Spaces:
Sleeping
Sleeping
Update README.md
Browse files
README.md
CHANGED
@@ -1,12 +1,66 @@
|
|
1 |
---
|
2 |
title: LinearRegression
|
3 |
emoji: 💻
|
4 |
-
colorFrom:
|
5 |
-
colorTo:
|
6 |
sdk: gradio
|
7 |
sdk_version: 3.23.0
|
8 |
app_file: app.py
|
9 |
pinned: false
|
10 |
---
|
11 |
|
12 |
-
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
1 |
---
|
2 |
title: LinearRegression
|
3 |
emoji: 💻
|
4 |
+
colorFrom: green
|
5 |
+
colorTo: gray
|
6 |
sdk: gradio
|
7 |
sdk_version: 3.23.0
|
8 |
app_file: app.py
|
9 |
pinned: false
|
10 |
---
|
11 |
|
12 |
+
#Linear Regression
|
13 |
+
Linear regression is a statistical method used to model the relationship between a dependent variable (often denoted as Y) and one or more independent variables (often denoted as X). The goal of linear regression is to find the line (or plane in higher dimensions) that best fits the data, by minimizing the distance between the predicted values and the actual values. The equation of the line can then be used to predict the value of the dependent variable for any given value of the independent variable. Linear regression is widely used in many fields, including economics, finance, and engineering, among others.
|
14 |
+
|
15 |
+
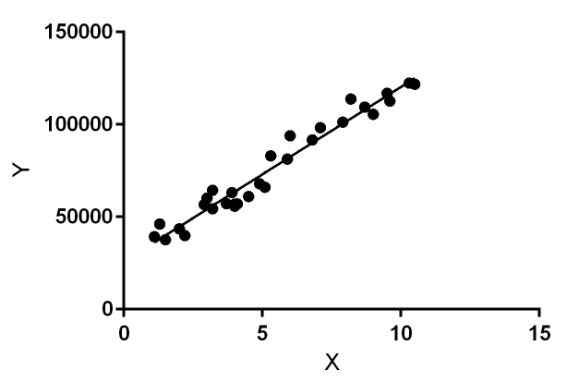
|
16 |
+
|
17 |
+
#Deployed
|
18 |
+
Created a Model that would take inputs and outputs and Train itself and adjust its weights and bias since The model only involves one node with bias it is easy to predict the weights and bias
|
19 |
+
|
20 |
+
#Code
|
21 |
+
```
|
22 |
+
import torch
|
23 |
+
import torch.nn as nn
|
24 |
+
from tqdm import tqdm
|
25 |
+
|
26 |
+
w = float(input("Enter weight : "))
|
27 |
+
b = float(input("Enter Bias : "))
|
28 |
+
|
29 |
+
x = torch.randn(1000)
|
30 |
+
y = (x*w)+b
|
31 |
+
|
32 |
+
class Lreg(nn.Module):
|
33 |
+
def __init__(self):
|
34 |
+
super(Lreg,self).__init__()
|
35 |
+
self.l = nn.Linear(1,1)
|
36 |
+
|
37 |
+
def forward(self,x):
|
38 |
+
y = self.l(x)
|
39 |
+
return y
|
40 |
+
|
41 |
+
model = Lreg()
|
42 |
+
crit = nn.MSELoss()
|
43 |
+
optim = torch.optim.SGD(model.parameters(),lr=0.01)
|
44 |
+
|
45 |
+
model.train()
|
46 |
+
for i in tqdm(range(1,5)):
|
47 |
+
for idx,ele in enumerate(x):
|
48 |
+
ele = ele.unsqueeze(0)
|
49 |
+
out = model(ele)
|
50 |
+
optim.zero_grad()
|
51 |
+
loss = crit(out,y[idx].unsqueeze(0))
|
52 |
+
loss.backward()
|
53 |
+
optim.step()
|
54 |
+
print(loss.item())
|
55 |
+
|
56 |
+
model.eval()
|
57 |
+
t = torch.tensor([7],dtype=torch.float32)
|
58 |
+
print("expected output ",t*w+b)
|
59 |
+
rout = model(t)
|
60 |
+
print(rout.item())
|
61 |
+
c = model.state_dict()
|
62 |
+
print(f'The wieght is {c["l.weight"].item()} and the bias is {c["l.bias"].item()}')
|
63 |
+
```
|
64 |
+
|
65 |
+
Made By Devic1 🖤
|
66 |
+
Check out the configuration reference at https://huggingface.co/docs/hub/spaces-config-reference
|