
refs/heads/master | <file_sep>import com.intellij.notification.Notification;
import com.intellij.notification.NotificationType;
import com.intellij.notification.Notifications;
import com.intellij.openapi.ide.CopyPasteManager;
import java.awt.datatransfer.StringSelection;
public class Copy extends FileAction {
@Override
void handleFileUri(String uri) {
// Copy file uri to clipboard
CopyPasteManager.getInstance().setContents(new StringSelection(uri));
// Display bubble
Notification notification = new Notification("Sourcegraph", "Sourcegraph",
"File URL copied to clipboard.", NotificationType.INFORMATION);
Notifications.Bus.notify(notification);
}
}<file_sep># Sourcegraph for JetBrains IDEs [](https://plugins.jetbrains.com/plugin/9682-sourcegraph)
- Search snippets of code on Sourcegraph.
- Copy and share a link to code on Sourcegraph.
- Quickly go from files in your editor to Sourcegraph.
The plugin works with all JetBrains IDEs including:
- IntelliJ IDEA
- IntelliJ IDEA Community Edition
- PhpStorm
- WebStorm
- PyCharm
- PyCharm Community Edition
- RubyMine
- AppCode
- CLion
- GoLand
- DataGrip
- Rider
- Android Studio
## Installation
- Select `IntelliJ IDEA` then `Preferences` (or use <kbd>⌘,</kbd>)
- Click `Plugins` in the left-hand pane.
- Choose `Browse repositories...`
- Search for `Sourcegraph` -> `Install`
- Restart your IDE if needed, then select some code and choose `Sourcegraph` in the right-click context menu to see actions and keyboard shortcuts.
## Configuring for use with a private Sourcegraph instance
The plugin is configurable _globally_ by creating a `sourcegraph-jetbrains.properties` in your home directory. For example, modify the following URL to match your on-premises Sourcegraph instance URL:
```
url = https://sourcegraph.example.com
defaultBranch = example-branch
remoteUrlReplacements = git.example.com, git-web.example.com
```
You may also choose to configure it _per repository_ using a `.idea/sourcegraph.xml` file in your repository like so:
```xml
<?xml version="1.0" encoding="UTF-8"?>
<project version="4">
<component name="Config">
<option name="url" value="https://sourcegraph.example.com" />
<option name="defaultBranch" value="example-branch" />
<option name="remoteUrlReplacements" value="git.example.com, git-web.example.com" />
</component>
</project>
```
By default, the plugin will use the `origin` git remote to determine which repository on Sourcegraph corresponds to your local repository. If your `origin` remote doesn't match Sourcegraph, you may instead configure a `sourcegraph` Git remote which will take priority.
## Questions & Feedback
Please file an issue: https://github.com/sourcegraph/sourcegraph-jetbrains/issues/new
## Uninstallation
- Select `IntelliJ IDEA` then `Preferences` (or use <kbd>⌘,</kbd>)
- Click `Plugins` in the left-hand pane.
- Search for `Sourcegraph` -> Right click -> `Uninstall` (or uncheck to disable)
## Development
- Start IntelliJ and choose `Check out from Version Control` -> `Git` -> `https://github.com/sourcegraph/sourcegraph-jetbrains`
- Develop as you would normally (hit Debug icon in top right of IntelliJ) or using gradlew commands:
1. `./gradlew runIde` to run an IDE instance with sourcegraph plugin installed
2. `./gradlew buildPlugin` to build plugin artifact (`build/distributions/Sourcegraph.zip`)
- To create `sourcegraph.jar`:
1. Update `plugin.xml` (change version AND describe changes in change notes).
2. Update `Util.java` (change `VERSION` constant).
3. Update `README.md` (copy changelog from plugin.xml).
4. choose `Build` -> `Prepare Plugin Module 'sourcegraph' For Deployment`
5. `git commit -m "all: release v<THE VERSION>"` and `git push` and `git tag v<THE VERSION>` and `git push --tags`
6. Upload the jar to the releases tab of this repository.
7. Publish according to http://www.jetbrains.org/intellij/sdk/docs/basics/getting_started/publishing_plugin.html (note: it takes ~2 business days for JetBrains support team to review the plugin).
## Version History
#### v1.2.1
- Added `Open In Sourcegraph` action to VCS History and Git Log to open a revision in the Sourcegraph diff view.
- Added `defaultBranch` configuration option that allows opening files in a specific branch on Sourcegraph.
- Added `remoteUrlReplacements` configuration option that allow users to replace specified values in the remote url with new strings.
#### v1.2.0
- The search menu entry is now no longer present when no text has been selected.
- When on a branch that does not exist remotely, `master` will now be used instead.
- Menu entries (Open file, etc.) are now under a Sourcegraph sub-menu.
- Added a "Copy link to file" action (alt+c / opt+c).
- Added a "Search in repository" action (alt+r / opt+r).
- It is now possible to configure the plugin per-repository using a `.idea/sourcegraph.xml` file. See the README for details.
- Special thanks: @oliviernotteghem for contributing the new features in this release!
#### v1.1.2
- Fixed an error that occurred when trying to search with no selection.
- The git remote used for repository detection is now `sourcegraph` and then `origin`, instead of the previously poor choice of just the first git remote.
#### v1.1.1
- Fixed search shortcut; Updated the search URL to reflect a recent Sourcegraph.com change.
#### v1.1.0
- Added support for using the plugin with on-premises Sourcegraph instances.
#### v1.0.0
- Initial Release; basic Open File & Search functionality.
<file_sep>import com.intellij.openapi.actionSystem.AnAction;
import com.intellij.openapi.actionSystem.AnActionEvent;
import com.intellij.openapi.editor.Document;
import com.intellij.openapi.editor.Editor;
import com.intellij.openapi.editor.SelectionModel;
import com.intellij.openapi.fileEditor.FileDocumentManager;
import com.intellij.openapi.fileEditor.FileEditorManager;
import com.intellij.openapi.project.Project;
import com.intellij.openapi.vfs.VirtualFile;
import com.intellij.openapi.diagnostic.Logger;
import com.intellij.openapi.application.ApplicationInfo;
import javax.annotation.Nullable;
import java.io.*;
import java.awt.Desktop;
import java.net.URI;
import java.net.URLEncoder;
public class SearchRepository extends SearchActionBase {
@Override
public void actionPerformed(AnActionEvent e) {
super.actionPerformedMode(e, "search.repository");
}
}<file_sep>plugins {
id 'java'
id 'org.jetbrains.intellij' version '0.7.2'
}
group 'com.sourcegraph.jetbrains'
sourceCompatibility = 1.8
repositories {
mavenCentral()
}
intellij {
version 'IC-2020.1'
pluginName 'Sourcegraph'
updateSinceUntilBuild false
sandboxDirectory 'idea-sandbox'
}
dependencies {
testImplementation(platform('org.junit:junit-bom:5.7.2'))
testImplementation('org.junit.jupiter:junit-jupiter')
}
test {
useJUnitPlatform()
}
runIde {
systemProperty("idea.is.internal", true)
}
| d995d71730a98ce848b729ac19eb414c03ff2b2f | [
"Markdown",
"Java",
"Gradle"
] | 4 | Java | abeatrix/sourcegraph-jetbrains | 939e2d12f69a439afee9748fef5b3e790a3bc467 | 6f72b22add836beeede2aa6ebb8f9ffeb6817d48 | |
refs/heads/develop | <file_sep>import './script/navbar';
import './script/orderClick';
import './style/main.scss';<file_sep>const button = document.querySelector("button[data-behavior='contact-button']")
const modal = document.querySelector("div[data-behavior='contact-modal']")
const close = document.querySelector("span[data-behavior='contact-close']")
const formOpen = () => {
modal.style.display = "block";
}
const formClose = () => {
modal.style.display = "none";
}
const formCloseWindow = (e) => {
if (e.target == modal) {
modal.style.display = "none";
}
}
window.addEventListener('click', formCloseWindow)
button.addEventListener('click', formOpen)
close.addEventListener('click', formClose)<file_sep># bluesky_interactive
tech test vehicle promotion page
| ffbedd70eccd41f949a5d330329272bb9b3ee798 | [
"JavaScript",
"Markdown"
] | 3 | JavaScript | davidliamwalsh/bluesky_interactive | 3d24e963322d7ce3515fc4c00f61b46b866283b3 | 4c6819626917c33f22fa631214554135e116b835 | |
refs/heads/master | <file_sep>
from flask import Flask
from flask_peewee.db import Database
import config
import grooveshark
app = Flask(__name__)
app.config.from_envvar("PIDJ_SETTINGS")
db = Database(app)
gs = grooveshark.Client(app)
<file_sep>
# PiDJ
A crowd-sourced DJ platform that allows anyone in the room to search for
and queue up songs.
Users visit a flask-powered website and can search (using the Grooveshark
api in the backend) and add a song of their choosing to the queue. Songs are played
in FIFO order using mpd. PiDJ is designed to be run on a raspberry pi that is connected
to speakers, although all software used is cross platform and can be run on x86 linux or osx.
## Technology
* Flask website
* gevent for IO and player control
* mpd for music control and streaming
* Facebook for user auth and identification
* Grooveshark api for song search and streaming
* peewee/sqlite for relational database
* redis for key-value store and song caching
<file_sep>Flask==0.9
Jinja2==2.6
WTForms==1.0.2
Werkzeug==0.8.3
distribute==0.6.27
facebook-sdk==0.4.0
flask-peewee==0.6.1
gevent==0.13.8
greenlet==0.4.0
peewee==2.0.4
pytz==2012h
requests==0.14.2
simplejson==2.6.2
wsgiref==0.1.2
wtf-peewee==0.2.0
<file_sep>
from app import app, db
from peewee import *
from pytz import timezone
import datetime
__all__ = ["Song", "Vote", "create_tables", "STATUS_PLAYED", "STATUS_PLAYING", "STATUS_QUEUED"]
STATUS_PLAYED, STATUS_PLAYING, STATUS_QUEUED = range(3)
mytz = timezone(app.config["TIMEZONE"])
class Song(db.Model):
user_id = CharField(max_length = 15)
user_name = CharField(max_length = 100)
song_name = CharField()
song_id = IntegerField()
album_name = CharField()
album_id = IntegerField()
artist_name = CharField()
added = DateTimeField(default = datetime.datetime.now)
status = IntegerField(default = STATUS_QUEUED)
finish = DateTimeField(default = datetime.datetime.now)
duration = IntegerField(default = 0)
def __unicode__(self):
return self.song_name
@staticmethod
def getPlaying():
try:
return Song.get(status = STATUS_PLAYING)
except Song.DoesNotExist:
return None
@staticmethod
def getNext():
try:
return Song.select().where(Song.status == STATUS_QUEUED).order_by(Song.added).get()
except Song.DoesNotExist:
return None
def serialize(self):
return {
"id": self.id,
"user_id": self.user_id,
"user_name": self.user_name,
"song_name": self.song_name,
"song_id": str(self.song_id),
"album_name": self.album_name,
"album_id": str(self.album_id),
"artist_name": self.artist_name,
"added": mytz.localize(self.added).isoformat(),
"status": self.status,
"finish": mytz.localize(self.finish).isoformat(),
"duration": self.duration
}
class Vote(db.Model):
user_id = CharField(max_length = 15, index = True)
song = ForeignKeyField(Song)
def create_tables():
Song.create_table()
Vote.create_table()
<file_sep>
__all__ = ["run", "models"]
from gevent import monkey
monkey.patch_all()
import gevent
from app import app
from views import *
import models
from gevent.pywsgi import WSGIServer
from werkzeug.wsgi import SharedDataMiddleware
from werkzeug.debug import DebuggedApplication
import os
def run_debug(port):
global app
app = DebuggedApplication(app, evalex = True)
WSGIServer(('', port), app).serve_forever()
def run_production(port):
global app
app = SharedDataMiddleware(app, {
'/': os.path.join(os.path.dirname(__file__), 'static')
})
WSGIServer(('', port), app).serve_forever()
def run(port):
import player
g1 = player.start()
if app.config["DEBUG"]:
g2 = gevent.spawn(run_debug, port)
else:
g2 = gevent.spawn(run_production, port)
gevent.joinall([g1, g2])
<file_sep>#!/usr/bin/env python
import sys; sys.dont_write_bytecode = True
import os
os.environ["PIDJ_SETTINGS"] = os.path.join(os.path.dirname(os.path.abspath(__file__)), "config.py")
import pidj
import sys
port = 5000
if len(sys.argv) >= 2:
port = int(sys.argv[1])
pidj.run(port)
<file_sep>
import functools
from flask import jsonify
from app import app
def api_route(action, **options):
def decorator(fn):
@functools.wraps(fn)
def wrapper(*args, **kwargs):
ret = fn(*args, **kwargs)
obj = {"status": "success"}
if ret:
obj.update(ret)
return jsonify(obj)
return app.route("/ajax/" + action, methods=["POST"])(wrapper)
return decorator
<file_sep>
from models import *
import gevent
from app import gs
import datetime
from gevent import socket
def log(s):
return
print s
class MPDClient(object):
def __init__(self):
self.socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
self.host = None
self.port = None
def close(self):
try:
self.socket.close()
except:
pass
def _reconn(self):
self.socket.connect((self.host, self.port))
self.read_until("\n")
def _tryfunc(self, f, *args, **kwargs):
try:
return f(*args, **kwargs)
except IOError:
self.close()
self.socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
self._reconn()
return f(*args, **kwargs)
def connect(self, host = "localhost", port = 6600):
self.host = host
self.port = int(port)
self._reconn()
def send(self, msg):
return self._tryfunc(self.socket.send, msg)
def recv(self, amt):
return self._tryfunc(self.socket.recv, amt)
def read_until(self, delim):
data = ""
while 1:
data += self.recv(1024)
if data.endswith(delim):
return data[:-len(delim)]
def status(self):
self.send("status\n")
data = self.read_until("OK\n")
d = {}
for line in data.split("\n"):
i = line.split(": ")
if len(i) < 2:
continue
d[i[0]] = i[1]
return d
def clear(self):
self.send("clear\n")
self.read_until("OK\n")
def consume(self, v):
self.send("consume " + str(v) + "\n")
self.read_until("OK\n")
def play(self):
self.send("play\n")
self.read_until("OK\n")
def add(self, song):
self.send("add " + song + "\n")
self.read_until("OK\n")
class Player(object):
def __init__(self):
self.client = None
def reconnect(self):
if self.client:
self.client.close()
self.client = MPDClient()
self.client.connect()
self.client.clear()
self.client.consume(1)
def addSongInner(self, r):
self.client.add(r["result"]["url"])
self.client.play()
def addSong(self, song):
if not gs.has_init:
gs.init()
r = gs.api("getSubscriberStreamKey", {"songID": song.song_id})
try:
self.addSongInner(r)
except ConnectionError:
self.reconnect()
self.addSongInner(r)
def start(self):
self.reconnect()
curSong = None
curUpdated = False
sp = Song.getPlaying()
if sp is not None:
sp.delete()
while 1:
if curSong == None:
log("curSong == none")
song = Song.getNext()
if song is not None:
log("found song")
self.addSong(song)
curUpdated = False
curSong = song
gevent.sleep(1.0)
else:
log("no next song found")
gevent.sleep(3.0)
else:
log("curSong != None")
if not curUpdated:
log("curUpdated = false")
s = self.client.status()
if "time" in s and s["playlistlength"] == "1" and s["time"] != "0:0":
duration = int(s["time"].split(":")[1])
print s
curSong.duration = duration
curSong.status = STATUS_PLAYING
curSong.finish = datetime.datetime.now() \
+ datetime.timedelta(seconds=duration) \
- datetime.timedelta(seconds=float(s["elapsed"]))
curSong.save()
curUpdated = True
sleep = (curSong.finish - datetime.datetime.now()).total_seconds() - 2
log("Sleeping: " + str(sleep))
gevent.sleep(sleep)
curSong.status = STATUS_PLAYED
curSong.save()
song = Song.getNext()
if song is not None:
log("Next song")
self.addSong(song)
curUpdated = False
curSong = song
else:
curSong = None
gevent.sleep(4.0)
else:
gevent.sleep(2.0)
else:
log("curUpdated = true")
gevent.sleep(5.0)
def start():
p = Player()
return gevent.spawn(p.start)
if __name__ == "__main__":
m = MPDClient()
m.connect("localhost", "6600")
print m.status()
<file_sep>
from app import app, gs
from models import *
from flask import render_template, request, session
from util import api_route
import requests
import facebook
def get_graph_api(reset = False):
if not reset and "fb_access_token" in session:
return facebook.GraphAPI(session["fb_access_token"])
user = facebook.get_user_from_cookie(
request.cookies,
app.config["FACEBOOK_APP_ID"],
app.config["FACEBOOK_APP_SECRET"]
)
if not user:
return None
session["fb_access_token"] = user["access_token"]
return facebook.GraphAPI(session["fb_access_token"])
@app.route('/')
def index():
return render_template("index.html", app_id = app.config["FACEBOOK_APP_ID"])
@api_route("search")
def ajax_search():
res = requests.get("http://tinysong.com/s/%s?format=json&limit=20&key=%s" %
(request.form["query"], app.config["TINYSONG_KEY"]))
return {"songs": res.json}
@api_route("queue")
def ajax_queue():
q = Song.select().where(Song.status == STATUS_QUEUED).order_by(Song.added)
obj = {"songs": [s.serialize() for s in q]}
try:
obj["playing"] = Song.select().where(Song.status == STATUS_PLAYING).order_by(Song.added.desc()).get().serialize()
except Song.DoesNotExist:
pass
return obj
@api_route("add")
def ajax_add():
songid = request.form["songid"]
graph = get_graph_api()
if not graph:
return {"status": "error", "error": "no facebook user"}
try:
profile = graph.get_object("me")
except facebook.GraphAPIError:
graph = get_graph_api(True)
profile = graph.get_object("me")
sinfo = gs.api("getSongsInfo", {"songIDs": [songid]})
songs = sinfo["result"]["songs"]
if len(songs) == 0:
return {"status": "error", "error": "song not found in database"}
song = songs[0]
song = Song(
user_id = profile["id"],
user_name = profile["name"],
song_name = song["SongName"],
song_id = song["SongID"],
album_name = song["AlbumName"],
album_id = song["AlbumID"],
artist_name = song["ArtistName"],
)
song.save()
return song.serialize()
<file_sep>#!/bin/sh
rm -f pidj.sqlite3
python -c "import pidj; pidj.models.create_tables()"
<file_sep>
import hashlib
import hmac
import simplejson
import requests
COUNTRY = {'ID': 221, 'CC1': 0, 'CC2': 0, 'CC3': 0, 'CC4': 0, 'DMA': 0, 'IPR': 0}
class Client(object):
def __init__(self, app):
self.key = app.config["GROOVESHARK_KEY"]
self.secret = app.config["GROOVESHARK_SECRET"]
self.user = app.config["GROOVESHARK_USER"]
self.passw = app.config["GROOVESHARK_PASS"]
self.session = None
self.has_init = False
app.before_first_request(lambda: self.init())
def signature(self, data):
return hmac.new(self.secret, data).hexdigest()
def api(self, method, params = {}):
params["country"] = COUNTRY
data = {
"method": method,
"parameters": params,
"header": {"wsKey": self.key}
}
if self.session is not None:
data["header"]["sessionID"] = self.session
data = simplejson.dumps(data)
sig = self.signature(data)
r = requests.post("https://api.grooveshark.com/ws3.php?sig=%s" % sig, data = data)
print r.json
return r.json
def init(self):
if self.has_init:
return
response = self.api("startSession")
if response["result"]["success"] == True:
self.session = response["result"]["sessionID"]
else:
raise Exception("Could not start session")
response = self.api("authenticate", {
"login": self.user,
"password": <PASSWORD>
})
self.has_init = True
<file_sep>
var userID;
var token;
var compiledTemplates = {};
var songData;
function getTemplate(tid) {
if (compiledTemplates[tid])
return compiledTemplates[tid];
var source = $("#" + tid).html();
var template = Handlebars.compile(source);
compiledTemplates[tid] = template;
return template;
}
function putTpl(tid, targ, params)
{
$(targ).html(getTemplate(tid)(params));
}
function loggedIn(auth) {
userID = auth.userID;
token = auth.accessToken;
putTpl("tpl-main", "#content");
setInterval(reloadQueue, 5000);
}
var stInterval = null;
var curSong = null;
function updateTime() {
var seconds = Number(curSong.duration) - Math.round((curSong.finish - new Date()) / 1000);
if (seconds >= curSong.duration) {
clearInterval(stInterval);
$("#playing").html("");
return;
}
var minutes = Math.floor(seconds / 60);
seconds = seconds % 60;
if (seconds < 10)
seconds = "0" + seconds;
$("#song-minutes").text(minutes);
$("#song-seconds").text(seconds);
}
var oldQueue = "";
function reloadQueue() {
$.post("/ajax/queue", {}, function(response) {
var str = JSON.stringify(response);
if (str == oldQueue)
return;
oldQueue = str;
putTpl("tpl-queue", "#queue", response);
if (response.playing) {
var song = response.playing;
song.added = new Date(song.added);
song.finish = new Date(song.finish);
if (Number(new Date()) > Number(song.finish))
return;
var mins = Math.floor(Number(song.duration) / 60);
var secs = song.duration % 60;
if (secs < 10)
secs = "0" + secs;
song.durationStr = mins + ":" + secs;
console.log(song);
curSong = song;
stInterval = setInterval(updateTime, 100);
putTpl("tpl-playing", "#playing", song);
}
});
}
$(function() {
FB.init({
appId: FB_APP_ID,
status: true,
cookie: true,
xfbml: true
});
FB.getLoginStatus(function(response) {
if (response.authResponse)
loggedIn(response.authResponse);
else
putTpl("tpl-login", "#content");
});
$(document).on("click", "#login-btn", function() {
FB.login(function (response) {
if (response.authResponse)
loggedIn(response.authResponse);
});
return false;
});
$(document).on("submit", "#search-form", function() {
var inpDiv = $("#search-form .search-input");
var val = inpDiv.val();
inpDiv.val("");
putTpl("tpl-modal", "#search-modal", {query: val});
$("#search-modal").modal("show");
$.post("/ajax/search", {query: val}, function(response) {
songData = response.songs;
for (var i = 0; i < songData.length; i++)
songData[i]["id"] = i;
putTpl("tpl-searchlist", "#search-modal .search-list", {songs: songData});
});
return false;
});
$(document).on("click", ".song-add-button", function() {
var idx = $(this).attr("data-id");
var sid = songData[idx].SongID;
$("#search-modal").modal("hide");
$.post("/ajax/add", {songid: sid}, function(response) {
reloadQueue();
});
return false;
});
$(document).on("click", "a[rel=external]", function() {
window.open(this.href, "pidjGsWindow", "width=800, height=600");
return false;
});
});
<file_sep>
# move this file to config.py
DEBUG = True
SECRET_KEY = "_none_"
FACEBOOK_APP_ID = ""
FACEBOOK_APP_SECRET = ""
GROOVESHARK_KEY = ""
GROOVESHARK_SECRET = ""
GROOVESHARK_USER = ""
GROOVESHARK_PASS = ""
TINYSONG_KEY = ""
TIMEZONE = "US/Central"
DATABASE = {
'name': 'pidj.sqlite3',
'engine': 'peewee.SqliteDatabase',
'check_same_thread': False
}
| 19972bfec26d98649a226e2a048b6265039bde11 | [
"Markdown",
"JavaScript",
"Python",
"Text",
"Shell"
] | 13 | Python | jamorton/pidj | 69be8d1d982df0db02cd0286e4d5df5a57ea5900 | 481143323ffea86a31d9ff537924befeb50ebbee | |
refs/heads/master | <file_sep>"""A script to detect and track faces in a video feed.
Author: <NAME>
"""
import cv2
faceCascade = cv2.CascadeClassifier('./haarcascade_frontalface_default.xml')
video = cv2.VideoCapture('./demo.mp4')
while True:
ret, frame = video.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
faces = faceCascade.detectMultiScale(
gray,
scaleFactor=1.1,
minNeighbors=5,
minSize=(30, 30)
)
for (x, y, w, h) in faces:
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.imshow('Trump', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
video.release()
cv2.destroyAllWindows()
<file_sep># Face-Tracker
A script to detect and track faces in a video feed.
<img src='anim.gif' />
# Dependencies
Python 2.7+
OpenCV 2.4+
# Running
python face_tracker.py
# Author
<NAME>
| e90b6c7973c0d6eaeabcf40bf803f8426b01eb70 | [
"Markdown",
"Python"
] | 2 | Python | NahomAgidew/Face-Tracker | 09699833b5463d6fa0c8e6c89822bab17ae94748 | b9d7b0850b792994a9c3609b7665fe5183c78f4a | |
refs/heads/master | <file_sep>#include <iostream>
uint8_t s[9],k,i,f,a[]=" \xff\xf9\xf1\xfe'r\xe9#\x9b%\x14\x1fI\xfd'\xff\xf1\x86\x17";
int main()
{
for(std::cin>>s;i<4;i++,puts(""))
for(int j:s)
for(k=0;j&&k<5;k++)
f=a[i*5+(j-48)/2]>>(j&1)*4,std::cout<<a[f<<k&8];
}
<file_sep># discord-challenge-6
One Lone Coder Discord Programming Challenge \#6
Write a program that takes a numeric input via stdin, and outputs it "enlarged" on stdout.
Example:
Input: 12345
<pre>
Output: # ##### ##### # # #####
# # # # # #
# ##### ##### ##### #####
# # # # #
# ##### ##### # #####
</pre>
Rules:
1) Must compile and run in ideone (www.ideone.com)
2) Input will only be 0..9, up to 8 digits
3) Output characters must be at least 3 lines high, and made up of '\#' symbols
4) Full code-golf rules apply, fight for your bytes!
5) No libraries allowed other than for input and output of character data
6) No \#defines
| d4482fae871ce4ef75a4b7ccccf29c3fb6218387 | [
"Markdown",
"C++"
] | 2 | C++ | drwonky/discord-challenge-6 | 024461ec64c0795a9ab7a7342f41f6fa5b7184f0 | d4cf88f55859e963e317dc62e90b58fe953455cb | |
refs/heads/master | <file_sep>import os
from allure_commons._allure import attach
from allure_commons.types import AttachmentType
from selenium import webdriver
from pages.drag_drop import drag_drop
def before_scenario(context,scenario):
dir = os.getcwd()
option=webdriver.ChromeOptions()
#option.add_argument("--start-maximized")
option.add_argument("headless")
config_file = "\config_files\chromedriver.exe"
context.browser = webdriver.Chrome(executable_path=dir + config_file, chrome_options=option)
#context.browser = webdriver.Chrome(executable_path=dir + config_file)
context.dd=drag_drop(context.browser)
def after_scenario(context,scenario):
context.browser.quit()
def after_step(context,step):
if step.status == "failed":
context.browser.save_screenshot('c://b/screenshot.png')
attach(
context.browser.get_screenshot_as_png(),
name="Screenshot",
attachment_type=AttachmentType.PNG)
<file_sep>from behave import when,then
from numpy.testing import assert_equal
expected_result="100"
@when(u'He drags the slide to the end')
def step_impl(context):
context.dd.slide()
@then(u'he sees the 100 value')
def step_impl(context):
v = context.dd.msg_slide()
assert_equal(v, expected_result)<file_sep>behave==1.2.6
numpy==1.19.5
selenium==3.141.0
<file_sep>from selenium.webdriver import ActionChains
from Browser import Browser
source_id = "draggable"
x_box_offset = 141
y_box_offset = 41
msg_id = "dropText"
slide = '/html[1]/body[1]/form[1]/fieldset[1]/div[1]/div[5]/input[1]'
x_slide_offset = 280
y_slide_offset = 16
value_id = "range"
class drag_drop(Browser):
def setup(self, link):
self.driver.get(link)
def drag(self):
source = self.driver.find_element_by_id(source_id)
action = ActionChains(self.driver)
action.drag_and_drop_by_offset(source, x_box_offset, y_box_offset).perform()
def slide(self):
slider = self.driver.find_element_by_xpath(slide)
action = ActionChains(self.driver)
action.drag_and_drop_by_offset(slider, x_slide_offset, y_slide_offset).perform()
def msg_box(self):
m = self.driver.find_element_by_id(msg_id).text
return m
def msg_slide(self):
v = self.driver.find_element_by_id(value_id).text
return v<file_sep>from behave import given,when,then
from numpy.testing import assert_equal
url="https://qavbox.github.io/demo/dragndrop/"
expected_result="Dropped!"
@given(u'The user is on the dragadrop home page')
def step_impl(context):
context.dd.setup(url)
@when(u'He drags the box to the target under "drop here" message')
def step_impl(context):
context.dd.drag()
@then(u'The user should see the message "dropped !"')
def step_impl(context):
msg=context.dd.msg_box()
assert_equal(msg,expected_result) | 797655a38b7941e64e83a74461d962ba71dbb3c6 | [
"Python",
"Text"
] | 5 | Python | mkrifa2021/Behave_jenkins | c3b4dee7075ed17439113f1bc786821f9ffe5d72 | 2a845e8dd310e8d3061b03f685016d8c783d2b09 | |
refs/heads/master | <repo_name>vict2586/Shoe_configurator<file_sep>/README.md
https://vict2586.github.io/Shoe_configurator/shoe1.html
<file_sep>/script.js
"use strict";
document.addEventListener("DOMContentLoaded", start);
let paint;
let link1 = "shoe_1.svg";
let link2 = "shoe_2.svg";
function start() {
getSvgInfo1();
getSvgInfo2();
};
async function getSvgInfo1() {
let response1 = await fetch(link1);
let object1 = await response1.text();
document.querySelector("#shoe1").innerHTML = object1;
interActivity();
};
async function getSvgInfo2() {
let response2 = await fetch(link2);
let object2 = await response2.text();
document.querySelector("#shoe2").innerHTML = object2;
interActivity();
};
function interActivity() {
document.querySelectorAll(".interact").forEach(eachG => {
eachG.addEventListener("click", theClick);
eachG.addEventListener("mouseover", theMouseover);
eachG.addEventListener("mouseout", theMouseout);
});
document.querySelectorAll(".color_btn").forEach(each_BTN => {
each_BTN.addEventListener("click", colorClick);
});
};
function theClick() {
paint = this;
this.style.fill = "grey";
};
function theMouseover() {
this.style.stroke = "blue";
};
function theMouseout() {
this.style.stroke = "none";
};
function colorClick() {
if (paint != undefined) {
paint.style.fill = this.getAttribute("fill");
}
};
| af9d94ec549e00666d18c1f82345a47e906cd6c8 | [
"Markdown",
"JavaScript"
] | 2 | Markdown | vict2586/Shoe_configurator | 2af702376abf4d0c4e6864acb265ff72becb6970 | cade4569d7f329e296a2b09935288b11aeea730f | |
refs/heads/main | <repo_name>acc4mei/acc4j<file_sep>/README.md
# acc4j
## java-gist
- Java
- JDK8
- **函数式接口**,Lambda表达式,方法引用
- Stream API
- LocalDateTime API
- Optional
- 注解,可重复注解,类型注解
- Jasypt
无侵入式数据加密,通常用于Spring配置文件的关键信息加密使用。
- Fel
轻量级表达式计算引擎,兼容Java语法。
- EasyExcel
基于Java的简单、节约内存的Excel读写工具。<file_sep>/java-gist/src/main/java/com/acc4j/jdk8/methodInterface/LambdaExpressionSample.java
package com.acc4j.jdk8.methodInterface;
import java.util.concurrent.Callable;
import java.util.function.Consumer;
import java.util.function.Function;
/**
* Lambda表达式:以匿名函数的形式“实现”函数式接口
* Lambda简化了匿名内部类形式,但实现原理并不同;匿名内部类在编译过程种会产生类;Lambda则是调用JVM的 invokedynamic 指令实现,不会产生类
*/
public class LambdaExpressionSample {
/**
* Lambda结构:(参数列表) -> {方法体}
* 语法:
* 1. 参数类型可省略,通常可以从上下文信息推断,只有一个参数时可省略小括号;当显式声明类型时,小括号不能省略
* 2. 方法体只有一行时可省略花括号;一行为返回值时可省略return;没有内容或者多行内容时花括号不能省略
* 3. 支持函数式接口调用对应方法实现
*/
public void sample() throws Exception {
System.out.println("--------------- LambdaExpression sample --------------");
// 不能省略{}
Runnable l0 = () -> {
};
Runnable l1 = () -> System.out.println("无参数,无返回值");
// 可以省略return
Callable<String> l2 = () -> "无参数,有返回值";
// 显式声明了类型,只有一个参数也不能省略小括号
Consumer<String> l3 = (String a) -> System.out.println("有参数,无返回值");
// 参数不声明类型,通过上下文信息推断,只有一个参数时可以省略小括号
Function<String, String> l4 = a -> "有参数,有返回值";
// 自定义函数式接口,多个参数,无返回值;用lambda来达成实现并调用
IFunctionSample<String, String> l5 = (String a, String b) -> System.out.println("自定义函数式接口,多个参数,无返回值");
l0.run();
l1.run();
System.out.println(l2.call());
l3.accept("l3");
System.out.println(l4.apply("l4"));
l5.sample("l", "5");
l5.desc();
System.out.println();
}
}
<file_sep>/java-gist/src/main/java/com/acc4j/jdk8/annotation/Sample.java
package com.acc4j.jdk8.annotation;
import java.lang.annotation.Documented;
import java.lang.annotation.ElementType;
import java.lang.annotation.Inherited;
import java.lang.annotation.Repeatable;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/*
@Documented 元注解,表示注释信息纳入JavaDoc
@Target 元注解,表示注解的目标类型,值类型 ElementType[];没有声明时支持所有类型
JDK8新增TYPE_USE,TYPE_PARAMETER的类型注解
@Retention 元注解,表示注解保留周期,值类型 RetentionPolicy;没有声明时,默认CLASS
SOURCE 只保留在源码中,纯标记
CLASS 保留到Class文件中,但是【JVM不会持有该类别信息,所以反射也无法获取】
RUNTIME 保留到Class文件,JVM会持有该类别信息,支持反射获取
@Inherited 元注解,表示注解信息可顺延着被标注元素的父子关系进行获取;注意,注解之间不存在继承关系
@Repeatable JDK8新增的元注解,表示注解可重复标注,实质上必须提供一个容器注解进行容纳。
容器注解注意事项:
① 必须含有目标注解类型数组的成员
② Target范围为目标注解的子集
③ Retention范围大于目标注解
④ 目标注解标注@Documented,@Inherited时,容器也需要对应标注
⑤ 被标注元素上的目标注解的值和@Inherited获取的值【不会被视为同组的值】,只有复数个目标注解才会纳入容器
参考资料:https://blog.csdn.net/javazejian/article/details/71860633
*/
@Documented
@Target({ElementType.TYPE, ElementType.METHOD, ElementType.PARAMETER})
@Retention(RetentionPolicy.RUNTIME)
@Inherited
@Repeatable(value = SampleContainer.class)
public @interface Sample {
/*
注解成员,可以定义多个,合法的类型有基本类型、String、Class、枚举、注解、以及这些类型的数组
在使用含有成员的注解时必须保证成员有值,显式赋值或默认值,默认值必须为常量内容
语法糖:成员名取为value并且只赋值该成员时,可直接 @Simple("xxx") 赋值
*/
String value() default "";
String other() default "";
}
<file_sep>/java-gist/src/test/java/com/acc4j/jdk8/optional/OptionalSampleTest.java
package com.acc4j.jdk8.optional;
import org.junit.Test;
public class OptionalSampleTest {
@Test
public void optionalSample() {
OptionalSample optionalSample = new OptionalSample();
optionalSample.create();
optionalSample.getOps();
optionalSample.valueOps();
}
}<file_sep>/java-gist/src/test/java/com/acc4j/easyExcel/EasyExcelSampleTest.java
package com.acc4j.easyExcel;
import com.acc4j.po.User;
import org.junit.Test;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
public class EasyExcelSampleTest {
@Test
public void easyExcelSample() {
EasyExcelSample.simpleWrite(getUserList(), "simple.xlsx", "simple");
EasyExcelSample.sheetWriter(getUserListList(), "sheet.xlsx");
List<User> r1 = EasyExcelSample.simpleRead("simple.xlsx", "simple");
List<User> r2 = EasyExcelSample.sheetRead("sheet.xlsx");
System.out.println(r1);
System.out.println(r2);
}
private List<User> getUserList() {
List<User> dataList = new ArrayList<>();
Random random = new Random();
int size = random.nextInt(10);
for (int i = 0; i < size; i++) {
User user = User.builder()
.name("tu" + i)
.age(i)
.sex(i > (size / 2) ? "男" : "女").build();
dataList.add(user);
}
return dataList;
}
private List<List<User>> getUserListList() {
List<List<User>> list = new ArrayList<>();
Random random = new Random();
int size = random.nextInt(10);
for (int i = 0; i < size; i++) {
list.add(getUserList());
}
return list;
}
}<file_sep>/java-gist/src/test/java/com/acc4j/jasypt/JasyptSampleTest.java
package com.acc4j.jasypt;
import org.junit.Test;
public class JasyptSampleTest {
@Test
public void jasyptSample() {
String e1 = JasyptSample.encrypt("simple message", "secret-password", "<PASSWORD>");
String e2 = JasyptSample.encrypt("simple message", "secret-password", "<PASSWORD>");
String e3 = JasyptSample.encrypt("simple message", "secret-password", "<PASSWORD>");
System.out.println("相同的原文多次加密后获取的密文并不一样:");
System.out.println("第1次加密:" + e1);
System.out.println("第2次加密:" + e2);
System.out.println("第3次加密:" + e3);
System.out.println();
String d1 = JasyptSample.decrypt(e1, "secret-password", "<PASSWORD>");
String d2 = JasyptSample.decrypt(e2, "secret-password", "<PASSWORD>");
String d3 = JasyptSample.decrypt(e3, "secret-password", "<PASSWORD>");
System.out.println("相同密钥和算法的情况下,即使原文多次加密产生的密文不一样也能解密恢复成原文:");
System.out.println("第1次解密:" + d1);
System.out.println("第2次解密:" + d2);
System.out.println("第3次解密:" + d3);
}
@Test
public void decryptSomething() {
System.out.println(JasyptSample.decrypt("LQrPL758gMeJ7Q89/zryriCLt6hJVBhC", "EWRREWRERWECCCXC", "PBEWithMD5AndDES"));
}
}<file_sep>/java-gist/src/main/java/com/acc4j/jdk8/methodInterface/MethodReferenceSample.java
package com.acc4j.jdk8.methodInterface;
import java.util.Arrays;
import java.util.Collection;
import java.util.concurrent.Callable;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
/**
* 方法引用:以具名函数的形式“实现”函数式接口
* 可以访问到的方法(包括构造方法)都能化作方法引用,从而作为函数式接口使用
*/
public class MethodReferenceSample {
/**
* 方法引用结构:类,接口,对象::方法名
* 1. 方法引用的参数是隐含的,与对应的函数式接口匹配
* 2. 支持函数式接口调用对应方法实现
*/
public void sample() throws Exception {
System.out.println("--------------- MethodReference sample --------------");
Consumer<String> m1 = System.out::println;
BiFunction<Collection<String>, Object, Boolean> m2 = Collection<String>::contains;
Callable<String> m3 = "a"::toUpperCase;
Function<String, String> m4 = String::new;
m1.accept("sout!");
Boolean apply = m2.apply(Arrays.asList("a", "b", "c"), "a");
System.out.println("判断元素是否存在:" + apply);
System.out.println("变换大写:" + m3.call());
System.out.println("创建字符串:" + m4.apply("new str"));
System.out.println();
}
}
<file_sep>/java-gist/src/main/java/com/acc4j/jdk8/methodInterface/IFunctionSample.java
package com.acc4j.jdk8.methodInterface;
/**
* 函数式接口:标注了@FunctionalInterface,有且仅有一个抽象方法的接口
*/
@FunctionalInterface
public interface IFunctionSample<A, B> {
void sample(A a, B b);
default void desc() {
System.out.println("函数式接口可以有default方法;default方法具备方法体,不会导致匿名实现产生歧义");
}
}
<file_sep>/java-gist/src/test/java/com/acc4j/jdk8/methodInterface/IFunctionSampleTest.java
package com.acc4j.jdk8.methodInterface;
import org.junit.Test;
public class IFunctionSampleTest {
@Test
public void functionInterface() throws Exception {
LambdaExpressionSample lambdaExpressionSample = new LambdaExpressionSample();
lambdaExpressionSample.sample();
MethodReferenceSample methodReferenceSample = new MethodReferenceSample();
methodReferenceSample.sample();
}
}<file_sep>/java-gist/src/main/java/com/acc4j/po/Parent.java
package com.acc4j.po;
import com.acc4j.jdk8.annotation.Sample;
import lombok.Data;
@Sample("on Parent")
@Data
public class Parent {
public void repeat(@Sample("on Parent Method Parameter") String msg) {
System.out.println(msg);
}
}
<file_sep>/java-gist/src/test/java/com/acc4j/jdk8/annotation/AnnotationSampleTest.java
package com.acc4j.jdk8.annotation;
import com.acc4j.po.Child;
import com.acc4j.po.Parent;
import org.junit.Test;
import java.lang.reflect.Method;
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class AnnotationSampleTest {
@Test
public void annotationSample() throws NoSuchMethodException {
String v1 = Parent.class.getAnnotation(Sample.class).value();
String v2 = Child.class.getAnnotation(Sample.class).value();
Sample[] samples = Child.class.getAnnotation(SampleContainer.class).value();
List<String> v3 = Arrays.stream(samples).map(Sample::value).collect(Collectors.toList());
// 获取类型注解,更加细粒度的进行代码元数据分析
Method repeat = Parent.class.getMethod("repeat", String.class);
System.out.println("获取Parent标注的@Sample:" + v1);
System.out.println("获取Child标注的@Inherited继承Parent获取的@Sample:" + v2);
System.out.println("获取Child重复标注的多个@Sample后自动装入容器注解@SampleContainer:" + v3);
System.out.println("获取Parent的方法上标注的@Sample:" + Arrays.deepToString(repeat.getParameterAnnotations()));
}
}
| d6eda768830ed20260d3f74ca4dc46cb181643d7 | [
"Markdown",
"Java"
] | 11 | Markdown | acc4mei/acc4j | 802023eb2656d65f2efee97a5198d04d2662c135 | d5e908abbd31d5f9bd6a1a2544edd5c1e9664cdc | |
refs/heads/master | <repo_name>alexandredagher/Metronome-<file_sep>/struct.h
/*
* stuct.h
*
* Created on: Apr 17, 2020
* Author: <NAME>
*/
#include <stdio.h>
#include <time.h>
#include <sys/netmgr.h>
#include <sys/neutrino.h>
#include <stdlib.h>
#include <sys/iofunc.h>
#include <sys/dispatch.h>
#include <string.h>
#include <unistd.h>
#include <sys/types.h>
#include <errno.h>
#include <unistd.h>
#include <pthread.h>
#include <sched.h>
#include <math.h>
#ifndef STRUCT_H_
#define STRUCT_H_
// Message that will be passed around
typedef union {
struct _pulse pulse;
char msg[255];
} my_message_t;
// Struct so that we can hold all of of data for a specific metronome into one object
struct DataTableRow
{
int timeSigTop;
int timeSigBot;
int numbOfIntervals;
char Pattern[32];
};
struct DataTableRow t[] = {
{2, 4, 4, "|1&2&"},
{3, 4, 6, "|1&2&3&"},
{4, 4, 8, "|1&2&3&4&"},
{5, 4, 10, "|1&2&3&4-5-"},
{3, 8, 6, "|1-2-3-"},
{6, 8, 6, "|1&a2&a"},
{9, 8, 9, "|1&a2&a3&a"},
{12, 8, 12, "|1&a2&a3&a4&a"}};
// Struct used for storing inputs from the command line easily into a object
typedef struct input {
int beatPerMin;
int timeSigTop;
int timeSigBot;
}input ;
#endif /* STRUCT_H_ */
<file_sep>/metronome.c
#include <stdio.h>
#include <time.h>
#include <sys/netmgr.h>
#include <sys/neutrino.h>
#include <stdlib.h>
#include <sys/iofunc.h>
#include <sys/dispatch.h>
#include <string.h>
#include <errno.h>
#include <unistd.h>
#include <pthread.h>
#include <unistd.h>
#include <sys/types.h>
#include <sched.h>
#include <math.h>
// This is my header file containing all the stucts used
#include "struct.h"
//Creating my Pulse Code Values and setting the Attach point name
#define MY_PULSE_CODE _PULSE_CODE_MINAVAIL
#define MY_PAUSE_CODE (MY_PULSE_CODE + 1)
#define MY_QUIT_CODE (MY_PAUSE_CODE + 1)
#define ATTACH_POINT "metronome"
// Declaring my global variables
char data[255];
int server_coid;
int beatPerMin = 0;
int timeSigTop = 0;
int timeSigBot = 0;
int secsPerInterval = 0;
int nanoSecs = 0;
//My attach pointer of type name_attach_t
name_attach_t * attach;
void *metronomeThread(void *arg) {
//Variables to be used
struct sigevent event;
struct itimerspec itime;
timer_t timer_id;
int rcvid;
my_message_t msg;
//Setting up my pulse
event.sigev_notify = SIGEV_PULSE;
event.sigev_coid = ConnectAttach(ND_LOCAL_NODE, 0, attach->chid,
_NTO_SIDE_CHANNEL, 0);
event.sigev_priority = SchedGet(0, 0, NULL);
event.sigev_code = MY_PULSE_CODE;
//This is what i will be using as my timer
timer_create(CLOCK_REALTIME, &event, &timer_id);
itime.it_value.tv_sec = 1;
itime.it_value.tv_nsec = 500000000;
//copying the inputs from the command line into my stuct of inputs
input * inputs = (input*) arg;
//Equations for the metronome
double beat = (double) 60 / inputs->beatPerMin;
double perMeasure = beat * 2;
double perInterval = (double) perMeasure / inputs->timeSigBot;
double fractional = perInterval - (int) perInterval;
// calculating the nano secconds
secsPerInterval = fractional;
nanoSecs = fractional * 1e+9;
//Setting
itime.it_interval.tv_sec = perInterval;
itime.it_interval.tv_nsec = (fractional * 1e+9);
timer_settime(timer_id, 0, &itime, NULL);
//Checking which pattern we need from the datarowTable and storing it index in the data row set
int index = -1;
for (int i = 0; i < 8; ++i)
if (t[i].timeSigTop == inputs->timeSigTop)
if (t[i].timeSigBot == inputs->timeSigBot)
index = i;
if (index == -1) {
printf("Incorrect pattern");
exit(EXIT_FAILURE);
}
while (1) {
//creating a temp char array with the values or the pattern so we can skip through each char in the pattern
char *ptr;
ptr = t[index].Pattern;
while (1) {
//Checking which pulse we recieved
rcvid = MsgReceive(attach->chid, &msg, sizeof(msg), NULL);
if (rcvid == 0) {
if (msg.pulse.code == MY_PULSE_CODE)
printf("%c", *ptr++);
else if (msg.pulse.code == MY_PAUSE_CODE) {
itime.it_value.tv_sec = msg.pulse.value.sival_int;
timer_settime(timer_id, 0, &itime, NULL);
} else if (msg.pulse.code == MY_QUIT_CODE) {
printf("\nQuiting\n");
TimerDestroy(timer_id);
exit(EXIT_SUCCESS);
}
// if we reach the end of the pattern then create a new line so that we can repeat the pattern
if (*ptr == '\0') {
printf("\n");
break;
}
}
//flushing the stdout so print statments can become visable
fflush( stdout);
}
}
return EXIT_SUCCESS;
}
int io_read(resmgr_context_t *ctp, io_read_t *msg, RESMGR_OCB_T *ocb) {
int nb;
nb = strlen(data);
if (data == NULL)
return 0;
//test to see if we have already sent the whole message.
if (ocb->offset == nb)
return 0;
//We will return which ever is smaller the size of our data or the size of the buffer
nb = min(nb, msg->i.nbytes);
//Set the number of bytes we will return
_IO_SET_READ_NBYTES(ctp, nb);
//Copy data into reply buffer.
SETIOV(ctp->iov, data, nb);
//update offset into our data used to determine start position for next read.
ocb->offset += nb;
//If we are going to send any bytes update the access time for this resource.
if (nb > 0)
ocb->attr->flags |= IOFUNC_ATTR_ATIME;
return (_RESMGR_NPARTS(1));
}
int io_write(resmgr_context_t *ctp, io_write_t *msg, RESMGR_OCB_T *ocb) {
int nb = 0;
if (msg->i.nbytes == ctp->info.msglen - (ctp->offset + sizeof(*msg))) {
/* have all the data */
char *buf;
char *alert_msg;
int i, small_integer;
buf = (char *) (msg + 1);
if (strstr(buf, "pause") != NULL) {
for (i = 0; i < 2; i++)
alert_msg = strsep(&buf, " ");
small_integer = atoi(alert_msg);
if (small_integer >= 1 && small_integer <= 9)
MsgSendPulse(server_coid, SchedGet(0, 0, NULL), MY_PAUSE_CODE,
small_integer);
else
printf("\nInteger is not between 1 and 9.\n");
} else if (strstr(buf, "quit") != NULL)
MsgSendPulse(server_coid, SchedGet(0, 0, NULL), MY_QUIT_CODE, 0);
else
printf("\nInvalid Command: %s\n", strsep(&buf, "\n"));
nb = msg->i.nbytes;
}
_IO_SET_WRITE_NBYTES(ctp, nb);
if (msg->i.nbytes > 0)
ocb->attr->flags |= IOFUNC_ATTR_MTIME | IOFUNC_ATTR_CTIME;
return (_RESMGR_NPARTS(0));
}
int io_open(resmgr_context_t *ctp, io_open_t *msg, RESMGR_HANDLE_T *handle,
void *extra) {
if ((server_coid = name_open(ATTACH_POINT, 0)) == -1) {
perror("name_open failed.");
return EXIT_FAILURE;
}
return (iofunc_open_default(ctp, msg, handle, extra));
}
int main(int argc, char *argv[]) {
dispatch_t *dpp;
resmgr_io_funcs_t io_funcs;
resmgr_connect_funcs_t connect_funcs;
iofunc_attr_t ioattr;
dispatch_context_t *ctp;
int id;
struct input inputs = { atoi(argv[1]), atoi(argv[2]), atoi(argv[3]), };
//Error checking for amount of command line arguments
if (argc != 4) {
perror("Not the correct amount of arguments.");
exit(EXIT_FAILURE);
}
// Attaching the attach point
if ((attach = name_attach(NULL, ATTACH_POINT, 0)) == NULL) {
perror("name attach failed");
return EXIT_FAILURE;
}
//Creating the dispatch
if ((dpp = dispatch_create()) == NULL) {
fprintf(stderr, "%s: Unable to allocate dispatch context.\n", argv[0]);
return (EXIT_FAILURE);
}
//Initializing the funtions and setting them to func_init
iofunc_func_init(_RESMGR_CONNECT_NFUNCS, &connect_funcs, _RESMGR_IO_NFUNCS,
&io_funcs);
connect_funcs.open = io_open;
io_funcs.read = io_read;
io_funcs.write = io_write;
iofunc_attr_init(&ioattr, S_IFCHR | 0666, NULL, NULL);
// Attaching the resouce manager to the metronome device
if ((id = resmgr_attach(dpp, NULL, "/dev/local/metronome", _FTYPE_ANY, 0,
&connect_funcs, &io_funcs, &ioattr)) == -1) {
fprintf(stderr, "%s: Unable to attach name.\n", argv[0]);
return (EXIT_FAILURE);
}
//Creating my thread and attaching the metronomeThread function to it
pthread_attr_t attr;
pthread_attr_init(&attr);
pthread_create(NULL, &attr, &metronomeThread, &inputs);
//Resource manager routine
ctp = dispatch_context_alloc(dpp);
while (1) {
ctp = dispatch_block(ctp);
dispatch_handler(ctp);
}
pthread_attr_destroy(&attr);
name_detach(attach, 0);
return EXIT_SUCCESS;
}
| 563b94a082446ea20abb7defb41d86e30e2f692a | [
"C"
] | 2 | C | alexandredagher/Metronome- | 03753a807ca3194b868e9585210d354da05c9dd5 | b1da55b04121d32ebc6133514b41341a90f8ba38 | |
refs/heads/master | <repo_name>jayKayEss/Tootr<file_sep>/sql/tootr.sql
-- MySQL dump 10.13 Distrib 5.5.11, for osx10.6 (i386)
--
-- Host: localhost Database: tootr
-- ------------------------------------------------------
-- Server version 5.5.11
/*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */;
/*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */;
/*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */;
/*!40101 SET NAMES utf8 */;
/*!40103 SET @OLD_TIME_ZONE=@@TIME_ZONE */;
/*!40103 SET TIME_ZONE='+00:00' */;
/*!40014 SET @OLD_UNIQUE_CHECKS=@@UNIQUE_CHECKS, UNIQUE_CHECKS=0 */;
/*!40014 SET @OLD_FOREIGN_KEY_CHECKS=@@FOREIGN_KEY_CHECKS, FOREIGN_KEY_CHECKS=0 */;
/*!40101 SET @OLD_SQL_MODE=@@SQL_MODE, SQL_MODE='NO_AUTO_VALUE_ON_ZERO' */;
/*!40111 SET @OLD_SQL_NOTES=@@SQL_NOTES, SQL_NOTES=0 */;
--
-- Table structure for table `edge`
--
DROP TABLE IF EXISTS `edge`;
/*!40101 SET @saved_cs_client = @@character_set_client */;
/*!40101 SET character_set_client = utf8 */;
CREATE TABLE `edge` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`updated` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP,
`stream_id` int(10) unsigned NOT NULL,
`from_id` int(10) unsigned NOT NULL,
`to_id` int(10) unsigned NOT NULL,
`rank` int(4) DEFAULT NULL,
`myrand` int(4) unsigned NOT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `stream_id` (`stream_id`,`from_id`,`to_id`),
KEY `updated` (`updated`)
) ENGINE=MyISAM AUTO_INCREMENT=63297 DEFAULT CHARSET=latin1;
/*!40101 SET character_set_client = @saved_cs_client */;
--
-- Table structure for table `node`
--
DROP TABLE IF EXISTS `node`;
/*!40101 SET @saved_cs_client = @@character_set_client */;
/*!40101 SET character_set_client = utf8 */;
CREATE TABLE `node` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`created` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
`term` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM AUTO_INCREMENT=17307 DEFAULT CHARSET=latin1;
/*!40101 SET character_set_client = @saved_cs_client */;
--
-- Table structure for table `stream`
--
DROP TABLE IF EXISTS `stream`;
/*!40101 SET @saved_cs_client = @@character_set_client */;
/*!40101 SET character_set_client = utf8 */;
CREATE TABLE `stream` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`created` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
`name` varchar(255) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=MyISAM AUTO_INCREMENT=9 DEFAULT CHARSET=latin1;
/*!40101 SET character_set_client = @saved_cs_client */;
/*!40103 SET TIME_ZONE=@OLD_TIME_ZONE */;
/*!40101 SET SQL_MODE=@OLD_SQL_MODE */;
/*!40014 SET FOREIGN_KEY_CHECKS=@OLD_FOREIGN_KEY_CHECKS */;
/*!40014 SET UNIQUE_CHECKS=@OLD_UNIQUE_CHECKS */;
/*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */;
/*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */;
/*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
/*!40111 SET SQL_NOTES=@OLD_SQL_NOTES */;
-- Dump completed on 2012-01-28 16:04:18
<file_sep>/srv/gettweet.php
<?php
require_once dirname(__FILE__).'/../lib/Consts.php';
require_once dirname(__FILE__).'/../lib/DB.php';
require_once dirname(__FILE__).'/../lib/Generator.php';
$stream = Tootr\Consts::$STREAMS[array_rand(Tootr\Consts::$STREAMS)];
$db = new Tootr\DB();
$streamId = $db->getStreamId($stream);
$generator = new Tootr\Generator($db, $stream);
$tweet = $generator->generate();
header('Content-type: application/json');
print json_encode(array(
'text' => $tweet,
'stream' => $stream
));
<file_sep>/assets/tootr.js
(function($){
$(document).ready(function(){
var tootr = new Tootr();
tootr.init();
});
function Tootr() {
this.colors = {
life: '#737d17',
death: '#333',
sex: '#9b6262',
money: '#4c8046'
};
}
Tootr.prototype = {
init: function() {
this.displayTweet();
var self = this;
setInterval(function(){
self.displayTweet();
}, 15000);
},
displayTweet: function() {
var self = this;
$.ajax({
dataType: 'json',
url: 'srv/gettweet.php',
success: function(data){
var stream = data.stream;
var text = data.text;
var elem = $('#thetweet');
elem.find('.container').fadeOut(function(){
$('body').animate({
'background-color': $.Color( self.colors[stream] )
});
elem.find('.user').html('@'+stream);
elem.find('.name').html(stream.charAt(0).toUpperCase() + stream.slice(1));
elem.find('.text').html(text);
elem.find('.avatar img').attr({src: 'img/'+stream+'.png'});
elem.find('.container').fadeIn();
});
}
});
},
};
})(jQuery);
<file_sep>/bin/test.php
<?php
namespace Tootr;
require_once 'lib/Consts.php';
require_once 'lib/DB.php';
require_once 'lib/Parser.php';
require_once 'lib/Generator.php';
$db = new DB();
foreach (Consts::$STREAMS as $stream) {
$streamId = $db->getStreamId($stream);
$generator = new Generator($db, $stream);
$tweet = $generator->generate() . "\n";
print "$stream [".strlen($tweet)."] $tweet\n";
}
<file_sep>/lib/DB.php
<?php
namespace Tootr;
class DB {
const USER = "tootr";
const PASS = "<PASSWORD>";
const GET_STREAM = "SELECT id FROM stream WHERE name=?";
const NEW_STREAM = "INSERT INTO stream (name) VALUES (?)";
const GET_NODE = "SELECT id FROM node WHERE term=?";
const GET_NODE_BY_ID = "SELECT * FROM node WHERE id=?";
const NEW_NODE = "INSERT INTO node (term) VALUES (?)";
const NEW_EDGE = <<<'EOL'
INSERT INTO edge (stream_id, from_id, to_id, rank, myrand) VALUES (?, ?, ?, 1, cast(rand()*10000 as unsigned))
ON DUPLICATE KEY UPDATE rank=rank+1
EOL;
const GET_EDGES = "SELECT * FROM edge WHERE stream_id=? AND from_id=?";
protected $dbh;
function __construct() {
$this->dbh = new \PDO('mysql:host=127.0.0.1;dbname=tootr', self::USER, self::PASS);
}
function getStreamId($name) {
$query = $this->dbh->prepare(self::GET_STREAM);
$query->execute(array($name));
if ($rec = $query->fetch(\PDO::FETCH_ASSOC)) {
return $rec['id'];
}
$query = $this->dbh->prepare(self::NEW_STREAM);
$query->execute(array($name));
return $this->dbh->lastInsertId();
}
function getNodeId($term) {
$query = $this->dbh->prepare(self::GET_NODE);
$query->execute(array($term));
if ($rec = $query->fetch(\PDO::FETCH_ASSOC)) {
return $rec['id'];
}
$query = $this->dbh->prepare(self::NEW_NODE);
$query->execute(array($term));
return $this->dbh->lastInsertId();
}
function getNode($id) {
$query = $this->dbh->prepare(self::GET_NODE_BY_ID);
$query->execute(array($id));
if ($rec = $query->fetch(\PDO::FETCH_ASSOC)) {
return $rec;
}
}
function addEdge($streamId, $fromId, $toId) {
$query = $this->dbh->prepare(self::NEW_EDGE);
$query->execute(array($streamId, $fromId, $toId));
}
function getRandomEdge($streamId, $fromId) {
$query = $this->dbh->prepare(self::GET_EDGES);
$query->execute(array($streamId, $fromId));
$picked = null;
$count = 1;
while ($rec = $query->fetch(\PDO::FETCH_ASSOC)) {
$max = $count + $rec['rank'];
$rand = rand(1, $max);
if ($rand > $count && $rand <= $max) {
$picked = $rec;
}
$count = $max;
}
if (isset($picked)) {
return $picked;
} else if (isset($rec)) {
return $rec;
} else {
return null;
}
}
}
<file_sep>/lib/Parser.php
<?php
namespace Tootr;
class Parser {
protected $db;
protected $streamId;
function __construct($db, $streamName) {
$this->db = $db;
$this->streamId = $db->getStreamId($streamName);
}
function parse($text) {
$words = preg_split('/\s+/', $text);
$lastWord = null;
$endOfSentence = false;
foreach ($words as $word) {
if (empty($word)) continue;
if (preg_match('/^http/', $word)) continue;
if (preg_match('/^@/', $word)) continue;
if (strtoupper($word) == 'RT') continue;
if (strtolower($word) == 'moneyblogs') continue;
if (strtolower($word) == '.info') continue;
if (preg_match('/[.?!]$/', $word)) {
$endOfSentence = true;
}
if ($lastWord) {
$this->addEdge($lastWord, $word);
} else {
$this->addEdge(null, $word); // headword
}
if ($endOfSentence) {
$lastWord = null;
$endOfSentence = false;
} else {
$lastWord = $word;
}
}
}
function addEdge($from, $to) {
if (!empty($from)) {
$fromId = $this->db->getNodeId($from);
} else {
$fromId = 0;
}
$toId = $this->db->getNodeId($to);
$this->db->addEdge($this->streamId, $fromId, $toId);
}
}
| d8ec08cf3f142de8bc6006e82d64a2430e8cacfb | [
"JavaScript",
"SQL",
"PHP"
] | 6 | SQL | jayKayEss/Tootr | 98a72561ec9c658fd247d1e37b81139c02dc7f78 | 097ca157bf094b0f655a93d476b4285728492a15 | |
refs/heads/master | <file_sep>#include "BoundingSphere.h"
#include <assert.h>
#include "Bounding.h"
#include "BoundingBox.h"
#include "BoundingFrustum.h"
#include "Ray.h"
#include "../Core/Plane.h"
#include "../Core/MathHelper.h"
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
//////////////////////////////////////////////////////////////////////////
BoundingSphere::BoundingSphere( void )
{
this->Center = Vector3::Zero;
this->Radius = 0.0f;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param center : 위치 벡터
///@param radius : 반지름 값
//////////////////////////////////////////////////////////////////////////
BoundingSphere::BoundingSphere( Vector3 center, float radius )
{
assert(radius >= 0.0f);
this->Center = center;
this->Radius = radius;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief ==
///@param value : 바운딩 스페어
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingSphere::operator==( const BoundingSphere& value ) const
{
if(this->Center == value.Center)
return (double)this->Radius == (double)value.Radius;
else
return false;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief !=
///@param value : 바운딩 스페어
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingSphere::operator!=( const BoundingSphere& value ) const
{
if (!(this->Center != value.Center))
return (double)this->Radius != (double)value.Radius;
else
return true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 서포트 매핑
///@param v : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 BoundingSphere::SupportMapping( Vector3 v )
{
float num = Radius / v.Length();
Vector3 result;
result.X = Center.X + v.X * num;
result.Y = Center.Y + v.Y * num;
result.Z = Center.Z + v.Z * num;
return result;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 개의 바운딩 스페어 합침
///@param original : 바운딩 스페어1
///@param additional : 바운딩 스페어2
///@return 결과 바운딩 스페어
//////////////////////////////////////////////////////////////////////////
BoundingSphere BoundingSphere::CreateMerged( BoundingSphere original, BoundingSphere additional )
{
Vector3 result = additional.Center - original.Center;
float num1 = result.Length();
float num2 = original.Radius;
float num3 = additional.Radius;
if((double)num2 + (double)num3 >= (double)num1)
{
if ((double)num2 - (double)num3 >= (double)num1)
return original;
else if ((double)num3 - (double)num2 >= (double)num1)
return additional;
}
Vector3 vector3 = result * (1.0f / num1);
float num4 = MathHelper::Min(-num2, num1 - num3);
float num5 = (float)(((double)MathHelper::Max(num2, num1 + num3) - (double)num4)* 0.5);
BoundingSphere boundingSphere;
boundingSphere.Center = original.Center + vector3 * (num5 + num4);
boundingSphere.Radius = num5;
return boundingSphere;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 박스로부터 바운딩 스페어 생성
///@param box : 바운딩 박스
///@return 결과 바운딩 스페어
//////////////////////////////////////////////////////////////////////////
BoundingSphere BoundingSphere::CreateFromBoundingBox( BoundingBox box )
{
BoundingSphere boundingSphere;
boundingSphere.Center = Vector3::Lerp(box.Min, box.Max, 0.5f);
float result;
result = Vector3::Distance(box.Min, box.Max);
boundingSphere.Radius = result * 0.5f;
return boundingSphere;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 박스와의 교차 검사
///@param box : 바운딩 박스
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingSphere::Intersects( BoundingBox box )
{
Vector3 result1 = Vector3::Clamp(Center, box.Min, box.Max);
float result2 = Vector3::DistanceSquared(Center, result1);
if((double)result2 <= (double)Radius * (double)Radius)
return true;
else
return false;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 프러스텀과의 교차 검사
///@param frustum : 바운딩 프러스텀
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingSphere::Intersects( BoundingFrustum frustum )
{
bool result;
frustum.Intersects(*this, result);
return result;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 평면과의 교차 검사
///@param plane : 평면
///@return 교차 결과
//////////////////////////////////////////////////////////////////////////
PlaneIntersectionType BoundingSphere::Intersects( Plane plane )
{
return plane.Intersects(*this);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 레이와의 교차 검사
///@param ray : 광선
///@return 교차 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingSphere::Intersects( Ray ray, float& result )
{
return ray.Intersects(*this, result);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 스페어와의 교차 검사
///@param sphere : 바운딩 스페어
///@return 교차 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingSphere::Intersects( BoundingSphere sphere )
{
float result = Vector3::DistanceSquared(Center, sphere.Center);
float num1 = Radius;
float num2 = sphere.Radius;
if((double)num1 * (double)num1 + 2.0 * (double)num1 * (double)num2 + (double)num2 * (double)num2 <= (double)result)
return false;
else
return true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 박스 포함 검사
///@param box : 바운<NAME>
///@return 포함 결과
//////////////////////////////////////////////////////////////////////////
ContainmentType BoundingSphere::Contains( BoundingBox box )
{
if(!box.Intersects(*this))
{
return ContainmentType_Disjoint;
}
else
{
float num = Radius * Radius;
Vector3 vector3;
vector3.X = Center.X - box.Min.X;
vector3.Y = Center.Y - box.Max.Y;
vector3.Z = Center.Z - box.Max.Z;
if((double)vector3.LengthSquared() > (double)num)
{
return ContainmentType_Intersects;
}
else
{
vector3.X = Center.X - box.Max.X;
vector3.Y = Center.Y - box.Max.Y;
vector3.Z = Center.Z - box.Max.Z;
if ((double)vector3.LengthSquared() > (double)num)
{
return ContainmentType_Intersects;
}
else
{
vector3.X = Center.X - box.Max.X;
vector3.Y = Center.Y - box.Min.Y;
vector3.Z = Center.Z - box.Max.Z;
if((double)vector3.LengthSquared() > (double)num)
{
return ContainmentType_Intersects;
}
else
{
vector3.X = Center.X - box.Min.X;
vector3.Y = Center.Y - box.Min.Y;
vector3.Z = Center.Z - box.Max.Z;
if((double)vector3.LengthSquared() > (double)num)
{
return ContainmentType_Intersects;
}
else
{
vector3.X = Center.X - box.Min.X;
vector3.Y = Center.Y - box.Max.Y;
vector3.Z = Center.Z - box.Min.Z;
if((double)vector3.LengthSquared() > (double)num)
{
return ContainmentType_Intersects;
}
else
{
vector3.X = Center.X - box.Max.X;
vector3.Y = Center.Y - box.Max.Y;
vector3.Z = Center.Z - box.Min.Z;
if((double)vector3.LengthSquared() > (double)num)
{
return ContainmentType_Intersects;
}
else
{
vector3.X = Center.X - box.Max.X;
vector3.Y = Center.Y - box.Min.Y;
vector3.Z = Center.Z - box.Min.Z;
if((double)vector3.LengthSquared() > (double)num)
{
return ContainmentType_Intersects;
}
else
{
vector3.X = Center.X - box.Min.X;
vector3.Y = Center.Y - box.Min.Y;
vector3.Z = Center.Z - box.Min.Z;
if((double)vector3.LengthSquared() > (double)num)
return ContainmentType_Intersects;
else
return ContainmentType_Contains;
}
}
}
}
}
}
}
}
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 프러스텀과의 포함 검사
///@param frustum : 바운딩 프러스텀
///@return 포함 결과
//////////////////////////////////////////////////////////////////////////
ContainmentType BoundingSphere::Contains( BoundingFrustum frustum )
{
if(!frustum.Intersects(*this))
{
return ContainmentType_Disjoint;
}
else
{
float num = Radius * Radius;
for(int i = 0; i < 8; i++)
{
Vector3 vector3_1 = frustum.cornerArray[i];
Vector3 vector3_2;
vector3_2.X = vector3_1.X - Center.X;
vector3_2.Y = vector3_1.Y - Center.Y;
vector3_2.Z = vector3_1.Z - Center.Z;
if((double)vector3_2.LengthSquared() > (double)num)
return ContainmentType_Intersects;
}
return ContainmentType_Contains;
}
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 위치 벡터의 포함 검사
///@param point : 위치 벡터
///@return 포함 결과
//////////////////////////////////////////////////////////////////////////
ContainmentType BoundingSphere::Contains( Vector3 point )
{
if ((double)Vector3::DistanceSquared(point, Center) >= (double)Radius * (double)Radius)
return ContainmentType_Disjoint;
else
return ContainmentType_Contains;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 스페어의 포함 여부 검사
///@param sphere : 바운딩 스페어
///@return 포함 결과
//////////////////////////////////////////////////////////////////////////
ContainmentType BoundingSphere::Contains( BoundingSphere sphere )
{
float result = Vector3::Distance(Center, sphere.Center);
float num1 = Radius;
float num2 = sphere.Radius;
if((double)num1 + (double)num2 < (double)result)
return ContainmentType_Disjoint;
else if((double)num1 - (double)num2 < (double)result)
return ContainmentType_Intersects;
else
return ContainmentType_Contains;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 매트릭스에 의한 바운딩 스페어 변환
///@param matrix : 매트릭스
///@return 결과 바운딩 스페어
//////////////////////////////////////////////////////////////////////////
BoundingSphere BoundingSphere::Transform( Matrix matrix )
{
BoundingSphere boundingSphere;
boundingSphere.Center = Vector3::Transform(Center, matrix);
float num = MathHelper::Max((float)(
(double)matrix.M11 * (double)matrix.M11 + (double)matrix.M12 * (double)matrix.M12 + (double)matrix.M13 * (double)matrix.M13),
MathHelper::Max((float)((double)matrix.M21 * (double)matrix.M21 + (double)matrix.M22 * (double)matrix.M22 + (double)matrix.M23 * (double)matrix.M23),
(float)((double)matrix.M31 * (double)matrix.M31 + (double)matrix.M32 * (double)matrix.M32 + (double)matrix.M33 * (double)matrix.M33)));
boundingSphere.Radius = Radius * (float)sqrt((double)num);
return boundingSphere;
}<file_sep>#pragma once
class ModelTool : public Execute
{
public:
ModelTool(ExecuteValues* values);
~ModelTool();
void Update();
void PreRender() {}
void Render();
void PostRender();
void ResizeScreen() {}
private:
class GameSkyBox* skyBox;
class GameWorld* world;
class GameAnimationModel* grund;
class GamePlayer* player;
};<file_sep>#include "stdafx.h"
#include "GizmoComponent.h"
#include "./Framework/Core/Matrix.h"
#include "./Framework/Core/Vector3.h"
#include "./Framework/Bounding/Ray.h"
#include "./Framework/Core/Plane.h"
#include "Gizmo.h"
Gizmo::GizmoComponent::GizmoComponent(ExecuteValues* values)
:values(values)
{
Init();
}
Gizmo::GizmoComponent::~GizmoComponent()
{
SAFE_RELEASE(rasterizer[0]);
SAFE_RELEASE(rasterizer[1]);
SAFE_RELEASE(depthStencilState[0]);
SAFE_RELEASE(depthStencilState[1]);
for (Gizmo* gizmo : quads)
SAFE_DELETE(gizmo);
for (Gizmo* gizmo : lines)
SAFE_DELETE(gizmo);
for (Gizmo* gizmo : scales)
SAFE_DELETE(gizmo);
for (Gizmo* gizmo : rotates)
SAFE_DELETE(gizmo);
for (Gizmo* gizmo : translates)
SAFE_DELETE(gizmo);
for (Gizmo* gizmo : boxes)
SAFE_DELETE(gizmo);
for (Gizmo* gizmo : spheres)
SAFE_DELETE(gizmo);
}
D3DXMATRIX Gizmo::GizmoComponent::TransformDelta()
{
scaleDelta *= SCALE_WEIGHT;
D3DXMATRIX S, T, T2;
D3DXMatrixIdentity(&S);
D3DXMatrixIdentity(&T);
D3DXMatrixIdentity(&T2);
//Inverse
D3DXMATRIX invRot;
D3DXMatrixInverse(&invRot, NULL, &rotMat);
D3DXMATRIX invPos;
D3DXMatrixTranslation(&T2, position.x, position.y, position.z);
D3DXMatrixInverse(&invPos, NULL, &T2);
D3DXVECTOR3 tempScale = { 1,1,1 };
if (scaleDelta != D3DXVECTOR3(0, 0, 0))
{
tempScale += scaleDelta;
D3DXMatrixScaling(&S, tempScale.x, tempScale.y, tempScale.z);
//축방향으로 스케일하기 위해
S = invPos*invRot*S*rotMat*T2;
}
D3DXMATRIX identity;
D3DXMatrixIdentity(&identity);
if (rotationDelta != identity)
{
//모델 중심으로 회전
rotationDelta = invPos*rotationDelta*T2;
}
if (translationDelta != D3DXVECTOR3(0, 0, 0))
{
//이동값은 방향구해서 가져왔으니 바로계산
D3DXMatrixTranslation(&T, translationDelta.x, translationDelta.y, translationDelta.z);
translationDelta = { 0,0,0 };
}
D3DXMATRIX ret = S* rotationDelta* T;
D3DXMatrixIdentity(&rotationDelta);
return ret;
}
void Gizmo::GizmoComponent::Init()
{
{
D3D11_RASTERIZER_DESC desc;
States::GetRasterizerDesc(&desc);
States::CreateRasterizer(&desc, &rasterizer[0]);
desc.CullMode = D3D11_CULL_NONE;
States::CreateRasterizer(&desc, &rasterizer[1]);
}
{
D3D11_DEPTH_STENCIL_DESC desc;
States::GetDepthStencilDesc(&desc);
States::CreateDepthStencil(&desc, &depthStencilState[0]);
desc.DepthEnable = false;
States::CreateDepthStencil(&desc, &depthStencilState[1]);
}
target = NULL;
D3DXMatrixIdentity(&gizmoWorld);
D3DXMatrixIdentity(&outsideWorld);
D3DXMatrixIdentity(&scaleMat);
D3DXMatrixIdentity(&rotMat);
D3DXMatrixIdentity(&posMat);
D3DXQuaternionIdentity(&rotQuat);
scale = { 1,1,1 };
rotate = { 0,0,0 };
position = { 0,0,0 };
ResetMouseValues();
ResetDeltas();
bMousePressed = false;
bDrawBounding = false;
activeMode = EGizmoMode::Translate;
activeAxis = EGizmoAxis::None;
colors[0] = D3DXCOLOR(1, 0, 0, 1);
colors[1] = D3DXCOLOR(0, 1, 0, 1);
colors[2] = D3DXCOLOR(0, 0, 1, 1);
geometryWorld[0] = Matrix::CreateWorld(Vector3(LINE_LENGTH, 0, 0), Vector3::Left, Vector3::Up).ToD3DXMATRIX();
geometryWorld[1] = Matrix::CreateWorld(Vector3(0, LINE_LENGTH, 0), Vector3::Down, Vector3::Left).ToD3DXMATRIX();
geometryWorld[2] = Matrix::CreateWorld(Vector3(0, 0, LINE_LENGTH), Vector3::Forward, Vector3::Up).ToD3DXMATRIX();
Create2D();
Create3D();
CreateBoundings();
}
void Gizmo::GizmoComponent::MouseCollision()
{
float* distance = NULL;
float sphereDist = 0;
Ray ray = ConvertMouseToRay(gizmoWorld);
if (xAxisBox->Intersects(ray, distance) || xSphere->Intersects(ray, sphereDist))
activeAxis = EGizmoAxis::X;
else if (yAxisBox->Intersects(ray, distance) || ySphere->Intersects(ray, sphereDist))
activeAxis = EGizmoAxis::Y;
else if (zAxisBox->Intersects(ray, distance) || zSphere->Intersects(ray, sphereDist))
activeAxis = EGizmoAxis::Z;
else if (xyAxisBox->Intersects(ray, distance))
activeAxis = EGizmoAxis::XY;
else if (xzAxisBox->Intersects(ray, distance))
activeAxis = EGizmoAxis::ZX;
else if (yzAxisBox->Intersects(ray, distance))
activeAxis = EGizmoAxis::YZ;
else
activeAxis = EGizmoAxis::None;
SAFE_DELETE(distance);
//highlight color
ResetHighlight();
switch (activeAxis)
{
case EGizmoAxis::None:
break;
case EGizmoAxis::X:
case EGizmoAxis::Y:
case EGizmoAxis::Z:
{
int index = (int)activeAxis;
lines[index]->bHighlight = true;
scales[index]->bHighlight = true;
rotates[index]->bHighlight = true;
translates[index]->bHighlight = true;
}
break;
case EGizmoAxis::XY:
quads[0]->bHighlight = true;
break;
case EGizmoAxis::ZX:
quads[1]->bHighlight = true;
break;
case EGizmoAxis::YZ:
quads[2]->bHighlight = true;
break;
}
}
void Gizmo::GizmoComponent::Pressing()
{
D3DXVECTOR3 tempPos;
D3DXMATRIX invRot;
D3DXMatrixInverse(&invRot, NULL, &rotMat);
D3DXVec3TransformCoord(&tempPos, &position, &invRot);
Vector3 tempPos2 = { tempPos.x, tempPos.y, tempPos.z };
//Collision Plane
Plane planeXY = Plane(Vector3::Forward, -tempPos2.Z);
Plane planeYZ = Plane(Vector3::Left, tempPos2.X);
Plane planeZX = Plane(Vector3::Down, tempPos2.Y);
Ray ray = ConvertMouseToRay(rotMat);
float value = 0;
lastIntersectPosition = intersectPosition;
switch (activeAxis)
{
case EGizmoAxis::None:
break;
case EGizmoAxis::XY:
case EGizmoAxis::X:
if (ray.Intersects(planeXY, value))
{
intersectPosition = (ray.Position + (ray.Direction * value));
if (lastIntersectPosition != Vector3::Zero)
{
delta = intersectPosition - lastIntersectPosition;
}
delta = activeAxis == EGizmoAxis::X ? Vector3(delta.X, 0, 0) : Vector3(delta.X, delta.Y, 0);
}
break;
case EGizmoAxis::Z:
case EGizmoAxis::YZ:
case EGizmoAxis::Y:
if (ray.Intersects(planeYZ, value))
{
intersectPosition = (ray.Position + (ray.Direction * value));
if (lastIntersectPosition != Vector3::Zero)
{
delta = intersectPosition - lastIntersectPosition;
}
switch (activeAxis)
{
case EGizmoAxis::Y:
delta = Vector3(0, delta.Y, 0);
break;
case EGizmoAxis::Z:
delta = Vector3(0, 0, delta.Z);
break;
default:
delta = Vector3(0, delta.Y, delta.Z);
break;
}
}
break;
case EGizmoAxis::ZX:
if (ray.Intersects(planeZX, value))
{
intersectPosition = (ray.Position + (ray.Direction * value));
if (lastIntersectPosition != Vector3::Zero)
{
delta = intersectPosition - lastIntersectPosition;
}
delta = Vector3(delta.X, 0, delta.Z);
}
break;
}
//ActiveMode
switch (activeMode)
{
case EGizmoMode::Scale:
{
scaleDelta += D3DXVECTOR3(delta.X, delta.Y, delta.Z);
D3DXMATRIX deltaTranslation;
D3DXMatrixTranslation(&deltaTranslation, delta.X, delta.Y, delta.Z);
switch (activeAxis)
{
case EGizmoAxis::X:
case EGizmoAxis::Y:
case EGizmoAxis::Z:
int index = (int)activeAxis;
lines[index]->vertexData[1].position += scaleDelta;
geometryWorld[index] *= deltaTranslation;
lines[index]->UpdateVertexData();
break;
}
}
break;
case EGizmoMode::Rotate:
{
lastMousePos = curMousePos;
curMousePos = Mouse::Get()->GetPosition();
float deltaX = 0;
if (lastMousePos != D3DXVECTOR3(0, 0, 0))
deltaX = curMousePos.x - lastMousePos.x;
D3DXVECTOR3 rotVec = D3DXVECTOR3(
Math::ToRadian(deltaX * ROTATE_WEIGHT), Math::ToRadian(deltaX * ROTATE_WEIGHT), Math::ToRadian(deltaX * ROTATE_WEIGHT));
D3DXMATRIX rot, tempRot;
D3DXMatrixIdentity(&rot);
rot = rotMat;
D3DXVECTOR3 vecX, vecY, vecZ;
vecX = D3DXVECTOR3(rot._11, rot._12, rot._13);
vecY = D3DXVECTOR3(rot._21, rot._22, rot._23);
vecZ = D3DXVECTOR3(rot._31, rot._32, rot._33);
D3DXQUATERNION q;
D3DXQuaternionIdentity(&q);
switch (activeAxis)
{
case EGizmoAxis::X:
D3DXMatrixRotationAxis(&tempRot, &vecX, rotVec.x);
D3DXQuaternionRotationAxis(&q, &vecX, rotVec.x);
break;
case EGizmoAxis::Y:
D3DXMatrixRotationAxis(&tempRot, &vecY, rotVec.y);
D3DXQuaternionRotationAxis(&q, &vecY, rotVec.y);
break;
case EGizmoAxis::Z:
D3DXMatrixRotationAxis(&tempRot, &vecZ, rotVec.z);
D3DXQuaternionRotationAxis(&q, &vecZ, rotVec.z);
break;
}
rotQuat *= q;
D3DXMatrixRotationQuaternion(&rotationDelta, &q);
D3DXMatrixRotationQuaternion(&rotMat, &rotQuat);
}
break;
case EGizmoMode::Translate:
{
D3DXVECTOR3 temp, temp2;
temp2 = { delta.X, delta.Y, delta.Z };
D3DXVec3TransformCoord(&temp, &temp2, &rotMat);
position += temp;
translationDelta += temp;
}
break;
}
}
void Gizmo::GizmoComponent::Update()
{
//TargetTransform
if (target != NULL)
{
D3DXMATRIX targetMat = *target;
D3DXMATRIX deltaMat = TransformDelta();
targetMat *= deltaMat;
*target = targetMat;
}
//ResizeWorldScale
//TODO: 모델거리에 비례해서 기즈모 크기조절
ResizeWorldScale();
if (Keyboard::Get()->Down('1'))
activeMode = EGizmoMode::Translate;
if (Keyboard::Get()->Down('2'))
activeMode = EGizmoMode::Rotate;
if (Keyboard::Get()->Down('3'))
activeMode = EGizmoMode::Scale;
//MouseInput
if (Mouse::Get()->Down(0))
{
MouseCollision();
bMousePressed = true;
}
if (bMousePressed)
{
ResetDeltas();
Pressing();
}
if (Mouse::Get()->Up(0))
{
bMousePressed = false;
ResetMouseValues();
ResetDeltas();
ResetHighlight();
ResetScaleGizmo();
}
//GizmoWorld
D3DXMatrixScaling(&scaleMat, scale.x, scale.y, scale.z);
D3DXMatrixTranslation(&posMat, position.x, position.y, position.z);
gizmoWorld = scaleMat*rotMat*posMat;
}
void Gizmo::GizmoComponent::Render()
{
D3D::GetDC()->OMSetDepthStencilState(depthStencilState[1], 1);
D3D::GetDC()->RSSetState(rasterizer[1]);
{
Draw2D();
Draw3D();
if (bDrawBounding == true)
DrawBounding();
}
D3D::GetDC()->RSSetState(rasterizer[0]);
D3D::GetDC()->OMSetDepthStencilState(depthStencilState[0], 1);
}
void Gizmo::GizmoComponent::PostRender()
{
ImGui::Begin("Gizmo");
ImGui::Checkbox("DrawBounding", &bDrawBounding);
ImGui::InputFloat3("Intersect Position", (float*)&intersectPosition);
ImGui::InputFloat3("LastIntersect Position", (float*)&lastIntersectPosition);
ImGui::InputFloat3("Delta", (float*)&delta);
ImGui::End();
}
void Gizmo::GizmoComponent::Draw2D()
{
for (size_t i = 0; i < quads.size(); i++)
{
if (activeMode == EGizmoMode::Translate)
{
quads[i]->rootWorld = gizmoWorld;
quads[i]->color = colors[i];
quads[i]->Render();
}
}
for (size_t i = 0; i < lines.size(); i++)
{
lines[i]->rootWorld = gizmoWorld;
lines[i]->color = colors[i];
lines[i]->Render();
}
}
void Gizmo::GizmoComponent::Draw3D()
{
for (int i = 0; i < 3; i++) //(order: x, y, z)
{
Gizmo* activeModel;
switch (activeMode)
{
case EGizmoMode::Translate:
activeModel = translates[i];
break;
case EGizmoMode::Rotate:
activeModel = rotates[i];
break;
case EGizmoMode::Scale:
activeModel = scales[i];
break;
}
activeModel->rootWorld = gizmoWorld;
activeModel->localWorld = geometryWorld[i];
activeModel->color = colors[i];
activeModel->Render();
}
}
void Gizmo::GizmoComponent::DrawBounding()
{
for (Gizmo* gizmo : boxes)
{
gizmo->rootWorld = gizmoWorld;
gizmo->Render();
}
for (Gizmo* gizmo : spheres)
{
gizmo->rootWorld = gizmoWorld;
gizmo->Render();
}
}
void Gizmo::GizmoComponent::Create2D()
{
//Quads
float halfLineOffset = LINE_OFFSET / 2;
quads.push_back(Gizmos::Quad(D3DXVECTOR3(halfLineOffset, halfLineOffset, 0), D3DXVECTOR3(0, 0, -1), D3DXVECTOR3(0, 1, 0), LINE_OFFSET,
LINE_OFFSET)); //XY
quads.push_back(Gizmos::Quad(D3DXVECTOR3(halfLineOffset, 0, halfLineOffset), D3DXVECTOR3(0, 1, 0), D3DXVECTOR3(1, 0, 0), LINE_OFFSET,
LINE_OFFSET)); //XZ
quads.push_back(Gizmos::Quad(D3DXVECTOR3(0, halfLineOffset, halfLineOffset), D3DXVECTOR3(1, 0, 0), D3DXVECTOR3(0, 1, 0), LINE_OFFSET,
LINE_OFFSET)); //ZY
//Lines
lines.push_back(Gizmos::Line({ 0,0,0 }, { LINE_LENGTH, 0,0 }));
lines.push_back(Gizmos::Line({ 0,0,0 }, { 0, LINE_LENGTH,0 }));
lines.push_back(Gizmos::Line({ 0,0,0 }, { 0, 0,LINE_LENGTH }));
}
void Gizmo::GizmoComponent::Create3D()
{
for (size_t i = 0; i < 3; i++)
{
scales.push_back(Gizmos::Scale());
rotates.push_back(Gizmos::Rotate());
translates.push_back(Gizmos::Translate());
}
}
void Gizmo::GizmoComponent::CreateBoundings()
{
//Boxes
const float MULTI_AXIS_THICKNESS = 0.05f;
const float SINGLE_AXIS_THICKNESS = 0.2f;
xAxisBox = new BoundingBox(Vector3(LINE_OFFSET, 0, 0),
Vector3(LINE_OFFSET + LINE_LENGTH, SINGLE_AXIS_THICKNESS, SINGLE_AXIS_THICKNESS));
boxes.push_back(Gizmos::WireCube({ LINE_OFFSET, 0, 0 },
{ LINE_OFFSET + LINE_LENGTH, SINGLE_AXIS_THICKNESS, SINGLE_AXIS_THICKNESS }));
yAxisBox = new BoundingBox(Vector3(0, LINE_OFFSET, 0),
Vector3(SINGLE_AXIS_THICKNESS, LINE_OFFSET + LINE_LENGTH, SINGLE_AXIS_THICKNESS));
boxes.push_back(Gizmos::WireCube({ 0, LINE_OFFSET, 0 },
{ SINGLE_AXIS_THICKNESS, LINE_OFFSET + LINE_LENGTH, SINGLE_AXIS_THICKNESS }));
zAxisBox = new BoundingBox(Vector3(0, 0, LINE_OFFSET),
Vector3(SINGLE_AXIS_THICKNESS, SINGLE_AXIS_THICKNESS, LINE_OFFSET + LINE_LENGTH));
boxes.push_back(Gizmos::WireCube({ 0, 0, LINE_OFFSET },
{ SINGLE_AXIS_THICKNESS, SINGLE_AXIS_THICKNESS, LINE_OFFSET + LINE_LENGTH }));
xzAxisBox = new BoundingBox(Vector3::Zero,
Vector3(LINE_OFFSET, MULTI_AXIS_THICKNESS, LINE_OFFSET));
boxes.push_back(Gizmos::WireCube({ 0, 0, 0 },
{ LINE_OFFSET, MULTI_AXIS_THICKNESS, LINE_OFFSET }));
xyAxisBox = new BoundingBox(Vector3::Zero,
Vector3(LINE_OFFSET, LINE_OFFSET, MULTI_AXIS_THICKNESS));
boxes.push_back(Gizmos::WireCube({ 0, 0, 0 },
{ LINE_OFFSET, LINE_OFFSET, MULTI_AXIS_THICKNESS }));
yzAxisBox = new BoundingBox(Vector3::Zero,
Vector3(MULTI_AXIS_THICKNESS, LINE_OFFSET, LINE_OFFSET));
boxes.push_back(Gizmos::WireCube({ 0, 0, 0 },
{ MULTI_AXIS_THICKNESS, LINE_OFFSET, LINE_OFFSET }));
//Spheres
const float RADIUS = 1.0f;
D3DXVECTOR3 position = lines[0]->vertexData[1].position;
Vector3 temp = { position.x, position.y, position.z };
xSphere = new BoundingSphere(temp, RADIUS);
spheres.push_back(Gizmos::WireSphere(position, RADIUS));
position = lines[1]->vertexData[1].position;
temp = { position.x, position.y, position.z };
ySphere = new BoundingSphere(temp, RADIUS);
spheres.push_back(Gizmos::WireSphere(position, RADIUS));
position = lines[2]->vertexData[1].position;
temp = { position.x, position.y, position.z };
zSphere = new BoundingSphere(temp, RADIUS);
spheres.push_back(Gizmos::WireSphere(position, RADIUS));
}
void Gizmo::GizmoComponent::ResetHighlight()
{
for (Gizmo* gizmo : quads)
gizmo->bHighlight = false;
for (Gizmo* gizmo : lines)
gizmo->bHighlight = false;
for (Gizmo* gizmo : scales)
gizmo->bHighlight = false;
for (Gizmo* gizmo : rotates)
gizmo->bHighlight = false;
for (Gizmo* gizmo : translates)
gizmo->bHighlight = false;
}
void Gizmo::GizmoComponent::ResetMouseValues()
{
intersectPosition = lastIntersectPosition = delta = Vector3::Zero;
curMousePos = lastMousePos = { 0,0,0 };
}
void Gizmo::GizmoComponent::ResetDeltas()
{
translationDelta = scaleDelta = { 0,0,0 };
D3DXMatrixIdentity(&rotationDelta);
}
void Gizmo::GizmoComponent::ResetScaleGizmo()
{
geometryWorld[0] = Matrix::CreateWorld(Vector3(LINE_LENGTH, 0, 0), Vector3::Left, Vector3::Up).ToD3DXMATRIX();
geometryWorld[1] = Matrix::CreateWorld(Vector3(0, LINE_LENGTH, 0), Vector3::Down, Vector3::Left).ToD3DXMATRIX();
geometryWorld[2] = Matrix::CreateWorld(Vector3(0, 0, LINE_LENGTH), Vector3::Forward, Vector3::Up).ToD3DXMATRIX();
lines[0]->vertexData[1].position = { LINE_LENGTH, 0,0 };
lines[1]->vertexData[1].position = { 0, LINE_LENGTH,0 };
lines[2]->vertexData[1].position = { 0, 0,LINE_LENGTH };
for (size_t i = 0; i < lines.size(); i++)
{
lines[i]->UpdateVertexData();
}
}
Ray Gizmo::GizmoComponent::ConvertMouseToRay(D3DXMATRIX& world)
{
//mouse pos, dir
D3DXVECTOR3 start;
values->MainCamera->GetPosition(&start);
D3DXVECTOR3 dir = values->MainCamera->GetDirection(values->Viewport, values->Perspective);
//inverse
D3DXMATRIX invMat;
D3DXMatrixInverse(&invMat, NULL, &world);
D3DXVec3TransformCoord(&start, &start, &invMat);
D3DXVec3TransformNormal(&dir, &dir, &invMat);
//ray
Ray ray = Ray(Vector3(start.x, start.y, start.z), Vector3(dir.x, dir.y, dir.z));
return ray;
}
void Gizmo::GizmoComponent::ResizeWorldScale()
{
if (target != NULL)
{
D3DXVECTOR3 targetPos, cameraPos, distance;
targetPos = { target->_41, target->_42, target->_43 };
values->MainCamera->GetPosition(&cameraPos);
distance = targetPos - cameraPos;
float scaleValue = D3DXVec3Length(&distance);
scaleValue /= RESIZE_RATIO;
scale = { scaleValue,scaleValue,scaleValue };
}
}
<file_sep>#include "stdafx.h"
#include "ModelTool.h"
#include "../Objects/GameAnimationModel.h"
#include "../Objects/ToolsModel.h"
#include "../Model/ModelAnimPlayer.h"
#include "../Objects/Gizmo.h"
#include "../Objects/GizmoComponent.h"
#include "../Fbx/Exporter.h"
ModelTool::ModelTool(ExecuteValues * values)
: Execute(values)
, player(NULL)
, currentSelectBone(-1)
{
wstring matFile = L"";
wstring meshFile = L"";
ExportModel();
//Grund
matFile = Models + L"RPG/Character.material";
meshFile = Models + L"RPG/Character.mesh";
player = new ToolsModel(Models + L"RPG/", matFile, meshFile);
player->AddClip(Models + L"RPG/Attack-Kick-L1.anim");
component = new Gizmo::GizmoComponent(values);
}
ModelTool::~ModelTool()
{
SAFE_DELETE(player);
}
void ModelTool::Update()
{
if (player != NULL) player->Update();
if (currentSelectBone != -1)component->Update();
}
void ModelTool::Render()
{
if (player != NULL) player->Render();
if (currentSelectBone != -1)component->Render();
}
void ModelTool::PostRender()
{
if (values->GuiSettings->bShowModelWindow == true)
{
ImGui::Begin("Model Info", &values->GuiSettings->bShowModelWindow);
if (ImGui::CollapsingHeader("ModelBone"))
{
ImGuiStyle& style = ImGui::GetStyle();
style.IndentSpacing = 15.0f;
CreateModelTrees(player->GetModel()->Bone(1));
}
if (ImGui::CollapsingHeader("SelectedBone"))
{
if (currentSelectBone != -1)
{
ModelBone* currentSelectedBone = player->GetModel()->Bone(currentSelectBone);
//string current = String::ToString(currentSelectedBone->Name());
ImGui::Text(String::ToString(currentSelectedBone->Name()).c_str());
}
}
if (ImGui::CollapsingHeader("Animation"))
{
ControlAnimPlayer();
}
ImGui::End();
}
}
void ModelTool::CreateModelTrees(ModelBone * bone)
{
string name = String::ToString(bone->Name());
UINT childCount = bone->ChildCount();
int index = bone->Index();
string sNumber = to_string(index) + ". ";
string sNumName = sNumber + name;
const char* cName = name.c_str();
const char* cNumber = sNumber.c_str();
const char* cNumberName = sNumName.c_str();
if (childCount == 0)
{
ImGui::Indent();
if (ImGui::Selectable(cNumberName))
currentSelectBone = index;
ImGui::Unindent();
}
else
{
if (ImGui::TreeNodeEx(cNumber, ImGuiTreeNodeFlags_OpenOnArrow))
{
ImGui::SameLine();
if (ImGui::Selectable(cName))
{
currentSelectBone = index;
targetMat = player->GetModel()->Bone(currentSelectBone)->AbsoluteTransform();
D3DXVECTOR3 pos = { targetMat._41,targetMat._42,targetMat._43 };
component->Position(pos);
component->SetTarget(&player->GetModel()->Bone(index)->Transform());
}
for (size_t i = 0; i < childCount; i++)
{
ModelBone* child = bone->Child(i);
CreateModelTrees(child);
}
ImGui::TreePop();
}
}
}
void ModelTool::ControlAnimPlayer()
{
}
D3DXMATRIX ModelTool::reculsiveBone_Transform(int index)
{
D3DXMATRIX result;
result = player->GetModel()->Bone(index)->Transform();
if (player->GetModel()->Bone(index)->Parent() != NULL)
{
result = result* player->GetModel()->Bone(index)->Parent()->Transform() ;
}
return result;
}
void ModelTool::ExportModel()
{
//Fbx::Exporter* exporter = new Fbx::Exporter(Assets + L"RPG_Character/RPG-Character.FBX");
//exporter->ExportMaterial(Models + L"RPG/", L"Character");
//exporter->ExportMeshData(Models + L"RPG/", L"Character");
Fbx::Exporter* exporter = new Fbx::Exporter(Assets + L"RPG_Character/[email protected]");
exporter->ExportAnimation(Models + L"RPG/", L"Attack-Kick-L1");
SAFE_DELETE(exporter);
exporter = new Fbx::Exporter(Assets + L"RPG_Character/[email protected]");
exporter->ExportAnimation(Models + L"RPG/", L"Attack-Kick-R1");
SAFE_DELETE(exporter);
}
<file_sep>#include "MathHelper.h"
#include <math.h>
#include "Vector3.h"
//////////////////////////////////////////////////////////////////////////
const float MathHelper::E = 2.718282f;
const float MathHelper::Log2E = 1.442695f;
const float MathHelper::Log10E = 0.4342945f;
const float MathHelper::Pi = 3.141593f;
const float MathHelper::TwoPi = 6.283185f;
const float MathHelper::PiOver2 = 1.570796f;
const float MathHelper::PiOver4 = 0.7853982f;
const float MathHelper::Epsilon = 1E-6f;
#pragma warning( disable : 4146)
const int MathHelper::IntMinValue = -2147483648;
const int MathHelper::IntMaxValue = 2147483647;
const float MathHelper::FloatMinValue = -3.402823E+38f;
const float MathHelper::FloatMaxValue = 3.402823E+38f;
#pragma warning( default : 4146)
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
//////////////////////////////////////////////////////////////////////////
MathHelper::MathHelper( void )
{
}
//////////////////////////////////////////////////////////////////////////
///////////////////////////////////////////////////////////////////////////
///@brief 반올림
///@param value : 값
///@return : 결과 값
//////////////////////////////////////////////////////////////////////////
double MathHelper::Round( double value )
{
return Round(value, 0);
}
//////////////////////////////////////////////////////////////////////////
///////////////////////////////////////////////////////////////////////////
///@brief 반올림
///@param value : 값
///@param position : 올림 자릿수
///@return : 결과
//////////////////////////////////////////////////////////////////////////
double MathHelper::Round( double value, int position )
{
double temp;
temp = value * pow(10.0, position); //원하는 소수점 자리만큼의 10의 누승
temp = floor(temp + 0.5); //0.5를 더해서 가장 가까운 낮은값을 찾음( ex=floor(0.2+0.5)->1.0, floor(0.7+0.5)->1.0f)
temp *= pow(10.0, -position); //역승해 원값으로 돌림
return temp;
}
//////////////////////////////////////////////////////////////////////////
///////////////////////////////////////////////////////////////////////////
///@brief 절대값
///@param value : 값
///@return 결과
//////////////////////////////////////////////////////////////////////////
int MathHelper::Abs( int value )
{
if(value >= 0)
return value;
else
return -value;
}
//////////////////////////////////////////////////////////////////////////
///////////////////////////////////////////////////////////////////////////
///@brief 절대값
///@param value : 값
///@return 결과
//////////////////////////////////////////////////////////////////////////
float MathHelper::Abs( float value )
{
if(value >= 0)
return value;
else
return -value;
}
//////////////////////////////////////////////////////////////////////////
///////////////////////////////////////////////////////////////////////////
///@brief 절대값
///@param value : 값
///@return 결과
//////////////////////////////////////////////////////////////////////////
double MathHelper::Abs( double value )
{
if(value >= 0)
return value;
else
return -value;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 각 -> 라디안
///@param degree : 각도
///@return 결과
//////////////////////////////////////////////////////////////////////////
float MathHelper::ToRadians( float degrees )
{
return degrees * 0.01745329f;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 라디안 -> 각
///@param radians : 라디안
///@return 결과
//////////////////////////////////////////////////////////////////////////
float MathHelper::ToDegrees( float radians )
{
return radians * 57.29578f;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 실수의 거리
///@param value1 : 값1
///@param value2 : 값2
///@return 결과
//////////////////////////////////////////////////////////////////////////
float MathHelper::Distance( float value1, float value2 )
{
return Abs(value1 - value2);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 실수 중 작은 값
///@param value1 : 값1
///@param value2 : 값2
///@return 결과
//////////////////////////////////////////////////////////////////////////
float MathHelper::Min( float value1, float value2 )
{
return value1 > value2 ? value2 : value1;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 실수 중 큰 값
///@param value1 : 값1
///@param value2 : 값2
///@return 결과
//////////////////////////////////////////////////////////////////////////
float MathHelper::Max( float value1, float value2 )
{
return value1 > value2 ? value1 : value2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 값 비교
///@param value : 값
///@param min : 최소값
///@param max : 최대값
///@return 값이 최대값보다 크면 최대값, 최소값보다 작으면 최소값, 아니면 원래 값
//////////////////////////////////////////////////////////////////////////
float MathHelper::Clamp( float value, float min, float max )
{
value = (double) value > (double) max ? max : value;
value = (double) value < (double) min ? min : value;
return value;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 선형보간
///@param value1 : 값1
///@param value12: 값2
///@param amount : 보간값
///@return 결과
//////////////////////////////////////////////////////////////////////////
float MathHelper::Lerp( float value1, float value2, float amount )
{
return value1 + (value2 - value1) * amount;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 부드럽게(점진적으로?) 보간
///@param value1 : 값1
///@param value12: 값2
///@param amount : 보간값
///@return 결과
//////////////////////////////////////////////////////////////////////////
float MathHelper::SmoothStep( float value1, float value2, float amount )
{
float num = Clamp(amount, 0.0f, 1.0f);
return Lerp(value1, value2, (float)((double)num * (double)num * (3.0 - 2.0 * (double)num)));
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 카트뮬-롬 보간
///@param value1 : 값1
///@param value2 : 값2
///@param value3 : 값3
///@param value4 : 값4
///@param amount : 보간값
///@return 결과
//////////////////////////////////////////////////////////////////////////
float MathHelper::CatmullRom( float value1, float value2, float value3, float value4, float amount )
{
float num1 = amount * amount;
float num2 = amount * num1;
return (float)(0.5 * (2.0 * (double)value2 + (-(double)value1 + (double)value3)* (double)amount + (2.0 * (double)value1 - 5.0 * (double)value2 + 4.0 * (double)value3 - (double)value4)* (double)num1 + (-(double)value1 + 3.0 * (double)value2 - 3.0 * (double)value3 + (double)value4)* (double)num2));
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 에르미트 보간
///@param value1 : 값1
///@param tangent1 : 탄젠트1
///@param value2 : 값2
///@param tangent2 : 탄젠트2
///@param amount : 보간값
///@return 결과
//////////////////////////////////////////////////////////////////////////
float MathHelper::Hermite( float value1, float tangent1, float value2, float tangent2, float amount )
{
float num1 = amount;
float num2 = num1 * num1;
float num3 = num1 * num2;
float num4 = (float)(2.0 * (double)num3 - 3.0 * (double)num2 + 1.0);
float num5 = (float)(-2.0 * (double)num3 + 3.0 * (double)num2);
float num6 = num3 - 2.0f * num2 + num1;
float num7 = num3 - num2;
return (float)((double)value1 * (double)num4 + (double)value2 * (double)num5 + (double)tangent1 * (double)num6 + (double)tangent2 * (double)num7);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 각이 360도를 넘어섰을때 각을 뒤집어줌(? 표현 진짜 거시기허네)
///@param angle : 각
///@return 결과
//////////////////////////////////////////////////////////////////////////
float MathHelper::WrapAngle( float angle )
{
angle = (float)fmod((double) angle, 6.28318548202515);
if ((double)angle <= -3.14159274101257)
angle += 6.283185f;
else if ((double)angle > 3.14159274101257)
angle -= 6.283185f;
return angle;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief y=0인 수직평면으로부터 value.Y에 대한 각도를 계산한다(아따, 요것도 표현이 쪼까 거시기허요잉)
///@param value : y축 값을 구할 벡터
//////////////////////////////////////////////////////////////////////////
float MathHelper::AngleOfInclineY( Vector3 value )
{
if(value == Vector3::Up)
return 90.0f;
return ToDegrees((float)acos(Vector3::Dot(value, Vector3(value.X, 0, value.Z))));
}<file_sep>#pragma once
#include "GameModel.h"
#include "AnimationClip.h"
class GameAnimationModel : public GameModel
{
public:
GameAnimationModel(wstring meshFile, wstring matFile);
virtual ~GameAnimationModel();
UINT AddClip(wstring file);
UINT AddClip(AnimationClip* clip);
void RemoveClip(UINT index);
void ClearClip();
AnimationClip* GetClip(UINT index);
bool Play(UINT index, AnimationPlayMode mode);
bool Play(UINT index, float startTime, float blendTime, float speed, AnimationPlayMode mode);
float GetPlayTime(wstring boneName);
Model* GetModel() { return model; }
virtual void Update();
virtual void Render();
protected:
struct AnimationBlender* GetBlenderFromBoneName(wstring name);
protected:
vector<AnimationClip *> clips;
vector<struct AnimationBlender *> blenders;
};<file_sep>#pragma once
#include <string>
#pragma warning( disable : 4996)
struct D3DXVECTOR3;
class Matrix;
class Quaternion;
//////////////////////////////////////////////////////////////////////////
///@brief 3D 벡터
//////////////////////////////////////////////////////////////////////////
class Vector3
{
public:
Vector3(void);
Vector3(float value);
Vector3(float x, float y, float z);
Vector3 operator -(void);
bool operator ==(const Vector3& value2) const;
bool operator !=(const Vector3& value2) const;
Vector3 operator +(const Vector3& value2) const;
Vector3 operator -(const Vector3& value2) const;
Vector3 operator *(const Vector3& value2) const;
Vector3 operator *(const float& scaleFactor) const;
Vector3 operator /(const Vector3& value2) const;
Vector3 operator /(const float& divider) const;
void operator +=(const Vector3& value2);
void operator -=(const Vector3& value2);
void operator *=(const Vector3& value2);
void operator *=(const float& scaleFactor);
void operator /=(const Vector3& value2);
void operator /=(const float& divider);
//////////////////////////////////////////////////////////////////////////
///@brief float형의 *연산 처리
///@param scalefactor : 값
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
friend Vector3 operator *(const float scaleFactor, const Vector3& value2)
{
return value2 * scaleFactor;
}
//////////////////////////////////////////////////////////////////////////
std::string ToString();
D3DXVECTOR3 ToD3DXVECTOR3();
float Length();
float LengthSquared();
void Normalize();
static float Distance(Vector3 value1, Vector3 value2);
static float DistanceSquared(Vector3 value1, Vector3 value2);
static float Dot(Vector3 value1, Vector3 value2);
static Vector3 Normalize(Vector3 value);
static Vector3 Cross(Vector3 vector1, Vector3 vector2);
static Vector3 Reflect(Vector3 vector, Vector3 normal);
static Vector3 Min(Vector3 value1, Vector3 value2);
static Vector3 Max(Vector3 value1, Vector3 value2);
static Vector3 Clamp(Vector3 value1, Vector3 min, Vector3 max);
static Vector3 Lerp(Vector3 value1, Vector3 value2, float amount);
static Vector3 SmoothStep(Vector3 value1, Vector3 value2, float amount);
static Vector3 CatmullRom(Vector3 value1, Vector3 value2, Vector3 value3, Vector3 value4, float amount);
static Vector3 Hermite(Vector3 value1, Vector3 tangent1, Vector3 value2, Vector3 tangent2, float amount);
static Vector3 Transform(Vector3 position, Matrix matrix);
static Vector3 Transform(Vector3 value, Quaternion rotation);
static Vector3 TransformNormal(Vector3 normal, Matrix matrix);
static Vector3 TransformCoord(Vector3 position, Matrix matrix);
public:
const static Vector3 Zero;///< 0.0f, 0.0f, 0.0f
const static Vector3 One;///< 1.0f, 1.0f, 1.0f
const static Vector3 UnitX;///< 1.0f, 0.0f, 0.0f
const static Vector3 UnitY;///< 0.0f, 1.0f, 0.0f
const static Vector3 UnitZ;///< 0.0f, 0.0f, 1.0f
const static Vector3 Up;///< 0.0f, 1.0f, 0.0f
const static Vector3 Down;///< 0.0f, -1.0f, 0.0f
const static Vector3 Right;///< 1.0f, 0.0f, 0.0f
const static Vector3 Left;///< -1.0f, 0.0f, 0.0f
const static Vector3 Forward;///< 0.0f, 0.0f, -1.0f
const static Vector3 Backward;///< 0.0f, 0.0f, 1.0f
float X;///< X
float Y;///< Y
float Z;///< Z
};<file_sep>#pragma once
#include "../Objects/GameAnimationModel.h"
class ToolsModel: public GameAnimationModel
{
public:
enum class LowerAction;
enum class UpperAction;
public:
ToolsModel(wstring animPath, wstring matFile, wstring meshFile);
~ToolsModel();
void Update();
void Render();
private:
};<file_sep>[Window][Debug##Default]
Pos=106,231
Size=400,400
Collapsed=0
[Window][System Info]
Pos=580,23
Size=336,155
Collapsed=0
[Window][Environment]
Pos=15,32
Size=538,336
Collapsed=0
[Window][Landscape]
Pos=142,156
Size=487,222
Collapsed=0
[Window][Model Info]
Pos=21,27
Size=383,698
Collapsed=0
[Window][ImGui Demo]
Pos=60,60
Size=550,680
Collapsed=0
<file_sep>#include "stdafx.h"
#include "ToolsModel.h"
ToolsModel::ToolsModel(wstring animPath, wstring matFile, wstring meshFile)
: GameAnimationModel(matFile, meshFile)
{
}
ToolsModel::~ToolsModel()
{
}
void ToolsModel::Update()
{
GameAnimationModel::Update();
}
void ToolsModel::Render()
{
GameAnimationModel::Render();
}
<file_sep>#pragma once
#include <string>
#pragma warning( disable : 4996)
struct D3DXQUATERNION;
class Vector3;
class Matrix;
//////////////////////////////////////////////////////////////////////////
///@brief 사원수
//////////////////////////////////////////////////////////////////////////
class Quaternion
{
public:
Quaternion(void);
Quaternion(float x, float y, float z, float w);
Quaternion(Vector3 vectorPart, float scalarPart);
Quaternion operator -(void);
bool operator ==(const Quaternion& quaternion2) const;
bool operator !=(const Quaternion& quaternion2) const;
Quaternion operator +(const Quaternion& quaternion2) const;
Quaternion operator -(const Quaternion& quaternion2) const;
Quaternion operator *(const Quaternion& quaternion2) const;
Quaternion operator *(const float& scaleFactor) const;
Quaternion operator /(const Quaternion& quaternion2) const;
void operator +=(const Quaternion& quaternion2);
void operator -=(const Quaternion& quaternion2);
void operator *=(const Quaternion& quaternion2);
void operator *=(const float& scaleFactor);
void operator /=(const Quaternion& quaternion2);
//////////////////////////////////////////////////////////////////////////
///@brief float형의 *연산 처리
///@param scalefactor : 값
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
friend Quaternion operator *(const float scaleFactor, const Quaternion& value2)
{
return value2 * scaleFactor;
}
//////////////////////////////////////////////////////////////////////////
std::string ToString();
D3DXQUATERNION ToD3DXQUATERNION();
float Length();
float LengthSquared();
void Normalize();
static Quaternion Normalize(Quaternion quaternion);
void Conjugate();
static Quaternion Conjugate(Quaternion value);
static Quaternion Inverse(Quaternion quaternion);
static Quaternion CreateFromAxisAngle(Vector3 axis, float angle);
static Quaternion CreateFromYawPitchRoll(float yaw, float pitch, float roll);
static Quaternion CreateFromRotationMatrix(Matrix matrix);
static float Dot(Quaternion quaternion1, Quaternion quaternion2);
static Quaternion Slerp(Quaternion quaternion1, Quaternion quaternion2, float amount);
static Quaternion Lerp(Quaternion quaternion1, Quaternion quaternion2, float amount);
static Quaternion Concatenate(Quaternion value1, Quaternion value2);
public:
const static Quaternion Identity;///< 0.0f, 0.0f, 0.0f, 1.0f
float X;///< X
float Y;///< Y
float Z;///< Z
float W;///< W
};<file_sep>#pragma once
#include "GameUnit.h"
class GamePlayer : public GameUnit
{
public:
enum class LowerAction;
enum class UpperAction;
public:
GamePlayer(wstring animPath, wstring matFile, wstring meshFile);
~GamePlayer();
void Update();
void Render();
private:
void HandleInput();
void LoadAnimation(wstring path);
void PlayLowerAction(LowerAction action);
void PlayLowerAction(LowerAction action, float startTime);
void PlayUpperAction(UpperAction action);
void PlayUpperAction(UpperAction action, float startTime);
void ActionMovement(D3DXVECTOR3 direction);
private:
struct GamePlayerSpec* specData;
struct GamePlayerInput* input;
bool bRunning;
D3DXVECTOR3 moveDirection;
float moveElapsedTime;
float actionElapsedTime;
float rootRotationAngle;
float rootElapsedAngle;
bool bActiveBooster;
float runDurationTime;
float walkDurationTime;
float boosterFinishDurationTime;
float boosterBreakDurationTime;
float weaponChangeDurationTime;
private:
enum class DebugMode
{
None = 0, NeverDie, Superman, God,
};
DebugMode debugMode;
enum class LowerAction
{
Unknown = 0,
Idle, Run, Damage, Walk, BackWalk, LeftTurn, RightTurn,
ForwardDead, BackwardDead, LeftDead, RightDead,
BoosterPrepare, BoosterActive, BoosterFinish, BoosterBreak,
BoosterLeftTurn, BoosterRightTurn,
Count,
};
enum class UpperAction
{
Unknown = 0,
Idle, Run, Damage, WeaponChange,
ForwardNonFire, LeftNonFire, RightNonFire,
ForwardMachineGunFire, LeftMachineGunFire, RightMachineGunFire, ReloadMachineGun,
ForwardShotgunFire, LeftShotgunFire, RightShotgunFire, ReloadShotgun,
ForwardHandgunFire, LeftHandgunFire, RightHandgunFire, ReloadHandgun,
ForwardDead, BackwardDead, LeftDead, RightDead,
BoosterPrepare, BoosterActive, BoosterFinish, BoosterBreak,
BoosterLeftTurn, BoosterRightTurn,
Count,
};
private:
LowerAction currentLowerAction;
UpperAction currentUpperAction;
LowerAction prepareLowerAction;
UpperAction prepareUpperAction;
bool bOverwriteLowerAction;
bool bOverwriteUpperAction;
vector<UINT> indexLowerAnimations;
vector<UINT> indexUpperAnimations;
};<file_sep>#pragma once
#include <string>
#pragma warning( disable : 4996)
struct D3DXVECTOR4;
class Vector2;
class Vector3;
class Matrix;
class Quaternion;
//////////////////////////////////////////////////////////////////////////
///@brief 4D 벡터
//////////////////////////////////////////////////////////////////////////
class Vector4
{
public:
Vector4(void);
Vector4(float x, float y, float z, float w);
Vector4(Vector2 value, float z, float w);
Vector4(Vector3 value, float w);
Vector4(float value);
Vector4 operator -(void);
bool operator ==(const Vector4& value2) const;
bool operator !=(const Vector4& value2) const;
Vector4 operator +(const Vector4& value2) const;
Vector4 operator -(const Vector4& value2) const;
Vector4 operator *(const Vector4& value2) const;
Vector4 operator *(const float& scaleFactor) const;
Vector4 operator /(const Vector4& value2) const;
Vector4 operator /(const float& divider) const;
void operator +=(const Vector4& value2);
void operator -=(const Vector4& value2);
void operator *=(const Vector4& value2);
void operator *=(const float& scaleFactor);
void operator /=(const Vector4& value2);
void operator /=(const float& divider);
//////////////////////////////////////////////////////////////////////////
///@brief float형의 *연산 처리
///@param scalefactor : 값
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
friend Vector4 operator *(const float scaleFactor, const Vector4& value2)
{
return value2 * scaleFactor;
}
//////////////////////////////////////////////////////////////////////////
std::string ToString();
D3DXVECTOR4 ToD3DXVECTOR4();
float Length();
float LengthSquared();
void Normalize();
static float Distance(Vector4 value1, Vector4 value2);
static float DistanceSquared(Vector4 value1, Vector4 value2);
static float Dot(Vector4 value1, Vector4 value2);
static Vector4 Normalize(Vector4 value);
static Vector4 Min(Vector4 value1, Vector4 value2);
static Vector4 Max(Vector4 value1, Vector4 value2);
static Vector4 Clamp(Vector4 value1, Vector4 min, Vector4 max);
static Vector4 Lerp(Vector4 value1, Vector4 value2, float amount);
static Vector4 SmoothStep(Vector4 value1, Vector4 value2, float amount);
static Vector4 CatmullRom(Vector4 value1, Vector4 value2, Vector4 value3, Vector4 value4, float amount);
static Vector4 Hermite(Vector4 value1, Vector4 tangent1, Vector4 value2, Vector4 tangent2, float amount);
static Vector4 Transform(Vector2 position, Matrix matrix);
static Vector4 Transform(Vector3 position, Matrix matrix);
static Vector4 Transform(Vector4 position, Matrix matrix);
static Vector4 Transform(Vector2 value, Quaternion rotation);
static Vector4 Transform(Vector3 value, Quaternion rotation);
static Vector4 Transform(Vector4 value, Quaternion rotation);
public:
const static Vector4 Zero;///< 0.0f, 0.0f, 0.0f, 0.0f
const static Vector4 One;///< 1.0f, 1.0f, 1.0f, 1.0f
const static Vector4 UnitX;///< 1.0f, 0.0f, 0.0f, 0.0f
const static Vector4 UnitY;///< 0.0f, 1.0f, 0.0f, 0.0f
const static Vector4 UnitZ;///< 0.0f, 0.0f, 1.0f, 0.0f
const static Vector4 UnitW;///< 0.0f, 0.0f, 1.0f, 1.0f
float X;///< X
float Y;///< Y
float Z;///< Z
float W;///< W
};<file_sep>#pragma once
#include "GameModel.h"
class GameSkyBox : public GameModel
{
public:
GameSkyBox(wstring matFile, wstring meshFile);
~GameSkyBox();
};<file_sep>
#pragma once
namespace Gizmo
{
class GizmoComponent;
}
class ModelTool : public Execute
{
public:
ModelTool(ExecuteValues* values);
~ModelTool();
void Update();
void PreRender() {}
void Render();
void PostRender();
void ResizeScreen() {}
private:
void CreateModelTrees(class ModelBone* bone);
void ControlAnimPlayer();
D3DXMATRIX reculsiveBone_Transform(int index);
void ExportModel();
private:
int currentSelectBone;
D3DXMATRIX targetMat;
class ToolsModel* player;
class Exporter* exporter;
Gizmo::GizmoComponent* component;
};<file_sep>#pragma once
#include "stdafx.h"
enum class UnitClassId
{
Unknown = 0,
LightMech, HeavyMech, Tank, Boss,
Count,
};
enum class UnitTypeId
{
Unknown = 0,
//Player
Grund, Kiev, Mark, Yager,
//Enemy
Cameleer, Maoming, Duskmas, Hammer, Tiger, PhantomBoss,
Count,
};
struct GameDataSpec
{
wstring file;
};
struct GamePlayerSpec : public GameDataSpec
{
UnitTypeId Type = UnitTypeId::Grund;
UnitClassId Class = UnitClassId::LightMech;
UINT Life = 3500;
float MechRadius = 2.0f;
float RunSpeed = 7.0f;
float WalkSpeed = 5.0f;
float WalkBackwardSpeed = 4.0f;
float TurnAngle = 45.0f;
float CriticalDamageTime = 1.0f;
float BoosterSpeed = 32.0f;
float BoosterActiveTime = 1.5f;
float BoosterPrepareTime = 0.5f;
float BoosterCoolTime = 8.0f;
float BoosterTurnAngle = 70.0f;
wstring MaterialFile = L"";
wstring MeshFile = L"";
wstring AnimationFolder = L"";
wstring DefaultWeaponFile = L"";
D3DXVECTOR3 CameraTargetOffset = D3DXVECTOR3(0, 2, 0);
D3DXVECTOR3 CameraPositionOffset = D3DXVECTOR3(0, 0, -5);
};
<file_sep>#pragma once
class ModelAnimPlayer
{
public:
enum class Mode
{
Play = 0, Pause, Stop,
};
ModelAnimPlayer(class Model* model);
~ModelAnimPlayer();
void SetMode(Mode value) { mode = value; }
Mode GetMode() { return mode; }
void Update();
void Render();
private:
void UpdateTime();
void UpdateBone();
private:
Shader* shader;
class Model* model;
class ModelAnimClip* currentClip;
Mode mode;
bool bUseSlerp;
int currentKeyframe;
int nextKeyframe;
float frameTime; //현재 프레임으로부터 경과시간
float frameFactor; //현재 프레임과 다음 프레임 사이의 보간값
vector<D3DXMATRIX> boneAnimation; //본이 애니메이션 계산된 행렬
vector<D3DXMATRIX> renderTransform; //스키닝이 계산된 렌더용 행렬
};<file_sep>#pragma once
#include <string>
#pragma warning( disable : 4996)
#include "Vector3.h"
enum PlaneIntersectionType;
struct D3DXPLANE;
class Vector4;
class Matrix;
class Quaternion;
class BoundingBox;
class BoundingFrustum;
class BoundingSphere;
//////////////////////////////////////////////////////////////////////////
///@brief 평면
//////////////////////////////////////////////////////////////////////////
class Plane
{
public:
Plane(void);
Plane(float a, float b, float c, float d);
Plane(Vector3 normal, float d);
Plane(Vector4 value);
Plane(Vector3 point1, Vector3 point2, Vector3 point3);
bool operator ==(const Plane& value) const;
bool operator !=(const Plane& value) const;
std::string ToString();
D3DXPLANE ToD3DXPLANE();
void Normalize();
float Dot(Vector4 value);
float DotCoordinate(Vector3 value);
float DotNormal(Vector3 value);
static Plane Normalize(Plane value);
static Plane Transform(Plane plane, Matrix matrix);
static Plane Transform(Plane plane, Quaternion rotation);
PlaneIntersectionType Intersects(BoundingBox box);
PlaneIntersectionType Intersects(BoundingFrustum frustum);
PlaneIntersectionType Intersects(BoundingSphere sphere);
public:
Vector3 Normal;///< 노멀 벡터
float D;///< 원점으로부터의 거리
};<file_sep>#pragma once
#include "../Core/Vector3.h"
enum PlaneIntersectionType;
enum ContainmentType;
class BoundingSphere;
class BoundingFrustum;
class Ray;
class Plane;
//////////////////////////////////////////////////////////////////////////
///@brief 충돌 박스
//////////////////////////////////////////////////////////////////////////
class BoundingBox
{
public:
BoundingBox(void);
BoundingBox(Vector3 min, Vector3 max);
bool operator ==(const BoundingBox& value) const;
bool operator !=(const BoundingBox& value) const;
void GetCorners(Vector3* pCorner);
std::string ToString();
Vector3 SupportMapping(Vector3 v);
static BoundingBox CreateMerged(BoundingBox original, BoundingBox additional);
static BoundingBox CreateFromSphere(BoundingSphere sphere);
bool Intersects(BoundingBox box);
bool Intersects(BoundingFrustum frustum);
PlaneIntersectionType Intersects(Plane plane);
bool Intersects(Ray ray, float* result);
bool Intersects(BoundingSphere sphere);
ContainmentType Contains(BoundingBox box);
ContainmentType Contains(BoundingFrustum frustum);
ContainmentType Contains(Vector3 point);
ContainmentType Contains(BoundingSphere sphere);
public:
const static int CornerCount;///< 모서리 갯수
Vector3 Min;///< 최소위치
Vector3 Max;///< 최대위치
};<file_sep>#include "Ray.h"
#include "BoundingBox.h"
#include "BoundingFrustum.h"
#include "BoundingSphere.h"
#include "../Core/Plane.h"
#include "../Core/MathHelper.h"
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
//////////////////////////////////////////////////////////////////////////
Ray::Ray( void )
{
this->Position = Vector3::Zero;
this->Direction = Vector3::Zero;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param Position : 위치
///@param Direction : 방향
//////////////////////////////////////////////////////////////////////////
Ray::Ray( Vector3 position, Vector3 direction )
{
this->Position = position;
this->Direction = direction;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief ==
///@param value : 레이
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Ray::operator==( const Ray& value ) const
{
if((double)Position.X == (double)value.Position.X && (double)Position.Y == (double)value.Position.Y && ((double)Position.Z == (double)value.Position.Z && (double)Direction.X == (double)value.Direction.X)&& (double)Direction.Y == (double)value.Direction.Y)
return (double)Direction.Z == (double)value.Direction.Z;
else
return false;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief !=
///@param value : 레이
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Ray::operator!=( const Ray& value ) const
{
if((double)Position.X == (double)value.Position.X && (double)Position.Y == (double)value.Position.Y && ((double)Position.Z == (double)value.Position.Z && (double)Direction.X == (double)value.Direction.X)&& (double)Direction.Y == (double)value.Direction.Y)
return (double)Direction.Z != (double)value.Direction.Z;
else
return true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 문자열로 변환
///@param 문자열
//////////////////////////////////////////////////////////////////////////
std::string Ray::ToString()
{
std::string temp;
temp += "{{Position:" + Position.ToString() + "}, ";
temp += "{Direction:" + Direction.ToString() + "}}";
return temp;
}
//////////////////////////////////////////////////////////////////////////
bool Ray::Intersects(Plane & plane, float& value)
{
float x = plane.Normal.X * this->Direction.X + plane.Normal.Y * this->Direction.Y + plane.Normal.Z * this->Direction.Z;
if (MathHelper::Abs(x) < 1E-05f)
{
value = 0.0f;
return false;
}
float single = plane.Normal.X * this->Position.X + plane.Normal.Y * this->Position.Y + plane.Normal.Z * this->Position.Z;
float d = (-plane.D - single) / x;
if (d < 0.0f)
{
if (d < -1E-05f)
{
value = 0.0f;
return false;
}
d = 0.0f;
}
value = d;
return true;
}
//////////////////////////////////////////////////////////////////////////
bool Ray::Intersects(BoundingSphere& sphere, float & value)
{
float x = sphere.Center.X - this->Position.X;
float y = sphere.Center.Y - this->Position.Y;
float z = sphere.Center.Z - this->Position.Z;
float single = x * x + y * y + z * z;
float radius = sphere.Radius * sphere.Radius;
if (single <= radius)
{
value = 0;
return true;
}
float x1 = x * this->Direction.X + y * this->Direction.Y + z * this->Direction.Z;
if (x1 < 0.0f)
{
value = 0;
return false;
}
float single1 = single - x1 * x1;
if (single1 > radius)
{
value = 0;
return false;
}
float single2 = (float)sqrt((double)(radius - single1));
value = x1 - single2;
return true;
}
//////////////////////////////////////////////////////////////////////////<file_sep>#pragma once
#include "../Core/Vector3.h"
//////////////////////////////////////////////////////////////////////////
///@brief GJK 충돌검출
//////////////////////////////////////////////////////////////////////////
class Gjk
{
public:
Gjk(void);
bool FullSimplex() const;
float MaxLengthSquared() const;
Vector3 ClosestPoint() const;
void Reset();
bool AddSupportPoint(Vector3 newPoint);
private:
void UpdateDeterminant(int index);
bool UpdateSimplex(int newIndex);
Vector3 ComputeClosestPoint();
bool IsSatisfiesRule(int xBits, int yBits);
static float Dot(Vector3 a, Vector3 b);
private:
const static int BitsToIndices[16];
Vector3 closestPoint; ///< 가까운 위치 벡터
Vector3 y[4];///< y축 벡터
float yLengthSq[4];///< y축 방향 크기
Vector3 edges[4][4];///< 모서리 벡터
float edgeLengthSq[4][4];///< 모서리 크기
float det[16][4];///< Determinant
int simplexBits;///< 심플렉스 비트(max:15)
float maxLengthSq;///< 제곱으로 계산한 크기 중 가장 큰 값
};<file_sep>#include "Matrix.h"
#include <assert.h>
#include <d3dx9math.h>
#include "Vector3.h"
#include "Quaternion.h"
#include "Plane.h"
//////////////////////////////////////////////////////////////////////////
const Matrix Matrix::Identity = Matrix(1.0f, 0.0f, 0.0f, 0.0f, 0.0f, 1.0f, 0.0f, 0.0f, 0.0f, 0.0f, 1.0f, 0.0f, 0.0f, 0.0f, 0.0f, 1.0f);
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
//////////////////////////////////////////////////////////////////////////
Matrix::Matrix( void )
{
this->M11 = 0.0f; this->M12 = 0.0f; this->M13 = 0.0f; this->M14 = 0.0f;
this->M21 = 0.0f; this->M22 = 0.0f; this->M23 = 0.0f; this->M24 = 0.0f;
this->M31 = 0.0f; this->M32 = 0.0f; this->M33 = 0.0f; this->M34 = 0.0f;
this->M41 = 0.0f; this->M42 = 0.0f; this->M43 = 0.0f; this->M44 = 0.0f;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param m11 : M11
///@param m12 : M12
///@param m13 : M13
///@param m14 : M14
///@param m21 : M21
///@param m22 : M22
///@param m23 : M23
///@param m24 : M24
///@param m31 : M31
///@param m32 : M32
///@param m33 : M33
///@param m34 : M34
///@param m41 : M41
///@param m42 : M42
///@param m43 : M43
///@param m44 : M44
//////////////////////////////////////////////////////////////////////////
Matrix::Matrix( float m11, float m12, float m13, float m14, float m21, float m22, float m23, float m24, float m31, float m32, float m33, float m34, float m41, float m42, float m43, float m44 )
{
this->M11 = m11; this->M12 = m12; this->M13 = m13; this->M14 = m14;
this->M21 = m21; this->M22 = m22; this->M23 = m23; this->M24 = m24;
this->M31 = m31; this->M32 = m32; this->M33 = m33; this->M34 = m34;
this->M41 = m41; this->M42 = m42; this->M43 = m43; this->M44 = m44;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 업 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Matrix::Up()
{
Vector3 vector3;
vector3.X = this->M21; vector3.Y = this->M22; vector3.Z = this->M23;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 업 벡터
///@param value : 벡터
//////////////////////////////////////////////////////////////////////////
void Matrix::Up( Vector3 value )
{
this->M21 = value.X; this->M22 = value.Y; this->M23 = value.Z;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 다운 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Matrix::Down()
{
Vector3 vector3;
vector3.X = -this->M21; vector3.Y = -this->M22; vector3.Z = -this->M23;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 다운 벡터
///@param value : 벡터
//////////////////////////////////////////////////////////////////////////
void Matrix::Down( Vector3 value )
{
this->M21 = -value.X; this->M22 = -value.Y; this->M23 = -value.Z;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 라이트 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Matrix::Right()
{
Vector3 vector3;
vector3.X = this->M11; vector3.Y = this->M12; vector3.Z = this->M13;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 다운 벡터
///@param value : 벡터
//////////////////////////////////////////////////////////////////////////
void Matrix::Right( Vector3 value )
{
this->M11 = value.X; this->M12 = value.Y; this->M13 = value.Z;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 레프트 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Matrix::Left()
{
Vector3 vector3;
vector3.X = -this->M11; vector3.Y = -this->M12; vector3.Z = -this->M13;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 레프트 벡터
///@param value : 벡터
//////////////////////////////////////////////////////////////////////////
void Matrix::Left( Vector3 value )
{
this->M11 = -value.X; this->M12 = -value.Y; this->M13 = -value.Z;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 포워드 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Matrix::Forward()
{
Vector3 vector3;
vector3.X = -this->M31; vector3.Y = -this->M32; vector3.Z = -this->M33;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 포워드 벡터
///@param value : 벡터
//////////////////////////////////////////////////////////////////////////
void Matrix::Forward( Vector3 value )
{
this->M31 = -value.X; this->M32 = -value.Y; this->M33 = -value.Z;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 백워드 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Matrix::Backward()
{
Vector3 vector3;
vector3.X = this->M31; vector3.Y = this->M32; vector3.Z = this->M33;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 백워드 벡터
///@param value : 벡터
//////////////////////////////////////////////////////////////////////////
void Matrix::Backward( Vector3 value )
{
this->M31 = value.X; this->M32 = value.Y; this->M33 = value.Z;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 이동 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Matrix::Translate()
{
Vector3 vector3;
vector3.X = this->M41; vector3.Y = this->M42; vector3.Z = this->M43;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 이동 벡터
///@param value : 벡터
//////////////////////////////////////////////////////////////////////////
void Matrix::Translate( Vector3 value )
{
this->M41 = value.X; this->M42 = value.Y; this->M43 = value.Z;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::operator-()
{
Matrix matrix;
matrix.M11 = -M11; matrix.M12 = -M12; matrix.M13 = -M13; matrix.M14 = -M14;
matrix.M21 = -M21; matrix.M22 = -M22; matrix.M23 = -M23; matrix.M24 = -M24;
matrix.M31 = -M31; matrix.M32 = -M32; matrix.M33 = -M33; matrix.M34 = -M34;
matrix.M41 = -M41; matrix.M42 = -M42; matrix.M43 = -M43; matrix.M44 = -M44;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief ==
///@param matrix2 : 매트릭스
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Matrix::operator==( const Matrix& matrix2 ) const
{
if ((double)this->M11 == (double)matrix2.M11 && (double)this->M22 == (double)matrix2.M22 && ((double)this->M33 == (double)matrix2.M33 && (double)this->M44 == (double)matrix2.M44)&& ((double)this->M12 == (double)matrix2.M12 && (double)this->M13 == (double)matrix2.M13 && ((double)this->M14 == (double)matrix2.M14 && (double)this->M21 == (double)matrix2.M21))&& ((double)this->M23 == (double)matrix2.M23 && (double)this->M24 == (double)matrix2.M24 && ((double)this->M31 == (double)matrix2.M31 && (double)this->M32 == (double)matrix2.M32)&& ((double)this->M34 == (double)matrix2.M34 && (double)this->M41 == (double)matrix2.M41 && (double)this->M42 == (double)matrix2.M42)))
return (double)this->M43 == (double)matrix2.M43;
else
return false;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief !=
///@param matrix2 : 매트릭스
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Matrix::operator!=( const Matrix& matrix2 ) const
{
if ((double)this->M11 == (double)matrix2.M11 && (double)this->M12 == (double)matrix2.M12 && ((double)this->M13 == (double)matrix2.M13 && (double)this->M14 == (double)matrix2.M14)&& ((double)this->M21 == (double)matrix2.M21 && (double)this->M22 == (double)matrix2.M22 && ((double)this->M23 == (double)matrix2.M23 && (double)this->M24 == (double)matrix2.M24))&& ((double)this->M31 == (double)matrix2.M31 && (double)this->M32 == (double)matrix2.M32 && ((double)this->M33 == (double)matrix2.M33 && (double)this->M34 == (double)matrix2.M34)&& ((double)this->M41 == (double)matrix2.M41 && (double)this->M42 == (double)matrix2.M42 && (double)this->M43 == (double)matrix2.M43)))
return (double)this->M44 != (double)matrix2.M44;
else
return true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief +
///@param matrix2 : 매트릭스
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::operator+( const Matrix& matrix2 ) const
{
Matrix matrix;
matrix.M11 = this->M11 + matrix2.M11; matrix.M12 = this->M12 + matrix2.M12; matrix.M13 = this->M13 + matrix2.M13; matrix.M14 = this->M14 + matrix2.M14;
matrix.M21 = this->M21 + matrix2.M21; matrix.M22 = this->M22 + matrix2.M22; matrix.M23 = this->M23 + matrix2.M23; matrix.M24 = this->M24 + matrix2.M24;
matrix.M31 = this->M31 + matrix2.M31; matrix.M32 = this->M32 + matrix2.M32; matrix.M33 = this->M33 + matrix2.M33; matrix.M34 = this->M34 + matrix2.M34;
matrix.M41 = this->M41 + matrix2.M41; matrix.M42 = this->M42 + matrix2.M42; matrix.M43 = this->M43 + matrix2.M43; matrix.M44 = this->M44 + matrix2.M44;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -
///@param matrix2 : 매트릭스
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::operator-( const Matrix& matrix2 ) const
{
Matrix matrix;
matrix.M11 = this->M11 - matrix2.M11; matrix.M12 = this->M12 - matrix2.M12; matrix.M13 = this->M13 - matrix2.M13; matrix.M14 = this->M14 - matrix2.M14;
matrix.M21 = this->M21 - matrix2.M21; matrix.M22 = this->M22 - matrix2.M22; matrix.M23 = this->M23 - matrix2.M23; matrix.M24 = this->M24 - matrix2.M24;
matrix.M31 = this->M31 - matrix2.M31; matrix.M32 = this->M32 - matrix2.M32; matrix.M33 = this->M33 - matrix2.M33; matrix.M34 = this->M34 - matrix2.M34;
matrix.M41 = this->M41 - matrix2.M41; matrix.M42 = this->M42 - matrix2.M42; matrix.M43 = this->M43 - matrix2.M43; matrix.M44 = this->M44 - matrix2.M44;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *
///@param matrix2 : 매트릭스
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::operator*( const Matrix& matrix2 ) const
{
Matrix matrix;
matrix.M11 = (float)((double)this->M11 * (double)matrix2.M11 + (double)this->M12 * (double)matrix2.M21 + (double)this->M13 * (double)matrix2.M31 + (double)this->M14 * (double)matrix2.M41);
matrix.M12 = (float)((double)this->M11 * (double)matrix2.M12 + (double)this->M12 * (double)matrix2.M22 + (double)this->M13 * (double)matrix2.M32 + (double)this->M14 * (double)matrix2.M42);
matrix.M13 = (float)((double)this->M11 * (double)matrix2.M13 + (double)this->M12 * (double)matrix2.M23 + (double)this->M13 * (double)matrix2.M33 + (double)this->M14 * (double)matrix2.M43);
matrix.M14 = (float)((double)this->M11 * (double)matrix2.M14 + (double)this->M12 * (double)matrix2.M24 + (double)this->M13 * (double)matrix2.M34 + (double)this->M14 * (double)matrix2.M44);
matrix.M21 = (float)((double)this->M21 * (double)matrix2.M11 + (double)this->M22 * (double)matrix2.M21 + (double)this->M23 * (double)matrix2.M31 + (double)this->M24 * (double)matrix2.M41);
matrix.M22 = (float)((double)this->M21 * (double)matrix2.M12 + (double)this->M22 * (double)matrix2.M22 + (double)this->M23 * (double)matrix2.M32 + (double)this->M24 * (double)matrix2.M42);
matrix.M23 = (float)((double)this->M21 * (double)matrix2.M13 + (double)this->M22 * (double)matrix2.M23 + (double)this->M23 * (double)matrix2.M33 + (double)this->M24 * (double)matrix2.M43);
matrix.M24 = (float)((double)this->M21 * (double)matrix2.M14 + (double)this->M22 * (double)matrix2.M24 + (double)this->M23 * (double)matrix2.M34 + (double)this->M24 * (double)matrix2.M44);
matrix.M31 = (float)((double)this->M31 * (double)matrix2.M11 + (double)this->M32 * (double)matrix2.M21 + (double)this->M33 * (double)matrix2.M31 + (double)this->M34 * (double)matrix2.M41);
matrix.M32 = (float)((double)this->M31 * (double)matrix2.M12 + (double)this->M32 * (double)matrix2.M22 + (double)this->M33 * (double)matrix2.M32 + (double)this->M34 * (double)matrix2.M42);
matrix.M33 = (float)((double)this->M31 * (double)matrix2.M13 + (double)this->M32 * (double)matrix2.M23 + (double)this->M33 * (double)matrix2.M33 + (double)this->M34 * (double)matrix2.M43);
matrix.M34 = (float)((double)this->M31 * (double)matrix2.M14 + (double)this->M32 * (double)matrix2.M24 + (double)this->M33 * (double)matrix2.M34 + (double)this->M34 * (double)matrix2.M44);
matrix.M41 = (float)((double)this->M41 * (double)matrix2.M11 + (double)this->M42 * (double)matrix2.M21 + (double)this->M43 * (double)matrix2.M31 + (double)this->M44 * (double)matrix2.M41);
matrix.M42 = (float)((double)this->M41 * (double)matrix2.M12 + (double)this->M42 * (double)matrix2.M22 + (double)this->M43 * (double)matrix2.M32 + (double)this->M44 * (double)matrix2.M42);
matrix.M43 = (float)((double)this->M41 * (double)matrix2.M13 + (double)this->M42 * (double)matrix2.M23 + (double)this->M43 * (double)matrix2.M33 + (double)this->M44 * (double)matrix2.M43);
matrix.M44 = (float)((double)this->M41 * (double)matrix2.M14 + (double)this->M42 * (double)matrix2.M24 + (double)this->M43 * (double)matrix2.M34 + (double)this->M44 * (double)matrix2.M44);
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *
///@param scaleFactor : 값
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::operator*( const float& scaleFactor ) const
{
float num = scaleFactor;
Matrix matrix1;
matrix1.M11 = this->M11 * num; matrix1.M12 = this->M12 * num; matrix1.M13 = this->M13 * num; matrix1.M14 = this->M14 * num;
matrix1.M21 = this->M21 * num; matrix1.M22 = this->M22 * num; matrix1.M23 = this->M23 * num; matrix1.M24 = this->M24 * num;
matrix1.M31 = this->M31 * num; matrix1.M32 = this->M32 * num; matrix1.M33 = this->M33 * num; matrix1.M34 = this->M34 * num;
matrix1.M41 = this->M41 * num; matrix1.M42 = this->M42 * num; matrix1.M43 = this->M43 * num; matrix1.M44 = this->M44 * num;
return matrix1;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /
///@param matrix2 : 매트릭스
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::operator/( const Matrix& matrix2 ) const
{
Matrix matrix;
matrix.M11 = this->M11 / matrix2.M11; matrix.M12 = this->M12 / matrix2.M12; matrix.M13 = this->M13 / matrix2.M13; matrix.M14 = this->M14 / matrix2.M14;
matrix.M21 = this->M21 / matrix2.M21; matrix.M22 = this->M22 / matrix2.M22; matrix.M23 = this->M23 / matrix2.M23; matrix.M24 = this->M24 / matrix2.M24;
matrix.M31 = this->M31 / matrix2.M31; matrix.M32 = this->M32 / matrix2.M32; matrix.M33 = this->M33 / matrix2.M33; matrix.M34 = this->M34 / matrix2.M34;
matrix.M41 = this->M41 / matrix2.M41; matrix.M42 = this->M42 / matrix2.M42; matrix.M43 = this->M43 / matrix2.M43; matrix.M44 = this->M44 / matrix2.M44;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /
///@param divider : 값
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::operator/( const float& divider ) const
{
float num = 1.0f / divider;
Matrix matrix;
matrix.M11 = this->M11 * num; matrix.M12 = this->M12 * num; matrix.M13 = this->M13 * num; matrix.M14 = this->M14 * num;
matrix.M21 = this->M21 * num; matrix.M22 = this->M22 * num; matrix.M23 = this->M23 * num; matrix.M24 = this->M24 * num;
matrix.M31 = this->M31 * num; matrix.M32 = this->M32 * num; matrix.M33 = this->M33 * num; matrix.M34 = this->M34 * num;
matrix.M41 = this->M41 * num; matrix.M42 = this->M42 * num; matrix.M43 = this->M43 * num; matrix.M44 = this->M44 * num;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief +=
///@param matrix2 : 매트릭스
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
void Matrix::operator+=( const Matrix& matrix2 )
{
*this = *this + matrix2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -=
///@param matrix2 : 매트릭스
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
void Matrix::operator-=( const Matrix& matrix2 )
{
*this = *this - matrix2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *=
///@param matrix2 : 매트릭스
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
void Matrix::operator*=( const Matrix& matrix2 )
{
*this = *this * matrix2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *=
///@param scaleFactor : 값
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
void Matrix::operator*=( const float& scaleFactor )
{
*this = *this * scaleFactor;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /=
///@param matrix2 : 매트릭스
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
void Matrix::operator/=( const Matrix& matrix2 )
{
*this = *this / matrix2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /=
///@param divider : 값
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
void Matrix::operator/=( const float& divider )
{
*this = *this / divider;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 문자열로 변환
///@return 문자열
//////////////////////////////////////////////////////////////////////////
std::string Matrix::ToString()
{
std::string temp;
char val[255];
sprintf(val, "{ {M11:%#f ", M11); temp += val;
sprintf(val, "M12:%#f ", M12); temp += val;
sprintf(val, "M13:%#f ", M13); temp += val;
sprintf(val, "M14:%#f} ", M14); temp += val;
sprintf(val, "{M21:%#f ", M21); temp += val;
sprintf(val, "M22:%#f ", M22); temp += val;
sprintf(val, "M23:%#f ", M23); temp += val;
sprintf(val, "M24:%#f} ", M24); temp += val;
sprintf(val, "{321:%#f ", M31); temp += val;
sprintf(val, "M32:%#f ", M32); temp += val;
sprintf(val, "M33:%#f ", M33); temp += val;
sprintf(val, "M34:%#f} ", M34); temp += val;
sprintf(val, "{M41:%#f ", M41); temp += val;
sprintf(val, "M42:%#f ", M42); temp += val;
sprintf(val, "M43:%#f ", M43); temp += val;
sprintf(val, "M44:%#f} }", M44); temp += val;
return temp;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief D3DXMATRIX로 변환
///@return D3DX 매트릭스
//////////////////////////////////////////////////////////////////////////
D3DXMATRIX Matrix::ToD3DXMATRIX()
{
D3DXMATRIX matrix;
matrix._11 = this->M11; matrix._12 = this->M12; matrix._13 = this->M13; matrix._14 = this->M14;
matrix._21 = this->M21; matrix._22 = this->M22; matrix._23 = this->M23; matrix._24 = this->M24;
matrix._31 = this->M31; matrix._32 = this->M32; matrix._33 = this->M33; matrix._34 = this->M34;
matrix._41 = this->M41; matrix._42 = this->M42; matrix._43 = this->M43; matrix._44 = this->M44;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 행렬식
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
float Matrix::Determinant()
{
float num1 = (float)((double)M33 * (double)M44 - (double)M34 * (double)M43);
float num2 = (float)((double)M32 * (double)M44 - (double)M34 * (double)M42);
float num3 = (float)((double)M32 * (double)M43 - (double)M33 * (double)M42);
float num4 = (float)((double)M31 * (double)M44 - (double)M34 * (double)M41);
float num5 = (float)((double)M31 * (double)M43 - (double)M33 * (double)M41);
float num6 = (float)((double)M31 * (double)M42 - (double)M32 * (double)M41);
float temp =
(float)((double)M11 * ((double)M22 * (double)num1 - (double)M23 * (double)num2 + (double)M24 * (double)num3) -
(double)M12 * ((double)M21 * (double)num1 - (double)M23 * (double)num4 + (double)M24 * (double)num5) +
(double)M13 * ((double)M21 * (double)num2 - (double)M22 * (double)num4 + (double)M24 * (double)num6) -
(double)M14 * ((double)M21 * (double)num3 - (double)M22 * (double)num5 + (double)M23 * (double)num6));
return temp;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 빌보드 매트릭스 생성
///@param objectPosition : 오브젝트 위치
///@param cameraPosition : 카메라 위치
///@param cameraUpVector : 카메라 업벡터
///@param cameraForwardVector : 카메라 포워드벡터
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateBillboard( Vector3 objectPosition, Vector3 cameraPosition, Vector3 cameraUpVector, Vector3* cameraForwardVector/*=NULL*/ )
{
Vector3 forwardVec;
forwardVec.X = objectPosition.X - cameraPosition.X;
forwardVec.Y = objectPosition.Y - cameraPosition.Y;
forwardVec.Z = objectPosition.Z - cameraPosition.Z;
float num = forwardVec.LengthSquared();
if ((double) num < 9.99999974737875E-05)
forwardVec = cameraForwardVector != NULL ? -(*cameraForwardVector) : Vector3::Forward;
else
forwardVec = forwardVec * (1.0f / (float)sqrt((double)num));
Vector3 rightVec;
rightVec = Vector3::Cross(cameraUpVector, forwardVec);
rightVec.Normalize();
Vector3 upVec;
upVec = Vector3::Cross(forwardVec, rightVec);
Matrix matrix;
matrix.M11 = rightVec.X; matrix.M12 = rightVec.Y; matrix.M13 = rightVec.Z; matrix.M14 = 0.0f;
matrix.M21 = upVec.X; matrix.M22 = upVec.Y; matrix.M23 = upVec.Z; matrix.M24 = 0.0f;
matrix.M31 = forwardVec.X; matrix.M32 = forwardVec.Y; matrix.M33 = forwardVec.Z; matrix.M34 = 0.0f;
matrix.M41 = objectPosition.X; matrix.M42 = objectPosition.Y; matrix.M43 = objectPosition.Z; matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 이동 매트릭스 생성
///@param position : 위치
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateTranslation( Vector3 position )
{
Matrix matrix;
matrix.M11 = 1.0f; matrix.M12 = 0.0f; matrix.M13 = 0.0f; matrix.M14 = 0.0f;
matrix.M21 = 0.0f; matrix.M22 = 1.0f; matrix.M23 = 0.0f; matrix.M24 = 0.0f;
matrix.M31 = 0.0f; matrix.M32 = 0.0f; matrix.M33 = 1.0f; matrix.M34 = 0.0f;
matrix.M41 = position.X;
matrix.M42 = position.Y;
matrix.M43 = position.Z;
matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 이동 매트릭스 생성
///@param xPosition : x 위치
///@param yPosition : y 위치
///@param zPosition : z 위치
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateTranslation( float xPosition, float yPosition, float zPosition )
{
Matrix matrix;
matrix.M11 = 1.0f; matrix.M12 = 0.0f; matrix.M13 = 0.0f; matrix.M14 = 0.0f;
matrix.M21 = 0.0f; matrix.M22 = 1.0f; matrix.M23 = 0.0f; matrix.M24 = 0.0f;
matrix.M31 = 0.0f; matrix.M32 = 0.0f; matrix.M33 = 1.0f; matrix.M34 = 0.0f;
matrix.M41 = xPosition;
matrix.M42 = yPosition;
matrix.M43 = zPosition;
matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 크기 매트릭스 생성
///@param scales : 크기
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateScale( Vector3 scales )
{
Matrix matrix;
matrix.M11 = scales.X; matrix.M12 = 0.0f; matrix.M13 = 0.0f; matrix.M14 = 0.0f;
matrix.M21 = 0.0f; matrix.M22 = scales.Y; matrix.M23 = 0.0f; matrix.M24 = 0.0f;
matrix.M31 = 0.0f; matrix.M32 = 0.0f; matrix.M33 = scales.Z; matrix.M34 = 0.0f;
matrix.M41 = 0.0f; matrix.M42 = 0.0f; matrix.M43 = 0.0f; matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 크기 매트릭스 생성
///@param xScale : x 크기
///@param yScale : y 크기
///@param zScale : z 크기
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateScale( float xScale, float yScale, float zScale )
{
Matrix matrix;
matrix.M11 = xScale; matrix.M12 = 0.0f; matrix.M13 = 0.0f; matrix.M14 = 0.0f;
matrix.M21 = 0.0f; matrix.M22 = yScale; matrix.M23 = 0.0f; matrix.M24 = 0.0f;
matrix.M31 = 0.0f; matrix.M32 = 0.0f; matrix.M33 = zScale; matrix.M34 = 0.0f;
matrix.M41 = 0.0f; matrix.M42 = 0.0f; matrix.M43 = 0.0f; matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 크기 매트릭스 생성
///@param scale : 크기값
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateScale( float scale )
{
Matrix matrix;
matrix.M11 = scale; matrix.M12 = 0.0f; matrix.M13 = 0.0f; matrix.M14 = 0.0f;
matrix.M21 = 0.0f; matrix.M22 = scale; matrix.M23 = 0.0f; matrix.M24 = 0.0f;
matrix.M31 = 0.0f; matrix.M32 = 0.0f; matrix.M33 = scale; matrix.M34 = 0.0f;
matrix.M41 = 0.0f; matrix.M42 = 0.0f; matrix.M43 = 0.0f; matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief X축 회전 매트릭스 생성
///@param radians : 라디안 각도값
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateRotationX( float radians )
{
float num1 = (float)cos((double) radians);
float num2 = (float)sin((double) radians);
Matrix matrix;
matrix.M11 = 1.0f; matrix.M12 = 0.0f; matrix.M13 = 0.0f; matrix.M14 = 0.0f;
matrix.M21 = 0.0f; matrix.M22 = num1; matrix.M23 = num2; matrix.M24 = 0.0f;
matrix.M31 = 0.0f; matrix.M32 = -num2; matrix.M33 = num1; matrix.M34 = 0.0f;
matrix.M41 = 0.0f; matrix.M42 = 0.0f; matrix.M43 = 0.0f; matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief Y축 회전 매트릭스 생성
///@param radians : 라디안 각도값
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateRotationY( float radians )
{
float num1 = (float)cos((double) radians);
float num2 = (float)sin((double) radians);
Matrix matrix;
matrix.M11 = num1; matrix.M12 = 0.0f; matrix.M13 = -num2; matrix.M14 = 0.0f;
matrix.M21 = 0.0f; matrix.M22 = 1.0f; matrix.M23 = 0.0f; matrix.M24 = 0.0f;
matrix.M31 = num2; matrix.M32 = 0.0f; matrix.M33 = num1; matrix.M34 = 0.0f;
matrix.M41 = 0.0f; matrix.M42 = 0.0f; matrix.M43 = 0.0f; matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief Z축 회전 매트릭스 생성
///@param radians : 라디안 각도값
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateRotationZ( float radians )
{
float num1 = (float)cos((double) radians);
float num2 = (float)sin((double) radians);
Matrix matrix;
matrix.M11 = num1; matrix.M12 = num2; matrix.M13 = 0.0f; matrix.M14 = 0.0f;
matrix.M21 = -num2; matrix.M22 = num1; matrix.M23 = 0.0f; matrix.M24 = 0.0f;
matrix.M31 = 0.0f; matrix.M32 = 0.0f; matrix.M33 = 1.0f; matrix.M34 = 0.0f;
matrix.M41 = 0.0f; matrix.M42 = 0.0f; matrix.M43 = 0.0f; matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 축 벡터 회전 매트릭스 생성
///@param axis : 축
///@param angle : 회전값
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateFromAxisAngle( Vector3 axis, float angle )
{
float sinValue = (float)sin((double)angle);
float cosValue = (float)cos((double)angle);
float xx = axis.X * axis.X;
float yy = axis.Y * axis.Y;
float zz = axis.Z * axis.Z;
float xy = axis.X * axis.Y;
float xz = axis.X * axis.Z;
float yz = axis.Y * axis.Z;
Matrix matrix;
matrix.M11 = xx + cosValue * (1.0f - xx);
matrix.M12 = (float)((double)xy - (double)cosValue * (double)xy + (double)sinValue * (double)axis.Z);
matrix.M13 = (float)((double)xz - (double)cosValue * (double)xz - (double)sinValue * (double)axis.Y);
matrix.M14 = 0.0f;
matrix.M21 = (float)((double)xy - (double)cosValue * (double)xy - (double)sinValue * (double)axis.Z);
matrix.M22 = yy + cosValue * (1.0f - yy);
matrix.M23 = (float)((double)yz - (double)cosValue * (double)yz + (double)sinValue * (double)axis.X);
matrix.M24 = 0.0f;
matrix.M31 = (float)((double)xz - (double)cosValue * (double)xz + (double)sinValue * (double)axis.Y);
matrix.M32 = (float)((double)yz - (double)cosValue * (double)yz - (double)sinValue * (double)axis.X);
matrix.M33 = zz + cosValue * (1.0f - zz);
matrix.M34 = 0.0f;
matrix.M41 = 0.0f;
matrix.M42 = 0.0f;
matrix.M43 = 0.0f;
matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 원근투영 매트릭스 생성
///@param width : 화면넓이
///@param height : 화면높이
///@param nearPlaneDistance : 근면거리
///@param farPlaneDistance : 원면거리
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreatePerspective( float width, float height, float nearPlaneDistance, float farPlaneDistance )
{
assert((double)nearPlaneDistance > 0.0);
assert((double)farPlaneDistance > 0.0);
assert((double)nearPlaneDistance < (double)farPlaneDistance);
Matrix matrix;
matrix.M11 = 2.0f * nearPlaneDistance / width;
matrix.M12 = matrix.M13 = matrix.M14 = 0.0f;
matrix.M22 = 2.0f * nearPlaneDistance / height;
matrix.M21 = matrix.M23 = matrix.M24 = 0.0f;
matrix.M33 = farPlaneDistance / (nearPlaneDistance - farPlaneDistance);
matrix.M31 = matrix.M32 = 0.0f;
matrix.M34 = -1.0f;
matrix.M41 = matrix.M42 = matrix.M44 = 0.0f;
matrix.M43 = (float)((double)nearPlaneDistance * (double)farPlaneDistance / ((double)nearPlaneDistance - (double)farPlaneDistance));
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief FOV를 기준으로 원근투영 매트릭스 생성
///@param fieldOfView : FOV
///@param aspectRatio : 화면비율
///@param nearPlaneDistance : 근면거리
///@param farPlaneDistance : 원면거리
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreatePerspectiveFieldOfView( float fieldOfView, float aspectRatio, float nearPlaneDistance, float farPlaneDistance )
{
assert((double)fieldOfView > 0.0 || (double)fieldOfView < 3.14159274101257);
assert((double)nearPlaneDistance > 0.0);
assert((double)farPlaneDistance > 0.0);
assert((double)nearPlaneDistance < (double)farPlaneDistance);
float num1 = 1.0f / (float)tan((double)fieldOfView * 0.5);
float num2 = num1 / aspectRatio;
Matrix matrix;
matrix.M11 = num2;
matrix.M12 = matrix.M13 = matrix.M14 = 0.0f;
matrix.M22 = num1;
matrix.M21 = matrix.M23 = matrix.M24 = 0.0f;
matrix.M31 = matrix.M32 = 0.0f;
matrix.M33 = farPlaneDistance / (nearPlaneDistance - farPlaneDistance);
matrix.M34 = -1.0f;
matrix.M41 = matrix.M42 = matrix.M44 = 0.0f;
matrix.M43 = (float)((double)nearPlaneDistance * (double)farPlaneDistance / ((double)nearPlaneDistance - (double)farPlaneDistance));
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 룩앳(뷰) 매트릭스 생성
///@param cameraPosition : 카메라 위치
///@param cameraTarget : 카메라 타켓
///@param cameraUpVector : 카메라 업벡터
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateLookAt( Vector3 cameraPosition, Vector3 cameraTarget, Vector3 cameraUpVector )
{
Vector3 vector3_1 = Vector3::Normalize(cameraPosition - cameraTarget);
Vector3 vector3_2 = Vector3::Normalize(Vector3::Cross(cameraUpVector, vector3_1));
Vector3 vector1 = Vector3::Cross(vector3_1, vector3_2);
Matrix matrix;
matrix.M11 = vector3_2.X; matrix.M12 = vector1.X; matrix.M13 = vector3_1.X; matrix.M14 = 0.0f;
matrix.M21 = vector3_2.Y; matrix.M22 = vector1.Y; matrix.M23 = vector3_1.Y; matrix.M24 = 0.0f;
matrix.M31 = vector3_2.Z; matrix.M32 = vector1.Z; matrix.M33 = vector3_1.Z; matrix.M34 = 0.0f;
matrix.M41 = -Vector3::Dot(vector3_2, cameraPosition);
matrix.M42 = -Vector3::Dot(vector1, cameraPosition);
matrix.M43 = -Vector3::Dot(vector3_1, cameraPosition);
matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 월드 매트릭스 생성
///@param position : 위치
///@param forward : 전방 방향
///@param up : 업
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateWorld( Vector3 position, Vector3 forward, Vector3 up )
{
Vector3 vector3_1 = Vector3::Normalize(-forward);
Vector3 vector2 = Vector3::Normalize(Vector3::Cross(up, vector3_1));
Vector3 vector3_2 = Vector3::Cross(vector3_1, vector2);
Matrix matrix;
matrix.M11 = vector2.X; matrix.M12 = vector2.Y; matrix.M13 = vector2.Z; matrix.M14 = 0.0f;
matrix.M21 = vector3_2.X; matrix.M22 = vector3_2.Y; matrix.M23 = vector3_2.Z; matrix.M24 = 0.0f;
matrix.M31 = vector3_1.X; matrix.M32 = vector3_1.Y; matrix.M33 = vector3_1.Z; matrix.M34 = 0.0f;
matrix.M41 = position.X; matrix.M42 = position.Y; matrix.M43 = position.Z; matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 쿼터니온으로부터 매트릭스 생성
///@param quaternion : 쿼터니온
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateFromQuaternion( Quaternion quaternion )
{
float xx = quaternion.X * quaternion.X;
float yy = quaternion.Y * quaternion.Y;
float zz = quaternion.Z * quaternion.Z;
float xy = quaternion.X * quaternion.Y;
float zw = quaternion.Z * quaternion.W;
float zx = quaternion.Z * quaternion.X;
float yw = quaternion.Y * quaternion.W;
float yz = quaternion.Y * quaternion.Z;
float xw = quaternion.X * quaternion.W;
Matrix matrix;
matrix.M11 = (float)(1.0 - 2.0 * ((double)yy + (double)zz));
matrix.M12 = (float)(2.0 * ((double)xy + (double)zw));
matrix.M13 = (float)(2.0 * ((double)zx - (double)yw));
matrix.M14 = 0.0f;
matrix.M21 = (float)(2.0 * ((double)xy - (double)zw));
matrix.M22 = (float)(1.0 - 2.0 * ((double)zz + (double)xx));
matrix.M23 = (float)(2.0 * ((double)yz + (double)xw));
matrix.M24 = 0.0f;
matrix.M31 = (float)(2.0 * ((double)zx + (double)yw));
matrix.M32 = (float)(2.0 * ((double)yz - (double)xw));
matrix.M33 = (float)(1.0 - 2.0 * ((double)yy + (double)xx));
matrix.M34 = 0.0f;
matrix.M41 = 0.0f;
matrix.M42 = 0.0f;
matrix.M43 = 0.0f;
matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 각 축값에 의한 회전 매트릭스 생성(쿼터니온 이용)
///@param yaw : Yaw
///@param pitch : Pitch
///@param roll : Roll
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateFromYawPitchRoll( float yaw, float pitch, float roll )
{
Quaternion result1;
result1 = Quaternion::CreateFromYawPitchRoll(yaw, pitch, roll);
Matrix result2;
result2 = CreateFromQuaternion(result1);
return result2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 그림자 매트릭스 생성
///@param lightDirection : 빛 방향 벡터
///@param plane : 면
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateShadow( Vector3 lightDirection, Plane plane )
{
Plane result = Plane::Normalize(plane);
float length = (float)((double)result.Normal.X * (double)lightDirection.X + (double)result.Normal.Y * (double)lightDirection.Y + (double)result.Normal.Z * (double)lightDirection.Z);
Matrix matrix;
matrix.M11 = -result.Normal.X * lightDirection.X + length;
matrix.M21 = -result.Normal.Y * lightDirection.X;
matrix.M31 = -result.Normal.Z * lightDirection.X;
matrix.M41 = -result.D * lightDirection.X;
matrix.M12 = -result.Normal.X * lightDirection.Y;
matrix.M22 = -result.Normal.Y * lightDirection.Y + length;
matrix.M32 = -result.Normal.Z * lightDirection.Y;
matrix.M42 = -result.D * lightDirection.Y;
matrix.M13 = -result.Normal.X * lightDirection.Z;
matrix.M23 = -result.Normal.Y * lightDirection.Z;
matrix.M33 = -result.Normal.Z * lightDirection.Z + length;
matrix.M43 = -result.D * lightDirection.Z;
matrix.M14 = 0.0f;
matrix.M24 = 0.0f;
matrix.M34 = 0.0f;
matrix.M44 = length;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 면의 반사 매트릭스 생성
///@param value : 면
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateReflection( Plane value )
{
value.Normalize();
Matrix matrix;
matrix.M11 = (float)((double)(-2.0f * value.Normal.X)* (double)value.Normal.X + 1.0);
matrix.M12 = (-2.0f * value.Normal.Y) * value.Normal.X;
matrix.M13 = (-2.0f * value.Normal.Z) * value.Normal.X;
matrix.M14 = 0.0f;
matrix.M21 = (-2.0f * value.Normal.X) * value.Normal.Y;
matrix.M22 = (float)((double)(-2.0f * value.Normal.Y)* (double)value.Normal.Y + 1.0);
matrix.M23 = (-2.0f * value.Normal.Z) * value.Normal.Y;
matrix.M24 = 0.0f;
matrix.M31 = (-2.0f * value.Normal.X) * value.Normal.Z;
matrix.M32 = (-2.0f * value.Normal.Y) * value.Normal.Z;
matrix.M33 = (float)((double)(-2.0f * value.Normal.Z)* (double)value.Normal.Z + 1.0);
matrix.M34 = 0.0f;
matrix.M41 = (-2.0f * value.Normal.X) * value.D;
matrix.M42 = (-2.0f * value.Normal.Y) * value.D;
matrix.M43 = (-2.0f * value.Normal.Z) * value.D;
matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 정사도법에 의한 행렬 생성
///@param width : 넓이
///@param height : 높이
///@param zNearPlane : z방향 근면
///@param zFarPlane : z방향 원면
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::CreateOrthographic( float width, float height, float zNearPlane, float zFarPlane )
{
Matrix matrix;
matrix.M11 = 2.0f / width;
matrix.M12 = matrix.M13 = matrix.M14 = 0.0f;
matrix.M22 = 2.0f / height;
matrix.M21 = matrix.M23 = matrix.M24 = 0.0f;
matrix.M33 = (float) (1.0 / ((double) zNearPlane - (double) zFarPlane));
matrix.M31 = matrix.M32 = matrix.M34 = 0.0f;
matrix.M41 = matrix.M42 = 0.0f;
matrix.M43 = zNearPlane / (zNearPlane - zFarPlane);
matrix.M44 = 1.0f;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 쿼터니온에 의한 매트릭스 변환
///@param value : 매트릭스
///@param rotation : 쿼터니온
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::Transform( Matrix value, Quaternion rotation )
{
float wxx = rotation.W * (rotation.X + rotation.X);
float wyy = rotation.W * (rotation.Y + rotation.Y);
float wzz = rotation.W * (rotation.Z + rotation.Z);
float xxx = rotation.X * (rotation.X + rotation.X);
float xyy = rotation.X * (rotation.Y + rotation.Y);
float xzz = rotation.X * (rotation.Z + rotation.Z);
float yyy = rotation.Y * (rotation.Y + rotation.Y);
float yzz = rotation.Y * (rotation.Z + rotation.Z);
float zzz = rotation.Z * (rotation.Z + rotation.Z);
float num1 = 1.0f - yyy - zzz;
float num2 = xyy - wzz;
float num3 = xzz + wyy;
float num4 = xyy + wzz;
float num5 = 1.0f - xxx - zzz;
float num6 = yzz - wxx;
float num7 = xzz - wyy;
float num8 = yzz + wxx;
float num9 = 1.0f - xxx - yyy;
Matrix matrix;
matrix.M11 = (float)((double)value.M11 * (double)num1 + (double)value.M12 * (double)num2 + (double)value.M13 * (double)num3);
matrix.M12 = (float)((double)value.M11 * (double)num4 + (double)value.M12 * (double)num5 + (double)value.M13 * (double)num6);
matrix.M13 = (float)((double)value.M11 * (double)num7 + (double)value.M12 * (double)num8 + (double)value.M13 * (double)num9);
matrix.M14 = value.M14;
matrix.M21 = (float)((double)value.M21 * (double)num1 + (double)value.M22 * (double)num2 + (double)value.M23 * (double)num3);
matrix.M22 = (float)((double)value.M21 * (double)num4 + (double)value.M22 * (double)num5 + (double)value.M23 * (double)num6);
matrix.M23 = (float)((double)value.M21 * (double)num7 + (double)value.M22 * (double)num8 + (double)value.M23 * (double)num9);
matrix.M24 = value.M24;
matrix.M31 = (float)((double)value.M31 * (double)num1 + (double)value.M32 * (double)num2 + (double)value.M33 * (double)num3);
matrix.M32 = (float)((double)value.M31 * (double)num4 + (double)value.M32 * (double)num5 + (double)value.M33 * (double)num6);
matrix.M33 = (float)((double)value.M31 * (double)num7 + (double)value.M32 * (double)num8 + (double)value.M33 * (double)num9);
matrix.M34 = value.M34;
matrix.M41 = (float)((double)value.M41 * (double)num1 + (double)value.M42 * (double)num2 + (double)value.M43 * (double)num3);
matrix.M42 = (float)((double)value.M41 * (double)num4 + (double)value.M42 * (double)num5 + (double)value.M43 * (double)num6);
matrix.M43 = (float)((double)value.M41 * (double)num7 + (double)value.M42 * (double)num8 + (double)value.M43 * (double)num9);
matrix.M44 = value.M44;
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 전치 매트릭스
///@param matrix : 행렬
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::Transpose( Matrix matrix )
{
Matrix matrix1;
matrix1.M11 = matrix.M11; matrix1.M12 = matrix.M21; matrix1.M13 = matrix.M31; matrix1.M14 = matrix.M41;
matrix1.M21 = matrix.M12; matrix1.M22 = matrix.M22; matrix1.M23 = matrix.M32; matrix1.M24 = matrix.M42;
matrix1.M31 = matrix.M13; matrix1.M32 = matrix.M23; matrix1.M33 = matrix.M33; matrix1.M34 = matrix.M43;
matrix1.M41 = matrix.M14; matrix1.M42 = matrix.M24; matrix1.M43 = matrix.M34; matrix1.M44 = matrix.M44;
return matrix1;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 역행렬
///@param matrix : 매트릭스
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::Invert( Matrix matrix )
{
Matrix matrix2;
float num5 = matrix.M11; float num4 = matrix.M12; float num3 = matrix.M13; float num2 = matrix.M14;
float num9 = matrix.M21; float num8 = matrix.M22; float num7 = matrix.M23; float num6 = matrix.M24;
float num17 = matrix.M31; float num16 = matrix.M32; float num15 = matrix.M33; float num14 = matrix.M34;
float num13 = matrix.M41; float num12 = matrix.M42; float num11 = matrix.M43; float num10 = matrix.M44;
float num23 = (num15 * num10) - (num14 * num11);
float num22 = (num16 * num10) - (num14 * num12);
float num21 = (num16 * num11) - (num15 * num12);
float num20 = (num17 * num10) - (num14 * num13);
float num19 = (num17 * num11) - (num15 * num13);
float num18 = (num17 * num12) - (num16 * num13);
float num39 = ((num8 * num23) - (num7 * num22)) + (num6 * num21);
float num38 = -(((num9 * num23) - (num7 * num20)) + (num6 * num19));
float num37 = ((num9 * num22) - (num8 * num20)) + (num6 * num18);
float num36 = -(((num9 * num21) - (num8 * num19)) + (num7 * num18));
float num = 1.0f / ((((num5 * num39) + (num4 * num38)) + (num3 * num37)) + (num2 * num36));
matrix2.M11 = num39 * num;
matrix2.M21 = num38 * num;
matrix2.M31 = num37 * num;
matrix2.M41 = num36 * num;
matrix2.M12 = -(((num4 * num23) - (num3 * num22)) + (num2 * num21)) * num;
matrix2.M22 = (((num5 * num23) - (num3 * num20)) + (num2 * num19)) * num;
matrix2.M32 = -(((num5 * num22) - (num4 * num20)) + (num2 * num18)) * num;
matrix2.M42 = (((num5 * num21) - (num4 * num19)) + (num3 * num18)) * num;
float num35 = (num7 * num10) - (num6 * num11);
float num34 = (num8 * num10) - (num6 * num12);
float num33 = (num8 * num11) - (num7 * num12);
float num32 = (num9 * num10) - (num6 * num13);
float num31 = (num9 * num11) - (num7 * num13);
float num30 = (num9 * num12) - (num8 * num13);
matrix2.M13 = (((num4 * num35) - (num3 * num34)) + (num2 * num33)) * num;
matrix2.M23 = -(((num5 * num35) - (num3 * num32)) + (num2 * num31)) * num;
matrix2.M33 = (((num5 * num34) - (num4 * num32)) + (num2 * num30)) * num;
matrix2.M43 = -(((num5 * num33) - (num4 * num31)) + (num3 * num30)) * num;
float num29 = (num7 * num14) - (num6 * num15);
float num28 = (num8 * num14) - (num6 * num16);
float num27 = (num8 * num15) - (num7 * num16);
float num26 = (num9 * num14) - (num6 * num17);
float num25 = (num9 * num15) - (num7 * num17);
float num24 = (num9 * num16) - (num8 * num17);
matrix2.M14 = -(((num4 * num29) - (num3 * num28)) + (num2 * num27)) * num;
matrix2.M24 = (((num5 * num29) - (num3 * num26)) + (num2 * num25)) * num;
matrix2.M34 = -(((num5 * num28) - (num4 * num26)) + (num2 * num24)) * num;
matrix2.M44 = (((num5 * num27) - (num4 * num25)) + (num3 * num24)) * num;
return matrix2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 매트릭스의 선형보간
///@param matrix1 : 매트릭스1
///@param matrix2 : 매트릭스2
///@param amount : 보간 값
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix Matrix::Lerp( Matrix matrix1, Matrix matrix2, float amount )
{
Matrix matrix;
matrix.M11 = matrix1.M11 + (matrix2.M11 - matrix1.M11) * amount;
matrix.M12 = matrix1.M12 + (matrix2.M12 - matrix1.M12) * amount;
matrix.M13 = matrix1.M13 + (matrix2.M13 - matrix1.M13) * amount;
matrix.M14 = matrix1.M14 + (matrix2.M14 - matrix1.M14) * amount;
matrix.M21 = matrix1.M21 + (matrix2.M21 - matrix1.M21) * amount;
matrix.M22 = matrix1.M22 + (matrix2.M22 - matrix1.M22) * amount;
matrix.M23 = matrix1.M23 + (matrix2.M23 - matrix1.M23) * amount;
matrix.M24 = matrix1.M24 + (matrix2.M24 - matrix1.M24) * amount;
matrix.M31 = matrix1.M31 + (matrix2.M31 - matrix1.M31) * amount;
matrix.M32 = matrix1.M32 + (matrix2.M32 - matrix1.M32) * amount;
matrix.M33 = matrix1.M33 + (matrix2.M33 - matrix1.M33) * amount;
matrix.M34 = matrix1.M34 + (matrix2.M34 - matrix1.M34) * amount;
matrix.M41 = matrix1.M41 + (matrix2.M41 - matrix1.M41) * amount;
matrix.M42 = matrix1.M42 + (matrix2.M42 - matrix1.M42) * amount;
matrix.M43 = matrix1.M43 + (matrix2.M43 - matrix1.M43) * amount;
matrix.M44 = matrix1.M44 + (matrix2.M44 - matrix1.M44) * amount;
return matrix;
}<file_sep>#pragma once
class Vector3;
//////////////////////////////////////////////////////////////////////////
///@brief 수학관련
//////////////////////////////////////////////////////////////////////////
class MathHelper
{
public:
MathHelper(void);
static double Round(double value);
static double Round(double value, int position);
static int Abs(int value);
static float Abs(float value);
static double Abs(double value);
static float ToRadians(float degrees);
static float ToDegrees(float radians);
static float Distance(float value1, float value2);
static float Min(float value1, float value2);
static float Max(float value1, float value2);
static float Clamp(float value, float min, float max);
static float Lerp(float value1, float value2, float amount);
static float SmoothStep(float value1, float value2, float amount);
static float CatmullRom(float value1, float value2, float value3, float value4, float amount);
static float Hermite(float value1, float tangent1, float value2, float tangent2, float amount);
static float WrapAngle(float angle);
static float AngleOfInclineY(Vector3 value);
public:
const static float E;///< 자연로그의 밑수
const static float Log2E;///< 자연로그의 밑수 2
const static float Log10E;///< 자연로그의 밑수 10
const static float Pi;///< PI
const static float TwoPi;///< PI * 2
const static float PiOver2;///< PI / 2
const static float PiOver4;///< PI / 4
const static float Epsilon;///< 0.000001f
const static int IntMinValue;///< Int형의 최소값
const static int IntMaxValue;///< Int형의 최대값
const static float FloatMinValue;///< Flaot형의 최소값
const static float FloatMaxValue;///< Float형의 최대값
};<file_sep>#include "stdafx.h"
#include "ModelAnimPlayer.h"
#include "Model.h"
#include "ModelMesh.h"
#include "ModelBone.h"
#include "ModelAnimClip.h"
ModelAnimPlayer::ModelAnimPlayer(Model * model)
: model(model), mode(Mode::Play)
, currentKeyframe(0), nextKeyframe(0)
, frameTime(0), frameFactor(0)
, bUseSlerp(true)
{
shader = new Shader(Shaders + L"999_Animation.hlsl");
vector<Material *>& materials = model->Materials();
for (Material* material : materials)
material->SetShader(shader);
currentClip = model->Clip(0);
boneAnimation.assign(model->BoneCount(), D3DXMATRIX());
renderTransform.assign(model->BoneCount(), D3DXMATRIX());
}
ModelAnimPlayer::~ModelAnimPlayer()
{
SAFE_DELETE(shader);
}
void ModelAnimPlayer::Update()
{
if (currentClip == NULL || mode != Mode::Play)
return;
UpdateTime();
UpdateBone();
model->Buffer()->SetBones(&renderTransform[0], renderTransform.size());
}
void ModelAnimPlayer::UpdateTime()
{
frameTime += Time::Delta();
float invFrameRate = 1.0f / currentClip->FrameRate();
while (frameTime > invFrameRate)
{
int keyframeCount = currentClip->TotalFrame();
currentKeyframe = (currentKeyframe + 1) % keyframeCount;
nextKeyframe = (currentKeyframe + 1) % keyframeCount;
frameTime -= invFrameRate;
}
frameFactor = frameTime / invFrameRate;
}
void ModelAnimPlayer::UpdateBone()
{
for (size_t i = 0; i < model->BoneCount(); i++)
{
ModelBone* bone = model->Bone(i);
D3DXMATRIX matAnimation;
D3DXMATRIX matParentAnimation;
D3DXMATRIX matInvBindPose = bone->AbsoluteTransform();
D3DXMatrixInverse(&matInvBindPose, NULL, &matInvBindPose);
ModelKeyframe* frame = currentClip->Keyframe(bone->Name());
if (frame == NULL) continue;
if (bUseSlerp == true)
{
D3DXMATRIX S, R, T;
ModelKeyframeData current = frame->Datas[currentKeyframe];
ModelKeyframeData next = frame->Datas[nextKeyframe];
D3DXVECTOR3 s1 = current.Scale;
D3DXVECTOR3 s2 = next.Scale;
D3DXVECTOR3 s;
D3DXVec3Lerp(&s, &s1, &s2, frameFactor);
D3DXMatrixScaling(&S, s.x, s.y, s.z);
D3DXQUATERNION q1 = current.Rotation;
D3DXQUATERNION q2 = next.Rotation;
D3DXQUATERNION q;
D3DXQuaternionSlerp(&q, &q1, &q2, frameFactor);
D3DXMatrixRotationQuaternion(&R, &q);
D3DXVECTOR3 t1 = current.Translation;
D3DXVECTOR3 t2 = next.Translation;
D3DXVECTOR3 t;
D3DXVec3Lerp(&t, &t1, &t2, frameFactor);
D3DXMatrixTranslation(&T, t.x, t.y, t.z);
matAnimation = S * R * T;
}
else
{
matAnimation = frame->Datas[currentKeyframe].Transform;
}
int parentIndex = bone->ParentIndex();
if (parentIndex < 0)
D3DXMatrixIdentity(&matParentAnimation);
else
matParentAnimation = boneAnimation[parentIndex];
boneAnimation[i] = matAnimation * matParentAnimation;
renderTransform[i] = matInvBindPose * boneAnimation[i];
}
}
void ModelAnimPlayer::Render()
{
model->Buffer()->SetVSBuffer(2);
for (ModelMesh* mesh : model->Meshes())
mesh->Render();
}<file_sep>#include "Vector2.h"
#include <d3dx9math.h>
#include "Matrix.h"
#include "Quaternion.h"
//////////////////////////////////////////////////////////////////////////
const Vector2 Vector2::Zero = Vector2(0.0f, 0.0f);
const Vector2 Vector2::One = Vector2(1.0f, 1.0f);
const Vector2 Vector2::UnitX = Vector2(1.0f, 0.0f);
const Vector2 Vector2::UnitY = Vector2(0.0f, 1.0f);
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
//////////////////////////////////////////////////////////////////////////
Vector2::Vector2( void )
{
this->X = 0.0f;
this->Y = 0.0f;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param value : x = y = value;
//////////////////////////////////////////////////////////////////////////
Vector2::Vector2( float value )
{
this->X = this->Y = value;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param x : X
///@param y : Y
//////////////////////////////////////////////////////////////////////////
Vector2::Vector2( float x, float y )
{
this->X = x;
this->Y = y;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::operator-( void )
{
Vector2 vector2;
vector2.X = -this->X;
vector2.Y = -this->Y;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief ==
///@param value2 : 벡터
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Vector2::operator==( const Vector2& value2 ) const
{
if((double)this->X == (double)value2.X)
return (double)this->Y == (double)value2.Y;
else
return false;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief !=
///@param value2 : 벡터
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Vector2::operator!=( const Vector2& value2 ) const
{
if((double)this->X == (double)value2.X)
return (double)this->Y != (double)value2.Y;
else
return true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief +
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::operator+( const Vector2& value2 ) const
{
Vector2 vector2;
vector2.X = this->X + value2.X;
vector2.Y = this->Y + value2.Y;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::operator-( const Vector2& value2 ) const
{
Vector2 vector2;
vector2.X = this->X - value2.X;
vector2.Y = this->Y - value2.Y;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::operator*( const Vector2& value2 ) const
{
Vector2 vector2;
vector2.X = this->X * value2.X;
vector2.Y = this->Y * value2.Y;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *
///@param scaleFactor : 값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::operator*( const float& scaleFactor ) const
{
Vector2 vector2;
vector2.X = this->X * scaleFactor;
vector2.Y = this->Y * scaleFactor;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::operator/( const Vector2& value2 ) const
{
Vector2 vector2;
vector2.X = this->X / value2.X;
vector2.Y = this->Y / value2.Y;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /
///@param divider : 값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::operator/( const float& divider ) const
{
float num = 1.0f / divider;
Vector2 vector2;
vector2.X = this->X * num;
vector2.Y = this->Y * num;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief +=
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector2::operator+=( const Vector2& value2 )
{
*this = *this + value2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -=
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector2::operator-=( const Vector2& value2 )
{
*this = *this - value2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *=
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector2::operator*=( const Vector2& value2 )
{
*this = *this * value2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /=
///@param scaleFactor : 값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector2::operator*=( const float& scaleFactor )
{
*this = *this * scaleFactor;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /=
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector2::operator/=( const Vector2& value2 )
{
*this = *this / value2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /=
///@param divider : 값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector2::operator/=( const float& divider )
{
*this = *this / divider;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 문자열로 변환
///@return 문자열
//////////////////////////////////////////////////////////////////////////
std::string Vector2::ToString()
{
std::string temp;
char val[255];
sprintf(val, "{X:%#f ", X); temp += val;
sprintf(val, "Y:%#f}", Y); temp += val;
return temp;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief D3DXVECTOR2로 변환
///@return D3DX 벡터
//////////////////////////////////////////////////////////////////////////
D3DXVECTOR2 Vector2::ToD3DXVECTOR2()
{
D3DXVECTOR2 vector2;
vector2.x = X;
vector2.y = Y;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 크기 계산
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector2::Length()
{
return (float)sqrt((double)this->X * (double)this->X + (double)this->Y * (double)this->Y);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 제곱으로 크기 계산
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector2::LengthSquared()
{
return (float)((double)this->X * (double)this->X + (double)this->Y * (double)this->Y);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 정규화
//////////////////////////////////////////////////////////////////////////
void Vector2::Normalize()
{
float num = 1.0f / (float)sqrt((double)this->X * (double)this->X + (double)this->Y * (double)this->Y);
this->X *= num;
this->Y *= num;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 거리계산
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector2::Distance( Vector2 value1, Vector2 value2 )
{
float num1 = value1.X - value2.X;
float num2 = value1.Y - value2.Y;
return (float)sqrt((double)num1 * (double)num1 + (double)num2 * (double)num2);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 제곱으로 거리계산
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector2::DistanceSquared( Vector2 value1, Vector2 value2 )
{
float num1 = value1.X - value2.X;
float num2 = value1.Y - value2.Y;
return (float)((double)num1 * (double)num1 + (double)num2 * (double)num2);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 내적 계산
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector2::Dot( Vector2 value1, Vector2 value2 )
{
return (float)((double)value1.X * (double)value2.X + (double)value1.Y * (double)value2.Y);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 정규화
///@param value : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::Normalize( Vector2 value )
{
float num = 1.0f / (float)sqrt((double)value.X * (double)value.X + (double)value.Y * (double)value.Y);
Vector2 vector2;
vector2.X = value.X * num;
vector2.Y = value.Y * num;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 반사값
///@param vector : 벡터
///@param normal : 노멀벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::Reflect( Vector2 vector, Vector2 normal )
{
float num = (float)((double)vector.X * (double)normal.X + (double)vector.Y * (double)normal.Y);
Vector2 vector2;
vector2.X = vector.X - 2.0f * num * normal.X;
vector2.Y = vector.Y - 2.0f * num * normal.Y;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 벡터 중 작은 벡터
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::Min( Vector2 value1, Vector2 value2 )
{
Vector2 vector2;
vector2.X = (double)value1.X < (double)value2.X ? value1.X : value2.X;
vector2.Y = (double)value1.Y < (double)value2.Y ? value1.Y : value2.Y;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 벡터 중 큰 벡터
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::Max( Vector2 value1, Vector2 value2 )
{
Vector2 vector2;
vector2.X = (double)value1.X > (double)value2.X ? value1.X : value2.X;
vector2.Y = (double)value1.Y > (double)value2.Y ? value1.Y : value2.Y;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 해당 벡터가 최소값보다 작을 경우 최소값, 반대의 경우 최대값
///@param value1 : 벡터
///@param min : 최소값 벡터
///@param max : 최대값 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::Clamp( Vector2 value1, Vector2 min, Vector2 max )
{
float num1 = value1.X;
float num2 = (double) num1 > (double) max.X ? max.X : num1;
float num3 = (double) num2 < (double) min.X ? min.X : num2;
float num4 = value1.Y;
float num5 = (double) num4 > (double) max.Y ? max.Y : num4;
float num6 = (double) num5 < (double) min.Y ? min.Y : num5;
Vector2 vector2;
vector2.X = num3;
vector2.Y = num6;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 벡터의 선형보간
///@param value1 : 벡터1
///@param value2 : 벡터2
///@param amount : 보간값
///@return 결과벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::Lerp( Vector2 value1, Vector2 value2, float amount )
{
Vector2 vector2;
vector2.X = value1.X + (value2.X - value1.X) * amount;
vector2.Y = value1.Y + (value2.Y - value1.Y) * amount;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 벡터를 부드럽게 보간
///@param value1 : 벡터1
///@param value2 : 벡터2
///@param amount : 보간값
///@return 결과벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::SmoothStep( Vector2 value1, Vector2 value2, float amount )
{
amount = (double)amount > 1.0 ? 1.0f : ((double)amount < 0.0 ? 0.0f : amount);
amount = (float)((double)amount * (double)amount * (3.0 - 2.0 * (double)amount));
Vector2 vector2;
vector2.X = value1.X + (value2.X - value1.X) * amount;
vector2.Y = value1.Y + (value2.Y - value1.Y) * amount;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 카트뮬-롬 보간
///@param value1 : 벡터1
///@param value2 : 벡터2
///@param value3 : 벡터3
///@param value4 : 벡터4
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::CatmullRom( Vector2 value1, Vector2 value2, Vector2 value3, Vector2 value4, float amount )
{
float num1 = amount * amount;
float num2 = amount * num1;
Vector2 vector2;
vector2.X = (float)(0.5 * (2.0 * (double)value2.X + (-(double)value1.X + (double)value3.X)* (double)amount + (2.0 * (double)value1.X - 5.0 * (double)value2.X + 4.0 * (double)value3.X - (double)value4.X)* (double)num1 + (-(double)value1.X + 3.0 * (double)value2.X - 3.0 * (double)value3.X + (double)value4.X)* (double)num2));
vector2.Y = (float)(0.5 * (2.0 * (double)value2.Y + (-(double)value1.Y + (double)value3.Y)* (double)amount + (2.0 * (double)value1.Y - 5.0 * (double)value2.Y + 4.0 * (double)value3.Y - (double)value4.Y)* (double)num1 + (-(double)value1.Y + 3.0 * (double)value2.Y - 3.0 * (double)value3.Y + (double)value4.Y)* (double)num2));
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 에르미트 보간
///@param value1 : 벡터1
///@param tagent1 : 벡터1의 탄젠트 벡터
///@param value2 : 벡터2
///@param tagent2 : 벡터2 탄젠트 벡터
///@param amount : 보간값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::Hermite( Vector2 value1, Vector2 tangent1, Vector2 value2, Vector2 tangent2, float amount )
{
float num1 = amount * amount;
float num2 = amount * num1;
float num3 = (float)(2.0 * (double)num2 - 3.0 * (double)num1 + 1.0);
float num4 = (float)(-2.0 * (double)num2 + 3.0 * (double)num1);
float num5 = num2 - 2.0f * num1 + amount;
float num6 = num2 - num1;
Vector2 vector2;
vector2.X = (float)((double)value1.X * (double)num3 + (double)value2.X * (double)num4 + (double)tangent1.X * (double)num5 + (double)tangent2.X * (double)num6);
vector2.Y = (float)((double)value1.Y * (double)num3 + (double)value2.Y * (double)num4 + (double)tangent1.Y * (double)num5 + (double)tangent2.Y * (double)num6);
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 매트릭스에 의한 벡터 변환
///@param position : 벡터
///@param matrix : 매트릭스
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::Transform( Vector2 position, Matrix matrix )
{
float num1 = (float)((double)position.X * (double)matrix.M11 + (double)position.Y * (double)matrix.M21) + matrix.M41;
float num2 = (float)((double)position.X * (double)matrix.M12 + (double)position.Y * (double)matrix.M22) + matrix.M42;
Vector2 vector2;
vector2.X = num1;
vector2.Y = num2;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 쿼터니온에 의한 벡터변환
///@param value : 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::Transform( Vector2 value, Quaternion rotation )
{
float num1 = rotation.X + rotation.X;
float num2 = rotation.Y + rotation.Y;
float num3 = rotation.Z + rotation.Z;
float num4 = rotation.W * num3;
float num5 = rotation.X * num1;
float num6 = rotation.X * num2;
float num7 = rotation.Y * num2;
float num8 = rotation.Z * num3;
float num9 = (float) ((double) value.X * (1.0 - (double) num7 - (double) num8) + (double) value.Y * ((double) num6 - (double) num4));
float num10 = (float) ((double) value.X * ((double) num6 + (double) num4) + (double) value.Y * (1.0 - (double) num5 - (double) num8));
Vector2 vector2;
vector2.X = num9;
vector2.Y = num10;
return vector2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 매트릭스에 의한 노멀벡터 변환
///@param normal : 법선 벡터
///@param matrix : 매트릭스
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector2 Vector2::TransformNormal( Vector2 normal, Matrix matrix )
{
float num1 = (float)((double)normal.X * (double)matrix.M11 + (double)normal.Y * (double)matrix.M21);
float num2 = (float)((double)normal.X * (double)matrix.M12 + (double)normal.Y * (double)matrix.M22);
Vector2 vector2;
vector2.X = num1;
vector2.Y = num2;
return vector2;
}<file_sep>#pragma once
#include "../Objects/GameAnimationModel.h"
class GameUnit : public GameAnimationModel
{
public:
GameUnit(wstring matFile, wstring meshFile);
virtual ~GameUnit();
virtual void Update();
virtual void Render();
};<file_sep>#include "Vector4.h"
#include <d3dx9math.h>
#include "Vector2.h"
#include "Vector3.h"
#include "Matrix.h"
#include "Quaternion.h"
//////////////////////////////////////////////////////////////////////////
const Vector4 Vector4::Zero = Vector4(0.0f, 0.0f, 0.0f, 0.0f);
const Vector4 Vector4::One = Vector4(1.0f, 1.0f, 1.0f, 1.0f);
const Vector4 Vector4::UnitX = Vector4(1.0f, 0.0f, 0.0f, 0.0f);
const Vector4 Vector4::UnitY = Vector4(0.0f, 1.0f, 0.0f, 0.0f);
const Vector4 Vector4::UnitZ = Vector4(0.0f, 0.0f, 1.0f, 0.0f);
const Vector4 Vector4::UnitW = Vector4(0.0f, 0.0f, 0.0f, 1.0f);
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
//////////////////////////////////////////////////////////////////////////
Vector4::Vector4( void )
{
this->X = 0.0f;
this->Y = 0.0f;
this->Z = 0.0f;
this->W = 0.0f;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param x : X
///@param y : Y
///@param z : Z
///@param w : W
//////////////////////////////////////////////////////////////////////////
Vector4::Vector4( float x, float y, float z, float w )
{
this->X = x;
this->Y = y;
this->Z = z;
this->W = w;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param value : x, y
///@param z : Z
///@param w : W
//////////////////////////////////////////////////////////////////////////
Vector4::Vector4( Vector2 value, float z, float w )
{
this->X = value.X;
this->Y = value.Y;
this->Z = z;
this->W = w;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param value : x, y, z
///@param w : W
//////////////////////////////////////////////////////////////////////////
Vector4::Vector4( Vector3 value, float w )
{
this->X = value.X;
this->Y = value.Y;
this->Z = value.Z;
this->W = w;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param x = y = z = w = value
//////////////////////////////////////////////////////////////////////////
Vector4::Vector4( float value )
{
this->X = this->Y = this->Z = this->W = value;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::operator-( void )
{
Vector4 vector4;
vector4.X = -this->X;
vector4.Y = -this->Y;
vector4.Z = -this->Z;
vector4.W = -this->W;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief ==
///@param value2 : 벡터
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Vector4::operator==( const Vector4& value2 ) const
{
if ((double)this->X == (double)value2.X && (double)this->Y == (double)value2.Y && (double)this->Z == (double)value2.Z)
return (double)this->W == (double)value2.W;
else
return false;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief !=
///@param value2 : 벡터
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Vector4::operator!=( const Vector4& value2 ) const
{
if ((double)this->X == (double)value2.X && (double)this->Y == (double)value2.Y && (double)this->Z == (double)value2.Z)
return (double)this->W != (double)value2.W;
else
return true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief +
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::operator+( const Vector4& value2 ) const
{
Vector4 vector4;
vector4.X = this->X + value2.X;
vector4.Y = this->Y + value2.Y;
vector4.Z = this->Z + value2.Z;
vector4.W = this->W + value2.W;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::operator-( const Vector4& value2 ) const
{
Vector4 vector4;
vector4.X = this->X - value2.X;
vector4.Y = this->Y - value2.Y;
vector4.Z = this->Z - value2.Z;
vector4.W = this->W - value2.W;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::operator*( const Vector4& value2 ) const
{
Vector4 vector4;
vector4.X = this->X * value2.X;
vector4.Y = this->Y * value2.Y;
vector4.Z = this->Z * value2.Z;
vector4.W = this->W * value2.W;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *
///@param scaleFactor : 값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::operator*( const float& scaleFactor ) const
{
Vector4 vector4;
vector4.X = this->X * scaleFactor;
vector4.Y = this->Y * scaleFactor;
vector4.Z = this->Z * scaleFactor;
vector4.W = this->W * scaleFactor;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::operator/( const Vector4& value2 ) const
{
Vector4 vector4;
vector4.X = this->X / value2.X;
vector4.Y = this->Y / value2.Y;
vector4.Z = this->Z / value2.Z;
vector4.W = this->W / value2.W;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /
///@param divider : 값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::operator/( const float& divider ) const
{
float num = 1.0f / divider;
Vector4 vector4;
vector4.X = this->X * num;
vector4.Y = this->Y * num;
vector4.Z = this->Z * num;
vector4.W = this->W * num;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief +=
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector4::operator+=( const Vector4& value2 )
{
*this = *this + value2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -=
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector4::operator-=( const Vector4& value2 )
{
*this = *this - value2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *=
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector4::operator*=( const Vector4& value2 )
{
*this = *this * value2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /=
///@param scaleFactor : 값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector4::operator*=( const float& scaleFactor )
{
*this = *this * scaleFactor;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /=
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector4::operator/=( const Vector4& value2 )
{
*this = *this / value2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /=
///@param divider : 값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector4::operator/=( const float& divider )
{
*this = *this / divider;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 문자열로 변환
///@return 문자열
//////////////////////////////////////////////////////////////////////////
std::string Vector4::ToString()
{
std::string temp;
char val[255];
sprintf(val, "{X:%#f ", X); temp += val;
sprintf(val, "Y:%#f ", Y); temp += val;
sprintf(val, "Z:%#f ", Z); temp += val;
sprintf(val, "W:%#f}", W); temp += val;
return temp;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief D3DXVECTOR4로 변환
///@return D3DX 벡터
//////////////////////////////////////////////////////////////////////////
D3DXVECTOR4 Vector4::ToD3DXVECTOR4()
{
D3DXVECTOR4 vector4;
vector4.x = X;
vector4.y = Y;
vector4.z = Z;
vector4.z = W;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 크기 계산
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector4::Length()
{
return (float)sqrt((double)this->X * (double)this->X + (double)this->Y * (double)this->Y + (double)this->Z * (double)this->Z + (double)this->W * (double)this->W);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 제곱으로 크기 계산
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector4::LengthSquared()
{
return (float)((double)this->X * (double)this->X + (double)this->Y * (double)this->Y + (double)this->Z * (double)this->Z + (double)this->W * (double)this->W);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 정규화
//////////////////////////////////////////////////////////////////////////
void Vector4::Normalize()
{
float num = 1.0f / (float)sqrt((double)this->X * (double)this->X + (double)this->Y * (double)this->Y + (double)this->Z * (double)this->Z + (double)this->W * (double)this->W);
this->X *= num;
this->Y *= num;
this->Z *= num;
this->W *= num;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 거리계산
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector4::Distance( Vector4 value1, Vector4 value2 )
{
float num1 = value1.X - value2.X;
float num2 = value1.Y - value2.Y;
float num3 = value1.Z - value2.Z;
float num4 = value1.W - value2.W;
return (float)sqrt((double)num1 * (double)num1 + (double)num2 * (double)num2 + (double)num3 * (double)num3 + (double)num4 * (double)num4);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 제곱으로 거리계산
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector4::DistanceSquared( Vector4 value1, Vector4 value2 )
{
float num1 = value1.X - value2.X;
float num2 = value1.Y - value2.Y;
float num3 = value1.Z - value2.Z;
float num4 = value1.W - value2.W;
return (float)((double)num1 * (double)num1 + (double)num2 * (double)num2 + (double)num3 * (double)num3 + (double)num4 * (double)num4);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 내적 계산
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector4::Dot( Vector4 value1, Vector4 value2 )
{
return (float)((double)value1.X * (double)value2.X + (double)value1.Y * (double)value2.Y + (double)value1.Z * (double)value2.Z + (double)value1.W * (double)value2.W);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 정규화
///@param value : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::Normalize( Vector4 value )
{
float num = 1.0f / (float)sqrt((double)value.X * (double)value.X + (double)value.Y * (double)value.Y + (double)value.Z * (double)value.Z + (double)value.W * (double)value.W);
Vector4 vector4;
vector4.X = value.X * num;
vector4.Y = value.Y * num;
vector4.Z = value.Z * num;
vector4.W = value.W * num;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 벡터 중 작은 벡터
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::Min( Vector4 value1, Vector4 value2 )
{
Vector4 vector4;
vector4.X = (double)value1.X < (double)value2.X ? value1.X : value2.X;
vector4.Y = (double)value1.Y < (double)value2.Y ? value1.Y : value2.Y;
vector4.Z = (double)value1.Z < (double)value2.Z ? value1.Z : value2.Z;
vector4.W = (double)value1.W < (double)value2.W ? value1.W : value2.W;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 벡터 중 큰 벡터
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::Max( Vector4 value1, Vector4 value2 )
{
Vector4 vector4;
vector4.X = (double)value1.X > (double)value2.X ? value1.X : value2.X;
vector4.Y = (double)value1.Y > (double)value2.Y ? value1.Y : value2.Y;
vector4.Z = (double)value1.Z > (double)value2.Z ? value1.Z : value2.Z;
vector4.W = (double)value1.W > (double)value2.W ? value1.W : value2.W;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 해당 벡터가 최소값보다 작을 경우 최소값, 반대의 경우 최대값
///@param value1 : 벡터
///@param min : 최소값 벡터
///@param max : 최대값 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::Clamp( Vector4 value1, Vector4 min, Vector4 max )
{
float num1 = value1.X;
float num2 = (double)num1 > (double)max.X ? max.X : num1;
float num3 = (double)num2 < (double)min.X ? min.X : num2;
float num4 = value1.Y;
float num5 = (double)num4 > (double)max.Y ? max.Y : num4;
float num6 = (double)num5 < (double)min.Y ? min.Y : num5;
float num7 = value1.Z;
float num8 = (double)num7 > (double)max.Z ? max.Z : num7;
float num9 = (double)num8 < (double)min.Z ? min.Z : num8;
float num10 = value1.W;
float num11 = (double)num10 > (double)max.W ? max.W : num10;
float num12 = (double)num11 < (double)min.W ? min.W : num11;
Vector4 vector4;
vector4.X = num3;
vector4.Y = num6;
vector4.Z = num9;
vector4.W = num12;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 벡터의 선형보간
///@param value1 : 벡터1
///@param value2 : 벡터2
///@param amount : 보간값
///@return 결과벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::Lerp( Vector4 value1, Vector4 value2, float amount )
{
Vector4 vector4;
vector4.X = value1.X + (value2.X - value1.X) * amount;
vector4.Y = value1.Y + (value2.Y - value1.Y) * amount;
vector4.Z = value1.Z + (value2.Z - value1.Z) * amount;
vector4.W = value1.W + (value2.W - value1.W) * amount;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 벡터를 부드럽게 보간
///@param value1 : 벡터1
///@param value2 : 벡터2
///@param amount : 보간값
///@return 결과벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::SmoothStep( Vector4 value1, Vector4 value2, float amount )
{
amount = (double)amount > 1.0 ? 1.0f : ((double)amount < 0.0 ? 0.0f : amount);
amount = (float)((double)amount * (double)amount * (3.0 - 2.0 * (double)amount));
Vector4 vector4;
vector4.X = value1.X + (value2.X - value1.X) * amount;
vector4.Y = value1.Y + (value2.Y - value1.Y) * amount;
vector4.Z = value1.Z + (value2.Z - value1.Z) * amount;
vector4.W = value1.W + (value2.W - value1.W) * amount;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 카트뮬-롬 보간
///@param value1 : 벡터1
///@param value2 : 벡터2
///@param value3 : 벡터3
///@param value4 : 벡터4
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::CatmullRom( Vector4 value1, Vector4 value2, Vector4 value3, Vector4 value4, float amount )
{
float num1 = amount * amount;
float num2 = amount * num1;
Vector4 vector4;
vector4.X = (float)(0.5 * (2.0 * (double)value2.X + (-(double)value1.X + (double)value3.X)* (double)amount + (2.0 * (double)value1.X - 5.0 * (double)value2.X + 4.0 * (double)value3.X - (double)value4.X)* (double)num1 + (-(double)value1.X + 3.0 * (double)value2.X - 3.0 * (double)value3.X + (double)value4.X)* (double)num2));
vector4.Y = (float)(0.5 * (2.0 * (double)value2.Y + (-(double)value1.Y + (double)value3.Y)* (double)amount + (2.0 * (double)value1.Y - 5.0 * (double)value2.Y + 4.0 * (double)value3.Y - (double)value4.Y)* (double)num1 + (-(double)value1.Y + 3.0 * (double)value2.Y - 3.0 * (double)value3.Y + (double)value4.Y)* (double)num2));
vector4.Z = (float)(0.5 * (2.0 * (double)value2.Z + (-(double)value1.Z + (double)value3.Z)* (double)amount + (2.0 * (double)value1.Z - 5.0 * (double)value2.Z + 4.0 * (double)value3.Z - (double)value4.Z)* (double)num1 + (-(double)value1.Z + 3.0 * (double)value2.Z - 3.0 * (double)value3.Z + (double)value4.Z)* (double)num2));
vector4.W = (float)(0.5 * (2.0 * (double)value2.W + (-(double)value1.W + (double)value3.W)* (double)amount + (2.0 * (double)value1.W - 5.0 * (double)value2.W + 4.0 * (double)value3.W - (double)value4.W)* (double)num1 + (-(double)value1.W + 3.0 * (double)value2.W - 3.0 * (double)value3.W + (double)value4.W)* (double)num2));
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 에르미트 보간
///@param value1 : 벡터1
///@param tagent1 : 벡터1의 탄젠트 벡터
///@param value2 : 벡터2
///@param tagent2 : 벡터2 탄젠트 벡터
///@param amount : 보간값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::Hermite( Vector4 value1, Vector4 tangent1, Vector4 value2, Vector4 tangent2, float amount )
{
float num1 = amount * amount;
float num2 = amount * num1;
float num3 = (float)(2.0 * (double)num2 - 3.0 * (double)num1 + 1.0);
float num4 = (float)(-2.0 * (double)num2 + 3.0 * (double)num1);
float num5 = num2 - 2.0f * num1 + amount;
float num6 = num2 - num1;
Vector4 vector4;
vector4.X = (float)((double)value1.X * (double)num3 + (double)value2.X * (double)num4 + (double)tangent1.X * (double)num5 + (double)tangent2.X * (double)num6);
vector4.Y = (float)((double)value1.Y * (double)num3 + (double)value2.Y * (double)num4 + (double)tangent1.Y * (double)num5 + (double)tangent2.Y * (double)num6);
vector4.Z = (float)((double)value1.Z * (double)num3 + (double)value2.Z * (double)num4 + (double)tangent1.Z * (double)num5 + (double)tangent2.Z * (double)num6);
vector4.W = (float)((double)value1.W * (double)num3 + (double)value2.W * (double)num4 + (double)tangent1.W * (double)num5 + (double)tangent2.W * (double)num6);
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 매트릭스에 의한 2D벡터 변환
///@param position : 벡터
///@param matrix : 매트릭스
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::Transform( Vector2 position, Matrix matrix )
{
float num1 = (float)((double)position.X * (double)matrix.M11 + (double)position.Y * (double)matrix.M21) + matrix.M41;
float num2 = (float)((double)position.X * (double)matrix.M12 + (double)position.Y * (double)matrix.M22) + matrix.M42;
float num3 = (float)((double)position.X * (double)matrix.M13 + (double)position.Y * (double)matrix.M23) + matrix.M43;
float num4 = (float)((double)position.X * (double)matrix.M14 + (double)position.Y * (double)matrix.M24) + matrix.M44;
Vector4 vector4;
vector4.X = num1;
vector4.Y = num2;
vector4.Z = num3;
vector4.W = num4;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 매트릭스에 의한 3D벡터 변환
///@param position : 벡터
///@param matrix : 매트릭스
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::Transform( Vector3 position, Matrix matrix )
{
float num1 = (float)((double)position.X * (double)matrix.M11 + (double)position.Y * (double)matrix.M21 + (double)position.Z * (double)matrix.M31) + matrix.M41;
float num2 = (float)((double)position.X * (double)matrix.M12 + (double)position.Y * (double)matrix.M22 + (double)position.Z * (double)matrix.M32) + matrix.M42;
float num3 = (float)((double)position.X * (double)matrix.M13 + (double)position.Y * (double)matrix.M23 + (double)position.Z * (double)matrix.M33) + matrix.M43;
float num4 = (float)((double)position.X * (double)matrix.M14 + (double)position.Y * (double)matrix.M24 + (double)position.Z * (double)matrix.M34) + matrix.M44;
Vector4 vector4;
vector4.X = num1;
vector4.Y = num2;
vector4.Z = num3;
vector4.W = num4;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 매트릭스에 의한 4D벡터 변환
///@param position : 벡터
///@param matrix : 매트릭스
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::Transform( Vector4 vector, Matrix matrix )
{
float num1 = (float)((double)vector.X * (double)matrix.M11 + (double)vector.Y * (double)matrix.M21 + (double)vector.Z * (double)matrix.M31 + (double)vector.W * (double)matrix.M41);
float num2 = (float)((double)vector.X * (double)matrix.M12 + (double)vector.Y * (double)matrix.M22 + (double)vector.Z * (double)matrix.M32 + (double)vector.W * (double)matrix.M42);
float num3 = (float)((double)vector.X * (double)matrix.M13 + (double)vector.Y * (double)matrix.M23 + (double)vector.Z * (double)matrix.M33 + (double)vector.W * (double)matrix.M43);
float num4 = (float)((double)vector.X * (double)matrix.M14 + (double)vector.Y * (double)matrix.M24 + (double)vector.Z * (double)matrix.M34 + (double)vector.W * (double)matrix.M44);
Vector4 vector4;
vector4.X = num1;
vector4.Y = num2;
vector4.Z = num3;
vector4.W = num4;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 쿼터니온에 의한 2D벡터 변환
///@param value : 벡터
///@param rotation : 쿼터니온
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::Transform( Vector2 value, Quaternion rotation )
{
float num1 = rotation.X + rotation.X;
float num2 = rotation.Y + rotation.Y;
float num3 = rotation.Z + rotation.Z;
float num4 = rotation.W * num1;
float num5 = rotation.W * num2;
float num6 = rotation.W * num3;
float num7 = rotation.X * num1;
float num8 = rotation.X * num2;
float num9 = rotation.X * num3;
float num10 = rotation.Y * num2;
float num11 = rotation.Y * num3;
float num12 = rotation.Z * num3;
float num13 = (float)((double)value.X * (1.0 - (double)num10 - (double)num12)+ (double)value.Y * ((double)num8 - (double)num6));
float num14 = (float)((double)value.X * ((double)num8 + (double)num6) + (double)value.Y * (1.0 - (double)num7 - (double)num12));
float num15 = (float)((double)value.X * ((double)num9 - (double)num5) + (double)value.Y * ((double)num11 + (double)num4));
Vector4 vector4;
vector4.X = num13;
vector4.Y = num14;
vector4.Z = num15;
vector4.W = 1.0f;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 쿼터니온에 의한 3D벡터 변환
///@param value : 벡터
///@param rotation : 쿼터니온
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::Transform( Vector3 value, Quaternion rotation )
{
float num1 = rotation.X + rotation.X;
float num2 = rotation.Y + rotation.Y;
float num3 = rotation.Z + rotation.Z;
float num4 = rotation.W * num1;
float num5 = rotation.W * num2;
float num6 = rotation.W * num3;
float num7 = rotation.X * num1;
float num8 = rotation.X * num2;
float num9 = rotation.X * num3;
float num10 = rotation.Y * num2;
float num11 = rotation.Y * num3;
float num12 = rotation.Z * num3;
float num13 = (float)((double)value.X * (1.0 - (double)num10 - (double)num12)+ (double)value.Y * ((double)num8 - (double)num6)+ (double)value.Z * ((double)num9 + (double)num5));
float num14 = (float)((double)value.X * ((double)num8 + (double)num6)+ (double)value.Y * (1.0 - (double)num7 - (double)num12)+ (double)value.Z * ((double)num11 - (double)num4));
float num15 = (float)((double)value.X * ((double)num9 - (double)num5)+ (double)value.Y * ((double)num11 + (double)num4)+ (double)value.Z * (1.0 - (double)num7 - (double)num10));
Vector4 vector4;
vector4.X = num13;
vector4.Y = num14;
vector4.Z = num15;
vector4.W = 1.0f;
return vector4;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 쿼터니온에 의한 4D벡터 변환
///@param value : 벡터
///@param rotation : 쿼터니온
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector4 Vector4::Transform( Vector4 value, Quaternion rotation )
{
float num1 = rotation.X + rotation.X;
float num2 = rotation.Y + rotation.Y;
float num3 = rotation.Z + rotation.Z;
float num4 = rotation.W * num1;
float num5 = rotation.W * num2;
float num6 = rotation.W * num3;
float num7 = rotation.X * num1;
float num8 = rotation.X * num2;
float num9 = rotation.X * num3;
float num10 = rotation.Y * num2;
float num11 = rotation.Y * num3;
float num12 = rotation.Z * num3;
float num13 = (float)((double)value.X * (1.0 - (double)num10 - (double)num12)+ (double)value.Y * ((double)num8 - (double)num6)+ (double)value.Z * ((double)num9 + (double)num5));
float num14 = (float)((double)value.X * ((double)num8 + (double)num6)+ (double)value.Y * (1.0 - (double)num7 - (double)num12)+ (double)value.Z * ((double)num11 - (double)num4));
float num15 = (float)((double)value.X * ((double)num9 - (double)num5)+ (double)value.Y * ((double)num11 + (double)num4)+ (double)value.Z * (1.0 - (double)num7 - (double)num10));
Vector4 vector4;
vector4.X = num13;
vector4.Y = num14;
vector4.Z = num15;
vector4.W = value.W;
return vector4;
}<file_sep>#pragma once
#include "stdafx.h"
template<typename T>
class Singleton
{
public:
static T* Get()
{
if (instance == nullptr)
instance = new T();
return instance;
}
static void Delete()
{
SAFE_DELETE(instance);
}
protected:
Singleton() {};
virtual ~Singleton() { SAFE_DELETE(instance); }
private:
static T* instance;
};
template <typename T> T* Singleton<T>::instance = nullptr;<file_sep>#include "stdafx.h"
#include "GameUnit.h"
GameUnit::GameUnit(wstring matFile, wstring meshFile)
: GameAnimationModel(matFile, meshFile)
{
}
GameUnit::~GameUnit()
{
}
void GameUnit::Update()
{
GameAnimationModel::Update();
}
void GameUnit::Render()
{
GameAnimationModel::Render();
}
<file_sep>#pragma once
#include <string>
#pragma warning( disable : 4996)
struct D3DXVECTOR2;
class Matrix;
class Quaternion;
//////////////////////////////////////////////////////////////////////////
///@brief 2D 벡터
//////////////////////////////////////////////////////////////////////////
class Vector2
{
public:
Vector2(void);
Vector2(float value);
Vector2(float x, float y);
Vector2 operator -(void);
bool operator ==(const Vector2& value2) const;
bool operator !=(const Vector2& value2) const;
Vector2 operator +(const Vector2& value2) const;
Vector2 operator -(const Vector2& value2) const;
Vector2 operator *(const Vector2& value2) const;
Vector2 operator *(const float& scaleFactor) const;
Vector2 operator /(const Vector2& value2) const;
Vector2 operator /(const float& divider) const;
void operator +=(const Vector2& value2);
void operator -=(const Vector2& value2);
void operator *=(const Vector2& value2);
void operator *=(const float& scaleFactor);
void operator /=(const Vector2& value2);
void operator /=(const float& divider);
//////////////////////////////////////////////////////////////////////////
///@brief float형의 *연산 처리
///@param scalefactor : 값
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
friend Vector2 operator *(const float scaleFactor, const Vector2& value2)
{
return value2 * scaleFactor;
}
//////////////////////////////////////////////////////////////////////////
std::string ToString();
D3DXVECTOR2 ToD3DXVECTOR2();
float Length();
float LengthSquared();
void Normalize();
static float Distance(Vector2 value1, Vector2 value2);
static float DistanceSquared(Vector2 value1, Vector2 value2);
static float Dot(Vector2 value1, Vector2 value2);
static Vector2 Normalize(Vector2 value);
static Vector2 Reflect(Vector2 vector, Vector2 normal);
static Vector2 Min(Vector2 value1, Vector2 value2);
static Vector2 Max(Vector2 value1, Vector2 value2);
static Vector2 Clamp(Vector2 value1, Vector2 min, Vector2 max);
static Vector2 Lerp(Vector2 value1, Vector2 value2, float amount);
static Vector2 SmoothStep(Vector2 value1, Vector2 value2, float amount);
static Vector2 CatmullRom(Vector2 value1, Vector2 value2, Vector2 value3, Vector2 value4, float amount);
static Vector2 Hermite(Vector2 value1, Vector2 tangent1, Vector2 value2, Vector2 tangent2, float amount);
static Vector2 Transform(Vector2 position, Matrix matrix);
static Vector2 Transform(Vector2 value, Quaternion rotation);
static Vector2 TransformNormal(Vector2 normal, Matrix matrix);
public:
const static Vector2 Zero;///< 0.0f, 0.0f
const static Vector2 One;///< 1.0f, 1.0f
const static Vector2 UnitX;///< 1.0f, 0.0f
const static Vector2 UnitY;///< 0.0f, 1.0f
float X;///< X
float Y;///< Y
};<file_sep>#include "Gjk.h"
#include "../Core/MathHelper.h"
//////////////////////////////////////////////////////////////////////////
const int Gjk::BitsToIndices[16] = {0, 1, 2, 17, 3, 25, 26, 209, 4, 33, 34, 273, 35, 281, 282, 2257};
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
//////////////////////////////////////////////////////////////////////////
Gjk::Gjk( void )
{
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 심플렉스가 가득찼는지 여부
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Gjk::FullSimplex() const
{
return simplexBits == 15;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 제곱으로 계산한 크기 중 가장 큰 값
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Gjk::MaxLengthSquared() const
{
return maxLengthSq;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 가까운 위치 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Gjk::ClosestPoint() const
{
return closestPoint;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 리셋
//////////////////////////////////////////////////////////////////////////
void Gjk::Reset()
{
simplexBits = 0;
maxLengthSq = 0.0f;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 검사할 포인트 추가
///@return 추가 결과
//////////////////////////////////////////////////////////////////////////
bool Gjk::AddSupportPoint( Vector3 newPoint )
{
int index1 = (BitsToIndices[simplexBits ^ 15] & 7) - 1;
y[index1] = newPoint;
yLengthSq[index1] = newPoint.LengthSquared();
int num = BitsToIndices[simplexBits];
while(num != 0)
{
int index2 = (num & 7) - 1;
Vector3 vector3 = y[index2] - newPoint;
edges[index2][index1] = vector3;
edges[index1][index2] = -vector3;
edgeLengthSq[index1][index2] = edgeLengthSq[index2][index1] = vector3.LengthSquared();
num >>= 3;
}
UpdateDeterminant(index1);
return UpdateSimplex(index1);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 행렬식 업데이트
///@param xmIdx : 갱신할 Determinant 번호
//////////////////////////////////////////////////////////////////////////
void Gjk::UpdateDeterminant( int index )
{
int index1 = 1 << index;
det[index1][index] = 1.0f;
int num1 = Gjk::BitsToIndices[simplexBits];
int num2 = num1;
int num3 = 0;
while(num2 != 0)
{
int index2 = (num2 & 7)- 1;
int num4 = 1 << index2;
int index3 = num4 | index1;
det[index3][index2] = Dot(edges[index][index2], y[index]);
det[index3][index] = Dot(edges[index2][index], y[index2]);
int num5 = num1;
for(int index4 = 0; index4 < num3; ++index4)
{
int index5 = (num5 & 7)- 1;
int num6 = 1 << index5;
int index6 = index3 | num6;
int index7 = (double)edgeLengthSq[index2][index5] < (double)edgeLengthSq[index][index5] ? index2 : index;
det[index6][index5] = (float)((double)det[index3][index2] * (double)Dot(edges[index7][index5], y[index2]) + (double)det[index3][index] * (double)Dot(edges[index7][index5], y[index]));
int index8 = (double)edgeLengthSq[index5][index2] < (double)edgeLengthSq[index][index2] ? index5 : index;
det[index6][index2] = (float)((double)det[num6 | index1][index5] * (double)Dot(edges[index8][index2], y[index5])+ (double)det[num6 | index1][index] * (double)Dot(edges[index8][index2], y[index]));
int index9 = (double)edgeLengthSq[index2][index] < (double)edgeLengthSq[index5][index] ? index2 : index5;
det[index6][index] = (float)((double)det[num4 | num6][index5] * (double)Dot(edges[index9][index], y[index5])+ (double)det[num4 | num6][index2] * (double)Dot(edges[index9][index], y[index2]));
num5 >>= 3;
}
num2 >>= 3;
++num3;
}
if((simplexBits | index1)== 15)
{
int index2 = (double)edgeLengthSq[1][0] < (double)edgeLengthSq[2][0] ? ((double)edgeLengthSq[1][0] < (double)edgeLengthSq[3][0] ? 1 : 3): ((double)edgeLengthSq[2][0] < (double)edgeLengthSq[3][0] ? 2 : 3);
det[15][0] = (float)((double)det[14][1] * (double)Dot(edges[index2][0], y[1])+ (double)det[14][2] * (double)Dot(edges[index2][0], y[2])+ (double)det[14][3] * (double)Dot(edges[index2][0], y[3]));
int index3 = (double)edgeLengthSq[0][1] < (double)edgeLengthSq[2][1] ? ((double)edgeLengthSq[0][1] < (double)edgeLengthSq[3][1] ? 0 : 3): ((double)edgeLengthSq[2][1] < (double)edgeLengthSq[3][1] ? 2 : 3);
det[15][1] = (float)((double)det[13][0] * (double)Dot(edges[index3][1], y[0])+ (double)det[13][2] * (double)Dot(edges[index3][1], y[2])+ (double)det[13][3] * (double)Dot(edges[index3][1], y[3]));
int index4 = (double)edgeLengthSq[0][2] < (double)edgeLengthSq[1][2] ? ((double)edgeLengthSq[0][2] < (double)edgeLengthSq[3][2] ? 0 : 3): ((double)edgeLengthSq[1][2] < (double)edgeLengthSq[3][2] ? 1 : 3);
det[15][2] = (float)((double)det[11][0] * (double)Dot(edges[index4][2], y[0])+ (double)det[11][1] * (double)Dot(edges[index4][2], y[1])+ (double)det[11][3] * (double)Dot(edges[index4][2], y[3]));
int index5 = (double)edgeLengthSq[0][3] < (double)edgeLengthSq[1][3] ? ((double)edgeLengthSq[0][3] < (double)edgeLengthSq[2][3] ? 0 : 2): ((double)edgeLengthSq[1][3] < (double)edgeLengthSq[2][3] ? 1 : 2);
det[15][3] = (float)((double)det[7][0] * (double)Dot(edges[index5][3], y[0])+ (double)det[7][1] * (double)Dot(edges[index5][3], y[1])+ (double)det[7][2] * (double)Dot(edges[index5][3], y[2]));
}
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 심플렉스 업데이트
///@param newIndex : 새로 업데이트할 인덱스
//////////////////////////////////////////////////////////////////////////
bool Gjk::UpdateSimplex( int newIndex )
{
int yBits = simplexBits | 1 << newIndex;
int xBits = 1 << newIndex;
for(int index = simplexBits; index != 0; --index)
{
if((index & yBits) == index && IsSatisfiesRule(index | xBits, yBits))
{
simplexBits = index | xBits;
closestPoint = ComputeClosestPoint();
return true;
}
}
bool flag = false;
if(IsSatisfiesRule(xBits, yBits))
{
simplexBits = xBits;
closestPoint = y[newIndex];
maxLengthSq = yLengthSq[newIndex];
flag = true;
}
return flag;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 가까운 위치 계산
///@return 결과 위치벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Gjk::ComputeClosestPoint()
{
float num1 = 0.0f;
int num2 = BitsToIndices[simplexBits];
Vector3 zero = Vector3::Zero;
maxLengthSq = 0.0f;
while (num2 != 0)
{
int index = (num2 & 7) - 1;
float num3 = det[simplexBits][index];
num1 += num3;
zero += y[index] * num3;
maxLengthSq = MathHelper::Max(maxLengthSq, yLengthSq[index]);
num2 >>= 3;
}
return zero / num1;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief Bits값이 규칙에 맞는지 검사
///@param xBits : XBits
///@param yBits : YBits
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Gjk::IsSatisfiesRule( int xBits, int yBits )
{
bool flag = true;
int num1 = BitsToIndices[yBits];
while(num1 != 0)
{
int index = (num1 & 7) - 1;
int num2 = 1 << index;
if((num2 & xBits) != 0)
{
if((double)det[xBits][index] <= 0.0)
{
flag = false;
break;
}
}
else if((double)det[xBits | num2][index] > 0.0)
{
flag = false;
break;
}
num1 >>= 3;
}
return flag;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 개의 벡터 내적
///@param a : 벡터1
///@param b : 벡터2
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Gjk::Dot( Vector3 a, Vector3 b )
{
return (float)((double)a.X * (double)b.X + (double)a.Y * (double)b.Y + (double)a.Z * (double)b.Z);
}<file_sep>#include "stdafx.h"
#include "ExportAnim.h"
#include "./Fbx/XmlToAnim.h"
ExportAnim::ExportAnim(ExecuteValues* values)
: Execute(values)
{
Fbx::XmlToAnim* anim = NULL;
vector<wstring> list;
Path::GetFiles(&list, Assets + L"Bone/Players/Grund/", L"*.Animation", false);
for (wstring file : list)
{
wstring name = Path::GetFileNameWithoutExtension(file);
anim = new Fbx::XmlToAnim(file);
anim->ExportAnimation(Models + L"Grund/", name);
SAFE_DELETE(anim);
}
}
ExportAnim::~ExportAnim()
{
}
void ExportAnim::Update()
{
}
void ExportAnim::Render()
{
}
void ExportAnim::PostRender()
{
}
<file_sep>#include "Quaternion.h"
#include <d3dx9math.h>
#include "Vector3.h"
#include "Matrix.h"
//////////////////////////////////////////////////////////////////////////
const Quaternion Quaternion::Identity = Quaternion(0.0f, 0.0f, 0.0f, 1.0f);
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
//////////////////////////////////////////////////////////////////////////
Quaternion::Quaternion( void )
{
this->X = 0.0f;
this->Y = 0.0f;
this->Z = 0.0f;
this->W = 0.0f;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param x : X
///@param y : Y
///@param z : Z
///@param w : W
//////////////////////////////////////////////////////////////////////////
Quaternion::Quaternion( float x, float y, float z, float w )
{
this->X = x;
this->Y = y;
this->Z = z;
this->W = w;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param vectorPart : 벡터(XYZ)
///@param scalarPart : 값(W)
//////////////////////////////////////////////////////////////////////////
Quaternion::Quaternion( Vector3 vectorPart, float scalarPart )
{
this->X = vectorPart.X;
this->Y = vectorPart.Y;
this->Z = vectorPart.Z;
this->W = scalarPart;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -
///@return 결과 쿼터니온
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::operator-( void )
{
Quaternion quaternion1;
quaternion1.X = -this->X;
quaternion1.Y = -this->Y;
quaternion1.Z = -this->Z;
quaternion1.W = -this->W;
return quaternion1;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief ==
///@param quaternion2 : 쿼터니온
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Quaternion::operator==( const Quaternion& quaternion2 ) const
{
if((double)X == (double)quaternion2.X && (double)Y == (double)quaternion2.Y && (double)Z == (double)quaternion2.Z)
return (double)W == (double)quaternion2.W;
else
return false;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief !=
///@param quaternion2 : 쿼터니온
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Quaternion::operator!=( const Quaternion& quaternion2 ) const
{
if((double)X == (double)quaternion2.X && (double)Y == (double)quaternion2.Y && (double)Z == (double)quaternion2.Z)
return (double)W != (double)quaternion2.W;
else
return true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief +
///@param quaternion2 : 쿼터니온
///@return 결과
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::operator+( const Quaternion& quaternion2 ) const
{
Quaternion quaternion;
quaternion.X = this->X + quaternion2.X;
quaternion.Y = this->Y + quaternion2.Y;
quaternion.Z = this->Z + quaternion2.Z;
quaternion.W = this->W + quaternion2.W;
return quaternion;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -
///@param quaternion2 : 쿼터니온
///@return 결과
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::operator-( const Quaternion& quaternion2 ) const
{
Quaternion quaternion;
quaternion.X = this->X - quaternion2.X;
quaternion.Y = this->Y - quaternion2.Y;
quaternion.Z = this->Z - quaternion2.Z;
quaternion.W = this->W - quaternion2.W;
return quaternion;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *
///@param quaternion2 : 쿼터니온
///@return 결과
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::operator*( const Quaternion& quaternion2 ) const
{
float num1 = (float)((double)this->Y * (double)quaternion2.Z - (double)this->Z * (double)quaternion2.Y);
float num2 = (float)((double)this->Z * (double)quaternion2.X - (double)this->X * (double)quaternion2.Z);
float num3 = (float)((double)this->X * (double)quaternion2.Y - (double)this->Y * (double)quaternion2.X);
float num4 = (float)((double)this->X * (double)quaternion2.X + (double)this->Y * (double)quaternion2.Y + (double)this->Z * (double)quaternion2.Z);
Quaternion quaternion;
quaternion.X = (float)((double)this->X * (double)quaternion2.W + (double)quaternion2.X * (double)this->W) + num1;
quaternion.Y = (float)((double)this->Y * (double)quaternion2.W + (double)quaternion2.Y * (double)this->W) + num2;
quaternion.Z = (float)((double)this->Z * (double)quaternion2.W + (double)quaternion2.Z * (double)this->W) + num3;
quaternion.W = this->W * quaternion2.W - num4;
return quaternion;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *
///@param scaleFactor : 값
///@return 결과
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::operator*( const float& scaleFactor ) const
{
Quaternion quaternion;
quaternion.X = this->X * scaleFactor;
quaternion.Y = this->Y * scaleFactor;
quaternion.Z = this->Z * scaleFactor;
quaternion.W = this->W * scaleFactor;
return quaternion;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *
///@param quaternion2 : 쿼터니온
///@return 결과
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::operator/( const Quaternion& quaternion2 ) const
{
float num1 = 1.0f / (float)((double)quaternion2.X * (double)quaternion2.X + (double)quaternion2.Y * (double)quaternion2.Y + (double)quaternion2.Z * (double)quaternion2.Z + (double)quaternion2.W * (double)quaternion2.W);
float num2 = -quaternion2.X * num1;
float num3 = -quaternion2.Y * num1;
float num4 = -quaternion2.Z * num1;
float num5 = quaternion2.W * num1;
float multiple1 = (float)((double)this->Y * (double)num4 - (double)this->Z * (double)num3);
float multiple2 = (float)((double)this->Z * (double)num2 - (double)this->X * (double)num4);
float multiple3 = (float)((double)this->X * (double)num3 - (double)this->Y * (double)num2);
float multiple4 = (float)((double)this->X * (double)num2 + (double)this->Y * (double)num3 + (double)this->Z * (double)num4);
Quaternion quaternion;
quaternion.X = (float)((double)this->X * (double)num5 + (double)num2 * (double)this->W) + multiple1;
quaternion.Y = (float)((double)this->Y * (double)num5 + (double)num3 * (double)this->W) + multiple2;
quaternion.Z = (float)((double)this->Z * (double)num5 + (double)num4 * (double)this->W) + multiple3;
quaternion.W = this->W * num5 - multiple4;
return quaternion;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief +=
///@param quaternion2 : 쿼터니온
///@return 결과
//////////////////////////////////////////////////////////////////////////
void Quaternion::operator+=( const Quaternion& quaternion2 )
{
*this = *this + quaternion2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -=
///@param quaternion2 : 쿼터니온
///@return 결과
//////////////////////////////////////////////////////////////////////////
void Quaternion::operator-=( const Quaternion& quaternion2 )
{
*this = *this - quaternion2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *=
///@param quaternion2 : 쿼터니온
///@return 결과
//////////////////////////////////////////////////////////////////////////
void Quaternion::operator*=( const Quaternion& quaternion2 )
{
*this = *this * quaternion2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *=
///@param scaleFactor : 값
///@return 결과
//////////////////////////////////////////////////////////////////////////
void Quaternion::operator*=( const float& scaleFactor )
{
*this = *this * scaleFactor;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /=
///@param quaternion2 : 쿼터니온
///@return 결과
//////////////////////////////////////////////////////////////////////////
void Quaternion::operator/=( const Quaternion& quaternion2 )
{
*this = *this / quaternion2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 문자열로 변환
///@param 문자열
//////////////////////////////////////////////////////////////////////////
std::string Quaternion::ToString()
{
std::string temp;
char val[255];
sprintf(val, "{X:%#f ", X); temp += val;
sprintf(val, "Y:%#f ", Y); temp += val;
sprintf(val, "Z:%#f ", Z); temp += val;
sprintf(val, "W:%#f}", W); temp += val;
return temp;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief D3DXQUATERNION으로 변환
///@param D3DX 쿼터니온
//////////////////////////////////////////////////////////////////////////
D3DXQUATERNION Quaternion::ToD3DXQUATERNION()
{
return D3DXQUATERNION(X, Y, Z, W);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 크기 계산
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Quaternion::Length()
{
return (float)sqrt((double)X * (double)X + (double)Y * (double)Y + (double)Z * (double)Z + (double)W * (double)W);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 제곱으로 크기 계산
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Quaternion::LengthSquared()
{
return (float)((double)X * (double)X + (double)Y * (double)Y + (double)Z * (double)Z + (double)W * (double)W);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 정규화
//////////////////////////////////////////////////////////////////////////
void Quaternion::Normalize()
{
float num = 1.0f / (float)sqrt((double)X * (double)X + (double)Y * (double)Y + (double)Z * (double)Z + (double)W * (double)W);
X *= num;
Y *= num;
Z *= num;
W *= num;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 정규화
///@param value : 쿼터니온
///@return 결과 쿼터니온
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::Normalize( Quaternion quaternion )
{
float num = 1.0f / (float)sqrt((double)quaternion.X * (double)quaternion.X + (double)quaternion.Y * (double)quaternion.Y + (double)quaternion.Z * (double)quaternion.Z + (double)quaternion.W * (double)quaternion.W);
Quaternion quaternion1;
quaternion1.X = quaternion.X * num;
quaternion1.Y = quaternion.Y * num;
quaternion1.Z = quaternion.Z * num;
quaternion1.W = quaternion.W * num;
return quaternion1;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 쿼터니온 켤레값
//////////////////////////////////////////////////////////////////////////
void Quaternion::Conjugate()
{
this->X = -this->X;
this->Y = -this->Y;
this->Z = -this->Z;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 쿼터니온 켤레값
///@param value : 쿼터니온
///@return 결과 쿼터니온
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::Conjugate( Quaternion value )
{
Quaternion quaternion;
quaternion.X = -value.X;
quaternion.Y = -value.Y;
quaternion.Z = -value.Z;
quaternion.W = value.W;
return quaternion;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 역 쿼터니온
///@param quaternion : 쿼터니온
///@return 결과 쿼터니온
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::Inverse( Quaternion quaternion )
{
float num = 1.0f / (float)((double)quaternion.X * (double)quaternion.X + (double)quaternion.Y * (double)quaternion.Y + (double)quaternion.Z * (double)quaternion.Z + (double)quaternion.W * (double)quaternion.W);
Quaternion quaternion1;
quaternion1.X = -quaternion.X * num;
quaternion1.Y = -quaternion.Y * num;
quaternion1.Z = -quaternion.Z * num;
quaternion1.W = quaternion.W * num;
return quaternion1;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 축과 각도에 의해 회전된 쿼터니온
///@param axis : 축 벡터
///@param angle : 각도
///@return 결과 쿼터니온
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::CreateFromAxisAngle( Vector3 axis, float angle )
{
float sinValue = (float)sin((double)(angle * 0.5f));
float cosValue = (float)cos((double)(angle * 0.5f));
Quaternion quaternion;
quaternion.X = axis.X * sinValue;
quaternion.Y = axis.Y * sinValue;
quaternion.Z = axis.Z * sinValue;
quaternion.W = cosValue;
return quaternion;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 각 각도에 의해 회전된 쿼터니온
///@param yaw : Yaw
///@param pitch : Pitch
///@param roll : Roll
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::CreateFromYawPitchRoll( float yaw, float pitch, float roll )
{
float roll1 = roll * 0.5f;
float roll2 = (float)sin((double)roll1);
float roll3 = (float)cos((double)roll1);
float pitch1 = pitch * 0.5f;
float pitch2 = (float)sin((double)pitch1);
float pitch3 = (float)cos((double)pitch1);
float yaw1 = yaw * 0.5f;
float yaw2 = (float)sin((double)yaw1);
float yaw3 = (float)cos((double)yaw1);
Quaternion quaternion;
quaternion.X = (float)((double)yaw3 * (double)pitch2 * (double)roll3 + (double)yaw2 * (double)pitch3 * (double)roll2);
quaternion.Y = (float)((double)yaw2 * (double)pitch3 * (double)roll3 - (double)yaw3 * (double)pitch2 * (double)roll2);
quaternion.Z = (float)((double)yaw3 * (double)pitch3 * (double)roll2 - (double)yaw2 * (double)pitch2 * (double)roll3);
quaternion.W = (float)((double)yaw3 * (double)pitch3 * (double)roll3 + (double)yaw2 * (double)pitch2 * (double)roll2);
return quaternion;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 매트릭스에 의해 회전된 쿼터니온
///@param matrix : 매트릭스
///@return 결과 쿼터니온
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::CreateFromRotationMatrix( Matrix matrix )
{
float num1 = matrix.M11 + matrix.M22 + matrix.M33;
Quaternion quaternion;
if((double)num1 > 0.0)
{
float num2 = (float)sqrt((double)num1 + 1.0);
quaternion.W = num2 * 0.5f;
float num3 = 0.5f / num2;
quaternion.X = (matrix.M23 - matrix.M32)* num3;
quaternion.Y = (matrix.M31 - matrix.M13)* num3;
quaternion.Z = (matrix.M12 - matrix.M21)* num3;
}
else if((double)matrix.M11 >= (double)matrix.M22 && (double)matrix.M11 >= (double)matrix.M33)
{
float num2 = (float)sqrt(1.0 + (double)matrix.M11 - (double)matrix.M22 - (double)matrix.M33);
float num3 = 0.5f / num2;
quaternion.X = 0.5f * num2;
quaternion.Y = (matrix.M12 + matrix.M21)* num3;
quaternion.Z = (matrix.M13 + matrix.M31)* num3;
quaternion.W = (matrix.M23 - matrix.M32)* num3;
}
else if((double)matrix.M22 > (double)matrix.M33)
{
float num2 = (float)sqrt(1.0 + (double)matrix.M22 - (double)matrix.M11 - (double)matrix.M33);
float num3 = 0.5f / num2;
quaternion.X = (matrix.M21 + matrix.M12)* num3;
quaternion.Y = 0.5f * num2;
quaternion.Z = (matrix.M32 + matrix.M23)* num3;
quaternion.W = (matrix.M31 - matrix.M13)* num3;
}
else
{
float num2 = (float)sqrt(1.0 + (double)matrix.M33 - (double)matrix.M11 - (double)matrix.M22);
float num3 = 0.5f / num2;
quaternion.X = (matrix.M31 + matrix.M13)* num3;
quaternion.Y = (matrix.M32 + matrix.M23)* num3;
quaternion.Z = 0.5f * num2;
quaternion.W = (matrix.M12 - matrix.M21)* num3;
}
return quaternion;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 내적
///@param quaternion1 : 쿼터니온1
///@param quaternion2 : 쿼터니온2
///@return 결과 쿼터니온
//////////////////////////////////////////////////////////////////////////
float Quaternion::Dot( Quaternion quaternion1, Quaternion quaternion2 )
{
return (float)((double)quaternion1.X * (double)quaternion2.X + (double)quaternion1.Y * (double)quaternion2.Y + (double)quaternion1.Z * (double)quaternion2.Z + (double)quaternion1.W * (double)quaternion2.W);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 구면보간
///@param quaternion1 : 쿼터니온1
///@param quaternion2 : 쿼터니온2
///@param amount : 보간값
///@return 결과 쿼터니온
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::Slerp( Quaternion quaternion1, Quaternion quaternion2, float amount )
{
float normal = (float)((double)quaternion1.X * (double)quaternion2.X + (double)quaternion1.Y * (double)quaternion2.Y + (double)quaternion1.Z * (double)quaternion2.Z + (double)quaternion1.W * (double)quaternion2.W);
bool flag = false;
if((double)normal < 0.0)
{
flag = true;
normal = -normal;
}
float num1, num2;
if((double)normal > 0.999998986721039)
{
num1 = 1.0f - amount;
num2 = flag ? -amount : amount;
}
else
{
float num3 = (float)acos((double)normal);
float num4 = (float)(1.0 / sin((double)num3));
num1 = (float)sin((1.0 - (double)amount)* (double)num3)* num4;
num2 = flag ? (float)-sin((double)amount * (double)num3)* num4 : (float)sin((double)amount * (double)num3)* num4;
}
Quaternion quaternion;
quaternion.X = (float)((double)num1 * (double)quaternion1.X + (double)num2 * (double)quaternion2.X);
quaternion.Y = (float)((double)num1 * (double)quaternion1.Y + (double)num2 * (double)quaternion2.Y);
quaternion.Z = (float)((double)num1 * (double)quaternion1.Z + (double)num2 * (double)quaternion2.Z);
quaternion.W = (float)((double)num1 * (double)quaternion1.W + (double)num2 * (double)quaternion2.W);
return quaternion;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 선형보간
///@param quaternion1 : 쿼터니온1
///@param quaternion2 : 쿼터니온2
///@param amount : 보간값
///@return 결과 쿼터니온
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::Lerp( Quaternion quaternion1, Quaternion quaternion2, float amount )
{
float amountInv = 1.0f - amount;
Quaternion quaternion;
if((double)quaternion1.X * (double)quaternion2.X + (double)quaternion1.Y * (double)quaternion2.Y + (double)quaternion1.Z * (double)quaternion2.Z + (double)quaternion1.W * (double)quaternion2.W >= 0.0)
{
quaternion.X = (float)((double)amountInv * (double)quaternion1.X + (double)amount * (double)quaternion2.X);
quaternion.Y = (float)((double)amountInv * (double)quaternion1.Y + (double)amount * (double)quaternion2.Y);
quaternion.Z = (float)((double)amountInv * (double)quaternion1.Z + (double)amount * (double)quaternion2.Z);
quaternion.W = (float)((double)amountInv * (double)quaternion1.W + (double)amount * (double)quaternion2.W);
}
else
{
quaternion.X = (float)((double)amountInv * (double)quaternion1.X - (double)amount * (double)quaternion2.X);
quaternion.Y = (float)((double)amountInv * (double)quaternion1.Y - (double)amount * (double)quaternion2.Y);
quaternion.Z = (float)((double)amountInv * (double)quaternion1.Z - (double)amount * (double)quaternion2.Z);
quaternion.W = (float)((double)amountInv * (double)quaternion1.W - (double)amount * (double)quaternion2.W);
}
float length = 1.0f / (float)sqrt((double)quaternion.X * (double)quaternion.X + (double)quaternion.Y * (double)quaternion.Y + (double)quaternion.Z * (double)quaternion.Z + (double)quaternion.W * (double)quaternion.W);
quaternion.X *= length;
quaternion.Y *= length;
quaternion.Z *= length;
quaternion.W *= length;
return quaternion;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 순서를 바꾸어 곱하기
///@param value1 : 쿼터니온1
///@param value2 : 쿼터니온2
///@return 결과 쿼터니온
//////////////////////////////////////////////////////////////////////////
Quaternion Quaternion::Concatenate( Quaternion value1, Quaternion value2 )
{
float num1 = (float)((double)value2.Y * (double)value1.Z - (double)value2.Z * (double)value1.Y);
float num2 = (float)((double)value2.Z * (double)value1.X - (double)value2.X * (double)value1.Z);
float num3 = (float)((double)value2.X * (double)value1.Y - (double)value2.Y * (double)value1.X);
float num4 = (float)((double)value2.X * (double)value1.X + (double)value2.Y * (double)value1.Y + (double)value2.Z * (double)value1.Z);
Quaternion quaternion;
quaternion.X = (float)((double)value2.X * (double)value1.W + (double)value1.X * (double)value2.W)+ num1;
quaternion.Y = (float)((double)value2.Y * (double)value1.W + (double)value1.Y * (double)value2.W)+ num2;
quaternion.Z = (float)((double)value2.Z * (double)value1.W + (double)value1.Z * (double)value2.W)+ num3;
quaternion.W = value2.W * value1.W - num4;
return quaternion;
}<file_sep>#pragma once
#include "../Core/Vector3.h"
class BoundingBox;
class BoundingFrustum;
class BoundingSphere;
class Plane;
//////////////////////////////////////////////////////////////////////////
///@brief 충돌 레이
//////////////////////////////////////////////////////////////////////////
class Ray
{
public:
Ray(void);
Ray(Vector3 position, Vector3 direction);
bool operator ==(const Ray& value) const;
bool operator !=(const Ray& value) const;
std::string ToString();
bool Intersects(Plane& plane, float& value);
bool Intersects(BoundingSphere& sphere, float& value);
public:
Vector3 Position;///< 위치
Vector3 Direction;///< 방향
};<file_sep>#pragma once
#include "./Framework/Bounding/BoundingBox.h"
#include "./Framework/Bounding/BoundingSphere.h"
namespace Gizmo
{
class Gizmo;
enum class EGizmoMode
{
Scale = 0, Rotate, Translate
};
enum class EGizmoAxis
{
None = -1, X, Y, Z,
XY, ZX, YZ,
};
class GizmoComponent
{
public:
GizmoComponent(ExecuteValues* values);
~GizmoComponent();
void Update();
void Render();
void PostRender();
void Position(const D3DXVECTOR3& value) { position = value; }
void SetTarget(D3DXMATRIX* target) { this->target = target; }
void Transform(D3DXMATRIX& val) { gizmoWorld = val; }
void OutsideWorld(D3DXMATRIX& val) { outsideWorld = val; }
D3DXMATRIX TransformDelta();
private:
//Initialize
void Init();
//Draw
void Draw2D();
void Draw3D();
void DrawBounding();
//Create
void Create2D();
void Create3D();
void CreateBoundings();
//Reset
void ResetHighlight();
void ResetMouseValues();
void ResetDeltas();
void ResetScaleGizmo();
//Mouse
void MouseCollision();
void Pressing();
Ray ConvertMouseToRay(D3DXMATRIX& world);
//ResizeWorldScale
void ResizeWorldScale();
private:
//Gizmos
D3DXMATRIX geometryWorld[3];
D3DXCOLOR colors[3];
vector<Gizmo *> quads;
vector<Gizmo *> lines;
vector<Gizmo *> scales, rotates, translates;
vector<Gizmo *> boxes;
vector<Gizmo *> spheres;
//Boundings
class BoundingBox* xAxisBox, *yAxisBox, *zAxisBox;
class BoundingBox* xyAxisBox, *yzAxisBox, *xzAxisBox;
class BoundingSphere* xSphere, *ySphere, *zSphere;
bool bDrawBounding;
//Line
const float LINE_LENGTH = 3.0f;
const float LINE_OFFSET = 1.0f;
//Enum
EGizmoMode activeMode;
EGizmoAxis activeAxis;
//MousePos
Vector3 intersectPosition, lastIntersectPosition, delta;
D3DXVECTOR3 curMousePos, lastMousePos;
bool bMousePressed;
//Transform
D3DXMATRIX outsideWorld;
D3DXMATRIX gizmoWorld;
D3DXVECTOR3 scale, rotate, position;
D3DXMATRIX scaleMat, rotMat, posMat;
D3DXQUATERNION rotQuat;
const float ROTATE_WEIGHT = 10.0f;
const float SCALE_WEIGHT = 0.1f;
const float RESIZE_RATIO = 30.0f;
//Deltas
D3DXVECTOR3 translationDelta, scaleDelta;
D3DXMATRIX rotationDelta;
//States
ID3D11RasterizerState* rasterizer[2];
ID3D11DepthStencilState* depthStencilState[2];
//GlobalValue
ExecuteValues* values;
//Target
D3DXMATRIX* target;
};
}<file_sep>#include "BoundingFrustum.h"
#include <assert.h>
#include "Bounding.h"
#include "Ray.h"
#include "BoundingBox.h"
#include "BoundingSphere.h"
#include "../Core/MathHelper.h"
//////////////////////////////////////////////////////////////////////////
const int BoundingFrustum::CornerCount = 8;
const int BoundingFrustum::NearPlaneIndex = 0;
const int BoundingFrustum::FarPlaneIndex = 1;
const int BoundingFrustum::LeftPlaneIndex = 2;
const int BoundingFrustum::RightPlaneIndex = 3;
const int BoundingFrustum::TopPlaneIndex = 4;
const int BoundingFrustum::BottomPlaneIndex = 5;
const int BoundingFrustum::NumPlanes = 6;
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
//////////////////////////////////////////////////////////////////////////
BoundingFrustum::BoundingFrustum( void )
{
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param value : 매트릭스
//////////////////////////////////////////////////////////////////////////
BoundingFrustum::BoundingFrustum( Matrix value )
{
SetMatrix(value);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief ==
///@param value : 바운딩 프러스텀
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingFrustum::operator==( const BoundingFrustum& value ) const
{
return Equals(value);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief ==
///@param value : 바운딩 프러스텀
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingFrustum::operator!=( const BoundingFrustum& value ) const
{
return !Equals(value);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 문자열로 변환
///@param 문자열
//////////////////////////////////////////////////////////////////////////
std::string BoundingFrustum::ToString()
{
std::string temp;
temp += "{{Near:" + planes[0].ToString() + "}, ";
temp += "{Far:" + planes[1].ToString() + "}, ";
temp += "{Left:" + planes[2].ToString() + "}, ";
temp += "{Right:" + planes[3].ToString() + "}, ";
temp += "{Top:" + planes[4].ToString() + "}, ";
temp += "{Bottom:" + planes[5].ToString() + "}}";
return temp;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 서포트 매핑
///@param v : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 BoundingFrustum::SupportMapping( Vector3 v )
{
float result1;
int index1 = 0;
result1 = Vector3::Dot(cornerArray[0], v);
for(int index2 = 1; index2 < 8; ++index2)
{
float result2;
result2 = Vector3::Dot(cornerArray[index2], v);
if((double)result2 > (double)result1)
{
index1 = index2;
result1 = result2;
}
}
return cornerArray[index1];
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 값이 같은 객체인지 검사
///@param value : <NAME>텀
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingFrustum::Equals( const BoundingFrustum& value ) const
{
bool flag = false;
flag = matrix == value.matrix;
return flag;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 근면
///@return 결과 면
//////////////////////////////////////////////////////////////////////////
Plane BoundingFrustum::Near() const
{
return planes[0];
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 원면
///@return 결과 면
//////////////////////////////////////////////////////////////////////////
Plane BoundingFrustum::Far() const
{
return planes[1];
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 좌측면
///@return 결과 면
//////////////////////////////////////////////////////////////////////////
Plane BoundingFrustum::Left() const
{
return planes[2];
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 우측면
///@return 결과 면
//////////////////////////////////////////////////////////////////////////
Plane BoundingFrustum::Right() const
{
return planes[3];
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 윗면
///@return 결과 면
//////////////////////////////////////////////////////////////////////////
Plane BoundingFrustum::Top() const
{
return planes[4];
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 아랫면
///@return 결과 면
//////////////////////////////////////////////////////////////////////////
Plane BoundingFrustum::Bottom() const
{
return planes[5];
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 매트릭스
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
Matrix BoundingFrustum::GetMatrix() const
{
return matrix;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 매트릭스 세팅
///@param value : 설정할 매트릭스
//////////////////////////////////////////////////////////////////////////
void BoundingFrustum::SetMatrix( const Matrix value )
{
matrix = value;
planes[2].Normal.X = -value.M14 - value.M11; planes[2].Normal.Y = -value.M24 - value.M21; planes[2].Normal.Z = -value.M34 - value.M31; planes[2].D = -value.M44 - value.M41;
planes[3].Normal.X = -value.M14 + value.M11; planes[3].Normal.Y = -value.M24 + value.M21; planes[3].Normal.Z = -value.M34 + value.M31; planes[3].D = -value.M44 + value.M41;
planes[4].Normal.X = -value.M14 + value.M12; planes[4].Normal.Y = -value.M24 + value.M22; planes[4].Normal.Z = -value.M34 + value.M32; planes[4].D = -value.M44 + value.M42;
planes[5].Normal.X = -value.M14 - value.M12; planes[5].Normal.Y = -value.M24 - value.M22; planes[5].Normal.Z = -value.M34 - value.M32; planes[5].D = -value.M44 - value.M42;
planes[0].Normal.X = -value.M13;
planes[0].Normal.Y = -value.M23;
planes[0].Normal.Z = -value.M33;
planes[0].D = -value.M43;
planes[1].Normal.X = -value.M14 + value.M13;
planes[1].Normal.Y = -value.M24 + value.M23;
planes[1].Normal.Z = -value.M34 + value.M33;
planes[1].D = -value.M44 + value.M43;
for(int index = 0; index < 6; ++index)
{
float num = planes[index].Normal.Length();
planes[index].Normal /= num;
planes[index].D /= num;
}
Ray intersectionLine1 = ComputeIntersectionLine(planes[0], planes[2]);
cornerArray[0] = ComputeIntersection(planes[4], intersectionLine1);
cornerArray[3] = ComputeIntersection(planes[5], intersectionLine1);
Ray intersectionLine2 = ComputeIntersectionLine(planes[3], planes[0]);
cornerArray[1] = ComputeIntersection(planes[4], intersectionLine2);
cornerArray[2] = ComputeIntersection(planes[5], intersectionLine2);
intersectionLine2 = ComputeIntersectionLine(planes[2], planes[1]);
cornerArray[4] = ComputeIntersection(planes[4], intersectionLine2);
cornerArray[7] = ComputeIntersection(planes[5], intersectionLine2);
intersectionLine2 = ComputeIntersectionLine(planes[1], planes[3]);
cornerArray[5] = ComputeIntersection(planes[4], intersectionLine2);
cornerArray[6] = ComputeIntersection(planes[5], intersectionLine2);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 코너 얻기
///@param pOut :
//////////////////////////////////////////////////////////////////////////
void BoundingFrustum::GetCorner( Vector3* pCorner )
{
assert(pCorner != NULL);
for(int i = 0; i < 8; i++)
pCorner[i] = cornerArray[i];
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 면 두개의 교차선 계산
///@return 결과 교차선
//////////////////////////////////////////////////////////////////////////
Ray BoundingFrustum::ComputeIntersectionLine( Plane p1, Plane p2 )
{
Ray ray;
ray.Direction = Vector3::Cross(p1.Normal, p2.Normal);
float num = ray.Direction.LengthSquared();
ray.Position = Vector3::Cross(-p1.D * p2.Normal + p2.D * p1.Normal, ray.Direction) / num;
return ray;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 면과 레이의 교차 위치 계산
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 BoundingFrustum::ComputeIntersection( Plane plane, Ray ray )
{
float num = (-plane.D - Vector3::Dot(plane.Normal, ray.Position)) / Vector3::Dot(plane.Normal, ray.Direction);
return ray.Position + ray.Direction * num;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 박스와 교차 검사
///@param box : 바운딩 박스
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingFrustum::Intersects( BoundingBox box )
{
bool result = false;
Intersects(box, result);
return result;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 박스와 교차 검사
///@param box : 바운딩 박스
///@param result : 결과
//////////////////////////////////////////////////////////////////////////
void BoundingFrustum::Intersects( BoundingBox box, bool& result )
{
gjk.Reset();
Vector3 result1;
result1 = cornerArray[0] - box.Min;
if((double)result1.LengthSquared() < 9.99999974737875E-06)
result1 = cornerArray[0] - box.Max;
float num1 = MathHelper::FloatMaxValue;
float num2;
result = false;
do
{
Vector3 v(-result1.X, -result1.Y, -result1.Z);
Vector3 result2 = SupportMapping(v);
Vector3 result3 = box.SupportMapping(result1);
Vector3 result4 = result2 - result3;
if((double)result1.X * (double)result4.X + (double)result1.Y * (double)result4.Y + (double)result1.Z * (double)result4.Z > 0.0)
{
return;
}
else
{
gjk.AddSupportPoint(result4);
result1 = gjk.ClosestPoint();
float num3 = num1;
num1 = result1.LengthSquared();
if ((double) num3 - (double)num1 <= 9.99999974737875E-06 * (double)num3)
return;
else
num2 = 4E-05f * gjk.MaxLengthSquared();
}
}
while(!gjk.FullSimplex() && (double)num1 >= (double)num2);
result = true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 프러스텀과 교차 검사
///@param frustum : 바운딩 프러스텀
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingFrustum::Intersects( BoundingFrustum frustum )
{
gjk.Reset();
Vector3 result1;
result1 = cornerArray[0] - frustum.cornerArray[0];
if((double) result1.LengthSquared() < 9.99999974737875E-06)
result1 = cornerArray[0] - frustum.cornerArray[1];
float num1 = MathHelper::FloatMaxValue;
float num2;
do
{
Vector3 v(-result1.X, -result1.Y, -result1.Z);
Vector3 result2 = SupportMapping(v);
Vector3 result3 = frustum.SupportMapping(result1);
Vector3 result4 = result2 - result3;
if((double)result1.X * (double)result4.X + (double)result1.Y * (double)result4.Y + (double)result1.Z * (double)result4.Z > 0.0)
{
return false;
}
else
{
gjk.AddSupportPoint(result4);
result1 = gjk.ClosestPoint();
float num3 = num1;
num1 = result1.LengthSquared();
num2 = 4E-05f * gjk.MaxLengthSquared();
if((double)num3 - (double)num1 <= 9.99999974737875E-06 * (double)num3)
return false;
}
}
while(!gjk.FullSimplex() && (double)num1 >= (double)num2);
return true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 면과 교차 검사
///@param plane : 면
///@return 결과
//////////////////////////////////////////////////////////////////////////
void BoundingFrustum::Intersects( Plane plane, PlaneIntersectionType& result )
{
int num = 0;
for(int index = 0; index < 8; ++index)
{
float result = Vector3::Dot(cornerArray[index], plane.Normal);
if((double)result + (double)plane.D > 0.0)
num |= 1;
else
num |= 2;
if(num == 3)
{
result = PlaneIntersectionType_Intersecting;
return ;
}
}
result = num == 1 ? PlaneIntersectionType_Front : PlaneIntersectionType_Back;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 레이와의 교차검사
///@param ray : 레이
///@param result : 교차 결과값
///@warning
/// 교차가 되지 않은 결과 result는 0.0f이 절대 아니다!
/// result == NULL 이다!!!!!
//////////////////////////////////////////////////////////////////////////
void BoundingFrustum::Intersects( Ray ray, float* result )
{
ContainmentType result1;
result1 = Contains(ray.Position);
if (result1 == ContainmentType_Contains)
{
*result = 0.0f;
}
else
{
float num1 = MathHelper::FloatMinValue;
float num2 = MathHelper::FloatMaxValue;
if(result != NULL)
{
delete result;
result = NULL;
}
result = new float();
for(int i = 0; i < 6; i++)
{
Plane plane = planes[i];
Vector3 vector2 = plane.Normal;
float result2 = Vector3::Dot(ray.Direction, vector2);
float result3 = Vector3::Dot(ray.Position, vector2);
result3 += plane.D;
if((double)MathHelper::Abs(result2) < 9.99999974737875E-06)
{
if ((double)result3 > 0.0)
return;
}
else
{
float num3 = -result3 / result2;
if((double)result2 < 0.0)
{
if((double) num3 > (double)num2)
return;
else if((double)num3 > (double)num1)
num1 = num3;
}
else if((double)num3 < (double)num1)
return;
else if((double)num3 < (double)num2)
num2 = num3;
}
}
float num4 = (double)num1 >= 0.0 ? num1 : num2;
if ((double) num4 >= 0.0)
*result = num4;
}
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 스페어와 교차검사
///@param sphere : 바운딩 스페어
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingFrustum::Intersects( BoundingSphere sphere )
{
bool result = false;
Intersects(sphere, result);
return result;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 스페어와 교차검사
///@param sphere : 바운딩 스페어
///@param result : 결과
//////////////////////////////////////////////////////////////////////////
void BoundingFrustum::Intersects( BoundingSphere sphere, bool& result )
{
gjk.Reset();
Vector3 result1 = cornerArray[0] - sphere.Center;
if((double)result1.LengthSquared() < 9.99999974737875E-06)
result1 = Vector3::UnitX;
float num1 = MathHelper::FloatMaxValue; //float.MaxValue
result = false;
float num2;
do
{
Vector3 v(-result1.X, -result1.Y, -result1.Z);
Vector3 result2 = SupportMapping(v);
Vector3 result3 = sphere.SupportMapping(result1);
Vector3 result4 = result2 - result3;
if((double)result1.X * (double)result4.X + (double)result1.Y * (double)result4.Y + (double)result1.Z * (double)result4.Z > 0.0)
{
return;
}
else
{
gjk.AddSupportPoint(result4);
result1 = gjk.ClosestPoint();
float num3 = num1;
num1 = result1.LengthSquared();
if((double)num3 - (double)num1 <= 9.99999974737875E-06 * (double)num3)
return;
else
num2 = 4E-05f * gjk.MaxLengthSquared();
}
}
while(!gjk.FullSimplex() && (double)num1 >= (double)num2);
result = true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 박스의 포함 여부 검사
///@param box : 바운딩 박스
///@return 포함 결과
//////////////////////////////////////////////////////////////////////////
ContainmentType BoundingFrustum::Contains( BoundingBox box )
{
bool flag = false;
for(int i = 0; i < 6; i++)
{
Plane plane = planes[i];
switch(box.Intersects(plane))
{
case PlaneIntersectionType_Front:
return ContainmentType_Disjoint;
case PlaneIntersectionType_Intersecting:
flag = true;
break;
}
}
if(!flag)
return ContainmentType_Contains;
else
return ContainmentType_Intersects;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 프러스텀의 포함여부 검사
///@param frustum : 바운딩 프러스텀
///@return 포함 결과
//////////////////////////////////////////////////////////////////////////
ContainmentType BoundingFrustum::Contains( BoundingFrustum frustum )
{
ContainmentType containmentType = ContainmentType_Disjoint;
if(Intersects(frustum))
{
containmentType = ContainmentType_Contains;
for(int index = 0; index < 8; ++index)
{
if(Contains(frustum.cornerArray[index]) == ContainmentType_Disjoint)
{
containmentType = ContainmentType_Intersects;
break;
}
}
}
return containmentType;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 위치 벡터의 포함여부 검사
///@param point : 위치 벡터
///@return 포함 결과
//////////////////////////////////////////////////////////////////////////
ContainmentType BoundingFrustum::Contains( Vector3 point )
{
for(int i = 0; i < 6; i++)
{
Plane plane = planes[i];
if((double)((float)((double)plane.Normal.X * (double)point.X + (double)plane.Normal.Y * (double)point.Y + (double)plane.Normal.Z * (double)point.Z)+ plane.D) > 9.99999974737875E-06)
return ContainmentType_Disjoint;
}
return ContainmentType_Contains;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 스페어의 포함여부 검사
///@param point : 위치 벡터
///@return 포함 결과
//////////////////////////////////////////////////////////////////////////
ContainmentType BoundingFrustum::Contains( BoundingSphere sphere )
{
Vector3 vector3 = sphere.Center;
float num1 = sphere.Radius;
int num2 = 0;
for(int i = 0; i < 6; i++)
{
Plane plane = planes[i];
float num3 = (float)((double)plane.Normal.X * (double)vector3.X + (double)plane.Normal.Y * (double)vector3.Y + (double)plane.Normal.Z * (double)vector3.Z) + plane.D;
if((double)num3 > (double)num1)
return ContainmentType_Disjoint;
else if ((double)num3 < -(double)num1)
++num2;
}
if(num2 != 6)
return ContainmentType_Intersects;
else
return ContainmentType_Contains;
}<file_sep>#include "stdafx.h"
#include "GameSkyBox.h"
GameSkyBox::GameSkyBox(wstring matFile, wstring meshFile)
: GameModel(matFile, meshFile)
{
}
GameSkyBox::~GameSkyBox()
{
}
<file_sep>#include "stdafx.h"
#include "GameModel.h"
GameModel::GameModel(wstring matFile, wstring meshFile)
: velocity(0, 0, 0)
{
model = new Model();
model->ReadMaterial(matFile);
model->ReadMesh(meshFile);
shader = new Shader(Shaders + L"999_GameModel.hlsl", "VS_Bone");
for (Material* material : model->Materials())
material->SetShader(shader);
boneBuffer = new BoneBuffer();
renderBuffer = new RenderBuffer();
}
GameModel::~GameModel()
{
SAFE_DELETE(renderBuffer);
SAFE_DELETE(boneBuffer);
SAFE_DELETE(shader);
SAFE_DELETE(model);
}
void GameModel::Velocity(D3DXVECTOR3 & vec)
{
velocity = vec;
}
D3DXVECTOR3 GameModel::Velocity()
{
return velocity;
}
void GameModel::AddPosition(D3DXVECTOR3 & vec)
{
D3DXVECTOR3 temp = Position() + vec;
Position(temp);
}
void GameModel::CalcPosition()
{
if (D3DXVec3Length(&velocity) <= 0.0f)
return;
D3DXVECTOR3 vec(0, 0, 0);
if (velocity.z != 0.0f)
vec += Direction() * velocity.z;
if (velocity.y != 0.0f)
vec += Up() * velocity.y;
if (velocity.x != 0.0f)
vec += Right() * velocity.x;
AddPosition(vec * Time::Delta());
}
void GameModel::Update()
{
CalcPosition();
D3DXMATRIX transformed = Transformed();
model->CopyAbsoluteBoneTo(transformed, boneTransforms);
}
void GameModel::Render()
{
if (Visible() == false) return;
if(boneTransforms.size() !=0)
boneBuffer->SetBones(&boneTransforms[0], boneTransforms.size());
boneBuffer->SetVSBuffer(2);
for (ModelMesh* mesh : model->Meshes())
{
int index = mesh->ParentBoneIndex();
renderBuffer->Data.BoneNumber = index;
renderBuffer->SetVSBuffer(3);
mesh->Render();
}
}
<file_sep>#include "stdafx.h"
#include "Model.h"
#include "ModelMesh.h"
#include "ModelBone.h"
#include "ModelAnimClip.h"
Model::Model()
{
buffer = new ModelBuffer();
}
Model::~Model()
{
SAFE_DELETE(buffer);
for (Material* material : materials)
SAFE_DELETE(material);
for (ModelBone* bone : bones)
SAFE_DELETE(bone);
for (ModelMesh* mesh : meshes)
SAFE_DELETE(mesh);
for (ModelAnimClip* clip : clips)
SAFE_DELETE(clip);
}
ModelMesh * Model::Mesh(wstring name)
{
for (ModelMesh* mesh : meshes)
{
if (mesh->name == name)
return mesh;
}
return NULL;
}
ModelBone * Model::Bone(wstring name)
{
for (ModelBone* bone : bones)
{
if (bone->name == name)
return bone;
}
return NULL;
}
ModelAnimClip * Model::Clip(wstring name)
{
for (ModelAnimClip* clip : clips)
{
if (clip->Name() == name)
return clip;
}
return NULL;
}
void Model::CopyAbsoluteBoneTo(vector<D3DXMATRIX>& transforms)
{
transforms.clear();
transforms.assign(bones.size(), D3DXMATRIX());
for (size_t i = 0; i < bones.size(); i++)
{
ModelBone* bone = bones[i];
if (bone->parent != NULL)
{
int index = bone->parent->index;
transforms[i] = bone->transform * transforms[index];
}
else
transforms[i] = bone->transform;
}
}
void Model::CopyAbsoluteBoneTo(D3DXMATRIX matrix, vector<D3DXMATRIX>& transforms)
{
transforms.clear();
transforms.assign(bones.size(), D3DXMATRIX());
for (size_t i = 0; i < bones.size(); i++)
{
ModelBone* bone = bones[i];
if (bone->parent != NULL)
{
int index = bone->parent->index;
transforms[i] = bone->transform * transforms[index];
}
else
transforms[i] = bone->transform * matrix;
}
}
void Models::Create()
{
}
void Models::Delete()
{
for (pair<wstring, vector<Material *>> temp : materialMap)
{
for (Material* material : temp.second)
SAFE_DELETE(material);
}
for (pair<wstring, MeshData> temp : meshDataMap)
{
MeshData data = temp.second;
for (ModelBone* bone : data.Bones)
SAFE_DELETE(bone);
for (ModelMesh* mesh : data.Meshes)
SAFE_DELETE(mesh);
}
}
<file_sep>#include "Vector3.h"
#include <d3dx9math.h>
#include "Matrix.h"
#include "Quaternion.h"
//////////////////////////////////////////////////////////////////////////
const Vector3 Vector3::Zero = Vector3(0.0f, 0.0f, 0.0f);
const Vector3 Vector3::One = Vector3(1.0f, 1.0f, 1.0f);
const Vector3 Vector3::UnitX = Vector3(1.0f, 0.0f, 0.0f);
const Vector3 Vector3::UnitY = Vector3(0.0f, 1.0f, 0.0f);
const Vector3 Vector3::UnitZ = Vector3(0.0f, 0.0f, 1.0f);
const Vector3 Vector3::Up = Vector3(0.0f, 1.0f, 0.0f);
const Vector3 Vector3::Down = Vector3(0.0f, -1.0f, 0.0f);
const Vector3 Vector3::Right = Vector3(1.0f, 0.0f, 0.0f);
const Vector3 Vector3::Left = Vector3(-1.0f, 0.0f, 0.0f);
const Vector3 Vector3::Forward = Vector3(0.0f, 0.0f, -1.0f);
const Vector3 Vector3::Backward = Vector3(0.0f, 0.0f, 1.0f);
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
//////////////////////////////////////////////////////////////////////////
Vector3::Vector3( void )
{
this->X = 0.0f;
this->Y = 0.0f;
this->Z = 0.0f;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param X = Y = Z = value
//////////////////////////////////////////////////////////////////////////
Vector3::Vector3( float value )
{
this->X = this->Y = this->Z = value;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param x : X
///@param y : Y
///@param z : Z
//////////////////////////////////////////////////////////////////////////
Vector3::Vector3( float x, float y, float z )
{
this->X = x;
this->Y = y;
this->Z = z;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::operator-( void )
{
Vector3 vector3;
vector3.X = -this->X;
vector3.Y = -this->Y;
vector3.Z = -this->Z;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief ==
///@param value2 : 벡터
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Vector3::operator==( const Vector3& value2 ) const
{
if ((double)this->X == (double)value2.X && (double)this->Y == (double)value2.Y)
return (double)this->Z == (double)value2.Z;
else
return false;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief !=
///@param value2 : 벡터
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool Vector3::operator!=( const Vector3& value2 ) const
{
if ((double)this->X == (double)value2.X && (double)this->Y == (double)value2.Y)
return (double)this->Z != (double)value2.Z;
else
return true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief +
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::operator+( const Vector3& value2 ) const
{
Vector3 vector3;
vector3.X = this->X + value2.X;
vector3.Y = this->Y + value2.Y;
vector3.Z = this->Z + value2.Z;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::operator-( const Vector3& value2 ) const
{
Vector3 vector3;
vector3.X = this->X - value2.X;
vector3.Y = this->Y - value2.Y;
vector3.Z = this->Z - value2.Z;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::operator*( const Vector3& value2 ) const
{
Vector3 vector3;
vector3.X = this->X * value2.X;
vector3.Y = this->Y * value2.Y;
vector3.Z = this->Z * value2.Z;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *
///@param scaleFactor : 값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::operator*( const float& scaleFactor ) const
{
Vector3 vector3;
vector3.X = this->X * scaleFactor;
vector3.Y = this->Y * scaleFactor;
vector3.Z = this->Z * scaleFactor;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::operator/( const Vector3& value2 ) const
{
Vector3 vector3;
vector3.X = this->X / value2.X;
vector3.Y = this->Y / value2.Y;
vector3.Z = this->Z / value2.Z;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /
///@param divider : 값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::operator/( const float& divider ) const
{
float num = 1.0f / divider;
Vector3 vector3;
vector3.X = this->X * num;
vector3.Y = this->Y * num;
vector3.Z = this->Z * num;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief +=
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector3::operator+=( const Vector3& value2 )
{
*this = *this + value2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief -=
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector3::operator-=( const Vector3& value2 )
{
*this = *this - value2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief *=
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector3::operator*=( const Vector3& value2 )
{
*this = *this * value2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /=
///@param scaleFactor : 값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector3::operator*=( const float& scaleFactor )
{
*this = *this * scaleFactor;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /=
///@param value2 : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector3::operator/=( const Vector3& value2 )
{
*this = *this / value2;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief /=
///@param divider : 값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
void Vector3::operator/=( const float& divider )
{
*this = *this / divider;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 문자열로 변환
///@return 문자열
//////////////////////////////////////////////////////////////////////////
std::string Vector3::ToString()
{
std::string temp;
char val[255];
sprintf(val, "{X:%#f ", X); temp += val;
sprintf(val, "Y:%#f ", Y); temp += val;
sprintf(val, "Z:%#f}", Z); temp += val;
return temp;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief D3DXVECTOR3로 변환
///@return D3DX 벡터
//////////////////////////////////////////////////////////////////////////
D3DXVECTOR3 Vector3::ToD3DXVECTOR3()
{
D3DXVECTOR3 vector3;
vector3.x = X;
vector3.y = Y;
vector3.z = Z;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 크기 계산
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector3::Length()
{
return (float)sqrt((double)this->X * (double)this->X + (double)this->Y * (double)this->Y + (double)this->Z * (double)this->Z);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 제곱으로 크기 계산
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector3::LengthSquared()
{
return (float)((double)this->X * (double)this->X + (double)this->Y * (double)this->Y + (double)this->Z * (double)this->Z);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 정규화
//////////////////////////////////////////////////////////////////////////
void Vector3::Normalize()
{
float num = 1.0f / (float)sqrt((double)this->X * (double)this->X + (double)this->Y * (double)this->Y + (double)this->Z * (double)this->Z);
this->X *= num;
this->Y *= num;
this->Z *= num;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 거리계산
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector3::Distance( Vector3 value1, Vector3 value2 )
{
float num1 = value1.X - value2.X;
float num2 = value1.Y - value2.Y;
float num3 = value1.Z - value2.Z;
return (float)sqrt((double)num1 * (double)num1 + (double)num2 * (double)num2 + (double)num3 * (double)num3);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 제곱으로 거리계산
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector3::DistanceSquared( Vector3 value1, Vector3 value2 )
{
float num1 = value1.X - value2.X;
float num2 = value1.Y - value2.Y;
float num3 = value1.Z - value2.Z;
return (float)((double)num1 * (double)num1 + (double)num2 * (double)num2 + (double)num3 * (double)num3);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 내적 계산
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Vector3::Dot( Vector3 value1, Vector3 value2 )
{
return (float)((double)value1.X * (double)value2.X + (double)value1.Y * (double)value2.Y + (double)value1.Z * (double)value2.Z);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 정규화
///@param value : 벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::Normalize( Vector3 value )
{
float num = 1.0f / (float)sqrt((double)value.X * (double)value.X + (double)value.Y * (double)value.Y + (double)value.Z * (double)value.Z);
Vector3 vector3;
vector3.X = value.X * num;
vector3.Y = value.Y * num;
vector3.Z = value.Z * num;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 외적 계산
///@param vector1 : 벡터1
///@param vector2 : 벡터2
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::Cross( Vector3 vector1, Vector3 vector2 )
{
Vector3 vector3;
vector3.X = (float)((double)vector1.Y * (double)vector2.Z - (double)vector1.Z * (double)vector2.Y);
vector3.Y = (float)((double)vector1.Z * (double)vector2.X - (double)vector1.X * (double)vector2.Z);
vector3.Z = (float)((double)vector1.X * (double)vector2.Y - (double)vector1.Y * (double)vector2.X);
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 반사값
///@param vector : 벡터
///@param normal : 노멀벡터
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::Reflect( Vector3 vector, Vector3 normal )
{
float num = (float)((double)vector.X * (double)normal.X + (double)vector.Y * (double)normal.Y + (double)vector.Z * (double)normal.Z);
Vector3 vector3;
vector3.X = vector.X - 2.0f * num * normal.X;
vector3.Y = vector.Y - 2.0f * num * normal.Y;
vector3.Z = vector.Z - 2.0f * num * normal.Z;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 벡터 중 작은 벡터
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::Min( Vector3 value1, Vector3 value2 )
{
Vector3 vector3;
vector3.X = (double)value1.X < (double)value2.X ? value1.X : value2.X;
vector3.Y = (double)value1.Y < (double)value2.Y ? value1.Y : value2.Y;
vector3.Z = (double)value1.Z < (double)value2.Z ? value1.Z : value2.Z;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 벡터 중 큰 벡터
///@param value1 : 벡터1
///@param value2 : 벡터2
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::Max( Vector3 value1, Vector3 value2 )
{
Vector3 vector3;
vector3.X = (double)value1.X > (double)value2.X ? value1.X : value2.X;
vector3.Y = (double)value1.Y > (double)value2.Y ? value1.Y : value2.Y;
vector3.Z = (double)value1.Z > (double)value2.Z ? value1.Z : value2.Z;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 해당 벡터가 최소값보다 작을 경우 최소값, 반대의 경우 최대값
///@param value1 : 벡터
///@param min : 최소값 벡터
///@param max : 최대값 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::Clamp( Vector3 value1, Vector3 min, Vector3 max )
{
float num1 = value1.X;
float num2 = (double)num1 > (double)max.X ? max.X : num1;
float num3 = (double)num2 < (double)min.X ? min.X : num2;
float num4 = value1.Y;
float num5 = (double)num4 > (double)max.Y ? max.Y : num4;
float num6 = (double)num5 < (double)min.Y ? min.Y : num5;
float num7 = value1.Z;
float num8 = (double)num7 > (double)max.Z ? max.Z : num7;
float num9 = (double)num8 < (double)min.Z ? min.Z : num8;
Vector3 vector3;
vector3.X = num3;
vector3.Y = num6;
vector3.Z = num9;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 벡터의 선형보간
///@param value1 : 벡터1
///@param value2 : 벡터2
///@param amount : 보간값
///@return 결과벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::Lerp( Vector3 value1, Vector3 value2, float amount )
{
Vector3 vector3;
vector3.X = value1.X + (value2.X - value1.X) * amount;
vector3.Y = value1.Y + (value2.Y - value1.Y) * amount;
vector3.Z = value1.Z + (value2.Z - value1.Z) * amount;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 두 벡터를 부드럽게 보간
///@param value1 : 벡터1
///@param value2 : 벡터2
///@param amount : 보간값
///@return 결과벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::SmoothStep( Vector3 value1, Vector3 value2, float amount )
{
amount = (double)amount > 1.0 ? 1.0f : ((double)amount < 0.0 ? 0.0f : amount);
amount = (float)((double)amount * (double)amount * (3.0 - 2.0 * (double)amount));
Vector3 vector3;
vector3.X = value1.X + (value2.X - value1.X)* amount;
vector3.Y = value1.Y + (value2.Y - value1.Y)* amount;
vector3.Z = value1.Z + (value2.Z - value1.Z)* amount;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 카트뮬-롬 보간
///@param value1 : 벡터1
///@param value2 : 벡터2
///@param value3 : 벡터3
///@param value4 : 벡터4
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::CatmullRom( Vector3 value1, Vector3 value2, Vector3 value3, Vector3 value4, float amount )
{
float num1 = amount * amount;
float num2 = amount * num1;
Vector3 vector3;
vector3.X = (float)(0.5 * (2.0 * (double)value2.X + (-(double)value1.X + (double)value3.X)* (double)amount + (2.0 * (double)value1.X - 5.0 * (double)value2.X + 4.0 * (double)value3.X - (double)value4.X)* (double)num1 + (-(double)value1.X + 3.0 * (double)value2.X - 3.0 * (double)value3.X + (double)value4.X)* (double)num2));
vector3.Y = (float)(0.5 * (2.0 * (double)value2.Y + (-(double)value1.Y + (double)value3.Y)* (double)amount + (2.0 * (double)value1.Y - 5.0 * (double)value2.Y + 4.0 * (double)value3.Y - (double)value4.Y)* (double)num1 + (-(double)value1.Y + 3.0 * (double)value2.Y - 3.0 * (double)value3.Y + (double)value4.Y)* (double)num2));
vector3.Z = (float)(0.5 * (2.0 * (double)value2.Z + (-(double)value1.Z + (double)value3.Z)* (double)amount + (2.0 * (double)value1.Z - 5.0 * (double)value2.Z + 4.0 * (double)value3.Z - (double)value4.Z)* (double)num1 + (-(double)value1.Z + 3.0 * (double)value2.Z - 3.0 * (double)value3.Z + (double)value4.Z)* (double)num2));
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 에르미트 보간
///@param value1 : 벡터1
///@param tagent1 : 벡터1의 탄젠트 벡터
///@param value2 : 벡터2
///@param tagent2 : 벡터2 탄젠트 벡터
///@param amount : 보간값
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::Hermite( Vector3 value1, Vector3 tangent1, Vector3 value2, Vector3 tangent2, float amount )
{
float num1 = amount * amount;
float num2 = amount * num1;
float num3 = (float)(2.0 * (double)num2 - 3.0 * (double)num1 + 1.0);
float num4 = (float)(-2.0 * (double)num2 + 3.0 * (double)num1);
float num5 = num2 - 2.0f * num1 + amount;
float num6 = num2 - num1;
Vector3 vector3;
vector3.X = (float)((double)value1.X * (double)num3 + (double)value2.X * (double)num4 + (double)tangent1.X * (double)num5 + (double)tangent2.X * (double)num6);
vector3.Y = (float)((double)value1.Y * (double)num3 + (double)value2.Y * (double)num4 + (double)tangent1.Y * (double)num5 + (double)tangent2.Y * (double)num6);
vector3.Z = (float)((double)value1.Z * (double)num3 + (double)value2.Z * (double)num4 + (double)tangent1.Z * (double)num5 + (double)tangent2.Z * (double)num6);
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 매트릭스에 의한 벡터 변환
///@param position : 벡터
///@param matrix : 매트릭스
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::Transform( Vector3 position, Matrix matrix )
{
float num1 = (float)((double)position.X * (double)matrix.M11 + (double)position.Y * (double)matrix.M21 + (double)position.Z * (double)matrix.M31)+ matrix.M41;
float num2 = (float)((double)position.X * (double)matrix.M12 + (double)position.Y * (double)matrix.M22 + (double)position.Z * (double)matrix.M32)+ matrix.M42;
float num3 = (float)((double)position.X * (double)matrix.M13 + (double)position.Y * (double)matrix.M23 + (double)position.Z * (double)matrix.M33)+ matrix.M43;
Vector3 vector3;
vector3.X = num1;
vector3.Y = num2;
vector3.Z = num3;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 쿼터니온에 의한 벡터 변환
///@param value : 벡터
///@param rotation : 쿼터니온
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::Transform( Vector3 value, Quaternion rotation )
{
float num1 = rotation.X + rotation.X;
float num2 = rotation.Y + rotation.Y;
float num3 = rotation.Z + rotation.Z;
float num4 = rotation.W * num1;
float num5 = rotation.W * num2;
float num6 = rotation.W * num3;
float num7 = rotation.X * num1;
float num8 = rotation.X * num2;
float num9 = rotation.X * num3;
float num10 = rotation.Y * num2;
float num11 = rotation.Y * num3;
float num12 = rotation.Z * num3;
float num13 = (float)((double)value.X * (1.0 - (double)num10 - (double)num12)+ (double)value.Y * ((double)num8 - (double)num6)+ (double)value.Z * ((double)num9 + (double)num5));
float num14 = (float)((double)value.X * ((double)num8 + (double)num6) + (double)value.Y * (1.0 - (double)num7 - (double)num12)+ (double)value.Z * ((double)num11 - (double)num4));
float num15 = (float)((double)value.X * ((double)num9 - (double)num5) + (double)value.Y * ((double)num11 + (double)num4)+ (double)value.Z * (1.0 - (double)num7 - (double)num10));
Vector3 vector3;
vector3.X = num13;
vector3.Y = num14;
vector3.Z = num15;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 매트릭스에 의한 노멀벡터 변환
///@param normal : 법선 벡터
///@param matrix : 매트릭스
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::TransformNormal( Vector3 normal, Matrix matrix )
{
float num1 = (float)((double)normal.X * (double)matrix.M11 + (double)normal.Y * (double)matrix.M21 + (double)normal.Z * (double)matrix.M31);
float num2 = (float)((double)normal.X * (double)matrix.M12 + (double)normal.Y * (double)matrix.M22 + (double)normal.Z * (double)matrix.M32);
float num3 = (float)((double)normal.X * (double)matrix.M13 + (double)normal.Y * (double)matrix.M23 + (double)normal.Z * (double)matrix.M33);
Vector3 vector3;
vector3.X = num1;
vector3.Y = num2;
vector3.Z = num3;
return vector3;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 매트릭스에 의한 위치(정점)벡터 변환
///@param position : 위치 벡터
///@param matrix : 매트릭스
///@return 결과 벡터
//////////////////////////////////////////////////////////////////////////
Vector3 Vector3::TransformCoord( Vector3 position, Matrix matrix )
{
float num1 = (matrix.M11 * position.X) + (matrix.M21 * position.Y) + (matrix.M31 * position.Z) + matrix.M41;
float num2 = (matrix.M12 * position.X) + (matrix.M22 * position.Y) + (matrix.M32 * position.Z) + matrix.M42;
float num3 = (matrix.M13 * position.X) + (matrix.M23 * position.Y) + (matrix.M33 * position.Z) + matrix.M43;
float num4 = (matrix.M14 * position.X) + (matrix.M24 * position.Y) + (matrix.M34 * position.Z) + matrix.M44;
return Vector3((num1 / num4), (num2 / num4), (num3 / num4));
}<file_sep>#pragma once
#include "../Core/Vector3.h"
enum PlaneIntersectionType;
enum ContainmentType;
class BoundingBox;
class BoundingFrustum;
class Ray;
class Plane;
//////////////////////////////////////////////////////////////////////////
///@brief 충돌 구
//////////////////////////////////////////////////////////////////////////
class BoundingSphere
{
public:
BoundingSphere(void);
BoundingSphere(Vector3 center, float radius);
bool operator ==(const BoundingSphere& value) const;
bool operator !=(const BoundingSphere& value) const;
Vector3 SupportMapping(Vector3 v);
static BoundingSphere CreateMerged(BoundingSphere original, BoundingSphere additional);
static BoundingSphere CreateFromBoundingBox(BoundingBox box);
bool Intersects(BoundingBox box);
bool Intersects(BoundingFrustum frustum);
PlaneIntersectionType Intersects(Plane plane);
bool Intersects(Ray ray, float& result);
bool Intersects(BoundingSphere sphere);
ContainmentType Contains(BoundingBox box);
ContainmentType Contains(BoundingFrustum frustum);
ContainmentType Contains(Vector3 point);
ContainmentType Contains(BoundingSphere sphere);
BoundingSphere Transform(Matrix matrix);
public:
Vector3 Center;///< 중심점 위치
float Radius;///< 반지름
};<file_sep>#include "BoundingBox.h"
#include <assert.h>
#include "Bounding.h"
#include "BoundingSphere.h"
#include "BoundingFrustum.h"
#include "Ray.h"
#include "../Core/Plane.h"
#include "../Core/MathHelper.h"
//////////////////////////////////////////////////////////////////////////
const int BoundingBox::CornerCount = 8;
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
//////////////////////////////////////////////////////////////////////////
BoundingBox::BoundingBox( void )
{
this->Min = Vector3::Zero;
this->Max = Vector3::Zero;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param min : 최소 위치벡터
///@param max : 최대 위치벡터
//////////////////////////////////////////////////////////////////////////
BoundingBox::BoundingBox( Vector3 min, Vector3 max )
{
this->Min = min;
this->Max = max;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief ==
///@param value : 바운딩 박스
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingBox::operator==( const BoundingBox& value ) const
{
if(this->Min == value.Min)
return this->Max == value.Max;
else
return false;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief !=
///@param value : 바운딩 박스
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingBox::operator!=( const BoundingBox& value ) const
{
if(!(this->Min != value.Min))
return this->Max != value.Max;
else
return true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 코너 위치
///@param pCorner : Vector[8]
//////////////////////////////////////////////////////////////////////////
void BoundingBox::GetCorners( Vector3* pCorner )
{
assert(pCorner != NULL);
pCorner[0] = Vector3(Min.X, Max.Y, Max.Z);
pCorner[1] = Vector3(Max.X, Max.Y, Max.Z);
pCorner[2] = Vector3(Max.X, Min.Y, Max.Z);
pCorner[3] = Vector3(Min.X, Min.Y, Max.Z);
pCorner[4] = Vector3(Min.X, Max.Y, Min.Z);
pCorner[5] = Vector3(Max.X, Max.Y, Min.Z);
pCorner[6] = Vector3(Max.X, Min.Y, Min.Z);
pCorner[7] = Vector3(Min.X, Min.Y, Min.Z);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 문자열로 변환
///@param 문자열
//////////////////////////////////////////////////////////////////////////
std::string BoundingBox::ToString()
{
std::string temp;
char val[255];
sprintf(val, "{ Min:{X:%#f ", Min.X); temp += val;
sprintf(val, "Y:%#f ", Min.Y); temp += val;
sprintf(val, "Z:%#f}, ", Min.Z); temp += val;
sprintf(val, "Max:{X:%#f ", Max.X); temp += val;
sprintf(val, "Y:%#f ", Max.Y); temp += val;
sprintf(val, "Z:%#f}}, ", Max.Z); temp += val;
return temp;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 서포트 매핑
///@param v : 벡터
///@return 결과
//////////////////////////////////////////////////////////////////////////
Vector3 BoundingBox::SupportMapping( Vector3 v )
{
Vector3 result;
result.X = (double)v.X >= 0.0 ? Max.X : Min.X;
result.Y = (double)v.Y >= 0.0 ? Max.Y : Min.Y;
result.Z = (double)v.Z >= 0.0 ? Max.Z : Min.Z;
return result;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 박스 2개를 합친다
///@param original : 바운딩 박스1
///@param additional : 바운딩 박스2
///@return 결과 바운딩박스
//////////////////////////////////////////////////////////////////////////
BoundingBox BoundingBox::CreateMerged( BoundingBox original, BoundingBox additional )
{
BoundingBox boundingBox;
boundingBox.Min = Vector3::Min(original.Min, additional.Min);
boundingBox.Max = Vector3::Max(original.Max, additional.Max);
return boundingBox;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 스페어로부터 바운딩 박스 생성
///@param sphere : 바운딩 스페어
///@return 결과 바운딩박스
//////////////////////////////////////////////////////////////////////////
BoundingBox BoundingBox::CreateFromSphere( BoundingSphere sphere )
{
BoundingBox boundingBox;
boundingBox.Min.X = sphere.Center.X - sphere.Radius;
boundingBox.Min.Y = sphere.Center.Y - sphere.Radius;
boundingBox.Min.Z = sphere.Center.Z - sphere.Radius;
boundingBox.Max.X = sphere.Center.X + sphere.Radius;
boundingBox.Max.Y = sphere.Center.Y + sphere.Radius;
boundingBox.Max.Z = sphere.Center.Z + sphere.Radius;
return boundingBox;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 박스와 교차 검사
///@param box : 바운딩 박스
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingBox::Intersects( BoundingBox box )
{
if ((double)Max.X < (double)box.Min.X || (double)Min.X > (double)box.Max.X || ((double)Max.Y < (double)box.Min.Y || (double)Min.Y > (double)box.Max.Y)|| ((double)Max.Z < (double)box.Min.Z || (double)Min.Z > (double)box.Max.Z))
return false;
else
return true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 프러스텀과 교차 검사
///@param frustum : 바운딩 프러스텀
//////////////////////////////////////////////////////////////////////////
bool BoundingBox::Intersects( BoundingFrustum frustum )
{
return frustum.Intersects(*this);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 평면과의 교차 검사
///@param plane : 평면
///@return 교차 결과
//////////////////////////////////////////////////////////////////////////
PlaneIntersectionType BoundingBox::Intersects( Plane plane )
{
Vector3 vector3_1;
vector3_1.X = (double)plane.Normal.X >= 0.0 ? Min.X : Max.X;
vector3_1.Y = (double)plane.Normal.Y >= 0.0 ? Min.Y : Max.Y;
vector3_1.Z = (double)plane.Normal.Z >= 0.0 ? Min.Z : Max.Z;
Vector3 vector3_2;
vector3_2.X = (double)plane.Normal.X >= 0.0 ? Max.X : Min.X;
vector3_2.Y = (double)plane.Normal.Y >= 0.0 ? Max.Y : Min.Y;
vector3_2.Z = (double)plane.Normal.Z >= 0.0 ? Max.Z : Min.Z;
if ((double)plane.Normal.X * (double)vector3_1.X + (double)plane.Normal.Y * (double)vector3_1.Y + (double)plane.Normal.Z * (double)vector3_1.Z + (double)plane.D > 0.0)
return PlaneIntersectionType_Front;
else if ((double)plane.Normal.X * (double)vector3_2.X + (double)plane.Normal.Y * (double)vector3_2.Y + (double)plane.Normal.Z * (double)vector3_2.Z + (double)plane.D < 0.0)
return PlaneIntersectionType_Back;
else
return PlaneIntersectionType_Intersecting;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 레이와의 교차 검사
///@param ray : 레이
///@param result : 결과
///@warning
/// 교차가 되지 않은 결과 result는 0.0f이 절대 아니다!
/// result == NULL 이다!!!!!
//////////////////////////////////////////////////////////////////////////
bool BoundingBox::Intersects( Ray ray, float* result )
{
if(result != NULL)
{
delete result;
result = NULL;
}
float num1 = 0.0f;
float num2 = MathHelper::FloatMaxValue;
//1. ray.Direction.X
if((double)MathHelper::Abs(ray.Direction.X) < 9.99999997475243E-07)
{
if((double)ray.Position.X < (double)Min.X || (double)ray.Position.X > (double)Max.X)
return false;
}
else
{
float num3 = 1.0f / ray.Direction.X;
float num4 = (Min.X - ray.Position.X)* num3;
float num5 = (Max.X - ray.Position.X)* num3;
if((double)num4 > (double)num5)
{
float num6 = num4;
num4 = num5;
num5 = num6;
}
num1 = MathHelper::Max(num4, num1);
num2 = MathHelper::Min(num5, num2);
if((double)num1 > (double)num2)
return false;
}
//2. ray.Direction.Y
if((double)MathHelper::Abs(ray.Direction.Y) < 9.99999997475243E-07)
{
if ((double)ray.Position.Y < (double)Min.Y || (double)ray.Position.Y >(double)Max.Y)
return false;
}
else
{
float num3 = 1.0f / ray.Direction.Y;
float num4 = (Min.Y - ray.Position.Y) * num3;
float num5 = (Max.Y - ray.Position.Y) * num3;
if((double)num4 > (double)num5)
{
float num6 = num4;
num4 = num5;
num5 = num6;
}
num1 = MathHelper::Max(num4, num1);
num2 = MathHelper::Min(num5, num2);
if((double)num1 > (double)num2)
return false;
}
//3. ray.Direction.Z
if((double)MathHelper::Abs(ray.Direction.Z) < 9.99999997475243E-07)
{
if((double)ray.Position.Z < (double)Min.Z || (double)ray.Position.Z > (double)Max.Z)
return false;
}
else
{
float num3 = 1.0f / ray.Direction.Z;
float num4 = (Min.Z - ray.Position.Z) * num3;
float num5 = (Max.Z - ray.Position.Z) * num3;
if((double)num4 > (double)num5)
{
float num6 = num4;
num4 = num5;
num5 = num6;
}
num1 = MathHelper::Max(num4, num1);
float num7 = MathHelper::Min(num5, num2);
if((double)num1 > (double)num7)
return false;
}
result = new float(num1);
return true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 스페어와 교차 검사
///@param sphere : 바운딩 스페어
///@return 결과
//////////////////////////////////////////////////////////////////////////
bool BoundingBox::Intersects( BoundingSphere sphere )
{
Vector3 result1 = Vector3::Clamp(sphere.Center, Min, Max);
float result2 = Vector3::DistanceSquared(sphere.Center, result1);
if((double)result2 <= (double)sphere.Radius * (double)sphere.Radius)
return true;
else
return false;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 박스 포함 검사
///@param box : 바운딩 박스
///@return 포함 결과
//////////////////////////////////////////////////////////////////////////
ContainmentType BoundingBox::Contains( BoundingBox box )
{
if ((double)Max.X < (double)box.Min.X || (double)Min.X > (double)box.Max.X || ((double)Max.Y < (double)box.Min.Y || (double)Min.Y > (double)box.Max.Y) || ((double)Max.Z < (double)box.Min.Z || (double)Min.Z > (double)box.Max.Z))
return ContainmentType_Disjoint;
else if ((double)Min.X > (double)box.Min.X || (double)box.Max.X > (double)Max.X || ((double)Min.Y > (double)box.Min.Y || (double)box.Max.Y > (double)Max.Y)|| ((double)Min.Z > (double)box.Min.Z || (double)box.Max.Z > (double)Max.Z))
return ContainmentType_Intersects;
else
return ContainmentType_Contains;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 프러스텀 포함 검사
///@param box : 바운딩 박스
///@return 포함 결과
//////////////////////////////////////////////////////////////////////////
ContainmentType BoundingBox::Contains( BoundingFrustum frustum )
{
if(!frustum.Intersects(*this))
{
return ContainmentType_Disjoint;
}
else
{
for(int i = 0; i < 8; i++)
{
Vector3 point = frustum.cornerArray[i];
if(Contains(point) == ContainmentType_Disjoint)
return ContainmentType_Intersects;
}
return ContainmentType_Contains;
}
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 위치 벡터 포함 검사
///@param point : 위치 벡터
///@return 포함 결과
//////////////////////////////////////////////////////////////////////////
ContainmentType BoundingBox::Contains( Vector3 point )
{
if ((double)Min.X > (double)point.X || (double)point.X > (double)Max.X || ((double)Min.Y > (double)point.Y || (double)point.Y > (double)Max.Y)|| ((double)Min.Z > (double)point.Z || (double)point.Z > (double)Max.Z))
return ContainmentType_Disjoint;
else
return ContainmentType_Contains;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 스페어 포함 검사
///@param point : 위치 벡터
///@return 포함 결과
//////////////////////////////////////////////////////////////////////////
ContainmentType BoundingBox::Contains( BoundingSphere sphere )
{
Vector3 result1 = Vector3::Clamp(sphere.Center, Min, Max);
float result2 = Vector3::DistanceSquared(sphere.Center, result1);
float num = sphere.Radius;
if((double)result2 > (double)num * (double)num)
return ContainmentType_Disjoint;
else if ((double)Min.X + (double)num > (double)sphere.Center.X || (double)sphere.Center.X > (double)Max.X - (double)num || ((double)Max.X - (double)Min.X <= (double)num || (double)Min.Y + (double)num > (double)sphere.Center.Y)|| ((double)sphere.Center.Y > (double)Max.Y - (double)num || (double)Max.Y - (double)Min.Y <= (double)num || ((double)Min.Z + (double)num > (double)sphere.Center.Z || (double)sphere.Center.Z > (double)Max.Z - (double)num))|| (double)Max.X - (double)Min.X <= (double)num)
return ContainmentType_Intersects;
else
return ContainmentType_Contains;
}<file_sep>#pragma once
#include <string>
#pragma warning( disable : 4996)
struct D3DXMATRIX;
class Vector3;
class Quaternion;
class Plane;
//////////////////////////////////////////////////////////////////////////
///@brief 행렬
//////////////////////////////////////////////////////////////////////////
class Matrix
{
public:
Matrix(void);
Matrix(float m11, float m12, float m13, float m14, float m21, float m22, float m23, float m24, float m31, float m32, float m33, float m34, float m41, float m42, float m43, float m44);
Vector3 Up(); void Up(Vector3 value);
Vector3 Down(); void Down(Vector3 value);
Vector3 Right(); void Right(Vector3 value);
Vector3 Left(); void Left(Vector3 value);
Vector3 Forward(); void Forward(Vector3 value);
Vector3 Backward(); void Backward(Vector3 value);
Vector3 Translate(); void Translate(Vector3 value);
Matrix operator -();
bool operator ==(const Matrix& matrix2) const;
bool operator !=(const Matrix& matrix2) const;
Matrix operator +(const Matrix& matrix2) const;
Matrix operator -(const Matrix& matrix2) const;
Matrix operator *(const Matrix& matrix2) const;
Matrix operator *(const float& scaleFactor) const;
Matrix operator /(const Matrix& matrix2) const;
Matrix operator /(const float& divider) const;
void operator +=(const Matrix& matrix2);
void operator -=(const Matrix& matrix2);
void operator *=(const Matrix& matrix2);
void operator *=(const float& scaleFactor);
void operator /=(const Matrix& matrix2);
void operator /=(const float& divider);
//////////////////////////////////////////////////////////////////////////
///@brief float형의 *연산 처리
///@param scalefactor : 값
///@param matrix2 : 매트릭스
///@return 결과 매트릭스
//////////////////////////////////////////////////////////////////////////
friend Matrix operator *(const float scaleFactor, const Matrix& matrix2)
{
return matrix2 * scaleFactor;
}
//////////////////////////////////////////////////////////////////////////
std::string ToString();
D3DXMATRIX ToD3DXMATRIX();
float Determinant();
static Matrix CreateBillboard(Vector3 objectPosition, Vector3 cameraPosition, Vector3 cameraUpVector, Vector3* cameraForwardVector=NULL);
static Matrix CreateTranslation(Vector3 position);
static Matrix CreateTranslation(float xPosition, float yPosition, float zPosition);
static Matrix CreateScale(Vector3 scales);
static Matrix CreateScale(float xScale, float yScale, float zScale);
static Matrix CreateScale(float scale);
static Matrix CreateRotationX(float radians);
static Matrix CreateRotationY(float radians);
static Matrix CreateRotationZ(float radians);
static Matrix CreateFromAxisAngle(Vector3 axis, float angle);
static Matrix CreatePerspective(float width, float height, float nearPlaneDistance, float farPlaneDistance);
static Matrix CreatePerspectiveFieldOfView(float fieldOfView, float aspectRatio, float nearPlaneDistance, float farPlaneDistance);
static Matrix CreateLookAt(Vector3 cameraPosition, Vector3 cameraTarget, Vector3 cameraUpVector);
static Matrix CreateWorld(Vector3 position, Vector3 forward, Vector3 up);
static Matrix CreateFromQuaternion(Quaternion quaternion);
static Matrix CreateFromYawPitchRoll(float yaw, float pitch, float roll);
static Matrix CreateShadow(Vector3 lightDirection, Plane plane);
static Matrix CreateReflection(Plane value);
static Matrix CreateOrthographic(float width, float height, float zNearPlane, float zFarPlane);
static Matrix Transform(Matrix value, Quaternion rotation);
static Matrix Transpose(Matrix matrix);
static Matrix Invert(Matrix matrix);
static Matrix Lerp(Matrix matrix1, Matrix matrix2, float amount);
public:
const static Matrix Identity;
float M11;
float M12;
float M13;
float M14;
float M21;
float M22;
float M23;
float M24;
float M31;
float M32;
float M33;
float M34;
float M41;
float M42;
float M43;
float M44;
};<file_sep>#pragma once
class BoundingFrustum;
namespace Gizmo
{
class Gizmo
{
public:
friend class Gizmos;
public:
class Buffer : public ShaderBuffer
{
public:
Buffer() : ShaderBuffer(&Data, sizeof(Data))
{
Data.Color = D3DXCOLOR(1, 1, 1, 1);
Data.HighlightColor = D3DXCOLOR(0, 0, 0, 1);
Data.IsHighlight = 0;
}
struct Struct
{
D3DXCOLOR Color;
D3DXCOLOR HighlightColor;
int IsHighlight;
int Padding[3];
}Data;
};
public:
~Gizmo();
void Render();
void UpdateVertexData();
public:
D3DXCOLOR color, highlightColor;
D3DXMATRIX rootWorld;
D3DXMATRIX localWorld;
vector<VertexColor> vertexData;
vector<UINT> indexData;
bool bHighlight;
private:
Gizmo();
Gizmo(vector<D3DXVECTOR3>&& positions, vector<UINT>&& indices);
void Init();
void CreateBuffer();
private:
D3D11_PRIMITIVE_TOPOLOGY topology;
WorldBuffer* worldBuffer;
ID3D11Buffer* vertexBuffer, *indexBuffer;
Shader* shader;
Buffer* buffer;
};
class Gizmos
{
public:
static Gizmo* Quad(D3DXVECTOR3 center, D3DXVECTOR3 normal, D3DXVECTOR3 up, float width, float height);
static Gizmo* Cube(D3DXVECTOR3 min, D3DXVECTOR3 max);
static Gizmo* Sphere(D3DXVECTOR3 center, float radius);
static Gizmo* Frustum(BoundingFrustum* frustum);
static Gizmo* Line(D3DXVECTOR3 start, D3DXVECTOR3 end);
static Gizmo* Ray(D3DXVECTOR3 start, D3DXVECTOR3 dir, float length = 10000.0f);
static Gizmo* WireCube(D3DXVECTOR3 min, D3DXVECTOR3 max);
static Gizmo* WireSphere(D3DXVECTOR3 center, float radius);
static Gizmo* Scale();
static Gizmo* Rotate();
static Gizmo* Translate();
};
}<file_sep>#include "Plane.h"
#include <d3dx9math.h>
#include "Vector4.h"
#include "Matrix.h"
#include "MathHelper.h"
#include "Quaternion.h"
#include "../Bounding/Bounding.h"
#include "../Bounding/BoundingBox.h"
#include "../Bounding/BoundingFrustum.h"
#include "../Bounding/BoundingSphere.h"
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
//////////////////////////////////////////////////////////////////////////
Plane::Plane( void )
{
this->D = 0.0f;
this->Normal = Vector3::Zero;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param a : 노멀축 X
///@param b : 노멀축 Y
///@param c : 노멀축 Z
///@param d : 거리값
//////////////////////////////////////////////////////////////////////////
Plane::Plane( float a, float b, float c, float d )
{
this->Normal.X = a;
this->Normal.Y = b;
this->Normal.Z = c;
this->D = d;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param normal : 노멀 벡터
///@param d : 거리값
//////////////////////////////////////////////////////////////////////////
Plane::Plane( Vector3 normal, float d )
{
this->Normal = normal;
this->D = d;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param value : 4D 벡터(XYZ, W=거리)
//////////////////////////////////////////////////////////////////////////
Plane::Plane( Vector4 value )
{
this->Normal.X = value.X;
this->Normal.Y = value.Y;
this->Normal.Z = value.Z;
this->D = value.W;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 생성자
///@param point1 : 위치 벡터1
///@param point2 : 위치 벡터2
///@param point3 : 위치 벡터3
//////////////////////////////////////////////////////////////////////////
Plane::Plane( Vector3 point1, Vector3 point2, Vector3 point3 )
{
float xValue1 = point2.X - point1.X;
float yValue1 = point2.Y - point1.Y;
float zValue1 = point2.Z - point1.Z;
float xValue2 = point3.X - point1.X;
float yValue2 = point3.Y - point1.Y;
float zValue2 = point3.Z - point1.Z;
float num1 = (float)((double)yValue1 * (double)zValue2 - (double)zValue1 * (double)yValue2);
float num2 = (float)((double)zValue1 * (double)xValue2 - (double)xValue1 * (double)zValue2);
float num3 = (float)((double)xValue1 * (double)yValue2 - (double)yValue1 * (double)xValue2);
float num4 = 1.0f / (float)sqrt((double)num1 * (double)num1 + (double)num2 * (double)num2 + (double)num3 * (double)num3);
this->Normal.X = num1 * num4;
this->Normal.Y = num2 * num4;
this->Normal.Z = num3 * num4;
this->D = (float)-((double)this->Normal.X * (double)point1.X + (double)this->Normal.Y * (double)point1.Y + (double)this->Normal.Z * (double)point1.Z);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief ==
///@param value : 평면
//////////////////////////////////////////////////////////////////////////
bool Plane::operator==( const Plane& value )const
{
if((double)Normal.X == (double)value.Normal.X && (double)Normal.Y == (double)value.Normal.Y && (double)Normal.Z == (double)value.Normal.Z)
return (double)D == (double)value.D;
else
return false;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief !=
///@param value : 평면
//////////////////////////////////////////////////////////////////////////
bool Plane::operator!=( const Plane& value )const
{
if((double)Normal.X == (double)value.Normal.X && (double)Normal.Y == (double)value.Normal.Y && (double)Normal.Z == (double)value.Normal.Z)
return (double)D != (double)value.D;
else
return true;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 문자열로 변환
///@param 문자열
//////////////////////////////////////////////////////////////////////////
std::string Plane::ToString()
{
std::string temp;
char val[255];
temp += "{Normal:" + Normal.ToString();
sprintf(val, " D:%#f}", D); temp += val;
return temp;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief
///@param
//////////////////////////////////////////////////////////////////////////
D3DXPLANE Plane::ToD3DXPLANE()
{
return D3DXPLANE(Normal.X, Normal.Y, Normal.Z, D);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 정규화
//////////////////////////////////////////////////////////////////////////
void Plane::Normalize()
{
float num1 = (float)((double)Normal.X * (double)Normal.X + (double)Normal.Y * (double)Normal.Y + (double)Normal.Z * (double)Normal.Z);
if((double)MathHelper::Abs(num1 - 1.0f) >= 1.19209289550781E-07)
{
float num2 = 1.0f / (float)sqrt((double)num1);
Normal.X *= num2;
Normal.Y *= num2;
Normal.Z *= num2;
D *= num2;
}
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 정규화
///@param value : 평면
///@return 결과 평면
//////////////////////////////////////////////////////////////////////////
Plane Plane::Normalize( Plane value )
{
float num1 = (float)((double)value.Normal.X * (double)value.Normal.X + (double)value.Normal.Y * (double)value.Normal.Y + (double)value.Normal.Z * (double)value.Normal.Z);
if((double)MathHelper::Abs(num1 - 1.0f) < 1.19209289550781E-07)
{
Plane plane;
plane.Normal = value.Normal;
plane.D = value.D;
return plane;
}
else
{
float num2 = 1.0f / (float)sqrt((double)num1);
Plane plane;
plane.Normal.X = value.Normal.X * num2;
plane.Normal.Y = value.Normal.Y * num2;
plane.Normal.Z = value.Normal.Z * num2;
plane.D = value.D * num2;
return plane;
}
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 매트릭스에 의한 평면 변환
///@param plane : 평면
///@param matrix : 매트릭스
///@return 결과 평면
//////////////////////////////////////////////////////////////////////////
Plane Plane::Transform( Plane plane, Matrix matrix )
{
Matrix result;
result = Matrix::Invert(matrix);
Plane plane1;
plane1.Normal.X = (float)((double)plane.Normal.X * (double)result.M11 + (double)plane.Normal.Y * (double)result.M12 + (double)plane.Normal.Z * (double)result.M13 + (double)plane.D * (double)result.M14);
plane1.Normal.Y = (float)((double)plane.Normal.X * (double)result.M21 + (double)plane.Normal.Y * (double)result.M22 + (double)plane.Normal.Z * (double)result.M23 + (double)plane.D * (double)result.M24);
plane1.Normal.Z = (float)((double)plane.Normal.X * (double)result.M31 + (double)plane.Normal.Y * (double)result.M32 + (double)plane.Normal.Z * (double)result.M33 + (double)plane.D * (double)result.M34);
plane1.D = (float)((double)plane.Normal.X * (double)result.M41 + (double)plane.Normal.Y * (double)result.M42 + (double)plane.Normal.Z * (double)result.M43 + (double)plane.D * (double)result.M44);
return plane1;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 쿼터니온에 의한 평면 변환
///@param plane : 평면
///@param rotation : 쿼터니온
///@return 결과 평면
//////////////////////////////////////////////////////////////////////////
Plane Plane::Transform( Plane plane, Quaternion rotation )
{
float wxx = rotation.W * (rotation.X + rotation.X);
float wyy = rotation.W * (rotation.Y + rotation.Y);
float wzz = rotation.W * (rotation.Z + rotation.Z);
float xxx = rotation.X * (rotation.X + rotation.X);
float xyy = rotation.X * (rotation.Y + rotation.Y);
float xzz = rotation.X * (rotation.Z + rotation.Z);
float yyy = rotation.Y * (rotation.Y + rotation.Y);
float yzz = rotation.Y * (rotation.Z + rotation.Z);
float zzz = rotation.Z * (rotation.Z + rotation.Z);
float num1 = 1.0f - yyy - zzz;
float num2 = xyy - wzz;
float num3 = xzz + wyy;
float num4 = xyy + wzz;
float num5 = 1.0f - xxx - zzz;
float num6 = yzz - wxx;
float num7 = xzz - wyy;
float num8 = yzz + wxx;
float num9 = 1.0f - xxx - yyy;
float num10 = plane.Normal.X;
float num11 = plane.Normal.Y;
float num12 = plane.Normal.Z;
Plane plane1;
plane1.Normal.X = (float)((double)num10 * (double)num1 + (double)num11 * (double)num2 + (double)num12 * (double)num3);
plane1.Normal.Y = (float)((double)num10 * (double)num4 + (double)num11 * (double)num5 + (double)num12 * (double)num6);
plane1.Normal.Z = (float)((double)num10 * (double)num7 + (double)num11 * (double)num8 + (double)num12 * (double)num9);
plane1.D = plane.D;
return plane1;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 평면과 4D 벡터의 내적
///@param value : 벡터
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Plane::Dot( Vector4 value )
{
return (float)((double)Normal.X * (double)value.X + (double)Normal.Y * (double)value.Y + (double)Normal.Z * (double)value.Z + (double)D * (double)value.W);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 평면과 3D 위치 벡터의 내적(W=1.0f)
///@param value : 벡터
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Plane::DotCoordinate( Vector3 value )
{
return (float)((double)Normal.X * (double)value.X + (double)Normal.Y * (double)value.Y + (double)Normal.Z * (double)value.Z)+ D;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 평면과 3D 방향 벡터의 내적(W=0.0f)
///@param value : 벡터
///@return 결과 값
//////////////////////////////////////////////////////////////////////////
float Plane::DotNormal( Vector3 value )
{
return (float)((double)Normal.X * (double)value.X + (double)Normal.Y * (double)value.Y + (double)Normal.Z * (double)value.Z);
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 박스와의 교차 검사
///@param box : 바운딩 박스
///@return 교차 결과
//////////////////////////////////////////////////////////////////////////
PlaneIntersectionType Plane::Intersects(BoundingBox box)
{
Vector3 vector3_1;
vector3_1.X = (double)Normal.X >= 0.0 ? box.Min.X : box.Max.X;
vector3_1.Y = (double)Normal.Y >= 0.0 ? box.Min.Y : box.Max.Y;
vector3_1.Z = (double)Normal.Z >= 0.0 ? box.Min.Z : box.Max.Z;
Vector3 vector3_2;
vector3_2.X = (double)Normal.X >= 0.0 ? box.Max.X : box.Min.X;
vector3_2.Y = (double)Normal.Y >= 0.0 ? box.Max.Y : box.Min.Y;
vector3_2.Z = (double)Normal.Z >= 0.0 ? box.Max.Z : box.Min.Z;
if((double)Normal.X * (double)vector3_1.X + (double)Normal.Y * (double)vector3_1.Y + (double)Normal.Z * (double)vector3_1.Z + (double)D > 0.0)
return PlaneIntersectionType_Front;
else if ((double)Normal.X * (double)vector3_2.X + (double)Normal.Y * (double)vector3_2.Y + (double)Normal.Z * (double)vector3_2.Z + (double)D < 0.0)
return PlaneIntersectionType_Back;
else
return PlaneIntersectionType_Intersecting;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 프러스텀과의 교차검사
///@param frustum : 바운딩 프러스텀
///@return 교차 결과
//////////////////////////////////////////////////////////////////////////
PlaneIntersectionType Plane::Intersects( BoundingFrustum frustum )
{
PlaneIntersectionType result;
frustum.Intersects(*this, result);
return result;
}
//////////////////////////////////////////////////////////////////////////
//////////////////////////////////////////////////////////////////////////
///@brief 바운딩 스페어와의 교차검사
///@param sphere : 바운딩 스페어
///@return 교차 결과
//////////////////////////////////////////////////////////////////////////
PlaneIntersectionType Plane::Intersects( BoundingSphere sphere )
{
float num = (float)((double)sphere.Center.X * (double)Normal.X + (double)sphere.Center.Y * (double)Normal.Y + (double)sphere.Center.Z * (double)Normal.Z) + D;
if ((double)num > (double)sphere.Radius)
return PlaneIntersectionType_Front;
else if ((double)num < -(double)sphere.Radius)
return PlaneIntersectionType_Back;
else
return PlaneIntersectionType_Intersecting;
}<file_sep>#include "stdafx.h"
#include "ExportMesh.h"
#include "./Fbx/Exporter.h"
ExportMesh::ExportMesh(ExecuteValues* values)
: Execute(values)
{
Fbx::Exporter* exporter = NULL;
//Grund
exporter = new Fbx::Exporter(Assets + L"Grund/Grund.fbx", true);
exporter->ExportMaterial(Models + L"Grund/", L"Grund");
exporter->ExportMeshData(Models + L"Grund/", L"Grund");
SAFE_DELETE(exporter);
//France
/*exporter = new Fbx::Exporter(Assets + L"Map/Stage1/France.fbx");
exporter->ExportMaterial(Models + L"Stage1/", L"France");
exporter->ExportMeshData(Models + L"Stage1/", L"France");
SAFE_DELETE(exporter);*/
//France_Sky
/*exporter = new Fbx::Exporter(Assets + L"Map/Stage1/France_Sky.fbx");
exporter->ExportMaterial(Models + L"Stage1/", L"France_Sky");
exporter->ExportMeshData(Models + L"Stage1/", L"France_Sky");
SAFE_DELETE(exporter);*/
}
ExportMesh::~ExportMesh()
{
}
void ExportMesh::Update()
{
}
void ExportMesh::Render()
{
}
void ExportMesh::PostRender()
{
}
<file_sep>#include "stdafx.h"
#include "DrawModel.h"
#include "../Objects/GameSkyBox.h"
#include "../Objects/GameWorld.h"
#include "../Objects/GameAnimationModel.h"
#include "../Units/GamePlayer.h"
DrawModel::DrawModel(ExecuteValues* values)
: Execute(values)
, skyBox(NULL), world(NULL), grund(NULL)
, player(NULL)
{
CreateEnvironment();
//CreateAnimationModel();
CreatePlayer();
}
DrawModel::~DrawModel()
{
SAFE_DELETE(player);
SAFE_DELETE(grund);
SAFE_DELETE(world);
SAFE_DELETE(skyBox);
}
void DrawModel::Update()
{
if (skyBox != NULL) skyBox->Update();
if (world != NULL) world->Update();
if (grund != NULL) grund->Update();
if (player != NULL) player->Update();
}
void DrawModel::Render()
{
if (skyBox != NULL) skyBox->Render();
if (world != NULL) world->Render();
if (grund != NULL) grund->Render();
if (player != NULL) player->Render();
}
void DrawModel::PostRender()
{
if (values->GuiSettings->bShowModelWindow == true)
{
ImGui::Begin("Model Info", &values->GuiSettings->bShowModelWindow);
int boneCount;
boneCount = player->GetModel()->BoneCount();
vector<string> boneNames;
for (int i = 0; i < boneCount; i++)
{
boneNames.push_back(String::ToString(player->GetModel()->Bone(i)->Name()));
}
static const char* current_Clip = NULL;
if (ImGui::BeginCombo("Anim Clip", current_Clip)) // The second parameter is the label previewed before opening the combo.
{
for (int n = 0; n < boneNames.size(); n++)
{
bool is_selected = (current_Clip == boneNames[n].c_str()); // You can store your selection however you want, outside or inside your objects
if (ImGui::Selectable(boneNames[n].c_str(), is_selected))
current_Clip = boneNames[n].c_str();
if (is_selected)
ImGui::SetItemDefaultFocus(); // Set the initial focus when opening the combo (scrolling + for keyboard navigation support in the upcoming navigation branch)
}
ImGui::EndCombo();
}
if (current_Clip != NULL)
{
}
ImGui::End();
}
}
void DrawModel::CreateEnvironment()
{
wstring matFile = L"";
wstring meshFile = L"";
//World
matFile = Models + L"Stage1/France.material";
meshFile = Models + L"Stage1/France.mesh";
world = new GameWorld(matFile, meshFile);
//SkyBox
matFile = Models + L"Stage1/France_Sky.material";
meshFile = Models + L"Stage1/France_Sky.mesh";
skyBox = new GameSkyBox(matFile, meshFile);
skyBox->Position(D3DXVECTOR3(0, 20, 0));
}
void DrawModel::CreateAnimationModel()
{
wstring matFile = L"";
wstring meshFile = L"";
//Grund
matFile = Models + L"Grund/Grund.material";
meshFile = Models + L"Grund/Grund.mesh";
grund = new GameAnimationModel(matFile, meshFile);
grund->AddClip(Models + L"Grund/Low_Idle.anim");
grund->AddClip(Models + L"Grund/Up_Idle.anim");
grund->AddClip(Models + L"Grund/Low_Run.anim");
grund->AddClip(Models + L"Grund/Up_Run.anim");
grund->AddClip(Models + L"Grund/Up_AttackFrontBase.anim");
grund->Play(0, 0, 0, 1, AnimationPlayMode::Repeat);
grund->Play(1, 0, 0, 1, AnimationPlayMode::Repeat);
}
void DrawModel::CreatePlayer()
{
wstring matFile = L"";
wstring meshFile = L"";
//Grund
matFile = Models + L"Grund/Grund.material";
meshFile = Models + L"Grund/Grund.mesh";
player = new GamePlayer(Models + L"Grund/", matFile, meshFile);
}
<file_sep>#pragma once
#include "Gjk.h"
#include "../Core/Vector3.h"
#include "../Core/Matrix.h"
#include "../Core/Plane.h"
enum PlaneIntersectionType;
enum ContainmentType;
class Ray;
class BoundingBox;
class BoundingSphere;
//////////////////////////////////////////////////////////////////////////
///@brief 충돌 프러스텀
//////////////////////////////////////////////////////////////////////////
class BoundingFrustum
{
public:
BoundingFrustum(void);
BoundingFrustum(Matrix value);
bool operator ==(const BoundingFrustum& value) const;
bool operator !=(const BoundingFrustum& value) const;
bool Equals(const BoundingFrustum& value) const;
std::string ToString();
Vector3 SupportMapping(Vector3 v);
Plane Near() const;
Plane Far() const;
Plane Left() const;
Plane Right() const;
Plane Top() const;
Plane Bottom() const;
Matrix GetMatrix() const;
void SetMatrix(const Matrix value);
void GetCorner(Vector3* pCorner);
static Ray ComputeIntersectionLine(Plane p1, Plane p2);
static Vector3 ComputeIntersection(Plane plane, Ray ray);
bool Intersects(BoundingBox box);
void Intersects(BoundingBox box, bool& result);
bool Intersects(BoundingFrustum frustum);
void Intersects(Plane plane, PlaneIntersectionType& result);
void Intersects(Ray ray, float* result);
bool Intersects(BoundingSphere sphere);
void Intersects(BoundingSphere sphere, bool& result);
ContainmentType Contains(BoundingBox box);
ContainmentType Contains(BoundingFrustum frustum);
ContainmentType Contains(Vector3 point);
ContainmentType Contains(BoundingSphere sphere);
public:
const static int CornerCount;
const static int NearPlaneIndex;
const static int FarPlaneIndex;
const static int LeftPlaneIndex;
const static int RightPlaneIndex;
const static int TopPlaneIndex;
const static int BottomPlaneIndex;
const static int NumPlanes;
Plane planes[6];
Vector3 cornerArray[8];
Matrix matrix;
Gjk gjk;
};<file_sep>#pragma once
class DrawModel : public Execute
{
public:
DrawModel(ExecuteValues* values);
~DrawModel();
void Update();
void PreRender() {}
void Render();
void PostRender();
void ResizeScreen() {}
private:
void CreateEnvironment();
void CreateAnimationModel();
void CreatePlayer();
private:
class GameSkyBox* skyBox;
class GameWorld* world;
class GameAnimationModel* grund;
class GamePlayer* player;
}; | cf29549a29824a4c6cbdebc34f684f88f3c66ef2 | [
"C",
"C++",
"INI"
] | 49 | C++ | choi92727/animationTool | 6236a20156a90dc0d195e9e16bb97cc8553ad24e | 8fdb91d6b82d0bc140a997b2d571706417d28908 | |
refs/heads/master | <repo_name>WiltonMontel/criptografia-cifra-de-cesar<file_sep>/cifra_cesar.py
# --------------- Códificação do trabalho Cifra de Cesar -------------------
# Variável utilizada para receber a entrada do usuário
mensagem = input('Digite a mensagem que deseja que seja criptografada ou descriptografada: ').upper()
# Define a quantidade de casas que cada letra vai transladar
chave = int(input('Digite o valor da chave: '))
# Modo de escolha do usuário para saber se deseja encriptar ou decriptar o texto
metodo = input('Escolha o modo ENCRIPTAR ou DECRIPTAR: ').upper()
# Alfabeto aceitavel no programa
alfabeto = 'ABCDEFGHIJKLMNOPQRSTUVWXYZ'
# Variavel criada para armazenar a mensagem criptografada ou descriptografada
modificado = ''
# Laço que sera executado para cada caractere
for caractere in mensagem:
if caractere in alfabeto:
# Retorna a posição do caractere dentro da constante e armazena na variável num
num = alfabeto.find(caractere)
# Obtem o numero do caractere
if metodo == 'ENCRIPTAR':
num = num + chave
elif metodo == 'DECRIPTAR':
num = num - chave
# Faz a verificação se o valor do caractere não ultrapassou a quantidade de letras do alfabeto ou se não é menor q 0
if num >= len(alfabeto):
num = num - len(alfabeto)
elif num < 0:
num = num + len(alfabeto)
# Concatena o caractere correspondente a num na variavel MODIFICADa no Alfabeto
modificado = modificado + alfabeto[num]
else:
# Concatenando o caractere sem efetuar criptografia ou decifragem
modificado = modificado + caractere
# Mostrar o texto encriptado ou decifrado na tela:
if metodo == 'ENCRIPTAR':
print('O texto criptografado é ', modificado)
if metodo == 'DECRIPTAR':
print('O texto decriptado é ', modificado)
| 4eb6d8153f8f7cdd170ffcc06277d6fa2a0da492 | [
"Python"
] | 1 | Python | WiltonMontel/criptografia-cifra-de-cesar | 31653af0cbc06f41ba10c8ea9e25e05fffe8c780 | 1d2057379bb65087be4bac40753c0fcc552414e1 | |
refs/heads/main | <repo_name>yester31/useful_code<file_sep>/18. visualize coco format dataset/visual_sample.py
import json
import cv2
import numpy as np
from PIL import ImageFont, ImageDraw, Image
# red , blue, green
color_map = [(0, 0, 255), (255, 0, 0), (0, 255, 0), (0, 255, 255), (255, 0, 255), (255, 255, 255),
(0, 0, 127), (127, 0, 0), (0, 127, 0), (0, 127, 127), (127, 0, 127), (127, 127, 127),
(0, 127, 255), (255, 127, 0)]
if __name__ == "__main__":
dataset_label_path = 'D:/dataset/coco2017/annotations/instances_val2017.json'
with open(dataset_label_path, 'rt', encoding='UTF-8') as annotations:
coco = json.load(annotations)
images = coco['images']
annotations = coco['annotations']
categories = coco['categories']
categ_dict = {}
for c_idx, categ in enumerate(categories):
categ_dict[categ["id"]] = categ["name"]
for i_idx, img in enumerate(images):
img_path = 'D:/dataset/coco2017/val2017/' + img['file_name']
# numpy 를 이용 하여 이미지 데이터 로드 (경로에 만약 한글이 포함 되어 있다면, cv2.imread()에서 파일을 못 읽음)
img_array = np.fromfile(img_path, np.uint8)
image = cv2.imdecode(img_array, cv2.IMREAD_COLOR)
for a_idx, anno in enumerate(annotations):
if img['id'] == anno['image_id']:
bbox = anno['bbox']
seg = anno['segmentation']
color = color_map[anno['category_id'] % len(color_map)]
# PIL을 통하여 이미지에 한글 출력 (cv2.putText()에서 한글 출력 못 함, 빌드 새로 해야함..)
pimg = Image.fromarray(image) # img 배열을 PIL이 처리 가능 하게 변환
name = categ_dict[anno['category_id']] # 해당 category_id의 이름 가져오기
ImageDraw.Draw(pimg).text((bbox[0] + 5, bbox[1] + 10), name,
font=ImageFont.truetype("fonts/gulim.ttc", 20),
fill=color) # 해당 라벨의 이름을 출력
# bounding box 출력
image = cv2.rectangle(np.array(pimg), (int(bbox[0]), int(bbox[1])),
(int(bbox[0] + bbox[2]), int(bbox[1] + bbox[3])),
color, 1)
# polygon 출력
if str(type(seg)) == '<class \'list\'>':
for pp_idx in range(len(seg)):
for p_idx in range(int(len(seg[pp_idx]) / 2)):
image = cv2.circle(image,
(int(seg[pp_idx][p_idx * 2]), int(seg[pp_idx][p_idx * 2 + 1])), 2,
color, -1)
cv2.imshow('results', image)
if cv2.waitKey() == 27:
cv2.destroyWindow("results")
break
print("done")
<file_sep>/04. simple websocket server node-express/app.js
"use strict";
const express = require('express'); // express라이브러리 호출
const app = express(); // express 객체 생성
const webMonitoringPort = 8080; // websocket test page web server port
const webSocketPort = 8000; // websocket server port
const fs = require('fs'); // 파일 처리 관련 라이브러리
const ejs = require('ejs'); // html파일에 JavaScript를 내장하여 서버에서 보낸 변수를 사용할 수 있음.
const path = require('path'); // 경로 관련 라이브러리
// 정적(static) 경로 설정
app.use(express.static(path.join(__dirname,'public'))); // public 폴더 내에 있는 파일들은 브라우저에서 접근 가능
app.use(express.static('public'));
// 예제로 만든 api client에서 /test로 호출하면 test.html 파일과 tt 변수를 리턴함.
app.get('/', (req, res) => {
fs.readFile('view/client.html', 'utf-8', function (error, data) {
if(error) {
console.log(error)
return;
} else {
let tt = 'text from sever'
res.send(
ejs.render(
data, {text : tt}
)
);
}
})
})
// 에러 처리 부분
app.use(function(req, res, next) {
res.status(404).send('Sorry cant find that!');
});
app.use(function (err, req, res, next) {
if(err){
console.error(err.stack);
console.log("error!!!!");
res.status(500).send('Something broke!');
}
});
app.listen(webMonitoringPort, () => {
console.log(`web server listening at : ${webMonitoringPort}`);
});
// 웹소켓 서버
const webSocket = require('./webSocketServer');
const server = app.listen(webSocketPort, () => {
console.log(`websocket server listening at : ${webSocketPort}`);
});
webSocket(server);<file_sep>/13. show deep learning result on Web Browser/server/public/webSocketClient.js
'use strict';
const wsid = '2'
const wstype = 'webc'
const url = 'ws://localhost:8000'
const wsk = new WebSocket(`${url}/wsid=${wsid}&wstype=${wstype}`); // ws
wsk.onopen = function () {
console.log('서버와 웹 소켓 연결됨');
wsk.send(JSON.stringify({
optype: "init_websocket", // optype : 동작 유형(init_websocket 클라이언트에서 연결 완료 후 서버로 확인 메시지 전달)
wsid: wsid, // wsid : 해당 소켓 아이디
wstype: wstype, // wstype : 소켓 접속 유형
msg : "Hello Server ? I am web client!!", // msg : 메시지
timestamp: timestamp() // timestamp : 현재 시간
}));
};
wsk.onmessage = function (event) {
let msgjson = JSON.parse(event.data);
switch (msgjson.optype) {
case 'output': // python으로부터 이미지 수신
let imaget = "data:image/jpg;base64," + msgjson.frame; // img tag의 소스로 전달하기 위해 frame 정보에 헤더를 붙임
document.getElementById("myimage").src = imaget; // (4) 화면에 출력
break;
default:
console.log('optype error : ' + msgjson.optype)
break;
}
}
wsk.onclose = function () {
console.log("disconnected websocket")
}
<file_sep>/12. image from browser to local with ws/websocket client py/client.py
import asyncio
import websockets
import cv2
import base64
import numpy as np
import json
from datetime import datetime
def timestamp() :
return datetime.now().strftime('%Y-%m-%d %H:%M:%S')
async def connect(ip, port, wsid, wstype):
# 웹 소켓에 접속
async with websockets.connect("ws://{}:{}/wsid={}&wstype={}".format(ip, port,wsid, wstype)) as websocket:
try:
if websocket.open == True:
init_websocket_msg = {
'optype': 'init_websocket',
'wsid': wsid,
'wstype': wstype,
'msg': 'Hello Server ? I am py',
'timestamp': timestamp()
}
jsonString = json.dumps(init_websocket_msg) # json 객체를 인코딩하여 string으로 만듬
await websocket.send(jsonString)
# 전달 받은 base64로 인코딩된 frame을 이미지로 변환하여 opencv로 영상 형태로 출력
while 1 :
data = await websocket.recv()
jsonObject = json.loads(data) # json 디코딩 (string 형태의 데이터를 json 객체로 만듬)
optype = jsonObject.get("optype") # dic 데이터 형식으로 호출
if optype == "input":
raw_frame = jsonObject.get("frame")
step1 = str(raw_frame.split(',')[1])
step2 = base64.b64decode(step1)
step5 = np.frombuffer(step2, np.uint8)
frame = cv2.imdecode(step5, cv2.IMREAD_COLOR)
cv2.imshow('(3) python_code', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
encode_param = [int(cv2.IMWRITE_JPEG_QUALITY), 100]
result, encimg = cv2.imencode('.jpg', frame, encode_param)
if False == result:
print('could not encode image!')
# 이미지를 String 형태로 변환(인코딩)시키는 과정
encoded_output = base64.b64encode(encimg).decode('utf-8')
output_msg = {
'optype': 'output',
'wsid': wsid,
'wstype': wstype,
'frame': encoded_output,
'timestamp': timestamp()
}
jsonString = json.dumps(output_msg) # json 객체를 인코딩하여 string으로 만듬
await websocket.send(jsonString)
else:
print("[ERROR] 메시지 : {}".format(data))
# python client 코드에서 웹소켓을 통해 영상을 전달하는 로직
'''
capture = cv2.VideoCapture(0) # 웹캠
while 0 :
ret, frame = capture.read()
frame = cv2.resize(frame, dsize=(350, 260), interpolation=cv2.INTER_AREA)
encode_param = [int(cv2.IMWRITE_JPEG_QUALITY), 100]
result, encimg = cv2.imencode('.jpg', frame, encode_param)
if False == result:
print('could not encode image!')
# 이미지를 String 형태로 변환(인코딩)시키는 과정
encoded = base64.b64encode(encimg).decode('utf-8')
input_msg = {
'optype': 'output',
'wsid': wsid,
'wstype': wstype,
'frame': encoded,
'timestamp': timestamp()
}
jsonString = json.dumps(input_msg) # json 객체를 인코딩하여 string으로 만듬
await websocket.send(jsonString)
'''
except:
print("error!!!")
return 0
if __name__ == "__main__":
ip = 'localhost'
port = 8000
wsid = 1
wstype = 'pyc'
asyncio.get_event_loop().run_until_complete(connect(ip, port, wsid, wstype))
asyncio.get_event_loop().run_forever()
<file_sep>/01. detected objects crop and save/tt.py
# --------------------------------------------------------
# Detected objects Crop and Save by class
# Licensed under The MIT License
# Written by Derek
# 2021-06-26
# --------------------------------------------------------'
import os, cv2, uuid, time
import numpy as np
# deep learning 모델에 결과로 detection된 객체들의 이미지를 파일로 클래스 별로 저장하는 예제 코드
# 각 frame에서 detection된 객체들의 객체 별 box, class id 이용하여 분류 저장
'''
생성되는 디렉토리 구조
- root dir - sub_root_dir - class_name0 - frame0_0_uuid
- frame0_1_uuid
- class_name1 - frame0_0_uuid
- class_name2 - frame0_0_uuid
- sub_root_dir - class_name1 - frame0_0_uuid
'''
class_name_table = ['person', 'bicycle', 'car', 'motorcycle', 'airplane', 'bus', 'train', 'truck', 'boat', 'traffic light', 'fire hydrant', 'stop sign', 'parking meter', 'bench', 'bird', 'cat', 'dog', 'horse', 'sheep', 'cow', 'elephant', 'bear', 'zebra', 'giraffe', 'backpack', 'umbrella', 'handbag', 'tie', 'suitcase', 'frisbee', 'skis', 'snowboard', 'sports ball', 'kite', 'baseball bat', 'baseball glove', 'skateboard', 'surfboard', 'tennis racket', 'bottle', 'wine glass', 'cup', 'fork', 'knife', 'spoon', 'bowl', 'banana', 'apple', 'sandwich', 'orange', 'broccoli', 'carrot', 'hot dog', 'pizza', 'donut', 'cake', 'chair', 'couch', 'potted plant', 'bed', 'dining table', 'toilet', 'tv', 'laptop', 'mouse', 'remote', 'keyboard', 'cell phone', 'microwave', 'oven', 'toaster', 'sink', 'refrigerator', 'book', 'clock', 'vase', 'scissors', 'teddy bear', 'hair drier', 'toothbrush']
def save_box_img(object_class_lsit, object_box_list, frame_id, frame, video_name, dir_root_path = './dataset') :
boc = time.time()
unique_number = str(uuid.uuid1()) # 유니크 숫자 생성
if os.path.exists(dir_root_path) is False: # 이미지를 저장할 최상위 폴더가 없다면
os.makedirs(dir_root_path) # 최상위 폴더 생성
print('make directory {} is done'.format(dir_root_path))
dir_sub_root_path = os.path.join(dir_root_path, video_name) # 해당 영상(video_name)에 대한 이미지를 저장할 폴더 경로
if os.path.exists(dir_sub_root_path) is False: # 해당 영상에 대한 폴더가 없다면
os.makedirs(dir_sub_root_path) # 해당 영상에 대한 폴더 생성
print('make directory {} is done'.format(dir_sub_root_path))
for oidx, class_id in enumerate(object_class_lsit): # 모델에서 찾은 객체 리스트
class_name = class_name_table[int(class_id)] # 해당 객체 클래스 이름
dir_class_path = os.path.join(dir_sub_root_path , class_name) # 클래스 폴더 경로
image_file_name = '{}_{}_{}.jpg'.format(frame_id, oidx, unique_number) # 이미지 파일 이름{프레임 번호}_{해당 프레임 객체 인식번호}_{유니크값}.jpg
image_file_path = os.path.join(dir_class_path, image_file_name) # 클래스 폴더 경로
x1, y1 = int(object_box_list[oidx, 0]), int(object_box_list[oidx, 1])
x2, y2 = int(object_box_list[oidx, 2]), int(object_box_list[oidx, 3])
croped_object_image = frame[y1:y2,x1:x2] # y1 y2 x1 x2
if os.path.exists(dir_class_path): # 해당 클래스 폴더가 있으면
cv2.imwrite(image_file_path, croped_object_image) # 그냥 저장
else : # 해당 클래스 폴더가 없으면
os.makedirs(dir_class_path) # 클래스 폴더 생성 후
print('make directory {} is done'.format(dir_class_path))
cv2.imwrite(image_file_path, croped_object_image) # 저장
print('({}/{}) save file {} is done'.format(oidx,len(object_class_lsit), image_file_path))
print('====================================================================================')
print('Detected object image crop and save process is done')
print('Duration time is {} [sec]'.format(time.time() - boc))
print('====================================================================================')
if __name__ == "__main__":
#yolov5s 결과 예시(원본 frame 이미지 사이즈로 리사이즈 및 패딩 처리 후)
# x1 y1 x2 y2 prob class_id
pred = np.array([[2.00000e+00, 3.47000e+02, 4.56000e+02, 6.82000e+02, 9.38516e-01, 2.00000e+00],
[5.88000e+02, 4.04000e+02, 8.81000e+02, 6.32000e+02, 9.18750e-01, 2.00000e+00],
[5.75000e+02, 3.99000e+02, 6.24000e+02, 4.41000e+02, 8.62566e-01, 2.00000e+00],
[6.11000e+02, 3.99000e+02, 6.91000e+02, 4.53000e+02, 7.66945e-01, 2.00000e+00],
[9.80000e+02, 3.90000e+02, 1.04700e+03, 4.23000e+02, 7.01548e-01, 2.00000e+00],
[4.37000e+02, 3.71000e+02, 5.59000e+02, 4.83000e+02, 6.38990e-01, 7.00000e+00],
[6.34000e+02, 3.28000e+02, 6.41000e+02, 3.45000e+02, 5.99452e-01, 9.00000e+00],
[5.45000e+02, 3.24000e+02, 5.59000e+02, 3.40000e+02, 5.74450e-01, 9.00000e+00],
[8.09000e+02, 3.94000e+02, 8.25000e+02, 4.32000e+02, 5.48406e-01, 0.00000e+00],
[5.52000e+02, 3.89000e+02, 5.79000e+02, 4.34000e+02, 5.12884e-01, 2.00000e+00],
[6.65000e+02, 3.40000e+02, 7.46000e+02, 4.10000e+02, 4.92299e-01, 7.00000e+00],
[7.93000e+02, 3.96000e+02, 8.06000e+02, 4.14000e+02, 4.88801e-01, 0.00000e+00],
[7.71000e+02, 3.90000e+02, 7.84000e+02, 4.09000e+02, 4.52478e-01, 0.00000e+00],
[9.04000e+02, 3.95000e+02, 9.23000e+02, 4.18000e+02, 4.35165e-01, 0.00000e+00],
[5.56000e+02, 3.25000e+02, 5.64000e+02, 3.40000e+02, 3.84715e-01, 9.00000e+00],
[8.24000e+02, 4.00000e+02, 8.37000e+02, 4.38000e+02, 3.59820e-01, 0.00000e+00],
[7.01000e+02, 4.29000e+02, 7.47000e+02, 4.64000e+02, 3.52281e-01, 0.00000e+00],
[6.65000e+02, 3.39000e+02, 7.46000e+02, 4.09000e+02, 3.46599e-01, 5.00000e+00],
[1.01900e+03, 3.89000e+02, 1.03900e+03, 4.11000e+02, 3.08561e-01, 0.00000e+00],
[7.57000e+02, 3.86000e+02, 7.70000e+02, 4.08000e+02, 2.78430e-01, 0.00000e+00],
[6.33000e+02, 3.73000e+02, 6.67000e+02, 4.02000e+02, 2.56180e-01, 7.00000e+00]])
frame = cv2.imread('NY_720x1280.jpg') # 해당 frame image (예시) NY_720x1280.jpg
object_class_lsit = pred[:,5] # class id list
object_box_list = pred[:,0:4] # box list (x1 y1 x2 y2)
video_name = 'video_name' # 영상 이름 (영상별로 구별하여 저장하고 싶은 경우 사용, 폴더명과 파일명에 포함됨)
frame_id = 0 # 해당 프레임 id (몇 번째 frame인지)
dir_root_path = './dataset' # 이미지를 저장할 최상위 폴더 경로 선택
save_box_img(object_class_lsit, object_box_list, frame_id, frame, video_name, dir_root_path)
<file_sep>/03. simple web server node-express/app.js
// --------------------------------------------------------
// Node Express Simple Web Server
// Licensed under The MIT License
// Written by Derek
// 2021-06-27
// --------------------------------------------------------
"use strict";
const express = require('express'); //express라이브러리 호출
const app = express(); // express 객체 생성
const webPort = 8000;
const fs = require('fs'); // 파일 처리 관련 라이브러리
const ejs = require('ejs'); // html파일에 JavaScript를 내장하여 서버에서 보낸 변수를 사용할 수 있음.
const path = require('path'); // 경로 관련 라이브러리
// 정적(static) 경로 설정
app.use(express.static(path.join(__dirname,'public'))); // public 폴더 내에 있는 파일들은 브라우저에서 접근 가능
// 예제로 만든 api client에서 /test로 호출하면 test.html 파일과 tt 변수를 리턴함.
app.get('/test', (req, res) => {
fs.readFile('public/test.html', 'utf-8', function (error, data) {
if(error) {
console.log(error)
return;
} else {
let tt = 'text from sever'
res.send(ejs.render(data, {
text : tt
}));
}
})
})
// 에러 처리 부분
app.use(function(req, res, next) {
res.status(404).send('Sorry cant find that!');
});
app.use(function (err, req, res, next) {
if(err){
console.error(err.stack);
console.log("error!!!!");
res.status(500).send('Something broke!');
}
});
app.listen(webPort, function(){
console.log(`listening on ${webPort}`)
}); // 객체 맴버 함수 listen(서버띄울 포트번호, 띄운 후 실행할 코드) 서버 열기 <file_sep>/16. produce a data path list file/splite_train_val.py
import os
import glob
import random
'''
3. train & val 나누기 (통합 파일에서 랜덤으로 나누기 or 각 폴더 별 랜덤으로 나누기)
output:
output/train/train.txt
output/train/val.txt
'''
# 해당 리스트 파일에서 클래스별 수 반환
def getClassCount(list_file_path, label):
print(list_file_path)
label_count_dict = {}
for i, v in enumerate(label):
label_count_dict[v] = 0
f = open(list_file_path, 'r', encoding='utf-8')
lines = f.readlines()
count = 0
for line in lines:
count += 1
label_count_dict[label[int(line.split(',')[-1].strip())]] += 1
f.close()
print(label_count_dict)
print('Total count : {}'.format(count))
print()
return label_count_dict, count
def showClassCount(save_path, label):
for paths in [x for x in glob.iglob(save_path + '/**', recursive=True)]:
paths = paths.replace('\\', '/')
if paths.split('.')[-1] == 'txt':
getClassCount(paths, label)
# tot_train.txt에서 입력한 비율로 train, val 리스트를 랜덤하게 나누기
def spliteDataset1(save_path, train, val):
if not os.path.exists(save_path + '/train/'): # 저장할 폴더가 없다면
os.makedirs(save_path + '/train/') # 폴더 생성
print('make directory {} is done'.format(save_path + '/train/'))
ratio = val / (train+val)
train_file = open(save_path + '/train/train.txt', 'w', encoding='utf-8')
val_file = open(save_path + '/train/val.txt', 'w', encoding='utf-8')
f = open(save_path + '/tot_train/tot_train.txt', 'r', encoding='utf-8')
data_path_list = f.readlines()
f.close()
count = len(data_path_list)
val_n = int(count * ratio + 0.5)
train_n = int(count - val_n)
val_loc = random.sample(range(1, count), val_n)
val_path = []
for n in range(val_n):
val_path.append(data_path_list[val_loc[n]])
train_path = data_path_list
for a in range(val_n):
train_path.remove(val_path[a])
for i, v in enumerate(train_path):
train_file.writelines(v)
for i, v in enumerate(val_path):
val_file.writelines(v)
train_file.close()
val_file.close()
# 차수 별로 (거의)동일하게 val 값 추출
def spliteDataset2(save_path, train, val):
if not os.path.exists(save_path + '/train/'): # 저장할 폴더가 없다면
os.makedirs(save_path + '/train/') # 폴더 생성
print('make directory {} is done'.format(save_path + '/train/'))
train_file = open(save_path + '/train/train.txt', 'w', encoding='utf-8')
val_file = open(save_path + '/train/val.txt', 'w', encoding='utf-8')
ratio = val / (train + val)
for txt in [x for x in glob.iglob(save_path + '/list_file/*.txt')]:
txt = txt.replace('\\', '/')
flag = txt.split('/')[-1].split('.')[0].split('_')[-1]
if flag == 'train':
f = open(txt, 'r', encoding='utf-8')
data_path_list = f.readlines()
f.close()
count = len(data_path_list)
val_n = int(count * ratio + 0.5)
train_n = int(count - val_n)
val_loc = random.sample(range(1, count), val_n)
val_path = []
for n in range(val_n):
val_path.append(data_path_list[val_loc[n]])
train_path = data_path_list
for a in range(val_n):
train_path.remove(val_path[a])
for i, v in enumerate(train_path):
train_file.writelines(v)
for i, v in enumerate(val_path):
val_file.writelines(v)
train_file.close()
val_file.close()
if __name__ == '__main__':
label = ['cat', 'dog']
save_path = 'output'
#spliteDataset1(save_path, 8, 2)
spliteDataset2(save_path, 8, 2)
showClassCount(save_path, label)
<file_sep>/05. simple webRTC using websocket/webSocketServer.js
"use strict";
const funcs = require('./public/libs'); // 커스텀 라이브러리 불러오기
const WebSocket = require('ws'); // 웹소켓 라이브러리
const Hashtable = require('jshashtable');// 해시테이블 라이브러리
const wshashtable = new Hashtable(); // 해시테이블 생성
global.wshashtable = wshashtable; // 해시테이블 전역 변수화 시키기
const { v4: uuidV4 } = require('uuid');
module.exports = (server) => {
// 웹소켓 서버 객체 생성
const wss = new WebSocket.Server({ server });
// 클라이언트 접속할 경우 그 클라이언트와 연결된 소켓객체(ws) 생성
wss.on('connection', (ws, req) => {
// 웹 소켓 연결 시 클라이언트 정보 수집
const ip = req.headers['x-forwarded-for'] || req.socket.remoteAddress;
const port = req.headers['x-forwarded-port'] || req.socket.remotePort;
console.log('새로운 클라이언트 접속', ip);
// 사전에 약속된 규칙으로 소켓서버 호출하여 변수 생성
let conuri = req.url; //wss://192.168.31.26:8000/wsid=1234&wstype=type1 <- 예시 소켓 호출 (로컬에서는 ws)
let wsid = conuri.replace(/.+wsid=([^&]+).*/,"$1");
let wstype= conuri.replace(/.+wstype=([^&]+).*/,"$1");
// 소켓 연결 정보 해시 테이블에 저장
wshashtable.put(wsid, ws);
wshashtable.keys().forEach(key =>{
console.log("접속중인 소켓 아이디 :: " + key);
wshashtable.get(key).send(JSON.stringify({'optype': 'check_ws_num' ,'ws_count': wshashtable.keys().length}))
console.log(key + " 로 업데이트된 웹소켓 접속수 전달")
})
// 클라이언트로부터 메시지 수신 시
ws.on('message', (fmessage) => {
let msgjson = JSON.parse(fmessage);
//console.log(msgjson)
switch (msgjson.optype) {
case 'send_ws_msg':
console.log("send_ws_msg 요청 :: ")
wshashtable.keys().forEach(key =>{
if (key != msgjson.wsid ){
wshashtable.get(key).send(fmessage)
}
})
break;
case 'initi_signal':
console.log("initi_signal 요청, ws_count ::" + wshashtable.keys().length);
if(wshashtable.keys().length >= 2){
ws.send(JSON.stringify({'optype': 'initi_signal' ,'ws_count': wshashtable.keys().length}))
}
break;
case 'check_ws_num':
console.log("check_ws_num 요청, ws_count ::" + wshashtable.keys().length);
ws.send(JSON.stringify({'optype': 'check_ws_num' ,'ws_count': wshashtable.keys().length}))
break;
case 'offer':
console.log("offer 요청 :: ")
//console.log(msgjson.offer);
wshashtable.keys().forEach(key =>{
if (key != msgjson.wsid ){
wshashtable.get(key).send(fmessage)
console.log(key + " 로 offer 전달")
}
})
break;
case 'answer':
console.log("answer 요청 :: ")
wshashtable.keys().forEach(key =>{
if (key != msgjson.wsid ){
wshashtable.get(key).send(fmessage)
console.log(key + " 로 answer 전달")
}
})
break;
case 'new-ice-candidate':
console.log("new-ice-candidate 요청 :: ")
wshashtable.keys().forEach(key =>{
if (key != msgjson.wsid ){
wshashtable.get(key).send(fmessage)
console.log(key + " 로 new-ice-candidate 전달")
}
})
break;
default:
console.log('optype error : ' + msgjson.optype)
}
});
// 에러시
ws.on('error', (error) => {
console.error(error);
});
// 연결 종료 시
ws.on('close', () => {
// 해시테이블에서 소켓 정보 제거 및 종료된 소켓 정보 출력
wshashtable.remove(wsid);
console.log(`[종료된 소켓 발생] ${funcs.timestamp()}`); // 현재 시간 출력
console.log(`[종료된 소켓 정보] 아이디 : ${wsid} / 접속 종류 : ${wstype} / 아이피 : ${ip} / 포트 : ${port}`);
console.log(`[현재 접속 리스트] : ${wshashtable.keys()}`);
wshashtable.keys().forEach(key =>{
wshashtable.get(key).send(JSON.stringify({'optype': 'check_ws_num' ,'ws_count': wshashtable.keys().length}))
console.log(key + " 로 업데이트된 웹소켓 접속수 전달")
})
});
});
};<file_sep>/11. simple python websocket server&client/client/client.py
# --------------------------------------------------------
# Simple Websocket Client
# Licensed under The MIT License
# Written by Derek
# 2021-06-27
# --------------------------------------------------------
import asyncio, websockets
'''
간단한 파이썬 웹소켓 클라이언트 구현
'''
async def connect():
# 웹 소켓에 접속을 합니다.
async with websockets.connect("ws://localhost:8000") as websocket:
# 웹 소켓 서버로 부터 메시지가 오면 콘솔에 출력
while True :
client_msg = input('> [client] ')
await websocket.send(client_msg)
server_msg = await websocket.recv()
print('< [server] {}'.format(server_msg))
asyncio.get_event_loop().run_until_complete(connect())<file_sep>/20. ensemble/ensemble.py
import os, json, time
import pandas as pd
import numpy as np
from ensemble_boxes import *
from tqdm import tqdm
from pycocotools.coco import COCO
from pycocotools.cocoeval import COCOeval
id_matching = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 27, 28, 31, 32,
33, 34, 35, 36, 37, 38, 39, 40, 41, 42, 43, 44, 46, 47, 48, 49, 50, 51, 52, 53, 54, 55, 56, 57, 58, 59,
60, 61, 62, 63, 64, 65, 67, 70, 72, 73, 74, 75, 76, 77, 78, 79, 80, 81, 82, 84, 85, 86, 87, 88, 89, 90]
def load_csv(path):
if os.path.isfile(path): # path 파일이 있다면
with open(path, 'r', encoding='UTF-8') as csvf:
data = pd.read_csv(csvf)
return data
else:
print('path is wrong')
def convert_format_step2(labels_list, scores_list, boxes_list, data, index, h, w):
pstring = data['PredictionString'][index]
if type(pstring) is float and np.isnan(pstring): # 해당 이미지에 대한 결과가 없다면 리턴
return
n_pstring = np.array(pstring.split(' '))
n_pstring = np.delete(n_pstring, len(n_pstring) - 1)
for idx in range(len(n_pstring) // 6):
idx6 = idx * 6
label = float(id_matching[int(n_pstring[idx6])])
score = float(n_pstring[idx6 + 1])
x1 = float(n_pstring[idx6 + 2]) / w
y1 = float(n_pstring[idx6 + 3]) / h
x2 = float(n_pstring[idx6 + 4]) / w
y2 = float(n_pstring[idx6 + 5]) / h
labels_list.append(label)
scores_list.append(score)
boxes_list.append([x1, y1, x2, y2])
def convert_format_step1(labels_lists, scores_lists, boxes_lists, weights, data, row_idx, model_idx, h, w, weights_size):
labels_list = []
scores_list = []
boxes_list = []
convert_format_step2(labels_list, scores_list, boxes_list, data, row_idx, h, w)
if len(labels_list) != 0:
weights.append(weights_size[model_idx])
labels_lists.append(labels_list)
scores_lists.append(scores_list)
boxes_lists.append(boxes_list)
def do_ensemble(save_path, datas, weights_size):
with open(save_path, 'wt', encoding='UTF-8') as coco:
ann = []
start0 = time.time()
for row_idx in tqdm(range(len(datas[0]))):
image_id = data0['image_id'][row_idx]
w = data0['width'][row_idx]
h = data0['height'][row_idx]
labels_lists = []
scores_lists = []
boxes_lists = []
weights = []
for model_idx in range(len(datas)):
convert_format_step1(labels_lists, scores_lists, boxes_lists, weights, datas[model_idx], row_idx,
model_idx, h, w, weights_size)
if len(labels_lists) == 0:
continue
else:
# boxes, scores, labels = nms(boxes_lists, scores_lists, labels_lists, weights=weights, iou_thr=0.5) # 0.439
# boxes, scores, labels = soft_nms(boxes_lists, scores_lists, labels_lists, weights=weights, iou_thr=0.5, sigma=0.1, thresh=0.0001) # 0.440
# boxes, scores, labels = non_maximum_weighted(boxes_lists, scores_lists, labels_lists, weights=weights, iou_thr=0.5, skip_box_thr=0.0001) # 0.447
# boxes, scores, labels = weighted_boxes_fusion(boxes_lists, scores_lists, labels_lists, weights=weights, iou_thr=0.5, skip_box_thr=0.0001) # 0.454
# boxes, scores, labels = weighted_boxes_fusion(boxes_lists, scores_lists, labels_lists, weights=weights, iou_thr=0.6, skip_box_thr=0.001) # 0.459
boxes, scores, labels = weighted_boxes_fusion(boxes_lists, scores_lists, labels_lists, weights=weights, iou_thr=0.7, skip_box_thr=0.001) # 0.460
# boxes, scores, labels = weighted_boxes_fusion(boxes_lists, scores_lists, labels_lists, weights=weights, iou_thr=0.8, skip_box_thr=0.001) # 0.459
for c_idx in range(len(labels)):
ann += [{'image_id': int(image_id),
'category_id': int(labels[c_idx]),
'bbox': [boxes[c_idx][0] * w,
boxes[c_idx][1] * h,
(boxes[c_idx][2] - boxes[c_idx][0]) * w,
(boxes[c_idx][3] - boxes[c_idx][1]) * h],
'score': float(scores[c_idx]), }]
json.dump(ann, coco)
print('{} images, duration time : {} [sec]'.format(len(data0), time.time() - start0))
if __name__ == '__main__':
# 1. file load
path0 = 'outputs/submission20.csv' # 40.631
path1 = 'outputs/submission21.csv' # 42.036
path2 = 'outputs/submission22.csv' # 43.047
data0 = load_csv(path0)
data1 = load_csv(path1)
data2 = load_csv(path2)
datas = []
datas.append(data0)
datas.append(data1)
datas.append(data2)
# 2. format convert & ensemble
weights_size = [1, 2, 3]
save_path = 'outputs/ensemble.json'
do_ensemble(save_path, datas, weights_size)
# 3. evaluate COCO AP
coco_gt = COCO('outputs/instances_val2017.json')
image_ids = sorted(coco_gt.getImgIds())
coco_dt = coco_gt.loadRes(save_path)
coco_eval = COCOeval(coco_gt, coco_dt, 'bbox')
coco_eval.params.imgIds = image_ids
coco_eval.evaluate()
coco_eval.accumulate()
coco_eval.summarize()
<file_sep>/16. produce a data path list file/main.py
from generate_path_list_file import *
from integrate_file_list import *
from splite_train_val import *
if __name__ == '__main__':
label = ['cat', 'dog']
dir_path = ['dataset/1차', 'dataset/2차', 'dataset/3차']
save_path = 'output'
# 1. 지정 경로의 데이터셋 리스트 파일 만들기
for i, v in enumerate(dir_path):
generateFileList(save_path, v, label)
# 2. 여러 리스트 파일을 통합
integrateFilelist(save_path)
# 3. train & val 나눠기 (통합 파일에서 랜덤으로 나눠기 or 각 폴더 별 랜덤으로 나눠기)
#spliteDataset1(save_path, 8, 2)
spliteDataset2(save_path, 8, 2)
# 4. 눠어진 클래스 별 수 출력
showClassCount(save_path, label)<file_sep>/04. simple websocket server node-express/webSocketServer.js
"use strict";
const funcs = require('./public/libs'); // 커스텀 라이브러리 불러오기
const WebSocket = require('ws'); // 웹소켓 라이브러리
const Hashtable = require('jshashtable');// 해시테이블 라이브러리
const wshashtable = new Hashtable(); // 해시테이블 생성
// global.wshashtable = wshashtable; // 해시테이블 전역 변수화 시키기
module.exports = (server) => {
// 웹소켓 서버 객체 생성
const wss = new WebSocket.Server({ server });
// 클라이언트 접속할 경우 그 클라이언트와 연결된 소켓객체(ws) 생성
wss.on('connection', (ws, req) => {
// 웹 소켓 연결 시 클라이언트 정보 수집
const ip = req.headers['x-forwarded-for'] || req.socket.remoteAddress;
const port = req.headers['x-forwarded-port'] || req.socket.remotePort;
console.log('새로운 클라이언트 접속', ip);
// 사전에 약속된 규칙으로 소켓서버 호출하여 변수 생성
let conuri = req.url; //wss://192.168.31.26:8000/wsid=1234&wstype=type1 <- 예시 소켓 호출 (로컬에서는 ws)
let wsid = conuri.replace(/.+wsid=([^&]+).*/,"$1");
let wstype= conuri.replace(/.+wstype=([^&]+).*/,"$1");
// 소켓 연결 정보 해시 테이블에 저장
wshashtable.put(wstype + wsid, ws);
if(wstype == "c++ws"){
ws.send(JSON.stringify({
optype:"server_msg",
wsid: "server1",
wstype:"server",
msg : "Hi? I am Server!!!",
count : 5,
timestamp:funcs.timestamp()
}))
}
// 클라이언트로부터 메시지 수신 시
ws.on('message', (fmessage) => {
let msgjson = null;
try {
// 전달 받은 문자열 메시지(fmessage)를 자바스크립트 객체로 변환
msgjson = JSON.parse(fmessage);
} catch (error) {
// 전달 받은 문자열이 JSON 형식이 아니라면 에러가 catch 부분으로 들어오게 됨.
//console.log(`[JSON 타입이 아닌 메시지 수신] error : ${error}`);
}
// JSON 형식의 문자열로 수신된 메시지만 처리 되도록 함.
if(msgjson){
// API 정의서 프로토콜을 정상적으로 지켰다면 모든 소켓 메시지들은 optype이라는 구분자로 각각의 처리를 구분 한다.
switch (msgjson.optype) {
// 클라이언트로 부터 받은 연결 확인 메시지 처리 부분
case 'init_websocket':
try {
console.log(`[init_websocket] 메시지 : ${JSON.stringify(msgjson.msg)}`);
}catch(error){
console.log(`[init_websocket] error : ${error}`);
}finally{
break;
}
// 클라이언트로부터 받은 메시지를 처리하고 다시 응답 전달
case 'client_msg':
try {
console.log(`[client_msg] 메시지 : ${JSON.stringify(msgjson)}`);
let count = msgjson.count - 1;
if (count > 0){
// 클라이언트로 응답 보내기
ws.send(JSON.stringify({
optype:"server_msg",
wsid: "server1",
wstype:"server",
count : count,
timestamp:funcs.timestamp()
}))
}
}catch(error){
console.log(`[msg_test] error : ${error}`);
}finally{
break;
}
default:
// 약속된 optype이 아닌 경우 오류 메시지를 응답으로 전달
console.log(`[잘못된 optype 수신] 전달 받은 optype과 매칭되는 api가 없습니다. : ${msgjson.optype}`);
ws.send(`[잘못된 optype 수신] 전달 받은 optype과 매칭되는 api가 없습니다. : ${msgjson.optype}`);
break;
}
}else{
// 전달 받은 메시지가 JSON 타입이 아닐 경우 오류 메시지를 응답으로 전달
console.log(`[JSON 타입이 아닌 메시지 수신]: (msg : ${fmessage}, ip : ${ip})`);
ws.send(`[JSON 타입이 아닌 메시지 수신]: Successfully, a message has arrived. But this msg type is not JSON format. PLEASE SEND JSON TYPE FORMAT MESSAGE. (msg : ${fmessage}, ip : ${ip})`);
}
});
// 에러시
ws.on('error', (error) => {
console.error(error);
});
// 연결 종료 시
ws.on('close', () => {
// 해시테이블에서 소켓 정보 제거 및 종료된 소켓 정보 출력
wshashtable.remove(wstype + wsid);
console.log(`[종료된 소켓 발생] ${funcs.timestamp()}`); // 현재 시간 출력
console.log(`[종료된 소켓 정보] 아이디 : ${wsid} / 접속 종류 : ${wstype} / 아이피 : ${ip} / 포트 : ${port}`);
console.log(`[현재 접속 리스트] : ${wshashtable.keys()}`);
});
});
};<file_sep>/20. ensemble/README.md
# Ensemble
- Detectron2 (git commit id 5e38c1...)
- Faster RCNN R101-DC5
- Faster RCNN R101-FPN
- Faster RCNN X101-FPN
- COCO 2017 val Dataset
- Recommend to run in Colab
## Algorithms
- NMS : 내림차순 정렬 후, 가장 높은 스코어의 바운딩 박스를 기준으로 IOU 임곗값을 초과하는 바운딩 박스를 제거.
- soft NMS : NMS와 달리, 비교 과정에서 IOU 임곗값을 초과하는 박스를 그냥 제거하는 것이 아니라 스코어 값을 기존 보다 낮게 변경 처리함.
- NMW : 동일한 객체에 대해서 IOU 임곗값을 초과하는 바운딩 박스들의 그룹을 만들고
각각의 IOU 값과 스코어 값으로 가중치를 만들어 그 그룹을 대표하는 값을 생성(라벨과 스코어는 그룹 중 스코어가 가장 높은 값 사용).
- WBF : 동일한 객체에 대해서 IOU 임곗값을 초과하는 바운딩 박스들의 그룹을 만들고
모델에 대한 가중치 값이 적용된 스코어 값을 그 그룹에서 가중치로 사용하여 대표하는 값을 생성(라벨은 그룹 중 스코어가 가장 높은 값, 스코어는 평균값 사용).
## Performance Evaluation
- box AP (Average Precision at IoU=0.50:0.95, area=all)
<table border="0" width="100%">
<tbody align="center">
<tr>
<td>Faster RCNN backbone</td>
<td>R101-DC5</td>
<td>R101-FPN</td>
<td>X101-FPN</td>
</tr>
<tr>
<td>box AP</td>
<td>40.631</td>
<td>42.036</td>
<td>43.047</td>
</tr>
</tbody>
</table>
<table border="0" width="100%">
<tbody align="center">
<tr>
<td>Ensemble Method</td>
<td>NMS</td>
<td>soft NMS</td>
<td>NMW</td>
<td><strong>WBF</strong></td>
</tr>
<tr>
<td>box AP</td>
<td>43.9</td>
<td>44.0</td>
<td>44.7</td>
<td><strong>46.0</strong></td>
</tr>
</tbody>
</table>
## Description
- 다양한 앙상블 기법들을 실제로 계산을 통해 결과를 추출하였고 그 값들에 대해 비교 평가함.
- 모든 기법들의 결과값은 각 모델의 결과 보다 나은 성능을 보임.
- NMS와 soft-NMS의 경우 가장 높은 스코어를 갖는 바운딩 박스 하나만을 기준으로 겹치는 정도에 따라 나머지 바운딩 박스를 제거하는 방식으로 이루어지지만,
NMW와 WBF의 경우 여러 바운딩 박스들의 정보를 종합하여 결과를 내기 때문에 더 우수한 것으로 보임.
- 특히, WBF의 경우 앙상블에서 쓰인 딥러닝 모델들의 가중치 값도 사용하기 때문에 NMW 보다 더 많은 정보를 기반으로 처리한다고 할 수 있음.
그렇기 때문에 더 나은 성능을 나타낸 것으로 보임.
## Reference
- Detectron2 : <https://github.com/facebookresearch/detectron2>
- WBF : <https://arxiv.org/abs/1910.13302>
- soft NMS : <https://arxiv.org/abs/1704.04503>
- NMW : <https://openaccess.thecvf.com/content_ICCV_2017_workshops/papers/w14/Zhou_CAD_Scale_Invariant_ICCV_2017_paper.pdf>
- pycocotools : <https://github.com/cocodataset/cocoapi>
- ensemble-boxes : <https://github.com/ZFTurbo/Weighted-Boxes-Fusion><file_sep>/15. connection with DB in c++/DB/DB.cpp
// 2021-8-17 by <NAME>
/***************************************************************************
Simple DB 예제
****************************************************************************/
#include <iostream>
#include <mysql.h>
int main()
{
MYSQL conn;
mysql_init(&conn);
MYSQL* connection = mysql_real_connect(&conn, "127.0.0.1", "root", "<PASSWORD>", "testdb", 3306, NULL, 0);
if (!connection)
std::cout << "mysql_real_connect() error" << std::endl;
else
std::cout << "mysql connection success" << std::endl;
if (mysql_query(connection, "SELECT * FROM testtable where test_id = 1") != 0)
{
std::cout << "mysql_query() error" << std::endl;
}
MYSQL_RES* sql_results = mysql_store_result(connection);
MYSQL_ROW sql_row;
for (int rdx = 0; rdx < sql_results->row_count; rdx++)
{
sql_row = mysql_fetch_row(sql_results);
for (int cdx = 0; cdx < sql_results->field_count; cdx++)
{
std::cout << sql_row[cdx];
if (cdx < sql_results->field_count - 1)std::cout << ", ";
}
std::cout << std::endl;
}
mysql_close(connection);
return 0;
}<file_sep>/19. timm/tutorial_timm.py
import timm
import torch
from pprint import pprint
# timm 에서 제공 하는 모델 zoo 리스트
model_names = timm.list_models(pretrained=True)
pprint(model_names)
# feature extractor 로 사용 하기
model = timm.create_model('efficientnetv2_rw_s',features_only=True, pretrained=True)
model.eval()
print(model)
print(f'Feature channels: {model.feature_info.channels()}')
o = model(torch.randn(2, 3, 224, 224))
for x in o:
print(x.shape)
<file_sep>/14. connection with DB in python/db.py
import pymysql # pip install pymysql 설치
# python 예제 코드 실행 전 필요한 DB 작업
# 1. 설치되어있는 mysql에 접속
# > mysql -u root -p
# 2. root 계정 비밀번호 1234로 설정
# m> ALTER USER 'root'@'localhost' IDENTIFIED BY '1234';
# 3. testdb 데이터 베이스 생성.
# m> CREATE DATABASE testdb;
# m> USE testdb;
# 4. testtable 생성 sql
# m>create table testtable ( test_id int NOT NULL, test_value varchar(10) NULL, PRIMARY KEY(test_id));
# 5. 테스트용 데이터 삽입 sql
# m>insert into testtable (test_id, test_value) values(1, 'test data');
conn = pymysql.connect(
user='root', # db 계정 아이디
password='<PASSWORD>', # root 계정 비밀번호
host='127.0.0.1', # ip주소
database='testdb', # Database name
port=3306, # port는 최초 설치 시 입력한 값(기본값은 3306)
charset='utf8'
)
#print(conn)
# db select, insert, update, delete 작업 객체
cursor = conn.cursor()
# 실행할 select 문 구성
sql = "SELECT * FROM testtable where test_id = 1"
# cursor 객체를 이용해서 수행한다.
cursor.execute(sql)
# select 된 결과 셋 얻어오기
resultList = cursor.fetchall() # tuple 이 들어있는 list
#print(len(resultList))
print(resultList) # db에서 가져온 값
# DB 에 저장된 rows 출력해보기
for result in resultList:
test_id = result[0] # test_id
test_value = result[1] # test_value
info = "test_id:{}, test_value :{}, ".format(test_id, test_value)
print(info)
<file_sep>/13. show deep learning result on Web Browser/deep_learing_model/websocket_client.py
import asyncio
import websockets
import cv2
import base64
import numpy as np
import json
import torch, torchvision
import time
from torchvision import transforms
from datetime import datetime
inst_classes = [
'__background__',
'person', 'bicycle', 'car', 'motorcycle', 'airplane', 'bus',
'train', 'truck', 'boat', 'traffic light', 'fire hydrant', 'N/A', 'stop sign',
'parking meter', 'bench', 'bird', 'cat', 'dog', 'horse', 'sheep', 'cow',
'elephant', 'bear', 'zebra', 'giraffe', 'N/A', 'backpack', 'umbrella', 'N/A', 'N/A',
'handbag', 'tie', 'suitcase', 'frisbee', 'skis', 'snowboard', 'sports ball',
'kite', 'baseball bat', 'baseball glove', 'skateboard', 'surfboard', 'tennis racket',
'bottle', 'N/A', 'wine glass', 'cup', 'fork', 'knife', 'spoon', 'bowl',
'banana', 'apple', 'sandwich', 'orange', 'broccoli', 'carrot', 'hot dog', 'pizza',
'donut', 'cake', 'chair', 'couch', 'potted plant', 'bed', 'N/A', 'dining table',
'N/A', 'N/A', 'toilet', 'N/A', 'tv', 'laptop', 'mouse', 'remote', 'keyboard', 'cell phone',
'microwave', 'oven', 'toaster', 'sink', 'refrigerator', 'N/A', 'book',
'clock', 'vase', 'scissors', 'teddy bear', 'hair drier', 'toothbrush'
]
transform = transforms.Compose([
transforms.ToTensor(),
])
def timestamp() :
return datetime.now().strftime('%Y-%m-%d %H:%M:%S')
async def connect(ip, port, wsid, wstype,model):
# 웹 소켓에 접속
async with websockets.connect("ws://{}:{}/wsid={}&wstype={}".format(ip, port,wsid, wstype)) as websocket:
try:
if websocket.open == True:
init_websocket_msg = {
'optype': 'init_websocket',
'wsid': wsid,
'wstype': wstype,
'msg': 'Hello Server ? I am py',
'timestamp': timestamp()
}
jsonString = json.dumps(init_websocket_msg) # json 객체를 인코딩하여 string으로 만듬
await websocket.send(jsonString)
# python client 코드에서 웹소켓을 통해 영상을 웹으로 전달하는 로직
capture = cv2.VideoCapture(0) # 웹캠
while 1 :
ret, frame = capture.read()
image_cv_rgb = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
image_tensor = transform(image_cv_rgb)
image_tensor_4d = torch.unsqueeze(image_tensor, 0) # 차원 추가 ex) [x,x,x] -> [1,x,x,x]
boc = time.time()
predictions = model(image_tensor_4d.to(device))
eoc = time.time()
fps = 1 / (eoc - boc)
boxes = predictions[0]['boxes'].cpu().detach().numpy()
scores = predictions[0]['scores'].cpu().detach().numpy()
labels = predictions[0]['labels'].cpu().detach().numpy()
for i, prob in enumerate(scores):
if prob > 0.99:
x0, y0, x1, y1 = boxes[i]
cv2.rectangle(frame, (int(x0), int(y0)), (int(x1), int(y1)), (0, 255, 0), 3)
cv2.putText(frame, inst_classes[labels[i]], (int(x0), int(y0)-5), cv2.FONT_HERSHEY_SIMPLEX, 1,
(0, 255, 0), 2)
cv2.putText(frame, f"fps = {fps:.2f}", (15, frame.shape[0] - 15), cv2.FONT_HERSHEY_SIMPLEX, 1,
(0, 0, 0), 2)
cv2.imshow("python", frame)
if cv2.waitKey(1) == 27:
break # esc to quit
encode_param = [int(cv2.IMWRITE_JPEG_QUALITY), 100]
result, encimg = cv2.imencode('.jpg', frame, encode_param)
if False == result:
print('could not encode image!')
# 이미지를 String 형태로 변환(인코딩)시키는 과정
encoded = base64.b64encode(encimg).decode('utf-8')
input_msg = {
'optype': 'output',
'wsid': wsid,
'wstype': wstype,
'frame': encoded,
'timestamp': timestamp()
}
jsonString = json.dumps(input_msg) # json 객체를 인코딩하여 string으로 만듬
await websocket.send(jsonString)
except:
print("error!!!")
return 0
if __name__ == "__main__":
device = torch.device('cuda') if torch.cuda.is_available() else torch.device('cpu')
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
model.to(device)
model.eval()
ip = 'localhost'
port = 8000
wsid = 1
wstype = 'pyc'
asyncio.get_event_loop().run_until_complete(connect(ip, port, wsid, wstype,model))
asyncio.get_event_loop().run_forever()
<file_sep>/12. image from browser to local with ws/websocket server nodejs/public/localVideo.js
'use strict';
// Video element where stream will be placed.
const localVideo = document.getElementById('local');
const Constraints = { video: true, audio: false };
navigator.mediaDevices.getUserMedia(Constraints) // 1. 호출 후 브라우저는 사용자에게 카메라에 액세스 할 수 있는 권한을 요청
.then(stream =>{ // 2. stream을 가져옴
localVideo.srcObject = stream; // 3. srcObject속성을 통해 로컬 스트림을 (1) 화면에 출력
})
.catch(error =>{ // 2. 실패 할경우 에러 메시지 출력
console.log('navigator.getUserMedia error: ', error);
});<file_sep>/13. show deep learning result on Web Browser/deep_learing_model/pytorch_model.py
import torchvision, torch
from torchvision import transforms
import cv2
import time
from PIL import Image
inst_classes = [
'__background__',
'person', 'bicycle', 'car', 'motorcycle', 'airplane', 'bus',
'train', 'truck', 'boat', 'traffic light', 'fire hydrant', 'N/A', 'stop sign',
'parking meter', 'bench', 'bird', 'cat', 'dog', 'horse', 'sheep', 'cow',
'elephant', 'bear', 'zebra', 'giraffe', 'N/A', 'backpack', 'umbrella', 'N/A', 'N/A',
'handbag', 'tie', 'suitcase', 'frisbee', 'skis', 'snowboard', 'sports ball',
'kite', 'baseball bat', 'baseball glove', 'skateboard', 'surfboard', 'tennis racket',
'bottle', 'N/A', 'wine glass', 'cup', 'fork', 'knife', 'spoon', 'bowl',
'banana', 'apple', 'sandwich', 'orange', 'broccoli', 'carrot', 'hot dog', 'pizza',
'donut', 'cake', 'chair', 'couch', 'potted plant', 'bed', 'N/A', 'dining table',
'N/A', 'N/A', 'toilet', 'N/A', 'tv', 'laptop', 'mouse', 'remote', 'keyboard', 'cell phone',
'microwave', 'oven', 'toaster', 'sink', 'refrigerator', 'N/A', 'book',
'clock', 'vase', 'scissors', 'teddy bear', 'hair drier', 'toothbrush'
]
if __name__ == '__main__':
# train on the GPU or on the CPU, if a GPU is not available
device = torch.device('cuda') if torch.cuda.is_available() else torch.device('cpu')
print(device)
model = torchvision.models.detection.fasterrcnn_resnet50_fpn(pretrained=True)
model.to(device)
model.eval()
transform = transforms.Compose([
transforms.ToTensor(),
])
if 0: # one test image run
file_path = "./dog.jpg"
#image_pil = Image.open(file_path)
#image = transform(image_pil)
image_cv = cv2.imread("./dog.jpg")
image_cv_rgb = cv2.cvtColor(image_cv, cv2.COLOR_BGR2RGB)
image_tensor = transform(image_cv_rgb)
image_tensor_4d = torch.unsqueeze(image_tensor, 0) # 차원 추가 ex) [x,x,x] -> [1,x,x,x]
predictions = model(image_tensor_4d.to(device)).cpu()
boxes = predictions[0]['boxes'].detach().numpy()
scores = predictions[0]['scores'].detach().numpy()
labels = predictions[0]['labels'].detach().numpy()
for i, prob in enumerate(scores):
if prob > 0.9:
x0, y0, x1, y1 = boxes[i]
cv2.rectangle(image_cv,(int(x0),int(y0)),(int(x1),int(y1)),(0,255,0),3)
cv2.imshow("test", image_cv)
cv2.waitKey(0)
else: # webcam run
video = cv2.VideoCapture(0)
while video.isOpened():
success, frame = video.read()
if success:
image_cv_rgb = cv2.cvtColor(frame, cv2.COLOR_BGR2RGB)
image_tensor = transform(image_cv_rgb)
image_tensor_4d = torch.unsqueeze(image_tensor, 0) # 차원 추가 ex) [x,x,x] -> [1,x,x,x]
boc = time.time()
predictions = model(image_tensor_4d.to(device))
eoc = time.time()
fps = 1 / (eoc-boc)
boxes = predictions[0]['boxes'].cpu().detach().numpy()
scores = predictions[0]['scores'].cpu().detach().numpy()
labels = predictions[0]['labels'].cpu().detach().numpy()
for i, prob in enumerate(scores):
if prob > 0.98:
x0, y0, x1, y1 = boxes[i]
cv2.rectangle(frame, (int(x0), int(y0)), (int(x1), int(y1)), (0, 255, 0), 3)
cv2.putText(frame, inst_classes[labels[i]], (int(x0), int(y0)), cv2.FONT_HERSHEY_SIMPLEX, 1, (0,255,0),2)
cv2.putText(frame, f"fps = {fps:.2f}", (15 , frame.shape[0] - 15), cv2.FONT_HERSHEY_SIMPLEX, 1, (255,255,255),2)
cv2.imshow("test", frame)
if cv2.waitKey(1) == 27:
break # esc to quit
else:
print("video is wrong!")
break
<file_sep>/11. simple python websocket server&client/server/server.py
# --------------------------------------------------------
# Simple Websocket Server
# Licensed under The MIT License
# Written by Derek
# 2021-06-27
# --------------------------------------------------------
import asyncio, websockets
'''
간단한 파이썬 웹소켓 서버 구현
클라이언트로 부터 메시지를 받으면 번갈아 가며 주고 받을수 있음.
'''
async def simpleServer(websocket, path):
while True:
client_msg = await websocket.recv()
print('< [client] {}'.format(client_msg))
resp = input('> [server] ')
await websocket.send(resp)
port = 8000
start_server = websockets.serve(simpleServer, "localhost", port) # 웹소켓 객체 생성
print('Server on localhost:{}'.format(port))
asyncio.get_event_loop().run_until_complete(start_server) # 비동기로 웹소켓 실행
asyncio.get_event_loop().run_forever()<file_sep>/12. image from browser to local with ws/websocket server nodejs/public/webSocketClient.js
'use strict';
const wsid = '2'
const wstype = 'webc'
const url = 'ws://localhost:8000'
const wsk = new WebSocket(`${url}/wsid=${wsid}&wstype=${wstype}`); // ws
let wsStatus = false;
wsk.onopen = function () {
console.log('서버와 웹 소켓 연결됨');
wsk.send(JSON.stringify({
optype: "init_websocket", // optype : 동작 유형(init_websocket 클라이언트에서 연결 완료 후 서버로 확인 메시지 전달)
wsid: wsid, // wsid : 해당 소켓 아이디
wstype: wstype, // wstype : 소켓 접속 유형
msg : "Hello Server ? I am web client!!", // msg : 메시지
timestamp: timestamp() // timestamp : 현재 시간
}));
wsStatus = true
};
wsk.onmessage = function (event) {
let msgjson = JSON.parse(event.data);
switch (msgjson.optype) {
case 'output': // python으로부터 이미지 수신
let imaget = "data:image/jpg;base64," + msgjson.frame; // img tag의 소스로 전달하기 위해 frame 정보에 헤더를 붙임
document.getElementById("myimage").src = imaget; // (4) 화면에 출력
break;
default:
console.log('optype error : ' + msgjson.optype)
break;
}
}
wsk.onclose = function () {
console.log("disconnected websocket")
wsStatus = false;
}
const canvas = document.getElementById('canvas');
const width = 350;
const height = 260;
function frame_capture() {
const context = canvas.getContext('2d'); // canvas에 그려질 이미지를 2d로 값 설정
context.drawImage(localVideo, 0, 0, width, height); // video tag의 영상을 canvas의 이미지로 갖고 온다. (2)출력
if(wsStatus){ // 웹소켓이 연결 되었을때만 전송
let frame = canvas.toDataURL('image/jpg', 1.0) // canvas에 그려진 이미지(base64 형태 소스)를 frame 변수에 담는다.
wsk.send(JSON.stringify({
optype: "input",
wsid: wsid,
wstype: wstype,
frame : frame,
timestamp: timestamp()
}))
}
}
setInterval(function() { frame_capture();}, 100); // 100ms마다 frame_capture() 함수 수행
<file_sep>/17. merge images in python/merge.py
import cv2
import numpy as np
output_image = cv2.imread('./result.png')
input_image = cv2.imread('./0030.jpg')
length = int(input_image.shape[0] / 1)
# crop piece from original image
small_image = input_image[:length:, :length]
big_image = output_image[:length * 4, :length * 4]
# Merge small image to big image
big_image[0:length, 0:length] = small_image
# draw outline
cv2.rectangle(big_image, (0, 0), (length, length), (0, 0, 255), 3)
# show
cv2.imshow('Merged_image', big_image)
cv2.waitKey(0)
# save
cv2.imwrite('./compare.png', big_image)
<file_sep>/16. produce a data path list file/generate_path_list_file.py
import os
import glob
'''
1. 지정 경로의 데이터셋 리스트 파일 만들기
*경로 리스트 파일 예시*
data path1, 클래스 인덱스
data path2, 클래스 인덱스
data path3, 클래스 인덱스
*로직
case1 -> 입력 폴더경로 아래 train or val 폴더가 존재 한다면, 그 안에 data들을 예시와 같은 형식으로 폴더명_train.txt 파일로 생성 (1차, 3차)
case2 -> 입력 폴더경로 아래 test 폴더가 존재 한다면, 그 안에 data들을 예시와 같은 형식으로 폴더명_test.txt 파일로 생성 (1차, 3차)
case3 -> 바로 클래스 폴더가 나오는 경우, 그 안에 data들을 아래 예시와 같은 형식으로 폴더명_train.txt 파일로 생성 (2차)
output:
output/list_file/1차_train.txt
output/list_file/2차_train.txt
output/list_file/3차_train.txt
output/list_file/1차_test.txt
output/list_file/3차_test.txt
'''
def generateFileList(save_path, dir_path, label):
label_dict = {}
for i, v in enumerate(label):
label_dict[v] = i
paths = [x for x in glob.iglob(dir_path + '/**') if os.path.isdir(x)]
train_count = 0
test_count = 0
if not os.path.exists(save_path + '/list_file/'): # 저장할 폴더가 없다면
os.makedirs(save_path + '/list_file/') # 폴더 생성
print('make directory {} is done'.format(save_path + '/list_file/'))
train_list_file = open(save_path + '/list_file/' + dir_path.split('/')[-1] + '_train.txt', 'w', encoding='utf-8')
test_list_file = open(save_path + '/list_file/' + dir_path.split('/')[-1] + '_test.txt', 'w', encoding='utf-8')
for elem in paths:
sub_dir_name = elem.split('\\')[-1]
# case1
if sub_dir_name == 'train' or sub_dir_name == 'val':
for jpg in [x for x in glob.iglob(elem + '/**', recursive=True)]:
jpg = jpg.replace('\\', '/')
if jpg.split('.')[-1] == 'jpg':
train_count += 1
train_list_file.writelines([f"{jpg}, {label_dict[jpg.split('/')[-2]]}\n"])
# case2
elif sub_dir_name == 'test':
for jpg in [x for x in glob.iglob(elem + '/**', recursive=True)]:
jpg = jpg.replace('\\', '/')
if jpg.split('.')[-1] == 'jpg':
test_count += 1
test_list_file.writelines([f"{jpg}, {label_dict[jpg.split('/')[-2]]}\n"])
# case3
else:
for jpg in [x for x in glob.iglob(elem + '/**', recursive=True)]:
jpg = jpg.replace('\\', '/')
if jpg.split('.')[-1] == 'jpg':
train_count += 1
train_list_file.writelines([f"{jpg}, {label_dict[jpg.split('/')[-2]]}\n"])
print('train_count : {}, test_count : {}'.format(train_count, test_count))
train_list_file.close()
test_list_file.close()
if train_count == 0:
if os.path.isfile(train_list_file.name):
os.remove(train_list_file.name)
if test_count == 0:
if os.path.isfile(test_list_file.name):
os.remove(test_list_file.name)
if __name__ == '__main__':
label = ['cat', 'dog']
dir_path = ['dataset/1차', 'dataset/2차', 'dataset/3차']
save_path = 'output'
for i, v in enumerate(dir_path):
generateFileList(save_path, v, label)<file_sep>/07. webRTC SFU server/webSocketServer.js
"use strict";
const funcs = require('./public/libs'); // 커스텀 라이브러리 불러오기
const WebSocket = require('ws'); // 웹소켓 라이브러리
const Hashtable = require('jshashtable');// 해시테이블 라이브러리
const wrtc = require('wrtc'); // webrtc 라이브러리
const wshashtable = new Hashtable(); // 웹소켓 정보 저장 해시테이블 생성
const streamtable = new Hashtable(); // 스트림 저장 해시테이블 생성
module.exports = (server) => {
// 웹소켓 서버 객체 생성
const wss = new WebSocket.Server({ server });
// 클라이언트 접속할 경우 그 클라이언트와 연결된 소켓객체(ws) 생성
wss.on('connection', (ws, req) => {
// 웹 소켓 연결 시 클라이언트 정보 수집
const ip = req.headers['x-forwarded-for'] || req.socket.remoteAddress;
const port = req.headers['x-forwarded-port'] || req.socket.remotePort;
console.log('새로운 클라이언트 접속', ip);
// 사전에 약속된 규칙으로 소켓서버 호출하여 변수 생성
let conuri = req.url; //wss://192.168.31.26:8000/wsid=1234&wstype=type1 <- 예시 소켓 호출 (로컬에서는 ws)
let wsid = conuri.replace(/.+wsid=([^&]+).*/,"$1");
let wstype= conuri.replace(/.+wstype=([^&]+).*/,"$1");
// 소켓 연결 정보 해시 테이블에 저장
wshashtable.put(wsid, ws);
wshashtable.keys().forEach(key =>{
console.log("접속중인 소켓 아이디 :: " + key);
wshashtable.get(key).send(JSON.stringify({'optype': 'check_ws_num' ,'ws_count': wshashtable.keys().length}))
console.log(key + " 로 업데이트된 웹소켓 접속수 전달")
})
const configuration = {'iceServers': [{'urls': 'stun:stun.l.google.com:19302'}]} // stun 서버 주소
var peerConnection = new wrtc.RTCPeerConnection(configuration); // RTCPeerConnection객체 생성
let remoteStream = null;
let localStream = null;
if (wstype == 'sub' && streamtable.keys().length > 0){
console.log('[Downstream] send pub stream to sub client')
localStream = streamtable.get('pub'); // 전달할 스트림이 있다면
}else{
console.log('[Downstream] no pub stream')
localStream = new wrtc.MediaStream(); // 전달할 스트림이 없다면 빈 스트림 임시로 전달
}
// sub 클라이언트에게 전달할 스트림을 track에 추가
localStream.getTracks().forEach(track => {
console.log(localStream);
peerConnection.addTrack(track, localStream);
});
// pub에서 전달 받은 스트림을 해시테이블에 저장
if (wstype == 'pub'){
peerConnection.addEventListener('track', async (event) => {
remoteStream = event.streams[0];
streamtable.put('pub', remoteStream);
console.log('[Upstream] find track and get pub stream !!')
console.log(remoteStream)
});
}
// 로컬의 ICE 후보를 수집 하기 위해 icecandidate를 이벤트로 등록
peerConnection.addEventListener('icecandidate', event => {
if (event.candidate) {
console.log('[Listener] find new icecandidate!!')
ws.send(JSON.stringify({optype : 'new-ice-candidate', icecandidate : event.candidate, 'wsid' : 'server'})); // 발견된 ICE를 클라이언트 피어로 전달
}else{
console.log('[Listener] All ICE candidates have been sent');
}
});
// 연결 완료 상황을 알기 위해 connectionstatechange 이벤트 등
peerConnection.addEventListener('connectionstatechange', event => {
if (event) {
console.log(`[Listener] 연결 상태 :: ${peerConnection.connectionState}`) // 연결 상태 체크
}
});
async function treatOffer(peerConnection, offer){
console.log("[수신측] 전달 받은 offer 내용을 수신측의 원격 정보로 등록.")
await peerConnection.setRemoteDescription(new wrtc.RTCSessionDescription(offer));// 전달 받은 호출 측 메타 정보를 이용하여 세션 객체로 생성하고 원격 설정 내용에 등록
const answer = await peerConnection.createAnswer(); // 수신 측 피어에 대한 정보를 담은 객체 생성
await peerConnection.setLocalDescription(answer); // 수신 측 피어 로컬 정보에 위 객체를 등록
return answer
}
async function treatAnswer(peerConnection, answer){
console.log("[호출측] 전달 받은 answer 내용을 호출측의 원격 정보로 등록")
//console.log(answer);
const remoteDesc = await new wrtc.RTCSessionDescription(answer); // 전달 받은 수신측 메타 정보로 세션 객체로 생성
await peerConnection.setRemoteDescription(remoteDesc); // 수신 측 세션 객체를 피어의 원격의 설정 내용에 등록
}
// 클라이언트로부터 메시지 수신 시
ws.on('message', (fmessage) => {
let msgjson = JSON.parse(fmessage);
//console.log(msgjson)
switch (msgjson.optype) {
case 'send_ws_msg':
console.log("send_ws_msg 요청 :: ")
wshashtable.keys().forEach(key =>{
if (key != msgjson.wsid ){
wshashtable.get(key).send(fmessage)
}
})
break;
case 'initi_signal':
console.log("initi_signal 요청, ws_count ::" + wshashtable.keys().length);
ws.send(JSON.stringify({'optype': 'initi_signal' ,'ws_count': wshashtable.keys().length}))
break;
case 'check_ws_num':
console.log("check_ws_num 요청, ws_count ::" + wshashtable.keys().length);
ws.send(JSON.stringify({'optype': 'check_ws_num' ,'ws_count': wshashtable.keys().length}))
break;
case 'offer':
console.log("offer 요청 :: ")
//console.log(msgjson.offer);
wshashtable.keys().forEach(key =>{
if (key == msgjson.wsid ){
treatOffer(peerConnection, msgjson.offer)
.then(answer =>{
console.log("[수신측] 호출측으로 수신측의 peerConnection 정보가 담긴 answer 전달 ");
ws.send(JSON.stringify({'optype': 'answer' ,'answer': answer, 'wsid' : wsid})); // 호출 측에 응답으로 수신측 피어에 대한 메타 정보를 전달
})
console.log(key + " 로 offer 전달")
}
})
break;
case 'new-ice-candidate':
console.log("new-ice-candidate 요청 :: ")
wshashtable.keys().forEach(key =>{
if (key == msgjson.wsid ){
peerConnection.addIceCandidate(msgjson.icecandidate); // 수신 받은 ICE를 등록
console.log(key + " 로 new-ice-candidate 전달")
}
})
break;
default:
console.log('optype error : ' + msgjson.optype)
}
});
// 에러시
ws.on('error', (error) => {
console.error(error);
});
// 연결 종료 시
ws.on('close', () => {
// 해시테이블에서 소켓 정보 제거 및 종료된 소켓 정보 출력
wshashtable.remove(wsid);
console.log(`[종료된 소켓 발생] ${funcs.timestamp()}`); // 현재 시간 출력
console.log(`[종료된 소켓 정보] 아이디 : ${wsid} / 접속 종류 : ${wstype} / 아이피 : ${ip} / 포트 : ${port}`);
console.log(`[현재 접속 리스트] : ${wshashtable.keys()}`);
wshashtable.keys().forEach(key =>{
wshashtable.get(key).send(JSON.stringify({'optype': 'check_ws_num' ,'ws_count': wshashtable.keys().length}))
console.log(key + " 로 업데이트된 웹소켓 접속수 전달")
})
});
});
};<file_sep>/07. webRTC SFU server/public/webRTC_sub.js
"use strict"
var wsk = null;
var wsid = null;
// 스트림을 전달 받을 비디오 요소
var localVideo = document.querySelector('#localVideo');
var wscount = document.querySelector('p#wscount');
var ws_button = document.querySelector('#ws_button');
const Constraints = { video: true, audio: false };
let localStream = null;
const remoteStream = new MediaStream(); //먼저 빈 인스턴스 생성
async function getUserMediaStream(Constraints){
console.log('0. 클라인언트의 카메라에서 localstream을 불러오기');
// 1. 호출 후 브라우저는 사용자에게 카메라에 액세스 할 수 있는 권한을 요청
await navigator.mediaDevices.getUserMedia(Constraints)
.then(stream =>{ // 2. stream을 가져옴
localVideo.srcObject = stream; // 3. srcObject속성을 통해 로컬 스트림을 화면에 출력
localStream = stream; // 4. 로컬의 스트림을 localStream 변수에 전달
})
.catch(error =>{ // 2. 실패 할경우 에러 메시지 출력
console.log('navigator.getUserMedia error: ', error);
});
}
// 시그널링을 위해 소켓 객체 생성
async function try_ws_connect(){
await getUserMediaStream(Constraints); // 로컬 스트림 처리 로직
const videoTracks = await localStream.getVideoTracks();
if(videoTracks.length > 0){
console.log(`1. 클라이언트 장치 확인 : ${videoTracks[0].label}`) // 사용하는 카메라 장치 확인
}
var wsid_html = await document.getElementById("wsid").value;
wsid = wsid_html;
const wstype = 'sub'
//const url = 'ws://192.168.31.77:8080' //https://0a810349c89b.ngrok.io
const url = 'wss://de93977e0955.ngrok.io'
wsk = new WebSocket(`${url}/wsid=${wsid}&wstype=${wstype}`); // 웹소켓 연결 및 소켓 객체 생성
console.log(`2. 웹소켓 연결 :: ${url}/wsid=${wsid}&wstype=${wstype}`)
const configuration = {'iceServers': [{'urls': 'stun:stun.l.google.com:19302'}]} // stun 서버 주소
const peerConnection = new RTCPeerConnection(configuration); // RTCPeerConnection객체 생성
// 로컬 스트림을 track에 추가
localStream.getTracks().forEach(track => {
console.log('add local stream to track!!')
peerConnection.addTrack(track, localStream);
console.log(localStream);
});
var remoteVideo = document.querySelector('#remoteVideo');
// 원격에서 전달되는 스트림 수신하기 위해 새로 추가된 track이 있는지 확인하는 리스너
peerConnection.addEventListener('track', async (event) => {
if(remoteVideo.srcObject !== event.streams[0]){
remoteVideo.srcObject = event.streams[0]; // 새로 발생된 track의 원격 스트림을 화면에 출력
console.log('[Listener] find track and get remote stream !!')
//console.log(event);
console.log(event.streams[0])
}
});
// 로컬의 ICE 후보를 수집 하기 위해 icecandidate를 이벤트로 등록
peerConnection.addEventListener('icecandidate', event => {
if (event.candidate) {
console.log('[Listener] find new icecandidate!!')
wsk.send(JSON.stringify({optype : 'new-ice-candidate', icecandidate : event.candidate, 'wsid' : wsid})); // 발견된 ICE를 원격 피어로 전달
}else{
console.log('[Listener] All ICE candidates have been sent');
}
});
// 연결 완료 상황을 알기 위해 connectionstatechange 이벤트 등
peerConnection.addEventListener('connectionstatechange', event => {
if (event) {
console.log(`[Listener] 연결 상태 :: ${peerConnection.connectionState}`) // 연결 상태 체크
}
});
async function getOffer(peerConnection){
console.log("4. [호출측] 정보 준비 offer")
const offer = await peerConnection.createOffer(); // 로컬에 대한 정보를 담은 객체 생성
await peerConnection.setLocalDescription(offer); // 이 객체를 로컬 설정에 등록
//console.log(offer)
return offer
}
async function treatOffer(peerConnection, offer){
console.log("7. [수신측] 전달 받은 offer 내용을 수신측의 원격 정보로 등록.")
await peerConnection.setRemoteDescription(new RTCSessionDescription(offer));// 전달 받은 호출 측 메타 정보를 이용하여 세션 객체로 생성하고 원격 설정 내용에 등록
const answer = await peerConnection.createAnswer(); // 수신 측 피어에 대한 정보를 담은 객체 생성
await peerConnection.setLocalDescription(answer); // 수신 측 피어 로컬 정보에 위 객체를 등록
return answer
}
async function treatAnswer(peerConnection, answer){
console.log("10. [호출측] 전달 받은 answer 내용을 호출측의 원격 정보로 등록")
//console.log(answer);
const remoteDesc = await new RTCSessionDescription(answer); // 전달 받은 수신측 메타 정보로 세션 객체로 생성
await peerConnection.setRemoteDescription(remoteDesc); // 수신 측 세션 객체를 피어의 원격의 설정 내용에 등록
}
// 웹소켓 연결시
wsk.onopen = function () {
console.log('3. [웹소켓 연결 성공] 웹 소켓 서버와 연결 됨');
wsk.send(JSON.stringify({'optype': 'initi_signal' ,'wsid' : wsid})); // 현재 접속해 있는 웹소켓 수 체크
};
// 웹소켓 메시지 처리
wsk.onmessage = function (event) {
let msgjson = JSON.parse(event.data);
switch (msgjson.optype) {
case 'initi_signal':
getOffer(peerConnection)
.then(offer =>{
console.log("5. [호출측] peerConnection 정보가 담긴 offer를 수신측으로 전달");
wsk.send(JSON.stringify({'optype': 'offer' ,'offer': offer, 'wsid' : wsid})); // 웹소켓을 통해 호출 측 피어에 대한 메타 정보를 수신 측으로 전달
})
break;
case 'check_ws_num':
console.log("웹소켓 접속자 수 업데이트");
wscount.innerHTML = "접속자 수 : " + msgjson.ws_count;
break;
case 'offer':
console.log("6. [수신측] offer 받음.")
treatOffer(peerConnection, msgjson.offer)
.then(answer =>{
console.log("8. [수신측] 호출측으로 수신측의 peerConnection 정보가 담긴 answer 전달 ");
wsk.send(JSON.stringify({'optype': 'answer' ,'answer': answer, 'wsid' : wsid})); // 호출 측에 응답으로 수신측 피어에 대한 메타 정보를 전달
})
break;
case 'answer':
console.log("9. [호출측] answer 받음.")
treatAnswer(peerConnection, msgjson.answer).then(()=>{
console.log("11. [호출측] 수신받은 answer 처리 끝. :: ");
})
break;
case 'new-ice-candidate':
console.log('새로운 new-ice-candidate를 받음.')
//console.log(msgjson.icecandidate)
try {
peerConnection.addIceCandidate(msgjson.icecandidate); // 수신 받은 ICE를 등록
console.log('전달 받은 new-ice-candidate를 등록 완료')
} catch (e) {
console.error('Error adding received ice candidate', e);
}
break;
default:
console.log('optype error : ' + msgjson.optype)
break;
}
};
// 웹소켓 종료시
wsk.onclose = function () {
console.log('[웹소켓 연결 종료] 웹 소켓 서버와 연결이 종료 됨');
};
}
<file_sep>/09. simple web socket server c++/simpleServer/simpleServer.cpp
#include <websocketpp/config/asio_no_tls.hpp>
#include <websocketpp/server.hpp>
#include <rapidjson/document.h>
#include <rapidjson/writer.h>
#include <rapidjson/stringbuffer.h>
#include <rapidjson/rapidjson.h>
#include <sstream>
#include <chrono>
#include <iostream>
#include <string>
using namespace std;
using namespace chrono;
using namespace rapidjson;
typedef websocketpp::server<websocketpp::config::asio> server;
using websocketpp::lib::placeholders::_1;
using websocketpp::lib::placeholders::_2;
using websocketpp::lib::bind;
string get_time_stamp()
{
system_clock::time_point now = system_clock::now();
system_clock::duration tp = now.time_since_epoch();
tp -= duration_cast<seconds>(tp);
time_t tt = system_clock::to_time_t(now);
char time_str[1000];
tm t = *localtime(&tt);
sprintf(time_str, "%04u-%02u-%02u %02u:%02u:%02u.%03u", t.tm_year + 1900,
t.tm_mon + 1, t.tm_mday, t.tm_hour, t.tm_min, t.tm_sec,
static_cast<unsigned>(tp / milliseconds(1)));
return time_str;
}
// pull out the type of messages sent by our config
typedef server::message_ptr message_ptr;
// Define a callback to handle incoming messages
void on_message(server* s, websocketpp::connection_hdl hdl, message_ptr msg) {
//std::cout << "on_message called with hdl: " << hdl.lock().get()
// << " and message: " << msg->get_payload()
// << std::endl;
// check for a special command to instruct the server to stop listening so
// it can be cleanly exited.
if (msg->get_payload() == "stop-listening") {
s->stop_listening();
return;
}
int count = 5;
std::string payload = msg->get_payload();
cout << "msg=[" << payload << "]" << endl;
Document d;
d.Parse(payload.c_str());
string optype = d["optype"].GetString();
std::cout << optype << std::endl;
if (optype == "client_msg") {
count = d["count"].GetInt();
count -= 1;
}
try {
stringstream ss;
ss << "{" << endl;
ss << "\"optype\" : \"" << "server_msg" << "\"," << endl;
ss << "\"wsid\" : \"" << "c++ server" << "\"," << endl;
ss << "\"wstype\" : \"" << "server" << "\"," << endl;
ss << "\"count\" : \"" << count << "\"," << endl;
ss << "\"timestamp\" : \"" << get_time_stamp() << "\"" << endl;
ss << "}" << endl;
string output_buf = ss.str();
s->send(hdl, output_buf, msg->get_opcode());
cout << "opcode??=[" << msg->get_opcode() << "]" << endl;
}
catch (websocketpp::exception const & e) {
std::cout << "Echo failed because: "
<< "(" << e.what() << ")" << std::endl;
}
}
int main() {
// Create a server endpoint
server echo_server;
try {
// Set logging settings
echo_server.set_access_channels(websocketpp::log::alevel::all);
echo_server.clear_access_channels(websocketpp::log::alevel::frame_payload);
// Initialize Asio
echo_server.init_asio();
// Register our message handler
echo_server.set_message_handler(bind(&on_message, &echo_server, ::_1, ::_2));
// Listen on port 9002
echo_server.listen(9002);
// Start the server accept loop
echo_server.start_accept();
// Start the ASIO io_service run loop
echo_server.run();
}
catch (websocketpp::exception const & e) {
std::cout << e.what() << std::endl;
}
catch (...) {
std::cout << "other exception" << std::endl;
}
}
<file_sep>/README.md
# Useful toy project
If you know it, maybe you use it somewhere?
Anyway, code that seems to be useful code are.
## index
1. Classify the detected object by class and save it as a file by python code
---
2. Making multiple images into video file by python code
---
3. Simple nodejs web server
---
4. Simple nodejs websocket server & client
---
5. Simple WebRTC Signal Server
---
6. WebRTC Signal Server
---
7. WebRTC SFU Server (pub -> server -> sub)
---
8. Simple C++ WebSocket Client
---
9. Simple C++ WebSocket Server
---
10. Base64 Encoding & Decoding with C++
---
11. Simple python WebSocket Server & Client
---
12. Sending and receiving webcam video by python web client
---
13. Output the python deep learning model results in a web browser
---
14. DB connection from python code
---
15. DB connection from C++ code
---
16. produce a data path list file with python
---
17. merge images with python
---
18. visualize coco format dataset
- using [COCO dataset](https://cocodataset.org)
---
19. timm example
---
20. detection model ensemble
---<file_sep>/16. produce a data path list file/integrate_file_list.py
import os
import glob
import random
'''
2. 여러 리스트 파일을 통합
(2-1. 만약 test가 없다면 위 train 리스트 파일을 이용하여 train & test 리스트 파일 재생성)
output:
output/tot_train/tot_train.txt (1차_train.txt + 2차_train.txt + 3차_train.txt)
output/test/test.txt (1차_test.txt + 3차_test.txt)
'''
def integrateFilelist(save_path, test_ratio=0.1):
if not os.path.exists(save_path + '/tot_train/'): # 저장할 폴더가 없다면
os.makedirs(save_path + '/tot_train/') # 폴더 생성
print('make directory {} is done'.format(save_path + '/tot_train/'))
if not os.path.exists(save_path + '/test/'): # 저장할 폴더가 없다면
os.makedirs(save_path + '/test/') # 폴더 생성
print('make directory {} is done'.format(save_path + '/test/'))
tot_train_file = open(save_path + '/tot_train/tot_train.txt', 'w', encoding='utf-8')
test_file = open(save_path + '/test/test.txt', 'w', encoding='utf-8')
count_test_file = 0
count_train_tot = 0
train_path = []
for txt in [x for x in glob.iglob(save_path + '/list_file/*.txt')]:
txt = txt.replace('\\', '/')
flag = txt.split('/')[-1].split('.')[0].split('_')[-1]
if flag == 'test':
count_test_file += 1
f = open(txt, 'r', encoding='utf-8')
lines = f.readlines()
for line in lines:
test_file.writelines(line)
f.close()
elif flag == 'train':
f = open(txt, 'r', encoding='utf-8')
lines = f.readlines()
for line in lines:
count_train_tot += 1
tot_train_file.writelines(line)
train_path.append(line)
f.close()
# 만약 train 데이터만 주어진다면 9:1 비율로 test 데이터 리스트 파일 생성
if count_test_file == 0:
print('There are no test list file. made test file {} ratio randomly'.format(test_ratio))
test = int(count_train_tot * test_ratio + 0.5)
test_loc = random.sample(range(1, count_train_tot), test)
for n in range(test):
test_file.writelines(train_path[test_loc[n]])
train_path.remove(train_path[test_loc[n]])
tot_train_file.close()
if os.path.isfile(tot_train_file.name):
os.remove(tot_train_file.name)
tot_train_file = open(save_path + '/tot_train/tot_train.txt', 'w', encoding='utf-8')
for i, v in enumerate(train_path):
tot_train_file.writelines(v)
tot_train_file.close()
test_file.close()
if __name__ == '__main__':
save_path = 'output'
integrateFilelist(save_path)
<file_sep>/02. squence image to video/tt.py
# --------------------------------------------------------
# squence images to video file
# Licensed under The MIT License
# Written by Derek
# 2021-06-27
# --------------------------------------------------------
import cv2, os, time
'''
연속 또는 무작위 다수 이미지 파일을 영상으로 만들기
데이터셋 출처 : https://motchallenge.net/data/MOT16/
'''
def imgs_to_video(output_dir_path, input_dir_path, video_name):
boc = time.time()
if not os.path.exists(output_dir_path): # 비디오를 저장할 폴더가 없다면
os.makedirs(output_dir_path) # 폴더 생성
print('make directory {} is done'.format(output_dir_path))
video_name_ext = '{}.mp4'.format(video_name) # 비디오 파일 이름{input folder name}.mp4
video_save_path = os.path.join(output_dir_path, video_name_ext) # 저장될 비디오 경로
images_list = os.listdir(input_dir_path) # 이미지 파일 이름 리스트 불러오기
first_image_path = os.path.join(input_dir_path, images_list[0]) # 첫번째 이미지 파일 경로
first_image = cv2.imread(first_image_path) # 첫번째 이미지 로드
height, width, layers = first_image.shape # 이미지 사이즈
size = (width, height) # 저장할 비디오 사이즈
fps = 30 # 저장할 비디오 fps
out = cv2.VideoWriter(video_save_path, cv2.VideoWriter_fourcc(*'DIVX'), fps, size) # 비디오로 쓰기 위해 비디오 객체 생성
for idx, image_name in enumerate(images_list): # 이미지 저장 폴더에서 하나씩 꺼내오기
img_path = os.path.join(input_dir_path, image_name) # 각 이미지 파일 경로
image = cv2.imread(img_path) # 이미지 로드
out.write(image) # 비디오 객체에 이미지 쓰기
print('({}/{}) image to {} video'.format(idx, len(images_list), video_name_ext))
out.release() # 비디오 객체 메모리 해제
print('====================================================================================')
print('{}th images to {} video done!'.format(len(images_list), video_name_ext))
print('Duration time is {} [sec]'.format(time.time() - boc))
print('====================================================================================')
if __name__ == "__main__":
input_dir_path = './MOT16-14/img1' # 이미지들 저장 경로
output_dir_path = './videos' # 저장될 비디오 경로
video_name = 'MOT16-14' # 생성할 비디오 이름
imgs_to_video(output_dir_path, input_dir_path, video_name) | c2f36d1806356e26bf0f7c743d6c4bcc7c269e69 | [
"JavaScript",
"Python",
"C++",
"Markdown"
] | 29 | Python | yester31/useful_code | 70ccd3f4efa2601181facc33d4b1375f0f41911f | 870cb56bcb04835feb3533a5593b0731e59f1d6b | |
refs/heads/main | <file_sep># Generated by Django 3.2.3 on 2021-05-19 19:08
from django.db import migrations
class Migration(migrations.Migration):
dependencies = [
('base', '0003_product_image'),
]
operations = [
migrations.RenameField(
model_name='product',
old_name='numReview',
new_name='numReviews',
),
]
| b803ce5a22ac506669ce063e507d789a0089f4c8 | [
"Python"
] | 1 | Python | alecaceres/proshop | 98c980b34421bc8d85556133ecd45538b59437ea | cf89c9ee8d5da5647176376c444e64dce8527f87 | |
refs/heads/main | <file_sep>import React, { useState } from 'react'
export const Sesion = ({ handleSubmited,handleStars}) => {
const [usuario, setUsuario] = useState('')
let jugador ={};
const [estrella, setEstrella] = useState("")
/* const handleStar = (e) =>{
e.preventDefault()
cantidad = estrella
handdleStars(cantidad)
}*/
const handleSubmit = (e) => {
e.preventDefault()
jugador.usuario=usuario
jugador.puntuacion=0
handleSubmited(jugador)
handleStars(estrella)
}
return (
<div>
<form onSubmit={handleSubmit}>
<input
id="dato"
label="Ingrese usuario"
value ={usuario}
onChange = {e => setUsuario(e.target.value)}
required
placeholder="usuario"
/>
<input
id="dato"
label="Ingrese usuario"
value ={estrella}
onChange = {e => setEstrella(e.target.value)}
required
placeholder="numero de estrellas"
/>
<button id="ingresar">Ingresar</button>
</form>
</div>
)
} | d87d7610cb5cd16d45acd955b011a61ee834f506 | [
"JavaScript"
] | 1 | JavaScript | JoelPapiREX/ESTRELLASJUEGO1 | 4b4200fabdc7f5417ddf4e7203609a74d70a2b5b | 07fc8f50f4f1ff16524491fe7b6ba58ace9557e4 | |
refs/heads/master | <repo_name>mrhdman/firstBootCampProject<file_sep>/pWORKINGd.py
# -------pWORKINGd-------
# This game was created by "mrhdman"
import os
import time
from sys import exit
from definitions import *
os.system("clear")
intro()
<file_sep>/definitions.py
import os
import time
def startGame():
os.system("clear")
print "\t\tpWORKINGd"
print """
The goal of this game is to make the
command line learning experience more enjoyable.
Enjoy!
"""
print "\n\nThis game is under construction. Come back later.\n\n"
exit()
def level3c():
print """
Nice! You're ready to play.
type 'exit' to leave the tutorial.
"""
answer3c = raw_input("$ ")
if answer3c == "exit":
startGame()
else:
print "type 'exit' and hit enter."
def level3b():
print "text.txt"
answer3b = raw_input("$ ")
if answer3b == "less text.txt":
level3c()
else:
print "type 'less text.txt' and hit enter."
def level3a():
print "pWORKINGd/tutorial/levelll"
answer3a = raw_input("$ ")
if answer3a == "ls":
level3b()
else:
print "type 'ls' and hit enter."
def level3():
os.system("clear")
print "\n\t\tTutorial: Level 3."
print """
\n\tDirections:
Type this in order. Hit enter after each command.
1. pwd
2. ls
3. less text.txt
4.
"""
answer3 = raw_input("$ ")
if answer3 == "pwd":
level3a()
else:
level3()
def level2():
os.system("clear")
print "\n\t\tTutorial: Level 2."
print "\n\tDIRECTIONS:"
print "-List the directory"
print "-Type 'ls' and hit enter to see what is here.\n\n"
print "$ pwd"
print "/pWORKINGd/tutorial/level_two"
right_answer = False
while True:
answer2 = raw_input("$ ")
if answer2 == "ls":
print "lvl1.txt\tlvl2.txt\tlvl3.txt"
print "\n\n\t\tType 'less lvl3.txt' to look at the lvl3 text file."
elif answer2 == "less lvl3.txt":
print "\n\n\t###tutorial/levelll###"
clock = 4
while clock >0:
time.sleep(1)
clock -=1
intro()
elif answer2 == "less lvl2.txt":
print "\n\n\t###tutorial/level_two###"
elif answer2 == "less lvl1.txt":
print "\n\n\t###tutorial/level1###"
else:
print "No"
level2()
def level1():
os.system("clear")
print "\t\tTutorial: Level 1."
print "$ pwd"
print "/pWORKINGd/tutorial/level1"
print "\n\t\tQuestion: How do you print the working directory?\n"
answer1 = raw_input("$ ")
if "pwd" in answer1:
os.system("clear")
print "Correct."
print "\n\n\n(READ THIS) (READ THIS) To access level 2 change the directory to 'tutorial/level_two'. (READ THIS) (READ THIS)"
clock = 3
while clock >-1:
time.sleep(1)
clock -=1
intro()
else:
print "type 'pwd' and hit enter"
level1()
def intro():
print "\n\t\t\t\tpWORKINGd\n"
print "\t KEY:"
print "pwd = print working directory"
print "cd = change directory"
print "\n\tDIRECTIONS:"
print "- To access level 1 change the directory to 'tutorial/level1'"
print "- Type 'tutorial/level1' and hit enter."
print "\n\n$ pwd"
print "/pWORKINGd/"
userinput = raw_input("cd ")
if userinput == "imready":
startGame()
elif userinput == "tutorial/levelll":
level3()
elif userinput == "tutorial/level_two":
level2()
elif userinput == "tutorial/level1":
level1()
else:
os.system("clear")
intro()
| 563ac105fe5ccc195c3c28408ab8c67f76e54dda | [
"Python"
] | 2 | Python | mrhdman/firstBootCampProject | 52dca049abcab62c4fb6df58eff2c33da72a439f | a7d3b55d2276af01bcddbeb2faaa6e51590466da | |
refs/heads/master | <file_sep>#!/usr/bin/env python
# -*- coding:utf-8 -*-
# Created by changjie.fan on 2016/6/28
"""
将RabbitMQ队列中需要发的邮件发出去
"""
import time
import simplejson as json
import os
import smtplib
# 多个MIME对象的集合
from email.mime.multipart import MIMEMultipart
# MIME文本对象
from email.mime.text import MIMEText
from email.header import Header
import pika
def send_mail(to_list=[], sub='', content=''):
""""send_mail("<EMAIL>","sub","content")
:param to_list:发给谁
:param sub:主题
:param content:内容
"""
# 设置服务器、用户名、口令及邮箱的后缀
mail_host = '192.168.10.8'
mail_user = '<EMAIL>'
mail_pass = '<PASSWORD>'
sender = "<EMAIL>"
msg_root = MIMEMultipart()
# 解决中文乱码
att_text = MIMEText(u'%s' % content, 'html', 'UTF-8')
# 添加邮件正文
msg_root.attach(att_text)
# 发件人
msg_root['from'] = sender
# 邮件标题
msg_root['Subject'] = Header(sub, 'utf-8')
# 设置时间
msg_root['Date'] = time.ctime(time.time())
msg_root['To'] = ';'.join(to_list)
# 构造附件(附件路径出现中文,会提示编码错误)
# if appendix_file:
# for rar_file_path in appendix_file:
# att = MIMEText(open(rar_file_path, 'rb').read(), 'gbk', 'UTF-8')
# att['Content-Type'] = 'application/octet-stream;name=%s' % Header(rar_file_path, 'UTF-8')
# att['Content-Disposition'] = 'attachment;filename=%s' % os.path.basename(rar_file_path)
# msg_root.attach(att)
# 群发邮件
smtp = smtplib.SMTP(mail_host, 25)
smtp.login(mail_user, mail_pass)
smtp.sendmail(sender, to_list, msg_root.as_string())
# 休眠5秒
time.sleep(5)
# 断开与服务器的连接
smtp.quit()
def send_mail_consumer():
"""
发送邮件的消费者
:return:
"""
# 建立连接connection到localhost
con = pika.BlockingConnection(pika.ConnectionParameters('192.168.10.207'))
# 创建虚拟连接channel
cha = con.channel()
# 创建队列anheng
result = cha.queue_declare(queue='send_email', durable=True)
# 创建名为yanfa,类型为fanout的交换机,其他类型还有direct和topic
cha.exchange_declare(durable=False,
exchange='tms',
type='direct', )
# 绑定exchange和queue,result.method.queue获取的是队列名称
cha.queue_bind(exchange='tms',
queue=result.method.queue,
routing_key='', )
# 公平分发,使每个consumer在同一时间最多处理一个message,收到ack前,不会分配新的message
cha.basic_qos(prefetch_count=1)
print ' [*] Waiting for messages. To exit press CTRL+C'
# 定义回调函数
def callback(ch, method, properties, body):
print " [x] Received %r" % (body,)
message = json.loads(body)
print send_mail([tmp for tmp in message['to_list'].split(';') if tmp], message['sub'], message['content'])
ch.basic_ack(delivery_tag=method.delivery_tag)
cha.basic_consume(callback,
queue='send_email',
no_ack=False, )
cha.start_consuming()
if __name__ == '__main__':
"""
系统异步发送邮件
"""
# consumer = SendMailConsumer()
# consumer.run()
send_mail_consumer()
<file_sep>#!/usr/bin/env python
# -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""
Git仓库实时备份
1. 从RabbitMQ中取出Push记录的数据库Id
2. 判断仓库在数据库中是否存在,存在的获取其在备份服务器上的路径,不存在的将其加入数据库中
2. 判断仓库在备份服务器上是否存在,不存在的即克隆,存在即更新
"""
import sys
import os
import time
import commands
import logging
from datetime import datetime
import smtplib
# 多个MIME对象的集合
from email.mime.multipart import MIMEMultipart
# MIME文本对象
from email.mime.text import MIMEText
from email.header import Header
from MySQLdb import connect, MySQLError
import amqp
reload(sys)
sys.setdefaultencoding('utf-8')
def send_mail(to_list=[], sub='', content='', appendix_file=''):
""""send_mail("<EMAIL>","sub","content")
:param to_list:发给谁
:param sub:主题
:param content:内容
:param appendix_file: 附件
"""
# 设置服务器、用户名、口令及邮箱的后缀
mail_host = '192.168.10.8'
mail_user = '<EMAIL>'
mail_pass = '<PASSWORD>'
sender = "<EMAIL>"
msg_root = MIMEMultipart()
# 解决中文乱码
att_text = MIMEText(u'%s' % content, 'html', 'UTF-8')
# 添加邮件正文
msg_root.attach(att_text)
# 发件人
msg_root['from'] = sender
# 邮件标题
msg_root['Subject'] = Header(sub, 'utf-8')
# 设置时间
msg_root['Date'] = time.ctime(time.time())
msg_root['To'] = ';'.join(to_list)
# 构造附件(附件路径出现中文,会提示编码错误)
if appendix_file != '':
rar_file_path = appendix_file
att = MIMEText(open(rar_file_path, 'rb').read(), 'gbk', 'UTF-8')
att['Content-Type'] = 'application/octet-stream;name=%s' % Header(rar_file_path, 'UTF-8')
att['Content-Disposition'] = 'attachment;filename=%s' % Header(rar_file_path, 'UTF-8')
msg_root.attach(att)
# 群发邮件
smtp = smtplib.SMTP(mail_host, 25)
smtp.login(mail_user, mail_pass)
smtp.sendmail(sender, to_list, msg_root.as_string())
# 休眠5秒
time.sleep(5)
# 断开与服务器的连接
smtp.quit()
def getLogger():
log_file = os.path.join('/var/log/git_backup', 'qm_'+datetime.now().strftime('%Y-%m-%d')+'.log')
logging.basicConfig(
level=logging.INFO,
format='',
datefmt='%Y-%m-%d/%H:%M:%S',
filename=log_file,
filemode='a'
)
file_handler = logging.FileHandler(log_file, 'a')
formatter = logging.Formatter('%(asctime)s %(name)s[line:%(lineno)d]:%(levelname)s %(message)s')
file_handler.setFormatter(formatter)
console_handler = logging.StreamHandler()
console_handler.setFormatter(formatter)
logging.root.addHandler(console_handler)
logging.root.addHandler(file_handler)
logger = logging.getLogger('Git')
logger.info('demo')
return logger
class Consumer:
"""
实时备份Git仓库
"""
def run(self):
conn = amqp.Connection(host='172.16.20.162:5672', userid='admin', password='<PASSWORD>', virtual_host='/', insist=False)
chan = conn.channel()
# durable=True持久化的队列 exclusive=True时表示是私有独立,只能创建者连接 auto_delete=Fals最后一个消费者断开也不删除队列
chan.queue_declare(queue='Git_backup', durable=True, exclusive=False, auto_delete=False)
chan.exchange_declare(exchange='sorting_room', type='direct', durable=True, auto_delete=False)
chan.queue_bind(queue='Git_backup', exchange='sorting_room', routing_key='Git')
chan.basic_consume(queue='Git_backup', no_ack=True, callback=self.commit_callback, consumer_tag="Git_consumer")
while True:
chan.wait()
chan.basic_cancel("Git_consumer")
chan.close()
conn.close()
def commit_callback(self, msg):
logger = getLogger()
row_id = msg.body
logger.info('row_id:%s' % row_id)
record = []
conn = None
cursor = None
try:
conn = connect(host='192.168.33.7', db='tms', user='tup', passwd='tup')
cursor = conn.cursor()
except MySQLError, e:
logger.info(e)
cursor.close()
conn.close()
send_mail(['<EMAIL>'], u'Git backup error', 'connect 192.168.31.91 db %s' % e)
sys.exit(1)
# 服务器配置
server_sql = "SELECT remote_name, root_path, backup_server_path, repository_path, reference_name, " \
"repo_server.id, push_type FROM repo_push_history history JOIN repo_server ON " \
"history.server_ip=repo_server.ip_address WHERE history.id=%s" % row_id
#logger.info("server_sql:%s" % server_sql)
cursor.execute(server_sql)
record = [tmp for tmp in cursor.fetchone()]
if record:
repo_url, root_path, backup_server_path, repository_path, reference_name, server_id, push_type = \
record[0], record[1], record[2], record[3], record[4], record[5], record[6]
repo_path = repository_path.replace(root_path, backup_server_path)
if os.path.basename(repo_path) == 'gitolite-admin.git':
update_sql = "UPDATE repo_push_history set status=1 WHERE id=%s" % row_id
logger.info('update_sql:%s' % update_sql)
cursor.execute(update_sql)
conn.commit()
return
if os.path.exists(repo_path):
status, info = commands.getstatusoutput('git --git-dir=%s remote' % repo_path)
logger.info(info)
if not status:
if 'origin' not in info.split():
if repo_url.endswith('29418'):
command = 'git --git-dir=%s remote add origin %s' % \
(repo_path, os.path.join(repo_url, repo_path.split(backup_server_path)[1].strip('/')))
else:
command = 'git --git-dir=%s remote add origin %s:%s' % \
(repo_path, repo_url, repo_path.split(backup_server_path)[1].strip('/'))
status, info = commands.getstatusoutput(command)
if status:
logger.info('command:%s' % command)
logger.info(info)
update_sql = "UPDATE repo_push_history SET status=-1, error_info='%s' WHERE id=%s" % \
(info.replace('\\n', '').replace('\'', '#').replace('"', '#'), row_id)
logger.info(update_sql)
cursor.execute(update_sql)
conn.commit()
return
if push_type == 'delete_reference':
status = False
else:
if reference_name.find('/tags/') > 0:
command = 'git --git-dir=%s fetch -f origin refs/tags/*:refs/tags/*' % repo_path
status, info = commands.getstatusoutput(command)
else:
command = 'git --git-dir=%s fetch -f origin refs/heads/*:refs/heads/*' % repo_path
status, info = commands.getstatusoutput(command)
if status:
logger.info('ERROR command:%s' % command)
logger.info(info)
update_sql = "UPDATE repo_push_history SET status=-1, error_info='%s' WHERE id=%s" % \
(info.replace('\\n', '').replace('\'', '#').replace('"', '#'), row_id)
logger.info('update_sql:%s' % update_sql)
cursor.execute(update_sql)
conn.commit()
return
else:
update_sql = "UPDATE repo_push_history set status=1 WHERE id=%s" % row_id
logger.info('update_sql:%s' % update_sql)
cursor.execute(update_sql)
conn.commit()
else:
current_path = os.getcwd()
if not os.path.exists(os.path.dirname(repo_path)):
os.makedirs(repo_path)
os.chdir(os.path.dirname(repo_path))
command = 'git clone %s:%s --bare' % (repo_url, repo_path.split(backup_server_path)[1].strip('/'))
status, info = commands.getstatusoutput(command)
os.chdir(current_path)
if status:
logger.info('command:%s' % command)
logger.info(info)
update_sql = "UPDATE repo_push_history set status=-1, error_info='%s' WHERE id=%s" % \
(info.replace('\\n', '').replace('\'', '#').replace('"', '#'), row_id)
logger.info(update_sql)
cursor.execute(update_sql)
conn.commit()
return
repo_sql = "SELECT id FROM repo_repo WHERE path='%s'" % repository_path
cursor.execute(repo_sql)
if not cursor.fetchone():
folders = repository_path.split(root_path)[1].strip('/').split('/')
platform_path = root_path
platform_id = ''
for name in folders:
platform_path = os.path.join(platform_path, name.strip())
platform_sql = "SELECT id, name FROM repo_platform WHERE path='%s' and server_id=%s" % \
(platform_path, server_id)
logger.info(update_sql)
cursor.execute(platform_sql)
platform = cursor.fetchone()
if platform:
platform_id = platform[0]
platform_path = platform[1]
break
if platform_id:
repo_name = repository_path.split(platform_path)[1].strip('/').split('.git')[0]
insert_sql = "INSERT INTO repo_repo(name, path, platform_id) VALUES('%s', '%s', '%s')" % \
(repo_name, repository_path, platform_id)
logger.info(update_sql)
cursor.execute(insert_sql)
conn.commit()
else:
send_mail(['<EMAIL>'], 'platform not exits',
'add new repo not exits platform in db<br>%s' % platform_path)
update_sql = "UPDATE repo_push_history set status=1, backup_time='%s' WHERE id=%s" % \
(time.strftime('%Y-%m-%d %H:%M:%S', time.localtime()), row_id)
logger.info(update_sql)
cursor.execute(update_sql)
conn.commit()
conn.close()
if __name__ == '__main__':
"""
需提供projectVersionManifest仓库工作区的路径
"""
consumer = Consumer()
consumer.run()
<file_sep># -*- encoding:utf-8 -*-
"""
项目及版本信息模块
"""
from tms import db
project_member = db.Table('tms_project_member', db.metadata,
db.Column('user_id', db.Integer, db.ForeignKey('tms_user.id')),
db.Column('project_id', db.Integer, db.ForeignKey('tms_project.id')))
project_followers = db.Table('tms_project_followers', db.metadata,
db.Column('user_id', db.Integer, db.ForeignKey('tms_user.id')),
db.Column('project_id', db.Integer, db.ForeignKey('tms_project.id'))
)
class Project(db.Model):
"""项目"""
__tablename__ = 'tms_project'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(200), unique=True, index=True)
# 项目在JIRA或其他任务管理工具中的KEY值或ID
project_key = db.Column(db.String(64), index=True)
# 1 工程项目具体的平台 2 app项目
category = db.Column(db.String(10))
description = db.Column(db.String(200))
# 状态 1开发中、2 迭代发布中、3 DCC、4 已关闭、5 暂停、6 取消
status = db.Column(db.String(20))
# 文件全称
apk_name = db.Column(db.String(64))
# 应用包名
apk_package = db.Column(db.String(300))
# 用例jar包路径
test_case_jar_url = db.Column(db.String(300))
# 最新版本号
last_version_number = db.Column(db.String(64))
# 最近发布时间
last_release_time = db.Column(db.DateTime)
# 版本通知邮件notice email address
to_email = db.Column(db.Text)
# 版本通知邮件cc email address
cc_email = db.Column(db.Text)
# 版本测试通过后通知邮件列表
test_pass_to_email = db.Column(db.Text)
# 版本测试失败后通知邮件列表
test_failure_to_email = db.Column(db.Text)
# 开发负责人
develop_leader_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
develop_leader = db.relationship("User", foreign_keys=[develop_leader_id],
backref=db.backref('developer_leader'), order_by=id)
# 测试负责人
test_leader_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
test_leader = db.relationship("User", foreign_keys=[test_leader_id],
backref=db.backref('test_leader'), order_by=id)
# 项目关注者
followers = db.relationship('User', secondary='tms_project_followers', backref='project_f')
def __repr__(self):
return u"<Project> %s" % self.name
class SpecialVersion(db.Model):
"""项目默认有ODM版本,特殊版本如Wiko专用版本, Sugar专用版本"""
__tablename__ = 'tms_project_special_version'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(50))
# myos配置的名称
app_name = db.Column(db.String(64))
description = db.Column(db.String(200))
# 存储版本的ftp路径
ftp_path = db.Column(db.String(300))
# 存储版本的SVN路径
svn_path = db.Column(db.String(300))
# release 路径
release_path = db.Column(db.String(300))
# 源码仓库,默认所有的版本使用一个仓库的不同分支进行管理
code_repo_id = db.Column(db.Integer, db.ForeignKey('repo_repo.id'))
code_repo = db.relationship('Repository', foreign_keys=[code_repo_id],
backref=db.backref('code_repo', order_by=id))
# 版本发布仓库,一类版一个仓库
release_repo_id = db.Column(db.Integer, db.ForeignKey('repo_repo.id'))
release_repo = db.relationship('Repository', foreign_keys=[release_repo_id],
backref=db.backref('release_repo', order_by=id))
# 版本所属项目
project_id = db.Column(db.Integer, db.ForeignKey('tms_project.id'))
project = db.relationship('Project', backref=db.backref('special_version', order_by=id))
def __repr__(self):
return "<SpecialVersion> %s" % '/'.join([self.project.name, self.name])
class Version(db.Model):
"""项目版本"""
__tablename__ = 'tms_project_version'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(200))
version_number = db.Column(db.String(64))
# 版本分类
# 0日构建版本、1正式版本
category = db.Column(db.String(20))
# 废弃、待自测、待测试、待发布、已发布
status = db.Column(db.String(20))
# 版本等级
level = db.Column(db.String(20))
# 版本完整的Ftp路径
ftp_path = db.Column(db.String(300))
# release 版本路径
release_path = db.Column(db.String(300))
# 版本SVN路径
svn_path = db.Column(db.String(300))
# 版本编译时间
compile_time = db.Column(db.DateTime())
# 版本编译者
compile_username = db.Column(db.String(64))
# 版本编译者邮箱地址
compile_email = db.Column(db.String(64))
# 版本JenkinsURL
jenkins_url = db.Column(db.String(300))
# 编译时Jenkins的配置
jenkins_config = db.Column(db.Text)
# 自动化测试配置
auto_test_config = db.Column(db.Text)
# 自动化测试结果
auto_test_result = db.Column(db.Boolean, default=False)
# 自动化测试报告
auto_test_report = db.Column(db.Text)
# 版本测试结果
testing_result = db.Column(db.Boolean, default=False)
# 版本测试报告
testing_reporter = db.Column(db.Text)
# 版测试时间
testing_time = db.Column(db.DateTime)
# 版本测试人
test_user_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
test_user = db.relationship('User', foreign_keys=[test_user_id], backref=db.backref('tester', order_by=id))
# 版本说明
version_note = db.Column(db.Text)
# 本次版本所有开发任务(JIRA任务) JSON格式
tasks = db.Column(db.Text)
# 本次版本的commits JSON格式
commits = db.Column(db.Text)
# 版本的SMI值
smi = db.Column(db.Integer)
# 版本发布时间
release_time = db.Column(db.DateTime())
# 版本发布人
release_user_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
release_user = db.relationship('User', foreign_keys=[release_user_id], backref=db.backref('publisher', order_by=id))
# 发布类型:迭代方法\DCC发放\特殊发放
release_type = db.Column(db.String(50))
# 发布说明
release_note = db.Column(db.Text)
# 遗留问题
unresolved_issues = db.Column(db.Text)
# 版本缺陷
version_issues = db.Column(db.Text)
# 版本附件
documents = db.Column(db.String(300))
# 本次版本上传到ftp的文件
ftp_file = db.Column(db.String(400))
# 本次需要更新到发布库的文件
release_file = db.Column(db.Text, default='')
# 版本适配信息
adaptation_note = db.Column(db.String(500))
# 导入注意事项
project_import_note = db.Column(db.String(500))
# 项目特殊版本,默默都是ODM版本
special_version_id = db.Column(db.Integer, db.ForeignKey('tms_project_special_version.id'))
special_version = db.relationship('SpecialVersion', backref=db.backref('special_version', order_by=id))
# 版本所属项目
project_id = db.Column(db.Integer, db.ForeignKey('tms_project.id'))
project = db.relationship('Project', backref=db.backref('version', order_by=id))
# 版本废弃时间
version_discard_time = db.Column(db.DateTime)
# 版本废弃人
discard_user_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
discard_user = db.relationship('User', foreign_keys=[release_user_id], backref=db.backref('discard', order_by=id))
def __repr__(self):
return "<Version> %s" % self.version_number
class VersionHistory(db.Model):
"""版本的操作历史"""
__tablename__ = 'tms_project_version_history'
id = db.Column(db.Integer, primary_key=True)
# 版本描述
description = db.Column(db.String(200))
# 版本编译时间
compile_time = db.Column(db.DateTime())
# 版本编译者
compile_username = db.Column(db.String(64))
# 版本编译者邮箱地址
compile_email = db.Column(db.String(64))
# 版本JenkinsURL
jenkins_url = db.Column(db.String(300))
# 编译时Jenkins的配置
jenkins_config = db.Column(db.Text)
# 自动化测试配置
auto_test_config = db.Column(db.Text)
# 自动化测试结果
auto_test_result = db.Column(db.Boolean, default=False)
# 自动化测试报告
auto_test_report = db.Column(db.Text)
# 版本测试结果
testing_result = db.Column(db.Boolean, default=False)
# 版本测试报告
testing_reporter = db.Column(db.Text)
# 版测试时间
testing_time = db.Column(db.DateTime)
# 版本测试人
test_user_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
test_user = db.relationship('User', foreign_keys=[test_user_id], backref=db.backref('tester_history', order_by=id))
# 版本自测人
self_test_user_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
self_test_user = db.relationship('User', foreign_keys=[self_test_user_id], backref=db.backref('self_tester_history', order_by=id))
# 版本自测结果
self_test_result = db.Column(db.Boolean, default=False)
# 版本自测时间
self_test_time = db.Column(db.DateTime)
# 本次版本所有开发任务(JIRA任务) JSON格式
tasks = db.Column(db.Text)
# 本次版本的commits JSON格式
commits = db.Column(db.Text)
# 版本
version_id = db.Column(db.Integer, db.ForeignKey('tms_project_version.id'))
version = db.relationship('Version', backref=db.backref('history', order_by=id))
def __repr__(self):
return "<VersionHistory> %s" % self.version.version_number
class ReleaseEmailAddress(db.Model):
"""版本发布通知邮件地址"""
__tablename__ = 'tms_release_email_address'
id = db.Column(db.Integer, primary_key=True)
email_address = db.Column(db.Text)
def __repr__(self):
return "<ReleaseEmailAddress> %r" % 'EmailAddress'
class ProjectTestCase(db.Model):
"""项目所用的测试用例"""
__tablename__ = 'tms_project_test_case'
id = db.Column(db.Integer, primary_key=True)
project_id = db.Column(db.Integer, db.ForeignKey('tms_project.id'))
project = db.relationship('Project', backref=db.backref('test_case', order_by=id))
test_case_id = db.Column(db.Integer, db.ForeignKey('tms_test_case.id'))
test_case = db.relationship('TestCase', backref=db.backref('project', order_by=id))
def __repr__(self):
return "<ProjectTestCase> %s" % self.project.name
<file_sep>#!/usr/bin/env python
# -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/7/4
"""
连接JIRA数据库
进行查询查询操作
"""
from MySQLdb import connect
class DBOption:
def __init__(self, db_host, db_name, db_user, db_passwd):
"""
数据连接
:param db_host:
:param db_name:
:param db_user:
:param db_passwd:
"""
self.conn = connect(host=db_host, db=db_name, user=db_user, passwd=db_passwd, charset='utf8')
self.cursor = self.conn.cursor()
def fetch_one(self, sql):
"""返回第一条结果"""
self.cursor.execute(sql)
r = self.cursor.fetchall()
if len(r) > 0:
return [r[0][e] for e in range(len(r[0]))]
return []
def fetch_all(self, sql):
"""返回第一条结果"""
self.cursor.execute(sql)
r = self.cursor.fetchall()
if len(r) > 0:
return [[r[p][e] for e in range(len(r[p]))] for p in range(len(r))]
return []
def insert_or_update_one(self, sql):
"""
插入一条记录
"""
self.cursor.execute(sql)
return self.conn.commit()
def insert_all(self, sql, data):
"""
插入多条纪录
"""
self.cursor.executemany(sql, data)
return self.conn.commit()
def close(self):
"""
关闭数据库连接
"""
self.cursor.close()
self.conn.close()
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""
report 模块为周报管理模块
定义项目模块的蓝图
"""
from flask import Blueprint
report = Blueprint('report', __name__)
from . import views
<file_sep># -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/6/12
"""
项目MyOS关联的Views
"""
import json
import os
from ConfigParser import ConfigParser, NoSectionError
from flask import render_template, redirect, url_for, request, flash, current_app, session, send_file
from flask.ext.login import login_user, logout_user, login_required, current_user
from flask.ext.mail import Mail, Message
from functools import update_wrapper
from . import project
from tms import db, mail
from androidProjectModules import *
from models import SpecialVersion, Version, Project
@project.route('/project_myos_config_page')
@login_required
def project_myos_config_page():
"""工程师项目MYOS配置页面"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '平台化MyOS项目', 'url': url_for('project.project_myos_config_page')})
session['menu_path'] = ['项目管理', '平台化MyOS项目']
return render_template('project/android/project_myos_config_page.html')
@project.route('/project_myos_config_data')
@login_required
def project_myos_config_data():
"""已导入平台化的项目"""
start = int(request.args.get('start', 0))
length = int(request.args.get('length', 10))
search = request.args.get('search[value]', '')
current_app.logger.info(search)
if search:
all_count = ProjectAndroid.query.filter(ProjectAndroid.myos_config_patch != '').filter(ProjectAndroid.name.like('%'+search+'%')).count()
else:
all_count = ProjectAndroid.query.count()
records = []
index = start + 1
if search:
all_record = ProjectAndroid.query.filter(ProjectAndroid.myos_config_patch != '').filter(ProjectAndroid.name.like('%'+search+'%')).all()
else:
all_record = ProjectAndroid.query.filter(ProjectAndroid.myos_config_patch != '').all()
for record in all_record[start: start + length]:
if record.parent:
records.append({
"id": record.id,
"index": index,
"name": '{0}_{1}'.format(record.parent.name, record.name),
"project_key": record.project_key,
"category": record.category,
"parent": record.parent.name ,
"myos_config_path": record.myos_config_patch,
})
else:
records.append({
"id": record.id,
"index": index,
"name": record.name,
"project_key": record.project_key,
"category": record.category,
"parent": '',
"myos_config_path": record.myos_config_patch,
})
index += 1
return '{"recordsTotal": %s ,"recordsFiltered": %s,"data":%s}' % (all_count, all_count, json.dumps(records))
@project.route('/project_myos_config_detail_page/<int:project_id>', methods=['GET', 'POST'])
@login_required
def project_myos_config_detail_page(project_id):
"""项目myos应用的具体配置信息"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '项目MyOS应用配置' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '项目MyOS应用配置', 'url': url_for('project.project_myos_config_detail_page', project_id=project_id)})
configs = ProjectMyOSConfig.query.filter(ProjectMyOSConfig.project_id == project_id).all()
project = ProjectAndroid.query.filter_by(id=project_id).first()
other_project = ProjectAndroid.query.filter(db.and_(ProjectAndroid.myos_config_patch != '',
ProjectAndroid.id != project_id)).all()
return render_template('/project/android/project_myos_config_detail_page.html',
project=project,
configs=configs,
other_project=other_project)
@project.route('/android_project_version/<int:project_id>')
@login_required
def android_project_version(project_id):
"""android项目的版本信息"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '项目版本' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '项目版本', 'url': url_for('project.android_project_version', project_id=project_id)})
project = ProjectAndroid.query.filter_by(id=project_id).first()
versions = ProjectVersion.query.filter(ProjectVersion.project_id == project_id).order_by(ProjectVersion.compile_time.desc()).all()
return render_template('/project/android/project_version_page.html', project=project, versions=versions)
@project.route('/project_version_myos_version_page/<int:project_version_id>', methods=['GET', 'POST'])
@login_required
def project_version_myos_version_page(project_version_id):
"""项目myos应用的具体配置信息"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '项目版本MyOS应用配置' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '项目版本MyOS应用配置',
'url': url_for('project.project_version_myos_version_page',
project_version_id=project_version_id)})
project_version = ProjectVersion.query.filter_by(id=project_version_id).first()
configs = ProjectVersionMyOSConfig.query.filter(ProjectVersionMyOSConfig.project_version_id == project_version_id).all()
return render_template('/project/android/project_version_myos_config_detail_page.html', project_version=project_version, configs=configs)
@project.route('/project_myos_app_search/<string:app_name>')
@login_required
def project_myos_app_search(app_name):
"""查找APP被哪些项目及哪些版本使用了"""
# 配置了此app且开关是打开的的项目
project_app = ProjectMyOSConfig.query.filter(db.and_(ProjectMyOSConfig.app_name.like('%{0}%'.format(app_name)),
ProjectMyOSConfig.support == 'yes')).all()
return render_template('/project/android/project_app_search_page.html', project_app=project_app)
@project.route('/app_project_version_page/<int:project_id>/<string:app_name>')
@login_required
def app_project_version_page(project_id, app_name):
"""项目中指定app在哪些版本里的版本信息"""
search_sql = "SELECT conf.id, conf.current_version, conf.last_version, conf.overrides, " \
"ver.version_number, ver.compile_time, pro.name FROM " \
"project_version_myos_app_config AS conf JOIN project_version AS ver ON " \
"conf.project_version_id=ver.id JOIN project_android AS pro ON ver.project_id= pro.id WHERE " \
"pro.id={0} AND conf.app_name='{1}'".format(project_id, app_name)
current_app.logger.info(search_sql)
records = db.session.execute(search_sql).fetchall()
result = []
for record in records:
result.append({
'id': record[0],
'current_version': record[1],
'last_version': record[2],
'overrides': record[3],
'version_number': record[4],
'compile_time': record[5]
})
return render_template('/project/android/project_app_version_page.html',
app_name=app_name,
project_version_app=result)
@project.route('/project_myos_app_edit/<int:project_id>/<int:project_app_id>', methods=['GET', 'POST'])
@project.route('/project_myos_app_edit/<int:project_id>', defaults={'project_app_id':None}, methods=['GET', 'POST'])
@login_required
def project_myos_app_edit(project_id, project_app_id):
"""
编辑项目的MYOS应用配置
:param project_id:
:param project_app_id:
:return:
"""
project_obj = ProjectAndroid.query.filter(ProjectAndroid.id == project_id).first()
if project_app_id:
project_app = ProjectMyOSConfig.query.filter(ProjectMyOSConfig.id == project_app_id).first()
versions = [tmp.version_number for tmp in Version.query.join(SpecialVersion).filter(
db.and_(SpecialVersion.app_name == project_app.app_name)).order_by(Version.id.desc()).all()]
else:
project_app = ProjectMyOSConfig()
versions = []
all_app = SpecialVersion.query.all()
if request.method == 'POST':
app_name = request.form.get('app_name')
other = ProjectMyOSConfig.query.filter(db.and_(ProjectMyOSConfig.project_id == project_id,
ProjectMyOSConfig.app_name == app_name)).first()
if other and other.id != project_app.id:
flash('%s:应用配置重复了' % app_name, 'warning')
else:
project_app.app_name = app_name
if request.form.get('support') == 'on':
project_app.support = 'yes'
else:
project_app.support = 'no'
project_app.overrides = request.form.get('overrides')
project_app.app_version = request.form.get('version')
project_app.project = project_obj
db.session.add(project_app)
db.session.commit()
return redirect(url_for('project.project_myos_config_detail_page', project_id=project_id))
return render_template('/project/android/project_myos_app_edit_page.html',
all_app=all_app,
project=project_obj,
app=project_app,
versions=versions)
@project.route('/get_myos_app_verion')
@login_required
def get_myos_app_verion():
"""
获取myos 应用所有已发布的版本
:return:
"""
app_name = request.args.get('app_name')
versions = [{'name': tmp.version_number}for tmp in Version.query.join(SpecialVersion).filter(
db.and_(SpecialVersion.app_name== app_name)).order_by(Version.id.desc()).all()]
return json.dumps(versions)
@project.route('/import_project_myos_config/<int:project_id>', methods=['GET', 'POST'])
@login_required
def import_project_myos_config(project_id):
"""
导入项目的myos配置文件
:param project_id:
:return:
"""
# 保存配置文件
new_config_file = request.files["app_config"]
current_app.logger.info(new_config_file)
upload_path = os.path.join(current_app.config['TMS_TEMP_DIR'], current_user.username, 'ApkVersion.ini')
if not os.path.exists(os.path.dirname(upload_path)):
os.makedirs(os.path.dirname(upload_path))
new_config_file.save(upload_path)
project_apk = []
cf = ConfigParser()
try:
cf.read(upload_path)
for section in cf.sections():
if section == 'ProjectInfo':
continue
project_apk.append(section)
except NoSectionError, e:
print e
print '[ERROR] apkVersion file config error'
# 项目当前的配置
current_config = ProjectMyOSConfig.query.filter(ProjectMyOSConfig.project_id == project_id).all()
if current_config:
for app in current_config:
if app.app_name in project_apk:
app.support = cf.get(app.app_name, 'support')
app.app_version = cf.get(app.app_name, 'version')
if cf.has_option(app.app_name, 'overrides'):
app.overrides = cf.get(app.app_name, 'overrides')
db.session.add(app)
project_apk.remove(app.app_name)
else:
db.session.delete(app)
for app_name in project_apk:
project_config = ProjectMyOSConfig()
project_config.app_name = app_name
project_config.support = cf.get(app_name, 'support')
project_config.app_version = cf.get(app_name, 'version')
if cf.has_option(app_name, 'overrides'):
project_config.overrides = cf.get(app_name, 'overrides')
project_config.project_id = project_id
db.session.add(project_config)
db.session.commit()
else:
for app_name in project_apk:
project_config = ProjectMyOSConfig()
project_config.app_name = app_name
project_config.support = cf.get(app_name, 'support')
project_config.app_version = cf.get(app_name, 'version')
if cf.has_option(app_name, 'overrides'):
project_config.overrides = cf.get(app_name, 'overrides')
project_config.project_id = project_id
db.session.add(project_config)
db.session.commit()
return redirect(url_for('project.project_myos_config_detail_page', project_id=project_id))
@project.route('/export_project_myos_config/<int:project_id>', methods=['GET', 'POST'])
@login_required
def export_project_myos_config(project_id):
"""
项目myos配置从数据库中导出到ApkVersion.ini文件
:param project_id:
:return:
"""
project_obj = ProjectAndroid.query.filter(ProjectAndroid.id == project_id).first()
current_config = ProjectMyOSConfig.query.filter(ProjectMyOSConfig.project_id == project_id).all()
cf = ConfigParser()
for app in current_config:
cf.add_section(app.app_name)
cf.set(app.app_name, 'support', app.support)
cf.set(app.app_name, 'app_version', app.app_version)
if app.overrides:
cf.set(app.app_name, 'overrides', app.overrides)
upload_path = os.path.join(current_app.config['TMS_TEMP_DIR'], current_user.username, 'ApkVersion.ini')
if not os.path.exists(os.path.dirname(upload_path)):
os.makedirs(os.path.dirname(upload_path))
fp = open(upload_path, 'w')
fp.write('[ProjectInfo]\n')
fp.write('#android version,optional value:L/M/N\n')
fp.write('ANDROID_VERSION=%s\n\n' % project_obj.android_version)
fp.write('#chip platform,optional value:MTK/QCOM/RDA\n')
fp.write('PLATFORM=%s\n\n' % project_obj.platform_name)
cf.write(fp)
fp.close()
return send_file(open(upload_path, 'rb'), as_attachment=True, attachment_filename=os.path.basename(upload_path))
@project.route('/project_myos_delete', methods=['GET', 'POST'])
@login_required
def project_myos_delete():
"""
删除项目应用配置
:return:
"""
app_ids = request.form.get('app_ids')
app_config = ProjectMyOSConfig.query.filter(ProjectMyOSConfig.id.in_(app_ids.split(','))).all()
for app in app_config:
db.session.delete(app)
db.session.commit()
return '{"result": true}'
@project.route('/project_myos_app_info/<string:app_name>/<string:version_number>')
@login_required
def project_myos_app_info(app_name, version_number):
"""
显示应用版本的详细信息
:param app_name:
:param version_number:
:return:
"""
app_version = Version.query.join(SpecialVersion).filter(db.and_(SpecialVersion.app_name == app_name,
Version.version_number == version_number)).first()
return redirect(url_for('developer.version_self_test', version_id=app_version.id))
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
import os
from datetime import datetime
import simplejson as json
from flask import render_template, redirect, url_for, request, flash, current_app, jsonify, g, session, send_file
from flask.ext.login import login_user, logout_user, login_required
from forms import LoginForm, ChangePasswordForm, ChangeEmailForm
from models import User, Role, SystemMenu, SystemParameter
from . import auth
from tms import db
from util import create_menu, getOrder
from tms.departmentMan.models import Department
@auth.route('/login', methods=['GET', 'POST'])
def login():
"""用户登录"""
if request.method == 'POST':
user = User.query.filter_by(username=request.form['username']).first()
if user is not None and user.verify_password(request.form['password']):
login_user(user, request.form.get('remember'))
user.last_seen = datetime.now()
role = user.role
if role and role.menu:
menu_ids = [menu_id for menu_id in role.menu.split(',') if id]
menus = [menu for menu in SystemMenu.query.filter(
db.and_(SystemMenu.activate == True,
SystemMenu.id.in_(menu_ids))).order_by(SystemMenu.order).all()]
current_page = role.index_page
else:
menus = []
current_page = ''
session['user'] = {
'id': user.id,
'username': user.username,
'display_name': user.display_name,
'email': user.email,
'role_name': role.name,
'role_id': user.role_id,
}
session['index_page'] = current_page
session['menu'] = create_menu(menus)
session['breadcrumbs'] = [{'text': 'Home', 'url': url_for(role.index_page)}]
return redirect(url_for(current_page, url_path=current_page))
flash('Invalid email or password.', 'danger')
return render_template('auth/login.html')
@auth.route('/logout')
@login_required
def logout():
"""用户登出"""
logout_user()
session.clear()
flash('You have been logged out.', 'info')
return redirect(url_for('auth.login'))
@auth.route('/settings', methods=['GET', 'POST'])
@login_required
def settings():
"""账号设置设置"""
password_form = ChangePasswordForm()
email_form = ChangeEmailForm()
return render_template('auth/settings.html', password_form=password_form, email_form=email_form)
@auth.route('/change_email', methods=['POST'])
@login_required
def change_email():
data = json.loads(request.form.get('data'))
return str(data['email'])
@auth.route('/account_page')
@login_required
def account_page():
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': u'账号管理', 'url': url_for('auth.account_page')})
session['menu_path'] = [u'系统管理', u'账号管理']
return render_template('admin/account/account_page.html', users=User.query.all())
@auth.route('/add_account', methods=['GET', 'POST'])
@login_required
def add_account():
"""新增账号"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '添加账号' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '添加账号', 'url': url_for('auth.add_account')})
new_user = None
if request.method == 'POST':
if request.form['password'] != request.form['password2']:
flash(u'两次输入的密码不相同', 'danger')
return redirect(url_for('auth.add_account'))
user = User.query.filter_by(email=request.form['email']).first()
if user is not None:
flash(u'此邮箱地址已注册过', 'danger')
return redirect(url_for('auth.add_account'))
new_user = User()
new_user.username = request.form['username']
new_user.email = request.form['email']
new_user.password = request.form['<PASSWORD>']
new_user.display_name = request.form['display_name']
new_user.role = Role.query.filter_by(id=request.form['role']).first()
new_user.department_id = request.form['department']
db.session.add(new_user)
db.session.commit()
flash(u'新增成功', 'info')
return redirect(url_for('auth.account_page'))
else:
#get请求
#一级部门
top_departments = Department.query.filter_by(level=1,activate=1).order_by(Department.id.asc()).all()
#二级部门
second_departments = Department.query.filter_by(level=2,activate=1).order_by(Department.id.asc()).all()
#三级部门
three_departments = Department.query.filter_by(level=3,activate=1).order_by(Department.id.asc()).all()
#四级部门
four_departments = Department.query.filter_by(level=4,activate=1).order_by(Department.id.asc()).all()
#五级部门
five_departments = Department.query.filter_by(level=5,activate=1).order_by(Department.id.asc()).all()
#六级部门
six_departments = Department.query.filter_by(level=6,activate=1).order_by(Department.id.asc()).all()
array = []
array.append('一级部门')
array.append('二级部门')
array.append('三级部门')
array.append('四级部门')
array.append('五级部门')
array.append('六级部门')
dict = {}
dict['一级部门'] = top_departments
dict['二级部门'] = second_departments
dict['三级部门'] = three_departments
dict['四级部门'] = four_departments
dict['五级部门'] = five_departments
dict['六级部门'] = six_departments
return render_template('admin/account/account_edit.html', user=new_user,
roles=Role.query.filter(db.and_(Role.name != 'translator',
Role.name != 'customer_manager')).all(),
current_page='auth.account_page',array=array,dict=dict,addflag=True)
@auth.route('/edit_account/<int:user_id>', methods=['GET', 'POST'])
@login_required
def edit_account(user_id):
"""编辑账号"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '编辑账号' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '编辑账号', 'url': url_for('auth.edit_account', user_id=user_id)})
user = user = User.query.filter_by(id=user_id).first()
if request.method == 'POST':
other_user = User.query.filter_by(email=request.form['email']).first()
if other_user and user.id != other_user.id:
flash(u'此邮箱地址已注册过', 'danger')
if request.form['password'] != request.form['password2']:
flash(u'两次输入的密码不相同', 'danger')
if user.password_hash != request.form['password']:
user.password = request.form['password']
user.username = request.form['username']
user.email = request.form['email']
user.display_name = request.form['display_name']
user.role = Role.query.filter_by(id=request.form['role']).first()
user.department_id = request.form['department']
db.session.add(user)
db.session.commit()
flash(u'更新成功!', 'info')
return redirect(url_for('auth.account_page'))
else:
#get请求
#一级部门
top_departments = Department.query.filter_by(level=1,activate=1).order_by(Department.id.asc()).all()
#二级部门
second_departments = Department.query.filter_by(level=2,activate=1).order_by(Department.id.asc()).all()
#三级部门
three_departments = Department.query.filter_by(level=3,activate=1).order_by(Department.id.asc()).all()
#四级部门
four_departments = Department.query.filter_by(level=4,activate=1).order_by(Department.id.asc()).all()
#五级部门
five_departments = Department.query.filter_by(level=5,activate=1).order_by(Department.id.asc()).all()
#六级部门
six_departments = Department.query.filter_by(level=6,activate=1).order_by(Department.id.asc()).all()
array = []
array.append('一级部门')
array.append('二级部门')
array.append('三级部门')
array.append('四级部门')
array.append('五级部门')
array.append('六级部门')
dict = {}
dict['一级部门'] = top_departments
dict['二级部门'] = second_departments
dict['三级部门'] = three_departments
dict['四级部门'] = four_departments
dict['五级部门'] = five_departments
dict['六级部门'] = six_departments
return render_template('admin/account/account_edit.html', user=user,
roles=Role.query.filter(db.and_(Role.name != 'translator', Role.name != 'customer_manager')).all(),
array=array,dict=dict,addflag=False)
@auth.route('/system_menu_page')
@login_required
def system_menu_page():
"""菜单清单"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': u'菜单管理', 'url': url_for('auth.system_menu_page')})
session['menu_path'] = [u'系统管理', u'菜单管理']
result = []
menus = SystemMenu.query.order_by(SystemMenu.order).all()
parents = []
result = {}
for menu in menus:
if menu.parent and menu.parent in result.keys():
result[menu.parent].append(menu)
elif menu.parent:
result.update({menu.parent:[menu]})
parents.append(menu.parent)
else:
result.update({menu.parent: []})
parents = sorted(parents, key=getOrder)
return render_template('admin/account/system_menu_page.html', parents=parents, menus=result)
@auth.route('/add_system_menu', methods=['GET', 'POST'])
@login_required
def add_system_menu():
"""新增菜单"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '添加菜单' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '添加菜单',
'url': url_for('auth.add_system_menu')})
if request.method == 'POST':
name = request.form['name']
action = request.form.get('action', '')
activate = request.form.get('activate')
note = request.form.get('note')
order = request.form['order']
parent_id = request.form['parent']
other_menu = SystemMenu.query.filter(db.and_(SystemMenu.action == action,
SystemMenu.parent_id == parent_id)).first()
if other_menu:
flash(u'同名菜单存在', 'danger')
else:
menu = SystemMenu()
menu.name = name
menu.action = action or ''
menu.note = note
if activate == 'on':
menu.activate = True
else:
menu.activate = False
menu.style = ''
menu.note = note
menu.order = order
if parent_id:
menu.parent_id = parent_id
db.session.add(menu)
db.session.commit()
flash(u'新增成功!', 'info')
return render_template('admin/account/system_menu_edit.html', menu=None,
parent_menus=SystemMenu.query.filter(
db.and_(SystemMenu.action == '', SystemMenu.activate == True)).all())
@auth.route('/_menu_activate')
@login_required
def set_menu_activate():
menu_id = request.args.get('id', 0, type=int)
activate = request.args.get('activate')
if activate == 'Enable':
db.session.execute('UPDATE tms_menu SET activate=1 WHERE id =%s or parent_id=%s' % (menu_id, menu_id))
elif activate == 'Disable':
db.session.execute('UPDATE tms_menu SET activate=0 WHERE id =%s or parent_id=%s' % (menu_id, menu_id))
db.session.commit()
return '{"result": true}'
@auth.route('/edit_system_menu/<int:menu_id>', methods=['GET', 'POST'])
@login_required
def edit_system_menu(menu_id):
"""修改菜单"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '编辑菜单' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '编辑菜单',
'url': url_for('auth.edit_system_menu', menu_id=menu_id)})
menu = SystemMenu.query.filter_by(id=menu_id).first()
if request.method == 'POST':
name = request.form['name']
action = request.form.get('action', '')
activate = request.form.get('activate')
note = request.form.get('note')
order = request.form['order']
parent_id = request.form['parent']
other_menu = SystemMenu.query.filter(db.and_(SystemMenu.action == action,
SystemMenu.parent_id == parent_id)).first()
if other_menu and other_menu.id != menu.id:
flash(u'同名菜单存在', 'danger')
else:
menu.name = name
menu.action = action or ''
menu.note = note
if activate == 'on':
menu.activate = True
else:
menu.activate = False
menu.style = ''
menu.note = note
menu.order = order
if parent_id:
menu.parent_id = parent_id
db.session.add(menu)
db.session.commit()
flash(u'修改成功!', 'info')
return render_template('admin/account/system_menu_edit.html', menu=menu,
parent_menus=SystemMenu.query.filter(
db.and_(SystemMenu.action == '', SystemMenu.activate == True)).all())
@auth.route('/role_page')
@login_required
def role_page():
"""系统角色管理"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': u'角色管理', 'url': url_for('auth.role_page')})
session['menu_path'] = [u'权限管理', u'角色管理']
roles = Role.query.all()
return render_template('admin/account/role_page.html', roles=roles,
current_page='auth.role_page')
@auth.route('/edit_role/<int:role_id>', methods=['GET', 'POST'])
@login_required
def edit_role(role_id):
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '编辑角色' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '编辑角色',
'url': url_for('auth.edit_role', role_id=role_id)})
role = Role.query.filter_by(id=role_id).first()
if request.method == 'POST':
all_id = [str(menu_id) for menu_id in request.form.getlist('role_menu') if menu_id]
if all_id:
query_sql = "SELECT DISTINCT parent_id FROM tms_menu WHERE id IN(%s)" % ','.join(all_id)
for row in db.engine.execute(query_sql):
if row[0] != 'None' and row[0] != None:
all_id.append(str(row[0]))
role.menu = ','.join(list(set(all_id)))
else:
role.menu = ''
role.name = request.form['name']
role.index_page = request.form['index_page']
db.session.add(role)
db.session.commit()
user_ids = request.form.getlist('role_remember')
remember = User.query.filter_by(role_id=role.id).all()
for user in remember:
if user.id in user_ids:
user_ids.remove(user.id)
else:
user.role = None
db.session.add(user)
users = User.query.filter(User.id.in_(user_ids))
for user in users:
user.role = role
db.session.add(user)
db.session.commit()
return redirect(url_for('auth.role_page'))
menus = SystemMenu.query.filter_by(activate=True).order_by(SystemMenu.order).all()
users = User.query.all()
if role.menu:
role_menu = [int(id) for id in role.menu.split(',') if id]
else:
role_menu = []
return render_template('admin/account/role_edit.html',
role=role, menus=menus, users=users, role_menu=role_menu)
@auth.route('/add_role/', methods=['GET', 'POST'])
@login_required
def add_role():
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '添加角色' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '添加角色', 'url': url_for('auth.add_role')})
if request.method == 'POST':
other_role = Role.query.filter_by(name=request.form['name']).first()
if other_role:
flash(u'同名角色已存在', 'danger')
else:
all_id = [str(menu_id) for menu_id in request.form.getlist('role_menu') if menu_id]
query_sql = "SELECT DISTINCT parent_id FROM tms_menu WHERE id IN(%s)" % ','.join(all_id)
for row in db.engine.execute(query_sql):
if row[0] != 'None' and row[0] != None:
current_app.logger.info(row[0])
all_id.append(str(row[0]))
role = Role()
role.menu = ','.join(list(set(all_id)))
role.name = request.form['name']
role.index_page = request.form['index_page']
db.session.add(role)
db.session.commit()
users = User.query.filter(User.id.in_(request.form.getlist('role_remember')))
for user in users:
user.role = role
db.session.add(user)
db.session.commit()
return redirect(url_for('auth.role_page'))
menus = SystemMenu.query.filter_by(activate=True).order_by(SystemMenu.order).all()
users = User.query.all()
return render_template('admin/account/role_edit.html', role=None, menus=menus, users=users,
current_page='auth.role_page')
@auth.route('/download_file/')
@login_required
def download_file():
"""文件下载"""
file_path = request.args.get('file_path', '')
return send_file(
open(file_path, 'rb'),
as_attachment=True, attachment_filename=os.path.basename(file_path))
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""任务模块的蓝图"""
from flask import Blueprint
task = Blueprint('task', __name__)
from . import views
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""Git 仓库路径导入数据库中"""
import sys
import os
from MySQLdb import connect
def main(repo_dir_path):
"""将指定目录下的repo仓库路径,添加到数据库中"""
repos = []
for dir_name in os.listdir(repo_dir_path):
if dir_name.endswith('.git') and os.path.isdir(os.path.join(repo_dir_path, dir_name)):
repos.append(os.path.join(repo_dir_path, dir_name))
print repos
con = connect(host='192.168.33.7', db='tms', user='gms', passwd='gms', charset='utf8')
cursor = con.cursor()
exits_sql = "SELECT path FROM repo_repo WHERE platform_id=89"
cursor.execute(exits_sql)
exists_repo = [tmp[0] for tmp in cursor.fetchall()]
print exists_repo
new_repo = []
for repo_path in repos:
if repo_path not in exists_repo:
new_repo.append((repo_path.split('/home/git/repositories/tinno/release/')[1].split('.git')[0].strip(),
repo_path, 89, 3))
print new_repo
insert_repo = "INSERT into repo_repo(name, path, platform_id, category) VALUES (%s, %s, %s, %s)"
cursor.executemany(insert_repo, new_repo)
con.commit()
con.close()
def update_repo_url():
con = connect(host='192.168.33.7', db='tms', user='gms', passwd='gms', charset='utf8')
cursor = con.cursor()
exits_sql = "SELECT id, name FROM repo_repo WHERE platform_id=89"
cursor.execute(exits_sql)
for tmp in cursor.fetchall():
update_sql = "UPDATE repo_repo set repo_url='%s' WHERE id=%s" % ('scm:tinno/release/'+tmp[1], tmp[0])
cursor.execute(update_sql)
con.commit()
con.close()
if __name__ == '__main__':
# main(sys.argv[1])
update_repo_url()<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""
将硬盘中平台信息
仓库路径导入到数据库中
"""
import os
from optparse import OptionParser
from DBOption import DBOption
def get_all_repo(platform_path):
"""
获取平台下所有的Git仓库路径
"""
repos = []
for root, dirs, files in os.walk(platform_path):
for dir_name in dirs:
if dir_name == '.repo' or dir_name == '.git':
continue
git_path = os.path.join(root, dir_name)
if git_path.find('/.repo/') > 0:
continue
if git_path.endswith('.git'):
repos.append(git_path)
return repos
def main():
"""
1. 导入服务器上的所有平台
2. 导入平台下的所有仓库
"""
des = u'import platform and Repository'
prog= u'AddRepository'
ver = u'%prog v0.0.1'
usage = u'%prog ip_address platform_path dir_path'
parse = OptionParser(description=des, prog=prog, version=ver, usage=usage, add_help_option=True)
parse.add_option('-t', '--server-ip', action='store', type='string', dest='ip_address',
help='repository server ip address')
parse.add_option('-p', '--platform-name', action='store', type='string', dest='platform_name',
help='repository platform name')
# 解析命令行参数
options, args = parse.parse_args()
ip_address = options.ip_address
platform_name = options.platform_name.strip('/')
# mysql
db_url = {'host': '192.168.31.91', 'db_name': 'tms', 'username': 'root', 'password': '<PASSWORD>'}
# sqlite
#db_url = '/home/roy/workspace/tms/tms-dev.sqlite'
db_option = DBOption(db_url, 'mysql')
db_option.db_connect()
server_sql = "SELECT id, root_path FROM repo_server WHERE ip_address='%s' " % ip_address
server = db_option.fetch_one(server_sql)
server_id = server[0]
server_root_path = server[1]
platform_path = os.path.join(server_root_path, platform_name)
platform_sql = "SELECT id FROM repo_platform WHERE path='%s' AND server_id=%s" % (platform_path, server_id)
platform = db_option.fetch_one(platform_sql)
if not platform:
platform_insert_sql = "INSERT INTO repo_platform (name, path, server_id) VALUES ('%s', '%s', %s)" % \
(platform_name, platform_path, server_id)
db_option.insert_one(platform_insert_sql)
platform = db_option.fetch_one(platform_sql)
platform_id = platform[0]
repo_paths = get_all_repo(platform_path)
repo_sql = "SELECT path FROM repo_repo WHERE platform_id=%s" % platform_id
exists_repo_paths = db_option.fetch_all(repo_sql)
if exists_repo_paths:
exists_repo_paths = [tmp[0] for tmp in exists_repo_paths]
repo_values = []
for repo_path in repo_paths:
if repo_path in exists_repo_paths:
continue
repo_name = repo_path.split(platform_path)[1].split('.git')[0].strip('/')
repo_values.append((repo_name, repo_path, platform_id))
if repo_values:
repo_insert_sql = "INSERT INTO repo_repo (name, path, platform_id) values (%s, %s, %s)"
for r in range(len(repo_values)/50+1):
db_option.insert_all(repo_insert_sql, repo_values[r*50:(r+1)*50])
if __name__ == '__main__':
main()
<file_sep>{% extends "developer/developer_base.html" %}
{% block header %}
{{ super() }}
<link href="{{ url_for('static', filename='assets/global/plugins/datatables/plugins/bootstrap/dataTables.bootstrap.css') }}"
rel="stylesheet" type="text/css"/>
{% endblock %}
{% block page_content %}
<!-- BEGIN CONTENT -->
<div class="row">
<div class="col-md-12">
<!-- BEGIN EXAMPLE TABLE PORTLET-->
<div class="portlet box grey-cascade">
<div class="portlet-title">
<div class="caption">
{% if all_project %}
<i class="fa fa-globe"></i>所有项目
{% else %}
<i class="fa fa-globe"></i>我的项目
{% endif %}
</div>
<div class="actions">
<a href="{{ url_for('developer.all_project') }}" class="btn btn-primary btn-sm">
显示所有项目</a>
</div>
</div>
<div class="portlet-body">
{% if session['user']['role_name'] == 'scm' %}
<div class="table-toolbar">
<div class="row">
<div class="col-md-6">
<div class="btn-group">
<a id="add_account_btn" class="btn green"
href="{{ url_for('developer.add_project') }}">
添加项目<i class="fa fa-plus"></i>
</a>
</div>
</div>
</div>
</div>
{% endif %}
<table class="table table-striped table-bordered table-hover" id="project_table">
<thead>
<tr>
<th width="20px;">No</th>
<th>项目名称</th>
<th>JIRA_KEY</th>
<th>项目类型</th>
<th>开发负责人</th>
<th>测试负责人</th>
<th>状态</th>
<th>最新版本</th>
<th>发布时间</th>
<th>Action</th>
</tr>
</thead>
<tbody>
{% for index, project, project_type in projects %}
<tr data="{{ project.id }}" type="">
<td>{{ index }}</td>
<td>{{ project.name }}</td>
<td>{{ project.project_key }}</td>
<td>{{ project.category }}</td>
<td>{{ project.develop_leader.display_name }}</td>
<td>{{ project.test_leader.display_name }}</td>
<td>{{ project.status }}</td>
<td>{{ project.last_version_number }}</td>
<td>{{ project.last_release_time }}</td>
<td>
<a class="btn btn-xs btn-primary"
href="{{ url_for('developer.project_version', project_id=project.id) }}">所有版本</a>
{% if all_project %}
{% if project_type == 'other' %}
<a id="as{{ project.id }}" class="btn btn-xs green" href="#"
onclick="start_project({{ project.id }}, 1);return false;">关注</a>
<a id="ac{{ project.id }}" class="btn btn-xs btn-warning" href="#"
style="display: none;"
onclick="start_project({{ project.id }}, -1);return false;">取消</a>
{% elif project_type == 'start' %}
<a id="as{{ project.id }}" class="btn btn-xs green" href="#"
style="display: none;"
onclick="start_project({{ project.id }}, 1);return false;">关注</a>
<a id="ac{{ project.id }}" class="btn btn-xs btn-warning" href="#"
onclick="start_project({{ project.id }}, -1);return false;">取消</a>
{% endif %}
{% endif %}
</td>
</tr>
{% endfor %}
{% for index, project, project_type, type in project_android %}
<tr data="{{ project.id }}" type="{{ type }}">
<td>{{ index }}</td>
<td>{{ project.name }}</td>
<td>{{ project.project_key }}</td>
<td>{{ project.category }}</td>
<td></td>
<td></td>
<td>{{ project.status }}</td>
<td></td>
<td></td>
<td>
<a id="ah{{ project.id }}" class="btn btn-xs blue" href="{{ url_for('project.project_code_history', project_id=project.id) }}">历史</a>
{% if project_type == 'other' %}
<a id="as{{ project.id }}" class="btn btn-xs green" href="#"
onclick="start_android_project({{ project.id }}, 1);return false;">关注</a>
<a id="ac{{ project.id }}" class="btn btn-xs btn-warning" href="#"
style="display: none;"
onclick="start_android_project({{ project.id }}, -1);return false;">取消</a>
{% elif project_type == 'start' %}
<a id="as{{ project.id }}" class="btn btn-xs green" href="#"
style="display: none;"
onclick="start_android_project({{ project.id }}, 1);return false;">关注</a>
<a id="ac{{ project.id }}" class="btn btn-xs btn-warning" href="#"
onclick="start_android_project({{ project.id }}, -1);return false;">取消</a>
{% endif %}
</td>
</tr>
{% endfor %}
</tbody>
</table>
</div>
</div>
<!-- END EXAMPLE TABLE PORTLET-->
</div>
</div>
<!-- END PAGE CONTENT-->
{% endblock %}
{% block script %}
<script src="{{ url_for('static', filename='assets/global/plugins/datatables/media/js/jquery.dataTables.min.js') }}"
type="text/javascript"></script>
<script src="{{ url_for('static', filename='assets/global/plugins/datatables/extensions/TableTools/js/dataTables.tableTools.min.js') }}"
type="text/javascript"></script>
<script src="{{ url_for('static', filename='assets/global/plugins/datatables/plugins/bootstrap/dataTables.bootstrap.js') }}"
type="text/javascript"></script>
<script src="{{ url_for('static', filename='assets/global/plugins/bootbox/bootbox.min.js') }}"
type="text/javascript"></script>
<script src="{{ url_for('static', filename='js/developer/developer_page.js') }}" type="text/javascript"></script>
<script type="text/javascript">
$SCRIPT_ROOT = {{ request.script_root|tojson|safe }};
</script>
{% endblock %}
<file_sep># -*- encoding:utf-8 -*-
"""
部门管理模块
"""
from tms import db
class Department(db.Model):
"""部门"""
__tablename__ = 'tms_department'
id = db.Column(db.Integer, primary_key=True)
#部门名称
name = db.Column(db.String(100))
#是否激活
activate = db.Column(db.Boolean, default=True)
#备注
note = db.Column(db.String(100))
#排序
order = db.Column(db.Integer)
#level 层级
level = db.Column(db.Integer)
parent_id = db.Column(db.Integer, db.ForeignKey('tms_department.id'), nullable=True)
parent = db.relation('Department', uselist=False, remote_side=[id],
backref=db.backref('children', order_by=order))
leader = db.Column(db.String(100))
def __repr__(self):
return '<Department %r>' % self.name
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""
数据库操作
"""
class DBOption:
def __init__(self, db_url, db_type='sqlite'):
self.db_type = db_type
self.db_url = db_url
self.conn = None
self.cursor = None
def db_connect(self):
"""
数据库连接、获取游标
"""
if self.db_type == 'sqlite':
import sqlite3
self.conn = sqlite3.connect(self.db_url)
self.cursor = self.conn.cursor()
else:
from MySQLdb import connect
self.conn = connect(host=self.db_url['host'], db=self.db_url['db_name'], user=self.db_url['username'],
passwd=self.db_url['password'], charset='utf8')
self.cursor = self.conn.cursor()
def fetch_one(self, sql):
"""返回第一条结果"""
print sql
self.cursor.execute(sql)
r = self.cursor.fetchall()
if len(r) > 0:
return [r[0][e]for e in range(len(r[0]))]
return []
def fetch_all(self, sql):
"""返回第一条结果"""
print sql
self.cursor.execute(sql)
r = self.cursor.fetchall()
if len(r) > 0:
return [[r[p][e] for e in range(len(r[p]))] for p in range(len(r))]
return []
def insert_one(self, sql):
"""
插入一条记录
"""
print sql
self.cursor.execute(sql)
return self.conn.commit()
def insert_all(self, sql, data):
"""
插入多条纪录
"""
print sql
self.cursor.executemany(sql, data)
return self.conn.commit()
def close(self):
"""
关闭数据库连接
"""
self.cursor.close()
self.conn.close()
<file_sep># -*- encoding:utf-8 -*-
"""
编译信息模块
"""
from tms import db
class CompileMode(db.Model):
"""编译模式"""
__tablename__ = 'jenkins_compilemode'
id = db.Column(db.Integer, primary_key=True)
compile_mode = db.Column(db.String(20), unique=True, index=True)
def __repr__(self):
return u"<CompileMode> %s" % self.compile_mode
class CompileOrder(db.Model):
"""编译命令"""
__tablename__ = 'jenkins_compileorder'
id = db.Column(db.Integer, primary_key=True)
compile_order = db.Column(db.String(20), unique=True, index=True)
def __repr__(self):
return u"<CompileOrder> %s" % self.compile_order
class CompileType(db.Model):
"""编译类型"""
__tablename__ = 'jenkins_compiletype'
id = db.Column(db.Integer, primary_key=True)
compile_type = db.Column(db.String(20), unique=True, index=True)
def __repr__(self):
return u"<CompileType> %s" % self.compile_type
class PlatForm(db.Model):
"""jenkins--编译平台"""
__tablename__ = 'jenkins_platform'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(20), unique=True, index=True)
def __repr__(self):
return u"<PlatForm> %s" % self.name
class Module(db.Model):
"""模块编译参数"""
__tablename__ = 'jenkins_module'
id = db.Column(db.Integer, primary_key=True)
pro_name = db.Column(db.String(20), index=True)
command = db.Column(db.Text())
path = db.Column(db.Text())
platform_id = db.Column(db.Integer,db.ForeignKey('jenkins_platform.id'))
platform = db.relationship("PlatForm", foreign_keys=[platform_id],
backref=db.backref('platform'), order_by=id)
module_name = db.Column(db.String(20), index=True)
def __repr__(self):
return u"<Module> %s" % self.module_name
<file_sep>var TableManaged = function () {
var initTable1 = function () {
var table = $('#project_table');
table.dataTable({
"language": {
"aria": {
"sortAscending": ": activate to sort column ascending",
"sortDescending": ": activate to sort column descending"
},
"emptyTable": "No data available in table",
"info": "Showing _START_ to _END_ of _TOTAL_ records",
"infoEmpty": "No records found",
"infoFiltered": "(filtered1 from _MAX_ total records)",
"lengthMenu": "Show _MENU_ records",
"search": "Search:",
"zeroRecords": "No matching records found",
"paginate": {
"previous":"Prev",
"next": "Next",
"last": "Last",
"first": "First"
}
},
"bStateSave": true, // save datatable state(pagination, sort, etc) in cookie.
"columns": [{
"orderable": false
}, {
"orderable": true
}, {
"orderable": true
}, {
"orderable": true
}, {
"orderable": true
}],
"lengthMenu": [
[12, 25, 50, -1],
[12, 25, 50, "All"] // change per page values here
],
// set the initial value
"pageLength": 12,
"pagingType": "bootstrap_full_number",
"columnDefs": [{ // set default column settings
'orderable': false,
'targets': [0]
}, {
"searchable": false,
"targets": [0]
}],
"order": [
[2, "asc"]
] // set first column as a default sort by asc
});
}
return {
//main function to initiate the module
init: function () {
if (!jQuery().dataTable) {
return;
}
initTable1();
}
};
}();
jQuery(document).ready(function() {
TableManaged.init();
$('#project_table tbody').on( 'dblclick', 'td', function () {
var tdSeq = $(this).parent().find("td").index($(this)[0]);
if(tdSeq == 8) {
return;
}else {
$(this).toggleClass('selected');
window.location.href = $SCRIPT_ROOT + '/qa/edit_project/' +$(this).parent().attr('data');
}
});
$("#project_table tr").live({
mouseover:function(){
$(this).css("cursor","pointer");
}
});
});<file_sep># -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/8/30
"""
项目共性问题
"""
import os
from datetime import datetime, date
import simplejson as json
from flask import render_template, redirect, url_for, request, flash, current_app, session, send_file
from flask.ext.login import login_user, logout_user, login_required, current_user
from flask.ext.mail import Mail, Message
from functools import update_wrapper
from jira import JIRAError
from . import project
from tms import db, mail
from tms.auth.models import User, Role
from androidProjectModules import *
from tms.auth.models import SystemParameter
from tms.baseline.models import BPlatform
from tms.utils.JiraClient import JiraClient
from tms.utils.excelOption import ExcelOptions
@project.route('/common_issue_page')
@login_required
def common_issue_page():
"""项目共性问题页面"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '共性问题', 'url': url_for('project.common_issue_page')})
session['menu_path'] = ['项目管理', '共性问题']
return render_template('project/android/common_issue/common_issue_page.html')
@project.route('/common_issue_data')
@login_required
def common_issue_data():
"""项目共性问题 测试工程师的界面"""
start = int(request.args.get('start', 0))
length = int(request.args.get('length', 10))
search = request.args.get('search[value]', '')
session['search'] = search
if search:
all_count = CommonIssue.query.filter(
db.or_(CommonIssue.name.like('%'+search+'%'),
CommonIssue.description.like('%' + search + '%'),
CommonIssue.baseline_name.like('%' + search + '%'),
CommonIssue.platform_name.like('%' + search + '%'))).count()
all_record = CommonIssue.query.filter(
db.or_(CommonIssue.name.like('%'+search+'%'),
CommonIssue.description.like('%' + search + '%'),
CommonIssue.baseline_name.like('%' + search + '%'),
CommonIssue.platform_name.like('%' + search + '%'))).order_by(CommonIssue.submit_issue_time.desc()).all()
else:
all_count = CommonIssue.query.count()
all_record = CommonIssue.query.order_by(CommonIssue.submit_issue_time.desc()).all()
records = []
index = start + 1
for record in all_record[start: start + length]:
enable_edit = False
if record.status == '1' and record.tester_id == current_user.id:
enable_edit = True
if record.status == 1:
status = '已创建'
elif record.status == 2:
status = '填写方案'
elif record.status == 3:
status = '等待确认'
elif record.status == 4:
status = '已确认'
else:
status = '已创建'
records.append({
"id": record.id,
"index": index,
"name": record.name,
"jira_key": record.jira_key,
"project_name": record.project.name,
"platform_name": record.platform_name,
"status": status,
"enable_edit": enable_edit
})
index += 1
return '{"recordsTotal": %s ,"recordsFiltered": %s,"data":%s}' % (all_count, all_count, json.dumps(records))
@project.route('/add_common_issue', methods=['GET', 'POST'])
@login_required
def add_common_issue():
"""添加项目共性问题"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '添加问题' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index + 1]
break
else:
session['breadcrumbs'].append(
{'text': '添加问题', 'url': url_for('project.add_common_issue')})
common_issue = CommonIssue()
all_project = [tmp for tmp in ProjectAndroid.query.order_by(ProjectAndroid.create_time.desc()).all() if tmp.status != '关闭']
all_platform = BPlatform.query.all()
all_developer = User.query.join(Role).filter(db.and_(User.activate==True, Role.name=='Android开发工程师')).all()
all_spm = User.query.join(Role).filter(db.and_(User.activate==True, Role.name=='spm')).all()
if request.method == 'POST':
name = request.form.get('name', '').strip()
project_id = request.form.get('project_id', '')
description = request.form.get('description', '').strip()
solutions = request.form.get('solutions', '').strip()
effect_scope = ','.join(request.form.getlist('effect_scope'))
platform_name = request.form.get('platform_name', '')
baseline_name = request.form.get('baseline_name', '').strip()
jira_key = request.form.get('jira_key', '').strip()
attachment = request.form.get('attachment', '')
developer_id = request.form.get('developer_id', '')
spm_id = request.form.get('spm_id', '')
category = request.form.get('category', '')
issue_from = request.form.get('issue_from', '')
other = CommonIssue.query.filter(CommonIssue.name == name).first()
if other:
flash(u'同名的共性问题已存在', 'warning')
else:
common_issue.name = name
common_issue.project_id = project_id
common_issue.description = description
common_issue.solutions = solutions
common_issue.effect_scope = effect_scope
common_issue.platform_name = platform_name
common_issue.baseline_name = baseline_name
common_issue.tester_id = current_user.id
if jira_key:
common_issue.jira_key = jira_key
if developer_id:
common_issue.developer_id = developer_id
common_issue.status = 2
if spm_id:
common_issue.spm_id = spm_id
common_issue.submit_issue_time = datetime.now()
common_issue.attachment = attachment
common_issue.category = category
common_issue.issue_from = issue_from
db.session.add(common_issue)
db.session.commit()
project_obj = ProjectAndroid.query.filter_by(id=project_id).first()
if common_issue.effect_scope == '平台':
msg = Message(
u'[TSDS] %s平台 共性问题——%s' % (common_issue.platform_name, common_issue.name),
sender='<EMAIL>',
recipients=[common_issue.developer.email, '<EMAIL>']
)
elif common_issue.effect_scope == '产品':
msg = Message(
u'[TSDS] %s产品 共性问题——%s' % (project_obj.name, common_issue.name),
sender='<EMAIL>',
recipients=[common_issue.developer.email, '<EMAIL>']
)
else:
msg = Message(
u'[TSDS] %s%s 共性问题——%s' % (project_obj.name, project_obj.customer_area, common_issue.name),
sender='<EMAIL>',
recipients=[common_issue.developer.email, common_issue.spm.email, common_issue.tester.email,
'<EMAIL>']
)
msg.html = '<h5>项目共性问题名称:</h5>{0}<br><br>' \
'<h5>问题创建人:</h5>{1}<{2}><br><br>' \
'<h5>提出问题项目:</h5>{3}<br><br>' \
'<h5>项目所属平台名称:</h5>{4}<br><br>' \
'<h5>项目当前基线:</h5>{5}<br><br>' \
'<h5>问题描述:</h5>{6}<br>'.format(common_issue.name, current_user.display_name, current_user.email,
common_issue.project.name, common_issue.platform_name,
common_issue.baseline_name, common_issue.description)
mail.send(msg)
return redirect(url_for('project.common_issue_page'))
issue_category_options = ['平台', '产品', '订单']
issue_from_options = []
issue_from_param = SystemParameter.query.filter_by(key='ISSUE_FROM').first()
if issue_from_param:
issue_from_options = [tmp for tmp in str(issue_from_param.value).split('\n') if tmp.strip()]
return render_template('project/android/common_issue/common_issue_edit.html',
common_issue=common_issue,
all_project=all_project,
all_developer=all_developer,
all_spm=all_spm,
all_platform=all_platform,
issue_category_options=issue_category_options,
issue_from_options=issue_from_options)
@project.route('/qa_edit_common_issue/<int:common_issue_id>', methods=['GET', 'POST'])
@login_required
def qa_edit_common_issue(common_issue_id):
"""测试工程师编辑项目共性问题"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '修改问题' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index + 1]
break
else:
session['breadcrumbs'].append(
{'text': '修改问题', 'url': url_for('project.qa_edit_common_issue', common_issue_id=common_issue_id)})
common_issue = CommonIssue.query.filter_by(id=common_issue_id).first()
if common_issue.attachment:
attachment = [(tmp, os.path.basename(tmp))for tmp in common_issue.attachment.split('#')]
else:
attachment = []
all_project = [tmp for tmp in ProjectAndroid.query.order_by(ProjectAndroid.create_time.desc()).all() if tmp.status != '关闭']
all_platform = BPlatform.query.all()
all_developer = User.query.join(Role).filter(db.and_(User.activate==True, Role.name=='Android开发工程师')).all()
all_spm = User.query.join(Role).filter(db.and_(User.activate==True, Role.name=='spm')).all()
if request.method == 'POST':
name = request.form.get('name', '').strip()
description = request.form.get('description', '').strip()
solutions = request.form.get('solutions', '').strip()
effect_scope = ','.join(request.form.getlist('effect_scope'))
platform_name = request.form.get('platform_name', '')
baseline_name = request.form.get('baseline_name', '').strip()
jira_key = request.form.get('jira_key', '').strip()
attachment = request.form.get('attachment', '')
developer_id = request.form.get('developer_id', '')
spm_id = request.form.get('spm_id', '')
category = request.form.get('category', '')
issue_from = request.form.get('issue_from', '')
other = CommonIssue.query.filter(CommonIssue.name == name).first()
if other and other.id != common_issue_id:
flash(u'同名的共性问题已存在', 'warning')
else:
common_issue.name = name
common_issue.description = description
common_issue.category = category
common_issue.solutions = solutions
if effect_scope != ',':
common_issue.effect_scope = effect_scope
common_issue.platform_name = platform_name
common_issue.baseline_name = baseline_name
if jira_key:
common_issue.jira_key = jira_key
if developer_id:
common_issue.developer_id = developer_id
common_issue.status = 2
if spm_id:
common_issue.spm_id = spm_id
common_issue.submit_issue_time = datetime.now()
if common_issue.attachment and common_issue.attachment.strip():
common_issue.attachment = '#'.join([tmp.strip() for tmp in common_issue.attachment.split('#') if tmp]
+ [tmp.strip() for tmp in attachment.split('#') if tmp.strip()])
else:
common_issue.attachment = attachment
common_issue.issue_from = issue_from
db.session.add(common_issue)
db.session.commit()
return redirect(url_for('project.common_issue_page'))
issue_category_options = ['平台', '产品', '订单']
issue_from_options = []
issue_from_param = SystemParameter.query.filter_by(key='ISSUE_FROM').first()
if issue_from_param:
issue_from_options = [tmp for tmp in str(issue_from_param.value).split('\n') if tmp.strip()]
return render_template('project/android/common_issue/common_issue_edit.html',
common_issue=common_issue,
all_project=all_project,
all_developer=all_developer,
all_spm=all_spm,
all_platform=all_platform,
attachment=attachment,
issue_category_options=issue_category_options,
issue_from_options=issue_from_options)
@project.route('/dev_edit_common_issue/<int:common_issue_id>', methods=['GET', 'POST'])
@login_required
def dev_edit_common_issue(common_issue_id):
"""开发工程师编辑项目共性问题"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '修改问题' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index + 1]
break
else:
session['breadcrumbs'].append(
{'text': '修改问题', 'url': url_for('project.dev_edit_common_issue', common_issue_id=common_issue_id)})
common_issue = CommonIssue.query.filter_by(id=common_issue_id).first()
if common_issue.attachment:
attachment = [(tmp, os.path.basename(tmp))for tmp in common_issue.attachment.split('#')]
else:
attachment = []
all_project = [tmp for tmp in ProjectAndroid.query.order_by(ProjectAndroid.create_time.desc()).all() if tmp.status != '关闭']
all_platform = BPlatform.query.all()
all_developer = User.query.join(Role).filter(db.and_(User.activate==True, Role.name=='Android开发工程师')).all()
all_spm = User.query.join(Role).filter(db.and_(User.activate==True, Role.name=='spm')).all()
if request.method == 'POST':
solutions = request.form.get('solutions', '').strip()
spm_id = request.form.get('spm_id', '')
attachment = request.form.get('attachment', '')
common_issue.solutions = solutions
if spm_id:
common_issue.spm_id = spm_id
common_issue.status = 3
common_issue.submit_solution_time = datetime.now()
if common_issue.attachment and common_issue.attachment.strip():
common_issue.attachment = '#'.join([tmp.strip() for tmp in common_issue.attachment.split('#') if tmp]
+ [tmp.strip() for tmp in attachment.split('#') if tmp.strip()])
else:
common_issue.attachment = attachment
db.session.add(common_issue)
db.session.commit()
project_obj = common_issue.project
if common_issue.effect_scope == '平台':
msg = Message(
u'[TSDS] %s平台 共性问题——%s(解决方案)' % (common_issue.platform_name, common_issue.name),
sender='<EMAIL>',
recipients=[common_issue.developer.email, '<EMAIL>']
)
elif common_issue.effect_scope == '产品':
msg = Message(
u'[TSDS] %s产品 共性问题——%s(解决方案)' % (project_obj.name, common_issue.name),
sender='<EMAIL>',
recipients=[common_issue.developer.email, '<EMAIL>']
)
else:
msg = Message(
u'[TSDS] %s%s 共性问题——%s(解决方案)' % (project_obj.name, project_obj.customer_area, common_issue.name),
sender='<EMAIL>',
recipients=[common_issue.developer.email, common_issue.spm.email, common_issue.tester.email,
'<EMAIL>']
)
msg.html = '<h5>项目共性问题名称:</h5>{0}<br><br>' \
'<h5>问题创建人:</h5>{1}<{2}><br><br>' \
'<h5>提出问题项目:</h5>{3}<br><br>' \
'<h5>项目所属平台名称:</h5>{4}<br><br>' \
'<h5>项目当前基线:</h5>{5}<br><br>' \
'<h5>问题描述:</h5>{6}<br>'\
'<h5>解决方案:</h5>{7}<br>'.format(common_issue.name, current_user.display_name, current_user.email,
common_issue.project.name, common_issue.platform_name,
common_issue.baseline_name, common_issue.description,
common_issue.solutions)
mail.send(msg)
return redirect(url_for('project.common_issue_page'))
issue_category_options = ['平台', '产品', '订单']
issue_from_options = []
issue_from_param = SystemParameter.query.filter_by(key='ISSUE_FROM').first()
if issue_from_param:
issue_from_options = [tmp for tmp in str(issue_from_param.value).split('\n') if tmp.strip()]
return render_template('project/android/common_issue/common_issue_edit.html',
common_issue=common_issue,
all_project=all_project,
all_developer=all_developer,
all_spm=all_spm,
all_platform=all_platform,
attachment=attachment,
issue_category_options=issue_category_options,
issue_from_options=issue_from_options)
@project.route('/spm_edit_common_issue/<int:common_issue_id>', methods=['GET', 'POST'])
@login_required
def spm_edit_common_issue(common_issue_id):
"""spm确认项目共性问题"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '修改问题' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index + 1]
break
else:
session['breadcrumbs'].append(
{'text': '修改问题', 'url': url_for('project.dev_edit_common_issue', common_issue_id=common_issue_id)})
common_issue = CommonIssue.query.filter_by(id=common_issue_id).first()
if common_issue.attachment:
attachment = [(tmp, os.path.basename(tmp))for tmp in common_issue.attachment.split('#')]
else:
attachment = []
all_project = [tmp for tmp in ProjectAndroid.query.order_by(ProjectAndroid.create_time.desc()).all() if tmp.status != '关闭']
all_platform = BPlatform.query.all()
all_developer = User.query.join(Role).filter(db.and_(User.activate==True, Role.name=='Android开发工程师')).all()
all_spm = User.query.join(Role).filter(db.and_(User.activate==True, Role.name=='spm')).all()
if request.method == 'POST':
name = request.form.get('name', '').strip()
description = request.form.get('description', '').strip()
solutions = request.form.get('solutions', '').strip()
effect_scope = ','.join(request.form.getlist('effect_scope'))
platform_name = request.form.get('platform_name', '')
baseline_name = request.form.get('baseline_name', '').strip()
attachment = request.form.get('attachment', '')
category = request.form.get('category', '')
issue_from = request.form.get('issue_from', '')
other = CommonIssue.query.filter(CommonIssue.name == name).first()
if other and other.id != common_issue_id:
flash(u'同名的共性问题已存在', 'warning')
else:
common_issue.name = name
common_issue.description = description
common_issue.category = category
common_issue.solutions = solutions
if effect_scope != ',':
common_issue.effect_scope = effect_scope
common_issue.platform_name = platform_name
common_issue.baseline_name = baseline_name
email_to_list = request.form.get('to_list', '')
common_issue.to_list = ';'.join([tmp.strip() for tmp in email_to_list.split(';') if tmp.strip()])
common_issue.status = 4
common_issue.issue_from = issue_from
common_issue.confirm_time = datetime.now()
if common_issue.attachment and common_issue.attachment.strip():
common_issue.attachment = '#'.join([tmp.strip() for tmp in common_issue.attachment.split('#') if tmp]
+ [tmp.strip() for tmp in attachment.split('#') if tmp.strip()])
else:
common_issue.attachment = attachment
# 在各个受影响的项目中创建JIRA任务
task_list = []
if common_issue.effect_scope != '':
jira_client = JiraClient()
# 在JIRA中直接给受影响的项目创建JIRA任务并分配给spm
effect_project_jira_key = [tmp.project_key for tmp in ProjectAndroid.query.filter(
ProjectAndroid.id.in_(common_issue.effect_scope.split(','))).all()]
for project_jira_key in effect_project_jira_key:
task_list.append(jira_client.create_issue(project_jira_key, common_issue.jira_key,
common_issue.solutions))
to_list = []
for tmp in common_issue.to_list.split(';'):
if tmp.strip() and tmp.endswith('@tinno.com'):
to_list.append(tmp)
# 添加创建者的邮箱
to_list.append(common_issue.tester.email)
to_list.append(common_issue.developer.email)
to_list.append(common_issue.spm.email)
to_list.append('<EMAIL>')
# 新增任务的Key
new_task_key = []
for task in task_list:
if task[1] and task[1] not in to_list and task[1].endswith('@tinno.com'):
to_list.append(task[1])
new_task_key.append('项目名称:{0} 负责人:{1} 新增任务KEY:{2}'.format(task[0], task[2], task[3]))
common_issue.effect_project_new_task_key = '\n'.join(new_task_key)
db.session.add(common_issue)
db.session.commit()
if current_user.email not in to_list:
to_list.append(current_user.email)
system_param = SystemParameter.query.filter_by(key='android_project_common_issue_email').first()
for email in system_param.value.split('\n'):
if email and email not in to_list:
to_list.append(email)
to_list = list(set(to_list))
# 邮件通知
if common_issue.effect_scope == '平台':
msg = Message(
u'[TSDS] %s平台 共性问题——%s' % (common_issue.platform_name, common_issue.name),
sender='<EMAIL>',
recipients=to_list
)
elif common_issue.effect_scope == '产品':
msg = Message(
u'[TSDS] %s产品 共性问题——%s' % (common_issue.project.name, common_issue.name),
sender='<EMAIL>',
recipients=to_list
)
else:
msg = Message(
u'[TSDS] %s%s 共性问题——%s' % (common_issue.project.name, common_issue.project.customer_area, common_issue.name),
sender='<EMAIL>',
recipients=to_list
)
msg.html = '<h5>项目共性问题名称:</h5>{0}<br><br>' \
'<h5>问题创建人:</h5>{1}<{2}><br><br>' \
'<h5>提出问题项目:</h5>{3}<br><br>' \
'<h5>项目所属平台名称:</h5>{4}<br><br>' \
'<h5>项目当前基线:</h5>{5}<br><br>' \
'<h5>问题描述:</h5>{6}<br><br>' \
'<h5>解决方案:</h5>{7}<br><br>' \
'<h5>已创建的任务:</h5>{8}<br><br>'.format(
common_issue.name, current_user.display_name,current_user.email,common_issue.project.name,
common_issue.platform_name, common_issue.baseline_name, common_issue.description.replace('\n', '<br>'),
'<br>'.join(['%s <a href="http://jira.tinno.com:8080/browse/%s">%s</a> %s' %
(task[3], task[0], task[0], task[2])for task in task_list]), common_issue.solutions)
mail.send(msg)
return redirect(url_for('project.common_issue_page'))
issue_category_options = ['平台', '产品', '订单']
issue_from_options = []
issue_from_param = SystemParameter.query.filter_by(key='ISSUE_FROM').first()
if issue_from_param:
issue_from_options = [tmp for tmp in str(issue_from_param.value).split('\n') if tmp.strip()]
return render_template('project/android/common_issue/common_issue_edit.html',
common_issue=common_issue,
all_project=all_project,
all_developer=all_developer,
all_spm=all_spm,
all_platform=all_platform,
attachment=attachment,
issue_category_options=issue_category_options,
issue_from_options=issue_from_options)
@project.route('/get_other_project')
@login_required
def get_other_project():
"""
获取会影响到的项目,获取范围规则是
平台问题,就获取同平台的所有项目;
产品问题,就获取此产品的所有订单项目;
订单问题,就获取同客户的所有项目(同一个Android版本)
:return:
"""
project_id = request.args.get('project_id', '')
platform_name = request.args.get('platform_name', '')
issue_category = request.args.get('issue_category', '')
results = []
# 提出共性问题的项目
current_project = ProjectAndroid.query.filter_by(id=project_id).first()
if issue_category == '平台' and platform_name:
for project in ProjectAndroid.query.filter(ProjectAndroid.platform_name == platform_name).all():
current_app.logger.info(project.id)
if project == current_project:
continue
if project.parent:
results.append({"id": project.id, "name": '%s_%s_%s' % (project.parent.name, project.name,
project.android_version)})
else:
results.append({"id": project.id, "name": '%s_%s' % (project.name, project.android_version)})
elif issue_category == '产品':
# 当前项目是产品项目时获取所有基于此项目的子项目
if current_project.customer_name and current_project.parent:
for project in ProjectAndroid.query.filter(ProjectAndroid.parent_id == current_project.parent_id).all():
if project == current_project:
continue
if project.parent:
results.append({"id": project.id, "name": '%s_%s_%s' % (project.parent.name, project.name,
project.android_version)})
else:
results.append({"id": project.id, "name": '%s_%s' % (project.name, project.android_version)})
else:
for project in ProjectAndroid.query.filter(ProjectAndroid.parent_id == project_id).all():
if project == current_project:
continue
if project.parent:
results.append({"id": project.id, "name": '%s_%s_%s' % (project.parent.name, project.name,
project.android_version)})
else:
results.append({"id": project.id, "name": '%s_%s' % (project.name, project.android_version)})
elif issue_category == '订单':
if current_project.customer_name:
for project in ProjectAndroid.query.filter(
db.and_(ProjectAndroid.customer_name == current_project.customer_name,
ProjectAndroid.android_version == current_project.android_version)).all():
if project == current_project:
continue
if project.parent:
results.append({"id": project.id, "name": '%s_%s_%s' % (project.parent.name, project.name,
project.android_version)})
else:
results.append({"id": project.id, "name": '%s_%s' % (project.name, project.android_version)})
return json.dumps(results)
@project.route('/common_issue_delete_file')
@login_required
def common_issue_delete_file():
"""删除项目共性问题附件"""
common_issue_id = request.args.get('common_issue_id', '')
file_path = request.args.get('file_path', '')
common_issue = CommonIssue.query.filter(CommonIssue.id == common_issue_id).first()
new_attachment = []
for tmp in common_issue.attachment.split('#'):
if tmp and tmp != file_path:
new_attachment.append(tmp)
common_issue.attachment = '#'.join(new_attachment)
db.session.add(common_issue)
db.session.commit()
return '{"result": true}'
@project.route('/common_issue_get_jira_key_info')
@login_required
def common_issue_get_jira_key_info():
"""获取原始JIRA_KEY的标题和描述"""
task_key = request.args.get('jira_key', '')
if task_key:
project = ProjectAndroid.query.filter_by(project_key=task_key.split('-')[0]).first()
if project:
project_id = project.id
try:
jira_client = JiraClient()
title, description = jira_client.get_issue_info(task_key)
except JIRAError, e:
current_app.logger.info(e)
title, description = '', ''
else:
project_id, title, description = '', '', ''
result = [{"title": title, "description": description, "project_id": project_id}]
return json.dumps(result)
@project.route('/export_common_issue')
@login_required
def export_common_issue():
"""导出共性问题"""
search = current_app.logger.info(session['search'])
if search:
all_record = CommonIssue.query.filter(
db.or_(CommonIssue.name.like('%' + search + '%'),
CommonIssue.description.like('%' + search + '%'),
CommonIssue.baseline_name.like('%' + search + '%'),
CommonIssue.platform_name.like('%' + search + '%'))).order_by(CommonIssue.submit_issue_time.desc()).all()
else:
all_record = CommonIssue.query.order_by(CommonIssue.submit_issue_time.desc()).all()
excel_file_path = os.path.join(current_app.config['TMS_TEMP_DIR'], "common_issue/%s.xls" % ('项目共性问题_' + str(date.today())))
excel_option = ExcelOptions( excel_file_path)
excel_option.export_common_issue_xls(all_record)
return '{"result": true, "filePath":"%s"}' % excel_file_path
<file_sep># -*- encoding:utf-8 -*-
"""
项目及版本信息模块
"""
from tms import db
class project_task_type(db.Model):
"""项目"""
__tablename__ = 'project_task_type'
id = db.Column(db.Integer, primary_key=True)
task_type = db.Column(db.String(20))
state = db.Column(db.Integer)
def __repr__(self):
return u"<project_task_type> %s" % self.task_type
class project_task_list(db.Model):
__tablename__ = 'project_task_list'
id = db.Column(db.Integer, primary_key=True)
task_type=db.Column(db.Integer, db.ForeignKey('project_task_type.id'))
type_1 = db.relationship('project_task_type', foreign_keys=[task_type], backref=db.backref('project_type1', order_by=id))
taskname=db.Column(db.String(50))
starttime=db.Column(db.DateTime())
endtime=db.Column(db.DateTime())
updatetime = db.Column(db.DateTime())
tdescribe=db.Column(db.String(500))
question_log=db.Column(db.String(1000))
state=db.Column(db.Integer)
tms_user=db.Column(db.Integer,db.ForeignKey('tms_user.id'))
user1 = db.relationship('User', foreign_keys=[tms_user], backref=db.backref('peoject_user1', order_by=id))
schedule=db.Column(db.Integer)
def __repr__(self):
return u"<project_mytask_page> %s" % self.taskname
<file_sep>#!/usr/bin/env python
# -*- coding: utf-8 -*-
# Created by <EMAIL> on 2016/11/24
"""
定时任务明天上午8点,将8点之前超过三天未关闭的Bug,逐条邮件通知到经办人.
三天是是从Bug创建开始到发邮件当前的时间
"""
import datetime
import sys
import time
from optparse import OptionParser
import smtplib
#多个MIME对象的集合
from email.mime.multipart import MIMEMultipart
#MIME文本对象
from email.mime.text import MIMEText
from email.header import Header
import MySQLdb
reload(sys)
sys.setdefaultencoding('utf-8')
def send_mail(to_list=[], cc_list=[], sub='', content='', appendix_file=''):
""""send_mail("<EMAIL>","sub","content")
:param to_list:发给谁
:param cc_list: 抄送给谁
:param sub:主题
:param content:内容
:param appendix_file: 附件
"""
# 设置服务器、用户名、口令及邮箱的后缀
mail_host = '192.168.10.8'
mail_user = '<EMAIL>'
mail_pass = '<PASSWORD>'
sender = "<EMAIL>"
msg_root = MIMEMultipart()
# 解决中文乱码
att_text = MIMEText(content, 'html', 'UTF-8')
# 添加邮件正文
msg_root.attach(att_text)
# 发件人
msg_root['from'] = sender
# 邮件标题
msg_root['Subject'] = Header(sub, 'utf-8')
# 设置时间
msg_root['Date'] = time.ctime(time.time())
msg_root['To'] = ';'.join(to_list)
if cc_list:
msg_root['Cc'] = ';'.join(cc_list)
# 构造附件(附件路径出现中文,会提示编码错误)
if appendix_file != '':
rar_file_path = appendix_file
att = MIMEText(open(rar_file_path, 'rb').read(), 'gbk', 'UTF-8')
att['Content-Type'] = 'application/octet-stream;name=%s' % Header(rar_file_path, 'UTF-8')
att['Content-Disposition'] = 'attachment;filename=%s' % Header(rar_file_path, 'UTF-8')
msg_root.attach(att)
# 群发邮件
smtp = smtplib.SMTP(mail_host, 25)
smtp.login(mail_user, mail_pass)
if cc_list:
smtp.sendmail(sender, [to_list, cc_list], msg_root.as_string())
else:
smtp.sendmail(sender, to_list, msg_root.as_string())
# 休眠5秒
time.sleep(5)
# 断开与服务器的连接
smtp.quit()
def get_time_out_bug(pkey, allow_date):
"""
获取指定项目中和当前时间相比超过三天的任务
:param pkey:
:param allow_date:
:return:
"""
current_time = datetime.datetime.now()
start_time = (current_time + datetime.timedelta(days=-allow_date)).strftime('%Y-%m-%d')
try:
conn = MySQLdb.connect(host='172.16.20.162', db='gms', user='tup', passwd='tup', charset='utf8')
cursor = conn.cursor()
query_sql = "SELECT issuenum, ASSIGNEE, SUMMARY, jiraissue.DESCRIPTION, CREATED, pname FROM jiraissue " \
"JOIN project ON jiraissue.PROJECT=project.ID WHERE project.pkey='{0}' " \
"AND jiraissue.issuetype !=2 AND jiraissue.issuestatus NOT IN(5,6) " \
"AND jiraissue.CREATED > '{1}'".format(pkey, start_time)
print query_sql
cursor.execute(query_sql)
bug_list = [tmp for tmp in cursor.fetchall()]
cursor.close()
conn.close()
return bug_list
except MySQLdb.Error, e:
send_mail(['<EMAIL>'], [], '查询超时Bug失败', str(e))
sys.exit(1)
def main():
des = u"JIRA Bug Timeout Email Notifier"
prog = u"JiraBugNotifier"
ver = "%prog v0.0.1"
usage = "%prog pkey timeout"
parse = OptionParser(description=des, prog=prog, version=ver, usage=usage, add_help_option=True)
parse.add_option('--pkey', action='store', type='string', dest='pkey', help="project jira key")
parse.add_option('--date', action='store', type='int', dest='allow_date', default=3, help="allow time out date")
options, args = parse.parse_args()
if not options.pkey:
print '项目JIRA Key不能为空'
sys.exit(1)
for bug in get_time_out_bug(options.pkey, options.allow_date):
print bug
if __name__ == '__main__':
main()<file_sep>
function table_init() {
var task_wording_table = $('#task_wording').DataTable({
"language": {
"aria": {
"sortAscending": ": activate to sort column ascending",
"sortDescending": ": activate to sort column descending"
},
"emptyTable": "No data available in table",
"info": "Showing _START_ to _END_ of _TOTAL_ records",
"infoEmpty": "No records found",
"infoFiltered": "(filtered1 from _MAX_ total records)",
"lengthMenu": "Show _MENU_ records",
"search": "Search:",
"zeroRecords": "No matching records found",
"paginate": {
"previous": "Prev",
"next": "Next",
"last": "Last",
"first": "First"
}
},
"bServerSide": true, // save datatable state(pagination, sort, etc) in cookie.
"bProcessing": true,
"ajax": {
url: '/task/sub_task_wording_data',
data: {
'sub_task_id': function () {
return window.sub_task_id;
}
},
},
"lengthMenu": [
[10, 50, 200],
[10, 50, 200] // change per page values here
],
// set the initial value
"pageLength": 10,
"pagingType": "bootstrap_full_number"
});
return task_wording_table;
}
var sub_task_id;
var task_wording_table;
$('#current_task tbody').on( 'click', 'tr', function () {
window.sub_task_id = $(this).attr('data');
if (task_wording_table) {
task_wording_table.ajax.reload();
} else {
task_wording_table = table_init();
}
$('#wording_title').text($(this).parent().find("td").eq(1).text()+"'s Wordings");
});
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""
从Gerrit中导所有用户进系统
"""
from MySQLdb import connect
from werkzeug.security import generate_password_hash
# 查询出现有数据
con = connect(host='192.168.33.7', db='tms', user='gms', passwd='gms', charset='utf8')
cursor = con.cursor()
user_sql = "SELECT email FROM tms_user"
cursor.execute(user_sql)
exists_user = [tmp[0].upper() for tmp in cursor.fetchall()]
cursor.close()
con.close()
print exists_user
# 导出数据
con = connect(host='192.168.10.207', db='reviewdb207', user='root', passwd='<PASSWORD>', charset='utf8')
cursor = con.cursor()
user_sql = "SELECT full_name, preferred_email FROM accounts WHERE full_name is not Null " \
"AND preferred_email is not NULL"
cursor.execute(user_sql)
records = []
for tmp in cursor.fetchall():
if tmp[1].upper() in exists_user:
print tmp[1]
else:
records.append((tmp[1].split('@')[0], generate_password_hash(tmp[1].split('@')[0]), tmp[0], tmp[1]))
cursor.close()
con.close()
# 导入数据
con = connect(host='192.168.33.7', db='tms', user='gms', passwd='gms', charset='utf8')
cursor = con.cursor()
insert_user = "INSERT into tms_user(username, password_hash, display_name, email) VALUES (%s, %s, %s, %s)"
for record in records:
print record
cursor.executemany(insert_user, [record])
con.commit()
con.close()
<file_sep># -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/9/8
"""
jira操作客户端
"""
from jira import JIRA
class JiraClient:
def __init__(self):
options = {
'server': 'http://jira.tinno.com:8080'
}
# jira client
self.jira_client = JIRA(options=options, basic_auth=('<PASSWORD>', '<PASSWORD>'))
# self.jira_client = JIRA(options=options, basic_auth=('changjie.fan', '<EMAIL>'))
def create_issue(self, project_key, task_key, comment):
"""
判断项目是否有共性问题模块,没有的创建
:param project_key: 项目jira_key
:param task_key: 原问题key
:param comment:备注中填写解决方案
:return:
"""
# 源问题及问题所属模块
src_issue = self.jira_client.issue(task_key)
src_issue_fields = src_issue.fields
src_issue_component_names = [component.name for component in src_issue_fields.components]
# 目的项目
dst_project = self.jira_client.project(project_key)
# 目的项目所有的模块
dst_issue_component_names = {}
for component in dst_project.components:
dst_issue_component_names.update({component.name: component})
dst_issue_component_ids = []
# 判断目的项目是否有共性问题模块,没有就创建
if u'共性问题' in dst_issue_component_names.keys():
dst_issue_component_ids.append(dst_issue_component_names[u'共性问题'])
else:
component = self.jira_client.create_component('共性问题', dst_project)
dst_issue_component_names.update({component.name: component})
dst_issue_component_ids.append(component)
# 挑选出源问题所在的模块,用于新问题的创建(新问题按源问题模块创建,并在共性问题模块下创建)
for src_name in src_issue_component_names:
if src_name in dst_issue_component_names.keys():
dst_issue_component_ids.append(dst_issue_component_names[src_name])
else:
component = self.jira_client.create_component(src_name, dst_project)
dst_issue_component_names.update({component.name: component})
dst_issue_component_ids.append(component)
print dst_issue_component_ids
dst_issue_dict = {
'project': {'key': project_key},
'summary': u'%s' % src_issue_fields.summary,
'description': u'%s' % src_issue_fields.description,
'issuetype': {'id': src_issue_fields.issuetype.id},
'components': [{'id': tmp.id } for tmp in dst_issue_component_ids],
'priority': {'id': src_issue_fields.priority.id}
}
print dst_issue_dict
# 创建问题
dst_issue = self.jira_client.create_issue(fields=dst_issue_dict)
# 添加问题备注填写解决方案
self.jira_client.add_comment(dst_issue.key, comment)
# 创建问题连接
self.jira_client.create_issue_link('Copy', src_issue, dst_issue)
# 将影响项目的jira负责人作为任务经办人分配
project = self.jira_client.project(project_key)
self.jira_client.assign_issue(dst_issue, project.lead.name)
print dir(project.lead)
print dir(dst_issue.fields)
return dst_issue.key, <EMAIL>" % project.lead.name, project.lead.displayName, project.name
def get_issue_info(self, jira_key):
issue = self.jira_client.issue(jira_key)
return issue.fields.summary, issue.fields.description
def delete_task(self, jira_key):
issue = self.jira_client.issue(jira_key)
issue.delete()
if __name__ == '__main__':
# jira_clent = JiraClient()
# print jira_clent.create_issue('SCM', 'PUBLIC-33', 'repo 2path:package/ap.git')
# JiraClient.create_issue('SCM', "PUBISSUE-1456")
# jira_client = JiraClient()
# print jira_client.get_issue_info('SCM-4')
pass
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
from tms import db
class RepositoryServer(db.Model):
"""
Git 服务器
"""
__tablename__ = 'repo_server'
id = db.Column(db.Integer, primary_key=True)
# 服务器名称
name = db.Column(db.String(64))
# 服务器ip地址
ip_address = db.Column(db.String(15))
# 服务器所有仓库的根目录, 通常是/home/git/repositories 主要在gerrit上可能会有不同
root_path = db.Column(db.String(200), default='/home/git/repositories')
# 在备份服务器上的地址
backup_server_path = db.Column(db.String(200))
# 克隆是的:前面的部分,主要也是为了兼容gerrit 通常是git@ip gerrit是ssh://ip:29418
repo_url = db.Column(db.String(200))
# 服务器状态 True表示正常, False表示停用
status = db.Column(db.Boolean, default=True)
# config的中远程名称
remote_name = db.Column(db.String(64))
def __repr__(self):
return "<RepoServer %r>" % self.name
class Platform(db.Model):
"""
仓库平台
"""
__tablename__ = 'repo_platform'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(500))
path = db.Column(db.String(700))
description = db.Column(db.String(200))
server_id = db.Column(db.Integer, db.ForeignKey('repo_server.id'))
server = db.relationship('RepositoryServer', backref=db.backref('repo_platform', order_by=id))
# 平台状态 True表示正常, False表示停用
status = db.Column(db.Boolean, default=True)
def __repr__(self):
return "<RepoPlatform %r>" % self.name
class Repository(db.Model):
"""
Git仓库
"""
__tablename__ = 'repo_repo'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(500))
path = db.Column(db.String(700))
# 1 project_code 2 app code 3 app release 4 manifest
category = db.Column(db.Integer, default=1)
description = db.Column(db.String(200))
repo_url = db.Column(db.String(300))
platform_id = db.Column(db.Integer, db.ForeignKey('repo_platform.id'))
platform = db.relationship('Platform', backref=db.backref('repo_repo', order_by=id))
# 仓库状态 True表示正常, False表示停用
status = db.Column(db.Boolean, default=True)
def __repr__(self):
return "<Repo %r>" % self.name
class Reference(db.Model):
"""
Git 仓库的应用表/分支或tag
"""
__tablename__ = 'repo_refs'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(200))
# 0 表示tag, 1表示分支
category = db.Column(db.Integer, default=0)
# 0 表示正常, 1 表示已删除
status = db.Column(db.Integer, default=0)
# 删除此引用的记录id
push_id = db.Column(db.Integer, nullable=True)
repository_id = db.Column(db.Integer, db.ForeignKey('repo_repo.id'))
repository = db.relationship('Repository', backref=db.backref('refs', order_by=id))
def __repr__(self):
return "<Reference %r>" % self.name
class Commit(db.Model):
"""
Git Commit 记录信息
"""
__tablename__ = 'repo_commit'
id = db.Column(db.Integer, primary_key=True)
# commit id的hash值
commit_hash = db.Column(db.String(40))
# 父commit的hash值,多个值用空格隔开
parent_hash = db.Column(db.String(200), nullable=True)
# tree对象
tree_hash = db.Column(db.String(40), nullable=True)
author_name = db.Column(db.String(64), nullable=True)
author_email = db.Column(db.String(64), nullable=True)
author_time = db.Column(db.DateTime, nullable=True)
committer_name = db.Column(db.String(64))
committer_email = db.Column(db.String(64))
commit_time = db.Column(db.DateTime)
message = db.Column(db.String(500), nullable=True)
repository_id = db.Column(db.Integer, db.ForeignKey('repo_repo.id'))
repository = db.relationship('Repository', backref=db.backref('repo_refs', order_by=id))
def __repr__(self):
return "<Commit %r>" % self.commit_hash
# 用户和组的多对多
repo_user_group = db.Table('repo_user_group', db.metadata,
db.Column('user_id', db.Integer, db.ForeignKey('repo_user.id')),
db.Column('group_id', db.Integer, db.ForeignKey('repo_group.id')))
# 用户和服务器的多对多
repo_user_server = db.Table('repo_user_server', db.metadata,
db.Column('user_id', db.Integer, db.ForeignKey('repo_user.id')),
db.Column('server_id', db.Integer, db.ForeignKey('repo_server.id')))
class GitUser(db.Model):
"""
Git 用户
"""
__tablename__ = 'repo_user'
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(64))
email_address = db.Column(db.String(64), unique=True)
ssh_key = db.Column(db.String(300))
# 用户状态True正常, False停用
activate = db.Column(db.Boolean, default=True)
group = db.relationship('GitUserGroup', secondary='repo_user_group', backref='repo_user')
server = db.relationship('RepositoryServer', secondary='repo_user_server', backref='repo_user')
def __repr__(self):
return "<GitUser %r>" % self.username
# 用户组和服务器的多对多
repo_group_server = db.Table('repo_group_server', db.metadata,
db.Column('group_id', db.Integer, db.ForeignKey('repo_group.id')),
db.Column('server_id', db.Integer, db.ForeignKey('repo_server.id')))
class GitUserGroup(db.Model):
"""
Git 用户组
1. 管理员组有最大的权限
2. read读权限
3。 write 写权限
"""
__tablename__ = 'repo_group'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(64), unique=True)
description = db.Column(db.String(300))
# 用户组状态True正常, False停用
status = db.Column(db.Boolean, default=True)
# 用户组可以在多台服务器上存在
server = db.relationship('RepositoryServer', secondary='repo_group_server', backref='repo_group')
def __repr__(self):
return "<GitUserGroup %r>" % self.name
class Permission(db.Model):
"""
Git仓库或平台权限
"""
__tablname__ = 'repo_permission'
id = db.Column(db.Integer, primary_key=True)
rights = db.Column(db.Text)
repository_id = db.Column(db.Integer, db.ForeignKey('repo_repo.id'))
repository = db.relationship('Repository', backref=db.backref('repo_permission', order_by=id))
platform_id = db.Column(db.Integer, db.ForeignKey('repo_platform.id'))
platform = db.relationship('Platform', backref=db.backref('repo_permission', order_by=id))
server_id = db.Column(db.Integer, db.ForeignKey('repo_server.id'))
server = db.relationship('RepositoryServer', backref=db.backref('repo_permission', order_by=id))
def __repr__(self):
return "<Permission %r>" % ('permission:'+self.id)
class PushHistory(db.Model):
"""
developer推送的历史记录
"""
__tablename__ = 'repo_push_history'
id = db.Column(db.Integer, primary_key=True)
# 当前Git服务器的Ip地址
server_ip = db.Column(db.String(15))
# Git仓库的完整路径
repository_path = db.Column(db.String(500))
# 推送的Tag
reference_name = db.Column(db.String(255))
# 更新的commits
commits = db.Column(db.Text)
# 推送时间
push_time = db.Column(db.DateTime())
# 推送人
push_user = db.Column(db.String(64))
# 推送类型 new branch/new tag/delete branch/delete tag/ update
push_type = db.Column(db.String(64))
# command
command = db.Column(db.String(255))
# 本次push所有commit的diff信息
diff_info = db.Column(db.Text)
# 处理状态0 表示未同步备份服务器,1表示已同步到备份服务器,2表示处理出错了
status = db.Column(db.Integer, default=0)
# 处理出错的信息
error_info = db.Column(db.String(200))
# 备注信息
note = db.Column(db.String(200))
# 备份时间
backup_time = db.Column(db.DateTime())
# commit和reference信息加入数据库时间
import_time = db.Column(db.DateTime())
# 实时备份状态 0表示未实时备份, 1 表示已实时备份
sync_status = db.Column(db.Integer, default=0)
# 实时备份的时间
sync_time = db.Column(db.DateTime)
def __repr__(self):
return '<PushHistory %r>' % self.reference_name
class PullHistory(db.Model):
"""
developer pull和clone历史记录
"""
__tablename__ = 'repo_pull_history'
id = db.Column(db.Integer, primary_key=True)
# 当前Git服务器的Ip地址
server_ip = db.Column(db.String(15))
# Git仓库的完整路径
repository_path = db.Column(db.String(500))
# 下载时间
pull_time = db.Column(db.DateTime())
# 下载人
pull_user = db.Column(db.String(64))
# 处理出错的信息
error_info = db.Column(db.String(200))
# 备注信息
note = db.Column(db.String(200))
def __repr__(self):
return '<PullHistory %r>' % self.reference_name
class GitServeBackupLog(db.Model):
"""Git服务器备份记录"""
__tablename__ = 'repo_serve_backup_log'
id = db.Column(db.Integer, primary_key=True)
# 备份Git服务器的ip地址
server_ip = db.Column(db.String(15))
# 平台路径
platform_path = db.Column(db.String(200))
# 备份完成时间
backup_time = db.Column(db.DateTime)
# 备份结果
result = db.Column(db.Boolean)
# 备份日志
info = db.Column(db.Text)
def __repr__(self):
return '<GitServeBackupLog %r>' % self.backup_time<file_sep>/**
* Created by changjie.fan on 2016/5/6.
*/
var is_all = false;
var test_case_table = $('#test_case_table').DataTable({
"language": {
"aria": {
"sortAscending": ": activate to sort column ascending",
"sortDescending": ": activate to sort column descending"
},
"emptyTable": "No data available in table",
"info": "Showing _START_ to _END_ of _TOTAL_ records",
"infoEmpty": "No records found",
"infoFiltered": "(filtered1 from _MAX_ total records)",
"lengthMenu": "Show _MENU_ records",
"search": "Search:",
"zeroRecords": "No matching records found",
"paginate": {
"previous": "Prev",
"next": "Next",
"last": "Last",
"first": "First"
}
},
"bFilter": false,
"bServerSide": true, // save datatable state(pagination, sort, etc) in cookie.
"bProcessing": true,
"ajax": {
url: '/qa/test_case_data',
data: {
is_all: function () {
return window.is_all;
}
},
},
"columns": [
{"data": 'index'},
{"data": 'number'},
{"data": 'level'},
{"data": 'test_type'},
{"data": 'module_name'},
{"data": 'standard_sdk'},
{"data": 'class_name'},
{"data": 'method_name'}
],
"lengthMenu": [
[10, 50, 200],
[10, 50, 200] // change per page values here
],
// set the initial value
"pageLength": 10,
"pagingType": "bootstrap_full_number"
});
function all_test_case() {
is_all = true;
test_case_table.ajax.reload();
}
$('#test_case_table tbody').on( 'dblclick', 'tr', function () {
$(this).toggleClass('selected');
window.location.href=$SCRIPT_ROOT + '/qa/edit_test_case/'+test_case_table.row($(this)).data().id;
});
$("#test_case_table tr").live({
mouseover:function(){
$(this).css("cursor","pointer");
}
});<file_sep>#!/usr/bin/env python
# -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/8/25
"""
"""
from tms import db
class BPlatform(db.Model):
"""厂商平台"""
__tablename__ = 'bs_platform'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(64))
# True正常、False取消
flag = db.Column(db.Boolean, default=True)
# 备注
note = db.Column(db.String(300))
def __repr__(self):
return '<Platform %r>' % self.name<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
from . import build
from flask.ext.login import login_user, logout_user, login_required, current_user
from flask import render_template, redirect, url_for, request, flash, current_app, session, send_file
from tms.project.androidProjectModules import *
from tms.auth.models import User, Role
from tms.build.models import *
@build.route('/module_compile_para_page')
@login_required
def module_compile_para_page():
"""
模块编译参数
:return:
"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '模块编译参数', 'url': url_for('build.module_compile_para_page')})
session['menu_path'] = ['编译管理', '模块编译参数']
query_type = 0
# 模块编译参数
modules = Module.query.order_by(Module.platform_id.asc()).all()
result = []
index = 1
for module in modules:
result.append((index, module))
index += 1
return render_template('build/module_compile_para_page.html', module_paras=result,query_type=query_type)
@build.route('/compile_mode_page')
@login_required
def compile_mode_page():
"""
编译模式
:return:
"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '模块编译参数', 'url': url_for('build.module_compile_para_page')})
session['menu_path'] = ['编译管理', '模块编译参数']
query_type = 1
# 模块编译参数
modules = CompileMode.query.all()
result = []
index = 1
for module in modules:
result.append((index, module))
index += 1
return render_template('build/module_compile_para_page.html', module_paras=result,query_type=query_type)
@build.route('/compile_order_page')
@login_required
def compile_order_page():
"""
编译命令
:return:
"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '模块编译参数', 'url': url_for('build.module_compile_para_page')})
session['menu_path'] = ['编译管理', '模块编译参数']
query_type = 2
# 模块编译参数
modules = CompileOrder.query.all()
result = []
index = 1
for module in modules:
result.append((index, module))
index += 1
return render_template('build/module_compile_para_page.html', module_paras=result,query_type=query_type)
@build.route('/compile_type_page')
@login_required
def compile_type_page():
"""
编译类型
:return:
"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '模块编译参数', 'url': url_for('build.module_compile_para_page')})
session['menu_path'] = ['编译管理', '模块编译参数']
query_type = 3
# 模块编译参数
modules = CompileType.query.all()
result = []
index = 1
for module in modules:
result.append((index, module))
index += 1
return render_template('build/module_compile_para_page.html', module_paras=result,query_type=query_type)
@build.route('/platform_page')
@login_required
def platform_page():
"""
平台名称
:return:
"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '模块编译参数', 'url': url_for('build.module_compile_para_page')})
session['menu_path'] = ['编译管理', '模块编译参数']
query_type = 4
# 模块编译参数
modules = PlatForm.query.all()
result = []
index = 1
for module in modules:
result.append((index, module))
index += 1
return render_template('build/module_compile_para_page.html', module_paras=result,query_type=query_type)
@build.route('/add_module_compile_para_page',methods=['GET', 'POST'])
@login_required
def add_module_compile_para_page():
"""
添加模块编译参数
:return:
"""
if request.method == 'GET':
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '模块编译参数', 'url': url_for('build.module_compile_para_page')})
session['menu_path'] = ['编译管理', '模块编译参数']
record = {}
operation_type = ['添加模块信息','添加平台信息','添加编译类型信息','添加编译命令信息','添加编译模式信息']
platforms = PlatForm.query.all()
return render_template('build/add_module_compile_para_page.html',record=record,operation_type=operation_type,platforms=platforms)
else:
operation_type = request.form['operation_type']
if operation_type == '添加模块信息':
pro_name = request.form['pro_name'].strip()
module_name = request.form['module_name'].strip()
platform_id = request.form['platform'].strip()
command = request.form['command'].strip().strip('\n')
path = request.form['path'].strip()
exists_module = Module.query.filter(Module.platform_id == platform_id,Module.module_name == module_name,Module.pro_name == pro_name).first()
if exists_module is not None:
flash(u'相同模块的命令"{0}"已存在'.format(exists_module.module_name), 'danger')
else:
module = Module()
module.module_name = module_name.strip()
module.platform_id = platform_id
module.pro_name = pro_name.strip()
module.command = command.strip().strip('\n')
module.path = path.strip()
db.session.add(module)
db.session.commit()
flash(u'新增成功!', 'info')
return redirect(url_for('build.module_compile_para_page'))
elif operation_type == '添加平台信息':
name = request.form['name'].strip()
exists_module = PlatForm.query.filter(PlatForm.name == name).first()
if exists_module is not None:
flash(u'相同名称的平台"{0}"已存在'.format(exists_module.name), 'danger')
else:
module = PlatForm()
module.name = name.strip()
db.session.add(module)
db.session.commit()
flash(u'新增成功!', 'info')
return redirect(url_for('build.module_compile_para_page'))
elif operation_type == '添加编译类型信息':
name = request.form['name'].strip()
exists_module = CompileType.query.filter(CompileType.compile_type == name).first()
if exists_module is not None:
flash(u'相同名称的编译类型"{0}"已存在'.format(exists_module.compile_type), 'danger')
else:
module = CompileType()
module.compile_type = name.strip()
db.session.add(module)
db.session.commit()
flash(u'新增成功!', 'info')
return redirect(url_for('build.module_compile_para_page'))
elif operation_type == '添加编译命令信息':
name = request.form['name'].strip()
exists_module = CompileOrder.query.filter(CompileOrder.compile_order == name).first()
if exists_module is not None:
flash(u'相同名称的编译命令"{0}"已存在'.format(exists_module.compile_order), 'danger')
else:
module = CompileOrder()
module.compile_order = name.strip()
db.session.add(module)
db.session.commit()
flash(u'新增成功!', 'info')
return redirect(url_for('build.module_compile_para_page'))
elif operation_type == '添加编译模式信息':
name = request.form['name'].strip()
exists_module = CompileMode.query.filter(CompileMode.compile_mode == name).first()
if exists_module is not None:
flash(u'相同名称的编译模式"{0}"已存在'.format(exists_module.compile_mode), 'danger')
else:
module = CompileMode()
module.compile_mode = name.strip()
db.session.add(module)
db.session.commit()
flash(u'新增成功!', 'info')
return redirect(url_for('build.module_compile_para_page'))
@build.route('/edit_module_compile_para_page/<string:id>',methods=['GET', 'POST'])
@login_required
def edit_module_compile_para_page(id):
"""
编辑模块编译参数
:return:
"""
if request.method == 'GET':
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '模块编译参数', 'url': url_for('build.module_compile_para_page')})
session['menu_path'] = ['编译管理', '模块编译参数']
if str(id).startswith('compile_mode_'):
#编译模式
array = str(id).split('_')
db_id = array[len(array) - 1]
compileMode = CompileMode.query.filter(CompileMode.id == db_id).first()
module = {}
module['id'] = compileMode.id
module['name'] = compileMode.compile_mode
query_type = 1
return render_template('build/edit_module_compile_para_page.html',query_type=query_type,module=module)
elif str(id).startswith('compile_order_'):
#编译命令
array = str(id).split('_')
db_id = array[len(array) - 1]
compileOrder = CompileOrder.query.filter(CompileOrder.id == db_id).first()
module = {}
module['id'] = compileOrder.id
module['name'] = compileOrder.compile_order
query_type = 2
return render_template('build/edit_module_compile_para_page.html',query_type=query_type,module=module)
elif str(id).startswith('compile_type_'):
#编译类型
array = str(id).split('_')
db_id = array[len(array) - 1]
compileType = CompileType.query.filter(CompileType.id == db_id).first()
module = {}
module['id'] = compileType.id
module['name'] = compileType.compile_type
query_type = 3
return render_template('build/edit_module_compile_para_page.html',query_type=query_type,module=module)
elif str(id).startswith('platform_'):
#平台
array = str(id).split('_')
db_id = array[len(array) - 1]
platForm = PlatForm.query.filter(PlatForm.id == db_id).first()
module = {}
module['id'] = platForm.id
module['name'] = platForm.name
query_type = 4
return render_template('build/edit_module_compile_para_page.html',query_type=query_type,module=module)
elif str(id).startswith('module_name_'):
#模块--命令路径
array = str(id).split('_')
db_id = array[len(array) - 1]
module = Module.query.filter(Module.id == db_id).first()
platForms = PlatForm.query.all()
query_type = 5
return render_template('build/edit_module_compile_para_page.html',query_type=query_type,module=module,platForms=platForms)
else:
query_type = request.form['query_type']
if query_type == '1':
#compileMode
name = request.form['name'].strip()
exists_module = CompileMode.query.filter(CompileMode.id != id,CompileMode.compile_mode == name).first()
if exists_module is not None:
flash(u'相同编译模式"{0}"已存在'.format(exists_module.compile_mode), 'danger')
else:
module = CompileMode.query.filter(CompileMode.id == id).first()
module.compile_mode = name
db.session.add(module)
db.session.commit()
flash(u'修改成功!', 'info')
return redirect(url_for('build.module_compile_para_page'))
elif query_type == '2':
#CompileOrder
name = request.form['name'].strip()
exists_module = CompileOrder.query.filter(CompileOrder.id != id,CompileOrder.compile_order == name).first()
if exists_module is not None:
flash(u'相同编译命令"{0}"已存在'.format(exists_module.compile_order), 'danger')
else:
module = CompileOrder.query.filter(CompileOrder.id == id).first()
module.compile_order = name
db.session.add(module)
db.session.commit()
flash(u'修改成功!', 'info')
return redirect(url_for('build.module_compile_para_page'))
elif query_type == '3':
#CompileType
name = request.form['name'].strip()
exists_module = CompileType.query.filter(CompileType.id != id,CompileType.compile_type == name).first()
if exists_module is not None:
flash(u'相同编译类型"{0}"已存在'.format(exists_module.compile_type), 'danger')
else:
module = CompileType.query.filter(CompileType.id == id).first()
module.compile_type = name
db.session.add(module)
db.session.commit()
flash(u'修改成功!', 'info')
return redirect(url_for('build.module_compile_para_page'))
elif query_type == '4':
#PlatForm
name = request.form['name'].strip()
exists_module = PlatForm.query.filter(PlatForm.id != id,PlatForm.name == name).first()
if exists_module is not None:
flash(u'相同平台名称"{0}"已存在'.format(exists_module.name), 'danger')
else:
module = PlatForm.query.filter(PlatForm.id == id).first()
module.name = name
db.session.add(module)
db.session.commit()
flash(u'修改成功!', 'info')
return redirect(url_for('build.module_compile_para_page'))
elif query_type == '5':
#module_name
pro_name = request.form['pro_name'].strip()
module_name = request.form['module_name'].strip()
platform_id = request.form['platform'].strip()
command = request.form['command'].strip().strip('\n')
path = request.form['path'].strip()
exists_module = Module.query.filter(Module.id != id,Module.module_name == module_name,Module.pro_name == pro_name,Module.platform_id == platform_id).first()
if exists_module is not None:
flash(u'相同项目名称,模块名称的模块"{0}"已存在'.format(exists_module.module_name), 'danger')
else:
module = Module.query.filter(Module.id == id).first()
module.module_name = module_name.strip()
module.platform_id = platform_id
module.pro_name = pro_name.strip()
module.command = command.strip().strip('\n')
module.path = path.strip()
db.session.add(module)
db.session.commit()
flash(u'修改成功!', 'info')
return redirect(url_for('build.module_compile_para_page'))
@build.route('/edit_module_compile_para_info/<int:id>/<int:query_type>')
@login_required
def edit_module_compile_para_info(id,query_type):
"""
编辑模块编译参数
:return:
"""
if request.method == 'GET':
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '模块编译参数', 'url': url_for('build.module_compile_para_page')})
session['menu_path'] = ['编译管理', '模块编译参数']
if query_type == 1:
#编译模式
compileMode = CompileMode.query.filter(CompileMode.id == id).first()
module = {}
module['id'] = compileMode.id
module['name'] = compileMode.compile_mode
query_type = 1
return render_template('build/edit_module_compile_para_page.html',query_type=query_type,module=module)
elif query_type == 2:
#编译命令
compileOrder = CompileOrder.query.filter(CompileOrder.id == id).first()
module = {}
module['id'] = compileOrder.id
module['name'] = compileOrder.compile_order
query_type = 2
return render_template('build/edit_module_compile_para_page.html',query_type=query_type,module=module)
elif query_type == 3:
#编译类型
compileType = CompileType.query.filter(CompileType.id == id).first()
module = {}
module['id'] = compileType.id
module['name'] = compileType.compile_type
query_type = 3
return render_template('build/edit_module_compile_para_page.html',query_type=query_type,module=module)
elif query_type == 4:
#平台
platForm = PlatForm.query.filter(PlatForm.id == id).first()
module = {}
module['id'] = platForm.id
module['name'] = platForm.name
query_type = 4
return render_template('build/edit_module_compile_para_page.html',query_type=query_type,module=module)
elif query_type == 5:
#模块--命令路径
module = Module.query.filter(Module.id == id).first()
platForms = PlatForm.query.all()
query_type = 5
return render_template('build/edit_module_compile_para_page.html',query_type=query_type,module=module,platForms=platForms)
<file_sep>#!/usr/bin/env python
# -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""
Gerrit 钩子
1. 检查提交说明
2. 记录push信息,进行实时同步
"""
import os
import sys
from optparse import OptionParser
import commands
import re
import time
import simplejson as json
import MySQLdb
from MySQLdb import connect
from post_updated import get_ip_address, push_pubisher, send_mail
reload(sys)
sys.setdefaultencoding('utf-8')
# myos配置数据库信息
db_host = '192.168.33.7'
db_user = 'tup'
db_passwd = 'tup'
db_name = 'tms'
# tsds配置数据库信息
jira_db_host = '172.16.5.95'
jira_db_name = 'jiradb6'
jira_db_user = 'jirauser'
jira_db_passwd = '<PASSWORD>'
def check_message_format(rp, msg):
"""检查提交说明格式和JIRA_KEY的有效性
:param rp: 检查提交说明格式的正则表达式
:param msg: 提交说明
"""
m = rp.match(msg)
format_error = False
key_error = False
if m:
task_key = m.groups()[1]
if task_key:
conn = connect(host=jira_db_host, db=jira_db_name, user=jira_db_user, passwd=jira_db_passwd, charset='utf8')
cursor = conn.cursor()
check_sql = "select issue.issuenum, issue.SUMMARY from jiraissue issue join project pro where " \
"issue.issuenum='%s' and issue.project=pro.id and pro.pkey='%s'" % (task_key.split('-')[1],
task_key.split('-')[0])
cursor.execute(check_sql)
row = cursor.fetchone()
if not row or not row[0]:
key_error = True
cursor.close()
conn.close()
else:
format_error = True
return format_error, key_error
def check_message(repo_path, old_rev, new_rev):
"""
检查提交说明的格式
JIRA key的真实性
JIRA title的完整性
"""
error_format_message = []
error_key_message = []
has_error = False
pattern = r'^<(REQ|BUG|FUNC|DEBUG|PATCH|BASELINE|MERGE)><([A-Z]{2,}\-\d+)><(.+)>$'
rp = re.compile(pattern)
if old_rev == '0000000000000000000000000000000000000000' or new_rev == '0000000000000000000000000000000000000000':
return True
else:
status, messages = commands.getstatusoutput('git --git-dir={0} log {1}..{2} '
'--pretty=format:"%s%b"'.format(repo_path, old_rev, new_rev))
if status:
print messages
sys.exit(1)
else:
messages = messages.split('\n')
for message in messages:
if message.startswith('Merge') or not message.strip():
continue
if message.find('Change-Id:') > 0:
message = message.split('Change-Id:')[0]
format_error, key_error = check_message_format(rp, message)
if format_error:
error_format_message.append(message)
if key_error:
error_key_message.append(message)
if error_format_message:
print '\n'.join(
[
'=' * 100,
"\033[43;31;1m推送失败\033[0m",
"如下提交说明格式书写出错了",
'\033[43;31;1m' + '\n'.join(error_format_message) + '\033[0m',
"\033[34m正确的格式是: <REQ|BUG|FUNC|DEBUG|PATCH|BASELINE|MERGE><Jira Key值><提交TAG:描述>\033[0m",
"\033[32m示例:<REQ><SQZ-22><S9201B_FLY_RU_4.2_V1.1:TP更新>\033[0m",
"\033[32m示例:<BUG><SZQ-114><S9201B_4.2_V1.1:TP无法使用和升级问题更新>\033[0m",
'=' * 100
])
has_error = True
if error_key_message:
print '\n'.join(
[
'=' * 100,
"\033[43;31;1m推送失败\033[0m\nJIRA_KEY不存在\n",
'\033[34m如下提交说明中的JIRA_KEY在JIRA数据库中不存在,请填写真实有效的JIRA_KEY\033[0m',
'\033[43;31;1m' + '\n'.join(error_key_message) + '\033[0m',
'=' * 100
])
has_error = True
return not has_error
def repository_push_log(git_path, ref_name, old_rev, new_rev, local_ip, uploader):
"""推送日志记入数据库中便于tsds解析
"""
date_format = '%Y-%m-%d %H:%M:%S'
now = time.strftime(date_format, time.localtime())
commits = []
if ref_name.find('/for/') > 0:
push_type = 'change push'
else:
push_type = 'update_reference'
if old_rev == '0000000000000000000000000000000000000000' or new_rev == '0000000000000000000000000000000000000000':
status, messages = commands.getstatusoutput('git --git-dir={0} log {1} -1 '
'--pretty=format:%H'.format(git_path, new_rev))
else:
status, messages = commands.getstatusoutput('git --git-dir={0} log {1}..{2} '
'--pretty=format:%H'.format(git_path, old_rev, new_rev))
commits = [tmp.strip() for tmp in messages.split()]
try:
conn = connect(host=db_host, db=db_name, user=db_user, passwd=<PASSWORD>)
cursor = conn.cursor()
sql = "INSERT INTO repo_push_history (server_ip, repository_path, reference_name, commits, push_time, " \
"push_user, push_type, status) VALUES('%s', '%s', '%s', '%s', '%s', '%s', '%s', 0 )" % \
(local_ip, git_path, ref_name, '/'.join(commits), now, uploader, push_type)
cursor.execute(sql)
conn.commit()
cursor.close()
conn.close()
except MySQLdb.Error, e:
send_mail(["<EMAIL>"], "Tup数据库记录Push history失败_{0}".format(local_ip),
'git仓库路径:{0}<br>git分支:{1}<br>更新时间:{2}<br>出错信息:{3}'.format(git_path, ref_name, now, e))
def main():
des = u"gerrit ref update hooks"
prog = u"ref-update"
ver = "%prog v0.0.1"
usage = "%prog ref-update --project <project name> --refname<refname> --uploader<uploader> --oldrev<shal> --newrev<shal>"
p = OptionParser(description=des, prog=prog, version=ver, usage=usage, add_help_option=True)
p.add_option('--project', action='store', type='string', dest='project', help="Gerrit project name")
p.add_option('--refname', action='store', type='string', dest='refname', help="git reference name")
p.add_option('--uploader', action='store', type='string', dest='uploader', help="Gerrit user and email")
p.add_option('--oldrev', action='store', type='string', dest='oldrev', help="old rev has")
p.add_option('--newrev', action='store', type='string', dest='newrev', help="new rev has")
option, args = p.parse_args()
repository = '/home/android/review-site/repositories/'
repo_path = option.project
git_path = os.path.join(repository,repo_path) + '.git'
old_rev = option.oldrev
new_rev = option.newrev
ref_name = option.refname
uploader = option.uploader
local_ip = get_ip_address('eth0')
if local_ip:
local_ip = get_ip_address('eth1')
# 检查提交说明信息
result = check_message(git_path, old_rev, new_rev)
if result:
# 添加push数据留着所实时备份
repository_push_log(git_path, ref_name, old_rev, new_rev, local_ip, uploader)
# 提交信息加入到rabbitmq队列中,等待后续处理
if old_rev != '0' * 40 and new_rev != '0' * 40:
message = {
'old_rev': old_rev,
'new_rev': new_rev,
'ref_name': ref_name,
'repo_path': repo_path,
'uploader': uploader,
'local_ip': local_ip
}
push_pubisher(json.dumps(message))
else:
sys.exit(1)
if __name__ == '__main__':
main()
<file_sep>/**
* Created by changjie.fan on 2016/7/12.
*/
// 项目JIRA任务
var android_project_jira_table = $('#android_project_jira_table').DataTable({
//dom: "Bfrtip",
"language": {
"aria": {
"sortAscending": ": activate to sort column ascending",
"sortDescending": ": activate to sort column descending"
},
"emptyTable": "No data available in table",
"info": "Showing _START_ to _END_ of _TOTAL_ records",
"infoEmpty": "No records found",
"infoFiltered": "(filtered1 from _MAX_ total records)",
"lengthMenu": "Show _MENU_ records",
"search": "Search:",
"zeroRecords": "No matching records found",
"paginate": {
"previous": "Prev",
"next": "Next",
"last": "Last",
"first": "First"
}
},
"bServerSide": true, // save datatable state(pagination, sort, etc) in cookie.
"bProcessing": true,
"ajax": {
url: '/project/project_code_jira_data',
data: {
'project_code_id': function () {
return $('#project_code_id').val();
},
'search_type': function(){
return $('#task_search_category').val();
},
'search_text': function(){
return $('#task_search_text').val();
}
}
},
"columns": [
{
'targets': 0,
'searchable': false,
'orderable': false,
'render': function (data, type, full, meta) {
return '<div class="checker"><span ><input type="checkbox" checked></span></div>';
}
},
{"data": 'index'},
{"data": 'task_key'},
{"data": 'summary'},
{"data": 'description'},
{"data": 'category'}
],
"fnRowCallback": function( nRow, aData, iDisplayIndex, iDisplayIndexFull ) {
if (aData.category == 'Critical') {
$('td', nRow).css('background-color', 'Orange');
}
},
"lengthMenu": [
[5, 20, 100, 200, 500],
[5, 20, 100, 200, 500] // change per page values here
],
// set the initial value
"pageLength": 5,
"pagingType": "bootstrap_full_number"
});
//任务查询
function task_search(){
$('#android_project_jira_table').DataTable().ajax.reload();
}
// 显示jira任务详细信息
$('#android_project_jira_table').on( 'dblclick', 'tr', function () {
var row = android_project_jira_table.row($(this)).data();
window.location.href = "/project/project_branch_task_detail/" + row.id;
});
//jira 任务导出Excel
function export_task_excel(){
var task_ids = new Array();
$('#android_project_jira_table td span').each(function () {
if ($(this).attr('class') && $(this).attr('class') == 'checked') {
task_ids.push(android_project_jira_table.row($($($(this).parent()).parent()).parent()).data().id);
}
});
if (task_ids.length == 0){
bootbox.alert('请至少选中一条记录');
}else {
$.ajax({
url: '/project/jira_task_export_excel',
type: 'POST',
data: {'task_ids': task_ids.join(',')},
success: function (data) {
window.location.href = '/auth/download_file/?file_path=' + data;
}
});
}
}
// select all
$('#task_select_all').on('click', function () {
var rows = android_project_jira_table.rows({'search': 'applied'}).nodes();
if ($('#task_select_all').parent().attr('class') == 'checked') {
$('input[type="checkbox"]', rows).each(function () {
$(this).parent().removeClass("checked");
});
} else {
$('input[type="checkbox"]', rows).each(function () {
$(this).parent().addClass("checked");
});
}
});
// 行选择
$('#android_project_jira_table tbody').on('change', 'input[type="checkbox"]', function () {
if($(this).parent().attr('class') == 'checked'){
$('#task_select_all').parent().removeClass("checked");
$(this).parent().removeClass("checked");
} else {
$(this).parent().addClass("checked");
}
});
// 提交commit
var android_project_commit_table = $('#android_project_commit_table').DataTable({
//dom: "Bfrtip",
"language": {
"aria": {
"sortAscending": ": activate to sort column ascending",
"sortDescending": ": activate to sort column descending"
},
"emptyTable": "No data available in table",
"info": "Showing _START_ to _END_ of _TOTAL_ records",
"infoEmpty": "No records found",
"infoFiltered": "(filtered1 from _MAX_ total records)",
"lengthMenu": "Show _MENU_ records",
"search": "Search:",
"zeroRecords": "No matching records found",
"paginate": {
"previous": "Prev",
"next": "Next",
"last": "Last",
"first": "First"
}
},
"bServerSide": true, // save datatable state(pagination, sort, etc) in cookie.
"bProcessing": true,
"ajax": {
url: '/project/project_code_commit_data',
data: {
'project_code_id': function () {
return $('#project_code_id').val();
},
'search_type': function(){
return $('#commit_search_category').val();
},
'search_text': function(){
return $('#commit_search_text').val();
}
}
},
"columns": [
{
'targets': 0,
'searchable': false,
'orderable': false,
'render': function (data, type, full, meta) {
return '<div class="checker"><span ><input type="checkbox" checked></span></div>';
}
},
{"data": 'index'},
{"data": 'repo_path'},
{"data": 'message'},
{"data": 'commit_email'},
{"data": 'commit_date'},
{"data": 'commit_id'}
],
"lengthMenu": [
[5, 20, 100, 200, 500],
[5, 20, 100, 200, 500] // change per page values here
],
// set the initial value
"pageLength": 5,
"pagingType": "bootstrap_full_number"
});
// 显示commit详细信息
$('#android_project_commit_table').on( 'dblclick', 'tr', function () {
var row = android_project_commit_table.row($(this)).data();
window.location.href = "/project/project_branch_commit_detail/" + row.id;
});
//commit 搜索
function commit_search(){
$('#android_project_commit_table').DataTable().ajax.reload();
}
// select all
$('#commit_select_all').on('click', function () {
var rows = android_project_commit_table.rows({'search': 'applied'}).nodes();
if ($('#commit_select_all').parent().attr('class') == 'checked') {
$('input[type="checkbox"]', rows).each(function () {
$(this).parent().removeClass("checked");
});
} else {
$('input[type="checkbox"]', rows).each(function () {
$(this).parent().addClass("checked");
});
}
});
// 行选择
$('#android_project_commit_table tbody').on('change', 'input[type="checkbox"]', function () {
if($(this).parent().attr('class') == 'checked'){
$('#commit_select_all').parent().removeClass("checked");
$(this).parent().removeClass("checked");
} else {
$(this).parent().addClass("checked");
}
});
//commit 导出Excel
function export_commit_excel(){
var commit_ids = new Array();
$('#android_project_commit_table td span').each(function () {
if ($(this).attr('class') && $(this).attr('class') == 'checked') {
commit_ids.push(android_project_commit_table.row($($($(this).parent()).parent()).parent()).data().id);
}
});
if (commit_ids.length == 0){
bootbox.alert('请至少选中一条记录');
}else {
$.ajax({
url: '/project/commit_export_excel',
type: 'POST',
data: {'commit_ids': commit_ids.join(',')},
success: function (data) {
window.location.href = '/auth/download_file/?file_path=' + data;
}
});
}
}
//分支比较
function compare_branch(){
if($('#first_project_code_id').val() && $('#second_project_code_id').val()) {
showMask();
window.location.href = '/project/project_branch_commit_compare/' + $('#first_project_code_id').val() +
'/' + $('#second_project_code_id').val();
}
}<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
from flask import Blueprint
build = Blueprint('build', __name__)
from . import views<file_sep># -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/6/12
"""
Android 项目的模型
"""
from tms import db
project_followers = db.Table('project_android_followers', db.metadata,
db.Column('user_id', db.Integer, db.ForeignKey('tms_user.id')),
db.Column('project_id', db.Integer, db.ForeignKey('project_android.id'))
)
class ProjectAndroid(db.Model):
""""Android工程项目"""
__tablename__ = 'project_android'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(64))
project_key = db.Column(db.String(64), nullable=False)
category = db.Column(db.String(64))
# Android版本号 N/M/L
android_version = db.Column(db.String(10))
# 平台厂商名称
platform_name = db.Column(db.String(64))
# 基础名称
baseline_name = db.Column(db.String(200))
# 版本在ftp服务器上的路径
version_ftp_path = db.Column(db.String(255))
# myos_configs库中ApkVersion.ini文件的路,路径存在表示导入了myos
myos_config_patch = db.Column(db.String(300))
# 提交通知邮件
commit_notice_email = db.Column(db.String(500))
# 默认货单
default_project_code = db.Column(db.Integer)
# 项目状态 开发、DCC、关闭
status = db.Column(db.String(20))
# spm
spm_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
spm = db.relation('User', backref=db.backref('project_spm'), foreign_keys='ProjectAndroid.spm_id')
# 测试负责人
tester_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
tester = db.relation('User', backref=db.backref('project_tester'), foreign_keys='ProjectAndroid.tester_id')
# SCM
scm_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
scm = db.relation('User', backref=db.backref('project_scm'), foreign_keys='ProjectAndroid.scm_id')
# 运营商项目才有的字段
operators = db.Column(db.String(64))
# 订单项目 出货地点
customer_area = db.Column(db.String(64))
# 订单项目可有的客户属性
customer_name = db.Column(db.String(64))
# 项目扩展字段
extended_field = db.Column(db.String(64))
parent_id = db.Column(db.Integer, db.ForeignKey('project_android.id'), nullable=True)
parent = db.relation('ProjectAndroid', uselist=False, remote_side=[id], backref=db.backref('children'))
# 项目关注者
followers = db.relationship('User', secondary='project_android_followers', backref='project_android_f')
# 项目创建时间
create_time = db.Column(db.DateTime)
# 备注信息
note = db.Column(db.String(200))
def __repr__(self):
return u"<AndroidProject>%s" % self.name
class ProjectAndroidInfo(db.Model):
""""Android工程项目的关联信息表"""
__tablename__ = 'project_android_info'
id = db.Column(db.Integer, primary_key=True)
jenkins_url = db.Column(db.Text)
coverity_url = db.Column(db.Text)
ftp_url = db.Column(db.Text)
svn_url = db.Column(db.String(255))
gerrit_url = db.Column(db.String(255))
push_command = db.Column(db.Text)
other_command = db.Column(db.Text)
project_android_id = db.Column(db.Integer, db.ForeignKey('project_android.id'))
project_android = db.relationship('ProjectAndroid', uselist=False, backref=db.backref('project_info'))
def __repr__(self):
return u"<ProjectAndroidInfo>%s" % self.project_android.name
class ProjectAndroidHistory(db.Model):
""""Android工程项目的修改历史"""
__tablename__ = 'project_android_history'
id = db.Column(db.Integer, primary_key=True)
project_android_id = db.Column(db.Integer, db.ForeignKey('project_android.id'))
project_android = db.relationship('ProjectAndroid', backref=db.backref('project_history'))
operate_time = db.Column(db.DateTime)
operate_user_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
operate_user = db.relation('User', backref=db.backref('project_android_history'))
operate_type = db.Column(db.String(10))
operate_content = db.Column(db.Text)
def __repr__(self):
return u"<ProjectAndroidHistory>%s" % self.project_android.name
class ProjectAndroidMemeber(db.Model):
"""项目成员"""
__tablename__ = 'project_android_memeber'
id = db.Column(db.Integer, primary_key=True)
user_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
user = db.relation('User', backref=db.backref('project_member'))
project_id = db.Column(db.Integer, db.ForeignKey('project_android.id'))
project = db.relation('ProjectAndroid', backref=db.backref('project_member'))
# 项目成员的角色SPM/dev_leader/scm/tester_leader
project_role = db.Column(db.String(64))
def __repr__(self):
return u"<ProjectAndroidMemeber>%s" % self.project.name
class ProjectMyOSConfig(db.Model):
"""项目MYOS应用配置表"""
__tablename__ = 'project_myos_app_config'
id = db.Column(db.Integer, primary_key=True)
app_name = db.Column(db.String(64))
app_version = db.Column(db.String(64))
overrides = db.Column(db.String(64))
support = db.Column(db.String(64))
project_id = db.Column(db.Integer, db.ForeignKey('project_android.id'))
project = db.relation('ProjectAndroid', backref=db.backref('myos_app'))
def __repr__(self):
return u"<ProjectMyOSConfig>%s" % self.app_name
class ProjectMyOSConfigLog(db.Model):
"""项目MYOS应用配置修改日志表"""
__tablename__ = 'project_myos_app_config_log'
id = db.Column(db.Integer, primary_key=True)
config_id = db.Column(db.Integer, db.ForeignKey('project_myos_app_config.id'))
config = db.relation('ProjectMyOSConfig', backref=db.backref('myos_app_config_log'))
old_version = db.Column(db.String(64))
new_version = db.Column(db.String(64))
# myos_configs仓库中的commit id
commit_id = db.Column(db.String(40))
# add/remove/modify
operate_type = db.Column(db.String(64))
operate_time = db.Column(db.DateTime)
operator_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
operator = db.relation('User', backref=db.backref('myos_config_modify_user'))
def __repr__(self):
return u"<ProjectMyOSConfigLog>%s" % self.config.app_name
class ProjectVersion(db.Model):
"""项目版本信息表"""
__tablename__ = 'project_version'
id = db.Column(db.Integer, primary_key=True)
version_number = db.Column(db.String(64))
# 旧的内部版本号
old_version = db.Column(db.String(64))
# 新的内部版本号
new_version = db.Column(db.String(64))
# 客户版本号
customer_version = db.Column(db.String(64))
# 外部的新版本号
out_new_version = db.Column(db.String(64))
# 版本目的
version_purpose = db.Column(db.String(300))
# 版本修改内容
version_modify = db.Column(db.Text)
# ftp服务器上的路径
remote_ftp_path = db.Column(db.String(300))
# 上传ftp的文件路径
ftp_files = db.Column(db.String(300))
# 编译者邮箱
user_email = db.Column(db.String(64))
# 编译人
user_name = db.Column(db.String(300))
# 编译时间
compile_time = db.Column(db.String(64))
# 项目JIRA_KEY
project_key = db.Column(db.String(300))
# 打包文件列表
package_content = db.Column(db.Text)
# 版本类型
version_category = db.Column(db.String(64))
# 编译结果
result = db.Column(db.Boolean, default=True)
# jenkins url
jenkins_url = db.Column(db.String(255))
# 变更集中的commit
commit_change_set = db.Column(db.Text)
# myos应用版本
myos_info = db.Column(db.Text)
# 关联项目
project_id = db.Column(db.Integer, db.ForeignKey('project_android.id'))
project = db.relation('ProjectAndroid', backref=db.backref('version'))
def __repr__(self):
return u"<ProjectVersion>%s" % self.version_number
class ProjectVersionMyOSConfig(db.Model):
"""项目版本中对应MYOS应用配置表和各应用当前最新版本"""
__tablename__ = 'project_version_myos_app_config'
id = db.Column(db.Integer, primary_key=True)
app_name = db.Column(db.String(64))
overrides = db.Column(db.String(64))
current_version = db.Column(db.String(64))
last_version = db.Column(db.String(64))
project_version_id = db.Column(db.Integer, db.ForeignKey('project_version.id'))
project_version = db.relation('ProjectVersion', backref=db.backref('version_myos_app'))
def __repr__(self):
return u"<ProjectVersionMyOSConfig>%s" % self.app_name
project_android_code = db.Table('project_android_code', db.metadata,
db.Column('project_android_id', db.Integer, db.ForeignKey('project_android.id')),
db.Column('project_code_id', db.Integer, db.ForeignKey('project_code.id')),
db.Column('description', db.String(64)))
class ProjectCode(db.Model):
"""
Android 项目的代码信息
"""
__tablename__ = 'project_code'
id = db.Column(db.Integer, primary_key=True)
# 提货单的路径
manifest_url = db.Column(db.String(255))
# 货单分支
manifest_branch = db.Column(db.String(64))
# 货单名称
manifest_name = db.Column(db.String(255))
# 货单仓库
manifest_repo = db.Column(db.String(255))
# 代码分支
branch_name = db.Column(db.String(255))
# 备份服务器上仓库的路径
local_repo_path = db.Column(db.String(255))
# 厂商平台名称
platform_name = db.Column(db.String(64))
# 邮件提醒功能的邮件地址,以分号间隔
commit_notice_email = db.Column(db.String(500))
# 项目
project_android = db.relationship('ProjectAndroid', secondary='project_android_code', backref='project_code')
# 状态 False 已废弃,True正常
activate = db.Column(db.Boolean, default=True)
def __repr__(self):
return u"<ProjectCode>%s" % self.manifest_name
class ProjectBranchCommit(db.Model):
"""
货单(分支)上的提交记录
"""
__tablename__ = 'project_branch_commit'
id = db.Column(db.Integer, primary_key=True)
# Git 仓库路径
repo_path = db.Column(db.String(255))
# id
commit_id = db.Column(db.String(40), index=True)
# tree id
tree_id = db.Column(db.String(40), index=True)
# auth 姓名
auth_name = db.Column(db.String(64), index=True)
# auth 邮箱
auth_email = db.Column(db.String(64), index=True)
# auth 时间
auth_date = db.Column(db.String(64))
# commit 姓名
commit_name = db.Column(db.String(64))
commit_email = db.Column(db.String(64))
commit_date = db.Column(db.String(64))
# 提交说明
message = db.Column(db.String(255))
# 修改的文件
change_file = db.Column(db.Text)
# push者
submitter = db.Column(db.String(64))
# 项目JIRA_KE
project_key = db.Column(db.String(64), index=True)
# JIRA任务KEY
task_key = db.Column(db.String(64), index=True)
# False表示已经此commit已经从分支上删除了
activate = db.Column(db.Boolean, default=True)
project_code_id = db.Column(db.Integer, db.ForeignKey('project_code.id'))
project_code = db.relation('ProjectCode', backref=db.backref('branch_commit'))
def __repr__(self):
return u"<ProjectBranchCommit>%s" % self.project_code.manifest_name
class ProjectBranchTask(db.Model):
"""
项目分支上修改的JIRA任务
"""
__tablename__ = 'project_branch_task'
id = db.Column(db.Integer, primary_key=True)
# jira数据库中issue的id
issue_id = db.Column(db.Integer)
# jira任务KEY
task_key = db.Column(db.String(64), index=True)
# 任务名称
summary = db.Column(db.String(255), index=True)
# 任务描述
description = db.Column(db.String(255))
# 任务类型Critical/Public
category = db.Column(db.String(64))
# False表示已经此commit已经从分支上删除了
activate = db.Column(db.Boolean, default=True)
project_code_id = db.Column(db.Integer, db.ForeignKey('project_code.id'))
project_code = db.relation('ProjectCode', backref=db.backref('branch_task'))
def __repr__(self):
return u"<ProjectBranchTask>%s" % self.task_key
class ProjectBranchTaskCommit(db.Model):
"""
任务关联的commit
"""
__tablename__ = 'project_branch_task_commit'
id = db.Column(db.Integer, primary_key=True)
project_branch_task_id = db.Column(db.Integer, db.ForeignKey('project_branch_task.id'))
project_branch_task = db.relation('ProjectBranchTask', backref=db.backref('branch_task_commit'))
project_branch_commit_id = db.Column(db.Integer, db.ForeignKey('project_branch_commit.id'))
project_branch_commit = db.relation('ProjectBranchCommit', backref=db.backref('branch_task_commit'))
def __repr__(self):
return u"<ProjectBranchTaskCommit>%s" % self.project_branch_task.name
class ProjectBranchMergeCommit(db.Model):
"""
项目分支上从其他分支合并来的提交
"""
__tabename__ = 'project_branch_merge_commit'
id = db.Column(db.Integer, primary_key=True)
commit_id = db.Column(db.String(40), index=True)
dest_project_code_id = db.Column(db.Integer, db.ForeignKey('project_code.id'))
dest_project_code = db.relation('ProjectCode', backref=db.backref('dest_project_code'),
foreign_keys='ProjectBranchMergeCommit.dest_project_code_id')
src_project_code_id = db.Column(db.Integer, db.ForeignKey('project_code.id'))
src_project_code = db.relation('ProjectCode', backref=db.backref('src_project_code'),
foreign_keys='ProjectBranchMergeCommit.src_project_code_id')
meger_author_email = db.Column(db.String(64))
def __repr__(self):
return u"<ProjectBranchMergeCommit>%s" % self.project_code.branch_name
class ProjectTag(db.Model):
"""
项目版本tag
"""
__tablename__ = 'project_tag'
id = db.Column(db.Integer, primary_key=True)
# 版本tag名称
tag_name = db.Column(db.String(255))
# False表示已经此tag已经删除了
activate = db.Column(db.Boolean, default=True)
project_id = db.Column(db.Integer, db.ForeignKey('project_android.id'))
project = db.relation('ProjectAndroid', backref=db.backref('project_tag'))
def __repr__(self):
return u"<ProjectTag>%s" % self.tag_name
class ProjectTagCommit(db.Model):
"""
项目版本tag的差异日志
"""
__tablename__ = 'project_tag_commit'
id = db.Column(db.Integer, primary_key=True)
project_tag_id = db.Column(db.Integer, db.ForeignKey('project_tag.id'))
project_tag = db.relation('ProjectTag', backref=db.backref('project_tag_commit'))
project_branch_commit_id = db.Column(db.Integer, db.ForeignKey('project_branch_commit.id'))
project_branch_commit = db.relation('ProjectBranchCommit', backref=db.backref('project_tag_commit'))
def __repr__(self):
return u"<ProjectTagCommit>%s" % self.project_tag.tag_name
class CommonIssue(db.Model):
"""项目共性问题"""
__tablename__ = 'project_common_issue'
id = db.Column(db.Integer, primary_key=True)
# 共性问题处理的项目
project_id = db.Column(db.Integer, db.ForeignKey('project_android.id'))
project = db.relation('ProjectAndroid', backref=db.backref('project_common_issue'))
# jira 中 原共性问题处理项目的任务key值
jira_key = db.Column(db.String(64))
# 问题名称
name = db.Column(db.String(255))
# 问题描述
description = db.Column(db.String(500))
# 解决方案
solutions = db.Column(db.Text)
# 附件
attachment = db.Column(db.String(500))
# 提交问题的测试工程师
tester_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
tester = db.relation('User', backref=db.backref('common_issue_tester'), foreign_keys='CommonIssue.tester_id')
submit_issue_time = db.Column(db.DateTime)
# 提供解决方案的开发工程师
developer_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
developer = db.relation('User', backref=db.backref('common_issue_developer'), foreign_keys='CommonIssue.developer_id')
submit_solution_time = db.Column(db.DateTime)
# 确认问题的spm
spm_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
spm = db.relation('User', backref=db.backref('common_issue_spm'), foreign_keys='CommonIssue.spm_id')
# spm确认时间
confirm_time = db.Column(db.DateTime)
# 影响范围(影响的项目)
effect_scope = db.Column(db.Text)
# 厂商平台
platform_name = db.Column(db.String(64))
# 厂商基线
baseline_name = db.Column(db.String(255))
# 已处理过的项目
processed_project = db.Column(db.String(255))
# 问题状态 1 测试录入问题 -> 2 待开发填写解决方案 -> 3 待spm确认问题 -> 4 Spm已确认 -1 问题取消(删除)
status = db.Column(db.Integer, default=1)
# 邮件通知列表
to_list = db.Column(db.String(500))
# 问题类别
category = db.Column(db.String(50))
# 在涉及项目中新建问题的JIRA_KEY
effect_project_new_task_key = db.Column(db.Text)
# 问题来源
issue_from = db.Column(db.String(50))
def __repr__(self):
return u"<CommonIssue> %r" % self.name
class ProjectTask(db.Model):
"""项目开发任务(和JIRA任务)"""
__tablename__ = 'project_task'
id = db.Column(db.Integer, primary_key=True)
# jira数据库中issue的id
issue_id = db.Column(db.Integer)
# jira任务KEY
task_key = db.Column(db.String(64), index=True)
# 任务名称
summary = db.Column(db.String(255), index=True)
# 任务描述
description = db.Column(db.String(255))
# 任务类型 客户需求、公共需求、缺陷、共性问题、安全补丁等
category = db.Column(db.String(64))
project_id = db.Column(db.Integer, db.ForeignKey('project_android.id'))
project = db.relation('ProjectAndroid', backref=db.backref('project_task'))
def __repr__(self):
return u"<ProjectTask>%s" % self.task_key
<file_sep># -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/6/28
"""
将发邮件的任务添加到Rabbitmq队列中
等待消费队列处理
"""
import simplejson as json
import pika
def send_mail_pubisher(message):
"""
向队列中添加邮件发送任务
:param message: json格式字串
:return:
"""
# 创建连接connection到localhost
con = pika.BlockingConnection(pika.ConnectionParameters('192.168.10.207'))
# 创建虚拟连接channel
cha = con.channel()
# 创建队列anheng,durable参数为真时,队列将持久化;exclusive为真时,建立临时队列
result = cha.queue_declare(queue='send_email', durable=True, exclusive=False)
# 创建名为yanfa,类型为fanout的exchange,其他类型还有direct和topic,如果指定durable为真,exchange将持久化
cha.exchange_declare(durable=False,
exchange='tms',
type='direct', )
# 绑定exchange和queue,result.method.queue获取的是队列名称
cha.queue_bind(exchange='tms',
queue=result.method.queue,
routing_key='', )
# 公平分发,使每个consumer在同一时间最多处理一个message,收到ack前,不会分配新的message
cha.basic_qos(prefetch_count=1)
# 消息持久化指定delivery_mode=2;
cha.basic_publish(exchange='',
routing_key='send_email',
body=message,
properties=pika.BasicProperties(
delivery_mode=2,
))
print '[x] Sent %r' % (message,)
# 关闭连接
con.close()
if __name__ == '__main__':
message = {
'to_list': '<EMAIL>',
'sub': 'Rabbitmq',
'content': 'Test RabbitMQ send Mail'
}
send_mail_pubisher(json.dumps(message))
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""测试工程师"""
import os
import json
from datetime import datetime
import commands
from flask import render_template, redirect, url_for, request, flash, current_app, session, send_file
from flask.ext.login import login_user, logout_user, login_required, current_user
from flask.ext.mail import Mail, Message
from functools import update_wrapper
from tms import db, mail
from tms.project.models import Version, VersionHistory, ReleaseEmailAddress
from tms.utils.MyFTP import MyFTP
from tms.utils.UpdateReleaseRepo import update_release_repository
from tms.utils.email_format import TEST_FAILURE_TEMPLATE, TEST_PASS_TEMPLATE, RELEASE_TEMPLATE, \
DCC_RELEASE_TEMPLATE, SPECIAL_VERSION_TEMPLATE, VERSION_TEST_TEMPLATE
from . import qa
from models import *
from tms.auth.models import User, Role
from tms.utils.SendMailPubisher import send_mail_pubisher
def need_test_version():
"""
待测试版本的装饰器
os测试用户登录时显示待测试的版本,其他角色不显示
"""
def decorator(fn):
def wrapped_function(*args, **kwargs):
if session['user']['role_name'] == 'os_test':
versions = Version.query.filter(Version.status == '测试').order_by(Version.compile_time.desc()).all()
session['need_test_version_count'] = len(versions)
session['need_test_version'] = [{"id": version.id, "name": '{0}_{1}'.format(version.name, version.version_number)}for version in versions]
return fn(*args, **kwargs)
return update_wrapper(wrapped_function, fn)
return decorator
@qa.route('/my_version')
@login_required
@need_test_version()
def my_version():
""" 待我测试的版本 """
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '我测试的版本', 'url': url_for('qa.my_version')})
session['menu_path'] = ['版本管理', '我测试的版本']
version_type = request.args.get('version_type', '')
current_app.logger.info(version_type)
if version_type == 'need_test':
versions = Version.query.filter(Version.status == '测试').order_by(Version.compile_time.desc()).all()
else:
# 当前用户测试过和待测试的版本
versions = Version.query.filter(Version.test_user_id == current_user.id).order_by(Version.compile_time.desc()).all()
return render_template('/qa/version_page.html', role_name=session['user']['role_name'], versions=versions,
version_type=version_type)
@qa.route('/all_version')
@login_required
@need_test_version()
def all_version():
"""所有版本"""
versions = Version.query.order_by(Version.compile_time.desc()).all()
return render_template('/qa/version_page.html', role_name=session['user']['role_name'], versions=versions)
@qa.route('/version_test/<int:version_id>', methods=['GET', 'POST'])
@login_required
@need_test_version()
def version_test(version_id):
"""版本测试结果"""
# 显示版本信息
if request.method == 'GET':
for index, breadcrumb in enumerate(session['breadcrumbs']):
if u'版本测试' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '版本测试',
'url': url_for('qa.version_test', version_id=version_id)})
version = Version.query.filter_by(id=version_id).first()
tasks = []
# 测试权限
test_permission = False
# 发布全新
release_permission = False
if session['user']['role_name'] == 'os_test' and version.status == '测试':
test_permission = True
if session['user']['role_name'] == 'os_test' and version.status == '测试通过':
release_permission = True
if version.tasks:
tasks = json.loads(version.tasks, strict=False)
if version.documents and version.documents.strip():
#documents = [(os.path.basename(tmp), os.path.getsize(tmp)/1024, tmp.replace('/', '<>')) for tmp in
# version.documents.split('#') if tmp.strip()]
documents = [(os.path.basename(tmp), '', tmp.replace('/', '<>')) for tmp in version.documents.split('#') if tmp.strip()]
else:
documents = []
version_level = ['Recommend', 'Must']
return render_template('/qa/version_test.html',
documents=documents,
version=version,
test_permission=test_permission,
release_permission=release_permission,
tasks=tasks,
version_level=version_level,
current_page='qa.my_version')
# 版本测试结果
elif request.method == 'POST':
version = Version.query.filter_by(id=version_id).first()
project = version.project
version.release_note = request.form.get('release_note', '')
version.version_issues = request.form.get('version_issues', '')
version.unresolved_issues = request.form.get('unresolved_issues', '')
version.testing_time = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
version.test_user = current_user
smi = request.form.get('smi', '')
if smi:
version.smi = int(smi)
version.level = request.form.get('level', 'Recommend')
result = request.form.get('result', '')
version.special_version.release_path = request.form.get('release_path', '')
# 测试通过后发送邮件地址
test_pass_to_email = request.form.get('test_pass_to_email', '')
if test_pass_to_email:
project.test_pass_to_email = ';'.join([tmp.strip() for tmp in test_pass_to_email.split(';') if tmp.strip()])
# 测试失败发送的邮件地址
test_failure_to_email = request.form.get('test_failure_to_email', '')
if test_failure_to_email:
project.test_failure_to_email = ';'.join([tmp.strip() for tmp in test_failure_to_email.split(';') if tmp.strip()])
# 选择发放版本,默认是全部
need_release_file = request.form.get('need_release_file', '')
if need_release_file:
version.release_file = '#'.join([tmp.strip() for tmp in need_release_file.split('#') if tmp.strip()])
else:
version.release_file = version.ftp_file
# 保存上传的附件
exits_documents = [tmp.strip() for tmp in version.documents.split('#') if tmp.strip()]
documents = request.files.getlist("file[]")
if documents:
upload_path = os.path.join(current_app.config['UPLOAD_FOLDER'], version.name, version.version_number)
if not os.path.exists(upload_path):
os.makedirs(upload_path)
for document in documents:
if document.filename:
current_app.logger.info(document.filename)
file_path = os.path.join(upload_path, document.filename)
# 删除已存在的
if os.path.exists(file_path) and os.path.isfile(file_path):
os.remove(file_path)
document.save(file_path)
if file_path not in exits_documents:
exits_documents.append(file_path)
version.documents = '#'.join(exits_documents)
# 通知邮件
to_list = []
cc_list = []
# 版本编译工程师
to_list.append(version.compile_email)
# 软件开发负责人
if project.develop_leader and project.develop_leader.email:
to_list.append(project.develop_leader.email)
# 软件的邮件记录
if project.to_email:
for email in project.to_email.split(';'):
if email.strip():
cc_list.append(email)
# 版本历史记录
version_history = VersionHistory()
# 测试不通过不能发放
if result == '-1':
if project.test_failure_to_email:
for email in project.test_failure_to_email.split(';'):
if email.strip():
to_list.append(email)
version.status = '测试不过'
version.testing_result = False
version_history.description = "{0}版本测试结果: 测试失败\n 测试人:{1}\n版本缺陷:{2}\n遗留问题{3}".format(
version.name, current_user.username, version.version_issues, version.unresolved_issues)
# 去重复
to_list = list(set(to_list))
cc_list = list(set(cc_list))
msg = Message(
'[TSDS] %s_%s 版本测试不通过' % (version.name, version.version_number),
sender='<EMAIL>',
# recipients=[version.project.test_leader.email, '<EMAIL>', '<EMAIL>']
recipients=to_list,
cc=cc_list
# recipients=['<EMAIL>']
)
msg.html = TEST_FAILURE_TEMPLATE.format(version.project.name,
version.name,
version.version_number,
version.level,
version.version_issues,
current_user.display_name,
current_user.email).replace('通讯录', '通 讯 录')
mail.send(msg)
flash(u'提交成功', 'info')
# 版本废弃
elif result == '4':
version.status = '已废弃'
version.version_discard_time = datetime.now()
version.discard_user_id = current_user.id
db.session.add(version)
# 研发版本测试结果通知
elif result == '5':
version.status = '测试通过'
version.test_user_id = current_user.id
version.testing_result = True
version.testing_time = datetime.now()
db.session.add(version)
# 版本测试通过后需要通知的人员
if project.test_pass_to_email:
for email in project.test_pass_to_email.split(';'):
if email.strip():
to_list.append(email)
# 配置组
to_list.append('<EMAIL>')
for scm in User.query.join(Role).filter(Role.name == 'scm').all():
cc_list.append(scm.email)
to_list = list(set(to_list + cc_list))
content = VERSION_TEST_TEMPLATE.format(project.name,
version.name,
version.version_number,
version.smi,
version.version_issues.replace('<', '<').replace('\n', '<br>'),
version.release_note.replace('<', '<').replace('\n', '<br>'),
current_user.display_name,
current_user.email).replace('通讯录', '通 讯 录')
message = {
'to_list': ';'.join([tmp for tmp in to_list if tmp]),
'sub': '[TSDS] {0}_{1}版本,测试结果'.format(project.name, version.version_number),
'content': content
}
send_mail_pubisher(json.dumps(message))
version_history.description = "{0}版本测试结果: 测试通过,测试通过\n 测试人:{1}\n版本缺陷:{2}\n遗留问题{3}" \
"\n发布说明{4}\n适配信息{5}\n导入注意事项{6}".format(version.name,
current_user.username,
version.version_issues,
version.unresolved_issues,
version.release_note,
version.adaptation_note,
version.project_import_note)
flash(u'提交成功', 'info')
# 测试通过进行版本发放
else:
# 版本测试通过后需要通知的人员
if project.test_pass_to_email:
for email in project.test_pass_to_email.split(';'):
if email.strip():
to_list.append(email)
# 配置组
to_list.append('<EMAIL>')
for scm in User.query.join(Role).filter(Role.name == 'scm').all():
cc_list.append(scm.email)
version.status = '已发布'
version.testing_result = True
version.release_time = datetime.now()
version.release_user_id = current_user.id
version.release_note = request.form.get('release_note', '')
version.adaptation_note = request.form.get('adaptation_note', '')
version.project_import_note = request.form.get('project_import_note', '')
project.last_release_time = version.release_time
project.last_version_number = version.version_number
if result == '1':
# version.release_type = '特殊发放'
# msg = Message(
# '[TSDS] {0}_{1} 特殊发放'.format(project.name, version.version_number),
# sender='<EMAIL>',
# recipients=to_list,
# cc=cc_list
# # recipients=['<EMAIL>']
# )
# if version.documents:
# for document in version.documents.split('#'):
# if document:
# file_name = os.path.basename(document)
# with current_app.open_resource(document) as fp:
# msg.attach(file_name.encode("utf-8"),
# current_app.config['MIME_TYPE'][os.path.splitext(file_name)[1]], fp.read())
#
# msg.html = SPECIAL_VERSION_TEMPLATE.format(project.name,
# version.name,
# version.version_number,
# version.smi,
# version.level,
# version.project_import_note,
# project.apk_name,
# project.apk_package,
# version.special_version.release_path,
# version.release_note.replace('\n', '<br>'),
# version.unresolved_issues.replace('\n', '<br>'),
# current_user.display_name,
# current_user.email)
# mail.send(msg)
content = SPECIAL_VERSION_TEMPLATE.format(project.name,
version.name,
version.version_number,
version.smi,
version.level,
version.project_import_note.replace('<', '<'),
project.apk_name,
project.apk_package,
version.special_version.release_path,
version.release_note.replace('<', '<').replace('\n', '<br>'),
version.unresolved_issues.replace('<', '<').replace('\n', '<br>'),
current_user.display_name,
current_user.email).replace('通讯录', '通 讯 录')
to_list = list(set(to_list + cc_list))
message = {
'to_list': ';'.join([tmp for tmp in to_list if tmp]),
'sub': '[TSDS] {0}_{1} 特殊发放'.format(project.name, version.version_number),
'content': content
}
send_mail_pubisher(json.dumps(message))
elif result == '2':
version.release_type = 'DCC发放'
# msg = Message(
# '[TSDS] {0}_{1}版本,DCC发放'.format(project.name, version.version_number),
# sender='<EMAIL>',
# recipients=to_list,
# cc=cc_list
# # recipients=['<EMAIL>']
# )
# if version.documents:
# for document in version.documents.split('#'):
# if document:
# file_name = os.path.basename(document)
# with current_app.open_resource(document) as fp:
# msg.attach(file_name.encode("utf-8"),
# current_app.config['MIME_TYPE'][os.path.splitext(file_name)[1]], fp.read())
# msg.html = DCC_RELEASE_TEMPLATE.format(project.name,
# version.name,
# version.version_number,
# version.smi,
# version.level,
# project.apk_name,
# project.apk_package,
# version.special_version.release_path,
# version.release_note.replace('\n', '<br>'),
# project.name,
# version.adaptation_note,
# version.unresolved_issues.replace('\n', '<br>'),
# current_user.display_name,
# current_user.email)
#
# mail.send(msg)
to_list = list(set(to_list + cc_list))
content = DCC_RELEASE_TEMPLATE.format(project.name,
version.name,
version.version_number,
version.smi,
version.level,
project.apk_name,
project.apk_package,
version.special_version.release_path,
version.release_note.replace('<', '<').replace('\n', '<br>'),
project.name,
version.adaptation_note.replace('<', '<'),
version.unresolved_issues.replace('<', '<').replace('\n', '<br>'),
current_user.display_name,
current_user.email).replace('通讯录', '通 讯 录')
message = {
'to_list': ';'.join([tmp for tmp in to_list if tmp]),
'sub': '[TSDS] {0}_{1}版本,DCC发放'.format(project.name, version.version_number),
'content': content
}
send_mail_pubisher(json.dumps(message))
elif result == '3':
version.release_type = '迭代发放'
# msg = Message(
# '[TSDS] {0}_{1}版本,迭代发放'.format(project.name, version.version_number),
# sender='<EMAIL>',
# recipients=to_list,
# cc=cc_list
# # recipients=['<EMAIL>']
# )
# if version.documents:
# for document in version.documents.split('#'):
# if document:
# file_name = os.path.basename(document)
# with current_app.open_resource(document) as fp:
# msg.attach(file_name.encode("utf-8"),
# current_app.config['MIME_TYPE'][os.path.splitext(file_name)[1]], fp.read())
# msg.html = RELEASE_TEMPLATE.format(project.name,
# version.name,
# version.version_number,
# version.smi,
# version.level,
# project.apk_name,
# project.apk_package,
# version.special_version.release_path,
# version.release_note.replace('\n', '<br>'),
# version.unresolved_issues.replace('\n', '<br>'),
# current_user.display_name,
# current_user.email)
# mail.send(msg)
to_list = list(set(to_list + cc_list))
content = RELEASE_TEMPLATE.format(project.name,
version.name,
version.version_number,
version.smi,
version.level,
project.apk_name,
project.apk_package,
version.special_version.release_path,
version.release_note.replace('<', '<').replace('\n', '<br>'),
version.unresolved_issues.replace('<', '<').replace('\n', '<br>'),
current_user.display_name,
current_user.email).replace('通讯录', '通 讯 录')
message = {
'to_list': ';'.join([tmp for tmp in to_list if tmp]),
'sub': '[TSDS] {0}_{1}版本,迭代发放'.format(project.name, version.version_number),
'content': content
}
send_mail_pubisher(json.dumps(message))
version_history.description = "{0}版本测试结果: 测试通过,已发布\n 测试人:{1}\n版本缺陷:{2}\n遗留问题{3}" \
"\n发布说明{4}\n适配信息{5}\n导入注意事项{6}".format(version.name,
current_user.username,
version.version_issues,
version.unresolved_issues,
version.release_note,
version.adaptation_note,
version.project_import_note)
flash(u'发布成功', 'info')
version_history.version_id = version_id
version_history.option_username = session['user']['username']
version_history.option_email = session['user']['email']
version_history.option_time = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
db.session.add(version)
db.session.add(version_history)
db.session.add(version.special_version)
db.session.add(project)
db.session.commit()
return redirect(url_for('qa.version_test', version_id=version_id))
@qa.route('/download_file/<string:file_path>')
@login_required
def download_file(file_path):
"""所有版本"""
file_path = file_path.replace('<>', '/')
return send_file(open(file_path, 'rb'), as_attachment=True, attachment_filename=os.path.basename(file_path))
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
from datetime import datetime
import json
import os
from flask import render_template, redirect, url_for, request, flash, current_app, session
from flask.ext.login import login_user, logout_user, login_required, current_user
from flask.ext.mail import Mail, Message
from werkzeug import secure_filename
from functools import update_wrapper
from tms import db, mail
from . import developer
from tms.project.models import Project, Version, VersionHistory, SpecialVersion
from tms.project.androidProjectModules import ProjectAndroid
from tms.auth.models import User, Role
from tms.repository.models import Repository
from tms.utils.email_format import VERSION_SELF_TEST_TEMPLATE
from tms.utils.SendMailPubisher import send_mail_pubisher
def need_self_test_version():
"""
待开发自测的版本装饰器
非开发工程师不显示
"""
def decorator(fn):
def wrapped_function(*args, **kwargs):
if session['user']['role_name'] == 'APK开发工程师':
# 当前用户所有待自测的版本
versions = Version.query.join(VersionHistory).filter(
db.and_(Version.compile_email == current_user.email, Version.status == '自测')).all()
session['need_test_version_count'] = len(versions)
session['need_test_version'] = [{"id": version.id, "name": '{0}_{1}'.format(version.name, version.version_number)}for version in versions]
return fn(*args, **kwargs)
return update_wrapper(wrapped_function, fn)
return decorator
@developer.route('/all_project')
@login_required
@need_self_test_version()
def all_project():
"""所有的项目"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '所有项目', 'url': url_for('developer.all_project')})
session['menu_path'] = ['项目管理', '所有项目']
projects = Project.query.all()
# 负责的项目
leader_project = Project.query.filter_by(develop_leader=current_user).all()
# 关注的项目
follower_project = Project.query.join(User.project_f).filter(User.id == current_user.id).all()
result = []
index = 1
for project_obj in projects:
if project_obj in leader_project:
result.append((index, project_obj, 'leader'))
elif project_obj in follower_project:
result.append((index, project_obj, 'start'))
else:
result.append((index, project_obj, 'other'))
index += 1
# 所有Android项目
# 已经关注的项目
follower_android_project = ProjectAndroid.query.join(User.project_android_f).filter(
User.id == current_user.id).all()
project_android = []
all_android_project = ProjectAndroid.query.all()
for project_obj in all_android_project:
if project_obj in follower_android_project:
project_android.append((index, project_obj, 'start', 'android'))
else:
project_android.append((index, project_obj, 'other', 'android'))
index += 1
return render_template('/developer/developer_page.html',
all_project=True,
role_name=session['user']['role_name'],
projects=result,
project_android=project_android
)
@developer.route('/all_apk_project_page')
@login_required
@need_self_test_version()
def all_apk_project_page():
"""所有APK的项目"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': 'APK项目', 'url': url_for('developer.all_apk_project_page')})
session['menu_path'] = ['项目管理', 'APK项目']
projects = Project.query.all()
# 负责的项目
leader_project = Project.query.filter_by(develop_leader=current_user).all()
# 关注的项目
follower_project = Project.query.join(User.project_f).filter(User.id == current_user.id).all()
result = []
index = 1
for project_obj in projects:
if project_obj in leader_project:
result.append((index, project_obj, 'leader'))
elif project_obj in follower_project:
result.insert(0, (index, project_obj, 'start'))
else:
result.append((index, project_obj, 'other'))
index += 1
return render_template('/developer/developer_page.html',
all_project=True,
role_name=session['user']['role_name'],
projects=result)
@developer.route('/os_dev_my_project')
@login_required
@need_self_test_version()
def os_dev_my_project():
"""os 开发工程师我负责的、参与、关注的项目"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '我的项目', 'url': url_for('developer.os_dev_my_project')})
session['menu_path'] = ['项目管理', '我的项目']
result = []
index = 1
# 负责的项目
leader_project = Project.query.filter_by(develop_leader=current_user).all()
for project_obj in leader_project:
result.append((index, project_obj, 'leader'))
index += 1
# 关注的APK项目
follower_project = Project.query.join(User.project_f).filter(User.id == current_user.id).all()
for project_obj in follower_project:
if project_obj not in leader_project:
result.append((index, project_obj, 'start'))
index += 1
# 关注的Android项目
project_android = []
follower_android_project = ProjectAndroid.query.join(User.project_android_f).filter(User.id == current_user.id).all()
for project_obj in follower_android_project:
project_android.append((index, project_obj, 'start', 'android'))
index += 1
# current_app.logger.info()
return render_template('/developer/developer_page.html',
role_name=session['user']['role_name'],
projects=result,
project_android=project_android
)
@developer.route('/start_project')
@login_required
def start_project():
"""关注项目"""
project_id = request.args.get('project_id', '')
start_option = request.args.get('start_option', '')
project = Project.query.filter_by(id=project_id).first()
if start_option == '1':
project.followers.append(current_user)
elif start_option == '-1':
project.followers.remove(current_user)
db.session.add(project)
db.session.commit()
return '{"result":true}'
@developer.route('/add_project', methods=['GET', 'POST'])
@login_required
@need_self_test_version()
def add_project():
"""新增项目"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '添加项目' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '添加项目', 'url': url_for('developer.add_project')})
if request.method == 'POST':
name = request.form['name']
apk_name = request.form['apk_name']
apk_package = request.form['apk_package']
project_key = request.form['project_key']
category = 'APP'
develop_leader_id = request.form['develop_leader']
test_leader_id = request.form.get('test_leader', '')
status = request.form['status']
last_version_number = request.form.get('last_version_number', '')
last_release_time = request.form.get('last_release_time')
to_email = request.form.get('to_email', '')
code_repo_id = request.form.get('code_repo', '')
release_repo_id = request.form.get('release_repo', '')
test_case_jar_url = request.form.get('test_case_jar_url', '')
other_project = Project.query.filter(Project.project_key == project_key).first()
if other_project is not None:
flash(u'相同JIRA_KEY项目"{0}"已存在'.format(other_project.name), 'danger')
else:
project_obj = Project()
project_obj.name = name
project_obj.apk_name = apk_name
project_obj.apk_package = apk_package
project_obj.category = category
project_obj.project_key = project_key
project_obj.test_case_jar_url = test_case_jar_url
project_obj.develop_leader = User.query.filter_by(id=develop_leader_id).first()
project_obj.test_leader = User.query.filter_by(id=test_leader_id).first()
project_obj.status = status
project_obj.last_version_number = last_version_number
project_obj.last_release_time = last_release_time
project_obj.to_email = to_email.strip().strip('\n')
db.session.add(project_obj)
db.session.commit()
special_version = SpecialVersion()
special_version.project = project_obj
special_version.name = 'ODM'
special_version.ftp_path = os.path.join('ftp://htsvr2/SW_PRJ/app', project_obj.name, 'ODM')
special_version.svn_path = os.path.join('https://version2.tinno.com/svn/release/APP', project_obj.name, 'ODM')
if code_repo_id:
special_version.code_repo_id = code_repo_id
if release_repo_id:
special_version.release_repo_id = release_repo_id
db.session.add(special_version)
db.session.commit()
flash(u'新增成功!', 'info')
return redirect(url_for('developer.all_project'))
# 超级管理员和配置管理员也可以编辑项目信息且能够编辑仓库信息
is_super_man = False
if session['user']['role_name'] == 'admin' or session['user']['role_name'] == 'scm':
is_super_man = True
developer_users = []
test_users = []
if is_super_man:
users = User.query.join(User.role).filter(db.or_(Role.name == 'APK开发工程师', Role.name == 'os_test')).all()
for user in users:
if user.role.name == 'APK开发工程师':
developer_users.append(user)
else:
test_users.append(user)
code_repo_list = Repository.query.filter(Repository.category == 2).all()
release_repo_list = Repository.query.filter(Repository.category == 3).all()
# 项目状态
project_category = ['MTK', 'QCM', 'RDA', 'APP']
# 项目类
project_status = ['开发中', '迭代发布中', '已关闭', '已暂停']
return render_template('/developer/project_add.html',
project=Project(),
is_super_man=is_super_man,
developer_users=developer_users,
test_users=test_users,
code_repo_list=code_repo_list,
release_repo_list=release_repo_list,
project_category=project_category,
project_status=project_status)
@developer.route('/edit_project/<int:project_id>', methods=['GET', 'POST'])
@login_required
@need_self_test_version()
def edit_project(project_id):
"""编辑"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '编辑项目' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '编辑项目',
'url': url_for('developer.edit_project', project_id=project_id)})
project_obj = Project.query.filter_by(id=project_id).first()
if request.method == 'POST':
name = request.form['name']
apk_name = request.form['apk_name']
apk_package = request.form['apk_package']
project_key = request.form['project_key']
category = 'APP'
develop_leader_id = request.form['develop_leader']
test_leader_id = request.form.get('test_leader', '')
status = request.form['status']
last_version_number = request.form.get('last_version_number', '')
last_release_time = request.form.get('last_release_time')
to_email = request.form.get('to_email', '')
test_case_jar_url = request.form.get('test_case_jar_url', '')
other_project = Project.query.filter_by(name=name).first()
if other_project is not None and other_project.id != project_id:
flash(u'相同名称的项目已存在', 'danger')
else:
project_obj.name = name
project_obj.apk_name = apk_name
project_obj.apk_package = apk_package
project_obj.category = category
project_obj.project_key = project_key
project_obj.test_case_jar_url = test_case_jar_url
project_obj.develop_leader = User.query.filter_by(id=develop_leader_id).first()
project_obj.test_leader = User.query.filter_by(id=test_leader_id).first()
project_obj.status = status
project_obj.last_version_number = last_version_number
project_obj.last_release_time = last_release_time
project_obj.to_email = ';'.join([tmp.strip() for tmp in to_email.strip().split(';') if tmp.strip()])
db.session.add(project_obj)
db.session.commit()
flash(u'修改成功!', 'info')
return redirect(url_for('developer.all_project'))
# 只有是项目开发负责才能编辑项目信息
is_developer_leader = False
if project_obj.develop_leader == current_user:
is_developer_leader = True
# 超级管理员和配置管理员也可以编辑项目信息且能够编辑仓库信息
is_super_man = False
if session['user']['role_name'] == 'admin' or session['user']['role_name'] == 'scm':
is_super_man = True
developer_users = []
test_users = []
if is_developer_leader or is_super_man:
users = User.query.join(User.role).filter(db.or_(Role.name == 'APK开发工程师', Role.name == 'os_test')).all()
for user in users:
if user.role.name == 'APK开发工程师':
developer_users.append(user)
else:
test_users.append(user)
# 项目最新的版本
last_version = Version.query.filter(db.and_(Version.project_id == project_obj.id,
Version.version_number == project_obj.last_version_number)).first()
last_version_id = ''
if last_version:
last_version_id = last_version.id
# 项目的发布对象(版本对象)
special_versions = SpecialVersion.query.filter(SpecialVersion.project_id == project_obj.id).all()
# 项目状态
project_category = ['MTK', 'QCM', 'RDA', 'APP']
# 项目类
project_status = ['开发中', '迭代发布中', '已关闭', '已暂停']
return render_template('/developer/project_edit.html',
project=project_obj,
last_version_id=last_version_id,
special_versions=special_versions,
is_super_man=is_super_man,
is_developer_leader=is_developer_leader,
developer_users=developer_users,
test_users=test_users,
project_status=project_status,
project_category=project_category)
@developer.route('/version_self_test/<int:version_id>', methods=['GET', 'POST'])
@login_required
@need_self_test_version()
def version_self_test(version_id):
"""自测版本的详细信息"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if u'版本信息' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': u'版本信息',
'url': url_for('developer.version_self_test', version_id=version_id)})
version = Version.query.filter_by(id=version_id).first()
# 显示版本信息
if request.method == 'GET':
tasks = []
if version.tasks:
tasks = json.loads(version.tasks, strict=False)
# 自测权限
permission = False
# 版本编译者和项目开发负责人有自测权限
if version.compile_email == session['user']['email'] and version.status == '自测':
permission = True
elif version.project.develop_leader_id == current_user.id and version.status == '自测':
permission = True
if version.documents and version.documents.strip():
documents = [(os.path.basename(tmp), os.path.getsize(tmp)/1024, tmp.replace('/', '<>')) for tmp in version.documents.split('#') if tmp]
else:
documents = []
version_level = ['Recommend', 'Must']
return render_template('/developer/version_self_test.html',
version=version,
documents=documents,
permission=permission,
tasks=tasks,
version_level=version_level)
# 版本自测结果
else:
version_history = VersionHistory.query.filter(VersionHistory.version_id == version.id).order_by(VersionHistory.compile_time.desc()).all()[0]
# 自测试结果
result = request.form.get('result', '')
# 版说明
version.version_note = request.form.get('version_note', '').replace('通讯录', '通u讯u录')
# 待发布的文件
need_release_file = request.form.get('need_release_file', '')
if request.form.get('need_release_file', ''):
version.release_file = '#'.join([tmp for tmp in need_release_file.split('#') if tmp.strip()])
else:
version.release_file = version.ftp_file
# 保存上传的附件
if version.documents:
exits_documents = [tmp.strip() for tmp in version.documents.split('#') if tmp.strip()]
else:
exits_documents = []
documents = request.files.getlist("file[]")
if documents:
upload_path = os.path.join(current_app.config['UPLOAD_FOLDER'], version.name, version.version_number)
if not os.path.exists(upload_path):
os.makedirs(upload_path)
for document in documents:
if document.filename:
current_app.logger.info(document.filename)
file_path = os.path.join(upload_path, document.filename)
# 删除已存在的
if os.path.exists(file_path) and os.path.isfile(file_path):
os.remove(file_path)
document.save(file_path)
if file_path not in exits_documents:
exits_documents.append(file_path)
version.documents = '#'.join(exits_documents)
if result == '1':
project = version.project
version.status = '测试'
version.level = request.form.get('level', 'C')
version_history.description = "{0}版本 自测通过".format(version.name)
to_list = ['<EMAIL>', version.compile_email]
cc_list = []
# 测试负责人
if version.project.test_leader and version.project.test_leader.email:
to_list.append(version.project.test_leader.email)
else:
to_list.append(session['user']['email'])
# 软件开发负责人
if version.project.develop_leader and version.project.develop_leader.email:
to_list.append(version.project.develop_leader.email)
# 版本发布通知邮件
if version.project.to_email:
for email in version.project.to_email.split(';'):
if email.strip():
to_list.append(email.strip())
# APK的版本发布邮件通知有的OS测试人员,由其内部进行测试任务的分配
for qa in User.query.join(Role).filter(Role.name == 'os_test').all():
to_list.append(qa.email)
# 配置组
for scm in User.query.join(Role).filter(Role.name == 'scm').all():
to_list.append(scm.email)
followers = project.followers
if followers:
for follower in followers:
cc_list.append(follower.email)
# 去重复
cc_list.append('<EMAIL>')
to_list = list(set(to_list + cc_list))
tasks = []
# msg = Message(
# u'[TSDS] %s_%s 版本需要测试' % (version.name, version.version_number),
# sender='<EMAIL>',
# # recipients=[version.project.test_leader.email, '<EMAIL>', '<EMAIL>']
# recipients=to_list,
# cc=cc_list
# )
# if exits_documents:
# for document in exits_documents:
# file_name = os.path.basename(document)
# with current_app.open_resource(document) as fp:
# msg.attach(file_name.encode("utf-8"), current_app.config['MIME_TYPE'][os.path.splitext(file_name)[1]], fp.read())
if version.tasks:
tasks = ['<table><thead><th>项目名称</th><th>JIRA_KEY</th><th>任务标题</th><th>任务创建人</th></thead><tbody>']
for task in json.loads(version.tasks, strict=False):
tasks.append(
'<tr>'
'<td>{0}</td>'
'<td><a href="http://jira.tinno.com:8080/browse/{1}">{2}</a></td>'
'<td>{3}</td>'
'<td>{4}</td>'
'</tr>'.format(task['project'], task['jira_key'], task['jira_key'], task['title'],
task['creator']))
tasks.append('</tbody></table>')
# msg.html = VERSION_SELF_TEST_TEMPLATE.format(project.name, version.name, version.version_number,
# version.level, version.ftp_path, version.jenkins_url,
# version.version_note, '\n'.join(tasks),
# current_user.display_name, current_user.email)
# mail.send(msg)
content = VERSION_SELF_TEST_TEMPLATE.format(project.name, version.name, version.version_number,
version.level, version.ftp_path, version.jenkins_url,
version.version_note, '\n'.join(tasks),
current_user.display_name, current_user.email)
message = {
'to_list': ';'.join([tmp for tmp in to_list if tmp]),
'sub': u'[TSDS] %s_%s 版本需要测试' % (version.name, version.version_number),
'content': content.replace('通讯录', '通 讯 录')
}
send_mail_pubisher(json.dumps(message))
else:
version.status = '自测不过'
version_history.description = "{0}版本 自测失败\n".format(version.name)
version_history.version_id = version_id
version_history.self_test_user = current_user
if request == '1':
version_history.self_test_result = True
else:
version_history.self_test_result = False
version_history.self_test_time = datetime.now().strftime('%Y-%m-%d %H:%M:%S')
db.session.add(version)
db.session.add(version_history)
db.session.commit()
return redirect(url_for('developer.my_version'))
@developer.route('/version_commit/<int:version_id>')
@login_required
def version_commit(version_id):
"""版本的commit信息"""
version = Version.query.filter_by(id=version_id).first()
if version.commits:
commits = json.loads(version.commits.replace('<', '<').replace('>', '>'), strict=False)
else:
commits = ''
return json.dumps({'data': commits})
@developer.route('/my_version')
@login_required
@need_self_test_version()
def my_version():
"""
我编译的版本
"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '我的版本', 'url': url_for('developer.my_version')})
session['menu_path'] = ['项目管理', '我的版本']
# 显示所有待自测的版本
version_type = request.args.get('version_type', '')
if version_type == 'need_test':
versions = Version.query.filter(
db.and_(Version.compile_email == session['user']['email'],
Version.status == '自测')).order_by(Version.compile_time.desc()).all()
else:
# 所有自己编译过的版本
versions = Version.query.filter(Version.compile_email == session['user']['email']).order_by(Version.compile_time.desc()).all()
return render_template('/developer/version_page.html',
role_name=session['user']['role_name'],
versions=versions,
version_type=version_type)
@developer.route('/project_version/<int:project_id>', methods=['GET', 'POST'])
@login_required
@need_self_test_version()
def project_version(project_id):
"""项目的所有版本"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if u'项目版本' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': u'项目版本',
'url': url_for('developer.project_version', project_id=project_id)})
project_special_versions = []
for special_version in SpecialVersion.query.filter(SpecialVersion.project_id == project_id).all():
project_special_versions.append(special_version)
return render_template('/developer/project_version_page.html',
project=Project.query.filter_by(id=project_id).first(),
project_special_versions=project_special_versions)
@developer.route('/project_version_data')
@login_required
@need_self_test_version()
def project_version_data():
start = int(request.args.get('start', 0))
length = int(request.args.get('length', 10))
project_id = request.args.get('project_id')
special_version_id = request.args.get('special_version_id')
version_sql = "SELECT p_v.id, p_v.version_number, p_v.status, p_v.compile_username, p_v.compile_time, " \
"p_v.ftp_path, p_v.svn_path, p_s_v.name, p_v.category FROM tms_project_version AS p_v JOIN " \
"tms_project_special_version AS p_s_v ON p_v.special_version_id=p_s_v.id JOIN tms_project AS " \
"pro ON p_s_v.project_id=pro.id WHERE pro.id={0} AND p_s_v.id={1} " \
"ORDER BY p_v.compile_time DESC".format(project_id, special_version_id)
all_version = db.session.execute(version_sql).fetchall()
versions = []
index = start + 1
for record in all_version[start:start+length]:
recpord = {}
recpord['index'] = index
recpord['id'] = record[0]
recpord['version_number'] = record[1]
recpord['status'] = record[2]
recpord['compile_username'] = record[3]
recpord['compile_time'] = str(record[4])
recpord['ftp_path'] = record[5]
recpord['svn_path'] = record[6]
recpord['special_version'] = record[7]
recpord['category'] = record[8]
versions.append(recpord)
index += 1
return '{"recordsTotal": %s ,"recordsFiltered": %s, "data":%s}' % (len(all_version), len(all_version),
json.dumps(versions))
@developer.route('/all_version')
@login_required
@need_self_test_version()
def all_version():
"""所有负责项目、参与项目、关注项目的版本"""
follower_project = Project.query.join(User.project_f).filter(User.id == current_user.id).all()
leader_project = Project.query.filter(Project.develop_leader_id == current_user.id).all()
project_id = []
for project_obj in follower_project:
project_id.append(project_obj.id)
for project_obj in leader_project:
project_id.append(project_obj.id)
versions = Version.query.filter(Version.project_id.in_(project_id)).order_by(Version.compile_time.desc())
return render_template('/developer/version_page.html', versions=versions)
@developer.route('/start_project_version')
@login_required
@need_self_test_version()
def start_project_version():
"""所有关注项目的版本"""
follower_project = Project.query.join(User.project_f).filter(User.id == current_user.id).all()
versions = Version.query.filter(Version.project_id.in_([project_obj.id for project_obj in follower_project])).order_by(Version.compile_time.desc()).all()
return render_template('/developer/version_page.html', versions=versions)
@developer.route('/delete_document')
@login_required
def delete_document():
"""删除附件"""
version_id = request.args.get('version_id', '')
document_path = request.args.get('document_path', '').replace('<>', '/')
version = Version.query.filter_by(id=version_id).first()
documents = version.documents.split('#')
result = []
for document in documents:
if document == document_path:
continue
else:
result.append(document)
version.documents = '#'.join(result)
db.session.add(version)
db.session.commit()
return '{"result": true}'
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
from datetime import datetime
import json
import os
from flask import render_template, redirect, url_for, request, flash, current_app, session
from flask.ext.login import login_user, logout_user, login_required, current_user
from flask.ext.mail import Mail, Message
from werkzeug import secure_filename
from tms import db, mail
from . import scm
from tms.project.models import Project, Version, VersionHistory, SpecialVersion, ReleaseEmailAddress
from tms.project.androidProjectModules import ProjectAndroid
from tms.auth.models import User, Role
from tms.repository.models import Repository, GitServeBackupLog
@scm.route('/all_project')
@login_required
def scm_page():
"""所有的项目"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '所有项目', 'url': url_for('scm.scm_page')})
session['menu_path'] = ['项目管理', '所有项目']
projects = Project.query.all()
# 负责的项目
leader_project = Project.query.filter_by(develop_leader=current_user).all()
# 关注的项目
follower_project = Project.query.join(User.project_f).filter(User.id == current_user.id).all()
result = []
index = 1
for project_obj in projects:
if project_obj in leader_project:
result.append((index, project_obj, 'leader'))
elif project_obj in follower_project:
result.append((index, project_obj, 'start'))
else:
result.append((index, project_obj, 'other'))
index += 1
# 所有Android项目
# 已经关注的项目
follower_android_project = ProjectAndroid.query.join(User.project_android_f).filter(
User.id == current_user.id).all()
project_android = []
all_android_project = ProjectAndroid.query.all()
for project_obj in all_android_project:
if project_obj in follower_android_project:
project_android.append((index, project_obj, 'start', 'android'))
else:
project_android.append((index, project_obj, 'other', 'android'))
index += 1
return render_template('/developer/developer_page.html',
all_project=True,
role_name=session['user']['role_name'],
projects=result,
project_android=project_android
)
@scm.route('/all_version')
@login_required
def all_version():
"""所有负责项目、参与项目、关注项目的版本"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': u'我的版本', 'url': url_for('scm.all_version')})
session['menu_path'] = [u'版本管理', u'我的版本']
versions = Version.query.all()
return render_template('/developer/version_page.html', versions=versions)
@scm.route('/add_special_version/<int:project_id>', methods=['GET', 'POST'])
@login_required
def add_special_version(project_id):
"""新增或编辑项目版本类型"""
project_obj = Project.query.filter(Project.id == project_id).first()
# 系统管理员和配置管理员才能修改项目的源码及发布仓库地址
code_repo_list = Repository.query.filter(Repository.category == 2).all()
release_repo_list = Repository.query.filter(Repository.category == 3).all()
if request.method == 'POST':
name = request.form.get('name')
description = request.form.get('description')
ftp_path = request.form.get('ftp_path')
svn_path = request.form.get('svn_path')
code_repo = request.form.get('code_repo')
release_repo = request.form.get('release_repo')
other_special_version = SpecialVersion.query.filter(
db.and_(SpecialVersion.name == name, SpecialVersion.project_id == project_id)).first()
if other_special_version:
flash(u'同名版本类型已存在', 'danger')
else:
special_version = SpecialVersion()
special_version.name = name
special_version.description = description
special_version.ftp_path = ftp_path
special_version.svn_path = svn_path
special_version.code_repo = Repository.query.filter(Repository.id == code_repo).first()
special_version.release_repo = Repository.query.filter(Repository.id == release_repo).first()
special_version.project = project_obj
db.session.add(special_version)
db.session.commit()
return redirect(url_for('developer.edit_project', project_id=project_id))
return render_template('/scm/edit_special_version.html',
project=project_obj,
special_version=None,
code_repo_list=code_repo_list,
special_version_id=0,
release_repo_list=release_repo_list
)
@scm.route('/edit_special_version/<int:special_version_id>', methods=['GET', 'POST'])
@login_required
def edit_special_version(special_version_id):
"""新增或编辑项目版本类型"""
special_version = SpecialVersion.query.filter(SpecialVersion.id == special_version_id).first()
project_obj = special_version.project
# 系统管理员和配置管理员才能修改项目的源码及发布仓库地址
code_repo_list = Repository.query.filter(Repository.category == 2).all()
release_repo_list = Repository.query.filter(Repository.category == 3).all()
# for repo in code_repo_list:
# repo.repo_url = '{0}:{1}/{2}'.format(repo.platform.server.remote_name, repo.platform.name, repo.name)
#
# for repo in release_repo_list:
# repo.repo_url = '{0}:{1}/{2}'.format(repo.platform.server.remote_name, repo.platform.name, repo.name)
if request.method == 'POST':
name = request.form.get('name')
app_name = request.form.get('app_name')
description = request.form.get('description')
ftp_path = request.form.get('ftp_path')
svn_path = request.form.get('svn_path')
code_repo = request.form.get('code_repo')
release_repo = request.form.get('release_repo')
other_special_version = SpecialVersion.query.filter(
db.and_(SpecialVersion.name == name, SpecialVersion.project_id == project_obj.id)).first()
if other_special_version and other_special_version.id != special_version_id:
flash(u'同名版本类型已存在', 'danger')
else:
special_version.name = name
special_version.app_name = app_name
special_version.description = description
special_version.ftp_path = ftp_path
special_version.svn_path = svn_path
special_version.code_repo = Repository.query.filter(Repository.id == code_repo).first()
special_version.release_repo = Repository.query.filter(Repository.id == release_repo).first()
db.session.add(special_version)
db.session.commit()
return redirect(url_for('developer.edit_project', project_id=project_obj.id))
return render_template('/scm/edit_special_version.html',
project=project_obj,
special_version=special_version,
special_version_id=special_version.id,
code_repo_list=code_repo_list,
release_repo_list=release_repo_list
)
@scm.route('/delete_special/<int:special_version_id>', methods=['GET', 'POST'])
@login_required
def delete_special(special_version_id):
"""删除特殊版本"""
special_version = SpecialVersion.query.filter(SpecialVersion.id == special_version_id).first()
project = special_version.project
db.session.delete(special_version)
db.session.commit()
flash(u'删除成功!', 'info')
return redirect(url_for('developer.edit_project', project_id=project.id))
@scm.route('/release_email_address', methods=['GET', 'POST'])
@login_required
def release_email_address():
"""维护版本发布通知邮件地址"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '版本发布通知邮件地址', 'url': url_for('scm.release_email_address')})
session['menu_path'] = ['项目管理', '版本发布通知邮件地址']
release_email_address_obj = ReleaseEmailAddress.query.first()
email_address = request.form.get('email_address', '')
if request.method == 'POST':
if release_email_address_obj:
release_email_address_obj.email_address = '\n'.join([tmp.strip() for tmp in email_address.split('\n') if tmp.strip()])
db.session.add(release_email_address_obj)
db.session.commit()
else:
release_email_address_obj = ReleaseEmailAddress()
release_email_address.email_address = email_address
db.session.add(release_email_address_obj)
db.session.commit()
return render_template('/scm/release_email_address_edit.html', release_email_address=release_email_address_obj)
@scm.route('/git_backup_log_page', methods=['GET', 'POST'])
@login_required
def git_backup_log_page():
"""Git服务器备份记录页面"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '代码管理', 'url': url_for('scm.git_backup_log_page')})
session['menu_path'] = ['代码管理', '备份管理']
return render_template('/scm/git_backup_log.html')
@scm.route('/git_backup_log_data', methods=['GET', 'POST'])
@login_required
def git_backup_log_data():
"""Git服务器备份记录数据"""
start = int(request.args.get('start', 0))
length = int(request.args.get('length', 10))
all_count = GitServeBackupLog.query.count()
records = []
index = start + 1
for record in GitServeBackupLog.query.order_by(GitServeBackupLog.backup_time.desc()).all()[start: start+length]:
records.append({
"id": record.id,
"index": index,
"server_ip": record.server_ip,
"platform_path": record.platform_path,
"backup_time": str(record.backup_time),
"result": record.result,
})
index += 1
return '{"recordsTotal": %s ,"recordsFiltered": %s,"data":%s}' % (all_count, all_count, json.dumps(records))
@scm.route('/git_backup_log_detail_info/<int:log_id>', methods=['GET', 'POST'])
@login_required
def git_backup_log_detail_info(log_id):
"""Git服务器备份记录详细信息"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if u'备份详情' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': u'备份详情',
'url': url_for('scm.git_backup_log_detail_info', log_id=log_id)})
log_record = GitServeBackupLog.query.filter(GitServeBackupLog.id == log_id).first()
return render_template('scm/git_backup_log_detail_info.html', log_record_info=log_record.info.replace('\\n', '<br>'))
<file_sep>#!/usr/bin/env python
# -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/6/12
"""
将平台化myos的项目配置信息导入数据库中
"""
import sys
import os
from ConfigParser import ConfigParser
from MySQLdb import connect
# myos配置数据库信息
db_host = '192.168.33.7'
db_user = 'tup'
db_passwd = 'tup'
db_name = 'tms'
def add_project(project_name, parent_id, myos_config_patch):
"""项目加入数据库中"""
con = connect(host=db_host, db=db_name, user=db_user, passwd=db_passwd, charset='utf8')
cursor = con.cursor()
if parent_id:
insert_project = "INSERT INTO project_android(name, category, parent_id, myos_config_patch) VALUES (%s, %s, %s, %s)"
cursor.executemany(insert_project, [(project_name, u'订单项目', parent_id, myos_config_patch)])
else:
insert_project = "INSERT INTO project_android(name, category, myos_config_patch) VALUES (%s, %s, %s)"
cursor.executemany(insert_project, [(project_name, u'产品项目', myos_config_patch)])
con.commit()
select_project = "SELECT id FROM project_android WHERE myos_config_patch='%s'" % myos_config_patch
print select_project
cursor.execute(select_project)
project_id = str(cursor.fetchone()[0])
con.close()
return project_id
def get_parent_project(myos_config_patch):
"""获取父项目的记录"""
con = connect(host=db_host, db=db_name, user=db_user, passwd=db_passwd, charset='utf8')
cursor = con.cursor()
select_project = "SELECT id FROM project_android WHERE myos_config_patch='%s' AND category='产品项目'" % myos_config_patch
cursor.execute(select_project)
project_id = cursor.fetchone()
if project_id:
project_id = project_id[0]
con.close()
return project_id
def add_config(project_id, config_path):
"""解析ApkVersion将其加入数据库中"""
cp = ConfigParser()
cp.read(config_path)
app_config = []
for app_name in cp.sections():
# print app_name
if app_name == 'ProjectInfo':
continue
if cp.get(app_name, 'support') == 'yes':
support = 'yes'
else:
support = 'no'
if cp.has_option(app_name, 'overrides') and cp.get(app_name, 'overrides') and cp.get(app_name, 'overrides') != 'null':
overrides = cp.get(app_name, 'overrides')
else:
overrides = ''
app_config.append((app_name, support, cp.get(app_name, 'version').strip(), overrides, project_id))
insert_myos_config = "INSERT INTO project_myos_app_config(app_name, support, app_version, overrides, project_id)" \
"VALUES (%s, %s, %s, %s, %s)"
con = connect(host=db_host, db=db_name, user=db_user, passwd=db_passwd, charset='utf8')
cursor = con.cursor()
cursor.executemany(insert_myos_config, app_config)
con.commit()
con.close()
def main(config_repo_path):
"""递归config仓库的所有目录找出ApkVersion.ini文件,然后解析ApkVersion.ini将其随产品名称、项目名称
一起添加进数据库
"""
project_config = []
for root, dirs, files in os.walk(config_repo_path):
for file_name in files:
if file_name == 'ApkVersion.ini':
project_config.append(os.path.join(root, file_name))
for project_config_path in project_config:
apk_version_path = project_config_path.split(config_repo_path)[1].strip('/')
if apk_version_path.find('trunk') > 0:
# 添加项目记录
product_name = [tmp for tmp in apk_version_path.split('/') if tmp.strip()][0]
parent_id = add_project(product_name, '', apk_version_path)
# 解析配置文件
add_config(parent_id, project_config_path)
elif apk_version_path.find('SUB_PROJECTS') > 0:
product_name = [tmp for tmp in apk_version_path.split('/') if tmp.strip()][0]
project_name = [tmp for tmp in apk_version_path.split('/SUB_PROJECTS/')[1].split('/') if tmp.strip()][0]
parent_id = get_parent_project(os.path.join(apk_version_path.split('/SUB_PROJECTS/')[0], 'ApkVersion.ini'))
project_id = add_project(project_name, parent_id, apk_version_path)
add_config(project_id, project_config_path)
else:
# 添加项目记录
product_name = [tmp for tmp in apk_version_path.split('/') if tmp.strip()][0]
project_name = [tmp for tmp in apk_version_path.split('/') if tmp.strip()][1]
parent_id = get_parent_project(os.path.join(product_name, 'trunk', 'ApkVersion.ini'))
project_id = add_project(project_name, parent_id, apk_version_path)
# 解析配置文件
add_config(project_id, project_config_path)
if __name__ == '__main__':
main(sys.argv[1])<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
import os
import sys
from optparse import OptionParser
import commands
def remove_remote(platform_path, remote_name):
"""
获取平台下所有的Git仓库的指定remote
"""
repos = []
for root, dirs, files in os.walk(platform_path):
for dir_name in dirs:
if dir_name == '.repo' or dir_name == '.git':
continue
git_path = os.path.join(root, dir_name)
if git_path.find('/.repo/') > 0:
continue
if git_path.endswith('.git'):
print git_path
status, info = commands.getstatusoutput('git --git-dir={0} remote'.format(git_path))
if status:
print info
sys.exit(1)
elif not info or remote_name not in [tmp for tmp in info.split('\n') if tmp.strip()]:
continue
status, info = commands.getstatusoutput('git --git-dir={0} remote rm {1}'.format(git_path, remote_name))
if status:
print info
sys.exit(1)
print info
return repos
def main():
"""
1. 导入服务器上的所有平台
2. 导入平台下的所有仓库
"""
des = u'import platform and Repository'
prog= u'AddRepository'
ver = u'%prog v0.0.1'
usage = u'%prog ip_address platform_path dir_path'
parse = OptionParser(description=des, prog=prog, version=ver, usage=usage, add_help_option=True)
parse.add_option('-p', '--platform-path', action='store', type='string', dest='platform_path',
help='repository platform name')
parse.add_option('-r', '--remote-name', action='store', type='string', dest='remote_name',
help='repository remote name', default='origin')
# 解析命令行参数
options, args = parse.parse_args()
platform_path = options.platform_path.strip('')
remote_name = options.remote_name
remove_remote(platform_path, remote_name)
if __name__ == '__main__':
main()
<file_sep>/**
* Created by changjie.fan on 2016/8/31.
*/
var common_issue_table = $('#common_issue_table').DataTable({
"language": {
"aria": {
"sortAscending": ": activate to sort column ascending",
"sortDescending": ": activate to sort column descending"
},
"emptyTable": "No data available in table",
"info": "Showing _START_ to _END_ of _TOTAL_ records",
"infoEmpty": "No records found",
"infoFiltered": "(filtered1 from _MAX_ total records)",
"lengthMenu": "Show _MENU_ records",
"search": "Search:",
"zeroRecords": "No matching records found",
"paginate": {
"previous": "Prev",
"next": "Next",
"last": "Last",
"first": "First"
}
},
"bFilter": true,
"bServerSide": true, // save datatable state(pagination, sort, etc) in cookie.
"bProcessing": true,
"ajax": {
url: '/project/common_issue_data',
data: {"is_all_issue": function(){
return window.is_all_issue;
}},
},
"columns": [
{"data": 'index'},
{"data": 'name'},
{"data": 'jira_key'},
{"data": 'project_name'},
{"data": 'platform_name'},
{"data": 'status'}
],
// "columnDefs": [
// {
// "render": function ( data, type, row ) {
// return "<a class='btn btn-primary btn-xs' href='"+$SCRIPT_ROOT+"/project/android_project_version/"+ row.id +"'>所有版本</a>";
// },
// "targets": -1
// },
// ],
// "createdRow": function ( row, data, index ) {
// if(!data['result']){
// $(row).css('background-color','red');
// }
// },
"lengthMenu": [
[10, 50, 200],
[10, 50, 200] // change per page values here
],
// set the initial value
"pageLength": 10,
"pagingType": "bootstrap_full_number"
});
$('#common_issue_table tbody').on( 'dblclick', 'tr', function () {
var row_data = common_issue_table.row(this).data().id;
if (role_name == 'Android测试' || role_name == 'scm'){
window.location.href = $SCRIPT_ROOT + '/project/qa_edit_common_issue/' + row_data;
}else if(role_name == 'Android开发工程师') {
window.location.href = $SCRIPT_ROOT + '/project/dev_edit_common_issue/' + row_data;
}else if(role_name == 'spm') {
window.location.href = $SCRIPT_ROOT + '/project/spm_edit_common_issue/' + row_data;
}
});
//导出Excel数据
function export_issue(){
$.ajax({
url: $SCRIPT_ROOT + '/project/export_common_issue',
success:function(data){
window.location.href = '/auth/download_file/?file_path=' + JSON.parse(data)["filePath"];
}
});
}<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""
项目信息管理的view
"""
from datetime import datetime,date
import json
import sys
import os
reload(sys)
sys.setdefaultencoding('utf8')
from flask import render_template, redirect, url_for, request, flash, current_app, session, send_file
from flask.ext.login import login_user, logout_user, login_required, current_user
from flask.ext.mail import Mail, Message
from functools import update_wrapper
from . import project
from tms import db, mail
from tms.auth.models import User, Role
from androidProjectModules import *
from models import Project
from tms.auth.models import SystemParameter
from tms.baseline.models import BPlatform
import MySQLdb
from MySQLdb import connect
from tms.utils.excelOption import ExcelOptions
@project.route('/android_project_page')
@login_required
def android_project_page():
"""
项目列表页面
:return:
"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '产品项目', 'url': url_for('project.android_project_page')})
session['menu_path'] = ['项目管理', '产品项目']
# 关注的项目
follower_project = ProjectAndroid.query.join(User.project_android_f).filter(User.id == current_user.id).all()
result = []
projects = ProjectAndroid.query.order_by(ProjectAndroid.id.desc()).all()
for project_obj in projects:
if project_obj in follower_project:
result.insert(0, (project_obj, True))
else:
result.append((project_obj, False))
return render_template('project/android/project_page.html', projects=result)
@project.route('/add_android_project_page/', methods=['GET', 'POST'])
@login_required
def add_android_project_page():
"""
新增项目
:return:
"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '新增项目信息' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index + 1]
break
else:
session['breadcrumbs'].append({'text': '新增项目信息', 'url': url_for('project.add_android_project_page')})
project_obj = ProjectAndroid()
if request.method == 'POST':
project_name = request.form.get('name', '').strip()
project_key = request.form.get('project_key', '').strip()
category = request.form.get('category', '').strip()
commit_notice_email = request.form.get('commit_notice_email', '').strip()
parent_id = request.form.get('parent_id', '')
android_version = request.form.get('android_version', '')
status = request.form.get('status', '')
platform_name = request.form.get('platform_name', '')
customer_name = request.form.get('customer_name', '')
customer_area = request.form.get('customer_area', '')
operators = request.form.get('operators', '')
extended_field = request.form.get('extended_field', '')
baseline_name = request.form.get('baseline_name', '')
scm = request.form.get('scm', '')
spm = request.form.get('spm', '')
myos_config_patch = request.form.get('myos_config_patch', '')
# 项目JIRA_KEY不能重复
other_project = ProjectAndroid.query.filter(ProjectAndroid.project_key == project_key).first()
if other_project:
flash(u'相同JIRA_KEY的项目已存在:%s' % other_project.name, 'warning')
else:
project_obj.name = project_name
project_obj.project_key = project_key
project_obj.category = category
project_obj.android_version = android_version
project_obj.status = status
project_obj.platform_name = platform_name
project_obj.customer_name = customer_name
project_obj.customer_area = customer_area
project_obj.operators = operators
project_obj.extended_field = extended_field
project_obj.baseline_name = baseline_name
project_obj.create_time = datetime.now()
project_obj.scm_id = scm
project_obj.spm_id = spm
project_obj.myos_config_patch = myos_config_patch
commit_notice_email_list = list(set([tmp.strip() for tmp in commit_notice_email.split(';') if tmp.strip()]))
project_obj.commit_notice_email = ';'.join(commit_notice_email_list)
if parent_id:
project_obj.parent_id = int(parent_id)
db.session.add(project_obj)
db.session.commit()
flash(u'新增', 'info')
return redirect(url_for('project.android_project_page'))
project_category = ['产品项目', '订单项目', '软件项目']
parent_projects = ProjectAndroid.query.filter(ProjectAndroid.category == '产品项目').all()
platforms = BPlatform.query.all()
# 软件项目经理
spm_options = User.query.join(Role).filter(db.and_(Role.name == 'spm', User.activate == True)).all()
# 配置管理工程师
scm_options = User.query.join(Role).filter(db.and_(Role.name == 'scm', User.activate == True)).all()
# 系统参数值
# 订单项目客户
customer_name_value = SystemParameter.query.filter_by(key='customer_name').first()
if customer_name_value:
customer_name_options = [tmp.strip() for tmp in customer_name_value.value.split('\n')]
customer_name_options.sort()
else:
customer_name_options = []
# 订单项目出货地点
customer_area_value = SystemParameter.query.filter_by(key='customer_area').first()
if customer_area_value:
customer_area_options = [tmp.strip() for tmp in customer_area_value.value.split('\n')]
customer_area_options.sort()
else:
customer_area_options = []
# 运营商参数
operators_value = SystemParameter.query.filter_by(key='operator').first()
if operators_value:
operators_options = [tmp.strip() for tmp in operators_value.value.split('\n')]
operators_options.sort()
else:
operators_options = []
# android 版本参数
android_version_value = SystemParameter.query.filter_by(key='android_version').first()
if android_version_value:
android_version = [tmp.strip() for tmp in android_version_value.value.split('\n')]
else:
android_version = []
# 项目状态参数
project_status_value = SystemParameter.query.filter_by(key='project_status').first()
if project_status_value:
project_status = [tmp.strip() for tmp in project_status_value.value.split('\n')]
else:
project_status = []
return render_template('project/android/add_project_page.html',
project=project_obj,
project_category=project_category,
parent_projects=parent_projects,
platforms=platforms,
customer_name_options=customer_name_options,
customer_area_options=customer_area_options,
operators_options=operators_options,
android_version=android_version,
project_status=project_status,
scm_options=scm_options,
spm_options=spm_options)
@project.route('/edit_android_project_page/<int:project_id>', methods=['GET', 'POST'])
@login_required
def edit_android_project_page(project_id):
"""
修改项目
:return:
"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '编辑项目信息' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index + 1]
break
else:
session['breadcrumbs'].append({'text': '编辑项目信息', 'url': url_for('project.edit_android_project_page',
project_id=project_id)})
project_obj = ProjectAndroid.query.filter(ProjectAndroid.id == project_id).first()
project_info_obj = ProjectAndroidInfo.query.filter(ProjectAndroidInfo.project_android_id == project_obj.id).first()
project_history_list = get_project_history(project_id)
if request.method == 'POST':
project_name = request.form.get('name', '').strip()
project_key = request.form.get('project_key', '').strip()
category = request.form.get('category', '').strip()
commit_notice_email = request.form.get('commit_notice_email', '').strip()
parent_id = request.form.get('parent_id', '')
android_version = request.form.get('android_version', '')
status = request.form.get('status', '')
platform_name = request.form.get('platform_name', '')
customer_name = request.form.get('customer_name', '')
customer_area = request.form.get('customer_area', '')
operators = request.form.get('operators', '')
extended_field = request.form.get('extended_field', '')
baseline_name = request.form.get('baseline_name', '')
scm_id = request.form.get('scm', '')
myos_config_patch = request.form.get('myos_config_patch', '')
spm_id = request.form.get('spm', '')
parent_id = request.form.get('parent_id', '')
# 项目JIRA_KEY不能重复
other_project = ProjectAndroid.query.filter(ProjectAndroid.project_key == project_key).first()
if other_project and other_project.id != project_obj.id:
flash(u'相同JIRA_KEY的项目已存在:%s' % other_project.name, 'warning')
else:
# 记录修改历史到project_android_history
history = {}
history['time'] = datetime.now().strftime("%Y-%m-%d %H:%M:%S")
history['user_id'] = current_user.id
history['project_id'] = project_id
history['type'] = 'edit'
history['content'] = []
if project_obj.name != project_name:
case = {}
case['name'] = '项目名称'
case['old'] = project_obj.name
case['new'] = project_name
history['content'].append(case)
project_obj.name = project_name
if project_obj.project_key != project_key:
case = {}
case['name'] = '项目JIRA_KEY'
case['old'] = project_obj.project_key
case['new'] = project_key
history['content'].append(case)
project_obj.project_key = project_key
if project_obj.parent_id != parent_id:
case = {}
case['name'] = '产品项目'
case['old'] = project_obj.parent_id
case['new'] = parent_id
history['content'].append(case)
if parent_id:
project_obj.parent_id = parent_id
else:
project_obj.parent = None
if project_obj.category != category:
case = {}
case['name'] = '项目类型'
case['old'] = project_obj.category
case['new'] = category
history['content'].append(case)
project_obj.category = category
if project_obj.android_version != android_version:
case = {}
case['name'] = 'Android版本'
case['old'] = project_obj.android_version
case['new'] = android_version
history['content'].append(case)
project_obj.android_version = android_version
if project_obj.status != status:
case = {}
case['name'] = '项目状态'
case['old'] = project_obj.status
case['new'] = status
history['content'].append(case)
project_obj.status = status
if project_obj.platform_name != platform_name:
case = {}
case['name'] = '平台厂商'
case['old'] = project_obj.platform_name
case['new'] = platform_name
history['content'].append(case)
project_obj.platform_name = platform_name
if project_obj.customer_name != customer_name:
case = {}
case['name'] = '订单项目客户名称'
case['old'] = project_obj.customer_name
case['new'] = customer_name
history['content'].append(case)
project_obj.customer_name = customer_name
if project_obj.customer_area != customer_area:
case = {}
case['name'] = '订单项目出货地点'
case['old'] = project_obj.customer_area
case['new'] = customer_area
history['content'].append(case)
project_obj.customer_area = customer_area
if project_obj.operators != operators:
case = {}
case['name'] = '运营商名称'
case['old'] = project_obj.operators
case['new'] = operators
history['content'].append(case)
project_obj.operators = operators
if project_obj.extended_field != extended_field:
case = {}
case['name'] = '扩展字段'
case['old'] = project_obj.extended_field
case['new'] = extended_field
history['content'].append(case)
project_obj.extended_field = extended_field
if project_obj.baseline_name != baseline_name:
case = {}
case['name'] = '基线名称'
case['old'] = project_obj.baseline_name
case['new'] = baseline_name
history['content'].append(case)
project_obj.baseline_name = baseline_name
if project_obj.myos_config_patch != myos_config_patch:
case = {}
case['name'] = 'MyOS配置路径'
case['old'] = project_obj.myos_config_patch
case['new'] = myos_config_patch
history['content'].append(case)
project_obj.myos_config_patch = myos_config_patch
if project_obj.scm_id != int(scm_id):
scm = User.query.filter_by(id=scm_id).first()
case = {}
case['name'] = '配置管理工程师'
if project_obj.scm:
case['old'] = project_obj.scm.display_name
else:
case['old'] = ''
case['new'] = scm.display_name
history['content'].append(case)
project_obj.scm = scm
if project_obj.spm_id != int(spm_id):
spm = User.query.filter_by(id=spm_id).first()
case = {}
case['name'] = '软件项目经理'
if project_obj.spm:
case['old'] = project_obj.spm.display_name
else:
case['old'] = ''
case['new'] = spm.display_name
history['content'].append(case)
project_obj.spm = spm
# 去除重复的邮箱地址
if commit_notice_email:
commit_notice_email_list = list(
set([tmp.strip() for tmp in commit_notice_email.split(';') if tmp.strip()]))
old_email_list = []
if project_obj.commit_notice_email:
old_email_list = project_obj.commit_notice_email.split(';')
if project_obj.commit_notice_email != ';'.join(commit_notice_email_list):
project_obj.commit_notice_email = ';'.join(commit_notice_email_list)
# 项目中删除的邮箱在分支中也要删除
need_delete_email = []
for old_email in old_email_list:
if old_email not in commit_notice_email_list:
need_delete_email.append(old_email)
# 更新所有分支的通知邮箱地址
project_code_list = project_obj.project_code
for project_code in project_code_list:
if project_code.commit_notice_email:
new_project_code_email = []
for email in project_code.commit_notice_email.split(';'):
if email not in need_delete_email:
new_project_code_email.append(email)
project_code.commit_notice_email = ';'.join(
list(set(new_project_code_email + commit_notice_email_list)))
else:
project_code.commit_notice_email = project_obj.commit_notice_email
db.session.add(project_code)
if parent_id and project_obj.parent_id != project_id:
if project_obj.parent_id != int(parent_id):
case = {}
case['name'] = '基础项目'
case['old'] = project_obj.parent_id
case['new'] = parent_id
history['content'].append(case)
project_obj.parent_id = int(parent_id)
add_project_history(history)
db.session.add(project_obj)
db.session.commit()
flash(u'修改成功', 'info')
return redirect(url_for('project.android_project_page'))
project_code = project_obj.project_code
project_category = ['产品项目', '订单项目', '软件项目']
parent_projects = ProjectAndroid.query.filter(ProjectAndroid.category == '产品项目').all()
platforms = BPlatform.query.all()
# 软件项目经理
spm_options = User.query.join(Role).filter(db.and_(Role.name == 'spm', User.activate == True)).all()
# 配置管理工程师
scm_options = User.query.join(Role).filter(db.and_(Role.name == 'scm', User.activate == True)).all()
# 系统参数
# 订单项目客户
customer_name_value = SystemParameter.query.filter_by(key='customer_name').first()
if customer_name_value:
customer_name_options = [tmp.strip() for tmp in customer_name_value.value.split('\n')]
customer_name_options.sort()
else:
customer_name_options = []
# 订单项目出货地点
customer_area_value = SystemParameter.query.filter_by(key='customer_area').first()
if customer_area_value:
customer_area_options = [tmp.strip() for tmp in customer_area_value.value.split('\n')]
customer_area_options.sort()
else:
customer_area_options = []
# 运营商参数
operators_value = SystemParameter.query.filter_by(key='operator').first()
if operators_value:
operators_options = [tmp.strip() for tmp in operators_value.value.split('\n')]
operators_options.sort()
else:
operators_options = []
# android 版本参数
android_version_value = SystemParameter.query.filter_by(key='android_version').first()
if android_version_value:
android_version = [tmp.strip() for tmp in android_version_value.value.split('\n')]
else:
android_version = []
# 项目状态参数
project_status_value = SystemParameter.query.filter_by(key='project_status').first()
if project_status_value:
project_status = [tmp.strip() for tmp in project_status_value.value.split('\n')]
else:
project_status = []
return render_template('project/android/edit_project_page.html',
project=project_obj,
project_info=project_info_obj,
project_history_list=project_history_list,
project_code=project_code,
project_category=project_category,
parent_projects=parent_projects,
platforms=platforms,
customer_name_options=customer_name_options,
customer_area_options=customer_area_options,
operators_options=operators_options,
android_version=android_version,
project_status=project_status,
scm_options=scm_options,
spm_options=spm_options)
@project.route('/android_project_jira')
@login_required
def android_project_jira():
# 项目Jira统计
jira_key = request.args.get('jira_key', '')
date_vrgs = request.args.get('date', '')
export_flag = request.args.get('export','')
# if not date_vrgs:
# date_vrgs = str(date.today())
jira_data = {}
# 任务每日更新,包括:按日查询新增任务,已关闭任务
tasks_daily_update_sql = "SELECT open_issue.Jdate,open_issue.openNum,close_issue.closedNum FROM " \
"(SELECT DATE(jiraissue.CREATED) as Jdate,COUNT(DATE(jiraissue.CREATED)) as openNum FROM jiraissue LEFT JOIN project ON project.ID = jiraissue.PROJECT " \
"WHERE project.pkey = '%s' AND jiraissue.issuetype = 1 GROUP BY DATE(jiraissue.CREATED)) open_issue " \
"LEFT JOIN " \
"(SELECT DATE(jiraissue.UPDATED) as Jdate,COUNT(DATE(jiraissue.UPDATED)) as closedNum FROM jiraissue LEFT JOIN project ON project.ID = jiraissue.PROJECT " \
"WHERE project.pkey = '%s' AND jiraissue.issuestatus = 6 AND jiraissue.issuetype = 1 GROUP BY DATE(jiraissue.UPDATED)) close_issue ON open_issue.Jdate = close_issue.Jdate " \
"UNION " \
"SELECT close_issue.Jdate,open_issue.openNum,close_issue.closedNum FROM " \
"(SELECT DATE(jiraissue.CREATED) as Jdate,COUNT(DATE(jiraissue.CREATED)) as openNum FROM jiraissue LEFT JOIN project ON project.ID = jiraissue.PROJECT " \
"WHERE project.pkey = '%s' AND jiraissue.issuetype = 1 GROUP BY DATE(jiraissue.CREATED)) open_issue " \
"RIGHT JOIN " \
"(SELECT DATE(jiraissue.UPDATED) as Jdate,COUNT(DATE(jiraissue.UPDATED)) as closedNum FROM jiraissue LEFT JOIN project ON project.ID = jiraissue.PROJECT " \
"WHERE project.pkey = '%s' AND jiraissue.issuestatus = 6 AND jiraissue.issuetype = 1 GROUP BY DATE(jiraissue.UPDATED)) close_issue ON open_issue.Jdate = close_issue.Jdate " \
"ORDER BY Jdate " % (jira_key, jira_key, jira_key, jira_key)
# # 每人负责的任务总数
# total_tasks_each_person_sql = "SELECT cu.display_name as assigner,COUNT(jiraissue.ASSIGNEE) as assignerNum FROM jiraissue LEFT JOIN project ON project.ID = jiraissue.PROJECT " \
# "LEFT JOIN cwd_user cu ON cu.user_name = jiraissue.ASSIGNEE OR cu.email_address = jiraissue.ASSIGNEE " \
# "WHERE project.pkey = '%s' GROUP BY jiraissue.ASSIGNEE ORDER BY COUNT(jiraissue.ASSIGNEE) DESC LIMIT 0,60 " % jira_key
# # 每人负责的正在进行中的任务数
total_tasks_developer_open_sql = "SELECT cu.display_name as assigner,COUNT(jiraissue.ASSIGNEE) as assignerNum FROM jiraissue LEFT JOIN project ON project.ID = jiraissue.PROJECT " \
"LEFT JOIN cwd_user cu ON cu.user_name = jiraissue.ASSIGNEE OR cu.email_address = jiraissue.ASSIGNEE " \
"WHERE project.pkey = '%s' AND jiraissue.issuestatus != 6 AND jiraissue.issuetype = 1 GROUP BY jiraissue.ASSIGNEE ORDER BY COUNT(jiraissue.ASSIGNEE) DESC LIMIT 0,60" % jira_key
# 全部未关闭的任务数
total_tasks_open_sql = "SELECT count(*) AS openNum FROM jiraissue LEFT JOIN project ON project.ID = jiraissue.PROJECT WHERE project.pkey = '%s' AND jiraissue.issuestatus != 6 AND jiraissue.issuetype = 1 " % jira_key
# 全部已关闭的任务数
total_tasks_closed_sql = "SELECT count(*) AS closeNum FROM jiraissue LEFT JOIN project ON project.ID = jiraissue.PROJECT WHERE project.pkey = '%s' AND jiraissue.issuestatus = 6 AND jiraissue.issuetype = 1 " % jira_key
#测试人员每个人提交的总任务数
total_tasks_tester_open_sql = "SELECT cu.display_name as creator,COUNT(*) as creatorNum FROM jiraissue LEFT JOIN project ON project.ID = jiraissue.PROJECT " \
"LEFT JOIN cwd_user cu ON cu.user_name = jiraissue.CREATOR OR cu.email_address = jiraissue.CREATOR " \
"WHERE project.pkey = '%s' AND jiraissue.issuetype = 1 GROUP BY jiraissue.CREATOR ORDER BY creatorNum desc" % jira_key
#测试人员当日每个人提交的任务数
daily_tasks_tester_open_sql = "SELECT cu.display_name as creator,COUNT(*) as creatorNum FROM jiraissue LEFT JOIN project ON project.ID = jiraissue.PROJECT " \
"LEFT JOIN cwd_user cu ON cu.user_name = jiraissue.CREATOR OR cu.email_address = jiraissue.CREATOR " \
"WHERE project.pkey = '%s' AND DATE(jiraissue.CREATED) = '%s' AND jiraissue.issuetype = 1 GROUP BY jiraissue.CREATOR ORDER BY creatorNum desc" % (jira_key,date.today() if not date_vrgs else date_vrgs)
#开发人员每个人关闭的总任务数
total_tasks_developer_closed_sql = "SELECT cu.display_name as assigner,COUNT(*) as assignerNum FROM jiraissue LEFT JOIN project ON project.ID = jiraissue.PROJECT " \
"LEFT JOIN cwd_user cu ON cu.user_name = jiraissue.ASSIGNEE OR cu.email_address = jiraissue.ASSIGNEE " \
"WHERE project.pkey = '%s' AND jiraissue.issuestatus = 6 AND jiraissue.issuetype = 1 GROUP BY jiraissue.ASSIGNEE ORDER BY assignerNum desc" % jira_key
#开发人员当日每个人关闭的任务数
daily_tasks_developer_closed_sql = "SELECT cu.display_name as assigner,COUNT(*) as assignerNum FROM jiraissue LEFT JOIN project ON project.ID = jiraissue.PROJECT " \
"LEFT JOIN cwd_user cu ON cu.user_name = jiraissue.ASSIGNEE OR cu.email_address = jiraissue.ASSIGNEE " \
"WHERE project.pkey = '%s' AND jiraissue.issuestatus = 6 AND DATE(jiraissue.UPDATED) = '%s' AND jiraissue.issuetype = 1 GROUP BY jiraissue.ASSIGNEE ORDER BY assignerNum desc" % (jira_key,date.today() if not date_vrgs else date_vrgs)
#按模块统计任务数,(存在同一个issue属于两个己以上模块)
total_tasks_by_module_sql = "SELECT total_talbe.module_name,total_talbe.total_num,open_table.open_num FROM " \
"(SELECT component.cname AS module_name,COUNT(*) AS total_num FROM jiraissue " \
"LEFT JOIN project ON project.ID = jiraissue.PROJECT " \
"LEFT JOIN nodeassociation ON jiraissue.ID = nodeassociation.SOURCE_NODE_ID " \
"LEFT JOIN component ON nodeassociation.SINK_NODE_ID = component.ID " \
"WHERE project.pkey = '%s' AND nodeassociation.ASSOCIATION_TYPE = 'IssueComponent' AND jiraissue.issuetype = 1 " \
"GROUP BY component.cname) total_talbe " \
"LEFT JOIN " \
"(SELECT component.cname AS module_name,COUNT(*) AS open_num FROM jiraissue " \
"LEFT JOIN project ON project.ID = jiraissue.PROJECT " \
"LEFT JOIN nodeassociation ON jiraissue.ID = nodeassociation.SOURCE_NODE_ID " \
"LEFT JOIN component ON nodeassociation.SINK_NODE_ID = component.ID " \
"WHERE project.pkey = '%s' AND nodeassociation.ASSOCIATION_TYPE = 'IssueComponent' AND jiraissue.issuetype = 1 AND jiraissue.issuestatus != 6 " \
"GROUP BY component.cname) open_table " \
"ON total_talbe.module_name = open_table.module_name ORDER BY total_talbe.total_num desc" % (jira_key,jira_key)
jira_db_host = current_app.config['JIRA_DB_HOST']
jira_db_name = current_app.config['JIRA_DB_NAME']
jira_db_user = current_app.config['JIRA_DB_USER']
jira_db_passwd = current_app.config['JIRA_DB_PASSWD']
conn = connect(host=jira_db_host, db=jira_db_name, user=jira_db_user, passwd=<PASSWORD>, charset='utf8')
cursor = conn.cursor()
cursor.execute(tasks_daily_update_sql)
tasks_daily_update_records = cursor.fetchall()
# cursor.execute(total_tasks_each_person_sql)
# total_tasks_each_person_records = cursor.fetchall()
#
cursor.execute(total_tasks_developer_open_sql)
total_tasks_developer_open_records = cursor.fetchall()
cursor.execute(total_tasks_open_sql)
total_tasks_open_records = cursor.fetchall()
cursor.execute(total_tasks_closed_sql)
total_tasks_closed_records = cursor.fetchall()
cursor.execute(total_tasks_tester_open_sql)
total_tasks_tester_open_records = cursor.fetchall()
cursor.execute(daily_tasks_tester_open_sql)
daily_tasks_tester_open_records = cursor.fetchall()
cursor.execute(total_tasks_developer_closed_sql)
total_tasks_developer_closed_records = cursor.fetchall()
cursor.execute(daily_tasks_developer_closed_sql)
daily_tasks_developer_closed_records = cursor.fetchall()
cursor.execute(total_tasks_by_module_sql)
total_tasks_by_module_records = cursor.fetchall()
cursor.close()
conn.close()
if not date_vrgs:
total_tasks = []
for total_tasks_open_record in total_tasks_open_records:
total_tasks_open_dic = {}
total_tasks_open_dic["status"] = "进行中"
total_tasks_open_dic["total_num"] = total_tasks_open_record[0]
total_tasks.append(total_tasks_open_dic)
for total_tasks_closed_record in total_tasks_closed_records:
total_tasks_closed_dic = {}
total_tasks_closed_dic["status"] = "已完成"
total_tasks_closed_dic["total_num"] = total_tasks_closed_record[0]
total_tasks.append(total_tasks_closed_dic)
jira_data['total_tasks'] = total_tasks
# tasks_daily_update = []
# for tasks_daily_update_record in tasks_daily_update_records:
# tasks_daily_update_dic = {}
# tasks_daily_update_dic["date"] = tasks_daily_update_record[0].strftime("%Y-%m-%d")
# tasks_daily_update_dic["new_open"] = 0 if tasks_daily_update_record[1] is None else int(
# tasks_daily_update_record[1])
# tasks_daily_update_dic["closed"] = 0 if tasks_daily_update_record[2] is None else int(
# tasks_daily_update_record[2])
# tasks_daily_update.append(tasks_daily_update_dic)
# jira_data['tasks_daily_update'] = tasks_daily_update
tasks_daily_summary = []
for index1,tasks_daily_update_record in enumerate(tasks_daily_update_records):
tasks_daily_summary_dic = {}
open_summary = 0
closed_summary = 0
tasks_daily_summary_dic["date"] = tasks_daily_update_record[0].strftime("%Y-%m-%d")
for index2,tasks_daily_update_sub in enumerate(tasks_daily_update_records):
if index1 >= index2:
open_summary += 0 if tasks_daily_update_sub[1] is None else int(tasks_daily_update_sub[1])
closed_summary += 0 if tasks_daily_update_sub[2] is None else int(tasks_daily_update_sub[2])
else:
break
tasks_daily_summary_dic["open_summary"] = open_summary
tasks_daily_summary_dic["closed_summary"] = closed_summary
tasks_daily_summary.append(tasks_daily_summary_dic)
jira_data['tasks_daily_summary'] = tasks_daily_summary
# total_tasks_each_person = []
# for total_tasks_each_person_record in total_tasks_each_person_records:
# total_tasks_each_person_dic = {}
# total_tasks_each_person_dic["assigner"] = total_tasks_each_person_record[0]
# total_tasks_each_person_dic["total_tasks"] = total_tasks_each_person_record[1]
# total_tasks_each_person.append(total_tasks_each_person_dic)
# jira_data['total_tasks_each_person'] = total_tasks_each_person
#
total_tasks_developer_open = []
for total_tasks_developer_open_record in total_tasks_developer_open_records:
total_tasks_developer_open_dic = {}
total_tasks_developer_open_dic["assigner"] = total_tasks_developer_open_record[0]
total_tasks_developer_open_dic["total_tasks"] = total_tasks_developer_open_record[1]
total_tasks_developer_open.append(total_tasks_developer_open_dic)
jira_data['total_tasks_developer_open'] = total_tasks_developer_open
total_tasks_by_module = []
for total_tasks_by_module_record in total_tasks_by_module_records:
total_tasks_by_module_dic = {}
total_tasks_by_module_dic['module_name'] = total_tasks_by_module_record[0]
total_tasks_by_module_dic['total_num'] = 0 if total_tasks_by_module_record[1] is None else int(total_tasks_by_module_record[1])
total_tasks_by_module_dic['open_num'] = 0 if total_tasks_by_module_record[2] is None else int(total_tasks_by_module_record[2])
total_tasks_by_module.append(total_tasks_by_module_dic)
jira_data['total_tasks_by_module'] = total_tasks_by_module
total_tasks_tester_open = []
for total_tasks_tester_open_record in total_tasks_tester_open_records:
total_tasks_tester_open_dic = {}
total_tasks_tester_open_dic['creator'] = total_tasks_tester_open_record[0]
total_tasks_tester_open_dic['creatorNum'] = total_tasks_tester_open_record[1]
total_tasks_tester_open.append(total_tasks_tester_open_dic)
jira_data['total_tasks_tester_open'] = total_tasks_tester_open
daily_tasks_tester_open = []
for daily_tasks_tester_open_record in daily_tasks_tester_open_records:
daily_tasks_tester_open_dic = {}
daily_tasks_tester_open_dic['creator'] = daily_tasks_tester_open_record[0]
daily_tasks_tester_open_dic['creatorNum'] = daily_tasks_tester_open_record[1]
daily_tasks_tester_open.append(daily_tasks_tester_open_dic)
jira_data['daily_tasks_tester_open'] = daily_tasks_tester_open
total_tasks_developer_closed = []
for total_tasks_developer_closed_record in total_tasks_developer_closed_records:
total_tasks_developer_closed_dic = {}
total_tasks_developer_closed_dic['assigner'] = total_tasks_developer_closed_record[0]
total_tasks_developer_closed_dic['assignerNum'] = total_tasks_developer_closed_record[1]
total_tasks_developer_closed.append(total_tasks_developer_closed_dic)
jira_data['total_tasks_developer_closed'] = total_tasks_developer_closed
daily_tasks_developer_closed = []
for daily_tasks_developer_closed_record in daily_tasks_developer_closed_records:
daily_tasks_developer_closed_dic = {}
daily_tasks_developer_closed_dic['assigner'] = daily_tasks_developer_closed_record[0]
daily_tasks_developer_closed_dic['assignerNum'] = daily_tasks_developer_closed_record[1]
daily_tasks_developer_closed.append(daily_tasks_developer_closed_dic)
jira_data['daily_tasks_developer_closed'] = daily_tasks_developer_closed
jira_data = json.dumps(jira_data)
if export_flag:
project_name = ProjectAndroid.query.filter(ProjectAndroid.project_key==jira_key).first().name
excel_name = project_name + '_jira_' + str(date.today())
excel_option = ExcelOptions(os.path.join(current_app.config['TMS_TEMP_DIR'], "jira/%s.xls" % excel_name))
excel_option.export_jira_xls(jira_data)
return excel_option.file_path
return jira_data
def add_project_history(history):
"""添加项目修改操作历史记录"""
project_history_obj = ProjectAndroidHistory()
project_history_obj.project_android_id = history['project_id']
project_history_obj.operate_time = history['time']
project_history_obj.operate_user_id = history['user_id']
project_history_obj.operate_type = history['type']
content = {}
content['content'] = history['content']
project_history_obj.operate_content = json.dumps(content, ensure_ascii=False)
db.session.add(project_history_obj)
db.session.commit()
def get_project_history(project_id):
"""
获取每个项目的修改历史记录,只有scm有权限查看
:param project_id:
:return:
"""
project_history_list_1 = ProjectAndroidHistory.query.filter(
ProjectAndroidHistory.project_android_id == project_id).order_by(
ProjectAndroidHistory.operate_time.desc()).all()
project_history_list = []
for project_history in project_history_list_1:
history = {}
history['id'] = project_history.id
history['project_android_id'] = project_history.project_android_id
history['operate_time'] = project_history.operate_time
history['operate_user'] = project_history.operate_user.display_name
history['operate_type'] = project_history.operate_type
content_obj = json.loads(project_history.operate_content)
history['operate_content'] = content_obj['content']
project_history_list.append(history)
return project_history_list
@project.route('/edit_android_project_info/<int:project_id>', methods=['GET', 'POST'])
@login_required
def edit_android_project_info(project_id):
"""
修改项目关联信息
:return:
"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '编辑项目信息' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index + 1]
break
else:
session['breadcrumbs'].append({'text': '编辑项目信息', 'url': url_for('project.edit_android_project_page',
project_id=project_id)})
project_info_obj = ProjectAndroidInfo.query.filter(ProjectAndroidInfo.project_android_id == project_id).first()
operate_type = 'edit'
if not project_info_obj:
project_info_obj = ProjectAndroidInfo()
project_info_obj.project_android_id = project_id
operate_type = 'add'
if request.method == 'POST':
jenkins_url = request.form.get('jenkins_url', '').strip()
coverity_url = request.form.get('coverity_url', '').strip()
ftp_url = request.form.get('ftp_url', '').strip()
svn_url = request.form.get('svn_url', '').strip()
gerrit_url = request.form.get('gerrit_url', '').strip()
push_command = request.form.get('push_command', '').strip()
other_command = request.form.get('other_command', '').strip()
parent_id = request.form.get('parent_id', '')
# 记录修改历史到project_android_history
history = {}
history['time'] = datetime.now().strftime("%Y-%m-%d %H:%M:%S")
history['user_id'] = current_user.id
history['project_id'] = project_id
history['type'] = operate_type
history['content'] = []
if project_info_obj.jenkins_url != jenkins_url:
case = {}
case['name'] = 'jenkins_url'
case['old'] = project_info_obj.jenkins_url
case['new'] = jenkins_url
history['content'].append(case)
if project_info_obj.coverity_url != coverity_url:
case = {}
case['name'] = 'coverity_url'
case['old'] = project_info_obj.coverity_url
case['new'] = coverity_url
history['content'].append(case)
if project_info_obj.ftp_url != ftp_url:
case = {}
case['name'] = 'ftp_url'
case['old'] = project_info_obj.ftp_url
case['new'] = ftp_url
history['content'].append(case)
if project_info_obj.svn_url != svn_url:
case = {}
case['name'] = 'svn_url'
case['old'] = project_info_obj.svn_url
case['new'] = svn_url
history['content'].append(case)
if project_info_obj.gerrit_url != gerrit_url:
case = {}
case['name'] = 'gerrit_url'
case['old'] = project_info_obj.gerrit_url
case['new'] = gerrit_url
history['content'].append(case)
if project_info_obj.push_command != push_command:
case = {}
case['name'] = 'push_command'
case['old'] = project_info_obj.push_command
case['new'] = push_command
history['content'].append(case)
if project_info_obj.other_command != other_command:
case = {}
case['name'] = 'other_command'
case['old'] = project_info_obj.other_command
case['new'] = other_command
history['content'].append(case)
if project_info_obj.project_android.parent_id != parent_id:
case = {}
case['name'] = 'parent project'
case['old'] = project_info_obj.project_android.parent_id
case['new'] = parent_id
history['content'].append(case)
# 记录修改历史到project_android_history 结束
project_info_obj.jenkins_url = jenkins_url
project_info_obj.coverity_url = coverity_url
project_info_obj.ftp_url = ftp_url
project_info_obj.svn_url = svn_url
project_info_obj.gerrit_url = gerrit_url
project_info_obj.push_command = push_command
project_info_obj.other_command = other_command
db.session.add(project_info_obj)
db.session.commit()
add_project_history(history)
flash(u'修改成功', 'info')
return redirect(url_for('project.android_project_page'))
@project.route('/start_project')
@login_required
def start_project():
"""关注项目"""
project_id = request.args.get('project_id', '')
start_option = request.args.get('start_option', '')
project_obj = ProjectAndroid.query.filter_by(id=project_id).first()
if start_option == '1':
project_obj.followers.append(current_user)
elif start_option == '-1':
project_obj.followers.remove(current_user)
db.session.add(project_obj)
db.session.commit()
return '{"result":true}'
@project.route('/spm_my_project')
@login_required
def spm_my_project():
"""spm 我负责的、参与、关注的项目"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '我的项目', 'url': url_for('project.spm_my_project')})
session['menu_path'] = ['项目管理', '我的项目']
# 关注的项目
follower_project = ProjectAndroid.query.join(User.project_android_f).filter(User.id == current_user.id).all()
result = []
projects = ProjectAndroid.query.order_by(ProjectAndroid.id.desc()).all()
for project_obj in projects:
if project_obj in follower_project:
result.insert(0, (project_obj, True))
else:
result.append((project_obj, False))
return render_template('project/android/project_page.html', projects=result)
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
import json
from datetime import datetime
from flask import render_template, redirect, url_for, request, flash, current_app, session
from flask.ext.login import login_required, current_user
from . import qa
from models import *
from tms.qa.views import need_test_version
@qa.route('/test_case_page')
@login_required
@need_test_version()
def test_case_page():
"""用例页面"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '我的用例', 'url': url_for('qa.test_case_page')})
session['menu_path'] = ['用例管理', '我的用例']
return render_template('qa/test_case_page.html')
@qa.route('/test_case_data')
@login_required
def test_case_data():
"""用例页面表格数据"""
start = int(request.args.get('start', 0))
length = int(request.args.get('length', 10))
is_all = request.args.get('is_all', 'false')
if is_all:
all_count = TestCase.query.filter(TestCase.activate == True).count()
test_case = TestCase.query.filter(TestCase.activate == True).all()[start: start+length]
else:
# 默认现在用例负责人是我的用例
all_count = TestCase.query.filter(
db.and_(TestCase.activate == True, TestCase.add_user_id == current_user.id)).count()
test_case = TestCase.query.filter(
db.and_(TestCase.activate == True,
TestCase.add_user_id == current_user.id)).all()[start: start+length]
records = []
index = start + 1
for record in test_case:
records.append({
"id": record.id,
"index": index,
"number": record.number,
"level": record.level,
"test_type": record.test_type,
"module_name": record.module_name,
"test_fun": record.test_fun,
"class_name": record.class_name,
"method_name": record.method_name,
"test_target": record.test_target,
"standard_sdk": record.standard_sdk,
"test_init": record.test_init,
"test_step": record.test_step,
"expect_result": record.expect_result,
"activate": record.activate,
"add_user": record.add_user.display_name,
"add_time": str(record.add_time),
"last_user": record.last_user.display_name,
"last_update_time": str(record.last_update_time),
})
index += 1
return '{"recordsTotal": %s ,"recordsFiltered": %s,"data":%s}' % (all_count, all_count, json.dumps(records))
@qa.route('/edit_test_case', defaults={'id': None}, methods=['GET', 'POST'])
@qa.route('/edit_test_case/<int:id>', methods=['GET', 'POST'])
@login_required
@need_test_version()
def edit_test_case(id):
"""添加或修改测试用例"""
if id:
test_case_obj = TestCase.query.filter(TestCase.id == id).first()
else:
test_case_obj = None
if request.method == 'POST':
number = request.form.get('number', '').strip()
other_test_case = TestCase.query.filter(TestCase.number == number).first()
if other_test_case and other_test_case.id != id:
flash(u'相同编号的测试用例已存在', 'dange')
else:
if id:
message = u'修改成功'
else:
test_case_obj = TestCase()
message = u'添加成功!'
test_case_history = TestCaseLog()
test_case_obj.number = number
test_case_obj.level = request.form.get('level', '').strip()
test_case_obj.test_type = request.form.get('test_type', '').strip()
test_case_obj.module_name = request.form.get('module_name', '').strip()
test_case_obj.class_name = request.form.get('class_name', '').strip()
test_case_obj.method_name = request.form.get('method_name', '').strip()
test_case_obj.test_fun = request.form.get('test_fun', '').strip()
test_case_obj.test_target = request.form.get('test_target', '').strip()
if request.form.get('standard_sdk', '') == 'on':
test_case_obj.standard_sdk = True
else:
test_case_obj.standard_sdk = False
test_case_obj.test_init = request.form.get('test_init', '').strip()
test_case_obj.test_step = request.form.get('test_step', '').strip()
test_case_obj.expect_result = request.form.get('expect_result', '').strip()
if request.form.get('activate', '') == 'on':
test_case_obj.activate = True
else:
test_case_obj.activate = False
if not id:
test_case_obj.add_user_id = current_user.id
test_case_obj.add_time = datetime.now()
test_case_history.content = u'新增测试用例,编号:{0},模块:{1},类名:{2},方法名:{3}' \
u''.format(test_case_obj.number, test_case_obj.module_name,
test_case_obj.class_name, test_case_obj.method_name)
else:
test_case_history.content = u'修改测试用例,编号:{0},模块:{1},类名:{2},方法名:{3}' \
u''.format(test_case_obj.number, test_case_obj.module_name,
test_case_obj.class_name, test_case_obj.method_name)
test_case_obj.last_user_id = current_user.id
test_case_obj.last_update_time = datetime.now()
db.session.add(test_case_obj)
db.session.commit()
test_case_history.test_case = test_case_obj
test_case_history.user_id = current_user.id
test_case_history.update_time = datetime.now()
db.session.add(test_case_history)
db.session.commit()
flash(message, 'info')
return redirect(url_for('qa.test_case_page'))
if session['user']['role_name'] == 'os_test':
permission = True
else:
permission = False
case_level = ['S', 'A', 'B', 'C']
test_type = [u'功能测试', u'冒烟测试']
return render_template('qa/edit_test_case.html', test_case=test_case_obj, permission=permission,
case_level=case_level, test_type=test_type)
<file_sep>#!/usr/bin/env python
# -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/8/25
"""
"""
import os
from datetime import datetime
import simplejson as json
from flask import render_template, redirect, url_for, request, flash, current_app, jsonify, g, session, send_file
from flask.ext.login import login_user, logout_user, login_required, current_user
from models import User, Role, SystemMenu, SystemParameter
from . import auth
from tms import db
@auth.route('/system_parameter_page')
@login_required
def system_parameter_page():
"""系统参数页面"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': u'系统参数', 'url': url_for('auth.system_parameter_page')})
session['menu_path'] = [u'系统管理', u'系统参数']
parameters = SystemParameter.query.all()
return render_template('admin/system/system_parameter_page.html', parameters=parameters)
@auth.route('/add_system_parameter', methods=['GET', 'POST'])
@login_required
def add_system_parameter():
"""新增系统参数"""
parameter = SystemParameter()
if request.method == 'POST':
key = request.form.get('key', '').strip()
value = request.form.get('value', '').strip().replace('\r', '\n')
flag = request.form.get('flag', 'off')
note = request.form.get('note', '').strip()
other = SystemParameter.query.filter(SystemParameter.key == key).first()
if other:
flash('相同的系统参数已存在', 'warning')
else:
parameter.key = key
parameter.value = '\n'.join(value.split('\n'))
if flag == 'on':
parameter.flag = True
else:
parameter.flag = False
parameter.note = note
db.session.add(parameter)
db.session.commit()
return redirect(url_for('auth.system_parameter_page'))
return render_template('admin/system/system_parameter_edit.html', parameter=parameter)
@auth.route('/edit_system_parameter/<int:parameter_id>', methods=['GET', 'POST'])
@login_required
def edit_system_parameter(parameter_id):
"""修改系统参数"""
parameter = SystemParameter.query.filter_by(id=parameter_id).first()
if request.method == 'POST':
key = request.form.get('key', '').strip()
value = request.form.get('value', '').strip().replace('\r', '\n')
flag = request.form.get('flag', 'off')
note = request.form.get('note', '').strip()
other = SystemParameter.query.filter(SystemParameter.key == key).first()
if other and other.id != parameter_id:
flash('相同的系统参数已存在', 'warning')
else:
parameter.key = key
parameter.value = '\n'.join(value.split('\n'))
if flag == 'on':
parameter.flag = True
else:
parameter.flag = False
parameter.note = note
db.session.add(parameter)
db.session.commit()
return redirect(url_for('auth.system_parameter_page'))
return render_template('admin/system/system_parameter_edit.html', parameter=parameter)
@auth.route('/upload_file', methods=['GET', 'POST'])
@login_required
def upload_file():
"""附件上传"""
# 保存附件
documents = request.files.getlist("file[]")
current_app.logger.info(documents)
attachment = []
if documents:
upload_path = os.path.join(current_app.config['UPLOAD_FOLDER'],
'%s_%s' % (current_user.username, datetime.now().strftime('%Y-%m-%d-%H-%M-%S')))
if not os.path.exists(upload_path):
os.makedirs(upload_path)
for document in documents:
current_app.logger.info(document.filename)
if document.filename:
file_path = os.path.join(upload_path, document.filename)
# 删除已存在的
if os.path.exists(file_path) and os.path.isfile(file_path):
os.remove(file_path)
document.save(file_path)
attachment.append(file_path)
return '{"success": "true", "data": "%s"}' % '#'.join(attachment)
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
from flask import render_template, redirect, url_for, request, flash, current_app
from flask.ext.login import login_user, logout_user, login_required, current_user
from . import repo
from tms import db
from models import RepositoryServer, Platform, Repository
@repo.route('/repo_server_page')
@login_required
def repo_server_page():
""""""
servers = RepositoryServer.query.filter_by(status=True).all()
current_app.logger.info(servers)
return render_template('repository/repository_server_page.html',
servers=[(index+1, server) for index, server in enumerate(servers)])
<file_sep>#!/usr/bin/env python
# -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/11/9
"""
Android 项目开发工程师
"""
from datetime import datetime
import json
import os
from flask import render_template, redirect, url_for, request, flash, current_app, session
from flask.ext.login import login_user, logout_user, login_required, current_user
from flask.ext.mail import Mail, Message
from werkzeug import secure_filename
from functools import update_wrapper
from tms import db, mail
from . import developer
from tms.project.models import Project, Version, VersionHistory, SpecialVersion
from tms.project.androidProjectModules import ProjectAndroid
from tms.auth.models import User, Role
from tms.repository.models import Repository
from tms.utils.email_format import VERSION_SELF_TEST_TEMPLATE
from tms.utils.SendMailPubisher import send_mail_pubisher
@developer.route('/android_dev_my_project')
@login_required
def android_dev_my_project():
"""
Android 开发工程师的我们的项目
:return:
"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '我的项目', 'url': url_for('developer.android_dev_my_project')})
session['menu_path'] = ['项目管理', '我的项目']
# 关注的项目
follower_project = ProjectAndroid.query.join(User.project_android_f).filter(User.id == current_user.id).all()
result = []
projects=ProjectAndroid.query.order_by(ProjectAndroid.id.desc()).all()
for project_obj in projects:
if project_obj in follower_project:
result.insert(0, (project_obj, True))
else:
result.append((project_obj, False))
return render_template('project/android/project_page.html', projects=result)<file_sep>#-*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""应用初始化"""
import json
from flask import Flask
from flask.sessions import SecureCookieSession, SecureCookieSessionInterface
from flask.ext.mail import Mail
from flask.ext.moment import Moment
from flask.ext.sqlalchemy import SQLAlchemy
from flask.ext.login import LoginManager
from flask.ext.migrate import Migrate
from config import config
mail = Mail()
moment = Moment()
db = SQLAlchemy()
login_manager = LoginManager()
login_manager.session_protection = 'strong'
login_manager.login_view = 'auth.login'
class JSONSecureCookieSession(SecureCookieSession):
serialization_method = json
class JSONSecureCookieSessionInterface(SecureCookieSessionInterface):
session_class = JSONSecureCookieSession
def create_app(config_name):
app = Flask(__name__)
app.config.from_object(config[config_name])
config[config_name].init_app(app)
app.session_interface = JSONSecureCookieSessionInterface()
mail.init_app(app)
moment.init_app(app)
db.init_app(app)
login_manager.init_app(app)
app.mail = mail
if not app.debug and not app.testing and not app.config['SSL_DISABLE']:
from flask.ext.sslify import SSLify
sslify = SSLify(app)
# 系统主入口
from tms.main import main as main_blueprint
app.register_blueprint(main_blueprint)
# 用户登陆/注销
from tms.auth import auth as auth_blueprint
app.register_blueprint(auth_blueprint, url_prefix='/auth')
# 项目管理
from tms.project import project as project_blueprint
app.register_blueprint(project_blueprint, url_prefix='/project')
# 工程师模块
from tms.developer import developer as developer_blueprint
app.register_blueprint(developer_blueprint, url_prefix='/developer')
# 任务模块
from tms.task import task as task_blueprint
app.register_blueprint(task_blueprint, url_prefix='/task')
# Git管理模块
from tms.repository import repo as repo_blueprint
app.register_blueprint(repo_blueprint, url_prefix='/repo')
# Qa管理模块
from tms.qa import qa as qa_blueprint
app.register_blueprint(qa_blueprint, url_prefix='/qa')
# scm管理模块
from tms.scm import scm as scm_blueprint
app.register_blueprint(scm_blueprint, url_prefix='/scm')
# 厂商平台、基线/补丁管理模块
from tms.baseline import baseline as baseline_blueprint
app.register_blueprint(baseline_blueprint, url_prefix='/baseline')
# 周报日报
from tms.report import report as report_blueprint
app.register_blueprint(report_blueprint, url_prefix='/report')
# build 编译管理模块
from tms.build import build as build_blueprint
app.register_blueprint(build_blueprint, url_prefix='/build')
# department 部门管理模块
from tms.departmentMan import department as department_blueprint
app.register_blueprint(department_blueprint, url_prefix='/department')
return app
<file_sep>#!/usr/bin/env python
# -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""
基于flask-script扩展的管理入口
"""
import os
import sys
reload(sys)
sys.setdefaultencoding('utf-8')
COV = None
if os.environ.get('tms_COVERAGE'):
import coverage
COV = coverage.coverage(branch=True, include='tms/*')
COV.start()
if os.path.exists('.env'):
print 'Importing environment from env...'
for line in open('.env'):
var = line.strip().stplit('=')
if len(var) == 2:
os.environ[var[0]] = var[1]
from tms import create_app, db
from tms.auth.models import Role, User
from flask.ext.script import Manager, Shell
from flask.ext.migrate import Migrate, MigrateCommand
app = create_app(os.getenv('tms') or 'default')
manager = Manager(app)
migrate = Migrate(app, db)
def make_shell_context():
return dict(app=app, db=db, User=User, Role=Role)
manager.add_command("shell", Shell(make_context=make_shell_context))
manager.add_command('db', MigrateCommand)
@manager.command
def test(coverage=False):
"""执行单元测试"""
if coverage and os.environ.get('tms_COVERAGE'):
os.environ['tms_COVERAGE'] = '1'
os.execvp(sys.executable, [sys.executable] + sys.argv)
import unittest
tests = unittest.TestLoader.discover('tests')
unittest.TextTestResult(verbosity=2).run(tests)
if COV:
COV.stop()
COV.start()
print 'Coverage Summary:'
COV.report()
base_dir = os.path.abspath(os.path.dirname(__file__))
cov_dir = os.path.join(base_dir, 'tmp/coverage')
COV.html_report(directory=cov_dir)
print 'HTML version: file://%s/index.html' % cov_dir
COV.erase()
@manager.command
def profile(length=25, profile_dir=None):
"""
Start the application under the code profiler.
在代码分析器中运行应用
"""
from werkzeug.contrib.profiler import ProfilerMiddleware
app.wsgi_app = ProfilerMiddleware(app.wsgi_app, restrictions=[length], profile_dir=profile_dir)
app.run()
@manager.command
def deploy():
"""执行部署任务"""
from flask.ext.migrate import upgrade
upgrade()
if __name__ == '__main__':
manager.run()
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
import sys
import commands
from git import Git, Repo, GitCmdObjectDB
class GitRepository:
"""
Git 仓库操作类
"""
def __init__(self, repo_path):
self.repo_path = repo_path
self.repo = Repo(repo_path, odbt=GitCmdObjectDB)
self.git = Git(self.repo_path)
@property
def heads(self):
"""仓库中的所有分支"""
return [head.name for head in self.repo.heads]
@property
def tags(self):
"""所有tag"""
return [tag.name for tag in self.repo.tags]
def log(self, reference_name='', log_format=''):
"""所有提交记录
默认格式是:
commit_id/tree/parents/author/author_email/author_date/committer/committer_email/committer_date/message
"""
if log_format:
format_str = log_format
else:
format_str = "%H<#br@>%P<#br@>%T<#br@>%an<#br@>%ae<#br@>%ad<#br@>%cn<#br@>%ce<#br@>%cd%<#br@>%s%b<####>"
if not reference_name:
reference_name = '--all'
command = 'git --git-dir={0} log --pretty="{1}" {2} --date=iso'.format(self.repo_path, format_str, reference_name)
print 'command:%s' % command
status, output = commands.getstatusoutput(command)
if status:
print output
sys.exit(1)
else:
commits = [[tmp.strip() for tmp in line.split('<#br@>') if tmp.strip()]
for line in output.split('<####>') if line.strip()]
return commits
def commits(self, rev_name):
"""
分支或tag上的所有commit对象
"""
return [commit_obj for commit_obj in self.repo.commit(rev_name).iter_parents()]
def diff_commit(self, old_rev, new_rev, log_format=''):
"""比较两个版本或tag获取其commit"""
if log_format:
format_str = log_format
else:
format_str = "%H<#br@>%P<#br@>%T<#br@>%an<#br@>%ae<#br@>%ad<#br@>%cn<#br@>%ce<#br@>%cd%<#br@>%s%b<####>"
command = 'git --git-dir={0} log --pretty="{1}" {2}..{3} --date=iso'.format(self.repo_path, format_str, old_rev, new_rev)
print 'command:%s' % command
status, output = commands.getstatusoutput(command)
if status:
print output
sys.exit(1)
else:
commits = [[tmp.strip() for tmp in line.split('<#br@>') if tmp.strip()]
for line in output.split('<####>') if line.strip()]
return commits
def log_commit(self, commit_hash, log_format=''):
"""
显示单个commit的信息
"""
if log_format:
format_str = log_format
else:
format_str = "%H<#br@>%P<#br@>%T<#br@>%an<#br@>%ae<#br@>%ad<#br@>%cn<#br@>%ce<#br@>%cd%<#br@>%s%b<####>"
command = 'git --git-dir={0} log -1 --pretty="{1}" {2} --date=iso'.format(self.repo_path, format_str, commit_hash)
print 'command:%s' % command
status, output = commands.getstatusoutput(command)
if status:
print output
sys.exit(1)
else:
commits = [[tmp.strip() for tmp in line.split('<#br@>') if tmp.strip()]
for line in output.split('<####>') if line.strip()]
return commits[0]
if __name__ == '__main__':
git_repo = GitRepository('/home/roy/workspace/tup')
print git_repo.heads
print git_repo.tags
for commit in git_repo.log():
print commit
<file_sep>#!/usr/bin/env python
# -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/7/6
"""
Gerrit 引用更新之后的钩子
1. 邮件通知
2. 解析本次push的commit信息
3. 将jira任务和commit 进行关联
"""
import time
import os
import sys
from optparse import OptionParser
import commands
import re
import simplejson as json
import smtplib
# 多个MIME对象的集合
from email.mime.multipart import MIMEMultipart
# MIME文本对象
from email.mime.text import MIMEText
from email.header import Header
# 本机ip地址
import socket
import fcntl
import struct
import MySQLdb
from MySQLdb import connect
import pika
reload(sys)
sys.setdefaultencoding('utf-8')
# tsds数据库信息
db_host = '192.168.33.7'
db_user = 'tup'
db_passwd = 'tup'
db_name = 'tms'
# tsds配置数据库信息
jira_db_host = '172.16.5.95'
jira_db_name = 'jiradb6'
jira_db_user = 'jirauser'
jira_db_passwd = '<PASSWORD>'
def get_ip_address(ifname):
"""获取本机ip地址"""
s = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
try:
return socket.inet_ntoa(fcntl.ioctl(
s.fileno(),
0x8915, # SIOCGIFADDR
struct.pack('256s', ifname[:15])
)[20:24])
except IOError, e:
return ''
def send_mail(to_list=[], sub='', content='', appendix_file=''):
""""send_mail("<EMAIL>","sub","content")
:param to_list:发给谁
:param sub:主题
:param content:内容
:param appendix_file: 附件
"""
# 设置服务器、用户名、口令及邮箱的后缀
mail_host = '192.168.10.8'
mail_user = '<EMAIL>'
mail_pass = '<PASSWORD>'
# sender = config_parser.get("email", "sender").strip("'")
sender = "<EMAIL>"
msg_root = MIMEMultipart()
# 解决中文乱码
att_text = MIMEText(content, 'html', 'UTF-8')
# 添加邮件正文
msg_root.attach(att_text)
# 发件人
msg_root['from'] = sender
# 邮件标题
msg_root['Subject'] = Header(sub, 'utf-8')
# 设置时间
msg_root['Date'] = time.ctime(time.time())
msg_root['To'] = ';'.join(to_list)
# 构造附件(附件路径出现中文,会提示编码错误)
if appendix_file != '':
rar_file_path = appendix_file
att = MIMEText(open(rar_file_path, 'rb').read(), 'gbk', 'UTF-8')
att['Content-Type'] = 'application/octet-stream;name=%s' % Header(rar_file_path, 'UTF-8')
att['Content-Disposition'] = 'attachment;filename=%s' % Header(rar_file_path, 'UTF-8')
msg_root.attach(att)
# 群发邮件
smtp = smtplib.SMTP(mail_host, 25)
smtp.login(mail_user, mail_pass)
smtp.sendmail(sender, to_list, msg_root.as_string())
# 休眠5秒
time.sleep(5)
# 断开与服务器的连接
smtp.quit()
def get_commit_info(git_path, old_rev, new_rev, local_ip):
"""
获取提交的详细信息
:param git_path: 仓库路径
:param old_rev: 基础Commit Id
:param new_rev: 当前最新的commit Id
"""
diff_info = ''
commit_info = ''
result = True
if old_rev != '0'*40 and new_rev != '0'*40:
command = 'git log -p {0}..{1}'.format(old_rev, new_rev)
os.chdir(git_path)
status, info = commands.getstatusoutput(command)
if status:
result = False
send_mail(["<EMAIL>"], "Get Commit Info Error{0}".format(local_ip),
"获取仓库的差异信息出错了<br>仓库地址:{0}<br>执行命令:{1}<br>出错信息:<br>Status:{2}<br>{3}".format(git_path, command, status, info))
else:
diff_info = info.replace('<', '<').split('\n')
if len(diff_info) > 2000:
command = 'git log -p --name-only {0}..{1}'.format(old_rev, new_rev)
status, info = commands.getstatusoutput(command)
diff_info = '<br>'.join(info.split('\n'))
else:
result = []
for tmp_line in diff_info:
if tmp_line.startswith('-'):
tmp_line = '<font color="red">' + tmp_line + '</font>'
elif tmp_line.startswith('+'):
tmp_line = '<font color="green">' + tmp_line + '</font>'
result.append(tmp_line)
diff_info = '<br>'.join(result)
# 格式化日志
command = 'git log --reverse --name-only --date=iso --pretty="<#GIT#>%H<#br#>%T<#br#>%an<#br#>%ae<#br#>%ad<#br#>%cn<#br#>%ce<#br#>%cd<#br#>%s%b</#GIT#>" {0}..{1}'.format(old_rev, new_rev)
status, info = commands.getstatusoutput(command)
if status:
result = False
print status
print info
send_mail(["<EMAIL>"], "Get Commit Info Error{0}".format(local_ip),
"获取仓库的差异信息出错了<br>仓库地址:{0}<br>执行命令:{1}<br>出错信息:<br>Status:{2}<br>{3}".format(git_path, command, status, info))
else:
commit_info = info
return result, diff_info, commit_info
def get_task_info(task_key):
"""从JIRA数据库中查询jira任务的信息"""
query_sql = "SELECT issue.ID, issue.SUMMARY, issue.DESCRIPTION FROM jiraissue issue JOIN project pro WHERE " \
"issue.issuenum='{0}' AND issue.project=pro.id AND pro.pkey='{1}'".format(task_key.split('-')[1],
task_key.split('-')[0])
record = ''
try:
conn = connect(host=jira_db_host, db=jira_db_name, user=jira_db_user, passwd=jira_db_passwd, charset='utf8')
cursor = conn.cursor()
cursor.execute(query_sql)
record = cursor.fetchone()
cursor.close()
conn.close()
except MySQLdb.Error, e:
send_mail(["<EMAIL>"], "查询JIRA数据库出错",
"post_update.py查询JIRA数据获取任务详细信息出错了<br>SQL:<br>{0}Error:<br>{1}".format(query_sql, e))
return record
def add_commit(repo_path, project_code_id, commit_info, submitter):
"""
将日志信息和JIRA任务信息,及任务和commit关联信息保存到数据库中
:param repo_path:
:param project_code_id:
:param commit_info:
:return:
"""
# 获取任务JIRA_KEY
pattern = r'^<(REQ|BUG|FUNC|DEBUG|PATCH|BASELINE|MERGE)><([A-Z]{2,}\-\d+)><(.+)>*'
rp = re.compile(pattern)
commit_info = [tmp.strip() for tmp in commit_info.split('<#GIT#>') if tmp.strip()]
commit_data = []
# 任务关联的commit
task_commit = {}
for commit in commit_info:
info, change_files = commit.split('</#GIT#>')
message = [MySQLdb.escape_string(tmp.strip()) for tmp in info.split('<#br#>') if tmp.strip()]
m = rp.match(message[8])
task_key = ''
if m:
task_key = m.groups()[1]
if task_key not in task_commit.keys():
task_commit[task_key] = []
change_files = '\n'.join([tmp.strip() for tmp in change_files.split('\n') if tmp.strip()])
commit_data.append([repo_path] + message + [change_files, task_key.split('-')[0], task_key, submitter, 1, project_code_id])
commit_insert_sql = "INSERT INTO project_branch_commit (repo_path, commit_id, tree_id, auth_name, auth_email, " \
"auth_date, commit_name, commit_email, commit_date, message, change_file, project_key, task_key, " \
"submitter, activate, project_code_id) VALUES(%s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s)"
# jira任务查询语句
query_task_sql = "SELECT id, task_key FROM project_branch_task WHERE task_key in({0}) AND " \
"project_code_id={1}".format(','.join(["'%s'" % tmp for tmp in task_commit.keys()]), project_code_id)
try:
conn = MySQLdb.connect(host=db_host, db=db_name, user=db_user, passwd=<PASSWORD>, charset='utf8')
cursor = conn.cursor()
# 向project_branch_commit表添加commit记录
for commit in commit_data:
cursor.executemany(commit_insert_sql, [commit])
row_id = cursor.lastrowid
conn.commit()
if not commit[9].strip().startswith('Merge '):
task_commit[commit[12]].append(row_id)
# 数据库中已存在task 任务
if task_commit.keys():
cursor.execute(query_task_sql)
exists_task = {}
for record in cursor.fetchall():
exists_task[record[1]] = record[0]
for task_key, commits in task_commit.items():
if task_key in exists_task.keys():
task_db_id = exists_task[task_key]
else:
record = get_task_info(task_key)
if record:
insert_task_sql = "INSERT INTO project_branch_task(issue_id, task_key, summary, description, " \
"project_code_id) VALUES (%s, %s, %s, %s, %s)"
cursor.executemany(insert_task_sql, [(record[0], task_key, record[1], record[2], project_code_id)])
else:
insert_task_sql = "INSERT INTO project_branch_task(task_key, project_code_id) VALUES (%s, %s)"
cursor.executemany(insert_task_sql, [(task_key, project_code_id)])
task_db_id = cursor.lastrowid
conn.commit()
insert_task_commit_sql = "INSERT INTO project_branch_task_commit(project_branch_task_id, project_branch_commit_id) VALUES (%s, %s)"
task_commits = []
for commit_id in commits:
task_commits.append((task_db_id, commit_id))
cursor.executemany(insert_task_commit_sql, task_commits)
conn.commit()
cursor.close()
conn.close()
except MySQLdb.Error, e:
send_mail(["<EMAIL>"], "添加{0}分支提交记录失败了".format(project_code_id),
"Error:{0}<br>Insert into Sql:{1}<br>Data:{2}".format(e, query_task_sql, "<br>".join(commit_info)))
def send_commit_notice_email(repo_path, ref_name, commit_diff_info, local_ip):
"""
将本次提交的信息邮件通知相关人员
:param ref_name:
:param commit_diff_info:
:return:
"""
# 查询此分支配置的邮件地址列表
project_code_id = ''
email_list = ''
branch_sql = ''
try:
conn = connect(host=db_host, db=db_name, user=db_user, passwd=db_passwd, charset='utf8')
cursor = conn.cursor()
branch_sql = "SELECT id, platform_name, commit_notice_email FROM project_code WHERE branch_name='{0}'".format(os.path.basename(ref_name))
cursor.execute(branch_sql)
rows = cursor.fetchall()
if rows and len(rows) > 0:
for row in rows:
project_code_id, platform_name, email_list = row[0], row[1], row[2]
if platform_name and repo_path.startswith(platform_name):
break
else:
email_list = ''
cursor.close()
conn.close()
except MySQLdb.Error, e:
send_mail(["<EMAIL>"], "获取{0}分支的通知邮件列表失败".format(ref_name),
"从数据库查询分支的邮件列表失败了<br>{0}<br>{1}<br>{2}".format(local_ip, branch_sql, e))
if email_list:
send_mail(email_list.split(';'), '{0}_代码提交通知邮件'.format(ref_name), commit_diff_info)
return project_code_id
def ref_post_update(old_rev, new_rev, ref_name, repo_path, submitter, local_ip):
# Git仓库的绝对路径
git_repo_path = os.path.join('/home/android/review-site/repositories/', repo_path)+'.git'
# 获取提交的差异日志详细信息
result, commit_diff_info, commit_info = get_commit_info(git_repo_path, old_rev, new_rev, local_ip)
if result:
# 发送提交提醒邮件
project_code_id = send_commit_notice_email(repo_path, ref_name,
'<br>'.join([repo_path, '='*80, commit_diff_info]), local_ip)
if project_code_id:
# 对提交记录解析,提取JIRA任务并录入数据
add_commit(repo_path, project_code_id, commit_info, submitter)
def push_consumer():
"""
发送邮件的消费者
:return:
"""
# 建立连接connection到localhost
con = pika.BlockingConnection(pika.ConnectionParameters('192.168.10.207'))
# 创建虚拟连接channel
cha = con.channel()
# 创建队列anheng
result = cha.queue_declare(queue='git207_push', durable=True)
# 创建名为yanfa,类型为fanout的交换机,其他类型还有direct和topic
cha.exchange_declare(durable=False,
exchange='git207',
type='direct', )
# 绑定exchange和queue,result.method.queue获取的是队列名称
cha.queue_bind(exchange='git207',
queue=result.method.queue,
routing_key='', )
# 公平分发,使每个consumer在同一时间最多处理一个message,收到ack前,不会分配新的message
cha.basic_qos(prefetch_count=1)
print ' [*] Waiting for messages. To exit press CTRL+C'
# 定义回调函数
def callback(ch, method, properties, body):
print " [x] Received %r" % (body,)
message = json.loads(body)
ref_post_update(message['old_rev'], message['new_rev'], message['ref_name'], message['repo_path'],
message['uploader'], message['local_ip'])
ch.basic_ack(delivery_tag=method.delivery_tag)
cha.basic_consume(callback,
queue='git207_push',
no_ack=False, )
cha.start_consuming()
def push_pubisher(message):
"""
向队列中添加邮件发送任务
:param message: json格式字串
:return:
"""
# 创建连接connection到localhost
con = pika.BlockingConnection(pika.ConnectionParameters('192.168.10.207'))
# 创建虚拟连接channel
cha = con.channel()
# 创建队列anheng,durable参数为真时,队列将持久化;exclusive为真时,建立临时队列
result = cha.queue_declare(queue='git207_push', durable=True, exclusive=False)
# 创建名为yanfa,类型为fanout的exchange,其他类型还有direct和topic,如果指定durable为真,exchange将持久化
cha.exchange_declare(durable=False,
exchange='git207',
type='direct', )
# 绑定exchange和queue,result.method.queue获取的是队列名称
cha.queue_bind(exchange='git207',
queue=result.method.queue,
routing_key='', )
# 公平分发,使每个consumer在同一时间最多处理一个message,收到ack前,不会分配新的message
cha.basic_qos(prefetch_count=1)
# 消息持久化指定delivery_mode=2;
cha.basic_publish(exchange='',
routing_key='git207_push',
body=message,
properties=pika.BasicProperties(
delivery_mode=2,
))
#print '[x] Sent %r' % (message,)
# 关闭连接
con.close()
if __name__ == '__main__':
push_consumer()
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""
project 模块为APK项目模块
定义项目模块的蓝图
"""
from flask import Blueprint
project = Blueprint('project', __name__)
from . import views
from . import projectMyOSViews
from . import androidProjectRepoView
from . import commonIssueView<file_sep>#!/usr/bin/env python
# -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/7/12
"""
将分支中的提交信息导入数据中
"""
import sys
import os
import re
import commands
from xml.etree.ElementTree import ElementTree
import MySQLdb
from MySQLdb import connect
reload(sys)
sys.setdefaultencoding('UTF-8')
# myos配置数据库信息
db_host = '192.168.33.7'
db_user = 'tup'
db_passwd = 'tup'
db_name = 'tms_dev'
# tsds配置数据库信息
jira_db_host = '172.16.5.95'
jira_db_name = 'jiradb6'
jira_db_user = 'jirauser'
jira_db_passwd = '<PASSWORD>'
def get_all_git(workspace):
""""
读取工程的manifest.xml操作其中的所有仓库
workspace: 是工程.repo/manifest.xml提货单所在的路径
comment: 提交说明
"""
tree = ElementTree()
element = tree.parse(workspace + '/.repo/manifest.xml')
default = element.find('default')
return element.findall('project')
def get_branch_log(git_path, branch_name):
"""
获取分支上所有的提交记录信息
:param git_path:
:param branch_name:
:return:
"""
os.chdir(git_path)
# 格式化日志
command = 'git log --reverse --name-only --date=iso --pretty="<#GIT#>%H<#br#>%T<#br#>%an<#br#>%ae<#br#>%ad<#br#>%cn<#br#>%ce<#br#>%cd<#br#>%s%b</#GIT#>" {0}'.format(
branch_name)
status, info = commands.getstatusoutput(command)
if status:
print command
print info
sys.exit(1)
else:
commit_info = info
return commit_info
def get_task_info(task_key):
"""从JIRA数据库中查询jira任务的信息"""
query_sql = "SELECT issue.ID, issue.SUMMARY, issue.DESCRIPTION FROM jiraissue issue JOIN project pro WHERE " \
"issue.issuenum='{0}' AND issue.project=pro.id AND pro.pkey='{1}'".format(task_key.split('-')[1],
task_key.split('-')[0])
record = ''
conn = connect(host=jira_db_host, db=jira_db_name, user=jira_db_user, passwd=jira_db_passwd, charset='utf8')
cursor = conn.cursor()
cursor.execute(query_sql)
record = cursor.fetchone()
cursor.close()
conn.close()
return record
def add_commit(repo_path, project_code_id, commit_info):
"""
将日志信息和JIRA任务信息,及任务和commit关联信息保存到数据库中
:param repo_path:
:param project_code_id:
:param commit_info:
:return:
"""
# 获取任务JIRA_KEY
pattern = r'^<(REQ|BUG|FUNC|DEBUG|PATCH|BASELINE|MERGE)><([A-Z]{2,}\-\d+)><(.+)>*'
rp = re.compile(pattern)
commit_info = [tmp.strip() for tmp in commit_info.split('<#GIT#>') if tmp.strip()]
commit_data = []
# 任务关联的commit
task_commit = {}
for commit in commit_info:
info, change_files = commit.split('</#GIT#>')
message = [MySQLdb.escape_string(tmp.strip()) for tmp in info.split('<#br#>') if tmp.strip()]
m = rp.match(message[8])
task_key = ''
if m:
task_key = m.groups()[1]
if task_key not in task_commit.keys():
task_commit[task_key] = []
change_files = '\n'.join([tmp.strip() for tmp in change_files.split('\n') if tmp.strip()])
commit_data.append(
[repo_path] + message + [change_files, task_key.split('-')[0], task_key, '{0} ({1})'.format(message[5], message[6]), 1, project_code_id])
commit_insert_sql = "INSERT INTO project_branch_commit (repo_path, commit_id, tree_id, auth_name, auth_email, " \
"auth_date, commit_name, commit_email, commit_date, message, change_file, project_key, task_key, " \
"submitter, activate, project_code_id) VALUES(%s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s)"
# jira任务查询语句
query_task_sql = "SELECT id, task_key FROM project_branch_task WHERE task_key in({0}) AND " \
"project_code_id={1}".format(','.join(["'%s'" % tmp for tmp in task_commit.keys()]),
project_code_id)
conn = MySQLdb.connect(host=db_host, db=db_name, user=db_user, passwd=db_passwd, charset='utf8')
cursor = conn.cursor()
# 向project_branch_commit表添加commit记录
for commit in commit_data:
# print commit_insert_sql
print commit
cursor.executemany(commit_insert_sql, [commit])
row_id = cursor.lastrowid
print row_id
conn.commit()
if commit[12]:
task_commit[commit[12]].append(row_id)
# 数据库中已存在task 任务
if task_commit.keys():
cursor.execute(query_task_sql)
exists_task = {}
for record in cursor.fetchall():
exists_task[record[1]] = record[0]
for task_key, commits in task_commit.items():
if task_key in exists_task.keys():
task_db_id = exists_task[task_key]
else:
record = get_task_info(task_key)
if record:
insert_task_sql = "INSERT INTO project_branch_task(issue_id, task_key, summary, description, " \
"project_code_id) VALUES (%s, %s, %s, %s, %s)"
cursor.executemany(insert_task_sql, [(record[0], task_key, record[1], record[2], project_code_id)])
else:
insert_task_sql = "INSERT INTO project_branch_task(task_key, project_code_id) VALUES (%s, %s)"
cursor.executemany(insert_task_sql, [(task_key, project_code_id)])
task_db_id = cursor.lastrowid
conn.commit()
insert_task_commit_sql = "INSERT INTO project_branch_task_commit(project_branch_task_id, project_branch_commit_id) VALUES (%s, %s)"
task_commits = []
for commit_id in commits:
task_commits.append((task_db_id, commit_id))
cursor.executemany(insert_task_commit_sql, task_commits)
conn.commit()
cursor.close()
conn.close()
def main(platform_name, branch_name, project_code_id):
current_path = os.getcwd()
projects = get_all_git(current_path)
for project in projects:
if project.attrib['name'].startswith(platform_name):
commit_info = get_branch_log(os.path.join(current_path, project.attrib['path']), branch_name)
add_commit(project.attrib['name'], project_code_id, commit_info)
if __name__ == '__main__':
"""
git服务器上平台名称
分支名称remotes/tinno/
数据库里货单的id
"""
main(sys.argv[1], sys.argv[2], sys.argv[3])<file_sep>{% extends "developer/developer_base.html" %}
{% block title %}提交记录详细信息{% endblock %}
{% block header %}
{{ super() }}
<link href="{{ url_for('static', filename='assets/global/plugins/bootstrap-select/bootstrap-select.min.css') }}"
rel="stylesheet" type="text/css"/>
<link href="{{ url_for('static', filename='assets/global/plugins/select2/select2.css') }}" rel="stylesheet"
type="text/css"/>
<link href="{{ url_for('static', filename='assets/global/plugins/bootstrap-duallistbox/bootstrap-duallistbox.min.css') }}"
rel="stylesheet" type="text/css"/>
<link href="{{ url_for('static', filename='assets/global/plugins/bootstrap-datetimepicker/css/bootstrap-datetimepicker.min.css') }} "
rel="stylesheet" type="text/css"/>
{% endblock %}
{% block page_content %}
<div class="row">
<div class="col-md-12">
<!-- BEGIN PORTLET-->
<div class="portlet box yellow-crusta">
<div class="portlet-title">
<div class="caption">
<i class="fa fa-gift"></i>Commit详细信息
</div>
</div>
<div class="portlet-body form">
<!-- BEGIN FORM-->
<form id="project_form" action="" class="horizontal-form" method="POST">
<div class="form-body">
<label><span class="label label-success"
style="font-size: medium">Repository Path:</span>{{ commit.repo_path }}</label><br>
<label><span class="label label-success"
style="font-size: medium">commit:</span>{{ commit.commit_id }}</label><br>
<label><span class="label label-primary"
style="font-size: medium">Author:</span> {{ commit.auth_name }}
<{{ commit.auth_email }}</label><br>
<label><span class="label label-primary"
style="font-size: medium">Auth Date:</span> {{ commit.auth_date }}</label><br>
<label><span class="label label-primary"
style="font-size: medium">Committer:</span> {{ commit.commit_name }}
<{{ commit.commit_email }}></label><br>
<label><span class="label label-primary"
style="font-size: medium">Commit Date:</span> {{ commit.commit_date }}
</label><br>
<label><span class="label label-info"
style="font-size: medium">Message:</span> {{ commit.message }}
</label><br>
<label><span class="label label-default"
style="font-size: medium">Change Files:</span><br>
{% for line in commit.change_file.split('\n') %}
{{ line }} <br>
{% endfor %}
</label><br>
</div>
</form>
</div>
</div>
<!-- END PORTLET-->
</div>
</div>
{% endblock %}
{% block script %}
<script src="{{ url_for('static', filename='assets/global/plugins/bootstrap-select/bootstrap-select.min.js') }}"></script>
<script src="{{ url_for('static', filename='assets/global/plugins/select2/select2.min.js') }}"></script>
<script src="{{ url_for('static', filename='assets/global/plugins/bootstrap-duallistbox/jquery.bootstrap-duallistbox.min.js') }}"></script>
<script src="{{ url_for('static', filename='assets/global/plugins/bootstrap-datetimepicker/js/bootstrap-datetimepicker.min.js') }}"
type="text/javascript"></script>
<script src="{{ url_for('static', filename='assets/global/plugins/bootbox/bootbox.min.js') }}"
type="text/javascript"></script>
<script type="text/javascript">
$SCRIPT_ROOT = {{ request.script_root|tojson|safe }};
</script>
{% endblock %}<file_sep>#!/usr/bin/env python
# -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/8/25
"""
"""
from flask import Blueprint
baseline = Blueprint('baseline', __name__)
from . import platformViews
<file_sep>#!/usr/bin/env python
# -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/8/25
"""
"""
from flask import render_template, redirect, url_for, request, flash, current_app, session
from flask.ext.login import login_user, logout_user, login_required, current_user
from flask.ext.mail import Mail, Message
from werkzeug import secure_filename
from functools import update_wrapper
from . import baseline
from tms import db
from models import BPlatform
@baseline.route('/platform_page')
@login_required
def platform_page():
"""系统参数页面"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': u'平台管理', 'url': url_for('baseline.platform_page')})
session['menu_path'] = [u'基线管理', u'平台管理']
platforms = BPlatform.query.all()
return render_template('baseline/platform/platform_page.html', platforms=platforms)
@baseline.route('/add_platform', methods=['GET', 'POST'])
@login_required
def add_platform():
"""添加平台"""
platform_obj = BPlatform()
if request.method == 'POST':
name = request.form.get('name', '').strip()
flag = request.form.get('flag', 'off')
note = request.form.get('note', '').strip()
other = BPlatform.query.filter(BPlatform.name == name).first()
if other:
flash('相同平台名称已存在', 'warning')
else:
platform_obj.name = name
if flag == 'on':
platform_obj.flag = True
else:
platform_obj.flag = False
platform_obj.note = note
db.session.add(platform_obj)
db.session.commit()
return redirect(url_for('baseline.platform_page'))
return render_template('baseline/platform/platform_edit.html', platform=platform_obj)
@baseline.route('/edit_platform/<int:platform_id>', methods=['GET', 'POST'])
@login_required
def edit_platform(platform_id):
"""修改平台信息"""
platform_obj = BPlatform.query.filter_by(id=platform_id).first()
if request.method == 'POST':
name = request.form.get('name', '').strip()
flag = request.form.get('flag', 'off')
note = request.form.get('note', '').strip()
other = BPlatform.query.filter(BPlatform.name == name).first()
if other and other.id != platform_id:
flash('相同平台名称已存在', 'warning')
else:
platform_obj.name = name
if flag == 'on':
platform_obj.flag = True
else:
platform_obj.flag = False
platform_obj.note = note
db.session.add(platform_obj)
db.session.commit()
return redirect(url_for('baseline.platform_page'))
return render_template('baseline/platform/platform_edit.html', platform=platform_obj)<file_sep>(function () {
var h = window.AmCharts;
h.AmPieChart = h.Class({inherits: h.AmSlicedChart, construct: function (d) {
this.type = "pie";
h.AmPieChart.base.construct.call(this, d);
this.cname = "AmPieChart";
this.pieBrightnessStep = 30;
this.minRadius = 10;
this.depth3D = 0;
this.startAngle = 90;
this.angle = this.innerRadius = 0;
this.startRadius = "500%";
this.pullOutRadius = "20%";
this.labelRadius = 20;
this.labelText = "[[title]]: [[percents]]%";
this.balloonText = "[[title]]: [[percents]]% ([[value]])\n[[description]]";
this.previousScale = 1;
this.adjustPrecision = !1;
h.applyTheme(this, d, this.cname)
}, drawChart: function () {
h.AmPieChart.base.drawChart.call(this);
var d = this.chartData;
if (h.ifArray(d)) {
if (0 < this.realWidth && 0 < this.realHeight) {
h.VML && (this.startAlpha = 1);
var f = this.startDuration, c = this.container, b = this.updateWidth();
this.realWidth = b;
var m = this.updateHeight();
this.realHeight = m;
var e = h.toCoordinate, k = e(this.marginLeft, b), a = e(this.marginRight, b), v = e(this.marginTop, m) + this.getTitleHeight(), n = e(this.marginBottom, m), y, z, g, x = h.toNumber(this.labelRadius), q =
this.measureMaxLabel();
q > this.maxLabelWidth && (q = this.maxLabelWidth);
this.labelText && this.labelsEnabled || (x = q = 0);
y = void 0 === this.pieX ? (b - k - a) / 2 + k : e(this.pieX, this.realWidth);
z = void 0 === this.pieY ? (m - v - n) / 2 + v : e(this.pieY, m);
g = e(this.radius, b, m);
g || (b = 0 <= x ? b - k - a - 2 * q : b - k - a, m = m - v - n, g = Math.min(b, m), m < b && (g /= 1 - this.angle / 90, g > b && (g = b)), m = h.toCoordinate(this.pullOutRadius, g), g = (0 <= x ? g - 1.8 * (x + m) : g - 1.8 * m) / 2);
g < this.minRadius && (g = this.minRadius);
m = e(this.pullOutRadius, g);
v = h.toCoordinate(this.startRadius, g);
e = e(this.innerRadius, g);
e >= g && (e = g - 1);
n = h.fitToBounds(this.startAngle, 0, 360);
0 < this.depth3D && (n = 270 <= n ? 270 : 90);
n -= 90;
b = g - g * this.angle / 90;
for (k = q = 0; k < d.length; k++)a = d[k], !0 !== a.hidden && (q += h.roundTo(a.percents, this.pf.precision));
q = h.roundTo(q, this.pf.precision);
this.tempPrec = NaN;
this.adjustPrecision && 100 != q && (this.tempPrec = this.pf.precision + 1);
for (k = 0; k < d.length; k++)if (a = d[k], !0 !== a.hidden && 0 < a.percents) {
var r = 360 * a.percents / 100, q = Math.sin((n + r / 2) / 180 * Math.PI), A = -Math.cos((n + r / 2) / 180 * Math.PI) * (b /
g), p = this.outlineColor;
p || (p = a.color);
var B = this.alpha;
isNaN(a.alpha) || (B = a.alpha);
p = {fill: a.color, stroke: p, "stroke-width": this.outlineThickness, "stroke-opacity": this.outlineAlpha, "fill-opacity": B};
a.url && (p.cursor = "pointer");
p = h.wedge(c, y, z, n, r, g, b, e, this.depth3D, p, this.gradientRatio, a.pattern);
h.setCN(this, p, "pie-item");
h.setCN(this, p.wedge, "pie-slice");
h.setCN(this, p, a.className, !0);
this.addEventListeners(p, a);
a.startAngle = n;
d[k].wedge = p;
0 < f && (this.chartCreated || p.setAttr("opacity", this.startAlpha));
a.ix = q;
a.iy = A;
a.wedge = p;
a.index = k;
if (this.labelsEnabled && this.labelText && a.percents >= this.hideLabelsPercent) {
var l = n + r / 2;
360 < l && (l -= 360);
var t = x;
isNaN(a.labelRadius) || (t = a.labelRadius);
var r = y + q * (g + t), B = z + A * (g + t), C, w = 0;
if (0 <= t) {
var D;
90 >= l && 0 <= l ? (D = 0, C = "start", w = 8) : 90 <= l && 180 > l ? (D = 1, C = "start", w = 8) : 180 <= l && 270 > l ? (D = 2, C = "end", w = -8) : 270 <= l && 360 > l && (D = 3, C = "end", w = -8);
a.labelQuarter = D
} else C = "middle";
var l = this.formatString(this.labelText, a), u = this.labelFunction;
u && (l = u(a, l));
u = a.labelColor;
u || (u = this.color);
"" !== l && (l = h.wrappedText(c, l, u, this.fontFamily, this.fontSize, C, !1, this.maxLabelWidth), h.setCN(this, l, "pie-label"), h.setCN(this, l, a.className, !0), l.translate(r + 1.5 * w, B), l.node.style.pointerEvents = "none", a.tx = r + 1.5 * w, a.ty = B, 0 <= t ? (t = l.getBBox(), u = h.rect(c, t.width + 5, t.height + 5, "#FFFFFF", .005), u.translate(r + 1.5 * w + t.x, B + t.y), a.hitRect = u, p.push(l), p.push(u)) : this.freeLabelsSet.push(l), a.label = l);
a.tx = r;
a.tx2 = r + w;
a.tx0 = y + q * g;
a.ty0 = z + A * g
}
r = e + (g - e) / 2;
a.pulled && (r += this.pullOutRadiusReal);
a.balloonX = q * r + y;
a.balloonY =
A * r + z;
a.startX = Math.round(q * v);
a.startY = Math.round(A * v);
a.pullX = Math.round(q * m);
a.pullY = Math.round(A * m);
this.graphsSet.push(p);
(0 === a.alpha || 0 < f && !this.chartCreated) && p.hide();
n += 360 * a.percents / 100
}
0 < x && !this.labelRadiusField && this.arrangeLabels();
this.pieXReal = y;
this.pieYReal = z;
this.radiusReal = g;
this.innerRadiusReal = e;
0 < x && this.drawTicks();
this.initialStart();
this.setDepths()
}
(d = this.legend) && d.invalidateSize()
} else this.cleanChart();
this.dispDUpd();
this.chartCreated = !0
}, setDepths: function () {
var d =
this.chartData, f;
for (f = 0; f < d.length; f++) {
var c = d[f], b = c.wedge, c = c.startAngle;
0 <= c && 180 > c ? b.toFront() : 180 <= c && b.toBack()
}
}, arrangeLabels: function () {
var d = this.chartData, f = d.length, c, b;
for (b = f - 1; 0 <= b; b--)c = d[b], 0 !== c.labelQuarter || c.hidden || this.checkOverlapping(b, c, 0, !0, 0);
for (b = 0; b < f; b++)c = d[b], 1 != c.labelQuarter || c.hidden || this.checkOverlapping(b, c, 1, !1, 0);
for (b = f - 1; 0 <= b; b--)c = d[b], 2 != c.labelQuarter || c.hidden || this.checkOverlapping(b, c, 2, !0, 0);
for (b = 0; b < f; b++)c = d[b], 3 != c.labelQuarter || c.hidden || this.checkOverlapping(b,
c, 3, !1, 0)
}, checkOverlapping: function (d, f, c, b, h) {
var e, k, a = this.chartData, v = a.length, n = f.label;
if (n) {
if (!0 === b)for (k = d + 1; k < v; k++)a[k].labelQuarter == c && (e = this.checkOverlappingReal(f, a[k], c)) && (k = v); else for (k = d - 1; 0 <= k; k--)a[k].labelQuarter == c && (e = this.checkOverlappingReal(f, a[k], c)) && (k = 0);
!0 === e && 100 > h && (e = f.ty + 3 * f.iy, f.ty = e, n.translate(f.tx2, e), f.hitRect && (n = n.getBBox(), f.hitRect.translate(f.tx2 + n.x, e + n.y)), this.checkOverlapping(d, f, c, b, h + 1))
}
}, checkOverlappingReal: function (d, f, c) {
var b = !1, m = d.label,
e = f.label;
d.labelQuarter != c || d.hidden || f.hidden || !e || (m = m.getBBox(), c = {}, c.width = m.width, c.height = m.height, c.y = d.ty, c.x = d.tx, d = e.getBBox(), e = {}, e.width = d.width, e.height = d.height, e.y = f.ty, e.x = f.tx, h.hitTest(c, e) && (b = !0));
return b
}})
})();<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""通知邮件模板"""
# 通过开发自测后的,版本通知邮件
VERSION_SELF_TEST_TEMPLATE = '<br>'.join([
'Dear All:<br>',
'{0}项目,{1}版本发布如下:<br>',
'<h4>版本号:</h4>{2}',
'<h4>升级建议:</h4>{3} 注 (修改重要问题,必须升级的话,该值为Must)',
'<h4>FTP地址:</h4>{4}',
'<h4>JenkinsURL:</h4>{5}',
'<h4>版本说明:</h4>{6}',
'<h4>版本变更内容:</h4>{7}',
'-'*100,
'<br>',
'Dear 袁成,',
'',
'请将版本上传至深圳SVN',
'',
'谢谢!',
'<br>',
'Best Regards',
'{8}|{9}',
'力争取胜 | 快速行动 | 团队精神',
])
# 测试失败后的,版本通知邮件
TEST_FAILURE_TEMPLATE = '<br>'.join([
'Dear All:',
'',
'{0}项目,{1}版本发布如下:',
'<br>',
'<h4>版本号:</h4>{2}',
'<h4>升级建议:</h4>{3} 注 (修改重要问题,必须升级的话,该值为Must)',
'<h4>测试结果:</h4><font color="red">未达到发放要求</font><br>'
'<h4>版本缺陷:</h4>{4}',
'<h4>',
'Best regards',
'{5}|{6}',
'测试部(南京)<br>',
'开放 | 尊重 | 分享 | 创新'
])
# 测试通过后的,版本通知邮件
TEST_PASS_TEMPLATE = '<br>'.join([
'Dear All:',
'',
'{0}项目,{1}版本发布如下:',
'<br>',
'<h4>版本号:</h4>{2}',
'<h4>升级建议:</h4>{3} 注 (修改重要问题,必须升级的话,该值为Must)',
'<h4>测试结果:</h4><font color="green">测试通过</font><br>'
'<h4>版本缺陷:</h4>{4}',
'<br>',
'Best regards',
'{5}|{6}',
'测试部(南京)<br>',
'开放 | 尊重 | 分享 | 创新'
])
# 版本迭代发放的通知邮件
RELEASE_TEMPLATE = '<br>'.join([
'Dear ALL:<br>',
'{0}项目, {1}的{2}版本经软件测试部验证和确认,已完成当前阶段功能的开发。通过公司独立应用测试标准,目前SMI为{3},',
'可交接给产品项目集成,谢谢。',
'<h4>升级建议:</h4>{4} 注 (修改重要问题,必须升级的话,该值为Must)',
'<h4>文件全称:</h4>{5}',
'<h4>应用包名:</h4>{6}',
'<h4>Release软件存放:</h4>{7}',
'<h4>发放声明:</h4>{8}',
'<h4>遗留问题列表:</h4>{9}<br><br>',
'Best regards',
'{10}|{11}',
'测试部(南京)<br>',
'开放 | 尊重 | 分享 | 创新'
])
# 版本DCC发放的通知邮件
DCC_RELEASE_TEMPLATE = '<br>'.join([
'Dear ALL:<br>',
'{0}项目, {1}的{2}版本经软件测试部验证和确认,已完成当前阶段功能的开发。通过公司独立应用测试标准,目前SMI为{3},',
'可交接给产品项目集成,谢谢。',
'<h4>升级建议</h4>{4} 注 (修改重要问题,必须升级的话,该值为Must)',
'<h4>文件全称:</h4>{5}',
'<h4>应用包名:</h4>{6}',
'<h4>Release软件存放:</h4>{7}',
'<h4>发放声明:</h4>{8}',
'<h4>{9}独立应用已做以下适配:</h4><br>{10}',
'<h4>遗留问题列表:</h4>{11}<br><br>',
'Best regards',
'{12}|{13}',
'测试部(南京)<br>',
'开放 | 尊重 | 分享 | 创新'
])
# 版本特别发放的通知邮件
SPECIAL_VERSION_TEMPLATE = '<br>'.join([
'Dear ALL:<br>',
'{0}项目, {1}的{2}版本经软件测试部验证和确认,已完成当前阶段功能的开发。通过公司独立应用测试标准,目前SMI为{3},',
'可交接给产品项目集成,谢谢。',
'<h4>升级建议:</h4>{4} 注 (修改重要问题,必须升级的话,该值为Must)',
'<h4><font color="red">注意: {5}</font></h4>'
'<h4>文件全称:</h4>{6}',
'<h4>应用包名:</h4>{7}',
'<h4>Release软件存放:</h4>{8}',
'<h4>发放声明:</h4>{9}',
'<h4>遗留问题列表:</h4>{10}<br><br>',
'Best regards',
'{11}|{12}',
'测试部(南京)<br>',
'开放 | 尊重 | 分享 | 创新'
])
# 研发版本通知邮件
VERSION_TEST_TEMPLATE = '<br>'.join([
'Dear All:',
'',
'{0}项目,{1}版本发布如下:',
'<br>',
'<h4>版本号:</h4>{2}',
'<h4>版本SMI值:</h4>{3}',
'<h4>版本缺陷:</h4>{4}',
'<h4>版本测试说明:</h4>{5}',
'<br>',
'Best regards',
'{6}|{7}',
'测试部(南京)<br>',
'开放 | 尊重 | 分享 | 创新'
])<file_sep>#-*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""
用户/角色/权限 数据库模块
"""
from datetime import datetime
import hashlib
from itsdangerous import TimedJSONWebSignatureSerializer as Serializer
from werkzeug.security import generate_password_hash, check_password_hash
from flask.ext.login import UserMixin, AnonymousUserMixin
from flask import current_app
from tms.exceptions import ValidationError
from tms import db, login_manager
class Role(db.Model):
"""角色"""
__tablename__ = 'tms_role'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(64), unique=True)
default = db.Column(db.Boolean, default=False, index=True)
permission = db.Column(db.Integer)
index_page = db.Column(db.String(64))
menu = db.Column(db.String(200))
@staticmethod
def insert_roles():
roles = {
'dev_engineer':(),
'test_engineer':(),
'scm': (),
'admin': (),
}
for r in roles:
role = Role.query.filter_by(name=r).first()
if role is None:
role = Role(name=r)
db.session.add(role)
db.session.commit()
def __repr__(self):
return '<Role %r>' % self.name
class User(UserMixin, db.Model):
"""用户"""
__tablename__ = 'tms_user'
id = db.Column(db.Integer, primary_key=True)
email = db.Column(db.String(64), unique=True, index=True)
username = db.Column(db.String(64), unique=True, index=True)
display_name = db.Column(db.String(64), nullable=True)
role_id = db.Column(db.Integer, db.ForeignKey('tms_role.id'))
role = db.relationship('Role', backref=db.backref('user', order_by=id))
password_hash = db.Column(db.String(128))
confirmed = db.Column(db.Boolean, default=False)
location = db.Column(db.String(64))
member_since = db.Column(db.DateTime(), default=datetime.now())
last_seen = db.Column(db.DateTime(), default=datetime.now())
avatar_hash = db.Column(db.String(32))
activate = db.Column(db.Boolean, default=True)
department_id = db.Column(db.Integer, db.ForeignKey('tms_department.id'))
department = db.relationship('Department', backref=db.backref('user', order_by=id))
@staticmethod
def generate_fake(count=100):
"""创建测试用户"""
from sqlalchemy.exc import IntegrityError
from random import seed
import forgery_py
seed()
for i in range(count):
u = User(
email=forgery_py.internet.email_address(),
username=forgery_py.internet.user_name(True),
password=<PASSWORD>(),
confirmed=True,
name=forgery_py.name.full_name(),
member_since=forgery_py.date.date(True)
)
db.session.add(u)
try:
db.session.commit()
except IntegrityError:
db.session.rollback()
@staticmethod
def __init__(self, **kwargs):
super(User, self).__init__(**kwargs)
if self.role is None:
if self.email == current_app.config['TMS_ADMIN']:
self.role = Role.query.filter_by(name='admin').first()
if self.role is None:
self.role = Role.query.filter_by(default=True).first()
if self.email is not None and self.avatar_hash is None:
self.avatar_hash = hashlib.md5(self.email.encode('utf-8')).hexdigest()
@property
def password(self):
raise AttributeError('password is not a readable attribute')
@password.setter
def password(self, password):
self.password_hash = generate_password_hash(password)
def reset_password(self, token, new_password):
s = Serializer(current_app.config['SECRET_KEY'])
try:
data = s.loads(s)
except Exception, e:
return False
if data.get('reset') != self.id:
return False
self.password = <PASSWORD>
db.session.add(self)
return True
def verify_password(self, password):
return check_password_hash(self.password_hash, password)
def generate_confirmation_token(self, expiration=3600):
"""生成验证连接有效期是1小时"""
s = Serializer(current_app.config['SECRET_KEY'], expiration)
return s.dumps({'reset': self.id})
def generate_email_change_token(self, new_email, expiration=3600):
s = Serializer(current_app.config['SECRET_KEY'], expiration)
return s.dumps({'change_email': self.id, 'new_email': new_email})
def change_email(self, token):
s = Serializer(current_app.config['SECRET_KEY'])
try:
data = s.loads(token)
except Exception, e:
return False
if data.get('change_email') != self.id:
return False
new_email = data.get('new_email')
if new_email is None:
return False
if self.query.filter_by(email=new_email) is not None:
return False
self.email = new_email
self.avatar_hash = hashlib.md5(self.email.encode('utf-8')).hexdigest()
db.session.add(self)
return True
def can(self, permissions):
pass
def is_administrator(self):
pass
def ping(self):
self.last_seen = datetime.now()
db.session.add(self)
def genrate_auth_token(self, expiration):
s = Serializer(current_app.config['SECRET_KEY'], expires_in=expiration)
return s.dumps({'id': self.id}).decode('ascii')
@staticmethod
def verify_auth_token(token):
s = Serializer(current_app.config['SECRET_KEY'])
try:
data = s.loads(token)
except Exception, e:
return None
return User.query.get(data['id'])
def __repr__(self):
return '<User %r>' % self.username
class AnonymousUser(AnonymousUserMixin):
def can(self, permissions):
return False
def is_administrator(self):
return False
login_manager.anonymous_user = AnonymousUser
@login_manager.user_loader
def load_user(user_id):
return User.query.get(int(user_id))
class SystemMenu(db.Model):
"""系统菜单"""
__tablename__ = 'tms_menu'
id = db.Column(db.Integer, primary_key=True)
name = db.Column(db.String(64))
action = db.Column(db.String(64))
activate = db.Column(db.Boolean, default=True)
note = db.Column(db.String(100))
order = db.Column(db.Integer)
# 菜单样式
style = db.Column(db.String(300), nullable=True)
parent_id = db.Column(db.Integer, db.ForeignKey('tms_menu.id'), nullable=True)
parent = db.relation('SystemMenu', uselist=False, remote_side=[id],
backref=db.backref('children', order_by=order))
def __repr__(self):
return '<SystemMenu %r>' % self.name
class SystemLog(db.Model):
"""系统日志"""
__tablename__ = 'tms_log'
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(50))
option = db.Column(db.String(300))
option_time = db.Column(db.DateTime)
def __repr__(self):
return '<SystemLog %r>' % self.username
class SystemParameter(db.Model):
"""系统参数表"""
__tablename__ = 'tms_paramter'
id = db.Column(db.Integer, primary_key=True)
key = db.Column(db.String(64))
value = db.Column(db.Text)
flag = db.Column(db.Boolean, default=True)
note = db.Column(db.String(255))
def __repr__(self):
return '<SystemParameter %r>' % self.key
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
import os
class MenuObject:
def __init__(self, id, name, url, style):
self.id = id
self.name = name
self.url = url
self.style = style
self.children = []
def create_menu(menus):
"""创建默认菜单"""
parents = []
for menu in menus:
if not menu.parent_id:
parents.append({'id': menu.id, 'name': menu.name, 'url': menu.action, 'style': menu.style,
'children': []})
for menu in menus:
if menu.parent_id:
for parent in parents:
if parent['id'] == menu.parent_id:
parent['children'].append({'id': menu.id, 'name': menu.name, 'url': menu.action,
'style': menu.style, 'children': []})
return parents
def getOrder(menu):
if menu:
return menu.order
else:
return 0<file_sep>#!/usr/bin/env python
# -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/7/6
"""
"""<file_sep>/**
* Created by changjie.fan on 2015/11/10.
*/
$(function(){
/*用户设置*/
$('#email_submit').bind('click',function(){
var data = {
data: JSON.stringify({
'email': $('#email').val()
})
};
$.ajax({
url: '/auth/change_email',
type: 'POST',
data: data,
success: function(msg){
alert(msg);
}
});
});
});<file_sep>#!/usr/bin/env python
# -*- coding:utf-8 -*-
"""
Created on 2016-2-27
@author: changjie.fan
将当天所有commit的信息导入到数据库中
"""
import logging
import sys
import os
import time
from datetime import datetime
from DBOption import DBOption
from GitRepository import GitRepository
def add_commit_reference(logger):
# mysql操作对象初始化
db_url = {'host': '192.168.33.7', 'db_name': 'tms', 'username': 'tup', 'password': 'tup'}
db_option = DBOption(db_url, 'mysql')
db_option.db_connect()
record_sql = "SELECT history.id, history.repository_path, history.push_type, history.commits, " \
"history.reference_name, repo_server.root_path, repo_server.backup_server_path, repo.id " \
"FROM repo_push_history AS history JOIN repo_server ON history.server_ip=repo_server.ip_address " \
"JOIN repo_repo AS repo ON repo.path=history.repository_path WHERE history.status=1 and " \
"history.server_ip IN('192.168.33.9', '192.168.33.13') LIMIT 100 "
new_references = []
new_commits = []
for record in db_option.fetch_all(record_sql):
logger.info(record[7])
row_id, repo_path, push_type, commits, reference_name, root_path, backup_server_path, repo_id = \
record[0], record[1], record[2], record[3], record[4], record[5], record[6], record[7]
if push_type == 'create_reference':
if reference_name.strip().startswith('refs/heads/'):
new_references.append((reference_name, 1, repo_id))
else:
new_references.append((reference_name, 0, repo_id))
elif push_type == 'update_reference':
repo_path = repo_path.replace(root_path, backup_server_path)
repo_obj = GitRepository(repo_path)
if commits.find('/') > 0:
for commit in repo_obj.diff_commit(commits.split('/')[0], commits.split('/')[1]):
if len(commit) == 9:
new_commits.append((commit[0], '', commit[1], commit[2], commit[3], commit[4],
commit[5].split('+')[0], commit[6], commit[7], commit[8].split('+')[0],
repo_id))
else:
new_commits.append((commit[0], commit[1], commit[2], commit[3], commit[4],
commit[5].split('+')[0], commit[6], commit[7], commit[8].split('+')[0],
commit[9], repo_id))
else:
commit = repo_obj.log_commit(commits)
if len(commit) == 9:
new_commits.append((commit[0], '', commit[1], commit[2], commit[3], commit[4],
commit[5].split('+')[0], commit[6], commit[7], commit[8].split('+')[0],
repo_id))
else:
new_commits.append((commit[0], commit[1], commit[2], commit[3], commit[4], commit[5].split('+')[0],
commit[6], commit[7], commit[8].split('+')[0], commit[9], repo_id))
elif push_type == 'delete_reference':
update_reference_sql = "UPDATE repo_refs set status=1, push_id={0} WHERE repository_id={1} " \
"and name='{2}'".format(row_id, repo_id, reference_name)
db_option.insert_one(update_reference_sql)
update_sql = "UPDATE repo_push_history set status=3, import_time='{0}' " \
"WHERE id={1}".format(time.strftime('%Y-%m-%d %H:%M:%S', time.localtime()), row_id)
db_option.insert_one(update_sql)
# 新增的分支和tag添加入数据库
if new_references:
insert_refs_sql = "INSERT INTO repo_refs (name, category, repository_id) VALUES (%s, %s, %s)"
db_option.insert_all(insert_refs_sql, new_references)
# 新增的commit添加入数据库
if new_commits:
insert_commit_sql = "INSERT INTO repo_commit (commit_hash, parent_hash, tree_hash, author_name, author_email, " \
"author_time, committer_name, committer_email, commit_time, message, repository_id) " \
"VALUES (%s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s)"
print new_commits
db_option.insert_all(insert_commit_sql, new_commits)
db_option.close()
if __name__ == "__main__":
log_file = os.path.join('/var/log/git_backup', datetime.now().strftime('%Y-%m-%d')+'.log')
logging.basicConfig(
level=logging.INFO,
format='',
datefmt='%Y-%m-%d/%H:%M:%S',
filename=log_file,
filemode='a'
)
file_handler = logging.FileHandler(log_file, 'a')
formatter = logging.Formatter('%(asctime)s %(name)s[line:%(lineno)d]:%(levelname)s %(message)s')
file_handler.setFormatter(formatter)
console_handler = logging.StreamHandler()
console_handler.setFormatter(formatter)
logging.root.addHandler(console_handler)
logging.root.addHandler(file_handler)
logger = logging.getLogger('Git')
add_commit_reference(logger)
while True:
add_commit_reference(logger)
time.sleep(200)
<file_sep>#!/bin/bash
python manage.py runserver -t 192.168.33.64 -p 5000
<file_sep>/**
* Created by changjie.fan on 2016/1/22.
*/
$("#department_manage_table").treetable({expandable: true});
// Highlight selected row
$("#department_manage_table tbody").on("mousedown", "tr", function () {
$(".selected").not(this).removeClass("selected");
$(this).toggleClass("selected");
});
// Drag & Drop Example Code
$("#department_manage_table .file, #department_manage_table .folder").draggable({
helper: "clone",
opacity: .75,
refreshPositions: true,
revert: "invalid",
revertDuration: 300,
scroll: true
});
//
$("#department_manage_table .folder").each(function () {
$(this).parents("#department_manage_table tr").droppable({
accept: ".file, .folder",
drop: function (e, ui) {
var droppedEl = ui.draggable.parents("tr");
$("#department_manage_table").treetable("move", droppedEl.data("ttId"), $(this).data("ttId"));
},
hoverClass: "accept",
over: function (e, ui) {
var droppedEl = ui.draggable.parents("tr");
if (this != droppedEl[0] && !$(this).is(".expanded")) {
$("#department_manage_table").treetable("expandNode", $(this).data("ttId"));
}
}
});
});
function setMenuActivate(activate){
var show_activate = 'Disable';
if(activate == 'Enable'){
show_activate = 'True';
}
var selected = $(".selected");
if(selected.length){
var department_id = $(".selected").data().ttId;
bootbox.confirm("Are you sure?", function(result) {
if(result){
$.getJSON($SCRIPT_ROOT+'/department/department_activate',{
id: department_id,
activate: activate
}, function(data){
bootbox.alert("Success");
if (!selected.data('ttParentId')) {
$("#department_manage_table tr").each(function (trindex, tritem) {
if($(tritem).data('ttParentId') == selected.data('ttId')){
$($(tritem).children()[1]).text(show_activate);
}
});
}else{
$(selected.children()[1]).text(show_activate);
}
});
}
});
}else{
bootbox.alert("You must selected department");
}
}
//
$("#department_manage_table tr").dblclick(function(){
window.location.href = $SCRIPT_ROOT + '/department/edit_department/' + $(this).attr('data-tt-id');
});<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""定义测试的蓝图"""
from flask import Blueprint
qa = Blueprint('qa', __name__)
from . import views
from . import testCaseViews
from . import projectViews
<file_sep>{% extends "qa/qa_base.html" %}
{% block page_content %}
<!-- BEGIN CONTENT -->
<div class="row">
<div class="col-md-12">
<!-- BEGIN EXAMPLE TABLE PORTLET-->
<div class="portlet box grey-cascade">
<div class="portlet-title">
<div class="caption">
{%if all_project %}
<i class="fa fa-globe"></i>所有项目
{% else %}
<i class="fa fa-globe"></i>我的项目
{% endif %}
</div>
<div class="actions">
<a href="{{ url_for('qa.all_project') }}" class="btn btn-primary btn-sm">所有项目</a>
</div>
</div>
<div class="portlet-body">
<table class="table table-striped table-bordered table-hover" id="project_table">
<thead>
<tr>
<th width="20px;">No</th>
<th>项目名称</th>
<th>JIRA_KEY</th>
<th>开发负责人</th>
<th>测试负责人</th>
<th>状态</th>
<th>最新版本</th>
<th>发布时间</th>
<th>项目用例</th>
</tr>
</thead>
<tbody>
{% for project in projects %}
<tr data="{{ project.id }}">
<td>{{ loop.index }}</td>
<td>{{project.name }}</td>
<td>{{ project.project_key }}</td>
<td>{{ project.develop_leader.display_name }}</td>
<td>{{ project.test_leader.display_name }}</td>
<td>{{ project.status }}</td>
<td>{{ project.last_version_number or '' }}</td>
<td>{{ project.last_release_time or ''}}</td>
{% if not all_project %}
<td>
<a class="btn btn-xs green"
href="{{ url_for('qa.project_test_case_page', project_id=project.id) }}">用例管理</a>
</td>
{% endif %}
</tr>
{% endfor %}
</tbody>
</table>
</div>
</div>
<!-- END EXAMPLE TABLE PORTLET-->
</div>
</div>
<!-- END PAGE CONTENT-->
{% endblock %}
{% block script %}
<script src="{{ url_for('static', filename='assets/global/plugins/bootbox/bootbox.min.js') }}"
type="text/javascript"></script>
<script src="{{url_for('static', filename='js/qa/project_page.js')}}" type="text/javascript"></script>
<script type="text/javascript">
$SCRIPT_ROOT = {{ request.script_root|tojson|safe }};
</script>
{% endblock %}
<file_sep>var ChartsAmcharts = function() {
var init_jira_total_tasks_chart = function(total_tasks) {
var data = [];
for(index in total_tasks){
data_dic = {};
data_dic['status'] = total_tasks[index]['status'];
data_dic['total_num'] = total_tasks[index]['total_num'];
data.push(data_dic);
}
var chart = AmCharts.makeChart("jira_total_tasks_chart", {
"type": "pie",
"theme": "light",
"fontFamily": 'Open Sans',
"color": '#888',
"dataProvider": data,
"valueField": "total_num",
"titleField": "status",
"balloonText": "[[title]]<br><span style='font-size:14px'><b>[[value]]</b> ([[percents]]%)</span>",
"legend": {
"alphaField": "alpha",
"useGraphSettings": true,
"valueAlign": "left",
"valueWidth": 100
},
"export": {
"enabled":true,
"fileName":"total_tasks_chart",
"libs":{
"path": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/plugins/export/libs/"
},
"position":"bottom-right",
"menu": [ {
"class": "export-main",
"menu": [ {
"label": "Download as image",
"menu": [ "PNG", "SVG" , "PDF"]
}, {
"label": "Save data",
"menu": [ "CSV", "XLSX" ]
}]
} ]
}
});
$('#jira_total_tasks_chart').closest('.portlet').find('.fullscreen').click(function() {
chart.invalidateSize();
});
}
var init_jira_tasks_daily_update_chart = function(tasks_daily_update) {
var data = [];
for(index in tasks_daily_update){
data_dic = {};
data_dic['date'] = tasks_daily_update[index]['date'];
data_dic['new_open'] = tasks_daily_update[index]['new_open'];
data_dic['closed'] = tasks_daily_update[index]['closed'];
data.push(data_dic);
}
var chart = AmCharts.makeChart("jira_tasks_daily_update_chart", {
"type": "serial",
"theme": "light",
"fontFamily": 'Open Sans',
"color": '#888888',
"pathToImages": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/images/",
"legend": {
"equalWidths": false,
"useGraphSettings": true,
"valueAlign": "left",
"valueWidth": 120
},
"dataProvider": data ,
"valueAxes": [{
"id": "distanceAxis",
"axisAlpha": 0,
"gridAlpha": 0,
"position": "left"
// "title": "distance"
}, {
"id": "new_openAxis",
"axisAlpha": 0,
"gridAlpha": 0,
"labelsEnabled": false,
"position": "right"
}, {
// "id": "durationAxis",
//// "duration": "mm",
//// "durationUnits": {
//// "hh": "h ",
//// "mm": "min"
//// },
// "axisAlpha": 0,
// "gridAlpha": 0,
// "inside": true,
// "position": "right",
// "title": "duration"
}],
"graphs": [
// {
// "alphaField": "alpha",
// "balloonText": "[[value]] miles",
// "dashLengthField": "dashLength",
// "fillAlphas": 0.7,
// "legendPeriodValueText": "total: [[value.sum]] mi",
// "legendValueText": "[[value]] mi",
// "title": "当日任务总数",
// "type": "column",
// "valueField": "distance",
// "valueAxis": "distanceAxis"
// },
{
"balloonText": "新增任务数:[[value]]",
"bullet": "round",
"bulletBorderAlpha": 1,
"useLineColorForBulletBorder": true,
"bulletColor": "#FFFFFF",
"bulletSizeField": "townSize",
"dashLengthField": "dashLength",
"descriptionField": "townName",
"labelPosition": "right",
"labelText": "[[townName2]]",
// "legendValueText": "[[description]]/[[value]]",
"legendValueText": "[[value]]",
"title": "新增任务数",
"fillAlphas": 0,
"valueField": "new_open",
"valueAxis": "distanceAxis"
}, {
"balloonText": "已关闭任务数:[[value]]",
"bullet": "square",
"bulletBorderAlpha": 1,
"bulletBorderThickness": 1,
"dashLengthField": "dashLength",
"legendValueText": "[[value]]",
"title": "已关闭任务数",
"fillAlphas": 0,
"valueField": "closed",
"valueAxis": "distanceAxis"
}],
"chartScrollbar": {},
"chartCursor": {
"categoryBalloonDateFormat": "DD",
"cursorAlpha": 0.1,
"cursorColor": "#000000",
"fullWidth": true,
"valueBalloonsEnabled": false,
"zoomable": false
},
"dataDateFormat": "YYYY-MM-DD",
"categoryField": "date",
"categoryAxis": {
"dateFormats": [{
"period": "DD",
"format": "DD"
}, {
// "period": "WW",
// "format": "MMM DD"
}, {
"period": "MM",
"format": "MMM"
}, {
"period": "YYYY",
"format": "YYYY"
}],
"parseDates": true,
"autoGridCount": false,
"axisColor": "#555555",
"gridAlpha": 0.1,
"gridColor": "#FFFFFF",
"gridCount": 50
},
"export": {
"enabled":true,
"libs":{
"path": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/plugins/export/libs/"
},
"position":"bottom-right",
"forceRemoveImages":true,
"fileName":"tasks_daily_update_chart",
"menu": [ {
"class": "export-main",
"menu": [ {
"label": "Download as image",
"menu": [ "PNG", "SVG" , "PDF"]
}, {
"label": "Save data",
"menu": [ "CSV", "XLSX" ]
} ]
} ]
}
});
$('#jira_tasks_daily_update_chart').closest('.portlet').find('.fullscreen').click(function() {
chart.invalidateSize();
});
}
var init_jira_tasks_daily_summary_chart = function(tasks_daily_summary) { //tasks_daily_update
var data = [];
for(index in tasks_daily_summary){
data_dic = {};
data_dic['date'] = tasks_daily_summary[index]['date'];
// if(index == 0){
data_dic['open_summary'] = tasks_daily_summary[index]['open_summary'];
data_dic['closed_summary'] = tasks_daily_summary[index]['closed_summary'];
// }else{
// tasks_daily_update[index]['new_open'] = tasks_daily_update[index]['new_open'] + tasks_daily_update[index-1]['new_open'];
// data_dic['open_summary'] = tasks_daily_update[index]['new_open'];
// tasks_daily_update[index]['closed'] = tasks_daily_update[index]['closed'] + tasks_daily_update[index-1]['closed'];
// data_dic['closed_summary'] = tasks_daily_update[index]['closed'];
// }
data.push(data_dic);
}
var chart = AmCharts.makeChart("jira_tasks_daily_summary_chart", {
"type": "serial",
"theme": "light",
"fontFamily": 'Open Sans',
"color": '#888888',
"pathToImages": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/images/",
"legend": {
"equalWidths": false,
"useGraphSettings": true,
"valueAlign": "left",
"valueWidth": 120
},
"dataProvider": data ,
"valueAxes": [{
"id": "distanceAxis",
"axisAlpha": 0,
"gridAlpha": 0,
"position": "left"
}],
"graphs": [
{
"balloonText": "新增任务汇总:[[value]]",
"bullet": "round",
"bulletBorderAlpha": 1,
"useLineColorForBulletBorder": true,
"bulletColor": "#FFFFFF",
"dashLengthField": "dashLength",
"labelPosition": "right",
"legendValueText": "[[value]]",
"title": "新增任务汇总",
"fillAlphas": 0,
"valueField": "open_summary",
"valueAxis": "distanceAxis"
}, {
"balloonText": "关闭任务汇总:[[value]]",
"bullet": "square",
"bulletBorderAlpha": 1,
"bulletBorderThickness": 1,
"dashLengthField": "dashLength",
"legendValueText": "[[value]]",
"title": "关闭任务汇总",
"fillAlphas": 0,
"valueField": "closed_summary",
"valueAxis": "distanceAxis"
}],
"chartScrollbar": {},
"chartCursor": {
"categoryBalloonDateFormat": "DD",
"cursorAlpha": 0.1,
"cursorColor": "#000000",
"fullWidth": true,
"valueBalloonsEnabled": false,
"zoomable": false
},
"dataDateFormat": "YYYY-MM-DD",
"categoryField": "date",
"categoryAxis": {
"dateFormats": [{
"period": "DD",
"format": "DD"
}, {
// "period": "WW",
// "format": "MMM DD"
}, {
"period": "MM",
"format": "MMM"
}, {
"period": "YYYY",
"format": "YYYY"
}],
"parseDates": true,
"autoGridCount": false,
"axisColor": "#555555",
"gridAlpha": 0.1,
"gridColor": "#FFFFFF",
"gridCount": 50
},
"export": {
"enabled":true,
"libs":{
"path": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/plugins/export/libs/"
},
"position":"bottom-right",
"fileName":"jira_tasks_daily_summary_chart",
"menu": [ {
"class": "export-main",
"menu": [ {
"label": "Download as image",
"menu": [ "PNG", "SVG" , "PDF"]
}, {
"label": "Save data",
"menu": [ "CSV", "XLSX" ]
} ]
} ]
}
});
$('#jira_tasks_daily_summary_chart').closest('.portlet').find('.fullscreen').click(function() {
chart.invalidateSize();
});
}
var init_jira_total_tasks_developer_open_chart = function(total_tasks_developer_open) {
var data = [];
for(index in total_tasks_developer_open){
data_dic = {};
data_dic['assigner'] = total_tasks_developer_open[index]['assigner'];
data_dic['assignerNum'] = total_tasks_developer_open[index]['total_tasks'];
data.push(data_dic);
}
var chart = AmCharts.makeChart("jira_total_tasks_developer_open_chart", {
"type": "serial",
"theme": "light",
"fontFamily": 'Open Sans',
"color": '#888',
"legend": {
"equalWidths": false,
"useGraphSettings": true,
"valueAlign": "left",
"valueWidth": 120
},
"dataProvider": data,
"pathToImages": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/images/",
"valueAxes": [{
"id": "assignerNumAxis",
"axisAlpha": 0,
"gridAlpha": 0,
"position": "left"
}],
"graphs": [{
"alphaField": "alpha",
"balloonText": "[[value]]",
"dashLengthField": "dashLength",
"fillAlphas": 0.7,
"legendPeriodValueText": "total: [[value.sum]]",
"legendValueText": "[[value]]",
"title": "开发人员进行中任务数:",
"type": "column",
"valueField": "assignerNum",
"valueAxis": "assignerNumAxis"
}],
"chartScrollbar": {},
"chartCursor": {
"cursorAlpha": 0.1,
"cursorColor": "#000000",
"fullWidth": true,
"valueBalloonsEnabled": false,
"zoomable": false
},
"categoryField": "assigner",
"categoryAxis": {
"autoGridCount": false,
"axisColor": "#555555",
"gridAlpha": 0.1,
"gridColor": "#FFFFFF",
"gridCount": 50
},
"export": {
"enabled":true,
"fileName":"total_tasks_developer_open_chart",
"libs":{
"path": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/plugins/export/libs/"
},
"position":"bottom-right",
"menu": [ {
"class": "export-main",
"menu": [ {
"label": "Download as image",
"menu": [ "PNG", "SVG" , "PDF"]
}, {
"label": "Save data",
"menu": [ "CSV", "XLSX" ]
} ]
} ]
}
});
$('#jira_total_tasks_developer_open_chart').closest('.portlet').find('.fullscreen').click(function() {
chart.invalidateSize();
});
}
var init_jira_total_tasks_each_person_chart = function(total_tasks_each_person) {
var data = [];
for(index in total_tasks_each_person){
data_dic = {};
data_dic['assigner'] = total_tasks_each_person[index]['assigner'];
data_dic['total_tasks'] = total_tasks_each_person[index]['total_tasks'];
data.push(data_dic);
}
var chart = AmCharts.makeChart("jira_total_tasks_each_person_chart", {
"type": "pie",
"theme": "light",
"fontFamily": 'Open Sans',
"color": '#888',
"dataProvider":data,
"valueField": "total_tasks",
"titleField": "assigner",
// "outlineAlpha": 0.4,
// "depth3D": 15,
"balloonText": "[[title]]<br><span style='font-size:14px'><b>[[value]]</b> ([[percents]]%)</span>",
// "angle": 30,
"export": {
"enabled":true,
"fileName":"total_tasks_each_person_chart",
"libs":{
"path": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/plugins/export/libs/"
},
"position":"bottom-right",
"menu": [ {
"class": "export-main",
"menu": [ {
"label": "Download as image",
"menu": [ "PNG", "SVG" , "PDF"]
}, {
"label": "Save data",
"menu": [ "CSV", "XLSX" ]
} ]
} ]
}
});
jQuery('.jira_total_tasks_each_person_chart_input').off().on('input change', function() {
var property = jQuery(this).data('property');
var target = chart;
var value = Number(this.value);
chart.startDuration = 0;
if (property == 'innerRadius') {
value += "%";
}
target[property] = value;
chart.validateNow();
});
$('#jira_total_tasks_each_person_chart').closest('.portlet').find('.fullscreen').click(function() {
chart.invalidateSize();
});
}
var init_jira_total_tasks_tester_open_chart = function(total_tasks_tester_open) {
var data = [];
for(index in total_tasks_tester_open){
data_dic = {};
data_dic['creator'] = total_tasks_tester_open[index]['creator'];
data_dic['creatorNum'] = total_tasks_tester_open[index]['creatorNum'];
data.push(data_dic);
}
var chart = AmCharts.makeChart("jira_total_tasks_tester_open_chart", {
"type": "serial",
"theme": "light",
"fontFamily": 'Open Sans',
"pathToImages": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/images/",
"color": '#888888',
"legend": {
"equalWidths": false,
"useGraphSettings": true,
"valueAlign": "left",
"valueWidth": 120
},
"dataProvider": data,
"valueAxes": [{
"id": "creatorNumAxis",
"axisAlpha": 0,
"gridAlpha": 0,
"position": "left"
}],
"graphs": [{
"alphaField": "alpha",
"balloonText": "[[value]]",
"dashLengthField": "dashLength",
"fillAlphas": 0.7,
"legendPeriodValueText": "total: [[value.sum]]",
"legendValueText": "[[value]]",
"title": "测试人员提交任务统计:",
"type": "column",
"valueField": "creatorNum",
"valueAxis": "creatorNumAxis"
}],
"chartScrollbar": {},
"chartCursor": {
"cursorAlpha": 0.1,
"cursorColor": "#000000",
"fullWidth": true,
"valueBalloonsEnabled": false,
"zoomable": false
},
"categoryField": "creator",
"categoryAxis": {
"autoGridCount": false,
"axisColor": "#555555",
"gridAlpha": 0.1,
"gridColor": "#FFFFFF",
"gridCount": 50
},
"export": {
"enabled":true,
"position":"bottom-right",
"fileName":"jira_total_tasks_tester_open_chart",
"libs":{
"path": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/plugins/export/libs/"
},
"position":"bottom-right",
"menu": [ {
"class": "export-main",
"menu": [ {
"label": "Download as image",
"menu": [ "PNG", "SVG" , "PDF"]
}, {
"label": "Save data",
"menu": [ "CSV", "XLSX" ]
}]
} ]
}
});
$('#jira_total_tasks_tester_open_chart').closest('.portlet').find('.fullscreen').click(function() {
chart.invalidateSize();
});
}
var init_jira_daily_tasks_tester_open_chart = function(daily_tasks_tester_open) {
var data = [];
for(index in daily_tasks_tester_open){
data_dic = {};
data_dic['creator'] = daily_tasks_tester_open[index]['creator'];
data_dic['creatorNum'] = daily_tasks_tester_open[index]['creatorNum'];
data.push(data_dic);
}
var chart = AmCharts.makeChart("jira_daily_tasks_tester_open_chart", {
"type": "serial",
"theme": "light",
"fontFamily": 'Open Sans',
"pathToImages": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/images/",
"color": '#888888',
"legend": {
"equalWidths": false,
"useGraphSettings": true,
"valueAlign": "left",
"valueWidth": 120
},
"dataProvider": data,
"valueAxes": [{
"id": "creatorNumAxis",
"axisAlpha": 0,
"gridAlpha": 0,
"position": "left"
}],
"graphs": [{
"alphaField": "alpha",
"balloonText": "[[value]]",
"dashLengthField": "dashLength",
"fillAlphas": 0.7,
"legendPeriodValueText": "total: [[value.sum]]",
"legendValueText": "[[value]]",
"title": "测试人员每日提交任务数:",
"type": "column",
"valueField": "creatorNum",
"valueAxis": "creatorNumAxis"
}],
"chartScrollbar": {},
"chartCursor": {
"cursorAlpha": 0.1,
"cursorColor": "#000000",
"fullWidth": true,
"valueBalloonsEnabled": false,
"zoomable": false
},
"categoryField": "creator",
"categoryAxis": {
"autoGridCount": false,
"axisColor": "#555555",
"gridAlpha": 0.1,
"gridColor": "#FFFFFF",
"gridCount": 50
},
"export": {
"enabled":true,
"fileName":"jira_daily_tasks_tester_open_chart",
"libs":{
"path": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/plugins/export/libs/"
},
"position":"bottom-right",
"menu": [ {
"class": "export-main",
"menu": [ {
"label": "Download as image",
"menu": [ "PNG", "SVG" , "PDF"]
}, {
"label": "Save data",
"menu": [ "CSV", "XLSX" ]
}]
} ]
}
});
$('#jira_daily_tasks_tester_open_chart').closest('.portlet').find('.fullscreen').click(function() {
chart.invalidateSize();
});
}
var init_jira_total_tasks_developer_closed_chart = function(total_tasks_developer_closed) {
var data = [];
for(index in total_tasks_developer_closed){
data_dic = {};
data_dic['assigner'] = total_tasks_developer_closed[index]['assigner'];
data_dic['assignerNum'] = total_tasks_developer_closed[index]['assignerNum'];
data.push(data_dic);
}
var chart = AmCharts.makeChart("jira_total_tasks_developer_closed_chart", {
"type": "serial",
"theme": "light",
"fontFamily": 'Open Sans',
"pathToImages": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/images/",
"color": '#888888',
"legend": {
"equalWidths": false,
"useGraphSettings": true,
"valueAlign": "left",
"valueWidth": 120
},
"dataProvider": data,
"valueAxes": [{
"id": "assignerNumAxis",
"axisAlpha": 0,
"gridAlpha": 0,
"position": "left"
}],
"graphs": [{
"alphaField": "alpha",
"balloonText": "[[value]]",
"dashLengthField": "dashLength",
"fillAlphas": 0.7,
"legendPeriodValueText": "total: [[value.sum]]",
"legendValueText": "[[value]]",
"title": "开发人员关闭任务统计:",
"type": "column",
"valueField": "assignerNum",
"valueAxis": "assignerNumAxis"
}],
"chartScrollbar": {},
"chartCursor": {
"cursorAlpha": 0.1,
"cursorColor": "#000000",
"fullWidth": true,
"valueBalloonsEnabled": false,
"zoomable": false
},
"categoryField": "assigner",
"categoryAxis": {
"autoGridCount": false,
"axisColor": "#555555",
"gridAlpha": 0.1,
"gridColor": "#FFFFFF",
"gridCount": 50
},
"export": {
"enabled":true,
"fileName":"jira_total_tasks_developer_closed_chart",
"libs":{
"path": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/plugins/export/libs/"
},
"position":"bottom-right",
"menu": [ {
"class": "export-main",
"menu": [ {
"label": "Download as image",
"menu": [ "PNG", "SVG" , "PDF"]
}, {
"label": "Save data",
"menu": [ "CSV", "XLSX" ]
}]
} ]
}
});
$('#jira_total_tasks_developer_closed_chart').closest('.portlet').find('.fullscreen').click(function() {
chart.invalidateSize();
});
}
var init_jira_daily_tasks_developer_closed_chart = function(daily_tasks_developer_closed) {
var data = [];
for(index in daily_tasks_developer_closed){
data_dic = {};
data_dic['assigner'] = daily_tasks_developer_closed[index]['assigner'];
data_dic['assignerNum'] = daily_tasks_developer_closed[index]['assignerNum'];
data.push(data_dic);
}
var chart = AmCharts.makeChart("jira_daily_tasks_developer_closed_chart", {
"type": "serial",
"theme": "light",
"fontFamily": 'Open Sans',
"pathToImages": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/images/",
"color": '#888888',
"legend": {
"equalWidths": false,
"useGraphSettings": true,
"valueAlign": "left",
"valueWidth": 120
},
"dataProvider": data,
"valueAxes": [{
"id": "assignerNumAxis",
"axisAlpha": 0,
"gridAlpha": 0,
"position": "left"
}],
"graphs": [{
"alphaField": "alpha",
"balloonText": "[[value]]",
"dashLengthField": "dashLength",
"fillAlphas": 0.7,
"legendPeriodValueText": "total: [[value.sum]]",
"legendValueText": "[[value]]",
"title": "开发人员每日关闭任务数:",
"type": "column",
"valueField": "assignerNum",
"valueAxis": "assignerNumAxis"
}],
"chartScrollbar": {},
"chartCursor": {
"cursorAlpha": 0.1,
"cursorColor": "#000000",
"fullWidth": true,
"valueBalloonsEnabled": false,
"zoomable": false
},
"categoryField": "assigner",
"categoryAxis": {
"autoGridCount": false,
"axisColor": "#555555",
"gridAlpha": 0.1,
"gridColor": "#FFFFFF",
"gridCount": 50
},
"export": {
"enabled":true,
"fileName":"jira_daily_tasks_developer_closed_chart",
"libs":{
"path": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/plugins/export/libs/"
},
"position":"bottom-right",
"menu": [ {
"class": "export-main",
"menu": [ {
"label": "Download as image",
"menu": [ "PNG", "SVG" , "PDF"]
}, {
"label": "Save data",
"menu": [ "CSV", "XLSX" ]
}]
} ]
}
});
$('#jira_daily_tasks_developer_closed_chart').closest('.portlet').find('.fullscreen').click(function() {
chart.invalidateSize();
});
}
var init_jira_total_tasks_by_module_chart = function(total_tasks_by_module) {
var data = [];
for(index in total_tasks_by_module){
data_dic = {};
data_dic['module_name'] = total_tasks_by_module[index]['module_name'];
data_dic['total_num'] = total_tasks_by_module[index]['total_num'];
data_dic['open_num'] = total_tasks_by_module[index]['open_num'];
data.push(data_dic);
}
var chart = AmCharts.makeChart("jira_total_tasks_by_module_chart", {
"type": "serial",
"theme": "light",
"fontFamily": 'Open Sans',
"pathToImages": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/images/",
"color": '#888888',
"legend": {
"equalWidths": false,
"useGraphSettings": true,
"valueAlign": "left",
"valueWidth": 120
},
"dataProvider":data,
"valueAxes": [{
"axisAlpha": 0,
"gridAlpha": 0,
"position": "left"
}],
"graphs": [{
"balloonText": "<b>[[value]]</b>",
"fillAlphas": 0.9,
"lineAlpha": 0.2,
"legendPeriodValueText": "total: [[value.sum]]",
"title": "该模块总数",
"type": "column",
"valueField": "total_num"
}, {
"balloonText": "<b>[[value]]</b>",
"fillAlphas": 0.9,
"lineAlpha": 0.2,
"legendPeriodValueText": "total: [[value.sum]]",
"title": "进行中",
"type": "column",
// "clustered":false,
// "columnWidth":0.5,
"valueField": "open_num"
}],
"chartScrollbar": {},
"chartCursor": {
"cursorAlpha": 0.1,
"cursorColor": "#000000",
"fullWidth": true,
"valueBalloonsEnabled": false,
"zoomable": false
},
"categoryField": "module_name",
"categoryAxis": {
"autoGridCount": false,
"axisColor": "#555555",
"gridAlpha": 0.1,
"gridColor": "#FFFFFF",
"gridCount": 50
},
"export": {
"enabled":true,
"fileName":"total_tasks_by_module_chart",
"libs":{
"path": Metronic.getGlobalPluginsPath() + "amcharts/amcharts/plugins/export/libs/"
},
"position":"bottom-right",
"menu": [ {
"class": "export-main",
"menu": [ {
"label": "Download as image",
"menu": [ "PNG", "SVG" , "PDF"]
}, {
"label": "Save data",
"menu": [ "CSV", "XLSX" ]
}]
} ]
}
});
$('#jira_total_tasks_by_module_chart').closest('.portlet').find('.fullscreen').click(function() {
chart.invalidateSize();
});
}
return {
//main function to initiate the module
init: function(jira_key,date,chart) {
if(date){
var el = $(chart).parent(".portlet-body");
Metronic.blockUI({
target: el,
animate: true,
overlayColor: 'none'
});
$.ajax({
type: "GET",
data:{jira_key:jira_key,date:date},
url: "/project/android_project_jira",
success: function(jira_data) {
Metronic.unblockUI(el);
var jira_datas = JSON.parse(jira_data.replace(/"/g,"\""));
if(chart.attr('id') == 'jira_daily_tasks_developer_closed_chart'){
console.log(date + ' daily_tasks_developer_closed:');
console.log(jira_datas['daily_tasks_developer_closed']);
init_jira_daily_tasks_developer_closed_chart(jira_datas['daily_tasks_developer_closed']);
}if(chart.attr('id') == 'jira_daily_tasks_tester_open_chart'){
console.log(date + ' daily_tasks_tester_open:');
console.log(jira_datas['daily_tasks_tester_open']);
init_jira_daily_tasks_tester_open_chart(jira_datas['daily_tasks_tester_open']);
}
},
error: function() {
Metronic.unblockUI(el);
}
});
}else{
showMask();
$.ajax({
type: "GET",
data:{jira_key:jira_key},
url: "/project/android_project_jira",
success: function(jira_data) {
unshowMask();
var jira_datas = JSON.parse(jira_data.replace(/"/g,"\""));
console.log(jira_datas);
init_jira_total_tasks_chart(jira_datas['total_tasks']);
// init_jira_tasks_daily_update_chart(jira_datas['tasks_daily_update']);
// init_jira_tasks_daily_summary_chart(jira_datas['tasks_daily_update']);
init_jira_tasks_daily_summary_chart(jira_datas['tasks_daily_summary']);
// init_jira_total_tasks_each_person_chart(jira_datas['total_tasks_each_person']);
init_jira_total_tasks_developer_open_chart(jira_datas['total_tasks_developer_open']);
init_jira_total_tasks_tester_open_chart(jira_datas['total_tasks_tester_open']);
init_jira_total_tasks_developer_closed_chart(jira_datas['total_tasks_developer_closed']);
init_jira_daily_tasks_tester_open_chart(jira_datas['daily_tasks_tester_open']);
init_jira_daily_tasks_developer_closed_chart(jira_datas['daily_tasks_developer_closed']);
init_jira_total_tasks_by_module_chart(jira_datas['total_tasks_by_module']);
},
error: function() {
bootbox.alert('Error on reloading the content. Please check your connection and try again.');
unshowMask();
}
});
}
}
};
}();<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""系统主入口"""
from flask import Blueprint
main = Blueprint('main', __name__)
from . import views
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
from flask.ext.wtf import Form
from wtforms import StringField, PasswordField, BooleanField, SubmitField
from wtforms.validators import Required, Length, Email, Regexp, EqualTo
from wtforms import ValidationError
from models import User
class LoginForm(Form):
email = StringField('Email', validators=[Required(), Length(1, 64), Email()])
password = PasswordField('<PASSWORD>', validators=[Required()])
remember_me = BooleanField('Remember me', default=False)
submit = SubmitField('Sign In')
class ChangePasswordForm(Form):
old_password = PasswordField('Old Password', validators=[Required()])
password = PasswordField('New Password', validators=[Required(), EqualTo('password2',
message='Passwords must match')])
password2 = PasswordField('Confirm password', validators=[Required()])
class ChangeEmailForm(Form):
email = StringField('New Email', validators=[Required(), Length(1, 64), Email()])
def validate_email(self, field):
if User.query.filter_by(email=field.data).first():
raise ValidationError('Email already registered.')
class AdministratorForm(Form):
"""管理员账号编辑"""
email = StringField('Email', validators=[Required(), Length(1, 64), Email()])
password = PasswordField('<PASSWORD>', validators=[Required()])
username = StringField('Username', validators=[Required(), Length(1, 64)])
submit = SubmitField('Submit')<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""定义auth模块的蓝图"""
from flask import Blueprint
auth = Blueprint('auth', __name__)
from . import views
from . import systemView<file_sep># -*- coding: utf-8 -*-
# Created by changjie.fan on 2016/7/5
"""
工程项目代码views
"""
import json
import os
from flask import render_template, redirect, url_for, request, flash, current_app, session, send_file
from flask.ext.login import login_user, logout_user, login_required, current_user
from flask.ext.mail import Mail, Message
from functools import update_wrapper
from . import project
from tms import db, mail
from androidProjectModules import *
from tms.utils.excelOption import ExcelOptions
@project.route('/project_add_manifest/<int:project_id>', methods=['GET', 'POST'])
@login_required
def project_add_manifest(project_id):
"""新增项目分支货单"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '新增项目分支货单' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '新增项目分支货单', 'url': url_for('project.project_add_manifest', project_id=project_id)})
project_obj = ProjectAndroid.query.filter(ProjectAndroid.id == project_id).first()
project_code = ProjectCode()
if request.method == 'POST':
manifest_url = request.form.get('manifest_url', '').strip()
manifest_branch = request.form.get('manifest_branch', '').strip()
manifest_name = request.form.get('manifest_name', '').strip()
manifest_repo = request.form.get('manifest_repo', '').strip()
branch_name = request.form.get('branch_name', '').strip()
# local_repo_path = request.form.get('local_repo_path', '').strip()
platform_name = request.form.get('platform_name', '').strip()
commit_notice_email = request.form.get('commit_notice_email', '').strip()
default_project_code = request.form.get('default_project_code', 'off').strip()
# 同一个项目不能添加重复的分支货单
project_code = ProjectCode.query.filter(ProjectCode.manifest_url == manifest_url).first()
if project_code:
project_code.project_android.append(project_obj)
if default_project_code == 'on':
project_obj.default_project_code = project_code.id
db.session.add(project_obj)
db.session.add(project_code)
db.session.commit()
else:
project_code = ProjectCode()
project_code.manifest_url = manifest_url
project_code.manifest_branch = manifest_branch
project_code.manifest_name = manifest_name
project_code.manifest_repo = manifest_repo
# project_code.local_repo_path = local_repo_path
project_code.branch_name = branch_name
project_code.platform_name = platform_name
# 项目配置的加上分支配置的邮件地址
if project_obj.commit_notice_email:
commit_notice_email_list = list(set([tmp.strip() for tmp in commit_notice_email.split(';') if tmp.strip()] + [tmp for tmp in project_obj.commit_notice_email.split(';') if tmp.strip()]))
else:
commit_notice_email_list = list(set([tmp.strip() for tmp in commit_notice_email.split(';') if tmp.strip()]))
project_code.commit_notice_email = ';'.join(commit_notice_email_list)
project_code.activate = True
project_code.project_id = project_obj.id
project_code.project_android.append(project_obj)
db.session.add(project_code)
db.session.commit()
if default_project_code == 'on':
project_obj.default_project_code = project_code.id
db.session.add(project_obj)
db.session.commit()
flash(u'新增成功!', 'info')
return redirect(url_for('project.edit_android_project_page', project_id=project_id))
return render_template('project/android/project_add_manifest.html',
project_code=project_code,
project=project_obj)
@project.route('/project_edit_manifest/<int:project_android_id>/<int:project_code_id>', methods=['GET', 'POST'])
@login_required
def project_edit_manifest(project_android_id, project_code_id):
"""
修改项目分支货单
:param project_code_id:
:return:
"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '编辑项目分支货单' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index + 1]
break
else:
session['breadcrumbs'].append(
{'text': '编辑项目分支货单', 'url': url_for('project.project_edit_manifest',
project_android_id=project_android_id,
project_code_id=project_code_id)})
project_obj = ProjectAndroid.query.filter(ProjectAndroid.id == project_android_id).first()
project_code = ProjectCode.query.filter(ProjectCode.id == project_code_id).first()
if request.method == 'POST':
manifest_url = request.form.get('manifest_url', '').strip()
manifest_branch = request.form.get('manifest_branch', '').strip()
manifest_name = request.form.get('manifest_name', '').strip()
manifest_repo = request.form.get('manifest_repo', '').strip()
branch_name = request.form.get('branch_name', '').strip()
# local_repo_path = request.form.get('local_repo_path', '').strip()
platform_name = request.form.get('platform_name', '').strip()
commit_notice_email = request.form.get('commit_notice_email', '').strip()
default_project_code = request.form.get('default_project_code', 'off').strip()
# 同一个项目不能添加重复的分支货单
other_project_code = ProjectCode.query.filter(ProjectCode.manifest_url == manifest_url).first()
if other_project_code and other_project_code.id != project_code_id:
flash(u'相同货单已存在!', 'warning')
else:
project_code.manifest_url = manifest_url
project_code.manifest_branch = manifest_branch
project_code.manifest_name = manifest_name
project_code.manifest_repo = manifest_repo
# project_code.local_repo_path = local_repo_path
project_code.branch_name = branch_name
project_code.platform_name = platform_name
commit_notice_email_list = list(set([tmp.strip() for tmp in commit_notice_email.split(';') if tmp.strip()]))
# 项目配置的加上分支配置的邮件地址
for project_tmp in project_code.project_android:
current_app.logger.info(project_tmp.commit_notice_email)
if project_tmp.commit_notice_email:
if commit_notice_email_list:
for tmp in project_tmp.commit_notice_email.split(';'):
if tmp.strip():
commit_notice_email_list.append(tmp)
project_code.commit_notice_email = ';'.join( list(set(commit_notice_email_list)))
project_code.activate = True
if default_project_code == 'on':
project_obj.default_project_code = project_code.id
db.session.add(project_obj)
elif default_project_code == 'off':
project_obj.default_project_code = 0
db.session.add(project_obj)
db.session.add(project_code)
db.session.commit()
flash(u'修改成功!', 'info')
return redirect(url_for('project.project_edit_manifest',
project_android_id=project_android_id,
project_code_id=project_code_id))
return render_template('project/android/project_add_manifest.html',
project_code=project_code,
project=project_obj)
@project.route('/project_code_history/<int:project_id>', defaults={'project_code_id': None}, methods=['GET', 'POST'])
@project.route('/project_code_history/<int:project_id>/<int:project_code_id>', methods=['GET', 'POST'])
@login_required
def project_code_history(project_id, project_code_id):
"""
项目项目指定分支上的所有提交记录,默认显示项目的默认分支
:param project_id:
:return:
"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '项目历史' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index + 1]
break
else:
session['breadcrumbs'].append(
{'text': '项目历史', 'url': url_for('project.project_code_history', project_id=project_id, project_code_id=project_code_id)})
project_obj = ProjectAndroid.query.filter(ProjectAndroid.id == project_id).first()
if not project_code_id:
project_code_id = project_obj.default_project_code
# 项目所有的提货单
project_code_list = project_obj.project_code
return render_template('project/android/project_code_history.html',
project=project_obj,
project_code_id=project_code_id,
project_code_list=project_code_list)
@project.route('/project_code_jira_data')
@login_required
def project_code_jira_data():
"""
项目提交记录的数据
:param project_code_id:
:return:
"""
start = int(request.args.get('start', 0))
length = int(request.args.get('length', 10))
search_type = request.args.get('search_type')
search_text = request.args.get('search_text', '').strip().replace('%', '')
project_code_id = request.args.get('project_code_id', '')
search_sql = "SELECT id, task_key, summary, description, category FROM project_branch_task WHERE " \
"project_code_id={0} ".format(project_code_id)
if search_type and search_text:
search_sql = search_sql + " AND {0} like '%{1}%'".format(search_type, search_text)
else:
search_sql = search_sql.format(project_code_id)
search_sql += ' ORDER BY id DESC'
jira_tasks = db.session.execute(search_sql).fetchall()
result = []
index = start + 1
for task in jira_tasks[start:start+length]:
record = {}
record['index'] = index
record['id'] = task[0]
record['task_key'] = task[1]
record['summary'] = task[2]
record['description'] = task[3]
record['category'] = task[4]
result.append(record)
index += 1
return '{"recordsTotal": %s ,"recordsFiltered": %s,"data":%s}' % (len(jira_tasks), len(jira_tasks), json.dumps(result))
@project.route('/project_code_commit_data')
@login_required
def project_code_commit_data():
"""
项目提交记录的数据
:param project_code_id:
:return:
"""
start = int(request.args.get('start', 0))
length = int(request.args.get('length', 10))
search_type = request.args.get('search_type')
search_text = request.args.get('search_text', '').strip().replace('%', '')
project_code_id = request.args.get('project_code_id', '')
search_sql = "SELECT id, repo_path, auth_name, auth_email, auth_date, commit_name, commit_email, commit_date, " \
"message, change_file, commit_id FROM project_branch_commit WHERE project_code_id={0} ".format(project_code_id)
if search_type and search_text:
if search_type == 'commit_date':
if not (search_text.startswith('=') or search_text.startswith('>=') or search_text.startswith('>') or
search_text.startswith('=<') or search_text.startswith('<')):
search_sql = search_sql + " AND {0} like '%{1}%'".format(search_type, search_text)
else:
search_sql = search_sql + " AND {0} {1}".format(search_type, search_text)
else:
search_sql = search_sql + " AND {0} like '%{1}%'".format(search_type, search_text)
else:
search_sql = search_sql.format(project_code_id)
search_sql += ' ORDER BY id DESC'
commits = db.session.execute(search_sql).fetchall()
result = []
index = start + 1
for commit in commits[start:start+length]:
record = {}
record['index'] = index
record['id'] = commit[0]
record['repo_path'] = commit[1]
record['auth_name'] = commit[2]
record['auth_email'] = commit[3]
record['auth_date'] = commit[4]
record['commit_name'] = commit[5]
record['commit_email'] = commit[6]
record['commit_date'] = commit[7]
record['message'] = commit[8].replace('<', '<')
record['change_file'] = commit[9]
record['commit_id'] = commit[10][:8]
result.append(record)
index += 1
return '{"recordsTotal": %s ,"recordsFiltered": %s,"data":%s}' % (len(commits), len(commits), json.dumps(result))
@project.route('/project_branch_commit_detail/<int:project_branch_commit_id>')
@login_required
def project_branch_commit_detail(project_branch_commit_id):
"""
显示commit的详细信息
:param project_branch_commit_id:
:return:
"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '提交详细信息' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index + 1]
break
else:
session['breadcrumbs'].append(
{'text': '提交详细信息', 'url': url_for('project.project_branch_commit_detail', project_branch_commit_id=project_branch_commit_id)})
commit = ProjectBranchCommit.query.filter(ProjectBranchCommit.id == project_branch_commit_id).first()
return render_template('project/android/project_branch_commit_detail.html', commit=commit)
@project.route('/project_branch_task_detail/<int:project_branch_task_id>', methods=['GET', 'POST'])
@login_required
def project_branch_task_detail(project_branch_task_id):
"""
显示jira任务和commit的详细信息
:param project_branch_task_id:
:return:
"""
task = ProjectBranchTask.query.filter(ProjectBranchTask.id == project_branch_task_id).first()
if request.method == 'POST':
task.category = request.form.get('category')
db.session.add(task)
db.session.commit()
return redirect(url_for('project.project_branch_task_detail', project_branch_task_id=project_branch_task_id))
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '任务详细信息' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index + 1]
break
else:
session['breadcrumbs'].append(
{'text': '任务详细信息', 'url': url_for('project.project_branch_task_detail', project_branch_task_id=project_branch_task_id)})
commits = [tmp.project_branch_commit for tmp in ProjectBranchTaskCommit.query.filter(ProjectBranchTaskCommit.project_branch_task_id == project_branch_task_id).all()]
category_option = ['Public', 'Critical']
return render_template('project/android/project_branch_task_detail.html', category_option=category_option, task=task, commits=commits)
@project.route('/project_branch_commit_compare/<int:first_project_code_id>/<int:second_project_code_id>')
@login_required
def project_branch_commit_compare(first_project_code_id, second_project_code_id):
"""
比较两个分支将first上比second多的内容显示出来
:param first_project_code_id:
:param second_project_id:
:return:
"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '分支比较' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index + 1]
break
else:
session['breadcrumbs'].append(
{'text': '分支比较', 'url': url_for('project.project_branch_commit_compare',
first_project_code_id=first_project_code_id,
second_project_code_id=second_project_code_id
)})
second_commits = [commit.commit_id for commit in
ProjectBranchCommit.query.filter(ProjectBranchCommit.project_code_id == second_project_code_id).all()]
difference_commits = ProjectBranchCommit.query.filter(
db.and_(ProjectBranchCommit.project_code_id == first_project_code_id,
~ProjectBranchCommit.commit_id.in_(second_commits)
)).order_by(ProjectBranchCommit.commit_date.desc()).all()
merge_commits = [tmp.commit_id for tmp in ProjectBranchMergeCommit.query.filter(
db.and_(ProjectBranchMergeCommit.dest_project_code_id == first_project_code_id,
ProjectBranchMergeCommit.src_project_code_id == second_project_code_id)).all()]
result = []
for commit in difference_commits:
if commit.commit_id not in merge_commits:
result.append(commit)
first_branch = ProjectCode.query.filter_by(id=first_project_code_id).first()
second_branch = ProjectCode.query.filter_by(id=second_project_code_id).first()
return render_template('project/android/project_branch_commit_compare.html',
difference_commits=result,
first_branch=first_branch,
second_branch=second_branch
)
@project.route('/set_project_branch_commit_merge_commit', methods=['POST'])
@login_required
def set_project_branch_commit_merge_commit():
"""
将commit标识为合并提交
:return:
"""
first_project_code_id = request.form.get('first_project_code')
second_project_code_id = request.form.get('second_project_code')
commit_id = request.form.get('commit_id')
option = request.form.get('option')
if option == 'on':
merge_commit = ProjectBranchMergeCommit()
merge_commit.commit_id = commit_id
merge_commit.dest_project_code_id = first_project_code_id
merge_commit.src_project_code_id = second_project_code_id
merge_commit.meger_author_email = current_user.email
db.session.add(merge_commit)
db.session.commit()
else:
merge_commit = ProjectBranchMergeCommit.query.filter(
db.and_(ProjectBranchMergeCommit.commit_id == commit_id,
ProjectBranchMergeCommit.dest_project_code_id == first_project_code_id,
ProjectBranchMergeCommit.src_project_code_id == second_project_code_id)).first()
if merge_commit:
db.session.delete(merge_commit)
db.session.commit()
return '{result: ture}'
@project.route('/jira_task_export_excel', methods=['POST'])
@login_required
def jira_task_export_excel():
"""
jira task 导出成Excel
:return:
"""
task_ids = request.form.get('task_ids')
project_tasks = ProjectBranchTask.query.filter(ProjectBranchTask.id.in_(task_ids.split(','))).all()
excel_option = ExcelOptions(os.path.join(current_app.config['TMS_TEMP_DIR'], "%s_jira.xls" % current_user.username))
excel_option.export_task_xls(project_tasks)
return excel_option.file_path
@project.route('/commit_export_excel', methods=['POST'])
@login_required
def commit_export_excel():
"""
jira task 导出成Excel
:return:
"""
commit_ids = request.form.get('commit_ids')
commits = ProjectBranchCommit.query.filter(ProjectBranchCommit.id.in_(commit_ids.split(','))).all()
excel_option = ExcelOptions(os.path.join(current_app.config['TMS_TEMP_DIR'], "%s_commits.xls" % current_user.username))
excel_option.export_commit_xls(commits)
return excel_option.file_path<file_sep>var project_table = $('#project_table').DataTable({
"language": {
"aria": {
"sortAscending": ": activate to sort column ascending",
"sortDescending": ": activate to sort column descending"
},
"emptyTable": "No data available in table",
"info": "Showing _START_ to _END_ of _TOTAL_ records",
"infoEmpty": "No records found",
"infoFiltered": "(filtered1 from _MAX_ total records)",
"lengthMenu": "Show _MENU_ records",
"search": "Search:",
"zeroRecords": "No matching records found",
"paginate": {
"previous": "Prev",
"next": "Next",
"last": "Last",
"first": "First"
}
},
"bFilter": true,
"lengthMenu": [
[10, 50, 200],
[10, 50, 200] // change per page values here
],
// set the initial value
"pageLength": 10,
"pagingType": "bootstrap_full_number"
});
// 关注项目
function start_project(project_id, start_option){
$.getJSON(
$SCRIPT_ROOT + "/developer/start_project",
{'project_id': project_id, 'start_option': start_option},
function(){
if(start_option == 1) {
$("#as"+project_id).hide();
$("#ac"+project_id).show();
}else{
$("#as"+project_id).show();
$("#ac"+project_id).hide();
}
}
);
}
//Android项目关注、取消
function start_android_project(project_id, start_option){
$.getJSON(
$SCRIPT_ROOT + "/project/start_project",
{'project_id': project_id, 'start_option': start_option},
function(){
if(start_option == 1) {
$("#as"+project_id).hide();
$("#ac"+project_id).show();
}else{
$("#as"+project_id).show();
$("#ac"+project_id).hide();
}
}
);
}
jQuery(document).ready(function() {
$('#project_table tbody').on( 'dblclick', 'td', function () {
var tdSeq = $(this).parent().find("td").index($(this)[0]);
if(tdSeq == 8) {
return;
}else {
$(this).toggleClass('selected');
if ($(this).parent().attr('type')){
window.location.href = $SCRIPT_ROOT + '/project/edit_android_project_page/' + $(this).parent().attr('data');
}else{
window.location.href = $SCRIPT_ROOT + '/developer/edit_project/' + $(this).parent().attr('data');
}
}
});
$("#project_table tr").live({
mouseover:function(){
$(this).css("cursor","pointer");
}
});
});<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""
将指定平台下所有的
Git仓库的分支、tag及commit信息导入到数据库中
"""
import sys
import os
from optparse import OptionParser
from GitRepository import GitRepository
from DBOption import DBOption
reload(sys)
sys.setdefaultencoding('utf-8')
def platform_repo(platform_path):
"""
获取平台目录下所有的git仓库
"""
repos = []
for root_path, dirs, files in os.walk(platform_path):
for dir_name in dirs:
if dir_name == 'repo.git' or dir_name == '.repo' or dir_name == '.git' or root_path.find('/.repo/') > 0:
continue
elif dir_name.endswith('.git'):
print os.path.join(root_path, dir_name)
repos.append(os.path.join(root_path, dir_name))
return repos
def add_refs(db_option, repo, repository_id):
"""
将repo中的分支和tag添加到数据库中
"""
insert_data = []
for head_name in repo.heads:
insert_data.append((head_name, 1, repository_id))
for tag_name in repo.tags:
print 'tag_name:%s' % tag_name
insert_data.append((tag_name, 0, repository_id))
insert_refs_sql = "INSERT INTO repo_refs (name, category, repository_id) VALUES (%s, %s, %s)"
db_option.insert_all(insert_refs_sql, insert_data)
def add_commits(db_option, repo, repository_id):
"""
将repo中的分支和tag添加到数据库中
"""
insert_data = []
for commit in repo.log():
print commit
# 仓库的第一个提交没有parent
if len(commit) == 9:
insert_data.append((commit[0], '', commit[1], commit[2], commit[3], commit[4], commit[5], commit[6], commit[7], commit[8], repository_id))
else:
insert_data.append((commit[0], commit[1], commit[2], commit[3], commit[4], commit[5], commit[6], commit[7], commit[8], commit[9], repository_id))
insert_commit_sql = "INSERT INTO repo_commit (commit_hash, parent_hash, tree_hash, author_name, author_email, " \
"author_time, committer_name, committer_email, commit_time, message, repository_id) " \
"VALUES (%s, %s, %s, %s, %s, %s, %s, %s, %s, %s, %s)"
db_option.insert_all(insert_commit_sql, insert_data)
def main():
des = u'import Repository info'
prog= u'AddRepository'
ver = u'%prog v0.0.1'
usage = u'%prog ip_address platform_path'
parse = OptionParser(description=des, prog=prog, version=ver, usage=usage, add_help_option=True)
parse.add_option('-t', '--server-ip', action='store', type='string', dest='ip_address',
help='repository server ip address')
parse.add_option('-p', '--platform-path', action='store', type='string', dest='platform_path',
help='repository platform name')
option, args = parse.parse_args()
ip_address = option.ip_address
platform_path = option.platform_path
server_sql = "SELECT id FROM repo_server WHERE ip_address='%s'" % ip_address
# mysql
db_url = {'host': '192.168.33.7', 'db_name': 'tms', 'username': 'tup', 'password': 'tup'}
db_option = DBOption(db_url, 'mysql')
db_option.db_connect()
server = db_option.fetch_one(server_sql)
server_id = server[0]
platform_sql = "SELECT id FROM repo_platform WHERE server_id=%s and path='%s'" % (server_id, platform_path)
print "platform_sql:%s" % platform_sql
platform = db_option.fetch_one(platform_sql)
if platform:
platform_id = platform[0]
else:
platform_insert_sql = "INSERT INTO repo_platform (name, path, server_id) VALUES ('%s', '%s', %s)" % \
(os.path.basename(platform_path), platform_path, server_id)
print "platform_insert_sql:%s" % platform_insert_sql
db_option.insert_one(platform_insert_sql)
platform_id = db_option.fetch_one(platform_sql)
for repo_path in platform_repo(platform_path):
# 获取仓库数据库的id或添加仓库入数据库
repo_sql = "SELECT id FROM repo_repo WHERE path='%s' and platform_id=%s" % (repo_path, platform_id)
print "repo_sql:%s" % repo_sql
repo_obj = db_option.fetch_one(repo_sql)
if repo_obj:
repo_id = repo_obj[0]
else:
insert_repo = "INSERT INTO repo_repo (name, path, platform_id) VALUE ('%s', '%s', %s)" % \
(os.path.basename(repo_path).split('.git')[0], repo_path, platform_id)
print "insert_repo:%s" % insert_repo
repo_id = db_option.insert_one(insert_repo)
# 初始化Git仓库操作对象
repo = GitRepository(repo_path)
# 添加分支或tag入数据库
add_refs(db_option, repo, repo_id)
# 添加commit记录入数据库
add_commits(db_option, repo, repo_id)
if __name__ == '__main__':
from time import time
start = time()
main()
print 'Total Time:%s' % str(time()-start)
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""Excel文件读写操作"""
import os
import xlwt
import json
from flask import current_app
import commands
from tms.project.androidProjectModules import ProjectAndroid
class ExcelOptions:
def __init__(self, file_path):
self.file_path = file_path
dir_path = os.path.dirname(self.file_path)
if not os.path.exists(dir_path):
os.makedirs(dir_path)
def export_task_xls(self, records):
"""导出项目JIRA任务"""
wb = xlwt.Workbook(encoding="utf8")
ws = wb.add_sheet(u"项目已提交代码的JIRA任务")
row = 0
ws.write(row, 0, 'JIRA_KEY')
ws.write(row, 1, 'Title')
ws.write(row, 2, 'Description')
ws.write(row, 3, 'Category')
for record in records:
row += 1
ws.write(row, 0, record.task_key)
ws.write(row, 1, record.summary)
ws.write(row, 2, record.description)
ws.write(row, 3, record.category)
wb.save(self.file_path)
def export_commit_xls(self, records):
"""导出项目提交记录"""
wb = xlwt.Workbook(encoding="utf8")
ws = wb.add_sheet(u"项目Commit")
row = 0
ws.write(row, 0, 'Repo')
ws.write(row, 1, 'Message')
ws.write(row, 2, 'Email')
ws.write(row, 3, 'Name')
ws.write(row, 4, 'Date')
ws.write(row, 5, 'ID')
for record in records:
row += 1
ws.write(row, 0, record.repo_path)
ws.write(row, 1, record.message)
ws.write(row, 2, record.commit_email)
ws.write(row, 3, record.commit_name)
ws.write(row, 4, record.commit_date)
ws.write(row, 5, record.commit_id)
wb.save(self.file_path)
def export_jira_xls(self,jira_datas):
"""导出项目Jira统计数据"""
temp=current_app.config['TMS_TEMP_DIR']
if not os.path.isdir(temp+'/jira'):
os.makedirs(os.path.join(str(commands.getoutput('pwd')), temp+'/jira'))
os.chdir(os.path.join(str(commands.getoutput('pwd')), temp+'/jira'))
wb = xlwt.Workbook(encoding="utf8")
jira_datas = json.loads(jira_datas)
ws = wb.add_sheet('total_tasks')
row = 0
ws.write(row, 0, 'status')
ws.write(row, 1, 'total_num')
for data in jira_datas['total_tasks']:
row += 1
ws.write(row, 0, data['status'])
ws.write(row, 1, data['total_num'])
# ws = wb.add_sheet('tasks_daily_update')
# row = 0
# ws.write(row, 0, 'date')
# ws.write(row, 1, 'new_open')
# ws.write(row, 2, 'closed')
# for data in jira_datas['tasks_daily_update']:
# row += 1
# ws.write(row, 0, data['date'])
# ws.write(row, 1, data['new_open'])
# ws.write(row, 2, data['closed'])
ws = wb.add_sheet('tasks_daily_summary')
row = 0
ws.write(row, 0, 'date')
ws.write(row, 1, 'open_summary')
ws.write(row, 2, 'closed_summary')
for data in jira_datas['tasks_daily_summary']:
row += 1
ws.write(row, 0, data['date'])
ws.write(row, 1, data['open_summary'])
ws.write(row, 2, data['closed_summary'])
ws = wb.add_sheet('daily_tasks_tester_open')
row = 0
ws.write(row, 0, 'creator')
ws.write(row, 1, 'creatorNum')
for data in jira_datas['daily_tasks_tester_open']:
row += 1
ws.write(row, 0, data['creator'])
ws.write(row, 1, data['creatorNum'])
ws = wb.add_sheet('daily_tasks_developer_closed')
row = 0
ws.write(row, 0, 'assigner')
ws.write(row, 1, 'assignerNum')
for data in jira_datas['daily_tasks_developer_closed']:
row += 1
ws.write(row, 0, data['assigner'])
ws.write(row, 1, data['assignerNum'])
ws = wb.add_sheet('total_tasks_tester_open')
row = 0
ws.write(row, 0, 'creator')
ws.write(row, 1, 'creatorNum')
for data in jira_datas['total_tasks_tester_open']:
row += 1
ws.write(row, 0, data['creator'])
ws.write(row, 1, data['creatorNum'])
ws = wb.add_sheet('total_tasks_developer_closed')
row = 0
ws.write(row, 0, 'assigner')
ws.write(row, 1, 'assignerNum')
for data in jira_datas['total_tasks_developer_closed']:
row += 1
ws.write(row, 0, data['assigner'])
ws.write(row, 1, data['assignerNum'])
wb.save(self.file_path)
def export_common_issue_xls(self, records):
"""导出项目提交记录"""
wb = xlwt.Workbook(encoding="utf8")
ws = wb.add_sheet(u"项目共性问题")
row = 0
ws.write(row, 0, '问题项目名称')
ws.write(row, 1, '平台名称')
ws.write(row, 2, '基线名称')
ws.write(row, 4, 'SPM')
ws.write(row, 5, '原问题JIRA_KEY')
ws.write(row, 6, '问题类别')
ws.write(row, 7, '问题来源')
ws.write(row, 8, '影响项目')
ws.write(row, 9, '问题名称')
ws.write(row, 10, '问题描述')
for record in records:
row += 1
effect_project_list = ProjectAndroid.query.filter(ProjectAndroid.id.in_([tmp for tmp in
record.effect_scope.split(',') if
tmp.strip()])).all()
effect_project = []
for tmp in effect_project_list:
if tmp.parent:
effect_project.append('%s_%s_%s' % (tmp.parent.name, tmp.android_version, tmp.name))
else:
effect_project.append('%s_%s' % (tmp.android_version, tmp.name))
project_obj = record.project
ws.write(row, 0, project_obj.name)
ws.write(row, 1, record.platform_name)
ws.write(row, 2, record.baseline_name)
ws.write(row, 3, record.spm.display_name)
ws.write(row, 4, record.jira_key)
ws.write(row, 5, record.category)
ws.write(row, 6, record.issue_from)
ws.write(row, 7, ','.join(effect_project))
ws.write(row, 8, record.name)
ws.write(row, 9, record.description)
wb.save(self.file_path)
<file_sep>Flask==0.10.1 # 基础框架jinja2/werkzeug
Flask-Script==0.6.6 # 执行脚本
Flask-WTF==0.9.4 # Form 验证
Flask-HTTPAuth==2.2.0
Flask-Login==0.3.1 # 登陆
Flask-SQLAlchemy==1.0 # sqlalchemy orm
Flask-Migrate==1.1.0 # model版本管理
Flask-Mail==0.9.0 # 邮件
Flask-Moment==0.2.1 #
Flask-SSLify # https 协议
Flask-excel #Excel 操作
#pandas # 解析从JIRA中导出的Excel
#html5lib
#BeautifulSoup4
ForgeryPy #
itsdangerous #
paramiko # ssh 连接
mysql-python # mysql连接
coverage #
simplejson
xlwt
pyexcel-xls # xls读库
pyexcel-xlsx # xlsx读库
jira #jira连接库
uwsgi
#celery # 任务异步处理队列
#redis # key-value 数据库
<file_sep>#!/usr/bin/python
# -*- coding:utf-8 -*-
import os.path
from ftplib import FTP
class MyFTP:
def __init__(self, host, username, password, post=21, buf_size=1024):
# 设置缓冲块大小
self.buf_size = buf_size
self.ftp = FTP()
# 打开调试级别2,显示详细信息;0为关闭调试信息
self.ftp.set_debuglevel(2)
# 连接
self.ftp.connect(host, post)
# 登录,如果匿名登录则用空串代替即可
self.ftp.login(username, password)
# print ftp.getwelcome()#显示ftp服务器欢迎信息
# ftp.cwd('xxx/xxx/') #选择操作目录
def ftp_up(self, file_path):
"""上传文件"""
# 以读模式在本地打开文件
file_handler = open(file_path, 'rb')
# 上传文件
self.ftp.storbinary('STOR %s' % os.path.basename(file_path), file_handler, self.buf_size)
self.ftp.set_debuglevel(0)
file_handler.close()
def ftp_down(self, local_path, ftp_path):
"""文件下载"""
file_name = os.path.basename(ftp_path)
# 以写模式在本地打开文件
file_handler = open(os.path.join(local_path, file_name), 'wb').write
# 接收服务器上文件并写入本地文件
self.ftp.retrbinary('RETR %s' % ftp_path, file_handler, self.buf_size)
self.ftp.set_debuglevel(0)
def quit(self):
self.ftp.quit()
print "ftp OK"
if __name__ == '__main__':
my_ftp = MyFTP('192.168.32.83', 'jenkins', 'jenkins')
my_ftp.ftp_down('/home/roy/tmp', '/应用开发/ApeAssistivePoint/基于8400小白点v4.rar')
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
from flask import Blueprint
department = Blueprint('department', __name__)
from . import views
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
def convert_to_dict(obj):
"""object对象转换成Dict对象"""
obj_dict = {}
obj_dict.update(obj.__dict__)
return obj_dict
def convert_to_dicts(objs):
"""把对象列表转换成字典列表"""
obj_arr = []
for o in objs:
obj_dict = {}
obj_dict.update(o.__dict__)
obj_arr.append(obj_dict)
return obj_arr
def class_to_dict(obj):
"""把对象(单个对象、list、set)转出字典"""
is_list = obj.__class__ == [].__class__
is_set = obj.__class__ == set().__class__
if is_list or is_set:
obj_arr = []
for o in obj:
obj_dict = {}
obj_dict.update(o.__dict__)
obj_arr.append(obj_dict)
return obj_arr
else:
obj_dict = {}
obj_dict.update(obj.__dict__)
return obj_dict
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
from flask import render_template, redirect, url_for, request, current_app
from flask.ext.login import login_required, current_user
from . import main
@main.route('/', methods=['GET', 'POST'])
@login_required
def index():
"""按不同的角色定位到不同的"""
return redirect(url_for(current_user.role.index_page))
<file_sep># -*- coding:utf-8 -*-
__author__ = 'android'
import json
from flask import render_template, redirect, url_for, request, flash, current_app, session, send_file
from flask.ext.login import login_user, logout_user, login_required, current_user
from flask.ext.mail import Mail, Message
from functools import update_wrapper
from datetime import datetime, timedelta
from .import report
from tms import db, mail
from tms.auth.models import User
from tms.project.androidProjectModules import *
from tms.auth.models import SystemParameter
from tms.baseline.models import BPlatform
from tms.report.models import project_task_type,project_task_list
import xlwt
import os
from simplejson import loads as json_loads, dumps as json_dumps
import commands
import flask_excel as excel
from tms.auth.models import User
import shutil
@report.route('/task_type_page')
@login_required
def task_type_page():
"""查询所有任务类型"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '我的任务', 'url': url_for('report.my_task_page')})
session['menu_path'] = ['周报日报', '类型管理']
project_task_types = project_task_type.query.all()
return render_template('report/task_type_page.html',project_task_types=project_task_types)
@report.route('/week_task_page')
@login_required
def week_task_page():
"""周报,查询当天前7天的任务"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '我的任务', 'url': url_for('report.my_task_page')})
session['menu_path'] = ['周报日报', '我的周报']
d = datetime.now()
d1 = d + timedelta(days=-7)
user_id=session['user']["id"]
project_task_lists=project_task_list.query.filter(db.and_(project_task_list.starttime>=d1),(project_task_list.starttime<=d),(project_task_list.tms_user==user_id)).order_by(project_task_list.id.desc()).all()
time=datetime.now()
for project_task_list1 in project_task_lists:
project_task_list1.state=(time-project_task_list1.updatetime).days
return render_template('report/week_task_page.html',project_task_lists=project_task_lists)
@report.route('/month_task_page')
@login_required
def month_task_page():
"""月报,查询当前月的任务"""
user_id=session['user']["id"]
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '我的任务', 'url': url_for('report.my_task_page')})
session['menu_path'] = ['周报日报', '我的月报']
i =datetime.now()
month=str(i.month)
minmonth=str(i.year)+"-"+str(i.month)+"-01"
maxmonth=str(i.year)+"-"+str(i.month)+"-31"
project_task_lists = project_task_list.query.filter(db.and_(project_task_list.starttime>=minmonth),(project_task_list.starttime<=maxmonth),(project_task_list.tms_user==user_id)).order_by(project_task_list.id.desc()).all()
time=datetime.now()
for project_task_list1 in project_task_lists:
project_task_list1.state=(time-project_task_list1.updatetime).days
# db.session.add(project_task_list1)
# db.session.commit()
return render_template('report/month_task_page.html',project_task_lists=project_task_lists,month=month)
@report.route('/all_task_page',methods=['GET', 'POST'])
@login_required
def all_task_page():
"""查询所有任务"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '我的任务', 'url': url_for('report.my_task_page')})
session['menu_path'] = ['周报日报', '全部任务']
users=get_users()
types=get_types()
project_task_lists = project_task_list.query.order_by(project_task_list.id.desc()).all()
time=datetime.now()
for project_task_list1 in project_task_lists:
project_task_list1.state=(time-project_task_list1.updatetime).days
return render_template('report/all_task_page.html',project_task_lists=project_task_lists,querytype=0,users=users,types=types)
@report.route('/preadd_task_type',methods=['GET', 'POST'])
@login_required
def preadd_task_type():
"""准备添加任务类型,实现页面跳转"""
return render_template('report/add_task_type.html')
@report.route('/add_task_type',methods=['GET', 'POST'])
@login_required
def add_task_type():
"""添加任务类型"""
task_type=project_task_type()
task_type.task_type=request.form.get('task_type')
task_type.state=request.form.get('state')
db.session.add(task_type)
db.session.commit()
project_task_types = project_task_type.query.all()
return render_template('report/task_type_page.html',project_task_types=project_task_types)
@report.route('/query_all_task_list',methods=['GET', 'POST'])
@login_required
def query_all_task_list():
"""全部任务,根据人物,类型,状态,时间 模糊查询"""
starttime=request.form.get('starttime').strip()
endtime=request.form.get('endtime').strip()
task_type=request.form.get('task_type').strip()
the_user=request.form.get('the_user').strip()
state=request.form.get('state').strip()
# print starttime+endtime+task_type+the_user+state
users=get_users()
types=get_types()
search_sql = " SELECT t.id,ptt.task_type,t.taskname,t.starttime,t.updatetime,t.question_log,t.SCHEDULE schedules,t.state,tu.display_name " \
"FROM project_task_list t ,project_task_type ptt,tms_user tu WHERE t.task_type = ptt.id AND t.tms_user = tu.id"
if(starttime!="" and endtime!=""):
search_sql+=" AND t.starttime >="+"'"+starttime+"'"+"AND t.starttime<="+"'"+endtime+"'"
if(task_type!='0' and task_type!=""):
search_sql+=" AND ptt.id ="+task_type
if(the_user!='0' and the_user!=""):
search_sql+=" AND tu.id="+the_user
if(state!='0' and state!=""):
if(state=='1'):
search_sql+=" AND t.state<=3 AND t.SCHEDULE!=100"
elif(state=='2'):
search_sql+=" AND t.state>3 AND t.SCHEDULE!=100"
else:
search_sql+=" AND t.schedule=100"
search_sql+=' ORDER BY t.id desc '
project_task_lists = db.session.execute(search_sql).fetchall()
return render_template('report/all_task_page.html',project_task_lists=project_task_lists,starttime=starttime,endtime=endtime,task_type=task_type,querytype=1,the_user=the_user,state=state,users=users,types=types)
@report.route('/query_week_task_list',methods=['GET', 'POST'])
@login_required
def query_week_task_list():
"""周报按照时间模糊查询"""
starttime=request.form.get('starttime','').strip()
endtime=request.form.get('endtime','').strip()
user_id=session['user']["id"]
d = datetime.now()
d1 = d + timedelta(days=-7)
if(starttime =='' or endtime==''):
project_task_lists=project_task_list.query.filter(db.and_(project_task_list.starttime>=d1),(project_task_list.starttime<=d),(project_task_list.tms_user==user_id)).order_by(project_task_list.id.desc()).all()
return render_template('report/week_task_page.html',project_task_lists=project_task_lists,starttime1=starttime,endtime1=endtime)
else:
project_task_lists = project_task_list.query.filter(db.and_(project_task_list.starttime>=starttime),(project_task_list.starttime<=endtime),(project_task_list.tms_user==user_id)).order_by(project_task_list.id.desc()).all()
return render_template('report/week_task_page.html',project_task_lists=project_task_lists,starttime1=starttime,endtime1=endtime)
@report.route('/query_month_task_list',methods=['GET', 'POST'])
@login_required
def query_month_task_list():
"""按照月模糊查询"""
select_month=request.form.get('select_month').strip()
i =datetime.now()
minmonth=str(i.year)+"-"+str(select_month)+"-01"
maxmonth=str(i.year)+"-"+str(select_month)+"-31"
user_id=session['user']["id"]
month=select_month;
project_task_lists = project_task_list.query.filter(db.and_(project_task_list.starttime>=minmonth),(project_task_list.starttime<=maxmonth),(project_task_list.tms_user==user_id)).order_by(project_task_list.id.desc()).all()
return render_template('report/month_task_page.html',project_task_lists=project_task_lists,month=month)
@report.route('/pre_update_my_task',methods=['GET', 'POST'])
@login_required
def pre_update_my_task():
"""获取要更新的任务"""
id1=request.form.get('id1')
state1=request.form.get('state1')
project_task_list1=project_task_list.query.filter(project_task_list.id == id1).first()
return render_template('report/update_task_page.html',project_task_list1=project_task_list1,state1=state1)
@report.route('/update_task_type',methods=['GET', 'POST'])
@login_required
def update_task_type():
"""修改类型"""
new_array = request.form['new_array']
array = str(new_array).split(";")
for arr in array :
if arr:
arr_array = str(arr).split(',')
id = arr_array[0]
id = str(id).replace('id_state_','')
state = arr_array[1]
task_type = project_task_type.query.filter(project_task_type.id == id).first()
task_type.state = state
db.session.add(task_type)
db.session.commit()
project_task_types = project_task_type.query.all()
return render_template('report/task_type_page.html',project_task_types=project_task_types)
@report.route('/update_my_task',methods=['GET', 'POST'])
@login_required
def update_my_task():
"""更新任务"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '我的任务', 'url': url_for('report.my_task_page')})
session['menu_path'] = ['周报日报', '我的任务']
id=request.form.get('id')
task_schedule=request.form.get('tdescribe')
question_log=request.form.get('question_log')
user_id=session['user']["id"]
my_task = project_task_list.query.filter(project_task_list.id == id).first()
my_task.schedule=task_schedule
my_task.question_log=question_log
my_task.updatetime=datetime.now()
db.session.add(my_task)
db.session.commit()
project_task_lists = project_task_list.query.filter(db.and_(project_task_list.tms_user==user_id),(project_task_list.schedule!=100)).all()
return render_template('report/my_task_page.html',project_task_lists=project_task_lists,state=2)
@report.route('/my_task_page')
@login_required
def my_task_page():
"""查询我的任务"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '我的任务', 'url': url_for('report.my_task_page')})
session['menu_path'] = ['周报日报', '我的任务']
time=datetime.now()
user_id=session['user']["id"]
project_task_lists = project_task_list.query.filter(db.and_(project_task_list.tms_user==user_id),(project_task_list.schedule!=100)).order_by(project_task_list.id.desc()).all()
for project_task_list1 in project_task_lists:
if(project_task_list1.state!=(time-project_task_list1.updatetime).days):
project_task_list1.state=(time-project_task_list1.updatetime).days
db.session.add(project_task_list1)
db.session.commit()
return render_template('report/my_task_page.html',project_task_lists=project_task_lists)
@report.route('/add_task_page',methods=['GET', 'POST'])
@login_required
def add_task_page():
"""增加任务"""
time=datetime.now()
id=session['user']["id"]
new_task =project_task_list()
new_task.task_type=request.form.get('task_type','').strip()
new_task.taskname=request.form.get('taskname','').strip()
new_task.schedule=request.form.get('schedule','').strip()
new_task.tdescribe=request.form.get('tdescribe','').strip()
new_task.question_log=request.form.get('question_log')
new_task.updatetime=time
new_task.starttime=time
new_task.state=(new_task.updatetime)-(new_task.starttime)
new_task.tms_user=id
db.session.add(new_task)
db.session.commit()
user_id=session['user']["id"]
project_task_lists = project_task_list.query.filter(db.and_(project_task_list.tms_user==user_id),(project_task_list.schedule!=100)).all()
return render_template('report/my_task_page.html',project_task_lists=project_task_lists,state=1)
@report.route('/preadd_task_page')
@login_required
def preadd_task_page():
"""准备增加任务需要的数据"""
project_task_types = project_task_type.query.filter(project_task_type.state==1).all()
return render_template('report/add_task_page.html',project_task_types=project_task_types)
def get_users():
"""获取登录用户所在组的成员"""
list = []
user_role_id=session['user']["role_id"]
users=User.query.filter(User.role_id==user_role_id)
for user in users:
list.append({'id': user.id, 'display_name': user.display_name})
return list
def get_types():
"""获取任务类型"""
types = []
obj1 = project_task_type.query.all()
for obj in obj1:
types.append({'id': obj.id, 'task_type': obj.task_type})
return types
@report.route('/download_week_list',methods=['GET', 'POST'])
@login_required
def download_week_list():
"""将周报的数据导出到Excle"""
starttime=request.form['starttime']
endtime=request.form['endtime']
user_id=session['user']["id"]
d = datetime.now()
d1 = d + timedelta(days=-7)
workbook=xlwt.Workbook(encoding='utf-8')
booksheet=workbook.add_sheet('Sheet 1', cell_overwrite_ok=True)
booksheet.col(1).width=400*20
booksheet.col(2).width=300*20
booksheet.col(3).width=400*20
booksheet.col(6).width=500*20
booksheet.col(7).width=500*20
if(starttime =='' or endtime==''):
project_task_lists=project_task_list.query.filter(db.and_(project_task_list.starttime>=d1),(project_task_list.starttime<=d),(project_task_list.tms_user==user_id))
else:
project_task_lists = project_task_list.query.filter(db.and_(project_task_list.starttime>=starttime),(project_task_list.starttime<=endtime),(project_task_list.tms_user==user_id)).all()
DATA=[('年份','开始时间','任务类型','任务名称','任务进度','任务人','描述','问题记录'),]
for list in project_task_lists:
DATA.append((str(d.year),str(list.starttime),str(list.type_1.task_type),list.taskname,str(list.schedule)+'%',list.user1.display_name,list.tdescribe,list.question_log))
for i,row in enumerate(DATA):
for j,col in enumerate(row):
booksheet.write(i,j,col)
user_name=User.query.filter(User.id==user_id).first().username
excel_name='my_task'+str(user_id)+'.xls'
workbook.save(excel_name)
file_name=current_app.config['TMS_TEMP_DIR']
basefile= str(commands.getoutput('pwd'))
if os.path.isdir(file_name):
pass
else:
os.mkdir(os.path.join(basefile, 'temp'))
if os.path.isdir(file_name+"/"+user_name):
pass
else:
os.mkdir(os.path.join(file_name, user_name))
if os.path.isfile(file_name+"/"+user_name+"/"+excel_name):
os.remove(file_name+"/"+user_name+"/"+excel_name)
shutil.move(basefile+"/"+excel_name,file_name+"/"+user_name+"/")
else:
shutil.move(basefile+"/"+excel_name,file_name+"/"+user_name+"/")
return file_name+'/'+user_name+'/'+excel_name
@report.route('/download_month_list',methods=['GET', 'POST'])
@login_required
def download_month_list():
"""将月报的数据导出到Excle"""
select_month=request.form['select_month']
user_id=session['user']["id"]
d = datetime.now()
minmonth=str(d.year)+"-"+str(select_month)+"-01"
maxmonth=str(d.year)+"-"+str(select_month)+"-31"
user_id=session['user']["id"]
project_task_lists = project_task_list.query.filter(db.and_(project_task_list.starttime>=minmonth),(project_task_list.starttime<=maxmonth),(project_task_list.tms_user==user_id))
workbook=xlwt.Workbook(encoding='utf-8')
booksheet=workbook.add_sheet('Sheet 1', cell_overwrite_ok=True)
booksheet.col(1).width=400*20
booksheet.col(2).width=300*20
booksheet.col(3).width=400*20
booksheet.col(6).width=500*20
booksheet.col(7).width=500*20
DATA=[('年份','开始时间','任务类型','任务名称','任务进度','任务人','描述','问题记录'),]
for list in project_task_lists:
DATA.append((str(d.year),str(list.starttime),str(list.type_1.task_type),list.taskname,str(list.schedule)+'%',list.user1.display_name,list.tdescribe,list.question_log))
for i,row in enumerate(DATA):
for j,col in enumerate(row):
booksheet.write(i,j,col)
user_name=User.query.filter(User.id==user_id).first().username
excel_name='my_task'+str(user_id)+'.xls'
workbook.save(excel_name)
file_name=current_app.config['TMS_TEMP_DIR']
basefile= str(commands.getoutput('pwd'))
if os.path.isdir(file_name):
pass
else:
os.mkdir(os.path.join(basefile, 'temp'))
if os.path.isdir(file_name+"/"+user_name):
pass
else:
os.mkdir(os.path.join(file_name, user_name))
if os.path.isfile(file_name+"/"+user_name+"/"+excel_name):
os.remove(file_name+"/"+user_name+"/"+excel_name)
shutil.move(basefile+"/"+excel_name,file_name+"/"+user_name+"/")
else:
shutil.move(basefile+"/"+excel_name,file_name+"/"+user_name+"/")
return file_name+'/'+user_name+'/'+excel_name
@report.route('/download_alltask_list',methods=['GET', 'POST'])
@login_required
def download_alltask_list():
"""将全部任务的数据导出到Excle"""
starttime=request.form['starttime']
endtime=request.form['endtime']
task_type=request.form['task_type']
the_user=request.form['the_user']
state=request.form['state']
user_id=session['user']["id"]
d = datetime.now()
d1 = d + timedelta(days=-7)
workbook=xlwt.Workbook(encoding='utf-8')
booksheet=workbook.add_sheet('Sheet 1', cell_overwrite_ok=True)
booksheet.col(1).width=400*20
booksheet.col(2).width=300*20
booksheet.col(3).width=400*20
booksheet.col(6).width=500*20
booksheet.col(7).width=500*20
search_sql = " SELECT t.id,ptt.task_type,t.taskname,t.starttime,t.updatetime,t.question_log,t.tdescribe,t.SCHEDULE schedules,t.state,tu.display_name " \
"FROM project_task_list t ,project_task_type ptt,tms_user tu WHERE t.task_type = ptt.id AND t.tms_user = tu.id"
if(starttime!="" and endtime!=""):
search_sql+=" AND t.starttime >="+"'"+starttime+"'"+"AND t.starttime<="+"'"+endtime+"'"
if(task_type!='0' and task_type!=""):
search_sql+=" AND ptt.id ="+task_type
if(the_user!='0' and the_user!=""):
search_sql+=" AND tu.id="+the_user
if(state!='0' and state!=""):
if(state=='1'):
search_sql+=" AND t.state<=3 AND t.SCHEDULE!=100"
elif(state=='2'):
search_sql+=" AND t.state>3 AND t.SCHEDULE!=100"
else:
search_sql+=" AND t.schedule=100"
# print search_sql
project_task_lists = db.session.execute(search_sql).fetchall()
DATA=[('年份','开始时间','任务类型','任务名称','任务进度','任务人','描述','问题记录'),]
for list in project_task_lists:
DATA.append((str(d.year),str(list.starttime),str(list.task_type),list.taskname,str(list.schedules)+'%',list.display_name,list.tdescribe,list.question_log))
for i,row in enumerate(DATA):
for j,col in enumerate(row):
booksheet.write(i,j,col)
user_name=User.query.filter(User.id==user_id).first().username
excel_name='my_task'+str(user_id)+'.xls'
workbook.save(excel_name)
file_name=current_app.config['TMS_TEMP_DIR']
basefile= str(commands.getoutput('pwd'))
if os.path.isdir(file_name):
pass
else:
os.mkdir(os.path.join(basefile, 'temp'))
if os.path.isdir(file_name+"/"+user_name):
pass
else:
os.mkdir(os.path.join(file_name, user_name))
if os.path.isfile(file_name+"/"+user_name+"/"+excel_name):
os.remove(file_name+"/"+user_name+"/"+excel_name)
shutil.move(basefile+"/"+excel_name,file_name+"/"+user_name+"/")
else:
shutil.move(basefile+"/"+excel_name,file_name+"/"+user_name+"/")
return file_name+'/'+user_name+'/'+excel_name
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
"""
工程配置
"""
import os
base_dir = os.path.abspath(os.path.dirname(__file__))
class Config:
SECRET_KEY = os.environ.get('SECRET_KEY') or 'tinno flask key'
SSL_DISABLE = False
SQLALCHEMY_COMMIT_ON_TEARDOWN = True
SQLALCHEMY_RECORD_QUERIES = True
SQLALCHEMY_TRACK_MODIFICATIONS = True
# 邮箱信息
MAIL_SERVER = '192.168.10.8'
MAIL_PORT = 25
MAIL_USER_TLS = False
MAIL_USERNAME = '<EMAIL>'
MAIL_PASSWORD = '<PASSWORD>'
TMS_MAIL_SUBJECT_PREFIX = '[TMS]'
TMS_MAIL_SENDER = 'Tinno SCM<<EMAIL>>'
TMS_ADMIN = os.environ.get('TMS_ADMIN') or '<EMAIL>'
# JIRA数据库信息
JIRA_DB_HOST = '172.16.5.95'
JIRA_DB_NAME = 'jiradb6'
JIRA_DB_USER = 'jirauser'
JIRA_DB_PASSWD = '<PASSWORD>'
TMS_POSTS_PER_PAGE = 20
TMS_FOLLOWERS_PRE_PAGE = 50
TMS_COMMENTS_PER_PAGE = 30
TMS_SLOW_DB_QUERY_TIM = 0.5
UPLOAD_FOLDER = os.path.join(base_dir, 'upload')
TMS_TEMP_DIR = os.path.join(base_dir, 'temp')
RELEASE_REPO_FOLDER = os.path.join(base_dir, 'tinno_release')
MIME_TYPE = {
'.xls': 'application/vnd.ms-excel',
'.xlsx': 'application/vnd.openxmlformats-officedocument.spreadsheetml.sheet',
'.txt': 'text/plain',
'.pdf': 'application/pdf',
'.doc': 'application/msword',
'.docx': 'application/vnd.openxmlformats-officedocument.wordprocessingml.document',
'.pptx': 'application/vnd.openxmlformats-officedocument.presentationml.presentation',
'.ppt': 'application/vnd.ms-powerpoint',
'.rar': 'application/rar',
'.zip': 'application/zip',
'.tar': 'application/x-tar',
'.gz': 'application/x-gzip'
}
JIRA_SERVER = 'http://172.16.5.95:8080'
@staticmethod
def init_app(app):
pass
class DevelopmentConfig(Config):
"""开发环境配置"""
DEBUG = True
SQLALCHEMY_DATABASE_URI = os.environ.get('DEV_DATABASE_URL') or \
'mysql://tup:[email protected]:3306/tms?charset=utf8'
@classmethod
def init_app(cls, app):
Config.init_app(app)
# 配置日志文件
import logging
import datetime
# 配置日志文件
formatter = logging.Formatter('%(asctime)s %(name)s[line:%(lineno)d]:%(levelname)s %(message)s')
# 输出到文件
log_file = os.path.join(base_dir, 'logs', datetime.datetime.now().strftime('%Y-%m-%d'), 'tms.log')
if not os.path.exists(os.path.dirname(log_file)):
os.makedirs(os.path.dirname(log_file))
file_handler = logging.FileHandler(log_file)
file_handler.setLevel(logging.DEBUG)
file_handler.setFormatter(formatter)
app.logger.addHandler(file_handler)
# 输出到控制台
console_handler = logging.StreamHandler()
console_handler.setLevel(logging.DEBUG)
console_handler.setFormatter(formatter)
app.logger.addHandler(console_handler)
app.logger.info('Developement')
class ProductionConfig(Config):
"""生产环境"""
DEBUG = False
SQLALCHEMY_DATABASE_URI = os.environ.get('DEV_DATABASE_URL') or 'mysql://tup:[email protected]:3306/tms?charset=utf8'
@classmethod
def init_app(cls, app):
Config.init_app(app)
cls.TMS_TEMP_DIR = '/var/www/tmp'
# 配置日志文件
import logging
import datetime
from logging.handlers import SMTPHandler
logging.basicConfig(
level=logging.DEBUG,
format='%(asctime)s %(name)s[line:%(lineno)d]:%(levelname)s %(message)s',
)
# 输出到文件
log_file = os.path.join(base_dir, 'logs', datetime.datetime.now().strftime('%Y-%m-%d'), 'tms.log')
if not os.path.exists(os.path.dirname(log_file)):
os.makedirs(os.path.dirname(log_file))
file_handler = logging.FileHandler(log_file)
file_handler.setLevel(logging.WARNING)
app.logger.addHandler(file_handler)
# 输出到控制台
console_handler = logging.StreamHandler()
console_handler.setLevel(logging.DEBUG)
app.logger.addHandler(console_handler)
# 将系统错误邮件通知给管理员
credentials = None
secure = None
if getattr(cls, 'MAIL_USERNAME', None) is not None:
credentials = (cls.MAIL_USERNAME, cls.MAIL_PASSWORD)
if getattr(cls, 'MAIL_USE_TLS', None):
secure = ()
mail_handler = SMTPHandler(
mailhost=(cls.MAIL_SERVER, cls.MAIL_PORT),
fromaddr=cls.TMS_MAIL_SENDER,
toaddrs=[cls.TMS_ADMIN],
subject=cls.TMS_MAIL_SUBJECT_PREFIX + 'Application Error',
credentials=credentials,
secure=secure,
)
mail_handler.setLevel(logging.ERROR)
app.logger.addHandler(mail_handler)
config = {
'production': ProductionConfig,
'default': DevelopmentConfig,
}
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
import commands
def update_release_repository(current_user, logger, version):
"""提交发布库"""
# 更新发布库
add_cmd = 'git add .'
result, output = commands.getstatusoutput(add_cmd)
# 非零就是出错了
if result:
return False, u'发布库执行git add .命令出错了\n{0}\n{1}'.format(result, output)
commit_cmd = 'git commit -m "<REQ><SCM-10><release {0} {1} by {2}>"'.format(version.name, version.version_number,
current_user.email)
result, output = commands.getstatusoutput(commit_cmd)
# 非零就是出错了
if result:
logger.info(commit_cmd)
logger.info(output)
return False, u'发布库执行\n{0}命令出错了\n{1}\n{2}'.format(commit_cmd, result, output)
create_tag_cmd = 'git tag {0}'.format(version.version_number)
result, output = commands.getstatusoutput(create_tag_cmd)
# 非零就是出错了
if result:
logger.info(commit_cmd)
logger.info(output)
return False, u'创建版本Tag\n {0} 命令出错了\n{1}\n{2}'.format(create_tag_cmd, result, output)
# push_master = 'git push origin master'
# result, output = commands.getstatusoutput(push_master)
# # 非零就是出错了
# if result:
# logger.info(commit_cmd)
# logger.info(output)
# return False, u'push master 分支命令出错了\n{0}\n{1}'.format(result, output)
#
# push_tag = 'git push origin {0}'.format(version.version_number)
# result, output = commands.getstatusoutput(push_tag)
# # 非零就是出错了
# if result:
# logger.info(commit_cmd)
# logger.info(output)
# return False, u'push 版本tag\n {0} 命令出错了\n{1}\n{2}'.format(push_tag, result, output)
return True, ''
<file_sep>[uwsgi]
socket=127.0.0.1:8001
chdir=/var/www/tms
wsgi-file=manage.py
callable=app
process=1
threads=2
stats=127.0.0.1:8181
buffer-size=55350
logdata=true
limit-as=6048
daemnize=/var/www/tms/logs/tms.log
uwsgi_connect_timeout=75
uwsgi_read_timeout=1200
uwsgi_send_timeout=1200
harakiri=2000
<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
from tms import db
class TestCase(db.Model):
"""测试用例"""
__tablename__ = 'tms_test_case'
id = db.Column(db.Integer, primary_key=True)
# 用例编号
number = db.Column(db.String(64), unique=True, index=True)
# 用例等级
level = db.Column(db.String(20), index=True)
# 测试类型
test_type = db.Column(db.String(64), default='功能')
# 功能模块(对应用例,用项目JIRA_KEY关联)
module_name = db.Column(db.String(64), index=True)
# 用例实现代码类名
class_name = db.Column(db.String(64))
# 方法名
method_name = db.Column(db.String(64))
# 测试功能
test_fun = db.Column(db.String(255))
# test目的
test_target = db.Column(db.String(255))
# 是否用标准SDK开发
standard_sdk = db.Column(db.Boolean, default=True)
# 初始条件
test_init = db.Column(db.String(255))
# 测试步骤
test_step = db.Column(db.String(255))
# 预期结果
expect_result = db.Column(db.String(255))
# 用例状态
activate = db.Column(db.Boolean, default=True)
# 添加人
add_user_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
add_user = db.relationship('User', foreign_keys=[add_user_id], backref=db.backref('case_add_user', order_by=id))
add_time = db.Column(db.DateTime())
# 最新修改人
last_user_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
last_user = db.relationship('User', foreign_keys=[last_user_id], backref=db.backref('last_last_user', order_by=id))
# 最新修改时间
last_update_time = db.Column(db.DateTime())
def __repr__(self):
return '<TestCase %r>' % self.number
class TestCaseLog(db.Model):
"""测试用例修改记录"""
__tablename__ = 'tms_test_case_log'
id = db.Column(db.Integer, primary_key=True)
user_id = db.Column(db.Integer, db.ForeignKey('tms_user.id'))
user = db.relationship('User')
test_case_id = db.Column(db.Integer, db.ForeignKey('tms_test_case.id'))
test_case = db.relationship('TestCase', backref=db.backref('modify_log', order_by=id))
update_time = db.Column(db.DateTime())
content = db.Column(db.String(500))
def __repr__(self):
return '<TestCaseLog %r>' % self.test_case.number
<file_sep>/**
* Created by changjie.fan on 2016/5/6.
*/
var project_myos_app_table = $('#project_myos_app_table').DataTable({
"language": {
"aria": {
"sortAscending": ": activate to sort column ascending",
"sortDescending": ": activate to sort column descending"
},
"emptyTable": "No data available in table",
"info": "Showing _START_ to _END_ of _TOTAL_ records",
"infoEmpty": "No records found",
"infoFiltered": "(filtered1 from _MAX_ total records)",
"lengthMenu": "Show _MENU_ records",
"search": "Search:",
"zeroRecords": "No matching records found",
"paginate": {
"previous": "Prev",
"next": "Next",
"last": "Last",
"first": "First"
}
},
"bFilter": true,
"lengthMenu": [
[10, 50, 200],
[10, 50, 200] // change per page values here
],
// set the initial value
"pageLength": 10,
"pagingType": "bootstrap_full_number"
});
$('#project_myos_app_table tbody').on('dblclick', 'tr', function () {
window.location.href = $SCRIPT_ROOT + '/project/project_myos_app_edit/' + project_id + '/' + $(this).attr('data');
});
// select all
$('#selectAll').on('click', function () {
if ($('#selectAll').parent().attr('class') == 'checked') {
$('input[type="checkbox"]', $('#project_myos_app_table tbody')).each(function () {
$(this).parent().removeClass("checked");
});
} else {
$('input[type="checkbox"]', $('#project_myos_app_table tbody')).each(function () {
$(this).parent().addClass("checked");
});
}
});
// 行选择
$('#project_myos_app_table tbody').on('change', 'input[type="checkbox"]', function () {
if($(this).parent().attr('class') == 'checked'){
$('#selectAll').parent().removeClass("checked");
$(this).parent().removeClass("checked");
} else {
$(this).parent().addClass("checked");
}
});
// 删除项目配置的myos应用
function delete_project_app_config(project_id){
var app_ids = new Array();
$('#project_myos_app_table td span').each(function () {
if ($(this).attr('class') && $(this).attr('class') == 'checked') {
app_ids.push($($($(this).parent()).parent()).parent().attr('data'));
}
});
if (!app_ids || app_ids.length == 0){
bootbox.alert('请先选择要删除的应用');
return;
}
bootbox.confirm("Are you sure?", function (result) {
if(result) {
$.ajax({
url: '/project/project_myos_delete',
type: 'post',
data: {project_id: project_id, app_ids: app_ids.join(',')},
success: function () {
window.location.reload();
}
});
}
});
}<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
from . import department
from flask.ext.login import login_user, logout_user, login_required, current_user
from flask import render_template, redirect, url_for, request, flash, current_app, session, send_file
from tms.project.androidProjectModules import *
from tms.auth.models import User, Role
from tms.auth.models import *
from tms.departmentMan.models import *
import json
DEPARTMENT_DEEP = 6
@department.route('/department_manage')
@login_required
def department_manage():
"""
部门管理
:return:
"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '部门管理', 'url': url_for('department.department_manage')})
session['menu_path'] = ['部门管理', '部门管理']
#一级部门
departments_1_list = Department.query.filter_by(level=1).order_by(Department.id.asc()).all()
result = {}
for department in departments_1_list:
#一级部门
#获取一级部门下面的子节点(部门)
array = search_childs(department)
department.leader_name = get_leader_name(department.leader)
result[department] = array
return render_template('departmentMan/department_manage_page.html', parents=departments_1_list, menus=result)
def search_childs(department1):
"""获取一级部门下面的子节点(部门)"""
#查找二级部门
departments2 = Department.query.filter_by(level=department1.level+1,parent_id=department1.id).order_by(Department.id.asc()).all()
array = []
for department2 in departments2:
dict = {}
dict['id'] = department2.id
dict['name'] = department2.name
dict['activate'] = department2.activate
dict['note'] = department2.note
dict['order'] = department2.order
leader_name = get_leader_name(department2.leader)
dict['leader_name'] = leader_name
dict['childs'] = search_childs(department2)
array.append(dict)
return array
def get_leader_name(leader):
"""获取leader名称"""
if leader:
leader_array = str(leader).split(',')
if len(leader_array) == 0:
return ''
leader_name_array = []
for arr in leader_array:
user = User.query.filter_by(id=arr).first()
leader_name_array.append(user.display_name)
return ','.join(leader_name_array)
else:
return ''
@department.route('/department_activate')
@login_required
def set_department_activate():
department_id = request.args.get('id', 0, type=int)
activate = request.args.get('activate')
if activate == 'Enable':
db.session.execute('update tms_department SET activate = 1 WHERE id in ( SELECT aa.id from ( SELECT id from tms_department t where FIND_IN_SET(id,getChildsByRootid(%s))) aa )' % (department_id))
elif activate == 'Disable':
db.session.execute('update tms_department SET activate = 0 WHERE id in ( SELECT aa.id from ( SELECT id from tms_department t where FIND_IN_SET(id,getChildsByRootid(%s))) aa )' % (department_id))
db.session.commit()
return '{"result": true}'
@department.route('/edit_department/<int:department_id>', methods=['GET', 'POST'])
@login_required
def edit_department(department_id):
"""修改部门"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '编辑部门' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '编辑部门','url': url_for('department.edit_department',
department_id=department_id)})
department = Department.query.filter_by(id=department_id).first()
origin_status = department.activate
if request.method == 'POST':
#更新数据
name = request.form['name']
activate = request.form.get('activate')
note = request.form.get('note')
order = request.form['order']
leader = request.form['leader_ids']
member = request.form['member_ids']
parent_department = request.form.get('parent_department', None)
# parent_id = request.form['parent']
other_department = Department.query.filter_by(name=str(name).strip()).first()
if other_department.id != department.id:
flash(u'同名部门存在', 'danger')
return redirect(url_for('department.department_manage'))
# else:
department.name = name
department.note = note
if activate == 'on':
department.activate = True
else:
department.activate = False
department.order = order
department.leader = leader
# if department.parent == None
#处理
flag,msg = dealwith_department(department.parent,parent_department,department)
if not flag:
flash(msg, 'danger')
return redirect(url_for('department.department_manage'))
if parent_department:
par_department = Department.query.filter_by(id=parent_department).first()
department.level = par_department.level + 1
department.parent_id = parent_department
else:
department.level = 1
department.parent_id = None
#处理状态
dealwith_status(origin_status,department)
db.session.add(department)
db.session.commit()
#修改成员所在的部门
before_users = User.query.filter_by(department_id=department.id).all()
for before_user in before_users:
before_user.department_id = None
db.session.add(before_user)
db.session.commit()
#修改成员所在的部门信息
if member:
member_array = str(member).split(',')
for arr in member_array:
if arr :
user = User.query.filter_by(id=arr).first()
user.department_id = department.id
db.session.add(user)
db.session.commit()
flash(u'修改成功!', 'info')
return redirect(url_for('department.department_manage'))
else:
#get请求-->展示数据
# department_list = []
#
# selected_department_dict = {}
#
# #当前部门所在的level
# current_level = department.level
#
# top_department = getParent(department)
#
# if current_level == 1:
# """"""
#
# #一级部门
# # top_departments = Department.query.filter_by(level=1,activate=1).order_by(Department.id.asc()).all()
# #
# # department_list.append((1,top_departments))
# #
# # selected_department_list.append(department.id)
#
# else:
#
# #获取父级部门
# for i in range(1,current_level):
#
# if i == 1:
# departments = Department.query.filter_by(level=i,activate=1).order_by(Department.id.asc()).all()
# else:
# departments = Department.query.filter_by(level=i,activate=1,parent_id=top_department[i-1]).order_by(Department.id.asc()).all()
#
# department_list.append((i,departments))
#
#
# #获取父级Id
#
# parent = department.parent
#
# while(current_level > 1):
# current_level = current_level - 1
#
# selected_department_dict[current_level] = parent.id
#
# parent = parent.parent
users = User.query.filter_by(activate=1).all()
#一级部门
top_departments = Department.query.filter_by(level=1,activate=1).order_by(Department.id.asc()).all()
#二级部门
second_departments = Department.query.filter_by(level=2,activate=1).order_by(Department.id.asc()).all()
#三级部门
three_departments = Department.query.filter_by(level=3,activate=1).order_by(Department.id.asc()).all()
#四级部门
four_departments = Department.query.filter_by(level=4,activate=1).order_by(Department.id.asc()).all()
#五级部门
five_departments = Department.query.filter_by(level=5,activate=1).order_by(Department.id.asc()).all()
array = []
array.append('一级部门')
array.append('二级部门')
array.append('三级部门')
array.append('四级部门')
array.append('五级部门')
dict = {}
dict['一级部门'] = top_departments
dict['二级部门'] = second_departments
dict['三级部门'] = three_departments
dict['四级部门'] = four_departments
dict['五级部门'] = five_departments
array_leader = department.leader.split(',')
department_members = User.query.filter_by(department_id=department.id).all()
member_page = []
for arr in department_members:
member_page.append(str(arr.id))
member_page = ','.join(member_page)
if len(department_members) == 0 :
member_page = ''
return render_template('departmentMan/department_edit.html', department=department,users=users,array=array,dict=dict,array_leader=array_leader,
member_page=member_page)
def dealwith_status(origin_status,department):
""""""
if department.activate == origin_status:
#如果状态一致,没有发生变更
""""""
else:
#状态发生变更
if department.activate:
# print 'True'
db.session.execute('update tms_department SET activate = 1 WHERE id in ( SELECT aa.id from '
'( SELECT id from tms_department t where FIND_IN_SET(id,getChildsByRootid(%s))) aa )' % (department.id))
else:
# print 'False'
db.session.execute('update tms_department SET activate = 0 WHERE id in ( SELECT aa.id from '
'( SELECT id from tms_department t where FIND_IN_SET(id,getChildsByRootid(%s))) aa )' % (department.id))
db.session.commit()
def dealwith_department(origin_parent_department,page_parent_department,current_department):
""""""
if page_parent_department and origin_parent_department:
#页面传过来的父级部门有值
if int(page_parent_department) == origin_parent_department.id:
#页面传过来的父级部门 等于 数据库里的父级部门 --》没有发生变更
return True,''
else:
if pageParentIfInChilds(page_parent_department,current_department):
#页面选择的父级部门是该部门或者该部门的子级别部门报错
return False,'页面选择的父级部门不能是该部门或者该部门的子级别部门,请重新选择'
page_parent_department_entity = Department.query.filter_by(id=page_parent_department).first()
if page_parent_department_entity.level == origin_parent_department.level:
#页面传过来的父级部门 级别 与 数据库里的父级部门的级别一致
return True,''
elif page_parent_department_entity.level < origin_parent_department.level:
#页面选择的父级级别 比 数据库的父界部门级别 小
#修改层级结构
update_childs(current_department,page_parent_department_entity.level,origin_parent_department.level)
return True,''
elif page_parent_department_entity.level > origin_parent_department.level:
#页面选择的父级部门的级别 大于 数据库中的父级部门级别
# 4 > 1
max_level = select_childs(current_department) #6
diff = max_level - origin_parent_department.level
if page_parent_department_entity.level + diff > DEPARTMENT_DEEP:
return False,'该操作会导致部门层次级别超过6级,请联系系统管理员'
else:
#修改层级结构
#页面选择的父级部门的级别 大于 数据库中的父级部门级别
# 3 > 2 当前:3
update_childs(current_department,page_parent_department_entity.level,origin_parent_department.level)
return True,''
else:
if origin_parent_department:
#页面传过来的父级部门没有值 --> 当前部门设置为一级部门
update_childs(current_department,0,origin_parent_department.level)
return True,''
elif page_parent_department:
#页面传过来的父级部门有值 --> 数据库里的父级部门没有值 --
if pageParentIfInChilds(page_parent_department,current_department):
#页面选择的父级部门是该部门或者该部门的子级别部门报错
return False,'页面选择的父级部门不能是该部门或者该部门的子级别部门,请重新选择'
page_parent_department_entity = Department.query.filter_by(id=page_parent_department).first()
max_level = select_childs(current_department) #6
diff = max_level - 0
if page_parent_department_entity.level + diff > DEPARTMENT_DEEP:
return False,'该操作会导致部门层次级别超过6级,请联系系统管理员'
update_childs(current_department,page_parent_department_entity.level,0)
return True,''
else:
#都没有父级部门
return True,''
def select_childs(current_department):
""""""
sql = 'SELECT MAX(t.level) from tms_department t where FIND_IN_SET(id,getChildsByRootid(%s))' % (current_department.id)
all_levels = db.session.execute(sql).fetchall()
max_level = all_levels[0][0]
return max_level
def pageParentIfInChilds(page_parent_department,current_department):
sql = ' SELECT t.id from tms_department t where FIND_IN_SET(id,getChildsByRootid(%s)) ' % (current_department.id)
all_departments = db.session.execute(sql).fetchall()
for department in all_departments:
if department[0] == int(page_parent_department):
return True
return False
def update_childs(current_department,page_parent_level,db_parent_level):
"""
page_parent_level: 1
db_parent_level:3
current_department.level:4
update_childs(current_department,3,2)
page_parent_level:3
db_parent_level:2
current_department.level:3
update_childs(current_department,0,2)
page_parent_level:0
db_parent_level:2
current_department.level:3
"""
diff = int(page_parent_level) - db_parent_level#-2
db.session.execute('update tms_department SET level = level + %s WHERE id in '
'( SELECT aa.id from ( SELECT id from tms_department t where FIND_IN_SET(id,getChildsByRootid(%s))) aa )' % (diff,current_department.id))
db.session.commit()
def getParent(department):
"""获取父级节点"""
dict = {}
while(department):
dict[department.level] = department.id
department = department.parent
return dict
@department.route('/add_department', methods=['GET', 'POST'])
@login_required
def add_department():
"""新增部门"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '新增部门' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '新增部门',
'url': url_for('department.add_department')})
if request.method == 'POST':
#新增部门到数据库
name = request.form['name']
activate = request.form.get('activate')
note = request.form.get('note')
order = request.form['order']
leader = request.form['leader_ids']
member = request.form['member_ids']
parent_department = request.form.get('parent_department', None)
# print 'leader',leader
# print 'parent_department',parent_department
# #一级部门
# select_department_1 = request.form.get('select_department_1', None)
# #二级部门
# select_department_2 = request.form.get('select_department_2', None)
# #三级部门
# select_department_3 = request.form.get('select_department_3', None)
# #四级部门
# select_department_4 = request.form.get('select_department_4', None)
# #五级部门
# select_department_5 = request.form.get('select_department_5', None)
#最多深度到6
other_department = Department.query.filter_by(name=str(name).strip()).first()
if other_department:
flash(u'同名部门存在', 'danger')
return redirect(url_for('department.department_manage'))
# else:
department = Department()
department.name = name
department.note = note
if activate == 'on':
department.activate = True
else:
department.activate = False
department.order = order
department.leader = leader
if parent_department:
par_department = Department.query.filter_by(id=parent_department).first()
department.level = par_department.level + 1
department.parent_id = parent_department
else:
department.level = 1
department.parent_id = None
# if select_department_1:
# #一级部门有值
#
# department.level = 2
# department.parent_id = select_department_1
#
# if select_department_2:
# #二级部门有值
# department.level = 3
# department.parent_id = select_department_2
#
# if select_department_3:
# #三级部门有值
# department.level = 4
# department.parent_id = select_department_3
#
# if select_department_4:
# #四级部门有值
# department.level = 5
# department.parent_id = select_department_4
#
# if select_department_5:
# #五级部门有值
# department.level = 6
# department.parent_id = select_department_5
#
# else:
# #一级部门没有值
# #该部门即为一级部门
#
# department.level = 1
db.session.add(department)
db.session.commit()
#修改成员所在的部门信息
if member:
member_array = str(member).split(',')
for arr in member_array:
if arr :
user = User.query.filter_by(id=arr).first()
user.department_id = department.id
db.session.add(user)
db.session.commit()
flash(u'新增成功!', 'info')
return redirect(url_for('department.department_manage'))
else:
#get请求 -- >进入新增部门页面
users = User.query.filter_by(activate=1).all()
#一级部门
top_departments = Department.query.filter_by(level=1,activate=1).order_by(Department.id.asc()).all()
#二级部门
second_departments = Department.query.filter_by(level=2,activate=1).order_by(Department.id.asc()).all()
#三级部门
three_departments = Department.query.filter_by(level=3,activate=1).order_by(Department.id.asc()).all()
#四级部门
four_departments = Department.query.filter_by(level=4,activate=1).order_by(Department.id.asc()).all()
#五级部门
five_departments = Department.query.filter_by(level=5,activate=1).order_by(Department.id.asc()).all()
array = []
array.append('一级部门')
array.append('二级部门')
array.append('三级部门')
array.append('四级部门')
array.append('五级部门')
dict = {}
dict['一级部门'] = top_departments
dict['二级部门'] = second_departments
dict['三级部门'] = three_departments
dict['四级部门'] = four_departments
dict['五级部门'] = five_departments
return render_template('departmentMan/department_add.html', department=None,top_departments=top_departments,users=users,
array=array,dict=dict)
@department.route('/department_childs', methods=['GET', 'POST'])
@login_required
def department_childs():
"""部门-->子部门"""
department_id = request.form.get('id')
level = request.form.get('level')
level = int(level) + 1
departments = Department.query.filter_by(parent_id=department_id,level=level,activate=1).order_by(Department.id.asc()).all()
department_list = []
for department in departments:
dict = {}
dict['id'] = department.id
dict['name'] = department.name
department_list.append(dict)
return json.dumps(department_list)<file_sep># -*- coding:utf-8 -*-
__author__ = 'changjie.fan'
""""""
import json
from datetime import datetime
from flask import render_template, redirect, url_for, request, flash, current_app, session
from flask.ext.login import login_required, current_user
from . import qa
from tms.project.models import Project, ProjectTestCase, Version, VersionHistory, SpecialVersion
from tms.auth.models import User, Role
from models import *
from tms.qa.views import need_test_version
@qa.route('/my_project')
@login_required
@need_test_version()
def my_project():
"""测试负责人时我的项目"""
session['breadcrumbs'] = [session['breadcrumbs'][0]]
session['breadcrumbs'].append({'text': '我的项目', 'url': url_for('qa.my_project')})
session['menu_path'] = ['项目管理', '我的项目']
projects = Project.query.filter(Project.test_leader_id == current_user.id).all()
return render_template('qa/project_page.html', projects=projects)
@qa.route('/all_project')
@login_required
@need_test_version()
def all_project():
"""所有的项目项目"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '所有项目' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '所有项目', 'url': url_for('qa.all_project')})
projects = Project.query.all()
return render_template('qa/project_page.html', projects=projects,all_project=True)
@qa.route('/project_test_case_page/<int:project_id>', methods=['GET', 'POST'])
@login_required
@need_test_version()
def project_test_case_page(project_id):
"""项目测试用例管理"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '项目用例管理' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '项目用例管理',
'url': url_for('qa.project_test_case_page', project_id=project_id)})
project = Project.query.filter(Project.id == project_id).first()
if request.method == 'POST':
test_case_ids = request.form.getlist('test_case_ids')
for case_id in test_case_ids:
project_case = ProjectTestCase()
project_case.project = project
project_case.test_case_id = int(case_id)
db.session.add(project_case)
db.session.commit()
project_test_cases = ProjectTestCase.query.filter(ProjectTestCase.project_id == project_id).all()
project_test_case_ids = [tmp.test_case.id for tmp in project_test_cases]
all_test_case = TestCase.query.filter(
db.and_(TestCase.activate == True,
TestCase.id.notin_(project_test_case_ids))).all()
flash(u'添加成功', 'info')
return redirect(url_for('qa.project_test_case_page', project_id=project_id))
else:
project_test_cases = ProjectTestCase.query.filter(ProjectTestCase.project_id == project_id).all()
project_test_case_ids = [tmp.test_case.id for tmp in project_test_cases]
all_test_case = TestCase.query.filter(
db.and_(TestCase.activate == True,
TestCase.id.notin_(project_test_case_ids))).all()
return render_template('qa/project_test_case_page.html',
project=project,
all_test_case=all_test_case,
project_test_cases=project_test_cases)
@qa.route('/delete_project_case', methods=['POST'])
@login_required
def delete_project_case():
"""删除项目指定的用例"""
project_case_ids = request.form.get('project_case_ids', '').split(',')
project_case = ProjectTestCase.query.filter(ProjectTestCase.id.in_(project_case_ids)).all()
for case in project_case:
db.session.delete(case)
db.session.commit()
flash(u'删除成功', 'info')
return '{"result": true}'
@qa.route('/edit_project/<int:project_id>', methods=['GET', 'POST'])
@login_required
@need_test_version()
def edit_project(project_id):
"""编辑"""
for index, breadcrumb in enumerate(session['breadcrumbs']):
if '编辑项目' == breadcrumb['text']:
session['breadcrumbs'] = session['breadcrumbs'][:index+1]
break
else:
session['breadcrumbs'].append({'text': '编辑项目',
'url': url_for('developer.edit_project', project_id=project_id)})
project_obj = Project.query.filter_by(id=project_id).first()
if request.method == 'POST':
project_key = request.form['project_key']
test_leader_id = request.form.get('test_leader', '')
status = request.form['status']
to_email = request.form.get('to_email', '')
test_case_jar_url = request.form.get('test_case_jar_url', '')
project_obj.project_key = project_key
project_obj.test_case_jar_url = test_case_jar_url
project_obj.test_leader_id = test_leader_id
project_obj.status = status
project_obj.to_email = ';'.join([tmp.strip() for tmp in to_email.strip().split(';') if tmp.strip()])
db.session.add(project_obj)
db.session.commit()
flash(u'修改成功!', 'info')
return redirect(url_for('qa.my_project'))
# 只有是项目开发负责才能编辑项目信息
is_developer_leader = False
if project_obj.develop_leader == current_user:
is_developer_leader = True
# 超级管理员和配置管理员也可以编辑项目信息且能够编辑仓库信息
is_super_man = False
if session['user']['role_name'] == 'admin' or session['user']['role_name'] == 'scm':
is_super_man = True
test_users = User.query.join(User.role).filter(Role.name == 'os_test').all()
# 项目最新的版本
last_version = Version.query.filter(db.and_(Version.project_id == project_obj.id,
Version.version_number == project_obj.last_version_number)).first()
last_version_id = ''
if last_version:
last_version_id = last_version.id
# 项目的发布对象(版本对象)
special_versions = SpecialVersion.query.filter(SpecialVersion.project_id == project_obj.id).all()
# 项目状态
project_category = ['MTK', 'QCM', 'RDA', 'APP']
# 项目类
project_status = ['开发中', '迭代发布中', '已关闭', '已暂停']
return render_template('/qa/project_edit.html',
project=project_obj,
last_version_id=last_version_id,
special_versions=special_versions,
is_super_man=is_super_man,
is_developer_leader=is_developer_leader,
test_users=test_users,
project_status=project_status,
project_category=project_category)
| 43faf650ce4ba39013715d0bcb941253b60e4bc8 | [
"HTML",
"JavaScript",
"INI",
"Python",
"Text",
"Shell"
] | 83 | Python | AKMFCJ/tms | 084fa38e675fa81554a2780d30c97da22a707ef1 | bd17a503c6fa33633d36e44aa335e27ab27faba9 | |
refs/heads/master | <repo_name>dhurtadorosales/HybridAppTemplate<file_sep>/src/pages/common/tabs/tabs.ts
import { Component } from '@angular/core';
import { HomePage } from '../../home/home';
import { UserListPage } from '../../user/user-list/user-list';
import { AliasListPage } from '../../alias/alias-list/alias-list';
import { AliasNewPage } from '../../alias/alias-new/alias-new';
@Component({
selector: 'page-tabs',
templateUrl: 'tabs.html',
})
export class TabsPage {
tab1: any;
tab2: any;
tab3: any;
tab4: any;
/**
*
*/
constructor() {
this.tab1 = HomePage;
this.tab2 = UserListPage;
this.tab3 = AliasListPage;
this.tab4 = AliasNewPage;
}
}<file_sep>/src/pages/alias/alias-list/alias-list.module.ts
import { NgModule } from '@angular/core';
import { IonicPageModule } from 'ionic-angular';
import { AliasListPage } from './alias-list';
@NgModule({
declarations: [
AliasListPage,
],
imports: [
IonicPageModule.forChild(AliasListPage),
],
})
export class AliasListPageModule {}
<file_sep>/src/providers/alias.provider.ts
import { Injectable } from '@angular/core';
import 'rxjs/add/operator/map';
import { Observable } from 'rxjs/Observable';
import { Http, Headers } from '@angular/http';
import { GLOBAL } from '../config/global';
@Injectable()
export class AliasProvider {
public url: string;
/**
* @param {Http} _http
*/
constructor(private _http: Http) {
this.url = GLOBAL.url;
this.getAliasAll();
}
/**
* @returns {Observable<any>}
*/
getAliasAll() {
return this
._http.get(this.url + '/alias/all')
.map(response => response.json());
}
/**
*
* @param token
* @param id
* @returns {Observable<any>}
*/
getAliasByUser(token, id) {
let params = 'authorization=' + token;
let headers = new Headers({'Content-Type' : 'application/x-www-form-urlencoded'});
return this
._http.post(this.url + '/alias/' + id, params, {headers: headers})
.map(response => response.json());
}
/**
* @param alias
* @returns {Observable<any>}
*/
newAlias(alias) {
let json = JSON.stringify(alias);
let params = 'json=' + json;
let headers = new Headers({'Content-Type' : 'application/x-www-form-urlencoded'});
return this
._http.post(this.url + '/alias/new', params, {headers: headers})
.map(response => response.json());
}
/**
*
* @param alias
* @returns {Observable<any>}
*/
editAlias(alias) {
let json = JSON.stringify(alias);
let params = 'json=' + json;
let headers = new Headers({'Content-Type' : 'application/x-www-form-urlencoded'});
return this
._http.post(this.url + '/alias/edit/' + alias.id, params, {headers: headers})
.map(response => response.json());
}
/**
*
* @param alias
* @returns {Observable<any>}
*/
deleteAlias(alias) {
let params = '';
let headers = new Headers({'Content-Type' : 'application/x-www-form-urlencoded'});
return this
._http.post(this.url + '/alias/delete/' + alias.id, params, {headers: headers})
.map(response => response.json());
}
}<file_sep>/src/pages/user/user-list/user-list.ts
import { Component } from '@angular/core';
import { NavController, NavParams } from 'ionic-angular';
import { UserProvider } from '../../../providers/user.provider';
import { User } from '../../../models/user.model';
@Component({
selector: 'page-user-list',
templateUrl: 'user-list.html',
providers: [
UserProvider
]
})
export class UserListPage {
public users: User;
/**
* @param {NavController} navCtrl
* @param {UserProvider} _userProvider
*/
constructor(
public navCtrl: NavController,
private _userProvider: UserProvider,
) {
this.getUsers();
}
/**
*
*/
getUsers() {
this._userProvider
.getUsersAll()
.subscribe(
response => {
if (!response.error) {
this.users = response;
}
else {
alert('error.connection');
}
},
error => {
alert(<any>error);
}
);
}
}
<file_sep>/src/pages/alias/alias-list/alias-list.ts
import { Component } from '@angular/core';
import { AlertController, LoadingController, NavController, NavParams, PopoverController} from 'ionic-angular';
import { Alias } from '../../../models/alias.model';
import { AliasProvider } from '../../../providers/alias.provider';
import { AliasEditPage } from '../alias-edit/alias-edit';
import { ToastProvider } from '../../../providers/toast.provider';
import { UserProvider } from '../../../providers/user.provider';
@Component({
selector: 'page-alias-list',
templateUrl: 'alias-list.html',
providers: [
AliasProvider,
ToastProvider
]
})
export class AliasListPage {
alias: Alias;
aliasEditPage: any;
loading: any;
popover: any;
token: any;
/**
*
* @param {NavController} navCtrl
* @param {NavParams} navParams
* @param {AlertController} alertCtrl
* @param {LoadingController} loadingCtrl
* @param {AliasProvider} _aliasProvider
* @param {ToastProvider} _toastProvider
* @param {UserProvider} _userProvider
*/
constructor(
public navCtrl: NavController,
public navParams: NavParams,
private alertCtrl: AlertController,
private loadingCtrl: LoadingController,
private _aliasProvider: AliasProvider,
private _toastProvider: ToastProvider,
private _userProvider: UserProvider
) {
this._userProvider.getToken();
this.loading = this.loadingCtrl.create({
content: 'message.loading',
dismissOnPageChange: true
});
this.aliasEditPage = AliasEditPage;
this.loading.present();
//this.getAliasAll();
this.getAliasByUser('<KEY>', 22);
}
/**
*
*/
getAliasAll() {
this._aliasProvider.getAliasAll().subscribe(
response => {
if (!response.error) {
this.alias = response;
if (response.length == 0) {
this._toastProvider.presentToast('message.alias.empty');
}
}
else {
this._toastProvider.presentToast('message.alias.get_all.error');
}
},
error => {
this._toastProvider.presentToast(error);
}
);
}
/**
*
* @param token
* @param id
*/
getAliasByUser(token, id) {
console.log(localStorage.getItem('token'));
this._aliasProvider.getAliasByUser(token, id).subscribe(
response => {
if (!response.error) {
this.alias = response;
if (response.length == 0) {
this._toastProvider.presentToast('message.alias.empty');
}
} else {
this._toastProvider.presentToast('message.alias.get_by_user.error');
}
},
error => {
this._toastProvider.presentToast(error);
}
);
}
/**
* @param alias
*/
deleteAlias(alias) {
this._aliasProvider.deleteAlias(alias)
.subscribe(
response => {
if (!response.error) {
this.refreshPage();
this._toastProvider.presentToast('message.alias.delete.success');
}
else {
this._toastProvider.presentToast('message.alias.delete.error');
}
},
error => {
this._toastProvider.presentToast(error);
}
);
}
/**
*
* @param alias
*/
showConfirm(alias) {
let alert = this.alertCtrl.create({
title: 'message.alias.delete.confirm.title',
message: 'message.alias.delete.confirm.question',
buttons: [
{
text: 'alert.cancel',
role: 'cancel',
handler: () => {
this.refreshPage();
}
},
{
text: 'alert.confirm',
handler: () => {
this.deleteAlias(alias);
}
}
]
});
alert.present();
}
/**
*
*/
refreshPage() {
this.getAliasAll();
}
/**
*
* @param refresher
*/
doRefresh(refresher) {
setTimeout(() => {
refresher.complete();
}, 2000);
}
}
<file_sep>/src/providers/user.provider.ts
import { Injectable } from '@angular/core';
import 'rxjs/add/operator/map';
import { Observable } from 'rxjs/Observable';
import { Http, Response, Headers } from '@angular/http';
import { GLOBAL } from '../config/global';
import { AlertController, Platform } from 'ionic-angular';
import { Storage } from '@ionic/storage';
@Injectable()
export class UserProvider {
url: string;
identity: string;
token: string;
/**
*
* @param {Http} _http
* @param {AlertController} alertCtrl
* @param {Platform} platform
* @param {Storage} storage
*/
constructor(
private _http: Http,
private alertCtrl: AlertController,
private platform: Platform,
private storage: Storage
) {
this.url = GLOBAL.url;
//this.chargeStorage();
}
/**
* @returns {Observable<any>}
*/
getUsersAll() {
return this
._http.get(this.url + '/user/all')
.map(response => response.json());
}
/**
*
* @param user
* @returns {Observable<any>}
*/
signIn(user) {
if (user.username === null || user.password === null) {
return Observable.throw('message.insert.credentials');
} else {
let json = JSON.stringify(user);
let params = "json=" + json;
let headers = new Headers({'Content-Type': 'application/x-www-form-urlencoded'});
return this
._http.post(this.url + '/login', params, {headers: headers})
.map(
response => response.json()
);
}
}
updateUser(userToUpdate) {
}
deleteUser(id) {
return this
._http.get(this.url)
.map(res => res.json());
}
searchUser(id) {
return this
._http.get(this.url)
.map(res => res.json());
}
/**
*
* @returns {string}
*/
getToken() {
let token;
if (this.platform.is('cordova')) {
//Mobile
token = this.storage.get('token');
} else {
//Desktop
if (this.token) {
token = localStorage.getItem('token');
} else {
token = localStorage.removeItem('token');
}
}
if (token != 'undefined') {
this.token = token;
}
else {
this.token = null;
}
//console.log(this.token);
return this.token;
}
}<file_sep>/src/pages/alias/alias-new/alias-new.ts
import { Component } from '@angular/core';
import { NavController, NavParams } from 'ionic-angular';
import { AliasProvider } from "../../../providers/alias.provider";
import { Alias } from '../../../models/alias.model';
import { FormBuilder } from '@angular/forms';
@Component({
selector: 'page-alias-new',
templateUrl: 'alias-new.html',
providers: [
AliasProvider
]
})
export class AliasNewPage {
public alias : Alias;
public statusAlias;
/**
*
* @param {NavController} navCtrl
* @param {FormBuilder} formBuilder
* @param {AliasProvider} _aliasProvider
*/
constructor(
public navCtrl: NavController,
private formBuilder: FormBuilder,
private _aliasProvider: AliasProvider,
) {
this.alias = new Alias(1, '', '', null);
}
/**
*
*/
newAlias() {
this._aliasProvider
.newAlias(this.alias)
.subscribe(
response => {
this.statusAlias = response.status;
if (this.statusAlias != 'success') {
this.statusAlias = 'error';
} else {
this.alias = response.data;
}
},
error => {
alert(<any>error);
}
);
}
}
<file_sep>/src/models/alias.model.ts
import { User } from './user.model';
export class Alias {
constructor(
public id: number,
public name: string,
public origin: string,
public user: User
) {}
}<file_sep>/README.md
# HybridAppTemplate
Hybrid App Template
<file_sep>/src/pages/home/home.ts
import { Component } from '@angular/core';
import { MenuController, NavController} from 'ionic-angular';
import { UserListPage } from '../user/user-list/user-list';
import { UserNewPage } from '../user/user-new/user-new';
import { AliasListPage } from '../alias/alias-list/alias-list';
@Component({
selector: 'page-home',
templateUrl: 'home.html'
})
export class HomePage {
usersPage: any;
aliasListPage: any;
userNewPage: any;
/**
*
* @param {NavController} navCtrl
* @param {MenuController} menuCtrl
*/
constructor(public navCtrl: NavController,
private menuCtrl: MenuController
) {
this.usersPage = UserListPage;
this.aliasListPage = AliasListPage;
this.userNewPage = UserNewPage;
}
/**
*
*/
showMenu(){
this.menuCtrl.toggle();
}
}
<file_sep>/src/config/global.ts
export const GLOBAL = {
url: 'http://localhost/phonegap_backend/web/app_dev.php/api'
};<file_sep>/src/pages/alias/alias-new/alias-new.module.ts
import { NgModule } from '@angular/core';
import { IonicPageModule } from 'ionic-angular';
import { AliasNewPage } from './alias-new';
@NgModule({
declarations: [
AliasNewPage,
],
imports: [
IonicPageModule.forChild(AliasNewPage),
],
})
export class AliasPageModule {}
<file_sep>/src/pages/user/user-new/user-new.ts
import { Component } from '@angular/core';
import { NavController, NavParams } from 'ionic-angular';
import { UserProvider } from "../../../providers/user.provider";
import { User } from '../../../models/user.model';
@Component({
selector: 'page-user-new',
templateUrl: 'user-new.html',
providers: [
UserProvider
]
})
export class UserNewPage {
public users: User;
constructor(
public navCtrl: NavController,
private _userProvider: UserProvider,
) {
this._userProvider.getUsersAll();
}
newUser() {
this._userProvider.getUsersAll().subscribe(
response => {
if (!response.error) {
this.users = response.users;
console.log(this.users);
}
else {
alert('Error connection!');
}
},
error => {
alert(<any>error);
}
);
}
}
<file_sep>/src/app/app.module.ts
import { BrowserModule } from '@angular/platform-browser';
import { ErrorHandler, NgModule } from '@angular/core';
import { IonicApp, IonicErrorHandler, IonicModule } from 'ionic-angular';
import { SplashScreen } from '@ionic-native/splash-screen';
import { StatusBar } from '@ionic-native/status-bar';
import { MyApp } from './app.component';
import { HttpModule } from '@angular/http';
import { FormsModule } from '@angular/forms';
import { LongPressModule } from 'ionic-long-press';
//Pages
import { HomePage } from '../pages/home/home';
import { UserListPage } from '../pages/user/user-list/user-list';
import { UserNewPage } from '../pages/user/user-new/user-new';
import { AliasListPage } from '../pages/alias/alias-list/alias-list';
import { TabsPage } from '../pages/common/tabs/tabs';
import { LoginPage } from '../pages/login/login';
import { AliasNewPage } from '../pages/alias/alias-new/alias-new';
import { AliasEditPage } from '../pages/alias/alias-edit/alias-edit';
//Providers
import { UserProvider } from '../providers/user.provider';
import { AliasProvider } from '../providers/alias.provider';
import { ToastProvider } from '../providers/toast.provider';
//Storage
import { IonicStorageModule } from '@ionic/storage';
@NgModule({
declarations: [
MyApp,
HomePage,
UserListPage,
UserNewPage,
AliasListPage,
AliasNewPage,
AliasEditPage,
TabsPage,
LoginPage
],
imports: [
BrowserModule,
IonicModule.forRoot(MyApp),
HttpModule,
IonicStorageModule.forRoot(),
FormsModule,
LongPressModule
],
bootstrap: [IonicApp],
entryComponents: [
MyApp,
HomePage,
UserListPage,
UserNewPage,
AliasNewPage,
AliasEditPage,
AliasListPage,
TabsPage,
LoginPage
],
providers: [
StatusBar,
SplashScreen,
UserProvider,
AliasProvider,
ToastProvider,
{provide: ErrorHandler, useClass: IonicErrorHandler}
]
})
export class AppModule {}
<file_sep>/src/pages/login/login.ts
import { Component } from '@angular/core';
import {
NavController, NavParams, ViewController, LoadingController, AlertController, Loading,
Platform
} from 'ionic-angular';
import { UserProvider } from '../../providers/user.provider';
import { HomePage } from '../home/home';
import { Storage } from '@ionic/storage';
import {AliasListPage} from "../alias/alias-list/alias-list";
@Component({
selector: 'page-login',
templateUrl: 'login.html',
providers: [UserProvider]
})
export class LoginPage {
public user;
public identity;
public token;
public loading;
public homePage: any;
/**
*
* @param {NavController} navCtrl
* @param {NavParams} navParams
* @param {ViewController} viewCtrl
* @param {AlertController} alertCtrl
* @param {LoadingController} loadingCtrl
* @param {Platform} platform
* @param {Storage} storage
* @param {UserProvider} _userProvider
*/
constructor(
public navCtrl: NavController,
public navParams: NavParams,
private viewCtrl: ViewController,
private alertCtrl: AlertController,
private loadingCtrl: LoadingController,
private platform: Platform,
private storage: Storage,
private _userProvider: UserProvider
) {
this.user = {
'username': '',
'password': ''
}
this.homePage = HomePage;
this.chargeStorage();
}
/**
*
*/
login(){
//this.showLoading();
this._userProvider
.signIn(this.user)
.subscribe(
response => {
if (response.error) {
this.showError(response.error)
} else {
this.token = response.access_token;
//Save localstorage
this.saveStorage();
this.navCtrl.push(HomePage);
}
},
error => {
this.showError(error);
}
);
}
/**
*
*/
private saveStorage() {
if (this.platform.is('cordova')) {
//Mobile
this.storage.set('token', this.token);
} else {
//Desktop
if (this.token) {
localStorage.setItem('token', JSON.stringify(this.token));
} else {
localStorage.removeItem('token');
}
}
}
/**
*
*/
logOut(){
this.token = null;
this.identity = null;
//Save localstorage
this.saveStorage();
}
/**
*
* @returns {Promise<any>}
*/
chargeStorage() {
let promise = new Promise(
(resolve, reject) => {
if (this.platform.is('cordova')) {
//mobile
this.storage
.ready()
.then(() => {
this.storage.get('token')
.then(token => {
if (token) {
this.token = token;
}
});
});
} else {
//desktop
if (localStorage.getItem('token')) {
//The item exists in localstorage
this.token = localStorage.getItem('token');
}
resolve();
}
});
return promise;
}
/**
*
*/
showLoading() {
this.loading = this.loadingCtrl.create({
content: 'message.wait',
dismissOnPageChange: true
});
this.loading.present();
}
/**
*
* @param text
*/
showError(text) {
let alert = this.alertCtrl.create({
title: 'message.fail',
subTitle: text,
buttons: ['OK']
});
alert.present(prompt);
}
}
<file_sep>/src/pages/alias/alias-edit/alias-edit.ts
import { Component } from '@angular/core';
import { NavController, NavParams } from 'ionic-angular';
import { AliasProvider } from '../../../providers/alias.provider';
import { FormBuilder } from '@angular/forms';
import { Alias } from '../../../models/alias.model';
@Component({
selector: 'page-alias-edit',
templateUrl: 'alias-edit.html',
})
export class AliasEditPage {
alias: Alias;
statusAlias: any;
/**
*
* @param {NavController} navCtrl
* @param {NavParams} navParams
* @param {FormBuilder} formBuilder
* @param {AliasProvider} _aliasProvider
*/
constructor(
public navCtrl: NavController,
public navParams: NavParams,
private formBuilder: FormBuilder,
private _aliasProvider: AliasProvider,
) {
this.alias = new Alias(
this.navParams.data.alias.id,
this.navParams.data.alias.name,
this.navParams.data.alias.origin,
this.navParams.data.alias.user
);
}
/**
*
*/
editAlias() {
this._aliasProvider
.editAlias(this.alias)
.subscribe(
response => {
this.statusAlias = response.status;
if (this.statusAlias != 'success') {
this.statusAlias = 'error';
} else {
this.alias = response.data;
}
},
error => {
alert(<any>error);
}
);
}
}
<file_sep>/src/pages/alias/alias-edit/alias-edit.module.ts
import { NgModule } from '@angular/core';
import { IonicPageModule } from 'ionic-angular';
import { AliasEditPage } from './alias-edit';
@NgModule({
declarations: [
AliasEditPage,
],
imports: [
IonicPageModule.forChild(AliasEditPage),
],
})
export class AliasEditPageModule {}
| f6127cc073e455342520cab0b156d46432b49cbc | [
"Markdown",
"TypeScript"
] | 17 | TypeScript | dhurtadorosales/HybridAppTemplate | b4329d8ca94692bf2cbefe2c06381e07e71d5fa5 | 3bc5d95daec9fc91cbcd17de9546b21fefbd85b0 | |
refs/heads/master | <file_sep>package coursera;
public class NewCeaserCipher {
/*public static String encrypt(String input, int key)
{
String alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
String shiftAlphabets = alphabet.substring(key) + alphabet.substring(0, key);
StringBuilder newStringB = new StringBuilder(input);
for(int i =0; i<newStringB.length(); i++)
{
char eachCharacter = newStringB.charAt(i);
char upperEachCharacter = Character.toUpperCase(eachCharacter);
int index = alphabet.indexOf(upperEachCharacter);
if(index != -1)
{
char newChar = shiftAlphabets.charAt(index);
if(Character.isLowerCase(eachCharacter))
{
newChar = Character.toLowerCase(newChar);
}
newStringB.setCharAt(i, newChar);
}
}
return newStringB.toString();
}*/
public static String encryptTwoWays(String input, int key1, int key2 )
{
String alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
String shiftAlphabets1 = alphabet.substring(key1) + alphabet.substring(0, key1);
String shiftAlphabets2 = alphabet.substring(key2) + alphabet.substring(0, key2);
StringBuilder newStringB = new StringBuilder(input);
for(int i =0; i<newStringB.length(); i++)
{
char eachCharacter = newStringB.charAt(i);
char upperEachCharacter = Character.toUpperCase(eachCharacter);
int index = alphabet.indexOf(upperEachCharacter);
char newChar; if(index != -1)
{
if(i%2 == 0)
{
newChar = shiftAlphabets1.charAt(index);
}
else {
newChar = shiftAlphabets2.charAt(index);
}
if(Character.isLowerCase(eachCharacter))
{
newChar = Character.toLowerCase(newChar);
}
newStringB.setCharAt(i, newChar);
}
}
return newStringB.toString();
}
public static void main(String[] args)
{
String newString = "Top ncmy qkff vi vguv vbg ycpx";
int key1 = 2, key2 = 20;
System.out.println(encryptTwoWays(newString, key1, key2));
}
}
<file_sep>package coursera;
public class WordPlay {
public static boolean isVowel(char ch)
{
char UpperCasech = Character.toUpperCase(ch);
if (UpperCasech == 'A' || UpperCasech == 'E'||UpperCasech == 'I'||UpperCasech == 'O'||UpperCasech == 'U')
{
return true;
}
else {
return false;
}
}
public static String replace(String phrase, char ch)
{
StringBuilder newString = new StringBuilder(phrase);
for(int i=0; i<newString.length(); i++)
{
char phraseChar = newString.charAt(i);
if(isVowel(phraseChar))
{
newString.setCharAt(i,ch);
}
}
return newString.toString();
}
public static String emphasize(String phrase, char ch)
{
StringBuilder newStringBuilder = new StringBuilder(phrase);
for(int i =0; i<newStringBuilder.length(); i++)
{
char eachCharacter = newStringBuilder.charAt(i);
int index = i + 1;
if(Character.toLowerCase(eachCharacter) == ch)
{
if(index%2 == 0)
{
newStringBuilder.setCharAt(i, '+');
}
else {
newStringBuilder.setCharAt(i, '*');
}
}
}
return newStringBuilder.toString();
}
public static void main(String[] args)
{
String newString = "<NAME>";
System.out.println(replace(newString, '*'));
System.out.println(emphasize(newString, 'a'));
}
}
<file_sep>package coursera;
public class DecryptCeaserCipher {
public static int[] countElements(String str)
{
int[] counter = new int[26];
//Writing down the alphabets
String alphabets = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
for(int i=0; i<str.length(); i++)
{
char currChar = str.charAt(i);
char upperCurrChar = Character.toUpperCase(currChar);
int index = alphabets.indexOf(upperCurrChar);
if(index != -1)
{
counter[index]++;
}
}
for(int i=0; i<counter.length; i++)
{
System.out.print(counter[i]+" ");
}
System.out.println();
return counter;
}
public static int maxElement(int[] counter)
{
// Initialize maximum element
int max = counter[0], maxIndex =0;
// Traverse array elements from second and
// compare every element with current max
for (int i = 1; i < counter.length; i++)
{ if (counter[i] > max)
{
max = counter[i];
maxIndex = i;
}
System.out.print(maxIndex+" ");
}
return maxIndex;
}
public static String decrypt(String str)
{
//CeaserCipher ccCeaserCipher = new CeaserCipher();
int[] count = countElements(str);
int max = maxElement(count);
System.out.println(max+" ");
int dKey = max - 4;
if(max<4)
{
dKey = 26 - (4-max);
}
System.out.println(dKey);
return encrypt(str, 26 - dKey);
}
public static String encrypt(String s, int key)
{
//Making a StringBuilder with a message(encrypted)
StringBuilder encrypted = new StringBuilder(s);
//Writing down the alphabets
String alphabets = "ABCDEFGHIJKLMNOPQRSTUVWXYZ";
//Compute the shifted alphabet
String shiftedAlphabets = alphabets.substring(key) + alphabets.substring(0, key);
for(int i=0; i<encrypted.length(); i++)
{
char currChar = encrypted.charAt(i);
char upperCurrChar = Character.toUpperCase(currChar);
int index = alphabets.indexOf(upperCurrChar);
if(index != -1)
{
char newChar = shiftedAlphabets.charAt(index);
if(Character.isLowerCase(currChar))
{
newChar = Character.toLowerCase(newChar);
}
encrypted.setCharAt(i, newChar);
}
}
return encrypted.toString();
}
public static void main(String[] args)
{
String testString = "<NAME>";
System.out.println(decrypt(testString));
}
}
| 3a84bf8230ef59326899c1827c472b326bb6db73 | [
"Java"
] | 3 | Java | Supriya090/Ceaser-Cipher | d8d9ad9a95c603be0367c218bcda294005fa758f | 0410966e6bcbcb55b4f2fda8d99751aab4f26e76 | |
refs/heads/main | <repo_name>yuma140902/line-echo<file_sep>/src/word-verifier.ts
import kuromoji from 'kuromoji'
import * as kana_util from './kana-util'
// ===== 形態素解析に関するモジュール =====
type ErrorReason = 'NOT_A_WORD' | 'COMPOUND_NOUN' | 'UNKNOWN_WORD';
type VerifyWordResult = Success | Fail;
type Success = {
succeeded: true,
tokens: kuromoji.IpadicFeatures[],
surface: string,
kana: string
}
type Fail = FailBase | FailNotANoun;
type FailBase = {
succeeded: false,
tokens: kuromoji.IpadicFeatures[],
error_reason: ErrorReason
}
type FailNotANoun = {
succeeded: false,
tokens: kuromoji.IpadicFeatures[],
error_reason: 'NOT_A_NOUN',
pos: string
}
// tokenが既知の単語であり、かつその品詞がposであるかどうか確認する
const posEqualsAndKnown = (token: kuromoji.IpadicFeatures, pos: string): boolean =>
token.word_type === 'KNOWN' && token.pos === pos;
export const friendlyPos = (token: kuromoji.IpadicFeatures): string =>
(token.word_type === 'UNKNOWN' ? '辞書に載っていない' : '') + token.pos;
const preprocess = (text: string): string =>
kana_util.han2zen(text.trim());
export const verifyWord = (tokenizer: kuromoji.Tokenizer<kuromoji.IpadicFeatures>, text: string): VerifyWordResult => {
text = preprocess(text);
const tokens = tokenizer.tokenize(text);
if (tokens.length === 1 && posEqualsAndKnown(tokens[0], '名詞')) {
return {
succeeded: true,
tokens: tokens,
surface: tokens[0].surface_form,
kana: tokens[0].reading || ''
};
}
else if (tokens.length === 1 && tokens[0].word_type === 'UNKNOWN') {
return {
succeeded: false,
tokens: tokens,
error_reason: 'NOT_A_WORD'
}
}
// 1単語だが名詞ではないとき
else if (tokens.length === 1) {
return {
succeeded: false,
tokens: tokens,
error_reason: 'NOT_A_NOUN',
pos: friendlyPos(tokens[0])
};
}
else {
const allNoun = tokens.every(token => (posEqualsAndKnown(token, '名詞') || posEqualsAndKnown(token, '接頭詞')));
// 入力が複合語と思われるとき
if (allNoun) {
return {
succeeded: false,
tokens: tokens,
error_reason: 'COMPOUND_NOUN'
};
}
// その他複数の単語
else {
return {
succeeded: false,
tokens: tokens,
error_reason: 'NOT_A_WORD'
};
}
}
}
<file_sep>/src/next-word.ts
import kuromoji from 'kuromoji'
import * as word_verifier from './word-verifier'
const freqlist = require('../rsc/freqlist_ja.json');
// ===== 次の言葉を考えるモジュール =====
function randomRanged(begin: number, end: number) {
begin = Math.ceil(begin);
end = Math.floor(end);
return Math.floor(Math.random() * (end - begin) + begin);
}
const MAX_TRIAL = 12;
interface NextWordInfo {
kana: string,
word: string
}
const nextWord = (tokenizer: kuromoji.Tokenizer<any>, lastKana: string): NextWordInfo | undefined => {
if (!freqlist[lastKana]) {
console.assert(false, `not implemented next-word (lastKana: ${lastKana})`);
return undefined;
}
let word;
const numWords = freqlist[lastKana].length;
let trial = 0;
do {
word = freqlist[lastKana][randomRanged(0, numWords)];
++trial;
if (trial > 12) {
word = undefined;
break;
}
} while (!word_verifier.verifyWord(tokenizer, word[1]).succeeded);
console.log("next-word trial", trial);
if (!word) return undefined;
return {
kana: word[0],
word: word[1]
};
}
export default nextWord;<file_sep>/src/index.ts
import path from 'path'
import express from 'express'
import * as line from '@line/bot-sdk'
import kuromoji from 'kuromoji'
import * as word_verifier from './word-verifier'
import * as kana_util from './kana-util'
import next_word from './next-word'
import * as db from './db'
const dic_path = path.join(__dirname, '../node_modules/kuromoji/dict') + '/'
const port = process.env.PORT || 3000;
const config = {
channelAccessToken: process.env.LINE_ACCESS_TOKEN || 'LINE ACCESS TOKEN IS NOT SET',
channelSecret: process.env.CHANNEL_SECRET || 'CHANNEL SECRET IS NOT SET'
};
const client = new line.Client(config);
let tokenizer: kuromoji.Tokenizer<kuromoji.IpadicFeatures>;
const app = express();
app.use(express.text());
app.get('/', (req, res) => {
res.sendStatus(200);
});
app.post('/webhook', line.middleware(config), (req, res) => {
Promise
.all(req.body.events.map(handleLineMessageEvent))
.then(result => res.json(result))
.catch(err => {
console.error(err);
res.status(500).end();
});
});
app.post('/api', (req, res) => {
console.log('api input:', req.body);
const message = req.body || '';
const userId = req.ip!;
getReplies(message, userId)
.then(responses => {
res.json(responses).end();
})
.catch(err => {
console.error(err);
res.status(500).end();
})
});
async function handleLineMessageEvent(event: line.MessageEvent) {
console.log('line msg event:', JSON.stringify(event));
const reply = (response: line.Message | line.Message[]) => client.replyMessage(event.replyToken, response);
if (event.type !== 'message' || event.message.type !== 'text' || event.source.type !== 'user') {
return Promise.resolve(null);
}
const responses = (await getReplies(event.message.text, event.source.userId))
.map(res => ({ 'type': 'text', 'text': res } as line.Message));
return client.replyMessage(event.replyToken, responses);
}
async function getReplies(message: string, userId: string): Promise<string[]> {
const result = word_verifier.verifyWord(tokenizer, message);
if (result.succeeded) {
const firstKana = kana_util.firstKana(result.kana);
const lastKana = kana_util.lastKana(result.kana);
const wordInfoResponse = `単語: ${result.surface}、よみ: ${result.kana}、最後の文字は${lastKana}`;
if (lastKana === 'ン') {
await db.removeUserLastKana(userId);
return [
wordInfoResponse,
'ンなので終了します',
'再開するには適当な単語を言ってください'
];
}
const botLastKana = await db.obtainUserLastKana(userId);
if (!botLastKana || firstKana === botLastKana) {
const nextWord = next_word(tokenizer, lastKana);
if (!nextWord) {
return ['参りました'];
}
const nextBotLastKana = kana_util.lastKana(nextWord.kana);
await db.updateUserLastKana(userId, nextBotLastKana);
return [
wordInfoResponse,
`${nextWord.word} (${nextWord.kana} : ${nextBotLastKana}) `
];
}
else {
return [
`前の単語は${botLastKana}で終わりましたが、${message} (${result.kana}) は${firstKana}から始まります`,
`${botLastKana}から始まる単語を入力してください`
];
}
}
else if (result.error_reason === 'COMPOUND_NOUN') {
const surfaces = result.tokens.map((token: any) => token.surface_form);
return [
`${message}は ${surfaces.join(' + ')} の複合語です。`,
'実在する言葉かどうか判定できないので、複合語は使えません。'
];
}
else if (result.error_reason === 'UNKNOWN_WORD') {
return [
`${message}は辞書に載っていません。`,
'辞書に載っている名詞しか使えません'
];
}
else if (result.error_reason === 'NOT_A_NOUN') {
return [
`「${message}」は${result.pos}です。`,
'名詞しか使えません。'
];
}
else if (result.error_reason === 'NOT_A_WORD') {
const tokens = result.tokens.map((token: any) => `${token.surface_form} : ${word_verifier.friendlyPos(token)}`);
return [
`形態素解析の結果、\n${tokens.join('\n')}\nとなりました。`,
`「${message}」は名詞ではないのでしりとりには使えません。`
];
}
else {
console.assert(false);
return [`[実績解除] 有能デバッガー\nあなたはこのBOTの開発者が気づかなかったバグを見つけ出した!`];
}
}
function getTokenizerPromise(): Promise<kuromoji.Tokenizer<kuromoji.IpadicFeatures>> {
console.log('Loading Kuromoji.js');
return new Promise((resolve) => {
kuromoji.builder({ dicPath: dic_path }).build(function (err, tokenizer) {
// tokenizer is ready
resolve(tokenizer);
console.log('Loaded Kuromoji.js');
if (err) {
console.error(err);
}
});
})
}
getTokenizerPromise().then(tokenizer_ => {
tokenizer = tokenizer_;
app.listen(port, () => console.log(`Listening on :${port}`));
});<file_sep>/src/db.ts
import { Pool } from 'pg'
// ===== データベースに関するモジュール =====
const pool = new Pool({
connectionString: process.env.DATABASE_URL,
ssl: { rejectUnauthorized: false }
});
export const updateUserLastKana = (userId: string, lastKana: string): Promise<void> => {
const sql = 'INSERT INTO "UserLastLetter" (user_id, last_letter) VALUES ($1, $2) ON CONFLICT (user_id) DO UPDATE SET last_letter = $2';
return pool
.query(sql, [userId, lastKana])
.then(res => {
// pass
})
.catch(err => {
console.error("エラー: updateUserLastKana()");
console.error(err);
});
}
export const obtainUserLastKana = (userId: string): Promise<string | undefined> => {
const sql = 'SELECT last_letter from "UserLastLetter" WHERE user_id = $1 LIMIT 1';
return pool
.query(sql, [userId])
.then(res => {
return res.rows[0].last_letter;
})
.catch(_ => {
// pass
// ゲーム開始時は必ずエラーになるのでエラーログは出力しない
});
}
export const removeUserLastKana = (userId: string): Promise<void> => {
const sql = 'DELETE FROM "UserLastLetter" WHERE user_id = $1';
return pool
.query(sql, [userId])
.then(_ => {
// pass
})
.catch(err => {
console.error("エラー: removeUserLastKana()");
console.error(err);
});
}
<file_sep>/src/kana-util.ts
// ===== 仮名に関するユーティリティモジュール =====
interface CharToCharMap {
[key: string]: string;
}
const han2zenMap: CharToCharMap = {
'ガ': 'ガ', 'ギ': 'ギ', 'グ': 'グ', 'ゲ': 'ゲ', 'ゴ': 'ゴ',
'ザ': 'ザ', 'ジ': 'ジ', 'ズ': 'ズ', 'ゼ': 'ゼ', 'ゾ': 'ゾ',
'ダ': 'ダ', 'ヂ': 'ヂ', 'ヅ': 'ヅ', 'デ': 'デ', 'ド': 'ド',
'バ': 'バ', 'ビ': 'ビ', 'ブ': 'ブ', 'ベ': 'ベ', 'ボ': 'ボ',
'パ': 'パ', 'ピ': 'ピ', 'プ': 'プ', 'ペ': 'ペ', 'ポ': 'ポ',
'ヴ': 'ヴ', 'ヷ': 'ヷ', 'ヺ': 'ヺ',
'ア': 'ア', 'イ': 'イ', 'ウ': 'ウ', 'エ': 'エ', 'オ': 'オ',
'カ': 'カ', 'キ': 'キ', 'ク': 'ク', 'ケ': 'ケ', 'コ': 'コ',
'サ': 'サ', 'シ': 'シ', 'ス': 'ス', 'セ': 'セ', 'ソ': 'ソ',
'タ': 'タ', 'チ': 'チ', 'ツ': 'ツ', 'テ': 'テ', 'ト': 'ト',
'ナ': 'ナ', 'ニ': 'ニ', 'ヌ': 'ヌ', 'ネ': 'ネ', 'ノ': 'ノ',
'ハ': 'ハ', 'ヒ': 'ヒ', 'フ': 'フ', 'ヘ': 'ヘ', 'ホ': 'ホ',
'マ': 'マ', 'ミ': 'ミ', 'ム': 'ム', 'メ': 'メ', 'モ': 'モ',
'ヤ': 'ヤ', 'ユ': 'ユ', 'ヨ': 'ヨ',
'ラ': 'ラ', 'リ': 'リ', 'ル': 'ル', 'レ': 'レ', 'ロ': 'ロ',
'ワ': 'ワ', 'ヲ': 'ヲ', 'ン': 'ン',
'ァ': 'ァ', 'ィ': 'ィ', 'ゥ': 'ゥ', 'ェ': 'ェ', 'ォ': 'ォ',
'ッ': 'ッ', 'ャ': 'ャ', 'ュ': 'ュ', 'ョ': 'ョ',
'。': '。', '、': '、', 'ー': 'ー', '「': '「', '」': '」', '・': '・'
};
const han2zenReg = new RegExp('(' + Object.keys(han2zenMap).join('|') + ')', 'g');
const small2bigMap: CharToCharMap = {
'ァ': 'ア', 'ィ': 'イ', 'ゥ': 'ウ', 'ェ': 'エ', 'ォ': 'オ',
'ヵ': 'カ', 'ヶ': 'ケ', 'ッ': 'ツ',
'ャ': 'ヤ', 'ュ': 'ユ', 'ョ': 'ヨ', 'ヮ': 'ワ'
};
const small2bigReg = new RegExp('(' + Object.keys(small2bigMap).join('|') + ')', 'g');
// textの中の半角の文字(英数字・カタカナ)を全角に変換する
export const han2zen = (text: string) => {
return text
.replace(han2zenReg, c => han2zenMap[c])
.replace(/[A-Za-z0-9]/g, c => String.fromCharCode(c.charCodeAt(0) + 0xFEE0))
.replace(/゙/g, '゛')
.replace(/゚/g, '゜');
}
// 小さいカタカナを大きいカタカナにする
export const small2big = (text: string) =>
text.replace(small2bigReg, c => small2bigMap[c]);
// 最初のカタカナを取得する
// kanaTextはカタカナと長音(ー)のみから成る文字列
// 最初のカタカナとは
// リンゴ -> リ
// ジャンプ -> ジ
export const firstKana = (kanaText: string) => kanaText[0];
// 最後のカタカナを取得する
// kanaTextはカタカナと長音(ー)のみから成る文字列
// 最後のカタカナとは
// バナナ -> ナ
// リンゴ -> ゴ
// トレーナー -> ナ
// ニンジャ -> ヤ
// ジンジャー -> ヤ
// ウワッ -> ツ
export const lastKana = (kanaText: string) => {
while (kanaText.slice(-1) === 'ー') {
kanaText = kanaText.slice(0, -1);
}
const lastKana = kanaText.slice(-1);
return small2big(lastKana);
}
| 99d272f768d4afef8d71114824ffa49271ccbd46 | [
"TypeScript"
] | 5 | TypeScript | yuma140902/line-echo | 71f0cc30caf4c2ed4bdd2451bda27850160127fb | 678ccbb52f5d072d5b89678d1b1c55415d2dfa1c | |
refs/heads/master | <repo_name>gatici/mano_monitoring<file_sep>/testSnmp/scale.sh
osm vnf-scale nss1 5 --scaling-group udr-vdu-manualscale --scale-out
<file_sep>/testSnmp/upload.sh
#for i in amf smf upf udm udr pcf ausf nrf
#do
#osm vnfd-create mslice1_$i'_vnfd.tar.gz'
#done
osm vnfd-create snmp_ee_vnf.tar.gz
osm nsd-create snmp_ee_ns.tar.gz
#osm nst-create mslice1_nst3.yaml
<file_sep>/jujumetric_sol06/charm_clean.sh
pushd simple
rm -rf manifest.yaml hooks src/ops venv *.charm
popd
<file_sep>/testSnmp/snmp_ee_vnf/helm-charts/eechart/source/install.sh
#!/bin/bash
##
# Licensed under the Apache License, Version 2.0 (the "License"); you may
# not use this file except in compliance with the License. You may obtain
# a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
# License for the specific language governing permissions and limitations
# under the License.
##
echo "installing libraries for snmp generator"
#apt-get install -y ...
apt-get update
apt-get install -y python3-pip unzip build-essential libsnmp-dev wget curl
curl -s https://storage.googleapis.com/golang/go1.11.8.linux-amd64.tar.gz | tar -v -C /usr/local -xz
export PATH=$PATH:/usr/local/go/bin
export GOPATH=/go
go get github.com/go-logfmt/logfmt && go get github.com/go-kit/kit/log
wget -q https://github.com/prometheus/snmp_exporter/archive/v0.17.0.tar.gz -P /tmp/ \
&& tar -C /tmp -xf /tmp/v0.17.0.tar.gz \
&& (cd /tmp/snmp_exporter-0.17.0/generator && go build) \
&& cp /tmp/snmp_exporter-0.17.0/generator/generator /usr/local/bin/snmp_generator
<file_sep>/jujumetric_sol06/replace.sh
osm nsd-delete native_charm-ns
osm vnfd-delete native_charm-vnf
osm vnfd-create native_charm_vnf.tar.gz
osm nsd-create native_charm_ns.tar.gz
<file_sep>/testSnmp/tar.sh
#for i in amf smf upf udm udr pcf ausf nrf
#do
# tar -czvf mslice1_$i'_vnfd.tar.gz' mslice1_$i'_vnfd'
#done
tar -czvf snmp_ee_ns.tar.gz snmp_ee_ns
tar -czvf snmp_ee_vnf.tar.gz snmp_ee_vnf
<file_sep>/simplehelm/create_ns.sh
osm ns-create --ns_name helmtest --nsd_name simple_ee-ns --vim_account osm_com115
<file_sep>/jujumetric_sol06/create_ns.sh
osm ns-create --ns_name jujumetrics1 --nsd_name native_charm-ns --vim_account osm_com115_v3
<file_sep>/simplehelm/simple_ee_vnf/helm-charts/eechart/source/vnf_ee.py
##
# Copyright 2019 Telefonica Investigacion y Desarrollo, S.A.U.
# This file is part of OSM
# All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
# implied.
# See the License for the specific language governing permissions and
# limitations under the License.
#
# For those usages not covered by the Apache License, Version 2.0 please
# contact with: <EMAIL>
##
import asyncio
import logging
import asyncssh
from osm_ee.exceptions import VnfException
class VnfEE:
def __init__(self, config_params):
self.logger = logging.getLogger('osm_ee.vnf')
self.config_params = config_params
async def config(self, id, params):
self.logger.debug("Execute action config params: {}".format(params))
# Config action is special, params are merged with previous config calls
self.config_params.update(params)
required_params = ["ssh-hostname"]
self._check_required_params(self.config_params, required_params)
yield "OK", "Configured"
async def touch(self, id, params):
self.logger.debug("Execute action touch params: '{}', type: {}".format(params, type(params)))
try:
self._check_required_params(params, ["file-path"])
# Check if filename is a single file or a list
file_list = params["file-path"] if isinstance(params["file-path"], list) else [params["file-path"]]
file_list_length = len(file_list)
async with asyncssh.connect(self.config_params["ssh-hostname"],
password=self.config_params.get("ssh-password"),
username=self.config_params.get("ssh-username"),
known_hosts=None) as conn:
for index, file_name in enumerate(file_list):
command = "touch {}".format(file_name)
self.logger.debug("Execute remote command: '{}'".format(command))
result = await conn.run(command)
self.logger.debug("Create command result: {}".format(result))
if result.exit_status != 0:
detailed_status = result.stderr
# TODO - ok but with some errors
else:
detailed_status = "Created file {}".format(file_name)
if index + 1 != file_list_length:
yield "PROCESSING", detailed_status
else:
yield "OK", detailed_status
except Exception as e:
self.logger.error("Error creating remote file: {}".format(repr(e)))
yield "ERROR", str(e)
async def sleep(self, id, params):
self.logger.debug("Execute action sleep, params: {}".format(params))
for i in range(3):
await asyncio.sleep(5)
self.logger.debug("Temporal result return, params: {}".format(params))
yield "PROCESSING", f"Processing {i} action id {id}"
yield "OK", f"Processed action id {id}"
@staticmethod
def _check_required_params(params, required_params):
for required_param in required_params:
if required_param not in params:
raise VnfException("Missing required param: {}".format(required_param))
<file_sep>/testSnmp/snmp_ee_vnf/helm-charts/eechart/source/vnf_ee.py
##
# All Rights Reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License");
# you may not use this file except in compliance with the License.
# You may obtain a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS,
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
# implied.
# See the License for the specific language governing permissions and
# limitations under the License.
##
import asyncio
import logging
import os
from osm_ee.exceptions import VnfException
import osm_ee.util.util_ee as util_ee
class VnfEE:
def __init__(self, config_params):
self.logger = logging.getLogger('osm_ee.vnf')
self.config_params = config_params
async def config(self, id, params):
self.logger.debug("Execute action config params: {}".format(params))
# Config action is special, params are merged with previous config calls
self.config_params.update(params)
required_params = ["ssh-hostname"]
self._check_required_params(self.config_params, required_params)
yield "OK", "Configured"
async def sleep(self, id, params):
self.logger.debug("Execute action sleep, params: {}".format(params))
for i in range(3):
await asyncio.sleep(5)
self.logger.debug("Temporal result return, params: {}".format(params))
yield "PROCESSING", f"Processing {i} action id {id}"
yield "OK", f"Processed action id {id}"
async def generate_snmp(self, id, params):
self.logger.debug("Executing SNMP exporter configuration generation\nWith params: {}".format(params))
commands = ("cp /app/vnf/generator/generator.yml ./",
"snmp_generator generate --output-path=/etc/snmp_exporter/snmp.yml",
"touch /etc/snmp_exporter/generator.yml")
for command in commands:
return_code, stdout, stderr = await util_ee.local_async_exec(command)
if return_code != 0:
yield "ERROR", "return code {}: {}".format(return_code, stderr.decode())
break
else:
yield "OK", stdout.decode()
@staticmethod
def _check_required_params(params, required_params):
for required_param in required_params:
if required_param not in params:
raise VnfException("Missing required param: {}".format(required_param))
<file_sep>/simplehelm/upload.sh
#for i in amf smf upf udm udr pcf ausf nrf
#do
#osm vnfd-create mslice1_$i'_vnfd.tar.gz'
#done
osm vnfd-create simple_ee_vnf.tar.gz
osm nsd-create simple_ee_ns.tar.gz
#osm nst-create mslice1_nst3.yaml
<file_sep>/jujumetric_sol06/native_charm_vnf/charms/simple/build/stage/src/charm.py
#!/usr/bin/env python3
##
# Copyright 2020 Canonical Ltd.
# All rights reserved.
#
# Licensed under the Apache License, Version 2.0 (the "License"); you may
# not use this file except in compliance with the License. You may obtain
# a copy of the License at
#
# http://www.apache.org/licenses/LICENSE-2.0
#
# Unless required by applicable law or agreed to in writing, software
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
# License for the specific language governing permissions and limitations
# under the License.
##
import sys
import subprocess
sys.path.append("lib")
import logging
from ops.charm import CharmBase
from ops.main import main
from ops.model import ActiveStatus
logger = logging.getLogger(__name__)
def get_metric(cmd):
out = subprocess.check_output(cmd, shell=True)
out = out.decode("utf-8").strip()
return float(out)
class MyNativeCharm(CharmBase):
def __init__(self, *args):
super().__init__(*args)
# Listen to charm events
self.framework.observe(self.on.install, self._on_install)
self.framework.observe(self.on.start, self._on_start)
self.framework.observe(self.on.config_changed, self._on_config_changed)
self.framework.observe(self.on.update_status, self._on_update_status)
self.framework.observe(self.on.collect_metrics, self.on_collect_metrics)
# Listen to the touch action event
self.framework.observe(self.on.touch_action, self.on_touch_action)
def _on_config_changed(self, _):
self.unit.status = ActiveStatus()
def _on_update_status(self, _):
self.unit.status = ActiveStatus()
def _on_install(self, event):
"""Called when the charm is being installed"""
self.model.unit.status = ActiveStatus()
def _on_start(self, event):
"""Called when the charm is being started"""
self.model.unit.status = ActiveStatus()
def on_touch_action(self, event):
"""Touch a file."""
filename = event.params["filename"]
try:
subprocess.run(["touch", filename], check=True)
event.set_results({"created": True, "filename": filename})
except subprocess.CalledProcessError as e:
event.fail("Action failed: {}".format(e))
self.model.unit.status = ActiveStatus()
def on_collect_metrics(self, event):
mem_free = get_metric("free | grep Mem | awk '{print 100*$3/$2}'")
cpu_util = get_metric("top -b -n2 | grep \"Cpu(s)\"|tail -n 1 | awk '{print $2 + $4}'")
logger.debug(f"Free memory: {mem_free}")
logger.debug(f"Cpu utilization: {cpu_util}")
event.add_metrics({"mem_free": int(mem_free), "cpu_util": int(cpu_util)})
if __name__ == "__main__":
main(MyNativeCharm)
<file_sep>/simplehelm/tar.sh
#for i in amf smf upf udm udr pcf ausf nrf
#do
tar -czvf mslice1_$i'_vnfd.tar.gz' mslice1_$i'_vnfd'
#done
tar -czvf simple_ee_ns.tar.gz simple_ee_ns
tar -czvf simple_ee_vnf.tar.gz simple_ee_vnf
<file_sep>/simplehelm/remove.sh
#osm nst-delete mslice1_nst
osm nsd-delete simple_ee-ns
#for i in amf smf upf udm udr pcf ausf nrf
#do
#osm vnfd-delete mslice1_$i'_vnfd'
#done
osm vnfd-delete simple_ee-vnf
<file_sep>/jujumetric_sol06/charm_build.sh
pushd simple
charmcraft build
cp -r build/prime/* .
cp -r venv/ops src/
popd
<file_sep>/jujumetric_sol06/simple/venv/ops-1.2.0.dist-info/DESCRIPTION.rst
# The Charmed Operator Framework
This Charmed Operator Framework simplifies [Kubernetes
operator](https://charmhub.io/about) development for
[model-driven application
management](https://juju.is/model-driven-operations).
A Kubernetes operator is a container that drives lifecycle management,
configuration, integration and daily actions for an application.
Operators simplify software management and operations. They capture
reusable app domain knowledge from experts in a software component that
can be shared.
This project extends the operator pattern to enable
[charmed operators](https://juju.is/universal-operators), not just
for Kubernetes but also operators for traditional Linux or Windows
application management.
Operators use a [Charmed Operator Lifecycle Manager
(Charmed OLM)](https://juju.is/operator-lifecycle-manager) to coordinate their
work in a cluster. The system uses Golang for concurrent event
processing under the hood, but enables the operators to be written in
Python.
## Simple, composable operators
Operators should 'do one thing and do it well'. Each operator drives a
single microservice and can be [composed with other
operators](https://juju.is/integration) to deliver a complex application.
It is better to have small, reusable operators that each drive a single
microservice very well. The operator handles instantiation, scaling,
configuration, optimisation, networking, service mesh, observability,
and day-2 operations specific to that microservice.
Operator composition takes place through declarative integration in
the OLM. Operators declare integration endpoints, and discover lines of
integration between those endpoints dynamically at runtime.
## Pure Python operators
The framework provides a standard Python library and object model that
represents the application graph, and an event distribution mechanism for
distributed system coordination and communication.
The OLM is written in Golang for efficient concurrency in event handling
and distribution. Operators can be written in any language. We recommend
this Python framework for ease of design, development and collaboration.
## Better collaboration
Operator developers publish Python libraries that make it easy to integrate
your operator with their operator. The framework includes standard tools
to distribute these integration libraries and keep them up to date.
Development collaboration happens at [Charmhub.io](https://charmhub.io/) where
operators are published along with integration libraries. Design and
code review discussions are hosted in the
[Charmhub forum](https://discourse.charmhub.io/). We recommend the
[Open Operator Manifesto](https://charmhub.io/manifesto) as a guideline for
high quality operator engineering.
## Event serialization and operator services
Distributed systems can be hard! So this framework exists to make it much
simpler to reason about operator behaviour, especially in complex deployments.
The Charmed OLM provides [operator services](https://juju.is/operator-services) such
as provisioning, event delivery, leader election and model management.
Coordination between operators is provided by a cluster-wide event
distribution system. Events are serialized to avoid race conditions in any
given container or machine. This greatly simplifies the development of
operators for high availability, scale-out and integrated applications.
## Model-driven Operator Lifecycle Manager
A key goal of the project is to improve the user experience for admins
working with multiple different operators.
We embrace [model-driven operations](https://juju.is/model-driven-operations)
in the Charmed Operator Lifecycle Manager. The model encompasses capacity,
storage, networking, the application graph and administrative access.
Admins describe the application graph of integrated microservices, and
the OLM then drives instantiation. A change in the model is propagated
to all affected operators, reducing the duplication of effort and
repetition normally found in operating a complex topology of services.
Administrative actions, updates, configuration and integration are all
driven through the OLM.
# Getting started
A package of operator code is called a charmed operator or “charm. You will use `charmcraft`
to register your operator name, and publish it when you are ready.
```
$ sudo snap install charmcraft --beta
charmcraft (beta) 0.6.0 from <NAME> (chipaca) installed
```
Charmed operators written using the Charmed Operator Framework are just Python code. The goal
is to feel natural for somebody used to coding in Python, and reasonably
easy to learn for somebody who is not a pythonista.
The dependencies of the operator framework are kept as minimal as possible;
currently that's Python 3.5 or greater, and `PyYAML` (both are included by
default in Ubuntu's cloud images from 16.04 on).
# A quick introduction
Make an empty directory `my-charm` and cd into it. Then start a new charmed operator
with:
```
$ charmcraft init
All done.
There are some notes about things we think you should do.
These are marked with ‘TODO:’, as is customary. Namely:
README.md: fill out the description
README.md: explain how to use the charm
metadata.yaml: fill out the charm's description
metadata.yaml: fill out the charm's summary
```
Charmed operators are just Python code. The entry point to your charmed operator can
be any filename, by default this is `src/charm.py` which must be executable
(and probably have `#!/usr/bin/env python3` on the first line).
You need a `metadata.yaml` to describe your charmed operator, and if you will support
configuration of your charmed operator then `config.yaml` files is required too. The
`requirements.txt` specifies any Python dependencies.
```
$ tree my-charm/
my-charm/
├── actions.yaml
├── config.yaml
├── LICENSE
├── metadata.yaml
├── README.md
├── requirements-dev.txt
├── requirements.txt
├── run_tests
├── src
│ └── charm.py
├── tests
│ ├── __init__.py
│ └── my_charm.py
```
`src/charm.py` here is the entry point to your charm code. At a minimum, it
needs to define a subclass of `CharmBase` and pass that into the framework
`main` function:
```python
from ops.charm import CharmBase
from ops.main import main
class MyCharm(CharmBase):
def __init__(self, *args):
super().__init__(*args)
self.framework.observe(self.on.start, self.on_start)
def on_start(self, event):
# Handle the start event here.
if __name__ == "__main__":
main(MyCharm)
```
That should be enough for you to be able to run
```
$ charmcraft build
Done, charm left in 'my-charm.charm'
$ juju deploy ./my-charm.charm
```
> 🛈 More information on [`charmcraft`](https://pypi.org/project/charmcraft/) can
> also be found on its [github page](https://github.com/canonical/charmcraft).
Happy charming!
# Testing your charmed operators
The operator framework provides a testing harness, so you can check your
charmed operator does the right thing in different scenarios, without having to create
a full deployment. `pydoc3 ops.testing` has the details, including this
example:
```python
harness = Harness(MyCharm)
# Do initial setup here
relation_id = harness.add_relation('db', 'postgresql')
# Now instantiate the charm to see events as the model changes
harness.begin()
harness.add_relation_unit(relation_id, 'postgresql/0')
harness.update_relation_data(relation_id, 'postgresql/0', {'key': 'val'})
# Check that charm has properly handled the relation_joined event for postgresql/0
self.assertEqual(harness.charm. ...)
```
## Talk to us
If you need help, have ideas, or would just like to chat with us, reach out on
IRC: we're in [#smooth-operator] on freenode (or try the [webchat]).
We also pay attention to [Charmhub discourse](https://discourse.charmhub.io/)
You can also deep dive into the [API docs] if that's your thing.
[webchat]: https://webchat.freenode.net/#smooth-operator
[#smooth-operator]: irc://chat.freenode.net/%23smooth-operator
[discourse]: https://discourse.juju.is/c/charming
[API docs]: https://ops.rtfd.io/
## Operator Framework development
To work in the framework itself you will need Python >= 3.5 and the
dependencies in `requirements-dev.txt` installed in your system, or a
virtualenv:
virtualenv --python=python3 env
source env/bin/activate
pip install -r requirements-dev.txt
Then you can try `./run_tests`, it should all go green.
For improved performance on the tests, ensure that you have PyYAML
installed with the correct extensions:
apt-get install libyaml-dev
pip install --force-reinstall --no-cache-dir pyyaml
If you want to build the documentation you'll need the requirements from
`docs/requirements.txt`, or in your virtualenv
pip install -r docs/requirements.txt
and then you can run `./build_docs`.
| 198dce95c19c21de8cd319904c3ba5c886602c50 | [
"Python",
"reStructuredText",
"Shell"
] | 16 | Shell | gatici/mano_monitoring | b3dc7cc842d50d2c8a5d0bb4adc98590c0c48820 | 13abded4b2b0f3a1a2dafde4e2ff11fb23246496 | |
refs/heads/master | <file_sep>click==6.6
dominate==2.2.1
Flask==0.11.1
Flask-Bootstrap==3.3.7.0
flask-script==2.0.5
gunicorn==19.6.0
itsdangerous==0.24
Jinja2==2.8
MarkupSafe==0.23
visitor==0.1.3
Werkzeug==0.11.11
<file_sep>from flask import Flask, render_template
from flask_bootstrap import Bootstrap
from flask_script import Manager
app = Flask(__name__)
bootstrap = Bootstrap(app)
manager = Manager(app)
@app.route('/')
def hello_world():
return render_template("index.html")
if __name__ == '__main__':
manager.run()
<file_sep># che.rocks
My personal website
| 4eb8e7961c6af3252807598a692615a7d10bca41 | [
"Markdown",
"Python",
"Text"
] | 3 | Text | Qumeric/che.rocks | fab9e00fed8b45e85b6faaae65cbf9fa97336817 | e1076f985dbd32344ef3f53c0f706ff34bc04d42 | |
refs/heads/master | <file_sep>package PACKAGE_NAME;
/**
* Created by haozhang on 3/5/17.
*/
public class Test {
}
<file_sep>package PACKAGE_NAME;
/**
* Created by haozhang on 3/5/17.
*/
public class FlyWithMouth {
}
| a982e60f067b2c95289c046f7dba113fc2426a7b | [
"Java"
] | 2 | Java | Howiezhang226/headfirstdesignpattern | b6313d64463e47eb07942475c5c58a0401a32555 | fdb857e46abdb6413834d61db3178c685a9f4dfb | |
refs/heads/master | <repo_name>JenyaIII/testRedux<file_sep>/src/Redux/reducers/cardReducers/cardsReducer.js
import {
ADD_USER,
GET_USERS,
GET_USERS_LOADING,
GET_USERS_ERROR,
DELETE_USER,
CHANGE_MODAL,
EDIT_FORM,
UPDATE_USER,
} from '../../actions/actionTypes';
const initialState = {
loading: false,
modalOpen: false,
editForm: false,
data: [],
currentUser: [],
};
export default function (state = initialState, action) {
switch (action.type) {
case ADD_USER: {
return {
...state,
data: [...state.data, action.payload],
};
}
case GET_USERS: {
return {
...state,
data: [...state.data, ...action.payload.data],
loading: false,
};
}
case GET_USERS_LOADING: {
return {
...state,
loading: true,
};
}
case GET_USERS_ERROR: {
return {
...state,
loading: false,
};
}
case DELETE_USER: {
return {
...state,
data: [
...state.data.filter((item) => item.id !== action.payload.id),
],
};
}
case CHANGE_MODAL: {
return {
...state,
modalOpen: action.payload,
};
}
case UPDATE_USER: {
return {
...state,
editForm: false,
modalOpen: false,
data: [
...state.data.filter((item) => item.id !== action.payload.id),
action.payload,
],
};
}
case EDIT_FORM: {
return {
...state,
currentUser: [
...state.currentUser, action.payload,
],
editForm: true,
modalOpen: true,
};
}
default:
return state;
}
}
<file_sep>/src/components/Card/Card.jsx
import React, { useEffect } from 'react';
import { useSelector, useDispatch } from 'react-redux';
import {
deleteUser,
getUsers,
getUsersLoading,
openOrCloseModal,
editUser,
} from '../../Redux/actions/actions';
import styles from './Card.module.scss';
import Loader from '../Loader/Loader';
const Card = () => {
const loading = useSelector((state) => state.cardsReducer.loading);
const users = useSelector((state) => state.cardsReducer.data);
const error = useSelector((state) => state.cardsReducer.usersError);
const dispatch = useDispatch();
useEffect(() => {
dispatch(getUsersLoading());
dispatch(getUsers());
}, [dispatch]);
if (error) {
return <p>{error}</p>;
}
const removeUser = (id, obj) => {
dispatch(deleteUser(id, obj));
};
const handleOpenEditForm = (item) => {
dispatch(editUser(item));
};
return (
<>
{loading ? (<Loader />) : ((users || users[0]).map((item) => (
<div className={styles.card} key={item.key}>
<div className={styles.cardTitle}>
<h3>{item.name}</h3>
</div>
<div className={styles.cardContent}>
Email:
{' '}
{item.email}
</div>
<div className={styles.cardContent}>
Telephone:
{' '}
{item.telephone}
</div>
<div className={styles.cardContent}>
Position:
{' '}
{item.position}
</div>
<div className={styles.cardButtons}>
<button type="button" onClick={() => handleOpenEditForm(item)}>
Edit
</button>
<button type="button" onClick={() => removeUser(item.id, item)}>
Delete
</button>
</div>
</div>
)))}
</>
);
};
export default Card;
<file_sep>/src/Redux/actions/actionTypes.js
export const ADD_USER = 'ADD_USER';
export const GET_USERS = 'GET_USERS';
export const GET_USERS_LOADING = 'GET_USERS_LOADING';
export const GET_USERS_ERROR = 'GET_USERS_ERROR';
export const DELETE_USER = 'DELETE_USER';
export const CHANGE_MODAL = 'CHANGE_MODAL';
export const EDIT_CARD = 'EDIT_CARD';
export const UPDATE_USER = 'UPDATE_USER';
export const EDIT_FORM = 'EDIT_FORM';
<file_sep>/src/components/ModalForm/AddUserForm.jsx
import React, { useState } from 'react';
import { useDispatch } from 'react-redux';
import { addUser, openOrCloseModal } from '../../Redux/actions/actions';
const AddUserForm = () => {
const [user, setUser] = useState({
id: null,
name: '',
email: '',
telephone: '',
position: '',
});
const dispatch = useDispatch();
const handleChange = (e) => {
setUser({ ...user, [e.target.name]: e.target.value });
};
const handleSubmit = (e) => {
e.preventDefault();
user.id = Date.now();
dispatch(addUser(user));
dispatch(openOrCloseModal(false));
setUser({
id: null,
name: '',
email: '',
telephone: '',
position: '',
});
};
const formList = [
{ name: 'name' },
{ name: 'email' },
{ name: 'telephone' },
{ name: 'position' },
];
return (
<div className="container">
<form onSubmit={handleSubmit}>
<div className="row">
{formList.map((item) => (
<div className="input-field" key={item.name}>
<div className="labelBlock">
<label>{item.name}</label>
</div>
<input
type="text"
name={item.name}
value={user[item.name]}
onChange={handleChange}
required
/>
</div>
))}
<div>
<button type="submit">
Add
</button>
</div>
</div>
</form>
</div>
);
};
export default AddUserForm;
<file_sep>/src/App.jsx
import React from 'react';
import './App.css';
import { useDispatch } from 'react-redux';
import { openOrCloseModal } from './Redux/actions/actions';
import Cards from './components/Cards/Cards';
import ModalForm from './components/ModalForm/Modal';
function App() {
const dispatch = useDispatch();
const handleOpenModal = (bool) => {
dispatch(openOrCloseModal(bool));
};
return (
<div className="App">
<div className="container">
<h1 style={{ color: 'grey' }}>Redux Cards</h1>
<Cards />
<div className="createCard">
<button
className="createButton"
type="button"
onClick={() => handleOpenModal(true)}
>
Create card
</button>
</div>
<div>
<ModalForm />
</div>
</div>
</div>
);
}
export default App;
<file_sep>/src/Redux/actions/actions.js
/* eslint-disable import/prefer-default-export */
import Axios from 'axios';
import {
ADD_USER,
GET_USERS,
GET_USERS_LOADING,
GET_USERS_ERROR,
DELETE_USER,
CHANGE_MODAL,
EDIT_FORM,
UPDATE_USER,
} from './actionTypes';
export const addUser = (obj) => async (dispatch) => {
try {
await Axios.post(
'http://localhost:5000/users', obj,
);
dispatch({
type: ADD_USER,
payload: obj,
});
} catch (err) {
dispatch({ type: GET_USERS_ERROR, payload: err });
console.log(`Error: ${err}`);
}
};
export const getUsers = () => async (dispatch) => {
// dispatch({ type: GET_USERS_LOADING });
try {
const userData = await Axios.get(
'http://localhost:5000/users',
);
console.log('USERDATA', userData);
dispatch({
type: GET_USERS,
payload: userData,
});
} catch (err) {
dispatch({ type: GET_USERS_ERROR, payload: err });
console.log(`Error: ${err}`);
}
};
export const getUsersLoading = () => ({
type: GET_USERS_LOADING,
});
export const deleteUser = (id, obj) => async (dispatch) => {
try {
await Axios.delete(
`http://localhost:5000/users/${id}`,
);
dispatch({
type: DELETE_USER,
payload: obj,
});
} catch (err) {
dispatch({ type: GET_USERS_ERROR, payload: err });
console.log(`Error: ${err}`);
}
};
export const openOrCloseModal = (bool) => ({
type: CHANGE_MODAL,
payload: bool,
});
export const updateUser = (item) => ({
type: UPDATE_USER,
payload: item,
});
export const editUser = (item) => ({
type: EDIT_FORM,
payload: item,
});
<file_sep>/src/components/ErrorScreen/ErrorScreen.jsx
import React from 'react';
import styles from './ErrorScreen.module.scss';
import background from '../../assets/error.jpeg';
const ErrorScreen = () => (
<>
<div className={styles.errorScreen}>
<div className={styles.errorTitle}>
<h3>Download users data ERROR !</h3>
</div>
<div className={styles.errorContent} />
</div>
</>
);
export default ErrorScreen;
| 66f9da8785136b359e68b0460d5dbc1b7a18dbf7 | [
"JavaScript"
] | 7 | JavaScript | JenyaIII/testRedux | 279db138427d959af7a261fb27b8597ff491ba47 | 8e4f888f92cb8ce313e307b5f2f2835480668caf | |
refs/heads/master | <repo_name>yaoxinmeng/PSA-Game-It<file_sep>/Assets/Scripts/CutScene6.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Video;
public class CutScene6 : MonoBehaviour
{
private GameObject game;
private VideoPlayer vp;
// Use this for initialization
void Start()
{
game = GameObject.Find("Game");
vp = GetComponent<VideoPlayer>();
if (PlayerPrefs.HasKey("Cutscene6"))
{
game.SetActive(true);
gameObject.SetActive(false);
}
else
{
game.SetActive(false);
}
}
// Update is called once per frame
void Update()
{
if (!vp.isPlaying)
{
PlayerPrefs.SetInt("Cutscene6", 1);
game.SetActive(true);
gameObject.SetActive(false);
}
}
}
<file_sep>/Assets/Scripts/StageSelection.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
// ========================================
// Unlock Stars Key Reference
// 0 - 0 star
// 1 - 1 stars
// 2 - 2 stars
// 3 - 3 stars
// 4 - locked
// ========================================
public class StageSelection : MonoBehaviour {
private UiController uiController;
private LevelManager levelManager;
private float timeTaken;
private int sceneIndex;
private string starsKey;
private GameObject oneStar;
private GameObject twoStar;
private GameObject threeStar;
private GameObject stage10;
private GameObject stage11;
private GameObject stage12;
private GameObject stage13;
private GameObject stage20;
private GameObject stage21;
private GameObject stage22;
private GameObject stage23;
private GameObject stage24;
private GameObject stage30;
private GameObject stage31;
private GameObject stage32;
private GameObject stage33;
private GameObject stage34;
private GameObject stage40;
private GameObject stage41;
private GameObject stage42;
private GameObject stage43;
private GameObject stage44;
private GameObject stage50;
private GameObject stage51;
private GameObject stage52;
private GameObject stage53;
private GameObject stage54;
private GameObject stage60;
private GameObject stage61;
private GameObject stage62;
private GameObject stage63;
private GameObject stage64;
private GameObject stage70;
private GameObject stage71;
private GameObject stage72;
private GameObject stage73;
private GameObject stage74;
private GameObject stageminilocked;
private GameObject stageminiunlocked;
void Start(){
uiController = GetComponent<UiController>();
levelManager = GetComponent<LevelManager>();
sceneIndex = SceneManager.GetActiveScene().buildIndex;
initialiseStars();
oneStar = GameObject.Find("GUI/Level Completed/Stars/1 Star");
twoStar = GameObject.Find("GUI/Level Completed/Stars/2 Star");
threeStar = GameObject.Find("GUI/Level Completed/Stars/3 Star");
stage10 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 1/Stage 1-0");
stage11 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 1/Stage 1-1");
stage12 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 1/Stage 1-2");
stage13 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 1/Stage 1-3");
stage20 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 2/Stage 2-0");
stage21 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 2/Stage 2-1");
stage22 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 2/Stage 2-2");
stage23 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 2/Stage 2-3");
stage24 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 2/Stage 2-4");
stage30 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 3/Stage 3-0");
stage31 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 3/Stage 3-1");
stage32 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 3/Stage 3-2");
stage33 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 3/Stage 3-3");
stage34 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 3/Stage 3-4");
stage40 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 4/Stage 4-0");
stage41 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 4/Stage 4-1");
stage42 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 4/Stage 4-2");
stage43 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 4/Stage 4-3");
stage44 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 4/Stage 4-4");
stage50 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 5/Stage 5-0");
stage51 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 5/Stage 5-1");
stage52 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 5/Stage 5-2");
stage53 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 5/Stage 5-3");
stage54 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 5/Stage 5-4");
stage60 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 6/Stage 6-0");
stage61 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 6/Stage 6-1");
stage62 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 6/Stage 6-2");
stage63 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 6/Stage 6-3");
stage64 = GameObject.Find("GUI/Stage Select Menu/Page One/Stage 6/Stage 6-4");
stage70 = GameObject.Find("GUI/Stage Select Menu/Page Two/Stage 7/Stage 7-0");
stage71 = GameObject.Find("GUI/Stage Select Menu/Page Two/Stage 7/Stage 7-1");
stage72 = GameObject.Find("GUI/Stage Select Menu/Page Two/Stage 7/Stage 7-2");
stage73 = GameObject.Find("GUI/Stage Select Menu/Page Two/Stage 7/Stage 7-3");
stage74 = GameObject.Find("GUI/Stage Select Menu/Page Two/Stage 7/Stage 7-4");
stageminilocked = GameObject.Find("GUI/Stage Select Menu/Page Two/Stage Mini/Locked");
stageminiunlocked = GameObject.Find("GUI/Stage Select Menu/Page Two/Stage Mini/Unlocked");
}
void Update(){
displayStages();
}
void displayStages(){
switch(PlayerPrefs.GetInt("SceneOneStars")){
case 0:
stage10.SetActive(true);
stage11.SetActive(false);
stage12.SetActive(false);
stage13.SetActive(false);
break;
case 1:
stage10.SetActive(false);
stage11.SetActive(true);
stage12.SetActive(false);
stage13.SetActive(false);
break;
case 2:
stage10.SetActive(false);
stage11.SetActive(false);
stage12.SetActive(true);
stage13.SetActive(false);
break;
case 3:
stage10.SetActive(false);
stage11.SetActive(false);
stage12.SetActive(false);
stage13.SetActive(true);
break;
}
switch(PlayerPrefs.GetInt("SceneTwoStars")){
case 0:
stage20.SetActive(true);
stage21.SetActive(false);
stage22.SetActive(false);
stage23.SetActive(false);
stage24.SetActive(false);
break;
case 1:
stage20.SetActive(false);
stage21.SetActive(true); //
stage22.SetActive(false);
stage23.SetActive(false);
stage24.SetActive(false);
break;
case 2:
stage20.SetActive(false);
stage21.SetActive(false);
stage22.SetActive(true); //
stage23.SetActive(false);
stage24.SetActive(false);
break;
case 3:
stage20.SetActive(false);
stage21.SetActive(false);
stage22.SetActive(false);
stage23.SetActive(true); //
stage24.SetActive(false);
break;
case 4:
stage20.SetActive(false);
stage21.SetActive(false);
stage22.SetActive(false);
stage23.SetActive(false);
stage24.SetActive(true); //
break;
}
switch(PlayerPrefs.GetInt("SceneThreeStars")){
case 0:
stage30.SetActive(true);
stage31.SetActive(false);
stage32.SetActive(false);
stage33.SetActive(false);
stage34.SetActive(false);
break;
case 1:
stage30.SetActive(false);
stage31.SetActive(true);
stage32.SetActive(false);
stage33.SetActive(false);
stage34.SetActive(false);
break;
case 2:
stage30.SetActive(false);
stage31.SetActive(false);
stage32.SetActive(true);
stage33.SetActive(false);
stage34.SetActive(false);
break;
case 3:
stage30.SetActive(false);
stage31.SetActive(false);
stage32.SetActive(false);
stage33.SetActive(true);
stage34.SetActive(false);
break;
case 4:
stage30.SetActive(false);
stage31.SetActive(false);
stage32.SetActive(false);
stage33.SetActive(false);
stage34.SetActive(true);
break;
}
switch(PlayerPrefs.GetInt("SceneFourStars")){
case 0:
stage40.SetActive(true);
stage41.SetActive(false);
stage42.SetActive(false);
stage43.SetActive(false);
stage44.SetActive(false);
break;
case 1:
stage40.SetActive(false);
stage41.SetActive(true);
stage42.SetActive(false);
stage43.SetActive(false);
stage44.SetActive(false);
break;
case 2:
stage40.SetActive(false);
stage41.SetActive(false);
stage42.SetActive(true);
stage43.SetActive(false);
stage44.SetActive(false);
break;
case 3:
stage40.SetActive(false);
stage41.SetActive(false);
stage42.SetActive(false);
stage43.SetActive(true);
stage44.SetActive(false);
break;
case 4:
stage40.SetActive(false);
stage41.SetActive(false);
stage42.SetActive(false);
stage43.SetActive(false);
stage44.SetActive(true);
break;
}
switch(PlayerPrefs.GetInt("SceneFiveStars")){
case 0:
stage50.SetActive(true);
stage51.SetActive(false);
stage52.SetActive(false);
stage53.SetActive(false);
stage54.SetActive(false);
break;
case 1:
stage50.SetActive(false);
stage51.SetActive(true);
stage52.SetActive(false);
stage53.SetActive(false);
stage54.SetActive(false);
break;
case 2:
stage50.SetActive(false);
stage51.SetActive(false);
stage52.SetActive(true);
stage53.SetActive(false);
stage54.SetActive(false);
break;
case 3:
stage50.SetActive(false);
stage51.SetActive(false);
stage52.SetActive(false);
stage53.SetActive(true);
stage54.SetActive(false);
break;
case 4:
stage50.SetActive(false);
stage51.SetActive(false);
stage52.SetActive(false);
stage53.SetActive(false);
stage54.SetActive(true);
break;
}
switch(PlayerPrefs.GetInt("SceneSixStars")){
case 0:
stage60.SetActive(true);
stage61.SetActive(false);
stage62.SetActive(false);
stage63.SetActive(false);
stage64.SetActive(false);
break;
case 1:
stage60.SetActive(false);
stage61.SetActive(true);
stage62.SetActive(false);
stage63.SetActive(false);
stage64.SetActive(false);
break;
case 2:
stage60.SetActive(false);
stage61.SetActive(false);
stage62.SetActive(true);
stage63.SetActive(false);
stage64.SetActive(false);
break;
case 3:
stage60.SetActive(false);
stage61.SetActive(false);
stage62.SetActive(false);
stage63.SetActive(true);
stage64.SetActive(false);
break;
case 4:
stage60.SetActive(false);
stage61.SetActive(false);
stage62.SetActive(false);
stage63.SetActive(false);
stage64.SetActive(true);
break;
}
switch(PlayerPrefs.GetInt("SceneSevenStars")){
case 0:
stage70.SetActive(true);
stage71.SetActive(false);
stage72.SetActive(false);
stage73.SetActive(false);
stage74.SetActive(false);
break;
case 1:
stage70.SetActive(false);
stage71.SetActive(true);
stage72.SetActive(false);
stage73.SetActive(false);
stage74.SetActive(false);
break;
case 2:
stage70.SetActive(false);
stage71.SetActive(false);
stage72.SetActive(true);
stage73.SetActive(false);
stage74.SetActive(false);
break;
case 3:
stage70.SetActive(false);
stage71.SetActive(false);
stage72.SetActive(false);
stage73.SetActive(true);
stage74.SetActive(false);
break;
case 4:
stage70.SetActive(false);
stage71.SetActive(false);
stage72.SetActive(false);
stage73.SetActive(false);
stage74.SetActive(true);
break;
}
switch(PlayerPrefs.GetInt("SceneMini")){
case 1: //1 means scene mini unlocked!
stageminiunlocked.SetActive(true);
stageminilocked.SetActive(false);
break;
case 4: //4 means scene mini locked!
stageminiunlocked.SetActive(false);
stageminilocked.SetActive(true);
break;
}
}
void initialiseStars() { //if player has not played the game before, initialise the stars for all stages to 0
if (PlayerPrefs.HasKey("SceneOneStars") == false){
PlayerPrefs.SetInt("SceneOneStars", 0);
PlayerPrefs.Save();
}
if (PlayerPrefs.HasKey("SceneTwoStars") == false){
PlayerPrefs.SetInt("SceneTwoStars", 4);
PlayerPrefs.Save();
}
if (PlayerPrefs.HasKey("SceneThreeStars") == false){
PlayerPrefs.SetInt("SceneThreeStars", 4);
PlayerPrefs.Save();
}
if (PlayerPrefs.HasKey("SceneFourStars") == false){
PlayerPrefs.SetInt("SceneFourStars", 4);
PlayerPrefs.Save();
}
if (PlayerPrefs.HasKey("SceneFiveStars") == false){
PlayerPrefs.SetInt("SceneFiveStars", 4);
PlayerPrefs.Save();
}
if (PlayerPrefs.HasKey("SceneSixStars") == false){
PlayerPrefs.SetInt("SceneSixStars", 4);
PlayerPrefs.Save();
}
if (PlayerPrefs.HasKey("SceneSevenStars") == false){
PlayerPrefs.SetInt("SceneSevenStars", 4);
PlayerPrefs.Save();
}
if (PlayerPrefs.HasKey("SceneMini") == false){
PlayerPrefs.SetInt("SceneMini", 4);
PlayerPrefs.Save();
}
}
public void checkStarsObtained(){
timeTaken = uiController.currentTime;
switch(sceneIndex){
//according to the scene index, each stage has different timing to get different stars
//adjust the conditions for acheiving the stars in the respective if statements
case 2:
if (timeTaken < 30f){
PlayerPrefs.SetInt("SceneOneStars", 3);
if (PlayerPrefs.GetInt("SceneTwoStars") == 4){
PlayerPrefs.SetInt("SceneTwoStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(true);
}
else if (timeTaken < 40f){
PlayerPrefs.SetInt("SceneOneStars", 2);
if (PlayerPrefs.GetInt("SceneTwoStars") == 4){
PlayerPrefs.SetInt("SceneTwoStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(false);
}
else {
PlayerPrefs.SetInt("SceneOneStars", 1);
if (PlayerPrefs.GetInt("SceneTwoStars") == 4){
PlayerPrefs.SetInt("SceneTwoStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(false);
threeStar.SetActive(false);
}
break;
case 3:
if (timeTaken < 30f){
PlayerPrefs.SetInt("SceneTwoStars", 3);
if (PlayerPrefs.GetInt("SceneThreeStars") == 4){
PlayerPrefs.SetInt("SceneThreeStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(true);
}
else if (timeTaken < 40f){
PlayerPrefs.SetInt("SceneTwoStars", 2);
if (PlayerPrefs.GetInt("SceneThreeStars") == 4){
PlayerPrefs.SetInt("SceneThreeStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(false);
}
else{
PlayerPrefs.SetInt("SceneTwoStars", 1);
if (PlayerPrefs.GetInt("SceneThreeStars") == 4){
PlayerPrefs.SetInt("SceneThreeStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(false);
threeStar.SetActive(false);
}
break;
case 4:
if (timeTaken < 30f){
PlayerPrefs.SetInt("SceneThreeStars", 3);
if (PlayerPrefs.GetInt("SceneFourStars") == 4){
PlayerPrefs.SetInt("SceneFourStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(true);
}
else if (timeTaken < 40f){
PlayerPrefs.SetInt("SceneThreeStars", 2);
if (PlayerPrefs.GetInt("SceneFourStars") == 4){
PlayerPrefs.SetInt("SceneFourStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(false);
}
else{
PlayerPrefs.SetInt("SceneThreeStars", 1);
if (PlayerPrefs.GetInt("SceneFourStars") == 4){
PlayerPrefs.SetInt("SceneFourStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(false);
threeStar.SetActive(false);
}
break;
case 5:
if (timeTaken < 55f){
PlayerPrefs.SetInt("SceneFourStars", 3);
if (PlayerPrefs.GetInt("SceneFiveStars") == 4){
PlayerPrefs.SetInt("SceneFiveStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(true);
}
else if (timeTaken < 65f){
PlayerPrefs.SetInt("SceneFourStars", 2);
if (PlayerPrefs.GetInt("SceneFiveStars") == 4){
PlayerPrefs.SetInt("SceneFiveStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(false);
}
else{
PlayerPrefs.SetInt("SceneFourStars", 1);
if (PlayerPrefs.GetInt("SceneFiveStars") == 4){
PlayerPrefs.SetInt("SceneFiveStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(false);
threeStar.SetActive(false);
}
break;
case 6:
if (timeTaken < 55f){
PlayerPrefs.SetInt("SceneFiveStars", 3);
if (PlayerPrefs.GetInt("SceneSixStars") == 4){
PlayerPrefs.SetInt("SceneSixStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(true);
}
else if (timeTaken < 65f){
PlayerPrefs.SetInt("SceneFiveStars", 2);
if (PlayerPrefs.GetInt("SceneSixStars") == 4){
PlayerPrefs.SetInt("SceneSixStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(false);
}
else{
PlayerPrefs.SetInt("SceneFiveStars", 1);
if (PlayerPrefs.GetInt("SceneSixStars") == 4){
PlayerPrefs.SetInt("SceneSixStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(false);
threeStar.SetActive(false);
}
break;
case 7:
if (timeTaken < 30f){
PlayerPrefs.SetInt("SceneSixStars", 3);
if (PlayerPrefs.GetInt("SceneSevenStars") == 4){
PlayerPrefs.SetInt("SceneSevenStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(true);
}
else if (timeTaken < 40f){
PlayerPrefs.SetInt("SceneSixStars", 2);
if (PlayerPrefs.GetInt("SceneSevenStars") == 4){
PlayerPrefs.SetInt("SceneSevenStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(false);
}
else{
PlayerPrefs.SetInt("SceneSixStars", 1);
if (PlayerPrefs.GetInt("SceneSevenStars") == 4){
PlayerPrefs.SetInt("SceneSevenStars", 0);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(false);
threeStar.SetActive(false);
}
break;
case 8:
if (timeTaken < 30f){
PlayerPrefs.SetInt("SceneSevenStars", 3);
if (PlayerPrefs.GetInt("SceneMini") == 4){
PlayerPrefs.SetInt("SceneMini", 1);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(true);
}
else if (timeTaken < 40f){
PlayerPrefs.SetInt("SceneSevenStars", 2);
if (PlayerPrefs.GetInt("SceneMini") == 4){
PlayerPrefs.SetInt("SceneMini", 1);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(true);
threeStar.SetActive(false);
}
else{
PlayerPrefs.SetInt("SceneSevenStars", 1);
if (PlayerPrefs.GetInt("SceneMini") == 4){
PlayerPrefs.SetInt("SceneMini", 1);
}
PlayerPrefs.Save();
oneStar.SetActive(true);
twoStar.SetActive(false);
threeStar.SetActive(false);
}
break;
}
}
public void loadStage1(){
levelManager.loadSelectedLevel(2);
}
public void loadStage2(){
if (PlayerPrefs.GetInt("SceneTwoStars") != 4){
levelManager.loadSelectedLevel(3);
}
}
public void loadStage3(){
if (PlayerPrefs.GetInt("SceneThreeStars") != 4){
levelManager.loadSelectedLevel(4);
}
}
public void loadStage4(){
if (PlayerPrefs.GetInt("SceneFourStars") != 4){
levelManager.loadSelectedLevel(5);
}
}
public void loadStage5(){
if (PlayerPrefs.GetInt("SceneFiveStars") != 4){
levelManager.loadSelectedLevel(6);
}
}
public void loadStage6(){
if (PlayerPrefs.GetInt("SceneSixStars") != 4){
levelManager.loadSelectedLevel(7);
}
}
public void loadStage7(){
if (PlayerPrefs.GetInt("SceneSevenStars") != 4){
levelManager.loadSelectedLevel(8);
}
}
public void loadFinalStage(){
if (PlayerPrefs.GetInt("SceneMini") != 4){
levelManager.loadSelectedLevel(9);
}
}
}
<file_sep>/Assets/Scripts/LevelManager.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class LevelManager : MonoBehaviour {
private int sceneIndex;
void Start() {
sceneIndex = SceneManager.GetActiveScene().buildIndex;
}
public void nextLevel () {
SceneManager.LoadScene (sceneIndex + 1);
UiController.nextLevel = false;
UiController.gameClear = false;
}
public void loadHomeScene(){
SceneManager.LoadScene (0); //assuming home scene still has build index of 0
UiController.nextLevel = false;
}
public void loadCurrentLevel(){
SceneManager.LoadScene(sceneIndex);
}
public void loadSelectedLevel(int scene){
SceneManager.LoadScene(scene);
UiController.stageSelect = false;
UiController.options = false;
UiController.pause = false;
}
}
<file_sep>/Assets/Scripts/RotatingSecurityCamera.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RotatingSecurityCamera : MonoBehaviour {
public float rotatingSpeed;
private bool flip = false;
public float leftLimitRotation = -45f;
public float rightLimitRotation = 45f;
private SpriteRenderer ren;
private float iniAlpha;
public bool isOn;
void Start()
{
ren = transform.GetChild(0).GetComponent<SpriteRenderer>();
iniAlpha = ren.color.a;
}
// Update is called once per frame
void Update ()
{
if (isOn)
{
float angle = transform.localEulerAngles.z;
angle = (angle > 180) ? angle - 360 : angle;
ren.color = new Vector4(ren.color.r, ren.color.g, ren.color.b, iniAlpha);
if (angle > rightLimitRotation)
{
rotatingSpeed = -1f * rotatingSpeed;
}
if (angle < leftLimitRotation)
{
rotatingSpeed = -1f * rotatingSpeed;
}
transform.Rotate(0f, 0f, -rotatingSpeed * Time.deltaTime);
tag = "Enemy";
transform.GetChild(0).tag = "Enemy";
}
else
{
tag = "Security Camera";
transform.GetChild(0).tag = "Security Camera";
transform.Rotate(0, 0, 0);
ren.color = new Vector4(ren.color.r, ren.color.g, ren.color.b, 0f);
}
}
}
<file_sep>/Assets/Scripts/Mini Game/LeftScreenMini.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class LeftScreenMini : MonoBehaviour {
public float forceOnPlayer;
// Use this for initialization
void Start () {
}
// Update is called once per frame
void Update () {
}
void OnTriggerExit2D(Collider2D collision)
{
GameObject obj = collision.gameObject;
if (obj.tag == "ContainerMini" || obj.tag == "Background" || obj.tag == "Ground")
Destroy(obj);
if (obj.tag == "Security Camera")
Destroy(obj.transform.parent.gameObject);
}
}
<file_sep>/Assets/Scripts/ATV.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ATV : MonoBehaviour {
[Range(0, 20f)]
public float patrolSpeed;
public bool isLeft; //check for direction
public bool isOn;
private float x; //initial x-coordinate
private bool changeDirection;
private Rigidbody2D rb;
private Animator anim;
void Start()
{
x = transform.position.x;
rb = GetComponent<Rigidbody2D>();
// anim = GetComponent<Animator>();
changeDirection = false;
anim = GetComponent<Animator>();
if (isLeft)
transform.Rotate(0, 180, 0); //if ATV moves left initially
}
void Update()
{
if (isOn)
{
gameObject.tag = "Enemy";
transform.Find("Top Surface").gameObject.tag = "Enemy";
if (isLeft)
rb.velocity = new Vector2(-patrolSpeed, 0);
else
rb.velocity = new Vector2(patrolSpeed, 0);
if (changeDirection)
{
if (transform.position.x < x)
{
isLeft = false;
}
else if (transform.position.x > x)
{
isLeft = true;
}
transform.Rotate(0, 180, 0);
changeDirection = false;
}
}
else
{
rb.velocity = Vector2.zero;
gameObject.tag = "Container";
transform.Find("Top Surface").gameObject.tag = "Container";
}
}
void OnTriggerEnter2D(Collider2D col)
{
GameObject obj = col.gameObject;
if (obj.gameObject.tag == "Checkpoint")
{
if (obj == transform.parent.GetChild(1).GetChild(0).gameObject || obj == transform.parent.GetChild(1).GetChild(1).gameObject)
{
changeDirection = true;
}
}
}
void OnTriggerExit2D(Collider2D col)
{
GameObject obj = col.gameObject;
if (obj.gameObject.tag == "Checkpoint")
{
if (obj == transform.parent.GetChild(1).GetChild(0).gameObject || obj == transform.parent.GetChild(1).GetChild(1).gameObject)
{
changeDirection = false;
}
}
}
}
<file_sep>/Assets/Scripts/MainChar2D.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MainChar2D : MonoBehaviour
{
public AudioClip jumpSFX;
public AudioClip landSFX;
public AudioClip dieSFX;
public AudioClip winSFX;
private AudioSource jumpSource;
private AudioSource landSource;
private AudioSource dieSource;
private AudioSource winSource;
public AudioSource[] audioList;
[Range(1, 10)]
public float walkingSpeed;
[Range(1, 10)]
public float jumpHeight;
[Range (0, 1)]
public float invisibilityBufferTime;
private Animator anim;
private LevelManager levelManager;
private Rigidbody2D rb;
private Spriter2UnityDX.EntityRenderer ren;
private float iniAlpha;
private bool faceLeft = false;
private bool isInvisible = false;
[HideInInspector]
public bool hitLWall = false;
[HideInInspector]
public bool hitRWall = false;
[HideInInspector]
public bool onCrane = false;
void Awake()
{
}
// Use this for initialization
void Start()
{
ren = GetComponent<Spriter2UnityDX.EntityRenderer>();
GetComponent<BoxCollider2D>().isTrigger = false;
anim = GetComponent<Animator>();
rb = GetComponent<Rigidbody2D>();
iniAlpha = ren.Color.a;
jumpSource = gameObject.AddComponent<AudioSource>();
jumpSource.clip = jumpSFX;
landSource = gameObject.AddComponent<AudioSource>();
landSource.clip = landSFX;
dieSource = gameObject.AddComponent<AudioSource>();
dieSource.clip = dieSFX;
winSource = gameObject.AddComponent<AudioSource>();
winSource.clip = winSFX;
audioList = GetComponents<AudioSource>();
foreach (AudioSource audio in audioList)
{
audio.volume = PlayerPrefs.GetFloat("SFXvolume");
audio.playOnAwake = false;
}
}
// Update is called once per frame
void Update ()
{
if (!anim.GetBool("isDead"))
{
if (!UiController.playerDied)
{
//for sounds
foreach (AudioSource audio in audioList)
{
audio.volume = PlayerPrefs.GetFloat("SFXvolume");
}
//for animation
if (GuiController.isTappedRight)
{
anim.SetBool("isRunningRight", true);
hitLWall = false;
if (!hitRWall)
transform.Translate(Vector3.right * walkingSpeed * Time.deltaTime);
}
else
{
anim.SetBool("isRunningRight", false);
}
if (GuiController.isTappedLeft)
{
anim.SetBool("isRunningLeft", true);
hitRWall = false;
if (!hitLWall)
transform.Translate(Vector3.left * walkingSpeed * Time.deltaTime);
}
else
{
anim.SetBool("isRunningLeft", false);
}
//for jump and drop
if (GuiController.isJumping && GuiController.isGrounded)
{
jumpSource.PlayOneShot(jumpSFX, 0.8f);
rb.velocity = new Vector2(rb.velocity.x, jumpHeight);
anim.SetTrigger("isJumping");
GuiController.isJumping = false;
}
else
anim.SetBool("isJumping", false);
if (GuiController.isDropping)
{
GetComponent<BoxCollider2D>().isTrigger = true;
rb.velocity = new Vector2(rb.velocity.x, -0.5f * jumpHeight);
}
//for inivisble container
if (isInvisible)
{
ren.Color = new Vector4(ren.Color.r, ren.Color.g, ren.Color.b, 0.5f);
gameObject.layer = 12;
}
else
{
ren.Color = new Vector4(ren.Color.r, ren.Color.g, ren.Color.b, iniAlpha);
gameObject.layer = 9;
}
}
else
{
dieSource.PlayOneShot(dieSFX, 1);
anim.SetBool("isDead", true);
}
}
else
{
gameObject.layer = 12;
}
//establishes isTrigger based on direction of travel
if (rb.velocity.y > 0)
{
if (!onCrane)
GetComponent<BoxCollider2D>().isTrigger = true;
GuiController.isGrounded = false;
GuiController.onGround = false;
}
else if (rb.velocity.y < 0)
{
if (!GuiController.isDropping)
GetComponent<BoxCollider2D>().isTrigger = false;
GuiController.isGrounded = false;
GuiController.onGround = false;
}
else
{
GetComponent<BoxCollider2D>().isTrigger = false;
}
}
void OnTriggerEnter2D(Collider2D collider)
{
GameObject obj = collider.gameObject;
// Leave the method if not colliding with defender
if (obj.tag == "GameController")
{
UiController.gameClear = true;
winSource.PlayOneShot(winSFX, 0.8f);
}
if (obj.tag == "Enemy")
{
UiController.playerDied = true;
}
if (obj.tag == "Invisible Container")
isInvisible = true;
}
void OnCollisionEnter2D(Collision2D col)
{
GameObject obj = col.gameObject;
if (obj.tag == "Wall")
{
if (obj.transform.position.x < transform.position.x)
hitLWall = true;
else
hitRWall = true;
rb.velocity = new Vector3(0, rb.velocity.y);
}
if (obj.tag == "Ground" || obj.tag == "Container")
{
GuiController.isGrounded = true;
landSource.PlayOneShot(landSFX, 0.5f);
}
if (obj.tag == "CraneContainer" && obj.transform.parent.Find("Crane").Find("Control Base").Find("Cable And Hook").GetComponent<Crane>().hasContainer)
{
landSource.PlayOneShot(landSFX, 0.5f);
GuiController.isGrounded = false;
}
if (obj.tag == "Ground")
GuiController.onGround = true;
if (obj.tag == "Enemy")
{
UiController.playerDied = true;
}
}
void OnTriggerExit2D(Collider2D collision)
{
GameObject obj = collision.gameObject;
if (obj.tag == "Invisible Container")
Invoke("becomeVisible", invisibilityBufferTime);
if (obj.tag == "Wall")
{
if (obj.transform.position.x < transform.position.x)
hitLWall = false;
else
hitRWall = false;
}
}
void OnTriggerStay2D(Collider2D collision)
{
GameObject obj = collision.gameObject;
if (obj.tag == "Wall")
{
if (obj.transform.position.x < transform.position.x)
hitLWall = true;
else
hitRWall = true;
rb.velocity = new Vector3(0, rb.velocity.y);
}
}
void OnCollisionStay2D(Collision2D col)
{
GameObject obj = col.gameObject;
if (obj.tag == "Ground" || obj.tag == "Container")
{
onCrane = false;
GuiController.isGrounded = true;
}
if (obj.tag == "Ground")
GuiController.onGround = true;
if (obj.tag == "CraneContainer")
{
if (obj.transform.parent.Find("Crane").Find("Control Base").Find("Cable And Hook").GetComponent<Crane>().hasContainer)
{
onCrane = true;
GuiController.isGrounded = false;
obj.transform.GetChild(0).GetComponent<CraneContainer>().hasPlayer = true;
}
else
{
GuiController.isGrounded = true;
onCrane = false;
obj.transform.GetChild(0).GetComponent<CraneContainer>().hasPlayer = true;
}
}
}
void OnCollisionExit2D(Collision2D collision)
{
GameObject obj = collision.gameObject;
if (obj.tag == "Wall")
{
if (obj.transform.position.x < transform.position.x)
hitLWall = false;
else
hitRWall = false;
}
if (obj.tag == "CraneContainer")
{
if (rb.velocity.y > 0)
rb.velocity = new Vector2(rb.velocity.x, 0);
else
rb.velocity = new Vector2(rb.velocity.x, -0.5f * jumpHeight);
}
}
void becomeVisible()
{
isInvisible = false;
}
}
<file_sep>/Assets/Scripts/Mini Game/ScoreMini.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ScoreMini : MonoBehaviour {
public GameObject screen;
public float screenVelocity;
public float score = 0;
// Use this for initialization
void Start () {
}
// Update is called once per frame
void Update () {
screenVelocity = screen.GetComponent<ScreenMini>().initialspeed;
if (!UiControllerMini.playerDied)
{
score += screenVelocity * Time.deltaTime;
GetComponent<Text>().text = "" + (int)score;
}
}
}
<file_sep>/Assets/Scripts/Mini Game/RightScreenMini.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class RightScreenMini : MonoBehaviour {
public GameObject container;
public GameObject securitycamera;
public GameObject securitycamera2;
public GameObject background;
public GameObject foreground;
public float yOffsetContainer;
public float xOffsetContainer;
public float yOffsetTopCamera;
public float yOffsetBotCamera;
public float iniMinCameraGap;
public float finMinCameraGap;
public float altCameraProb;
public float xOffsetBg;
public float yOffsetBg;
public float xOffsetFore;
public float yOffsetFore;
public int cameraTime;
private float decreaseGap;
// Use this for initialization
void Start () {
if (iniMinCameraGap > finMinCameraGap)
decreaseGap = (iniMinCameraGap - finMinCameraGap) / cameraTime;
}
// Update is called once per frame
void Update () {
}
void OnTriggerEnter2D(Collider2D other)
{
if(other.gameObject.tag == "ContainerMini")
{
Instantiate(container, new Vector2(transform.position.x + xOffsetContainer, transform.position.y + yOffsetContainer), Quaternion.identity);
}
if (other.gameObject.tag == "Security Camera")
{
float x = Random.Range(iniMinCameraGap, 2 * iniMinCameraGap);
if (iniMinCameraGap > finMinCameraGap)
iniMinCameraGap = iniMinCameraGap - decreaseGap;
if(Random.value > altCameraProb)
{
if (Random.value < 0.5)
{
Instantiate(securitycamera, new Vector2(transform.position.x + x, transform.position.y + yOffsetBotCamera), Quaternion.identity);
}
else
{
Instantiate(securitycamera, new Vector2(transform.position.x + x, transform.position.y + yOffsetTopCamera), Quaternion.identity);
}
}
else
{
Instantiate(securitycamera2, new Vector2(transform.position.x + x, transform.position.y + yOffsetTopCamera), Quaternion.identity);
}
}
if (other.gameObject.tag == "Background")
{
Instantiate(background, new Vector2(transform.position.x + xOffsetBg, transform.position.y + yOffsetBg), Quaternion.identity);
}
if (other.gameObject.tag == "Ground")
{
Instantiate(foreground, new Vector2(transform.position.x + xOffsetFore, transform.position.y + yOffsetFore), Quaternion.identity);
}
}
}
<file_sep>/Assets/Scripts/Mini Game/ScreenMini.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class ScreenMini : MonoBehaviour {
private Rigidbody2D rb;
[Range(0, 2)]
public float initialspeed;
[Range(0, 10)]
public float finalspeed;
[Range(0, 1)]
public float acceleration;
[Range(0, 10)]
public float timedelay;
private float accumtime = 0f;
// Use this for initialization
void Start ()
{
rb = GetComponent<Rigidbody2D>();
rb.velocity = new Vector2(initialspeed, 0f);
}
// Update is called once per frame
void Update ()
{
if (UiControllerMini.playerDied || !UiControllerMini.gameStart)
rb.velocity = Vector2.zero;
else
{
accumtime += Time.deltaTime;
rb.velocity = new Vector2(initialspeed, 0f);
if (accumtime > timedelay)
{
if (initialspeed < finalspeed)
{
initialspeed += acceleration;
rb.velocity = new Vector2(initialspeed, 0f);
}
accumtime = 0f;
}
}
}
}
<file_sep>/Assets/Scripts/Scene2Tutorial.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Scene2Tutorial : MonoBehaviour {
// Use this for initialization
void Start () {
//checks if tutorial has been completed previously
if (PlayerPrefs.GetInt("Tutorial 2") == 1)
transform.GetChild(0).gameObject.SetActive(false);
else
{
transform.GetChild(0).gameObject.SetActive(true);
UiController.tutorialOn = true;
}
transform.GetChild(1).gameObject.SetActive(false);
}
// Update is called once per frame
void Update () {
if (transform.GetChild(0).gameObject.activeInHierarchy)
{
if (!UiController.tutorialOn)
{
transform.GetChild(0).gameObject.SetActive(false);
PlayerPrefs.SetInt("Tutorial 2", 1);
}
}
if (GuiController.canDismiss)
transform.GetChild(1).gameObject.SetActive(true);
else
transform.GetChild(1).gameObject.SetActive(false);
}
}
<file_sep>/Assets/Scripts/CameraController.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CameraController : MonoBehaviour {
private Transform cam_position;
public GameObject main_char;
// Use this for initialization
void Start () {
cam_position = GetComponent<Transform>();
cam_position.position = new Vector3(main_char.GetComponent<Transform>().position.x, main_char.GetComponent<Transform>().position.y, -10);
}
// Update is called once per frame
void Update () {
cam_position.position = new Vector3(main_char.GetComponent<Transform>().position.x, main_char.GetComponent<Transform>().position.y, -10);
}
}
<file_sep>/Assets/Scripts/Crane.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Crane : MonoBehaviour {
[Range (0, 10)]
public float speed;
private GameObject craneContainer;
private Rigidbody2D rbBase;
private Rigidbody2D rbHook;
private bool up = false;
private bool down = false;
private bool left = false;
private bool right = false;
[HideInInspector]
public bool hasContainer = false;
public bool isOn;
// Use this for initialization
void Start () {
rbBase = transform.parent.gameObject.GetComponent<Rigidbody2D>();
rbHook = GetComponent<Rigidbody2D>();
craneContainer = transform.parent.parent.parent.Find("CraneContainer").gameObject;
left = true;
untrapPlayer();
}
// Update is called once per frame
void Update () {
if (UiController.sceneIndex == 1)
GetComponent<AudioSource>().volume = 0;
else
GetComponent<AudioSource>().volume = PlayerPrefs.GetFloat("SFXvolume") * GetComponent<AudioSource>().volume;
if (isOn)
{
if (up)
{
rbHook.velocity = new Vector2(0, speed);
rbBase.velocity = Vector2.zero;
}
else if (down)
{
rbBase.velocity = Vector2.zero;
rbHook.velocity = new Vector2(0, -speed);
}
else if (left)
{
rbHook.velocity = new Vector2(-speed, 0);
rbBase.velocity = new Vector2(-speed, 0);
}
else if (right)
{
rbHook.velocity = new Vector2(speed, 0);
rbBase.velocity = new Vector2(speed, 0);
}
else
{
rbBase.velocity = Vector2.zero;
rbHook.velocity = Vector2.zero;
}
}
else
{
rbBase.velocity = Vector2.zero;
rbHook.velocity = Vector2.zero;
}
if (hasContainer)
craneContainer.GetComponent<Rigidbody2D>().velocity = rbHook.velocity;
if (craneContainer.GetComponent<BoxCollider2D>().isTrigger)
untrapPlayer();
}
void OnTriggerEnter2D(Collider2D other)
{
GameObject obj = other.gameObject;
if (obj.tag == "Bottom Left Anchor" || obj.tag == "Bottom Right Anchor")
{
if(obj == transform.parent.parent.parent.Find("Crane BLeft Anchor").gameObject || obj == transform.parent.parent.parent.Find("Crane BRight Anchor").gameObject)
{
up = true;
down = false;
rbHook.velocity = new Vector2(0, speed);
if (hasContainer)
{
craneContainer.GetComponent<Rigidbody2D>().velocity = Vector2.zero;
hasContainer = false;
untrapPlayer();
}
else
{
craneContainer.GetComponent<Rigidbody2D>().velocity = rbHook.velocity;
hasContainer = true;
trapPlayer();
}
GetComponent<Collider2D>().enabled = false;
Invoke("resetcollider", 0.5f);
}
}
else if (obj.tag == "Top Left Anchor" && obj == transform.parent.parent.parent.Find("Crane TLeft Anchor").gameObject)
{
if (up)
{
if(hasContainer)
{
right = true;
up = false;
}
else
{
down = true;
up = false;
}
}
if (left)
{
down = true;
left = false;
}
}
else if (obj.tag == "Top Right Anchor" && obj == transform.parent.parent.parent.Find("Crane TRight Anchor").gameObject)
{
if (up)
{
if (hasContainer)
{
left = true;
up = false;
}
else
{
down = true;
up = false;
}
}
if (right)
{
down = true;
right = false;
}
}
}
void resetcollider()
{
GetComponent<Collider2D>().enabled = true;
}
void trapPlayer()
{
transform.Find("Left Sub Arm").GetComponent<BoxCollider2D>().enabled = true;
transform.Find("Right Sub Arm").GetComponent<BoxCollider2D>().enabled = true;
}
void untrapPlayer()
{
transform.Find("Left Sub Arm").GetComponent<BoxCollider2D>().enabled = false;
transform.Find("Right Sub Arm").GetComponent<BoxCollider2D>().enabled = false;
}
}
<file_sep>/Library/Collab/Original/Assets/Scripts/UiController.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class UiController : MonoBehaviour {
public static bool playerDied = false; //toggles true on death, maybe can show cutscene
public static bool pause = false; // used to pause game
public static bool nextLevel = false; //checks if level passed
public static bool stageSelect = false;
public static bool options = false;
public static bool gameClear = false;
public static bool dying = false;
//For all tutorials
public static bool tutorialOn = false;
// public static bool surePrompt = false;
private bool confirm = false;
private bool deathmenu = false;
private LevelManager levelManager;
private StageSelection stageSelection;
private GameObject startMenu;
private GameObject pauseMenu;
private GameObject optionsMenu;
private GameObject stageSelectMenu;
private GameObject sureBoPrompt;
private GameObject pauseButton;
private GameObject completionScreen;
private GameObject dieMenu;
private GameObject zoomButton;
private GameObject movementControls;
public GameObject stagePageOne;
public GameObject stagePageTwo;
public GameObject timingTopLeft;
public Text pauseTiming;
public Text levelCompletionTiming;
public Text deadTiming;
public static int sceneIndex;
private float startTime;
public float currentTime;
void Start() {
levelManager = GetComponent<LevelManager>();
stageSelection = GetComponent<StageSelection>();
completionScreen = GameObject.Find("GUI/Level Completed");
startMenu = GameObject.Find("GUI/Start Menu");
pauseButton = GameObject.Find("GUI/Pause Button");
pauseMenu = GameObject.Find("GUI/Pause Menu");
movementControls = GameObject.Find("GUI/Movement Controls");
stageSelectMenu = GameObject.Find("GUI/Stage Select Menu");
dieMenu = GameObject.Find("GUI/Die Screen");
optionsMenu = GameObject.Find("GUI/Options Menu");
zoomButton = GameObject.Find("GUI/Zoom Button");
//stagePageOne = GameObject.Find("GUI/Stage Select Menu/Page One");
//stagePageTwo = GameObject.Find("GUI/Stage Select Menu/Page Two");
sceneIndex = SceneManager.GetActiveScene().buildIndex;
pauseTiming = GameObject.Find("Time Taken").GetComponent<Text>();
startTime = Time.time;
if (sceneIndex == 1)
{
startMenu.SetActive(true);
pauseButton.SetActive(false);
timingTopLeft.SetActive(false);
movementControls.SetActive(false);
stageSelectMenu.SetActive(false);
pauseMenu.SetActive(false);
optionsMenu.SetActive(false);
completionScreen.SetActive(false);
dieMenu.SetActive(false);
zoomButton.SetActive(false);
}
else
{
pauseButton.SetActive(true);
timingTopLeft.SetActive(true);
startMenu.SetActive(false);
movementControls.SetActive(true);
stageSelectMenu.SetActive(false);
pauseMenu.SetActive(false);
optionsMenu.SetActive(false);
completionScreen.SetActive(false);
dieMenu.SetActive(false);
zoomButton.SetActive(true);
}
}
// Update is called once per frame
void Update () {
if (playerDied)
{
if (!deathmenu)
{
Invoke("deathMenu", 2f);
deathmenu = true;
}
//for BGM
GetComponent<AudioSource>().volume = 0;
GetComponent<Image>().color = new Vector4(GetComponent<Image>().color.r, GetComponent<Image>().color.g, GetComponent<Image>().color.b, 0.2f);
}
else
{
//Track the time taken for each stage.
showTimeTaken();
GetComponent<AudioSource>().volume = PlayerPrefs.GetFloat("BGMvolume");
if (sceneIndex != 1)
{
if (tutorialOn)
{
Time.timeScale = 0f;
GetComponent<Image>().color = new Vector4(GetComponent<Image>().color.r, GetComponent<Image>().color.g, GetComponent<Image>().color.b, 0.3f);
timingTopLeft.SetActive(false);
movementControls.SetActive(false);
}
else
{
Time.timeScale = 1f;
GetComponent<Image>().color = new Vector4(GetComponent<Image>().color.r, GetComponent<Image>().color.g, GetComponent<Image>().color.b, 0f);
timingTopLeft.SetActive(true);
movementControls.SetActive(true);
}
}
else
{
GetComponent<Image>().color = new Vector4(GetComponent<Image>().color.r, GetComponent<Image>().color.g, GetComponent<Image>().color.b, 0.3f);
}
if (pause)
{
Time.timeScale = 0f;
pauseMenu.SetActive(true);
}
else
{
Time.timeScale = 1.0f;
pauseMenu.SetActive(false);
}
if (gameClear)
{
Time.timeScale = 0;
stageSelection.checkStarsObtained();
completionScreen.SetActive(true);
}
if (nextLevel)
{
Time.timeScale = 1;
completionScreen.SetActive(false);
print("Load Next level!");
levelManager.nextLevel();
}
if (stageSelect)
{
startMenu.SetActive(false);
pauseButton.SetActive(true);
movementControls.SetActive(false);
stageSelectMenu.SetActive(true);
stagePageOne.SetActive(true);
pauseMenu.SetActive(false);
optionsMenu.SetActive(false);
completionScreen.SetActive(false);
dieMenu.SetActive(false);
}
if (options)
{
startMenu.SetActive(false);
pauseButton.SetActive(true);
movementControls.SetActive(false);
stageSelectMenu.SetActive(false);
stagePageOne.SetActive(true);
pauseMenu.SetActive(false);
optionsMenu.SetActive(true);
completionScreen.SetActive(false);
dieMenu.SetActive(false);
}
}
// if (surePrompt){
// startMenu.SetActive(false);
// pauseButton.SetActive(false);
// movementControls.SetActive(false);
// stageSelectMenu.SetActive(false);
// stagePageOne.SetActive(false);
// pauseMenu.SetActive(false);
// optionsMenu.SetActive(false);
// completionScreen.SetActive(false);
// }
}
public void closeScreen()
{
if (options)
{
options = false;
optionsMenu.SetActive(false);
}
if (stageSelect)
{
stageSelect = false;
stageSelectMenu.SetActive(false);
}
if (sceneIndex == 1)
startMenu.SetActive(true);
else
pause = true;
}
public void pauseGame() {
if (pause) {
pause = false;
print("Resume Game!");
} else {
pause = true;
print("Pause Game!");
}
}
public void startGame() {
options = false;
nextLevel = true;
print("Start Game!");
}
public void toggleSelectStage(){
if(stageSelect){
stageSelect = false;
} else {
dying = false;
options = false;
stageSelect = true;
print("Select Stage");
}
}
public void returnToHome(){
options = false;
stageSelect = false;
pause = false;
playerDied = false;
levelManager.loadHomeScene();
print("Return to Home View!");
}
//for controlling the stage select menu
public void previousPage(){
stagePageOne.SetActive(!stagePageOne.activeInHierarchy);
print("show page 1!");
stagePageTwo.SetActive(false);
}
public void nextPage(){
if (stagePageTwo.activeInHierarchy == false){
stagePageOne.SetActive(!stagePageOne.activeInHierarchy);
print("show page 2!");
stagePageTwo.SetActive(true);
}
}
public void toggleOptions(){
if (options){
options = false;
print("Close Options");
} else {
stageSelect = false;
options = true;
print("Options");
}
}
public void reloadLevel(){
if (sceneIndex == 1){
print("Do not do anything when it's in the main screen. Why would you want to restart when you are in the main screen?!");
} else {
pause = false;
options = false;
gameClear = false;
Time.timeScale = 1;
playerDied = false;
levelManager.loadCurrentLevel();
print("Reload level!");
}
}
private void showTimeTaken(){
currentTime = Time.time - startTime;
currentTime = Mathf.Round(currentTime * 100f) / 100f;
pauseTiming.text = currentTime.ToString();
levelCompletionTiming.text = currentTime.ToString();
timingTopLeft.GetComponentInChildren<Text>().text = currentTime.ToString();
deadTiming.text = currentTime.ToString();
}
public void playButton(){
if (sceneIndex == 1){
startGame();
} else {
if (options){
options = false;
optionsMenu.SetActive(false);
}
pauseGame();
}
}
public void nextStage(){
nextLevel = true;
}
public void deathMenu()
{
dieMenu.SetActive(true);
}
// For Debugging purposes //
public void eraseAllData(){
PlayerPrefs.DeleteAll();
returnToHome();
print("Cleared all data! Stages back to square 1");
}
public void unlock2(){
PlayerPrefs.SetInt("SceneTwoStars", 1);
returnToHome();
}
public void unlock6(){
PlayerPrefs.SetInt("SceneSixStars", 1);
returnToHome();
}
public void loadFinalCut(){
levelManager.loadSelectedLevel(10);
}
}
<file_sep>/Assets/Scripts/CraneTutorial.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class CraneTutorial : MonoBehaviour {
List<GameObject> list = new List<GameObject>();
private GameObject[] tutorialList;
private float accumtime = 0;
public static int n;
public float displayTime;
// Use this for initialization
void Start () {
foreach(Transform child in transform)
{
if (child != transform)
list.Add(child.gameObject);
}
tutorialList = list.ToArray();
for (int x = 0; x < transform.childCount; x++)
{
if (x == 0)
tutorialList[x].SetActive(true);
else
tutorialList[x].SetActive(false);
}
n = 0;
}
// Update is called once per frame
void Update () {
if (n == 0)
tutorialList[n].SetActive(true);
if (n < transform.childCount)
{
accumtime += Time.deltaTime;
if (accumtime > displayTime)
{
tutorialList[n].SetActive(false);
accumtime = 0;
n++;
if (n < transform.childCount)
tutorialList[n].SetActive(true);
}
}
}
}
<file_sep>/Assets/Scripts/FinalScene.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FinalScene : MonoBehaviour {
private LevelManager levelManager;
private UnityEngine.Video.VideoPlayer vp;
// Use this for initialization
void Awake () {
levelManager = GetComponent<LevelManager>();
vp = GetComponent<UnityEngine.Video.VideoPlayer>();
}
// Update is called once per frame
void Update () {
Invoke("checkIfFinished", 1f);
}
void checkIfFinished(){
if (vp.isPlaying == false){
levelManager.loadSelectedLevel(0);
}
}
}
<file_sep>/Assets/Scripts/Scene1Tutorial.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Scene1Tutorial : MonoBehaviour {
public GameObject tutorialWall;
public GameObject arrow1;
public GameObject arrow2;
public GameObject arrow3;
private float accumtime;
private int n = 0;
//Tutorial 1
private bool tutorial1 = true;
//Tutorial 2
private bool tutorial2 = false;
//Tutorial 3
private bool tutorial3 = false;
//Tutorial 4
private bool zoomedIn = false;
private bool zoomedOut = false;
private bool tutorial4 = false;
//Tutorial 5
private bool tutorial5 = false;
//Tutorial 6
private bool tutorial6 = false;
// Use this for initialization
void Start () {
//checks if tutorial has been completed previously
if (PlayerPrefs.GetInt("Tutorial 1") == 1)
{
tutorialWall.SetActive(false);
gameObject.SetActive(false);
}
else
{
transform.GetChild(0).gameObject.SetActive(true);
for (int x = 1; x < transform.childCount; x++)
{
transform.GetChild(x).gameObject.SetActive(false);
}
arrow1.SetActive(true);
arrow2.SetActive(false);
arrow3.SetActive(false);
UiController.tutorialOn = true;
}
}
// Update is called once per frame
void Update () {
if (!UiController.tutorialOn)
{
for (int x = 0; x < transform.childCount; x++)
{
transform.GetChild(x).gameObject.SetActive(false);
}
}
// For Tutorial 1
if (!arrow1.activeInHierarchy && tutorial1 && !tutorial2)
{
UiController.tutorialOn = true;
transform.GetChild(1).gameObject.SetActive(true);
arrow2.SetActive(true);
tutorial2 = true;
}
// For Tutorial 2
if (!arrow2.activeInHierarchy && tutorial2 && !tutorial3)
{
UiController.tutorialOn = true;
transform.GetChild(2).gameObject.SetActive(true);
arrow3.SetActive(true);
tutorial3 = true;
}
// For Tutorial 3
if (!arrow3.activeInHierarchy && tutorial3 && !tutorial4)
{
UiController.tutorialOn = true;
transform.GetChild(3).gameObject.SetActive(true);
tutorial4 = true;
}
// For Tutorial 4
if (GuiController.zoomed)
zoomedIn = true;
if (zoomedIn && !GuiController.zoomed)
zoomedOut = true;
if (zoomedOut && zoomedIn && tutorial4 && !tutorial5)
{
UiController.tutorialOn = true;
GuiController.zoomed = false;
transform.GetChild(4).gameObject.SetActive(true);
tutorialWall.SetActive(false);
tutorial5 = true;
}
//For Tutorial 5
if (!UiController.tutorialOn && tutorial5 && !tutorial6)
{
UiController.tutorialOn = true;
transform.GetChild(5).gameObject.SetActive(true);
tutorial6 = true;
}
//For Tutorial 6
if (tutorial6 && !UiController.tutorialOn)
{
transform.GetChild(5).gameObject.SetActive(false);
}
if (GuiController.canDismiss)
transform.GetChild(6).gameObject.SetActive(true);
else
transform.GetChild(6).gameObject.SetActive(false);
}
}
<file_sep>/Assets/Scripts/CharDialogs.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CharDialogs : MonoBehaviour {
private float startTime;
private float currentTime;
[Tooltip("Select a prime number for better effect e.g 7, 11, 13, 17...")]
public float intervalToShowDialog = 11f;
public float durationToShowDialog = 3f;
private int choiceOfDialog;
private GameObject currentDialog;
private bool showDialog = true;
// Use this for initialization
void Start () {
for (int n = 0; n < transform.childCount; n++)
{
transform.GetChild(n).gameObject.SetActive(false);
}
startTime = Time.time;
}
// Update is called once per frame
void Update () {
decideShowDialog();
}
void decideShowDialog(){
currentTime = Time.time - startTime;
if (Mathf.Round(currentTime) % Mathf.Round(intervalToShowDialog) == 0 && showDialog){
showDialog = false;
choiceOfDialog = Random.Range(0, transform.childCount);
currentDialog = transform.GetChild(choiceOfDialog).gameObject;
currentDialog.SetActive(true);
StartCoroutine(closeDialog());
}
}
IEnumerator closeDialog()
{
yield return new WaitForSeconds(durationToShowDialog);
currentDialog.SetActive(false);
showDialog = true;
}
}
<file_sep>/Assets/Scripts/SecurityCamera.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SecurityCamera : MonoBehaviour {
public bool isOn;
private SpriteRenderer ren;
private float iniAlpha;
// Use this for initialization
void Start () {
ren = GetComponent<SpriteRenderer>();
iniAlpha = ren.color.a;
}
// Update is called once per frame
void Update () {
if (isOn)
{
tag = "Enemy";
ren.color = new Vector4(ren.color.r, ren.color.g, ren.color.b, iniAlpha);
}
else
{
ren.color = new Vector4(ren.color.r, ren.color.g, ren.color.b, 0f);
tag = "Security Camera";
}
}
}
<file_sep>/Assets/Scripts/CutScene.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CutScene : MonoBehaviour {
private LevelManager levelManager;
private UnityEngine.Video.VideoPlayer vp;
// Use this for initialization
void Awake () {
levelManager = GetComponent<LevelManager>();
vp = GetComponent<UnityEngine.Video.VideoPlayer>();
if (PlayerPrefs.HasKey("watchedIntro") == false){
PlayerPrefs.SetInt("watchedIntro", 1);
} else {
levelManager.loadSelectedLevel(2);
}
}
// Update is called once per frame
void Update () {
Invoke("checkIfFinished", 1f);
}
void checkIfFinished(){
if (vp.isPlaying == false){
levelManager.loadSelectedLevel(2);
}
}
}
<file_sep>/Assets/Scripts/GuiController.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine.UI;
using UnityEngine;
public class GuiController : MonoBehaviour
{
public static bool canDismiss = false;
public static bool isTappedLeft = false;
public static bool isTappedRight = false;
public Camera playercamera;
[Range(0, 1)]
public float orthoZoomSpeed;
[Range(5, 15)]
public float maxCameraSize;
[Range(0, 5)]
public float tutorialTime;
private float initialCameraSize;
private float accumtime = 0;
public static bool isJumping = false;
public static bool isGrounded = true;
public static bool isDropping = false;
public static bool onGround = false;
public static bool downIsPressed = false;
public static bool zoomed = false;
private bool debugTap = false; //for debugging
void Start () {
initialCameraSize = playercamera.orthographicSize;
}
void Awake () {
isTappedLeft = false;
isTappedRight = false;
}
void Update()
{
if (UiController.tutorialOn)
{
stopLeft(); stopRight();
accumtime += Time.deltaTime;
if (accumtime > tutorialTime)
{
canDismiss = true;
if (Input.touchCount > 0)
{
UiController.tutorialOn = false;
canDismiss = false;
accumtime = 0;
}
if (debugTap) //for debug
{
UiController.tutorialOn = false;
canDismiss = false;
accumtime = 0;
debugTap = false;
}
}
}
if (zoomed)
playercamera.orthographicSize = maxCameraSize;
else
playercamera.orthographicSize = initialCameraSize;
}
public void detectJump ()
{
if (isGrounded)
isJumping = true;
else
isJumping = false;
}
public void detectDrop()
{
if (isGrounded && !onGround && !downIsPressed)
{
isDropping = true;
downIsPressed = true;
Invoke("stopDrop", 0.4f);
Invoke("stopPress", 0.7f);
}
}
void stopPress()
{
downIsPressed = false;
}
void stopDrop ()
{
isDropping = false;
}
public void detectLeft()
{
isTappedLeft = true;
}
public void stopLeft()
{
isTappedLeft = false;
}
public void detectRight()
{
isTappedRight = true;
}
public void stopRight()
{
isTappedRight = false;
}
public void zoomOut()
{
zoomed = true;
}
public void zoomIn()
{
zoomed = false;
}
public void tap() //for debug
{
debugTap = true;
}
}
<file_sep>/Assets/Scripts/Checkpoint.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Checkpoint : MonoBehaviour {
private float accumTime = 0;
public float displacement;
public float period;
// Use this for initialization
void Start () {
}
// Update is called once per frame
void Update () {
accumTime += Time.deltaTime;
if (accumTime < period)
transform.Translate(Time.deltaTime * displacement, 0, 0);
else
{
accumTime = 0;
displacement = -displacement;
}
}
void OnTriggerEnter2D(Collider2D other)
{
if (other.gameObject.tag == "Player")
{
gameObject.SetActive(false);
}
}
}
<file_sep>/Assets/Scripts/Scene3Tutorial.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Scene3Tutorial : MonoBehaviour {
//Crane tutorial
public GameObject mainChar;
// Use this for initialization
void Start()
{
//checks if tutorial has been completed previously
if (PlayerPrefs.GetInt("Tutorial 3") == 1)
{
transform.GetChild(0).gameObject.SetActive(false);
transform.GetChild(1).gameObject.SetActive(false);
}
else
{
transform.GetChild(0).gameObject.SetActive(true);
transform.GetChild(1).gameObject.SetActive(false);
UiController.tutorialOn = true;
}
transform.GetChild(2).gameObject.SetActive(false);
//Crane trivia
if (transform.Find("Crane Tutorial") != null)
transform.Find("Crane Tutorial").gameObject.SetActive(false);
}
// Update is called once per frame
void Update()
{
//For Tutorial 1
if (transform.GetChild(0).gameObject.activeInHierarchy)
{
if (!UiController.tutorialOn)
{
transform.GetChild(0).gameObject.SetActive(false);
transform.GetChild(1).gameObject.SetActive(true);
UiController.tutorialOn = true;
}
}
//For Tutorial 2
if (transform.GetChild(1).gameObject.activeInHierarchy)
{
if (!UiController.tutorialOn)
{
transform.GetChild(1).gameObject.SetActive(false);
PlayerPrefs.SetInt("Tutorial 3", 1);
}
}
if (GuiController.canDismiss)
transform.GetChild(2).gameObject.SetActive(true);
else
transform.GetChild(2).gameObject.SetActive(false);
//For crane trivia
if (mainChar.GetComponent<MainChar2D>().onCrane)
transform.Find("Crane Tutorial").gameObject.SetActive(true);
else
{
transform.Find("Crane Tutorial").gameObject.SetActive(false);
CraneTutorial.n = 0;
}
}
}
<file_sep>/Assets/Scripts/Mini Game/MainCharMini.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class MainCharMini : MonoBehaviour
{
public AudioClip jumpSFX;
public AudioClip landSFX;
public AudioClip dieSFX;
private AudioSource jumpSource;
private AudioSource landSource;
private AudioSource dieSource;
[Range(1, 10)]
public float walkingSpeed;
[Range(1, 10)]
public float jumpHeight;
private Animator anim;
private LevelManager levelManager;
private Rigidbody2D rb;
private Spriter2UnityDX.EntityRenderer ren;
private float iniAlpha;
private bool faceLeft = false;
//[HideInInspector]
public bool hitLWall = false;
//[HideInInspector]
public bool hitRWall = false;
void Awake()
{
jumpSource = gameObject.AddComponent<AudioSource>();
jumpSource.clip = jumpSFX;
landSource = gameObject.AddComponent<AudioSource>();
landSource.clip = landSFX;
dieSource = gameObject.AddComponent<AudioSource>();
dieSource.clip = dieSFX;
}
// Use this for initialization
void Start()
{
ren = GetComponent<Spriter2UnityDX.EntityRenderer>();
GetComponent<BoxCollider2D>().isTrigger = false;
anim = GetComponent<Animator>();
rb = GetComponent<Rigidbody2D>();
iniAlpha = ren.Color.a;
}
// Update is called once per frame
void Update()
{
if (!anim.GetBool("isDead"))
{
if (!UiControllerMini.playerDied)
{
//for animation
if (GuiController.isTappedRight && !hitRWall)
{
anim.SetBool("isRunningRight", true);
transform.Translate(Vector3.right * walkingSpeed * Time.deltaTime);
hitLWall = false;
}
else
anim.SetBool("isRunningRight", false);
if (GuiController.isTappedLeft)
{
anim.SetBool("isRunningLeft", true);
if (!hitLWall)
{
transform.Translate(Vector3.left * walkingSpeed * Time.deltaTime);
}
hitRWall = false;
}
else
{
anim.SetBool("isRunningLeft", false);
hitRWall = false;
}
//for jump and drop
if (GuiController.isJumping && GuiController.isGrounded)
{
jumpSource.PlayOneShot(jumpSFX, 0.8f);
anim.SetTrigger("isJumping");
rb.velocity = new Vector3(0, jumpHeight, 0);
GuiController.isJumping = false;
}
else
anim.SetBool("isJumping", false);
if (GuiController.isDropping)
{
gameObject.GetComponent<BoxCollider2D>().isTrigger = true;
rb.velocity = new Vector2(rb.velocity.x, -0.5f * jumpHeight);
}
}
else
{
dieSource.PlayOneShot(dieSFX, 1);
anim.SetBool("isDead", true);
}
}
//establishes isTrigger based on direction of travel
if (rb.velocity.y > 0)
{
gameObject.GetComponent<BoxCollider2D>().isTrigger = true;
GuiController.isGrounded = false;
GuiController.onGround = false;
}
else if (rb.velocity.y < 0)
{
if (!GuiController.isDropping)
gameObject.GetComponent<BoxCollider2D>().isTrigger = false;
GuiController.isGrounded = false;
GuiController.onGround = false;
}
else
gameObject.GetComponent<BoxCollider2D>().isTrigger = false;
}
void OnTriggerEnter2D(Collider2D collider)
{
GameObject obj = collider.gameObject;
if (obj.tag == "Enemy")
UiControllerMini.playerDied = true;
if (obj.tag == "Wall")
{
if (obj.transform.position.x < transform.position.x)
{
transform.Translate(Vector3.right * obj.transform.parent.gameObject.GetComponent<Rigidbody2D>().velocity.x * Time.deltaTime);
hitLWall = true;
}
else
{
rb.velocity = new Vector2(0, rb.velocity.y);
hitRWall = true;
}
}
}
void OnCollisionEnter2D(Collision2D col)
{
GameObject obj = col.gameObject;
if (obj.tag == "Ground" || obj.tag == "Container")
{
GuiController.isGrounded = true;
landSource.PlayOneShot(landSFX, 0.5f);
}
if (obj.tag == "Ground")
GuiController.onGround = true;
if (obj.tag == "Enemy")
UiControllerMini.playerDied = true;
}
void OnTriggerStay2D(Collider2D collision)
{
GameObject obj = collision.gameObject;
if (obj.tag == "Wall")
{
if (obj.transform.position.x < transform.position.x)
{
transform.Translate(Vector3.right * obj.transform.parent.gameObject.GetComponent<Rigidbody2D>().velocity.x * Time.deltaTime);
hitLWall = true;
}
else
{
rb.velocity = new Vector2(0, rb.velocity.y);
hitRWall = true;
}
}
}
void OnTriggerExit(Collider other)
{
if(other.gameObject.tag == "Wall")
{
hitRWall = false;
hitLWall = false;
}
}
void OnCollisionStay2D(Collision2D col)
{
GameObject obj = col.gameObject;
if (obj.tag == "Ground" || obj.tag == "Container")
GuiController.isGrounded = true;
if (obj.tag == "Ground")
GuiController.onGround = true;
}
}
<file_sep>/Assets/Scripts/Mini Game/UiControllerMini.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class UiControllerMini : MonoBehaviour
{
public static bool gameStart = false;
public static bool playerDied = false; //toggles true on death, maybe can show cutscene
public static bool pause = false; // used to pause game
public static bool nextLevel = false; //checks if level passed
public static bool options = false;
// public static bool surePrompt = false;
private bool confirm = false;
private float accumtime = 0f;
private LevelManager levelManager;
private GameObject optionsMenu;
private GameObject sureBoPrompt;
private GameObject pauseButton;
private GameObject levelComplete;
private GameObject score;
private GameObject tutorial;
private GameObject movementControls;
private int sceneIndex;
void Start()
{
levelManager = GetComponent<LevelManager>();
pauseButton = GameObject.Find("GUI/Pause Button");
movementControls = GameObject.Find("GUI/Movement Controls");
levelComplete = GameObject.Find("GUI/Level Complete");
score = GameObject.Find("GUI/Score");
optionsMenu = GameObject.Find("GUI/Options Menu");
tutorial = GameObject.Find("GUI/Tutorial");
//stagePageOne = GameObject.Find("GUI/Stage Select Menu/Page One");
//stagePageTwo = GameObject.Find("GUI/Stage Select Menu/Page Two");
sceneIndex = SceneManager.GetActiveScene().buildIndex;
levelComplete.SetActive(false);
optionsMenu.SetActive(false);
movementControls.SetActive(false);
score.SetActive(false);
pauseButton.SetActive(false);
gameStart = false;
playerDied = false;
pause = false;
nextLevel = false;
options = false;
}
// Update is called once per frame
void Update()
{
//pre-game instructions
if (!gameStart)
{
//for BGM
GetComponent<AudioSource>().volume = 0.2f * PlayerPrefs.GetFloat("BGMvolume");
accumtime += Time.deltaTime;
if (tutorial.transform.GetChild(0).gameObject.activeInHierarchy && accumtime > 2f)
{
tutorial.transform.GetChild(0).gameObject.SetActive(false);
tutorial.transform.GetChild(1).gameObject.SetActive(true);
accumtime = 0f;
}
else if (tutorial.transform.GetChild(1).gameObject.activeInHierarchy && accumtime > 1f)
{
tutorial.transform.GetChild(1).gameObject.SetActive(false);
tutorial.transform.GetChild(2).gameObject.SetActive(true);
accumtime = 0f;
}
else if (tutorial.transform.GetChild(2).gameObject.activeInHierarchy && accumtime > 1f)
{
tutorial.transform.GetChild(2).gameObject.SetActive(false);
tutorial.transform.GetChild(3).gameObject.SetActive(true);
accumtime = 0f;
}
else if (tutorial.transform.GetChild(3).gameObject.activeInHierarchy && accumtime > 1f)
{
tutorial.transform.GetChild(3).gameObject.SetActive(false);
gameStart = true;
}
}
else if (!playerDied)
{
//for BGM
GetComponent<AudioSource>().volume = PlayerPrefs.GetFloat("BGMvolume");
pauseButton.SetActive(true);
movementControls.SetActive(true);
score.SetActive(true);
optionsMenu.SetActive(false);
GetComponent<Image>().color = new Vector4(GetComponent<Image>().color.r, GetComponent<Image>().color.g, GetComponent<Image>().color.b, 0f);
}
else
{
//for BGM
GetComponent<AudioSource>().volume = 0;
levelComplete.SetActive(true);
pauseButton.SetActive(false);
GetComponent<Image>().color = new Vector4(GetComponent<Image>().color.r, GetComponent<Image>().color.g, GetComponent<Image>().color.b, 0.2f);
score.SetActive(false);
movementControls.SetActive(false);
Invoke("nextStage", 3.5f);
}
if (pause)
{
Time.timeScale = 0f;
optionsMenu.SetActive(true);
}
else
{
Time.timeScale = 1.0f;
optionsMenu.SetActive(false);
}
if (nextLevel)
{
Time.timeScale = 1;
print("Load Next level!");
levelManager.nextLevel();
}
if (options)
{
pauseButton.SetActive(false);
movementControls.SetActive(false);
optionsMenu.SetActive(true);
}
// if (surePrompt){
// pauseButton.SetActive(false);
// movementControls.SetActive(false);
// stageSelectMenu.SetActive(false);
// stagePageOne.SetActive(false);
// optionsMenu.SetActive(false);
// }
}
// void initialiseVolume(){
// if (PlayerPrefs.HasKey("MusicVol") == false){
// PlayerPrefs.SetFloat("MusicVol", 0.9f);
// }
// if (PlayerPrefs.HasKey("SFXVol") == false){
// PlayerPrefs.SetFloat("SFXVol", 0.9f);
// }
// }
public void pauseGame()
{
if (pause)
{
pause = false;
print("Resume Game!");
}
else
{
pause = true;
print("Pause Game!");
}
}
public void startGame()
{
options = false;
nextLevel = true;
print("Start Game!");
}
public void returnToHome()
{
options = false;
pause = false;
levelManager.loadHomeScene();
print("Return to Home View!");
}
public void toggleOptions()
{
if (options)
{
options = false;
print("Close Options");
}
else
{
options = true;
print("Options");
}
}
public void reloadLevel()
{
if (sceneIndex == 0)
{
print("Do not do anything when it's in the main screen. Why would you want to restart when you are in the main screen?!");
}
else
{
pause = false;
options = false;
gameStart = false;
Time.timeScale = 1;
levelManager.loadCurrentLevel();
print("Reload level!");
}
}
public void playButton()
{
if (sceneIndex == 0)
{
if (PlayerPrefs.GetInt("SceneOneStars") != 4)
levelManager.loadSelectedLevel(1); //goes to video cutscene
if (PlayerPrefs.GetInt("SceneTwoStars") != 4)
levelManager.loadSelectedLevel(3);
if (PlayerPrefs.GetInt("SceneThreeStars") != 4)
levelManager.loadSelectedLevel(4);
if (PlayerPrefs.GetInt("SceneFourStars") != 4)
levelManager.loadSelectedLevel(5);
if (PlayerPrefs.GetInt("SceneFiveStars") != 4)
levelManager.loadSelectedLevel(6);
if (PlayerPrefs.GetInt("SceneSixStars") != 4)
levelManager.loadSelectedLevel(7);
if (PlayerPrefs.GetInt("SceneSevenStars") != 4)
levelManager.loadSelectedLevel(8);
if (PlayerPrefs.GetInt("SceneMini") != 4)
levelManager.loadSelectedLevel(9);
}
else
{
if (options)
{
options = false;
optionsMenu.SetActive(false);
}
pauseGame();
}
}
public void nextStage()
{
playerDied = false;
nextLevel = true;
}
// For Debugging purposes //
public void eraseAllData()
{
PlayerPrefs.DeleteAll();
returnToHome();
print("Cleared all data! Stages back to square 1");
}
public void unlock2()
{
PlayerPrefs.SetInt("SceneTwoStars", 1);
returnToHome();
}
public void unlock6()
{
PlayerPrefs.SetInt("SceneSixStars", 1);
returnToHome();
}
public void loadFinalCut()
{
levelManager.loadSelectedLevel(9);
}
}
<file_sep>/Assets/Scripts/Mini Game/SecurityCameraMini.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SecurityCameraMini : MonoBehaviour {
private bool isOn = false;
private SpriteRenderer ren;
private float iniAlpha;
private float accumtime = 0;
public float onTime;
public float offTime;
// Use this for initialization
void Start () {
ren = GetComponent<SpriteRenderer>();
iniAlpha = ren.color.a;
}
// Update is called once per frame
void Update () {
accumtime += Time.deltaTime;
if(accumtime > offTime && !isOn)
{
isOn = true;
accumtime = 0;
}
if (accumtime > onTime && isOn)
{
isOn = false;
accumtime = 0;
}
if (isOn)
{
tag = "Enemy";
ren.color = new Vector4(ren.color.r, ren.color.g, ren.color.b, iniAlpha);
}
else
{
ren.color = new Vector4(ren.color.r, ren.color.g, ren.color.b, 0.1f);
tag = "Untagged";
}
}
}
<file_sep>/Assets/Scripts/CraneContainer.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CraneContainer : MonoBehaviour {
private Rigidbody2D rb;
public bool hasPlayer = false;
// Use this for initialization
void Start () {
rb = GetComponentInParent<Rigidbody2D>();
}
// Update is called once per frame
void Update () {
if (rb.velocity == Vector2.zero || hasPlayer)
transform.parent.GetComponent<BoxCollider2D>().isTrigger = false;
else
transform.parent.GetComponent<BoxCollider2D>().isTrigger = true;
}
void OnTriggerEnter2D(Collider2D other)
{
if(rb.velocity.y < 0 && other.gameObject.tag == "Player")
{
UiController.playerDied = true;
print("Player died");
}
}
}
<file_sep>/Assets/Scripts/Mini Game/LevelCompletion.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class LevelCompletion : MonoBehaviour {
public GameObject score;
private float accumtime = 0;
private float a = 0.01f;
private bool wasOff = true;
// Use this for initialization
void Start () {
}
// Update is called once per frame
void Update () {
if(isActiveAndEnabled && wasOff)
{
GetComponent<Text>().text = GetComponent<Text>().text + " Score: " + score.GetComponent<Text>().text;
wasOff = false;
}
accumtime += Time.deltaTime;
if (accumtime > 0.1 && GetComponent<Text>().fontSize < 90)
{
GetComponent<Text>().fontSize += 6;
a += a;
accumtime = a;
}
}
}
<file_sep>/Assets/Scripts/Switch.cs
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Switch : MonoBehaviour {
List<GameObject> objList = new List<GameObject>();
private bool triggerswitch = false;
[Range (0f, 90f)]
public float switchAngle;
// Use this for initialization
void Start () {
//gets all children that are to be switched on/off
foreach (Transform child in transform.parent)
{
if (child != transform && child != transform.Find("Switch Sprite"))
objList.Add(child.gameObject);
}
}
// Update is called once per frame
void Update () {
if (triggerswitch)
{
foreach (GameObject obj in objList)
{
switch (obj.tag)
{
case "Crane":
obj.transform.Find("Crane").Find("Control Base").Find("Cable And Hook").GetComponent<Crane>().isOn = !obj.transform.Find("Crane").Find("Control Base").Find("Cable And Hook").GetComponent<Crane>().isOn;
break;
case "Security Camera":
obj.GetComponentInChildren<SecurityCamera>().isOn = !obj.GetComponentInChildren<SecurityCamera>().isOn;
break;
case "Rotating Camera":
obj.transform.Find("Pivot").GetChild(0).GetComponent<RotatingSecurityCamera>().isOn = !obj.transform.Find("Pivot").GetChild(0).GetComponent<RotatingSecurityCamera>().isOn;
break;
case "ATV":
obj.transform.Find("ATV").GetComponent<ATV>().isOn = !obj.transform.Find("ATV").GetComponent<ATV>().isOn;
break;
default:
break;
}
}
triggerswitch = false;
}
}
void OnTriggerEnter2D(Collider2D collision)
{
GameObject obj = collision.gameObject;
Rigidbody2D rb = obj.GetComponent<Rigidbody2D>();
if (obj.tag == "Player")
{
if (obj.transform.position.x < transform.position.x && transform.rotation.z >= 0)
{
transform.Rotate(0, 0, -switchAngle);
triggerswitch = true;
switchAngle = -switchAngle;
}
if (obj.transform.position.x > transform.position.x && transform.rotation.z < 0)
{
transform.Rotate(0, 0, -switchAngle);
triggerswitch = true;
switchAngle = -switchAngle;
}
}
}
}
| 89043af1f3093f3095b381c39082a8009a0deeb8 | [
"C#"
] | 29 | C# | yaoxinmeng/PSA-Game-It | 83b6ed844a53e52af53bd078a9c267765e9accb8 | 1d83f6bdf68db8bdff319f45eef64bd37f7d8d53 | |
refs/heads/master | <repo_name>AndreyAntipov/meteor-accounts-guest<file_sep>/accounts-guest-client.js
/*****************
* special anonymous behavior so that visitors can
* manipulate their work
*
*/
// If our app has a Blaze, override the {{currentUser}} helper to deal guest logins
if (Package.blaze) {
/**
* @global
* @name currentUser
* @isHelper true
* @summary Calls [Meteor.user()](#meteor_user). Use `{{#if currentUser}}` to check whether the user is logged in.
* Where "logged in" means: The user has has authenticated (e.g. through providing credentials)
*/
Package.blaze.Blaze.Template.registerHelper('currentUser', function () {
var user = Meteor.user();
if (user &&
typeof user.profile !== 'undefined' &&
typeof user.profile.guest !== 'undefined' &&
user.profile.guest &&
AccountsGuest.name === false){
// a guest login is not a real login where the user is authenticated.
// This allows the account-base "Sign-in" to still appear
return null;
} else {
return Meteor.user();
}
});
}
//no non-logged in users
/* you might need to limit this to avoid flooding the user db */
Meteor.loginVisitor = function (email, callback) {
if (!Meteor.userId()) {
Meteor.call('createGuest', email, function (error, result) {
if (error) {
return callback && callback(error);
}
/* if a simple "true" is returned, we are in a disabled mode */
if(result === true) return callback && callback();
Meteor.loginWithPassword(result.email, result.password, function(error) {
if(error) {
callback && callback(error);
} else {
callback && callback();
}
});
});
}
}
Meteor.startup(function(){
Deps.autorun(function () {
if (!Meteor.userId()) {
if (AccountsGuest.forced === true) {
Meteor.loginVisitor();
}
}
});
});
<file_sep>/accounts-guest-server.js
Moniker = Npm.require('moniker');
Accounts.removeOldGuests = function (before) {
if (typeof before === 'undefined') {
before = new Date();
before.setHours(before.getHours() - 1);
}
res = Meteor.users.remove({createdAt: {$lte: before}, 'profile.guest': true});
//res = Meteor.users.remove( {'profile.guest': true});
return res;
};
/* adapted from pull-request https://github.com/dcsan
* See https://github.com/artwells/meteor-accounts-guest/commit/28cbbf0eca2d80f78925ac619abf53d0769c0d9d
*/
Meteor.methods({
createGuest: function (email)
{
check(email, Match.OneOf(String, null, undefined));
/* if explicitly disabled, happily do nothing */
if (AccountsGuest.enabled === false){
return true;
}
// count = Meteor.users.find().count() + 1
if (AccountsGuest.name === true) {
guestname = Moniker.choose();
// Just in case, let's make sure this name isn't taken
while (Meteor.users.find({username:guestname}).count() > 0) {
guestname = Moniker.choose();
}
} else {
guestname = "guest-#" + Random.id()
}
if (!email) {
email = guestname + "@example.com";
}
guest = {
username: guestname,
email: email,
profile: {guest: true},
password: <PASSWORD>(),
};
Accounts.createUser(guest);
// console.log("createGuest" + guestname);
return guest;
}
});
| b44bf31e6483b324930fbcde843e373e5bf0e208 | [
"JavaScript"
] | 2 | JavaScript | AndreyAntipov/meteor-accounts-guest | e41e80e4d92eeeb6cded13870420a64b5377a1f6 | 8927c8d061337064ed6a9cf8eb943ffbbbd225d8 | |
refs/heads/master | <repo_name>YuraPelehatiy/internet-store-app<file_sep>/src/layouts/App.js
import React from 'react';
import { Route, Switch } from 'react-router-dom';
import { routes } from '../routes';
import Desktop from './Desktop';
import AuthPage from '../pages/Auth/AuthPage';
const App = () => (
<Switch>
<Route path={routes.authLogin} component={AuthPage} />
<Route path={routes.authRegister} component={AuthPage} />
<Route path={routes.authRemember} component={AuthPage} />
<Route component={Desktop} />
</Switch>
);
export default App;<file_sep>/src/components/ProductLinkAdmin/ProductLinkAdmin.js
import React from 'react';
import { Link } from 'react-router-dom';
import { formatRoute } from 'react-router-named-routes';
import { routes } from '../../routes';
import s from './ProductLinkAdmin.module.css';
import ActionButton from '../ActionButton/ActionButton';
const ProductLinkAdmin = ({
id,
title,
showModalToUpdateProduct,
showModalToRemoveProduct,
users,
}) => (
<div className={s.ProductLinkAdmin}>
<Link to={formatRoute((users && routes.adminUser) || routes.adminProduct, { id })}>
{title}
</Link>
<div className={s.ProductLinkAdminButtons}>
<ActionButton
mediumSize
onClick={() => showModalToUpdateProduct(id)}
>
Edit
</ActionButton>
<ActionButton
mediumSize
onClick={() => showModalToRemoveProduct(id)}
>
Remove
</ActionButton>
</div>
</div>
);
export default ProductLinkAdmin;<file_sep>/src/modules/products/productsSelectors.js
import { createSelector } from 'reselect';
const getProductsIds = state => state.products.products;
const getProductById = (state, id) => state.entities.products[id];
const getProductsEntities = state => state.entities.products;
export const getProducst = createSelector(
[getProductsIds, getProductsEntities],
(products, entities) => products.map(id => entities[id]),
);
export const getProduct = createSelector(getProductById, result => result);<file_sep>/src/pages/NotFound/NotFoundPage.js
import React from 'react';
const NotFoundPage = () => (
<h2>Page is Not Found</h2>
);
export default NotFoundPage;<file_sep>/src/modules/products/productsReducer.js
import { handleActions } from 'redux-actions';
import * as constants from './productsConstants';
const initialState = {
products: [],
isLoading: false,
error: null,
limit: 12,
page: 0,
offset: 0,
};
export default handleActions(
{
// Fetch all products
[constants.FETCH_PRODUCTS_START]: state => ({
...state,
isLoading: true,
error: null,
}),
[constants.FETCH_PRODUCTS_OK]: (state, action) => ({
...state,
isLoading: false,
products: action.payload.ids,
}),
[constants.FETCH_PRODUCTS_ERROR]: (state, action) => ({
...state,
isLoading: false,
error: action.payload,
}),
// Get one product
[constants.GET_PRODUCT_START]: state => ({
...state,
isLoading: true,
error: null,
}),
[constants.GET_PRODUCT_OK]: (state, action) => ({
...state,
isLoading: false,
products: [action.payload.id[0]].concat(state.products),
}),
[constants.GET_PRODUCT_ERROR]: (state, action) => ({
...state,
isLoading: false,
error: action.payload,
}),
[constants.SET_PAGE]: (state, action) => ({
...state,
page: action.payload.page,
offset: action.payload.page * state.limit,
}),
},
initialState,
);<file_sep>/src/modules/app/appOperations.js
import * as Api from '../../api/Api';
import * as appActions from './appActions';
export const init = () => async (dispatch) => {
try {
Api.initApi();
const res = await Api.User.getCurrent();
dispatch(appActions.addUser({
user: res.data.user,
}));
} catch (err) {
Api.setToken(undefined);
}
};
export const logout = () => (dispatch) => {
Api.removeToken();
dispatch(appActions.removeUser());
};
export const login = values => async (dispatch) => {
try {
const res = await Api.Auth.login(values);
Api.setToken(res.data.token);
const resUser = await Api.User.getCurrent();
dispatch(appActions.addUser({
user: resUser.data.user,
}));
} catch (err) {
throw new Error();
}
};
export const register = values => async () => {
try {
const res = await Api.Auth.register(values);
if (!res.data.success) {
throw new Error();
}
} catch (err) {
throw new Error();
}
};
export const remember = values => async () => {
try {
const res = await Api.Auth.remember(values);
if (!res.data.success) {
throw new Error();
}
} catch (err) {
throw new Error();
}
};<file_sep>/src/modules/app/appReducer.js
import { handleActions } from 'redux-actions';
import * as constants from './appConstants';
const initialState = {
user: {},
};
export default handleActions(
{
[constants.ADD_USER]: (state, action) => ({
...state,
user: action.payload.user,
}),
[constants.REMOVE_USER]: state => ({
...state,
user: {},
}),
},
initialState,
);<file_sep>/src/modules/cart/cartConstants.js
export const ADD = 'cart/ADD';
export const REMOVE = 'cart/REMOVE';
export const FETCH_PRODUCTS_START = 'cart/FETCH_PRODUCTS_START';
export const FETCH_PRODUCTS_OK = 'cart/FETCH_PRODUCTS_OK';
export const FETCH_PRODUCTS_ERROR = 'cart/FETCH_PRODUCTS_ERROR';
export const INCREASE = 'cart/INCREASE';
export const DECREASE = 'cart/DECREASE';
export const ENTER_VALUE = 'cart/ENTER_VALUE';<file_sep>/src/modules/cart/cartActions.js
import { createAction } from 'redux-actions';
import * as constants from './cartConstants';
export const add = createAction(constants.ADD);
export const remove = createAction(constants.REMOVE);
export const fetchProductsStart = createAction(constants.FETCH_PRODUCTS_START);
export const fetchProductsOk = createAction(constants.FETCH_PRODUCTS_OK);
export const fetchProductsError = createAction(constants.FETCH_PRODUCTS_ERROR);
export const increase = createAction(constants.INCREASE);
export const decrease = createAction(constants.DECREASE);
export const enterValue = createAction(constants.ENTER_VALUE);<file_sep>/src/modules/cart/cartOperation.js
import { normalize } from 'normalizr';
import * as schemes from '../../api/schemes';
import * as actions from './cartActions';
import * as Api from '../../api/Api';
export const fetchProducts = () => async (dispatch, getState) => {
try {
const products = getState().entities.products;
const items = getState().cart.items;
if (Object.keys(products).length > 1 || Object.keys(items).length === 0) {
return;
}
const ids = Object.keys(items);
dispatch(actions.fetchProductsStart());
const res = await Api.Cart.getProductsByIds(ids);
const { entities } = normalize(res.data, schemes.ProductCollection);
dispatch(actions.fetchProductsOk({
entities,
}));
} catch (err) {
dispatch(actions.fetchProductsError(err.message));
}
};<file_sep>/src/components/InputForm/InputForm.js
import React from 'react';
import classNames from 'classnames/bind';
import s from './InputForm.module.css';
const cx = classNames.bind(s);
const InputForm = ({
meta,
...props
}) => (
<div className={s.InputContainer}>
<input
{...props}
className={cx({
InputField: true,
InputFieldError: (meta && meta.error && meta.touched),
})}
/>
{meta && meta.error && meta.touched && <span className={s.ErrorField}>{meta.error}</span>}
</div>
);
export default InputForm;<file_sep>/src/modules/cart/cartSelectors.js
import { createSelector } from 'reselect';
const getProductsItems = (state, isLoading) => {
// We use this condition and the isLoading parameter to force
// the getProducts selector to recalculate after fetching data
// from the server.
if (isLoading) {
return [];
}
return state.cart.items;
};
const getProductsEntities = state => state.entities.products;
export const getProducts = createSelector(
[getProductsItems, getProductsEntities],
(items, entities) => Object.keys(items).map((id) => {
if (entities[id] === undefined) {
return ({
id,
title: 'Loading...',
price: 0,
});
}
return entities[id];
}),
);
export const getTotalPrice = createSelector(
[getProducts, getProductsItems],
(products, items) => (
products.reduce((acc, product) => acc + product.price * items[product.id].count, 0)
),
);
export const getCountItems = createSelector(
[getProductsItems],
(items) => {
const ids = Object.keys(items);
return ids.reduce((acc, id) => acc + items[id].count, 0);
},
);<file_sep>/src/pages/Cart/CartPageComponent.js
import React from 'react';
import s from './CartPage.module.css';
import ActionButton from '../../components/ActionButton/ActionButton';
import CartList from './CartList/CartList';
const CartPageComponent = ({
products,
renderProductLink,
countTotalPrice,
itemsCart,
}) => (
<div className={s.CartPage}>
<h2 className={s.CartPageTop}>Cart list</h2>
<hr />
<CartList
products={products}
renderProductLink={renderProductLink}
itemsCart={itemsCart}
/>
<hr />
<div className={s.CartPageBottom}>
<div className={s.CartPageTotalPrice}>
Total Price: {countTotalPrice}
</div>
<ActionButton>Checkout</ActionButton>
</div>
</div>
);
export default CartPageComponent;<file_sep>/src/pages/ProductAdmin/ProductPageAdminContainer.js
import { connect } from 'react-redux';
import {
compose, lifecycle, branch, renderComponent, mapProps,
} from 'recompose';
import ProductPageAdminComponent from './ProductPageAdminComponent';
import * as adminOperations from '../../modules/admin/adminOperations';
import * as adminSelectors from '../../modules/admin/adminSelectors';
import Loader from '../../components/Loader/Loader';
import ErrorLoadign from '../../components/ErrorLoading/ErrorLoading';
const mapStateToProps = (state, props) => ({
product: adminSelectors.getProduct(state, props.match.params.id),
isLoading: state.admin.isLoading,
isError: !!state.admin.error,
error: state.admin.error,
});
const mapStateToDispatch = {
getProduct: adminOperations.getProduct,
removeProduct: adminOperations.removeProduct,
updateProduct: adminOperations.updateProduct,
};
export default compose(
connect(
mapStateToProps,
mapStateToDispatch,
),
lifecycle({
componentDidMount() {
this.props.getProduct(this.props.match.params.id);
},
}),
branch(
props => props.isLoading,
renderComponent(Loader),
branch(
props => props.isError,
renderComponent(ErrorLoadign),
),
),
mapProps(props => ({
updateProduct: props.updateProduct,
removeProduct: props.removeProduct,
product: props.product,
history: props.history,
})),
)(ProductPageAdminComponent);<file_sep>/src/modules/app/appActions.js
import { createAction } from 'redux-actions';
import * as constants from './appConstants';
export const addUser = createAction(constants.ADD_USER);
export const removeUser = createAction(constants.REMOVE_USER);<file_sep>/src/pages/Home/ProductList/ProductListContainer.js
import React from 'react';
import { connect } from 'react-redux';
import {
compose, branch, renderComponent, mapProps,
} from 'recompose';
import * as productsSelectors from '../../../modules/products/productsSelectors';
import * as cartActions from '../../../modules/cart/cartActions';
import ProdutcListComponent from './ProdutcListComponent';
import ProductLink from '../../../components/ProductLink/ProductLink';
import Loader from '../../../components/Loader/Loader';
import ErrorLoadign from '../../../components/ErrorLoading/ErrorLoading';
const mapStateToProps = state => ({
products: productsSelectors.getProducst(state),
isLoading: state.products.isLoading,
isError: !!state.products.error,
error: state.products.error,
});
const mapStateToDispatch = {
addItemToCart: cartActions.add,
};
export default compose(
connect(
mapStateToProps,
mapStateToDispatch,
),
branch(
props => props.isLoading,
renderComponent(Loader),
branch(
props => props.isError,
renderComponent(ErrorLoadign),
),
),
mapProps(props => ({
renderProductLink: item => (
<ProductLink
key={item.id}
id={item.id}
title={item.title}
image={item.image}
price={item.price}
onActionButtonClick={props.addItemToCart}
actionButtonTitle="Add to Cart"
/>
),
products: props.products,
})),
)(ProdutcListComponent);<file_sep>/src/modules/admin/adminConstants.js
export const SET_PAGE_PRODUCTS = 'admin/SET_PAGE_PRODUCTS';
export const SET_PAGE_USERS = 'admin/SET_PAGE_USERS';
//--------------------------------------------------------------------------
// Constants for products
//--------------------------------------------------------------------------
export const FETCH_PRODUCTS_START = 'admin/FETCH_PRODUCTS_START';
export const FETCH_PRODUCTS_OK = 'admin/FETCH_PRODUCTS_OK';
export const FETCH_PRODUCTS_ERROR = 'admin/FETCH_PRODUCTS_ERROR';
export const CREATE_PRODUCT_START = 'admin/CREATE_PRODUCT_START';
export const CREATE_PRODUCT_OK = 'admin/CREATE_PRODUCT_OK';
export const CREATE_PRODUCT_ERROR = 'admin/CREATE_PRODUCT_ERROR';
export const UPDATE_PRODUCT_START = 'admin/UPDATE_PRODUCT_START';
export const UPDATE_PRODUCT_OK = 'admin/UPDATE_PRODUCT_OK';
export const UPDATE_PRODUCT_ERROR = 'admin/UPDATE_PRODUCT_ERROR';
export const REMOVE_PRODUCT_START = 'admin/REMOVE_PRODUCT_START';
export const REMOVE_PRODUCT_OK = 'admin/REMOVE_PRODUCT_OK';
export const REMOVE_PRODUCT_ERROR = 'admin/REMOVE_PRODUCT_ERROR';
export const GET_PRODUCT_START = 'admin/GET_PRODUCT_START';
export const GET_PRODUCT_OK = 'admin/GET_PRODUCT_OK';
export const GET_PRODUCT_ERROR = 'admin/GET_PRODUCT_ERROR';
//--------------------------------------------------------------------------
// Constants for users
//--------------------------------------------------------------------------
export const FETCH_USERS_START = 'admin/FETCH_USERS_START';
export const FETCH_USERS_OK = 'admin/FETCH_USERS_OK';
export const FETCH_USERS_ERROR = 'admin/FETCH_USERS_ERROR';
export const UPDATE_USER_START = 'admin/UPDATE_USER_START';
export const UPDATE_USER_OK = 'admin/UPDATE_USER_OK';
export const UPDATE_USER_ERROR = 'admin/UPDATE_USER_ERROR';
export const REMOVE_USER_START = 'admin/REMOVE_USER_START';
export const REMOVE_USER_OK = 'admin/REMOVE_USER_OK';
export const REMOVE_USER_ERROR = 'admin/REMOVE_USER_ERROR';
export const GET_USER_START = 'admin/GET_USER_START';
export const GET_USER_OK = 'admin/GET_USER_OK';
export const GET_USER_ERROR = 'admin/GET_USER_ERROR';<file_sep>/src/pages/Auth/Remember/RememberPage.js
import React from 'react';
import { Form, Field } from 'react-final-form';
import { FORM_ERROR } from 'final-form';
import { connect } from 'react-redux';
import * as appOperations from '../../../modules/app/appOperations';
import InputForm from '../../../components/InputForm/InputForm';
import ActionButton from '../../../components/ActionButton/ActionButton';
import ErrorSubmiting from '../../../components/ErrorSubmiting/ErrorSubmiting';
const validate = (values) => {
const errors = {};
if (!values.email || values.email.trim().length === 0 || !values.email.includes('@')) {
errors.email = 'Please enter a valid email address';
}
return errors;
};
const RememberPage = ({ remember }) => {
const onSubmit = async (values, form) => { // eslint-disable-line
try {
await remember(values);
form.reset();
} catch (err) {
return {
[FORM_ERROR]: 'Something went wrong',
};
}
};
return (
<div>
<Form
onSubmit={onSubmit}
validate={validate}
render={({ handleSubmit, submitError, submitSucceeded }) => (
<>
<Field name="email">
{({ input, meta }) => (
<InputForm {...input} type="text" placeholder="Email" meta={meta} />
)}
</Field>
<div>
<ActionButton onClick={handleSubmit}>Remember</ActionButton>
</div>
{submitError && <ErrorSubmiting>{submitError}</ErrorSubmiting>}
{submitSucceeded && <div>We have sent instructions for your email</div>}
</>
)}
/>
</div>
);
};
const mapStateToDispatch = {
remember: appOperations.remember,
};
export default connect(
undefined,
mapStateToDispatch,
)(RememberPage);<file_sep>/src/components/ProductForm/ProductForm.js
import React from 'react';
import { Form, Field } from 'react-final-form';
import { FORM_ERROR } from 'final-form';
import InputForm from '../InputForm/InputForm';
import ActionButton from '../ActionButton/ActionButton';
import TextAreaForm from '../TextAreaForm/TextAreaForm';
import ErrorSubmiting from '../ErrorSubmiting/ErrorSubmiting';
const validate = (values) => {
const errors = {};
if (!values.title || values.title.trim().length === 0) {
errors.title = 'Requared';
}
if (!values.description || values.description.trim().length === 0) {
errors.description = 'Requared';
}
if (!values.image || values.image.trim().length === 0) {
errors.image = 'Requared';
}
if (!values.price) {
errors.price = 'Requared';
}
return errors;
};
const ProductForm = ({
onClose,
onSubmitAction,
onSubmitButtonTitle,
product,
}) => {
const onSubmit = async (values, form) => { // eslint-disable-line
try {
await onSubmitAction(values);
form.reset();
onClose();
} catch (err) {
return {
[FORM_ERROR]: 'Something went wrong',
};
}
};
return (
<>
<Form
initialValues={{ ...product }}
onSubmit={onSubmit}
validate={validate}
render={({
handleSubmit, submitError, submitting, submitSucceeded,
}) => (
<>
<Field name="title">
{({ input, meta }) => (
<InputForm {...input} type="text" placeholder="Title" meta={meta} />
)}
</Field>
<Field name="description">
{({ input, meta }) => (
<TextAreaForm {...input} placeholder="Description" meta={meta} />
)}
</Field>
<Field name="image">
{({ input, meta }) => (
<InputForm {...input} type="text" placeholder="Image URL" meta={meta} />
)}
</Field>
<Field name="price">
{({ input, meta }) => (
<InputForm {...input} type="number" placeholder="Price" meta={meta} />
)}
</Field>
<div>
<ActionButton onClick={handleSubmit}>
{onSubmitButtonTitle}
</ActionButton>
</div>
{submitting && <h2>Submiting...</h2>}
{submitSucceeded && <h2>Complete</h2>}
{submitError && <ErrorSubmiting>{submitError}</ErrorSubmiting>}
</>
)}
/>
</>
);
};
export default ProductForm;<file_sep>/src/pages/Home/ProductList/ProdutcListComponent.js
import React from 'react';
import s from './ProductList.module.css';
const ProdutcListComponent = ({
products,
renderProductLink,
}) => (
<div className={s.ProductList}>
{products.map(item => renderProductLink(item))}
</div>
);
export default ProdutcListComponent;<file_sep>/src/pages/Auth/Login/LoginPage.js
import React from 'react';
import { Form, Field } from 'react-final-form';
import { FORM_ERROR } from 'final-form';
import { Redirect } from 'react-router-dom';
import { connect } from 'react-redux';
import * as appOperations from '../../../modules/app/appOperations';
import { routes } from '../../../routes';
import InputForm from '../../../components/InputForm/InputForm';
import ActionButton from '../../../components/ActionButton/ActionButton';
import ErrorSubmiting from '../../../components/ErrorSubmiting/ErrorSubmiting';
const validate = (values) => {
const errors = {};
if (!values.email || values.email.trim().length < 0 || !values.email.includes('@')) {
errors.email = 'Please enter a valid email address';
}
if (!values.password || values.password.trim().length < 8) {
errors.password = 'Please enter a valid password, password must be 8 or more symbols';
}
return errors;
};
const LoginPage = ({ login }) => {
const onSubmit = async (values, form) => { // eslint-disable-line
try {
await login(values);
form.reset();
} catch (err) {
return {
[FORM_ERROR]: 'Wrong email or password',
};
}
};
return (
<div>
<Form
onSubmit={onSubmit}
validate={validate}
render={({ handleSubmit, submitError, submitSucceeded }) => (
<>
<Field name="email">
{({ input, meta }) => (
<InputForm {...input} type="text" placeholder="Email" meta={meta} />
)}
</Field>
<Field name="password">
{({ input, meta }) => (
<InputForm {...input} type="password" placeholder="Password" meta={meta} />
)}
</Field>
<div>
<ActionButton onClick={handleSubmit}>Login</ActionButton>
</div>
{submitError && <ErrorSubmiting>{submitError}</ErrorSubmiting>}
{submitSucceeded && <Redirect to={routes.home} />}
</>
)}
/>
</div>
);
};
const mapStateToDispatch = {
login: appOperations.login,
};
export default connect(
undefined,
mapStateToDispatch,
)(LoginPage);<file_sep>/src/modules/admin/adminOperations.js
import { normalize } from 'normalizr';
import * as schemes from '../../api/schemes';
import * as actions from './adminActions';
import * as Api from '../../api/Api';
// Poructs operations
export const fetchProducts = () => async (dispatch, getState) => {
try {
const offset = getState().admin.offsetProducts;
const limit = getState().admin.limit;
dispatch(actions.fetchProductsStart());
const res = await Api.AdminProducts.fetchProducts(offset, limit);
const { result: ids, entities } = normalize(res.data, schemes.AdminProductCollection);
dispatch(actions.fetchProductsOk({
ids,
entities,
}));
} catch (err) {
dispatch(actions.fetchProductsError(err.message));
}
};
export const createProduct = newProduct => async (dispatch) => {
try {
dispatch(actions.createProductStart());
const res = await Api.AdminProducts.createProduct(newProduct);
const { result: ids, entities } = normalize(res.data, schemes.AdminProductCollection);
dispatch(actions.createProductOk({
ids,
entities,
}));
} catch (err) {
dispatch(actions.createProductError(err.message));
}
};
export const updateProduct = productData => async (dispatch) => {
try {
dispatch(actions.updateProductStart());
const res = await Api.AdminProducts.updateProductById(productData.id, productData);
const { result: ids, entities } = normalize(res.data, schemes.AdminProductCollection);
dispatch(actions.updateProductOk({
ids,
entities,
}));
} catch (err) {
dispatch(actions.updateProductError(err.message));
}
};
export const removeProduct = id => async (dispatch) => {
try {
dispatch(actions.removeProductStart());
const res = await Api.AdminProducts.removeProductById(id);
if (res && res.data && res.data.success) {
const ids = [id];
dispatch(actions.removeProductOk({
ids,
}));
}
} catch (err) {
dispatch(actions.removeProductError(err.message));
}
};
export const getProduct = id => async (dispatch, getState) => {
try {
const product = getState().entities.products[id];
if (product) {
return;
}
dispatch(actions.getProductStart());
const res = await Api.AdminProducts.getProductsById(id);
const { result, entities } = normalize(res.data, schemes.AdminProductCollection);
dispatch(actions.getProductOk({
id: result,
entities,
}));
} catch (err) {
dispatch(actions.getProductError(err.message));
}
};
// Users operations
export const fetchUsers = () => async (dispatch, getState) => {
try {
const offset = getState().admin.offsetUsers;
const limit = getState().admin.limit;
dispatch(actions.fetchUsersStart());
const res = await Api.AdminUsers.fetchUsers(offset, limit);
const { result: ids, entities } = normalize(res.data, schemes.AdminUserCollection);
dispatch(actions.fetchUsersOk({
ids,
entities,
}));
} catch (err) {
dispatch(actions.fetchUsersError(err.message));
}
};
export const updateUser = userData => async (dispatch) => {
try {
dispatch(actions.updateUserStart());
const res = await Api.AdminUsers.updateUserById(userData.id, userData);
const { result: ids, entities } = normalize(res.data, schemes.AdminUserCollection);
dispatch(actions.updateUserOk({
ids,
entities,
}));
} catch (err) {
dispatch(actions.updateUserError(err.message));
}
};
export const removeUser = id => async (dispatch) => {
try {
dispatch(actions.removeUserStart());
const res = await Api.AdminUsers.removeUserById(id);
if (res && res.data && res.data.success) {
const ids = [id];
dispatch(actions.removeUserOk({
ids,
}));
}
} catch (err) {
dispatch(actions.removeUserError(err.message));
}
};
export const getUser = id => async (dispatch, getState) => {
try {
const user = getState().entities.users[id];
if (user) {
return;
}
dispatch(actions.getUserStart());
const res = await Api.AdminUsers.getUserById(id);
const { result, entities } = normalize(res.data, schemes.AdminUserCollection);
dispatch(actions.getUserOk({
id: result,
entities,
}));
} catch (err) {
dispatch(actions.getUserError(err.message));
}
};<file_sep>/src/pages/Admin/UserListPageAdmin/UserListPageAdminComponent.js
import React from 'react';
import PaginatePanel from '../../../components/PaginatePanel/PaginatePanel';
import UserListAdminContainer from '../UserListAdmin/UserListAdminContainer';
const UserListPageAdminComponent = ({
handleOnPageChange,
selectedPage,
}) => (
<div>
<UserListAdminContainer />
<PaginatePanel
onPageChange={handleOnPageChange}
initialPage={selectedPage}
pageCount={10}
pageRangeDisplayed={5}
marginRangeDisplayed={3}
/>
</div>
);
export default UserListPageAdminComponent;<file_sep>/src/pages/Home/HomePage.js
import React from 'react';
import { Switch, Route } from 'react-router-dom';
import { routes } from '../../routes';
import ProductPageContainer from '../Product/ProductPageContainer';
import ProdutcListPageComponent from './ProductListPage/ProductListPageContainer';
import NotFoundPage from '../NotFound/NotFoundPage';
const HomePage = ({
match,
}) => (
<div>
<Switch>
<Route
exact
path={match.path}
component={ProdutcListPageComponent}
/>
<Route
path={routes.product}
component={ProductPageContainer}
/>
<Route component={NotFoundPage} />
</Switch>
</div>
);
HomePage.propTypes = {
};
export default HomePage;<file_sep>/src/pages/Contact/ContactPage.js
import React from 'react';
const ContactPage = () => (
<h1>
Contact page
</h1>
);
export default ContactPage;<file_sep>/src/modules/admin/adminReducerHandlers/adminReducerProducts.js
import * as constants from '../adminConstants';
export const adminReducerProducts = {
// Fetch all products
[constants.FETCH_PRODUCTS_START]: state => ({
...state,
isLoading: true,
error: null,
}),
[constants.FETCH_PRODUCTS_OK]: (state, action) => ({
...state,
isLoading: false,
products: action.payload.ids,
}),
[constants.FETCH_PRODUCTS_ERROR]: (state, action) => ({
...state,
isLoading: false,
error: action.payload,
}),
// Create product
[constants.CREATE_PRODUCT_START]: state => ({
...state,
error: null,
}),
[constants.CREATE_PRODUCT_OK]: (state, action) => ({
...state,
products: action.payload.ids.concat(state.products),
}),
[constants.CREATE_PRODUCT_ERROR]: (state, action) => ({
...state,
error: action.payload,
}),
// Update product
[constants.UPDATE_PRODUCT_START]: state => ({
...state,
error: null,
}),
[constants.UPDATE_PRODUCT_OK]: (state, action) => ({
...state,
products: state.products.map((product) => {
if (product === action.payload.ids[0]) {
return action.payload.ids[0];
}
return product;
}),
}),
[constants.UPDATE_PRODUCT_ERROR]: (state, action) => ({
...state,
error: action.payload,
}),
// Remove product
[constants.REMOVE_PRODUCT_START]: state => ({
...state,
error: null,
}),
[constants.REMOVE_PRODUCT_OK]: (state, action) => ({
...state,
products: state.products.filter(product => product !== action.payload.ids[0]),
}),
[constants.REMOVE_PRODUCT_ERROR]: (state, action) => ({
...state,
error: action.payload,
}),
// Get one product
[constants.GET_PRODUCT_START]: state => ({
...state,
isLoading: true,
error: null,
}),
[constants.GET_PRODUCT_OK]: (state, action) => ({
...state,
isLoading: false,
products: [action.payload.id[0]].concat(state.products),
}),
[constants.GET_PRODUCT_ERROR]: (state, action) => ({
...state,
isLoading: false,
error: action.payload,
}),
};<file_sep>/src/api/Api.js
import axios from 'axios';
// ---------------------------------------------
// Provides API for work with products, users,
// auth and token
// ---------------------------------------------
// Provides API for work with token and localStorage
let _token = null;
export const setToken = (token) => {
_token = token;
localStorage.setItem('token', token); // eslint-disable-line
if (_token === 'undefined') {
_token = null;
}
axios.defaults.headers.Authorization = _token
? `Bearer ${_token}`
: null;
};
export const removeToken = () => {
localStorage.removeItem('token'); // eslint-disable-line
_token = null;
axios.defaults.headers.Authorization = null;
};
export const isAuthenticated = () => {
if (_token === 'undefined') {
return false;
}
return !!_token;
};
export const initApi = () => {
const token = localStorage.getItem('token'); // eslint-disable-line
setToken(token);
};
// Provides API for work with proucts on admin side
export const AdminProducts = {
fetchProducts(offset, limit) {
const query = `?offset=${offset}&limit=${limit}`;
return axios.get(`/api/v3/products${query}`);
},
getProductsById(id) {
return axios.get(`/api/v3/products/${id}`);
},
createProduct(body) {
return axios.post('/api/v3/products', body);
},
updateProductById(id, body) {
return axios.patch(`/api/v3/products/${id}`, body);
},
removeProductById(id) {
return axios.delete(`/api/v3/products/${id}`);
},
};
// Provides API for work with users on admin side
export const AdminUsers = {
fetchUsers(offset, limit) {
const query = `?offset=${offset}&limit=${limit}`;
return axios.get(`/api/v3/users${query}`);
},
getUserById(id) {
return axios.get(`/api/v3/users/${id}`);
},
updateUserById(id, body) {
return axios.patch(`/api/v3/users/${id}`, body);
},
removeUserById(id) {
return axios.delete(`/api/v3/users/${id}`);
},
};
// Provides API for work with proucts on users side
export const Products = {
fetchProducts(offset, limit) {
const query = `?offset=${offset}&limit=${limit}`;
return axios.get(`/api/v3/products${query}`);
},
getProductsById(id) {
return axios.get(`/api/v3/products/${id}`);
},
};
// Provides API for work with proucts on cart page
export const Cart = {
getProductsByIds(ids) {
const query = 'ids[]=' + ids.join('&ids[]='); // eslint-disable-line
return axios.get(`/api/v3/products?${query}`);
},
};
// Provides API for Authenticate
export const Auth = {
login(body) {
return axios.post('/api/v3/auth/login', body);
},
register(body) {
return axios.post('/api/v3/auth/register', body);
},
remember(body) {
return axios.post('/api/v3/auth/remember', body);
},
};
// Provides API for work wtih users on user side
export const User = {
getCurrent() {
return axios.get('/api/v3/users/current');
},
};<file_sep>/src/pages/Home/ProductListPage/ProdutcListPageComponent.js
import React from 'react';
import PaginatePanel from '../../../components/PaginatePanel/PaginatePanel';
import ProdutcListContainer from '../ProductList/ProductListContainer';
const ProdutcListPageComponent = ({
handleOnPageChange,
selectedPage,
}) => (
<div>
<ProdutcListContainer />
<PaginatePanel
onPageChange={handleOnPageChange}
initialPage={selectedPage}
pageCount={10}
pageRangeDisplayed={5}
marginRangeDisplayed={3}
/>
</div>
);
export default ProdutcListPageComponent;<file_sep>/src/components/ActionButton/ActionButton.js
import React from 'react';
import classNames from 'classnames/bind';
import s from './ActionButton.module.css';
const cx = classNames.bind(s);
const ActionButton = ({
smallSize,
mediumSize,
children,
...props
}) => (
<button
className={cx({
ActionButton,
ActionButtonLarge: (!smallSize && !mediumSize),
ActionButtonMedium: mediumSize,
ActionButtonSmall: smallSize,
})}
{...props}
>
{children}
</button>
);
export default ActionButton;<file_sep>/src/pages/Auth/AuthPage.js
import React from 'react';
import {
NavLink, Link, Route, Switch,
} from 'react-router-dom';
import { routes } from '../../routes';
import s from './AuthPage.module.css';
import LoginPage from './Login/LoginPage';
import RegisterPage from './Register/RegisterPage';
import RememberPage from './Remember/RememberPage';
const AuthPage = () => (
<div className={s.AuthPage}>
<div className={s.Logo}>
<Link to={routes.home}>Internet Store</Link>
</div>
<div className={s.AuthForm}>
<ul className={s.AuthFormNav}>
<li className={s.AuthFormNavItem}>
<NavLink
to={routes.authLogin}
className={s.AuthFormLink}
activeClassName={s.AuthFormActiveLink}
>
Login
</NavLink>
</li>
<li className={s.AuthFormNavItem}>
<NavLink
to={routes.authRegister}
className={s.AuthFormLink}
activeClassName={s.AuthFormActiveLink}
>
Register
</NavLink>
</li>
</ul>
<Switch>
<Route path={routes.authLogin} component={LoginPage} />
<Route path={routes.authRegister} component={RegisterPage} />
<Route path={routes.authRemember} component={RememberPage} />
</Switch>
<div>
<Link to={routes.authRemember}>Forgot password?</Link>
</div>
</div>
</div>
);
export default AuthPage;<file_sep>/src/pages/Admin/AdminPage.js
import React from 'react';
import { Route, Switch } from 'react-router-dom';
import { routes } from '../../routes';
import AdminNav from './AdminNav/AdminNav';
import ProductListAdminContainer from './ProductListPageAdmin/ProductListPageAdminContainer';
import ProductPageAdminContainer from '../ProductAdmin/ProductPageAdminContainer';
import UserListAdminContainer from './UserListPageAdmin/UserListPageAdminContainer';
import UsersPageAdminContainer from '../UsersAdmin/UsersPageAdminContainer';
import NotFoundPage from '../NotFound/NotFoundPage';
const AdminPage = ({
match,
}) => (
<div>
<AdminNav />
<Switch>
<Route
exact
path={match.path}
component={ProductListAdminContainer}
/>
<Route
path={routes.adminProduct}
component={ProductPageAdminContainer}
/>
<Route
path={routes.adminUsers}
component={UserListAdminContainer}
/>
<Route
path={routes.adminUser}
component={UsersPageAdminContainer}
/>
<Route component={NotFoundPage} />
</Switch>
</div>
);
export default AdminPage;<file_sep>/src/components/SearchForm/SearchForm.js
import React from 'react';
import { Form, Field } from 'react-final-form';
/* import InputForm from '../InputForm/InputForm';
import ActionButton from '../ActionButton/ActionButton'; */
import s from './SearchForm.module.css';
const validate = (values) => {
const errors = {};
if (!values.search || values.search.trim().length === 0) {
errors.search = 'Field is empty';
}
return errors;
};
const SearchForm = () => {
const onSubmit = (values) => { // eslint-disable-line
};
return (
<div className={s.SearchContainer}>
<Form
onSubmit={onSubmit}
validate={validate}
render={({ handleSubmit }) => ( // eslint-disable-line
<>
<Field name="search">
{({ input }) => (
<input
className={s.SearchField}
{...input}
type="search"
placeholder="What are you looking for..."
/>
)}
</Field>
<button
className={s.SearchButton}
>
Search
</button>
</>
)}
/>
</div>
);
};
export default SearchForm;<file_sep>/src/pages/Home/ProductListPage/ProductListPageContainer.js
import { connect } from 'react-redux';
import {
compose, lifecycle, branch, renderComponent, mapProps, withHandlers,
} from 'recompose';
import * as productsOperations from '../../../modules/products/productsOperations';
import * as productsActions from '../../../modules/products/productsActions';
import ProdutcListPageComponent from './ProdutcListPageComponent';
import ErrorLoadign from '../../../components/ErrorLoading/ErrorLoading';
const mapStateToProps = state => ({
isError: !!state.products.error,
error: state.products.error,
selectedPage: state.products.page,
offset: state.products.offset,
});
const mapStateToDispatch = {
fetchProducts: productsOperations.fetchProducts,
setPage: productsActions.setPage,
};
export default compose(
connect(
mapStateToProps,
mapStateToDispatch,
),
branch(
props => props.isError,
renderComponent(ErrorLoadign),
),
withHandlers({
handleOnPageChange: props => ({ selected }) => {
props.setPage({ page: selected });
},
}),
lifecycle({
componentDidUpdate(nextProps) {
if (this.props.offset !== nextProps.offset) {
this.props.fetchProducts(true);
}
},
componentDidMount() {
this.props.fetchProducts();
},
}),
mapProps(props => ({
handleOnPageChange: props.handleOnPageChange,
selectedPage: props.selectedPage,
})),
)(ProdutcListPageComponent);<file_sep>/src/modules/admin/adminSelectors.js
import { createSelector } from 'reselect';
const getProductsIds = state => state.admin.products;
const getProductById = (state, id) => state.entities.products[id];
const getProductsEntities = state => state.entities.products;
export const getProducst = createSelector(
[getProductsIds, getProductsEntities],
(products, entities) => products.map(id => entities[id]),
);
export const getProduct = createSelector(getProductById, result => result);
const getUsersIds = state => state.admin.users;
const getUserById = (state, id) => state.entities.users[id];
const getUsersEntities = state => state.entities.users;
export const getUsers = createSelector(
[getUsersIds, getUsersEntities],
(products, entities) => products.map(id => entities[id]),
);
export const getUser = createSelector(getUserById, result => result);<file_sep>/src/components/UserForm/UserForm.js
import React from 'react';
import { Form, Field } from 'react-final-form';
import { FORM_ERROR } from 'final-form';
import InputForm from '../InputForm/InputForm';
import ActionButton from '../ActionButton/ActionButton';
import ErrorSubmiting from '../ErrorSubmiting/ErrorSubmiting';
import s from './UserForm.module.css';
const validate = (values) => {
const errors = {};
if (!values.firstName || values.firstName.trim().length === 0) {
errors.firstName = 'Please enter a valid first name';
}
if (!values.lastName || values.lastName.trim().length === 0) {
errors.lastName = 'Please enter a valid last name';
}
if (!values.email || values.email.trim().length === 0 || !values.email.includes('@')) {
errors.email = 'Please enter a valid email address';
}
return errors;
};
const UserForm = ({
user,
onSubmitAction,
onSubmitButtonTitle,
actionAfterSucceeded,
}) => {
const onSubmit = async (values) => { // eslint-disable-line
try {
await onSubmitAction(values);
if (actionAfterSucceeded) {
actionAfterSucceeded();
}
} catch (err) {
return {
[FORM_ERROR]: 'Something went wrong',
};
}
};
return (
<>
<Form
initialValues={{ ...user }}
onSubmit={onSubmit}
validate={validate}
render={({
handleSubmit, submitError, submitting, submitSucceeded,
}) => (
<>
<Field name="firstName">
{({ input, meta }) => (
<InputForm {...input} type="text" placeholder="<NAME>" meta={meta} />
)}
</Field>
<Field name="lastName">
{({ input, meta }) => (
<InputForm {...input} type="text" placeholder="<NAME>" meta={meta} />
)}
</Field>
<Field name="email">
{({ input, meta }) => (
<InputForm {...input} type="text" placeholder="Email" meta={meta} />
)}
</Field>
<div className={s.userRoleSwitch}>
<div className={s.roleSwitch}>
<Field name="role" type="radio" component="input" value="user" />
{' '}User{' '}
</div>
<div className={s.roleSwitch}>
<Field name="role" type="radio" component="input" value="admin" />
{' '}Admin{' '}
</div>
</div>
<div>
<ActionButton
onClick={handleSubmit}
>
{onSubmitButtonTitle}
</ActionButton>
</div>
{submitting && <h2>Submiting...</h2>}
{submitSucceeded && <h2>Complete</h2>}
{submitError && <ErrorSubmiting>{submitError}</ErrorSubmiting>}
</>
)}
/>
</>
);
};
export default UserForm;<file_sep>/src/pages/TermsAndConditions/TermsAndConditionsPage.js
import React from 'react';
const TermsAndConditionsPage = () => (
<h1>
Terms and Conditions page
</h1>
);
export default TermsAndConditionsPage;<file_sep>/src/components/ProductLink/ProductLink.js
import React from 'react';
import { Link } from 'react-router-dom';
import { formatRoute } from 'react-router-named-routes';
import classNames from 'classnames/bind';
import s from './ProductLink.module.css';
import { routes } from '../../routes';
import ActionButton from '../ActionButton/ActionButton';
import Counter from '../Counter/Counter';
const cx = classNames.bind(s);
const ProductLink = ({
title,
id,
image,
price,
onActionButtonClick,
actionButtonTitle,
cart,
count,
increment,
decrement,
onEnterValueCounter,
}) => (
<div className={cx({ ProductLink: true, ProductLinkCart: cart })}>
<div className={cx({ LeftHandSide: cart })}>
{cart && (
<Counter
value={count}
increment={increment}
decrement={decrement}
onEnterValue={onEnterValueCounter}
id={id}
/>
)
}
<Link
to={formatRoute(routes.product, { id })}
className={s.linkAnchor}
>
<div className={cx({ ProductVisibleCart: cart })}>
<div>
<img src={image} alt={title} className={s.productImage} title={title} />
</div>
<div>
<h3 className={s.productTitle}>
{title}
</h3>
<div className={s.productPrice}>
{price} грн
</div>
</div>
</div>
</Link>
</div>
<ActionButton
onClick={() => onActionButtonClick({ id, value: 1 })}
>
{actionButtonTitle}
</ActionButton>
</div>
);
export default ProductLink;<file_sep>/src/pages/Admin/UserListAdmin/UserListAdminComponent.js
import React from 'react';
import ProductLinkAdmin from '../../../components/ProductLinkAdmin/ProductLinkAdmin';
import s from './UserListAdmin.module.css';
const UserListAdminComponent = ({
users,
openModalForm,
openModalAsk,
ModalForm,
ModalAsk,
}) => (
<div className={s.ProductListAdmin}>
{ModalForm()}
{ModalAsk()}
{users.map(({ id, firstName, lastName }) => (
<ProductLinkAdmin
users
key={id}
id={id}
title={`${firstName} ${lastName}`}
showModalToUpdateProduct={openModalForm}
showModalToRemoveProduct={openModalAsk}
/>
))}
</div>
);
export default UserListAdminComponent;<file_sep>/src/pages/User/UserPageContainer.js
import { connect } from 'react-redux';
import { compose, mapProps } from 'recompose';
import ProductPageComponent from './UserPageComponent';
const mapStateToProps = state => ({
user: state.app.user,
});
export default compose(
connect(
mapStateToProps,
),
mapProps(props => ({
...props.user,
})),
)(ProductPageComponent);<file_sep>/src/modules/admin/adminReducerHandlers/adminReducerUsers.js
import * as constants from '../adminConstants';
export const adminReducerUsers = {
// Fetch all users
[constants.FETCH_USERS_START]: state => ({
...state,
isLoading: true,
error: null,
}),
[constants.FETCH_USERS_OK]: (state, action) => ({
...state,
isLoading: false,
users: action.payload.ids,
}),
[constants.FETCH_USERS_ERROR]: (state, action) => ({
...state,
isLoading: false,
error: action.payload,
}),
// Update user
[constants.UPDATE_USER_START]: state => ({
...state,
error: null,
}),
[constants.UPDATE_USER_OK]: (state, action) => ({
...state,
users: state.users.map((user) => {
if (user === action.payload.ids[0]) {
return action.payload.ids[0];
}
return user;
}),
}),
[constants.UPDATE_USER_ERROR]: (state, action) => ({
...state,
error: action.payload,
}),
// Remove user
[constants.REMOVE_USER_START]: state => ({
...state,
error: null,
}),
[constants.REMOVE_USER_OK]: (state, action) => ({
...state,
users: state.users.filter(user => user !== action.payload.ids[0]),
}),
[constants.REMOVE_USER_ERROR]: (state, action) => ({
...state,
error: action.payload,
}),
// Get one user
[constants.GET_USER_START]: state => ({
...state,
isLoading: true,
error: null,
}),
[constants.GET_USER_OK]: (state, action) => ({
...state,
isLoading: false,
users: [action.payload.id[0]].concat(state.users),
}),
[constants.GET_USER_ERROR]: (state, action) => ({
...state,
isLoading: false,
error: action.payload,
}),
};<file_sep>/src/pages/UsersAdmin/UsersPageAdminContainer.js
import { connect } from 'react-redux';
import {
compose, lifecycle, branch, renderComponent, mapProps,
} from 'recompose';
import UsersPageAdminComponent from './UsersPageAdminComponent';
import * as adminOperations from '../../modules/admin/adminOperations';
import * as adminSelectors from '../../modules/admin/adminSelectors';
import Loader from '../../components/Loader/Loader';
import ErrorLoadign from '../../components/ErrorLoading/ErrorLoading';
const mapStateToProps = (state, props) => ({
user: adminSelectors.getUser(state, props.match.params.id),
isLoading: state.admin.isLoading,
isError: !!state.admin.error,
error: state.admin.error,
});
const mapStateToDispatch = {
getUser: adminOperations.getUser,
removeUser: adminOperations.removeUser,
updateUser: adminOperations.updateUser,
};
export default compose(
connect(
mapStateToProps,
mapStateToDispatch,
),
lifecycle({
componentDidMount() {
this.props.getUser(this.props.match.params.id);
},
}),
branch(
props => props.isLoading,
renderComponent(Loader),
branch(
props => props.isError,
renderComponent(ErrorLoadign),
),
),
mapProps(props => ({
updateUser: props.updateUser,
removeUser: props.removeUser,
user: props.user,
history: props.history,
})),
)(UsersPageAdminComponent);<file_sep>/src/components/Header/Header.js
import React from 'react';
import { Link } from 'react-router-dom';
import { connect } from 'react-redux';
import { routes } from '../../routes';
import s from './Header.module.css';
import * as appOperations from '../../modules/app/appOperations';
import * as cartSelectors from '../../modules/cart/cartSelectors';
import ActionButton from '../ActionButton/ActionButton';
import SearchForm from '../SearchForm/SearchForm';
const Header = ({
cartItemsCount,
user,
logout,
}) => (
<div className={s.Header}>
<div className={s.HeaderTop}>
<ul className={s.HeaderLinks}>
<li className={s.HeaderLinkItem}>
<Link to={routes.about}>About Us</Link>
</li>
<li className={s.HeaderLinkItem}>
<Link to={routes.contact}>Contact</Link>
</li>
<li className={s.HeaderLinkItem}>
<Link to={routes.privacypolicy}>Privacy Policy</Link>
</li>
<li className={s.HeaderLinkItem}>
<Link to={routes.termsandconditions}>Terms and Conditions</Link>
</li>
{user
&& user.id !== undefined
&& (
<li className={s.HeaderLinkItem}>
<Link to={routes.user}>
User: {`${user.firstName} ${user.lastName}`}
</Link>
</li>
)}
<li className={s.HeaderLinkItem}>
{user && user.id !== undefined
? (
<ActionButton
onClick={logout}
smallSize
>
Logout
</ActionButton>
)
: (
<Link to={routes.authLogin}>
<ActionButton smallSize>Login/Register</ActionButton>
</Link>
)
}
</li>
</ul>
</div>
<div className={s.HeaderMain}>
<div className={s.Logo}>
<Link to={routes.home}>Internet Store</Link>
</div>
<div className={s.SearchBox}>
<SearchForm />
</div>
<div>
<Link to={{ pathname: routes.cart, state: { modal: true } }}>
Cart ({cartItemsCount})
</Link>
</div>
</div>
</div>
);
const mapStateToProps = state => ({
user: state.app.user,
cartItemsCount: cartSelectors.getCountItems(state),
});
const mapStateToDispatch = {
logout: appOperations.logout,
};
export default connect(mapStateToProps, mapStateToDispatch)(Header);<file_sep>/src/layouts/Desktop.js
import React from 'react';
import { Route, Switch } from 'react-router-dom';
import {
compose, lifecycle, mapProps, withProps,
} from 'recompose';
import s from './Desktop.module.css';
import { routes } from '../routes';
import AdminPage from '../pages/Admin/AdminPage';
import HomePage from '../pages/Home/HomePage';
import CartPage from '../pages/Cart/CartPageContainer';
import CartPageModal from '../pages/Cart/CartModal/CartModal';
import AboutPage from '../pages/About/AboutPage';
import ContactPage from '../pages/Contact/ContactPage';
import PrivacyPolicyPage from '../pages/PrivacyPolicy/PrivacyPolicyPage';
import TermsAndConditionsPage from '../pages/TermsAndConditions/TermsAndConditionsPage';
import NotFoundPage from '../pages/NotFound/NotFoundPage';
import Header from '../components/Header/Header';
import Footer from '../components/Footer/Footer';
import ProtectedRoute from '../components/ProtectedRoute/ProtectedRoute';
import ProtectedRouteUser from '../components/ProtectedRouteUser/ProtectedRouteUser';
import UserPage from '../pages/User/UserPageContainer';
const App = ({ location, previousLocation }) => {
const isModal = !!(
location.state
&& location.state.modal
&& previousLocation !== location
);
return (
<div className={s.App}>
<Header />
<div className={s.Content}>
<Switch location={isModal ? previousLocation : location}>
<ProtectedRoute path={routes.admin} component={AdminPage} />
<Route path={routes.cart} component={CartPage} />
<Route path={routes.about} component={AboutPage} />
<Route path={routes.contact} component={ContactPage} />
<Route path={routes.privacypolicy} component={PrivacyPolicyPage} />
<Route path={routes.termsandconditions} component={TermsAndConditionsPage} />
<ProtectedRouteUser path={routes.user} component={UserPage} />
<Route path={routes.home} component={HomePage} />
<Route component={NotFoundPage} />
</Switch>
</div>
{isModal ? <Route path={routes.cart} component={CartPageModal} /> : null}
<Footer />
</div>
);
};
// This logic allows you to open the cart page in the modal window
// when we click on the cart link and on a separate page when we
// go to the cart page directly by the link or reload the page with
// the open modal box of the cart
let previousLocation;
const getPreviousLocation = () => previousLocation;
const setPreviousLocation = (newPreviousLocation) => {
previousLocation = newPreviousLocation;
};
export default compose(
withProps(
(props) => {
setPreviousLocation(props.location);
},
),
lifecycle({
componentWillUpdate(nextProps) {
const { location } = this.props;
// Set previousLocation if props.location is not modal
if (
nextProps.history.action !== 'POP'
&& (!location.state || !location.state.modal)
) {
setPreviousLocation(this.props.location);
}
},
}),
mapProps(props => ({
...props,
previousLocation: getPreviousLocation(),
})),
)(App);<file_sep>/src/modules/cart/cartReducer.js
import { handleActions } from 'redux-actions';
import * as constants from './cartConstants';
const initialState = {
items: {},
isLoading: false,
error: null,
};
export default handleActions(
{
[constants.ADD]: (state, action) => ({
...state,
items: addItem(state, action),
}),
[constants.REMOVE]: (state, action) => ({
...state,
items: removeItem(state, action),
}),
[constants.FETCH_PRODUCTS_START]: state => ({
...state,
isLoading: true,
error: null,
}),
[constants.FETCH_PRODUCTS_OK]: state => ({
...state,
isLoading: false,
}),
[constants.FETCH_PRODUCTS_ERROR]: (state, action) => ({
...state,
isLoading: false,
error: action.payload,
}),
[constants.INCREASE]: (state, action) => ({
...state,
items: {
...state.items,
[action.payload.id]: {
...state.items[action.payload.id],
count: state.items[action.payload.id].count + 1,
},
},
}),
[constants.DECREASE]: (state, action) => ({
...state,
items: decrease(state, action),
}),
[constants.ENTER_VALUE]: (state, action) => ({
...state,
items: enterValue(state, action),
}),
},
initialState,
);
function addItem(state, action) {
if (!state.items[action.payload.id]) {
return {
...state.items,
[action.payload.id]: {
id: action.payload.id,
count: action.payload.value,
},
};
}
return {
...state.items,
[action.payload.id]: {
id: action.payload.id,
count: state.items[action.payload.id].count + action.payload.value,
},
};
}
function removeItem(state, action) {
const items = state.items;
delete items[action.payload.id];
return {
...items,
};
}
function decrease(state, action) {
if (state.items[action.payload.id].count - 1 < 0) {
return state.items;
}
return {
...state.items,
[action.payload.id]: {
...state.items[action.payload.id],
count: state.items[action.payload.id].count - 1,
},
};
}
function enterValue(state, action) {
if (action.payload.value < 0) {
return {
...state.items,
[action.payload.id]: {
...state.items[action.payload.id],
count: 0,
},
};
}
return {
...state.items,
[action.payload.id]: {
...state.items[action.payload.id],
count: action.payload.value,
},
};
}<file_sep>/src/components/Footer/Footer.js
import React from 'react';
import { Link } from 'react-router-dom';
import s from './Footer.module.css';
import { routes } from '../../routes';
const Footer = () => (
<div className={s.Footer}>
<div className={s.FooterMain}>
<ul className={s.FooterLinks}>
<h4>Store information</h4>
<li className={s.FooterLinkItem}>
<Link to={routes.about}>About Us</Link>
</li>
<li className={s.FooterLinkItem}>
<Link to={routes.contact}>Contact</Link>
</li>
<li className={s.FooterLinkItem}>
<Link to={routes.privacypolicy}>Privacy Policy</Link>
</li>
<li className={s.FooterLinkItem}>
<Link to={routes.termsandconditions}>Terms and Conditions</Link>
</li>
</ul>
</div>
<h3 className={s.FooterS}>Internet Store 2018</h3>
</div>
);
export default Footer;<file_sep>/src/routes.js
export const routes = {
admin: '/admin',
adminProduct: '/admin/products/:id',
adminUser: '/admin/user/:id',
adminUsers: '/admin/users',
home: '/',
product: '/products/:id',
user: '/user',
cart: '/cart',
cartCheckout: '/cart/checkout',
about: '/about',
contact: '/contact',
termsandconditions: '/termsandconditions',
privacypolicy: '/privacypolicy',
auth: '/auth',
authRemember: '/auth/remember',
authLogin: '/auth/login',
authRegister: '/auth/register',
}<file_sep>/src/modules/products/productsConstants.js
export const FETCH_PRODUCTS_START = 'products/FETCH_PRODUCTS_START';
export const FETCH_PRODUCTS_OK = 'products/FETCH_PRODUCTS_OK';
export const FETCH_PRODUCTS_ERROR = 'products/FETCH_PRODUCTS_ERROR';
export const GET_PRODUCT_START = 'products/GET_PRODUCT_START';
export const GET_PRODUCT_OK = 'products/GET_PRODUCT_OK';
export const GET_PRODUCT_ERROR = 'products/GET_PRODUCT_ERROR';
export const SET_PAGE = 'products/SET_PAGE';<file_sep>/src/modules/entities/entitiesReducer.js
const initialState = {
products: {
// [product.id]: {
// ...product
// }
},
users: {
// [user.id]: {
// ...user
// }
},
};
export default function entitiesReducer(state = initialState, action) {
if (action.payload && action.payload.entities) {
const newState = { ...state };
Object.keys(action.payload.entities).forEach((key) => {
Object.assign(newState[key], action.payload.entities[key]);
});
return newState;
}
return state;
}<file_sep>/src/schemes/products.js
import T from 'prop-types';
export const productPropTypes = T.shape({
id: T.string.isRequired,
title: T.string.isRequired,
description: T.string.isRequired,
image: T.string.isRequired,
price: T.number.isRequired,
}).isRequired;<file_sep>/src/pages/Cart/CartList/CartList.js
import React from 'react';
import s from './CartList.module.css';
const CartList = ({
products,
itemsCart,
renderProductLink,
}) => {
if (products.length === 0) {
return (
<h2 className={s.EmptyCart}>Your cart is empty</h2>
);
}
return (
<div className={s.CartList}>
{products.map(item => renderProductLink(item, itemsCart))}
</div>
);
};
export default CartList;<file_sep>/src/modules/rootModule.js
import { combineReducers } from 'redux';
import products from './products/productsReducer';
import cart from './cart/cartReducer';
import entities from './entities/entitiesReducer';
import admin from './admin/adminReducer';
import app from './app/appReducer';
export default combineReducers({
products,
cart,
entities,
admin,
app,
});<file_sep>/src/api/schemes.js
import { schema } from 'normalizr';
// Provides schemes to normalizate data
export const Product = new schema.Entity('products');
export const ProductCollection = [Product];
export const AdminProduct = new schema.Entity('products');
export const AdminProductCollection = [Product];
export const AdminUser = new schema.Entity('users');
export const AdminUserCollection = [AdminUser];<file_sep>/src/modules/products/productsOperations.js
import { normalize } from 'normalizr';
import * as schemes from '../../api/schemes';
import * as actions from './productsActions';
import * as Api from '../../api/Api';
export const fetchProducts = refresh => async (dispatch, getState) => {
try {
const limit = getState().products.limit;
const offset = getState().products.offset;
const ids = getState().products.products;
// When we go straight through the link to the product page,
// we can already have one product
if (ids.length > 1 && !refresh) {
return;
}
dispatch(actions.fetchProductsStart());
const res = await Api.Products.fetchProducts(offset, limit);
const { result, entities } = normalize(res.data, schemes.ProductCollection);
dispatch(actions.fetchProductsOk({
ids: result,
entities,
}));
} catch (err) {
dispatch(actions.fetchProductsError(err.message));
}
};
export const getProduct = id => async (dispatch, getState) => {
try {
const product = getState().entities.products[id];
if (product) {
return;
}
dispatch(actions.getProductStart());
const res = await Api.Products.getProductsById(id);
const { result, entities } = normalize(res.data, schemes.ProductCollection);
dispatch(actions.getProductOk({
id: result,
entities,
}));
} catch (err) {
dispatch(actions.getProductError(err.message));
}
};<file_sep>/src/pages/Admin/AdminNav/AdminNav.js
import React from 'react';
import { NavLink } from 'react-router-dom';
import s from './AdminNav.module.css';
import { routes } from '../../../routes';
const AdminNav = () => (
<div className={s.AdminNav}>
<NavLink
exact
to={routes.admin}
className={s.AdminNavLink}
activeClassName={s.AdminNavLinkActive}
>
Products
</NavLink>
<NavLink
to={routes.adminUsers}
className={s.AdminNavLink}
activeClassName={s.AdminNavLinkActive}
>
Users
</NavLink>
</div>
);
export default AdminNav;<file_sep>/src/pages/UsersAdmin/UsersPageAdminComponent.js
import React from 'react';
import { Form } from 'react-final-form';
import { FORM_ERROR } from 'final-form';
import ActionButton from '../../components/ActionButton/ActionButton';
import UserForm from '../../components/UserForm/UserForm';
import ErrorSubmiting from '../../components/ErrorSubmiting/ErrorSubmiting';
const UserPageAdminComponent = ({
user,
updateUser,
removeUser,
history,
}) => {
const onSubmitRemove = async ({ id }) => { // eslint-disable-line
try {
await removeUser(id);
history.go(-1);
} catch (err) {
return {
[FORM_ERROR]: 'Somethings went wrogn',
};
}
};
return (
<div>
<UserForm
user={user}
onSubmitAction={updateUser}
onSubmitButtonTitle="Update"
actionAfterSucceeded={history.goBack}
/>
<Form
initialValues={{ ...user }}
onSubmit={onSubmitRemove}
render={({
handleSubmit, submitError, submitting, submitSucceeded,
}) => (
<>
<ActionButton onClick={handleSubmit}>Remove</ActionButton>
{submitting && <h2>Submiting...</h2>}
{submitSucceeded && <h2>Complete</h2>}
{submitError && <ErrorSubmiting>{submitError}</ErrorSubmiting>}
</>
)}
/>
</div>
);
};
export default UserPageAdminComponent; | 3c1c1157229bead29dc3b3a992a48c962918de69 | [
"JavaScript"
] | 55 | JavaScript | YuraPelehatiy/internet-store-app | ccc1d1686f138e78cb76ce7fef847612baf02f32 | ce466031ad3f9909d9fe389372e366be877d9c2d | |
refs/heads/master | <file_sep>import React from 'react'
import Title from '../../../components/Title'
import { Flex, Box } from '@rebass/grid'
import ilustraAtena from '../../../assets/ilustra-atena.svg'
const About = () => {
return (
<section className="container about">
<Flex alignItems="center" flexWrap="wrap">
<Box width={[1, 1 / 2]}>
<Title large color="primary">
Mas afinal,
<br />o que é a <span className="red">Atena</span> ?
</Title>
<p className="super">
Inspirada na deusa grega da sabedoria, a <strong>Atena</strong> é
uma iniciativa <strong>open source</strong> de gamificação da
Impulso, que tem como objetivos promover o engajamento e premiar os
esforços das pessoas que pertencem à Impulso Network.
</p>
<br />
<p className="super ifdesktop">
Assim que você entra na comunidade, automaticamente se tornará um(a)
jogador(a) e poderá pontuar por meio da execução de diversas{' '}
<strong>atividades</strong>, alcançar <strong>Níveis</strong> e
obter <strong>conquistas</strong> como reconhecimento pelos seus
esforços.
</p>
</Box>
<Box width={[1, 1 / 2]}>
<img
src={ilustraAtena}
alt="Ilustração da Atena"
className="ilustra"
/>
</Box>
</Flex>
</section>
)
}
export default About
<file_sep>import React, { Component } from 'react'
import InfoCards from './InfoCards'
import TeamAccordion from './TeamAccordion'
import AdminRanking from './AdminRanking'
import Chart from './Chart'
import Users from './Users'
import EditExperience from './EditExperience'
import EditAchievements from './EditAchievements'
import CreateAchievements from './CreateAchievements'
import { Container, Aside, Section, Option } from './styles'
class Admin extends Component {
state = {
active: 'players',
achievementsType: null,
options: [
{ key: 'generalReports', name: 'Relatórios Gerais' },
{ key: 'ranking', name: 'Rankings' },
{ key: 'editExperience', name: 'Experiência' },
{ key: 'achievements', name: 'Conquistas' }
]
}
handleClick = active => {
active === 'editAchievements' || active === 'createAchievements'
? this.setState({ achievementsType: active })
: active === 'achievements'
? this.setState({ active, achievementsType: 'editAchievements' })
: this.setState({ active, achievementsType: null })
}
renderOptions = title => {
const { active, options } = this.state
return (
<>
<h3>{title}</h3>
{options.map(option => (
<Option
key={option.key}
active={active === option.key}
onClick={() => this.handleClick(option.key)}
>
{option.name}
</Option>
))}
</>
)
}
render() {
const { active, achievementsType } = this.state
return (
<Container>
<Aside>
{this.renderOptions('Jogo')}
{active === 'achievements' && (
<>
<Option
marginLeft="15px"
key="editAchievements"
active={achievementsType === 'editAchievements'}
onClick={() => this.handleClick('editAchievements')}
>
Editar
</Option>
<Option
marginLeft="15px"
key="createAchievements"
active={achievementsType === 'createAchievements'}
onClick={() => this.handleClick('createAchievements')}
>
Criar
</Option>
</>
)}
<small />
<h3>Jogadores</h3>
<Option
key="players"
active={active === 'players'}
onClick={() => this.handleClick('players')}
>
Informações do jogador
</Option>
</Aside>
<Section>
{active === 'generalReports' ? (
<>
<h2>Relatórios Gerais</h2>
<Chart />
<InfoCards />
<TeamAccordion />
</>
) : active === 'editExperience' ? (
<>
<h2>Experiência(XP)</h2>
<EditExperience />
</>
) : active === 'ranking' ? (
<>
<h2>Ranking</h2>
<AdminRanking />
</>
) : active === 'players' ? (
<>
<h2>Informações do Jogador</h2>
<Users />
</>
) : active === 'achievements' ? null : null}
{achievementsType === 'editAchievements' ? (
<>
<h2>Editar Conquistas</h2>
<EditAchievements />
</>
) : achievementsType === 'createAchievements' ? (
<>
<h2>Criar Conquistas</h2>
<CreateAchievements />
</>
) : null}
</Section>
</Container>
)
}
}
export default Admin
<file_sep>import styled from 'styled-components'
import theme from '../../styles/theme'
export const StyledUserCard = styled.div`
background-color: ${theme.color.white};
height: 413px;
width: 325px;
width: ${props => props.width};
margin-right: 8px;
padding: 0 12px 18px;
box-shadow: 8px 8px 60px 0px rgba(0, 0, 0, 0.08);
margin-top: ${({ first }) => (first ? null : ` 70px;`)};
order: ${({ order }) => order};
border-radius: 10px;
position: relative;
@media (max-width: 998px) {
margin-top: 70px;
&:first-child {
order: 2;
}
}
@media (max-width: 649px) {
margin-right: 0;
&:first-child {
margin-top: 0;
}
}
`
export const Container = styled.div`
background-color: #fff;
height: 413px;
border-radius: 10px;
display: flex;
justify-content: space-between;
align-items: center;
flex-direction: column;
figure {
margin-top: 0;
background-color: #6567a9;
padding-top: 30px;
display: flex;
width: 232px;
align-items: center;
justify-content: center;
border-bottom-right-radius: 50%;
border-bottom-left-radius: 50%;
}
img {
width: 216px;
height: 216px;
border-radius: 50%;
padding: 0 5px 8px 5px;
}
`
export const Position = styled.div`
background-color: #fff;
width: 64px;
height: 64px;
border-radius: 50%;
border-width: 5px;
border-style: solid;
border-color: #6567a9;
display: flex;
position: absolute;
transform: translateY(330%);
text-align: center;
font-size: 20px;
font-weight: bold;
justify-content: center;
align-items: center;
`
export const Info = styled.div`
margin-top: 30px;
padding-bottom: 14px;
width: 100%;
h1 {
text-align: center;
text-transform: uppercase;
font-size: 20px;
color: #595b98;
}
`
export const Point = styled.div`
flex: 1;
text-transform: uppercase;
font-size: 16px;
font-weight: bold;
flex-direction: column;
text-align: center;
border-right: ${props => (props.border ? 'solid 1px #e2e2e2' : 'none')};
p {
color: #666c71;
font-size: 16px;
font-weight: bold;
padding: 6px;
margin: 0;
}
`
<file_sep>import styled from 'styled-components'
import theme from '../../styles/theme'
export const StyledScreenError = styled.section`
main {
padding-bottom: 100px;
background: ${({ background }) => (background ? 'white' : 'transparent')};
}
._inner {
color: ${theme.color.gray};
flex: 1;
min-height: 400px;
}
._inner > p {
margin-top: 60px;
}
.super {
max-width: 782px;
margin: 0 auto;
b {
font-weight: 600;
}
}
p.super {
font-weight: 300;
}
.month {
color: ${theme.color.secondary};
}
@media (max-width: 760px) {
main {
padding-top: 160px;
padding-bottom: 60px;
}
._inner > p {
margin-top: 30px;
}
}
`
export const Header = styled.div`
background: ${theme.color.primary};
height: 100px;
`
export const Loading = styled.div`
align-items: center;
display: flex;
height: ${({ height }) => height || 'initial'};
justify-content: center;
padding: ${({ paddingSize }) => paddingSize || '0'};
width: ${({ width }) => width || '100%'};
img {
height: ${({ imgSize }) => imgSize || '10rem'};
}
`
export const SmallLoadingWrapper = styled.div`
align-items: center;
background: rgba(0, 0, 0, 0.2);
border-radius: 10px;
display: flex;
height: ${({ height }) => height || '100%'};
justify-content: center;
position: absolute;
right: 0;
top: 0;
width: ${({ width }) => width || '100%'};
z-index: 10;
img {
height: 40%;
margin: 0 auto;
}
`
export const SmallErrorWrapper = styled.div`
align-items: center;
background: ${theme.color.white};
border-radius: 10px;
color: ${theme.color.primary};
cursor: pointer;
display: flex;
flex-direction: column;
font-weight: bold;
height: ${({ height }) => height || '100%'};
justify-content: center;
padding: 14px;
position: absolute;
right: 0;
text-align: center;
top: 0;
width: ${({ width }) => width || '100%'};
z-index: 1000;
p {
color: ${theme.color.gray};
padding-bottom: 40px;
}
`
<file_sep>import React from 'react'
import PropTypes from 'prop-types'
import StyledSubTitle from './SubTitle.style'
const SubTitle = props => {
const { children } = props
return <StyledSubTitle>{children}</StyledSubTitle>
}
SubTitle.propTypes = {
children: PropTypes.string.isRequired
}
export default SubTitle
<file_sep>import { delay, call, put } from 'redux-saga/effects'
import api from '../../services/api'
import { Creators as RankingActions } from '../ducks/ranking'
export function* getRanking(action) {
try {
const { data } = yield call(api.get, `/ranking/${action.selected}?limit=20`)
const rankingData = {
data,
loading: false,
error: data.message || ''
}
yield put(RankingActions.getRankingResponse(rankingData))
} catch (error) {
yield put(
RankingActions.getRankingResponse({
loading: false,
error: error.message,
data: []
})
)
}
}
export function* getRankingUsers() {
try {
yield delay(1000)
// throw new Error("Servidor indisponivel");
const data = [
{
name: '<NAME>',
xp: 1234,
position: 4,
level: 5,
isCoreTeam: true,
teams: ['atari', 'flamengo', 'Chiefs'],
avatar:
'https://avatars.slack-edge.com/2018-08-01/409144328290_81d8f97e55540e84881c_72.png'
},
{
name: '<NAME>',
xp: 1234,
position: 4,
level: 5,
isCoreTeam: true,
teams: ['atari', 'flamengo', 'Chiefs'],
avatar:
'https://avatars.slack-edge.com/2018-08-01/409144328290_81d8f97e55540e84881c_72.png'
},
{
name: '<NAME>',
xp: 1234,
position: 4,
level: 5,
isCoreTeam: true,
teams: ['atari', 'flamengo', 'Chiefs'],
avatar:
'https://avatars.slack-edge.com/2018-08-01/409144328290_81d8f97e55540e84881c_72.png'
},
{
name: '<NAME>',
xp: 1234,
position: 4,
level: 5,
isCoreTeam: true,
teams: ['atari', 'flamengo', 'Chiefs'],
avatar:
'https://avatars.slack-edge.com/2018-08-01/409144328290_81d8f97e55540e84881c_72.png'
},
{
name: '<NAME>',
xp: 1234,
position: 4,
level: 5,
isCoreTeam: true,
teams: ['atari', 'flamengo', 'Chiefs'],
avatar:
'https://avatars.slack-edge.com/2018-08-01/409144328290_81d8f97e55540e84881c_72.png'
}
]
yield put(
RankingActions.getRankingUsersResponse({
data,
loading: false,
error: null
})
)
} catch (error) {
yield put(
RankingActions.getRankingUsersResponse({
data: null,
loading: false,
error: error.message
})
)
}
}
<file_sep>import React from 'react'
import PropTypes from 'prop-types'
import { Route, Redirect } from 'react-router-dom'
import { store } from '../store'
export default function RouteWrapper({
component: Component,
isPrivate,
coreTeam,
...rest
}) {
const { uuid, isCoreTeam } = store.getState().auth
if (!uuid && isPrivate) {
return <Redirect to="/" />
}
if (uuid && coreTeam && !isCoreTeam) {
return <Redirect to="/" />
}
return <Route {...rest} component={Component} />
}
RouteWrapper.propTypes = {
isPrivate: PropTypes.bool,
coreTeam: PropTypes.bool,
component: PropTypes.any
}
RouteWrapper.defaultProps = {
component: null,
isPrivate: false,
coreTeam: false
}
<file_sep>import React, { Component } from 'react'
import PropTypes from 'prop-types'
import Select from 'react-select'
import { Flex } from '@rebass/grid'
import { bindActionCreators } from 'redux'
import { connect } from 'react-redux'
import { Creators as RankingActions } from '../../../store/ducks/ranking'
import { Creators as UserActions } from '../../../store/ducks/user'
import { PageLoading, PageError, SmallError } from '../../../components/utils'
import RankingRow from '../../Ranking/RankingRow'
import SearchBar from '../../../components/SearchBar'
import InfoCards from '../InfoCards'
import TeamAccordion from '../TeamAccordion'
import {
Container,
RankingHeader,
UserSection,
CardWrapper,
Portrait,
Card,
ButtonWrapper,
Button,
Info
} from './styles'
class Users extends Component {
static propTypes = {
actions: PropTypes.object,
user: PropTypes.object,
userInfo: PropTypes.object,
getUserInfo: PropTypes.func.isRequired,
ranking: PropTypes.object
}
state = { user: null }
getUserInfo = name => {
if (name) {
this.props.actions.getUserInfo(name)
this.setState({ user: name })
}
this.props.actions.getUserInfo(this.state.user)
}
renderUsersList = users => {
const { loading, error, data } = users
if (error) return <PageError message={error} />
if (!data && !loading) return
if (!data && loading) return <PageLoading />
return (
<Flex justifyContent="space-around" mt={50} mb={50} flexWrap="wrap">
<RankingHeader>
<div className="ranking">RANKING</div>
<div className="userInfo" />
<div className="level">LEVEL</div>
<div className="xp">XP</div>
</RankingHeader>
{data.map((card, index) => (
<RankingRow getUserInfo={this.getUserInfo} key={index} {...card} />
))}
</Flex>
)
}
renderUserInfos = userInfo => {
const { loading, error, data } = userInfo
if (error)
return (
<UserSection>
<SmallError
refresh={() => this.getUserInfo()}
height="100px"
message={error}
/>
</UserSection>
)
if (!data) return !loading ? null : <PageLoading />
return (
<UserSection>
<CardWrapper>
<Portrait>
<figure>
<img src={data.avatar} alt="" />
<small>{`${data.monthlyPosition}º`}</small>
</figure>
<p>{data.name}</p>
</Portrait>
<Card>
<Info width="30%">
<h4>ranking geral</h4>
<p>{data.generalPosition}</p>
</Info>
<Info>
<h4>level</h4>
<p>{data.level}</p>
</Info>
<Info>
<h4>xp</h4>
<p>{data.score}</p>
</Info>
<Info width="280px">
<h4>times</h4>
<Select
isMulti
placeholder="Selecione..."
defaultValue={data.teams}
options={data.availableTeams}
/>
</Info>
</Card>
</CardWrapper>
<ButtonWrapper>
<Button>atualizar</Button>
</ButtonWrapper>
<InfoCards user={data.name} />
<TeamAccordion user={data.name} />
</UserSection>
)
}
render() {
const { users } = this.props.ranking
const { userInfo } = this.props.user
return (
<Container>
<SearchBar />
{this.renderUsersList(users)}
{this.renderUserInfos(userInfo)}
</Container>
)
}
}
const mapStateToProps = state => ({
ranking: state.ranking,
user: state.user
})
const mapDispatchToProps = dispatch => {
return {
actions: bindActionCreators(
Object.assign({}, RankingActions, UserActions),
dispatch
)
}
}
export default connect(
mapStateToProps,
mapDispatchToProps
)(Users)
<file_sep><img src="https://s3-sa-east-1.amazonaws.com/assets.impulso.network/images/impulsonetwork-logo.svg" style="width: 350px">
[](https://www.codacy.com/app/impulsonetwork/atena-frontend?utm_source=github.com&utm_medium=referral&utm_content=universoimpulso/atena-frontend&utm_campaign=Badge_Grade)
[](https://dev.azure.com/universoimpulso/Atena/_build/latest?definitionId=5&branchName=master)
[](http://atena.impulso.network)
[](LICENSE)
[](https://twitter.com/UniversoImpulso)
[](https://app.netlify.com/sites/atena/deploys)
## Front - Atena v.0.2
A Atena é uma iniciativa da Impulso Network em colaboração com vários Impulsers, com o objetivo de promover o engajamento e recompensar as atividades e esforços de cada pessoa na Impulso. Nele você poderá adquirir Pontos de Reputação através da execução de diversas atividades e com base nesses dois fatores os Impulsers receberão níveis e conquistas, reconhecendo o esforço despendido nas atividades.
## Configuração
Um passo-a-passo da configuração mínima que você precisa para obter o Atena em execução.
### Desenvolvimento
- Faça um `fork` do projeto para a tua conta, e então faça o `clone`
```sh
> git clone https://github.com/[sua-conta]/atena-frontend
```
- Navegue até a pasta de destino onde fez o clone do projeto
```sh
> cd atena-frontend/
```
- Instale o `yarn` a partir do `npm` (ser global é opcional)
```sh
> npm i yarn -g
```
- instalar os repositorios utilizando o `yarn`
```sh
> yarn -i
```
- Adicionar referências remotas
```sh
> git remote add upstream https://github.com/universoimpulso/atena-frontend
```
### Executando
- Inicie o servidor utilizando o seguinte comando:
```sh
> yarn start
```
### [Integração com o Github](GITHUB.md)
O Atena possui integração com o Github, onde o usuário ganha pontos ao criar pull requests, efetuar reviews, e ter seu pull request aprovado.
## Contribuindo
O principal objetivo deste repositório é continuar a evoluir o Atena, tornando-o mais rápido e fácil de usar.
O desenvolvimento da Atena acontece a céu aberto no GitHub, e somos gratos à comunidade por contribuir com correções de bugs e melhorias. Leia abaixo para saber como você pode participar da melhoria da Atena e da Impulso network.
## Comunidade
Todos os comentários e sugestões são bem-vindas e podem ser feitas via Issues no Github ou lá no RocketChat!
💬 Junte-se a comunidade em [Impulso Network](https://impulso.network)
<file_sep>import { delay, put } from 'redux-saga/effects'
import { Creators as ExperienceCardActions } from '../ducks/experienceCard'
import { toast } from 'react-toastify'
const data = {
general: [
{
key: 'limitPerDay',
name: 'limite de experiência diária',
text: 'até:',
values: [
{
key: 'limitPerDay',
initialValue: 20
}
]
},
{
key: 'inactivityPeriod',
name: 'período de inatividade',
text: 'até:',
values: [
{
key: 'inactivityPeriod',
initialValue: 8
}
]
},
{
key: 'inactivityLost',
name: 'XP perdido por inatividade',
text: 'até:',
values: [
{
key: 'inactivityLost',
initialValue: 2
}
]
}
],
activity: [
{
key: 'rocketChat',
name: 'rocket chat',
values: [
{
key: 'message',
text: 'mensagens',
initialValue: 2
},
{
key: 'reactions',
text: 'dar reactions',
initialValue: 1
},
{
key: 'receiveReaction',
text: 'receber reactions',
initialValue: 2
}
]
},
{
key: 'meetup',
name: 'meetup',
values: [
{
key: 'participant',
text: 'participante',
initialValue: 5
},
{
key: 'mediator',
text: 'mediador',
initialValue: 3
},
{
key: 'facilitator',
text: 'facilitador',
initialValue: 3
}
]
},
{
key: 'blog',
name: 'blog',
values: [
{ key: 'post', text: 'postagem realizada', initialValue: 3 },
{
key: 'comment',
text: 'comentário realizado',
initialValue: 2
}
]
},
{
key: 'github',
name: 'open source',
values: [
{ key: 'issue', text: 'issues', initialValue: 3 },
{
key: 'review',
text: 'dar reactions',
initialValue: 2
},
{
key: 'pullRequest',
text: 'pull request',
initialValue: 2
},
{
key: 'approvedPullRequest',
text: 'PR aprovada',
initialValue: 5
}
]
},
{
key: 'referral',
name: 'referral',
values: [
{
key: 'indication',
text: 'impulser indicado',
initialValue: 3
},
{
key: 'allocation',
text: 'alocado via indicação',
initialValue: 2
}
]
},
{
key: 'research',
name: 'research',
values: [
{
key: 'validation',
text: 'passar pela validação',
initialValue: 2
},
{
key: 'skill',
text: 'skill assessments',
initialValue: 2
}
]
},
{
key: 'allocation',
name: 'alocação',
values: [
{
key: 'interview',
text: 'entrevistado',
initialValue: 2
},
{
key: 'approved',
text: 'aprovado',
initialValue: 3
},
{
key: 'allocated',
text: 'alocado',
initialValue: 2
}
]
},
{
key: 'linkedin',
name: 'linkedin',
values: [
{
key: 'impulser',
text: 'trabalhando na impulso',
initialValue: 2
}
]
}
]
}
const data2 = {
general: [
{
key: 'limitPerDay',
name: 'limite de experiência diária',
text: 'até:',
values: [
{
key: 'limitPerDay',
initialValue: 23
}
]
},
{
key: 'inactivityPeriod',
name: 'período de inatividade',
text: 'até:',
values: [
{
key: 'inactivityPeriod',
initialValue: 8
}
]
},
{
key: 'inactivityLost',
name: 'XP perdido por inatividade',
text: 'até:',
values: [
{
key: 'inactivityLost',
initialValue: 2
}
]
}
],
activity: [
{
key: 'rocketChat',
name: 'rocket chat',
values: [
{
key: 'message',
text: 'mensagens',
initialValue: 2
},
{
key: 'reactions',
text: 'dar reactions',
initialValue: 1
},
{
key: 'receiveReaction',
text: 'receber reactions',
initialValue: 2
}
]
},
{
key: 'meetup',
name: 'meetup',
values: [
{
key: 'participant',
text: 'participante',
initialValue: 5
},
{
key: 'mediator',
text: 'mediador',
initialValue: 3
},
{
key: 'facilitator',
text: 'facilitador',
initialValue: 3
}
]
},
{
key: 'blog',
name: 'blog',
values: [
{ key: 'post', text: 'postagem realizada', initialValue: 3 },
{
key: 'comment',
text: 'comentário realizado',
initialValue: 2
}
]
},
{
key: 'github',
name: 'open source',
values: [
{ key: 'issue', text: 'issues', initialValue: 3 },
{
key: 'review',
text: 'dar reactions',
initialValue: 2
},
{
key: 'pullRequest',
text: 'pull request',
initialValue: 2
},
{
key: 'approvedPullRequest',
text: 'PR aprovada',
initialValue: 5
}
]
},
{
key: 'referral',
name: 'referral',
values: [
{
key: 'indication',
text: 'impulser indicado',
initialValue: 3
},
{
key: 'allocation',
text: 'alocado via indicação',
initialValue: 2
}
]
},
{
key: 'research',
name: 'research',
values: [
{
key: 'validation',
text: 'passar pela validação',
initialValue: 2
},
{
key: 'skill',
text: 'skill assessments',
initialValue: 2
}
]
},
{
key: 'allocation',
name: 'alocação',
values: [
{
key: 'interview',
text: 'entrevistado',
initialValue: 2
},
{
key: 'approved',
text: 'aprovado',
initialValue: 3
},
{
key: 'allocated',
text: 'alocado',
initialValue: 2
}
]
},
{
key: 'linkedin',
name: 'linkedin',
values: [
{
key: 'impulser',
text: 'trabalhando na impulso',
initialValue: 2
}
]
}
]
}
export function* getExperience() {
try {
yield delay(1000)
// throw new Error("Não foi possível buscar os dados");
yield put(ExperienceCardActions.getExperienceSuccess(data))
} catch (error) {
yield put(ExperienceCardActions.getExperienceFailure(error.message))
}
}
export function* putExperience(action) {
try {
yield delay(1000)
// throw new Error("Não foi possível atualizar a pontuação");
yield put(ExperienceCardActions.putExperienceSuccess(data2))
toast.success('Pontuação atualizada com sucesso!')
} catch (error) {
toast.error(error.message)
yield put(ExperienceCardActions.putExperienceFailure())
}
}
<file_sep>import React from 'react'
import { StyledFooter, StyledCopyright } from './style'
import impulsoIcon from '../../assets/impulso.svg'
import SocialLinks from './SocialLinks'
const Footer = () => (
<StyledFooter>
<section>
<img className="impulsoIcon" src={impulsoIcon} alt="Logo da Impulso" />
<SocialLinks />
<hr />
<StyledCopyright>
2019 © Todos os direitos reservados. Impulso.
</StyledCopyright>
</section>
</StyledFooter>
)
export default Footer
<file_sep>import React from 'react'
import { Flex, Box } from '@rebass/grid'
import Menu from './Menu/'
import atena from '../../assets/atena.svg'
import StyledHeader from './styles'
const Header = props => (
<StyledHeader>
<Flex justifyContent="space-between" alignItems="center">
<Box flex="1">
<a href="/">
<img alt="Logo atena" src={atena} />
</a>
</Box>
<Box>
<Menu {...props} />
</Box>
</Flex>
</StyledHeader>
)
export default Header
<file_sep>import { combineReducers } from 'redux'
import auth from './auth'
import user from './user'
import ranking from './ranking'
import generalReports from './generalReports'
import experienceCard from './experienceCard'
import achievements from './achievements'
export default combineReducers({
auth,
user,
ranking,
generalReports,
experienceCard,
achievements
})
<file_sep>const Theme = {
gridSize: '1280px',
spacing: {
unit: 8
},
fontFamily: {
primary: 'proxima-nova',
secondary: 'essonnes-display'
},
fontSize: {
default: '14px',
medium: '20px',
large: '36px',
veryLarge: '48px',
super: '100px',
extraLarge: '48px'
},
color: {
white: '#FFFFFF',
background: '#F0F0F7',
black: '#000000',
gray: '#666C71',
lightGray: '#C9C9D8',
primary: '#595B98',
primaryLight: '#8688D9',
primaryHover: '#9598ED',
primaryFocus: '#414287',
scampi: '#6567A9',
secondary: '#DC3C2C',
bg: '#FDFDFD',
green: '#279949',
linkedin: '#1061AC',
linkedinHover: '#007bb7'
}
}
export default Theme
<file_sep>import React, { Component } from 'react'
import { Formik } from 'formik'
import { toast } from 'react-toastify'
import PropTypes from 'prop-types'
import { bindActionCreators } from 'redux'
import { connect } from 'react-redux'
import { Creators as AchievementsActions } from '../../../store/ducks/achievements'
import { SmallLoading } from '../../../components/utils'
import { Card, StyledInput } from './styles'
class EditAchievements extends Component {
static propTypes = {
editAchievement: PropTypes.func.isRequired,
data: PropTypes.array,
achievements: PropTypes.object
}
putValues = values => {
const { AchievementName, type, value } = this.props.data
const formatedValues = []
for (let [key, value] of Object.entries(values.tiers)) {
formatedValues.push({ name: key, value })
}
values.tiers = formatedValues
const sameValues = value.tiers.filter(
(v, index) => v.value === values.tiers[index].value
)
if (sameValues.length === 5) {
toast.warn('Nenhum valor alterado, sua requisição nao foi enviada!')
return
}
const data = { AchievementName, type, values }
this.props.editAchievement(data)
}
notify = type => {
if (type === 'negativeNumber') {
if (toast.isActive('negativeNumber')) return
toast.error('Não é possível submeter valores negativos', {
toastId: 'negativeNumber'
})
}
}
getValues = data => {
const values = {}
data.forEach(data => Object.assign(values, { [data.name]: data.value }))
return values
}
validate = value => {
const validate = value && value > 0 ? undefined : true
if (validate) this.notify('negativeNumber')
return validate
}
render() {
const { type } = this.props.data
const { name, tiers } = this.props.data.value
const { editLoading } = this.props.achievements
return (
<Card>
<p>{name}</p>
<Formik
onSubmit={tiers => this.putValues({ tiers, name })}
initialValues={this.getValues(tiers)}
>
{({ handleSubmit, handleChange, errors, touched }) => (
<form onSubmit={handleSubmit}>
<ul>
{tiers.map(tier => (
<li key={tier.name}>
<span>{tier.name}</span>
<StyledInput
name={tier.name}
validate={value => this.validate(value)}
type="number"
onChange={handleChange}
error={errors[tier.name] && touched[tier.name]}
/>
</li>
))}
</ul>
<button type="submit">atualizar</button>
</form>
)}
</Formik>
{editLoading === type.concat(name) && <SmallLoading />}
</Card>
)
}
}
const mapStateToProps = state => ({ achievements: state.achievements })
const mapDispatchToProps = dispatch =>
bindActionCreators(AchievementsActions, dispatch)
export default connect(
mapStateToProps,
mapDispatchToProps
)(EditAchievements)
<file_sep>import styled from 'styled-components'
const StyledHeader = styled.header`
left: 0;
margin: 0 auto;
max-width: ${props => props.theme.gridSize};
padding: 30px 50px;
position: absolute;
right: 0;
top: 0;
z-index: 999;
@media (max-width: 760px) {
padding: 14px 20px;
img {
display: block;
height: 40px;
margin: auto;
}
}
`
export default StyledHeader
<file_sep>import React from 'react'
import PropTypes from 'prop-types'
import { ThemeProvider } from 'styled-components'
import theme from '../styles/theme'
import StyledScreenGithub from './Github.style'
import hiwlpc from '../assets/hiwlpc.png'
import Card from '../components/Card'
import Title from '../components/Title'
const ScreenGithub = ({ match, location }) => {
let type = 'retry'
const { status } = match.params
const { search } = location
let url = search.replace('?url=', '') || 'https://impulso.network/'
const getStatus = () => {
if (status === 'success' || status === 'error') type = status
}
getStatus()
const messages = {
success: {
title: 'Olá novamente, nobre Impulser!',
subtitle: 'Sua dedicação foi posta a prova e você passou com honrarias!',
text:
"Quer entender um pouco mais? Não tem problema, dá uma olhadinha aqui neste papiro: <a href='https://github.com/universoimpulso/atena'>https://github.com/universoimpulso/atena</a>"
},
retry: {
title: 'Olá, nobre Impulser!',
subtitle: 'Ops! parece que você entrou na caverna errada.',
text: `Que falta faz um GPS, não é? :P <br> Para tentar novamente, siga esse caminho e não vai errar: <a href='${url}'>${url}</a>`
},
error: {
title: 'Olá, nobre Impulser!',
subtitle:
'Não conseguimos localizar seu e-mail público ou privado na API do GITHUB.',
text:
'Esse recurso da sua armadura de cavaleiro não está pronto para ganhar bonificações na contribuição do projeto Atena!'
}
}
return (
<ThemeProvider theme={theme}>
<StyledScreenGithub background={`url(${hiwlpc})`}>
<div className="_inner">
<Card large>
<span className="cardIcon">
<i className="fab fa-github" />
</span>
<p className="title">
<strong>{messages[type].title}</strong>
</p>
<Title align="center">{messages[type].subtitle}</Title>
<p
className="super"
dangerouslySetInnerHTML={{ __html: messages[type].text }}
/>
</Card>
</div>
<div className="help">
Ainda está em dúvida de como funciona? <br />
Não tem problema, dá uma olhadinha aqui: <br />
<a href="https://impulso.network/">https://impulso.network</a>
</div>
</StyledScreenGithub>
</ThemeProvider>
)
}
ScreenGithub.propTypes = {
location: PropTypes.string,
match: PropTypes.string
}
export default ScreenGithub
<file_sep>import styled from 'styled-components'
import Circle from '../../assets/circulos.png'
import Lines from '../../assets/lines.png'
import Imgbg from '../../assets/img-bg.svg'
const StyledScreenHowItWorks = styled.section`
.layout {
padding-top: 0;
background-color: #fdfdfd;
color: #666c71;
padding-bottom: 80px;
}
.container {
padding: 100px calc(15% + 1vw);
}
.about {
position: relative;
z-index: 10;
img {
@media (max-width: 760px) {
transform: scale(0.8);
}
}
&::before {
content: url("${Lines}");
display: block;
position: absolute;
top: 0;
left: 0;
right: 0;
margin: auto;
z-index: -1;
opacity: 0.6;
width: 90vw;
left: 50%;
transform: translateX(-50%);
max-width: 1280px;
}
p {
text-align: left;
}
}
.xprules {
text-align: center;
position: relative;
z-index: 10;
.align-content {
align-items: center;
justify-content: center;
align-title {
text-align: center;
}
}
&::before {
content: url("${Imgbg}");
display: block;
position: absolute;
z-index: -1;
right: 0;
right: 0;
transform: translateX(60%);
}
.rules {
margin: 70px 0 80px;
background: white;
height: 100px;
font-size: 20px;
font-weight: bold;
border-radius: 50px;
box-shadow: 8px 8px 60px rgba(0, 0, 0, 0.2);
overflow: hidden;
button {
display: flex;
align-items: center;
height: 100px;
justify-content: center;
padding: 0 30px;
text-decoration: none;
color: #595b98;
background: #fff;
width: 100%;
cursor: pointer;
span::after {
content: "";
display: block;
width: 0px;
height: 3px;
background: #c9ced2;
border-radius: 3px;
position: absolute;
transform: translateY(6px);
transition: 0.2s all ease-in;
}
&:hover {
span::after {
width: 30px;
background: #595b98;
}
}
&.selected {
background: #595b98;
color: white;
span::after {
width: 30px;
background: #ffffff;
}
}
}
}
.rules__inner {
text-align: left;
margin-bottom: 60px;
opacity: 0;
visibility: 0;
display: none;
&.selected {
opacity: 1;
visibility: visible;
display: flex;
}
h1 {
margin-top: 0;
}
p {
text-align: left;
}
}
}
.rules__table {
margin-bottom: 20px;
p {
margin: 0;
display: flex;
justify-content: space-between;
line-height: 3;
border-bottom: 1px solid #e2e2e2;
}
.value {
color: #595b98;
font-weight: bold;
}
}
.roles__navigation {
display: flex;
margin-bottom: 40px;
a {
font-size: 20px;
font-weight: bold;
color: #c9ced2;
text-decoration: none;
& + a::before {
content: "";
display: inline-block;
width: 4px;
height: 4px;
background: #dc3c2c;
border-radius: 3px;
margin-bottom: 3px;
margin-left: 20px;
margin-right: 20px;
}
&::after {
content: "";
display: block;
width: 0px;
height: 3px;
background: #c9ced2;
border-radius: 3px;
position: absolute;
transform: translateY(6px);
transition: 0.2s all ease-in;
}
&:not(:first-child)::after {
transform: translateX(44px) translateY(6px);
}
&:hover::after {
width: 24px;
}
&.selected {
color: #595b98;
&::after {
width: 24px;
background: #595b98;
}
}
}
}
.cards {
div {
flex: 1;
}
&::before {
display: block;
content: url("${Circle}");
position: absolute;
z-index: 0;
left: 0;
transform: translateX(-50%) translateY(-38%);
}
p {
text-align: left;
}
button {
margin-top: 50px;
}
img {
position: absolute;
top: 30px;
right: 30px;
}
}
.ilustra {
float: right;
}
@media (max-width: 760px) {
.container {
padding: 60px 15px;
}
.about {
padding: 0 15px;
}
.xprules {
width: 100%;
padding: 0;
text-align: left;
.align-content {
padding-bottom: 30px;
p {
text-align: left;
}
}
.rules {
font-size: 16px;
margin: 20px;
width: 90%;
button.selected span::after {
margin-left: 5px;
}
}
}
.roles__navigation {
margin-top: 20px;
a {
font-size: 16px;
&::before {
margin-left: 10px;
}
}
}
.cards {
padding: 0;
&::before {
zoom: 0.5;
}
.cards-box {
margin-bottom: 50px;
}
}
.question-title {
font-size: 18px;
}
}
`
export default StyledScreenHowItWorks
<file_sep>import styled from 'styled-components'
import Select from 'react-select'
import theme from '../../../styles/theme'
import { Field } from 'formik'
export const StyledForm = styled.form`
display: flex;
flex-direction: column;
font-weight: bold;
justify-content: space-between;
h3 {
color: ${theme.color.primary};
font-size: 16px;
margin: 30px 0;
text-transform: uppercase;
}
p {
color: ${theme.color.gray};
font-size: 14px;
margin: 25px 0 5px 0;
}
span {
color: ${theme.color.secondary};
font-size: 12px;
font-weight: normal;
padding-top: 4px;
}
`
export const StyledSelect = styled(Select)`
border: none;
box-shadow: 0 3px 15px rgba(0, 0, 0, 0.1);
color: ${theme.color.gray};
max-width: 25%;
`
export const StyledSmallInput = styled(Field)`
border: ${({ error }) => (error ? '1px solid red' : '1px solid gray')};
border-radius: 6px;
color: ${theme.color.gray};
font-size: 14px;
font-weight: bold;
height: 50px;
margin-bottom: 20px;
padding: 0 4px;
width: 100px;
`
export const DateWrapper = styled.section`
display: flex;
flex-wrap: wrap;
justify-content: space-between;
width: 50%;
span {
color: ${theme.color.gray};
font-size: 14px;
margin: 25px 0 5px 0;
}
input {
color: ${theme.color.gray};
border: none;
border-radius: 6px;
box-shadow: 0 3px 15px rgba(0, 0, 0, 0.1);
font-weight: bold;
height: 50px;
padding: 0 16px;
width: 95%;
}
`
export const Box = styled.div`
display: flex;
flex-direction: column;
width: 50%;
`
export const StyledInput = styled.input`
border: none;
border-radius: 6px;
box-shadow: 0 3px 15px rgba(0, 0, 0, 0.1);
color: ${theme.color.gray};
font-weight: bold;
height: 50px;
padding: 0 16px;
width: ${({ width }) => width || '50%'};
`
export const Wrapper = styled.div`
display: flex;
flex-wrap: wrap;
justify-content: space-between;
`
export const Card = styled.div`
background: ${theme.color.white};
border: ${({ cardError }) => (cardError ? '1px solid red' : 'none')};
border-radius: 10px;
box-shadow: 0 3px 15px rgba(0, 0, 0, 0.1);
color: ${theme.color.gray};
display: flex;
flex-direction: column;
font-size: 12px;
height: 182px;
justify-content: space-between;
margin-top: 30px;
min-width: 48%;
text-transform: uppercase;
padding: 20px;
div {
display: flex;
flex-direction: column;
margin-bottom: 20px;
ul {
display: flex;
justify-content: space-between;
li {
display: flex;
flex-direction: column;
p {
color: ${theme.color.primaryHover};
font-weight: bold;
margin: 0 auto;
padding-bottom: 15px;
}
}
input {
height: 50px;
width: 70px;
}
}
}
`
export const Title = styled.p`
border-bottom: 1px solid ${theme.color.lightGray};
font-size: 12px;
height: 20px;
padding-bottom: 20px;
`
export const Button = styled.button`
align-self: flex-end;
background: ${theme.color.white};
border: 2px solid ${theme.color.primary};
border-radius: 100px;
color: ${theme.color.primary};
cursor: pointer;
font-weight: bold;
height: 46px;
margin: 50px 0;
outline: none;
text-transform: uppercase;
transition: all 0.3s linear;
width: 30%;
&:hover {
color: ${theme.color.white};
background: ${theme.color.primary};
}
&:active {
transform: scale(0.95);
}
`
<file_sep>import styled from 'styled-components'
const StyledTab = styled.div`
display: flex;
min-height: 120px;
.tab__content {
position: absolute;
opacity: 0;
visibility: hidden;
transition: 0.2s all ease-in;
}
a:focus .tab__content {
opacity: 1;
visibility: visible;
}
`
export default StyledTab
<file_sep>import React, { Fragment } from 'react'
import PropTypes from 'prop-types'
import StyledTab from './style'
const renderItem = ({ title, content }, index) => (
<a key={index} href="javascript:;" className="tab__item">
<span className="tab__title">{title}</span>
<div className="tab__content">{content}</div>
</a>
)
const renderItems = data => data.map((item, index) => renderItem(item, index))
const Tab = ({ data }) => (
<StyledTab>
<Fragment>{renderItems(data)}</Fragment>
</StyledTab>
)
renderItem.propTypes = {
title: PropTypes.string.isRequired,
content: PropTypes.element.isRequired
}
Tab.propTypes = {
data: PropTypes.array.isRequired
}
export default Tab
<file_sep>import React from 'react'
import { Switch } from 'react-router-dom'
import Route from '../routes/Route'
import HowItWorks from '../pages/HowItWorks'
import Ranking from '../pages/Ranking'
import Admin from '../pages/admin'
import UserInfo from '../pages/UserInfo'
import Github from '../pages/Github'
import { LinkedInPopUp } from 'react-linkedin-login-oauth2'
export default function Routes() {
return (
<Switch>
<Route exact path="/" component={HowItWorks} />
<Route path="/ranking" component={Ranking} />
<Route path="/github/:status" component={Github} />
<Route path="/linkedin" component={LinkedInPopUp} />
<Route path="/admin" component={Admin} isPrivate coreTeam />
<Route path="/userInfo" component={UserInfo} isPrivate />
</Switch>
)
}
<file_sep>import React from 'react'
import PropTypes from 'prop-types'
import { Flex } from '@rebass/grid'
import Title from '../Title'
import {
StyledScreenError,
Loading,
SmallLoadingWrapper,
SmallErrorWrapper,
Header
} from './styles'
import loading from '../../assets/loading.svg'
export const PageError = ({ message, background, withHeader }) => (
<StyledScreenError background={background}>
{withHeader && <Header />}
<main className="layout">
<div className="_inner">
<Flex justifyContent="center">
<Title align={'center'} extraLarge>
Opa! algo deu errado...
</Title>
</Flex>
<p className="super">
Caro(a) cavaleiro(a), aconteceu um erro. Por favor tente novamente.
</p>
<p>{message}</p>
</div>
</main>
</StyledScreenError>
)
export const SmallError = ({ refresh, height, width, message }) => (
<SmallErrorWrapper height={height} width={width} onClick={refresh}>
<p>Algo deu errado! clique aqui para atualizar</p>
{message}
</SmallErrorWrapper>
)
export const PageLoading = ({ paddingSize, imgSize, width, height }) => (
<Loading
width={width}
height={height}
paddingSize={paddingSize}
imgSize={imgSize}
>
<img src={loading} alt="" />
</Loading>
)
export const SmallLoading = ({ width, height }) => (
<SmallLoadingWrapper width={width} height={height}>
<img src={loading} alt="" />
</SmallLoadingWrapper>
)
PageError.propTypes = {
message: PropTypes.string.isRequired,
background: PropTypes.string,
withHeader: PropTypes.bool
}
SmallError.propTypes = {
refresh: PropTypes.func,
height: PropTypes.string,
width: PropTypes.string,
message: PropTypes.string
}
PageLoading.propTypes = {
paddingSize: PropTypes.string,
imgSize: PropTypes.string,
width: PropTypes.string,
height: PropTypes.string
}
SmallLoading.propTypes = {
width: PropTypes.string,
height: PropTypes.string
}
<file_sep>import styled from 'styled-components'
import Select from 'react-select'
import theme from '../../../styles/theme'
export const Container = styled.div`
display: flex;
height: 410px;
margin: 60px 0 30px 0;
width: 100%;
`
export const CardsWrapper = styled.div`
flex: 1;
position: relative;
&:not(:last-child) {
margin-right: 30px;
}
h4 {
color: ${theme.color.primary};
font-size: 16px;
margin-bottom: 30px;
text-transform: uppercase;
}
`
export const Card = styled.div`
background: ${theme.color.white};
border-radius: 10px;
display: flex;
flex-direction: column;
font-size: 12px;
justify-content: space-between;
height: 110px;
margin-bottom: 10px;
padding: 15px;
position: relative;
text-transform: uppercase;
width: 100%;
p {
display: inline-block;
color: ${theme.color.gray};
font-weight: bold;
}
h1 {
font-size: 25px;
color: ${theme.color.primaryHover};
font-weight: bold;
margin: 0;
}
header {
display: flex;
justify-content: space-between;
align-items: center;
}
`
export const StyledSelect = styled(Select)`
color: ${theme.color.primaryHover};
font-weight: bold;
margin-left: 5px;
text-transform: uppercase;
width: 40%;
`
export const Percentage = styled.span`
color: ${props =>
props.positive ? theme.color.green : theme.color.secondary};
font-weight: bold;
margin-left: 3px;
`
<file_sep>import styled from 'styled-components'
import theme from '../../styles/theme'
export const Container = styled.div`
background: ${theme.color.background};
display: flex;
font-size: 16px;
height: 100%;
margin-top: 100px;
padding-top: 40px;
width: 100%;
`
export const Aside = styled.aside`
margin: 0 30px;
height: 600px;
width: 320px;
h3 {
color: ${theme.color.gray};
font-weight: bold;
margin-bottom: 30px;
text-transform: uppercase;
}
small {
border-bottom: solid 1px ${theme.color.lightGray};
display: block;
margin: 30px 0;
width: 236px;
}
`
export const Option = styled.p`
cursor: pointer;
line-height: 100%;
margin: 30px 0;
margin-left: ${props => props.marginLeft || 0};
${({ active }) =>
active
? `color: ${theme.color.primary}
font-weight: bold;`
: `color: ${theme.color.gray}`};
&:after {
content: '';
display: block;
width: ${props => (props.active ? '30px' : 0)};
height: 3px;
background: ${theme.color.primary};
border-radius: 3px;
position: absolute;
transform: translateY(6px);
transition: 0.2s all ease-in;
}
&:hover {
color: ${theme.color.primary};
font-weight: bold;
:after {
width: 30px;
}
}
`
export const Section = styled.section`
width: 100%;
margin: 0 50px 0 20px;
h2 {
font-size: 36px;
font-weight: bold;
color: ${theme.color.primary};
margin-bottom: 20px;
}
`
<file_sep>import React from 'react'
import PropTypes from 'prop-types'
import StyledButton from './style'
const Button = ({ children }) => <StyledButton>{children}</StyledButton>
Button.propTypes = {
children: PropTypes.element.isRequired
}
export default Button
<file_sep>import React from 'react'
import PropTypes from 'prop-types'
import { StyledRankingRow } from './style'
import avatarSvg from '../../../assets/avatar.svg'
const RankingRow = ({ position, name, avatar, level, score }) => {
return (
<StyledRankingRow>
<div className="ranking">{position}º</div>
<div className="userInfo">
{avatar ? (
<img
src={avatar || avatarSvg}
alt={`Foto de ${name}`}
onError={e => {
e.target.onerror = null
e.target.src = avatarSvg
}}
/>
) : (
<img src={avatarSvg} alt={name} />
)}
<p>{name}</p>
</div>
<div className="level">{level}</div>
<div className="xp">{score}</div>
</StyledRankingRow>
)
}
RankingRow.propTypes = {
position: PropTypes.number.isRequired,
name: PropTypes.string.isRequired,
avatar: PropTypes.string.isRequired,
level: PropTypes.number.isRequired,
score: PropTypes.number.isRequired
}
export default RankingRow
<file_sep>import { decrypt } from './crypto'
import { store } from '../store'
const TOKEN_KEY = '@at:atpin'
export const isAuthenticated = async () => {
const token = localStorage.getItem(TOKEN_KEY)
const data = await decrypt(token)
return token !== null && data !== null
}
export const isCoreTeam = async () => {
const token = localStorage.getItem(TOKEN_KEY)
const data = await decrypt(token)
return token !== null && data !== null && data.isCoreTeam
}
export const getToken = () => store.getState().auth.token
<file_sep>import React, { Component } from 'react'
import PropTypes from 'prop-types'
import { bindActionCreators } from 'redux'
import { connect } from 'react-redux'
import { Creators as UserActions } from '../../store/ducks/user'
import { PageError, PageLoading } from '../../components/utils'
import avatarSvg from '../../assets/avatarWhite.svg'
import {
Container,
Header,
ImageWrapper,
UserName,
Info,
Title,
Icon,
Accordion,
AccordionBody,
BadgeWrapper,
Stars,
Star,
Badge,
Score,
ScoreBar,
Achievement
} from './styles'
class UserInfo extends Component {
static propTypes = {
getProfile: PropTypes.func.isRequired,
uuid: PropTypes.string.isRequired,
profile: PropTypes.shape({
error: PropTypes.string.isRequired,
loading: PropTypes.bool.isRequired,
userInfo: PropTypes.shape({
avatar: PropTypes.string,
name: PropTypes.string,
monthlyPosition: PropTypes.number,
generalPosition: PropTypes.number,
score: PropTypes.number,
level: PropTypes.number
}).isRequired,
userAchievements: PropTypes.arrayOf(
PropTypes.shape({
name: PropTypes.string.isRequired,
achievements: PropTypes.arrayOf(
PropTypes.shape({
type: PropTypes.string.isRequired,
name: PropTypes.string.isRequired,
medal: PropTypes.number.isRequired,
tier: PropTypes.number.isRequired,
maxScore: PropTypes.number.isRequired,
score: PropTypes.number.isRequired
})
).isRequired
})
)
})
}
state = {
selected: null,
colors: ['#EF7C1E', '#A3A2A2', '#F7AA22', '#9DC2D6', '#BBE2ED']
}
componentDidMount() {
this.props.getProfile(this.props.uuid)
}
handleClick = index => {
this.setState({ selected: index === this.state.selected ? null : index })
}
formatTitle = data => {
const title = data.split(' ')
return (
<>
{title[0]} <span>{title[1]}</span>
</>
)
}
renderAccordion = data => {
const { selected } = this.state
return data.map((data, index) => (
<Accordion key={index}>
<header onClick={() => this.handleClick(index)}>
{this.formatTitle(data.name)}
<Icon className="fas fa-sort-down" selected={selected === index} />
</header>
<AccordionBody selected={selected === index}>
{data.achievements.map(achievement =>
this.renderAchievement(achievement)
)}
</AccordionBody>
</Accordion>
))
}
renderAchievement = data => {
const { colors } = this.state
const { type, name, medal, tier, maxScore, score } = data
const reqStars = require.context('../../assets/stars', true, /\.svg$/)
const starPath = reqStars.keys()
const stars = starPath.map(path => reqStars(path))
const reqBadges =
type === 'network.message.sended'
? require.context('../../assets/badges/messageSend', true, /\.svg$/)
: type === 'network.reaction.sended'
? require.context('../../assets/badges/reactionSend', true, /\.svg$/)
: type === 'network.reaction.received'
? require.context('../../assets/badges/reactionGiven', true, /\.svg$/)
: type === 'issueCreated'
? require.context('../../assets/badges/issueCreated', true, /\.svg$/)
: type === 'pullRequestApproved'
? require.context('../../assets/badges/PRApproved', true, /\.svg$/)
: type === 'pullRequestCreated'
? require.context('../../assets/badges/PRCreated', true, /\.svg$/)
: type === 'review'
? require.context('../../assets/badges/review', true, /\.svg$/)
: type === 'comments'
? require.context('../../assets/badges/comments', true, /\.svg$/)
: type === 'posts'
? require.context('../../assets/badges/posts', true, /\.svg$/)
: null
const badgePath = reqBadges.keys()
const badges = badgePath.map(path => reqBadges(path))
return (
<BadgeWrapper key={type}>
<Stars>
<Star align={100} src={tier <= 0 ? stars[5] : stars[medal]} />
<Star align={50} src={tier <= 1 ? stars[5] : stars[medal]} />
<Star align={0} src={tier <= 2 ? stars[5] : stars[medal]} />
<Star align={50} src={tier <= 3 ? stars[5] : stars[medal]} />
<Star align={100} src={tier <= 4 ? stars[5] : stars[medal]} />
</Stars>
<Badge>
<img src={badges[medal]} alt="badge" />
</Badge>
<Score color={colors[medal]}>
{score} / {maxScore}
</Score>
<ScoreBar status={(score / maxScore) * 100} color={colors[medal]} />
<Achievement>{name.split('|')[1]}</Achievement>
</BadgeWrapper>
)
}
render() {
const { error, loading, userInfo, userAchievements } = this.props.profile
if (!!error)
return <PageError message={error} background="white" withHeader />
if (loading) return <PageLoading width="100%" height="800px" />
const {
avatar,
name,
monthlyPosition,
generalPosition,
score,
level
} = userInfo
return (
<Container>
<Header>
<ImageWrapper>
<div>
<img
src={avatar || avatarSvg}
onError={e => {
e.target.onerror = null
e.target.src = avatarSvg
}}
alt={`Foto de ${name}`}
/>
</div>
<small>
{monthlyPosition === 'coreTeam' ? '-' : `${monthlyPosition}º`}
</small>
</ImageWrapper>
<UserName>{name}</UserName>
<Info>
<div>
<p>level</p>
<span>{level}</span>
</div>
<div>
<p>reputação</p>
<span>{score}</span>
</div>
<div>
<p>ranking geral</p>
<span>
{generalPosition === 'coreTeam' ? '-' : `${generalPosition}º`}
</span>
</div>
</Info>
</Header>
{/* <Title>suas conquistas</Title> */}
{/* {this.renderAccordion(userAchievements)} */}
</Container>
)
}
}
const mapStateToProps = state => ({
profile: state.user.profile,
uuid: state.auth.uuid
})
const mapDispatchToProps = dispatch => bindActionCreators(UserActions, dispatch)
export default connect(
mapStateToProps,
mapDispatchToProps
)(UserInfo)
<file_sep>import React from 'react'
import PropTypes from 'prop-types'
import StyledCard from './style'
import { Flex, Box } from '@rebass/grid'
const Card = props => {
const { children, large } = props
return (
<StyledCard large={large}>
<Flex justifyContent="space-between" alignItems="center">
<Box width={1}>{children}</Box>
</Flex>
</StyledCard>
)
}
Card.propTypes = {
children: PropTypes.element.isRequired,
large: PropTypes.bool.isRequired
}
export default Card
<file_sep>import React, { Component, Fragment } from 'react'
import PropTypes from 'prop-types'
import { Creators as achievementsActions } from '../../../store/ducks/achievements'
import { bindActionCreators } from 'redux'
import { connect } from 'react-redux'
import { Container, Header, Icon, Wrapper } from './styles'
import Card from './Card'
class EditAchievements extends Component {
static propTypes = {
getAchievements: PropTypes.func.isRequired,
achievements: PropTypes.object.isRequired,
achievementsValues: PropTypes.array
}
state = {
active: null
}
componentDidMount() {
this.props.getAchievements()
}
handleClick = index => {
this.setState({ active: index === this.state.active ? null : index })
}
render() {
const { active } = this.state
const { achievementsValues } = this.props.achievements
return (
<Container>
{achievementsValues &&
achievementsValues.map((achievement, index) => (
<Fragment key={index}>
<Header onClick={() => this.handleClick(index)}>
<p>
{achievement.name}
<Icon
selected={active === index}
className="fas fa-sort-down"
/>
</p>
</Header>
<Wrapper selected={active === index}>
{achievement.data.map((data, index) => (
<Fragment key={index}>
<h3>{data.type}</h3>
{data.values.map((value, index) => (
<Card
key={index}
data={{
value,
AchievementName: achievement.name,
type: data.type
}}
/>
))}
</Fragment>
))}
</Wrapper>
</Fragment>
))}
</Container>
)
}
}
const mapStateToProps = state => ({ achievements: state.achievements })
const mapDispatchToProps = dispatch =>
bindActionCreators(achievementsActions, dispatch)
export default connect(
mapStateToProps,
mapDispatchToProps
)(EditAchievements)
<file_sep>import React, { Component } from 'react'
import { connect } from 'react-redux'
import PropTypes from 'prop-types'
import { bindActionCreators } from 'redux'
import { Flex, Box } from '@rebass/grid'
import moment from 'moment'
import 'moment/locale/pt-br'
import { Creators as RankingActions } from '../../store/ducks/ranking'
import RankingRow from './RankingRow'
import Title from '../../components/Title'
import FullPage from '../../components/FullPage'
import Podium from '../../components/Podium'
import { PageError, PageLoading } from '../../components/utils'
import BgRanking from '../../assets/bg_ranking.png'
import {
StyledScreenRanking,
StyledRectangleGroup,
StyledRectangle,
UsersRanking,
RankingHeader
} from './styles'
class ScreenRanking extends Component {
static propTypes = {
getRanking: PropTypes.func.isRequired,
ranking: PropTypes.shape({
loading: PropTypes.bool,
error: PropTypes.string,
data: PropTypes.array
}).isRequired
}
state = {
selected: 'general'
}
componentDidMount() {
this.props.getRanking(this.state.selected)
}
toggleRanking = selected => {
this.setState({ selected })
this.props.getRanking(selected)
}
currentMouth = () => {
moment().locale('pt-br')
return moment().format('MMMM')
}
generatePosition = data => {
data.forEach((user, index) => (user.position = index + 1))
const podium = data.splice(0, 3)
return { podium, users: data }
}
render() {
const { selected } = this.state
const { error, loading, data } = this.props.ranking
const { podium, users } = this.generatePosition(data)
if (!!error)
return (
<StyledScreenRanking>
<main className="layout">
<FullPage background={`url(${BgRanking})`} height="40" overlay>
<Flex alignItems="baseline" justifyContent="center" flex="1">
<Box>
<Title large color="white" align="center">
Ranking
</Title>
</Box>
</Flex>
</FullPage>
<div className="_inner">
<Flex
justifyContent="center"
alignItems="center"
mt={100}
mb={100}>
<PageError message={error} />
</Flex>
</div>
</main>
</StyledScreenRanking>
)
return (
<StyledScreenRanking>
<main className="layout">
<FullPage background={`url(${BgRanking})`} height="40" overlay>
<Flex alignItems="baseline" justifyContent="center" flex="1">
<Box>
<Title large color="white" align="center">
Ranking
</Title>
</Box>
</Flex>
</FullPage>
{
<div className="_inner">
<p className="super">
Confira aqui a sua colocação no ranking da Atena. Vale lembrar
que o<b> Ranking Mensal</b> exibe o <b>saldo</b> de Reputação
que você obteve
<b> durante um mês</b>. Já o <b>Ranking Geral</b> exibe o saldo
de Reputação de toda sua jornada na Impulso!
</p>
<Flex justifyContent="center" alignItems="center">
<StyledRectangleGroup>
<StyledRectangle
onClick={() => this.toggleRanking('monthly')}
active={selected === 'monthly'}
left>
<p>Ranking Mensal</p>
</StyledRectangle>
<StyledRectangle
onClick={() => this.toggleRanking('general')}
active={selected === 'general'}
right>
<p>Ranking Geral</p>
</StyledRectangle>
</StyledRectangleGroup>
</Flex>
{loading || !users ? (
<PageLoading />
) : (
<>
<Flex justifyContent="center">
<Title align={'center'} extraLarge>
RANKING
{selected === 'general' ? (
<>
<br />
<span className="month"> GERAL</span>
</>
) : (
<>
<br />
DO MÊS DE
<span className="month"> {this.currentMouth()}</span>
</>
)}
</Title>
</Flex>
{<Podium firstUsers={podium} />}
<UsersRanking>
<RankingHeader>
<div className="ranking">RANKING</div>
<div className="userInfo" />
<div className="level">LEVEL</div>
<div className="xp">REPUTAÇÃO</div>
</RankingHeader>
{users.map((card, index) => (
<RankingRow key={index} {...card} />
))}
</UsersRanking>
</>
)}
</div>
}
</main>
</StyledScreenRanking>
)
}
}
const mapStateToProps = state => ({
ranking: state.ranking.ranking
})
const mapDispatchToProps = dispatch =>
bindActionCreators(RankingActions, dispatch)
export default connect(
mapStateToProps,
mapDispatchToProps
)(ScreenRanking)
<file_sep>import React from 'react'
import badge from '../../../assets/badge.svg'
import stars from '../../../assets/stars.svg'
import Card from '../../../components/Card'
import { Flex, Box } from '@rebass/grid'
import Title from '../../../components/Title'
import Button from '../../../components/Button'
const QuestionCards = () => {
return (
<section className="container cards">
<Flex css={{ margin: '0 -25px' }} flexWrap="wrap">
<Box width={[1, 1 / 2]} px={25} className="cards-box">
<Card>
<img alt="estrelas" src={stars} />
<Title className="question-title">
O QUE SÃO E<br /> COMO GANHAR <br />
<span className="red">NÍVEIS</span>?
</Title>
<p className="super">
Os <strong>Níveis</strong> são representações simplificadas do seu
avanço na Atena e são atingidos mediante uma determinada
quantidade de <strong>reputação</strong> ganha. Além disso, eles
conferem o acesso à novas missões, cargos e recompensas.
</p>
<Button>
<a
target="_blank"
rel="noopener noreferrer"
href="https://www.notion.so/impulso/N-veis-Como-ganhar-6d67592b078f49b3879ce4db91081be4">
mais detalhes
</a>
</Button>
</Card>
</Box>
<Box
width={[1, 1 / 2]}
px={25}
css={{
display: 'flex',
flexDirection: 'column'
}}>
<Card>
<img alt="badge" src={badge} />
<Title className="question-title">
O QUE SÃO E <br /> COMO GANHAR <br />{' '}
<span className="red">CONQUISTAS</span>?
</Title>
<p className="super">
<strong>Conquistas</strong> são formas de reconhecer o seu
esforço. Após realizar algo que mereça esse reconhecimento, você
será condecorado(a) com uma <strong>medalha</strong> que
representa este marco.
</p>
<Button>
<a
target="_blank"
rel="noopener noreferrer"
href="https://www.notion.so/impulso/Conquistas-Como-ganhar-f8cded2569e7411ebabb78c8e99a2f94">
mais detalhes
</a>
</Button>
</Card>
</Box>
</Flex>
</section>
)
}
export default QuestionCards
<file_sep>import { persistStore } from 'redux-persist'
import { createStore, compose, applyMiddleware } from 'redux'
import createSagaMiddleware from 'redux-saga'
import persistReducers from './persistReducers'
import reducers from './ducks'
import sagas from './sagas'
const sagaMonitor =
process.env.NODE_ENV === 'development'
? console.tron.createSagaMonitor()
: null
const sagaMiddleware = createSagaMiddleware({ sagaMonitor })
const getError = store => next => action => {
if (action.data) {
if (action.data.errorType && action.data.errorType === 'sessionExpired') {
store.dispatch({ type: 'FORCE_SIGN_OUT' })
}
}
next(action)
}
const middlewares = [sagaMiddleware, getError]
const composer =
process.env.NODE_ENV === 'development'
? compose(
applyMiddleware(...middlewares),
console.tron.createEnhancer()
)
: applyMiddleware(...middlewares)
const store = createStore(persistReducers(reducers), composer)
const persistor = persistStore(store)
sagaMiddleware.run(sagas)
export { store, persistor }
<file_sep>import { createActions, createReducer } from 'reduxsauce'
const INITIAL_STATE = {
activeModal: false,
loading: false,
token: '',
avatar: '',
uuid: '',
isCoreTeam: false
}
export const { Types, Creators } = createActions({
toggleModal: ['data'],
signInRequest: ['data'],
signInSuccess: ['data'],
signInFailure: ['data'],
forceSignOut: ['data'],
signOut: ['data']
})
const toggleModal = (state = INITIAL_STATE) => ({
...state,
activeModal: !state.activeModal
})
const signInRequest = (state = INITIAL_STATE) => ({
...state,
loading: true
})
const signInSuccess = (state = INITIAL_STATE, action) => {
const { token, uuid, isCoreTeam, avatar } = action.data
return {
...state,
activeModal: false,
loading: false,
token,
uuid,
avatar,
isCoreTeam
}
}
const signInFailure = (state = INITIAL_STATE) => ({
...state,
loading: false,
activeModal: false
})
const signOut = (state = INITIAL_STATE) => {
return {
...state,
token: '',
uuid: '',
avatar: '',
isCoreTeam: false
}
}
const forceSignOut = (state = INITIAL_STATE) => {
return {
...state
}
}
export default createReducer(INITIAL_STATE, {
[Types.TOGGLE_MODAL]: toggleModal,
[Types.SIGN_IN_REQUEST]: signInRequest,
[Types.SIGN_IN_SUCCESS]: signInSuccess,
[Types.SIGN_IN_FAILURE]: signInFailure,
[Types.FORCE_SIGN_OUT]: forceSignOut,
[Types.SIGN_OUT]: signOut
})
<file_sep>import React, { Component } from 'react'
import PropTypes from 'prop-types'
import { bindActionCreators } from 'redux'
import { connect } from 'react-redux'
import { Formik } from 'formik'
import DatePicker from 'react-datepicker'
import 'react-datepicker/dist/react-datepicker.css'
import { Creators as achievementsActions } from '../../../store/ducks/achievements'
import {
StyledForm,
StyledSelect,
StyledInput,
StyledSmallInput,
DateWrapper,
Box,
Wrapper,
Card,
Title,
Button
} from './styles'
class CreateAchievements extends Component {
static propTypes = {
getAchievements: PropTypes.func.isRequired,
achievements: PropTypes.object.isRequired,
achievementsValues: PropTypes.array
}
state = {
startDate: null,
endDate: null,
limitDate: null,
achievementTypeOptions: [
{ value: 'permanent', label: 'Permanente', isDisabled: true },
{ value: 'temporary', label: 'Temporária' }
],
category: [
{ value: 'rocketchat', label: 'RocketChat' },
{ value: 'github', label: 'Github' }
],
activity: [
{ value: 'Mensagens Enviadas', label: 'Mensagens Enviadas' },
{ value: 'Reactions Dados', label: 'Reactions Dados' },
{ value: 'Reactions Recebidos', label: 'Reactions Recebidos' }
],
achievement: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
}
componentDidMount() {
this.props.getAchievements()
}
handleCategoryChange = event => {}
handleActivityChange = event => {}
handleChangeStart = date => {
this.setState({
startDate: date
})
}
handleChangeEnd = date => {
this.setState({
endDate: date
})
}
handleChangeLimit = date => {
this.setState({
limitDate: date
})
}
getValues = () => {
const { achievement } = this.state
let tiers = {}
achievement.forEach(value =>
value.tiers.forEach(tier => {
Object.assign(tiers, { [`${value.name}-${tier.name}`]: tier.value })
})
)
return tiers
}
validateTier = value => (!value || value <= 0 ? true : null)
handleSubmit = (values, actions) => {
actions.setSubmitting(false)
}
render() {
const { achievement } = this.state
return (
<Formik
onSubmit={(values, actions) => this.handleSubmit(values, actions)}
initialValues={this.getValues()}
>
{({ handleSubmit, handleChange, setFieldValue, errors, touched }) => (
<StyledForm onSubmit={handleSubmit}>
<h3>Criar nova conquista</h3>
<p>Tipo de conquista</p>
<StyledSelect
placeholder="Selecione..."
name="achievementType"
options={this.state.achievementTypeOptions}
onChange={value => setFieldValue('achievementType', value.value)}
/>
<p>Categoria</p>
<StyledSelect
placeholder="Selecione..."
name="category"
options={this.state.category}
onChange={value => setFieldValue('category', value.value)}
/>
<p>Atividade</p>
<StyledSelect
placeholder="Selecione..."
name="activity"
options={this.state.activity}
onChange={value => setFieldValue('activity', value.value)}
/>
<p>Nome da conquista</p>
<StyledInput
placeholder="Nome"
name="achievementName"
type="text"
onChange={handleChange}
/>
<DateWrapper>
<Box>
<p>Inicío</p>
<DatePicker
selectsStart
placeholderText="Inicio"
name="initialDate"
minDate={new Date()}
dateFormat="dd/MM/yyyy"
selected={this.state.startDate}
startDate={this.state.startDate}
endDate={this.state.endDate}
onChange={data => {
this.handleChangeStart(data)
setFieldValue('initialDate', data)
}}
/>
</Box>
<Box>
<p>Término</p>
<DatePicker
selectsEnd
placeholderText="Término"
name="finalDate"
disabled={this.state.startDate === null}
minDate={new Date()}
input
dateFormat="dd/MM/yyyy"
selected={this.state.endDate}
startDate={this.state.startDate}
endDate={this.state.endDate}
onChange={data => {
this.handleChangeEnd(data)
setFieldValue('finalDate', data)
}}
/>
</Box>
<Box>
<p>Data limite</p>
<DatePicker
name="limitDate"
placeholderText="Limite"
minDate={new Date()}
disabled={this.state.endDate === null}
maxDate={this.state.endDate}
dateFormat="dd/MM/yyyy"
selected={this.state.limitDate}
onChange={data => {
this.handleChangeLimit(data)
setFieldValue('limitDate', data)
}}
/>
</Box>
</DateWrapper>
<p>Quantidade de ações</p>
<Wrapper>
{achievement.map(value => (
<Card key={value.name}>
<Title>{value.name}</Title>
<div>
<ul>
{value.tiers.map((tier, index) => {
const tierName = `${value.name}-${tier.name}`
return (
<li key={index}>
<p>{tier.name}</p>
<StyledSmallInput
validate={value => this.validateTier(value)}
name={tierName}
onChange={handleChange}
type="number"
error={errors[tierName] && touched[tierName]}
/>
</li>
)
})}
</ul>
</div>
</Card>
))}
</Wrapper>
<Button type="submit">concluir</Button>
</StyledForm>
)}
</Formik>
)
}
}
const mapStateToProps = state => ({ achievements: state.achievements })
const mapDispatchToProps = dispatch =>
bindActionCreators(achievementsActions, dispatch)
export default connect(
mapStateToProps,
mapDispatchToProps
)(CreateAchievements)
<file_sep>import styled from 'styled-components'
import theme from '../../styles/theme'
export const StyledFooter = styled.footer`
background-color: ${theme.color.primary};
color: ${theme.color.white};
padding: 50px 0 40px;
> section {
max-width: 380px;
margin: 0 auto;
hr {
width: 85%;
margin: 40px auto;
}
}
.impulsoIcon {
display: flex;
margin: 0 auto 40px;
}
`
export const StyledCopyright = styled.p`
text-align: center;
color: ${theme.color.primaryLight};
margin: 0;
`
<file_sep>import React, { Component } from 'react'
import PropTypes from 'prop-types'
import { bindActionCreators } from 'redux'
import { connect } from 'react-redux'
import { Creators as RankingActions } from '../../store/ducks/ranking'
import { Container, SearchBar } from './styles'
class SearchUserBar extends Component {
static propTypes = {
getRankingUsers: PropTypes.func.isRequired,
ranking: PropTypes.object
}
state = {
inputValue: '',
options: [
{ value: 'level', label: 'Nível' },
{ value: 'position', label: 'Posição' }
]
}
handleChange = event => this.setState({ inputValue: event.target.value })
handleSubmit = event => {
if (event.key === 'Enter') {
this.props.getRankingUsers(this.state.inputValue)
this.setState({ inputValue: '' })
}
}
render() {
return (
<Container>
<SearchBar
type="text"
value={this.state.inputValue}
onChange={this.handleChange}
onKeyPress={this.handleSubmit}
placeholder=" Busca por jogador"
/>
</Container>
)
}
}
const mapStateToProps = state => ({
ranking: state.ranking
})
const mapDispatchToProps = dispatch =>
bindActionCreators(RankingActions, dispatch)
export default connect(
mapStateToProps,
mapDispatchToProps
)(SearchUserBar)
<file_sep>import styled from 'styled-components'
import theme from '../../../styles/theme'
export const Container = styled.section`
width: 100%;
h4 {
color: ${theme.color.primary};
font-size: 16px;
margin-bottom: 30px;
text-transform: uppercase;
}
`
export const Wrapper = styled.div`
display: flex;
flex: 1;
height: 85%;
`
export const Info = styled.div`
color: ${theme.color.gray};
display: flex;
flex-grow: 1;
flex-direction: column;
flex-wrap: wrap;
font-size: 14px;
font-weight: bold;
height: 100%;
margin-left: 50px;
text-transform: uppercase;
`
export const Card = styled.div`
background: ${theme.color.white};
border-radius: 10px;
height: ${props => props.height};
margin-bottom: 10px;
padding: 20px;
width: ${props => props.width};
h5 {
color: ${theme.color.gray};
font-size: 14px;
margin-bottom: 20px;
}
`
export const Level = styled.ul`
display: flex;
flex-direction: column;
flex-wrap: wrap;
max-height: calc(100% - 40px);
padding-left: 25px;
width: 100%;
li {
display: flex;
padding-bottom: 24px;
width: 45%;
span {
border-bottom: 2px dotted ${theme.color.gray};
flex: 1;
margin: 0 6px;
width: 25%;
}
div {
border-radius: 100%;
display: inline-block;
height: 1em;
margin: auto 4px;
width: 1em;
}
}
`
export const Total = styled.div`
align-items: baseline;
background: ${theme.color.background};
border-radius: 5px;
color: ${theme.color.primaryHover};
display: flex;
font-size: 12px;
font-weight: bold;
height: 40px;
line-height: 40px;
overflow: hidden;
padding: 0 20px;
text-transform: uppercase;
white-space: nowrap;
width: 100%;
span {
border-bottom: 2px dotted ${theme.color.primaryHover};
flex: 1;
margin: 0 6px;
}
`
<file_sep>import { createActions, createReducer } from 'reduxsauce'
const INITIAL_STATE = {
loading: null,
cardLoading: null,
experienceValues: {},
getError: null
}
export const { Types, Creators } = createActions({
getExperience: ['data'],
getExperienceSuccess: ['data'],
getExperienceFailure: ['data'],
putExperience: ['data'],
putExperienceSuccess: ['data'],
putExperienceFailure: ['data']
})
const getExperience = (state = INITIAL_STATE, action) => ({
...state,
getError: '',
loading: true
})
const getExperienceSuccess = (state = INITIAL_STATE, action) => ({
...state,
loading: false,
experienceValues: action.data
})
const getExperienceFailure = (state = INITIAL_STATE, action) => ({
...state,
loading: false,
getError: action.error
})
const putExperience = (state = INITIAL_STATE, action) => {
const { key } = action.data
return {
...state,
cardLoading: key
}
}
const putExperienceSuccess = (state = INITIAL_STATE, action) => ({
...state,
cardLoading: null,
experienceValues: action.data
})
const putExperienceFailure = (state = INITIAL_STATE, action) => ({
...state,
cardLoading: null
})
export default createReducer(INITIAL_STATE, {
[Types.GET_EXPERIENCE]: getExperience,
[Types.GET_EXPERIENCE_SUCCESS]: getExperienceSuccess,
[Types.GET_EXPERIENCE_FAILURE]: getExperienceFailure,
[Types.PUT_EXPERIENCE]: putExperience,
[Types.PUT_EXPERIENCE_SUCCESS]: putExperienceSuccess,
[Types.PUT_EXPERIENCE_FAILURE]: putExperienceFailure
})
<file_sep>import React, { Component } from 'react'
import { AccordionWrapper, Awnser, Icon } from './style'
class Accordion extends Component {
state = {
active: null,
questions: [
{
content: 'Como me comunico com a Atena?',
awnser:
'Para centralizar as informações a respeito da sua Reputação, Níveis e Conquistas criamos a bot ATENA. Através de comandos é possível pedir à Atena para exibir sua reputação (!meuspontos), Ranking Mensal (!ranking), Ranking Geral (!rankinggeral) e Conquistas (!minhasconquistas).'
},
{
content: 'Existirão atualizações?',
awnser:
'Sim! A Impulso Network é muito dinâmica e não para de crescer. O surgimento de novos canais, atividades e práticas corriqueiras e positivas na comunidade serão mapeadas para que novas features sejam criadas.'
},
{
content: 'Existe alguma premiação?',
awnser:
'Claro! Além da Reputação obtida através da sua participação na comunidade (representada por Reputação e Níveis), Atena poderá te premiar com acesso a atividades especiais da comunidade, cupons de desconto para serviços, além de produtos e brindes exclusivos.'
},
{
content: 'Qual a diferença entre ranking mensal e geral?',
awnser:
'Enquanto o Ranking Geral valoriza o esforço durante todo o seu percurso com a Atena e mostra o acumulado de Reputação e seu Nível, o Ranking Mensal foca no que foi realizado e recompensa o primeiro colocado naquele mês.'
},
{
content: 'Como faço para participar do projeto?',
awnser: [
`Qualquer pessoa pode ajudar Atena a crescer, basta entrar no canal <strong>#projeto-atena</strong> no nosso Rocket.chat ou acessar diretamente o nosso repositório no
<a
target="_blank"
rel="noopener noreferrer"
href="https://github.com/universoimpulso/atena"
>
Github
</a> .
Ainda não faz parte da Impulso Network? Basta acessar o
<a
target="_blank"
rel="noopener noreferrer"
href="https://app.impulso.network/"
>
Rocket.chat
</a>
e fazer o seu cadastro, é rapidinho.`
]
}
]
}
handleClick = index => {
this.setState({ active: index === this.state.active ? null : index })
}
render() {
const { active, questions } = this.state
return (
<AccordionWrapper>
{questions.map((question, index) => (
<section key={index} onClick={() => this.handleClick(index)}>
<span className="accordion__link">
{question.content}
<Icon selected={active === index} className="fas fa-sort-down" />
</span>
<Awnser selected={active === index} className="accordion_content">
<p dangerouslySetInnerHTML={{ __html: question.awnser }} />
</Awnser>
</section>
))}
</AccordionWrapper>
)
}
}
export default Accordion
<file_sep>import React from 'react'
import { Flex } from '@rebass/grid'
import PropTypes from 'prop-types'
import UserCard from '../UserCard'
const Podium = ({ firstUsers }) => {
return (
<Flex justifyContent="center" mt={50} mb={80} flexWrap="wrap">
{firstUsers.map((card, index) => (
<UserCard key={index} first={index === 0} {...card} />
))}
</Flex>
)
}
Podium.propTypes = {
firstUsers: PropTypes.array
}
export default Podium
<file_sep>import React, { Component } from 'react'
import hiwlpc from '../../assets/hiwlpc.png'
import StyledScreenHowItWorks from './styles'
import { Flex, Box } from '@rebass/grid'
import XpRules from './XpRules'
import FullPage from '../../components/FullPage'
import Title from '../../components/Title'
import SubTitle from '../../components/Title/SubTitle'
import Faq from './Faq'
import About from './About'
import QuestionCards from './QuestionCards'
class ScreenHowItWorks extends Component {
render() {
return (
<StyledScreenHowItWorks>
<main className="layout">
<FullPage background={`url(${hiwlpc})`}>
<Flex alignItems="center" justifyContent="center" flex="1">
<Box>
<Title large color="white" align="center">
Bem vindo(a)
<br />à atena!
</Title>
<SubTitle>
Participe da comunidade, compartilhe ideias, construa projetos
e ganhe pontos baseados em suas ações.
</SubTitle>
</Box>
</Flex>
</FullPage>
<About />
<XpRules />
<QuestionCards />
<Faq />
</main>
</StyledScreenHowItWorks>
)
}
}
export default ScreenHowItWorks
<file_sep>import React, { Component } from 'react'
import { Link } from 'react-router-dom'
import PropTypes from 'prop-types'
import { connect } from 'react-redux'
import { bindActionCreators } from 'redux'
import { Creators as AuthActions } from '../../../store/ducks/auth'
import Auth from '../../auth'
import avatarSvg from '../../../assets/avatarWhite.svg'
import StyledMenu from './styles'
class Menu extends Component {
static propTypes = {
signOut: PropTypes.func.isRequired,
toggleModal: PropTypes.func.isRequired,
auth: PropTypes.shape({
activeModal: PropTypes.bool.isRequired,
avatar: PropTypes.string,
uuid: PropTypes.string,
isCoreTeam: PropTypes.bool
}).isRequired
}
toggleModal = () => {
this.props.toggleModal()
}
logout = () => {
this.props.signOut()
}
render() {
const { uuid, isCoreTeam, activeModal, avatar } = this.props.auth
return (
<>
<StyledMenu>
<li key="index">
<Link to="/">
<button>como funciona</button>
</Link>
</li>
<li key="ranking">
<Link to="/ranking">
<button>ranking</button>
</Link>
</li>
{uuid ? (
<>
{isCoreTeam && (
<li key="admin">
<Link to="/admin">
<button>Admin</button>
</Link>
</li>
)}
<li key="logout">
<button onClick={this.logout}>logout</button>
</li>
<li className="user">
<Link to="/userInfo">
<button className="profile">
<img
src={avatar || avatarSvg}
onError={e => {
e.target.onerror = null
e.target.src = avatarSvg
}}
alt={`Foto do usuário`}
className="avatar"
/>
</button>
</Link>
</li>
</>
) : (
<li key="login">
<button onClick={this.toggleModal}>login</button>
</li>
)}
</StyledMenu>
{activeModal && <Auth />}
</>
)
}
}
const mapStateToProps = state => ({
auth: state.auth
})
const mapDispatchToProps = dispatch => bindActionCreators(AuthActions, dispatch)
export default connect(
mapStateToProps,
mapDispatchToProps
)(Menu)
<file_sep>import styled from 'styled-components'
const StyledFaq = styled.section`
button {
margin-top: 50px;
}
@media (max-width: 760px) {
h1 {
br {
display: none;
}
}
i {
padding-left: 20px;
}
}
`
export default StyledFaq
<file_sep>import { delay, put } from 'redux-saga/effects'
import { Creators as GeneralReportsActions } from '../ducks/generalReports'
export function* getUsers(action) {
try {
yield delay(1000)
const generalReportsData = {
users: [
{ name: 'Nível 1', value: 335 },
{ name: 'Nível 2', value: 310 },
{ name: 'Nível 3', value: 234 },
{ name: 'Nível 4', value: 535 },
{ name: 'Nível 5', value: 154 },
{ name: 'Nível 6', value: 754 },
{ name: 'Nível 7', value: 354 },
{ name: 'Nível 8', value: 854 },
{ name: 'Nível 9', value: 154 },
{ name: 'Nível 10', value: 554 }
],
totalUsers: 12043,
loading: false,
error: null
}
// throw new Error("Não foi possível buscar os dados");
yield put(GeneralReportsActions.getUsersResponse(generalReportsData))
} catch (error) {
yield put(
GeneralReportsActions.getUsersResponse({
users: null,
totalUsers: null,
loading: false,
error: error.message
})
)
}
}
export function* getUsersAchievements(action) {
try {
yield delay(1000)
const data = {
name: 'conquistas',
byMonth: 865,
byMonthPercentage: 13.8,
byYear: 3587,
byYearPercentage: -13.8,
total: 12987
}
// throw new Error("Não foi possível buscar os dados");
yield put(
GeneralReportsActions.getUsersAchievementsResponse({
type: 'achievements',
loading: false,
error: null,
data
})
)
} catch (error) {
yield put(
GeneralReportsActions.getUsersAchievementsResponse({
type: 'achievements',
loading: false,
error: error.message,
data: null
})
)
}
}
export function* getMissions(action) {
try {
yield delay(2000)
const data = {
name: 'missões',
byMonth: 565,
byMonthPercentage: -14.8,
byYear: 2587,
byYearPercentage: 11.8,
total: 22987
}
if (action.data) throw new Error('Você nao tem acesso')
// throw new Error("Não foi possível buscar os dados");
yield put(
GeneralReportsActions.getMissionsResponse({
type: 'missions',
loading: false,
error: null,
data
})
)
} catch (error) {
const data = {
name: 'missões',
byMonth: 565,
byMonthPercentage: -14.8,
byYear: 2587,
byYearPercentage: 11.8,
total: 22987
}
yield put(
GeneralReportsActions.getMissionsResponse({
type: 'missions',
loading: false,
data,
error: error.message
})
)
}
}
export function* getXp(action) {
try {
yield delay(3000)
const data = {
name: 'experiência',
byMonth: 165,
byMonthPercentage: -6.8,
byYear: 587,
byYearPercentage: 16.8,
total: 2987
}
// throw new Error("Não foi possível buscar os dados");
yield put(
GeneralReportsActions.getXpResponse({
type: 'xp',
loading: false,
error: null,
data
})
)
} catch (error) {
yield put(
GeneralReportsActions.getXpResponse({
type: 'xp',
loading: false,
error: error.message,
data: null
})
)
}
}
export function* getTeams() {
try {
yield delay(2000)
const data = [
{
name: 'network',
total: 1837,
message: {
send: 1452,
receive: 7489
},
reactions: {
send: 759,
receive: 785
},
response: {
send: 784,
receive: 695
},
blog: {
posts: 452,
comments: 745
},
github: {
issues: 548,
reviews: 475,
pullRequests: {
created: 125,
approved: 98
}
},
meetups: {
participants: 236,
mediators: 65,
facilitators: 74
},
referral: {
allocated: 74,
indications: 745
}
},
{
name: 'atari',
total: 54837,
message: {
send: 1452,
receive: 7489
},
reactions: {
send: 759,
receive: 785
},
response: {
send: 784,
receive: 695
},
blog: {
posts: 452,
comments: 745
},
github: {
issues: 548,
reviews: 475,
pullRequests: {
created: 125,
approved: 98
}
},
meetups: {
participants: 236,
mediators: 65,
facilitators: 74
},
referral: {
allocated: 74,
indications: 745
}
},
{
name: 'corinthians',
total: 26837,
message: {
send: 1452,
receive: 7489
},
reactions: {
send: 759,
receive: 785
},
response: {
send: 784,
receive: 695
},
blog: {
posts: 452,
comments: 745
},
github: {
issues: 548,
reviews: 475,
pullRequests: {
created: 125,
approved: 98
}
},
meetups: {
participants: 236,
mediators: 65,
facilitators: 74
},
referral: {
allocated: 74,
indications: 745
}
}
]
// throw new Error("Não foi possível buscar times");
yield put(
GeneralReportsActions.getTeamsResponse({
data,
loading: false,
error: null
})
)
} catch (error) {
yield put(
GeneralReportsActions.getTeamsResponse({
data: null,
loading: false,
error: error.message
})
)
}
}
<file_sep>import React, { Component } from 'react'
import PropTypes from 'prop-types'
import moment from 'moment'
import 'moment/locale/pt-br'
import { bindActionCreators } from 'redux'
import { connect } from 'react-redux'
import { SmallLoading, SmallError } from '../../../components/utils'
import { Creators as GeneralReportsActions } from '../../../store/ducks/generalReports'
import {
Container,
CardsWrapper,
Card,
Percentage,
StyledSelect
} from './styles'
class InfoCards extends Component {
static propTypes = {
getUsersAchievements: PropTypes.func.isRequired,
getMissions: PropTypes.func.isRequired,
getXp: PropTypes.func.isRequired,
generalReports: PropTypes.shape({
achievements: PropTypes.shape({
byMonth: PropTypes.number,
byMonthPercentage: PropTypes.number,
byYear: PropTypes.number,
byYearPercentage: PropTypes.number,
total: PropTypes.number
}).isRequired,
missions: PropTypes.shape({
byMonth: PropTypes.number,
byMonthPercentage: PropTypes.number,
byYear: PropTypes.number,
byYearPercentage: PropTypes.number,
total: PropTypes.number
}).isRequired,
xp: PropTypes.shape({
byMonth: PropTypes.number,
byMonthPercentage: PropTypes.number,
byYear: PropTypes.number,
byYearPercentage: PropTypes.number,
total: PropTypes.number
}).isRequired
}).isRequired
}
state = {}
getFormatedMonth = month => {
return moment()
.locale('pt-BR')
.subtract(month, 'month')
.format('MMM/YY')
}
getMonth = month => {
return moment()
.subtract(month, 'month')
.format('MM/YY')
}
renderOptions = type => {
let options = []
for (let i = 0; i < 7; i++) {
options.push({
value: { type, data: this.getMonth(i) },
label: this.getFormatedMonth(i)
})
}
return options
}
handleChange = value => {
const { type, data } = value.value
if (type === 'achievements') {
this.props.getUsersAchievements(data)
} else if (type === 'missions') {
this.props.getMissions(data)
} else if (type === 'xp') {
this.props.getXp(data)
}
}
componentDidMount() {
const { achievements, missions, xp } = this.props.generalReports
!achievements.data && this.props.getUsersAchievements()
!missions.data && this.props.getMissions()
!xp.data && this.props.getXp()
}
renderCards = ({ type, loading, error, data }) => {
if (!data && loading)
return (
<CardsWrapper>
<SmallLoading width="100%" height="100%" />
</CardsWrapper>
)
if (!data && error)
return (
<CardsWrapper>
<SmallError
width="100%"
height="100%"
message={error}
refresh={() => this.handleChange(null, type)}
/>
</CardsWrapper>
)
const {
name,
byMonth,
byMonthPercentage,
byYear,
byYearPercentage,
total
} = data
return (
<CardsWrapper>
<h4>{name}</h4>
<Card>
{loading && <SmallLoading width="100%" height="100%" />}
{error && (
<SmallError
width="100%"
height="100%"
message={error}
refresh={() => this.handleChange(null, type)}
/>
)}
<header>
<p>total {name} mês</p>
<StyledSelect
defaultValue={{
value: { type, data: this.getMonth(0) },
label: this.getFormatedMonth(0)
}}
options={this.renderOptions(type)}
onChange={this.handleChange}
/>
</header>
<h1>{byMonth}</h1>
<Percentage positive={byMonthPercentage > 0}>
<i
className={`fa fa-long-arrow-alt-${
byMonthPercentage < 0 ? 'down' : 'up'
}`}
/>
{byMonthPercentage} %
</Percentage>
</Card>
<Card>
<p>total {name} do ano</p>
<h1>{byYear}</h1>
<Percentage positive={byYearPercentage > 0}>
<i
className={`fa fa-long-arrow-alt-${
byYearPercentage < 1 ? 'down' : 'up'
}`}
/>
{byYearPercentage} %
</Percentage>
</Card>
<Card>
<p>total de {name}</p>
<h1>{total}</h1>
</Card>
</CardsWrapper>
)
}
render() {
const { achievements, missions, xp } = this.props.generalReports
return (
<Container>
{this.renderCards(achievements)}
{this.renderCards(missions)}
{this.renderCards(xp)}
</Container>
)
}
}
const mapStateToProps = state => ({ generalReports: state.generalReports })
const mapDispatchToProps = dispatch =>
bindActionCreators(GeneralReportsActions, dispatch)
export default connect(
mapStateToProps,
mapDispatchToProps
)(InfoCards)
<file_sep>REACT_APP_API_URL=
REACT_APP_ATENA_CRYPTO_SALT=
<file_sep>import styled from 'styled-components'
const StyledScreenHowItWorks = styled.section`
.layout {
padding-top: 0;
background-color: #fdfdfd;
color: #666c71;
}
`
export default StyledScreenHowItWorks
<file_sep>import React, { Component } from 'react'
import hiwlpc from '../assets/hiwlpc.png'
import StyledScreenError from './Error.style'
import { Flex, Box } from '@rebass/grid'
import FullPage from '../components'
import Title from '../components/Title'
import SubTitle from '../components/Title/SubTitle'
class ScreenError extends Component {
render() {
return (
<StyledScreenError>
<main className="layout">
<FullPage background={`url(${hiwlpc})`}>
<Flex alignItems="center" justifyContent="center" flex="1">
<Box>
<Title large color="white" align="center">
404
</Title>
<SubTitle>Ops! Página não encontrada :/</SubTitle>
</Box>
</Flex>
</FullPage>
</main>
</StyledScreenError>
)
}
}
export default ScreenError
<file_sep>import { all, takeLatest } from 'redux-saga/effects'
import { Types as AuthTypes } from '../ducks/auth'
import { Types as UserTypes } from '../ducks/user'
import { Types as RankingTypes } from '../ducks/ranking'
import { Types as GeneralReportsTypes } from '../ducks/generalReports'
import { Types as ExperienceCardTypes } from '../ducks/experienceCard'
import { Types as AchievementsTypes } from '../ducks/achievements'
import { signIn, logout, forceLogout } from './auth'
import { getProfile, getUserInfo, putUserInfo } from './user'
import { getRanking, getRankingUsers } from './ranking'
import {
getUsers,
getUsersAchievements,
getMissions,
getXp,
getTeams
} from './generalReports'
import { getExperience, putExperience } from './experienceCard'
import {
getAchievements,
editAchievement,
createAchievement
} from './achievements'
export default function* rootSaga() {
yield all([
takeLatest(AuthTypes.SIGN_IN_REQUEST, signIn),
takeLatest(AuthTypes.SIGN_OUT, logout),
takeLatest(AuthTypes.FORCE_SIGN_OUT, forceLogout),
takeLatest(UserTypes.GET_USER_INFO, getUserInfo),
takeLatest(UserTypes.PUT_USER_INFO, putUserInfo),
takeLatest(UserTypes.GET_PROFILE, getProfile),
takeLatest(RankingTypes.GET_RANKING, getRanking),
takeLatest(RankingTypes.GET_RANKING_USERS, getRankingUsers),
takeLatest(GeneralReportsTypes.GET_USERS, getUsers),
takeLatest(
GeneralReportsTypes.GET_USERS_ACHIEVEMENTS,
getUsersAchievements
),
takeLatest(GeneralReportsTypes.GET_MISSIONS, getMissions),
takeLatest(GeneralReportsTypes.GET_XP, getXp),
takeLatest(GeneralReportsTypes.GET_TEAMS, getTeams),
takeLatest(ExperienceCardTypes.GET_EXPERIENCE, getExperience),
takeLatest(ExperienceCardTypes.PUT_EXPERIENCE, putExperience),
takeLatest(AchievementsTypes.GET_ACHIEVEMENTS, getAchievements),
takeLatest(AchievementsTypes.EDIT_ACHIEVEMENT, editAchievement),
takeLatest(AchievementsTypes.CREATE_ACHIEVEMENT, createAchievement)
])
}
<file_sep>import styled from 'styled-components'
const StyledScreenGithub = styled.section`
background: ${props => props.background};
background-size: cover;
background-position: center;
padding: 100px 0;
.title {
font-size: 20px;
line-height: 1.5;
font-family: ${props => props.theme.fontFamily.primary};
}
.super {
font-size: 20px;
line-height: 1.5;
margin-top: 60px;
a {
color: ${props => props.theme.color.primary};
}
}
.help {
font-size: 16px;
line-height: 1.5;
color: ${props => props.theme.color.white};
text-align: center;
margin: 60px auto 0;
flex: 1;
a {
color: ${props => props.theme.color.primaryHover};
&:hover {
color: ${props => props.theme.color.white};
}
}
}
a {
font-weight: bold;
text-decoration: none;
margin-top: 10px;
display: block;
word-break: break-all;
&:hover {
color: ${props => props.theme.color.primaryHover};
}
}
._inner {
flex: 1;
max-height: 590px
}
._inner > p {
margin-top: 60px;
}
@media (max-width: 760px) {
main {
padding-top: 160px;
padding-bottom: 60px;
}
._inner > p {
margin-top: 30px;
}
}
`
export default StyledScreenGithub
<file_sep>import styled from 'styled-components'
import theme from '../../styles/theme'
const StyledButton = styled.div`
color: ${theme.color.primary};
display: inline-block;
text-transform: uppercase;
font-weight: bold;
border-radius: 100px;
padding: 12px 20px;
border: 2px solid;
margin: 20px 0 10px;
cursor: pointer;
background-color: transparent;
& a {
text-decoration: none;
color: ${theme.color.primary};
}
&:hover {
color: ${theme.color.primary};
background-color: ${theme.color.primary};
& a {
color: white;
}
}
&:focus {
outline: none;
color: ${theme.color.primaryFocus};
background-color: ${theme.color.primaryFocus};
& a {
color: white;
}
}
`
export default StyledButton
<file_sep>import styled from 'styled-components'
import { Field } from 'formik'
import theme from '../../../styles/theme'
export const Container = styled.div`
align-items: flex-start;
display: flex;
flex-wrap: wrap;
justify-content: space-evenly;
width: 100%;
`
export const Title = styled.h3`
color: ${theme.color.primary};
font-size: 16px;
font-weight: bold;
margin-top: 30px;
text-transform: uppercase;
width: 100%;
`
export const Card = styled.div`
background: ${theme.color.white};
border-radius: 10px;
color: ${theme.color.gray};
display: flex;
flex-direction: column;
font-size: 12px;
font-weight: bold;
margin: 2% 3% 2% 0;
min-height: 258px;
padding: 20px;
position: relative;
text-transform: uppercase;
width: 30%;
p {
margin-bottom: 20px;
text-align: center;
}
small {
border-bottom: solid 1px ${theme.color.lightGray};
display: block;
margin: 0 auto;
width: 100%;
}
form {
display: flex;
flex-direction: column;
margin-top: 10px;
width: 100%;
span {
color: ${theme.color.primaryHover};
margin: 10px 0;
}
}
button {
background: ${theme.color.white};
border: 2px solid ${theme.color.primary};
border-radius: 100px;
color: ${theme.color.primary};
cursor: pointer;
font-weight: bold;
height: 46px;
margin-top: 20px;
outline: none;
text-transform: uppercase;
transition: all 0.3s linear;
:hover {
background: ${theme.color.primary};
color: ${theme.color.white};
}
:active {
transform: scale(0.95);
}
:focus {
outline: none;
}
::-moz-focus-inner {
border: 0;
}
}
`
export const StyledInput = styled(Field)`
border: ${({ error }) => (error ? '1px solid red' : '1px solid gray')};
border-radius: 6px;
color: ${theme.color.gray};
font-size: 16px;
font-weight: bold;
height: 50px;
margin-bottom: 20px;
padding: 0 6px 0 18px;
width: 100px;
`
<file_sep>import styled from 'styled-components'
import theme from '../../styles/theme'
export const AccordionWrapper = styled.div`
& > section {
text-decoration: none;
border-bottom: 1px solid #e2e2e2;
display: block;
cursor: pointer;
&:focus {
outline: none;
.accordion__link {
color: ${props => theme.color.primary};
}
}
}
.accordion__link {
color: ${props => theme.color.gray};
font-size: ${props => theme.fontSize.medium};
display: flex;
width: 100%;
justify-content: space-between;
padding: 30px 0px;
&:hover {
color: ${props => theme.color.primary};
}
}
`
export const Awnser = styled.div`
${({ selected }) =>
selected
? `opacity: 1;
visibility: visible;
height: auto;
transition: 0.2s all ease-in;`
: `height: 0;
opacity: 0;
visibility: hidden;`}
& > p {
color: ${props => theme.color.gray};
text-align: left;
margin-bottom: 30px;
cursor: auto;
}
`
export const Icon = styled.i`
${({ selected }) =>
selected
? `transition: 0.2s all ease-in;
transform: rotate(180deg);`
: `transition: 0.2s all ease-in;
transform: rotate(0);`}
`
<file_sep>import { put, delay } from 'redux-saga/effects'
import { toast } from 'react-toastify'
import { Creators as achievementsActions } from '../ducks/achievements'
const data = [
{
name: 'RocketChat',
data: [
{
type: 'Mensagens enviadas',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Reações Dadas',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Reações Recebidas',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Reactions enviados',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Reactions recebidos',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
}
]
},
{
name: 'Github',
data: [
{
type: 'Pull Request',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Review',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Pull Request aprovada',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Issue',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
}
]
}
]
const data2 = [
{
name: 'RocketChat',
data: [
{
type: 'Mensagens enviadas',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1511 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Reações Dadas',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Reações Recebidas',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Reactions enviados',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Reactions recebidos',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
}
]
},
{
name: 'Github',
data: [
{
type: 'Pull Request',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Review',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Pull Request aprovada',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
},
{
type: 'Issue',
values: [
{
name: 'bronze',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'silver',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'gold',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'platinum',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
},
{
name: 'diamond',
tiers: [
{ name: 'I', value: 1500 },
{ name: 'II', value: 1600 },
{ name: 'III', value: 1700 },
{ name: 'IV', value: 1800 },
{ name: 'V', value: 2000 }
]
}
]
}
]
}
]
export function* getAchievements(action) {
try {
// throw new Error("Servidor indisponivel");
yield put(achievementsActions.getAchievementsSuccess(data))
} catch (error) {
yield put(
achievementsActions.getAchievementsFailure('Erro ao buscar cards')
)
}
}
export function* editAchievement(action) {
try {
yield delay(2000)
// throw new Error("Servidor indisponivel");
yield put(achievementsActions.editAchievementSuccess(data2))
toast.success('Conquista alterada com sucesso!')
} catch (error) {
toast.error(`Não foi possível alterar conquista. ${error.message}`)
yield put(achievementsActions.editAchievementFailure())
}
}
export function* createAchievement(action) {
try {
yield put(achievementsActions.createAchievementSuccess())
} catch (error) {
yield put(
achievementsActions.createAchievementFailure('Erro ao buscar cards')
)
}
}
<file_sep>/* eslint-disable jsx-a11y/anchor-is-valid */
import React, { Component } from 'react'
import { Flex, Box } from '@rebass/grid'
import Title from '../../../components/Title'
import Button from '../../../components/Button'
class XpRules extends Component {
state = {
selected: 'gain'
}
changeInfos = selected => {
this.setState({ selected })
}
render() {
const { selected } = this.state
return (
<section className="container xprules">
<Flex justifyContent className="align-content">
<Box width={4 / 5}>
<Title large className="align-title">
O que é e como <br />
ganhar <span className="red">reputação</span>?
</Title>
<p className="super">
Sua pontuação será medida em <strong>Pontos de Reputação</strong>.
Esse recurso é muito utilizado nos jogos como uma representação
numérica do esforço e aprendizado obtido por um(a) personagem.
Aqui na Impulso, a quantidade de reputação será baseada nas
atividades que você realizar na nossa comunidade.
</p>
</Box>
</Flex>
<Flex className="rules">
<Box width={1 / 2} px={0}>
<button
onClick={() => this.changeInfos('gain')}
className={`${
selected === 'gain' ? 'selected' : ''
} a--rules--button`}>
<span>01. Obtendo Reputação</span>
</button>
</Box>
<Box width={1 / 2} px={0}>
<button
onClick={() => this.changeInfos('losing')}
className={`${
selected === 'losing' ? 'selected' : ''
} a--rules--button`}>
<span>02. Perdendo Reputação</span>
</button>
</Box>
</Flex>
{selected === 'gain' ? (
<Flex
css={{ margin: '0 -30px' }}
className={`rules__inner rules__inner-1 ${
selected === 'gain' ? 'selected' : ''
} `}
flexWrap="wrap">
<Box width={[1, 1 / 2]} px={30}>
<Title>
<span className="red">01.</span>
<br />
OBTENDO REPUTAÇÃO
</Title>
<p>
A obtenção de pontos é feita através da{' '}
<strong>realização de atividades</strong> dentro da Impulso. Por
exemplo: participar de um curso da Impulso Academy, reagir à uma
mensagem no Rocket.chat e até criar uma postagem no nosso Blog.
</p>
</Box>
<Box width={[1, 1 / 2]} px={30}>
<div className="roles__navigation">
<a className="selected">Rocket.Chat</a>
</div>
<div className="rules__table">
<p>
Mensagem postada <span className="value">+3</span>
</p>
<p>
Reaction dado <span className="value">+2</span>
</p>
<p>
Reaction recebido <span className="value">+3</span>
</p>
</div>
<Button>
<a
href="https://www.notion.so/impulso/Reputa-o-Como-ganhar-372bc91f3e404b418b50267ddcadce6f"
target="_blank"
rel="noopener noreferrer">
mais detalhes
</a>
</Button>
</Box>
</Flex>
) : selected === 'losing' ? (
<Flex
css={{ margin: '0 -30px' }}
className={`rules__inner rules__inner-2 ${
selected === 'losing' ? 'selected' : ''
} `}
flexWrap="wrap">
<Box width={1} px={30}>
<Title>
<span className="red">02.</span>
<br />
PERDENDO REPUTAÇÃO
</Title>
<p>
Em oposição às atividades que promovem a obtenção de Reputação,
a única forma de perder pontos é através da{' '}
<strong>Inatividade Completa</strong> na comunidade Impulso. A
inatividade começa a contar a partir de <strong>14 dias</strong>{' '}
após à sua última participação. Apos este período, você perde{' '}
<strong>dois pontos por dia</strong>, até voltar a participar na
comunidade novamente.
</p>
</Box>
</Flex>
) : null}
</section>
)
}
}
export default XpRules
| 30cb86e772ef8fb77c70f982e6f2dd8759f72024 | [
"JavaScript",
"Markdown",
"Shell"
] | 57 | JavaScript | universoimpulso/atena-frontend | 22981c043957824627dce1035db44e6c5603aa2c | 088e0aed37e059149f4f5d23fd6d7323b2781446 | |
refs/heads/master | <file_sep>class CreateJobApps < ActiveRecord::Migration[5.2]
def change
create_table :job_apps do |t|
t.string :First
t.string :Name
t.string :Last
t.string :Name
t.numeric :Phone
t.string :Job
t.text :Role
t.text :Place
t.timestamps
end
end
end
<file_sep>json.extract! job_app, :id, :First, :Name, :Last, :Name, :Phone, :Job, :Role, :Place, :created_at, :updated_at
json.url job_app_url(job_app, format: :json)
<file_sep>module PacecomTechnologiesHelper
def show_errors(object,first_Name)
if object.errors.any?
if !object.errors.messages[first_Name].blank?
object.errors.add(:first_Name, '*cannot be blank, *should be unique').join(',')
end
end
end
def show_errorsa(object,last_Name)
if object.errors.any?
if !object.errors.messages[last_Name].blank?
#object.errors.messages[last_Name].join(", ")
object.errors.add(:last_Name, '*cannot be blank.').join('')
end
end
end
def show_errorsb(object,phone)
if object.errors.any?
if !object.errors.messages[phone].blank?
#object.errors.messages[phone].join(", ")
object.errors.add(:phone, '*cannot be blank, *only numbers').join(',')
end
end
end
def show_errorsc(object,job_Role)
if object.errors.any?
if !object.errors.messages[job_Role].blank?
# object.errors.messages[job_Role].join(", ")
object.errors.add(:job_Role, '*cannot be blank.').join('')
end
end
end
def show_errorsd(object,place)
if object.errors.any?
if !object.errors.messages[place].blank?
#object.errors.messages[place].join(", ")
object.errors.add(:place, '*cannot be blank.').join('')
end
end
end
end
<file_sep>require "application_system_test_case"
class PacecomTechnologiesTest < ApplicationSystemTestCase
setup do
@pacecom_technology = pacecom_technologies(:one)
end
test "visiting the index" do
visit pacecom_technologies_url
assert_selector "h1", text: "Pacecom Technologies"
end
test "creating a Pacecom technology" do
visit pacecom_technologies_url
click_on "New Pacecom Technology"
fill_in "First name", with: @pacecom_technology.First_Name
fill_in "Job role", with: @pacecom_technology.Job_Role
fill_in "Last name", with: @pacecom_technology.Last_Name
fill_in "Phone", with: @pacecom_technology.Phone
fill_in "Place", with: @pacecom_technology.Place
click_on "Create Pacecom technology"
assert_text "Pacecom technology was successfully created"
click_on "Back"
end
test "updating a Pacecom technology" do
visit pacecom_technologies_url
click_on "Edit", match: :first
fill_in "First name", with: @pacecom_technology.First_Name
fill_in "Job role", with: @pacecom_technology.Job_Role
fill_in "Last name", with: @pacecom_technology.Last_Name
fill_in "Phone", with: @pacecom_technology.Phone
fill_in "Place", with: @pacecom_technology.Place
click_on "Update Pacecom technology"
assert_text "Pacecom technology was successfully updated"
click_on "Back"
end
test "destroying a Pacecom technology" do
visit pacecom_technologies_url
page.accept_confirm do
click_on "Destroy", match: :first
end
assert_text "Pacecom technology was successfully destroyed"
end
end
<file_sep>json.extract! pacecom_technology, :id, :First_Name, :Last_Name, :Phone, :Job_Role, :Place, :created_at, :updated_at
json.url pacecom_technology_url(pacecom_technology, format: :json)
<file_sep>class CreatePacecomTechnologies < ActiveRecord::Migration[5.2]
def change
create_table :pacecom_technologies do |t|
t.string :First_Name
t.string :Last_Name
t.integer :Phone
t.text :Job_Role
t.text :Place
t.timestamps
end
end
end
<file_sep>class PacecomTechnologiesController < ApplicationController
before_action :set_pacecom_technology, only: [:show, :edit, :update, :destroy]
# GET /pacecom_technologies
# GET /pacecom_technologies.json
def index
@pacecom_technologies = PacecomTechnology.all
end
# GET /pacecom_technologies/1
# GET /pacecom_technologies/1.json
def show
end
# GET /pacecom_technologies/new
def new
@pacecom_technology = PacecomTechnology.new
end
# GET /pacecom_technologies/1/edit
def edit
end
# POST /pacecom_technologies
# POST /pacecom_technologies.json
def create
@pacecom_technology = PacecomTechnology.new(pacecom_technology_params)
respond_to do |format|
if @pacecom_technology.save
format.html { redirect_to @pacecom_technology, notice: 'New user was successfully created.' }
format.json { render :show, status: :created, location: @pacecom_technology }
else
format.html { render :new }
format.json { render json: @pacecom_technology.errors, status: :unprocessable_entity }
end
end
end
# PATCH/PUT /pacecom_technologies/1
# PATCH/PUT /pacecom_technologies/1.json
def update
respond_to do |format|
if @pacecom_technology.update(pacecom_technology_params)
format.html { redirect_to @pacecom_technology, notice: 'User was successfully updated.' }
format.json { render :show, status: :ok, location: @pacecom_technology }
else
format.html { render :edit }
format.json { render json: @pacecom_technology.errors, status: :unprocessable_entity }
end
end
end
# DELETE /pacecom_technologies/1
# DELETE /pacecom_technologies/1.json
def destroy
@pacecom_technology.destroy
respond_to do |format|
format.html { redirect_to pacecom_technologies_url, notice: 'User was successfully destroyed.' }
format.json { head :no_content }
end
end
private
# Use callbacks to share common setup or constraints between actions.
def set_pacecom_technology
@pacecom_technology = PacecomTechnology.find(params[:id])
end
# Only allow a list of trusted parameters through.
def pacecom_technology_params
params.require(:pacecom_technology).permit(:First_Name, :Last_Name, :Phone, :Job_Role, :Place, :image)
end
end
<file_sep>class JobApp < ApplicationRecord
end
<file_sep>class PacecomTechnology < ApplicationRecord
has_one_attached :image
validates :First_Name, :Last_Name, :Phone, :Job_Role, :Place, :presence => true
validates :Phone, presence: true
validate :correct_image_type
# validates_uniqueness_of :First_Name, :message =>'should be unique'
validates_uniqueness_of :First_Name, message:"should be unique"
#validates_uniqueness_of :First_Name, scope: :Last_Name
#add_index :pacecom_technologies, [ :First_Name, :Last_Name ], :unique => true, message 'noooo'
private
def correct_image_type
if image.attached? && !image.content_type.in?(%w(image/jpeg image/png))
errors.add(:image, 'must be jpeg or png.')
elsif image.attached? == false
errors.add(:image, 'Not attached')
end
end
end
<file_sep>json.partial! "pacecom_technologies/pacecom_technology", pacecom_technology: @pacecom_technology
<file_sep>class JobAppsController < ApplicationController
before_action :set_job_app, only: [:show, :edit, :update, :destroy]
# GET /job_apps
# GET /job_apps.json
def index
@job_apps = JobApp.all
end
# GET /job_apps/1
# GET /job_apps/1.json
def show
end
# GET /job_apps/new
def new
@job_app = JobApp.new
end
# GET /job_apps/1/edit
def edit
end
# POST /job_apps
# POST /job_apps.json
def create
@job_app = JobApp.new(job_app_params)
respond_to do |format|
if @job_app.save
format.html { redirect_to @job_app, notice: 'Job app was successfully created.' }
format.json { render :show, status: :created, location: @job_app }
else
format.html { render :new }
format.json { render json: @job_app.errors, status: :unprocessable_entity }
end
end
end
# PATCH/PUT /job_apps/1
# PATCH/PUT /job_apps/1.json
def update
respond_to do |format|
if @job_app.update(job_app_params)
format.html { redirect_to @job_app, notice: 'Job app was successfully updated.' }
format.json { render :show, status: :ok, location: @job_app }
else
format.html { render :edit }
format.json { render json: @job_app.errors, status: :unprocessable_entity }
end
end
end
# DELETE /job_apps/1
# DELETE /job_apps/1.json
def destroy
@job_app.destroy
respond_to do |format|
format.html { redirect_to job_apps_url, notice: 'Job app was successfully destroyed.' }
format.json { head :no_content }
end
end
private
# Use callbacks to share common setup or constraints between actions.
def set_job_app
@job_app = JobApp.find(params[:id])
end
# Only allow a list of trusted parameters through.
def job_app_params
params.require(:job_app).permit(:First, :Name, :Last, :Name, :Phone, :Job, :Role, :Place)
end
end
<file_sep>require 'test_helper'
class PacecomTechnologiesControllerTest < ActionDispatch::IntegrationTest
setup do
@pacecom_technology = pacecom_technologies(:one)
end
test "should get index" do
get pacecom_technologies_url
assert_response :success
end
test "should get new" do
get new_pacecom_technology_url
assert_response :success
end
test "should create pacecom_technology" do
assert_difference('PacecomTechnology.count') do
post pacecom_technologies_url, params: { pacecom_technology: { First_Name: @pacecom_technology.First_Name, Job_Role: @pacecom_technology.Job_Role, Last_Name: @pacecom_technology.Last_Name, Phone: @pacecom_technology.Phone, Place: @pacecom_technology.Place } }
end
assert_redirected_to pacecom_technology_url(PacecomTechnology.last)
end
test "should show pacecom_technology" do
get pacecom_technology_url(@pacecom_technology)
assert_response :success
end
test "should get edit" do
get edit_pacecom_technology_url(@pacecom_technology)
assert_response :success
end
test "should update pacecom_technology" do
patch pacecom_technology_url(@pacecom_technology), params: { pacecom_technology: { First_Name: @pacecom_technology.First_Name, Job_Role: @pacecom_technology.Job_Role, Last_Name: @pacecom_technology.Last_Name, Phone: @pacecom_technology.Phone, Place: @pacecom_technology.Place } }
assert_redirected_to pacecom_technology_url(@pacecom_technology)
end
test "should destroy pacecom_technology" do
assert_difference('PacecomTechnology.count', -1) do
delete pacecom_technology_url(@pacecom_technology)
end
assert_redirected_to pacecom_technologies_url
end
end
<file_sep>json.array! @job_apps, partial: "job_apps/job_app", as: :job_app
<file_sep>require "application_system_test_case"
class JobAppsTest < ApplicationSystemTestCase
setup do
@job_app = job_apps(:one)
end
test "visiting the index" do
visit job_apps_url
assert_selector "h1", text: "Job Apps"
end
test "creating a Job app" do
visit job_apps_url
click_on "New Job App"
fill_in "First", with: @job_app.First
fill_in "Job", with: @job_app.Job
fill_in "Last", with: @job_app.Last
fill_in "Name", with: @job_app.Name
fill_in "Phone", with: @job_app.Phone
fill_in "Place", with: @job_app.Place
fill_in "Role", with: @job_app.Role
click_on "Create Job app"
assert_text "Job app was successfully created"
click_on "Back"
end
test "updating a Job app" do
visit job_apps_url
click_on "Edit", match: :first
fill_in "First", with: @job_app.First
fill_in "Job", with: @job_app.Job
fill_in "Last", with: @job_app.Last
fill_in "Name", with: @job_app.Name
fill_in "Phone", with: @job_app.Phone
fill_in "Place", with: @job_app.Place
fill_in "Role", with: @job_app.Role
click_on "Update Job app"
assert_text "Job app was successfully updated"
click_on "Back"
end
test "destroying a Job app" do
visit job_apps_url
page.accept_confirm do
click_on "Destroy", match: :first
end
assert_text "Job app was successfully destroyed"
end
end
<file_sep>require 'test_helper'
class JobAppsControllerTest < ActionDispatch::IntegrationTest
setup do
@job_app = job_apps(:one)
end
test "should get index" do
get job_apps_url
assert_response :success
end
test "should get new" do
get new_job_app_url
assert_response :success
end
test "should create job_app" do
assert_difference('JobApp.count') do
post job_apps_url, params: { job_app: { First: @job_app.First, Job: @job_app.Job, Last: @job_app.Last, Name: @job_app.Name, Phone: @job_app.Phone, Place: @job_app.Place, Role: @job_app.Role } }
end
assert_redirected_to job_app_url(JobApp.last)
end
test "should show job_app" do
get job_app_url(@job_app)
assert_response :success
end
test "should get edit" do
get edit_job_app_url(@job_app)
assert_response :success
end
test "should update job_app" do
patch job_app_url(@job_app), params: { job_app: { First: @job_app.First, Job: @job_app.Job, Last: @job_app.Last, Name: @job_app.Name, Phone: @job_app.Phone, Place: @job_app.Place, Role: @job_app.Role } }
assert_redirected_to job_app_url(@job_app)
end
test "should destroy job_app" do
assert_difference('JobApp.count', -1) do
delete job_app_url(@job_app)
end
assert_redirected_to job_apps_url
end
end
<file_sep>json.partial! "job_apps/job_app", job_app: @job_app
| f86c2091e7ab0bd5f91cd62b32d7f8fa735fae58 | [
"Ruby"
] | 16 | Ruby | NakulBharadwaj123/CrudOnRails | 419ab5d0f03f84bba72671e428cb46f72c6c6668 | bf9bc80406eabe9e9581660a8362bebf74647534 | |
refs/heads/master | <file_sep>package com.example.transmissoriotparacegos.providers
import com.example.transmissoriotparacegos.models.IoTDevice
import com.google.gson.GsonBuilder
import okhttp3.OkHttpClient
import okhttp3.logging.HttpLoggingInterceptor
import retrofit2.Retrofit
import retrofit2.adapter.rxjava.RxJavaCallAdapterFactory
import retrofit2.converter.gson.GsonConverterFactory
import rx.Observable
class IoTApi(val ip: String) {
var service: IoTServiceDef
init {
val logging = HttpLoggingInterceptor()
logging.level = HttpLoggingInterceptor.Level.BODY
val httpClient = OkHttpClient.Builder()
httpClient.addInterceptor(logging)
val gson = GsonBuilder().setLenient().create()
val retrofit = Retrofit.Builder().apply {
baseUrl("$ip/")
addCallAdapterFactory(RxJavaCallAdapterFactory.create())
addConverterFactory((GsonConverterFactory.create(gson)))
client(httpClient.build())
}.build()
service = retrofit.create<IoTServiceDef>(IoTServiceDef::class.java)
}
fun getVariaveis() : Observable<IoTDevice> {
return service.getVariaveis()
.map {
IoTDevice(it.nome, it.descricao, it.capacidades)
}
}
}<file_sep>package com.example.transmissoriotparacegos.providers
import com.example.transmissoriotparacegos.models.IoTDevice
import retrofit2.http.GET
import rx.Observable
interface IoTServiceDef {
@GET("/variavel")
fun getVariaveis(): Observable<IoTDevice>
}<file_sep>package com.example.transmissoriotparacegos.ui.home
import androidx.lifecycle.LiveData
import androidx.lifecycle.MutableLiveData
import androidx.lifecycle.ViewModel
import com.example.transmissoriotparacegos.models.IoTDevice
import com.example.transmissoriotparacegos.providers.IoTApi
import rx.android.schedulers.AndroidSchedulers
import rx.schedulers.Schedulers
class HomeViewModel(val ip: String) : ViewModel() {
private val api = IoTApi(ip)
lateinit var iotDevice: MutableLiveData<IoTDevice>
init {
api.getVariaveis()
.subscribeOn(Schedulers.io())
.observeOn(AndroidSchedulers.mainThread())
.subscribe {
iotDevice.postValue(it)
}
}
}
| aa1c4ce907eda5c404e63e28a94d1731a8717eb3 | [
"Kotlin"
] | 3 | Kotlin | crislmfroes/TransmissorAutomacaoIoT | c6308dabac34e1bd72aeffee6d87e86cf61f7076 | 571ae727a81cb7788b48e73eecc8a0d64ea1a91b | |
refs/heads/master | <repo_name>anon05/FiveM-FSN-Framework<file_sep>/fsn_store/client.lua
local stores = {
{id = 'liquorace', xyz = vector3(1392.3483886719, 3604.0036621094, 34.980926513672), blip=true, busy = false},
{id = 'ltdgas', xyz = vector3(1698.4125976563, 4924.998046875, 42.063678741455), blip=true, busy = false},
{id = 'ltdgas', xyz = vector3(-707.94940185547, -914.28442382813, 19.215589523315), blip=true, busy = false},
{id = 'ltdgas', xyz = vector3(1163.341796875, -323.94161987305, 69.205139160156), blip=true, busy = false},
{id = 'ltdgas', xyz = vector3(-48.42569732666, -1757.5212402344, 29.420993804932), blip=true, busy = false},
{id = 'robsliquor', xyz = vector3(-1487.6705322266, -379.61917114258, 40.163394927979), blip=true, busy = false},
{id = 'robsliquor', xyz = vector3(-2968.5520019531, 390.56546020508, 15.043312072754), blip=true, busy = false},
{id = 'robsliquor', xyz = vector3(1166.4477539063, 2708.3881835938, 38.157699584961), blip=true, busy = false},
{id = 'robsliquor', xyz = vector3(1136.1823730469, -981.75445556641, 46.415802001953), blip=true, busy = false},
{id = 'robsliquor', xyz = vector3(-1223.4569091797, -906.90423583984, 12.3263463974), blip=true, busy = false},
{id = 'twentyfourseven', xyz = vector3(25.816429138184, -1347.3413085938, 29.497024536133), blip=true, busy = false},
{id = 'twentyfourseven', xyz = vector3(374.22787475586, 326.03570556641, 103.56636810303), blip=true, busy = false},
{id = 'twentyfourseven', xyz = vector3(-3242.7116699219, 1001.4896240234, 12.830704689026), blip=true, busy = false},
{id = 'twentyfourseven', xyz = vector3(1961.62890625, 3741.0764160156, 32.343776702881), blip=true, busy = false},
{id = 'twentyfourseven', xyz = vector3(1730.2172851563, 6415.9599609375, 35.037227630615), blip=true, busy = false},
{id = 'twentyfourseven', xyz = vector3(2678.9938964844, 3281.0151367188, 55.241138458252), blip=true, busy = false},
{id = 'twentyfourseven', xyz = vector3(2557.4074707031, 382.74633789063, 108.62294769287), blip=true, busy = false},
{id = 'pillbox', xyz = vector3(316.72573852539, -588.17431640625, 43.291801452637), blip=false, busy = false}
}
Util.Tick(function()
for k, s in pairs(stores) do
local playerPed = GetPlayerPed(-1)
local playerPos = GetEntityCoords(playerPed)
local storeCoords = s.xyz
local dist = Util.GetVecDist(playerPos, storeCoords)
if dist < 10.0 then
DrawMarker(25, s.xyz.x,s.xyz.y,s.xyz.z - 0.95, 0, 0, 0, 0, 0, 0, 1.0, 1.0, 1.0, 255, 255, 255, 150, 0, 0, 2, 0, 0, 0, 0)
if dist < 2.0 then
if not s.busy then
Util.DrawText3D(s.xyz.x, s.xyz.y, s.xyz.z, '[ E ] Access Store',{255,255,255,200}, 0.25)
if IsControlJustReleased(0, Util.GetKeyNumber('E')) then
TriggerServerEvent('fsn_store:request', s.id)
end
else
Util.DrawText3D(s.xyz.x, s.xyz.y, s.xyz.z, '~r~ Store in use\n Please try again later',{255,255,255,200}, 0.25)
end
end
end
end
end)
Citizen.CreateThread( function()
for k, store in pairs(stores) do
if store.blip then
local blip = AddBlipForCoord(store.xyz.x, store.xyz.y, store.xyz.z)
SetBlipSprite(blip, 52)
SetBlipAsShortRange(blip, true)
BeginTextCommandSetBlipName("STRING")
AddTextComponentString("Store")
EndTextCommandSetBlipName(blip)
end
end
end )
local storekeepers = {
{x = -705.81408691406, y = -914.63317871094, z = 19.215587615967, h = 88.849708557129, ped = false},
{x = -46.755832672119, y = -1758.6920166016, z = 29.421007156372, h = 50.685424804688, ped = false},
{x = 24.2922706604, y = -1347.4547119141, z = 29.497022628784, h = 270.94165039063, ped = false},
{x = -1222.3319091797, y = -908.91625976563, z = 12.326347351074, h = 26.143705368042, ped = false},
{x = -1486.1457519531, y = -377.66079711914, z = 40.163425445557, h = 139.18792724609, ped = false},
{x = 372.76062011719, y = 328.07223510742, z = 103.56637573242, h = 249.64683532715, ped = false},
{x = 1164.9409179688, y = -323.45886230469, z = 69.205146789551, h = 97.392929077148, ped = false},
{x = 1133.9039306641, y = -982.02099609375, z = 46.415802001953, h = 271.70544433594, ped = false},
{x = 1959.0269775391, y = 3741.3435058594, z = 32.343746185303, h = 299.91790771484, ped = false},
{x = 1166.1110839844, y = 2710.9475097656, z = 38.157703399658, h = 176.98136901855, ped = false},
{x = 1392.1517333984, y = 3606.2194824219, z = 34.980926513672, h = 201.22804260254, ped = false},
{x = 1697.9591064453, y = 4922.5615234375, z = 42.063674926758, h = 326.3063659668, ped = false},
{x = 316.4407043457, y = -587.11462402344, z = 43.291835784912, h = 184.49844360352, ped = false}
}
local robbing = false
local robbingstart = 0
local lastrob = 0
local curtime = 0
Citizen.CreateThread(function()
while true do
Citizen.Wait(1000)
curtime = curtime+1
end
end)
Citizen.CreateThread(function()
Citizen.Wait(10000)
while true do
Citizen.Wait(0)
for k,v in pairs(storekeepers) do
if GetDistanceBetweenCoords(v.x, v.y, v.z, GetEntityCoords(GetPlayerPed(-1)), true) < 10 then
if v.ped then
if IsPlayerFreeAiming(PlayerId()) and IsPlayerFreeAimingAtEntity(PlayerId(), v.ped) then
if robbing then
TaskStandStill(v.ped, 3000)
TaskCower(v.ped, 3000)
if not IsEntityPlayingAnim(v.ped, 'random@mugging3', "handsup_standing_base", 3) then
RequestAnimDict('random@mugging3')
TaskPlayAnim(v.ped, "random@mugging3", "handsup_standing_base", 4.0, -4, -1, 49, 0, 0, 0, 0)
end
local maff = robbingstart + 30
if maff < curtime then
robbing = false
TriggerEvent('fsn_bank:change:walletAdd', math.random(100, 600))
TriggerEvent('fsn_inventory:item:add', 'dirty_money', math.random(500,1000))
Citizen.Wait(4000)
end
elseif not IsPedInCombat(v.ped) then
local quickmaff = lastrob + 1800
if quickmaff < curtime or lastrob == 0 then
if math.random(0,100) > 20 then
TaskStandStill(v.ped, 3000)
TaskCower(v.ped, 3000)
SetPedSweat(v.ped, 100.0)
robbing = true
TriggerEvent('fsn_notify:displayNotification', 'Robbing...', 'centerLeft', 6000, 'info')
exports["fsn_progress"]:fsn_ProgressBar(58, 133, 255,'ROBBING',30)
robbingstart = curtime
lastrob = curtime
if not IsEntityPlayingAnim(v.ped, 'random@mugging3', "handsup_standing_base", 3) then
RequestAnimDict('random@mugging3')
TaskPlayAnim(v.ped, "random@mugging3", "handsup_standing_base", 4.0, -4, -1, 49, 0, 0, 0, 0)
end
if math.random(0,100) > 30 then
local pos = GetEntityCoords(GetPlayerPed(-1))
local coords = {
x = pos.x,
y = pos.y,
z = pos.z
}
TriggerServerEvent('fsn_police:dispatch', coords, 12, '10-31b | ARMED ROBBERY IN PROGRESS')
end
else
TaskCombatPed(v.ped, GetPlayerPed(-1), 0, 16)
if math.random(0,100) > 30 then
local pos = GetEntityCoords(GetPlayerPed(-1))
local coords = {
x = pos.x,
y = pos.y,
z = pos.z
}
TriggerServerEvent('fsn_police:dispatch', coords, 12, '10-31b | ARMED ROBBERY IN PROGRESS')
end
robbing = false
end
else
TriggerEvent('fsn_notify:displayNotification', 'You can\'t do that yet!', 'centerLeft', 6000, 'info')
local pos = GetEntityCoords(GetPlayerPed(-1))
local coords = {
x = pos.x,
y = pos.y,
z = pos.z
}
TriggerServerEvent('fsn_police:dispatch', coords, 12, '10-31b | Attempted armed store robbery')
Citizen.Wait(6000)
end
end
else
if robbing then
exports["fsn_progress"]:removeBar()
TriggerEvent('fsn_notify:displayNotification', 'You failed...', 'centerLeft', 6000, 'error')
robbing = false
Citizen.Wait(6000)
end
end
end
end
end
end
end)
Citizen.CreateThread(function()
Citizen.Wait(10000)
while true do Citizen.Wait(0)
for k,v in pairs(storekeepers) do
if GetDistanceBetweenCoords(v.x, v.y, v.z, GetEntityCoords(GetPlayerPed(-1)), true) < 10 then
if exports["fsn_entfinder"]:getPedNearCoords(v.x, v.y, v.z,5) then
if GetEntityModel(exports["fsn_entfinder"]:getPedNearCoords(v.x, v.y, v.z,5)) == 416176080 then
v.ped = exports["fsn_entfinder"]:getPedNearCoords(v.x, v.y, v.z,5)
fsn_drawText3D(GetEntityCoords(v.ped).x, GetEntityCoords(v.ped).y, GetEntityCoords(v.ped).z, '~w~ ')
else
if v.ped then
if not IsPedFleeing(v.ped) and not IsPedFleeing(v.ped) then
v.ped = false
end
end
end
--v.ped = exports['fsn_main']:fsn_FindPedNearbyCoords(v.x, v.y, v.z,5)
else
if not v.ped then
RequestModel(416176080)
while not HasModelLoaded(416176080) do
Wait(1)
end
v.ped = CreatePed(5, 416176080, v.x, v.y, v.z, true, true)
SetEntityHeading(v.ped, v.h)
elseif GetDistanceBetweenCoords(v.x, v.y, v.z, GetEntityCoords(v.ped, true)) < 50 then
if not IsPedInCombat(v.ped) and not IsPedFleeing(v.ped) then
RequestModel(416176080)
while not HasModelLoaded(416176080) do
Wait(1)
end
v.ped = CreatePed(5, 416176080, v.x, v.y, v.z, true, true)
SetEntityHeading(v.ped, v.h)
end
end
end
end
end
end
end)
<file_sep>/fsn_store/server.lua
-- These will restock every restart, unless you make something so it doesn't.
local stores = {
['liquorace'] = {
busy = false,
stock = {
beef_jerky = {amt=999,price=4},
cupcake = {amt = 999, price = 1},
microwave_burrito = {amt = 999, price = 8},
panini = {amt = 999, price = 6},
pepsi = {amt = 999, price = 5},
phone = {amt = 999, price = 250},
bandage = {amt = 1500, price = 250},
binoculars = {amt = 999, price = 250}
}
},
['ltdgas'] = {
busy = false,
stock = {
beef_jerky = {amt=999,price=4},
cupcake = {amt = 999, price = 1},
microwave_burrito = {amt = 999, price = 8},
panini = {amt = 999, price = 6},
pepsi = {amt = 999, price = 5},
phone = {amt = 999, price = 250},
bandage = {amt = 1500, price = 250},
binoculars = {amt = 999, price = 250}
}
},
['robsliquor'] = {
busy = false,
stock = {
beef_jerky = {amt=999,price=4},
cupcake = {amt = 999, price = 1},
microwave_burrito = {amt = 999, price = 8},
panini = {amt = 999, price = 6},
pepsi = {amt = 999, price = 5},
phone = {amt = 999, price = 250},
bandage = {amt = 1500, price = 250},
binoculars = {amt = 999, price = 250}
}
},
['twentyfourseven'] = {
busy = false,
stock = {
beef_jerky = {amt=999,price=4},
cupcake = {amt = 999, price = 1},
microwave_burrito = {amt = 999, price = 8},
panini = {amt = 999, price = 6},
pepsi = {amt = 999, price = 5},
phone = {amt = 999, price = 250},
bandage = {amt = 1500, price = 250},
binoculars = {amt = 999, price = 250}
}
},
['pillbox'] = {
busy = false,
stock = {
beef_jerky = {amt=999,price=4},
cupcake = {amt = 999, price = 1},
microwave_burrito = {amt = 999, price = 8},
panini = {amt = 999, price = 6},
pepsi = {amt = 999, price = 5},
phone = {amt = 999, price = 250},
bandage = {amt = 1500, price = 250},
binoculars = {amt = 999, price = 250}
}
},
}
local items = {
beef_jerky = {
index = 'beef_jerky',
name = '<NAME>',
data = {
weight = 0.5
},
},
cupcake = {
index = 'cupcake',
name = 'Cupcake',
data = {
weight = 0.5
}
},
microwave_burrito = {
index = 'microwave_burrito',
name = 'Microwave Burrito',
data = {
weight = 1.5
}
},
panini = {
index = 'panini',
name = 'Panini',
data = {
weight = 1
}
},
pepsi = {
index = 'pepsi',
name = 'Pepsi',
data = {
weight = 1
}
},
pepsi_max = {
index = 'pepsi_max',
name = 'Pepsi Max',
data = {
weight = 1
}
},
water = {
index = 'water',
name = 'Water',
data = {
weight = 1
}
},
coffee = {
index = 'coffee',
name = 'Coffee',
data = {
weight = 1
}
},
lockpick = {
index = 'lockpick',
name = 'Lockpick',
data = {
weight = 1.5
}
},
zipties = {
index = 'zipties',
name = 'Zip Ties',
data = {
weight = 3
}
},
phone = {
index = 'phone',
name = 'Phone',
data = {
weight = 1
}
},
bandage = {
index = 'bandage',
name = 'Bandage',
data = {
weight = 0.4
}
},
binoculars = {
index = 'binoculars',
name = 'Binoculars',
data ={
weight = 3
}
}
}
--[[
Hint: you could add some code here to make players own stores and put stock in??
]]--
RegisterServerEvent('fsn_store:request')
AddEventHandler('fsn_store:request', function(store_id)
local s = stores[store_id]
if s then
if s.busy == false then
s.busy = source
local inv = {}
for k, v in pairs(s.stock) do
if items[k] then
local item = items[k]
if item.data then
item.data.price = s.stock[k].price
else
item.data = {price=s.stock[k].price}
end
item.amt = s.stock[k].amt
table.insert(inv, #inv+1, item)
else
print('ERROR (fsn_store) :: Item '..k..' is not defined in server.lua')
end
end
TriggerClientEvent('fsn_inventory:store:recieve', source, store_id, inv)
else
TriggerClientEvent('fsn_notify:displayNotification', source, 'This store is in use by another player: '..s.busy, 'centerRight', 8000, 'error')
end
else
TriggerClientEvent('fsn_notify:displayNotification', source, 'ERROR: This store does not seem to exist', 'centerRight', 8000, 'error')
end
end)
RegisterServerEvent('fsn_store:boughtOne')
AddEventHandler('fsn_store:boughtOne', function(store_id, item)
local s = stores[store_id]
if s then
if stores[store_id]['stock'][item] then
stores[store_id]['stock'][item].amt = stores[store_id]['stock'][item].amt - 1
TriggerEvent('fsn_main:logging:addLog', source, 'weapons', '[GUNSTORE] Player('..source..') purchased '..item..' from '..store_id)
else
TriggerClientEvent('fsn_notify:displayNotification', source, 'ERROR: This store does not have that item - please speak to a developer', 'centerRight', 8000, 'error')
end
else
TriggerClientEvent('fsn_notify:displayNotification', source, 'ERROR: This store does not seem to exist', 'centerRight', 8000, 'error')
end
end)
RegisterServerEvent('fsn_store:closedStore')
AddEventHandler('fsn_store:closedStore', function(store_id)
local s = stores[store_id]
if s then
s.busy = false
else
TriggerClientEvent('fsn_notify:displayNotification', source, 'ERROR: This store does not seem to exist', 'centerRight', 8000, 'error')
end
end)
AddEventHandler('playerDropped', function()
for k,v in pairs(stores) do
if v.busy == source then
v.busy = false
end
end
end) | aae876aa2c9b2637a7537f6f6612cdaa1c49f870 | [
"Lua"
] | 2 | Lua | anon05/FiveM-FSN-Framework | 72a580a368e8f51f07bbe14f2c3b0827e1632c7e | a03e9e504bb3185f1dd8b5a62370bc3c32821f42 | |
refs/heads/master | <file_sep>package weblab.http.header;
import weblab.http.statuscode.StatusCode;
/**
* Header to define the Http Version in use
*
* @author <NAME> <<EMAIL>>
*/
public class HttpHeader implements Header {
private String mHttpVersion;
private StatusCode mStatusCode;
public HttpHeader(String httpVersion, StatusCode statusCode) {
mHttpVersion = httpVersion;
mStatusCode = statusCode;
}
@Override
public String toString() {
return mHttpVersion + " " + mStatusCode.getStatusCode() + " " + mStatusCode.getMessage() + EOL;
}
}
<file_sep># Simple Http Server Express.js Style #
This is a simple implementation of a Http Server in Java. Currently it only serves static files like HTML, Css, Javascript and Images and enables some simple routing mechanisms.
## Execution ##
The server.jar in the dist folder can be used to execute the server. It serves all files from the public_html.
## Routing ##
The server is written similar to express.js. The only things needed to instantiate the server is a config and a base server.
```
#!java
Config config = ConfigFactory.parseFile(...);
Server server = new MultithreadedServer(config.getInt("server.port"), config);
```
After that we are good to go and can start attaching middlewares and routes to the server. Like in express.js the server itself is also a router.
```
#!java
// express.js style routing, pretty neat right?
server
// this middleware attaches the Server: NDS Http Server header to each request, just for fun
.use((request, response, next) -> {
response.addHeader(new ServerHeader("NDS Http Server"));
next.apply();
})
// serve static files
.use(new FileMiddleware(config.getString("server.static")))
// no file found? let's try these routes then
.use("/", (request, response) -> {
// listens to /
// let's return only hello world
response.setStatusCode(StatusCode.OK);
response.addHeader(new ContentTypeHeader("text/html"));
response.setContent("Hello World");
});
/*
* ok that is neat, but what about nested routers?
* oh thats possible too? cool! let's try that.
*/
Router router = new Router();
router.use("/test", (request, response) -> {
response.setStatusCode(StatusCode.OK);
response.addHeader(new ContentTypeHeader("text/html"));
response.setContent("I am the nested route /test");
});
// let's add the sub router
// makes the /test route available under /workspace/text
server.use("/workspace", router);
// no route matched till now apparently, so let's return 404
server.use((request, response, next) -> {
if(response.getStatusCode() == null) {
response.setStatusCode(StatusCode.NOT_FOUND);
}
});
```
Ok after setting up the server all that is left, is to start it
```
#!java
server.start();
```
The Configuration File looks like this
```
#!json
"server": {
"port": 9090,
"static": "./public_html"
},
"mime": {
"extension": {
"js": "application/javascript",
"css": "text/css",
"html": "text/html",
"jpg": "image/jpeg",
"jpeg": "image/jpeg",
"png": "image/png",
"ico": "image/x-icon"
}
}
```
<file_sep>package weblab.server;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.net.ServerSocket;
import java.net.Socket;
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import com.typesafe.config.Config;
/**
* Abstract Server implementation, which handles incoming connections, logging,
* cleanup and middlewares.
*
* @author <NAME> <<EMAIL>>
*/
public abstract class Server extends Router {
/**
* logging file
*/
private FileWriter mLog;
/**
* logging format
*/
private DateFormat mFormat;
/**
* defines if logging is enabled or not
*/
private boolean _DEBUG_ = false;
/**
* socket the server is listening to
*/
private ServerSocket mServerSocket;
/**
* the connection handler for new connections
*/
private ConnectionHandler mConnectionHandler;
/**
* server configuration (e.g. allowed mime types)
*/
private Config mConfig;
/**
* custom constructor, needs to be called by subclasses
*
* @param port
* the port on which the server will listen
* @throws IOException
* when the ServerSocket can not be opened
*/
public Server(final int port, final Config config) throws IOException {
mServerSocket = new ServerSocket(port);
mConnectionHandler = new ConnectionHandler(this);
mFormat = new SimpleDateFormat("HH:mm:ss:SSS");
mConfig = config;
try {
mLog = new FileWriter(new File("log.txt"));
} catch (IOException e) {
_DEBUG_ = false;
System.err.println("[" + mFormat.format(new Date()) + "] could not open logfile");
}
System.out.println("Server now running on port " + port);
}
/**
* get the server's configuration
*
* @return the server configuration
*/
public Config getConfig() {
return mConfig;
}
/**
* starts the server and initializes the ConnectionHandler waiting for new
* Clients trying to connect calling {@link #clientConnected(Socket)} on the
* server
*/
public void start() {
System.out.println("starting server ...");
if (running()) {
mConnectionHandler.start();
}
}
/**
* shuts down the server by closing the {@link ServerSocket} and calls
* {@link #cleanUp()} on the server
*/
public synchronized void shutdown() {
System.out.println("shutting down server ...");
if (running()) {
log("initializing server-shutdown ...");
try {
mServerSocket.close();
} catch (IOException e) {
log("error while closing the server-socket");
}
cleanUp();
}
}
/**
* get the {@link ServerSocket} of the server
*
* @return the {@link ServerSocket} on which the server is accepting
* connections
*/
public ServerSocket getSocket() {
return mServerSocket;
}
/**
* check if the server is running or not
*
* @return true if the server is running, false otherwise
*/
public synchronized boolean running() {
if (mServerSocket == null) {
return false;
}
return !mServerSocket.isClosed();
}
/**
* called by the {@link ConnectionHandler} when a client connected
*
* @param socket
* which has been opened by ServerSocket
*/
public abstract void clientConnected(final Socket socket);
/**
* called by {@link Connection} when the connection is being closed
*
* @param connection
* the connection which was closed
*/
public abstract void clientDisconnected(Connection connection);
/**
* called after closing the ServerSocket to clean up anyhting necessary
*/
public abstract void cleanUp();
/**
* write a given message to the log-file
*
* @param message
* the message to be written
*/
public synchronized void log(final String message) {
if (_DEBUG_ && mLog != null) {
try {
String log = "[" + mFormat.format(new Date()) + "] " + message + "\n\n";
System.out.println(log);
mLog.append(log);
mLog.flush();
} catch (IOException e) {
System.err
.println("[" + mFormat.format(new Date()) + "] something went wrong while writing to log-file");
}
}
}
}
<file_sep>package weblab.server;
import java.util.UUID;
/**
* Interface connections and sessions implement.
*
* @author <NAME> <<EMAIL>>
*/
public interface Session {
/**
* get the server on which the session is running
*
* @return the actual server
*/
public Server getServer();
/**
* get the session's id
*
* @return the session id
*/
public UUID getSessionId();
}
<file_sep>package weblab.request;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStream;
import java.io.InputStreamReader;
import java.util.LinkedList;
import java.util.List;
import java.util.StringTokenizer;
import com.typesafe.config.Config;
import weblab.http.method.Method;
import weblab.http.request.Query;
public class RequestFactory {
public static Request build(InputStream in, Config config) throws IOException {
// let's read what the client sent
BufferedReader reader = new BufferedReader(new InputStreamReader(in));
String http;
try {
// first line gives information about the Http protocol
http = reader.readLine();
if (http != null) {
// the request looks something like this:
// GET|POST /theUrl HTTP_VERSION
// let's get the needed information (token == " ")
StringTokenizer tokenizer = new StringTokenizer(http);
// get the method
String method = tokenizer.nextToken();
// get the query url (also extract parameters)
Query query = new Query(tokenizer.nextToken());
String httpVersion = tokenizer.nextToken();
// TODO maybe include headers later for caching etc.
List<String> headers = new LinkedList<>();
String header;
while ((header = reader.readLine()) != null && !header.equals("")) {
headers.add(header);
}
Request request = new Request(config, in, Method.fromSlug(method), query, httpVersion, headers);
return request;
}
} catch (IOException e) {
e.printStackTrace();
}
return null;
}
}
<file_sep>package weblab.http.request;
import java.util.HashMap;
/**
* Represents a Query for a request, query string and parameters
*
* @author <NAME> <<EMAIL>>
*/
public class Query {
/**
* the query string
*/
private String mQuery;
/**
* the passed parameters
*/
private HashMap<String, String> mParameters;
/**
* create an empty query
*/
public Query() {
this("", new HashMap<String, String>());
}
/**
* create a query from a query string and extract the parameters
*
* @param queryString
* the query string
*/
public Query(String queryString) {
String[] queryParts = queryString.split("\\?");
try {
mQuery = queryParts[0];
mParameters = Query.buildParameters(queryParts[1]);
} catch (Exception e) {
// no query parameters
}
}
/**
* create a query by passing the query string and the parameters
*
* @param query
* @param parameters
*/
public Query(String query, HashMap<String, String> parameters) {
mQuery = query;
mParameters = parameters;
}
/**
* get the query string
*
* @return the query string
*/
public String getQuery() {
return mQuery;
}
/**
* set the query string
*
* @param query
* the query string
*/
public void setQuery(String query) {
mQuery = query;
}
/**
* get the parameters
*
* @return the parameters
*/
public HashMap<String, String> getParameters() {
return mParameters;
}
/**
* set the parameters
*
* @param parameters
* the parameters
*/
public void setParameters(HashMap<String, String> parameters) {
mParameters = parameters;
}
/**
* add a paramter to the query
*
* @param key
* the key
* @param value
* the value
*/
public void addParameter(String key, String value) {
mParameters.put(key, value);
}
/**
* build parameters from a query string
*
* @param parameterString
* the query string
*
* @return the extracted parameters
*/
public static HashMap<String, String> buildParameters(String parameterString) {
HashMap<String, String> parameters = new HashMap<>();
String[] parameterParts = parameterString.split("\\&");
for (String parameter : parameterParts) {
try {
String[] parameterPair = parameter.split("\\=");
parameters.put(parameterPair[0], parameterPair[1]);
} catch (Exception e) {
// ignore invalid parameter Strings
}
}
return parameters;
}
}
<file_sep>package weblab.http.header;
/**
* Header to define the type of the content being sent
*
* @author <NAME> <<EMAIL>>
*/
public class ContentTypeHeader implements Header {
public static final String IDENTIFIER = "Content-Type";
private String mContentType;
public ContentTypeHeader(String contentType) {
mContentType = contentType;
}
@Override
public String toString() {
return IDENTIFIER + ": " + mContentType + EOL;
}
}
<file_sep>package weblab.request;
public class MiddlewareUsable implements Usable {
private Middleware mMiddleware;
public MiddlewareUsable(Middleware middleware) {
mMiddleware = middleware;
}
@Override
public boolean applicable(Request request) {
return true;
}
@Override
public void use(Request request, Response response, Nextable next) {
mMiddleware.execute(request, response, next);
}
}
<file_sep>package weblab.http.header;
/**
* Not supported in current version
*
* @author <NAME> <<EMAIL>>
*/
public class ReferrerHeader implements Header {
public static final String IDENTIFIER = "Referer";
private String mReferer;
public ReferrerHeader(String referer) {
mReferer = referer;
}
@Override
public String toString() {
return IDENTIFIER + ": " + mReferer + EOL;
}
}
<file_sep>(function() {
console.log('hello world');
var p = document.createElement('p');
p.innerHTML = 'I was appended through Javascript';
var img = document.getElementsByTagName('img')[0];
img.parentNode.insertBefore(p, img);
})();<file_sep>package weblab.server;
import java.io.IOException;
import java.net.Socket;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
import com.typesafe.config.Config;
/**
* Multithreaded server implementation for resolving requests.
*
* @author <NAME> <<EMAIL>>
*/
public class MultithreadedServer extends Server {
private List<Connection> mConnections;
/**
* Create a new instance of MultithreadedServer
*
* @param port
* the port the server will listen to
*
* @throws IOException
* if the port is already in use
*/
public MultithreadedServer(int port, Config config) throws IOException {
super(port, config);
if (running()) {
mConnections = new ArrayList<Connection>();
}
}
/**
* called if the ConnectionHandler opened a new connection
*
* @param socket
* the socket of the new connection
*/
@Override
public void clientConnected(Socket socket) {
// create a new connection
Connection connection = new Connection(UUID.randomUUID(), this, socket);
// add the connection so it can be terminated if the server is shut down
mConnections.add(connection);
// resolve the connection
connection.start();
}
@Override
public synchronized void clientDisconnected(Connection connection) {
mConnections.remove(connection);
}
/**
* close all open connections
*/
@Override
public void cleanUp() {
for (Connection connection : mConnections) {
try {
connection.close();
} catch (IOException e) {
// nothing to do here
}
}
mConnections.clear();
}
}<file_sep>package weblab.request;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import weblab.http.file.FileStreamer;
import weblab.http.header.Header;
import weblab.http.header.HttpHeader;
import weblab.http.statuscode.StatusCode;
public class Response extends HeaderHolder {
private OutputStream mOut;
private StatusCode mStatusCode;
private String mContent;
private FileStreamer mFileStreamer;
public Response(OutputStream out) {
mOut = out;
}
public OutputStream getOutputStream() {
return mOut;
}
public void setStatusCode(StatusCode statusCode) {
mStatusCode = statusCode;
}
public StatusCode getStatusCode() {
return mStatusCode;
}
public void setFileStreamer(FileStreamer fileStreamer) {
mFileStreamer = fileStreamer;
}
public void setContent(String content) {
mContent = content;
}
public void sendBack(Request request) throws IOException {
// create a data stream
DataOutputStream out = new DataOutputStream(getOutputStream());
out.writeBytes(new HttpHeader(request.getHttpVersion(), getStatusCode()).toString());
// send the headers back to the client
for (Header header : getHeaders()) {
out.writeBytes(header.toString());
}
// empty line as delimiter of headers and content
out.writeBytes(Header.EOL);
// write the content back to the client
if (mContent != null) {
out.writeBytes(mContent);
} else if(mFileStreamer != null){
mFileStreamer.stream(out);
}
}
}
<file_sep>package weblab.request;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import weblab.http.header.Header;
public class HeaderHolder {
private List<Header> mHeaders = new LinkedList<>();
public List<Header> getHeaders() {
return mHeaders;
}
public void addHeader(Header header) {
Iterator<Header> iterator = mHeaders.iterator();
while(iterator.hasNext()) {
if(iterator.next().getClass().equals(header.getClass())) {
iterator.remove();
break;
}
}
mHeaders.add(header);
}
public Header getHeader(Class<? extends Header> header) {
Iterator<Header> iterator = mHeaders.iterator();
Header current;
while(iterator.hasNext()) {
current = iterator.next();
if(header.isAssignableFrom(current.getClass())) {
iterator.remove();
return current;
}
}
return null;
}
}
<file_sep>package weblab.http.header;
/**
* Header to define the server name
*
* @author <NAME> <<EMAIL>>
*/
public class ServerHeader implements Header {
private String mServerName;
public ServerHeader(String serverName) {
mServerName = serverName;
}
@Override
public String toString() {
return "Server: " + mServerName + EOL;
}
}
| a2318efda28cd79afb8b9146b12d8be8f306957e | [
"Markdown",
"Java",
"JavaScript"
] | 14 | Java | daves-weblab/NDS-Http-Server | 962102ee134c85e2a33cbab2c9427d183699e66f | 72a57fa10de9e840fb2896f779f1771ae6487fd6 | |
refs/heads/master | <file_sep># QuickSnippet for Visual Studio Code
> Quickly create custom snippets for any language from selected text.
## Install the extension
1. Open Visual Studio Code
2. Press `Ctrl+P` to open the Quick Open dialog
3. Type `ext install quicksnippet` to find the extension.
4. Press `Enter` or click the cloud icon to install it
5. Restart Visual Studio Code if prompted
## Get started creating *so many* snippets!
1. Select text from a file.
2. Press `Ctrl+P` to open the Quick Open dialog
3. Search for `Create Snippet`, and press enter. The selected text will be added as a snippet in the user snippets file for the current language.
4. Edit the snippet properties (name, shortcut, description), and save your changes.
5. Flip back to your original file, and start typing - your snippet should now appear in the completions list.
** Enjoy!**<file_sep>var vscode = require('vscode');
var path = require('path');
var fs = require('fs');
var json = require('comment-json');
var prependFile = require('prepend-file');
var DEFAULT_SNIPPET_NAME = 'My Snippet Name: REPLACE OR IT WILL BE OVERWRITTEN';
var SNIPPET_TEMPLATE_NAME = 'snippet-comment-template.txt';
// this method is called when the extension is activated
exports.activate = function activate(context) {
console.log('Extension "create-snippet" is now active!');
SNIPPET_TEMPLATE_NAME = path.join(context.extensionPath, SNIPPET_TEMPLATE_NAME);
var disposable = vscode.commands.registerCommand(
'extension.createSnippet',
function() {
var editor = vscode.window.activeTextEditor;
if (!editor) {
// Don't open text editor
return;
}
var snippetFilename = getSnippetFilename(editor);
fs.exists(snippetFilename, function(exists) {
if (!exists) {
fs.writeFileSync(snippetFilename, '{ }', {encoding: 'utf8'});
}
createSnippet(snippetFilename, editor);
});
});
context.subscriptions.push(disposable);
};
function createSnippet(snippetFilename, editor) {
fs.readFile(snippetFilename, 'utf8', function(e, data) {
console.log(data);
var jsonData = json.parse(data, null, false);
jsonData = addSelectedSnippet(jsonData, editor);
updateSnippetFile(snippetFilename, jsonData);
});
}
function addSelectedSnippet(jsonData, editor) {
var newJsonData = {};
var snippetBody = getSnippetBody(editor);
if (typeof (jsonData['//$']) !== 'undefined') {
// newJsonData['//$'] = jsonData['//$'];
}
newJsonData[DEFAULT_SNIPPET_NAME] = {
prefix: 'yourPrefixHere',
body: snippetBody,
description: 'Your snippet description here.'
};
for (var key in jsonData) {
if (key !== DEFAULT_SNIPPET_NAME && key !== '//$') {
newJsonData[key] = jsonData[key];
}
}
console.log(newJsonData);
return newJsonData;
}
function getSnippetFilename(editor) {
var userDataPath = process.env.APPDATA ||
(process.platform === 'darwin' ?
process.env.HOME + 'Library/Preference' : '/var/local');
return path.join(
userDataPath,
'Code', 'User', 'snippets',
editor.document.languageId + '.json');
}
function getSnippetBody(editor) {
var selection = editor.document.getText(editor.selection);
var snippetParts = (selection + '$1').split(/\r?\n/);
snippetParts[0] = snippetParts[0].trimLeft();
var trimLast = snippetParts[snippetParts.length - 1].trimLeft();
var trimLastLength = snippetParts[snippetParts.length - 1].length - trimLast.length;
snippetParts[snippetParts.length - 1] = trimLast;
for (var i = 0; i < snippetParts.length; i++) {
snippetParts[i] = snippetParts[i].trimRight();
if (i === 0 || i === snippetParts.length - 1) {
continue;
}
var trim = snippetParts[i].trimLeft();
var trimLength = snippetParts[i].length - trim.length;
var trimCharacter = snippetParts[i][0];
snippetParts[i] =
Array(Math.max(trimLength - trimLastLength + 1, 0))
.join(trimCharacter) + trim;
}
return snippetParts;
}
function updateSnippetFile(snippetFilename, jsonData) {
console.log(jsonData);
fs.writeFile(
snippetFilename,
json.stringify(jsonData, null, ' '), {encoding: 'utf8'},
function(err) {
if (err) {
throw err;
}
var snippetComment = fs.readFileSync(SNIPPET_TEMPLATE_NAME, 'utf8').replace('$(languageId)', path.basename(snippetFilename));
var current = fs.readFileSync(snippetFilename, 'utf8');
if (!current.startsWith(snippetComment)) {
prependFile.sync(snippetFilename, snippetComment, {encoding: 'utf8'});
}
vscode.workspace.openTextDocument(snippetFilename).then(function(doc) {
vscode.window.showTextDocument(doc, vscode.ViewColumn.Two)
.then(selectDefaultSnippet);
});
}
);
}
function selectDefaultSnippet() {
var editor = vscode.window.activeTextEditor;
var index = editor.document.getText().indexOf(DEFAULT_SNIPPET_NAME);
editor.selection = new vscode.Selection(
editor.document.positionAt(index),
editor.document.positionAt(index + DEFAULT_SNIPPET_NAME.length)
);
}
| ecce58c915768e15f8c72d3d31c198469e8c2924 | [
"Markdown",
"JavaScript"
] | 2 | Markdown | mousetraps/vscode-quicksnippet | 3fb6b4dab96b801a352dac880daba2feed9cbc7f | e30f2499ff8f6ed32206a066278de7cc20aee159 | |
refs/heads/master | <repo_name>david-estevez-62/expandSearch-page<file_sep>/main.js
var status = 'retract';
function expandSearch(){
// $('#searchPg').toggle(200);
// $('#searchBar').toggleClass('fullscreen');
// var searchBar = $(this)[0];
if(status === 'retract'){
var searchBar = $(this).children()[0];
$(this).animate({height: "100%", width: "100%"}, 175);
searchBar.className = 'class2';
// $(this).children.removeClass('class1')
var span = document.createElement('span');
var text = document.createTextNode('X');
span.appendChild(text);
span.addEventListener("click", retractSearch)
$(this).append(span);
status = 'expand';
}
// else if(status === 'expand'){
// $(this).animate({height: "60px", width: "575px"}, 175);
// status = 'retract';
// }
// $("#searchPg input").focus();
}
function retractSearch(e){
e.stopPropagation();
// $('#searchPg').toggle(200);
// $('#searchBar').toggleClass('fullscreen');
console.log('hi')
if(status === 'expand'){
var searchBar = $(this).siblings()[0];
console.log(searchBar)
searchBar.className = 'class1';
$(this).parent().animate({height: "60px", width: "575px"}, 175);
$(this)[0].remove();
status = 'retract';
}
}
$('#searchBar').on('click', expandSearch);
// $('#searchBar span').on('click', retractSearch)
// $('#searchBar input[type="search"]').on('click', function(e){
// e.stopPropagation();
// console.log('hi');
// }); | 64de3d7725a08dd46d45bce4862977fd255b1eb1 | [
"JavaScript"
] | 1 | JavaScript | david-estevez-62/expandSearch-page | f671628c23131a190c7ade792a0e2ecfc95ff222 | 3679786939f8251648149e30911a558d1f3fb3f8 | |
refs/heads/master | <repo_name>Jonas228/WPFGehirnJogging<file_sep>/WPFGehirnJogging/Vokabeltrainer.xaml.cs
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.CompilerServices;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Forms;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using WPFGehirnJogging.Klassen;
using MessageBox = System.Windows.MessageBox;
namespace WPFGehirnJogging
{
/// <summary>
/// Interaktionslogik für Vokabeltrainer.xaml
/// </summary>
public partial class Vokabeltrainer : Page
{
public Vokabeltrainer()
{
InitializeComponent();
}
private void Button_Click(object sender, RoutedEventArgs e)
{
NavigationService?.Navigate(new Uri("Vokabeltrainer2.xaml", UriKind.Relative));
}
}
}
<file_sep>/WPFGehirnJogging/Vokabeltrainer2.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using WPFGehirnJogging.Klassen;
using MessageBox = System.Windows.MessageBox;
namespace WPFGehirnJogging
{
/// <summary>
/// Interaktionslogik für Vokabeltrainer2.xaml
/// </summary>
public partial class Vokabeltrainer2 : Page
{
private Dictionary<string, string[]> _dicVokabel;
private int pZuffi;
public Vokabeltrainer2()
{
InitializeComponent();
//Eventhandler der das Event von dem Button abfängt
CVokabeltest test = new CVokabeltest();
//Einlesen des Vokabulars
_dicVokabel = new Dictionary<string, string[]>();
_dicVokabel = test.CsvEinlesen();
CVokabel first = new CVokabel();
first.DeutschesWort = "auto";
first.UserEingabe = "car";
string[] foo = new string[3];
//sucht alle Lösungen aus dem Dictionary für die Vokabel und speichert es in die Vokabel
_dicVokabel.TryGetValue(first.DeutschesWort, out foo);
//speichert alle Englischen Wörter in das EnglischeWort Array
first.EnglischesWort = foo;
for (int i = 1; i < foo.Length - 1; i++)
{
first.EnglischesWort[i - 1] = foo[i];
}
ZufäligVokabelnAusgeben();
//Return ob richtig oder falsch
if (first.CheckResult(first.UserEingabe))
{
MessageBox.Show("Richtig");
first.AnzahlRichtig++;
}
else
{
MessageBox.Show("Falsch");
first.AnzahlFalsch++;
}
}
void ZufäligVokabelnAusgeben()
{
Random rnd = new Random();
pZuffi = rnd.Next(_dicVokabel.Count);
//return _dicVokabel.ElementAt(rnd.Next(0, _dicVokabel.Count)).Value;
string xs = _dicVokabel.ElementAt(pZuffi).Key;
//schreibe string in das Label
}
}
}
<file_sep>/WPFGehirnJogging/MainWindow.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
using WPFGehirnJogging.Klassen;
namespace WPFGehirnJogging
{
/// <summary>
/// Interaktionslogik für MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
double Width = SystemParameters.PrimaryScreenWidth;
double Height = SystemParameters.PrimaryScreenHeight;
this.Width = Width;
this.Height = Height;
this.ResizeMode = ResizeMode.NoResize;
//Iniatilisierung von NavigationService
NavigationService navigationService = NavigationService.GetNavigationService(this);
Loaded += MainWindow_Loaded;
}
private void MainWindow_Loaded(object sender, RoutedEventArgs e)
{
//Beim Starten wird das Menü in das MainFrame geladen
MainFrame.NavigationService.Navigate(new Uri("Menü.xaml", UriKind.Relative));
}
}
}
<file_sep>/WPFGehirnJogging/Klassen/Aufgaben/CMatheAufgabe.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace WPFGehirnJogging.Klassen.Aufgaben
{
class CMatheAufgabe:CAufgaben
{
}
}
<file_sep>/WPFGehirnJogging/Klassen/CVokabelübung.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace WPFGehirnJogging.Klassen
{
class CVokabelübung
{
}
}
<file_sep>/WPFGehirnJogging/Klassen/Test/CTest.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace WPFGehirnJogging
{
class CTest
{
//ToDo Struct Siehe Foto iPhone
private string _beschreibung;
private int _minuten;
private int _anzahlAufgaben;
private int _anzahlFalsch, _anzahlRichtig;
public CTest()
{
}
}
}
<file_sep>/WPFGehirnJogging/Klassen/Aufgaben/CAufgaben.cs
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Linq;
using System.Security.RightsManagement;
using System.Text;
using System.Threading.Tasks;
namespace WPFGehirnJogging.Klassen
{
class CAufgaben
{
public void DisplayAufgabe()
{
}
public virtual void DisplayResult()
{
}
public virtual bool CheckResult(string Result)
{
return true;
}
}
}
<file_sep>/WPFGehirnJogging/Klassen/Aufgaben/CVokabel.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace WPFGehirnJogging.Klassen
{
internal class CVokabel : CAufgaben
{
private string _deutscheWort, _userEingabe;
private string[] _englischesWort;
private int _anzahlRichtig, _anzahlFalsch;
public CVokabel()
{
_deutscheWort="";
_userEingabe = "";
_anzahlFalsch = 0;
_anzahlRichtig = 0;
}
public string DeutschesWort { get => _deutscheWort; set => _deutscheWort = value; }
public string UserEingabe { get => _userEingabe; set => _userEingabe = value; }
public string[] EnglischesWort { get => _englischesWort; set => _englischesWort = value; }
public int AnzahlFalsch { get => _anzahlFalsch; set => _anzahlFalsch = value; }
public int AnzahlRichtig { get => _anzahlRichtig; set => _anzahlRichtig = value; }
public override void DisplayResult()
{
}
public override bool CheckResult(string Result)
{
return EnglischesWort.Contains(Result);
}
}
}
<file_sep>/WPFGehirnJogging/Klassen/Test/CVokabeltest.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.IO;
namespace WPFGehirnJogging.Klassen
{
class CVokabeltest:CTest
{
public CVokabeltest()
{
}
public Dictionary<string, string[]> CsvEinlesen()
{
//Einlesen der CSV Datei und speichern der Vokabeln in ein Dictionary
string filesource = "C:\\Users\\jonaspenner\\source\\repos\\WPFGehirnJogging\\vl.csv";
if (File.Exists(filesource))
{
Dictionary<string, string[]> dic = new Dictionary<string, string[]>();
string[] lines = File.ReadAllLines(filesource);
StreamReader streamReader = new StreamReader(filesource);
var rowValue = streamReader.ReadLine();
var values = rowValue.Split(';');
for (var i = 0; i < lines.Length; i++)
{
values = lines[i].Split(separator: ';');
//Deklaration von der Vokabel
var vocab = new CVokabel
{
DeutschesWort = values[0],
EnglischesWort = values
};
dic.Add(vocab.DeutschesWort, vocab.EnglischesWort);
}
return dic;
}
else
{
throw new FileNotFoundException();
}
}
}
}
<file_sep>/WPFGehirnJogging/Menü.xaml.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace WPFGehirnJogging
{
/// <summary>
/// Interaktionslogik für Menü.xaml
/// </summary>
public partial class Menü : Page
{
public Menü()
{
InitializeComponent();
}
private void BtnVokabeltrainer_Click(object sender, RoutedEventArgs e)
{
NavigationService?.Navigate(new Uri("Vokabeltrainer.xaml", UriKind.Relative));
}
}
}
<file_sep>/WPFGehirnJogging/Klassen/CBenutzer.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace WPFGehirnJogging.Klassen
{
class CBenutzer
{
private string _vorname, _nachname;
private int _sucessindicator;
public CBenutzer(string Vorname, string Nachname)
{
_vorname = Vorname;
_nachname = Nachname;
_sucessindicator = 0;
}
}
}
| e3a21f411cc0619ebe810fdcb82e899467935c2c | [
"C#"
] | 11 | C# | Jonas228/WPFGehirnJogging | 40668c1c7f4bfa4f6fa60cd33ae5fefa229865b3 | 93b059603fe59685fc998774302668ffc10d7530 | |
refs/heads/master | <file_sep>import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class Welcome extends JFrame{
public Welcome(){
super("Pokemon Game Beta");
Trainer t = new Trainer("sulton");
Container c = getContentPane();
//label for background
JLabel bg = new JLabel();
ImageIcon background = new ImageIcon("D://pokemon_welcome.jpg");
bg.setIcon(background);
bg.setBounds(0, -75, 500, 550);
bg.setAlignmentX(Component.BOTTOM_ALIGNMENT);
c.add(bg);
c.setLayout(null);
//add button
JButton btn1 = new JButton("PLAY");
btn1.setBounds(200, 240, 100, 30);
btn1.setAlignmentX(Component.BOTTOM_ALIGNMENT);
btn1.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
MainGame mg = new MainGame(t);
}
});
c.add(btn1);
JButton btn2 = new JButton("ABOUT US");
btn2.setBounds(200, 280, 100, 30);
btn2.setAlignmentX(Component.BOTTOM_ALIGNMENT);
btn2.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
JOptionPane.showMessageDialog(null, " Hi I'm <NAME> 6110110107");
}
});
c.add(btn2);
JButton btn3 = new JButton("QUIT");
btn3.setBounds(200, 320, 100, 30);
btn3.setAlignmentX(Component.BOTTOM_ALIGNMENT);
btn3.addActionListener(new ActionListener(){
public void actionPerformed(ActionEvent e){
System.exit(0);
}
});
c.add(btn3);
//system
setSize(500,450);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setVisible(true);
}
}<file_sep>import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class ImageGallary extends JFrame{
private Trainer trainer;
Pokemon pokemon;
public ImageGallary(Pokemon pokemon){
super("Image Gallary");
this.pokemon = pokemon;
Container c = getContentPane();
//layout
c.setLayout(null);
JLabel bgall = new JLabel();
ImageIcon backgroundall = new ImageIcon("D://all.jpg");
bgall.setIcon(backgroundall);
bgall.setBounds(0, -50, 400, 200);
bgall.setAlignmentX(Component.BOTTOM_ALIGNMENT);
c.add(bgall);
JLabel bgall1 = new JLabel();
ImageIcon backgroundall1 = new ImageIcon("D://1.jpg");
bgall1.setIcon(backgroundall1);
bgall1.setBounds(200, 0, 100, 100);
bgall1.setAlignmentX(Component.BOTTOM_ALIGNMENT);
c.add(bgall1);
JLabel bgall2 = new JLabel();
ImageIcon backgroundall2 = new ImageIcon("D://2.jpg");
bgall2.setIcon(backgroundall2);
bgall2.setBounds(200, 100, 100, 100);
bgall2.setAlignmentX(Component.BOTTOM_ALIGNMENT);
c.add(bgall2);
JLabel bgall3 = new JLabel();
ImageIcon backgroundall3 = new ImageIcon("D://3.jpg");
bgall3.setIcon(backgroundall3);
bgall3.setBounds(0, 100, 100, 100);
bgall3.setAlignmentX(Component.BOTTOM_ALIGNMENT);
c.add(bgall3);
JLabel bgall4 = new JLabel();
ImageIcon backgroundall4 = new ImageIcon("D://4.jpg");
bgall4.setIcon(backgroundall4);
bgall4.setBounds(100, 100, 100, 100);
bgall4.setAlignmentX(Component.BOTTOM_ALIGNMENT);
c.add(bgall4);
setSize(350, 300);
setVisible(true);
setLocationRelativeTo(null);
}
}<file_sep>import java.util.*;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class Catch extends JFrame{
Pokemon pokemon;
Trainer t = new Trainer("");
public Catch(Pokemon pokemon){
super("Catch pokemon");
this.pokemon = pokemon;
Container c = getContentPane();
//layout
JLabel bgcatch = new JLabel();
ImageIcon backgroundcatch = new ImageIcon("D://pokeoncc.png");
bgcatch.setIcon(backgroundcatch);
bgcatch.setBounds(-10, 0, 400, 200);
bgcatch.setAlignmentX(Component.BOTTOM_ALIGNMENT);
c.add(bgcatch);
c.setLayout(null);
setSize(400, 300);
setVisible(true);
setLocationRelativeTo(null);
t.play();
}
}
<file_sep>public class Raichu extends Pikachu{ //mutate of pikachu
public Raichu(String name){
super(name,500); //can change
}
public void attack(Pokemon enemy){
System.out.println("Pokemon " + name + " attack " + enemy.getName());
enemy.damage(100); // can change
}
} | 5d800dc28ae46b7c7796091dfe19931afe1b1344 | [
"Java"
] | 4 | Java | sultonza24/pokemon-game | 6879a0337f559a789446701fbcfdf25a838f038c | 0883bdd92ff29138612e7bc2f8c6915d59d7d946 | |
refs/heads/master | <file_sep># GurtWest
A static file list
<file_sep>var feed = new Instafeed({
//clientId: '<KEY>',
accessToken: "<KEY>",
get: "user",
userId: "7464627426",
resolution: "standard_resolution",
template:
'<a target="_blank" href="{{link}}" title="{{caption}}"><img src="{{image}}" alt="{{caption}}" /></a>'
});
feed.run();
<file_sep>var project = require("./_project.js");
var gulp = require("gulp");
// Copy Images to build folder
gulp.task("images", function() {
return gulp
.src(project.buildSrc + "/images/*")
.pipe(gulp.dest(project.buildDest + "/images"));
});
| 9ed73ea791791f4d9ee4e77705f1fc83f7d33035 | [
"Markdown",
"JavaScript"
] | 3 | Markdown | discoliam/gurtwest | 9c56955a0fd0f6c0c599a157f23404d801b63e80 | 45689da8f1b0a74b0e88b866751240854a0e274a | |
refs/heads/master | <repo_name>leonelvelez314/BGCarritoOld<file_sep>/www/js/controllers.js
angular.module('starter.controllers', [])
.controller('AppCtrl', function($scope, $ionicModal, $timeout,$http) {
// With the new view caching in Ionic, Controllers are only called
// when they are recreated or on app start, instead of every page change.
// To listen for when this page is active (for example, to refresh data),
// listen for the $ionicView.enter event:
//$scope.$on('$ionicView.enter', function(e) {
//});
// Form data for the login modal
// Create the login modal that we will use later
$ionicModal.fromTemplateUrl('templates/login.html', {
scope: $scope
}).then(function(modal) {
$scope.modal = modal;
});
// Triggered in the login modal to close it
$scope.closeLogin = function() {
$scope.modal.hide();
};
// Open the login modal
$scope.login = function() {
$scope.modal.show();
};
})
.controller('ProductsCtrl', function($scope, $http) {
console.log(localStorage.getItem( 'token'));
$http.post('https://rolimapp.com:3000/productos', {transaccion:'generico', tipo:'4'}).then(function(respuesta){
$scope.productos = respuesta.data.data;
}, function(errorResponse){
console.log(errorResponse);
});
})
.controller('DetalleCtrl', function($scope, $stateParams, $http, $window) {
$http.post('https://rolimapp.com:3000/productos', {transaccion:'generico', tipo:'4'}).then(function(respuesta){
$scope.existe = false;
$scope.productos = respuesta.data.data;
let productosSelecc = JSON.parse(localStorage.getItem('productosSeleccionados'));
var listProductosSelec = new Array();
listProductosSelec = productosSelecc;
if(productosSelecc !== null)
{
if(productosSelecc.length >= 0)
{
let existe = false
angular.forEach(listProductosSelec, function(obj, key){
if(Number(obj.id) === Number($stateParams.productid))
{
existe = true;
}
});
if(existe)
{
$scope.existe = true;
}
}
}
angular.forEach($scope.productos, function(value, key){
if(Number(value.id) === Number($stateParams.productid))
{
$scope.producto = value;
}
});
$scope.addCar = function()
{
let productosSelecc = JSON.parse(localStorage.getItem('productosSeleccionados'));
var listProductosSelec = new Array();
listProductosSelec = productosSelecc;
if(productosSelecc !== null)
{
if(productosSelecc.length === 0)
{
listProductosSelec.push($scope.producto);
localStorage.setItem('productosSeleccionados', JSON.stringify(listProductosSelec) );
}else{
let existe = false
angular.forEach(listProductosSelec, function(obj, key){
if(Number(obj.id) === Number($stateParams.productid))
{
existe = true;
}
});
if(!existe)
{
listProductosSelec.push($scope.producto);
localStorage.setItem('productosSeleccionados', JSON.stringify(listProductosSelec));
}
}
}else{
listProductosSelec.push($scope.producto);
localStorage.setItem('productosSeleccionados', JSON.stringify(listProductosSelec));
}
$window.location.href = '#/app/products'
}
}, function(errorResponse){
console.log(errorResponse);
});
})
.controller('LoginCtrl', function($scope, $timeout,$http, $window) {
$scope.loginData = {username:'', password:''};
// Perform the login action when the user submits the login form
$scope.doLogin = function() {
console.log('Doing login', $scope.loginData);
let request = {
transaccion : 'autenticarUsuario',
datosUsuario : {
email : $scope.loginData.username,
password : $scope.loginData.password
}
}
$http.post('https://rolimapp.com:3000/usuarios', request).then(function(respuesta){
console.log(respuesta)
if(respuesta.data.codigoRetorno === '0001')
{
$timeout(function() {
localStorage.setItem( 'token', respuesta.data.token);
localStorage.setItem( 'email', respuesta.data.usuario.email);
localStorage.setItem( 'nombre', respuesta.data.usuario.nombre);
$window.location.href = '#/app/products'
}, 1000);
}
}, function(errorResponse){
console.log(errorResponse);
});
};
})
.controller('CarCtrl', function($scope, $http) {
$scope.productosSelecc = JSON.parse(localStorage.getItem( 'productosSeleccionados'))
$scope.quitar = function(key)
{
$scope.productosSelecc.splice(key, 1)
localStorage.setItem( 'productosSeleccionados', JSON.stringify($scope.productosSelecc) )
}
$scope.countItems = function()
{
let valDinero = 0;
angular.forEach($scope.productosSelecc, function(value, key){
valDinero = valDinero + Number(value.precio);
});
let cantidad = `$${Number(valDinero)}`
return cantidad;
}
let token = localStorage.getItem( 'token')
let config = {'x-token': token}
var req = {
method: 'POST',
url: 'https://rolimapp.com:3000/planes',
headers: config,
data: {transaccion: 'consultarPlanes'}
}
$scope.comprar = function()
{
$http(req).then(function(respuesta){
console.log(respuesta)
}, function(errorResponse){
console.log(errorResponse);
});
}
});
<file_sep>/README.md
# BGCarritoOld
Pruebas de BG
| ae5617aefc9de52cc9410ac8a7fbdfc9ddfc70db | [
"JavaScript",
"Markdown"
] | 2 | JavaScript | leonelvelez314/BGCarritoOld | 1c96a90ff2ec6cb2abebfc93eda810ab7c649a2d | 28c9a6380b1d4950d7706822dfc38fb185638e02 | |
refs/heads/master | <repo_name>blythe104/looking<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/main/fragment/MusicFragment.java
package com.looking.classicalparty.moudles.main.fragment;
import android.graphics.Color;
import android.os.AsyncTask;
import android.os.Bundle;
import android.util.Log;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageButton;
import android.widget.LinearLayout;
import android.widget.ListView;
import android.widget.TextView;
import com.google.gson.Gson;
import com.handmark.pulltorefresh.library.PullToRefreshBase;
import com.handmark.pulltorefresh.library.PullToRefreshListView;
import com.looking.classicalparty.R;
import com.looking.classicalparty.lib.base.fragment.BaseFragment;
import com.looking.classicalparty.lib.constants.ConstantApi;
import com.looking.classicalparty.lib.constants.StringContants;
import com.looking.classicalparty.lib.http.HttpUtils;
import com.looking.classicalparty.lib.http.Param;
import com.looking.classicalparty.lib.http.ResultCallback;
import com.looking.classicalparty.lib.utils.SharedPreUtils;
import com.looking.classicalparty.moudles.music.adapter.MusicAdapter;
import com.looking.classicalparty.moudles.music.bean.MusicDetailBean;
import com.looking.classicalparty.moudles.music.dialog.MusicDetailDialog;
import com.looking.classicalparty.moudles.music.dialog.MusicDialog;
import com.squareup.okhttp.Request;
import java.util.ArrayList;
import java.util.List;
/**
* Created by xin on 2016/10/19.
*/
public class MusicFragment extends BaseFragment implements View.OnClickListener {
private ListView lv_music;
private List<MusicDetailBean.ActivityEntity> musicDatas;
private MusicAdapter musicAdapter;
private int curPage = 1;
private View loadMoreFoot;
private LinearLayout loadMore;
private TextView mTvDesc;
private int totalPage;
private ImageButton btnListener;
private TextView music_name;
private PullToRefreshListView plistview_music;
@Override
public View initView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.music_fragment_layout, null);
lv_music = (ListView) view.findViewById(R.id.listview_music);
plistview_music = (PullToRefreshListView) view.findViewById(R.id.plistview_music);
music_name = (TextView) view.findViewById(R.id.music_name);
btnListener = (ImageButton) view.findViewById(R.id.btn_listener);
loadMoreFoot = View.inflate(getActivity(), R.layout.ll_foot_layout, null);
loadMore = (LinearLayout) loadMoreFoot.findViewById(R.id.ll_load_more);
mTvDesc = (TextView) loadMoreFoot.findViewById(R.id.tv_desc);
lv_music.addFooterView(loadMoreFoot);
musicDatas = new ArrayList<>();
musicAdapter = new MusicAdapter(getActivity(), musicDatas);
lv_music.setAdapter(musicAdapter);
plistview_music.setAdapter(musicAdapter);
plistview_music.setOnRefreshListener(new PullToRefreshBase.OnRefreshListener2<ListView>() {
@Override
public void onPullDownToRefresh(PullToRefreshBase<ListView> pullToRefreshBase) {
musicDatas.clear();
getMusicDatas(1);
new FinishRefresh().execute();
}
@Override
public void onPullUpToRefresh(PullToRefreshBase<ListView> pullToRefreshBase) {
if (curPage < totalPage) {
getMusicDatas(++curPage);
}
musicAdapter.notifyDataSetChanged();
new FinishRefresh().execute();
}
});
loadMore.setOnClickListener(this);
musicAdapter.setMusicListener(new MusicAdapter.MusicListener() {
@Override
public void showListenerDialog(int position) {
musicDialog("http://www.jingdian.party/" + musicDatas.get(position).getCover_path(), "http://www" +
"" + ".jingdian.party/" + musicDatas.get(position).getV_path(), musicDatas.get(position)
.getTitle(), musicDatas.get(position).getDirector());
}
@Override
public void showMusicDetail(int position) {
musicDetail(musicDatas.get(position).getTitle(),musicDatas.get(position).getDirector(),musicDatas.get(position).getSummary());
}
});
return view;
}
private void musicDetail(String title,String singer,String summary) {
MusicDetailDialog musicDetailDialog = new MusicDetailDialog(getActivity());
musicDetailDialog.show();
musicDetailDialog.initMusicData(title,singer,summary);
}
private void musicDialog(String imgPath, String path, String title, String singer) {
MusicDialog musicDialog = new MusicDialog(getActivity());
musicDialog.show();
musicDialog.initMusicData(imgPath, path, title, singer);
}
@Override
public void loadData() {
super.loadData();
//首次加载第一页的数据
getMusicDatas(1);
}
/**
* 获取音乐列表数据
*/
private void getMusicDatas(int curPages) {
List<Param> paramList = new ArrayList<>();
Param key = new Param("key", SharedPreUtils.getString(StringContants.KEY));
Param page = new Param("page", curPages + "");
Param pageCount = new Param("pageCount", 10 + "");
paramList.add(key);
paramList.add(page);
paramList.add(pageCount);
HttpUtils.post(ConstantApi.MUSIC, paramList, new ResultCallback() {
@Override
public void onSuccess(Object response) {
MusicDetailBean musicDetailBean = new Gson().fromJson(response.toString(), MusicDetailBean.class);
Log.d("musicDetail---", musicDetailBean.toString());
if (musicDetailBean.getResult() == 200) {
musicDatas.addAll(musicDetailBean.getActivity());
}
totalPage = musicDetailBean.getTotalPage();
curPage = Integer.parseInt(musicDetailBean.getCurrPage());
musicAdapter.notifyDataSetChanged();
plistview_music.onRefreshComplete();
}
@Override
public void onFailure(Request request, Exception e) {
}
@Override
public void onNoNetWork(String resultMsg) {
}
});
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.ll_load_more:
curPage++;
if (curPage <= totalPage) {
getMusicDatas(curPage);
} else {
mTvDesc.setText("已加载全部");
mTvDesc.setTextColor(Color.parseColor("#8c909d"));
}
break;
}
}
private class FinishRefresh extends AsyncTask<Void, Void, Void> {
@Override
protected Void doInBackground(Void... params) {
return null;
}
@Override
protected void onPreExecute() {
plistview_music.onRefreshComplete();
super.onPreExecute();
}
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/main/fragment/MovieFragment.java
package com.looking.classicalparty.moudles.main.fragment;
import android.os.Bundle;
import android.support.design.widget.TabLayout;
import android.support.v4.view.ViewPager;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import com.google.gson.Gson;
import com.looking.classicalparty.R;
import com.looking.classicalparty.lib.base.fragment.BaseFragment;
import com.looking.classicalparty.lib.constants.ConstantApi;
import com.looking.classicalparty.lib.constants.StringContants;
import com.looking.classicalparty.lib.http.HttpUtils;
import com.looking.classicalparty.lib.http.Param;
import com.looking.classicalparty.lib.http.ResultCallback;
import com.looking.classicalparty.lib.utils.SharedPreUtils;
import com.looking.classicalparty.moudles.movie.adapter.SimpleFragmentPagerAdapter;
import com.looking.classicalparty.moudles.movie.bean.CategoryBean;
import com.squareup.okhttp.Request;
import java.util.ArrayList;
import java.util.List;
/**
* Created by xin on 2016/10/19.
*/
public class MovieFragment extends BaseFragment implements TabLayout.OnTabSelectedListener {
List<CategoryBean.CategoryEntity> categoryBeen;
private TabLayout mTab;
private ViewPager mViewPager;
private SimpleFragmentPagerAdapter fragmentPagerAdapter;
@Override
public View initView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.movie_fragment_layout, null);
mTab = (TabLayout) view.findViewById(R.id.tab);
mViewPager = (ViewPager) view.findViewById(R.id.viewpager);
categoryBeen = new ArrayList<>();
fragmentPagerAdapter = new SimpleFragmentPagerAdapter(getFragmentManager(), getActivity(), categoryBeen);
mViewPager.setAdapter(fragmentPagerAdapter);
mTab.setOnTabSelectedListener(this);
mTab.setupWithViewPager(mViewPager);
return view;
}
@Override
protected void loadData() {
super.loadData();
getCategory();
}
@Override
public void onTabSelected(TabLayout.Tab tab) {
//设置viewpager与tab的位置同步变化
mViewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(TabLayout.Tab tab) {
}
@Override
public void onTabReselected(TabLayout.Tab tab) {
}
/**
* 获取类别列表
*/
private void getCategory() {
List<Param> paramList = new ArrayList<>();
Param key = new Param("key", SharedPreUtils.getString(StringContants.KEY));
paramList.add(key);
HttpUtils.post(ConstantApi.CATEGORY, paramList, new ResultCallback() {
@Override
public void onSuccess(Object response) {
CategoryBean categoryBean = new Gson().fromJson(response.toString(), CategoryBean.class);
if (categoryBean.getResult() == 200) {
categoryBeen.addAll(categoryBean.getCategory());
}
fragmentPagerAdapter.notifyDataSetChanged();
}
@Override
public void onFailure(Request request, Exception e) {
}
@Override
public void onNoNetWork(String resultMsg) {
}
});
}
}
<file_sep>/app/build.gradle
apply plugin: 'com.android.application'
android {
useLibrary 'org.apache.http.legacy'
compileSdkVersion 'Google Inc.:Google APIs:24'
buildToolsVersion '25.0.0'
defaultConfig {
applicationId "com.looking.classicalparty"
minSdkVersion 18
targetSdkVersion 23
versionCode 1
versionName "1.0"
jackOptions {
enabled true
}
ndk {
// 设置支持的 SO 库构架,一般而言,取你所有的库支持的构架的`交集`。
abiFilters 'armeabi' // 'armeabi-v7a', 'arm64-v8a', 'x86', 'x86_64', 'mips', 'mips64'
}
}
//应用 Bugtags 插件
apply plugin: 'com.bugtags.library.plugin'
bugtags {
appKey "<KEY>" //这里是你的 appKey
appSecret "d7ef6550ecafbe1a5e943dada811aeb6" //这里是你的 appSecret,管理员在设置页可以查看
mappingUploadEnabled true
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
signingConfigs {
debugConfig {
keyAlias 'classical'
keyPassword '<PASSWORD>' //System.console().readLine(" :input keyPassword : ")
storeFile file('../classical.jks')
storePassword '<PASSWORD>' //System.console().readLine(" :input storePassword : ");
}
releaseConfig {
keyAlias 'classical'
keyPassword '<PASSWORD>' //System.console().readLine(" :input keyPassword : ")
storeFile file('../classical.jks')
storePassword '<PASSWORD>' //System.console().readLine(" :input storePassword : ");
}
}
//修改生成的apk名字
applicationVariants.all { variant ->
variant.outputs.each { output ->
def oldFile = output.outputFile
if (variant.buildType.name.equals('release')) {
def releaseApkName = 'classical-v' + defaultConfig.versionName + '-' + productFlavors.name + '.apk'
output.outputFile = new File(oldFile.parent, releaseApkName)
} else if (variant.buildType.name.equals('debug')) {
// def releaseApkName = getDebugApkName()
// output.outputFile = new File(oldFile.parent, releaseApkName)
}
}
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
resValue("string", "app_name", "classical")
// buildConfigField "String", "BASE_URL", "http://www.jingdian.party/api/"
}
//修改生成的apk名字
applicationVariants.all { variant ->
variant.outputs.each { output ->
def oldFile = output.outputFile
if (variant.buildType.name.equals('release')) {
def releaseApkName = 'classical-v' + defaultConfig.versionName + '-' + productFlavors.name + '.apk'
output.outputFile = new File(oldFile.parent, releaseApkName)
} else if (variant.buildType.name.equals('debug')) {
def releaseApkName = getDebugApkName()
output.outputFile = new File(oldFile.parent, releaseApkName)
}
}
}
debug {
minifyEnabled false
resValue("string", "app_name", "classical")
// buildConfigField "String", "BASE_URL", "http://www.jingdian.party/api/"
signingConfig signingConfigs.debugConfig
}
}
}
def releaseTime() {
new Date().format("yyyy-MM-dd", TimeZone.getTimeZone("UTC"));
}
def getDebugApkName() {
'classicalParty-v' + android.defaultConfig.versionName + '-' + "(" + releaseTime() + ")" + '-' + '01' + '.apk'
}
dependencies {
compile fileTree(include: ['*.jar'], dir: 'libs')
compile('de.keyboardsurfer.android.widget:crouton:1.8.4@aar') {
exclude group: 'com.google.android', module: 'support-v4'
}
//时间选择控件
//二维码生成
compile files('libs/zxing.jar')
//banner依赖库
compile 'com.squareup.okhttp:okhttp:2.7.0'
compile 'com.google.code.gson:gson:2.6.2'
compile 'com.android.support:design:25.1.0'
compile 'com.github.flavienlaurent.datetimepicker:library:0.0.2'
compile 'com.youth.banner:banner:1.4.9'
compile 'com.github.bumptech.glide:glide:3.7.0'
compile 'com.github.tgioihan:imageloader:1.0.3'
compile 'com.bugtags.library:bugtags-lib:latest.integration'
compile 'me.wcy:lrcview:1.4.1'
compile 'com.android.support:appcompat-v7:25.1.0'
compile 'com.github.userswlwork:pull-to-refresh:1.0.0'
compile 'com.android.support.constraint:constraint-layout:1.0.2'
//日历控件
compile 'com.joybar.calendar:librarycalendar:1.0.5'
testCompile 'junit:junit:4.12'
}
//配置lambda表达式
buildscript {
repositories {
mavenCentral()
}
dependencies {
classpath 'me.tatarka:gradle-retrolambda:3.2.5'
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/login/observer/SubjectListener.java
package com.looking.classicalparty.moudles.login.observer;
/**
* Created by xin on 2017/4/12.
*/
public interface SubjectListener {
void add(ObserverListener observerListener);
void notifyObserver(int status);
void remove(ObserverListener observerListener);
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/mine/dialog/ChooiseDateDialog.java
package com.looking.classicalparty.moudles.mine.dialog;
import android.app.Dialog;
import android.content.Context;
import android.os.Bundle;
import android.widget.DatePicker;
import com.looking.classicalparty.R;
import java.util.Calendar;
/**
* Created by xin on 2016/10/28.
*/
public class ChooiseDateDialog extends Dialog {
private DatePicker datePicker;
private Calendar calendar;
private int year;
private int month;
private int day;
private onDateSetListener listener;
public ChooiseDateDialog(Context context) {
this(context, R.style.base_dialog_theme);
}
public ChooiseDateDialog(Context context, int themeResId) {
super(context, themeResId);
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.chooise_date_layout);
datePicker = (DatePicker) findViewById(R.id.datePicker);
calendar = Calendar.getInstance();
year = calendar.get(Calendar.YEAR);
month = calendar.get(Calendar.MONTH) + 1;
day = calendar.get(Calendar.DAY_OF_MONTH);
}
public void setDateListener(onDateSetListener listener){
this.listener=listener;
}
public interface onDateSetListener{
void setDate(int year,int month,int day);
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/movie/fragment/PageFragment.java
package com.looking.classicalparty.moudles.movie.fragment;
import android.content.Intent;
import android.graphics.Color;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.AdapterView;
import android.widget.LinearLayout;
import android.widget.ListView;
import android.widget.TextView;
import com.google.gson.Gson;
import com.looking.classicalparty.R;
import com.looking.classicalparty.lib.base.fragment.BaseFragment;
import com.looking.classicalparty.lib.constants.ConstantApi;
import com.looking.classicalparty.lib.constants.StringContants;
import com.looking.classicalparty.lib.http.HttpUtils;
import com.looking.classicalparty.lib.http.Param;
import com.looking.classicalparty.lib.http.ResultCallback;
import com.looking.classicalparty.lib.utils.SharedPreUtils;
import com.looking.classicalparty.moudles.movie.adapter.VideoAdapter;
import com.looking.classicalparty.moudles.movie.bean.GetVideoBean;
import com.looking.classicalparty.moudles.movie.view.MovieDetailActivity;
import com.squareup.okhttp.Request;
import java.util.ArrayList;
import java.util.List;
public class PageFragment extends BaseFragment implements View.OnClickListener {
public static final String ARG_PAGE = "ARG_PAGE";
List<GetVideoBean.ActivityEntity> videoDatas;
int curPage = 1;
private String mVid;
private ListView llVideo;
private int totalPage;
private VideoAdapter videoAdapter;
private View loadMoreFoot;
private LinearLayout loadMore;
private TextView mTvDesc;
public static PageFragment newInstance(int page, String vid) {
Bundle args = new Bundle();
args.putString(ARG_PAGE, vid);
PageFragment pageFragment = new PageFragment();
pageFragment.setArguments(args);
return pageFragment;
}
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
mVid = getArguments().getString(ARG_PAGE);
}
@Override
protected View initView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_page, container, false);
llVideo = (ListView) view.findViewById(R.id.ll_video);
loadMoreFoot = View.inflate(getActivity(), R.layout.ll_foot_layout, null);
loadMore = (LinearLayout) loadMoreFoot.findViewById(R.id.ll_load_more);
mTvDesc = (TextView) loadMoreFoot.findViewById(R.id.tv_desc);
llVideo.addFooterView(loadMoreFoot);
videoDatas = new ArrayList<>();
videoAdapter = new VideoAdapter(getActivity(), videoDatas);
llVideo.setAdapter(videoAdapter);
loadMore.setOnClickListener(this);
llVideo.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent detailIntent = new Intent(getActivity(), MovieDetailActivity.class);
detailIntent.putExtra("id", videoDatas.get(position).getId());
startActivity(detailIntent);
}
});
return view;
}
@Override
protected void loadData() {
super.loadData();
getVideoDatas(curPage, mVid);
}
/**
* 获取音乐列表数据
*/
private void getVideoDatas(int curPage, String vid) {
List<Param> paramList = new ArrayList<>();
Param key = new Param("key", SharedPreUtils.getString(StringContants.KEY));
Param page = new Param("page", curPage + "");
Param videoid = new Param("cate_id", vid);
Param pageCount = new Param("pageCount", 10 + "");
paramList.add(key);
paramList.add(page);
paramList.add(pageCount);
paramList.add(videoid);
HttpUtils.post(ConstantApi.VIDEO, paramList, new ResultCallback() {
@Override
public void onSuccess(Object response) {
GetVideoBean getVideoBean = new Gson().fromJson(response.toString(), GetVideoBean.class);
if (getVideoBean.getResult() == 200) {
videoDatas.addAll(getVideoBean.getActivity());
}
totalPage = getVideoBean.getTotalPage();
videoAdapter.notifyDataSetChanged();
}
@Override
public void onFailure(Request request, Exception e) {
}
@Override
public void onNoNetWork(String resultMsg) {
}
});
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.ll_load_more:
curPage++;
if (curPage <= totalPage) {
getVideoDatas(curPage, mVid);
} else {
mTvDesc.setText("已加载全部");
mTvDesc.setTextColor(Color.parseColor("#8c909d"));
}
break;
}
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/login/bean/UserBean.java
package com.looking.classicalparty.moudles.login.bean;
import com.looking.classicalparty.lib.base.Bean.BaseBean;
/**
* Created by xin on 2016/10/19.
*/
public class UserBean extends BaseBean {
/**
* token :
* user :
*/
private String token;
/**
* mid : 11
* musername : blythe
*/
private UserEntity user;
public String getToken() {
return token;
}
public void setToken(String token) {
this.token = token;
}
public UserEntity getUser() {
return user;
}
public void setUser(UserEntity user) {
this.user = user;
}
public static class UserEntity {
private String mid;
private String musername;
public String getMid() {
return mid;
}
public void setMid(String mid) {
this.mid = mid;
}
public String getMusername() {
return musername;
}
public void setMusername(String musername) {
this.musername = musername;
}
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/lib/ui/BaseUi.java
package com.looking.classicalparty.lib.ui;
import android.content.Context;
import android.util.AttributeSet;
import android.view.LayoutInflater;
import android.view.View;
import android.widget.LinearLayout;
import com.looking.classicalparty.lib.listener.IUIListener;
/**
* Created by chuangtou on 16/7/18.
* 国丞创投
*/
public abstract class BaseUi extends LinearLayout implements IUIListener {
protected LayoutInflater mInflater;
protected View rootView;
public BaseUi(Context context) {
super(context);
mInflater = LayoutInflater.from(getContext());
initView();
}
public BaseUi(Context context, AttributeSet attrs) {
super(context, attrs);
mInflater = LayoutInflater.from(getContext());
initView();
}
public BaseUi(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
mInflater = LayoutInflater.from(getContext());
initView();
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/lib/widget/CustomBaseInterface.java
package com.looking.classicalparty.lib.widget;
/**
* Created by xin on 2016/10/20.
*/
public interface CustomBaseInterface {
public void setMenuName(String menuName);
public void setIcon(int rid);
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/find/bean/ReviewsBean.java
package com.looking.classicalparty.moudles.find.bean;
/**
* Created by xin on 2017/3/29.
*/
public class ReviewsBean {
private String id;
private String title;
private String director;
private String cover_path;
private String summary;
private String vscreate_time;
private String musername;
private String url;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getDirector() {
return director;
}
public void setDirector(String director) {
this.director = director;
}
public String getCover_path() {
return cover_path;
}
public void setCover_path(String cover_path) {
this.cover_path = cover_path;
}
public String getSummary() {
return summary;
}
public void setSummary(String summary) {
this.summary = summary;
}
public String getVscreate_time() {
return vscreate_time;
}
public void setVscreate_time(String vscreate_time) {
this.vscreate_time = vscreate_time;
}
public String getMusername() {
return musername;
}
public void setMusername(String musername) {
this.musername = musername;
}
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/mine/dialog/ChooiseGenderDialog.java
package com.looking.classicalparty.moudles.mine.dialog;
import android.app.Dialog;
import android.content.Context;
import android.os.Bundle;
import com.looking.classicalparty.R;
/**
* Created by xin on 2016/10/28.
*/
public class ChooiseGenderDialog extends Dialog {
public ChooiseGenderDialog(Context context) {
this(context, R.style.base_dialog_theme);
}
public ChooiseGenderDialog(Context context, int themeResId) {
super(context, themeResId);
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.change_gender_layout);
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/movie/adapter/VideoAdapter.java
package com.looking.classicalparty.moudles.movie.adapter;
import android.content.Context;
import android.text.TextUtils;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import com.looking.classicalparty.R;
import com.looking.classicalparty.lib.utils.ImageLoaderUtils;
import com.looking.classicalparty.moudles.movie.bean.GetVideoBean;
import java.util.List;
/**
* Created by xin on 2017/3/29.
*/
public class VideoAdapter extends BaseAdapter {
private Context context;
private List<GetVideoBean.ActivityEntity> musicDatas;
public VideoAdapter(Context context, List<GetVideoBean.ActivityEntity> musicDatas) {
this.context = context;
this.musicDatas = musicDatas;
}
@Override
public int getCount() {
if (null == musicDatas) {
return 0;
}
return musicDatas.size();
}
@Override
public GetVideoBean.ActivityEntity getItem(int position) {
return musicDatas.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder mViewHolder = null;
if (null == convertView) {
mViewHolder = new ViewHolder();
convertView = View.inflate(context, R.layout.item_movie_layout, null);
mViewHolder.iv_movie = (ImageView) convertView.findViewById(R.id.iv_movie);
mViewHolder.movie = (TextView) convertView.findViewById(R.id.movie);
mViewHolder.score = (TextView) convertView.findViewById(R.id.score);
mViewHolder.director = (TextView) convertView.findViewById(R.id.director);
mViewHolder.actors = (TextView) convertView.findViewById(R.id.actors);
mViewHolder.musername = (TextView) convertView.findViewById(R.id.musername);
convertView.setTag(mViewHolder);
} else {
mViewHolder = (ViewHolder) convertView.getTag();
}
ImageLoaderUtils.display(context, "http://www.jingdian.party/" + musicDatas.get(position).getCover_path(),
mViewHolder.iv_movie);
mViewHolder.movie.setText(musicDatas.get(position).getTitle());
mViewHolder.director.setText("导演:" + musicDatas.get(position).getDirector());
mViewHolder.score.setText(musicDatas.get(position).getScores());
mViewHolder.actors.setText("演员:" + musicDatas.get(position).getActors());
String musername;
if (TextUtils.isEmpty(musicDatas.get(position).getMusername())) {
musername = "classical";
} else {
musername = musicDatas.get(position).getMusername();
}
mViewHolder.musername.setText("推荐者:" + musername);
return convertView;
}
static class ViewHolder {
public TextView movie;
public ImageView iv_movie;
public TextView score;
public TextView director;
public TextView actors;
public TextView musername;
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/mine/bean/VersionBean.java
package com.looking.classicalparty.moudles.mine.bean;
/**
* Created by xin on 2017/4/11.
*/
public class VersionBean {
/**
* versionName : 经典party-安卓版-1.0
* versionCode : 1.0
* versionRemark : 版本描述
* versionFile : 暂无
*/
private String versionName;
private String versionCode;
private String versionRemark;
private String versionFile;
public String getVersionName() {
return versionName;
}
public void setVersionName(String versionName) {
this.versionName = versionName;
}
public String getVersionCode() {
return versionCode;
}
public void setVersionCode(String versionCode) {
this.versionCode = versionCode;
}
public String getVersionRemark() {
return versionRemark;
}
public void setVersionRemark(String versionRemark) {
this.versionRemark = versionRemark;
}
public String getVersionFile() {
return versionFile;
}
public void setVersionFile(String versionFile) {
this.versionFile = versionFile;
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/lib/common/UserInfo.java
package com.looking.classicalparty.lib.common;
import android.content.Context;
import com.looking.classicalparty.lib.constants.StringContants;
import com.looking.classicalparty.lib.utils.SharedPreUtils;
/**
* Created by xin on 2016/10/24.
*/
public class UserInfo {
String token = "";
private Context context;
public UserInfo() {
token = SharedPreUtils.getString(StringContants.TOKEN,"");
}
public void clear() {
SharedPreUtils.saveString(StringContants.TOKEN, "");
}
public boolean isLogin() {
if (null == token) {
return false;
}
if ("".equals(token)) {
return false;
}
if (token.isEmpty()) {
return false;
}
return true;
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/register/view/RegisterActivity.java
package com.looking.classicalparty.moudles.register.view;
import android.content.Intent;
import android.text.TextUtils;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import com.google.gson.Gson;
import com.looking.classicalparty.R;
import com.looking.classicalparty.lib.base.Bean.BaseBean;
import com.looking.classicalparty.lib.base.activity.BaseActivity;
import com.looking.classicalparty.lib.constants.ConstantApi;
import com.looking.classicalparty.lib.constants.StringContants;
import com.looking.classicalparty.lib.http.HttpUtils;
import com.looking.classicalparty.lib.http.Param;
import com.looking.classicalparty.lib.http.ResultCallback;
import com.looking.classicalparty.lib.ui.TitleBar;
import com.looking.classicalparty.lib.utils.SharedPreUtils;
import com.looking.classicalparty.moudles.login.view.LoginActivity;
import com.looking.classicalparty.moudles.register.bean.RegisterBean;
import com.squareup.okhttp.Request;
import java.util.ArrayList;
import java.util.List;
import de.keyboardsurfer.android.widget.crouton.Crouton;
import de.keyboardsurfer.android.widget.crouton.Style;
public class RegisterActivity extends BaseActivity {
private Button btn_Register;
private EditText mEtUserName;
private EditText mEtPassword;
private TitleBar mTitle;
@Override
public void initView() {
setContentView(R.layout.activity_register);
mTitle = (TitleBar) findViewById(R.id.title_bar);
btn_Register = (Button) findViewById(R.id.btn_register);
mEtUserName = (EditText) findViewById(R.id.username);
mEtPassword = (EditText) findViewById(R.id.password);
btn_Register.setOnClickListener(this);
}
@Override
public void initListener() {
mTitle.setOnClickListener(new TitleBar.OnClickListener() {
@Override
public void OnLeftClick() {
finish();
}
@Override
public void OnRightClick() {
}
});
}
@Override
public void initData() {
mTitle.setTitle("注册");
}
/**
* 检测用户名是否可用
*
* @param username
*/
public void checkUser(String username, String pwd) {
List<Param> paramList = new ArrayList<>();
Param key = new Param("key", SharedPreUtils.getString(StringContants.KEY, ""));
Param musername = new Param("musername", username);
paramList.add(key);
paramList.add(musername);
HttpUtils.post(ConstantApi.checkUser, paramList, new ResultCallback() {
@Override
public void onSuccess(Object response) {
BaseBean baseBean = new Gson().fromJson(response.toString(), BaseBean.class);
Log.d("check----", baseBean.toString());
if (baseBean.getResult() == 200) {
//调用注册接口
userRegister(username, pwd);
} else {
//该用户名存在
Toast.makeText(RegisterActivity.this, "该用户名已经存在", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onFailure(Request request, Exception e) {
}
@Override
public void onNoNetWork(String resultMsg) {
}
});
}
/**
* 注册接口
*
* @param username
* @param pwd
*/
private void userRegister(String username, String pwd) {
List<Param> paramlist = new ArrayList<>();
Param key = new Param("key", SharedPreUtils.getString(StringContants.KEY));
Param name = new Param("musername", username);
Param password = new Param("mpassword", pwd);
Param mmobile = new Param("mmobile", "");
paramlist.add(mmobile);
paramlist.add(name);
paramlist.add(key);
paramlist.add(password);
HttpUtils.post(ConstantApi.register, paramlist, new ResultCallback() {
@Override
public void onSuccess(Object response) {
Log.d("register---", response.toString());
RegisterBean registerBean = new Gson().fromJson(response.toString(), RegisterBean.class);
if (registerBean.getResult() == 200) {
Toast.makeText(RegisterActivity.this, "注册成功,请登录", Toast.LENGTH_SHORT).show();
startActivity(new Intent(RegisterActivity.this, LoginActivity.class));
} else {
Toast.makeText(RegisterActivity.this, "注册失败", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onFailure(Request request, Exception e) {
}
@Override
public void onNoNetWork(String resultMsg) {
}
});
}
@Override
public void processClick(View v) {
switch (v.getId()) {
case R.id.btn_register:
//检测用户名是否可用
String username = mEtUserName.getText().toString().trim();
String password = <PASSWORD>().<PASSWORD>();
if (!TextUtils.isEmpty(username)) {
if (TextUtils.isEmpty(password)) {
Crouton.makeText(RegisterActivity.this, "密码不能为空", Style.CONFIRM).show();
} else {
checkUser(username, password);
}
} else {
Crouton.makeText(RegisterActivity.this, "用户名不能为空", Style.CONFIRM).show();
}
break;
}
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/mine/view/PersonalActivity.java
package com.looking.classicalparty.moudles.mine.view;
import android.Manifest;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.graphics.Bitmap;
import android.hardware.Camera;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.provider.MediaStore;
import android.support.annotation.NonNull;
import android.support.v4.app.ActivityCompat;
import android.support.v4.app.Fragment;
import android.support.v4.app.FragmentManager;
import android.support.v4.app.FragmentTransaction;
import android.support.v4.content.ContextCompat;
import android.util.Log;
import android.view.View;
import android.widget.EditText;
import android.widget.FrameLayout;
import android.widget.LinearLayout;
import android.widget.TextView;
import android.widget.Toast;
import com.fourmob.datetimepicker.date.DatePickerDialog;
import com.google.gson.Gson;
import com.joybar.librarycalendar.data.CalendarDate;
import com.joybar.librarycalendar.fragment.CalendarViewFragment;
import com.joybar.librarycalendar.fragment.CalendarViewPagerFragment;
import com.looking.classicalparty.R;
import com.looking.classicalparty.lib.base.activity.BaseActivity;
import com.looking.classicalparty.lib.constants.ConstantApi;
import com.looking.classicalparty.lib.constants.StringContants;
import com.looking.classicalparty.lib.http.HttpUtils;
import com.looking.classicalparty.lib.http.Param;
import com.looking.classicalparty.lib.http.ResultCallback;
import com.looking.classicalparty.lib.utils.FileSizeUtil;
import com.looking.classicalparty.lib.utils.GetPathUri4kitk;
import com.looking.classicalparty.lib.utils.ImageLoaderUtils;
import com.looking.classicalparty.lib.utils.LogUtils;
import com.looking.classicalparty.lib.utils.SDCardUtils;
import com.looking.classicalparty.lib.utils.SharedPreUtils;
import com.looking.classicalparty.lib.widget.CircleImageView;
import com.looking.classicalparty.moudles.mine.PersonBean;
import com.looking.classicalparty.moudles.mine.bean.MemberBean;
import com.looking.classicalparty.moudles.mine.bean.UploadAvaBean;
import com.looking.classicalparty.moudles.mine.dialog.ChooiseDateDialog;
import com.looking.classicalparty.moudles.mine.dialog.ChooiseGenderDialog;
import com.looking.classicalparty.moudles.mine.dialog.ChooisePhotoDialog;
import com.looking.classicalparty.moudles.mine.dialog.NicknameDialog;
import com.looking.classicalparty.moudles.mine.observer.ConcreteSubject;
import com.looking.classicalparty.moudles.mine.observer.Observer;
import com.squareup.okhttp.Request;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.List;
import de.keyboardsurfer.android.widget.crouton.Crouton;
import de.keyboardsurfer.android.widget.crouton.Style;
public class PersonalActivity extends BaseActivity implements CalendarViewPagerFragment.OnPageChangeListener,
CalendarViewFragment.OnDateClickListener, CalendarViewFragment.OnDateCancelListener {
private static final int CAMERA_REQUEST_CODE = 1001;
private static final int PHOTO_REQUEST_CODE = 1002;
private static final int CROPPHOTO = 1003;
private static String path = "/sdcard/myHead/";
private FrameLayout mFrBack;
private TextView mTvTitle;
private CircleImageView circleImageView;
private LinearLayout mLlChangePhoto;
private ChooisePhotoDialog chooisePhotoDialog;
private DatePickerDialog datePickerDialog;
private ChooiseGenderDialog genderDialog;
private ChangeDescObserver changeDescObserver;
private NicknameDialog nicknameDialog;
private EditText etNickName;
private EditText etSexy;
private EditText etSign;
private TextView tvBirthday;
private PersonBean.User user;
private TextView updatePerson;
private ChooiseDateDialog chooiseDateDialog;
private boolean isChoiceModelSingle = true;
private List<CalendarDate> mListDate = new ArrayList<>();
private File updatefile;
private File file;
private File filepath;
private static String listToString(List<CalendarDate> list) {
StringBuffer stringBuffer = new StringBuffer();
for (CalendarDate date : list) {
stringBuffer.append(date.getSolar().solarYear + "-" + date.getSolar().solarMonth + "-" + date.getSolar()
.solarDay).append(" ");
}
return stringBuffer.toString();
}
@Override
public void initView() {
setContentView(R.layout.activity_person);
initTitle();
// initFragment();
user = (PersonBean.User) getIntent().getSerializableExtra("user");
final Calendar calendar = Calendar.getInstance();
etNickName = (EditText) findViewById(R.id.et_nickname);
etSexy = (EditText) findViewById(R.id.et_sexy);
etSign = (EditText) findViewById(R.id.et_sign);
tvBirthday = (TextView) findViewById(R.id.tv_birthday);
updatePerson = (TextView) findViewById(R.id.tv_update_person);
genderDialog = new ChooiseGenderDialog(this);
nicknameDialog = new NicknameDialog(this);
changeDescObserver = new ChangeDescObserver();
ConcreteSubject.getInstance().register(changeDescObserver);
updatePerson.setOnClickListener(this);
tvBirthday.setOnClickListener(this);
datePickerDialog = DatePickerDialog.newInstance((ddg, year, month, day) -> {
}, //
calendar.get(Calendar.YEAR), calendar.get(Calendar.MONTH), calendar.get(Calendar.DAY_OF_MONTH));
chooisePhotoDialog.setPhotoListener(new ChooisePhotoDialog.PhotoListener() {
@Override
public void takePhoto() {
requstPermission(CAMERA_REQUEST_CODE);
}
@Override
public void chooisePhoto() {
requstPermission(PHOTO_REQUEST_CODE);
}
});
}
private void nicknameDialog() {
nicknameDialog.show();
}
@Override
protected void onDestroy() {
ConcreteSubject.getInstance().unRegister(changeDescObserver);
super.onDestroy();
}
private void loadAvatarImg(String res) {
ImageLoaderUtils.display(getApplication(), res, R.mipmap.mine_two, circleImageView);
}
/**
* 提交用户更新信息
*/
private void updatePersonInfo() {
List<Param> paramList = new ArrayList<>();
Param key = new Param("key", SharedPreUtils.getString(StringContants.KEY));
Param token = new Param("Token", SharedPreUtils.getString(StringContants.TOKEN));
Param nickname = new Param("mnickname", etNickName.getText().toString().trim());
Param birthday = new Param("mbirthday", tvBirthday.getText().toString().trim());
Param gender = new Param("mgender", etSexy.getText().toString().trim());
Param sign = new Param("msignature", etSign.getText().toString().trim());
paramList.add(key);
paramList.add(token);
paramList.add(nickname);
paramList.add(birthday);
paramList.add(gender);
paramList.add(sign);
HttpUtils.post(ConstantApi.UPDATEINFO, paramList, new ResultCallback() {
@Override
public void onSuccess(Object response) {
MemberBean memberBean = new Gson().fromJson(response.toString(), MemberBean.class);
if (200 == memberBean.getResult()) {
Toast.makeText(PersonalActivity.this, "更新个人信息成功", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(PersonalActivity.this, "信息更新失败", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onFailure(Request request, Exception e) {
}
@Override
public void onNoNetWork(String resultMsg) {
}
});
}
/**
* 选择性别
*/
private void chooiseGender() {
genderDialog.show();
}
/**
* 选择出生日期
*/
private void chooiseBirthday() {
datePickerDialog.setYearRange(1985, 2028);
}
private void requstPermission(int code) {
switch (code) {
case 1001:
//没有相机权限
if (PackageManager.PERMISSION_GRANTED != ContextCompat.checkSelfPermission(this, android.Manifest
.permission.CAMERA)) {
if (ActivityCompat.shouldShowRequestPermissionRationale(this, Manifest.permission.CAMERA)) {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle(R.string.app_name).setMessage("申请相机权限").setPositiveButton("确定", (dialog, v)
-> {
//申请相机权限
ActivityCompat.requestPermissions(PersonalActivity.this, new String[]{Manifest.permission
.CAMERA}, CAMERA_REQUEST_CODE);
// 跳转到系统的界面
Intent intent = new Intent();
intent.setAction("android.intent.action.MAIN");
intent.setClassName("com.android.settings", "com.android.settings.ManageApplications");
startActivity(intent);
}).setNegativeButton("暂不申请", null).show();
} else {
//申请相机权限
Crouton.makeText(PersonalActivity.this, "没有相机权限", Style.CONFIRM).show();
ActivityCompat.requestPermissions(PersonalActivity.this, new String[]{Manifest.permission
.CAMERA}, CAMERA_REQUEST_CODE);
}
} else {
//调用相机拍照
// Toast.makeText(this, "相机权限已经开启", Toast.LENGTH_SHORT).show();
if (isCameraCanUse()) {
Intent camareIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
camareIntent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(new File(Environment
.getExternalStorageDirectory(), "head.jpg")));
startActivityForResult(camareIntent, CAMERA_REQUEST_CODE);
}
}
break;
case 1002:
if (PackageManager.PERMISSION_GRANTED == ContextCompat.checkSelfPermission(this, Manifest.permission
.WRITE_EXTERNAL_STORAGE)) {
Intent choiceIntent = new Intent(Intent.ACTION_PICK, null);
choiceIntent.setDataAndType(MediaStore.Images.Media.EXTERNAL_CONTENT_URI, "image/*");
startActivityForResult(choiceIntent, PHOTO_REQUEST_CODE);
} else {
AlertDialog.Builder builder = new AlertDialog.Builder(PersonalActivity.this);
builder.setTitle(R.string.app_name).setMessage("当前无读写权限").setPositiveButton("去设置", new
DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// 跳转到系统的设置界面
Intent intent = new Intent();
intent.setAction("android.intent.action.MAIN");
intent.setClassName("com.android.settings", "com.android.settings.ManageApplications");
startActivity(intent);
}
}).setNegativeButton("暂不设置", null).show();
}
break;
}
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
switch (requestCode) {
case CAMERA_REQUEST_CODE:
if (SDCardUtils.isSDCardEnable()) {
updatefile = new File(Environment.getExternalStorageDirectory() + "/head.jpg");
//裁剪图片
cropPhoto(Uri.fromFile(updatefile));
LogUtils.d("updatefile----TAKEPHOTOCODE-", updatefile + "");
} else {
Toast.makeText(PersonalActivity.this, "未找到SD卡", Toast.LENGTH_SHORT).show();
}
break;
case CROPPHOTO:
Bundle extras = data.getExtras();
if (extras != null) {
Bitmap head = (Bitmap) extras.get("data");
LogUtils.d("--head-", head + "--");
if (head != null) {
setPicToView(head);//保存在SD卡中
// TODO: 2016/7/1 上传裁剪过后的图片
// mAvatarImg.setImageBitmap(head);
upUserHeader(filepath);
//用ImageView显示出来
if (chooisePhotoDialog != null) {
chooisePhotoDialog.dismiss();
}
}
}
break;
//在本地选择图片
case PHOTO_REQUEST_CODE:
if (null == data) {
return;
}
Uri uri = data.getData();
if (null != uri) {
//将图片发送到服务器
String ImgData = GetPathUri4kitk.getPath(PersonalActivity.this, uri);
double mFileSize = FileSizeUtil.getFileOrFilesSize(ImgData, 3);
LogUtils.d("---filesize-", mFileSize + "");
if (mFileSize > 5) {
Toast.makeText(PersonalActivity.this, "请选择5M以内的图片", Toast.LENGTH_SHORT).show();
} else {
cropPhoto(uri);//裁剪图片
}
}
break;
}
super.onActivityResult(requestCode, resultCode, data);
}
/**
* 更新头像
*/
// TODO: 2017/4/26 还需要修改,有问题,需要调试
private void upUserHeader(File updatefile) {
List<Param> paramList = new ArrayList<>();
Param key = new Param("key", SharedPreUtils.getString(StringContants.KEY));
Param token = new Param("Token", SharedPreUtils.getString(StringContants.TOKEN));
Param file = new Param("file", updatefile);
paramList.add(key);
paramList.add(token);
paramList.add(file);
HttpUtils.post(ConstantApi.UPLOADAVA, paramList, new ResultCallback() {
@Override
public void onSuccess(Object response) {
UploadAvaBean uploadAvaBean = new Gson().fromJson(response.toString(), UploadAvaBean.class);
Log.d("updateAva---", uploadAvaBean.toString());
if (uploadAvaBean.getResult() == 200) {
Toast.makeText(PersonalActivity.this, "头像上传成功", Toast.LENGTH_SHORT).show();
} else {
Toast.makeText(PersonalActivity.this, "头像上传失败", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onFailure(Request request, Exception e) {
}
@Override
public void onNoNetWork(String resultMsg) {
}
});
}
//将图片设置到视图
private void setPicToView(Bitmap mBitmap) {
String sdStatus = Environment.getExternalStorageState();
if (!sdStatus.equals(Environment.MEDIA_MOUNTED)) {
// 检测sd是否可用
return;
}
FileOutputStream b = null;
file = new File(path);
file.mkdirs();// 创建文件夹
String fileName = path + "head.jpg";//图片名字
filepath = new File(fileName);
try {
b = new FileOutputStream(filepath);
mBitmap.compress(Bitmap.CompressFormat.JPEG, 80, b);
// 把数据写入文件
} catch (FileNotFoundException e) {
e.printStackTrace();
} finally {
try {//关闭流
if (b != null) {
b.flush();
b.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
private void cropPhoto(Uri uri) {
Intent intent = new Intent("com.android.camera.action.CROP");
intent.setDataAndType(uri, "image/*");
intent.putExtra("crop", "true");
// aspectX aspectY 是宽高的比例
intent.putExtra("aspectX", 1);
intent.putExtra("aspectY", 1);
// outputX outputY 是裁剪图片宽高
intent.putExtra("outputX", 150);
intent.putExtra("outputY", 150);
intent.putExtra("return-data", true);
startActivityForResult(intent, CROPPHOTO);
}
/**
* 测试当前摄像头能否被使用
*
* @return
*/
public boolean isCameraCanUse() {
boolean canUse = true;
Camera mCamera = null;
try {
mCamera = Camera.open(0);
mCamera.setDisplayOrientation(90);
} catch (Exception e) {
canUse = false;
}
if (canUse) {
mCamera.release();
mCamera = null;
}
return canUse;
}
/**
* 出来权限申请成功或者失败的操作
*
* @param requestCode
* @param permissions
* @param grantResults
*/
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[]
grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
if (requestCode == CAMERA_REQUEST_CODE) {
if (grantResults[0] == PackageManager.PERMISSION_GRANTED) {
Intent camareIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
camareIntent.putExtra(MediaStore.EXTRA_OUTPUT, Uri.fromFile(new File(Environment
.getExternalStorageDirectory(), "head.jpg")));
startActivityForResult(camareIntent, CAMERA_REQUEST_CODE);
} else {
if (!ActivityCompat.shouldShowRequestPermissionRationale(this, Manifest.permission.CAMERA)) {
Crouton.makeText(this, "相机权限已经被禁止", Style.CONFIRM).show();
}
}
}
}
private void initTitle() {
mFrBack = (FrameLayout) findViewById(R.id.fr_back);
mTvTitle = (TextView) findViewById(R.id.tv_title);
chooisePhotoDialog = new ChooisePhotoDialog(this);
chooiseDateDialog = new ChooiseDateDialog(this);
circleImageView = (CircleImageView) findViewById(R.id.circle_image);
mLlChangePhoto = (LinearLayout) findViewById(R.id.ll_change_photo);
mTvTitle.setText("个人资料");
}
@Override
public void initListener() {
mFrBack.setOnClickListener(this);
mLlChangePhoto.setOnClickListener(this);
circleImageView.setOnClickListener(this);
}
@Override
public void initData() {
// getPersonDetail();
etNickName.setText(user.getMusername());
etSexy.setText(user.getMsex() == "1" ? "男" : "女");
etSign.setText(user.getMsignature());
loadAvatarImg(user.getMavatar());
}
@Override
public void processClick(View v) {
switch (v.getId()) {
case R.id.fr_back:
finish();
break;
case R.id.circle_image:
chooisePhotoDialog.show();
break;
case R.id.tv_update_person:
updatePersonInfo();
break;
case R.id.tv_birthday:
// isChoiceModelSingle = true;
// initFragment();
// chooiseDateDialog.show();
break;
}
}
private void initFragment() {
FragmentManager fm = getSupportFragmentManager();
FragmentTransaction tx = fm.beginTransaction();
Fragment fragment = CalendarViewPagerFragment.newInstance(isChoiceModelSingle);
tx.replace(R.id.fl_content, fragment);
tx.commit();
}
@Override
public void onDateCancel(CalendarDate calendarDate) {
int count = mListDate.size();
for (int i = 0; i < count; i++) {
CalendarDate date = mListDate.get(i);
if (date.getSolar().solarDay == calendarDate.getSolar().solarDay) {
mListDate.remove(i);
break;
}
}
tvBirthday.setText(listToString(mListDate));
}
@Override
public void onDateClick(CalendarDate calendarDate) {
int year = calendarDate.getSolar().solarYear;
int month = calendarDate.getSolar().solarMonth;
int day = calendarDate.getSolar().solarDay;
if (isChoiceModelSingle) {
tvBirthday.setText(year + "-" + month + "-" + day);
} else {
//System.out.println(calendarDate.getSolar().solarDay);
mListDate.add(calendarDate);
tvBirthday.setText(listToString(mListDate));
}
}
@Override
public void onPageChange(int year, int month) {
tvBirthday.setText(year + "-" + month);
mListDate.clear();
}
private class ChangeDescObserver implements Observer {
private String getDesc;
@Override
public void update(String desc) {
getDesc = desc;
LogUtils.d("desc------", getDesc);
SharedPreUtils.saveString("sign", getDesc);
}
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/lib/common/PullToRefreshListView.java
package com.looking.classicalparty.lib.common;
import android.content.Context;
import android.graphics.drawable.AnimationDrawable;
import android.util.AttributeSet;
import android.view.MotionEvent;
import android.view.View;
import android.view.ViewGroup;
import android.view.animation.Animation;
import android.view.animation.LinearInterpolator;
import android.view.animation.RotateAnimation;
import android.widget.AdapterView;
import android.widget.ImageView;
import android.widget.ListView;
import android.widget.TextView;
import com.looking.classicalparty.R;
import com.nineoldandroids.animation.Animator;
import com.nineoldandroids.animation.ValueAnimator;
public class PullToRefreshListView extends ListView {
private View mHeaderView;
private View mFooterView;
private int mHeaderViewHeight;
private int mFooterViewHeight;
private boolean isHeaderViewEnable = true;// 头布局是否可用,默认可用
private boolean isFooterViewEnable = true;// 脚布局是否可用,默认可用
private TextView tv_header_title;
private ImageView iv_arrow;
private ImageView iv_refresh;
private ImageView iv_refresh_complete;
private TextView tv_footer_title;
private ImageView iv_footer_arrow;
private ImageView iv_footer_refresh;
private int startY = -1;
private boolean isOnRefresh = false;// 当前是否正在刷新
private boolean isOnLoadingMore = false;// 当前是否加载更多
private final static int RATIO = 3; // 实际的padding的距离与界面上偏移距离的比例
private static final int STATE_PULL_TO_REFRESH = 1;// 下拉刷新
private static final int STATE_RELEASE_TO_REFRESH = 2;// 松开刷新
private static final int STATE_REFRESHING = 3;// 正在刷新
private int mCurrentRefreshState = STATE_PULL_TO_REFRESH;// 当前状态初始值为下拉刷新
private static final int STATE_PULL_TO_LOADMORE = 4;// 上拉加载更多
private static final int STATE_RELEASE_TO_LOADMORE = 5;// 松开载入更多
private static final int STATE_LOADMOREING = 6;// 正在加载更多
private int mCurrentFooterState = STATE_PULL_TO_LOADMORE;// 当前状态初始值为上拉加载更多
private RotateAnimation animUp;// 箭头向上动画
private RotateAnimation animDown;// 箭头向下动画
private RotateAnimation animRefresh;// 正在刷新动画
private OnRefreshListener mListener;
public PullToRefreshListView(Context context, AttributeSet attrs, int defStyle) {
super(context, attrs, defStyle);
initView();
}
public PullToRefreshListView(Context context, AttributeSet attrs) {
super(context, attrs);
initView();
}
public PullToRefreshListView(Context context) {
super(context);
initView();
}
private void initView() {
// initAnim();// 初始化动画效果
initHeaderView();
initFooterView();
}
/**
* 设置头布局刷新是否可用,默认true
*/
public void setHeaderViewEnable(boolean isHeaderViewEnable) {
this.isHeaderViewEnable = isHeaderViewEnable;
}
/**
* 设置脚布局加载更多是否可用,默认true
*/
public void setFooterViewEnable(boolean isFooterViewEnable) {
this.isFooterViewEnable = isFooterViewEnable;
}
/**
* 设置刷新头布局的背景颜色
*/
public void setHeaderBackGround(int color) {
mHeaderView.setBackgroundColor(color);
}
/**
* 设置加载教布局的背景颜色
*/
public void setFooterBackGround(int color) {
mFooterView.setBackgroundColor(color);
}
/**
* 初始化头布局
*/
private void initHeaderView() {
mHeaderView = View.inflate(getContext(), R.layout.item_refresh_header, null);
// 在activity创建时,手动测量布局,获取测量布局的高度
measureView(mHeaderView);
mHeaderViewHeight = mHeaderView.getMeasuredHeight();
// 设置padding隐藏头布局
mHeaderView.setPadding(0, -mHeaderViewHeight, 0, 0);
mHeaderView.invalidate();
iv_refresh_complete = (ImageView) mHeaderView.findViewById(R.id.iv_refresh_complete);
tv_header_title = (TextView) mHeaderView.findViewById(R.id.tv_header_title);
final AnimationDrawable anim = (AnimationDrawable) iv_refresh.getDrawable();
iv_refresh.post(new Runnable() {
@Override
public void run() {
anim.stop();
anim.start();
}
});
this.addHeaderView(mHeaderView);// 添加头布局
}
/**
* 初始化脚布局
*/
private void initFooterView() {
mFooterView = View.inflate(getContext(), R.layout.item_refresh_footer, null);
// 在activity创建时,手动测量布局,获取测量布局的高度
measureView(mFooterView);
mFooterViewHeight = mFooterView.getMeasuredHeight();
// 设置padding隐藏脚布局
mFooterView.setPadding(0, 0, 0, -mFooterViewHeight);
mFooterView.invalidate();
tv_footer_title = (TextView) mFooterView.findViewById(R.id.tv_footer_title);
iv_footer_refresh = (ImageView) mFooterView.findViewById(R.id.iv_footer_refresh);
final AnimationDrawable anim = (AnimationDrawable) iv_footer_refresh.getDrawable();
iv_footer_refresh.post(new Runnable() {
@Override
public void run() {
anim.stop();
anim.start();
}
});
this.addFooterView(mFooterView);// 添加脚布局
}
/**
* 初始化箭头的动画效果
*/
private void initAnim() {
// 向上转动的动画效果
animUp = new RotateAnimation(0, -180, Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f);
animUp.setDuration(500);
animUp.setFillAfter(true);
// 向下转动的动画效果
animDown = new RotateAnimation(-180, 0, Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f);
animDown.setDuration(500);
animDown.setFillAfter(true);
// 正在刷新的动画效果
animRefresh = new RotateAnimation(0, 360, Animation.RELATIVE_TO_SELF, 0.5f, Animation.RELATIVE_TO_SELF, 0.5f);
animRefresh.setDuration(500);
animRefresh.setInterpolator(new LinearInterpolator());
animRefresh.setRepeatCount(-1);
animRefresh.setRepeatMode(Animation.RESTART);
}
@Override
public boolean onTouchEvent(MotionEvent ev) {
switch (ev.getAction()) {
case MotionEvent.ACTION_DOWN:
startY = (int) ev.getY(); // 设置初始值为-1
break;
case MotionEvent.ACTION_MOVE:
// 触摸按下时的事件有可能没有响应到,再次获取开始时的坐标
if (startY == -1) {
startY = (int) ev.getY();
}
// 滑动时当前状态为正在刷新,什么都不用做
if (mCurrentRefreshState == STATE_REFRESHING || mCurrentFooterState == STATE_LOADMOREING) {
break;
}
int endY = (int) ev.getY();
int dY = endY - startY;
/******** 向下划并且当前在第一个条目位置 ********/
if (dY > 0 && getFirstVisiblePosition() == 0 && !isOnRefresh && !isOnLoadingMore && isHeaderViewEnable) {
int paddingTop = dY / RATIO - mHeaderViewHeight;
// 控制下拉的居上的间距不能超过控件高度
if (paddingTop > mHeaderViewHeight) {
paddingTop = mHeaderViewHeight;
}
if (paddingTop >= 0 && mCurrentRefreshState != STATE_RELEASE_TO_REFRESH) {
// 切换到松开刷新
mCurrentRefreshState = STATE_RELEASE_TO_REFRESH;
refreshHeaderState();
} else if (paddingTop < 0 && mCurrentRefreshState != STATE_PULL_TO_REFRESH) {
// 切换到下拉刷新
mCurrentRefreshState = STATE_PULL_TO_REFRESH;
refreshHeaderState();
}
mHeaderView.setPadding(0, paddingTop, 0, 0);
setSelection(0);
}
/******** 向上划且当前在最后一个条目位置 ********/
if (dY < 0 && getLastVisiblePosition() == getCount() - 1 && !isOnLoadingMore && !isOnRefresh
&& isFooterViewEnable && isFillScreenItem()) {
int paddingBottom = Math.abs(dY) / RATIO - mFooterViewHeight;
if (paddingBottom > mFooterViewHeight) {
paddingBottom = mFooterViewHeight;
}
if (paddingBottom >= 0 && mCurrentFooterState != STATE_RELEASE_TO_LOADMORE) {
// 切换到松开载入更多
mCurrentFooterState = STATE_RELEASE_TO_LOADMORE;
refreshFooterState();
} else if (paddingBottom <= 0 && mCurrentFooterState != STATE_PULL_TO_LOADMORE) {
// 切换到上拉加载更多
mCurrentFooterState = STATE_PULL_TO_LOADMORE;
refreshFooterState();
}
mFooterView.setPadding(0, 0, 0, paddingBottom);
setSelection(getCount() - 1);// 显示最后一个位置
}
break;
case MotionEvent.ACTION_UP:
startY = -1;// 起始坐标归零
/******** 刷新操作 ********/
if (isHeaderViewEnable) {
if (mCurrentRefreshState == STATE_RELEASE_TO_REFRESH) {
// 如果当前是松开刷新, 就要切换为正在刷新
mCurrentRefreshState = STATE_REFRESHING;
isOnRefresh = true;
// 显示头布局
mHeaderView.setPadding(0, 0, 0, 0);
refreshHeaderState();
// 下拉刷新回调
if (mListener != null) {
mListener.onrefresh();
}
} else if (mCurrentRefreshState == STATE_PULL_TO_REFRESH) {
// 隐藏头布局
mHeaderView.setPadding(0, 0, 0, -mHeaderViewHeight);
}
}
/******** 加载更多操作 ********/
if (isFooterViewEnable) {
if (mCurrentFooterState == STATE_RELEASE_TO_LOADMORE) {
// 如果当前是松开载入更多, 就要切换为正在加载更多
mCurrentFooterState = STATE_LOADMOREING;
isOnLoadingMore = true;
// 显示脚布局
mFooterView.setPadding(0, 0, 0, 0);
refreshFooterState();
// 加载更多回调
if (mListener != null) {
mListener.onLoadMore();
}
} else if (mCurrentFooterState == STATE_PULL_TO_LOADMORE) {
// 隐藏脚布局
mFooterView.setPadding(0, 0, 0, -mFooterViewHeight);
}
}
break;
default:
break;
}
return super.onTouchEvent(ev);
}
/**
* 根据当前的刷新状态刷新头布局
*/
private void refreshHeaderState() {
switch (mCurrentRefreshState) {
case STATE_PULL_TO_REFRESH:
tv_header_title.setText("下拉刷新↓");
iv_refresh.clearAnimation();
iv_refresh.setVisibility(View.INVISIBLE);
iv_arrow.clearAnimation();
iv_arrow.setVisibility(View.INVISIBLE);
// iv_arrow.startAnimation(animDown);
break;
case STATE_RELEASE_TO_REFRESH:
tv_header_title.setText("松开立即刷新↑");
iv_arrow.setVisibility(View.INVISIBLE);
iv_refresh.setVisibility(View.VISIBLE);
final AnimationDrawable anim = (AnimationDrawable) iv_refresh.getDrawable();
anim.start();
break;
case STATE_REFRESHING:
tv_header_title.setText("努力加载中...");
iv_refresh.setVisibility(View.VISIBLE);
iv_arrow.clearAnimation();// 必须清除动画,才能隐藏控件
iv_arrow.setVisibility(View.INVISIBLE);
break;
default:
break;
}
}
/**
* 根据当前加载更多的状态刷新脚布局
*/
private void refreshFooterState() {
switch (mCurrentFooterState) {
case STATE_PULL_TO_LOADMORE:
tv_footer_title.setText("上拉加载更多↑");
iv_footer_refresh.clearAnimation();
iv_footer_refresh.setVisibility(View.INVISIBLE);
iv_footer_arrow.setVisibility(View.VISIBLE);
break;
case STATE_RELEASE_TO_LOADMORE:
tv_footer_title.setText("松开立即加载↓");
iv_footer_refresh.clearAnimation();
iv_footer_refresh.setVisibility(View.INVISIBLE);
iv_footer_arrow.setVisibility(View.VISIBLE);
break;
case STATE_LOADMOREING:
tv_footer_title.setText("努力加载中...");
iv_footer_refresh.setVisibility(View.VISIBLE);
iv_footer_arrow.clearAnimation();
iv_footer_arrow.setVisibility(View.INVISIBLE);
break;
}
}
/**
* 刷新完成调用的方法
*/
public void onRefreshAndLoadComplete() {
if (isOnRefresh) {
// 隐藏头布局,恢复初始状态
isOnRefresh = false;
final ValueAnimator va = ValueAnimator.ofInt(0, mHeaderViewHeight);
va.addUpdateListener(new ValueAnimator.AnimatorUpdateListener() {
@Override
public void onAnimationUpdate(ValueAnimator animation) {
int height = (Integer) va.getAnimatedValue();
mHeaderView.setPadding(0, -height, 0, 0);
}
});
va.addListener(new Animator.AnimatorListener() {
@Override
public void onAnimationStart(Animator animation) {
}
@Override
public void onAnimationEnd(Animator animation) {
mCurrentRefreshState = STATE_PULL_TO_REFRESH;
tv_header_title.setText("下拉刷新↓");
iv_refresh_complete.setVisibility(View.INVISIBLE);
iv_arrow.setVisibility(View.VISIBLE);
}
@Override
public void onAnimationCancel(Animator animation) {
}
@Override
public void onAnimationRepeat(Animator animation) {
}
});
va.setDuration(500);
va.start();
tv_header_title.setText("刷新完成");
iv_refresh.clearAnimation();
iv_refresh.setVisibility(View.INVISIBLE);
iv_refresh_complete.setVisibility(View.VISIBLE);
}
if (isOnLoadingMore) {
// 隐藏脚布局,恢复初始状态
isOnLoadingMore = false;
mFooterView.setPadding(0, 0, 0, -mFooterViewHeight);
mCurrentFooterState = STATE_PULL_TO_LOADMORE;
tv_footer_title.setText("上拉加载更多");
iv_footer_refresh.clearAnimation();
iv_footer_refresh.setVisibility(INVISIBLE);
iv_footer_arrow.setVisibility(View.VISIBLE);
}
}
/**
* 条目是否填满整个屏幕
*/
private boolean isFillScreenItem() {
final int firstVisiblePosition = this.getFirstVisiblePosition();
final int lastVisiblePostion = this.getLastVisiblePosition();
final int visibleItemCount = lastVisiblePostion - firstVisiblePosition + 1;
final int totalItemCount = this.getCount();
if (visibleItemCount < totalItemCount) {
return true;
} else {
return false;
}
}
/**
* 测量View的宽高
*/
private void measureView(View child) {
ViewGroup.LayoutParams p = child.getLayoutParams();
if (p == null) {
p = new ViewGroup.LayoutParams(ViewGroup.LayoutParams.MATCH_PARENT, ViewGroup.LayoutParams.WRAP_CONTENT);
}
int childWidthSpec = ViewGroup.getChildMeasureSpec(0, 0 + 0, p.width);
int lpHeight = p.height;
int childHeightSpec;
if (lpHeight > 0) {
childHeightSpec = MeasureSpec.makeMeasureSpec(lpHeight, MeasureSpec.EXACTLY);
} else {
childHeightSpec = MeasureSpec.makeMeasureSpec(0, MeasureSpec.UNSPECIFIED);
}
child.measure(childWidthSpec, childHeightSpec);
}
/**
* 重写条目item点击事件
*/
private OnItemClickListener mItemListener;
public void setOnItemClickListener(OnItemClickListener listener) {
mItemListener = listener;
super.setOnItemClickListener(new OnItemClickListener() {// 将点击事件由当前类实现
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
if (mItemListener != null) {
mItemListener.onItemClick(parent, view, position - getHeaderViewsCount(), id);
}
}
});
}
/**
* 重写条目item长按事件
*/
private OnItemLongClickListener mItemLongClickListener;
public void setOnItemLongClickListener(OnItemLongClickListener listener) {
mItemLongClickListener = listener;
super.setOnItemLongClickListener(new OnItemLongClickListener() {
@Override
public boolean onItemLongClick(AdapterView<?> parent, View view, int position, long id) {
if (mItemLongClickListener != null) {
mItemLongClickListener.onItemLongClick(parent, view, position - getHeaderViewsCount(), id);
}
return true;
}
});
}
/**
* 设置回调接口
*/
public void setOnRefreshListener(OnRefreshListener listener) {
mListener = listener;
}
public interface OnRefreshListener {
// 下拉刷新的回调用法
public void onrefresh();
// 上拉加载更多回调方法
public void onLoadMore();
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/register/bean/RegisterBean.java
package com.looking.classicalparty.moudles.register.bean;
import com.looking.classicalparty.lib.base.Bean.BaseBean;
/**
* Created by xin on 2016/10/24.
*/
public class RegisterBean extends BaseBean{
/**
* user : {"mid":20}
*/
private UserEntity user;
public UserEntity getUser() {
return user;
}
public void setUser(UserEntity user) {
this.user = user;
}
public static class UserEntity {
/**
* mid : 20
*/
private int mid;
public int getMid() {
return mid;
}
public void setMid(int mid) {
this.mid = mid;
}
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/music/adapter/MusicAdapter.java
package com.looking.classicalparty.moudles.music.adapter;
import android.content.Context;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.TextView;
import com.looking.classicalparty.R;
import com.looking.classicalparty.lib.utils.ImageLoaderUtils;
import com.looking.classicalparty.moudles.music.bean.MusicDetailBean;
import java.util.List;
/**
* Created by xin on 2017/3/29.
*/
public class MusicAdapter extends BaseAdapter {
private Context context;
private MusicListener listener;
private List<MusicDetailBean.ActivityEntity> musicDatas;
public MusicAdapter(Context context, List<MusicDetailBean.ActivityEntity> musicDatas) {
this.context = context;
this.musicDatas = musicDatas;
}
@Override
public int getCount() {
if (null == musicDatas) {
return 0;
}
return musicDatas.size();
}
@Override
public MusicDetailBean.ActivityEntity getItem(int position) {
return musicDatas.get(position);
}
@Override
public long getItemId(int position) {
return position;
}
@Override
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder mViewHolder = null;
if (null == convertView) {
mViewHolder = new ViewHolder();
convertView = View.inflate(context, R.layout.item_music_layout, null);
mViewHolder.iv_music = (ImageView) convertView.findViewById(R.id.iv_music);
mViewHolder.tv_musicName = (TextView) convertView.findViewById(R.id.tv_musicName);
mViewHolder.tv_singer = (TextView) convertView.findViewById(R.id.tv_singer);
mViewHolder.iv_more = (ImageView) convertView.findViewById(R.id.iv_more);
mViewHolder.ll_listener_music = (LinearLayout) convertView.findViewById(R.id.ll_listener_music);
convertView.setTag(mViewHolder);
} else {
mViewHolder = (ViewHolder) convertView.getTag();
}
ImageLoaderUtils.display(context, "http://www.jingdian.party/" + musicDatas.get(position).getCover_path(),
mViewHolder.iv_music);
mViewHolder.tv_musicName.setText(musicDatas.get(position).getTitle());
mViewHolder.tv_singer.setText(musicDatas.get(position).getDirector());
mViewHolder.ll_listener_music.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
listener.showListenerDialog(position);
}
});
mViewHolder.iv_more.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
listener.showMusicDetail(position);
}
});
return convertView;
}
public void setMusicListener(MusicListener listener) {
this.listener = listener;
}
public interface MusicListener {
public void showListenerDialog(int position);
public void showMusicDetail(int position);
}
static class ViewHolder {
public TextView tv_musicName;
public TextView tv_singer;
public ImageView iv_music;
public ImageView iv_more;
public LinearLayout ll_listener_music;
}
}
<file_sep>/README.md
# looking
music or video
关于经典的视频和音乐
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/find/bean/MusicBean.java
package com.looking.classicalparty.moudles.find.bean;
/**
* Created by xin on 2017/3/29.
*/
public class MusicBean {
private String id;
private String title;
private String director;
private String cover_path;
private String v_path;
private Object vscreate_time;
private String musername;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getDirector() {
return director;
}
public void setDirector(String director) {
this.director = director;
}
public String getCover_path() {
return cover_path;
}
public void setCover_path(String cover_path) {
this.cover_path = cover_path;
}
public String getV_path() {
return v_path;
}
public void setV_path(String v_path) {
this.v_path = v_path;
}
public Object getVscreate_time() {
return vscreate_time;
}
public void setVscreate_time(Object vscreate_time) {
this.vscreate_time = vscreate_time;
}
public String getMusername() {
return musername;
}
public void setMusername(String musername) {
this.musername = musername;
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/lib/common/UpdateDialog.java
package com.looking.classicalparty.lib.common;
import android.app.Dialog;
import android.content.Context;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import com.looking.classicalparty.R;
/**
* Created by xin on 2016/10/24.
*/
public class UpdateDialog extends Dialog implements View.OnClickListener {
private Button btnUpdate;
private Button btnCancel;
private UpdateListener listener;
public UpdateDialog(Context context) {
this(context, R.style.base_dialog_theme);
}
public UpdateDialog(Context context, int themeResId) {
super(context, themeResId);
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.tips_dialog_layout);
btnUpdate = (Button) findViewById(R.id.btn_update);
btnCancel = (Button) findViewById(R.id.btn_cancel);
btnUpdate.setOnClickListener(this);
btnCancel.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.btn_update:
if (listener != null) {
listener.update();
}
break;
case R.id.btn_cancel:
dismiss();
break;
}
}
public void setUpdateListener(UpdateListener listener) {
this.listener = listener;
}
public interface tipsListener {
void getAppData();
}
public interface UpdateListener {
public void update();
public void closeDialog();
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/find/adapter/ReviewAdapter.java
package com.looking.classicalparty.moudles.find.adapter;
import android.content.Context;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import android.widget.TextView;
import com.looking.classicalparty.R;
import com.looking.classicalparty.lib.utils.ImageLoaderUtils;
import com.looking.classicalparty.moudles.find.bean.ReviewsBean;
import java.util.List;
/**
* Created by xin on 2016/10/25.
*/
public class ReviewAdapter extends RecyclerView.Adapter<RecyclerView.ViewHolder> {
public List<ReviewsBean> mDatas;
private OnItemClickListener mOnItemClickListener;
private Context context;
public ReviewAdapter(Context context, List<ReviewsBean> datas) {
this.context = context;
this.mDatas = datas;
}
public void setOnItemClickListener(OnItemClickListener listener) {
this.mOnItemClickListener = listener;
}
@Override
public RecyclerView.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
if (viewType == ITEM_TYPE.ITEM_TYPE_THEME.ordinal()) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.theme_desc_layout, parent, false);
return new ThemeViewHolder(view);
} else if (viewType == ITEM_TYPE.ITEM_TYPE_MOVIE.ordinal()) {
View view = LayoutInflater.from(parent.getContext()).inflate(R.layout.movie_detail_layout, parent, false);
return new MovieViewHolder(view);
}
return null;
}
@Override
public void onBindViewHolder(final RecyclerView.ViewHolder holder, final int position) {
if (holder instanceof ThemeViewHolder) {
((ThemeViewHolder) holder).mTvTitle.setText("MOVIE");
((ThemeViewHolder) holder).mIvTitle.setImageResource(R.mipmap.img_music);
} else if (holder instanceof MovieViewHolder) {
ImageLoaderUtils.display(context, mDatas.get(position).getCover_path(), ((MovieViewHolder) holder)
.mIvPhoto);
((MovieViewHolder) holder).mTvName.setText(mDatas.get(position).getTitle());
((MovieViewHolder) holder).mTvWriter.setText(mDatas.get(position).getDirector());
((MovieViewHolder) holder).mRecommer.setText(mDatas.get(position).getMusername());
if (mOnItemClickListener != null) {
holder.itemView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
mOnItemClickListener.onItemClick(((MovieViewHolder) holder).mIvPhoto, position);
}
});
}
}
}
@Override
public int getItemViewType(int position) {
return position < 0 ? ITEM_TYPE.ITEM_TYPE_THEME.ordinal() : ITEM_TYPE.ITEM_TYPE_MOVIE.ordinal();
}
@Override
public int getItemCount() {
return mDatas.size();
}
// 定义类型
public static enum ITEM_TYPE {
ITEM_TYPE_THEME,
ITEM_TYPE_MOVIE,
}
public static interface OnItemClickListener {
void onItemClick(View view, int positon);
}
/**
* 定义的主题ViewHolder
*/
public class ThemeViewHolder extends RecyclerView.ViewHolder {
private ImageView mIvTitle;
private TextView mTvTitle;
public ThemeViewHolder(View itemView) {
super(itemView);
mIvTitle = (ImageView) itemView.findViewById(R.id.iv_title);
mTvTitle = (TextView) itemView.findViewById(R.id.tv_title);
}
}
public class MovieViewHolder extends RecyclerView.ViewHolder {
private ImageView mIvPhoto;
private TextView mTvName;
private TextView mTvWriter;
private TextView mRecommer;
public MovieViewHolder(View itemView) {
super(itemView);
mIvPhoto = (ImageView) itemView.findViewById(R.id.iv_photo);
mTvName = (TextView) itemView.findViewById(R.id.tv_name);
mTvWriter = (TextView) itemView.findViewById(R.id.tv_writer);
mRecommer = (TextView) itemView.findViewById(R.id.tv_recommer);
}
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/about/view/AboutUsActivity.java
package com.looking.classicalparty.moudles.about.view;
import android.view.View;
import android.widget.ImageView;
import com.looking.classicalparty.R;
import com.looking.classicalparty.lib.base.activity.BaseActivity;
import com.looking.classicalparty.lib.ui.TitleBar;
public class AboutUsActivity extends BaseActivity {
private TitleBar mTitleBar;
private ImageView mImgCode;
@Override
public void initView() {
setContentView(R.layout.activity_about_us);
mTitleBar = (TitleBar) findViewById(R.id.title_bar);
//二维码位置
mImgCode = (ImageView) findViewById(R.id.img_code);
}
@Override
public void initListener() {
}
@Override
public void initData() {
mTitleBar.setTitle("关于我们");
mTitleBar.setOnClickListener(new TitleBar.OnClickListener() {
@Override
public void OnLeftClick() {
finish();
}
@Override
public void OnRightClick() {
}
});
// setImgCode();
}
// /**
// * 设置二维码到指定位置
// */
// private void setImgCode() {
//
// String content = "hello welcome!";
// try {
// if (!TextUtils.isEmpty(content)) {
// LogoConfig logoConfig = new LogoConfig();
// Bitmap logoBitmap = logoConfig.modifyLogo(BitmapFactory.decodeResource(getResources(), R.mipmap
// .img_logo), BitmapFactory.decodeResource(getResources(), R.mipmap.img_logo));
// Bitmap codeBitmap = logoConfig.createCode(content, logoBitmap);
// mImgCode.setImageBitmap(codeBitmap);
// } else {
// Crouton.makeText(this, "请输入要生成的字符串", Style.CONFIRM).show();
// }
// } catch (Exception e) {
// e.printStackTrace();
// }
// }
@Override
public void processClick(View v) {
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/launch/LaunchActivity.java
package com.looking.classicalparty.moudles.launch;
import android.content.Intent;
import android.os.Handler;
import android.os.Message;
import android.text.TextUtils;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
import com.google.gson.Gson;
import com.looking.classicalparty.R;
import com.looking.classicalparty.lib.base.activity.BaseActivity;
import com.looking.classicalparty.lib.common.TipDialog;
import com.looking.classicalparty.lib.common.UpdateDialog;
import com.looking.classicalparty.lib.constants.ConstantApi;
import com.looking.classicalparty.lib.constants.StringContants;
import com.looking.classicalparty.lib.http.HttpUtils;
import com.looking.classicalparty.lib.http.Param;
import com.looking.classicalparty.lib.http.ResultCallback;
import com.looking.classicalparty.lib.utils.LogUtils;
import com.looking.classicalparty.lib.utils.PackageManagerUtils;
import com.looking.classicalparty.lib.utils.SharedPreUtils;
import com.looking.classicalparty.moudles.launch.bean.AppKeyBean;
import com.looking.classicalparty.moudles.main.MainActivity;
import com.looking.classicalparty.moudles.mine.bean.VersionBean;
import com.looking.classicalparty.moudles.mine.bean.VersionResponse;
import com.squareup.okhttp.Request;
import java.util.ArrayList;
import java.util.List;
public class LaunchActivity extends BaseActivity {
private static final String TAG = "SplashActivity";
private static final int GO_MAIN = 100;
private static final int GO_GUIDE = 200;
private static final int GO_UPDATE = 300;
// 延迟3秒
private static final long SPLASH_DELAY_MILLIS = 3000;
private TipDialog tipDialog;
private VersionBean mVersion;
private Button inApp;
private boolean isDownload;
private Handler mHandler = new Handler() {
@Override
public void handleMessage(Message msg) {
super.handleMessage(msg);
switch (msg.what) {
case GO_MAIN:
goMain();
break;
case GO_GUIDE:
goGuide();
break;
case GO_UPDATE:
showUpdateDialog();
break;
}
}
};
/**
* 获取AppKey
*/
public void getAppKey() {
List<Param> paramList = new ArrayList<>();
Param key = new Param("", "");
paramList.add(key);
HttpUtils.post(ConstantApi.getkey, paramList, new ResultCallback() {
@Override
public void onSuccess(Object response) {
LogUtils.d("appkey", response.toString());
AppKeyBean keyBean = new Gson().fromJson(response.toString(), AppKeyBean.class);
if (keyBean.getResult() == 200) {
SharedPreUtils.saveString(StringContants.KEY, keyBean.getKey());
}
}
@Override
public void onFailure(Request request, Exception e) {
}
@Override
public void onNoNetWork(String resultMsg) {
}
});
}
public void goGuide() {
}
/**
* 判断弹出框是否出现
*/
private void isHasTips() {
if (TextUtils.isEmpty(SharedPreUtils.getString(StringContants.KEY, ""))) {
tipDialog = new TipDialog(this);
tipDialog.setTipsListener(new TipDialog.tipsListener() {
@Override
public void getAppData() {
getAppKey();
}
});
tipDialog.show();
}
}
/**
* 跳转到主页面
*/
private void goMain() {
startActivity(new Intent(this, MainActivity.class));
}
@Override
public void initView() {
setContentView(R.layout.activity_launch);
inApp = (Button) findViewById(R.id.inapp);
}
@Override
public void initListener() {
inApp.setOnClickListener(this);
}
@Override
public void initData() {
getAppKey();
getAppVersion();
//判断是否有key
//isHasTips();
}
@Override
public void processClick(View v) {
switch (v.getId()) {
case R.id.inapp:
mHandler.sendEmptyMessage(GO_MAIN);
break;
}
}
/**
* 获取AppVersion
*/
private void getAppVersion() {
//跳转到主界面
List<Param> paramList = new ArrayList<>();
Param key = new Param("key", SharedPreUtils.getString(StringContants.KEY));
Param apptype = new Param("apptype", "android");
paramList.add(key);
paramList.add(apptype);
HttpUtils.post(ConstantApi.VERSION, paramList, new ResultCallback() {
@Override
public void onSuccess(Object response) {
VersionResponse versionResponse = new Gson().fromJson(response.toString(), VersionResponse.class);
Log.d("version---", versionResponse.toString());
if (200 == versionResponse.getResult()) {
mVersion = versionResponse.getVersion();
if (mVersion == null) {
//版本信息为空
} else {
//判断版本信息 版本高的话弹窗提示是否更新
if (PackageManagerUtils.getVersionCode(LaunchActivity.this) < Double.parseDouble(mVersion
.getVersionCode())) {
Toast.makeText(LaunchActivity.this, "更新", Toast.LENGTH_SHORT).show();
mHandler.sendEmptyMessage(GO_UPDATE);
}
}
}
}
@Override
public void onFailure(Request request, Exception e) {
}
@Override
public void onNoNetWork(String resultMsg) {
}
});
}
/**
* 显示更新对话框
*/
protected void showUpdateDialog() {
UpdateDialog updateDialog = new UpdateDialog(this);
updateDialog.setCanceledOnTouchOutside(false);
updateDialog.setUpdateListener(new UpdateDialog.UpdateListener() {
@Override
public void update() {
downloadApk();
isDownload = true;
updateDialog.dismiss();
}
@Override
public void closeDialog() {
finish();
}
});
updateDialog.show();
}
private void downloadApk() {
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/login/observer/ObserverListener.java
package com.looking.classicalparty.moudles.login.observer;
/**
* Created by xin on 2017/4/12.
* 使用观察者模式,实现登录和退出的同步
*/
public interface ObserverListener {
void observerIsLogin(int isLogin);
}
<file_sep>/app/src/main/java/com/looking/classicalparty/lib/utils/PlayOnlineMusic.java
package com.looking.classicalparty.lib.utils;
import android.app.Activity;
import android.text.TextUtils;
import com.looking.classicalparty.moudles.find.bean.MusicBean;
import java.io.File;
public abstract class PlayOnlineMusic extends PlayMusic {
private MusicBean mOnlineMusic;
public PlayOnlineMusic(Activity activity, MusicBean onlineMusic) {
super(activity, 3);
mOnlineMusic = onlineMusic;
}
@Override
protected void getPlayInfo() {
String artist = mOnlineMusic.getMusername();
String title = mOnlineMusic.getTitle();
// 下载封面
String albumFileName = FileUtils.getAlbumFileName(artist, title);
File albumFile = new File(FileUtils.getAlbumDir(), albumFileName);
String picUrl = "http://www.jingdian.party/" + mOnlineMusic.getCover_path();
if (!albumFile.exists() && !TextUtils.isEmpty(picUrl)) {
downloadAlbum(picUrl, albumFileName);
} else {
mCounter++;
}
music = new MusicBean();
music.setTitle(title);
music.setMusername(artist);
}
private void downloadAlbum(String picUrl, String fileName) {
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/moudles/mine/observer/ConcreteObserver.java
package com.looking.classicalparty.moudles.mine.observer;
/**
* Created by xin on 2016/11/2.
* 实体观察者对象
*/
public class ConcreteObserver implements Observer {
private String desco;
@Override
public void update(String desc) {
this.desco = desc;
}
}
<file_sep>/app/src/main/java/com/looking/classicalparty/lib/common/TipDialog.java
package com.looking.classicalparty.lib.common;
import android.app.Dialog;
import android.content.Context;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import com.looking.classicalparty.R;
/**
* Created by xin on 2016/10/24.
*/
public class TipDialog extends Dialog implements View.OnClickListener {
private tipsListener listener;
private Button mBtnTipsOne;
private Button mBtnTipTwo;
public TipDialog(Context context) {
this(context, R.style.base_dialog_theme);
}
public TipDialog(Context context, int themeResId) {
super(context, themeResId);
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.tips_dialog_layout);
mBtnTipsOne = (Button) findViewById(R.id.btn_tips_one);
mBtnTipTwo = (Button) findViewById(R.id.btn_tips_two);
mBtnTipsOne.setOnClickListener(this);
mBtnTipTwo.setOnClickListener(this);
}
public void setTipsListener(tipsListener listener) {
this.listener = listener;
}
@Override
public void onClick(View v) {
switch (v.getId()) {
case R.id.btn_tips_one:
if (listener != null) {
listener.getAppData();
}
break;
case R.id.btn_tips_two:
dismiss();
break;
}
}
public interface tipsListener {
void getAppData();
}
}
| a2ef8dafc752ddd6b3a5eb3b36c37e66b0f3b85d | [
"Markdown",
"Java",
"Gradle"
] | 29 | Java | blythe104/looking | 0a96bbc695397a50c6471d87de73446798631dff | ea2a897e7f19850c5a13cf21c0444ac93c72fc72 | |
refs/heads/master | <file_sep>package ramsden.ryan.GUI;
import java.awt.Canvas;
import java.awt.Color;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics2D;
import java.awt.Point;
import java.awt.Rectangle;
import java.awt.event.KeyEvent;
import ramsden.ryan.Control;
public class TextInput extends Interface {
public String text;
public Font font = new Font("Verdana", Font.PLAIN, 12);
public FontMetrics fm;
public int caratPosition, caratX;
public TextInput(Interface i, String text, Rectangle r) {
super(i, r);
setText(text);
setColor(new Color(0xe2e2e2));
fm = new Canvas().getFontMetrics(font);
}
@Override
public void drawInterface(Graphics2D g2d, int offsetX, int offsetY) {
g2d.setColor(getColor());
g2d.setFont(font);
Rectangle r = getContainer();
g2d.fillRect(r.x+offsetX, r.y+offsetY, r.width, r.height);
g2d.setColor(Color.BLACK);
g2d.drawString(getText(), r.x+2+offsetX, 8+r.y+fm.getHeight()/2+offsetY);
//Not too happy with this.
if(Control.selectedTextBox == this && System.currentTimeMillis()%1000<700)
g2d.drawLine(caratX+offsetX+r.x+2, r.y+offsetY+3, caratX+offsetX+r.x+2, r.y+offsetY+r.height-4);
}
@Override
public void click(Point p, boolean onClickDown) {
Control.selectedTextBox = this;
p.translate(-4, 0); //This is so we dont have to round to the nearest letter.
int caratPosition = 0;
while(fm.stringWidth(getText().substring(0, caratPosition)) < p.getX())
if(++caratPosition >= getText().length()) break;
updateCarat(caratPosition);
}
public void updateCarat(int position)
{
caratPosition = Math.max(Math.min(position, getText().length()),0);
caratX = fm.stringWidth(getText().substring(0, caratPosition));
}
public void enter()
{
System.out.println("Enter");
}
public void setText(String text)
{
if(text == null) text = "";
this.text = text;
if(text.length() > caratPosition) updateCarat(text.length());
}
public void setFont(Font font)
{
this.font = font;
}
public void updateContainer()
{
int hgt = fm.getHeight();
int adv = fm.stringWidth(getText());
getContainer().setSize(adv+2 , hgt-2);
}
public String getText() {
if(text == null) return "";
return text;
}
public boolean validate(char c)
{
return Character.isAlphabetic(c) || Character.isDigit(c);
}
public void addCharacter(int i, char c) {
if(i == KeyEvent.VK_LEFT) updateCarat(caratPosition-1);
else if(i == KeyEvent.VK_RIGHT) updateCarat(caratPosition+1);
else if(i == 8 && text.length() > 0 && caratPosition > 0) {
text = text.substring(0, caratPosition-1) + text.substring(caratPosition);
updateCarat(caratPosition-1);
} else if(i == 127 && text.length() > caratPosition) {
text = text.substring(0, caratPosition) + text.substring(caratPosition+1);
} else if(i == 10) {
enter();
} else if(validate(c)) {
text = text.substring(0, caratPosition) + c + text.substring(caratPosition);
updateCarat(caratPosition+1);
}
}
}
<file_sep>package ramsden.ryan.GUI;
import java.awt.Canvas;
import java.awt.Color;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics2D;
import java.awt.Point;
import java.awt.Rectangle;
public class TextComponent extends Interface {
public String text;
public Font font = new Font("Verdana", Font.PLAIN, 12);
public TextComponent(Interface i, String text, Point p) {
super(i, new Rectangle(p.x, p.y, 0, 0));
setText(text);
setStyle(Font.BOLD);
if(i instanceof Dialog) setColor(Color.LIGHT_GRAY);
else setColor(Color.BLACK);
click = false;
}
public TextComponent(Interface i, String text, Point p, int size) {
this(i, text, p);
setSize(size);
}
public TextComponent(Interface i, String text, Point p, int size, int style) {
this(i, text, p, size);
setStyle(style);
}
public TextComponent(Interface i, String text, Point p, int size, int style, Color c) {
this(i, text, p, size, style);
setColor(c);
}
@Override
public void drawInterface(Graphics2D g2d, int offsetX, int offsetY) {
g2d.setColor(getColor());
g2d.setFont(font);
Rectangle r = getContainer();
g2d.drawString(text, r.x+offsetX, r.y+offsetY+r.height);
}
@Override
public void click(Point p, boolean onClickDown) {}
public void setText(String text)
{
this.text = text;
updateContainer();
}
public void setFont(Font font)
{
this.font = font;
}
public void setStyle(int style)
{
setFont(font.deriveFont(style, font.getSize()));
}
public void setSize(int px)
{
setFont(font.deriveFont(font.getStyle(), px));
}
public void updateContainer()
{
FontMetrics fm = new Canvas().getFontMetrics(font);
int hgt = fm.getHeight();
int adv = fm.stringWidth(text);
getContainer().setSize(adv+2 , hgt-2);
}
public String getText() {
return text;
}
}
<file_sep>package ramsden.ryan;
import java.util.ArrayList;
import ramsden.ryan.GUI.DisplayPanel;
public class Engine {
public static final int MAX_ATOMS = 20;
private ArrayList<Particle> atoms;
private Vector p1 = new Vector(); // position
private Vector p2 = new Vector();
private Vector v1 = new Vector(); // velocity
private Vector v2 = new Vector();
private Vector n = new Vector(); // normal vector
private Vector un = new Vector(); // unit normal
private Vector ut = new Vector(); // unit tangent
public int collisions, gameTicks, maxGameTicks = 0, collideAtTicks = 0;
private int width = 0, height = 0;
/** Construct a container of atoms. */
public Engine(int width, int height) {
atoms = new ArrayList<Particle>(MAX_ATOMS);
this.width = width;
this.height = height;
}
public Engine(Engine copy) {
setState(copy);
}
public void setState(Engine copy) {
atoms = new ArrayList<Particle>(MAX_ATOMS);
collisions = 0;
maxGameTicks = 0;
gameTicks = 0;
collideAtTicks = 0;
for(Particle p : copy.atoms) atoms.add(new Particle(p));
if(this != Control.model) {
setWidth(copy.getWidth());
setHeight(copy.getHeight());
} else {
scale(copy.getWidth(), copy.getHeight(), Control.width, Control.height);
}
}
public void scale(int widthOld, int heightOld, int width, int height) {
double scaleW = 1;
double scaleH = 1;
if(Control.currentQuestion == null) {
scaleW = (double)width/widthOld;
scaleH = (double)height/heightOld;
}
for(Particle p : atoms) {
Vector pos = p.getPosition();
p.setPosition(width/2 + (pos.x - widthOld/2)*scaleW, height/2 + (pos.y - heightOld/2)*scaleH);
}
}
public void updateImages() {
for (Particle atom : getAtoms()) {atom.updateImage(); atom.updateDrawPosition();}
}
public void updateDrawPositions() {
for (Particle atom : getAtoms()) {atom.updateDrawPosition();}
}
public void updateGame() {
gameTicks++;
if(Control.currentQuestion != null && maxGameTicks != 0 && gameTicks >= maxGameTicks) {
Control.setPlaying(false);
if(gameTicks > maxGameTicks) {
Control.model.setState(Control.currentQuestion.generateQuestionEngine());
Control.model.updateImages();
DisplayPanel.collided1=null;
DisplayPanel.collided2=null;
}
return;
}
for (Particle atom : getAtoms()) iterate(atom);
for (Particle atom : getAtoms()) checkCollisions(atom);
}
/** Advance each atom. */
public void iterate(Particle atom1) {
if(this == Control.model) atom1.updateDrawPosition();
if(DisplayPanel.currentDrag == atom1) return;
p1 = atom1.getPosition();
v1 = atom1.getVelocity();
p1.add(v1);
atom1.setPosition(p1);
}
public void checkCollisions(Particle atom1)
{
ArrayList<Particle> collisions = new ArrayList<Particle>();
for (int i = atoms.indexOf(atom1); i < atoms.size(); i++) {
Particle atom2 = atoms.get(i);
if(atom1 == atom2) continue;
if(willCollide(atom1, atom2)) collisions.add(atom2);
}
for(Particle atom2 : collisions)
{
collideAtoms(atom1, atom2);
// atom1.updateDrawPosition();
// atom2.updateDrawPosition();
}
collideWalls(atom1);
}
public boolean willCollide(Particle a1, Particle a2) {
double radius = a1.getR() + a2.getR();
p1 = a1.getPosition(new Vector());
p2 = a2.getPosition(new Vector());
n = n.set(p1).subtract(p2);
double norm = n.norm();
if(norm > radius - 5 && a1.getVelocity().isZero() && a2.getVelocity().isZero()) return false;
return (norm < radius);
}
private void collideAtoms(Particle a1, Particle a2) {
collisions++;
if(!(Control.stepMode && this == Control.model && collideAtTicks > gameTicks)) {
double radius = a1.getR() + a2.getR();
//This is so the collision is more accurate when velocity is large
//It moves the particles so they have a smaller intersection upon collision, making it more accurate.
//
double divideBy = Math.max(a1.getVelocity().norm(), a2.getVelocity().norm());
if(divideBy == 0) divideBy = 5;
for(int i = 0;i < Math.ceil(divideBy); i++) {
a1.setPosition(a1.getPosition().subtract(a1.getVelocity().divide(divideBy)));
a2.setPosition(a2.getPosition().subtract(a2.getVelocity().divide(divideBy)));
if(!willCollide(a1, a2)) break;
}
p1 = a1.getPosition();
p2 = a2.getPosition();
n = n.set(p1).subtract(p2);
if(!willCollide(a1, a2)) {
a1.setPosition(a1.getPosition().add(a1.getVelocity().divide(divideBy)));
a2.setPosition(a2.getPosition().add(a2.getVelocity().divide(divideBy)));
}
if(willCollide(a1, a2)) {
double dr = (radius - n.norm()) / 2;
un = un.set(n).unitVector();
p1.add(un.scale(dr));
un = un.set(n).unitVector();
p2.add(un.scale(-dr));
a1.setPosition(p1);
a2.setPosition(p2);
}
}
if(Control.stepMode && this == Control.model) {
Control.setPlaying(false);
DisplayPanel.collided1 = a1;
DisplayPanel.collided2 = a2;
if(collideAtTicks < gameTicks) {
collideAtTicks = gameTicks+1;
return;
}
}
if(Control.currentQuestion != null && collisions >= 1) {
for(int i = 0; i < 8; i++) {
a1.view[i] = false;
a2.view[i] = false;
}
maxGameTicks = (int) (gameTicks + Control.GAME_HERTZ/4);
}
p1 = a1.getPosition(new Vector());
p2 = a2.getPosition(new Vector());
// Find normal and tangential components of v1/v2
n = n.set(p1).subtract(p2);
un = un.set(n).unitVector();
ut = ut.set(-un.y, un.x);
v1 = a1.getVelocity(v1);
v2 = a2.getVelocity(v2);
double v1n = un.dot(v1);
double v1t = ut.dot(v1);
double v2n = un.dot(v2);
double v2t = ut.dot(v2);
// Calculate new v1/v2 in normal direction
double m1 = a1.getM();
double m2 = a2.getM();
double v1nNew = Control.efficiency*(v1n * (m1 - m2) + 2d * m2 * v2n) / (m1 + m2);
double v2nNew = Control.efficiency*(v2n * (m2 - m1) + 2d * m1 * v1n) / (m1 + m2);
// Update velocities with sum of normal & tangential components
v1 = v1.set(un).scale(v1nNew);
v2 = v2.set(ut).scale(v1t);
a1.setVelocity(v1.add(v2));
v1 = v1.set(un).scale(v2nNew);
v2 = v2.set(ut).scale(v2t);
a2.setVelocity(v1.add(v2));
//Control.outputValues();
}
public boolean willCollideWhenPlaced(Particle atom1)
{
for (int i = 0; i < getAtoms().size(); i++) {
Particle atom2 = getAtoms().get(i);
if(atom1 == atom2) continue;
if(willCollide(atom1, atom2)) return true;
}
return false;
}
// Check for collision with wall
private void collideWalls(Particle atom) {
double radius = atom.getR();
boolean hitWall = false;
p1 = atom.getPosition(p1);
v1 = atom.getVelocity(v1);
if (p1.x < radius) {
p1.x = radius;
v1.x = -v1.x;
hitWall = true;
}
if (p1.y < radius) {
p1.y = radius;
v1.y = -v1.y;
hitWall = true;
}
if (p1.x > getWidth() - radius) {
p1.x = getWidth() - radius;
v1.x = -v1.x;
hitWall = true;
}
if (p1.y > getHeight() - radius) {
p1.y = getHeight() - radius;
v1.y = -v1.y;
hitWall = true;
}
if(hitWall){
if(maxGameTicks != 0) maxGameTicks = gameTicks-1; //Walls will bug everything out
else {
atom.setVelocity(v1);
atom.setPosition(p1);
}
}
}
public void clear()
{
atoms.clear();
gameTicks = 0;
collideAtTicks = 0;
maxGameTicks = 0;
}
public ArrayList<Particle> getAtoms() { return atoms; }
public void addAtom(Particle p) {
atoms.add(p);
}
public Particle getNewAtom()
{
Particle p = new Particle();
p.setR(100);
p.updateImage();
return p;
}
public int getWidth() {
return (this == Control.model ? Control.width : width);
}
public void setWidth(int width) {
this.width = width;
}
public int getHeight() {
return (this == Control.model ? Control.height : height);
}
public void setHeight(int height) {
this.height = height;
}
}
<file_sep>package ramsden.ryan;
import java.awt.Color;
import java.awt.Toolkit;
import java.awt.image.BufferedImage;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.PrintWriter;
import java.io.UnsupportedEncodingException;
import java.util.Random;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.imageio.ImageIO;
import ramsden.ryan.GUI.DisplayPanel;
import ramsden.ryan.GUI.Information;
import ramsden.ryan.GUI.TextComponent;
import ramsden.ryan.GUI.TextInput;
public class Control {
public static final double GAME_HERTZ = 30;
public static DisplayPanel view;
public static Engine model;
public static Question currentQuestion;
private static boolean playing = true;
public static boolean questionFinished = false;
public static boolean running = true;
public static boolean stepMode = false;
public static double efficiency = 1.00;
public static int sqSize = 50;
public static int width = 1000; //same ratio as 1920/1058 (Maximised on 1080p)
public static int height = 551; //Minimum height and width
public static int currentSeed = 0;
public static Control manager;
public static Color bgColor = new Color(0xc9c9c9);//0xb3b3b3);
public static Color lineColor = new Color(0xb3b3b3);//0xa1a1a1);
public static int fps = 60;
public static Particle selected;
private int frameCount = 0;
private static int studentID = 0;
private static String username;
public static TextInput selectedTextBox;
public static TextComponent clickedAnswer;
private SQL sql;
//TODO REMOVE THIS
public static void takeSnapShot() {
System.out.println("Taking screenshot");
BufferedImage bufImage = new BufferedImage(view.getSize().width, view.getSize().height,BufferedImage.TYPE_INT_RGB);
view.paint(bufImage.createGraphics());
String filename = "" + (System.currentTimeMillis()/1000);
File imageFile = new File("screenshots"+File.separator+filename+".png");
try{
System.out.println("Saved at "+imageFile.getAbsolutePath());
imageFile.createNewFile();
ImageIO.write(bufImage, "png", imageFile);
}catch(Exception ex){ ex.printStackTrace();}
PrintWriter writer;
try {
writer = new PrintWriter("screenshots"+File.separator+filename+".txt", "UTF-8");
double totalKE =0, totalMx=0, totalMy=0; int pindex = 0;
for(Particle p : model.getAtoms()) {
pindex++;
double ke = p.getConvertedKE();
double mx = p.getConvertedMomentumX();
double my = p.getConvertedMomentumY();
totalKE += ke;
totalMx += mx;
totalMy += my;
writer.println("Particle "+pindex+": K.E = "+Information.format(ke)+" Mx = "+Information.format(mx)+" My = "+Information.format(my)+" Mass = "+Information.format(p.getConvertedMass())+" Velocity = "+Information.format(p.getConvertedVelocity())+" Vx = "+Information.format(p.getConvertedVelocityX())+" Vy = "+Information.format(p.getConvertedVelocityY()));
}
writer.println("Total K.E. : "+Information.format(totalKE));
writer.println("Total X Momentum : "+Information.format(totalMx));
writer.println("Total Y Momentum : "+Information.format(totalMy));
writer.close();
} catch (FileNotFoundException | UnsupportedEncodingException e) {
e.printStackTrace();
}
//outputValues();
}
public Control(DisplayPanel view, Engine model) {
Control.view = view;
Control.model = model;
}
public static boolean isPlaying() {
return playing;
}
public static void setPlaying(boolean playing) {
if(playing) {
DisplayPanel.collided1 = null;
DisplayPanel.collided2 = null;
}
model.updateDrawPositions();
Control.playing = playing;
}
public void runGameLoop()
{
Thread loop = new Thread()
{
public void run()
{
gameLoop();
}
};
loop.start();
}
private void gameLoop()
{
final double TIME_BETWEEN_UPDATES = 1000000000 / GAME_HERTZ;
final int MAX_UPDATES_BEFORE_RENDER = 1;
double lastUpdateTime = System.nanoTime();
double lastRenderTime = System.nanoTime();
final double TARGET_FPS = 60;
final double TARGET_TIME_BETWEEN_RENDERS = 1000000000 / TARGET_FPS;
//Simple way of finding FPS.
int lastSecondTime = (int) (lastUpdateTime / 1000000000);
while (running)
{
double now = System.nanoTime();
int updateCount = 0;
//Do as many game updates as we need to, potentially playing catchup.
if(isPlaying()) {
while(now - lastUpdateTime > TIME_BETWEEN_UPDATES && updateCount < MAX_UPDATES_BEFORE_RENDER)
{
model.updateGame();
lastUpdateTime += TIME_BETWEEN_UPDATES;
updateCount++;
}
} else lastUpdateTime = now;
//Render. To do so, we need to calculate interpolation for a smooth render.
float interpolation = Math.min(1.0f, (float) ((now - lastUpdateTime) / TIME_BETWEEN_UPDATES));
view.drawGame(interpolation);
Toolkit.getDefaultToolkit().sync();
frameCount++;
lastRenderTime = now;
//Update the frames we got.
int thisSecond = (int) (lastUpdateTime / 1000000000);
if (thisSecond > lastSecondTime)
{
fps = frameCount;
frameCount = 0;
lastSecondTime = thisSecond;
Information.toggleCursor();
}
DisplayPanel.fps.text = "FPS: "+fps; //We dont want to do settext, because it will update the container
//Yield until it has been at least the target time between renders. This saves the CPU from hogging.
while (now - lastRenderTime < TARGET_TIME_BETWEEN_RENDERS && now - lastUpdateTime < TIME_BETWEEN_UPDATES)
{
Thread.yield();
try {Thread.sleep(1);} catch(Exception e) {}
now = System.nanoTime();
}
}
}
public static void setQuestion(Question question) {
currentQuestion = question;
Particle.r = new Random(question.seed);
view.questionText.setText(Control.currentQuestion.question);
view.questionInput.setText(question.myAnswer);
view.questionButton.setText("Mark");
view.questionButton.setSize(40, 20);
view.questionDialog.setSize(Math.max(view.questionText.getContainer().width+16, 160), 80);
view.questionDialog.setHidden(false);
view.add.setHidden(true);
DisplayPanel.collided1=null;DisplayPanel.collided2=null;
model.setState(Control.currentQuestion.generateQuestionEngine());
model.updateImages();
}
public SQL getSQL() {
if(sql == null) {
System.out.println("Connecting to server");
sql = new SQL();
// Thread connectThread = new Thread(){
// @Override
// public void run()
// {
if(!sql.connect()) {
if(view.sid.getText().equals("Connecting")) view.connectionError.setHidden(false);
view.loggedinAs.setText("Failed to connect");
sql = null;
}
// }
// };
// connectThread.start();
}
return sql;
}
public void closeSQL() {
sql.close();
}
public boolean isConnected()
{
return (sql != null && sql.isConnected());
}
public static String getUsername() {
if(username == null || username.trim().isEmpty()) {
if(studentID != 0 && username == null) return ""+studentID;
else return "";
} else return username.trim();
}
public static int getStudentID() {
return studentID;
}
public static void setStudentID(int id) {
studentID = id;
}
public static void setStudentID(String studentID) {
if(studentID.trim().isEmpty()) {
Control.setStudentID(0);
return;
}
if(validate(studentID)) {
if(studentID.length() == 6) {
studentID = studentID.substring(1);
}
}
Control.setStudentID(Integer.parseInt(studentID));
}
public static boolean validate(String studentID)
{
if(studentID.length() == 5) {
Pattern p = Pattern.compile("\\d{5}$");
Matcher matcher = p.matcher(studentID);
return matcher.find();
} else if(studentID.length() == 6){
Pattern p = Pattern.compile("s\\d{5}$");
Matcher matcher = p.matcher(studentID);
return matcher.find();
}
return false;
}
public static void setUsername(String user) {
username = user;
}
}<file_sep>package ramsden.ryan.GUI;
import java.awt.Color;
import java.awt.Font;
import java.awt.Point;
import java.awt.Rectangle;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import ramsden.ryan.Control;
import ramsden.ryan.Question;
import ramsden.ryan.SQL;
public class ResultsViewer {
private TableDialog answerViewer;
public HashMap<Integer, ArrayList<Answer>> seedStorage = new HashMap<Integer, ArrayList<Answer>>();
private ScrollBar sb;
private TextComponent results;
private TextInput textInput;
private Comparator<String> comparator;
private String searchQuery;
public ArrayList<Button> currAnswers = new ArrayList<Button>();
public String currStudentName;
public int currStudentID;
private TextComponent currViewing;
public ResultsViewer(Rectangle r) {
init(r);
comparator = new Comparator<String>() {
public int compare(String s1, String s2) {
if(!s1.substring(0, searchQuery.length()).equals(s2.substring(0, searchQuery.length()))) {
if(s1.startsWith(searchQuery)) return -1;
if(s2.startsWith(searchQuery)) return 1;
}
return s1.compareTo(s2);
}
};
}
public void search(String text) {
if(Control.manager == null) return;
SQL sql = Control.manager.getSQL();
if(sql == null || !sql.isConnected() || answerViewer.isHidden()) return;
searchQuery = text;
answerViewer.clear();
seedStorage.clear();
ResultSet rs;
ArrayList<String> students = new ArrayList<String>();
if(text.matches("[A-z]+")) rs = sql.query("SELECT * FROM `students` WHERE ((`firstname` LIKE '%"+text+"%') OR (`surname` LIKE '%"+text+"%'))");
else rs = sql.query("SELECT * FROM `students` WHERE `studentID` LIKE '%"+text.replaceFirst("s", "")+"%'");
try {
while(rs.next()) {
//Check if this user has any answers;
students.add(rs.getString(2)+" "+rs.getString(3)+":"+rs.getString(1));
}
} catch (SQLException e) {}
Collections.sort(students, comparator);
for(String s : students) {
int i=0, correct=0, id = Integer.parseInt(s.split(":")[1]);
ResultSet ans = sql.query("SELECT * FROM `answers` WHERE `studentID`="+id);
try {
ArrayList<Answer> seed = new ArrayList<Answer>();
while(ans.next() || i == 0) {
i++;
if(Integer.parseInt(ans.getString(4)) == 1) correct++;
seed.add(new Answer(ans.getInt(2), ans.getString(3), Integer.parseInt(ans.getString(4)) == 1));
};
seedStorage.put(id, seed);
answerViewer.addRow(new String[]{s.split(":")[0],s.split(":")[1], correct+"/"+i+" "+(int)(100*(double)correct/i)+"%"});
} catch (SQLException e) {e.printStackTrace();}
}
if(answerViewer.getText(1, answerViewer.selectedRow) != null) {
viewAnswers(answerViewer.getText(0, answerViewer.selectedRow),Integer.parseInt(answerViewer.getText(1, answerViewer.selectedRow)));
}
results.setText(answerViewer.rowArray.size() + " Results");
}
public void sort()
{
}
public void init(Rectangle r)
{
answerViewer = new TableDialog(null, r, 3, 20, 50);
answerViewer.parent = this;
answerViewer.setCloseable(true);
textInput = new TextInput(answerViewer, "", new Rectangle(20, 255, answerViewer.columnWidth[1], 20)) {
@Override
public void addCharacter(int keyChar, char key)
{
super.addCharacter(keyChar, key);
search(text);
}
@Override
public boolean validate(char c)
{
return (Character.isLetterOrDigit(c) || (c+"").equals(" "));
}
};
Color c = Color.LIGHT_GRAY;
new TextComponent(answerViewer, "Student Answers", new Point(10, 8), 18, Font.BOLD,c);
new TextComponent(answerViewer, "Name", new Point(20, 30), 14, Font.BOLD,c);
new TextComponent(answerViewer, "ID", new Point(35+answerViewer.columnWidth[1], 30), 14, Font.BOLD,c);
new TextComponent(answerViewer, "Questions", new Point(20+answerViewer.columnWidth[2], 30), 14, Font.BOLD,c);
results = new TextComponent(answerViewer, "", new Point(answerViewer.columnWidth[1]+25, 255), 14);
currViewing = new TextComponent(answerViewer, "", new Point(20, 285), 14,Font.BOLD, c);
sb = new ScrollBar(answerViewer, new Rectangle(answerViewer.columnWidth[3]+20, 50, 20, 201), 40, this);
sb.setColor(TableDialog.bd);
newScroll(0);
}
public void newScroll(double amount)
{
answerViewer.scroll = amount;
}
public ScrollBar getScrollBar() {
return sb;
}
public boolean isHidden()
{
return answerViewer.isHidden();
}
public void setHidden(boolean b) {
if(b && Control.manager != null && !Control.manager.isConnected()) return;
answerViewer.setHidden(b);
if(!isHidden()) {
search(""); //This will just get the initial results.
Control.selectedTextBox = textInput;
}
}
public void toggle() {
//answerViewer.centerOnScreen();
setHidden(!answerViewer.isHidden());
}
public void viewAnswers(String studentName, final int studentID) {
for(Button b : currAnswers) b.remove();
currAnswers.clear();
currStudentName = studentName;
currStudentID = studentID;
currViewing.setText(studentName);
for (int i = 0; i < seedStorage.get(studentID).size(); i++) {
final int seed = seedStorage.get(studentID).get(i).seed;
final String ans = seedStorage.get(studentID).get(i).answer;
Button b = new Button(answerViewer, new Rectangle(20+(i%5)*54, 310+(i/5)*54, 50, 50), (seed%2==0 ? "2D" : "1D")) {
@Override
public void click(Point p, boolean onClickDown) {
super.click(p, onClickDown);
if(!onClickDown) {
Question q = new Question(seed);
q.myAnswer = ans;
Control.setQuestion(q);
}
}
};
new TextComponent(b, (1+i)+"." ,new Point(2,-4), 8, Font.PLAIN);
b.getTextComponent().setSize(16);
b.getTextComponent().setStyle(Font.BOLD);
b.setColor(seedStorage.get(studentID).get(i).correct ? Question.Colour.GREEN.getColor() : Question.Colour.RED.getColor());
b.centerText();
currAnswers.add(b);
}
}
class Answer {
int seed;
String answer;
boolean correct;
public Answer(int s, String string, boolean c) {
seed = s;
answer = string;
correct = c;
}
}
}
<file_sep>package ramsden.ryan.GUI;
import java.awt.Color;
import java.awt.Graphics2D;
import java.awt.Point;
import java.awt.Rectangle;
public class Dialog extends Interface {
public Color border = new Color(0xb2b2b2);
public Color inside = new Color(0x4F5A69);
public int borderSize = 4;
public Dialog(Interface i, Rectangle r) {
super(i, r);
setColor(inside);
}
@Override
public void drawInterface(Graphics2D g2d, int offsetX, int offsetY) {
Rectangle r = getContainer();
g2d.setColor(border);
g2d.fillRect(r.x+offsetX, r.y+offsetY, r.width, r.height);
g2d.setColor(getColor());
g2d.fillRect(r.x+offsetX+borderSize, r.y+offsetY+borderSize, r.width-borderSize*2, r.height-borderSize*2);
drawChildren(g2d, offsetX, offsetY);
}
@Override
public void click(Point p, boolean isClickDown) {
//We dont need to do anything
}
}
<file_sep>import os
files = os.listdir('.')
index = 0
for filename in files:
os.rename(filename, 'Image '+str(index+1)+'.png')
index += 1 | b13ca5fa7d548f36942824e856baccf682b49e93 | [
"Java",
"Python"
] | 7 | Java | Draesia/ParticleCollisionSimulation | 96ed4f576bdd32f58acab76207d5dbf352b5559b | 0fb6a657378c1927f83642e8f50e936ef4f9c80a | |
refs/heads/master | <file_sep>// SVELTE THEME FOR GLITTER
// Coyright 2009(c) <NAME>
// this removes the default twitter styles
$('style').remove();
var stylesheets = $('link[type="text/css"]')
$.each(stylesheets, function() {
// make sure not to remove the custom style we added
if($(this).attr('id') != 'customStyle') {
$(this).remove();
}
});
// move the profile info into the left column for svelte
$('#side #profile').insertAfter($('#status_update_box'));
$('.profile-head').append($('#profile .stats'));
$('#side').wrapAll('<div id="side_wrap"></div>');
// start autorefreshing
refreshTimeline();
// this moves the ellipsis into the status message when it's length exceeds 140 chars
// TODO - need to find more efficient way of doing this, this fires too many times
$('#timeline').bind('DOMSubtreeModified', function() {
$.each($('.entry-content'), function() {
$(this).append($(this).siblings('a'));
});
});
// this is the svelte specific sliding menu
$('#side_base').hover(
function() {
$('#side_base').css('width','250px');
$('#side_wrap').stop().animate({'width': '250px'}, 200);
},
function() {
$('#side_wrap').stop().animate({'width': '17px'}, 200);
$('#side_base').css('width','auto');
}
);
function refreshTimeline() {
// ensure autorefreshing only occurs for your timeline
if(window.location.hash == '' || window.location.hash == '#home') {
if(window.location.pathname == '/') {
var currDate = new Date();
currDate.toLocaleTimeString();
$('#timeline').load("/ #timeline li");
}
}
// be polite to twitter and don't refresh the page more than 2 times a minute
window.setTimeout(refreshTimeline,30000);
} | 9349c11c8ee1ebac8e9ac545e7ba513d8a481601 | [
"JavaScript"
] | 1 | JavaScript | mmilo/glitter | 48f542218f5a0022446eb237b9c3097ca59ce631 | 44031be0f7dc28313d4f7db3155d33e94c8e61bf | |
refs/heads/master | <repo_name>deepanshu79/Pulsar-prediction<file_sep>/README.md
# Pulsar-prediction
This project predicts target class of pulsars(neutron star) using SVM , Kernel-SVM and Naive bayes models of machine learning.
<file_sep>/SVM.py
# SVM
# Importing the libraries
import numpy as np
import matplotlib.pyplot as plt
import pandas as pd
# Importing the dataset
dataset = pd.read_csv('pulsar_stars.csv')
X = dataset.iloc[:, :-1].values
y = dataset.iloc[:, -1].values
# Data visualization
men = X[:, 0]
plt.scatter(men, y)
plt.title('Mean of the integrated profile vs target_class')
plt.xlabel('Mean of the integrated profile')
plt.ylabel('target_class')
plt.show()
glu = X[:, 1]
plt.scatter(glu, y)
plt.title('Standard deviation of the integrated profile vs target_class')
plt.xlabel('Standard deviation of the integrated profile')
plt.ylabel('target_class')
plt.show()
bld = X[:, 2]
plt.scatter(bld, y)
plt.title('Excess kurtosis of the integrated profile vs target_class')
plt.xlabel('Excess kurtosis of the integrated profile')
plt.ylabel('target_class')
plt.show()
skn = X[:, 3]
plt.scatter(skn, y)
plt.title('Skewness of the integrated profile vs target_class')
plt.xlabel('Skewness of the integrated profile')
plt.ylabel('target_class')
plt.show()
ins = X[:, 4]
plt.scatter(ins, y)
plt.title('Mean of the DM-SNR curve vs target_class')
plt.xlabel( 'Mean of the DM-SNR curve')
plt.ylabel('target_class')
plt.show()
bmi = X[:, 5]
plt.scatter(bmi, y)
plt.title('Standard deviation of the DM-SNR curve vs target_class')
plt.xlabel('Standard deviation of the DM-SNR curve')
plt.ylabel('target_class')
plt.show()
dpf = X[:, 6]
plt.scatter(dpf, y)
plt.title('Excess kurtosis of the DM-SNR curve vs target_class')
plt.xlabel('Excess kurtosis of the DM-SNR curve')
plt.ylabel('target_class')
plt.show()
age = X[:, 7]
plt.scatter(age, y)
plt.title('Skewness of the DM-SNR curve vs target_class')
plt.xlabel('Skewness of the DM-SNR curve')
plt.ylabel('target_class')
plt.show()
# Splitting the dataset into the Training set and Test set
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size = 0.15, random_state = 0)
# Feature Scaling
from sklearn.preprocessing import StandardScaler
sc = StandardScaler()
X_train = sc.fit_transform(X_train)
X_test = sc.transform(X_test)
# Training the SVM model on the Training set
from sklearn.svm import SVC
classifier = SVC(kernel = 'linear', random_state = 0)
classifier.fit(X_train, y_train)
# Predicting the Test set results
y_pred = classifier.predict(X_test)
# Making the Confusion Matrix
from sklearn.metrics import confusion_matrix
cm = confusion_matrix(y_test, y_pred)
print(cm)
# R-Squared and Adjusted R-Squared values
from sklearn.metrics import r2_score
R2 = r2_score(y_test, y_pred)
print("R-square value: "+str(R2))
n = 2685
p = 8
AR2 = 1-(1-R2)*(n-1)/(n-p-1)
print("Adjusted R-square value: "+str(AR2))
| 1bcce46d5de70a13613e8c61473b439872474a44 | [
"Markdown",
"Python"
] | 2 | Markdown | deepanshu79/Pulsar-prediction | 250911fad08fac8a3cc404a9cccfc412e376c944 | 09768000f50a4cf5bca994550bc4ac56c7cff7df | |
refs/heads/master | <file_sep>import React from 'react';
import { Link } from 'react-router-dom';
import LoginForm from '../../components/LoginForm/LoginForm'
import './LoginPage.css'
import HeaderContext from '../../contexts/HeaderContext';
class LoginPage extends React.Component {
static defaultProps = {
history: {
push: () => {},
},
}
static contextType = HeaderContext
handleLoginSuccess = (token) => {
const { history } = this.props;
this.context.setAuthToken(token)
history.push('/posts')
}
render(){
return (
<>
<Link to={'/posts'}>
<h1 className='title-header'>BandBridge</h1>
</Link>
<section className='login-page'>
<h1 className='login-header'>Login</h1>
<LoginForm
onLoginSuccess={this.handleLoginSuccess}
/>
</section>
</>
)
}
}
export default LoginPage;<file_sep>import React from 'react';
import { Link } from 'react-router-dom';
import TokenService from '../../services/TokenService';
import HeaderContext from '../../contexts/HeaderContext';
import './Header.css'
class Header extends React.Component{
static contextType = HeaderContext;
handleLogoutClick = () => {
TokenService.clearAuthToken()
this.context.setAuthToken(TokenService.getAuthToken())
}
renderUserName = () => {
if(this.context.authToken){
return <h3 className='user-name'>{TokenService.getUserName()}</h3>
}
else {
return <h3 className='user-name'>Guest</h3>
}
}
renderLogoutLink() {
return (
<div className='header-login'>
{this.renderUserName()}
<Link to='/' onClick={this.handleLogoutClick}><button className='login-button'>Logout</button></Link>
</div>
)
}
renderLoginLink(){
return (
<div className='header-login'>
{this.renderUserName()}
<Link to='/login'><button className='login-button'>Login</button></Link>
{' '}
<Link to='signup'><button className='login-button'>Sign up</button></Link>
</div>
)
}
render(){
return (
<>
<Link to={'/posts'}>
<h1 className='title-header'>BandBridge</h1>
</Link>
<header className='app-header'>
{this.context.authToken
? this.renderLogoutLink()
: this.renderLoginLink()}
</header>
</>
)
}
}
export default Header;
<file_sep>import React from 'react';
import Lists from '../../Utils/Lists';
import AuthApiService from '../../services/AuthApiService';
class RegistrationForm extends React.Component {
handleSubmit = (ev) => {
ev.preventDefault();
const {user_name, password, location, instrument, styles, commitment} = ev.target;
console.log(styles.value)
this.setState({error: null});
AuthApiService.postUser({
user_name: user_name.value,
password: <PASSWORD>,
location: location.value,
instrument: instrument.value,
styles: styles.value,
commitment: commitment.value,
})
.then(() => {
user_name.value = ''
password.value = ''
this.props.onRegistrationSuccess()
})
.catch(res => {
this.setState({error: res.error})
})
}
handleInputOptions = inputs => {
return inputs.map((input, key )=> <option key={key}value={input}>{input}</option>)
}
render() {
let instruments = this.handleInputOptions(Lists.instrumentOptions);
let locations = this.handleInputOptions(Lists.locationOptions);
let styles = this.handleInputOptions(Lists.styles.sort());
let commitment = this.handleInputOptions(Lists.commitment);
return (
<form className='registraion-form' onSubmit={this.handleSubmit}>
<div className='reg-form-row'>
<label htmlFor='user_name'>Username: </label>
<input name='user_name' id='user_name' type='text' placeholder='username...' required />
</div>
<div className='reg-form-row'>
<label htmlFor='password'>Password: </label>
<input name='password' id='password' type='password' placeholder='password...' required />
</div>
<div className='reg-form-row'>
<label htmlFor='location'>Location: </label>
<select name='location' id='location' required>
{locations}
</select>
</div>
<div className='reg-form-row'>
<label htmlFor='instrument'>Instrument: </label>
<select name='instrument' id='instrument' required>
{instruments}
</select>
</div>
<div className='reg-form-row'>
<label htmlFor='styles'>Styles (Genres): </label>
<select name='styles' className='reg-form-multi' id='styles' multiple size='5' required>
{styles}
</select>
</div>
<div className='reg-form-row'>
<label htmlFor='commitment'>Hours Per Week (Availability): </label>
<select name='commitment' id='commitment' required>
{commitment}
</select>
</div>
<button type='submit' className='reg-form-button'>Register</button>
</form>
)
}
}
export default RegistrationForm;
<file_sep>import React from 'react';
import TokenService from '../services/TokenService';
const HeaderContext = React.createContext({
setAuthToken: () => {}
})
export default HeaderContext;
export class HeaderProvider extends React.Component {
state = {
authToken: TokenService.getAuthToken()
}
setAuthToken = authToken => {
this.setState({
authToken
})
}
render(){
const value = {
authToken: this.state.authToken,
setAuthToken: this.setAuthToken,
}
return (
<HeaderContext.Provider value={value}>
{this.props.children}
</HeaderContext.Provider>
)
}
}
<file_sep>import React from 'react';
const Lists = {
instrumentOptions: [
'Guitar(6)',
'Guitar(7)',
'Guiter(8)',
'Acoustic-Guitar',
'Bass(4)',
'Bass(5)',
'Acoustic-Bass',
'Double-Bass',
'Drums',
'Vocals',
'Piano',
'Keyboard',
'Violin',
'Saxophone',
'Cello',
'Flute'
],
locationOptions: [
'Montgomery (AL)',
'Juneau (AK)',
'Phoenix (AZ)',
'Little Rock (AR)',
'Sacramento (CA)',
'Denver (CO)',
'Hartford (CT)',
'Dover (DE)',
'Tallahassee (FL)',
'Atlanta (GA)',
'Honolulu (HI)',
'Boise (ID)',
'Springfield (IL)',
'Indianapolis (IN)',
'Des Moines (IA)',
'Topeka (KS)',
'Frankfort (KY)',
'Baton Rouge (LA)',
'Augusta (ME)',
'Annapolis (MD)',
'Boston (MA)',
'Lansing (MI)',
'Saint Paul (MN)',
'Jackson (MS)',
'Jefferson City (MO)',
'Helena (MT)',
'Lincoln (NE)',
'Carson City (NV)',
'Concord (NH)',
'Trenton (NJ)',
'Santa Fe (NM)',
'Albany (NY)',
'Raleigh (NC)',
'Bismarck (ND)',
'Columbus (OH)',
'Oklahoma City (OK)',
'Salem (OR)',
'Harrisburg (PA)',
'Providence (RI)',
'Columbia (SC)',
'Pierre (SD)',
'Nashville (TN)',
'Austin (TX)',
'Salt Lake City (UT)',
'Montpelier (VT)',
'Richmond (VA)',
'Olympia (WA)',
'Charleston (WV)',
'Madison (WI)',
'Cheyenne (WY)'
],
skillLvls: [
'Beginner',
'Hobbyist',
'Intermediate',
'Advanced',
'Professional'
],
styles: [
'Blues','Classic-Rock','Country','Dance','Disco','Funk','Grunge',
'Hip-Hop','Jazz','Metal','New-Age','Oldies','Other','Pop','R&B',
'Rap','Reggae','Rock','Techno','Industrial','Alternative','Ska',
'Death-Metal','Soundtrack','Euro-Techno','Ambient',
'Trip-Hop','Vocal','Fusion','Trance','Classical',
'Instrumental','Acid','House','Gospel',
'Noise','Alt-Rock','Bass','Soul','Punk','Space','Meditative',
'Instrumental-Pop','Instrumental Rock','Gothic',
'Darkwave','Techno-Industrial','Electronic','Pop-Folk',
'Eurodance','Dream','Southern-Rock','Comedy','Cult','Pop/Funk','Jungle',
'Cabaret','New-Wave','Psychadelic','Rave','Showtunes',
'Lo-Fi','Tribal','Acid-Punk','Acid Jazz','Polka','Retro',
'Musical','Rock','Hard-Rock','Folk','Folk-Rock',
'National Folk','Swing','Fast-Fusion','Bebob','Latin','Revival',
'Celtic','Bluegrass','Avantgarde','Gothic-Rock','Progressive-Rock',
'Psychedelic Rock','Symphonic Rock','Slow-Rock','Big-Band',
'Chorus','Easy-Listening','Acoustic','Chanson',
'Opera','Chamber-Music','Sonata','Symphony','Satire','Slow-Jam','Club','Tango','Samba',
'Folklore','Ballad','Power Ballad','Rhythmic Soul','Freestyle',
'Duet','Punk-Rock','Acapella','Euro-House','Dance-Hall', 'Pop-Punk',
'Alt-Metal', 'Post-Hardcore', 'Hardcore', 'Math-Rock'
],
commitment: [
'Low(1-3)',
'Mid-low(3-6)',
'Mid(6-10)',
'Mid-High(10-14)',
'High(14+)'
],
post_type: [
'Band',
'Side-Project',
'Recording',
'One-off (gig)',
'Shed'
],
makeOptions(arr){
return arr.map((item, key) => <option key={key} value={item}>{item}</option>)
},
}
export default Lists;<file_sep>import React from 'react';
import { Link } from 'react-router-dom';
import './PostListItem.css';
import { FontAwesomeIcon } from '@fortawesome/react-fontawesome'
class PostListItem extends React.Component {
state = {
isCollapsed: true
}
toggleCollapse = (ev) => {
ev.preventDefault();
this.setState({
isCollapsed: !this.state.isCollapsed
})
}
classByIndex = index => {
if (index === 0 || index % 2 === 0) {
return 'odd-item';
} else {
return 'even-item';
}
}
determineContent = post => {
let buttonText = this.buttonText();
let indexClass = this.classByIndex(this.props.index);
if(this.state.isCollapsed){
return (
<div className={indexClass}>
<Link to={`/posts/${post.id}`}>
<h2 className='item-header'>{post.style}</h2>
</Link>
<p><strong>Location: </strong>{post.location}</p>
<p><strong>Need: </strong>{post.instruments_need.split(' ').join(', ')}</p>
<p>{this.truncDesc(post.description)}</p>
<button onClick={this.toggleCollapse} className='toggle'>{buttonText}</button>
</div>
)
}
else return (
<div className={indexClass}>
<Link to={`/posts/${post.id}`}>
<h2 className='item-header'>{post.style}</h2>
</Link>
<p>Posted by: <em>{post.author.user_name}</em> on {new Date(post.author.date_created).toLocaleDateString('en-US')}</p>
<ul className='post-item-attr'>
<li><strong>Location: </strong>{post.location}</li>
<li><strong>Need: </strong>{post.instruments_need.split(' ').join(', ')}</li>
<li><strong>Style:</strong> {post.style}</li>
<li><strong>Availability:</strong> {post.commitment}</li>
<li><strong>Desired Skill:</strong> {post.skill_lvl}</li>
</ul>
<p>{post.description}</p>
<button onClick={this.toggleCollapse} className='toggle'>{buttonText}</button>
</div>
)
}
buttonText = () => {
if(this.state.isCollapsed){
return <FontAwesomeIcon size='lg' icon='arrow-alt-circle-down' />
}
else return <FontAwesomeIcon size='lg' icon='arrow-alt-circle-up' />
}
truncDesc = description => {
const newDesc = description.split(' ').splice(0, 8).join(' ').concat('...')
return newDesc;
}
render(){
const { post } = this.props;
let content = this.determineContent(post);
return (
<>
{content}
</>
)
}
}
export default PostListItem;
<file_sep>import React from 'react';
import ReactDOM from 'react-dom';
import { BrowserRouter } from 'react-router-dom';
import './index.css';
import { PostProvider } from './contexts/PostContext';
import { PostListProvider } from './contexts/PostListContext';
import {HeaderProvider} from './contexts/HeaderContext';
import App from './components/App/App';
import { library } from '@fortawesome/fontawesome-svg-core';
import {faArrowAltCircleDown, faArrowAltCircleUp} from '@fortawesome/free-solid-svg-icons';
library.add(faArrowAltCircleDown, faArrowAltCircleUp);
ReactDOM.render(
<BrowserRouter>
<PostListProvider>
<PostProvider>
<HeaderProvider>
<App />
</HeaderProvider>
</PostProvider>
</PostListProvider>
</BrowserRouter>
,
document.getElementById('root'));
<file_sep>import React from 'react';
import Header from '../../components/Header/Header';
import PostForm from '../../components/PostForm/PostForm';
class PostFormPage extends React.Component {
static defaultProps = {
histroy: {
push: () => {},
}
}
handlePostSuccess = () => {
const { history } = this.props
history.push('/posts')
}
render(){
return (
<>
<Header />
<div>
<PostForm onPostSuccess={this.handlePostSuccess} />
</div>
</>
)
}
}
export default PostFormPage;<file_sep>import React from 'react';
import ReactDOM from 'react-dom';
import CommentForm from '../components/CommentForm/CommentForm';
import renderer from 'react-test-renderer';
it('renders without crashing', () => {
const div = document.createElement('div');
ReactDOM.render(<CommentForm />, div);
ReactDOM.unmountComponentAtNode(div);
});
it('renders UI as expected', () => {
const tree = renderer
.create(<CommentForm />)
.toJSON();
expect(tree).toMatchSnapshot();
})<file_sep>import React from 'react';
import { Switch, Route } from 'react-router-dom';
import PrivateRoute from '../../Utils/PrivateRoute';
import PublicOnlyRoute from '../../Utils/PublicOnlyRoute';
import LandingPage from '../../routes/LandingPage/LandingPage';
import SignupPage from '../../routes/SignupPage/SignupPage'
import PostListPage from '../../routes/PostListPage/PostListPage'
import LoginPage from '../../routes/LoginPage/LoginPage';
import PostFormPage from '../../routes/PostFormPage/PostFormPage';
import PostPage from '../../routes/PostPage/PostPage';
import './App.css';
class App extends React.Component {
state = {
hasError: false
}
render(){
return (
<div className="App">
<Switch>
<PublicOnlyRoute
exact path={'/'}
component={LandingPage}
/>
<Route
exact path={'/posts'}
component={PostListPage}
/>
<PublicOnlyRoute
exact path={'/login'}
component={LoginPage}
/>
<PublicOnlyRoute
exact path={'/signup'}
component={SignupPage}
/>
<PrivateRoute
exact path={'/postform'}
component={PostFormPage}
/>
<PrivateRoute
path={'/posts/:postId'}
component={PostPage}
/>
</Switch>
</div>
);
}
}
export default App;
<file_sep>import React from 'react';
import AuthApiService from '../../services/AuthApiService';
class LoginForm extends React.Component {
static defaultProps = {
onLoginSuccess: () => {}
}
state = {error: null}
handleSubmitJwtAuth = ev => {
ev.preventDefault();
this.setState({error: null});
const {user_name, password} = ev.target;
AuthApiService.postLogin({
user_name: user_name.value,
password: <PASSWORD>,
})
.then(res => {
user_name.value = '';
password.value = '';
this.props.onLoginSuccess(res.authToken);
})
.catch(res => {
this.setState({error: res.error})
})
}
render(){
const {error} = this.state;
return (
<form className='login-form' onSubmit={this.handleSubmitJwtAuth}>
<div role='alert'>
{error && <p style={{color: 'red'}}>{error}</p>}
</div>
<div className='user_name form-row'>
<label htmlFor='login-user'>User name: </label>
<input className='input' type='text' name='user_name' id='login-user' required/>
</div>
<div className='password form-row'>
<label htmlFor='login-password'>Password: </label>
<input className='input' type='password' name='password' id='login-password' required/>
</div>
<button type='submit' className='login-page-button'>Login</button>
</form>
)
}
}
export default LoginForm;<file_sep>import React from 'react';
import ReactDOM from 'react-dom';
import PostListItem from '../components/PostListItem/PostListItem';
import {MemoryRouter} from 'react-router-dom';
import renderer from 'react-test-renderer';
const dummyPost = {
id: 1,
style: 'Rock',
location: 'Atlanta (GA)',
instruments_need: 'Drums Piano',
commitment: 'Low(1-3)',
skill_lvl: 'Hobbyist',
description: 'hello'
}
it('renders without crashing', () => {
const div = document.createElement('div');
ReactDOM.render(<MemoryRouter><PostListItem post={dummyPost} index={1} /></MemoryRouter>, div);
ReactDOM.unmountComponentAtNode(div);
});
it('renders UI as expected', () => {
const tree = renderer
.create(<MemoryRouter><PostListItem post={dummyPost} index={1}/></MemoryRouter>)
.toJSON();
expect(tree).toMatchSnapshot();
})<file_sep>import React from 'react';
import './LandingPage.css';
import { Link } from 'react-router-dom';
function LandingPage() {
return (
<div className='landing-page'>
<h1 className='lp-header'>BandBridge</h1>
<section className='lp-info'>
<h2>Find your band</h2>
<p className='bb-desc'>BandBridge helps connect you with artists that share your musical interests.
Make an account to join the conversation or continue as a guest to check it out.</p>
</section>
<section className='lp-links-container'>
<div className='lp-links'>
<Link to='/signup'><button className='lp-button'>Sign up</button></Link>
<Link to='/login'><button className='lp-button'>Login</button></Link>
<Link to='/posts'><button className='lp-button'>Guest</button></Link>
</div>
</section>
</div>
)
}
export default LandingPage;<file_sep>export default {
API_ENDPOINT: process.env.REACT_APP_API_ENDPOINT || 'http://localhost:8000/api',
TOKEN_KEY: 'bandbridge-key',
USER_KEY: 'bandbridge-user',
}
<file_sep>import config from '../config';
import TokenService from './TokenService';
const PostApiService = {
getAllPosts(){
return fetch(`${config.API_ENDPOINT}/posts`)
.then(res =>
(!res.ok)
? res.json().then(e => Promise.reject(e))
: res.json()
)
},
getPost(postId){
return fetch(`${config.API_ENDPOINT}/posts/${postId}`, {
headers: {
'authorization': `bearer ${TokenService.getAuthToken()}`
},
})
.then(res =>
(!res.ok)
? res.json().then(e => Promise.reject(e))
: res.json()
)
},
makePost(post){
return fetch(`${config.API_ENDPOINT}/posts`, {
method: 'POST',
headers: {
'content-type': 'application/json',
'authorization': `bearer ${TokenService.getAuthToken()}`
},
body: JSON.stringify(post),
})
.then(res =>
(!res.ok)
? res.json().then(e => Promise.reject(e))
: res.json()
)
},
getPostComments(postId){
return fetch(`${config.API_ENDPOINT}/posts/${postId}/comments`, {
headers: {
'authorization': `bearer ${TokenService.getAuthToken()}`
},
})
.then(res =>
(!res.ok)
? res.json().then(e => Promise.reject(e))
: res.json()
)
},
getPostsByLocation(location){
return fetch(`${config.API_ENDPOINT}/posts?location=${location}`, {
header: {
'authorization': `bearer ${TokenService.getAuthToken()}`
},
})
.then(res =>
(!res.ok)
? res.json().then(e => Promise.reject(e))
: res.json())
},
getFilteredPosts(...args){
console.log('args', args)
let instrument = args[0];
let location = args[1];
let queryString= `${config.API_ENDPOINT}/posts?`;
if(instrument){
queryString += `instrument=` + instrument;
}
if (location){
queryString += `&location=` + location;
}
return fetch(queryString, {
header: {
'authorization': `bearer ${TokenService.getAuthToken()}`
},
})
.then(res =>
(!res.ok)
? res.json().then(e => Promise.reject(e))
: res.json())
},
getPostsByInstrument(instrument){
return fetch(`${config.API_ENDPOINT}/posts?instrument=${instrument}`, {
header: {
'authorization': `bearer ${TokenService.getAuthToken()}`
},
})
.then(res =>
(!res.ok)
? res.json().then(e => Promise.reject(e))
: res.json())
},
postComment(postId, text){
return fetch(`${config.API_ENDPOINT}/posts/${postId}/comments`, {
method: 'POST',
headers: {
'content-type': 'application/json',
'authorization': `bearer ${TokenService.getAuthToken()}`
},
body: JSON.stringify({
post_id: postId,
text,
}),
})
.then(res =>
(!res.ok)
? res.json().then(e => Promise.reject(e))
: res.json()
)
},
}
export default PostApiService;<file_sep>import React from 'react';
import { Link } from 'react-router-dom';
import Header from '../../components/Header/Header';
import PostListItem from '../../components/PostListItem/PostListItem';
import PostListContext from '../../contexts/PostListContext';
import PostApiService from '../../services/PostApiService';
import Lists from '../../Utils/Lists';
import './PostListPage.css';
class PostListPage extends React.Component {
static contextType = PostListContext;
componentDidMount(){
this.context.clearError()
PostApiService.getAllPosts()
.then(this.context.setPostList)
.catch(this.context.setError)
}
handleClearFilter = ev => {
ev.preventDefault();
PostApiService.getAllPosts()
.then(this.context.setPostList)
.catch(this.context.setError)
}
handleFilter = ev => {
ev.preventDefault();
const {instrument_filter, location_filter } = ev.target;
PostApiService.getFilteredPosts(instrument_filter.value, location_filter.value)
.then(this.context.setPostList)
.catch(this.context.setError)
}
renderPosts() {
const { postList = []} = this.context;
return postList.map((post, index) =>
<PostListItem
index={index}
key={post.id}
post={post}/>
)
}
render() {
const {error} = this.context;
let locations = Lists.makeOptions(Lists.locationOptions);
let instruments = Lists.makeOptions(Lists.instrumentOptions)
return (
<>
<Header />
<div className='post-list-filters'>
<form onSubmit={this.handleFilter} className='filter-form'>
<select name='location_filter' defaultValue='' id='location_filter' className='filter-select'>
<option value='' disabled >Choose location</option>
{locations}
</select>
<select name='instrument_filter' defaultValue='' id='instrument_filter' className='filter-select'>
<option value='' disabled >Choose instrument</option>
{instruments}
</select>
<button type='submit' className='filter-button'>Filter</button>
<button type='button' onClick={this.handleClearFilter} className='filter-button'>Clear</button>
</form>
<Link to={'/postform'}><button className='create-post-button'>Create Post</button></Link>
</div>
{error && <div className='error'><p>Something Went Wrong</p></div>}
<div className='post-list'>
{this.renderPosts()}
</div>
</>
)
}
}
export default PostListPage;<file_sep>BandBridge Client
=================
> ## Summary
BandBridge connects musicians based on location, style, skill and availability. Users are able to make posts specifying their musical needs or aspirations and can filter their feed of posts to find projects and bands that they might be interested in. Users can also make comments on posts to start a conversation and hopefully start a band.
[Live App](https://band-bridge.ygnick.now.sh/)
Landing Page

Registration Page

Post List Page

Post Form Page

Post Page

Technologies used
* React
* react-dom
* react-router-dom
* jwt-decode
To run development(local) server - npm start
To deploy
1. npm run build
2. now ./build
| 9e097435a179563369f3feed6b205e14c178952f | [
"JavaScript",
"Markdown"
] | 17 | JavaScript | thinkful-ei-emu/nick-capstone1-client | cc79ca0b35c75c78d49c999f8d0603645bd5f11b | cf9ebb2d3d01b7d081aca3086406a44afa43607b | |
refs/heads/master | <file_sep><?php
namespace Naoned\PhpConvention;
use Composer\Script\Event;
use Symfony\Component\Process\Process;
class GitHook
{
const GIT_HOOK_PATH = '.git/hooks';
const GIT_HOOK_SCRIPTS_PATH = '/githooks';
public static function install(Event $event)
{
if (!is_dir(self::GIT_HOOK_PATH)) {
$event->getIO()->write('Ignore GitHook Script because it isn\'t launch in a git repository.');
return;
}
// Install pre-commit git hook
$cmd = sprintf(
'cp %1$s/%3$s %2$s/ && chmod +x %2$s/%3$s',
__DIR__ . self::GIT_HOOK_SCRIPTS_PATH,
self::GIT_HOOK_PATH,
'*'
);
$process = new Process($cmd);
$process->run(function ($type, $buffer) use ($event) { $event->getIO()->write($buffer, false); });
if (!$process->isSuccessful()) {
throw new \RuntimeException('CMD failed: '. $cmd);
}
}
}
<file_sep># php-convention
FROM php:5.6-cli
MAINTAINER <NAME> "<EMAIL>"
# Install git
RUN apt-get update && apt-get install -y \
git \
unzip
# Install composer
RUN curl -sS https://getcomposer.org/installer | php \
&& mv composer.phar /usr/local/bin/composer
RUN mkdir -p /usr/src/app
WORKDIR /usr/src/app
COPY composer.json /usr/src/app/
RUN composer install
COPY . /usr/src/app
<file_sep>#!/bin/bash
# Naoned - Php Convention pre-commit hook for git
#
# @author <NAME> <<EMAIL>>
# @author <NAME> <<EMAIL>>
#
function commit_valid {
# Ascii generator : http://patorjk.com/software/taag/#p=display&h=0&f=ANSI%20Shadow&t=YEAH%20BABY%2C%20You%20rock%20!%0A%0A
# echo -e "${COLOR_VALID}----------------------------------------------------------------------------------------------------------------------------------------------------\033[0m"
echo -e "${COLOR_VALID}██╗ ██╗███████╗ █████╗ ██╗ ██╗ ██████╗ █████╗ ██████╗ ██╗ ██╗ ██╗ ██╗ ██████╗ ██╗ ██╗ ██████╗ ██████╗ ██████╗██╗ ██╗ ██╗\033[0m"
echo -e "${COLOR_VALID}╚██╗ ██╔╝██╔════╝██╔══██╗██║ ██║ ██╔══██╗██╔══██╗██╔══██╗╚██╗ ██╔╝ ╚██╗ ██╔╝██╔═══██╗██║ ██║ ██╔══██╗██╔═══██╗██╔════╝██║ ██╔╝ ██║\033[0m"
echo -e "${COLOR_VALID} ╚████╔╝ █████╗ ███████║███████║ ██████╔╝███████║██████╔╝ ╚████╔╝ ╚████╔╝ ██║ ██║██║ ██║ ██████╔╝██║ ██║██║ █████╔╝ ██║\033[0m"
echo -e "${COLOR_VALID} ╚██╔╝ ██╔══╝ ██╔══██║██╔══██║ ██╔══██╗██╔══██║██╔══██╗ ╚██╔╝ ╚██╔╝ ██║ ██║██║ ██║ ██╔══██╗██║ ██║██║ ██╔═██╗ ╚═╝\033[0m"
echo -e "${COLOR_VALID} ██║ ███████╗██║ ██║██║ ██║ ██████╔╝██║ ██║██████╔╝ ██║ ▄█╗ ██║ ╚██████╔╝╚██████╔╝ ██║ ██║╚██████╔╝╚██████╗██║ ██╗ ██╗\033[0m"
echo -e "${COLOR_VALID} ╚═╝ ╚══════╝╚═╝ ╚═╝╚═╝ ╚═╝ ╚═════╝ ╚═╝ ╚═╝╚═════╝ ╚═╝ ╚═╝ ╚═╝ ╚═════╝ ╚═════╝ ╚═╝ ╚═╝ ╚═════╝ ╚═════╝╚═╝ ╚═╝ ╚═╝\033[0m"
exit 0
}
function commit_not_valid {
# Ascii generator : http://patorjk.com/software/taag/#p=display&h=0&f=ANSI%20Shadow&t=you%20shall%20not%20pass%20!%0A
# echo -e "${COLOR_NOT_VALID}--------------------------------------------------------------------------------------------------------------------------------------------------\033[0m"
echo -e "${COLOR_NOT_VALID}██╗ ██╗ ██████╗ ██╗ ██╗ ███████╗██╗ ██╗ █████╗ ██╗ ██╗ ███╗ ██╗ ██████╗ ████████╗ ██████╗ █████╗ ███████╗███████╗ ██╗\033[0m"
echo -e "${COLOR_NOT_VALID}╚██╗ ██╔╝██╔═══██╗██║ ██║ ██╔════╝██║ ██║██╔══██╗██║ ██║ ████╗ ██║██╔═══██╗╚══██╔══╝ ██╔══██╗██╔══██╗██╔════╝██╔════╝ ██║\033[0m"
echo -e "${COLOR_NOT_VALID} ╚████╔╝ ██║ ██║██║ ██║ ███████╗███████║███████║██║ ██║ ██╔██╗ ██║██║ ██║ ██║ ██████╔╝███████║███████╗███████╗ ██║\033[0m"
echo -e "${COLOR_NOT_VALID} ╚██╔╝ ██║ ██║██║ ██║ ╚════██║██╔══██║██╔══██║██║ ██║ ██║╚██╗██║██║ ██║ ██║ ██╔═══╝ ██╔══██║╚════██║╚════██║ ╚═╝\033[0m"
echo -e "${COLOR_NOT_VALID} ██║ ╚██████╔╝╚██████╔╝ ███████║██║ ██║██║ ██║███████╗███████╗ ██║ ╚████║╚██████╔╝ ██║ ██║ ██║ ██║███████║███████║ ██╗\033[0m"
echo -e "${COLOR_NOT_VALID} ╚═╝ ╚═════╝ ╚═════╝ ╚══════╝╚═╝ ╚═╝╚═╝ ╚═╝╚══════╝╚══════╝ ╚═╝ ╚═══╝ ╚═════╝ ╚═╝ ╚═╝ ╚═╝ ╚═╝╚══════╝╚══════╝ ╚═╝\033[0m"
echo ""
echo -e "\e[41m\e[97mYour commit can not be validated. Fix the errors above and try to commit again.\033[0m"
exit 1
}
TMP_STAGING=".tmp_staging"
COLOR_VALID="\e[32m"
COLOR_ERROR="\e[37m\e[43m"
COLOR_NOT_VALID="\e[31m"
PHP_FILE_PATTERN="\.(php|phtml)$"
PHPCS_BIN=bin/phpcs
PHPCS_CODING_STANDARD="$PWD/vendor/naoned/php-convention/phpcs/Iadvize/ruleset.xml"
PHPCS_IGNORE=
PHPCS_IGNORE_WARNINGS=1
PHPCS_ENCODING=utf-8
PHPMD_BIN=bin/phpmd
PHPMD_RULESET="$PWD/vendor/naoned/php-convention/phpmd/phpmd.xml"
PHPMD_FORMAT="text"
# check if phpcs binary exist
if [ ! -x $PHPCS_BIN ]; then
echo -e "${COLOR_ERROR}PHP CodeSniffer bin not found or executable -> $PHPCS_BIN\033[0m"
exit 1
fi
# check if phpmd binary exist
if [ ! -x $PHPMD_BIN ]; then
echo -e "${COLOR_ERROR}PHP CodeSniffer bin not found or executable -> $PHPCS_BIN\033[0m"
exit 1
fi
# stolen from template file
if git rev-parse --verify HEAD
then
against=HEAD
else
# Initial commit: diff against an empty tree object
against=4b825dc642cb6eb9a060e54bf8d69288fbee4904
fi
# this is the magic:
# retrieve all files in staging area that are added, modified or renamed
# but no deletions etc
FILES=$(git diff-index --name-only --cached --diff-filter=ACMR $against -- )
if [ "$FILES" == "" ]; then
exit 0
fi
# match files against whitelist
FILES_TO_CHECK=""
for FILE in $FILES
do
echo "$FILE" | egrep -q "$PHP_FILE_PATTERN"
RETVAL=$?
if [ "$RETVAL" -eq "0" ]
then
FILES_TO_CHECK="$FILES_TO_CHECK $FILE"
fi
done
if [ "$FILES_TO_CHECK" == "" ]; then
exit 0
fi
# create temporary copy of staging area
if [ -e $TMP_STAGING ]; then
rm -rf $TMP_STAGING
fi
mkdir $TMP_STAGING
# Copy contents of staged version of files to temporary staging area
# because we only want the staged version that will be commited and not
# the version in the working directory
STAGED_FILES=""
for FILE in $FILES_TO_CHECK
do
ID=$(git diff-index --cached HEAD $FILE | cut -d " " -f4)
# create staged version of file in temporary staging area with the same
# path as the original file so that the phpcs ignore filters can be applied
mkdir -p "$TMP_STAGING/$(dirname $FILE)"
git cat-file blob $ID > "$TMP_STAGING/$FILE"
STAGED_FILES="$STAGED_FILES $TMP_STAGING/$FILE"
done
# Test PHPCS
# Install PhpCS Symfony 2 code standard
PHPCS_CODE_STANDARD_SYMFONY="$PWD/vendor/squizlabs/php_codesniffer/CodeSniffer.conf"
if [ ! -f "$PHPCS_CODE_STANDARD_SYMFONY" ]; then
$PHPCS_BIN --config-set installed_paths $PWD/vendor/escapestudios/symfony2-coding-standard
fi
# execute the code sniffer
if [ "$PHPCS_IGNORE" != "" ]; then
IGNORE="--ignore=$PHPCS_IGNORE"
else
IGNORE=""
fi
if [ "$PHPCS_ENCODING" != "" ]; then
ENCODING="--encoding=$PHPCS_ENCODING"
else
ENCODING=""
fi
if [ "$PHPCS_IGNORE_WARNINGS" == "1" ]; then
IGNORE_WARNINGS="-n"
else
IGNORE_WARNINGS=""
fi
OUTPUT_PHPCS=$($PHPCS_BIN -s --colors $IGNORE_WARNINGS --standard=$PHPCS_CODING_STANDARD $ENCODING $IGNORE $TMP_STAGING)
RETVAL_PHPCS=$?
# Test PHPMD
OUTPUT_PHPMD=$($PHPMD_BIN $TMP_STAGING $PHPMD_FORMAT $PHPMD_RULESET)
RETVAL_PHPMD=$?
# delete temporary copy of staging area
rm -rf $TMP_STAGING
if [ $RETVAL_PHPCS -ne 0 ] || [ $RETVAL_PHPMD -ne 0 ]; then
if [ $RETVAL_PHPCS -ne 0 ]; then
echo -e "${COLOR_ERROR} You have PHPCS errors. Fix them ! \033[0m"
echo "$OUTPUT_PHPCS"
echo ""
fi
if [ $RETVAL_PHPMD -ne 0 ]; then
echo -e "${COLOR_ERROR} You have PHPMD errors. Fix them ! \033[0m"
echo "$OUTPUT_PHPMD"
echo ""
fi
commit_not_valid
fi
exit 0
| 21dde328e4d0bd0597ad85de2cb675140385eed8 | [
"Dockerfile",
"PHP",
"Shell"
] | 3 | PHP | naoned/php-convention | 6f587f0b3532998c0f988ec441f944f296a4b62c | 938c0d37d8f32b05fc9950e20f21b3e789c9726c | |
refs/heads/main | <repo_name>lopezbraian/api-task<file_sep>/app.js
const express = require('express')
const app = express()
const cors = require('cors')
const bodyParser = require('body-parser')
const route = require('./routes')
const { errorHandler, wrapwithBoom, routeNoFound } = require('./middlewares/errorHandlers')
//Middlwares
app.use(cors())
app.use(bodyParser.json())
app.use(express.urlencoded({ extended: false }))
//Route
app.use('/api', route)
// Handle Route No found
app.use(routeNoFound)
app.use(wrapwithBoom)
app.use(errorHandler)
module.exports = app;<file_sep>/docker-compose.yml
version: "3.9"
services:
api:
container_name: api-task
build: .
volumes:
- .:/usr/src/app
ports:
- "3000:3000"
links:
- db
db:
container_name: db_task
image: mongo
volumes:
- ./db:/data/db
ports:
- "27017:27017"<file_sep>/Dockerfile
FROM node:lts-alpine3.10
RUN mkdir -p /usr/src/app
WORKDIR /usr/src/app
COPY ./package.json ./package.json
RUN npm install
RUN npm install -g nodemon
COPY . /usr/src/app
EXPOSE 3000
CMD ["npm" ,"run", "dev"]
<file_sep>/components/tasks/controller/index.js
const TaskService = require("../../../services/task");
class TaskController {
constructor() {
this.taskService = new TaskService();
}
async getAll(req, res, next) {
try {
const Task = await this.taskService.getAll();
return res.status(200).json({
data: Task,
message: "Todas las tareas",
});
} catch (err) {
next(err);
}
}
async create(req, res, next) {
const { content, title } = req.body;
const newProduct = {
content,
title,
};
try {
const task = await this.taskService.create(newProduct);
return res.status(201).json({
data: task,
message: "Task creada",
});
} catch (err) {
next(err);
}
}
async getBy(req, res, next) {
const { id } = req.params;
try {
const task = await this.taskService.getBy(id);
return res.status(200).json({
data: task,
});
} catch (err) {
next(err);
}
}
async delete(req, res, next) {
const { id } = req.params;
try {
const resp = await this.taskService.delete(id);
if (resp.deletedCount !== 0) {
return res.status(200).json({
message: "Borrado correctamente",
});
}
} catch (err) {
next(err);
}
}
async edit(req, res, next) {
const { newTask, id } = req.body;
try {
const resp = await this.taskService.edit(id, newTask);
return res.status(200).json({
data: resp,
message: "Task actualizada",
});
} catch (err) {
next(err);
}
}
}
module.exports = TaskController;
<file_sep>/README.md
# api-task
## Routes
<strong>GET</strong> api/task
<strong>GET</strong> api/task/:id
<strong>POST</strong> api/task
<strong>DELETE</strong> api/task/:id
<strong>PUT</strong> api/task/
<file_sep>/config/index.js
require('dotenv').config()
const uriDb = process.env.URI_DB
const mode = process.env.NODE_ENV
module.exports = {
uriDb,
mode
}<file_sep>/utils/schema/task.js
const joi = require("joi");
const createTask = joi.object({
content: joi.string().max(40).required(),
title: joi.string().max(20).required(),
done: joi.boolean(),
});
const idTask = joi.object({
id: joi.string().regex(/^[0-9a-fA-F]{24}$/),
});
const updateTask = joi.object({
newTask: {
content: joi.string().max(40),
title: joi.string().max(20),
done: joi.boolean(),
},
id: joi.string().regex(/^[0-9a-fA-F]{24}$/),
});
module.exports = {
createTask,
idTask,
updateTask,
};
<file_sep>/middlewares/errorHandlers.js
const boom = require("@hapi/boom");
function wrapwithBoom(err, req, res, next) {
if (!err.isBoom) {
next(boom.badImplementation(err));
}
next(err);
}
function errorHandler(err, req, res, next) {
const {
output: { statusCode, payload },
} = err;
res.status(statusCode).json(payload);
}
function routeNoFound(req, res) {
const { output } = boom.notFound();
res.status(output.statusCode).json(output.payload);
}
module.exports = { errorHandler, wrapwithBoom, routeNoFound };
| afc362821906ecdfb5ce4796674e81a9ca502331 | [
"JavaScript",
"Markdown",
"YAML",
"Dockerfile"
] | 8 | JavaScript | lopezbraian/api-task | 49c1e051cb29a40081596d28f7eff83e79c6b4b2 | 6742fd02ba770fba321b35cce1c3354966fb358a | |
refs/heads/master | <file_sep>/* Hello World Example
This example code is in the Public Domain (or CC0 licensed, at your option.)
Unless required by applicable law or agreed to in writing, this
software is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
CONDITIONS OF ANY KIND, either express or implied.
*/
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "esp_system.h"
#include "esp_spi_flash.h"
#include <sodium.h>
#include <string.h>
#define PASSWORD "<PASSWORD>"
void app_main()
{
// Bree: This is for troubleshooting / error-handling for libsodium (i.e. argon2)
if (sodium_init() < 0)
{
printf("Sodium failed!\n");
return;
}
char hashed_password[crypto_pwhash_STRBYTES];
unsigned char salt[crypto_pwhash_SALTBYTES];
unsigned char key[crypto_box_SEEDBYTES];
randombytes_buf(salt, sizeof salt);
// Bree: All print statements for error handling tests for argon2 functionality....
// Bree: Remember!!!!
// In menuconfig: set MAIN TASK SIZE >= 8192 and set XTAL FREQ = 26 MHz
if (crypto_pwhash_str(hashed_password, PASSWORD, strlen(PASSWORD), 3, 3) == -1) {
printf("FAILED1!\n");
}
if (crypto_pwhash_str(hashed_password, PASSWORD, strlen(PASSWORD), 3, 10) == -1) {
printf("FAILED2!\n");
}
if (crypto_pwhash_str(hashed_password, PASSWORD, strlen(PASSWORD), 3, 50) == -1) {
printf("FAILED3!\n");
}
if (crypto_pwhash_str(hashed_password, PASSWORD, strlen(PASSWORD), 3, 150) == -1) {
printf("FAILED4!\n");
}
if (crypto_pwhash_str(hashed_password, PASSWORD, strlen(PASSWORD), crypto_pwhash_OPSLIMIT_SENSITIVE, 512) == -1) {
printf("FAILED5!\n");
}
if (crypto_pwhash_str(hashed_password, PASSWORD, strlen(PASSWORD), 3, 1024) == -1) {
printf("FAILED6!\n");
}
if (crypto_pwhash_str(hashed_password, PASSWORD, strlen(PASSWORD), 3, 4096) == -1) {
printf("FAILED7!\n");
}
if (crypto_pwhash_str(hashed_password, PASSWORD, strlen(PASSWORD), 3, 500000) == -1) {
printf("FAILED8!\n");
}
if (crypto_pwhash_str(hashed_password, <PASSWORD>, strlen(PASSWORD), 3, crypto_pwhash_MEMLIMIT_INTERACTIVE) == -1) {
printf("FAILED9!\n");
}
if (crypto_pwhash(key, sizeof key, PASSWORD, strlen(PASSWORD), salt, 3, 100, crypto_pwhash_ALG_DEFAULT) != 0)
{
printf("FAILED10!\n");
}
if (crypto_pwhash(key, sizeof key, PASSWORD, strlen(PASSWORD), salt, crypto_pwhash_OPSLIMIT_INTERACTIVE, crypto_pwhash_MEMLIMIT_INTERACTIVE, crypto_pwhash_ALG_DEFAULT) != 0)
{
printf("FAILED11!\n");
}
//return;
// Bree: Here is the basic Hello World program (no more libsodium / argon2 error finding)
printf("Hello world!\n");
printf("\nWe are:\nBree\nDamon\nKouma\nSohin\n\n");
/* Print chip information */
esp_chip_info_t chip_info;
esp_chip_info(&chip_info);
printf("This is ESP32 chip with %d CPU cores, WiFi%s%s, ",
chip_info.cores,
(chip_info.features & CHIP_FEATURE_BT) ? "/BT" : "",
(chip_info.features & CHIP_FEATURE_BLE) ? "/BLE" : "");
printf("\nsilicon revision %d, ", chip_info.revision);
printf("\n%dMB %s flash\n", spi_flash_get_chip_size() / (1024 * 1024),
(chip_info.features & CHIP_FEATURE_EMB_FLASH) ? "embedded" : "external");
for (int i = 10; i >= 0; i--) {
printf("\nRestarting in %d seconds...\n", i);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
printf("Restarting now.\n");
fflush(stdout);
esp_restart();
}
<file_sep>[X] Implement vTaskDelayUntil() to make the working sensors periodic.
[ ] Get dust sensor working
[ ] Get gas sensor working
[X] MQTT stuff
[ ] Output to LEDs from Manager
[ ] Write to OLED
[ ] Change Manager to require a password on startup
[ ] Check for USB input periodically in manager and have manager require a password then
[ ] Check 5V(?) voltage via GPIO, it is apparently connected directly to the USB connection
[ ] Implement user controls after password put in
[ ] Check a flag to output read values to screen only after password input
[ ] Install a battery so code can continue after disconnection<file_sep>//Example static (known stack size) task creation:
// Dimensions the buffer that the task being created will use as its stack.
// NOTE: This is the number of words the stack will hold, not the number of
// bytes. For example, if each stack item is 32-bits, and this is set to 100,
// then 400 bytes (100 * 32-bits) will be allocated.
#define STACK_SIZE 200
// Structure that will hold the TCB of the task being created.
StaticTask_t xTaskBuffer;
// Buffer that the task being created will use as its stack. Note this is
// an array of StackType_t variables. The size of StackType_t is dependent on
// the RTOS port.
StackType_t xStack[ STACK_SIZE ];
// Function that implements the task being created.
void vTaskCode( void * pvParameters )
{
// The parameter value is expected to be 1 as 1 is passed in the
// pvParameters value in the call to xTaskCreateStatic().
configASSERT( ( uint32_t ) pvParameters == 1UL );
for( ;; )
{
// Task code goes here.
}
}
// Function that creates a task.
void vOtherFunction( void )
{
TaskHandle_t xHandle = NULL;
// Create the task without using any dynamic memory allocation.
xHandle = xTaskCreateStatic(
vTaskCode, // Function that implements the task.
"NAME", // Text name for the task.
STACK_SIZE, // Stack size in words, not bytes.
( void * ) 1, // Parameter passed into the task.
tskIDLE_PRIORITY,// Priority at which the task is created.
xStack, // Array to use as the task's stack.
&xTaskBuffer ); // Variable to hold the task's data structure.
// puxStackBuffer and pxTaskBuffer were not NULL, so the task will have
// been created, and xHandle will be the task's handle. Use the handle
// to suspend the task.
vTaskSuspend( xHandle );
//vTaskResume( xHandle ); to resume the task and it will run in accordance to its priority
//INCLUDE_vTaskSuspend must be set to 1 otherwise can't supsend and resume tasks
}
//relative delay for a task
void vTaskFunction( void * pvParameters )
{
// Block for 500ms.
const TickType_t xDelay = 500 / portTICK_PERIOD_MS;
for( ;; )
{
// Simply toggle the LED every 500ms, blocking between each toggle.
vToggleLED();
vTaskDelay( xDelay );
}
}
//takes taskhandle and gives the human-readable task name
char *pcTaskGetTaskName(TaskHandle_t xTaskToQuery)
//returns start of stack for xTask
uint8_t *pxTaskGetStackStart(TaskHandle_t xTask) //use NULL for xTask to return start stack of current task
<file_sep>#include <string.h>
#include <stdlib.h>
#include "sdkconfig.h"
#include "MQTTClient.h"
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "freertos/event_groups.h"
#include "esp_wifi.h"
#include "esp_event_loop.h"
#include "esp_system.h"
#include "esp_task_wdt.h"
#include "esp_attr.h"
#include "nvs_flash.h"
#include "driver/gpio.h"
#include "driver/ledc.h"
#include "lwip/err.h"
#include "lwip/sockets.h"
#include "lwip/sys.h"
#include "lwip/netdb.h"
#include "lwip/dns.h"
#include "mbedtls/platform.h"
#include "mbedtls/net.h"
#include "mbedtls/debug.h"
#include "mbedtls/ssl.h"
#include "mbedtls/entropy.h"
#include "mbedtls/ctr_drbg.h"
#include "mbedtls/error.h"
#include "mbedtls/certs.h"
#include "fileTable.h"
#include "qExample.c"
/* The examples use simple WiFi configuration that you can set via
'make menuconfig'.
If you'd rather not, just change the below entries to strings with
the config you want - ie #define EXAMPLE_WIFI_SSID "mywifissid"
*/
/* The event group allows multiple bits for each event,
but we only care about one event - are we connected
to the AP with an IP? */
const int CONNECTED_BIT = BIT0;
/* Constants that aren't configurable in menuconfig */
#define MQTT_SERVER "m15.cloudmqtt.com"
#define MQTT_USER "afhnaorh"
#define MQTT_PASS "<PASSWORD>"
#define MQTT_PORT 33521
#define MQTT_CLIENTID "GROUP2"
#define MQTT_WEBSOCKET 1 // 0=no 1=yes
#define MQTT_BUF_SIZE 512
xSemaphoreHandle print_mux;
static unsigned char mqtt_sendBuf[MQTT_BUF_SIZE];
static unsigned char mqtt_readBuf[MQTT_BUF_SIZE];
//static unsigned char mqtt_sendBuf[MQTT_BUF_SIZE];
//static unsigned char mqtt_readBuf[MQTT_BUF_SIZE];
static const char *CTAG = "MQTTS";
/* FreeRTOS event group to signal when we are connected & ready to make a request */
EventGroupHandle_t wifi_event_group;
static esp_err_t event_handler(void *ctx, system_event_t *event)
{
switch(event->event_id) {
case SYSTEM_EVENT_STA_START:
esp_wifi_connect();
break;
case SYSTEM_EVENT_STA_GOT_IP:
xEventGroupSetBits(wifi_event_group, CONNECTED_BIT);
break;
case SYSTEM_EVENT_STA_DISCONNECTED:
/* This is a workaround as ESP32 WiFi libs don't currently
auto-reassociate. */
esp_wifi_connect();
xEventGroupClearBits(wifi_event_group, CONNECTED_BIT);
break;
default:
break;
}
return ESP_OK;
}
void mqtt_task(void *pvParameters)
{
int ret;
//define values used in vTaskDelayUntil() to make task periodic
TickType_t xLastWakeTime;
//portTICK_RATE_MS converts from ms to ticks, allowing me to say
//"10000 milliseconds" and not care about my board's tick rate
const TickType_t xFrequency = 5000*portTICK_RATE_MS;
while(1){
xLastWakeTime = xTaskGetTickCount();
Network network;
MQTTClient client;
NetworkInit(&network);
network.websocket = MQTT_WEBSOCKET;
xEventGroupWaitBits(wifi_event_group, CONNECTED_BIT, false, true, portMAX_DELAY);
ret = NetworkConnect(&network, MQTT_SERVER, MQTT_PORT);
MQTTClientInit(&client, &network,
2000, // command_timeout_ms
mqtt_sendBuf, //sendbuf,
MQTT_BUF_SIZE, //sendbuf_size,
mqtt_readBuf, //readbuf,
MQTT_BUF_SIZE //readbuf_size
);
char buf[30];
MQTTString clientId = MQTTString_initializer;
sprintf(buf, MQTT_CLIENTID);
clientId.cstring = buf;
MQTTString username = MQTTString_initializer;
username.cstring = MQTT_USER;
MQTTString password = MQTTString_initializer;
password.cstring = MQTT_PASS;
MQTTPacket_connectData data = MQTTPacket_connectData_initializer;
data.clientID = clientId;
data.willFlag = 0;
data.MQTTVersion = 4; // 3 = 3.1 4 = 3.1.1
data.keepAliveInterval = 5;
data.cleansession = 1;
data.username = username;
data.password = <PASSWORD>;
ret = MQTTConnect(&client, &data);
if (ret != SUCCESS) {
printf("\n\nError I think: %d\n\n",ret);
goto exit;
}
printf("\n\nError I thinkn't: %d\n\n",ret);
char msgbuf[200];
MQTTMessage message;
message.qos = QOS0;
message.retained = false;
message.dup = false;
message.payload = (void*)msgbuf;
message.payloadlen = strlen(msgbuf)+1;
toComm = popW();
switch(toComm%10){
case 0:
//publish to iupui/group2/temperature
printf("\n\nPublish to temp\n\n");
sprintf(msgbuf, "{\"temperature\":%d,}", toComm);
printf("\n\n%s\n\n",msgbuf);
ret = MQTTPublish(&client, "iupui/group2/", &message);
//toComm = -1234;
if (ret != SUCCESS) {
printf("MQTTPublish NOT: %d", ret);
}else{
printf("MQTTPublish wait I think it did: %d",ret);
}
break;
case 1:
//publish to iupui/group2/humidity
printf("\n\nPublish to humidity\n\n");
sprintf(msgbuf, "{\"humidity\":%d,}", toComm);
printf("\n\n%s\n\n",msgbuf);
ret = MQTTPublish(&client, "iupui/group2/", &message);
toComm = -1234;
if (ret != SUCCESS) {
printf("MQTTPublish NOT: %d", ret);
}else{
printf("MQTTPublish wait I think it did: %d",ret);
}
break;
case 2:
//publish to iupui/group2/light
printf("\n\nPublish to light\n\n");
sprintf(msgbuf, "{\"light\":%d,}", toComm);
printf("\n\n%s\n\n",msgbuf);
ret = MQTTPublish(&client, "iupui/group2/", &message);
toComm = -1234;
if (ret != SUCCESS) {
printf("MQTTPublish NOT: %d", ret);
}else{
printf("MQTTPublish wait I think it did: %d",ret);
}
break;
default:
//no publish
printf("\n\n | COMM TASK ERROR | Out-of-bounds value put into toComm\n\n");
break;
}//end switch case
//printf("\n\n| COMM TASK | Before delay\n\n");
vTaskDelayUntil(&xLastWakeTime, xFrequency);
//printf("\n\n| COMM TASK | After delay\n\n");
exit:
MQTTDisconnect(&client);
NetworkDisconnect(&network);
}
}
static void initialise_wifi(void)
{
tcpip_adapter_init();
wifi_event_group = xEventGroupCreate();
ESP_ERROR_CHECK( esp_event_loop_init(event_handler, NULL) );
wifi_init_config_t cfg = WIFI_INIT_CONFIG_DEFAULT();
ESP_ERROR_CHECK( esp_wifi_init(&cfg) );
ESP_ERROR_CHECK( esp_wifi_set_storage(WIFI_STORAGE_RAM) );
wifi_config_t wifi_config = {
.sta = {
.ssid = "AndroidAP",
.password = "<PASSWORD>",
},
};
ESP_LOGI(CTAG, "Setting WiFi configuration SSID %s...", wifi_config.sta.ssid);
ESP_ERROR_CHECK( esp_wifi_set_mode(WIFI_MODE_STA) );
ESP_ERROR_CHECK( esp_wifi_set_config(ESP_IF_WIFI_STA, &wifi_config) );
ESP_ERROR_CHECK( esp_wifi_start() );
}
void comms_open()
{
nvs_flash_init();
initialise_wifi();
// MQTT and TLS needs a deep stack, 12288 seems to work, anything
// less will get us a stack overflow error
xTaskCreate(&mqtt_task, "mqtt_task", 12288, NULL, 5, NULL);
}
<file_sep>/*
* MIT License
*
* Copyright (c) 2017 <NAME>
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* @file tsl2561.h
* @brief Interface definitions for the ESP32-compatible TSL2561 Light to Digital Converter component.
*
* This component provides structures and functions that are useful for communicating with the device.
*
* Technically, the TSL2561 device is an I2C not SMBus device, however the datasheet makes it clear
* that most SMBus operations are compatible with this device, so it makes sense to use an SMBus interface
* to manage communication.
*/
#ifndef TSL2561_H
#define TSL2561_H
#include <stdbool.h>
#include "../../esp32-smbus/include/smbus.h"
#ifdef __cplusplus
extern "C" {
#endif
/**
* @brief Enum for recognised TSL256x devices
*/
typedef enum
{
TSL2561_DEVICE_TYPE_INVALID = 0b1111, ///< Invalid device
TSL2561_DEVICE_TYPE_TSL2560CS = 0b0000, ///< TSL2560CS (Chipscale)
TSL2561_DEVICE_TYPE_TSL2561CS = 0b0001, ///< TSL2561CS (Chipscale)
TSL2561_DEVICE_TYPE_TSL2560T_FN_CL = 0b0100, ///< TSL2560T/FN/CL (TMB-6 or Dual Flat No-Lead-6 or ChipLED-6)
TSL2561_DEVICE_TYPE_TSL2561T_FN_CL = 0b0101, ///< TSL2561T/FN/CL (TMB-6 or Dual Flat No-Lead-6 or ChipLED-6)
} tsl2561_device_type_t;
/**
* @brief Enum for supported integration durations.
* These durations assume the default internal oscillator frequency of 735 kHz.
*/
typedef enum
{
TSL2561_INTEGRATION_TIME_13MS = 0x00, ///< Integrate over 13.7 milliseconds
TSL2561_INTEGRATION_TIME_101MS = 0x01, ///< Integrate over 101 milliseconds
TSL2561_INTEGRATION_TIME_402MS = 0x02, ///< Integrate over 402 milliseconds
} tsl2561_integration_time_t;
/**
* @brief Enum for supported gain values.
*/
typedef enum
{
TSL2561_GAIN_1X = 0x00,
TSL2561_GAIN_16X = 0x10,
} tsl2561_gain_t;
typedef uint8_t tsl2561_revision_t; ///< The type of the IC's revision value
typedef uint16_t tsl2561_visible_t; ///< The type of a visible light measurement value
typedef uint16_t tsl2561_infrared_t; ///< The type of an infrared light measurement value
/**
* @brief Structure containing information related to the SMBus protocol.
*/
typedef struct
{
bool init; ///< True if struct has been initialised, otherwise false
bool powered; ///< True if the device has been powered up
smbus_info_t * smbus_info; ///< Pointer to associated SMBus info
tsl2561_device_type_t device_type; ///< Detected type of device (Chipscale vs T/FN/CL)
tsl2561_integration_time_t integration_time; ///< Current integration time for measurements
tsl2561_gain_t gain; ///< Current gain for measurements
} tsl2561_info_t;
/**
* @brief Construct a new TSL2561 info instance.
* New instance should be initialised before calling other functions.
* @return Pointer to new device info instance, or NULL if it cannot be created.
*/
tsl2561_info_t * tsl2561_malloc(void);
/**
* @brief Delete an existing TSL2561 info instance.
* @param[in,out] tsl2561_info Pointer to TSL2561 info instance that will be freed and set to NULL.
*/
void tsl2561_free(tsl2561_info_t ** tsl2561_info);
/**
* @brief Initialise a TSL2561 info instance with the specified SMBus information.
* @param[in] tsl2561_info Pointer to TSL2561 info instance.
* @param[in] smbus_info Pointer to SMBus info instance.
*/
esp_err_t tsl2561_init(tsl2561_info_t * tsl2561_info, smbus_info_t * smbus_info);
/**
* @brief Retrieve the Device Type ID and Revision number from the device.
* @param[in] tsl2561_info Pointer to initialised TSL2561 info instance.
* @param[out] device The retrieved Device Type ID.
* @param[out] revision The retrieved Device Revision number.
* @return ESP_OK if successful, ESP_FAIL or ESP_ERR_* if an error occurred.
*/
esp_err_t tsl2561_device_id(const tsl2561_info_t * tsl2561_info, tsl2561_device_type_t * device, tsl2561_revision_t * revision);
/**
* @brief Set the integration time and gain. These values are set together
* as they are programmed via the same register.
* @param[in] tsl2561_info Pointer to initialised TSL2561 info instance.
* @param[out] integration_time The integration time to use for the next measurement.
* @param[out] infrared The gain setting to use for the next measurement.
* @return ESP_OK if successful, ESP_FAIL or ESP_ERR_* if an error occurred.
*/
esp_err_t tsl2561_set_integration_time_and_gain(tsl2561_info_t * tsl2561_info, tsl2561_integration_time_t integration_time, tsl2561_gain_t gain);
/**
* @brief Retrieve a visible and infrared light measurement from the device.
* This function will sleep until the integration time has passed.
* @param[in] tsl2561_info Pointer to initialised TSL2561 info instance.
* @param[out] visible The resultant visible light measurement.
* @param[out] infrared The resultant infrared light measurement.
* @return ESP_OK if successful, ESP_FAIL or ESP_ERR_* if an error occurred.
*/
esp_err_t tsl2561_read(tsl2561_info_t * tsl2561_info, tsl2561_visible_t * visible, tsl2561_infrared_t * infrared);
/**
* @brief Compute the Lux approximation from a visible and infrared light measurement.
* The calculation is performed according to the procedure given in the datasheet.
* @param[in] tsl2561_info Pointer to initialised TSL2561 info instance.
* @param[in] visible The visible light measurement.
* @param[in] infrared The infrared light measurement.
* @return The resulting approximation of the light measurement in Lux.
*/
uint32_t tsl2561_compute_lux(const tsl2561_info_t * tsl2561_info, tsl2561_visible_t visible, tsl2561_infrared_t infrared);
#ifdef __cplusplus
}
#endif
#endif // TSL2561_H
<file_sep>/*
ECE-566 RTOS - FALL 2018
Adapted by <NAME>
For: DHT22 Temp & Humidity Sensor + LED
*/
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "esp_system.h"
#include "rom/ets_sys.h"
#include "nvs_flash.h"
#include "driver/gpio.h"
#include "sdkconfig.h"
#include "DHT22.h"
#include "qExample.c"
#include "fileTable.h"
#ifndef DHT_MAIN
#define DHT_MAIN
//#define BLINK_GPIO CONFIG_BLINK_GPIO // Bree: These are for setting via menuconfig but we don't need
//Damon: Create a task handle that's very visible to allow sensor.c to view it in the most unsafe manner possible
TaskHandle_t handleDHT = NULL;
TaskHandle_t handleHumid = NULL;
int temp_read();
int humid_read();
int tempValue = 0; //read updates these values, write puts them onto the queue
int humidValue = 0;
float SSt = 0; //to find the standard deviation of temperature task, take sum of values and sum of squared values, then divide by n
float St = 0;
int nt = 0;
float SSh = 0;
float Sh = 0;
int nh = 0;
int TEMP_TASK_PERIOD = 10000;
int HUMID_TASK_PERIOD = 10000;
// Bree: define DHT22 task function
void temp_task(void *pvParameter)
{
//define values used in vTaskDelayUntil() to make task periodic
TickType_t xLastWakeTime;
TickType_t x2DoneWithTask;
const TickType_t xFrequency = TEMP_TASK_PERIOD*portTICK_RATE_MS;
while(1){
xLastWakeTime = xTaskGetTickCount();
temp_read(); //temp_task periodically updates the value
//For dev use only. Used in determining task characteristics
x2DoneWithTask = xTaskGetTickCount();
St += x2DoneWithTask - xLastWakeTime;
SSt += (x2DoneWithTask - xLastWakeTime)*(x2DoneWithTask - xLastWakeTime);
nt++;
printf("\n\nTemp Task Info: %d Ticks to complete task",(x2DoneWithTask - xLastWakeTime));
printf("\nTemp Task Info: %f is Standard Deviation", ((SSt/nt) - (St/nt)*(St/nt))); //this is 0 because DHT22 takes 3 ticks to read by design
printf("\nTemp Task Info: %d is Temperature Slack Time", 100 - (x2DoneWithTask - xLastWakeTime));
printf("\nTemp Task Info: %d is utilization", (x2DoneWithTask - xLastWakeTime)/TEMP_TASK_PERIOD);
printf("\nTemp Task Info: %d is Task Period", TEMP_TASK_PERIOD);
//
vTaskDelayUntil(&xLastWakeTime, xFrequency);
}
}
void humid_task(void *pvParameter)
{
//see temp_task for explanation of periodic task control
TickType_t hLastWakeTime;
TickType_t h2DoneWithTask;
const TickType_t hFrequency = 10000*portTICK_RATE_MS;
while(1){
hLastWakeTime = xTaskGetTickCount();
humid_read(); //temp_task periodically updates the value
//For dev use only. Used in determining task characteristics
h2DoneWithTask = xTaskGetTickCount();
Sh += h2DoneWithTask - hLastWakeTime;
SSh += (h2DoneWithTask - hLastWakeTime)*(h2DoneWithTask - hLastWakeTime);
nh++;
printf("\n\nTemp Task Info: %d Ticks to complete task",(h2DoneWithTask - hLastWakeTime));
printf("\nTemp Task Info: %f is Standard Deviation", ((SSh/nh) - (Sh/nh)*(Sh/nh))); //this is 0 because DHT22 takes 3 ticks to read by design
printf("\nTemp Task Info: %d is Temperature Slack Time", 100 - (h2DoneWithTask - hLastWakeTime));
printf("\nTemp Task Info: %d is utilization", (h2DoneWithTask - hLastWakeTime)/HUMID_TASK_PERIOD);
printf("\nTemp Task Info: %d is Task Period", HUMID_TASK_PERIOD);
//
vTaskDelayUntil(&hLastWakeTime, hFrequency);
}
}
//Bree: define LED Blink task function
void blink_task(void *pvParameter)
{
/* Configure the IOMUX register for pad BLINK_GPIO (some pads are
muxed to GPIO on reset already, but some default to other
functions and need to be switched to GPIO. Consult the
Technical Reference for a list of pads and their default
functions.)
*/
//gpio_pad_select_gpio(LEDgpio);
setLEDgpio( 2 );
/* Set the GPIO as a push/pull output */
gpio_set_direction(2, GPIO_MODE_OUTPUT);
while(1) {
/* Blink off (output low) */
gpio_set_level(2, 0);
vTaskDelay(1000 / portTICK_PERIOD_MS);
/* Blink on (output high) */
gpio_set_level(2, 1);
vTaskDelay(1000 / portTICK_PERIOD_MS);
}
}
int temp_read(){
setDHTgpio( 23 ); // Bree: 23 is what I chose to set my default gpio for DHT
int ret = readDHT();
errorHandler(ret);
tempValue = getTemperature();
return getTemperature();
}
void temp_ioctl(int input){ //change values of temp
TEMP_TASK_PERIOD = input;
}
int temp_write(){
int ret = tempValue;
ret = ret*10; //the "name" for temperature is put into the ones' place, so value needs to be multiplied by 10 to make room
ret = ret+0; //name for temperature is 0
pushQ(&ret);
return ret;
}
void humid_ioctl(int input){
HUMID_TASK_PERIOD = input;
}
void humid_close(){
vTaskSuspend(handleHumid);
}
void temp_close(){
vTaskSuspend(handleDHT);
}
void temp_open()
{
if(handleDHT == NULL){ //on first call, define this task
// DHT22 Read task code (from original DHT22 program)
nvs_flash_init();
vTaskDelay( 1000 / portTICK_RATE_MS );
xTaskCreate( &temp_task, "TempTask", 2048, NULL, 5, &handleDHT );
struct row tempRow;
tempRow.read = temp_read;
tempRow.name = 1;
tempRow.write = temp_write;
tempRow.close = temp_close;
tempRow.open = temp_open;
devTable[rowsInUse++] = tempRow;
}else{ //on every other call, resume this task
vTaskResume(handleDHT);
}
// LED Blink task code (from original LED Blink program)
//xTaskCreate(&blink_task, "blink_task", configMINIMAL_STACK_SIZE, NULL, 5, NULL);
}
int humid_read(){
setDHTgpio( 23 ); // Bree: 23 is what I chose to set my default gpio for DHT
int ret = readDHT();
errorHandler(ret);
//printf("\n| Humid_Read | Humidity Task has this name: %d",devTable[1].name);
return getHumidity();
}
int humid_write(){
// Initialise the xLastWakeTime variable with the current time.
int ret = (int)getHumidity();
ret = ret*10; //the "name" for humidity is put into the ones place of the read value
ret += 1; //humidity = 1
pushQ(&ret); //puts humidity value onto queue
return ret;
}
void humid_open()
{
if(handleHumid == NULL){ //on first call, define this task
// DHT22 Read task code (from original DHT22 program)
nvs_flash_init();
vTaskDelay( 1000 / portTICK_RATE_MS );
//Damon: These task definitions mean it only looks at humidity side of things
xTaskCreate( &humid_task, "HumidTask", 2048, NULL, 5, &handleHumid );
struct row humidRow;
humidRow.read = humid_read;
humidRow.write = humid_write;
humidRow.open = humid_open;
humidRow.close = humid_close;
humidRow.name = 2;
devTable[rowsInUse++] = humidRow;
}else{ //on every other call, resume this task
vTaskResume(handleHumid);
}
}
#endif
<file_sep>/* queues.c */
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "freertos/queue.h"
#ifndef QUEUE_MAIN
#define QUEUE_MAIN
QueueHandle_t q=NULL;
QueueHandle_t w=NULL;
/*
void consumer_task(void *pvParameter)
{
unsigned long counter;
if(q == NULL){
printf("Queue is not ready");
return;
}
while(1){
xQueueReceive(q,&counter,(TickType_t )(1000/portTICK_PERIOD_MS));
printf("value received on queue: %lu \n",counter);
vTaskDelay(500/portTICK_PERIOD_MS); //wait for 500 ms
}
}
*/
/*
void producer_task(void *pvParameter){
unsigned long counter=1;
if(q == NULL){
printf("Queue is not ready \n");
return;
}
while(1){
printf("value sent on queue: %lu \n",counter);
xQueueSend(q,(void *)&counter,(TickType_t )0); // add the counter value to the queue
counter++;
vTaskDelay(1000/portTICK_PERIOD_MS); //wait for a second
}
}
*/
void pushQ(int* input){
xQueueSend(q,input,(TickType_t)0);
//printf("\nValue sent to queue: %d\n",*input);
vTaskDelay(1000/portTICK_PERIOD_MS);
}
int popQ(){
unsigned long storage;
xQueueReceive(q,&storage,(TickType_t )(1000/portTICK_PERIOD_MS));
//printf("\nValue read off queue: %lu \n",storage);
return (int)storage;
}
int popW(){ //w holds the values manager wants communicated
unsigned long storage;
xQueueReceive(w,&storage,(TickType_t )(1000/portTICK_PERIOD_MS));
//printf("\nValue read off queue: %lu \n",storage);
return (int)storage;
}
void pushW(int* input){
xQueueSend(w,input,(TickType_t)0);
//printf("\nValue sent to queue: %d\n",*input);
vTaskDelay(1000/portTICK_PERIOD_MS);
}
void q_app_main()
{
q=xQueueCreate(20,sizeof(unsigned long));
w=xQueueCreate(20,sizeof(unsigned long));
if(q != NULL && w != NULL){
printf("Queues are created\n");
vTaskDelay(1000/portTICK_PERIOD_MS); //wait for a second
//xTaskCreate(&producer_task,"producer_task",2048,NULL,5,NULL);
//printf("producer task started\n");
//xTaskCreate(&consumer_task,"consumer_task",2048,NULL,5,NULL);
//printf("consumer task started\n");
}else{
printf("Queue creation failed");
}
}
#endif
<file_sep>#ifndef FILETABLE_SEEN
#define FILETABLE_SEEN
struct row{
int name;
int (*read)();
int (*write)();
void (*close)();
void (*open)();
};
int rowsInUse = 0;
struct row devTable[6];
int toComm = -1234; //the data being sent to comm
int signature[6] = {0,0,0,0,0,0}; //devTable[i] has its signature put into signature[i]. Values in signature[] range from -2 to +2 to represent VERY LOW, LOW, NORMAL, HIGH, and VERY HIGH, respectively.
int passFlag = 0; //check if password has been implemented. If not, don't print anything to screen
#endif //FILETABLE_SEEN
<file_sep>#include <stdio.h>
#include "fileTable.h"
#include "DHT_main.c"
TaskHandle_t handleCommand = NULL;
gpio_num_t FIRE_ALARM_GPIO = GPIO_NUM_14; //variables to make them easier to change
gpio_num_t FURNACE_GPIO = GPIO_NUM_15;
gpio_num_t AC_GPIO = GPIO_NUM_16;
gpio_num_t HUMIDIFIER_GPIO = GPIO_NUM_17;
gpio_num_t GAS_LEAK_GPIO = GPIO_NUM_18;
void command_task(void* pvParameter){ //go through the signature array to see if any values or combination of values are dangerous, then set various LEDs to represent system responses
TickType_t CommandLastWakeTime;
const TickType_t CommandFrequency = 2000*portTICK_RATE_MS; //period of two seconds because things like fire and the need to turn on AC are important
while(1){ //task goes through signature[] and sets output values based on dangerous scenarios
CommandLastWakeTime = xTaskGetTickCount();
if(signature[0] == 2 && signature[4] == 2){ //heat and particle sensors both going nuts
gpio_set_level(FIRE_ALARM_GPIO, 1);
if(passFlag == 1){ printf("\n\nFIRE!\n\n"); }
}else
gpio_set_level(FIRE_ALARM_GPIO, 0);
if(signature[0] < 0 && signature[2] > -1){ //if lights are on (i.e., employees in the building) and temperature is below 20C, power furnace
gpio_set_level(FURNACE_GPIO, 1);
if(passFlag == 1){ printf("\n\nTurning on furnace %d\n\n", passFlag); }
}else
gpio_set_level(FURNACE_GPIO, 0);
if(signature[0] > 0 && signature[2] > -1){ //if lights are on and temperature is above 25C, power AC
gpio_set_level(AC_GPIO, 1);
if(passFlag == 1){ printf("\n\nTurning on air conditioning\n\n"); }
}else
gpio_set_level(AC_GPIO, 1);
if(signature[1] > 0 && signature[2] > -1){ //if lights are on and humidity is up, use dehumidifier
gpio_set_level(HUMIDIFIER_GPIO, 1);
if(passFlag == 1){ printf("\n\nTurning on dehumidifier"); }
}else
gpio_set_level(HUMIDIFIER_GPIO, 0);
if(signature[3] > 0){ //if gas leak detected, raise an alarm regardless of the time of day
gpio_set_level(GAS_LEAK_GPIO, 1);
if(passFlag == 1) {printf("\n\nPOSSIBLE GAS LEAK\n\n"); }
}else
gpio_set_level(GAS_LEAK_GPIO, 0);
vTaskDelayUntil(&CommandLastWakeTime, CommandFrequency);
}
}
void command_open(){
xTaskCreate(&command_task, "command_task", 1024, NULL, 5, handleCommand);
}
<file_sep>/********************************************************************************************************
By: <NAME>
For: TSL2561 Light-to-Digital sensor, for use with ESP32 with ESP-IDF
Adapted from: David Antliff
( see https://github.com/DavidAntliff/esp32-tsl2561-example.git )
// BREE NOTES: I ran ESP32's I2C example prgrogram first to configure / choose
which of ESP32's GPIO pins to set as SDA (data) master and SCL (clock) master.
For my board, the best choice was GPIO 18 (SDA) ad GPIO 19(SCL) but your
board may very well be different so check the pinout in your datasheet!
Also, it's important to understand the I2C is a Master-Slave connection,
and you must wire your Master SDA and SCL pins to your Slave SDA and SCL
pins, respectively. Finally, you also connect the sensor's SDA and SCL pins
to the Master SDA and SCL pins.
Be sure to only connect the sensor's VCC to 3.3V! 5V will damage it.
//
//
* MIT License
*
* Copyright (c) 2017 <NAME>
*
* Permission is hereby granted, free of charge, to any person obtaining a copy
* of this software and associated documentation files (the "Software"), to deal
* in the Software without restriction, including without limitation the rights
* to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
* copies of the Software, and to permit persons to whom the Software is
* furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all
* copies or substantial portions of the Software.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
* IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
* FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
* AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
* LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
* OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
* SOFTWARE.
*/
/**
* @file app_main.c
* @brief Example application for the TSL2561 Light-to-Digital Converter.
*/
#include <stdio.h>
#include "freertos/FreeRTOS.h"
#include "freertos/task.h"
#include "esp_system.h"
#include "driver/gpio.h"
#include "driver/i2c.h"
#include "esp_log.h"
#include "sdkconfig.h"
#include "../components/esp32-smbus/include/smbus.h"
#include "../components/esp32-tsl2561/include/tsl2561.h"
#include "qExample.c"
#include "fileTable.h"
#ifndef LIGHT_MAIN
#define LIGHT_MAIN
#define TAG *"app"
#define I2C_MASTER_NUM I2C_NUM_0
#define I2C_MASTER_TX_BUF_LEN 0 // disabled
#define I2C_MASTER_RX_BUF_LEN 0 // disabled
#define I2C_MASTER_FREQ_HZ 100000
#define I2C_MASTER_SDA_IO CONFIG_I2C_MASTER_SDA
#define I2C_MASTER_SCL_IO CONFIG_I2C_MASTER_SCL
void tsl2561_output();
int lightValueRead = 0;
TaskHandle_t handleLight = NULL;
static void i2c_master_init(void)
{
int i2c_master_port = I2C_MASTER_NUM;
i2c_config_t conf;
conf.mode = I2C_MODE_MASTER;
conf.sda_io_num = I2C_MASTER_SDA_IO;
conf.sda_pullup_en = GPIO_PULLUP_DISABLE; // GY-2561 provides 10kΩ pullups
conf.scl_io_num = I2C_MASTER_SCL_IO;
conf.scl_pullup_en = GPIO_PULLUP_DISABLE; // GY-2561 provides 10kΩ pullups
conf.master.clk_speed = I2C_MASTER_FREQ_HZ;
i2c_param_config(i2c_master_port, &conf);
i2c_driver_install(i2c_master_port, conf.mode,
I2C_MASTER_RX_BUF_LEN,
I2C_MASTER_TX_BUF_LEN, 0);
}
int light_read(){
return lightValueRead;
}
void tsl2561_task(void * pvParameter)
{
//Every 10 seconds, update the read value
TickType_t lLastWakeTime;
const TickType_t lFrequency = 10000*portTICK_RATE_MS;
// Set up I2C
i2c_master_init();
i2c_port_t i2c_num = I2C_MASTER_NUM;
uint8_t address = CONFIG_TSL2561_I2C_ADDRESS;
// Set up the SMBus
smbus_info_t * smbus_info = smbus_malloc();
smbus_init(smbus_info, i2c_num, address);
smbus_set_timeout(smbus_info, 1000 / portTICK_RATE_MS);
// Set up the TSL2561 device
tsl2561_info_t * tsl2561_info = tsl2561_malloc();
tsl2561_init(tsl2561_info, smbus_info);
// Set sensor integration time and gain
tsl2561_set_integration_time_and_gain(tsl2561_info, TSL2561_INTEGRATION_TIME_402MS, TSL2561_GAIN_1X);
//tsl2561_set_integration_time_and_gain(tsl2561_info, TSL2561_INTEGRATION_TIME_402MS, TSL2561_GAIN_16X);
while (1)
{
lLastWakeTime = xTaskGetTickCount();
tsl2561_visible_t visible = 0;
tsl2561_infrared_t infrared = 0;
tsl2561_read(tsl2561_info, &visible, &infrared);
lightValueRead = tsl2561_compute_lux(tsl2561_info, visible, infrared);
/*printf("\nFull spectrum: %d\n", visible + infrared);
printf("Infrared: %d\n", infrared);
printf("Visible: %d\n", visible);
printf("Lux: %d\n", tsl2561_compute_lux(tsl2561_info, visible, infrared));*/
light_read();
vTaskDelayUntil(&lLastWakeTime,lFrequency);
}
}
int light_write(){
int send;
send = lightValueRead;
send = send*10;//the "name" for light is put into the ones' place, so need to multiply the read value by 10 to make room
send = send+2; //the name of light is 2
pushQ(&send);
//printf("\n |Light Write| Visible Light Value: %d\n", lightValueRead); //global variables are still bad practice
return lightValueRead;
}
void light_close(){
vTaskSuspend(handleLight);
}
void light_open()
{
if(handleLight == NULL){ //on first call, define this task
xTaskCreate(&tsl2561_task, "tsl2561_task", 2048, NULL, 5, handleLight);
struct row lightRow;
lightRow.read = light_read;
lightRow.write = light_write;
lightRow.name = 2;
lightRow.open = light_open;
lightRow.close = light_close;
devTable[rowsInUse++] = lightRow;
// I2C/SMBus Test application
//extern void test_smbus_task(void * pvParameter);
//xTaskCreate(&test_smbus_task, "test_smbus_task", 2048, NULL, 5, NULL);
}else{ //on every other call, resume this task
vTaskResume(handleLight);
}
}
#endif
<file_sep>#include "DHT_main.c"
#include "light_main.c"
#include "qExample.c"
#include "sensor.c"
#include "fileTable.h"
#include "communications.c"
#include "command.c"
TaskHandle_t handleManage = NULL;
void manage_task();
int tempAvg;
int humidAvg;
int lightAvg;
gpio_num_t SUPERUSER_GPIO = GPIO_NUM_27; //variables to make them easier to change
char input[20];
char password[] = "<PASSWORD>";
int sort; //sort looks at the first digit off of top of queue, then sends it to correct averaging value
void app_main(){
temp_open();
humid_open(); //open all of the tasks we'll need and put them on the stack. We implemented it this way to make the Mnager more modular, but same idea as example code in class.
light_open();
q_app_main(); //these are data structures used by the manager to control the rest of the system.
command_open();
sense_open();
comms_open();
command_open();
tempAvg = temp_write()/10; //then set initial values
humidAvg = humid_write()/10;
lightAvg = light_write()/10;
nvs_flash_init();
xTaskCreate( &manage_task, "ManageTask", 2048, NULL, 5, &handleManage );
}
void updateTempAvg(int toAdd){
int tempTempAvg;
toAdd = toAdd/10;
tempAvg = 6*tempAvg; //weighted average: 60% current value, 40% new value
toAdd = 4*toAdd;
tempAvg += toAdd;
tempAvg = tempAvg/10;
tempTempAvg = tempAvg*10;
pushW(&tempTempAvg);
// printf("\nWeighted Temperature Average: %d\n",tempAvg);
//set temperature's signature value for command task
if(tempAvg > 40){
signature[0] = 2; //probably fire
}else if(tempAvg > 25){
signature[0] = 1; //hot summer day
}else if(tempAvg > 20){
signature[0] = 0; //20-25C is about room temperature
}else if(tempAvg > 15){
signature[0] = -1; //cold winter day outside
}else
signature[0] = -2; //terrifying apocalyptic event
}
void updateHumidAvg(int toAdd){
int tempHumidAvg;
toAdd = toAdd/10;
humidAvg = 6*humidAvg; //weighted average: 60% current value, 40% new value
toAdd = 4*toAdd;
humidAvg += toAdd;
humidAvg = humidAvg/10;
tempHumidAvg = (humidAvg*10)+1;
pushW(&tempHumidAvg);;
// printf("\nWeighted Humidity Average: %d\n",humidAvg);
if(humidAvg > 50){
signature[1] = 2; //way too humid
}else if(humidAvg > 40){
signature[1] = 1; //still too humid but a believable amount
}else if(humidAvg > 25){
signature[1] = 0; //20-25C is about room temperature
}else if(humidAvg > 15){
signature[1] = -1; //dry day
}else
signature[1] = -2; //terrifying apocalyptic event
}
void updateLightAvg(int toAdd){
int tempLightAvg;
toAdd = toAdd/10;
lightAvg = 6*(lightAvg)*(lightAvg); //weighted average: 60% current value, 40% new value
toAdd = 4*toAdd;
lightAvg += toAdd;
lightAvg = lightAvg/10;
tempLightAvg = (lightAvg*10)+2;
pushW(&tempLightAvg);
// printf("\nWeighted Light Average: %d\n",lightAvg);
if(lightAvg > 400){
signature[2] = 2; //possible that the sun exploded
}else if(lightAvg > 300){
signature[2] = 1; //still too bright
}else if(lightAvg > 200){
signature[2] = 0; //I think this is normal? Need to calibrate this
}else if(lightAvg > 100){
signature[2] = -1; //normal night darkness
}else
signature[2] = -2; //terrifying apocalyptic event
}
void noUpdates(){
printf("\nNo values found on queue this period.\n");
}
void manage_task(){
int name; //name is the "name" of the sensor, put into the ones place for ease of reading
int tableIndex;
TickType_t xLastWakeTime;
//every ten seconds, read off from the queue
const TickType_t xFrequency = 15000*portTICK_RATE_MS;
while(1){
//to test that the functions are working properly
xLastWakeTime = xTaskGetTickCount();
for(tableIndex = 0; tableIndex < rowsInUse; tableIndex++){
sort = popQ();
printf("\nManager Task: %d is popQ\n", sort);
name = sort%10; //name is stored in ones' place of sort, so this works I think
switch(name){
case 0:
updateTempAvg(sort);
break;
case 1:
updateHumidAvg(sort);
break;
case 2:
updateLightAvg(sort);
break;
default:
noUpdates();
break;
}//end switch case
}//end tableIndexing
vTaskDelayUntil(&xLastWakeTime,xFrequency);
}//end whileLoop for manager task
}//end manager task
<file_sep>/* Create_DHT_Task.c */
#include "DHT_main.c"
// Buffer that the task being created will use as its stack. Note this is
// an array of StackType_t variables. The size of StackType_t is dependent on
// the RTOS port.
//Damon:I don't know how much stack DHT will need so this should go up/down as needed for different task implementations
int STACK_SIZE = 63;
StackType_t xStack[ STACK_SIZE ];
// Damon: These all start with the same priority I think, but maybe the ctl command is supposed to change that so I made it a variable
int DHT_priority = 10;
// Damon: Function that creates a task. Each task needs its own unique creation function I think, unless we want to mess around with adding parameters to these.
// Damon: From what I understand, Manager task should create/destroy tasks as needed, and this is an example that should create a task using DHT_task()
void createDHTTask( void )
{
TaskHandle_t xHandle = NULL;
// Create the task without using any dynamic memory allocation.
//Damon: This can be ported to the dynamic-memory task creation function if you guys want, I just chose the simpler one
xHandle = xTaskCreateStatic(
DHT_task, // Function that implements the task.
"DHT_Name", // Text name for the task.
STACK_SIZE, // Stack size in bytes, not words.
( void * ) 1, // Parameter passed into the task.
DHT_priority, // Priority at which the task is created.
xStack, // Array to use as the task's stack.
&xTaskBuffer ); // Variable to hold the task's data structure.
// puxStackBuffer and pxTaskBuffer were not NULL, so the task will have
// been created, and xHandle will be the task's handle. Use the handle
// to suspend the task.
vTaskSuspend( xHandle );
}<file_sep># ece566
ESP-32 Projects as featured in ECE566 Course at IUPUI
<file_sep>/* This is the CA certificate for the CA trust chain of
www.howsmyssl.com in PEM format, as dumped via:
openssl s_client -showcerts -connect mqtt.iotcebu.com:8083 </dev/null
The CA cert is the last cert in the chain output by the server.
*/
#include <stdio.h>
#include <stdint.h>
#include <string.h>
/*
1 s:/C=US/O=Let's Encrypt/CN=Let's Encrypt Authority X3
i:/O=Digital Signature Trust Co./CN=DST Root CA X3
*/
const char *server_root_cert = "-----BEGIN CERTIFICATE-----\r\n"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>keh<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"<KEY>"
"pu/xO28QOG8=\r\n"
"-----END CERTIFICATE-----\r\n";
<file_sep>#include "DHT_main.c"
#include "light_main.c"
#include "qExample.c"
TaskHandle_t handleSense = NULL;
void sense_task();
void sense_open(){
nvs_flash_init();
vTaskDelay( 1000 / portTICK_RATE_MS );
xTaskCreate( &sense_task, "SenseTask", 2048, NULL, 5, &handleSense );
}
void sense_task(){
int tableIndex;
TickType_t sLastWakeTime;
const TickType_t sFrequency = 15000*portTICK_RATE_MS;
while(1){
//sensor task just calls the write() functions, which push read values onto the shared queue
sLastWakeTime = xTaskGetTickCount();
for(tableIndex = 0; tableIndex < rowsInUse; tableIndex++){
printf("\n|SENSE TASK| tableIndex = %d and read value is %d ", tableIndex, devTable[tableIndex].write()/10);
}
vTaskDelayUntil(&sLastWakeTime, sFrequency);
}
}
<file_sep>/*
DHT22 temperature sensor driver
*/
#ifndef DHT22_H_
#define DHT22_H_
#define DHT_OK 0
#define DHT_CHECKSUM_ERROR -1
#define DHT_TIMEOUT_ERROR -2
// == function prototypes =======================================
void setDHTgpio(int gpio);
void setLEDgpio(int gpio); // Bree created to integrate her LED blinking for fun
void errorHandler(int response);
int readDHT();
float getHumidity();
float getTemperature();
int getSignalLevel( int usTimeOut, bool state );
float temps[10];
int usedtemps;
int tempRead;
int tempOpen;
#endif
| f6be713afbe9f14e830d805fa0e5abdd9ee5d2c9 | [
"Markdown",
"C",
"Text"
] | 16 | C | dkmyers/ece566 | 31a0ae2876304eea807fb54198e0334990dfc238 | 3211f1ae38aac88b906eb32c7c3b8b418c6ca031 | |
refs/heads/main | <repo_name>belegz/HRCGroup<file_sep>/Robot.java
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.Map;
/**
Represents an intelligent agent moving through a particular room.
The robot only has one sensor - the ability to get the status of any
tile in the environment through the command env.getTileStatus(row, col).
@author <NAME>, <NAME>
*/
public class Robot {
private Environment env;
private int posRow;
private int posCol;
private boolean toCleanOrNotToClean;
private ArrayList<Position> dirtyTiles = new ArrayList<>();
private boolean isFirst = true;
private ArrayList<Action> path = new ArrayList<>();
private boolean pathFound = false;
private int pathLength;
private boolean isFinding = false;
private ArrayList<Action> plan = new ArrayList<>();
private static ArrayList<Position> remainingDirtyTiles = new ArrayList<>();
private static boolean isFirstRobot = true;
private static int order = 0;
private int myOrder;
private static Map<Integer,Boolean> RobotList = new HashMap<>();
private static Map<Integer,Position> currentTargets = new HashMap<>();
private static Map<Integer,Position> nextPositions = new HashMap<>();
/**
Initializes a Robot on a specific tile in the environment.
*/
public Robot (Environment env, int posRow, int posCol) {
this.env = env;
this.posRow = posRow;
this.posCol = posCol;
this.toCleanOrNotToClean = false;
order++;
this.myOrder = order;
this.RobotList.put(this.myOrder, false);
}
public int getPosRow() { return posRow; }
public int getPosCol() { return posCol; }
public void incPosRow() { posRow++; }
public void decPosRow() { posRow--; }
public void incPosCol() { posCol++; }
public void decPosCol() { posCol--; }
/**
Returns the next action to be taken by the robot. A support function
that processes the path LinkedList that has been populates by the
search functions.
*/
public Action getMatrix() {
double[][] matrix = new double[this.env.getRows()][this.env.getCols()];
Tile[][] map = this.env.getTiles();
for(int i = 0;i<this.env.getRows();i++) {
for(int j= 0;j<this.env.getCols();j++) {
if(map[i][j].getStatus() == TileStatus.DIRTY) {
matrix[i][j] = 1;
}else if(map[i][j].getStatus() == TileStatus.CLEAN) {
matrix[i][j] = -0.4;
}else if(map[i][j].getStatus() == TileStatus.IMPASSABLE) {
//TODO: determine wall's value
matrix[i][j] = 0;
}
}
}
//TODO: find next action with argmax
//action up
//moving up successfully
for(int i = 0;i<this.env.getRows();i++) {
for(int j= 0;j<this.env.getCols();j++) {
if(map[i][j].getStatus() == TileStatus.DIRTY) {
matrix[i][j] = 1;
}else if(map[i][j].getStatus() == TileStatus.CLEAN) {
matrix[i][j] = -0.4;
}else if(map[i][j].getStatus() == TileStatus.IMPASSABLE) {
//TODO: determine wall's value
matrix[i][j] = 0;
}
}
}
return Action.DO_NOTHING;
}
public Action getAction() {
// // Part 1
// this.dirtyTiles = getDirtyTiles();
// if(this.dirtyTiles.size() == 0) {
// return Action.DO_NOTHING;
// }
// int mindis_x = this.env.getCols();
// int mindis_y = this.env.getRows();
// int mindis = mindis_x + mindis_y;
// for(Position target:this.dirtyTiles) {
// int new_x = target.col - this.getPosCol();
// int new_y = target.row - this.getPosRow();
// if(new_x == 0 && new_y == 0) {
// System.out.println("Cleaned pos: "+target.col+","+target.row);
// return Action.CLEAN;
// }
// int new_dis = Math.abs(new_x )+ Math.abs(new_y);
// if( new_dis < mindis) {
// mindis = new_dis;
// mindis_x = new_x;
// mindis_y = new_y;
// }
// }
// if(mindis_x == 0) {
// if(mindis_y > 0) {
// return Action.MOVE_DOWN;
// }else{
// return Action.MOVE_UP;
// }
// }else if(mindis_y == 0) {
// if(mindis_x > 0) {
// return Action.MOVE_RIGHT;
// }else{
// return Action.MOVE_LEFT;
// }
// }
//
// if(mindis_x <= mindis_y) {
// // move on x
// if(mindis_x > 0) {
// return Action.MOVE_RIGHT;
// }else{
// return Action.MOVE_LEFT;
// }
// }else if(mindis_x > mindis_y) {
// // move on y
// if(mindis_y > 0) {
// return Action.MOVE_DOWN;
// }else{
// return Action.MOVE_UP;
// }
// }
// return Action.DO_NOTHING;
// // Part 2: bfs search
// if (this.env.getTiles()[this.posRow][this.posCol].getStatus() == TileStatus.DIRTY) {
// return Action.CLEAN;
// }
//
// if(this.isFirst) {
// bfs();
// this.isFirst = false;
// }
//
//
// if(this.plan.size() == 0) {
// return Action.DO_NOTHING;
// }
//
// Action currentAction = this.plan.get(0);
// this.plan.remove(0);
// return currentAction;
// // Part 3: communicate
// if (this.env.getTiles()[this.posRow][this.posCol].getStatus() == TileStatus.DIRTY) {
// return Action.CLEAN;
// }
// if(this.isFirstRobot) {
// this.remainingDirtyTiles = this.getDirtyTiles();
// this.isFirstRobot = false;
// }
//
// if(this.remainingDirtyTiles.size()!=0 && this.plan.size() == 0) {
// bfs();
// }
// if(this.plan.size() == 0) {
// return Action.DO_NOTHING;
// }
//
// Action currentAction = this.plan.get(0);
// this.plan.remove(0);
// return currentAction;
// // Part 4: remove target
// int currentCount = this.env.getRobots().size();
// if(currentCount < this.order) {
// for(Integer order:this.currentTargets.keySet()) {
// boolean isContained = false;
// for(Robot r:this.env.getRobots()) {
// if(r.myOrder == order) {
// isContained = true;
// break;
// }
// }
//
// if(!isContained) {
// Position toBeAdded = this.currentTargets.get(order);
// this.remainingDirtyTiles.add(toBeAdded);
// this.order = currentCount;
// continue;
// }
// }
// }
//
// if (this.env.getTiles()[this.posRow][this.posCol].getStatus() == TileStatus.DIRTY) {
// return Action.CLEAN;
// }
// if(this.isFirstRobot) {
// this.remainingDirtyTiles = this.getDirtyTiles();
// this.isFirstRobot = false;
// }
//
// if(this.remainingDirtyTiles.size()!=0 && this.plan.size() == 0) {
// bfs();
// }
// if(this.plan.size() == 0) {
// return Action.DO_NOTHING;
// }
//
// Action currentAction = this.plan.get(0);
// this.plan.remove(0);
// return currentAction;
// Part 5: personal space
int currentCount = this.env.getRobots().size();
if(currentCount < this.order) {
for(Integer order:this.currentTargets.keySet()) {
boolean isContained = false;
for(Robot r:this.env.getRobots()) {
if(r.myOrder == order) {
isContained = true;
break;
}
}
if(!isContained) {
Position toBeAdded = this.currentTargets.get(order);
this.remainingDirtyTiles.add(toBeAdded);
this.order = currentCount;
continue;
}
}
}
if (this.env.getTiles()[this.posRow][this.posCol].getStatus() == TileStatus.DIRTY) {
return Action.CLEAN;
}
if(this.isFirstRobot) {
this.remainingDirtyTiles = this.getDirtyTiles();
this.isFirstRobot = false;
}
if(this.remainingDirtyTiles.size()!=0 && this.plan.size() == 0) {
bfs();
}
if(this.plan.size() == 0) {
return Action.DO_NOTHING;
}
Action currentAction = this.plan.get(0);
Position nextPos = null;
boolean isInNext = false;
if(currentAction.equals(Action.MOVE_UP)) {
isInNext = this.checkInNextPos(this.posRow - 1, this.posCol);
nextPos = new Position(this.posRow - 1,this.posCol);
}else if(currentAction.equals(Action.MOVE_DOWN)) {
isInNext = this.checkInNextPos(this.posRow + 1, this.posCol);
nextPos = new Position(this.posRow + 1,this.posCol);
}else if(currentAction.equals(Action.MOVE_LEFT)) {
isInNext = this.checkInNextPos(this.posRow, this.posCol - 1);
nextPos = new Position(this.posRow,this.posCol - 1);
}else if(currentAction.equals(Action.MOVE_RIGHT)) {
isInNext = this.checkInNextPos(this.posRow, this.posCol + 1);
nextPos = new Position(this.posRow,this.posCol + 1);
}
if(isInNext) {
// System.out.println("Is in!");
bfs();
if(this.plan.size() == 0) {
return Action.DO_NOTHING;
}
Action nextAction = this.plan.remove(0);
this.removeFromNextPos(this.posRow, this.posCol);
return nextAction;
}else {
this.removeFromNextPos(this.posRow, this.posCol);
this.nextPositions.put(this.myOrder, nextPos);
}
this.plan.remove(0);
return currentAction;
}
public ArrayList<Position> getDirtyTiles() {
ArrayList<Position> dirtyTiles = new ArrayList<>();
for (int i = 0; i < this.env.getRows(); i++) {
for (int j = 0; j < this.env.getCols(); j++) {
Tile currentTile = this.env.getTiles()[i][j];
if (currentTile.getStatus() == TileStatus.DIRTY) {
Position dirtyPos = new Position(i,j);
dirtyTiles.add(dirtyPos);
}
}
}
return dirtyTiles;
}
// bfs
public class State {
public int row;
public int column;
public ArrayList<Action> path;
public ArrayList<Position> targets;
State(int row, int column, ArrayList<Action> currentA, ArrayList<Position> currentT) {
this.row = row;
this.column = column;
this.path = currentA;
this.targets = currentT;
}
}
public void bfs() {
ArrayList<State> foundPath = new ArrayList<>();
ArrayList<State> visitedStates = new ArrayList<>();
// ArrayList<Position> destination = getDirtyTiles();
ArrayList<Position> destination = this.remainingDirtyTiles;
ArrayList<Action> minPath = new ArrayList<>();
State currentState = new State(this.posRow,this.posCol,new ArrayList<>(),destination);
foundPath.add(currentState);
while(!foundPath.isEmpty()) {
State currentPos = foundPath.remove(0);
if(!isVisited(currentPos.row,currentPos.column,currentPos,visitedStates) && !this.checkInNextPos(currentPos.row, currentPos.column)) {
visitedStates.add(currentPos);
if(isInTargets(currentPos)) {
this.addToCurrent(currentPos.row, currentPos.column);
this.removeFromDirtyTiles(currentPos.row, currentPos.column);
this.removeFromTargets(currentPos);
this.pathFound = true;
minPath = currentPos.path;
this.plan = currentPos.path;
this.pathLength = this.plan.size();
return;
}
directionSearch(currentPos,visitedStates,foundPath);
}
}
}
public void directionSingleSearch(int row, int col, State currentPos,ArrayList<State> foundPath,Action act) {
// System.out.println("single search");
ArrayList<Action> currentA = (ArrayList) currentPos.path.clone();
ArrayList<Position> currentT = (ArrayList<Position>) currentPos.targets.clone();
currentA.add(act);
State nextState = new State(row,col,currentA,currentT);
foundPath.add(nextState);
}
public boolean isVisited(int row,int col, State currentState, ArrayList<State> visitedStates) {
for(State currS:visitedStates) {
int targetNumber = 0;
if(currS.row == row && currS.column == col) {
for(Position target:currS.targets) {
for(Position currT:currentState.targets) {
if(target.row == currT.row && target.col == currT.col) {
targetNumber++;
if(targetNumber == currS.targets.size()) {
return true;
}
}
}
}
}
}
return false;
}
private void directionSearch(State currentPos, ArrayList<State> visitedStates, ArrayList<State> foundPath) {
// System.out.println("search");
if(this.isReadyForSearch(currentPos,visitedStates,foundPath,"up")) {
this.directionSingleSearch(currentPos.row-1, currentPos.column, currentPos, foundPath, Action.MOVE_UP);
}
if(this.isReadyForSearch(currentPos, visitedStates, foundPath, "down")) {
this.directionSingleSearch(currentPos.row+1, currentPos.column, currentPos, foundPath, Action.MOVE_DOWN);
}
if(this.isReadyForSearch(currentPos, visitedStates, foundPath, "left")) {
this.directionSingleSearch(currentPos.row, currentPos.column-1, currentPos, foundPath, Action.MOVE_LEFT);
}
if(this.isReadyForSearch(currentPos, visitedStates, foundPath, "right")) {
this.directionSingleSearch(currentPos.row, currentPos.column+1, currentPos, foundPath, Action.MOVE_RIGHT);
}
}
private void removeFromTargets(State currentPos) {
Position currtarget = null;
for(Position currT:currentPos.targets) {
if(currT.row == currentPos.row && currT.col == currentPos.column) {
currtarget = currT;
}
}
currentPos.targets.remove(currtarget);
}
private void removeFromDirtyTiles(int row, int col) {
Position currtarget = null;
for(Position currT:this.remainingDirtyTiles) {
if(currT.row == row && currT.col == col) {
currtarget = currT;
}
}
this.remainingDirtyTiles.remove(currtarget);
}
private boolean checkInNextPos(int row, int col) {
for(int key:this.nextPositions.keySet()) {
if(key != this.myOrder &&
(this.nextPositions.get(key).row == row && this.nextPositions.get(key).col == col)) {
return true;
}
}
return false;
}
private void removeFromNextPos(int row, int col) {
int toRemove = -1;
for(int key:this.nextPositions.keySet()) {
if(this.nextPositions.get(key).row == row && this.nextPositions.get(key).col == col) {
toRemove = key;
break;
}
}
// System.out.println(this.nextPositions.toString());
this.nextPositions.remove(toRemove);
// System.out.println(this.nextPositions.toString());
}
private void addToCurrent(int row, int col) {
Position currPos = null;
for(Position currT:this.remainingDirtyTiles) {
if(currT.row == row && currT.col == col) {
currPos = currT;
}
}
this.currentTargets.put(this.myOrder, currPos);
}
public boolean isInTargets(State currentPos) {
for(Position currT:currentPos.targets) {
if(currT.row == currentPos.row && currT.col == currentPos.column) {
return true;
}
}
return false;
}
private boolean isReadyForSearch(State currentPos, ArrayList<State> visitedStates, ArrayList<State> foundPath, String direction) {
if(direction == "up") {
return this.env.validPos(currentPos.row-1,currentPos.column)
&& !isVisited(currentPos.row-1,currentPos.column,currentPos,visitedStates)
&& !isVisited(currentPos.row-1,currentPos.column,currentPos,foundPath);
}else if(direction == "down") {
return this.env.validPos(currentPos.row+1,currentPos.column)
&& !isVisited(currentPos.row+1,currentPos.column,currentPos,visitedStates)
&& !isVisited(currentPos.row+1,currentPos.column,currentPos,foundPath);
}else if(direction == "left") {
return this.env.validPos(currentPos.row,currentPos.column-1)
&& !isVisited(currentPos.row,currentPos.column-1,currentPos,visitedStates)
&& !isVisited(currentPos.row,currentPos.column-1,currentPos,foundPath);
}else if(direction == "right") {
return this.env.validPos(currentPos.row,currentPos.column+1)
&& !isVisited(currentPos.row,currentPos.column+1,currentPos,visitedStates)
&& !isVisited(currentPos.row,currentPos.column+1,currentPos,foundPath);
}else {
return false;
}
}
} | dc4df9ebb75d6546124e86a3e4810b41fa68bbcb | [
"Java"
] | 1 | Java | belegz/HRCGroup | 8213a6b2b3117129013e37cfa91e10d1bad5122c | b5945ccaf6d710d025acde05c8a50cc631b0573c | |
refs/heads/master | <file_sep>#!/usr/bin/env sh
SCHEMA_FILE=/etc/pdns/schema.sql
if [ -f ${SCHEMA_FILE} ]; then
sqlite3 /srv/pdns.sqlite < ${SCHEMA_FILE}
rm ${SCHEMA_FILE}
fi
pdns_server \
--api=${PDNS_API:-yes} \
--api-key=${PDNS_API_KEY:-<KEY>} \
--webserver-address=${PDNS_WEBSERVER_ADDRESS:-0.0.0.0} \
--webserver-port=${PDNS_WEBSERVER_PORT:-42902} \
--webserver-allow-from=${PDNS_WEBSERVER_ALLOW_FROM:-0.0.0.0/0,::/0} \
--dnsupdate=yes \
--master=yes \
--setgid=pdns \
--setuid=pdns \
--daemon=no \
--disable-tcp=no \
--launch=gsqlite3 \
--gsqlite3-database=/srv/pdns.sqlite<file_sep>
FROM alpine:3.10
MAINTAINER <NAME> <<EMAIL>>
COPY assets/schema.sql /etc/pdns/schema.sql
COPY assets/entrypoint.sh /root/entrypoint.sh
VOLUME ["/srv/pdns"]
EXPOSE 53/tcp 53/udp
RUN apk --no-cache add pdns pdns-backend-sqlite3 sqlite \
&& rm /etc/pdns/pdns.conf \
&& mkdir -p /var/empty/var/run/ \
&& chmod u+x /root/entrypoint.sh
ENTRYPOINT [ "/root/entrypoint.sh" ]
<file_sep># PowerDNS with DNS-Update
This repository provides a PowerDNS authorative DNS server installation with support for DNS UPDATE ([RFC 2136](https://tools.ietf.org/html/rfc2136) and [RFC 3007](https://tools.ietf.org/html/rfc3007)) configured.
## Building the Image
docker build -t pdns-dnsupdate .
or
make
## Running the Image
docker run -d -p 42902:42902/tcp -p 53:53/udp -p 53:53/tcp pdns-dnsupdate
To start a shell session inside the container:
docker exec -it <CONTAINER-ID> /bin/bash
## PowerDNS Configuration
The following environment variables can be set:
* `PDNS_API`
* `PDNS_API_KEY`
* `PDNS_WEBSERVER_ADDRESS`
* `PDNS_WEBSERVER_PORT`
* `PDNS_WEBSERVER_ALLOW_FROM`
When running the container, environment variables can be set like this:
docker run -d -e PDNS_API_KEY=test -p 42902:42902/tcp -p 53:53/udp -p 53:53/tcp pdns
## Configuring a Zone
On the container, you can use pdnsutil to configure a zone to test your setup. In order to add a zone *example.com* with a nameserver *ns1* at 192.168.1.2 and a hostname *newhost* at 192.168.1.3, execute the following commands:
pdnsutil create-zone example.com ns1.example.com
pdnsutil add-record example.com ns1 A 192.168.1.2
pdnsutil add-record example.com new-host A 192.168.1.3
To test your setup, run from the Docker host machine:
dig +noall +answer newhost.example.com @127.0.0.1
## DNS UPDATE
### Configuration
In order to use DNS UPDATE securely, a shared secret must be configured. To do so, execute the following command inside the container:
pdnsutil generate-tsig-key nsupdate-key hmac-md5
To furthermore configure DNS-UPDATE using our new key for the domain *example.com*, run:
pdnsutil activate-tsig-key example.com nsupdate-key master
pdnsutil set-meta example.com TSIG-ALLOW-DNSUPDATE nsupdate-key
pdnsutil set-meta example.com ALLOW-DNSUPDATE-FROM 0.0.0.0/0
You can then retrieve the generated key using:
pdnsutil list-tsig-keys
### Sending an Update Request
Use the `nsupdate` tool to send DNS UPDATE requests:
nsupdate -y nsupdate-key:<SHARED-SECRET>
Then, in order to set the IP address for newhost to 172.16.0.1, type
server 127.0.0.1
update delete newhost.example.com
update add newhost.example.com 500 IN A 172.16.0.1
send
quit
## PDNS API
Information about the API can be found [here](https://doc.powerdns.com/md/httpapi/README/).
<file_sep>build: Dockerfile assets/entrypoint.sh assets/schema.sql
docker build -t olivermichel/pdns-dnsupdate .
push:
docker push olivermichel/pdns-dnsupdate
.PHONY: build push | 06e1489426063271f53266a4b976e8d07edb84c0 | [
"Markdown",
"Makefile",
"Dockerfile",
"Shell"
] | 4 | Shell | olivermichel/pdns-dnsupdate | 195ba37566f5b4c34d8deadd7dd170f6cc5428c2 | f87e80345f580de9ded97e9de6c46d1122dc3451 | |
refs/heads/master | <repo_name>Thomasan1999/memsource-assignment<file_sep>/.eslintrc.js
module.exports = {
root: true,
env: {
node: true
},
overrides: [
{
files: '*.vue',
rules: {
'max-len': 'off'
}
}
],
rules: {
'arrow-body-style': ['error', 'as-needed', {requireReturnForObjectLiteral: true}],
'arrow-parens': ['error', 'always'],
'arrow-spacing': ['error', {after: true, before: true}],
'brace-style': ['error', 'allman'],
camelcase: 'off',
'class-methods-use-this': 'off',
'comma-dangle': ['error', 'never'],
'consistent-return': 'off',
'global-require': 'off',
indent: ['error', 4, {SwitchCase: 1}],
'indent-legacy': 'off',
'import/extensions': 'off',
'import/no-dynamic-require': 'off',
'import/prefer-default-export': 'off',
'newline-per-chained-call': 'off',
'import/no-cycle': 'off',
'import/no-extraneous-dependencies': 'off',
'linebreak-style': ['error', 'windows'],
'lines-between-class-members': 'off',
'max-len': ['error', 120],
'new-cap': 'off',
'no-console': 'off',
'no-debugger': process.env.NODE_ENV === 'production' ? 'error' : 'off',
'no-floating-decimal': 'off',
'no-multi-assign': ['error', {ignoreNonDeclaration: true}],
'no-multi-spaces': 'off',
'no-param-reassign': ['error', {props: false}],
'no-undef': 'off',
'no-unused-vars': 'off',
'no-use-before-define': 'off',
'no-restricted-globals': 'off',
'no-template-curly-in-string': 'off',
'no-trailing-spaces': 'off',
'no-underscore-dangle': 'off',
'object-curly-newline': 'off',
'object-curly-spacing': ['error', 'never'],
'object-shorthand': ['error', 'always'],
'prefer-destructuring': 'off',
'prefer-promise-reject-errors': 'off',
'quote-props': 'off',
quotes: ['error', 'single', {allowTemplateLiterals: true}],
semi: ['error', 'always'],
'space-before-function-paren': ['error', {
anonymous: 'always',
asyncArrow: 'always',
named: 'never'
}],
'vue/experimental-script-setup-vars': 'off',
'vue/script-indent': ['off', 4, {
baseIndent: 1
}]
},
parser: 'vue-eslint-parser',
parserOptions: {
parser: '@typescript-eslint/parser'
},
settings: {
'import/resolver': {
typescript: {}
}
}
};
<file_sep>/src/models/ProjectModel.ts
import Model from '@/models/Model';
export default class ProjectModel extends Model
{
public readonly name!: string;
public readonly sourceLanguage!: string;
public readonly status!: 'NEW' | 'COMPLETED' | 'DELIVERED';
public readonly targetLanguages!: string[];
constructor(attrs)
{
super();
this.set(attrs);
}
}
<file_sep>/src/store/index.ts
import {defineStore} from 'pinia';
import {ProjectGetData, ProjectModelFormData, ProjectPostData} from '@/types/project';
import axios from 'axios';
import ProjectModel from '@/models/ProjectModel';
import {cloneDeep} from 'lodash';
import {ModelRaw} from '@/models/Model';
import {ApiResponseData} from '@/types';
const useStore = defineStore('main', {
state()
{
return {
idCounter: {} as Record<string, number>,
initialized: false,
projects: [] as ProjectModel[],
projectsUrl: '/projects'
};
},
actions: {
async addProject(formData: ProjectModelFormData): Promise<ProjectModel>
{
const dataToSend = {
...formData,
status: 'NEW' as ProjectModel['status']
};
const res = await axios.post<ProjectPostData>(this.projectsUrl, dataToSend);
const resDataParsed = this.makeResponseDataStorable(res.data);
const projectModel = new ProjectModel(resDataParsed);
this.projects.push(projectModel);
return projectModel;
},
async deleteProject(projectToDelete: ProjectModel): Promise<void>
{
await axios.delete(this.getProjectUrl(projectToDelete));
this.projects = this.projects.filter((project) => project.id !== projectToDelete.id);
},
getProjectUrl(project: ProjectModel): string
{
return `${this.projectsUrl}/${project.id}`;
},
async fetchProjects(): Promise<void>
{
const res = await axios.get<ProjectGetData>(this.projectsUrl);
this.projects = res.data._embedded.projects.map((project) => new ProjectModel(project));
},
incrementIdCounter(counterName: string): void
{
this.idCounter[counterName] ??= 0;
this.idCounter[counterName]++;
},
async init(): Promise<void>
{
if (this.initialized)
{
throw new Error('State has been already initialized');
}
await this.fetchProjects();
},
makeResponseDataStorable(resData: ApiResponseData): ModelRaw
{
const resDataCopy: any = cloneDeep(resData);
delete resDataCopy._links;
return resDataCopy;
},
async saveProject(projectToSave: ProjectModel, formData: ProjectModelFormData): Promise<ProjectModel>
{
const res = await axios.patch<ProjectPostData>(this.getProjectUrl(projectToSave), formData);
const projectModel = this.projects.find((project) => project.id === res.data.id)! as ProjectModel;
const resDataParsed = this.makeResponseDataStorable(res.data);
projectModel.set(resDataParsed);
return projectModel;
}
}
});
export default useStore;
<file_sep>/src/models/Model.ts
import {DataType, ExtractPropertyNames, PartialRecord} from '@/types';
import {Merge} from 'ts-essentials';
import dayjs, {Dayjs} from 'dayjs';
export type ModelRaw<T extends Model = Model> = Merge<
T,
{[s in ExtractPropertyNames<T, Dayjs | null>]: string | null}
>;
export default class Model
{
public readonly id!: number;
public readonly dateCreated!: Dayjs | null;
public readonly dateDue!: Dayjs | null;
public readonly dateUpdated!: Dayjs | null;
public set(attrs: Record<keyof Model, any>): void
{
Object.entries(attrs).forEach(([key, value]) =>
{
this[key] = this.transforms[key] ? this.transformValue(key, value) : value;
});
}
protected get transforms(): PartialRecord<keyof Model, DataType>
{
return {
dateCreated: 'date',
dateDue: 'date',
dateUpdated: 'date'
};
}
private transformValue(key: string, value: any): any
{
if (value === null || value === undefined)
{
return value;
}
switch (this.transforms[key] as DataType)
{
case 'date':
return dayjs(value);
}
}
}
<file_sep>/vite.config.ts
import vue from '@vitejs/plugin-vue';
import * as path from 'path';
import {defineConfig} from 'vite';
import eslintPlugin from 'vite-plugin-eslint';
import {minifyHtml} from 'vite-plugin-html';
export default defineConfig({
plugins: [
eslintPlugin({
cache: false
}),
vue(),
minifyHtml()
],
resolve: {
alias: {
'@': path.resolve(__dirname, './src')
}
},
server: {
port: 8080
}
});
<file_sep>/src/types/index.d.ts
import {Merge} from 'ts-essentials';
import {ModelRaw} from '@/models/Model';
export type ApiResponseData<T extends ModelRaw = ModelRaw> = Merge<T, {_links: ApiResponseLinks}>;
export type ApiResponseLinks = Record<string, {href: string}>;
export type DataType = 'boolean' | 'date' | 'number' | 'string' | 'string[]';
type ExtractPropertyNames<Object, Type> = {
[K in keyof Object]: Object[K] extends Type ? K : never
}[keyof Object]
export type PartialRecord<K extends keyof any, T> = {
[P in K]?: T;
};
export type SortOrder = 1 | -1;
export type TableColumn = {name: string, sortable?: boolean, type: DataType};
<file_sep>/src/types/project.d.ts
import ProjectModel from '@/models/ProjectModel';
import {ModelRaw} from '@/models/Model';
import {ApiResponseData} from '@/types/index';
export type ProjectModelFormData = Pick<ProjectModel, 'name' | 'sourceLanguage' | 'targetLanguages'>;
export type ProjectGetData = ApiResponseData<{
_embedded: {projects: ModelRaw<ProjectModel>[]}
}>;
export type ProjectPostData = ApiResponseData<ModelRaw<ProjectModel>>;
<file_sep>/src/locales/en.ts
export default {
add: 'Add',
form: {
deleteInput: 'Delete Input',
submit: {
add: 'Submit',
edit: 'Save'
}
},
goBack: 'Go back',
project: {
id: 'Id',
actions: {
add: 'Create new project',
delete: 'Delete project',
edit: 'Edit project',
reset: 'Reset form'
},
dateDue: 'Date Due',
name: 'Name',
saveSuccessful: 'Project has been successfully saved.',
sourceLanguage: 'Source Language',
status: 'Status',
targetLanguages: 'Target Languages'
},
projects: 'Projects',
totalOverview: {
dueDateInPast: 'No. of projects with due date in the past',
heading: 'Total Overview',
mostProminent: 'Most prominent source languages',
projectsByStatus: 'No. of projects by the status'
}
};
<file_sep>/src/main.ts
import {createApp} from 'vue';
import {createPinia} from 'pinia';
import App from '@/App.vue';
import {createI18n} from 'vue-i18n';
import en from '@/locales/en';
import router from '@/router';
import VueAxios from 'vue-axios';
import axios from 'axios';
import dayjs from 'dayjs';
const app = createApp(App);
const i18n = createI18n({
locale: 'en',
messages: {
en
}
});
app.use(createPinia()).use(i18n);
app.use(router);
app.use(VueAxios, axios);
app.config.globalProperties.$dayjs = dayjs;
axios.defaults.baseURL = import.meta.env.VITE_API_URL;
app.mount('#app');
<file_sep>/src/router/index.ts
import {createRouter, createWebHistory} from 'vue-router';
import ProjectCreate from '@/components/projects/ProjectCreate.vue';
import ProjectEdit from '@/components/projects/ProjectEdit.vue';
import Projects from '@/components/projects/Projects.vue';
import App from '@/App.vue';
const router = createRouter({
history: createWebHistory(),
routes: [
{
path: '/projects',
component: App,
children: [
{
path: 'create',
name: 'ProjectCreate',
component: ProjectCreate
},
{
path: ':id',
name: 'ProjectEdit',
component: ProjectEdit
},
{
path: '',
name: 'Projects',
component: Projects
}
]
},
{
path: '/',
redirect: '/projects'
}
]
});
export default router;
| 4d43b5e973e84df9e8fe632426910612ddda6169 | [
"JavaScript",
"TypeScript"
] | 10 | JavaScript | Thomasan1999/memsource-assignment | 58275e9a4a2b7f7366db0d2ca2f1ab983d9e4aed | da1fd7ee28ae46c2d67e520d8ca1258dfb46db42 | |
refs/heads/master | <file_sep>## Generate ICONS for Cordova/Electron
Output will be in root folder **/res**, res copy to your root cordova project.
How import icons to cordova config read file **config.xml** in root
## Setup - first install all npm packagist
You must have **NPM and NODE** in your computer.
```bash
npm install
```
## Bash script for generating icons
```bash
node index.js path/to/icon.png
```
<file_sep>const Jimp = require('jimp');
const path = require('path');
const fs = require('fs');
const cli_args = process.argv.slice(2);
const image_path = path.join(__dirname, cli_args[0]);
const dir = './res';
const dir_ios = path.join(dir, 'ios');
const dir_android = path.join(dir, 'android');
function prepareFolder() {
if (!fs.existsSync(dir)) {
fs.mkdirSync(dir);
fs.mkdirSync(dir_ios);
fs.mkdirSync(dir_android);
} else {
deleteFolder(dir);
prepareFolder();
}
}
function deleteFolder(folderPath) {
if (fs.existsSync(folderPath)) {
fs.readdirSync(folderPath).forEach((file, index) => {
const curPath = path.join(folderPath, file);
if (fs.lstatSync(curPath).isDirectory()) { // recurse
deleteFolder(curPath);
} else { // delete file
fs.unlinkSync(curPath);
}
});
fs.rmdirSync(folderPath);
}
}
function createImage(device, loadImagePath, fileName, width = 256, height = 256, format = 'jpg', quality = 80) {
Jimp.read(loadImagePath)
.then(lenna => {
let output = null;
if (!fileName) fileName = width + 'x' + height;
fileName = fileName + '.' + format;
if (device === 'ios') output = path.join(dir_ios, fileName);
if (device === 'android') output = path.join(dir_android, fileName);
console.log(output); // print what will be generated
if (!output) return false;
return lenna
.resize(width, height) // resize
.quality(quality) // set JPEG quality
.write(output); // save
})
.catch(err => {
console.error(err);
});
}
prepareFolder();
/** iOS 8.0+ **/
// iPhone 6 Plus
createImage('ios', image_path, 'icon-60@3x', 180, 180);
/** iOS 7.0+ **/
// iPhone / iPod Touch
createImage('ios', image_path, 'icon-60', 60, 60);
createImage('ios', image_path, 'icon-60@2x', 120, 120);
// iPad
createImage('ios', image_path, 'icon-76', 76, 76);
createImage('ios', image_path, 'icon-76@2x', 152, 152);
// Spotlight Icon
createImage('ios', image_path, 'icon-40', 40, 40);
createImage('ios', image_path, 'icon-40@2x', 80, 80);
/** iOS 6.1 **/
// iPhone / iPod Touch
createImage('ios', image_path, 'icon', 57, 57);
createImage('ios', image_path, 'icon@2x', 144, 114);
// iPad
createImage('ios', image_path, 'icon-72', 72, 72);
createImage('ios', image_path, 'icon-72@2x', 144, 114);
// iPad Pro
createImage('ios', image_path, 'icon-167', 167, 167);
// iPhone Spotlight and Settings Icon
createImage('ios', image_path, 'icon-small', 29, 29);
createImage('ios', image_path, 'icon-small@2x', 58, 58);
createImage('ios', image_path, 'icon-small@3x', 87, 87);
// iPad Spotlight and Settings Icon
createImage('ios', image_path, 'icon-50', 50, 50);
createImage('ios', image_path, 'icon-50@2x', 100, 100);
// iPad Pro
createImage('ios', image_path, 'icon-83.5@2x', 167, 167);
/** Android **/
// ldpi 36x36
createImage('android', image_path, 'ldpi', 36, 36);
// mdpi 48x48
createImage('android', image_path, 'mdpi', 48, 48);
// hdpi 72x72
createImage('android', image_path, 'hdpi', 72, 72);
// xhdpi 96x96
createImage('android', image_path, 'xhdpi', 96, 96);
// xxhdpi 144x144
createImage('android', image_path, 'xxhdpi', 144, 144);
// xxxhdpi 192x192
createImage('android', image_path, 'xxxhdpi', 192, 192);
| 18cd54cf115e67e15cd3dbc4cfa5a8fbd05cb81e | [
"Markdown",
"JavaScript"
] | 2 | Markdown | mafikes/generate-cordova-icons | 61789f29c5c9cf2d924a74270cdbfbe66b171244 | 3bac73bb6e3ca7ae9b5b28005f6796611ca57e4b | |
refs/heads/master | <repo_name>AriBishop/GeometryTool<file_sep>/GeometryTool.java
/*
* Author: <NAME>, <EMAIL>
* Course: CSE 1001, Section 02, Spring 2016
* Project: geometrytool
*/
import java.util.Scanner;
public class GeometryTool{
public static void main(String[] args){
Scanner kb = new Scanner(System.in);
double rectLength = 0, rectWidth = 0, squaLength = 0, circRadius = 0, numDeg = 0, cylRadius = 0,
cylHeight = 0;
double rectArea = 0, rectPerimeter = 0, rectDiagonal = 0;
double squaArea = 0, squaPerimeter = 0, squaDiagonal = 0;
double circArea = 0, circCircumference = 0, sectArea = 0;
double cylVolume = 0, cylSA = 0;
//Calculate the Area, Perimeter and Diagonal of the rectangle
rectArea = rectLength * rectWidth;
rectPerimeter = (2*rectLength) + (2*rectWidth);
rectDiagonal = Math.sqrt((rectLength * rectLength) + (rectWidth*rectWidth));
//Print the rectangle Area, Perimeter and Diagonal to the screen
System.out.println("Area of Rectangle is: " + rectArea);
System.out.println("Perimeter of Rectangle is: " + rectPerimeter);
System.out.println("Diagonals of Rectangle is: " + rectDiagonal);
//Calculate the square Area, Perimeter, and Diagonal
squaArea = Math.pow(squaLength, 2);
squaPerimeter = squaLength * 4;
squaDiagonal = Math.sqrt((Math.pow(squaLength, 2) * 2));
//Print square Area, Perimeter and Diagonal to screen
System.out.println("Area of Square is: " + squaArea);
System.out.println("Perimeter of Square is: " + squaPerimeter);
System.out.println("Diagonals of Square: " + squaDiagonal);
//Calculate circle Area/Circumference and sector Area
circArea = Math.PI * (Math.pow(circRadius, 2));
circCircumference = Math.PI * (circRadius * 2);
sectArea = (numDeg/360) * Math.PI * (circRadius * 2);
//Print circle Area/Circumference and sector Area
System.out.println("Area of Circle is: " + circArea);
System.out.println("Circumference of circle is: " + circCircumference);
System.out.println("Area of a sector is: " + sectArea);
//Calculate cylinder Volume and Surface Area
cylVolume = Math.PI * (Math.pow(cylRadius, 2)) * cylHeight;
cylSA = (2*Math.PI*(Math.pow(cylRadius, 2))) + ((2*Math.PI*cylRadius)*cylHeight);
//Print cylinder Volume and SA
System.out.println("Volume of cylinder is: " + cylVolume);
System.out.println("Surface are of cylinder is: " + cylSA);
}
}<file_sep>/README.md
# GeometryTool
A java 1 assignment that focused on the use of the Math API and number manipulation. Calculates: area, perimeter, and diagonal of rectangle; area, perimeter, and diagonal of a square; area, circumference, and sector area of a circle; and cylinder volume and surface area.
NOTE: Sector of a circle needs to be fixed.
| d0bd53b5d92300b07c6a30dea3dc0f54400881b3 | [
"Markdown",
"Java"
] | 2 | Java | AriBishop/GeometryTool | f000fb808ccd61b0ecb7de3760866872f386362b | 30ef63530d6056cdfd73d04e5d956b18a15b876d |
Subsets and Splits
No community queries yet
The top public SQL queries from the community will appear here once available.